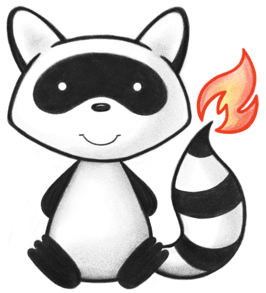
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A set of related requests that can be used to capture intended activities that have inter-dependencies such as "give this medication after that one". 052 */ 053@ResourceDef(name="RequestOrchestration", profile="http://hl7.org/fhir/StructureDefinition/RequestOrchestration") 054public class RequestOrchestration extends DomainResource { 055 056 @Block() 057 public static class RequestOrchestrationActionComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * The linkId of the action from the PlanDefinition that corresponds to this action in the RequestOrchestration resource. 060 */ 061 @Child(name = "linkId", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="Pointer to specific item from the PlanDefinition", formalDefinition="The linkId of the action from the PlanDefinition that corresponds to this action in the RequestOrchestration resource." ) 063 protected StringType linkId; 064 065 /** 066 * A user-visible prefix for the action. For example a section or item numbering such as 1. or A. 067 */ 068 @Child(name = "prefix", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 069 @Description(shortDefinition="User-visible prefix for the action (e.g. 1. or A.)", formalDefinition="A user-visible prefix for the action. For example a section or item numbering such as 1. or A." ) 070 protected StringType prefix; 071 072 /** 073 * The title of the action displayed to a user. 074 */ 075 @Child(name = "title", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 076 @Description(shortDefinition="User-visible title", formalDefinition="The title of the action displayed to a user." ) 077 protected StringType title; 078 079 /** 080 * A short description of the action used to provide a summary to display to the user. 081 */ 082 @Child(name = "description", type = {MarkdownType.class}, order=4, min=0, max=1, modifier=false, summary=true) 083 @Description(shortDefinition="Short description of the action", formalDefinition="A short description of the action used to provide a summary to display to the user." ) 084 protected MarkdownType description; 085 086 /** 087 * A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically. 088 */ 089 @Child(name = "textEquivalent", type = {MarkdownType.class}, order=5, min=0, max=1, modifier=false, summary=true) 090 @Description(shortDefinition="Static text equivalent of the action, used if the dynamic aspects cannot be interpreted by the receiving system", formalDefinition="A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically." ) 091 protected MarkdownType textEquivalent; 092 093 /** 094 * Indicates how quickly the action should be addressed with respect to other actions. 095 */ 096 @Child(name = "priority", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 097 @Description(shortDefinition="routine | urgent | asap | stat", formalDefinition="Indicates how quickly the action should be addressed with respect to other actions." ) 098 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 099 protected Enumeration<RequestPriority> priority; 100 101 /** 102 * A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a section of a documentation template. 103 */ 104 @Child(name = "code", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 105 @Description(shortDefinition="Code representing the meaning of the action or sub-actions", formalDefinition="A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a section of a documentation template." ) 106 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-code") 107 protected List<CodeableConcept> code; 108 109 /** 110 * Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources. 111 */ 112 @Child(name = "documentation", type = {RelatedArtifact.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 113 @Description(shortDefinition="Supporting documentation for the intended performer of the action", formalDefinition="Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources." ) 114 protected List<RelatedArtifact> documentation; 115 116 /** 117 * Goals that are intended to be achieved by following the requests in this action. 118 */ 119 @Child(name = "goal", type = {Goal.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 120 @Description(shortDefinition="What goals", formalDefinition="Goals that are intended to be achieved by following the requests in this action." ) 121 protected List<Reference> goal; 122 123 /** 124 * An expression that describes applicability criteria, or start/stop conditions for the action. 125 */ 126 @Child(name = "condition", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 127 @Description(shortDefinition="Whether or not the action is applicable", formalDefinition="An expression that describes applicability criteria, or start/stop conditions for the action." ) 128 protected List<RequestOrchestrationActionConditionComponent> condition; 129 130 /** 131 * Defines input data requirements for the action. 132 */ 133 @Child(name = "input", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 134 @Description(shortDefinition="Input data requirements", formalDefinition="Defines input data requirements for the action." ) 135 protected List<RequestOrchestrationActionInputComponent> input; 136 137 /** 138 * Defines the outputs of the action, if any. 139 */ 140 @Child(name = "output", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 141 @Description(shortDefinition="Output data definition", formalDefinition="Defines the outputs of the action, if any." ) 142 protected List<RequestOrchestrationActionOutputComponent> output; 143 144 /** 145 * A relationship to another action such as "before" or "30-60 minutes after start of". 146 */ 147 @Child(name = "relatedAction", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 148 @Description(shortDefinition="Relationship to another action", formalDefinition="A relationship to another action such as \"before\" or \"30-60 minutes after start of\"." ) 149 protected List<RequestOrchestrationActionRelatedActionComponent> relatedAction; 150 151 /** 152 * An optional value describing when the action should be performed. 153 */ 154 @Child(name = "timing", type = {DateTimeType.class, Age.class, Period.class, Duration.class, Range.class, Timing.class}, order=14, min=0, max=1, modifier=false, summary=false) 155 @Description(shortDefinition="When the action should take place", formalDefinition="An optional value describing when the action should be performed." ) 156 protected DataType timing; 157 158 /** 159 * Identifies the facility where the action will occur; e.g. home, hospital, specific clinic, etc. 160 */ 161 @Child(name = "location", type = {CodeableReference.class}, order=15, min=0, max=1, modifier=false, summary=false) 162 @Description(shortDefinition="Where it should happen", formalDefinition="Identifies the facility where the action will occur; e.g. home, hospital, specific clinic, etc." ) 163 protected CodeableReference location; 164 165 /** 166 * The participant that should perform or be responsible for this action. 167 */ 168 @Child(name = "participant", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 169 @Description(shortDefinition="Who should perform the action", formalDefinition="The participant that should perform or be responsible for this action." ) 170 protected List<RequestOrchestrationActionParticipantComponent> participant; 171 172 /** 173 * The type of action to perform (create, update, remove). 174 */ 175 @Child(name = "type", type = {CodeableConcept.class}, order=17, min=0, max=1, modifier=false, summary=false) 176 @Description(shortDefinition="create | update | remove | fire-event", formalDefinition="The type of action to perform (create, update, remove)." ) 177 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-type") 178 protected CodeableConcept type; 179 180 /** 181 * Defines the grouping behavior for the action and its children. 182 */ 183 @Child(name = "groupingBehavior", type = {CodeType.class}, order=18, min=0, max=1, modifier=false, summary=false) 184 @Description(shortDefinition="visual-group | logical-group | sentence-group", formalDefinition="Defines the grouping behavior for the action and its children." ) 185 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-grouping-behavior") 186 protected Enumeration<ActionGroupingBehavior> groupingBehavior; 187 188 /** 189 * Defines the selection behavior for the action and its children. 190 */ 191 @Child(name = "selectionBehavior", type = {CodeType.class}, order=19, min=0, max=1, modifier=false, summary=false) 192 @Description(shortDefinition="any | all | all-or-none | exactly-one | at-most-one | one-or-more", formalDefinition="Defines the selection behavior for the action and its children." ) 193 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-selection-behavior") 194 protected Enumeration<ActionSelectionBehavior> selectionBehavior; 195 196 /** 197 * Defines expectations around whether an action is required. 198 */ 199 @Child(name = "requiredBehavior", type = {CodeType.class}, order=20, min=0, max=1, modifier=false, summary=false) 200 @Description(shortDefinition="must | could | must-unless-documented", formalDefinition="Defines expectations around whether an action is required." ) 201 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-required-behavior") 202 protected Enumeration<ActionRequiredBehavior> requiredBehavior; 203 204 /** 205 * Defines whether the action should usually be preselected. 206 */ 207 @Child(name = "precheckBehavior", type = {CodeType.class}, order=21, min=0, max=1, modifier=false, summary=false) 208 @Description(shortDefinition="yes | no", formalDefinition="Defines whether the action should usually be preselected." ) 209 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-precheck-behavior") 210 protected Enumeration<ActionPrecheckBehavior> precheckBehavior; 211 212 /** 213 * Defines whether the action can be selected multiple times. 214 */ 215 @Child(name = "cardinalityBehavior", type = {CodeType.class}, order=22, min=0, max=1, modifier=false, summary=false) 216 @Description(shortDefinition="single | multiple", formalDefinition="Defines whether the action can be selected multiple times." ) 217 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-cardinality-behavior") 218 protected Enumeration<ActionCardinalityBehavior> cardinalityBehavior; 219 220 /** 221 * The resource that is the target of the action (e.g. CommunicationRequest). 222 */ 223 @Child(name = "resource", type = {Reference.class}, order=23, min=0, max=1, modifier=false, summary=false) 224 @Description(shortDefinition="The target of the action", formalDefinition="The resource that is the target of the action (e.g. CommunicationRequest)." ) 225 protected Reference resource; 226 227 /** 228 * A reference to an ActivityDefinition that describes the action to be taken in detail, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured. 229 */ 230 @Child(name = "definition", type = {CanonicalType.class, UriType.class}, order=24, min=0, max=1, modifier=false, summary=false) 231 @Description(shortDefinition="Description of the activity to be performed", formalDefinition="A reference to an ActivityDefinition that describes the action to be taken in detail, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured." ) 232 protected DataType definition; 233 234 /** 235 * A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input. 236 */ 237 @Child(name = "transform", type = {CanonicalType.class}, order=25, min=0, max=1, modifier=false, summary=false) 238 @Description(shortDefinition="Transform to apply the template", formalDefinition="A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input." ) 239 protected CanonicalType transform; 240 241 /** 242 * Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result. 243 */ 244 @Child(name = "dynamicValue", type = {}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 245 @Description(shortDefinition="Dynamic aspects of the definition", formalDefinition="Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result." ) 246 protected List<RequestOrchestrationActionDynamicValueComponent> dynamicValue; 247 248 /** 249 * Sub actions. 250 */ 251 @Child(name = "action", type = {RequestOrchestrationActionComponent.class}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 252 @Description(shortDefinition="Sub action", formalDefinition="Sub actions." ) 253 protected List<RequestOrchestrationActionComponent> action; 254 255 private static final long serialVersionUID = -1292193514L; 256 257 /** 258 * Constructor 259 */ 260 public RequestOrchestrationActionComponent() { 261 super(); 262 } 263 264 /** 265 * @return {@link #linkId} (The linkId of the action from the PlanDefinition that corresponds to this action in the RequestOrchestration resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 266 */ 267 public StringType getLinkIdElement() { 268 if (this.linkId == null) 269 if (Configuration.errorOnAutoCreate()) 270 throw new Error("Attempt to auto-create RequestOrchestrationActionComponent.linkId"); 271 else if (Configuration.doAutoCreate()) 272 this.linkId = new StringType(); // bb 273 return this.linkId; 274 } 275 276 public boolean hasLinkIdElement() { 277 return this.linkId != null && !this.linkId.isEmpty(); 278 } 279 280 public boolean hasLinkId() { 281 return this.linkId != null && !this.linkId.isEmpty(); 282 } 283 284 /** 285 * @param value {@link #linkId} (The linkId of the action from the PlanDefinition that corresponds to this action in the RequestOrchestration resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 286 */ 287 public RequestOrchestrationActionComponent setLinkIdElement(StringType value) { 288 this.linkId = value; 289 return this; 290 } 291 292 /** 293 * @return The linkId of the action from the PlanDefinition that corresponds to this action in the RequestOrchestration resource. 294 */ 295 public String getLinkId() { 296 return this.linkId == null ? null : this.linkId.getValue(); 297 } 298 299 /** 300 * @param value The linkId of the action from the PlanDefinition that corresponds to this action in the RequestOrchestration resource. 301 */ 302 public RequestOrchestrationActionComponent setLinkId(String value) { 303 if (Utilities.noString(value)) 304 this.linkId = null; 305 else { 306 if (this.linkId == null) 307 this.linkId = new StringType(); 308 this.linkId.setValue(value); 309 } 310 return this; 311 } 312 313 /** 314 * @return {@link #prefix} (A user-visible prefix for the action. For example a section or item numbering such as 1. or A.). This is the underlying object with id, value and extensions. The accessor "getPrefix" gives direct access to the value 315 */ 316 public StringType getPrefixElement() { 317 if (this.prefix == null) 318 if (Configuration.errorOnAutoCreate()) 319 throw new Error("Attempt to auto-create RequestOrchestrationActionComponent.prefix"); 320 else if (Configuration.doAutoCreate()) 321 this.prefix = new StringType(); // bb 322 return this.prefix; 323 } 324 325 public boolean hasPrefixElement() { 326 return this.prefix != null && !this.prefix.isEmpty(); 327 } 328 329 public boolean hasPrefix() { 330 return this.prefix != null && !this.prefix.isEmpty(); 331 } 332 333 /** 334 * @param value {@link #prefix} (A user-visible prefix for the action. For example a section or item numbering such as 1. or A.). This is the underlying object with id, value and extensions. The accessor "getPrefix" gives direct access to the value 335 */ 336 public RequestOrchestrationActionComponent setPrefixElement(StringType value) { 337 this.prefix = value; 338 return this; 339 } 340 341 /** 342 * @return A user-visible prefix for the action. For example a section or item numbering such as 1. or A. 343 */ 344 public String getPrefix() { 345 return this.prefix == null ? null : this.prefix.getValue(); 346 } 347 348 /** 349 * @param value A user-visible prefix for the action. For example a section or item numbering such as 1. or A. 350 */ 351 public RequestOrchestrationActionComponent setPrefix(String value) { 352 if (Utilities.noString(value)) 353 this.prefix = null; 354 else { 355 if (this.prefix == null) 356 this.prefix = new StringType(); 357 this.prefix.setValue(value); 358 } 359 return this; 360 } 361 362 /** 363 * @return {@link #title} (The title of the action displayed to a user.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 364 */ 365 public StringType getTitleElement() { 366 if (this.title == null) 367 if (Configuration.errorOnAutoCreate()) 368 throw new Error("Attempt to auto-create RequestOrchestrationActionComponent.title"); 369 else if (Configuration.doAutoCreate()) 370 this.title = new StringType(); // bb 371 return this.title; 372 } 373 374 public boolean hasTitleElement() { 375 return this.title != null && !this.title.isEmpty(); 376 } 377 378 public boolean hasTitle() { 379 return this.title != null && !this.title.isEmpty(); 380 } 381 382 /** 383 * @param value {@link #title} (The title of the action displayed to a user.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 384 */ 385 public RequestOrchestrationActionComponent setTitleElement(StringType value) { 386 this.title = value; 387 return this; 388 } 389 390 /** 391 * @return The title of the action displayed to a user. 392 */ 393 public String getTitle() { 394 return this.title == null ? null : this.title.getValue(); 395 } 396 397 /** 398 * @param value The title of the action displayed to a user. 399 */ 400 public RequestOrchestrationActionComponent setTitle(String value) { 401 if (Utilities.noString(value)) 402 this.title = null; 403 else { 404 if (this.title == null) 405 this.title = new StringType(); 406 this.title.setValue(value); 407 } 408 return this; 409 } 410 411 /** 412 * @return {@link #description} (A short description of the action used to provide a summary to display to the user.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 413 */ 414 public MarkdownType getDescriptionElement() { 415 if (this.description == null) 416 if (Configuration.errorOnAutoCreate()) 417 throw new Error("Attempt to auto-create RequestOrchestrationActionComponent.description"); 418 else if (Configuration.doAutoCreate()) 419 this.description = new MarkdownType(); // bb 420 return this.description; 421 } 422 423 public boolean hasDescriptionElement() { 424 return this.description != null && !this.description.isEmpty(); 425 } 426 427 public boolean hasDescription() { 428 return this.description != null && !this.description.isEmpty(); 429 } 430 431 /** 432 * @param value {@link #description} (A short description of the action used to provide a summary to display to the user.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 433 */ 434 public RequestOrchestrationActionComponent setDescriptionElement(MarkdownType value) { 435 this.description = value; 436 return this; 437 } 438 439 /** 440 * @return A short description of the action used to provide a summary to display to the user. 441 */ 442 public String getDescription() { 443 return this.description == null ? null : this.description.getValue(); 444 } 445 446 /** 447 * @param value A short description of the action used to provide a summary to display to the user. 448 */ 449 public RequestOrchestrationActionComponent setDescription(String value) { 450 if (Utilities.noString(value)) 451 this.description = null; 452 else { 453 if (this.description == null) 454 this.description = new MarkdownType(); 455 this.description.setValue(value); 456 } 457 return this; 458 } 459 460 /** 461 * @return {@link #textEquivalent} (A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically.). This is the underlying object with id, value and extensions. The accessor "getTextEquivalent" gives direct access to the value 462 */ 463 public MarkdownType getTextEquivalentElement() { 464 if (this.textEquivalent == null) 465 if (Configuration.errorOnAutoCreate()) 466 throw new Error("Attempt to auto-create RequestOrchestrationActionComponent.textEquivalent"); 467 else if (Configuration.doAutoCreate()) 468 this.textEquivalent = new MarkdownType(); // bb 469 return this.textEquivalent; 470 } 471 472 public boolean hasTextEquivalentElement() { 473 return this.textEquivalent != null && !this.textEquivalent.isEmpty(); 474 } 475 476 public boolean hasTextEquivalent() { 477 return this.textEquivalent != null && !this.textEquivalent.isEmpty(); 478 } 479 480 /** 481 * @param value {@link #textEquivalent} (A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically.). This is the underlying object with id, value and extensions. The accessor "getTextEquivalent" gives direct access to the value 482 */ 483 public RequestOrchestrationActionComponent setTextEquivalentElement(MarkdownType value) { 484 this.textEquivalent = value; 485 return this; 486 } 487 488 /** 489 * @return A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically. 490 */ 491 public String getTextEquivalent() { 492 return this.textEquivalent == null ? null : this.textEquivalent.getValue(); 493 } 494 495 /** 496 * @param value A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically. 497 */ 498 public RequestOrchestrationActionComponent setTextEquivalent(String value) { 499 if (Utilities.noString(value)) 500 this.textEquivalent = null; 501 else { 502 if (this.textEquivalent == null) 503 this.textEquivalent = new MarkdownType(); 504 this.textEquivalent.setValue(value); 505 } 506 return this; 507 } 508 509 /** 510 * @return {@link #priority} (Indicates how quickly the action should be addressed with respect to other actions.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 511 */ 512 public Enumeration<RequestPriority> getPriorityElement() { 513 if (this.priority == null) 514 if (Configuration.errorOnAutoCreate()) 515 throw new Error("Attempt to auto-create RequestOrchestrationActionComponent.priority"); 516 else if (Configuration.doAutoCreate()) 517 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 518 return this.priority; 519 } 520 521 public boolean hasPriorityElement() { 522 return this.priority != null && !this.priority.isEmpty(); 523 } 524 525 public boolean hasPriority() { 526 return this.priority != null && !this.priority.isEmpty(); 527 } 528 529 /** 530 * @param value {@link #priority} (Indicates how quickly the action should be addressed with respect to other actions.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 531 */ 532 public RequestOrchestrationActionComponent setPriorityElement(Enumeration<RequestPriority> value) { 533 this.priority = value; 534 return this; 535 } 536 537 /** 538 * @return Indicates how quickly the action should be addressed with respect to other actions. 539 */ 540 public RequestPriority getPriority() { 541 return this.priority == null ? null : this.priority.getValue(); 542 } 543 544 /** 545 * @param value Indicates how quickly the action should be addressed with respect to other actions. 546 */ 547 public RequestOrchestrationActionComponent setPriority(RequestPriority value) { 548 if (value == null) 549 this.priority = null; 550 else { 551 if (this.priority == null) 552 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 553 this.priority.setValue(value); 554 } 555 return this; 556 } 557 558 /** 559 * @return {@link #code} (A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a section of a documentation template.) 560 */ 561 public List<CodeableConcept> getCode() { 562 if (this.code == null) 563 this.code = new ArrayList<CodeableConcept>(); 564 return this.code; 565 } 566 567 /** 568 * @return Returns a reference to <code>this</code> for easy method chaining 569 */ 570 public RequestOrchestrationActionComponent setCode(List<CodeableConcept> theCode) { 571 this.code = theCode; 572 return this; 573 } 574 575 public boolean hasCode() { 576 if (this.code == null) 577 return false; 578 for (CodeableConcept item : this.code) 579 if (!item.isEmpty()) 580 return true; 581 return false; 582 } 583 584 public CodeableConcept addCode() { //3 585 CodeableConcept t = new CodeableConcept(); 586 if (this.code == null) 587 this.code = new ArrayList<CodeableConcept>(); 588 this.code.add(t); 589 return t; 590 } 591 592 public RequestOrchestrationActionComponent addCode(CodeableConcept t) { //3 593 if (t == null) 594 return this; 595 if (this.code == null) 596 this.code = new ArrayList<CodeableConcept>(); 597 this.code.add(t); 598 return this; 599 } 600 601 /** 602 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist {3} 603 */ 604 public CodeableConcept getCodeFirstRep() { 605 if (getCode().isEmpty()) { 606 addCode(); 607 } 608 return getCode().get(0); 609 } 610 611 /** 612 * @return {@link #documentation} (Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.) 613 */ 614 public List<RelatedArtifact> getDocumentation() { 615 if (this.documentation == null) 616 this.documentation = new ArrayList<RelatedArtifact>(); 617 return this.documentation; 618 } 619 620 /** 621 * @return Returns a reference to <code>this</code> for easy method chaining 622 */ 623 public RequestOrchestrationActionComponent setDocumentation(List<RelatedArtifact> theDocumentation) { 624 this.documentation = theDocumentation; 625 return this; 626 } 627 628 public boolean hasDocumentation() { 629 if (this.documentation == null) 630 return false; 631 for (RelatedArtifact item : this.documentation) 632 if (!item.isEmpty()) 633 return true; 634 return false; 635 } 636 637 public RelatedArtifact addDocumentation() { //3 638 RelatedArtifact t = new RelatedArtifact(); 639 if (this.documentation == null) 640 this.documentation = new ArrayList<RelatedArtifact>(); 641 this.documentation.add(t); 642 return t; 643 } 644 645 public RequestOrchestrationActionComponent addDocumentation(RelatedArtifact t) { //3 646 if (t == null) 647 return this; 648 if (this.documentation == null) 649 this.documentation = new ArrayList<RelatedArtifact>(); 650 this.documentation.add(t); 651 return this; 652 } 653 654 /** 655 * @return The first repetition of repeating field {@link #documentation}, creating it if it does not already exist {3} 656 */ 657 public RelatedArtifact getDocumentationFirstRep() { 658 if (getDocumentation().isEmpty()) { 659 addDocumentation(); 660 } 661 return getDocumentation().get(0); 662 } 663 664 /** 665 * @return {@link #goal} (Goals that are intended to be achieved by following the requests in this action.) 666 */ 667 public List<Reference> getGoal() { 668 if (this.goal == null) 669 this.goal = new ArrayList<Reference>(); 670 return this.goal; 671 } 672 673 /** 674 * @return Returns a reference to <code>this</code> for easy method chaining 675 */ 676 public RequestOrchestrationActionComponent setGoal(List<Reference> theGoal) { 677 this.goal = theGoal; 678 return this; 679 } 680 681 public boolean hasGoal() { 682 if (this.goal == null) 683 return false; 684 for (Reference item : this.goal) 685 if (!item.isEmpty()) 686 return true; 687 return false; 688 } 689 690 public Reference addGoal() { //3 691 Reference t = new Reference(); 692 if (this.goal == null) 693 this.goal = new ArrayList<Reference>(); 694 this.goal.add(t); 695 return t; 696 } 697 698 public RequestOrchestrationActionComponent addGoal(Reference t) { //3 699 if (t == null) 700 return this; 701 if (this.goal == null) 702 this.goal = new ArrayList<Reference>(); 703 this.goal.add(t); 704 return this; 705 } 706 707 /** 708 * @return The first repetition of repeating field {@link #goal}, creating it if it does not already exist {3} 709 */ 710 public Reference getGoalFirstRep() { 711 if (getGoal().isEmpty()) { 712 addGoal(); 713 } 714 return getGoal().get(0); 715 } 716 717 /** 718 * @return {@link #condition} (An expression that describes applicability criteria, or start/stop conditions for the action.) 719 */ 720 public List<RequestOrchestrationActionConditionComponent> getCondition() { 721 if (this.condition == null) 722 this.condition = new ArrayList<RequestOrchestrationActionConditionComponent>(); 723 return this.condition; 724 } 725 726 /** 727 * @return Returns a reference to <code>this</code> for easy method chaining 728 */ 729 public RequestOrchestrationActionComponent setCondition(List<RequestOrchestrationActionConditionComponent> theCondition) { 730 this.condition = theCondition; 731 return this; 732 } 733 734 public boolean hasCondition() { 735 if (this.condition == null) 736 return false; 737 for (RequestOrchestrationActionConditionComponent item : this.condition) 738 if (!item.isEmpty()) 739 return true; 740 return false; 741 } 742 743 public RequestOrchestrationActionConditionComponent addCondition() { //3 744 RequestOrchestrationActionConditionComponent t = new RequestOrchestrationActionConditionComponent(); 745 if (this.condition == null) 746 this.condition = new ArrayList<RequestOrchestrationActionConditionComponent>(); 747 this.condition.add(t); 748 return t; 749 } 750 751 public RequestOrchestrationActionComponent addCondition(RequestOrchestrationActionConditionComponent t) { //3 752 if (t == null) 753 return this; 754 if (this.condition == null) 755 this.condition = new ArrayList<RequestOrchestrationActionConditionComponent>(); 756 this.condition.add(t); 757 return this; 758 } 759 760 /** 761 * @return The first repetition of repeating field {@link #condition}, creating it if it does not already exist {3} 762 */ 763 public RequestOrchestrationActionConditionComponent getConditionFirstRep() { 764 if (getCondition().isEmpty()) { 765 addCondition(); 766 } 767 return getCondition().get(0); 768 } 769 770 /** 771 * @return {@link #input} (Defines input data requirements for the action.) 772 */ 773 public List<RequestOrchestrationActionInputComponent> getInput() { 774 if (this.input == null) 775 this.input = new ArrayList<RequestOrchestrationActionInputComponent>(); 776 return this.input; 777 } 778 779 /** 780 * @return Returns a reference to <code>this</code> for easy method chaining 781 */ 782 public RequestOrchestrationActionComponent setInput(List<RequestOrchestrationActionInputComponent> theInput) { 783 this.input = theInput; 784 return this; 785 } 786 787 public boolean hasInput() { 788 if (this.input == null) 789 return false; 790 for (RequestOrchestrationActionInputComponent item : this.input) 791 if (!item.isEmpty()) 792 return true; 793 return false; 794 } 795 796 public RequestOrchestrationActionInputComponent addInput() { //3 797 RequestOrchestrationActionInputComponent t = new RequestOrchestrationActionInputComponent(); 798 if (this.input == null) 799 this.input = new ArrayList<RequestOrchestrationActionInputComponent>(); 800 this.input.add(t); 801 return t; 802 } 803 804 public RequestOrchestrationActionComponent addInput(RequestOrchestrationActionInputComponent t) { //3 805 if (t == null) 806 return this; 807 if (this.input == null) 808 this.input = new ArrayList<RequestOrchestrationActionInputComponent>(); 809 this.input.add(t); 810 return this; 811 } 812 813 /** 814 * @return The first repetition of repeating field {@link #input}, creating it if it does not already exist {3} 815 */ 816 public RequestOrchestrationActionInputComponent getInputFirstRep() { 817 if (getInput().isEmpty()) { 818 addInput(); 819 } 820 return getInput().get(0); 821 } 822 823 /** 824 * @return {@link #output} (Defines the outputs of the action, if any.) 825 */ 826 public List<RequestOrchestrationActionOutputComponent> getOutput() { 827 if (this.output == null) 828 this.output = new ArrayList<RequestOrchestrationActionOutputComponent>(); 829 return this.output; 830 } 831 832 /** 833 * @return Returns a reference to <code>this</code> for easy method chaining 834 */ 835 public RequestOrchestrationActionComponent setOutput(List<RequestOrchestrationActionOutputComponent> theOutput) { 836 this.output = theOutput; 837 return this; 838 } 839 840 public boolean hasOutput() { 841 if (this.output == null) 842 return false; 843 for (RequestOrchestrationActionOutputComponent item : this.output) 844 if (!item.isEmpty()) 845 return true; 846 return false; 847 } 848 849 public RequestOrchestrationActionOutputComponent addOutput() { //3 850 RequestOrchestrationActionOutputComponent t = new RequestOrchestrationActionOutputComponent(); 851 if (this.output == null) 852 this.output = new ArrayList<RequestOrchestrationActionOutputComponent>(); 853 this.output.add(t); 854 return t; 855 } 856 857 public RequestOrchestrationActionComponent addOutput(RequestOrchestrationActionOutputComponent t) { //3 858 if (t == null) 859 return this; 860 if (this.output == null) 861 this.output = new ArrayList<RequestOrchestrationActionOutputComponent>(); 862 this.output.add(t); 863 return this; 864 } 865 866 /** 867 * @return The first repetition of repeating field {@link #output}, creating it if it does not already exist {3} 868 */ 869 public RequestOrchestrationActionOutputComponent getOutputFirstRep() { 870 if (getOutput().isEmpty()) { 871 addOutput(); 872 } 873 return getOutput().get(0); 874 } 875 876 /** 877 * @return {@link #relatedAction} (A relationship to another action such as "before" or "30-60 minutes after start of".) 878 */ 879 public List<RequestOrchestrationActionRelatedActionComponent> getRelatedAction() { 880 if (this.relatedAction == null) 881 this.relatedAction = new ArrayList<RequestOrchestrationActionRelatedActionComponent>(); 882 return this.relatedAction; 883 } 884 885 /** 886 * @return Returns a reference to <code>this</code> for easy method chaining 887 */ 888 public RequestOrchestrationActionComponent setRelatedAction(List<RequestOrchestrationActionRelatedActionComponent> theRelatedAction) { 889 this.relatedAction = theRelatedAction; 890 return this; 891 } 892 893 public boolean hasRelatedAction() { 894 if (this.relatedAction == null) 895 return false; 896 for (RequestOrchestrationActionRelatedActionComponent item : this.relatedAction) 897 if (!item.isEmpty()) 898 return true; 899 return false; 900 } 901 902 public RequestOrchestrationActionRelatedActionComponent addRelatedAction() { //3 903 RequestOrchestrationActionRelatedActionComponent t = new RequestOrchestrationActionRelatedActionComponent(); 904 if (this.relatedAction == null) 905 this.relatedAction = new ArrayList<RequestOrchestrationActionRelatedActionComponent>(); 906 this.relatedAction.add(t); 907 return t; 908 } 909 910 public RequestOrchestrationActionComponent addRelatedAction(RequestOrchestrationActionRelatedActionComponent t) { //3 911 if (t == null) 912 return this; 913 if (this.relatedAction == null) 914 this.relatedAction = new ArrayList<RequestOrchestrationActionRelatedActionComponent>(); 915 this.relatedAction.add(t); 916 return this; 917 } 918 919 /** 920 * @return The first repetition of repeating field {@link #relatedAction}, creating it if it does not already exist {3} 921 */ 922 public RequestOrchestrationActionRelatedActionComponent getRelatedActionFirstRep() { 923 if (getRelatedAction().isEmpty()) { 924 addRelatedAction(); 925 } 926 return getRelatedAction().get(0); 927 } 928 929 /** 930 * @return {@link #timing} (An optional value describing when the action should be performed.) 931 */ 932 public DataType getTiming() { 933 return this.timing; 934 } 935 936 /** 937 * @return {@link #timing} (An optional value describing when the action should be performed.) 