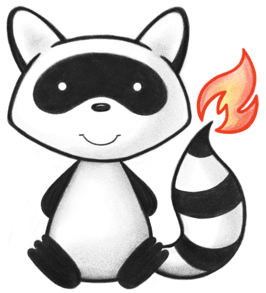
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.ChildOrder; 045import ca.uhn.fhir.model.api.annotation.DatatypeDef; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.Block; 048 049/** 050 * SampledData Type: A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data. 051 */ 052@DatatypeDef(name="SampledData") 053public class SampledData extends DataType implements ICompositeType { 054 055 /** 056 * The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series. 057 */ 058 @Child(name = "origin", type = {Quantity.class}, order=0, min=1, max=1, modifier=false, summary=true) 059 @Description(shortDefinition="Zero value and units", formalDefinition="The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series." ) 060 protected Quantity origin; 061 062 /** 063 * Amount of intervalUnits between samples, e.g. milliseconds for time-based sampling. 064 */ 065 @Child(name = "interval", type = {DecimalType.class}, order=1, min=0, max=1, modifier=false, summary=true) 066 @Description(shortDefinition="Number of intervalUnits between samples", formalDefinition="Amount of intervalUnits between samples, e.g. milliseconds for time-based sampling." ) 067 protected DecimalType interval; 068 069 /** 070 * The measurement unit in which the sample interval is expressed. 071 */ 072 @Child(name = "intervalUnit", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 073 @Description(shortDefinition="The measurement unit of the interval between samples", formalDefinition="The measurement unit in which the sample interval is expressed." ) 074 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ucum-units") 075 protected CodeType intervalUnit; 076 077 /** 078 * A correction factor that is applied to the sampled data points before they are added to the origin. 079 */ 080 @Child(name = "factor", type = {DecimalType.class}, order=3, min=0, max=1, modifier=false, summary=true) 081 @Description(shortDefinition="Multiply data by this before adding to origin", formalDefinition="A correction factor that is applied to the sampled data points before they are added to the origin." ) 082 protected DecimalType factor; 083 084 /** 085 * The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit). 086 */ 087 @Child(name = "lowerLimit", type = {DecimalType.class}, order=4, min=0, max=1, modifier=false, summary=true) 088 @Description(shortDefinition="Lower limit of detection", formalDefinition="The lower limit of detection of the measured points. This is needed if any of the data points have the value \"L\" (lower than detection limit)." ) 089 protected DecimalType lowerLimit; 090 091 /** 092 * The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit). 093 */ 094 @Child(name = "upperLimit", type = {DecimalType.class}, order=5, min=0, max=1, modifier=false, summary=true) 095 @Description(shortDefinition="Upper limit of detection", formalDefinition="The upper limit of detection of the measured points. This is needed if any of the data points have the value \"U\" (higher than detection limit)." ) 096 protected DecimalType upperLimit; 097 098 /** 099 * The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once. 100 */ 101 @Child(name = "dimensions", type = {PositiveIntType.class}, order=6, min=1, max=1, modifier=false, summary=true) 102 @Description(shortDefinition="Number of sample points at each time point", formalDefinition="The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once." ) 103 protected PositiveIntType dimensions; 104 105 /** 106 * Reference to ConceptMap that defines the codes used in the data. 107 */ 108 @Child(name = "codeMap", type = {CanonicalType.class}, order=7, min=0, max=1, modifier=false, summary=false) 109 @Description(shortDefinition="Defines the codes used in the data", formalDefinition="Reference to ConceptMap that defines the codes used in the data." ) 110 protected CanonicalType codeMap; 111 112 /** 113 * A series of data points which are decimal values separated by a single space (character u20). The units in which the offsets are expressed are found in intervalUnit. The absolute point at which the measurements begin SHALL be conveyed outside the scope of this datatype, e.g. Observation.effectiveDateTime for a timing offset. 114 */ 115 @Child(name = "offsets", type = {StringType.class}, order=8, min=0, max=1, modifier=false, summary=false) 116 @Description(shortDefinition="Offsets, typically in time, at which data values were taken", formalDefinition="A series of data points which are decimal values separated by a single space (character u20). The units in which the offsets are expressed are found in intervalUnit. The absolute point at which the measurements begin SHALL be conveyed outside the scope of this datatype, e.g. Observation.effectiveDateTime for a timing offset." ) 117 protected StringType offsets; 118 119 /** 120 * A series of data points which are decimal values or codes separated by a single space (character u20). The special codes "E" (error), "L" (below detection limit) and "U" (above detection limit) are also defined for used in place of decimal values. 