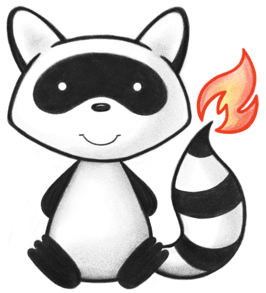
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A search parameter that defines a named search item that can be used to search/filter on a resource. 052 */ 053@ResourceDef(name="SearchParameter", profile="http://hl7.org/fhir/StructureDefinition/SearchParameter") 054public class SearchParameter extends CanonicalResource { 055 056 public enum SearchProcessingModeType { 057 /** 058 * The search parameter is derived directly from the selected nodes based on the type definitions. 059 */ 060 NORMAL, 061 /** 062 * The search parameter is derived by a phonetic transform from the selected nodes. 063 */ 064 PHONETIC, 065 /** 066 * The interpretation of the xpath statement is unknown (and can't be automated). 067 */ 068 OTHER, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static SearchProcessingModeType fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("normal".equals(codeString)) 077 return NORMAL; 078 if ("phonetic".equals(codeString)) 079 return PHONETIC; 080 if ("other".equals(codeString)) 081 return OTHER; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown SearchProcessingModeType code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case NORMAL: return "normal"; 090 case PHONETIC: return "phonetic"; 091 case OTHER: return "other"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case NORMAL: return "http://hl7.org/fhir/search-processingmode"; 099 case PHONETIC: return "http://hl7.org/fhir/search-processingmode"; 100 case OTHER: return "http://hl7.org/fhir/search-processingmode"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case NORMAL: return "The search parameter is derived directly from the selected nodes based on the type definitions."; 108 case PHONETIC: return "The search parameter is derived by a phonetic transform from the selected nodes."; 109 case OTHER: return "The interpretation of the xpath statement is unknown (and can't be automated)."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case NORMAL: return "Normal"; 117 case PHONETIC: return "Phonetic"; 118 case OTHER: return "Other"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class SearchProcessingModeTypeEnumFactory implements EnumFactory<SearchProcessingModeType> { 126 public SearchProcessingModeType fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("normal".equals(codeString)) 131 return SearchProcessingModeType.NORMAL; 132 if ("phonetic".equals(codeString)) 133 return SearchProcessingModeType.PHONETIC; 134 if ("other".equals(codeString)) 135 return SearchProcessingModeType.OTHER; 136 throw new IllegalArgumentException("Unknown SearchProcessingModeType code '"+codeString+"'"); 137 } 138 public Enumeration<SearchProcessingModeType> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<SearchProcessingModeType>(this, SearchProcessingModeType.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<SearchProcessingModeType>(this, SearchProcessingModeType.NULL, code); 146 if ("normal".equals(codeString)) 147 return new Enumeration<SearchProcessingModeType>(this, SearchProcessingModeType.NORMAL, code); 148 if ("phonetic".equals(codeString)) 149 return new Enumeration<SearchProcessingModeType>(this, SearchProcessingModeType.PHONETIC, code); 150 if ("other".equals(codeString)) 151 return new Enumeration<SearchProcessingModeType>(this, SearchProcessingModeType.OTHER, code); 152 throw new FHIRException("Unknown SearchProcessingModeType code '"+codeString+"'"); 153 } 154 public String toCode(SearchProcessingModeType code) { 155 if (code == SearchProcessingModeType.NORMAL) 156 return "normal"; 157 if (code == SearchProcessingModeType.PHONETIC) 158 return "phonetic"; 159 if (code == SearchProcessingModeType.OTHER) 160 return "other"; 161 return "?"; 162 } 163 public String toSystem(SearchProcessingModeType code) { 164 return code.getSystem(); 165 } 166 } 167 168 @Block() 169 public static class SearchParameterComponentComponent extends BackboneElement implements IBaseBackboneElement { 170 /** 171 * The definition of the search parameter that describes this part. 172 */ 173 @Child(name = "definition", type = {CanonicalType.class}, order=1, min=1, max=1, modifier=false, summary=false) 174 @Description(shortDefinition="Defines how the part works", formalDefinition="The definition of the search parameter that describes this part." ) 175 protected CanonicalType definition; 176 177 /** 178 * A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression. 179 */ 180 @Child(name = "expression", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 181 @Description(shortDefinition="Subexpression relative to main expression", formalDefinition="A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression." ) 182 protected StringType expression; 183 184 private static final long serialVersionUID = -1469435618L; 185 186 /** 187 * Constructor 188 */ 189 public SearchParameterComponentComponent() { 190 super(); 191 } 192 193 /** 194 * Constructor 195 */ 196 public SearchParameterComponentComponent(String definition, String expression) { 197 super(); 198 this.setDefinition(definition); 199 this.setExpression(expression); 200 } 201 202 /** 203 * @return {@link #definition} (The definition of the search parameter that describes this part.