938 */ 939 public DateTimeType getTimingDateTimeType() throws FHIRException { 940 if (this.timing == null) 941 this.timing = new DateTimeType(); 942 if (!(this.timing instanceof DateTimeType)) 943 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.timing.getClass().getName()+" was encountered"); 944 return (DateTimeType) this.timing; 945 } 946 947 public boolean hasTimingDateTimeType() { 948 return this != null && this.timing instanceof DateTimeType; 949 } 950 951 /** 952 * @return {@link #timing} (An optional value describing when the action should be performed.) 953 */ 954 public Age getTimingAge() throws FHIRException { 955 if (this.timing == null) 956 this.timing = new Age(); 957 if (!(this.timing instanceof Age)) 958 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.timing.getClass().getName()+" was encountered"); 959 return (Age) this.timing; 960 } 961 962 public boolean hasTimingAge() { 963 return this != null && this.timing instanceof Age; 964 } 965 966 /** 967 * @return {@link #timing} (An optional value describing when the action should be performed.) 968 */ 969 public Period getTimingPeriod() throws FHIRException { 970 if (this.timing == null) 971 this.timing = new Period(); 972 if (!(this.timing instanceof Period)) 973 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.timing.getClass().getName()+" was encountered"); 974 return (Period) this.timing; 975 } 976 977 public boolean hasTimingPeriod() { 978 return this != null && this.timing instanceof Period; 979 } 980 981 /** 982 * @return {@link #timing} (An optional value describing when the action should be performed.) 983 */ 984 public Duration getTimingDuration() throws FHIRException { 985 if (this.timing == null) 986 this.timing = new Duration(); 987 if (!(this.timing instanceof Duration)) 988 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.timing.getClass().getName()+" was encountered"); 989 return (Duration) this.timing; 990 } 991 992 public boolean hasTimingDuration() { 993 return this != null && this.timing instanceof Duration; 994 } 995 996 /** 997 * @return {@link #timing} (An optional value describing when the action should be performed.) 998 */ 999 public Range getTimingRange() throws FHIRException { 1000 if (this.timing == null) 1001 this.timing = new Range(); 1002 if (!(this.timing instanceof Range)) 1003 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.timing.getClass().getName()+" was encountered"); 1004 return (Range) this.timing; 1005 } 1006 1007 public boolean hasTimingRange() { 1008 return this != null && this.timing instanceof Range; 1009 } 1010 1011 /** 1012 * @return {@link #timing} (An optional value describing when the action should be performed.) 1013 */ 1014 public Timing getTimingTiming() throws FHIRException { 1015 if (this.timing == null) 1016 this.timing = new Timing(); 1017 if (!(this.timing instanceof Timing)) 1018 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.timing.getClass().getName()+" was encountered"); 1019 return (Timing) this.timing; 1020 } 1021 1022 public boolean hasTimingTiming() { 1023 return this != null && this.timing instanceof Timing; 1024 } 1025 1026 public boolean hasTiming() { 1027 return this.timing != null && !this.timing.isEmpty(); 1028 } 1029 1030 /** 1031 * @param value {@link #timing} (An optional value describing when the action should be performed.) 1032 */ 1033 public RequestOrchestrationActionComponent setTiming(DataType value) { 1034 if (value != null && !(value instanceof DateTimeType || value instanceof Age || value instanceof Period || value instanceof Duration || value instanceof Range || value instanceof Timing)) 1035 throw new FHIRException("Not the right type for RequestOrchestration.action.timing[x]: "+value.fhirType()); 1036 this.timing = value; 1037 return this; 1038 } 1039 1040 /** 1041 * @return {@link #location} (Identifies the facility where the action will occur; e.g. home, hospital, specific clinic, etc.) 1042 */ 1043 public CodeableReference getLocation() { 1044 if (this.location == null) 1045 if (Configuration.errorOnAutoCreate()) 1046 throw new Error("Attempt to auto-create RequestOrchestrationActionComponent.location"); 1047 else if (Configuration.doAutoCreate()) 1048 this.location = new CodeableReference(); // cc 1049 return this.location; 1050 } 1051 1052 public boolean hasLocation() { 1053 return this.location != null && !this.location.isEmpty(); 1054 } 1055 1056 /** 1057 * @param value {@link #location} (Identifies the facility where the action will occur; e.g. home, hospital, specific clinic, etc.) 1058 */ 1059 public RequestOrchestrationActionComponent setLocation(CodeableReference value) { 1060 this.location = value; 1061 return this; 1062 } 1063 1064 /** 1065 * @return {@link #participant} (The participant that should perform or be responsible for this action.) 1066 */ 1067 public List<RequestOrchestrationActionParticipantComponent> getParticipant() { 1068 if (this.participant == null) 1069 this.participant = new ArrayList<RequestOrchestrationActionParticipantComponent>(); 1070 return this.participant; 1071 } 1072 1073 /** 1074 * @return Returns a reference to <code>this</code> for easy method chaining 1075 */ 1076 public RequestOrchestrationActionComponent setParticipant(List<RequestOrchestrationActionParticipantComponent> theParticipant) { 1077 this.participant = theParticipant; 1078 return this; 1079 } 1080 1081 public boolean hasParticipant() { 1082 if (this.participant == null) 1083 return false; 1084 for (RequestOrchestrationActionParticipantComponent item : this.participant) 1085 if (!item.isEmpty()) 1086 return true; 1087 return false; 1088 } 1089 1090 public RequestOrchestrationActionParticipantComponent addParticipant() { //3 1091 RequestOrchestrationActionParticipantComponent t = new RequestOrchestrationActionParticipantComponent(); 1092 if (this.participant == null) 1093 this.participant = new ArrayList<RequestOrchestrationActionParticipantComponent>(); 1094 this.participant.add(t); 1095 return t; 1096 } 1097 1098 public RequestOrchestrationActionComponent addParticipant(RequestOrchestrationActionParticipantComponent t) { //3 1099 if (t == null) 1100 return this; 1101 if (this.participant == null) 1102 this.participant = new ArrayList<RequestOrchestrationActionParticipantComponent>(); 1103 this.participant.add(t); 1104 return this; 1105 } 1106 1107 /** 1108 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist {3} 1109 */ 1110 public RequestOrchestrationActionParticipantComponent getParticipantFirstRep() { 1111 if (getParticipant().isEmpty()) { 1112 addParticipant(); 1113 } 1114 return getParticipant().get(0); 1115 } 1116 1117 /** 1118 * @return {@link #type} (The type of action to perform (create, update, remove).) 1119 */ 1120 public CodeableConcept getType() { 1121 if (this.type == null) 1122 if (Configuration.errorOnAutoCreate()) 1123 throw new Error("Attempt to auto-create RequestOrchestrationActionComponent.type"); 1124 else if (Configuration.doAutoCreate()) 1125 this.type = new CodeableConcept(); // cc 1126 return this.type; 1127 } 1128 1129 public boolean hasType() { 1130 return this.type != null && !this.type.isEmpty(); 1131 } 1132 1133 /** 1134 * @param value {@link #type} (The type of action to perform (create, update, remove).) 1135 */ 1136 public RequestOrchestrationActionComponent setType(CodeableConcept value) { 1137 this.type = value; 1138 return this; 1139 } 1140 1141 /** 1142 * @return {@link #groupingBehavior} (Defines the grouping behavior for the action and its children.). This is the underlying object with id, value and extensions. The accessor "getGroupingBehavior" gives direct access to the value 1143 */ 1144 public Enumeration<ActionGroupingBehavior> getGroupingBehaviorElement() { 1145 if (this.groupingBehavior == null) 1146 if (Configuration.errorOnAutoCreate()) 1147 throw new Error("Attempt to auto-create RequestOrchestrationActionComponent.groupingBehavior"); 1148 else if (Configuration.doAutoCreate()) 1149 this.groupingBehavior = new Enumeration<ActionGroupingBehavior>(new ActionGroupingBehaviorEnumFactory()); // bb 1150 return this.groupingBehavior; 1151 } 1152 1153 public boolean hasGroupingBehaviorElement() { 1154 return this.groupingBehavior != null && !this.groupingBehavior.isEmpty(); 1155 } 1156 1157 public boolean hasGroupingBehavior() { 1158 return this.groupingBehavior != null && !this.groupingBehavior.isEmpty(); 1159 } 1160 1161 /** 1162 * @param value {@link #groupingBehavior} (Defines the grouping behavior for the action and its children.). This is the underlying object with id, value and extensions. The accessor "getGroupingBehavior" gives direct access to the value 1163 */ 1164 public RequestOrchestrationActionComponent setGroupingBehaviorElement(Enumeration<ActionGroupingBehavior> value) { 1165 this.groupingBehavior = value; 1166 return this; 1167 } 1168 1169 /** 1170 * @return Defines the grouping behavior for the action and its children. 1171 */ 1172 public ActionGroupingBehavior getGroupingBehavior() { 1173 return this.groupingBehavior == null ? null : this.groupingBehavior.getValue(); 1174 } 1175 1176 /** 1177 * @param value Defines the grouping behavior for the action and its children. 1178 */ 1179 public RequestOrchestrationActionComponent setGroupingBehavior(ActionGroupingBehavior value) { 1180 if (value == null) 1181 this.groupingBehavior = null; 1182 else { 1183 if (this.groupingBehavior == null) 1184 this.groupingBehavior = new Enumeration<ActionGroupingBehavior>(new ActionGroupingBehaviorEnumFactory()); 1185 this.groupingBehavior.setValue(value); 1186 } 1187 return this; 1188 } 1189 1190 /** 1191 * @return {@link #selectionBehavior} (Defines the selection behavior for the action and its children.). This is the underlying object with id, value and extensions. The accessor "getSelectionBehavior" gives direct access to the value 1192 */ 1193 public Enumeration<ActionSelectionBehavior> getSelectionBehaviorElement() { 1194 if (this.selectionBehavior == null) 1195 if (Configuration.errorOnAutoCreate()) 1196 throw new Error("Attempt to auto-create RequestOrchestrationActionComponent.selectionBehavior"); 1197 else if (Configuration.doAutoCreate()) 1198 this.selectionBehavior = new Enumeration<ActionSelectionBehavior>(new ActionSelectionBehaviorEnumFactory()); // bb 1199 return this.selectionBehavior; 1200 } 1201 1202 public boolean hasSelectionBehaviorElement() { 1203 return this.selectionBehavior != null && !this.selectionBehavior.isEmpty(); 1204 } 1205 1206 public boolean hasSelectionBehavior() { 1207 return this.selectionBehavior != null && !this.selectionBehavior.isEmpty(); 1208 } 1209 1210 /** 1211 * @param value {@link #selectionBehavior} (Defines the selection behavior for the action and its children.). This is the underlying object with id, value and extensions. The accessor "getSelectionBehavior" gives direct access to the value 1212 */ 1213 public RequestOrchestrationActionComponent setSelectionBehaviorElement(Enumeration<ActionSelectionBehavior> value) { 1214 this.selectionBehavior = value; 1215 return this; 1216 } 1217 1218 /** 1219 * @return Defines the selection behavior for the action and its children. 1220 */ 1221 public ActionSelectionBehavior getSelectionBehavior() { 1222 return this.selectionBehavior == null ? null : this.selectionBehavior.getValue(); 1223 } 1224 1225 /** 1226 * @param value Defines the selection behavior for the action and its children. 1227 */ 1228 public RequestOrchestrationActionComponent setSelectionBehavior(ActionSelectionBehavior value) { 1229 if (value == null) 1230 this.selectionBehavior = null; 1231 else { 1232 if (this.selectionBehavior == null) 1233 this.selectionBehavior = new Enumeration<ActionSelectionBehavior>(new ActionSelectionBehaviorEnumFactory()); 1234 this.selectionBehavior.setValue(value); 1235 } 1236 return this; 1237 } 1238 1239 /** 1240 * @return {@link #requiredBehavior} (Defines expectations around whether an action is required.). This is the underlying object with id, value and extensions. The accessor "getRequiredBehavior" gives direct access to the value 1241 */ 1242 public Enumeration<ActionRequiredBehavior> getRequiredBehaviorElement() { 1243 if (this.requiredBehavior == null) 1244 if (Configuration.errorOnAutoCreate()) 1245 throw new Error("Attempt to auto-create RequestOrchestrationActionComponent.requiredBehavior"); 1246 else if (Configuration.doAutoCreate()) 1247 this.requiredBehavior = new Enumeration<ActionRequiredBehavior>(new ActionRequiredBehaviorEnumFactory()); // bb 1248 return this.requiredBehavior; 1249 } 1250 1251 public boolean hasRequiredBehaviorElement() { 1252 return this.requiredBehavior != null && !this.requiredBehavior.isEmpty(); 1253 } 1254 1255 public boolean hasRequiredBehavior() { 1256 return this.requiredBehavior != null && !this.requiredBehavior.isEmpty(); 1257 } 1258 1259 /** 1260 * @param value {@link #requiredBehavior} (Defines expectations around whether an action is required.). This is the underlying object with id, value and extensions. The accessor "getRequiredBehavior" gives direct access to the value 1261 */ 1262 public RequestOrchestrationActionComponent setRequiredBehaviorElement(Enumeration<ActionRequiredBehavior> value) { 1263 this.requiredBehavior = value; 1264 return this; 1265 } 1266 1267 /** 1268 * @return Defines expectations around whether an action is required. 1269 */ 1270 public ActionRequiredBehavior getRequiredBehavior() { 1271 return this.requiredBehavior == null ? null : this.requiredBehavior.getValue(); 1272 } 1273 1274 /** 1275 * @param value Defines expectations around whether an action is required. 1276 */ 1277 public RequestOrchestrationActionComponent setRequiredBehavior(ActionRequiredBehavior value) { 1278 if (value == null) 1279 this.requiredBehavior = null; 1280 else { 1281 if (this.requiredBehavior == null) 1282 this.requiredBehavior = new Enumeration<ActionRequiredBehavior>(new ActionRequiredBehaviorEnumFactory()); 1283 this.requiredBehavior.setValue(value); 1284 } 1285 return this; 1286 } 1287 1288 /** 1289 * @return {@link #precheckBehavior} (Defines whether the action should usually be preselected.). This is the underlying object with id, value and extensions. The accessor "getPrecheckBehavior" gives direct access to the value 1290 */ 1291 public Enumeration<ActionPrecheckBehavior> getPrecheckBehaviorElement() { 1292 if (this.precheckBehavior == null) 1293 if (Configuration.errorOnAutoCreate()) 1294 throw new Error("Attempt to auto-create RequestOrchestrationActionComponent.precheckBehavior"); 1295 else if (Configuration.doAutoCreate()) 1296 this.precheckBehavior = new Enumeration<ActionPrecheckBehavior>(new ActionPrecheckBehaviorEnumFactory()); // bb 1297 return this.precheckBehavior; 1298 } 1299 1300 public boolean hasPrecheckBehaviorElement() { 1301 return this.precheckBehavior != null && !this.precheckBehavior.isEmpty(); 1302 } 1303 1304 public boolean hasPrecheckBehavior() { 1305 return this.precheckBehavior != null && !this.precheckBehavior.isEmpty(); 1306 } 1307 1308 /** 1309 * @param value {@link #precheckBehavior} (Defines whether the action should usually be preselected.). This is the underlying object with id, value and extensions. The accessor "getPrecheckBehavior" gives direct access to the value 1310 */ 1311 public RequestOrchestrationActionComponent setPrecheckBehaviorElement(Enumeration<ActionPrecheckBehavior> value) { 1312 this.precheckBehavior = value; 1313 return this; 1314 } 1315 1316 /** 1317 * @return Defines whether the action should usually be preselected. 1318 */ 1319 public ActionPrecheckBehavior getPrecheckBehavior() { 1320 return this.precheckBehavior == null ? null : this.precheckBehavior.getValue(); 1321 } 1322 1323 /** 1324 * @param value Defines whether the action should usually be preselected. 1325 */ 1326 public RequestOrchestrationActionComponent setPrecheckBehavior(ActionPrecheckBehavior value) { 1327 if (value == null) 1328 this.precheckBehavior = null; 1329 else { 1330 if (this.precheckBehavior == null) 1331 this.precheckBehavior = new Enumeration<ActionPrecheckBehavior>(new ActionPrecheckBehaviorEnumFactory()); 1332 this.precheckBehavior.setValue(value); 1333 } 1334 return this; 1335 } 1336 1337 /** 1338 * @return {@link #cardinalityBehavior} (Defines whether the action can be selected multiple times.). This is the underlying object with id, value and extensions. The accessor "getCardinalityBehavior" gives direct access to the value 1339 */ 1340 public Enumeration<ActionCardinalityBehavior> getCardinalityBehaviorElement() { 1341 if (this.cardinalityBehavior == null) 1342 if (Configuration.errorOnAutoCreate()) 1343 throw new Error("Attempt to auto-create RequestOrchestrationActionComponent.cardinalityBehavior"); 1344 else if (Configuration.doAutoCreate()) 1345 this.cardinalityBehavior = new Enumeration<ActionCardinalityBehavior>(new ActionCardinalityBehaviorEnumFactory()); // bb 1346 return this.cardinalityBehavior; 1347 } 1348 1349 public boolean hasCardinalityBehaviorElement() { 1350 return this.cardinalityBehavior != null && !this.cardinalityBehavior.isEmpty(); 1351 } 1352 1353 public boolean hasCardinalityBehavior() { 1354 return this.cardinalityBehavior != null && !this.cardinalityBehavior.isEmpty(); 1355 } 1356 1357 /** 1358 * @param value {@link #cardinalityBehavior} (Defines whether the action can be selected multiple times.). This is the underlying object with id, value and extensions. The accessor "getCardinalityBehavior" gives direct access to the value 1359 */ 1360 public RequestOrchestrationActionComponent setCardinalityBehaviorElement(Enumeration<ActionCardinalityBehavior> value) { 1361 this.cardinalityBehavior = value; 1362 return this; 1363 } 1364 1365 /** 1366 * @return Defines whether the action can be selected multiple times. 1367 */ 1368 public ActionCardinalityBehavior getCardinalityBehavior() { 1369 return this.cardinalityBehavior == null ? null : this.cardinalityBehavior.getValue(); 1370 } 1371 1372 /** 1373 * @param value Defines whether the action can be selected multiple times. 1374 */ 1375 public RequestOrchestrationActionComponent setCardinalityBehavior(ActionCardinalityBehavior value) { 1376 if (value == null) 1377 this.cardinalityBehavior = null; 1378 else { 1379 if (this.cardinalityBehavior == null) 1380 this.cardinalityBehavior = new Enumeration<ActionCardinalityBehavior>(new ActionCardinalityBehaviorEnumFactory()); 1381 this.cardinalityBehavior.setValue(value); 1382 } 1383 return this; 1384 } 1385 1386 /** 1387 * @return {@link #resource} (The resource that is the target of the action (e.g. CommunicationRequest).) 1388 */ 1389 public Reference getResource() { 1390 if (this.resource == null) 1391 if (Configuration.errorOnAutoCreate()) 1392 throw new Error("Attempt to auto-create RequestOrchestrationActionComponent.resource"); 1393 else if (Configuration.doAutoCreate()) 1394 this.resource = new Reference(); // cc 1395 return this.resource; 1396 } 1397 1398 public boolean hasResource() { 1399 return this.resource != null && !this.resource.isEmpty(); 1400 } 1401 1402 /** 1403 * @param value {@link #resource} (The resource that is the target of the action (e.g. CommunicationRequest).) 1404 */ 1405 public RequestOrchestrationActionComponent setResource(Reference value) { 1406 this.resource = value; 1407 return this; 1408 } 1409 1410 /** 1411 * @return {@link #definition} (A reference to an ActivityDefinition that describes the action to be taken in detail, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured.) 1412 */ 1413 public DataType getDefinition() { 1414 return this.definition; 1415 } 1416 1417 /** 1418 * @return {@link #definition} (A reference to an ActivityDefinition that describes the action to be taken in detail, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured.) 1419 */ 1420 public CanonicalType getDefinitionCanonicalType() throws FHIRException { 1421 if (this.definition == null) 1422 this.definition = new CanonicalType(); 1423 if (!(this.definition instanceof CanonicalType)) 1424 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.definition.getClass().getName()+" was encountered"); 1425 return (CanonicalType) this.definition; 1426 } 1427 1428 public boolean hasDefinitionCanonicalType() { 1429 return this != null && this.definition instanceof CanonicalType; 1430 } 1431 1432 /** 1433 * @return {@link #definition} (A reference to an ActivityDefinition that describes the action to be taken in detail, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured.) 1434 */ 1435 public UriType getDefinitionUriType() throws FHIRException { 1436 if (this.definition == null) 1437 this.definition = new UriType(); 1438 if (!(this.definition instanceof UriType)) 1439 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.definition.getClass().getName()+" was encountered"); 1440 return (UriType) this.definition; 1441 } 1442 1443 public boolean hasDefinitionUriType() { 1444 return this != null && this.definition instanceof UriType; 1445 } 1446 1447 public boolean hasDefinition() { 1448 return this.definition != null && !this.definition.isEmpty(); 1449 } 1450 1451 /** 1452 * @param value {@link #definition} (A reference to an ActivityDefinition that describes the action to be taken in detail, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured.) 1453 */ 1454 public RequestOrchestrationActionComponent setDefinition(DataType value) { 1455 if (value != null && !(value instanceof CanonicalType || value instanceof UriType)) 1456 throw new FHIRException("Not the right type for RequestOrchestration.action.definition[x]: "+value.fhirType()); 1457 this.definition = value; 1458 return this; 1459 } 1460 1461 /** 1462 * @return {@link #transform} (A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.). This is the underlying object with id, value and extensions. The accessor "getTransform" gives direct access to the value 1463 */ 1464 public CanonicalType getTransformElement() { 1465 if (this.transform == null) 1466 if (Configuration.errorOnAutoCreate()) 1467 throw new Error("Attempt to auto-create RequestOrchestrationActionComponent.transform"); 1468 else if (Configuration.doAutoCreate()) 1469 this.transform = new CanonicalType(); // bb 1470 return this.transform; 1471 } 1472 1473 public boolean hasTransformElement() { 1474 return this.transform != null && !this.transform.isEmpty(); 1475 } 1476 1477 public boolean hasTransform() { 1478 return this.transform != null && !this.transform.isEmpty(); 1479 } 1480 1481 /** 1482 * @param value {@link #transform} (A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.). This is the underlying object with id, value and extensions. The accessor "getTransform" gives direct access to the value 1483 */ 1484 public RequestOrchestrationActionComponent setTransformElement(CanonicalType value) { 1485 this.transform = value; 1486 return this; 1487 } 1488 1489 /** 1490 * @return A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input. 1491 */ 1492 public String getTransform() { 1493 return this.transform == null ? null : this.transform.getValue(); 1494 } 1495 1496 /** 1497 * @param value A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input. 1498 */ 1499 public RequestOrchestrationActionComponent setTransform(String value) { 1500 if (Utilities.noString(value)) 1501 this.transform = null; 1502 else { 1503 if (this.transform == null) 1504 this.transform = new CanonicalType(); 1505 this.transform.setValue(value); 1506 } 1507 return this; 1508 } 1509 1510 /** 1511 * @return {@link #dynamicValue} (Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result.) 1512 */ 1513 public List<RequestOrchestrationActionDynamicValueComponent> getDynamicValue() { 1514 if (this.dynamicValue == null) 1515 this.dynamicValue = new ArrayList<RequestOrchestrationActionDynamicValueComponent>(); 1516 return this.dynamicValue; 1517 } 1518 1519 /** 1520 * @return Returns a reference to <code>this</code> for easy method chaining 1521 */ 1522 public RequestOrchestrationActionComponent setDynamicValue(List<RequestOrchestrationActionDynamicValueComponent> theDynamicValue) { 1523 this.dynamicValue = theDynamicValue; 1524 return this; 1525 } 1526 1527 public boolean hasDynamicValue() { 1528 if (this.dynamicValue == null) 1529 return false; 1530 for (RequestOrchestrationActionDynamicValueComponent item : this.dynamicValue) 1531 if (!item.isEmpty()) 1532 return true; 1533 return false; 1534 } 1535 1536 public RequestOrchestrationActionDynamicValueComponent addDynamicValue() { //3 1537 RequestOrchestrationActionDynamicValueComponent t = new RequestOrchestrationActionDynamicValueComponent(); 1538 if (this.dynamicValue == null) 1539 this.dynamicValue = new ArrayList<RequestOrchestrationActionDynamicValueComponent>(); 1540 this.dynamicValue.add(t); 1541 return t; 1542 } 1543 1544 public RequestOrchestrationActionComponent addDynamicValue(RequestOrchestrationActionDynamicValueComponent t) { //3 1545 if (t == null) 1546 return this; 1547 if (this.dynamicValue == null) 1548 this.dynamicValue = new ArrayList<RequestOrchestrationActionDynamicValueComponent>(); 1549 this.dynamicValue.add(t); 1550 return this; 1551 } 1552 1553 /** 1554 * @return The first repetition of repeating field {@link #dynamicValue}, creating it if it does not already exist {3} 1555 */ 1556 public RequestOrchestrationActionDynamicValueComponent getDynamicValueFirstRep() { 1557 if (getDynamicValue().isEmpty()) { 1558 addDynamicValue(); 1559 } 1560 return getDynamicValue().get(0); 1561 } 1562 1563 /** 1564 * @return {@link #action} (Sub actions.) 1565 */ 1566 public List<RequestOrchestrationActionComponent> getAction() { 1567 if (this.action == null) 1568 this.action = new ArrayList<RequestOrchestrationActionComponent>(); 1569 return this.action; 1570 } 1571 1572 /** 1573 * @return Returns a reference to <code>this</code> for easy method chaining 1574 */ 1575 public RequestOrchestrationActionComponent setAction(List<RequestOrchestrationActionComponent> theAction) { 1576 this.action = theAction; 1577 return this; 1578 } 1579 1580 public boolean hasAction() { 1581 if (this.action == null) 1582 return false; 1583 for (RequestOrchestrationActionComponent item : this.action) 1584 if (!item.isEmpty()) 1585 return true; 1586 return false; 1587 } 1588 1589 public RequestOrchestrationActionComponent addAction() { //3 1590 RequestOrchestrationActionComponent t = new RequestOrchestrationActionComponent(); 1591 if (this.action == null) 1592 this.action = new ArrayList<RequestOrchestrationActionComponent>(); 1593 this.action.add(t); 1594 return t; 1595 } 1596 1597 public RequestOrchestrationActionComponent addAction(RequestOrchestrationActionComponent t) { //3 1598 if (t == null) 1599 return this; 1600 if (this.action == null) 1601 this.action = new ArrayList<RequestOrchestrationActionComponent>(); 1602 this.action.add(t); 1603 return this; 1604 } 1605 1606 /** 1607 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist {3} 1608 */ 1609 public RequestOrchestrationActionComponent getActionFirstRep() { 1610 if (getAction().isEmpty()) { 1611 addAction(); 1612 } 1613 return getAction().get(0); 1614 } 1615 1616 protected void listChildren(List<Property> children) { 1617 super.listChildren(children); 1618 children.add(new Property("linkId", "string", "The linkId of the action from the PlanDefinition that corresponds to this action in the RequestOrchestration resource.", 0, 1, linkId)); 1619 children.add(new Property("prefix", "string", "A user-visible prefix for the action. For example a section or item numbering such as 1. or A.", 0, 1, prefix)); 1620 children.add(new Property("title", "string", "The title of the action displayed to a user.", 0, 1, title)); 1621 children.add(new Property("description", "markdown", "A short description of the action used to provide a summary to display to the user.", 0, 1, description)); 1622 children.add(new Property("textEquivalent", "markdown", "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically.", 0, 1, textEquivalent)); 1623 children.add(new Property("priority", "code", "Indicates how quickly the action should be addressed with respect to other actions.", 0, 1, priority)); 1624 children.add(new Property("code", "CodeableConcept", "A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a section of a documentation template.", 0, java.lang.Integer.MAX_VALUE, code)); 1625 children.add(new Property("documentation", "RelatedArtifact", "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.", 0, java.lang.Integer.MAX_VALUE, documentation)); 1626 children.add(new Property("goal", "Reference(Goal)", "Goals that are intended to be achieved by following the requests in this action.", 0, java.lang.Integer.MAX_VALUE, goal)); 1627 children.add(new Property("condition", "", "An expression that describes applicability criteria, or start/stop conditions for the action.", 0, java.lang.Integer.MAX_VALUE, condition)); 1628 children.add(new Property("input", "", "Defines input data requirements for the action.", 0, java.lang.Integer.MAX_VALUE, input)); 1629 children.add(new Property("output", "", "Defines the outputs of the action, if any.", 0, java.lang.Integer.MAX_VALUE, output)); 1630 children.add(new Property("relatedAction", "", "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".", 0, java.lang.Integer.MAX_VALUE, relatedAction)); 1631 children.add(new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing)); 1632 children.add(new Property("location", "CodeableReference(Location)", "Identifies the facility where the action will occur; e.g. home, hospital, specific clinic, etc.", 0, 1, location)); 1633 children.add(new Property("participant", "", "The participant that should perform or be responsible for this action.", 0, java.lang.Integer.MAX_VALUE, participant)); 1634 children.add(new Property("type", "CodeableConcept", "The type of action to perform (create, update, remove).", 0, 1, type)); 1635 children.add(new Property("groupingBehavior", "code", "Defines the grouping behavior for the action and its children.", 0, 1, groupingBehavior)); 1636 children.add(new Property("selectionBehavior", "code", "Defines the selection behavior for the action and its children.", 0, 1, selectionBehavior)); 1637 children.add(new Property("requiredBehavior", "code", "Defines expectations around whether an action is required.", 0, 1, requiredBehavior)); 1638 children.add(new Property("precheckBehavior", "code", "Defines whether the action should usually be preselected.", 0, 1, precheckBehavior)); 1639 children.add(new Property("cardinalityBehavior", "code", "Defines whether the action can be selected multiple times.", 0, 1, cardinalityBehavior)); 1640 children.add(new Property("resource", "Reference(Any)", "The resource that is the target of the action (e.g. CommunicationRequest).", 0, 1, resource)); 1641 children.add(new Property("definition[x]", "canonical(ActivityDefinition|ObservationDefinition|PlanDefinition|Questionnaire|SpecimenDefinition)|uri", "A reference to an ActivityDefinition that describes the action to be taken in detail, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured.", 0, 1, definition)); 1642 children.add(new Property("transform", "canonical(StructureMap)", "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.", 0, 1, transform)); 1643 children.add(new Property("dynamicValue", "", "Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result.", 0, java.lang.Integer.MAX_VALUE, dynamicValue)); 1644 children.add(new Property("action", "@RequestOrchestration.action", "Sub actions.", 0, java.lang.Integer.MAX_VALUE, action)); 1645 } 1646 1647 @Override 1648 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1649 switch (_hash) { 1650 case -1102667083: /*linkId*/ return new Property("linkId", "string", "The linkId of the action from the PlanDefinition that corresponds to this action in the RequestOrchestration resource.", 0, 1, linkId); 1651 case -980110702: /*prefix*/ return new Property("prefix", "string", "A user-visible prefix for the action. For example a section or item numbering such as 1. or A.", 0, 1, prefix); 1652 case 110371416: /*title*/ return new Property("title", "string", "The title of the action displayed to a user.", 0, 1, title); 1653 case -1724546052: /*description*/ return new Property("description", "markdown", "A short description of the action used to provide a summary to display to the user.", 0, 1, description); 1654 case -900391049: /*textEquivalent*/ return new Property("textEquivalent", "markdown", "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically.", 0, 1, textEquivalent); 1655 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly the action should be addressed with respect to other actions.", 0, 1, priority); 1656 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a section of a documentation template.", 0, java.lang.Integer.MAX_VALUE, code); 1657 case 1587405498: /*documentation*/ return new Property("documentation", "RelatedArtifact", "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.", 0, java.lang.Integer.MAX_VALUE, documentation); 1658 case 3178259: /*goal*/ return new Property("goal", "Reference(Goal)", "Goals that are intended to be achieved by following the requests in this action.", 0, java.lang.Integer.MAX_VALUE, goal); 1659 case -861311717: /*condition*/ return new Property("condition", "", "An expression that describes applicability criteria, or start/stop conditions for the action.", 0, java.lang.Integer.MAX_VALUE, condition); 1660 case 100358090: /*input*/ return new Property("input", "", "Defines input data requirements for the action.", 0, java.lang.Integer.MAX_VALUE, input); 1661 case -1005512447: /*output*/ return new Property("output", "", "Defines the outputs of the action, if any.", 0, java.lang.Integer.MAX_VALUE, output); 1662 case -384107967: /*relatedAction*/ return new Property("relatedAction", "", "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".", 0, java.lang.Integer.MAX_VALUE, relatedAction); 1663 case 164632566: /*timing[x]*/ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 1664 case -873664438: /*timing*/ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 1665 case -1837458939: /*timingDateTime*/ return new Property("timing[x]", "dateTime", "An optional value describing when the action should be performed.", 0, 1, timing); 1666 case 164607061: /*timingAge*/ return new Property("timing[x]", "Age", "An optional value describing when the action should be performed.", 0, 1, timing); 1667 case -615615829: /*timingPeriod*/ return new Property("timing[x]", "Period", "An optional value describing when the action should be performed.", 0, 1, timing); 1668 case -1327253506: /*timingDuration*/ return new Property("timing[x]", "Duration", "An optional value describing when the action should be performed.", 0, 1, timing); 1669 case -710871277: /*timingRange*/ return new Property("timing[x]", "Range", "An optional value describing when the action should be performed.", 0, 1, timing); 1670 case -497554124: /*timingTiming*/ return new Property("timing[x]", "Timing", "An optional value describing when the action should be performed.", 0, 1, timing); 1671 case 1901043637: /*location*/ return new Property("location", "CodeableReference(Location)", "Identifies the facility where the action will