121 */ 122 @Child(name = "data", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=false) 123 @Description(shortDefinition="Decimal values with spaces, or \"E\" | \"U\" | \"L\", or another code", formalDefinition="A series of data points which are decimal values or codes separated by a single space (character u20). The special codes \"E\" (error), \"L\" (below detection limit) and \"U\" (above detection limit) are also defined for used in place of decimal values." ) 124 protected StringType data; 125 126 private static final long serialVersionUID = 1859118926L; 127 128 /** 129 * Constructor 130 */ 131 public SampledData() { 132 super(); 133 } 134 135 /** 136 * Constructor 137 */ 138 public SampledData(Quantity origin, String intervalUnit, int dimensions) { 139 super(); 140 this.setOrigin(origin); 141 this.setIntervalUnit(intervalUnit); 142 this.setDimensions(dimensions); 143 } 144 145 /** 146 * @return {@link #origin} (The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series.) 147 */ 148 public Quantity getOrigin() { 149 if (this.origin == null) 150 if (Configuration.errorOnAutoCreate()) 151 throw new Error("Attempt to auto-create SampledData.origin"); 152 else if (Configuration.doAutoCreate()) 153 this.origin = new Quantity(); // cc 154 return this.origin; 155 } 156 157 public boolean hasOrigin() { 158 return this.origin != null && !this.origin.isEmpty(); 159 } 160 161 /** 162 * @param value {@link #origin} (The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series.) 163 */ 164 public SampledData setOrigin(Quantity value) { 165 this.origin = value; 166 return this; 167 } 168 169 /** 170 * @return {@link #interval} (Amount of intervalUnits between samples, e.g. milliseconds for time-based sampling.). This is the underlying object with id, value and extensions. The accessor "getInterval" gives direct access to the value 171 */ 172 public DecimalType getIntervalElement() { 173 if (this.interval == null) 174 if (Configuration.errorOnAutoCreate()) 175 throw new Error("Attempt to auto-create SampledData.interval"); 176 else if (Configuration.doAutoCreate()) 177 this.interval = new DecimalType(); // bb 178 return this.interval; 179 } 180 181 public boolean hasIntervalElement() { 182 return this.interval != null && !this.interval.isEmpty(); 183 } 184 185 public boolean hasInterval() { 186 return this.interval != null && !this.interval.isEmpty(); 187 } 188 189 /** 190 * @param value {@link #interval} (Amount of intervalUnits between samples, e.g. milliseconds for time-based sampling.). This is the underlying object with id, value and extensions. The accessor "getInterval" gives direct access to the value 191 */ 192 public SampledData setIntervalElement(DecimalType value) { 193 this.interval = value; 194 return this; 195 } 196 197 /** 198 * @return Amount of intervalUnits between samples, e.g. milliseconds for time-based sampling. 199 */ 200 public BigDecimal getInterval() { 201 return this.interval == null ? null : this.interval.getValue(); 202 } 203 204 /** 205 * @param value Amount of intervalUnits between samples, e.g. milliseconds for time-based sampling. 206 */ 207 public SampledData setInterval(BigDecimal value) { 208 if (value == null) 209 this.interval = null; 210 else { 211 if (this.interval == null) 212 this.interval = new DecimalType(); 213 this.interval.setValue(value); 214 } 215 return this; 216 } 217 218 /** 219 * @param value Amount of intervalUnits between samples, e.g. milliseconds for time-based sampling. 220 */ 221 public SampledData setInterval(long value) { 222 this.interval = new DecimalType(); 223 this.interval.setValue(value); 224 return this; 225 } 226 227 /** 228 * @param value Amount of intervalUnits between samples, e.g. milliseconds for time-based sampling. 229 */ 230 public SampledData setInterval(double value) { 231 this.interval = new DecimalType(); 232 this.interval.setValue(value); 233 return this; 234 } 235 236 /** 237 * @return {@link #intervalUnit} (The measurement unit in which the sample interval is expressed.). This is the underlying object with id, value and extensions. The accessor "getIntervalUnit" gives direct access to the value 238 */ 239 public CodeType getIntervalUnitElement() { 240 if (this.intervalUnit == null) 241 if (Configuration.errorOnAutoCreate()) 242 throw new Error("Attempt to auto-create SampledData.intervalUnit"); 243 else if (Configuration.doAutoCreate()) 244 this.intervalUnit = new CodeType(); // bb 245 return this.intervalUnit; 246 } 247 248 public boolean hasIntervalUnitElement() { 249 return this.intervalUnit != null && !this.intervalUnit.isEmpty(); 250 } 251 252 public boolean hasIntervalUnit() { 253 return this.intervalUnit != null && !this.intervalUnit.isEmpty(); 254 } 255 256 /** 257 * @param value {@link #intervalUnit} (The measurement unit in which the sample interval is expressed.). This is the underlying object with id, value and extensions. The accessor "getIntervalUnit" gives direct access to the value 258 */ 259 public SampledData setIntervalUnitElement(CodeType value) { 260 this.intervalUnit = value; 261 return this; 262 } 263 264 /** 265 * @return The measurement unit in which the sample interval is expressed. 