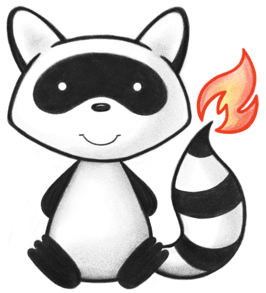
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Information about a person that is involved in a patient's health or the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process. 052 */ 053@ResourceDef(name="RelatedPerson", profile="http://hl7.org/fhir/StructureDefinition/RelatedPerson") 054public class RelatedPerson extends DomainResource { 055 056 @Block() 057 public static class RelatedPersonCommunicationComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-AU" for Australian English. 060 */ 061 @Child(name = "language", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="The language which can be used to communicate with the related person about the patient's health", formalDefinition="The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-AU\" for Australian English." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/all-languages") 064 protected CodeableConcept language; 065 066 /** 067 * Indicates whether or not the related person prefers this language (over other languages he or she masters up a certain level). 068 */ 069 @Child(name = "preferred", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 070 @Description(shortDefinition="Language preference indicator", formalDefinition="Indicates whether or not the related person prefers this language (over other languages he or she masters up a certain level)." ) 071 protected BooleanType preferred; 072 073 private static final long serialVersionUID = 633792918L; 074 075 /** 076 * Constructor 077 */ 078 public RelatedPersonCommunicationComponent() { 079 super(); 080 } 081 082 /** 083 * Constructor 084 */ 085 public RelatedPersonCommunicationComponent(CodeableConcept language) { 086 super(); 087 this.setLanguage(language); 088 } 089 090 /** 091 * @return {@link #language} (The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-AU" for Australian English.) 092 */ 093 public CodeableConcept getLanguage() { 094 if (this.language == null) 095 if (Configuration.errorOnAutoCreate()) 096 throw new Error("Attempt to auto-create RelatedPersonCommunicationComponent.language"); 097 else if (Configuration.doAutoCreate()) 098 this.language = new CodeableConcept(); // cc 099 return this.language; 100 } 101 102 public boolean hasLanguage() { 103 return this.language != null && !this.language.isEmpty(); 104 } 105 106 /** 107 * @param value {@link #language} (The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-AU" for Australian English.) 108 */ 109 public RelatedPersonCommunicationComponent setLanguage(CodeableConcept value) { 110 this.language = value; 111 return this; 112 } 113 114 /** 115 * @return {@link #preferred} (Indicates whether or not the related person prefers this language (over other languages he or she masters up a certain level).). This is the underlying object with id, value and extensions. The accessor "getPreferred" gives direct access to the value 116 */ 117 public BooleanType getPreferredElement() { 118 if (this.preferred == null) 119 if (Configuration.errorOnAutoCreate()) 120 throw new Error("Attempt to auto-create RelatedPersonCommunicationComponent.preferred"); 121 else if (Configuration.doAutoCreate()) 122 this.preferred = new BooleanType(); // bb 123 return this.preferred; 124 } 125 126 public boolean hasPreferredElement() { 127 return this.preferred != null && !this.preferred.isEmpty(); 128 } 129 130 public boolean hasPreferred() { 131 return this.preferred != null && !this.preferred.isEmpty(); 132 } 133 134 /** 135 * @param value {@link #preferred} (Indicates whether or not the related person prefers this language (over other languages he or she masters up a certain level).). This is the underlying object with id, value and extensions. The accessor "getPreferred" gives direct access to the value 136 */ 137 public RelatedPersonCommunicationComponent setPreferredElement(BooleanType value) { 138 this.preferred = value; 139 return this; 140 } 141 142 /** 143 * @return Indicates whether or not the related person prefers this language (over other languages he or she masters up a certain level). 144 */ 145 public boolean getPreferred() { 146 return this.preferred == null || this.preferred.isEmpty() ? false : this.preferred.getValue(); 147 } 148 149 /** 150 * @param value Indicates whether or not the related person prefers this language (over other languages he or she masters up a certain level). 151 */ 152 public RelatedPersonCommunicationComponent setPreferred(boolean value) { 153 if (this.preferred == null) 154 this.preferred = new BooleanType(); 155 this.preferred.setValue(value); 156 return this; 157 } 158 159 protected void listChildren(List<Property> children) { 160 super.listChildren(children); 161 children.add(new Property("language", "CodeableConcept", "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-AU\" for Australian English.", 0, 1, language)); 162 children.add(new Property("preferred", "boolean", "Indicates whether or not the related person prefers this language (over other languages he or she masters up a certain level).", 0, 1, preferred)); 163 } 164 165 @Override 166 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 167 switch (_hash) { 168 case -1613589672: /*language*/ return new Property("language", "CodeableConcept", "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-AU\" for Australian English.", 0, 1, language); 169 case -1294005119: /*preferred*/ return new Property("preferred", "boolean", "Indicates whether or not the related person prefers this language (over other languages he or she masters up a certain level).", 0, 1, preferred); 170 default: return super.getNamedProperty(_hash, _name, _checkValid); 171 } 172 173 } 174 175 @Override 176 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 177 switch (hash) { 178 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeableConcept 179 case -1294005119: /*preferred*/ return this.preferred == null ? new Base[0] : new Base[] {this.preferred}; // BooleanType 180 default: return super.getProperty(hash, name, checkValid); 181 } 182 183 } 184 185 @Override 186 public Base setProperty(int hash, String name, Base value) throws FHIRException { 187 switch (hash) { 188 case -1613589672: // language 189 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 190 return value; 191 case -1294005119: // preferred 192 this.preferred = TypeConvertor.castToBoolean(value); // BooleanType 193 return value; 194 default: return super.setProperty(hash, name, value); 195 } 196 197 } 198 199 @Override 200 public Base setProperty(String name, Base value) throws FHIRException { 201 if (name.equals("language")) { 202 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 203 } else if (name.equals("preferred")) { 204 this.preferred = TypeConvertor.castToBoolean(value); // BooleanType 205 } else 206 return super.setProperty(name, value); 207 return value; 208 } 209 210 @Override 211 public void removeChild(String name, Base value) throws FHIRException { 212 if (name.equals("language")) { 213 this.language = null; 214 } else if (name.equals("preferred")) { 215 this.preferred = null; 216 } else 217 super.removeChild(name, value); 218 219 } 220 221 @Override 222 public Base makeProperty(int hash, String name) throws FHIRException { 223 switch (hash) { 224 case -1613589672: return getLanguage(); 225 case -1294005119: return getPreferredElement(); 226 default: return super.makeProperty(hash, name); 227 } 228 229 } 230 231 @Override 232 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 233 switch (hash) { 234 case -1613589672: /*language*/ return new String[] {"CodeableConcept"}; 235 case -1294005119: /*preferred*/ return new String[] {"boolean"}; 236 default: return super.getTypesForProperty(hash, name); 237 } 238 239 } 240 241 @Override 242 public Base addChild(String name) throws FHIRException { 243 if (name.equals("language")) { 244 this.language = new CodeableConcept(); 245 return this.language; 246 } 247 else if (name.equals("preferred")) { 248 throw new FHIRException("Cannot call addChild on a singleton property RelatedPerson.communication.preferred"); 249 } 250 else 251 return super.addChild(name); 252 } 253 254 public RelatedPersonCommunicationComponent copy() { 255 RelatedPersonCommunicationComponent dst = new RelatedPersonCommunicationComponent(); 256 copyValues(dst); 257 return dst; 258 } 259 260 public void copyValues(RelatedPersonCommunicationComponent dst) { 261 super.copyValues(dst); 262 dst.language = language == null ? null : language.copy(); 263 dst.preferred = preferred == null ? null : preferred.copy(); 264 } 265 266 @Override 267 public boolean equalsDeep(Base other_) { 268 if (!super.equalsDeep(other_)) 269 return false; 270 if (!(other_ instanceof RelatedPersonCommunicationComponent)) 271 return false; 272 RelatedPersonCommunicationComponent o = (RelatedPersonCommunicationComponent) other_; 273 return compareDeep(language, o.language, true) && compareDeep(preferred, o.preferred, true); 274 } 275 276 @Override 277 public boolean equalsShallow(Base other_) { 278 if (!super.equalsShallow(other_)) 279 return false; 280 if (!(other_ instanceof RelatedPersonCommunicationComponent)) 281 return false; 282 RelatedPersonCommunicationComponent o = (RelatedPersonCommunicationComponent) other_; 283 return compareValues(preferred, o.preferred, true); 284 } 285 286 public boolean isEmpty() { 287 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, preferred); 288 } 289 290 public String fhirType() { 291 return "RelatedPerson.communication"; 292 293 } 294 295 } 296 297 /** 298 * Identifier for a person within a particular scope. 299 */ 300 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 301 @Description(shortDefinition="A human identifier for this person", formalDefinition="Identifier for a person within a particular scope." ) 302 protected List<Identifier> identifier; 303 304 /** 305 * Whether this related person record is in active use. 306 */ 307 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=true, summary=true) 308 @Description(shortDefinition="Whether this related person's record is in active use", formalDefinition="Whether this related person record is in active use." ) 309 protected BooleanType active; 310 311 /** 312 * The patient this person is related to. 313 */ 314 @Child(name = "patient", type = {Patient.class}, order=2, min=1, max=1, modifier=false, summary=true) 315 @Description(shortDefinition="The patient this person is related to", formalDefinition="The patient this person is related to." ) 316 protected Reference patient; 317 318 /** 319 * The nature of the relationship between the related person and the patient. 320 */ 321 @Child(name = "relationship", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 322 @Description(shortDefinition="The relationship of the related person to the patient", formalDefinition="The nature of the relationship between the related person and the patient." ) 323 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/relatedperson-relationshiptype") 324 protected List<CodeableConcept> relationship; 325 326 /** 327 * A name associated with the person. 328 */ 329 @Child(name = "name", type = {HumanName.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 330 @Description(shortDefinition="A name associated with the person", formalDefinition="A name associated with the person." ) 331 protected List<HumanName> name; 332 333 /** 334 * A contact detail for the person, e.g. a telephone number or an email address. 335 */ 336 @Child(name = "telecom", type = {ContactPoint.