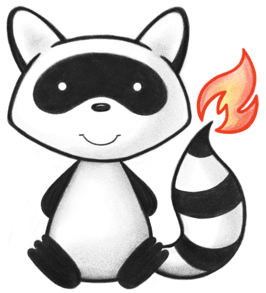
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.Block; 050 051/** 052 * An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome. 053 */ 054@ResourceDef(name="RiskAssessment", profile="http://hl7.org/fhir/StructureDefinition/RiskAssessment") 055public class RiskAssessment extends DomainResource { 056 057 @Block() 058 public static class RiskAssessmentPredictionComponent extends BackboneElement implements IBaseBackboneElement { 059 /** 060 * One of the potential outcomes for the patient (e.g. remission, death, a particular condition). 061 */ 062 @Child(name = "outcome", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 063 @Description(shortDefinition="Possible outcome for the subject", formalDefinition="One of the potential outcomes for the patient (e.g. remission, death, a particular condition)." ) 064 protected CodeableConcept outcome; 065 066 /** 067 * Indicates how likely the outcome is (in the specified timeframe). 068 */ 069 @Child(name = "probability", type = {DecimalType.class, Range.class}, order=2, min=0, max=1, modifier=false, summary=false) 070 @Description(shortDefinition="Likelihood of specified outcome", formalDefinition="Indicates how likely the outcome is (in the specified timeframe)." ) 071 protected DataType probability; 072 073 /** 074 * Indicates how likely the outcome is (in the specified timeframe), expressed as a qualitative value (e.g. low, medium, or high). 075 */ 076 @Child(name = "qualitativeRisk", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 077 @Description(shortDefinition="Likelihood of specified outcome as a qualitative value", formalDefinition="Indicates how likely the outcome is (in the specified timeframe), expressed as a qualitative value (e.g. low, medium, or high)." ) 078 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/risk-probability") 079 protected CodeableConcept qualitativeRisk; 080 081 /** 082 * Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.). 083 */ 084 @Child(name = "relativeRisk", type = {DecimalType.class}, order=4, min=0, max=1, modifier=false, summary=false) 085 @Description(shortDefinition="Relative likelihood", formalDefinition="Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.)." ) 086 protected DecimalType relativeRisk; 087 088 /** 089 * Indicates the period of time or age range of the subject to which the specified probability applies. 090 */ 091 @Child(name = "when", type = {Period.class, Range.class}, order=5, min=0, max=1, modifier=false, summary=false) 092 @Description(shortDefinition="Timeframe or age range", formalDefinition="Indicates the period of time or age range of the subject to which the specified probability applies." ) 093 protected DataType when; 094 095 /** 096 * Additional information explaining the basis for the prediction. 097 */ 098 @Child(name = "rationale", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 099 @Description(shortDefinition="Explanation of prediction", formalDefinition="Additional information explaining the basis for the prediction." ) 100 protected StringType rationale; 101 102 private static final long serialVersionUID = -1559504257L; 103 104 /** 105 * Constructor 106 */ 107 public RiskAssessmentPredictionComponent() { 108 super(); 109 } 110 111 /** 112 * @return {@link #outcome} (One of the potential outcomes for the patient (e.g. remission, death, a particular condition).) 113 */ 114 public CodeableConcept getOutcome() { 115 if (this.outcome == null) 116 if (Configuration.errorOnAutoCreate()) 117 throw new Error("Attempt to auto-create RiskAssessmentPredictionComponent.outcome"); 118 else if (Configuration.doAutoCreate()) 119 this.outcome = new CodeableConcept(); // cc 120 return this.outcome; 121 } 122 123 public boolean hasOutcome() { 124 return this.outcome != null && !this.outcome.isEmpty(); 125 } 126 127 /** 128 * @param value {@link #outcome} (One of the potential outcomes for the patient (e.g. remission, death, a particular condition).) 129 */ 130 public RiskAssessmentPredictionComponent setOutcome(CodeableConcept value) { 131 this.outcome = value; 132 return this; 133 } 134 135 /** 136 * @return {@link #probability} (Indicates how likely the outcome is (in the specified timeframe).) 137 */ 138 public DataType getProbability() { 139 return this.probability; 140 } 141 142 /** 143 * @return {@link #probability} (Indicates how likely the outcome is (in the specified timeframe).) 144 */ 145 public DecimalType getProbabilityDecimalType() throws FHIRException { 146 if (this.probability == null) 147 this.probability = new DecimalType(); 148 if (!(this.probability instanceof DecimalType)) 149 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.probability.getClass().getName()+" was encountered"); 150 return (DecimalType) this.probability; 151 } 152 153 public boolean hasProbabilityDecimalType() { 154 return this != null && this.probability instanceof DecimalType; 155 } 156 157 /** 158 * @return {@link #probability} (Indicates how likely the outcome is (in the specified timeframe).) 159 */ 160 public Range getProbabilityRange() throws FHIRException { 161 if (this.probability == null) 162 this.probability = new Range(); 163 if (!(this.probability instanceof Range)) 164 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.probability.getClass().getName()+" was encountered"); 165 return (Range) this.probability; 166 } 167 168 public boolean hasProbabilityRange() { 169 return this != null && this.probability instanceof Range; 170 } 171 172 public boolean hasProbability() { 173 return this.probability != null && !this.probability.isEmpty(); 174 } 175 176 /** 177 * @param value {@link #probability} (Indicates how likely the outcome is (in the specified timeframe).) 178 */ 179 public RiskAssessmentPredictionComponent setProbability(DataType value) { 180 if (value != null && !(value instanceof DecimalType || value instanceof Range)) 181 throw new FHIRException("Not the right type for RiskAssessment.prediction.probability[x]: "+value.fhirType()); 182 this.probability = value; 183 return this; 184 } 185 186 /** 187 * @return {@link #qualitativeRisk} (Indicates how likely the outcome is (in the specified timeframe), expressed as a qualitative value (e.g. low, medium, or high).) 188 */ 189 public CodeableConcept getQualitativeRisk() { 190 if (this.qualitativeRisk == null) 191 if (Configuration.errorOnAutoCreate()) 192 throw new Error("Attempt to auto-create RiskAssessmentPredictionComponent.qualitativeRisk"); 193 else if (Configuration.doAutoCreate()) 194 this.qualitativeRisk = new CodeableConcept(); // cc 195 return this.qualitativeRisk; 196 } 197 198 public boolean hasQualitativeRisk() { 199 return this.qualitativeRisk != null && !this.qualitativeRisk.isEmpty(); 200 } 201 202 /** 203 * @param value {@link #qualitativeRisk} (Indicates how likely the outcome is (in the specified timeframe), expressed as a qualitative value (e.g. low, medium, or high).) 204 */ 205 public RiskAssessmentPredictionComponent setQualitativeRisk(CodeableConcept value) { 206 this.qualitativeRisk = value; 207 return this; 208 } 209 210 /** 211 * @return {@link #relativeRisk} (Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.).). This is the underlying object with id, value and extensions. The accessor "getRelativeRisk" gives direct access to the value 212 */ 213 public DecimalType getRelativeRiskElement() { 214 if (this.relativeRisk == null) 215 if (Configuration.errorOnAutoCreate()) 216 throw new Error("Attempt to auto-create RiskAssessmentPredictionComponent.relativeRisk"); 217 else if (Configuration.doAutoCreate()) 218 this.relativeRisk = new DecimalType(); // bb 219 return this.relativeRisk; 220 } 221 222 public boolean hasRelativeRiskElement() { 223 return this.relativeRisk != null && !this.relativeRisk.isEmpty(); 224 } 225 226 public boolean hasRelativeRisk() { 227 return this.relativeRisk != null && !this.relativeRisk.isEmpty(); 228 } 229 230 /** 231 * @param value {@link #relativeRisk} (Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.).). This is the underlying object with id, value and extensions. The accessor "getRelativeRisk" gives direct access to the value 232 */ 233 public RiskAssessmentPredictionComponent setRelativeRiskElement(DecimalType value) { 234 this.relativeRisk = value; 235 return this; 236 } 237 238 /** 239 * @return Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.). 240 */ 241 public BigDecimal getRelativeRisk() { 242 return this.relativeRisk == null ? null : this.relativeRisk.getValue(); 243 } 244 245 /** 246 * @param value Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.). 247 */ 248 public RiskAssessmentPredictionComponent setRelativeRisk(BigDecimal value) { 249 if (value == null) 250 this.relativeRisk = null; 251 else { 252 if (this.relativeRisk == null) 253 this.relativeRisk = new DecimalType(); 254 this.relativeRisk.setValue(value); 255 } 256 return this; 257 } 258 259 /** 260 * @param value Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.). 261 */ 262 public RiskAssessmentPredictionComponent setRelativeRisk(long value) { 263 this.relativeRisk = new DecimalType(); 264 this.relativeRisk.setValue(value); 265 return this; 266 } 267 268 /** 269 * @param value Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.). 270 */ 271 public RiskAssessmentPredictionComponent setRelativeRisk(double value) { 272 this.relativeRisk = new DecimalType(); 273 this.relativeRisk.setValue(value); 274 return this; 275 } 276 277 /** 278 * @return {@link #when} (Indicates the period of time or age range of the subject to which the specified probability applies.) 279 */ 280 public DataType getWhen() { 281 return this.when; 282 } 283 284 /** 285 * @return {@link #when} (Indicates the period of time or age range of the subject to which the specified probability applies.) 286 */ 287 public Period getWhenPeriod() throws FHIRException { 288 if (this.when == null) 289 this.when = new Period(); 290 if (!(this.when instanceof Period)) 291 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.when.getClass().getName()+" was encountered"); 292 return (Period) this.when; 293 } 294 295 public boolean hasWhenPeriod() { 296 return this != null && this.when instanceof Period; 297 } 298 299 /** 300 * @return {@link #when} (Indicates the period of time or age range of the subject to which the specified probability applies.) 301 */ 302 public Range getWhenRange() throws FHIRException { 303 if (this.when == null) 304 this.when = new Range(); 305 if (!(this.when instanceof Range)) 306 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.when.getClass().getName()+" was encountered"); 307 return (Range) this.when; 308 } 309 310 public boolean hasWhenRange() { 311 return this != null && this.when instanceof Range; 312 } 313 314 public boolean hasWhen() { 315 return this.when != null && !this.when.isEmpty(); 316 } 317 318 /** 319 * @param value {@link #when} (Indicates the period of time or age range of the subject to which the specified probability applies.) 320 */ 321 public RiskAssessmentPredictionComponent setWhen(DataType value) { 322 if (value != null && !(value instanceof Period || value instanceof Range)) 323 throw new FHIRException("Not the right type for RiskAssessment.prediction.when[x]: "+value.fhirType()); 324 this.when = value; 325 return this; 326 } 327 328 /** 329 * @return {@link #rationale} (Additional information explaining the basis for the prediction.). This is the underlying object with id, value and extensions. The accessor "getRationale" gives direct access to the value 330 */ 331 public StringType getRationaleElement() { 332 if (this.rationale == null) 333 if (Configuration.errorOnAutoCreate()) 334 throw new Error("Attempt to auto-create RiskAssessmentPredictionComponent.rationale"); 335 else if (Configuration.doAutoCreate()) 336 this.rationale = new StringType(); // bb 337 return this.rationale; 338 } 339 340 public boolean hasRationaleElement() { 341 return this.rationale != null && !this.rationale.isEmpty(); 342 } 343 344 public boolean hasRationale() { 345 return this.rationale != null && !this.rationale.isEmpty(); 346 } 347 348 /** 349 * @param value {@link #rationale} (Additional information explaining the basis for the prediction.). This is the underlying object with id, value and extensions. The accessor "getRationale" gives direct access to the value 350 */ 351 public RiskAssessmentPredictionComponent setRationaleElement(StringType value) { 352 this.rationale = value; 353 return this; 354 } 355 356 /** 357 * @return Additional information explaining the basis for the prediction. 358 */ 359 public String getRationale() { 360 return this.rationale == null ? null : this.rationale.getValue(); 361 } 362 363 /** 364 * @param value Additional information explaining the basis for the prediction. 