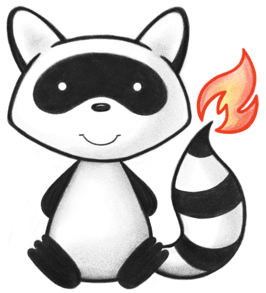
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.model.Enumerations.*; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.Block; 048 049import org.hl7.fhir.utilities.Utilities; 050/** 051 * Regulatory approval, clearance or licencing related to a regulated product, treatment, facility or activity that is cited in a guidance, regulation, rule or legislative act. An example is Market Authorization relating to a Medicinal Product. 052 */ 053@ResourceDef(name="RegulatedAuthorization", profile="http://hl7.org/fhir/StructureDefinition/RegulatedAuthorization") 054public class RegulatedAuthorization extends DomainResource { 055 056 @Block() 057 public static class RegulatedAuthorizationCaseComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Identifier by which this case can be referenced. 060 */ 061 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="Identifier by which this case can be referenced", formalDefinition="Identifier by which this case can be referenced." ) 063 protected Identifier identifier; 064 065 /** 066 * The defining type of case. 067 */ 068 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 069 @Description(shortDefinition="The defining type of case", formalDefinition="The defining type of case." ) 070 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/regulated-authorization-case-type") 071 protected CodeableConcept type; 072 073 /** 074 * The status associated with the case. 075 */ 076 @Child(name = "status", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 077 @Description(shortDefinition="The status associated with the case", formalDefinition="The status associated with the case." ) 078 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 079 protected CodeableConcept status; 080 081 /** 082 * Relevant date for this case. 083 */ 084 @Child(name = "date", type = {Period.class, DateTimeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 085 @Description(shortDefinition="Relevant date for this case", formalDefinition="Relevant date for this case." ) 086 protected DataType date; 087 088 /** 089 * A regulatory submission from an organization to a regulator, as part of an assessing case. Multiple applications may occur over time, with more or different information to support or modify the submission or the authorization. The applications can be considered as steps within the longer running case or procedure for this authorization process. 090 */ 091 @Child(name = "application", type = {RegulatedAuthorizationCaseComponent.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 092 @Description(shortDefinition="Applications submitted to obtain a regulated authorization. Steps within the longer running case or procedure", formalDefinition="A regulatory submission from an organization to a regulator, as part of an assessing case. Multiple applications may occur over time, with more or different information to support or modify the submission or the authorization. The applications can be considered as steps within the longer running case or procedure for this authorization process." ) 093 protected List<RegulatedAuthorizationCaseComponent> application; 094 095 private static final long serialVersionUID = 2052202113L; 096 097 /** 098 * Constructor 099 */ 100 public RegulatedAuthorizationCaseComponent() { 101 super(); 102 } 103 104 /** 105 * @return {@link #identifier} (Identifier by which this case can be referenced.) 106 */ 107 public Identifier getIdentifier() { 108 if (this.identifier == null) 109 if (Configuration.errorOnAutoCreate()) 110 throw new Error("Attempt to auto-create RegulatedAuthorizationCaseComponent.identifier"); 111 else if (Configuration.doAutoCreate()) 112 this.identifier = new Identifier(); // cc 113 return this.identifier; 114 } 115 116 public boolean hasIdentifier() { 117 return this.identifier != null && !this.identifier.isEmpty(); 118 } 119 120 /** 121 * @param value {@link #identifier} (Identifier by which this case can be referenced.) 122 */ 123 public RegulatedAuthorizationCaseComponent setIdentifier(Identifier value) { 124 this.identifier = value; 125 return this; 126 } 127 128 /** 129 * @return {@link #type} (The defining type of case.) 130 */ 131 public CodeableConcept getType() { 132 if (this.type == null) 133 if (Configuration.errorOnAutoCreate()) 134 throw new Error("Attempt to auto-create RegulatedAuthorizationCaseComponent.type"); 135 else if (Configuration.doAutoCreate()) 136 this.type = new CodeableConcept(); // cc 137 return this.type; 138 } 139 140 public boolean hasType() { 141 return this.type != null && !this.type.isEmpty(); 142 } 143 144 /** 145 * @param value {@link #type} (The defining type of case.) 146 */ 147 public RegulatedAuthorizationCaseComponent setType(CodeableConcept value) { 148 this.type = value; 149 return this; 150 } 151 152 /** 153 * @return {@link #status} (The status associated with the case.) 154 */ 155 public CodeableConcept getStatus() { 156 if (this.status == null) 157 if (Configuration.errorOnAutoCreate()) 158 throw new Error("Attempt to auto-create RegulatedAuthorizationCaseComponent.status"); 159 else if (Configuration.doAutoCreate()) 160 this.status = new CodeableConcept(); // cc 161 return this.status; 162 } 163 164 public boolean hasStatus() { 165 return this.status != null && !this.status.isEmpty(); 166 } 167 168 /** 169 * @param value {@link #status} (The status associated with the case.) 170 */ 171 public RegulatedAuthorizationCaseComponent setStatus(CodeableConcept value) { 172 this.status = value; 173 return this; 174 } 175 176 /** 177 * @return {@link #date} (Relevant date for this case.) 178 */ 179 public DataType getDate() { 180 return this.date; 181 } 182 183 /** 184 * @return {@link #date} (Relevant date for this case.) 185 */ 186 public Period getDatePeriod() throws FHIRException { 187 if (this.date == null) 188 this.date = new Period(); 189 if (!(this.date instanceof Period)) 190 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.date.getClass().getName()+" was encountered"); 191 return (Period) this.date; 192 } 193 194 public boolean hasDatePeriod() { 195 return this != null && this.date instanceof Period; 196 } 197 198 /** 199 * @return {@link #date} (Relevant date for this case.) 200 */ 201 public DateTimeType getDateDateTimeType() throws FHIRException { 202 if (this.date == null) 203 this.date = new DateTimeType(); 204 if (!(this.date instanceof DateTimeType)) 205 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.date.getClass().getName()+" was encountered"); 206 return (DateTimeType) this.date; 207 } 208 209 public boolean hasDateDateTimeType() { 210 return this != null && this.date instanceof DateTimeType; 211 } 212 213 public boolean hasDate() { 214 return this.date != null && !this.date.isEmpty(); 215 } 216 217 /** 218 * @param value {@link #date} (Relevant date for this case.) 219 */ 220 public RegulatedAuthorizationCaseComponent setDate(DataType value) { 221 if (value != null && !(value instanceof Period || value instanceof DateTimeType)) 222 throw new FHIRException("Not the right type for RegulatedAuthorization.case.date[x]: "+value.fhirType()); 223 this.date = value; 224 return this; 225 } 226 227 /** 228 * @return {@link #application} (A regulatory submission from an organization to a regulator, as part of an assessing case. Multiple applications may occur over time, with more or different information to support or modify the submission or the authorization. The applications can be considered as steps within the longer running case or procedure for this authorization process.) 229 */ 230 public List<RegulatedAuthorizationCaseComponent> getApplication() { 231 if (this.application == null) 232 this.application = new ArrayList<RegulatedAuthorizationCaseComponent>(); 233 return this.application; 234 } 235 236 /** 237 * @return Returns a reference to <code>this</code> for easy method chaining 238 */ 239 public RegulatedAuthorizationCaseComponent setApplication(List<RegulatedAuthorizationCaseComponent> theApplication) { 240 this.application = theApplication; 241 return this; 242 } 243 244 public boolean hasApplication() { 245 if (this.application == null) 246 return false; 247 for (RegulatedAuthorizationCaseComponent item : this.application) 248 if (!item.isEmpty()) 249 return true; 250 return false; 251 } 252 253 public RegulatedAuthorizationCaseComponent addApplication() { //3 254 RegulatedAuthorizationCaseComponent t = new RegulatedAuthorizationCaseComponent(); 255 if (this.application == null) 256 this.application = new ArrayList<RegulatedAuthorizationCaseComponent>(); 257 this.application.add(t); 258 return t; 259 } 260 261 public RegulatedAuthorizationCaseComponent addApplication(RegulatedAuthorizationCaseComponent t) { //3 262 if (t == null) 263 return this; 264 if (this.application == null) 265 this.application = new ArrayList<RegulatedAuthorizationCaseComponent>(); 266 this.application.add(t); 267 return this; 268 } 269 270 /** 271 * @return The first repetition of repeating field {@link #application}, creating it if it does not already exist {3} 272 */ 273 public RegulatedAuthorizationCaseComponent getApplicationFirstRep() { 274 if (getApplication().isEmpty()) { 275 addApplication(); 276 } 277 return getApplication().get(0); 278 } 279 280 protected void listChildren(List<Property> children) { 281 super.listChildren(children); 282 children.add(new Property("identifier", "Identifier", "Identifier by which this case can be referenced.", 0, 1, identifier)); 