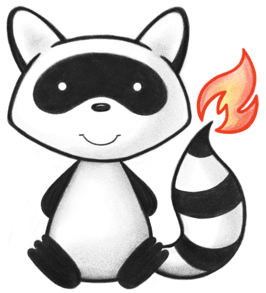
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A scientific study of nature that sometimes includes processes involved in health and disease. For example, clinical trials are research studies that involve people. These studies may be related to new ways to screen, prevent, diagnose, and treat disease. They may also study certain outcomes and certain groups of people by looking at data collected in the past or future. 052 */ 053@ResourceDef(name="ResearchStudy", profile="http://hl7.org/fhir/StructureDefinition/ResearchStudy") 054public class ResearchStudy extends DomainResource { 055 056 @Block() 057 public static class ResearchStudyLabelComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Kind of name. 060 */ 061 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="primary | official | scientific | plain-language | subtitle | short-title | acronym | earlier-title | language | auto-translated | human-use | machine-use | duplicate-uid", formalDefinition="Kind of name." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/title-type") 064 protected CodeableConcept type; 065 066 /** 067 * The name. 068 */ 069 @Child(name = "value", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 070 @Description(shortDefinition="The name", formalDefinition="The name." ) 071 protected StringType value; 072 073 private static final long serialVersionUID = 944223389L; 074 075 /** 076 * Constructor 077 */ 078 public ResearchStudyLabelComponent() { 079 super(); 080 } 081 082 /** 083 * @return {@link #type} (Kind of name.) 084 */ 085 public CodeableConcept getType() { 086 if (this.type == null) 087 if (Configuration.errorOnAutoCreate()) 088 throw new Error("Attempt to auto-create ResearchStudyLabelComponent.type"); 089 else if (Configuration.doAutoCreate()) 090 this.type = new CodeableConcept(); // cc 091 return this.type; 092 } 093 094 public boolean hasType() { 095 return this.type != null && !this.type.isEmpty(); 096 } 097 098 /** 099 * @param value {@link #type} (Kind of name.) 100 */ 101 public ResearchStudyLabelComponent setType(CodeableConcept value) { 102 this.type = value; 103 return this; 104 } 105 106 /** 107 * @return {@link #value} (The name.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 108 */ 109 public StringType getValueElement() { 110 if (this.value == null) 111 if (Configuration.errorOnAutoCreate()) 112 throw new Error("Attempt to auto-create ResearchStudyLabelComponent.value"); 113 else if (Configuration.doAutoCreate()) 114 this.value = new StringType(); // bb 115 return this.value; 116 } 117 118 public boolean hasValueElement() { 119 return this.value != null && !this.value.isEmpty(); 120 } 121 122 public boolean hasValue() { 123 return this.value != null && !this.value.isEmpty(); 124 } 125 126 /** 127 * @param value {@link #value} (The name.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 128 */ 129 public ResearchStudyLabelComponent setValueElement(StringType value) { 130 this.value = value; 131 return this; 132 } 133 134 /** 135 * @return The name. 136 */ 137 public String getValue() { 138 return this.value == null ? null : this.value.getValue(); 139 } 140 141 /** 142 * @param value The name. 143 */ 144 public ResearchStudyLabelComponent setValue(String value) { 145 if (Utilities.noString(value)) 146 this.value = null; 147 else { 148 if (this.value == null) 149 this.value = new StringType(); 150 this.value.setValue(value); 151 } 152 return this; 153 } 154 155 protected void listChildren(List<Property> children) { 156 super.listChildren(children); 157 children.add(new Property("type", "CodeableConcept", "Kind of name.", 0, 1, type)); 158 children.add(new Property("value", "string", "The name.", 0, 1, value)); 159 } 160 161 @Override 162 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 163 switch (_hash) { 164 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Kind of name.", 0, 1, type); 165 case 111972721: /*value*/ return new Property("value", "string", "The name.", 0, 1, value); 166 default: return super.getNamedProperty(_hash, _name, _checkValid); 167 } 168 169 } 170 171 @Override 172 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 173 switch (hash) { 174 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 175 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 176 default: return super.getProperty(hash, name, checkValid); 177 } 178 179 } 180 181 @Override 182 public Base setProperty(int hash, String name, Base value) throws FHIRException { 183 switch (hash) { 184 case 3575610: // type 185 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 186 return value; 187 case 111972721: // value 188 this.value = TypeConvertor.castToString(value); // StringType 189 return value; 190 default: return super.setProperty(hash, name, value); 191 } 192 193 } 194 195 @Override 196 public Base setProperty(String name, Base value) throws FHIRException { 197 if (name.equals("type")) { 198 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 199 } else if (name.equals("value")) { 200 this.value = TypeConvertor.castToString(value); // StringType 201 } else 202 return super.setProperty(name, value); 203 return value; 204 } 205 206 @Override 207 public void removeChild(String name, Base value) throws FHIRException { 208 if (name.equals("type")) { 209 this.type = null; 210 } else if (name.equals("value")) { 211 this.value = null; 212 } else 213 super.removeChild(name, value); 214 215 } 216 217 @Override 218 public Base makeProperty(int hash, String name) throws FHIRException { 219 switch (hash) { 220 case 3575610: return getType(); 221 case 111972721: return getValueElement(); 222 default: return super.makeProperty(hash, name); 223 } 224 225 } 226 227 @Override 228 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 229 switch (hash) { 230 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 231 case 111972721: /*value*/ return new String[] {"string"}; 232 default: return super.getTypesForProperty(hash, name); 233 } 234 235 } 236 237 @Override 238 public Base addChild(String name) throws FHIRException { 239 if (name.equals("type")) { 240 this.type = new CodeableConcept(); 241 return this.type; 242 } 243 else if (name.equals("value")) { 244 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.label.value"); 245 } 246 else 247 return super.addChild(name); 248 } 249 250 public ResearchStudyLabelComponent copy() { 251 ResearchStudyLabelComponent dst = new ResearchStudyLabelComponent(); 252 copyValues(dst); 253 return dst; 254 } 255 256 public void copyValues(ResearchStudyLabelComponent dst) { 257 super.copyValues(dst); 258 dst.type = type == null ? null : type.copy(); 259 dst.value = value == null ? null : value.copy(); 260 } 261 262 @Override 263 public boolean equalsDeep(Base other_) { 264 if (!super.equalsDeep(other_)) 265 return false; 266 if (!(other_ instanceof ResearchStudyLabelComponent)) 267 return false; 268 ResearchStudyLabelComponent o = (ResearchStudyLabelComponent) other_; 269 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 270 } 271 272 @Override 273 public boolean equalsShallow(Base other_) { 274 if (!super.equalsShallow(other_)) 275 return false; 276 if (!(other_ instanceof ResearchStudyLabelComponent)) 277 return false; 278 ResearchStudyLabelComponent o = (ResearchStudyLabelComponent) other_; 279 return compareValues(value, o.value, true); 280 } 281 282 public boolean isEmpty() { 283 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 284 } 285 286 public String fhirType() { 287 return "ResearchStudy.label"; 288 289 } 290 291 } 292 293 @Block() 294 public static class ResearchStudyAssociatedPartyComponent extends BackboneElement implements IBaseBackboneElement { 295 /** 296 * Name of associated party. 297 */ 298 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 299 @Description(shortDefinition="Name of associated party", formalDefinition="Name of associated party." ) 300 protected StringType name; 301 302 /** 303 * Type of association. 304 */ 305 @Child(name = "role", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 306 @Description(shortDefinition="sponsor | lead-sponsor | sponsor-investigator | primary-investigator | collaborator | funding-source | general-contact | recruitment-contact | sub-investigator | study-director | study-chair", formalDefinition="Type of association." ) 307 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-party-role") 308 protected CodeableConcept role; 309 310 /** 311 * Identifies the start date and the end date of the associated party in the role. 312 */ 313 @Child(name = "period", type = {Period.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 314 @Description(shortDefinition="When active in the role", formalDefinition="Identifies the start date and the end date of the associated party in the role." ) 315 protected List<Period> period; 316 317 /** 318 * A categorization other than role for the associated party. 319 */ 320 @Child(name = "classifier", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 321 @Description(shortDefinition="nih | fda | government | nonprofit | academic | industry", formalDefinition="A categorization other than role for the associated party." ) 322 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-party-organization-type") 323 protected List<CodeableConcept> classifier; 324 325 /** 326 * Individual or organization associated with study (use practitionerRole to specify their organisation). 327 */ 328 @Child(name = "party", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=5, min=0, max=1, modifier=false, summary=false) 329 @Description(shortDefinition="Individual or organization associated with study (use practitionerRole to specify their organisation)", formalDefinition="Individual or organization associated with study (use practitionerRole to specify their organisation)." ) 330 protected Reference party; 331 332 private static final long serialVersionUID = -1418550998L; 333 334 /** 335 * Constructor 336 */ 337 public ResearchStudyAssociatedPartyComponent() { 338 super(); 339 } 340 341 /** 342 * Constructor 343 */ 344 public ResearchStudyAssociatedPartyComponent(CodeableConcept role) { 345 super(); 346 this.setRole(role); 347 } 348 349 /** 350 * @return {@link #name} (Name of associated party.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 351 */ 352 public StringType getNameElement() { 353 if (this.name == null) 354 if (Configuration.errorOnAutoCreate()) 355 throw new Error("Attempt to auto-create ResearchStudyAssociatedPartyComponent.name"); 356 else if (Configuration.doAutoCreate()) 357 this.name = new StringType(); // bb 358 return this.name; 359 } 360 361 public boolean hasNameElement() { 362 return this.name != null && !this.name.isEmpty(); 363 } 364 365 public boolean hasName() { 366 return this.name != null && !this.name.isEmpty(); 367 } 368 369 /** 370 * @param value {@link #name} (Name of associated party.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 371 */ 372 public ResearchStudyAssociatedPartyComponent setNameElement(StringType value) { 373 this.name = value; 374 return this; 375 } 376 377 /** 378 * @return Name of associated party. 379 */ 380 public String getName() { 381 return this.name == null ? null : this.name.getValue(); 382 } 383 384 /** 385 * @param value Name of associated party. 386 */ 387 public ResearchStudyAssociatedPartyComponent setName(String value) { 388 if (Utilities.noString(value)) 389 this.name = null; 390 else { 391 if (this.name == null) 392 this.name = new StringType(); 393 this.name.setValue(value); 394 } 395 return this; 396 } 397 398 /** 399 * @return {@link #role} (Type of association.) 400 */ 401 public CodeableConcept getRole() { 402 if (this.role == null) 403 if (Configuration.errorOnAutoCreate()) 404 throw new Error("Attempt to auto-create ResearchStudyAssociatedPartyComponent.role"); 405 else if (Configuration.doAutoCreate()) 406 this.role = new CodeableConcept(); // cc 407 return this.role; 408 } 409 410 public boolean hasRole() { 411 return this.role != null && !this.role.isEmpty(); 412 } 413 414 /** 415 * @param value {@link #role} (Type of association.) 416 */ 417 public ResearchStudyAssociatedPartyComponent setRole(CodeableConcept value) { 418 this.role = value; 419 return this; 420 } 421 422 /** 423 * @return {@link #period} (Identifies the start date and the end date of the associated party in the role.) 424 */ 425 public List<Period> getPeriod() { 426 if (this.period == null) 427 this.period = new ArrayList<Period>(); 428 return this.period; 429 } 430 431 /** 432 * @return Returns a reference to <code>this</code> for easy method chaining 433 */ 434 public ResearchStudyAssociatedPartyComponent setPeriod(List<Period> thePeriod) { 435 this.period = thePeriod; 436 return this; 437 } 438 439 public boolean hasPeriod() { 440 if (this.period == null) 441 return false; 442 for (Period item : this.period) 443 if (!item.isEmpty()) 444 return true; 445 return false; 446 } 447 448 public Period addPeriod() { //3 449 Period t = new Period(); 450 if (this.period == null) 451 this.period = new ArrayList<Period>(); 452 this.period.add(t); 453 return t; 454 } 455 456 public ResearchStudyAssociatedPartyComponent addPeriod(Period t) { //3 457 if (t == null) 458 return this; 459 if (this.period == null) 460 this.period = new ArrayList<Period>(); 461 this.period.add(t); 462 return this; 463 } 464 465 /** 466 * @return The first repetition of repeating field {@link #period}, creating it if it does not already exist {3} 467 */ 468 public Period getPeriodFirstRep() { 469 if (getPeriod().isEmpty()) { 470 addPeriod(); 471 } 472 return getPeriod().get(0); 473 } 474 475 /** 476 * @return {@link #classifier} (A categorization other than role for the associated party.) 477 */ 478 public List<CodeableConcept> getClassifier() { 479 if (this.classifier == null) 480 this.classifier = new ArrayList<CodeableConcept>(); 481 return this.classifier; 482 } 483 484 /** 485 * @return Returns a reference to <code>this</code> for easy method chaining 486 */ 487 public ResearchStudyAssociatedPartyComponent setClassifier(List<CodeableConcept> theClassifier) { 488 this.classifier = theClassifier; 489 return this; 490 } 491 492 public boolean hasClassifier() { 493 if (this.classifier == null) 494 return false; 495 for (CodeableConcept item : this.classifier) 496 if (!item.isEmpty()) 497 return true; 498 return false; 499 } 500 501 public CodeableConcept addClassifier() { //3 502 CodeableConcept t = new CodeableConcept(); 503 if (this.classifier == null) 504 this.classifier = new ArrayList<CodeableConcept>(); 505 this.classifier.add(t); 506 return t; 507 } 508 509 public ResearchStudyAssociatedPartyComponent addClassifier(CodeableConcept t) { //3 510 if (t == null) 511 return this; 512 if (this.classifier == null) 513 this.classifier = new ArrayList<CodeableConcept>(); 514 this.classifier.add(t); 515 return this; 516 } 517 518 /** 519 * @return The first repetition of repeating field {@link #classifier}, creating it if it does not already exist {3} 520 */ 521 public CodeableConcept getClassifierFirstRep() { 522 if (getClassifier().isEmpty()) { 523 addClassifier(); 524 } 525 return getClassifier().get(0); 526 } 527 528 /** 529 * @return {@link #party} (Individual or organization associated with study (use practitionerRole to specify their organisation).) 530 */ 531 public Reference getParty() { 532 if (this.party == null) 533 if (Configuration.errorOnAutoCreate()) 534 throw new Error("Attempt to auto-create ResearchStudyAssociatedPartyComponent.party"); 535 else if (Configuration.doAutoCreate()) 536 this.party = new Reference(); // cc 537 return this.party; 538 } 539 540 public boolean hasParty() { 541 return this.party != null && !this.party.isEmpty(); 542 } 543 544 /** 545 * @param value {@link #party} (Individual or organization associated with study (use practitionerRole to specify their organisation).) 546 */ 547 public ResearchStudyAssociatedPartyComponent setParty(Reference value) { 548 this.party = value; 549 return this; 550 } 551 552 protected void listChildren(List<Property> children) { 553 super.listChildren(children); 554 children.add(new Property("name", "string", "Name of associated party.", 0, 1, name)); 555 children.add(new Property("role", "CodeableConcept", "Type of association.", 0, 1, role)); 556 children.add(new Property("period", "Period", "Identifies the start date and the end date of the associated party in the role.", 0, java.lang.Integer.MAX_VALUE, period)); 557 children.add(new Property("classifier", "CodeableConcept", "A categorization other than role for the associated party.", 0, java.lang.Integer.MAX_VALUE, classifier)); 558 children.add(new Property("party", "Reference(Practitioner|PractitionerRole|Organization)", "Individual or organization associated with study (use practitionerRole to specify their organisation).", 0, 1, party)); 559 } 560 561 @Override 562 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 563 switch (_hash) { 564 case 3373707: /*name*/ return new Property("name", "string", "Name of associated party.", 0, 1, name); 565 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Type of association.", 0, 1, role); 566 case -991726143: /*period*/ return new Property("period", "Period", "Identifies the start date and the end date of the associated party in the role.", 0, java.lang.Integer.MAX_VALUE, period); 567 case -281470431: /*classifier*/ return new Property("classifier", "CodeableConcept", "A categorization other than role for the associated party.", 0, java.lang.Integer.MAX_VALUE, classifier); 568 case 106437350: /*party*/ return new Property("party", "Reference(Practitioner|PractitionerRole|Organization)", "Individual or organization associated with study (use practitionerRole to specify their organisation).", 0, 1, party); 569 default: return super.getNamedProperty(_hash, _name, _checkValid); 570 } 571 572 } 573 574 @Override 575 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 576 switch (hash) { 577 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 578 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 579 case -991726143: /*period*/ return this.period == null ? new Base[0] : this.period.toArray(new Base[this.period.size()]); // Period 580 case -281470431: /*classifier*/ return this.classifier == null ? new Base[0] : this.classifier.toArray(new Base[this.classifier.size()]); // CodeableConcept 581 case 106437350: /*party*/ return this.party == null ? new Base[0] : new Base[] {this.party}; // Reference 582 default: return super.getProperty(hash, name, checkValid); 583 } 584 585 } 586 587 @Override 588 public Base setProperty(int hash, String name, Base value) throws FHIRException { 589 switch (hash) { 590 case 3373707: // name 591 this.name = TypeConvertor.castToString(value); // StringType 592 return value; 593 case 3506294: // role 594 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 595 return value; 596 case -991726143: // period 597 this.getPeriod().add(TypeConvertor.castToPeriod(value)); // Period 598 return value; 599 case -281470431: // classifier 600 this.getClassifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 601 return value; 602 case 106437350: // party 603 this.party = TypeConvertor.castToReference(value); // Reference 604 return value; 605 default: return super.setProperty(hash, name, value); 606 } 607 608 } 609 610 @Override 611 public Base setProperty(String name, Base value) throws FHIRException { 612 if (name.equals("name")) { 613 this.name = TypeConvertor.castToString(value); // StringType 614 } else if (name.equals("role")) { 615 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 616 } else if (name.equals("period")) { 617 this.getPeriod().add(TypeConvertor.castToPeriod(value)); 618 } else if (name.equals("classifier")) { 619 this.getClassifier().add(TypeConvertor.castToCodeableConcept(value)); 620 } else if (name.equals("party")) { 621 this.party = TypeConvertor.castToReference(value); // Reference 622 } else 623 return super.setProperty(name, value); 624 return value; 625 } 626 627 @Override 628 public void removeChild(String name, Base value) throws FHIRException { 629 if (name.equals("name")) { 630 this.name = null; 631 } else if (name.equals("role")) { 632 this.role = null; 633 } else if (name.equals("period")) { 634 this.getPeriod().remove(value); 635 } else if (name.equals("classifier")) { 636 this.getClassifier().remove(value); 637 } else if (name.equals("party")) { 638 this.party = null; 639 } else 640 super.removeChild(name, value); 641 642 } 643 644 @Override 645 public Base makeProperty(int hash, String name) throws FHIRException { 646 switch (hash) { 647 case 3373707: return getNameElement(); 648 case 3506294: return getRole(); 649 case -991726143: return addPeriod(); 650 case -281470431: return addClassifier(); 651 case 106437350: return getParty(); 652 default: return super.makeProperty(hash, name); 653 } 654 655 } 656 657 @Override 658 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 659 switch (hash) { 660 case 3373707: /*name*/ return new String[] {"string"}; 661 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 662 case -991726143: /*period*/ return new String[] {"Period"}; 663 case -281470431: /*classifier*/ return new String[] {"CodeableConcept"}; 664 case 106437350: /*party*/ return new String[] {"Reference"}; 665 default: return super.getTypesForProperty(hash, name); 666 } 667 668 } 669 670 @Override 671 public Base addChild(String name) throws FHIRException { 672 if (name.equals("name")) { 673 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.associatedParty.name"); 674 } 675 else if (name.equals("role")) { 676 this.role = new CodeableConcept(); 677 return this.role; 678 } 679 else if (name.equals("period")) { 680 return addPeriod(); 681 } 682 else if (name.equals("classifier")) { 683 return addClassifier(); 684 } 685 else if (name.equals("party")) { 686 this.party = new Reference(); 687 return this.party; 688 } 689 else 690 return super.addChild(name); 691 } 692 693 public ResearchStudyAssociatedPartyComponent copy() { 694 ResearchStudyAssociatedPartyComponent dst = new ResearchStudyAssociatedPartyComponent(); 695 copyValues(dst); 696 return dst; 697 } 698 699 public void copyValues(ResearchStudyAssociatedPartyComponent dst) { 700 super.copyValues(dst); 701 dst.name = name == null ? null : name.copy(); 702 dst.role = role == null ? null : role.copy(); 703 if (period != null) { 704 dst.period = new ArrayList<Period>(); 705 for (Period i : period) 706 dst.period.add(i.copy()); 707 }; 708 if (classifier != null) { 709 dst.classifier = new ArrayList<CodeableConcept>(); 710 for (CodeableConcept i : classifier) 711 dst.classifier.add(i.copy()); 712 }; 713 dst.party = party == null ? null : party.copy(); 714 } 715 716 @Override 717 public boolean equalsDeep(Base other_) { 718 if (!super.equalsDeep(other_)) 719 return false; 720 if (!(other_ instanceof ResearchStudyAssociatedPartyComponent)) 721 return false; 722 ResearchStudyAssociatedPartyComponent o = (ResearchStudyAssociatedPartyComponent) other_; 723 return compareDeep(name, o.name, true) && compareDeep(role, o.role, true) && compareDeep(period, o.period, true) 724 && compareDeep(classifier, o.classifier, true) && compareDeep(party, o.party, true); 725 } 726 727 @Override 728 public boolean equalsShallow(Base other_) { 729 if (!super.equalsShallow(other_)) 730 return false; 731 if (!(other_ instanceof ResearchStudyAssociatedPartyComponent)) 732 return false; 733 ResearchStudyAssociatedPartyComponent o = (ResearchStudyAssociatedPartyComponent) other_; 734 return compareValues(name, o.name, true); 735 } 736 737 public boolean isEmpty() { 738 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, role, period, classifier 739 , party); 740 } 741 742 public String fhirType() { 743 return "ResearchStudy.associatedParty"; 744 745 } 746 747 } 748 749 @Block() 750 public static class ResearchStudyProgressStatusComponent extends BackboneElement implements IBaseBackboneElement { 751 /** 752 * Label for status or state (e.g. recruitment status). 753 */ 754 @Child(name = "state", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 755 @Description(shortDefinition="Label for status or state (e.g. recruitment status)", formalDefinition="Label for status or state (e.g. recruitment status)." ) 756 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-status") 757 protected CodeableConcept state; 758 759 /** 760 * An indication of whether or not the date is a known date when the state changed or will change. A value of true indicates a known date. A value of false indicates an estimated date. 761 */ 762 @Child(name = "actual", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 763 @Description(shortDefinition="Actual if true else anticipated", formalDefinition="An indication of whether or not the date is a known date when the state changed or will change. A value of true indicates a known date. A value of false indicates an estimated date." ) 764 protected BooleanType actual; 765 766 /** 767 * Date range. 768 */ 769 @Child(name = "period", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=false) 770 @Description(shortDefinition="Date range", formalDefinition="Date range." ) 771 protected Period period; 772 773 private static final long serialVersionUID = 1232680620L; 774 775 /** 776 * Constructor 777 */ 778 public ResearchStudyProgressStatusComponent() { 779 super(); 780 } 781 782 /** 783 * Constructor 784 */ 785 public ResearchStudyProgressStatusComponent(CodeableConcept state) { 786 super(); 787 this.setState(state); 788 } 789 790 /** 791 * @return {@link #state} (Label for status or state (e.g. recruitment status).) 792 */ 793 public CodeableConcept getState() { 794 if (this.state == null) 795 if (Configuration.errorOnAutoCreate()) 796 throw new Error("Attempt to auto-create ResearchStudyProgressStatusComponent.state"); 797 else if (Configuration.doAutoCreate()) 798 this.state = new CodeableConcept(); // cc 799 return this.state; 800 } 801 802 public boolean hasState() { 803 return this.state != null && !this.state.isEmpty(); 804 } 805 806 /** 807 * @param value {@link #state} (Label for status or state (e.g. recruitment status).) 808 */ 809 public ResearchStudyProgressStatusComponent setState(CodeableConcept value) { 810 this.state = value; 811 return this; 812 } 813 814 /** 815 * @return {@link #actual} (An indication of whether or not the date is a known date when the state changed or will change. A value of true indicates a known date. A value of false indicates an estimated date.). This is the underlying object with id, value and extensions. The accessor "getActual" gives direct access to the value 816 */ 817 public BooleanType getActualElement() { 818 if (this.actual == null) 819 if (Configuration.errorOnAutoCreate()) 820 throw new Error("Attempt to auto-create ResearchStudyProgressStatusComponent.actual"); 821 else if (Configuration.doAutoCreate()) 822 this.actual = new BooleanType(); // bb 823 return this.actual; 824 } 825 826 public boolean hasActualElement() { 827 return this.actual != null && !this.actual.isEmpty(); 828 } 829 830 public boolean hasActual() { 831 return this.actual != null && !this.actual.isEmpty(); 832 } 833 834 /** 835 * @param value {@link #actual} (An indication of whether or not the date is a known date when the state changed or will change. A value of true indicates a known date. A value of false indicates an estimated date.). This is the underlying object with id, value and extensions. The accessor "getActual" gives direct access to the value 836 */ 837 public ResearchStudyProgressStatusComponent setActualElement(BooleanType value) { 838 this.actual = value; 839 return this; 840 } 841 842 /** 843 * @return An indication of whether or not the date is a known date when the state changed or will change. A value of true indicates a known date. A value of false indicates an estimated date. 844 */ 845 public boolean getActual() { 846 return this.actual == null || this.actual.isEmpty() ? false : this.actual.getValue(); 847 } 848 849 /** 850 * @param value An indication of whether or not the date is a known date when the state changed or will change. A value of true indicates a known date. A value of false indicates an estimated date. 851 */ 852 public ResearchStudyProgressStatusComponent setActual(boolean value) { 853 if (this.actual == null) 854 this.actual = new BooleanType(); 855 this.actual.setValue(value); 856 return this; 857 } 858 859 /** 860 * @return {@link #period} (Date range.) 