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 337 @Description(shortDefinition="A contact detail for the person", formalDefinition="A contact detail for the person, e.g. a telephone number or an email address." ) 338 protected List<ContactPoint> telecom; 339 340 /** 341 * Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes. 342 */ 343 @Child(name = "gender", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 344 @Description(shortDefinition="male | female | other | unknown", formalDefinition="Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes." ) 345 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/administrative-gender") 346 protected Enumeration<AdministrativeGender> gender; 347 348 /** 349 * The date on which the related person was born. 350 */ 351 @Child(name = "birthDate", type = {DateType.class}, order=7, min=0, max=1, modifier=false, summary=true) 352 @Description(shortDefinition="The date on which the related person was born", formalDefinition="The date on which the related person was born." ) 353 protected DateType birthDate; 354 355 /** 356 * Address where the related person can be contacted or visited. 357 */ 358 @Child(name = "address", type = {Address.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 359 @Description(shortDefinition="Address where the related person can be contacted or visited", formalDefinition="Address where the related person can be contacted or visited." ) 360 protected List<Address> address; 361 362 /** 363 * Image of the person. 364 */ 365 @Child(name = "photo", type = {Attachment.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 366 @Description(shortDefinition="Image of the person", formalDefinition="Image of the person." ) 367 protected List<Attachment> photo; 368 369 /** 370 * The period of time during which this relationship is or was active. If there are no dates defined, then the interval is unknown. 371 */ 372 @Child(name = "period", type = {Period.class}, order=10, min=0, max=1, modifier=false, summary=false) 373 @Description(shortDefinition="Period of time that this relationship is considered valid", formalDefinition="The period of time during which this relationship is or was active. If there are no dates defined, then the interval is unknown." ) 374 protected Period period; 375 376 /** 377 * A language which may be used to communicate with the related person about the patient's health. 378 */ 379 @Child(name = "communication", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 380 @Description(shortDefinition="A language which may be used to communicate with the related person about the patient's health", formalDefinition="A language which may be used to communicate with the related person about the patient's health." ) 381 protected List<RelatedPersonCommunicationComponent> communication; 382 383 private static final long serialVersionUID = -857475397L; 384 385 /** 386 * Constructor 387 */ 388 public RelatedPerson() { 389 super(); 390 } 391 392 /** 393 * Constructor 394 */ 395 public RelatedPerson(Reference patient) { 396 super(); 397 this.setPatient(patient); 398 } 399 400 /** 401 * @return {@link #identifier} (Identifier for a person within a particular scope.) 402 */ 403 public List<Identifier> getIdentifier() { 404 if (this.identifier == null) 405 this.identifier = new ArrayList<Identifier>(); 406 return this.identifier; 407 } 408 409 /** 410 * @return Returns a reference to <code>this</code> for easy method chaining 411 */ 412 public RelatedPerson setIdentifier(List<Identifier> theIdentifier) { 413 this.identifier = theIdentifier; 414 return this; 415 } 416 417 public boolean hasIdentifier() { 418 if (this.identifier == null) 419 return false; 420 for (Identifier item : this.identifier) 421 if (!item.isEmpty()) 422 return true; 423 return false; 424 } 425 426 public Identifier addIdentifier() { //3 427 Identifier t = new Identifier(); 428 if (this.identifier == null) 429 this.identifier = new ArrayList<Identifier>(); 430 this.identifier.add(t); 431 return t; 432 } 433 434 public RelatedPerson addIdentifier(Identifier t) { //3 435 if (t == null) 436 return this; 437 if (this.identifier == null) 438 this.identifier = new ArrayList<Identifier>(); 439 this.identifier.add(t); 440 return this; 441 } 442 443 /** 444 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 445 */ 446 public Identifier getIdentifierFirstRep() { 447 if (getIdentifier().isEmpty()) { 448 addIdentifier(); 449 } 450 return getIdentifier().get(0); 451 } 452 453 /** 454 * @return {@link #active} (Whether this related person record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 455 */ 456 public BooleanType getActiveElement() { 457 if (this.active == null) 458 if (Configuration.errorOnAutoCreate()) 459 throw new Error("Attempt to auto-create RelatedPerson.active"); 460 else if (Configuration.doAutoCreate()) 461 this.active = new BooleanType(); // bb 462 return this.active; 463 } 464 465 public boolean hasActiveElement() { 466 return this.active != null && !this.active.isEmpty(); 467 } 468 469 public boolean hasActive() { 470 return this.active != null && !this.active.isEmpty(); 471 } 472 473 /** 474 * @param value {@link #active} (Whether this related person record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 475 */ 476 public RelatedPerson setActiveElement(BooleanType value) { 477 this.active = value; 478 return this; 479 } 480 481 /** 482 * @return Whether this related person record is in active use. 483 */ 484 public boolean getActive() { 485 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 486 } 487 488 /** 489 * @param value Whether this related person record is in active use. 490 */ 491 public RelatedPerson setActive(boolean value) { 492 if (this.active == null) 493 this.active = new BooleanType(); 494 this.active.setValue(value); 495 return this; 496 } 497 498 /** 499 * @return {@link #patient} (The patient this person is related to.) 500 */ 501 public Reference getPatient() { 502 if (this.patient == null) 503 if (Configuration.errorOnAutoCreate()) 504 throw new Error("Attempt to auto-create RelatedPerson.patient"); 505 else if (Configuration.doAutoCreate()) 506 this.patient = new Reference(); // cc 507 return this.patient; 508 } 509 510 public boolean hasPatient() { 511 return this.patient != null && !this.patient.isEmpty(); 512 } 513 514 /** 515 * @param value {@link #patient} (The patient this person is related to.) 516 */ 517 public RelatedPerson setPatient(Reference value) { 518 this.patient = value; 519 return this; 520 } 521 522 /** 523 * @return {@link #relationship} (The nature of the relationship between the related person and the patient.) 524 */ 525 public List<CodeableConcept> getRelationship() { 526 if (this.relationship == null) 527 this.relationship = new ArrayList<CodeableConcept>(); 528 return this.relationship; 529 } 530 531 /** 532 * @return Returns a reference to <code>this</code> for easy method chaining 533 */ 534 public RelatedPerson setRelationship(List<CodeableConcept> theRelationship) { 535 this.relationship = theRelationship; 536 return this; 537 } 538 539 public boolean hasRelationship() { 540 if (this.relationship == null) 541 return false; 542 for (CodeableConcept item : this.relationship) 543 if (!item.isEmpty()) 544 return true; 545 return false; 546 } 547 548 public CodeableConcept addRelationship() { //3 549 CodeableConcept t = new CodeableConcept(); 550 if (this.relationship == null) 551 this.relationship = new ArrayList<CodeableConcept>(); 552 this.relationship.add(t); 553 return t; 554 } 555 556 public RelatedPerson addRelationship(CodeableConcept t) { //3 557 if (t == null) 558 return this; 559 if (this.relationship == null) 560 this.relationship = new ArrayList<CodeableConcept>(); 561 this.relationship.add(t); 562 return this; 563 } 564 565 /** 566 * @return The first repetition of repeating field {@link #relationship}, creating it if it does not already exist {3} 567 */ 568 public CodeableConcept getRelationshipFirstRep() { 569 if (getRelationship().isEmpty()) { 570 addRelationship(); 571 } 572 return getRelationship().get(0); 573 } 574 575 /** 576 * @return {@link #name} (A name associated with the person.) 577 */ 578 public List<HumanName> getName() { 579 if (this.name == null) 580 this.name = new ArrayList<HumanName>(); 581 return this.name; 582 } 583 584 /** 585 * @return Returns a reference to <code>this</code> for easy method chaining 586 */ 587 public RelatedPerson setName(List<HumanName> theName) { 588 this.name = theName; 589 return this; 590 } 591 592 public boolean hasName() { 593 if (this.name == null) 594 return false; 595 for (HumanName item : this.name) 596 if (!item.isEmpty()) 597 return true; 598 return false; 599 } 600 601 public HumanName addName() { //3 602 HumanName t = new HumanName(); 603 if (this.name == null) 604 this.name = new ArrayList<HumanName>(); 605 this.name.add(t); 606 return t; 607 } 608 609 public RelatedPerson addName(HumanName t) { //3 610 if (t == null) 611 return this; 612 if (this.name == null) 613 this.name = new ArrayList<HumanName>(); 614 this.name.add(t); 615 return this; 616 } 617 618 /** 619 * @return The first repetition of repeating field {@link #name}, creating it if it does not already exist {3} 620 */ 621 public HumanName getNameFirstRep() { 622 if (getName().isEmpty()) { 623 addName(); 624 } 625 return getName().get(0); 626 } 627 628 /** 629 * @return {@link #telecom} (A contact detail for the person, e.g. a telephone number or an email address.) 630 */ 631 public List<ContactPoint> getTelecom() { 632 if (this.telecom == null) 633 this.telecom = new ArrayList<ContactPoint>(); 634 return this.telecom; 635 } 636 637 /** 638 * @return Returns a reference to <code>this</code> for easy method chaining 639 */ 640 public RelatedPerson setTelecom(List<ContactPoint> theTelecom) { 641 this.telecom = theTelecom; 642 return this; 643 } 644 645 public boolean hasTelecom() { 646 if (this.telecom == null) 647 return false; 648 for (ContactPoint item : this.telecom) 649 if (!item.isEmpty()) 650 return true; 651 return false; 652 } 653 654 public ContactPoint addTelecom() { //3 655 ContactPoint t = new ContactPoint(); 656 if (this.telecom == null) 657 this.telecom = new ArrayList<ContactPoint>(); 658 this.telecom.add(t); 659 return t; 660 } 661 662 public RelatedPerson addTelecom(ContactPoint t) { //3 663 if (t == null) 664 return this; 665 if (this.telecom == null) 666 this.telecom = new ArrayList<ContactPoint>(); 667 this.telecom.add(t); 668 return this; 669 } 670 671 /** 672 * @return The first repetition of repeating field {@link #telecom}, creating it if it does not already exist {3} 673 */ 674 public ContactPoint getTelecomFirstRep() { 675 if (getTelecom().isEmpty()) { 676 addTelecom(); 677 } 678 return getTelecom().get(0); 679 } 680 681 /** 682 * @return {@link #gender} (Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 683 */ 684 public Enumeration<AdministrativeGender> getGenderElement() { 685 if (this.gender == null) 686 if (Configuration.errorOnAutoCreate()) 687 throw new Error("Attempt to auto-create RelatedPerson.gender"); 688 else if (Configuration.doAutoCreate()) 689 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 690 return this.gender; 691 } 692 693 public boolean hasGenderElement() { 694 return this.gender != null && !this.gender.isEmpty(); 695 } 696 697 public boolean hasGender() { 698 return this.gender != null && !this.gender.isEmpty(); 699 } 700 701 /** 702 * @param value {@link #gender} (Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 703 */ 704 public RelatedPerson setGenderElement(Enumeration<AdministrativeGender> value) { 705 this.gender = value; 706 return this; 707 } 708 709 /** 710 * @return Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes. 711 */ 712 public AdministrativeGender getGender() { 713 return this.gender == null ? null : this.gender.getValue(); 714 } 715 716 /** 717 * @param value Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes. 718 */ 719 public RelatedPerson setGender(AdministrativeGender value) { 720 if (value == null) 721 this.gender = null; 722 else { 723 if (this.gender == null) 724 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 725 this.gender.setValue(value); 726 } 727 return this; 728 } 729 730 /** 731 * @return {@link #birthDate} (The date on which the related person was born.). This is the underlying object with id, value and extensions. The accessor "getBirthDate" gives direct access to the value 732 */ 733 public DateType getBirthDateElement() { 734 if (this.birthDate == null) 735 if (Configuration.errorOnAutoCreate()) 736 throw new Error("Attempt to auto-create RelatedPerson.birthDate"); 737 else if (Configuration.doAutoCreate()) 738 this.birthDate = new DateType(); // bb 739 return this.birthDate; 740 } 741 742 public boolean hasBirthDateElement() { 743 return this.birthDate != null && !this.birthDate.isEmpty(); 744 } 745 746 public boolean hasBirthDate() { 747 return this.birthDate != null && !this.birthDate.isEmpty(); 748 } 749 750 /** 751 * @param value {@link #birthDate} (The date on which the related person was born.). This is the underlying object with id, value and extensions. The accessor "getBirthDate" gives direct access to the value 752 */ 753 public RelatedPerson setBirthDateElement(DateType value) { 754 this.birthDate = value; 755 return this; 756 } 757 758 /** 759 * @return The date on which the related person was born. 760 */ 761 public Date getBirthDate() { 762 return this.birthDate == null ? null : this.birthDate.getValue(); 763 } 764 765 /** 766 * @param value The date on which the related person was born. 767 */ 768 public RelatedPerson setBirthDate(Date value) { 769 if (value == null) 770 this.birthDate = null; 771 else { 772 if (this.birthDate == null) 773 this.birthDate = new DateType(); 774 this.birthDate.setValue(value); 775 } 776 return this; 777 } 778 779 /** 780 * @return {@link #address} (Address where the related person can be contacted or visited.) 781 */ 782 public List<Address> getAddress() { 783 if (this.address == null) 784 this.address = new ArrayList<Address>(); 785 return this.address; 786 } 787 788 /** 789 * @return Returns a reference to <code>this</code> for easy method chaining 790 */ 791 public RelatedPerson setAddress(List<Address> theAddress) { 792 this.address = theAddress; 793 return this; 794 } 795 796 public boolean hasAddress() { 797 if (this.address == null) 798 return false; 799 for (Address item : this.address) 800 if (!item.isEmpty()) 801 return true; 802 return false; 803 } 804 805 public Address addAddress() { //3 806 Address t = new Address(); 807 if (this.address == null) 808 this.address = new ArrayList<Address>(); 809 this.address.add(t); 810 return t; 811 } 812 813 public RelatedPerson addAddress(Address t) { //3 814 if (t == null) 815 return this; 816 if (this.address == null) 817 this.address = new ArrayList<Address>(); 818 this.address.add(t); 819 return this; 820 } 821 822 /** 823 * @return The first repetition of repeating field {@link #address}, creating it if it does not already exist {3} 824 */ 825 public Address getAddressFirstRep() { 826 if (getAddress().isEmpty()) { 827 addAddress(); 828 } 829 return getAddress().get(0); 830 } 831 832 /** 833 * @return {@link #photo} (Image of the person.) 834 */ 835 public List<Attachment> getPhoto() { 836 if (this.photo == null) 837 this.photo = new ArrayList<Attachment>(); 838 return this.photo; 839 } 840 841 /** 842 * @return Returns a reference to <code>this</code> for easy method chaining 843 */ 844 public RelatedPerson setPhoto(List<Attachment> thePhoto) { 845 this.photo = thePhoto; 846 return this; 847 } 848 849 public boolean hasPhoto() { 850 if (this.photo == null) 851 return false; 852 for (Attachment item : this.photo) 853 if (!item.isEmpty()) 854 return true; 855 return false; 856 } 857 858 public Attachment addPhoto() { //3 859 Attachment t = new Attachment(); 860 if (this.photo == null) 861 this.photo = new ArrayList<Attachment>(); 862 this.photo.add(t); 863 return t; 864 } 865 866 public RelatedPerson addPhoto(Attachment t) { //3 867 if (t == null) 868 return this; 869 if (this.photo == null) 870 this.photo = new ArrayList<Attachment>(); 871 this.photo.add(t); 872 return this; 873 } 874 875 /** 876 * @return The first repetition of repeating field {@link #photo}, creating it if it does not already exist {3} 877 */ 878 public Attachment getPhotoFirstRep() { 879 if (getPhoto().isEmpty()) { 880 addPhoto(); 881 } 882 return getPhoto().get(0); 883 } 884 885 /** 886 * @return {@link #period} (The period of time during which this relationship is or was active. If there are no dates defined, then the interval is unknown.) 887 */ 888 public Period getPeriod() { 889 if (this.period == null) 890 if (Configuration.errorOnAutoCreate()) 891 throw new Error("Attempt to auto-create RelatedPerson.period"); 892 else if (Configuration.doAutoCreate()) 893 this.period = new Period(); // cc 894 return this.period; 895 } 896 897 public boolean hasPeriod() { 898 return this.period != null && !this.period.isEmpty(); 899 } 900 901 /** 902 * @param value {@link #period} (The period of time during which this relationship is or was active. If there are no dates defined, then the interval is unknown.) 903 */ 904 public RelatedPerson setPeriod(Period value) { 905 this.period = value; 906 return this; 907 } 908 909 /** 910 * @return {@link #communication} (A language which may be used to communicate with the related person about the patient's health.) 911 */ 912 public List<RelatedPersonCommunicationComponent> getCommunication() { 913 if (this.communication == null) 914 this.communication = new ArrayList<RelatedPersonCommunicationComponent>(); 915 return this.communication; 916 } 917 918 /** 919 * @return Returns a reference to <code>this</code> for easy method chaining 920 */ 921 public RelatedPerson setCommunication(List<RelatedPersonCommunicationComponent> theCommunication) { 922 this.communication = theCommunication; 923 return this; 924 } 925 926 public boolean hasCommunication() { 927 if (this.communication == null) 928 return false; 929 for (RelatedPersonCommunicationComponent item : this.communication) 930 if (!item.isEmpty()) 931 return true; 932 return false; 933 } 934 935 public RelatedPersonCommunicationComponent addCommunication() { //3 936 RelatedPersonCommunicationComponent t = new RelatedPersonCommunicationComponent(); 937 if (this.communication == null) 938 this.communication = new ArrayList<RelatedPersonCommunicationComponent>(); 939 this.communication.add(t); 940 return t; 941 } 942 943 public RelatedPerson addCommunication(RelatedPersonCommunicationComponent t) { //3 944 if (t == null) 945 return this; 946 if (this.communication == null) 947 this.communication = new ArrayList<RelatedPersonCommunicationComponent>(); 948 this.communication.add(t); 949 return this; 950 } 951 952 /** 953 * @return The first repetition of repeating field {@link #communication}, creating it if it does not already exist {3} 954 */ 955 public RelatedPersonCommunicationComponent getCommunicationFirstRep() { 956 if (getCommunication().isEmpty()) { 957 addCommunication(); 958 } 959 return getCommunication().get(0); 960 } 961 962 protected void listChildren(List<Property> children) { 963 super.listChildren(children); 964 children.add(new Property("identifier", "Identifier", "Identifier for a person within a particular scope.", 0, java.lang.Integer.MAX_VALUE, identifier)); 965 children.add(new Property("active", "boolean", "Whether this related person record is in active use.", 0, 1, active)); 966 children.add(new Property("patient", "Reference(Patient)", "The patient this person is related to.", 0, 1, patient)); 967 children.add(new Property("relationship", "CodeableConcept", "The nature of the relationship between the related person and the patient.", 0, java.lang.Integer.MAX_VALUE, relationship)); 968 children.add(new Property("name", "HumanName", "A name associated with the person.", 0, java.lang.Integer.MAX_VALUE, name)); 969 children.add(new Property("telecom", "ContactPoint", "A contact detail for the person, e.g. a telephone number or an email address.", 0, java.lang.Integer.MAX_VALUE, telecom)); 970 children.add(new Property("gender", "code", "Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.", 0, 1, gender)); 971 children.add(new Property("birthDate", "date", "The date on which the related person was born.", 0, 1, birthDate)); 972 children.add(new Property("address", "Address", "Address where the related person can be contacted or visited.", 0, java.lang.Integer.MAX_VALUE, address)); 973 children.add(new Property("photo", "Attachment", "Image of the person.", 0, java.lang.Integer.MAX_VALUE, photo)); 974 children.add(new Property("period", "Period", "The period of time during which this relationship is or was active. If there are no dates defined, then the interval is unknown.", 0, 1, period)); 975 children.add(new Property("communication", "", "A language which may be used to communicate with the related person about the patient's health.", 0, java.lang.Integer.MAX_VALUE, communication)); 976 } 977 978 @Override 979 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 980 switch (_hash) { 981 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier for a person within a particular scope.", 0, java.lang.Integer.MAX_VALUE, identifier); 982 case -1422950650: /*active*/ return new Property("active", "boolean", "Whether this related person record is in active use.", 0, 1, active); 983 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The patient this person is related to.", 0, 1, patient); 984 case -261851592: /*relationship*/ return new Property("relationship", "CodeableConcept", "The nature of the relationship between the related person and the patient.", 0, java.lang.Integer.MAX_VALUE, relationship); 985 case 3373707: /*name*/ return new Property("name", "HumanName", "A name associated with the person.", 0, java.lang.Integer.MAX_VALUE, name); 986 case -1429363305: /*telecom*/ return new Property("telecom", "ContactPoint", "A contact detail for the person, e.g. a telephone number or an email address.", 0, java.lang.Integer.MAX_VALUE, telecom); 987 case -1249512767: /*gender*/ return new Property("gender", "code", "Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.", 0, 1, gender); 988 case -1210031859: /*birthDate*/ return new Property("birthDate", "date", "The date on which the related person was born.", 0, 1, birthDate); 989 case -1147692044: /*address*/ return new Property("address", "Address", "Address where the related person can be contacted or visited.", 0, java.lang.Integer.MAX_VALUE, address); 990 case 106642994: /*photo*/ return new Property("photo", "Attachment", "Image of the person.", 0, java.lang.Integer.MAX_VALUE, photo); 991 case -991726143: /*period*/ return new Property("period", "Period", "The period of time during which this relationship is or was active. If there are no dates defined, then the interval is unknown.", 0, 1, period); 992 case -1035284522: /*communication*/ return new Property("communication", "", "A language which may be used to communicate with the related person about the patient's health.", 0, java.lang.Integer.MAX_VALUE, communication); 993 default: return super.getNamedProperty(_hash, _name, _checkValid); 