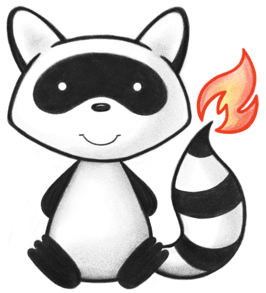
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A scientific study of nature that sometimes includes processes involved in health and disease. For example, clinical trials are research studies that involve people. These studies may be related to new ways to screen, prevent, diagnose, and treat disease. They may also study certain outcomes and certain groups of people by looking at data collected in the past or future. 052 */ 053@ResourceDef(name="ResearchStudy", profile="http://hl7.org/fhir/StructureDefinition/ResearchStudy") 054public class ResearchStudy extends DomainResource { 055 056 @Block() 057 public static class ResearchStudyLabelComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Kind of name. 060 */ 061 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="primary | official | scientific | plain-language | subtitle | short-title | acronym | earlier-title | language | auto-translated | human-use | machine-use | duplicate-uid", formalDefinition="Kind of name." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/title-type") 064 protected CodeableConcept type; 065 066 /** 067 * The name. 068 */ 069 @Child(name = "value", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 070 @Description(shortDefinition="The name", formalDefinition="The name." ) 071 protected StringType value; 072 073 private static final long serialVersionUID = 944223389L; 074 075 /** 076 * Constructor 077 */ 078 public ResearchStudyLabelComponent() { 079 super(); 080 } 081 082 /** 083 * @return {@link #type} (Kind of name.) 084 */ 085 public CodeableConcept getType() { 086 if (this.type == null) 087 if (Configuration.errorOnAutoCreate()) 088 throw new Error("Attempt to auto-create ResearchStudyLabelComponent.type"); 089 else if (Configuration.doAutoCreate()) 090 this.type = new CodeableConcept(); // cc 091 return this.type; 092 } 093 094 public boolean hasType() { 095 return this.type != null && !this.type.isEmpty(); 096 } 097 098 /** 099 * @param value {@link #type} (Kind of name.) 100 */ 101 public ResearchStudyLabelComponent setType(CodeableConcept value) { 102 this.type = value; 103 return this; 104 } 105 106 /** 107 * @return {@link #value} (The name.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 108 */ 109 public StringType getValueElement() { 110 if (this.value == null) 111 if (Configuration.errorOnAutoCreate()) 112 throw new Error("Attempt to auto-create ResearchStudyLabelComponent.value"); 113 else if (Configuration.doAutoCreate()) 114 this.value = new StringType(); // bb 115 return this.value; 116 } 117 118 public boolean hasValueElement() { 119 return this.value != null && !this.value.isEmpty(); 120 } 121 122 public boolean hasValue() { 123 return this.value != null && !this.value.isEmpty(); 124 } 125 126 /** 127 * @param value {@link #value} (The name.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 128 */ 129 public ResearchStudyLabelComponent setValueElement(StringType value) { 130 this.value = value; 131 return this; 132 } 133 134 /** 135 * @return The name. 136 */ 137 public String getValue() { 138 return this.value == null ? null : this.value.getValue(); 139 } 140 141 /** 142 * @param value The name. 143 */ 144 public ResearchStudyLabelComponent setValue(String value) { 145 if (Utilities.noString(value)) 146 this.value = null; 147 else { 148 if (this.value == null) 149 this.value = new StringType(); 150 this.value.setValue(value); 151 } 152 return this; 153 } 154 155 protected void listChildren(List<Property> children) { 156 super.listChildren(children); 157 children.add(new Property("type", "CodeableConcept", "Kind of name.", 0, 1, type)); 158 children.add(new Property("value", "string", "The name.", 0, 1, value)); 159 } 160 161 @Override 162 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 163 switch (_hash) { 164 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Kind of name.", 0, 1, type); 165 case 111972721: /*value*/ return new Property("value", "string", "The name.", 0, 1, value); 166 default: return super.getNamedProperty(_hash, _name, _checkValid); 167 } 168 169 } 170 171 @Override 172 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 173 switch (hash) { 174 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 175 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 176 default: return super.getProperty(hash, name, checkValid); 177 } 178 179 } 180 181 @Override 182 public Base setProperty(int hash, String name, Base value) throws FHIRException { 183 switch (hash) { 184 case 3575610: // type 185 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 186 return value; 187 case 111972721: // value 188 this.value = TypeConvertor.castToString(value); // StringType 189 return value; 190 default: return super.setProperty(hash, name, value); 191 } 192 193 } 194 195 @Override 196 public Base setProperty(String name, Base value) throws FHIRException { 197 if (name.equals("type")) { 198 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 199 } else if (name.equals("value")) { 200 this.value = TypeConvertor.castToString(value); // StringType 201 } else 202 return super.setProperty(name, value); 203 return value; 204 } 205 206 @Override 207 public Base makeProperty(int hash, String name) throws FHIRException { 208 switch (hash) { 209 case 3575610: return getType(); 210 case 111972721: return getValueElement(); 211 default: return super.makeProperty(hash, name); 212 } 213 214 } 215 216 @Override 217 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 218 switch (hash) { 219 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 220 case 111972721: /*value*/ return new String[] {"string"}; 221 default: return super.getTypesForProperty(hash, name); 222 } 223 224 } 225 226 @Override 227 public Base addChild(String name) throws FHIRException { 228 if (name.equals("type")) { 229 this.type = new CodeableConcept(); 230 return this.type; 231 } 232 else if (name.equals("value")) { 233 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.label.value"); 234 } 235 else 236 return super.addChild(name); 237 } 238 239 public ResearchStudyLabelComponent copy() { 240 ResearchStudyLabelComponent dst = new ResearchStudyLabelComponent(); 241 copyValues(dst); 242 return dst; 243 } 244 245 public void copyValues(ResearchStudyLabelComponent dst) { 246 super.copyValues(dst); 247 dst.type = type == null ? null : type.copy(); 248 dst.value = value == null ? null : value.copy(); 249 } 250 251 @Override 252 public boolean equalsDeep(Base other_) { 253 if (!super.equalsDeep(other_)) 254 return false; 255 if (!(other_ instanceof ResearchStudyLabelComponent)) 256 return false; 257 ResearchStudyLabelComponent o = (ResearchStudyLabelComponent) other_; 258 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 259 } 260 261 @Override 262 public boolean equalsShallow(Base other_) { 263 if (!super.equalsShallow(other_)) 264 return false; 265 if (!(other_ instanceof ResearchStudyLabelComponent)) 266 return false; 267 ResearchStudyLabelComponent o = (ResearchStudyLabelComponent) other_; 268 return compareValues(value, o.value, true); 269 } 270 271 public boolean isEmpty() { 272 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 273 } 274 275 public String fhirType() { 276 return "ResearchStudy.label"; 277 278 } 279 280 } 281 282 @Block() 283 public static class ResearchStudyAssociatedPartyComponent extends BackboneElement implements IBaseBackboneElement { 284 /** 285 * Name of associated party. 286 */ 287 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 288 @Description(shortDefinition="Name of associated party", formalDefinition="Name of associated party." ) 289 protected StringType name; 290 291 /** 292 * Type of association. 293 */ 294 @Child(name = "role", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 295 @Description(shortDefinition="sponsor | lead-sponsor | sponsor-investigator | primary-investigator | collaborator | funding-source | general-contact | recruitment-contact | sub-investigator | study-director | study-chair", formalDefinition="Type of association." ) 296 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-party-role") 297 protected CodeableConcept role; 298 299 /** 300 * Identifies the start date and the end date of the associated party in the role. 301 */ 302 @Child(name = "period", type = {Period.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 303 @Description(shortDefinition="When active in the role", formalDefinition="Identifies the start date and the end date of the associated party in the role." ) 304 protected List<Period> period; 305 306 /** 307 * A categorization other than role for the associated party. 308 */ 309 @Child(name = "classifier", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 310 @Description(shortDefinition="nih | fda | government | nonprofit | academic | industry", formalDefinition="A categorization other than role for the associated party." ) 311 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-party-organization-type") 312 protected List<CodeableConcept> classifier; 313 314 /** 315 * Individual or organization associated with study (use practitionerRole to specify their organisation). 316 */ 317 @Child(name = "party", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=5, min=0, max=1, modifier=false, summary=false) 318 @Description(shortDefinition="Individual or organization associated with study (use practitionerRole to specify their organisation)", formalDefinition="Individual or organization associated with study (use practitionerRole to specify their organisation)." ) 319 protected Reference party; 320 321 private static final long serialVersionUID = -1418550998L; 322 323 /** 324 * Constructor 325 */ 326 public ResearchStudyAssociatedPartyComponent() { 327 super(); 328 } 329 330 /** 331 * Constructor 332 */ 333 public ResearchStudyAssociatedPartyComponent(CodeableConcept role) { 334 super(); 335 this.setRole(role); 336 } 337 338 /** 339 * @return {@link #name} (Name of associated party.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 340 */ 341 public StringType getNameElement() { 342 if (this.name == null) 343 if (Configuration.errorOnAutoCreate()) 344 throw new Error("Attempt to auto-create ResearchStudyAssociatedPartyComponent.name"); 345 else if (Configuration.doAutoCreate()) 346 this.name = new StringType(); // bb 347 return this.name; 348 } 349 350 public boolean hasNameElement() { 351 return this.name != null && !this.name.isEmpty(); 352 } 353 354 public boolean hasName() { 355 return this.name != null && !this.name.isEmpty(); 356 } 357 358 /** 359 * @param value {@link #name} (Name of associated party.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 360 */ 361 public ResearchStudyAssociatedPartyComponent setNameElement(StringType value) { 362 this.name = value; 363 return this; 364 } 365 366 /** 367 * @return Name of associated party. 368 */ 369 public String getName() { 370 return this.name == null ? null : this.name.getValue(); 371 } 372 373 /** 374 * @param value Name of associated party. 375 */ 376 public ResearchStudyAssociatedPartyComponent setName(String value) { 377 if (Utilities.noString(value)) 378 this.name = null; 379 else { 380 if (this.name == null) 381 this.name = new StringType(); 382 this.name.setValue(value); 383 } 384 return this; 385 } 386 387 /** 388 * @return {@link #role} (Type of association.) 389 */ 390 public CodeableConcept getRole() { 391 if (this.role == null) 392 if (Configuration.errorOnAutoCreate()) 393 throw new Error("Attempt to auto-create ResearchStudyAssociatedPartyComponent.role"); 394 else if (Configuration.doAutoCreate()) 395 this.role = new CodeableConcept(); // cc 396 return this.role; 397 } 398 399 public boolean hasRole() { 400 return this.role != null && !this.role.isEmpty(); 401 } 402 403 /** 404 * @param value {@link #role} (Type of association.) 405 */ 406 public ResearchStudyAssociatedPartyComponent setRole(CodeableConcept value) { 407 this.role = value; 408 return this; 409 } 410 411 /** 412 * @return {@link #period} (Identifies the start date and the end date of the associated party in the role.) 413 */ 414 public List<Period> getPeriod() { 415 if (this.period == null) 416 this.period = new ArrayList<Period>(); 417 return this.period; 418 } 419 420 /** 421 * @return Returns a reference to <code>this</code> for easy method chaining 422 */ 423 public ResearchStudyAssociatedPartyComponent setPeriod(List<Period> thePeriod) { 424 this.period = thePeriod; 425 return this; 426 } 427 428 public boolean hasPeriod() { 429 if (this.period == null) 430 return false; 431 for (Period item : this.period) 432 if (!item.isEmpty()) 433 return true; 434 return false; 435 } 436 437 public Period addPeriod() { //3 438 Period t = new Period(); 439 if (this.period == null) 440 this.period = new ArrayList<Period>(); 441 this.period.add(t); 442 return t; 443 } 444 445 public ResearchStudyAssociatedPartyComponent addPeriod(Period t) { //3 446 if (t == null) 447 return this; 448 if (this.period == null) 449 this.period = new ArrayList<Period>(); 450 this.period.add(t); 451 return this; 452 } 453 454 /** 455 * @return The first repetition of repeating field {@link #period}, creating it if it does not already exist {3} 456 */ 457 public Period getPeriodFirstRep() { 458 if (getPeriod().isEmpty()) { 459 addPeriod(); 460 } 461 return getPeriod().get(0); 462 } 463 464 /** 465 * @return {@link #classifier} (A categorization other than role for the associated party.) 466 */ 467 public List<CodeableConcept> getClassifier() { 468 if (this.classifier == null) 469 this.classifier = new ArrayList<CodeableConcept>(); 470 return this.classifier; 471 } 472 473 /** 474 * @return Returns a reference to <code>this</code> for easy method chaining 475 */ 476 public ResearchStudyAssociatedPartyComponent setClassifier(List<CodeableConcept> theClassifier) { 477 this.classifier = theClassifier; 478 return this; 479 } 480 481 public boolean hasClassifier() { 482 if (this.classifier == null) 483 return false; 484 for (CodeableConcept item : this.classifier) 485 if (!item.isEmpty()) 486 return true; 487 return false; 488 } 489 490 public CodeableConcept addClassifier() { //3 491 CodeableConcept t = new CodeableConcept(); 492 if (this.classifier == null) 493 this.classifier = new ArrayList<CodeableConcept>(); 494 this.classifier.add(t); 495 return t; 496 } 497 498 public ResearchStudyAssociatedPartyComponent addClassifier(CodeableConcept t) { //3 499 if (t == null) 500 return this; 501 if (this.classifier == null) 502 this.classifier = new ArrayList<CodeableConcept>(); 503 this.classifier.add(t); 504 return this; 505 } 506 507 /** 508 * @return The first repetition of repeating field {@link #classifier}, creating it if it does not already exist {3} 509 */ 510 public CodeableConcept getClassifierFirstRep() { 511 if (getClassifier().isEmpty()) { 512 addClassifier(); 513 } 514 return getClassifier().get(0); 515 } 516 517 /** 518 * @return {@link #party} (Individual or organization associated with study (use practitionerRole to specify their organisation).) 519 */ 520 public Reference getParty() { 521 if (this.party == null) 522 if (Configuration.errorOnAutoCreate()) 523 throw new Error("Attempt to auto-create ResearchStudyAssociatedPartyComponent.party"); 524 else if (Configuration.doAutoCreate()) 525 this.party = new Reference(); // cc 526 return this.party; 527 } 528 529 public boolean hasParty() { 530 return this.party != null && !this.party.isEmpty(); 531 } 532 533 /** 534 * @param value {@link #party} (Individual or organization associated with study (use practitionerRole to specify their organisation).) 535 */ 536 public ResearchStudyAssociatedPartyComponent setParty(Reference value) { 537 this.party = value; 538 return this; 539 } 540 541 protected void listChildren(List<Property> children) { 542 super.listChildren(children); 543 children.add(new Property("name", "string", "Name of associated party.", 0, 1, name)); 544 children.add(new Property("role", "CodeableConcept", "Type of association.", 0, 1, role)); 545 children.add(new Property("period", "Period", "Identifies the start date and the end date of the associated party in the role.", 0, java.lang.Integer.MAX_VALUE, period)); 546 children.add(new Property("classifier", "CodeableConcept", "A categorization other than role for the associated party.", 0, java.lang.Integer.MAX_VALUE, classifier)); 547 children.add(new Property("party", "Reference(Practitioner|PractitionerRole|Organization)", "Individual or organization associated with study (use practitionerRole to specify their organisation).", 0, 1, party)); 548 } 549 550 @Override 551 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 552 switch (_hash) { 553 case 3373707: /*name*/ return new Property("name", "string", "Name of associated party.", 0, 1, name); 554 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Type of association.", 0, 1, role); 555 case -991726143: /*period*/ return new Property("period", "Period", "Identifies the start date and the end date of the associated party in the role.", 0, java.lang.Integer.MAX_VALUE, period); 556 case -281470431: /*classifier*/ return new Property("classifier", "CodeableConcept", "A categorization other than role for the associated party.", 0, java.lang.Integer.MAX_VALUE, classifier); 557 case 106437350: /*party*/ return new Property("party", "Reference(Practitioner|PractitionerRole|Organization)", "Individual or organization associated with study (use practitionerRole to specify their organisation).", 0, 1, party); 558 default: return super.getNamedProperty(_hash, _name, _checkValid); 559 } 560 561 } 562 563 @Override 564 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 565 switch (hash) { 566 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 567 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 568 case -991726143: /*period*/ return this.period == null ? new Base[0] : this.period.toArray(new Base[this.period.size()]); // Period 569 case -281470431: /*classifier*/ return this.classifier == null ? new Base[0] : this.classifier.toArray(new Base[this.classifier.size()]); // CodeableConcept 570 case 106437350: /*party*/ return this.party == null ? new Base[0] : new Base[] {this.party}; // Reference 571 default: return super.getProperty(hash, name, checkValid); 572 } 573 574 } 575 576 @Override 577 public Base setProperty(int hash, String name, Base value) throws FHIRException { 578 switch (hash) { 579 case 3373707: // name 580 this.name = TypeConvertor.castToString(value); // StringType 581 return value; 582 case 3506294: // role 583 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 584 return value; 585 case -991726143: // period 586 this.getPeriod().add(TypeConvertor.castToPeriod(value)); // Period 587 return value; 588 case -281470431: // classifier 589 this.getClassifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 590 return value; 591 case 106437350: // party 592 this.party = TypeConvertor.castToReference(value); // Reference 593 return value; 594 default: return super.setProperty(hash, name, value); 595 } 596 597 } 598 599 @Override 600 public Base setProperty(String name, Base value) throws FHIRException { 601 if (name.equals("name")) { 602 this.name = TypeConvertor.castToString(value); // StringType 603 } else if (name.equals("role")) { 604 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 605 } else if (name.equals("period")) { 606 this.getPeriod().add(TypeConvertor.castToPeriod(value)); 607 } else if (name.equals("classifier")) { 608 this.getClassifier().add(TypeConvertor.castToCodeableConcept(value)); 609 } else if (name.equals("party")) { 610 this.party = TypeConvertor.castToReference(value); // Reference 611 } else 612 return super.setProperty(name, value); 613 return value; 614 } 615 616 @Override 617 public Base makeProperty(int hash, String name) throws FHIRException { 618 switch (hash) { 619 case 3373707: return getNameElement(); 620 case 3506294: return getRole(); 621 case -991726143: return addPeriod(); 622 case -281470431: return addClassifier(); 623 case 106437350: return getParty(); 624 default: return super.makeProperty(hash, name); 625 } 626 627 } 628 629 @Override 630 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 631 switch (hash) { 632 case 3373707: /*name*/ return new String[] {"string"}; 633 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 634 case -991726143: /*period*/ return new String[] {"Period"}; 635 case -281470431: /*classifier*/ return new String[] {"CodeableConcept"}; 636 case 106437350: /*party*/ return new String[] {"Reference"}; 637 default: return super.getTypesForProperty(hash, name); 638 } 639 640 } 641 642 @Override 643 public Base addChild(String name) throws FHIRException { 644 if (name.equals("name")) { 645 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.associatedParty.name"); 646 } 647 else if (name.equals("role")) { 648 this.role = new CodeableConcept(); 649 return this.role; 650 } 651 else if (name.equals("period")) { 652 return addPeriod(); 653 } 654 else if (name.equals("classifier")) { 655 return addClassifier(); 656 } 657 else if (name.equals("party")) { 658 this.party = new Reference(); 659 return this.party; 660 } 661 else 662 return super.addChild(name); 663 } 664 665 public ResearchStudyAssociatedPartyComponent copy() { 666 ResearchStudyAssociatedPartyComponent dst = new ResearchStudyAssociatedPartyComponent(); 667 copyValues(dst); 668 return dst; 669 } 670 671 public void copyValues(ResearchStudyAssociatedPartyComponent dst) { 672 super.copyValues(dst); 673 dst.name = name == null ? null : name.copy(); 674 dst.role = role == null ? null : role.copy(); 675 if (period != null) { 676 dst.period = new ArrayList<Period>(); 677 for (Period i : period) 678 dst.period.add(i.copy()); 679 }; 680 if (classifier != null) { 681 dst.classifier = new ArrayList<CodeableConcept>(); 682 for (CodeableConcept i : classifier) 683 dst.classifier.add(i.copy()); 684 }; 685 dst.party = party == null ? null : party.copy(); 686 } 687 688 @Override 689 public boolean equalsDeep(Base other_) { 690 if (!super.equalsDeep(other_)) 691 return false; 692 if (!(other_ instanceof ResearchStudyAssociatedPartyComponent)) 693 return false; 694 ResearchStudyAssociatedPartyComponent o = (ResearchStudyAssociatedPartyComponent) other_; 695 return compareDeep(name, o.name, true) && compareDeep(role, o.role, true) && compareDeep(period, o.period, true) 696 && compareDeep(classifier, o.classifier, true) && compareDeep(party, o.party, true); 697 } 698 699 @Override 700 public boolean equalsShallow(Base other_) { 701 if (!super.equalsShallow(other_)) 702 return false; 703 if (!(other_ instanceof ResearchStudyAssociatedPartyComponent)) 704 return false; 705 ResearchStudyAssociatedPartyComponent o = (ResearchStudyAssociatedPartyComponent) other_; 706 return compareValues(name, o.name, true); 707 } 708 709 public boolean isEmpty() { 710 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, role, period, classifier 711 , party); 712 } 713 714 public String fhirType() { 715 return "ResearchStudy.associatedParty"; 716 717 } 718 719 } 720 721 @Block() 722 public static class ResearchStudyProgressStatusComponent extends BackboneElement implements IBaseBackboneElement { 723 /** 724 * Label for status or state (e.g. recruitment status). 725 */ 726 @Child(name = "state", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 727 @Description(shortDefinition="Label for status or state (e.g. recruitment status)", formalDefinition="Label for status or state (e.g. recruitment status)." ) 728 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-status") 729 protected CodeableConcept state; 730 731 /** 732 * An indication of whether or not the date is a known date when the state changed or will change. A value of true indicates a known date. A value of false indicates an estimated date. 733 */ 734 @Child(name = "actual", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 735 @Description(shortDefinition="Actual if true else anticipated", formalDefinition="An indication of whether or not the date is a known date when the state changed or will change. A value of true indicates a known date. A value of false indicates an estimated date." ) 736 protected BooleanType actual; 737 738 /** 739 * Date range. 740 */ 741 @Child(name = "period", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=false) 742 @Description(shortDefinition="Date range", formalDefinition="Date range." ) 743 protected Period period; 744 745 private static final long serialVersionUID = 1232680620L; 746 747 /** 748 * Constructor 749 */ 750 public ResearchStudyProgressStatusComponent() { 751 super(); 752 } 753 754 /** 755 * Constructor 756 */ 757 public ResearchStudyProgressStatusComponent(CodeableConcept state) { 758 super(); 759 this.setState(state); 760 } 761 762 /** 763 * @return {@link #state} (Label for status or state (e.g. recruitment status).) 764 */ 765 public CodeableConcept getState() { 766 if (this.state == null) 767 if (Configuration.errorOnAutoCreate()) 768 throw new Error("Attempt to auto-create ResearchStudyProgressStatusComponent.state"); 769 else if (Configuration.doAutoCreate()) 770 this.state = new CodeableConcept(); // cc 771 return this.state; 772 } 773 774 public boolean hasState() { 775 return this.state != null && !this.state.isEmpty(); 776 } 777 778 /** 779 * @param value {@link #state} (Label for status or state (e.g. recruitment status).) 780 */ 781 public ResearchStudyProgressStatusComponent setState(CodeableConcept value) { 782 this.state = value; 783 return this; 784 } 785 786 /** 787 * @return {@link #actual} (An indication of whether or not the date is a known date when the state changed or will change. A value of true indicates a known date. A value of false indicates an estimated date.). This is the underlying object with id, value and extensions. The accessor "getActual" gives direct access to the value 788 */ 789 public BooleanType getActualElement() { 790 if (this.actual == null) 791 if (Configuration.errorOnAutoCreate()) 792 throw new Error("Attempt to auto-create ResearchStudyProgressStatusComponent.actual"); 793 else if (Configuration.doAutoCreate()) 794 this.actual = new BooleanType(); // bb 795 return this.actual; 796 } 797 798 public boolean hasActualElement() { 799 return this.actual != null && !this.actual.isEmpty(); 800 } 801 802 public boolean hasActual() { 803 return this.actual != null && !this.actual.isEmpty(); 804 } 805 806 /** 807 * @param value {@link #actual} (An indication of whether or not the date is a known date when the state changed or will change. A value of true indicates a known date. A value of false indicates an estimated date.). This is the underlying object with id, value and extensions. The accessor "getActual" gives direct access to the value 808 */ 809 public ResearchStudyProgressStatusComponent setActualElement(BooleanType value) { 810 this.actual = value; 811 return this; 812 } 813 814 /** 815 * @return An indication of whether or not the date is a known date when the state changed or will change. A value of true indicates a known date. A value of false indicates an estimated date. 816 */ 817 public boolean getActual() { 818 return this.actual == null || this.actual.isEmpty() ? false : this.actual.getValue(); 819 } 820 821 /** 822 * @param value An indication of whether or not the date is a known date when the state changed or will change. A value of true indicates a known date. A value of false indicates an estimated date. 823 */ 824 public ResearchStudyProgressStatusComponent setActual(boolean value) { 825 if (this.actual == null) 826 this.actual = new BooleanType(); 827 this.actual.setValue(value); 828 return this; 829 } 830 831 /** 832 * @return {@link #period} (Date range.) 