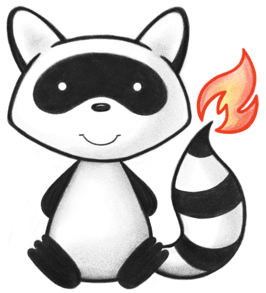
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The Requirements resource is used to describe an actor - a human or an application that plays a role in data exchange, and that may have obligations associated with the role the actor plays. 052 */ 053@ResourceDef(name="Requirements", profile="http://hl7.org/fhir/StructureDefinition/Requirements") 054public class Requirements extends CanonicalResource { 055 056 public enum ConformanceExpectation { 057 /** 058 * Support for the specified capability is required to be considered conformant. 059 */ 060 SHALL, 061 /** 062 * Support for the specified capability is strongly encouraged, and failure to support it should only occur after careful consideration. 063 */ 064 SHOULD, 065 /** 066 * Support for the specified capability is not necessary to be considered conformant, and the requirement should be considered strictly optional. 067 */ 068 MAY, 069 /** 070 * Support for the specified capability is strongly discouraged and should occur only after careful consideration. 071 */ 072 SHOULDNOT, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static ConformanceExpectation fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("SHALL".equals(codeString)) 081 return SHALL; 082 if ("SHOULD".equals(codeString)) 083 return SHOULD; 084 if ("MAY".equals(codeString)) 085 return MAY; 086 if ("SHOULD-NOT".equals(codeString)) 087 return SHOULDNOT; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown ConformanceExpectation code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case SHALL: return "SHALL"; 096 case SHOULD: return "SHOULD"; 097 case MAY: return "MAY"; 098 case SHOULDNOT: return "SHOULD-NOT"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case SHALL: return "http://hl7.org/fhir/conformance-expectation"; 106 case SHOULD: return "http://hl7.org/fhir/conformance-expectation"; 107 case MAY: return "http://hl7.org/fhir/conformance-expectation"; 108 case SHOULDNOT: return "http://hl7.org/fhir/conformance-expectation"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case SHALL: return "Support for the specified capability is required to be considered conformant."; 116 case SHOULD: return "Support for the specified capability is strongly encouraged, and failure to support it should only occur after careful consideration."; 117 case MAY: return "Support for the specified capability is not necessary to be considered conformant, and the requirement should be considered strictly optional."; 118 case SHOULDNOT: return "Support for the specified capability is strongly discouraged and should occur only after careful consideration."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case SHALL: return "SHALL"; 126 case SHOULD: return "SHOULD"; 127 case MAY: return "MAY"; 128 case SHOULDNOT: return "SHOULD-NOT"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class ConformanceExpectationEnumFactory implements EnumFactory<ConformanceExpectation> { 136 public ConformanceExpectation fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("SHALL".equals(codeString)) 141 return ConformanceExpectation.SHALL; 142 if ("SHOULD".equals(codeString)) 143 return ConformanceExpectation.SHOULD; 144 if ("MAY".equals(codeString)) 145 return ConformanceExpectation.MAY; 146 if ("SHOULD-NOT".equals(codeString)) 147 return ConformanceExpectation.SHOULDNOT; 148 throw new IllegalArgumentException("Unknown ConformanceExpectation code '"+codeString+"'"); 149 } 150 public Enumeration<ConformanceExpectation> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<ConformanceExpectation>(this, ConformanceExpectation.NULL, code); 155 String codeString = ((PrimitiveType) code).asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<ConformanceExpectation>(this, ConformanceExpectation.NULL, code); 158 if ("SHALL".equals(codeString)) 159 return new Enumeration<ConformanceExpectation>(this, ConformanceExpectation.SHALL, code); 160 if ("SHOULD".equals(codeString)) 161 return new Enumeration<ConformanceExpectation>(this, ConformanceExpectation.SHOULD, code); 162 if ("MAY".equals(codeString)) 163 return new Enumeration<ConformanceExpectation>(this, ConformanceExpectation.MAY, code); 164 if ("SHOULD-NOT".equals(codeString)) 165 return new Enumeration<ConformanceExpectation>(this, ConformanceExpectation.SHOULDNOT, code); 166 throw new FHIRException("Unknown ConformanceExpectation code '"+codeString+"'"); 167 } 168 public String toCode(ConformanceExpectation code) { 169 if (code == ConformanceExpectation.NULL) 170 return null; 171 if (code == ConformanceExpectation.SHALL) 172 return "SHALL"; 173 if (code == ConformanceExpectation.SHOULD) 174 return "SHOULD"; 175 if (code == ConformanceExpectation.MAY) 176 return "MAY"; 177 if (code == ConformanceExpectation.SHOULDNOT) 178 return "SHOULD-NOT"; 179 return "?"; 180 } 181 public String toSystem(ConformanceExpectation code) { 182 return code.getSystem(); 183 } 184 } 185 186 @Block() 187 public static class RequirementsStatementComponent extends BackboneElement implements IBaseBackboneElement { 188 /** 189 * Key that identifies this statement (unique within this resource). 190 */ 191 @Child(name = "key", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=false) 192 @Description(shortDefinition="Key that identifies this statement", formalDefinition="Key that identifies this statement (unique within this resource)." ) 193 protected IdType key; 194 195 /** 196 * A short human usable label for this statement. 197 */ 198 @Child(name = "label", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 199 @Description(shortDefinition="Short Human label for this statement", formalDefinition="A short human usable label for this statement." ) 200 protected StringType label; 201 202 /** 203 * A short human usable label for this statement. 204 */ 205 @Child(name = "conformance", type = {CodeType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 206 @Description(shortDefinition="SHALL | SHOULD | MAY | SHOULD-NOT", formalDefinition="A short human usable label for this statement." ) 207 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/conformance-expectation") 208 protected List<Enumeration<ConformanceExpectation>> conformance; 209 210 /** 211 * This boolean flag is set to true of the text of the requirement is conditional on something e.g. it includes lanauage like 'if x then y'. This conditionality flag is introduced for purposes of filtering and colour highlighting etc. 212 */ 213 @Child(name = "conditionality", type = {BooleanType.class}, order=4, min=0, max=1, modifier=false, summary=false) 214 @Description(shortDefinition="Set to true if requirements statement is conditional", formalDefinition="This boolean flag is set to true of the text of the requirement is conditional on something e.g. it includes lanauage like 'if x then y'. This conditionality flag is introduced for purposes of filtering and colour highlighting etc." ) 215 protected BooleanType conditionality; 216 217 /** 218 * The actual requirement for human consumption. 219 */ 220 @Child(name = "requirement", type = {MarkdownType.class}, order=5, min=1, max=1, modifier=false, summary=false) 221 @Description(shortDefinition="The actual requirement", formalDefinition="The actual requirement for human consumption." ) 222 protected MarkdownType requirement; 223 224 /** 225 * Another statement on one of the requirements that this requirement clarifies or restricts. 226 */ 227 @Child(name = "derivedFrom", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 228 @Description(shortDefinition="Another statement this clarifies/restricts ([url#]key)", formalDefinition="Another statement on one of the requirements that this requirement clarifies or restricts." ) 229 protected StringType derivedFrom; 230 231 /** 232 * A larger requirement that this requirement helps to refine and enable. 233 */ 234 @Child(name = "parent", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=false) 235 @Description(shortDefinition="A larger requirement that this requirement helps to refine and enable", formalDefinition="A larger requirement that this requirement helps to refine and enable." ) 236 protected StringType parent; 237 238 /** 239 * A reference to another artifact that satisfies this requirement. This could be a Profile, extension, or an element in one of those, or a CapabilityStatement, OperationDefinition, SearchParameter, CodeSystem(/code), ValueSet, Libary etc. 240 */ 241 @Child(name = "satisfiedBy", type = {UrlType.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 242 @Description(shortDefinition="Design artifact that satisfies this requirement", formalDefinition="A reference to another artifact that satisfies this requirement. This could be a Profile, extension, or an element in one of those, or a CapabilityStatement, OperationDefinition, SearchParameter, CodeSystem(/code), ValueSet, Libary etc." ) 243 protected List<UrlType> satisfiedBy; 244 245 /** 246 * A reference to another artifact that created this requirement. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere. 247 */ 248 @Child(name = "reference", type = {UrlType.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 249 @Description(shortDefinition="External artifact (rule/document etc. that) created this requirement", formalDefinition="A reference to another artifact that created this requirement. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere." ) 250 protected List<UrlType> reference; 251 252 /** 253 * Who asked for this statement to be a requirement. By default, it's assumed that the publisher knows who it is if it matters. 254 */ 255 @Child(name = "source", type = {CareTeam.class, Device.class, Group.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 256 @Description(shortDefinition="Who asked for this statement", formalDefinition="Who asked for this statement to be a requirement. By default, it's assumed that the publisher knows who it is if it matters." ) 257 protected List<Reference> source; 258 259 private static final long serialVersionUID = 1299503021L; 260 261 /** 262 * Constructor 263 */ 264 public RequirementsStatementComponent() { 265 super(); 266 } 267 268 /** 269 * Constructor 270 */ 271 public RequirementsStatementComponent(String key, String requirement) { 272 super(); 273 this.setKey(key); 274 this.setRequirement(requirement); 275 } 276 277 /** 278 * @return {@link #key} (Key that identifies this statement (unique within this resource).). This is the underlying object with id, value and extensions. The accessor "getKey" gives direct access to the value 279 */ 280 public IdType getKeyElement() { 281 if (this.key == null) 282 if (Configuration.errorOnAutoCreate()) 283 throw new Error("Attempt to auto-create RequirementsStatementComponent.key"); 284 else if (Configuration.doAutoCreate()) 285 this.key = new IdType(); // bb 286 return this.key; 287 } 288 289 public boolean hasKeyElement() { 290 return this.key != null && !this.key.isEmpty(); 291 } 292 293 public boolean hasKey() { 294 return this.key != null && !this.key.isEmpty(); 295 } 296 297 /** 298 * @param value {@link #key} (Key that identifies this statement (unique within this resource).). This is the underlying object with id, value and extensions. The accessor "getKey" gives direct access to the value 299 */ 300 public RequirementsStatementComponent setKeyElement(IdType value) { 301 this.key = value; 302 return this; 303 } 304 305 /** 306 * @return Key that identifies this statement (unique within this resource). 307 */ 308 public String getKey() { 309 return this.key == null ? null : this.key.getValue(); 310 } 311 312 /** 313 * @param value Key that identifies this statement (unique within this resource). 314 */ 315 public RequirementsStatementComponent setKey(String value) { 316 if (this.key == null) 317 this.key = new IdType(); 318 this.key.setValue(value); 319 return this; 320 } 321 322 /** 323 * @return {@link #label} (A short human usable label for this statement.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 324 */ 325 public StringType getLabelElement() { 326 if (this.label == null) 327 if (Configuration.errorOnAutoCreate()) 328 throw new Error("Attempt to auto-create RequirementsStatementComponent.label"); 329 else if (Configuration.doAutoCreate()) 330 this.label = new StringType(); // bb 331 return this.label; 332 } 333 334 public boolean hasLabelElement() { 335 return this.label != null && !this.label.isEmpty(); 336 } 337 338 public boolean hasLabel() { 339 return this.label != null && !this.label.isEmpty(); 340 } 341 342 /** 343 * @param value {@link #label} (A short human usable label for this statement.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 344 */ 345 public RequirementsStatementComponent setLabelElement(StringType value) { 346 this.label = value; 347 return this; 348 } 349 350 /** 351 * @return A short human usable label for this statement. 352 */ 353 public String getLabel() { 354 return this.label == null ? null : this.label.getValue(); 355 } 356 357 /** 358 * @param value A short human usable label for this statement. 359 */ 360 public RequirementsStatementComponent setLabel(String value) { 361 if (Utilities.noString(value)) 362 this.label = null; 363 else { 364 if (this.label == null) 365 this.label = new StringType(); 366 this.label.setValue(value); 367 } 368 return this; 369 } 370 371 /** 372 * @return {@link #conformance} (A short human usable label for this statement.) 373 */ 374 public List<Enumeration<ConformanceExpectation>> getConformance() { 375 if (this.conformance == null) 376 this.conformance = new ArrayList<Enumeration<ConformanceExpectation>>(); 377 return this.conformance; 378 } 379 380 /** 381 * @return Returns a reference to <code>this</code> for easy method chaining 382 */ 383 public RequirementsStatementComponent setConformance(List<Enumeration<ConformanceExpectation>> theConformance) { 384 this.conformance = theConformance; 385 return this; 386 } 387 388 public boolean hasConformance() { 389 if (this.conformance == null) 390 return false; 391 for (Enumeration<ConformanceExpectation> item : this.conformance) 392 if (!item.isEmpty()) 393 return true; 394 return false; 395 } 396 397 /** 398 * @return {@link #conformance} (A short human usable label for this statement.) 399 */ 400 public Enumeration<ConformanceExpectation> addConformanceElement() {//2 401 Enumeration<ConformanceExpectation> t = new Enumeration<ConformanceExpectation>(new ConformanceExpectationEnumFactory()); 402 if (this.conformance == null) 403 this.conformance = new ArrayList<Enumeration<ConformanceExpectation>>(); 404 this.conformance.add(t); 405 return t; 406 } 407 408 /** 409 * @param value {@link #conformance} (A short human usable label for this statement.) 410 */ 411 public RequirementsStatementComponent addConformance(ConformanceExpectation value) { //1 412 Enumeration<ConformanceExpectation> t = new Enumeration<ConformanceExpectation>(new ConformanceExpectationEnumFactory()); 413 t.setValue(value); 414 if (this.conformance == null) 415 this.conformance = new ArrayList<Enumeration<ConformanceExpectation>>(); 416 this.conformance.add(t); 417 return this; 418 } 419 420 /** 421 * @param value {@link #conformance} (A short human usable label for this statement.) 422 */ 423 public boolean hasConformance(ConformanceExpectation value) { 424 if (this.conformance == null) 425 return false; 426 for (Enumeration<ConformanceExpectation> v : this.conformance) 427 if (v.getValue().equals(value)) // code 428 return true; 429 return false; 430 } 431 432 /** 433 * @return {@link #conditionality} (This boolean flag is set to true of the text of the requirement is conditional on something e.g. it includes lanauage like 'if x then y'. This conditionality flag is introduced for purposes of filtering and colour highlighting etc.). This is the underlying object with id, value and extensions. The accessor "getConditionality" gives direct access to the value 434 */ 435 public BooleanType getConditionalityElement() { 436 if (this.conditionality == null) 437 if (Configuration.errorOnAutoCreate()) 438 throw new Error("Attempt to auto-create RequirementsStatementComponent.conditionality"); 439 else if (Configuration.doAutoCreate()) 440 this.conditionality = new BooleanType(); // bb 441 return this.conditionality; 442 } 443 444 public boolean hasConditionalityElement() { 445 return this.conditionality != null && !this.conditionality.isEmpty(); 446 } 447 448 public boolean hasConditionality() { 449 return this.conditionality != null && !this.conditionality.isEmpty(); 450 } 451 452 /** 453 * @param value {@link #conditionality} (This boolean flag is set to true of the text of the requirement is conditional on something e.g. it includes lanauage like 'if x then y'. This conditionality flag is introduced for purposes of filtering and colour highlighting etc.). This is the underlying object with id, value and extensions. The accessor "getConditionality" gives direct access to the value 454 */ 455 public RequirementsStatementComponent setConditionalityElement(BooleanType value) { 456 this.conditionality = value; 457 return this; 458 } 459 460 /** 461 * @return This boolean flag is set to true of the text of the requirement is conditional on something e.g. it includes lanauage like 'if x then y'. This conditionality flag is introduced for purposes of filtering and colour highlighting etc. 462 */ 463 public boolean getConditionality() { 464 return this.conditionality == null || this.conditionality.isEmpty() ? false : this.conditionality.getValue(); 465 } 466 467 /** 468 * @param value This boolean flag is set to true of the text of the requirement is conditional on something e.g. it includes lanauage like 'if x then y'. This conditionality flag is introduced for purposes of filtering and colour highlighting etc. 469 */ 470 public RequirementsStatementComponent setConditionality(boolean value) { 471 if (this.conditionality == null) 472 this.conditionality = new BooleanType(); 473 this.conditionality.setValue(value); 474 return this; 475 } 476 477 /** 478 * @return {@link #requirement} (The actual requirement for human consumption.). This is the underlying object with id, value and extensions. The accessor "getRequirement" gives direct access to the value 479 */ 480 public MarkdownType getRequirementElement() { 481 if (this.requirement == null) 482 if (Configuration.errorOnAutoCreate()) 483 throw new Error("Attempt to auto-create RequirementsStatementComponent.requirement"); 484 else if (Configuration.doAutoCreate()) 485 this.requirement = new MarkdownType(); // bb 486 return this.requirement; 487 } 488 489 public boolean hasRequirementElement() { 490 return this.requirement != null && !this.requirement.isEmpty(); 491 } 492 493 public boolean hasRequirement() { 494 return this.requirement != null && !this.requirement.isEmpty(); 495 } 496 497 /** 498 * @param value {@link #requirement} (The actual requirement for human consumption.). This is the underlying object with id, value and extensions. The accessor "getRequirement" gives direct access to the value 499 */ 500 public RequirementsStatementComponent setRequirementElement(MarkdownType value) { 501 this.requirement = value; 502 return this; 503 } 504 505 /** 506 * @return The actual requirement for human consumption. 507 */ 508 public String getRequirement() { 509 return this.requirement == null ? null : this.requirement.getValue(); 510 } 511 512 /** 513 * @param value The actual requirement for human consumption. 514 */ 515 public RequirementsStatementComponent setRequirement(String value) { 516 if (this.requirement == null) 517 this.requirement = new MarkdownType(); 518 this.requirement.setValue(value); 519 return this; 520 } 521 522 /** 523 * @return {@link #derivedFrom} (Another statement on one of the requirements that this requirement clarifies or restricts.). This is the underlying object with id, value and extensions. The accessor "getDerivedFrom" gives direct access to the value 524 */ 525 public StringType getDerivedFromElement() { 526 if (this.derivedFrom == null) 527 if (Configuration.errorOnAutoCreate()) 528 throw new Error("Attempt to auto-create RequirementsStatementComponent.derivedFrom"); 529 else if (Configuration.doAutoCreate()) 530 this.derivedFrom = new StringType(); // bb 531 return this.derivedFrom; 532 } 533 534 public boolean hasDerivedFromElement() { 535 return this.derivedFrom != null && !this.derivedFrom.isEmpty(); 536 } 537 538 public boolean hasDerivedFrom() { 539 return this.derivedFrom != null && !this.derivedFrom.isEmpty(); 540 } 541 542 /** 543 * @param value {@link #derivedFrom} (Another statement on one of the requirements that this requirement clarifies or restricts.). This is the underlying object with id, value and extensions. The accessor "getDerivedFrom" gives direct access to the value 544 */ 545 public RequirementsStatementComponent setDerivedFromElement(StringType value) { 546 this.derivedFrom = value; 547 return this; 548 } 549 550 /** 551 * @return Another statement on one of the requirements that this requirement clarifies or restricts. 552 */ 553 public String getDerivedFrom() { 554 return this.derivedFrom == null ? null : this.derivedFrom.getValue(); 555 } 556 557 /** 558 * @param value Another statement on one of the requirements that this requirement clarifies or restricts. 559 */ 560 public RequirementsStatementComponent setDerivedFrom(String value) { 561 if (Utilities.noString(value)) 562 this.derivedFrom = null; 563 else { 564 if (this.derivedFrom == null) 565 this.derivedFrom = new StringType(); 566 this.derivedFrom.setValue(value); 567 } 568 return this; 569 } 570 571 /** 572 * @return {@link #parent} (A larger requirement that this requirement helps to refine and enable.). This is the underlying object with id, value and extensions. The accessor "getParent" gives direct access to the value 573 */ 574 public StringType getParentElement() { 575 if (this.parent == null) 576 if (Configuration.errorOnAutoCreate()) 577 throw new Error("Attempt to auto-create RequirementsStatementComponent.parent"); 578 else if (Configuration.doAutoCreate()) 579 this.parent = new StringType(); // bb 580 return this.parent; 581 } 582 583 public boolean hasParentElement() { 584 return this.parent != null && !this.parent.isEmpty(); 585 } 586 587 public boolean hasParent() { 588 return this.parent != null && !this.parent.isEmpty(); 589 } 590 591 /** 592 * @param value {@link #parent} (A larger requirement that this requirement helps to refine and enable.). This is the underlying object with id, value and extensions. The accessor "getParent" gives direct access to the value 593 */ 594 public RequirementsStatementComponent setParentElement(StringType value) { 595 this.parent = value; 596 return this; 597 } 598 599 /** 600 * @return A larger requirement that this requirement helps to refine and enable. 601 */ 602 public String getParent() { 603 return this.parent == null ? null : this.parent.getValue(); 604 } 605 606 /** 607 * @param value A larger requirement that this requirement helps to refine and enable. 