994 } 995 996 } 997 998 @Override 999 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1000 switch (hash) { 1001 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1002 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 1003 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 1004 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : this.relationship.toArray(new Base[this.relationship.size()]); // CodeableConcept 1005 case 3373707: /*name*/ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // HumanName 1006 case -1429363305: /*telecom*/ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 1007 case -1249512767: /*gender*/ return this.gender == null ? new Base[0] : new Base[] {this.gender}; // Enumeration<AdministrativeGender> 1008 case -1210031859: /*birthDate*/ return this.birthDate == null ? new Base[0] : new Base[] {this.birthDate}; // DateType 1009 case -1147692044: /*address*/ return this.address == null ? new Base[0] : this.address.toArray(new Base[this.address.size()]); // Address 1010 case 106642994: /*photo*/ return this.photo == null ? new Base[0] : this.photo.toArray(new Base[this.photo.size()]); // Attachment 1011 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1012 case -1035284522: /*communication*/ return this.communication == null ? new Base[0] : this.communication.toArray(new Base[this.communication.size()]); // RelatedPersonCommunicationComponent 1013 default: return super.getProperty(hash, name, checkValid); 1014 } 1015 1016 } 1017 1018 @Override 1019 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1020 switch (hash) { 1021 case -1618432855: // identifier 1022 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1023 return value; 1024 case -1422950650: // active 1025 this.active = TypeConvertor.castToBoolean(value); // BooleanType 1026 return value; 1027 case -791418107: // patient 1028 this.patient = TypeConvertor.castToReference(value); // Reference 1029 return value; 1030 case -261851592: // relationship 1031 this.getRelationship().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1032 return value; 1033 case 3373707: // name 1034 this.getName().add(TypeConvertor.castToHumanName(value)); // HumanName 1035 return value; 1036 case -1429363305: // telecom 1037 this.getTelecom().add(TypeConvertor.castToContactPoint(value)); // ContactPoint 1038 return value; 1039 case -1249512767: // gender 1040 value = new AdministrativeGenderEnumFactory().fromType(TypeConvertor.castToCode(value)); 1041 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1042 return value; 1043 case -1210031859: // birthDate 1044 this.birthDate = TypeConvertor.castToDate(value); // DateType 1045 return value; 1046 case -1147692044: // address 1047 this.getAddress().add(TypeConvertor.castToAddress(value)); // Address 1048 return value; 1049 case 106642994: // photo 1050 this.getPhoto().add(TypeConvertor.castToAttachment(value)); // Attachment 1051 return value; 1052 case -991726143: // period 1053 this.period = TypeConvertor.castToPeriod(value); // Period 1054 return value; 1055 case -1035284522: // communication 1056 this.getCommunication().add((RelatedPersonCommunicationComponent) value); // RelatedPersonCommunicationComponent 1057 return value; 1058 default: return super.setProperty(hash, name, value); 1059 } 1060 1061 } 1062 1063 @Override 1064 public Base setProperty(String name, Base value) throws FHIRException { 1065 if (name.equals("identifier")) { 1066 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1067 } else if (name.equals("active")) { 1068 this.active = TypeConvertor.castToBoolean(value); // BooleanType 1069 } else if (name.equals("patient")) { 1070 this.patient = TypeConvertor.castToReference(value); // Reference 1071 } else if (name.equals("relationship")) { 1072 this.getRelationship().add(TypeConvertor.castToCodeableConcept(value)); 1073 } else if (name.equals("name")) { 1074 this.getName().add(TypeConvertor.castToHumanName(value)); 1075 } else if (name.equals("telecom")) { 1076 this.getTelecom().add(TypeConvertor.castToContactPoint(value)); 1077 } else if (name.equals("gender")) { 1078 value = new AdministrativeGenderEnumFactory().fromType(TypeConvertor.castToCode(value)); 1079 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1080 } else if (name.equals("birthDate")) { 1081 this.birthDate = TypeConvertor.castToDate(value); // DateType 1082 } else if (name.equals("address")) { 1083 this.getAddress().add(TypeConvertor.castToAddress(value)); 1084 } else if (name.equals("photo")) { 1085 this.getPhoto().add(TypeConvertor.castToAttachment(value)); 1086 } else if (name.equals("period")) { 1087 this.period = TypeConvertor.castToPeriod(value); // Period 1088 } else if (name.equals("communication")) { 1089 this.getCommunication().add((RelatedPersonCommunicationComponent) value); 1090 } else 1091 return super.setProperty(name, value); 1092 return value; 1093 } 1094 1095 @Override 1096 public void removeChild(String name, Base value) throws FHIRException { 1097 if (name.equals("identifier")) { 1098 this.getIdentifier().remove(value); 1099 } else if (name.equals("active")) { 1100 this.active = null; 1101 } else if (name.equals("patient")) { 1102 this.patient = null; 1103 } else if (name.equals("relationship")) { 1104 this.getRelationship().remove(value); 1105 } else if (name.equals("name")) { 1106 this.getName().remove(value); 1107 } else if (name.equals("telecom")) { 1108 this.getTelecom().remove(value); 1109 } else if (name.equals("gender")) { 1110 value = new AdministrativeGenderEnumFactory().fromType(TypeConvertor.castToCode(value)); 1111 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1112 } else if (name.equals("birthDate")) { 1113 this.birthDate = null; 1114 } else if (name.equals("address")) { 1115 this.getAddress().remove(value); 1116 } else if (name.equals("photo")) { 1117 this.getPhoto().remove(value); 1118 } else if (name.equals("period")) { 1119 this.period = null; 1120 } else if (name.equals("communication")) { 1121 this.getCommunication().remove((RelatedPersonCommunicationComponent) value); 1122 } else 1123 super.removeChild(name, value); 1124 1125 } 1126 1127 @Override 1128 public Base makeProperty(int hash, String name) throws FHIRException { 1129 switch (hash) { 1130 case -1618432855: return addIdentifier(); 1131 case -1422950650: return getActiveElement(); 1132 case -791418107: return getPatient(); 1133 case -261851592: return addRelationship(); 1134 case 3373707: return addName(); 1135 case -1429363305: return addTelecom(); 1136 case -1249512767: return getGenderElement(); 1137 case -1210031859: return getBirthDateElement(); 1138 case -1147692044: return addAddress(); 1139 case 106642994: return addPhoto(); 1140 case -991726143: return getPeriod(); 1141 case -1035284522: return addCommunication(); 1142 default: return super.makeProperty(hash, name); 1143 } 1144 1145 } 1146 1147 @Override 1148 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1149 switch (hash) { 1150 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1151 case -1422950650: /*active*/ return new String[] {"boolean"}; 1152 case -791418107: /*patient*/ return new String[] {"Reference"}; 1153 case -261851592: /*relationship*/ return new String[] {"CodeableConcept"}; 1154 case 3373707: /*name*/ return new String[] {"HumanName"}; 1155 case -1429363305: /*telecom*/ return new String[] {"ContactPoint"}; 1156 case -1249512767: /*gender*/ return new String[] {"code"}; 1157 case -1210031859: /*birthDate*/ return new String[] {"date"}; 1158 case -1147692044: /*address*/ return new String[] {"Address"}; 1159 case 106642994: /*photo*/ return new String[] {"Attachment"}; 1160 case -991726143: /*period*/ return new String[] {"Period"}; 1161 case -1035284522: /*communication*/ return new String[] {}; 1162 default: return super.getTypesForProperty(hash, name); 1163 } 1164 1165 } 1166 1167 @Override 1168 public Base addChild(String name) throws FHIRException { 1169 if (name.equals("identifier")) { 1170 return addIdentifier(); 1171 } 1172 else if (name.equals("active")) { 1173 throw new FHIRException("Cannot call addChild on a singleton property RelatedPerson.active"); 1174 } 1175 else if (name.equals("patient")) { 1176 this.patient = new Reference(); 1177 return this.patient; 1178 } 1179 else if (name.equals("relationship")) { 1180 return addRelationship(); 1181 } 1182 else if (name.equals("name")) { 1183 return addName(); 1184 } 1185 else if (name.equals("telecom")) { 1186 return addTelecom(); 1187 } 1188 else if (name.equals("gender")) { 1189 throw new FHIRException("Cannot call addChild on a singleton property RelatedPerson.gender"); 1190 } 1191 else if (name.equals("birthDate")) { 1192 throw new FHIRException("Cannot call addChild on a singleton property RelatedPerson.birthDate"); 1193 } 1194 else if (name.equals("address")) { 1195 return addAddress(); 1196 } 1197 else if (name.equals("photo")) { 1198 return addPhoto(); 1199 } 1200 else if (name.equals("period")) { 1201 this.period = new Period(); 1202 return this.period; 1203 } 1204 else if (name.equals("communication")) { 1205 return addCommunication(); 1206 } 1207 else 1208 return super.addChild(name); 1209 } 1210 1211 public String fhirType() { 1212 return "RelatedPerson"; 1213 1214 } 1215 1216 public RelatedPerson copy() { 1217 RelatedPerson dst = new RelatedPerson(); 1218 copyValues(dst); 1219 return dst; 1220 } 1221 1222 public void copyValues(RelatedPerson dst) { 1223 super.copyValues(dst); 1224 if (identifier != null) { 1225 dst.identifier = new ArrayList<Identifier>(); 1226 for (Identifier i : identifier) 1227 dst.identifier.add(i.copy()); 1228 }; 1229 dst.active = active == null ? null : active.copy(); 1230 dst.patient = patient == null ? null : patient.copy(); 1231 if (relationship != null) { 1232 dst.relationship = new ArrayList<CodeableConcept>(); 1233 for (CodeableConcept i : relationship) 1234 dst.relationship.add(i.copy()); 1235 }; 1236 if (name != null) { 1237 dst.name = new ArrayList<HumanName>(); 1238 for (HumanName i : name) 1239 dst.name.add(i.copy()); 1240 }; 1241 if (telecom != null) { 1242 dst.telecom = new ArrayList<ContactPoint>(); 1243 for (ContactPoint i : telecom) 1244 dst.telecom.add(i.copy()); 1245 }; 1246 dst.gender = gender == null ? null : gender.copy(); 1247 dst.birthDate = birthDate == null ? null : birthDate.copy(); 1248 if (address != null) { 1249 dst.address = new ArrayList<Address>(); 1250 for (Address i : address) 1251 dst.address.add(i.copy()); 1252 }; 1253 if (photo != null) { 1254 dst.photo = new ArrayList<Attachment>(); 1255 for (Attachment i : photo) 1256 dst.photo.add(i.copy()); 1257 }; 1258 dst.period = period == null ? null : period.copy(); 1259 if (communication != null) { 1260 dst.communication = new ArrayList<RelatedPersonCommunicationComponent>(); 1261 for (RelatedPersonCommunicationComponent i : communication) 1262 dst.communication.add(i.copy()); 1263 }; 1264 } 1265 1266 protected RelatedPerson typedCopy() { 1267 return copy(); 1268 } 1269 1270 @Override 1271 public boolean equalsDeep(Base other_) { 1272 if (!super.equalsDeep(other_)) 1273 return false; 1274 if (!(other_ instanceof RelatedPerson)) 1275 return false; 1276 RelatedPerson o = (RelatedPerson) other_; 1277 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(patient, o.patient, true) 1278 && compareDeep(relationship, o.relationship, true) && compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true) 1279 && compareDeep(gender, o.gender, true) && compareDeep(birthDate, o.birthDate, true) && compareDeep(address, o.address, true) 1280 && compareDeep(photo, o.photo, true) && compareDeep(period, o.period, true) && compareDeep(communication, o.communication, true) 1281 ; 1282 } 1283 1284 @Override 1285 public boolean equalsShallow(Base other_) { 1286 if (!super.equalsShallow(other_)) 1287 return false; 1288 if (!