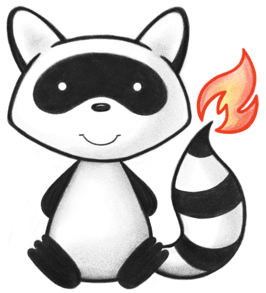
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.model.Enumerations.*; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.Block; 048 049import org.hl7.fhir.utilities.Utilities; 050/** 051 * Regulatory approval, clearance or licencing related to a regulated product, treatment, facility or activity that is cited in a guidance, regulation, rule or legislative act. An example is Market Authorization relating to a Medicinal Product. 052 */ 053@ResourceDef(name="RegulatedAuthorization", profile="http://hl7.org/fhir/StructureDefinition/RegulatedAuthorization") 054public class RegulatedAuthorization extends DomainResource { 055 056 @Block() 057 public static class RegulatedAuthorizationCaseComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Identifier by which this case can be referenced. 060 */ 061 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="Identifier by which this case can be referenced", formalDefinition="Identifier by which this case can be referenced." ) 063 protected Identifier identifier; 064 065 /** 066 * The defining type of case. 067 */ 068 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 069 @Description(shortDefinition="The defining type of case", formalDefinition="The defining type of case." ) 070 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/regulated-authorization-case-type") 071 protected CodeableConcept type; 072 073 /** 074 * The status associated with the case. 075 */ 076 @Child(name = "status", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 077 @Description(shortDefinition="The status associated with the case", formalDefinition="The status associated with the case." ) 078 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 079 protected CodeableConcept status; 080 081 /** 082 * Relevant date for this case. 083 */ 084 @Child(name = "date", type = {Period.class, DateTimeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 085 @Description(shortDefinition="Relevant date for this case", formalDefinition="Relevant date for this case." ) 086 protected DataType date; 087 088 /** 089 * A regulatory submission from an organization to a regulator, as part of an assessing case. Multiple applications may occur over time, with more or different information to support or modify the submission or the authorization. The applications can be considered as steps within the longer running case or procedure for this authorization process. 090 */ 091 @Child(name = "application", type = {RegulatedAuthorizationCaseComponent.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 092 @Description(shortDefinition="Applications submitted to obtain a regulated authorization. Steps within the longer running case or procedure", formalDefinition="A regulatory submission from an organization to a regulator, as part of an assessing case. Multiple applications may occur over time, with more or different information to support or modify the submission or the authorization. The applications can be considered as steps within the longer running case or procedure for this authorization process." ) 093 protected List<RegulatedAuthorizationCaseComponent> application; 094 095 private static final long serialVersionUID = 2052202113L; 096 097 /** 098 * Constructor 099 */ 100 public RegulatedAuthorizationCaseComponent() { 101 super(); 102 } 103 104 /** 105 * @return {@link #identifier} (Identifier by which this case can be referenced.) 106 */ 107 public Identifier getIdentifier() { 108 if (this.identifier == null) 109 if (Configuration.errorOnAutoCreate()) 110 throw new Error("Attempt to auto-create RegulatedAuthorizationCaseComponent.identifier"); 111 else if (Configuration.doAutoCreate()) 112 this.identifier = new Identifier(); // cc 113 return this.identifier; 114 } 115 116 public boolean hasIdentifier() { 117 return this.identifier != null && !this.identifier.isEmpty(); 118 } 119 120 /** 121 * @param value {@link #identifier} (Identifier by which this case can be referenced.) 122 */ 123 public RegulatedAuthorizationCaseComponent setIdentifier(Identifier value) { 124 this.identifier = value; 125 return this; 126 } 127 128 /** 129 * @return {@link #type} (The defining type of case.) 130 */ 131 public CodeableConcept getType() { 132 if (this.type == null) 133 if (Configuration.errorOnAutoCreate()) 134 throw new Error("Attempt to auto-create RegulatedAuthorizationCaseComponent.type"); 135 else if (Configuration.doAutoCreate()) 136 this.type = new CodeableConcept(); // cc 137 return this.type; 138 } 139 140 public boolean hasType() { 141 return this.type != null && !this.type.isEmpty(); 142 } 143 144 /** 145 * @param value {@link #type} (The defining type of case.) 146 */ 147 public RegulatedAuthorizationCaseComponent setType(CodeableConcept value) { 148 this.type = value; 149 return this; 150 } 151 152 /** 153 * @return {@link #status} (The status associated with the case.) 154 */ 155 public CodeableConcept getStatus() { 156 if (this.status == null) 157 if (Configuration.errorOnAutoCreate()) 158 throw new Error("Attempt to auto-create RegulatedAuthorizationCaseComponent.status"); 159 else if (Configuration.doAutoCreate()) 160 this.status = new CodeableConcept(); // cc 161 return this.status; 162 } 163 164 public boolean hasStatus() { 165 return this.status != null && !this.status.isEmpty(); 166 } 167 168 /** 169 * @param value {@link #status} (The status associated with the case.) 170 */ 171 public RegulatedAuthorizationCaseComponent setStatus(CodeableConcept value) { 172 this.status = value; 173 return this; 174 } 175 176 /** 177 * @return {@link #date} (Relevant date for this case.) 178 */ 179 public DataType getDate() { 180 return this.date; 181 } 182 183 /** 184 * @return {@link #date} (Relevant date for this case.) 185 */ 186 public Period getDatePeriod() throws FHIRException { 187 if (this.date == null) 188 this.date = new Period(); 189 if (!(this.date instanceof Period)) 190 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.date.getClass().getName()+" was encountered"); 191 return (Period) this.date; 192 } 193 194 public boolean hasDatePeriod() { 195 return this != null && this.date instanceof Period; 196 } 197 198 /** 199 * @return {@link #date} (Relevant date for this case.) 200 */ 201 public DateTimeType getDateDateTimeType() throws FHIRException { 202 if (this.date == null) 203 this.date = new DateTimeType(); 204 if (!(this.date instanceof DateTimeType)) 205 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.date.getClass().getName()+" was encountered"); 206 return (DateTimeType) this.date; 207 } 208 209 public boolean hasDateDateTimeType() { 210 return this != null && this.date instanceof DateTimeType; 211 } 212 213 public boolean hasDate() { 214 return this.date != null && !this.date.isEmpty(); 215 } 216 217 /** 218 * @param value {@link #date} (Relevant date for this case.) 219 */ 220 public RegulatedAuthorizationCaseComponent setDate(DataType value) { 221 if (value != null && !(value instanceof Period || value instanceof DateTimeType)) 222 throw new FHIRException("Not the right type for RegulatedAuthorization.case.date[x]: "+value.fhirType()); 223 this.date = value; 224 return this; 225 } 226 227 /** 228 * @return {@link #application} (A regulatory submission from an organization to a regulator, as part of an assessing case. Multiple applications may occur over time, with more or different information to support or modify the submission or the authorization. The applications can be considered as steps within the longer running case or procedure for this authorization process.) 229 */ 230 public List<RegulatedAuthorizationCaseComponent> getApplication() { 231 if (this.application == null) 232 this.application = new ArrayList<RegulatedAuthorizationCaseComponent>(); 233 return this.application; 234 } 235 236 /** 237 * @return Returns a reference to <code>this</code> for easy method chaining 238 */ 239 public RegulatedAuthorizationCaseComponent setApplication(List<RegulatedAuthorizationCaseComponent> theApplication) { 240 this.application = theApplication; 241 return this; 242 } 243 244 public boolean hasApplication() { 245 if (this.application == null) 246 return false; 247 for (RegulatedAuthorizationCaseComponent item : this.application) 248 if (!item.isEmpty()) 249 return true; 250 return false; 251 } 252 253 public RegulatedAuthorizationCaseComponent addApplication() { //3 254 RegulatedAuthorizationCaseComponent t = new RegulatedAuthorizationCaseComponent(); 255 if (this.application == null) 256 this.application = new ArrayList<RegulatedAuthorizationCaseComponent>(); 257 this.application.add(t); 258 return t; 259 } 260 261 public RegulatedAuthorizationCaseComponent addApplication(RegulatedAuthorizationCaseComponent t) { //3 262 if (t == null) 263 return this; 264 if (this.application == null) 265 this.application = new ArrayList<RegulatedAuthorizationCaseComponent>(); 266 this.application.add(t); 267 return this; 268 } 269 270 /** 271 * @return The first repetition of repeating field {@link #application}, creating it if it does not already exist {3} 272 */ 273 public RegulatedAuthorizationCaseComponent getApplicationFirstRep() { 274 if (getApplication().isEmpty()) { 275 addApplication(); 276 } 277 return getApplication().get(0); 