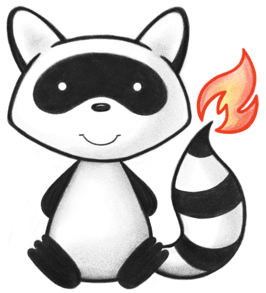
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037 038import org.hl7.fhir.utilities.FhirPublication; 039import org.hl7.fhir.utilities.Utilities; 040import org.hl7.fhir.r5.model.Enumerations.*; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.exceptions.FHIRException; 043import org.hl7.fhir.instance.model.api.ICompositeType; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 047import ca.uhn.fhir.model.api.annotation.Child; 048import ca.uhn.fhir.model.api.annotation.ChildOrder; 049import ca.uhn.fhir.model.api.annotation.Description; 050import ca.uhn.fhir.model.api.annotation.Block; 051 052import org.hl7.fhir.instance.model.api.IAnyResource; 053/** 054 * This is the base resource type for everything. 055 */ 056public abstract class Resource extends BaseResource implements IAnyResource { 057 058 /** 059 * The logical id of the resource, as used in the URL for the resource. Once assigned, this value never changes. 060 */ 061 @Child(name = "id", type = {IdType.class}, order=0, min=0, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="Logical id of this artifact", formalDefinition="The logical id of the resource, as used in the URL for the resource. Once assigned, this value never changes." ) 063 protected IdType id; 064 065 /** 066 * The metadata about the resource. This is content that is maintained by the infrastructure. Changes to the content might not always be associated with version changes to the resource. 067 */ 068 @Child(name = "meta", type = {Meta.class}, order=1, min=0, max=1, modifier=false, summary=true) 069 @Description(shortDefinition="Metadata about the resource", formalDefinition="The metadata about the resource. This is content that is maintained by the infrastructure. Changes to the content might not always be associated with version changes to the resource." ) 070 protected Meta meta; 071 072 /** 073 * A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content. Often, this is a reference to an implementation guide that defines the special rules along with other profiles etc. 074 */ 075 @Child(name = "implicitRules", type = {UriType.class}, order=2, min=0, max=1, modifier=true, summary=true) 076 @Description(shortDefinition="A set of rules under which this content was created", formalDefinition="A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content. Often, this is a reference to an implementation guide that defines the special rules along with other profiles etc." ) 077 protected UriType implicitRules; 078 079 /** 080 * The base language in which the resource is written. 081 */ 082 @Child(name = "language", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 083 @Description(shortDefinition="Language of the resource content", formalDefinition="The base language in which the resource is written." ) 084 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/all-languages") 085 protected CodeType language; 086 087 private static final long serialVersionUID = -559462759L; 088 089 /** 090 * Constructor 091 */ 092 public Resource() { 093 super(); 094 } 095 096 /** 097 * @return {@link #id} (The logical id of the resource, as used in the URL for the resource. Once assigned, this value never changes.). This is the underlying object with id, value and extensions. The accessor "getId" gives direct access to the value 098 */ 099 public IdType getIdElement() { 100 if (this.id == null) 101 if (Configuration.errorOnAutoCreate()) 102 throw new Error("Attempt to auto-create Resource.id"); 103 else if (Configuration.doAutoCreate()) 104 this.id = new IdType(); // bb 105 return this.id; 106 } 107 108 public boolean hasIdElement() { 109 return this.id != null && !this.id.isEmpty(); 110 } 111 112 public boolean hasId() { 113 return this.id != null && !this.id.isEmpty(); 114 } 115 116 /** 117 * @param value {@link #id} (The logical id of the resource, as used in the URL for the resource. Once assigned, this value never changes.). This is the underlying object with id, value and extensions. The accessor "getId" gives direct access to the value 118 */ 119 public Resource setIdElement(IdType value) { 120 this.id = value; 121 return this; 122 } 123 124 /** 125 * @return The logical id of the resource, as used in the URL for the resource. Once assigned, this value never changes. 126 */ 127 public String getId() { 128 return this.id == null ? null : this.id.getValue(); 129 } 130 131 /** 132 * @param value The logical id of the resource, as used in the URL for the resource. Once assigned, this value never changes. 