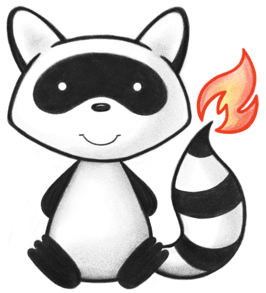
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A ResearchSubject is a participant or object which is the recipient of investigative activities in a research study. 052 */ 053@ResourceDef(name="ResearchSubject", profile="http://hl7.org/fhir/StructureDefinition/ResearchSubject") 054public class ResearchSubject extends DomainResource { 055 056 @Block() 057 public static class ResearchSubjectProgressComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Identifies the aspect of the subject's journey that the state refers to. 060 */ 061 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="state | milestone", formalDefinition="Identifies the aspect of the subject's journey that the state refers to." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-subject-state-type") 064 protected CodeableConcept type; 065 066 /** 067 * The current state of the subject. 068 */ 069 @Child(name = "subjectState", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 070 @Description(shortDefinition="candidate | eligible | follow-up | ineligible | not-registered | off-study | on-study | on-study-intervention | on-study-observation | pending-on-study | potential-candidate | screening | withdrawn", formalDefinition="The current state of the subject." ) 071 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-subject-state") 072 protected CodeableConcept subjectState; 073 074 /** 075 * The milestones the subject has passed through. 076 */ 077 @Child(name = "milestone", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 078 @Description(shortDefinition="SignedUp | Screened | Randomized", formalDefinition="The milestones the subject has passed through." ) 079 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/research-subject-milestone") 080 protected CodeableConcept milestone; 081 082 /** 083 * The reason for the state change. If coded it should follow the formal subject state model. 084 */ 085 @Child(name = "reason", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 086 @Description(shortDefinition="State change reason", formalDefinition="The reason for the state change. If coded it should follow the formal subject state model." ) 087 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/state-change-reason") 088 protected CodeableConcept reason; 089 090 /** 091 * The date when the new status started. 092 */ 093 @Child(name = "startDate", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 094 @Description(shortDefinition="State change date", formalDefinition="The date when the new status started." ) 095 protected DateTimeType startDate; 096 097 /** 098 * The date when the state ended. 099 */ 100 @Child(name = "endDate", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 101 @Description(shortDefinition="State change date", formalDefinition="The date when the state ended." ) 102 protected DateTimeType endDate; 103 104 private static final long serialVersionUID = -330838916L; 105 106 /** 107 * Constructor 108 */ 109 public ResearchSubjectProgressComponent() { 110 super(); 111 } 112 113 /** 114 * @return {@link #type} (Identifies the aspect of the subject's journey that the state refers to.) 115 */ 116 public CodeableConcept getType() { 117 if (this.type == null) 118 if (Configuration.errorOnAutoCreate()) 119 throw new Error("Attempt to auto-create ResearchSubjectProgressComponent.type"); 120 else if (Configuration.doAutoCreate()) 121 this.type = new CodeableConcept(); // cc 122 return this.type; 123 } 124 125 public boolean hasType() { 126 return this.type != null && !this.type.isEmpty(); 127 } 128 129 /** 130 * @param value {@link #type} (Identifies the aspect of the subject's journey that the state refers to.) 131 */ 132 public ResearchSubjectProgressComponent setType(CodeableConcept value) { 133 this.type = value; 134 return this; 135 } 136 137 /** 138 * @return {@link #subjectState} (The current state of the subject.) 139 */ 140 public CodeableConcept getSubjectState() { 141 if (this.subjectState == null) 142 if (Configuration.errorOnAutoCreate()) 143 throw new Error("Attempt to auto-create ResearchSubjectProgressComponent.subjectState"); 144 else if (Configuration.doAutoCreate()) 145 this.subjectState = new CodeableConcept(); // cc 146 return this.subjectState; 147 } 148 149 public boolean hasSubjectState() { 150 return this.subjectState != null && !this.subjectState.isEmpty(); 151 } 152 153 /** 154 * @param value {@link #subjectState} (The current state of the subject.) 155 */ 156 public ResearchSubjectProgressComponent setSubjectState(CodeableConcept value) { 157 this.subjectState = value; 158 return this; 159 } 160 161 /** 162 * @return {@link #milestone} (The milestones the subject has passed through.) 163 */ 164 public CodeableConcept getMilestone() { 165 if (this.milestone == null) 166 if (Configuration.errorOnAutoCreate()) 167 throw new Error("Attempt to auto-create ResearchSubjectProgressComponent.milestone"); 168 else if (Configuration.doAutoCreate()) 169 this.milestone = new CodeableConcept(); // cc 170 return this.milestone; 171 } 172 173 public boolean hasMilestone() { 174 return this.milestone != null && !this.milestone.isEmpty(); 175 } 176 177 /** 178 * @param value {@link #milestone} (The milestones the subject has passed through.) 179 */ 180 public ResearchSubjectProgressComponent setMilestone(CodeableConcept value) { 181 this.milestone = value; 182 return this; 183 } 184 185 /** 186 * @return {@link #reason} (The reason for the state change. If coded it should follow the formal subject state model.) 187 */ 188 public CodeableConcept getReason() { 189 if (this.reason == null) 190 if (Configuration.errorOnAutoCreate()) 191 throw new Error("Attempt to auto-create ResearchSubjectProgressComponent.reason"); 192 else if (Configuration.doAutoCreate()) 193 this.reason = new CodeableConcept(); // cc 194 return this.reason; 195 } 196 197 public boolean hasReason() { 198 return this.reason != null && !this.reason.isEmpty(); 199 } 200 201 /** 202 * @param value {@link #reason} (The reason for the state change. If coded it should follow the formal subject state model.) 203 */ 204 public ResearchSubjectProgressComponent setReason(CodeableConcept value) { 205 this.reason = value; 206 return this; 207 } 208 209 /** 210 * @return {@link #startDate} (The date when the new status started.). This is the underlying object with id, value and extensions. The accessor "getStartDate" gives direct access to the value 211 */ 212 public DateTimeType getStartDateElement() { 213 if (this.startDate == null) 214 if (Configuration.errorOnAutoCreate()) 215 throw new Error("Attempt to auto-create ResearchSubjectProgressComponent.startDate"); 216 else if (Configuration.doAutoCreate()) 217 this.startDate = new DateTimeType(); // bb 218 return this.startDate; 219 } 220 221 public boolean hasStartDateElement() { 222 return this.startDate != null && !this.startDate.isEmpty(); 223 } 224 225 public boolean hasStartDate() { 226 return this.startDate != null && !this.startDate.isEmpty(); 227 } 228 229 /** 230 * @param value {@link #startDate} (The date when the new status started.). This is the underlying object with id, value and extensions. The accessor "getStartDate" gives direct access to the value 231 */ 232 public ResearchSubjectProgressComponent setStartDateElement(DateTimeType value) { 233 this.startDate = value; 234 return this; 235 } 236 237 /** 238 * @return The date when the new status started. 239 */ 240 public Date getStartDate() { 241 return this.startDate == null ? null : this.startDate.getValue(); 242 } 243 244 /** 245 * @param value The date when the new status started. 246 */ 247 public ResearchSubjectProgressComponent setStartDate(Date value) { 248 if (value == null) 249 this.startDate = null; 250 else { 251 if (this.startDate == null) 252 this.startDate = new DateTimeType(); 253 this.startDate.setValue(value); 254 } 255 return this; 256 } 257 258 /** 259 * @return {@link #endDate} (The date when the state ended.). This is the underlying object with id, value and extensions. The accessor "getEndDate" gives direct access to the value 260 */ 261 public DateTimeType getEndDateElement() { 262 if (this.endDate == null) 263 if (Configuration.errorOnAutoCreate()) 264 throw new Error("Attempt to auto-create ResearchSubjectProgressComponent.endDate"); 265 else if (Configuration.doAutoCreate()) 266 this.endDate = new DateTimeType(); // bb 267 return this.endDate; 268 } 269 270 public boolean hasEndDateElement() { 271 return this.endDate != null && !this.endDate.isEmpty(); 272 } 273 274 public boolean hasEndDate() { 275 return this.endDate != null && !this.endDate.isEmpty(); 276 } 277 278 /** 279 * @param value {@link #endDate} (The date when the state ended.). This is the underlying object with id, value and extensions. The accessor "getEndDate" gives direct access to the value 280 */ 281 public ResearchSubjectProgressComponent setEndDateElement(DateTimeType value) { 282 this.endDate = value; 283 return this; 284 } 285 286 /** 287 * @return The date when the state ended. 288 */ 289 public Date getEndDate() { 290 return this.endDate == null ? null : this.endDate.getValue(); 291 } 292 293 /** 294 * @param value The date when the state ended. 