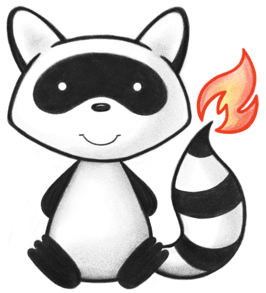
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * RelatedArtifact Type: Related artifacts such as additional documentation, justification, or bibliographic references. 050 */ 051@DatatypeDef(name="RelatedArtifact") 052public class RelatedArtifact extends DataType implements ICompositeType { 053 054 public enum RelatedArtifactType { 055 /** 056 * Additional documentation for the knowledge resource. This would include additional instructions on usage as well as additional information on clinical context or appropriateness. 057 */ 058 DOCUMENTATION, 059 /** 060 * The target artifact is a summary of the justification for the knowledge resource including supporting evidence, relevant guidelines, or other clinically important information. This information is intended to provide a way to make the justification for the knowledge resource available to the consumer of interventions or results produced by the knowledge resource. 061 */ 062 JUSTIFICATION, 063 /** 064 * Bibliographic citation for papers, references, or other relevant material for the knowledge resource. This is intended to allow for citation of related material, but that was not necessarily specifically prepared in connection with this knowledge resource. 065 */ 066 CITATION, 067 /** 068 * The previous version of the knowledge artifact, used to establish an ordering of versions of an artifact, independent of the status of each version. 069 */ 070 PREDECESSOR, 071 /** 072 * The subsequent version of the knowledge artfact, used to establish an ordering of versions of an artifact, independent of the status of each version. 073 */ 074 SUCCESSOR, 075 /** 076 * This artifact is derived from the target artifact. This is intended to capture the relationship in which a particular knowledge resource is based on the content of another artifact, but is modified to capture either a different set of overall requirements, or a more specific set of requirements such as those involved in a particular institution or clinical setting. The artifact may be derived from one or more target artifacts. 077 */ 078 DERIVEDFROM, 079 /** 080 * This artifact depends on the target artifact. There is a requirement to use the target artifact in the creation or interpretation of this artifact. 081 */ 082 DEPENDSON, 083 /** 084 * This artifact is composed of the target artifact. This artifact is constructed with the target artifact as a component. The target artifact is a part of this artifact. (A dataset is composed of data.). 085 */ 086 COMPOSEDOF, 087 /** 088 * This artifact is a part of the target artifact. The target artifact is composed of this artifact (and possibly other artifacts). 089 */ 090 PARTOF, 091 /** 092 * This artifact amends or changes the target artifact. This artifact adds additional information that is functionally expected to replace information in the target artifact. This artifact replaces a part but not all of the target artifact. 093 */ 094 AMENDS, 095 /** 096 * This artifact is amended with or changed by the target artifact. There is information in this artifact that should be functionally replaced with information in the target artifact. 097 */ 098 AMENDEDWITH, 099 /** 100 * This artifact adds additional information to the target artifact. The additional information does not replace or change information in the target artifact. 101 */ 102 APPENDS, 103 /** 104 * This artifact has additional information in the target artifact. 105 */ 106 APPENDEDWITH, 107 /** 108 * This artifact cites the target artifact. This may be a bibliographic citation for papers, references, or other relevant material for the knowledge resource. This is intended to allow for citation of related material, but that was not necessarily specifically prepared in connection with this knowledge resource. 109 */ 110 CITES, 111 /** 112 * This artifact is cited by the target artifact. 113 */ 114 CITEDBY, 115 /** 116 * This artifact contains comments about the target artifact. 117 */ 118 COMMENTSON, 119 /** 120 * This artifact has comments about it in the target artifact. The type of comments may be expressed in the targetClassifier element such as reply, review, editorial, feedback, solicited, unsolicited, structured, unstructured. 121 */ 122 COMMENTIN, 123 /** 124 * This artifact is a container in which the target artifact is contained. A container is a data structure whose instances are collections of other objects. (A database contains the dataset.). 125 */ 126 CONTAINS, 127 /** 128 * This artifact is contained in the target artifact. The target artifact is a data structure whose instances are collections of other objects. 129 */ 130 CONTAINEDIN, 131 /** 132 * This artifact identifies errors and replacement content for the target artifact. 133 */ 134 CORRECTS, 135 /** 136 * This artifact has corrections to it in the target artifact. The target artifact identifies errors and replacement content for this artifact. 137 */ 138 CORRECTIONIN, 139 /** 140 * This artifact replaces or supersedes the target artifact. The target artifact may be considered deprecated. 141 */ 142 REPLACES, 143 /** 144 * This artifact is replaced with or superseded by the target artifact. This artifact may be considered deprecated. 145 */ 146 REPLACEDWITH, 147 /** 148 * This artifact retracts the target artifact. The content that was published in the target artifact should be considered removed from publication and should no longer be considered part of the public record. 149 */ 150 RETRACTS, 151 /** 152 * This artifact is retracted by the target artifact. The content that was published in this artifact should be considered removed from publication and should no longer be considered part of the public record. 153 */ 154 RETRACTEDBY, 155 /** 156 * This artifact is a signature of the target artifact. 157 */ 158 SIGNS, 159 /** 160 * This artifact has characteristics in common with the target artifact. This relationship may be used in systems to ?deduplicate? knowledge artifacts from different sources, or in systems to show ?similar items?. 161 */ 162 SIMILARTO, 163 /** 164 * This artifact provides additional support for the target artifact. The type of support is not documentation as it does not describe, explain, or instruct regarding the target artifact. 165 */ 166 SUPPORTS, 167 /** 168 * The target artifact contains additional information related to the knowledge artifact but is not documentation as the additional information does not describe, explain, or instruct regarding the knowledge artifact content or application. This could include an associated dataset. 169 */ 170 SUPPORTEDWITH, 171 /** 172 * This artifact was generated by transforming the target artifact (e.g., format or language conversion). This is intended to capture the relationship in which a particular knowledge resource is based on the content of another artifact, but changes are only apparent in form and there is only one target artifact with the ?transforms? relationship type. 173 */ 174 TRANSFORMS, 175 /** 176 * This artifact was transformed into the target artifact (e.g., by format or language conversion). 177 */ 178 TRANSFORMEDINTO, 179 /** 180 * This artifact was generated by transforming a related artifact (e.g., format or language conversion), noted separately with the ?transforms? relationship type. This transformation used the target artifact to inform the transformation. The target artifact may be a conversion script or translation guide. 181 */ 182 TRANSFORMEDWITH, 183 /** 184 * This artifact provides additional documentation for the target artifact. This could include additional instructions on usage as well as additional information on clinical context or appropriateness. 185 */ 186 DOCUMENTS, 187 /** 188 * The target artifact is a precise description of a concept in this artifact. This may be used when the RelatedArtifact datatype is used in elements contained in this artifact. 189 */ 190 SPECIFICATIONOF, 191 /** 192 * This artifact was created with the target artifact. The target artifact is a tool or support material used in the creation of the artifact, and not content that the artifact was derived from. 193 */ 194 CREATEDWITH, 195 /** 196 * The related artifact is the citation for this artifact. 