(other_ instanceof RelatedPerson)) 1289 return false; 1290 RelatedPerson o = (RelatedPerson) other_; 1291 return compareValues(active, o.active, true) && compareValues(gender, o.gender, true) && compareValues(birthDate, o.birthDate, true) 1292 ; 1293 } 1294 1295 public boolean isEmpty() { 1296 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, patient 1297 , relationship, name, telecom, gender, birthDate, address, photo, period, communication 1298 ); 1299 } 1300 1301 @Override 1302 public ResourceType getResourceType() { 1303 return ResourceType.RelatedPerson; 1304 } 1305 1306 /** 1307 * Search parameter: <b>active</b> 1308 * <p> 1309 * Description: <b>Indicates if the related person record is active</b><br> 1310 * Type: <b>token</b><br> 1311 * Path: <b>RelatedPerson.active</b><br> 1312 * </p> 1313 */ 1314 @SearchParamDefinition(name="active", path="RelatedPerson.active", description="Indicates if the related person record is active", type="token" ) 1315 public static final String SP_ACTIVE = "active"; 1316 /** 1317 * <b>Fluent Client</b> search parameter constant for <b>active</b> 1318 * <p> 1319 * Description: <b>Indicates if the related person record is active</b><br> 1320 * Type: <b>token</b><br> 1321 * Path: <b>RelatedPerson.active</b><br> 1322 * </p> 1323 */ 1324 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTIVE); 1325 1326 /** 1327 * Search parameter: <b>family</b> 1328 * <p> 1329 * Description: <b>A portion of the family name of the related person</b><br> 1330 * Type: <b>string</b><br> 1331 * Path: <b>RelatedPerson.name.family</b><br> 1332 * </p> 1333 */ 1334 @SearchParamDefinition(name="family", path="RelatedPerson.name.family", description="A portion of the family name of the related person", type="string" ) 1335 public static final String SP_FAMILY = "family"; 1336 /** 1337 * <b>Fluent Client</b> search parameter constant for <b>family</b> 1338 * <p> 1339 * Description: <b>A portion of the family name of the related person</b><br> 1340 * Type: <b>string</b><br> 1341 * Path: <b>RelatedPerson.name.family</b><br> 1342 * </p> 1343 */ 1344 public static final ca.uhn.fhir.rest.gclient.StringClientParam FAMILY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_FAMILY); 1345 1346 /** 1347 * Search parameter: <b>given</b> 1348 * <p> 1349 * Description: <b>A portion of the given name of the related person</b><br> 1350 * Type: <b>string</b><br> 1351 * Path: <b>RelatedPerson.name.given</b><br> 1352 * </p> 1353 */ 1354 @SearchParamDefinition(name="given", path="RelatedPerson.name.given", description="A portion of the given name of the related person", type="string" ) 1355 public static final String SP_GIVEN = "given"; 1356 /** 1357 * <b>Fluent Client</b> search parameter constant for <b>given</b> 1358 * <p> 1359 * Description: <b>A portion of the given name of the related person</b><br> 1360 * Type: <b>string</b><br> 1361 * Path: <b>RelatedPerson.name.given</b><br> 1362 * </p> 1363 */ 1364 public static final ca.uhn.fhir.rest.gclient.StringClientParam GIVEN = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_GIVEN); 1365 1366 /** 1367 * Search parameter: <b>name</b> 1368 * <p> 1369 * Description: <b>A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text</b><br> 1370 * Type: <b>string</b><br> 1371 * Path: <b>RelatedPerson.name</b><br> 1372 * </p> 1373 */ 1374 @SearchParamDefinition(name="name", path="RelatedPerson.name", description="A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text", type="string" ) 1375 public static final String SP_NAME = "name"; 1376 /** 1377 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1378 * <p> 1379 * Description: <b>A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text</b><br> 1380 * Type: <b>string</b><br> 1381 * Path: <b>RelatedPerson.name</b><br> 1382 * </p> 1383 */ 1384 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 1385 1386 /** 1387 * Search parameter: <b>relationship</b> 1388 * <p> 1389 * Description: <b>The relationship between the patient and the relatedperson</b><br> 1390 * Type: <b>token</b><br> 1391 * Path: <b>RelatedPerson.relationship</b><br> 1392 * </p> 1393 */ 1394 @SearchParamDefinition(name="relationship", path="RelatedPerson.relationship", description="The relationship between the patient and the relatedperson", type="token" ) 1395 public static final String SP_RELATIONSHIP = "relationship"; 1396 /** 1397 * <b>Fluent Client</b> search parameter constant for <b>relationship</b> 1398 * <p> 1399 * Description: <b>The relationship between the patient and the relatedperson</b><br> 1400 * Type: <b>token</b><br> 1401 * Path: <b>RelatedPerson.relationship</b><br> 1402 * </p> 1403 */ 1404 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RELATIONSHIP = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_RELATIONSHIP); 1405 1406 /** 1407 * Search parameter: <b>identifier</b> 1408 * <p> 1409 * Description: <b>Multiple Resources: 1410 1411* [Account](account.html): Account number 1412* [AdverseEvent](adverseevent.html): Business identifier for the event 1413* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1414* [Appointment](appointment.html): An Identifier of the Appointment 1415* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1416* [Basic](basic.html): Business identifier 1417* [BodyStructure](bodystructure.html): Bodystructure identifier 1418* [CarePlan](careplan.html): External Ids for this plan 1419* [CareTeam](careteam.html): External Ids for this team 1420* [ChargeItem](chargeitem.html): Business Identifier for item 1421* [Claim](claim.html): The primary identifier of the financial resource 1422* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1423* [ClinicalImpression](clinicalimpression.html): Business identifier 1424* [Communication](communication.html): Unique identifier 1425* [CommunicationRequest](communicationrequest.html): Unique identifier 1426* [Composition](composition.html): Version-independent identifier for the Composition 1427* [Condition](condition.html): A unique identifier of the condition record 1428* [Consent](consent.html): Identifier for this record (external references) 1429* [Contract](contract.html): The identity of the contract 1430* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1431* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1432* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1433* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1434* [DeviceRequest](devicerequest.html): Business identifier for request/order 1435* [DeviceUsage](deviceusage.html): Search by identifier 1436* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1437* [DocumentReference](documentreference.html): Identifier of the attachment binary 1438* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1439* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1440* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1441* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1442* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1443* [Flag](flag.html): Business identifier 1444* [Goal](goal.html): External Ids for this goal 1445* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1446* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1447* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1448* [Immunization](immunization.html): Business identifier 1449* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1450* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1451* [Invoice](invoice.html): Business Identifier for item 1452* [List](list.html): Business identifier 1453* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1454* [Medication](medication.html): Returns medications with this external identifier 1455* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1456* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1457* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1458* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1459* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1460* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1461* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1462* [Observation](observation.html): The unique id for a particular observation 1463* [Person](person.html): A person Identifier 1464* [Procedure](procedure.html): A unique identifier for a procedure 1465* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1466* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1467* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1468* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1469* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1470* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1471* [Specimen](specimen.html): The unique identifier associated with the specimen 1472* [SupplyDelivery](supplydelivery.html): External identifier 1473* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1474* [Task](task.html): Search for a task instance by its business identifier 1475* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1476</b><br> 1477 * Type: <b>token</b><br> 1478 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1479 * </p> 1480 */ 1481 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 1482 public static final String SP_IDENTIFIER = "identifier"; 1483 /** 1484 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1485 * <p> 1486 * Description: <b>Multiple Resources: 1487 1488* [Account](account.html): Account number 1489* [AdverseEvent](adverseevent.html): Business identifier for the event 1490* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1491* [Appointment](appointment.html): An Identifier of the Appointment 1492* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1493* [Basic](basic.html): Business identifier 1494* [BodyStructure](bodystructure.html): Bodystructure identifier 1495* [CarePlan](careplan.html): External Ids for this plan 1496* [CareTeam](careteam.html): External Ids for this team 1497* [ChargeItem](chargeitem.html): Business Identifier for item 1498* [Claim](claim.html): The primary identifier of the financial resource 1499* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1500* [ClinicalImpression](clinicalimpression.html): Business identifier 1501* [Communication](communication.html): Unique identifier 1502* [CommunicationRequest](communicationrequest.html): Unique identifier 1503* [Composition](composition.html): Version-independent identifier for the Composition 1504* [Condition](condition.html): A unique identifier of the condition record 1505* [Consent](consent.html): Identifier for this record (external references) 1506* [Contract](contract.html): The identity of the contract 1507* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1508* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1509* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1510* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1511* [DeviceRequest](devicerequest.html): Business identifier for request/order 1512* [DeviceUsage](deviceusage.html): Search by identifier 1513* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1514* [DocumentReference](documentreference.html): Identifier of the attachment binary 1515* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1516* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1517* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1518* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1519* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1520* [Flag](flag.html): Business