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 204 */ 205 public CanonicalType getDefinitionElement() { 206 if (this.definition == null) 207 if (Configuration.errorOnAutoCreate()) 208 throw new Error("Attempt to auto-create SearchParameterComponentComponent.definition"); 209 else if (Configuration.doAutoCreate()) 210 this.definition = new CanonicalType(); // bb 211 return this.definition; 212 } 213 214 public boolean hasDefinitionElement() { 215 return this.definition != null && !this.definition.isEmpty(); 216 } 217 218 public boolean hasDefinition() { 219 return this.definition != null && !this.definition.isEmpty(); 220 } 221 222 /** 223 * @param value {@link #definition} (The definition of the search parameter that describes this part.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 224 */ 225 public SearchParameterComponentComponent setDefinitionElement(CanonicalType value) { 226 this.definition = value; 227 return this; 228 } 229 230 /** 231 * @return The definition of the search parameter that describes this part. 232 */ 233 public String getDefinition() { 234 return this.definition == null ? null : this.definition.getValue(); 235 } 236 237 /** 238 * @param value The definition of the search parameter that describes this part. 239 */ 240 public SearchParameterComponentComponent setDefinition(String value) { 241 if (this.definition == null) 242 this.definition = new CanonicalType(); 243 this.definition.setValue(value); 244 return this; 245 } 246 247 /** 248 * @return {@link #expression} (A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 249 */ 250 public StringType getExpressionElement() { 251 if (this.expression == null) 252 if (Configuration.errorOnAutoCreate()) 253 throw new Error("Attempt to auto-create SearchParameterComponentComponent.expression"); 254 else if (Configuration.doAutoCreate()) 255 this.expression = new StringType(); // bb 256 return this.expression; 257 } 258 259 public boolean hasExpressionElement() { 260 return this.expression != null && !this.expression.isEmpty(); 261 } 262 263 public boolean hasExpression() { 264 return this.expression != null && !this.expression.isEmpty(); 265 } 266 267 /** 268 * @param value {@link #expression} (A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 269 */ 270 public SearchParameterComponentComponent setExpressionElement(StringType value) { 271 this.expression = value; 272 return this; 273 } 274 275 /** 276 * @return A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression. 277 */ 278 public String getExpression() { 279 return this.expression == null ? null : this.expression.getValue(); 280 } 281 282 /** 283 * @param value A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression. 284 */ 285 public SearchParameterComponentComponent setExpression(String value) { 286 if (this.expression == null) 287 this.expression = new StringType(); 288 this.expression.setValue(value); 289 return this; 290 } 291 292 protected void listChildren(List<Property> children) { 293 super.listChildren(children); 294 children.add(new Property("definition", "canonical(SearchParameter)", "The definition of the search parameter that describes this part.", 0, 1, definition)); 295 children.add(new Property("expression", "string", "A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.", 0, 1, expression)); 296 } 297 298 @Override 299 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 300 switch (_hash) { 301 case -1014418093: /*definition*/ return new Property("definition", "canonical(SearchParameter)", "The definition of the search parameter that describes this part.", 0, 1, definition); 302 case -1795452264: /*expression*/ return new Property("expression", "string", "A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.", 0, 1, expression); 303 default: return super.getNamedProperty(_hash, _name, _checkValid); 304 } 305 306 } 307 308 @Override 309 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 310 switch (hash) { 311 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // CanonicalType 312 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 313 default: return super.getProperty(hash, name, checkValid); 314 } 315 316 } 317 318 @Override 319 public Base setProperty(int hash, String name, Base value) throws FHIRException { 320 switch (hash) { 321 case -1014418093: // definition 322 this.definition = TypeConvertor.castToCanonical(value); // CanonicalType 323 return value; 324 case -1795452264: // expression 325 this.expression = TypeConvertor.castToString(value); // StringType 326 return value; 327 default: return super.setProperty(hash, name, value); 328 } 329 330 } 331 332 @Override 333 public Base setProperty(String name, Base value) throws FHIRException { 334 if (name.equals("definition")) { 335 this.definition = TypeConvertor.castToCanonical(value); // CanonicalType 