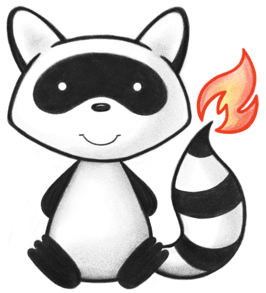
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048import org.hl7.fhir.instance.model.api.IAnyResource; 049import org.hl7.fhir.instance.model.api.IBaseReference; 050import org.hl7.fhir.instance.model.api.ICompositeType; 051import org.hl7.fhir.instance.model.api.IIdType; 052/** 053 * Reference Type: A reference from one resource to another. 054 */ 055@DatatypeDef(name="Reference") 056public class Reference extends BaseReference implements IBaseReference, ICompositeType { 057 058 /** 059 * A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources. 060 */ 061 @Child(name = "reference", type = {StringType.class}, order=0, min=0, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="Literal reference, Relative, internal or absolute URL", formalDefinition="A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources." ) 063 protected StringType reference; 064 065 /** 066 * The expected type of the target of the reference. If both Reference.type and Reference.reference are populated and Reference.reference is a FHIR URL, both SHALL be consistent. 067 068The type is the Canonical URL of Resource Definition that is the type this reference refers to. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition/ e.g. "Patient" is a reference to http://hl7.org/fhir/StructureDefinition/Patient. Absolute URLs are only allowed for logical models (and can only be used in references in logical models, not resources). 069 */ 070 @Child(name = "type", type = {UriType.class}, order=1, min=0, max=1, modifier=false, summary=true) 071 @Description(shortDefinition="Type the reference refers to (e.g. \"Patient\") - must be a resource in resources", formalDefinition="The expected type of the target of the reference. If both Reference.type and Reference.reference are populated and Reference.reference is a FHIR URL, both SHALL be consistent.\n\nThe type is the Canonical URL of Resource Definition that is the type this reference refers to. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition/ e.g. \"Patient\" is a reference to http://hl7.org/fhir/StructureDefinition/Patient. Absolute URLs are only allowed for logical models (and can only be used in references in logical models, not resources)." ) 072 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 073 protected UriType type; 074 075 /** 076 * An identifier for the target resource. This is used when there is no way to reference the other resource directly, either because the entity it represents is not available through a FHIR server, or because there is no way for the author of the resource to convert a known identifier to an actual location. There is no requirement that a Reference.identifier point to something that is actually exposed as a FHIR instance, but it SHALL point to a business concept that would be expected to be exposed as a FHIR instance, and that instance would need to be of a FHIR resource type allowed by the reference. 077 */ 078 @Child(name = "identifier", type = {Identifier.class}, order=2, min=0, max=1, modifier=false, summary=true) 079 @Description(shortDefinition="Logical reference, when literal reference is not known", formalDefinition="An identifier for the target resource. This is used when there is no way to reference the other resource directly, either because the entity it represents is not available through a FHIR server, or because there is no way for the author of the resource to convert a known identifier to an actual location. There is no requirement that a Reference.identifier point to something that is actually exposed as a FHIR instance, but it SHALL point to a business concept that would be expected to be exposed as a FHIR instance, and that instance would need to be of a FHIR resource type allowed by the reference." ) 080 protected Identifier identifier; 081 082 /** 083 * Plain text narrative that identifies the resource in addition to the resource reference. 084 */ 085 @Child(name = "display", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 086 @Description(shortDefinition="Text alternative for the resource", formalDefinition="Plain text narrative that identifies the resource in addition to the resource reference." ) 087 protected StringType display; 088 089 private static final long serialVersionUID = 784245805L; 090 091 /** 092 * Constructor 093 */ 094 public Reference() { 095 super(); 096 } 097 098 /** 099 * Constructor 100 * 101 * @param theReference The given reference string (e.g. "Patient/123" or "http://example.com/Patient/123") 102 */ 103 public Reference(String theReference) { 104 super(theReference); 105 } 106 107 /** 108 * Constructor 