833 */ 834 public Period getPeriod() { 835 if (this.period == null) 836 if (Configuration.errorOnAutoCreate()) 837 throw new Error("Attempt to auto-create ResearchStudyProgressStatusComponent.period"); 838 else if (Configuration.doAutoCreate()) 839 this.period = new Period(); // cc 840 return this.period; 841 } 842 843 public boolean hasPeriod() { 844 return this.period != null && !this.period.isEmpty(); 845 } 846 847 /** 848 * @param value {@link #period} (Date range.) 849 */ 850 public ResearchStudyProgressStatusComponent setPeriod(Period value) { 851 this.period = value; 852 return this; 853 } 854 855 protected void listChildren(List<Property> children) { 856 super.listChildren(children); 857 children.add(new Property("state", "CodeableConcept", "Label for status or state (e.g. recruitment status).", 0, 1, state)); 858 children.add(new Property("actual", "boolean", "An indication of whether or not the date is a known date when the state changed or will change. A value of true indicates a known date. A value of false indicates an estimated date.", 0, 1, actual)); 859 children.add(new Property("period", "Period", "Date range.", 0, 1, period)); 860 } 861 862 @Override 863 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 864 switch (_hash) { 865 case 109757585: /*state*/ return new Property("state", "CodeableConcept", "Label for status or state (e.g. recruitment status).", 0, 1, state); 866 case -1422939762: /*actual*/ return new Property("actual", "boolean", "An indication of whether or not the date is a known date when the state changed or will change. A value of true indicates a known date. A value of false indicates an estimated date.", 0, 1, actual); 867 case -991726143: /*period*/ return new Property("period", "Period", "Date range.", 0, 1, period); 868 default: return super.getNamedProperty(_hash, _name, _checkValid); 869 } 870 871 } 872 873 @Override 874 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 875 switch (hash) { 876 case 109757585: /*state*/ return this.state == null ? new Base[0] : new Base[] {this.state}; // CodeableConcept 877 case -1422939762: /*actual*/ return this.actual == null ? new Base[0] : new Base[] {this.actual}; // BooleanType 878 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 879 default: return super.getProperty(hash, name, checkValid); 880 } 881 882 } 883 884 @Override 885 public Base setProperty(int hash, String name, Base value) throws FHIRException { 886 switch (hash) { 887 case 109757585: // state 888 this.state = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 889 return value; 890 case -1422939762: // actual 891 this.actual = TypeConvertor.castToBoolean(value); // BooleanType 892 return value; 893 case -991726143: // period 894 this.period = TypeConvertor.castToPeriod(value); // Period 895 return value; 896 default: return super.setProperty(hash, name, value); 897 } 898 899 } 900 901 @Override 902 public Base setProperty(String name, Base value) throws FHIRException { 903 if (name.equals("state")) { 904 this.state = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 905 } else if (name.equals("actual")) { 906 this.actual = TypeConvertor.castToBoolean(value); // BooleanType 907 } else if (name.equals("period")) { 908 this.period = TypeConvertor.castToPeriod(value); // Period 909 } else 910 return super.setProperty(name, value); 911 return value; 912 } 913 914 @Override 915 public Base makeProperty(int hash, String name) throws FHIRException { 916 switch (hash) { 917 case 109757585: return getState(); 918 case -1422939762: return getActualElement(); 919 case -991726143: return getPeriod(); 920 default: return super.makeProperty(hash, name); 921 } 922 923 } 924 925 @Override 926 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 927 switch (hash) { 928 case 109757585: /*state*/ return new String[] {"CodeableConcept"}; 929 case -1422939762: /*actual*/ return new String[] {"boolean"}; 930 case -991726143: /*period*/ return new String[] {"Period"}; 931 default: return super.getTypesForProperty(hash, name); 932 } 933 934 } 935 936 @Override 937 public Base addChild(String name) throws FHIRException { 938 if (name.equals("state")) { 939 this.state = new CodeableConcept(); 940 return this.state; 941 } 942 else if (name.equals("actual")) { 943 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.progressStatus.actual"); 944 } 945 else if (name.equals("period")) { 946 this.period = new Period(); 947 return this.period; 948 } 949 else 950 return super.addChild(name); 951 } 952 953 public ResearchStudyProgressStatusComponent copy() { 954 ResearchStudyProgressStatusComponent dst = new ResearchStudyProgressStatusComponent(); 955 copyValues(dst); 956 return dst; 957 } 958 959 public void copyValues(ResearchStudyProgressStatusComponent dst) { 960 super.copyValues(dst); 961 dst.state = state == null ? null : state.copy(); 962 dst.actual = actual == null ? null : actual.copy(); 963 dst.period = period == null ? null : period.copy(); 964 } 965 966 @Override 967 public boolean equalsDeep(Base other_) { 968 if (!super.equalsDeep(other_)) 969 return false; 970 if (!(other_ instanceof ResearchStudyProgressStatusComponent)) 971 return false; 972 ResearchStudyProgressStatusComponent o = (ResearchStudyProgressStatusComponent) other_; 973 return compareDeep(state, o.state, true) && compareDeep(actual, o.actual, true) && compareDeep(period, o.period, true) 974 ; 975 } 976 977 @Override 978 public boolean equalsShallow(Base other_) { 979 if (!super.equalsShallow(other_)) 980 return false; 981 if (!(other_ instanceof ResearchStudyProgressStatusComponent)) 982 return false; 983 ResearchStudyProgressStatusComponent o = (ResearchStudyProgressStatusComponent) other_; 984 return compareValues(actual, o.actual, true); 985 } 986 987 public boolean isEmpty() { 988 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(state, actual, period); 989 } 990 991 public String fhirType() { 992 return "ResearchStudy.progressStatus"; 993 994 } 995 996 } 997 998 @Block() 999 public static class ResearchStudyRecruitmentComponent extends BackboneElement implements IBaseBackboneElement { 1000 /** 1001 * Estimated total number of participants to be enrolled. 1002 */ 1003 @Child(name = "targetNumber", type = {UnsignedIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1004 @Description(shortDefinition="Estimated total number of participants to be enrolled", formalDefinition="Estimated total number of participants to be enrolled." ) 1005 protected UnsignedIntType targetNumber; 1006 1007 /** 1008 * Actual total number of participants enrolled in study. 1009 */ 1010 @Child(name = "actualNumber", type = {UnsignedIntType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1011 @Description(shortDefinition="Actual total number of participants enrolled in study", formalDefinition="Actual total number of participants enrolled in study." ) 1012 protected UnsignedIntType actualNumber; 1013 1014 /** 1015 * Inclusion and exclusion criteria. 1016 */ 1017 @Child(name = "eligibility", type = {Group.class, EvidenceVariable.class}, order=3, min=0, max=1, modifier=false, summary=false) 1018 @Description(shortDefinition="Inclusion and exclusion criteria", formalDefinition="Inclusion and exclusion criteria." ) 1019 protected Reference eligibility; 1020 1021 /** 1022 * Group of participants who were enrolled in study. 1023 */ 1024 @Child(name = "actualGroup", type = {Group.class}, order=4, min=0, max=1, modifier=false, summary=true) 1025 @Description(shortDefinition="Group of participants who were enrolled in study", formalDefinition="Group of participants who were enrolled in study." ) 1026 protected Reference actualGroup; 1027 1028 private static final long serialVersionUID = 1483229827L; 1029 1030 /** 1031 * Constructor 1032 */ 1033 public ResearchStudyRecruitmentComponent() { 1034 super(); 1035 } 1036 1037 /** 1038 * @return {@link #targetNumber} (Estimated total number of participants to be enrolled.). This is the underlying object with id, value and extensions. The accessor "getTargetNumber" gives direct access to the value 1039 */ 1040 public UnsignedIntType getTargetNumberElement() { 1041 if (this.targetNumber == null) 1042 if (Configuration.errorOnAutoCreate()) 1043 throw new Error("Attempt to auto-create ResearchStudyRecruitmentComponent.targetNumber"); 1044 else if (Configuration.doAutoCreate()) 1045 this.targetNumber = new UnsignedIntType(); // bb 1046 return this.targetNumber; 1047 } 1048 1049 public boolean hasTargetNumberElement() { 1050 return this.targetNumber != null && !this.targetNumber.isEmpty(); 1051 } 1052 1053 public boolean hasTargetNumber() { 1054 return this.targetNumber != null && !this.targetNumber.isEmpty(); 1055 } 1056 1057 /** 1058 * @param value {@link #targetNumber} (Estimated total number of participants to be enrolled.). This is the underlying object with id, value and extensions. The accessor "getTargetNumber" gives direct access to the value 1059 */ 1060 public ResearchStudyRecruitmentComponent setTargetNumberElement(UnsignedIntType value) { 1061 this.targetNumber = value; 1062 return this; 1063 } 1064 1065 /** 1066 * @return Estimated total number of participants to be enrolled. 1067 */ 1068 public int getTargetNumber() { 1069 return this.targetNumber == null || this.targetNumber.isEmpty() ? 0 : this.targetNumber.getValue(); 1070 } 1071 1072 /** 1073 * @param value Estimated total number of participants to be enrolled. 1074 */ 1075 public ResearchStudyRecruitmentComponent setTargetNumber(int value) { 1076 if (this.targetNumber == null) 1077 this.targetNumber = new UnsignedIntType(); 1078 this.targetNumber.setValue(value); 1079 return this; 1080 } 1081 1082 /** 1083 * @return {@link #actualNumber} (Actual total number of participants enrolled in study.). This is the underlying object with id, value and extensions. The accessor "getActualNumber" gives direct access to the value 1084 */ 1085 public UnsignedIntType getActualNumberElement() { 1086 if (this.actualNumber == null) 1087 if (Configuration.errorOnAutoCreate()) 1088 throw new Error("Attempt to auto-create ResearchStudyRecruitmentComponent.actualNumber"); 1089 else if (Configuration.doAutoCreate()) 1090 this.actualNumber = new UnsignedIntType(); // bb 1091 return this.actualNumber; 1092 } 1093 1094 public boolean hasActualNumberElement() { 1095 return this.actualNumber != null && !this.actualNumber.isEmpty(); 1096 } 1097 1098 public boolean hasActualNumber() { 1099 return this.actualNumber != null && !this.actualNumber.isEmpty(); 1100 } 1101 1102 /** 1103 * @param value {@link #actualNumber} (Actual total number of participants enrolled in study.). This is the underlying object with id, value and extensions. The accessor "getActualNumber" gives direct access to the value 1104 */ 1105 public ResearchStudyRecruitmentComponent setActualNumberElement(UnsignedIntType value) { 1106 this.actualNumber = value; 1107 return this; 1108 } 1109 1110 /** 1111 * @return Actual total number of participants enrolled in study. 1112 */ 1113 public int getActualNumber() { 1114 return this.actualNumber == null || this.actualNumber.isEmpty() ? 0 : this.actualNumber.getValue(); 1115 } 1116 1117 /** 1118 * @param value Actual total number of participants enrolled in study. 1119 */ 1120 public ResearchStudyRecruitmentComponent setActualNumber(int value) { 1121 if (this.actualNumber == null) 1122 this.actualNumber = new UnsignedIntType(); 1123 this.actualNumber.setValue(value); 1124 return this; 1125 } 1126 1127 /** 1128 * @return {@link #eligibility} (Inclusion and exclusion criteria.) 1129 */ 1130 public Reference getEligibility() { 1131 if (this.eligibility == null) 1132 if (Configuration.errorOnAutoCreate()) 1133 throw new Error("Attempt to auto-create ResearchStudyRecruitmentComponent.eligibility"); 1134 else if (Configuration.doAutoCreate()) 1135 this.eligibility = new Reference(); // cc 1136 return this.eligibility; 1137 } 1138 1139 public boolean hasEligibility() { 1140 return this.eligibility != null && !this.eligibility.isEmpty(); 1141 } 1142 1143 /** 1144 * @param value {@link #eligibility} (Inclusion and exclusion criteria.) 1145 */ 1146 public ResearchStudyRecruitmentComponent setEligibility(Reference value) { 1147 this.eligibility = value; 1148 return this; 1149 } 1150 1151 /** 1152 * @return {@link #actualGroup} (Group of participants who were enrolled in study.) 1153 */ 1154 public Reference getActualGroup() { 1155 if (this.actualGroup == null) 1156 if (Configuration.errorOnAutoCreate()) 1157 throw new Error("Attempt to auto-create ResearchStudyRecruitmentComponent.actualGroup"); 1158 else if (Configuration.doAutoCreate()) 1159 this.actualGroup = new Reference(); // cc 1160 return this.actualGroup; 1161 } 1162 1163 public boolean hasActualGroup() { 1164 return this.actualGroup != null && !this.actualGroup.isEmpty(); 1165 } 1166 1167 /** 1168 * @param value {@link #actualGroup} (Group of participants who were enrolled in study.) 1169 */ 1170 public ResearchStudyRecruitmentComponent setActualGroup(Reference value) { 1171 this.actualGroup = value; 1172 return this; 1173 } 1174 1175 protected void listChildren(List<Property> children) { 1176 super.listChildren(children); 1177 children.add(new Property("targetNumber", "unsignedInt", "Estimated total number of participants to be enrolled.", 0, 1, targetNumber)); 1178 children.add(new Property("actualNumber", "unsignedInt", "Actual total number of participants enrolled in study.", 0, 1, actualNumber)); 1179 children.add(new Property("eligibility", "Reference(Group|EvidenceVariable)", "Inclusion and exclusion criteria.", 0, 1, eligibility)); 1180 children.add(new Property("actualGroup", "Reference(Group)", "Group of participants who were enrolled in study.", 0, 1, actualGroup)); 1181 } 1182 1183 @Override 1184 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1185 switch (_hash) { 1186 case -682948550: /*targetNumber*/ return new Property("targetNumber", "unsignedInt", "Estimated total number of participants to be enrolled.", 0, 1, targetNumber); 1187 case 746557047: /*actualNumber*/ return new Property("actualNumber", "unsignedInt", "Actual total number of participants enrolled in study.", 0, 1, actualNumber); 1188 case -930847859: /*eligibility*/ return new Property("eligibility", "Reference(Group|EvidenceVariable)", "Inclusion and exclusion criteria.", 0, 1, eligibility); 1189 case 1403004305: /*actualGroup*/ return new Property("actualGroup", "Reference(Group)", "Group of participants who were enrolled in study.", 0, 1, actualGroup); 1190 default: return super.getNamedProperty(_hash, _name, _checkValid); 1191 } 1192 1193 } 1194 1195 @Override 1196 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1197 switch (hash) { 1198 case -682948550: /*targetNumber*/ return this.targetNumber == null ? new Base[0] : new Base[] {this.targetNumber}; // UnsignedIntType 1199 case 746557047: /*actualNumber*/ return this.actualNumber == null ? new Base[0] : new Base[] {this.actualNumber}; // UnsignedIntType 1200 case -930847859: /*eligibility*/ return this.eligibility == null ? new Base[0] : new Base[] {this.eligibility}; // Reference 1201 case 1403004305: /*actualGroup*/ return this.actualGroup == null ? new Base[0] : new Base[] {this.actualGroup}; // Reference 1202 default: return super.getProperty(hash, name, checkValid); 1203 } 1204 1205 } 1206 1207 @Override 1208 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1209 switch (hash) { 1210 case -682948550: // targetNumber 1211 this.targetNumber = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1212 return value; 1213 case 746557047: // actualNumber 1214 this.actualNumber = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1215 return value; 1216 case -930847859: // eligibility 1217 this.eligibility = TypeConvertor.castToReference(value); // Reference 1218 return value; 1219 case 1403004305: // actualGroup 1220 this.actualGroup = TypeConvertor.castToReference(value); // Reference 1221 return value; 1222 default: return super.setProperty(hash, name, value); 1223 } 1224 1225 } 1226 1227 @Override 1228 public Base setProperty(String name, Base value) throws FHIRException { 1229 if (name.equals("targetNumber")) { 1230 this.targetNumber = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1231 } else if (name.equals("actualNumber")) { 1232 this.actualNumber = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1233 } else if (name.equals("eligibility")) { 1234 this.eligibility = TypeConvertor.castToReference(value); // Reference 1235 } else if (name.equals("actualGroup")) { 1236 this.actualGroup = TypeConvertor.castToReference(value); // Reference 1237 } else 1238 return super.setProperty(name, value); 1239 return value; 1240 } 1241 1242 @Override 1243 public Base makeProperty(int hash, String name) throws FHIRException { 1244 switch (hash) { 1245 case -682948550: return getTargetNumberElement(); 1246 case 746557047: return getActualNumberElement(); 1247 case -930847859: return getEligibility(); 1248 case 1403004305: return getActualGroup(); 1249 default: return super.makeProperty(hash, name); 1250 } 1251 1252 } 1253 1254 @Override 1255 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1256 switch (hash) { 1257 case -682948550: /*targetNumber*/ return new String[] {"unsignedInt"}; 1258 case 746557047: /*actualNumber*/ return new String[] {"unsignedInt"}; 1259 case -930847859: /*eligibility*/ return new String[] {"Reference"}; 1260 case 1403004305: /*actualGroup*/ return new String[] {"Reference"}; 1261 default: return super.getTypesForProperty(hash, name); 1262 } 1263 1264 } 1265 1266 @Override 1267 public Base addChild(String name) throws FHIRException { 1268 if (name.equals("targetNumber")) { 1269 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.recruitment.targetNumber"); 1270 } 1271 else if (name.equals("actualNumber")) { 1272 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.recruitment.actualNumber"); 1273 } 1274 else if (name.equals("eligibility")) { 1275 this.eligibility = new Reference(); 1276 return this.eligibility; 1277 } 1278 else if (name.equals("actualGroup")) { 1279 this.actualGroup = new Reference(); 1280 return this.actualGroup; 1281 } 1282 else 1283 return super.addChild(name); 1284 } 1285 1286 public ResearchStudyRecruitmentComponent copy() { 1287 ResearchStudyRecruitmentComponent dst = new ResearchStudyRecruitmentComponent(); 1288 copyValues(dst); 1289 return dst; 1290 } 1291 1292 public void copyValues(ResearchStudyRecruitmentComponent dst) { 1293 super.copyValues(dst); 1294 dst.targetNumber = targetNumber == null ? null : targetNumber.copy(); 1295 dst.actualNumber = actualNumber == null ? null : actualNumber.copy(); 1296 dst.eligibility = eligibility == null ? null : eligibility.copy(); 1297 dst.actualGroup = actualGroup == null ? null : actualGroup.copy(); 1298 } 1299 1300 @Override 1301 public boolean equalsDeep(Base other_) { 1302 if (!super.equalsDeep(other_)) 1303 return false; 1304 if (!(other_ instanceof ResearchStudyRecruitmentComponent)) 1305 return false; 1306 ResearchStudyRecruitmentComponent o = (ResearchStudyRecruitmentComponent) other_; 1307 return compareDeep(targetNumber, o.targetNumber, true) && compareDeep(actualNumber, o.actualNumber, true) 1308 && compareDeep(eligibility, o.eligibility, true) && compareDeep(actualGroup, o.actualGroup, true) 1309 ; 1310 } 1311 1312 @Override 1313 public boolean equalsShallow(Base other_) { 1314 if (!super.equalsShallow(other_)) 1315 return false; 1316 if (!(other_ instanceof ResearchStudyRecruitmentComponent)) 1317 return false; 1318 ResearchStudyRecruitmentComponent o = (ResearchStudyRecruitmentComponent) other_; 1319 return compareValues(targetNumber, o.targetNumber, true) && compareValues(actualNumber, o.actualNumber, true) 1320 ; 1321 } 1322 1323 public boolean isEmpty() { 1324 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(targetNumber, actualNumber 1325 , eligibility, actualGroup); 1326 } 1327 1328 public String fhirType() { 1329 return "ResearchStudy.recruitment"; 1330 1331 } 1332 1333 } 1334 1335 @Block() 1336 public static class ResearchStudyComparisonGroupComponent extends BackboneElement implements IBaseBackboneElement { 1337 /** 1338 * Allows the comparisonGroup for the study and the comparisonGroup for the subject to be linked easily. 1339 */ 1340 @Child(name = "linkId", type = {IdType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1341 @Description(shortDefinition="Allows the comparisonGroup for the study and the comparisonGroup for the subject to be linked easily", formalDefinition="Allows the comparisonGroup for the study and the comparisonGroup for the subject to be linked easily." ) 1342 protected IdType linkId; 1343 1344 /** 1345 * Unique, human-readable label for this comparisonGroup of the study. 1346 */ 1347 @Child(name = "name", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 1348 @Description(shortDefinition="Label for study comparisonGroup", formalDefinition="Unique, human-readable label for this comparisonGroup of the study." ) 1349 protected StringType name; 1350 1351 /** 1352 * Categorization of study comparisonGroup, e.g. experimental, active comparator, placebo comparater. 1353 */ 1354 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1355 @Description(shortDefinition="Categorization of study comparisonGroup", formalDefinition="Categorization of study comparisonGroup, e.g. experimental, active comparator, placebo comparater." ) 1356 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-arm-type") 1357 protected CodeableConcept type; 1358 1359 /** 1360 * A succinct description of the path through the study that would be followed by a subject adhering to this comparisonGroup. 1361 */ 1362 @Child(name = "description", type = {MarkdownType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1363 @Description(shortDefinition="Short explanation of study path", formalDefinition="A succinct description of the path through the study that would be followed by a subject adhering to this comparisonGroup." ) 1364 protected MarkdownType description; 1365 1366 /** 1367 * Interventions or exposures in this comparisonGroup or cohort. 1368 */ 1369 @Child(name = "intendedExposure", type = {EvidenceVariable.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1370 @Description(shortDefinition="Interventions or exposures in this comparisonGroup or cohort", formalDefinition="Interventions or exposures in this comparisonGroup or cohort." ) 1371 protected List<Reference> intendedExposure; 1372 1373 /** 1374 * Group of participants who were enrolled in study comparisonGroup. 1375 */ 1376 @Child(name = "observedGroup", type = {Group.class}, order=6, min=0, max=1, modifier=false, summary=false) 1377 @Description(shortDefinition="Group of participants who were enrolled in study comparisonGroup", formalDefinition="Group of participants who were enrolled in study comparisonGroup." ) 1378 protected Reference observedGroup; 1379 1380 private static final long serialVersionUID = 1107310853L; 1381 1382 /** 1383 * Constructor 1384 */ 1385 public ResearchStudyComparisonGroupComponent() { 1386 super(); 1387 } 1388 1389 /** 1390 * Constructor 1391 */ 1392 public ResearchStudyComparisonGroupComponent(String name) { 1393 super(); 1394 this.setName(name); 1395 } 1396 1397 /** 1398 * @return {@link #linkId} (Allows the comparisonGroup for the study and the comparisonGroup for the subject to be linked easily.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 1399 */ 1400 public IdType getLinkIdElement() { 1401 if (this.linkId == null) 1402 if (Configuration.errorOnAutoCreate()) 1403 throw new Error("Attempt to auto-create ResearchStudyComparisonGroupComponent.linkId"); 1404 else if (Configuration.doAutoCreate()) 1405 this.linkId = new IdType(); // bb 1406 return this.linkId; 1407 } 1408 1409 public boolean hasLinkIdElement() { 1410 return this.linkId != null && !this.linkId.isEmpty(); 1411 } 1412 1413 public boolean hasLinkId() { 1414 return this.linkId != null && !this.linkId.isEmpty(); 1415 } 1416 1417 /** 1418 * @param value {@link #linkId} (Allows the comparisonGroup for the study and the comparisonGroup for the subject to be linked easily.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 1419 */ 1420 public ResearchStudyComparisonGroupComponent setLinkIdElement(IdType value) { 1421 this.linkId = value; 1422 return this; 1423 } 1424 1425 /** 1426 * @return Allows the comparisonGroup for the study and the comparisonGroup for the subject to be linked easily. 1427 */ 1428 public String getLinkId() { 1429 return this.linkId == null ? null : this.linkId.getValue(); 1430 } 1431 1432 /** 1433 * @param value Allows the comparisonGroup for the study and the comparisonGroup for the subject to be linked easily. 1434 */ 1435 public ResearchStudyComparisonGroupComponent setLinkId(String value) { 1436 if (Utilities.noString(value)) 1437 this.linkId = null; 1438 else { 1439 if (this.linkId == null) 1440 this.linkId = new IdType(); 1441 this.linkId.setValue(value); 1442 } 1443 return this; 1444 } 1445 1446 /** 1447 * @return {@link #name} (Unique, human-readable label for this comparisonGroup of the study.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1448 */ 1449 public StringType getNameElement() { 1450 if (this.name == null) 1451 if (Configuration.errorOnAutoCreate()) 1452 throw new Error("Attempt to auto-create ResearchStudyComparisonGroupComponent.name"); 1453 else if (Configuration.doAutoCreate()) 1454 this.name = new StringType(); // bb 1455 return this.name; 1456 } 1457 1458 public boolean hasNameElement() { 1459 return this.name != null && !this.name.isEmpty(); 1460 } 1461 1462 public boolean hasName() { 1463 return this.name != null && !this.name.isEmpty(); 1464 } 1465 1466 /** 1467 * @param value {@link #name} (Unique, human-readable label for this comparisonGroup of the study.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1468 */ 1469 public ResearchStudyComparisonGroupComponent setNameElement(StringType value) { 1470 this.name = value; 1471 return this; 1472 } 1473 1474 /** 1475 * @return Unique, human-readable label for this comparisonGroup of the study. 1476 */ 1477 public String getName() { 1478 return this.name == null ? null : this.name.getValue(); 1479 } 1480 1481 /** 1482 * @param value Unique, human-readable label for this comparisonGroup of the study. 1483 */ 1484 public ResearchStudyComparisonGroupComponent setName(String value) { 1485 if (this.name == null) 1486 this.name = new StringType(); 1487 this.name.setValue(value); 1488 return this; 1489 } 1490 1491 /** 1492 * @return {@link #type} (Categorization of study comparisonGroup, e.g. experimental, active comparator, placebo comparater.) 1493 */ 1494 public CodeableConcept getType() { 1495 if (this.type == null) 1496 if (Configuration.errorOnAutoCreate()) 1497 throw new Error("Attempt to auto-create ResearchStudyComparisonGroupComponent.type"); 1498 else if (Configuration.doAutoCreate()) 1499 this.type = new CodeableConcept(); // cc 1500 return this.type; 1501 } 1502 1503 public boolean hasType() { 1504 return this.type != null && !this.type.isEmpty(); 1505 } 1506 1507 /** 1508 * @param value {@link #type} (Categorization of study comparisonGroup, e.g. experimental, active comparator, placebo comparater.) 1509 */ 1510 public ResearchStudyComparisonGroupComponent setType(CodeableConcept value) { 1511 this.type = value; 1512 return this; 1513 } 1514 1515 /** 1516 * @return {@link #description} (A succinct description of the path through the study that would be followed by a subject adhering to this comparisonGroup.