283 children.add(new Property("type", "CodeableConcept", "The defining type of case.", 0, 1, type)); 284 children.add(new Property("status", "CodeableConcept", "The status associated with the case.", 0, 1, status)); 285 children.add(new Property("date[x]", "Period|dateTime", "Relevant date for this case.", 0, 1, date)); 286 children.add(new Property("application", "@RegulatedAuthorization.case", "A regulatory submission from an organization to a regulator, as part of an assessing case. Multiple applications may occur over time, with more or different information to support or modify the submission or the authorization. The applications can be considered as steps within the longer running case or procedure for this authorization process.", 0, java.lang.Integer.MAX_VALUE, application)); 287 } 288 289 @Override 290 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 291 switch (_hash) { 292 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier by which this case can be referenced.", 0, 1, identifier); 293 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The defining type of case.", 0, 1, type); 294 case -892481550: /*status*/ return new Property("status", "CodeableConcept", "The status associated with the case.", 0, 1, status); 295 case 1443311122: /*date[x]*/ return new Property("date[x]", "Period|dateTime", "Relevant date for this case.", 0, 1, date); 296 case 3076014: /*date*/ return new Property("date[x]", "Period|dateTime", "Relevant date for this case.", 0, 1, date); 297 case 432297743: /*datePeriod*/ return new Property("date[x]", "Period", "Relevant date for this case.", 0, 1, date); 298 case 185136489: /*dateDateTime*/ return new Property("date[x]", "dateTime", "Relevant date for this case.", 0, 1, date); 299 case 1554253136: /*application*/ return new Property("application", "@RegulatedAuthorization.case", "A regulatory submission from an organization to a regulator, as part of an assessing case. Multiple applications may occur over time, with more or different information to support or modify the submission or the authorization. The applications can be considered as steps within the longer running case or procedure for this authorization process.", 0, java.lang.Integer.MAX_VALUE, application); 300 default: return super.getNamedProperty(_hash, _name, _checkValid); 301 } 302 303 } 304 305 @Override 306 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 307 switch (hash) { 308 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 309 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 310 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // CodeableConcept 311 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DataType 312 case 1554253136: /*application*/ return this.application == null ? new Base[0] : this.application.toArray(new Base[this.application.size()]); // RegulatedAuthorizationCaseComponent 313 default: return super.getProperty(hash, name, checkValid); 314 } 315 316 } 317 318 @Override 319 public Base setProperty(int hash, String name, Base value) throws FHIRException { 320 switch (hash) { 321 case -1618432855: // identifier 322 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 323 return value; 324 case 3575610: // type 325 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 326 return value; 327 case -892481550: // status 328 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 329 return value; 330 case 3076014: // date 331 this.date = TypeConvertor.castToType(value); // DataType 332 return value; 333 case 1554253136: // application 334 this.getApplication().add((RegulatedAuthorizationCaseComponent) value); // RegulatedAuthorizationCaseComponent 335 return value; 336 default: return super.setProperty(hash, name, value); 337 } 338 339 } 340 341 @Override 342 public Base setProperty(String name, Base value) throws FHIRException { 343 if (name.equals("identifier")) { 344 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 345 } else if (name.equals("type")) { 346 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 347 } else if (name.equals("status")) { 348 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 349 } else if (name.equals("date[x]")) { 350 this.date = TypeConvertor.castToType(value); // DataType 351 } else if (name.equals("application")) { 352 this.getApplication().add((RegulatedAuthorizationCaseComponent) value); 353 } else 354 return super.setProperty(name, value); 355 return value; 356 } 357 358 @Override 359 public void removeChild(String name, Base value) throws FHIRException { 360 if (name.equals("identifier")) { 361 this.identifier = null; 362 } else if (name.equals("type")) { 363 this.type = null; 364 } else if (name.equals("status")) { 365 this.status = null; 366 } else if (name.equals("date[x]")) { 367 this.date = null; 368 } else if (name.equals("application")) { 369 this.getApplication().remove((RegulatedAuthorizationCaseComponent) value); 370 } else 371 super.removeChild(name, value); 372 373 } 374 375 @Override 376 public Base makeProperty(int hash, String name) throws FHIRException { 377 switch (hash) { 378 case -1618432855: return getIdentifier(); 379 case 3575610: return getType(); 380 case -892481550: return getStatus(); 381 case 1443311122: return getDate(); 382 case 3076014: return getDate(); 383 case 1554253136: return addApplication(); 384 default: return super.makeProperty(hash, name); 385 } 386 387 } 388 389 @Override 390 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 391 switch (hash) { 392 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 393 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 394 case -892481550: /*status*/ return new String[] {"CodeableConcept"}; 395 case 3076014: /*date*/ return new String[] {"Period", "dateTime"}; 396 case 1554253136: /*application*/ return new String[] {"@RegulatedAuthorization.case"}; 397 default: return super.getTypesForProperty(hash, name); 398 } 399 400 } 401 402 @Override 403 public Base addChild(String name) throws FHIRException { 404 if (name.equals("identifier")) { 405 this.identifier = new Identifier(); 406 return this.identifier; 407 } 408 else if (name.equals("type")) { 409 this.type = new CodeableConcept(); 410 return this.type; 411 } 412 else if (name.equals("status")) { 413 this.status = new CodeableConcept(); 414 return this.status; 415 } 416 else if (name.equals("datePeriod")) { 417 this.date = new Period(); 418 return this.date; 419 } 420 else if (name.equals("dateDateTime")) { 421 this.date = new DateTimeType(); 422 return this.date; 423 } 424 else if (name.equals("application")) { 425 return addApplication(); 426 } 427 else 428 return super.addChild(name); 429 } 430 431 public RegulatedAuthorizationCaseComponent copy() { 432 RegulatedAuthorizationCaseComponent dst = new RegulatedAuthorizationCaseComponent(); 433 copyValues(dst); 434 return dst; 435 } 436 437 public void copyValues(RegulatedAuthorizationCaseComponent dst) { 438 super.copyValues(dst); 439 dst.identifier = identifier == null ? null : identifier.copy(); 440 dst.type = type == null ? null : type.copy(); 441 dst.status = status == null ? null : status.copy(); 442 dst.date = date == null ? null : date.copy(); 443 if (application != null) { 444 dst.application = new ArrayList<RegulatedAuthorizationCaseComponent>(); 445 for (RegulatedAuthorizationCaseComponent i : application) 446 dst.application.add(i.copy()); 447 }; 448 } 449 450 @Override 451 public boolean equalsDeep(Base other_) { 452 if (!super.equalsDeep(other_)) 453 return false; 454 if (!(other_ instanceof RegulatedAuthorizationCaseComponent)) 455 return false; 456 RegulatedAuthorizationCaseComponent o = (RegulatedAuthorizationCaseComponent) other_; 457 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) && compareDeep(status, o.status, true) 458 && compareDeep(date, o.date, true) && compareDeep(application, o.application, true); 459 } 460 461 @Override 462 public boolean equalsShallow(Base other_) { 463 if (!super.equalsShallow(other_)) 464 return false; 465 if (!(other_ instanceof RegulatedAuthorizationCaseComponent)) 466 return false; 467 RegulatedAuthorizationCaseComponent o = (RegulatedAuthorizationCaseComponent) other_; 468 return true; 469 } 470 471 public boolean isEmpty() { 472 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, status 473 , date, application); 474 } 475 476 public String fhirType() { 477 return "RegulatedAuthorization.case"; 478 479 } 480 481 } 482 483 /** 484 * Business identifier for the authorization, typically assigned by the authorizing body. 485 */ 486 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 487 @Description(shortDefinition="Business identifier for the authorization, typically assigned by the authorizing body", formalDefinition="Business identifier for the authorization, typically assigned by the authorizing body." ) 488 protected List<Identifier> identifier; 489 490 /** 491 * The product type, treatment, facility or activity that is being authorized. 492 */ 493 @Child(name = "subject", type = {MedicinalProductDefinition.class, BiologicallyDerivedProduct.class, NutritionProduct.class, PackagedProductDefinition.class, ManufacturedItemDefinition.class, Ingredient.class, SubstanceDefinition.class, DeviceDefinition.class, ResearchStudy.class, ActivityDefinition.class, PlanDefinition.class, ObservationDefinition.class, Practitioner.class, Organization.class, Location.