197 */ 198 CITEAS, 199 /** 200 * added to help the parsers with the generic types 201 */ 202 NULL; 203 public static RelatedArtifactType fromCode(String codeString) throws FHIRException { 204 if (codeString == null || "".equals(codeString)) 205 return null; 206 if ("documentation".equals(codeString)) 207 return DOCUMENTATION; 208 if ("justification".equals(codeString)) 209 return JUSTIFICATION; 210 if ("citation".equals(codeString)) 211 return CITATION; 212 if ("predecessor".equals(codeString)) 213 return PREDECESSOR; 214 if ("successor".equals(codeString)) 215 return SUCCESSOR; 216 if ("derived-from".equals(codeString)) 217 return DERIVEDFROM; 218 if ("depends-on".equals(codeString)) 219 return DEPENDSON; 220 if ("composed-of".equals(codeString)) 221 return COMPOSEDOF; 222 if ("part-of".equals(codeString)) 223 return PARTOF; 224 if ("amends".equals(codeString)) 225 return AMENDS; 226 if ("amended-with".equals(codeString)) 227 return AMENDEDWITH; 228 if ("appends".equals(codeString)) 229 return APPENDS; 230 if ("appended-with".equals(codeString)) 231 return APPENDEDWITH; 232 if ("cites".equals(codeString)) 233 return CITES; 234 if ("cited-by".equals(codeString)) 235 return CITEDBY; 236 if ("comments-on".equals(codeString)) 237 return COMMENTSON; 238 if ("comment-in".equals(codeString)) 239 return COMMENTIN; 240 if ("contains".equals(codeString)) 241 return CONTAINS; 242 if ("contained-in".equals(codeString)) 243 return CONTAINEDIN; 244 if ("corrects".equals(codeString)) 245 return CORRECTS; 246 if ("correction-in".equals(codeString)) 247 return CORRECTIONIN; 248 if ("replaces".equals(codeString)) 249 return REPLACES; 250 if ("replaced-with".equals(codeString)) 251 return REPLACEDWITH; 252 if ("retracts".equals(codeString)) 253 return RETRACTS; 254 if ("retracted-by".equals(codeString)) 255 return RETRACTEDBY; 256 if ("signs".equals(codeString)) 257 return SIGNS; 258 if ("similar-to".equals(codeString)) 259 return SIMILARTO; 260 if ("supports".equals(codeString)) 261 return SUPPORTS; 262 if ("supported-with".equals(codeString)) 263 return SUPPORTEDWITH; 264 if ("transforms".equals(codeString)) 265 return TRANSFORMS; 266 if ("transformed-into".equals(codeString)) 267 return TRANSFORMEDINTO; 268 if ("transformed-with".equals(codeString)) 269 return TRANSFORMEDWITH; 270 if ("documents".equals(codeString)) 271 return DOCUMENTS; 272 if ("specification-of".equals(codeString)) 273 return SPECIFICATIONOF; 274 if ("created-with".equals(codeString)) 275 return CREATEDWITH; 276 if ("cite-as".equals(codeString)) 277 return CITEAS; 278 if (Configuration.isAcceptInvalidEnums()) 279 return null; 280 else 281 throw new FHIRException("Unknown RelatedArtifactType code '"+codeString+"'"); 282 } 283 public String toCode() { 284 switch (this) { 285 case DOCUMENTATION: return "documentation"; 286 case JUSTIFICATION: return "justification"; 287 case CITATION: return "citation"; 288 case PREDECESSOR: return "predecessor"; 289 case SUCCESSOR: return "successor"; 290 case DERIVEDFROM: return "derived-from"; 291 case DEPENDSON: return "depends-on"; 292 case COMPOSEDOF: return "composed-of"; 293 case PARTOF: return "part-of"; 294 case AMENDS: return "amends"; 295 case AMENDEDWITH: return "amended-with"; 296 case APPENDS: return "appends"; 297 case APPENDEDWITH: return "appended-with"; 298 case CITES: return "cites"; 299 case CITEDBY: return "cited-by"; 300 case COMMENTSON: return "comments-on"; 301 case COMMENTIN: return "comment-in"; 302 case CONTAINS: return "contains"; 303 case CONTAINEDIN: return "contained-in"; 304 case CORRECTS: return "corrects"; 305 case CORRECTIONIN: return "correction-in"; 306 case REPLACES: return "replaces"; 307 case REPLACEDWITH: return "replaced-with"; 308 case RETRACTS: return "retracts"; 309 case RETRACTEDBY: return "retracted-by"; 310 case SIGNS: return "signs"; 311 case SIMILARTO: return "similar-to"; 312 case SUPPORTS: return "supports"; 313 case SUPPORTEDWITH: return "supported-with"; 314 case TRANSFORMS: return "transforms"; 315 case TRANSFORMEDINTO: return "transformed-into"; 316 case TRANSFORMEDWITH: return "transformed-with"; 317 case DOCUMENTS: return "documents"; 318 case SPECIFICATIONOF: return "specification-of"; 319 case CREATEDWITH: return "created-with"; 320 case CITEAS: return "cite-as"; 321 case NULL: return null; 322 default: return "?"; 323 } 324 } 325 public String getSystem() { 326 switch (this) { 327 case DOCUMENTATION: return "http://hl7.org/fhir/related-artifact-type"; 328 case JUSTIFICATION: return "http://hl7.org/fhir/related-artifact-type"; 329 case CITATION: return "http://hl7.org/fhir/related-artifact-type"; 330 case PREDECESSOR: return "http://hl7.org/fhir/related-artifact-type"; 331 case SUCCESSOR: return "http://hl7.org/fhir/related-artifact-type"; 332 case DERIVEDFROM: return "http://hl7.org/fhir/related-artifact-type"; 333 case DEPENDSON: return "http://hl7.org/fhir/related-artifact-type"; 334 case COMPOSEDOF: return "http://hl7.org/fhir/related-artifact-type"; 335 case PARTOF: return "http://hl7.org/fhir/related-artifact-type"; 336 case AMENDS: return "http://hl7.org/fhir/related-artifact-type"; 337 case AMENDEDWITH: return "http://hl7.org/fhir/related-artifact-type"; 338 case APPENDS: return "http://hl7.org/fhir/related-artifact-type"; 339 case APPENDEDWITH: return "http://hl7.org/fhir/related-artifact-type"; 340 case CITES: return "http://hl7.org/fhir/related-artifact-type"; 341 case CITEDBY: return "http://hl7.org/fhir/related-artifact-type"; 342 case COMMENTSON: return "http://hl7.org/fhir/related-artifact-type"; 343 case COMMENTIN: return "http://hl7.org/fhir/related-artifact-type"; 344 case CONTAINS: return "http://hl7.org/fhir/related-artifact-type"; 345 case CONTAINEDIN: return "http://hl7.org/fhir/related-artifact-type"; 346 case CORRECTS: return "http://hl7.org/fhir/related-artifact-type"; 347 case CORRECTIONIN: return "http://hl7.org/fhir/related-artifact-type"; 348 case REPLACES: return "http://hl7.org/fhir/related-artifact-type"; 349 case REPLACEDWITH: return "http://hl7.org/fhir/related-artifact-type"; 350 case RETRACTS: return "http://hl7.org/fhir/related-artifact-type"; 351 case RETRACTEDBY: return "http://hl7.org/fhir/related-artifact-type"; 352 case SIGNS: return "http://hl7.org/fhir/related-artifact-type"; 353 case SIMILARTO: return "http://hl7.org/fhir/related-artifact-type"; 354 case SUPPORTS: return "http://hl7.org/fhir/related-artifact-type"; 355 case SUPPORTEDWITH: return "http://hl7.org/fhir/related-artifact-type"; 356 case TRANSFORMS: return "http://hl7.org/fhir/related-artifact-type"; 357 case TRANSFORMEDINTO: return "http://hl7.org/fhir/related-artifact-type"; 358 case TRANSFORMEDWITH: return "http://hl7.org/fhir/related-artifact-type"; 359 case DOCUMENTS: return "http://hl7.org/fhir/related-artifact-type"; 360 case SPECIFICATIONOF: return "http://hl7.org/fhir/related-artifact-type"; 361 case CREATEDWITH: return "http://hl7.org/fhir/related-artifact-type"; 362 case CITEAS: return "http://hl7.org/fhir/related-artifact-type"; 363 case NULL: return null; 364 default: return "?"; 365 } 366 } 367 public String getDefinition() { 368 switch (this) { 369 case DOCUMENTATION: return "Additional documentation for the knowledge resource. This would include additional instructions on usage as well as additional information on clinical context or appropriateness."; 370 case JUSTIFICATION: return "The target artifact is a summary of the justification for the knowledge resource including supporting evidence, relevant guidelines, or other clinically important information. This information is intended to provide a way to make the justification for the knowledge resource available to the consumer of interventions or results produced by the knowledge resource."; 371 case CITATION: return "Bibliographic citation for papers, references, or other relevant material for the knowledge resource. This is intended to allow for citation of related material, but that was not necessarily specifically prepared in connection with this knowledge resource."; 372 case PREDECESSOR: return "The previous version of the knowledge artifact, used to establish an ordering of versions of an artifact, independent of the status of each version."; 373 case SUCCESSOR: return "The subsequent version of the knowledge artfact, used to establish an ordering of versions of an artifact, independent of the status of each version."; 374 case DERIVEDFROM: return "This artifact is derived from the target artifact. This is intended to capture the relationship in which a particular knowledge resource is based on the content of another artifact, but is modified to capture either a different set of overall requirements, or a more specific set of requirements such as those involved in a particular institution or clinical setting. The artifact may be derived from one or more target artifacts."; 375 case DEPENDSON: return "This artifact depends on the target artifact. There is a requirement to use the target artifact in the creation or interpretation of this artifact."; 376 case COMPOSEDOF: return "This artifact is composed of the target artifact. This artifact is constructed with the target artifact as a component. The target artifact is a part of this artifact. (A dataset is composed of data.)