133 */ 134 public Resource setId(String value) { 135 if (Utilities.noString(value)) 136 this.id = null; 137 else { 138 if (this.id == null) 139 this.id = new IdType(); 140 this.id.setValue(value); 141 } 142 return this; 143 } 144 145 /** 146 * @return {@link #meta} (The metadata about the resource. This is content that is maintained by the infrastructure. Changes to the content might not always be associated with version changes to the resource.) 147 */ 148 public Meta getMeta() { 149 if (this.meta == null) 150 if (Configuration.errorOnAutoCreate()) 151 throw new Error("Attempt to auto-create Resource.meta"); 152 else if (Configuration.doAutoCreate()) 153 this.meta = new Meta(); // cc 154 return this.meta; 155 } 156 157 public boolean hasMeta() { 158 return this.meta != null && !this.meta.isEmpty(); 159 } 160 161 /** 162 * @param value {@link #meta} (The metadata about the resource. This is content that is maintained by the infrastructure. Changes to the content might not always be associated with version changes to the resource.) 163 */ 164 public Resource setMeta(Meta value) { 165 this.meta = value; 166 return this; 167 } 168 169 /** 170 * @return {@link #implicitRules} (A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content. Often, this is a reference to an implementation guide that defines the special rules along with other profiles etc.). This is the underlying object with id, value and extensions. The accessor "getImplicitRules" gives direct access to the value 171 */ 172 public UriType getImplicitRulesElement() { 173 if (this.implicitRules == null) 174 if (Configuration.errorOnAutoCreate()) 175 throw new Error("Attempt to auto-create Resource.implicitRules"); 176 else if (Configuration.doAutoCreate()) 177 this.implicitRules = new UriType(); // bb 178 return this.implicitRules; 179 } 180 181 public boolean hasImplicitRulesElement() { 182 return this.implicitRules != null && !this.implicitRules.isEmpty(); 183 } 184 185 public boolean hasImplicitRules() { 186 return this.implicitRules != null && !this.implicitRules.isEmpty(); 187 } 188 189 /** 190 * @param value {@link #implicitRules} (A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content. Often, this is a reference to an implementation guide that defines the special rules along with other profiles etc.). This is the underlying object with id, value and extensions. The accessor "getImplicitRules" gives direct access to the value 191 */ 192 public Resource setImplicitRulesElement(UriType value) { 193 this.implicitRules = value; 194 return this; 195 } 196 197 /** 198 * @return A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content. Often, this is a reference to an implementation guide that defines the special rules along with other profiles etc. 199 */ 200 public String getImplicitRules() { 201 return this.implicitRules == null ? null : this.implicitRules.getValue(); 202 } 203 204 /** 205 * @param value A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content. Often, this is a reference to an implementation guide that defines the special rules along with other profiles etc. 206 */ 207 public Resource setImplicitRules(String value) { 208 if (Utilities.noString(value)) 209 this.implicitRules = null; 210 else { 211 if (this.implicitRules == null) 212 this.implicitRules = new UriType(); 213 this.implicitRules.setValue(value); 214 } 215 return this; 216 } 217 218 /** 219 * @return {@link #language} (The base language in which the resource is written.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 220 */ 221 public CodeType getLanguageElement() { 222 if (this.language == null) 223 if (Configuration.errorOnAutoCreate()) 224 throw new Error("Attempt to auto-create Resource.language"); 225 else if (Configuration.doAutoCreate()) 226 this.language = new CodeType(); // bb 227 return this.language; 228 } 229 230 public boolean hasLanguageElement() { 231 return this.language != null && !this.language.isEmpty(); 232 } 233 234 public boolean hasLanguage() { 235 return this.language != null && !this.language.isEmpty(); 236 } 237 238 /** 239 * @param value {@link #language} (The base language in which the resource is written.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 240 */ 241 public Resource setLanguageElement(CodeType value) { 242 this.language = value; 243 return this; 244 } 245 246 /** 247 * @return The base language in which the resource is written. 