identifier 1521* [Goal](goal.html): External Ids for this goal 1522* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1523* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1524* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1525* [Immunization](immunization.html): Business identifier 1526* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1527* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1528* [Invoice](invoice.html): Business Identifier for item 1529* [List](list.html): Business identifier 1530* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1531* [Medication](medication.html): Returns medications with this external identifier 1532* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1533* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1534* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1535* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1536* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1537* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1538* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1539* [Observation](observation.html): The unique id for a particular observation 1540* [Person](person.html): A person Identifier 1541* [Procedure](procedure.html): A unique identifier for a procedure 1542* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1543* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1544* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1545* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1546* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1547* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1548* [Specimen](specimen.html): The unique identifier associated with the specimen 1549* [SupplyDelivery](supplydelivery.html): External identifier 1550* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1551* [Task](task.html): Search for a task instance by its business identifier 1552* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1553</b><br> 1554 * Type: <b>token</b><br> 1555 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1556 * </p> 1557 */ 1558 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1559 1560 /** 1561 * Search parameter: <b>patient</b> 1562 * <p> 1563 * Description: <b>Multiple Resources: 1564 1565* [Account](account.html): The entity that caused the expenses 1566* [AdverseEvent](adverseevent.html): Subject impacted by event 1567* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 1568* [Appointment](appointment.html): One of the individuals of the appointment is this patient 1569* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 1570* [AuditEvent](auditevent.html): Where the activity involved patient data 1571* [Basic](basic.html): Identifies the focus of this resource 1572* [BodyStructure](bodystructure.html): Who this is about 1573* [CarePlan](careplan.html): Who the care plan is for 1574* [CareTeam](careteam.html): Who care team is for 1575* [ChargeItem](chargeitem.html): Individual service was done for/to 1576* [Claim](claim.html): Patient receiving the products or services 1577* [ClaimResponse](claimresponse.html): The subject of care 1578* [ClinicalImpression](clinicalimpression.html): Patient assessed 1579* [Communication](communication.html): Focus of message 1580* [CommunicationRequest](communicationrequest.html): Focus of message 1581* [Composition](composition.html): Who and/or what the composition is about 1582* [Condition](condition.html): Who has the condition? 1583* [Consent](consent.html): Who the consent applies to 1584* [Contract](contract.html): The identity of the subject of the contract (if a patient) 1585* [Coverage](coverage.html): Retrieve coverages for a patient 1586* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 1587* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 1588* [DetectedIssue](detectedissue.html): Associated patient 1589* [DeviceRequest](devicerequest.html): Individual the service is ordered for 1590* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 1591* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 1592* [DocumentReference](documentreference.html): Who/what is the subject of the document 1593* [Encounter](encounter.html): The patient present at the encounter 1594* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 1595* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 1596* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 1597* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 1598* [Flag](flag.html): The identity of a subject to list flags for 1599* [Goal](goal.html): Who this goal is intended for 1600* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 1601* [ImagingSelection](imagingselection.html): Who the study is about 1602* [ImagingStudy](imagingstudy.html): Who the study is about 1603* [Immunization](immunization.html): The patient for the vaccination record 1604* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 1605* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 1606* [Invoice](invoice.html): Recipient(s) of goods and services 1607* [List](list.html): If all resources have the same subject 1608* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 1609* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 1610* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 1611* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 1612* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 1613* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 1614* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 1615* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 1616* [Observation](observation.html): The subject that the observation is about (if patient) 1617* [Person](person.html): The Person links to this Patient 1618* [Procedure](procedure.html): Search by subject - a patient 1619* [Provenance](provenance.html): Where the activity involved patient data 1620* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 1621* [RelatedPerson](relatedperson.html): The patient this related person is related to 1622* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 1623* [ResearchSubject](researchsubject.html): Who or what is part of study 1624* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 1625* [ServiceRequest](servicerequest.html): Search by subject - a patient 1626* [Specimen](specimen.html): The patient the specimen comes from 1627* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 1628* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 1629* [Task](task.html): Search by patient 1630* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 1631</b><br> 1632 * Type: <b>reference</b><br> 1633 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 1634 * </p> 1635 */ 1636 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 1637 public static final String SP_PATIENT = "patient"; 1638 /** 1639 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1640 * <p> 1641 * Description: <b>Multiple Resources: 1642 1643* [Account](account.html): The entity that caused the expenses 1644* [AdverseEvent](adverseevent.html): Subject impacted by event 1645* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 1646* [Appointment](appointment.html): One of the individuals of the appointment is this patient 1647* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 1648* [AuditEvent](auditevent.html): Where the activity involved patient data 1649* [Basic](basic.html): Identifies the focus of this resource 1650* [BodyStructure](bodystructure.html): Who this is about 1651* [CarePlan](careplan.html): Who the care plan is for 1652* [CareTeam](careteam.html): Who care team is for 1653* [ChargeItem](chargeitem.html): Individual service was done for/to 1654* [Claim](claim.html): Patient receiving the products or services 1655* [ClaimResponse](claimresponse.html): The subject of care 1656* [ClinicalImpression](clinicalimpression.html): Patient assessed 1657* [Communication](communication.html): Focus of message 1658* [CommunicationRequest](communicationrequest.html): Focus of message 1659* [Composition](composition.html): Who and/or what the composition is about 1660* [Condition](condition.html): Who has the condition? 1661* [Consent](consent.html): Who the consent applies to 1662* [Contract](contract.html): The identity of the subject of the contract (if a patient) 1663* [Coverage](coverage.html): Retrieve coverages for a patient 1664* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 1665* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 1666* [DetectedIssue](detectedissue.html): Associated patient 1667* [DeviceRequest](devicerequest.html): Individual the service is ordered for 1668* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 1669* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 1670* [DocumentReference](documentreference.html): Who/what is the subject of the document 1671* [Encounter](encounter.html): The patient present at the encounter 1672* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 1673* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 1674* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 1675* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 1676* [Flag](flag.html): The identity of a subject to list flags for 1677* [Goal](goal.html): Who this goal is intended for 1678* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 1679* [ImagingSelection](imagingselection.html): Who the study is about 1680* [ImagingStudy](imagingstudy.html): Who the study is about 1681* [Immunization](immunization.html): The patient for the vaccination record 1682* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 1683* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 1684* [Invoice](invoice.html): Recipient(s) of goods and services 1685* [List](list.html): If all resources have the same subject 1686* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 1687* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 1688* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 1689* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 1690* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 1691* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 1692* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 1693* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 1694* [Observation](observation.html): The subject that the observation is about (if patient) 1695* [Person](person.html): The Person links to this Patient 1696* [Procedure](procedure.html): Search by subject - a patient 1697* [Provenance](provenance.html): Where the activity involved patient data 1698* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 1699* [RelatedPerson](relatedperson.html): The patient this related person is related to 1700* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 1701* [ResearchSubject](researchsubject.html): Who or what is part of study 1702* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 1703* [ServiceRequest](servicerequest.html): Search by subject - a patient 1704* [Specimen](specimen.html): The patient the specimen comes from 1705* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 1706* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 1707* [Task](task.html): Search by patient 1708* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 1709</b><br> 1710 * Type: <b>reference</b><br> 1711 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 1712 * </p> 1713 */ 1714 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1715 1716/** 1717 * Constant for fluent queries to be used to add include statements. Specifies 1718 * the path value of "<b>RelatedPerson:patient</b>". 