336 } else if (name.equals("expression")) { 337 this.expression = TypeConvertor.castToString(value); // StringType 338 } else 339 return super.setProperty(name, value); 340 return value; 341 } 342 343 @Override 344 public Base makeProperty(int hash, String name) throws FHIRException { 345 switch (hash) { 346 case -1014418093: return getDefinitionElement(); 347 case -1795452264: return getExpressionElement(); 348 default: return super.makeProperty(hash, name); 349 } 350 351 } 352 353 @Override 354 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 355 switch (hash) { 356 case -1014418093: /*definition*/ return new String[] {"canonical"}; 357 case -1795452264: /*expression*/ return new String[] {"string"}; 358 default: return super.getTypesForProperty(hash, name); 359 } 360 361 } 362 363 @Override 364 public Base addChild(String name) throws FHIRException { 365 if (name.equals("definition")) { 366 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.component.definition"); 367 } 368 else if (name.equals("expression")) { 369 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.component.expression"); 370 } 371 else 372 return super.addChild(name); 373 } 374 375 public SearchParameterComponentComponent copy() { 376 SearchParameterComponentComponent dst = new SearchParameterComponentComponent(); 377 copyValues(dst); 378 return dst; 379 } 380 381 public void copyValues(SearchParameterComponentComponent dst) { 382 super.copyValues(dst); 383 dst.definition = definition == null ? null : definition.copy(); 384 dst.expression = expression == null ? null : expression.copy(); 385 } 386 387 @Override 388 public boolean equalsDeep(Base other_) { 389 if (!super.equalsDeep(other_)) 390 return false; 391 if (!(other_ instanceof SearchParameterComponentComponent)) 392 return false; 393 SearchParameterComponentComponent o = (SearchParameterComponentComponent) other_; 394 return compareDeep(definition, o.definition, true) && compareDeep(expression, o.expression, true) 395 ; 396 } 397 398 @Override 399 public boolean equalsShallow(Base other_) { 400 if (!super.equalsShallow(other_)) 401 return false; 402 if (!(other_ instanceof SearchParameterComponentComponent)) 403 return false; 404 SearchParameterComponentComponent o = (SearchParameterComponentComponent) other_; 405 return compareValues(definition, o.definition, true) && compareValues(expression, o.expression, true) 406 ; 407 } 408 409 public boolean isEmpty() { 410 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(definition, expression); 411 } 412 413 public String fhirType() { 414 return "SearchParameter.component"; 415 416 } 417 418 } 419 420 /** 421 * An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this search parameter is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the search parameter is stored on different servers. 422 */ 423 @Child(name = "url", type = {UriType.class}, order=0, min=1, max=1, modifier=false, summary=true) 424 @Description(shortDefinition="Canonical identifier for this search parameter, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this search parameter is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the search parameter is stored on different servers." ) 425 protected UriType url; 426 427 /** 428 * A formal identifier that is used to identify this search parameter when it is represented in other formats, or referenced in a specification, model, design or an instance. 429 */ 430 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 431 @Description(shortDefinition="Additional identifier for the search parameter (business identifier)", formalDefinition="A formal identifier that is used to identify this search parameter when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 432 protected List<Identifier> identifier; 433 434 /** 435 * The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 436 */ 437 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 438 @Description(shortDefinition="Business version of the search parameter", formalDefinition="The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 439 protected StringType version; 440 441 /** 442 * Indicates the mechanism used to compare versions to determine which is more current. 443 */ 444 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 445 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 446 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 447 protected DataType versionAlgorithm; 448 449 /** 450 * A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation. 451 */ 452 @Child(name = "name", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=true) 453 @Description(shortDefinition="Name for this search parameter (computer friendly)", formalDefinition="A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 454 protected StringType name; 455 456 /** 457 * A short, descriptive, user-friendly title for the search parameter. 