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 494 @Description(shortDefinition="The product type, treatment, facility or activity that is being authorized", formalDefinition="The product type, treatment, facility or activity that is being authorized." ) 495 protected List<Reference> subject; 496 497 /** 498 * Overall type of this authorization, for example drug marketing approval, orphan drug designation. 499 */ 500 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 501 @Description(shortDefinition="Overall type of this authorization, for example drug marketing approval, orphan drug designation", formalDefinition="Overall type of this authorization, for example drug marketing approval, orphan drug designation." ) 502 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/regulated-authorization-type") 503 protected CodeableConcept type; 504 505 /** 506 * General textual supporting information. 507 */ 508 @Child(name = "description", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=true) 509 @Description(shortDefinition="General textual supporting information", formalDefinition="General textual supporting information." ) 510 protected MarkdownType description; 511 512 /** 513 * The territory (e.g., country, jurisdiction etc.) in which the authorization has been granted. 514 */ 515 @Child(name = "region", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 516 @Description(shortDefinition="The territory in which the authorization has been granted", formalDefinition="The territory (e.g., country, jurisdiction etc.) in which the authorization has been granted." ) 517 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 518 protected List<CodeableConcept> region; 519 520 /** 521 * The status that is authorised e.g. approved. Intermediate states and actions can be tracked with cases and applications. 522 */ 523 @Child(name = "status", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 524 @Description(shortDefinition="The status that is authorised e.g. approved. Intermediate states can be tracked with cases and applications", formalDefinition="The status that is authorised e.g. approved. Intermediate states and actions can be tracked with cases and applications." ) 525 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 526 protected CodeableConcept status; 527 528 /** 529 * The date at which the current status was assigned. 530 */ 531 @Child(name = "statusDate", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 532 @Description(shortDefinition="The date at which the current status was assigned", formalDefinition="The date at which the current status was assigned." ) 533 protected DateTimeType statusDate; 534 535 /** 536 * The time period in which the regulatory approval, clearance or licencing is in effect. As an example, a Marketing Authorization includes the date of authorization and/or an expiration date. 537 */ 538 @Child(name = "validityPeriod", type = {Period.class}, order=7, min=0, max=1, modifier=false, summary=true) 539 @Description(shortDefinition="The time period in which the regulatory approval etc. is in effect, e.g. a Marketing Authorization includes the date of authorization and/or expiration date", formalDefinition="The time period in which the regulatory approval, clearance or licencing is in effect. As an example, a Marketing Authorization includes the date of authorization and/or an expiration date." ) 540 protected Period validityPeriod; 541 542 /** 543 * Condition for which the use of the regulated product applies. 544 */ 545 @Child(name = "indication", type = {CodeableReference.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 546 @Description(shortDefinition="Condition for which the use of the regulated product applies", formalDefinition="Condition for which the use of the regulated product applies." ) 547 protected List<CodeableReference> indication; 548 549 /** 550 * The intended use of the product, e.g. prevention, treatment, diagnosis. 551 */ 552 @Child(name = "intendedUse", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=true) 553 @Description(shortDefinition="The intended use of the product, e.g. prevention, treatment", formalDefinition="The intended use of the product, e.g. prevention, treatment, diagnosis." ) 554 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/product-intended-use") 555 protected CodeableConcept intendedUse; 556 557 /** 558 * The legal or regulatory framework against which this authorization is granted, or other reasons for it. 559 */ 560 @Child(name = "basis", type = {CodeableConcept.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 561 @Description(shortDefinition="The legal/regulatory framework or reasons under which this authorization is granted", formalDefinition="The legal or regulatory framework against which this authorization is granted, or other reasons for it." ) 562 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/regulated-authorization-basis") 563 protected List<CodeableConcept> basis; 564 565 /** 566 * The organization that has been granted this authorization, by some authoritative body (the 'regulator'). 567 */ 568 @Child(name = "holder", type = {Organization.class}, order=11, min=0, max=1, modifier=false, summary=true) 569 @Description(shortDefinition="The organization that has been granted this authorization, by the regulator", formalDefinition="The organization that has been granted this authorization, by some authoritative body (the 'regulator')." ) 570 protected Reference holder; 571 572 /** 573 * The regulatory authority or authorizing body granting the authorization. For example, European Medicines Agency (EMA), Food and Drug Administration (FDA), Health Canada (HC), etc. 574 */ 575 @Child(name = "regulator", type = {Organization.class}, order=12, min=0, max=1, modifier=false, summary=true) 576 @Description(shortDefinition="The regulatory authority or authorizing body granting the authorization", formalDefinition="The regulatory authority or authorizing body granting the authorization. For example, European Medicines Agency (EMA), Food and Drug Administration (FDA), Health Canada (HC), etc." ) 577 protected Reference regulator; 578 579 /** 580 * Additional information or supporting documentation about the authorization. 581 */ 582 @Child(name = "attachedDocument", type = {DocumentReference.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 583 @Description(shortDefinition="Additional information or supporting documentation about the authorization", formalDefinition="Additional information or supporting documentation about the authorization." ) 584 protected List<Reference> attachedDocument; 585 586 /** 587 * The case or regulatory procedure for granting or amending a regulated authorization. An authorization is granted in response to submissions/applications by those seeking authorization. A case is the administrative process that deals with the application(s) that relate to this and assesses them. Note: This area is subject to ongoing review and the workgroup is seeking implementer feedback on its use (see link at bottom of page). 588 */ 589 @Child(name = "case", type = {}, order=14, min=0, max=1, modifier=false, summary=true) 590 @Description(shortDefinition="The case or regulatory procedure for granting or amending a regulated authorization. Note: This area is subject to ongoing review and the workgroup is seeking implementer feedback on its use (see link at bottom of page)", formalDefinition="The case or regulatory procedure for granting or amending a regulated authorization. An authorization is granted in response to submissions/applications by those seeking authorization. A case is the administrative process that deals with the application(s) that relate to this and assesses them. Note: This area is subject to ongoing review and the workgroup is seeking implementer feedback on its use (see link at bottom of page)." ) 591 protected RegulatedAuthorizationCaseComponent case_; 592 593 private static final long serialVersionUID = 1227409639L; 594 595 /** 596 * Constructor 597 */ 598 public RegulatedAuthorization() { 599 super(); 600 } 601 602 /** 603 * @return {@link #identifier} (Business identifier for the authorization, typically assigned by the authorizing body.) 604 */ 605 public List<Identifier> getIdentifier() { 606 if (this.identifier == null) 607 this.identifier = new ArrayList<Identifier>(); 608 return this.identifier; 609 } 610 611 /** 612 * @return Returns a reference to <code>this</code> for easy method chaining 613 */ 614 public RegulatedAuthorization setIdentifier(List<Identifier> theIdentifier) { 615 this.identifier = theIdentifier; 616 return this; 617 } 618 619 public boolean hasIdentifier() { 620 if (this.identifier == null) 621 return false; 622 for (Identifier item : this.identifier) 623 if (!item.isEmpty()) 624 return true; 625 return false; 626 } 627 628 public Identifier addIdentifier() { //3 629 Identifier t = new Identifier(); 630 if (this.identifier == null) 631 this.identifier = new ArrayList<Identifier>(); 632 this.identifier.add(t); 633 return t; 634 } 635 636 public RegulatedAuthorization addIdentifier(Identifier t) { //3 637 if (t == null) 638 return this; 639 if (this.identifier == null) 640 this.identifier = new ArrayList<Identifier>(); 641 this.identifier.add(t); 642 return this; 643 } 644 645 /** 646 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 647 */ 648 public Identifier getIdentifierFirstRep() { 649 if (getIdentifier().isEmpty()) { 650 addIdentifier(); 651 } 652 return getIdentifier().get(0); 653 } 654 655 /** 656 * @return {@link #subject} (The product type, treatment, facility or activity that is being authorized.) 