295 */ 296 public ResearchSubjectProgressComponent setEndDate(Date value) { 297 if (value == null) 298 this.endDate = null; 299 else { 300 if (this.endDate == null) 301 this.endDate = new DateTimeType(); 302 this.endDate.setValue(value); 303 } 304 return this; 305 } 306 307 protected void listChildren(List<Property> children) { 308 super.listChildren(children); 309 children.add(new Property("type", "CodeableConcept", "Identifies the aspect of the subject's journey that the state refers to.", 0, 1, type)); 310 children.add(new Property("subjectState", "CodeableConcept", "The current state of the subject.", 0, 1, subjectState)); 311 children.add(new Property("milestone", "CodeableConcept", "The milestones the subject has passed through.", 0, 1, milestone)); 312 children.add(new Property("reason", "CodeableConcept", "The reason for the state change. If coded it should follow the formal subject state model.", 0, 1, reason)); 313 children.add(new Property("startDate", "dateTime", "The date when the new status started.", 0, 1, startDate)); 314 children.add(new Property("endDate", "dateTime", "The date when the state ended.", 0, 1, endDate)); 315 } 316 317 @Override 318 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 319 switch (_hash) { 320 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Identifies the aspect of the subject's journey that the state refers to.", 0, 1, type); 321 case -1520444731: /*subjectState*/ return new Property("subjectState", "CodeableConcept", "The current state of the subject.", 0, 1, subjectState); 322 case -1065084560: /*milestone*/ return new Property("milestone", "CodeableConcept", "The milestones the subject has passed through.", 0, 1, milestone); 323 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "The reason for the state change. If coded it should follow the formal subject state model.", 0, 1, reason); 324 case -2129778896: /*startDate*/ return new Property("startDate", "dateTime", "The date when the new status started.", 0, 1, startDate); 325 case -1607727319: /*endDate*/ return new Property("endDate", "dateTime", "The date when the state ended.", 0, 1, endDate); 326 default: return super.getNamedProperty(_hash, _name, _checkValid); 327 } 328 329 } 330 331 @Override 332 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 333 switch (hash) { 334 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 335 case -1520444731: /*subjectState*/ return this.subjectState == null ? new Base[0] : new Base[] {this.subjectState}; // CodeableConcept 336 case -1065084560: /*milestone*/ return this.milestone == null ? new Base[0] : new Base[] {this.milestone}; // CodeableConcept 337 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableConcept 338 case -2129778896: /*startDate*/ return this.startDate == null ? new Base[0] : new Base[] {this.startDate}; // DateTimeType 339 case -1607727319: /*endDate*/ return this.endDate == null ? new Base[0] : new Base[] {this.endDate}; // DateTimeType 340 default: return super.getProperty(hash, name, checkValid); 341 } 342 343 } 344 345 @Override 346 public Base setProperty(int hash, String name, Base value) throws FHIRException { 347 switch (hash) { 348 case 3575610: // type 349 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 350 return value; 351 case -1520444731: // subjectState 352 this.subjectState = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 353 return value; 354 case -1065084560: // milestone 355 this.milestone = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 356 return value; 357 case -934964668: // reason 358 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 359 return value; 360 case -2129778896: // startDate 361 this.startDate = TypeConvertor.castToDateTime(value); // DateTimeType 362 return value; 363 case -1607727319: // endDate 364 this.endDate = TypeConvertor.castToDateTime(value); // DateTimeType 365 return value; 366 default: return super.setProperty(hash, name, value); 367 } 368 369 } 370 371 @Override 372 public Base setProperty(String name, Base value) throws FHIRException { 373 if (name.equals("type")) { 374 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 375 } else if (name.equals("subjectState")) { 376 this.subjectState = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 377 } else if (name.equals("milestone")) { 378 this.milestone = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 379 } else if (name.equals("reason")) { 380 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 381 } else if (name.equals("startDate")) { 382 this.startDate = TypeConvertor.castToDateTime(value); // DateTimeType 383 } else if (name.equals("endDate")) { 384 this.endDate = TypeConvertor.castToDateTime(value); // DateTimeType 385 } else 386 return super.setProperty(name, value); 387 return value; 388 } 389 390 @Override 391 public void removeChild(String name, Base value) throws FHIRException { 392 if (name.equals("type")) { 393 this.type = null; 394 } else if (name.equals("subjectState")) { 395 this.subjectState = null; 396 } else if (name.equals("milestone")) { 397 this.milestone = null; 398 } else if (name.equals("reason")) { 399 this.reason = null; 400 } else if (name.equals("startDate")) { 401 this.startDate = null; 402 } else if (name.equals("endDate")) { 403 this.endDate = null; 404 } else 405 super.removeChild(name, value); 406 407 } 408 409 @Override 410 public Base makeProperty(int hash, String name) throws FHIRException { 411 switch (hash) { 412 case 3575610: return getType(); 413 case -1520444731: return getSubjectState(); 414 case -1065084560: return getMilestone(); 415 case -934964668: return getReason(); 416 case -2129778896: return getStartDateElement(); 417 case -1607727319: return getEndDateElement(); 418 default: return super.makeProperty(hash, name); 419 } 420 421 } 422 423 @Override 424 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 425 switch (hash) { 426 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 427 case -1520444731: /*subjectState*/ return new String[] {"CodeableConcept"}; 428 case -1065084560: /*milestone*/ return new String[] {"CodeableConcept"}; 429 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 430 case -2129778896: /*startDate*/ return new String[] {"dateTime"}; 431 case -1607727319: /*endDate*/ return new String[] {"dateTime"}; 432 default: return super.getTypesForProperty(hash, name); 433 } 434 435 } 436 437 @Override 438 public Base addChild(String name) throws FHIRException { 439 if (name.equals("type")) { 440 this.type = new CodeableConcept(); 441 return this.type; 442 } 443 else if (name.equals("subjectState")) { 444 this.subjectState = new CodeableConcept(); 445 return this.subjectState; 446 } 447 else if (name.equals("milestone")) { 448 this.milestone = new CodeableConcept(); 449 return this.milestone; 450 } 451 else if (name.equals("reason")) { 452 this.reason = new CodeableConcept(); 453 return this.reason; 454 } 455 else if (name.equals("startDate")) { 456 throw new FHIRException("Cannot call addChild on a singleton property ResearchSubject.progress.startDate"); 457 } 458 else if (name.equals("endDate")) { 459 throw new FHIRException("Cannot call addChild on a singleton property ResearchSubject.progress.endDate"); 460 } 461 else 462 return super.addChild(name); 463 } 464 465 public ResearchSubjectProgressComponent copy() { 466 ResearchSubjectProgressComponent dst = new ResearchSubjectProgressComponent(); 467 copyValues(dst); 468 return dst; 469 } 470 471 public void copyValues(ResearchSubjectProgressComponent dst) { 472 super.copyValues(dst); 473 dst.type = type == null ? null : type.copy(); 474 dst.subjectState = subjectState == null ? null : subjectState.copy(); 475 dst.milestone = milestone == null ? null : milestone.copy(); 476 dst.reason = reason == null ? null : reason.copy(); 477 dst.startDate = startDate == null ? null : startDate.copy(); 478 dst.endDate = endDate == null ? null : endDate.copy(); 479 } 480 481 @Override 482 public boolean equalsDeep(Base other_) { 483 if (!super.equalsDeep(other_)) 484 return false; 485 if (!(other_ instanceof ResearchSubjectProgressComponent)) 486 return false; 487 ResearchSubjectProgressComponent o = (ResearchSubjectProgressComponent) other_; 488 return compareDeep(type, o.type, true) && compareDeep(subjectState, o.subjectState, true) && compareDeep(milestone, o.milestone, true) 489 && compareDeep(reason, o.reason, true) && compareDeep(startDate, o.startDate, true) && compareDeep(endDate, o.endDate, true) 490 ; 491 } 492 493 @Override 494 public boolean equalsShallow(Base other_) { 495 if (!super.equalsShallow(other_)) 496 return false; 497 if (!(other_ instanceof ResearchSubjectProgressComponent)) 498 return false; 499 ResearchSubjectProgressComponent o = (ResearchSubjectProgressComponent) other_; 500 return compareValues(startDate, o.startDate, true) && compareValues(endDate, o.endDate, true); 501 } 502 503 public boolean isEmpty() { 504 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, subjectState, milestone 505 , reason, startDate, endDate); 506 } 507 508 public String fhirType() { 509 return "ResearchSubject.progress"; 510 511 } 512 513 } 514 515 /** 516 * Identifiers assigned to this research subject for a study. 517 */ 518 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 519 @Description(shortDefinition="Business Identifier for research subject in a study", formalDefinition="Identifiers assigned to this research subject for a study." ) 520 protected List<Identifier> identifier; 521 522 /** 523 * The publication state of the resource (not of the subject). 