458 */ 459 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 460 @Description(shortDefinition="Name for this search parameter (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the search parameter." ) 461 protected StringType title; 462 463 /** 464 * Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter. 465 */ 466 @Child(name = "derivedFrom", type = {CanonicalType.class}, order=6, min=0, max=1, modifier=false, summary=false) 467 @Description(shortDefinition="Original definition for the search parameter", formalDefinition="Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter." ) 468 protected CanonicalType derivedFrom; 469 470 /** 471 * The status of this search parameter. Enables tracking the life-cycle of the content. 472 */ 473 @Child(name = "status", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 474 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this search parameter. Enables tracking the life-cycle of the content." ) 475 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 476 protected Enumeration<PublicationStatus> status; 477 478 /** 479 * A Boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 480 */ 481 @Child(name = "experimental", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=true) 482 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 483 protected BooleanType experimental; 484 485 /** 486 * The date (and optionally time) when the search parameter was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes. 487 */ 488 @Child(name = "date", type = {DateTimeType.class}, order=9, min=0, max=1, modifier=false, summary=true) 489 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the search parameter was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes." ) 490 protected DateTimeType date; 491 492 /** 493 * The name of the organization or individual tresponsible for the release and ongoing maintenance of the search parameter. 494 */ 495 @Child(name = "publisher", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 496 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual tresponsible for the release and ongoing maintenance of the search parameter." ) 497 protected StringType publisher; 498 499 /** 500 * Contact details to assist a user in finding and communicating with the publisher. 501 */ 502 @Child(name = "contact", type = {ContactDetail.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 503 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 504 protected List<ContactDetail> contact; 505 506 /** 507 * And how it used. 508 */ 509 @Child(name = "description", type = {MarkdownType.class}, order=12, min=1, max=1, modifier=false, summary=true) 510 @Description(shortDefinition="Natural language description of the search parameter", formalDefinition="And how it used." ) 511 protected MarkdownType description; 512 513 /** 514 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate search parameter instances. 515 */ 516 @Child(name = "useContext", type = {UsageContext.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 517 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate search parameter instances." ) 518 protected List<UsageContext> useContext; 519 520 /** 521 * A legal or geographic region in which the search parameter is intended to be used. 522 */ 523 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 524 @Description(shortDefinition="Intended jurisdiction for search parameter (if applicable)", formalDefinition="A legal or geographic region in which the search parameter is intended to be used." ) 525 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 526 protected List<CodeableConcept> jurisdiction; 527 528 /** 529 * Explanation of why this search parameter is needed and why it has been designed as it has. 530 */ 531 @Child(name = "purpose", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 532 @Description(shortDefinition="Why this search parameter is defined", formalDefinition="Explanation of why this search parameter is needed and why it has been designed as it has." ) 533 protected MarkdownType purpose; 534 535 /** 536 * A copyright statement relating to the search parameter and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the search parameter. 537 */ 538 @Child(name = "copyright", type = {MarkdownType.class}, order=16, min=0, max=1, modifier=false, summary=false) 539 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the search parameter and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the search parameter." ) 540 protected MarkdownType copyright; 541 542 /** 543 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 544 */ 545 @Child(name = "copyrightLabel", type = {StringType.class}, order=17, min=0, max=1, modifier=false, summary=false) 546 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 547 protected StringType copyrightLabel; 548 549 /** 550 * The label that is recommended to be used in the URL or the parameter name in a parameters resource for this search parameter. In some cases, servers may need to use a different CapabilityStatement searchParam.name to differentiate between multiple SearchParameters that happen to have the same code. 551 */ 552 @Child(name = "code", type = {CodeType.class}, order=18, min=1, max=1, modifier=false, summary=true) 553 @Description(shortDefinition="Recommended name for parameter in search url", formalDefinition="The label that is recommended to be used in the URL or the parameter name in a parameters resource for this search parameter. In some cases, servers may need to use a different CapabilityStatement searchParam.name to differentiate between multiple SearchParameters that happen to have the same code." ) 554 protected CodeType code; 555 556 /** 557 * The base resource type(s) that this search parameter can be used against. 