657 */ 658 public List<Reference> getSubject() { 659 if (this.subject == null) 660 this.subject = new ArrayList<Reference>(); 661 return this.subject; 662 } 663 664 /** 665 * @return Returns a reference to <code>this</code> for easy method chaining 666 */ 667 public RegulatedAuthorization setSubject(List<Reference> theSubject) { 668 this.subject = theSubject; 669 return this; 670 } 671 672 public boolean hasSubject() { 673 if (this.subject == null) 674 return false; 675 for (Reference item : this.subject) 676 if (!item.isEmpty()) 677 return true; 678 return false; 679 } 680 681 public Reference addSubject() { //3 682 Reference t = new Reference(); 683 if (this.subject == null) 684 this.subject = new ArrayList<Reference>(); 685 this.subject.add(t); 686 return t; 687 } 688 689 public RegulatedAuthorization addSubject(Reference t) { //3 690 if (t == null) 691 return this; 692 if (this.subject == null) 693 this.subject = new ArrayList<Reference>(); 694 this.subject.add(t); 695 return this; 696 } 697 698 /** 699 * @return The first repetition of repeating field {@link #subject}, creating it if it does not already exist {3} 700 */ 701 public Reference getSubjectFirstRep() { 702 if (getSubject().isEmpty()) { 703 addSubject(); 704 } 705 return getSubject().get(0); 706 } 707 708 /** 709 * @return {@link #type} (Overall type of this authorization, for example drug marketing approval, orphan drug designation.) 710 */ 711 public CodeableConcept getType() { 712 if (this.type == null) 713 if (Configuration.errorOnAutoCreate()) 714 throw new Error("Attempt to auto-create RegulatedAuthorization.type"); 715 else if (Configuration.doAutoCreate()) 716 this.type = new CodeableConcept(); // cc 717 return this.type; 718 } 719 720 public boolean hasType() { 721 return this.type != null && !this.type.isEmpty(); 722 } 723 724 /** 725 * @param value {@link #type} (Overall type of this authorization, for example drug marketing approval, orphan drug designation.) 726 */ 727 public RegulatedAuthorization setType(CodeableConcept value) { 728 this.type = value; 729 return this; 730 } 731 732 /** 733 * @return {@link #description} (General textual supporting information.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 734 */ 735 public MarkdownType getDescriptionElement() { 736 if (this.description == null) 737 if (Configuration.errorOnAutoCreate()) 738 throw new Error("Attempt to auto-create RegulatedAuthorization.description"); 739 else if (Configuration.doAutoCreate()) 740 this.description = new MarkdownType(); // bb 741 return this.description; 742 } 743 744 public boolean hasDescriptionElement() { 745 return this.description != null && !this.description.isEmpty(); 746 } 747 748 public boolean hasDescription() { 749 return this.description != null && !this.description.isEmpty(); 750 } 751 752 /** 753 * @param value {@link #description} (General textual supporting information.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 754 */ 755 public RegulatedAuthorization setDescriptionElement(MarkdownType value) { 756 this.description = value; 757 return this; 758 } 759 760 /** 761 * @return General textual supporting information. 762 */ 763 public String getDescription() { 764 return this.description == null ? null : this.description.getValue(); 765 } 766 767 /** 768 * @param value General textual supporting information. 769 */ 770 public RegulatedAuthorization setDescription(String value) { 771 if (Utilities.noString(value)) 772 this.description = null; 773 else { 774 if (this.description == null) 775 this.description = new MarkdownType(); 776 this.description.setValue(value); 777 } 778 return this; 779 } 780 781 /** 782 * @return {@link #region} (The territory (e.g., country, jurisdiction etc.) in which the authorization has been granted.) 783 */ 784 public List<CodeableConcept> getRegion() { 785 if (this.region == null) 786 this.region = new ArrayList<CodeableConcept>(); 787 return this.region; 788 } 789 790 /** 791 * @return Returns a reference to <code>this</code> for easy method chaining 792 */ 793 public RegulatedAuthorization setRegion(List<CodeableConcept> theRegion) { 794 this.region = theRegion; 795 return this; 796 } 797 798 public boolean hasRegion() { 799 if (this.region == null) 800 return false; 801 for (CodeableConcept item : this.region) 802 if (!item.isEmpty()) 803 return true; 804 return false; 805 } 806 807 public CodeableConcept addRegion() { //3 808 CodeableConcept t = new CodeableConcept(); 809 if (this.region == null) 810 this.region = new ArrayList<CodeableConcept>(); 811 this.region.add(t); 812 return t; 813 } 814 815 public RegulatedAuthorization addRegion(CodeableConcept t) { //3 816 if (t == null) 817 return this; 818 if (this.region == null) 819 this.region = new ArrayList<CodeableConcept>(); 820 this.region.add(t); 821 return this; 822 } 823 824 /** 825 * @return The first repetition of repeating field {@link #region}, creating it if it does not already exist {3} 826 */ 827 public CodeableConcept getRegionFirstRep() { 828 if (getRegion().isEmpty()) { 829 addRegion(); 830 } 831 return getRegion().get(0); 832 } 833 834 /** 835 * @return {@link #status} (The status that is authorised e.g. approved. Intermediate states and actions can be tracked with cases and applications.) 836 */ 837 public CodeableConcept getStatus() { 838 if (this.status == null) 839 if (Configuration.errorOnAutoCreate()) 840 throw new Error("Attempt to auto-create RegulatedAuthorization.status"); 841 else if (Configuration.doAutoCreate()) 842 this.status = new CodeableConcept(); // cc 843 return this.status; 844 } 845 846 public boolean hasStatus() { 847 return this.status != null && !this.status.isEmpty(); 848 } 849 850 /** 851 * @param value {@link #status} (The status that is authorised e.g. approved. Intermediate states and actions can be tracked with cases and applications.) 852 */ 853 public RegulatedAuthorization setStatus(CodeableConcept value) { 854 this.status = value; 855 return this; 856 } 857 858 /** 859 * @return {@link #statusDate} (The date at which the current status was assigned.). This is the underlying object with id, value and extensions. The accessor "getStatusDate" gives direct access to the value 860 */ 861 public DateTimeType getStatusDateElement() { 862 if (this.statusDate == null) 863 if (Configuration.errorOnAutoCreate()) 864 throw new Error("Attempt to auto-create RegulatedAuthorization.statusDate"); 865 else if (Configuration.doAutoCreate()) 866 this.statusDate = new DateTimeType(); // bb 867 return this.statusDate; 868 } 869 870 public boolean hasStatusDateElement() { 871 return this.statusDate != null && !this.statusDate.isEmpty(); 872 } 873 874 public boolean hasStatusDate() { 875 return this.statusDate != null && !this.statusDate.isEmpty(); 876 } 877 878 /** 879 * @param value {@link #statusDate} (The date at which the current status was assigned.). This is the underlying object with id, value and extensions. The accessor "getStatusDate" gives direct access to the value 880 */ 881 public RegulatedAuthorization setStatusDateElement(DateTimeType value) { 882 this.statusDate = value; 883 return this; 884 } 885 886 /** 887 * @return The date at which the current status was assigned. 888 */ 889 public Date getStatusDate() { 890 return this.statusDate == null ? null : this.statusDate.getValue(); 891 } 892 893 /** 894 * @param value The date at which the current status was assigned. 895 */ 896 public RegulatedAuthorization setStatusDate(Date value) { 897 if (value == null) 898 this.statusDate = null; 899 else { 900 if (this.statusDate == null) 901 this.statusDate = new DateTimeType(); 902 this.statusDate.setValue(value); 903 } 904 return this; 905 } 906 907 /** 908 * @return {@link #validityPeriod} (The time period in which the regulatory approval, clearance or licencing is in effect. As an example, a Marketing Authorization includes the date of authorization and/or an expiration date.) 909 */ 910 public Period getValidityPeriod() { 911 if (this.validityPeriod == null) 912 if (Configuration.errorOnAutoCreate()) 913 throw new Error("Attempt to auto-create RegulatedAuthorization.validityPeriod"); 914 else if (Configuration.doAutoCreate()) 915 this.validityPeriod = new Period(); // cc 916 return this.validityPeriod; 917 } 918 919 public boolean hasValidityPeriod() { 920 return this.validityPeriod != null && !this.validityPeriod.isEmpty(); 921 } 922 923 /** 924 * @param value {@link #validityPeriod} (The time period in which the regulatory approval, clearance or licencing is in effect. As an example, a Marketing Authorization includes the date of authorization and/or an expiration date.) 925 */ 926 public RegulatedAuthorization setValidityPeriod(Period value) { 927 this.validityPeriod = value; 928 return this; 929 } 930 931 /** 932 * @return {@link #indication} (Condition for which the use of the regulated product applies.) 