266 */ 267 public String getIntervalUnit() { 268 return this.intervalUnit == null ? null : this.intervalUnit.getValue(); 269 } 270 271 /** 272 * @param value The measurement unit in which the sample interval is expressed. 273 */ 274 public SampledData setIntervalUnit(String value) { 275 if (this.intervalUnit == null) 276 this.intervalUnit = new CodeType(); 277 this.intervalUnit.setValue(value); 278 return this; 279 } 280 281 /** 282 * @return {@link #factor} (A correction factor that is applied to the sampled data points before they are added to the origin.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 283 */ 284 public DecimalType getFactorElement() { 285 if (this.factor == null) 286 if (Configuration.errorOnAutoCreate()) 287 throw new Error("Attempt to auto-create SampledData.factor"); 288 else if (Configuration.doAutoCreate()) 289 this.factor = new DecimalType(); // bb 290 return this.factor; 291 } 292 293 public boolean hasFactorElement() { 294 return this.factor != null && !this.factor.isEmpty(); 295 } 296 297 public boolean hasFactor() { 298 return this.factor != null && !this.factor.isEmpty(); 299 } 300 301 /** 302 * @param value {@link #factor} (A correction factor that is applied to the sampled data points before they are added to the origin.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 303 */ 304 public SampledData setFactorElement(DecimalType value) { 305 this.factor = value; 306 return this; 307 } 308 309 /** 310 * @return A correction factor that is applied to the sampled data points before they are added to the origin. 311 */ 312 public BigDecimal getFactor() { 313 return this.factor == null ? null : this.factor.getValue(); 314 } 315 316 /** 317 * @param value A correction factor that is applied to the sampled data points before they are added to the origin. 318 */ 319 public SampledData setFactor(BigDecimal value) { 320 if (value == null) 321 this.factor = null; 322 else { 323 if (this.factor == null) 324 this.factor = new DecimalType(); 325 this.factor.setValue(value); 326 } 327 return this; 328 } 329 330 /** 331 * @param value A correction factor that is applied to the sampled data points before they are added to the origin. 332 */ 333 public SampledData setFactor(long value) { 334 this.factor = new DecimalType(); 335 this.factor.setValue(value); 336 return this; 337 } 338 339 /** 340 * @param value A correction factor that is applied to the sampled data points before they are added to the origin. 341 */ 342 public SampledData setFactor(double value) { 343 this.factor = new DecimalType(); 344 this.factor.setValue(value); 345 return this; 346 } 347 348 /** 349 * @return {@link #lowerLimit} (The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit).). This is the underlying object with id, value and extensions. The accessor "getLowerLimit" gives direct access to the value 350 */ 351 public DecimalType getLowerLimitElement() { 352 if (this.lowerLimit == null) 353 if (Configuration.errorOnAutoCreate()) 354 throw new Error("Attempt to auto-create SampledData.lowerLimit"); 355 else if (Configuration.doAutoCreate()) 356 this.lowerLimit = new DecimalType(); // bb 357 return this.lowerLimit; 358 } 359 360 public boolean hasLowerLimitElement() { 361 return this.lowerLimit != null && !this.lowerLimit.isEmpty(); 362 } 363 364 public boolean hasLowerLimit() { 365 return this.lowerLimit != null && !this.lowerLimit.isEmpty(); 366 } 367 368 /** 369 * @param value {@link #lowerLimit} (The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit).). This is the underlying object with id, value and extensions. The accessor "getLowerLimit" gives direct access to the value 370 */ 371 public SampledData setLowerLimitElement(DecimalType value) { 372 this.lowerLimit = value; 373 return this; 374 } 375 376 /** 377 * @return The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit). 378 */ 379 public BigDecimal getLowerLimit() { 380 return this.lowerLimit == null ? null : this.lowerLimit.getValue(); 381 } 382 383 /** 384 * @param value The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit). 385 */ 386 public SampledData setLowerLimit(BigDecimal value) { 387 if (value == null) 388 this.lowerLimit = null; 389 else { 390 if (this.lowerLimit == null) 391 this.lowerLimit = new DecimalType(); 392 this.lowerLimit.setValue(value); 393 } 394 return this; 395 } 396 397 /** 398 * @param value The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit). 399 */ 400 public SampledData setLowerLimit(long value) { 401 this.lowerLimit = new DecimalType(); 402 this.lowerLimit.setValue(value); 403 return this; 404 } 405 406 /** 407 * @param value The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit). 