occur; e.g. home, hospital, specific clinic, etc.", 0, 1, location); 1672 case 767422259: /*participant*/ return new Property("participant", "", "The participant that should perform or be responsible for this action.", 0, java.lang.Integer.MAX_VALUE, participant); 1673 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of action to perform (create, update, remove).", 0, 1, type); 1674 case 586678389: /*groupingBehavior*/ return new Property("groupingBehavior", "code", "Defines the grouping behavior for the action and its children.", 0, 1, groupingBehavior); 1675 case 168639486: /*selectionBehavior*/ return new Property("selectionBehavior", "code", "Defines the selection behavior for the action and its children.", 0, 1, selectionBehavior); 1676 case -1163906287: /*requiredBehavior*/ return new Property("requiredBehavior", "code", "Defines expectations around whether an action is required.", 0, 1, requiredBehavior); 1677 case -1174249033: /*precheckBehavior*/ return new Property("precheckBehavior", "code", "Defines whether the action should usually be preselected.", 0, 1, precheckBehavior); 1678 case -922577408: /*cardinalityBehavior*/ return new Property("cardinalityBehavior", "code", "Defines whether the action can be selected multiple times.", 0, 1, cardinalityBehavior); 1679 case -341064690: /*resource*/ return new Property("resource", "Reference(Any)", "The resource that is the target of the action (e.g. CommunicationRequest).", 0, 1, resource); 1680 case -1139422643: /*definition[x]*/ return new Property("definition[x]", "canonical(ActivityDefinition|ObservationDefinition|PlanDefinition|Questionnaire|SpecimenDefinition)|uri", "A reference to an ActivityDefinition that describes the action to be taken in detail, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured.", 0, 1, definition); 1681 case -1014418093: /*definition*/ return new Property("definition[x]", "canonical(ActivityDefinition|ObservationDefinition|PlanDefinition|Questionnaire|SpecimenDefinition)|uri", "A reference to an ActivityDefinition that describes the action to be taken in detail, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured.", 0, 1, definition); 1682 case 933485793: /*definitionCanonical*/ return new Property("definition[x]", "canonical(ActivityDefinition|ObservationDefinition|PlanDefinition|Questionnaire|SpecimenDefinition)", "A reference to an ActivityDefinition that describes the action to be taken in detail, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured.", 0, 1, definition); 1683 case -1139428583: /*definitionUri*/ return new Property("definition[x]", "uri", "A reference to an ActivityDefinition that describes the action to be taken in detail, a PlanDefinition that describes a series of actions to be taken, a Questionnaire that should be filled out, a SpecimenDefinition describing a specimen to be collected, or an ObservationDefinition that specifies what observation should be captured.", 0, 1, definition); 1684 case 1052666732: /*transform*/ return new Property("transform", "canonical(StructureMap)", "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.", 0, 1, transform); 1685 case 572625010: /*dynamicValue*/ return new Property("dynamicValue", "", "Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result.", 0, java.lang.Integer.MAX_VALUE, dynamicValue); 1686 case -1422950858: /*action*/ return new Property("action", "@RequestOrchestration.action", "Sub actions.", 0, java.lang.Integer.MAX_VALUE, action); 1687 default: return super.getNamedProperty(_hash, _name, _checkValid); 1688 } 1689 1690 } 1691 1692 @Override 1693 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1694 switch (hash) { 1695 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // StringType 1696 case -980110702: /*prefix*/ return this.prefix == null ? new Base[0] : new Base[] {this.prefix}; // StringType 1697 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 1698 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1699 case -900391049: /*textEquivalent*/ return this.textEquivalent == null ? new Base[0] : new Base[] {this.textEquivalent}; // MarkdownType 1700 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<RequestPriority> 1701 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 1702 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : this.documentation.toArray(new Base[this.documentation.size()]); // RelatedArtifact 1703 case 3178259: /*goal*/ return this.goal == null ? new Base[0] : this.goal.toArray(new Base[this.goal.size()]); // Reference 1704 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : this.condition.toArray(new Base[this.condition.size()]); // RequestOrchestrationActionConditionComponent 1705 case 100358090: /*input*/ return this.input == null ? new Base[0] : this.input.toArray(new Base[this.input.size()]); // RequestOrchestrationActionInputComponent 1706 case -1005512447: /*output*/ return this.output == null ? new Base[0] : this.output.toArray(new Base[this.output.size()]); // RequestOrchestrationActionOutputComponent 1707 case -384107967: /*relatedAction*/ return this.relatedAction == null ? new Base[0] : this.relatedAction.toArray(new Base[this.relatedAction.size()]); // RequestOrchestrationActionRelatedActionComponent 1708 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // DataType 1709 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // CodeableReference 1710 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // RequestOrchestrationActionParticipantComponent 1711 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1712 case 586678389: /*groupingBehavior*/ return this.groupingBehavior == null ? new Base[0] : new Base[] {this.groupingBehavior}; // Enumeration<ActionGroupingBehavior> 1713 case 168639486: /*selectionBehavior*/ return this.selectionBehavior == null ? new Base[0] : new Base[] {this.selectionBehavior}; // Enumeration<ActionSelectionBehavior> 1714 case -1163906287: /*requiredBehavior*/ return this.requiredBehavior == null ? new Base[0] : new Base[] {this.requiredBehavior}; // Enumeration<ActionRequiredBehavior> 1715 case -1174249033: /*precheckBehavior*/ return this.precheckBehavior == null ? new Base[0] : new Base[] {this.precheckBehavior}; // Enumeration<ActionPrecheckBehavior> 1716 case -922577408: /*cardinalityBehavior*/ return this.cardinalityBehavior == null ? new Base[0] : new Base[] {this.cardinalityBehavior}; // Enumeration<ActionCardinalityBehavior> 1717 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // Reference 1718 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // DataType 1719 case 1052666732: /*transform*/ return this.transform == null ? new Base[0] : new Base[] {this.transform}; // CanonicalType 1720 case 572625010: /*dynamicValue*/ return this.dynamicValue == null ? new Base[0] : this.dynamicValue.toArray(new Base[this.dynamicValue.size()]); // RequestOrchestrationActionDynamicValueComponent 1721 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // RequestOrchestrationActionComponent 1722 default: return super.getProperty(hash, name, checkValid); 1723 } 1724 1725 } 1726 1727 @Override 1728 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1729 switch (hash) { 1730 case -1102667083: // linkId 1731 this.linkId = TypeConvertor.castToString(value); // StringType 1732 return value; 1733 case -980110702: // prefix 1734 this.prefix = TypeConvertor.castToString(value); // StringType 1735 return value; 1736 case 110371416: // title 1737 this.title = TypeConvertor.castToString(value); // StringType 1738 return value; 1739 case -1724546052: // description 1740 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1741 return value; 1742 case -900391049: // textEquivalent 1743 this.textEquivalent = TypeConvertor.castToMarkdown(value); // MarkdownType 1744 return value; 1745 case -1165461084: // priority 1746 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1747 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1748 return value; 1749 case 3059181: // code 1750 this.getCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1751 return value; 1752 case 1587405498: // documentation 1753 this.getDocumentation().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 1754 return value; 1755 case 3178259: // goal 1756 this.getGoal().add(TypeConvertor.castToReference(value)); // Reference 1757 return value; 1758 case -861311717: // condition 1759 this.getCondition().add((RequestOrchestrationActionConditionComponent) value); // RequestOrchestrationActionConditionComponent 1760 return value; 1761 case 100358090: // input 1762 this.getInput().add((RequestOrchestrationActionInputComponent) value); // RequestOrchestrationActionInputComponent 1763 return value; 1764 case -1005512447: // output 1765 this.getOutput().add((RequestOrchestrationActionOutputComponent) value); // RequestOrchestrationActionOutputComponent 1766 return value; 1767 case -384107967: // relatedAction 1768 this.getRelatedAction().add((RequestOrchestrationActionRelatedActionComponent) value); // RequestOrchestrationActionRelatedActionComponent 1769 return value; 1770 case -873664438: // timing 1771 this.timing = TypeConvertor.castToType(value); // DataType 1772 return value; 1773 case 1901043637: // location 1774 this.location = TypeConvertor.castToCodeableReference(value); // CodeableReference 1775 return value; 1776 case 767422259: // participant 1777 this.getParticipant().add((RequestOrchestrationActionParticipantComponent) value); // RequestOrchestrationActionParticipantComponent 1778 return value; 1779 case 3575610: // type 1780 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1781 return value; 1782 case 586678389: // groupingBehavior 1783 value = new ActionGroupingBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1784 this.groupingBehavior = (Enumeration) value; // Enumeration<ActionGroupingBehavior> 1785 return value; 1786 case 168639486: // selectionBehavior 1787 value = new ActionSelectionBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1788 this.selectionBehavior = (Enumeration) value; // Enumeration<ActionSelectionBehavior> 1789 return value; 1790 case -1163906287: // requiredBehavior 1791 value = new ActionRequiredBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1792 this.requiredBehavior = (Enumeration) value; // Enumeration<ActionRequiredBehavior> 1793 return value; 1794 case -1174249033: // precheckBehavior 1795 value = new ActionPrecheckBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1796 this.precheckBehavior = (Enumeration) value; // Enumeration<ActionPrecheckBehavior> 1797 return value; 1798 case -922577408: // cardinalityBehavior 1799 value = new ActionCardinalityBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1800 this.cardinalityBehavior = (Enumeration) value; // Enumeration<ActionCardinalityBehavior> 1801 return value; 1802 case -341064690: // resource 1803 this.resource = TypeConvertor.castToReference(value); // Reference 1804 return value; 1805 case -1014418093: // definition 1806 this.definition = TypeConvertor.castToType(value); // DataType 1807 return value; 1808 case 1052666732: // transform 1809 this.transform = TypeConvertor.castToCanonical(value); // CanonicalType 1810 return value; 1811 case 572625010: // dynamicValue 1812 this.getDynamicValue().add((RequestOrchestrationActionDynamicValueComponent) value); // RequestOrchestrationActionDynamicValueComponent 1813 return value; 1814 case -1422950858: // action 1815 this.getAction().add((RequestOrchestrationActionComponent) value); // RequestOrchestrationActionComponent 1816 return value; 1817 default: return super.setProperty(hash, name, value); 1818 } 1819 1820 } 1821 1822 @Override 1823 public Base setProperty(String name, Base value) throws FHIRException { 1824 if (name.equals("linkId")) { 1825 this.linkId = TypeConvertor.castToString(value); // StringType 1826 } else if (name.equals("prefix")) { 1827 this.prefix = TypeConvertor.castToString(value); // StringType 1828 } else if (name.equals("title")) { 1829 this.title = TypeConvertor.castToString(value); // StringType 1830 } else if (name.equals("description")) { 1831 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1832 } else if (name.equals("textEquivalent")) { 1833 this.textEquivalent = TypeConvertor.castToMarkdown(value); // MarkdownType 1834 } else if (name.equals("priority")) { 1835 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1836 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1837 } else if (name.equals("code")) { 1838 this.getCode().add(TypeConvertor.castToCodeableConcept(value)); 1839 } else if (name.equals("documentation")) { 1840 this.getDocumentation().add(TypeConvertor.castToRelatedArtifact(value)); 1841 } else if (name.equals("goal")) { 1842 this.getGoal().add(TypeConvertor.castToReference(value)); 1843 } else if (name.equals("condition")) { 1844 this.getCondition().add((RequestOrchestrationActionConditionComponent) value); 1845 } else if (name.equals("input")) { 1846 this.getInput().add((RequestOrchestrationActionInputComponent) value); 1847 } else if (name.equals("output")) { 1848 this.getOutput().add((RequestOrchestrationActionOutputComponent) value); 1849 } else if (name.equals("relatedAction")) { 1850 this.getRelatedAction().add((RequestOrchestrationActionRelatedActionComponent) value); 1851 } else if (name.equals("timing[x]")) { 1852 this.timing = TypeConvertor.castToType(value); // DataType 1853 } else if (name.equals("location")) { 1854 this.location = TypeConvertor.castToCodeableReference(value); // CodeableReference 1855 } else if (name.equals("participant")) { 1856 this.getParticipant().add((RequestOrchestrationActionParticipantComponent) value); 1857 } else if (name.equals("type")) { 1858 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1859 } else if (name.equals("groupingBehavior")) { 1860 value = new ActionGroupingBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1861 this.groupingBehavior = (Enumeration) value; // Enumeration<ActionGroupingBehavior> 1862 } else if (name.equals("selectionBehavior")) { 1863 value = new ActionSelectionBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1864 this.selectionBehavior = (Enumeration) value; // Enumeration<ActionSelectionBehavior> 1865 } else if (name.equals("requiredBehavior")) { 1866 value = new ActionRequiredBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1867 this.requiredBehavior = (Enumeration) value; // Enumeration<ActionRequiredBehavior> 1868 } else if (name.equals("precheckBehavior")) { 1869 value = new ActionPrecheckBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1870 this.precheckBehavior = (Enumeration) value; // Enumeration<ActionPrecheckBehavior> 1871 } else if (name.equals("cardinalityBehavior")) { 1872 value = new ActionCardinalityBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1873 this.cardinalityBehavior = (Enumeration) value; // Enumeration<ActionCardinalityBehavior> 1874 } else if (name.equals("resource")) { 1875 this.resource = TypeConvertor.castToReference(value); // Reference 1876 } else if (name.equals("definition[x]")) { 1877 this.definition = TypeConvertor.castToType(value); // DataType 1878 } else if (name.equals("transform")) { 1879 this.transform = TypeConvertor.castToCanonical(value); // CanonicalType 1880 } else if (name.equals("dynamicValue")) { 1881 this.getDynamicValue().add((RequestOrchestrationActionDynamicValueComponent) value); 1882 } else if (name.equals("action")) { 1883 this.getAction().add((RequestOrchestrationActionComponent) value); 1884 } else 1885 return super.setProperty(name, value); 1886 return value; 1887 } 1888 1889 @Override 1890 public void removeChild(String name, Base value) throws FHIRException { 1891 if (name.equals("linkId")) { 1892 this.linkId = null; 1893 } else if (name.equals("prefix")) { 1894 this.prefix = null; 1895 } else if (name.equals("title")) { 1896 this.title = null; 1897 } else if (name.equals("description")) { 1898 this.description = null; 1899 } else if (name.equals("textEquivalent")) { 1900 this.textEquivalent = null; 1901 } else if (name.equals("priority")) { 1902 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1903 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1904 } else if (name.equals("code")) { 1905 this.getCode().remove(value); 1906 } else if (name.equals("documentation")) { 1907 this.getDocumentation().remove(value); 1908 } else if (name.equals("goal")) { 1909 this.getGoal().remove(value); 1910 } else if (name.equals("condition")) { 1911 this.getCondition().remove((RequestOrchestrationActionConditionComponent) value); 1912 } else if (name.equals("input")) { 1913 this.getInput().remove((RequestOrchestrationActionInputComponent) value); 1914 } else if (name.equals("output")) { 1915 this.getOutput().remove((RequestOrchestrationActionOutputComponent) value); 1916 } else if (name.equals("relatedAction")) { 1917 this.getRelatedAction().remove((RequestOrchestrationActionRelatedActionComponent) value); 1918 } else if (name.equals("timing[x]")) { 1919 this.timing = null; 1920 } else if (name.equals("location")) { 1921 this.location = null; 1922 } else if (name.equals("participant")) { 1923 this.getParticipant().remove((RequestOrchestrationActionParticipantComponent) value); 1924 } else if (name.equals("type")) { 1925 this.type = null; 1926 } else if (name.equals("groupingBehavior")) { 1927 value = new ActionGroupingBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1928 this.groupingBehavior = (Enumeration) value; // Enumeration<ActionGroupingBehavior> 1929 } else if (name.equals("selectionBehavior")) { 1930 value = new ActionSelectionBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1931 this.selectionBehavior = (Enumeration) value; // Enumeration<ActionSelectionBehavior> 1932 } else if (name.equals("requiredBehavior")) { 1933 value = new ActionRequiredBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1934 this.requiredBehavior = (Enumeration) value; // Enumeration<ActionRequiredBehavior> 1935 } else if (name.equals("precheckBehavior")) { 1936 value = new ActionPrecheckBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1937 this.precheckBehavior = (Enumeration) value; // Enumeration<ActionPrecheckBehavior> 1938 } else if (name.equals("cardinalityBehavior")) { 1939 value = new ActionCardinalityBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1940 this.cardinalityBehavior = (Enumeration) value; // Enumeration<ActionCardinalityBehavior> 1941 } else if (name.equals("resource")) { 1942 this.resource = null; 1943 } else if (name.equals("definition[x]")) { 1944 this.definition = null; 1945 } else if (name.equals("transform")) { 1946 this.transform = null; 1947 } else if (name.equals("dynamicValue")) { 1948 this.getDynamicValue().remove((RequestOrchestrationActionDynamicValueComponent) value); 1949 } else if (name.equals("action")) { 1950 this.getAction().remove((RequestOrchestrationActionComponent) value); 1951 } else 1952 super.removeChild(name, value); 1953 1954 } 1955 1956 @Override 1957 public Base makeProperty(int hash, String name) throws FHIRException { 1958 switch (hash) { 1959 case -1102667083: return getLinkIdElement(); 1960 case -980110702: return getPrefixElement(); 1961 case 110371416: return getTitleElement(); 1962 case -1724546052: return getDescriptionElement(); 1963 case -900391049: return getTextEquivalentElement(); 1964 case -1165461084: return getPriorityElement(); 1965 case 3059181: return addCode(); 1966 case 1587405498: return addDocumentation(); 1967 case 3178259: return addGoal(); 1968 case -861311717: return addCondition(); 1969 case 100358090: return addInput(); 1970 case -1005512447: return addOutput(); 1971 case -384107967: return addRelatedAction(); 1972 case 164632566: return getTiming(); 1973 case -873664438: return getTiming(); 1974 case 1901043637: return getLocation(); 1975 case 767422259: return addParticipant(); 1976 case 3575610: return getType(); 1977 case 586678389: return getGroupingBehaviorElement(); 1978 case 168639486: return getSelectionBehaviorElement(); 1979 case -1163906287: return getRequiredBehaviorElement(); 1980 case -1174249033: return getPrecheckBehaviorElement(); 1981 case -922577408: return getCardinalityBehaviorElement(); 1982 case -341064690: return getResource(); 1983 case -1139422643: return getDefinition(); 1984 case -1014418093: return getDefinition(); 1985 case 1052666732: return getTransformElement(); 1986 case 572625010: return addDynamicValue(); 1987 case -1422950858: return addAction(); 1988 default: return super.makeProperty(hash, name); 1989 } 1990 1991 } 1992 1993 @Override 1994 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1995 switch (hash) { 1996 case -1102667083: /*linkId*/ return new String[] {"string"}; 1997 case -980110702: /*prefix*/ return new String[] {"string"}; 1998 case 110371416: /*title*/ return new String[] {"string"}; 1999 case -1724546052: /*description*/ return new String[] {"markdown"}; 2000 case -900391049: /*textEquivalent*/ return new String[] {"markdown"}; 2001 case -1165461084: /*priority*/ return new String[] {"code"}; 2002 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2003 case 1587405498: /*documentation*/ return new String[] {"RelatedArtifact"}; 2004 case 3178259: /*goal*/ return new String[] {"Reference"}; 2005 case -861311717: /*condition*/ return new String[] {}; 2006 case 100358090: /*input*/ return new String[] {}; 2007 case -1005512447: /*output*/ return new String[] {}; 2008 case -384107967: /*relatedAction*/ return new String[] {}; 2009 case -873664438: /*timing*/ return new String[] {"dateTime", "Age", "Period", "Duration", "Range", "Timing"}; 2010 case 1901043637: /*location*/ return new String[] {"CodeableReference"}; 2011 case 767422259: /*participant*/ return new String[] {}; 2012 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2013 case 586678389: /*groupingBehavior*/ return new String[] {"code"}; 2014 case 168639486: /*selectionBehavior*/ return new String[] {"code"}; 2015 case -1163906287: /*requiredBehavior*/ return new String[] {"code"}; 2016 case -1174249033: /*precheckBehavior*/ return new String[] {"code"}; 2017 case -922577408: /*cardinalityBehavior*/ return new String[] {"code"}; 2018 case -341064690: /*resource*/ return new String[] {"Reference"}; 2019 case -1014418093: /*definition*/ return new String[] {"canonical", "uri"}; 2020 case 1052666732: /*transform*/ return new String[] {"canonical"}; 2021 case 572625010: /*dynamicValue*/ return new String[] {}; 2022 case -1422950858: /*action*/ return new String[] {"@RequestOrchestration.action"}; 2023 default: return super.getTypesForProperty(hash, name); 2024 } 2025 2026 } 2027 2028 @Override 2029 public Base addChild(String name) throws FHIRException { 2030 if (name.equals("linkId")) { 2031 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.linkId"); 2032 } 2033 else if (name.equals("prefix")) { 2034 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.prefix"); 2035 } 2036 else if (name.equals("title")) { 2037 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.title"); 2038 } 2039 else if (name.equals("description")) { 2040 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.description"); 2041 } 2042 else if (name.equals("textEquivalent")) { 2043 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.textEquivalent"); 2044 } 2045 else if (name.equals("priority")) { 2046 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.priority"); 2047 } 2048 else if (name.equals("code")) { 2049 return addCode(); 2050 } 2051 else if (name.equals("documentation")) { 2052 return addDocumentation(); 2053 } 2054 else if (name.equals("goal")) { 2055 return addGoal(); 2056 } 2057 else if (name.equals("condition")) { 2058 return addCondition(); 2059 } 2060 else if (name.equals("input")) { 2061 return addInput(); 2062 } 2063 else if (name.equals("output")) { 2064 return addOutput(); 2065 } 2066 else if (name.equals("relatedAction")) { 2067 return addRelatedAction(); 2068 } 2069 else if (name.equals("timingDateTime")) { 2070 this.timing = new DateTimeType(); 2071 return this.timing; 2072 } 2073 else if (name.equals("timingAge")) { 2074 this.timing = new Age(); 2075 return this.timing; 2076 } 2077 else if (name.equals("timingPeriod")) { 2078 this.timing = new Period(); 2079 return this.timing; 2080 } 2081 else if (name.equals("timingDuration")) { 2082 this.timing = new Duration(); 2083 return this.timing; 2084 } 2085 else if (name.equals("timingRange")) { 2086 this.timing = new Range(); 2087 return this.timing; 2088 } 2089 else if (name.equals("timingTiming")) { 2090 this.timing = new Timing(); 2091 return this.timing; 2092 } 2093 else if (name.equals("location")) { 2094 this.location = new CodeableReference(); 2095 return this.location; 2096 } 2097 else if (name.equals("participant")) { 2098 return addParticipant(); 2099 } 2100 else if (name.equals("type")) { 2101 this.type = new CodeableConcept(); 2102 return this.type; 2103 } 2104 else if (name.equals("groupingBehavior")) { 2105 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.groupingBehavior"); 2106 } 2107 else if (name.equals("selectionBehavior")) { 2108 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.selectionBehavior"); 2109 } 2110 else if (name.equals("requiredBehavior")) { 2111 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.requiredBehavior"); 2112 } 2113 else if (name.equals("precheckBehavior")) { 2114 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.precheckBehavior"); 2115 } 2116 else if (name.equals("cardinalityBehavior")) { 2117 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.cardinalityBehavior"); 2118 } 2119 else if (name.equals("resource")) { 2120 this.resource = new Reference(); 2121 return this.resource; 2122 } 2123 else if (name.equals("definitionCanonical")) { 2124 this.definition = new CanonicalType(); 2125 return this.definition; 2126 } 2127 else if (name.equals("definitionUri")) { 2128 this.definition = new UriType(); 2129 return this.definition; 2130 } 2131 else if (name.equals("transform")) { 2132 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.transform"); 2133 } 2134 else if (name.equals("dynamicValue")) { 2135 return addDynamicValue(); 2136 } 2137 else if (name.equals("action")) { 2138 return addAction(); 2139 } 2140 else 2141 return super.addChild(name); 2142 } 2143 2144 public RequestOrchestrationActionComponent copy() { 2145 RequestOrchestrationActionComponent dst = new RequestOrchestrationActionComponent(); 2146 copyValues(dst); 2147 return dst; 2148 } 2149 2150 public void copyValues(RequestOrchestrationActionComponent dst) { 2151 super.copyValues(dst); 2152 dst.linkId = linkId == null ? null : linkId.copy(); 2153 dst.prefix = prefix == null ? null : prefix.copy(); 2154 dst.title = title == null ? null : title.copy(); 2155 dst.description = description == null ? null : description.copy(); 2156 dst.textEquivalent = textEquivalent == null ? null : textEquivalent.copy(); 2157 dst.priority = priority == null ? null : priority.copy(); 2158 if (code != null) { 2159 dst.code = new ArrayList<CodeableConcept>(); 2160 for (CodeableConcept i : code) 2161 dst.code.add(i.copy()); 2162 }; 2163 if (documentation != null) { 2164 dst.documentation = new ArrayList<RelatedArtifact>(); 2165 for (RelatedArtifact i : documentation) 2166 dst.documentation.add(i.copy()); 2167 }; 2168 if (goal != null) { 2169 dst.goal = new ArrayList<Reference>(); 2170 for (Reference i : goal) 2171 dst.goal.add(i.copy()); 2172 }; 2173 if (condition != null) { 2174 dst.condition = new ArrayList<RequestOrchestrationActionConditionComponent>(); 2175 for (RequestOrchestrationActionConditionComponent i : condition) 2176 dst.condition.add(i.copy()); 2177 }; 2178 if (input != null) { 2179 dst.input = new ArrayList<RequestOrchestrationActionInputComponent>(); 2180 for (RequestOrchestrationActionInputComponent i : input) 2181 dst.input.add(i.copy()); 2182 }; 2183 if (output != null) { 2184 dst.output = new ArrayList<RequestOrchestrationActionOutputComponent>(); 2185 for (RequestOrchestrationActionOutputComponent i : output) 2186 dst.output.add(i.copy()); 2187 }; 2188 if (relatedAction != null) { 2189 dst.relatedAction = new ArrayList<RequestOrchestrationActionRelatedActionComponent>(); 2190 for (RequestOrchestrationActionRelatedActionComponent i : relatedAction) 2191 dst.relatedAction.add(i.copy()); 2192 }; 2193 dst.timing = timing == null ? null : timing.copy(); 2194 dst.location = location == null ? null : location.copy(); 2195 if (participant != null) { 2196 dst.participant = new ArrayList<RequestOrchestrationActionParticipantComponent>(); 2197 for (RequestOrchestrationActionParticipantComponent i : participant) 2198 dst.participant.add(i.copy()); 2199 }; 2200 dst.type = type == null ? null : type.copy(); 2201 dst.groupingBehavior = groupingBehavior == null ? null : groupingBehavior.copy(); 2202 dst.selectionBehavior = selectionBehavior == null ? null : selectionBehavior.copy(); 2203 dst.requiredBehavior = requiredBehavior == null ? null : requiredBehavior.copy(); 2204 dst.precheckBehavior = precheckBehavior == null ? null : precheckBehavior.copy(); 2205 dst.cardinalityBehavior = cardinalityBehavior == null ? null : cardinalityBehavior.copy(); 2206 dst.resource = resource == null ? null : resource.copy(); 2207 dst.definition = definition == null ? null : definition.copy(); 2208 dst.transform = transform == null ? null : transform.copy(); 2209 if (dynamicValue != null) { 2210 dst.dynamicValue = new ArrayList<RequestOrchestrationActionDynamicValueComponent>(); 2211 for (RequestOrchestrationActionDynamicValueComponent i : dynamicValue) 2212 dst.dynamicValue.add(i.copy()); 2213 }; 2214 if (action != null) { 2215 dst.action = new ArrayList<RequestOrchestrationActionComponent>(); 2216 for (RequestOrchestrationActionComponent i : action) 2217 dst.action.add(i.copy()); 2218 }; 2219 } 2220 2221 @Override 2222 public boolean equalsDeep(Base other_) { 2223 if (!super.equalsDeep(other_)) 2224 return false; 2225 if (!(other_ instanceof RequestOrchestrationActionComponent)) 2226 return false; 2227 RequestOrchestrationActionComponent o = (RequestOrchestrationActionComponent) other_; 2228 return compareDeep(linkId, o.linkId, true) && compareDeep(prefix, o.prefix, true) && compareDeep(title, o.title, true) 2229 && compareDeep(description, o.description, true) && compareDeep(textEquivalent, o.textEquivalent, true) 2230 && compareDeep(priority, o.priority, true) && compareDeep(code, o.code, true) && compareDeep(documentation, o.documentation, true) 2231 && compareDeep(goal, o.goal, true) && compareDeep(condition, o.condition, true) && compareDeep(input, o.input, true) 2232 && compareDeep(output, o.output, true) && compareDeep(relatedAction, o.relatedAction, true) && compareDeep(timing, o.timing, true) 2233 && compareDeep(location, o.location, true) && compareDeep(participant, o.participant, true) && compareDeep(type, o.type, true) 2234 && compareDeep(groupingBehavior, o.groupingBehavior, true) && compareDeep(selectionBehavior, o.selectionBehavior, true) 2235 && compareDeep(requiredBehavior, o.requiredBehavior, true) && compareDeep(precheckBehavior, o.precheckBehavior, true) 2236 && compareDeep(cardinalityBehavior, o.cardinalityBehavior, true) && compareDeep(resource, o.resource, true) 2237 && compareDeep(definition, o.definition, true) && compareDeep(transform, o.transform, true) && compareDeep(dynamicValue, o.dynamicValue, true) 2238 && compareDeep(action, o.action, true); 2239 } 2240 2241 @Override 2242 public boolean equalsShallow(Base other_) { 2243 if (!super.equalsShallow(other_)) 2244 return false; 2245 if (!(other_ instanceof RequestOrchestrationActionComponent)) 2246 return false; 2247 RequestOrchestrationActionComponent o = (RequestOrchestrationActionComponent) other_; 2248 return compareValues(linkId, o.linkId, true) && compareValues(prefix, o.prefix, true) && compareValues(title, o.title, true) 2249 && compareValues(description, o.description, true) && compareValues(textEquivalent, o.textEquivalent, true) 2250 && compareValues(priority, o.priority, true) && compareValues(groupingBehavior, o.groupingBehavior, true) 2251 && compareValues(selectionBehavior, o.selectionBehavior, true) && compareValues(requiredBehavior, o.requiredBehavior, true) 2252 && compareValues(precheckBehavior, o.precheckBehavior, true) && compareValues(cardinalityBehavior, o.cardinalityBehavior, true) 2253 && compareValues(transform, o.transform, true); 2254 } 2255 2256 public boolean isEmpty() { 2257 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, prefix, title, description 2258 , textEquivalent, priority, code, documentation, goal, condition, input, output 2259 , relatedAction, timing, location, participant, type, groupingBehavior, selectionBehavior 2260 , requiredBehavior, precheckBehavior, cardinalityBehavior, resource, definition, transform 2261 , dynamicValue, action); 2262 } 2263 2264 public String fhirType() { 2265 return "RequestOrchestration.action"; 2266 2267 } 2268 2269 } 2270 2271 @Block() 2272 public static class RequestOrchestrationActionConditionComponent extends BackboneElement implements IBaseBackboneElement { 2273 /** 2274 * The kind of condition. 2275 */ 2276 @Child(name = "kind", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2277 @Description(shortDefinition="applicability | start | stop", formalDefinition="The kind of condition." ) 2278 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-condition-kind") 2279 protected Enumeration<ActionConditionKind> kind; 2280 2281 /** 2282 * An expression that returns true or false, indicating whether or not the condition is satisfied. 2283 */ 2284 @Child(name = "expression", type = {Expression.class}, order=2, min=0, max=1, modifier=false, summary=false) 2285 @Description(shortDefinition="Boolean-valued expression", formalDefinition="An expression that returns true or false, indicating whether or not the condition is satisfied." ) 2286 protected Expression expression; 2287 2288 private static final long serialVersionUID = -455150438L; 2289 2290 /** 2291 * Constructor 2292 */ 2293 public RequestOrchestrationActionConditionComponent() { 2294 super(); 2295 } 2296 2297 /** 2298 * Constructor 2299 */ 2300 public RequestOrchestrationActionConditionComponent(ActionConditionKind kind) { 2301 super(); 2302 this.setKind(kind); 2303 } 2304 2305 /** 2306 * @return {@link #kind} (The kind of condition.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 2307 */ 2308 public Enumeration<ActionConditionKind> getKindElement() { 2309 if (this.kind == null) 2310 if (Configuration.errorOnAutoCreate()) 2311 throw new Error("Attempt to auto-create RequestOrchestrationActionConditionComponent.kind"); 2312 else if (Configuration.doAutoCreate()) 2313 this.kind = new Enumeration<ActionConditionKind>(new ActionConditionKindEnumFactory()); // bb 2314 return this.kind; 2315 } 2316 2317 public boolean hasKindElement() { 2318 return this.kind != null && !this.kind.isEmpty(); 2319 } 2320 2321 public boolean hasKind() { 2322 return this.kind != null && !this.kind.isEmpty(); 2323 } 2324 2325 /** 2326 * @param value {@link #kind} (The kind of condition.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 2327 */ 2328 public RequestOrchestrationActionConditionComponent setKindElement(Enumeration<ActionConditionKind> value) { 2329 this.kind = value; 2330 return this; 2331 } 2332 2333 /** 2334 * @return The kind of condition. 