933 */ 934 public List<CodeableReference> getIndication() { 935 if (this.indication == null) 936 this.indication = new ArrayList<CodeableReference>(); 937 return this.indication; 938 } 939 940 /** 941 * @return Returns a reference to <code>this</code> for easy method chaining 942 */ 943 public RegulatedAuthorization setIndication(List<CodeableReference> theIndication) { 944 this.indication = theIndication; 945 return this; 946 } 947 948 public boolean hasIndication() { 949 if (this.indication == null) 950 return false; 951 for (CodeableReference item : this.indication) 952 if (!item.isEmpty()) 953 return true; 954 return false; 955 } 956 957 public CodeableReference addIndication() { //3 958 CodeableReference t = new CodeableReference(); 959 if (this.indication == null) 960 this.indication = new ArrayList<CodeableReference>(); 961 this.indication.add(t); 962 return t; 963 } 964 965 public RegulatedAuthorization addIndication(CodeableReference t) { //3 966 if (t == null) 967 return this; 968 if (this.indication == null) 969 this.indication = new ArrayList<CodeableReference>(); 970 this.indication.add(t); 971 return this; 972 } 973 974 /** 975 * @return The first repetition of repeating field {@link #indication}, creating it if it does not already exist {3} 976 */ 977 public CodeableReference getIndicationFirstRep() { 978 if (getIndication().isEmpty()) { 979 addIndication(); 980 } 981 return getIndication().get(0); 982 } 983 984 /** 985 * @return {@link #intendedUse} (The intended use of the product, e.g. prevention, treatment, diagnosis.) 986 */ 987 public CodeableConcept getIntendedUse() { 988 if (this.intendedUse == null) 989 if (Configuration.errorOnAutoCreate()) 990 throw new Error("Attempt to auto-create RegulatedAuthorization.intendedUse"); 991 else if (Configuration.doAutoCreate()) 992 this.intendedUse = new CodeableConcept(); // cc 993 return this.intendedUse; 994 } 995 996 public boolean hasIntendedUse() { 997 return this.intendedUse != null && !this.intendedUse.isEmpty(); 998 } 999 1000 /** 1001 * @param value {@link #intendedUse} (The intended use of the product, e.g. prevention, treatment, diagnosis.) 1002 */ 1003 public RegulatedAuthorization setIntendedUse(CodeableConcept value) { 1004 this.intendedUse = value; 1005 return this; 1006 } 1007 1008 /** 1009 * @return {@link #basis} (The legal or regulatory framework against which this authorization is granted, or other reasons for it.) 1010 */ 1011 public List<CodeableConcept> getBasis() { 1012 if (this.basis == null) 1013 this.basis = new ArrayList<CodeableConcept>(); 1014 return this.basis; 1015 } 1016 1017 /** 1018 * @return Returns a reference to <code>this</code> for easy method chaining 1019 */ 1020 public RegulatedAuthorization setBasis(List<CodeableConcept> theBasis) { 1021 this.basis = theBasis; 1022 return this; 1023 } 1024 1025 public boolean hasBasis() { 1026 if (this.basis == null) 1027 return false; 1028 for (CodeableConcept item : this.basis) 1029 if (!item.isEmpty()) 1030 return true; 1031 return false; 1032 } 1033 1034 public CodeableConcept addBasis() { //3 1035 CodeableConcept t = new CodeableConcept(); 1036 if (this.basis == null) 1037 this.basis = new ArrayList<CodeableConcept>(); 1038 this.basis.add(t); 1039 return t; 1040 } 1041 1042 public RegulatedAuthorization addBasis(CodeableConcept t) { //3 1043 if (t == null) 1044 return this; 1045 if (this.basis == null) 1046 this.basis = new ArrayList<CodeableConcept>(); 1047 this.basis.add(t); 1048 return this; 1049 } 1050 1051 /** 1052 * @return The first repetition of repeating field {@link #basis}, creating it if it does not already exist {3} 1053 */ 1054 public CodeableConcept getBasisFirstRep() { 1055 if (getBasis().isEmpty()) { 1056 addBasis(); 1057 } 1058 return getBasis().get(0); 1059 } 1060 1061 /** 1062 * @return {@link #holder} (The organization that has been granted this authorization, by some authoritative body (the 'regulator').) 1063 */ 1064 public Reference getHolder() { 1065 if (this.holder == null) 1066 if (Configuration.errorOnAutoCreate()) 1067 throw new Error("Attempt to auto-create RegulatedAuthorization.holder"); 1068 else if (Configuration.doAutoCreate()) 1069 this.holder = new Reference(); // cc 1070 return this.holder; 1071 } 1072 1073 public boolean hasHolder() { 1074 return this.holder != null && !this.holder.isEmpty(); 1075 } 1076 1077 /** 1078 * @param value {@link #holder} (The organization that has been granted this authorization, by some authoritative body (the 'regulator').) 1079 */ 1080 public RegulatedAuthorization setHolder(Reference value) { 1081 this.holder = value; 1082 return this; 1083 } 1084 1085 /** 1086 * @return {@link #regulator} (The regulatory authority or authorizing body granting the authorization. For example, European Medicines Agency (EMA), Food and Drug Administration (FDA), Health Canada (HC), etc.) 1087 */ 1088 public Reference getRegulator() { 1089 if (this.regulator == null) 1090 if (Configuration.errorOnAutoCreate()) 1091 throw new Error("Attempt to auto-create RegulatedAuthorization.regulator"); 1092 else if (Configuration.doAutoCreate()) 1093 this.regulator = new Reference(); // cc 1094 return this.regulator; 1095 } 1096 1097 public boolean hasRegulator() { 1098 return this.regulator != null && !this.regulator.isEmpty(); 1099 } 1100 1101 /** 1102 * @param value {@link #regulator} (The regulatory authority or authorizing body granting the authorization. For example, European Medicines Agency (EMA), Food and Drug Administration (FDA), Health Canada (HC), etc.) 1103 */ 1104 public RegulatedAuthorization setRegulator(Reference value) { 1105 this.regulator = value; 1106 return this; 1107 } 1108 1109 /** 1110 * @return {@link #attachedDocument} (Additional information or supporting documentation about the authorization.) 1111 */ 1112 public List<Reference> getAttachedDocument() { 1113 if (this.attachedDocument == null) 1114 this.attachedDocument = new ArrayList<Reference>(); 1115 return this.attachedDocument; 1116 } 1117 1118 /** 1119 * @return Returns a reference to <code>this</code> for easy method chaining 1120 */ 1121 public RegulatedAuthorization setAttachedDocument(List<Reference> theAttachedDocument) { 1122 this.attachedDocument = theAttachedDocument; 1123 return this; 1124 } 1125 1126 public boolean hasAttachedDocument() { 1127 if (this.attachedDocument == null) 1128 return false; 1129 for (Reference item : this.attachedDocument) 1130 if (!item.isEmpty()) 1131 return true; 1132 return false; 1133 } 1134 1135 public Reference addAttachedDocument() { //3 1136 Reference t = new Reference(); 1137 if (this.attachedDocument == null) 1138 this.attachedDocument = new ArrayList<Reference>(); 1139 this.attachedDocument.add(t); 1140 return t; 1141 } 1142 1143 public RegulatedAuthorization addAttachedDocument(Reference t) { //3 1144 if (t == null) 1145 return this; 1146 if (this.attachedDocument == null) 1147 this.attachedDocument = new ArrayList<Reference>(); 1148 this.attachedDocument.add(t); 1149 return this; 1150 } 1151 1152 /** 1153 * @return The first repetition of repeating field {@link #attachedDocument}, creating it if it does not already exist {3} 1154 */ 1155 public Reference getAttachedDocumentFirstRep() { 1156 if (getAttachedDocument().isEmpty()) { 1157 addAttachedDocument(); 1158 } 1159 return getAttachedDocument().get(0); 1160 } 1161 1162 /** 1163 * @return {@link #case_} (The case or regulatory procedure for granting or amending a regulated authorization. An authorization is granted in response to submissions/applications by those seeking authorization. A case is the administrative process that deals with the application(s) that relate to this and assesses them. Note: This area is subject to ongoing review and the workgroup is seeking implementer feedback on its use (see link at bottom of page).) 1164 */ 1165 public RegulatedAuthorizationCaseComponent getCase() { 1166 if (this.case_ == null) 1167 if (Configuration.errorOnAutoCreate()) 1168 throw new Error("Attempt to auto-create RegulatedAuthorization.case_"); 1169 else if (Configuration.doAutoCreate()) 1170 this.case_ = new RegulatedAuthorizationCaseComponent(); // cc 1171 return this.case_; 1172 } 1173 1174 public boolean hasCase() { 1175 return this.case_ != null && !this.case_.isEmpty(); 1176 } 1177 1178 /** 1179 * @param value {@link #case_} (The case or regulatory procedure for granting or amending a regulated authorization. An authorization is granted in response to submissions/applications by those seeking authorization. A case is the administrative process that deals with the application(s) that relate to this and assesses them. Note: This area is subject to ongoing review and the workgroup is seeking implementer feedback on its use (see link at bottom of page).) 1180 */ 1181 public RegulatedAuthorization setCase(RegulatedAuthorizationCaseComponent value) { 1182 this.case_ = value; 1183 return this; 1184 } 1185 1186 protected void listChildren(List<Property> children) { 1187 super.listChildren(children); 1188 children.add(new Property("identifier", "Identifier", "Business identifier for the authorization, typically assigned by the authorizing body.