408 */ 409 public SampledData setLowerLimit(double value) { 410 this.lowerLimit = new DecimalType(); 411 this.lowerLimit.setValue(value); 412 return this; 413 } 414 415 /** 416 * @return {@link #upperLimit} (The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit).). This is the underlying object with id, value and extensions. The accessor "getUpperLimit" gives direct access to the value 417 */ 418 public DecimalType getUpperLimitElement() { 419 if (this.upperLimit == null) 420 if (Configuration.errorOnAutoCreate()) 421 throw new Error("Attempt to auto-create SampledData.upperLimit"); 422 else if (Configuration.doAutoCreate()) 423 this.upperLimit = new DecimalType(); // bb 424 return this.upperLimit; 425 } 426 427 public boolean hasUpperLimitElement() { 428 return this.upperLimit != null && !this.upperLimit.isEmpty(); 429 } 430 431 public boolean hasUpperLimit() { 432 return this.upperLimit != null && !this.upperLimit.isEmpty(); 433 } 434 435 /** 436 * @param value {@link #upperLimit} (The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit).). This is the underlying object with id, value and extensions. The accessor "getUpperLimit" gives direct access to the value 437 */ 438 public SampledData setUpperLimitElement(DecimalType value) { 439 this.upperLimit = value; 440 return this; 441 } 442 443 /** 444 * @return The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit). 445 */ 446 public BigDecimal getUpperLimit() { 447 return this.upperLimit == null ? null : this.upperLimit.getValue(); 448 } 449 450 /** 451 * @param value The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit). 452 */ 453 public SampledData setUpperLimit(BigDecimal value) { 454 if (value == null) 455 this.upperLimit = null; 456 else { 457 if (this.upperLimit == null) 458 this.upperLimit = new DecimalType(); 459 this.upperLimit.setValue(value); 460 } 461 return this; 462 } 463 464 /** 465 * @param value The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit). 466 */ 467 public SampledData setUpperLimit(long value) { 468 this.upperLimit = new DecimalType(); 469 this.upperLimit.setValue(value); 470 return this; 471 } 472 473 /** 474 * @param value The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit). 475 */ 476 public SampledData setUpperLimit(double value) { 477 this.upperLimit = new DecimalType(); 478 this.upperLimit.setValue(value); 479 return this; 480 } 481 482 /** 483 * @return {@link #dimensions} (The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once.). This is the underlying object with id, value and extensions. The accessor "getDimensions" gives direct access to the value 484 */ 485 public PositiveIntType getDimensionsElement() { 486 if (this.dimensions == null) 487 if (Configuration.errorOnAutoCreate()) 488 throw new Error("Attempt to auto-create SampledData.dimensions"); 489 else if (Configuration.doAutoCreate()) 490 this.dimensions = new PositiveIntType(); // bb 491 return this.dimensions; 492 } 493 494 public boolean hasDimensionsElement() { 495 return this.dimensions != null && !this.dimensions.isEmpty(); 496 } 497 498 public boolean hasDimensions() { 499 return this.dimensions != null && !this.dimensions.isEmpty(); 500 } 501 502 /** 503 * @param value {@link #dimensions} (The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once.). This is the underlying object with id, value and extensions. The accessor "getDimensions" gives direct access to the value 504 */ 505 public SampledData setDimensionsElement(PositiveIntType value) { 506 this.dimensions = value; 507 return this; 508 } 509 510 /** 511 * @return The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once. 512 */ 513 public int getDimensions() { 514 return this.dimensions == null || this.dimensions.isEmpty() ? 0 : this.dimensions.getValue(); 515 } 516 517 /** 518 * @param value The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once. 519 */ 520 public SampledData setDimensions(int value) { 521 if (this.dimensions == null) 522 this.dimensions = new PositiveIntType(); 523 this.dimensions.setValue(value); 524 return this; 525 } 526 527 /** 528 * @return {@link #codeMap} (Reference to ConceptMap that defines the codes used in the data.). This is the underlying object with id, value and extensions. The accessor "getCodeMap" gives direct access to the value 529 */ 530 public CanonicalType getCodeMapElement() { 531 if (this.codeMap == null) 532 if (Configuration.errorOnAutoCreate()) 533 throw new Error("Attempt to auto-create SampledData.codeMap"); 534 else if (Configuration.doAutoCreate()) 535 this.codeMap = new CanonicalType(); // bb 536 return this.codeMap; 537 } 538 539 public boolean hasCodeMapElement() { 540 return this.codeMap != null && !this.codeMap.isEmpty(); 541 } 542 543 public boolean hasCodeMap() { 544 return this.codeMap != null && !this.codeMap.isEmpty(); 545 } 546 547 /** 548 * @param value {@link #codeMap} (Reference to ConceptMap that defines the codes used in the data.). This is the underlying object with id, value and extensions. The accessor "getCodeMap" gives direct access to the value 549 */ 550 public SampledData setCodeMapElement(CanonicalType value) { 551 this.codeMap = value; 552 return this; 553 } 554 555 /** 556 * @return Reference to ConceptMap that defines the codes used in the data. 557 */ 558 public String getCodeMap() { 559 return this.codeMap == null ? null : this.codeMap.getValue(); 560 } 561 562 /** 563 * @param