109 * 110 * @param theReference The given reference as an IdType (e.g. "Patient/123" or "http://example.com/Patient/123") 111 */ 112 public Reference(IIdType theReference) { 113 super(theReference); 114 } 115 116 /** 117 * Constructor 118 * 119 * @param theResource The resource represented by this reference 120 */ 121 public Reference(IAnyResource theResource) { 122 super(theResource); 123 } 124 125 /** 126 * @return {@link #reference} (A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value 127 */ 128 public StringType getReferenceElement_() { 129 if (this.reference == null) 130 if (Configuration.errorOnAutoCreate()) 131 throw new Error("Attempt to auto-create Reference.reference"); 132 else if (Configuration.doAutoCreate()) 133 this.reference = new StringType(); // bb 134 return this.reference; 135 } 136 137 public boolean hasReferenceElement() { 138 return this.reference != null && !this.reference.isEmpty(); 139 } 140 141 public boolean hasReference() { 142 return this.reference != null && !this.reference.isEmpty(); 143 } 144 145 /** 146 * @param value {@link #reference} (A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value 147 */ 148 public Reference setReferenceElement(StringType value) { 149 this.reference = value; 150 return this; 151 } 152 153 /** 154 * @return A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources. 155 */ 156 public String getReference() { 157 return this.reference == null ? null : this.reference.getValue(); 158 } 159 160 /** 161 * @param value A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources. 162 */ 163 public Reference setReference(String value) { 164 if (Utilities.noString(value)) 165 this.reference = null; 166 else { 167 if (this.reference == null) 168 this.reference = new StringType(); 169 this.reference.setValue(value); 170 } 171 return this; 172 } 173 174 /** 175 * @return {@link #type} (The expected type of the target of the reference. If both Reference.type and Reference.reference are populated and Reference.reference is a FHIR URL, both SHALL be consistent. 176 177The type is the Canonical URL of Resource Definition that is the type this reference refers to. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition/ e.g. "Patient" is a reference to http://hl7.org/fhir/StructureDefinition/Patient. Absolute URLs are only allowed for logical models (and can only be used in references in logical models, not resources).). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 178 */ 179 public UriType getTypeElement() { 180 if (this.type == null) 181 if (Configuration.errorOnAutoCreate()) 182 throw new Error("Attempt to auto-create Reference.type"); 183 else if (Configuration.doAutoCreate()) 184 this.type = new UriType(); // bb 185 return this.type; 186 } 187 188 public boolean hasTypeElement() { 189 return this.type != null && !this.type.isEmpty(); 190 } 191 192 public boolean hasType() { 193 return this.type != null && !this.type.isEmpty(); 194 } 195 196 /** 197 * @param value {@link #type} (The expected type of the target of the reference. If both Reference.type and Reference.reference are populated and Reference.reference is a FHIR URL, both SHALL be consistent. 198 199The type is the Canonical URL of Resource Definition that is the type this reference refers to. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition/ e.g. "Patient" is a reference to http://hl7.org/fhir/StructureDefinition/Patient. Absolute URLs are only allowed for logical models (and can only be used in references in logical models, not resources).). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 200 */ 201 public Reference setTypeElement(UriType value) { 202 this.type = value; 203 return this; 204 } 205 206 /** 207 * @return The expected type of the target of the reference. If both Reference.type and Reference.reference are populated and Reference.reference is a FHIR URL, both SHALL be consistent. 208 209The type is the Canonical URL of Resource Definition that is the type this reference refers to. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition/ e.g. "Patient" is a reference to http://hl7.org/fhir/StructureDefinition/Patient. Absolute URLs are only allowed for logical models (and can only be used in references in logical models, not resources). 210 */ 211 public String getType() { 212 return this.type == null ? null : this.type.getValue(); 213 } 214 215 /** 216 * @param value The expected type of the target of the reference. If both Reference.type and Reference.reference are populated and Reference.reference is a FHIR URL, both SHALL be consistent. 217 218The type is the Canonical URL of Resource Definition that is the type this reference refers to. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition/ e.g. "Patient" is a reference to http://hl7.org/fhir/StructureDefinition/Patient. Absolute URLs are only allowed for logical models (and can only be used in references in logical models, not resources). 219 */ 220 public Reference setType(String value) { 221 if (Utilities.noString(value)) 222 this.type = null; 223 else { 224 if (this.type == null) 225 this.type = new UriType(); 226 this.type.setValue(value); 227 } 228 return this; 229 } 230 231 /** 232 * @return {@link #identifier} (An identifier for the target resource. This is used when there is no way to reference the other resource directly, either because the entity it represents is not available through a FHIR server, or because there is no way for the author of the resource to convert a known identifier to an actual location. There is no requirement that a Reference.identifier point to something that is actually exposed as a FHIR instance, but it SHALL point to a business concept that would be expected to be exposed as a FHIR instance, and that instance would need to be of a FHIR resource type allowed by the reference.) 233 */ 234 public Identifier getIdentifier() { 235 if (this.identifier == null) 236 if (Configuration.errorOnAutoCreate()) 237 throw new Error("Attempt to auto-create Reference.identifier"); 238 else if (Configuration.doAutoCreate()) 239 this.identifier = new Identifier(); // cc 240 return this.identifier; 241 } 242 243 public boolean hasIdentifier() { 244 return this.identifier != null && !this.identifier.isEmpty(); 245 } 246 247 /** 248 * @param value {@link #identifier} (An identifier for the target resource. This is used when there is no way to reference the other resource directly, either because the entity it represents is not available through a FHIR server, or because there is no way for the author of the resource to convert a known identifier to an actual location. There is no requirement that a Reference.identifier point to something that is actually exposed as a FHIR instance, but it SHALL point to a business concept that would be expected to be exposed as a FHIR instance, and that instance would need to be of a FHIR resource type allowed by the reference.) 249 */ 250 public Reference setIdentifier(Identifier value) { 251 this.identifier = value; 252 return this; 253 } 254 255 /** 256 * @return {@link #display} (Plain text narrative that identifies the resource in addition to the resource reference.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 257 */ 258 public StringType getDisplayElement() { 259 if (this.display == null) 260 if (Configuration.errorOnAutoCreate()) 261 throw new Error("Attempt to auto-create Reference.display"); 262 else if (Configuration.doAutoCreate()) 263 this.display = new StringType(); // bb 264 return this.display; 265 } 266 267 public boolean hasDisplayElement() { 268 return this.display != null && !this.display.isEmpty(); 269 } 270 271 public boolean hasDisplay() { 272 return this.display != null && !this.display.isEmpty(); 273 } 274 275 /** 276 * @param value {@link #display} (Plain text narrative that identifies the resource in addition to the resource reference.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 277 */ 278 public Reference setDisplayElement(StringType value) { 279 this.display = value; 280 return this; 281 } 282 283 /** 284 * @return Plain text narrative that identifies the resource in addition to the resource reference. 285 */ 286 public String getDisplay() { 287 return this.display == null ? null : this.display.getValue(); 288 } 289 290 /** 291 * @param value Plain text narrative that identifies the resource in addition to the resource reference. 292 */ 293 public Reference setDisplay(String value) { 294 if (Utilities.noString(value)) 295 this.display = null; 296 else { 297 if (this.display == null) 298 this.display = new StringType(); 299 this.display.setValue(value); 300 } 301 return this; 302 } 303 304 protected void listChildren(List<Property> children) { 305 super.listChildren(children); 306 children.add(new Property("reference", "string", "A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources.", 0, 1, reference)); 307 children.add(new Property("type", "uri", "The expected type of the target of the reference. If both Reference.type and Reference.reference are populated and Reference.reference is a FHIR URL, both SHALL be consistent.\n\nThe type is the Canonical URL of Resource Definition that is the type this reference refers to. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition/ e.g. \"Patient\" is a reference to http://hl7.org/fhir/StructureDefinition/Patient. Absolute URLs are only allowed for logical models (and can only be used in references in logical models, not resources).", 0, 1, type)); 308 children.add(new Property("identifier", "Identifier", "An identifier for the target resource. This is used when there is no way to reference the other resource directly, either because the entity it represents is not available through a FHIR server, or because there is no way for the author of the resource to convert a known identifier to an actual location. There is no requirement that a Reference.identifier point to something that is actually exposed as a FHIR instance, but it SHALL point to a business concept that would be expected to be exposed as a FHIR instance, and that instance would need to be of a FHIR resource type allowed by the reference.", 0, 1, identifier)); 309 children.add(new Property("display", "string", "Plain text narrative that identifies the resource in addition to the resource reference.", 0, 1, display)); 310 } 311 312 @Override 313 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 314 switch (_hash) { 315 case -925155509: /*reference*/ return new Property("reference", "string", "A reference to a location at which the other resource is found. The reference may be a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources.", 0, 1, reference); 316 case 3575610: /*type*/ return new Property("type", "uri", "The expected type of the target of the reference. If both Reference.type and Reference.reference are populated and Reference.reference is a FHIR URL, both SHALL be consistent.\n\nThe type is the Canonical URL of Resource Definition that is the type this reference refers to. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition/ e.g. \"Patient\" is a reference to http://hl7.org/fhir/StructureDefinition/Patient. Absolute URLs are only allowed for logical models (and can only be used in references in logical models, not resources).", 0, 1, type); 317 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "An identifier for the target resource. This is used when there is no way to reference the other resource directly, either because the entity it represents is not available through a FHIR server, or because there is no way for the author of the resource to convert a known identifier to an actual location. There is no requirement that a Reference.identifier point to something that is actually exposed as a FHIR instance, but it SHALL point to a business concept that would be expected to be exposed as a FHIR instance, and that instance would need to be of a FHIR resource type allowed by the reference.", 0, 1, identifier); 318 case 1671764162: /*display*/ return new Property("display", "string", "Plain text narrative that identifies the resource in addition to the resource reference.", 0, 1, display); 319 default: return super.getNamedProperty(_hash, _name, _checkValid); 320 } 321 322 } 323 324 @Override 325 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 326 switch (hash) { 327 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // StringType 328 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // UriType 329 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 330 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 331 default: return super.getProperty(hash, name, checkValid); 332 } 333 334 } 335 336 @Override 337 public Base setProperty(int hash, String name, Base value) throws FHIRException { 338 switch (hash) { 339 case -925155509: // reference 340 this.reference = TypeConvertor.castToString(value); // StringType 341 return value; 342 case 3575610: // type 343 this.type = TypeConvertor.castToUri(value); // UriType 344 return value; 345 case -1618432855: // identifier 346 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 347 return value; 348 case 1671764162: // display 349 this.display = TypeConvertor.castToString(value); // StringType 350 return value; 351 default: return super.setProperty(hash, name, value); 352 } 353 354 } 355 356 @Override 357 public Base setProperty(String name, Base value) throws FHIRException { 358 if (name.equals("reference")) { 359 this.reference = TypeConvertor.castToString(value); // StringType 360 } else if (name.equals("type")) { 361 this.type = TypeConvertor.castToUri(value); // UriType 362 } else if (name.equals("identifier")) { 363 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 364 } else if (name.equals("display")) { 365 this.display = TypeConvertor.castToString(value); // StringType 366 } else 367 return super.setProperty(name, value); 368 return value; 369 } 370 371 @Override 372 