278 } 279 280 protected void listChildren(List<Property> children) { 281 super.listChildren(children); 282 children.add(new Property("identifier", "Identifier", "Identifier by which this case can be referenced.", 0, 1, identifier)); 283 children.add(new Property("type", "CodeableConcept", "The defining type of case.", 0, 1, type)); 284 children.add(new Property("status", "CodeableConcept", "The status associated with the case.", 0, 1, status)); 285 children.add(new Property("date[x]", "Period|dateTime", "Relevant date for this case.", 0, 1, date)); 286 children.add(new Property("application", "@RegulatedAuthorization.case", "A regulatory submission from an organization to a regulator, as part of an assessing case. Multiple applications may occur over time, with more or different information to support or modify the submission or the authorization. The applications can be considered as steps within the longer running case or procedure for this authorization process.", 0, java.lang.Integer.MAX_VALUE, application)); 287 } 288 289 @Override 290 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 291 switch (_hash) { 292 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier by which this case can be referenced.", 0, 1, identifier); 293 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The defining type of case.", 0, 1, type); 294 case -892481550: /*status*/ return new Property("status", "CodeableConcept", "The status associated with the case.", 0, 1, status); 295 case 1443311122: /*date[x]*/ return new Property("date[x]", "Period|dateTime", "Relevant date for this case.", 0, 1, date); 296 case 3076014: /*date*/ return new Property("date[x]", "Period|dateTime", "Relevant date for this case.", 0, 1, date); 297 case 432297743: /*datePeriod*/ return new Property("date[x]", "Period", "Relevant date for this case.", 0, 1, date); 298 case 185136489: /*dateDateTime*/ return new Property("date[x]", "dateTime", "Relevant date for this case.", 0, 1, date); 299 case 1554253136: /*application*/ return new Property("application", "@RegulatedAuthorization.case", "A regulatory submission from an organization to a regulator, as part of an assessing case. Multiple applications may occur over time, with more or different information to support or modify the submission or the authorization. The applications can be considered as steps within the longer running case or procedure for this authorization process.", 0, java.lang.Integer.MAX_VALUE, application); 300 default: return super.getNamedProperty(_hash, _name, _checkValid); 301 } 302 303 } 304 305 @Override 306 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 307 switch (hash) { 308 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 309 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 310 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // CodeableConcept 311 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DataType 312 case 1554253136: /*application*/ return this.application == null ? new Base[0] : this.application.toArray(new Base[this.application.size()]); // RegulatedAuthorizationCaseComponent 313 default: return super.getProperty(hash, name, checkValid); 314 } 315 316 } 317 318 @Override 319 public Base setProperty(int hash, String name, Base value) throws FHIRException { 320 switch (hash) { 321 case -1618432855: // identifier 322 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 323 return value; 324 case 3575610: // type 325 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 326 return value; 327 case -892481550: // status 328 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 329 return value; 330 case 3076014: // date 331 this.date = TypeConvertor.castToType(value); // DataType 332 return value; 333 case 1554253136: // application 334 this.getApplication().add((RegulatedAuthorizationCaseComponent) value); // RegulatedAuthorizationCaseComponent 335 return value; 336 default: return super.setProperty(hash, name, value); 337 } 338 339 } 340 341 @Override 342 public Base setProperty(String name, Base value) throws FHIRException { 343 if (name.equals("identifier")) { 344 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 345 } else if (name.equals("type")) { 346 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 347 } else if (name.equals("status")) { 348 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 349 } else if (name.equals("date[x]")) { 350 this.date = TypeConvertor.castToType(value); // DataType 351 } else if (name.equals("application")) { 352 this.getApplication().add((RegulatedAuthorizationCaseComponent) value); 353 } else 354 return super.setProperty(name, value); 355 return value; 356 } 357 358 @Override 359 public Base makeProperty(int hash, String name) throws FHIRException { 360 switch (hash) { 361 case -1618432855: return getIdentifier(); 362 case 3575610: return getType(); 363 case -892481550: return getStatus(); 364 case 1443311122: return getDate(); 365 case 3076014: return getDate(); 366 case 1554253136: return addApplication(); 367 default: return super.makeProperty(hash, name); 368 } 369 370 } 371 372 @Override 373 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 374 switch (hash) { 375 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 376 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 377 case -892481550: /*status*/ return new String[] {"CodeableConcept"}; 378 case 3076014: /*date*/ return new String[] {"Period", "dateTime"}; 379 case 1554253136: /*application*/ return new String[] {"@RegulatedAuthorization.case"}; 380 default: return super.getTypesForProperty(hash, name); 381 } 382 383 } 384 385 @Override 386 public Base addChild(String name) throws FHIRException { 387 if (name.equals("identifier")) { 388 this.identifier = new Identifier(); 389 return this.identifier; 390 } 391 else if (name.equals("type")) { 392 this.type = new CodeableConcept(); 393 return this.type; 394 } 395 else if (name.equals("status")) { 396 this.status = new CodeableConcept(); 397 return this.status; 398 } 399 else if (name.equals("datePeriod")) { 400 this.date = new Period(); 401 return this.date; 402 } 403 else if (name.equals("dateDateTime")) { 404 this.date = new DateTimeType(); 405 return this.date; 406 } 407 else if (name.equals("application")) { 408 return addApplication(); 409 } 410 else 411 return super.addChild(name); 412 } 413 414 public RegulatedAuthorizationCaseComponent copy() { 415 RegulatedAuthorizationCaseComponent dst = new RegulatedAuthorizationCaseComponent(); 416 copyValues(dst); 417 return dst; 418 } 419 420 public void copyValues(RegulatedAuthorizationCaseComponent dst) { 421 super.copyValues(dst); 422 dst.identifier = identifier == null ? null : identifier.copy(); 423 dst.type = type == null ? null : type.copy(); 424 dst.status = status == null ? null : status.copy(); 425 dst.date = date == null ? null : date.copy(); 426 if (application != null) { 427 dst.application = new ArrayList<RegulatedAuthorizationCaseComponent>(); 428 for (RegulatedAuthorizationCaseComponent i : application) 429 dst.application.add(i.copy()); 430 }; 431 } 432 433 @Override 434 public boolean equalsDeep(Base other_) { 435 if (!super.equalsDeep(other_)) 436 return false; 437 if (!(other_ instanceof RegulatedAuthorizationCaseComponent)) 438 return false; 439 RegulatedAuthorizationCaseComponent o = (RegulatedAuthorizationCaseComponent) other_; 440 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) && compareDeep(status, o.status, true) 441 && compareDeep(date, o.date, true) && compareDeep(application, o.application, true); 442 } 443 444 @Override 445 public boolean equalsShallow(Base other_) { 446 if (!super.equalsShallow(other_)) 447 return false; 448 if (!(other_ instanceof RegulatedAuthorizationCaseComponent)) 449 return false; 450 RegulatedAuthorizationCaseComponent o = (RegulatedAuthorizationCaseComponent) other_; 451 return true; 452 } 453 454 public boolean isEmpty() { 455 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, status 456 , date, application); 457 } 458 459 public String fhirType() { 460 return "RegulatedAuthorization.case"; 461 462 } 463 464 } 465 466 /** 467 * Business identifier for the authorization, typically assigned by the authorizing body. 468 */ 469 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 470 @Description(shortDefinition="Business identifier for the authorization, typically assigned by the authorizing body", formalDefinition="Business identifier for the authorization, typically assigned by the authorizing body." ) 471 protected List<Identifier> identifier; 472 473 /** 474 * The product type, treatment, facility or activity that is being authorized. 475 */ 476 @Child(name = "subject", type = {MedicinalProductDefinition.class, BiologicallyDerivedProduct.class, NutritionProduct.class, PackagedProductDefinition.class, ManufacturedItemDefinition.class, Ingredient.class, SubstanceDefinition.class, DeviceDefinition.class, ResearchStudy.class, ActivityDefinition.class, PlanDefinition.class, ObservationDefinition.class, Practitioner.class, Organization.class, Location.