value Reference to ConceptMap that defines the codes used in the data. 564 */ 565 public SampledData setCodeMap(String value) { 566 if (Utilities.noString(value)) 567 this.codeMap = null; 568 else { 569 if (this.codeMap == null) 570 this.codeMap = new CanonicalType(); 571 this.codeMap.setValue(value); 572 } 573 return this; 574 } 575 576 /** 577 * @return {@link #offsets} (A series of data points which are decimal values separated by a single space (character u20). The units in which the offsets are expressed are found in intervalUnit. The absolute point at which the measurements begin SHALL be conveyed outside the scope of this datatype, e.g. Observation.effectiveDateTime for a timing offset.). This is the underlying object with id, value and extensions. The accessor "getOffsets" gives direct access to the value 578 */ 579 public StringType getOffsetsElement() { 580 if (this.offsets == null) 581 if (Configuration.errorOnAutoCreate()) 582 throw new Error("Attempt to auto-create SampledData.offsets"); 583 else if (Configuration.doAutoCreate()) 584 this.offsets = new StringType(); // bb 585 return this.offsets; 586 } 587 588 public boolean hasOffsetsElement() { 589 return this.offsets != null && !this.offsets.isEmpty(); 590 } 591 592 public boolean hasOffsets() { 593 return this.offsets != null && !this.offsets.isEmpty(); 594 } 595 596 /** 597 * @param value {@link #offsets} (A series of data points which are decimal values separated by a single space (character u20). The units in which the offsets are expressed are found in intervalUnit. The absolute point at which the measurements begin SHALL be conveyed outside the scope of this datatype, e.g. Observation.effectiveDateTime for a timing offset.). This is the underlying object with id, value and extensions. The accessor "getOffsets" gives direct access to the value 598 */ 599 public SampledData setOffsetsElement(StringType value) { 600 this.offsets = value; 601 return this; 602 } 603 604 /** 605 * @return A series of data points which are decimal values separated by a single space (character u20). The units in which the offsets are expressed are found in intervalUnit. The absolute point at which the measurements begin SHALL be conveyed outside the scope of this datatype, e.g. Observation.effectiveDateTime for a timing offset. 606 */ 607 public String getOffsets() { 608 return this.offsets == null ? null : this.offsets.getValue(); 609 } 610 611 /** 612 * @param value A series of data points which are decimal values separated by a single space (character u20). The units in which the offsets are expressed are found in intervalUnit. The absolute point at which the measurements begin SHALL be conveyed outside the scope of this datatype, e.g. Observation.effectiveDateTime for a timing offset. 613 */ 614 public SampledData setOffsets(String value) { 615 if (Utilities.noString(value)) 616 this.offsets = null; 617 else { 618 if (this.offsets == null) 619 this.offsets = new StringType(); 620 this.offsets.setValue(value); 621 } 622 return this; 623 } 624 625 /** 626 * @return {@link #data} (A series of data points which are decimal values or codes separated by a single space (character u20). The special codes "E" (error), "L" (below detection limit) and "U" (above detection limit) are also defined for used in place of decimal values.). This is the underlying object with id, value and extensions. The accessor "getData" gives direct access to the value 627 */ 628 public StringType getDataElement() { 629 if (this.data == null) 630 if (Configuration.errorOnAutoCreate()) 631 throw new Error("Attempt to auto-create SampledData.data"); 632 else if (Configuration.doAutoCreate()) 633 this.data = new StringType(); // bb 634 return this.data; 635 } 636 637 public boolean hasDataElement() { 638 return this.data != null && !this.data.isEmpty(); 639 } 640 641 public boolean hasData() { 642 return this.data != null && !this.data.isEmpty(); 643 } 644 645 /** 646 * @param value {@link #data} (A series of data points which are decimal values or codes separated by a single space (character u20). The special codes "E" (error), "L" (below detection limit) and "U" (above detection limit) are also defined for used in place of decimal values.). This is the underlying object with id, value and extensions. The accessor "getData" gives direct access to the value 647 */ 648 public SampledData setDataElement(StringType value) { 649 this.data = value; 650 return this; 651 } 652 653 /** 654 * @return A series of data points which are decimal values or codes separated by a single space (character u20). The special codes "E" (error), "L" (below detection limit) and "U" (above detection limit) are also defined for used in place of decimal values. 655 */ 656 public String getData() { 657 return this.data == null ? null : this.data.getValue(); 658 } 659 660 /** 661 * @param value A series of data points which are decimal values or codes separated by a single space (character u20). The special codes "E" (error), "L" (below detection limit) and "U" (above detection limit) are also defined for used in place of decimal values. 