524 */ 525 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 526 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The publication state of the resource (not of the subject)." ) 527 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 528 protected Enumeration<PublicationStatus> status; 529 530 /** 531 * The current state (status) of the subject and resons for status change where appropriate. 532 */ 533 @Child(name = "progress", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 534 @Description(shortDefinition="Subject status", formalDefinition="The current state (status) of the subject and resons for status change where appropriate." ) 535 protected List<ResearchSubjectProgressComponent> progress; 536 537 /** 538 * The dates the subject began and ended their participation in the study. 539 */ 540 @Child(name = "period", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=true) 541 @Description(shortDefinition="Start and end of participation", formalDefinition="The dates the subject began and ended their participation in the study." ) 542 protected Period period; 543 544 /** 545 * Reference to the study the subject is participating in. 546 */ 547 @Child(name = "study", type = {ResearchStudy.class}, order=4, min=1, max=1, modifier=false, summary=true) 548 @Description(shortDefinition="Study subject is part of", formalDefinition="Reference to the study the subject is participating in." ) 549 protected Reference study; 550 551 /** 552 * The record of the person, animal or other entity involved in the study. 553 */ 554 @Child(name = "subject", type = {Patient.class, Group.class, Specimen.class, Device.class, Medication.class, Substance.class, BiologicallyDerivedProduct.class}, order=5, min=1, max=1, modifier=false, summary=true) 555 @Description(shortDefinition="Who or what is part of study", formalDefinition="The record of the person, animal or other entity involved in the study." ) 556 protected Reference subject; 557 558 /** 559 * The name of the arm in the study the subject is expected to follow as part of this study. 560 */ 561 @Child(name = "assignedComparisonGroup", type = {IdType.class}, order=6, min=0, max=1, modifier=false, summary=false) 562 @Description(shortDefinition="What path should be followed", formalDefinition="The name of the arm in the study the subject is expected to follow as part of this study." ) 563 protected IdType assignedComparisonGroup; 564 565 /** 566 * The name of the arm in the study the subject actually followed as part of this study. 567 */ 568 @Child(name = "actualComparisonGroup", type = {IdType.class}, order=7, min=0, max=1, modifier=false, summary=false) 569 @Description(shortDefinition="What path was followed", formalDefinition="The name of the arm in the study the subject actually followed as part of this study." ) 570 protected IdType actualComparisonGroup; 571 572 /** 573 * A record of the patient's informed agreement to participate in the study. 574 */ 575 @Child(name = "consent", type = {Consent.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 576 @Description(shortDefinition="Agreement to participate in study", formalDefinition="A record of the patient's informed agreement to participate in the study." ) 577 protected List<Reference> consent; 578 579 private static final long serialVersionUID = -1058527147L; 580 581 /** 582 * Constructor 583 */ 584 public ResearchSubject() { 585 super(); 586 } 587 588 /** 589 * Constructor 590 */ 591 public ResearchSubject(PublicationStatus status, Reference study, Reference subject) { 592 super(); 593 this.setStatus(status); 594 this.setStudy(study); 595 this.setSubject(subject); 596 } 597 598 /** 599 * @return {@link #identifier} (Identifiers assigned to this research subject for a study.) 600 */ 601 public List<Identifier> getIdentifier() { 602 if (this.identifier == null) 603 this.identifier = new ArrayList<Identifier>(); 604 return this.identifier; 605 } 606 607 /** 608 * @return Returns a reference to <code>this</code> for easy method chaining 609 */ 610 public ResearchSubject setIdentifier(List<Identifier> theIdentifier) { 611 this.identifier = theIdentifier; 612 return this; 613 } 614 615 public boolean hasIdentifier() { 616 if (this.identifier == null) 617 return false; 618 for (Identifier item : this.identifier) 619 if (!item.isEmpty()) 620 return true; 621 return false; 622 } 623 624 public Identifier addIdentifier() { //3 625 Identifier t = new Identifier(); 626 if (this.identifier == null) 627 this.identifier = new ArrayList<Identifier>(); 628 this.identifier.add(t); 629 return t; 630 } 631 632 public ResearchSubject addIdentifier(Identifier t) { //3 633 if (t == null) 634 return this; 635 if (this.identifier == null) 636 this.identifier = new ArrayList<Identifier>(); 637 this.identifier.add(t); 638 return this; 639 } 640 641 /** 642 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 643 */ 644 public Identifier getIdentifierFirstRep() { 645 if (getIdentifier().isEmpty()) { 646 addIdentifier(); 647 } 648 return getIdentifier().get(0); 649 } 650 651 /** 652 * @return {@link #status} (The publication state of the resource (not of the subject).). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 653 */ 654 public Enumeration<PublicationStatus> getStatusElement() { 655 if (this.status == null) 656 if (Configuration.errorOnAutoCreate()) 657 throw new Error("Attempt to auto-create ResearchSubject.status"); 658 else if (Configuration.doAutoCreate()) 659 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 660 return this.status; 661 } 662 663 public boolean hasStatusElement() { 664 return this.status != null && !this.status.isEmpty(); 665 } 666 667 public boolean hasStatus() { 668 return this.status != null && !this.status.isEmpty(); 669 } 670 671 /** 672 * @param value {@link #status} (The publication state of the resource (not of the subject).). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 673 */ 674 public ResearchSubject setStatusElement(Enumeration<PublicationStatus> value) { 675 this.status = value; 676 return this; 677 } 678 679 /** 680 * @return The publication state of the resource (not of the subject). 681 */ 682 public PublicationStatus getStatus() { 683 return this.status == null ? null : this.status.getValue(); 684 } 685 686 /** 687 * @param value The publication state of the resource (not of the subject). 688 */ 689 public ResearchSubject setStatus(PublicationStatus value) { 690 if (this.status == null) 691 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 692 this.status.setValue(value); 693 return this; 694 } 695 696 /** 697 * @return {@link #progress} (The current state (status) of the subject and resons for status change where appropriate.) 698 */ 699 public List<ResearchSubjectProgressComponent> getProgress() { 700 if (this.progress == null) 701 this.progress = new ArrayList<ResearchSubjectProgressComponent>(); 702 return this.progress; 703 } 704 705 /** 706 * @return Returns a reference to <code>this</code> for easy method chaining 707 */ 708 public ResearchSubject setProgress(List<ResearchSubjectProgressComponent> theProgress) { 709 this.progress = theProgress; 710 return this; 711 } 712 713 public boolean hasProgress() { 714 if (this.progress == null) 715 return false; 716 for (ResearchSubjectProgressComponent item : this.progress) 717 if (!item.isEmpty()) 718 return true; 719 return false; 720 } 721 722 public ResearchSubjectProgressComponent addProgress() { //3 723 ResearchSubjectProgressComponent t = new ResearchSubjectProgressComponent(); 724 if (this.progress == null) 725 this.progress = new ArrayList<ResearchSubjectProgressComponent>(); 726 this.progress.add(t); 727 return t; 728 } 729 730 public ResearchSubject addProgress(ResearchSubjectProgressComponent t) { //3 731 if (t == null) 732 return this; 733 if (this.progress == null) 734 this.progress = new ArrayList<ResearchSubjectProgressComponent>(); 735 this.progress.add(t); 736 return this; 737 } 738 739 /** 740 * @return The first repetition of repeating field {@link #progress}, creating it if it does not already exist {3} 741 */ 742 public ResearchSubjectProgressComponent getProgressFirstRep() { 743 if (getProgress().isEmpty()) { 744 addProgress(); 745 } 746 return getProgress().get(0); 747 } 748 749 /** 750 * @return {@link #period} (The dates the subject began and ended their participation in the study.) 751 */ 752 public Period getPeriod() { 753 if (this.period == null) 754 if (Configuration.errorOnAutoCreate()) 755 throw new Error("Attempt to auto-create ResearchSubject.period"); 756 else if (Configuration.doAutoCreate()) 757 this.period = new Period(); // cc 758 return this.period; 759 } 760 761 public boolean hasPeriod() { 762 return this.period != null && !this.period.isEmpty(); 763 } 764 765 /** 766 * @param value {@link #period} (The dates the subject began and ended their participation in the study.) 767 */ 768 public ResearchSubject setPeriod(Period value) { 769 this.period = value; 770 return this; 771 } 772 773 /** 774 * @return {@link #study} (Reference to the study the subject is participating in.) 775 */ 776 public Reference getStudy() { 777 if (this.study == null) 778 if (Configuration.errorOnAutoCreate()) 779 throw new Error("Attempt to auto-create ResearchSubject.study"); 780 else if (Configuration.doAutoCreate()) 781 this.study = new Reference(); // cc 782 return this.study; 783 } 784 785 public boolean hasStudy() { 786 return this.study != null && !this.study.isEmpty(); 787 } 788 789 /** 790 * @param value {@link #study} (Reference to the study the subject is participating in.) 791 */ 792 public ResearchSubject setStudy(Reference value) { 793 this.study = value; 794 return this; 795 } 796 797 /** 798 * @return {@link #subject} (The record of the person, animal or other entity involved in the study.) 