608 */ 609 public RequirementsStatementComponent setParent(String value) { 610 if (Utilities.noString(value)) 611 this.parent = null; 612 else { 613 if (this.parent == null) 614 this.parent = new StringType(); 615 this.parent.setValue(value); 616 } 617 return this; 618 } 619 620 /** 621 * @return {@link #satisfiedBy} (A reference to another artifact that satisfies this requirement. This could be a Profile, extension, or an element in one of those, or a CapabilityStatement, OperationDefinition, SearchParameter, CodeSystem(/code), ValueSet, Libary etc.) 622 */ 623 public List<UrlType> getSatisfiedBy() { 624 if (this.satisfiedBy == null) 625 this.satisfiedBy = new ArrayList<UrlType>(); 626 return this.satisfiedBy; 627 } 628 629 /** 630 * @return Returns a reference to <code>this</code> for easy method chaining 631 */ 632 public RequirementsStatementComponent setSatisfiedBy(List<UrlType> theSatisfiedBy) { 633 this.satisfiedBy = theSatisfiedBy; 634 return this; 635 } 636 637 public boolean hasSatisfiedBy() { 638 if (this.satisfiedBy == null) 639 return false; 640 for (UrlType item : this.satisfiedBy) 641 if (!item.isEmpty()) 642 return true; 643 return false; 644 } 645 646 /** 647 * @return {@link #satisfiedBy} (A reference to another artifact that satisfies this requirement. This could be a Profile, extension, or an element in one of those, or a CapabilityStatement, OperationDefinition, SearchParameter, CodeSystem(/code), ValueSet, Libary etc.) 648 */ 649 public UrlType addSatisfiedByElement() {//2 650 UrlType t = new UrlType(); 651 if (this.satisfiedBy == null) 652 this.satisfiedBy = new ArrayList<UrlType>(); 653 this.satisfiedBy.add(t); 654 return t; 655 } 656 657 /** 658 * @param value {@link #satisfiedBy} (A reference to another artifact that satisfies this requirement. This could be a Profile, extension, or an element in one of those, or a CapabilityStatement, OperationDefinition, SearchParameter, CodeSystem(/code), ValueSet, Libary etc.) 659 */ 660 public RequirementsStatementComponent addSatisfiedBy(String value) { //1 661 UrlType t = new UrlType(); 662 t.setValue(value); 663 if (this.satisfiedBy == null) 664 this.satisfiedBy = new ArrayList<UrlType>(); 665 this.satisfiedBy.add(t); 666 return this; 667 } 668 669 /** 670 * @param value {@link #satisfiedBy} (A reference to another artifact that satisfies this requirement. This could be a Profile, extension, or an element in one of those, or a CapabilityStatement, OperationDefinition, SearchParameter, CodeSystem(/code), ValueSet, Libary etc.) 671 */ 672 public boolean hasSatisfiedBy(String value) { 673 if (this.satisfiedBy == null) 674 return false; 675 for (UrlType v : this.satisfiedBy) 676 if (v.getValue().equals(value)) // url 677 return true; 678 return false; 679 } 680 681 /** 682 * @return {@link #reference} (A reference to another artifact that created this requirement. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.) 683 */ 684 public List<UrlType> getReference() { 685 if (this.reference == null) 686 this.reference = new ArrayList<UrlType>(); 687 return this.reference; 688 } 689 690 /** 691 * @return Returns a reference to <code>this</code> for easy method chaining 692 */ 693 public RequirementsStatementComponent setReference(List<UrlType> theReference) { 694 this.reference = theReference; 695 return this; 696 } 697 698 public boolean hasReference() { 699 if (this.reference == null) 700 return false; 701 for (UrlType item : this.reference) 702 if (!item.isEmpty()) 703 return true; 704 return false; 705 } 706 707 /** 708 * @return {@link #reference} (A reference to another artifact that created this requirement. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.) 709 */ 710 public UrlType addReferenceElement() {//2 711 UrlType t = new UrlType(); 712 if (this.reference == null) 713 this.reference = new ArrayList<UrlType>(); 714 this.reference.add(t); 715 return t; 716 } 717 718 /** 719 * @param value {@link #reference} (A reference to another artifact that created this requirement. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.) 720 */ 721 public RequirementsStatementComponent addReference(String value) { //1 722 UrlType t = new UrlType(); 723 t.setValue(value); 724 if (this.reference == null) 725 this.reference = new ArrayList<UrlType>(); 726 this.reference.add(t); 727 return this; 728 } 729 730 /** 731 * @param value {@link #reference} (A reference to another artifact that created this requirement. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.) 732 */ 733 public boolean hasReference(String value) { 734 if (this.reference == null) 735 return false; 736 for (UrlType v : this.reference) 737 if (v.getValue().equals(value)) // url 738 return true; 739 return false; 740 } 741 742 /** 743 * @return {@link #source} (Who asked for this statement to be a requirement. By default, it's assumed that the publisher knows who it is if it matters.) 744 */ 745 public List<Reference> getSource() { 746 if (this.source == null) 747 this.source = new ArrayList<Reference>(); 748 return this.source; 749 } 750 751 /** 752 * @return Returns a reference to <code>this</code> for easy method chaining 753 */ 754 public RequirementsStatementComponent setSource(List<Reference> theSource) { 755 this.source = theSource; 756 return this; 757 } 758 759 public boolean hasSource() { 760 if (this.source == null) 761 return false; 762 for (Reference item : this.source) 763 if (!item.isEmpty()) 764 return true; 765 return false; 766 } 767 768 public Reference addSource() { //3 769 Reference t = new Reference(); 770 if (this.source == null) 771 this.source = new ArrayList<Reference>(); 772 this.source.add(t); 773 return t; 774 } 775 776 public RequirementsStatementComponent addSource(Reference t) { //3 777 if (t == null) 778 return this; 779 if (this.source == null) 780 this.source = new ArrayList<Reference>(); 781 this.source.add(t); 782 return this; 783 } 784 785 /** 786 * @return The first repetition of repeating field {@link #source}, creating it if it does not already exist {3} 787 */ 788 public Reference getSourceFirstRep() { 789 if (getSource().isEmpty()) { 790 addSource(); 791 } 792 return getSource().get(0); 793 } 794 795 protected void listChildren(List<Property> children) { 796 super.listChildren(children); 797 children.add(new Property("key", "id", "Key that identifies this statement (unique within this resource).", 0, 1, key)); 798 children.add(new Property("label", "string", "A short human usable label for this statement.", 0, 1, label)); 799 children.add(new Property("conformance", "code", "A short human usable label for this statement.", 0, java.lang.Integer.MAX_VALUE, conformance)); 800 children.add(new Property("conditionality", "boolean", "This boolean flag is set to true of the text of the requirement is conditional on something e.g. it includes lanauage like 'if x then y'. This conditionality flag is introduced for purposes of filtering and colour highlighting etc.", 0, 1, conditionality)); 801 children.add(new Property("requirement", "markdown", "The actual requirement for human consumption.", 0, 1, requirement)); 802 children.add(new Property("derivedFrom", "string", "Another statement on one of the requirements that this requirement clarifies or restricts.", 0, 1, derivedFrom)); 803 children.add(new Property("parent", "string", "A larger requirement that this requirement helps to refine and enable.", 0, 1, parent)); 804 children.add(new Property("satisfiedBy", "url", "A reference to another artifact that satisfies this requirement. This could be a Profile, extension, or an element in one of those, or a CapabilityStatement, OperationDefinition, SearchParameter, CodeSystem(/code), ValueSet, Libary etc.", 0, java.lang.Integer.MAX_VALUE, satisfiedBy)); 805 children.add(new Property("reference", "url", "A reference to another artifact that created this requirement. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.", 0, java.lang.Integer.MAX_VALUE, reference)); 806 children.add(new Property("source", "Reference(CareTeam|Device|Group|HealthcareService|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "Who asked for this statement to be a requirement. By default, it's assumed that the publisher knows who it is if it matters.", 0, java.lang.Integer.MAX_VALUE, source)); 807 } 808 809 @Override 810 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 811 switch (_hash) { 812 case 106079: /*key*/ return new Property("key", "id", "Key that identifies this statement (unique within this resource).", 0, 1, key); 813 case 102727412: /*label*/ return new Property("label", "string", "A short human usable label for this statement.", 0, 1, label); 814 case 1374858133: /*conformance*/ return new Property("conformance", "code", "A short human usable label for this statement.", 0, java.lang.Integer.MAX_VALUE, conformance); 815 case -389077912: /*conditionality*/ return new Property("conditionality", "boolean", "This boolean flag is set to true of the text of the requirement is conditional on something e.g. it includes lanauage like 'if x then y'. This conditionality flag is introduced for purposes of filtering and colour highlighting etc.", 0, 1, conditionality); 816 case 363387971: /*requirement*/ return new Property("requirement", "markdown", "The actual requirement for human consumption.", 0, 1, requirement); 817 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "string", "Another statement on one of the requirements that this requirement clarifies or restricts.", 0, 1, derivedFrom); 818 case -995424086: /*parent*/ return new Property("parent", "string", "A larger requirement that this requirement helps to refine and enable.", 0, 1, parent); 819 case -1268787159: /*satisfiedBy*/ return new Property("satisfiedBy", "url", "A reference to another artifact that satisfies this requirement. This could be a Profile, extension, or an element in one of those, or a CapabilityStatement, OperationDefinition, SearchParameter, CodeSystem(/code), ValueSet, Libary etc.", 0, java.lang.Integer.MAX_VALUE, satisfiedBy); 820 case -925155509: /*reference*/ return new Property("reference", "url", "A reference to another artifact that created this requirement. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.", 0, java.lang.Integer.MAX_VALUE, reference); 821 case -896505829: /*source*/ return new Property("source", "Reference(CareTeam|Device|Group|HealthcareService|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "Who asked for this statement to be a requirement. By default, it's assumed that the publisher knows who it is if it matters.", 0, java.lang.Integer.MAX_VALUE, source); 822 default: return super.getNamedProperty(_hash, _name, _checkValid); 823 } 824 825 } 826 827 @Override 828 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 829 switch (hash) { 830 case 106079: /*key*/ return this.key == null ? new Base[0] : new Base[] {this.key}; // IdType 831 case 102727412: /*label*/ return this.label == null ? new Base[0] : new Base[] {this.label}; // StringType 832 case 1374858133: /*conformance*/ return this.conformance == null ? new Base[0] : this.conformance.toArray(new Base[this.conformance.size()]); // Enumeration<ConformanceExpectation> 833 case -389077912: /*conditionality*/ return this.conditionality == null ? new Base[0] : new Base[] {this.conditionality}; // BooleanType 834 case 363387971: /*requirement*/ return this.requirement == null ? new Base[0] : new Base[] {this.requirement}; // MarkdownType 835 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : new Base[] {this.derivedFrom}; // StringType 836 case -995424086: /*parent*/ return this.parent == null ? new Base[0] : new Base[] {this.parent}; // StringType 837 case -1268787159: /*satisfiedBy*/ return this.satisfiedBy == null ? new Base[0] : this.satisfiedBy.toArray(new Base[this.satisfiedBy.size()]); // UrlType 838 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : this.reference.toArray(new Base[this.reference.size()]); // UrlType 839 case -896505829: /*source*/ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 840 default: return super.getProperty(hash, name, checkValid); 841 } 842 843 } 844 845 @Override 846 public Base setProperty(int hash, String name, Base value) throws FHIRException { 847 switch (hash) { 848 case 106079: // key 849 this.key = TypeConvertor.castToId(value); // IdType 850 return value; 851 case 102727412: // label 852 this.label = TypeConvertor.castToString(value); // StringType 853 return value; 854 case 1374858133: // conformance 855 value = new ConformanceExpectationEnumFactory().fromType(TypeConvertor.castToCode(value)); 856 this.getConformance().add((Enumeration) value); // Enumeration<ConformanceExpectation> 857 return value; 858 case -389077912: // conditionality 859 this.conditionality = TypeConvertor.castToBoolean(value); // BooleanType 860 return value; 861 case 363387971: // requirement 862 this.requirement = TypeConvertor.castToMarkdown(value); // MarkdownType 863 return value; 864 case 1077922663: // derivedFrom 865 this.derivedFrom = TypeConvertor.castToString(value); // StringType 866 return value; 867 case -995424086: // parent 868 this.parent = TypeConvertor.castToString(value); // StringType 869 return value; 870 case -1268787159: // satisfiedBy 871 this.getSatisfiedBy().add(TypeConvertor.castToUrl(value)); // UrlType 872 return value; 873 case -925155509: // reference 874 this.getReference().add(TypeConvertor.castToUrl(value)); // UrlType 875 return value; 876 case -896505829: // source 877 this.getSource().add(TypeConvertor.castToReference(value)); // Reference 878 return value; 879 default: return super.setProperty(hash, name, value); 880 } 881 882 } 883 884 @Override 885 public Base setProperty(String name, Base value) throws FHIRException { 886 if (name.equals("key")) { 887 this.key = TypeConvertor.castToId(value); // IdType 888 } else if (name.equals("label")) { 889 this.label = TypeConvertor.castToString(value); // StringType 890 } else if (name.equals("conformance")) { 891 value = new ConformanceExpectationEnumFactory().fromType(TypeConvertor.castToCode(value)); 892 this.getConformance().add((Enumeration) value); 893 } else if (name.equals("conditionality")) { 894 this.conditionality = TypeConvertor.castToBoolean(value); // BooleanType 895 } else if (name.equals("requirement")) { 896 this.requirement = TypeConvertor.castToMarkdown(value); // MarkdownType 897 } else if (name.equals("derivedFrom")) { 898 this.derivedFrom = TypeConvertor.castToString(value); // StringType 899 } else if (name.equals("parent")) { 900 this.parent = TypeConvertor.castToString(value); // StringType 901 } else if (name.equals("satisfiedBy")) { 902 this.getSatisfiedBy().add(TypeConvertor.castToUrl(value)); 903 } else if (name.equals("reference")) { 904 this.getReference().add(TypeConvertor.castToUrl(value)); 905 } else if (name.equals("source")) { 906 this.getSource().add(TypeConvertor.castToReference(value)); 907 } else 908 return super.setProperty(name, value); 909 return value; 910 } 911 912 @Override 913 public void removeChild(String name, Base value) throws FHIRException { 914 if (name.equals("key")) { 915 this.key = null; 916 } else if (name.equals("label")) { 917 this.label = null; 918 } else if (name.equals("conformance")) { 919 value = new ConformanceExpectationEnumFactory().fromType(TypeConvertor.castToCode(value)); 920 this.getConformance().remove((Enumeration) value); 921 } else if (name.equals("conditionality")) { 922 this.conditionality = null; 923 } else if (name.equals("requirement")) { 924 this.requirement = null; 925 } else if (name.equals("derivedFrom")) { 926 this.derivedFrom = null; 927 } else if (name.equals("parent")) { 928 this.parent = null; 929 } else if (name.equals("satisfiedBy")) { 930 this.getSatisfiedBy().remove(value); 931 } else if (name.equals("reference")) { 932 this.getReference().remove(value); 933 } else if (name.equals("source")) { 934 this.getSource().remove(value); 935 } else 936 super.removeChild(name, value); 937 938 } 939 940 @Override 941 public Base makeProperty(int hash, String name) throws FHIRException { 942 switch (hash) { 943 case 106079: return getKeyElement(); 944 case 102727412: return getLabelElement(); 945 case 1374858133: return addConformanceElement(); 946 case -389077912: return getConditionalityElement(); 947 case 363387971: return getRequirementElement(); 948 case 1077922663: return getDerivedFromElement(); 949 case -995424086: return getParentElement(); 950 case -1268787159: return addSatisfiedByElement(); 951 case -925155509: return addReferenceElement(); 952 case -896505829: return addSource(); 953 default: return super.makeProperty(hash, name); 954 } 955 956 } 957 958 @Override 959 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 960 switch (hash) { 961 case 106079: /*key*/ return new String[] {"id"}; 962 case 102727412: /*label*/ return new String[] {"string"}; 963 case 1374858133: /*conformance*/ return new String[] {"code"}; 964 case -389077912: /*conditionality*/ return new String[] {"boolean"}; 965 case 363387971: /*requirement*/ return new String[] {"markdown"}; 966 case 1077922663: /*derivedFrom*/ return new String[] {"string"}; 967 case -995424086: /*parent*/ return new String[] {"string"}; 968 case -1268787159: /*satisfiedBy*/ return new String[] {"url"}; 969 case -925155509: /*reference*/ return new String[] {"url"}; 970 case -896505829: /*source*/ return new String[] {"Reference"}; 971 default: return super.getTypesForProperty(hash, name); 972 } 973 974 } 975 976 @Override 977 public Base addChild(String name) throws FHIRException { 978 if (name.equals("key")) { 979 throw new FHIRException("Cannot call addChild on a singleton property Requirements.statement.key"); 980 } 981 else if (name.equals("label")) { 982 throw new FHIRException("Cannot call addChild on a singleton property Requirements.statement.label"); 983 } 984 else if (name.equals("conformance")) { 985 throw new FHIRException("Cannot call addChild on a singleton property Requirements.statement.conformance"); 986 } 987 else if (name.equals("conditionality")) { 988 throw new FHIRException("Cannot call addChild on a singleton property Requirements.statement.conditionality"); 989 } 990 else if (name.equals("requirement")) { 991 throw new FHIRException("Cannot call addChild on a singleton property Requirements.statement.requirement"); 992 } 993 else if (name.equals("derivedFrom")) { 994 throw new FHIRException("Cannot call addChild on a singleton property Requirements.statement.derivedFrom"); 995 } 996 else if (name.equals("parent")) { 997 throw new FHIRException("Cannot call addChild on a singleton property Requirements.statement.parent"); 998 } 999 else if (name.equals("satisfiedBy")) { 1000 throw new FHIRException("Cannot call addChild on a singleton property Requirements.statement.satisfiedBy"); 1001 } 1002 else if (name.equals("reference")) { 1003 throw new FHIRException("Cannot call addChild on a singleton property Requirements.statement.reference"); 1004 } 1005 else if (name.equals("source")) { 1006 return addSource(); 1007 } 1008 else 1009 return super.addChild(name); 1010 } 1011 1012 public RequirementsStatementComponent copy() { 1013 RequirementsStatementComponent dst = new RequirementsStatementComponent(); 1014 copyValues(dst); 1015 return dst; 1016 } 1017 1018 public void copyValues(RequirementsStatementComponent dst) { 1019 super.copyValues(dst); 1020 dst.key = key == null ? null : key.copy(); 1021 dst.label = label == null ? null : label.copy(); 1022 if (conformance != null) { 1023 dst.conformance = new ArrayList<Enumeration<ConformanceExpectation>>(); 1024 for (Enumeration<ConformanceExpectation> i : conformance) 1025 dst.conformance.add(i.copy()); 1026 }; 1027 dst.conditionality = conditionality == null ? null : conditionality.copy(); 1028 dst.requirement = requirement == null ? null : requirement.copy(); 1029 dst.derivedFrom = derivedFrom == null ? null : derivedFrom.copy(); 1030 dst.parent = parent == null ? null : parent.copy(); 1031 if (satisfiedBy != null) { 1032 dst.satisfiedBy = new ArrayList<UrlType>(); 1033 for (UrlType i : satisfiedBy) 1034 dst.satisfiedBy.add(i.copy()); 1035 }; 1036 if (reference != null) { 1037 dst.reference = new ArrayList<UrlType>(); 1038 for (UrlType i : reference) 1039 dst.reference.add(i.copy()); 1040 }; 1041 if (source != null) { 1042 dst.source = new ArrayList<Reference>(); 1043 for (Reference i : source) 1044 dst.source.add(i.copy()); 1045 }; 1046 } 1047 1048 @Override 1049 public boolean equalsDeep(Base other_) { 1050 if (!super.equalsDeep(other_)) 1051 return false; 1052 if (!(other_ instanceof RequirementsStatementComponent)) 1053 return false; 1054 RequirementsStatementComponent o = (RequirementsStatementComponent) other_; 1055 return compareDeep(key, o.key, true) && compareDeep(label, o.label, true) && compareDeep(conformance, o.conformance, true) 1056 && compareDeep(conditionality, o.conditionality, true) && compareDeep(requirement, o.requirement, true) 1057 && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(parent, o.parent, true) && compareDeep(satisfiedBy, o.satisfiedBy, true) 1058 && compareDeep(reference, o.reference, true) && compareDeep(source, o.source, true); 1059 } 1060 1061 @Override 1062 public boolean equalsShallow(Base other_) { 1063 if (!super.equalsShallow(other_)) 1064 return false; 1065 if (!(other_ instanceof RequirementsStatementComponent)) 1066 return false; 1067 RequirementsStatementComponent o = (RequirementsStatementComponent) other_; 1068 return compareValues(key, o.key, true) && compareValues(label, o.label, true) && compareValues(conformance, o.conformance, true) 1069 && compareValues(conditionality, o.conditionality, true) && compareValues(requirement, o.requirement, true) 1070 && compareValues(derivedFrom, o.derivedFrom, true) && compareValues(parent, o.parent, true) && compareValues(satisfiedBy, o.satisfiedBy, true) 1071 && compareValues(reference, o.reference, true); 1072 } 1073 1074 public boolean isEmpty() { 1075 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(key, label, conformance 1076 , conditionality, requirement, derivedFrom, parent, satisfiedBy, reference, source 1077 ); 1078 } 1079 1080 public String fhirType() { 1081 return "Requirements.statement"; 1082 1083 } 1084 1085 } 1086 1087 /** 1088 * An absolute URI that is used to identify this Requirements when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Requirements is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Requirements is stored on different servers. 1089 */ 1090 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 1091 @Description(shortDefinition="Canonical identifier for this Requirements, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this Requirements when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Requirements is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Requirements is stored on different servers." ) 1092 protected UriType url; 1093 1094 /** 1095 * A formal identifier that is used to identify this Requirements when it is represented in other formats, or referenced in a specification, model, design or an instance. 