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 477 @Description(shortDefinition="The product type, treatment, facility or activity that is being authorized", formalDefinition="The product type, treatment, facility or activity that is being authorized." ) 478 protected List<Reference> subject; 479 480 /** 481 * Overall type of this authorization, for example drug marketing approval, orphan drug designation. 482 */ 483 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 484 @Description(shortDefinition="Overall type of this authorization, for example drug marketing approval, orphan drug designation", formalDefinition="Overall type of this authorization, for example drug marketing approval, orphan drug designation." ) 485 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/regulated-authorization-type") 486 protected CodeableConcept type; 487 488 /** 489 * General textual supporting information. 490 */ 491 @Child(name = "description", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=true) 492 @Description(shortDefinition="General textual supporting information", formalDefinition="General textual supporting information." ) 493 protected MarkdownType description; 494 495 /** 496 * The territory (e.g., country, jurisdiction etc.) in which the authorization has been granted. 497 */ 498 @Child(name = "region", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 499 @Description(shortDefinition="The territory in which the authorization has been granted", formalDefinition="The territory (e.g., country, jurisdiction etc.) in which the authorization has been granted." ) 500 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 501 protected List<CodeableConcept> region; 502 503 /** 504 * The status that is authorised e.g. approved. Intermediate states and actions can be tracked with cases and applications. 505 */ 506 @Child(name = "status", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 507 @Description(shortDefinition="The status that is authorised e.g. approved. Intermediate states can be tracked with cases and applications", formalDefinition="The status that is authorised e.g. approved. Intermediate states and actions can be tracked with cases and applications." ) 508 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 509 protected CodeableConcept status; 510 511 /** 512 * The date at which the current status was assigned. 513 */ 514 @Child(name = "statusDate", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 515 @Description(shortDefinition="The date at which the current status was assigned", formalDefinition="The date at which the current status was assigned." ) 516 protected DateTimeType statusDate; 517 518 /** 519 * The time period in which the regulatory approval, clearance or licencing is in effect. As an example, a Marketing Authorization includes the date of authorization and/or an expiration date. 520 */ 521 @Child(name = "validityPeriod", type = {Period.class}, order=7, min=0, max=1, modifier=false, summary=true) 522 @Description(shortDefinition="The time period in which the regulatory approval etc. is in effect, e.g. a Marketing Authorization includes the date of authorization and/or expiration date", formalDefinition="The time period in which the regulatory approval, clearance or licencing is in effect. As an example, a Marketing Authorization includes the date of authorization and/or an expiration date." ) 523 protected Period validityPeriod; 524 525 /** 526 * Condition for which the use of the regulated product applies. 527 */ 528 @Child(name = "indication", type = {CodeableReference.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 529 @Description(shortDefinition="Condition for which the use of the regulated product applies", formalDefinition="Condition for which the use of the regulated product applies." ) 530 protected List<CodeableReference> indication; 531 532 /** 533 * The intended use of the product, e.g. prevention, treatment, diagnosis. 534 */ 535 @Child(name = "intendedUse", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=true) 536 @Description(shortDefinition="The intended use of the product, e.g. prevention, treatment", formalDefinition="The intended use of the product, e.g. prevention, treatment, diagnosis." ) 537 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/product-intended-use") 538 protected CodeableConcept intendedUse; 539 540 /** 541 * The legal or regulatory framework against which this authorization is granted, or other reasons for it. 542 */ 543 @Child(name = "basis", type = {CodeableConcept.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 544 @Description(shortDefinition="The legal/regulatory framework or reasons under which this authorization is granted", formalDefinition="The legal or regulatory framework against which this authorization is granted, or other reasons for it." ) 545 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/regulated-authorization-basis") 546 protected List<CodeableConcept> basis; 547 548 /** 549 * The organization that has been granted this authorization, by some authoritative body (the 'regulator'). 550 */ 551 @Child(name = "holder", type = {Organization.class}, order=11, min=0, max=1, modifier=false, summary=true) 552 @Description(shortDefinition="The organization that has been granted this authorization, by the regulator", formalDefinition="The organization that has been granted this authorization, by some authoritative body (the 'regulator')." ) 553 protected Reference holder; 554 555 /** 556 * The regulatory authority or authorizing body granting the authorization. For example, European Medicines Agency (EMA), Food and Drug Administration (FDA), Health Canada (HC), etc. 557 */ 558 @Child(name = "regulator", type = {Organization.class}, order=12, min=0, max=1, modifier=false, summary=true) 559 @Description(shortDefinition="The regulatory authority or authorizing body granting the authorization", formalDefinition="The regulatory authority or authorizing body granting the authorization. For example, European Medicines Agency (EMA), Food and Drug Administration (FDA), Health Canada (HC), etc." ) 560 protected Reference regulator; 561 562 /** 563 * Additional information or supporting documentation about the authorization. 564 */ 565 @Child(name = "attachedDocument", type = {DocumentReference.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 566 @Description(shortDefinition="Additional information or supporting documentation about the authorization", formalDefinition="Additional information or supporting documentation about the authorization." ) 567 protected List<Reference> attachedDocument; 568 569 /** 570 * The case or regulatory procedure for granting or amending a regulated authorization. An authorization is granted in response to submissions/applications by those seeking authorization. A case is the administrative process that deals with the application(s) that relate to this and assesses them. Note: This area is subject to ongoing review and the workgroup is seeking implementer feedback on its use (see link at bottom of page). 571 */ 572 @Child(name = "case", type = {}, order=14, min=0, max=1, modifier=false, summary=true) 573 @Description(shortDefinition="The case or regulatory procedure for granting or amending a regulated authorization. Note: This area is subject to ongoing review and the workgroup is seeking implementer feedback on its use (see link at bottom of page)", formalDefinition="The case or regulatory procedure for granting or amending a regulated authorization. An authorization is granted in response to submissions/applications by those seeking authorization. A case is the administrative process that deals with the application(s) that relate to this and assesses them. Note: This area is subject to ongoing review and the workgroup is seeking implementer feedback on its use (see link at bottom of page)." ) 574 protected RegulatedAuthorizationCaseComponent case_; 575 576 private static final long serialVersionUID = 1227409639L; 577 578 /** 579 * Constructor 580 */ 581 public RegulatedAuthorization() { 582 super(); 583 } 584 585 /** 586 * @return {@link #identifier} (Business identifier for the authorization, typically assigned by the authorizing body.) 587 */ 588 public List<Identifier> getIdentifier() { 589 if (this.identifier == null) 590 this.identifier = new ArrayList<Identifier>(); 591 return this.identifier; 592 } 593 594 /** 595 * @return Returns a reference to <code>this</code> for easy method chaining 596 */ 597 public RegulatedAuthorization setIdentifier(List<Identifier> theIdentifier) { 598 this.identifier = theIdentifier; 599 return this; 600 } 601 602 public boolean hasIdentifier() { 603 if (this.identifier == null) 604 return false; 605 for (Identifier item : this.identifier) 606 if (!item.isEmpty()) 607 return true; 608 return false; 609 } 610 611 public Identifier addIdentifier() { //3 612 Identifier t = new Identifier(); 613 if (this.identifier == null) 614 this.identifier = new ArrayList<Identifier>(); 615 this.identifier.add(t); 616 return t; 617 } 618 619 public RegulatedAuthorization addIdentifier(Identifier t) { //3 620 if (t == null) 621 return this; 622 if (this.identifier == null) 623 this.identifier = new ArrayList<Identifier>(); 624 this.identifier.add(t); 625 return this; 626 } 627 628 /** 629 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 630 */ 631 public Identifier getIdentifierFirstRep() { 632 if (getIdentifier().isEmpty()) { 633 addIdentifier(); 634 } 635 return getIdentifier().get(0); 636 } 637 638 /** 639 * @return {@link #subject} (The product type, treatment, facility or activity that is being authorized.) 