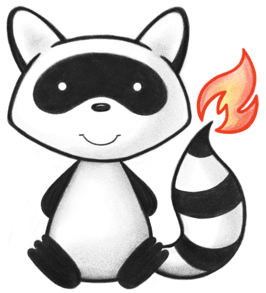
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The Requirements resource is used to describe an actor - a human or an application that plays a role in data exchange, and that may have obligations associated with the role the actor plays. 052 */ 053@ResourceDef(name="Requirements", profile="http://hl7.org/fhir/StructureDefinition/Requirements") 054public class Requirements extends CanonicalResource { 055 056 public enum ConformanceExpectation { 057 /** 058 * Support for the specified capability is required to be considered conformant. 059 */ 060 SHALL, 061 /** 062 * Support for the specified capability is strongly encouraged, and failure to support it should only occur after careful consideration. 063 */ 064 SHOULD, 065 /** 066 * Support for the specified capability is not necessary to be considered conformant, and the requirement should be considered strictly optional. 067 */ 068 MAY, 069 /** 070 * Support for the specified capability is strongly discouraged and should occur only after careful consideration. 071 */ 072 SHOULDNOT, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static ConformanceExpectation fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("SHALL".equals(codeString)) 081 return SHALL; 082 if ("SHOULD".equals(codeString)) 083 return SHOULD; 084 if ("MAY".equals(codeString)) 085 return MAY; 086 if ("SHOULD-NOT".equals(codeString)) 087 return SHOULDNOT; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown ConformanceExpectation code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case SHALL: return "SHALL"; 096 case SHOULD: return "SHOULD"; 097 case MAY: return "MAY"; 098 case SHOULDNOT: return "SHOULD-NOT"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case SHALL: return "http://hl7.org/fhir/conformance-expectation"; 106 case SHOULD: return "http://hl7.org/fhir/conformance-expectation"; 107 case MAY: return "http://hl7.org/fhir/conformance-expectation"; 108 case SHOULDNOT: return "http://hl7.org/fhir/conformance-expectation"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case SHALL: return "Support for the specified capability is required to be considered conformant."; 116 case SHOULD: return "Support for the specified capability is strongly encouraged, and failure to support it should only occur after careful consideration."; 117 case MAY: return "Support for the specified capability is not necessary to be considered conformant, and the requirement should be considered strictly optional."; 118 case SHOULDNOT: return "Support for the specified capability is strongly discouraged and should occur only after careful consideration."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case SHALL: return "SHALL"; 126 case SHOULD: return "SHOULD"; 127 case MAY: return "MAY"; 128 case SHOULDNOT: return "SHOULD-NOT"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class ConformanceExpectationEnumFactory implements EnumFactory<ConformanceExpectation> { 136 public ConformanceExpectation fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("SHALL".equals(codeString)) 141 return ConformanceExpectation.SHALL; 142 if ("SHOULD".equals(codeString)) 143 return ConformanceExpectation.SHOULD; 144 if ("MAY".equals(codeString)) 145 return ConformanceExpectation.MAY; 146 if ("SHOULD-NOT".equals(codeString)) 147 return ConformanceExpectation.SHOULDNOT; 148 throw new IllegalArgumentException("Unknown ConformanceExpectation code '"+codeString+"'"); 149 } 150 public Enumeration<ConformanceExpectation> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<ConformanceExpectation>(this, ConformanceExpectation.NULL, code); 155 String codeString = ((PrimitiveType) code).asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<ConformanceExpectation>(this, ConformanceExpectation.NULL, code); 158 if ("SHALL".equals(codeString)) 159 return new Enumeration<ConformanceExpectation>(this, ConformanceExpectation.SHALL, code); 160 if ("SHOULD".equals(codeString)) 161 return new Enumeration<ConformanceExpectation>(this, ConformanceExpectation.SHOULD, code); 162 if ("MAY".equals(codeString)) 163 return new Enumeration<ConformanceExpectation>(this, ConformanceExpectation.MAY, code); 164 if ("SHOULD-NOT".equals(codeString)) 165 return new Enumeration<ConformanceExpectation>(this, ConformanceExpectation.SHOULDNOT, code); 166 throw new FHIRException("Unknown ConformanceExpectation code '"+codeString+"'"); 167 } 168 public String toCode(ConformanceExpectation code) { 169 if (code == ConformanceExpectation.SHALL) 170 return "SHALL"; 171 if (code == ConformanceExpectation.SHOULD) 172 return "SHOULD"; 173 if (code == ConformanceExpectation.MAY) 174 return "MAY"; 175 if (code == ConformanceExpectation.SHOULDNOT) 176 return "SHOULD-NOT"; 177 return "?"; 178 } 179 public String toSystem(ConformanceExpectation code) { 180 return code.getSystem(); 181 } 182 } 183 184 @Block() 185 public static class RequirementsStatementComponent extends BackboneElement implements IBaseBackboneElement { 186 /** 187 * Key that identifies this statement (unique within this resource). 188 */ 189 @Child(name = "key", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=false) 190 @Description(shortDefinition="Key that identifies this statement", formalDefinition="Key that identifies this statement (unique within this resource)." ) 191 protected IdType key; 192 193 /** 194 * A short human usable label for this statement. 195 */ 196 @Child(name = "label", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 197 @Description(shortDefinition="Short Human label for this statement", formalDefinition="A short human usable label for this statement." ) 198 protected StringType label; 199 200 /** 201 * A short human usable label for this statement. 202 */ 203 @Child(name = "conformance", type = {CodeType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 204 @Description(shortDefinition="SHALL | SHOULD | MAY | SHOULD-NOT", formalDefinition="A short human usable label for this statement." ) 205 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/conformance-expectation") 206 protected List<Enumeration<ConformanceExpectation>> conformance; 207 208 /** 209 * This boolean flag is set to true of the text of the requirement is conditional on something e.g. it includes lanauage like 'if x then y'. This conditionality flag is introduced for purposes of filtering and colour highlighting etc. 210 */ 211 @Child(name = "conditionality", type = {BooleanType.class}, order=4, min=0, max=1, modifier=false, summary=false) 212 @Description(shortDefinition="Set to true if requirements statement is conditional", formalDefinition="This boolean flag is set to true of the text of the requirement is conditional on something e.g. it includes lanauage like 'if x then y'. This conditionality flag is introduced for purposes of filtering and colour highlighting etc." ) 213 protected BooleanType conditionality; 214 215 /** 216 * The actual requirement for human consumption. 217 */ 218 @Child(name = "requirement", type = {MarkdownType.class}, order=5, min=1, max=1, modifier=false, summary=false) 219 @Description(shortDefinition="The actual requirement", formalDefinition="The actual requirement for human consumption." ) 220 protected MarkdownType requirement; 221 222 /** 223 * Another statement on one of the requirements that this requirement clarifies or restricts. 224 */ 225 @Child(name = "derivedFrom", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 226 @Description(shortDefinition="Another statement this clarifies/restricts ([url#]key)", formalDefinition="Another statement on one of the requirements that this requirement clarifies or restricts." ) 227 protected StringType derivedFrom; 228 229 /** 230 * A larger requirement that this requirement helps to refine and enable. 231 */ 232 @Child(name = "parent", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=false) 233 @Description(shortDefinition="A larger requirement that this requirement helps to refine and enable", formalDefinition="A larger requirement that this requirement helps to refine and enable." ) 234 protected StringType parent; 235 236 /** 237 * A reference to another artifact that satisfies this requirement. This could be a Profile, extension, or an element in one of those, or a CapabilityStatement, OperationDefinition, SearchParameter, CodeSystem(/code), ValueSet, Libary etc. 238 */ 239 @Child(name = "satisfiedBy", type = {UrlType.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 240 @Description(shortDefinition="Design artifact that satisfies this requirement", formalDefinition="A reference to another artifact that satisfies this requirement. This could be a Profile, extension, or an element in one of those, or a CapabilityStatement, OperationDefinition, SearchParameter, CodeSystem(/code), ValueSet, Libary etc." ) 241 protected List<UrlType> satisfiedBy; 242 243 /** 244 * A reference to another artifact that created this requirement. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere. 245 */ 246 @Child(name = "reference", type = {UrlType.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 247 @Description(shortDefinition="External artifact (rule/document etc. that) created this requirement", formalDefinition="A reference to another artifact that created this requirement. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere." ) 248 protected List<UrlType> reference; 249 250 /** 251 * Who asked for this statement to be a requirement. By default, it's assumed that the publisher knows who it is if it matters. 252 */ 253 @Child(name = "source", type = {CareTeam.class, Device.class, Group.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 254 @Description(shortDefinition="Who asked for this statement", formalDefinition="Who asked for this statement to be a requirement. By default, it's assumed that the publisher knows who it is if it matters." ) 255 protected List<Reference> source; 256 257 private static final long serialVersionUID = 1299503021L; 258 259 /** 260 * Constructor 261 */ 262 public RequirementsStatementComponent() { 263 super(); 264 } 265 266 /** 267 * Constructor 268 */ 269 public RequirementsStatementComponent(String key, String requirement) { 270 super(); 271 this.setKey(key); 272 this.setRequirement(requirement); 273 } 274 275 /** 276 * @return {@link #key} (Key that identifies this statement (unique within this resource).). This is the underlying object with id, value and extensions. The accessor "getKey" gives direct access to the value 277 */ 278 public IdType getKeyElement() { 279 if (this.key == null) 280 if (Configuration.errorOnAutoCreate()) 281 throw new Error("Attempt to auto-create RequirementsStatementComponent.key"); 282 else if (Configuration.doAutoCreate()) 283 this.key = new IdType(); // bb 284 return this.key; 285 } 286 287 public boolean hasKeyElement() { 288 return this.key != null && !this.key.isEmpty(); 289 } 290 291 public boolean hasKey() { 292 return this.key != null && !this.key.isEmpty(); 293 } 294 295 /** 296 * @param value {@link #key} (Key that identifies this statement (unique within this resource).). This is the underlying object with id, value and extensions. The accessor "getKey" gives direct access to the value 297 */ 298 public RequirementsStatementComponent setKeyElement(IdType value) { 299 this.key = value; 300 return this; 301 } 302 303 /** 304 * @return Key that identifies this statement (unique within this resource). 305 */ 306 public String getKey() { 307 return this.key == null ? null : this.key.getValue(); 308 } 309 310 /** 311 * @param value Key that identifies this statement (unique within this resource). 312 */ 313 public RequirementsStatementComponent setKey(String value) { 314 if (this.key == null) 315 this.key = new IdType(); 316 this.key.setValue(value); 317 return this; 318 } 319 320 /** 321 * @return {@link #label} (A short human usable label for this statement.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 322 */ 323 public StringType getLabelElement() { 324 if (this.label == null) 325 if (Configuration.errorOnAutoCreate()) 326 throw new Error("Attempt to auto-create RequirementsStatementComponent.label"); 327 else if (Configuration.doAutoCreate()) 328 this.label = new StringType(); // bb 329 return this.label; 330 } 331 332 public boolean hasLabelElement() { 333 return this.label != null && !this.label.isEmpty(); 334 } 335 336 public boolean hasLabel() { 337 return this.label != null && !this.label.isEmpty(); 338 } 339 340 /** 341 * @param value {@link #label} (A short human usable label for this statement.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 342 */ 343 public RequirementsStatementComponent setLabelElement(StringType value) { 344 this.label = value; 345 return this; 346 } 347 348 /** 349 * @return A short human usable label for this statement. 350 */ 351 public String getLabel() { 352 return this.label == null ? null : this.label.getValue(); 353 } 354 355 /** 356 * @param value A short human usable label for this statement. 357 */ 358 public RequirementsStatementComponent setLabel(String value) { 359 if (Utilities.noString(value)) 360 this.label = null; 361 else { 362 if (this.label == null) 363 this.label = new StringType(); 364 this.label.setValue(value); 365 } 366 return this; 367 } 368 369 /** 370 * @return {@link #conformance} (A short human usable label for this statement.) 371 */ 372 public List<Enumeration<ConformanceExpectation>> getConformance() { 373 if (this.conformance == null) 374 this.conformance = new ArrayList<Enumeration<ConformanceExpectation>>(); 375 return this.conformance; 376 } 377 378 /** 379 * @return Returns a reference to <code>this</code> for easy method chaining 380 */ 381 public RequirementsStatementComponent setConformance(List<Enumeration<ConformanceExpectation>> theConformance) { 382 this.conformance = theConformance; 383 return this; 384 } 385 386 public boolean hasConformance() { 387 if (this.conformance == null) 388 return false; 389 for (Enumeration<ConformanceExpectation> item : this.conformance) 390 if (!item.isEmpty()) 391 return true; 392 return false; 393 } 394 395 /** 396 * @return {@link #conformance} (A short human usable label for this statement.) 397 */ 398 public Enumeration<ConformanceExpectation> addConformanceElement() {//2 399 Enumeration<ConformanceExpectation> t = new Enumeration<ConformanceExpectation>(new ConformanceExpectationEnumFactory()); 400 if (this.conformance == null) 401 this.conformance = new ArrayList<Enumeration<ConformanceExpectation>>(); 402 this.conformance.add(t); 403 return t; 404 } 405 406 /** 407 * @param value {@link #conformance} (A short human usable label for this statement.) 408 */ 409 public RequirementsStatementComponent addConformance(ConformanceExpectation value) { //1 410 Enumeration<ConformanceExpectation> t = new Enumeration<ConformanceExpectation>(new ConformanceExpectationEnumFactory()); 411 t.setValue(value); 412 if (this.conformance == null) 413 this.conformance = new ArrayList<Enumeration<ConformanceExpectation>>(); 414 this.conformance.add(t); 415 return this; 416 } 417 418 /** 419 * @param value {@link #conformance} (A short human usable label for this statement.) 420 */ 421 public boolean hasConformance(ConformanceExpectation value) { 422 if (this.conformance == null) 423 return false; 424 for (Enumeration<ConformanceExpectation> v : this.conformance) 425 if (v.getValue().equals(value)) // code 426 return true; 427 return false; 428 } 429 430 /** 431 * @return {@link #conditionality} (This boolean flag is set to true of the text of the requirement is conditional on something e.g. it includes lanauage like 'if x then y'. This conditionality flag is introduced for purposes of filtering and colour highlighting etc.). This is the underlying object with id, value and extensions. The accessor "getConditionality" gives direct access to the value 432 */ 433 public BooleanType getConditionalityElement() { 434 if (this.conditionality == null) 435 if (Configuration.errorOnAutoCreate()) 436 throw new Error("Attempt to auto-create RequirementsStatementComponent.conditionality"); 437 else if (Configuration.doAutoCreate()) 438 this.conditionality = new BooleanType(); // bb 439 return this.conditionality; 440 } 441 442 public boolean hasConditionalityElement() { 443 return this.conditionality != null && !this.conditionality.isEmpty(); 444 } 445 446 public boolean hasConditionality() { 447 return this.conditionality != null && !this.conditionality.isEmpty(); 448 } 449 450 /** 451 * @param value {@link #conditionality} (This boolean flag is set to true of the text of the requirement is conditional on something e.g. it includes lanauage like 'if x then y'. This conditionality flag is introduced for purposes of filtering and colour highlighting etc.). This is the underlying object with id, value and extensions. The accessor "getConditionality" gives direct access to the value 452 */ 453 public RequirementsStatementComponent setConditionalityElement(BooleanType value) { 454 this.conditionality = value; 455 return this; 456 } 457 458 /** 459 * @return This boolean flag is set to true of the text of the requirement is conditional on something e.g. it includes lanauage like 'if x then y'. This conditionality flag is introduced for purposes of filtering and colour highlighting etc. 460 */ 461 public boolean getConditionality() { 462 return this.conditionality == null || this.conditionality.isEmpty() ? false : this.conditionality.getValue(); 463 } 464 465 /** 466 * @param value This boolean flag is set to true of the text of the requirement is conditional on something e.g. it includes lanauage like 'if x then y'. This conditionality flag is introduced for purposes of filtering and colour highlighting etc. 467 */ 468 public RequirementsStatementComponent setConditionality(boolean value) { 469 if (this.conditionality == null) 470 this.conditionality = new BooleanType(); 471 this.conditionality.setValue(value); 472 return this; 473 } 474 475 /** 476 * @return {@link #requirement} (The actual requirement for human consumption.). This is the underlying object with id, value and extensions. The accessor "getRequirement" gives direct access to the value 477 */ 478 public MarkdownType getRequirementElement() { 479 if (this.requirement == null) 480 if (Configuration.errorOnAutoCreate()) 481 throw new Error("Attempt to auto-create RequirementsStatementComponent.requirement"); 482 else if (Configuration.doAutoCreate()) 483 this.requirement = new MarkdownType(); // bb 484 return this.requirement; 485 } 486 487 public boolean hasRequirementElement() { 488 return this.requirement != null && !this.requirement.isEmpty(); 489 } 490 491 public boolean hasRequirement() { 492 return this.requirement != null && !this.requirement.isEmpty(); 493 } 494 495 /** 496 * @param value {@link #requirement} (The actual requirement for human consumption.). This is the underlying object with id, value and extensions. The accessor "getRequirement" gives direct access to the value 497 */ 498 public RequirementsStatementComponent setRequirementElement(MarkdownType value) { 499 this.requirement = value; 500 return this; 501 } 502 503 /** 504 * @return The actual requirement for human consumption. 505 */ 506 public String getRequirement() { 507 return this.requirement == null ? null : this.requirement.getValue(); 508 } 509 510 /** 511 * @param value The actual requirement for human consumption. 512 */ 513 public RequirementsStatementComponent setRequirement(String value) { 514 if (this.requirement == null) 515 this.requirement = new MarkdownType(); 516 this.requirement.setValue(value); 517 return this; 518 } 519 520 /** 521 * @return {@link #derivedFrom} (Another statement on one of the requirements that this requirement clarifies or restricts.). This is the underlying object with id, value and extensions. The accessor "getDerivedFrom" gives direct access to the value 522 */ 523 public StringType getDerivedFromElement() { 524 if (this.derivedFrom == null) 525 if (Configuration.errorOnAutoCreate()) 526 throw new Error("Attempt to auto-create RequirementsStatementComponent.derivedFrom"); 527 else if (Configuration.doAutoCreate()) 528 this.derivedFrom = new StringType(); // bb 529 return this.derivedFrom; 530 } 531 532 public boolean hasDerivedFromElement() { 533 return this.derivedFrom != null && !this.derivedFrom.isEmpty(); 534 } 535 536 public boolean hasDerivedFrom() { 537 return this.derivedFrom != null && !this.derivedFrom.isEmpty(); 538 } 539 540 /** 541 * @param value {@link #derivedFrom} (Another statement on one of the requirements that this requirement clarifies or restricts.). This is the underlying object with id, value and extensions. The accessor "getDerivedFrom" gives direct access to the value 542 */ 543 public RequirementsStatementComponent setDerivedFromElement(StringType value) { 544 this.derivedFrom = value; 545 return this; 546 } 547 548 /** 549 * @return Another statement on one of the requirements that this requirement clarifies or restricts. 550 */ 551 public String getDerivedFrom() { 552 return this.derivedFrom == null ? null : this.derivedFrom.getValue(); 553 } 554 555 /** 556 * @param value Another statement on one of the requirements that this requirement clarifies or restricts. 557 */ 558 public RequirementsStatementComponent setDerivedFrom(String value) { 559 if (Utilities.noString(value)) 560 this.derivedFrom = null; 561 else { 562 if (this.derivedFrom == null) 563 this.derivedFrom = new StringType(); 564 this.derivedFrom.setValue(value); 565 } 566 return this; 567 } 568 569 /** 570 * @return {@link #parent} (A larger requirement that this requirement helps to refine and enable.). This is the underlying object with id, value and extensions. The accessor "getParent" gives direct access to the value 571 */ 572 public StringType getParentElement() { 573 if (this.parent == null) 574 if (Configuration.errorOnAutoCreate()) 575 throw new Error("Attempt to auto-create RequirementsStatementComponent.parent"); 576 else if (Configuration.doAutoCreate()) 577 this.parent = new StringType(); // bb 578 return this.parent; 579 } 580 581 public boolean hasParentElement() { 582 return this.parent != null && !this.parent.isEmpty(); 583 } 584 585 public boolean hasParent() { 586 return this.parent != null && !this.parent.isEmpty(); 587 } 588 589 /** 590 * @param value {@link #parent} (A larger requirement that this requirement helps to refine and enable.). This is the underlying object with id, value and extensions. The accessor "getParent" gives direct access to the value 591 */ 592 public RequirementsStatementComponent setParentElement(StringType value) { 593 this.parent = value; 594 return this; 595 } 596 597 /** 598 * @return A larger requirement that this requirement helps to refine and enable. 599 */ 600 public String getParent() { 601 return this.parent == null ? null : this.parent.getValue(); 602 } 603 604 /** 605 * @param value A larger requirement that this requirement helps to refine and enable. 