861 */ 862 public Period getPeriod() { 863 if (this.period == null) 864 if (Configuration.errorOnAutoCreate()) 865 throw new Error("Attempt to auto-create ResearchStudyProgressStatusComponent.period"); 866 else if (Configuration.doAutoCreate()) 867 this.period = new Period(); // cc 868 return this.period; 869 } 870 871 public boolean hasPeriod() { 872 return this.period != null && !this.period.isEmpty(); 873 } 874 875 /** 876 * @param value {@link #period} (Date range.) 877 */ 878 public ResearchStudyProgressStatusComponent setPeriod(Period value) { 879 this.period = value; 880 return this; 881 } 882 883 protected void listChildren(List<Property> children) { 884 super.listChildren(children); 885 children.add(new Property("state", "CodeableConcept", "Label for status or state (e.g. recruitment status).", 0, 1, state)); 886 children.add(new Property("actual", "boolean", "An indication of whether or not the date is a known date when the state changed or will change. A value of true indicates a known date. A value of false indicates an estimated date.", 0, 1, actual)); 887 children.add(new Property("period", "Period", "Date range.", 0, 1, period)); 888 } 889 890 @Override 891 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 892 switch (_hash) { 893 case 109757585: /*state*/ return new Property("state", "CodeableConcept", "Label for status or state (e.g. recruitment status).", 0, 1, state); 894 case -1422939762: /*actual*/ return new Property("actual", "boolean", "An indication of whether or not the date is a known date when the state changed or will change. A value of true indicates a known date. A value of false indicates an estimated date.", 0, 1, actual); 895 case -991726143: /*period*/ return new Property("period", "Period", "Date range.", 0, 1, period); 896 default: return super.getNamedProperty(_hash, _name, _checkValid); 897 } 898 899 } 900 901 @Override 902 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 903 switch (hash) { 904 case 109757585: /*state*/ return this.state == null ? new Base[0] : new Base[] {this.state}; // CodeableConcept 905 case -1422939762: /*actual*/ return this.actual == null ? new Base[0] : new Base[] {this.actual}; // BooleanType 906 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 907 default: return super.getProperty(hash, name, checkValid); 908 } 909 910 } 911 912 @Override 913 public Base setProperty(int hash, String name, Base value) throws FHIRException { 914 switch (hash) { 915 case 109757585: // state 916 this.state = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 917 return value; 918 case -1422939762: // actual 919 this.actual = TypeConvertor.castToBoolean(value); // BooleanType 920 return value; 921 case -991726143: // period 922 this.period = TypeConvertor.castToPeriod(value); // Period 923 return value; 924 default: return super.setProperty(hash, name, value); 925 } 926 927 } 928 929 @Override 930 public Base setProperty(String name, Base value) throws FHIRException { 931 if (name.equals("state")) { 932 this.state = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 933 } else if (name.equals("actual")) { 934 this.actual = TypeConvertor.castToBoolean(value); // BooleanType 935 } else if (name.equals("period")) { 936 this.period = TypeConvertor.castToPeriod(value); // Period 937 } else 938 return super.setProperty(name, value); 939 return value; 940 } 941 942 @Override 943 public void removeChild(String name, Base value) throws FHIRException { 944 if (name.equals("state")) { 945 this.state = null; 946 } else if (name.equals("actual")) { 947 this.actual = null; 948 } else if (name.equals("period")) { 949 this.period = null; 950 } else 951 super.removeChild(name, value); 952 953 } 954 955 @Override 956 public Base makeProperty(int hash, String name) throws FHIRException { 957 switch (hash) { 958 case 109757585: return getState(); 959 case -1422939762: return getActualElement(); 960 case -991726143: return getPeriod(); 961 default: return super.makeProperty(hash, name); 962 } 963 964 } 965 966 @Override 967 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 968 switch (hash) { 969 case 109757585: /*state*/ return new String[] {"CodeableConcept"}; 970 case -1422939762: /*actual*/ return new String[] {"boolean"}; 971 case -991726143: /*period*/ return new String[] {"Period"}; 972 default: return super.getTypesForProperty(hash, name); 973 } 974 975 } 976 977 @Override 978 public Base addChild(String name) throws FHIRException { 979 if (name.equals("state")) { 980 this.state = new CodeableConcept(); 981 return this.state; 982 } 983 else if (name.equals("actual")) { 984 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.progressStatus.actual"); 985 } 986 else if (name.equals("period")) { 987 this.period = new Period(); 988 return this.period; 989 } 990 else 991 return super.addChild(name); 992 } 993 994 public ResearchStudyProgressStatusComponent copy() { 995 ResearchStudyProgressStatusComponent dst = new ResearchStudyProgressStatusComponent(); 996 copyValues(dst); 997 return dst; 998 } 999 1000 public void copyValues(ResearchStudyProgressStatusComponent dst) { 1001 super.copyValues(dst); 1002 dst.state = state == null ? null : state.copy(); 1003 dst.actual = actual == null ? null : actual.copy(); 1004 dst.period = period == null ? null : period.copy(); 1005 } 1006 1007 @Override 1008 public boolean equalsDeep(Base other_) { 1009 if (!super.equalsDeep(other_)) 1010 return false; 1011 if (!(other_ instanceof ResearchStudyProgressStatusComponent)) 1012 return false; 1013 ResearchStudyProgressStatusComponent o = (ResearchStudyProgressStatusComponent) other_; 1014 return compareDeep(state, o.state, true) && compareDeep(actual, o.actual, true) && compareDeep(period, o.period, true) 1015 ; 1016 } 1017 1018 @Override 1019 public boolean equalsShallow(Base other_) { 1020 if (!super.equalsShallow(other_)) 1021 return false; 1022 if (!(other_ instanceof ResearchStudyProgressStatusComponent)) 1023 return false; 1024 ResearchStudyProgressStatusComponent o = (ResearchStudyProgressStatusComponent) other_; 1025 return compareValues(actual, o.actual, true); 1026 } 1027 1028 public boolean isEmpty() { 1029 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(state, actual, period); 1030 } 1031 1032 public String fhirType() { 1033 return "ResearchStudy.progressStatus"; 1034 1035 } 1036 1037 } 1038 1039 @Block() 1040 public static class ResearchStudyRecruitmentComponent extends BackboneElement implements IBaseBackboneElement { 1041 /** 1042 * Estimated total number of participants to be enrolled. 1043 */ 1044 @Child(name = "targetNumber", type = {UnsignedIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1045 @Description(shortDefinition="Estimated total number of participants to be enrolled", formalDefinition="Estimated total number of participants to be enrolled." ) 1046 protected UnsignedIntType targetNumber; 1047 1048 /** 1049 * Actual total number of participants enrolled in study. 1050 */ 1051 @Child(name = "actualNumber", type = {UnsignedIntType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1052 @Description(shortDefinition="Actual total number of participants enrolled in study", formalDefinition="Actual total number of participants enrolled in study." ) 1053 protected UnsignedIntType actualNumber; 1054 1055 /** 1056 * Inclusion and exclusion criteria. 1057 */ 1058 @Child(name = "eligibility", type = {Group.class, EvidenceVariable.class}, order=3, min=0, max=1, modifier=false, summary=false) 1059 @Description(shortDefinition="Inclusion and exclusion criteria", formalDefinition="Inclusion and exclusion criteria." ) 1060 protected Reference eligibility; 1061 1062 /** 1063 * Group of participants who were enrolled in study. 1064 */ 1065 @Child(name = "actualGroup", type = {Group.class}, order=4, min=0, max=1, modifier=false, summary=true) 1066 @Description(shortDefinition="Group of participants who were enrolled in study", formalDefinition="Group of participants who were enrolled in study." ) 1067 protected Reference actualGroup; 1068 1069 private static final long serialVersionUID = 1483229827L; 1070 1071 /** 1072 * Constructor 1073 */ 1074 public ResearchStudyRecruitmentComponent() { 1075 super(); 1076 } 1077 1078 /** 1079 * @return {@link #targetNumber} (Estimated total number of participants to be enrolled.). This is the underlying object with id, value and extensions. The accessor "getTargetNumber" gives direct access to the value 1080 */ 1081 public UnsignedIntType getTargetNumberElement() { 1082 if (this.targetNumber == null) 1083 if (Configuration.errorOnAutoCreate()) 1084 throw new Error("Attempt to auto-create ResearchStudyRecruitmentComponent.targetNumber"); 1085 else if (Configuration.doAutoCreate()) 1086 this.targetNumber = new UnsignedIntType(); // bb 1087 return this.targetNumber; 1088 } 1089 1090 public boolean hasTargetNumberElement() { 1091 return this.targetNumber != null && !this.targetNumber.isEmpty(); 1092 } 1093 1094 public boolean hasTargetNumber() { 1095 return this.targetNumber != null && !this.targetNumber.isEmpty(); 1096 } 1097 1098 /** 1099 * @param value {@link #targetNumber} (Estimated total number of participants to be enrolled.). This is the underlying object with id, value and extensions. The accessor "getTargetNumber" gives direct access to the value 1100 */ 1101 public ResearchStudyRecruitmentComponent setTargetNumberElement(UnsignedIntType value) { 1102 this.targetNumber = value; 1103 return this; 1104 } 1105 1106 /** 1107 * @return Estimated total number of participants to be enrolled. 1108 */ 1109 public int getTargetNumber() { 1110 return this.targetNumber == null || this.targetNumber.isEmpty() ? 0 : this.targetNumber.getValue(); 1111 } 1112 1113 /** 1114 * @param value Estimated total number of participants to be enrolled. 1115 */ 1116 public ResearchStudyRecruitmentComponent setTargetNumber(int value) { 1117 if (this.targetNumber == null) 1118 this.targetNumber = new UnsignedIntType(); 1119 this.targetNumber.setValue(value); 1120 return this; 1121 } 1122 1123 /** 1124 * @return {@link #actualNumber} (Actual total number of participants enrolled in study.). This is the underlying object with id, value and extensions. The accessor "getActualNumber" gives direct access to the value 1125 */ 1126 public UnsignedIntType getActualNumberElement() { 1127 if (this.actualNumber == null) 1128 if (Configuration.errorOnAutoCreate()) 1129 throw new Error("Attempt to auto-create ResearchStudyRecruitmentComponent.actualNumber"); 1130 else if (Configuration.doAutoCreate()) 1131 this.actualNumber = new UnsignedIntType(); // bb 1132 return this.actualNumber; 1133 } 1134 1135 public boolean hasActualNumberElement() { 1136 return this.actualNumber != null && !this.actualNumber.isEmpty(); 1137 } 1138 1139 public boolean hasActualNumber() { 1140 return this.actualNumber != null && !this.actualNumber.isEmpty(); 1141 } 1142 1143 /** 1144 * @param value {@link #actualNumber} (Actual total number of participants enrolled in study.). This is the underlying object with id, value and extensions. The accessor "getActualNumber" gives direct access to the value 1145 */ 1146 public ResearchStudyRecruitmentComponent setActualNumberElement(UnsignedIntType value) { 1147 this.actualNumber = value; 1148 return this; 1149 } 1150 1151 /** 1152 * @return Actual total number of participants enrolled in study. 1153 */ 1154 public int getActualNumber() { 1155 return this.actualNumber == null || this.actualNumber.isEmpty() ? 0 : this.actualNumber.getValue(); 1156 } 1157 1158 /** 1159 * @param value Actual total number of participants enrolled in study. 1160 */ 1161 public ResearchStudyRecruitmentComponent setActualNumber(int value) { 1162 if (this.actualNumber == null) 1163 this.actualNumber = new UnsignedIntType(); 1164 this.actualNumber.setValue(value); 1165 return this; 1166 } 1167 1168 /** 1169 * @return {@link #eligibility} (Inclusion and exclusion criteria.) 1170 */ 1171 public Reference getEligibility() { 1172 if (this.eligibility == null) 1173 if (Configuration.errorOnAutoCreate()) 1174 throw new Error("Attempt to auto-create ResearchStudyRecruitmentComponent.eligibility"); 1175 else if (Configuration.doAutoCreate()) 1176 this.eligibility = new Reference(); // cc 1177 return this.eligibility; 1178 } 1179 1180 public boolean hasEligibility() { 1181 return this.eligibility != null && !this.eligibility.isEmpty(); 1182 } 1183 1184 /** 1185 * @param value {@link #eligibility} (Inclusion and exclusion criteria.) 1186 */ 1187 public ResearchStudyRecruitmentComponent setEligibility(Reference value) { 1188 this.eligibility = value; 1189 return this; 1190 } 1191 1192 /** 1193 * @return {@link #actualGroup} (Group of participants who were enrolled in study.) 1194 */ 1195 public Reference getActualGroup() { 1196 if (this.actualGroup == null) 1197 if (Configuration.errorOnAutoCreate()) 1198 throw new Error("Attempt to auto-create ResearchStudyRecruitmentComponent.actualGroup"); 1199 else if (Configuration.doAutoCreate()) 1200 this.actualGroup = new Reference(); // cc 1201 return this.actualGroup; 1202 } 1203 1204 public boolean hasActualGroup() { 1205 return this.actualGroup != null && !this.actualGroup.isEmpty(); 1206 } 1207 1208 /** 1209 * @param value {@link #actualGroup} (Group of participants who were enrolled in study.) 1210 */ 1211 public ResearchStudyRecruitmentComponent setActualGroup(Reference value) { 1212 this.actualGroup = value; 1213 return this; 1214 } 1215 1216 protected void listChildren(List<Property> children) { 1217 super.listChildren(children); 1218 children.add(new Property("targetNumber", "unsignedInt", "Estimated total number of participants to be enrolled.", 0, 1, targetNumber)); 1219 children.add(new Property("actualNumber", "unsignedInt", "Actual total number of participants enrolled in study.", 0, 1, actualNumber)); 1220 children.add(new Property("eligibility", "Reference(Group|EvidenceVariable)", "Inclusion and exclusion criteria.", 0, 1, eligibility)); 1221 children.add(new Property("actualGroup", "Reference(Group)", "Group of participants who were enrolled in study.", 0, 1, actualGroup)); 1222 } 1223 1224 @Override 1225 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1226 switch (_hash) { 1227 case -682948550: /*targetNumber*/ return new Property("targetNumber", "unsignedInt", "Estimated total number of participants to be enrolled.", 0, 1, targetNumber); 1228 case 746557047: /*actualNumber*/ return new Property("actualNumber", "unsignedInt", "Actual total number of participants enrolled in study.", 0, 1, actualNumber); 1229 case -930847859: /*eligibility*/ return new Property("eligibility", "Reference(Group|EvidenceVariable)", "Inclusion and exclusion criteria.", 0, 1, eligibility); 1230 case 1403004305: /*actualGroup*/ return new Property("actualGroup", "Reference(Group)", "Group of participants who were enrolled in study.", 0, 1, actualGroup); 1231 default: return super.getNamedProperty(_hash, _name, _checkValid); 1232 } 1233 1234 } 1235 1236 @Override 1237 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1238 switch (hash) { 1239 case -682948550: /*targetNumber*/ return this.targetNumber == null ? new Base[0] : new Base[] {this.targetNumber}; // UnsignedIntType 1240 case 746557047: /*actualNumber*/ return this.actualNumber == null ? new Base[0] : new Base[] {this.actualNumber}; // UnsignedIntType 1241 case -930847859: /*eligibility*/ return this.eligibility == null ? new Base[0] : new Base[] {this.eligibility}; // Reference 1242 case 1403004305: /*actualGroup*/ return this.actualGroup == null ? new Base[0] : new Base[] {this.actualGroup}; // Reference 1243 default: return super.getProperty(hash, name, checkValid); 1244 } 1245 1246 } 1247 1248 @Override 1249 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1250 switch (hash) { 1251 case -682948550: // targetNumber 1252 this.targetNumber = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1253 return value; 1254 case 746557047: // actualNumber 1255 this.actualNumber = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1256 return value; 1257 case -930847859: // eligibility 1258 this.eligibility = TypeConvertor.castToReference(value); // Reference 1259 return value; 1260 case 1403004305: // actualGroup 1261 this.actualGroup = TypeConvertor.castToReference(value); // Reference 1262 return value; 1263 default: return super.setProperty(hash, name, value); 1264 } 1265 1266 } 1267 1268 @Override 1269 public Base setProperty(String name, Base value) throws FHIRException { 1270 if (name.equals("targetNumber")) { 1271 this.targetNumber = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1272 } else if (name.equals("actualNumber")) { 1273 this.actualNumber = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1274 } else if (name.equals("eligibility")) { 1275 this.eligibility = TypeConvertor.castToReference(value); // Reference 1276 } else if (name.equals("actualGroup")) { 1277 this.actualGroup = TypeConvertor.castToReference(value); // Reference 1278 } else 1279 return super.setProperty(name, value); 1280 return value; 1281 } 1282 1283 @Override 1284 public void removeChild(String name, Base value) throws FHIRException { 1285 if (name.equals("targetNumber")) { 1286 this.targetNumber = null; 1287 } else if (name.equals("actualNumber")) { 1288 this.actualNumber = null; 1289 } else if (name.equals("eligibility")) { 1290 this.eligibility = null; 1291 } else if (name.equals("actualGroup")) { 1292 this.actualGroup = null; 1293 } else 1294 super.removeChild(name, value); 1295 1296 } 1297 1298 @Override 1299 public Base makeProperty(int hash, String name) throws FHIRException { 1300 switch (hash) { 1301 case -682948550: return getTargetNumberElement(); 1302 case 746557047: return getActualNumberElement(); 1303 case -930847859: return getEligibility(); 1304 case 1403004305: return getActualGroup(); 1305 default: return super.makeProperty(hash, name); 1306 } 1307 1308 } 1309 1310 @Override 1311 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1312 switch (hash) { 1313 case -682948550: /*targetNumber*/ return new String[] {"unsignedInt"}; 1314 case 746557047: /*actualNumber*/ return new String[] {"unsignedInt"}; 1315 case -930847859: /*eligibility*/ return new String[] {"Reference"}; 1316 case 1403004305: /*actualGroup*/ return new String[] {"Reference"}; 1317 default: return super.getTypesForProperty(hash, name); 1318 } 1319 1320 } 1321 1322 @Override 1323 public Base addChild(String name) throws FHIRException { 1324 if (name.equals("targetNumber")) { 1325 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.recruitment.targetNumber"); 1326 } 1327 else if (name.equals("actualNumber")) { 1328 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.recruitment.actualNumber"); 1329 } 1330 else if (name.equals("eligibility")) { 1331 this.eligibility = new Reference(); 1332 return this.eligibility; 1333 } 1334 else if (name.equals("actualGroup")) { 1335 this.actualGroup = new Reference(); 1336 return this.actualGroup; 1337 } 1338 else 1339 return super.addChild(name); 1340 } 1341 1342 public ResearchStudyRecruitmentComponent copy() { 1343 ResearchStudyRecruitmentComponent dst = new ResearchStudyRecruitmentComponent(); 1344 copyValues(dst); 1345 return dst; 1346 } 1347 1348 public void copyValues(ResearchStudyRecruitmentComponent dst) { 1349 super.copyValues(dst); 1350 dst.targetNumber = targetNumber == null ? null : targetNumber.copy(); 1351 dst.actualNumber = actualNumber == null ? null : actualNumber.copy(); 1352 dst.eligibility = eligibility == null ? null : eligibility.copy(); 1353 dst.actualGroup = actualGroup == null ? null : actualGroup.copy(); 1354 } 1355 1356 @Override 1357 public boolean equalsDeep(Base other_) { 1358 if (!super.equalsDeep(other_)) 1359 return false; 1360 if (!(other_ instanceof ResearchStudyRecruitmentComponent)) 1361 return false; 1362 ResearchStudyRecruitmentComponent o = (ResearchStudyRecruitmentComponent) other_; 1363 return compareDeep(targetNumber, o.targetNumber, true) && compareDeep(actualNumber, o.actualNumber, true) 1364 && compareDeep(eligibility, o.eligibility, true) && compareDeep(actualGroup, o.actualGroup, true) 1365 ; 1366 } 1367 1368 @Override 1369 public boolean equalsShallow(Base other_) { 1370 if (!super.equalsShallow(other_)) 1371 return false; 1372 if (!(other_ instanceof ResearchStudyRecruitmentComponent)) 1373 return false; 1374 ResearchStudyRecruitmentComponent o = (ResearchStudyRecruitmentComponent) other_; 1375 return compareValues(targetNumber, o.targetNumber, true) && compareValues(actualNumber, o.actualNumber, true) 1376 ; 1377 } 1378 1379 public boolean isEmpty() { 1380 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(targetNumber, actualNumber 1381 , eligibility, actualGroup); 1382 } 1383 1384 public String fhirType() { 1385 return "ResearchStudy.recruitment"; 1386 1387 } 1388 1389 } 1390 1391 @Block() 1392 public static class ResearchStudyComparisonGroupComponent extends BackboneElement implements IBaseBackboneElement { 1393 /** 1394 * Allows the comparisonGroup for the study and the comparisonGroup for the subject to be linked easily. 1395 */ 1396 @Child(name = "linkId", type = {IdType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1397 @Description(shortDefinition="Allows the comparisonGroup for the study and the comparisonGroup for the subject to be linked easily", formalDefinition="Allows the comparisonGroup for the study and the comparisonGroup for the subject to be linked easily." ) 1398 protected IdType linkId; 1399 1400 /** 1401 * Unique, human-readable label for this comparisonGroup of the study. 1402 */ 1403 @Child(name = "name", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 1404 @Description(shortDefinition="Label for study comparisonGroup", formalDefinition="Unique, human-readable label for this comparisonGroup of the study." ) 1405 protected StringType name; 1406 1407 /** 1408 * Categorization of study comparisonGroup, e.g. experimental, active comparator, placebo comparater. 1409 */ 1410 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1411 @Description(shortDefinition="Categorization of study comparisonGroup", formalDefinition="Categorization of study comparisonGroup, e.g. experimental, active comparator, placebo comparater." ) 1412 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-arm-type") 1413 protected CodeableConcept type; 1414 1415 /** 1416 * A succinct description of the path through the study that would be followed by a subject adhering to this comparisonGroup. 1417 */ 1418 @Child(name = "description", type = {MarkdownType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1419 @Description(shortDefinition="Short explanation of study path", formalDefinition="A succinct description of the path through the study that would be followed by a subject adhering to this comparisonGroup." ) 1420 protected MarkdownType description; 1421 1422 /** 1423 * Interventions or exposures in this comparisonGroup or cohort. 1424 */ 1425 @Child(name = "intendedExposure", type = {EvidenceVariable.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1426 @Description(shortDefinition="Interventions or exposures in this comparisonGroup or cohort", formalDefinition="Interventions or exposures in this comparisonGroup or cohort." ) 1427 protected List<Reference> intendedExposure; 1428 1429 /** 1430 * Group of participants who were enrolled in study comparisonGroup. 1431 */ 1432 @Child(name = "observedGroup", type = {Group.class}, order=6, min=0, max=1, modifier=false, summary=false) 1433 @Description(shortDefinition="Group of participants who were enrolled in study comparisonGroup", formalDefinition="Group of participants who were enrolled in study comparisonGroup." ) 1434 protected Reference observedGroup; 1435 1436 private static final long serialVersionUID = 1107310853L; 1437 1438 /** 1439 * Constructor 1440 */ 1441 public ResearchStudyComparisonGroupComponent() { 1442 super(); 1443 } 1444 1445 /** 1446 * Constructor 1447 */ 1448 public ResearchStudyComparisonGroupComponent(String name) { 1449 super(); 1450 this.setName(name); 1451 } 1452 1453 /** 1454 * @return {@link #linkId} (Allows the comparisonGroup for the study and the comparisonGroup for the subject to be linked easily.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 1455 */ 1456 public IdType getLinkIdElement() { 1457 if (this.linkId == null) 1458 if (Configuration.errorOnAutoCreate()) 1459 throw new Error("Attempt to auto-create ResearchStudyComparisonGroupComponent.linkId"); 1460 else if (Configuration.doAutoCreate()) 1461 this.linkId = new IdType(); // bb 1462 return this.linkId; 1463 } 1464 1465 public boolean hasLinkIdElement() { 1466 return this.linkId != null && !this.linkId.isEmpty(); 1467 } 1468 1469 public boolean hasLinkId() { 1470 return this.linkId != null && !this.linkId.isEmpty(); 1471 } 1472 1473 /** 1474 * @param value {@link #linkId} (Allows the comparisonGroup for the study and the comparisonGroup for the subject to be linked easily.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 1475 */ 1476 public ResearchStudyComparisonGroupComponent setLinkIdElement(IdType value) { 1477 this.linkId = value; 1478 return this; 1479 } 1480 1481 /** 1482 * @return Allows the comparisonGroup for the study and the comparisonGroup for the subject to be linked easily. 1483 */ 1484 public String getLinkId() { 1485 return this.linkId == null ? null : this.linkId.getValue(); 1486 } 1487 1488 /** 1489 * @param value Allows the comparisonGroup for the study and the comparisonGroup for the subject to be linked easily. 1490 */ 1491 public ResearchStudyComparisonGroupComponent setLinkId(String value) { 1492 if (Utilities.noString(value)) 1493 this.linkId = null; 1494 else { 1495 if (this.linkId == null) 1496 this.linkId = new IdType(); 1497 this.linkId.setValue(value); 1498 } 1499 return this; 1500 } 1501 1502 /** 1503 * @return {@link #name} (Unique, human-readable label for this comparisonGroup of the study.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1504 */ 1505 public StringType getNameElement() { 1506 if (this.name == null) 1507 if (Configuration.errorOnAutoCreate()) 1508 throw new Error("Attempt to auto-create ResearchStudyComparisonGroupComponent.name"); 1509 else if (Configuration.doAutoCreate()) 1510 this.name = new StringType(); // bb 1511 return this.name; 1512 } 1513 1514 public boolean hasNameElement() { 1515 return this.name != null && !this.name.isEmpty(); 1516 } 1517 1518 public boolean hasName() { 1519 return this.name != null && !this.name.isEmpty(); 1520 } 1521 1522 /** 1523 * @param value {@link #name} (Unique, human-readable label for this comparisonGroup of the study.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1524 */ 1525 public ResearchStudyComparisonGroupComponent setNameElement(StringType value) { 1526 this.name = value; 1527 return this; 1528 } 1529 1530 /** 1531 * @return Unique, human-readable label for this comparisonGroup of the study. 1532 */ 1533 public String getName() { 1534 return this.name == null ? null : this.name.getValue(); 1535 } 1536 1537 /** 1538 * @param value Unique, human-readable label for this comparisonGroup of the study. 1539 */ 1540 public ResearchStudyComparisonGroupComponent setName(String value) { 1541 if (this.name == null) 1542 this.name = new StringType(); 1543 this.name.setValue(value); 1544 return this; 1545 } 1546 1547 /** 1548 * @return {@link #type} (Categorization of study comparisonGroup, e.g. experimental, active comparator, placebo comparater.) 1549 */ 1550 public CodeableConcept getType() { 1551 if (this.type == null) 1552 if (Configuration.errorOnAutoCreate()) 1553 throw new Error("Attempt to auto-create ResearchStudyComparisonGroupComponent.type"); 1554 else if (Configuration.doAutoCreate()) 1555 this.type = new CodeableConcept(); // cc 1556 return this.type; 1557 } 1558 1559 public boolean hasType() { 1560 return this.type != null && !this.type.isEmpty(); 1561 } 1562 1563 /** 1564 * @param value {@link #type} (Categorization of study comparisonGroup, e.g. experimental, active comparator, placebo comparater.) 1565 */ 1566 public ResearchStudyComparisonGroupComponent setType(CodeableConcept value) { 1567 this.type = value; 1568 return this; 1569 } 1570 1571 /** 1572 * @return {@link #description} (A succinct description of the path through the study that would be followed by a subject adhering to this comparisonGroup.