."; 377 case PARTOF: return "This artifact is a part of the target artifact. The target artifact is composed of this artifact (and possibly other artifacts)."; 378 case AMENDS: return "This artifact amends or changes the target artifact. This artifact adds additional information that is functionally expected to replace information in the target artifact. This artifact replaces a part but not all of the target artifact."; 379 case AMENDEDWITH: return "This artifact is amended with or changed by the target artifact. There is information in this artifact that should be functionally replaced with information in the target artifact."; 380 case APPENDS: return "This artifact adds additional information to the target artifact. The additional information does not replace or change information in the target artifact."; 381 case APPENDEDWITH: return "This artifact has additional information in the target artifact."; 382 case CITES: return "This artifact cites the target artifact. This may be a bibliographic citation for papers, references, or other relevant material for the knowledge resource. This is intended to allow for citation of related material, but that was not necessarily specifically prepared in connection with this knowledge resource."; 383 case CITEDBY: return "This artifact is cited by the target artifact."; 384 case COMMENTSON: return "This artifact contains comments about the target artifact."; 385 case COMMENTIN: return "This artifact has comments about it in the target artifact. The type of comments may be expressed in the targetClassifier element such as reply, review, editorial, feedback, solicited, unsolicited, structured, unstructured."; 386 case CONTAINS: return "This artifact is a container in which the target artifact is contained. A container is a data structure whose instances are collections of other objects. (A database contains the dataset.)."; 387 case CONTAINEDIN: return "This artifact is contained in the target artifact. The target artifact is a data structure whose instances are collections of other objects."; 388 case CORRECTS: return "This artifact identifies errors and replacement content for the target artifact."; 389 case CORRECTIONIN: return "This artifact has corrections to it in the target artifact. The target artifact identifies errors and replacement content for this artifact."; 390 case REPLACES: return "This artifact replaces or supersedes the target artifact. The target artifact may be considered deprecated."; 391 case REPLACEDWITH: return "This artifact is replaced with or superseded by the target artifact. This artifact may be considered deprecated."; 392 case RETRACTS: return "This artifact retracts the target artifact. The content that was published in the target artifact should be considered removed from publication and should no longer be considered part of the public record."; 393 case RETRACTEDBY: return "This artifact is retracted by the target artifact. The content that was published in this artifact should be considered removed from publication and should no longer be considered part of the public record."; 394 case SIGNS: return "This artifact is a signature of the target artifact."; 395 case SIMILARTO: return "This artifact has characteristics in common with the target artifact. This relationship may be used in systems to ?deduplicate? knowledge artifacts from different sources, or in systems to show ?similar items?."; 396 case SUPPORTS: return "This artifact provides additional support for the target artifact. The type of support is not documentation as it does not describe, explain, or instruct regarding the target artifact."; 397 case SUPPORTEDWITH: return "The target artifact contains additional information related to the knowledge artifact but is not documentation as the additional information does not describe, explain, or instruct regarding the knowledge artifact content or application. This could include an associated dataset."; 398 case TRANSFORMS: return "This artifact was generated by transforming the target artifact (e.g., format or language conversion). This is intended to capture the relationship in which a particular knowledge resource is based on the content of another artifact, but changes are only apparent in form and there is only one target artifact with the ?transforms? relationship type."; 399 case TRANSFORMEDINTO: return "This artifact was transformed into the target artifact (e.g., by format or language conversion)."; 400 case TRANSFORMEDWITH: return "This artifact was generated by transforming a related artifact (e.g., format or language conversion), noted separately with the ?transforms? relationship type. This transformation used the target artifact to inform the transformation. The target artifact may be a conversion script or translation guide."; 401 case DOCUMENTS: return "This artifact provides additional documentation for the target artifact. This could include additional instructions on usage as well as additional information on clinical context or appropriateness."; 402 case SPECIFICATIONOF: return "The target artifact is a precise description of a concept in this artifact. This may be used when the RelatedArtifact datatype is used in elements contained in this artifact."; 403 case CREATEDWITH: return "This artifact was created with the target artifact. The target artifact is a tool or support material used in the creation of the artifact, and not content that the artifact was derived from."; 404 case CITEAS: return "The related artifact is the citation for this artifact."; 405 case NULL: return null; 406 default: return "?"; 407 } 408 } 409 public String getDisplay() { 410 switch (this) { 411 case DOCUMENTATION: return "Documentation"; 412 case JUSTIFICATION: return "Justification"; 413 case CITATION: return "Citation"; 414 case PREDECESSOR: return "Predecessor"; 415 case SUCCESSOR: return "Successor"; 416 case DERIVEDFROM: return "Derived From"; 417 case DEPENDSON: return "Depends On"; 418 case COMPOSEDOF: return "Composed Of"; 419 case PARTOF: return "Part Of"; 420 case AMENDS: return "Amends"; 421 case AMENDEDWITH: return "Amended With"; 422 case APPENDS: return "Appends"; 423 case APPENDEDWITH: return "Appended With"; 424 case CITES: return "Cites"; 425 case CITEDBY: return "Cited By"; 426 case COMMENTSON: return "Is Comment On"; 427 case COMMENTIN: return "Has Comment In"; 428 case CONTAINS: return "Contains"; 429 case CONTAINEDIN: return "Contained In"; 430 case CORRECTS: return "Corrects"; 431 case CORRECTIONIN: return "Correction In"; 432 case REPLACES: return "Replaces"; 433 case REPLACEDWITH: return "Replaced With"; 434 case RETRACTS: return "Retracts"; 435 case RETRACTEDBY: return "Retracted By"; 436 case SIGNS: return "Signs"; 437 case SIMILARTO: return "Similar To"; 438 case SUPPORTS: return "Supports"; 439 case SUPPORTEDWITH: return "Supported With"; 440 case TRANSFORMS: return "Transforms"; 441 case TRANSFORMEDINTO: return "Transformed Into"; 442 case TRANSFORMEDWITH: return "Transformed With"; 443 case DOCUMENTS: return "Documents"; 444 case SPECIFICATIONOF: return "Specification Of"; 445 case CREATEDWITH: return "Created With"; 446 case CITEAS: return "Cite As"; 447 case NULL: return null; 448 default: return "?"; 449 } 450 } 451 } 452 453 public static class RelatedArtifactTypeEnumFactory implements EnumFactory<RelatedArtifactType> { 454 public RelatedArtifactType fromCode(String codeString) throws IllegalArgumentException { 455 if (codeString == null || "".equals(codeString)) 456 if (codeString == null || "".equals(codeString)) 457 return null; 458 if ("documentation".equals(codeString)) 459 return RelatedArtifactType.DOCUMENTATION; 460 if ("justification".equals(codeString)) 461 return RelatedArtifactType.JUSTIFICATION; 462 if ("citation".equals(codeString)) 463 return RelatedArtifactType.CITATION; 464 if ("predecessor".equals(codeString)) 465 return RelatedArtifactType.PREDECESSOR; 466 if ("successor".equals(codeString)) 467 return RelatedArtifactType.SUCCESSOR; 468 if ("derived-from".equals(codeString)) 469 return RelatedArtifactType.DERIVEDFROM; 470 if ("depends-on".equals(codeString)) 471 return RelatedArtifactType.DEPENDSON; 472 if ("composed-of".equals(codeString)) 473 return RelatedArtifactType.COMPOSEDOF; 474 if ("part-of".equals(codeString)) 475 return RelatedArtifactType.PARTOF; 476 if ("amends".equals(codeString)) 477 return RelatedArtifactType.AMENDS; 478 if ("amended-with".equals(codeString)) 479 return RelatedArtifactType.AMENDEDWITH; 480 if ("appends".equals(codeString)) 481 return RelatedArtifactType.APPENDS; 482 if ("appended-with".equals(codeString)) 483 return RelatedArtifactType.APPENDEDWITH; 484 if ("cites".equals(codeString)) 485 return RelatedArtifactType.CITES; 486 if ("cited-by".equals(codeString)) 487 return RelatedArtifactType.CITEDBY; 488 if ("comments-on".equals(codeString)) 489 return RelatedArtifactType.COMMENTSON; 490 if ("comment-in".equals(codeString)) 491 return RelatedArtifactType.COMMENTIN; 492 if ("contains".equals(codeString)) 493 return RelatedArtifactType.CONTAINS; 494 if ("contained-in".equals(codeString)) 495 return RelatedArtifactType.CONTAINEDIN; 496 if ("corrects".equals(codeString)) 497 return RelatedArtifactType.CORRECTS; 498 if ("correction-in".equals(codeString)) 499 return RelatedArtifactType.CORRECTIONIN; 500 if ("replaces".equals(codeString)) 501 return RelatedArtifactType.REPLACES; 502 if ("replaced-with".equals(codeString)) 503 return RelatedArtifactType.REPLACEDWITH; 504 if ("retracts".equals(codeString)) 505 return RelatedArtifactType.RETRACTS; 506 if ("retracted-by".equals(codeString)) 507 return RelatedArtifactType.RETRACTEDBY; 508 if ("signs".equals(codeString)) 509 return RelatedArtifactType.SIGNS; 510 if ("similar-to".equals(codeString)) 511 return RelatedArtifactType.SIMILARTO; 512 if ("supports".equals(codeString)) 513 return RelatedArtifactType.SUPPORTS; 514 if ("supported-with".equals(codeString)) 515 return RelatedArtifactType.SUPPORTEDWITH; 516 if ("transforms".equals(codeString)) 517 return RelatedArtifactType.TRANSFORMS; 518 if ("transformed-into".equals(codeString)) 519 return RelatedArtifactType.TRANSFORMEDINTO; 520 if ("transformed-with".equals(codeString)) 521 return RelatedArtifactType.TRANSFORMEDWITH; 522 if ("documents".equals(codeString)) 523 return RelatedArtifactType.DOCUMENTS; 524 if ("specification-of".equals(codeString)) 525 return RelatedArtifactType.SPECIFICATIONOF; 526 if ("created-with".equals(codeString)) 527 return RelatedArtifactType.CREATEDWITH; 528 if ("cite-as".equals(codeString)) 529 return RelatedArtifactType.CITEAS; 530 throw new IllegalArgumentException("Unknown RelatedArtifactType code '"+codeString+"'"); 531 } 532 public Enumeration<RelatedArtifactType> fromType(PrimitiveType<?