606 */ 607 public RequirementsStatementComponent setParent(String value) { 608 if (Utilities.noString(value)) 609 this.parent = null; 610 else { 611 if (this.parent == null) 612 this.parent = new StringType(); 613 this.parent.setValue(value); 614 } 615 return this; 616 } 617 618 /** 619 * @return {@link #satisfiedBy} (A reference to another artifact that satisfies this requirement. This could be a Profile, extension, or an element in one of those, or a CapabilityStatement, OperationDefinition, SearchParameter, CodeSystem(/code), ValueSet, Libary etc.) 620 */ 621 public List<UrlType> getSatisfiedBy() { 622 if (this.satisfiedBy == null) 623 this.satisfiedBy = new ArrayList<UrlType>(); 624 return this.satisfiedBy; 625 } 626 627 /** 628 * @return Returns a reference to <code>this</code> for easy method chaining 629 */ 630 public RequirementsStatementComponent setSatisfiedBy(List<UrlType> theSatisfiedBy) { 631 this.satisfiedBy = theSatisfiedBy; 632 return this; 633 } 634 635 public boolean hasSatisfiedBy() { 636 if (this.satisfiedBy == null) 637 return false; 638 for (UrlType item : this.satisfiedBy) 639 if (!item.isEmpty()) 640 return true; 641 return false; 642 } 643 644 /** 645 * @return {@link #satisfiedBy} (A reference to another artifact that satisfies this requirement. This could be a Profile, extension, or an element in one of those, or a CapabilityStatement, OperationDefinition, SearchParameter, CodeSystem(/code), ValueSet, Libary etc.) 646 */ 647 public UrlType addSatisfiedByElement() {//2 648 UrlType t = new UrlType(); 649 if (this.satisfiedBy == null) 650 this.satisfiedBy = new ArrayList<UrlType>(); 651 this.satisfiedBy.add(t); 652 return t; 653 } 654 655 /** 656 * @param value {@link #satisfiedBy} (A reference to another artifact that satisfies this requirement. This could be a Profile, extension, or an element in one of those, or a CapabilityStatement, OperationDefinition, SearchParameter, CodeSystem(/code), ValueSet, Libary etc.) 657 */ 658 public RequirementsStatementComponent addSatisfiedBy(String value) { //1 659 UrlType t = new UrlType(); 660 t.setValue(value); 661 if (this.satisfiedBy == null) 662 this.satisfiedBy = new ArrayList<UrlType>(); 663 this.satisfiedBy.add(t); 664 return this; 665 } 666 667 /** 668 * @param value {@link #satisfiedBy} (A reference to another artifact that satisfies this requirement. This could be a Profile, extension, or an element in one of those, or a CapabilityStatement, OperationDefinition, SearchParameter, CodeSystem(/code), ValueSet, Libary etc.) 669 */ 670 public boolean hasSatisfiedBy(String value) { 671 if (this.satisfiedBy == null) 672 return false; 673 for (UrlType v : this.satisfiedBy) 674 if (v.getValue().equals(value)) // url 675 return true; 676 return false; 677 } 678 679 /** 680 * @return {@link #reference} (A reference to another artifact that created this requirement. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.) 681 */ 682 public List<UrlType> getReference() { 683 if (this.reference == null) 684 this.reference = new ArrayList<UrlType>(); 685 return this.reference; 686 } 687 688 /** 689 * @return Returns a reference to <code>this</code> for easy method chaining 690 */ 691 public RequirementsStatementComponent setReference(List<UrlType> theReference) { 692 this.reference = theReference; 693 return this; 694 } 695 696 public boolean hasReference() { 697 if (this.reference == null) 698 return false; 699 for (UrlType item : this.reference) 700 if (!item.isEmpty()) 701 return true; 702 return false; 703 } 704 705 /** 706 * @return {@link #reference} (A reference to another artifact that created this requirement. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.) 707 */ 708 public UrlType addReferenceElement() {//2 709 UrlType t = new UrlType(); 710 if (this.reference == null) 711 this.reference = new ArrayList<UrlType>(); 712 this.reference.add(t); 713 return t; 714 } 715 716 /** 717 * @param value {@link #reference} (A reference to another artifact that created this requirement. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.) 718 */ 719 public RequirementsStatementComponent addReference(String value) { //1 720 UrlType t = new UrlType(); 721 t.setValue(value); 722 if (this.reference == null) 723 this.reference = new ArrayList<UrlType>(); 724 this.reference.add(t); 725 return this; 726 } 727 728 /** 729 * @param value {@link #reference} (A reference to another artifact that created this requirement. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.) 730 */ 731 public boolean hasReference(String value) { 732 if (this.reference == null) 733 return false; 734 for (UrlType v : this.reference) 735 if (v.getValue().equals(value)) // url 736 return true; 737 return false; 738 } 739 740 /** 741 * @return {@link #source} (Who asked for this statement to be a requirement. By default, it's assumed that the publisher knows who it is if it matters.) 742 */ 743 public List<Reference> getSource() { 744 if (this.source == null) 745 this.source = new ArrayList<Reference>(); 746 return this.source; 747 } 748 749 /** 750 * @return Returns a reference to <code>this</code> for easy method chaining 751 */ 752 public RequirementsStatementComponent setSource(List<Reference> theSource) { 753 this.source = theSource; 754 return this; 755 } 756 757 public boolean hasSource() { 758 if (this.source == null) 759 return false; 760 for (Reference item : this.source) 761 if (!item.isEmpty()) 762 return true; 763 return false; 764 } 765 766 public Reference addSource() { //3 767 Reference t = new Reference(); 768 if (this.source == null) 769 this.source = new ArrayList<Reference>(); 770 this.source.add(t); 771 return t; 772 } 773 774 public RequirementsStatementComponent addSource(Reference t) { //3 775 if (t == null) 776 return this; 777 if (this.source == null) 778 this.source = new ArrayList<Reference>(); 779 this.source.add(t); 780 return this; 781 } 782 783 /** 784 * @return The first repetition of repeating field {@link #source}, creating it if it does not already exist {3} 785 */ 786 public Reference getSourceFirstRep() { 787 if (getSource().isEmpty()) { 788 addSource(); 789 } 790 return getSource().get(0); 791 } 792 793 protected void listChildren(List<Property> children) { 794 super.listChildren(children); 795 children.add(new Property("key", "id", "Key that identifies this statement (unique within this resource).", 0, 1, key)); 796 children.add(new Property("label", "string", "A short human usable label for this statement.", 0, 1, label)); 797 children.add(new Property("conformance", "code", "A short human usable label for this statement.", 0, java.lang.Integer.MAX_VALUE, conformance)); 798 children.add(new Property("conditionality", "boolean", "This boolean flag is set to true of the text of the requirement is conditional on something e.g. it includes lanauage like 'if x then y'. This conditionality flag is introduced for purposes of filtering and colour highlighting etc.", 0, 1, conditionality)); 799 children.add(new Property("requirement", "markdown", "The actual requirement for human consumption.", 0, 1, requirement)); 800 children.add(new Property("derivedFrom", "string", "Another statement on one of the requirements that this requirement clarifies or restricts.", 0, 1, derivedFrom)); 801 children.add(new Property("parent", "string", "A larger requirement that this requirement helps to refine and enable.", 0, 1, parent)); 802 children.add(new Property("satisfiedBy", "url", "A reference to another artifact that satisfies this requirement. This could be a Profile, extension, or an element in one of those, or a CapabilityStatement, OperationDefinition, SearchParameter, CodeSystem(/code), ValueSet, Libary etc.", 0, java.lang.Integer.MAX_VALUE, satisfiedBy)); 803 children.add(new Property("reference", "url", "A reference to another artifact that created this requirement. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.", 0, java.lang.Integer.MAX_VALUE, reference)); 804 children.add(new Property("source", "Reference(CareTeam|Device|Group|HealthcareService|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "Who asked for this statement to be a requirement. By default, it's assumed that the publisher knows who it is if it matters.", 0, java.lang.Integer.MAX_VALUE, source)); 805 } 806 807 @Override 808 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 809 switch (_hash) { 810 case 106079: /*key*/ return new Property("key", "id", "Key that identifies this statement (unique within this resource).", 0, 1, key); 811 case 102727412: /*label*/ return new Property("label", "string", "A short human usable label for this statement.", 0, 1, label); 812 case 1374858133: /*conformance*/ return new Property("conformance", "code", "A short human usable label for this statement.", 0, java.lang.Integer.MAX_VALUE, conformance); 813 case -389077912: /*conditionality*/ return new Property("conditionality", "boolean", "This boolean flag is set to true of the text of the requirement is conditional on something e.g. it includes lanauage like 'if x then y'. This conditionality flag is introduced for purposes of filtering and colour highlighting etc.", 0, 1, conditionality); 814 case 363387971: /*requirement*/ return new Property("requirement", "markdown", "The actual requirement for human consumption.", 0, 1, requirement); 815 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "string", "Another statement on one of the requirements that this requirement clarifies or restricts.", 0, 1, derivedFrom); 816 case -995424086: /*parent*/ return new Property("parent", "string", "A larger requirement that this requirement helps to refine and enable.", 0, 1, parent); 817 case -1268787159: /*satisfiedBy*/ return new Property("satisfiedBy", "url", "A reference to another artifact that satisfies this requirement. This could be a Profile, extension, or an element in one of those, or a CapabilityStatement, OperationDefinition, SearchParameter, CodeSystem(/code), ValueSet, Libary etc.", 0, java.lang.Integer.MAX_VALUE, satisfiedBy); 818 case -925155509: /*reference*/ return new Property("reference", "url", "A reference to another artifact that created this requirement. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.", 0, java.lang.Integer.MAX_VALUE, reference); 819 case -896505829: /*source*/ return new Property("source", "Reference(CareTeam|Device|Group|HealthcareService|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "Who asked for this statement to be a requirement. By default, it's assumed that the publisher knows who it is if it matters.", 0, java.lang.Integer.MAX_VALUE, source); 820 default: return super.getNamedProperty(_hash, _name, _checkValid); 821 } 822 823 } 824 825 @Override 826 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 827 switch (hash) { 828 case 106079: /*key*/ return this.key == null ? new Base[0] : new Base[] {this.key}; // IdType 829 case 102727412: /*label*/ return this.label == null ? new Base[0] : new Base[] {this.label}; // StringType 830 case 1374858133: /*conformance*/ return this.conformance == null ? new Base[0] : this.conformance.toArray(new Base[this.conformance.size()]); // Enumeration<ConformanceExpectation> 831 case -389077912: /*conditionality*/ return this.conditionality == null ? new Base[0] : new Base[] {this.conditionality}; // BooleanType 832 case 363387971: /*requirement*/ return this.requirement == null ? new Base[0] : new Base[] {this.requirement}; // MarkdownType 833 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : new Base[] {this.derivedFrom}; // StringType 834 case -995424086: /*parent*/ return this.parent == null ? new Base[0] : new Base[] {this.parent}; // StringType 835 case -1268787159: /*satisfiedBy*/ return this.satisfiedBy == null ? new Base[0] : this.satisfiedBy.toArray(new Base[this.satisfiedBy.size()]); // UrlType 836 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : this.reference.toArray(new Base[this.reference.size()]); // UrlType 837 case -896505829: /*source*/ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 838 default: return super.getProperty(hash, name, checkValid); 839 } 840 841 } 842 843 @Override 844 public Base setProperty(int hash, String name, Base value) throws FHIRException { 845 switch (hash) { 846 case 106079: // key 847 this.key = TypeConvertor.castToId(value); // IdType 848 return value; 849 case 102727412: // label 850 this.label = TypeConvertor.castToString(value); // StringType 851 return value; 852 case 1374858133: // conformance 853 value = new ConformanceExpectationEnumFactory().fromType(TypeConvertor.castToCode(value)); 854 this.getConformance().add((Enumeration) value); // Enumeration<ConformanceExpectation> 855 return value; 856 case -389077912: // conditionality 857 this.conditionality = TypeConvertor.castToBoolean(value); // BooleanType 858 return value; 859 case 363387971: // requirement 860 this.requirement = TypeConvertor.castToMarkdown(value); // MarkdownType 861 return value; 862 case 1077922663: // derivedFrom 863 this.derivedFrom = TypeConvertor.castToString(value); // StringType 864 return value; 865 case -995424086: // parent 866 this.parent = TypeConvertor.castToString(value); // StringType 867 return value; 868 case -1268787159: // satisfiedBy 869 this.getSatisfiedBy().add(TypeConvertor.castToUrl(value)); // UrlType 870 return value; 871 case -925155509: // reference 872 this.getReference().add(TypeConvertor.castToUrl(value)); // UrlType 873 return value; 874 case -896505829: // source 875 this.getSource().add(TypeConvertor.castToReference(value)); // Reference 876 return value; 877 default: return super.setProperty(hash, name, value); 878 } 879 880 } 881 882 @Override 883 public Base setProperty(String name, Base value) throws FHIRException { 884 if (name.equals("key")) { 885 this.key = TypeConvertor.castToId(value); // IdType 886 } else if (name.equals("label")) { 887 this.label = TypeConvertor.castToString(value); // StringType 888 } else if (name.equals("conformance")) { 889 value = new ConformanceExpectationEnumFactory().fromType(TypeConvertor.castToCode(value)); 890 this.getConformance().add((Enumeration) value); 891 } else if (name.equals("conditionality")) { 892 this.conditionality = TypeConvertor.castToBoolean(value); // BooleanType 893 } else if (name.equals("requirement")) { 894 this.requirement = TypeConvertor.castToMarkdown(value); // MarkdownType 895 } else if (name.equals("derivedFrom")) { 896 this.derivedFrom = TypeConvertor.castToString(value); // StringType 897 } else if (name.equals("parent")) { 898 this.parent = TypeConvertor.castToString(value); // StringType 899 } else if (name.equals("satisfiedBy")) { 900 this.getSatisfiedBy().add(TypeConvertor.castToUrl(value)); 901 } else if (name.equals("reference")) { 902 this.getReference().add(TypeConvertor.castToUrl(value)); 903 } else if (name.equals("source")) { 904 this.getSource().add(TypeConvertor.castToReference(value)); 905 } else 906 return super.setProperty(name, value); 907 return value; 908 } 909 910 @Override 911 public Base makeProperty(int hash, String name) throws FHIRException { 912 switch (hash) { 913 case 106079: return getKeyElement(); 914 case 102727412: return getLabelElement(); 915 case 1374858133: return addConformanceElement(); 916 case -389077912: return getConditionalityElement(); 917 case 363387971: return getRequirementElement(); 918 case 1077922663: return getDerivedFromElement(); 919 case -995424086: return getParentElement(); 920 case -1268787159: return addSatisfiedByElement(); 921 case -925155509: return addReferenceElement(); 922 case -896505829: return addSource(); 923 default: return super.makeProperty(hash, name); 924 } 925 926 } 927 928 @Override 929 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 930 switch (hash) { 931 case 106079: /*key*/ return new String[] {"id"}; 932 case 102727412: /*label*/ return new String[] {"string"}; 933 case 1374858133: /*conformance*/ return new String[] {"code"}; 934 case -389077912: /*conditionality*/ return new String[] {"boolean"}; 935 case 363387971: /*requirement*/ return new String[] {"markdown"}; 936 case 1077922663: /*derivedFrom*/ return new String[] {"string"}; 937 case -995424086: /*parent*/ return new String[] {"string"}; 938 case -1268787159: /*satisfiedBy*/ return new String[] {"url"}; 939 case -925155509: /*reference*/ return new String[] {"url"}; 940 case -896505829: /*source*/ return new String[] {"Reference"}; 941 default: return super.getTypesForProperty(hash, name); 942 } 943 944 } 945 946 @Override 947 public Base addChild(String name) throws FHIRException { 948 if (name.equals("key")) { 949 throw new FHIRException("Cannot call addChild on a singleton property Requirements.statement.key"); 950 } 951 else if (name.equals("label")) { 952 throw new FHIRException("Cannot call addChild on a singleton property Requirements.statement.label"); 953 } 954 else if (name.equals("conformance")) { 955 throw new FHIRException("Cannot call addChild on a singleton property Requirements.statement.conformance"); 956 } 957 else if (name.equals("conditionality")) { 958 throw new FHIRException("Cannot call addChild on a singleton property Requirements.statement.conditionality"); 959 } 960 else if (name.equals("requirement")) { 961 throw new FHIRException("Cannot call addChild on a singleton property Requirements.statement.requirement"); 962 } 963 else if (name.equals("derivedFrom")) { 964 throw new FHIRException("Cannot call addChild on a singleton property Requirements.statement.derivedFrom"); 965 } 966 else if (name.equals("parent")) { 967 throw new FHIRException("Cannot call addChild on a singleton property Requirements.statement.parent"); 968 } 969 else if (name.equals("satisfiedBy")) { 970 throw new FHIRException("Cannot call addChild on a singleton property Requirements.statement.satisfiedBy"); 971 } 972 else if (name.equals("reference")) { 973 throw new FHIRException("Cannot call addChild on a singleton property Requirements.statement.reference"); 974 } 975 else if (name.equals("source")) { 976 return addSource(); 977 } 978 else 979 return super.addChild(name); 980 } 981 982 public RequirementsStatementComponent copy() { 983 RequirementsStatementComponent dst = new RequirementsStatementComponent(); 984 copyValues(dst); 985 return dst; 986 } 987 988 public void copyValues(RequirementsStatementComponent dst) { 989 super.copyValues(dst); 990 dst.key = key == null ? null : key.copy(); 991 dst.label = label == null ? null : label.copy(); 992 if (conformance != null) { 993 dst.conformance = new ArrayList<Enumeration<ConformanceExpectation>>(); 994 for (Enumeration<ConformanceExpectation> i : conformance) 995 dst.conformance.add(i.copy()); 996 }; 997 dst.conditionality = conditionality == null ? null : conditionality.copy(); 998 dst.requirement = requirement == null ? null : requirement.copy(); 999 dst.derivedFrom = derivedFrom == null ? null : derivedFrom.copy(); 1000 dst.parent = parent == null ? null : parent.copy(); 1001 if (satisfiedBy != null) { 1002 dst.satisfiedBy = new ArrayList<UrlType>(); 1003 for (UrlType i : satisfiedBy) 1004 dst.satisfiedBy.add(i.copy()); 1005 }; 1006 if (reference != null) { 1007 dst.reference = new ArrayList<UrlType>(); 1008 for (UrlType i : reference) 1009 dst.reference.add(i.copy()); 1010 }; 1011 if (source != null) { 1012 dst.source = new ArrayList<Reference>(); 1013 for (Reference i : source) 1014 dst.source.add(i.copy()); 1015 }; 1016 } 1017 1018 @Override 1019 public boolean equalsDeep(Base other_) { 1020 if (!super.equalsDeep(other_)) 1021 return false; 1022 if (!(other_ instanceof RequirementsStatementComponent)) 1023 return false; 1024 RequirementsStatementComponent o = (RequirementsStatementComponent) other_; 1025 return compareDeep(key, o.key, true) && compareDeep(label, o.label, true) && compareDeep(conformance, o.conformance, true) 1026 && compareDeep(conditionality, o.conditionality, true) && compareDeep(requirement, o.requirement, true) 1027 && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(parent, o.parent, true) && compareDeep(satisfiedBy, o.satisfiedBy, true) 1028 && compareDeep(reference, o.reference, true) && compareDeep(source, o.source, true); 1029 } 1030 1031 @Override 1032 public boolean equalsShallow(Base other_) { 1033 if (!super.equalsShallow(other_)) 1034 return false; 1035 if (!(other_ instanceof RequirementsStatementComponent)) 1036 return false; 1037 RequirementsStatementComponent o = (RequirementsStatementComponent) other_; 1038 return compareValues(key, o.key, true) && compareValues(label, o.label, true) && compareValues(conformance, o.conformance, true) 1039 && compareValues(conditionality, o.conditionality, true) && compareValues(requirement, o.requirement, true) 1040 && compareValues(derivedFrom, o.derivedFrom, true) && compareValues(parent, o.parent, true) && compareValues(satisfiedBy, o.satisfiedBy, true) 1041 && compareValues(reference, o.reference, true); 1042 } 1043 1044 public boolean isEmpty() { 1045 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(key, label, conformance 1046 , conditionality, requirement, derivedFrom, parent, satisfiedBy, reference, source 1047 ); 1048 } 1049 1050 public String fhirType() { 1051 return "Requirements.statement"; 1052 1053 } 1054 1055 } 1056 1057 /** 1058 * An absolute URI that is used to identify this Requirements when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Requirements is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Requirements is stored on different servers. 1059 */ 1060 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 1061 @Description(shortDefinition="Canonical identifier for this Requirements, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this Requirements when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Requirements is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Requirements is stored on different servers." ) 1062 protected UriType url; 1063 1064 /** 1065 * A formal identifier that is used to identify this Requirements when it is represented in other formats, or referenced in a specification, model, design or an instance. 1066 */ 1067 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1068 @Description(shortDefinition="Additional identifier for the Requirements (business identifier)", formalDefinition="A formal identifier that is used to identify this Requirements when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 1069 protected List<Identifier> identifier; 1070 1071 /** 1072 * The identifier that is used to identify this version of the Requirements when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Requirements author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1073 */ 1074 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1075 @Description(shortDefinition="Business version of the Requirements", formalDefinition="The identifier that is used to identify this version of the Requirements when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Requirements author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 1076 protected StringType version; 1077 1078 /** 1079 * Indicates the mechanism used to compare versions to determine which is more current. 