558 */ 559 @Child(name = "base", type = {CodeType.class}, order=19, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 560 @Description(shortDefinition="The resource type(s) this search parameter applies to", formalDefinition="The base resource type(s) that this search parameter can be used against." ) 561 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-independent-all-resource-types") 562 protected List<Enumeration<VersionIndependentResourceTypesAll>> base; 563 564 /** 565 * The type of value that a search parameter may contain, and how the content is interpreted. 566 */ 567 @Child(name = "type", type = {CodeType.class}, order=20, min=1, max=1, modifier=false, summary=true) 568 @Description(shortDefinition="number | date | string | token | reference | composite | quantity | uri | special", formalDefinition="The type of value that a search parameter may contain, and how the content is interpreted." ) 569 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-param-type") 570 protected Enumeration<SearchParamType> type; 571 572 /** 573 * A FHIRPath expression that returns a set of elements for the search parameter. 574 */ 575 @Child(name = "expression", type = {StringType.class}, order=21, min=0, max=1, modifier=false, summary=false) 576 @Description(shortDefinition="FHIRPath expression that extracts the values", formalDefinition="A FHIRPath expression that returns a set of elements for the search parameter." ) 577 protected StringType expression; 578 579 /** 580 * How the search parameter relates to the set of elements returned by evaluating the expression query. 581 */ 582 @Child(name = "processingMode", type = {CodeType.class}, order=22, min=0, max=1, modifier=false, summary=false) 583 @Description(shortDefinition="normal | phonetic | other", formalDefinition="How the search parameter relates to the set of elements returned by evaluating the expression query." ) 584 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-processingmode") 585 protected Enumeration<SearchProcessingModeType> processingMode; 586 587 /** 588 * FHIRPath expression that defines/sets a complex constraint for when this SearchParameter is applicable. 589 */ 590 @Child(name = "constraint", type = {StringType.class}, order=23, min=0, max=1, modifier=false, summary=false) 591 @Description(shortDefinition="FHIRPath expression that constraints the usage of this SearchParamete", formalDefinition="FHIRPath expression that defines/sets a complex constraint for when this SearchParameter is applicable." ) 592 protected StringType constraint; 593 594 /** 595 * Types of resource (if a resource is referenced). 596 */ 597 @Child(name = "target", type = {CodeType.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 598 @Description(shortDefinition="Types of resource (if a resource reference)", formalDefinition="Types of resource (if a resource is referenced)." ) 599 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-independent-all-resource-types") 600 protected List<Enumeration<VersionIndependentResourceTypesAll>> target; 601 602 /** 603 * Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match. 604 */ 605 @Child(name = "multipleOr", type = {BooleanType.class}, order=25, min=0, max=1, modifier=false, summary=false) 606 @Description(shortDefinition="Allow multiple values per parameter (or)", formalDefinition="Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match." ) 607 protected BooleanType multipleOr; 608 609 /** 610 * Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match. 611 */ 612 @Child(name = "multipleAnd", type = {BooleanType.class}, order=26, min=0, max=1, modifier=false, summary=false) 613 @Description(shortDefinition="Allow multiple parameters (and)", formalDefinition="Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match." ) 614 protected BooleanType multipleAnd; 615 616 /** 617 * Comparators supported for the search parameter. 618 */ 619 @Child(name = "comparator", type = {CodeType.class}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 620 @Description(shortDefinition="eq | ne | gt | lt | ge | le | sa | eb | ap", formalDefinition="Comparators supported for the search parameter." ) 621 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-comparator") 622 protected List<Enumeration<SearchComparator>> comparator; 623 624 /** 625 * A modifier supported for the search parameter. 