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1189 children.add(new Property("subject", "Reference(MedicinalProductDefinition|BiologicallyDerivedProduct|NutritionProduct|PackagedProductDefinition|ManufacturedItemDefinition|Ingredient|SubstanceDefinition|DeviceDefinition|ResearchStudy|ActivityDefinition|PlanDefinition|ObservationDefinition|Practitioner|Organization|Location)", "The product type, treatment, facility or activity that is being authorized.", 0, java.lang.Integer.MAX_VALUE, subject)); 1190 children.add(new Property("type", "CodeableConcept", "Overall type of this authorization, for example drug marketing approval, orphan drug designation.", 0, 1, type)); 1191 children.add(new Property("description", "markdown", "General textual supporting information.", 0, 1, description)); 1192 children.add(new Property("region", "CodeableConcept", "The territory (e.g., country, jurisdiction etc.) in which the authorization has been granted.", 0, java.lang.Integer.MAX_VALUE, region)); 1193 children.add(new Property("status", "CodeableConcept", "The status that is authorised e.g. approved. Intermediate states and actions can be tracked with cases and applications.", 0, 1, status)); 1194 children.add(new Property("statusDate", "dateTime", "The date at which the current status was assigned.", 0, 1, statusDate)); 1195 children.add(new Property("validityPeriod", "Period", "The time period in which the regulatory approval, clearance or licencing is in effect. As an example, a Marketing Authorization includes the date of authorization and/or an expiration date.", 0, 1, validityPeriod)); 1196 children.add(new Property("indication", "CodeableReference(ClinicalUseDefinition)", "Condition for which the use of the regulated product applies.", 0, java.lang.Integer.MAX_VALUE, indication)); 1197 children.add(new Property("intendedUse", "CodeableConcept", "The intended use of the product, e.g. prevention, treatment, diagnosis.", 0, 1, intendedUse)); 1198 children.add(new Property("basis", "CodeableConcept", "The legal or regulatory framework against which this authorization is granted, or other reasons for it.", 0, java.lang.Integer.MAX_VALUE, basis)); 1199 children.add(new Property("holder", "Reference(Organization)", "The organization that has been granted this authorization, by some authoritative body (the 'regulator').", 0, 1, holder)); 1200 children.add(new Property("regulator", "Reference(Organization)", "The regulatory authority or authorizing body granting the authorization. For example, European Medicines Agency (EMA), Food and Drug Administration (FDA), Health Canada (HC), etc.", 0, 1, regulator)); 1201 children.add(new Property("attachedDocument", "Reference(DocumentReference)", "Additional information or supporting documentation about the authorization.", 0, java.lang.Integer.MAX_VALUE, attachedDocument)); 1202 children.add(new Property("case", "", "The case or regulatory procedure for granting or amending a regulated authorization. An authorization is granted in response to submissions/applications by those seeking authorization. A case is the administrative process that deals with the application(s) that relate to this and assesses them. Note: This area is subject to ongoing review and the workgroup is seeking implementer feedback on its use (see link at bottom of page).", 0, 1, case_)); 1203 } 1204 1205 @Override 1206 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1207 switch (_hash) { 1208 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifier for the authorization, typically assigned by the authorizing body.", 0, java.lang.Integer.MAX_VALUE, identifier); 1209 case -1867885268: /*subject*/ return new Property("subject", "Reference(MedicinalProductDefinition|BiologicallyDerivedProduct|NutritionProduct|PackagedProductDefinition|ManufacturedItemDefinition|Ingredient|SubstanceDefinition|DeviceDefinition|ResearchStudy|ActivityDefinition|PlanDefinition|ObservationDefinition|Practitioner|Organization|Location)", "The product type, treatment, facility or activity that is being authorized.", 0, java.lang.Integer.MAX_VALUE, subject); 1210 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Overall type of this authorization, for example drug marketing approval, orphan drug designation.", 0, 1, type); 1211 case -1724546052: /*description*/ return new Property("description", "markdown", "General textual supporting information.", 0, 1, description); 1212 case -934795532: /*region*/ return new Property("region", "CodeableConcept", "The territory (e.g., country, jurisdiction etc.) in which the authorization has been granted.", 0, java.lang.Integer.MAX_VALUE, region); 1213 case -892481550: /*status*/ return new Property("status", "CodeableConcept", "The status that is authorised e.g. approved. Intermediate states and actions can be tracked with cases and applications.", 0, 1, status); 1214 case 247524032: /*statusDate*/ return new Property("statusDate", "dateTime", "The date at which the current status was assigned.", 0, 1, statusDate); 1215 case -1434195053: /*validityPeriod*/ return new Property("validityPeriod", "Period", "The time period in which the regulatory approval, clearance or licencing is in effect. As an example, a Marketing Authorization includes the date of authorization and/or an expiration date.", 0, 1, validityPeriod); 1216 case -597168804: /*indication*/ return new Property("indication", "CodeableReference(ClinicalUseDefinition)", "Condition for which the use of the regulated product applies.", 0, java.lang.Integer.MAX_VALUE, indication); 1217 case -1618671268: /*intendedUse*/ return new Property("intendedUse", "CodeableConcept", "The intended use of the product, e.g. prevention, treatment, diagnosis.", 0, 1, intendedUse); 1218 case 93508670: /*basis*/ return new Property("basis", "CodeableConcept", "The legal or regulatory framework against which this authorization is granted, or other reasons for it.", 0, java.lang.Integer.MAX_VALUE, basis); 1219 case -1211707988: /*holder*/ return new Property("holder", "Reference(Organization)", "The organization that has been granted this authorization, by some authoritative body (the 'regulator').", 0, 1, holder); 1220 case 414760449: /*regulator*/ return new Property("regulator", "Reference(Organization)", "The regulatory authority or authorizing body granting the authorization. For example, European Medicines Agency (EMA), Food and Drug Administration (FDA), Health Canada (HC), etc.", 0, 1, regulator); 1221 case -513945889: /*attachedDocument*/ return new Property("attachedDocument", "Reference(DocumentReference)", "Additional information or supporting documentation about the authorization.", 0, java.lang.Integer.MAX_VALUE, attachedDocument); 1222 case 3046192: /*case*/ return new Property("case", "", "The case or regulatory procedure for granting or amending a regulated authorization. An authorization is granted in response to submissions/applications by those seeking authorization. A case is the administrative process that deals with the application(s) that relate to this and assesses them. Note: This area is subject to ongoing review and the workgroup is seeking implementer feedback on its use (see link at bottom of page).", 0, 1, case_); 1223 default: return super.getNamedProperty(_hash, _name, _checkValid); 1224 } 1225 1226 } 1227 1228 @Override 1229 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1230 switch (hash) { 1231 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1232 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // Reference 1233 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1234 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1235 case -934795532: /*region*/ return this.region == null ? new Base[0] : this.region.toArray(new Base[this.region.size()]); // CodeableConcept 1236 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // CodeableConcept 1237 case 247524032: /*statusDate*/ return this.statusDate == null ? new Base[0] : new Base[] {this.statusDate}; // DateTimeType 1238 case -1434195053: /*validityPeriod*/ return this.validityPeriod == null ? new Base[0] : new Base[] {this.validityPeriod}; // Period 1239 case -597168804: /*indication*/ return this.indication == null ? new Base[0] : this.indication.toArray(new Base[this.indication.size()]); // CodeableReference 1240 case -1618671268: /*intendedUse*/ return this.intendedUse == null ? new Base[0] : new Base[] {this.intendedUse}; // CodeableConcept 1241 case 93508670: /*basis*/ return this.basis == null ? new Base[0] : this.basis.toArray(new Base[this.basis.size()]); // CodeableConcept 1242 case -1211707988: /*holder*/ return this.holder == null ? new Base[0] : new Base[] {this.holder}; // Reference 1243 case 414760449: /*regulator*/ return this.regulator == null ? new Base[0] : new Base[] {this.regulator}; // Reference 1244 case -513945889: /*attachedDocument*/ return this.attachedDocument == null ? new Base[0] : this.attachedDocument.toArray(new Base[this.attachedDocument.size()]); // Reference 1245 case 3046192: /*case*/ return this.case_ == null ? new Base[0] : new Base[] {this.case_}; // RegulatedAuthorizationCaseComponent 1246 default: return super.getProperty(hash, name, checkValid); 1247 } 1248 1249 } 1250 1251 @Override 1252 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1253 switch (hash) { 1254 case -1618432855: // identifier 1255 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1256 return value; 1257 case -1867885268: // subject 1258 this.getSubject().add(TypeConvertor.castToReference(value)); // Reference 1259 return value; 1260 case 3575610: // type 1261 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1262 return value; 1263 case -1724546052: // description 1264 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1265 return value; 1266 case -934795532: // region 1267 this.getRegion().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1268 return value; 1269 case -892481550: // status 1270 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1271 return value; 1272 case 247524032: // statusDate 1273 this.statusDate = TypeConvertor.castToDateTime(value); // DateTimeType 1274 return value; 1275 case -1434195053: // validityPeriod 1276 this.validityPeriod = TypeConvertor.castToPeriod(value); // Period 1277 return value; 1278 case -597168804: // indication 1279 this.getIndication().