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1517 */ 1518 public MarkdownType getDescriptionElement() { 1519 if (this.description == null) 1520 if (Configuration.errorOnAutoCreate()) 1521 throw new Error("Attempt to auto-create ResearchStudyComparisonGroupComponent.description"); 1522 else if (Configuration.doAutoCreate()) 1523 this.description = new MarkdownType(); // bb 1524 return this.description; 1525 } 1526 1527 public boolean hasDescriptionElement() { 1528 return this.description != null && !this.description.isEmpty(); 1529 } 1530 1531 public boolean hasDescription() { 1532 return this.description != null && !this.description.isEmpty(); 1533 } 1534 1535 /** 1536 * @param value {@link #description} (A succinct description of the path through the study that would be followed by a subject adhering to this comparisonGroup.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1537 */ 1538 public ResearchStudyComparisonGroupComponent setDescriptionElement(MarkdownType value) { 1539 this.description = value; 1540 return this; 1541 } 1542 1543 /** 1544 * @return A succinct description of the path through the study that would be followed by a subject adhering to this comparisonGroup. 1545 */ 1546 public String getDescription() { 1547 return this.description == null ? null : this.description.getValue(); 1548 } 1549 1550 /** 1551 * @param value A succinct description of the path through the study that would be followed by a subject adhering to this comparisonGroup. 1552 */ 1553 public ResearchStudyComparisonGroupComponent setDescription(String value) { 1554 if (Utilities.noString(value)) 1555 this.description = null; 1556 else { 1557 if (this.description == null) 1558 this.description = new MarkdownType(); 1559 this.description.setValue(value); 1560 } 1561 return this; 1562 } 1563 1564 /** 1565 * @return {@link #intendedExposure} (Interventions or exposures in this comparisonGroup or cohort.) 1566 */ 1567 public List<Reference> getIntendedExposure() { 1568 if (this.intendedExposure == null) 1569 this.intendedExposure = new ArrayList<Reference>(); 1570 return this.intendedExposure; 1571 } 1572 1573 /** 1574 * @return Returns a reference to <code>this</code> for easy method chaining 1575 */ 1576 public ResearchStudyComparisonGroupComponent setIntendedExposure(List<Reference> theIntendedExposure) { 1577 this.intendedExposure = theIntendedExposure; 1578 return this; 1579 } 1580 1581 public boolean hasIntendedExposure() { 1582 if (this.intendedExposure == null) 1583 return false; 1584 for (Reference item : this.intendedExposure) 1585 if (!item.isEmpty()) 1586 return true; 1587 return false; 1588 } 1589 1590 public Reference addIntendedExposure() { //3 1591 Reference t = new Reference(); 1592 if (this.intendedExposure == null) 1593 this.intendedExposure = new ArrayList<Reference>(); 1594 this.intendedExposure.add(t); 1595 return t; 1596 } 1597 1598 public ResearchStudyComparisonGroupComponent addIntendedExposure(Reference t) { //3 1599 if (t == null) 1600 return this; 1601 if (this.intendedExposure == null) 1602 this.intendedExposure = new ArrayList<Reference>(); 1603 this.intendedExposure.add(t); 1604 return this; 1605 } 1606 1607 /** 1608 * @return The first repetition of repeating field {@link #intendedExposure}, creating it if it does not already exist {3} 1609 */ 1610 public Reference getIntendedExposureFirstRep() { 1611 if (getIntendedExposure().isEmpty()) { 1612 addIntendedExposure(); 1613 } 1614 return getIntendedExposure().get(0); 1615 } 1616 1617 /** 1618 * @return {@link #observedGroup} (Group of participants who were enrolled in study comparisonGroup.) 1619 */ 1620 public Reference getObservedGroup() { 1621 if (this.observedGroup == null) 1622 if (Configuration.errorOnAutoCreate()) 1623 throw new Error("Attempt to auto-create ResearchStudyComparisonGroupComponent.observedGroup"); 1624 else if (Configuration.doAutoCreate()) 1625 this.observedGroup = new Reference(); // cc 1626 return this.observedGroup; 1627 } 1628 1629 public boolean hasObservedGroup() { 1630 return this.observedGroup != null && !this.observedGroup.isEmpty(); 1631 } 1632 1633 /** 1634 * @param value {@link #observedGroup} (Group of participants who were enrolled in study comparisonGroup.) 1635 */ 1636 public ResearchStudyComparisonGroupComponent setObservedGroup(Reference value) { 1637 this.observedGroup = value; 1638 return this; 1639 } 1640 1641 protected void listChildren(List<Property> children) { 1642 super.listChildren(children); 1643 children.add(new Property("linkId", "id", "Allows the comparisonGroup for the study and the comparisonGroup for the subject to be linked easily.", 0, 1, linkId)); 1644 children.add(new Property("name", "string", "Unique, human-readable label for this comparisonGroup of the study.", 0, 1, name)); 1645 children.add(new Property("type", "CodeableConcept", "Categorization of study comparisonGroup, e.g. experimental, active comparator, placebo comparater.", 0, 1, type)); 1646 children.add(new Property("description", "markdown", "A succinct description of the path through the study that would be followed by a subject adhering to this comparisonGroup.", 0, 1, description)); 1647 children.add(new Property("intendedExposure", "Reference(EvidenceVariable)", "Interventions or exposures in this comparisonGroup or cohort.", 0, java.lang.Integer.MAX_VALUE, intendedExposure)); 1648 children.add(new Property("observedGroup", "Reference(Group)", "Group of participants who were enrolled in study comparisonGroup.", 0, 1, observedGroup)); 1649 } 1650 1651 @Override 1652 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1653 switch (_hash) { 1654 case -1102667083: /*linkId*/ return new Property("linkId", "id", "Allows the comparisonGroup for the study and the comparisonGroup for the subject to be linked easily.", 0, 1, linkId); 1655 case 3373707: /*name*/ return new Property("name", "string", "Unique, human-readable label for this comparisonGroup of the study.", 0, 1, name); 1656 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Categorization of study comparisonGroup, e.g. experimental, active comparator, placebo comparater.", 0, 1, type); 1657 case -1724546052: /*description*/ return new Property("description", "markdown", "A succinct description of the path through the study that would be followed by a subject adhering to this comparisonGroup.", 0, 1, description); 1658 case -407218606: /*intendedExposure*/ return new Property("intendedExposure", "Reference(EvidenceVariable)", "Interventions or exposures in this comparisonGroup or cohort.", 0, java.lang.Integer.MAX_VALUE, intendedExposure); 1659 case 375599255: /*observedGroup*/ return new Property("observedGroup", "Reference(Group)", "Group of participants who were enrolled in study comparisonGroup.", 0, 1, observedGroup); 1660 default: return super.getNamedProperty(_hash, _name, _checkValid); 1661 } 1662 1663 } 1664 1665 @Override 1666 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1667 switch (hash) { 1668 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // IdType 1669 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1670 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1671 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1672 case -407218606: /*intendedExposure*/ return this.intendedExposure == null ? new Base[0] : this.intendedExposure.toArray(new Base[this.intendedExposure.size()]); // Reference 1673 case 375599255: /*observedGroup*/ return this.observedGroup == null ? new Base[0] : new Base[] {this.observedGroup}; // Reference 1674 default: return super.getProperty(hash, name, checkValid); 1675 } 1676 1677 } 1678 1679 @Override 1680 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1681 switch (hash) { 1682 case -1102667083: // linkId 1683 this.linkId = TypeConvertor.castToId(value); // IdType 1684 return value; 1685 case 3373707: // name 1686 this.name = TypeConvertor.castToString(value); // StringType 1687 return value; 1688 case 3575610: // type 1689 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1690 return value; 1691 case -1724546052: // description 1692 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1693 return value; 1694 case -407218606: // intendedExposure 1695 this.getIntendedExposure().add(TypeConvertor.castToReference(value)); // Reference 1696 return value; 1697 case 375599255: // observedGroup 1698 this.observedGroup = TypeConvertor.castToReference(value); // Reference 1699 return value; 1700 default: return super.setProperty(hash, name, value); 1701 } 1702 1703 } 1704 1705 @Override 1706 public Base setProperty(String name, Base value) throws FHIRException { 1707 if (name.equals("linkId")) { 1708 this.linkId = TypeConvertor.castToId(value); // IdType 1709 } else if (name.equals("name")) { 1710 this.name = TypeConvertor.castToString(value); // StringType 1711 } else if (name.equals("type")) { 1712 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1713 } else if (name.equals("description")) { 1714 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1715 } else if (name.equals("intendedExposure")) { 1716 this.getIntendedExposure().add(TypeConvertor.castToReference(value)); 1717 } else if (name.equals("observedGroup")) { 1718 this.observedGroup = TypeConvertor.castToReference(value); // Reference 1719 } else 1720 return super.setProperty(name, value); 1721 return value; 1722 } 1723 1724 @Override 1725 public Base makeProperty(int hash, String name) throws FHIRException { 1726 switch (hash) { 1727 case -1102667083: return getLinkIdElement(); 1728 case 3373707: return getNameElement(); 1729 case 3575610: return getType(); 1730 case -1724546052: return getDescriptionElement(); 1731 case -407218606: return addIntendedExposure(); 1732 case 375599255: return getObservedGroup(); 1733 default: return super.makeProperty(hash, name); 1734 } 1735 1736 } 1737 1738 @Override 1739 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1740 switch (hash) { 1741 case -1102667083: /*linkId*/ return new String[] {"id"}; 1742 case 3373707: /*name*/ return new String[] {"string"}; 1743 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1744 case -1724546052: /*description*/ return new String[] {"markdown"}; 1745 case -407218606: /*intendedExposure*/ return new String[] {"Reference"}; 1746 case 375599255: /*observedGroup*/ return new String[] {"Reference"}; 1747 default: return super.getTypesForProperty(hash, name); 1748 } 1749 1750 } 1751 1752 @Override 1753 public Base addChild(String name) throws FHIRException { 1754 if (name.equals("linkId")) { 1755 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.comparisonGroup.linkId"); 1756 } 1757 else if (name.equals("name")) { 1758 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.comparisonGroup.name"); 1759 } 1760 else if (name.equals("type")) { 1761 this.type = new CodeableConcept(); 1762 return this.type; 1763 } 1764 else if (name.equals("description")) { 1765 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.comparisonGroup.description"); 1766 } 1767 else if (name.equals("intendedExposure")) { 1768 return addIntendedExposure(); 1769 } 1770 else if (name.equals("observedGroup")) { 1771 this.observedGroup = new Reference(); 1772 return this.observedGroup; 1773 } 1774 else 1775 return super.addChild(name); 1776 } 1777 1778 public ResearchStudyComparisonGroupComponent copy() { 1779 ResearchStudyComparisonGroupComponent dst = new ResearchStudyComparisonGroupComponent(); 1780 copyValues(dst); 1781 return dst; 1782 } 1783 1784 public void copyValues(ResearchStudyComparisonGroupComponent dst) { 1785 super.copyValues(dst); 1786 dst.linkId = linkId == null ? null : linkId.copy(); 1787 dst.name = name == null ? null : name.copy(); 1788 dst.type = type == null ? null : type.copy(); 1789 dst.description = description == null ? null : description.copy(); 1790 if (intendedExposure != null) { 1791 dst.intendedExposure = new ArrayList<Reference>(); 1792 for (Reference i : intendedExposure) 1793 dst.intendedExposure.add(i.copy()); 1794 }; 1795 dst.observedGroup = observedGroup == null ? null : observedGroup.copy(); 1796 } 1797 1798 @Override 1799 public boolean equalsDeep(Base other_) { 1800 if (!super.equalsDeep(other_)) 1801 return false; 1802 if (!(other_ instanceof ResearchStudyComparisonGroupComponent)) 1803 return false; 1804 ResearchStudyComparisonGroupComponent o = (ResearchStudyComparisonGroupComponent) other_; 1805 return compareDeep(linkId, o.linkId, true) && compareDeep(name, o.name, true) && compareDeep(type, o.type, true) 1806 && compareDeep(description, o.description, true) && compareDeep(intendedExposure, o.intendedExposure, true) 1807 && compareDeep(observedGroup, o.observedGroup, true); 1808 } 1809 1810 @Override 1811 public boolean equalsShallow(Base other_) { 1812 if (!super.equalsShallow(other_)) 1813 return false; 1814 if (!(other_ instanceof ResearchStudyComparisonGroupComponent)) 1815 return false; 1816 ResearchStudyComparisonGroupComponent o = (ResearchStudyComparisonGroupComponent) other_; 1817 return compareValues(linkId, o.linkId, true) && compareValues(name, o.name, true) && compareValues(description, o.description, true) 1818 ; 1819 } 1820 1821 public boolean isEmpty() { 1822 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, name, type, description 1823 , intendedExposure, observedGroup); 1824 } 1825 1826 public String fhirType() { 1827 return "ResearchStudy.comparisonGroup"; 1828 1829 } 1830 1831 } 1832 1833 @Block() 1834 public static class ResearchStudyObjectiveComponent extends BackboneElement implements IBaseBackboneElement { 1835 /** 1836 * Unique, human-readable label for this objective of the study. 1837 */ 1838 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1839 @Description(shortDefinition="Label for the objective", formalDefinition="Unique, human-readable label for this objective of the study." ) 1840 protected StringType name; 1841 1842 /** 1843 * The kind of study objective. 1844 */ 1845 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1846 @Description(shortDefinition="primary | secondary | exploratory", formalDefinition="The kind of study objective." ) 1847 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-objective-type") 1848 protected CodeableConcept type; 1849 1850 /** 1851 * Free text description of the objective of the study. This is what the study is trying to achieve rather than how it is going to achieve it (see ResearchStudy.description). 1852 */ 1853 @Child(name = "description", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1854 @Description(shortDefinition="Description of the objective", formalDefinition="Free text description of the objective of the study. This is what the study is trying to achieve rather than how it is going to achieve it (see ResearchStudy.description)." ) 1855 protected MarkdownType description; 1856 1857 private static final long serialVersionUID = -1976083810L; 1858 1859 /** 1860 * Constructor 1861 */ 1862 public ResearchStudyObjectiveComponent() { 1863 super(); 1864 } 1865 1866 /** 1867 * @return {@link #name} (Unique, human-readable label for this objective of the study.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1868 */ 1869 public StringType getNameElement() { 1870 if (this.name == null) 1871 if (Configuration.errorOnAutoCreate()) 1872 throw new Error("Attempt to auto-create ResearchStudyObjectiveComponent.name"); 1873 else if (Configuration.doAutoCreate()) 1874 this.name = new StringType(); // bb 1875 return this.name; 1876 } 1877 1878 public boolean hasNameElement() { 1879 return this.name != null && !this.name.isEmpty(); 1880 } 1881 1882 public boolean hasName() { 1883 return this.name != null && !this.name.isEmpty(); 1884 } 1885 1886 /** 1887 * @param value {@link #name} (Unique, human-readable label for this objective of the study.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1888 */ 1889 public ResearchStudyObjectiveComponent setNameElement(StringType value) { 1890 this.name = value; 1891 return this; 1892 } 1893 1894 /** 1895 * @return Unique, human-readable label for this objective of the study. 1896 */ 1897 public String getName() { 1898 return this.name == null ? null : this.name.getValue(); 1899 } 1900 1901 /** 1902 * @param value Unique, human-readable label for this objective of the study. 1903 */ 1904 public ResearchStudyObjectiveComponent setName(String value) { 1905 if (Utilities.noString(value)) 1906 this.name = null; 1907 else { 1908 if (this.name == null) 1909 this.name = new StringType(); 1910 this.name.setValue(value); 1911 } 1912 return this; 1913 } 1914 1915 /** 1916 * @return {@link #type} (The kind of study objective.) 1917 */ 1918 public CodeableConcept getType() { 1919 if (this.type == null) 1920 if (Configuration.errorOnAutoCreate()) 1921 throw new Error("Attempt to auto-create ResearchStudyObjectiveComponent.type"); 1922 else if (Configuration.doAutoCreate()) 1923 this.type = new CodeableConcept(); // cc 1924 return this.type; 1925 } 1926 1927 public boolean hasType() { 1928 return this.type != null && !this.type.isEmpty(); 1929 } 1930 1931 /** 1932 * @param value {@link #type} (The kind of study objective.) 1933 */ 1934 public ResearchStudyObjectiveComponent setType(CodeableConcept value) { 1935 this.type = value; 1936 return this; 1937 } 1938 1939 /** 1940 * @return {@link #description} (Free text description of the objective of the study. This is what the study is trying to achieve rather than how it is going to achieve it (see ResearchStudy.description).). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1941 */ 1942 public MarkdownType getDescriptionElement() { 1943 if (this.description == null) 1944 if (Configuration.errorOnAutoCreate()) 1945 throw new Error("Attempt to auto-create ResearchStudyObjectiveComponent.description"); 1946 else if (Configuration.doAutoCreate()) 1947 this.description = new MarkdownType(); // bb 1948 return this.description; 1949 } 1950 1951 public boolean hasDescriptionElement() { 1952 return this.description != null && !this.description.isEmpty(); 1953 } 1954 1955 public boolean hasDescription() { 1956 return this.description != null && !this.description.isEmpty(); 1957 } 1958 1959 /** 1960 * @param value {@link #description} (Free text description of the objective of the study. This is what the study is trying to achieve rather than how it is going to achieve it (see ResearchStudy.description).). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1961 */ 1962 public ResearchStudyObjectiveComponent setDescriptionElement(MarkdownType value) { 1963 this.description = value; 1964 return this; 1965 } 1966 1967 /** 1968 * @return Free text description of the objective of the study. This is what the study is trying to achieve rather than how it is going to achieve it (see ResearchStudy.description). 1969 */ 1970 public String getDescription() { 1971 return this.description == null ? null : this.description.getValue(); 1972 } 1973 1974 /** 1975 * @param value Free text description of the objective of the study. This is what the study is trying to achieve rather than how it is going to achieve it (see ResearchStudy.description). 1976 */ 1977 public ResearchStudyObjectiveComponent setDescription(String value) { 1978 if (Utilities.noString(value)) 1979 this.description = null; 1980 else { 1981 if (this.description == null) 1982 this.description = new MarkdownType(); 1983 this.description.setValue(value); 1984 } 1985 return this; 1986 } 1987 1988 protected void listChildren(List<Property> children) { 1989 super.listChildren(children); 1990 children.add(new Property("name", "string", "Unique, human-readable label for this objective of the study.", 0, 1, name)); 1991 children.add(new Property("type", "CodeableConcept", "The kind of study objective.", 0, 1, type)); 1992 children.add(new Property("description", "markdown", "Free text description of the objective of the study. This is what the study is trying to achieve rather than how it is going to achieve it (see ResearchStudy.description).", 0, 1, description)); 1993 } 1994 1995 @Override 1996 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1997 switch (_hash) { 1998 case 3373707: /*name*/ return new Property("name", "string", "Unique, human-readable label for this objective of the study.", 0, 1, name); 1999 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The kind of study objective.", 0, 1, type); 2000 case -1724546052: /*description*/ return new Property("description", "markdown", "Free text description of the objective of the study. This is what the study is trying to achieve rather than how it is going to achieve it (see ResearchStudy.description).", 0, 1, description); 2001 default: return super.getNamedProperty(_hash, _name, _checkValid); 2002 } 2003 2004 } 2005 2006 @Override 2007 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2008 switch (hash) { 2009 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2010 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2011 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2012 default: return super.getProperty(hash, name, checkValid); 2013 } 2014 2015 } 2016 2017 @Override 2018 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2019 switch (hash) { 2020 case 3373707: // name 2021 this.name = TypeConvertor.castToString(value); // StringType 2022 return value; 2023 case 3575610: // type 2024 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2025 return value; 2026 case -1724546052: // description 2027 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2028 return value; 2029 default: return super.setProperty(hash, name, value); 2030 } 2031 2032 } 2033 2034 @Override 2035 public Base setProperty(String name, Base value) throws FHIRException { 2036 if (name.equals("name")) { 2037 this.name = TypeConvertor.castToString(value); // StringType 2038 } else if (name.equals("type")) { 2039 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2040 } else if (name.equals("description")) { 2041 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2042 } else 2043 return super.setProperty(name, value); 2044 return value; 2045 } 2046 2047 @Override 2048 public Base makeProperty(int hash, String name) throws FHIRException { 2049 switch (hash) { 2050 case 3373707: return getNameElement(); 2051 case 3575610: return getType(); 2052 case -1724546052: return getDescriptionElement(); 2053 default: return super.makeProperty(hash, name); 2054 } 2055 2056 } 2057 2058 @Override 2059 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2060 switch (hash) { 2061 case 3373707: /*name*/ return new String[] {"string"}; 2062 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2063 case -1724546052: /*description*/ return new String[] {"markdown"}; 2064 default: return super.getTypesForProperty(hash, name); 2065 } 2066 2067 } 2068 2069 @Override 2070 public Base addChild(String name) throws FHIRException { 2071 if (name.equals("name")) { 2072 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.objective.name"); 2073 } 2074 else if (name.equals("type")) { 2075 this.type = new CodeableConcept(); 2076 return this.type; 2077 } 2078 else if (name.equals("description")) { 2079 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.objective.description"); 2080 } 2081 else 2082 return super.addChild(name); 2083 } 2084 2085 public ResearchStudyObjectiveComponent copy() { 2086 ResearchStudyObjectiveComponent dst = new ResearchStudyObjectiveComponent(); 2087 copyValues(dst); 2088 return dst; 2089 } 2090 2091 public void copyValues(ResearchStudyObjectiveComponent dst) { 2092 super.copyValues(dst); 2093 dst.name = name == null ? null : name.copy(); 2094 dst.type = type == null ? null : type.copy(); 2095 dst.description = description == null ? null : description.copy(); 2096 } 2097 2098 @Override 2099 public boolean equalsDeep(Base other_) { 2100 if (!super.equalsDeep(other_)) 2101 return false; 2102 if (!(other_ instanceof ResearchStudyObjectiveComponent)) 2103 return false; 2104 ResearchStudyObjectiveComponent o = (ResearchStudyObjectiveComponent) other_; 2105 return compareDeep(name, o.name, true) && compareDeep(type, o.type, true) && compareDeep(description, o.description, true) 2106 ; 2107 } 2108 2109 @Override 2110 public boolean equalsShallow(Base other_) { 2111 if (!super.equalsShallow(other_)) 2112 return false; 2113 if (!(other_ instanceof ResearchStudyObjectiveComponent)) 2114 return false; 2115 ResearchStudyObjectiveComponent o = (ResearchStudyObjectiveComponent) other_; 2116 return compareValues(name, o.name, true) && compareValues(description, o.description, true); 2117 } 2118 2119 public boolean isEmpty() { 2120 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, type, description 2121 ); 2122 } 2123 2124 public String fhirType() { 2125 return "ResearchStudy.objective"; 2126 2127 } 2128 2129 } 2130 2131 @Block() 2132 public static class ResearchStudyOutcomeMeasureComponent extends BackboneElement implements IBaseBackboneElement { 2133 /** 2134 * Label for the outcome. 2135 */ 2136 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2137 @Description(shortDefinition="Label for the outcome", formalDefinition="Label for the outcome." ) 2138 protected StringType name; 2139 2140 /** 2141 * The parameter or characteristic being assessed as one of the values by which the study is assessed. 2142 */ 2143 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2144 @Description(shortDefinition="primary | secondary | exploratory", formalDefinition="The parameter or characteristic being assessed as one of the values by which the study is assessed." ) 2145 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-objective-type") 2146 protected List<CodeableConcept> type; 2147 2148 /** 2149 * Description of the outcome. 2150 */ 2151 @Child(name = "description", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2152 @Description(shortDefinition="Description of the outcome", formalDefinition="Description of the outcome." ) 2153 protected MarkdownType description; 2154 2155 /** 2156 * Structured outcome definition. 2157 */ 2158 @Child(name = "reference", type = {EvidenceVariable.class}, order=4, min=0, max=1, modifier=false, summary=false) 2159 @Description(shortDefinition="Structured outcome definition", formalDefinition="Structured outcome definition." ) 2160 protected Reference reference; 2161 2162 private static final long serialVersionUID = 1786559672L; 2163 2164 /** 2165 * Constructor 2166 */ 2167 public ResearchStudyOutcomeMeasureComponent() { 2168 super(); 2169 } 2170 2171 /** 2172 * @return {@link #name} (Label for the outcome.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2173 */ 2174 public StringType getNameElement() { 2175 if (this.name == null) 2176 if (Configuration.errorOnAutoCreate()) 2177 throw new Error("Attempt to auto-create ResearchStudyOutcomeMeasureComponent.name"); 2178 else if (Configuration.doAutoCreate()) 2179 this.name = new StringType(); // bb 2180 return this.name; 2181 } 2182 2183 public boolean hasNameElement() { 2184 return this.name != null && !this.name.isEmpty(); 2185 } 2186 2187 public boolean hasName() { 2188 return this.name != null && !this.name.isEmpty(); 2189 } 2190 2191 /** 2192 * @param value {@link #name} (Label for the outcome.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2193 */ 2194 public ResearchStudyOutcomeMeasureComponent setNameElement(StringType value) { 2195 this.name = value; 2196 return this; 2197 } 2198 2199 /** 2200 * @return Label for the outcome. 2201 */ 2202 public String getName() { 2203 return this.name == null ? null : this.name.getValue(); 2204 } 2205 2206 /** 2207 * @param value Label for the outcome. 2208 */ 2209 public ResearchStudyOutcomeMeasureComponent setName(String value) { 2210 if (Utilities.noString(value)) 2211 this.name = null; 2212 else { 2213 if (this.name == null) 2214 this.name = new StringType(); 2215 this.name.setValue(value); 2216 } 2217 return this; 2218 } 2219 2220 /** 2221 * @return {@link #type} (The parameter or characteristic being assessed as one of the values by which the study is assessed.) 