> code) throws FHIRException { 533 if (code == null) 534 return null; 535 if (code.isEmpty()) 536 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.NULL, code); 537 String codeString = ((PrimitiveType) code).asStringValue(); 538 if (codeString == null || "".equals(codeString)) 539 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.NULL, code); 540 if ("documentation".equals(codeString)) 541 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.DOCUMENTATION, code); 542 if ("justification".equals(codeString)) 543 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.JUSTIFICATION, code); 544 if ("citation".equals(codeString)) 545 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.CITATION, code); 546 if ("predecessor".equals(codeString)) 547 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.PREDECESSOR, code); 548 if ("successor".equals(codeString)) 549 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.SUCCESSOR, code); 550 if ("derived-from".equals(codeString)) 551 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.DERIVEDFROM, code); 552 if ("depends-on".equals(codeString)) 553 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.DEPENDSON, code); 554 if ("composed-of".equals(codeString)) 555 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.COMPOSEDOF, code); 556 if ("part-of".equals(codeString)) 557 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.PARTOF, code); 558 if ("amends".equals(codeString)) 559 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.AMENDS, code); 560 if ("amended-with".equals(codeString)) 561 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.AMENDEDWITH, code); 562 if ("appends".equals(codeString)) 563 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.APPENDS, code); 564 if ("appended-with".equals(codeString)) 565 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.APPENDEDWITH, code); 566 if ("cites".equals(codeString)) 567 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.CITES, code); 568 if ("cited-by".equals(codeString)) 569 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.CITEDBY, code); 570 if ("comments-on".equals(codeString)) 571 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.COMMENTSON, code); 572 if ("comment-in".equals(codeString)) 573 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.COMMENTIN, code); 574 if ("contains".equals(codeString)) 575 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.CONTAINS, code); 576 if ("contained-in".equals(codeString)) 577 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.CONTAINEDIN, code); 578 if ("corrects".equals(codeString)) 579 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.CORRECTS, code); 580 if ("correction-in".equals(codeString)) 581 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.CORRECTIONIN, code); 582 if ("replaces".equals(codeString)) 583 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.REPLACES, code); 584 if ("replaced-with".equals(codeString)) 585 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.REPLACEDWITH, code); 586 if ("retracts".equals(codeString)) 587 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.RETRACTS, code); 588 if ("retracted-by".equals(codeString)) 589 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.RETRACTEDBY, code); 590 if ("signs".equals(codeString)) 591 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.SIGNS, code); 592 if ("similar-to".equals(codeString)) 593 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.SIMILARTO, code); 594 if ("supports".equals(codeString)) 595 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.SUPPORTS, code); 596 if ("supported-with".equals(codeString)) 597 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.SUPPORTEDWITH, code); 598 if ("transforms".equals(codeString)) 599 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.TRANSFORMS, code); 600 if ("transformed-into".equals(codeString)) 601 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.TRANSFORMEDINTO, code); 602 if ("transformed-with".equals(codeString)) 603 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.TRANSFORMEDWITH, code); 604 if ("documents".equals(codeString)) 605 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.DOCUMENTS, code); 606 if ("specification-of".equals(codeString)) 607 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.SPECIFICATIONOF, code); 608 if ("created-with".equals(codeString)) 609 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.CREATEDWITH, code); 610 if ("cite-as".equals(codeString)) 611 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.CITEAS, code); 612 throw new FHIRException("Unknown RelatedArtifactType code '"+codeString+"'"); 613 } 614 public String toCode(RelatedArtifactType code) { 615 if (code == RelatedArtifactType.NULL) 616 return null; 617 if (code == RelatedArtifactType.DOCUMENTATION) 618 return "documentation"; 619 if (code == RelatedArtifactType.JUSTIFICATION) 620 return "justification"; 621 if (code == RelatedArtifactType.CITATION) 622 return "citation"; 623 if (code == RelatedArtifactType.PREDECESSOR) 624 return "predecessor"; 625 if (code == RelatedArtifactType.SUCCESSOR) 626 return "successor"; 627 if (code == RelatedArtifactType.DERIVEDFROM) 628 return "derived-from"; 629 if (code == RelatedArtifactType.DEPENDSON) 630 return "depends-on"; 631 if (code == RelatedArtifactType.COMPOSEDOF) 632 return "composed-of"; 633 if (code == RelatedArtifactType.PARTOF) 634 return "part-of"; 635 if (code == RelatedArtifactType.AMENDS) 636 return "amends"; 637 if (code == RelatedArtifactType.AMENDEDWITH) 638 return "amended-with"; 639 if (code == RelatedArtifactType.APPENDS) 640 return "appends"; 641 if (code == RelatedArtifactType.APPENDEDWITH) 642 return "appended-with"; 643 if (code == RelatedArtifactType.CITES) 644 return "cites"; 645 if (code == RelatedArtifactType.CITEDBY) 646 return "cited-by"; 647 if (code == RelatedArtifactType.COMMENTSON) 648 return "comments-on"; 649 if (code == RelatedArtifactType.COMMENTIN) 650 return "comment-in"; 651 if (code == RelatedArtifactType.CONTAINS) 652 return "contains"; 653 if (code == RelatedArtifactType.CONTAINEDIN) 654 return "contained-in"; 655 if (code == RelatedArtifactType.CORRECTS) 656 return "corrects"; 657 if (code == RelatedArtifactType.CORRECTIONIN) 658 return "correction-in"; 659 if (code == RelatedArtifactType.REPLACES) 660 return "replaces"; 661 if (code == RelatedArtifactType.REPLACEDWITH) 662 return "replaced-with"; 663 if (code == RelatedArtifactType.RETRACTS) 664 return "retracts"; 665 if (code == RelatedArtifactType.RETRACTEDBY) 666 return "retracted-by"; 667 if (code == RelatedArtifactType.SIGNS) 668 return "signs"; 669 if (code == RelatedArtifactType.SIMILARTO) 670 return "similar-to"; 671 if (code == RelatedArtifactType.SUPPORTS) 672 return "supports"; 673 if (code == RelatedArtifactType.SUPPORTEDWITH) 674 return "supported-with"; 675 if (code == RelatedArtifactType.TRANSFORMS) 676 return "transforms"; 677 if (code == RelatedArtifactType.TRANSFORMEDINTO) 678 return "transformed-into"; 679 if (code == RelatedArtifactType.TRANSFORMEDWITH) 680 return "transformed-with"; 681 if (code == RelatedArtifactType.DOCUMENTS) 682 return "documents"; 683 if (code == RelatedArtifactType.SPECIFICATIONOF) 684 return "specification-of"; 685 if (code == RelatedArtifactType.CREATEDWITH) 686 return "created-with"; 687 if (code == RelatedArtifactType.CITEAS) 688 return "cite-as"; 689 return "?"; 690 } 691 public String toSystem(RelatedArtifactType code) { 692 return code.getSystem(); 693 } 694 } 695 696 /** 697 * The type of relationship to the related artifact. 