1080 */ 1081 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 1082 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 1083 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 1084 protected DataType versionAlgorithm; 1085 1086 /** 1087 * A natural language name identifying the Requirements. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1088 */ 1089 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1090 @Description(shortDefinition="Name for this Requirements (computer friendly)", formalDefinition="A natural language name identifying the Requirements. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 1091 protected StringType name; 1092 1093 /** 1094 * A short, descriptive, user-friendly title for the Requirements. 1095 */ 1096 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1097 @Description(shortDefinition="Name for this Requirements (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the Requirements." ) 1098 protected StringType title; 1099 1100 /** 1101 * The status of this Requirements. Enables tracking the life-cycle of the content. 1102 */ 1103 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 1104 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this Requirements. Enables tracking the life-cycle of the content." ) 1105 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 1106 protected Enumeration<PublicationStatus> status; 1107 1108 /** 1109 * A Boolean value to indicate that this Requirements is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1110 */ 1111 @Child(name = "experimental", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1112 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this Requirements is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 1113 protected BooleanType experimental; 1114 1115 /** 1116 * The date (and optionally time) when the Requirements was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the Requirements changes. 1117 */ 1118 @Child(name = "date", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1119 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the Requirements was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the Requirements changes." ) 1120 protected DateTimeType date; 1121 1122 /** 1123 * The name of the organization or individual responsible for the release and ongoing maintenance of the Requirements. 1124 */ 1125 @Child(name = "publisher", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1126 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the Requirements." ) 1127 protected StringType publisher; 1128 1129 /** 1130 * Contact details to assist a user in finding and communicating with the publisher. 1131 */ 1132 @Child(name = "contact", type = {ContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1133 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 1134 protected List<ContactDetail> contact; 1135 1136 /** 1137 * A free text natural language description of the requirements. 1138 */ 1139 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 1140 @Description(shortDefinition="Natural language description of the requirements", formalDefinition="A free text natural language description of the requirements." ) 1141 protected MarkdownType description; 1142 1143 /** 1144 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate Requirements instances. 1145 */ 1146 @Child(name = "useContext", type = {UsageContext.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1147 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate Requirements instances." ) 1148 protected List<UsageContext> useContext; 1149 1150 /** 1151 * A legal or geographic region in which the Requirements is intended to be used. 1152 */ 1153 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1154 @Description(shortDefinition="Intended jurisdiction for Requirements (if applicable)", formalDefinition="A legal or geographic region in which the Requirements is intended to be used." ) 1155 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 1156 protected List<CodeableConcept> jurisdiction; 1157 1158 /** 1159 * Explanation of why this Requirements is needed and why it has been designed as it has. 1160 */ 1161 @Child(name = "purpose", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 1162 @Description(shortDefinition="Why this Requirements is defined", formalDefinition="Explanation of why this Requirements is needed and why it has been designed as it has." ) 1163 protected MarkdownType purpose; 1164 1165 /** 1166 * A copyright statement relating to the Requirements and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Requirements. 1167 */ 1168 @Child(name = "copyright", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 1169 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the Requirements and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Requirements." ) 1170 protected MarkdownType copyright; 1171 1172 /** 1173 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1174 */ 1175 @Child(name = "copyrightLabel", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 1176 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 1177 protected StringType copyrightLabel; 1178 1179 /** 1180 * Another set of Requirements that this set of Requirements builds on and updates. 1181 */ 1182 @Child(name = "derivedFrom", type = {CanonicalType.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1183 @Description(shortDefinition="Other set of Requirements this builds on", formalDefinition="Another set of Requirements that this set of Requirements builds on and updates." ) 1184 protected List<CanonicalType> derivedFrom; 1185 1186 /** 1187 * A reference to another artifact that created this set of requirements. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere. 1188 */ 1189 @Child(name = "reference", type = {UrlType.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1190 @Description(shortDefinition="External artifact (rule/document etc. that) created this set of requirements", formalDefinition="A reference to another artifact that created this set of requirements. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere." ) 1191 protected List<UrlType> reference; 1192 1193 /** 1194 * An actor these requirements are in regard to. 1195 */ 1196 @Child(name = "actor", type = {CanonicalType.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1197 @Description(shortDefinition="Actor for these requirements", formalDefinition="An actor these requirements are in regard to." ) 1198 protected List<CanonicalType> actor; 1199 1200 /** 1201 * The actual statement of requirement, in markdown format. 1202 */ 1203 @Child(name = "statement", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1204 @Description(shortDefinition="Actual statement as markdown", formalDefinition="The actual statement of requirement, in markdown format." ) 1205 protected List<RequirementsStatementComponent> statement; 1206 1207 private static final long serialVersionUID = -395151267L; 1208 1209 /** 1210 * Constructor 1211 */ 1212 public Requirements() { 1213 super(); 1214 } 1215 1216 /** 1217 * Constructor 1218 */ 1219 public Requirements(PublicationStatus status) { 1220 super(); 1221 this.setStatus(status); 1222 } 1223 1224 /** 1225 * @return {@link #url} (An absolute URI that is used to identify this Requirements when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Requirements is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Requirements is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1226 */ 1227 public UriType getUrlElement() { 1228 if (this.url == null) 1229 if (Configuration.errorOnAutoCreate()) 1230 throw new Error("Attempt to auto-create Requirements.url"); 1231 else if (Configuration.doAutoCreate()) 1232 this.url = new UriType(); // bb 1233 return this.url; 1234 } 1235 1236 public boolean hasUrlElement() { 1237 return this.url != null && !this.url.isEmpty(); 1238 } 1239 1240 public boolean hasUrl() { 1241 return this.url != null && !this.url.isEmpty(); 1242 } 1243 1244 /** 1245 * @param value {@link #url} (An absolute URI that is used to identify this Requirements when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Requirements is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Requirements is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1246 */ 1247 public Requirements setUrlElement(UriType value) { 1248 this.url = value; 1249 return this; 1250 } 1251 1252 /** 1253 * @return An absolute URI that is used to identify this Requirements when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Requirements is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Requirements is stored on different servers. 1254 */ 1255 public String getUrl() { 1256 return this.url == null ? null : this.url.getValue(); 1257 } 1258 1259 /** 1260 * @param value An absolute URI that is used to identify this Requirements when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Requirements is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Requirements is stored on different servers. 1261 */ 1262 public Requirements setUrl(String value) { 1263 if (Utilities.noString(value)) 1264 this.url = null; 1265 else { 1266 if (this.url == null) 1267 this.url = new UriType(); 1268 this.url.setValue(value); 1269 } 1270 return this; 1271 } 1272 1273 /** 1274 * @return {@link #identifier} (A formal identifier that is used to identify this Requirements when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1275 */ 1276 public List<Identifier> getIdentifier() { 1277 if (this.identifier == null) 1278 this.identifier = new ArrayList<Identifier>(); 1279 return this.identifier; 1280 } 1281 1282 /** 1283 * @return Returns a reference to <code>this</code> for easy method chaining 1284 */ 1285 public Requirements setIdentifier(List<Identifier> theIdentifier) { 1286 this.identifier = theIdentifier; 1287 return this; 1288 } 1289 1290 public boolean hasIdentifier() { 1291 if (this.identifier == null) 1292 return false; 1293 for (Identifier item : this.identifier) 1294 if (!item.isEmpty()) 1295 return true; 1296 return false; 1297 } 1298 1299 public Identifier addIdentifier() { //3 1300 Identifier t = new Identifier(); 1301 if (this.identifier == null) 1302 this.identifier = new ArrayList<Identifier>(); 1303 this.identifier.add(t); 1304 return t; 1305 } 1306 1307 public Requirements addIdentifier(Identifier t) { //3 1308 if (t == null) 1309 return this; 1310 if (this.identifier == null) 1311 this.identifier = new ArrayList<Identifier>(); 1312 this.identifier.add(t); 1313 return this; 1314 } 1315 1316 /** 1317 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1318 */ 1319 public Identifier getIdentifierFirstRep() { 1320 if (getIdentifier().isEmpty()) { 1321 addIdentifier(); 1322 } 1323 return getIdentifier().get(0); 1324 } 1325 1326 /** 1327 * @return {@link #version} (The identifier that is used to identify this version of the Requirements when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Requirements author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1328 */ 1329 public StringType getVersionElement() { 1330 if (this.version == null) 1331 if (Configuration.errorOnAutoCreate()) 1332 throw new Error("Attempt to auto-create Requirements.version"); 1333 else if (Configuration.doAutoCreate()) 1334 this.version = new StringType(); // bb 1335 return this.version; 1336 } 1337 1338 public boolean hasVersionElement() { 1339 return this.version != null && !this.version.isEmpty(); 1340 } 1341 1342 public boolean hasVersion() { 1343 return this.version != null && !this.version.isEmpty(); 1344 } 1345 1346 /** 1347 * @param value {@link #version} (The identifier that is used to identify this version of the Requirements when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Requirements author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1348 */ 1349 public Requirements setVersionElement(StringType value) { 1350 this.version = value; 1351 return this; 1352 } 1353 1354 /** 1355 * @return The identifier that is used to identify this version of the Requirements when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Requirements author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1356 */ 1357 public String getVersion() { 1358 return this.version == null ? null : this.version.getValue(); 1359 } 1360 1361 /** 1362 * @param value The identifier that is used to identify this version of the Requirements when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Requirements author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1363 */ 1364 public Requirements setVersion(String value) { 1365 if (Utilities.noString(value)) 1366 this.version = null; 1367 else { 1368 if (this.version == null) 1369 this.version = new StringType(); 1370 this.version.setValue(value); 1371 } 1372 return this; 1373 } 1374 1375 /** 1376 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1377 */ 1378 public DataType getVersionAlgorithm() { 1379 return this.versionAlgorithm; 1380 } 1381 1382 /** 1383 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1384 */ 1385 public StringType getVersionAlgorithmStringType() throws FHIRException { 1386 if (this.versionAlgorithm == null) 1387 this.versionAlgorithm = new StringType(); 1388 if (!(this.versionAlgorithm instanceof StringType)) 1389 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1390 return (StringType) this.versionAlgorithm; 1391 } 1392 1393 public boolean hasVersionAlgorithmStringType() { 1394 return this != null && this.versionAlgorithm instanceof StringType; 1395 } 1396 1397 /** 1398 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1399 */ 1400 public Coding getVersionAlgorithmCoding() throws FHIRException { 1401 if (this.versionAlgorithm == null) 1402 this.versionAlgorithm = new Coding(); 1403 if (!(this.versionAlgorithm instanceof Coding)) 1404 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1405 return (Coding) this.versionAlgorithm; 1406 } 1407 1408 public boolean hasVersionAlgorithmCoding() { 1409 return this != null && this.versionAlgorithm instanceof Coding; 1410 } 1411 1412 public boolean hasVersionAlgorithm() { 1413 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 1414 } 1415 1416 /** 1417 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1418 */ 1419 public Requirements setVersionAlgorithm(DataType value) { 1420 if (value != null && !(value instanceof StringType || value instanceof Coding)) 1421 throw new FHIRException("Not the right type for Requirements.versionAlgorithm[x]: "+value.fhirType()); 1422 this.versionAlgorithm = value; 1423 return this; 1424 } 1425 1426 /** 1427 * @return {@link #name} (A natural language name identifying the Requirements. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1428 */ 1429 public StringType getNameElement() { 1430 if (this.name == null) 1431 if (Configuration.errorOnAutoCreate()) 1432 throw new Error("Attempt to auto-create Requirements.name"); 1433 else if (Configuration.doAutoCreate()) 1434 this.name = new StringType(); // bb 1435 return this.name; 1436 } 1437 1438 public boolean hasNameElement() { 1439 return this.name != null && !this.name.isEmpty(); 1440 } 1441 1442 public boolean hasName() { 1443 return this.name != null && !this.name.isEmpty(); 1444 } 1445 1446 /** 1447 * @param value {@link #name} (A natural language name identifying the Requirements. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1448 */ 1449 public Requirements setNameElement(StringType value) { 1450 this.name = value; 1451 return this; 1452 } 1453 1454 /** 1455 * @return A natural language name identifying the Requirements. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1456 */ 1457 public String getName() { 1458 return this.name == null ? null : this.name.getValue(); 1459 } 1460 1461 /** 1462 * @param value A natural language name identifying the Requirements. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1463 */ 1464 public Requirements setName(String value) { 1465 if (Utilities.noString(value)) 1466 this.name = null; 1467 else { 1468 if (this.name == null) 1469 this.name = new StringType(); 1470 this.name.setValue(value); 1471 } 1472 return this; 1473 } 1474 1475 /** 1476 * @return {@link #title} (A short, descriptive, user-friendly title for the Requirements.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1477 */ 1478 public StringType getTitleElement() { 1479 if (this.title == null) 1480 if (Configuration.errorOnAutoCreate()) 1481 throw new Error("Attempt to auto-create Requirements.title"); 1482 else if (Configuration.doAutoCreate()) 1483 this.title = new StringType(); // bb 1484 return this.title; 1485 } 1486 1487 public boolean hasTitleElement() { 1488 return this.title != null && !this.title.isEmpty(); 1489 } 1490 1491 public boolean hasTitle() { 1492 return this.title != null && !this.title.isEmpty(); 1493 } 1494 1495 /** 1496 * @param value {@link #title} (A short, descriptive, user-friendly title for the Requirements.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1497 */ 1498 public Requirements setTitleElement(StringType value) { 1499 this.title = value; 1500 return this; 1501 } 1502 1503 /** 1504 * @return A short, descriptive, user-friendly title for the Requirements. 1505 */ 1506 public String getTitle() { 1507 return this.title == null ? null : this.title.getValue(); 1508 } 1509 1510 /** 1511 * @param value A short, descriptive, user-friendly title for the Requirements. 1512 */ 1513 public Requirements setTitle(String value) { 1514 if (Utilities.noString(value)) 1515 this.title = null; 1516 else { 1517 if (this.title == null) 1518 this.title = new StringType(); 1519 this.title.setValue(value); 1520 } 1521 return this; 1522 } 1523 1524 /** 1525 * @return {@link #status} (The status of this Requirements. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1526 */ 1527 public Enumeration<PublicationStatus> getStatusElement() { 1528 if (this.status == null) 1529 if (Configuration.errorOnAutoCreate()) 1530 throw new Error("Attempt to auto-create Requirements.status"); 1531 else if (Configuration.doAutoCreate()) 1532 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1533 return this.status; 1534 } 1535 1536 public boolean hasStatusElement() { 1537 return this.status != null && !this.status.isEmpty(); 1538 } 1539 1540 public boolean hasStatus() { 1541 return this.status != null && !this.status.isEmpty(); 1542 } 1543 1544 /** 1545 * @param value {@link #status} (The status of this Requirements. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1546 */ 1547 public Requirements setStatusElement(Enumeration<PublicationStatus> value) { 1548 this.status = value; 1549 return this; 1550 } 1551 1552 /** 1553 * @return The status of this Requirements. Enables tracking the life-cycle of the content. 1554 */ 1555 public PublicationStatus getStatus() { 1556 return this.status == null ? null : this.status.getValue(); 1557 } 1558 1559 /** 1560 * @param value The status of this Requirements. Enables tracking the life-cycle of the content. 1561 */ 1562 public Requirements setStatus(PublicationStatus value) { 1563 if (this.status == null) 1564 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1565 this.status.setValue(value); 1566 return this; 1567 } 1568 1569 /** 1570 * @return {@link #experimental} (A Boolean value to indicate that this Requirements is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1571 */ 1572 public BooleanType getExperimentalElement() { 1573 if (this.experimental == null) 1574 if (Configuration.errorOnAutoCreate()) 1575 throw new Error("Attempt to auto-create Requirements.experimental"); 1576 else if (Configuration.doAutoCreate()) 1577 this.experimental = new BooleanType(); // bb 1578 return this.experimental; 1579 } 1580 1581 public boolean hasExperimentalElement() { 1582 return this.experimental != null && !this.experimental.isEmpty(); 1583 } 1584 1585 public boolean hasExperimental() { 1586 return this.experimental != null && !this.experimental.isEmpty(); 1587 } 1588 1589 /** 1590 * @param value {@link #experimental} (A Boolean value to indicate that this Requirements is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1591 */ 1592 public Requirements setExperimentalElement(BooleanType value) { 1593 this.experimental = value; 1594 return this; 1595 } 1596 1597 /** 1598 * @return A Boolean value to indicate that this Requirements is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1599 */ 1600 public boolean getExperimental() { 1601 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1602 } 1603 1604 /** 1605 * @param value A Boolean value to indicate that this Requirements is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1606 */ 1607 public Requirements setExperimental(boolean value) { 1608 if (this.experimental == null) 1609 this.experimental = new BooleanType(); 1610 this.experimental.setValue(value); 1611 return this; 1612 } 1613 1614 /** 1615 * @return {@link #date} (The date (and optionally time) when the Requirements was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the Requirements changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1616 */ 1617 public DateTimeType getDateElement() { 1618 if (this.date == null) 1619 if (Configuration.errorOnAutoCreate()) 1620 throw new Error("Attempt to auto-create Requirements.date"); 1621 else if (Configuration.doAutoCreate()) 1622 this.date = new DateTimeType(); // bb 1623 return this.date; 1624 } 1625 1626 public boolean hasDateElement() { 1627 return this.date != null && !this.date.isEmpty(); 1628 } 1629 1630 public boolean hasDate() { 1631 return this.date != null && !this.date.isEmpty(); 1632 } 1633 1634 /** 1635 * @param value {@link #date} (The date (and optionally time) when the Requirements was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the Requirements changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1636 */ 1637 public Requirements setDateElement(DateTimeType value) { 1638 this.date = value; 1639 return this; 1640 } 1641 1642 /** 1643 * @return The date (and optionally time) when the Requirements was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the Requirements changes. 1644 */ 1645 public Date getDate() { 1646 return this.date == null ? null : this.date.getValue(); 1647 } 1648 1649 /** 1650 * @param value The date (and optionally time) when the Requirements was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the Requirements changes. 1651 */ 1652 public Requirements setDate(Date value) { 1653 if (value == null) 1654 this.date = null; 1655 else { 1656 if (this.date == null) 1657 this.date = new DateTimeType(); 1658 this.date.setValue(value); 1659 } 1660 return this; 1661 } 1662 1663 /** 1664 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the Requirements.