799 */ 800 public Reference getSubject() { 801 if (this.subject == null) 802 if (Configuration.errorOnAutoCreate()) 803 throw new Error("Attempt to auto-create ResearchSubject.subject"); 804 else if (Configuration.doAutoCreate()) 805 this.subject = new Reference(); // cc 806 return this.subject; 807 } 808 809 public boolean hasSubject() { 810 return this.subject != null && !this.subject.isEmpty(); 811 } 812 813 /** 814 * @param value {@link #subject} (The record of the person, animal or other entity involved in the study.) 815 */ 816 public ResearchSubject setSubject(Reference value) { 817 this.subject = value; 818 return this; 819 } 820 821 /** 822 * @return {@link #assignedComparisonGroup} (The name of the arm in the study the subject is expected to follow as part of this study.). This is the underlying object with id, value and extensions. The accessor "getAssignedComparisonGroup" gives direct access to the value 823 */ 824 public IdType getAssignedComparisonGroupElement() { 825 if (this.assignedComparisonGroup == null) 826 if (Configuration.errorOnAutoCreate()) 827 throw new Error("Attempt to auto-create ResearchSubject.assignedComparisonGroup"); 828 else if (Configuration.doAutoCreate()) 829 this.assignedComparisonGroup = new IdType(); // bb 830 return this.assignedComparisonGroup; 831 } 832 833 public boolean hasAssignedComparisonGroupElement() { 834 return this.assignedComparisonGroup != null && !this.assignedComparisonGroup.isEmpty(); 835 } 836 837 public boolean hasAssignedComparisonGroup() { 838 return this.assignedComparisonGroup != null && !this.assignedComparisonGroup.isEmpty(); 839 } 840 841 /** 842 * @param value {@link #assignedComparisonGroup} (The name of the arm in the study the subject is expected to follow as part of this study.). This is the underlying object with id, value and extensions. The accessor "getAssignedComparisonGroup" gives direct access to the value 843 */ 844 public ResearchSubject setAssignedComparisonGroupElement(IdType value) { 845 this.assignedComparisonGroup = value; 846 return this; 847 } 848 849 /** 850 * @return The name of the arm in the study the subject is expected to follow as part of this study. 851 */ 852 public String getAssignedComparisonGroup() { 853 return this.assignedComparisonGroup == null ? null : this.assignedComparisonGroup.getValue(); 854 } 855 856 /** 857 * @param value The name of the arm in the study the subject is expected to follow as part of this study. 858 */ 859 public ResearchSubject setAssignedComparisonGroup(String value) { 860 if (Utilities.noString(value)) 861 this.assignedComparisonGroup = null; 862 else { 863 if (this.assignedComparisonGroup == null) 864 this.assignedComparisonGroup = new IdType(); 865 this.assignedComparisonGroup.setValue(value); 866 } 867 return this; 868 } 869 870 /** 871 * @return {@link #actualComparisonGroup} (The name of the arm in the study the subject actually followed as part of this study.). This is the underlying object with id, value and extensions. The accessor "getActualComparisonGroup" gives direct access to the value 872 */ 873 public IdType getActualComparisonGroupElement() { 874 if (this.actualComparisonGroup == null) 875 if (Configuration.errorOnAutoCreate()) 876 throw new Error("Attempt to auto-create ResearchSubject.actualComparisonGroup"); 877 else if (Configuration.doAutoCreate()) 878 this.actualComparisonGroup = new IdType(); // bb 879 return this.actualComparisonGroup; 880 } 881 882 public boolean hasActualComparisonGroupElement() { 883 return this.actualComparisonGroup != null && !this.actualComparisonGroup.isEmpty(); 884 } 885 886 public boolean hasActualComparisonGroup() { 887 return this.actualComparisonGroup != null && !this.actualComparisonGroup.isEmpty(); 888 } 889 890 /** 891 * @param value {@link #actualComparisonGroup} (The name of the arm in the study the subject actually followed as part of this study.). This is the underlying object with id, value and extensions. The accessor "getActualComparisonGroup" gives direct access to the value 892 */ 893 public ResearchSubject setActualComparisonGroupElement(IdType value) { 894 this.actualComparisonGroup = value; 895 return this; 896 } 897 898 /** 899 * @return The name of the arm in the study the subject actually followed as part of this study. 900 */ 901 public String getActualComparisonGroup() { 902 return this.actualComparisonGroup == null ? null : this.actualComparisonGroup.getValue(); 903 } 904 905 /** 906 * @param value The name of the arm in the study the subject actually followed as part of this study. 907 */ 908 public ResearchSubject setActualComparisonGroup(String value) { 909 if (Utilities.noString(value)) 910 this.actualComparisonGroup = null; 911 else { 912 if (this.actualComparisonGroup == null) 913 this.actualComparisonGroup = new IdType(); 914 this.actualComparisonGroup.setValue(value); 915 } 916 return this; 917 } 918 919 /** 920 * @return {@link #consent} (A record of the patient's informed agreement to participate in the study.) 921 */ 922 public List<Reference> getConsent() { 923 if (this.consent == null) 924 this.consent = new ArrayList<Reference>(); 925 return this.consent; 926 } 927 928 /** 929 * @return Returns a reference to <code>this</code> for easy method chaining 930 */ 931 public ResearchSubject setConsent(List<Reference> theConsent) { 932 this.consent = theConsent; 933 return this; 934 } 935 936 public boolean hasConsent() { 937 if (this.consent == null) 938 return false; 939 for (Reference item : this.consent) 940 if (!item.isEmpty()) 941 return true; 942 return false; 943 } 944 945 public Reference addConsent() { //3 946 Reference t = new Reference(); 947 if (this.consent == null) 948 this.consent = new ArrayList<Reference>(); 949 this.consent.add(t); 950 return t; 951 } 952 953 public ResearchSubject addConsent(Reference t) { //3 954 if (t == null) 955 return this; 956 if (this.consent == null) 957 this.consent = new ArrayList<Reference>(); 958 this.consent.add(t); 959 return this; 960 } 961 962 /** 963 * @return The first repetition of repeating field {@link #consent}, creating it if it does not already exist {3} 964 */ 965 public Reference getConsentFirstRep() { 966 if (getConsent().isEmpty()) { 967 addConsent(); 968 } 969 return getConsent().get(0); 970 } 971 972 protected void listChildren(List<Property> children) { 973 super.listChildren(children); 974 children.add(new Property("identifier", "Identifier", "Identifiers assigned to this research subject for a study.", 0, java.lang.Integer.MAX_VALUE, identifier)); 975 children.add(new Property("status", "code", "The publication state of the resource (not of the subject).", 0, 1, status)); 976 children.add(new Property("progress", "", "The current state (status) of the subject and resons for status change where appropriate.", 0, java.lang.Integer.MAX_VALUE, progress)); 977 children.add(new Property("period", "Period", "The dates the subject began and ended their participation in the study.", 0, 1, period)); 978 children.add(new Property("study", "Reference(ResearchStudy)", "Reference to the study the subject is participating in.", 0, 1, study)); 979 children.add(new Property("subject", "Reference(Patient|Group|Specimen|Device|Medication|Substance|BiologicallyDerivedProduct)", "The record of the person, animal or other entity involved in the study.", 0, 1, subject)); 980 children.add(new Property("assignedComparisonGroup", "id", "The name of the arm in the study the subject is expected to follow as part of this study.", 0, 1, assignedComparisonGroup)); 981 children.add(new Property("actualComparisonGroup", "id", "The name of the arm in the study the subject actually followed as part of this study.", 0, 1, actualComparisonGroup)); 982 children.add(new Property("consent", "Reference(Consent)", "A record of the patient's informed agreement to participate in the study.", 0, java.lang.Integer.MAX_VALUE, consent)); 983 } 984 985 @Override 986 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 987 switch (_hash) { 988 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers assigned to this research subject for a study.", 0, java.lang.Integer.MAX_VALUE, identifier); 989 case -892481550: /*status*/ return new Property("status", "code", "The publication state of the resource (not of the subject).", 0, 1, status); 990 case -1001078227: /*progress*/ return new Property("progress", "", "The current state (status) of the subject and resons for status change where appropriate.", 0, java.lang.Integer.MAX_VALUE, progress); 991 case -991726143: /*period*/ return new Property("period", "Period", "The dates the subject began and ended their participation in the study.", 0, 1, period); 992 case 109776329: /*study*/ return new Property("study", "Reference(ResearchStudy)", "Reference to the study the subject is participating in.", 0, 1, study); 993 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Specimen|Device|Medication|Substance|BiologicallyDerivedProduct)", "The record of the person, animal or other entity involved in the study.", 0, 1, subject); 994 case 394644552: /*assignedComparisonGroup*/ return new