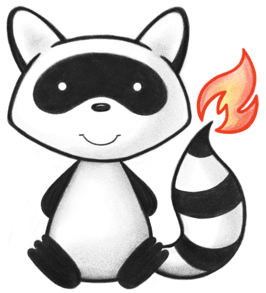
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A container for slots of time that may be available for booking appointments. 052 */ 053@ResourceDef(name="Schedule", profile="http://hl7.org/fhir/StructureDefinition/Schedule") 054public class Schedule extends DomainResource { 055 056 /** 057 * External Ids for this item. 058 */ 059 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 060 @Description(shortDefinition="External Ids for this item", formalDefinition="External Ids for this item." ) 061 protected List<Identifier> identifier; 062 063 /** 064 * Whether this schedule record is in active use or should not be used (such as was entered in error). 065 */ 066 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=true, summary=true) 067 @Description(shortDefinition="Whether this schedule is in active use", formalDefinition="Whether this schedule record is in active use or should not be used (such as was entered in error)." ) 068 protected BooleanType active; 069 070 /** 071 * A broad categorization of the service that is to be performed during this appointment. 072 */ 073 @Child(name = "serviceCategory", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 074 @Description(shortDefinition="High-level category", formalDefinition="A broad categorization of the service that is to be performed during this appointment." ) 075 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-category") 076 protected List<CodeableConcept> serviceCategory; 077 078 /** 079 * The specific service that is to be performed during this appointment. 080 */ 081 @Child(name = "serviceType", type = {CodeableReference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 082 @Description(shortDefinition="Specific service", formalDefinition="The specific service that is to be performed during this appointment." ) 083 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-type") 084 protected List<CodeableReference> serviceType; 085 086 /** 087 * The specialty of a practitioner that would be required to perform the service requested in this appointment. 088 */ 089 @Child(name = "specialty", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 090 @Description(shortDefinition="Type of specialty needed", formalDefinition="The specialty of a practitioner that would be required to perform the service requested in this appointment." ) 091 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/c80-practice-codes") 092 protected List<CodeableConcept> specialty; 093 094 /** 095 * Further description of the schedule as it would be presented to a consumer while searching. 096 */ 097 @Child(name = "name", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 098 @Description(shortDefinition="Human-readable label", formalDefinition="Further description of the schedule as it would be presented to a consumer while searching." ) 099 protected StringType name; 100 101 /** 102 * Slots that reference this schedule resource provide the availability details to these referenced resource(s). 103 */ 104 @Child(name = "actor", type = {Patient.class, Practitioner.class, PractitionerRole.class, CareTeam.class, RelatedPerson.class, Device.class, HealthcareService.class, Location.class}, order=6, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 105 @Description(shortDefinition="Resource(s) that availability information is being provided for", formalDefinition="Slots that reference this schedule resource provide the availability details to these referenced resource(s)." ) 106 protected List<Reference> actor; 107 108 /** 109 * The period of time that the slots that reference this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a "template" for planning outside these dates. 110 */ 111 @Child(name = "planningHorizon", type = {Period.class}, order=7, min=0, max=1, modifier=false, summary=true) 112 @Description(shortDefinition="Period of time covered by schedule", formalDefinition="The period of time that the slots that reference this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a \"template\" for planning outside these dates." ) 113 protected Period planningHorizon; 114 115 /** 116 * Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated. 117 */ 118 @Child(name = "comment", type = {MarkdownType.class}, order=8, min=0, max=1, modifier=false, summary=false) 119 @Description(shortDefinition="Comments on availability", formalDefinition="Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated." ) 120 protected MarkdownType comment; 121 122 private static final long serialVersionUID = -370559713L; 123 124 /** 125 * Constructor 126 */ 127 public Schedule() { 128 super(); 129 } 130 131 /** 132 * Constructor 133 */ 134 public Schedule(Reference actor) { 135 super(); 136 this.addActor(actor); 137 } 138 139 /** 140 * @return {@link #identifier} (External Ids for this item.) 141 */ 142 public List<Identifier> getIdentifier() { 