365 */ 366 public RiskAssessmentPredictionComponent setRationale(String value) { 367 if (Utilities.noString(value)) 368 this.rationale = null; 369 else { 370 if (this.rationale == null) 371 this.rationale = new StringType(); 372 this.rationale.setValue(value); 373 } 374 return this; 375 } 376 377 protected void listChildren(List<Property> children) { 378 super.listChildren(children); 379 children.add(new Property("outcome", "CodeableConcept", "One of the potential outcomes for the patient (e.g. remission, death, a particular condition).", 0, 1, outcome)); 380 children.add(new Property("probability[x]", "decimal|Range", "Indicates how likely the outcome is (in the specified timeframe).", 0, 1, probability)); 381 children.add(new Property("qualitativeRisk", "CodeableConcept", "Indicates how likely the outcome is (in the specified timeframe), expressed as a qualitative value (e.g. low, medium, or high).", 0, 1, qualitativeRisk)); 382 children.add(new Property("relativeRisk", "decimal", "Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.).", 0, 1, relativeRisk)); 383 children.add(new Property("when[x]", "Period|Range", "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 1, when)); 384 children.add(new Property("rationale", "string", "Additional information explaining the basis for the prediction.", 0, 1, rationale)); 385 } 386 387 @Override 388 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 389 switch (_hash) { 390 case -1106507950: /*outcome*/ return new Property("outcome", "CodeableConcept", "One of the potential outcomes for the patient (e.g. remission, death, a particular condition).", 0, 1, outcome); 391 case 1430185003: /*probability[x]*/ return new Property("probability[x]", "decimal|Range", "Indicates how likely the outcome is (in the specified timeframe).", 0, 1, probability); 392 case -1290561483: /*probability*/ return new Property("probability[x]", "decimal|Range", "Indicates how likely the outcome is (in the specified timeframe).", 0, 1, probability); 393 case 888495452: /*probabilityDecimal*/ return new Property("probability[x]", "decimal", "Indicates how likely the outcome is (in the specified timeframe).", 0, 1, probability); 394 case 9275912: /*probabilityRange*/ return new Property("probability[x]", "Range", "Indicates how likely the outcome is (in the specified timeframe).", 0, 1, probability); 395 case 123308730: /*qualitativeRisk*/ return new Property("qualitativeRisk", "CodeableConcept", "Indicates how likely the outcome is (in the specified timeframe), expressed as a qualitative value (e.g. low, medium, or high).", 0, 1, qualitativeRisk); 396 case -70741061: /*relativeRisk*/ return new Property("relativeRisk", "decimal", "Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.).", 0, 1, relativeRisk); 397 case 1312831238: /*when[x]*/ return new Property("when[x]", "Period|Range", "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 1, when); 398 case 3648314: /*when*/ return new Property("when[x]", "Period|Range", "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 1, when); 399 case 251476379: /*whenPeriod*/ return new Property("when[x]", "Period", "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 1, when); 400 case -1098542557: /*whenRange*/ return new Property("when[x]", "Range", "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 1, when); 401 case 345689335: /*rationale*/ return new Property("rationale", "string", "Additional information explaining the basis for the prediction.", 0, 1, rationale); 402 default: return super.getNamedProperty(_hash, _name, _checkValid); 403 } 404 405 } 406 407 @Override 408 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 409 switch (hash) { 410 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // CodeableConcept 411 case -1290561483: /*probability*/ return this.probability == null ? new Base[0] : new Base[] {this.probability}; // DataType 412 case 123308730: /*qualitativeRisk*/ return this.qualitativeRisk == null ? new Base[0] : new Base[] {this.qualitativeRisk}; // CodeableConcept 413 case -70741061: /*relativeRisk*/ return this.relativeRisk == null ? new Base[0] : new Base[] {this.relativeRisk}; // DecimalType 414 case 3648314: /*when*/ return this.when == null ? new Base[0] : new Base[] {this.when}; // DataType 415 case 345689335: /*rationale*/ return this.rationale == null ? new Base[0] : new Base[] {this.rationale}; // StringType 416 default: return super.getProperty(hash, name, checkValid); 417 } 418 419 } 420 421 @Override 422 public Base setProperty(int hash, String name, Base value) throws FHIRException { 423 switch (hash) { 424 case -1106507950: // outcome 425 this.outcome = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 426 return value; 427 case -1290561483: // probability 428 this.probability = TypeConvertor.castToType(value); // DataType 429 return value; 430 case 123308730: // qualitativeRisk 431 this.qualitativeRisk = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 432 return value; 433 case -70741061: // relativeRisk 434 this.relativeRisk = TypeConvertor.castToDecimal(value); // DecimalType 435 return value; 436 case 3648314: // when 437 this.when = TypeConvertor.castToType(value); // DataType 438 return value; 439 case 345689335: // rationale 440 this.rationale = TypeConvertor.castToString(value); // StringType 441 return value; 442 default: return super.setProperty(hash, name, value); 443 } 444 445 } 446 447 @Override 448 public Base setProperty(String name, Base value) throws FHIRException { 449 if (name.equals("outcome")) { 450 this.outcome = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 451 } else if (name.equals("probability[x]")) { 452 this.probability = TypeConvertor.castToType(value); // DataType 453 } else if (name.equals("qualitativeRisk")) { 454 this.qualitativeRisk = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 455 } else if (name.equals("relativeRisk")) { 456 this.relativeRisk = TypeConvertor.castToDecimal(value); // DecimalType 457 } else if (name.equals("when[x]")) { 458 this.when = TypeConvertor.castToType(value); // DataType 459 } else if (name.equals("rationale")) { 460 this.rationale = TypeConvertor.castToString(value); // StringType 461 } else 462 return super.setProperty(name, value); 463 return value; 464 } 465 466 @Override 467 public void removeChild(String name, Base value) throws FHIRException { 468 if (name.equals("outcome")) { 469 this.outcome = null; 470 } else if (name.equals("probability[x]")) { 471 this.probability = null; 472 } else if (name.equals("qualitativeRisk")) { 473 this.qualitativeRisk = null; 474 } else if (name.equals("relativeRisk")) { 475 this.relativeRisk = null; 476 } else if (name.equals("when[x]")) { 477 this.when = null; 478 } else if (name.equals("rationale")) { 479 this.rationale = null; 480 } else 481 super.removeChild(name, value); 482 483 } 484 485 @Override 486 public Base makeProperty(int hash, String name) throws FHIRException { 487 switch (hash) { 488 case -1106507950: return getOutcome(); 489 case 1430185003: return getProbability(); 490 case -1290561483: return getProbability(); 491 case 123308730: return getQualitativeRisk(); 492 case -70741061: return getRelativeRiskElement(); 493 case 1312831238: return getWhen(); 494 case 3648314: return getWhen(); 495 case 345689335: return getRationaleElement(); 496 default: return super.makeProperty(hash, name); 497 } 498 499 } 500 501 @Override 502 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 503 switch (hash) { 504 case -1106507950: /*outcome*/ return new String[] {"CodeableConcept"}; 505 case -1290561483: /*probability*/ return new String[] {"decimal", "Range"}; 506 case 123308730: /*qualitativeRisk*/ return new String[] {"CodeableConcept"}; 507 case -70741061: /*relativeRisk*/ return new String[] {"decimal"}; 508 case 3648314: /*when*/ return new String[] {"Period", "Range"}; 509 case 345689335: /*rationale*/ return new String[] {"string"}; 510 default: return super.getTypesForProperty(hash, name); 511 } 512 513 } 514 515 @Override 516 public Base addChild(String name) throws FHIRException { 517 if (name.equals("outcome")) { 518 this.outcome = new CodeableConcept(); 519 return this.outcome; 520 } 521 else if (name.equals("probabilityDecimal")) { 522 this.probability = new DecimalType(); 523 return this.probability; 524 } 525 else if (name.equals("probabilityRange")) { 526 this.probability = new Range(); 527 return this.probability; 528 } 529 else if (name.equals("qualitativeRisk")) { 530 this.qualitativeRisk = new CodeableConcept(); 531 return this.qualitativeRisk; 532 } 533 else if (name.equals("relativeRisk")) { 534 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.prediction.relativeRisk"); 535 } 536 else if (name.equals("whenPeriod")) { 537 this.when = new Period(); 538 return this.when; 539 } 540 else if (name.equals("whenRange")) { 541 this.when = new Range(); 542 return this.when; 543 } 544 else if (name.equals("rationale")) { 545 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.prediction.rationale"); 546 } 547 else 548 return super.addChild(name); 549 } 550 551 public RiskAssessmentPredictionComponent copy() { 552 RiskAssessmentPredictionComponent dst = new RiskAssessmentPredictionComponent(); 553 copyValues(dst); 554 return dst; 555 } 556 557 public void copyValues(RiskAssessmentPredictionComponent dst) { 558 super.copyValues(dst); 559 dst.outcome = outcome == null ? null : outcome.copy(); 560 dst.probability = probability == null ? null : probability.copy(); 561 dst.qualitativeRisk = qualitativeRisk == null ? null : qualitativeRisk.copy(); 562 dst.relativeRisk = relativeRisk == null ? null : relativeRisk.copy(); 563 dst.when = when == null ? null : when.copy(); 564 dst.rationale = rationale == null ? null : rationale.copy(); 565 } 566 567 @Override 568 public boolean equalsDeep(Base other_) { 569 if (!super.equalsDeep(other_)) 570 return false; 571 if (!(other_ instanceof RiskAssessmentPredictionComponent)) 572 return false; 573 RiskAssessmentPredictionComponent o = (RiskAssessmentPredictionComponent) other_; 574 return compareDeep(outcome, o.outcome, true) && compareDeep(probability, o.probability, true) && compareDeep(qualitativeRisk, o.qualitativeRisk, true) 575 && compareDeep(relativeRisk, o.relativeRisk, true) && compareDeep(when, o.when, true) && compareDeep(rationale, o.rationale, true) 576 ; 577 } 578 579 @Override 580 public boolean equalsShallow(Base other_) { 581 if (!super.equalsShallow(other_)) 582 return false; 583 if (!(other_ instanceof RiskAssessmentPredictionComponent)) 584 return false; 585 RiskAssessmentPredictionComponent o = (RiskAssessmentPredictionComponent) other_; 586 return compareValues(relativeRisk, o.relativeRisk, true) && compareValues(rationale, o.rationale, true) 587 ; 588 } 589 590 public boolean isEmpty() { 591 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(outcome, probability, qualitativeRisk 592 , relativeRisk, when, rationale); 593 } 594 595 public String fhirType() { 596 return "RiskAssessment.prediction"; 597 598 } 599 600 } 601 602 /** 603 * Business identifier assigned to the risk assessment. 604 */ 605 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 606 @Description(shortDefinition="Unique identifier for the assessment", formalDefinition="Business identifier assigned to the risk assessment." ) 607 protected List<Identifier> identifier; 608 609 /** 610 * A reference to the request that is fulfilled by this risk assessment. 611 */ 612 @Child(name = "basedOn", type = {Reference.class}, order=1, min=0, max=1, modifier=false, summary=false) 613 @Description(shortDefinition="Request fulfilled by this assessment", formalDefinition="A reference to the request that is fulfilled by this risk assessment." ) 614 protected Reference basedOn; 615 616 /** 617 * A reference to a resource that this risk assessment is part of, such as a Procedure. 618 */ 619 @Child(name = "parent", type = {Reference.class}, order=2, min=0, max=1, modifier=false, summary=false) 620 @Description(shortDefinition="Part of this occurrence", formalDefinition="A reference to a resource that this risk assessment is part of, such as a Procedure." ) 621 protected Reference parent; 622 623 /** 624 * The status of the RiskAssessment, using the same statuses as an Observation. 625 */ 626 @Child(name = "status", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=true) 627 @Description(shortDefinition="registered | preliminary | final | amended +", formalDefinition="The status of the RiskAssessment, using the same statuses as an Observation." ) 628 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-status") 629 protected Enumeration<ObservationStatus> status; 630 631 /** 632 * The algorithm, process or mechanism used to evaluate the risk. 633 */ 634 @Child(name = "method", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 635 @Description(shortDefinition="Evaluation mechanism", formalDefinition="The algorithm, process or mechanism used to evaluate the risk." ) 636 protected CodeableConcept method; 637 638 /** 639 * The type of the risk assessment performed. 