2335 */ 2336 public ActionConditionKind getKind() { 2337 return this.kind == null ? null : this.kind.getValue(); 2338 } 2339 2340 /** 2341 * @param value The kind of condition. 2342 */ 2343 public RequestOrchestrationActionConditionComponent setKind(ActionConditionKind value) { 2344 if (this.kind == null) 2345 this.kind = new Enumeration<ActionConditionKind>(new ActionConditionKindEnumFactory()); 2346 this.kind.setValue(value); 2347 return this; 2348 } 2349 2350 /** 2351 * @return {@link #expression} (An expression that returns true or false, indicating whether or not the condition is satisfied.) 2352 */ 2353 public Expression getExpression() { 2354 if (this.expression == null) 2355 if (Configuration.errorOnAutoCreate()) 2356 throw new Error("Attempt to auto-create RequestOrchestrationActionConditionComponent.expression"); 2357 else if (Configuration.doAutoCreate()) 2358 this.expression = new Expression(); // cc 2359 return this.expression; 2360 } 2361 2362 public boolean hasExpression() { 2363 return this.expression != null && !this.expression.isEmpty(); 2364 } 2365 2366 /** 2367 * @param value {@link #expression} (An expression that returns true or false, indicating whether or not the condition is satisfied.) 2368 */ 2369 public RequestOrchestrationActionConditionComponent setExpression(Expression value) { 2370 this.expression = value; 2371 return this; 2372 } 2373 2374 protected void listChildren(List<Property> children) { 2375 super.listChildren(children); 2376 children.add(new Property("kind", "code", "The kind of condition.", 0, 1, kind)); 2377 children.add(new Property("expression", "Expression", "An expression that returns true or false, indicating whether or not the condition is satisfied.", 0, 1, expression)); 2378 } 2379 2380 @Override 2381 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2382 switch (_hash) { 2383 case 3292052: /*kind*/ return new Property("kind", "code", "The kind of condition.", 0, 1, kind); 2384 case -1795452264: /*expression*/ return new Property("expression", "Expression", "An expression that returns true or false, indicating whether or not the condition is satisfied.", 0, 1, expression); 2385 default: return super.getNamedProperty(_hash, _name, _checkValid); 2386 } 2387 2388 } 2389 2390 @Override 2391 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2392 switch (hash) { 2393 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // Enumeration<ActionConditionKind> 2394 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // Expression 2395 default: return super.getProperty(hash, name, checkValid); 2396 } 2397 2398 } 2399 2400 @Override 2401 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2402 switch (hash) { 2403 case 3292052: // kind 2404 value = new ActionConditionKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 2405 this.kind = (Enumeration) value; // Enumeration<ActionConditionKind> 2406 return value; 2407 case -1795452264: // expression 2408 this.expression = TypeConvertor.castToExpression(value); // Expression 2409 return value; 2410 default: return super.setProperty(hash, name, value); 2411 } 2412 2413 } 2414 2415 @Override 2416 public Base setProperty(String name, Base value) throws FHIRException { 2417 if (name.equals("kind")) { 2418 value = new ActionConditionKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 2419 this.kind = (Enumeration) value; // Enumeration<ActionConditionKind> 2420 } else if (name.equals("expression")) { 2421 this.expression = TypeConvertor.castToExpression(value); // Expression 2422 } else 2423 return super.setProperty(name, value); 2424 return value; 2425 } 2426 2427 @Override 2428 public void removeChild(String name, Base value) throws FHIRException { 2429 if (name.equals("kind")) { 2430 value = new ActionConditionKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 2431 this.kind = (Enumeration) value; // Enumeration<ActionConditionKind> 2432 } else if (name.equals("expression")) { 2433 this.expression = null; 2434 } else 2435 super.removeChild(name, value); 2436 2437 } 2438 2439 @Override 2440 public Base makeProperty(int hash, String name) throws FHIRException { 2441 switch (hash) { 2442 case 3292052: return getKindElement(); 2443 case -1795452264: return getExpression(); 2444 default: return super.makeProperty(hash, name); 2445 } 2446 2447 } 2448 2449 @Override 2450 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2451 switch (hash) { 2452 case 3292052: /*kind*/ return new String[] {"code"}; 2453 case -1795452264: /*expression*/ return new String[] {"Expression"}; 2454 default: return super.getTypesForProperty(hash, name); 2455 } 2456 2457 } 2458 2459 @Override 2460 public Base addChild(String name) throws FHIRException { 2461 if (name.equals("kind")) { 2462 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.condition.kind"); 2463 } 2464 else if (name.equals("expression")) { 2465 this.expression = new Expression(); 2466 return this.expression; 2467 } 2468 else 2469 return super.addChild(name); 2470 } 2471 2472 public RequestOrchestrationActionConditionComponent copy() { 2473 RequestOrchestrationActionConditionComponent dst = new RequestOrchestrationActionConditionComponent(); 2474 copyValues(dst); 2475 return dst; 2476 } 2477 2478 public void copyValues(RequestOrchestrationActionConditionComponent dst) { 2479 super.copyValues(dst); 2480 dst.kind = kind == null ? null : kind.copy(); 2481 dst.expression = expression == null ? null : expression.copy(); 2482 } 2483 2484 @Override 2485 public boolean equalsDeep(Base other_) { 2486 if (!super.equalsDeep(other_)) 2487 return false; 2488 if (!(other_ instanceof RequestOrchestrationActionConditionComponent)) 2489 return false; 2490 RequestOrchestrationActionConditionComponent o = (RequestOrchestrationActionConditionComponent) other_; 2491 return compareDeep(kind, o.kind, true) && compareDeep(expression, o.expression, true); 2492 } 2493 2494 @Override 2495 public boolean equalsShallow(Base other_) { 2496 if (!super.equalsShallow(other_)) 2497 return false; 2498 if (!(other_ instanceof RequestOrchestrationActionConditionComponent)) 2499 return false; 2500 RequestOrchestrationActionConditionComponent o = (RequestOrchestrationActionConditionComponent) other_; 2501 return compareValues(kind, o.kind, true); 2502 } 2503 2504 public boolean isEmpty() { 2505 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(kind, expression); 2506 } 2507 2508 public String fhirType() { 2509 return "RequestOrchestration.action.condition"; 2510 2511 } 2512 2513 } 2514 2515 @Block() 2516 public static class RequestOrchestrationActionInputComponent extends BackboneElement implements IBaseBackboneElement { 2517 /** 2518 * A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers. 2519 */ 2520 @Child(name = "title", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2521 @Description(shortDefinition="User-visible title", formalDefinition="A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers." ) 2522 protected StringType title; 2523 2524 /** 2525 * Defines the data that is to be provided as input to the action. 2526 */ 2527 @Child(name = "requirement", type = {DataRequirement.class}, order=2, min=0, max=1, modifier=false, summary=false) 2528 @Description(shortDefinition="What data is provided", formalDefinition="Defines the data that is to be provided as input to the action." ) 2529 protected DataRequirement requirement; 2530 2531 /** 2532 * Points to an existing input or output element that provides data to this input. 2533 */ 2534 @Child(name = "relatedData", type = {IdType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2535 @Description(shortDefinition="What data is provided", formalDefinition="Points to an existing input or output element that provides data to this input." ) 2536 protected IdType relatedData; 2537 2538 private static final long serialVersionUID = -1064046709L; 2539 2540 /** 2541 * Constructor 2542 */ 2543 public RequestOrchestrationActionInputComponent() { 2544 super(); 2545 } 2546 2547 /** 2548 * @return {@link #title} (A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2549 */ 2550 public StringType getTitleElement() { 2551 if (this.title == null) 2552 if (Configuration.errorOnAutoCreate()) 2553 throw new Error("Attempt to auto-create RequestOrchestrationActionInputComponent.title"); 2554 else if (Configuration.doAutoCreate()) 2555 this.title = new StringType(); // bb 2556 return this.title; 2557 } 2558 2559 public boolean hasTitleElement() { 2560 return this.title != null && !this.title.isEmpty(); 2561 } 2562 2563 public boolean hasTitle() { 2564 return this.title != null && !this.title.isEmpty(); 2565 } 2566 2567 /** 2568 * @param value {@link #title} (A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2569 */ 2570 public RequestOrchestrationActionInputComponent setTitleElement(StringType value) { 2571 this.title = value; 2572 return this; 2573 } 2574 2575 /** 2576 * @return A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers. 2577 */ 2578 public String getTitle() { 2579 return this.title == null ? null : this.title.getValue(); 2580 } 2581 2582 /** 2583 * @param value A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers. 2584 */ 2585 public RequestOrchestrationActionInputComponent setTitle(String value) { 2586 if (Utilities.noString(value)) 2587 this.title = null; 2588 else { 2589 if (this.title == null) 2590 this.title = new StringType(); 2591 this.title.setValue(value); 2592 } 2593 return this; 2594 } 2595 2596 /** 2597 * @return {@link #requirement} (Defines the data that is to be provided as input to the action.) 2598 */ 2599 public DataRequirement getRequirement() { 2600 if (this.requirement == null) 2601 if (Configuration.errorOnAutoCreate()) 2602 throw new Error("Attempt to auto-create RequestOrchestrationActionInputComponent.requirement"); 2603 else if (Configuration.doAutoCreate()) 2604 this.requirement = new DataRequirement(); // cc 2605 return this.requirement; 2606 } 2607 2608 public boolean hasRequirement() { 2609 return this.requirement != null && !this.requirement.isEmpty(); 2610 } 2611 2612 /** 2613 * @param value {@link #requirement} (Defines the data that is to be provided as input to the action.) 2614 */ 2615 public RequestOrchestrationActionInputComponent setRequirement(DataRequirement value) { 2616 this.requirement = value; 2617 return this; 2618 } 2619 2620 /** 2621 * @return {@link #relatedData} (Points to an existing input or output element that provides data to this input.). This is the underlying object with id, value and extensions. The accessor "getRelatedData" gives direct access to the value 2622 */ 2623 public IdType getRelatedDataElement() { 2624 if (this.relatedData == null) 2625 if (Configuration.errorOnAutoCreate()) 2626 throw new Error("Attempt to auto-create RequestOrchestrationActionInputComponent.relatedData"); 2627 else if (Configuration.doAutoCreate()) 2628 this.relatedData = new IdType(); // bb 2629 return this.relatedData; 2630 } 2631 2632 public boolean hasRelatedDataElement() { 2633 return this.relatedData != null && !this.relatedData.isEmpty(); 2634 } 2635 2636 public boolean hasRelatedData() { 2637 return this.relatedData != null && !this.relatedData.isEmpty(); 2638 } 2639 2640 /** 2641 * @param value {@link #relatedData} (Points to an existing input or output element that provides data to this input.). This is the underlying object with id, value and extensions. The accessor "getRelatedData" gives direct access to the value 2642 */ 2643 public RequestOrchestrationActionInputComponent setRelatedDataElement(IdType value) { 2644 this.relatedData = value; 2645 return this; 2646 } 2647 2648 /** 2649 * @return Points to an existing input or output element that provides data to this input. 2650 */ 2651 public String getRelatedData() { 2652 return this.relatedData == null ? null : this.relatedData.getValue(); 2653 } 2654 2655 /** 2656 * @param value Points to an existing input or output element that provides data to this input. 2657 */ 2658 public RequestOrchestrationActionInputComponent setRelatedData(String value) { 2659 if (Utilities.noString(value)) 2660 this.relatedData = null; 2661 else { 2662 if (this.relatedData == null) 2663 this.relatedData = new IdType(); 2664 this.relatedData.setValue(value); 2665 } 2666 return this; 2667 } 2668 2669 protected void listChildren(List<Property> children) { 2670 super.listChildren(children); 2671 children.add(new Property("title", "string", "A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers.", 0, 1, title)); 2672 children.add(new Property("requirement", "DataRequirement", "Defines the data that is to be provided as input to the action.", 0, 1, requirement)); 2673 children.add(new Property("relatedData", "id", "Points to an existing input or output element that provides data to this input.", 0, 1, relatedData)); 2674 } 2675 2676 @Override 2677 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2678 switch (_hash) { 2679 case 110371416: /*title*/ return new Property("title", "string", "A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers.", 0, 1, title); 2680 case 363387971: /*requirement*/ return new Property("requirement", "DataRequirement", "Defines the data that is to be provided as input to the action.", 0, 1, requirement); 2681 case 1112535669: /*relatedData*/ return new Property("relatedData", "id", "Points to an existing input or output element that provides data to this input.", 0, 1, relatedData); 2682 default: return super.getNamedProperty(_hash, _name, _checkValid); 2683 } 2684 2685 } 2686 2687 @Override 2688 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2689 switch (hash) { 2690 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2691 case 363387971: /*requirement*/ return this.requirement == null ? new Base[0] : new Base[] {this.requirement}; // DataRequirement 2692 case 1112535669: /*relatedData*/ return this.relatedData == null ? new Base[0] : new Base[] {this.relatedData}; // IdType 2693 default: return super.getProperty(hash, name, checkValid); 2694 } 2695 2696 } 2697 2698 @Override 2699 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2700 switch (hash) { 2701 case 110371416: // title 2702 this.title = TypeConvertor.castToString(value); // StringType 2703 return value; 2704 case 363387971: // requirement 2705 this.requirement = TypeConvertor.castToDataRequirement(value); // DataRequirement 2706 return value; 2707 case 1112535669: // relatedData 2708 this.relatedData = TypeConvertor.castToId(value); // IdType 2709 return value; 2710 default: return super.setProperty(hash, name, value); 2711 } 2712 2713 } 2714 2715 @Override 2716 public Base setProperty(String name, Base value) throws FHIRException { 2717 if (name.equals("title")) { 2718 this.title = TypeConvertor.castToString(value); // StringType 2719 } else if (name.equals("requirement")) { 2720 this.requirement = TypeConvertor.castToDataRequirement(value); // DataRequirement 2721 } else if (name.equals("relatedData")) { 2722 this.relatedData = TypeConvertor.castToId(value); // IdType 2723 } else 2724 return super.setProperty(name, value); 2725 return value; 2726 } 2727 2728 @Override 2729 public void removeChild(String name, Base value) throws FHIRException { 2730 if (name.equals("title")) { 2731 this.title = null; 2732 } else if (name.equals("requirement")) { 2733 this.requirement = null; 2734 } else if (name.equals("relatedData")) { 2735 this.relatedData = null; 2736 } else 2737 super.removeChild(name, value); 2738 2739 } 2740 2741 @Override 2742 public Base makeProperty(int hash, String name) throws FHIRException { 2743 switch (hash) { 2744 case 110371416: return getTitleElement(); 2745 case 363387971: return getRequirement(); 2746 case 1112535669: return getRelatedDataElement(); 2747 default: return super.makeProperty(hash, name); 2748 } 2749 2750 } 2751 2752 @Override 2753 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2754 switch (hash) { 2755 case 110371416: /*title*/ return new String[] {"string"}; 2756 case 363387971: /*requirement*/ return new String[] {"DataRequirement"}; 2757 case 1112535669: /*relatedData*/ return new String[] {"id"}; 2758 default: return super.getTypesForProperty(hash, name); 2759 } 2760 2761 } 2762 2763 @Override 2764 public Base addChild(String name) throws FHIRException { 2765 if (name.equals("title")) { 2766 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.input.title"); 2767 } 2768 else if (name.equals("requirement")) { 2769 this.requirement = new DataRequirement(); 2770 return this.requirement; 2771 } 2772 else if (name.equals("relatedData")) { 2773 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.input.relatedData"); 2774 } 2775 else 2776 return super.addChild(name); 2777 } 2778 2779 public RequestOrchestrationActionInputComponent copy() { 2780 RequestOrchestrationActionInputComponent dst = new RequestOrchestrationActionInputComponent(); 2781 copyValues(dst); 2782 return dst; 2783 } 2784 2785 public void copyValues(RequestOrchestrationActionInputComponent dst) { 2786 super.copyValues(dst); 2787 dst.title = title == null ? null : title.copy(); 2788 dst.requirement = requirement == null ? null : requirement.copy(); 2789 dst.relatedData = relatedData == null ? null : relatedData.copy(); 2790 } 2791 2792 @Override 2793 public boolean equalsDeep(Base other_) { 2794 if (!super.equalsDeep(other_)) 2795 return false; 2796 if (!(other_ instanceof RequestOrchestrationActionInputComponent)) 2797 return false; 2798 RequestOrchestrationActionInputComponent o = (RequestOrchestrationActionInputComponent) other_; 2799 return compareDeep(title, o.title, true) && compareDeep(requirement, o.requirement, true) && compareDeep(relatedData, o.relatedData, true) 2800 ; 2801 } 2802 2803 @Override 2804 public boolean equalsShallow(Base other_) { 2805 if (!super.equalsShallow(other_)) 2806 return false; 2807 if (!(other_ instanceof RequestOrchestrationActionInputComponent)) 2808 return false; 2809 RequestOrchestrationActionInputComponent o = (RequestOrchestrationActionInputComponent) other_; 2810 return compareValues(title, o.title, true) && compareValues(relatedData, o.relatedData, true); 2811 } 2812 2813 public boolean isEmpty() { 2814 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(title, requirement, relatedData 2815 ); 2816 } 2817 2818 public String fhirType() { 2819 return "RequestOrchestration.action.input"; 2820 2821 } 2822 2823 } 2824 2825 @Block() 2826 public static class RequestOrchestrationActionOutputComponent extends BackboneElement implements IBaseBackboneElement { 2827 /** 2828 * A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers. 2829 */ 2830 @Child(name = "title", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2831 @Description(shortDefinition="User-visible title", formalDefinition="A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers." ) 2832 protected StringType title; 2833 2834 /** 2835 * Defines the data that results as output from the action. 2836 */ 2837 @Child(name = "requirement", type = {DataRequirement.class}, order=2, min=0, max=1, modifier=false, summary=false) 2838 @Description(shortDefinition="What data is provided", formalDefinition="Defines the data that results as output from the action." ) 2839 protected DataRequirement requirement; 2840 2841 /** 2842 * Points to an existing input or output element that is results as output from the action. 2843 */ 2844 @Child(name = "relatedData", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2845 @Description(shortDefinition="What data is provided", formalDefinition="Points to an existing input or output element that is results as output from the action." ) 2846 protected StringType relatedData; 2847 2848 private static final long serialVersionUID = 1822414421L; 2849 2850 /** 2851 * Constructor 2852 */ 2853 public RequestOrchestrationActionOutputComponent() { 2854 super(); 2855 } 2856 2857 /** 2858 * @return {@link #title} (A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2859 */ 2860 public StringType getTitleElement() { 2861 if (this.title == null) 2862 if (Configuration.errorOnAutoCreate()) 2863 throw new Error("Attempt to auto-create RequestOrchestrationActionOutputComponent.title"); 2864 else if (Configuration.doAutoCreate()) 2865 this.title = new StringType(); // bb 2866 return this.title; 2867 } 2868 2869 public boolean hasTitleElement() { 2870 return this.title != null && !this.title.isEmpty(); 2871 } 2872 2873 public boolean hasTitle() { 2874 return this.title != null && !this.title.isEmpty(); 2875 } 2876 2877 /** 2878 * @param value {@link #title} (A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2879 */ 2880 public RequestOrchestrationActionOutputComponent setTitleElement(StringType value) { 2881 this.title = value; 2882 return this; 2883 } 2884 2885 /** 2886 * @return A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers. 2887 */ 2888 public String getTitle() { 2889 return this.title == null ? null : this.title.getValue(); 2890 } 2891 2892 /** 2893 * @param value A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers. 2894 */ 2895 public RequestOrchestrationActionOutputComponent setTitle(String value) { 2896 if (Utilities.noString(value)) 2897 this.title = null; 2898 else { 2899 if (this.title == null) 2900 this.title = new StringType(); 2901 this.title.setValue(value); 2902 } 2903 return this; 2904 } 2905 2906 /** 2907 * @return {@link #requirement} (Defines the data that results as output from the action.) 2908 */ 2909 public DataRequirement getRequirement() { 2910 if (this.requirement == null) 2911 if (Configuration.errorOnAutoCreate()) 2912 throw new Error("Attempt to auto-create RequestOrchestrationActionOutputComponent.requirement"); 2913 else if (Configuration.doAutoCreate()) 2914 this.requirement = new DataRequirement(); // cc 2915 return this.requirement; 2916 } 2917 2918 public boolean hasRequirement() { 2919 return this.requirement != null && !this.requirement.isEmpty(); 2920 } 2921 2922 /** 2923 * @param value {@link #requirement} (Defines the data that results as output from the action.) 2924 */ 2925 public RequestOrchestrationActionOutputComponent setRequirement(DataRequirement value) { 2926 this.requirement = value; 2927 return this; 2928 } 2929 2930 /** 2931 * @return {@link #relatedData} (Points to an existing input or output element that is results as output from the action.). This is the underlying object with id, value and extensions. The accessor "getRelatedData" gives direct access to the value 2932 */ 2933 public StringType getRelatedDataElement() { 2934 if (this.relatedData == null) 2935 if (Configuration.errorOnAutoCreate()) 2936 throw new Error("Attempt to auto-create RequestOrchestrationActionOutputComponent.relatedData"); 2937 else if (Configuration.doAutoCreate()) 2938 this.relatedData = new StringType(); // bb 2939 return this.relatedData; 2940 } 2941 2942 public boolean hasRelatedDataElement() { 2943 return this.relatedData != null && !this.relatedData.isEmpty(); 2944 } 2945 2946 public boolean hasRelatedData() { 2947 return this.relatedData != null && !this.relatedData.isEmpty(); 2948 } 2949 2950 /** 2951 * @param value {@link #relatedData} (Points to an existing input or output element that is results as output from the action.). This is the underlying object with id, value and extensions. The accessor "getRelatedData" gives direct access to the value 2952 */ 2953 public RequestOrchestrationActionOutputComponent setRelatedDataElement(StringType value) { 2954 this.relatedData = value; 2955 return this; 2956 } 2957 2958 /** 2959 * @return Points to an existing input or output element that is results as output from the action. 2960 */ 2961 public String getRelatedData() { 2962 return this.relatedData == null ? null : this.relatedData.getValue(); 2963 } 2964 2965 /** 2966 * @param value Points to an existing input or output element that is results as output from the action. 2967 */ 2968 public RequestOrchestrationActionOutputComponent setRelatedData(String value) { 2969 if (Utilities.noString(value)) 2970 this.relatedData = null; 2971 else { 2972 if (this.relatedData == null) 2973 this.relatedData = new StringType(); 2974 this.relatedData.setValue(value); 2975 } 2976 return this; 2977 } 2978 2979 protected void listChildren(List<Property> children) { 2980 super.listChildren(children); 2981 children.add(new Property("title", "string", "A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers.", 0, 1, title)); 2982 children.add(new Property("requirement", "DataRequirement", "Defines the data that results as output from the action.", 0, 1, requirement)); 2983 children.add(new Property("relatedData", "string", "Points to an existing input or output element that is results as output from the action.", 0, 1, relatedData)); 2984 } 2985 2986 @Override 2987 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2988 switch (_hash) { 2989 case 110371416: /*title*/ return new Property("title", "string", "A human-readable label for the data requirement used to label data flows in BPMN or similar diagrams. Also provides a human readable label when rendering the data requirement that conveys its purpose to human readers.", 0, 1, title); 2990 case 363387971: /*requirement*/ return new Property("requirement", "DataRequirement", "Defines the data that results as output from the action.", 0, 1, requirement); 2991 case 1112535669: /*relatedData*/ return new Property("relatedData", "string", "Points to an existing input or output element that is results as output from the action.", 0, 1, relatedData); 2992 default: return super.getNamedProperty(_hash, _name, _checkValid); 2993 } 2994 2995 } 2996 2997 @Override 2998 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2999 switch (hash) { 3000 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 3001 case 363387971: /*requirement*/ return this.requirement == null ? new Base[0] : new Base[] {this.requirement}; // DataRequirement 3002 case 1112535669: /*relatedData*/ return this.relatedData == null ? new Base[0] : new Base[] {this.relatedData}; // StringType 3003 default: return super.getProperty(hash, name, checkValid); 3004 } 3005 3006 } 3007 3008 @Override 3009 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3010 switch (hash) { 3011 case 110371416: // title 3012 this.title = TypeConvertor.castToString(value); // StringType 3013 return value; 3014 case 363387971: // requirement 3015 this.requirement = TypeConvertor.castToDataRequirement(value); // DataRequirement 3016 return value; 3017 case 1112535669: // relatedData 3018 this.relatedData = TypeConvertor.castToString(value); // StringType 3019 return value; 3020 default: return super.setProperty(hash, name, value); 3021 } 3022 3023 } 3024 3025 @Override 3026 public Base setProperty(String name, Base value) throws FHIRException { 3027 if (name.equals("title")) { 3028 this.title = TypeConvertor.castToString(value); // StringType 3029 } else if (name.equals("requirement")) { 3030 this.requirement = TypeConvertor.castToDataRequirement(value); // DataRequirement 3031 } else if (name.equals("relatedData")) { 3032 this.relatedData = TypeConvertor.castToString(value); // StringType 3033 } else 3034 return super.setProperty(name, value); 3035 return value; 3036 } 3037 3038 @Override 3039 public void removeChild(String name, Base value) throws FHIRException { 3040 if (name.equals("title")) { 3041 this.title = null; 3042 } else if (name.equals("requirement")) { 3043 this.requirement = null; 3044 } else if (name.equals("relatedData")) { 3045 this.relatedData = null; 3046 } else 3047 super.removeChild(name, value); 3048 3049 } 3050 3051 @Override 3052 public Base makeProperty(int hash, String name) throws FHIRException { 3053 switch (hash) { 3054 case 110371416: return getTitleElement(); 3055 case 363387971: return getRequirement(); 3056 case 1112535669: return getRelatedDataElement(); 3057 default: return super.makeProperty(hash, name); 3058 } 3059 3060 } 3061 3062 @Override 3063 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3064 switch (hash) { 3065 case 110371416: /*title*/ return new String[] {"string"}; 3066 case 363387971: /*requirement*/ return new String[] {"DataRequirement"}; 3067 case 1112535669: /*relatedData*/ return new String[] {"string"}; 3068 default: return super.getTypesForProperty(hash, name); 3069 } 3070 3071 } 3072 3073 @Override 3074 public Base addChild(String name) throws FHIRException { 3075 if (name.equals("title")) { 3076 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.output.title"); 3077 } 3078 else if (name.equals("requirement")) { 3079 this.requirement = new DataRequirement(); 3080 return this.requirement; 3081 } 3082 else if (name.equals("relatedData")) { 3083 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.output.relatedData"); 3084 } 3085 else 3086 return super.addChild(name); 3087 } 3088 3089 public RequestOrchestrationActionOutputComponent copy() { 3090 RequestOrchestrationActionOutputComponent dst = new RequestOrchestrationActionOutputComponent(); 3091 copyValues(dst); 3092 return dst; 3093 } 3094 3095 public void copyValues(RequestOrchestrationActionOutputComponent dst) { 3096 super.copyValues(dst); 3097 dst.title = title == null ? null : title.copy(); 3098 dst.requirement = requirement == null ? null : requirement.copy(); 3099 dst.relatedData = relatedData == null ? null : relatedData.copy(); 3100 } 3101 3102 @Override 3103 public boolean equalsDeep(Base other_) { 3104 if (!super.equalsDeep(other_)) 3105 return false; 3106 if (!(other_ instanceof RequestOrchestrationActionOutputComponent)) 3107 return false; 3108 RequestOrchestrationActionOutputComponent o = (RequestOrchestrationActionOutputComponent) other_; 3109 return compareDeep(title, o.title, true) && compareDeep(requirement, o.requirement, true) && compareDeep(relatedData, o.relatedData, true) 3110 ; 3111 } 3112 3113 @Override 3114 public boolean equalsShallow(Base other_) { 3115 if (!super.equalsShallow(other_)) 3116 return false; 3117 if (!(other_ instanceof RequestOrchestrationActionOutputComponent)) 3118 return false; 3119 RequestOrchestrationActionOutputComponent o = (RequestOrchestrationActionOutputComponent) other_; 3120 return compareValues(title, o.title, true) && compareValues(relatedData, o.relatedData, true); 3121 } 3122 3123 public boolean isEmpty() { 3124 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(title, requirement, relatedData 3125 ); 3126 } 3127 3128 public String fhirType() { 3129 return "RequestOrchestration.action.output"; 3130 3131 } 3132 3133 } 3134 3135 @Block() 3136 public static class RequestOrchestrationActionRelatedActionComponent extends BackboneElement implements IBaseBackboneElement { 3137 /** 3138 * The element id of the target related action. 3139 */ 3140 @Child(name = "targetId", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3141 @Description(shortDefinition="What action this is related to", formalDefinition="The element id of the target related action." ) 3142 protected IdType targetId; 3143 3144 /** 3145 * The relationship of this action to the related action. 3146 */ 3147 @Child(name = "relationship", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=false) 3148 @Description(shortDefinition="before | before-start | before-end | concurrent | concurrent-with-start | concurrent-with-end | after | after-start | after-end", formalDefinition="The relationship of this action to the related action." ) 3149 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-relationship-type") 3150 protected Enumeration<ActionRelationshipType> relationship; 3151 3152 /** 3153 * The relationship of the end of this action to the related action. 3154 */ 3155 @Child(name = "endRelationship", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 3156 @Description(shortDefinition="before | before-start | before-end | concurrent | concurrent-with-start | concurrent-with-end | after | after-start | after-end", formalDefinition="The relationship of the end of this action to the related action." ) 3157 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-relationship-type") 3158 protected Enumeration<ActionRelationshipType> endRelationship; 3159 3160 /** 3161 * A duration or range of durations to apply to the relationship. For example, 30-60 minutes before. 3162 */ 3163 @Child(name = "offset", type = {Duration.class, Range.class}, order=4, min=0, max=1, modifier=false, summary=false) 3164 @Description(shortDefinition="Time offset for the relationship", formalDefinition="A duration or range of durations to apply to the relationship. For example, 30-60 minutes before." ) 3165 protected DataType offset; 3166 3167 private static final long serialVersionUID = 1997058061L; 3168 3169 /** 3170 * Constructor 3171 */ 3172 public RequestOrchestrationActionRelatedActionComponent() { 3173 super(); 3174 } 3175 3176 /** 3177 * Constructor 3178 */ 3179 public RequestOrchestrationActionRelatedActionComponent(String targetId, ActionRelationshipType relationship) { 3180 super(); 3181 this.setTargetId(targetId); 3182 this.setRelationship(relationship); 3183 } 3184 3185 /** 3186 * @return {@link #targetId} (The element id of the target related action.). This is the underlying object with id, value and extensions. The accessor "getTargetId" gives direct access to the value 3187 */ 3188 public IdType getTargetIdElement() { 3189 if (this.targetId == null) 3190 if (Configuration.errorOnAutoCreate()) 3191 throw new Error("Attempt to auto-create RequestOrchestrationActionRelatedActionComponent.targetId"); 3192 else if (Configuration.doAutoCreate()) 3193 this.targetId = new IdType(); // bb 3194 return this.targetId; 3195 } 3196 3197 public boolean hasTargetIdElement() { 3198 return this.targetId != null && !this.targetId.isEmpty(); 3199 } 3200 3201 public boolean hasTargetId() { 3202 return this.targetId != null && !this.targetId.isEmpty(); 3203 } 3204 3205 /** 3206 * @param value {@link #targetId} (The element id of the target related action.). This is the underlying object with id, value and extensions. The accessor "getTargetId" gives direct access to the value 3207 */ 3208 public RequestOrchestrationActionRelatedActionComponent setTargetIdElement(IdType value) { 3209 this.targetId = value; 3210 return this; 3211 } 3212 3213 /** 3214 * @return The element id of the target related action. 3215 */ 3216 public String getTargetId() { 3217 return this.targetId == null ? null : this.targetId.getValue(); 3218 } 3219 3220 /** 3221 * @param value The element id of the target related action. 