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1573 */ 1574 public MarkdownType getDescriptionElement() { 1575 if (this.description == null) 1576 if (Configuration.errorOnAutoCreate()) 1577 throw new Error("Attempt to auto-create ResearchStudyComparisonGroupComponent.description"); 1578 else if (Configuration.doAutoCreate()) 1579 this.description = new MarkdownType(); // bb 1580 return this.description; 1581 } 1582 1583 public boolean hasDescriptionElement() { 1584 return this.description != null && !this.description.isEmpty(); 1585 } 1586 1587 public boolean hasDescription() { 1588 return this.description != null && !this.description.isEmpty(); 1589 } 1590 1591 /** 1592 * @param value {@link #description} (A succinct description of the path through the study that would be followed by a subject adhering to this comparisonGroup.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1593 */ 1594 public ResearchStudyComparisonGroupComponent setDescriptionElement(MarkdownType value) { 1595 this.description = value; 1596 return this; 1597 } 1598 1599 /** 1600 * @return A succinct description of the path through the study that would be followed by a subject adhering to this comparisonGroup. 1601 */ 1602 public String getDescription() { 1603 return this.description == null ? null : this.description.getValue(); 1604 } 1605 1606 /** 1607 * @param value A succinct description of the path through the study that would be followed by a subject adhering to this comparisonGroup. 1608 */ 1609 public ResearchStudyComparisonGroupComponent setDescription(String value) { 1610 if (Utilities.noString(value)) 1611 this.description = null; 1612 else { 1613 if (this.description == null) 1614 this.description = new MarkdownType(); 1615 this.description.setValue(value); 1616 } 1617 return this; 1618 } 1619 1620 /** 1621 * @return {@link #intendedExposure} (Interventions or exposures in this comparisonGroup or cohort.) 1622 */ 1623 public List<Reference> getIntendedExposure() { 1624 if (this.intendedExposure == null) 1625 this.intendedExposure = new ArrayList<Reference>(); 1626 return this.intendedExposure; 1627 } 1628 1629 /** 1630 * @return Returns a reference to <code>this</code> for easy method chaining 1631 */ 1632 public ResearchStudyComparisonGroupComponent setIntendedExposure(List<Reference> theIntendedExposure) { 1633 this.intendedExposure = theIntendedExposure; 1634 return this; 1635 } 1636 1637 public boolean hasIntendedExposure() { 1638 if (this.intendedExposure == null) 1639 return false; 1640 for (Reference item : this.intendedExposure) 1641 if (!item.isEmpty()) 1642 return true; 1643 return false; 1644 } 1645 1646 public Reference addIntendedExposure() { //3 1647 Reference t = new Reference(); 1648 if (this.intendedExposure == null) 1649 this.intendedExposure = new ArrayList<Reference>(); 1650 this.intendedExposure.add(t); 1651 return t; 1652 } 1653 1654 public ResearchStudyComparisonGroupComponent addIntendedExposure(Reference t) { //3 1655 if (t == null) 1656 return this; 1657 if (this.intendedExposure == null) 1658 this.intendedExposure = new ArrayList<Reference>(); 1659 this.intendedExposure.add(t); 1660 return this; 1661 } 1662 1663 /** 1664 * @return The first repetition of repeating field {@link #intendedExposure}, creating it if it does not already exist {3} 1665 */ 1666 public Reference getIntendedExposureFirstRep() { 1667 if (getIntendedExposure().isEmpty()) { 1668 addIntendedExposure(); 1669 } 1670 return getIntendedExposure().get(0); 1671 } 1672 1673 /** 1674 * @return {@link #observedGroup} (Group of participants who were enrolled in study comparisonGroup.) 1675 */ 1676 public Reference getObservedGroup() { 1677 if (this.observedGroup == null) 1678 if (Configuration.errorOnAutoCreate()) 1679 throw new Error("Attempt to auto-create ResearchStudyComparisonGroupComponent.observedGroup"); 1680 else if (Configuration.doAutoCreate()) 1681 this.observedGroup = new Reference(); // cc 1682 return this.observedGroup; 1683 } 1684 1685 public boolean hasObservedGroup() { 1686 return this.observedGroup != null && !this.observedGroup.isEmpty(); 1687 } 1688 1689 /** 1690 * @param value {@link #observedGroup} (Group of participants who were enrolled in study comparisonGroup.) 1691 */ 1692 public ResearchStudyComparisonGroupComponent setObservedGroup(Reference value) { 1693 this.observedGroup = value; 1694 return this; 1695 } 1696 1697 protected void listChildren(List<Property> children) { 1698 super.listChildren(children); 1699 children.add(new Property("linkId", "id", "Allows the comparisonGroup for the study and the comparisonGroup for the subject to be linked easily.", 0, 1, linkId)); 1700 children.add(new Property("name", "string", "Unique, human-readable label for this comparisonGroup of the study.", 0, 1, name)); 1701 children.add(new Property("type", "CodeableConcept", "Categorization of study comparisonGroup, e.g. experimental, active comparator, placebo comparater.", 0, 1, type)); 1702 children.add(new Property("description", "markdown", "A succinct description of the path through the study that would be followed by a subject adhering to this comparisonGroup.", 0, 1, description)); 1703 children.add(new Property("intendedExposure", "Reference(EvidenceVariable)", "Interventions or exposures in this comparisonGroup or cohort.", 0, java.lang.Integer.MAX_VALUE, intendedExposure)); 1704 children.add(new Property("observedGroup", "Reference(Group)", "Group of participants who were enrolled in study comparisonGroup.", 0, 1, observedGroup)); 1705 } 1706 1707 @Override 1708 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1709 switch (_hash) { 1710 case -1102667083: /*linkId*/ return new Property("linkId", "id", "Allows the comparisonGroup for the study and the comparisonGroup for the subject to be linked easily.", 0, 1, linkId); 1711 case 3373707: /*name*/ return new Property("name", "string", "Unique, human-readable label for this comparisonGroup of the study.", 0, 1, name); 1712 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Categorization of study comparisonGroup, e.g. experimental, active comparator, placebo comparater.", 0, 1, type); 1713 case -1724546052: /*description*/ return new Property("description", "markdown", "A succinct description of the path through the study that would be followed by a subject adhering to this comparisonGroup.", 0, 1, description); 1714 case -407218606: /*intendedExposure*/ return new Property("intendedExposure", "Reference(EvidenceVariable)", "Interventions or exposures in this comparisonGroup or cohort.", 0, java.lang.Integer.MAX_VALUE, intendedExposure); 1715 case 375599255: /*observedGroup*/ return new Property("observedGroup", "Reference(Group)", "Group of participants who were enrolled in study comparisonGroup.", 0, 1, observedGroup); 1716 default: return super.getNamedProperty(_hash, _name, _checkValid); 1717 } 1718 1719 } 1720 1721 @Override 1722 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1723 switch (hash) { 1724 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // IdType 1725 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1726 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1727 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1728 case -407218606: /*intendedExposure*/ return this.intendedExposure == null ? new Base[0] : this.intendedExposure.toArray(new Base[this.intendedExposure.size()]); // Reference 1729 case 375599255: /*observedGroup*/ return this.observedGroup == null ? new Base[0] : new Base[] {this.observedGroup}; // Reference 1730 default: return super.getProperty(hash, name, checkValid); 1731 } 1732 1733 } 1734 1735 @Override 1736 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1737 switch (hash) { 1738 case -1102667083: // linkId 1739 this.linkId = TypeConvertor.castToId(value); // IdType 1740 return value; 1741 case 3373707: // name 1742 this.name = TypeConvertor.castToString(value); // StringType 1743 return value; 1744 case 3575610: // type 1745 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1746 return value; 1747 case -1724546052: // description 1748 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1749 return value; 1750 case -407218606: // intendedExposure 1751 this.getIntendedExposure().add(TypeConvertor.castToReference(value)); // Reference 1752 return value; 1753 case 375599255: // observedGroup 1754 this.observedGroup = TypeConvertor.castToReference(value); // Reference 1755 return value; 1756 default: return super.setProperty(hash, name, value); 1757 } 1758 1759 } 1760 1761 @Override 1762 public Base setProperty(String name, Base value) throws FHIRException { 1763 if (name.equals("linkId")) { 1764 this.linkId = TypeConvertor.castToId(value); // IdType 1765 } else if (name.equals("name")) { 1766 this.name = TypeConvertor.castToString(value); // StringType 1767 } else if (name.equals("type")) { 1768 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1769 } else if (name.equals("description")) { 1770 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1771 } else if (name.equals("intendedExposure")) { 1772 this.getIntendedExposure().add(TypeConvertor.castToReference(value)); 1773 } else if (name.equals("observedGroup")) { 1774 this.observedGroup = TypeConvertor.castToReference(value); // Reference 1775 } else 1776 return super.setProperty(name, value); 1777 return value; 1778 } 1779 1780 @Override 1781 public void removeChild(String name, Base value) throws FHIRException { 1782 if (name.equals("linkId")) { 1783 this.linkId = null; 1784 } else if (name.equals("name")) { 1785 this.name = null; 1786 } else if (name.equals("type")) { 1787 this.type = null; 1788 } else if (name.equals("description")) { 1789 this.description = null; 1790 } else if (name.equals("intendedExposure")) { 1791 this.getIntendedExposure().remove(value); 1792 } else if (name.equals("observedGroup")) { 1793 this.observedGroup = null; 1794 } else 1795 super.removeChild(name, value); 1796 1797 } 1798 1799 @Override 1800 public Base makeProperty(int hash, String name) throws FHIRException { 1801 switch (hash) { 1802 case -1102667083: return getLinkIdElement(); 1803 case 3373707: return getNameElement(); 1804 case 3575610: return getType(); 1805 case -1724546052: return getDescriptionElement(); 1806 case -407218606: return addIntendedExposure(); 1807 case 375599255: return getObservedGroup(); 1808 default: return super.makeProperty(hash, name); 1809 } 1810 1811 } 1812 1813 @Override 1814 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1815 switch (hash) { 1816 case -1102667083: /*linkId*/ return new String[] {"id"}; 1817 case 3373707: /*name*/ return new String[] {"string"}; 1818 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1819 case -1724546052: /*description*/ return new String[] {"markdown"}; 1820 case -407218606: /*intendedExposure*/ return new String[] {"Reference"}; 1821 case 375599255: /*observedGroup*/ return new String[] {"Reference"}; 1822 default: return super.getTypesForProperty(hash, name); 1823 } 1824 1825 } 1826 1827 @Override 1828 public Base addChild(String name) throws FHIRException { 1829 if (name.equals("linkId")) { 1830 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.comparisonGroup.linkId"); 1831 } 1832 else if (name.equals("name")) { 1833 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.comparisonGroup.name"); 1834 } 1835 else if (name.equals("type")) { 1836 this.type = new CodeableConcept(); 1837 return this.type; 1838 } 1839 else if (name.equals("description")) { 1840 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.comparisonGroup.description"); 1841 } 1842 else if (name.equals("intendedExposure")) { 1843 return addIntendedExposure(); 1844 } 1845 else if (name.equals("observedGroup")) { 1846 this.observedGroup = new Reference(); 1847 return this.observedGroup; 1848 } 1849 else 1850 return super.addChild(name); 1851 } 1852 1853 public ResearchStudyComparisonGroupComponent copy() { 1854 ResearchStudyComparisonGroupComponent dst = new ResearchStudyComparisonGroupComponent(); 1855 copyValues(dst); 1856 return dst; 1857 } 1858 1859 public void copyValues(ResearchStudyComparisonGroupComponent dst) { 1860 super.copyValues(dst); 1861 dst.linkId = linkId == null ? null : linkId.copy(); 1862 dst.name = name == null ? null : name.copy(); 1863 dst.type = type == null ? null : type.copy(); 1864 dst.description = description == null ? null : description.copy(); 1865 if (intendedExposure != null) { 1866 dst.intendedExposure = new ArrayList<Reference>(); 1867 for (Reference i : intendedExposure) 1868 dst.intendedExposure.add(i.copy()); 1869 }; 1870 dst.observedGroup = observedGroup == null ? null : observedGroup.copy(); 1871 } 1872 1873 @Override 1874 public boolean equalsDeep(Base other_) { 1875 if (!super.equalsDeep(other_)) 1876 return false; 1877 if (!(other_ instanceof ResearchStudyComparisonGroupComponent)) 1878 return false; 1879 ResearchStudyComparisonGroupComponent o = (ResearchStudyComparisonGroupComponent) other_; 1880 return compareDeep(linkId, o.linkId, true) && compareDeep(name, o.name, true) && compareDeep(type, o.type, true) 1881 && compareDeep(description, o.description, true) && compareDeep(intendedExposure, o.intendedExposure, true) 1882 && compareDeep(observedGroup, o.observedGroup, true); 1883 } 1884 1885 @Override 1886 public boolean equalsShallow(Base other_) { 1887 if (!super.equalsShallow(other_)) 1888 return false; 1889 if (!(other_ instanceof ResearchStudyComparisonGroupComponent)) 1890 return false; 1891 ResearchStudyComparisonGroupComponent o = (ResearchStudyComparisonGroupComponent) other_; 1892 return compareValues(linkId, o.linkId, true) && compareValues(name, o.name, true) && compareValues(description, o.description, true) 1893 ; 1894 } 1895 1896 public boolean isEmpty() { 1897 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, name, type, description 1898 , intendedExposure, observedGroup); 1899 } 1900 1901 public String fhirType() { 1902 return "ResearchStudy.comparisonGroup"; 1903 1904 } 1905 1906 } 1907 1908 @Block() 1909 public static class ResearchStudyObjectiveComponent extends BackboneElement implements IBaseBackboneElement { 1910 /** 1911 * Unique, human-readable label for this objective of the study. 1912 */ 1913 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1914 @Description(shortDefinition="Label for the objective", formalDefinition="Unique, human-readable label for this objective of the study." ) 1915 protected StringType name; 1916 1917 /** 1918 * The kind of study objective. 1919 */ 1920 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1921 @Description(shortDefinition="primary | secondary | exploratory", formalDefinition="The kind of study objective." ) 1922 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-objective-type") 1923 protected CodeableConcept type; 1924 1925 /** 1926 * Free text description of the objective of the study. This is what the study is trying to achieve rather than how it is going to achieve it (see ResearchStudy.description). 1927 */ 1928 @Child(name = "description", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1929 @Description(shortDefinition="Description of the objective", formalDefinition="Free text description of the objective of the study. This is what the study is trying to achieve rather than how it is going to achieve it (see ResearchStudy.description)." ) 1930 protected MarkdownType description; 1931 1932 private static final long serialVersionUID = -1976083810L; 1933 1934 /** 1935 * Constructor 1936 */ 1937 public ResearchStudyObjectiveComponent() { 1938 super(); 1939 } 1940 1941 /** 1942 * @return {@link #name} (Unique, human-readable label for this objective of the study.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1943 */ 1944 public StringType getNameElement() { 1945 if (this.name == null) 1946 if (Configuration.errorOnAutoCreate()) 1947 throw new Error("Attempt to auto-create ResearchStudyObjectiveComponent.name"); 1948 else if (Configuration.doAutoCreate()) 1949 this.name = new StringType(); // bb 1950 return this.name; 1951 } 1952 1953 public boolean hasNameElement() { 1954 return this.name != null && !this.name.isEmpty(); 1955 } 1956 1957 public boolean hasName() { 1958 return this.name != null && !this.name.isEmpty(); 1959 } 1960 1961 /** 1962 * @param value {@link #name} (Unique, human-readable label for this objective of the study.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1963 */ 1964 public ResearchStudyObjectiveComponent setNameElement(StringType value) { 1965 this.name = value; 1966 return this; 1967 } 1968 1969 /** 1970 * @return Unique, human-readable label for this objective of the study. 1971 */ 1972 public String getName() { 1973 return this.name == null ? null : this.name.getValue(); 1974 } 1975 1976 /** 1977 * @param value Unique, human-readable label for this objective of the study. 1978 */ 1979 public ResearchStudyObjectiveComponent setName(String value) { 1980 if (Utilities.noString(value)) 1981 this.name = null; 1982 else { 1983 if (this.name == null) 1984 this.name = new StringType(); 1985 this.name.setValue(value); 1986 } 1987 return this; 1988 } 1989 1990 /** 1991 * @return {@link #type} (The kind of study objective.) 1992 */ 1993 public CodeableConcept getType() { 1994 if (this.type == null) 1995 if (Configuration.errorOnAutoCreate()) 1996 throw new Error("Attempt to auto-create ResearchStudyObjectiveComponent.type"); 1997 else if (Configuration.doAutoCreate()) 1998 this.type = new CodeableConcept(); // cc 1999 return this.type; 2000 } 2001 2002 public boolean hasType() { 2003 return this.type != null && !this.type.isEmpty(); 2004 } 2005 2006 /** 2007 * @param value {@link #type} (The kind of study objective.) 2008 */ 2009 public ResearchStudyObjectiveComponent setType(CodeableConcept value) { 2010 this.type = value; 2011 return this; 2012 } 2013 2014 /** 2015 * @return {@link #description} (Free text description of the objective of the study. This is what the study is trying to achieve rather than how it is going to achieve it (see ResearchStudy.description).). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2016 */ 2017 public MarkdownType getDescriptionElement() { 2018 if (this.description == null) 2019 if (Configuration.errorOnAutoCreate()) 2020 throw new Error("Attempt to auto-create ResearchStudyObjectiveComponent.description"); 2021 else if (Configuration.doAutoCreate()) 2022 this.description = new MarkdownType(); // bb 2023 return this.description; 2024 } 2025 2026 public boolean hasDescriptionElement() { 2027 return this.description != null && !this.description.isEmpty(); 2028 } 2029 2030 public boolean hasDescription() { 2031 return this.description != null && !this.description.isEmpty(); 2032 } 2033 2034 /** 2035 * @param value {@link #description} (Free text description of the objective of the study. This is what the study is trying to achieve rather than how it is going to achieve it (see ResearchStudy.description).). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2036 */ 2037 public ResearchStudyObjectiveComponent setDescriptionElement(MarkdownType value) { 2038 this.description = value; 2039 return this; 2040 } 2041 2042 /** 2043 * @return Free text description of the objective of the study. This is what the study is trying to achieve rather than how it is going to achieve it (see ResearchStudy.description). 2044 */ 2045 public String getDescription() { 2046 return this.description == null ? null : this.description.getValue(); 2047 } 2048 2049 /** 2050 * @param value Free text description of the objective of the study. This is what the study is trying to achieve rather than how it is going to achieve it (see ResearchStudy.description). 2051 */ 2052 public ResearchStudyObjectiveComponent setDescription(String value) { 2053 if (Utilities.noString(value)) 2054 this.description = null; 2055 else { 2056 if (this.description == null) 2057 this.description = new MarkdownType(); 2058 this.description.setValue(value); 2059 } 2060 return this; 2061 } 2062 2063 protected void listChildren(List<Property> children) { 2064 super.listChildren(children); 2065 children.add(new Property("name", "string", "Unique, human-readable label for this objective of the study.", 0, 1, name)); 2066 children.add(new Property("type", "CodeableConcept", "The kind of study objective.", 0, 1, type)); 2067 children.add(new Property("description", "markdown", "Free text description of the objective of the study. This is what the study is trying to achieve rather than how it is going to achieve it (see ResearchStudy.description).", 0, 1, description)); 2068 } 2069 2070 @Override 2071 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2072 switch (_hash) { 2073 case 3373707: /*name*/ return new Property("name", "string", "Unique, human-readable label for this objective of the study.", 0, 1, name); 2074 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The kind of study objective.", 0, 1, type); 2075 case -1724546052: /*description*/ return new Property("description", "markdown", "Free text description of the objective of the study. This is what the study is trying to achieve rather than how it is going to achieve it (see ResearchStudy.description).", 0, 1, description); 2076 default: return super.getNamedProperty(_hash, _name, _checkValid); 2077 } 2078 2079 } 2080 2081 @Override 2082 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2083 switch (hash) { 2084 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2085 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2086 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2087 default: return super.getProperty(hash, name, checkValid); 2088 } 2089 2090 } 2091 2092 @Override 2093 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2094 switch (hash) { 2095 case 3373707: // name 2096 this.name = TypeConvertor.castToString(value); // StringType 2097 return value; 2098 case 3575610: // type 2099 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2100 return value; 2101 case -1724546052: // description 2102 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2103 return value; 2104 default: return super.setProperty(hash, name, value); 2105 } 2106 2107 } 2108 2109 @Override 2110 public Base setProperty(String name, Base value) throws FHIRException { 2111 if (name.equals("name")) { 2112 this.name = TypeConvertor.castToString(value); // StringType 2113 } else if (name.equals("type")) { 2114 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2115 } else if (name.equals("description")) { 2116 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2117 } else 2118 return super.setProperty(name, value); 2119 return value; 2120 } 2121 2122 @Override 2123 public void removeChild(String name, Base value) throws FHIRException { 2124 if (name.equals("name")) { 2125 this.name = null; 2126 } else if (name.equals("type")) { 2127 this.type = null; 2128 } else if (name.equals("description")) { 2129 this.description = null; 2130 } else 2131 super.removeChild(name, value); 2132 2133 } 2134 2135 @Override 2136 public Base makeProperty(int hash, String name) throws FHIRException { 2137 switch (hash) { 2138 case 3373707: return getNameElement(); 2139 case 3575610: return getType(); 2140 case -1724546052: return getDescriptionElement(); 2141 default: return super.makeProperty(hash, name); 2142 } 2143 2144 } 2145 2146 @Override 2147 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2148 switch (hash) { 2149 case 3373707: /*name*/ return new String[] {"string"}; 2150 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2151 case -1724546052: /*description*/ return new String[] {"markdown"}; 2152 default: return super.getTypesForProperty(hash, name); 2153 } 2154 2155 } 2156 2157 @Override 2158 public Base addChild(String name) throws FHIRException { 2159 if (name.equals("name")) { 2160 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.objective.name"); 2161 } 2162 else if (name.equals("type")) { 2163 this.type = new CodeableConcept(); 2164 return this.type; 2165 } 2166 else if (name.equals("description")) { 2167 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.objective.description"); 2168 } 2169 else 2170 return super.addChild(name); 2171 } 2172 2173 public ResearchStudyObjectiveComponent copy() { 2174 ResearchStudyObjectiveComponent dst = new ResearchStudyObjectiveComponent(); 2175 copyValues(dst); 2176 return dst; 2177 } 2178 2179 public void copyValues(ResearchStudyObjectiveComponent dst) { 2180 super.copyValues(dst); 2181 dst.name = name == null ? null : name.copy(); 2182 dst.type = type == null ? null : type.copy(); 2183 dst.description = description == null ? null : description.copy(); 2184 } 2185 2186 @Override 2187 public boolean equalsDeep(Base other_) { 2188 if (!super.equalsDeep(other_)) 2189 return false; 2190 if (!(other_ instanceof ResearchStudyObjectiveComponent)) 2191 return false; 2192 ResearchStudyObjectiveComponent o = (ResearchStudyObjectiveComponent) other_; 2193 return compareDeep(name, o.name, true) && compareDeep(type, o.type, true) && compareDeep(description, o.description, true) 2194 ; 2195 } 2196 2197 @Override 2198 public boolean equalsShallow(Base other_) { 2199 if (!super.equalsShallow(other_)) 2200 return false; 2201 if (!(other_ instanceof ResearchStudyObjectiveComponent)) 2202 return false; 2203 ResearchStudyObjectiveComponent o = (ResearchStudyObjectiveComponent) other_; 2204 return compareValues(name, o.name, true) && compareValues(description, o.description, true); 2205 } 2206 2207 public boolean isEmpty() { 2208 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, type, description 2209 ); 2210 } 2211 2212 public String fhirType() { 2213 return "ResearchStudy.objective"; 2214 2215 } 2216 2217 } 2218 2219 @Block() 2220 public static class ResearchStudyOutcomeMeasureComponent extends BackboneElement implements IBaseBackboneElement { 2221 /** 2222 * Label for the outcome. 2223 */ 2224 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2225 @Description(shortDefinition="Label for the outcome", formalDefinition="Label for the outcome." ) 2226 protected StringType name; 2227 2228 /** 2229 * The parameter or characteristic being assessed as one of the values by which the study is assessed. 2230 */ 2231 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2232 @Description(shortDefinition="primary | secondary | exploratory", formalDefinition="The parameter or characteristic being assessed as one of the values by which the study is assessed." ) 2233 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-objective-type") 2234 protected List<CodeableConcept> type; 2235 2236 /** 2237 * Description of the outcome. 2238 */ 2239 @Child(name = "description", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2240 @Description(shortDefinition="Description of the outcome", formalDefinition="Description of the outcome." ) 2241 protected MarkdownType description; 2242 2243 /** 2244 * Structured outcome definition. 2245 */ 2246 @Child(name = "reference", type = {EvidenceVariable.class}, order=4, min=0, max=1, modifier=false, summary=false) 2247 @Description(shortDefinition="Structured outcome definition", formalDefinition="Structured outcome definition." ) 2248 protected Reference reference; 2249 2250 private static final long serialVersionUID = 1786559672L; 2251 2252 /** 2253 * Constructor 2254 */ 2255 public ResearchStudyOutcomeMeasureComponent() { 2256 super(); 2257 } 2258 2259 /** 2260 * @return {@link #name} (Label for the outcome.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2261 */ 2262 public StringType getNameElement() { 2263 if (this.name == null) 2264 if (Configuration.errorOnAutoCreate()) 2265 throw new Error("Attempt to auto-create ResearchStudyOutcomeMeasureComponent.name"); 2266 else if (Configuration.doAutoCreate()) 2267 this.name = new StringType(); // bb 2268 return this.name; 2269 } 2270 2271 public boolean hasNameElement() { 2272 return this.name != null && !this.name.isEmpty(); 2273 } 2274 2275 public boolean hasName() { 2276 return this.name != null && !this.name.isEmpty(); 2277 } 2278 2279 /** 2280 * @param value {@link #name} (Label for the outcome.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2281 */ 2282 public ResearchStudyOutcomeMeasureComponent setNameElement(StringType value) { 2283 this.name = value; 2284 return this; 2285 } 2286 2287 /** 2288 * @return Label for the outcome. 2289 */ 2290 public String getName() { 2291 return this.name == null ? null : this.name.getValue(); 2292 } 2293 2294 /** 2295 * @param value Label for the outcome. 