640 */ 641 public List<Reference> getSubject() { 642 if (this.subject == null) 643 this.subject = new ArrayList<Reference>(); 644 return this.subject; 645 } 646 647 /** 648 * @return Returns a reference to <code>this</code> for easy method chaining 649 */ 650 public RegulatedAuthorization setSubject(List<Reference> theSubject) { 651 this.subject = theSubject; 652 return this; 653 } 654 655 public boolean hasSubject() { 656 if (this.subject == null) 657 return false; 658 for (Reference item : this.subject) 659 if (!item.isEmpty()) 660 return true; 661 return false; 662 } 663 664 public Reference addSubject() { //3 665 Reference t = new Reference(); 666 if (this.subject == null) 667 this.subject = new ArrayList<Reference>(); 668 this.subject.add(t); 669 return t; 670 } 671 672 public RegulatedAuthorization addSubject(Reference t) { //3 673 if (t == null) 674 return this; 675 if (this.subject == null) 676 this.subject = new ArrayList<Reference>(); 677 this.subject.add(t); 678 return this; 679 } 680 681 /** 682 * @return The first repetition of repeating field {@link #subject}, creating it if it does not already exist {3} 683 */ 684 public Reference getSubjectFirstRep() { 685 if (getSubject().isEmpty()) { 686 addSubject(); 687 } 688 return getSubject().get(0); 689 } 690 691 /** 692 * @return {@link #type} (Overall type of this authorization, for example drug marketing approval, orphan drug designation.) 693 */ 694 public CodeableConcept getType() { 695 if (this.type == null) 696 if (Configuration.errorOnAutoCreate()) 697 throw new Error("Attempt to auto-create RegulatedAuthorization.type"); 698 else if (Configuration.doAutoCreate()) 699 this.type = new CodeableConcept(); // cc 700 return this.type; 701 } 702 703 public boolean hasType() { 704 return this.type != null && !this.type.isEmpty(); 705 } 706 707 /** 708 * @param value {@link #type} (Overall type of this authorization, for example drug marketing approval, orphan drug designation.) 709 */ 710 public RegulatedAuthorization setType(CodeableConcept value) { 711 this.type = value; 712 return this; 713 } 714 715 /** 716 * @return {@link #description} (General textual supporting information.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 717 */ 718 public MarkdownType getDescriptionElement() { 719 if (this.description == null) 720 if (Configuration.errorOnAutoCreate()) 721 throw new Error("Attempt to auto-create RegulatedAuthorization.description"); 722 else if (Configuration.doAutoCreate()) 723 this.description = new MarkdownType(); // bb 724 return this.description; 725 } 726 727 public boolean hasDescriptionElement() { 728 return this.description != null && !this.description.isEmpty(); 729 } 730 731 public boolean hasDescription() { 732 return this.description != null && !this.description.isEmpty(); 733 } 734 735 /** 736 * @param value {@link #description} (General textual supporting information.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 737 */ 738 public RegulatedAuthorization setDescriptionElement(MarkdownType value) { 739 this.description = value; 740 return this; 741 } 742 743 /** 744 * @return General textual supporting information. 745 */ 746 public String getDescription() { 747 return this.description == null ? null : this.description.getValue(); 748 } 749 750 /** 751 * @param value General textual supporting information. 752 */ 753 public RegulatedAuthorization setDescription(String value) { 754 if (Utilities.noString(value)) 755 this.description = null; 756 else { 757 if (this.description == null) 758 this.description = new MarkdownType(); 759 this.description.setValue(value); 760 } 761 return this; 762 } 763 764 /** 765 * @return {@link #region} (The territory (e.g., country, jurisdiction etc.) in which the authorization has been granted.) 766 */ 767 public List<CodeableConcept> getRegion() { 768 if (this.region == null) 769 this.region = new ArrayList<CodeableConcept>(); 770 return this.region; 771 } 772 773 /** 774 * @return Returns a reference to <code>this</code> for easy method chaining 775 */ 776 public RegulatedAuthorization setRegion(List<CodeableConcept> theRegion) { 777 this.region = theRegion; 778 return this; 779 } 780 781 public boolean hasRegion() { 782 if (this.region == null) 783 return false; 784 for (CodeableConcept item : this.region) 785 if (!item.isEmpty()) 786 return true; 787 return false; 788 } 789 790 public CodeableConcept addRegion() { //3 791 CodeableConcept t = new CodeableConcept(); 792 if (this.region == null) 793 this.region = new ArrayList<CodeableConcept>(); 794 this.region.add(t); 795 return t; 796 } 797 798 public RegulatedAuthorization addRegion(CodeableConcept t) { //3 799 if (t == null) 800 return this; 801 if (this.region == null) 802 this.region = new ArrayList<CodeableConcept>(); 803 this.region.add(t); 804 return this; 805 } 806 807 /** 808 * @return The first repetition of repeating field {@link #region}, creating it if it does not already exist {3} 809 */ 810 public CodeableConcept getRegionFirstRep() { 811 if (getRegion().isEmpty()) { 812 addRegion(); 813 } 814 return getRegion().get(0); 815 } 816 817 /** 818 * @return {@link #status} (The status that is authorised e.g. approved. Intermediate states and actions can be tracked with cases and applications.) 819 */ 820 public CodeableConcept getStatus() { 821 if (this.status == null) 822 if (Configuration.errorOnAutoCreate()) 823 throw new Error("Attempt to auto-create RegulatedAuthorization.status"); 824 else if (Configuration.doAutoCreate()) 825 this.status = new CodeableConcept(); // cc 826 return this.status; 827 } 828 829 public boolean hasStatus() { 830 return this.status != null && !this.status.isEmpty(); 831 } 832 833 /** 834 * @param value {@link #status} (The status that is authorised e.g. approved. Intermediate states and actions can be tracked with cases and applications.) 835 */ 836 public RegulatedAuthorization setStatus(CodeableConcept value) { 837 this.status = value; 838 return this; 839 } 840 841 /** 842 * @return {@link #statusDate} (The date at which the current status was assigned.). This is the underlying object with id, value and extensions. The accessor "getStatusDate" gives direct access to the value 843 */ 844 public DateTimeType getStatusDateElement() { 845 if (this.statusDate == null) 846 if (Configuration.errorOnAutoCreate()) 847 throw new Error("Attempt to auto-create RegulatedAuthorization.statusDate"); 848 else if (Configuration.doAutoCreate()) 849 this.statusDate = new DateTimeType(); // bb 850 return this.statusDate; 851 } 852 853 public boolean hasStatusDateElement() { 854 return this.statusDate != null && !this.statusDate.isEmpty(); 855 } 856 857 public boolean hasStatusDate() { 858 return this.statusDate != null && !this.statusDate.isEmpty(); 859 } 860 861 /** 862 * @param value {@link #statusDate} (The date at which the current status was assigned.). This is the underlying object with id, value and extensions. The accessor "getStatusDate" gives direct access to the value 863 */ 864 public RegulatedAuthorization setStatusDateElement(DateTimeType value) { 865 this.statusDate = value; 866 return this; 867 } 868 869 /** 870 * @return The date at which the current status was assigned. 871 */ 872 public Date getStatusDate() { 873 return this.statusDate == null ? null : this.statusDate.getValue(); 874 } 875 876 /** 877 * @param value The date at which the current status was assigned. 878 */ 879 public RegulatedAuthorization setStatusDate(Date value) { 880 if (value == null) 881 this.statusDate = null; 882 else { 883 if (this.statusDate == null) 884 this.statusDate = new DateTimeType(); 885 this.statusDate.setValue(value); 886 } 887 return this; 888 } 889 890 /** 891 * @return {@link #validityPeriod} (The time period in which the regulatory approval, clearance or licencing is in effect. As an example, a Marketing Authorization includes the date of authorization and/or an expiration date.) 892 */ 893 public Period getValidityPeriod() { 894 if (this.validityPeriod == null) 895 if (Configuration.errorOnAutoCreate()) 896 throw new Error("Attempt to auto-create RegulatedAuthorization.validityPeriod"); 897 else if (Configuration.doAutoCreate()) 898 this.validityPeriod = new Period(); // cc 899 return this.validityPeriod; 900 } 901 902 public boolean hasValidityPeriod() { 903 return this.validityPeriod != null && !this.validityPeriod.isEmpty(); 904 } 905 906 /** 907 * @param value {@link #validityPeriod} (The time period in which the regulatory approval, clearance or licencing is in effect. As an example, a Marketing Authorization includes the date of authorization and/or an expiration date.) 908 */ 909 public RegulatedAuthorization setValidityPeriod(Period value) { 910 this.validityPeriod = value; 911 return this; 912 } 913 914 /** 915 * @return {@link #indication} (Condition for which the use of the regulated product applies.) 