1096 */ 1097 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1098 @Description(shortDefinition="Additional identifier for the Requirements (business identifier)", formalDefinition="A formal identifier that is used to identify this Requirements when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 1099 protected List<Identifier> identifier; 1100 1101 /** 1102 * The identifier that is used to identify this version of the Requirements when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Requirements author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1103 */ 1104 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1105 @Description(shortDefinition="Business version of the Requirements", formalDefinition="The identifier that is used to identify this version of the Requirements when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Requirements author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 1106 protected StringType version; 1107 1108 /** 1109 * Indicates the mechanism used to compare versions to determine which is more current. 1110 */ 1111 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 1112 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 1113 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 1114 protected DataType versionAlgorithm; 1115 1116 /** 1117 * A natural language name identifying the Requirements. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1118 */ 1119 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1120 @Description(shortDefinition="Name for this Requirements (computer friendly)", formalDefinition="A natural language name identifying the Requirements. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 1121 protected StringType name; 1122 1123 /** 1124 * A short, descriptive, user-friendly title for the Requirements. 1125 */ 1126 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1127 @Description(shortDefinition="Name for this Requirements (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the Requirements." ) 1128 protected StringType title; 1129 1130 /** 1131 * The status of this Requirements. Enables tracking the life-cycle of the content. 1132 */ 1133 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 1134 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this Requirements. Enables tracking the life-cycle of the content." ) 1135 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 1136 protected Enumeration<PublicationStatus> status; 1137 1138 /** 1139 * A Boolean value to indicate that this Requirements is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1140 */ 1141 @Child(name = "experimental", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1142 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this Requirements is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 1143 protected BooleanType experimental; 1144 1145 /** 1146 * The date (and optionally time) when the Requirements was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the Requirements changes. 1147 */ 1148 @Child(name = "date", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1149 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the Requirements was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the Requirements changes." ) 1150 protected DateTimeType date; 1151 1152 /** 1153 * The name of the organization or individual responsible for the release and ongoing maintenance of the Requirements. 1154 */ 1155 @Child(name = "publisher", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1156 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the Requirements." ) 1157 protected StringType publisher; 1158 1159 /** 1160 * Contact details to assist a user in finding and communicating with the publisher. 1161 */ 1162 @Child(name = "contact", type = {ContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1163 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 1164 protected List<ContactDetail> contact; 1165 1166 /** 1167 * A free text natural language description of the requirements. 1168 */ 1169 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 1170 @Description(shortDefinition="Natural language description of the requirements", formalDefinition="A free text natural language description of the requirements." ) 1171 protected MarkdownType description; 1172 1173 /** 1174 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate Requirements instances. 1175 */ 1176 @Child(name = "useContext", type = {UsageContext.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1177 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate Requirements instances." ) 1178 protected List<UsageContext> useContext; 1179 1180 /** 1181 * A legal or geographic region in which the Requirements is intended to be used. 1182 */ 1183 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1184 @Description(shortDefinition="Intended jurisdiction for Requirements (if applicable)", formalDefinition="A legal or geographic region in which the Requirements is intended to be used." ) 1185 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 1186 protected List<CodeableConcept> jurisdiction; 1187 1188 /** 1189 * Explanation of why this Requirements is needed and why it has been designed as it has. 1190 */ 1191 @Child(name = "purpose", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 1192 @Description(shortDefinition="Why this Requirements is defined", formalDefinition="Explanation of why this Requirements is needed and why it has been designed as it has." ) 1193 protected MarkdownType purpose; 1194 1195 /** 1196 * A copyright statement relating to the Requirements and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Requirements. 1197 */ 1198 @Child(name = "copyright", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 1199 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the Requirements and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Requirements." ) 1200 protected MarkdownType copyright; 1201 1202 /** 1203 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1204 */ 1205 @Child(name = "copyrightLabel", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 1206 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 1207 protected StringType copyrightLabel; 1208 1209 /** 1210 * Another set of Requirements that this set of Requirements builds on and updates. 1211 */ 1212 @Child(name = "derivedFrom", type = {CanonicalType.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1213 @Description(shortDefinition="Other set of Requirements this builds on", formalDefinition="Another set of Requirements that this set of Requirements builds on and updates." ) 1214 protected List<CanonicalType> derivedFrom; 1215 1216 /** 1217 * A reference to another artifact that created this set of requirements. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere. 1218 */ 1219 @Child(name = "reference", type = {UrlType.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1220 @Description(shortDefinition="External artifact (rule/document etc. that) created this set of requirements", formalDefinition="A reference to another artifact that created this set of requirements. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere." ) 1221 protected List<UrlType> reference; 1222 1223 /** 1224 * An actor these requirements are in regard to. 1225 */ 1226 @Child(name = "actor", type = {CanonicalType.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1227 @Description(shortDefinition="Actor for these requirements", formalDefinition="An actor these requirements are in regard to." ) 1228 protected List<CanonicalType> actor; 1229 1230 /** 1231 * The actual statement of requirement, in markdown format. 1232 */ 1233 @Child(name = "statement", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1234 @Description(shortDefinition="Actual statement as markdown", formalDefinition="The actual statement of requirement, in markdown format." ) 1235 protected List<RequirementsStatementComponent> statement; 1236 1237 private static final long serialVersionUID = -395151267L; 1238 1239 /** 1240 * Constructor 1241 */ 1242 public Requirements() { 1243 super(); 1244 } 1245 1246 /** 1247 * Constructor 1248 */ 1249 public Requirements(PublicationStatus status) { 1250 super(); 1251 this.setStatus(status); 1252 } 1253 1254 /** 1255 * @return {@link #url} (An absolute URI that is used to identify this Requirements when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Requirements is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Requirements is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1256 */ 1257 public UriType getUrlElement() { 1258 if (this.url == null) 1259 if (Configuration.errorOnAutoCreate()) 1260 throw new Error("Attempt to auto-create Requirements.url"); 1261 else if (Configuration.doAutoCreate()) 1262 this.url = new UriType(); // bb 1263 return this.url; 1264 } 1265 1266 public boolean hasUrlElement() { 1267 return this.url != null && !this.url.isEmpty(); 1268 } 1269 1270 public boolean hasUrl() { 1271 return this.url != null && !this.url.isEmpty(); 1272 } 1273 1274 /** 1275 * @param value {@link #url} (An absolute URI that is used to identify this Requirements when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Requirements is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Requirements is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1276 */ 1277 public Requirements setUrlElement(UriType value) { 1278 this.url = value; 1279 return this; 1280 } 1281 1282 /** 1283 * @return An absolute URI that is used to identify this Requirements when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Requirements is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Requirements is stored on different servers. 1284 */ 1285 public String getUrl() { 1286 return this.url == null ? null : this.url.getValue(); 1287 } 1288 1289 /** 1290 * @param value An absolute URI that is used to identify this Requirements when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Requirements is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Requirements is stored on different servers. 1291 */ 1292 public Requirements setUrl(String value) { 1293 if (Utilities.noString(value)) 1294 this.url = null; 1295 else { 1296 if (this.url == null) 1297 this.url = new UriType(); 1298 this.url.setValue(value); 1299 } 1300 return this; 1301 } 1302 1303 /** 1304 * @return {@link #identifier} (A formal identifier that is used to identify this Requirements when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1305 */ 1306 public List<Identifier> getIdentifier() { 1307 if (this.identifier == null) 1308 this.identifier = new ArrayList<Identifier>(); 1309 return this.identifier; 1310 } 1311 1312 /** 1313 * @return Returns a reference to <code>this</code> for easy method chaining 1314 */ 1315 public Requirements setIdentifier(List<Identifier> theIdentifier) { 1316 this.identifier = theIdentifier; 1317 return this; 1318 } 1319 1320 public boolean hasIdentifier() { 1321 if (this.identifier == null) 1322 return false; 1323 for (Identifier item : this.identifier) 1324 if (!item.isEmpty()) 1325 return true; 1326 return false; 1327 } 1328 1329 public Identifier addIdentifier() { //3 1330 Identifier t = new Identifier(); 1331 if (this.identifier == null) 1332 this.identifier = new ArrayList<Identifier>(); 1333 this.identifier.add(t); 1334 return t; 1335 } 1336 1337 public Requirements addIdentifier(Identifier t) { //3 1338 if (t == null) 1339 return this; 1340 if (this.identifier == null) 1341 this.identifier = new ArrayList<Identifier>(); 1342 this.identifier.add(t); 1343 return this; 1344 } 1345 1346 /** 1347 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1348 */ 1349 public Identifier getIdentifierFirstRep() { 1350 if (getIdentifier().isEmpty()) { 1351 addIdentifier(); 1352 } 1353 return getIdentifier().get(0); 1354 } 1355 1356 /** 1357 * @return {@link #version} (The identifier that is used to identify this version of the Requirements when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Requirements author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1358 */ 1359 public StringType getVersionElement() { 1360 if (this.version == null) 1361 if (Configuration.errorOnAutoCreate()) 1362 throw new Error("Attempt to auto-create Requirements.version"); 1363 else if (Configuration.doAutoCreate()) 1364 this.version = new StringType(); // bb 1365 return this.version; 1366 } 1367 1368 public boolean hasVersionElement() { 1369 return this.version != null && !this.version.isEmpty(); 1370 } 1371 1372 public boolean hasVersion() { 1373 return this.version != null && !this.version.isEmpty(); 1374 } 1375 1376 /** 1377 * @param value {@link #version} (The identifier that is used to identify this version of the Requirements when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Requirements author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1378 */ 1379 public Requirements setVersionElement(StringType value) { 1380 this.version = value; 1381 return this; 1382 } 1383 1384 /** 1385 * @return The identifier that is used to identify this version of the Requirements when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Requirements author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1386 */ 1387 public String getVersion() { 1388 return this.version == null ? null : this.version.getValue(); 1389 } 1390 1391 /** 1392 * @param value The identifier that is used to identify this version of the Requirements when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Requirements author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1393 */ 1394 public Requirements setVersion(String value) { 1395 if (Utilities.noString(value)) 1396 this.version = null; 1397 else { 1398 if (this.version == null) 1399 this.version = new StringType(); 1400 this.version.setValue(value); 1401 } 1402 return this; 1403 } 1404 1405 /** 1406 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1407 */ 1408 public DataType getVersionAlgorithm() { 1409 return this.versionAlgorithm; 1410 } 1411 1412 /** 1413 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1414 */ 1415 public StringType getVersionAlgorithmStringType() throws FHIRException { 1416 if (this.versionAlgorithm == null) 1417 this.versionAlgorithm = new StringType(); 1418 if (!(this.versionAlgorithm instanceof StringType)) 1419 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1420 return (StringType) this.versionAlgorithm; 1421 } 1422 1423 public boolean hasVersionAlgorithmStringType() { 1424 return this != null && this.versionAlgorithm instanceof StringType; 1425 } 1426 1427 /** 1428 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1429 */ 1430 public Coding getVersionAlgorithmCoding() throws FHIRException { 1431 if (this.versionAlgorithm == null) 1432 this.versionAlgorithm = new Coding(); 1433 if (!(this.versionAlgorithm instanceof Coding)) 1434 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1435 return (Coding) this.versionAlgorithm; 1436 } 1437 1438 public boolean hasVersionAlgorithmCoding() { 1439 return this != null && this.versionAlgorithm instanceof Coding; 1440 } 1441 1442 public boolean hasVersionAlgorithm() { 1443 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 1444 } 1445 1446 /** 1447 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1448 */ 1449 public Requirements setVersionAlgorithm(DataType value) { 1450 if (value != null && !(value instanceof StringType || value instanceof Coding)) 1451 throw new FHIRException("Not the right type for Requirements.versionAlgorithm[x]: "+value.fhirType()); 1452 this.versionAlgorithm = value; 1453 return this; 1454 } 1455 1456 /** 1457 * @return {@link #name} (A natural language name identifying the Requirements. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1458 */ 1459 public StringType getNameElement() { 1460 if (this.name == null) 1461 if (Configuration.errorOnAutoCreate()) 1462 throw new Error("Attempt to auto-create Requirements.name"); 1463 else if (Configuration.doAutoCreate()) 1464 this.name = new StringType(); // bb 1465 return this.name; 1466 } 1467 1468 public boolean hasNameElement() { 1469 return this.name != null && !this.name.isEmpty(); 1470 } 1471 1472 public boolean hasName() { 1473 return this.name != null && !this.name.isEmpty(); 1474 } 1475 1476 /** 1477 * @param value {@link #name} (A natural language name identifying the Requirements. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1478 */ 1479 public Requirements setNameElement(StringType value) { 1480 this.name = value; 1481 return this; 1482 } 1483 1484 /** 1485 * @return A natural language name identifying the Requirements. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1486 */ 1487 public String getName() { 1488 return this.name == null ? null : this.name.getValue(); 1489 } 1490 1491 /** 1492 * @param value A natural language name identifying the Requirements. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1493 */ 1494 public Requirements setName(String value) { 1495 if (Utilities.noString(value)) 1496 this.name = null; 1497 else { 1498 if (this.name == null) 1499 this.name = new StringType(); 1500 this.name.setValue(value); 1501 } 1502 return this; 1503 } 1504 1505 /** 1506 * @return {@link #title} (A short, descriptive, user-friendly title for the Requirements.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1507 */ 1508 public StringType getTitleElement() { 1509 if (this.title == null) 1510 if (Configuration.errorOnAutoCreate()) 1511 throw new Error("Attempt to auto-create Requirements.title"); 1512 else if (Configuration.doAutoCreate()) 1513 this.title = new StringType(); // bb 1514 return this.title; 1515 } 1516 1517 public boolean hasTitleElement() { 1518 return this.title != null && !this.title.isEmpty(); 1519 } 1520 1521 public boolean hasTitle() { 1522 return this.title != null && !this.title.isEmpty(); 1523 } 1524 1525 /** 1526 * @param value {@link #title} (A short, descriptive, user-friendly title for the Requirements.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1527 */ 1528 public Requirements setTitleElement(StringType value) { 1529 this.title = value; 1530 return this; 1531 } 1532 1533 /** 1534 * @return A short, descriptive, user-friendly title for the Requirements. 1535 */ 1536 public String getTitle() { 1537 return this.title == null ? null : this.title.getValue(); 1538 } 1539 1540 /** 1541 * @param value A short, descriptive, user-friendly title for the Requirements. 1542 */ 1543 public Requirements setTitle(String value) { 1544 if (Utilities.noString(value)) 1545 this.title = null; 1546 else { 1547 if (this.title == null) 1548 this.title = new StringType(); 1549 this.title.setValue(value); 1550 } 1551 return this; 1552 } 1553 1554 /** 1555 * @return {@link #status} (The status of this Requirements. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1556 */ 1557 public Enumeration<PublicationStatus> getStatusElement() { 1558 if (this.status == null) 1559 if (Configuration.errorOnAutoCreate()) 1560 throw new Error("Attempt to auto-create Requirements.status"); 1561 else if (Configuration.doAutoCreate()) 1562 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1563 return this.status; 1564 } 1565 1566 public boolean hasStatusElement() { 1567 return this.status != null && !this.status.isEmpty(); 1568 } 1569 1570 public boolean hasStatus() { 1571 return this.status != null && !this.status.isEmpty(); 1572 } 1573 1574 /** 1575 * @param value {@link #status} (The status of this Requirements. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1576 */ 1577 public Requirements setStatusElement(Enumeration<PublicationStatus> value) { 1578 this.status = value; 1579 return this; 1580 } 1581 1582 /** 1583 * @return The status of this Requirements. Enables tracking the life-cycle of the content. 1584 */ 1585 public PublicationStatus getStatus() { 1586 return this.status == null ? null : this.status.getValue(); 1587 } 1588 1589 /** 1590 * @param value The status of this Requirements. Enables tracking the life-cycle of the content. 1591 */ 1592 public Requirements setStatus(PublicationStatus value) { 1593 if (this.status == null) 1594 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1595 this.status.setValue(value); 1596 return this; 1597 } 1598 1599 /** 1600 * @return {@link #experimental} (A Boolean value to indicate that this Requirements is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1601 */ 1602 public BooleanType getExperimentalElement() { 1603 if (this.experimental == null) 1604 if (Configuration.errorOnAutoCreate()) 1605 throw new Error("Attempt to auto-create Requirements.experimental"); 1606 else if (Configuration.doAutoCreate()) 1607 this.experimental = new BooleanType(); // bb 1608 return this.experimental; 1609 } 1610 1611 public boolean hasExperimentalElement() { 1612 return this.experimental != null && !this.experimental.isEmpty(); 1613 } 1614 1615 public boolean hasExperimental() { 1616 return this.experimental != null && !this.experimental.isEmpty(); 1617 } 1618 1619 /** 1620 * @param value {@link #experimental} (A Boolean value to indicate that this Requirements is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1621 */ 1622 public Requirements setExperimentalElement(BooleanType value) { 1623 this.experimental = value; 1624 return this; 1625 } 1626 1627 /** 1628 * @return A Boolean value to indicate that this Requirements is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1629 */ 1630 public boolean getExperimental() { 1631 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1632 } 1633 1634 /** 1635 * @param value A Boolean value to indicate that this Requirements is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1636 */ 1637 public Requirements setExperimental(boolean value) { 1638 if (this.experimental == null) 1639 this.experimental = new BooleanType(); 1640 this.experimental.setValue(value); 1641 return this; 1642 } 1643 1644 /** 1645 * @return {@link #date} (The date (and optionally time) when the Requirements was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the Requirements changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1646 */ 1647 public DateTimeType getDateElement() { 1648 if (this.date == null) 1649 if (Configuration.errorOnAutoCreate()) 1650 throw new Error("Attempt to auto-create Requirements.date"); 1651 else if (Configuration.doAutoCreate()) 1652 this.date = new DateTimeType(); // bb 1653 return this.date; 1654 } 1655 1656 public boolean hasDateElement() { 1657 return this.date != null && !this.date.isEmpty(); 1658 } 1659 1660 public boolean hasDate() { 1661 return this.date != null && !this.date.isEmpty(); 1662 } 1663 1664 /** 1665 * @param value {@link #date} (The date (and optionally time) when the Requirements was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the Requirements changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1666 */ 1667 public Requirements setDateElement(DateTimeType value) { 1668 this.date = value; 1669 return this; 1670 } 1671 1672 /** 1673 * @return The date (and optionally time) when the Requirements was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the Requirements changes. 1674 */ 1675 public Date getDate() { 1676 return this.date == null ? null : this.date.getValue(); 1677 } 1678 1679 /** 1680 * @param value The date (and optionally time) when the Requirements was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the Requirements changes. 1681 */ 1682 public Requirements setDate(Date value) { 1683 if (value == null) 1684 this.date = null; 1685 else { 1686 if (this.date == null) 1687 this.date = new DateTimeType(); 1688 this.date.setValue(value); 1689 } 1690 return this; 1691 } 1692 1693 /** 1694 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the Requirements.