143 if (this.identifier == null) 144 this.identifier = new ArrayList<Identifier>(); 145 return this.identifier; 146 } 147 148 /** 149 * @return Returns a reference to <code>this</code> for easy method chaining 150 */ 151 public Schedule setIdentifier(List<Identifier> theIdentifier) { 152 this.identifier = theIdentifier; 153 return this; 154 } 155 156 public boolean hasIdentifier() { 157 if (this.identifier == null) 158 return false; 159 for (Identifier item : this.identifier) 160 if (!item.isEmpty()) 161 return true; 162 return false; 163 } 164 165 public Identifier addIdentifier() { //3 166 Identifier t = new Identifier(); 167 if (this.identifier == null) 168 this.identifier = new ArrayList<Identifier>(); 169 this.identifier.add(t); 170 return t; 171 } 172 173 public Schedule addIdentifier(Identifier t) { //3 174 if (t == null) 175 return this; 176 if (this.identifier == null) 177 this.identifier = new ArrayList<Identifier>(); 178 this.identifier.add(t); 179 return this; 180 } 181 182 /** 183 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 184 */ 185 public Identifier getIdentifierFirstRep() { 186 if (getIdentifier().isEmpty()) { 187 addIdentifier(); 188 } 189 return getIdentifier().get(0); 190 } 191 192 /** 193 * @return {@link #active} (Whether this schedule record is in active use or should not be used (such as was entered in error).). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 194 */ 195 public BooleanType getActiveElement() { 196 if (this.active == null) 197 if (Configuration.errorOnAutoCreate()) 198 throw new Error("Attempt to auto-create Schedule.active"); 199 else if (Configuration.doAutoCreate()) 200 this.active = new BooleanType(); // bb 201 return this.active; 202 } 203 204 public boolean hasActiveElement() { 205 return this.active != null && !this.active.isEmpty(); 206 } 207 208 public boolean hasActive() { 209 return this.active != null && !this.active.isEmpty(); 210 } 211 212 /** 213 * @param value {@link #active} (Whether this schedule record is in active use or should not be used (such as was entered in error).). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 214 */ 215 public Schedule setActiveElement(BooleanType value) { 216 this.active = value; 217 return this; 218 } 219 220 /** 221 * @return Whether this schedule record is in active use or should not be used (such as was entered in error). 222 */ 223 public boolean getActive() { 224 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 225 } 226 227 /** 228 * @param value Whether this schedule record is in active use or should not be used (such as was entered in error). 229 */ 230 public Schedule setActive(boolean value) { 231 if (this.active == null) 232 this.active = new BooleanType(); 233 this.active.setValue(value); 234 return this; 235 } 236 237 /** 238 * @return {@link #serviceCategory} (A broad categorization of the service that is to be performed during this appointment.) 239 */ 240 public List<CodeableConcept> getServiceCategory() { 241 if (this.serviceCategory == null) 242 this.serviceCategory = new ArrayList<CodeableConcept>(); 243 return this.serviceCategory; 244 } 245 246 /** 247 * @return Returns a reference to <code>this</code> for easy method chaining 248 */ 249 public Schedule setServiceCategory(List<CodeableConcept> theServiceCategory) { 250 this.serviceCategory = theServiceCategory; 251 return this; 252 } 253 254 public boolean hasServiceCategory() { 255 if (this.serviceCategory == null) 256 return false; 257 for (CodeableConcept item : this.serviceCategory) 258 if (!item.isEmpty()) 259 return true; 260 return false; 261 } 262 263 public CodeableConcept addServiceCategory() { //3 264 CodeableConcept t = new CodeableConcept(); 265 if (this.serviceCategory == null) 266 this.serviceCategory = new ArrayList<CodeableConcept>(); 267 this.serviceCategory.add(t); 268 return t; 269 } 270 271 public Schedule addServiceCategory(CodeableConcept t) { //3 272 if (t == null) 273 return this; 274 if (this.serviceCategory == null) 275 this.serviceCategory = new ArrayList<CodeableConcept>(); 276 this.serviceCategory.add(t); 277 return this; 278 } 279 280 /** 281 * @return The first repetition of repeating field {@link #serviceCategory}, creating it if it does not already exist {3} 282 */ 283 public CodeableConcept getServiceCategoryFirstRep() { 284 if (getServiceCategory().isEmpty()) { 285 addServiceCategory(); 286 } 287 return getServiceCategory().get(0); 288 } 289 290 /** 291 * @return {@link #serviceType} (The specific service that is to be performed during this appointment.) 