Property("assignedComparisonGroup", "id", "The name of the arm in the study the subject is expected to follow as part of this study.", 0, 1, assignedComparisonGroup); 995 case -676906872: /*actualComparisonGroup*/ return new Property("actualComparisonGroup", "id", "The name of the arm in the study the subject actually followed as part of this study.", 0, 1, actualComparisonGroup); 996 case 951500826: /*consent*/ return new Property("consent", "Reference(Consent)", "A record of the patient's informed agreement to participate in the study.", 0, java.lang.Integer.MAX_VALUE, consent); 997 default: return super.getNamedProperty(_hash, _name, _checkValid); 998 } 999 1000 } 1001 1002 @Override 1003 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1004 switch (hash) { 1005 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1006 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 1007 case -1001078227: /*progress*/ return this.progress == null ? new Base[0] : this.progress.toArray(new Base[this.progress.size()]); // ResearchSubjectProgressComponent 1008 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1009 case 109776329: /*study*/ return this.study == null ? new Base[0] : new Base[] {this.study}; // Reference 1010 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1011 case 394644552: /*assignedComparisonGroup*/ return this.assignedComparisonGroup == null ? new Base[0] : new Base[] {this.assignedComparisonGroup}; // IdType 1012 case -676906872: /*actualComparisonGroup*/ return this.actualComparisonGroup == null ? new Base[0] : new Base[] {this.actualComparisonGroup}; // IdType 1013 case 951500826: /*consent*/ return this.consent == null ? new Base[0] : this.consent.toArray(new Base[this.consent.size()]); // Reference 1014 default: return super.getProperty(hash, name, checkValid); 1015 } 1016 1017 } 1018 1019 @Override 1020 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1021 switch (hash) { 1022 case -1618432855: // identifier 1023 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1024 return value; 1025 case -892481550: // status 1026 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1027 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1028 return value; 1029 case -1001078227: // progress 1030 this.getProgress().add((ResearchSubjectProgressComponent) value); // ResearchSubjectProgressComponent 1031 return value; 1032 case -991726143: // period 1033 this.period = TypeConvertor.castToPeriod(value); // Period 1034 return value; 1035 case 109776329: // study 1036 this.study = TypeConvertor.castToReference(value); // Reference 1037 return value; 1038 case -1867885268: // subject 1039 this.subject = TypeConvertor.castToReference(value); // Reference 1040 return value; 1041 case 394644552: // assignedComparisonGroup 1042 this.assignedComparisonGroup = TypeConvertor.castToId(value); // IdType 1043 return value; 1044 case -676906872: // actualComparisonGroup 1045 this.actualComparisonGroup = TypeConvertor.castToId(value); // IdType 1046 return value; 1047 case 951500826: // consent 1048 this.getConsent().add(TypeConvertor.castToReference(value)); // Reference 1049 return value; 1050 default: return super.setProperty(hash, name, value); 1051 } 1052 1053 } 1054 1055 @Override 1056 public Base setProperty(String name, Base value) throws FHIRException { 1057 if (name.equals("identifier")) { 1058 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1059 } else if (name.equals("status")) { 1060 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1061 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1062 } else if (name.equals("progress")) { 1063 this.getProgress().add((ResearchSubjectProgressComponent) value); 1064 } else if (name.equals("period")) { 1065 this.period = TypeConvertor.castToPeriod(value); // Period 1066 } else if (name.equals("study")) { 1067 this.study = TypeConvertor.castToReference(value); // Reference 1068 } else if (name.equals("subject")) { 1069 this.subject = TypeConvertor.castToReference(value); // Reference 1070 } else if (name.equals("assignedComparisonGroup")) { 1071 this.assignedComparisonGroup = TypeConvertor.castToId(value); // IdType 1072 } else if (name.equals("actualComparisonGroup")) { 1073 this.actualComparisonGroup = TypeConvertor.castToId(value); // IdType 1074 } else if (name.equals("consent")) { 1075 this.getConsent().add(TypeConvertor.castToReference(value)); 1076 } else 1077 return super.setProperty(name, value); 1078 return value; 1079 } 1080 1081 @Override 1082 public void removeChild(String name, Base value) throws FHIRException { 1083 if (name.equals("identifier")) { 1084 this.getIdentifier().remove(value); 1085 } else if (name.equals("status")) { 1086 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1087 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1088 } else if (name.equals("progress")) { 1089 this.getProgress().remove((ResearchSubjectProgressComponent) value); 1090 } else if (name.equals("period")) { 1091 this.period = null; 1092 } else if (name.equals("study")) { 1093 this.study = null; 1094 } else if (name.equals("subject")) { 1095 this.subject = null; 1096 } else if (name.equals("assignedComparisonGroup")) { 1097 this.assignedComparisonGroup = null; 1098 } else if (name.equals("actualComparisonGroup")) { 1099 this.actualComparisonGroup = null; 1100 } else if (name.equals("consent")) { 1101 this.getConsent().remove(value); 1102 } else 1103 super.removeChild(name, value); 1104 1105 } 1106 1107 @Override 1108 public Base makeProperty(int hash, String name) throws FHIRException { 1109 switch (hash) { 1110 case -1618432855: return addIdentifier(); 1111 case -892481550: return getStatusElement(); 1112 case -1001078227: return addProgress(); 1113 case -991726143: return getPeriod(); 1114 case 109776329: return getStudy(); 1115 case -1867885268: return getSubject(); 1116 case 394644552: return getAssignedComparisonGroupElement(); 1117 case -676906872: return getActualComparisonGroupElement(); 1118 case 951500826: return addConsent(); 1119 default: return super.makeProperty(hash, name); 1120 } 1121 1122 } 1123 1124 @Override 1125 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1126 switch (hash) { 1127 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1128 case -892481550: /*status*/ return new String[] {"code"}; 1129 case -1001078227: /*progress*/ return new String[] {}; 1130 case -991726143: /*period*/ return new String[] {"Period"}; 1131 case 109776329: /*study*/ return new String[] {"Reference"}; 1132 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1133 case 394644552: /*assignedComparisonGroup*/ return new String[] {"id"}; 1134 case -676906872: /*actualComparisonGroup*/ return new String[] {"id"}; 1135 case 951500826: /*consent*/ return new String[] {"Reference"}; 1136 default: return super.getTypesForProperty(hash, name); 1137 } 1138 1139 } 1140 1141 @Override 1142 public Base addChild(String name) throws FHIRException { 1143 if (name.equals("identifier")) { 1144 return addIdentifier(); 1145 } 1146 else if (name.equals("status")) { 1147 throw new FHIRException("Cannot call addChild on a singleton property ResearchSubject.status"); 1148 } 1149 else if (name.equals("progress")) { 1150 return addProgress(); 1151 } 1152 else if (name.equals("period")) { 1153 this.period = new Period(); 1154 return this.period; 1155 } 1156 else if (name.equals("study")) { 1157 this.study = new Reference(); 1158 return this.study; 1159 } 1160 else if (name.equals("subject")) { 1161 this.subject = new Reference(); 1162 return this.subject; 1163 } 1164 else if (name.equals("assignedComparisonGroup")) { 1165 throw new FHIRException("Cannot call addChild on a singleton property ResearchSubject.assignedComparisonGroup"); 1166 } 1167 else if (name.equals("actualComparisonGroup")) { 1168 throw new FHIRException("Cannot call addChild on a singleton property ResearchSubject.actualComparisonGroup"); 1169 } 1170 else if (name.equals("consent")) { 1171 return addConsent(); 1172 } 1173 else 1174 return super.addChild(name); 1175 } 1176 1177 public String fhirType() { 1178 return "ResearchSubject"; 1179 1180 } 1181 1182 public ResearchSubject copy() { 1183 ResearchSubject dst = new ResearchSubject(); 1184 copyValues(dst); 1185 return dst; 1186 } 1187 1188 public void copyValues(ResearchSubject dst) { 1189 super.copyValues(dst); 1190 if (identifier != null) { 1191 dst.identifier = new ArrayList<Identifier>(); 1192 for (Identifier i : identifier) 1193 dst.identifier.add(i.copy()); 1194 }; 1195 dst.status = status == null ? null : status.copy(); 1196 if (progress != null) { 1197 dst.progress = new ArrayList<ResearchSubjectProgressComponent>(); 1198 for (ResearchSubjectProgressComponent i : progress) 1199 dst.progress.add(i.copy()); 1200 }; 1201 dst.period = period == null ? null : period.copy(); 1202 dst.study = study == null ? null : study.copy(); 1203 dst.subject = subject == null ? null : subject.copy(); 1204 dst.assignedComparisonGroup = assignedComparisonGroup == null ? null : assignedComparisonGroup.copy(); 1205 dst.actualComparisonGroup = actualComparisonGroup == null ? null : actualComparisonGroup.copy(); 1206 if (consent != null) { 1207 dst.consent = new ArrayList<Reference>(); 1208 for (Reference i : consent) 1209 dst.consent.add(i.copy()); 1210 }; 1211 } 1212 1213 protected ResearchSubject typedCopy() { 1214 return copy(); 1215 } 1216 1217 @Override 1218 public boolean equalsDeep(Base other_) { 1219 if (!super.equalsDeep(other_)) 1220 return false; 1221 if (!