2222 */ 2223 public List<CodeableConcept> getType() { 2224 if (this.type == null) 2225 this.type = new ArrayList<CodeableConcept>(); 2226 return this.type; 2227 } 2228 2229 /** 2230 * @return Returns a reference to <code>this</code> for easy method chaining 2231 */ 2232 public ResearchStudyOutcomeMeasureComponent setType(List<CodeableConcept> theType) { 2233 this.type = theType; 2234 return this; 2235 } 2236 2237 public boolean hasType() { 2238 if (this.type == null) 2239 return false; 2240 for (CodeableConcept item : this.type) 2241 if (!item.isEmpty()) 2242 return true; 2243 return false; 2244 } 2245 2246 public CodeableConcept addType() { //3 2247 CodeableConcept t = new CodeableConcept(); 2248 if (this.type == null) 2249 this.type = new ArrayList<CodeableConcept>(); 2250 this.type.add(t); 2251 return t; 2252 } 2253 2254 public ResearchStudyOutcomeMeasureComponent addType(CodeableConcept t) { //3 2255 if (t == null) 2256 return this; 2257 if (this.type == null) 2258 this.type = new ArrayList<CodeableConcept>(); 2259 this.type.add(t); 2260 return this; 2261 } 2262 2263 /** 2264 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 2265 */ 2266 public CodeableConcept getTypeFirstRep() { 2267 if (getType().isEmpty()) { 2268 addType(); 2269 } 2270 return getType().get(0); 2271 } 2272 2273 /** 2274 * @return {@link #description} (Description of the outcome.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2275 */ 2276 public MarkdownType getDescriptionElement() { 2277 if (this.description == null) 2278 if (Configuration.errorOnAutoCreate()) 2279 throw new Error("Attempt to auto-create ResearchStudyOutcomeMeasureComponent.description"); 2280 else if (Configuration.doAutoCreate()) 2281 this.description = new MarkdownType(); // bb 2282 return this.description; 2283 } 2284 2285 public boolean hasDescriptionElement() { 2286 return this.description != null && !this.description.isEmpty(); 2287 } 2288 2289 public boolean hasDescription() { 2290 return this.description != null && !this.description.isEmpty(); 2291 } 2292 2293 /** 2294 * @param value {@link #description} (Description of the outcome.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2295 */ 2296 public ResearchStudyOutcomeMeasureComponent setDescriptionElement(MarkdownType value) { 2297 this.description = value; 2298 return this; 2299 } 2300 2301 /** 2302 * @return Description of the outcome. 2303 */ 2304 public String getDescription() { 2305 return this.description == null ? null : this.description.getValue(); 2306 } 2307 2308 /** 2309 * @param value Description of the outcome. 2310 */ 2311 public ResearchStudyOutcomeMeasureComponent setDescription(String value) { 2312 if (Utilities.noString(value)) 2313 this.description = null; 2314 else { 2315 if (this.description == null) 2316 this.description = new MarkdownType(); 2317 this.description.setValue(value); 2318 } 2319 return this; 2320 } 2321 2322 /** 2323 * @return {@link #reference} (Structured outcome definition.) 2324 */ 2325 public Reference getReference() { 2326 if (this.reference == null) 2327 if (Configuration.errorOnAutoCreate()) 2328 throw new Error("Attempt to auto-create ResearchStudyOutcomeMeasureComponent.reference"); 2329 else if (Configuration.doAutoCreate()) 2330 this.reference = new Reference(); // cc 2331 return this.reference; 2332 } 2333 2334 public boolean hasReference() { 2335 return this.reference != null && !this.reference.isEmpty(); 2336 } 2337 2338 /** 2339 * @param value {@link #reference} (Structured outcome definition.) 2340 */ 2341 public ResearchStudyOutcomeMeasureComponent setReference(Reference value) { 2342 this.reference = value; 2343 return this; 2344 } 2345 2346 protected void listChildren(List<Property> children) { 2347 super.listChildren(children); 2348 children.add(new Property("name", "string", "Label for the outcome.", 0, 1, name)); 2349 children.add(new Property("type", "CodeableConcept", "The parameter or characteristic being assessed as one of the values by which the study is assessed.", 0, java.lang.Integer.MAX_VALUE, type)); 2350 children.add(new Property("description", "markdown", "Description of the outcome.", 0, 1, description)); 2351 children.add(new Property("reference", "Reference(EvidenceVariable)", "Structured outcome definition.", 0, 1, reference)); 2352 } 2353 2354 @Override 2355 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2356 switch (_hash) { 2357 case 3373707: /*name*/ return new Property("name", "string", "Label for the outcome.", 0, 1, name); 2358 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The parameter or characteristic being assessed as one of the values by which the study is assessed.", 0, java.lang.Integer.MAX_VALUE, type); 2359 case -1724546052: /*description*/ return new Property("description", "markdown", "Description of the outcome.", 0, 1, description); 2360 case -925155509: /*reference*/ return new Property("reference", "Reference(EvidenceVariable)", "Structured outcome definition.", 0, 1, reference); 2361 default: return super.getNamedProperty(_hash, _name, _checkValid); 2362 } 2363 2364 } 2365 2366 @Override 2367 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2368 switch (hash) { 2369 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2370 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 2371 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2372 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 2373 default: return super.getProperty(hash, name, checkValid); 2374 } 2375 2376 } 2377 2378 @Override 2379 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2380 switch (hash) { 2381 case 3373707: // name 2382 this.name = TypeConvertor.castToString(value); // StringType 2383 return value; 2384 case 3575610: // type 2385 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2386 return value; 2387 case -1724546052: // description 2388 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2389 return value; 2390 case -925155509: // reference 2391 this.reference = TypeConvertor.castToReference(value); // Reference 2392 return value; 2393 default: return super.setProperty(hash, name, value); 2394 } 2395 2396 } 2397 2398 @Override 2399 public Base setProperty(String name, Base value) throws FHIRException { 2400 if (name.equals("name")) { 2401 this.name = TypeConvertor.castToString(value); // StringType 2402 } else if (name.equals("type")) { 2403 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 2404 } else if (name.equals("description")) { 2405 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2406 } else if (name.equals("reference")) { 2407 this.reference = TypeConvertor.castToReference(value); // Reference 2408 } else 2409 return super.setProperty(name, value); 2410 return value; 2411 } 2412 2413 @Override 2414 public Base makeProperty(int hash, String name) throws FHIRException { 2415 switch (hash) { 2416 case 3373707: return getNameElement(); 2417 case 3575610: return addType(); 2418 case -1724546052: return getDescriptionElement(); 2419 case -925155509: return getReference(); 2420 default: return super.makeProperty(hash, name); 2421 } 2422 2423 } 2424 2425 @Override 2426 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2427 switch (hash) { 2428 case 3373707: /*name*/ return new String[] {"string"}; 2429 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2430 case -1724546052: /*description*/ return new String[] {"markdown"}; 2431 case -925155509: /*reference*/ return new String[] {"Reference"}; 2432 default: return super.getTypesForProperty(hash, name); 2433 } 2434 2435 } 2436 2437 @Override 2438 public Base addChild(String name) throws FHIRException { 2439 if (name.equals("name")) { 2440 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.outcomeMeasure.name"); 2441 } 2442 else if (name.equals("type")) { 2443 return addType(); 2444 } 2445 else if (name.equals("description")) { 2446 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.outcomeMeasure.description"); 2447 } 2448 else if (name.equals("reference")) { 2449 this.reference = new Reference(); 2450 return this.reference; 2451 } 2452 else 2453 return super.addChild(name); 2454 } 2455 2456 public ResearchStudyOutcomeMeasureComponent copy() { 2457 ResearchStudyOutcomeMeasureComponent dst = new ResearchStudyOutcomeMeasureComponent(); 2458 copyValues(dst); 2459 return dst; 2460 } 2461 2462 public void copyValues(ResearchStudyOutcomeMeasureComponent dst) { 2463 super.copyValues(dst); 2464 dst.name = name == null ? null : name.copy(); 2465 if (type != null) { 2466 dst.type = new ArrayList<CodeableConcept>(); 2467 for (CodeableConcept i : type) 2468 dst.type.add(i.copy()); 2469 }; 2470 dst.description = description == null ? null : description.copy(); 2471 dst.reference = reference == null ? null : reference.copy(); 2472 } 2473 2474 @Override 2475 public boolean equalsDeep(Base other_) { 2476 if (!super.equalsDeep(other_)) 2477 return false; 2478 if (!(other_ instanceof ResearchStudyOutcomeMeasureComponent)) 2479 return false; 2480 ResearchStudyOutcomeMeasureComponent o = (ResearchStudyOutcomeMeasureComponent) other_; 2481 return compareDeep(name, o.name, true) && compareDeep(type, o.type, true) && compareDeep(description, o.description, true) 2482 && compareDeep(reference, o.reference, true); 2483 } 2484 2485 @Override 2486 public boolean equalsShallow(Base other_) { 2487 if (!super.equalsShallow(other_)) 2488 return false; 2489 if (!(other_ instanceof ResearchStudyOutcomeMeasureComponent)) 2490 return false; 2491 ResearchStudyOutcomeMeasureComponent o = (ResearchStudyOutcomeMeasureComponent) other_; 2492 return compareValues(name, o.name, true) && compareValues(description, o.description, true); 2493 } 2494 2495 public boolean isEmpty() { 2496 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, type, description 2497 , reference); 2498 } 2499 2500 public String fhirType() { 2501 return "ResearchStudy.outcomeMeasure"; 2502 2503 } 2504 2505 } 2506 2507 /** 2508 * Canonical identifier for this study resource, represented as a globally unique URI. 2509 */ 2510 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=false) 2511 @Description(shortDefinition="Canonical identifier for this study resource", formalDefinition="Canonical identifier for this study resource, represented as a globally unique URI." ) 2512 protected UriType url; 2513 2514 /** 2515 * Identifiers assigned to this research study by the sponsor or other systems. 2516 */ 2517 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2518 @Description(shortDefinition="Business Identifier for study", formalDefinition="Identifiers assigned to this research study by the sponsor or other systems." ) 2519 protected List<Identifier> identifier; 2520 2521 /** 2522 * The business version for the study record. 2523 */ 2524 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2525 @Description(shortDefinition="The business version for the study record", formalDefinition="The business version for the study record." ) 2526 protected StringType version; 2527 2528 /** 2529 * Name for this study (computer friendly). 2530 */ 2531 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2532 @Description(shortDefinition="Name for this study (computer friendly)", formalDefinition="Name for this study (computer friendly)." ) 2533 protected StringType name; 2534 2535 /** 2536 * The human readable name of the research study. 2537 */ 2538 @Child(name = "title", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 2539 @Description(shortDefinition="Human readable name of the study", formalDefinition="The human readable name of the research study." ) 2540 protected StringType title; 2541 2542 /** 2543 * Additional names for the study. 2544 */ 2545 @Child(name = "label", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2546 @Description(shortDefinition="Additional names for the study", formalDefinition="Additional names for the study." ) 2547 protected List<ResearchStudyLabelComponent> label; 2548 2549 /** 2550 * The set of steps expected to be performed as part of the execution of the study. 2551 */ 2552 @Child(name = "protocol", type = {PlanDefinition.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2553 @Description(shortDefinition="Steps followed in executing study", formalDefinition="The set of steps expected to be performed as part of the execution of the study." ) 2554 protected List<Reference> protocol; 2555 2556 /** 2557 * A larger research study of which this particular study is a component or step. 2558 */ 2559 @Child(name = "partOf", type = {ResearchStudy.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2560 @Description(shortDefinition="Part of larger study", formalDefinition="A larger research study of which this particular study is a component or step." ) 2561 protected List<Reference> partOf; 2562 2563 /** 2564 * Citations, references, URLs and other related documents. When using relatedArtifact to share URLs, the relatedArtifact.type will often be set to one of "documentation" or "supported-with" and the URL value will often be in relatedArtifact.document.url but another possible location is relatedArtifact.resource when it is a canonical URL. 2565 */ 2566 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2567 @Description(shortDefinition="References, URLs, and attachments", formalDefinition="Citations, references, URLs and other related documents. When using relatedArtifact to share URLs, the relatedArtifact.type will often be set to one of \"documentation\" or \"supported-with\" and the URL value will often be in relatedArtifact.document.url but another possible location is relatedArtifact.resource when it is a canonical URL." ) 2568 protected List<RelatedArtifact> relatedArtifact; 2569 2570 /** 2571 * The date (and optionally time) when the ResearchStudy Resource was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ResearchStudy Resource changes. 2572 */ 2573 @Child(name = "date", type = {DateTimeType.class}, order=9, min=0, max=1, modifier=false, summary=false) 2574 @Description(shortDefinition="Date the resource last changed", formalDefinition="The date (and optionally time) when the ResearchStudy Resource was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ResearchStudy Resource changes." ) 2575 protected DateTimeType date; 2576 2577 /** 2578 * The publication state of the resource (not of the study). 2579 */ 2580 @Child(name = "status", type = {CodeType.class}, order=10, min=1, max=1, modifier=true, summary=true) 2581 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The publication state of the resource (not of the study)." ) 2582 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 2583 protected Enumeration<PublicationStatus> status; 2584 2585 /** 2586 * The type of study based upon the intent of the study activities. A classification of the intent of the study. 2587 */ 2588 @Child(name = "primaryPurposeType", type = {CodeableConcept.class}, order=11, min=0, max=1, modifier=false, summary=true) 2589 @Description(shortDefinition="treatment | prevention | diagnostic | supportive-care | screening | health-services-research | basic-science | device-feasibility", formalDefinition="The type of study based upon the intent of the study activities. A classification of the intent of the study." ) 2590 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-prim-purp-type") 2591 protected CodeableConcept primaryPurposeType; 2592 2593 /** 2594 * The stage in the progression of a therapy from initial experimental use in humans in clinical trials to post-market evaluation. 2595 */ 2596 @Child(name = "phase", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=false, summary=true) 2597 @Description(shortDefinition="n-a | early-phase-1 | phase-1 | phase-1-phase-2 | phase-2 | phase-2-phase-3 | phase-3 | phase-4", formalDefinition="The stage in the progression of a therapy from initial experimental use in humans in clinical trials to post-market evaluation." ) 2598 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-phase") 2599 protected CodeableConcept phase; 2600 2601 /** 2602 * Codes categorizing the type of study such as investigational vs. observational, type of blinding, type of randomization, safety vs. efficacy, etc. 2603 */ 2604 @Child(name = "studyDesign", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2605 @Description(shortDefinition="Classifications of the study design characteristics", formalDefinition="Codes categorizing the type of study such as investigational vs. observational, type of blinding, type of randomization, safety vs. efficacy, etc." ) 2606 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/study-design") 2607 protected List<CodeableConcept> studyDesign; 2608 2609 /** 2610 * The medication(s), food(s), therapy(ies), device(s) or other concerns or interventions that the study is seeking to gain more information about. 2611 */ 2612 @Child(name = "focus", type = {CodeableReference.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2613 @Description(shortDefinition="Drugs, devices, etc. under study", formalDefinition="The medication(s), food(s), therapy(ies), device(s) or other concerns or interventions that the study is seeking to gain more information about." ) 2614 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-focus-type") 2615 protected List<CodeableReference> focus; 2616 2617 /** 2618 * The condition that is the focus of the study. For example, In a study to examine risk factors for Lupus, might have as an inclusion criterion "healthy volunteer", but the target condition code would be a Lupus SNOMED code. 2619 */ 2620 @Child(name = "condition", type = {CodeableConcept.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2621 @Description(shortDefinition="Condition being studied", formalDefinition="The condition that is the focus of the study. For example, In a study to examine risk factors for Lupus, might have as an inclusion criterion \"healthy volunteer\", but the target condition code would be a Lupus SNOMED code." ) 2622 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 2623 protected List<CodeableConcept> condition; 2624 2625 /** 2626 * Key terms to aid in searching for or filtering the study. 2627 */ 2628 @Child(name = "keyword", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2629 @Description(shortDefinition="Used to search for the study", formalDefinition="Key terms to aid in searching for or filtering the study." ) 2630 protected List<CodeableConcept> keyword; 2631 2632 /** 2633 * A country, state or other area where the study is taking place rather than its precise geographic location or address. 2634 */ 2635 @Child(name = "region", type = {CodeableConcept.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2636 @Description(shortDefinition="Geographic area for the study", formalDefinition="A country, state or other area where the study is taking place rather than its precise geographic location or address." ) 2637 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 2638 protected List<CodeableConcept> region; 2639 2640 /** 2641 * A brief text for explaining the study. 2642 */ 2643 @Child(name = "descriptionSummary", type = {MarkdownType.class}, order=18, min=0, max=1, modifier=false, summary=false) 2644 @Description(shortDefinition="Brief text explaining the study", formalDefinition="A brief text for explaining the study." ) 2645 protected MarkdownType descriptionSummary; 2646 2647 /** 2648 * A detailed and human-readable narrative of the study. E.g., study abstract. 2649 */ 2650 @Child(name = "description", type = {MarkdownType.class}, order=19, min=0, max=1, modifier=false, summary=false) 2651 @Description(shortDefinition="Detailed narrative of the study", formalDefinition="A detailed and human-readable narrative of the study. E.g., study abstract." ) 2652 protected MarkdownType description; 2653 2654 /** 2655 * Identifies the start date and the expected (or actual, depending on status) end date for the study. 2656 */ 2657 @Child(name = "period", type = {Period.class}, order=20, min=0, max=1, modifier=false, summary=true) 2658 @Description(shortDefinition="When the study began and ended", formalDefinition="Identifies the start date and the expected (or actual, depending on status) end date for the study." ) 2659 protected Period period; 2660 2661 /** 2662 * A facility in which study activities are conducted. 2663 */ 2664 @Child(name = "site", type = {Location.class, ResearchStudy.class, Organization.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2665 @Description(shortDefinition="Facility where study activities are conducted", formalDefinition="A facility in which study activities are conducted." ) 2666 protected List<Reference> site; 2667 2668 /** 2669 * Comments made about the study by the performer, subject or other participants. 2670 */ 2671 @Child(name = "note", type = {Annotation.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2672 @Description(shortDefinition="Comments made about the study", formalDefinition="Comments made about the study by the performer, subject or other participants." ) 2673 protected List<Annotation> note; 2674 2675 /** 2676 * Additional grouping mechanism or categorization of a research study. Example: FDA regulated device, FDA regulated drug, MPG Paragraph 23b (a German legal requirement), IRB-exempt, etc. Implementation Note: do not use the classifier element to support existing semantics that are already supported thru explicit elements in the resource. 2677 */ 2678 @Child(name = "classifier", type = {CodeableConcept.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2679 @Description(shortDefinition="Classification for the study", formalDefinition="Additional grouping mechanism or categorization of a research study. Example: FDA regulated device, FDA regulated drug, MPG Paragraph 23b (a German legal requirement), IRB-exempt, etc. Implementation Note: do not use the classifier element to support existing semantics that are already supported thru explicit elements in the resource." ) 2680 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-classifiers") 2681 protected List<CodeableConcept> classifier; 2682 2683 /** 2684 * Sponsors, collaborators, and other parties. 2685 */ 2686 @Child(name = "associatedParty", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2687 @Description(shortDefinition="Sponsors, collaborators, and other parties", formalDefinition="Sponsors, collaborators, and other parties." ) 2688 protected List<ResearchStudyAssociatedPartyComponent> associatedParty; 2689 2690 /** 2691 * Status of study with time for that status. 2692 */ 2693 @Child(name = "progressStatus", type = {}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2694 @Description(shortDefinition="Status of study with time for that status", formalDefinition="Status of study with time for that status." ) 2695 protected List<ResearchStudyProgressStatusComponent> progressStatus; 2696 2697 /** 2698 * A description and/or code explaining the premature termination of the study. 2699 */ 2700 @Child(name = "whyStopped", type = {CodeableConcept.class}, order=26, min=0, max=1, modifier=false, summary=true) 2701 @Description(shortDefinition="accrual-goal-met | closed-due-to-toxicity | closed-due-to-lack-of-study-progress | temporarily-closed-per-study-design", formalDefinition="A description and/or code explaining the premature termination of the study." ) 2702 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-reason-stopped") 2703 protected CodeableConcept whyStopped; 2704 2705 /** 2706 * Target or actual group of participants enrolled in study. 2707 */ 2708 @Child(name = "recruitment", type = {}, order=27, min=0, max=1, modifier=false, summary=true) 2709 @Description(shortDefinition="Target or actual group of participants enrolled in study", formalDefinition="Target or actual group of participants enrolled in study." ) 2710 protected ResearchStudyRecruitmentComponent recruitment; 2711 2712 /** 2713 * Describes an expected event or sequence of events for one of the subjects of a study. E.g. for a living subject: exposure to drug A, wash-out, exposure to drug B, wash-out, follow-up. E.g. for a stability study: {store sample from lot A at 25 degrees for 1 month}, {store sample from lot A at 40 degrees for 1 month}. 2714 */ 2715 @Child(name = "comparisonGroup", type = {}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2716 @Description(shortDefinition="Defined path through the study for a subject", formalDefinition="Describes an expected event or sequence of events for one of the subjects of a study. E.g. for a living subject: exposure to drug A, wash-out, exposure to drug B, wash-out, follow-up. E.g. for a stability study: {store sample from lot A at 25 degrees for 1 month}, {store sample from lot A at 40 degrees for 1 month}." ) 2717 protected List<ResearchStudyComparisonGroupComponent> comparisonGroup; 2718 2719 /** 2720 * A goal that the study is aiming to achieve in terms of a scientific question to be answered by the analysis of data collected during the study. 2721 */ 2722 @Child(name = "objective", type = {}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2723 @Description(shortDefinition="A goal for the study", formalDefinition="A goal that the study is aiming to achieve in terms of a scientific question to be answered by the analysis of data collected during the study." ) 2724 protected List<ResearchStudyObjectiveComponent> objective; 2725 2726 /** 2727 * An "outcome measure", "endpoint", "effect measure" or "measure of effect" is a specific measurement or observation used to quantify the effect of experimental variables on the participants in a study, or for observational studies, to describe patterns of diseases or traits or associations with exposures, risk factors or treatment. 2728 */ 2729 @Child(name = "outcomeMeasure", type = {}, order=30, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2730 @Description(shortDefinition="A variable measured during the study", formalDefinition="An \"outcome measure\", \"endpoint\", \"effect measure\" or \"measure of effect\" is a specific measurement or observation used to quantify the effect of experimental variables on the participants in a study, or for observational studies, to describe patterns of diseases or traits or associations with exposures, risk factors or treatment." ) 2731 protected List<ResearchStudyOutcomeMeasureComponent> outcomeMeasure; 2732 2733 /** 2734 * Link to one or more sets of results generated by the study. Could also link to a research registry holding the results such as ClinicalTrials.gov. 2735 */ 2736 @Child(name = "result", type = {EvidenceReport.class, Citation.class, DiagnosticReport.class}, order=31, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2737 @Description(shortDefinition="Link to results generated during the study", formalDefinition="Link to one or more sets of results generated by the study. Could also link to a research registry holding the results such as ClinicalTrials.gov." ) 2738 protected List<Reference> result; 2739 2740 private static final long serialVersionUID = -1217395129L; 2741 2742 /** 2743 * Constructor 2744 */ 2745 public ResearchStudy() { 2746 super(); 2747 } 2748 2749 /** 2750 * Constructor 2751 */ 2752 public ResearchStudy(PublicationStatus status) { 2753 super(); 2754 this.setStatus(status); 2755 } 2756 2757 /** 2758 * @return {@link #url} (Canonical identifier for this study resource, represented as a globally unique URI.