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1695 */ 1696 public StringType getPublisherElement() { 1697 if (this.publisher == null) 1698 if (Configuration.errorOnAutoCreate()) 1699 throw new Error("Attempt to auto-create Requirements.publisher"); 1700 else if (Configuration.doAutoCreate()) 1701 this.publisher = new StringType(); // bb 1702 return this.publisher; 1703 } 1704 1705 public boolean hasPublisherElement() { 1706 return this.publisher != null && !this.publisher.isEmpty(); 1707 } 1708 1709 public boolean hasPublisher() { 1710 return this.publisher != null && !this.publisher.isEmpty(); 1711 } 1712 1713 /** 1714 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the Requirements.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1715 */ 1716 public Requirements setPublisherElement(StringType value) { 1717 this.publisher = value; 1718 return this; 1719 } 1720 1721 /** 1722 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the Requirements. 1723 */ 1724 public String getPublisher() { 1725 return this.publisher == null ? null : this.publisher.getValue(); 1726 } 1727 1728 /** 1729 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the Requirements. 1730 */ 1731 public Requirements setPublisher(String value) { 1732 if (Utilities.noString(value)) 1733 this.publisher = null; 1734 else { 1735 if (this.publisher == null) 1736 this.publisher = new StringType(); 1737 this.publisher.setValue(value); 1738 } 1739 return this; 1740 } 1741 1742 /** 1743 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 1744 */ 1745 public List<ContactDetail> getContact() { 1746 if (this.contact == null) 1747 this.contact = new ArrayList<ContactDetail>(); 1748 return this.contact; 1749 } 1750 1751 /** 1752 * @return Returns a reference to <code>this</code> for easy method chaining 1753 */ 1754 public Requirements setContact(List<ContactDetail> theContact) { 1755 this.contact = theContact; 1756 return this; 1757 } 1758 1759 public boolean hasContact() { 1760 if (this.contact == null) 1761 return false; 1762 for (ContactDetail item : this.contact) 1763 if (!item.isEmpty()) 1764 return true; 1765 return false; 1766 } 1767 1768 public ContactDetail addContact() { //3 1769 ContactDetail t = new ContactDetail(); 1770 if (this.contact == null) 1771 this.contact = new ArrayList<ContactDetail>(); 1772 this.contact.add(t); 1773 return t; 1774 } 1775 1776 public Requirements addContact(ContactDetail t) { //3 1777 if (t == null) 1778 return this; 1779 if (this.contact == null) 1780 this.contact = new ArrayList<ContactDetail>(); 1781 this.contact.add(t); 1782 return this; 1783 } 1784 1785 /** 1786 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 1787 */ 1788 public ContactDetail getContactFirstRep() { 1789 if (getContact().isEmpty()) { 1790 addContact(); 1791 } 1792 return getContact().get(0); 1793 } 1794 1795 /** 1796 * @return {@link #description} (A free text natural language description of the requirements.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1797 */ 1798 public MarkdownType getDescriptionElement() { 1799 if (this.description == null) 1800 if (Configuration.errorOnAutoCreate()) 1801 throw new Error("Attempt to auto-create Requirements.description"); 1802 else if (Configuration.doAutoCreate()) 1803 this.description = new MarkdownType(); // bb 1804 return this.description; 1805 } 1806 1807 public boolean hasDescriptionElement() { 1808 return this.description != null && !this.description.isEmpty(); 1809 } 1810 1811 public boolean hasDescription() { 1812 return this.description != null && !this.description.isEmpty(); 1813 } 1814 1815 /** 1816 * @param value {@link #description} (A free text natural language description of the requirements.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1817 */ 1818 public Requirements setDescriptionElement(MarkdownType value) { 1819 this.description = value; 1820 return this; 1821 } 1822 1823 /** 1824 * @return A free text natural language description of the requirements. 1825 */ 1826 public String getDescription() { 1827 return this.description == null ? null : this.description.getValue(); 1828 } 1829 1830 /** 1831 * @param value A free text natural language description of the requirements. 1832 */ 1833 public Requirements setDescription(String value) { 1834 if (Utilities.noString(value)) 1835 this.description = null; 1836 else { 1837 if (this.description == null) 1838 this.description = new MarkdownType(); 1839 this.description.setValue(value); 1840 } 1841 return this; 1842 } 1843 1844 /** 1845 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate Requirements instances.) 1846 */ 1847 public List<UsageContext> getUseContext() { 1848 if (this.useContext == null) 1849 this.useContext = new ArrayList<UsageContext>(); 1850 return this.useContext; 1851 } 1852 1853 /** 1854 * @return Returns a reference to <code>this</code> for easy method chaining 1855 */ 1856 public Requirements setUseContext(List<UsageContext> theUseContext) { 1857 this.useContext = theUseContext; 1858 return this; 1859 } 1860 1861 public boolean hasUseContext() { 1862 if (this.useContext == null) 1863 return false; 1864 for (UsageContext item : this.useContext) 1865 if (!item.isEmpty()) 1866 return true; 1867 return false; 1868 } 1869 1870 public UsageContext addUseContext() { //3 1871 UsageContext t = new UsageContext(); 1872 if (this.useContext == null) 1873 this.useContext = new ArrayList<UsageContext>(); 1874 this.useContext.add(t); 1875 return t; 1876 } 1877 1878 public Requirements addUseContext(UsageContext t) { //3 1879 if (t == null) 1880 return this; 1881 if (this.useContext == null) 1882 this.useContext = new ArrayList<UsageContext>(); 1883 this.useContext.add(t); 1884 return this; 1885 } 1886 1887 /** 1888 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 1889 */ 1890 public UsageContext getUseContextFirstRep() { 1891 if (getUseContext().isEmpty()) { 1892 addUseContext(); 1893 } 1894 return getUseContext().get(0); 1895 } 1896 1897 /** 1898 * @return {@link #jurisdiction} (A legal or geographic region in which the Requirements is intended to be used.) 1899 */ 1900 public List<CodeableConcept> getJurisdiction() { 1901 if (this.jurisdiction == null) 1902 this.jurisdiction = new ArrayList<CodeableConcept>(); 1903 return this.jurisdiction; 1904 } 1905 1906 /** 1907 * @return Returns a reference to <code>this</code> for easy method chaining 1908 */ 1909 public Requirements setJurisdiction(List<CodeableConcept> theJurisdiction) { 1910 this.jurisdiction = theJurisdiction; 1911 return this; 1912 } 1913 1914 public boolean hasJurisdiction() { 1915 if (this.jurisdiction == null) 1916 return false; 1917 for (CodeableConcept item : this.jurisdiction) 1918 if (!item.isEmpty()) 1919 return true; 1920 return false; 1921 } 1922 1923 public CodeableConcept addJurisdiction() { //3 1924 CodeableConcept t = new CodeableConcept(); 1925 if (this.jurisdiction == null) 1926 this.jurisdiction = new ArrayList<CodeableConcept>(); 1927 this.jurisdiction.add(t); 1928 return t; 1929 } 1930 1931 public Requirements addJurisdiction(CodeableConcept t) { //3 1932 if (t == null) 1933 return this; 1934 if (this.jurisdiction == null) 1935 this.jurisdiction = new ArrayList<CodeableConcept>(); 1936 this.jurisdiction.add(t); 1937 return this; 1938 } 1939 1940 /** 1941 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 1942 */ 1943 public CodeableConcept getJurisdictionFirstRep() { 1944 if (getJurisdiction().isEmpty()) { 1945 addJurisdiction(); 1946 } 1947 return getJurisdiction().get(0); 1948 } 1949 1950 /** 1951 * @return {@link #purpose} (Explanation of why this Requirements is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1952 */ 1953 public MarkdownType getPurposeElement() { 1954 if (this.purpose == null) 1955 if (Configuration.errorOnAutoCreate()) 1956 throw new Error("Attempt to auto-create Requirements.purpose"); 1957 else if (Configuration.doAutoCreate()) 1958 this.purpose = new MarkdownType(); // bb 1959 return this.purpose; 1960 } 1961 1962 public boolean hasPurposeElement() { 1963 return this.purpose != null && !this.purpose.isEmpty(); 1964 } 1965 1966 public boolean hasPurpose() { 1967 return this.purpose != null && !this.purpose.isEmpty(); 1968 } 1969 1970 /** 1971 * @param value {@link #purpose} (Explanation of why this Requirements is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1972 */ 1973 public Requirements setPurposeElement(MarkdownType value) { 1974 this.purpose = value; 1975 return this; 1976 } 1977 1978 /** 1979 * @return Explanation of why this Requirements is needed and why it has been designed as it has. 1980 */ 1981 public String getPurpose() { 1982 return this.purpose == null ? null : this.purpose.getValue(); 1983 } 1984 1985 /** 1986 * @param value Explanation of why this Requirements is needed and why it has been designed as it has. 1987 */ 1988 public Requirements setPurpose(String value) { 1989 if (Utilities.noString(value)) 1990 this.purpose = null; 1991 else { 1992 if (this.purpose == null) 1993 this.purpose = new MarkdownType(); 1994 this.purpose.setValue(value); 1995 } 1996 return this; 1997 } 1998 1999 /** 2000 * @return {@link #copyright} (A copyright statement relating to the Requirements and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Requirements.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 2001 */ 2002 public MarkdownType getCopyrightElement() { 2003 if (this.copyright == null) 2004 if (Configuration.errorOnAutoCreate()) 2005 throw new Error("Attempt to auto-create Requirements.copyright"); 2006 else if (Configuration.doAutoCreate()) 2007 this.copyright = new MarkdownType(); // bb 2008 return this.copyright; 2009 } 2010 2011 public boolean hasCopyrightElement() { 2012 return this.copyright != null && !this.copyright.isEmpty(); 2013 } 2014 2015 public boolean hasCopyright() { 2016 return this.copyright != null && !this.copyright.isEmpty(); 2017 } 2018 2019 /** 2020 * @param value {@link #copyright} (A copyright statement relating to the Requirements and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Requirements.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 2021 */ 2022 public Requirements setCopyrightElement(MarkdownType value) { 2023 this.copyright = value; 2024 return this; 2025 } 2026 2027 /** 2028 * @return A copyright statement relating to the Requirements and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Requirements. 2029 */ 2030 public String getCopyright() { 2031 return this.copyright == null ? null : this.copyright.getValue(); 2032 } 2033 2034 /** 2035 * @param value A copyright statement relating to the Requirements and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Requirements. 2036 */ 2037 public Requirements setCopyright(String value) { 2038 if (Utilities.noString(value)) 2039 this.copyright = null; 2040 else { 2041 if (this.copyright == null) 2042 this.copyright = new MarkdownType(); 2043 this.copyright.setValue(value); 2044 } 2045 return this; 2046 } 2047 2048 /** 2049 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2050 */ 2051 public StringType getCopyrightLabelElement() { 2052 if (this.copyrightLabel == null) 2053 if (Configuration.errorOnAutoCreate()) 2054 throw new Error("Attempt to auto-create Requirements.copyrightLabel"); 2055 else if (Configuration.doAutoCreate()) 2056 this.copyrightLabel = new StringType(); // bb 2057 return this.copyrightLabel; 2058 } 2059 2060 public boolean hasCopyrightLabelElement() { 2061 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2062 } 2063 2064 public boolean hasCopyrightLabel() { 2065 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2066 } 2067 2068 /** 2069 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2070 */ 2071 public Requirements setCopyrightLabelElement(StringType value) { 2072 this.copyrightLabel = value; 2073 return this; 2074 } 2075 2076 /** 2077 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2078 */ 2079 public String getCopyrightLabel() { 2080 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 2081 } 2082 2083 /** 2084 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2085 */ 2086 public Requirements setCopyrightLabel(String value) { 2087 if (Utilities.noString(value)) 2088 this.copyrightLabel = null; 2089 else { 2090 if (this.copyrightLabel == null) 2091 this.copyrightLabel = new StringType(); 2092 this.copyrightLabel.setValue(value); 2093 } 2094 return this; 2095 } 2096 2097 /** 2098 * @return {@link #derivedFrom} (Another set of Requirements that this set of Requirements builds on and updates.) 2099 */ 2100 public List<CanonicalType> getDerivedFrom() { 2101 if (this.derivedFrom == null) 2102 this.derivedFrom = new ArrayList<CanonicalType>(); 2103 return this.derivedFrom; 2104 } 2105 2106 /** 2107 * @return Returns a reference to <code>this</code> for easy method chaining 2108 */ 2109 public Requirements setDerivedFrom(List<CanonicalType> theDerivedFrom) { 2110 this.derivedFrom = theDerivedFrom; 2111 return this; 2112 } 2113 2114 public boolean hasDerivedFrom() { 2115 if (this.derivedFrom == null) 2116 return false; 2117 for (CanonicalType item : this.derivedFrom) 2118 if (!item.isEmpty()) 2119 return true; 2120 return false; 2121 } 2122 2123 /** 2124 * @return {@link #derivedFrom} (Another set of Requirements that this set of Requirements builds on and updates.) 2125 */ 2126 public CanonicalType addDerivedFromElement() {//2 2127 CanonicalType t = new CanonicalType(); 2128 if (this.derivedFrom == null) 2129 this.derivedFrom = new ArrayList<CanonicalType>(); 2130 this.derivedFrom.add(t); 2131 return t; 2132 } 2133 2134 /** 2135 * @param value {@link #derivedFrom} (Another set of Requirements that this set of Requirements builds on and updates.) 2136 */ 2137 public Requirements addDerivedFrom(String value) { //1 2138 CanonicalType t = new CanonicalType(); 2139 t.setValue(value); 2140 if (this.derivedFrom == null) 2141 this.derivedFrom = new ArrayList<CanonicalType>(); 2142 this.derivedFrom.add(t); 2143 return this; 2144 } 2145 2146 /** 2147 * @param value {@link #derivedFrom} (Another set of Requirements that this set of Requirements builds on and updates.) 2148 */ 2149 public boolean hasDerivedFrom(String value) { 2150 if (this.derivedFrom == null) 2151 return false; 2152 for (CanonicalType v : this.derivedFrom) 2153 if (v.getValue().equals(value)) // canonical 2154 return true; 2155 return false; 2156 } 2157 2158 /** 2159 * @return {@link #reference} (A reference to another artifact that created this set of requirements. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.) 2160 */ 2161 public List<UrlType> getReference() { 2162 if (this.reference == null) 2163 this.reference = new ArrayList<UrlType>(); 2164 return this.reference; 2165 } 2166 2167 /** 2168 * @return Returns a reference to <code>this</code> for easy method chaining 2169 */ 2170 public Requirements setReference(List<UrlType> theReference) { 2171 this.reference = theReference; 2172 return this; 2173 } 2174 2175 public boolean hasReference() { 2176 if (this.reference == null) 2177 return false; 2178 for (UrlType item : this.reference) 2179 if (!item.isEmpty()) 2180 return true; 2181 return false; 2182 } 2183 2184 /** 2185 * @return {@link #reference} (A reference to another artifact that created this set of requirements. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.) 2186 */ 2187 public UrlType addReferenceElement() {//2 2188 UrlType t = new UrlType(); 2189 if (this.reference == null) 2190 this.reference = new ArrayList<UrlType>(); 2191 this.reference.add(t); 2192 return t; 2193 } 2194 2195 /** 2196 * @param value {@link #reference} (A reference to another artifact that created this set of requirements. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.) 2197 */ 2198 public Requirements addReference(String value) { //1 2199 UrlType t = new UrlType(); 2200 t.setValue(value); 2201 if (this.reference == null) 2202 this.reference = new ArrayList<UrlType>(); 2203 this.reference.add(t); 2204 return this; 2205 } 2206 2207 /** 2208 * @param value {@link #reference} (A reference to another artifact that created this set of requirements. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.) 2209 */ 2210 public boolean hasReference(String value) { 2211 if (this.reference == null) 2212 return false; 2213 for (UrlType v : this.reference) 2214 if (v.getValue().equals(value)) // url 2215 return true; 2216 return false; 2217 } 2218 2219 /** 2220 * @return {@link #actor} (An actor these requirements are in regard to.) 2221 */ 2222 public List<CanonicalType> getActor() { 2223 if (this.actor == null) 2224 this.actor = new ArrayList<CanonicalType>(); 2225 return this.actor; 2226 } 2227 2228 /** 2229 * @return Returns a reference to <code>this</code> for easy method chaining 2230 */ 2231 public Requirements setActor(List<CanonicalType> theActor) { 2232 this.actor = theActor; 2233 return this; 2234 } 2235 2236 public boolean hasActor() { 2237 if (this.actor == null) 2238 return false; 2239 for (CanonicalType item : this.actor) 2240 if (!item.isEmpty()) 2241 return true; 2242 return false; 2243 } 2244 2245 /** 2246 * @return {@link #actor} (An actor these requirements are in regard to.) 2247 */ 2248 public CanonicalType addActorElement() {//2 2249 CanonicalType t = new CanonicalType(); 2250 if (this.actor == null) 2251 this.actor = new ArrayList<CanonicalType>(); 2252 this.actor.add(t); 2253 return t; 2254 } 2255 2256 /** 2257 * @param value {@link #actor} (An actor these requirements are in regard to.) 2258 */ 2259 public Requirements addActor(String value) { //1 2260 CanonicalType t = new CanonicalType(); 2261 t.setValue(value); 2262 if (this.actor == null) 2263 this.actor = new ArrayList<CanonicalType>(); 2264 this.actor.add(t); 2265 return this; 2266 } 2267 2268 /** 2269 * @param value {@link #actor} (An actor these requirements are in regard to.) 2270 */ 2271 public boolean hasActor(String value) { 2272 if (this.actor == null) 2273 return false; 2274 for (CanonicalType v : this.actor) 2275 if (v.getValue().equals(value)) // canonical 2276 return true; 2277 return false; 2278 } 2279 2280 /** 2281 * @return {@link #statement} (The actual statement of requirement, in markdown format.) 2282 */ 2283 public List<RequirementsStatementComponent> getStatement() { 2284 if (this.statement == null) 2285 this.statement = new ArrayList<RequirementsStatementComponent>(); 2286 return this.statement; 2287 } 2288 2289 /** 2290 * @return Returns a reference to <code>this</code> for easy method chaining 2291 */ 2292 public Requirements setStatement(List<RequirementsStatementComponent> theStatement) { 2293 this.statement = theStatement; 2294 return this; 2295 } 2296 2297 public boolean hasStatement() { 2298 if (this.statement == null) 2299 return false; 2300 for (RequirementsStatementComponent item : this.statement) 2301 if (!item.isEmpty()) 2302 return true; 2303 return false; 2304 } 2305 2306 public RequirementsStatementComponent addStatement() { //3 2307 RequirementsStatementComponent t = new RequirementsStatementComponent(); 2308 if (this.statement == null) 2309 this.statement = new ArrayList<RequirementsStatementComponent>(); 2310 this.statement.add(t); 2311 return t; 2312 } 2313 2314 public Requirements addStatement(RequirementsStatementComponent t) { //3 2315 if (t == null) 2316 return this; 2317 if (this.statement == null) 2318 this.statement = new ArrayList<RequirementsStatementComponent>(); 2319 this.statement.add(t); 2320 return this; 2321 } 2322 2323 /** 2324 * @return The first repetition of repeating field {@link #statement}, creating it if it does not already exist {3} 2325 */ 2326 public RequirementsStatementComponent getStatementFirstRep() { 2327 if (getStatement().isEmpty()) { 2328 addStatement(); 2329 } 2330 return getStatement().get(0); 2331 } 2332 2333 protected void listChildren(List<Property> children) { 2334 super.listChildren(children); 2335 children.add(new Property("url", "uri", "An absolute URI that is used to identify this Requirements when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Requirements is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Requirements is stored on different servers.", 0, 1, url)); 2336 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this Requirements when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2337 children.add(new Property("version", "string", "The identifier that is used to identify this version of the Requirements when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Requirements author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 2338 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 2339 children.add(new Property("name", "string", "A natural language name identifying the Requirements. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 2340 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the Requirements.", 0, 1, title)); 2341 children.add(new Property("status", "code", "The status of this Requirements. Enables tracking the life-cycle of the content.", 0, 1, status)); 2342 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this Requirements is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 2343 children.add(new Property("date", "dateTime", "The date (and optionally time) when the Requirements was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the Requirements changes.", 0, 1, date)); 2344 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the Requirements.", 0, 1, publisher)); 2345 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 2346 children.add(new Property("description", "markdown", "A free text natural language description of the requirements.", 0, 1, description)); 2347 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate Requirements instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 2348 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the Requirements is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 2349 children.add(new Property("purpose", "markdown", "Explanation of why this Requirements is needed and why it has been designed as it has.", 0, 1, purpose)); 2350 children.add(new Property("copyright", "markdown", "A copyright statement relating to the Requirements and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Requirements.", 0, 1, copyright)); 2351 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 2352 children.add(new Property("derivedFrom", "canonical(Requirements)", "Another set of Requirements that this set of Requirements builds on and updates.", 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 2353 children.add(new Property("reference", "url", "A reference to another artifact that created this set of requirements. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.", 0, java.lang.Integer.MAX_VALUE, reference)); 2354 children.add(new Property("actor", "canonical(ActorDefinition)", "An actor these requirements are in regard to.", 0, java.lang.Integer.MAX_VALUE, actor)); 2355 children.add(new Property("statement", "", "The actual statement of requirement, in markdown format.", 0, java.lang.Integer.MAX_VALUE, statement)); 2356 } 2357 2358 @Override 2359 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2360 switch (_hash) { 2361 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this Requirements when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Requirements is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Requirements is stored on different servers.", 0, 1, url); 2362 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this Requirements when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 2363 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the Requirements when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Requirements author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 2364 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2365 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2366 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2367 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2368 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the Requirements. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 2369 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the Requirements.", 0, 1, title); 2370 case -892481550: /*status*/ return new Property("status", "code", "The status of this Requirements. Enables tracking the life-cycle of the content.", 0, 1, status); 2371 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this Requirements is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 2372 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the Requirements was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the Requirements changes.", 0, 1, date); 2373 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the Requirements.", 0, 1, publisher); 2374 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 2375 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the requirements.", 0, 1, description); 2376 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate Requirements instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 2377 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the Requirements is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 2378 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this Requirements is needed and why it has been designed as it has.", 0, 1, purpose); 2379 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the Requirements and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Requirements.", 0, 1, copyright); 2380 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 2381 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "canonical(Requirements)", "Another set of Requirements that this set of Requirements builds on and updates.", 0, java.lang.Integer.MAX_VALUE, derivedFrom); 2382 case -925155509: /*reference*/ return new Property("reference", "url", "A reference to another artifact that created this set of requirements. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.", 0, java.lang.Integer.MAX_VALUE, reference); 2383 case 92645877: /*actor*/ return new Property("actor", "canonical(ActorDefinition)", "An actor these requirements are in regard to.", 0, java.lang.Integer.MAX_VALUE, actor); 2384 case -2085148305: /*statement*/ return new Property("statement", "", "The actual statement of requirement, in markdown format.", 0, java.lang.Integer.MAX_VALUE, statement); 2385 default: return super.getNamedProperty(_hash, _name, _checkValid); 2386 } 2387 2388 } 2389 2390 @Override 2391 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2392 switch (hash) { 2393 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2394 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2395 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2396 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 2397 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2398 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2399 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2400 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 2401 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2402 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 2403 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2404 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2405 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2406 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2407 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 2408 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 2409 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 2410 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // CanonicalType 2411 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : this.reference.toArray(new Base[this.reference.size()]); // UrlType 2412 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : this.actor.toArray(new Base[this.actor.size()]); // CanonicalType 2413 case -2085148305: /*statement*/ return this.statement == null ? new Base[0] : this.statement.toArray(new Base[this.statement.size()]); // RequirementsStatementComponent 2414 default: return super.getProperty(hash, name, checkValid); 2415 } 2416 2417 } 2418 2419 @Override 2420 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2421 switch (hash) { 2422 case 116079: // url 2423 this.url = TypeConvertor.castToUri(value); // UriType 2424 return value; 2425 case -1618432855: // identifier 2426 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2427 return value; 2428 case 351608024: // version 2429 this.version = TypeConvertor.castToString(value); // StringType 2430 return value; 2431 case 1508158071: // versionAlgorithm 2432 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2433 return value; 2434 case 3373707: // name 2435 this.name = TypeConvertor.castToString(value); // StringType 2436 return value; 2437 case 110371416: // title 2438 this.title = TypeConvertor.castToString(value); // StringType 2439 return value; 2440 case -892481550: // status 2441 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2442 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2443 return value; 2444 case -404562712: // experimental 2445 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2446 return value; 2447 case 3076014: // date 2448 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2449 return value; 2450 case 1447404028: // publisher 2451 this.publisher = TypeConvertor.castToString(value); // StringType 2452 return value; 2453 case 951526432: // contact 2454 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2455 return value; 2456 case -1724546052: // description 2457 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2458 return value; 2459 case -669707736: // useContext 2460 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 2461 return value; 2462 case -507075711: // jurisdiction 2463 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2464 return value; 2465 case -220463842: // purpose 2466 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2467 return value; 2468 case 1522889671: // copyright 2469 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2470 return value; 2471 case 765157229: // copyrightLabel 2472 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2473 return value; 2474 case 1077922663: // derivedFrom 2475 this.getDerivedFrom().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2476 return value; 2477 case -925155509: // reference 2478 this.getReference().add(TypeConvertor.castToUrl(value)); // UrlType 2479 return value; 2480 case 92645877: // actor 2481 this.getActor().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2482 return value; 2483 case -2085148305: // statement 2484 this.getStatement().add((RequirementsStatementComponent) value); // RequirementsStatementComponent 2485 return value; 2486 default: return super.setProperty(hash, name, value); 2487 } 2488 2489 } 2490 2491 @Override 2492 public Base setProperty(String name, Base value) throws FHIRException { 2493 if (name.equals("url")) { 2494 this.url = TypeConvertor.castToUri(value); // UriType 2495 } else if (name.equals("identifier")) { 2496 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2497 } else if (name.equals("version")) { 2498 this.version = TypeConvertor.castToString(value); // StringType 2499 } else if (name.equals("versionAlgorithm[x]")) { 2500 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2501 } else if (name.equals("name")) { 2502 this.name = TypeConvertor.castToString(value); // StringType 2503 } else if (name.equals("title")) { 2504 this.title = TypeConvertor.castToString(value); // StringType 2505 } else if (name.equals("status")) { 2506 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2507 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2508 } else if (name.equals("experimental")) { 2509 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2510 } else if (name.equals("date")) { 2511 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2512 } else if (name.equals("publisher")) { 2513 this.publisher = TypeConvertor.castToString(value); // StringType 2514 } else if (name.equals("contact")) { 2515 this.getContact().add(TypeConvertor.castToContactDetail(value)); 2516 } else if (name.equals("description")) { 2517 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2518 } else if (name.equals("useContext")) { 2519 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 2520 } else if (name.equals("jurisdiction")) { 2521 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 2522 } else if (name.equals("purpose")) { 2523 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2524 } else if (name.equals("copyright")) { 2525 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2526 } else if (name.equals("copyrightLabel")) { 2527 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2528 } else if (name.equals("derivedFrom")) { 2529 this.getDerivedFrom().add(TypeConvertor.castToCanonical(value)); 2530 } else if (name.equals("reference")) { 2531 this.getReference().add(TypeConvertor.castToUrl(value)); 2532 } else if (name.equals("actor")) { 2533 this.getActor().add(TypeConvertor.castToCanonical(value)); 2534 } else if (name.equals("statement")) { 2535 this.getStatement().add((RequirementsStatementComponent) value); 2536 } else 2537 return super.setProperty(name, value); 2538 return value; 2539 } 2540 2541 @Override 2542 public void removeChild(String name, Base value) throws FHIRException { 2543 if (name.equals("url")) { 2544 this.url = null; 2545 } else if (name.equals("identifier")) { 2546 this.getIdentifier().remove(value); 2547 } else if (name.equals("version")) { 2548 this.version = null; 2549 } else if (name.equals("versionAlgorithm[x]")) { 2550 this.versionAlgorithm = null; 2551 } else if (name.equals("name")) { 2552 this.name = null; 2553 } else if (name.equals("title")) { 2554 this.title = null; 2555 } else if (name.equals("status")) { 2556 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2557 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2558 } else if (name.equals("experimental")) { 2559 this.experimental = null; 2560 } else if (name.equals("date")) { 2561 this.date = null; 2562 } else if (name.equals("publisher")) { 2563 this.publisher = null; 2564 } else if (name.equals("contact")) { 2565 this.getContact().remove(value); 2566 } else if (name.equals("description")) { 2567 this.description = null; 2568 } else if (name.equals("useContext")) { 2569 this.getUseContext().remove(value); 2570 } else if (name.equals("jurisdiction")) { 2571 this.getJurisdiction().remove(value); 2572 } else if (name.equals("purpose")) { 2573 this.purpose = null; 2574 } else if (name.equals("copyright")) { 2575 this.copyright = null; 2576 } else if (name.equals("copyrightLabel")) { 2577 this.copyrightLabel = null; 2578 } else if (name.equals("derivedFrom")) { 2579 this.getDerivedFrom().remove(value); 2580 } else if (name.equals("reference")) { 2581 this.getReference().remove(value); 2582 } else if (name.equals("actor")) { 2583 this.getActor().remove(value); 2584 } else if (name.equals("statement")) { 2585 this.getStatement().remove((RequirementsStatementComponent) value); 2586 } else 2587 super.removeChild(name, value); 2588 2589 } 2590 2591 @Override 2592 public Base makeProperty(int hash, String name) throws FHIRException { 2593 switch (hash) { 2594 case 116079: return getUrlElement(); 2595 case -1618432855: return addIdentifier(); 2596 case 351608024: return getVersionElement(); 2597 case -115699031: return getVersionAlgorithm(); 2598 case 1508158071: return getVersionAlgorithm(); 2599 case 3373707: return getNameElement(); 2600 case 110371416: return getTitleElement(); 2601 case -892481550: return getStatusElement(); 2602 case -404562712: return getExperimentalElement(); 2603 case 3076014: return getDateElement(); 2604 case 1447404028: return getPublisherElement(); 2605 case 951526432: return addContact(); 2606 case -1724546052: return getDescriptionElement(); 2607 case -669707736: return addUseContext(); 2608 case -507075711: return addJurisdiction(); 2609 case -220463842: return getPurposeElement(); 2610 case 1522889671: return getCopyrightElement(); 2611 case 765157229: return getCopyrightLabelElement(); 2612 case 1077922663: return addDerivedFromElement(); 2613 case -925155509: return addReferenceElement(); 2614 case 92645877: return addActorElement(); 2615 case -2085148305: return addStatement(); 2616 default: return super.makeProperty(hash, name); 2617 } 2618 2619 } 2620 2621 @Override 2622 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2623 switch (hash) { 2624 case 116079: /*url*/ return new String[] {"uri"}; 2625 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2626 case 351608024: /*version*/ return new String[] {"string"}; 2627 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 2628 case 3373707: /*name*/ return new String[] {"string"}; 2629 case 110371416: /*title*/ return new String[] {"string"}; 2630 case -892481550: /*status*/ return new String[] {"code"}; 2631 case -404562712: /*experimental*/ return new String[] {"boolean"}; 2632 case 3076014: /*date*/ return new String[] {"dateTime"}; 2633 case 1447404028: /*publisher*/ return new String[] {"string"}; 2634 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 2635 case -1724546052: /*description*/ return new String[] {"markdown"}; 2636 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 2637 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 2638 case -220463842: /*purpose*/ return new String[] {"markdown"}; 2639 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 2640 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 2641 case 1077922663: /*derivedFrom*/ return new String[] {"canonical"}; 2642 case -925155509: /*reference*/ return new String[] {"url"}; 2643 case 92645877: /*actor*/ return new String[] {"canonical"}; 2644 case -2085148305: /*statement*/ return new String[] {}; 2645 default: return super.getTypesForProperty(hash, name); 2646 } 2647 2648 } 2649 2650 @Override 2651 public Base addChild(String name) throws FHIRException { 2652 if (name.equals("url")) { 2653 throw new FHIRException("Cannot call addChild on a singleton property Requirements.url"); 2654 } 2655 else if (name.equals("identifier")) { 2656 return addIdentifier(); 2657 } 2658 else if (name.equals("version")) { 2659 throw new FHIRException("Cannot call addChild on a singleton property Requirements.version"); 2660 } 2661 else if (name.equals("versionAlgorithmString")) { 2662 this.versionAlgorithm = new StringType(); 2663 return this.versionAlgorithm; 2664 } 2665 else if (name.equals("versionAlgorithmCoding")) { 2666 this.versionAlgorithm = new Coding(); 2667 return this.versionAlgorithm; 2668 } 2669 else if (name.equals("name")) { 2670 throw new FHIRException("Cannot call addChild on a singleton property Requirements.name"); 2671 } 2672 else if (name.equals("title")) { 2673 throw new FHIRException("Cannot call addChild on a singleton property Requirements.title"); 2674 } 2675 else if (name.equals("status")) { 2676 throw new FHIRException("Cannot call addChild on a singleton property Requirements.status"); 2677 } 2678 else if (name.equals("experimental")) { 2679 throw new FHIRException("Cannot call addChild on a singleton property Requirements.experimental"); 2680 } 2681 else if (name.equals("date")) { 2682 throw new FHIRException("Cannot call addChild on a singleton property Requirements.date"); 2683 } 2684 else if (name.equals("publisher")) { 2685 throw new FHIRException("Cannot call addChild on a singleton property Requirements.publisher"); 2686 } 2687 else if (name.equals("contact")) { 2688 return addContact(); 2689 } 2690 else if (name.equals("description")) { 2691 throw new FHIRException("Cannot call addChild on a singleton property Requirements.description"); 2692 } 2693 else if (name.equals("useContext")) { 2694 return addUseContext(); 2695 } 2696 else if (name.equals("jurisdiction")) { 2697 return addJurisdiction(); 2698 } 2699 else if (name.equals("purpose")) { 2700 throw new FHIRException("Cannot call addChild on a singleton property Requirements.purpose"); 2701 } 2702 else if (name.equals("copyright")) { 2703 throw new FHIRException("Cannot call addChild on a singleton property Requirements.copyright"); 2704 } 2705 else if (name.equals("copyrightLabel")) { 2706 throw new FHIRException("Cannot call addChild on a singleton property Requirements.copyrightLabel"); 2707 } 2708 else if (name.equals("derivedFrom")) { 2709 throw new FHIRException("Cannot call addChild on a singleton property Requirements.derivedFrom"); 2710 } 2711 else if (name.equals("reference")) { 2712 throw new FHIRException("Cannot call addChild on a singleton property Requirements.reference"); 2713 } 2714 else if (name.equals("actor")) { 2715 throw new FHIRException("Cannot call addChild on a singleton property Requirements.actor"); 2716 } 2717 else if (name.equals("statement")) { 2718 return addStatement(); 2719 } 2720 else 2721 return super.addChild(name); 2722 } 2723 2724 public String fhirType() { 2725 return "Requirements"; 2726 2727 } 2728 2729 public Requirements copy() { 2730 Requirements dst = new Requirements(); 2731 copyValues(dst); 2732 return dst; 2733 } 2734 2735 public void copyValues(Requirements dst) { 2736 super.copyValues(dst); 2737 dst.url = url == null ? null : url.copy(); 2738 if (identifier != null) { 2739 dst.identifier = new ArrayList<Identifier>(); 2740 for (Identifier i : identifier) 2741 dst.identifier.add(i.copy()); 2742 }; 2743 dst.version = version == null ? null : version.copy(); 2744 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 2745 dst.name = name == null ? null : name.copy(); 2746 dst.title = title == null ? null : title.copy(); 2747 dst.status = status == null ? null : status.copy(); 2748 dst.experimental = experimental == null ? null : experimental.copy(); 2749 dst.date = date == null ? null : date.copy(); 2750 dst.publisher = publisher == null ? null : publisher.copy(); 2751 if (contact != null) { 2752 dst.contact = new ArrayList<ContactDetail>(); 2753 for (ContactDetail i : contact) 2754 dst.contact.add(i.copy()); 2755 }; 2756 dst.description = description == null ? null : description.copy(); 2757 if (useContext != null) { 2758 dst.useContext = new ArrayList<UsageContext>(); 2759 for (UsageContext i : useContext) 2760 dst.useContext.add(i.copy()); 2761 }; 2762 if (jurisdiction != null) { 2763 dst.jurisdiction = new ArrayList<CodeableConcept>(); 2764 for (CodeableConcept i : jurisdiction) 2765 dst.jurisdiction.add(i.copy()); 2766 }; 2767 dst.purpose = purpose == null ? null : purpose.copy(); 2768 dst.copyright = copyright == null ? null : copyright.copy(); 2769 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 2770 if (derivedFrom != null) { 2771 dst.derivedFrom = new ArrayList<CanonicalType>(); 2772 for (CanonicalType i : derivedFrom) 2773 dst.derivedFrom.add(i.copy()); 2774 }; 2775 if (reference != null) { 2776 dst.reference = new ArrayList<UrlType>(); 2777 for (UrlType i : reference) 2778 dst.reference.add(i.copy()); 2779 }; 2780 if (actor != null) { 2781 dst.actor = new ArrayList<CanonicalType>(); 2782 for (CanonicalType i : actor) 2783 dst.actor.add(i.copy()); 2784 }; 2785 if (statement != null) { 2786 dst.statement = new ArrayList<RequirementsStatementComponent>(); 2787 for (RequirementsStatementComponent i : statement) 2788 dst.statement.add(i.copy()); 2789 }; 2790 } 2791 2792 protected Requirements typedCopy() { 2793 return copy(); 2794 } 2795 2796 @Override 2797 public boolean equalsDeep(Base other_) { 2798 if (!super.equalsDeep(other_)) 2799 return false; 2800 if (!(other_ instanceof Requirements)) 2801 return false; 2802 Requirements o = (Requirements) other_; 2803 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 2804 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 2805 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 2806 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 2807 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 2808 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 2809 && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(reference, o.reference, true) && compareDeep(actor, o.actor, true) 2810 && compareDeep(statement, o.statement, true); 2811 } 2812 2813 @Override 2814 public boolean equalsShallow(Base other_) { 2815 if (!super.equalsShallow(other_)) 2816 return false; 2817 if (!