(other_ instanceof ResearchSubject)) 1222 return false; 1223 ResearchSubject o = (ResearchSubject) other_; 1224 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(progress, o.progress, true) 1225 && compareDeep(period, o.period, true) && compareDeep(study, o.study, true) && compareDeep(subject, o.subject, true) 1226 && compareDeep(assignedComparisonGroup, o.assignedComparisonGroup, true) && compareDeep(actualComparisonGroup, o.actualComparisonGroup, true) 1227 && compareDeep(consent, o.consent, true); 1228 } 1229 1230 @Override 1231 public boolean equalsShallow(Base other_) { 1232 if (!super.equalsShallow(other_)) 1233 return false; 1234 if (!(other_ instanceof ResearchSubject)) 1235 return false; 1236 ResearchSubject o = (ResearchSubject) other_; 1237 return compareValues(status, o.status, true) && compareValues(assignedComparisonGroup, o.assignedComparisonGroup, true) 1238 && compareValues(actualComparisonGroup, o.actualComparisonGroup, true); 1239 } 1240 1241 public boolean isEmpty() { 1242 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, progress 1243 , period, study, subject, assignedComparisonGroup, actualComparisonGroup, consent 1244 ); 1245 } 1246 1247 @Override 1248 public ResourceType getResourceType() { 1249 return ResourceType.ResearchSubject; 1250 } 1251 1252 /** 1253 * Search parameter: <b>status</b> 1254 * <p> 1255 * Description: <b>draft | active | retired | unknown</b><br> 1256 * Type: <b>token</b><br> 1257 * Path: <b>ResearchSubject.status</b><br> 1258 * </p> 1259 */ 1260 @SearchParamDefinition(name="status", path="ResearchSubject.status", description="draft | active | retired | unknown", type="token" ) 1261 public static final String SP_STATUS = "status"; 1262 /** 1263 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1264 * <p> 1265 * Description: <b>draft | active | retired | unknown</b><br> 1266 * Type: <b>token</b><br> 1267 * Path: <b>ResearchSubject.status</b><br> 1268 * </p> 1269 */ 1270 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1271 1272 /** 1273 * Search parameter: <b>study</b> 1274 * <p> 1275 * Description: <b>Study subject is part of</b><br> 1276 * Type: <b>reference</b><br> 1277 * Path: <b>ResearchSubject.study</b><br> 1278 * </p> 1279 */ 1280 @SearchParamDefinition(name="study", path="ResearchSubject.study", description="Study subject is part of", type="reference", target={ResearchStudy.class } ) 1281 public static final String SP_STUDY = "study"; 1282 /** 1283 * <b>Fluent Client</b> search parameter constant for <b>study</b> 1284 * <p> 1285 * Description: <b>Study subject is part of</b><br> 1286 * Type: <b>reference</b><br> 1287 * Path: <b>ResearchSubject.study</b><br> 1288 * </p> 1289 */ 1290 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam STUDY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_STUDY); 1291 1292/** 1293 * Constant for fluent queries to be used to add include statements. Specifies 1294 * the path value of "<b>ResearchSubject:study</b>". 1295 */ 1296 public static final ca.uhn.fhir.model.api.Include INCLUDE_STUDY = new ca.uhn.fhir.model.api.Include("ResearchSubject:study").toLocked(); 1297 1298 /** 1299 * Search parameter: <b>subject</b> 1300 * <p> 1301 * Description: <b>Who or what is part of study</b><br> 1302 * Type: <b>reference</b><br> 1303 * Path: <b>ResearchSubject.subject</b><br> 1304 * </p> 1305 */ 1306 @SearchParamDefinition(name="subject", path="ResearchSubject.subject", description="Who or what is part of study", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={BiologicallyDerivedProduct.class, Device.class, Group.class, Medication.class, Patient.class, Specimen.class, Substance.class } ) 1307 public static final String SP_SUBJECT = "subject"; 1308 /** 1309 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1310 * <p> 1311 * Description: <b>Who or what is part of study</b><br> 1312 * Type: <b>reference</b><br> 1313 * Path: <b>ResearchSubject.subject</b><br> 1314 * </p> 1315 */ 1316 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1317 1318/** 1319 * Constant for fluent queries to be used to add include statements. Specifies 1320 * the path value of "<b>ResearchSubject:subject</b>". 1321 */ 1322 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("ResearchSubject:subject").toLocked(); 1323 1324 /** 1325 * Search parameter: <b>subject_state</b> 1326 * <p> 1327 * Description: <b>candidate | eligible | follow-up | ineligible | not-registered | off-study | on-study | on-study-intervention | on-study-observation | pending-on-study | potential-candidate | screening | withdrawn</b><br> 1328 * Type: <b>token</b><br> 1329 * Path: <b>ResearchSubject.progress.subjectState</b><br> 1330 * </p> 1331 */ 1332 @SearchParamDefinition(name="subject_state", path="ResearchSubject.progress.subjectState", description="candidate | eligible | follow-up | ineligible | not-registered | off-study | on-study | on-study-intervention | on-study-observation | pending-on-study | potential-candidate | screening | withdrawn", type="token" ) 1333 public static final String SP_SUBJECTSTATE = "subject_state"; 1334 /** 1335 * <b>Fluent Client</b> search parameter constant for <b>subject_state</b> 1336 * <p> 1337 * Description: <b>candidate | eligible | follow-up | ineligible | not-registered | off-study | on-study | on-study-intervention | on-study-observation | pending-on-study | potential-candidate | screening | withdrawn</b><br> 1338 * Type: <b>token</b><br> 1339 * Path: <b>ResearchSubject.progress.subjectState</b><br> 1340 * </p> 1341 */ 1342 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SUBJECTSTATE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SUBJECTSTATE); 1343 1344 /** 1345 * Search parameter: <b>date</b> 1346 * <p> 1347 * Description: <b>Multiple Resources: 1348 1349* [AdverseEvent](adverseevent.html): When the event occurred 1350* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 1351* [Appointment](appointment.html): Appointment date/time. 1352* [AuditEvent](auditevent.html): Time when the event was recorded 1353* [CarePlan](careplan.html): Time period plan covers 1354* [CareTeam](careteam.html): A date within the coverage time period. 1355* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 1356* [Composition](composition.html): Composition editing time 1357* [Consent](consent.html): When consent was agreed to 1358* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 1359* [DocumentReference](documentreference.html): When this document reference was created 1360* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 1361* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 1362* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 1363* [Flag](flag.html): Time period when flag is active 1364* [Immunization](immunization.html): Vaccination (non)-Administration Date 1365* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 1366* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 1367* [Invoice](invoice.html): Invoice date / posting date 1368* [List](list.html): When the list was prepared 1369* [MeasureReport](measurereport.html): The date of the measure report 1370* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 1371* [Observation](observation.html): Clinically relevant time/time-period for observation 1372* [Procedure](procedure.html): When the procedure occurred or is occurring 1373* [ResearchSubject](researchsubject.html): Start and end of participation 1374* [RiskAssessment](riskassessment.html): When was assessment made? 1375* [SupplyRequest](supplyrequest.html): When the request was made 1376</b><br> 1377 * Type: <b>date</b><br> 1378 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 1379 * </p> 1380 */ 1381 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 1382 public static final String SP_DATE = "date"; 1383 /** 1384 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1385 * <p> 1386 * Description: <b>Multiple Resources: 1387 1388* [AdverseEvent](adverseevent.html): When the event occurred 1389* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 1390* [Appointment](appointment.html): Appointment date/time. 1391* [AuditEvent](auditevent.html): Time when the event was recorded 1392* [CarePlan](careplan.html): Time period plan covers 1393* [CareTeam](careteam.html): A date within the coverage time period. 