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2759 */ 2760 public UriType getUrlElement() { 2761 if (this.url == null) 2762 if (Configuration.errorOnAutoCreate()) 2763 throw new Error("Attempt to auto-create ResearchStudy.url"); 2764 else if (Configuration.doAutoCreate()) 2765 this.url = new UriType(); // bb 2766 return this.url; 2767 } 2768 2769 public boolean hasUrlElement() { 2770 return this.url != null && !this.url.isEmpty(); 2771 } 2772 2773 public boolean hasUrl() { 2774 return this.url != null && !this.url.isEmpty(); 2775 } 2776 2777 /** 2778 * @param value {@link #url} (Canonical identifier for this study resource, represented as a globally unique URI.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2779 */ 2780 public ResearchStudy setUrlElement(UriType value) { 2781 this.url = value; 2782 return this; 2783 } 2784 2785 /** 2786 * @return Canonical identifier for this study resource, represented as a globally unique URI. 2787 */ 2788 public String getUrl() { 2789 return this.url == null ? null : this.url.getValue(); 2790 } 2791 2792 /** 2793 * @param value Canonical identifier for this study resource, represented as a globally unique URI. 2794 */ 2795 public ResearchStudy setUrl(String value) { 2796 if (Utilities.noString(value)) 2797 this.url = null; 2798 else { 2799 if (this.url == null) 2800 this.url = new UriType(); 2801 this.url.setValue(value); 2802 } 2803 return this; 2804 } 2805 2806 /** 2807 * @return {@link #identifier} (Identifiers assigned to this research study by the sponsor or other systems.) 2808 */ 2809 public List<Identifier> getIdentifier() { 2810 if (this.identifier == null) 2811 this.identifier = new ArrayList<Identifier>(); 2812 return this.identifier; 2813 } 2814 2815 /** 2816 * @return Returns a reference to <code>this</code> for easy method chaining 2817 */ 2818 public ResearchStudy setIdentifier(List<Identifier> theIdentifier) { 2819 this.identifier = theIdentifier; 2820 return this; 2821 } 2822 2823 public boolean hasIdentifier() { 2824 if (this.identifier == null) 2825 return false; 2826 for (Identifier item : this.identifier) 2827 if (!item.isEmpty()) 2828 return true; 2829 return false; 2830 } 2831 2832 public Identifier addIdentifier() { //3 2833 Identifier t = new Identifier(); 2834 if (this.identifier == null) 2835 this.identifier = new ArrayList<Identifier>(); 2836 this.identifier.add(t); 2837 return t; 2838 } 2839 2840 public ResearchStudy addIdentifier(Identifier t) { //3 2841 if (t == null) 2842 return this; 2843 if (this.identifier == null) 2844 this.identifier = new ArrayList<Identifier>(); 2845 this.identifier.add(t); 2846 return this; 2847 } 2848 2849 /** 2850 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2851 */ 2852 public Identifier getIdentifierFirstRep() { 2853 if (getIdentifier().isEmpty()) { 2854 addIdentifier(); 2855 } 2856 return getIdentifier().get(0); 2857 } 2858 2859 /** 2860 * @return {@link #version} (The business version for the study record.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2861 */ 2862 public StringType getVersionElement() { 2863 if (this.version == null) 2864 if (Configuration.errorOnAutoCreate()) 2865 throw new Error("Attempt to auto-create ResearchStudy.version"); 2866 else if (Configuration.doAutoCreate()) 2867 this.version = new StringType(); // bb 2868 return this.version; 2869 } 2870 2871 public boolean hasVersionElement() { 2872 return this.version != null && !this.version.isEmpty(); 2873 } 2874 2875 public boolean hasVersion() { 2876 return this.version != null && !this.version.isEmpty(); 2877 } 2878 2879 /** 2880 * @param value {@link #version} (The business version for the study record.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2881 */ 2882 public ResearchStudy setVersionElement(StringType value) { 2883 this.version = value; 2884 return this; 2885 } 2886 2887 /** 2888 * @return The business version for the study record. 2889 */ 2890 public String getVersion() { 2891 return this.version == null ? null : this.version.getValue(); 2892 } 2893 2894 /** 2895 * @param value The business version for the study record. 2896 */ 2897 public ResearchStudy setVersion(String value) { 2898 if (Utilities.noString(value)) 2899 this.version = null; 2900 else { 2901 if (this.version == null) 2902 this.version = new StringType(); 2903 this.version.setValue(value); 2904 } 2905 return this; 2906 } 2907 2908 /** 2909 * @return {@link #name} (Name for this study (computer friendly).). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2910 */ 2911 public StringType getNameElement() { 2912 if (this.name == null) 2913 if (Configuration.errorOnAutoCreate()) 2914 throw new Error("Attempt to auto-create ResearchStudy.name"); 2915 else if (Configuration.doAutoCreate()) 2916 this.name = new StringType(); // bb 2917 return this.name; 2918 } 2919 2920 public boolean hasNameElement() { 2921 return this.name != null && !this.name.isEmpty(); 2922 } 2923 2924 public boolean hasName() { 2925 return this.name != null && !this.name.isEmpty(); 2926 } 2927 2928 /** 2929 * @param value {@link #name} (Name for this study (computer friendly).). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2930 */ 2931 public ResearchStudy setNameElement(StringType value) { 2932 this.name = value; 2933 return this; 2934 } 2935 2936 /** 2937 * @return Name for this study (computer friendly). 2938 */ 2939 public String getName() { 2940 return this.name == null ? null : this.name.getValue(); 2941 } 2942 2943 /** 2944 * @param value Name for this study (computer friendly). 2945 */ 2946 public ResearchStudy setName(String value) { 2947 if (Utilities.noString(value)) 2948 this.name = null; 2949 else { 2950 if (this.name == null) 2951 this.name = new StringType(); 2952 this.name.setValue(value); 2953 } 2954 return this; 2955 } 2956 2957 /** 2958 * @return {@link #title} (The human readable name of the research study.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2959 */ 2960 public StringType getTitleElement() { 2961 if (this.title == null) 2962 if (Configuration.errorOnAutoCreate()) 2963 throw new Error("Attempt to auto-create ResearchStudy.title"); 2964 else if (Configuration.doAutoCreate()) 2965 this.title = new StringType(); // bb 2966 return this.title; 2967 } 2968 2969 public boolean hasTitleElement() { 2970 return this.title != null && !this.title.isEmpty(); 2971 } 2972 2973 public boolean hasTitle() { 2974 return this.title != null && !this.title.isEmpty(); 2975 } 2976 2977 /** 2978 * @param value {@link #title} (The human readable name of the research study.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2979 */ 2980 public ResearchStudy setTitleElement(StringType value) { 2981 this.title = value; 2982 return this; 2983 } 2984 2985 /** 2986 * @return The human readable name of the research study. 2987 */ 2988 public String getTitle() { 2989 return this.title == null ? null : this.title.getValue(); 2990 } 2991 2992 /** 2993 * @param value The human readable name of the research study. 2994 */ 2995 public ResearchStudy setTitle(String value) { 2996 if (Utilities.noString(value)) 2997 this.title = null; 2998 else { 2999 if (this.title == null) 3000 this.title = new StringType(); 3001 this.title.setValue(value); 3002 } 3003 return this; 3004 } 3005 3006 /** 3007 * @return {@link #label} (Additional names for the study.) 3008 */ 3009 public List<ResearchStudyLabelComponent> getLabel() { 3010 if (this.label == null) 3011 this.label = new ArrayList<ResearchStudyLabelComponent>(); 3012 return this.label; 3013 } 3014 3015 /** 3016 * @return Returns a reference to <code>this</code> for easy method chaining 3017 */ 3018 public ResearchStudy setLabel(List<ResearchStudyLabelComponent> theLabel) { 3019 this.label = theLabel; 3020 return this; 3021 } 3022 3023 public boolean hasLabel() { 3024 if (this.label == null) 3025 return false; 3026 for (ResearchStudyLabelComponent item : this.label) 3027 if (!item.isEmpty()) 3028 return true; 3029 return false; 3030 } 3031 3032 public ResearchStudyLabelComponent addLabel() { //3 3033 ResearchStudyLabelComponent t = new ResearchStudyLabelComponent(); 3034 if (this.label == null) 3035 this.label = new ArrayList<ResearchStudyLabelComponent>(); 3036 this.label.add(t); 3037 return t; 3038 } 3039 3040 public ResearchStudy addLabel(ResearchStudyLabelComponent t) { //3 3041 if (t == null) 3042 return this; 3043 if (this.label == null) 3044 this.label = new ArrayList<ResearchStudyLabelComponent>(); 3045 this.label.add(t); 3046 return this; 3047 } 3048 3049 /** 3050 * @return The first repetition of repeating field {@link #label}, creating it if it does not already exist {3} 3051 */ 3052 public ResearchStudyLabelComponent getLabelFirstRep() { 3053 if (getLabel().isEmpty()) { 3054 addLabel(); 3055 } 3056 return getLabel().get(0); 3057 } 3058 3059 /** 3060 * @return {@link #protocol} (The set of steps expected to be performed as part of the execution of the study.) 3061 */ 3062 public List<Reference> getProtocol() { 3063 if (this.protocol == null) 3064 this.protocol = new ArrayList<Reference>(); 3065 return this.protocol; 3066 } 3067 3068 /** 3069 * @return Returns a reference to <code>this</code> for easy method chaining 3070 */ 3071 public ResearchStudy setProtocol(List<Reference> theProtocol) { 3072 this.protocol = theProtocol; 3073 return this; 3074 } 3075 3076 public boolean hasProtocol() { 3077 if (this.protocol == null) 3078 return false; 3079 for (Reference item : this.protocol) 3080 if (!item.isEmpty()) 3081 return true; 3082 return false; 3083 } 3084 3085 public Reference addProtocol() { //3 3086 Reference t = new Reference(); 3087 if (this.protocol == null) 3088 this.protocol = new ArrayList<Reference>(); 3089 this.protocol.add(t); 3090 return t; 3091 } 3092 3093 public ResearchStudy addProtocol(Reference t) { //3 3094 if (t == null) 3095 return this; 3096 if (this.protocol == null) 3097 this.protocol = new ArrayList<Reference>(); 3098 this.protocol.add(t); 3099 return this; 3100 } 3101 3102 /** 3103 * @return The first repetition of repeating field {@link #protocol}, creating it if it does not already exist {3} 3104 */ 3105 public Reference getProtocolFirstRep() { 3106 if (getProtocol().isEmpty()) { 3107 addProtocol(); 3108 } 3109 return getProtocol().get(0); 3110 } 3111 3112 /** 3113 * @return {@link #partOf} (A larger research study of which this particular study is a component or step.) 3114 */ 3115 public List<Reference> getPartOf() { 3116 if (this.partOf == null) 3117 this.partOf = new ArrayList<Reference>(); 3118 return this.partOf; 3119 } 3120 3121 /** 3122 * @return Returns a reference to <code>this</code> for easy method chaining 3123 */ 3124 public ResearchStudy setPartOf(List<Reference> thePartOf) { 3125 this.partOf = thePartOf; 3126 return this; 3127 } 3128 3129 public boolean hasPartOf() { 3130 if (this.partOf == null) 3131 return false; 3132 for (Reference item : this.partOf) 3133 if (!item.isEmpty()) 3134 return true; 3135 return false; 3136 } 3137 3138 public Reference addPartOf() { //3 3139 Reference t = new Reference(); 3140 if (this.partOf == null) 3141 this.partOf = new ArrayList<Reference>(); 3142 this.partOf.add(t); 3143 return t; 3144 } 3145 3146 public ResearchStudy addPartOf(Reference t) { //3 3147 if (t == null) 3148 return this; 3149 if (this.partOf == null) 3150 this.partOf = new ArrayList<Reference>(); 3151 this.partOf.add(t); 3152 return this; 3153 } 3154 3155 /** 3156 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 3157 */ 3158 public Reference getPartOfFirstRep() { 3159 if (getPartOf().isEmpty()) { 3160 addPartOf(); 3161 } 3162 return getPartOf().get(0); 3163 } 3164 3165 /** 3166 * @return {@link #relatedArtifact} (Citations, references, URLs and other related documents. When using relatedArtifact to share URLs, the relatedArtifact.type will often be set to one of "documentation" or "supported-with" and the URL value will often be in relatedArtifact.document.url but another possible location is relatedArtifact.resource when it is a canonical URL.) 3167 */ 3168 public List<RelatedArtifact> getRelatedArtifact() { 3169 if (this.relatedArtifact == null) 3170 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3171 return this.relatedArtifact; 3172 } 3173 3174 /** 3175 * @return Returns a reference to <code>this</code> for easy method chaining 3176 */ 3177 public ResearchStudy setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 3178 this.relatedArtifact = theRelatedArtifact; 3179 return this; 3180 } 3181 3182 public boolean hasRelatedArtifact() { 3183 if (this.relatedArtifact == null) 3184 return false; 3185 for (RelatedArtifact item : this.relatedArtifact) 3186 if (!item.isEmpty()) 3187 return true; 3188 return false; 3189 } 3190 3191 public RelatedArtifact addRelatedArtifact() { //3 3192 RelatedArtifact t = new RelatedArtifact(); 3193 if (this.relatedArtifact == null) 3194 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3195 this.relatedArtifact.add(t); 3196 return t; 3197 } 3198 3199 public ResearchStudy addRelatedArtifact(RelatedArtifact t) { //3 3200 if (t == null) 3201 return this; 3202 if (this.relatedArtifact == null) 3203 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3204 this.relatedArtifact.add(t); 3205 return this; 3206 } 3207 3208 /** 3209 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {3} 3210 */ 3211 public RelatedArtifact getRelatedArtifactFirstRep() { 3212 if (getRelatedArtifact().isEmpty()) { 3213 addRelatedArtifact(); 3214 } 3215 return getRelatedArtifact().get(0); 3216 } 3217 3218 /** 3219 * @return {@link #date} (The date (and optionally time) when the ResearchStudy Resource was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ResearchStudy Resource changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3220 */ 3221 public DateTimeType getDateElement() { 3222 if (this.date == null) 3223 if (Configuration.errorOnAutoCreate()) 3224 throw new Error("Attempt to auto-create ResearchStudy.date"); 3225 else if (Configuration.doAutoCreate()) 3226 this.date = new DateTimeType(); // bb 3227 return this.date; 3228 } 3229 3230 public boolean hasDateElement() { 3231 return this.date != null && !this.date.isEmpty(); 3232 } 3233 3234 public boolean hasDate() { 3235 return this.date != null && !this.date.isEmpty(); 3236 } 3237 3238 /** 3239 * @param value {@link #date} (The date (and optionally time) when the ResearchStudy Resource was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ResearchStudy Resource changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3240 */ 3241 public ResearchStudy setDateElement(DateTimeType value) { 3242 this.date = value; 3243 return this; 3244 } 3245 3246 /** 3247 * @return The date (and optionally time) when the ResearchStudy Resource was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ResearchStudy Resource changes. 3248 */ 3249 public Date getDate() { 3250 return this.date == null ? null : this.date.getValue(); 3251 } 3252 3253 /** 3254 * @param value The date (and optionally time) when the ResearchStudy Resource was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ResearchStudy Resource changes. 3255 */ 3256 public ResearchStudy setDate(Date value) { 3257 if (value == null) 3258 this.date = null; 3259 else { 3260 if (this.date == null) 3261 this.date = new DateTimeType(); 3262 this.date.setValue(value); 3263 } 3264 return this; 3265 } 3266 3267 /** 3268 * @return {@link #status} (The publication state of the resource (not of the study).). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3269 */ 3270 public Enumeration<PublicationStatus> getStatusElement() { 3271 if (this.status == null) 3272 if (Configuration.errorOnAutoCreate()) 3273 throw new Error("Attempt to auto-create ResearchStudy.status"); 3274 else if (Configuration.doAutoCreate()) 3275 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 3276 return this.status; 3277 } 3278 3279 public boolean hasStatusElement() { 3280 return this.status != null && !this.status.isEmpty(); 3281 } 3282 3283 public boolean hasStatus() { 3284 return this.status != null && !this.status.isEmpty(); 3285 } 3286 3287 /** 3288 * @param value {@link #status} (The publication state of the resource (not of the study).). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3289 */ 3290 public ResearchStudy setStatusElement(Enumeration<PublicationStatus> value) { 3291 this.status = value; 3292 return this; 3293 } 3294 3295 /** 3296 * @return The publication state of the resource (not of the study). 3297 */ 3298 public PublicationStatus getStatus() { 3299 return this.status == null ? null : this.status.getValue(); 3300 } 3301 3302 /** 3303 * @param value The publication state of the resource (not of the study). 3304 */ 3305 public ResearchStudy setStatus(PublicationStatus value) { 3306 if (this.status == null) 3307 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 3308 this.status.setValue(value); 3309 return this; 3310 } 3311 3312 /** 3313 * @return {@link #primaryPurposeType} (The type of study based upon the intent of the study activities. A classification of the intent of the study.) 3314 */ 3315 public CodeableConcept getPrimaryPurposeType() { 3316 if (this.primaryPurposeType == null) 3317 if (Configuration.errorOnAutoCreate()) 3318 throw new Error("Attempt to auto-create ResearchStudy.primaryPurposeType"); 3319 else if (Configuration.doAutoCreate()) 3320 this.primaryPurposeType = new CodeableConcept(); // cc 3321 return this.primaryPurposeType; 3322 } 3323 3324 public boolean hasPrimaryPurposeType() { 3325 return this.primaryPurposeType != null && !this.primaryPurposeType.isEmpty(); 3326 } 3327 3328 /** 3329 * @param value {@link #primaryPurposeType} (The type of study based upon the intent of the study activities. A classification of the intent of the study.) 3330 */ 3331 public ResearchStudy setPrimaryPurposeType(CodeableConcept value) { 3332 this.primaryPurposeType = value; 3333 return this; 3334 } 3335 3336 /** 3337 * @return {@link #phase} (The stage in the progression of a therapy from initial experimental use in humans in clinical trials to post-market evaluation.) 3338 */ 3339 public CodeableConcept getPhase() { 3340 if (this.phase == null) 3341 if (Configuration.errorOnAutoCreate()) 3342 throw new Error("Attempt to auto-create ResearchStudy.phase"); 3343 else if (Configuration.doAutoCreate()) 3344 this.phase = new CodeableConcept(); // cc 3345 return this.phase; 3346 } 3347 3348 public boolean hasPhase() { 3349 return this.phase != null && !this.phase.isEmpty(); 3350 } 3351 3352 /** 3353 * @param value {@link #phase} (The stage in the progression of a therapy from initial experimental use in humans in clinical trials to post-market evaluation.) 3354 */ 3355 public ResearchStudy setPhase(CodeableConcept value) { 3356 this.phase = value; 3357 return this; 3358 } 3359 3360 /** 3361 * @return {@link #studyDesign} (Codes categorizing the type of study such as investigational vs. observational, type of blinding, type of randomization, safety vs. efficacy, etc.) 3362 */ 3363 public List<CodeableConcept> getStudyDesign() { 3364 if (this.studyDesign == null) 3365 this.studyDesign = new ArrayList<CodeableConcept>(); 3366 return this.studyDesign; 3367 } 3368 3369 /** 3370 * @return Returns a reference to <code>this</code> for easy method chaining 3371 */ 3372 public ResearchStudy setStudyDesign(List<CodeableConcept> theStudyDesign) { 3373 this.studyDesign = theStudyDesign; 3374 return this; 3375 } 3376 3377 public boolean hasStudyDesign() { 3378 if (this.studyDesign == null) 3379 return false; 3380 for (CodeableConcept item : this.studyDesign) 3381 if (!item.isEmpty()) 3382 return true; 3383 return false; 3384 } 3385 3386 public CodeableConcept addStudyDesign() { //3 3387 CodeableConcept t = new CodeableConcept(); 3388 if (this.studyDesign == null) 3389 this.studyDesign = new ArrayList<CodeableConcept>(); 3390 this.studyDesign.add(t); 3391 return t; 3392 } 3393 3394 public ResearchStudy addStudyDesign(CodeableConcept t) { //3 3395 if (t == null) 3396 return this; 3397 if (this.studyDesign == null) 3398 this.studyDesign = new ArrayList<CodeableConcept>(); 3399 this.studyDesign.add(t); 3400 return this; 3401 } 3402 3403 /** 3404 * @return The first repetition of repeating field {@link #studyDesign}, creating it if it does not already exist {3} 3405 */ 3406 public CodeableConcept getStudyDesignFirstRep() { 3407 if (getStudyDesign().isEmpty()) { 3408 addStudyDesign(); 3409 } 3410 return getStudyDesign().get(0); 3411 } 3412 3413 /** 3414 * @return {@link #focus} (The medication(s), food(s), therapy(ies), device(s) or other concerns or interventions that the study is seeking to gain more information about.) 3415 */ 3416 public List<CodeableReference> getFocus() { 3417 if (this.focus == null) 3418 this.focus = new ArrayList<CodeableReference>(); 3419 return this.focus; 3420 } 3421 3422 /** 3423 * @return Returns a reference to <code>this</code> for easy method chaining 3424 */ 3425 public ResearchStudy setFocus(List<CodeableReference> theFocus) { 3426 this.focus = theFocus; 3427 return this; 3428 } 3429 3430 public boolean hasFocus() { 3431 if (this.focus == null) 3432 return false; 3433 for (CodeableReference item : this.focus) 3434 if (!item.isEmpty()) 3435 return true; 3436 return false; 3437 } 3438 3439 public CodeableReference addFocus() { //3 3440 CodeableReference t = new CodeableReference(); 3441 if (this.focus == null) 3442 this.focus = new ArrayList<CodeableReference>(); 3443 this.focus.add(t); 3444 return t; 3445 } 3446 3447 public ResearchStudy addFocus(CodeableReference t) { //3 3448 if (t == null) 3449 return this; 3450 if (this.focus == null) 3451 this.focus = new ArrayList<CodeableReference>(); 3452 this.focus.add(t); 3453 return this; 3454 } 3455 3456 /** 3457 * @return The first repetition of repeating field {@link #focus}, creating it if it does not already exist {3} 3458 */ 3459 public CodeableReference getFocusFirstRep() { 3460 if (getFocus().isEmpty()) { 3461 addFocus(); 3462 } 3463 return getFocus().get(0); 3464 } 3465 3466 /** 3467 * @return {@link #condition} (The condition that is the focus of the study. For example, In a study to examine risk factors for Lupus, might have as an inclusion criterion "healthy volunteer", but the target condition code would be a Lupus SNOMED code.) 3468 */ 3469 public List<CodeableConcept> getCondition() { 3470 if (this.condition == null) 3471 this.condition = new ArrayList<CodeableConcept>(); 3472 return this.condition; 3473 } 3474 3475 /** 3476 * @return Returns a reference to <code>this</code> for easy method chaining 3477 */ 3478 public ResearchStudy setCondition(List<CodeableConcept> theCondition) { 3479 this.condition = theCondition; 3480 return this; 3481 } 3482 3483 public boolean hasCondition() { 3484 if (this.condition == null) 3485 return false; 3486 for (CodeableConcept item : this.condition) 3487 if (!item.isEmpty()) 3488 return true; 3489 return false; 3490 } 3491 3492 public CodeableConcept addCondition() { //3 3493 CodeableConcept t = new CodeableConcept(); 3494 if (this.condition == null) 3495 this.condition = new ArrayList<CodeableConcept>(); 3496 this.condition.add(t); 3497 return t; 3498 } 3499 3500 public ResearchStudy addCondition(CodeableConcept t) { //3 3501 if (t == null) 3502 return this; 3503 if (this.condition == null) 3504 this.condition = new ArrayList<CodeableConcept>(); 3505 this.condition.add(t); 3506 return this; 3507 } 3508 3509 /** 3510 * @return The first repetition of repeating field {@link #condition}, creating it if it does not already exist {3} 3511 */ 3512 public CodeableConcept getConditionFirstRep() { 3513 if (getCondition().isEmpty()) { 3514 addCondition(); 3515 } 3516 return getCondition().get(0); 3517 } 3518 3519 /** 3520 * @return {@link #keyword} (Key terms to aid in searching for or filtering the study.) 3521 */ 3522 public List<CodeableConcept> getKeyword() { 3523 if (this.keyword == null) 3524 this.keyword = new ArrayList<CodeableConcept>(); 3525 return this.keyword; 3526 } 3527 3528 /** 3529 * @return Returns a reference to <code>this</code> for easy method chaining 3530 */ 3531 public ResearchStudy setKeyword(List<CodeableConcept> theKeyword) { 3532 this.keyword = theKeyword; 3533 return this; 3534 } 3535 3536 public boolean hasKeyword() { 3537 if (this.keyword == null) 3538 return false; 3539 for (CodeableConcept item : this.keyword) 3540 if (!item.isEmpty()) 3541 return true; 3542 return false; 3543 } 3544 3545 public CodeableConcept addKeyword() { //3 3546 CodeableConcept t = new CodeableConcept(); 3547 if (this.keyword == null) 3548 this.keyword = new ArrayList<CodeableConcept>(); 3549 this.keyword.add(t); 3550 return t; 3551 } 3552 3553 public ResearchStudy addKeyword(CodeableConcept t) { //3 3554 if (t == null) 3555 return this; 3556 if (this.keyword == null) 3557 this.keyword = new ArrayList<CodeableConcept>(); 3558 this.keyword.add(t); 3559 return this; 3560 } 3561 3562 /** 3563 * @return The first repetition of repeating field {@link #keyword}, creating it if it does not already exist {3} 3564 */ 3565 public CodeableConcept getKeywordFirstRep() { 3566 if (getKeyword().isEmpty()) { 3567 addKeyword(); 3568 } 3569 return getKeyword().get(0); 3570 } 3571 3572 /** 3573 * @return {@link #region} (A country, state or other area where the study is taking place rather than its precise geographic location or address.) 3574 */ 3575 public List<CodeableConcept> getRegion() { 3576 if (this.region == null) 3577 this.region = new ArrayList<CodeableConcept>(); 3578 return this.region; 3579 } 3580 3581 /** 3582 * @return Returns a reference to <code>this</code> for easy method chaining 3583 */ 3584 public ResearchStudy setRegion(List<CodeableConcept> theRegion) { 3585 this.region = theRegion; 3586 return this; 3587 } 3588 3589 public boolean hasRegion() { 3590 if (this.region == null) 3591 return false; 3592 for (CodeableConcept item : this.region) 3593 if (!item.isEmpty()) 3594 return true; 3595 return false; 3596 } 3597 3598 public CodeableConcept addRegion() { //3 3599 CodeableConcept t = new CodeableConcept(); 3600 if (this.region == null) 3601 this.region = new ArrayList<CodeableConcept>(); 3602 this.region.add(t); 3603 return t; 3604 } 3605 3606 public ResearchStudy addRegion(CodeableConcept t) { //3 3607 if (t == null) 3608 return this; 3609 if (this.region == null) 3610 this.region = new ArrayList<CodeableConcept>(); 3611 this.region.add(t); 3612 return this; 3613 } 3614 3615 /** 3616 * @return The first repetition of repeating field {@link #region}, creating it if it does not already exist {3} 3617 */ 3618 public CodeableConcept getRegionFirstRep() { 3619 if (getRegion().isEmpty()) { 3620 addRegion(); 3621 } 3622 return getRegion().get(0); 3623 } 3624 3625 /** 3626 * @return {@link #descriptionSummary} (A brief text for explaining the study.). This is the underlying object with id, value and extensions. The accessor "getDescriptionSummary" gives direct access to the value 3627 */ 3628 public MarkdownType getDescriptionSummaryElement() { 3629 if (this.descriptionSummary == null) 3630 if (Configuration.errorOnAutoCreate()) 3631 throw new Error("Attempt to auto-create ResearchStudy.descriptionSummary"); 3632 else if (Configuration.doAutoCreate()) 3633 this.descriptionSummary = new MarkdownType(); // bb 3634 return this.descriptionSummary; 3635 } 3636 3637 public boolean hasDescriptionSummaryElement() { 3638 return this.descriptionSummary != null && !this.descriptionSummary.isEmpty(); 3639 } 3640 3641 public boolean hasDescriptionSummary() { 3642 return this.descriptionSummary != null && !this.descriptionSummary.isEmpty(); 3643 } 3644 3645 /** 3646 * @param value {@link #descriptionSummary} (A brief text for explaining the study.). This is the underlying object with id, value and extensions. The accessor "getDescriptionSummary" gives direct access to the value 3647 */ 3648 public ResearchStudy setDescriptionSummaryElement(MarkdownType value) { 3649 this.descriptionSummary = value; 3650 return this; 3651 } 3652 3653 /** 3654 * @return A brief text for explaining the study. 