916 */ 917 public List<CodeableReference> getIndication() { 918 if (this.indication == null) 919 this.indication = new ArrayList<CodeableReference>(); 920 return this.indication; 921 } 922 923 /** 924 * @return Returns a reference to <code>this</code> for easy method chaining 925 */ 926 public RegulatedAuthorization setIndication(List<CodeableReference> theIndication) { 927 this.indication = theIndication; 928 return this; 929 } 930 931 public boolean hasIndication() { 932 if (this.indication == null) 933 return false; 934 for (CodeableReference item : this.indication) 935 if (!item.isEmpty()) 936 return true; 937 return false; 938 } 939 940 public CodeableReference addIndication() { //3 941 CodeableReference t = new CodeableReference(); 942 if (this.indication == null) 943 this.indication = new ArrayList<CodeableReference>(); 944 this.indication.add(t); 945 return t; 946 } 947 948 public RegulatedAuthorization addIndication(CodeableReference t) { //3 949 if (t == null) 950 return this; 951 if (this.indication == null) 952 this.indication = new ArrayList<CodeableReference>(); 953 this.indication.add(t); 954 return this; 955 } 956 957 /** 958 * @return The first repetition of repeating field {@link #indication}, creating it if it does not already exist {3} 959 */ 960 public CodeableReference getIndicationFirstRep() { 961 if (getIndication().isEmpty()) { 962 addIndication(); 963 } 964 return getIndication().get(0); 965 } 966 967 /** 968 * @return {@link #intendedUse} (The intended use of the product, e.g. prevention, treatment, diagnosis.) 969 */ 970 public CodeableConcept getIntendedUse() { 971 if (this.intendedUse == null) 972 if (Configuration.errorOnAutoCreate()) 973 throw new Error("Attempt to auto-create RegulatedAuthorization.intendedUse"); 974 else if (Configuration.doAutoCreate()) 975 this.intendedUse = new CodeableConcept(); // cc 976 return this.intendedUse; 977 } 978 979 public boolean hasIntendedUse() { 980 return this.intendedUse != null && !this.intendedUse.isEmpty(); 981 } 982 983 /** 984 * @param value {@link #intendedUse} (The intended use of the product, e.g. prevention, treatment, diagnosis.) 985 */ 986 public RegulatedAuthorization setIntendedUse(CodeableConcept value) { 987 this.intendedUse = value; 988 return this; 989 } 990 991 /** 992 * @return {@link #basis} (The legal or regulatory framework against which this authorization is granted, or other reasons for it.) 993 */ 994 public List<CodeableConcept> getBasis() { 995 if (this.basis == null) 996 this.basis = new ArrayList<CodeableConcept>(); 997 return this.basis; 998 } 999 1000 /** 1001 * @return Returns a reference to <code>this</code> for easy method chaining 1002 */ 1003 public RegulatedAuthorization setBasis(List<CodeableConcept> theBasis) { 1004 this.basis = theBasis; 1005 return this; 1006 } 1007 1008 public boolean hasBasis() { 1009 if (this.basis == null) 1010 return false; 1011 for (CodeableConcept item : this.basis) 1012 if (!item.isEmpty()) 1013 return true; 1014 return false; 1015 } 1016 1017 public CodeableConcept addBasis() { //3 1018 CodeableConcept t = new CodeableConcept(); 1019 if (this.basis == null) 1020 this.basis = new ArrayList<CodeableConcept>(); 1021 this.basis.add(t); 1022 return t; 1023 } 1024 1025 public RegulatedAuthorization addBasis(CodeableConcept t) { //3 1026 if (t == null) 1027 return this; 1028 if (this.basis == null) 1029 this.basis = new ArrayList<CodeableConcept>(); 1030 this.basis.add(t); 1031 return this; 1032 } 1033 1034 /** 1035 * @return The first repetition of repeating field {@link #basis}, creating it if it does not already exist {3} 1036 */ 1037 public CodeableConcept getBasisFirstRep() { 1038 if (getBasis().isEmpty()) { 1039 addBasis(); 1040 } 1041 return getBasis().get(0); 1042 } 1043 1044 /** 1045 * @return {@link #holder} (The organization that has been granted this authorization, by some authoritative body (the 'regulator').) 1046 */ 1047 public Reference getHolder() { 1048 if (this.holder == null) 1049 if (Configuration.errorOnAutoCreate()) 1050 throw new Error("Attempt to auto-create RegulatedAuthorization.holder"); 1051 else if (Configuration.doAutoCreate()) 1052 this.holder = new Reference(); // cc 1053 return this.holder; 1054 } 1055 1056 public boolean hasHolder() { 1057 return this.holder != null && !this.holder.isEmpty(); 1058 } 1059 1060 /** 1061 * @param value {@link #holder} (The organization that has been granted this authorization, by some authoritative body (the 'regulator').) 1062 */ 1063 public RegulatedAuthorization setHolder(Reference value) { 1064 this.holder = value; 1065 return this; 1066 } 1067 1068 /** 1069 * @return {@link #regulator} (The regulatory authority or authorizing body granting the authorization. For example, European Medicines Agency (EMA), Food and Drug Administration (FDA), Health Canada (HC), etc.) 1070 */ 1071 public Reference getRegulator() { 1072 if (this.regulator == null) 1073 if (Configuration.errorOnAutoCreate()) 1074 throw new Error("Attempt to auto-create RegulatedAuthorization.regulator"); 1075 else if (Configuration.doAutoCreate()) 1076 this.regulator = new Reference(); // cc 1077 return this.regulator; 1078 } 1079 1080 public boolean hasRegulator() { 1081 return this.regulator != null && !this.regulator.isEmpty(); 1082 } 1083 1084 /** 1085 * @param value {@link #regulator} (The regulatory authority or authorizing body granting the authorization. For example, European Medicines Agency (EMA), Food and Drug Administration (FDA), Health Canada (HC), etc.) 1086 */ 1087 public RegulatedAuthorization setRegulator(Reference value) { 1088 this.regulator = value; 1089 return this; 1090 } 1091 1092 /** 1093 * @return {@link #attachedDocument} (Additional information or supporting documentation about the authorization.) 1094 */ 1095 public List<Reference> getAttachedDocument() { 1096 if (this.attachedDocument == null) 1097 this.attachedDocument = new ArrayList<Reference>(); 1098 return this.attachedDocument; 1099 } 1100 1101 /** 1102 * @return Returns a reference to <code>this</code> for easy method chaining 1103 */ 1104 public RegulatedAuthorization setAttachedDocument(List<Reference> theAttachedDocument) { 1105 this.attachedDocument = theAttachedDocument; 1106 return this; 1107 } 1108 1109 public boolean hasAttachedDocument() { 1110 if (this.attachedDocument == null) 1111 return false; 1112 for (Reference item : this.attachedDocument) 1113 if (!item.isEmpty()) 1114 return true; 1115 return false; 1116 } 1117 1118 public Reference addAttachedDocument() { //3 1119 Reference t = new Reference(); 1120 if (this.attachedDocument == null) 1121 this.attachedDocument = new ArrayList<Reference>(); 1122 this.attachedDocument.add(t); 1123 return t; 1124 } 1125 1126 public RegulatedAuthorization addAttachedDocument(Reference t) { //3 1127 if (t == null) 1128 return this; 1129 if (this.attachedDocument == null) 1130 this.attachedDocument = new ArrayList<Reference>(); 1131 this.attachedDocument.add(t); 1132 return this; 1133 } 1134 1135 /** 1136 * @return The first repetition of repeating field {@link #attachedDocument}, creating it if it does not already exist {3} 1137 */ 1138 public Reference getAttachedDocumentFirstRep() { 1139 if (getAttachedDocument().isEmpty()) { 1140 addAttachedDocument(); 1141 } 1142 return getAttachedDocument().get(0); 1143 } 1144 1145 /** 1146 * @return {@link #case_} (The case or regulatory procedure for granting or amending a regulated authorization. An authorization is granted in response to submissions/applications by those seeking authorization. A case is the administrative process that deals with the application(s) that relate to this and assesses them. Note: This area is subject to ongoing review and the workgroup is seeking implementer feedback on its use (see link at bottom of page).) 1147 */ 1148 public RegulatedAuthorizationCaseComponent getCase() { 1149 if (this.case_ == null) 1150 if (Configuration.errorOnAutoCreate()) 1151 throw new Error("Attempt to auto-create RegulatedAuthorization.case_"); 1152 else if (Configuration.doAutoCreate()) 1153 this.case_ = new RegulatedAuthorizationCaseComponent(); // cc 1154 return this.case_; 1155 } 1156 1157 public boolean hasCase() { 1158 return this.case_ != null && !this.case_.isEmpty(); 1159 } 1160 1161 /** 1162 * @param value {@link #case_} (The case or regulatory procedure for granting or amending a regulated authorization. An authorization is granted in response to submissions/applications by those seeking authorization. A case is the administrative process that deals with the application(s) that relate to this and assesses them. Note: This area is subject to ongoing review and the workgroup is seeking implementer feedback on its use (see link at bottom of page).) 1163 */ 1164 public RegulatedAuthorization setCase(RegulatedAuthorizationCaseComponent value) { 1165 this.case_ = value; 1166 return this; 1167 } 1168 1169 protected void listChildren(List<Property> children) { 1170 super.listChildren(children); 1171 children.add(new Property("identifier", "Identifier", "Business identifier for the authorization, typically assigned by the authorizing body.