292 */ 293 public List<CodeableReference> getServiceType() { 294 if (this.serviceType == null) 295 this.serviceType = new ArrayList<CodeableReference>(); 296 return this.serviceType; 297 } 298 299 /** 300 * @return Returns a reference to <code>this</code> for easy method chaining 301 */ 302 public Schedule setServiceType(List<CodeableReference> theServiceType) { 303 this.serviceType = theServiceType; 304 return this; 305 } 306 307 public boolean hasServiceType() { 308 if (this.serviceType == null) 309 return false; 310 for (CodeableReference item : this.serviceType) 311 if (!item.isEmpty()) 312 return true; 313 return false; 314 } 315 316 public CodeableReference addServiceType() { //3 317 CodeableReference t = new CodeableReference(); 318 if (this.serviceType == null) 319 this.serviceType = new ArrayList<CodeableReference>(); 320 this.serviceType.add(t); 321 return t; 322 } 323 324 public Schedule addServiceType(CodeableReference t) { //3 325 if (t == null) 326 return this; 327 if (this.serviceType == null) 328 this.serviceType = new ArrayList<CodeableReference>(); 329 this.serviceType.add(t); 330 return this; 331 } 332 333 /** 334 * @return The first repetition of repeating field {@link #serviceType}, creating it if it does not already exist {3} 335 */ 336 public CodeableReference getServiceTypeFirstRep() { 337 if (getServiceType().isEmpty()) { 338 addServiceType(); 339 } 340 return getServiceType().get(0); 341 } 342 343 /** 344 * @return {@link #specialty} (The specialty of a practitioner that would be required to perform the service requested in this appointment.) 345 */ 346 public List<CodeableConcept> getSpecialty() { 347 if (this.specialty == null) 348 this.specialty = new ArrayList<CodeableConcept>(); 349 return this.specialty; 350 } 351 352 /** 353 * @return Returns a reference to <code>this</code> for easy method chaining 354 */ 355 public Schedule setSpecialty(List<CodeableConcept> theSpecialty) { 356 this.specialty = theSpecialty; 357 return this; 358 } 359 360 public boolean hasSpecialty() { 361 if (this.specialty == null) 362 return false; 363 for (CodeableConcept item : this.specialty) 364 if (!item.isEmpty()) 365 return true; 366 return false; 367 } 368 369 public CodeableConcept addSpecialty() { //3 370 CodeableConcept t = new CodeableConcept(); 371 if (this.specialty == null) 372 this.specialty = new ArrayList<CodeableConcept>(); 373 this.specialty.add(t); 374 return t; 375 } 376 377 public Schedule addSpecialty(CodeableConcept t) { //3 378 if (t == null) 379 return this; 380 if (this.specialty == null) 381 this.specialty = new ArrayList<CodeableConcept>(); 382 this.specialty.add(t); 383 return this; 384 } 385 386 /** 387 * @return The first repetition of repeating field {@link #specialty}, creating it if it does not already exist {3} 388 */ 389 public CodeableConcept getSpecialtyFirstRep() { 390 if (getSpecialty().isEmpty()) { 391 addSpecialty(); 392 } 393 return getSpecialty().get(0); 394 } 395 396 /** 397 * @return {@link #name} (Further description of the schedule as it would be presented to a consumer while searching.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 398 */ 399 public StringType getNameElement() { 400 if (this.name == null) 401 if (Configuration.errorOnAutoCreate()) 402 throw new Error("Attempt to auto-create Schedule.name"); 403 else if (Configuration.doAutoCreate()) 404 this.name = new StringType(); // bb 405 return this.name; 406 } 407 408 public boolean hasNameElement() { 409 return this.name != null && !this.name.isEmpty(); 410 } 411 412 public boolean hasName() { 413 return this.name != null && !this.name.isEmpty(); 414 } 415 416 /** 417 * @param value {@link #name} (Further description of the schedule as it would be presented to a consumer while searching.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 418 */ 419 public Schedule setNameElement(StringType value) { 420 this.name = value; 421 return this; 422 } 423 424 /** 425 * @return Further description of the schedule as it would be presented to a consumer while searching. 426 */ 427 public String getName() { 428 return this.name == null ? null : this.name.getValue(); 429 } 430 431 /** 432 * @param value Further description of the schedule as it would be presented to a consumer while searching. 433 */ 434 public Schedule setName(String value) { 435 if (Utilities.noString(value)) 436 this.name = null; 437 else { 438 if (this.name == null) 439 this.name = new StringType(); 440 this.name.setValue(value); 441 } 442 return this; 443 } 444 445 /** 446 * @return {@link #actor} (Slots that reference this schedule resource provide the availability details to these referenced resource(s).) 