662 */ 663 public SampledData setData(String value) { 664 if (Utilities.noString(value)) 665 this.data = null; 666 else { 667 if (this.data == null) 668 this.data = new StringType(); 669 this.data.setValue(value); 670 } 671 return this; 672 } 673 674 protected void listChildren(List<Property> children) { 675 super.listChildren(children); 676 children.add(new Property("origin", "Quantity", "The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series.", 0, 1, origin)); 677 children.add(new Property("interval", "decimal", "Amount of intervalUnits between samples, e.g. milliseconds for time-based sampling.", 0, 1, interval)); 678 children.add(new Property("intervalUnit", "code", "The measurement unit in which the sample interval is expressed.", 0, 1, intervalUnit)); 679 children.add(new Property("factor", "decimal", "A correction factor that is applied to the sampled data points before they are added to the origin.", 0, 1, factor)); 680 children.add(new Property("lowerLimit", "decimal", "The lower limit of detection of the measured points. This is needed if any of the data points have the value \"L\" (lower than detection limit).", 0, 1, lowerLimit)); 681 children.add(new Property("upperLimit", "decimal", "The upper limit of detection of the measured points. This is needed if any of the data points have the value \"U\" (higher than detection limit).", 0, 1, upperLimit)); 682 children.add(new Property("dimensions", "positiveInt", "The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once.", 0, 1, dimensions)); 683 children.add(new Property("codeMap", "canonical(ConceptMap)", "Reference to ConceptMap that defines the codes used in the data.", 0, 1, codeMap)); 684 children.add(new Property("offsets", "string", "A series of data points which are decimal values separated by a single space (character u20). The units in which the offsets are expressed are found in intervalUnit. The absolute point at which the measurements begin SHALL be conveyed outside the scope of this datatype, e.g. Observation.effectiveDateTime for a timing offset.", 0, 1, offsets)); 685 children.add(new Property("data", "string", "A series of data points which are decimal values or codes separated by a single space (character u20). The special codes \"E\" (error), \"L\" (below detection limit) and \"U\" (above detection limit) are also defined for used in place of decimal values.", 0, 1, data)); 686 } 687 688 @Override 689 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 690 switch (_hash) { 691 case -1008619738: /*origin*/ return new Property("origin", "Quantity", "The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series.", 0, 1, origin); 692 case 570418373: /*interval*/ return new Property("interval", "decimal", "Amount of intervalUnits between samples, e.g. milliseconds for time-based sampling.", 0, 1, interval); 693 case -1569830935: /*intervalUnit*/ return new Property("intervalUnit", "code", "The measurement unit in which the sample interval is expressed.", 0, 1, intervalUnit); 694 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A correction factor that is applied to the sampled data points before they are added to the origin.", 0, 1, factor); 695 case 1209133370: /*lowerLimit*/ return new Property("lowerLimit", "decimal", "The lower limit of detection of the measured points. This is needed if any of the data points have the value \"L\" (lower than detection limit).", 0, 1, lowerLimit); 696 case -1681713095: /*upperLimit*/ return new Property("upperLimit", "decimal", "The upper limit of detection of the measured points. This is needed if any of the data points have the value \"U\" (higher than detection limit).", 0, 1, upperLimit); 697 case 414334925: /*dimensions*/ return new Property("dimensions", "positiveInt", "The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once.", 0, 1, dimensions); 698 case 941825071: /*codeMap*/ return new Property("codeMap", "canonical(ConceptMap)", "Reference to ConceptMap that defines the codes used in the data.", 0, 1, codeMap); 699 case -1548407232: /*offsets*/ return new Property("offsets", "string", "A series of data points which are decimal values separated by a single space (character u20). The units in which the offsets are expressed are found in intervalUnit. The absolute point at which the measurements begin SHALL be conveyed outside the scope of this datatype, e.g. Observation.effectiveDateTime for a timing offset.", 0, 1, offsets); 700 case 3076010: /*data*/ return new Property("data", "string", "A series of data points which are decimal values or codes separated by a single space (character u20). The special codes \"E\" (error), \"L\" (below detection limit) and \"U\" (above detection limit) are also defined for used in place of decimal values.", 0, 1, data); 701 default: return super.getNamedProperty(_hash, _name, _checkValid); 702 } 703 704 } 705 706 @Override 707 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 708 switch (hash) { 709 case -1008619738: /*origin*/ return this.origin == null ? new Base[0] : new Base[] {this.origin}; // Quantity 