3222 */ 3223 public RequestOrchestrationActionRelatedActionComponent setTargetId(String value) { 3224 if (this.targetId == null) 3225 this.targetId = new IdType(); 3226 this.targetId.setValue(value); 3227 return this; 3228 } 3229 3230 /** 3231 * @return {@link #relationship} (The relationship of this action to the related action.). This is the underlying object with id, value and extensions. The accessor "getRelationship" gives direct access to the value 3232 */ 3233 public Enumeration<ActionRelationshipType> getRelationshipElement() { 3234 if (this.relationship == null) 3235 if (Configuration.errorOnAutoCreate()) 3236 throw new Error("Attempt to auto-create RequestOrchestrationActionRelatedActionComponent.relationship"); 3237 else if (Configuration.doAutoCreate()) 3238 this.relationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); // bb 3239 return this.relationship; 3240 } 3241 3242 public boolean hasRelationshipElement() { 3243 return this.relationship != null && !this.relationship.isEmpty(); 3244 } 3245 3246 public boolean hasRelationship() { 3247 return this.relationship != null && !this.relationship.isEmpty(); 3248 } 3249 3250 /** 3251 * @param value {@link #relationship} (The relationship of this action to the related action.). This is the underlying object with id, value and extensions. The accessor "getRelationship" gives direct access to the value 3252 */ 3253 public RequestOrchestrationActionRelatedActionComponent setRelationshipElement(Enumeration<ActionRelationshipType> value) { 3254 this.relationship = value; 3255 return this; 3256 } 3257 3258 /** 3259 * @return The relationship of this action to the related action. 3260 */ 3261 public ActionRelationshipType getRelationship() { 3262 return this.relationship == null ? null : this.relationship.getValue(); 3263 } 3264 3265 /** 3266 * @param value The relationship of this action to the related action. 3267 */ 3268 public RequestOrchestrationActionRelatedActionComponent setRelationship(ActionRelationshipType value) { 3269 if (this.relationship == null) 3270 this.relationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); 3271 this.relationship.setValue(value); 3272 return this; 3273 } 3274 3275 /** 3276 * @return {@link #endRelationship} (The relationship of the end of this action to the related action.). This is the underlying object with id, value and extensions. The accessor "getEndRelationship" gives direct access to the value 3277 */ 3278 public Enumeration<ActionRelationshipType> getEndRelationshipElement() { 3279 if (this.endRelationship == null) 3280 if (Configuration.errorOnAutoCreate()) 3281 throw new Error("Attempt to auto-create RequestOrchestrationActionRelatedActionComponent.endRelationship"); 3282 else if (Configuration.doAutoCreate()) 3283 this.endRelationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); // bb 3284 return this.endRelationship; 3285 } 3286 3287 public boolean hasEndRelationshipElement() { 3288 return this.endRelationship != null && !this.endRelationship.isEmpty(); 3289 } 3290 3291 public boolean hasEndRelationship() { 3292 return this.endRelationship != null && !this.endRelationship.isEmpty(); 3293 } 3294 3295 /** 3296 * @param value {@link #endRelationship} (The relationship of the end of this action to the related action.). This is the underlying object with id, value and extensions. The accessor "getEndRelationship" gives direct access to the value 3297 */ 3298 public RequestOrchestrationActionRelatedActionComponent setEndRelationshipElement(Enumeration<ActionRelationshipType> value) { 3299 this.endRelationship = value; 3300 return this; 3301 } 3302 3303 /** 3304 * @return The relationship of the end of this action to the related action. 3305 */ 3306 public ActionRelationshipType getEndRelationship() { 3307 return this.endRelationship == null ? null : this.endRelationship.getValue(); 3308 } 3309 3310 /** 3311 * @param value The relationship of the end of this action to the related action. 3312 */ 3313 public RequestOrchestrationActionRelatedActionComponent setEndRelationship(ActionRelationshipType value) { 3314 if (value == null) 3315 this.endRelationship = null; 3316 else { 3317 if (this.endRelationship == null) 3318 this.endRelationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); 3319 this.endRelationship.setValue(value); 3320 } 3321 return this; 3322 } 3323 3324 /** 3325 * @return {@link #offset} (A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.) 3326 */ 3327 public DataType getOffset() { 3328 return this.offset; 3329 } 3330 3331 /** 3332 * @return {@link #offset} (A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.) 3333 */ 3334 public Duration getOffsetDuration() throws FHIRException { 3335 if (this.offset == null) 3336 this.offset = new Duration(); 3337 if (!(this.offset instanceof Duration)) 3338 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.offset.getClass().getName()+" was encountered"); 3339 return (Duration) this.offset; 3340 } 3341 3342 public boolean hasOffsetDuration() { 3343 return this != null && this.offset instanceof Duration; 3344 } 3345 3346 /** 3347 * @return {@link #offset} (A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.) 3348 */ 3349 public Range getOffsetRange() throws FHIRException { 3350 if (this.offset == null) 3351 this.offset = new Range(); 3352 if (!(this.offset instanceof Range)) 3353 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.offset.getClass().getName()+" was encountered"); 3354 return (Range) this.offset; 3355 } 3356 3357 public boolean hasOffsetRange() { 3358 return this != null && this.offset instanceof Range; 3359 } 3360 3361 public boolean hasOffset() { 3362 return this.offset != null && !this.offset.isEmpty(); 3363 } 3364 3365 /** 3366 * @param value {@link #offset} (A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.) 3367 */ 3368 public RequestOrchestrationActionRelatedActionComponent setOffset(DataType value) { 3369 if (value != null && !(value instanceof Duration || value instanceof Range)) 3370 throw new FHIRException("Not the right type for RequestOrchestration.action.relatedAction.offset[x]: "+value.fhirType()); 3371 this.offset = value; 3372 return this; 3373 } 3374 3375 protected void listChildren(List<Property> children) { 3376 super.listChildren(children); 3377 children.add(new Property("targetId", "id", "The element id of the target related action.", 0, 1, targetId)); 3378 children.add(new Property("relationship", "code", "The relationship of this action to the related action.", 0, 1, relationship)); 3379 children.add(new Property("endRelationship", "code", "The relationship of the end of this action to the related action.", 0, 1, endRelationship)); 3380 children.add(new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset)); 3381 } 3382 3383 @Override 3384 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3385 switch (_hash) { 3386 case -441951604: /*targetId*/ return new Property("targetId", "id", "The element id of the target related action.", 0, 1, targetId); 3387 case -261851592: /*relationship*/ return new Property("relationship", "code", "The relationship of this action to the related action.", 0, 1, relationship); 3388 case -1506024781: /*endRelationship*/ return new Property("endRelationship", "code", "The relationship of the end of this action to the related action.", 0, 1, endRelationship); 3389 case -1960684787: /*offset[x]*/ return new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset); 3390 case -1019779949: /*offset*/ return new Property("offset[x]", "Duration|Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset); 3391 case 134075207: /*offsetDuration*/ return new Property("offset[x]", "Duration", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset); 3392 case 1263585386: /*offsetRange*/ return new Property("offset[x]", "Range", "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, offset); 3393 default: return super.getNamedProperty(_hash, _name, _checkValid); 3394 } 3395 3396 } 3397 3398 @Override 3399 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3400 switch (hash) { 3401 case -441951604: /*targetId*/ return this.targetId == null ? new Base[0] : new Base[] {this.targetId}; // IdType 3402 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // Enumeration<ActionRelationshipType> 3403 case -1506024781: /*endRelationship*/ return this.endRelationship == null ? new Base[0] : new Base[] {this.endRelationship}; // Enumeration<ActionRelationshipType> 3404 case -1019779949: /*offset*/ return this.offset == null ? new Base[0] : new Base[] {this.offset}; // DataType 3405 default: return super.getProperty(hash, name, checkValid); 3406 } 3407 3408 } 3409 3410 @Override 3411 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3412 switch (hash) { 3413 case -441951604: // targetId 3414 this.targetId = TypeConvertor.castToId(value); // IdType 3415 return value; 3416 case -261851592: // relationship 3417 value = new ActionRelationshipTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3418 this.relationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 3419 return value; 3420 case -1506024781: // endRelationship 3421 value = new ActionRelationshipTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3422 this.endRelationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 3423 return value; 3424 case -1019779949: // offset 3425 this.offset = TypeConvertor.castToType(value); // DataType 3426 return value; 3427 default: return super.setProperty(hash, name, value); 3428 } 3429 3430 } 3431 3432 @Override 3433 public Base setProperty(String name, Base value) throws FHIRException { 3434 if (name.equals("targetId")) { 3435 this.targetId = TypeConvertor.castToId(value); // IdType 3436 } else if (name.equals("relationship")) { 3437 value = new ActionRelationshipTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3438 this.relationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 3439 } else if (name.equals("endRelationship")) { 3440 value = new ActionRelationshipTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3441 this.endRelationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 3442 } else if (name.equals("offset[x]")) { 3443 this.offset = TypeConvertor.castToType(value); // DataType 3444 } else 3445 return super.setProperty(name, value); 3446 return value; 3447 } 3448 3449 @Override 3450 public void removeChild(String name, Base value) throws FHIRException { 3451 if (name.equals("targetId")) { 3452 this.targetId = null; 3453 } else if (name.equals("relationship")) { 3454 value = new ActionRelationshipTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3455 this.relationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 3456 } else if (name.equals("endRelationship")) { 3457 value = new ActionRelationshipTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3458 this.endRelationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 3459 } else if (name.equals("offset[x]")) { 3460 this.offset = null; 3461 } else 3462 super.removeChild(name, value); 3463 3464 } 3465 3466 @Override 3467 public Base makeProperty(int hash, String name) throws FHIRException { 3468 switch (hash) { 3469 case -441951604: return getTargetIdElement(); 3470 case -261851592: return getRelationshipElement(); 3471 case -1506024781: return getEndRelationshipElement(); 3472 case -1960684787: return getOffset(); 3473 case -1019779949: return getOffset(); 3474 default: return super.makeProperty(hash, name); 3475 } 3476 3477 } 3478 3479 @Override 3480 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3481 switch (hash) { 3482 case -441951604: /*targetId*/ return new String[] {"id"}; 3483 case -261851592: /*relationship*/ return new String[] {"code"}; 3484 case -1506024781: /*endRelationship*/ return new String[] {"code"}; 3485 case -1019779949: /*offset*/ return new String[] {"Duration", "Range"}; 3486 default: return super.getTypesForProperty(hash, name); 3487 } 3488 3489 } 3490 3491 @Override 3492 public Base addChild(String name) throws FHIRException { 3493 if (name.equals("targetId")) { 3494 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.relatedAction.targetId"); 3495 } 3496 else if (name.equals("relationship")) { 3497 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.relatedAction.relationship"); 3498 } 3499 else if (name.equals("endRelationship")) { 3500 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.relatedAction.endRelationship"); 3501 } 3502 else if (name.equals("offsetDuration")) { 3503 this.offset = new Duration(); 3504 return this.offset; 3505 } 3506 else if (name.equals("offsetRange")) { 3507 this.offset = new Range(); 3508 return this.offset; 3509 } 3510 else 3511 return super.addChild(name); 3512 } 3513 3514 public RequestOrchestrationActionRelatedActionComponent copy() { 3515 RequestOrchestrationActionRelatedActionComponent dst = new RequestOrchestrationActionRelatedActionComponent(); 3516 copyValues(dst); 3517 return dst; 3518 } 3519 3520 public void copyValues(RequestOrchestrationActionRelatedActionComponent dst) { 3521 super.copyValues(dst); 3522 dst.targetId = targetId == null ? null : targetId.copy(); 3523 dst.relationship = relationship == null ? null : relationship.copy(); 3524 dst.endRelationship = endRelationship == null ? null : endRelationship.copy(); 3525 dst.offset = offset == null ? null : offset.copy(); 3526 } 3527 3528 @Override 3529 public boolean equalsDeep(Base other_) { 3530 if (!super.equalsDeep(other_)) 3531 return false; 3532 if (!(other_ instanceof RequestOrchestrationActionRelatedActionComponent)) 3533 return false; 3534 RequestOrchestrationActionRelatedActionComponent o = (RequestOrchestrationActionRelatedActionComponent) other_; 3535 return compareDeep(targetId, o.targetId, true) && compareDeep(relationship, o.relationship, true) 3536 && compareDeep(endRelationship, o.endRelationship, true) && compareDeep(offset, o.offset, true) 3537 ; 3538 } 3539 3540 @Override 3541 public boolean equalsShallow(Base other_) { 3542 if (!super.equalsShallow(other_)) 3543 return false; 3544 if (!(other_ instanceof RequestOrchestrationActionRelatedActionComponent)) 3545 return false; 3546 RequestOrchestrationActionRelatedActionComponent o = (RequestOrchestrationActionRelatedActionComponent) other_; 3547 return compareValues(targetId, o.targetId, true) && compareValues(relationship, o.relationship, true) 3548 && compareValues(endRelationship, o.endRelationship, true); 3549 } 3550 3551 public boolean isEmpty() { 3552 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(targetId, relationship, endRelationship 3553 , offset); 3554 } 3555 3556 public String fhirType() { 3557 return "RequestOrchestration.action.relatedAction"; 3558 3559 } 3560 3561 } 3562 3563 @Block() 3564 public static class RequestOrchestrationActionParticipantComponent extends BackboneElement implements IBaseBackboneElement { 3565 /** 3566 * The type of participant in the action. 3567 */ 3568 @Child(name = "type", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 3569 @Description(shortDefinition="careteam | device | group | healthcareservice | location | organization | patient | practitioner | practitionerrole | relatedperson", formalDefinition="The type of participant in the action." ) 3570 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-participant-type") 3571 protected Enumeration<ActionParticipantType> type; 3572 3573 /** 3574 * The type of participant in the action. 3575 */ 3576 @Child(name = "typeCanonical", type = {CanonicalType.class}, order=2, min=0, max=1, modifier=false, summary=false) 3577 @Description(shortDefinition="Who or what can participate", formalDefinition="The type of participant in the action." ) 3578 protected CanonicalType typeCanonical; 3579 3580 /** 3581 * The type of participant in the action. 3582 */ 3583 @Child(name = "typeReference", type = {CareTeam.class, Device.class, DeviceDefinition.class, Endpoint.class, Group.class, HealthcareService.class, Location.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class}, order=3, min=0, max=1, modifier=false, summary=false) 3584 @Description(shortDefinition="Who or what can participate", formalDefinition="The type of participant in the action." ) 3585 protected Reference typeReference; 3586 3587 /** 3588 * The role the participant should play in performing the described action. 3589 */ 3590 @Child(name = "role", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 3591 @Description(shortDefinition="E.g. Nurse, Surgeon, Parent, etc", formalDefinition="The role the participant should play in performing the described action." ) 3592 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/action-participant-role") 3593 protected CodeableConcept role; 3594 3595 /** 3596 * Indicates how the actor will be involved in the action - author, reviewer, witness, etc. 3597 */ 3598 @Child(name = "function", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 3599 @Description(shortDefinition="E.g. Author, Reviewer, Witness, etc", formalDefinition="Indicates how the actor will be involved in the action - author, reviewer, witness, etc." ) 3600 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-participant-function") 3601 protected CodeableConcept function; 3602 3603 /** 3604 * A reference to the actual participant. 3605 */ 3606 @Child(name = "actor", type = {CanonicalType.class, CareTeam.class, Device.class, DeviceDefinition.class, Endpoint.class, Group.class, HealthcareService.class, Location.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class}, order=6, min=0, max=1, modifier=false, summary=false) 3607 @Description(shortDefinition="Who/what is participating?", formalDefinition="A reference to the actual participant." ) 3608 protected DataType actor; 3609 3610 private static final long serialVersionUID = -147206285L; 3611 3612 /** 3613 * Constructor 3614 */ 3615 public RequestOrchestrationActionParticipantComponent() { 3616 super(); 3617 } 3618 3619 /** 3620 * @return {@link #type} (The type of participant in the action.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3621 */ 3622 public Enumeration<ActionParticipantType> getTypeElement() { 3623 if (this.type == null) 3624 if (Configuration.errorOnAutoCreate()) 3625 throw new Error("Attempt to auto-create RequestOrchestrationActionParticipantComponent.type"); 3626 else if (Configuration.doAutoCreate()) 3627 this.type = new Enumeration<ActionParticipantType>(new ActionParticipantTypeEnumFactory()); // bb 3628 return this.type; 3629 } 3630 3631 public boolean hasTypeElement() { 3632 return this.type != null && !this.type.isEmpty(); 3633 } 3634 3635 public boolean hasType() { 3636 return this.type != null && !this.type.isEmpty(); 3637 } 3638 3639 /** 3640 * @param value {@link #type} (The type of participant in the action.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3641 */ 3642 public RequestOrchestrationActionParticipantComponent setTypeElement(Enumeration<ActionParticipantType> value) { 3643 this.type = value; 3644 return this; 3645 } 3646 3647 /** 3648 * @return The type of participant in the action. 3649 */ 3650 public ActionParticipantType getType() { 3651 return this.type == null ? null : this.type.getValue(); 3652 } 3653 3654 /** 3655 * @param value The type of participant in the action. 3656 */ 3657 public RequestOrchestrationActionParticipantComponent setType(ActionParticipantType value) { 3658 if (value == null) 3659 this.type = null; 3660 else { 3661 if (this.type == null) 3662 this.type = new Enumeration<ActionParticipantType>(new ActionParticipantTypeEnumFactory()); 3663 this.type.setValue(value); 3664 } 3665 return this; 3666 } 3667 3668 /** 3669 * @return {@link #typeCanonical} (The type of participant in the action.). This is the underlying object with id, value and extensions. The accessor "getTypeCanonical" gives direct access to the value 3670 */ 3671 public CanonicalType getTypeCanonicalElement() { 3672 if (this.typeCanonical == null) 3673 if (Configuration.errorOnAutoCreate()) 3674 throw new Error("Attempt to auto-create RequestOrchestrationActionParticipantComponent.typeCanonical"); 3675 else if (Configuration.doAutoCreate()) 3676 this.typeCanonical = new CanonicalType(); // bb 3677 return this.typeCanonical; 3678 } 3679 3680 public boolean hasTypeCanonicalElement() { 3681 return this.typeCanonical != null && !this.typeCanonical.isEmpty(); 3682 } 3683 3684 public boolean hasTypeCanonical() { 3685 return this.typeCanonical != null && !this.typeCanonical.isEmpty(); 3686 } 3687 3688 /** 3689 * @param value {@link #typeCanonical} (The type of participant in the action.). This is the underlying object with id, value and extensions. The accessor "getTypeCanonical" gives direct access to the value 3690 */ 3691 public RequestOrchestrationActionParticipantComponent setTypeCanonicalElement(CanonicalType value) { 3692 this.typeCanonical = value; 3693 return this; 3694 } 3695 3696 /** 3697 * @return The type of participant in the action. 3698 */ 3699 public String getTypeCanonical() { 3700 return this.typeCanonical == null ? null : this.typeCanonical.getValue(); 3701 } 3702 3703 /** 3704 * @param value The type of participant in the action. 3705 */ 3706 public RequestOrchestrationActionParticipantComponent setTypeCanonical(String value) { 3707 if (Utilities.noString(value)) 3708 this.typeCanonical = null; 3709 else { 3710 if (this.typeCanonical == null) 3711 this.typeCanonical = new CanonicalType(); 3712 this.typeCanonical.setValue(value); 3713 } 3714 return this; 3715 } 3716 3717 /** 3718 * @return {@link #typeReference} (The type of participant in the action.) 3719 */ 3720 public Reference getTypeReference() { 3721 if (this.typeReference == null) 3722 if (Configuration.errorOnAutoCreate()) 3723 throw new Error("Attempt to auto-create RequestOrchestrationActionParticipantComponent.typeReference"); 3724 else if (Configuration.doAutoCreate()) 3725 this.typeReference = new Reference(); // cc 3726 return this.typeReference; 3727 } 3728 3729 public boolean hasTypeReference() { 3730 return this.typeReference != null && !this.typeReference.isEmpty(); 3731 } 3732 3733 /** 3734 * @param value {@link #typeReference} (The type of participant in the action.) 3735 */ 3736 public RequestOrchestrationActionParticipantComponent setTypeReference(Reference value) { 3737 this.typeReference = value; 3738 return this; 3739 } 3740 3741 /** 3742 * @return {@link #role} (The role the participant should play in performing the described action.) 3743 */ 3744 public CodeableConcept getRole() { 3745 if (this.role == null) 3746 if (Configuration.errorOnAutoCreate()) 3747 throw new Error("Attempt to auto-create RequestOrchestrationActionParticipantComponent.role"); 3748 else if (Configuration.doAutoCreate()) 3749 this.role = new CodeableConcept(); // cc 3750 return this.role; 3751 } 3752 3753 public boolean hasRole() { 3754 return this.role != null && !this.role.isEmpty(); 3755 } 3756 3757 /** 3758 * @param value {@link #role} (The role the participant should play in performing the described action.) 3759 */ 3760 public RequestOrchestrationActionParticipantComponent setRole(CodeableConcept value) { 3761 this.role = value; 3762 return this; 3763 } 3764 3765 /** 3766 * @return {@link #function} (Indicates how the actor will be involved in the action - author, reviewer, witness, etc.) 3767 */ 3768 public CodeableConcept getFunction() { 3769 if (this.function == null) 3770 if (Configuration.errorOnAutoCreate()) 3771 throw new Error("Attempt to auto-create RequestOrchestrationActionParticipantComponent.function"); 3772 else if (Configuration.doAutoCreate()) 3773 this.function = new CodeableConcept(); // cc 3774 return this.function; 3775 } 3776 3777 public boolean hasFunction() { 3778 return this.function != null && !this.function.isEmpty(); 3779 } 3780 3781 /** 3782 * @param value {@link #function} (Indicates how the actor will be involved in the action - author, reviewer, witness, etc.) 3783 */ 3784 public RequestOrchestrationActionParticipantComponent setFunction(CodeableConcept value) { 3785 this.function = value; 3786 return this; 3787 } 3788 3789 /** 3790 * @return {@link #actor} (A reference to the actual participant.) 3791 */ 3792 public DataType getActor() { 3793 return this.actor; 3794 } 3795 3796 /** 3797 * @return {@link #actor} (A reference to the actual participant.) 3798 */ 3799 public CanonicalType getActorCanonicalType() throws FHIRException { 3800 if (this.actor == null) 3801 this.actor = new CanonicalType(); 3802 if (!(this.actor instanceof CanonicalType)) 3803 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.actor.getClass().getName()+" was encountered"); 3804 return (CanonicalType) this.actor; 3805 } 3806 3807 public boolean hasActorCanonicalType() { 3808 return this != null && this.actor instanceof CanonicalType; 3809 } 3810 3811 /** 3812 * @return {@link #actor} (A reference to the actual participant.) 3813 */ 3814 public Reference getActorReference() throws FHIRException { 3815 if (this.actor == null) 3816 this.actor = new Reference(); 3817 if (!(this.actor instanceof Reference)) 3818 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.actor.getClass().getName()+" was encountered"); 3819 return (Reference) this.actor; 3820 } 3821 3822 public boolean hasActorReference() { 3823 return this != null && this.actor instanceof Reference; 3824 } 3825 3826 public boolean hasActor() { 3827 return this.actor != null && !this.actor.isEmpty(); 3828 } 3829 3830 /** 3831 * @param value {@link #actor} (A reference to the actual participant.) 3832 */ 3833 public RequestOrchestrationActionParticipantComponent setActor(DataType value) { 3834 if (value != null && !(value instanceof CanonicalType || value instanceof Reference)) 3835 throw new FHIRException("Not the right type for RequestOrchestration.action.participant.actor[x]: "+value.fhirType()); 3836 this.actor = value; 3837 return this; 3838 } 3839 3840 protected void listChildren(List<Property> children) { 3841 super.listChildren(children); 3842 children.add(new Property("type", "code", "The type of participant in the action.", 0, 1, type)); 3843 children.add(new Property("typeCanonical", "canonical(CapabilityStatement)", "The type of participant in the action.", 0, 1, typeCanonical)); 3844 children.add(new Property("typeReference", "Reference(CareTeam|Device|DeviceDefinition|Endpoint|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "The type of participant in the action.", 0, 1, typeReference)); 3845 children.add(new Property("role", "CodeableConcept", "The role the participant should play in performing the described action.", 0, 1, role)); 3846 children.add(new Property("function", "CodeableConcept", "Indicates how the actor will be involved in the action - author, reviewer, witness, etc.", 0, 1, function)); 3847 children.add(new Property("actor[x]", "canonical(CapabilityStatement)|Reference(CareTeam|Device|DeviceDefinition|Endpoint|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "A reference to the actual participant.", 0, 1, actor)); 3848 } 3849 3850 @Override 3851 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3852 switch (_hash) { 3853 case 3575610: /*type*/ return new Property("type", "code", "The type of participant in the action.", 0, 1, type); 3854 case -466635046: /*typeCanonical*/ return new Property("typeCanonical", "canonical(CapabilityStatement)", "The type of participant in the action.", 0, 1, typeCanonical); 3855 case 2074825009: /*typeReference*/ return new Property("typeReference", "Reference(CareTeam|Device|DeviceDefinition|Endpoint|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "The type of participant in the action.", 0, 1, typeReference); 3856 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The role the participant should play in performing the described action.", 0, 1, role); 3857 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "Indicates how the actor will be involved in the action - author, reviewer, witness, etc.", 0, 1, function); 3858 case -1650558357: /*actor[x]*/ return new Property("actor[x]", "canonical(CapabilityStatement)|Reference(CareTeam|Device|DeviceDefinition|Endpoint|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "A reference to the actual participant.", 0, 1, actor); 3859 case 92645877: /*actor*/ return new Property("actor[x]", "canonical(CapabilityStatement)|Reference(CareTeam|Device|DeviceDefinition|Endpoint|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "A reference to the actual participant.", 0, 1, actor); 3860 case 1323531903: /*actorCanonical*/ return new Property("actor[x]", "canonical(CapabilityStatement)", "A reference to the actual participant.", 0, 1, actor); 3861 case -429975338: /*actorReference*/ return new Property("actor[x]", "Reference(CareTeam|Device|DeviceDefinition|Endpoint|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "A reference to the actual participant.", 0, 1, actor); 3862 default: return super.getNamedProperty(_hash, _name, _checkValid); 3863 } 3864 3865 } 3866 3867 @Override 3868 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3869 switch (hash) { 3870 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ActionParticipantType> 3871 case -466635046: /*typeCanonical*/ return this.typeCanonical == null ? new Base[0] : new Base[] {this.typeCanonical}; // CanonicalType 3872 case 2074825009: /*typeReference*/ return this.typeReference == null ? new Base[0] : new Base[] {this.typeReference}; // Reference 3873 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 3874 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 3875 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // DataType 3876 default: return super.getProperty(hash, name, checkValid); 3877 } 3878 3879 } 3880 3881 @Override 3882 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3883 switch (hash) { 3884 case 3575610: // type 3885 value = new ActionParticipantTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3886 this.type = (Enumeration) value; // Enumeration<ActionParticipantType> 3887 return value; 3888 case -466635046: // typeCanonical 3889 this.typeCanonical = TypeConvertor.castToCanonical(value); // CanonicalType 3890 return value; 3891 case 2074825009: // typeReference 3892 this.typeReference = TypeConvertor.castToReference(value); // Reference 3893 return value; 3894 case 3506294: // role 3895 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3896 return value; 3897 case 1380938712: // function 3898 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3899 return value; 3900 case 92645877: // actor 3901 this.actor = TypeConvertor.castToType(value); // DataType 3902 return value; 3903 default: return super.setProperty(hash, name, value); 3904 } 3905 3906 } 3907 3908 @Override 3909 public Base setProperty(String name, Base value) throws FHIRException { 3910 if (name.equals("type")) { 3911 value = new ActionParticipantTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3912 this.type = (Enumeration) value; // Enumeration<ActionParticipantType> 3913 } else if (name.equals("typeCanonical")) { 3914 this.typeCanonical = TypeConvertor.castToCanonical(value); // CanonicalType 3915 } else if (name.equals("typeReference")) { 3916 this.typeReference = TypeConvertor.castToReference(value); // Reference 3917 } else if (name.equals("role")) { 3918 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3919 } else if (name.equals("function")) { 3920 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3921 } else if (name.equals("actor[x]")) { 3922 this.actor = TypeConvertor.castToType(value); // DataType 3923 } else 3924 return super.setProperty(name, value); 3925 return value; 3926 } 3927 3928 @Override 3929 public void removeChild(String name, Base value) throws FHIRException { 3930 if (name.equals("type")) { 3931 value = new ActionParticipantTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3932 this.type = (Enumeration) value; // Enumeration<ActionParticipantType> 3933 } else if (name.equals("typeCanonical")) { 3934 this.typeCanonical = null; 3935 } else if (name.equals("typeReference")) { 3936 this.typeReference = null; 3937 } else if (name.equals("role")) { 3938 this.role = null; 3939 } else if (name.equals("function")) { 3940 this.function = null; 3941 } else if (name.equals("actor[x]")) { 3942 this.actor = null; 3943 } else 3944 super.removeChild(name, value); 3945 3946 } 3947 3948 @Override 3949 public Base makeProperty(int hash, String name) throws FHIRException { 3950 switch (hash) { 3951 case 3575610: return getTypeElement(); 3952 case -466635046: return getTypeCanonicalElement(); 3953 case 2074825009: return getTypeReference(); 3954 case 3506294: return getRole(); 3955 case 1380938712: return getFunction(); 3956 case -1650558357: return getActor(); 3957 case 92645877: return getActor(); 3958 default: return super.makeProperty(hash, name); 3959 } 3960 3961 } 3962 3963 @Override 3964 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3965 switch (hash) { 3966 case 3575610: /*type*/ return new String[] {"code"}; 3967 case -466635046: /*typeCanonical*/ return new String[] {"canonical"}; 3968 case 2074825009: /*typeReference*/ return new String[] {"Reference"}; 3969 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 3970 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 3971 case 92645877: /*actor*/ return new String[] {"canonical", "Reference"}; 3972 default: return super.getTypesForProperty(hash, name); 3973 } 3974 3975 } 3976 3977 @Override 3978 public Base addChild(String name) throws FHIRException { 3979 if (name.equals("type")) { 3980 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.participant.type"); 3981 } 3982 else if (name.equals("typeCanonical")) { 3983 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.participant.typeCanonical"); 3984 } 3985 else if (name.equals("typeReference")) { 3986 this.typeReference = new Reference(); 3987 return this.typeReference; 3988 } 3989 else if (name.equals("role")) { 3990 this.role = new CodeableConcept(); 3991 return this.role; 3992 } 3993 else if (name.equals("function")) { 3994 this.function = new CodeableConcept(); 3995 return this.function; 3996 } 3997 else if (name.equals("actorCanonical")) { 3998 this.actor = new CanonicalType(); 3999 return this.actor; 4000 } 4001 else if (name.equals("actorReference")) { 4002 this.actor = new Reference(); 4003 return this.actor; 4004 } 4005 else 4006 return super.addChild(name); 4007 } 4008 4009 public RequestOrchestrationActionParticipantComponent copy() { 4010 RequestOrchestrationActionParticipantComponent dst = new RequestOrchestrationActionParticipantComponent(); 4011 copyValues(dst); 4012 return dst; 4013 } 4014 4015 public void copyValues(RequestOrchestrationActionParticipantComponent dst) { 4016 super.copyValues(dst); 4017 dst.type = type == null ? null : type.copy(); 4018 dst.typeCanonical = typeCanonical == null ? null : typeCanonical.copy(); 4019 dst.typeReference = typeReference == null ? null : typeReference.copy(); 4020 dst.role = role == null ? null : role.copy(); 4021 dst.function = function == null ? null : function.copy(); 4022 dst.actor = actor == null ? null : actor.copy(); 4023 } 4024 4025 @Override 4026 public boolean equalsDeep(Base other_) { 4027 if (!super.equalsDeep(other_)) 4028 return false; 4029 if (!(other_ instanceof RequestOrchestrationActionParticipantComponent)) 4030 return false; 4031 RequestOrchestrationActionParticipantComponent o = (RequestOrchestrationActionParticipantComponent) other_; 4032 return compareDeep(type, o.type, true) && compareDeep(typeCanonical, o.typeCanonical, true) && compareDeep(typeReference, o.typeReference, true) 4033 && compareDeep(role, o.role, true) && compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true) 4034 ; 4035 } 4036 4037 @Override 4038 public boolean equalsShallow(Base other_) { 4039 if (!super.equalsShallow(other_)) 4040 return false; 4041 if (!