1394* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 1395* [Composition](composition.html): Composition editing time 1396* [Consent](consent.html): When consent was agreed to 1397* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 1398* [DocumentReference](documentreference.html): When this document reference was created 1399* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 1400* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 1401* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 1402* [Flag](flag.html): Time period when flag is active 1403* [Immunization](immunization.html): Vaccination (non)-Administration Date 1404* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 1405* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 1406* [Invoice](invoice.html): Invoice date / posting date 1407* [List](list.html): When the list was prepared 1408* [MeasureReport](measurereport.html): The date of the measure report 1409* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 1410* [Observation](observation.html): Clinically relevant time/time-period for observation 1411* [Procedure](procedure.html): When the procedure occurred or is occurring 1412* [ResearchSubject](researchsubject.html): Start and end of participation 1413* [RiskAssessment](riskassessment.html): When was assessment made? 1414* [SupplyRequest](supplyrequest.html): When the request was made 1415</b><br> 1416 * Type: <b>date</b><br> 1417 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 1418 * </p> 1419 */ 1420 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1421 1422 /** 1423 * Search parameter: <b>identifier</b> 1424 * <p> 1425 * Description: <b>Multiple Resources: 1426 1427* [Account](account.html): Account number 1428* [AdverseEvent](adverseevent.html): Business identifier for the event 1429* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1430* [Appointment](appointment.html): An Identifier of the Appointment 1431* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1432* [Basic](basic.html): Business identifier 1433* [BodyStructure](bodystructure.html): Bodystructure identifier 1434* [CarePlan](careplan.html): External Ids for this plan 1435* [CareTeam](careteam.html): External Ids for this team 1436* [ChargeItem](chargeitem.html): Business Identifier for item 1437* [Claim](claim.html): The primary identifier of the financial resource 1438* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1439* [ClinicalImpression](clinicalimpression.html): Business identifier 1440* [Communication](communication.html): Unique identifier 1441* [CommunicationRequest](communicationrequest.html): Unique identifier 1442* [Composition](composition.html): Version-independent identifier for the Composition 1443* [Condition](condition.html): A unique identifier of the condition record 1444* [Consent](consent.html): Identifier for this record (external references) 1445* [Contract](contract.html): The identity of the contract 1446* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1447* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1448* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1449* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1450* [DeviceRequest](devicerequest.html): Business identifier for request/order 1451* [DeviceUsage](deviceusage.html): Search by identifier 1452* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1453* [DocumentReference](documentreference.html): Identifier of the attachment binary 1454* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1455* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1456* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1457* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1458* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1459* [Flag](flag.html): Business identifier 1460* [Goal](goal.html): External Ids for this goal 1461* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1462* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1463* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1464* [Immunization](immunization.html): Business identifier 1465* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1466* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1467* [Invoice](invoice.html): Business Identifier for item 1468* [List](list.html): Business identifier 1469* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1470* [Medication](medication.html): Returns medications with this external identifier 1471* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1472* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1473* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1474* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1475* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1476* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1477* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1478* [Observation](observation.html): The unique id for a particular observation 1479* [Person](person.html): A person Identifier 1480* [Procedure](procedure.html): A unique identifier for a procedure 1481* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1482* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1483* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1484* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1485* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1486* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1487* [Specimen](specimen.html): The unique identifier associated with the specimen 1488* [SupplyDelivery](supplydelivery.html): External identifier 1489* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1490* [Task](task.html): Search for a task instance by its business identifier 1491* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1492</b><br> 1493 * Type: <b>token</b><br> 1494 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1495 * </p> 1496 */ 1497 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 1498 public static final String SP_IDENTIFIER = "identifier"; 1499 /** 1500 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1501 * <p> 1502 * Description: <b>Multiple Resources: 1503 1504* [Account](account.html): Account number 1505* [AdverseEvent](adverseevent.html): Business identifier for the event 1506* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1507* [Appointment](appointment.html): An Identifier of the Appointment 1508* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1509* [Basic](basic.html): Business identifier 1510* [BodyStructure](bodystructure.html): Bodystructure identifier 1511* [CarePlan](careplan.html): External Ids for this plan 1512* [CareTeam](careteam.html): External Ids for this team 1513* [ChargeItem](chargeitem.html): Business Identifier for item 1514* [Claim](claim.html): The primary identifier of the financial resource 1515* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1516* [ClinicalImpression](clinicalimpression.html): Business identifier 1517* [Communication](communication.html): Unique identifier 1518* [CommunicationRequest](communicationrequest.html): Unique identifier 1519* [Composition](composition.html): Version-independent identifier for the Composition 1520* [Condition](condition.html): A unique identifier of the condition record 1521* [Consent](consent.html): Identifier for this record (external references) 1522* [Contract](contract.html): The identity of the contract 1523* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1524* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1525* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1526* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1527* [DeviceRequest](devicerequest.html): Business identifier for request/order 1528* [DeviceUsage](deviceusage.html): Search by identifier 1529* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1530* [DocumentReference](documentreference.html): Identifier of the attachment binary 1531* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1532* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1533* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1534* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1535* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1536* [Flag](flag.html): Business identifier 1537* [Goal](goal.html): External Ids for this goal 1538* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1539* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1540* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1541* [Immunization](immunization.html): Business identifier 1542* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1543* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1544* [Invoice](invoice.html): Business Identifier for item 1545* [List](list.html): Business identifier 1546* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1547* [Medication](medication.html): Returns medications with this external identifier 1548* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1549* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1550* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1551* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1552* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1553* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1554* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1555* [Observation](observation.html): The unique id for a particular observation 1556* [Person](person.html): A person Identifier 1557* [Procedure](procedure.html): A unique identifier for a procedure 1558* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1559* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1560* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1561* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1562* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1563* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1564* [Specimen](specimen.html): The unique identifier associated with the specimen 1565* [SupplyDelivery](supplydelivery.html): External identifier 1566* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1567* [Task](task.html): Search for a task instance by its business identifier 1568* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1569</b><br> 1570 * Type: <b>token</b><br> 1571 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1572 * </p> 1573 */ 1574 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1575 1576 /** 1577 * Search parameter: <b>patient</b> 1578 * <p> 1579 * Description: <b>Multiple Resources: 1580 1581* [Account](account.html): The entity that caused the expenses 1582* [AdverseEvent](adverseevent.html): Subject impacted by event 1583* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 1584* [Appointment](appointment.html): One of the individuals of the appointment is this patient 1585* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 1586* [AuditEvent](auditevent.html): Where the activity involved patient data 1587* [Basic](basic.html): Identifies the focus of this resource 1588* [BodyStructure](bodystructure.html): Who this is about 1589* [CarePlan](careplan.html): Who the care plan is for 1590* [CareTeam](careteam.html): Who care team is for 1591* [ChargeItem](chargeitem.html): Individual service was done for/to 1592* [Claim](claim.html): Patient receiving the products or services 1593* [ClaimResponse](claimresponse.html): The subject of care 1594* [ClinicalImpression](clinicalimpression.html): Patient assessed 1595* [Communication](communication.html): Focus of message 1596* [CommunicationRequest](communicationrequest.html): Focus of message 1597* [Composition](composition.html): Who and/or what the composition is about 1598* [Condition](condition.html): Who has the condition? 