447 */ 448 public List<Reference> getActor() { 449 if (this.actor == null) 450 this.actor = new ArrayList<Reference>(); 451 return this.actor; 452 } 453 454 /** 455 * @return Returns a reference to <code>this</code> for easy method chaining 456 */ 457 public Schedule setActor(List<Reference> theActor) { 458 this.actor = theActor; 459 return this; 460 } 461 462 public boolean hasActor() { 463 if (this.actor == null) 464 return false; 465 for (Reference item : this.actor) 466 if (!item.isEmpty()) 467 return true; 468 return false; 469 } 470 471 public Reference addActor() { //3 472 Reference t = new Reference(); 473 if (this.actor == null) 474 this.actor = new ArrayList<Reference>(); 475 this.actor.add(t); 476 return t; 477 } 478 479 public Schedule addActor(Reference t) { //3 480 if (t == null) 481 return this; 482 if (this.actor == null) 483 this.actor = new ArrayList<Reference>(); 484 this.actor.add(t); 485 return this; 486 } 487 488 /** 489 * @return The first repetition of repeating field {@link #actor}, creating it if it does not already exist {3} 490 */ 491 public Reference getActorFirstRep() { 492 if (getActor().isEmpty()) { 493 addActor(); 494 } 495 return getActor().get(0); 496 } 497 498 /** 499 * @return {@link #planningHorizon} (The period of time that the slots that reference this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a "template" for planning outside these dates.) 500 */ 501 public Period getPlanningHorizon() { 502 if (this.planningHorizon == null) 503 if (Configuration.errorOnAutoCreate()) 504 throw new Error("Attempt to auto-create Schedule.planningHorizon"); 505 else if (Configuration.doAutoCreate()) 506 this.planningHorizon = new Period(); // cc 507 return this.planningHorizon; 508 } 509 510 public boolean hasPlanningHorizon() { 511 return this.planningHorizon != null && !this.planningHorizon.isEmpty(); 512 } 513 514 /** 515 * @param value {@link #planningHorizon} (The period of time that the slots that reference this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a "template" for planning outside these dates.) 516 */ 517 public Schedule setPlanningHorizon(Period value) { 518 this.planningHorizon = value; 519 return this; 520 } 521 522 /** 523 * @return {@link #comment} (Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 524 */ 525 public MarkdownType getCommentElement() { 526 if (this.comment == null) 527 if (Configuration.errorOnAutoCreate()) 528 throw new Error("Attempt to auto-create Schedule.comment"); 529 else if (Configuration.doAutoCreate()) 530 this.comment = new MarkdownType(); // bb 531 return this.comment; 532 } 533 534 public boolean hasCommentElement() { 535 return this.comment != null && !this.comment.isEmpty(); 536 } 537 538 public boolean hasComment() { 539 return this.comment != null && !this.comment.isEmpty(); 540 } 541 542 /** 543 * @param value {@link #comment} (Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 544 */ 545 public Schedule setCommentElement(MarkdownType value) { 546 this.comment = value; 547 return this; 548 } 549 550 /** 551 * @return Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated. 552 */ 553 public String getComment() { 554 return this.comment == null ? null : this.comment.getValue(); 555 } 556 557 /** 558 * @param value Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated. 559 */ 560 public Schedule setComment(String value) { 561 if (Utilities.noString(value)) 562 this.comment = null; 563 else { 564 if (this.comment == null) 565 this.comment = new MarkdownType(); 566 this.comment.setValue(value); 567 } 568 return this; 569 } 570 571 protected void listChildren(List<Property> children) { 572 super.listChildren(children); 573 children.add(new Property("identifier", "Identifier", "External Ids for this item.", 0, java.lang.Integer.MAX_VALUE, identifier)); 574 children.add(new Property("active", "boolean", "Whether this schedule record is in active use or should not be used (such as was entered in error).", 0, 1, active)); 575 children.add(new Property("serviceCategory", "CodeableConcept", "A broad categorization of the service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, serviceCategory)); 576 children.add(new Property("serviceType", "CodeableReference(HealthcareService)", "The specific service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, serviceType)); 577 children.add(new Property("specialty", "CodeableConcept", "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 0, java.lang.Integer.MAX_VALUE, specialty)); 578 children.add(new Property("name", "string", "Further description of the schedule as it would be presented to a consumer while searching.", 0, 1, name)); 579 children.add(new Property("actor", "Reference(Patient|Practitioner|PractitionerRole|CareTeam|RelatedPerson|Device|HealthcareService|Location)", "Slots that reference this schedule resource provide the availability details to these referenced resource(s).", 0, java.lang.Integer.MAX_VALUE, actor)); 580 children.add(new Property("planningHorizon", "Period", "The period of time that the slots that reference this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a \"template\" for planning outside these dates.", 0, 1, planningHorizon)); 581 children.add(new Property("comment", "markdown", "Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated.", 0, 1, comment)); 582 } 583 584 @Override 585 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 586 switch (_hash) { 