(other_ instanceof RequestOrchestrationActionParticipantComponent)) 4042 return false; 4043 RequestOrchestrationActionParticipantComponent o = (RequestOrchestrationActionParticipantComponent) other_; 4044 return compareValues(type, o.type, true) && compareValues(typeCanonical, o.typeCanonical, true); 4045 } 4046 4047 public boolean isEmpty() { 4048 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, typeCanonical, typeReference 4049 , role, function, actor); 4050 } 4051 4052 public String fhirType() { 4053 return "RequestOrchestration.action.participant"; 4054 4055 } 4056 4057 } 4058 4059 @Block() 4060 public static class RequestOrchestrationActionDynamicValueComponent extends BackboneElement implements IBaseBackboneElement { 4061 /** 4062 * The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). 4063 */ 4064 @Child(name = "path", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 4065 @Description(shortDefinition="The path to the element to be set dynamically", formalDefinition="The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details)." ) 4066 protected StringType path; 4067 4068 /** 4069 * An expression specifying the value of the customized element. 4070 */ 4071 @Child(name = "expression", type = {Expression.class}, order=2, min=0, max=1, modifier=false, summary=false) 4072 @Description(shortDefinition="An expression that provides the dynamic value for the customization", formalDefinition="An expression specifying the value of the customized element." ) 4073 protected Expression expression; 4074 4075 private static final long serialVersionUID = 1064529082L; 4076 4077 /** 4078 * Constructor 4079 */ 4080 public RequestOrchestrationActionDynamicValueComponent() { 4081 super(); 4082 } 4083 4084 /** 4085 * @return {@link #path} (The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 4086 */ 4087 public StringType getPathElement() { 4088 if (this.path == null) 4089 if (Configuration.errorOnAutoCreate()) 4090 throw new Error("Attempt to auto-create RequestOrchestrationActionDynamicValueComponent.path"); 4091 else if (Configuration.doAutoCreate()) 4092 this.path = new StringType(); // bb 4093 return this.path; 4094 } 4095 4096 public boolean hasPathElement() { 4097 return this.path != null && !this.path.isEmpty(); 4098 } 4099 4100 public boolean hasPath() { 4101 return this.path != null && !this.path.isEmpty(); 4102 } 4103 4104 /** 4105 * @param value {@link #path} (The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 4106 */ 4107 public RequestOrchestrationActionDynamicValueComponent setPathElement(StringType value) { 4108 this.path = value; 4109 return this; 4110 } 4111 4112 /** 4113 * @return The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). 4114 */ 4115 public String getPath() { 4116 return this.path == null ? null : this.path.getValue(); 4117 } 4118 4119 /** 4120 * @param value The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). 4121 */ 4122 public RequestOrchestrationActionDynamicValueComponent setPath(String value) { 4123 if (Utilities.noString(value)) 4124 this.path = null; 4125 else { 4126 if (this.path == null) 4127 this.path = new StringType(); 4128 this.path.setValue(value); 4129 } 4130 return this; 4131 } 4132 4133 /** 4134 * @return {@link #expression} (An expression specifying the value of the customized element.) 4135 */ 4136 public Expression getExpression() { 4137 if (this.expression == null) 4138 if (Configuration.errorOnAutoCreate()) 4139 throw new Error("Attempt to auto-create RequestOrchestrationActionDynamicValueComponent.expression"); 4140 else if (Configuration.doAutoCreate()) 4141 this.expression = new Expression(); // cc 4142 return this.expression; 4143 } 4144 4145 public boolean hasExpression() { 4146 return this.expression != null && !this.expression.isEmpty(); 4147 } 4148 4149 /** 4150 * @param value {@link #expression} (An expression specifying the value of the customized element.) 4151 */ 4152 public RequestOrchestrationActionDynamicValueComponent setExpression(Expression value) { 4153 this.expression = value; 4154 return this; 4155 } 4156 4157 protected void listChildren(List<Property> children) { 4158 super.listChildren(children); 4159 children.add(new Property("path", "string", "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).", 0, 1, path)); 4160 children.add(new Property("expression", "Expression", "An expression specifying the value of the customized element.", 0, 1, expression)); 4161 } 4162 4163 @Override 4164 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4165 switch (_hash) { 4166 case 3433509: /*path*/ return new Property("path", "string", "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolvable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).", 0, 1, path); 4167 case -1795452264: /*expression*/ return new Property("expression", "Expression", "An expression specifying the value of the customized element.", 0, 1, expression); 4168 default: return super.getNamedProperty(_hash, _name, _checkValid); 4169 } 4170 4171 } 4172 4173 @Override 4174 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4175 switch (hash) { 4176 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 4177 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // Expression 4178 default: return super.getProperty(hash, name, checkValid); 4179 } 4180 4181 } 4182 4183 @Override 4184 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4185 switch (hash) { 4186 case 3433509: // path 4187 this.path = TypeConvertor.castToString(value); // StringType 4188 return value; 4189 case -1795452264: // expression 4190 this.expression = TypeConvertor.castToExpression(value); // Expression 4191 return value; 4192 default: return super.setProperty(hash, name, value); 4193 } 4194 4195 } 4196 4197 @Override 4198 public Base setProperty(String name, Base value) throws FHIRException { 4199 if (name.equals("path")) { 4200 this.path = TypeConvertor.castToString(value); // StringType 4201 } else if (name.equals("expression")) { 4202 this.expression = TypeConvertor.castToExpression(value); // Expression 4203 } else 4204 return super.setProperty(name, value); 4205 return value; 4206 } 4207 4208 @Override 4209 public void removeChild(String name, Base value) throws FHIRException { 4210 if (name.equals("path")) { 4211 this.path = null; 4212 } else if (name.equals("expression")) { 4213 this.expression = null; 4214 } else 4215 super.removeChild(name, value); 4216 4217 } 4218 4219 @Override 4220 public Base makeProperty(int hash, String name) throws FHIRException { 4221 switch (hash) { 4222 case 3433509: return getPathElement(); 4223 case -1795452264: return getExpression(); 4224 default: return super.makeProperty(hash, name); 4225 } 4226 4227 } 4228 4229 @Override 4230 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4231 switch (hash) { 4232 case 3433509: /*path*/ return new String[] {"string"}; 4233 case -1795452264: /*expression*/ return new String[] {"Expression"}; 4234 default: return super.getTypesForProperty(hash, name); 4235 } 4236 4237 } 4238 4239 @Override 4240 public Base addChild(String name) throws FHIRException { 4241 if (name.equals("path")) { 4242 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.action.dynamicValue.path"); 4243 } 4244 else if (name.equals("expression")) { 4245 this.expression = new Expression(); 4246 return this.expression; 4247 } 4248 else 4249 return super.addChild(name); 4250 } 4251 4252 public RequestOrchestrationActionDynamicValueComponent copy() { 4253 RequestOrchestrationActionDynamicValueComponent dst = new RequestOrchestrationActionDynamicValueComponent(); 4254 copyValues(dst); 4255 return dst; 4256 } 4257 4258 public void copyValues(RequestOrchestrationActionDynamicValueComponent dst) { 4259 super.copyValues(dst); 4260 dst.path = path == null ? null : path.copy(); 4261 dst.expression = expression == null ? null : expression.copy(); 4262 } 4263 4264 @Override 4265 public boolean equalsDeep(Base other_) { 4266 if (!super.equalsDeep(other_)) 4267 return false; 4268 if (!(other_ instanceof RequestOrchestrationActionDynamicValueComponent)) 4269 return false; 4270 RequestOrchestrationActionDynamicValueComponent o = (RequestOrchestrationActionDynamicValueComponent) other_; 4271 return compareDeep(path, o.path, true) && compareDeep(expression, o.expression, true); 4272 } 4273 4274 @Override 4275 public boolean equalsShallow(Base other_) { 4276 if (!super.equalsShallow(other_)) 4277 return false; 4278 if (!(other_ instanceof RequestOrchestrationActionDynamicValueComponent)) 4279 return false; 4280 RequestOrchestrationActionDynamicValueComponent o = (RequestOrchestrationActionDynamicValueComponent) other_; 4281 return compareValues(path, o.path, true); 4282 } 4283 4284 public boolean isEmpty() { 4285 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, expression); 4286 } 4287 4288 public String fhirType() { 4289 return "RequestOrchestration.action.dynamicValue"; 4290 4291 } 4292 4293 } 4294 4295 /** 4296 * Allows a service to provide a unique, business identifier for the request. 4297 */ 4298 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4299 @Description(shortDefinition="Business identifier", formalDefinition="Allows a service to provide a unique, business identifier for the request." ) 4300 protected List<Identifier> identifier; 4301 4302 /** 4303 * A canonical URL referencing a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request. 4304 */ 4305 @Child(name = "instantiatesCanonical", type = {CanonicalType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4306 @Description(shortDefinition="Instantiates FHIR protocol or definition", formalDefinition="A canonical URL referencing a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request." ) 4307 protected List<CanonicalType> instantiatesCanonical; 4308 4309 /** 4310 * A URL referencing an externally defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request. 4311 */ 4312 @Child(name = "instantiatesUri", type = {UriType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4313 @Description(shortDefinition="Instantiates external protocol or definition", formalDefinition="A URL referencing an externally defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request." ) 4314 protected List<UriType> instantiatesUri; 4315 4316 /** 4317 * A plan, proposal or order that is fulfilled in whole or in part by this request. 4318 */ 4319 @Child(name = "basedOn", type = {Reference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4320 @Description(shortDefinition="Fulfills plan, proposal, or order", formalDefinition="A plan, proposal or order that is fulfilled in whole or in part by this request." ) 4321 protected List<Reference> basedOn; 4322 4323 /** 4324 * Completed or terminated request(s) whose function is taken by this new request. 4325 */ 4326 @Child(name = "replaces", type = {Reference.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4327 @Description(shortDefinition="Request(s) replaced by this request", formalDefinition="Completed or terminated request(s) whose function is taken by this new request." ) 4328 protected List<Reference> replaces; 4329 4330 /** 4331 * A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time. 4332 */ 4333 @Child(name = "groupIdentifier", type = {Identifier.class}, order=5, min=0, max=1, modifier=false, summary=true) 4334 @Description(shortDefinition="Composite request this is part of", formalDefinition="A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time." ) 4335 protected Identifier groupIdentifier; 4336 4337 /** 4338 * The current state of the request. For request orchestrations, the status reflects the status of all the requests in the orchestration. 4339 */ 4340 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 4341 @Description(shortDefinition="draft | active | on-hold | revoked | completed | entered-in-error | unknown", formalDefinition="The current state of the request. For request orchestrations, the status reflects the status of all the requests in the orchestration." ) 4342 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-status") 4343 protected Enumeration<RequestStatus> status; 4344 4345 /** 4346 * Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain. 4347 */ 4348 @Child(name = "intent", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 4349 @Description(shortDefinition="proposal | plan | directive | order | original-order | reflex-order | filler-order | instance-order | option", formalDefinition="Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain." ) 4350 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-intent") 4351 protected Enumeration<RequestIntent> intent; 4352 4353 /** 4354 * Indicates how quickly the request should be addressed with respect to other requests. 4355 */ 4356 @Child(name = "priority", type = {CodeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 4357 @Description(shortDefinition="routine | urgent | asap | stat", formalDefinition="Indicates how quickly the request should be addressed with respect to other requests." ) 4358 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 4359 protected Enumeration<RequestPriority> priority; 4360 4361 /** 4362 * A code that identifies what the overall request orchestration is. 4363 */ 4364 @Child(name = "code", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=true) 4365 @Description(shortDefinition="What's being requested/ordered", formalDefinition="A code that identifies what the overall request orchestration is." ) 4366 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-code") 4367 protected CodeableConcept code; 4368 4369 /** 4370 * The subject for which the request orchestration was created. 4371 */ 4372 @Child(name = "subject", type = {CareTeam.class, Device.class, Group.class, HealthcareService.class, Location.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class}, order=10, min=0, max=1, modifier=false, summary=false) 4373 @Description(shortDefinition="Who the request orchestration is about", formalDefinition="The subject for which the request orchestration was created." ) 4374 protected Reference subject; 4375 4376 /** 4377 * Describes the context of the request orchestration, if any. 4378 */ 4379 @Child(name = "encounter", type = {Encounter.class}, order=11, min=0, max=1, modifier=false, summary=false) 4380 @Description(shortDefinition="Created as part of", formalDefinition="Describes the context of the request orchestration, if any." ) 4381 protected Reference encounter; 4382 4383 /** 4384 * Indicates when the request orchestration was created. 4385 */ 4386 @Child(name = "authoredOn", type = {DateTimeType.class}, order=12, min=0, max=1, modifier=false, summary=false) 4387 @Description(shortDefinition="When the request orchestration was authored", formalDefinition="Indicates when the request orchestration was created." ) 4388 protected DateTimeType authoredOn; 4389 4390 /** 4391 * Provides a reference to the author of the request orchestration. 4392 */ 4393 @Child(name = "author", type = {Device.class, Practitioner.class, PractitionerRole.class}, order=13, min=0, max=1, modifier=false, summary=false) 4394 @Description(shortDefinition="Device or practitioner that authored the request orchestration", formalDefinition="Provides a reference to the author of the request orchestration." ) 4395 protected Reference author; 4396 4397 /** 4398 * Describes the reason for the request orchestration in coded or textual form. 4399 */ 4400 @Child(name = "reason", type = {CodeableReference.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4401 @Description(shortDefinition="Why the request orchestration is needed", formalDefinition="Describes the reason for the request orchestration in coded or textual form." ) 4402 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/action-reason-code") 4403 protected List<CodeableReference> reason; 4404 4405 /** 4406 * Goals that are intended to be achieved by following the requests in this RequestOrchestration. 4407 */ 4408 @Child(name = "goal", type = {Goal.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4409 @Description(shortDefinition="What goals", formalDefinition="Goals that are intended to be achieved by following the requests in this RequestOrchestration." ) 4410 protected List<Reference> goal; 4411 4412 /** 4413 * Provides a mechanism to communicate additional information about the response. 4414 */ 4415 @Child(name = "note", type = {Annotation.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4416 @Description(shortDefinition="Additional notes about the response", formalDefinition="Provides a mechanism to communicate additional information about the response." ) 4417 protected List<Annotation> note; 4418 4419 /** 4420 * The actions, if any, produced by the evaluation of the artifact. 4421 */ 4422 @Child(name = "action", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4423 @Description(shortDefinition="Proposed actions, if any", formalDefinition="The actions, if any, produced by the evaluation of the artifact." ) 4424 protected List<RequestOrchestrationActionComponent> action; 4425 4426 private static final long serialVersionUID = -683989911L; 4427 4428 /** 4429 * Constructor 4430 */ 4431 public RequestOrchestration() { 4432 super(); 4433 } 4434 4435 /** 4436 * Constructor 4437 */ 4438 public RequestOrchestration(RequestStatus status, RequestIntent intent) { 4439 super(); 4440 this.setStatus(status); 4441 this.setIntent(intent); 4442 } 4443 4444 /** 4445 * @return {@link #identifier} (Allows a service to provide a unique, business identifier for the request.) 4446 */ 4447 public List<Identifier> getIdentifier() { 4448 if (this.identifier == null) 4449 this.identifier = new ArrayList<Identifier>(); 4450 return this.identifier; 4451 } 4452 4453 /** 4454 * @return Returns a reference to <code>this</code> for easy method chaining 4455 */ 4456 public RequestOrchestration setIdentifier(List<Identifier> theIdentifier) { 4457 this.identifier = theIdentifier; 4458 return this; 4459 } 4460 4461 public boolean hasIdentifier() { 4462 if (this.identifier == null) 4463 return false; 4464 for (Identifier item : this.identifier) 4465 if (!item.isEmpty()) 4466 return true; 4467 return false; 4468 } 4469 4470 public Identifier addIdentifier() { //3 4471 Identifier t = new Identifier(); 4472 if (this.identifier == null) 4473 this.identifier = new ArrayList<Identifier>(); 4474 this.identifier.add(t); 4475 return t; 4476 } 4477 4478 public RequestOrchestration addIdentifier(Identifier t) { //3 4479 if (t == null) 4480 return this; 4481 if (this.identifier == null) 4482 this.identifier = new ArrayList<Identifier>(); 4483 this.identifier.add(t); 4484 return this; 4485 } 4486 4487 /** 4488 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 4489 */ 4490 public Identifier getIdentifierFirstRep() { 4491 if (getIdentifier().isEmpty()) { 4492 addIdentifier(); 4493 } 4494 return getIdentifier().get(0); 4495 } 4496 4497 /** 4498 * @return {@link #instantiatesCanonical} (A canonical URL referencing a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.) 4499 */ 4500 public List<CanonicalType> getInstantiatesCanonical() { 4501 if (this.instantiatesCanonical == null) 4502 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 4503 return this.instantiatesCanonical; 4504 } 4505 4506 /** 4507 * @return Returns a reference to <code>this</code> for easy method chaining 4508 */ 4509 public RequestOrchestration setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 4510 this.instantiatesCanonical = theInstantiatesCanonical; 4511 return this; 4512 } 4513 4514 public boolean hasInstantiatesCanonical() { 4515 if (this.instantiatesCanonical == null) 4516 return false; 4517 for (CanonicalType item : this.instantiatesCanonical) 4518 if (!item.isEmpty()) 4519 return true; 4520 return false; 4521 } 4522 4523 /** 4524 * @return {@link #instantiatesCanonical} (A canonical URL referencing a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.) 4525 */ 4526 public CanonicalType addInstantiatesCanonicalElement() {//2 4527 CanonicalType t = new CanonicalType(); 4528 if (this.instantiatesCanonical == null) 4529 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 4530 this.instantiatesCanonical.add(t); 4531 return t; 4532 } 4533 4534 /** 4535 * @param value {@link #instantiatesCanonical} (A canonical URL referencing a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.) 4536 */ 4537 public RequestOrchestration addInstantiatesCanonical(String value) { //1 4538 CanonicalType t = new CanonicalType(); 4539 t.setValue(value); 4540 if (this.instantiatesCanonical == null) 4541 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 4542 this.instantiatesCanonical.add(t); 4543 return this; 4544 } 4545 4546 /** 4547 * @param value {@link #instantiatesCanonical} (A canonical URL referencing a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.) 4548 */ 4549 public boolean hasInstantiatesCanonical(String value) { 4550 if (this.instantiatesCanonical == null) 4551 return false; 4552 for (CanonicalType v : this.instantiatesCanonical) 4553 if (v.getValue().equals(value)) // canonical 4554 return true; 4555 return false; 4556 } 4557 4558 /** 4559 * @return {@link #instantiatesUri} (A URL referencing an externally defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.) 4560 */ 4561 public List<UriType> getInstantiatesUri() { 4562 if (this.instantiatesUri == null) 4563 this.instantiatesUri = new ArrayList<UriType>(); 4564 return this.instantiatesUri; 4565 } 4566 4567 /** 4568 * @return Returns a reference to <code>this</code> for easy method chaining 4569 */ 4570 public RequestOrchestration setInstantiatesUri(List<UriType> theInstantiatesUri) { 4571 this.instantiatesUri = theInstantiatesUri; 4572 return this; 4573 } 4574 4575 public boolean hasInstantiatesUri() { 4576 if (this.instantiatesUri == null) 4577 return false; 4578 for (UriType item : this.instantiatesUri) 4579 if (!item.isEmpty()) 4580 return true; 4581 return false; 4582 } 4583 4584 /** 4585 * @return {@link #instantiatesUri} (A URL referencing an externally defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.) 4586 */ 4587 public UriType addInstantiatesUriElement() {//2 4588 UriType t = new UriType(); 4589 if (this.instantiatesUri == null) 4590 this.instantiatesUri = new ArrayList<UriType>(); 4591 this.instantiatesUri.add(t); 4592 return t; 4593 } 4594 4595 /** 4596 * @param value {@link #instantiatesUri} (A URL referencing an externally defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.) 4597 */ 4598 public RequestOrchestration addInstantiatesUri(String value) { //1 4599 UriType t = new UriType(); 4600 t.setValue(value); 4601 if (this.instantiatesUri == null) 4602 this.instantiatesUri = new ArrayList<UriType>(); 4603 this.instantiatesUri.add(t); 4604 return this; 4605 } 4606 4607 /** 4608 * @param value {@link #instantiatesUri} (A URL referencing an externally defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.) 4609 */ 4610 public boolean hasInstantiatesUri(String value) { 4611 if (this.instantiatesUri == null) 4612 return false; 4613 for (UriType v : this.instantiatesUri) 4614 if (v.getValue().equals(value)) // uri 4615 return true; 4616 return false; 4617 } 4618 4619 /** 4620 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in whole or in part by this request.) 4621 */ 4622 public List<Reference> getBasedOn() { 4623 if (this.basedOn == null) 4624 this.basedOn = new ArrayList<Reference>(); 4625 return this.basedOn; 4626 } 4627 4628 /** 4629 * @return Returns a reference to <code>this</code> for easy method chaining 4630 */ 4631 public RequestOrchestration setBasedOn(List<Reference> theBasedOn) { 4632 this.basedOn = theBasedOn; 4633 return this; 4634 } 4635 4636 public boolean hasBasedOn() { 4637 if (this.basedOn == null) 4638 return false; 4639 for (Reference item : this.basedOn) 4640 if (!item.isEmpty()) 4641 return true; 4642 return false; 4643 } 4644 4645 public Reference addBasedOn() { //3 4646 Reference t = new Reference(); 4647 if (this.basedOn == null) 4648 this.basedOn = new ArrayList<Reference>(); 4649 this.basedOn.add(t); 4650 return t; 4651 } 4652 4653 public RequestOrchestration addBasedOn(Reference t) { //3 4654 if (t == null) 4655 return this; 4656 if (this.basedOn == null) 4657 this.basedOn = new ArrayList<Reference>(); 4658 this.basedOn.add(t); 4659 return this; 4660 } 4661 4662 /** 4663 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 4664 */ 4665 public Reference getBasedOnFirstRep() { 4666 if (getBasedOn().isEmpty()) { 4667 addBasedOn(); 4668 } 4669 return getBasedOn().get(0); 4670 } 4671 4672 /** 4673 * @return {@link #replaces} (Completed or terminated request(s) whose function is taken by this new request.) 4674 */ 4675 public List<Reference> getReplaces() { 4676 if (this.replaces == null) 4677 this.replaces = new ArrayList<Reference>(); 4678 return this.replaces; 4679 } 4680 4681 /** 4682 * @return Returns a reference to <code>this</code> for easy method chaining 4683 */ 4684 public RequestOrchestration setReplaces(List<Reference> theReplaces) { 4685 this.replaces = theReplaces; 4686 return this; 4687 } 4688 4689 public boolean hasReplaces() { 4690 if (this.replaces == null) 4691 return false; 4692 for (Reference item : this.replaces) 4693 if (!item.isEmpty()) 4694 return true; 4695 return false; 4696 } 4697 4698 public Reference addReplaces() { //3 4699 Reference t = new Reference(); 4700 if (this.replaces == null) 4701 this.replaces = new ArrayList<Reference>(); 4702 this.replaces.add(t); 4703 return t; 4704 } 4705 4706 public RequestOrchestration addReplaces(Reference t) { //3 4707 if (t == null) 4708 return this; 4709 if (this.replaces == null) 4710 this.replaces = new ArrayList<Reference>(); 4711 this.replaces.add(t); 4712 return this; 4713 } 4714 4715 /** 4716 * @return The first repetition of repeating field {@link #replaces}, creating it if it does not already exist {3} 4717 */ 4718 public Reference getReplacesFirstRep() { 4719 if (getReplaces().isEmpty()) { 4720 addReplaces(); 4721 } 4722 return getReplaces().get(0); 4723 } 4724 4725 /** 4726 * @return {@link #groupIdentifier} (A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.) 4727 */ 4728 public Identifier getGroupIdentifier() { 4729 if (this.groupIdentifier == null) 4730 if (Configuration.errorOnAutoCreate()) 4731 throw new Error("Attempt to auto-create RequestOrchestration.groupIdentifier"); 4732 else if (Configuration.doAutoCreate()) 4733 this.groupIdentifier = new Identifier(); // cc 4734 return this.groupIdentifier; 4735 } 4736 4737 public boolean hasGroupIdentifier() { 4738 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 4739 } 4740 4741 /** 4742 * @param value {@link #groupIdentifier} (A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.) 4743 */ 4744 public RequestOrchestration setGroupIdentifier(Identifier value) { 4745 this.groupIdentifier = value; 4746 return this; 4747 } 4748 4749 /** 4750 * @return {@link #status} (The current state of the request. For request orchestrations, the status reflects the status of all the requests in the orchestration.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4751 */ 4752 public Enumeration<RequestStatus> getStatusElement() { 4753 if (this.status == null) 4754 if (Configuration.errorOnAutoCreate()) 4755 throw new Error("Attempt to auto-create RequestOrchestration.status"); 4756 else if (Configuration.doAutoCreate()) 4757 this.status = new Enumeration<RequestStatus>(new RequestStatusEnumFactory()); // bb 4758 return this.status; 4759 } 4760 4761 public boolean hasStatusElement() { 4762 return this.status != null && !this.status.isEmpty(); 4763 } 4764 4765 public boolean hasStatus() { 4766 return this.status != null && !this.status.isEmpty(); 4767 } 4768 4769 /** 4770 * @param value {@link #status} (The current state of the request. For request orchestrations, the status reflects the status of all the requests in the orchestration.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4771 */ 4772 public RequestOrchestration setStatusElement(Enumeration<RequestStatus> value) { 4773 this.status = value; 4774 return this; 4775 } 4776 4777 /** 4778 * @return The current state of the request. For request orchestrations, the status reflects the status of all the requests in the orchestration. 4779 */ 4780 public RequestStatus getStatus() { 4781 return this.status == null ? null : this.status.getValue(); 4782 } 4783 4784 /** 4785 * @param value The current state of the request. For request orchestrations, the status reflects the status of all the requests in the orchestration. 4786 */ 4787 public RequestOrchestration setStatus(RequestStatus value) { 4788 if (this.status == null) 4789 this.status = new Enumeration<RequestStatus>(new RequestStatusEnumFactory()); 4790 this.status.setValue(value); 4791 return this; 4792 } 4793 4794 /** 4795 * @return {@link #intent} (Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 4796 */ 4797 public Enumeration<RequestIntent> getIntentElement() { 4798 if (this.intent == null) 4799 if (Configuration.errorOnAutoCreate()) 4800 throw new Error("Attempt to auto-create RequestOrchestration.intent"); 4801 else if (Configuration.doAutoCreate()) 4802 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); // bb 4803 return this.intent; 4804 } 4805 4806 public boolean hasIntentElement() { 4807 return this.intent != null && !this.intent.isEmpty(); 4808 } 4809 4810 public boolean hasIntent() { 4811 return this.intent != null && !this.intent.isEmpty(); 4812 } 4813 4814 /** 4815 * @param value {@link #intent} (Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 4816 */ 4817 public RequestOrchestration setIntentElement(Enumeration<RequestIntent> value) { 4818 this.intent = value; 4819 return this; 4820 } 4821 4822 /** 4823 * @return Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain. 4824 */ 4825 public RequestIntent getIntent() { 4826 return this.intent == null ? null : this.intent.getValue(); 4827 } 4828 4829 /** 4830 * @param value Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain. 4831 */ 4832 public RequestOrchestration setIntent(RequestIntent value) { 4833 if (this.intent == null) 4834 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); 4835 this.intent.setValue(value); 4836 return this; 4837 } 4838 4839 /** 4840 * @return {@link #priority} (Indicates how quickly the request should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 4841 */ 4842 public Enumeration<RequestPriority> getPriorityElement() { 4843 if (this.priority == null) 4844 if (Configuration.errorOnAutoCreate()) 4845 throw new Error("Attempt to auto-create RequestOrchestration.priority"); 4846 else if (Configuration.doAutoCreate()) 4847 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 4848 return this.priority; 4849 } 4850 4851 public boolean hasPriorityElement() { 4852 return this.priority != null && !this.priority.isEmpty(); 4853 } 4854 4855 public boolean hasPriority() { 4856 return this.priority != null && !this.priority.isEmpty(); 4857 } 4858 4859 /** 4860 * @param value {@link #priority} (Indicates how quickly the request should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 4861 */ 4862 public RequestOrchestration setPriorityElement(Enumeration<RequestPriority> value) { 4863 this.priority = value; 4864 return this; 4865 } 4866 4867 /** 4868 * @return Indicates how quickly the request should be addressed with respect to other requests. 4869 */ 4870 public RequestPriority getPriority() { 4871 return this.priority == null ? null : this.priority.getValue(); 4872 } 4873 4874 /** 4875 * @param value Indicates how quickly the request should be addressed with respect to other requests. 4876 */ 4877 public RequestOrchestration setPriority(RequestPriority value) { 4878 if (value == null) 4879 this.priority = null; 4880 else { 4881 if (this.priority == null) 4882 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 4883 this.priority.setValue(value); 4884 } 4885 return this; 4886 } 4887 4888 /** 4889 * @return {@link #code} (A code that identifies what the overall request orchestration is.) 4890 */ 4891 public CodeableConcept getCode() { 4892 if (this.code == null) 4893 if (Configuration.errorOnAutoCreate()) 4894 throw new Error("Attempt to auto-create RequestOrchestration.code"); 4895 else if (Configuration.doAutoCreate()) 4896 this.code = new CodeableConcept(); // cc 4897 return this.code; 4898 } 4899 4900 public boolean hasCode() { 4901 return this.code != null && !this.code.isEmpty(); 4902 } 4903 4904 /** 4905 * @param value {@link #code} (A code that identifies what the overall request orchestration is.) 4906 */ 4907 public RequestOrchestration setCode(CodeableConcept value) { 4908 this.code = value; 4909 return this; 4910 } 4911 4912 /** 4913 * @return {@link #subject} (The subject for which the request orchestration was created.) 4914 */ 4915 public Reference getSubject() { 4916 if (this.subject == null) 4917 if (Configuration.errorOnAutoCreate()) 4918 throw new Error("Attempt to auto-create RequestOrchestration.subject"); 4919 else if (Configuration.doAutoCreate()) 4920 this.subject = new Reference(); // cc 4921 return this.subject; 4922 } 4923 4924 public boolean hasSubject() { 4925 return this.subject != null && !this.subject.isEmpty(); 4926 } 4927 4928 /** 4929 * @param value {@link #subject} (The subject for which the request orchestration was created.) 4930 */ 4931 public RequestOrchestration setSubject(Reference value) { 4932 this.subject = value; 4933 return this; 4934 } 4935 4936 /** 4937 * @return {@link #encounter} (Describes the context of the request orchestration, if any.) 4938 */ 4939 public Reference getEncounter() { 4940 if (this.encounter == null) 4941 if (Configuration.errorOnAutoCreate()) 4942 throw new Error("Attempt to auto-create RequestOrchestration.encounter"); 4943 else if (Configuration.doAutoCreate()) 4944 this.encounter = new Reference(); // cc 4945 return this.encounter; 4946 } 4947 4948 public boolean hasEncounter() { 4949 return this.encounter != null && !this.encounter.isEmpty(); 4950 } 4951 4952 /** 4953 * @param value {@link #encounter} (Describes the context of the request orchestration, if any.) 4954 */ 4955 public RequestOrchestration setEncounter(Reference value) { 4956 this.encounter = value; 4957 return this; 4958 } 4959 4960 /** 4961 * @return {@link #authoredOn} (Indicates when the request orchestration was created.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 4962 */ 4963 public DateTimeType getAuthoredOnElement() { 4964 if (this.authoredOn == null) 4965 if (Configuration.errorOnAutoCreate()) 4966 throw new Error("Attempt to auto-create RequestOrchestration.authoredOn"); 4967 else if (Configuration.doAutoCreate()) 4968 this.authoredOn = new DateTimeType(); // bb 4969 return this.authoredOn; 4970 } 4971 4972 public boolean hasAuthoredOnElement() { 4973 return this.authoredOn != null && !this.authoredOn.isEmpty(); 4974 } 4975 4976 public boolean hasAuthoredOn() { 4977 return this.authoredOn != null && !this.authoredOn.isEmpty(); 4978 } 4979 4980 /** 4981 * @param value {@link #authoredOn} (Indicates when the request orchestration was created.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 4982 */ 4983 public RequestOrchestration setAuthoredOnElement(DateTimeType value) { 4984 this.authoredOn = value; 4985 return this; 4986 } 4987 4988 /** 4989 * @return Indicates when the request orchestration was created. 