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1665 */ 1666 public StringType getPublisherElement() { 1667 if (this.publisher == null) 1668 if (Configuration.errorOnAutoCreate()) 1669 throw new Error("Attempt to auto-create Requirements.publisher"); 1670 else if (Configuration.doAutoCreate()) 1671 this.publisher = new StringType(); // bb 1672 return this.publisher; 1673 } 1674 1675 public boolean hasPublisherElement() { 1676 return this.publisher != null && !this.publisher.isEmpty(); 1677 } 1678 1679 public boolean hasPublisher() { 1680 return this.publisher != null && !this.publisher.isEmpty(); 1681 } 1682 1683 /** 1684 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the Requirements.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1685 */ 1686 public Requirements setPublisherElement(StringType value) { 1687 this.publisher = value; 1688 return this; 1689 } 1690 1691 /** 1692 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the Requirements. 1693 */ 1694 public String getPublisher() { 1695 return this.publisher == null ? null : this.publisher.getValue(); 1696 } 1697 1698 /** 1699 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the Requirements. 1700 */ 1701 public Requirements setPublisher(String value) { 1702 if (Utilities.noString(value)) 1703 this.publisher = null; 1704 else { 1705 if (this.publisher == null) 1706 this.publisher = new StringType(); 1707 this.publisher.setValue(value); 1708 } 1709 return this; 1710 } 1711 1712 /** 1713 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 1714 */ 1715 public List<ContactDetail> getContact() { 1716 if (this.contact == null) 1717 this.contact = new ArrayList<ContactDetail>(); 1718 return this.contact; 1719 } 1720 1721 /** 1722 * @return Returns a reference to <code>this</code> for easy method chaining 1723 */ 1724 public Requirements setContact(List<ContactDetail> theContact) { 1725 this.contact = theContact; 1726 return this; 1727 } 1728 1729 public boolean hasContact() { 1730 if (this.contact == null) 1731 return false; 1732 for (ContactDetail item : this.contact) 1733 if (!item.isEmpty()) 1734 return true; 1735 return false; 1736 } 1737 1738 public ContactDetail addContact() { //3 1739 ContactDetail t = new ContactDetail(); 1740 if (this.contact == null) 1741 this.contact = new ArrayList<ContactDetail>(); 1742 this.contact.add(t); 1743 return t; 1744 } 1745 1746 public Requirements addContact(ContactDetail t) { //3 1747 if (t == null) 1748 return this; 1749 if (this.contact == null) 1750 this.contact = new ArrayList<ContactDetail>(); 1751 this.contact.add(t); 1752 return this; 1753 } 1754 1755 /** 1756 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 1757 */ 1758 public ContactDetail getContactFirstRep() { 1759 if (getContact().isEmpty()) { 1760 addContact(); 1761 } 1762 return getContact().get(0); 1763 } 1764 1765 /** 1766 * @return {@link #description} (A free text natural language description of the requirements.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1767 */ 1768 public MarkdownType getDescriptionElement() { 1769 if (this.description == null) 1770 if (Configuration.errorOnAutoCreate()) 1771 throw new Error("Attempt to auto-create Requirements.description"); 1772 else if (Configuration.doAutoCreate()) 1773 this.description = new MarkdownType(); // bb 1774 return this.description; 1775 } 1776 1777 public boolean hasDescriptionElement() { 1778 return this.description != null && !this.description.isEmpty(); 1779 } 1780 1781 public boolean hasDescription() { 1782 return this.description != null && !this.description.isEmpty(); 1783 } 1784 1785 /** 1786 * @param value {@link #description} (A free text natural language description of the requirements.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1787 */ 1788 public Requirements setDescriptionElement(MarkdownType value) { 1789 this.description = value; 1790 return this; 1791 } 1792 1793 /** 1794 * @return A free text natural language description of the requirements. 1795 */ 1796 public String getDescription() { 1797 return this.description == null ? null : this.description.getValue(); 1798 } 1799 1800 /** 1801 * @param value A free text natural language description of the requirements. 1802 */ 1803 public Requirements setDescription(String value) { 1804 if (Utilities.noString(value)) 1805 this.description = null; 1806 else { 1807 if (this.description == null) 1808 this.description = new MarkdownType(); 1809 this.description.setValue(value); 1810 } 1811 return this; 1812 } 1813 1814 /** 1815 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate Requirements instances.) 1816 */ 1817 public List<UsageContext> getUseContext() { 1818 if (this.useContext == null) 1819 this.useContext = new ArrayList<UsageContext>(); 1820 return this.useContext; 1821 } 1822 1823 /** 1824 * @return Returns a reference to <code>this</code> for easy method chaining 1825 */ 1826 public Requirements setUseContext(List<UsageContext> theUseContext) { 1827 this.useContext = theUseContext; 1828 return this; 1829 } 1830 1831 public boolean hasUseContext() { 1832 if (this.useContext == null) 1833 return false; 1834 for (UsageContext item : this.useContext) 1835 if (!item.isEmpty()) 1836 return true; 1837 return false; 1838 } 1839 1840 public UsageContext addUseContext() { //3 1841 UsageContext t = new UsageContext(); 1842 if (this.useContext == null) 1843 this.useContext = new ArrayList<UsageContext>(); 1844 this.useContext.add(t); 1845 return t; 1846 } 1847 1848 public Requirements addUseContext(UsageContext t) { //3 1849 if (t == null) 1850 return this; 1851 if (this.useContext == null) 1852 this.useContext = new ArrayList<UsageContext>(); 1853 this.useContext.add(t); 1854 return this; 1855 } 1856 1857 /** 1858 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 1859 */ 1860 public UsageContext getUseContextFirstRep() { 1861 if (getUseContext().isEmpty()) { 1862 addUseContext(); 1863 } 1864 return getUseContext().get(0); 1865 } 1866 1867 /** 1868 * @return {@link #jurisdiction} (A legal or geographic region in which the Requirements is intended to be used.) 1869 */ 1870 public List<CodeableConcept> getJurisdiction() { 1871 if (this.jurisdiction == null) 1872 this.jurisdiction = new ArrayList<CodeableConcept>(); 1873 return this.jurisdiction; 1874 } 1875 1876 /** 1877 * @return Returns a reference to <code>this</code> for easy method chaining 1878 */ 1879 public Requirements setJurisdiction(List<CodeableConcept> theJurisdiction) { 1880 this.jurisdiction = theJurisdiction; 1881 return this; 1882 } 1883 1884 public boolean hasJurisdiction() { 1885 if (this.jurisdiction == null) 1886 return false; 1887 for (CodeableConcept item : this.jurisdiction) 1888 if (!item.isEmpty()) 1889 return true; 1890 return false; 1891 } 1892 1893 public CodeableConcept addJurisdiction() { //3 1894 CodeableConcept t = new CodeableConcept(); 1895 if (this.jurisdiction == null) 1896 this.jurisdiction = new ArrayList<CodeableConcept>(); 1897 this.jurisdiction.add(t); 1898 return t; 1899 } 1900 1901 public Requirements addJurisdiction(CodeableConcept t) { //3 1902 if (t == null) 1903 return this; 1904 if (this.jurisdiction == null) 1905 this.jurisdiction = new ArrayList<CodeableConcept>(); 1906 this.jurisdiction.add(t); 1907 return this; 1908 } 1909 1910 /** 1911 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 1912 */ 1913 public CodeableConcept getJurisdictionFirstRep() { 1914 if (getJurisdiction().isEmpty()) { 1915 addJurisdiction(); 1916 } 1917 return getJurisdiction().get(0); 1918 } 1919 1920 /** 1921 * @return {@link #purpose} (Explanation of why this Requirements is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1922 */ 1923 public MarkdownType getPurposeElement() { 1924 if (this.purpose == null) 1925 if (Configuration.errorOnAutoCreate()) 1926 throw new Error("Attempt to auto-create Requirements.purpose"); 1927 else if (Configuration.doAutoCreate()) 1928 this.purpose = new MarkdownType(); // bb 1929 return this.purpose; 1930 } 1931 1932 public boolean hasPurposeElement() { 1933 return this.purpose != null && !this.purpose.isEmpty(); 1934 } 1935 1936 public boolean hasPurpose() { 1937 return this.purpose != null && !this.purpose.isEmpty(); 1938 } 1939 1940 /** 1941 * @param value {@link #purpose} (Explanation of why this Requirements is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1942 */ 1943 public Requirements setPurposeElement(MarkdownType value) { 1944 this.purpose = value; 1945 return this; 1946 } 1947 1948 /** 1949 * @return Explanation of why this Requirements is needed and why it has been designed as it has. 1950 */ 1951 public String getPurpose() { 1952 return this.purpose == null ? null : this.purpose.getValue(); 1953 } 1954 1955 /** 1956 * @param value Explanation of why this Requirements is needed and why it has been designed as it has. 1957 */ 1958 public Requirements setPurpose(String value) { 1959 if (Utilities.noString(value)) 1960 this.purpose = null; 1961 else { 1962 if (this.purpose == null) 1963 this.purpose = new MarkdownType(); 1964 this.purpose.setValue(value); 1965 } 1966 return this; 1967 } 1968 1969 /** 1970 * @return {@link #copyright} (A copyright statement relating to the Requirements and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Requirements.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1971 */ 1972 public MarkdownType getCopyrightElement() { 1973 if (this.copyright == null) 1974 if (Configuration.errorOnAutoCreate()) 1975 throw new Error("Attempt to auto-create Requirements.copyright"); 1976 else if (Configuration.doAutoCreate()) 1977 this.copyright = new MarkdownType(); // bb 1978 return this.copyright; 1979 } 1980 1981 public boolean hasCopyrightElement() { 1982 return this.copyright != null && !this.copyright.isEmpty(); 1983 } 1984 1985 public boolean hasCopyright() { 1986 return this.copyright != null && !this.copyright.isEmpty(); 1987 } 1988 1989 /** 1990 * @param value {@link #copyright} (A copyright statement relating to the Requirements and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Requirements.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1991 */ 1992 public Requirements setCopyrightElement(MarkdownType value) { 1993 this.copyright = value; 1994 return this; 1995 } 1996 1997 /** 1998 * @return A copyright statement relating to the Requirements and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Requirements. 1999 */ 2000 public String getCopyright() { 2001 return this.copyright == null ? null : this.copyright.getValue(); 2002 } 2003 2004 /** 2005 * @param value A copyright statement relating to the Requirements and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Requirements. 2006 */ 2007 public Requirements setCopyright(String value) { 2008 if (Utilities.noString(value)) 2009 this.copyright = null; 2010 else { 2011 if (this.copyright == null) 2012 this.copyright = new MarkdownType(); 2013 this.copyright.setValue(value); 2014 } 2015 return this; 2016 } 2017 2018 /** 2019 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2020 */ 2021 public StringType getCopyrightLabelElement() { 2022 if (this.copyrightLabel == null) 2023 if (Configuration.errorOnAutoCreate()) 2024 throw new Error("Attempt to auto-create Requirements.copyrightLabel"); 2025 else if (Configuration.doAutoCreate()) 2026 this.copyrightLabel = new StringType(); // bb 2027 return this.copyrightLabel; 2028 } 2029 2030 public boolean hasCopyrightLabelElement() { 2031 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2032 } 2033 2034 public boolean hasCopyrightLabel() { 2035 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2036 } 2037 2038 /** 2039 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2040 */ 2041 public Requirements setCopyrightLabelElement(StringType value) { 2042 this.copyrightLabel = value; 2043 return this; 2044 } 2045 2046 /** 2047 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2048 */ 2049 public String getCopyrightLabel() { 2050 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 2051 } 2052 2053 /** 2054 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2055 */ 2056 public Requirements setCopyrightLabel(String value) { 2057 if (Utilities.noString(value)) 2058 this.copyrightLabel = null; 2059 else { 2060 if (this.copyrightLabel == null) 2061 this.copyrightLabel = new StringType(); 2062 this.copyrightLabel.setValue(value); 2063 } 2064 return this; 2065 } 2066 2067 /** 2068 * @return {@link #derivedFrom} (Another set of Requirements that this set of Requirements builds on and updates.) 2069 */ 2070 public List<CanonicalType> getDerivedFrom() { 2071 if (this.derivedFrom == null) 2072 this.derivedFrom = new ArrayList<CanonicalType>(); 2073 return this.derivedFrom; 2074 } 2075 2076 /** 2077 * @return Returns a reference to <code>this</code> for easy method chaining 2078 */ 2079 public Requirements setDerivedFrom(List<CanonicalType> theDerivedFrom) { 2080 this.derivedFrom = theDerivedFrom; 2081 return this; 2082 } 2083 2084 public boolean hasDerivedFrom() { 2085 if (this.derivedFrom == null) 2086 return false; 2087 for (CanonicalType item : this.derivedFrom) 2088 if (!item.isEmpty()) 2089 return true; 2090 return false; 2091 } 2092 2093 /** 2094 * @return {@link #derivedFrom} (Another set of Requirements that this set of Requirements builds on and updates.) 2095 */ 2096 public CanonicalType addDerivedFromElement() {//2 2097 CanonicalType t = new CanonicalType(); 2098 if (this.derivedFrom == null) 2099 this.derivedFrom = new ArrayList<CanonicalType>(); 2100 this.derivedFrom.add(t); 2101 return t; 2102 } 2103 2104 /** 2105 * @param value {@link #derivedFrom} (Another set of Requirements that this set of Requirements builds on and updates.) 2106 */ 2107 public Requirements addDerivedFrom(String value) { //1 2108 CanonicalType t = new CanonicalType(); 2109 t.setValue(value); 2110 if (this.derivedFrom == null) 2111 this.derivedFrom = new ArrayList<CanonicalType>(); 2112 this.derivedFrom.add(t); 2113 return this; 2114 } 2115 2116 /** 2117 * @param value {@link #derivedFrom} (Another set of Requirements that this set of Requirements builds on and updates.) 2118 */ 2119 public boolean hasDerivedFrom(String value) { 2120 if (this.derivedFrom == null) 2121 return false; 2122 for (CanonicalType v : this.derivedFrom) 2123 if (v.getValue().equals(value)) // canonical 2124 return true; 2125 return false; 2126 } 2127 2128 /** 2129 * @return {@link #reference} (A reference to another artifact that created this set of requirements. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.) 2130 */ 2131 public List<UrlType> getReference() { 2132 if (this.reference == null) 2133 this.reference = new ArrayList<UrlType>(); 2134 return this.reference; 2135 } 2136 2137 /** 2138 * @return Returns a reference to <code>this</code> for easy method chaining 2139 */ 2140 public Requirements setReference(List<UrlType> theReference) { 2141 this.reference = theReference; 2142 return this; 2143 } 2144 2145 public boolean hasReference() { 2146 if (this.reference == null) 2147 return false; 2148 for (UrlType item : this.reference) 2149 if (!item.isEmpty()) 2150 return true; 2151 return false; 2152 } 2153 2154 /** 2155 * @return {@link #reference} (A reference to another artifact that created this set of requirements. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.) 2156 */ 2157 public UrlType addReferenceElement() {//2 2158 UrlType t = new UrlType(); 2159 if (this.reference == null) 2160 this.reference = new ArrayList<UrlType>(); 2161 this.reference.add(t); 2162 return t; 2163 } 2164 2165 /** 2166 * @param value {@link #reference} (A reference to another artifact that created this set of requirements. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.) 2167 */ 2168 public Requirements addReference(String value) { //1 2169 UrlType t = new UrlType(); 2170 t.setValue(value); 2171 if (this.reference == null) 2172 this.reference = new ArrayList<UrlType>(); 2173 this.reference.add(t); 2174 return this; 2175 } 2176 2177 /** 2178 * @param value {@link #reference} (A reference to another artifact that created this set of requirements. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.) 2179 */ 2180 public boolean hasReference(String value) { 2181 if (this.reference == null) 2182 return false; 2183 for (UrlType v : this.reference) 2184 if (v.getValue().equals(value)) // url 2185 return true; 2186 return false; 2187 } 2188 2189 /** 2190 * @return {@link #actor} (An actor these requirements are in regard to.) 2191 */ 2192 public List<CanonicalType> getActor() { 2193 if (this.actor == null) 2194 this.actor = new ArrayList<CanonicalType>(); 2195 return this.actor; 2196 } 2197 2198 /** 2199 * @return Returns a reference to <code>this</code> for easy method chaining 2200 */ 2201 public Requirements setActor(List<CanonicalType> theActor) { 2202 this.actor = theActor; 2203 return this; 2204 } 2205 2206 public boolean hasActor() { 2207 if (this.actor == null) 2208 return false; 2209 for (CanonicalType item : this.actor) 2210 if (!item.isEmpty()) 2211 return true; 2212 return false; 2213 } 2214 2215 /** 2216 * @return {@link #actor} (An actor these requirements are in regard to.) 2217 */ 2218 public CanonicalType addActorElement() {//2 2219 CanonicalType t = new CanonicalType(); 2220 if (this.actor == null) 2221 this.actor = new ArrayList<CanonicalType>(); 2222 this.actor.add(t); 2223 return t; 2224 } 2225 2226 /** 2227 * @param value {@link #actor} (An actor these requirements are in regard to.) 2228 */ 2229 public Requirements addActor(String value) { //1 2230 CanonicalType t = new CanonicalType(); 2231 t.setValue(value); 2232 if (this.actor == null) 2233 this.actor = new ArrayList<CanonicalType>(); 2234 this.actor.add(t); 2235 return this; 2236 } 2237 2238 /** 2239 * @param value {@link #actor} (An actor these requirements are in regard to.) 2240 */ 2241 public boolean hasActor(String value) { 2242 if (this.actor == null) 2243 return false; 2244 for (CanonicalType v : this.actor) 2245 if (v.getValue().equals(value)) // canonical 2246 return true; 2247 return false; 2248 } 2249 2250 /** 2251 * @return {@link #statement} (The actual statement of requirement, in markdown format.) 2252 */ 2253 public List<RequirementsStatementComponent> getStatement() { 2254 if (this.statement == null) 2255 this.statement = new ArrayList<RequirementsStatementComponent>(); 2256 return this.statement; 2257 } 2258 2259 /** 2260 * @return Returns a reference to <code>this</code> for easy method chaining 2261 */ 2262 public Requirements setStatement(List<RequirementsStatementComponent> theStatement) { 2263 this.statement = theStatement; 2264 return this; 2265 } 2266 2267 public boolean hasStatement() { 2268 if (this.statement == null) 2269 return false; 2270 for (RequirementsStatementComponent item : this.statement) 2271 if (!item.isEmpty()) 2272 return true; 2273 return false; 2274 } 2275 2276 public RequirementsStatementComponent addStatement() { //3 2277 RequirementsStatementComponent t = new RequirementsStatementComponent(); 2278 if (this.statement == null) 2279 this.statement = new ArrayList<RequirementsStatementComponent>(); 2280 this.statement.add(t); 2281 return t; 2282 } 2283 2284 public Requirements addStatement(RequirementsStatementComponent t) { //3 2285 if (t == null) 2286 return this; 2287 if (this.statement == null) 2288 this.statement = new ArrayList<RequirementsStatementComponent>(); 2289 this.statement.add(t); 2290 return this; 2291 } 2292 2293 /** 2294 * @return The first repetition of repeating field {@link #statement}, creating it if it does not already exist {3} 2295 */ 2296 public RequirementsStatementComponent getStatementFirstRep() { 2297 if (getStatement().isEmpty()) { 2298 addStatement(); 2299 } 2300 return getStatement().get(0); 2301 } 2302 2303 protected void listChildren(List<Property> children) { 2304 super.listChildren(children); 2305 children.add(new Property("url", "uri", "An absolute URI that is used to identify this Requirements when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Requirements is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Requirements is stored on different servers.", 0, 1, url)); 2306 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this Requirements when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2307 children.add(new Property("version", "string", "The identifier that is used to identify this version of the Requirements when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Requirements author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 2308 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 2309 children.add(new Property("name", "string", "A natural language name identifying the Requirements. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 2310 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the Requirements.", 0, 1, title)); 2311 children.add(new Property("status", "code", "The status of this Requirements. Enables tracking the life-cycle of the content.", 0, 1, status)); 2312 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this Requirements is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 2313 children.add(new Property("date", "dateTime", "The date (and optionally time) when the Requirements was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the Requirements changes.", 0, 1, date)); 2314 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the Requirements.", 0, 1, publisher)); 2315 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 2316 children.add(new Property("description", "markdown", "A free text natural language description of the requirements.", 0, 1, description)); 2317 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate Requirements instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 2318 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the Requirements is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 2319 children.add(new Property("purpose", "markdown", "Explanation of why this Requirements is needed and why it has been designed as it has.", 0, 1, purpose)); 2320 children.add(new Property("copyright", "markdown", "A copyright statement relating to the Requirements and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Requirements.", 0, 1, copyright)); 2321 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 2322 children.add(new Property("derivedFrom", "canonical(Requirements)", "Another set of Requirements that this set of Requirements builds on and updates.", 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 2323 children.add(new Property("reference", "url", "A reference to another artifact that created this set of requirements. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.", 0, java.lang.Integer.MAX_VALUE, reference)); 2324 children.add(new Property("actor", "canonical(ActorDefinition)", "An actor these requirements are in regard to.", 0, java.lang.Integer.MAX_VALUE, actor)); 2325 children.add(new Property("statement", "", "The actual statement of requirement, in markdown format.", 0, java.lang.Integer.MAX_VALUE, statement)); 2326 } 2327 2328 @Override 2329 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2330 switch (_hash) { 2331 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this Requirements when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this Requirements is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the Requirements is stored on different servers.", 0, 1, url); 2332 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this Requirements when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 2333 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the Requirements when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Requirements author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 2334 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2335 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2336 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2337 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2338 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the Requirements. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 2339 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the Requirements.", 0, 1, title); 2340 case -892481550: /*status*/ return new Property("status", "code", "The status of this Requirements. Enables tracking the life-cycle of the content.", 0, 1, status); 2341 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this Requirements is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 2342 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the Requirements was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the Requirements changes.", 0, 1, date); 2343 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the Requirements.", 0, 1, publisher); 2344 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 2345 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the requirements.", 0, 1, description); 2346 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate Requirements instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 2347 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the Requirements is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 2348 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this Requirements is needed and why it has been designed as it has.", 0, 1, purpose); 2349 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the Requirements and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the Requirements.", 0, 1, copyright); 2350 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 2351 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "canonical(Requirements)", "Another set of Requirements that this set of Requirements builds on and updates.", 0, java.lang.Integer.MAX_VALUE, derivedFrom); 2352 case -925155509: /*reference*/ return new Property("reference", "url", "A reference to another artifact that created this set of requirements. This could be a Profile, etc., or external regulation, or business requirements expressed elsewhere.", 0, java.lang.Integer.MAX_VALUE, reference); 2353 case 92645877: /*actor*/ return new Property("actor", "canonical(ActorDefinition)", "An actor these requirements are in regard to.", 0, java.lang.Integer.MAX_VALUE, actor); 2354 case -2085148305: /*statement*/ return new Property("statement", "", "The actual statement of requirement, in markdown format.", 0, java.lang.Integer.MAX_VALUE, statement); 2355 default: return super.getNamedProperty(_hash, _name, _checkValid); 2356 } 2357 2358 } 2359 2360 @Override 2361 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2362 switch (hash) { 2363 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2364 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2365 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2366 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 2367 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2368 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2369 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2370 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 2371 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2372 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 2373 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2374 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2375 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2376 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2377 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 2378 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 2379 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 2380 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // CanonicalType 2381 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : this.reference.toArray(new Base[this.reference.size()]); // UrlType 2382 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : this.actor.toArray(new Base[this.actor.size()]); // CanonicalType 2383 case -2085148305: /*statement*/ return this.statement == null ? new Base[0] : this.statement.toArray(new Base[this.statement.size()]); // RequirementsStatementComponent 2384 default: return super.getProperty(hash, name, checkValid); 2385 } 2386 2387 } 2388 2389 @Override 2390 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2391 switch (hash) { 2392 case 116079: // url 2393 this.url = TypeConvertor.castToUri(value); // UriType 2394 return value; 2395 case -1618432855: // identifier 2396 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2397 return value; 2398 case 351608024: // version 2399 this.version = TypeConvertor.castToString(value); // StringType 2400 return value; 2401 case 1508158071: // versionAlgorithm 2402 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2403 return value; 2404 case 3373707: // name 2405 this.name = TypeConvertor.castToString(value); // StringType 2406 return value; 2407 case 110371416: // title 2408 this.title = TypeConvertor.castToString(value); // StringType 2409 return value; 2410 case -892481550: // status 2411 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2412 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2413 return value; 2414 case -404562712: // experimental 2415 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2416 return value; 2417 case 3076014: // date 2418 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2419 return value; 2420 case 1447404028: // publisher 2421 this.publisher = TypeConvertor.castToString(value); // StringType 2422 return value; 2423 case 951526432: // contact 2424 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2425 return value; 2426 case -1724546052: // description 2427 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2428 return value; 2429 case -669707736: // useContext 2430 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 2431 return value; 2432 case -507075711: // jurisdiction 2433 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2434 return value; 2435 case -220463842: // purpose 2436 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2437 return value; 2438 case 1522889671: // copyright 2439 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2440 return value; 2441 case 765157229: // copyrightLabel 2442 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2443 return value; 2444 case 1077922663: // derivedFrom 2445 this.getDerivedFrom().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2446 return value; 2447 case -925155509: // reference 2448 this.getReference().add(TypeConvertor.castToUrl(value)); // UrlType 2449 return value; 2450 case 92645877: // actor 2451 this.getActor().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2452 return value; 2453 case -2085148305: // statement 2454 this.getStatement().add((RequirementsStatementComponent) value); // RequirementsStatementComponent 2455 return value; 2456 default: return super.setProperty(hash, name, value); 2457 } 2458 2459 } 2460 2461 @Override 2462 public Base setProperty(String name, Base value) throws FHIRException { 2463 if (name.equals("url")) { 2464 this.url = TypeConvertor.castToUri(value); // UriType 2465 } else if (name.equals("identifier")) { 2466 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2467 } else if (name.equals("version")) { 2468 this.version = TypeConvertor.castToString(value); // StringType 2469 } else if (name.equals("versionAlgorithm[x]")) { 2470 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2471 } else if (name.equals("name")) { 2472 this.name = TypeConvertor.castToString(value); // StringType 2473 } else if (name.equals("title")) { 2474 this.title = TypeConvertor.castToString(value); // StringType 2475 } else if (name.equals("status")) { 2476 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2477 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2478 } else if (name.equals("experimental")) { 2479 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2480 } else if (name.equals("date")) { 2481 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2482 } else if (name.equals("publisher")) { 2483 this.publisher = TypeConvertor.castToString(value); // StringType 2484 } else if (name.equals("contact")) { 2485 this.getContact().add(TypeConvertor.castToContactDetail(value)); 2486 } else if (name.equals("description")) { 2487 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2488 } else if (name.equals("useContext")) { 2489 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 2490 } else if (name.equals("jurisdiction")) { 2491 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 2492 } else if (name.equals("purpose")) { 2493 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2494 } else if (name.equals("copyright")) { 2495 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2496 } else if (name.equals("copyrightLabel")) { 2497 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2498 } else if (name.equals("derivedFrom")) { 2499 this.getDerivedFrom().add(TypeConvertor.castToCanonical(value)); 2500 } else if (name.equals("reference")) { 2501 this.getReference().add(TypeConvertor.castToUrl(value)); 2502 } else if (name.equals("actor")) { 2503 this.getActor().add(TypeConvertor.castToCanonical(value)); 2504 } else if (name.equals("statement")) { 2505 this.getStatement().add((RequirementsStatementComponent) value); 2506 } else 2507 return super.setProperty(name, value); 2508 return value; 2509 } 2510 2511 @Override 2512 public Base makeProperty(int hash, String name) throws FHIRException { 2513 switch (hash) { 2514 case 116079: return getUrlElement(); 2515 case -1618432855: return addIdentifier(); 2516 case 351608024: return getVersionElement(); 2517 case -115699031: return getVersionAlgorithm(); 2518 case 1508158071: return getVersionAlgorithm(); 2519 case 3373707: return getNameElement(); 2520 case 110371416: return getTitleElement(); 2521 case -892481550: return getStatusElement(); 2522 case -404562712: return getExperimentalElement(); 2523 case 3076014: return getDateElement(); 2524 case 1447404028: return getPublisherElement(); 2525 case 951526432: return addContact(); 2526 case -1724546052: return getDescriptionElement(); 2527 case -669707736: return addUseContext(); 2528 case -507075711: return addJurisdiction(); 2529 case -220463842: return getPurposeElement(); 2530 case 1522889671: return getCopyrightElement(); 2531 case 765157229: return getCopyrightLabelElement(); 2532 case 1077922663: return addDerivedFromElement(); 2533 case -925155509: return addReferenceElement(); 2534 case 92645877: return addActorElement(); 2535 case -2085148305: return addStatement(); 2536 default: return super.makeProperty(hash, name); 2537 } 2538 2539 } 2540 2541 @Override 2542 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2543 switch (hash) { 2544 case 116079: /*url*/ return new String[] {"uri"}; 2545 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2546 case 351608024: /*version*/ return new String[] {"string"}; 2547 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 2548 case 3373707: /*name*/ return new String[] {"string"}; 2549 case 110371416: /*title*/ return new String[] {"string"}; 2550 case -892481550: /*status*/ return new String[] {"code"}; 2551 case -404562712: /*experimental*/ return new String[] {"boolean"}; 2552 case 3076014: /*date*/ return new String[] {"dateTime"}; 2553 case 1447404028: /*publisher*/ return new String[] {"string"}; 2554 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 2555 case -1724546052: /*description*/ return new String[] {"markdown"}; 2556 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 2557 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 2558 case -220463842: /*purpose*/ return new String[] {"markdown"}; 2559 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 2560 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 2561 case 1077922663: /*derivedFrom*/ return new String[] {"canonical"}; 2562 case -925155509: /*reference*/ return new String[] {"url"}; 2563 case 92645877: /*actor*/ return new String[] {"canonical"}; 2564 case -2085148305: /*statement*/ return new String[] {}; 2565 default: return super.getTypesForProperty(hash, name); 2566 } 2567 2568 } 2569 2570 @Override 2571 public Base addChild(String name) throws FHIRException { 2572 if (name.equals("url")) { 2573 throw new FHIRException("Cannot call addChild on a singleton property Requirements.url"); 2574 } 2575 else if (name.equals("identifier")) { 2576 return addIdentifier(); 2577 } 2578 else if (name.equals("version")) { 2579 throw new FHIRException("Cannot call addChild on a singleton property Requirements.version"); 2580 } 2581 else if (name.equals("versionAlgorithmString")) { 2582 this.versionAlgorithm = new StringType(); 2583 return this.versionAlgorithm; 2584 } 2585 else if (name.equals("versionAlgorithmCoding")) { 2586 this.versionAlgorithm = new Coding(); 2587 return this.versionAlgorithm; 2588 } 2589 else if (name.equals("name")) { 2590 throw new FHIRException("Cannot call addChild on a singleton property Requirements.name"); 2591 } 2592 else if (name.equals("title")) { 2593 throw new FHIRException("Cannot call addChild on a singleton property Requirements.title"); 2594 } 2595 else if (name.equals("status")) { 2596 throw new FHIRException("Cannot call addChild on a singleton property Requirements.status"); 2597 } 2598 else if (name.equals("experimental")) { 2599 throw new FHIRException("Cannot call addChild on a singleton property Requirements.experimental"); 2600 } 2601 else if (name.equals("date")) { 2602 throw new FHIRException("Cannot call addChild on a singleton property Requirements.date"); 2603 } 2604 else if (name.equals("publisher")) { 2605 throw new FHIRException("Cannot call addChild on a singleton property Requirements.publisher"); 2606 } 2607 else if (name.equals("contact")) { 2608 return addContact(); 2609 } 2610 else if (name.equals("description")) { 2611 throw new FHIRException("Cannot call addChild on a singleton property Requirements.description"); 2612 } 2613 else if (name.equals("useContext")) { 2614 return addUseContext(); 2615 } 2616 else if (name.equals("jurisdiction")) { 2617 return addJurisdiction(); 2618 } 2619 else if (name.equals("purpose")) { 2620 throw new FHIRException("Cannot call addChild on a singleton property Requirements.purpose"); 2621 } 2622 else if (name.equals("copyright")) { 2623 throw new FHIRException("Cannot call addChild on a singleton property Requirements.copyright"); 2624 } 2625 else if (name.equals("copyrightLabel")) { 2626 throw new FHIRException("Cannot call addChild on a singleton property Requirements.copyrightLabel"); 2627 } 2628 else if (name.equals("derivedFrom")) { 2629 throw new FHIRException("Cannot call addChild on a singleton property Requirements.derivedFrom"); 2630 } 2631 else if (name.equals("reference")) { 2632 throw new FHIRException("Cannot call addChild on a singleton property Requirements.reference"); 2633 } 2634 else if (name.equals("actor")) { 2635 throw new FHIRException("Cannot call addChild on a singleton property Requirements.actor"); 2636 } 2637 else if (name.equals("statement")) { 2638 return addStatement(); 2639 } 2640 else 2641 return super.addChild(name); 2642 } 2643 2644 public String fhirType() { 2645 return "Requirements"; 2646 2647 } 2648 2649 public Requirements copy() { 2650 Requirements dst = new Requirements(); 2651 copyValues(dst); 2652 return dst; 2653 } 2654 2655 public void copyValues(Requirements dst) { 2656 super.copyValues(dst); 2657 dst.url = url == null ? null : url.copy(); 2658 if (identifier != null) { 2659 dst.identifier = new ArrayList<Identifier>(); 2660 for (Identifier i : identifier) 2661 dst.identifier.add(i.copy()); 2662 }; 2663 dst.version = version == null ? null : version.copy(); 2664 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 2665 dst.name = name == null ? null : name.copy(); 2666 dst.title = title == null ? null : title.copy(); 2667 dst.status = status == null ? null : status.copy(); 2668 dst.experimental = experimental == null ? null : experimental.copy(); 2669 dst.date = date == null ? null : date.copy(); 2670 dst.publisher = publisher == null ? null : publisher.copy(); 2671 if (contact != null) { 2672 dst.contact = new ArrayList<ContactDetail>(); 2673 for (ContactDetail i : contact) 2674 dst.contact.add(i.copy()); 2675 }; 2676 dst.description = description == null ? null : description.copy(); 2677 if (useContext != null) { 2678 dst.useContext = new ArrayList<UsageContext>(); 2679 for (UsageContext i : useContext) 2680 dst.useContext.add(i.copy()); 2681 }; 2682 if (jurisdiction != null) { 2683 dst.jurisdiction = new ArrayList<CodeableConcept>(); 2684 for (CodeableConcept i : jurisdiction) 2685 dst.jurisdiction.add(i.copy()); 2686 }; 2687 dst.purpose = purpose == null ? null : purpose.copy(); 2688 dst.copyright = copyright == null ? null : copyright.copy(); 2689 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 2690 if (derivedFrom != null) { 2691 dst.derivedFrom = new ArrayList<CanonicalType>(); 2692 for (CanonicalType i : derivedFrom) 2693 dst.derivedFrom.add(i.copy()); 2694 }; 2695 if (reference != null) { 2696 dst.reference = new ArrayList<UrlType>(); 2697 for (UrlType i : reference) 2698 dst.reference.add(i.copy()); 2699 }; 2700 if (actor != null) { 2701 dst.actor = new ArrayList<CanonicalType>(); 2702 for (CanonicalType i : actor) 2703 dst.actor.add(i.copy()); 2704 }; 2705 if (statement != null) { 2706 dst.statement = new ArrayList<RequirementsStatementComponent>(); 2707 for (RequirementsStatementComponent i : statement) 2708 dst.statement.add(i.copy()); 2709 }; 2710 } 2711 2712 protected Requirements typedCopy() { 2713 return copy(); 2714 } 2715 2716 @Override 2717 public boolean equalsDeep(Base other_) { 2718 if (!super.equalsDeep(other_)) 2719 return false; 2720 if (!(other_ instanceof Requirements)) 2721 return false; 2722 Requirements o = (Requirements) other_; 2723 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 2724 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 2725 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 2726 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 2727 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 2728 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 2729 && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(reference, o.reference, true) && compareDeep(actor, o.actor, true) 2730 && compareDeep(statement, o.statement, true); 2731 } 2732 2733 @Override 2734 public boolean equalsShallow(Base other_) { 2735 if (!super.equalsShallow(other_)) 2736 return false; 2737 if (!(other_ instanceof Requirements)) 2738 return false; 2739 Requirements o = (Requirements) other_; 2740 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 2741 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 2742 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 2743 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 2744 && compareValues(derivedFrom, o.derivedFrom, true) && compareValues(reference, o.reference, true) && compareValues(actor, o.actor, true) 2745 ; 2746 } 2747 2748 public boolean isEmpty() { 2749 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 2750 , versionAlgorithm, name, title, status, experimental, date, publisher, contact 2751 , description, useContext, jurisdiction, purpose, copyright, copyrightLabel, derivedFrom 2752 , reference, actor, statement); 2753 } 2754 2755 @Override 2756 public ResourceType getResourceType() { 2757 return ResourceType.Requirements; 2758 } 2759 2760 /** 2761 * Search parameter: <b>context-quantity</b> 2762 * <p> 2763 * Description: <b>Multiple Resources: 2764 2765* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 2766* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 2767* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 2768* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 2769* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 2770* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 2771* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 2772* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 2773* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 2774* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 2775* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 2776* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 2777* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 2778* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 2779* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 2780* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 2781* [Library](library.html): A quantity- or range-valued use context assigned to the library 2782* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 2783* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 2784* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 2785* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 2786* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 2787* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 2788* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 2789* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 2790* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 2791* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 2792* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 2793* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 2794* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 2795</b><br> 2796 * Type: <b>quantity</b><br> 2797 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 2798 * </p> 2799 */ 2800 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 2801 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 2802 /** 2803 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 2804 * <p> 2805 * Description: <b>Multiple Resources: 2806 2807* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 2808* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 2809* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 2810* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 2811* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 2812* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 2813* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 