2296 */ 2297 public ResearchStudyOutcomeMeasureComponent setName(String value) { 2298 if (Utilities.noString(value)) 2299 this.name = null; 2300 else { 2301 if (this.name == null) 2302 this.name = new StringType(); 2303 this.name.setValue(value); 2304 } 2305 return this; 2306 } 2307 2308 /** 2309 * @return {@link #type} (The parameter or characteristic being assessed as one of the values by which the study is assessed.) 2310 */ 2311 public List<CodeableConcept> getType() { 2312 if (this.type == null) 2313 this.type = new ArrayList<CodeableConcept>(); 2314 return this.type; 2315 } 2316 2317 /** 2318 * @return Returns a reference to <code>this</code> for easy method chaining 2319 */ 2320 public ResearchStudyOutcomeMeasureComponent setType(List<CodeableConcept> theType) { 2321 this.type = theType; 2322 return this; 2323 } 2324 2325 public boolean hasType() { 2326 if (this.type == null) 2327 return false; 2328 for (CodeableConcept item : this.type) 2329 if (!item.isEmpty()) 2330 return true; 2331 return false; 2332 } 2333 2334 public CodeableConcept addType() { //3 2335 CodeableConcept t = new CodeableConcept(); 2336 if (this.type == null) 2337 this.type = new ArrayList<CodeableConcept>(); 2338 this.type.add(t); 2339 return t; 2340 } 2341 2342 public ResearchStudyOutcomeMeasureComponent addType(CodeableConcept t) { //3 2343 if (t == null) 2344 return this; 2345 if (this.type == null) 2346 this.type = new ArrayList<CodeableConcept>(); 2347 this.type.add(t); 2348 return this; 2349 } 2350 2351 /** 2352 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 2353 */ 2354 public CodeableConcept getTypeFirstRep() { 2355 if (getType().isEmpty()) { 2356 addType(); 2357 } 2358 return getType().get(0); 2359 } 2360 2361 /** 2362 * @return {@link #description} (Description of the outcome.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2363 */ 2364 public MarkdownType getDescriptionElement() { 2365 if (this.description == null) 2366 if (Configuration.errorOnAutoCreate()) 2367 throw new Error("Attempt to auto-create ResearchStudyOutcomeMeasureComponent.description"); 2368 else if (Configuration.doAutoCreate()) 2369 this.description = new MarkdownType(); // bb 2370 return this.description; 2371 } 2372 2373 public boolean hasDescriptionElement() { 2374 return this.description != null && !this.description.isEmpty(); 2375 } 2376 2377 public boolean hasDescription() { 2378 return this.description != null && !this.description.isEmpty(); 2379 } 2380 2381 /** 2382 * @param value {@link #description} (Description of the outcome.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2383 */ 2384 public ResearchStudyOutcomeMeasureComponent setDescriptionElement(MarkdownType value) { 2385 this.description = value; 2386 return this; 2387 } 2388 2389 /** 2390 * @return Description of the outcome. 2391 */ 2392 public String getDescription() { 2393 return this.description == null ? null : this.description.getValue(); 2394 } 2395 2396 /** 2397 * @param value Description of the outcome. 2398 */ 2399 public ResearchStudyOutcomeMeasureComponent setDescription(String value) { 2400 if (Utilities.noString(value)) 2401 this.description = null; 2402 else { 2403 if (this.description == null) 2404 this.description = new MarkdownType(); 2405 this.description.setValue(value); 2406 } 2407 return this; 2408 } 2409 2410 /** 2411 * @return {@link #reference} (Structured outcome definition.) 2412 */ 2413 public Reference getReference() { 2414 if (this.reference == null) 2415 if (Configuration.errorOnAutoCreate()) 2416 throw new Error("Attempt to auto-create ResearchStudyOutcomeMeasureComponent.reference"); 2417 else if (Configuration.doAutoCreate()) 2418 this.reference = new Reference(); // cc 2419 return this.reference; 2420 } 2421 2422 public boolean hasReference() { 2423 return this.reference != null && !this.reference.isEmpty(); 2424 } 2425 2426 /** 2427 * @param value {@link #reference} (Structured outcome definition.) 2428 */ 2429 public ResearchStudyOutcomeMeasureComponent setReference(Reference value) { 2430 this.reference = value; 2431 return this; 2432 } 2433 2434 protected void listChildren(List<Property> children) { 2435 super.listChildren(children); 2436 children.add(new Property("name", "string", "Label for the outcome.", 0, 1, name)); 2437 children.add(new Property("type", "CodeableConcept", "The parameter or characteristic being assessed as one of the values by which the study is assessed.", 0, java.lang.Integer.MAX_VALUE, type)); 2438 children.add(new Property("description", "markdown", "Description of the outcome.", 0, 1, description)); 2439 children.add(new Property("reference", "Reference(EvidenceVariable)", "Structured outcome definition.", 0, 1, reference)); 2440 } 2441 2442 @Override 2443 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2444 switch (_hash) { 2445 case 3373707: /*name*/ return new Property("name", "string", "Label for the outcome.", 0, 1, name); 2446 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The parameter or characteristic being assessed as one of the values by which the study is assessed.", 0, java.lang.Integer.MAX_VALUE, type); 2447 case -1724546052: /*description*/ return new Property("description", "markdown", "Description of the outcome.", 0, 1, description); 2448 case -925155509: /*reference*/ return new Property("reference", "Reference(EvidenceVariable)", "Structured outcome definition.", 0, 1, reference); 2449 default: return super.getNamedProperty(_hash, _name, _checkValid); 2450 } 2451 2452 } 2453 2454 @Override 2455 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2456 switch (hash) { 2457 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2458 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 2459 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2460 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 2461 default: return super.getProperty(hash, name, checkValid); 2462 } 2463 2464 } 2465 2466 @Override 2467 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2468 switch (hash) { 2469 case 3373707: // name 2470 this.name = TypeConvertor.castToString(value); // StringType 2471 return value; 2472 case 3575610: // type 2473 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2474 return value; 2475 case -1724546052: // description 2476 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2477 return value; 2478 case -925155509: // reference 2479 this.reference = TypeConvertor.castToReference(value); // Reference 2480 return value; 2481 default: return super.setProperty(hash, name, value); 2482 } 2483 2484 } 2485 2486 @Override 2487 public Base setProperty(String name, Base value) throws FHIRException { 2488 if (name.equals("name")) { 2489 this.name = TypeConvertor.castToString(value); // StringType 2490 } else if (name.equals("type")) { 2491 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 2492 } else if (name.equals("description")) { 2493 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2494 } else if (name.equals("reference")) { 2495 this.reference = TypeConvertor.castToReference(value); // Reference 2496 } else 2497 return super.setProperty(name, value); 2498 return value; 2499 } 2500 2501 @Override 2502 public void removeChild(String name, Base value) throws FHIRException { 2503 if (name.equals("name")) { 2504 this.name = null; 2505 } else if (name.equals("type")) { 2506 this.getType().remove(value); 2507 } else if (name.equals("description")) { 2508 this.description = null; 2509 } else if (name.equals("reference")) { 2510 this.reference = null; 2511 } else 2512 super.removeChild(name, value); 2513 2514 } 2515 2516 @Override 2517 public Base makeProperty(int hash, String name) throws FHIRException { 2518 switch (hash) { 2519 case 3373707: return getNameElement(); 2520 case 3575610: return addType(); 2521 case -1724546052: return getDescriptionElement(); 2522 case -925155509: return getReference(); 2523 default: return super.makeProperty(hash, name); 2524 } 2525 2526 } 2527 2528 @Override 2529 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2530 switch (hash) { 2531 case 3373707: /*name*/ return new String[] {"string"}; 2532 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2533 case -1724546052: /*description*/ return new String[] {"markdown"}; 2534 case -925155509: /*reference*/ return new String[] {"Reference"}; 2535 default: return super.getTypesForProperty(hash, name); 2536 } 2537 2538 } 2539 2540 @Override 2541 public Base addChild(String name) throws FHIRException { 2542 if (name.equals("name")) { 2543 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.outcomeMeasure.name"); 2544 } 2545 else if (name.equals("type")) { 2546 return addType(); 2547 } 2548 else if (name.equals("description")) { 2549 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.outcomeMeasure.description"); 2550 } 2551 else if (name.equals("reference")) { 2552 this.reference = new Reference(); 2553 return this.reference; 2554 } 2555 else 2556 return super.addChild(name); 2557 } 2558 2559 public ResearchStudyOutcomeMeasureComponent copy() { 2560 ResearchStudyOutcomeMeasureComponent dst = new ResearchStudyOutcomeMeasureComponent(); 2561 copyValues(dst); 2562 return dst; 2563 } 2564 2565 public void copyValues(ResearchStudyOutcomeMeasureComponent dst) { 2566 super.copyValues(dst); 2567 dst.name = name == null ? null : name.copy(); 2568 if (type != null) { 2569 dst.type = new ArrayList<CodeableConcept>(); 2570 for (CodeableConcept i : type) 2571 dst.type.add(i.copy()); 2572 }; 2573 dst.description = description == null ? null : description.copy(); 2574 dst.reference = reference == null ? null : reference.copy(); 2575 } 2576 2577 @Override 2578 public boolean equalsDeep(Base other_) { 2579 if (!super.equalsDeep(other_)) 2580 return false; 2581 if (!(other_ instanceof ResearchStudyOutcomeMeasureComponent)) 2582 return false; 2583 ResearchStudyOutcomeMeasureComponent o = (ResearchStudyOutcomeMeasureComponent) other_; 2584 return compareDeep(name, o.name, true) && compareDeep(type, o.type, true) && compareDeep(description, o.description, true) 2585 && compareDeep(reference, o.reference, true); 2586 } 2587 2588 @Override 2589 public boolean equalsShallow(Base other_) { 2590 if (!super.equalsShallow(other_)) 2591 return false; 2592 if (!(other_ instanceof ResearchStudyOutcomeMeasureComponent)) 2593 return false; 2594 ResearchStudyOutcomeMeasureComponent o = (ResearchStudyOutcomeMeasureComponent) other_; 2595 return compareValues(name, o.name, true) && compareValues(description, o.description, true); 2596 } 2597 2598 public boolean isEmpty() { 2599 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, type, description 2600 , reference); 2601 } 2602 2603 public String fhirType() { 2604 return "ResearchStudy.outcomeMeasure"; 2605 2606 } 2607 2608 } 2609 2610 /** 2611 * Canonical identifier for this study resource, represented as a globally unique URI. 2612 */ 2613 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=false) 2614 @Description(shortDefinition="Canonical identifier for this study resource", formalDefinition="Canonical identifier for this study resource, represented as a globally unique URI." ) 2615 protected UriType url; 2616 2617 /** 2618 * Identifiers assigned to this research study by the sponsor or other systems. 2619 */ 2620 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2621 @Description(shortDefinition="Business Identifier for study", formalDefinition="Identifiers assigned to this research study by the sponsor or other systems." ) 2622 protected List<Identifier> identifier; 2623 2624 /** 2625 * The business version for the study record. 2626 */ 2627 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2628 @Description(shortDefinition="The business version for the study record", formalDefinition="The business version for the study record." ) 2629 protected StringType version; 2630 2631 /** 2632 * Name for this study (computer friendly). 2633 */ 2634 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2635 @Description(shortDefinition="Name for this study (computer friendly)", formalDefinition="Name for this study (computer friendly)." ) 2636 protected StringType name; 2637 2638 /** 2639 * The human readable name of the research study. 2640 */ 2641 @Child(name = "title", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 2642 @Description(shortDefinition="Human readable name of the study", formalDefinition="The human readable name of the research study." ) 2643 protected StringType title; 2644 2645 /** 2646 * Additional names for the study. 2647 */ 2648 @Child(name = "label", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2649 @Description(shortDefinition="Additional names for the study", formalDefinition="Additional names for the study." ) 2650 protected List<ResearchStudyLabelComponent> label; 2651 2652 /** 2653 * The set of steps expected to be performed as part of the execution of the study. 2654 */ 2655 @Child(name = "protocol", type = {PlanDefinition.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2656 @Description(shortDefinition="Steps followed in executing study", formalDefinition="The set of steps expected to be performed as part of the execution of the study." ) 2657 protected List<Reference> protocol; 2658 2659 /** 2660 * A larger research study of which this particular study is a component or step. 2661 */ 2662 @Child(name = "partOf", type = {ResearchStudy.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2663 @Description(shortDefinition="Part of larger study", formalDefinition="A larger research study of which this particular study is a component or step." ) 2664 protected List<Reference> partOf; 2665 2666 /** 2667 * Citations, references, URLs and other related documents. When using relatedArtifact to share URLs, the relatedArtifact.type will often be set to one of "documentation" or "supported-with" and the URL value will often be in relatedArtifact.document.url but another possible location is relatedArtifact.resource when it is a canonical URL. 2668 */ 2669 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2670 @Description(shortDefinition="References, URLs, and attachments", formalDefinition="Citations, references, URLs and other related documents. When using relatedArtifact to share URLs, the relatedArtifact.type will often be set to one of \"documentation\" or \"supported-with\" and the URL value will often be in relatedArtifact.document.url but another possible location is relatedArtifact.resource when it is a canonical URL." ) 2671 protected List<RelatedArtifact> relatedArtifact; 2672 2673 /** 2674 * The date (and optionally time) when the ResearchStudy Resource was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ResearchStudy Resource changes. 2675 */ 2676 @Child(name = "date", type = {DateTimeType.class}, order=9, min=0, max=1, modifier=false, summary=false) 2677 @Description(shortDefinition="Date the resource last changed", formalDefinition="The date (and optionally time) when the ResearchStudy Resource was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ResearchStudy Resource changes." ) 2678 protected DateTimeType date; 2679 2680 /** 2681 * The publication state of the resource (not of the study). 2682 */ 2683 @Child(name = "status", type = {CodeType.class}, order=10, min=1, max=1, modifier=true, summary=true) 2684 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The publication state of the resource (not of the study)." ) 2685 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 2686 protected Enumeration<PublicationStatus> status; 2687 2688 /** 2689 * The type of study based upon the intent of the study activities. A classification of the intent of the study. 2690 */ 2691 @Child(name = "primaryPurposeType", type = {CodeableConcept.class}, order=11, min=0, max=1, modifier=false, summary=true) 2692 @Description(shortDefinition="treatment | prevention | diagnostic | supportive-care | screening | health-services-research | basic-science | device-feasibility", formalDefinition="The type of study based upon the intent of the study activities. A classification of the intent of the study." ) 2693 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-prim-purp-type") 2694 protected CodeableConcept primaryPurposeType; 2695 2696 /** 2697 * The stage in the progression of a therapy from initial experimental use in humans in clinical trials to post-market evaluation. 2698 */ 2699 @Child(name = "phase", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=false, summary=true) 2700 @Description(shortDefinition="n-a | early-phase-1 | phase-1 | phase-1-phase-2 | phase-2 | phase-2-phase-3 | phase-3 | phase-4", formalDefinition="The stage in the progression of a therapy from initial experimental use in humans in clinical trials to post-market evaluation." ) 2701 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-phase") 2702 protected CodeableConcept phase; 2703 2704 /** 2705 * Codes categorizing the type of study such as investigational vs. observational, type of blinding, type of randomization, safety vs. efficacy, etc. 2706 */ 2707 @Child(name = "studyDesign", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2708 @Description(shortDefinition="Classifications of the study design characteristics", formalDefinition="Codes categorizing the type of study such as investigational vs. observational, type of blinding, type of randomization, safety vs. efficacy, etc." ) 2709 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/study-design") 2710 protected List<CodeableConcept> studyDesign; 2711 2712 /** 2713 * The medication(s), food(s), therapy(ies), device(s) or other concerns or interventions that the study is seeking to gain more information about. 2714 */ 2715 @Child(name = "focus", type = {CodeableReference.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2716 @Description(shortDefinition="Drugs, devices, etc. under study", formalDefinition="The medication(s), food(s), therapy(ies), device(s) or other concerns or interventions that the study is seeking to gain more information about." ) 2717 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-focus-type") 2718 protected List<CodeableReference> focus; 2719 2720 /** 2721 * The condition that is the focus of the study. For example, In a study to examine risk factors for Lupus, might have as an inclusion criterion "healthy volunteer", but the target condition code would be a Lupus SNOMED code. 2722 */ 2723 @Child(name = "condition", type = {CodeableConcept.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2724 @Description(shortDefinition="Condition being studied", formalDefinition="The condition that is the focus of the study. For example, In a study to examine risk factors for Lupus, might have as an inclusion criterion \"healthy volunteer\", but the target condition code would be a Lupus SNOMED code." ) 2725 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 2726 protected List<CodeableConcept> condition; 2727 2728 /** 2729 * Key terms to aid in searching for or filtering the study. 2730 */ 2731 @Child(name = "keyword", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2732 @Description(shortDefinition="Used to search for the study", formalDefinition="Key terms to aid in searching for or filtering the study." ) 2733 protected List<CodeableConcept> keyword; 2734 2735 /** 2736 * A country, state or other area where the study is taking place rather than its precise geographic location or address. 2737 */ 2738 @Child(name = "region", type = {CodeableConcept.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2739 @Description(shortDefinition="Geographic area for the study", formalDefinition="A country, state or other area where the study is taking place rather than its precise geographic location or address." ) 2740 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 2741 protected List<CodeableConcept> region; 2742 2743 /** 2744 * A brief text for explaining the study. 2745 */ 2746 @Child(name = "descriptionSummary", type = {MarkdownType.class}, order=18, min=0, max=1, modifier=false, summary=false) 2747 @Description(shortDefinition="Brief text explaining the study", formalDefinition="A brief text for explaining the study." ) 2748 protected MarkdownType descriptionSummary; 2749 2750 /** 2751 * A detailed and human-readable narrative of the study. E.g., study abstract. 2752 */ 2753 @Child(name = "description", type = {MarkdownType.class}, order=19, min=0, max=1, modifier=false, summary=false) 2754 @Description(shortDefinition="Detailed narrative of the study", formalDefinition="A detailed and human-readable narrative of the study. E.g., study abstract." ) 2755 protected MarkdownType description; 2756 2757 /** 2758 * Identifies the start date and the expected (or actual, depending on status) end date for the study. 2759 */ 2760 @Child(name = "period", type = {Period.class}, order=20, min=0, max=1, modifier=false, summary=true) 2761 @Description(shortDefinition="When the study began and ended", formalDefinition="Identifies the start date and the expected (or actual, depending on status) end date for the study." ) 2762 protected Period period; 2763 2764 /** 2765 * A facility in which study activities are conducted. 2766 */ 2767 @Child(name = "site", type = {Location.class, ResearchStudy.class, Organization.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2768 @Description(shortDefinition="Facility where study activities are conducted", formalDefinition="A facility in which study activities are conducted." ) 2769 protected List<Reference> site; 2770 2771 /** 2772 * Comments made about the study by the performer, subject or other participants. 2773 */ 2774 @Child(name = "note", type = {Annotation.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2775 @Description(shortDefinition="Comments made about the study", formalDefinition="Comments made about the study by the performer, subject or other participants." ) 2776 protected List<Annotation> note; 2777 2778 /** 2779 * Additional grouping mechanism or categorization of a research study. Example: FDA regulated device, FDA regulated drug, MPG Paragraph 23b (a German legal requirement), IRB-exempt, etc. Implementation Note: do not use the classifier element to support existing semantics that are already supported thru explicit elements in the resource. 2780 */ 2781 @Child(name = "classifier", type = {CodeableConcept.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2782 @Description(shortDefinition="Classification for the study", formalDefinition="Additional grouping mechanism or categorization of a research study. Example: FDA regulated device, FDA regulated drug, MPG Paragraph 23b (a German legal requirement), IRB-exempt, etc. Implementation Note: do not use the classifier element to support existing semantics that are already supported thru explicit elements in the resource." ) 2783 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-classifiers") 2784 protected List<CodeableConcept> classifier; 2785 2786 /** 2787 * Sponsors, collaborators, and other parties. 2788 */ 2789 @Child(name = "associatedParty", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2790 @Description(shortDefinition="Sponsors, collaborators, and other parties", formalDefinition="Sponsors, collaborators, and other parties." ) 2791 protected List<ResearchStudyAssociatedPartyComponent> associatedParty; 2792 2793 /** 2794 * Status of study with time for that status. 2795 */ 2796 @Child(name = "progressStatus", type = {}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2797 @Description(shortDefinition="Status of study with time for that status", formalDefinition="Status of study with time for that status." ) 2798 protected List<ResearchStudyProgressStatusComponent> progressStatus; 2799 2800 /** 2801 * A description and/or code explaining the premature termination of the study. 2802 */ 2803 @Child(name = "whyStopped", type = {CodeableConcept.class}, order=26, min=0, max=1, modifier=false, summary=true) 2804 @Description(shortDefinition="accrual-goal-met | closed-due-to-toxicity | closed-due-to-lack-of-study-progress | temporarily-closed-per-study-design", formalDefinition="A description and/or code explaining the premature termination of the study." ) 2805 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-study-reason-stopped") 2806 protected CodeableConcept whyStopped; 2807 2808 /** 2809 * Target or actual group of participants enrolled in study. 2810 */ 2811 @Child(name = "recruitment", type = {}, order=27, min=0, max=1, modifier=false, summary=true) 2812 @Description(shortDefinition="Target or actual group of participants enrolled in study", formalDefinition="Target or actual group of participants enrolled in study." ) 2813 protected ResearchStudyRecruitmentComponent recruitment; 2814 2815 /** 2816 * Describes an expected event or sequence of events for one of the subjects of a study. E.g. for a living subject: exposure to drug A, wash-out, exposure to drug B, wash-out, follow-up. E.g. for a stability study: {store sample from lot A at 25 degrees for 1 month}, {store sample from lot A at 40 degrees for 1 month}. 2817 */ 2818 @Child(name = "comparisonGroup", type = {}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2819 @Description(shortDefinition="Defined path through the study for a subject", formalDefinition="Describes an expected event or sequence of events for one of the subjects of a study. E.g. for a living subject: exposure to drug A, wash-out, exposure to drug B, wash-out, follow-up. E.g. for a stability study: {store sample from lot A at 25 degrees for 1 month}, {store sample from lot A at 40 degrees for 1 month}." ) 2820 protected List<ResearchStudyComparisonGroupComponent> comparisonGroup; 2821 2822 /** 2823 * A goal that the study is aiming to achieve in terms of a scientific question to be answered by the analysis of data collected during the study. 2824 */ 2825 @Child(name = "objective", type = {}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2826 @Description(shortDefinition="A goal for the study", formalDefinition="A goal that the study is aiming to achieve in terms of a scientific question to be answered by the analysis of data collected during the study." ) 2827 protected List<ResearchStudyObjectiveComponent> objective; 2828 2829 /** 2830 * An "outcome measure", "endpoint", "effect measure" or "measure of effect" is a specific measurement or observation used to quantify the effect of experimental variables on the participants in a study, or for observational studies, to describe patterns of diseases or traits or associations with exposures, risk factors or treatment. 2831 */ 2832 @Child(name = "outcomeMeasure", type = {}, order=30, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2833 @Description(shortDefinition="A variable measured during the study", formalDefinition="An \"outcome measure\", \"endpoint\", \"effect measure\" or \"measure of effect\" is a specific measurement or observation used to quantify the effect of experimental variables on the participants in a study, or for observational studies, to describe patterns of diseases or traits or associations with exposures, risk factors or treatment." ) 2834 protected List<ResearchStudyOutcomeMeasureComponent> outcomeMeasure; 2835 2836 /** 2837 * Link to one or more sets of results generated by the study. Could also link to a research registry holding the results such as ClinicalTrials.gov. 2838 */ 2839 @Child(name = "result", type = {EvidenceReport.class, Citation.class, DiagnosticReport.class}, order=31, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2840 @Description(shortDefinition="Link to results generated during the study", formalDefinition="Link to one or more sets of results generated by the study. Could also link to a research registry holding the results such as ClinicalTrials.gov." ) 2841 protected List<Reference> result; 2842 2843 private static final long serialVersionUID = -1217395129L; 2844 2845 /** 2846 * Constructor 2847 */ 2848 public ResearchStudy() { 2849 super(); 2850 } 2851 2852 /** 2853 * Constructor 2854 */ 2855 public ResearchStudy(PublicationStatus status) { 2856 super(); 2857 this.setStatus(status); 2858 } 2859 2860 /** 2861 * @return {@link #url} (Canonical identifier for this study resource, represented as a globally unique URI.