(other_ instanceof Requirements)) 2818 return false; 2819 Requirements o = (Requirements) other_; 2820 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 2821 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 2822 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 2823 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 2824 && compareValues(derivedFrom, o.derivedFrom, true) && compareValues(reference, o.reference, true) && compareValues(actor, o.actor, true) 2825 ; 2826 } 2827 2828 public boolean isEmpty() { 2829 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 2830 , versionAlgorithm, name, title, status, experimental, date, publisher, contact 2831 , description, useContext, jurisdiction, purpose, copyright, copyrightLabel, derivedFrom 2832 , reference, actor, statement); 2833 } 2834 2835 @Override 2836 public ResourceType getResourceType() { 2837 return ResourceType.Requirements; 2838 } 2839 2840 /** 2841 * Search parameter: <b>context-quantity</b> 2842 * <p> 2843 * Description: <b>Multiple Resources: 2844 2845* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 2846* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 2847* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 2848* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 2849* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 2850* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 2851* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 2852* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 2853* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 2854* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 2855* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 2856* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 2857* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 2858* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 2859* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 2860* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 2861* [Library](library.html): A quantity- or range-valued use context assigned to the library 2862* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 2863* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 2864* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 2865* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 2866* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 2867* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 2868* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 2869* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 2870* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 2871* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 2872* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 2873* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 2874* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 2875</b><br> 2876 * Type: <b>quantity</b><br> 2877 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 2878 * </p> 2879 */ 2880 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 2881 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 2882 /** 2883 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 2884 * <p> 2885 * Description: <b>Multiple Resources: 2886 2887* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 2888* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 2889* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 2890* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 2891* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 2892* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 2893* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 2894* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 2895* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 2896* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 2897* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 2898* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 2899* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 2900* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 2901* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 2902* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 2903* [Library](library.html): A quantity- or range-valued use context assigned to the library 2904* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 2905* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 2906* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 2907* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 2908* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 2909* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 2910* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 2911* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 2912* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 2913* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 2914* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 2915* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 2916* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 2917</b><br> 2918 * Type: <b>quantity</b><br> 2919 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 2920 * </p> 2921 */ 2922 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 2923 2924 /** 2925 * Search parameter: <b>context-type-quantity</b> 2926 * <p> 2927 * Description: <b>Multiple Resources: 2928 2929* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 2930* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 2931* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 2932* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 2933* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 2934* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 2935* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 2936* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 2937* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 2938* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 2939* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 2940* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 2941* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 2942* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 2943* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 2944* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 2945* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 2946* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 2947* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 2948* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 2949* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 2950* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 2951* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 2952* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 2953* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 2954* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 2955* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 2956* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 2957* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 2958* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 2959</b><br> 2960 * Type: <b>composite</b><br> 2961 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2962 * </p> 2963 */ 2964 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 2965 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 2966 /** 2967 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 2968 * <p> 2969 * Description: <b>Multiple Resources: 2970 2971* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 2972* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 2973* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 2974* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 2975* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 2976* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 2977* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 2978* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 2979* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 2980* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 2981* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 2982* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 2983* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 2984* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 2985* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 2986* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 2987* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 2988* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 2989* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 2990* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 2991* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 2992* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 2993* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 2994* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 2995* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 2996* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 2997* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 2998* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 2999* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3000* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3001</b><br> 3002 * Type: <b>composite</b><br> 3003 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3004 * </p> 3005 */ 3006 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 3007 3008 /** 3009 * Search parameter: <b>context-type-value</b> 3010 * <p> 3011 * Description: <b>Multiple Resources: 3012 3013* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3014* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3015* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3016* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3017* [Citation](citation.html): A use context type and value assigned to the citation 3018* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3019* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3020* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3021* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3022* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3023* [Evidence](evidence.html): A use context type and value assigned to the evidence 3024* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3025* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3026* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3027* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 3028* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 3029* [Library](library.html): A use context type and value assigned to the library 3030* [Measure](measure.html): A use context type and value assigned to the measure 3031* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 3032* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 3033* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 3034* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 3035* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 3036* [Requirements](requirements.html): A use context type and value assigned to the requirements 3037* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3038* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3039* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3040* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3041* [TestScript](testscript.html): A use context type and value assigned to the test script 3042* [ValueSet](valueset.html): A use context type and value assigned to the value set 3043</b><br> 3044 * Type: <b>composite</b><br> 3045 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3046 * </p> 3047 */ 3048 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 3049 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3050 /** 3051 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3052 * <p> 3053 * Description: <b>Multiple Resources: 3054 3055* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3056* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3057* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3058* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3059* [Citation](citation.html): A use context type and value assigned to the citation 3060* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3061* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3062* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3063* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3064* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3065* [Evidence](evidence.html): A use context type and value assigned to the evidence 3066* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3067* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3068* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3069* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 3070* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 3071* [Library](library.html): A use context type and value assigned to the library 3072* [Measure](measure.html): A use context type and value assigned to the measure 3073* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 3074* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 3075* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 3076* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 3077* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 3078* [Requirements](requirements.html): A use context type and value assigned to the requirements 3079* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3080* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3081* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3082* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3083* [TestScript](testscript.html): A use context type and value assigned to the test script 3084* [ValueSet](valueset.html): A use context type and value assigned to the value set 3085</b><br> 3086 * Type: <b>composite</b><br> 3087 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3088 * </p> 3089 */ 3090 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 3091 3092 /** 3093 * Search parameter: <b>context-type</b> 3094 * <p> 3095 * Description: <b>Multiple Resources: 3096 3097* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3098* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3099* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3100* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3101* [Citation](citation.html): A type of use context assigned to the citation 3102* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3103* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3104* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3105* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3106* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3107* [Evidence](evidence.html): A type of use context assigned to the evidence 3108* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3109* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3110* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3111* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3112* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3113* [Library](library.html): A type of use context assigned to the library 3114* [Measure](measure.html): A type of use context assigned to the measure 3115* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3116* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3117* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3118* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3119* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3120* [Requirements](requirements.html): A type of use context assigned to the requirements 3121* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3122* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3123* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3124* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3125* [TestScript](testscript.html): A type of use context assigned to the test script 3126* [ValueSet](valueset.html): A type of use context assigned to the value set 3127</b><br> 3128 * Type: <b>token</b><br> 3129 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3130 * </p> 3131 */ 3132 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 3133 public static final String SP_CONTEXT_TYPE = "context-type"; 3134 /** 3135 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3136 * <p> 3137 * Description: <b>Multiple Resources: 3138 3139* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3140* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3141* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3142* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3143* [Citation](citation.html): A type of use context assigned to the citation 3144* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3145* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3146* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3147* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3148* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3149* [Evidence](evidence.html): A type of use context assigned to the evidence 3150* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3151* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3152* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3153* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3154* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3155* [Library](library.html): A type of use context assigned to the library 3156* [Measure](measure.html): A type of use context assigned to the measure 3157* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3158* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3159* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3160* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3161* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3162* [Requirements](requirements.html): A type of use context assigned to the requirements 3163* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3164* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3165* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3166* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3167* [TestScript](testscript.html): A type of use context assigned to the test script 3168* [ValueSet](valueset.html): A type of use context assigned to the value set 3169</b><br> 3170 * Type: <b>token</b><br> 3171 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3172 * </p> 3173 */ 3174 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 3175 3176 /** 3177 * Search parameter: <b>context</b> 3178 * <p> 3179 * Description: <b>Multiple Resources: 3180 3181* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3182* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3183* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3184* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3185* [Citation](citation.html): A use context assigned to the citation 3186* [CodeSystem](codesystem.html): A use context assigned to the code system 3187* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3188* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3189* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3190* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3191* [Evidence](evidence.html): A use context assigned to the evidence 3192* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3193* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3194* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3195* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3196* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3197* [Library](library.html): A use context assigned to the library 3198* [Measure](measure.html): A use context assigned to the measure 3199* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3200* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3201* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3202* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3203* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3204* [Requirements](requirements.html): A use context assigned to the requirements 3205* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3206* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3207* [StructureMap](structuremap.html): A use context assigned to the structure map 3208* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3209* [TestScript](testscript.html): A use context assigned to the test script 3210* [ValueSet](valueset.html): A use context assigned to the value set 3211</b><br> 3212 * Type: <b>token</b><br> 3213 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3214 * </p> 3215 */ 3216 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 3217 public static final String SP_CONTEXT = "context"; 3218 /** 3219 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3220 * <p> 3221 * Description: <b>Multiple Resources: 3222 3223* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3224* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3225* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3226* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3227* [Citation](citation.html): A use context assigned to the citation 3228* [CodeSystem](codesystem.html): A use context assigned to the code system 3229* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3230* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3231* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3232* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3233* [Evidence](evidence.html): A use context assigned to the evidence 3234* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3235* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3236* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3237* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3238* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3239* [Library](library.html): A use context assigned to the library 3240* [Measure](measure.html): A use context assigned to the measure 3241* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3242* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3243* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3244* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3245* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3246* [Requirements](requirements.html): A use context assigned to the requirements 3247* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3248* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3249* [StructureMap](structuremap.html): A use context assigned to the structure map 3250* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3251* [TestScript](testscript.html): A use context assigned to the test script 3252* [ValueSet](valueset.html): A use context assigned to the value set 3253</b><br> 3254 * Type: <b>token</b><br> 3255 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3256 * </p> 3257 */ 3258 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 3259 3260 /** 3261 * Search parameter: <b>date</b> 3262 * <p> 3263 * Description: <b>Multiple Resources: 3264 3265* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3266* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3267* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3268* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3269* [Citation](citation.html): The citation publication date 3270* [CodeSystem](codesystem.html): The code system publication date 3271* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3272* [ConceptMap](conceptmap.html): The concept map publication date 3273* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3274* [EventDefinition](eventdefinition.html): The event definition publication date 3275* [Evidence](evidence.html): The evidence publication date 3276* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3277* [ExampleScenario](examplescenario.html): The example scenario publication date 3278* [GraphDefinition](graphdefinition.html): The graph definition publication date 3279* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3280* [Library](library.html): The library publication date 3281* [Measure](measure.html): The measure publication date 3282* [MessageDefinition](messagedefinition.html): The message definition publication date 3283* [NamingSystem](namingsystem.html): The naming system publication date 3284* [OperationDefinition](operationdefinition.html): The operation definition publication date 3285* [PlanDefinition](plandefinition.html): The plan definition publication date 3286* [Questionnaire](questionnaire.html): The questionnaire publication date 3287* [Requirements](requirements.html): The requirements publication date 3288* [SearchParameter](searchparameter.html): The search parameter publication date 3289* [StructureDefinition](structuredefinition.html): The structure definition publication date 3290* [StructureMap](structuremap.html): The structure map publication date 3291* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3292* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3293* [TestScript](testscript.html): The test script publication date 3294* [ValueSet](valueset.html): The value set publication date 3295</b><br> 3296 * Type: <b>date</b><br> 3297 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3298 * </p> 3299 */ 3300 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 3301 public static final String SP_DATE = "date"; 3302 /** 3303 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3304 * <p> 3305 * Description: <b>Multiple Resources: 3306 3307* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3308* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3309* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3310* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3311* [Citation](citation.html): The citation publication date 3312* [CodeSystem](codesystem.html): The