640 */ 641 @Child(name = "code", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 642 @Description(shortDefinition="Type of assessment", formalDefinition="The type of the risk assessment performed." ) 643 protected CodeableConcept code; 644 645 /** 646 * The patient or group the risk assessment applies to. 647 */ 648 @Child(name = "subject", type = {Patient.class, Group.class}, order=6, min=1, max=1, modifier=false, summary=true) 649 @Description(shortDefinition="Who/what does assessment apply to?", formalDefinition="The patient or group the risk assessment applies to." ) 650 protected Reference subject; 651 652 /** 653 * The encounter where the assessment was performed. 654 */ 655 @Child(name = "encounter", type = {Encounter.class}, order=7, min=0, max=1, modifier=false, summary=true) 656 @Description(shortDefinition="Where was assessment performed?", formalDefinition="The encounter where the assessment was performed." ) 657 protected Reference encounter; 658 659 /** 660 * The date (and possibly time) the risk assessment was performed. 661 */ 662 @Child(name = "occurrence", type = {DateTimeType.class, Period.class}, order=8, min=0, max=1, modifier=false, summary=true) 663 @Description(shortDefinition="When was assessment made?", formalDefinition="The date (and possibly time) the risk assessment was performed." ) 664 protected DataType occurrence; 665 666 /** 667 * For assessments or prognosis specific to a particular condition, indicates the condition being assessed. 668 */ 669 @Child(name = "condition", type = {Condition.class}, order=9, min=0, max=1, modifier=false, summary=true) 670 @Description(shortDefinition="Condition assessed", formalDefinition="For assessments or prognosis specific to a particular condition, indicates the condition being assessed." ) 671 protected Reference condition; 672 673 /** 674 * The provider, patient, related person, or software application that performed the assessment. 675 */ 676 @Child(name = "performer", type = {Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, Device.class}, order=10, min=0, max=1, modifier=false, summary=true) 677 @Description(shortDefinition="Who did assessment?", formalDefinition="The provider, patient, related person, or software application that performed the assessment." ) 678 protected Reference performer; 679 680 /** 681 * The reason the risk assessment was performed. 682 */ 683 @Child(name = "reason", type = {CodeableReference.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 684 @Description(shortDefinition="Why the assessment was necessary?", formalDefinition="The reason the risk assessment was performed." ) 685 protected List<CodeableReference> reason; 686 687 /** 688 * Indicates the source data considered as part of the assessment (for example, FamilyHistory, Observations, Procedures, Conditions, etc.). 689 */ 690 @Child(name = "basis", type = {Reference.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 691 @Description(shortDefinition="Information used in assessment", formalDefinition="Indicates the source data considered as part of the assessment (for example, FamilyHistory, Observations, Procedures, Conditions, etc.)." ) 692 protected List<Reference> basis; 693 694 /** 695 * Describes the expected outcome for the subject. 696 */ 697 @Child(name = "prediction", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 698 @Description(shortDefinition="Outcome predicted", formalDefinition="Describes the expected outcome for the subject." ) 699 protected List<RiskAssessmentPredictionComponent> prediction; 700 701 /** 702 * A description of the steps that might be taken to reduce the identified risk(s). 703 */ 704 @Child(name = "mitigation", type = {StringType.class}, order=14, min=0, max=1, modifier=false, summary=false) 705 @Description(shortDefinition="How to reduce risk", formalDefinition="A description of the steps that might be taken to reduce the identified risk(s)." ) 706 protected StringType mitigation; 707 708 /** 709 * Additional comments about the risk assessment. 710 */ 711 @Child(name = "note", type = {Annotation.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 712 @Description(shortDefinition="Comments on the risk assessment", formalDefinition="Additional comments about the risk assessment." ) 713 protected List<Annotation> note; 714 715 private static final long serialVersionUID = 1076114228L; 716 717 /** 718 * Constructor 719 */ 720 public RiskAssessment() { 721 super(); 722 } 723 724 /** 725 * Constructor 726 */ 727 public RiskAssessment(ObservationStatus status, Reference subject) { 728 super(); 729 this.setStatus(status); 730 this.setSubject(subject); 731 } 732 733 /** 734 * @return {@link #identifier} (Business identifier assigned to the risk assessment.) 735 */ 736 public List<Identifier> getIdentifier() { 737 if (this.identifier == null) 738 this.identifier = new ArrayList<Identifier>(); 739 return this.identifier; 740 } 741 742 /** 743 * @return Returns a reference to <code>this</code> for easy method chaining 744 */ 745 public RiskAssessment setIdentifier(List<Identifier> theIdentifier) { 746 this.identifier = theIdentifier; 747 return this; 748 } 749 750 public boolean hasIdentifier() { 751 if (this.identifier == null) 752 return false; 753 for (Identifier item : this.identifier) 754 if (!item.isEmpty()) 755 return true; 756 return false; 757 } 758 759 public Identifier addIdentifier() { //3 760 Identifier t = new Identifier(); 761 if (this.identifier == null) 762 this.identifier = new ArrayList<Identifier>(); 763 this.identifier.add(t); 764 return t; 765 } 766 767 public RiskAssessment addIdentifier(Identifier t) { //3 768 if (t == null) 769 return this; 770 if (this.identifier == null) 771 this.identifier = new ArrayList<Identifier>(); 772 this.identifier.add(t); 773 return this; 774 } 775 776 /** 777 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 778 */ 779 public Identifier getIdentifierFirstRep() { 780 if (getIdentifier().isEmpty()) { 781 addIdentifier(); 782 } 783 return getIdentifier().get(0); 784 } 785 786 /** 787 * @return {@link #basedOn} (A reference to the request that is fulfilled by this risk assessment.) 788 */ 789 public Reference getBasedOn() { 790 if (this.basedOn == null) 791 if (Configuration.errorOnAutoCreate()) 792 throw new Error("Attempt to auto-create RiskAssessment.basedOn"); 793 else if (Configuration.doAutoCreate()) 794 this.basedOn = new Reference(); // cc 795 return this.basedOn; 796 } 797 798 public boolean hasBasedOn() { 799 return this.basedOn != null && !this.basedOn.isEmpty(); 800 } 801 802 /** 803 * @param value {@link #basedOn} (A reference to the request that is fulfilled by this risk assessment.) 804 */ 805 public RiskAssessment setBasedOn(Reference value) { 806 this.basedOn = value; 807 return this; 808 } 809 810 /** 811 * @return {@link #parent} (A reference to a resource that this risk assessment is part of, such as a Procedure.) 812 */ 813 public Reference getParent() { 814 if (this.parent == null) 815 if (Configuration.errorOnAutoCreate()) 816 throw new Error("Attempt to auto-create RiskAssessment.parent"); 817 else if (Configuration.doAutoCreate()) 818 this.parent = new Reference(); // cc 819 return this.parent; 820 } 821 822 public boolean hasParent() { 823 return this.parent != null && !this.parent.isEmpty(); 824 } 825 826 /** 827 * @param value {@link #parent} (A reference to a resource that this risk assessment is part of, such as a Procedure.) 828 */ 829 public RiskAssessment setParent(Reference value) { 830 this.parent = value; 831 return this; 832 } 833 834 /** 835 * @return {@link #status} (The status of the RiskAssessment, using the same statuses as an Observation.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 836 */ 837 public Enumeration<ObservationStatus> getStatusElement() { 838 if (this.status == null) 839 if (Configuration.errorOnAutoCreate()) 840 throw new Error("Attempt to auto-create RiskAssessment.status"); 841 else if (Configuration.doAutoCreate()) 842 this.status = new Enumeration<ObservationStatus>(new ObservationStatusEnumFactory()); // bb 843 return this.status; 844 } 845 846 public boolean hasStatusElement() { 847 return this.status != null && !this.status.isEmpty(); 848 } 849 850 public boolean hasStatus() { 851 return this.status != null && !this.status.isEmpty(); 852 } 853 854 /** 855 * @param value {@link #status} (The status of the RiskAssessment, using the same statuses as an Observation.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 856 */ 857 public RiskAssessment setStatusElement(Enumeration<ObservationStatus> value) { 858 this.status = value; 859 return this; 860 } 861 862 /** 863 * @return The status of the RiskAssessment, using the same statuses as an Observation. 864 */ 865 public ObservationStatus getStatus() { 866 return this.status == null ? null : this.status.getValue(); 867 } 868 869 /** 870 * @param value The status of the RiskAssessment, using the same statuses as an Observation. 871 */ 872 public RiskAssessment setStatus(ObservationStatus value) { 873 if (this.status == null) 874 this.status = new Enumeration<ObservationStatus>(new ObservationStatusEnumFactory()); 875 this.status.setValue(value); 876 return this; 877 } 878 879 /** 880 * @return {@link #method} (The algorithm, process or mechanism used to evaluate the risk.) 881 */ 882 public CodeableConcept getMethod() { 883 if (this.method == null) 884 if (Configuration.errorOnAutoCreate()) 885 throw new Error("Attempt to auto-create RiskAssessment.method"); 886 else if (Configuration.doAutoCreate()) 887 this.method = new CodeableConcept(); // cc 888 return this.method; 889 } 890 891 public boolean hasMethod() { 892 return this.method != null && !this.method.isEmpty(); 893 } 894 895 /** 896 * @param value {@link #method} (The algorithm, process or mechanism used to evaluate the risk.) 897 */ 898 public RiskAssessment setMethod(CodeableConcept value) { 899 this.method = value; 900 return this; 901 } 902 903 /** 904 * @return {@link #code} (The type of the risk assessment performed.) 905 */ 906 public CodeableConcept getCode() { 907 if (this.code == null) 908 if (Configuration.errorOnAutoCreate()) 909 throw new Error("Attempt to auto-create RiskAssessment.code"); 910 else if (Configuration.doAutoCreate()) 911 this.code = new CodeableConcept(); // cc 912 return this.code; 913 } 914 915 public boolean hasCode() { 916 return this.code != null && !this.code.isEmpty(); 917 } 918 919 /** 920 * @param value {@link #code} (The type of the risk assessment performed.) 921 */ 922 public RiskAssessment setCode(CodeableConcept value) { 923 this.code = value; 924 return this; 925 } 926 927 /** 928 * @return {@link #subject} (The patient or group the risk assessment applies to.) 929 */ 930 public Reference getSubject() { 931 if (this.subject == null) 932 if (Configuration.errorOnAutoCreate()) 933 throw new Error("Attempt to auto-create RiskAssessment.subject"); 934 else if (Configuration.doAutoCreate()) 935 this.subject = new Reference(); // cc 936 return this.subject; 937 } 938 939 public boolean hasSubject() { 940 return this.subject != null && !this.subject.isEmpty(); 941 } 942 943 /** 944 * @param value {@link #subject} (The patient or group the risk assessment applies to.) 945 */ 946 public RiskAssessment setSubject(Reference value) { 947 this.subject = value; 948 return this; 949 } 950 951 /** 952 * @return {@link #encounter} (The encounter where the assessment was performed.) 953 */ 954 public Reference getEncounter() { 955 if (this.encounter == null) 956 if (Configuration.errorOnAutoCreate()) 957 throw new Error("Attempt to auto-create RiskAssessment.encounter"); 958 else if (Configuration.doAutoCreate()) 959 this.encounter = new Reference(); // cc 960 return this.encounter; 961 } 962 963 public boolean hasEncounter() { 964 return this.encounter != null && !this.encounter.isEmpty(); 965 } 966 967 /** 968 * @param value {@link #encounter} (The encounter where the assessment was performed.) 969 */ 970 public RiskAssessment setEncounter(Reference value) { 971 this.encounter = value; 972 return this; 973 } 974 975 /** 976 * @return {@link #occurrence} (The date (and possibly time) the risk assessment was performed.) 977 */ 978 public DataType getOccurrence() { 979 return this.occurrence; 980 } 981 982 /** 983 * @return {@link #occurrence} (The date (and possibly time) the risk assessment was performed.) 984 */ 985 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 986 if (this.occurrence == null) 987 this.occurrence = new DateTimeType(); 988 if (!(this.occurrence instanceof DateTimeType)) 989 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 990 return (DateTimeType) this.occurrence; 991 } 992 993 public boolean hasOccurrenceDateTimeType() { 994 return this != null && this.occurrence instanceof DateTimeType; 995 } 996 997 /** 998 * @return {@link #occurrence} (The date (and possibly time) the risk assessment was performed.) 