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1172 children.add(new Property("subject", "Reference(MedicinalProductDefinition|BiologicallyDerivedProduct|NutritionProduct|PackagedProductDefinition|ManufacturedItemDefinition|Ingredient|SubstanceDefinition|DeviceDefinition|ResearchStudy|ActivityDefinition|PlanDefinition|ObservationDefinition|Practitioner|Organization|Location)", "The product type, treatment, facility or activity that is being authorized.", 0, java.lang.Integer.MAX_VALUE, subject)); 1173 children.add(new Property("type", "CodeableConcept", "Overall type of this authorization, for example drug marketing approval, orphan drug designation.", 0, 1, type)); 1174 children.add(new Property("description", "markdown", "General textual supporting information.", 0, 1, description)); 1175 children.add(new Property("region", "CodeableConcept", "The territory (e.g., country, jurisdiction etc.) in which the authorization has been granted.", 0, java.lang.Integer.MAX_VALUE, region)); 1176 children.add(new Property("status", "CodeableConcept", "The status that is authorised e.g. approved. Intermediate states and actions can be tracked with cases and applications.", 0, 1, status)); 1177 children.add(new Property("statusDate", "dateTime", "The date at which the current status was assigned.", 0, 1, statusDate)); 1178 children.add(new Property("validityPeriod", "Period", "The time period in which the regulatory approval, clearance or licencing is in effect. As an example, a Marketing Authorization includes the date of authorization and/or an expiration date.", 0, 1, validityPeriod)); 1179 children.add(new Property("indication", "CodeableReference(ClinicalUseDefinition)", "Condition for which the use of the regulated product applies.", 0, java.lang.Integer.MAX_VALUE, indication)); 1180 children.add(new Property("intendedUse", "CodeableConcept", "The intended use of the product, e.g. prevention, treatment, diagnosis.", 0, 1, intendedUse)); 1181 children.add(new Property("basis", "CodeableConcept", "The legal or regulatory framework against which this authorization is granted, or other reasons for it.", 0, java.lang.Integer.MAX_VALUE, basis)); 1182 children.add(new Property("holder", "Reference(Organization)", "The organization that has been granted this authorization, by some authoritative body (the 'regulator').", 0, 1, holder)); 1183 children.add(new Property("regulator", "Reference(Organization)", "The regulatory authority or authorizing body granting the authorization. For example, European Medicines Agency (EMA), Food and Drug Administration (FDA), Health Canada (HC), etc.", 0, 1, regulator)); 1184 children.add(new Property("attachedDocument", "Reference(DocumentReference)", "Additional information or supporting documentation about the authorization.", 0, java.lang.Integer.MAX_VALUE, attachedDocument)); 1185 children.add(new Property("case", "", "The case or regulatory procedure for granting or amending a regulated authorization. An authorization is granted in response to submissions/applications by those seeking authorization. A case is the administrative process that deals with the application(s) that relate to this and assesses them. Note: This area is subject to ongoing review and the workgroup is seeking implementer feedback on its use (see link at bottom of page).", 0, 1, case_)); 1186 } 1187 1188 @Override 1189 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1190 switch (_hash) { 1191 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifier for the authorization, typically assigned by the authorizing body.", 0, java.lang.Integer.MAX_VALUE, identifier); 1192 case -1867885268: /*subject*/ return new Property("subject", "Reference(MedicinalProductDefinition|BiologicallyDerivedProduct|NutritionProduct|PackagedProductDefinition|ManufacturedItemDefinition|Ingredient|SubstanceDefinition|DeviceDefinition|ResearchStudy|ActivityDefinition|PlanDefinition|ObservationDefinition|Practitioner|Organization|Location)", "The product type, treatment, facility or activity that is being authorized.", 0, java.lang.Integer.MAX_VALUE, subject); 1193 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Overall type of this authorization, for example drug marketing approval, orphan drug designation.", 0, 1, type); 1194 case -1724546052: /*description*/ return new Property("description", "markdown", "General textual supporting information.", 0, 1, description); 1195 case -934795532: /*region*/ return new Property("region", "CodeableConcept", "The territory (e.g., country, jurisdiction etc.) in which the authorization has been granted.", 0, java.lang.Integer.MAX_VALUE, region); 1196 case -892481550: /*status*/ return new Property("status", "CodeableConcept", "The status that is authorised e.g. approved. Intermediate states and actions can be tracked with cases and applications.", 0, 1, status); 1197 case 247524032: /*statusDate*/ return new Property("statusDate", "dateTime", "The date at which the current status was assigned.", 0, 1, statusDate); 1198 case -1434195053: /*validityPeriod*/ return new Property("validityPeriod", "Period", "The time period in which the regulatory approval, clearance or licencing is in effect. As an example, a Marketing Authorization includes the date of authorization and/or an expiration date.", 0, 1, validityPeriod); 1199 case -597168804: /*indication*/ return new Property("indication", "CodeableReference(ClinicalUseDefinition)", "Condition for which the use of the regulated product applies.", 0, java.lang.Integer.MAX_VALUE, indication); 1200 case -1618671268: /*intendedUse*/ return new Property("intendedUse", "CodeableConcept", "The intended use of the product, e.g. prevention, treatment, diagnosis.", 0, 1, intendedUse); 1201 case 93508670: /*basis*/ return new Property("basis", "CodeableConcept", "The legal or regulatory framework against which this authorization is granted, or other reasons for it.", 0, java.lang.Integer.MAX_VALUE, basis); 1202 case -1211707988: /*holder*/ return new Property("holder", "Reference(Organization)", "The organization that has been granted this authorization, by some authoritative body (the 'regulator').", 0, 1, holder); 1203 case 414760449: /*regulator*/ return new Property("regulator", "Reference(Organization)", "The regulatory authority or authorizing body granting the authorization. For example, European Medicines Agency (EMA), Food and Drug Administration (FDA), Health Canada (HC), etc.", 0, 1, regulator); 1204 case -513945889: /*attachedDocument*/ return new Property("attachedDocument", "Reference(DocumentReference)", "Additional information or supporting documentation about the authorization.", 0, java.lang.Integer.MAX_VALUE, attachedDocument); 1205 case 3046192: /*case*/ return new Property("case", "", "The case or regulatory procedure for granting or amending a regulated authorization. An authorization is granted in response to submissions/applications by those seeking authorization. A case is the administrative process that deals with the application(s) that relate to this and assesses them. Note: This area is subject to ongoing review and the workgroup is seeking implementer feedback on its use (see link at bottom of page).", 0, 1, case_); 1206 default: return super.getNamedProperty(_hash, _name, _checkValid); 1207 } 1208 1209 } 1210 1211 @Override 1212 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1213 switch (hash) { 1214 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1215 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // Reference 1216 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1217 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1218 case -934795532: /*region*/ return this.region == null ? new Base[0] : this.region.toArray(new Base[this.region.size()]); // CodeableConcept 1219 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // CodeableConcept 1220 case 247524032: /*statusDate*/ return this.statusDate == null ? new Base[0] : new Base[] {this.statusDate}; // DateTimeType 1221 case -1434195053: /*validityPeriod*/ return this.validityPeriod == null ? new Base[0] : new Base[] {this.validityPeriod}; // Period 1222 case -597168804: /*indication*/ return this.indication == null ? new Base[0] : this.indication.toArray(new Base[this.indication.size()]); // CodeableReference 1223 case -1618671268: /*intendedUse*/ return this.intendedUse == null ? new Base[0] : new Base[] {this.intendedUse}; // CodeableConcept 1224 case 93508670: /*basis*/ return this.basis == null ? new Base[0] : this.basis.toArray(new Base[this.basis.size()]); // CodeableConcept 1225 case -1211707988: /*holder*/ return this.holder == null ? new Base[0] : new Base[] {this.holder}; // Reference 1226 case 414760449: /*regulator*/ return this.regulator == null ? new Base[0] : new Base[] {this.regulator}; // Reference 1227 case -513945889: /*attachedDocument*/ return this.attachedDocument == null ? new Base[0] : this.attachedDocument.toArray(new Base[this.attachedDocument.size()]); // Reference 1228 case 3046192: /*case*/ return this.case_ == null ? new Base[0] : new Base[] {this.case_}; // RegulatedAuthorizationCaseComponent 1229 default: return super.getProperty(hash, name, checkValid); 1230 } 1231 1232 } 1233 1234 @Override 1235 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1236 switch (hash) { 1237 case -1618432855: // identifier 1238 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1239 return value; 1240 case -1867885268: // subject 1241 this.getSubject().add(TypeConvertor.castToReference(value)); // Reference 1242 return value; 1243 case 3575610: // type 1244 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1245 return value; 1246 case -1724546052: // description 1247 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1248 return value; 1249 case -934795532: // region 1250 this.getRegion().