698 */ 699 @Child(name = "type", type = {CodeType.class}, order=0, min=1, max=1, modifier=false, summary=true) 700 @Description(shortDefinition="documentation | justification | citation | predecessor | successor | derived-from | depends-on | composed-of | part-of | amends | amended-with | appends | appended-with | cites | cited-by | comments-on | comment-in | contains | contained-in | corrects | correction-in | replaces | replaced-with | retracts | retracted-by | signs | similar-to | supports | supported-with | transforms | transformed-into | transformed-with | documents | specification-of | created-with | cite-as", formalDefinition="The type of relationship to the related artifact." ) 701 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/related-artifact-type") 702 protected Enumeration<RelatedArtifactType> type; 703 704 /** 705 * Provides additional classifiers of the related artifact. 706 */ 707 @Child(name = "classifier", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 708 @Description(shortDefinition="Additional classifiers", formalDefinition="Provides additional classifiers of the related artifact." ) 709 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/citation-artifact-classifier") 710 protected List<CodeableConcept> classifier; 711 712 /** 713 * A short label that can be used to reference the citation from elsewhere in the containing artifact, such as a footnote index. 714 */ 715 @Child(name = "label", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 716 @Description(shortDefinition="Short label", formalDefinition="A short label that can be used to reference the citation from elsewhere in the containing artifact, such as a footnote index." ) 717 protected StringType label; 718 719 /** 720 * A brief description of the document or knowledge resource being referenced, suitable for display to a consumer. 721 */ 722 @Child(name = "display", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 723 @Description(shortDefinition="Brief description of the related artifact", formalDefinition="A brief description of the document or knowledge resource being referenced, suitable for display to a consumer." ) 724 protected StringType display; 725 726 /** 727 * A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format. 728 */ 729 @Child(name = "citation", type = {MarkdownType.class}, order=4, min=0, max=1, modifier=false, summary=true) 730 @Description(shortDefinition="Bibliographic citation for the artifact", formalDefinition="A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format." ) 731 protected MarkdownType citation; 732 733 /** 734 * The document being referenced, represented as an attachment. This is exclusive with the resource element. 735 */ 736 @Child(name = "document", type = {Attachment.class}, order=5, min=0, max=1, modifier=false, summary=true) 737 @Description(shortDefinition="What document is being referenced", formalDefinition="The document being referenced, represented as an attachment. This is exclusive with the resource element." ) 738 protected Attachment document; 739 740 /** 741 * The related artifact, such as a library, value set, profile, or other knowledge resource. 742 */ 743 @Child(name = "resource", type = {CanonicalType.class}, order=6, min=0, max=1, modifier=false, summary=true) 744 @Description(shortDefinition="What artifact is being referenced", formalDefinition="The related artifact, such as a library, value set, profile, or other knowledge resource." ) 745 protected CanonicalType resource; 746 747 /** 748 * The related artifact, if the artifact is not a canonical resource, or a resource reference to a canonical resource. 749 */ 750 @Child(name = "resourceReference", type = {Reference.class}, order=7, min=0, max=1, modifier=false, summary=true) 751 @Description(shortDefinition="What artifact, if not a conformance resource", formalDefinition="The related artifact, if the artifact is not a canonical resource, or a resource reference to a canonical resource." ) 752 protected Reference resourceReference; 753 754 /** 755 * The publication status of the artifact being referred to. 756 */ 757 @Child(name = "publicationStatus", type = {CodeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 758 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The publication status of the artifact being referred to." ) 759 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 760 protected Enumeration<PublicationStatus> publicationStatus; 761 762 /** 763 * The date of publication of the artifact being referred to. 764 */ 765 @Child(name = "publicationDate", type = {DateType.class}, order=9, min=0, max=1, modifier=false, summary=true) 766 @Description(shortDefinition="Date of publication of the artifact being referred to", formalDefinition="The date of publication of the artifact being referred to." ) 767 protected DateType publicationDate; 768 769 private static final long serialVersionUID = 556640693L; 770 771 /** 772 * Constructor 773 */ 774 public RelatedArtifact() { 775 super(); 776 } 777 778 /** 779 * Constructor 780 */ 781 public RelatedArtifact(RelatedArtifactType type) { 782 super(); 783 this.setType(type); 784 } 785 786 /** 787 * @return {@link #type} (The type of relationship to the related artifact.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 788 */ 789 public Enumeration<RelatedArtifactType> getTypeElement() { 790 if (this.type == null) 791 if (Configuration.errorOnAutoCreate()) 792 throw new Error("Attempt to auto-create RelatedArtifact.type"); 793 else if (Configuration.doAutoCreate()) 794 this.type = new Enumeration<RelatedArtifactType>(new RelatedArtifactTypeEnumFactory()); // bb 795 return this.type; 796 } 797 798 public boolean hasTypeElement() { 799 return this.type != null && !this.type.isEmpty(); 800 } 801 802 public boolean hasType() { 803 return this.type != null && !this.type.isEmpty(); 804 } 805 806 /** 807 * @param value {@link #type} (The type of relationship to the related artifact.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 808 */ 809 public RelatedArtifact setTypeElement(Enumeration<RelatedArtifactType> value) { 810 this.type = value; 811 return this; 812 } 813 814 /** 815 * @return The type of relationship to the related artifact. 816 */ 817 public RelatedArtifactType getType() { 818 return this.type == null ? null : this.type.getValue(); 819 } 820 821 /** 822 * @param value The type of relationship to the related artifact. 823 */ 824 public RelatedArtifact setType(RelatedArtifactType value) { 825 if (this.type == null) 826 this.type = new Enumeration<RelatedArtifactType>(new RelatedArtifactTypeEnumFactory()); 827 this.type.setValue(value); 828 return this; 829 } 830 831 /** 832 * @return {@link #classifier} (Provides additional classifiers of the related artifact.) 833 */ 834 public List<CodeableConcept> getClassifier() { 835 if (this.classifier == null) 836 this.classifier = new ArrayList<CodeableConcept>(); 837 return this.classifier; 838 } 839 840 /** 841 * @return Returns a reference to <code>this</code> for easy method chaining 842 */ 843 public RelatedArtifact setClassifier(List<CodeableConcept> theClassifier) { 844 this.classifier = theClassifier; 845 return this; 846 } 847 848 public boolean hasClassifier() { 849 if (this.classifier == null) 850 return false; 851 for (CodeableConcept item : this.classifier) 852 if (!item.isEmpty()) 853 return true; 854 return false; 855 } 856 857 public CodeableConcept addClassifier() { //3 858 CodeableConcept t = new CodeableConcept(); 859 if (this.classifier == null) 860 this.classifier = new ArrayList<CodeableConcept>(); 861 this.classifier.add(t); 862 return t; 863 } 864 865 public RelatedArtifact addClassifier(CodeableConcept t) { //3 866 if (t == null) 867 return this; 868 if (this.classifier == null) 869 this.classifier = new ArrayList<CodeableConcept>(); 870 this.classifier.add(t); 871 return this; 872 } 873 874 /** 875 * @return The first repetition of repeating field {@link #classifier}, creating it if it does not already exist {3} 876 */ 877 public CodeableConcept getClassifierFirstRep() { 878 if (getClassifier().isEmpty()) { 879 addClassifier(); 880 } 881 return getClassifier().get(0); 882 } 883 884 /** 885 * @return {@link #label} (A short label that can be used to reference the citation from elsewhere in the containing artifact, such as a footnote index.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 886 */ 887 public StringType getLabelElement() { 888 if (this.label == null) 889 if (Configuration.errorOnAutoCreate()) 890 throw new Error("Attempt to auto-create RelatedArtifact.label"); 891 else if (Configuration.doAutoCreate()) 892 this.label = new StringType(); // bb 893 return this.label; 894 } 895 896 public boolean hasLabelElement() { 897 return this.label != null && !this.label.isEmpty(); 898 } 899 900 public boolean hasLabel() { 901 return this.label != null && !this.label.isEmpty(); 902 } 903 904 /** 905 * @param value {@link #label} (A short label that can be used to reference the citation from elsewhere in the containing artifact, such as a footnote index.