999 */ 1000 public Period getOccurrencePeriod() throws FHIRException { 1001 if (this.occurrence == null) 1002 this.occurrence = new Period(); 1003 if (!(this.occurrence instanceof Period)) 1004 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1005 return (Period) this.occurrence; 1006 } 1007 1008 public boolean hasOccurrencePeriod() { 1009 return this != null && this.occurrence instanceof Period; 1010 } 1011 1012 public boolean hasOccurrence() { 1013 return this.occurrence != null && !this.occurrence.isEmpty(); 1014 } 1015 1016 /** 1017 * @param value {@link #occurrence} (The date (and possibly time) the risk assessment was performed.) 1018 */ 1019 public RiskAssessment setOccurrence(DataType value) { 1020 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1021 throw new FHIRException("Not the right type for RiskAssessment.occurrence[x]: "+value.fhirType()); 1022 this.occurrence = value; 1023 return this; 1024 } 1025 1026 /** 1027 * @return {@link #condition} (For assessments or prognosis specific to a particular condition, indicates the condition being assessed.) 1028 */ 1029 public Reference getCondition() { 1030 if (this.condition == null) 1031 if (Configuration.errorOnAutoCreate()) 1032 throw new Error("Attempt to auto-create RiskAssessment.condition"); 1033 else if (Configuration.doAutoCreate()) 1034 this.condition = new Reference(); // cc 1035 return this.condition; 1036 } 1037 1038 public boolean hasCondition() { 1039 return this.condition != null && !this.condition.isEmpty(); 1040 } 1041 1042 /** 1043 * @param value {@link #condition} (For assessments or prognosis specific to a particular condition, indicates the condition being assessed.) 1044 */ 1045 public RiskAssessment setCondition(Reference value) { 1046 this.condition = value; 1047 return this; 1048 } 1049 1050 /** 1051 * @return {@link #performer} (The provider, patient, related person, or software application that performed the assessment.) 1052 */ 1053 public Reference getPerformer() { 1054 if (this.performer == null) 1055 if (Configuration.errorOnAutoCreate()) 1056 throw new Error("Attempt to auto-create RiskAssessment.performer"); 1057 else if (Configuration.doAutoCreate()) 1058 this.performer = new Reference(); // cc 1059 return this.performer; 1060 } 1061 1062 public boolean hasPerformer() { 1063 return this.performer != null && !this.performer.isEmpty(); 1064 } 1065 1066 /** 1067 * @param value {@link #performer} (The provider, patient, related person, or software application that performed the assessment.) 1068 */ 1069 public RiskAssessment setPerformer(Reference value) { 1070 this.performer = value; 1071 return this; 1072 } 1073 1074 /** 1075 * @return {@link #reason} (The reason the risk assessment was performed.) 1076 */ 1077 public List<CodeableReference> getReason() { 1078 if (this.reason == null) 1079 this.reason = new ArrayList<CodeableReference>(); 1080 return this.reason; 1081 } 1082 1083 /** 1084 * @return Returns a reference to <code>this</code> for easy method chaining 1085 */ 1086 public RiskAssessment setReason(List<CodeableReference> theReason) { 1087 this.reason = theReason; 1088 return this; 1089 } 1090 1091 public boolean hasReason() { 1092 if (this.reason == null) 1093 return false; 1094 for (CodeableReference item : this.reason) 1095 if (!item.isEmpty()) 1096 return true; 1097 return false; 1098 } 1099 1100 public CodeableReference addReason() { //3 1101 CodeableReference t = new CodeableReference(); 1102 if (this.reason == null) 1103 this.reason = new ArrayList<CodeableReference>(); 1104 this.reason.add(t); 1105 return t; 1106 } 1107 1108 public RiskAssessment addReason(CodeableReference t) { //3 1109 if (t == null) 1110 return this; 1111 if (this.reason == null) 1112 this.reason = new ArrayList<CodeableReference>(); 1113 this.reason.add(t); 1114 return this; 1115 } 1116 1117 /** 1118 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 1119 */ 1120 public CodeableReference getReasonFirstRep() { 1121 if (getReason().isEmpty()) { 1122 addReason(); 1123 } 1124 return getReason().get(0); 1125 } 1126 1127 /** 1128 * @return {@link #basis} (Indicates the source data considered as part of the assessment (for example, FamilyHistory, Observations, Procedures, Conditions, etc.).) 1129 */ 1130 public List<Reference> getBasis() { 1131 if (this.basis == null) 1132 this.basis = new ArrayList<Reference>(); 1133 return this.basis; 1134 } 1135 1136 /** 1137 * @return Returns a reference to <code>this</code> for easy method chaining 1138 */ 1139 public RiskAssessment setBasis(List<Reference> theBasis) { 1140 this.basis = theBasis; 1141 return this; 1142 } 1143 1144 public boolean hasBasis() { 1145 if (this.basis == null) 1146 return false; 1147 for (Reference item : this.basis) 1148 if (!item.isEmpty()) 1149 return true; 1150 return false; 1151 } 1152 1153 public Reference addBasis() { //3 1154 Reference t = new Reference(); 1155 if (this.basis == null) 1156 this.basis = new ArrayList<Reference>(); 1157 this.basis.add(t); 1158 return t; 1159 } 1160 1161 public RiskAssessment addBasis(Reference t) { //3 1162 if (t == null) 1163 return this; 1164 if (this.basis == null) 1165 this.basis = new ArrayList<Reference>(); 1166 this.basis.add(t); 1167 return this; 1168 } 1169 1170 /** 1171 * @return The first repetition of repeating field {@link #basis}, creating it if it does not already exist {3} 1172 */ 1173 public Reference getBasisFirstRep() { 1174 if (getBasis().isEmpty()) { 1175 addBasis(); 1176 } 1177 return getBasis().get(0); 1178 } 1179 1180 /** 1181 * @return {@link #prediction} (Describes the expected outcome for the subject.) 1182 */ 1183 public List<RiskAssessmentPredictionComponent> getPrediction() { 1184 if (this.prediction == null) 1185 this.prediction = new ArrayList<RiskAssessmentPredictionComponent>(); 1186 return this.prediction; 1187 } 1188 1189 /** 1190 * @return Returns a reference to <code>this</code> for easy method chaining 1191 */ 1192 public RiskAssessment setPrediction(List<RiskAssessmentPredictionComponent> thePrediction) { 1193 this.prediction = thePrediction; 1194 return this; 1195 } 1196 1197 public boolean hasPrediction() { 1198 if (this.prediction == null) 1199 return false; 1200 for (RiskAssessmentPredictionComponent item : this.prediction) 1201 if (!item.isEmpty()) 1202 return true; 1203 return false; 1204 } 1205 1206 public RiskAssessmentPredictionComponent addPrediction() { //3 1207 RiskAssessmentPredictionComponent t = new RiskAssessmentPredictionComponent(); 1208 if (this.prediction == null) 1209 this.prediction = new ArrayList<RiskAssessmentPredictionComponent>(); 1210 this.prediction.add(t); 1211 return t; 1212 } 1213 1214 public RiskAssessment addPrediction(RiskAssessmentPredictionComponent t) { //3 1215 if (t == null) 1216 return this; 1217 if (this.prediction == null) 1218 this.prediction = new ArrayList<RiskAssessmentPredictionComponent>(); 1219 this.prediction.add(t); 1220 return this; 1221 } 1222 1223 /** 1224 * @return The first repetition of repeating field {@link #prediction}, creating it if it does not already exist {3} 1225 */ 1226 public RiskAssessmentPredictionComponent getPredictionFirstRep() { 1227 if (getPrediction().isEmpty()) { 1228 addPrediction(); 1229 } 1230 return getPrediction().get(0); 1231 } 1232 1233 /** 1234 * @return {@link #mitigation} (A description of the steps that might be taken to reduce the identified risk(s).). This is the underlying object with id, value and extensions. The accessor "getMitigation" gives direct access to the value 1235 */ 1236 public StringType getMitigationElement() { 1237 if (this.mitigation == null) 1238 if (Configuration.errorOnAutoCreate()) 1239 throw new Error("Attempt to auto-create RiskAssessment.mitigation"); 1240 else if (Configuration.doAutoCreate()) 1241 this.mitigation = new StringType(); // bb 1242 return this.mitigation; 1243 } 1244 1245 public boolean hasMitigationElement() { 1246 return this.mitigation != null && !this.mitigation.isEmpty(); 1247 } 1248 1249 public boolean hasMitigation() { 1250 return this.mitigation != null && !this.mitigation.isEmpty(); 1251 } 1252 1253 /** 1254 * @param value {@link #mitigation} (A description of the steps that might be taken to reduce the identified risk(s).). This is the underlying object with id, value and extensions. The accessor "getMitigation" gives direct access to the value 1255 */ 1256 public RiskAssessment setMitigationElement(StringType value) { 1257 this.mitigation = value; 1258 return this; 1259 } 1260 1261 /** 1262 * @return A description of the steps that might be taken to reduce the identified risk(s). 1263 */ 1264 public String getMitigation() { 1265 return this.mitigation == null ? null : this.mitigation.getValue(); 1266 } 1267 1268 /** 1269 * @param value A description of the steps that might be taken to reduce the identified risk(s). 1270 */ 1271 public RiskAssessment setMitigation(String value) { 1272 if (Utilities.noString(value)) 1273 this.mitigation = null; 1274 else { 1275 if (this.mitigation == null) 1276 this.mitigation = new StringType(); 1277 this.mitigation.setValue(value); 1278 } 1279 return this; 1280 } 1281 1282 /** 1283 * @return {@link #note} (Additional comments about the risk assessment.) 1284 */ 1285 public List<Annotation> getNote() { 1286 if (this.note == null) 1287 this.note = new ArrayList<Annotation>(); 1288 return this.note; 1289 } 1290 1291 /** 1292 * @return Returns a reference to <code>this</code> for easy method chaining 1293 */ 1294 public RiskAssessment setNote(List<Annotation> theNote) { 1295 this.note = theNote; 1296 return this; 1297 } 1298 1299 public boolean hasNote() { 1300 if (this.note == null) 1301 return false; 1302 for (Annotation item : this.note) 1303 if (!item.isEmpty()) 1304 return true; 1305 return false; 1306 } 1307 1308 public Annotation addNote() { //3 1309 Annotation t = new Annotation(); 1310 if (this.note == null) 1311 this.note = new ArrayList<Annotation>(); 1312 this.note.add(t); 1313 return t; 1314 } 1315 1316 public RiskAssessment addNote(Annotation t) { //3 1317 if (t == null) 1318 return this; 1319 if (this.note == null) 1320 this.note = new ArrayList<Annotation>(); 1321 this.note.add(t); 1322 return this; 1323 } 1324 1325 /** 1326 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1327 */ 1328 public Annotation getNoteFirstRep() { 1329 if (getNote().isEmpty()) { 1330 addNote(); 1331 } 1332 return getNote().get(0); 1333 } 1334 1335 protected void listChildren(List<Property> children) { 1336 super.listChildren(children); 1337 children.add(new Property("identifier", "Identifier", "Business identifier assigned to the risk assessment.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1338 children.add(new Property("basedOn", "Reference(Any)", "A reference to the request that is fulfilled by this risk assessment.", 0, 1, basedOn)); 1339 children.add(new Property("parent", "Reference(Any)", "A reference to a resource that this risk assessment is part of, such as a Procedure.", 0, 1, parent)); 1340 children.add(new Property("status", "code", "The status of the RiskAssessment, using the same statuses as an Observation.", 0, 1, status)); 1341 children.add(new Property("method", "CodeableConcept", "The algorithm, process or mechanism used to evaluate the risk.", 0, 1, method)); 1342 children.add(new Property("code", "CodeableConcept", "The type of the risk assessment performed.", 0, 1, code)); 1343 children.add(new Property("subject", "Reference(Patient|Group)", "The patient or group the risk assessment applies to.", 0, 1, subject)); 1344 children.add(new Property("encounter", "Reference(Encounter)", "The encounter where the assessment was performed.", 0, 1, encounter)); 1345 children.add(new Property("occurrence[x]", "dateTime|Period", "The date (and possibly time) the risk assessment was performed.", 0, 1, occurrence)); 1346 children.add(new Property("condition", "Reference(Condition)", "For assessments or prognosis specific to a particular condition, indicates the condition being assessed.", 0, 1, condition)); 1347 children.add(new Property("performer", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device)", "The provider, patient, related person, or software application that performed the assessment.", 0, 1, performer)); 1348 children.add(new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport|DocumentReference)", "The reason the risk assessment was performed.", 0, java.lang.Integer.MAX_VALUE, reason)); 1349 children.add(new Property("basis", "Reference(Any)", "Indicates the source data considered as part of the assessment (for example, FamilyHistory, Observations, Procedures, Conditions, etc.).", 0, java.lang.Integer.MAX_VALUE, basis)); 1350 children.add(new Property("prediction", "", "Describes the expected