code system publication date 3313* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3314* [ConceptMap](conceptmap.html): The concept map publication date 3315* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3316* [EventDefinition](eventdefinition.html): The event definition publication date 3317* [Evidence](evidence.html): The evidence publication date 3318* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3319* [ExampleScenario](examplescenario.html): The example scenario publication date 3320* [GraphDefinition](graphdefinition.html): The graph definition publication date 3321* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3322* [Library](library.html): The library publication date 3323* [Measure](measure.html): The measure publication date 3324* [MessageDefinition](messagedefinition.html): The message definition publication date 3325* [NamingSystem](namingsystem.html): The naming system publication date 3326* [OperationDefinition](operationdefinition.html): The operation definition publication date 3327* [PlanDefinition](plandefinition.html): The plan definition publication date 3328* [Questionnaire](questionnaire.html): The questionnaire publication date 3329* [Requirements](requirements.html): The requirements publication date 3330* [SearchParameter](searchparameter.html): The search parameter publication date 3331* [StructureDefinition](structuredefinition.html): The structure definition publication date 3332* [StructureMap](structuremap.html): The structure map publication date 3333* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3334* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3335* [TestScript](testscript.html): The test script publication date 3336* [ValueSet](valueset.html): The value set publication date 3337</b><br> 3338 * Type: <b>date</b><br> 3339 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3340 * </p> 3341 */ 3342 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3343 3344 /** 3345 * Search parameter: <b>description</b> 3346 * <p> 3347 * Description: <b>Multiple Resources: 3348 3349* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3350* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3351* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3352* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3353* [Citation](citation.html): The description of the citation 3354* [CodeSystem](codesystem.html): The description of the code system 3355* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3356* [ConceptMap](conceptmap.html): The description of the concept map 3357* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3358* [EventDefinition](eventdefinition.html): The description of the event definition 3359* [Evidence](evidence.html): The description of the evidence 3360* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3361* [GraphDefinition](graphdefinition.html): The description of the graph definition 3362* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3363* [Library](library.html): The description of the library 3364* [Measure](measure.html): The description of the measure 3365* [MessageDefinition](messagedefinition.html): The description of the message definition 3366* [NamingSystem](namingsystem.html): The description of the naming system 3367* [OperationDefinition](operationdefinition.html): The description of the operation definition 3368* [PlanDefinition](plandefinition.html): The description of the plan definition 3369* [Questionnaire](questionnaire.html): The description of the questionnaire 3370* [Requirements](requirements.html): The description of the requirements 3371* [SearchParameter](searchparameter.html): The description of the search parameter 3372* [StructureDefinition](structuredefinition.html): The description of the structure definition 3373* [StructureMap](structuremap.html): The description of the structure map 3374* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3375* [TestScript](testscript.html): The description of the test script 3376* [ValueSet](valueset.html): The description of the value set 3377</b><br> 3378 * Type: <b>string</b><br> 3379 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3380 * </p> 3381 */ 3382 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 3383 public static final String SP_DESCRIPTION = "description"; 3384 /** 3385 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3386 * <p> 3387 * Description: <b>Multiple Resources: 3388 3389* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3390* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3391* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3392* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3393* [Citation](citation.html): The description of the citation 3394* [CodeSystem](codesystem.html): The description of the code system 3395* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3396* [ConceptMap](conceptmap.html): The description of the concept map 3397* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3398* [EventDefinition](eventdefinition.html): The description of the event definition 3399* [Evidence](evidence.html): The description of the evidence 3400* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3401* [GraphDefinition](graphdefinition.html): The description of the graph definition 3402* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3403* [Library](library.html): The description of the library 3404* [Measure](measure.html): The description of the measure 3405* [MessageDefinition](messagedefinition.html): The description of the message definition 3406* [NamingSystem](namingsystem.html): The description of the naming system 3407* [OperationDefinition](operationdefinition.html): The description of the operation definition 3408* [PlanDefinition](plandefinition.html): The description of the plan definition 3409* [Questionnaire](questionnaire.html): The description of the questionnaire 3410* [Requirements](requirements.html): The description of the requirements 3411* [SearchParameter](searchparameter.html): The description of the search parameter 3412* [StructureDefinition](structuredefinition.html): The description of the structure definition 3413* [StructureMap](structuremap.html): The description of the structure map 3414* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3415* [TestScript](testscript.html): The description of the test script 3416* [ValueSet](valueset.html): The description of the value set 3417</b><br> 3418 * Type: <b>string</b><br> 3419 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3420 * </p> 3421 */ 3422 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 3423 3424 /** 3425 * Search parameter: <b>identifier</b> 3426 * <p> 3427 * Description: <b>Multiple Resources: 3428 3429* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3430* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3431* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3432* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3433* [Citation](citation.html): External identifier for the citation 3434* [CodeSystem](codesystem.html): External identifier for the code system 3435* [ConceptMap](conceptmap.html): External identifier for the concept map 3436* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3437* [EventDefinition](eventdefinition.html): External identifier for the event definition 3438* [Evidence](evidence.html): External identifier for the evidence 3439* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3440* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3441* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3442* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3443* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3444* [Library](library.html): External identifier for the library 3445* [Measure](measure.html): External identifier for the measure 3446* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3447* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3448* [NamingSystem](namingsystem.html): External identifier for the naming system 3449* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3450* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3451* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3452* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3453* [Requirements](requirements.html): External identifier for the requirements 3454* [SearchParameter](searchparameter.html): External identifier for the search parameter 3455* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3456* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3457* [StructureMap](structuremap.html): External identifier for the structure map 3458* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3459* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3460* [TestPlan](testplan.html): An identifier for the test plan 3461* [TestScript](testscript.html): External identifier for the test script 3462* [ValueSet](valueset.html): External identifier for the value set 3463</b><br> 3464 * Type: <b>token</b><br> 3465 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3466 * </p> 3467 */ 3468 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 3469 public static final String SP_IDENTIFIER = "identifier"; 3470 /** 3471 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3472 * <p> 3473 * Description: <b>Multiple Resources: 3474 3475* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3476* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3477* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3478* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3479* [Citation](citation.html): External identifier for the citation 3480* [CodeSystem](codesystem.html): External identifier for the code system 3481* [ConceptMap](conceptmap.html): External identifier for the concept map 3482* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3483* [EventDefinition](eventdefinition.html): External identifier for the event definition 3484* [Evidence](evidence.html): External identifier for the evidence 3485* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3486* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3487* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3488* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3489* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3490* [Library](library.html): External identifier for the library 3491* [Measure](measure.html): External identifier for the measure 3492* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3493* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3494* [NamingSystem](namingsystem.html): External identifier for the naming system 3495* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3496* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3497* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3498* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3499* [Requirements](requirements.html): External identifier for the requirements 3500* [SearchParameter](searchparameter.html): External identifier for the search parameter 3501* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3502* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3503* [StructureMap](structuremap.html): External identifier for the structure map 3504* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3505* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3506* [TestPlan](testplan.html): An identifier for the test plan 3507* [TestScript](testscript.html): External identifier for the test script 3508* [ValueSet](valueset.html): External identifier for the value set 3509</b><br> 3510 * Type: <b>token</b><br> 3511 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3512 * </p> 3513 */ 3514 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3515 3516 /** 3517 * Search parameter: <b>jurisdiction</b> 3518 * <p> 3519 * Description: <b>Multiple Resources: 3520 3521* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3522* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3523* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3524* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3525* [Citation](citation.html): Intended jurisdiction for the citation 3526* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3527* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3528* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3529* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3530* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3531* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3532* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3533* [Library](library.html): Intended jurisdiction for the library 3534* [Measure](measure.html): Intended jurisdiction for the measure 3535* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3536* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3537* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3538* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3539* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3540* [Requirements](requirements.html): Intended jurisdiction for the requirements 3541* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3542* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3543* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3544* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3545* [TestScript](testscript.html): Intended jurisdiction for the test script 3546* [ValueSet](valueset.html): Intended jurisdiction for the value set 3547</b><br> 3548 * Type: <b>token</b><br> 3549 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3550 * </p> 3551 */ 3552 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 3553 public static final String SP_JURISDICTION = "jurisdiction"; 3554 /** 3555 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3556 * <p> 3557 * Description: <b>Multiple Resources: 3558 3559* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3560* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3561* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3562* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3563* [Citation](citation.html): Intended jurisdiction for the citation 3564* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3565* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3566* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3567* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3568* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3569* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3570* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3571* [Library](library.html): Intended jurisdiction for the library 3572* [Measure](measure.html): Intended jurisdiction for the measure 3573* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3574* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3575* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3576* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3577* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3578* [Requirements](requirements.html): Intended jurisdiction for the requirements 3579* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3580* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3581* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3582* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3583* [TestScript](testscript.html): Intended jurisdiction for the test script 3584* [ValueSet](valueset.html): Intended jurisdiction for the value set 3585</b><br> 3586 * Type: <b>token</b><br> 3587 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3588 * </p> 3589 */ 3590 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 3591 3592 /** 3593 * Search parameter: <b>name</b> 3594 * <p> 3595 * Description: <b>Multiple Resources: 3596 3597* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 3598* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 3599* [Citation](citation.html): Computationally friendly name of the citation 3600* [CodeSystem](codesystem.html): Computationally friendly name of the code system 3601* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 3602* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 3603* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 3604* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 3605* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 3606* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 3607* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 3608* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 3609* [Library](library.html): Computationally friendly name of the library 3610* [Measure](measure.html): Computationally friendly name of the measure 3611* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 3612* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 3613* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 3614* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 3615* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 3616* [Requirements](requirements.html): Computationally friendly name of the requirements 3617* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 3618* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 3619* [StructureMap](structuremap.html): Computationally friendly name of the structure map 3620* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 3621* [TestScript](testscript.html): Computationally friendly name of the test script 3622* [ValueSet](valueset.html): Computationally friendly name of the value set 3623</b><br> 3624 * Type: <b>string</b><br> 3625 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 3626 * </p> 3627 */ 3628 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 3629 public static final String SP_NAME = "name"; 3630 /** 3631 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3632 * <p> 3633 * Description: <b>Multiple Resources: 3634 3635* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 3636* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 3637* [Citation](citation.html): Computationally friendly name of the citation 3638* [CodeSystem](codesystem.html): Computationally friendly name of the code system 3639* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 3640* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 3641* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 3642* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 3643* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 3644* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 3645* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 3646* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 3647* [Library](library.html): Computationally friendly name of the library 3648* [Measure](measure.html): Computationally friendly name of the measure 3649* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 3650* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 3651* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 3652* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 3653* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 3654* [Requirements](requirements.html): Computationally friendly name of the requirements 3655* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 3656* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 3657* [StructureMap](structuremap.html): Computationally friendly name of the structure map 3658* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 3659* [TestScript](testscript.html): Computationally friendly name of the test script 3660* [ValueSet](valueset.html): Computationally friendly name of the value set 3661</b><br> 3662 * Type: <b>string</b><br> 3663 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 3664 * </p> 3665 */ 3666 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 3667 3668 /** 3669 * Search parameter: <b>publisher</b> 3670 * <p> 3671 * Description: <b>Multiple Resources: 3672 3673* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 3674* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 3675* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 3676* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 3677* [Citation](citation.html): Name of the publisher of the citation 3678* [CodeSystem](codesystem.html): Name of the publisher of the code system 3679* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 3680* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 3681* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 3682* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 3683* [Evidence](evidence.html): Name of the publisher of the evidence 3684* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 3685* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 3686* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 3687* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 3688* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 3689* [Library](library.html): Name of the publisher of the library 3690* [Measure](measure.html): Name of the publisher of the measure 3691* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 3692* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 3693* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 3694* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 3695* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 3696* [Requirements](requirements.html): Name of the publisher of the requirements 3697* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 3698* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 3699* [StructureMap](structuremap.html): Name of the publisher of the structure map 3700* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 3701* [TestScript](testscript.html): Name of the publisher of the test script 3702* [ValueSet](valueset.html): Name of the publisher of the value set 3703</b><br> 3704 * Type: <b>string</b><br> 3705 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 3706 * </p> 3707 */ 3708 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 3709 public static final String SP_PUBLISHER = "publisher"; 3710 /** 3711 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3712 * <p> 3713 * Description: <b>Multiple Resources: 3714 3715* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 3716* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 3717* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 3718* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 3719* [Citation](citation.html): Name of the publisher of the citation 3720* [CodeSystem](codesystem.html): Name of the publisher of the code system 3721* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 3722* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 3723* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 3724* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 3725* [Evidence](evidence.html): Name of the publisher of the evidence 3726* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 3727* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 3728* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 3729* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 3730* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 3731* [Library](library.html): Name of the publisher of the library 3732* [Measure](measure.html): Name of the publisher of the measure 3733* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 3734* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 