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 906 */ 907 public RelatedArtifact setLabelElement(StringType value) { 908 this.label = value; 909 return this; 910 } 911 912 /** 913 * @return A short label that can be used to reference the citation from elsewhere in the containing artifact, such as a footnote index. 914 */ 915 public String getLabel() { 916 return this.label == null ? null : this.label.getValue(); 917 } 918 919 /** 920 * @param value A short label that can be used to reference the citation from elsewhere in the containing artifact, such as a footnote index. 921 */ 922 public RelatedArtifact setLabel(String value) { 923 if (Utilities.noString(value)) 924 this.label = null; 925 else { 926 if (this.label == null) 927 this.label = new StringType(); 928 this.label.setValue(value); 929 } 930 return this; 931 } 932 933 /** 934 * @return {@link #display} (A brief description of the document or knowledge resource being referenced, suitable for display to a consumer.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 935 */ 936 public StringType getDisplayElement() { 937 if (this.display == null) 938 if (Configuration.errorOnAutoCreate()) 939 throw new Error("Attempt to auto-create RelatedArtifact.display"); 940 else if (Configuration.doAutoCreate()) 941 this.display = new StringType(); // bb 942 return this.display; 943 } 944 945 public boolean hasDisplayElement() { 946 return this.display != null && !this.display.isEmpty(); 947 } 948 949 public boolean hasDisplay() { 950 return this.display != null && !this.display.isEmpty(); 951 } 952 953 /** 954 * @param value {@link #display} (A brief description of the document or knowledge resource being referenced, suitable for display to a consumer.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 955 */ 956 public RelatedArtifact setDisplayElement(StringType value) { 957 this.display = value; 958 return this; 959 } 960 961 /** 962 * @return A brief description of the document or knowledge resource being referenced, suitable for display to a consumer. 963 */ 964 public String getDisplay() { 965 return this.display == null ? null : this.display.getValue(); 966 } 967 968 /** 969 * @param value A brief description of the document or knowledge resource being referenced, suitable for display to a consumer. 970 */ 971 public RelatedArtifact setDisplay(String value) { 972 if (Utilities.noString(value)) 973 this.display = null; 974 else { 975 if (this.display == null) 976 this.display = new StringType(); 977 this.display.setValue(value); 978 } 979 return this; 980 } 981 982 /** 983 * @return {@link #citation} (A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format.). This is the underlying object with id, value and extensions. The accessor "getCitation" gives direct access to the value 984 */ 985 public MarkdownType getCitationElement() { 986 if (this.citation == null) 987 if (Configuration.errorOnAutoCreate()) 988 throw new Error("Attempt to auto-create RelatedArtifact.citation"); 989 else if (Configuration.doAutoCreate()) 990 this.citation = new MarkdownType(); // bb 991 return this.citation; 992 } 993 994 public boolean hasCitationElement() { 995 return this.citation != null && !this.citation.isEmpty(); 996 } 997 998 public boolean hasCitation() { 999 return this.citation != null && !this.citation.isEmpty(); 1000 } 1001 1002 /** 1003 * @param value {@link #citation} (A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format.). This is the underlying object with id, value and extensions. The accessor "getCitation" gives direct access to the value 1004 */ 1005 public RelatedArtifact setCitationElement(MarkdownType value) { 1006 this.citation = value; 1007 return this; 1008 } 1009 1010 /** 1011 * @return A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format. 1012 */ 1013 public String getCitation() { 1014 return this.citation == null ? null : this.citation.getValue(); 1015 } 1016 1017 /** 1018 * @param value A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format. 1019 */ 1020 public RelatedArtifact setCitation(String value) { 1021 if (Utilities.noString(value)) 1022 this.citation = null; 1023 else { 1024 if (this.citation == null) 1025 this.citation = new MarkdownType(); 1026 this.citation.setValue(value); 1027 } 1028 return this; 1029 } 1030 1031 /** 1032 * @return {@link #document} (The document being referenced, represented as an attachment. This is exclusive with the resource element.) 1033 */ 1034 public Attachment getDocument() { 1035 if (this.document == null) 1036 if (Configuration.errorOnAutoCreate()) 1037 throw new Error("Attempt to auto-create RelatedArtifact.document"); 1038 else if (Configuration.doAutoCreate()) 1039 this.document = new Attachment(); // cc 1040 return this.document; 1041 } 1042 1043 public boolean hasDocument() { 1044 return this.document != null && !this.document.isEmpty(); 1045 } 1046 1047 /** 1048 * @param value {@link #document} (The document being referenced, represented as an attachment. This is exclusive with the resource element.) 1049 */ 1050 public RelatedArtifact setDocument(Attachment value) { 1051 this.document = value; 1052 return this; 1053 } 1054 1055 /** 1056 * @return {@link #resource} (The related artifact, such as a library, value set, profile, or other knowledge resource.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 1057 */ 1058 public CanonicalType getResourceElement() { 1059 if (this.resource == null) 1060 if (Configuration.errorOnAutoCreate()) 1061 throw new Error("Attempt to auto-create RelatedArtifact.resource"); 1062 else if (Configuration.doAutoCreate()) 1063 this.resource = new CanonicalType(); // bb 1064 return this.resource; 1065 } 1066 1067 public boolean hasResourceElement() { 1068 return this.resource != null && !this.resource.isEmpty(); 1069 } 1070 1071 public boolean hasResource() { 1072 return this.resource != null && !this.resource.isEmpty(); 1073 } 1074 1075 /** 1076 * @param value {@link #resource} (The related artifact, such as a library, value set, profile, or other knowledge resource.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 1077 */ 1078 public RelatedArtifact setResourceElement(CanonicalType value) { 1079 this.resource = value; 1080 return this; 1081 } 1082 1083 /** 1084 * @return The related artifact, such as a library, value set, profile, or other knowledge resource. 1085 */ 1086 public String getResource() { 1087 return this.resource == null ? null : this.resource.getValue(); 1088 } 1089 1090 /** 1091 * @param value The related artifact, such as a library, value set, profile, or other knowledge resource. 1092 */ 1093 public RelatedArtifact setResource(String value) { 1094 if (Utilities.noString(value)) 1095 this.resource = null; 1096 else { 1097 if (this.resource == null) 1098 this.resource = new CanonicalType(); 1099 this.resource.setValue(value); 1100 } 1101 return this; 1102 } 1103 1104 /** 1105 * @return {@link #resourceReference} (The related artifact, if the artifact is not a canonical resource, or a resource reference to a canonical resource.) 1106 */ 1107 public Reference getResourceReference() { 1108 if (this.resourceReference == null) 1109 if (Configuration.errorOnAutoCreate()) 1110 throw new Error("Attempt to auto-create RelatedArtifact.resourceReference"); 1111 else if (Configuration.doAutoCreate()) 1112 this.resourceReference = new Reference(); // cc 1113 return this.resourceReference; 1114 } 1115 1116 public boolean hasResourceReference() { 1117 return this.resourceReference != null && !this.resourceReference.isEmpty(); 1118 } 1119 1120 /** 1121 * @param value {@link #resourceReference} (The related artifact, if the artifact is not a canonical resource, or a resource reference to a canonical resource.) 