1719 */ 1720 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("RelatedPerson:patient").toLocked(); 1721 1722 /** 1723 * Search parameter: <b>address-city</b> 1724 * <p> 1725 * Description: <b>Multiple Resources: 1726 1727* [Patient](patient.html): A city specified in an address 1728* [Person](person.html): A city specified in an address 1729* [Practitioner](practitioner.html): A city specified in an address 1730* [RelatedPerson](relatedperson.html): A city specified in an address 1731</b><br> 1732 * Type: <b>string</b><br> 1733 * Path: <b>Patient.address.city | Person.address.city | Practitioner.address.city | RelatedPerson.address.city</b><br> 1734 * </p> 1735 */ 1736 @SearchParamDefinition(name="address-city", path="Patient.address.city | Person.address.city | Practitioner.address.city | RelatedPerson.address.city", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A city specified in an address\r\n* [Person](person.html): A city specified in an address\r\n* [Practitioner](practitioner.html): A city specified in an address\r\n* [RelatedPerson](relatedperson.html): A city specified in an address\r\n", type="string" ) 1737 public static final String SP_ADDRESS_CITY = "address-city"; 1738 /** 1739 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 1740 * <p> 1741 * Description: <b>Multiple Resources: 1742 1743* [Patient](patient.html): A city specified in an address 1744* [Person](person.html): A city specified in an address 1745* [Practitioner](practitioner.html): A city specified in an address 1746* [RelatedPerson](relatedperson.html): A city specified in an address 1747</b><br> 1748 * Type: <b>string</b><br> 1749 * Path: <b>Patient.address.city | Person.address.city | Practitioner.address.city | RelatedPerson.address.city</b><br> 1750 * </p> 1751 */ 1752 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_CITY); 1753 1754 /** 1755 * Search parameter: <b>address-country</b> 1756 * <p> 1757 * Description: <b>Multiple Resources: 1758 1759* [Patient](patient.html): A country specified in an address 1760* [Person](person.html): A country specified in an address 1761* [Practitioner](practitioner.html): A country specified in an address 1762* [RelatedPerson](relatedperson.html): A country specified in an address 1763</b><br> 1764 * Type: <b>string</b><br> 1765 * Path: <b>Patient.address.country | Person.address.country | Practitioner.address.country | RelatedPerson.address.country</b><br> 1766 * </p> 1767 */ 1768 @SearchParamDefinition(name="address-country", path="Patient.address.country | Person.address.country | Practitioner.address.country | RelatedPerson.address.country", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A country specified in an address\r\n* [Person](person.html): A country specified in an address\r\n* [Practitioner](practitioner.html): A country specified in an address\r\n* [RelatedPerson](relatedperson.html): A country specified in an address\r\n", type="string" ) 1769 public static final String SP_ADDRESS_COUNTRY = "address-country"; 1770 /** 1771 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 1772 * <p> 1773 * Description: <b>Multiple Resources: 1774 1775* [Patient](patient.html): A country specified in an address 1776* [Person](person.html): A country specified in an address 1777* [Practitioner](practitioner.html): A country specified in an address 1778* [RelatedPerson](relatedperson.html): A country specified in an address 1779</b><br> 1780 * Type: <b>string</b><br> 1781 * Path: <b>Patient.address.country | Person.address.country | Practitioner.address.country | RelatedPerson.address.country</b><br> 1782 * </p> 1783 */ 1784 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_COUNTRY); 1785 1786 /** 1787 * Search parameter: <b>address-postalcode</b> 1788 * <p> 1789 * Description: <b>Multiple Resources: 1790 1791* [Patient](patient.html): A postalCode specified in an address 1792* [Person](person.html): A postal code specified in an address 1793* [Practitioner](practitioner.html): A postalCode specified in an address 1794* [RelatedPerson](relatedperson.html): A postal code specified in an address 1795</b><br> 1796 * Type: <b>string</b><br> 1797 * Path: <b>Patient.address.postalCode | Person.address.postalCode | Practitioner.address.postalCode | RelatedPerson.address.postalCode</b><br> 1798 * </p> 1799 */ 1800 @SearchParamDefinition(name="address-postalcode", path="Patient.address.postalCode | Person.address.postalCode | Practitioner.address.postalCode | RelatedPerson.address.postalCode", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A postalCode specified in an address\r\n* [Person](person.html): A postal code specified in an address\r\n* [Practitioner](practitioner.html): A postalCode specified in an address\r\n* [RelatedPerson](relatedperson.html): A postal code specified in an address\r\n", type="string" ) 1801 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 1802 /** 1803 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 1804 * <p> 1805 * Description: <b>Multiple Resources: 1806 1807* [Patient](patient.html): A postalCode specified in an address 1808* [Person](person.html): A postal code specified in an address 1809* [Practitioner](practitioner.html): A postalCode specified in an address 1810* [RelatedPerson](relatedperson.html): A postal code specified in an address 1811</b><br> 1812 * Type: <b>string</b><br> 1813 * Path: <b>Patient.address.postalCode | Person.address.postalCode | Practitioner.address.postalCode | RelatedPerson.address.postalCode</b><br> 1814 * </p> 1815 */ 1816 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_POSTALCODE); 1817 1818 /** 1819 * Search parameter: <b>address-state</b> 1820 * <p> 1821 * Description: <b>Multiple Resources: 1822 1823* [Patient](patient.html): A state specified in an address 1824* [Person](person.html): A state specified in an address 1825* [Practitioner](practitioner.html): A state specified in an address 1826* [RelatedPerson](relatedperson.html): A state specified in an address 1827</b><br> 1828 * Type: <b>string</b><br> 1829 * Path: <b>Patient.address.state | Person.address.state | Practitioner.address.state | RelatedPerson.address.state</b><br> 1830 * </p> 1831 */ 1832 @SearchParamDefinition(name="address-state", path="Patient.address.state | Person.address.state | Practitioner.address.state | RelatedPerson.address.state", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A state specified in an address\r\n* [Person](person.html): A state specified in an address\r\n* [Practitioner](practitioner.html): A state specified in an address\r\n* [RelatedPerson](relatedperson.html): A state specified in an address\r\n", type="string" ) 1833 public static final String SP_ADDRESS_STATE = "address-state"; 1834 /** 1835 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 1836 * <p> 1837 * Description: <b>Multiple Resources: 1838 1839* [Patient](patient.html): A state specified in an address 1840* [Person](person.html): A state specified in an address 1841* [Practitioner](practitioner.html): A state specified in an address 1842* [RelatedPerson](relatedperson.html): A state specified in an address 1843</b><br> 1844 * Type: <b>string</b><br> 1845 * Path: <b>Patient.address.state | Person.address.state | Practitioner.address.state | RelatedPerson.address.state</b><br> 1846 * </p> 1847 */ 1848 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_STATE); 1849 1850 /** 1851 * Search parameter: <b>address-use</b> 1852 * <p> 1853 * Description: <b>Multiple Resources: 1854 1855* [Patient](patient.html): A use code specified in an address 1856* [Person](person.html): A use code specified in an address 1857* [Practitioner](practitioner.html): A use code specified in an address 1858* [RelatedPerson](relatedperson.html): A use code specified in an address 1859</b><br> 1860 * Type: <b>token</b><br> 1861 * Path: <b>Patient.address.use | Person.address.use | Practitioner.address.use | RelatedPerson.address.use</b><br> 1862 * </p> 1863 */ 1864 @SearchParamDefinition(name="address-use", path="Patient.address.use | Person.address.use | Practitioner.address.use | RelatedPerson.address.use", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A use code specified in an address\r\n* [Person](person.html): A use code specified in an address\r\n* [Practitioner](practitioner.html): A use code specified in an address\r\n* [RelatedPerson](relatedperson.html): A use code specified in an address\r\n", type="token" ) 1865 public static final String SP_ADDRESS_USE = "address-use"; 1866 /** 1867 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 1868 * <p> 1869 * Description: <b>Multiple Resources: 1870 1871* [Patient](patient.html): A use code specified in an address 1872* [Person](person.html): A use code specified in an address 1873* [Practitioner](practitioner.html): A use code specified in an address 1874* [RelatedPerson](relatedperson.html): A use code specified in an address 1875</b><br> 1876 * Type: <b>token</b><br> 1877 * Path: <b>Patient.address.use | Person.address.use | Practitioner.address.use | RelatedPerson.address.use</b><br> 1878 * </p> 1879 */ 1880 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ADDRESS_USE); 1881 1882 /** 1883 * Search parameter: <b>address</b> 1884 * <p> 1885 * Description: <b>Multiple Resources: 1886 1887* [Patient](patient.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 1888* [Person](person.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 1889* [Practitioner](practitioner.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 1890* [RelatedPerson](relatedperson.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 1891</b><br> 1892 * Type: <b>string</b><br> 1893 * Path: <b>Patient.address | Person.address | Practitioner.address | RelatedPerson.address</b><br> 1894 * </p> 1895 */ 1896 @SearchParamDefinition(name="address", path="Patient.address | Person.address | Practitioner.address | RelatedPerson.address", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text\r\n* [Person](person.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text\r\n* [Practitioner](practitioner.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text\r\n* [RelatedPerson](relatedperson.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text\r\n", type="string" ) 1897 public static final String SP_ADDRESS = "address"; 1898 /** 1899 * <b>Fluent Client</b> search parameter constant for <b>address</b> 1900 * <p> 1901 * Description: <b>Multiple Resources: 1902 1903* [Patient](patient.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 1904* [Person](person.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 1905* [Practitioner](practitioner.