3735* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 3736* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 3737* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 3738* [Requirements](requirements.html): Name of the publisher of the requirements 3739* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 3740* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 3741* [StructureMap](structuremap.html): Name of the publisher of the structure map 3742* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 3743* [TestScript](testscript.html): Name of the publisher of the test script 3744* [ValueSet](valueset.html): Name of the publisher of the value set 3745</b><br> 3746 * Type: <b>string</b><br> 3747 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 3748 * </p> 3749 */ 3750 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 3751 3752 /** 3753 * Search parameter: <b>status</b> 3754 * <p> 3755 * Description: <b>Multiple Resources: 3756 3757* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 3758* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 3759* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 3760* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 3761* [Citation](citation.html): The current status of the citation 3762* [CodeSystem](codesystem.html): The current status of the code system 3763* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 3764* [ConceptMap](conceptmap.html): The current status of the concept map 3765* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 3766* [EventDefinition](eventdefinition.html): The current status of the event definition 3767* [Evidence](evidence.html): The current status of the evidence 3768* [EvidenceReport](evidencereport.html): The current status of the evidence report 3769* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 3770* [ExampleScenario](examplescenario.html): The current status of the example scenario 3771* [GraphDefinition](graphdefinition.html): The current status of the graph definition 3772* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 3773* [Library](library.html): The current status of the library 3774* [Measure](measure.html): The current status of the measure 3775* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 3776* [MessageDefinition](messagedefinition.html): The current status of the message definition 3777* [NamingSystem](namingsystem.html): The current status of the naming system 3778* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 3779* [OperationDefinition](operationdefinition.html): The current status of the operation definition 3780* [PlanDefinition](plandefinition.html): The current status of the plan definition 3781* [Questionnaire](questionnaire.html): The current status of the questionnaire 3782* [Requirements](requirements.html): The current status of the requirements 3783* [SearchParameter](searchparameter.html): The current status of the search parameter 3784* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 3785* [StructureDefinition](structuredefinition.html): The current status of the structure definition 3786* [StructureMap](structuremap.html): The current status of the structure map 3787* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 3788* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 3789* [TestPlan](testplan.html): The current status of the test plan 3790* [TestScript](testscript.html): The current status of the test script 3791* [ValueSet](valueset.html): The current status of the value set 3792</b><br> 3793 * Type: <b>token</b><br> 3794 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 3795 * </p> 3796 */ 3797 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 3798 public static final String SP_STATUS = "status"; 3799 /** 3800 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3801 * <p> 3802 * Description: <b>Multiple Resources: 3803 3804* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 3805* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 3806* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 3807* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 3808* [Citation](citation.html): The current status of the citation 3809* [CodeSystem](codesystem.html): The current status of the code system 3810* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 3811* [ConceptMap](conceptmap.html): The current status of the concept map 3812* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 3813* [EventDefinition](eventdefinition.html): The current status of the event definition 3814* [Evidence](evidence.html): The current status of the evidence 3815* [EvidenceReport](evidencereport.html): The current status of the evidence report 3816* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 3817* [ExampleScenario](examplescenario.html): The current status of the example scenario 3818* [GraphDefinition](graphdefinition.html): The current status of the graph definition 3819* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 3820* [Library](library.html): The current status of the library 3821* [Measure](measure.html): The current status of the measure 3822* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 3823* [MessageDefinition](messagedefinition.html): The current status of the message definition 3824* [NamingSystem](namingsystem.html): The current status of the naming system 3825* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 3826* [OperationDefinition](operationdefinition.html): The current status of the operation definition 3827* [PlanDefinition](plandefinition.html): The current status of the plan definition 3828* [Questionnaire](questionnaire.html): The current status of the questionnaire 3829* [Requirements](requirements.html): The current status of the requirements 3830* [SearchParameter](searchparameter.html): The current status of the search parameter 3831* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 3832* [StructureDefinition](structuredefinition.html): The current status of the structure definition 3833* [StructureMap](structuremap.html): The current status of the structure map 3834* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 3835* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 3836* [TestPlan](testplan.html): The current status of the test plan 3837* [TestScript](testscript.html): The current status of the test script 3838* [ValueSet](valueset.html): The current status of the value set 3839</b><br> 3840 * Type: <b>token</b><br> 3841 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 3842 * </p> 3843 */ 3844 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3845 3846 /** 3847 * Search parameter: <b>title</b> 3848 * <p> 3849 * Description: <b>Multiple Resources: 3850 3851* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 3852* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 3853* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 3854* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 3855* [Citation](citation.html): The human-friendly name of the citation 3856* [CodeSystem](codesystem.html): The human-friendly name of the code system 3857* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 3858* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 3859* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 3860* [Evidence](evidence.html): The human-friendly name of the evidence 3861* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 3862* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 3863* [Library](library.html): The human-friendly name of the library 3864* [Measure](measure.html): The human-friendly name of the measure 3865* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 3866* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 3867* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 3868* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 3869* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 3870* [Requirements](requirements.html): The human-friendly name of the requirements 3871* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 3872* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 3873* [StructureMap](structuremap.html): The human-friendly name of the structure map 3874* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 3875* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 3876* [TestScript](testscript.html): The human-friendly name of the test script 3877* [ValueSet](valueset.html): The human-friendly name of the value set 3878</b><br> 3879 * Type: <b>string</b><br> 3880 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 3881 * </p> 3882 */ 3883 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 3884 public static final String SP_TITLE = "title"; 3885 /** 3886 * <b>Fluent Client</b> search parameter constant for <b>title</b> 3887 * <p> 3888 * Description: <b>Multiple Resources: 3889 3890* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 3891* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 3892* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 3893* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 3894* [Citation](citation.html): The human-friendly name of the citation 3895* [CodeSystem](codesystem.html): The human-friendly name of the code system 3896* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 3897* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 3898* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 3899* [Evidence](evidence.html): The human-friendly name of the evidence 3900* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 3901* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 3902* [Library](library.html): The human-friendly name of the library 3903* [Measure](measure.html): The human-friendly name of the measure 3904* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 3905* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 3906* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 3907* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 3908* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 3909* [Requirements](requirements.html): The human-friendly name of the requirements 3910* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 3911* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 3912* [StructureMap](structuremap.html): The human-friendly name of the structure map 3913* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 3914* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 3915* [TestScript](testscript.html): The human-friendly name of the test script 3916* [ValueSet](valueset.html): The human-friendly name of the value set 3917</b><br> 3918 * Type: <b>string</b><br> 3919 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 3920 * </p> 3921 */ 3922 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 3923 3924 /** 3925 * Search parameter: <b>url</b> 3926 * <p> 3927 * Description: <b>Multiple Resources: 3928 3929* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 3930* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 3931* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 3932* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 3933* [Citation](citation.html): The uri that identifies the citation 3934* [CodeSystem](codesystem.html): The uri that identifies the code system 3935* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 3936* [ConceptMap](conceptmap.html): The URI that identifies the concept map 3937* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 3938* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 3939* [Evidence](evidence.html): The uri that identifies the evidence 3940* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 3941* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 3942* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 3943* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 3944* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 3945* [Library](library.html): The uri that identifies the library 3946* [Measure](measure.html): The uri that identifies the measure 3947* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 3948* [NamingSystem](namingsystem.html): The uri that identifies the naming system 3949* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 3950* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 3951* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 3952* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 3953* [Requirements](requirements.html): The uri that identifies the requirements 3954* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 3955* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 3956* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 3957* [StructureMap](structuremap.html): The uri that identifies the structure map 3958* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 3959* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 3960* [TestPlan](testplan.html): The uri that identifies the test plan 3961* [TestScript](testscript.html): The uri that identifies the test script 3962* [ValueSet](valueset.html): The uri that identifies the value set 3963</b><br> 3964 * Type: <b>uri</b><br> 3965 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 3966 * </p> 3967 */ 3968 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 3969 public static final String SP_URL = "url"; 3970 /** 3971 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3972 * <p> 3973 * Description: <b>Multiple Resources: 3974 3975* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 3976* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 3977* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 3978* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 3979* [Citation](citation.html): The uri that identifies the citation 3980* [CodeSystem](codesystem.html): The uri that identifies the code system 3981* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 3982* [ConceptMap](conceptmap.html): The URI that identifies the concept map 3983* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 3984* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 3985* [Evidence](evidence.html): The uri that identifies the evidence 3986* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 3987* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 3988* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 3989* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 3990* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 3991* [Library](library.html): The uri that identifies the library 3992* [Measure](measure.html): The uri that identifies the measure 3993* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 3994* [NamingSystem](namingsystem.html): The uri that identifies the naming system 3995* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 3996* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 3997* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 3998* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 3999* [Requirements](requirements.html): The uri that identifies the requirements 4000* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4001* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4002* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4003* [StructureMap](structuremap.html): The uri that identifies the structure map 4004* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4005* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4006* [TestPlan](testplan.html): The uri that identifies the test plan 4007* [TestScript](testscript.html): The uri that identifies the test script 4008* [ValueSet](valueset.html): The uri that identifies the value set 4009</b><br> 4010 * Type: <b>uri</b><br> 4011 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4012 * </p> 4013 */ 4014 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4015 4016 /** 4017 * Search parameter: <b>version</b> 4018 * <p> 4019 * Description: <b>Multiple Resources: 4020 4021* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4022* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4023* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4024* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4025* [Citation](citation.html): The business version of the citation 4026* [CodeSystem](codesystem.html): The business version of the code system 4027* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 4028* [ConceptMap](conceptmap.html): The business version of the concept map 4029* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 4030* [EventDefinition](eventdefinition.html): The business version of the event definition 4031* [Evidence](evidence.html): The business version of the evidence 4032* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 4033* [ExampleScenario](examplescenario.html): The business version of the example scenario 4034* [GraphDefinition](graphdefinition.html): The business version of the graph definition 4035* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 4036* [Library](library.html): The business version of the library 4037* [Measure](measure.html): The business version of the measure 4038* [MessageDefinition](messagedefinition.html): The business version of the message definition 4039* [NamingSystem](namingsystem.html): The business version of the naming system 4040* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4041* [PlanDefinition](plandefinition.html): The business version of the plan definition 4042* [Questionnaire](questionnaire.html): The business version of the questionnaire 4043* [Requirements](requirements.html): The business version of the requirements 4044* [SearchParameter](searchparameter.html): The business version of the search parameter 4045* [StructureDefinition](structuredefinition.html): The business version of the structure definition 4046* [StructureMap](structuremap.html): The business version of the structure map 4047* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 4048* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 4049* [TestScript](testscript.html): The business version of the test script 4050* [ValueSet](valueset.html): The business version of the value set 4051</b><br> 4052 * Type: <b>token</b><br> 4053 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 4054 * </p> 4055 */ 4056 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 4057 public static final String SP_VERSION = "version"; 4058 /** 4059 * <b>Fluent Client</b> search parameter constant for <b>version</b> 4060 * <p> 4061 * Description: <b>Multiple Resources: 4062 4063* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4064* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4065* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4066* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4067* [Citation](citation.html): The business version of the citation 4068* [CodeSystem](codesystem.html): The business version of the code system 4069* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 4070* [ConceptMap](conceptmap.html): The business version of the concept map 4071* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 4072* [EventDefinition](eventdefinition.html): The business version of the event definition 4073* [Evidence](evidence.html): The business version of the evidence 4074* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 4075* [ExampleScenario](examplescenario.html): The business version of the example scenario 4076* [GraphDefinition](graphdefinition.html): The business version of the graph definition 4077* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 4078* [Library](library.html): The business version of the library 4079* [Measure](measure.html): The business version of the measure 4080* [MessageDefinition](messagedefinition.html): The business version of the message definition 4081* [NamingSystem](namingsystem.html): The business version of the naming system 4082* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4083* [PlanDefinition](plandefinition.html): The business version of the plan definition 4084* [Questionnaire](questionnaire.html): The business version of the questionnaire 4085* [Requirements](requirements.html): The business version of the requirements 4086* [SearchParameter](searchparameter.html): The business version of the search parameter 4087* [StructureDefinition](structuredefinition.html): The business version of the structure definition 4088* [StructureMap](structuremap.html): The business version of the structure map 4089* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 4090* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 4091* [TestScript](testscript.html): The business version of the test script 4092* [ValueSet](valueset.html): The business version of the value set 4093</b><br> 4094 * Type: <b>token</b><br> 4095 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 4096 * </p> 4097 */ 4098 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 4099 4100 /** 4101 * Search parameter: <b>actor</b> 4102 * <p> 4103 * Description: <b>An actor these requirements are for</b><br> 4104 * Type: <b>reference</b><br> 4105 * Path: <b>Requirements.actor</b><br> 4106 * </p> 4107 */ 4108 @SearchParamDefinition(name="actor", path="Requirements.actor", description="An actor these requirements are for", type="reference", target={ActorDefinition.class } ) 4109 public static final String SP_ACTOR = "actor"; 4110 /** 4111 * <b>Fluent Client</b> search parameter constant for <b>actor</b> 4112 * <p> 4113 * Description: <b>An actor these requirements are for</b><br> 4114 * Type: <b>reference</b><br> 4115 * Path: <b>Requirements.actor</b><br> 4116 * </p> 4117 */ 4118 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACTOR); 4119 4120/** 4121 * Constant for fluent queries to be used to add include statements. Specifies 4122 * the path value of "<b>Requirements:actor</b>". 4123 */ 4124 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTOR = new ca.uhn.fhir.model.api.Include("Requirements:actor").toLocked(); 4125 4126 /** 4127 * Search parameter: <b>derived-from</b> 4128 * <p> 4129 * Description: <b>The requirements these are derived from</b><br> 4130 * Type: <b>reference</b><br> 4131 * Path: <b>Requirements.derivedFrom</b><br> 4132 * </p> 4133 */ 4134 @SearchParamDefinition(name="derived-from", path="Requirements.derivedFrom", description="The requirements these are derived from", type="reference", target={Requirements.class } ) 4135 public static final String SP_DERIVED_FROM = "derived-from"; 4136 /** 4137 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 4138 * <p> 4139 * Description: <b>The requirements these are derived from</b><br> 4140 * Type: <b>reference</b><br> 4141 * Path: <b>Requirements.derivedFrom</b><br> 4142 * </p> 4143 */ 4144 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 4145 4146/** 4147 * Constant for fluent queries to be used to add include statements. Specifies 4148 * the path value of "<b>Requirements:derived-from</b>". 4149 */ 4150 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("Requirements:derived-from").toLocked(); 4151 4152// Manual code (from Configuration.txt): 4153 4154 public RequirementsStatementComponent findStatement(String key) { 4155 for (RequirementsStatementComponent t : getStatement()) { 4156 if (key.equals(t.getKey())) { 4157 return t; 4158 } 4159 } 4160 return null; 4161 } 4162 4163// end addition 4164 4165} 4166