public void removeChild(String name, Base value) throws FHIRException { 373 if (name.equals("reference")) { 374 this.reference = null; 375 } else if (name.equals("type")) { 376 this.type = null; 377 } else if (name.equals("identifier")) { 378 this.identifier = null; 379 } else if (name.equals("display")) { 380 this.display = null; 381 } else 382 super.removeChild(name, value); 383 384 } 385 386 @Override 387 public Base makeProperty(int hash, String name) throws FHIRException { 388 switch (hash) { 389 case -925155509: return getReferenceElement_(); 390 case 3575610: return getTypeElement(); 391 case -1618432855: return getIdentifier(); 392 case 1671764162: return getDisplayElement(); 393 default: return super.makeProperty(hash, name); 394 } 395 396 } 397 398 @Override 399 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 400 switch (hash) { 401 case -925155509: /*reference*/ return new String[] {"string"}; 402 case 3575610: /*type*/ return new String[] {"uri"}; 403 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 404 case 1671764162: /*display*/ return new String[] {"string"}; 405 default: return super.getTypesForProperty(hash, name); 406 } 407 408 } 409 410 @Override 411 public Base addChild(String name) throws FHIRException { 412 if (name.equals("reference")) { 413 throw new FHIRException("Cannot call addChild on a singleton property Reference.reference"); 414 } 415 else if (name.equals("type")) { 416 throw new FHIRException("Cannot call addChild on a singleton property Reference.type"); 417 } 418 else if (name.equals("identifier")) { 419 this.identifier = new Identifier(); 420 return this.identifier; 421 } 422 else if (name.equals("display")) { 423 throw new FHIRException("Cannot call addChild on a singleton property Reference.display"); 424 } 425 else 426 return super.addChild(name); 427 } 428 429 public String fhirType() { 430 return "Reference"; 431 432 } 433 434 public Reference copy() { 435 Reference dst = new Reference(); 436 copyValues(dst); 437 return dst; 438 } 439 440 public void copyValues(Reference dst) { 441 super.copyValues(dst); 442 dst.reference = reference == null ? null : reference.copy(); 443 dst.type = type == null ? null : type.copy(); 444 dst.identifier = identifier == null ? null : identifier.copy(); 445 dst.display = display == null ? null : display.copy(); 446 } 447 448 protected Reference typedCopy() { 449 return copy(); 450 } 451 452 @Override 453 public boolean equalsDeep(Base other_) { 454 if (!super.equalsDeep(other_)) 455 return false; 456 if (!(other_ instanceof Reference)) 457 return false; 458 Reference o = (Reference) other_; 459 return compareDeep(reference, o.reference, true) && compareDeep(type, o.type, true) && compareDeep(identifier, o.identifier, true) 460 && compareDeep(display, o.display, true); 461 } 462 463 @Override 464 public boolean equalsShallow(Base other_) { 465 if (!super.equalsShallow(other_)) 466 return false; 467 if (!(other_ instanceof Reference)) 468 return false; 469 Reference o = (Reference) other_; 470 return compareValues(reference, o.reference, true) && compareValues(type, o.type, true) && compareValues(display, o.display, true) 471 ; 472 } 473 474 public boolean isEmpty() { 475 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, type, identifier 476 , display); 477 } 478 479// Manual code (from Configuration.txt): 480@Override 481 public String toString() { 482 if (hasReference()) 483 return "Reference["+getReference()+"]"; 484 if (hasIdentifier()) 485 return "Reference[id:"+getIdentifier()+"]"; 486 if (hasDisplay()) 487 return "Reference['"+getDisplay()+"']"; 488 return "Reference[??]"; 489 } 490 491 /** 492 * Convenience setter which sets the reference to the complete {@link IIdType#getValue() value} of the given 493 * reference. 494 * 495 * @param theReference The reference, or <code>null</code> 496 * @return 497 * @return Returns a reference to this 498 */ 499 public Reference setReferenceElement(IIdType theReference) { 500 if (theReference != null) { 501 setReference(theReference.getValue()); 502 } else { 503 setReference(null); 504 } 505 return this; 506 } 507 508 509 public boolean matches(Reference value) { 510 if (value.hasReference() || hasReference()) { 511 if (!(value.hasReference() && hasReference())) { 512 return false; 513 } 514 if (!reference.matches(value.getReference())) { 515 return true; 516 } 517 } 518 if (value.hasIdentifier() || hasIdentifier()) { 519 if (!(value.hasIdentifier() && hasIdentifier())) { 520 return false; 521 } 522 if (!identifier.matches(value.getIdentifier())) { 523 return true; 524 } 525 } 526 return false; 527 } 528 529 530// end addition 531 532} 533