3655 */ 3656 public String getDescriptionSummary() { 3657 return this.descriptionSummary == null ? null : this.descriptionSummary.getValue(); 3658 } 3659 3660 /** 3661 * @param value A brief text for explaining the study. 3662 */ 3663 public ResearchStudy setDescriptionSummary(String value) { 3664 if (Utilities.noString(value)) 3665 this.descriptionSummary = null; 3666 else { 3667 if (this.descriptionSummary == null) 3668 this.descriptionSummary = new MarkdownType(); 3669 this.descriptionSummary.setValue(value); 3670 } 3671 return this; 3672 } 3673 3674 /** 3675 * @return {@link #description} (A detailed and human-readable narrative of the study. E.g., study abstract.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3676 */ 3677 public MarkdownType getDescriptionElement() { 3678 if (this.description == null) 3679 if (Configuration.errorOnAutoCreate()) 3680 throw new Error("Attempt to auto-create ResearchStudy.description"); 3681 else if (Configuration.doAutoCreate()) 3682 this.description = new MarkdownType(); // bb 3683 return this.description; 3684 } 3685 3686 public boolean hasDescriptionElement() { 3687 return this.description != null && !this.description.isEmpty(); 3688 } 3689 3690 public boolean hasDescription() { 3691 return this.description != null && !this.description.isEmpty(); 3692 } 3693 3694 /** 3695 * @param value {@link #description} (A detailed and human-readable narrative of the study. E.g., study abstract.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3696 */ 3697 public ResearchStudy setDescriptionElement(MarkdownType value) { 3698 this.description = value; 3699 return this; 3700 } 3701 3702 /** 3703 * @return A detailed and human-readable narrative of the study. E.g., study abstract. 3704 */ 3705 public String getDescription() { 3706 return this.description == null ? null : this.description.getValue(); 3707 } 3708 3709 /** 3710 * @param value A detailed and human-readable narrative of the study. E.g., study abstract. 3711 */ 3712 public ResearchStudy setDescription(String value) { 3713 if (Utilities.noString(value)) 3714 this.description = null; 3715 else { 3716 if (this.description == null) 3717 this.description = new MarkdownType(); 3718 this.description.setValue(value); 3719 } 3720 return this; 3721 } 3722 3723 /** 3724 * @return {@link #period} (Identifies the start date and the expected (or actual, depending on status) end date for the study.) 3725 */ 3726 public Period getPeriod() { 3727 if (this.period == null) 3728 if (Configuration.errorOnAutoCreate()) 3729 throw new Error("Attempt to auto-create ResearchStudy.period"); 3730 else if (Configuration.doAutoCreate()) 3731 this.period = new Period(); // cc 3732 return this.period; 3733 } 3734 3735 public boolean hasPeriod() { 3736 return this.period != null && !this.period.isEmpty(); 3737 } 3738 3739 /** 3740 * @param value {@link #period} (Identifies the start date and the expected (or actual, depending on status) end date for the study.) 3741 */ 3742 public ResearchStudy setPeriod(Period value) { 3743 this.period = value; 3744 return this; 3745 } 3746 3747 /** 3748 * @return {@link #site} (A facility in which study activities are conducted.) 3749 */ 3750 public List<Reference> getSite() { 3751 if (this.site == null) 3752 this.site = new ArrayList<Reference>(); 3753 return this.site; 3754 } 3755 3756 /** 3757 * @return Returns a reference to <code>this</code> for easy method chaining 3758 */ 3759 public ResearchStudy setSite(List<Reference> theSite) { 3760 this.site = theSite; 3761 return this; 3762 } 3763 3764 public boolean hasSite() { 3765 if (this.site == null) 3766 return false; 3767 for (Reference item : this.site) 3768 if (!item.isEmpty()) 3769 return true; 3770 return false; 3771 } 3772 3773 public Reference addSite() { //3 3774 Reference t = new Reference(); 3775 if (this.site == null) 3776 this.site = new ArrayList<Reference>(); 3777 this.site.add(t); 3778 return t; 3779 } 3780 3781 public ResearchStudy addSite(Reference t) { //3 3782 if (t == null) 3783 return this; 3784 if (this.site == null) 3785 this.site = new ArrayList<Reference>(); 3786 this.site.add(t); 3787 return this; 3788 } 3789 3790 /** 3791 * @return The first repetition of repeating field {@link #site}, creating it if it does not already exist {3} 3792 */ 3793 public Reference getSiteFirstRep() { 3794 if (getSite().isEmpty()) { 3795 addSite(); 3796 } 3797 return getSite().get(0); 3798 } 3799 3800 /** 3801 * @return {@link #note} (Comments made about the study by the performer, subject or other participants.) 3802 */ 3803 public List<Annotation> getNote() { 3804 if (this.note == null) 3805 this.note = new ArrayList<Annotation>(); 3806 return this.note; 3807 } 3808 3809 /** 3810 * @return Returns a reference to <code>this</code> for easy method chaining 3811 */ 3812 public ResearchStudy setNote(List<Annotation> theNote) { 3813 this.note = theNote; 3814 return this; 3815 } 3816 3817 public boolean hasNote() { 3818 if (this.note == null) 3819 return false; 3820 for (Annotation item : this.note) 3821 if (!item.isEmpty()) 3822 return true; 3823 return false; 3824 } 3825 3826 public Annotation addNote() { //3 3827 Annotation t = new Annotation(); 3828 if (this.note == null) 3829 this.note = new ArrayList<Annotation>(); 3830 this.note.add(t); 3831 return t; 3832 } 3833 3834 public ResearchStudy addNote(Annotation t) { //3 3835 if (t == null) 3836 return this; 3837 if (this.note == null) 3838 this.note = new ArrayList<Annotation>(); 3839 this.note.add(t); 3840 return this; 3841 } 3842 3843 /** 3844 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 3845 */ 3846 public Annotation getNoteFirstRep() { 3847 if (getNote().isEmpty()) { 3848 addNote(); 3849 } 3850 return getNote().get(0); 3851 } 3852 3853 /** 3854 * @return {@link #classifier} (Additional grouping mechanism or categorization of a research study. Example: FDA regulated device, FDA regulated drug, MPG Paragraph 23b (a German legal requirement), IRB-exempt, etc. Implementation Note: do not use the classifier element to support existing semantics that are already supported thru explicit elements in the resource.) 3855 */ 3856 public List<CodeableConcept> getClassifier() { 3857 if (this.classifier == null) 3858 this.classifier = new ArrayList<CodeableConcept>(); 3859 return this.classifier; 3860 } 3861 3862 /** 3863 * @return Returns a reference to <code>this</code> for easy method chaining 3864 */ 3865 public ResearchStudy setClassifier(List<CodeableConcept> theClassifier) { 3866 this.classifier = theClassifier; 3867 return this; 3868 } 3869 3870 public boolean hasClassifier() { 3871 if (this.classifier == null) 3872 return false; 3873 for (CodeableConcept item : this.classifier) 3874 if (!item.isEmpty()) 3875 return true; 3876 return false; 3877 } 3878 3879 public CodeableConcept addClassifier() { //3 3880 CodeableConcept t = new CodeableConcept(); 3881 if (this.classifier == null) 3882 this.classifier = new ArrayList<CodeableConcept>(); 3883 this.classifier.add(t); 3884 return t; 3885 } 3886 3887 public ResearchStudy addClassifier(CodeableConcept t) { //3 3888 if (t == null) 3889 return this; 3890 if (this.classifier == null) 3891 this.classifier = new ArrayList<CodeableConcept>(); 3892 this.classifier.add(t); 3893 return this; 3894 } 3895 3896 /** 3897 * @return The first repetition of repeating field {@link #classifier}, creating it if it does not already exist {3} 3898 */ 3899 public CodeableConcept getClassifierFirstRep() { 3900 if (getClassifier().isEmpty()) { 3901 addClassifier(); 3902 } 3903 return getClassifier().get(0); 3904 } 3905 3906 /** 3907 * @return {@link #associatedParty} (Sponsors, collaborators, and other parties.) 3908 */ 3909 public List<ResearchStudyAssociatedPartyComponent> getAssociatedParty() { 3910 if (this.associatedParty == null) 3911 this.associatedParty = new ArrayList<ResearchStudyAssociatedPartyComponent>(); 3912 return this.associatedParty; 3913 } 3914 3915 /** 3916 * @return Returns a reference to <code>this</code> for easy method chaining 3917 */ 3918 public ResearchStudy setAssociatedParty(List<ResearchStudyAssociatedPartyComponent> theAssociatedParty) { 3919 this.associatedParty = theAssociatedParty; 3920 return this; 3921 } 3922 3923 public boolean hasAssociatedParty() { 3924 if (this.associatedParty == null) 3925 return false; 3926 for (ResearchStudyAssociatedPartyComponent item : this.associatedParty) 3927 if (!item.isEmpty()) 3928 return true; 3929 return false; 3930 } 3931 3932 public ResearchStudyAssociatedPartyComponent addAssociatedParty() { //3 3933 ResearchStudyAssociatedPartyComponent t = new ResearchStudyAssociatedPartyComponent(); 3934 if (this.associatedParty == null) 3935 this.associatedParty = new ArrayList<ResearchStudyAssociatedPartyComponent>(); 3936 this.associatedParty.add(t); 3937 return t; 3938 } 3939 3940 public ResearchStudy addAssociatedParty(ResearchStudyAssociatedPartyComponent t) { //3 3941 if (t == null) 3942 return this; 3943 if (this.associatedParty == null) 3944 this.associatedParty = new ArrayList<ResearchStudyAssociatedPartyComponent>(); 3945 this.associatedParty.add(t); 3946 return this; 3947 } 3948 3949 /** 3950 * @return The first repetition of repeating field {@link #associatedParty}, creating it if it does not already exist {3} 3951 */ 3952 public ResearchStudyAssociatedPartyComponent getAssociatedPartyFirstRep() { 3953 if (getAssociatedParty().isEmpty()) { 3954 addAssociatedParty(); 3955 } 3956 return getAssociatedParty().get(0); 3957 } 3958 3959 /** 3960 * @return {@link #progressStatus} (Status of study with time for that status.) 3961 */ 3962 public List<ResearchStudyProgressStatusComponent> getProgressStatus() { 3963 if (this.progressStatus == null) 3964 this.progressStatus = new ArrayList<ResearchStudyProgressStatusComponent>(); 3965 return this.progressStatus; 3966 } 3967 3968 /** 3969 * @return Returns a reference to <code>this</code> for easy method chaining 3970 */ 3971 public ResearchStudy setProgressStatus(List<ResearchStudyProgressStatusComponent> theProgressStatus) { 3972 this.progressStatus = theProgressStatus; 3973 return this; 3974 } 3975 3976 public boolean hasProgressStatus() { 3977 if (this.progressStatus == null) 3978 return false; 3979 for (ResearchStudyProgressStatusComponent item : this.progressStatus) 3980 if (!item.isEmpty()) 3981 return true; 3982 return false; 3983 } 3984 3985 public ResearchStudyProgressStatusComponent addProgressStatus() { //3 3986 ResearchStudyProgressStatusComponent t = new ResearchStudyProgressStatusComponent(); 3987 if (this.progressStatus == null) 3988 this.progressStatus = new ArrayList<ResearchStudyProgressStatusComponent>(); 3989 this.progressStatus.add(t); 3990 return t; 3991 } 3992 3993 public ResearchStudy addProgressStatus(ResearchStudyProgressStatusComponent t) { //3 3994 if (t == null) 3995 return this; 3996 if (this.progressStatus == null) 3997 this.progressStatus = new ArrayList<ResearchStudyProgressStatusComponent>(); 3998 this.progressStatus.add(t); 3999 return this; 4000 } 4001 4002 /** 4003 * @return The first repetition of repeating field {@link #progressStatus}, creating it if it does not already exist {3} 4004 */ 4005 public ResearchStudyProgressStatusComponent getProgressStatusFirstRep() { 4006 if (getProgressStatus().isEmpty()) { 4007 addProgressStatus(); 4008 } 4009 return getProgressStatus().get(0); 4010 } 4011 4012 /** 4013 * @return {@link #whyStopped} (A description and/or code explaining the premature termination of the study.) 4014 */ 4015 public CodeableConcept getWhyStopped() { 4016 if (this.whyStopped == null) 4017 if (Configuration.errorOnAutoCreate()) 4018 throw new Error("Attempt to auto-create ResearchStudy.whyStopped"); 4019 else if (Configuration.doAutoCreate()) 4020 this.whyStopped = new CodeableConcept(); // cc 4021 return this.whyStopped; 4022 } 4023 4024 public boolean hasWhyStopped() { 4025 return this.whyStopped != null && !this.whyStopped.isEmpty(); 4026 } 4027 4028 /** 4029 * @param value {@link #whyStopped} (A description and/or code explaining the premature termination of the study.) 4030 */ 4031 public ResearchStudy setWhyStopped(CodeableConcept value) { 4032 this.whyStopped = value; 4033 return this; 4034 } 4035 4036 /** 4037 * @return {@link #recruitment} (Target or actual group of participants enrolled in study.) 4038 */ 4039 public ResearchStudyRecruitmentComponent getRecruitment() { 4040 if (this.recruitment == null) 4041 if (Configuration.errorOnAutoCreate()) 4042 throw new Error("Attempt to auto-create ResearchStudy.recruitment"); 4043 else if (Configuration.doAutoCreate()) 4044 this.recruitment = new ResearchStudyRecruitmentComponent(); // cc 4045 return this.recruitment; 4046 } 4047 4048 public boolean hasRecruitment() { 4049 return this.recruitment != null && !this.recruitment.isEmpty(); 4050 } 4051 4052 /** 4053 * @param value {@link #recruitment} (Target or actual group of participants enrolled in study.) 4054 */ 4055 public ResearchStudy setRecruitment(ResearchStudyRecruitmentComponent value) { 4056 this.recruitment = value; 4057 return this; 4058 } 4059 4060 /** 4061 * @return {@link #comparisonGroup} (Describes an expected event or sequence of events for one of the subjects of a study. E.g. for a living subject: exposure to drug A, wash-out, exposure to drug B, wash-out, follow-up. E.g. for a stability study: {store sample from lot A at 25 degrees for 1 month}, {store sample from lot A at 40 degrees for 1 month}.) 4062 */ 4063 public List<ResearchStudyComparisonGroupComponent> getComparisonGroup() { 4064 if (this.comparisonGroup == null) 4065 this.comparisonGroup = new ArrayList<ResearchStudyComparisonGroupComponent>(); 4066 return this.comparisonGroup; 4067 } 4068 4069 /** 4070 * @return Returns a reference to <code>this</code> for easy method chaining 4071 */ 4072 public ResearchStudy setComparisonGroup(List<ResearchStudyComparisonGroupComponent> theComparisonGroup) { 4073 this.comparisonGroup = theComparisonGroup; 4074 return this; 4075 } 4076 4077 public boolean hasComparisonGroup() { 4078 if (this.comparisonGroup == null) 4079 return false; 4080 for (ResearchStudyComparisonGroupComponent item : this.comparisonGroup) 4081 if (!item.isEmpty()) 4082 return true; 4083 return false; 4084 } 4085 4086 public ResearchStudyComparisonGroupComponent addComparisonGroup() { //3 4087 ResearchStudyComparisonGroupComponent t = new ResearchStudyComparisonGroupComponent(); 4088 if (this.comparisonGroup == null) 4089 this.comparisonGroup = new ArrayList<ResearchStudyComparisonGroupComponent>(); 4090 this.comparisonGroup.add(t); 4091 return t; 4092 } 4093 4094 public ResearchStudy addComparisonGroup(ResearchStudyComparisonGroupComponent t) { //3 4095 if (t == null) 4096 return this; 4097 if (this.comparisonGroup == null) 4098 this.comparisonGroup = new ArrayList<ResearchStudyComparisonGroupComponent>(); 4099 this.comparisonGroup.add(t); 4100 return this; 4101 } 4102 4103 /** 4104 * @return The first repetition of repeating field {@link #comparisonGroup}, creating it if it does not already exist {3} 4105 */ 4106 public ResearchStudyComparisonGroupComponent getComparisonGroupFirstRep() { 4107 if (getComparisonGroup().isEmpty()) { 4108 addComparisonGroup(); 4109 } 4110 return getComparisonGroup().get(0); 4111 } 4112 4113 /** 4114 * @return {@link #objective} (A goal that the study is aiming to achieve in terms of a scientific question to be answered by the analysis of data collected during the study.) 4115 */ 4116 public List<ResearchStudyObjectiveComponent> getObjective() { 4117 if (this.objective == null) 4118 this.objective = new ArrayList<ResearchStudyObjectiveComponent>(); 4119 return this.objective; 4120 } 4121 4122 /** 4123 * @return Returns a reference to <code>this</code> for easy method chaining 4124 */ 4125 public ResearchStudy setObjective(List<ResearchStudyObjectiveComponent> theObjective) { 4126 this.objective = theObjective; 4127 return this; 4128 } 4129 4130 public boolean hasObjective() { 4131 if (this.objective == null) 4132 return false; 4133 for (ResearchStudyObjectiveComponent item : this.objective) 4134 if (!item.isEmpty()) 4135 return true; 4136 return false; 4137 } 4138 4139 public ResearchStudyObjectiveComponent addObjective() { //3 4140 ResearchStudyObjectiveComponent t = new ResearchStudyObjectiveComponent(); 4141 if (this.objective == null) 4142 this.objective = new ArrayList<ResearchStudyObjectiveComponent>(); 4143 this.objective.add(t); 4144 return t; 4145 } 4146 4147 public ResearchStudy addObjective(ResearchStudyObjectiveComponent t) { //3 4148 if (t == null) 4149 return this; 4150 if (this.objective == null) 4151 this.objective = new ArrayList<ResearchStudyObjectiveComponent>(); 4152 this.objective.add(t); 4153 return this; 4154 } 4155 4156 /** 4157 * @return The first repetition of repeating field {@link #objective}, creating it if it does not already exist {3} 4158 */ 4159 public ResearchStudyObjectiveComponent getObjectiveFirstRep() { 4160 if (getObjective().isEmpty()) { 4161 addObjective(); 4162 } 4163 return getObjective().get(0); 4164 } 4165 4166 /** 4167 * @return {@link #outcomeMeasure} (An "outcome measure", "endpoint", "effect measure" or "measure of effect" is a specific measurement or observation used to quantify the effect of experimental variables on the participants in a study, or for observational studies, to describe patterns of diseases or traits or associations with exposures, risk factors or treatment.) 4168 */ 4169 public List<ResearchStudyOutcomeMeasureComponent> getOutcomeMeasure() { 4170 if (this.outcomeMeasure == null) 4171 this.outcomeMeasure = new ArrayList<ResearchStudyOutcomeMeasureComponent>(); 4172 return this.outcomeMeasure; 4173 } 4174 4175 /** 4176 * @return Returns a reference to <code>this</code> for easy method chaining 4177 */ 4178 public ResearchStudy setOutcomeMeasure(List<ResearchStudyOutcomeMeasureComponent> theOutcomeMeasure) { 4179 this.outcomeMeasure = theOutcomeMeasure; 4180 return this; 4181 } 4182 4183 public boolean hasOutcomeMeasure() { 4184 if (this.outcomeMeasure == null) 4185 return false; 4186 for (ResearchStudyOutcomeMeasureComponent item : this.outcomeMeasure) 4187 if (!item.isEmpty()) 4188 return true; 4189 return false; 4190 } 4191 4192 public ResearchStudyOutcomeMeasureComponent addOutcomeMeasure() { //3 4193 ResearchStudyOutcomeMeasureComponent t = new ResearchStudyOutcomeMeasureComponent(); 4194 if (this.outcomeMeasure == null) 4195 this.outcomeMeasure = new ArrayList<ResearchStudyOutcomeMeasureComponent>(); 4196 this.outcomeMeasure.add(t); 4197 return t; 4198 } 4199 4200 public ResearchStudy addOutcomeMeasure(ResearchStudyOutcomeMeasureComponent t) { //3 4201 if (t == null) 4202 return this; 4203 if (this.outcomeMeasure == null) 4204 this.outcomeMeasure = new ArrayList<ResearchStudyOutcomeMeasureComponent>(); 4205 this.outcomeMeasure.add(t); 4206 return this; 4207 } 4208 4209 /** 4210 * @return The first repetition of repeating field {@link #outcomeMeasure}, creating it if it does not already exist {3} 4211 */ 4212 public ResearchStudyOutcomeMeasureComponent getOutcomeMeasureFirstRep() { 4213 if (getOutcomeMeasure().isEmpty()) { 4214 addOutcomeMeasure(); 4215 } 4216 return getOutcomeMeasure().get(0); 4217 } 4218 4219 /** 4220 * @return {@link #result} (Link to one or more sets of results generated by the study. Could also link to a research registry holding the results such as ClinicalTrials.gov.) 4221 */ 4222 public List<Reference> getResult() { 4223 if (this.result == null) 4224 this.result = new ArrayList<Reference>(); 4225 return this.result; 4226 } 4227 4228 /** 4229 * @return Returns a reference to <code>this</code> for easy method chaining 4230 */ 4231 public ResearchStudy setResult(List<Reference> theResult) { 4232 this.result = theResult; 4233 return this; 4234 } 4235 4236 public boolean hasResult() { 4237 if (this.result == null) 4238 return false; 4239 for (Reference item : this.result) 4240 if (!item.isEmpty()) 4241 return true; 4242 return false; 4243 } 4244 4245 public Reference addResult() { //3 4246 Reference t = new Reference(); 4247 if (this.result == null) 4248 this.result = new ArrayList<Reference>(); 4249 this.result.add(t); 4250 return t; 4251 } 4252 4253 public ResearchStudy addResult(Reference t) { //3 4254 if (t == null) 4255 return this; 4256 if (this.result == null) 4257 this.result = new ArrayList<Reference>(); 4258 this.result.add(t); 4259 return this; 4260 } 4261 4262 /** 4263 * @return The first repetition of repeating field {@link #result}, creating it if it does not already exist {3} 4264 */ 4265 public Reference getResultFirstRep() { 4266 if (getResult().isEmpty()) { 4267 addResult(); 4268 } 4269 return getResult().get(0); 4270 } 4271 4272 protected void listChildren(List<Property> children) { 4273 super.listChildren(children); 4274 children.add(new Property("url", "uri", "Canonical identifier for this study resource, represented as a globally unique URI.", 0, 1, url)); 4275 children.add(new Property("identifier", "Identifier", "Identifiers assigned to this research study by the sponsor or other systems.", 0, java.lang.Integer.MAX_VALUE, identifier)); 4276 children.add(new Property("version", "string", "The business version for the study record.", 0, 1, version)); 4277 children.add(new Property("name", "string", "Name for this study (computer friendly).", 0, 1, name)); 4278 children.add(new Property("title", "string", "The human readable name of the research study.", 0, 1, title)); 4279 children.add(new Property("label", "", "Additional names for the study.", 0, java.lang.Integer.MAX_VALUE, label)); 4280 children.add(new Property("protocol", "Reference(PlanDefinition)", "The set of steps expected to be performed as part of the execution of the study.", 0, java.lang.Integer.MAX_VALUE, protocol)); 4281 children.add(new Property("partOf", "Reference(ResearchStudy)", "A larger research study of which this particular study is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 4282 children.add(new Property("relatedArtifact", "RelatedArtifact", "Citations, references, URLs and other related documents. When using relatedArtifact to share URLs, the relatedArtifact.type will often be set to one of \"documentation\" or \"supported-with\" and the URL value will often be in relatedArtifact.document.url but another possible location is relatedArtifact.resource when it is a canonical URL.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 4283 children.add(new Property("date", "dateTime", "The date (and optionally time) when the ResearchStudy Resource was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ResearchStudy Resource changes.", 0, 1, date)); 4284 children.add(new Property("status", "code", "The publication state of the resource (not of the study).", 0, 1, status)); 4285 children.add(new Property("primaryPurposeType", "CodeableConcept", "The type of study based upon the intent of the study activities. A classification of the intent of the study.", 0, 1, primaryPurposeType)); 4286 children.add(new Property("phase", "CodeableConcept", "The stage in the progression of a therapy from initial experimental use in humans in clinical trials to post-market evaluation.", 0, 1, phase)); 4287 children.add(new Property("studyDesign", "CodeableConcept", "Codes categorizing the type of study such as investigational vs. observational, type of blinding, type of randomization, safety vs. efficacy, etc.", 0, java.lang.Integer.MAX_VALUE, studyDesign)); 4288 children.add(new Property("focus", "CodeableReference(Medication|MedicinalProductDefinition|SubstanceDefinition|EvidenceVariable)", "The medication(s), food(s), therapy(ies), device(s) or other concerns or interventions that the study is seeking to gain more information about.", 0, java.lang.Integer.MAX_VALUE, focus)); 4289 children.add(new Property("condition", "CodeableConcept", "The condition that is the focus of the study. For example, In a study to examine risk factors for Lupus, might have as an inclusion criterion \"healthy volunteer\", but the target condition code would be a Lupus SNOMED code.", 0, java.lang.Integer.MAX_VALUE, condition)); 4290 children.add(new Property("keyword", "CodeableConcept", "Key terms to aid in searching for or filtering the study.", 0, java.lang.Integer.MAX_VALUE, keyword)); 4291 children.add(new Property("region", "CodeableConcept", "A country, state or other area where the study is taking place rather than its precise geographic location or address.", 0, java.lang.Integer.MAX_VALUE, region)); 4292 children.add(new Property("descriptionSummary", "markdown", "A brief text for explaining the study.", 0, 1, descriptionSummary)); 4293 children.add(new Property("description", "markdown", "A detailed and human-readable narrative of the study. E.g., study abstract.", 0, 1, description)); 4294 children.add(new Property("period", "Period", "Identifies the start date and the expected (or actual, depending on status) end date for the study.", 0, 1, period)); 4295 children.add(new Property("site", "Reference(Location|ResearchStudy|Organization)", "A facility in which study activities are conducted.", 0, java.lang.Integer.MAX_VALUE, site)); 4296 children.add(new Property("note", "Annotation", "Comments made about the study by the performer, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note)); 4297 children.add(new Property("classifier", "CodeableConcept", "Additional grouping mechanism or categorization of a research study. Example: FDA regulated device, FDA regulated drug, MPG Paragraph 23b (a German legal requirement), IRB-exempt, etc. Implementation Note: do not use the classifier element to support existing semantics that are already supported thru explicit elements in the resource.", 0, java.lang.Integer.MAX_VALUE, classifier)); 4298 children.add(new Property("associatedParty", "", "Sponsors, collaborators, and other parties.", 0, java.lang.Integer.MAX_VALUE, associatedParty)); 4299 children.add(new Property("progressStatus", "", "Status of study with time for that status.", 0, java.lang.Integer.MAX_VALUE, progressStatus)); 4300 children.add(new Property("whyStopped", "CodeableConcept", "A description and/or code explaining the premature termination of the study.", 0, 1, whyStopped)); 4301 children.add(new Property("recruitment", "", "Target or actual group of participants enrolled in study.", 0, 1, recruitment)); 4302 children.add(new Property("comparisonGroup", "", "Describes an expected event or sequence of events for one of the subjects of a study. E.g. for a living subject: exposure to drug A, wash-out, exposure to drug B, wash-out, follow-up. E.g. for a stability study: {store sample from lot A at 25 degrees for 1 month}, {store sample from lot A at 40 degrees for 1 month}.", 0, java.lang.Integer.MAX_VALUE, comparisonGroup)); 4303 children.add(new Property("objective", "", "A goal that the study is aiming to achieve in terms of a scientific question to be answered by the analysis of data collected during the study.", 0, java.lang.Integer.MAX_VALUE, objective)); 4304 children.add(new Property("outcomeMeasure", "", "An \"outcome measure\", \"endpoint\", \"effect measure\" or \"measure of effect\" is a specific measurement or observation used to quantify the effect of experimental variables on the participants in a study, or for observational studies, to describe patterns of diseases or traits or associations with exposures, risk factors or treatment.", 0, java.lang.Integer.MAX_VALUE, outcomeMeasure)); 4305 children.add(new Property("result", "Reference(EvidenceReport|Citation|DiagnosticReport)", "Link to one or more sets of results generated by the study. Could also link to a research registry holding the results such as ClinicalTrials.gov.", 0, java.lang.Integer.MAX_VALUE, result)); 4306 } 4307 4308 @Override 4309 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4310 switch (_hash) { 4311 case 116079: /*url*/ return new Property("url", "uri", "Canonical identifier for this study resource, represented as a globally unique URI.", 0, 1, url); 4312 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers assigned to this research study by the sponsor or other systems.", 0, java.lang.Integer.MAX_VALUE, identifier); 4313 case 351608024: /*version*/ return new Property("version", "string", "The business version for the study record.", 0, 1, version); 4314 case 3373707: /*name*/ return new Property("name", "string", "Name for this study (computer friendly).", 0, 1, name); 4315 case 110371416: /*title*/ return new Property("title", "string", "The human readable name of the research study.", 0, 1, title); 4316 case 102727412: /*label*/ return new Property("label", "", "Additional names for the study.", 0, java.lang.Integer.MAX_VALUE, label); 4317 case -989163880: /*protocol*/ return new Property("protocol", "Reference(PlanDefinition)", "The set of steps expected to be performed as part of the execution of the study.", 0, java.lang.Integer.MAX_VALUE, protocol); 4318 case -995410646: /*partOf*/ return new Property("partOf", "Reference(ResearchStudy)", "A larger research study of which this particular study is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 4319 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Citations, references, URLs and other related documents. When using relatedArtifact to share URLs, the relatedArtifact.type will often be set to one of \"documentation\" or \"supported-with\" and the URL value will often be in relatedArtifact.document.url but another possible location is relatedArtifact.resource when it is a canonical URL.