587 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "External Ids for this item.", 0, java.lang.Integer.MAX_VALUE, identifier); 588 case -1422950650: /*active*/ return new Property("active", "boolean", "Whether this schedule record is in active use or should not be used (such as was entered in error).", 0, 1, active); 589 case 1281188563: /*serviceCategory*/ return new Property("serviceCategory", "CodeableConcept", "A broad categorization of the service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, serviceCategory); 590 case -1928370289: /*serviceType*/ return new Property("serviceType", "CodeableReference(HealthcareService)", "The specific service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, serviceType); 591 case -1694759682: /*specialty*/ return new Property("specialty", "CodeableConcept", "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 0, java.lang.Integer.MAX_VALUE, specialty); 592 case 3373707: /*name*/ return new Property("name", "string", "Further description of the schedule as it would be presented to a consumer while searching.", 0, 1, name); 593 case 92645877: /*actor*/ return new Property("actor", "Reference(Patient|Practitioner|PractitionerRole|CareTeam|RelatedPerson|Device|HealthcareService|Location)", "Slots that reference this schedule resource provide the availability details to these referenced resource(s).", 0, java.lang.Integer.MAX_VALUE, actor); 594 case -1718507650: /*planningHorizon*/ return new Property("planningHorizon", "Period", "The period of time that the slots that reference this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a \"template\" for planning outside these dates.", 0, 1, planningHorizon); 595 case 950398559: /*comment*/ return new Property("comment", "markdown", "Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated.", 0, 1, comment); 596 default: return super.getNamedProperty(_hash, _name, _checkValid); 597 } 598 599 } 600 601 @Override 602 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 603 switch (hash) { 604 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 605 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 606 case 1281188563: /*serviceCategory*/ return this.serviceCategory == null ? new Base[0] : this.serviceCategory.toArray(new Base[this.serviceCategory.size()]); // CodeableConcept 607 case -1928370289: /*serviceType*/ return this.serviceType == null ? new Base[0] : this.serviceType.toArray(new Base[this.serviceType.size()]); // CodeableReference 608 case -1694759682: /*specialty*/ return this.specialty == null ? new Base[0] : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 609 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 610 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : this.actor.toArray(new Base[this.actor.size()]); // Reference 611 case -1718507650: /*planningHorizon*/ return this.planningHorizon == null ? new Base[0] : new Base[] {this.planningHorizon}; // Period 612 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // MarkdownType 613 default: return super.getProperty(hash, name, checkValid); 614 } 615 616 } 617 618 @Override 619 public Base setProperty(int hash, String name, Base value) throws FHIRException { 620 switch (hash) { 621 case -1618432855: // identifier 622 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 623 return value; 624 case -1422950650: // active 625 this.active = TypeConvertor.castToBoolean(value); // BooleanType 626 return value; 627 case 1281188563: // serviceCategory 628 this.getServiceCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 629 return value; 630 case -1928370289: // serviceType 631 this.getServiceType().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 632 return value; 633 case -1694759682: // specialty 634 this.getSpecialty().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 635 return value; 636 case 3373707: // name 637 this.name = TypeConvertor.castToString(value); // StringType 638 return value; 639 case 92645877: // actor 640 this.getActor().add(TypeConvertor.castToReference(value)); // Reference 641 return value; 642 case -1718507650: // planningHorizon 643 this.planningHorizon = TypeConvertor.castToPeriod(value); // Period 644 return value; 645 case 950398559: // comment 646 this.comment = TypeConvertor.castToMarkdown(value); // MarkdownType 647 return value; 648 default: return super.setProperty(hash, name, value); 649 } 650 651 } 652 653 @Override 654 public Base setProperty(String name, Base value) throws FHIRException { 655 if (name.equals("identifier")) { 656 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 657 } else if (name.equals("active")) { 658 this.active = TypeConvertor.castToBoolean(value); // BooleanType 659 } else if (name.equals("serviceCategory")) { 660 this.getServiceCategory().add(TypeConvertor.castToCodeableConcept(value)); 661 } else if (name.equals("serviceType")) { 662 