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1251 return value; 1252 case -892481550: // status 1253 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1254 return value; 1255 case 247524032: // statusDate 1256 this.statusDate = TypeConvertor.castToDateTime(value); // DateTimeType 1257 return value; 1258 case -1434195053: // validityPeriod 1259 this.validityPeriod = TypeConvertor.castToPeriod(value); // Period 1260 return value; 1261 case -597168804: // indication 1262 this.getIndication().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1263 return value; 1264 case -1618671268: // intendedUse 1265 this.intendedUse = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1266 return value; 1267 case 93508670: // basis 1268 this.getBasis().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1269 return value; 1270 case -1211707988: // holder 1271 this.holder = TypeConvertor.castToReference(value); // Reference 1272 return value; 1273 case 414760449: // regulator 1274 this.regulator = TypeConvertor.castToReference(value); // Reference 1275 return value; 1276 case -513945889: // attachedDocument 1277 this.getAttachedDocument().add(TypeConvertor.castToReference(value)); // Reference 1278 return value; 1279 case 3046192: // case 1280 this.case_ = (RegulatedAuthorizationCaseComponent) value; // RegulatedAuthorizationCaseComponent 1281 return value; 1282 default: return super.setProperty(hash, name, value); 1283 } 1284 1285 } 1286 1287 @Override 1288 public Base setProperty(String name, Base value) throws FHIRException { 1289 if (name.equals("identifier")) { 1290 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1291 } else if (name.equals("subject")) { 1292 this.getSubject().add(TypeConvertor.castToReference(value)); 1293 } else if (name.equals("type")) { 1294 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1295 } else if (name.equals("description")) { 1296 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1297 } else if (name.equals("region")) { 1298 this.getRegion().add(TypeConvertor.castToCodeableConcept(value)); 1299 } else if (name.equals("status")) { 1300 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1301 } else if (name.equals("statusDate")) { 1302 this.statusDate = TypeConvertor.castToDateTime(value); // DateTimeType 1303 } else if (name.equals("validityPeriod")) { 1304 this.validityPeriod = TypeConvertor.castToPeriod(value); // Period 1305 } else if (name.equals("indication")) { 1306 this.getIndication().add(TypeConvertor.castToCodeableReference(value)); 1307 } else if (name.equals("intendedUse")) { 1308 this.intendedUse = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1309 } else if (name.equals("basis")) { 1310 this.getBasis().add(TypeConvertor.castToCodeableConcept(value)); 1311 } else if (name.equals("holder")) { 1312 this.holder = TypeConvertor.castToReference(value); // Reference 1313 } else if (name.equals("regulator")) { 1314 this.regulator = TypeConvertor.castToReference(value); // Reference 1315 } else if (name.equals("attachedDocument")) { 1316 this.getAttachedDocument().add(TypeConvertor.castToReference(value)); 1317 } else if (name.equals("case")) { 1318 this.case_ = (RegulatedAuthorizationCaseComponent) value; // RegulatedAuthorizationCaseComponent 1319 } else 1320 return super.setProperty(name, value); 1321 return value; 1322 } 1323 1324 @Override 1325 public Base makeProperty(int hash, String name) throws FHIRException { 1326 switch (hash) { 1327 case -1618432855: return addIdentifier(); 1328 case -1867885268: return addSubject(); 1329 case 3575610: return getType(); 1330 case -1724546052: return getDescriptionElement(); 1331 case -934795532: return addRegion(); 1332 case -892481550: return getStatus(); 1333 case 247524032: return getStatusDateElement(); 1334 case -1434195053: return getValidityPeriod(); 1335 case -597168804: return addIndication(); 1336 case -1618671268: return getIntendedUse(); 1337 case 93508670: return addBasis(); 1338 case -1211707988: return getHolder(); 1339 case 414760449: return getRegulator(); 1340 case -513945889: return addAttachedDocument(); 1341 case 3046192: return getCase(); 1342 default: return super.makeProperty(hash, name); 1343 } 1344 1345 } 1346 1347 @Override 1348 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1349 switch (hash) { 1350 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1351 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1352 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1353 case -1724546052: /*description*/ return new String[] {"markdown"}; 1354 case -934795532: /*region*/ return new String[] {"CodeableConcept"}; 1355 case -892481550: /*status*/ return new String[] {"CodeableConcept"}; 1356 case 247524032: /*statusDate*/ return new String[] {"dateTime"}; 1357 case -1434195053: /*validityPeriod*/ return new String[] {"Period"}; 1358 case -597168804: /*indication*/ return new String[] {"CodeableReference"}; 1359 case -1618671268: /*intendedUse*/ return new String[] {"CodeableConcept"}; 1360 case 93508670: /*basis*/ return new String[] {"CodeableConcept"}; 1361 case -1211707988: /*holder*/ return new String[] {"Reference"}; 1362 case 414760449: /*regulator*/ return new String[] {"Reference"}; 1363 case -513945889: /*attachedDocument*/ return new String[] {"Reference"}; 1364 case 3046192: /*case*/ return new String[] {}; 1365 default: return super.getTypesForProperty(hash, name); 1366 } 1367 1368 } 1369 1370 @Override 1371 public Base addChild(String name) throws FHIRException { 1372 if (name.equals("identifier")) { 1373 return addIdentifier(); 1374 } 1375 else if (name.equals("subject")) { 1376 return addSubject(); 1377 } 1378 else if (name.equals("type")) { 1379 this.type = new CodeableConcept(); 1380 return this.type; 1381 } 1382 else if (name.equals("description")) { 1383 throw new FHIRException("Cannot call addChild on a singleton property RegulatedAuthorization.description"); 1384 } 1385 else if (name.equals("region")) { 1386 return addRegion(); 1387 } 1388 else if (name.equals("status")) { 1389 this.status = new CodeableConcept(); 1390 return this.status; 1391 } 1392 else if (name.equals("statusDate")) { 1393 throw new FHIRException("Cannot call addChild on a singleton property RegulatedAuthorization.statusDate"); 1394 } 1395 else if (name.equals("validityPeriod")) { 1396 this.validityPeriod = new Period(); 1397 return this.validityPeriod; 1398 } 1399 else if (name.equals("indication")) { 1400 return addIndication(); 1401 } 1402 else if (name.equals("intendedUse")) { 1403 this.intendedUse = new CodeableConcept(); 1404 return this.intendedUse; 1405 } 1406 else if (name.equals("basis")) { 1407 return addBasis(); 1408 } 1409 else if (name.equals("holder")) { 1410 this.holder = new Reference(); 1411 return this.holder; 1412 } 1413 else if (name.equals("regulator")) { 1414 this.regulator = new Reference(); 1415 return this.regulator; 1416 } 1417 else if (name.equals("attachedDocument")) { 1418 return addAttachedDocument(); 1419 } 1420 else if (name.equals("case")) { 1421 this.case_ = new RegulatedAuthorizationCaseComponent(); 1422 return this.case_; 1423 } 1424 else 1425 return super.addChild(name); 1426 } 1427 1428 public String fhirType() { 1429 return "RegulatedAuthorization"; 1430 1431 } 1432 1433 public RegulatedAuthorization copy() { 1434 RegulatedAuthorization dst = new RegulatedAuthorization(); 1435 copyValues(dst); 1436 return dst; 1437 } 1438 1439 public void copyValues(RegulatedAuthorization dst) { 1440 super.copyValues(dst); 1441 if (identifier != null) { 1442 dst.identifier = new ArrayList<Identifier>(); 1443 for (Identifier i : identifier) 1444 dst.identifier.add(i.copy()); 1445 }; 1446 if (subject != null) { 1447 dst.subject = new ArrayList<Reference>(); 1448 for (Reference i : subject) 1449 dst.subject.add(i.copy()); 1450 }; 1451 dst.type = type == null ? null : type.copy(); 1452 dst.description = description == null ? null : description.copy(); 1453 if (region != null) { 1454 dst.region = new ArrayList<CodeableConcept>(); 1455 for (CodeableConcept i : region) 1456 dst.region.add(i.copy()); 1457 }; 1458 dst.status = status == null ? null : status.copy(); 1459 dst.statusDate = statusDate == null ? null : statusDate.copy(); 1460 dst.validityPeriod = validityPeriod == null ? null : validityPeriod.copy(); 1461 if (indication != null) { 1462 dst.indication = new ArrayList<CodeableReference>(); 1463 for (CodeableReference i : indication) 1464 dst.indication.add(i.copy()); 1465 }; 1466 dst.intendedUse = intendedUse == null ? null : intendedUse.copy(); 1467 if (basis != null) { 1468 dst.basis = new ArrayList<CodeableConcept>(); 1469 for (CodeableConcept i : basis) 1470 dst.basis.add(i.copy()); 1471 }; 1472 dst.holder = holder == null ? null : holder.copy(); 1473 dst.regulator = regulator == null ? null : regulator.copy(); 1474 if (attachedDocument != null) { 1475 dst.attachedDocument = new ArrayList<Reference>(); 1476 for (Reference i : attachedDocument) 1477 dst.attachedDocument.add(i.copy()); 1478 }; 1479 dst.case_ = case_ == null ? null : case_.copy(); 1480 } 1481 1482 protected RegulatedAuthorization typedCopy() { 1483 return copy(); 1484 } 1485 1486 @Override 1487 public boolean equalsDeep(Base other_) { 1488 if (!super.equalsDeep(other_)) 1489 return false; 1490 if (!