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 4320 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the ResearchStudy Resource was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ResearchStudy Resource changes.", 0, 1, date); 4321 case -892481550: /*status*/ return new Property("status", "code", "The publication state of the resource (not of the study).", 0, 1, status); 4322 case -2132842986: /*primaryPurposeType*/ return new Property("primaryPurposeType", "CodeableConcept", "The type of study based upon the intent of the study activities. A classification of the intent of the study.", 0, 1, primaryPurposeType); 4323 case 106629499: /*phase*/ return new Property("phase", "CodeableConcept", "The stage in the progression of a therapy from initial experimental use in humans in clinical trials to post-market evaluation.", 0, 1, phase); 4324 case 1709211879: /*studyDesign*/ return new Property("studyDesign", "CodeableConcept", "Codes categorizing the type of study such as investigational vs. observational, type of blinding, type of randomization, safety vs. efficacy, etc.", 0, java.lang.Integer.MAX_VALUE, studyDesign); 4325 case 97604824: /*focus*/ return new Property("focus", "CodeableReference(Medication|MedicinalProductDefinition|SubstanceDefinition|EvidenceVariable)", "The medication(s), food(s), therapy(ies), device(s) or other concerns or interventions that the study is seeking to gain more information about.", 0, java.lang.Integer.MAX_VALUE, focus); 4326 case -861311717: /*condition*/ return new Property("condition", "CodeableConcept", "The condition that is the focus of the study. For example, In a study to examine risk factors for Lupus, might have as an inclusion criterion \"healthy volunteer\", but the target condition code would be a Lupus SNOMED code.", 0, java.lang.Integer.MAX_VALUE, condition); 4327 case -814408215: /*keyword*/ return new Property("keyword", "CodeableConcept", "Key terms to aid in searching for or filtering the study.", 0, java.lang.Integer.MAX_VALUE, keyword); 4328 case -934795532: /*region*/ return new Property("region", "CodeableConcept", "A country, state or other area where the study is taking place rather than its precise geographic location or address.", 0, java.lang.Integer.MAX_VALUE, region); 4329 case 21530634: /*descriptionSummary*/ return new Property("descriptionSummary", "markdown", "A brief text for explaining the study.", 0, 1, descriptionSummary); 4330 case -1724546052: /*description*/ return new Property("description", "markdown", "A detailed and human-readable narrative of the study. E.g., study abstract.", 0, 1, description); 4331 case -991726143: /*period*/ return new Property("period", "Period", "Identifies the start date and the expected (or actual, depending on status) end date for the study.", 0, 1, period); 4332 case 3530567: /*site*/ return new Property("site", "Reference(Location|ResearchStudy|Organization)", "A facility in which study activities are conducted.", 0, java.lang.Integer.MAX_VALUE, site); 4333 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments made about the study by the performer, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note); 4334 case -281470431: /*classifier*/ return new Property("classifier", "CodeableConcept", "Additional grouping mechanism or categorization of a research study. Example: FDA regulated device, FDA regulated drug, MPG Paragraph 23b (a German legal requirement), IRB-exempt, etc. Implementation Note: do not use the classifier element to support existing semantics that are already supported thru explicit elements in the resource.", 0, java.lang.Integer.MAX_VALUE, classifier); 4335 case -1841460864: /*associatedParty*/ return new Property("associatedParty", "", "Sponsors, collaborators, and other parties.", 0, java.lang.Integer.MAX_VALUE, associatedParty); 4336 case -1897502593: /*progressStatus*/ return new Property("progressStatus", "", "Status of study with time for that status.", 0, java.lang.Integer.MAX_VALUE, progressStatus); 4337 case -699986715: /*whyStopped*/ return new Property("whyStopped", "CodeableConcept", "A description and/or code explaining the premature termination of the study.", 0, 1, whyStopped); 4338 case 780783004: /*recruitment*/ return new Property("recruitment", "", "Target or actual group of participants enrolled in study.", 0, 1, recruitment); 4339 case -138266634: /*comparisonGroup*/ return new Property("comparisonGroup", "", "Describes an expected event or sequence of events for one of the subjects of a study. E.g. for a living subject: exposure to drug A, wash-out, exposure to drug B, wash-out, follow-up. E.g. for a stability study: {store sample from lot A at 25 degrees for 1 month}, {store sample from lot A at 40 degrees for 1 month}.", 0, java.lang.Integer.MAX_VALUE, comparisonGroup); 4340 case -1489585863: /*objective*/ return new Property("objective", "", "A goal that the study is aiming to achieve in terms of a scientific question to be answered by the analysis of data collected during the study.", 0, java.lang.Integer.MAX_VALUE, objective); 4341 case -1510689364: /*outcomeMeasure*/ return new Property("outcomeMeasure", "", "An \"outcome measure\", \"endpoint\", \"effect measure\" or \"measure of effect\" is a specific measurement or observation used to quantify the effect of experimental variables on the participants in a study, or for observational studies, to describe patterns of diseases or traits or associations with exposures, risk factors or treatment.", 0, java.lang.Integer.MAX_VALUE, outcomeMeasure); 4342 case -934426595: /*result*/ return new Property("result", "Reference(EvidenceReport|Citation|DiagnosticReport)", "Link to one or more sets of results generated by the study. Could also link to a research registry holding the results such as ClinicalTrials.gov.", 0, java.lang.Integer.MAX_VALUE, result); 4343 default: return super.getNamedProperty(_hash, _name, _checkValid); 4344 } 4345 4346 } 4347 4348 @Override 4349 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4350 switch (hash) { 4351 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 4352 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4353 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 4354 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 4355 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 4356 case 102727412: /*label*/ return this.label == null ? new Base[0] : this.label.toArray(new Base[this.label.size()]); // ResearchStudyLabelComponent 4357 case -989163880: /*protocol*/ return this.protocol == null ? new Base[0] : this.protocol.toArray(new Base[this.protocol.size()]); // Reference 4358 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 4359 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 4360 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 4361 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 4362 case -2132842986: /*primaryPurposeType*/ return this.primaryPurposeType == null ? new Base[0] : new Base[] {this.primaryPurposeType}; // CodeableConcept 4363 case 106629499: /*phase*/ return this.phase == null ? new Base[0] : new Base[] {this.phase}; // CodeableConcept 4364 case 1709211879: /*studyDesign*/ return this.studyDesign == null ? new Base[0] : this.studyDesign.toArray(new Base[this.studyDesign.size()]); // CodeableConcept 4365 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // CodeableReference 4366 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : this.condition.toArray(new Base[this.condition.size()]); // CodeableConcept 4367 case -814408215: /*keyword*/ return this.keyword == null ? new Base[0] : this.keyword.toArray(new Base[this.keyword.size()]); // CodeableConcept 4368 case -934795532: /*region*/ return this.region == null ? new Base[0] : this.region.toArray(new Base[this.region.size()]); // CodeableConcept 4369 case 21530634: /*descriptionSummary*/ return this.descriptionSummary == null ? new Base[0] : new Base[] {this.descriptionSummary}; // MarkdownType 4370 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 4371 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 4372 case 3530567: /*site*/ return this.site == null ? new Base[0] : this.site.toArray(new Base[this.site.size()]); // Reference 4373 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 4374 case -281470431: /*classifier*/ return this.classifier == null ? new Base[0] : this.classifier.toArray(new Base[this.classifier.size()]); // CodeableConcept 4375 case -1841460864: /*associatedParty*/ return this.associatedParty == null ? new Base[0] : this.associatedParty.toArray(new Base[this.associatedParty.size()]); // ResearchStudyAssociatedPartyComponent 4376 case -1897502593: /*progressStatus*/ return this.progressStatus == null ? new Base[0] : this.progressStatus.toArray(new Base[this.progressStatus.size()]); // ResearchStudyProgressStatusComponent 4377 case -699986715: /*whyStopped*/ return this.whyStopped == null ? new Base[0] : new Base[] {this.whyStopped}; // CodeableConcept 4378 case 780783004: /*recruitment*/ return this.recruitment == null ? new Base[0] : new Base[] {this.recruitment}; // ResearchStudyRecruitmentComponent 4379 case -138266634: /*comparisonGroup*/ return this.comparisonGroup == null ? new Base[0] : this.comparisonGroup.toArray(new Base[this.comparisonGroup.size()]); // ResearchStudyComparisonGroupComponent 4380 case -1489585863: /*objective*/ return this.objective == null ? new Base[0] : this.objective.toArray(new Base[this.objective.size()]); // ResearchStudyObjectiveComponent 4381 case -1510689364: /*outcomeMeasure*/ return this.outcomeMeasure == null ? new Base[0] : this.outcomeMeasure.toArray(new Base[this.outcomeMeasure.size()]); // ResearchStudyOutcomeMeasureComponent 4382 case -934426595: /*result*/ return this.result == null ? new Base[0] : this.result.toArray(new Base[this.result.size()]); // Reference 4383 default: return super.getProperty(hash, name, checkValid); 4384 } 4385 4386 } 4387 4388 @Override 4389 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4390 switch (hash) { 4391 case 116079: // url 4392 this.url = TypeConvertor.castToUri(value); // UriType 4393 return value; 4394 case -1618432855: // identifier 4395 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 4396 return value; 4397 case 351608024: // version 4398 this.version = TypeConvertor.castToString(value); // StringType 4399 return value; 4400 case 3373707: // name 4401 this.name = TypeConvertor.castToString(value); // StringType 4402 return value; 4403 case 110371416: // title 4404 this.title = TypeConvertor.castToString(value); // StringType 4405 return value; 4406 case 102727412: // label 4407 this.getLabel().add((ResearchStudyLabelComponent) value); // ResearchStudyLabelComponent 4408 return value; 4409 case -989163880: // protocol 4410 this.getProtocol().add(TypeConvertor.castToReference(value)); // Reference 4411 return value; 4412 case -995410646: // partOf 4413 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 4414 return value; 4415 case 666807069: // relatedArtifact 4416 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 4417 return value; 4418 case 3076014: // date 4419 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4420 return value; 4421 case -892481550: // status 4422 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4423 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4424 return value; 4425 case -2132842986: // primaryPurposeType 4426 this.primaryPurposeType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4427 return value; 4428 case 106629499: // phase 4429 this.phase = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4430 return value; 4431 case 1709211879: // studyDesign 4432 this.getStudyDesign().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4433 return value; 4434 case 97604824: // focus 4435 this.getFocus().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 4436 return value; 4437 case -861311717: // condition 4438 this.getCondition().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4439 return value; 4440 case -814408215: // keyword 4441 this.getKeyword().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4442 return value; 4443 case -934795532: // region 4444 this.getRegion().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4445 return value; 4446 case 21530634: // descriptionSummary 4447 this.descriptionSummary = TypeConvertor.castToMarkdown(value); // MarkdownType 4448 return value; 4449 case -1724546052: // description 4450 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4451 return value; 4452 case -991726143: // period 4453 this.period = TypeConvertor.castToPeriod(value); // Period 4454 return value; 4455 case 3530567: // site 4456 this.getSite().add(TypeConvertor.castToReference(value)); // Reference 4457 return value; 4458 case 3387378: // note 4459 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 4460 return value; 4461 case -281470431: // classifier 4462 this.getClassifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4463 return value; 4464 case -1841460864: // associatedParty 4465 this.getAssociatedParty().add((ResearchStudyAssociatedPartyComponent) value); // ResearchStudyAssociatedPartyComponent 4466 return value; 4467 case -1897502593: // progressStatus 4468 this.getProgressStatus().add((ResearchStudyProgressStatusComponent) value); // ResearchStudyProgressStatusComponent 4469 return value; 4470 case -699986715: // whyStopped 4471 this.whyStopped = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4472 return value; 4473 case 780783004: // recruitment 4474 this.recruitment = (ResearchStudyRecruitmentComponent) value; // ResearchStudyRecruitmentComponent 4475 return value; 4476 case -138266634: // comparisonGroup 4477 this.getComparisonGroup().add((ResearchStudyComparisonGroupComponent) value); // ResearchStudyComparisonGroupComponent 4478 return value; 4479 case -1489585863: // objective 4480 this.getObjective().add((ResearchStudyObjectiveComponent) value); // ResearchStudyObjectiveComponent 4481 return value; 4482 case -1510689364: // outcomeMeasure 4483 this.getOutcomeMeasure().add((ResearchStudyOutcomeMeasureComponent) value); // ResearchStudyOutcomeMeasureComponent 4484 return value; 4485 case -934426595: // result 4486 this.getResult().add(TypeConvertor.castToReference(value)); // Reference 4487 return value; 4488 default: return super.setProperty(hash, name, value); 4489 } 4490 4491 } 4492 4493 @Override 4494 public Base setProperty(String name, Base value) throws FHIRException { 4495 if (name.equals("url")) { 4496 this.url = TypeConvertor.castToUri(value); // UriType 4497 } else if (name.equals("identifier")) { 4498 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 4499 } else if (name.equals("version")) { 4500 this.version = TypeConvertor.castToString(value); // StringType 4501 } else if (name.equals("name")) { 4502 this.name = TypeConvertor.castToString(value); // StringType 4503 } else if (name.equals("title")) { 4504 this.title = TypeConvertor.castToString(value); // StringType 4505 } else if (name.equals("label")) { 4506 this.getLabel().add((ResearchStudyLabelComponent) value); 4507 } else if (name.equals("protocol")) { 4508 this.getProtocol().add(TypeConvertor.castToReference(value)); 4509 } else if (name.equals("partOf")) { 4510 this.getPartOf().add(TypeConvertor.castToReference(value)); 4511 } else if (name.equals("relatedArtifact")) { 4512 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); 4513 } else if (name.equals("date")) { 4514 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4515 } else if (name.equals("status")) { 4516 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4517 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4518 } else if (name.equals("primaryPurposeType")) { 4519 this.primaryPurposeType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4520 } else if (name.equals("phase")) { 4521 this.phase = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4522 } else if (name.equals("studyDesign")) { 4523 this.getStudyDesign().add(TypeConvertor.castToCodeableConcept(value)); 4524 } else if (name.equals("focus")) { 4525 this.getFocus().add(TypeConvertor.castToCodeableReference(value)); 4526 } else if (name.equals("condition")) { 4527 this.getCondition().add(TypeConvertor.castToCodeableConcept(value)); 4528 } else if (name.equals("keyword")) { 4529 this.getKeyword().add(TypeConvertor.castToCodeableConcept(value)); 4530 } else if (name.equals("region")) { 4531 this.getRegion().add(TypeConvertor.castToCodeableConcept(value)); 4532 } else if (name.equals("descriptionSummary")) { 4533 this.descriptionSummary = TypeConvertor.castToMarkdown(value); // MarkdownType 4534 } else if (name.equals("description")) { 4535 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4536 } else if (name.equals("period")) { 4537 this.period = TypeConvertor.castToPeriod(value); // Period 4538 } else if (name.equals("site")) { 4539 this.getSite().add(TypeConvertor.castToReference(value)); 4540 } else if (name.equals("note")) { 4541 this.getNote().add(TypeConvertor.castToAnnotation(value)); 4542 } else if (name.equals("classifier")) { 4543 this.getClassifier().add(TypeConvertor.castToCodeableConcept(value)); 4544 } else if (name.equals("associatedParty")) { 4545 this.getAssociatedParty().add((ResearchStudyAssociatedPartyComponent) value); 4546 } else if (name.equals("progressStatus")) { 4547 this.getProgressStatus().add((ResearchStudyProgressStatusComponent) value); 4548 } else if (name.equals("whyStopped")) { 4549 this.whyStopped = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4550 } else if (name.equals("recruitment")) { 4551 this.recruitment = (ResearchStudyRecruitmentComponent) value; // ResearchStudyRecruitmentComponent 4552 } else if (name.equals("comparisonGroup")) { 4553 this.getComparisonGroup().add((ResearchStudyComparisonGroupComponent) value); 4554 } else if (name.equals("objective")) { 4555 this.getObjective().add((ResearchStudyObjectiveComponent) value); 4556 } else if (name.equals("outcomeMeasure")) { 4557 this.getOutcomeMeasure().add((ResearchStudyOutcomeMeasureComponent) value); 4558 } else if (name.equals("result")) { 4559 this.getResult().add(TypeConvertor.castToReference(value)); 4560 } else 4561 return super.setProperty(name, value); 4562 return value; 4563 } 4564 4565 @Override 4566 public Base makeProperty(int hash, String name) throws FHIRException { 4567 switch (hash) { 4568 case 116079: return getUrlElement(); 4569 case -1618432855: return addIdentifier(); 4570 case 351608024: return getVersionElement(); 4571 case 3373707: return getNameElement(); 4572 case 110371416: return getTitleElement(); 4573 case 102727412: return addLabel(); 4574 case -989163880: return addProtocol(); 4575 case -995410646: return addPartOf(); 4576 case 666807069: return addRelatedArtifact(); 4577 case 3076014: return getDateElement(); 4578 case -892481550: return getStatusElement(); 4579 case -2132842986: return getPrimaryPurposeType(); 4580 case 106629499: return getPhase(); 4581 case 1709211879: return addStudyDesign(); 4582 case 97604824: return addFocus(); 4583 case -861311717: return addCondition(); 4584 case -814408215: return addKeyword(); 4585 case -934795532: return addRegion(); 4586 case 21530634: return getDescriptionSummaryElement(); 4587 case -1724546052: return getDescriptionElement(); 4588 case -991726143: return getPeriod(); 4589 case 3530567: return addSite(); 4590 case 3387378: return addNote(); 4591 case -281470431: return addClassifier(); 4592 case -1841460864: return addAssociatedParty(); 4593 case -1897502593: return addProgressStatus(); 4594 case -699986715: return getWhyStopped(); 4595 case 780783004: return getRecruitment(); 4596 case -138266634: return addComparisonGroup(); 4597 case -1489585863: return addObjective(); 4598 case -1510689364: return addOutcomeMeasure(); 4599 case -934426595: return addResult(); 4600 default: return super.makeProperty(hash, name); 4601 } 4602 4603 } 4604 4605 @Override 4606 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4607 switch (hash) { 4608 case 116079: /*url*/ return new String[] {"uri"}; 4609 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4610 case 351608024: /*version*/ return new String[] {"string"}; 4611 case 3373707: /*name*/ return new String[] {"string"}; 4612 case 110371416: /*title*/ return new String[] {"string"}; 4613 case 102727412: /*label*/ return new String[] {}; 4614 case -989163880: /*protocol*/ return new String[] {"Reference"}; 4615 case -995410646: /*partOf*/ return new String[] {"Reference"}; 4616 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 4617 case 3076014: /*date*/ return new String[] {"dateTime"}; 4618 case -892481550: /*status*/ return new String[] {"code"}; 4619 case -2132842986: /*primaryPurposeType*/ return new String[] {"CodeableConcept"}; 4620 case 106629499: /*phase*/ return new String[] {"CodeableConcept"}; 4621 case 1709211879: /*studyDesign*/ return new String[] {"CodeableConcept"}; 4622 case 97604824: /*focus*/ return new String[] {"CodeableReference"}; 4623 case -861311717: /*condition*/ return new String[] {"CodeableConcept"}; 4624 case -814408215: /*keyword*/ return new String[] {"CodeableConcept"}; 4625 case -934795532: /*region*/ return new String[] {"CodeableConcept"}; 4626 case 21530634: /*descriptionSummary*/ return new String[] {"markdown"}; 4627 case -1724546052: /*description*/ return new String[] {"markdown"}; 4628 case -991726143: /*period*/ return new String[] {"Period"}; 4629 case 3530567: /*site*/ return new String[] {"Reference"}; 4630 case 3387378: /*note*/ return new String[] {"Annotation"}; 4631 case -281470431: /*classifier*/ return new String[] {"CodeableConcept"}; 4632 case -1841460864: /*associatedParty*/ return new String[] {}; 4633 case -1897502593: /*progressStatus*/ return new String[] {}; 4634 case -699986715: /*whyStopped*/ return new String[] {"CodeableConcept"}; 4635 case 780783004: /*recruitment*/ return new String[] {}; 4636 case -138266634: /*comparisonGroup*/ return new String[] {}; 4637 case -1489585863: /*objective*/ return new String[] {}; 4638 case -1510689364: /*outcomeMeasure*/ return new String[] {}; 4639 case -934426595: /*result*/ return new String[] {"Reference"}; 4640 default: return super.getTypesForProperty(hash, name); 4641 } 4642 4643 } 4644 4645 @Override 4646 public Base addChild(String name) throws FHIRException { 4647 if (name.equals("url")) { 4648 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.url"); 4649 } 4650 else if (name.equals("identifier")) { 4651 return addIdentifier(); 4652 } 4653 else if (name.equals("version")) { 4654 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.version"); 4655 } 4656 else if (name.equals("name")) { 4657 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.name"); 4658 } 4659 else if (name.equals("title")) { 4660 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.title"); 4661 } 4662 else if (name.equals("label")) { 4663 return addLabel(); 4664 } 4665 else if (name.equals("protocol")) { 4666 return addProtocol(); 4667 } 4668 else if (name.equals("partOf")) { 4669 return addPartOf(); 4670 } 4671 else if (name.equals("relatedArtifact")) { 4672 return addRelatedArtifact(); 4673 } 4674 else if (name.equals("date")) { 4675 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.date"); 4676 } 4677 else if (name.equals("status")) { 4678 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.status"); 4679 } 4680 else if (name.equals("primaryPurposeType")) { 4681 this.primaryPurposeType = new CodeableConcept(); 4682 return this.primaryPurposeType; 4683 } 4684 else if (name.equals("phase")) { 4685 this.phase = new CodeableConcept(); 4686 return this.phase; 4687 } 4688 else if (name.equals("studyDesign")) { 4689 return addStudyDesign(); 4690 } 4691 else if (name.equals("focus")) { 4692 return addFocus(); 4693 } 4694 else if (name.equals("condition")) { 4695 return addCondition(); 4696 } 4697 else if (name.equals("keyword")) { 4698 return addKeyword(); 4699 } 4700 else if (name.equals("region")) { 4701 return addRegion(); 4702 } 4703 else if (name.equals("descriptionSummary")) { 4704 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.descriptionSummary"); 4705 } 4706 else if (name.equals("description")) { 4707 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.description"); 4708 } 4709 else if (name.equals("period")) { 4710 this.period = new Period(); 4711 return this.period; 4712 } 4713 else if (name.equals("site")) { 4714 return addSite(); 4715 } 4716 else if (name.equals("note")) { 4717 return addNote(); 4718 } 4719 else if (name.equals("classifier")) { 4720 return addClassifier(); 4721 } 4722 else if (name.equals("associatedParty")) { 4723 return addAssociatedParty(); 4724 } 4725 else if (name.equals("progressStatus")) { 4726 return addProgressStatus(); 4727 } 4728 else if (name.equals("whyStopped")) { 4729 this.whyStopped = new CodeableConcept(); 4730 return this.whyStopped; 4731 } 4732 else if (name.equals("recruitment")) { 4733 this.recruitment = new ResearchStudyRecruitmentComponent(); 4734 return this.recruitment; 4735 } 4736 else if (name.equals("comparisonGroup")) { 4737 return addComparisonGroup(); 4738 } 4739 else if (name.equals("objective")) { 4740 return addObjective(); 4741 } 4742 else if (name.equals("outcomeMeasure")) { 4743 return addOutcomeMeasure(); 4744 } 4745 else if (name.equals("result")) { 4746 return addResult(); 4747 } 4748 else 4749 return super.addChild(name); 4750 } 4751 4752 public String fhirType() { 4753 return "ResearchStudy"; 4754 4755 } 4756 4757 public ResearchStudy copy() { 4758 ResearchStudy dst = new ResearchStudy(); 4759 copyValues(dst); 4760 return dst; 4761 } 4762 4763 public void copyValues(ResearchStudy dst) { 4764 super.copyValues(dst); 4765 dst.url = url == null ? null : url.copy(); 4766 if (identifier != null) { 4767 dst.identifier = new