2814* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 2815* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 2816* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 2817* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 2818* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 2819* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 2820* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 2821* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 2822* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 2823* [Library](library.html): A quantity- or range-valued use context assigned to the library 2824* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 2825* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 2826* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 2827* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 2828* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 2829* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 2830* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 2831* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 2832* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 2833* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 2834* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 2835* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 2836* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 2837</b><br> 2838 * Type: <b>quantity</b><br> 2839 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 2840 * </p> 2841 */ 2842 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 2843 2844 /** 2845 * Search parameter: <b>context-type-quantity</b> 2846 * <p> 2847 * Description: <b>Multiple Resources: 2848 2849* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 2850* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 2851* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 2852* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 2853* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 2854* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 2855* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 2856* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 2857* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 2858* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 2859* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 2860* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 2861* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 2862* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 2863* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 2864* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 2865* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 2866* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 2867* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 2868* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 2869* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 2870* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 2871* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 2872* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 2873* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 2874* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 2875* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 2876* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 2877* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 2878* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 2879</b><br> 2880 * Type: <b>composite</b><br> 2881 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2882 * </p> 2883 */ 2884 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 2885 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 2886 /** 2887 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 2888 * <p> 2889 * Description: <b>Multiple Resources: 2890 2891* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 2892* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 2893* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 2894* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 2895* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 2896* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 2897* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 2898* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 2899* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 2900* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 2901* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 2902* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 2903* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 2904* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 2905* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 2906* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 2907* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 2908* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 2909* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 2910* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 2911* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 2912* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 2913* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 2914* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 2915* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 2916* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 2917* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 2918* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 2919* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 2920* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 2921</b><br> 2922 * Type: <b>composite</b><br> 2923 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2924 * </p> 2925 */ 2926 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 2927 2928 /** 2929 * Search parameter: <b>context-type-value</b> 2930 * <p> 2931 * Description: <b>Multiple Resources: 2932 2933* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 2934* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 2935* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 2936* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 2937* [Citation](citation.html): A use context type and value assigned to the citation 2938* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 2939* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 2940* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 2941* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 2942* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 2943* [Evidence](evidence.html): A use context type and value assigned to the evidence 2944* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 2945* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 2946* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 2947* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 2948* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 2949* [Library](library.html): A use context type and value assigned to the library 2950* [Measure](measure.html): A use context type and value assigned to the measure 2951* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 2952* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 2953* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 2954* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 2955* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 2956* [Requirements](requirements.html): A use context type and value assigned to the requirements 2957* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 2958* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 2959* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 2960* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 2961* [TestScript](testscript.html): A use context type and value assigned to the test script 2962* [ValueSet](valueset.html): A use context type and value assigned to the value set 2963</b><br> 2964 * Type: <b>composite</b><br> 2965 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2966 * </p> 2967 */ 2968 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 2969 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 2970 /** 2971 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 2972 * <p> 2973 * Description: <b>Multiple Resources: 2974 2975* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 2976* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 2977* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 2978* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 2979* [Citation](citation.html): A use context type and value assigned to the citation 2980* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 2981* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 2982* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 2983* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 2984* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 2985* [Evidence](evidence.html): A use context type and value assigned to the evidence 2986* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 2987* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 2988* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 2989* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 2990* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 2991* [Library](library.html): A use context type and value assigned to the library 2992* [Measure](measure.html): A use context type and value assigned to the measure 2993* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 2994* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 2995* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 2996* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 2997* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 2998* [Requirements](requirements.html): A use context type and value assigned to the requirements 2999* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3000* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3001* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3002* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3003* [TestScript](testscript.html): A use context type and value assigned to the test script 3004* [ValueSet](valueset.html): A use context type and value assigned to the value set 3005</b><br> 3006 * Type: <b>composite</b><br> 3007 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3008 * </p> 3009 */ 3010 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 3011 3012 /** 3013 * Search parameter: <b>context-type</b> 3014 * <p> 3015 * Description: <b>Multiple Resources: 3016 3017* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3018* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3019* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3020* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3021* [Citation](citation.html): A type of use context assigned to the citation 3022* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3023* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3024* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3025* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3026* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3027* [Evidence](evidence.html): A type of use context assigned to the evidence 3028* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3029* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3030* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3031* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3032* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3033* [Library](library.html): A type of use context assigned to the library 3034* [Measure](measure.html): A type of use context assigned to the measure 3035* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3036* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3037* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3038* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3039* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3040* [Requirements](requirements.html): A type of use context assigned to the requirements 3041* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3042* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3043* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3044* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3045* [TestScript](testscript.html): A type of use context assigned to the test script 3046* [ValueSet](valueset.html): A type of use context assigned to the value set 3047</b><br> 3048 * Type: <b>token</b><br> 3049 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3050 * </p> 3051 */ 3052 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 3053 public static final String SP_CONTEXT_TYPE = "context-type"; 3054 /** 3055 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3056 * <p> 3057 * Description: <b>Multiple Resources: 3058 3059* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3060* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3061* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3062* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3063* [Citation](citation.html): A type of use context assigned to the citation 3064* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3065* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3066* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3067* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3068* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3069* [Evidence](evidence.html): A type of use context assigned to the evidence 3070* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3071* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3072* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3073* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3074* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3075* [Library](library.html): A type of use context assigned to the library 3076* [Measure](measure.html): A type of use context assigned to the measure 3077* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3078* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3079* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3080* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3081* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3082* [Requirements](requirements.html): A type of use context assigned to the requirements 3083* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3084* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3085* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3086* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3087* [TestScript](testscript.html): A type of use context assigned to the test script 3088* [ValueSet](valueset.html): A type of use context assigned to the value set 3089</b><br> 3090 * Type: <b>token</b><br> 3091 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3092 * </p> 3093 */ 3094 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 3095 3096 /** 3097 * Search parameter: <b>context</b> 3098 * <p> 3099 * Description: <b>Multiple Resources: 3100 3101* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3102* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3103* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3104* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3105* [Citation](citation.html): A use context assigned to the citation 3106* [CodeSystem](codesystem.html): A use context assigned to the code system 3107* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3108* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3109* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3110* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3111* [Evidence](evidence.html): A use context assigned to the evidence 3112* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3113* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3114* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3115* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3116* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3117* [Library](library.html): A use context assigned to the library 3118* [Measure](measure.html): A use context assigned to the measure 3119* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3120* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3121* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3122* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3123* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3124* [Requirements](requirements.html): A use context assigned to the requirements 3125* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3126* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3127* [StructureMap](structuremap.html): A use context assigned to the structure map 3128* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3129* [TestScript](testscript.html): A use context assigned to the test script 3130* [ValueSet](valueset.html): A use context assigned to the value set 3131</b><br> 3132 * Type: <b>token</b><br> 3133 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3134 * </p> 3135 */ 3136 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 3137 public static final String SP_CONTEXT = "context"; 3138 /** 3139 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3140 * <p> 3141 * Description: <b>Multiple Resources: 3142 3143* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3144* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3145* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3146* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3147* [Citation](citation.html): A use context assigned to the citation 3148* [CodeSystem](codesystem.html): A use context assigned to the code system 3149* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3150* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3151* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3152* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3153* [Evidence](evidence.html): A use context assigned to the evidence 3154* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3155* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3156* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3157* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3158* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3159* [Library](library.html): A use context assigned to the library 3160* [Measure](measure.html): A use context assigned to the measure 3161* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3162* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3163* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3164* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3165* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3166* [Requirements](requirements.html): A use context assigned to the requirements 3167* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3168* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3169* [StructureMap](structuremap.html): A use context assigned to the structure map 3170* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3171* [TestScript](testscript.html): A use context assigned to the test script 3172* [ValueSet](valueset.html): A use context assigned to the value set 3173</b><br> 3174 * Type: <b>token</b><br> 3175 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3176 * </p> 3177 */ 3178 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 3179 3180 /** 3181 * Search parameter: <b>date</b> 3182 * <p> 3183 * Description: <b>Multiple Resources: 3184 3185* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3186* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3187* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3188* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3189* [Citation](citation.html): The citation publication date 3190* [CodeSystem](codesystem.html): The code system publication date 3191* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3192* [ConceptMap](conceptmap.html): The concept map publication date 3193* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3194* [EventDefinition](eventdefinition.html): The event definition publication date 3195* [Evidence](evidence.html): The evidence publication date 3196* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3197* [ExampleScenario](examplescenario.html): The example scenario publication date 3198* [GraphDefinition](graphdefinition.html): The graph definition publication date 3199* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3200* [Library](library.html): The library publication date 3201* [Measure](measure.html): The measure publication date 3202* [MessageDefinition](messagedefinition.html): The message definition publication date 3203* [NamingSystem](namingsystem.html): The naming system publication date 3204* [OperationDefinition](operationdefinition.html): The operation definition publication date 3205* [PlanDefinition](plandefinition.html): The plan definition publication date 3206* [Questionnaire](questionnaire.html): The questionnaire publication date 3207* [Requirements](requirements.html): The requirements publication date 3208* [SearchParameter](searchparameter.html): The search parameter publication date 3209* [StructureDefinition](structuredefinition.html): The structure definition publication date 3210* [StructureMap](structuremap.html): The structure map publication date 3211* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3212* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3213* [TestScript](testscript.html): The test script publication date 3214* [ValueSet](valueset.html): The value set publication date 3215</b><br> 3216 * Type: <b>date</b><br> 3217 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3218 * </p> 3219 */ 3220 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 3221 public static final String SP_DATE = "date"; 3222 /** 3223 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3224 * <p> 3225 * Description: <b>Multiple Resources: 3226 3227* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3228* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3229* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3230* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3231* [Citation](citation.html): The citation publication date 3232* [CodeSystem](codesystem.html): The code system publication date 3233* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3234* [ConceptMap](conceptmap.html): The concept map publication date 3235* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3236* [EventDefinition](eventdefinition.html): The event definition publication date 3237* [Evidence](evidence.html): The evidence publication date 3238* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3239* [ExampleScenario](examplescenario.html): The example scenario publication date 3240* [GraphDefinition](graphdefinition.html): The graph definition publication date 3241* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3242* [Library](library.html): The library publication date 3243* [Measure](measure.html): The