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2862 */ 2863 public UriType getUrlElement() { 2864 if (this.url == null) 2865 if (Configuration.errorOnAutoCreate()) 2866 throw new Error("Attempt to auto-create ResearchStudy.url"); 2867 else if (Configuration.doAutoCreate()) 2868 this.url = new UriType(); // bb 2869 return this.url; 2870 } 2871 2872 public boolean hasUrlElement() { 2873 return this.url != null && !this.url.isEmpty(); 2874 } 2875 2876 public boolean hasUrl() { 2877 return this.url != null && !this.url.isEmpty(); 2878 } 2879 2880 /** 2881 * @param value {@link #url} (Canonical identifier for this study resource, represented as a globally unique URI.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2882 */ 2883 public ResearchStudy setUrlElement(UriType value) { 2884 this.url = value; 2885 return this; 2886 } 2887 2888 /** 2889 * @return Canonical identifier for this study resource, represented as a globally unique URI. 2890 */ 2891 public String getUrl() { 2892 return this.url == null ? null : this.url.getValue(); 2893 } 2894 2895 /** 2896 * @param value Canonical identifier for this study resource, represented as a globally unique URI. 2897 */ 2898 public ResearchStudy setUrl(String value) { 2899 if (Utilities.noString(value)) 2900 this.url = null; 2901 else { 2902 if (this.url == null) 2903 this.url = new UriType(); 2904 this.url.setValue(value); 2905 } 2906 return this; 2907 } 2908 2909 /** 2910 * @return {@link #identifier} (Identifiers assigned to this research study by the sponsor or other systems.) 2911 */ 2912 public List<Identifier> getIdentifier() { 2913 if (this.identifier == null) 2914 this.identifier = new ArrayList<Identifier>(); 2915 return this.identifier; 2916 } 2917 2918 /** 2919 * @return Returns a reference to <code>this</code> for easy method chaining 2920 */ 2921 public ResearchStudy setIdentifier(List<Identifier> theIdentifier) { 2922 this.identifier = theIdentifier; 2923 return this; 2924 } 2925 2926 public boolean hasIdentifier() { 2927 if (this.identifier == null) 2928 return false; 2929 for (Identifier item : this.identifier) 2930 if (!item.isEmpty()) 2931 return true; 2932 return false; 2933 } 2934 2935 public Identifier addIdentifier() { //3 2936 Identifier t = new Identifier(); 2937 if (this.identifier == null) 2938 this.identifier = new ArrayList<Identifier>(); 2939 this.identifier.add(t); 2940 return t; 2941 } 2942 2943 public ResearchStudy addIdentifier(Identifier t) { //3 2944 if (t == null) 2945 return this; 2946 if (this.identifier == null) 2947 this.identifier = new ArrayList<Identifier>(); 2948 this.identifier.add(t); 2949 return this; 2950 } 2951 2952 /** 2953 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2954 */ 2955 public Identifier getIdentifierFirstRep() { 2956 if (getIdentifier().isEmpty()) { 2957 addIdentifier(); 2958 } 2959 return getIdentifier().get(0); 2960 } 2961 2962 /** 2963 * @return {@link #version} (The business version for the study record.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2964 */ 2965 public StringType getVersionElement() { 2966 if (this.version == null) 2967 if (Configuration.errorOnAutoCreate()) 2968 throw new Error("Attempt to auto-create ResearchStudy.version"); 2969 else if (Configuration.doAutoCreate()) 2970 this.version = new StringType(); // bb 2971 return this.version; 2972 } 2973 2974 public boolean hasVersionElement() { 2975 return this.version != null && !this.version.isEmpty(); 2976 } 2977 2978 public boolean hasVersion() { 2979 return this.version != null && !this.version.isEmpty(); 2980 } 2981 2982 /** 2983 * @param value {@link #version} (The business version for the study record.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2984 */ 2985 public ResearchStudy setVersionElement(StringType value) { 2986 this.version = value; 2987 return this; 2988 } 2989 2990 /** 2991 * @return The business version for the study record. 2992 */ 2993 public String getVersion() { 2994 return this.version == null ? null : this.version.getValue(); 2995 } 2996 2997 /** 2998 * @param value The business version for the study record. 2999 */ 3000 public ResearchStudy setVersion(String value) { 3001 if (Utilities.noString(value)) 3002 this.version = null; 3003 else { 3004 if (this.version == null) 3005 this.version = new StringType(); 3006 this.version.setValue(value); 3007 } 3008 return this; 3009 } 3010 3011 /** 3012 * @return {@link #name} (Name for this study (computer friendly).). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3013 */ 3014 public StringType getNameElement() { 3015 if (this.name == null) 3016 if (Configuration.errorOnAutoCreate()) 3017 throw new Error("Attempt to auto-create ResearchStudy.name"); 3018 else if (Configuration.doAutoCreate()) 3019 this.name = new StringType(); // bb 3020 return this.name; 3021 } 3022 3023 public boolean hasNameElement() { 3024 return this.name != null && !this.name.isEmpty(); 3025 } 3026 3027 public boolean hasName() { 3028 return this.name != null && !this.name.isEmpty(); 3029 } 3030 3031 /** 3032 * @param value {@link #name} (Name for this study (computer friendly).). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3033 */ 3034 public ResearchStudy setNameElement(StringType value) { 3035 this.name = value; 3036 return this; 3037 } 3038 3039 /** 3040 * @return Name for this study (computer friendly). 3041 */ 3042 public String getName() { 3043 return this.name == null ? null : this.name.getValue(); 3044 } 3045 3046 /** 3047 * @param value Name for this study (computer friendly). 3048 */ 3049 public ResearchStudy setName(String value) { 3050 if (Utilities.noString(value)) 3051 this.name = null; 3052 else { 3053 if (this.name == null) 3054 this.name = new StringType(); 3055 this.name.setValue(value); 3056 } 3057 return this; 3058 } 3059 3060 /** 3061 * @return {@link #title} (The human readable name of the research study.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3062 */ 3063 public StringType getTitleElement() { 3064 if (this.title == null) 3065 if (Configuration.errorOnAutoCreate()) 3066 throw new Error("Attempt to auto-create ResearchStudy.title"); 3067 else if (Configuration.doAutoCreate()) 3068 this.title = new StringType(); // bb 3069 return this.title; 3070 } 3071 3072 public boolean hasTitleElement() { 3073 return this.title != null && !this.title.isEmpty(); 3074 } 3075 3076 public boolean hasTitle() { 3077 return this.title != null && !this.title.isEmpty(); 3078 } 3079 3080 /** 3081 * @param value {@link #title} (The human readable name of the research study.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3082 */ 3083 public ResearchStudy setTitleElement(StringType value) { 3084 this.title = value; 3085 return this; 3086 } 3087 3088 /** 3089 * @return The human readable name of the research study. 3090 */ 3091 public String getTitle() { 3092 return this.title == null ? null : this.title.getValue(); 3093 } 3094 3095 /** 3096 * @param value The human readable name of the research study. 3097 */ 3098 public ResearchStudy setTitle(String value) { 3099 if (Utilities.noString(value)) 3100 this.title = null; 3101 else { 3102 if (this.title == null) 3103 this.title = new StringType(); 3104 this.title.setValue(value); 3105 } 3106 return this; 3107 } 3108 3109 /** 3110 * @return {@link #label} (Additional names for the study.) 3111 */ 3112 public List<ResearchStudyLabelComponent> getLabel() { 3113 if (this.label == null) 3114 this.label = new ArrayList<ResearchStudyLabelComponent>(); 3115 return this.label; 3116 } 3117 3118 /** 3119 * @return Returns a reference to <code>this</code> for easy method chaining 3120 */ 3121 public ResearchStudy setLabel(List<ResearchStudyLabelComponent> theLabel) { 3122 this.label = theLabel; 3123 return this; 3124 } 3125 3126 public boolean hasLabel() { 3127 if (this.label == null) 3128 return false; 3129 for (ResearchStudyLabelComponent item : this.label) 3130 if (!item.isEmpty()) 3131 return true; 3132 return false; 3133 } 3134 3135 public ResearchStudyLabelComponent addLabel() { //3 3136 ResearchStudyLabelComponent t = new ResearchStudyLabelComponent(); 3137 if (this.label == null) 3138 this.label = new ArrayList<ResearchStudyLabelComponent>(); 3139 this.label.add(t); 3140 return t; 3141 } 3142 3143 public ResearchStudy addLabel(ResearchStudyLabelComponent t) { //3 3144 if (t == null) 3145 return this; 3146 if (this.label == null) 3147 this.label = new ArrayList<ResearchStudyLabelComponent>(); 3148 this.label.add(t); 3149 return this; 3150 } 3151 3152 /** 3153 * @return The first repetition of repeating field {@link #label}, creating it if it does not already exist {3} 3154 */ 3155 public ResearchStudyLabelComponent getLabelFirstRep() { 3156 if (getLabel().isEmpty()) { 3157 addLabel(); 3158 } 3159 return getLabel().get(0); 3160 } 3161 3162 /** 3163 * @return {@link #protocol} (The set of steps expected to be performed as part of the execution of the study.) 3164 */ 3165 public List<Reference> getProtocol() { 3166 if (this.protocol == null) 3167 this.protocol = new ArrayList<Reference>(); 3168 return this.protocol; 3169 } 3170 3171 /** 3172 * @return Returns a reference to <code>this</code> for easy method chaining 3173 */ 3174 public ResearchStudy setProtocol(List<Reference> theProtocol) { 3175 this.protocol = theProtocol; 3176 return this; 3177 } 3178 3179 public boolean hasProtocol() { 3180 if (this.protocol == null) 3181 return false; 3182 for (Reference item : this.protocol) 3183 if (!item.isEmpty()) 3184 return true; 3185 return false; 3186 } 3187 3188 public Reference addProtocol() { //3 3189 Reference t = new Reference(); 3190 if (this.protocol == null) 3191 this.protocol = new ArrayList<Reference>(); 3192 this.protocol.add(t); 3193 return t; 3194 } 3195 3196 public ResearchStudy addProtocol(Reference t) { //3 3197 if (t == null) 3198 return this; 3199 if (this.protocol == null) 3200 this.protocol = new ArrayList<Reference>(); 3201 this.protocol.add(t); 3202 return this; 3203 } 3204 3205 /** 3206 * @return The first repetition of repeating field {@link #protocol}, creating it if it does not already exist {3} 3207 */ 3208 public Reference getProtocolFirstRep() { 3209 if (getProtocol().isEmpty()) { 3210 addProtocol(); 3211 } 3212 return getProtocol().get(0); 3213 } 3214 3215 /** 3216 * @return {@link #partOf} (A larger research study of which this particular study is a component or step.) 3217 */ 3218 public List<Reference> getPartOf() { 3219 if (this.partOf == null) 3220 this.partOf = new ArrayList<Reference>(); 3221 return this.partOf; 3222 } 3223 3224 /** 3225 * @return Returns a reference to <code>this</code> for easy method chaining 3226 */ 3227 public ResearchStudy setPartOf(List<Reference> thePartOf) { 3228 this.partOf = thePartOf; 3229 return this; 3230 } 3231 3232 public boolean hasPartOf() { 3233 if (this.partOf == null) 3234 return false; 3235 for (Reference item : this.partOf) 3236 if (!item.isEmpty()) 3237 return true; 3238 return false; 3239 } 3240 3241 public Reference addPartOf() { //3 3242 Reference t = new Reference(); 3243 if (this.partOf == null) 3244 this.partOf = new ArrayList<Reference>(); 3245 this.partOf.add(t); 3246 return t; 3247 } 3248 3249 public ResearchStudy addPartOf(Reference t) { //3 3250 if (t == null) 3251 return this; 3252 if (this.partOf == null) 3253 this.partOf = new ArrayList<Reference>(); 3254 this.partOf.add(t); 3255 return this; 3256 } 3257 3258 /** 3259 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 3260 */ 3261 public Reference getPartOfFirstRep() { 3262 if (getPartOf().isEmpty()) { 3263 addPartOf(); 3264 } 3265 return getPartOf().get(0); 3266 } 3267 3268 /** 3269 * @return {@link #relatedArtifact} (Citations, references, URLs and other related documents. When using relatedArtifact to share URLs, the relatedArtifact.type will often be set to one of "documentation" or "supported-with" and the URL value will often be in relatedArtifact.document.url but another possible location is relatedArtifact.resource when it is a canonical URL.) 3270 */ 3271 public List<RelatedArtifact> getRelatedArtifact() { 3272 if (this.relatedArtifact == null) 3273 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3274 return this.relatedArtifact; 3275 } 3276 3277 /** 3278 * @return Returns a reference to <code>this</code> for easy method chaining 3279 */ 3280 public ResearchStudy setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 3281 this.relatedArtifact = theRelatedArtifact; 3282 return this; 3283 } 3284 3285 public boolean hasRelatedArtifact() { 3286 if (this.relatedArtifact == null) 3287 return false; 3288 for (RelatedArtifact item : this.relatedArtifact) 3289 if (!item.isEmpty()) 3290 return true; 3291 return false; 3292 } 3293 3294 public RelatedArtifact addRelatedArtifact() { //3 3295 RelatedArtifact t = new RelatedArtifact(); 3296 if (this.relatedArtifact == null) 3297 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3298 this.relatedArtifact.add(t); 3299 return t; 3300 } 3301 3302 public ResearchStudy addRelatedArtifact(RelatedArtifact t) { //3 3303 if (t == null) 3304 return this; 3305 if (this.relatedArtifact == null) 3306 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3307 this.relatedArtifact.add(t); 3308 return this; 3309 } 3310 3311 /** 3312 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {3} 3313 */ 3314 public RelatedArtifact getRelatedArtifactFirstRep() { 3315 if (getRelatedArtifact().isEmpty()) { 3316 addRelatedArtifact(); 3317 } 3318 return getRelatedArtifact().get(0); 3319 } 3320 3321 /** 3322 * @return {@link #date} (The date (and optionally time) when the ResearchStudy Resource was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ResearchStudy Resource changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3323 */ 3324 public DateTimeType getDateElement() { 3325 if (this.date == null) 3326 if (Configuration.errorOnAutoCreate()) 3327 throw new Error("Attempt to auto-create ResearchStudy.date"); 3328 else if (Configuration.doAutoCreate()) 3329 this.date = new DateTimeType(); // bb 3330 return this.date; 3331 } 3332 3333 public boolean hasDateElement() { 3334 return this.date != null && !this.date.isEmpty(); 3335 } 3336 3337 public boolean hasDate() { 3338 return this.date != null && !this.date.isEmpty(); 3339 } 3340 3341 /** 3342 * @param value {@link #date} (The date (and optionally time) when the ResearchStudy Resource was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ResearchStudy Resource changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3343 */ 3344 public ResearchStudy setDateElement(DateTimeType value) { 3345 this.date = value; 3346 return this; 3347 } 3348 3349 /** 3350 * @return The date (and optionally time) when the ResearchStudy Resource was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ResearchStudy Resource changes. 3351 */ 3352 public Date getDate() { 3353 return this.date == null ? null : this.date.getValue(); 3354 } 3355 3356 /** 3357 * @param value The date (and optionally time) when the ResearchStudy Resource was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ResearchStudy Resource changes. 3358 */ 3359 public ResearchStudy setDate(Date value) { 3360 if (value == null) 3361 this.date = null; 3362 else { 3363 if (this.date == null) 3364 this.date = new DateTimeType(); 3365 this.date.setValue(value); 3366 } 3367 return this; 3368 } 3369 3370 /** 3371 * @return {@link #status} (The publication state of the resource (not of the study).). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3372 */ 3373 public Enumeration<PublicationStatus> getStatusElement() { 3374 if (this.status == null) 3375 if (Configuration.errorOnAutoCreate()) 3376 throw new Error("Attempt to auto-create ResearchStudy.status"); 3377 else if (Configuration.doAutoCreate()) 3378 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 3379 return this.status; 3380 } 3381 3382 public boolean hasStatusElement() { 3383 return this.status != null && !this.status.isEmpty(); 3384 } 3385 3386 public boolean hasStatus() { 3387 return this.status != null && !this.status.isEmpty(); 3388 } 3389 3390 /** 3391 * @param value {@link #status} (The publication state of the resource (not of the study).). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3392 */ 3393 public ResearchStudy setStatusElement(Enumeration<PublicationStatus> value) { 3394 this.status = value; 3395 return this; 3396 } 3397 3398 /** 3399 * @return The publication state of the resource (not of the study). 3400 */ 3401 public PublicationStatus getStatus() { 3402 return this.status == null ? null : this.status.getValue(); 3403 } 3404 3405 /** 3406 * @param value The publication state of the resource (not of the study). 3407 */ 3408 public ResearchStudy setStatus(PublicationStatus value) { 3409 if (this.status == null) 3410 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 3411 this.status.setValue(value); 3412 return this; 3413 } 3414 3415 /** 3416 * @return {@link #primaryPurposeType} (The type of study based upon the intent of the study activities. A classification of the intent of the study.) 3417 */ 3418 public CodeableConcept getPrimaryPurposeType() { 3419 if (this.primaryPurposeType == null) 3420 if (Configuration.errorOnAutoCreate()) 3421 throw new Error("Attempt to auto-create ResearchStudy.primaryPurposeType"); 3422 else if (Configuration.doAutoCreate()) 3423 this.primaryPurposeType = new CodeableConcept(); // cc 3424 return this.primaryPurposeType; 3425 } 3426 3427 public boolean hasPrimaryPurposeType() { 3428 return this.primaryPurposeType != null && !this.primaryPurposeType.isEmpty(); 3429 } 3430 3431 /** 3432 * @param value {@link #primaryPurposeType} (The type of study based upon the intent of the study activities. A classification of the intent of the study.) 3433 */ 3434 public ResearchStudy setPrimaryPurposeType(CodeableConcept value) { 3435 this.primaryPurposeType = value; 3436 return this; 3437 } 3438 3439 /** 3440 * @return {@link #phase} (The stage in the progression of a therapy from initial experimental use in humans in clinical trials to post-market evaluation.) 3441 */ 3442 public CodeableConcept getPhase() { 3443 if (this.phase == null) 3444 if (Configuration.errorOnAutoCreate()) 3445 throw new Error("Attempt to auto-create ResearchStudy.phase"); 3446 else if (Configuration.doAutoCreate()) 3447 this.phase = new CodeableConcept(); // cc 3448 return this.phase; 3449 } 3450 3451 public boolean hasPhase() { 3452 return this.phase != null && !this.phase.isEmpty(); 3453 } 3454 3455 /** 3456 * @param value {@link #phase} (The stage in the progression of a therapy from initial experimental use in humans in clinical trials to post-market evaluation.) 3457 */ 3458 public ResearchStudy setPhase(CodeableConcept value) { 3459 this.phase = value; 3460 return this; 3461 } 3462 3463 /** 3464 * @return {@link #studyDesign} (Codes categorizing the type of study such as investigational vs. observational, type of blinding, type of randomization, safety vs. efficacy, etc.) 3465 */ 3466 public List<CodeableConcept> getStudyDesign() { 3467 if (this.studyDesign == null) 3468 this.studyDesign = new ArrayList<CodeableConcept>(); 3469 return this.studyDesign; 3470 } 3471 3472 /** 3473 * @return Returns a reference to <code>this</code> for easy method chaining 3474 */ 3475 public ResearchStudy setStudyDesign(List<CodeableConcept> theStudyDesign) { 3476 this.studyDesign = theStudyDesign; 3477 return this; 3478 } 3479 3480 public boolean hasStudyDesign() { 3481 if (this.studyDesign == null) 3482 return false; 3483 for (CodeableConcept item : this.studyDesign) 3484 if (!item.isEmpty()) 3485 return true; 3486 return false; 3487 } 3488 3489 public CodeableConcept addStudyDesign() { //3 3490 CodeableConcept t = new CodeableConcept(); 3491 if (this.studyDesign == null) 3492 this.studyDesign = new ArrayList<CodeableConcept>(); 3493 this.studyDesign.add(t); 3494 return t; 3495 } 3496 3497 public ResearchStudy addStudyDesign(CodeableConcept t) { //3 3498 if (t == null) 3499 return this; 3500 if (this.studyDesign == null) 3501 this.studyDesign = new ArrayList<CodeableConcept>(); 3502 this.studyDesign.add(t); 3503 return this; 3504 } 3505 3506 /** 3507 * @return The first repetition of repeating field {@link #studyDesign}, creating it if it does not already exist {3} 3508 */ 3509 public CodeableConcept getStudyDesignFirstRep() { 3510 if (getStudyDesign().isEmpty()) { 3511 addStudyDesign(); 3512 } 3513 return getStudyDesign().get(0); 3514 } 3515 3516 /** 3517 * @return {@link #focus} (The medication(s), food(s), therapy(ies), device(s) or other concerns or interventions that the study is seeking to gain more information about.) 3518 */ 3519 public List<CodeableReference> getFocus() { 3520 if (this.focus == null) 3521 this.focus = new ArrayList<CodeableReference>(); 3522 return this.focus; 3523 } 3524 3525 /** 3526 * @return Returns a reference to <code>this</code> for easy method chaining 3527 */ 3528 public ResearchStudy setFocus(List<CodeableReference> theFocus) { 3529 this.focus = theFocus; 3530 return this; 3531 } 3532 3533 public boolean hasFocus() { 3534 if (this.focus == null) 3535 return false; 3536 for (CodeableReference item : this.focus) 3537 if (!item.isEmpty()) 3538 return true; 3539 return false; 3540 } 3541 3542 public CodeableReference addFocus() { //3 3543 CodeableReference t = new CodeableReference(); 3544 if (this.focus == null) 3545 this.focus = new ArrayList<CodeableReference>(); 3546 this.focus.add(t); 3547 return t; 3548 } 3549 3550 public ResearchStudy addFocus(CodeableReference t) { //3 3551 if (t == null) 3552 return this; 3553 if (this.focus == null) 3554 this.focus = new ArrayList<CodeableReference>(); 3555 this.focus.add(t); 3556 return this; 3557 } 3558 3559 /** 3560 * @return The first repetition of repeating field {@link #focus}, creating it if it does not already exist {3} 3561 */ 3562 public CodeableReference getFocusFirstRep() { 3563 if (getFocus().isEmpty()) { 3564 addFocus(); 3565 } 3566 return getFocus().get(0); 3567 } 3568 3569 /** 3570 * @return {@link #condition} (The condition that is the focus of the study. For example, In a study to examine risk factors for Lupus, might have as an inclusion criterion "healthy volunteer", but the target condition code would be a Lupus SNOMED code.) 3571 */ 3572 public List<CodeableConcept> getCondition() { 3573 if (this.condition == null) 3574 this.condition = new ArrayList<CodeableConcept>(); 3575 return this.condition; 3576 } 3577 3578 /** 3579 * @return Returns a reference to <code>this</code> for easy method chaining 3580 */ 3581 public ResearchStudy setCondition(List<CodeableConcept> theCondition) { 3582 this.condition = theCondition; 3583 return this; 3584 } 3585 3586 public boolean hasCondition() { 3587 if (this.condition == null) 3588 return false; 3589 for (CodeableConcept item : this.condition) 3590 if (!item.isEmpty()) 3591 return true; 3592 return false; 3593 } 3594 3595 public CodeableConcept addCondition() { //3 3596 CodeableConcept t = new CodeableConcept(); 3597 if (this.condition == null) 3598 this.condition = new ArrayList<CodeableConcept>(); 3599 this.condition.add(t); 3600 return t; 3601 } 3602 3603 public ResearchStudy addCondition(CodeableConcept t) { //3 3604 if (t == null) 3605 return this; 3606 if (this.condition == null) 3607 this.condition = new ArrayList<CodeableConcept>(); 3608 this.condition.add(t); 3609 return this; 3610 } 3611 3612 /** 3613 * @return The first repetition of repeating field {@link #condition}, creating it if it does not already exist {3} 3614 */ 3615 public CodeableConcept getConditionFirstRep() { 3616 if (getCondition().isEmpty()) { 3617 addCondition(); 3618 } 3619 return getCondition().get(0); 3620 } 3621 3622 /** 3623 * @return {@link #keyword} (Key terms to aid in searching for or filtering the study.) 3624 */ 3625 public List<CodeableConcept> getKeyword() { 3626 if (this.keyword == null) 3627 this.keyword = new ArrayList<CodeableConcept>(); 3628 return this.keyword; 3629 } 3630 3631 /** 3632 * @return Returns a reference to <code>this</code> for easy method chaining 3633 */ 3634 public ResearchStudy setKeyword(List<CodeableConcept> theKeyword) { 3635 this.keyword = theKeyword; 3636 return this; 3637 } 3638 3639 public boolean hasKeyword() { 3640 if (this.keyword == null) 3641 return false; 3642 for (CodeableConcept item : this.keyword) 3643 if (!item.isEmpty()) 3644 return true; 3645 return false; 3646 } 3647 3648 public CodeableConcept addKeyword() { //3 3649 CodeableConcept t = new CodeableConcept(); 3650 if (this.keyword == null) 3651 this.keyword = new ArrayList<CodeableConcept>(); 3652 this.keyword.add(t); 3653 return t; 3654 } 3655 3656 public ResearchStudy addKeyword(CodeableConcept t) { //3 3657 if (t == null) 3658 return this; 3659 if (this.keyword == null) 3660 this.keyword = new ArrayList<CodeableConcept>(); 3661 this.keyword.add(t); 3662 return this; 3663 } 3664 3665 /** 3666 * @return The first repetition of repeating field {@link #keyword}, creating it if it does not already exist {3} 3667 */ 3668 public CodeableConcept getKeywordFirstRep() { 3669 if (getKeyword().isEmpty()) { 3670 addKeyword(); 3671 } 3672 return getKeyword().get(0); 3673 } 3674 3675 /** 3676 * @return {@link #region} (A country, state or other area where the study is taking place rather than its precise geographic location or address.) 3677 */ 3678 public List<CodeableConcept> getRegion() { 3679 if (this.region == null) 3680 this.region = new ArrayList<CodeableConcept>(); 3681 return this.region; 3682 } 3683 3684 /** 3685 * @return Returns a reference to <code>this</code> for easy method chaining 3686 */ 3687 public ResearchStudy setRegion(List<CodeableConcept> theRegion) { 3688 this.region = theRegion; 3689 return this; 3690 } 3691 3692 public boolean hasRegion() { 3693 if (this.region == null) 3694 return false; 3695 for (CodeableConcept item : this.region) 3696 if (!item.isEmpty()) 3697 return true; 3698 return false; 3699 } 3700 3701 public CodeableConcept addRegion() { //3 3702 CodeableConcept t = new CodeableConcept(); 3703 if (this.region == null) 3704 this.region = new ArrayList<CodeableConcept>(); 3705 this.region.add(t); 3706 return t; 3707 } 3708 3709 public ResearchStudy addRegion(CodeableConcept t) { //3 3710 if (t == null) 3711 return this; 3712 if (this.region == null) 3713 this.region = new ArrayList<CodeableConcept>(); 3714 this.region.add(t); 3715 return this; 3716 } 3717 3718 /** 3719 * @return The first repetition of repeating field {@link #region}, creating it if it does not already exist {3} 3720 */ 3721 public CodeableConcept getRegionFirstRep() { 3722 if (getRegion().isEmpty()) { 3723 addRegion(); 3724 } 3725 return getRegion().get(0); 3726 } 3727 3728 /** 3729 * @return {@link #descriptionSummary} (A brief text for explaining the study.). This is the underlying object with id, value and extensions. The accessor "getDescriptionSummary" gives direct access to the value 3730 */ 3731 public MarkdownType getDescriptionSummaryElement() { 3732 if (this.descriptionSummary == null) 3733 if (Configuration.errorOnAutoCreate()) 3734 throw new Error("Attempt to auto-create ResearchStudy.descriptionSummary"); 3735 else if (Configuration.doAutoCreate()) 3736 this.descriptionSummary = new MarkdownType(); // bb 3737 return this.descriptionSummary; 3738 } 3739 3740 public boolean hasDescriptionSummaryElement() { 3741 return this.descriptionSummary != null && !this.descriptionSummary.isEmpty(); 3742 } 3743 3744 public boolean hasDescriptionSummary() { 3745 return this.descriptionSummary != null && !this.descriptionSummary.isEmpty(); 3746 } 3747 3748 /** 3749 * @param value {@link #descriptionSummary} (A brief text for explaining the study.). This is the underlying object with id, value and extensions. The accessor "getDescriptionSummary" gives direct access to the value 3750 */ 3751 public ResearchStudy setDescriptionSummaryElement(MarkdownType value) { 3752 this.descriptionSummary = value; 3753 return this; 3754 } 3755 3756 /** 3757 * @return A brief text for explaining the study. 3758 */ 3759 public String getDescriptionSummary() { 3760 return this.descriptionSummary == null ? null : this.descriptionSummary.getValue(); 3761 } 3762 3763 /** 3764 * @param value A brief text for explaining the study. 3765 */ 3766 public ResearchStudy setDescriptionSummary(String value) { 3767 if (Utilities.noString(value)) 3768 this.descriptionSummary = null; 3769 else { 3770 if (this.descriptionSummary == null) 3771 this.descriptionSummary = new MarkdownType(); 3772 this.descriptionSummary.setValue(value); 3773 } 3774 return this; 3775 } 3776 3777 /** 3778 * @return {@link #description} (A detailed and human-readable narrative of the study. E.g., study abstract.