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1280 return value; 1281 case -1618671268: // intendedUse 1282 this.intendedUse = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1283 return value; 1284 case 93508670: // basis 1285 this.getBasis().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1286 return value; 1287 case -1211707988: // holder 1288 this.holder = TypeConvertor.castToReference(value); // Reference 1289 return value; 1290 case 414760449: // regulator 1291 this.regulator = TypeConvertor.castToReference(value); // Reference 1292 return value; 1293 case -513945889: // attachedDocument 1294 this.getAttachedDocument().add(TypeConvertor.castToReference(value)); // Reference 1295 return value; 1296 case 3046192: // case 1297 this.case_ = (RegulatedAuthorizationCaseComponent) value; // RegulatedAuthorizationCaseComponent 1298 return value; 1299 default: return super.setProperty(hash, name, value); 1300 } 1301 1302 } 1303 1304 @Override 1305 public Base setProperty(String name, Base value) throws FHIRException { 1306 if (name.equals("identifier")) { 1307 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1308 } else if (name.equals("subject")) { 1309 this.getSubject().add(TypeConvertor.castToReference(value)); 1310 } else if (name.equals("type")) { 1311 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1312 } else if (name.equals("description")) { 1313 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1314 } else if (name.equals("region")) { 1315 this.getRegion().add(TypeConvertor.castToCodeableConcept(value)); 1316 } else if (name.equals("status")) { 1317 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1318 } else if (name.equals("statusDate")) { 1319 this.statusDate = TypeConvertor.castToDateTime(value); // DateTimeType 1320 } else if (name.equals("validityPeriod")) { 1321 this.validityPeriod = TypeConvertor.castToPeriod(value); // Period 1322 } else if (name.equals("indication")) { 1323 this.getIndication().add(TypeConvertor.castToCodeableReference(value)); 1324 } else if (name.equals("intendedUse")) { 1325 this.intendedUse = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1326 } else if (name.equals("basis")) { 1327 this.getBasis().add(TypeConvertor.castToCodeableConcept(value)); 1328 } else if (name.equals("holder")) { 1329 this.holder = TypeConvertor.castToReference(value); // Reference 1330 } else if (name.equals("regulator")) { 1331 this.regulator = TypeConvertor.castToReference(value); // Reference 1332 } else if (name.equals("attachedDocument")) { 1333 this.getAttachedDocument().add(TypeConvertor.castToReference(value)); 1334 } else if (name.equals("case")) { 1335 this.case_ = (RegulatedAuthorizationCaseComponent) value; // RegulatedAuthorizationCaseComponent 1336 } else 1337 return super.setProperty(name, value); 1338 return value; 1339 } 1340 1341 @Override 1342 public void removeChild(String name, Base value) throws FHIRException { 1343 if (name.equals("identifier")) { 1344 this.getIdentifier().remove(value); 1345 } else if (name.equals("subject")) { 1346 this.getSubject().remove(value); 1347 } else if (name.equals("type")) { 1348 this.type = null; 1349 } else if (name.equals("description")) { 1350 this.description = null; 1351 } else if (name.equals("region")) { 1352 this.getRegion().remove(value); 1353 } else if (name.equals("status")) { 1354 this.status = null; 1355 } else if (name.equals("statusDate")) { 1356 this.statusDate = null; 1357 } else if (name.equals("validityPeriod")) { 1358 this.validityPeriod = null; 1359 } else if (name.equals("indication")) { 1360 this.getIndication().remove(value); 1361 } else if (name.equals("intendedUse")) { 1362 this.intendedUse = null; 1363 } else if (name.equals("basis")) { 1364 this.getBasis().remove(value); 1365 } else if (name.equals("holder")) { 1366 this.holder = null; 1367 } else if (name.equals("regulator")) { 1368 this.regulator = null; 1369 } else if (name.equals("attachedDocument")) { 1370 this.getAttachedDocument().remove(value); 1371 } else if (name.equals("case")) { 1372 this.case_ = (RegulatedAuthorizationCaseComponent) value; // RegulatedAuthorizationCaseComponent 1373 } else 1374 super.removeChild(name, value); 1375 1376 } 1377 1378 @Override 1379 public Base makeProperty(int hash, String name) throws FHIRException { 1380 switch (hash) { 1381 case -1618432855: return addIdentifier(); 1382 case -1867885268: return addSubject(); 1383 case 3575610: return getType(); 1384 case -1724546052: return getDescriptionElement(); 1385 case -934795532: return addRegion(); 1386 case -892481550: return getStatus(); 1387 case 247524032: return getStatusDateElement(); 1388 case -1434195053: return getValidityPeriod(); 1389 case -597168804: return addIndication(); 1390 case -1618671268: return getIntendedUse(); 1391 case 93508670: return addBasis(); 1392 case -1211707988: return getHolder(); 1393 case 414760449: return getRegulator(); 1394 case -513945889: return addAttachedDocument(); 1395 case 3046192: return getCase(); 1396 default: return super.makeProperty(hash, name); 1397 } 1398 1399 } 1400 1401 @Override 1402 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1403 switch (hash) { 1404 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1405 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1406 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1407 case -1724546052: /*description*/ return new String[] {"markdown"}; 1408 case -934795532: /*region*/ return new String[] {"CodeableConcept"}; 1409 case -892481550: /*status*/ return new String[] {"CodeableConcept"}; 1410 case 247524032: /*statusDate*/ return new String[] {"dateTime"}; 1411 case -1434195053: /*validityPeriod*/ return new String[] {"Period"}; 1412 case -597168804: /*indication*/ return new String[] {"CodeableReference"}; 1413 case -1618671268: /*intendedUse*/ return new String[] {"CodeableConcept"}; 1414 case 93508670: /*basis*/ return new String[] {"CodeableConcept"}; 1415 case -1211707988: /*holder*/ return new String[] {"Reference"}; 1416 case 414760449: /*regulator*/ return new String[] {"Reference"}; 1417 case -513945889: /*attachedDocument*/ return new String[] {"Reference"}; 1418 case 3046192: /*case*/ return new String[] {}; 1419 default: return super.getTypesForProperty(hash, name); 1420 } 1421 1422 } 1423 1424 @Override 1425 public Base addChild(String name) throws FHIRException { 1426 if (name.equals("identifier")) { 1427 return addIdentifier(); 1428 } 1429 else if (name.equals("subject")) { 1430 return addSubject(); 1431 } 1432 else if (name.equals("type")) { 1433 this.type = new CodeableConcept(); 1434 return this.type; 1435 } 1436 else if (name.equals("description")) { 1437 throw new FHIRException("Cannot call addChild on a singleton property RegulatedAuthorization.description"); 1438 } 1439 else if (name.equals("region")) { 1440 return addRegion(); 1441 } 1442 else if (name.equals("status")) { 1443 this.status = new CodeableConcept(); 1444 return this.status; 1445 } 1446 else if (name.equals("statusDate")) { 1447 throw new FHIRException("Cannot call addChild on a singleton property RegulatedAuthorization.statusDate"); 1448 } 1449 else if (name.equals("validityPeriod")) { 1450 this.validityPeriod = new Period(); 1451 return this.validityPeriod; 1452 } 1453 else if (name.equals("indication")) { 1454 return addIndication(); 1455 } 1456 else if (name.equals("intendedUse")) { 1457 this.intendedUse = new CodeableConcept(); 1458 return this.intendedUse; 1459 } 1460 else if (name.equals("basis")) { 1461 return addBasis(); 1462 } 1463 else if (name.equals("holder")) { 1464 this.holder = new Reference(); 1465 return this.holder; 1466 } 1467 else if (name.equals("regulator")) { 1468 this.regulator = new Reference(); 1469 return this.regulator; 1470 } 1471 else if (name.equals("attachedDocument")) { 1472 return addAttachedDocument(); 1473 } 1474 else if (name.equals("case")) { 1475 this.case_ = new RegulatedAuthorizationCaseComponent(); 1476 return this.case_; 1477 } 1478 else 1479 return super.addChild(name); 1480 } 1481 1482 public String fhirType() { 1483 return "RegulatedAuthorization"; 1484 1485 } 1486 1487 public RegulatedAuthorization copy() { 1488 RegulatedAuthorization dst = new RegulatedAuthorization(); 1489 copyValues(dst); 1490 return dst; 1491 } 1492 1493 public void copyValues(RegulatedAuthorization dst) { 1494 super.copyValues(dst); 1495 if (identifier != null) { 1496 dst.identifier = new ArrayList<Identifier>(); 1497 for (Identifier i : identifier) 1498 dst.identifier.add(i.copy()); 1499 }; 1500 if (subject != null) { 1501 dst.subject = new ArrayList<Reference>(); 1502 for (Reference i : subject) 1503 dst.subject.add(i.copy()); 1504 }; 1505 dst.type = type == null ? null : type.copy(); 1506 dst.description = description == null ? null : description.copy(); 1507 if (region != null) { 1508 dst.region = new ArrayList<CodeableConcept>(); 1509 for (CodeableConcept i : region) 1510 dst.region.add(i.copy()); 1511 }; 1512 dst.status = status == null ? null : status.copy(); 1513 dst.statusDate = statusDate == null ? null : statusDate.copy(); 1514 dst.validityPeriod = validityPeriod == null ? null : validityPeriod.copy(); 1515 if (indication != null) { 1516 dst.indication = new ArrayList<CodeableReference>(); 1517 for (CodeableReference i : indication) 1518 dst.indication.add(i.copy()); 1519 }; 1520 dst.intendedUse = intendedUse == null ? null : intendedUse.copy(); 1521 if (basis != null) { 1522 dst.basis = new ArrayList<CodeableConcept>(); 1523 for (CodeableConcept i : basis) 1524 dst.basis.add(i.copy()); 1525 }; 1526 dst.holder = holder == null ? null : holder.copy(); 1527 dst.regulator = regulator == null ? null : regulator.copy(); 1528 if (attachedDocument != null) { 1529 dst.attachedDocument = new ArrayList<Reference>(); 1530 for (Reference i : attachedDocument) 1531 dst.attachedDocument.add(i.copy()); 1532 }; 1533 dst.case_ = case_ == null ? null : case_.copy(); 1534 } 1535 1536 protected RegulatedAuthorization typedCopy() { 1537 return copy(); 1538 } 1539 1540 @Override 1541 public boolean equalsDeep(Base other_) { 1542 if (!super.equalsDeep(other_)) 1543 return false; 1544 if (!