this.getServiceType().add(TypeConvertor.castToCodeableReference(value)); 663 } else if (name.equals("specialty")) { 664 this.getSpecialty().add(TypeConvertor.castToCodeableConcept(value)); 665 } else if (name.equals("name")) { 666 this.name = TypeConvertor.castToString(value); // StringType 667 } else if (name.equals("actor")) { 668 this.getActor().add(TypeConvertor.castToReference(value)); 669 } else if (name.equals("planningHorizon")) { 670 this.planningHorizon = TypeConvertor.castToPeriod(value); // Period 671 } else if (name.equals("comment")) { 672 this.comment = TypeConvertor.castToMarkdown(value); // MarkdownType 673 } else 674 return super.setProperty(name, value); 675 return value; 676 } 677 678 @Override 679 public void removeChild(String name, Base value) throws FHIRException { 680 if (name.equals("identifier")) { 681 this.getIdentifier().remove(value); 682 } else if (name.equals("active")) { 683 this.active = null; 684 } else if (name.equals("serviceCategory")) { 685 this.getServiceCategory().remove(value); 686 } else if (name.equals("serviceType")) { 687 this.getServiceType().remove(value); 688 } else if (name.equals("specialty")) { 689 this.getSpecialty().remove(value); 690 } else if (name.equals("name")) { 691 this.name = null; 692 } else if (name.equals("actor")) { 693 this.getActor().remove(value); 694 } else if (name.equals("planningHorizon")) { 695 this.planningHorizon = null; 696 } else if (name.equals("comment")) { 697 this.comment = null; 698 } else 699 super.removeChild(name, value); 700 701 } 702 703 @Override 704 public Base makeProperty(int hash, String name) throws FHIRException { 705 switch (hash) { 706 case -1618432855: return addIdentifier(); 707 case -1422950650: return getActiveElement(); 708 case 1281188563: return addServiceCategory(); 709 case -1928370289: return addServiceType(); 710 case -1694759682: return addSpecialty(); 711 case 3373707: return getNameElement(); 712 case 92645877: return addActor(); 713 case -1718507650: return getPlanningHorizon(); 714 case 950398559: return getCommentElement(); 715 default: return super.makeProperty(hash, name); 716 } 717 718 } 719 720 @Override 721 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 722 switch (hash) { 723 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 724 case -1422950650: /*active*/ return new String[] {"boolean"}; 725 case 1281188563: /*serviceCategory*/ return new String[] {"CodeableConcept"}; 726 case -1928370289: /*serviceType*/ return new String[] {"CodeableReference"}; 727 case -1694759682: /*specialty*/ return new String[] {"CodeableConcept"}; 728 case 3373707: /*name*/ return new String[] {"string"}; 729 case 92645877: /*actor*/ return new String[] {"Reference"}; 730 case -1718507650: /*planningHorizon*/ return new String[] {"Period"}; 731 case 950398559: /*comment*/ return new String[] {"markdown"}; 732 default: return super.getTypesForProperty(hash, name); 733 } 734 735 } 736 737 @Override 738 public Base addChild(String name) throws FHIRException { 739 if (name.equals("identifier")) { 740 return addIdentifier(); 741 } 742 else if (name.equals("active")) { 743 throw new FHIRException("Cannot call addChild on a singleton property Schedule.active"); 744 } 745 else if (name.equals("serviceCategory")) { 746 return addServiceCategory(); 747 } 748 else if (name.equals("serviceType")) { 749 return addServiceType(); 750 } 751 else if (name.equals("specialty")) { 752 return addSpecialty(); 753 } 754 else if (name.equals("name")) { 755 throw new FHIRException("Cannot call addChild on a singleton property Schedule.name"); 756 } 757 else if (name.equals("actor")) { 758 return addActor(); 759 } 760 else if (name.equals("planningHorizon")) { 761 this.planningHorizon = new Period(); 762 return this.planningHorizon; 763 } 764 else if (name.equals("comment")) { 765 throw new FHIRException("Cannot call addChild on a singleton property Schedule.comment"); 766 } 767 else 768 return super.addChild(name); 769 } 770 771 public String fhirType() { 772 return "Schedule"; 773 774 } 775 776 public Schedule copy() { 777 Schedule dst = new Schedule(); 778 copyValues(dst); 779 return dst; 780 } 781 782 public void copyValues(Schedule dst) { 783 super.copyValues(dst); 784 if (identifier != null) { 785 dst.identifier = new ArrayList<Identifier>(); 786 for (Identifier i : identifier) 787 dst.identifier.add(i.copy()); 788 }; 789 dst.active = active == null ? null : active.copy(); 790 if (serviceCategory != null) { 791 dst.serviceCategory = new ArrayList<CodeableConcept>(); 792 for (CodeableConcept i : serviceCategory) 793 dst.serviceCategory.add(i.copy()); 794 }; 795 if (serviceType != null) { 796 dst.serviceType = new ArrayList<CodeableReference>(); 797 for (CodeableReference i : serviceType) 798 dst.serviceType.add(i.copy()); 799 }; 800 if (specialty != null) { 801 dst.specialty = new ArrayList<CodeableConcept>(); 802 for (CodeableConcept i : specialty) 803 dst.specialty.add(i.copy()); 804 }; 805 dst.name = name == null ? null : name.copy(); 806 if (actor != null) { 807 dst.actor = new ArrayList<Reference>(); 808 for (Reference i : actor) 809 dst.actor.add(i.copy()); 810 }; 811 dst.planningHorizon = planningHorizon == null ? null : planningHorizon.copy(); 812 dst.comment = comment == null ? null : comment.copy(); 813 } 814 815 protected Schedule typedCopy() { 816 return copy(); 817 } 818 819 @Override 820 public boolean equalsDeep(Base other_) { 821 if (!super.equalsDeep(other_)) 822 return false; 823 if (!