710 case 570418373: /*interval*/ return this.interval == null ? new Base[0] : new Base[] {this.interval}; // DecimalType 711 case -1569830935: /*intervalUnit*/ return this.intervalUnit == null ? new Base[0] : new Base[] {this.intervalUnit}; // CodeType 712 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 713 case 1209133370: /*lowerLimit*/ return this.lowerLimit == null ? new Base[0] : new Base[] {this.lowerLimit}; // DecimalType 714 case -1681713095: /*upperLimit*/ return this.upperLimit == null ? new Base[0] : new Base[] {this.upperLimit}; // DecimalType 715 case 414334925: /*dimensions*/ return this.dimensions == null ? new Base[0] : new Base[] {this.dimensions}; // PositiveIntType 716 case 941825071: /*codeMap*/ return this.codeMap == null ? new Base[0] : new Base[] {this.codeMap}; // CanonicalType 717 case -1548407232: /*offsets*/ return this.offsets == null ? new Base[0] : new Base[] {this.offsets}; // StringType 718 case 3076010: /*data*/ return this.data == null ? new Base[0] : new Base[] {this.data}; // StringType 719 default: return super.getProperty(hash, name, checkValid); 720 } 721 722 } 723 724 @Override 725 public Base setProperty(int hash, String name, Base value) throws FHIRException { 726 switch (hash) { 727 case -1008619738: // origin 728 this.origin = TypeConvertor.castToQuantity(value); // Quantity 729 return value; 730 case 570418373: // interval 731 this.interval = TypeConvertor.castToDecimal(value); // DecimalType 732 return value; 733 case -1569830935: // intervalUnit 734 this.intervalUnit = TypeConvertor.castToCode(value); // CodeType 735 return value; 736 case -1282148017: // factor 737 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 738 return value; 739 case 1209133370: // lowerLimit 740 this.lowerLimit = TypeConvertor.castToDecimal(value); // DecimalType 741 return value; 742 case -1681713095: // upperLimit 743 this.upperLimit = TypeConvertor.castToDecimal(value); // DecimalType 744 return value; 745 case 414334925: // dimensions 746 this.dimensions = TypeConvertor.castToPositiveInt(value); // PositiveIntType 747 return value; 748 case 941825071: // codeMap 749 this.codeMap = TypeConvertor.castToCanonical(value); // CanonicalType 750 return value; 751 case -1548407232: // offsets 752 this.offsets = TypeConvertor.castToString(value); // StringType 753 return value; 754 case 3076010: // data 755 this.data = TypeConvertor.castToString(value); // StringType 756 return value; 757 default: return super.setProperty(hash, name, value); 758 } 759 760 } 761 762 @Override 763 public Base setProperty(String name, Base value) throws FHIRException { 764 if (name.equals("origin")) { 765 this.origin = TypeConvertor.castToQuantity(value); // Quantity 766 } else if (name.equals("interval")) { 767 this.interval = TypeConvertor.castToDecimal(value); // DecimalType 768 } else if (name.equals("intervalUnit")) { 769 this.intervalUnit = TypeConvertor.castToCode(value); // CodeType 770 } else if (name.equals("factor")) { 771 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 772 } else if (name.equals("lowerLimit")) { 773 this.lowerLimit = TypeConvertor.castToDecimal(value); // DecimalType 774 } else if (name.equals("upperLimit")) { 775 this.upperLimit = TypeConvertor.castToDecimal(value); // DecimalType 776 } else if (name.equals("dimensions")) { 777 this.dimensions = TypeConvertor.castToPositiveInt(value); // PositiveIntType 778 } else if (name.equals("codeMap")) { 779 this.codeMap = TypeConvertor.castToCanonical(value); // CanonicalType 780 } else if (name.equals("offsets")) { 781 this.offsets = TypeConvertor.castToString(value); // StringType 782 } else if (name.equals("data")) { 783 this.data = TypeConvertor.castToString(value); // StringType 784 } else 785 return super.setProperty(name, value); 786 return value; 787 } 788 789 @Override 790 public void removeChild(String name, Base value) throws FHIRException { 791 if (name.equals("origin")) { 792 this.origin = null; 793 } else if (name.equals("interval")) { 794 this.interval = null; 795 } else if (name.equals("intervalUnit")) { 796 this.intervalUnit = null; 797 } else if (name.equals("factor")) { 798 this.factor = null; 799 } else if (name.equals("lowerLimit")) { 800 this.lowerLimit = null; 801 } else if (name.equals("upperLimit")) { 802 this.upperLimit = null; 803 } else if (name.equals("dimensions")) { 804 this.dimensions = null; 805 } else if (name.equals("codeMap")) { 806 this.codeMap = null; 807 } else if (name.equals("offsets")) { 808 this.offsets = null; 809 } else if (name.equals("data")) { 810 this.data = null; 811 } else 812 super.removeChild(name, value); 813 814 } 815 816 @Override 817 public Base makeProperty(int hash, String name) throws FHIRException { 818 switch (hash) { 819 case -1008619738: return getOrigin(); 820 case 570418373: return getIntervalElement(); 821 case -1569830935: return getIntervalUnitElement(); 822 case -1282148017: return getFactorElement(); 823 case 1209133370: return getLowerLimitElement(); 824 case -1681713095: return getUpperLimitElement(); 825 case 414334925: return getDimensionsElement(); 