measure publication date 3244* [MessageDefinition](messagedefinition.html): The message definition publication date 3245* [NamingSystem](namingsystem.html): The naming system publication date 3246* [OperationDefinition](operationdefinition.html): The operation definition publication date 3247* [PlanDefinition](plandefinition.html): The plan definition publication date 3248* [Questionnaire](questionnaire.html): The questionnaire publication date 3249* [Requirements](requirements.html): The requirements publication date 3250* [SearchParameter](searchparameter.html): The search parameter publication date 3251* [StructureDefinition](structuredefinition.html): The structure definition publication date 3252* [StructureMap](structuremap.html): The structure map publication date 3253* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3254* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3255* [TestScript](testscript.html): The test script publication date 3256* [ValueSet](valueset.html): The value set publication date 3257</b><br> 3258 * Type: <b>date</b><br> 3259 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3260 * </p> 3261 */ 3262 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3263 3264 /** 3265 * Search parameter: <b>description</b> 3266 * <p> 3267 * Description: <b>Multiple Resources: 3268 3269* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3270* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3271* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3272* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3273* [Citation](citation.html): The description of the citation 3274* [CodeSystem](codesystem.html): The description of the code system 3275* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3276* [ConceptMap](conceptmap.html): The description of the concept map 3277* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3278* [EventDefinition](eventdefinition.html): The description of the event definition 3279* [Evidence](evidence.html): The description of the evidence 3280* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3281* [GraphDefinition](graphdefinition.html): The description of the graph definition 3282* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3283* [Library](library.html): The description of the library 3284* [Measure](measure.html): The description of the measure 3285* [MessageDefinition](messagedefinition.html): The description of the message definition 3286* [NamingSystem](namingsystem.html): The description of the naming system 3287* [OperationDefinition](operationdefinition.html): The description of the operation definition 3288* [PlanDefinition](plandefinition.html): The description of the plan definition 3289* [Questionnaire](questionnaire.html): The description of the questionnaire 3290* [Requirements](requirements.html): The description of the requirements 3291* [SearchParameter](searchparameter.html): The description of the search parameter 3292* [StructureDefinition](structuredefinition.html): The description of the structure definition 3293* [StructureMap](structuremap.html): The description of the structure map 3294* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3295* [TestScript](testscript.html): The description of the test script 3296* [ValueSet](valueset.html): The description of the value set 3297</b><br> 3298 * Type: <b>string</b><br> 3299 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3300 * </p> 3301 */ 3302 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 3303 public static final String SP_DESCRIPTION = "description"; 3304 /** 3305 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3306 * <p> 3307 * Description: <b>Multiple Resources: 3308 3309* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3310* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3311* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3312* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3313* [Citation](citation.html): The description of the citation 3314* [CodeSystem](codesystem.html): The description of the code system 3315* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3316* [ConceptMap](conceptmap.html): The description of the concept map 3317* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3318* [EventDefinition](eventdefinition.html): The description of the event definition 3319* [Evidence](evidence.html): The description of the evidence 3320* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3321* [GraphDefinition](graphdefinition.html): The description of the graph definition 3322* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3323* [Library](library.html): The description of the library 3324* [Measure](measure.html): The description of the measure 3325* [MessageDefinition](messagedefinition.html): The description of the message definition 3326* [NamingSystem](namingsystem.html): The description of the naming system 3327* [OperationDefinition](operationdefinition.html): The description of the operation definition 3328* [PlanDefinition](plandefinition.html): The description of the plan definition 3329* [Questionnaire](questionnaire.html): The description of the questionnaire 3330* [Requirements](requirements.html): The description of the requirements 3331* [SearchParameter](searchparameter.html): The description of the search parameter 3332* [StructureDefinition](structuredefinition.html): The description of the structure definition 3333* [StructureMap](structuremap.html): The description of the structure map 3334* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3335* [TestScript](testscript.html): The description of the test script 3336* [ValueSet](valueset.html): The description of the value set 3337</b><br> 3338 * Type: <b>string</b><br> 3339 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3340 * </p> 3341 */ 3342 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 3343 3344 /** 3345 * Search parameter: <b>identifier</b> 3346 * <p> 3347 * Description: <b>Multiple Resources: 3348 3349* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3350* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3351* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3352* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3353* [Citation](citation.html): External identifier for the citation 3354* [CodeSystem](codesystem.html): External identifier for the code system 3355* [ConceptMap](conceptmap.html): External identifier for the concept map 3356* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3357* [EventDefinition](eventdefinition.html): External identifier for the event definition 3358* [Evidence](evidence.html): External identifier for the evidence 3359* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3360* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3361* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3362* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3363* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3364* [Library](library.html): External identifier for the library 3365* [Measure](measure.html): External identifier for the measure 3366* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3367* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3368* [NamingSystem](namingsystem.html): External identifier for the naming system 3369* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3370* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3371* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3372* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3373* [Requirements](requirements.html): External identifier for the requirements 3374* [SearchParameter](searchparameter.html): External identifier for the search parameter 3375* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3376* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3377* [StructureMap](structuremap.html): External identifier for the structure map 3378* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3379* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3380* [TestPlan](testplan.html): An identifier for the test plan 3381* [TestScript](testscript.html): External identifier for the test script 3382* [ValueSet](valueset.html): External identifier for the value set 3383</b><br> 3384 * Type: <b>token</b><br> 3385 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3386 * </p> 3387 */ 3388 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 3389 public static final String SP_IDENTIFIER = "identifier"; 3390 /** 3391 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3392 * <p> 3393 * Description: <b>Multiple Resources: 3394 3395* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3396* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3397* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3398* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3399* [Citation](citation.html): External identifier for the citation 3400* [CodeSystem](codesystem.html): External identifier for the code system 3401* [ConceptMap](conceptmap.html): External identifier for the concept map 3402* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3403* [EventDefinition](eventdefinition.html): External identifier for the event definition 3404* [Evidence](evidence.html): External identifier for the evidence 3405* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3406* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3407* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3408* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3409* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3410* [Library](library.html): External identifier for the library 3411* [Measure](measure.html): External identifier for the measure 3412* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3413* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3414* [NamingSystem](namingsystem.html): External identifier for the naming system 3415* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3416* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3417* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3418* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3419* [Requirements](requirements.html): External identifier for the requirements 3420* [SearchParameter](searchparameter.html): External identifier for the search parameter 3421* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3422* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3423* [StructureMap](structuremap.html): External identifier for the structure map 3424* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3425* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3426* [TestPlan](testplan.html): An identifier for the test plan 3427* [TestScript](testscript.html): External identifier for the test script 3428* [ValueSet](valueset.html): External identifier for the value set 3429</b><br> 3430 * Type: <b>token</b><br> 3431 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3432 * </p> 3433 */ 3434 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3435 3436 /** 3437 * Search parameter: <b>jurisdiction</b> 3438 * <p> 3439 * Description: <b>Multiple Resources: 3440 3441* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3442* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3443* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3444* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3445* [Citation](citation.html): Intended jurisdiction for the citation 3446* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3447* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3448* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3449* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3450* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3451* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3452* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3453* [Library](library.html): Intended jurisdiction for the library 3454* [Measure](measure.html): Intended jurisdiction for the measure 3455* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3456* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3457* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3458* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3459* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3460* [Requirements](requirements.html): Intended jurisdiction for the requirements 3461* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3462* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3463* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3464* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3465* [TestScript](testscript.html): Intended jurisdiction for the test script 3466* [ValueSet](valueset.html): Intended jurisdiction for the value set 3467</b><br> 3468 * Type: <b>token</b><br> 3469 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3470 * </p> 3471 */ 3472 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 3473 public static final String SP_JURISDICTION = "jurisdiction"; 3474 /** 3475 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3476 * <p> 3477 * Description: <b>Multiple Resources: 3478 3479* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3480* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3481* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3482* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3483* [Citation](citation.html): Intended jurisdiction for the citation 3484* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3485* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3486* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3487* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3488* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3489* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3490* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3491* [Library](library.html): Intended jurisdiction for the library 3492* [Measure](measure.html): Intended jurisdiction for the measure 3493* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3494* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3495* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3496* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3497* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3498* [Requirements](requirements.html): Intended jurisdiction for the requirements 3499* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3500* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3501* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3502* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3503* [TestScript](testscript.html): Intended jurisdiction for the test script 3504* [ValueSet](valueset.html): Intended jurisdiction for the value set 3505</b><br> 3506 * Type: <b>token</b><br> 3507 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3508 * </p> 3509 */ 3510 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 3511 3512 /** 3513 * Search parameter: <b>name</b> 3514 * <p> 3515 * Description: <b>Multiple Resources: 3516 3517* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 3518* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 3519* [Citation](citation.html): Computationally friendly name of the citation 3520* [CodeSystem](codesystem.html): Computationally friendly name of the code system 3521* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 3522* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 3523* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 3524* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 3525* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 3526* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 3527* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 3528* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 3529* [Library](library.html): Computationally friendly name of the library 3530* [Measure](measure.html): Computationally friendly name of the measure 3531* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 3532* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 3533* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 3534* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 3535* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 3536* [Requirements](requirements.html): Computationally friendly name of the requirements 3537* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 3538* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 3539* [StructureMap](structuremap.html): Computationally friendly name of the structure map 3540* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 3541* [TestScript](testscript.html): Computationally friendly name of the test script 3542* [ValueSet](valueset.html): Computationally friendly name of the value set 3543</b><br> 3544 * Type: <b>string</b><br> 3545 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 3546 * </p> 3547 */ 3548 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 3549 public static final String SP_NAME = "name"; 3550 /** 3551 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3552 * <p> 3553 * Description: <b>Multiple Resources: 3554 3555* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 3556* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 3557* [Citation](citation.html): Computationally friendly name of the citation 3558* [CodeSystem](codesystem.html): Computationally friendly name of the code system 3559* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 3560* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 3561* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 3562* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 3563* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 3564* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 3565* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 3566* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 3567* [Library](library.html): Computationally friendly name of the library 3568* [Measure](measure.html): Computationally friendly name of the measure 3569* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 3570* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 3571* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 3572* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 3573* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 3574* [Requirements](requirements.html): Computationally friendly name of the requirements 3575* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 3576* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 3577* [StructureMap](structuremap.html): Computationally friendly name of the structure map 3578* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 3579* [TestScript](testscript.html): Computationally friendly name of the test script 3580* [ValueSet](valueset.html): Computationally friendly name of the value set 3581</b><br> 3582 * Type: <b>string</b><br> 3583 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 3584 * </p> 3585 */ 3586 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 3587 3588 /** 3589 * Search parameter: <b>publisher</b> 3590 * <p> 3591 * Description: <b>Multiple Resources: 3592 3593* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 3594* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 3595* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 3596* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 3597* [Citation](citation.html): Name of the publisher of the citation 3598* [CodeSystem](codesystem.html): Name of the publisher of the code system 3599* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 3600* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 3601* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 3602* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 3603* [Evidence](evidence.html): Name of the publisher of the evidence 3604* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 3605* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 3606* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 3607* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 3608* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 3609* [Library](library.html): Name of the publisher of the library 3610* [Measure](measure.html): Name of the publisher of the measure 3611* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 3612* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 3613* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 3614* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 3615* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 3616* [Requirements](requirements.html): Name of the publisher of the requirements 3617* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 3618* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 3619* [StructureMap](structuremap.html): Name of the publisher of the structure map 3620* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 3621* [TestScript](testscript.html): Name of the publisher of the test script 3622* [ValueSet](valueset.html): Name of the publisher of the value set 3623</b><br> 3624 * Type: <b>string</b><br> 3625 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 3626 * </p> 3627 */ 3628 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 3629 public static final String SP_PUBLISHER = "publisher"; 3630 /** 3631 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3632 * <p> 3633 * Description: <b>Multiple Resources: 3634 3635* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 3636* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 3637* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 3638* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 3639* [Citation](citation.html): Name of the publisher of the citation 3640* [CodeSystem](codesystem.html): Name of the publisher of the code system 3641* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 3642* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 3643* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 3644* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 3645* [Evidence](evidence.html): Name of the publisher of the evidence 3646* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 3647* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 3648* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 3649* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 3650* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 3651* [Library](library.html): Name of the publisher of the library 3652* [Measure](measure.html): Name of the publisher of the measure 3653* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 3654* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 3655* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 3656* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 3657* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 3658* [Requirements](requirements.html): Name of the publisher of the requirements 3659* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 3660* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 