1122 */ 1123 public RelatedArtifact setResourceReference(Reference value) { 1124 this.resourceReference = value; 1125 return this; 1126 } 1127 1128 /** 1129 * @return {@link #publicationStatus} (The publication status of the artifact being referred to.). This is the underlying object with id, value and extensions. The accessor "getPublicationStatus" gives direct access to the value 1130 */ 1131 public Enumeration<PublicationStatus> getPublicationStatusElement() { 1132 if (this.publicationStatus == null) 1133 if (Configuration.errorOnAutoCreate()) 1134 throw new Error("Attempt to auto-create RelatedArtifact.publicationStatus"); 1135 else if (Configuration.doAutoCreate()) 1136 this.publicationStatus = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1137 return this.publicationStatus; 1138 } 1139 1140 public boolean hasPublicationStatusElement() { 1141 return this.publicationStatus != null && !this.publicationStatus.isEmpty(); 1142 } 1143 1144 public boolean hasPublicationStatus() { 1145 return this.publicationStatus != null && !this.publicationStatus.isEmpty(); 1146 } 1147 1148 /** 1149 * @param value {@link #publicationStatus} (The publication status of the artifact being referred to.). This is the underlying object with id, value and extensions. The accessor "getPublicationStatus" gives direct access to the value 1150 */ 1151 public RelatedArtifact setPublicationStatusElement(Enumeration<PublicationStatus> value) { 1152 this.publicationStatus = value; 1153 return this; 1154 } 1155 1156 /** 1157 * @return The publication status of the artifact being referred to. 1158 */ 1159 public PublicationStatus getPublicationStatus() { 1160 return this.publicationStatus == null ? null : this.publicationStatus.getValue(); 1161 } 1162 1163 /** 1164 * @param value The publication status of the artifact being referred to. 1165 */ 1166 public RelatedArtifact setPublicationStatus(PublicationStatus value) { 1167 if (value == null) 1168 this.publicationStatus = null; 1169 else { 1170 if (this.publicationStatus == null) 1171 this.publicationStatus = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1172 this.publicationStatus.setValue(value); 1173 } 1174 return this; 1175 } 1176 1177 /** 1178 * @return {@link #publicationDate} (The date of publication of the artifact being referred to.). This is the underlying object with id, value and extensions. The accessor "getPublicationDate" gives direct access to the value 1179 */ 1180 public DateType getPublicationDateElement() { 1181 if (this.publicationDate == null) 1182 if (Configuration.errorOnAutoCreate()) 1183 throw new Error("Attempt to auto-create RelatedArtifact.publicationDate"); 1184 else if (Configuration.doAutoCreate()) 1185 this.publicationDate = new DateType(); // bb 1186 return this.publicationDate; 1187 } 1188 1189 public boolean hasPublicationDateElement() { 1190 return this.publicationDate != null && !this.publicationDate.isEmpty(); 1191 } 1192 1193 public boolean hasPublicationDate() { 1194 return this.publicationDate != null && !this.publicationDate.isEmpty(); 1195 } 1196 1197 /** 1198 * @param value {@link #publicationDate} (The date of publication of the artifact being referred to.). This is the underlying object with id, value and extensions. The accessor "getPublicationDate" gives direct access to the value 1199 */ 1200 public RelatedArtifact setPublicationDateElement(DateType value) { 1201 this.publicationDate = value; 1202 return this; 1203 } 1204 1205 /** 1206 * @return The date of publication of the artifact being referred to. 1207 */ 1208 public Date getPublicationDate() { 1209 return this.publicationDate == null ? null : this.publicationDate.getValue(); 1210 } 1211 1212 /** 1213 * @param value The date of publication of the artifact being referred to. 1214 */ 1215 public RelatedArtifact setPublicationDate(Date value) { 1216 if (value == null) 1217 this.publicationDate = null; 1218 else { 1219 if (this.publicationDate == null) 1220 this.publicationDate = new DateType(); 1221 this.publicationDate.setValue(value); 1222 } 1223 return this; 1224 } 1225 1226 protected void listChildren(List<Property> children) { 1227 super.listChildren(children); 1228 children.add(new Property("type", "code", "The type of relationship to the related artifact.", 0, 1, type)); 1229 children.add(new Property("classifier", "CodeableConcept", "Provides additional classifiers of the related artifact.", 0, java.lang.Integer.MAX_VALUE, classifier)); 1230 children.add(new Property("label", "string", "A short label that can be used to reference the citation from elsewhere in the containing artifact, such as a footnote index.", 0, 1, label)); 1231 children.add(new Property("display", "string", "A brief description of the document or knowledge resource being referenced, suitable for display to a consumer.", 0, 1, display)); 1232 children.add(new Property("citation", "markdown", "A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format.", 0, 1, citation)); 1233 children.add(new Property("document", "Attachment", "The document being referenced, represented as an attachment. This is exclusive with the resource element.", 0, 1, document)); 1234 children.add(new Property("resource", "canonical(Any)", "The related artifact, such as a library, value set, profile, or other knowledge resource.", 0, 1, resource)); 1235 children.add(new Property("resourceReference", "Reference(Any)", "The related artifact, if the artifact is not a canonical resource, or a resource reference to a canonical resource.", 0, 1, resourceReference)); 1236 children.add(new Property("publicationStatus", "code", "The publication status of the artifact being referred to.", 0, 1, publicationStatus)); 1237 children.add(new Property("publicationDate", "date", "The date of publication of the artifact being referred to.", 0, 1, publicationDate)); 1238 } 1239 1240 @Override 1241 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1242 switch (_hash) { 1243 case 3575610: /*type*/ return new Property("type", "code", "The type of relationship to the related artifact.", 0, 1, type); 1244 case -281470431: /*classifier*/ return new Property("classifier", "CodeableConcept", "Provides additional classifiers of the related artifact.", 0, java.lang.Integer.MAX_VALUE, classifier); 1245 case 102727412: /*label*/ return new Property("label", "string", "A short label that can be used to reference the citation from elsewhere in the containing artifact, such as a footnote index.", 0, 1, label); 1246 case 1671764162: /*display*/ return new Property("display", "string", "A brief description of the document or knowledge resource being referenced, suitable for display to a consumer.", 0, 1, display); 1247 case -1442706713: /*citation*/ return new Property("citation", "markdown", "A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format.", 0, 1, citation); 1248 case 861720859: /*document*/ return new Property("document", "Attachment", "The document being referenced, represented as an attachment. This is exclusive with the resource element.", 0, 1, document); 1249 case -341064690: /*resource*/ return new Property("resource", "canonical(Any)", "The related artifact, such as a library, value set, profile, or other knowledge resource.", 0, 1, resource); 1250 case -610120995: /*resourceReference*/ return new Property("resourceReference", "Reference(Any)", "The related artifact, if the artifact is not a canonical resource, or a resource reference to a canonical resource.", 0, 1, resourceReference); 1251 case 616500542: /*publicationStatus*/ return new Property("publicationStatus", "code", "The publication status of the artifact being referred to.", 0, 1, publicationStatus); 1252 case 1470566394: /*publicationDate*/ return new Property("publicationDate", "date", "The date of publication of the artifact being referred to.", 0, 1, publicationDate); 1253 default: return super.getNamedProperty(_hash, _name, _checkValid); 1254 } 1255 1256 } 1257 1258 @Override 1259 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1260 switch (hash) { 1261 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<RelatedArtifactType> 1262 case -281470431: /*classifier*/ return this.classifier == null ? new Base[0] : this.classifier.toArray(new Base[this.classifier.size()]); // CodeableConcept 1263 case 102727412: /*label*/ return this.label == null ? new Base[0] : new Base[] {this.label}; // StringType 1264 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 1265 case -1442706713: /*citation*/ return this.citation == null ? new Base[0] : new Base[] {this.citation}; // MarkdownType 1266 case 861720859: /*document*/ return this.document == null ? new Base[0] : new Base[] {this.document}; // Attachment 1267 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // CanonicalType 1268 case -610120995: /*resourceReference*/ return this.resourceReference == null ? new Base[0] : new Base[] {this.resourceReference}; // Reference 1269 case 616500542: /*publicationStatus*/ return this.publicationStatus == null ? new Base[0] : new Base[] {this.publicationStatus}; // Enumeration<PublicationStatus> 1270 case 1470566394: /*publicationDate*/ return this.publicationDate == null ? new Base[0] : new Base[] {this.publicationDate}; // DateType 1271 default: return super.getProperty(hash, name, checkValid); 1272 } 1273 1274 } 1275 1276 @Override 1277 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1278 switch (hash) { 1279 case 3575610: // type 1280 value = new RelatedArtifactTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1281 this.type = (Enumeration) value; // Enumeration<RelatedArtifactType> 1282 return value; 1283 case -281470431: // classifier 1284 this.getClassifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1285 return value; 1286 case 102727412: // label 1287 this.label = TypeConvertor.castToString(value); // StringType 1288 return value; 1289 case 1671764162: // display 1290 this.display = TypeConvertor.castToString(value); // StringType 1291 return value; 1292 case -1442706713: // citation 1293 this.citation = TypeConvertor.castToMarkdown(value); // MarkdownType 1294 return value; 1295 case 861720859: // document 1296 this.document = TypeConvertor.castToAttachment(value); // Attachment 1297 return value; 