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 1906* [RelatedPerson](relatedperson.html): A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text 1907</b><br> 1908 * Type: <b>string</b><br> 1909 * Path: <b>Patient.address | Person.address | Practitioner.address | RelatedPerson.address</b><br> 1910 * </p> 1911 */ 1912 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS); 1913 1914 /** 1915 * Search parameter: <b>birthdate</b> 1916 * <p> 1917 * Description: <b>Multiple Resources: 1918 1919* [Patient](patient.html): The patient's date of birth 1920* [Person](person.html): The person's date of birth 1921* [RelatedPerson](relatedperson.html): The Related Person's date of birth 1922</b><br> 1923 * Type: <b>date</b><br> 1924 * Path: <b>Patient.birthDate | Person.birthDate | RelatedPerson.birthDate</b><br> 1925 * </p> 1926 */ 1927 @SearchParamDefinition(name="birthdate", path="Patient.birthDate | Person.birthDate | RelatedPerson.birthDate", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): The patient's date of birth\r\n* [Person](person.html): The person's date of birth\r\n* [RelatedPerson](relatedperson.html): The Related Person's date of birth\r\n", type="date" ) 1928 public static final String SP_BIRTHDATE = "birthdate"; 1929 /** 1930 * <b>Fluent Client</b> search parameter constant for <b>birthdate</b> 1931 * <p> 1932 * Description: <b>Multiple Resources: 1933 1934* [Patient](patient.html): The patient's date of birth 1935* [Person](person.html): The person's date of birth 1936* [RelatedPerson](relatedperson.html): The Related Person's date of birth 1937</b><br> 1938 * Type: <b>date</b><br> 1939 * Path: <b>Patient.birthDate | Person.birthDate | RelatedPerson.birthDate</b><br> 1940 * </p> 1941 */ 1942 public static final ca.uhn.fhir.rest.gclient.DateClientParam BIRTHDATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_BIRTHDATE); 1943 1944 /** 1945 * Search parameter: <b>email</b> 1946 * <p> 1947 * Description: <b>Multiple Resources: 1948 1949* [Patient](patient.html): A value in an email contact 1950* [Person](person.html): A value in an email contact 1951* [Practitioner](practitioner.html): A value in an email contact 1952* [PractitionerRole](practitionerrole.html): A value in an email contact 1953* [RelatedPerson](relatedperson.html): A value in an email contact 1954</b><br> 1955 * Type: <b>token</b><br> 1956 * Path: <b>Patient.telecom.where(system='email') | Person.telecom.where(system='email') | Practitioner.telecom.where(system='email') | PractitionerRole.contact.telecom.where(system='email') | RelatedPerson.telecom.where(system='email')</b><br> 1957 * </p> 1958 */ 1959 @SearchParamDefinition(name="email", path="Patient.telecom.where(system='email') | Person.telecom.where(system='email') | Practitioner.telecom.where(system='email') | PractitionerRole.contact.telecom.where(system='email') | RelatedPerson.telecom.where(system='email')", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A value in an email contact\r\n* [Person](person.html): A value in an email contact\r\n* [Practitioner](practitioner.html): A value in an email contact\r\n* [PractitionerRole](practitionerrole.html): A value in an email contact\r\n* [RelatedPerson](relatedperson.html): A value in an email contact\r\n", type="token" ) 1960 public static final String SP_EMAIL = "email"; 1961 /** 1962 * <b>Fluent Client</b> search parameter constant for <b>email</b> 1963 * <p> 1964 * Description: <b>Multiple Resources: 1965 1966* [Patient](patient.html): A value in an email contact 1967* [Person](person.html): A value in an email contact 1968* [Practitioner](practitioner.html): A value in an email contact 1969* [PractitionerRole](practitionerrole.html): A value in an email contact 1970* [RelatedPerson](relatedperson.html): A value in an email contact 1971</b><br> 1972 * Type: <b>token</b><br> 1973 * Path: <b>Patient.telecom.where(system='email') | Person.telecom.where(system='email') | Practitioner.telecom.where(system='email') | PractitionerRole.contact.telecom.where(system='email') | RelatedPerson.telecom.where(system='email')</b><br> 1974 * </p> 1975 */ 1976 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EMAIL); 1977 1978 /** 1979 * Search parameter: <b>gender</b> 1980 * <p> 1981 * Description: <b>Multiple Resources: 1982 1983* [Patient](patient.html): Gender of the patient 1984* [Person](person.html): The gender of the person 1985* [Practitioner](practitioner.html): Gender of the practitioner 1986* [RelatedPerson](relatedperson.html): Gender of the related person 1987</b><br> 1988 * Type: <b>token</b><br> 1989 * Path: <b>Patient.gender | Person.gender | Practitioner.gender | RelatedPerson.gender</b><br> 1990 * </p> 1991 */ 1992 @SearchParamDefinition(name="gender", path="Patient.gender | Person.gender | Practitioner.gender | RelatedPerson.gender", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): Gender of the patient\r\n* [Person](person.html): The gender of the person\r\n* [Practitioner](practitioner.html): Gender of the practitioner\r\n* [RelatedPerson](relatedperson.html): Gender of the related person\r\n", type="token" ) 1993 public static final String SP_GENDER = "gender"; 1994 /** 1995 * <b>Fluent Client</b> search parameter constant for <b>gender</b> 1996 * <p> 1997 * Description: <b>Multiple Resources: 1998 1999* [Patient](patient.html): Gender of the patient 2000* [Person](person.html): The gender of the person 2001* [Practitioner](practitioner.html): Gender of the practitioner 2002* [RelatedPerson](relatedperson.html): Gender of the related person 2003</b><br> 2004 * Type: <b>token</b><br> 2005 * Path: <b>Patient.gender | Person.gender | Practitioner.gender | RelatedPerson.gender</b><br> 2006 * </p> 2007 */ 2008 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GENDER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GENDER); 2009 2010 /** 2011 * Search parameter: <b>phone</b> 2012 * <p> 2013 * Description: <b>Multiple Resources: 2014 2015* [Patient](patient.html): A value in a phone contact 2016* [Person](person.html): A value in a phone contact 2017* [Practitioner](practitioner.html): A value in a phone contact 2018* [PractitionerRole](practitionerrole.html): A value in a phone contact 2019* [RelatedPerson](relatedperson.html): A value in a phone contact 2020</b><br> 2021 * Type: <b>token</b><br> 2022 * Path: <b>Patient.telecom.where(system='phone') | Person.telecom.where(system='phone') | Practitioner.telecom.where(system='phone') | PractitionerRole.contact.telecom.where(system='phone') | RelatedPerson.telecom.where(system='phone')</b><br> 2023 * </p> 2024 */ 2025 @SearchParamDefinition(name="phone", path="Patient.telecom.where(system='phone') | Person.telecom.where(system='phone') | Practitioner.telecom.where(system='phone') | PractitionerRole.contact.telecom.where(system='phone') | RelatedPerson.telecom.where(system='phone')", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A value in a phone contact\r\n* [Person](person.html): A value in a phone contact\r\n* [Practitioner](practitioner.html): A value in a phone contact\r\n* [PractitionerRole](practitionerrole.html): A value in a phone contact\r\n* [RelatedPerson](relatedperson.html): A value in a phone contact\r\n", type="token" ) 2026 public static final String SP_PHONE = "phone"; 2027 /** 2028 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 2029 * <p> 2030 * Description: <b>Multiple Resources: 2031 2032* [Patient](patient.html): A value in a phone contact 2033* [Person](person.html): A value in a phone contact 2034* [Practitioner](practitioner.html): A value in a phone contact 2035* [PractitionerRole](practitionerrole.html): A value in a phone contact 2036* [RelatedPerson](relatedperson.html): A value in a phone contact 2037</b><br> 2038 * Type: <b>token</b><br> 2039 * Path: <b>Patient.telecom.where(system='phone') | Person.telecom.where(system='phone') | Practitioner.telecom.where(system='phone') | PractitionerRole.contact.telecom.where(system='phone') | RelatedPerson.telecom.where(system='phone')</b><br> 2040 * </p> 2041 */ 2042 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PHONE); 2043 2044 /** 2045 * Search parameter: <b>phonetic</b> 2046 * <p> 2047 * Description: <b>Multiple Resources: 2048 2049* [Patient](patient.html): A portion of either family or given name using some kind of phonetic matching algorithm 2050* [Person](person.html): A portion of name using some kind of phonetic matching algorithm 2051* [Practitioner](practitioner.html): A portion of either family or given name using some kind of phonetic matching algorithm 2052* [RelatedPerson](relatedperson.html): A portion of name using some kind of phonetic matching algorithm 2053</b><br> 2054 * Type: <b>string</b><br> 2055 * Path: <b>Patient.name | Person.name | Practitioner.name | RelatedPerson.name</b><br> 2056 * </p> 2057 */ 2058 @SearchParamDefinition(name="phonetic", path="Patient.name | Person.name | Practitioner.name | RelatedPerson.name", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): A portion of either family or given name using some kind of phonetic matching algorithm\r\n* [Person](person.html): A portion of name using some kind of phonetic matching algorithm\r\n* [Practitioner](practitioner.html): A portion of either family or given name using some kind of phonetic matching algorithm\r\n* [RelatedPerson](relatedperson.html): A portion of name using some kind of phonetic matching algorithm\r\n", type="string" ) 2059 public static final String SP_PHONETIC = "phonetic"; 2060 /** 2061 * <b>Fluent Client</b> search parameter constant for <b>phonetic</b> 2062 * <p> 2063 * Description: <b>Multiple Resources: 2064 2065* [Patient](patient.html): A portion of either family or given name using some kind of phonetic matching algorithm 2066* [Person](person.html): A portion of name using some kind of phonetic matching algorithm 2067* [Practitioner](practitioner.html): A portion of either family or given name using some kind of phonetic matching algorithm 2068* [RelatedPerson](relatedperson.html): A portion of name using some kind of phonetic matching algorithm 2069</b><br> 2070 * Type: <b>string</b><br> 2071 * Path: <b>Patient.name | Person.name | Practitioner.name | RelatedPerson.name</b><br> 2072 * </p> 2073 */ 2074 public static final ca.uhn.fhir.rest.gclient.StringClientParam PHONETIC = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PHONETIC); 2075 2076 /** 2077 * Search parameter: <b>telecom</b> 2078 * <p> 2079 * Description: <b>Multiple Resources: 2080 2081* [Patient](patient.html): The value in any kind of telecom details of the patient 2082* [Person](person.html): The value in any kind of contact 2083* [Practitioner](practitioner.html): The value in any kind of contact 2084* [PractitionerRole](practitionerrole.html): The value in any kind of contact 2085* [RelatedPerson](relatedperson.html): The value in any kind of contact 2086</b><br> 2087 * Type: <b>token</b><br> 2088 * Path: <b>Patient.telecom | Person.telecom | Practitioner.telecom | PractitionerRole.contact.telecom | RelatedPerson.telecom</b><br> 2089 * </p> 2090 */ 2091 @SearchParamDefinition(name="telecom", path="Patient.telecom | Person.telecom | Practitioner.telecom | PractitionerRole.contact.telecom | RelatedPerson.telecom", description="Multiple Resources: \r\n\r\n* [Patient](patient.html): The value in any kind of telecom details of the patient\r\n* [Person](person.html): The value in any kind of contact\r\n* [Practitioner](practitioner.html): The value in any kind of contact\r\n* [PractitionerRole](practitionerrole.html): The value in any kind of contact\r\n* [RelatedPerson](relatedperson.html): The value in any kind of contact\r\n", type="token" ) 2092 public static final String SP_TELECOM = "telecom"; 2093 /** 2094 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 2095 * <p> 2096 * Description: <b>Multiple Resources: 2097 2098* [Patient](patient.html): The value in any kind of telecom details of the patient 2099* [Person](person.html): The value in any kind of contact 2100* [Practitioner](practitioner.html): The value in any kind of contact 2101* [PractitionerRole](practitionerrole.html): The value in any kind of contact 2102* [RelatedPerson](relatedperson.html): The value in any kind of contact 2103</b><br> 2104 * Type: <b>token</b><br> 2105 * Path: <b>Patient.telecom | Person.telecom | Practitioner.telecom | PractitionerRole.contact.telecom | RelatedPerson.telecom</b><br> 2106 * </p> 2107 */ 2108 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TELECOM); 2109 2110 2111} 2112