3661* [StructureMap](structuremap.html): Name of the publisher of the structure map 3662* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 3663* [TestScript](testscript.html): Name of the publisher of the test script 3664* [ValueSet](valueset.html): Name of the publisher of the value set 3665</b><br> 3666 * Type: <b>string</b><br> 3667 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 3668 * </p> 3669 */ 3670 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 3671 3672 /** 3673 * Search parameter: <b>status</b> 3674 * <p> 3675 * Description: <b>Multiple Resources: 3676 3677* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 3678* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 3679* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 3680* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 3681* [Citation](citation.html): The current status of the citation 3682* [CodeSystem](codesystem.html): The current status of the code system 3683* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 3684* [ConceptMap](conceptmap.html): The current status of the concept map 3685* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 3686* [EventDefinition](eventdefinition.html): The current status of the event definition 3687* [Evidence](evidence.html): The current status of the evidence 3688* [EvidenceReport](evidencereport.html): The current status of the evidence report 3689* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 3690* [ExampleScenario](examplescenario.html): The current status of the example scenario 3691* [GraphDefinition](graphdefinition.html): The current status of the graph definition 3692* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 3693* [Library](library.html): The current status of the library 3694* [Measure](measure.html): The current status of the measure 3695* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 3696* [MessageDefinition](messagedefinition.html): The current status of the message definition 3697* [NamingSystem](namingsystem.html): The current status of the naming system 3698* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 3699* [OperationDefinition](operationdefinition.html): The current status of the operation definition 3700* [PlanDefinition](plandefinition.html): The current status of the plan definition 3701* [Questionnaire](questionnaire.html): The current status of the questionnaire 3702* [Requirements](requirements.html): The current status of the requirements 3703* [SearchParameter](searchparameter.html): The current status of the search parameter 3704* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 3705* [StructureDefinition](structuredefinition.html): The current status of the structure definition 3706* [StructureMap](structuremap.html): The current status of the structure map 3707* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 3708* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 3709* [TestPlan](testplan.html): The current status of the test plan 3710* [TestScript](testscript.html): The current status of the test script 3711* [ValueSet](valueset.html): The current status of the value set 3712</b><br> 3713 * Type: <b>token</b><br> 3714 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 3715 * </p> 3716 */ 3717 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 3718 public static final String SP_STATUS = "status"; 3719 /** 3720 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3721 * <p> 3722 * Description: <b>Multiple Resources: 3723 3724* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 3725* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 3726* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 3727* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 3728* [Citation](citation.html): The current status of the citation 3729* [CodeSystem](codesystem.html): The current status of the code system 3730* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 3731* [ConceptMap](conceptmap.html): The current status of the concept map 3732* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 3733* [EventDefinition](eventdefinition.html): The current status of the event definition 3734* [Evidence](evidence.html): The current status of the evidence 3735* [EvidenceReport](evidencereport.html): The current status of the evidence report 3736* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 3737* [ExampleScenario](examplescenario.html): The current status of the example scenario 3738* [GraphDefinition](graphdefinition.html): The current status of the graph definition 3739* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 3740* [Library](library.html): The current status of the library 3741* [Measure](measure.html): The current status of the measure 3742* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 3743* [MessageDefinition](messagedefinition.html): The current status of the message definition 3744* [NamingSystem](namingsystem.html): The current status of the naming system 3745* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 3746* [OperationDefinition](operationdefinition.html): The current status of the operation definition 3747* [PlanDefinition](plandefinition.html): The current status of the plan definition 3748* [Questionnaire](questionnaire.html): The current status of the questionnaire 3749* [Requirements](requirements.html): The current status of the requirements 3750* [SearchParameter](searchparameter.html): The current status of the search parameter 3751* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 3752* [StructureDefinition](structuredefinition.html): The current status of the structure definition 3753* [StructureMap](structuremap.html): The current status of the structure map 3754* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 3755* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 3756* [TestPlan](testplan.html): The current status of the test plan 3757* [TestScript](testscript.html): The current status of the test script 3758* [ValueSet](valueset.html): The current status of the value set 3759</b><br> 3760 * Type: <b>token</b><br> 3761 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 3762 * </p> 3763 */ 3764 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3765 3766 /** 3767 * Search parameter: <b>title</b> 3768 * <p> 3769 * Description: <b>Multiple Resources: 3770 3771* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 3772* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 3773* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 3774* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 3775* [Citation](citation.html): The human-friendly name of the citation 3776* [CodeSystem](codesystem.html): The human-friendly name of the code system 3777* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 3778* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 3779* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 3780* [Evidence](evidence.html): The human-friendly name of the evidence 3781* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 3782* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 3783* [Library](library.html): The human-friendly name of the library 3784* [Measure](measure.html): The human-friendly name of the measure 3785* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 3786* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 3787* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 3788* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 3789* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 3790* [Requirements](requirements.html): The human-friendly name of the requirements 3791* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 3792* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 3793* [StructureMap](structuremap.html): The human-friendly name of the structure map 3794* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 3795* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 3796* [TestScript](testscript.html): The human-friendly name of the test script 3797* [ValueSet](valueset.html): The human-friendly name of the value set 3798</b><br> 3799 * Type: <b>string</b><br> 3800 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 3801 * </p> 3802 */ 3803 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 3804 public static final String SP_TITLE = "title"; 3805 /** 3806 * <b>Fluent Client</b> search parameter constant for <b>title</b> 3807 * <p> 3808 * Description: <b>Multiple Resources: 3809 3810* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 3811* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 3812* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 3813* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 3814* [Citation](citation.html): The human-friendly name of the citation 3815* [CodeSystem](codesystem.html): The human-friendly name of the code system 3816* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 3817* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 3818* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 3819* [Evidence](evidence.html): The human-friendly name of the evidence 3820* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 3821* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 3822* [Library](library.html): The human-friendly name of the library 3823* [Measure](measure.html): The human-friendly name of the measure 3824* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 3825* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 3826* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 3827* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 3828* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 3829* [Requirements](requirements.html): The human-friendly name of the requirements 3830* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 3831* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 3832* [StructureMap](structuremap.html): The human-friendly name of the structure map 3833* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 3834* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 3835* [TestScript](testscript.html): The human-friendly name of the test script 3836* [ValueSet](valueset.html): The human-friendly name of the value set 3837</b><br> 3838 * Type: <b>string</b><br> 3839 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 3840 * </p> 3841 */ 3842 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 3843 3844 /** 3845 * Search parameter: <b>url</b> 3846 * <p> 3847 * Description: <b>Multiple Resources: 3848 3849* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 3850* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 3851* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 3852* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 3853* [Citation](citation.html): The uri that identifies the citation 3854* [CodeSystem](codesystem.html): The uri that identifies the code system 3855* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 3856* [ConceptMap](conceptmap.html): The URI that identifies the concept map 3857* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 3858* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 3859* [Evidence](evidence.html): The uri that identifies the evidence 3860* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 3861* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 3862* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 3863* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 3864* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 3865* [Library](library.html): The uri that identifies the library 3866* [Measure](measure.html): The uri that identifies the measure 3867* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 3868* [NamingSystem](namingsystem.html): The uri that identifies the naming system 3869* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 3870* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 3871* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 3872* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 3873* [Requirements](requirements.html): The uri that identifies the requirements 3874* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 3875* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 3876* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 3877* [StructureMap](structuremap.html): The uri that identifies the structure map 3878* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 3879* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 3880* [TestPlan](testplan.html): The uri that identifies the test plan 3881* [TestScript](testscript.html): The uri that identifies the test script 3882* [ValueSet](valueset.html): The uri that identifies the value set 3883</b><br> 3884 * Type: <b>uri</b><br> 3885 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 3886 * </p> 3887 */ 3888 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 3889 public static final String SP_URL = "url"; 3890 /** 3891 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3892 * <p> 3893 * Description: <b>Multiple Resources: 3894 3895* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 3896* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 3897* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 3898* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 3899* [Citation](citation.html): The uri that identifies the citation 3900* [CodeSystem](codesystem.html): The uri that identifies the code system 3901* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 3902* [ConceptMap](conceptmap.html): The URI that identifies the concept map 3903* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 3904* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 3905* [Evidence](evidence.html): The uri that identifies the evidence 3906* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 3907* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 3908* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 3909* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 3910* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 3911* [Library](library.html): The uri that identifies the library 3912* [Measure](measure.html): The uri that identifies the measure 3913* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 3914* [NamingSystem](namingsystem.html): The uri that identifies the naming system 3915* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 3916* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 3917* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 3918* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 3919* [Requirements](requirements.html): The uri that identifies the requirements 3920* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 3921* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 3922* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 3923* [StructureMap](structuremap.html): The uri that identifies the structure map 3924* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 3925* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 3926* [TestPlan](testplan.html): The uri that identifies the test plan 3927* [TestScript](testscript.html): The uri that identifies the test script 3928* [ValueSet](valueset.html): The uri that identifies the value set 3929</b><br> 3930 * Type: <b>uri</b><br> 3931 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 3932 * </p> 3933 */ 3934 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3935 3936 /** 3937 * Search parameter: <b>version</b> 3938 * <p> 3939 * Description: <b>Multiple Resources: 3940 3941* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 3942* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 3943* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 3944* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 3945* [Citation](citation.html): The business version of the citation 3946* [CodeSystem](codesystem.html): The business version of the code system 3947* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 3948* [ConceptMap](conceptmap.html): The business version of the concept map 3949* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 3950* [EventDefinition](eventdefinition.html): The business version of the event definition 3951* [Evidence](evidence.html): The business version of the evidence 3952* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 3953* [ExampleScenario](examplescenario.html): The business version of the example scenario 3954* [GraphDefinition](graphdefinition.html): The business version of the graph definition 3955* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 3956* [Library](library.html): The business version of the library 3957* [Measure](measure.html): The business version of the measure 3958* [MessageDefinition](messagedefinition.html): The business version of the message definition 3959* [NamingSystem](namingsystem.html): The business version of the naming system 3960* [OperationDefinition](operationdefinition.html): The business version of the operation definition 3961* [PlanDefinition](plandefinition.html): The business version of the plan definition 3962* [Questionnaire](questionnaire.html): The business version of the questionnaire 3963* [Requirements](requirements.html): The business version of the requirements 3964* [SearchParameter](searchparameter.html): The business version of the search parameter 3965* [StructureDefinition](structuredefinition.html): The business version of the structure definition 3966* [StructureMap](structuremap.html): The business version of the structure map 3967* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 3968* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 3969* [TestScript](testscript.html): The business version of the test script 3970* [ValueSet](valueset.html): The business version of the value set 3971</b><br> 3972 * Type: <b>token</b><br> 3973 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 3974 * </p> 3975 */ 3976 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 3977 public static final String SP_VERSION = "version"; 3978 /** 3979 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3980 * <p> 3981 * Description: <b>Multiple Resources: 3982 3983* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 3984* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 3985* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 3986* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 3987* [Citation](citation.html): The business version of the citation 3988* [CodeSystem](codesystem.html): The business version of the code system 3989* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 3990* [ConceptMap](conceptmap.html): The business version of the concept map 3991* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 3992* [EventDefinition](eventdefinition.html): The business version of the event definition 3993* [Evidence](evidence.html): The business version of the evidence 3994* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 3995* [ExampleScenario](examplescenario.html): The business version of the example scenario 3996* [GraphDefinition](graphdefinition.html): The business version of the graph definition 3997* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 3998* [Library](library.html): The business version of the library 3999* [Measure](measure.html): The business version of the measure 4000* [MessageDefinition](messagedefinition.html): The business version of the message definition 4001* [NamingSystem](namingsystem.html): The business version of the naming system 4002* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4003* [PlanDefinition](plandefinition.html): The business version of the plan definition 4004* [Questionnaire](questionnaire.html): The business version of the questionnaire 4005* [Requirements](requirements.html): The business version of the requirements 4006* [SearchParameter](searchparameter.html): The business version of the search parameter 4007* [StructureDefinition](structuredefinition.html): The business version of the structure definition 4008* [StructureMap](structuremap.html): The business version of the structure map 4009* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 4010* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 4011* [TestScript](testscript.html): The business version of the test script 4012* [ValueSet](valueset.html): The business version of the value set 4013</b><br> 4014 * Type: <b>token</b><br> 4015 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 4016 * </p> 4017 */ 4018 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 4019 4020 /** 4021 * Search parameter: <b>actor</b> 4022 * <p> 4023 * Description: <b>An actor these requirements are for</b><br> 4024 * Type: <b>reference</b><br> 4025 * Path: <b>Requirements.actor</b><br> 4026 * </p> 4027 */ 4028 @SearchParamDefinition(name="actor", path="Requirements.actor", description="An actor these requirements are for", type="reference", target={ActorDefinition.class } ) 4029 public static final String SP_ACTOR = "actor"; 4030 /** 4031 * <b>Fluent Client</b> search parameter constant for <b>actor</b> 4032 * <p> 4033 * Description: <b>An actor these requirements are for</b><br> 4034 * Type: <b>reference</b><br> 4035 * Path: <b>Requirements.actor</b><br> 4036 * </p> 4037 */ 4038 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACTOR); 4039 4040/** 4041 * Constant for fluent queries to be used to add include statements. Specifies 4042 * the path value of "<b>Requirements:actor</b>". 4043 */ 4044 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTOR = new ca.uhn.fhir.model.api.Include("Requirements:actor").toLocked(); 4045 4046 /** 4047 * Search parameter: <b>derived-from</b> 4048 * <p> 4049 * Description: <b>The requirements these are derived from</b><br> 4050 * Type: <b>reference</b><br> 4051 * Path: <b>Requirements.derivedFrom</b><br> 4052 * </p> 4053 */ 4054 @SearchParamDefinition(name="derived-from", path="Requirements.derivedFrom", description="The requirements these are derived from", type="reference", target={Requirements.class } ) 4055 public static final String SP_DERIVED_FROM = "derived-from"; 4056 /** 4057 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 4058 * <p> 4059 * Description: <b>The requirements these are derived from</b><br> 4060 * Type: <b>reference</b><br> 4061 * Path: <b>Requirements.derivedFrom</b><br> 4062 * </p> 4063 */ 4064 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 4065 4066/** 4067 * Constant for fluent queries to be used to add include statements. Specifies 4068 * the path value of "<b>Requirements:derived-from</b>". 4069 */ 4070 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("Requirements:derived-from").toLocked(); 4071 4072// Manual code (from Configuration.txt): 4073 4074 public RequirementsStatementComponent findStatement(String key) { 4075 for (RequirementsStatementComponent t : getStatement()) { 4076 if (key.equals(t.getKey())) { 4077 return t; 4078 } 4079 } 4080 return null; 4081 } 4082 4083// end addition 4084 4085} 4086