1298 case -341064690: // resource 1299 this.resource = TypeConvertor.castToCanonical(value); // CanonicalType 1300 return value; 1301 case -610120995: // resourceReference 1302 this.resourceReference = TypeConvertor.castToReference(value); // Reference 1303 return value; 1304 case 616500542: // publicationStatus 1305 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1306 this.publicationStatus = (Enumeration) value; // Enumeration<PublicationStatus> 1307 return value; 1308 case 1470566394: // publicationDate 1309 this.publicationDate = TypeConvertor.castToDate(value); // DateType 1310 return value; 1311 default: return super.setProperty(hash, name, value); 1312 } 1313 1314 } 1315 1316 @Override 1317 public Base setProperty(String name, Base value) throws FHIRException { 1318 if (name.equals("type")) { 1319 value = new RelatedArtifactTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1320 this.type = (Enumeration) value; // Enumeration<RelatedArtifactType> 1321 } else if (name.equals("classifier")) { 1322 this.getClassifier().add(TypeConvertor.castToCodeableConcept(value)); 1323 } else if (name.equals("label")) { 1324 this.label = TypeConvertor.castToString(value); // StringType 1325 } else if (name.equals("display")) { 1326 this.display = TypeConvertor.castToString(value); // StringType 1327 } else if (name.equals("citation")) { 1328 this.citation = TypeConvertor.castToMarkdown(value); // MarkdownType 1329 } else if (name.equals("document")) { 1330 this.document = TypeConvertor.castToAttachment(value); // Attachment 1331 } else if (name.equals("resource")) { 1332 this.resource = TypeConvertor.castToCanonical(value); // CanonicalType 1333 } else if (name.equals("resourceReference")) { 1334 this.resourceReference = TypeConvertor.castToReference(value); // Reference 1335 } else if (name.equals("publicationStatus")) { 1336 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1337 this.publicationStatus = (Enumeration) value; // Enumeration<PublicationStatus> 1338 } else if (name.equals("publicationDate")) { 1339 this.publicationDate = TypeConvertor.castToDate(value); // DateType 1340 } else 1341 return super.setProperty(name, value); 1342 return value; 1343 } 1344 1345 @Override 1346 public void removeChild(String name, Base value) throws FHIRException { 1347 if (name.equals("type")) { 1348 value = new RelatedArtifactTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1349 this.type = (Enumeration) value; // Enumeration<RelatedArtifactType> 1350 } else if (name.equals("classifier")) { 1351 this.getClassifier().remove(value); 1352 } else if (name.equals("label")) { 1353 this.label = null; 1354 } else if (name.equals("display")) { 1355 this.display = null; 1356 } else if (name.equals("citation")) { 1357 this.citation = null; 1358 } else if (name.equals("document")) { 1359 this.document = null; 1360 } else if (name.equals("resource")) { 1361 this.resource = null; 1362 } else if (name.equals("resourceReference")) { 1363 this.resourceReference = null; 1364 } else if (name.equals("publicationStatus")) { 1365 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1366 this.publicationStatus = (Enumeration) value; // Enumeration<PublicationStatus> 1367 } else if (name.equals("publicationDate")) { 1368 this.publicationDate = null; 1369 } else 1370 super.removeChild(name, value); 1371 1372 } 1373 1374 @Override 1375 public Base makeProperty(int hash, String name) throws FHIRException { 1376 switch (hash) { 1377 case 3575610: return getTypeElement(); 1378 case -281470431: return addClassifier(); 1379 case 102727412: return getLabelElement(); 1380 case 1671764162: return getDisplayElement(); 1381 case -1442706713: return getCitationElement(); 1382 case 861720859: return getDocument(); 1383 case -341064690: return getResourceElement(); 1384 case -610120995: return getResourceReference(); 1385 case 616500542: return getPublicationStatusElement(); 1386 case 1470566394: return getPublicationDateElement(); 1387 default: return super.makeProperty(hash, name); 1388 } 1389 1390 } 1391 1392 @Override 1393 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1394 switch (hash) { 1395 case 3575610: /*type*/ return new String[] {"code"}; 1396 case -281470431: /*classifier*/ return new String[] {"CodeableConcept"}; 1397 case 102727412: /*label*/ return new String[] {"string"}; 1398 case 1671764162: /*display*/ return new String[] {"string"}; 1399 case -1442706713: /*citation*/ return new String[] {"markdown"}; 1400 case 861720859: /*document*/ return new String[] {"Attachment"}; 1401 case -341064690: /*resource*/ return new String[] {"canonical"}; 1402 case -610120995: /*resourceReference*/ return new String[] {"Reference"}; 1403 case 616500542: /*publicationStatus*/ return new String[] {"code"}; 1404 case 1470566394: /*publicationDate*/ return new String[] {"date"}; 1405 default: return super.getTypesForProperty(hash, name); 1406 } 1407 1408 } 1409 1410 @Override 1411 public Base addChild(String name) throws FHIRException { 1412 if (name.equals("type")) { 1413 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.type"); 1414 } 1415 else if (name.equals("classifier")) { 1416 return addClassifier(); 1417 } 1418 else if (name.equals("label")) { 1419 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.label"); 1420 } 1421 else if (name.equals("display")) { 1422 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.display"); 1423 } 1424 else if (name.equals("citation")) { 1425 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.citation"); 1426 } 1427 else if (name.equals("document")) { 1428 this.document = new Attachment(); 1429 return this.document; 1430 } 1431 else if (name.equals("resource")) { 1432 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.resource"); 1433 } 1434 else if (name.equals("resourceReference")) { 1435 this.resourceReference = new Reference(); 1436 return this.resourceReference; 1437 } 1438 else if (name.equals("publicationStatus")) { 1439 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.publicationStatus"); 1440 } 1441 else if (name.equals("publicationDate")) { 1442 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.publicationDate"); 1443 } 1444 else 1445 return super.addChild(name); 1446 } 1447 1448 public String fhirType() { 1449 return "RelatedArtifact"; 1450 1451 } 1452 1453 public RelatedArtifact copy() { 1454 RelatedArtifact dst = new RelatedArtifact(); 1455 copyValues(dst); 1456 return dst; 1457 } 1458 1459 public void copyValues(RelatedArtifact dst) { 1460 super.copyValues(dst); 1461 dst.type = type == null ? null : type.copy(); 1462 if (classifier != null) { 1463 dst.classifier = new ArrayList<CodeableConcept>(); 1464 for (CodeableConcept i : classifier) 1465 dst.classifier.add(i.copy()); 1466 }; 1467 dst.label = label == null ? null : label.copy(); 1468 dst.display = display == null ? null : display.copy(); 1469 dst.citation = citation == null ? null : citation.copy(); 1470 dst.document = document == null ? null : document.copy(); 1471 dst.resource = resource == null ? null : resource.copy(); 1472 dst.resourceReference = resourceReference == null ? null : resourceReference.copy(); 1473 dst.publicationStatus = publicationStatus == null ? null : publicationStatus.copy(); 1474 dst.publicationDate = publicationDate == null ? null : publicationDate.copy(); 1475 } 1476 1477 protected RelatedArtifact typedCopy() { 1478 return copy(); 1479 } 1480 1481 @Override 1482 public boolean equalsDeep(Base other_) { 1483 if (!super.equalsDeep(other_)) 1484 return false; 1485 if (!(other_ instanceof RelatedArtifact)) 1486 return false; 1487 RelatedArtifact o = (RelatedArtifact) other_; 1488 return compareDeep(type, o.type, true) && compareDeep(classifier, o.classifier, true) && compareDeep(label, o.label, true) 1489 && compareDeep(display, o.display, true) && compareDeep(citation, o.citation, true) && compareDeep(document, o.document, true) 1490 && compareDeep(resource, o.resource, true) && compareDeep(resourceReference, o.resourceReference, true) 1491 && compareDeep(publicationStatus, o.publicationStatus, true) && compareDeep(publicationDate, o.publicationDate, true) 1492 ; 1493 } 1494 1495 @Override 1496 public boolean equalsShallow(Base other_) { 1497 if (!super.equalsShallow(other_)) 1498 return false; 1499 if (!(other_ instanceof RelatedArtifact)) 1500 return false; 1501 RelatedArtifact o = (RelatedArtifact) other_; 1502 return compareValues(type, o.type, true) && compareValues(label, o.label, true) && compareValues(display, o.display, true) 1503 && compareValues(citation, o.citation, true) && compareValues(resource, o.resource, true) && compareValues(publicationStatus, o.publicationStatus, true) 1504 && compareValues(publicationDate, o.publicationDate, true); 1505 } 1506 1507 public boolean isEmpty() { 1508 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, classifier, label 1509 , display, citation, document, resource, resourceReference, publicationStatus, publicationDate 1510 ); 1511 } 1512 1513 1514} 1515