(other_ instanceof Schedule)) 824 return false; 825 Schedule o = (Schedule) other_; 826 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(serviceCategory, o.serviceCategory, true) 827 && compareDeep(serviceType, o.serviceType, true) && compareDeep(specialty, o.specialty, true) && compareDeep(name, o.name, true) 828 && compareDeep(actor, o.actor, true) && compareDeep(planningHorizon, o.planningHorizon, true) && compareDeep(comment, o.comment, true) 829 ; 830 } 831 832 @Override 833 public boolean equalsShallow(Base other_) { 834 if (!super.equalsShallow(other_)) 835 return false; 836 if (!(other_ instanceof Schedule)) 837 return false; 838 Schedule o = (Schedule) other_; 839 return compareValues(active, o.active, true) && compareValues(name, o.name, true) && compareValues(comment, o.comment, true) 840 ; 841 } 842 843 public boolean isEmpty() { 844 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, serviceCategory 845 , serviceType, specialty, name, actor, planningHorizon, comment); 846 } 847 848 @Override 849 public ResourceType getResourceType() { 850 return ResourceType.Schedule; 851 } 852 853 /** 854 * Search parameter: <b>active</b> 855 * <p> 856 * Description: <b>Is the schedule in active use</b><br> 857 * Type: <b>token</b><br> 858 * Path: <b>Schedule.active</b><br> 859 * </p> 860 */ 861 @SearchParamDefinition(name="active", path="Schedule.active", description="Is the schedule in active use", type="token" ) 862 public static final String SP_ACTIVE = "active"; 863 /** 864 * <b>Fluent Client</b> search parameter constant for <b>active</b> 865 * <p> 866 * Description: <b>Is the schedule in active use</b><br> 867 * Type: <b>token</b><br> 868 * Path: <b>Schedule.active</b><br> 869 * </p> 870 */ 871 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTIVE); 872 873 /** 874 * Search parameter: <b>actor</b> 875 * <p> 876 * Description: <b>The individual(HealthcareService, Practitioner, Location, ...) to find a Schedule for</b><br> 877 * Type: <b>reference</b><br> 878 * Path: <b>Schedule.actor</b><br> 879 * </p> 880 */ 881 @SearchParamDefinition(name="actor", path="Schedule.actor", description="The individual(HealthcareService, Practitioner, Location, ...) to find a Schedule for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={CareTeam.class, Device.class, HealthcareService.class, Location.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 882 public static final String SP_ACTOR = "actor"; 883 /** 884 * <b>Fluent Client</b> search parameter constant for <b>actor</b> 885 * <p> 886 * Description: <b>The individual(HealthcareService, Practitioner, Location, ...) to find a Schedule for</b><br> 887 * Type: <b>reference</b><br> 888 * Path: <b>Schedule.actor</b><br> 889 * </p> 890 */ 891 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACTOR); 892 893/** 894 * Constant for fluent queries to be used to add include statements. Specifies 895 * the path value of "<b>Schedule:actor</b>". 896 */ 897 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTOR = new ca.uhn.fhir.model.api.Include("Schedule:actor").toLocked(); 898 899 /** 900 * Search parameter: <b>date</b> 901 * <p> 902 * Description: <b>Search for Schedule resources that have a period that contains this date specified</b><br> 903 * Type: <b>date</b><br> 904 * Path: <b>Schedule.planningHorizon</b><br> 905 * </p> 906 */ 907 @SearchParamDefinition(name="date", path="Schedule.planningHorizon", description="Search for Schedule resources that have a period that contains this date specified", type="date" ) 908 public static final String SP_DATE = "date"; 909 /** 910 * <b>Fluent Client</b> search parameter constant for <b>date</b> 911 * <p> 912 * Description: <b>Search for Schedule resources that have a period that contains this date specified</b><br> 913 * Type: <b>date</b><br> 914 * Path: <b>Schedule.planningHorizon</b><br> 915 * </p> 916 */ 917 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 918 919 /** 920 * Search parameter: <b>identifier</b> 921 * <p> 922 * Description: <b>A Schedule Identifier</b><br> 923 * Type: <b>token</b><br> 924 * Path: <b>Schedule.identifier</b><br> 925 * </p> 926 */ 927 @SearchParamDefinition(name="identifier", path="Schedule.identifier", description="A Schedule Identifier", type="token" ) 928 public static final String SP_IDENTIFIER = "identifier"; 929 /** 930 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 931 * <p> 932 * Description: <b>A Schedule Identifier</b><br> 933 * Type: <b>token</b><br> 934 * Path: <b>Schedule.identifier</b><br> 935 * </p> 936 */ 937 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 938 939 /** 940 * Search parameter: <b>name</b> 941 * <p> 942 * Description: <b>A portion of the Schedule name</b><br> 943 * Type: <b>string</b><br> 944 * Path: <b>Schedule.name</b><br> 945 * </p> 946 */ 947 @SearchParamDefinition(name="name", path="Schedule.name", description="A portion of the Schedule name", type="string" ) 948 public static final String SP_NAME = "name"; 949 /** 950 * <b>Fluent Client</b> search parameter constant for <b>name</b> 951 * <p> 952 * Description: <b>A portion of the Schedule name</b><br> 953 * Type: <b>string</b><br> 954 * Path: <b>Schedule.name</b><br> 955 * </p> 956 */ 957 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 958 959 /** 960 * Search parameter: <b>service-category</b> 961 * <p> 962 * Description: <b>High-level category</b><br> 963 * Type: <b>token</b><br> 964 * Path: <b>Schedule.serviceCategory</b><br> 965 * </p> 966 */ 967 @SearchParamDefinition(name="service-category", path="Schedule.serviceCategory", description="High-level category", type="token" ) 968 public static final String SP_SERVICE_CATEGORY = "service-category"; 969 /** 970 * <b>Fluent Client</b> search parameter constant for <b>service-category</b> 971 * <p> 972 * Description: <b>High-level category</b><br> 973 * Type: <b>token</b><br> 974 * Path: <b>Schedule.serviceCategory</b><br> 975 * </p> 976 */ 977 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SERVICE_CATEGORY); 978 979 /** 980 * Search parameter: <b>service-type-reference</b> 981 * <p> 982 * Description: <b>The type (by HealthcareService) of appointments that can be booked into associated slot(s)</b><br> 983 * Type: <b>reference</b><br> 984 * Path: <b>Schedule.serviceType.reference</b><br> 985 * </p> 986 */ 987 @SearchParamDefinition(name="service-type-reference", path="Schedule.serviceType.reference", description="The type (by HealthcareService) of appointments that can be booked into associated slot(s)", type="reference", target={HealthcareService.class } ) 988 public static final String SP_SERVICE_TYPE_REFERENCE = "service-type-reference"; 989 /** 990 * <b>Fluent Client</b> search parameter constant for <b>service-type-reference</b> 991 * <p> 992 * Description: <b>The type (by HealthcareService) of appointments that can be booked into associated slot(s)</b><br> 993 * Type: <b>reference</b><br> 994 * Path: <b>Schedule.serviceType.reference</b><br> 995 * </p> 996 */ 997 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SERVICE_TYPE_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SERVICE_TYPE_REFERENCE); 998 999/** 1000 * Constant for fluent queries to be used to add include statements. Specifies 1001 * the path value of "<b>Schedule:service-type-reference</b>". 1002 */ 1003 public static final ca.uhn.fhir.model.api.Include INCLUDE_SERVICE_TYPE_REFERENCE = new ca.uhn.fhir.model.api.Include("Schedule:service-type-reference").toLocked(); 1004 1005 /** 1006 * Search parameter: <b>service-type</b> 1007 * <p> 1008 * Description: <b>The type (by coding) of appointments that can be booked into associated slot(s)</b><br> 1009 * Type: <b>token</b><br> 1010 * Path: <b>Schedule.serviceType.concept</b><br> 1011 * </p> 1012 */ 1013 @SearchParamDefinition(name="service-type", path="Schedule.serviceType.concept", description="The type (by coding) of appointments that can be booked into associated slot(s)", type="token" ) 1014 public static final String SP_SERVICE_TYPE = "service-type"; 1015 /** 1016 * <b>Fluent Client</b> search parameter constant for <b>service-type</b> 1017 * <p> 1018 * Description: <b>The type (by coding) of appointments that can be booked into associated slot(s)</b><br> 1019 * Type: <b>token</b><br> 1020 * Path: <b>Schedule.serviceType.concept</b><br> 1021 * </p> 1022 */ 1023 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SERVICE_TYPE); 1024 1025 /** 1026 * Search parameter: <b>specialty</b> 1027 * <p> 1028 * Description: <b>Type of specialty needed</b><br> 1029 * Type: <b>token</b><br> 1030 * Path: <b>Schedule.specialty</b><br> 1031 * </p> 1032 */ 1033 @SearchParamDefinition(name="specialty", path="Schedule.specialty", description="Type of specialty needed", type="token" ) 1034 public static final String SP_SPECIALTY = "specialty"; 1035 /** 1036 * <b>Fluent Client</b> search parameter constant for <b>specialty</b> 1037 * <p> 1038 * Description: <b>Type of specialty needed</b><br> 1039 * Type: <b>token</b><br> 1040 * Path: <b>Schedule.specialty</b><br> 1041 * </p> 1042 */ 1043 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIALTY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SPECIALTY); 1044 1045 1046} 1047