826 case 941825071: return getCodeMapElement(); 827 case -1548407232: return getOffsetsElement(); 828 case 3076010: return getDataElement(); 829 default: return super.makeProperty(hash, name); 830 } 831 832 } 833 834 @Override 835 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 836 switch (hash) { 837 case -1008619738: /*origin*/ return new String[] {"Quantity"}; 838 case 570418373: /*interval*/ return new String[] {"decimal"}; 839 case -1569830935: /*intervalUnit*/ return new String[] {"code"}; 840 case -1282148017: /*factor*/ return new String[] {"decimal"}; 841 case 1209133370: /*lowerLimit*/ return new String[] {"decimal"}; 842 case -1681713095: /*upperLimit*/ return new String[] {"decimal"}; 843 case 414334925: /*dimensions*/ return new String[] {"positiveInt"}; 844 case 941825071: /*codeMap*/ return new String[] {"canonical"}; 845 case -1548407232: /*offsets*/ return new String[] {"string"}; 846 case 3076010: /*data*/ return new String[] {"string"}; 847 default: return super.getTypesForProperty(hash, name); 848 } 849 850 } 851 852 @Override 853 public Base addChild(String name) throws FHIRException { 854 if (name.equals("origin")) { 855 this.origin = new Quantity(); 856 return this.origin; 857 } 858 else if (name.equals("interval")) { 859 throw new FHIRException("Cannot call addChild on a singleton property SampledData.interval"); 860 } 861 else if (name.equals("intervalUnit")) { 862 throw new FHIRException("Cannot call addChild on a singleton property SampledData.intervalUnit"); 863 } 864 else if (name.equals("factor")) { 865 throw new FHIRException("Cannot call addChild on a singleton property SampledData.factor"); 866 } 867 else if (name.equals("lowerLimit")) { 868 throw new FHIRException("Cannot call addChild on a singleton property SampledData.lowerLimit"); 869 } 870 else if (name.equals("upperLimit")) { 871 throw new FHIRException("Cannot call addChild on a singleton property SampledData.upperLimit"); 872 } 873 else if (name.equals("dimensions")) { 874 throw new FHIRException("Cannot call addChild on a singleton property SampledData.dimensions"); 875 } 876 else if (name.equals("codeMap")) { 877 throw new FHIRException("Cannot call addChild on a singleton property SampledData.codeMap"); 878 } 879 else if (name.equals("offsets")) { 880 throw new FHIRException("Cannot call addChild on a singleton property SampledData.offsets"); 881 } 882 else if (name.equals("data")) { 883 throw new FHIRException("Cannot call addChild on a singleton property SampledData.data"); 884 } 885 else 886 return super.addChild(name); 887 } 888 889 public String fhirType() { 890 return "SampledData"; 891 892 } 893 894 public SampledData copy() { 895 SampledData dst = new SampledData(); 896 copyValues(dst); 897 return dst; 898 } 899 900 public void copyValues(SampledData dst) { 901 super.copyValues(dst); 902 dst.origin = origin == null ? null : origin.copy(); 903 dst.interval = interval == null ? null : interval.copy(); 904 dst.intervalUnit = intervalUnit == null ? null : intervalUnit.copy(); 905 dst.factor = factor == null ? null : factor.copy(); 906 dst.lowerLimit = lowerLimit == null ? null : lowerLimit.copy(); 907 dst.upperLimit = upperLimit == null ? null : upperLimit.copy(); 908 dst.dimensions = dimensions == null ? null : dimensions.copy(); 909 dst.codeMap = codeMap == null ? null : codeMap.copy(); 910 dst.offsets = offsets == null ? null : offsets.copy(); 911 dst.data = data == null ? null : data.copy(); 912 } 913 914 protected SampledData typedCopy() { 915 return copy(); 916 } 917 918 @Override 919 public boolean equalsDeep(Base other_) { 920 if (!super.equalsDeep(other_)) 921 return false; 922 if (!(other_ instanceof SampledData)) 923 return false; 924 SampledData o = (SampledData) other_; 925 return compareDeep(origin, o.origin, true) && compareDeep(interval, o.interval, true) && compareDeep(intervalUnit, o.intervalUnit, true) 926 && compareDeep(factor, o.factor, true) && compareDeep(lowerLimit, o.lowerLimit, true) && compareDeep(upperLimit, o.upperLimit, true) 927 && compareDeep(dimensions, o.dimensions, true) && compareDeep(codeMap, o.codeMap, true) && compareDeep(offsets, o.offsets, true) 928 && compareDeep(data, o.data, true); 929 } 930 931 @Override 932 public boolean equalsShallow(Base other_) { 933 if (!super.equalsShallow(other_)) 934 return false; 935 if (!(other_ instanceof SampledData)) 936 return false; 937 SampledData o = (SampledData) other_; 938 return compareValues(interval, o.interval, true) && compareValues(intervalUnit, o.intervalUnit, true) 939 && compareValues(factor, o.factor, true) && compareValues(lowerLimit, o.lowerLimit, true) && compareValues(upperLimit, o.upperLimit, true) 940 && compareValues(dimensions, o.dimensions, true) && compareValues(codeMap, o.codeMap, true) && compareValues(offsets, o.offsets, true) 941 && compareValues(data, o.data, true); 942 } 943 944 public boolean isEmpty() { 945 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(origin, interval, intervalUnit 946 , factor, lowerLimit, upperLimit, dimensions, codeMap, offsets, data); 947 } 948 949 950} 951