ArrayList<Identifier>(); 4768 for (Identifier i : identifier) 4769 dst.identifier.add(i.copy()); 4770 }; 4771 dst.version = version == null ? null : version.copy(); 4772 dst.name = name == null ? null : name.copy(); 4773 dst.title = title == null ? null : title.copy(); 4774 if (label != null) { 4775 dst.label = new ArrayList<ResearchStudyLabelComponent>(); 4776 for (ResearchStudyLabelComponent i : label) 4777 dst.label.add(i.copy()); 4778 }; 4779 if (protocol != null) { 4780 dst.protocol = new ArrayList<Reference>(); 4781 for (Reference i : protocol) 4782 dst.protocol.add(i.copy()); 4783 }; 4784 if (partOf != null) { 4785 dst.partOf = new ArrayList<Reference>(); 4786 for (Reference i : partOf) 4787 dst.partOf.add(i.copy()); 4788 }; 4789 if (relatedArtifact != null) { 4790 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 4791 for (RelatedArtifact i : relatedArtifact) 4792 dst.relatedArtifact.add(i.copy()); 4793 }; 4794 dst.date = date == null ? null : date.copy(); 4795 dst.status = status == null ? null : status.copy(); 4796 dst.primaryPurposeType = primaryPurposeType == null ? null : primaryPurposeType.copy(); 4797 dst.phase = phase == null ? null : phase.copy(); 4798 if (studyDesign != null) { 4799 dst.studyDesign = new ArrayList<CodeableConcept>(); 4800 for (CodeableConcept i : studyDesign) 4801 dst.studyDesign.add(i.copy()); 4802 }; 4803 if (focus != null) { 4804 dst.focus = new ArrayList<CodeableReference>(); 4805 for (CodeableReference i : focus) 4806 dst.focus.add(i.copy()); 4807 }; 4808 if (condition != null) { 4809 dst.condition = new ArrayList<CodeableConcept>(); 4810 for (CodeableConcept i : condition) 4811 dst.condition.add(i.copy()); 4812 }; 4813 if (keyword != null) { 4814 dst.keyword = new ArrayList<CodeableConcept>(); 4815 for (CodeableConcept i : keyword) 4816 dst.keyword.add(i.copy()); 4817 }; 4818 if (region != null) { 4819 dst.region = new ArrayList<CodeableConcept>(); 4820 for (CodeableConcept i : region) 4821 dst.region.add(i.copy()); 4822 }; 4823 dst.descriptionSummary = descriptionSummary == null ? null : descriptionSummary.copy(); 4824 dst.description = description == null ? null : description.copy(); 4825 dst.period = period == null ? null : period.copy(); 4826 if (site != null) { 4827 dst.site = new ArrayList<Reference>(); 4828 for (Reference i : site) 4829 dst.site.add(i.copy()); 4830 }; 4831 if (note != null) { 4832 dst.note = new ArrayList<Annotation>(); 4833 for (Annotation i : note) 4834 dst.note.add(i.copy()); 4835 }; 4836 if (classifier != null) { 4837 dst.classifier = new ArrayList<CodeableConcept>(); 4838 for (CodeableConcept i : classifier) 4839 dst.classifier.add(i.copy()); 4840 }; 4841 if (associatedParty != null) { 4842 dst.associatedParty = new ArrayList<ResearchStudyAssociatedPartyComponent>(); 4843 for (ResearchStudyAssociatedPartyComponent i : associatedParty) 4844 dst.associatedParty.add(i.copy()); 4845 }; 4846 if (progressStatus != null) { 4847 dst.progressStatus = new ArrayList<ResearchStudyProgressStatusComponent>(); 4848 for (ResearchStudyProgressStatusComponent i : progressStatus) 4849 dst.progressStatus.add(i.copy()); 4850 }; 4851 dst.whyStopped = whyStopped == null ? null : whyStopped.copy(); 4852 dst.recruitment = recruitment == null ? null : recruitment.copy(); 4853 if (comparisonGroup != null) { 4854 dst.comparisonGroup = new ArrayList<ResearchStudyComparisonGroupComponent>(); 4855 for (ResearchStudyComparisonGroupComponent i : comparisonGroup) 4856 dst.comparisonGroup.add(i.copy()); 4857 }; 4858 if (objective != null) { 4859 dst.objective = new ArrayList<ResearchStudyObjectiveComponent>(); 4860 for (ResearchStudyObjectiveComponent i : objective) 4861 dst.objective.add(i.copy()); 4862 }; 4863 if (outcomeMeasure != null) { 4864 dst.outcomeMeasure = new ArrayList<ResearchStudyOutcomeMeasureComponent>(); 4865 for (ResearchStudyOutcomeMeasureComponent i : outcomeMeasure) 4866 dst.outcomeMeasure.add(i.copy()); 4867 }; 4868 if (result != null) { 4869 dst.result = new ArrayList<Reference>(); 4870 for (Reference i : result) 4871 dst.result.add(i.copy()); 4872 }; 4873 } 4874 4875 protected ResearchStudy typedCopy() { 4876 return copy(); 4877 } 4878 4879 @Override 4880 public boolean equalsDeep(Base other_) { 4881 if (!super.equalsDeep(other_)) 4882 return false; 4883 if (!(other_ instanceof ResearchStudy)) 4884 return false; 4885 ResearchStudy o = (ResearchStudy) other_; 4886 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 4887 && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) && compareDeep(label, o.label, true) 4888 && compareDeep(protocol, o.protocol, true) && compareDeep(partOf, o.partOf, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 4889 && compareDeep(date, o.date, true) && compareDeep(status, o.status, true) && compareDeep(primaryPurposeType, o.primaryPurposeType, true) 4890 && compareDeep(phase, o.phase, true) && compareDeep(studyDesign, o.studyDesign, true) && compareDeep(focus, o.focus, true) 4891 && compareDeep(condition, o.condition, true) && compareDeep(keyword, o.keyword, true) && compareDeep(region, o.region, true) 4892 && compareDeep(descriptionSummary, o.descriptionSummary, true) && compareDeep(description, o.description, true) 4893 && compareDeep(period, o.period, true) && compareDeep(site, o.site, true) && compareDeep(note, o.note, true) 4894 && compareDeep(classifier, o.classifier, true) && compareDeep(associatedParty, o.associatedParty, true) 4895 && compareDeep(progressStatus, o.progressStatus, true) && compareDeep(whyStopped, o.whyStopped, true) 4896 && compareDeep(recruitment, o.recruitment, true) && compareDeep(comparisonGroup, o.comparisonGroup, true) 4897 && compareDeep(objective, o.objective, true) && compareDeep(outcomeMeasure, o.outcomeMeasure, true) 4898 && compareDeep(result, o.result, true); 4899 } 4900 4901 @Override 4902 public boolean equalsShallow(Base other_) { 4903 if (!super.equalsShallow(other_)) 4904 return false; 4905 if (!(other_ instanceof ResearchStudy)) 4906 return false; 4907 ResearchStudy o = (ResearchStudy) other_; 4908 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 4909 && compareValues(title, o.title, true) && compareValues(date, o.date, true) && compareValues(status, o.status, true) 4910 && compareValues(descriptionSummary, o.descriptionSummary, true) && compareValues(description, o.description, true) 4911 ; 4912 } 4913 4914 public boolean isEmpty() { 4915 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 4916 , name, title, label, protocol, partOf, relatedArtifact, date, status, primaryPurposeType 4917 , phase, studyDesign, focus, condition, keyword, region, descriptionSummary, description 4918 , period, site, note, classifier, associatedParty, progressStatus, whyStopped 4919 , recruitment, comparisonGroup, objective, outcomeMeasure, result); 4920 } 4921 4922 @Override 4923 public ResourceType getResourceType() { 4924 return ResourceType.ResearchStudy; 4925 } 4926 4927 /** 4928 * Search parameter: <b>classifier</b> 4929 * <p> 4930 * Description: <b>Classification for the study</b><br> 4931 * Type: <b>token</b><br> 4932 * Path: <b>ResearchStudy.classifier</b><br> 4933 * </p> 4934 */ 4935 @SearchParamDefinition(name="classifier", path="ResearchStudy.classifier", description="Classification for the study", type="token" ) 4936 public static final String SP_CLASSIFIER = "classifier"; 4937 /** 4938 * <b>Fluent Client</b> search parameter constant for <b>classifier</b> 4939 * <p> 4940 * Description: <b>Classification for the study</b><br> 4941 * Type: <b>token</b><br> 4942 * Path: <b>ResearchStudy.classifier</b><br> 4943 * </p> 4944 */ 4945 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASSIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CLASSIFIER); 4946 4947 /** 4948 * Search parameter: <b>condition</b> 4949 * <p> 4950 * Description: <b>Condition being studied</b><br> 4951 * Type: <b>token</b><br> 4952 * Path: <b>ResearchStudy.condition</b><br> 4953 * </p> 4954 */ 4955 @SearchParamDefinition(name="condition", path="ResearchStudy.condition", description="Condition being studied", type="token" ) 4956 public static final String SP_CONDITION = "condition"; 4957 /** 4958 * <b>Fluent Client</b> search parameter constant for <b>condition</b> 4959 * <p> 4960 * Description: <b>Condition being studied</b><br> 4961 * Type: <b>token</b><br> 4962 * Path: <b>ResearchStudy.condition</b><br> 4963 * </p> 4964 */ 4965 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONDITION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONDITION); 4966 4967 /** 4968 * Search parameter: <b>date</b> 4969 * <p> 4970 * Description: <b>When the study began and ended</b><br> 4971 * Type: <b>date</b><br> 4972 * Path: <b>ResearchStudy.period</b><br> 4973 * </p> 4974 */ 4975 @SearchParamDefinition(name="date", path="ResearchStudy.period", description="When the study began and ended", type="date" ) 4976 public static final String SP_DATE = "date"; 4977 /** 4978 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4979 * <p> 4980 * Description: <b>When the study began and ended</b><br> 4981 * Type: <b>date</b><br> 4982 * Path: <b>ResearchStudy.period</b><br> 4983 * </p> 4984 */ 4985 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4986 4987 /** 4988 * Search parameter: <b>description</b> 4989 * <p> 4990 * Description: <b>Detailed narrative of the study</b><br> 4991 * Type: <b>string</b><br> 4992 * Path: <b>ResearchStudy.description</b><br> 4993 * </p> 4994 */ 4995 @SearchParamDefinition(name="description", path="ResearchStudy.description", description="Detailed narrative of the study", type="string" ) 4996 public static final String SP_DESCRIPTION = "description"; 4997 /** 4998 * <b>Fluent Client</b> search parameter constant for <b>description</b> 4999 * <p> 5000 * Description: <b>Detailed narrative of the study</b><br> 5001 * Type: <b>string</b><br> 5002 * Path: <b>ResearchStudy.description</b><br> 5003 * </p> 5004 */ 5005 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 5006 5007 /** 5008 * Search parameter: <b>eligibility</b> 5009 * <p> 5010 * Description: <b>Inclusion and exclusion criteria</b><br> 5011 * Type: <b>reference</b><br> 5012 * Path: <b>ResearchStudy.recruitment.eligibility</b><br> 5013 * </p> 5014 */ 5015 @SearchParamDefinition(name="eligibility", path="ResearchStudy.recruitment.eligibility", description="Inclusion and exclusion criteria", type="reference", target={EvidenceVariable.class, Group.class } ) 5016 public static final String SP_ELIGIBILITY = "eligibility"; 5017 /** 5018 * <b>Fluent Client</b> search parameter constant for <b>eligibility</b> 5019 * <p> 5020 * Description: <b>Inclusion and exclusion criteria</b><br> 5021 * Type: <b>reference</b><br> 5022 * Path: <b>ResearchStudy.recruitment.eligibility</b><br> 5023 * </p> 5024 */ 5025 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ELIGIBILITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ELIGIBILITY); 5026 5027/** 5028 * Constant for fluent queries to be used to add include statements. Specifies 5029 * the path value of "<b>ResearchStudy:eligibility</b>". 5030 */ 5031 public static final ca.uhn.fhir.model.api.Include INCLUDE_ELIGIBILITY = new ca.uhn.fhir.model.api.Include("ResearchStudy:eligibility").toLocked(); 5032 5033 /** 5034 * Search parameter: <b>focus-code</b> 5035 * <p> 5036 * Description: <b>Drugs, devices, etc. under study, as a code</b><br> 5037 * Type: <b>token</b><br> 5038 * Path: <b>ResearchStudy.focus.concept</b><br> 5039 * </p> 5040 */ 5041 @SearchParamDefinition(name="focus-code", path="ResearchStudy.focus.concept", description="Drugs, devices, etc. under study, as a code", type="token" ) 5042 public static final String SP_FOCUS_CODE = "focus-code"; 5043 /** 5044 * <b>Fluent Client</b> search parameter constant for <b>focus-code</b> 5045 * <p> 5046 * Description: <b>Drugs, devices, etc. under study, as a code</b><br> 5047 * Type: <b>token</b><br> 5048 * Path: <b>ResearchStudy.focus.concept</b><br> 5049 * </p> 5050 */ 5051 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FOCUS_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FOCUS_CODE); 5052 5053 /** 5054 * Search parameter: <b>focus-reference</b> 5055 * <p> 5056 * Description: <b>Drugs, devices, etc. under study, as a reference</b><br> 5057 * Type: <b>reference</b><br> 5058 * Path: <b>ResearchStudy.focus.reference</b><br> 5059 * </p> 5060 */ 5061 @SearchParamDefinition(name="focus-reference", path="ResearchStudy.focus.reference", description="Drugs, devices, etc. under study, as a reference", type="reference", target={EvidenceVariable.class, Medication.class, MedicinalProductDefinition.class, SubstanceDefinition.class } ) 5062 public static final String SP_FOCUS_REFERENCE = "focus-reference"; 5063 /** 5064 * <b>Fluent Client</b> search parameter constant for <b>focus-reference</b> 5065 * <p> 5066 * Description: <b>Drugs, devices, etc. under study, as a reference</b><br> 5067 * Type: <b>reference</b><br> 5068 * Path: <b>ResearchStudy.focus.reference</b><br> 5069 * </p> 5070 */ 5071 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FOCUS_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FOCUS_REFERENCE); 5072 5073/** 5074 * Constant for fluent queries to be used to add include statements. Specifies 5075 * the path value of "<b>ResearchStudy:focus-reference</b>". 5076 */ 5077 public static final ca.uhn.fhir.model.api.Include INCLUDE_FOCUS_REFERENCE = new ca.uhn.fhir.model.api.Include("ResearchStudy:focus-reference").toLocked(); 5078 5079 /** 5080 * Search parameter: <b>identifier</b> 5081 * <p> 5082 * Description: <b>Business Identifier for study</b><br> 5083 * Type: <b>token</b><br> 5084 * Path: <b>ResearchStudy.identifier</b><br> 5085 * </p> 5086 */ 5087 @SearchParamDefinition(name="identifier", path="ResearchStudy.identifier", description="Business Identifier for study", type="token" ) 5088 public static final String SP_IDENTIFIER = "identifier"; 5089 /** 5090 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5091 * <p> 5092 * Description: <b>Business Identifier for study</b><br> 5093 * Type: <b>token</b><br> 5094 * Path: <b>ResearchStudy.identifier</b><br> 5095 * </p> 5096 */ 5097 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 5098 5099 /** 5100 * Search parameter: <b>keyword</b> 5101 * <p> 5102 * Description: <b>Used to search for the study</b><br> 5103 * Type: <b>token</b><br> 5104 * Path: <b>ResearchStudy.keyword</b><br> 5105 * </p> 5106 */ 5107 @SearchParamDefinition(name="keyword", path="ResearchStudy.keyword", description="Used to search for the study", type="token" ) 5108 public static final String SP_KEYWORD = "keyword"; 5109 /** 5110 * <b>Fluent Client</b> search parameter constant for <b>keyword</b> 5111 * <p> 5112 * Description: <b>Used to search for the study</b><br> 5113 * Type: <b>token</b><br> 5114 * Path: <b>ResearchStudy.keyword</b><br> 5115 * </p> 5116 */ 5117 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KEYWORD = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_KEYWORD); 5118 5119 /** 5120 * Search parameter: <b>name</b> 5121 * <p> 5122 * Description: <b>Name for this study</b><br> 5123 * Type: <b>string</b><br> 5124 * Path: <b>ResearchStudy.name</b><br> 5125 * </p> 5126 */ 5127 @SearchParamDefinition(name="name", path="ResearchStudy.name", description="Name for this study", type="string" ) 5128 public static final String SP_NAME = "name"; 5129 /** 5130 * <b>Fluent Client</b> search parameter constant for <b>name</b> 5131 * <p> 5132 * Description: <b>Name for this study</b><br> 5133 * Type: <b>string</b><br> 5134 * Path: <b>ResearchStudy.name</b><br> 5135 * </p> 5136 */ 5137 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 5138 5139 /** 5140 * Search parameter: <b>objective-description</b> 5141 * <p> 5142 * Description: <b>Free text description of the objective of the study</b><br> 5143 * Type: <b>string</b><br> 5144 * Path: <b>ResearchStudy.objective.description</b><br> 5145 * </p> 5146 */ 5147 @SearchParamDefinition(name="objective-description", path="ResearchStudy.objective.description", description="Free text description of the objective of the study", type="string" ) 5148 public static final String SP_OBJECTIVE_DESCRIPTION = "objective-description"; 5149 /** 5150 * <b>Fluent Client</b> search parameter constant for <b>objective-description</b> 5151 * <p> 5152 * Description: <b>Free text description of the objective of the study</b><br> 5153 * Type: <b>string</b><br> 5154 * Path: <b>ResearchStudy.objective.description</b><br> 5155 * </p> 5156 */ 5157 public static final ca.uhn.fhir.rest.gclient.StringClientParam OBJECTIVE_DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_OBJECTIVE_DESCRIPTION); 5158 5159 /** 5160 * Search parameter: <b>objective-type</b> 5161 * <p> 5162 * Description: <b>The kind of study objective</b><br> 5163 * Type: <b>token</b><br> 5164 * Path: <b>ResearchStudy.objective.type</b><br> 5165 * </p> 5166 */ 5167 @SearchParamDefinition(name="objective-type", path="ResearchStudy.objective.type", description="The kind of study objective", type="token" ) 5168 public static final String SP_OBJECTIVE_TYPE = "objective-type"; 5169 /** 5170 * <b>Fluent Client</b> search parameter constant for <b>objective-type</b> 5171 * <p> 5172 * Description: <b>The kind of study objective</b><br> 5173 * Type: <b>token</b><br> 5174 * Path: <b>ResearchStudy.objective.type</b><br> 5175 * </p> 5176 */ 5177 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OBJECTIVE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_OBJECTIVE_TYPE); 5178 5179 /** 5180 * Search parameter: <b>part-of</b> 5181 * <p> 5182 * Description: <b>Part of larger study</b><br> 5183 * Type: <b>reference</b><br> 5184 * Path: <b>ResearchStudy.partOf</b><br> 5185 * </p> 5186 */ 5187 @SearchParamDefinition(name="part-of", path="ResearchStudy.partOf", description="Part of larger study", type="reference", target={ResearchStudy.class } ) 5188 public static final String SP_PART_OF = "part-of"; 5189 /** 5190 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 5191 * <p> 5192 * Description: <b>Part of larger study</b><br> 5193 * Type: <b>reference</b><br> 5194 * Path: <b>ResearchStudy.partOf</b><br> 5195 * </p> 5196 */ 5197 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PART_OF); 5198 5199/** 5200 * Constant for fluent queries to be used to add include statements. Specifies 5201 * the path value of "<b>ResearchStudy:part-of</b>". 5202 */ 5203 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("ResearchStudy:part-of").toLocked(); 5204 5205 /** 5206 * Search parameter: <b>phase</b> 5207 * <p> 5208 * Description: <b>The stage in the progression of a study</b><br> 5209 * Type: <b>token</b><br> 5210 * Path: <b>ResearchStudy.phase</b><br> 5211 * </p> 5212 */ 5213 @SearchParamDefinition(name="phase", path="ResearchStudy.phase", description="The stage in the progression of a study", type="token" ) 5214 public static final String SP_PHASE = "phase"; 5215 /** 5216 * <b>Fluent Client</b> search parameter constant for <b>phase</b> 5217 * <p> 5218 * Description: <b>The stage in the progression of a study</b><br> 5219 * Type: <b>token</b><br> 5220 * Path: <b>ResearchStudy.phase</b><br> 5221 * </p> 5222 */ 5223 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHASE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PHASE); 5224 5225 /** 5226 * Search parameter: <b>protocol</b> 5227 * <p> 5228 * Description: <b>Steps followed in executing study</b><br> 5229 * Type: <b>reference</b><br> 5230 * Path: <b>ResearchStudy.protocol</b><br> 5231 * </p> 5232 */ 5233 @SearchParamDefinition(name="protocol", path="ResearchStudy.protocol", description="Steps followed in executing study", type="reference", target={PlanDefinition.class } ) 5234 public static final String SP_PROTOCOL = "protocol"; 5235 /** 5236 * <b>Fluent Client</b> search parameter constant for <b>protocol</b> 5237 * <p> 5238 * Description: <b>Steps followed in executing study</b><br> 5239 * Type: <b>reference</b><br> 5240 * Path: <b>ResearchStudy.protocol</b><br> 5241 * </p> 5242 */ 5243 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROTOCOL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROTOCOL); 5244 5245/** 5246 * Constant for fluent queries to be used to add include statements. Specifies 5247 * the path value of "<b>ResearchStudy:protocol</b>". 5248 */ 5249 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROTOCOL = new ca.uhn.fhir.model.api.Include("ResearchStudy:protocol").toLocked(); 5250 5251 /** 5252 * Search parameter: <b>recruitment-actual</b> 5253 * <p> 5254 * Description: <b>Actual number of participants enrolled in study across all groups</b><br> 5255 * Type: <b>number</b><br> 5256 * Path: <b>ResearchStudy.recruitment.actualNumber</b><br> 5257 * </p> 5258 */ 5259 @SearchParamDefinition(name="recruitment-actual", path="ResearchStudy.recruitment.actualNumber", description="Actual number of participants enrolled in study across all groups", type="number" ) 5260 public static final String SP_RECRUITMENT_ACTUAL = "recruitment-actual"; 5261 /** 5262 * <b>Fluent Client</b> search parameter constant for <b>recruitment-actual</b> 5263 * <p> 5264 * Description: <b>Actual number of participants enrolled in study across all groups</b><br> 5265 * Type: <b>number</b><br> 5266 * Path: <b>ResearchStudy.recruitment.actualNumber</b><br> 5267 * </p> 5268 */ 5269 public static final ca.uhn.fhir.rest.gclient.NumberClientParam RECRUITMENT_ACTUAL = new ca.uhn.fhir.rest.gclient.NumberClientParam(SP_RECRUITMENT_ACTUAL); 5270 5271 /** 5272 * Search parameter: <b>recruitment-target</b> 5273 * <p> 5274 * Description: <b>Target number of participants enrolled in study across all groups</b><br> 5275 * Type: <b>number</b><br> 5276 * Path: <b>ResearchStudy.recruitment.targetNumber</b><br> 5277 * </p> 5278 */ 5279 @SearchParamDefinition(name="recruitment-target", path="ResearchStudy.recruitment.targetNumber", description="Target number of participants enrolled in study across all groups", type="number" ) 5280 public static final String SP_RECRUITMENT_TARGET = "recruitment-target"; 5281 /** 5282 * <b>Fluent Client</b> search parameter constant for <b>recruitment-target</b> 5283 * <p> 5284 * Description: <b>Target number of participants enrolled in study across all groups</b><br> 5285 * Type: <b>number</b><br> 5286 * Path: <b>ResearchStudy.recruitment.targetNumber</b><br> 5287 * </p> 5288 */ 5289 public static final ca.uhn.fhir.rest.gclient.NumberClientParam RECRUITMENT_TARGET = new ca.uhn.fhir.rest.gclient.NumberClientParam(SP_RECRUITMENT_TARGET); 5290 5291 /** 5292 * Search parameter: <b>region</b> 5293 * <p> 5294 * Description: <b>Geographic area for the study</b><br> 5295 * Type: <b>token</b><br> 5296 * Path: <b>ResearchStudy.region</b><br> 5297 * </p> 5298 */ 5299 @SearchParamDefinition(name="region", path="ResearchStudy.region", description="Geographic area for the study", type="token" ) 5300 public static final String SP_REGION = "region"; 5301 /** 5302 * <b>Fluent Client</b> search parameter constant for <b>region</b> 5303 * <p> 5304 * Description: <b>Geographic area for the study</b><br> 5305 * Type: <b>token</b><br> 5306 * Path: <b>ResearchStudy.region</b><br> 5307 * </p> 5308 */ 5309 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REGION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REGION); 5310 5311 /** 5312 * Search parameter: <b>site</b> 5313 * <p> 5314 * Description: <b>Facility where study activities are conducted</b><br> 5315 * Type: <b>reference</b><br> 5316 * Path: <b>ResearchStudy.site</b><br> 5317 * </p> 5318 */ 5319 @SearchParamDefinition(name="site", path="ResearchStudy.site", description="Facility where study activities are conducted", type="reference", target={Location.class, Organization.class, ResearchStudy.class } ) 5320 public static final String SP_SITE = "site"; 5321 /** 5322 * <b>Fluent Client</b> search parameter constant for <b>site</b> 5323 * <p> 5324 * Description: <b>Facility where study activities are conducted</b><br> 5325 * Type: <b>reference</b><br> 5326 * Path: <b>ResearchStudy.site</b><br> 5327 * </p> 5328 */ 5329 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SITE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SITE); 5330 5331/** 5332 * Constant for fluent queries to be used to add include statements. Specifies 5333 * the path value of "<b>ResearchStudy:site</b>". 5334 */ 5335 public static final ca.uhn.fhir.model.api.Include INCLUDE_SITE = new ca.uhn.fhir.model.api.Include("ResearchStudy:site").toLocked(); 5336 5337 /** 5338 * Search parameter: <b>status</b> 5339 * <p> 5340 * Description: <b>active | active-but-not-recruiting | administratively-completed | approved | closed-to-accrual | closed-to-accrual-and-intervention | completed | disapproved | enrolling-by-invitation | in-review | not-yet-recruiting | recruiting | temporarily-closed-to-accrual | temporarily-closed-to-accrual-and-intervention | terminated | withdrawn</b><br> 5341 * Type: <b>token</b><br> 5342 * Path: <b>ResearchStudy.status</b><br> 5343 * </p> 5344 */ 5345 @SearchParamDefinition(name="status", path="ResearchStudy.status", description="active | active-but-not-recruiting | administratively-completed | approved | closed-to-accrual | closed-to-accrual-and-intervention | completed | disapproved | enrolling-by-invitation | in-review | not-yet-recruiting | recruiting | temporarily-closed-to-accrual | temporarily-closed-to-accrual-and-intervention | terminated | withdrawn", type="token" ) 5346 public static final String SP_STATUS = "status"; 5347 /** 5348 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5349 * <p> 5350 * Description: <b>active | active-but-not-recruiting | administratively-completed | approved | closed-to-accrual | closed-to-accrual-and-intervention | completed | disapproved | enrolling-by-invitation | in-review | not-yet-recruiting | recruiting | temporarily-closed-to-accrual | temporarily-closed-to-accrual-and-intervention | terminated | withdrawn</b><br> 5351 * Type: <b>token</b><br> 5352 * Path: <b>ResearchStudy.status</b><br> 5353 * </p> 5354 */ 5355 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 5356 5357 /** 5358 * Search parameter: <b>study-design</b> 5359 * <p> 5360 * Description: <b>Classifications of the study design characteristics</b><br> 5361 * Type: <b>token</b><br> 5362 * Path: <b>ResearchStudy.studyDesign</b><br> 5363 * </p> 5364 */ 5365 @SearchParamDefinition(name="study-design", path="ResearchStudy.studyDesign", description="Classifications of the study design characteristics", type="token" ) 5366 public static final String SP_STUDY_DESIGN = "study-design"; 5367 /** 5368 * <b>Fluent Client</b> search parameter constant for <b>study-design</b> 5369 * <p> 5370 * Description: <b>Classifications of the study design characteristics</b><br> 5371 * Type: <b>token</b><br> 5372 * Path: <b>ResearchStudy.studyDesign</b><br> 5373 * </p> 5374 */ 5375 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STUDY_DESIGN = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STUDY_DESIGN); 5376 5377 /** 5378 * Search parameter: <b>title</b> 5379 * <p> 5380 * Description: <b>The human readable name of the research study</b><br> 5381 * Type: <b>string</b><br> 5382 * Path: <b>ResearchStudy.title</b><br> 5383 * </p> 5384 */ 5385 @SearchParamDefinition(name="title", path="ResearchStudy.title", description="The human readable name of the research study", type="string" ) 5386 public static final String SP_TITLE = "title"; 5387 /** 5388 * <b>Fluent Client</b> search parameter constant for <b>title</b> 5389 * <p> 5390 * Description: <b>The human readable name of the research study</b><br> 5391 * Type: <b>string</b><br> 5392 * Path: <b>ResearchStudy.title</b><br> 5393 * </p> 5394 */ 5395 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 5396 5397 5398} 5399