248 */ 249 public String getLanguage() { 250 return this.language == null ? null : this.language.getValue(); 251 } 252 253 /** 254 * @param value The base language in which the resource is written. 255 */ 256 public Resource setLanguage(String value) { 257 if (Utilities.noString(value)) 258 this.language = null; 259 else { 260 if (this.language == null) 261 this.language = new CodeType(); 262 this.language.setValue(value); 263 } 264 return this; 265 } 266 267 protected void listChildren(List<Property> children) { 268 super.listChildren(children); 269 children.add(new Property("id", "id", "The logical id of the resource, as used in the URL for the resource. Once assigned, this value never changes.", 0, 1, id)); 270 children.add(new Property("meta", "Meta", "The metadata about the resource. This is content that is maintained by the infrastructure. Changes to the content might not always be associated with version changes to the resource.", 0, 1, meta)); 271 children.add(new Property("implicitRules", "uri", "A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content. Often, this is a reference to an implementation guide that defines the special rules along with other profiles etc.", 0, 1, implicitRules)); 272 children.add(new Property("language", "code", "The base language in which the resource is written.", 0, 1, language)); 273 } 274 275 @Override 276 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 277 switch (_hash) { 278 case 3355: /*id*/ return new Property("id", "id", "The logical id of the resource, as used in the URL for the resource. Once assigned, this value never changes.", 0, 1, id); 279 case 3347973: /*meta*/ return new Property("meta", "Meta", "The metadata about the resource. This is content that is maintained by the infrastructure. Changes to the content might not always be associated with version changes to the resource.", 0, 1, meta); 280 case -961826286: /*implicitRules*/ return new Property("implicitRules", "uri", "A reference to a set of rules that were followed when the resource was constructed, and which must be understood when processing the content. Often, this is a reference to an implementation guide that defines the special rules along with other profiles etc.", 0, 1, implicitRules); 281 case -1613589672: /*language*/ return new Property("language", "code", "The base language in which the resource is written.", 0, 1, language); 282 default: return super.getNamedProperty(_hash, _name, _checkValid); 283 } 284 285 } 286 287 @Override 288 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 289 switch (hash) { 290 case 3355: /*id*/ return this.id == null ? new Base[0] : new Base[] {this.id}; // IdType 291 case 3347973: /*meta*/ return this.meta == null ? new Base[0] : new Base[] {this.meta}; // Meta 292 case -961826286: /*implicitRules*/ return this.implicitRules == null ? new Base[0] : new Base[] {this.implicitRules}; // UriType 293 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeType 294 default: return super.getProperty(hash, name, checkValid); 295 } 296 297 } 298 299 @Override 300 public Base setProperty(int hash, String name, Base value) throws FHIRException { 301 switch (hash) { 302 case 3355: // id 303 this.id = TypeConvertor.castToId(value); // IdType 304 return value; 305 case 3347973: // meta 306 this.meta = TypeConvertor.castToMeta(value); // Meta 307 return value; 308 case -961826286: // implicitRules 309 this.implicitRules = TypeConvertor.castToUri(value); // UriType 310 return value; 311 case -1613589672: // language 312 this.language = TypeConvertor.castToCode(value); // CodeType 313 return value; 314 default: return super.setProperty(hash, name, value); 315 } 316 317 } 318 319 @Override 320 public Base setProperty(String name, Base value) throws FHIRException { 321 if (name.equals("id")) { 322 this.id = TypeConvertor.castToId(value); // IdType 323 } else if (name.equals("meta")) { 324 this.meta = TypeConvertor.castToMeta(value); // Meta 325 } else if (name.equals("implicitRules")) { 326 this.implicitRules = TypeConvertor.castToUri(value); // UriType 327 } else if (name.equals("language")) { 328 this.language = TypeConvertor.castToCode(value); // CodeType 329 } else 330 return super.setProperty(name, value); 331 return value; 332 } 333 334 @Override 335 public void removeChild(String name, Base