outcome for the subject.", 0, java.lang.Integer.MAX_VALUE, prediction)); 1351 children.add(new Property("mitigation", "string", "A description of the steps that might be taken to reduce the identified risk(s).", 0, 1, mitigation)); 1352 children.add(new Property("note", "Annotation", "Additional comments about the risk assessment.", 0, java.lang.Integer.MAX_VALUE, note)); 1353 } 1354 1355 @Override 1356 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1357 switch (_hash) { 1358 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifier assigned to the risk assessment.", 0, java.lang.Integer.MAX_VALUE, identifier); 1359 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(Any)", "A reference to the request that is fulfilled by this risk assessment.", 0, 1, basedOn); 1360 case -995424086: /*parent*/ return new Property("parent", "Reference(Any)", "A reference to a resource that this risk assessment is part of, such as a Procedure.", 0, 1, parent); 1361 case -892481550: /*status*/ return new Property("status", "code", "The status of the RiskAssessment, using the same statuses as an Observation.", 0, 1, status); 1362 case -1077554975: /*method*/ return new Property("method", "CodeableConcept", "The algorithm, process or mechanism used to evaluate the risk.", 0, 1, method); 1363 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The type of the risk assessment performed.", 0, 1, code); 1364 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The patient or group the risk assessment applies to.", 0, 1, subject); 1365 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The encounter where the assessment was performed.", 0, 1, encounter); 1366 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period", "The date (and possibly time) the risk assessment was performed.", 0, 1, occurrence); 1367 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period", "The date (and possibly time) the risk assessment was performed.", 0, 1, occurrence); 1368 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime", "The date (and possibly time) the risk assessment was performed.", 0, 1, occurrence); 1369 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "Period", "The date (and possibly time) the risk assessment was performed.", 0, 1, occurrence); 1370 case -861311717: /*condition*/ return new Property("condition", "Reference(Condition)", "For assessments or prognosis specific to a particular condition, indicates the condition being assessed.", 0, 1, condition); 1371 case 481140686: /*performer*/ return new Property("performer", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device)", "The provider, patient, related person, or software application that performed the assessment.", 0, 1, performer); 1372 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport|DocumentReference)", "The reason the risk assessment was performed.", 0, java.lang.Integer.MAX_VALUE, reason); 1373 case 93508670: /*basis*/ return new Property("basis", "Reference(Any)", "Indicates the source data considered as part of the assessment (for example, FamilyHistory, Observations, Procedures, Conditions, etc.).", 0, java.lang.Integer.MAX_VALUE, basis); 1374 case 1161234575: /*prediction*/ return new Property("prediction", "", "Describes the expected outcome for the subject.", 0, java.lang.Integer.MAX_VALUE, prediction); 1375 case 1293793087: /*mitigation*/ return new Property("mitigation", "string", "A description of the steps that might be taken to reduce the identified risk(s).", 0, 1, mitigation); 1376 case 3387378: /*note*/ return new Property("note", "Annotation", "Additional comments about the risk assessment.", 0, java.lang.Integer.MAX_VALUE, note); 1377 default: return super.getNamedProperty(_hash, _name, _checkValid); 1378 } 1379 1380 } 1381 1382 @Override 1383 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1384 switch (hash) { 1385 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1386 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : new Base[] {this.basedOn}; // Reference 1387 case -995424086: /*parent*/ return this.parent == null ? new Base[0] : new Base[] {this.parent}; // Reference 1388 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ObservationStatus> 1389 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // CodeableConcept 1390 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1391 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1392 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 1393 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // DataType 1394 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : new Base[] {this.condition}; // Reference 1395 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : new Base[] {this.performer}; // Reference 1396 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 1397 case 93508670: /*basis*/ return this.basis == null ? new Base[0] : this.basis.toArray(new Base[this.basis.size()]); // Reference 1398 case 1161234575: /*prediction*/ return this.prediction == null ? new Base[0] : this.prediction.toArray(new Base[this.prediction.size()]); // RiskAssessmentPredictionComponent 1399 case 1293793087: /*mitigation*/ return this.mitigation == null ? new Base[0] : new Base[] {this.mitigation}; // StringType 1400 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1401 default: return super.getProperty(hash, name, checkValid); 1402 } 1403 1404 } 1405 1406 @Override 1407 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1408 switch (hash) { 1409 case -1618432855: // identifier 1410 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1411 return value; 1412 case -332612366: // basedOn 1413 this.basedOn = TypeConvertor.castToReference(value); // Reference 1414 return value; 1415 case -995424086: // parent 1416 this.parent = TypeConvertor.castToReference(value); // Reference 1417 return value; 1418 case -892481550: // status 1419 value = new ObservationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1420 this.status = (Enumeration) value; // Enumeration<ObservationStatus> 1421 return value; 1422 case -1077554975: // method 1423 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1424 return value; 1425 case 3059181: // code 1426 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1427 return value; 1428 case -1867885268: // subject 1429 this.subject = TypeConvertor.castToReference(value); // Reference 1430 return value; 1431 case 1524132147: // encounter 1432 this.encounter = TypeConvertor.castToReference(value); // Reference 1433 return value; 1434 case 1687874001: // occurrence 1435 this.occurrence = TypeConvertor.castToType(value); // DataType 1436 return value; 1437 case -861311717: // condition 1438 this.condition = TypeConvertor.castToReference(value); // Reference 1439 return value; 1440 case 481140686: // performer 1441 this.performer = TypeConvertor.castToReference(value); // Reference 1442 return value; 1443 case -934964668: // reason 1444 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1445 return value; 1446 case 93508670: // basis 1447 this.getBasis().add(TypeConvertor.castToReference(value)); // Reference 1448 return value; 1449 case 1161234575: // prediction 1450 this.getPrediction().add((RiskAssessmentPredictionComponent) value); // RiskAssessmentPredictionComponent 1451 return value; 1452 case 1293793087: // mitigation 1453 this.mitigation = TypeConvertor.castToString(value); // StringType 1454 return value; 1455 case 3387378: // note 1456 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1457 return value; 1458 default: return super.setProperty(hash, name, value); 1459 } 1460 1461 } 1462 1463 @Override 1464 public Base setProperty(String name, Base value) throws FHIRException { 1465 if (name.equals("identifier")) { 1466 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1467 } else if (name.equals("basedOn")) { 1468 this.basedOn = TypeConvertor.castToReference(value); // Reference 1469 } else if (name.equals("parent")) { 1470 this.parent = TypeConvertor.castToReference(value); // Reference 1471 } else if (name.equals("status")) { 1472 value = new ObservationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1473 this.status = (Enumeration) value; // Enumeration<ObservationStatus> 1474 } else if (name.equals("method")) { 1475 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1476 } else if (name.equals("code")) { 1477 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1478 } else if (name.equals("subject")) { 1479 this.subject = TypeConvertor.castToReference(value); // Reference 1480 } else if (name.equals("encounter")) { 1481 this.encounter = TypeConvertor.castToReference(value); // Reference 1482 } else if (name.equals("occurrence[x]")) { 1483 this.occurrence = TypeConvertor.castToType(value); // DataType 1484 } else if (name.equals("condition")) { 1485 this.condition = TypeConvertor.castToReference(value); // Reference 1486 } else if (name.equals("performer")) { 1487 this.performer = TypeConvertor.castToReference(value); // Reference 1488 } else if (name.equals("reason")) { 1489 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 1490 } else if (name.equals("basis")) { 1491 this.getBasis().add(TypeConvertor.castToReference(value)); 1492 } else if (name.equals("prediction")) { 1493 this.getPrediction().add((RiskAssessmentPredictionComponent) value); 1494 } else if (name.equals("mitigation")) { 1495 this.mitigation = TypeConvertor.castToString(value); // StringType 1496 } else if (name.equals("note")) { 1497 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1498 } else 1499 return super.setProperty(name, value); 1500 return value; 1501 } 1502 1503 @Override 1504 public void removeChild(String name, Base value) throws FHIRException { 1505 if (name.equals("identifier")) { 1506 this.getIdentifier().remove(value); 1507 } else if (name.equals("basedOn")) { 1508 this.basedOn = null; 1509 } else if (name.equals("parent")) { 1510 this.parent = null; 1511 } else if (name.equals("status")) { 1512 value = new ObservationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1513 this.status = (Enumeration) value; // Enumeration<ObservationStatus> 1514 } else if (name.equals("method")) { 1515 this.method = null; 1516 } else if (name.equals("code")) { 1517 this.code = null; 1518 } else if (name.equals("subject")) { 1519 this.subject = null; 1520 } else if (name.equals("encounter")) { 1521 this.encounter = null; 1522 } else if (name.equals("occurrence[x]")) { 1523 this.occurrence = null; 1524 } else if (name.equals("condition")) { 1525 this.condition = null; 1526 } else if (name.equals("performer")) { 1527 this.performer = null; 1528 } else if (name.equals("reason")) { 1529 this.getReason().remove(value); 1530 } else if (name.equals("basis")) { 1531 this.getBasis().remove(value); 1532 } else if (name.equals("prediction")) { 1533 this.getPrediction().remove((RiskAssessmentPredictionComponent) value); 1534 } else if (name.equals("mitigation")) { 1535 this.mitigation = null; 1536 } else if (name.equals("note")) { 1537 this.getNote().remove(value); 1538 } else 1539 super.removeChild(name, value); 1540 1541 } 1542 1543 @Override 1544 public Base makeProperty(int hash, String name) throws FHIRException { 1545 switch (hash) { 1546 case -1618432855: return addIdentifier(); 1547 case -332612366: return getBasedOn(); 1548 case -995424086: return getParent(); 1549 case -892481550: return getStatusElement(); 1550 case -1077554975: return getMethod(); 1551 case 3059181: return getCode(); 1552 case -1867885268: return getSubject(); 1553 case 1524132147: return getEncounter(); 1554 case -2022646513: return getOccurrence(); 1555 case 1687874001: return getOccurrence(); 1556 case -861311717: return getCondition(); 1557 case 481140686: return getPerformer(); 1558 case -934964668: return addReason(); 1559 case 93508670: return addBasis(); 1560 case 1161234575: return addPrediction(); 1561 case 1293793087: return getMitigationElement(); 1562 case 3387378: return addNote(); 1563 default: return super.makeProperty(hash, name); 1564 } 1565 1566 } 1567 1568 @Override 1569 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1570 switch (hash) { 1571 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1572 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1573 case -995424086: /*parent*/ return new String[] {"Reference"}; 1574 case -892481550: /*status*/ return new String[] {"code"}; 1575 case -1077554975: /*method*/ return new String[] {"CodeableConcept"}; 1576 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1577 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1578 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 1579 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period"}; 1580 case -861311717: /*condition*/ return new String[] {"Reference"}; 1581 case 481140686: /*performer*/ return new String[] {"Reference"}; 1582 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 