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3779 */ 3780 public MarkdownType getDescriptionElement() { 3781 if (this.description == null) 3782 if (Configuration.errorOnAutoCreate()) 3783 throw new Error("Attempt to auto-create ResearchStudy.description"); 3784 else if (Configuration.doAutoCreate()) 3785 this.description = new MarkdownType(); // bb 3786 return this.description; 3787 } 3788 3789 public boolean hasDescriptionElement() { 3790 return this.description != null && !this.description.isEmpty(); 3791 } 3792 3793 public boolean hasDescription() { 3794 return this.description != null && !this.description.isEmpty(); 3795 } 3796 3797 /** 3798 * @param value {@link #description} (A detailed and human-readable narrative of the study. E.g., study abstract.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3799 */ 3800 public ResearchStudy setDescriptionElement(MarkdownType value) { 3801 this.description = value; 3802 return this; 3803 } 3804 3805 /** 3806 * @return A detailed and human-readable narrative of the study. E.g., study abstract. 3807 */ 3808 public String getDescription() { 3809 return this.description == null ? null : this.description.getValue(); 3810 } 3811 3812 /** 3813 * @param value A detailed and human-readable narrative of the study. E.g., study abstract. 3814 */ 3815 public ResearchStudy setDescription(String value) { 3816 if (Utilities.noString(value)) 3817 this.description = null; 3818 else { 3819 if (this.description == null) 3820 this.description = new MarkdownType(); 3821 this.description.setValue(value); 3822 } 3823 return this; 3824 } 3825 3826 /** 3827 * @return {@link #period} (Identifies the start date and the expected (or actual, depending on status) end date for the study.) 3828 */ 3829 public Period getPeriod() { 3830 if (this.period == null) 3831 if (Configuration.errorOnAutoCreate()) 3832 throw new Error("Attempt to auto-create ResearchStudy.period"); 3833 else if (Configuration.doAutoCreate()) 3834 this.period = new Period(); // cc 3835 return this.period; 3836 } 3837 3838 public boolean hasPeriod() { 3839 return this.period != null && !this.period.isEmpty(); 3840 } 3841 3842 /** 3843 * @param value {@link #period} (Identifies the start date and the expected (or actual, depending on status) end date for the study.) 3844 */ 3845 public ResearchStudy setPeriod(Period value) { 3846 this.period = value; 3847 return this; 3848 } 3849 3850 /** 3851 * @return {@link #site} (A facility in which study activities are conducted.) 3852 */ 3853 public List<Reference> getSite() { 3854 if (this.site == null) 3855 this.site = new ArrayList<Reference>(); 3856 return this.site; 3857 } 3858 3859 /** 3860 * @return Returns a reference to <code>this</code> for easy method chaining 3861 */ 3862 public ResearchStudy setSite(List<Reference> theSite) { 3863 this.site = theSite; 3864 return this; 3865 } 3866 3867 public boolean hasSite() { 3868 if (this.site == null) 3869 return false; 3870 for (Reference item : this.site) 3871 if (!item.isEmpty()) 3872 return true; 3873 return false; 3874 } 3875 3876 public Reference addSite() { //3 3877 Reference t = new Reference(); 3878 if (this.site == null) 3879 this.site = new ArrayList<Reference>(); 3880 this.site.add(t); 3881 return t; 3882 } 3883 3884 public ResearchStudy addSite(Reference t) { //3 3885 if (t == null) 3886 return this; 3887 if (this.site == null) 3888 this.site = new ArrayList<Reference>(); 3889 this.site.add(t); 3890 return this; 3891 } 3892 3893 /** 3894 * @return The first repetition of repeating field {@link #site}, creating it if it does not already exist {3} 3895 */ 3896 public Reference getSiteFirstRep() { 3897 if (getSite().isEmpty()) { 3898 addSite(); 3899 } 3900 return getSite().get(0); 3901 } 3902 3903 /** 3904 * @return {@link #note} (Comments made about the study by the performer, subject or other participants.) 3905 */ 3906 public List<Annotation> getNote() { 3907 if (this.note == null) 3908 this.note = new ArrayList<Annotation>(); 3909 return this.note; 3910 } 3911 3912 /** 3913 * @return Returns a reference to <code>this</code> for easy method chaining 3914 */ 3915 public ResearchStudy setNote(List<Annotation> theNote) { 3916 this.note = theNote; 3917 return this; 3918 } 3919 3920 public boolean hasNote() { 3921 if (this.note == null) 3922 return false; 3923 for (Annotation item : this.note) 3924 if (!item.isEmpty()) 3925 return true; 3926 return false; 3927 } 3928 3929 public Annotation addNote() { //3 3930 Annotation t = new Annotation(); 3931 if (this.note == null) 3932 this.note = new ArrayList<Annotation>(); 3933 this.note.add(t); 3934 return t; 3935 } 3936 3937 public ResearchStudy addNote(Annotation t) { //3 3938 if (t == null) 3939 return this; 3940 if (this.note == null) 3941 this.note = new ArrayList<Annotation>(); 3942 this.note.add(t); 3943 return this; 3944 } 3945 3946 /** 3947 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 3948 */ 3949 public Annotation getNoteFirstRep() { 3950 if (getNote().isEmpty()) { 3951 addNote(); 3952 } 3953 return getNote().get(0); 3954 } 3955 3956 /** 3957 * @return {@link #classifier} (Additional grouping mechanism or categorization of a research study. Example: FDA regulated device, FDA regulated drug, MPG Paragraph 23b (a German legal requirement), IRB-exempt, etc. Implementation Note: do not use the classifier element to support existing semantics that are already supported thru explicit elements in the resource.) 3958 */ 3959 public List<CodeableConcept> getClassifier() { 3960 if (this.classifier == null) 3961 this.classifier = new ArrayList<CodeableConcept>(); 3962 return this.classifier; 3963 } 3964 3965 /** 3966 * @return Returns a reference to <code>this</code> for easy method chaining 3967 */ 3968 public ResearchStudy setClassifier(List<CodeableConcept> theClassifier) { 3969 this.classifier = theClassifier; 3970 return this; 3971 } 3972 3973 public boolean hasClassifier() { 3974 if (this.classifier == null) 3975 return false; 3976 for (CodeableConcept item : this.classifier) 3977 if (!item.isEmpty()) 3978 return true; 3979 return false; 3980 } 3981 3982 public CodeableConcept addClassifier() { //3 3983 CodeableConcept t = new CodeableConcept(); 3984 if (this.classifier == null) 3985 this.classifier = new ArrayList<CodeableConcept>(); 3986 this.classifier.add(t); 3987 return t; 3988 } 3989 3990 public ResearchStudy addClassifier(CodeableConcept t) { //3 3991 if (t == null) 3992 return this; 3993 if (this.classifier == null) 3994 this.classifier = new ArrayList<CodeableConcept>(); 3995 this.classifier.add(t); 3996 return this; 3997 } 3998 3999 /** 4000 * @return The first repetition of repeating field {@link #classifier}, creating it if it does not already exist {3} 4001 */ 4002 public CodeableConcept getClassifierFirstRep() { 4003 if (getClassifier().isEmpty()) { 4004 addClassifier(); 4005 } 4006 return getClassifier().get(0); 4007 } 4008 4009 /** 4010 * @return {@link #associatedParty} (Sponsors, collaborators, and other parties.) 4011 */ 4012 public List<ResearchStudyAssociatedPartyComponent> getAssociatedParty() { 4013 if (this.associatedParty == null) 4014 this.associatedParty = new ArrayList<ResearchStudyAssociatedPartyComponent>(); 4015 return this.associatedParty; 4016 } 4017 4018 /** 4019 * @return Returns a reference to <code>this</code> for easy method chaining 4020 */ 4021 public ResearchStudy setAssociatedParty(List<ResearchStudyAssociatedPartyComponent> theAssociatedParty) { 4022 this.associatedParty = theAssociatedParty; 4023 return this; 4024 } 4025 4026 public boolean hasAssociatedParty() { 4027 if (this.associatedParty == null) 4028 return false; 4029 for (ResearchStudyAssociatedPartyComponent item : this.associatedParty) 4030 if (!item.isEmpty()) 4031 return true; 4032 return false; 4033 } 4034 4035 public ResearchStudyAssociatedPartyComponent addAssociatedParty() { //3 4036 ResearchStudyAssociatedPartyComponent t = new ResearchStudyAssociatedPartyComponent(); 4037 if (this.associatedParty == null) 4038 this.associatedParty = new ArrayList<ResearchStudyAssociatedPartyComponent>(); 4039 this.associatedParty.add(t); 4040 return t; 4041 } 4042 4043 public ResearchStudy addAssociatedParty(ResearchStudyAssociatedPartyComponent t) { //3 4044 if (t == null) 4045 return this; 4046 if (this.associatedParty == null) 4047 this.associatedParty = new ArrayList<ResearchStudyAssociatedPartyComponent>(); 4048 this.associatedParty.add(t); 4049 return this; 4050 } 4051 4052 /** 4053 * @return The first repetition of repeating field {@link #associatedParty}, creating it if it does not already exist {3} 4054 */ 4055 public ResearchStudyAssociatedPartyComponent getAssociatedPartyFirstRep() { 4056 if (getAssociatedParty().isEmpty()) { 4057 addAssociatedParty(); 4058 } 4059 return getAssociatedParty().get(0); 4060 } 4061 4062 /** 4063 * @return {@link #progressStatus} (Status of study with time for that status.) 4064 */ 4065 public List<ResearchStudyProgressStatusComponent> getProgressStatus() { 4066 if (this.progressStatus == null) 4067 this.progressStatus = new ArrayList<ResearchStudyProgressStatusComponent>(); 4068 return this.progressStatus; 4069 } 4070 4071 /** 4072 * @return Returns a reference to <code>this</code> for easy method chaining 4073 */ 4074 public ResearchStudy setProgressStatus(List<ResearchStudyProgressStatusComponent> theProgressStatus) { 4075 this.progressStatus = theProgressStatus; 4076 return this; 4077 } 4078 4079 public boolean hasProgressStatus() { 4080 if (this.progressStatus == null) 4081 return false; 4082 for (ResearchStudyProgressStatusComponent item : this.progressStatus) 4083 if (!item.isEmpty()) 4084 return true; 4085 return false; 4086 } 4087 4088 public ResearchStudyProgressStatusComponent addProgressStatus() { //3 4089 ResearchStudyProgressStatusComponent t = new ResearchStudyProgressStatusComponent(); 4090 if (this.progressStatus == null) 4091 this.progressStatus = new ArrayList<ResearchStudyProgressStatusComponent>(); 4092 this.progressStatus.add(t); 4093 return t; 4094 } 4095 4096 public ResearchStudy addProgressStatus(ResearchStudyProgressStatusComponent t) { //3 4097 if (t == null) 4098 return this; 4099 if (this.progressStatus == null) 4100 this.progressStatus = new ArrayList<ResearchStudyProgressStatusComponent>(); 4101 this.progressStatus.add(t); 4102 return this; 4103 } 4104 4105 /** 4106 * @return The first repetition of repeating field {@link #progressStatus}, creating it if it does not already exist {3} 4107 */ 4108 public ResearchStudyProgressStatusComponent getProgressStatusFirstRep() { 4109 if (getProgressStatus().isEmpty()) { 4110 addProgressStatus(); 4111 } 4112 return getProgressStatus().get(0); 4113 } 4114 4115 /** 4116 * @return {@link #whyStopped} (A description and/or code explaining the premature termination of the study.) 4117 */ 4118 public CodeableConcept getWhyStopped() { 4119 if (this.whyStopped == null) 4120 if (Configuration.errorOnAutoCreate()) 4121 throw new Error("Attempt to auto-create ResearchStudy.whyStopped"); 4122 else if (Configuration.doAutoCreate()) 4123 this.whyStopped = new CodeableConcept(); // cc 4124 return this.whyStopped; 4125 } 4126 4127 public boolean hasWhyStopped() { 4128 return this.whyStopped != null && !this.whyStopped.isEmpty(); 4129 } 4130 4131 /** 4132 * @param value {@link #whyStopped} (A description and/or code explaining the premature termination of the study.) 4133 */ 4134 public ResearchStudy setWhyStopped(CodeableConcept value) { 4135 this.whyStopped = value; 4136 return this; 4137 } 4138 4139 /** 4140 * @return {@link #recruitment} (Target or actual group of participants enrolled in study.) 4141 */ 4142 public ResearchStudyRecruitmentComponent getRecruitment() { 4143 if (this.recruitment == null) 4144 if (Configuration.errorOnAutoCreate()) 4145 throw new Error("Attempt to auto-create ResearchStudy.recruitment"); 4146 else if (Configuration.doAutoCreate()) 4147 this.recruitment = new ResearchStudyRecruitmentComponent(); // cc 4148 return this.recruitment; 4149 } 4150 4151 public boolean hasRecruitment() { 4152 return this.recruitment != null && !this.recruitment.isEmpty(); 4153 } 4154 4155 /** 4156 * @param value {@link #recruitment} (Target or actual group of participants enrolled in study.) 4157 */ 4158 public ResearchStudy setRecruitment(ResearchStudyRecruitmentComponent value) { 4159 this.recruitment = value; 4160 return this; 4161 } 4162 4163 /** 4164 * @return {@link #comparisonGroup} (Describes an expected event or sequence of events for one of the subjects of a study. E.g. for a living subject: exposure to drug A, wash-out, exposure to drug B, wash-out, follow-up. E.g. for a stability study: {store sample from lot A at 25 degrees for 1 month}, {store sample from lot A at 40 degrees for 1 month}.) 4165 */ 4166 public List<ResearchStudyComparisonGroupComponent> getComparisonGroup() { 4167 if (this.comparisonGroup == null) 4168 this.comparisonGroup = new ArrayList<ResearchStudyComparisonGroupComponent>(); 4169 return this.comparisonGroup; 4170 } 4171 4172 /** 4173 * @return Returns a reference to <code>this</code> for easy method chaining 4174 */ 4175 public ResearchStudy setComparisonGroup(List<ResearchStudyComparisonGroupComponent> theComparisonGroup) { 4176 this.comparisonGroup = theComparisonGroup; 4177 return this; 4178 } 4179 4180 public boolean hasComparisonGroup() { 4181 if (this.comparisonGroup == null) 4182 return false; 4183 for (ResearchStudyComparisonGroupComponent item : this.comparisonGroup) 4184 if (!item.isEmpty()) 4185 return true; 4186 return false; 4187 } 4188 4189 public ResearchStudyComparisonGroupComponent addComparisonGroup() { //3 4190 ResearchStudyComparisonGroupComponent t = new ResearchStudyComparisonGroupComponent(); 4191 if (this.comparisonGroup == null) 4192 this.comparisonGroup = new ArrayList<ResearchStudyComparisonGroupComponent>(); 4193 this.comparisonGroup.add(t); 4194 return t; 4195 } 4196 4197 public ResearchStudy addComparisonGroup(ResearchStudyComparisonGroupComponent t) { //3 4198 if (t == null) 4199 return this; 4200 if (this.comparisonGroup == null) 4201 this.comparisonGroup = new ArrayList<ResearchStudyComparisonGroupComponent>(); 4202 this.comparisonGroup.add(t); 4203 return this; 4204 } 4205 4206 /** 4207 * @return The first repetition of repeating field {@link #comparisonGroup}, creating it if it does not already exist {3} 4208 */ 4209 public ResearchStudyComparisonGroupComponent getComparisonGroupFirstRep() { 4210 if (getComparisonGroup().isEmpty()) { 4211 addComparisonGroup(); 4212 } 4213 return getComparisonGroup().get(0); 4214 } 4215 4216 /** 4217 * @return {@link #objective} (A goal that the study is aiming to achieve in terms of a scientific question to be answered by the analysis of data collected during the study.) 4218 */ 4219 public List<ResearchStudyObjectiveComponent> getObjective() { 4220 if (this.objective == null) 4221 this.objective = new ArrayList<ResearchStudyObjectiveComponent>(); 4222 return this.objective; 4223 } 4224 4225 /** 4226 * @return Returns a reference to <code>this</code> for easy method chaining 4227 */ 4228 public ResearchStudy setObjective(List<ResearchStudyObjectiveComponent> theObjective) { 4229 this.objective = theObjective; 4230 return this; 4231 } 4232 4233 public boolean hasObjective() { 4234 if (this.objective == null) 4235 return false; 4236 for (ResearchStudyObjectiveComponent item : this.objective) 4237 if (!item.isEmpty()) 4238 return true; 4239 return false; 4240 } 4241 4242 public ResearchStudyObjectiveComponent addObjective() { //3 4243 ResearchStudyObjectiveComponent t = new ResearchStudyObjectiveComponent(); 4244 if (this.objective == null) 4245 this.objective = new ArrayList<ResearchStudyObjectiveComponent>(); 4246 this.objective.add(t); 4247 return t; 4248 } 4249 4250 public ResearchStudy addObjective(ResearchStudyObjectiveComponent t) { //3 4251 if (t == null) 4252 return this; 4253 if (this.objective == null) 4254 this.objective = new ArrayList<ResearchStudyObjectiveComponent>(); 4255 this.objective.add(t); 4256 return this; 4257 } 4258 4259 /** 4260 * @return The first repetition of repeating field {@link #objective}, creating it if it does not already exist {3} 4261 */ 4262 public ResearchStudyObjectiveComponent getObjectiveFirstRep() { 4263 if (getObjective().isEmpty()) { 4264 addObjective(); 4265 } 4266 return getObjective().get(0); 4267 } 4268 4269 /** 4270 * @return {@link #outcomeMeasure} (An "outcome measure", "endpoint", "effect measure" or "measure of effect" is a specific measurement or observation used to quantify the effect of experimental variables on the participants in a study, or for observational studies, to describe patterns of diseases or traits or associations with exposures, risk factors or treatment.) 4271 */ 4272 public List<ResearchStudyOutcomeMeasureComponent> getOutcomeMeasure() { 4273 if (this.outcomeMeasure == null) 4274 this.outcomeMeasure = new ArrayList<ResearchStudyOutcomeMeasureComponent>(); 4275 return this.outcomeMeasure; 4276 } 4277 4278 /** 4279 * @return Returns a reference to <code>this</code> for easy method chaining 4280 */ 4281 public ResearchStudy setOutcomeMeasure(List<ResearchStudyOutcomeMeasureComponent> theOutcomeMeasure) { 4282 this.outcomeMeasure = theOutcomeMeasure; 4283 return this; 4284 } 4285 4286 public boolean hasOutcomeMeasure() { 4287 if (this.outcomeMeasure == null) 4288 return false; 4289 for (ResearchStudyOutcomeMeasureComponent item : this.outcomeMeasure) 4290 if (!item.isEmpty()) 4291 return true; 4292 return false; 4293 } 4294 4295 public ResearchStudyOutcomeMeasureComponent addOutcomeMeasure() { //3 4296 ResearchStudyOutcomeMeasureComponent t = new ResearchStudyOutcomeMeasureComponent(); 4297 if (this.outcomeMeasure == null) 4298 this.outcomeMeasure = new ArrayList<ResearchStudyOutcomeMeasureComponent>(); 4299 this.outcomeMeasure.add(t); 4300 return t; 4301 } 4302 4303 public ResearchStudy addOutcomeMeasure(ResearchStudyOutcomeMeasureComponent t) { //3 4304 if (t == null) 4305 return this; 4306 if (this.outcomeMeasure == null) 4307 this.outcomeMeasure = new ArrayList<ResearchStudyOutcomeMeasureComponent>(); 4308 this.outcomeMeasure.add(t); 4309 return this; 4310 } 4311 4312 /** 4313 * @return The first repetition of repeating field {@link #outcomeMeasure}, creating it if it does not already exist {3} 4314 */ 4315 public ResearchStudyOutcomeMeasureComponent getOutcomeMeasureFirstRep() { 4316 if (getOutcomeMeasure().isEmpty()) { 4317 addOutcomeMeasure(); 4318 } 4319 return getOutcomeMeasure().get(0); 4320 } 4321 4322 /** 4323 * @return {@link #result} (Link to one or more sets of results generated by the study. Could also link to a research registry holding the results such as ClinicalTrials.gov.) 4324 */ 4325 public List<Reference> getResult() { 4326 if (this.result == null) 4327 this.result = new ArrayList<Reference>(); 4328 return this.result; 4329 } 4330 4331 /** 4332 * @return Returns a reference to <code>this</code> for easy method chaining 4333 */ 4334 public ResearchStudy setResult(List<Reference> theResult) { 4335 this.result = theResult; 4336 return this; 4337 } 4338 4339 public boolean hasResult() { 4340 if (this.result == null) 4341 return false; 4342 for (Reference item : this.result) 4343 if (!item.isEmpty()) 4344 return true; 4345 return false; 4346 } 4347 4348 public Reference addResult() { //3 4349 Reference t = new Reference(); 4350 if (this.result == null) 4351 this.result = new ArrayList<Reference>(); 4352 this.result.add(t); 4353 return t; 4354 } 4355 4356 public ResearchStudy addResult(Reference t) { //3 4357 if (t == null) 4358 return this; 4359 if (this.result == null) 4360 this.result = new ArrayList<Reference>(); 4361 this.result.add(t); 4362 return this; 4363 } 4364 4365 /** 4366 * @return The first repetition of repeating field {@link #result}, creating it if it does not already exist {3} 4367 */ 4368 public Reference getResultFirstRep() { 4369 if (getResult().isEmpty()) { 4370 addResult(); 4371 } 4372 return getResult().get(0); 4373 } 4374 4375 protected void listChildren(List<Property> children) { 4376 super.listChildren(children); 4377 children.add(new Property("url", "uri", "Canonical identifier for this study resource, represented as a globally unique URI.", 0, 1, url)); 4378 children.add(new Property("identifier", "Identifier", "Identifiers assigned to this research study by the sponsor or other systems.", 0, java.lang.Integer.MAX_VALUE, identifier)); 4379 children.add(new Property("version", "string", "The business version for the study record.", 0, 1, version)); 4380 children.add(new Property("name", "string", "Name for this study (computer friendly).", 0, 1, name)); 4381 children.add(new Property("title", "string", "The human readable name of the research study.", 0, 1, title)); 4382 children.add(new Property("label", "", "Additional names for the study.", 0, java.lang.Integer.MAX_VALUE, label)); 4383 children.add(new Property("protocol", "Reference(PlanDefinition)", "The set of steps expected to be performed as part of the execution of the study.", 0, java.lang.Integer.MAX_VALUE, protocol)); 4384 children.add(new Property("partOf", "Reference(ResearchStudy)", "A larger research study of which this particular study is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 4385 children.add(new Property("relatedArtifact", "RelatedArtifact", "Citations, references, URLs and other related documents. When using relatedArtifact to share URLs, the relatedArtifact.type will often be set to one of \"documentation\" or \"supported-with\" and the URL value will often be in relatedArtifact.document.url but another possible location is relatedArtifact.resource when it is a canonical URL.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 4386 children.add(new Property("date", "dateTime", "The date (and optionally time) when the ResearchStudy Resource was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ResearchStudy Resource changes.", 0, 1, date)); 4387 children.add(new Property("status", "code", "The publication state of the resource (not of the study).", 0, 1, status)); 4388 children.add(new Property("primaryPurposeType", "CodeableConcept", "The type of study based upon the intent of the study activities. A classification of the intent of the study.", 0, 1, primaryPurposeType)); 4389 children.add(new Property("phase", "CodeableConcept", "The stage in the progression of a therapy from initial experimental use in humans in clinical trials to post-market evaluation.", 0, 1, phase)); 4390 children.add(new Property("studyDesign", "CodeableConcept", "Codes categorizing the type of study such as investigational vs. observational, type of blinding, type of randomization, safety vs. efficacy, etc.", 0, java.lang.Integer.MAX_VALUE, studyDesign)); 4391 children.add(new Property("focus", "CodeableReference(Medication|MedicinalProductDefinition|SubstanceDefinition|EvidenceVariable)", "The medication(s), food(s), therapy(ies), device(s) or other concerns or interventions that the study is seeking to gain more information about.", 0, java.lang.Integer.MAX_VALUE, focus)); 4392 children.add(new Property("condition", "CodeableConcept", "The condition that is the focus of the study. For example, In a study to examine risk factors for Lupus, might have as an inclusion criterion \"healthy volunteer\", but the target condition code would be a Lupus SNOMED code.", 0, java.lang.Integer.MAX_VALUE, condition)); 4393 children.add(new Property("keyword", "CodeableConcept", "Key terms to aid in searching for or filtering the study.", 0, java.lang.Integer.MAX_VALUE, keyword)); 4394 children.add(new Property("region", "CodeableConcept", "A country, state or other area where the study is taking place rather than its precise geographic location or address.", 0, java.lang.Integer.MAX_VALUE, region)); 4395 children.add(new Property("descriptionSummary", "markdown", "A brief text for explaining the study.", 0, 1, descriptionSummary)); 4396 children.add(new Property("description", "markdown", "A detailed and human-readable narrative of the study. E.g., study abstract.", 0, 1, description)); 4397 children.add(new Property("period", "Period", "Identifies the start date and the expected (or actual, depending on status) end date for the study.", 0, 1, period)); 4398 children.add(new Property("site", "Reference(Location|ResearchStudy|Organization)", "A facility in which study activities are conducted.", 0, java.lang.Integer.MAX_VALUE, site)); 4399 children.add(new Property("note", "Annotation", "Comments made about the study by the performer, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note)); 4400 children.add(new Property("classifier", "CodeableConcept", "Additional grouping mechanism or categorization of a research study. Example: FDA regulated device, FDA regulated drug, MPG Paragraph 23b (a German legal requirement), IRB-exempt, etc. Implementation Note: do not use the classifier element to support existing semantics that are already supported thru explicit elements in the resource.", 0, java.lang.Integer.MAX_VALUE, classifier)); 4401 children.add(new Property("associatedParty", "", "Sponsors, collaborators, and other parties.", 0, java.lang.Integer.MAX_VALUE, associatedParty)); 4402 children.add(new Property("progressStatus", "", "Status of study with time for that status.", 0, java.lang.Integer.MAX_VALUE, progressStatus)); 4403 children.add(new Property("whyStopped", "CodeableConcept", "A description and/or code explaining the premature termination of the study.", 0, 1, whyStopped)); 4404 children.add(new Property("recruitment", "", "Target or actual group of participants enrolled in study.", 0, 1, recruitment)); 4405 children.add(new Property("comparisonGroup", "", "Describes an expected event or sequence of events for one of the subjects of a study. E.g. for a living subject: exposure to drug A, wash-out, exposure to drug B, wash-out, follow-up. E.g. for a stability study: {store sample from lot A at 25 degrees for 1 month}, {store sample from lot A at 40 degrees for 1 month}.", 0, java.lang.Integer.MAX_VALUE, comparisonGroup)); 4406 children.add(new Property("objective", "", "A goal that the study is aiming to achieve in terms of a scientific question to be answered by the analysis of data collected during the study.", 0, java.lang.Integer.MAX_VALUE, objective)); 4407 children.add(new Property("outcomeMeasure", "", "An \"outcome measure\", \"endpoint\", \"effect measure\" or \"measure of effect\" is a specific measurement or observation used to quantify the effect of experimental variables on the participants in a study, or for observational studies, to describe patterns of diseases or traits or associations with exposures, risk factors or treatment.", 0, java.lang.Integer.MAX_VALUE, outcomeMeasure)); 4408 children.add(new Property("result", "Reference(EvidenceReport|Citation|DiagnosticReport)", "Link to one or more sets of results generated by the study. Could also link to a research registry holding the results such as ClinicalTrials.gov.", 0, java.lang.Integer.MAX_VALUE, result)); 4409 } 4410 4411 @Override 4412 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4413 switch (_hash) { 4414 case 116079: /*url*/ return new Property("url", "uri", "Canonical identifier for this study resource, represented as a globally unique URI.", 0, 1, url); 4415 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers assigned to this research study by the sponsor or other systems.", 0, java.lang.Integer.MAX_VALUE, identifier); 4416 case 351608024: /*version*/ return new Property("version", "string", "The business version for the study record.", 0, 1, version); 4417 case 3373707: /*name*/ return new Property("name", "string", "Name for this study (computer friendly).", 0, 1, name); 4418 case 110371416: /*title*/ return new Property("title", "string", "The human readable name of the research study.", 0, 1, title); 4419 case 102727412: /*label*/ return new Property("label", "", "Additional names for the study.", 0, java.lang.Integer.MAX_VALUE, label); 4420 case -989163880: /*protocol*/ return new Property("protocol", "Reference(PlanDefinition)", "The set of steps expected to be performed as part of the execution of the study.", 0, java.lang.Integer.MAX_VALUE, protocol); 4421 case -995410646: /*partOf*/ return new Property("partOf", "Reference(ResearchStudy)", "A larger research study of which this particular study is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 4422 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Citations, references, URLs and other related documents. When using relatedArtifact to share URLs, the relatedArtifact.type will often be set to one of \"documentation\" or \"supported-with\" and the URL value will often be in relatedArtifact.document.url but another possible location is relatedArtifact.resource when it is a canonical URL.