1599* [Consent](consent.html): Who the consent applies to 1600* [Contract](contract.html): The identity of the subject of the contract (if a patient) 1601* [Coverage](coverage.html): Retrieve coverages for a patient 1602* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 1603* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 1604* [DetectedIssue](detectedissue.html): Associated patient 1605* [DeviceRequest](devicerequest.html): Individual the service is ordered for 1606* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 1607* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 1608* [DocumentReference](documentreference.html): Who/what is the subject of the document 1609* [Encounter](encounter.html): The patient present at the encounter 1610* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 1611* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 1612* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 1613* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 1614* [Flag](flag.html): The identity of a subject to list flags for 1615* [Goal](goal.html): Who this goal is intended for 1616* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 1617* [ImagingSelection](imagingselection.html): Who the study is about 1618* [ImagingStudy](imagingstudy.html): Who the study is about 1619* [Immunization](immunization.html): The patient for the vaccination record 1620* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 1621* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 1622* [Invoice](invoice.html): Recipient(s) of goods and services 1623* [List](list.html): If all resources have the same subject 1624* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 1625* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 1626* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 1627* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 1628* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 1629* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 1630* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 1631* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 1632* [Observation](observation.html): The subject that the observation is about (if patient) 1633* [Person](person.html): The Person links to this Patient 1634* [Procedure](procedure.html): Search by subject - a patient 1635* [Provenance](provenance.html): Where the activity involved patient data 1636* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 1637* [RelatedPerson](relatedperson.html): The patient this related person is related to 1638* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 1639* [ResearchSubject](researchsubject.html): Who or what is part of study 1640* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 1641* [ServiceRequest](servicerequest.html): Search by subject - a patient 1642* [Specimen](specimen.html): The patient the specimen comes from 1643* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 1644* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 1645* [Task](task.html): Search by patient 1646* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 1647</b><br> 1648 * Type: <b>reference</b><br> 1649 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 1650 * </p> 1651 */ 1652 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 1653 public static final String SP_PATIENT = "patient"; 1654 /** 1655 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1656 * <p> 1657 * Description: <b>Multiple Resources: 1658 1659* [Account](account.html): The entity that caused the expenses 1660* [AdverseEvent](adverseevent.html): Subject impacted by event 1661* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 1662* [Appointment](appointment.html): One of the individuals of the appointment is this patient 1663* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 1664* [AuditEvent](auditevent.html): Where the activity involved patient data 1665* [Basic](basic.html): Identifies the focus of this resource 1666* [BodyStructure](bodystructure.html): Who this is about 1667* [CarePlan](careplan.html): Who the care plan is for 1668* [CareTeam](careteam.html): Who care team is for 1669* [ChargeItem](chargeitem.html): Individual service was done for/to 1670* [Claim](claim.html): Patient receiving the products or services 1671* [ClaimResponse](claimresponse.html): The subject of care 1672* [ClinicalImpression](clinicalimpression.html): Patient assessed 1673* [Communication](communication.html): Focus of message 1674* [CommunicationRequest](communicationrequest.html): Focus of message 1675* [Composition](composition.html): Who and/or what the composition is about 1676* [Condition](condition.html): Who has the condition? 1677* [Consent](consent.html): Who the consent applies to 1678* [Contract](contract.html): The identity of the subject of the contract (if a patient) 1679* [Coverage](coverage.html): Retrieve coverages for a patient 1680* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 1681* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 1682* [DetectedIssue](detectedissue.html): Associated patient 1683* [DeviceRequest](devicerequest.html): Individual the service is ordered for 1684* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 1685* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 1686* [DocumentReference](documentreference.html): Who/what is the subject of the document 1687* [Encounter](encounter.html): The patient present at the encounter 1688* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 1689* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 1690* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 1691* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 1692* [Flag](flag.html): The identity of a subject to list flags for 1693* [Goal](goal.html): Who this goal is intended for 1694* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 1695* [ImagingSelection](imagingselection.html): Who the study is about 1696* [ImagingStudy](imagingstudy.html): Who the study is about 1697* [Immunization](immunization.html): The patient for the vaccination record 1698* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 1699* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 1700* [Invoice](invoice.html): Recipient(s) of goods and services 1701* [List](list.html): If all resources have the same subject 1702* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 1703* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 1704* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 1705* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 1706* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 1707* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 1708* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 1709* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 1710* [Observation](observation.html): The subject that the observation is about (if patient) 1711* [Person](person.html): The Person links to this Patient 1712* [Procedure](procedure.html): Search by subject - a patient 1713* [Provenance](provenance.html): Where the activity involved patient data 1714* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 1715* [RelatedPerson](relatedperson.html): The patient this related person is related to 1716* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 1717* [ResearchSubject](researchsubject.html): Who or what is part of study 1718* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 1719* [ServiceRequest](servicerequest.html): Search by subject - a patient 1720* [Specimen](specimen.html): The patient the specimen comes from 1721* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 1722* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 1723* [Task](task.html): Search by patient 1724* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 1725</b><br> 1726 * Type: <b>reference</b><br> 1727 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 1728 * </p> 1729 */ 1730 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1731 1732/** 1733 * Constant for fluent queries to be used to add include statements. Specifies 1734 * the path value of "<b>ResearchSubject:patient</b>". 1735 */ 1736 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ResearchSubject:patient").toLocked(); 1737 1738 1739} 1740