1583 case 93508670: /*basis*/ return new String[] {"Reference"}; 1584 case 1161234575: /*prediction*/ return new String[] {}; 1585 case 1293793087: /*mitigation*/ return new String[] {"string"}; 1586 case 3387378: /*note*/ return new String[] {"Annotation"}; 1587 default: return super.getTypesForProperty(hash, name); 1588 } 1589 1590 } 1591 1592 @Override 1593 public Base addChild(String name) throws FHIRException { 1594 if (name.equals("identifier")) { 1595 return addIdentifier(); 1596 } 1597 else if (name.equals("basedOn")) { 1598 this.basedOn = new Reference(); 1599 return this.basedOn; 1600 } 1601 else if (name.equals("parent")) { 1602 this.parent = new Reference(); 1603 return this.parent; 1604 } 1605 else if (name.equals("status")) { 1606 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.status"); 1607 } 1608 else if (name.equals("method")) { 1609 this.method = new CodeableConcept(); 1610 return this.method; 1611 } 1612 else if (name.equals("code")) { 1613 this.code = new CodeableConcept(); 1614 return this.code; 1615 } 1616 else if (name.equals("subject")) { 1617 this.subject = new Reference(); 1618 return this.subject; 1619 } 1620 else if (name.equals("encounter")) { 1621 this.encounter = new Reference(); 1622 return this.encounter; 1623 } 1624 else if (name.equals("occurrenceDateTime")) { 1625 this.occurrence = new DateTimeType(); 1626 return this.occurrence; 1627 } 1628 else if (name.equals("occurrencePeriod")) { 1629 this.occurrence = new Period(); 1630 return this.occurrence; 1631 } 1632 else if (name.equals("condition")) { 1633 this.condition = new Reference(); 1634 return this.condition; 1635 } 1636 else if (name.equals("performer")) { 1637 this.performer = new Reference(); 1638 return this.performer; 1639 } 1640 else if (name.equals("reason")) { 1641 return addReason(); 1642 } 1643 else if (name.equals("basis")) { 1644 return addBasis(); 1645 } 1646 else if (name.equals("prediction")) { 1647 return addPrediction(); 1648 } 1649 else if (name.equals("mitigation")) { 1650 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.mitigation"); 1651 } 1652 else if (name.equals("note")) { 1653 return addNote(); 1654 } 1655 else 1656 return super.addChild(name); 1657 } 1658 1659 public String fhirType() { 1660 return "RiskAssessment"; 1661 1662 } 1663 1664 public RiskAssessment copy() { 1665 RiskAssessment dst = new RiskAssessment(); 1666 copyValues(dst); 1667 return dst; 1668 } 1669 1670 public void copyValues(RiskAssessment dst) { 1671 super.copyValues(dst); 1672 if (identifier != null) { 1673 dst.identifier = new ArrayList<Identifier>(); 1674 for (Identifier i : identifier) 1675 dst.identifier.add(i.copy()); 1676 }; 1677 dst.basedOn = basedOn == null ? null : basedOn.copy(); 1678 dst.parent = parent == null ? null : parent.copy(); 1679 dst.status = status == null ? null : status.copy(); 1680 dst.method = method == null ? null : method.copy(); 1681 dst.code = code == null ? null : code.copy(); 1682 dst.subject = subject == null ? null : subject.copy(); 1683 dst.encounter = encounter == null ? null : encounter.copy(); 1684 dst.occurrence = occurrence == null ? null : occurrence.copy(); 1685 dst.condition = condition == null ? null : condition.copy(); 1686 dst.performer = performer == null ? null : performer.copy(); 1687 if (reason != null) { 1688 dst.reason = new ArrayList<CodeableReference>(); 1689 for (CodeableReference i : reason) 1690 dst.reason.add(i.copy()); 1691 }; 1692 if (basis != null) { 1693 dst.basis = new ArrayList<Reference>(); 1694 for (Reference i : basis) 1695 dst.basis.add(i.copy()); 1696 }; 1697 if (prediction != null) { 1698 dst.prediction = new ArrayList<RiskAssessmentPredictionComponent>(); 1699 for (RiskAssessmentPredictionComponent i : prediction) 1700 dst.prediction.add(i.copy()); 1701 }; 1702 dst.mitigation = mitigation == null ? null : mitigation.copy(); 1703 if (note != null) { 1704 dst.note = new ArrayList<Annotation>(); 1705 for (Annotation i : note) 1706 dst.note.add(i.copy()); 1707 }; 1708 } 1709 1710 protected RiskAssessment typedCopy() { 1711 return copy(); 1712 } 1713 1714 @Override 1715 public boolean equalsDeep(Base other_) { 1716 if (!super.equalsDeep(other_)) 1717 return false; 1718 if (!(other_ instanceof RiskAssessment)) 1719 return false; 1720 RiskAssessment o = (RiskAssessment) other_; 1721 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(parent, o.parent, true) 1722 && compareDeep(status, o.status, true) && compareDeep(method, o.method, true) && compareDeep(code, o.code, true) 1723 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) && compareDeep(occurrence, o.occurrence, true) 1724 && compareDeep(condition, o.condition, true) && compareDeep(performer, o.performer, true) && compareDeep(reason, o.reason, true) 1725 && compareDeep(basis, o.basis, true) && compareDeep(prediction, o.prediction, true) && compareDeep(mitigation, o.mitigation, true) 1726 && compareDeep(note, o.note, true); 1727 } 1728 1729 @Override 1730 public boolean equalsShallow(Base other_) { 1731 if (!super.equalsShallow(other_)) 1732 return false; 1733 if (!(other_ instanceof RiskAssessment)) 1734 return false; 1735 RiskAssessment o = (RiskAssessment) other_; 1736 return compareValues(status, o.status, true) && compareValues(mitigation, o.mitigation, true); 1737 } 1738 1739 public boolean isEmpty() { 1740 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, parent 1741 , status, method, code, subject, encounter, occurrence, condition, performer 1742 , reason, basis, prediction, mitigation, note); 1743 } 1744 1745 @Override 1746 public ResourceType getResourceType() { 1747 return ResourceType.RiskAssessment; 1748 } 1749 1750 /** 1751 * Search parameter: <b>condition</b> 1752 * <p> 1753 * Description: <b>Condition assessed</b><br> 1754 * Type: <b>reference</b><br> 1755 * Path: <b>RiskAssessment.condition</b><br> 1756 * </p> 1757 */ 1758 @SearchParamDefinition(name="condition", path="RiskAssessment.condition", description="Condition assessed", type="reference", target={Condition.class } ) 1759 public static final String SP_CONDITION = "condition"; 1760 /** 1761 * <b>Fluent Client</b> search parameter constant for <b>condition</b> 1762 * <p> 1763 * Description: <b>Condition assessed</b><br> 1764 * Type: <b>reference</b><br> 1765 * Path: <b>RiskAssessment.condition</b><br> 1766 * </p> 1767 */ 1768 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONDITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONDITION); 1769 1770/** 1771 * Constant for fluent queries to be used to add include statements. Specifies 1772 * the path value of "<b>RiskAssessment:condition</b>". 1773 */ 1774 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONDITION = new ca.uhn.fhir.model.api.Include("RiskAssessment:condition").toLocked(); 1775 1776 /** 1777 * Search parameter: <b>method</b> 1778 * <p> 1779 * Description: <b>Evaluation mechanism</b><br> 1780 * Type: <b>token</b><br> 1781 * Path: <b>RiskAssessment.method</b><br> 1782 * </p> 1783 */ 1784 @SearchParamDefinition(name="method", path="RiskAssessment.method", description="Evaluation mechanism", type="token" ) 1785 public static final String SP_METHOD = "method"; 1786 /** 1787 * <b>Fluent Client</b> search parameter constant for <b>method</b> 1788 * <p> 1789 * Description: <b>Evaluation mechanism</b><br> 1790 * Type: <b>token</b><br> 1791 * Path: <b>RiskAssessment.method</b><br> 1792 * </p> 1793 */ 1794 public static final ca.uhn.fhir.rest.gclient.TokenClientParam METHOD = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_METHOD); 1795 1796 /** 1797 * Search parameter: <b>performer</b> 1798 * <p> 1799 * Description: <b>Who did assessment?</b><br> 1800 * Type: <b>reference</b><br> 1801 * Path: <b>RiskAssessment.performer</b><br> 1802 * </p> 1803 */ 1804 @SearchParamDefinition(name="performer", path="RiskAssessment.performer", description="Who did assessment?", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Device.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 1805 public static final String SP_PERFORMER = "performer"; 1806 /** 1807 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 1808 * <p> 1809 * Description: <b>Who did assessment?</b><br> 1810 * Type: <b>reference</b><br> 1811 * Path: <b>RiskAssessment.performer</b><br> 1812 * </p> 1813 */ 1814 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 1815 1816/** 1817 * Constant for fluent queries to be used to add include statements. Specifies 1818 * the path value of "<b>RiskAssessment:performer</b>". 1819 */ 1820 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("RiskAssessment:performer").toLocked(); 1821 1822 /** 1823 * Search parameter: <b>probability</b> 1824 * <p> 1825 * Description: <b>Likelihood of specified outcome</b><br> 1826 * Type: <b>number</b><br> 1827 * Path: <b>RiskAssessment.prediction.probability.ofType(decimal)</b><br> 1828 * </p> 1829 */ 1830 @SearchParamDefinition(name="probability", path="RiskAssessment.prediction.probability.ofType(decimal)", description="Likelihood of specified outcome", type="number" ) 1831 public static final String SP_PROBABILITY = "probability"; 1832 /** 1833 * <b>Fluent Client</b> search parameter constant for <b>probability</b> 1834 * <p> 1835 * Description: <b>Likelihood of specified outcome</b><br> 1836 * Type: <b>number</b><br> 1837 * Path: <b>RiskAssessment.prediction.probability.ofType(decimal)</b><br> 1838 * </p> 1839 */ 1840 public static final ca.uhn.fhir.rest.gclient.NumberClientParam PROBABILITY = new ca.uhn.fhir.rest.gclient.NumberClientParam(SP_PROBABILITY); 1841 1842 /** 1843 * Search parameter: <b>risk</b> 1844 * <p> 1845 * Description: <b>Likelihood of specified outcome as a qualitative value</b><br> 1846 * Type: <b>token</b><br> 1847 * Path: <b>RiskAssessment.prediction.qualitativeRisk</b><br> 1848 * </p> 1849 */ 1850 @SearchParamDefinition(name="risk", path="RiskAssessment.prediction.qualitativeRisk", description="Likelihood of specified outcome as a qualitative value", type="token" ) 1851 public static final String SP_RISK = "risk"; 1852 /** 1853 * <b>Fluent Client</b> search parameter constant for <b>risk</b> 1854 * <p> 1855 * Description: <b>Likelihood of specified outcome as a qualitative value</b><br> 1856 * Type: <b>token</b><br> 1857 * Path: <b>RiskAssessment.prediction.qualitativeRisk</b><br> 1858 * </p> 1859 */ 1860 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RISK = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_RISK); 1861 1862 /** 1863 * Search parameter: <b>subject</b> 1864 * <p> 1865 * Description: <b>Who/what does assessment apply to?</b><br> 1866 * Type: <b>reference</b><br> 1867 * Path: <b>RiskAssessment.subject</b><br> 1868 * </p> 1869 */ 1870 @SearchParamDefinition(name="subject", path="RiskAssessment.subject", description="Who/what does assessment apply to?", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Group.class, Patient.class } ) 1871 public static final String SP_SUBJECT = "subject"; 1872 /** 1873 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1874 * <p> 1875 * Description: <b>Who/what does assessment apply to?</b><br> 1876 * Type: <b>reference</b><br> 1877 * Path: <b>RiskAssessment.subject</b><br> 1878 * </p> 1879 */ 1880 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1881 1882/** 1883 * Constant for fluent queries to be used to add include statements. Specifies 1884 * the path value of "<b>RiskAssessment:subject</b>". 1885 */ 1886 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("RiskAssessment:subject").toLocked(); 1887 1888 /** 1889 * Search parameter: <b>date</b> 1890 * <p> 1891 * Description: <b>Multiple Resources: 1892 1893* [AdverseEvent](adverseevent.html): When the event occurred 1894* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 1895* [Appointment](appointment.html): Appointment date/time. 1896* [AuditEvent](auditevent.html): Time when the event was recorded 1897* [CarePlan](careplan.html): Time period plan covers 1898* [CareTeam](careteam.html): A date within the coverage time period. 