4990 */ 4991 public Date getAuthoredOn() { 4992 return this.authoredOn == null ? null : this.authoredOn.getValue(); 4993 } 4994 4995 /** 4996 * @param value Indicates when the request orchestration was created. 4997 */ 4998 public RequestOrchestration setAuthoredOn(Date value) { 4999 if (value == null) 5000 this.authoredOn = null; 5001 else { 5002 if (this.authoredOn == null) 5003 this.authoredOn = new DateTimeType(); 5004 this.authoredOn.setValue(value); 5005 } 5006 return this; 5007 } 5008 5009 /** 5010 * @return {@link #author} (Provides a reference to the author of the request orchestration.) 5011 */ 5012 public Reference getAuthor() { 5013 if (this.author == null) 5014 if (Configuration.errorOnAutoCreate()) 5015 throw new Error("Attempt to auto-create RequestOrchestration.author"); 5016 else if (Configuration.doAutoCreate()) 5017 this.author = new Reference(); // cc 5018 return this.author; 5019 } 5020 5021 public boolean hasAuthor() { 5022 return this.author != null && !this.author.isEmpty(); 5023 } 5024 5025 /** 5026 * @param value {@link #author} (Provides a reference to the author of the request orchestration.) 5027 */ 5028 public RequestOrchestration setAuthor(Reference value) { 5029 this.author = value; 5030 return this; 5031 } 5032 5033 /** 5034 * @return {@link #reason} (Describes the reason for the request orchestration in coded or textual form.) 5035 */ 5036 public List<CodeableReference> getReason() { 5037 if (this.reason == null) 5038 this.reason = new ArrayList<CodeableReference>(); 5039 return this.reason; 5040 } 5041 5042 /** 5043 * @return Returns a reference to <code>this</code> for easy method chaining 5044 */ 5045 public RequestOrchestration setReason(List<CodeableReference> theReason) { 5046 this.reason = theReason; 5047 return this; 5048 } 5049 5050 public boolean hasReason() { 5051 if (this.reason == null) 5052 return false; 5053 for (CodeableReference item : this.reason) 5054 if (!item.isEmpty()) 5055 return true; 5056 return false; 5057 } 5058 5059 public CodeableReference addReason() { //3 5060 CodeableReference t = new CodeableReference(); 5061 if (this.reason == null) 5062 this.reason = new ArrayList<CodeableReference>(); 5063 this.reason.add(t); 5064 return t; 5065 } 5066 5067 public RequestOrchestration addReason(CodeableReference t) { //3 5068 if (t == null) 5069 return this; 5070 if (this.reason == null) 5071 this.reason = new ArrayList<CodeableReference>(); 5072 this.reason.add(t); 5073 return this; 5074 } 5075 5076 /** 5077 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 5078 */ 5079 public CodeableReference getReasonFirstRep() { 5080 if (getReason().isEmpty()) { 5081 addReason(); 5082 } 5083 return getReason().get(0); 5084 } 5085 5086 /** 5087 * @return {@link #goal} (Goals that are intended to be achieved by following the requests in this RequestOrchestration.) 5088 */ 5089 public List<Reference> getGoal() { 5090 if (this.goal == null) 5091 this.goal = new ArrayList<Reference>(); 5092 return this.goal; 5093 } 5094 5095 /** 5096 * @return Returns a reference to <code>this</code> for easy method chaining 5097 */ 5098 public RequestOrchestration setGoal(List<Reference> theGoal) { 5099 this.goal = theGoal; 5100 return this; 5101 } 5102 5103 public boolean hasGoal() { 5104 if (this.goal == null) 5105 return false; 5106 for (Reference item : this.goal) 5107 if (!item.isEmpty()) 5108 return true; 5109 return false; 5110 } 5111 5112 public Reference addGoal() { //3 5113 Reference t = new Reference(); 5114 if (this.goal == null) 5115 this.goal = new ArrayList<Reference>(); 5116 this.goal.add(t); 5117 return t; 5118 } 5119 5120 public RequestOrchestration addGoal(Reference t) { //3 5121 if (t == null) 5122 return this; 5123 if (this.goal == null) 5124 this.goal = new ArrayList<Reference>(); 5125 this.goal.add(t); 5126 return this; 5127 } 5128 5129 /** 5130 * @return The first repetition of repeating field {@link #goal}, creating it if it does not already exist {3} 5131 */ 5132 public Reference getGoalFirstRep() { 5133 if (getGoal().isEmpty()) { 5134 addGoal(); 5135 } 5136 return getGoal().get(0); 5137 } 5138 5139 /** 5140 * @return {@link #note} (Provides a mechanism to communicate additional information about the response.) 5141 */ 5142 public List<Annotation> getNote() { 5143 if (this.note == null) 5144 this.note = new ArrayList<Annotation>(); 5145 return this.note; 5146 } 5147 5148 /** 5149 * @return Returns a reference to <code>this</code> for easy method chaining 5150 */ 5151 public RequestOrchestration setNote(List<Annotation> theNote) { 5152 this.note = theNote; 5153 return this; 5154 } 5155 5156 public boolean hasNote() { 5157 if (this.note == null) 5158 return false; 5159 for (Annotation item : this.note) 5160 if (!item.isEmpty()) 5161 return true; 5162 return false; 5163 } 5164 5165 public Annotation addNote() { //3 5166 Annotation t = new Annotation(); 5167 if (this.note == null) 5168 this.note = new ArrayList<Annotation>(); 5169 this.note.add(t); 5170 return t; 5171 } 5172 5173 public RequestOrchestration addNote(Annotation t) { //3 5174 if (t == null) 5175 return this; 5176 if (this.note == null) 5177 this.note = new ArrayList<Annotation>(); 5178 this.note.add(t); 5179 return this; 5180 } 5181 5182 /** 5183 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 5184 */ 5185 public Annotation getNoteFirstRep() { 5186 if (getNote().isEmpty()) { 5187 addNote(); 5188 } 5189 return getNote().get(0); 5190 } 5191 5192 /** 5193 * @return {@link #action} (The actions, if any, produced by the evaluation of the artifact.) 5194 */ 5195 public List<RequestOrchestrationActionComponent> getAction() { 5196 if (this.action == null) 5197 this.action = new ArrayList<RequestOrchestrationActionComponent>(); 5198 return this.action; 5199 } 5200 5201 /** 5202 * @return Returns a reference to <code>this</code> for easy method chaining 5203 */ 5204 public RequestOrchestration setAction(List<RequestOrchestrationActionComponent> theAction) { 5205 this.action = theAction; 5206 return this; 5207 } 5208 5209 public boolean hasAction() { 5210 if (this.action == null) 5211 return false; 5212 for (RequestOrchestrationActionComponent item : this.action) 5213 if (!item.isEmpty()) 5214 return true; 5215 return false; 5216 } 5217 5218 public RequestOrchestrationActionComponent addAction() { //3 5219 RequestOrchestrationActionComponent t = new RequestOrchestrationActionComponent(); 5220 if (this.action == null) 5221 this.action = new ArrayList<RequestOrchestrationActionComponent>(); 5222 this.action.add(t); 5223 return t; 5224 } 5225 5226 public RequestOrchestration addAction(RequestOrchestrationActionComponent t) { //3 5227 if (t == null) 5228 return this; 5229 if (this.action == null) 5230 this.action = new ArrayList<RequestOrchestrationActionComponent>(); 5231 this.action.add(t); 5232 return this; 5233 } 5234 5235 /** 5236 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist {3} 5237 */ 5238 public RequestOrchestrationActionComponent getActionFirstRep() { 5239 if (getAction().isEmpty()) { 5240 addAction(); 5241 } 5242 return getAction().get(0); 5243 } 5244 5245 protected void listChildren(List<Property> children) { 5246 super.listChildren(children); 5247 children.add(new Property("identifier", "Identifier", "Allows a service to provide a unique, business identifier for the request.", 0, java.lang.Integer.MAX_VALUE, identifier)); 5248 children.add(new Property("instantiatesCanonical", "canonical", "A canonical URL referencing a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 5249 children.add(new Property("instantiatesUri", "uri", "A URL referencing an externally defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 5250 children.add(new Property("basedOn", "Reference(Any)", "A plan, proposal or order that is fulfilled in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 5251 children.add(new Property("replaces", "Reference(Any)", "Completed or terminated request(s) whose function is taken by this new request.", 0, java.lang.Integer.MAX_VALUE, replaces)); 5252 children.add(new Property("groupIdentifier", "Identifier", "A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.", 0, 1, groupIdentifier)); 5253 children.add(new Property("status", "code", "The current state of the request. For request orchestrations, the status reflects the status of all the requests in the orchestration.", 0, 1, status)); 5254 children.add(new Property("intent", "code", "Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain.", 0, 1, intent)); 5255 children.add(new Property("priority", "code", "Indicates how quickly the request should be addressed with respect to other requests.", 0, 1, priority)); 5256 children.add(new Property("code", "CodeableConcept", "A code that identifies what the overall request orchestration is.", 0, 1, code)); 5257 children.add(new Property("subject", "Reference(CareTeam|Device|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "The subject for which the request orchestration was created.", 0, 1, subject)); 5258 children.add(new Property("encounter", "Reference(Encounter)", "Describes the context of the request orchestration, if any.", 0, 1, encounter)); 5259 children.add(new Property("authoredOn", "dateTime", "Indicates when the request orchestration was created.", 0, 1, authoredOn)); 5260 children.add(new Property("author", "Reference(Device|Practitioner|PractitionerRole)", "Provides a reference to the author of the request orchestration.", 0, 1, author)); 5261 children.add(new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport|DocumentReference)", "Describes the reason for the request orchestration in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, reason)); 5262 children.add(new Property("goal", "Reference(Goal)", "Goals that are intended to be achieved by following the requests in this RequestOrchestration.", 0, java.lang.Integer.MAX_VALUE, goal)); 5263 children.add(new Property("note", "Annotation", "Provides a mechanism to communicate additional information about the response.", 0, java.lang.Integer.MAX_VALUE, note)); 5264 children.add(new Property("action", "", "The actions, if any, produced by the evaluation of the artifact.", 0, java.lang.Integer.MAX_VALUE, action)); 5265 } 5266 5267 @Override 5268 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5269 switch (_hash) { 5270 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Allows a service to provide a unique, business identifier for the request.", 0, java.lang.Integer.MAX_VALUE, identifier); 5271 case 8911915: /*instantiatesCanonical*/ return new Property("instantiatesCanonical", "canonical", "A canonical URL referencing a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 5272 case -1926393373: /*instantiatesUri*/ return new Property("instantiatesUri", "uri", "A URL referencing an externally defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 5273 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(Any)", "A plan, proposal or order that is fulfilled in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn); 5274 case -430332865: /*replaces*/ return new Property("replaces", "Reference(Any)", "Completed or terminated request(s) whose function is taken by this new request.", 0, java.lang.Integer.MAX_VALUE, replaces); 5275 case -445338488: /*groupIdentifier*/ return new Property("groupIdentifier", "Identifier", "A shared identifier common to multiple independent Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.", 0, 1, groupIdentifier); 5276 case -892481550: /*status*/ return new Property("status", "code", "The current state of the request. For request orchestrations, the status reflects the status of all the requests in the orchestration.", 0, 1, status); 5277 case -1183762788: /*intent*/ return new Property("intent", "code", "Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain.", 0, 1, intent); 5278 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly the request should be addressed with respect to other requests.", 0, 1, priority); 5279 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code that identifies what the overall request orchestration is.", 0, 1, code); 5280 case -1867885268: /*subject*/ return new Property("subject", "Reference(CareTeam|Device|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "The subject for which the request orchestration was created.", 0, 1, subject); 5281 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "Describes the context of the request orchestration, if any.", 0, 1, encounter); 5282 case -1500852503: /*authoredOn*/ return new Property("authoredOn", "dateTime", "Indicates when the request orchestration was created.", 0, 1, authoredOn); 5283 case -1406328437: /*author*/ return new Property("author", "Reference(Device|Practitioner|PractitionerRole)", "Provides a reference to the author of the request orchestration.", 0, 1, author); 5284 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport|DocumentReference)", "Describes the reason for the request orchestration in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, reason); 5285 case 3178259: /*goal*/ return new Property("goal", "Reference(Goal)", "Goals that are intended to be achieved by following the requests in this RequestOrchestration.", 0, java.lang.Integer.MAX_VALUE, goal); 5286 case 3387378: /*note*/ return new Property("note", "Annotation", "Provides a mechanism to communicate additional information about the response.", 0, java.lang.Integer.MAX_VALUE, note); 5287 case -1422950858: /*action*/ return new Property("action", "", "The actions, if any, produced by the evaluation of the artifact.", 0, java.lang.Integer.MAX_VALUE, action); 5288 default: return super.getNamedProperty(_hash, _name, _checkValid); 5289 } 5290 5291 } 5292 5293 @Override 5294 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5295 switch (hash) { 5296 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 5297 case 8911915: /*instantiatesCanonical*/ return this.instantiatesCanonical == null ? new Base[0] : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 5298 case -1926393373: /*instantiatesUri*/ return this.instantiatesUri == null ? new Base[0] : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 5299 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 5300 case -430332865: /*replaces*/ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 5301 case -445338488: /*groupIdentifier*/ return this.groupIdentifier == null ? new Base[0] : new Base[] {this.groupIdentifier}; // Identifier 5302 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<RequestStatus> 5303 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // Enumeration<RequestIntent> 5304 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<RequestPriority> 5305 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 5306 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 5307 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 5308 case -1500852503: /*authoredOn*/ return this.authoredOn == null ? new Base[0] : new Base[] {this.authoredOn}; // DateTimeType 5309 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 5310 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 5311 case 3178259: /*goal*/ return this.goal == null ? new Base[0] : this.goal.toArray(new Base[this.goal.size()]); // Reference 5312 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 5313 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // RequestOrchestrationActionComponent 5314 default: return super.getProperty(hash, name, checkValid); 5315 } 5316 5317 } 5318 5319 @Override 5320 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5321 switch (hash) { 5322 case -1618432855: // identifier 5323 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 5324 return value; 5325 case 8911915: // instantiatesCanonical 5326 this.getInstantiatesCanonical().add(TypeConvertor.castToCanonical(value)); // CanonicalType 5327 return value; 5328 case -1926393373: // instantiatesUri 5329 this.getInstantiatesUri().add(TypeConvertor.castToUri(value)); // UriType 5330 return value; 5331 case -332612366: // basedOn 5332 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 5333 return value; 5334 case -430332865: // replaces 5335 this.getReplaces().add(TypeConvertor.castToReference(value)); // Reference 5336 return value; 5337 case -445338488: // groupIdentifier 5338 this.groupIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 5339 return value; 5340 case -892481550: // status 5341 value = new RequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5342 this.status = (Enumeration) value; // Enumeration<RequestStatus> 5343 return value; 5344 case -1183762788: // intent 5345 value = new RequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 5346 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 5347 return value; 5348 case -1165461084: // priority 5349 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 5350 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 5351 return value; 5352 case 3059181: // code 5353 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5354 return value; 5355 case -1867885268: // subject 5356 this.subject = TypeConvertor.castToReference(value); // Reference 5357 return value; 5358 case 1524132147: // encounter 5359 this.encounter = TypeConvertor.castToReference(value); // Reference 5360 return value; 5361 case -1500852503: // authoredOn 5362 this.authoredOn = TypeConvertor.castToDateTime(value); // DateTimeType 5363 return value; 5364 case -1406328437: // author 5365 this.author = TypeConvertor.castToReference(value); // Reference 5366 return value; 5367 case -934964668: // reason 5368 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 5369 return value; 5370 case 3178259: // goal 5371 this.getGoal().add(TypeConvertor.castToReference(value)); // Reference 5372 return value; 5373 case 3387378: // note 5374 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 5375 return value; 5376 case -1422950858: // action 5377 this.getAction().add((RequestOrchestrationActionComponent) value); // RequestOrchestrationActionComponent 5378 return value; 5379 default: return super.setProperty(hash, name, value); 5380 } 5381 5382 } 5383 5384 @Override 5385 public Base setProperty(String name, Base value) throws FHIRException { 5386 if (name.equals("identifier")) { 5387 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 5388 } else if (name.equals("instantiatesCanonical")) { 5389 this.getInstantiatesCanonical().add(TypeConvertor.castToCanonical(value)); 5390 } else if (name.equals("instantiatesUri")) { 5391 this.getInstantiatesUri().add(TypeConvertor.castToUri(value)); 5392 } else if (name.equals("basedOn")) { 5393 this.getBasedOn().add(TypeConvertor.castToReference(value)); 5394 } else if (name.equals("replaces")) { 5395 this.getReplaces().add(TypeConvertor.castToReference(value)); 5396 } else if (name.equals("groupIdentifier")) { 5397 this.groupIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 5398 } else if (name.equals("status")) { 5399 value = new RequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5400 this.status = (Enumeration) value; // Enumeration<RequestStatus> 5401 } else if (name.equals("intent")) { 5402 value = new RequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 5403 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 5404 } else if (name.equals("priority")) { 5405 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 5406 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 5407 } else if (name.equals("code")) { 5408 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5409 } else if (name.equals("subject")) { 5410 this.subject = TypeConvertor.castToReference(value); // Reference 5411 } else if (name.equals("encounter")) { 5412 this.encounter = TypeConvertor.castToReference(value); // Reference 5413 } else if (name.equals("authoredOn")) { 5414 this.authoredOn = TypeConvertor.castToDateTime(value); // DateTimeType 5415 } else if (name.equals("author")) { 5416 this.author = TypeConvertor.castToReference(value); // Reference 5417 } else if (name.equals("reason")) { 5418 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 5419 } else if (name.equals("goal")) { 5420 this.getGoal().add(TypeConvertor.castToReference(value)); 5421 } else if (name.equals("note")) { 5422 this.getNote().add(TypeConvertor.castToAnnotation(value)); 5423 } else if (name.equals("action")) { 5424 this.getAction().add((RequestOrchestrationActionComponent) value); 5425 } else 5426 return super.setProperty(name, value); 5427 return value; 5428 } 5429 5430 @Override 5431 public void removeChild(String name, Base value) throws FHIRException { 5432 if (name.equals("identifier")) { 5433 this.getIdentifier().remove(value); 5434 } else if (name.equals("instantiatesCanonical")) { 5435 this.getInstantiatesCanonical().remove(value); 5436 } else if (name.equals("instantiatesUri")) { 5437 this.getInstantiatesUri().remove(value); 5438 } else if (name.equals("basedOn")) { 5439 this.getBasedOn().remove(value); 5440 } else if (name.equals("replaces")) { 5441 this.getReplaces().remove(value); 5442 } else if (name.equals("groupIdentifier")) { 5443 this.groupIdentifier = null; 5444 } else if (name.equals("status")) { 5445 value = new RequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5446 this.status = (Enumeration) value; // Enumeration<RequestStatus> 5447 } else if (name.equals("intent")) { 5448 value = new RequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 5449 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 5450 } else if (name.equals("priority")) { 5451 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 5452 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 5453 } else if (name.equals("code")) { 5454 this.code = null; 5455 } else if (name.equals("subject")) { 5456 this.subject = null; 5457 } else if (name.equals("encounter")) { 5458 this.encounter = null; 5459 } else if (name.equals("authoredOn")) { 5460 this.authoredOn = null; 5461 } else if (name.equals("author")) { 5462 this.author = null; 5463 } else if (name.equals("reason")) { 5464 this.getReason().remove(value); 5465 } else if (name.equals("goal")) { 5466 this.getGoal().remove(value); 5467 } else if (name.equals("note")) { 5468 this.getNote().remove(value); 5469 } else if (name.equals("action")) { 5470 this.getAction().remove((RequestOrchestrationActionComponent) value); 5471 } else 5472 super.removeChild(name, value); 5473 5474 } 5475 5476 @Override 5477 public Base makeProperty(int hash, String name) throws FHIRException { 5478 switch (hash) { 5479 case -1618432855: return addIdentifier(); 5480 case 8911915: return addInstantiatesCanonicalElement(); 5481 case -1926393373: return addInstantiatesUriElement(); 5482 case -332612366: return addBasedOn(); 5483 case -430332865: return addReplaces(); 5484 case -445338488: return getGroupIdentifier(); 5485 case -892481550: return getStatusElement(); 5486 case -1183762788: return getIntentElement(); 5487 case -1165461084: return getPriorityElement(); 5488 case 3059181: return getCode(); 5489 case -1867885268: return getSubject(); 5490 case 1524132147: return getEncounter(); 5491 case -1500852503: return getAuthoredOnElement(); 5492 case -1406328437: return getAuthor(); 5493 case -934964668: return addReason(); 5494 case 3178259: return addGoal(); 5495 case 3387378: return addNote(); 5496 case -1422950858: return addAction(); 5497 default: return super.makeProperty(hash, name); 5498 } 5499 5500 } 5501 5502 @Override 5503 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5504 switch (hash) { 5505 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 5506 case 8911915: /*instantiatesCanonical*/ return new String[] {"canonical"}; 5507 case -1926393373: /*instantiatesUri*/ return new String[] {"uri"}; 5508 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 5509 case -430332865: /*replaces*/ return new String[] {"Reference"}; 5510 case -445338488: /*groupIdentifier*/ return new String[] {"Identifier"}; 5511 case -892481550: /*status*/ return new String[] {"code"}; 5512 case -1183762788: /*intent*/ return new String[] {"code"}; 5513 case -1165461084: /*priority*/ return new String[] {"code"}; 5514 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 5515 case -1867885268: /*subject*/ return new String[] {"Reference"}; 5516 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 5517 case -1500852503: /*authoredOn*/ return new String[] {"dateTime"}; 5518 case -1406328437: /*author*/ return new String[] {"Reference"}; 5519 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 5520 case 3178259: /*goal*/ return new String[] {"Reference"}; 5521 case 3387378: /*note*/ return new String[] {"Annotation"}; 5522 case -1422950858: /*action*/ return new String[] {}; 5523 default: return super.getTypesForProperty(hash, name); 5524 } 5525 5526 } 5527 5528 @Override 5529 public Base addChild(String name) throws FHIRException { 5530 if (name.equals("identifier")) { 5531 return addIdentifier(); 5532 } 5533 else if (name.equals("instantiatesCanonical")) { 5534 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.instantiatesCanonical"); 5535 } 5536 else if (name.equals("instantiatesUri")) { 5537 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.instantiatesUri"); 5538 } 5539 else if (name.equals("basedOn")) { 5540 return addBasedOn(); 5541 } 5542 else if (name.equals("replaces")) { 5543 return addReplaces(); 5544 } 5545 else if (name.equals("groupIdentifier")) { 5546 this.groupIdentifier = new Identifier(); 5547 return this.groupIdentifier; 5548 } 5549 else if (name.equals("status")) { 5550 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.status"); 5551 } 5552 else if (name.equals("intent")) { 5553 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.intent"); 5554 } 5555 else if (name.equals("priority")) { 5556 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.priority"); 5557 } 5558 else if (name.equals("code")) { 5559 this.code = new CodeableConcept(); 5560 return this.code; 5561 } 5562 else if (name.equals("subject")) { 5563 this.subject = new Reference(); 5564 return this.subject; 5565 } 5566 else if (name.equals("encounter")) { 5567 this.encounter = new Reference(); 5568 return this.encounter; 5569 } 5570 else if (name.equals("authoredOn")) { 5571 throw new FHIRException("Cannot call addChild on a singleton property RequestOrchestration.authoredOn"); 5572 } 5573 else if (name.equals("author")) { 5574 this.author = new Reference(); 5575 return this.author; 5576 } 5577 else if (name.equals("reason")) { 5578 return addReason(); 5579 } 5580 else if (name.equals("goal")) { 5581 return addGoal(); 5582 } 5583 else if (name.equals("note")) { 5584 return addNote(); 5585 } 5586 else if (name.equals("action")) { 5587 return addAction(); 5588 } 5589 else 5590 return super.addChild(name); 5591 } 5592 5593 public String fhirType() { 5594 return "RequestOrchestration"; 5595 5596 } 5597 5598 public RequestOrchestration copy() { 5599 RequestOrchestration dst = new RequestOrchestration(); 5600 copyValues(dst); 5601 return dst; 5602 } 5603 5604 public void copyValues(RequestOrchestration dst) { 5605 super.copyValues(dst); 5606 if (identifier != null) { 5607 dst.identifier = new ArrayList<Identifier>(); 5608 for (Identifier i : identifier) 5609 dst.identifier.add(i.copy()); 5610 }; 5611 if (instantiatesCanonical != null) { 5612 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 5613 for (CanonicalType i : instantiatesCanonical) 5614 dst.instantiatesCanonical.add(i.copy()); 5615 }; 5616 if (instantiatesUri != null) { 5617 dst.instantiatesUri = new ArrayList<UriType>(); 5618 for (UriType i : instantiatesUri) 5619 dst.instantiatesUri.add(i.copy()); 5620 }; 5621 if (basedOn != null) { 5622 dst.basedOn = new ArrayList<Reference>(); 5623 for (Reference i : basedOn) 5624 dst.basedOn.add(i.copy()); 5625 }; 5626 if (replaces != null) { 5627 dst.replaces = new ArrayList<Reference>(); 5628 for (Reference i : replaces) 5629 dst.replaces.add(i.copy()); 5630 }; 5631 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 5632 dst.status = status == null ? null : status.copy(); 5633 dst.intent = intent == null ? null : intent.copy(); 5634 dst.priority = priority == null ? null : priority.copy(); 5635 dst.code = code == null ? null : code.copy(); 5636 dst.subject = subject == null ? null : subject.copy(); 5637 dst.encounter = encounter == null ? null : encounter.copy(); 5638 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 5639 dst.author = author == null ? null : author.copy(); 5640 if (reason != null) { 5641 dst.reason = new ArrayList<CodeableReference>(); 5642 for (CodeableReference i : reason) 5643 dst.reason.add(i.copy()); 5644 }; 5645 if (goal != null) { 5646 dst.goal = new ArrayList<Reference>(); 5647 for (Reference i : goal) 5648 dst.goal.add(i.copy()); 5649 }; 5650 if (note != null) { 5651 dst.note = new ArrayList<Annotation>(); 5652 for (Annotation i : note) 5653 dst.note.add(i.copy()); 5654 }; 5655 if (action != null) { 5656 dst.action = new ArrayList<RequestOrchestrationActionComponent>(); 5657 for (RequestOrchestrationActionComponent i : action) 5658 dst.action.add(i.copy()); 5659 }; 5660 } 5661 5662 protected RequestOrchestration typedCopy() { 5663 return copy(); 5664 } 5665 5666 @Override 5667 public boolean equalsDeep(Base other_) { 5668 if (!super.equalsDeep(other_)) 5669 return false; 5670 if (!(other_ instanceof RequestOrchestration)) 5671 return false; 5672 RequestOrchestration o = (RequestOrchestration) other_; 5673 return compareDeep(identifier, o.identifier, true) && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 5674 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(basedOn, o.basedOn, true) 5675 && compareDeep(replaces, o.replaces, true) && compareDeep(groupIdentifier, o.groupIdentifier, true) 5676 && compareDeep(status, o.status, true) && compareDeep(intent, o.intent, true) && compareDeep(priority, o.priority, true) 5677 && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 5678 && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(author, o.author, true) && compareDeep(reason, o.reason, true) 5679 && compareDeep(goal, o.goal, true) && compareDeep(note, o.note, true) && compareDeep(action, o.action, true) 5680 ; 5681 } 5682 5683 @Override 5684 public boolean equalsShallow(Base other_) { 5685 if (!super.equalsShallow(other_)) 5686 return false; 5687 if (!(other_ instanceof RequestOrchestration)) 5688 return false; 5689 RequestOrchestration o = (RequestOrchestration) other_; 5690 return compareValues(instantiatesCanonical, o.instantiatesCanonical, true) && compareValues(instantiatesUri, o.instantiatesUri, true) 5691 && compareValues(status, o.status, true) && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) 5692 && compareValues(authoredOn, o.authoredOn, true); 5693 } 5694 5695 public boolean isEmpty() { 5696 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical 5697 , instantiatesUri, basedOn, replaces, groupIdentifier, status, intent, priority 5698 , code, subject, encounter, authoredOn, author, reason, goal, note, action 5699 ); 5700 } 5701 5702 @Override 5703 public ResourceType getResourceType() { 5704 return ResourceType.RequestOrchestration; 5705 } 5706 5707 /** 5708 * Search parameter: <b>author</b> 5709 * <p> 5710 * Description: <b>The author of the request orchestration</b><br> 5711 * Type: <b>reference</b><br> 5712 * Path: <b>RequestOrchestration.author</b><br> 5713 * </p> 5714 */ 5715 @SearchParamDefinition(name="author", path="RequestOrchestration.author", description="The author of the request orchestration", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Device.class, Practitioner.class, PractitionerRole.class } ) 5716 public static final String SP_AUTHOR = "author"; 5717 /** 5718 * <b>Fluent Client</b> search parameter constant for <b>author</b> 5719 * <p> 5720 * Description: <b>The author of the request orchestration</b><br> 5721 * Type: <b>reference</b><br> 5722 * Path: <b>RequestOrchestration.author</b><br> 5723 * </p> 5724 */ 5725 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 5726 5727/** 5728 * Constant for fluent queries to be used to add include statements. Specifies 5729 * the path value of "<b>RequestOrchestration:author</b>". 5730 */ 5731 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("RequestOrchestration:author").toLocked(); 5732 5733 /** 5734 * Search parameter: <b>authored</b> 5735 * <p> 5736 * Description: <b>The date the request orchestration was authored</b><br> 5737 * Type: <b>date</b><br> 5738 * Path: <b>RequestOrchestration.authoredOn</b><br> 5739 * </p> 5740 */ 5741 @SearchParamDefinition(name="authored", path="RequestOrchestration.authoredOn", description="The date the request orchestration was authored", type="date" ) 5742 public static final String SP_AUTHORED = "authored"; 5743 /** 5744 * <b>Fluent Client</b> search parameter constant for <b>authored</b> 5745 * <p> 5746 * Description: <b>The date the request orchestration was authored</b><br> 5747 * Type: <b>date</b><br> 5748 * Path: <b>RequestOrchestration.authoredOn</b><br> 5749 * </p> 5750 */ 5751 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHORED); 5752 5753 /** 5754 * Search parameter: <b>based-on</b> 5755 * <p> 5756 * Description: <b>What this request fullfills.</b><br> 5757 * Type: <b>reference</b><br> 5758 * Path: <b>RequestOrchestration.basedOn</b><br> 5759 * </p> 5760 */ 5761 @SearchParamDefinition(name="based-on", path="RequestOrchestration.basedOn", description="What this request fullfills.", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 5762 public static final String SP_BASED_ON = "based-on"; 5763 /** 5764 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 5765 * <p> 5766 * Description: <b>What this request fullfills.</b><br> 5767 * Type: <b>reference</b><br> 5768 * Path: <b>RequestOrchestration.basedOn</b><br> 5769 * </p> 5770 */ 5771 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 5772 5773/** 5774 * Constant for fluent queries to be used to add include statements. Specifies 5775 * the path value of "<b>RequestOrchestration:based-on</b>". 5776 */ 5777 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("RequestOrchestration:based-on").toLocked(); 5778 5779 /** 5780 * Search parameter: <b>group-identifier</b> 5781 * <p> 5782 * Description: <b>The group identifier for the request orchestration</b><br> 5783 * Type: <b>token</b><br> 5784 * Path: <b>RequestOrchestration.groupIdentifier</b><br> 5785 * </p> 5786 */ 5787 @SearchParamDefinition(name="group-identifier", path="RequestOrchestration.groupIdentifier", description="The group identifier for the request orchestration", type="token" ) 5788 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 5789 /** 5790 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 5791 * <p> 5792 * Description: <b>The group identifier for the request orchestration</b><br> 5793 * Type: <b>token</b><br> 5794 * Path: <b>RequestOrchestration.groupIdentifier</b><br> 5795 * </p> 5796 */ 5797 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GROUP_IDENTIFIER); 5798 5799 /** 5800 * Search parameter: <b>instantiates-canonical</b> 5801 * <p> 5802 * Description: <b>The FHIR-based definition from which the request orchestration is realized</b><br> 5803 * Type: <b>reference</b><br> 5804 * Path: <b>RequestOrchestration.instantiatesCanonical</b><br> 5805 * </p> 5806 */ 5807 @SearchParamDefinition(name="instantiates-canonical", path="RequestOrchestration.instantiatesCanonical", description="The FHIR-based definition from which the request orchestration is realized", type="reference", target={ActivityDefinition.class, ActorDefinition.class, CapabilityStatement.class, ChargeItemDefinition.class, Citation.class, CodeSystem.