(other_ instanceof RegulatedAuthorization)) 1491 return false; 1492 RegulatedAuthorization o = (RegulatedAuthorization) other_; 1493 return compareDeep(identifier, o.identifier, true) && compareDeep(subject, o.subject, true) && compareDeep(type, o.type, true) 1494 && compareDeep(description, o.description, true) && compareDeep(region, o.region, true) && compareDeep(status, o.status, true) 1495 && compareDeep(statusDate, o.statusDate, true) && compareDeep(validityPeriod, o.validityPeriod, true) 1496 && compareDeep(indication, o.indication, true) && compareDeep(intendedUse, o.intendedUse, true) 1497 && compareDeep(basis, o.basis, true) && compareDeep(holder, o.holder, true) && compareDeep(regulator, o.regulator, true) 1498 && compareDeep(attachedDocument, o.attachedDocument, true) && compareDeep(case_, o.case_, true) 1499 ; 1500 } 1501 1502 @Override 1503 public boolean equalsShallow(Base other_) { 1504 if (!super.equalsShallow(other_)) 1505 return false; 1506 if (!(other_ instanceof RegulatedAuthorization)) 1507 return false; 1508 RegulatedAuthorization o = (RegulatedAuthorization) other_; 1509 return compareValues(description, o.description, true) && compareValues(statusDate, o.statusDate, true) 1510 ; 1511 } 1512 1513 public boolean isEmpty() { 1514 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, subject, type 1515 , description, region, status, statusDate, validityPeriod, indication, intendedUse 1516 , basis, holder, regulator, attachedDocument, case_); 1517 } 1518 1519 @Override 1520 public ResourceType getResourceType() { 1521 return ResourceType.RegulatedAuthorization; 1522 } 1523 1524 /** 1525 * Search parameter: <b>case-type</b> 1526 * <p> 1527 * Description: <b>The defining type of case</b><br> 1528 * Type: <b>token</b><br> 1529 * Path: <b>RegulatedAuthorization.case.type</b><br> 1530 * </p> 1531 */ 1532 @SearchParamDefinition(name="case-type", path="RegulatedAuthorization.case.type", description="The defining type of case", type="token" ) 1533 public static final String SP_CASE_TYPE = "case-type"; 1534 /** 1535 * <b>Fluent Client</b> search parameter constant for <b>case-type</b> 1536 * <p> 1537 * Description: <b>The defining type of case</b><br> 1538 * Type: <b>token</b><br> 1539 * Path: <b>RegulatedAuthorization.case.type</b><br> 1540 * </p> 1541 */ 1542 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CASE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CASE_TYPE); 1543 1544 /** 1545 * Search parameter: <b>case</b> 1546 * <p> 1547 * Description: <b>The case or procedure number</b><br> 1548 * Type: <b>token</b><br> 1549 * Path: <b>RegulatedAuthorization.case.identifier</b><br> 1550 * </p> 1551 */ 1552 @SearchParamDefinition(name="case", path="RegulatedAuthorization.case.identifier", description="The case or procedure number", type="token" ) 1553 public static final String SP_CASE = "case"; 1554 /** 1555 * <b>Fluent Client</b> search parameter constant for <b>case</b> 1556 * <p> 1557 * Description: <b>The case or procedure number</b><br> 1558 * Type: <b>token</b><br> 1559 * Path: <b>RegulatedAuthorization.case.identifier</b><br> 1560 * </p> 1561 */ 1562 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CASE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CASE); 1563 1564 /** 1565 * Search parameter: <b>holder</b> 1566 * <p> 1567 * Description: <b>The organization that holds the granted authorization</b><br> 1568 * Type: <b>reference</b><br> 1569 * Path: <b>RegulatedAuthorization.holder</b><br> 1570 * </p> 1571 */ 1572 @SearchParamDefinition(name="holder", path="RegulatedAuthorization.holder", description="The organization that holds the granted authorization", type="reference", target={Organization.class } ) 1573 public static final String SP_HOLDER = "holder"; 1574 /** 1575 * <b>Fluent Client</b> search parameter constant for <b>holder</b> 1576 * <p> 1577 * Description: <b>The organization that holds the granted authorization</b><br> 1578 * Type: <b>reference</b><br> 1579 * Path: <b>RegulatedAuthorization.holder</b><br> 1580 * </p> 1581 */ 1582 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam HOLDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_HOLDER); 1583 1584/** 1585 * Constant for fluent queries to be used to add include statements. Specifies 1586 * the path value of "<b>RegulatedAuthorization:holder</b>". 1587 */ 1588 public static final ca.uhn.fhir.model.api.Include INCLUDE_HOLDER = new ca.uhn.fhir.model.api.Include("RegulatedAuthorization:holder").toLocked(); 1589 1590 /** 1591 * Search parameter: <b>identifier</b> 1592 * <p> 1593 * Description: <b>Business identifier for the authorization, typically assigned by the authorizing body</b><br> 1594 * Type: <b>token</b><br> 1595 * Path: <b>RegulatedAuthorization.identifier</b><br> 1596 * </p> 1597 */ 1598 @SearchParamDefinition(name="identifier", path="RegulatedAuthorization.identifier", description="Business identifier for the authorization, typically assigned by the authorizing body", type="token" ) 1599 public static final String SP_IDENTIFIER = "identifier"; 1600 /** 1601 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1602 * <p> 1603 * Description: <b>Business identifier for the authorization, typically assigned by the authorizing body</b><br> 1604 * Type: <b>token</b><br> 1605 * Path: <b>RegulatedAuthorization.identifier</b><br> 1606 * </p> 1607 */ 1608 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1609 1610 /** 1611 * Search parameter: <b>region</b> 1612 * <p> 1613 * Description: <b>The territory (e.g., country, jurisdiction etc.) in which the authorization has been granted</b><br> 1614 * Type: <b>token</b><br> 1615 * Path: <b>RegulatedAuthorization.region</b><br> 1616 * </p> 1617 */ 1618 @SearchParamDefinition(name="region", path="RegulatedAuthorization.region", description="The territory (e.g., country, jurisdiction etc.) in which the authorization has been granted", type="token" ) 1619 public static final String SP_REGION = "region"; 1620 /** 1621 * <b>Fluent Client</b> search parameter constant for <b>region</b> 1622 * <p> 1623 * Description: <b>The territory (e.g., country, jurisdiction etc.) in which the authorization has been granted</b><br> 1624 * Type: <b>token</b><br> 1625 * Path: <b>RegulatedAuthorization.region</b><br> 1626 * </p> 1627 */ 1628 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REGION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REGION); 1629 1630 /** 1631 * Search parameter: <b>status</b> 1632 * <p> 1633 * Description: <b>The status that is authorised e.g. approved. Intermediate states can be tracked with cases and applications</b><br> 1634 * Type: <b>token</b><br> 1635 * Path: <b>RegulatedAuthorization.status</b><br> 1636 * </p> 1637 */ 1638 @SearchParamDefinition(name="status", path="RegulatedAuthorization.status", description="The status that is authorised e.g. approved. Intermediate states can be tracked with cases and applications", type="token" ) 1639 public static final String SP_STATUS = "status"; 1640 /** 1641 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1642 * <p> 1643 * Description: <b>The status that is authorised e.g. approved. Intermediate states can be tracked with cases and applications</b><br> 1644 * Type: <b>token</b><br> 1645 * Path: <b>RegulatedAuthorization.status</b><br> 1646 * </p> 1647 */ 1648 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1649 1650 /** 1651 * Search parameter: <b>subject</b> 1652 * <p> 1653 * Description: <b>The type of regulated product, treatment, facility or activity that is being authorized</b><br> 1654 * Type: <b>reference</b><br> 1655 * Path: <b>RegulatedAuthorization.subject</b><br> 1656 * </p> 1657 */ 1658 @SearchParamDefinition(name="subject", path="RegulatedAuthorization.subject", description="The type of regulated product, treatment, facility or activity that is being authorized", type="reference", target={ActivityDefinition.class, BiologicallyDerivedProduct.class, DeviceDefinition.class, Ingredient.class, Location.class, ManufacturedItemDefinition.class, MedicinalProductDefinition.class, NutritionProduct.class, ObservationDefinition.class, Organization.class, PackagedProductDefinition.class, PlanDefinition.class, Practitioner.class, ResearchStudy.class, SubstanceDefinition.class } ) 1659 public static final String SP_SUBJECT = "subject"; 1660 /** 1661 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1662 * <p> 1663 * Description: <b>The type of regulated product, treatment, facility or activity that is being authorized</b><br> 1664 * Type: <b>reference</b><br> 1665 * Path: <b>RegulatedAuthorization.subject</b><br> 1666 * </p> 1667 */ 1668 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1669 1670/** 1671 * Constant for fluent queries to be used to add include statements. Specifies 1672 * the path value of "<b>RegulatedAuthorization:subject</b>". 1673 */ 1674 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("RegulatedAuthorization:subject").toLocked(); 1675 1676 1677} 1678