1899* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 1900* [Composition](composition.html): Composition editing time 1901* [Consent](consent.html): When consent was agreed to 1902* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 1903* [DocumentReference](documentreference.html): When this document reference was created 1904* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 1905* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 1906* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 1907* [Flag](flag.html): Time period when flag is active 1908* [Immunization](immunization.html): Vaccination (non)-Administration Date 1909* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 1910* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 1911* [Invoice](invoice.html): Invoice date / posting date 1912* [List](list.html): When the list was prepared 1913* [MeasureReport](measurereport.html): The date of the measure report 1914* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 1915* [Observation](observation.html): Clinically relevant time/time-period for observation 1916* [Procedure](procedure.html): When the procedure occurred or is occurring 1917* [ResearchSubject](researchsubject.html): Start and end of participation 1918* [RiskAssessment](riskassessment.html): When was assessment made? 1919* [SupplyRequest](supplyrequest.html): When the request was made 1920</b><br> 1921 * Type: <b>date</b><br> 1922 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 1923 * </p> 1924 */ 1925 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 1926 public static final String SP_DATE = "date"; 1927 /** 1928 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1929 * <p> 1930 * Description: <b>Multiple Resources: 1931 1932* [AdverseEvent](adverseevent.html): When the event occurred 1933* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 1934* [Appointment](appointment.html): Appointment date/time. 1935* [AuditEvent](auditevent.html): Time when the event was recorded 1936* [CarePlan](careplan.html): Time period plan covers 1937* [CareTeam](careteam.html): A date within the coverage time period. 1938* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 1939* [Composition](composition.html): Composition editing time 1940* [Consent](consent.html): When consent was agreed to 1941* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 1942* [DocumentReference](documentreference.html): When this document reference was created 1943* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 1944* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 1945* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 1946* [Flag](flag.html): Time period when flag is active 1947* [Immunization](immunization.html): Vaccination (non)-Administration Date 1948* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 1949* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 1950* [Invoice](invoice.html): Invoice date / posting date 1951* [List](list.html): When the list was prepared 1952* [MeasureReport](measurereport.html): The date of the measure report 1953* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 1954* [Observation](observation.html): Clinically relevant time/time-period for observation 1955* [Procedure](procedure.html): When the procedure occurred or is occurring 1956* [ResearchSubject](researchsubject.html): Start and end of participation 1957* [RiskAssessment](riskassessment.html): When was assessment made? 1958* [SupplyRequest](supplyrequest.html): When the request was made 1959</b><br> 1960 * Type: <b>date</b><br> 1961 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 1962 * </p> 1963 */ 1964 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1965 1966 /** 1967 * Search parameter: <b>encounter</b> 1968 * <p> 1969 * Description: <b>Multiple Resources: 1970 1971* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 1972* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 1973* [ChargeItem](chargeitem.html): Encounter associated with event 1974* [Claim](claim.html): Encounters associated with a billed line item 1975* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 1976* [Communication](communication.html): The Encounter during which this Communication was created 1977* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 1978* [Composition](composition.html): Context of the Composition 1979* [Condition](condition.html): The Encounter during which this Condition was created 1980* [DeviceRequest](devicerequest.html): Encounter during which request was created 1981* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 1982* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 1983* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 1984* [Flag](flag.html): Alert relevant during encounter 1985* [ImagingStudy](imagingstudy.html): The context of the study 1986* [List](list.html): Context in which list created 1987* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 1988* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 1989* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 1990* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 1991* [Observation](observation.html): Encounter related to the observation 1992* [Procedure](procedure.html): The Encounter during which this Procedure was created 1993* [Provenance](provenance.html): Encounter related to the Provenance 1994* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 1995* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 1996* [RiskAssessment](riskassessment.html): Where was assessment performed? 1997* [ServiceRequest](servicerequest.html): An encounter in which this request is made 1998* [Task](task.html): Search by encounter 1999* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2000</b><br> 2001 * Type: <b>reference</b><br> 2002 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2003 * </p> 2004 */ 2005 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", target={Encounter.class } ) 2006 public static final String SP_ENCOUNTER = "encounter"; 2007 /** 2008 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2009 * <p> 2010 * Description: <b>Multiple Resources: 2011 2012* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 2013* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 2014* [ChargeItem](chargeitem.html): Encounter associated with event 2015* [Claim](claim.html): Encounters associated with a billed line item 2016* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 2017* [Communication](communication.html): The Encounter during which this Communication was created 2018* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 2019* [Composition](composition.html): Context of the Composition 2020* [Condition](condition.html): The Encounter during which this Condition was created 2021* [DeviceRequest](devicerequest.html): Encounter during which request was created 2022* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 2023* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 2024* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 2025* [Flag](flag.html): Alert relevant during encounter 2026* [ImagingStudy](imagingstudy.html): The context of the study 2027* [List](list.html): Context in which list created 2028* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 2029* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 2030* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 2031* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 2032* [Observation](observation.html): Encounter related to the observation 2033* [Procedure](procedure.html): The Encounter during which this Procedure was created 2034* [Provenance](provenance.html): Encounter related to the Provenance 2035* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 2036* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 2037* [RiskAssessment](riskassessment.html): Where was assessment performed? 2038* [ServiceRequest](servicerequest.html): An encounter in which this request is made 2039* [Task](task.html): Search by encounter 2040* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 2041</b><br> 2042 * Type: <b>reference</b><br> 2043 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 2044 * </p> 2045 */ 2046 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2047 2048/** 2049 * Constant for fluent queries to be used to add include statements. Specifies 2050 * the path value of "<b>RiskAssessment:encounter</b>". 2051 */ 2052 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("RiskAssessment:encounter").toLocked(); 2053 2054 /** 2055 * Search parameter: <b>identifier</b> 2056 * <p> 2057 * Description: <b>Multiple Resources: 2058 2059* [Account](account.html): Account number 2060* [AdverseEvent](adverseevent.html): Business identifier for the event 2061* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2062* [Appointment](appointment.html): An Identifier of the Appointment 2063* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2064* [Basic](basic.html): Business identifier 2065* [BodyStructure](bodystructure.html): Bodystructure identifier 2066* [CarePlan](careplan.html): External Ids for this plan 2067* [CareTeam](careteam.html): External Ids for this team 2068* [ChargeItem](chargeitem.html): Business Identifier for item 2069* [Claim](claim.html): The primary identifier of the financial resource 2070* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2071* [ClinicalImpression](clinicalimpression.html): Business identifier 2072* [Communication](communication.html): Unique identifier 2073* [CommunicationRequest](communicationrequest.html): Unique identifier 2074* [Composition](composition.html): Version-independent identifier for the Composition 2075* [Condition](condition.html): A unique identifier of the condition record 2076* [Consent](consent.html): Identifier for this record (external references) 2077* [Contract](contract.html): The identity of the contract 2078* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2079* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2080* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2081* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2082* [DeviceRequest](devicerequest.html): Business identifier for request/order 2083* [DeviceUsage](deviceusage.html): Search by identifier 2084* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2085* [DocumentReference](documentreference.html): Identifier of the attachment binary 2086* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2087* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2088* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2089* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2090* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2091* [Flag](flag.html): Business identifier 2092* [Goal](goal.html): External Ids for this goal 2093* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2094* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2095* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2096* [Immunization](immunization.html): Business identifier 2097* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2098* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2099* [Invoice](invoice.html): Business Identifier for item 2100* [List](list.html): Business identifier 2101* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2102* [Medication](medication.html): Returns medications with this external identifier 2103* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2104* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2105* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2106* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2107* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2108* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2109* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2110* [Observation](observation.html): The unique id for a particular observation 2111* [Person](person.html): A person Identifier 2112* [Procedure](procedure.html): A unique identifier for a procedure 2113* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2114* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2115* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2116* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2117* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2118* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2119* [Specimen](specimen.html): The unique identifier associated with the specimen 2120* [SupplyDelivery](supplydelivery.html): External identifier 2121* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2122* [Task](task.html): Search for a task instance by its business identifier 2123* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2124</b><br> 2125 * Type: <b>token</b><br> 2126 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2127 * </p> 2128 */ 2129 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2130 public static final String SP_IDENTIFIER = "identifier"; 2131 /** 2132 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2133 * <p> 2134 * Description: <b>Multiple Resources: 2135 2136* [Account](account.html): Account number 2137* [AdverseEvent](adverseevent.html): Business identifier for the event 2138* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2139* [Appointment](appointment.html): An Identifier of the Appointment 2140* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2141* [Basic](basic.html): Business identifier 2142* [BodyStructure](bodystructure.html): Bodystructure identifier 2143* [CarePlan](careplan.html): External Ids for this plan 2144* [CareTeam](careteam.html): External Ids for this team 2145* [ChargeItem](chargeitem.html): Business Identifier for item 2146* [Claim](claim.html): The primary identifier of the financial resource 