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, ConditionDefinition.class, Contract.class, Device.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, GraphDefinition.class, ImplementationGuide.class, Library.class, Measure.class, MessageDefinition.class, NamingSystem.class, ObservationDefinition.class, OperationDefinition.class, PlanDefinition.class, Questionnaire.class, Requirements.class, ResearchStudy.class, SearchParameter.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, SubscriptionTopic.class, TerminologyCapabilities.class, TestPlan.class, TestScript.class, ValueSet.class } ) 5808 public static final String SP_INSTANTIATES_CANONICAL = "instantiates-canonical"; 5809 /** 5810 * <b>Fluent Client</b> search parameter constant for <b>instantiates-canonical</b> 5811 * <p> 5812 * Description: <b>The FHIR-based definition from which the request orchestration is realized</b><br> 5813 * Type: <b>reference</b><br> 5814 * Path: <b>RequestOrchestration.instantiatesCanonical</b><br> 5815 * </p> 5816 */ 5817 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSTANTIATES_CANONICAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INSTANTIATES_CANONICAL); 5818 5819/** 5820 * Constant for fluent queries to be used to add include statements. Specifies 5821 * the path value of "<b>RequestOrchestration:instantiates-canonical</b>". 5822 */ 5823 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSTANTIATES_CANONICAL = new ca.uhn.fhir.model.api.Include("RequestOrchestration:instantiates-canonical").toLocked(); 5824 5825 /** 5826 * Search parameter: <b>instantiates-uri</b> 5827 * <p> 5828 * Description: <b>The external definition from which the request orchestration is realized</b><br> 5829 * Type: <b>uri</b><br> 5830 * Path: <b>RequestOrchestration.instantiatesUri</b><br> 5831 * </p> 5832 */ 5833 @SearchParamDefinition(name="instantiates-uri", path="RequestOrchestration.instantiatesUri", description="The external definition from which the request orchestration is realized", type="uri" ) 5834 public static final String SP_INSTANTIATES_URI = "instantiates-uri"; 5835 /** 5836 * <b>Fluent Client</b> search parameter constant for <b>instantiates-uri</b> 5837 * <p> 5838 * Description: <b>The external definition from which the request orchestration is realized</b><br> 5839 * Type: <b>uri</b><br> 5840 * Path: <b>RequestOrchestration.instantiatesUri</b><br> 5841 * </p> 5842 */ 5843 public static final ca.uhn.fhir.rest.gclient.UriClientParam INSTANTIATES_URI = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_INSTANTIATES_URI); 5844 5845 /** 5846 * Search parameter: <b>intent</b> 5847 * <p> 5848 * Description: <b>The intent of the request orchestration</b><br> 5849 * Type: <b>token</b><br> 5850 * Path: <b>RequestOrchestration.intent</b><br> 5851 * </p> 5852 */ 5853 @SearchParamDefinition(name="intent", path="RequestOrchestration.intent", description="The intent of the request orchestration", type="token" ) 5854 public static final String SP_INTENT = "intent"; 5855 /** 5856 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 5857 * <p> 5858 * Description: <b>The intent of the request orchestration</b><br> 5859 * Type: <b>token</b><br> 5860 * Path: <b>RequestOrchestration.intent</b><br> 5861 * </p> 5862 */ 5863 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INTENT); 5864 5865 /** 5866 * Search parameter: <b>participant</b> 5867 * <p> 5868 * Description: <b>The participant in the requests in the orchestration</b><br> 5869 * Type: <b>reference</b><br> 5870 * Path: <b>RequestOrchestration.action.participant.actor.ofType(Reference) | RequestOrchestration.action.participant.actor.ofType(canonical)</b><br> 5871 * </p> 5872 */ 5873 @SearchParamDefinition(name="participant", path="RequestOrchestration.action.participant.actor.ofType(Reference) | RequestOrchestration.action.participant.actor.ofType(canonical)", description="The participant in the requests in the orchestration", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={CapabilityStatement.class, CareTeam.class, Device.class, DeviceDefinition.class, Endpoint.class, Group.class, HealthcareService.class, Location.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 5874 public static final String SP_PARTICIPANT = "participant"; 5875 /** 5876 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 5877 * <p> 5878 * Description: <b>The participant in the requests in the orchestration</b><br> 5879 * Type: <b>reference</b><br> 5880 * Path: <b>RequestOrchestration.action.participant.actor.ofType(Reference) | RequestOrchestration.action.participant.actor.ofType(canonical)</b><br> 5881 * </p> 5882 */ 5883 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARTICIPANT); 5884 5885/** 5886 * Constant for fluent queries to be used to add include statements. Specifies 5887 * the path value of "<b>RequestOrchestration:participant</b>". 5888 */ 5889 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT = new ca.uhn.fhir.model.api.Include("RequestOrchestration:participant").toLocked(); 5890 5891 /** 5892 * Search parameter: <b>priority</b> 5893 * <p> 5894 * Description: <b>The priority of the request orchestration</b><br> 5895 * Type: <b>token</b><br> 5896 * Path: <b>RequestOrchestration.priority</b><br> 5897 * </p> 5898 */ 5899 @SearchParamDefinition(name="priority", path="RequestOrchestration.priority", description="The priority of the request orchestration", type="token" ) 5900 public static final String SP_PRIORITY = "priority"; 5901 /** 5902 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 5903 * <p> 5904 * Description: <b>The priority of the request orchestration</b><br> 5905 * Type: <b>token</b><br> 5906 * Path: <b>RequestOrchestration.priority</b><br> 5907 * </p> 5908 */ 5909 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRIORITY); 5910 5911 /** 5912 * Search parameter: <b>status</b> 5913 * <p> 5914 * Description: <b>The status of the request orchestration</b><br> 5915 * Type: <b>token</b><br> 5916 * Path: <b>RequestOrchestration.status</b><br> 5917 * </p> 5918 */ 5919 @SearchParamDefinition(name="status", path="RequestOrchestration.status", description="The status of the request orchestration", type="token" ) 5920 public static final String SP_STATUS = "status"; 5921 /** 5922 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5923 * <p> 5924 * Description: <b>The status of the request orchestration</b><br> 5925 * Type: <b>token</b><br> 5926 * Path: <b>RequestOrchestration.status</b><br> 5927 * </p> 5928 */ 5929 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 5930 5931 /** 5932 * Search parameter: <b>subject</b> 5933 * <p> 5934 * Description: <b>The subject that the request orchestration is about</b><br> 5935 * Type: <b>reference</b><br> 5936 * Path: <b>RequestOrchestration.subject</b><br> 5937 * </p> 5938 */ 5939 @SearchParamDefinition(name="subject", path="RequestOrchestration.subject", description="The subject that the request orchestration is about", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={CareTeam.class, Device.class, Group.class, HealthcareService.class, Location.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 5940 public static final String SP_SUBJECT = "subject"; 5941 /** 5942 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 5943 * <p> 5944 * Description: <b>The subject that the request orchestration is about</b><br> 5945 * Type: <b>reference</b><br> 5946 * Path: <b>RequestOrchestration.subject</b><br> 5947 * </p> 5948 */ 5949 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 5950 5951/** 5952 * Constant for fluent queries to be used to add include statements. Specifies 5953 * the path value of "<b>RequestOrchestration:subject</b>". 5954 */ 5955 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("RequestOrchestration:subject").toLocked(); 5956 5957 /** 5958 * Search parameter: <b>code</b> 5959 * <p> 5960 * Description: <b>Multiple Resources: 5961 5962* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 5963* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 5964* [AuditEvent](auditevent.html): More specific code for the event 5965* [Basic](basic.html): Kind of Resource 5966* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 5967* [Condition](condition.html): Code for the condition 5968* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 5969* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 5970* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 5971* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 5972* [ImagingSelection](imagingselection.html): The imaging selection status 5973* [List](list.html): What the purpose of this list is 5974* [Medication](medication.html): Returns medications for a specific code 5975* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 5976* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 5977* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 5978* [MedicationStatement](medicationstatement.html): Return statements of this medication code 5979* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 5980* [Observation](observation.html): The code of the observation type 5981* [Procedure](procedure.html): A code to identify a procedure 5982* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 5983* [Task](task.html): Search by task code 5984</b><br> 5985 * Type: <b>token</b><br> 5986 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 5987 * </p> 5988 */ 5989 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 5990 public static final String SP_CODE = "code"; 5991 /** 5992 * <b>Fluent Client</b> search parameter constant for <b>code</b> 5993 * <p> 5994 * Description: <b>Multiple Resources: 5995 5996* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 5997* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 5998* [AuditEvent](auditevent.html): More specific code for the event 5999* [Basic](basic.html): Kind of Resource 6000* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 6001* [Condition](condition.html): Code for the condition 6002* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 6003* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 6004* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 6005* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 6006* [ImagingSelection](imagingselection.html): The imaging selection status 6007* [List](list.html): What the purpose of this list is 6008* [Medication](medication.html): Returns medications for a specific code 6009* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 6010* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 6011* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 6012* [MedicationStatement](medicationstatement.html): Return statements of this medication code 6013* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 6014* [Observation](observation.html): The code of the observation type 6015* [Procedure](procedure.html): A code to identify a procedure 6016* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 6017* [Task](task.html): Search by task code 6018</b><br> 6019 * Type: <b>token</b><br> 6020 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 6021 * </p> 6022 */ 6023 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 6024 6025 /** 6026 * Search parameter: <b>encounter</b> 6027 * <p> 6028 * Description: <b>Multiple Resources: 6029 6030* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 6031* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 6032* [ChargeItem](chargeitem.html): Encounter associated with event 6033* [Claim](claim.html): Encounters associated with a billed line item 6034* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 6035* [Communication](communication.html): The Encounter during which this Communication was created 6036* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 6037* [Composition](composition.html): Context of the Composition 6038* [Condition](condition.html): The Encounter during which this Condition was created 6039* [DeviceRequest](devicerequest.html): Encounter during which request was created 6040* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 6041* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 6042* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 6043* [Flag](flag.html): Alert relevant during encounter 6044* [ImagingStudy](imagingstudy.html): The context of the study 6045* [List](list.html): Context in which list created 6046* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 6047* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 6048* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 6049* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 6050* [Observation](observation.html): Encounter related to the observation 6051* [Procedure](procedure.html): The Encounter during which this Procedure was created 6052* [Provenance](provenance.html): Encounter related to the Provenance 6053* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 6054* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 6055* [RiskAssessment](riskassessment.html): Where was assessment performed? 6056* [ServiceRequest](servicerequest.html): An encounter in which this request is made 6057* [Task](task.html): Search by encounter 6058* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 6059</b><br> 6060 * Type: <b>reference</b><br> 6061 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 6062 * </p> 6063 */ 6064 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 6065 public static final String SP_ENCOUNTER = "encounter"; 6066 /** 6067 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 6068 * <p> 6069 * Description: <b>Multiple Resources: 6070 6071* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 6072* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 6073* [ChargeItem](chargeitem.html): Encounter associated with event 6074* [Claim](claim.html): Encounters associated with a billed line item 6075* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 6076* [Communication](communication.html): The Encounter during which this Communication was created 6077* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 6078* [Composition](composition.html): Context of the Composition 6079* [Condition](condition.html): The Encounter during which this Condition was created 6080* [DeviceRequest](devicerequest.html): Encounter during which request was created 6081* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 6082* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 6083* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 6084* [Flag](flag.html): Alert relevant during encounter 6085* [ImagingStudy](imagingstudy.html): The context of the study 6086* [List](list.html): Context in which list created 6087* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 6088* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 6089* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 6090* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 6091* [Observation](observation.html): Encounter related to the observation 6092* [Procedure](procedure.html): The Encounter during which this Procedure was created 6093* [Provenance](provenance.html): Encounter related to the Provenance 6094* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 6095* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 6096* [RiskAssessment](riskassessment.html): Where was assessment performed? 6097* [ServiceRequest](servicerequest.html): An encounter in which this request is made 6098* [Task](task.html): Search by encounter 6099* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 6100</b><br> 6101 * Type: <b>reference</b><br> 6102 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 6103 * </p> 6104 */ 6105 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 6106 6107/** 6108 * Constant for fluent queries to be used to add include statements. Specifies 6109 * the path value of "<b>RequestOrchestration:encounter</b>". 6110 */ 6111 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("RequestOrchestration:encounter").toLocked(); 6112 6113 /** 6114 * Search parameter: <b>identifier</b> 6115 * <p> 6116 * Description: <b>Multiple Resources: 6117 6118* [Account](account.html): Account number 6119* [AdverseEvent](adverseevent.html): Business identifier for the event 6120* [AllergyIntolerance](allergyintolerance.html): External ids for this item 6121* [Appointment](appointment.html): An Identifier of the Appointment 6122* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 6123* [Basic](basic.html): Business identifier 6124* [BodyStructure](bodystructure.html): Bodystructure identifier 6125* [CarePlan](careplan.html): External Ids for this plan 6126* [CareTeam](careteam.html): External Ids for this team 6127* [ChargeItem](chargeitem.html): Business Identifier for item 6128* [Claim](claim.html): The primary identifier of the financial resource 6129* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 6130* [ClinicalImpression](clinicalimpression.html): Business identifier 6131* [Communication](communication.html): Unique identifier 6132* [CommunicationRequest](communicationrequest.html): Unique identifier 6133* [Composition](composition.html): Version-independent identifier for the Composition 6134* [Condition](condition.html): A unique identifier of the condition record 6135* [Consent](consent.html): Identifier for this record (external references) 6136* [Contract](contract.html): The identity of the contract 6137* [Coverage](coverage.html): The primary identifier of the insured and the coverage 6138* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 6139* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 6140* [DetectedIssue](detectedissue.html): Unique id for the detected issue 6141* [DeviceRequest](devicerequest.html): Business identifier for request/order 6142* [DeviceUsage](deviceusage.html): Search by identifier 6143* [DiagnosticReport](diagnosticreport.html): An identifier for the report 6144* [DocumentReference](documentreference.html): Identifier of the attachment binary 6145* [Encounter](encounter.html): Identifier(s) by which this encounter is known 6146* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 6147* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 6148* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 6149* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 6150* [Flag](flag.html): Business identifier 6151* [Goal](goal.html): External Ids for this goal 6152* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 6153* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 6154* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 6155* [Immunization](immunization.html): Business identifier 6156* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 6157* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 6158* [Invoice](invoice.html): Business Identifier for item 6159* [List](list.html): Business identifier 6160* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 6161* [Medication](medication.html): Returns medications with this external identifier 6162* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 6163* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 6164* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 6165* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 6166* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 6167* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 6168* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 6169* [Observation](observation.html): The unique id for a particular observation 6170* [Person](person.html): A person Identifier 6171* [Procedure](procedure.html): A unique identifier for a procedure 6172* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 6173* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 6174* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 6175* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 6176* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 6177* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 6178* [Specimen](specimen.html): The unique identifier associated with the specimen 6179* [SupplyDelivery](supplydelivery.html): External identifier 6180* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 6181* [Task](task.html): Search for a task instance by its business identifier 6182* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 6183</b><br> 6184 * Type: <b>token</b><br> 6185 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 6186 * </p> 6187 */ 6188 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 6189 public static final String SP_IDENTIFIER = "identifier"; 6190 /** 6191 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 6192 * <p> 6193 * Description: <b>Multiple Resources: 6194 6195* [Account](account.html): Account number 6196* [AdverseEvent](adverseevent.html): Business identifier for the event 6197* [AllergyIntolerance](allergyintolerance.html): External ids for this item 6198* [Appointment](appointment.html): An Identifier of the Appointment 6199* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 6200* [Basic](basic.html): Business identifier 6201* [BodyStructure](bodystructure.html): Bodystructure identifier 6202* [CarePlan](careplan.html): External Ids for this plan 6203* [CareTeam](careteam.html): External Ids for this team 6204* [ChargeItem](chargeitem.html): Business Identifier for item 6205* [Claim](claim.html): The primary identifier of the financial resource 6206* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 6207* [ClinicalImpression](clinicalimpression.html): Business identifier 6208* [Communication](communication.html): Unique identifier 6209* [CommunicationRequest](communicationrequest.html): Unique identifier 6210* [Composition](composition.html): Version-independent identifier for the Composition 6211* [Condition](condition.html): A unique identifier of the condition record 6212* [Consent](consent.html): Identifier for this record (external references) 6213* [Contract](contract.html): The identity of the contract 6214* [Coverage](coverage.html): The primary identifier of the insured and the coverage 6215* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 6216* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 6217* [DetectedIssue](detectedissue.html): Unique id for the detected issue 6218* [DeviceRequest](devicerequest.html): Business identifier for request/order 6219* [DeviceUsage](deviceusage.html): Search by identifier 6220* [DiagnosticReport](diagnosticreport.html): An identifier for the report 6221* [DocumentReference](documentreference.html): Identifier of the attachment binary 6222* [Encounter](encounter.html): Identifier(s) by which this encounter is known 6223* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 6224* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 6225* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 6226* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 6227* [Flag](flag.html): Business identifier 6228* [Goal](goal.html): External Ids for this goal 6229* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 6230* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 6231* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 6232* [Immunization](immunization.html): Business identifier 6233* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 6234* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 6235* [Invoice](invoice.html): Business Identifier for item 6236* [List](list.html): Business identifier 6237* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 6238* [Medication](medication.html): Returns medications with this external identifier 6239* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 6240* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 6241* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 6242* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 6243* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 6244* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 6245* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 6246* [Observation](observation.html): The unique id for a particular observation 6247* [Person](person.html): A person Identifier 6248* [Procedure](procedure.html): A unique identifier for a procedure 6249* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 6250* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 6251* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 6252* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 6253* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 6254* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 6255* [Specimen](specimen.html): The unique identifier associated with the specimen 6256* [SupplyDelivery](supplydelivery.html): External identifier 6257* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 6258* [Task](task.html): Search for a task instance by its business identifier 6259* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 6260</b><br> 6261 * Type: <b>token</b><br> 6262 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 6263 * </p> 6264 */ 6265 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 6266 6267 /** 6268 * Search parameter: <b>patient</b> 6269 * <p> 6270 * Description: <b>Multiple Resources: 6271 6272* [Account](account.html): The entity that caused the expenses 6273* [AdverseEvent](adverseevent.html): Subject impacted by event 6274* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 6275* [Appointment](appointment.html): One of the individuals of the appointment is this patient 6276* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 6277* [AuditEvent](auditevent.html): Where the activity involved patient data 6278* [Basic](basic.html): Identifies the focus of this resource 6279* [BodyStructure](bodystructure.html): Who this is about 6280* [CarePlan](careplan.html): Who the care plan is for 6281* [CareTeam](careteam.html): Who care team is for 6282* [ChargeItem](chargeitem.html): Individual service was done for/to 6283* [Claim](claim.html): Patient receiving the products or services 6284* [ClaimResponse](claimresponse.html): The subject of care 6285* [ClinicalImpression](clinicalimpression.html): Patient assessed 6286* [Communication](communication.html): Focus of message 6287* [CommunicationRequest](communicationrequest.html): Focus of message 6288* [Composition](composition.html): Who and/or what the composition is about 6289* [Condition](condition.html): Who has the condition? 6290* [Consent](consent.html): Who the consent applies to 6291* [Contract](contract.html): The identity of the subject of the contract (if a patient) 6292* [Coverage](coverage.html): Retrieve coverages for a patient 6293* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 6294* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 6295* [DetectedIssue](detectedissue.html): Associated patient 6296* [DeviceRequest](devicerequest.html): Individual the service is ordered for 6297* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 6298* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 6299* [DocumentReference](documentreference.html): Who/what is the subject of the document 6300* [Encounter](encounter.html): The patient present at the encounter 6301* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 6302* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 6303* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 6304* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 6305* [Flag](flag.html): The identity of a subject to list flags for 6306* [Goal](goal.html): Who this goal is intended for 6307* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 6308* [ImagingSelection](imagingselection.html): Who the study is about 6309* [ImagingStudy](imagingstudy.html): Who the study is about 6310* [Immunization](immunization.html): The patient for the vaccination record 6311* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 6312* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 6313* [Invoice](invoice.html): Recipient(s) of goods and services 6314* [List](list.html): If all resources have the same subject 6315* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 6316* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 6317* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 6318* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 6319* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 6320* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 6321* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 6322* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 6323* [Observation](observation.html): The subject that the observation is about (if patient) 6324* [Person](person.html): The Person links to this Patient 6325* [Procedure](procedure.html): Search by subject - a patient 6326* [Provenance](provenance.html): Where the activity involved patient data 6327* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 6328* [RelatedPerson](relatedperson.html): The patient this related person is related to 6329* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 6330* [ResearchSubject](researchsubject.html): Who or what is part of study 6331* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 6332* [ServiceRequest](servicerequest.html): Search by subject - a patient 6333* [Specimen](specimen.html): The patient the specimen comes from 6334* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 6335* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 6336* [Task](task.html): Search by patient 6337* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 6338</b><br> 6339 * Type: <b>reference</b><br> 6340 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 6341 * </p> 6342 */ 6343 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 6344 public static final String SP_PATIENT = "patient"; 6345 /** 6346 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 6347 * <p> 6348 * Description: <b>Multiple Resources: 6349 6350* [Account](account.html): The entity that caused the expenses 6351* [AdverseEvent](adverseevent.html): Subject impacted by event 6352* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 6353* [Appointment](appointment.html): One of the individuals of the appointment is this patient 6354* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 6355* [AuditEvent](auditevent.html): Where the activity involved patient data 6356* [Basic](basic.html): Identifies the focus of this resource 6357* [BodyStructure](bodystructure.html): Who this is about 6358* [CarePlan](careplan.html): Who the care plan is for 6359* [CareTeam](careteam.html): Who care team is for 6360* [ChargeItem](chargeitem.html): Individual service was done for/to 6361* [Claim](claim.html): Patient receiving the products or services 6362* [ClaimResponse](claimresponse.html): The subject of care 6363* [ClinicalImpression](clinicalimpression.html): Patient assessed 6364* [Communication](communication.html): Focus of message 6365* [CommunicationRequest](communicationrequest.html): Focus of message 6366* [Composition](composition.html): Who and/or what the composition is about 6367* [Condition](condition.html): Who has the condition? 6368* [Consent](consent.html): Who the consent applies to 6369* [Contract](contract.html): The identity of the subject of the contract (if a patient) 6370* [Coverage](coverage.html): Retrieve coverages for a patient 6371* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 6372* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 6373* [DetectedIssue](detectedissue.html): Associated patient 6374* [DeviceRequest](devicerequest.html): Individual the service is ordered for 6375* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 6376* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 6377* [DocumentReference](documentreference.html): Who/what is the subject of the document 6378* [Encounter](encounter.html): The patient present at the encounter 6379* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 6380* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 6381* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 6382* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 6383* [Flag](flag.html): The identity of a subject to list flags for 6384* [Goal](goal.html): Who this goal is intended for 6385* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 6386* [ImagingSelection](imagingselection.html): Who the study is about 6387* [ImagingStudy](imagingstudy.html): Who the study is about 6388* [Immunization](immunization.html): The patient for the vaccination record 6389* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 6390* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 6391* [Invoice](invoice.html): Recipient(s) of goods and services 6392* [List](list.html): If all resources have the same subject 6393* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 6394* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 6395* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 6396* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 6397* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 6398* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 6399* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 6400* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 6401* [Observation](observation.html): The subject that the observation is about (if patient) 6402* [Person](person.html): The Person links to this Patient 6403* [Procedure](procedure.html): Search by subject - a patient 6404* [Provenance](provenance.html): Where the activity involved patient data 6405* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 6406* [RelatedPerson](relatedperson.html): The patient this related person is related to 6407* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 6408* [ResearchSubject](researchsubject.html): Who or what is part of study 6409* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 6410* [ServiceRequest](servicerequest.html): Search by subject - a patient 6411* [Specimen](specimen.html): The patient the specimen comes from 6412* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 6413* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 6414* [Task](task.html): Search by patient 6415* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 6416</b><br> 6417 * Type: <b>reference</b><br> 6418 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 6419 * </p> 6420 */ 6421 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 6422 6423/** 6424 * Constant for fluent queries to be used to add include statements. Specifies 6425 * the path value of "<b>RequestOrchestration:patient</b>". 6426 */ 6427 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("RequestOrchestration:patient").toLocked(); 6428 6429 6430} 6431