626 */ 627 @Child(name = "modifier", type = {CodeType.class}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 628 @Description(shortDefinition="missing | exact | contains | not | text | in | not-in | below | above | type | identifier | of-type | code-text | text-advanced | iterate", formalDefinition="A modifier supported for the search parameter." ) 629 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-modifier-code") 630 protected List<Enumeration<SearchModifierCode>> modifier; 631 632 /** 633 * Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type. 634 */ 635 @Child(name = "chain", type = {StringType.class}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 636 @Description(shortDefinition="Chained names supported", formalDefinition="Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type." ) 637 protected List<StringType> chain; 638 639 /** 640 * Used to define the parts of a composite search parameter. 641 */ 642 @Child(name = "component", type = {}, order=30, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 643 @Description(shortDefinition="For Composite resources to define the parts", formalDefinition="Used to define the parts of a composite search parameter." ) 644 protected List<SearchParameterComponentComponent> component; 645 646 private static final long serialVersionUID = -327665794L; 647 648 /** 649 * Constructor 650 */ 651 public SearchParameter() { 652 super(); 653 } 654 655 /** 656 * Constructor 657 */ 658 public SearchParameter(String url, String name, PublicationStatus status, String description, String code, VersionIndependentResourceTypesAll base, SearchParamType type) { 659 super(); 660 this.setUrl(url); 661 this.setName(name); 662 this.setStatus(status); 663 this.setDescription(description); 664 this.setCode(code); 665 this.addBase(base); 666 this.setType(type); 667 } 668 669 /** 670 * @return {@link #url} (An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this search parameter is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the search parameter is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 671 */ 672 public UriType getUrlElement() { 673 if (this.url == null) 674 if (Configuration.errorOnAutoCreate()) 675 throw new Error("Attempt to auto-create SearchParameter.url"); 676 else if (Configuration.doAutoCreate()) 677 this.url = new UriType(); // bb 678 return this.url; 679 } 680 681 public boolean hasUrlElement() { 682 return this.url != null && !this.url.isEmpty(); 683 } 684 685 public boolean hasUrl() { 686 return this.url != null && !this.url.isEmpty(); 687 } 688 689 /** 690 * @param value {@link #url} (An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this search parameter is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the search parameter is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 691 */ 692 public SearchParameter setUrlElement(UriType value) { 693 this.url = value; 694 return this; 695 } 696 697 /** 698 * @return An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this search parameter is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the search parameter is stored on different servers. 699 */ 700 public String getUrl() { 701 return this.url == null ? null : this.url.getValue(); 702 } 703 704 /** 705 * @param value An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this search parameter is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the search parameter is stored on different servers. 706 */ 707 public SearchParameter setUrl(String value) { 708 if (this.url == null) 709 this.url = new UriType(); 710 this.url.setValue(value); 711 return this; 712 } 713 714 /** 715 * @return {@link #identifier} (A formal identifier that is used to identify this search parameter when it is represented in other formats, or referenced in a specification, model, design or an instance.) 716 */ 717 public List<Identifier> getIdentifier() { 718 if (this.identifier == null) 719 this.identifier = new ArrayList<Identifier>(); 720 return this.identifier; 721 } 722 723 /** 724 * @return Returns a reference to <code>this</code> for easy method chaining 725 */ 726 public SearchParameter setIdentifier(List<Identifier> theIdentifier) { 727 this.identifier = theIdentifier; 728 return this; 729 } 730 731 public boolean hasIdentifier() { 732 if (this.identifier == null) 733 return false; 734 for (Identifier item : this.identifier) 735 if (!item.isEmpty()) 736 return true; 737 return false; 738 } 739 740 public Identifier addIdentifier() { //3 741 Identifier t = new Identifier(); 742 if (this.identifier == null) 743 this.identifier = new ArrayList<Identifier>(); 744 this.identifier.add(t); 745 return t; 746 } 747 748 public SearchParameter addIdentifier(Identifier t) { //3 749 if (t == null) 750 return this; 751 if (this.identifier == null) 752 this.identifier = new ArrayList<Identifier>(); 753 this.identifier.add(t); 754 return this; 755 } 756 757 /** 758 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 759 */ 760 public Identifier getIdentifierFirstRep() { 761 if (getIdentifier().isEmpty()) { 762 addIdentifier(); 763 } 764 return