value) throws FHIRException { 336 if (name.equals("id")) { 337 this.id = null; 338 } else if (name.equals("meta")) { 339 this.meta = null; 340 } else if (name.equals("implicitRules")) { 341 this.implicitRules = null; 342 } else if (name.equals("language")) { 343 this.language = null; 344 } else 345 super.removeChild(name, value); 346 347 } 348 349 @Override 350 public Base makeProperty(int hash, String name) throws FHIRException { 351 switch (hash) { 352 case 3355: return getIdElement(); 353 case 3347973: return getMeta(); 354 case -961826286: return getImplicitRulesElement(); 355 case -1613589672: return getLanguageElement(); 356 default: return super.makeProperty(hash, name); 357 } 358 359 } 360 361 @Override 362 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 363 switch (hash) { 364 case 3355: /*id*/ return new String[] {"id"}; 365 case 3347973: /*meta*/ return new String[] {"Meta"}; 366 case -961826286: /*implicitRules*/ return new String[] {"uri"}; 367 case -1613589672: /*language*/ return new String[] {"code"}; 368 default: return super.getTypesForProperty(hash, name); 369 } 370 371 } 372 373 @Override 374 public Base addChild(String name) throws FHIRException { 375 if (name.equals("id")) { 376 throw new FHIRException("Cannot call addChild on a singleton property Resource.id"); 377 } 378 else if (name.equals("meta")) { 379 this.meta = new Meta(); 380 return this.meta; 381 } 382 else if (name.equals("implicitRules")) { 383 throw new FHIRException("Cannot call addChild on a singleton property Resource.implicitRules"); 384 } 385 else if (name.equals("language")) { 386 throw new FHIRException("Cannot call addChild on a singleton property Resource.language"); 387 } 388 else 389 return super.addChild(name); 390 } 391 392 public String fhirType() { 393 return "Resource"; 394 395 } 396 397 public abstract Resource copy(); 398 399 public void copyValues(Resource dst) { 400 super.copyValues(dst); 401 dst.id = id == null ? null : id.copy(); 402 dst.meta = meta == null ? null : meta.copy(); 403 dst.implicitRules = implicitRules == null ? null : implicitRules.copy(); 404 dst.language = language == null ? null : language.copy(); 405 } 406 407 @Override 408 public boolean equalsDeep(Base other_) { 409 if (!super.equalsDeep(other_)) 410 return false; 411 if (!(other_ instanceof Resource)) 412 return false; 413 Resource o = (Resource) other_; 414 return compareDeep(id, o.id, true) && compareDeep(meta, o.meta, true) && compareDeep(implicitRules, o.implicitRules, true) 415 && compareDeep(language, o.language, true); 416 } 417 418 @Override 419 public boolean equalsShallow(Base other_) { 420 if (!super.equalsShallow(other_)) 421 return false; 422 if (!(other_ instanceof Resource)) 423 return false; 424 Resource o = (Resource) other_; 425 return compareValues(id, o.id, true) && compareValues(implicitRules, o.implicitRules, true) && compareValues(language, o.language, true) 426 ; 427 } 428 429 public boolean isEmpty() { 430 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(id, meta, implicitRules 431 , language); 432 } 433 434// Manual code (from Configuration.txt): 435@Override 436 public String getIdBase() { 437 return getId(); 438 } 439 440 @Override 441 public void setIdBase(String value) { 442 setId(value); 443 } 444 public abstract ResourceType getResourceType(); 445 446 public String getLanguage(String defValue) { 447 return hasLanguage() ? getLanguage() : defValue; 448 } 449 450 private String webPath; 451 public boolean hasWebPath() { 452 return webPath != null; 453 } 454 public String getWebPath() { 455 return webPath; 456 } 457 public void setWebPath(String webPath) { 458 this.webPath = webPath; 459 } 460 461 // when possible, the source package is considered when performing reference resolution. 462 463 464 465 private PackageInformation sourcePackage; 466 467 public boolean hasSourcePackage() { 468 return sourcePackage != null; 469 } 470 471 public PackageInformation getSourcePackage() { 472 return sourcePackage; 473 } 474 475 public void setSourcePackage(PackageInformation sourcePackage) { 476 this.sourcePackage = sourcePackage; 477 } 478 479 /** 480 * @return the logical ID part of this resource's id 481 * @see IdType#getIdPart() 482 */ 483 public String getIdPart() { 484 return getIdElement().getIdPart(); 485 } 486 487 488 public FhirPublication getFHIRPublicationVersion() { 489 return FhirPublication.R5; 490 } 491 492// end addition 493 494} 495