(other_ instanceof RegulatedAuthorization)) 1545 return false; 1546 RegulatedAuthorization o = (RegulatedAuthorization) other_; 1547 return compareDeep(identifier, o.identifier, true) && compareDeep(subject, o.subject, true) && compareDeep(type, o.type, true) 1548 && compareDeep(description, o.description, true) && compareDeep(region, o.region, true) && compareDeep(status, o.status, true) 1549 && compareDeep(statusDate, o.statusDate, true) && compareDeep(validityPeriod, o.validityPeriod, true) 1550 && compareDeep(indication, o.indication, true) && compareDeep(intendedUse, o.intendedUse, true) 1551 && compareDeep(basis, o.basis, true) && compareDeep(holder, o.holder, true) && compareDeep(regulator, o.regulator, true) 1552 && compareDeep(attachedDocument, o.attachedDocument, true) && compareDeep(case_, o.case_, true) 1553 ; 1554 } 1555 1556 @Override 1557 public boolean equalsShallow(Base other_) { 1558 if (!super.equalsShallow(other_)) 1559 return false; 1560 if (!(other_ instanceof RegulatedAuthorization)) 1561 return false; 1562 RegulatedAuthorization o = (RegulatedAuthorization) other_; 1563 return compareValues(description, o.description, true) && compareValues(statusDate, o.statusDate, true) 1564 ; 1565 } 1566 1567 public boolean isEmpty() { 1568 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, subject, type 1569 , description, region, status, statusDate, validityPeriod, indication, intendedUse 1570 , basis, holder, regulator, attachedDocument, case_); 1571 } 1572 1573 @Override 1574 public ResourceType getResourceType() { 1575 return ResourceType.RegulatedAuthorization; 1576 } 1577 1578 /** 1579 * Search parameter: <b>case-type</b> 1580 * <p> 1581 * Description: <b>The defining type of case</b><br> 1582 * Type: <b>token</b><br> 1583 * Path: <b>RegulatedAuthorization.case.type</b><br> 1584 * </p> 1585 */ 1586 @SearchParamDefinition(name="case-type", path="RegulatedAuthorization.case.type", description="The defining type of case", type="token" ) 1587 public static final String SP_CASE_TYPE = "case-type"; 1588 /** 1589 * <b>Fluent Client</b> search parameter constant for <b>case-type</b> 1590 * <p> 1591 * Description: <b>The defining type of case</b><br> 1592 * Type: <b>token</b><br> 1593 * Path: <b>RegulatedAuthorization.case.type</b><br> 1594 * </p> 1595 */ 1596 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CASE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CASE_TYPE); 1597 1598 /** 1599 * Search parameter: <b>case</b> 1600 * <p> 1601 * Description: <b>The case or procedure number</b><br> 1602 * Type: <b>token</b><br> 1603 * Path: <b>RegulatedAuthorization.case.identifier</b><br> 1604 * </p> 1605 */ 1606 @SearchParamDefinition(name="case", path="RegulatedAuthorization.case.identifier", description="The case or procedure number", type="token" ) 1607 public static final String SP_CASE = "case"; 1608 /** 1609 * <b>Fluent Client</b> search parameter constant for <b>case</b> 1610 * <p> 1611 * Description: <b>The case or procedure number</b><br> 1612 * Type: <b>token</b><br> 1613 * Path: <b>RegulatedAuthorization.case.identifier</b><br> 1614 * </p> 1615 */ 1616 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CASE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CASE); 1617 1618 /** 1619 * Search parameter: <b>holder</b> 1620 * <p> 1621 * Description: <b>The organization that holds the granted authorization</b><br> 1622 * Type: <b>reference</b><br> 1623 * Path: <b>RegulatedAuthorization.holder</b><br> 1624 * </p> 1625 */ 1626 @SearchParamDefinition(name="holder", path="RegulatedAuthorization.holder", description="The organization that holds the granted authorization", type="reference", target={Organization.class } ) 1627 public static final String SP_HOLDER = "holder"; 1628 /** 1629 * <b>Fluent Client</b> search parameter constant for <b>holder</b> 1630 * <p> 1631 * Description: <b>The organization that holds the granted authorization</b><br> 1632 * Type: <b>reference</b><br> 1633 * Path: <b>RegulatedAuthorization.holder</b><br> 1634 * </p> 1635 */ 1636 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam HOLDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_HOLDER); 1637 1638/** 1639 * Constant for fluent queries to be used to add include statements. Specifies 1640 * the path value of "<b>RegulatedAuthorization:holder</b>". 1641 */ 1642 public static final ca.uhn.fhir.model.api.Include INCLUDE_HOLDER = new ca.uhn.fhir.model.api.Include("RegulatedAuthorization:holder").toLocked(); 1643 1644 /** 1645 * Search parameter: <b>identifier</b> 1646 * <p> 1647 * Description: <b>Business identifier for the authorization, typically assigned by the authorizing body</b><br> 1648 * Type: <b>token</b><br> 1649 * Path: <b>RegulatedAuthorization.identifier</b><br> 1650 * </p> 1651 */ 1652 @SearchParamDefinition(name="identifier", path="RegulatedAuthorization.identifier", description="Business identifier for the authorization, typically assigned by the authorizing body", type="token" ) 1653 public static final String SP_IDENTIFIER = "identifier"; 1654 /** 1655 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1656 * <p> 1657 * Description: <b>Business identifier for the authorization, typically assigned by the authorizing body</b><br> 1658 * Type: <b>token</b><br> 1659 * Path: <b>RegulatedAuthorization.identifier</b><br> 1660 * </p> 1661 */ 1662 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1663 1664 /** 1665 * Search parameter: <b>region</b> 1666 * <p> 1667 * Description: <b>The territory (e.g., country, jurisdiction etc.) in which the authorization has been granted</b><br> 1668 * Type: <b>token</b><br> 1669 * Path: <b>RegulatedAuthorization.region</b><br> 1670 * </p> 1671 */ 1672 @SearchParamDefinition(name="region", path="RegulatedAuthorization.region", description="The territory (e.g., country, jurisdiction etc.) in which the authorization has been granted", type="token" ) 1673 public static final String SP_REGION = "region"; 1674 /** 1675 * <b>Fluent Client</b> search parameter constant for <b>region</b> 1676 * <p> 1677 * Description: <b>The territory (e.g., country, jurisdiction etc.) in which the authorization has been granted</b><br> 1678 * Type: <b>token</b><br> 1679 * Path: <b>RegulatedAuthorization.region</b><br> 1680 * </p> 1681 */ 1682 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REGION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REGION); 1683 1684 /** 1685 * Search parameter: <b>status</b> 1686 * <p> 1687 * Description: <b>The status that is authorised e.g. approved. Intermediate states can be tracked with cases and applications</b><br> 1688 * Type: <b>token</b><br> 1689 * Path: <b>RegulatedAuthorization.status</b><br> 1690 * </p> 1691 */ 1692 @SearchParamDefinition(name="status", path="RegulatedAuthorization.status", description="The status that is authorised e.g. approved. Intermediate states can be tracked with cases and applications", type="token" ) 1693 public static final String SP_STATUS = "status"; 1694 /** 1695 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1696 * <p> 1697 * Description: <b>The status that is authorised e.g. approved. Intermediate states can be tracked with cases and applications</b><br> 1698 * Type: <b>token</b><br> 1699 * Path: <b>RegulatedAuthorization.status</b><br> 1700 * </p> 1701 */ 1702 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1703 1704 /** 1705 * Search parameter: <b>subject</b> 1706 * <p> 1707 * Description: <b>The type of regulated product, treatment, facility or activity that is being authorized</b><br> 1708 * Type: <b>reference</b><br> 1709 * Path: <b>RegulatedAuthorization.subject</b><br> 1710 * </p> 1711 */ 1712 @SearchParamDefinition(name="subject", path="RegulatedAuthorization.subject", description="The type of regulated product, treatment, facility or activity that is being authorized", type="reference", target={ActivityDefinition.class, BiologicallyDerivedProduct.class, DeviceDefinition.class, Ingredient.class, Location.class, ManufacturedItemDefinition.class, MedicinalProductDefinition.class, NutritionProduct.class, ObservationDefinition.class, Organization.class, PackagedProductDefinition.class, PlanDefinition.class, Practitioner.class, ResearchStudy.class, SubstanceDefinition.class } ) 1713 public static final String SP_SUBJECT = "subject"; 1714 /** 1715 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1716 * <p> 1717 * Description: <b>The type of regulated product, treatment, facility or activity that is being authorized</b><br> 1718 * Type: <b>reference</b><br> 1719 * Path: <b>RegulatedAuthorization.subject</b><br> 1720 * </p> 1721 */ 1722 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1723 1724/** 1725 * Constant for fluent queries to be used to add include statements. Specifies 1726 * the path value of "<b>RegulatedAuthorization:subject</b>". 1727 */ 1728 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("RegulatedAuthorization:subject").toLocked(); 1729 1730 1731} 1732