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 4423 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the ResearchStudy Resource was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the ResearchStudy Resource changes.", 0, 1, date); 4424 case -892481550: /*status*/ return new Property("status", "code", "The publication state of the resource (not of the study).", 0, 1, status); 4425 case -2132842986: /*primaryPurposeType*/ return new Property("primaryPurposeType", "CodeableConcept", "The type of study based upon the intent of the study activities. A classification of the intent of the study.", 0, 1, primaryPurposeType); 4426 case 106629499: /*phase*/ return new Property("phase", "CodeableConcept", "The stage in the progression of a therapy from initial experimental use in humans in clinical trials to post-market evaluation.", 0, 1, phase); 4427 case 1709211879: /*studyDesign*/ return new Property("studyDesign", "CodeableConcept", "Codes categorizing the type of study such as investigational vs. observational, type of blinding, type of randomization, safety vs. efficacy, etc.", 0, java.lang.Integer.MAX_VALUE, studyDesign); 4428 case 97604824: /*focus*/ return new Property("focus", "CodeableReference(Medication|MedicinalProductDefinition|SubstanceDefinition|EvidenceVariable)", "The medication(s), food(s), therapy(ies), device(s) or other concerns or interventions that the study is seeking to gain more information about.", 0, java.lang.Integer.MAX_VALUE, focus); 4429 case -861311717: /*condition*/ return new Property("condition", "CodeableConcept", "The condition that is the focus of the study. For example, In a study to examine risk factors for Lupus, might have as an inclusion criterion \"healthy volunteer\", but the target condition code would be a Lupus SNOMED code.", 0, java.lang.Integer.MAX_VALUE, condition); 4430 case -814408215: /*keyword*/ return new Property("keyword", "CodeableConcept", "Key terms to aid in searching for or filtering the study.", 0, java.lang.Integer.MAX_VALUE, keyword); 4431 case -934795532: /*region*/ return new Property("region", "CodeableConcept", "A country, state or other area where the study is taking place rather than its precise geographic location or address.", 0, java.lang.Integer.MAX_VALUE, region); 4432 case 21530634: /*descriptionSummary*/ return new Property("descriptionSummary", "markdown", "A brief text for explaining the study.", 0, 1, descriptionSummary); 4433 case -1724546052: /*description*/ return new Property("description", "markdown", "A detailed and human-readable narrative of the study. E.g., study abstract.", 0, 1, description); 4434 case -991726143: /*period*/ return new Property("period", "Period", "Identifies the start date and the expected (or actual, depending on status) end date for the study.", 0, 1, period); 4435 case 3530567: /*site*/ return new Property("site", "Reference(Location|ResearchStudy|Organization)", "A facility in which study activities are conducted.", 0, java.lang.Integer.MAX_VALUE, site); 4436 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments made about the study by the performer, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note); 4437 case -281470431: /*classifier*/ return new Property("classifier", "CodeableConcept", "Additional grouping mechanism or categorization of a research study. Example: FDA regulated device, FDA regulated drug, MPG Paragraph 23b (a German legal requirement), IRB-exempt, etc. Implementation Note: do not use the classifier element to support existing semantics that are already supported thru explicit elements in the resource.", 0, java.lang.Integer.MAX_VALUE, classifier); 4438 case -1841460864: /*associatedParty*/ return new Property("associatedParty", "", "Sponsors, collaborators, and other parties.", 0, java.lang.Integer.MAX_VALUE, associatedParty); 4439 case -1897502593: /*progressStatus*/ return new Property("progressStatus", "", "Status of study with time for that status.", 0, java.lang.Integer.MAX_VALUE, progressStatus); 4440 case -699986715: /*whyStopped*/ return new Property("whyStopped", "CodeableConcept", "A description and/or code explaining the premature termination of the study.", 0, 1, whyStopped); 4441 case 780783004: /*recruitment*/ return new Property("recruitment", "", "Target or actual group of participants enrolled in study.", 0, 1, recruitment); 4442 case -138266634: /*comparisonGroup*/ return new Property("comparisonGroup", "", "Describes an expected event or sequence of events for one of the subjects of a study. E.g. for a living subject: exposure to drug A, wash-out, exposure to drug B, wash-out, follow-up. E.g. for a stability study: {store sample from lot A at 25 degrees for 1 month}, {store sample from lot A at 40 degrees for 1 month}.", 0, java.lang.Integer.MAX_VALUE, comparisonGroup); 4443 case -1489585863: /*objective*/ return new Property("objective", "", "A goal that the study is aiming to achieve in terms of a scientific question to be answered by the analysis of data collected during the study.", 0, java.lang.Integer.MAX_VALUE, objective); 4444 case -1510689364: /*outcomeMeasure*/ return new Property("outcomeMeasure", "", "An \"outcome measure\", \"endpoint\", \"effect measure\" or \"measure of effect\" is a specific measurement or observation used to quantify the effect of experimental variables on the participants in a study, or for observational studies, to describe patterns of diseases or traits or associations with exposures, risk factors or treatment.", 0, java.lang.Integer.MAX_VALUE, outcomeMeasure); 4445 case -934426595: /*result*/ return new Property("result", "Reference(EvidenceReport|Citation|DiagnosticReport)", "Link to one or more sets of results generated by the study. Could also link to a research registry holding the results such as ClinicalTrials.gov.", 0, java.lang.Integer.MAX_VALUE, result); 4446 default: return super.getNamedProperty(_hash, _name, _checkValid); 4447 } 4448 4449 } 4450 4451 @Override 4452 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4453 switch (hash) { 4454 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 4455 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4456 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 4457 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 4458 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 4459 case 102727412: /*label*/ return this.label == null ? new Base[0] : this.label.toArray(new Base[this.label.size()]); // ResearchStudyLabelComponent 4460 case -989163880: /*protocol*/ return this.protocol == null ? new Base[0] : this.protocol.toArray(new Base[this.protocol.size()]); // Reference 4461 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 4462 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 4463 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 4464 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 4465 case -2132842986: /*primaryPurposeType*/ return this.primaryPurposeType == null ? new Base[0] : new Base[] {this.primaryPurposeType}; // CodeableConcept 4466 case 106629499: /*phase*/ return this.phase == null ? new Base[0] : new Base[] {this.phase}; // CodeableConcept 4467 case 1709211879: /*studyDesign*/ return this.studyDesign == null ? new Base[0] : this.studyDesign.toArray(new Base[this.studyDesign.size()]); // CodeableConcept 4468 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // CodeableReference 4469 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : this.condition.toArray(new Base[this.condition.size()]); // CodeableConcept 4470 case -814408215: /*keyword*/ return this.keyword == null ? new Base[0] : this.keyword.toArray(new Base[this.keyword.size()]); // CodeableConcept 4471 case -934795532: /*region*/ return this.region == null ? new Base[0] : this.region.toArray(new Base[this.region.size()]); // CodeableConcept 4472 case 21530634: /*descriptionSummary*/ return this.descriptionSummary == null ? new Base[0] : new Base[] {this.descriptionSummary}; // MarkdownType 4473 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 4474 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 4475 case 3530567: /*site*/ return this.site == null ? new Base[0] : this.site.toArray(new Base[this.site.size()]); // Reference 4476 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 4477 case -281470431: /*classifier*/ return this.classifier == null ? new Base[0] : this.classifier.toArray(new Base[this.classifier.size()]); // CodeableConcept 4478 case -1841460864: /*associatedParty*/ return this.associatedParty == null ? new Base[0] : this.associatedParty.toArray(new Base[this.associatedParty.size()]); // ResearchStudyAssociatedPartyComponent 4479 case -1897502593: /*progressStatus*/ return this.progressStatus == null ? new Base[0] : this.progressStatus.toArray(new Base[this.progressStatus.size()]); // ResearchStudyProgressStatusComponent 4480 case -699986715: /*whyStopped*/ return this.whyStopped == null ? new Base[0] : new Base[] {this.whyStopped}; // CodeableConcept 4481 case 780783004: /*recruitment*/ return this.recruitment == null ? new Base[0] : new Base[] {this.recruitment}; // ResearchStudyRecruitmentComponent 4482 case -138266634: /*comparisonGroup*/ return this.comparisonGroup == null ? new Base[0] : this.comparisonGroup.toArray(new Base[this.comparisonGroup.size()]); // ResearchStudyComparisonGroupComponent 4483 case -1489585863: /*objective*/ return this.objective == null ? new Base[0] : this.objective.toArray(new Base[this.objective.size()]); // ResearchStudyObjectiveComponent 4484 case -1510689364: /*outcomeMeasure*/ return this.outcomeMeasure == null ? new Base[0] : this.outcomeMeasure.toArray(new Base[this.outcomeMeasure.size()]); // ResearchStudyOutcomeMeasureComponent 4485 case -934426595: /*result*/ return this.result == null ? new Base[0] : this.result.toArray(new Base[this.result.size()]); // Reference 4486 default: return super.getProperty(hash, name, checkValid); 4487 } 4488 4489 } 4490 4491 @Override 4492 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4493 switch (hash) { 4494 case 116079: // url 4495 this.url = TypeConvertor.castToUri(value); // UriType 4496 return value; 4497 case -1618432855: // identifier 4498 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 4499 return value; 4500 case 351608024: // version 4501 this.version = TypeConvertor.castToString(value); // StringType 4502 return value; 4503 case 3373707: // name 4504 this.name = TypeConvertor.castToString(value); // StringType 4505 return value; 4506 case 110371416: // title 4507 this.title = TypeConvertor.castToString(value); // StringType 4508 return value; 4509 case 102727412: // label 4510 this.getLabel().add((ResearchStudyLabelComponent) value); // ResearchStudyLabelComponent 4511 return value; 4512 case -989163880: // protocol 4513 this.getProtocol().add(TypeConvertor.castToReference(value)); // Reference 4514 return value; 4515 case -995410646: // partOf 4516 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 4517 return value; 4518 case 666807069: // relatedArtifact 4519 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 4520 return value; 4521 case 3076014: // date 4522 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4523 return value; 4524 case -892481550: // status 4525 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4526 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4527 return value; 4528 case -2132842986: // primaryPurposeType 4529 this.primaryPurposeType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4530 return value; 4531 case 106629499: // phase 4532 this.phase = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4533 return value; 4534 case 1709211879: // studyDesign 4535 this.getStudyDesign().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4536 return value; 4537 case 97604824: // focus 4538 this.getFocus().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 4539 return value; 4540 case -861311717: // condition 4541 this.getCondition().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4542 return value; 4543 case -814408215: // keyword 4544 this.getKeyword().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4545 return value; 4546 case -934795532: // region 4547 this.getRegion().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4548 return value; 4549 case 21530634: // descriptionSummary 4550 this.descriptionSummary = TypeConvertor.castToMarkdown(value); // MarkdownType 4551 return value; 4552 case -1724546052: // description 4553 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4554 return value; 4555 case -991726143: // period 4556 this.period = TypeConvertor.castToPeriod(value); // Period 4557 return value; 4558 case 3530567: // site 4559 this.getSite().add(TypeConvertor.castToReference(value)); // Reference 4560 return value; 4561 case 3387378: // note 4562 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 4563 return value; 4564 case -281470431: // classifier 4565 this.getClassifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4566 return value; 4567 case -1841460864: // associatedParty 4568 this.getAssociatedParty().add((ResearchStudyAssociatedPartyComponent) value); // ResearchStudyAssociatedPartyComponent 4569 return value; 4570 case -1897502593: // progressStatus 4571 this.getProgressStatus().add((ResearchStudyProgressStatusComponent) value); // ResearchStudyProgressStatusComponent 4572 return value; 4573 case -699986715: // whyStopped 4574 this.whyStopped = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4575 return value; 4576 case 780783004: // recruitment 4577 this.recruitment = (ResearchStudyRecruitmentComponent) value; // ResearchStudyRecruitmentComponent 4578 return value; 4579 case -138266634: // comparisonGroup 4580 this.getComparisonGroup().add((ResearchStudyComparisonGroupComponent) value); // ResearchStudyComparisonGroupComponent 4581 return value; 4582 case -1489585863: // objective 4583 this.getObjective().add((ResearchStudyObjectiveComponent) value); // ResearchStudyObjectiveComponent 4584 return value; 4585 case -1510689364: // outcomeMeasure 4586 this.getOutcomeMeasure().add((ResearchStudyOutcomeMeasureComponent) value); // ResearchStudyOutcomeMeasureComponent 4587 return value; 4588 case -934426595: // result 4589 this.getResult().add(TypeConvertor.castToReference(value)); // Reference 4590 return value; 4591 default: return super.setProperty(hash, name, value); 4592 } 4593 4594 } 4595 4596 @Override 4597 public Base setProperty(String name, Base value) throws FHIRException { 4598 if (name.equals("url")) { 4599 this.url = TypeConvertor.castToUri(value); // UriType 4600 } else if (name.equals("identifier")) { 4601 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 4602 } else if (name.equals("version")) { 4603 this.version = TypeConvertor.castToString(value); // StringType 4604 } else if (name.equals("name")) { 4605 this.name = TypeConvertor.castToString(value); // StringType 4606 } else if (name.equals("title")) { 4607 this.title = TypeConvertor.castToString(value); // StringType 4608 } else if (name.equals("label")) { 4609 this.getLabel().add((ResearchStudyLabelComponent) value); 4610 } else if (name.equals("protocol")) { 4611 this.getProtocol().add(TypeConvertor.castToReference(value)); 4612 } else if (name.equals("partOf")) { 4613 this.getPartOf().add(TypeConvertor.castToReference(value)); 4614 } else if (name.equals("relatedArtifact")) { 4615 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); 4616 } else if (name.equals("date")) { 4617 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4618 } else if (name.equals("status")) { 4619 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4620 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4621 } else if (name.equals("primaryPurposeType")) { 4622 this.primaryPurposeType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4623 } else if (name.equals("phase")) { 4624 this.phase = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4625 } else if (name.equals("studyDesign")) { 4626 this.getStudyDesign().add(TypeConvertor.castToCodeableConcept(value)); 4627 } else if (name.equals("focus")) { 4628 this.getFocus().add(TypeConvertor.castToCodeableReference(value)); 4629 } else if (name.equals("condition")) { 4630 this.getCondition().add(TypeConvertor.castToCodeableConcept(value)); 4631 } else if (name.equals("keyword")) { 4632 this.getKeyword().add(TypeConvertor.castToCodeableConcept(value)); 4633 } else if (name.equals("region")) { 4634 this.getRegion().add(TypeConvertor.castToCodeableConcept(value)); 4635 } else if (name.equals("descriptionSummary")) { 4636 this.descriptionSummary = TypeConvertor.castToMarkdown(value); // MarkdownType 4637 } else if (name.equals("description")) { 4638 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4639 } else if (name.equals("period")) { 4640 this.period = TypeConvertor.castToPeriod(value); // Period 4641 } else if (name.equals("site")) { 4642 this.getSite().add(TypeConvertor.castToReference(value)); 4643 } else if (name.equals("note")) { 4644 this.getNote().add(TypeConvertor.castToAnnotation(value)); 4645 } else if (name.equals("classifier")) { 4646 this.getClassifier().add(TypeConvertor.castToCodeableConcept(value)); 4647 } else if (name.equals("associatedParty")) { 4648 this.getAssociatedParty().add((ResearchStudyAssociatedPartyComponent) value); 4649 } else if (name.equals("progressStatus")) { 4650 this.getProgressStatus().add((ResearchStudyProgressStatusComponent) value); 4651 } else if (name.equals("whyStopped")) { 4652 this.whyStopped = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4653 } else if (name.equals("recruitment")) { 4654 this.recruitment = (ResearchStudyRecruitmentComponent) value; // ResearchStudyRecruitmentComponent 4655 } else if (name.equals("comparisonGroup")) { 4656 this.getComparisonGroup().add((ResearchStudyComparisonGroupComponent) value); 4657 } else if (name.equals("objective")) { 4658 this.getObjective().add((ResearchStudyObjectiveComponent) value); 4659 } else if (name.equals("outcomeMeasure")) { 4660 this.getOutcomeMeasure().add((ResearchStudyOutcomeMeasureComponent) value); 4661 } else if (name.equals("result")) { 4662 this.getResult().add(TypeConvertor.castToReference(value)); 4663 } else 4664 return super.setProperty(name, value); 4665 return value; 4666 } 4667 4668 @Override 4669 public void removeChild(String name, Base value) throws FHIRException { 4670 if (name.equals("url")) { 4671 this.url = null; 4672 } else if (name.equals("identifier")) { 4673 this.getIdentifier().remove(value); 4674 } else if (name.equals("version")) { 4675 this.version = null; 4676 } else if (name.equals("name")) { 4677 this.name = null; 4678 } else if (name.equals("title")) { 4679 this.title = null; 4680 } else if (name.equals("label")) { 4681 this.getLabel().remove((ResearchStudyLabelComponent) value); 4682 } else if (name.equals("protocol")) { 4683 this.getProtocol().remove(value); 4684 } else if (name.equals("partOf")) { 4685 this.getPartOf().remove(value); 4686 } else if (name.equals("relatedArtifact")) { 4687 this.getRelatedArtifact().remove(value); 4688 } else if (name.equals("date")) { 4689 this.date = null; 4690 } else if (name.equals("status")) { 4691 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4692 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4693 } else if (name.equals("primaryPurposeType")) { 4694 this.primaryPurposeType = null; 4695 } else if (name.equals("phase")) { 4696 this.phase = null; 4697 } else if (name.equals("studyDesign")) { 4698 this.getStudyDesign().remove(value); 4699 } else if (name.equals("focus")) { 4700 this.getFocus().remove(value); 4701 } else if (name.equals("condition")) { 4702 this.getCondition().remove(value); 4703 } else if (name.equals("keyword")) { 4704 this.getKeyword().remove(value); 4705 } else if (name.equals("region")) { 4706 this.getRegion().remove(value); 4707 } else if (name.equals("descriptionSummary")) { 4708 this.descriptionSummary = null; 4709 } else if (name.equals("description")) { 4710 this.description = null; 4711 } else if (name.equals("period")) { 4712 this.period = null; 4713 } else if (name.equals("site")) { 4714 this.getSite().remove(value); 4715 } else if (name.equals("note")) { 4716 this.getNote().remove(value); 4717 } else if (name.equals("classifier")) { 4718 this.getClassifier().remove(value); 4719 } else if (name.equals("associatedParty")) { 4720 this.getAssociatedParty().remove((ResearchStudyAssociatedPartyComponent) value); 4721 } else if (name.equals("progressStatus")) { 4722 this.getProgressStatus().remove((ResearchStudyProgressStatusComponent) value); 4723 } else if (name.equals("whyStopped")) { 4724 this.whyStopped = null; 4725 } else if (name.equals("recruitment")) { 4726 this.recruitment = (ResearchStudyRecruitmentComponent) value; // ResearchStudyRecruitmentComponent 4727 } else if (name.equals("comparisonGroup")) { 4728 this.getComparisonGroup().remove((ResearchStudyComparisonGroupComponent) value); 4729 } else if (name.equals("objective")) { 4730 this.getObjective().remove((ResearchStudyObjectiveComponent) value); 4731 } else if (name.equals("outcomeMeasure")) { 4732 this.getOutcomeMeasure().remove((ResearchStudyOutcomeMeasureComponent) value); 4733 } else if (name.equals("result")) { 4734 this.getResult().remove(value); 4735 } else 4736 super.removeChild(name, value); 4737 4738 } 4739 4740 @Override 4741 public Base makeProperty(int hash, String name) throws FHIRException { 4742 switch (hash) { 4743 case 116079: return getUrlElement(); 4744 case -1618432855: return addIdentifier(); 4745 case 351608024: return getVersionElement(); 4746 case 3373707: return getNameElement(); 4747 case 110371416: return getTitleElement(); 4748 case 102727412: return addLabel(); 4749 case -989163880: return addProtocol(); 4750 case -995410646: return addPartOf(); 4751 case 666807069: return addRelatedArtifact(); 4752 case 3076014: return getDateElement(); 4753 case -892481550: return getStatusElement(); 4754 case -2132842986: return getPrimaryPurposeType(); 4755 case 106629499: return getPhase(); 4756 case 1709211879: return addStudyDesign(); 4757 case 97604824: return addFocus(); 4758 case -861311717: return addCondition(); 4759 case -814408215: return addKeyword(); 4760 case -934795532: return addRegion(); 4761 case 21530634: return getDescriptionSummaryElement(); 4762 case -1724546052: return getDescriptionElement(); 4763 case -991726143: return getPeriod(); 4764 case 3530567: return addSite(); 4765 case 3387378: return addNote(); 4766 case -281470431: return addClassifier(); 4767 case -1841460864: return addAssociatedParty(); 4768 case -1897502593: return addProgressStatus(); 4769 case -699986715: return getWhyStopped(); 4770 case 780783004: return getRecruitment(); 4771 case -138266634: return addComparisonGroup(); 4772 case -1489585863: return addObjective(); 4773 case -1510689364: return addOutcomeMeasure(); 4774 case -934426595: return addResult(); 4775 default: return super.makeProperty(hash, name); 4776 } 4777 4778 } 4779 4780 @Override 4781 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4782 switch (hash) { 4783 case 116079: /*url*/ return new String[] {"uri"}; 4784 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4785 case 351608024: /*version*/ return new String[] {"string"}; 4786 case 3373707: /*name*/ return new String[] {"string"}; 4787 case 110371416: /*title*/ return new String[] {"string"}; 4788 case 102727412: /*label*/ return new String[] {}; 4789 case -989163880: /*protocol*/ return new String[] {"Reference"}; 4790 case -995410646: /*partOf*/ return new String[] {"Reference"}; 4791 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 4792 case 3076014: /*date*/ return new String[] {"dateTime"}; 4793 case -892481550: /*status*/ return new String[] {"code"}; 4794 case -2132842986: /*primaryPurposeType*/ return new String[] {"CodeableConcept"}; 4795 case 106629499: /*phase*/ return new String[] {"CodeableConcept"}; 4796 case 1709211879: /*studyDesign*/ return new String[] {"CodeableConcept"}; 4797 case 97604824: /*focus*/ return new String[] {"CodeableReference"}; 4798 case -861311717: /*condition*/ return new String[] {"CodeableConcept"}; 4799 case -814408215: /*keyword*/ return new String[] {"CodeableConcept"}; 4800 case -934795532: /*region*/ return new String[] {"CodeableConcept"}; 4801 case 21530634: /*descriptionSummary*/ return new String[] {"markdown"}; 4802 case -1724546052: /*description*/ return new String[] {"markdown"}; 4803 case -991726143: /*period*/ return new String[] {"Period"}; 4804 case 3530567: /*site*/ return new String[] {"Reference"}; 4805 case 3387378: /*note*/ return new String[] {"Annotation"}; 4806 case -281470431: /*classifier*/ return new String[] {"CodeableConcept"}; 4807 case -1841460864: /*associatedParty*/ return new String[] {}; 4808 case -1897502593: /*progressStatus*/ return new String[] {}; 4809 case -699986715: /*whyStopped*/ return new String[] {"CodeableConcept"}; 4810 case 780783004: /*recruitment*/ return new String[] {}; 4811 case -138266634: /*comparisonGroup*/ return new String[] {}; 4812 case -1489585863: /*objective*/ return new String[] {}; 4813 case -1510689364: /*outcomeMeasure*/ return new String[] {}; 4814 case -934426595: /*result*/ return new String[] {"Reference"}; 4815 default: return super.getTypesForProperty(hash, name); 4816 } 4817 4818 } 4819 4820 @Override 4821 public Base addChild(String name) throws FHIRException { 4822 if (name.equals("url")) { 4823 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.url"); 4824 } 4825 else if (name.equals("identifier")) { 4826 return addIdentifier(); 4827 } 4828 else if (name.equals("version")) { 4829 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.version"); 4830 } 4831 else if (name.equals("name")) { 4832 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.name"); 4833 } 4834 else if (name.equals("title")) { 4835 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.title"); 4836 } 4837 else if (name.equals("label")) { 4838 return addLabel(); 4839 } 4840 else if (name.equals("protocol")) { 4841 return addProtocol(); 4842 } 4843 else if (name.equals("partOf")) { 4844 return addPartOf(); 4845 } 4846 else if (name.equals("relatedArtifact")) { 4847 return addRelatedArtifact(); 4848 } 4849 else if (name.equals("date")) { 4850 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.date"); 4851 } 4852 else if (name.equals("status")) { 4853 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.status"); 4854 } 4855 else if (name.equals("primaryPurposeType")) { 4856 this.primaryPurposeType = new CodeableConcept(); 4857 return this.primaryPurposeType; 4858 } 4859 else if (name.equals("phase")) { 4860 this.phase = new CodeableConcept(); 4861 return this.phase; 4862 } 4863 else if (name.equals("studyDesign")) { 4864 return addStudyDesign(); 4865 } 4866 else if (name.equals("focus")) { 4867 return addFocus(); 4868 } 4869 else if (name.equals("condition")) { 4870 return addCondition(); 4871 } 4872 else if (name.equals("keyword")) { 4873 return addKeyword(); 4874 } 4875 else if (name.equals("region")) { 4876 return addRegion(); 4877 } 4878 else if (name.equals("descriptionSummary")) { 4879 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.descriptionSummary"); 4880 } 4881 else if (name.equals("description")) { 4882 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.description"); 4883 } 4884 else if (name.equals("period")) { 4885 this.period = new Period(); 4886 return this.period; 4887 } 4888 else if (name.equals("site")) { 4889 return addSite(); 4890 } 4891 else if (name.equals("note")) { 4892 return addNote(); 4893 } 4894 else if (name.equals("classifier")) { 4895 return addClassifier(); 4896 } 4897 else if (name.equals("associatedParty")) { 4898 return addAssociatedParty(); 4899 } 4900 else if (name.equals("progressStatus")) { 4901 return addProgressStatus(); 4902 } 4903 else if (name.equals("whyStopped")) { 4904 this.whyStopped = new CodeableConcept(); 4905 return this.whyStopped; 4906 } 4907 else if (name.equals("recruitment")) { 4908 this.recruitment = new ResearchStudyRecruitmentComponent(); 4909 return this.recruitment; 4910 } 4911 else if (name.equals("comparisonGroup")) { 4912 return addComparisonGroup(); 4913 } 4914 else if (name.equals("objective")) { 4915 return addObjective(); 4916 } 4917 else if (name.equals("outcomeMeasure")) { 4918 return addOutcomeMeasure(); 4919 } 4920 else if (name.equals("result")) { 4921 return addResult(); 4922 } 4923 else 4924 return super.addChild(name); 4925 } 4926 4927 public String fhirType() { 4928 return "ResearchStudy"; 4929 4930 } 4931 4932 public ResearchStudy copy() { 4933 ResearchStudy dst = new ResearchStudy(); 4934 copyValues(dst); 4935 return dst; 4936 } 4937 4938 public void copyValues(ResearchStudy dst) { 4939 super.copyValues(dst); 4940 dst.url = url == null ? null : url.copy(); 4941 if (identifier != null) { 4942 dst.identifier = new ArrayList<Identifier>(); 4943 for (Identifier i : identifier) 4944 dst.identifier.add(i.copy()); 4945 }; 4946 dst.version = version == null ? null : version.copy(); 4947 dst.name = name == null ? null : name.copy(); 4948 dst.title = title == null ? null : title.copy(); 4949 if (label != null) { 4950 dst.label = new ArrayList<ResearchStudyLabelComponent>(); 4951 for (ResearchStudyLabelComponent i : label) 4952 dst.label.add(i.copy()); 4953 }; 4954 if (protocol != null) { 4955 dst.protocol = new ArrayList<Reference>(); 4956 for (Reference i : protocol) 4957 dst.protocol.add(i.copy()); 4958 }; 4959 if (partOf != null) { 4960 dst.partOf = new ArrayList<Reference>(); 4961 for (Reference i : partOf) 4962 dst.partOf.add(i.copy()); 4963 }; 4964 if (relatedArtifact != null) { 4965 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 4966 for (RelatedArtifact i : relatedArtifact) 4967 dst.relatedArtifact.add(i.copy()); 4968 }; 4969 dst.date = date == null ? null : date.copy(); 4970 dst.status = status == null ? null : status.copy(); 4971 dst.primaryPurposeType = primaryPurposeType == null ? null : primaryPurposeType.copy(); 4972 dst.phase = phase == null ? null : phase.copy(); 4973 if (studyDesign != null) { 4974 dst.studyDesign = new ArrayList<CodeableConcept>(); 4975 for (CodeableConcept i : studyDesign) 4976 dst.studyDesign.add(i.copy()); 4977 }; 4978 if (focus != null) { 4979 dst.focus = new ArrayList<CodeableReference>(); 4980 for (CodeableReference i : focus) 4981 dst.focus.add(i.copy()); 4982 }; 4983 if (condition != null) { 4984 dst.condition = new ArrayList<CodeableConcept>(); 4985 for (CodeableConcept i : condition) 4986 dst.condition.add(i.copy()); 4987 }; 4988 if (keyword != null) { 4989 dst.keyword = new ArrayList<CodeableConcept>(); 4990 for (CodeableConcept i : keyword) 4991 dst.keyword.add(i.copy()); 4992 }; 4993 if (region != null) { 4994 dst.region = new ArrayList<CodeableConcept>(); 4995 for (CodeableConcept i : region) 4996 dst.region.add(i.copy()); 4997 }; 4998 dst.descriptionSummary = descriptionSummary == null ? null : descriptionSummary.copy(); 4999 dst.description = description == null ? null : description.copy(); 5000 dst.period = period == null ? null : period.copy(); 5001 if (site != null) { 5002 dst.site = new ArrayList<Reference>(); 5003 for (Reference i : site) 5004 dst.site.add(i.copy()); 5005 }; 5006 if (note != null) { 5007 dst.note = new ArrayList<Annotation>(); 5008 for (Annotation i : note) 5009 dst.note.add(i.copy()); 5010 }; 5011 if (classifier != null) { 5012 dst.classifier = new ArrayList<CodeableConcept>(); 5013 for (CodeableConcept i : classifier) 5014 dst.classifier.add(i.copy()); 5015 }; 5016 if (associatedParty != null) { 5017 dst.associatedParty = new ArrayList<ResearchStudyAssociatedPartyComponent>(); 5018 for (ResearchStudyAssociatedPartyComponent i : associatedParty) 5019 dst.associatedParty.add(i.copy()); 5020 }; 5021 if (progressStatus != null) { 5022 dst.progressStatus = new ArrayList<ResearchStudyProgressStatusComponent>(); 5023 for (ResearchStudyProgressStatusComponent i : progressStatus) 5024 dst.progressStatus.add(i.copy()); 5025 }; 5026 dst.whyStopped = whyStopped == null ? null : whyStopped.copy(); 5027 dst.recruitment = recruitment == null ? null : recruitment.copy(); 5028 if (comparisonGroup != null) { 5029 dst.comparisonGroup = new ArrayList<ResearchStudyComparisonGroupComponent>(); 5030 for (ResearchStudyComparisonGroupComponent i : comparisonGroup) 5031 dst.comparisonGroup.add(i.copy()); 5032 }; 5033 if (objective != null) { 5034 dst.objective = new ArrayList<ResearchStudyObjectiveComponent>(); 5035 for (ResearchStudyObjectiveComponent i : objective) 5036 dst.objective.add(i.copy()); 5037 }; 5038 if (outcomeMeasure != null) { 5039 dst.outcomeMeasure = new ArrayList<ResearchStudyOutcomeMeasureComponent>(); 5040 for (ResearchStudyOutcomeMeasureComponent i : outcomeMeasure) 5041 dst.outcomeMeasure.add(i.copy()); 5042 }; 5043 if (result != null) { 5044 dst.result = new ArrayList<Reference>(); 5045 for (Reference i : result) 5046 dst.result.add(i.copy()); 5047 }; 5048 } 5049 5050 protected ResearchStudy typedCopy() { 5051 return copy(); 5052 } 5053 5054 @Override 5055 public boolean equalsDeep(Base other_) { 5056 if (!super.equalsDeep(other_)) 5057 return false; 5058 if (!(other_ instanceof ResearchStudy)) 5059 return false; 5060 ResearchStudy o = (ResearchStudy) other_; 5061 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 5062 && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) && compareDeep(label, o.label, true) 5063 && compareDeep(protocol, o.protocol, true) && compareDeep(partOf, o.partOf, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 5064 && compareDeep(date, o.date, true) && compareDeep(status, o.status, true) && compareDeep(primaryPurposeType, o.primaryPurposeType, true) 5065 && compareDeep(phase, o.phase, true) && compareDeep(studyDesign, o.studyDesign, true) && compareDeep(focus, o.focus, true) 5066 && compareDeep(condition, o.condition, true) && compareDeep(keyword, o.keyword, true) && compareDeep(region, o.region, true) 5067 && compareDeep(descriptionSummary, o.descriptionSummary, true) && compareDeep(description, o.description, true) 5068 && compareDeep(period, o.period, true) && compareDeep(site, o.site, true) && compareDeep(note, o.note, true) 5069 && compareDeep(classifier, o.classifier, true) && compareDeep(associatedParty, o.associatedParty, true) 5070 && compareDeep(progressStatus, o.progressStatus, true) && compareDeep(whyStopped, o.whyStopped, true) 5071 && compareDeep(recruitment, o.recruitment, true) && compareDeep(comparisonGroup, o.comparisonGroup, true) 5072 && compareDeep(objective, o.objective, true) && compareDeep(outcomeMeasure, o.outcomeMeasure, true) 5073 && compareDeep(result, o.result, true); 5074 } 5075 5076 @Override 5077 public boolean equalsShallow(Base other_) { 5078 if (!super.equalsShallow(other_)) 5079 return false; 5080 if (!(other_ instanceof ResearchStudy)) 5081 return false; 5082 ResearchStudy o = (ResearchStudy) other_; 5083 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 5084 && compareValues(title, o.title, true) && compareValues(date, o.date, true) && compareValues(status, o.status, true) 5085 && compareValues(descriptionSummary, o.descriptionSummary, true) && compareValues(description, o.description, true) 5086 ; 5087 } 5088 5089 public boolean isEmpty() { 5090 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 5091 , name, title, label, protocol, partOf, relatedArtifact, date, status, primaryPurposeType 5092 , phase, studyDesign, focus, condition, keyword, region, descriptionSummary, description 5093 , period, site, note, classifier, associatedParty, progressStatus, whyStopped 5094 , recruitment, comparisonGroup, objective, outcomeMeasure, result); 5095 } 5096 5097 @Override 5098 public ResourceType getResourceType() { 5099 return ResourceType.ResearchStudy; 5100 } 5101 5102 /** 5103 * Search parameter: <b>classifier</b> 5104 * <p> 5105 * Description: <b>Classification for the study</b><br> 5106 * Type: <b>token</b><br> 5107 * Path: <b>ResearchStudy.classifier</b><br> 5108 * </p> 5109 */ 5110 @SearchParamDefinition(name="classifier", path="ResearchStudy.classifier", description="Classification for the study", type="token" ) 5111 public static final String SP_CLASSIFIER = "classifier"; 5112 /** 5113 * <b>Fluent Client</b> search parameter constant for <b>classifier</b> 5114 * <p> 5115 * Description: <b>Classification for the study</b><br> 5116 * Type: <b>token</b><br> 5117 * Path: <b>ResearchStudy.classifier</b><br> 5118 * </p> 5119 */ 5120 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASSIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CLASSIFIER); 5121 5122 /** 5123 * Search parameter: <b>condition</b> 5124 * <p> 5125 * Description: <b>Condition being studied</b><br> 5126 * Type: <b>token</b><br> 5127 * Path: <b>ResearchStudy.condition</b><br> 5128 * </p> 5129 */ 5130 @SearchParamDefinition(name="condition", path="ResearchStudy.condition", description="Condition being studied", type="token" ) 5131 public static final String SP_CONDITION = "condition"; 5132 /** 5133 * <b>Fluent Client</b> search parameter constant for <b>condition</b> 5134 * <p> 5135 * Description: <b>Condition being studied</b><br> 5136 * Type: <b>token</b><br> 5137 * Path: <b>ResearchStudy.condition</b><br> 5138 * </p> 5139 */ 5140 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONDITION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONDITION); 5141 5142 /** 5143 * Search parameter: <b>date</b> 5144 * <p> 5145 * Description: <b>When the study began and ended</b><br> 5146 * Type: <b>date</b><br> 5147 * Path: <b>ResearchStudy.period</b><br> 5148 * </p> 5149 */ 5150 @SearchParamDefinition(name="date", path="ResearchStudy.period", description="When the study began and ended", type="date" ) 5151 public static final String SP_DATE = "date"; 5152 /** 5153 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5154 * <p> 5155 * Description: <b>When the study began and ended</b><br> 5156 * Type: <b>date</b><br> 5157 * Path: <b>ResearchStudy.period</b><br> 5158 * </p> 5159 */ 5160 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 5161 5162 /** 5163 * Search parameter: <b>description</b> 5164 * <p> 5165 * Description: <b>Detailed narrative of the study</b><br> 5166 * Type: <b>string</b><br> 5167 * Path: <b>ResearchStudy.description</b><br> 5168 * </p> 5169 */ 5170 @SearchParamDefinition(name="description", path="ResearchStudy.description", description="Detailed narrative of the study", type="string" ) 5171 public static final String SP_DESCRIPTION = "description"; 5172 /** 5173 * <b>Fluent Client</b> search parameter constant for <b>description</b> 5174 * <p> 5175 * Description: <b>Detailed narrative of the study</b><br> 5176 * Type: <b>string</b><br> 5177 * Path: <b>ResearchStudy.description</b><br> 5178 * </p> 5179 */ 5180 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 5181 5182 /** 5183 * Search parameter: <b>eligibility</b> 5184 * <p> 5185 * Description: <b>Inclusion and exclusion criteria</b><br> 5186 * Type: <b>reference</b><br> 5187 * Path: <b>ResearchStudy.recruitment.eligibility</b><br> 5188 * </p> 5189 */ 5190 @SearchParamDefinition(name="eligibility", path="ResearchStudy.recruitment.eligibility", description="Inclusion and exclusion criteria", type="reference", target={EvidenceVariable.class, Group.class } ) 5191 public static final String SP_ELIGIBILITY = "eligibility"; 5192 /** 5193 * <b>Fluent Client</b> search parameter constant for <b>eligibility</b> 5194 * <p> 5195 * Description: <b>Inclusion and exclusion criteria</b><br> 5196 * Type: <b>reference</b><br> 5197 * Path: <b>ResearchStudy.recruitment.eligibility</b><br> 5198 * </p> 5199 */ 5200 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ELIGIBILITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ELIGIBILITY); 5201 5202/** 5203 * Constant for fluent queries to be used to add include statements. Specifies 5204 * the path value of "<b>ResearchStudy:eligibility</b>". 5205 */ 5206 public static final ca.uhn.fhir.model.api.Include INCLUDE_ELIGIBILITY = new ca.uhn.fhir.model.api.Include("ResearchStudy:eligibility").toLocked(); 5207 5208 /** 5209 * Search parameter: <b>focus-code</b> 5210 * <p> 5211 * Description: <b>Drugs, devices, etc. under study, as a code</b><br> 5212 * Type: <b>token</b><br> 5213 * Path: <b>ResearchStudy.focus.concept</b><br> 5214 * </p> 5215 */ 5216 @SearchParamDefinition(name="focus-code", path="ResearchStudy.focus.concept", description="Drugs, devices, etc. under study, as a code", type="token" ) 5217 public static final String SP_FOCUS_CODE = "focus-code"; 5218 /** 5219 * <b>Fluent Client</b> search parameter constant for <b>focus-code</b> 5220 * <p> 5221 * Description: <b>Drugs, devices, etc. under study, as a code</b><br> 5222 * Type: <b>token</b><br> 5223 * Path: <b>ResearchStudy.focus.concept</b><br> 5224 * </p> 5225 */ 5226 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FOCUS_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FOCUS_CODE); 5227 5228 /** 5229 * Search parameter: <b>focus-reference</b> 5230 * <p> 5231 * Description: <b>Drugs, devices, etc. under study, as a reference</b><br> 5232 * Type: <b>reference</b><br> 5233 * Path: <b>ResearchStudy.focus.reference</b><br> 5234 * </p> 5235 */ 5236 @SearchParamDefinition(name="focus-reference", path="ResearchStudy.focus.reference", description="Drugs, devices, etc. under study, as a reference", type="reference", target={EvidenceVariable.class, Medication.class, MedicinalProductDefinition.class, SubstanceDefinition.class } ) 5237 public static final String SP_FOCUS_REFERENCE = "focus-reference"; 5238 /** 5239 * <b>Fluent Client</b> search parameter constant for <b>focus-reference</b> 5240 * <p> 5241 * Description: <b>Drugs, devices, etc. under study, as a reference</b><br> 5242 * Type: <b>reference</b><br> 5243 * Path: <b>ResearchStudy.focus.reference</b><br> 5244 * </p> 5245 */ 5246 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FOCUS_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FOCUS_REFERENCE); 5247 5248/** 5249 * Constant for fluent queries to be used to add include statements. Specifies 5250 * the path value of "<b>ResearchStudy:focus-reference</b>". 5251 */ 5252 public static final ca.uhn.fhir.model.api.Include INCLUDE_FOCUS_REFERENCE = new ca.uhn.fhir.model.api.Include("ResearchStudy:focus-reference").toLocked(); 5253 5254 /** 5255 * Search parameter: <b>identifier</b> 5256 * <p> 5257 * Description: <b>Business Identifier for study</b><br> 5258 * Type: <b>token</b><br> 5259 * Path: <b>ResearchStudy.identifier</b><br> 5260 * </p> 5261 */ 5262 @SearchParamDefinition(name="identifier", path="ResearchStudy.identifier", description="Business Identifier for study", type="token" ) 5263 public static final String SP_IDENTIFIER = "identifier"; 5264 /** 5265 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5266 * <p> 5267 * Description: <b>Business Identifier for study</b><br> 5268 * Type: <b>token</b><br> 5269 * Path: <b>ResearchStudy.identifier</b><br> 5270 * </p> 5271 */ 5272 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 5273 5274 /** 5275 * Search parameter: <b>keyword</b> 5276 * <p> 5277 * Description: <b>Used to search for the study</b><br> 5278 * Type: <b>token</b><br> 5279 * Path: <b>ResearchStudy.keyword</b><br> 5280 * </p> 5281 */ 5282 @SearchParamDefinition(name="keyword", path="ResearchStudy.keyword", description="Used to search for the study", type="token" ) 5283 public static final String SP_KEYWORD = "keyword"; 5284 /** 5285 * <b>Fluent Client</b> search parameter constant for <b>keyword</b> 5286 * <p> 5287 * Description: <b>Used to search for the study</b><br> 5288 * Type: <b>token</b><br> 5289 * Path: <b>ResearchStudy.keyword</b><br> 5290 * </p> 5291 */ 5292 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KEYWORD = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_KEYWORD); 5293 5294 /** 5295 * Search parameter: <b>name</b> 5296 * <p> 5297 * Description: <b>Name for this study</b><br> 5298 * Type: <b>string</b><br> 5299 * Path: <b>ResearchStudy.name</b><br> 5300 * </p> 5301 */ 5302 @SearchParamDefinition(name="name", path="ResearchStudy.name", description="Name for this study", type="string" ) 5303 public static final String SP_NAME = "name"; 5304 /** 5305 * <b>Fluent Client</b> search parameter constant for <b>name</b> 5306 * <p> 5307 * Description: <b>Name for this study</b><br> 5308 * Type: <b>string</b><br> 5309 * Path: <b>ResearchStudy.name</b><br> 5310 * </p> 5311 */ 5312 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 5313 5314 /** 5315 * Search parameter: <b>objective-description</b> 5316 * <p> 5317 * Description: <b>Free text description of the objective of the study</b><br> 5318 * Type: <b>string</b><br> 5319 * Path: <b>ResearchStudy.objective.description</b><br> 5320 * </p> 5321 */ 5322 @SearchParamDefinition(name="objective-description", path="ResearchStudy.objective.description", description="Free text description of the objective of the study", type="string" ) 5323 public static final String SP_OBJECTIVE_DESCRIPTION = "objective-description"; 5324 /** 5325 * <b>Fluent Client</b> search parameter constant for <b>objective-description</b> 5326 * <p> 5327 * Description: <b>Free text description of the objective of the study</b><br> 5328 * Type: <b>string</b><br> 5329 * Path: <b>ResearchStudy.objective.description</b><br> 5330 * </p> 5331 */ 5332 public static final ca.uhn.fhir.rest.gclient.StringClientParam OBJECTIVE_DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_OBJECTIVE_DESCRIPTION); 5333 5334 /** 5335 * Search parameter: <b>objective-type</b> 5336 * <p> 5337 * Description: <b>The kind of study objective</b><br> 5338 * Type: <b>token</b><br> 5339 * Path: <b>ResearchStudy.objective.type</b><br> 5340 * </p> 5341 */ 5342 @SearchParamDefinition(name="objective-type", path="ResearchStudy.objective.type", description="The kind of study objective", type="token" ) 5343 public static final String SP_OBJECTIVE_TYPE = "objective-type"; 5344 /** 5345 * <b>Fluent Client</b> search parameter constant for <b>objective-type</b> 5346 * <p> 5347 * Description: <b>The kind of study objective</b><br> 5348 * Type: <b>token</b><br> 5349 * Path: <b>ResearchStudy.objective.type</b><br> 5350 * </p> 5351 */ 5352 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OBJECTIVE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_OBJECTIVE_TYPE); 5353 5354 /** 5355 * Search parameter: <b>part-of</b> 5356 * <p> 5357 * Description: <b>Part of larger study</b><br> 5358 * Type: <b>reference</b><br> 5359 * Path: <b>ResearchStudy.partOf</b><br> 5360 * </p> 5361 */ 5362 @SearchParamDefinition(name="part-of", path="ResearchStudy.partOf", description="Part of larger study", type="reference", target={ResearchStudy.class } ) 5363 public static final String SP_PART_OF = "part-of"; 5364 /** 5365 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 5366 * <p> 5367 * Description: <b>Part of larger study</b><br> 5368 * Type: <b>reference</b><br> 5369 * Path: <b>ResearchStudy.partOf</b><br> 5370 * </p> 5371 */ 5372 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PART_OF); 5373 5374/** 5375 * Constant for fluent queries to be used to add include statements. Specifies 5376 * the path value of "<b>ResearchStudy:part-of</b>". 5377 */ 5378 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("ResearchStudy:part-of").toLocked(); 5379 5380 /** 5381 * Search parameter: <b>phase</b> 5382 * <p> 5383 * Description: <b>The stage in the progression of a study</b><br> 5384 * Type: <b>token</b><br> 5385 * Path: <b>ResearchStudy.phase</b><br> 5386 * </p> 5387 */ 5388 @SearchParamDefinition(name="phase", path="ResearchStudy.phase", description="The stage in the progression of a study", type="token" ) 5389 public static final String SP_PHASE = "phase"; 5390 /** 5391 * <b>Fluent Client</b> search parameter constant for <b>phase</b> 5392 * <p> 5393 * Description: <b>The stage in the progression of a study</b><br> 5394 * Type: <b>token</b><br> 5395 * Path: <b>ResearchStudy.phase</b><br> 5396 * </p> 5397 */ 5398 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHASE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PHASE); 5399 5400 /** 5401 * Search parameter: <b>protocol</b> 5402 * <p> 5403 * Description: <b>Steps followed in executing study</b><br> 5404 * Type: <b>reference</b><br> 5405 * Path: <b>ResearchStudy.protocol</b><br> 5406 * </p> 5407 */ 5408 @SearchParamDefinition(name="protocol", path="ResearchStudy.protocol", description="Steps followed in executing study", type="reference", target={PlanDefinition.class } ) 5409 public static final String SP_PROTOCOL = "protocol"; 5410 /** 5411 * <b>Fluent Client</b> search parameter constant for <b>protocol</b> 5412 * <p> 5413 * Description: <b>Steps followed in executing study</b><br> 5414 * Type: <b>reference</b><br> 5415 * Path: <b>ResearchStudy.protocol</b><br> 5416 * </p> 5417 */ 5418 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROTOCOL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROTOCOL); 5419 5420/** 5421 * Constant for fluent queries to be used to add include statements. Specifies 5422 * the path value of "<b>ResearchStudy:protocol</b>". 5423 */ 5424 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROTOCOL = new ca.uhn.fhir.model.api.Include("ResearchStudy:protocol").toLocked(); 5425 5426 /** 5427 * Search parameter: <b>recruitment-actual</b> 5428 * <p> 5429 * Description: <b>Actual number of participants enrolled in study across all groups</b><br> 5430 * Type: <b>number</b><br> 5431 * Path: <b>ResearchStudy.recruitment.actualNumber</b><br> 5432 * </p> 5433 */ 5434 @SearchParamDefinition(name="recruitment-actual", path="ResearchStudy.recruitment.actualNumber", description="Actual number of participants enrolled in study across all groups", type="number" ) 5435 public static final String SP_RECRUITMENT_ACTUAL = "recruitment-actual"; 5436 /** 5437 * <b>Fluent Client</b> search parameter constant for <b>recruitment-actual</b> 5438 * <p> 5439 * Description: <b>Actual number of participants enrolled in study across all groups</b><br> 5440 * Type: <b>number</b><br> 5441 * Path: <b>ResearchStudy.recruitment.actualNumber</b><br> 5442 * </p> 5443 */ 5444 public static final ca.uhn.fhir.rest.gclient.NumberClientParam RECRUITMENT_ACTUAL = new ca.uhn.fhir.rest.gclient.NumberClientParam(SP_RECRUITMENT_ACTUAL); 5445 5446 /** 5447 * Search parameter: <b>recruitment-target</b> 5448 * <p> 5449 * Description: <b>Target number of participants enrolled in study across all groups</b><br> 5450 * Type: <b>number</b><br> 5451 * Path: <b>ResearchStudy.recruitment.targetNumber</b><br> 5452 * </p> 5453 */ 5454 @SearchParamDefinition(name="recruitment-target", path="ResearchStudy.recruitment.targetNumber", description="Target number of participants enrolled in study across all groups", type="number" ) 5455 public static final String SP_RECRUITMENT_TARGET = "recruitment-target"; 5456 /** 5457 * <b>Fluent Client</b> search parameter constant for <b>recruitment-target</b> 5458 * <p> 5459 * Description: <b>Target number of participants enrolled in study across all groups</b><br> 5460 * Type: <b>number</b><br> 5461 * Path: <b>ResearchStudy.recruitment.targetNumber</b><br> 5462 * </p> 5463 */ 5464 public static final ca.uhn.fhir.rest.gclient.NumberClientParam RECRUITMENT_TARGET = new ca.uhn.fhir.rest.gclient.NumberClientParam(SP_RECRUITMENT_TARGET); 5465 5466 /** 5467 * Search parameter: <b>region</b> 5468 * <p> 5469 * Description: <b>Geographic area for the study</b><br> 5470 * Type: <b>token</b><br> 5471 * Path: <b>ResearchStudy.region</b><br> 5472 * </p> 5473 */ 5474 @SearchParamDefinition(name="region", path="ResearchStudy.region", description="Geographic area for the study", type="token" ) 5475 public static final String SP_REGION = "region"; 5476 /** 5477 * <b>Fluent Client</b> search parameter constant for <b>region</b> 5478 * <p> 5479 * Description: <b>Geographic area for the study</b><br> 5480 * Type: <b>token</b><br> 5481 * Path: <b>ResearchStudy.region</b><br> 5482 * </p> 5483 */ 5484 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REGION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REGION); 5485 5486 /** 5487 * Search parameter: <b>site</b> 5488 * <p> 5489 * Description: <b>Facility where study activities are conducted</b><br> 5490 * Type: <b>reference</b><br> 5491 * Path: <b>ResearchStudy.site</b><br> 5492 * </p> 5493 */ 5494 @SearchParamDefinition(name="site", path="ResearchStudy.site", description="Facility where study activities are conducted", type="reference", target={Location.class, Organization.class, ResearchStudy.class } ) 5495 public static final String SP_SITE = "site"; 5496 /** 5497 * <b>Fluent Client</b> search parameter constant for <b>site</b> 5498 * <p> 5499 * Description: <b>Facility where study activities are conducted</b><br> 5500 * Type: <b>reference</b><br> 5501 * Path: <b>ResearchStudy.site</b><br> 5502 * </p> 5503 */ 5504 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SITE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SITE); 5505 5506/** 5507 * Constant for fluent queries to be used to add include statements. Specifies 5508 * the path value of "<b>ResearchStudy:site</b>". 5509 */ 5510 public static final ca.uhn.fhir.model.api.Include INCLUDE_SITE = new ca.uhn.fhir.model.api.Include("ResearchStudy:site").toLocked(); 5511 5512 /** 5513 * Search parameter: <b>status</b> 5514 * <p> 5515 * Description: <b>active | active-but-not-recruiting | administratively-completed | approved | closed-to-accrual | closed-to-accrual-and-intervention | completed | disapproved | enrolling-by-invitation | in-review | not-yet-recruiting | recruiting | temporarily-closed-to-accrual | temporarily-closed-to-accrual-and-intervention | terminated | withdrawn</b><br> 5516 * Type: <b>token</b><br> 5517 * Path: <b>ResearchStudy.status</b><br> 5518 * </p> 5519 */ 5520 @SearchParamDefinition(name="status", path="ResearchStudy.status", description="active | active-but-not-recruiting | administratively-completed | approved | closed-to-accrual | closed-to-accrual-and-intervention | completed | disapproved | enrolling-by-invitation | in-review | not-yet-recruiting | recruiting | temporarily-closed-to-accrual | temporarily-closed-to-accrual-and-intervention | terminated | withdrawn", type="token" ) 5521 public static final String SP_STATUS = "status"; 5522 /** 5523 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5524 * <p> 5525 * Description: <b>active | active-but-not-recruiting | administratively-completed | approved | closed-to-accrual | closed-to-accrual-and-intervention | completed | disapproved | enrolling-by-invitation | in-review | not-yet-recruiting | recruiting | temporarily-closed-to-accrual | temporarily-closed-to-accrual-and-intervention | terminated | withdrawn</b><br> 5526 * Type: <b>token</b><br> 5527 * Path: <b>ResearchStudy.status</b><br> 5528 * </p> 5529 */ 5530 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 5531 5532 /** 5533 * Search parameter: <b>study-design</b> 5534 * <p> 5535 * Description: <b>Classifications of the study design characteristics</b><br> 5536 * Type: <b>token</b><br> 5537 * Path: <b>ResearchStudy.studyDesign</b><br> 5538 * </p> 5539 */ 5540 @SearchParamDefinition(name="study-design", path="ResearchStudy.studyDesign", description="Classifications of the study design characteristics", type="token" ) 5541 public static final String SP_STUDY_DESIGN = "study-design"; 5542 /** 5543 * <b>Fluent Client</b> search parameter constant for <b>study-design</b> 5544 * <p> 5545 * Description: <b>Classifications of the study design characteristics</b><br> 5546 * Type: <b>token</b><br> 5547 * Path: <b>ResearchStudy.studyDesign</b><br> 5548 * </p> 5549 */ 5550 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STUDY_DESIGN = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STUDY_DESIGN); 5551 5552 /** 5553 * Search parameter: <b>title</b> 5554 * <p> 5555 * Description: <b>The human readable name of the research study</b><br> 5556 * Type: <b>string</b><br> 5557 * Path: <b>ResearchStudy.title</b><br> 5558 * </p> 5559 */ 5560 @SearchParamDefinition(name="title", path="ResearchStudy.title", description="The human readable name of the research study", type="string" ) 5561 public static final String SP_TITLE = "title"; 5562 /** 5563 * <b>Fluent Client</b> search parameter constant for <b>title</b> 5564 * <p> 5565 * Description: <b>The human readable name of the research study</b><br> 5566 * Type: <b>string</b><br> 5567 * Path: <b>ResearchStudy.title</b><br> 5568 * </p> 5569 */ 5570 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 5571 5572 5573} 5574