2147* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2148* [ClinicalImpression](clinicalimpression.html): Business identifier 2149* [Communication](communication.html): Unique identifier 2150* [CommunicationRequest](communicationrequest.html): Unique identifier 2151* [Composition](composition.html): Version-independent identifier for the Composition 2152* [Condition](condition.html): A unique identifier of the condition record 2153* [Consent](consent.html): Identifier for this record (external references) 2154* [Contract](contract.html): The identity of the contract 2155* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2156* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2157* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2158* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2159* [DeviceRequest](devicerequest.html): Business identifier for request/order 2160* [DeviceUsage](deviceusage.html): Search by identifier 2161* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2162* [DocumentReference](documentreference.html): Identifier of the attachment binary 2163* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2164* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2165* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2166* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2167* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2168* [Flag](flag.html): Business identifier 2169* [Goal](goal.html): External Ids for this goal 2170* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2171* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2172* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2173* [Immunization](immunization.html): Business identifier 2174* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2175* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2176* [Invoice](invoice.html): Business Identifier for item 2177* [List](list.html): Business identifier 2178* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2179* [Medication](medication.html): Returns medications with this external identifier 2180* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2181* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2182* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2183* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2184* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2185* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2186* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2187* [Observation](observation.html): The unique id for a particular observation 2188* [Person](person.html): A person Identifier 2189* [Procedure](procedure.html): A unique identifier for a procedure 2190* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2191* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2192* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2193* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2194* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2195* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2196* [Specimen](specimen.html): The unique identifier associated with the specimen 2197* [SupplyDelivery](supplydelivery.html): External identifier 2198* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2199* [Task](task.html): Search for a task instance by its business identifier 2200* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2201</b><br> 2202 * Type: <b>token</b><br> 2203 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2204 * </p> 2205 */ 2206 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2207 2208 /** 2209 * Search parameter: <b>patient</b> 2210 * <p> 2211 * Description: <b>Multiple Resources: 2212 2213* [Account](account.html): The entity that caused the expenses 2214* [AdverseEvent](adverseevent.html): Subject impacted by event 2215* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2216* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2217* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2218* [AuditEvent](auditevent.html): Where the activity involved patient data 2219* [Basic](basic.html): Identifies the focus of this resource 2220* [BodyStructure](bodystructure.html): Who this is about 2221* [CarePlan](careplan.html): Who the care plan is for 2222* [CareTeam](careteam.html): Who care team is for 2223* [ChargeItem](chargeitem.html): Individual service was done for/to 2224* [Claim](claim.html): Patient receiving the products or services 2225* [ClaimResponse](claimresponse.html): The subject of care 2226* [ClinicalImpression](clinicalimpression.html): Patient assessed 2227* [Communication](communication.html): Focus of message 2228* [CommunicationRequest](communicationrequest.html): Focus of message 2229* [Composition](composition.html): Who and/or what the composition is about 2230* [Condition](condition.html): Who has the condition? 2231* [Consent](consent.html): Who the consent applies to 2232* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2233* [Coverage](coverage.html): Retrieve coverages for a patient 2234* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2235* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2236* [DetectedIssue](detectedissue.html): Associated patient 2237* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2238* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2239* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2240* [DocumentReference](documentreference.html): Who/what is the subject of the document 2241* [Encounter](encounter.html): The patient present at the encounter 2242* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2243* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2244* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2245* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2246* [Flag](flag.html): The identity of a subject to list flags for 2247* [Goal](goal.html): Who this goal is intended for 2248* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2249* [ImagingSelection](imagingselection.html): Who the study is about 2250* [ImagingStudy](imagingstudy.html): Who the study is about 2251* [Immunization](immunization.html): The patient for the vaccination record 2252* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2253* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2254* [Invoice](invoice.html): Recipient(s) of goods and services 2255* [List](list.html): If all resources have the same subject 2256* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2257* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2258* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2259* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2260* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2261* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2262* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2263* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2264* [Observation](observation.html): The subject that the observation is about (if patient) 2265* [Person](person.html): The Person links to this Patient 2266* [Procedure](procedure.html): Search by subject - a patient 2267* [Provenance](provenance.html): Where the activity involved patient data 2268* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2269* [RelatedPerson](relatedperson.html): The patient this related person is related to 2270* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2271* [ResearchSubject](researchsubject.html): Who or what is part of study 2272* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2273* [ServiceRequest](servicerequest.html): Search by subject - a patient 2274* [Specimen](specimen.html): The patient the specimen comes from 2275* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2276* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2277* [Task](task.html): Search by patient 2278* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2279</b><br> 2280 * Type: <b>reference</b><br> 2281 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2282 * </p> 2283 */ 2284 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 2285 public static final String SP_PATIENT = "patient"; 2286 /** 2287 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2288 * <p> 2289 * Description: <b>Multiple Resources: 2290 2291* [Account](account.html): The entity that caused the expenses 2292* [AdverseEvent](adverseevent.html): Subject impacted by event 2293* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2294* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2295* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2296* [AuditEvent](auditevent.html): Where the activity involved patient data 2297* [Basic](basic.html): Identifies the focus of this resource 2298* [BodyStructure](bodystructure.html): Who this is about 2299* [CarePlan](careplan.html): Who the care plan is for 2300* [CareTeam](careteam.html): Who care team is for 2301* [ChargeItem](chargeitem.html): Individual service was done for/to 2302* [Claim](claim.html): Patient receiving the products or services 2303* [ClaimResponse](claimresponse.html): The subject of care 2304* [ClinicalImpression](clinicalimpression.html): Patient assessed 2305* [Communication](communication.html): Focus of message 2306* [CommunicationRequest](communicationrequest.html): Focus of message 2307* [Composition](composition.html): Who and/or what the composition is about 2308* [Condition](condition.html): Who has the condition? 2309* [Consent](consent.html): Who the consent applies to 2310* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2311* [Coverage](coverage.html): Retrieve coverages for a patient 2312* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2313* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2314* [DetectedIssue](detectedissue.html): Associated patient 2315* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2316* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2317* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2318* [DocumentReference](documentreference.html): Who/what is the subject of the document 2319* [Encounter](encounter.html): The patient present at the encounter 2320* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2321* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2322* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2323* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2324* [Flag](flag.html): The identity of a subject to list flags for 2325* [Goal](goal.html): Who this goal is intended for 2326* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2327* [ImagingSelection](imagingselection.html): Who the study is about 2328* [ImagingStudy](imagingstudy.html): Who the study is about 2329* [Immunization](immunization.html): The patient for the vaccination record 2330* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2331* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2332* [Invoice](invoice.html): Recipient(s) of goods and services 2333* [List](list.html): If all resources have the same subject 2334* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2335* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2336* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2337* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2338* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2339* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2340* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2341* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2342* [Observation](observation.html): The subject that the observation is about (if patient) 2343* [Person](person.html): The Person links to this Patient 2344* [Procedure](procedure.html): Search by subject - a patient 2345* [Provenance](provenance.html): Where the activity involved patient data 2346* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2347* [RelatedPerson](relatedperson.html): The patient this related person is related to 2348* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2349* [ResearchSubject](researchsubject.html): Who or what is part of study 2350* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2351* [ServiceRequest](servicerequest.html): Search by subject - a patient 2352* [Specimen](specimen.html): The patient the specimen comes from 2353* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2354* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2355* [Task](task.html): Search by patient 2356* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2357</b><br> 2358 * Type: <b>reference</b><br> 2359 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2360 * </p> 2361 */ 2362 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2363 2364/** 2365 * Constant for fluent queries to be used to add include statements. Specifies 2366 * the path value of "<b>RiskAssessment:patient</b>". 2367 */ 2368 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("RiskAssessment:patient").toLocked(); 2369 2370 2371} 2372