getIdentifier().get(0); 765 } 766 767 /** 768 * @return {@link #version} (The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 769 */ 770 public StringType getVersionElement() { 771 if (this.version == null) 772 if (Configuration.errorOnAutoCreate()) 773 throw new Error("Attempt to auto-create SearchParameter.version"); 774 else if (Configuration.doAutoCreate()) 775 this.version = new StringType(); // bb 776 return this.version; 777 } 778 779 public boolean hasVersionElement() { 780 return this.version != null && !this.version.isEmpty(); 781 } 782 783 public boolean hasVersion() { 784 return this.version != null && !this.version.isEmpty(); 785 } 786 787 /** 788 * @param value {@link #version} (The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 789 */ 790 public SearchParameter setVersionElement(StringType value) { 791 this.version = value; 792 return this; 793 } 794 795 /** 796 * @return The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 797 */ 798 public String getVersion() { 799 return this.version == null ? null : this.version.getValue(); 800 } 801 802 /** 803 * @param value The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 804 */ 805 public SearchParameter setVersion(String value) { 806 if (Utilities.noString(value)) 807 this.version = null; 808 else { 809 if (this.version == null) 810 this.version = new StringType(); 811 this.version.setValue(value); 812 } 813 return this; 814 } 815 816 /** 817 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 818 */ 819 public DataType getVersionAlgorithm() { 820 return this.versionAlgorithm; 821 } 822 823 /** 824 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 825 */ 826 public StringType getVersionAlgorithmStringType() throws FHIRException { 827 if (this.versionAlgorithm == null) 828 this.versionAlgorithm = new StringType(); 829 if (!(this.versionAlgorithm instanceof StringType)) 830 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 831 return (StringType) this.versionAlgorithm; 832 } 833 834 public boolean hasVersionAlgorithmStringType() { 835 return this != null && this.versionAlgorithm instanceof StringType; 836 } 837 838 /** 839 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 840 */ 841 public Coding getVersionAlgorithmCoding() throws FHIRException { 842 if (this.versionAlgorithm == null) 843 this.versionAlgorithm = new Coding(); 844 if (!(this.versionAlgorithm instanceof Coding)) 845 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 846 return (Coding) this.versionAlgorithm; 847 } 848 849 public boolean hasVersionAlgorithmCoding() { 850 return this != null && this.versionAlgorithm instanceof Coding; 851 } 852 853 public boolean hasVersionAlgorithm() { 854 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 855 } 856 857 /** 858 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 859 */ 860 public SearchParameter setVersionAlgorithm(DataType value) { 861 if (value != null && !(value instanceof StringType || value instanceof Coding)) 862 throw new FHIRException("Not the right type for SearchParameter.versionAlgorithm[x]: "+value.fhirType()); 863 this.versionAlgorithm = value; 864 return this; 865 } 866 867 /** 868 * @return {@link #name} (A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 869 */ 870 public StringType getNameElement() { 871 if (this.name == null) 872 if (Configuration.errorOnAutoCreate()) 873 throw new Error("Attempt to auto-create SearchParameter.name"); 874 else if (Configuration.doAutoCreate()) 875 this.name = new StringType(); // bb 876 return this.name; 877 } 878 879 public boolean hasNameElement() { 880 return this.name != null && !this.name.isEmpty(); 881 } 882 883 public boolean hasName() { 884 return this.name != null && !this.name.isEmpty(); 885 } 886 887 /** 888 * @param value {@link #name} (A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 889 */ 890 public SearchParameter setNameElement(StringType value) { 891 this.name = value; 892 return this; 893 } 894 895 /** 896 * @return A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation. 897 */ 898 public String getName() { 899 return this.name == null ? null : this.name.getValue(); 900 } 901 902 /** 903 * @param value A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation. 904 */ 905 public SearchParameter setName(String value) { 906 if (this.name == null) 907 this.name = new StringType(); 908 this.name.setValue(value); 909 return this; 910 } 911 912 /** 913 * @return {@link #title} (A short, descriptive, user-friendly title for the search parameter.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 914 */ 915 public StringType getTitleElement() { 916 if (this.title == null) 917 if (Configuration.errorOnAutoCreate()) 918 throw new Error("Attempt to auto-create SearchParameter.title"); 919 else if (Configuration.doAutoCreate()) 920 this.title = new StringType(); // bb 921 return this.title; 922 } 923 924 public boolean hasTitleElement() { 925 return this.title != null && !this.title.isEmpty(); 926 } 927 928 public boolean hasTitle() { 929 return this.title != null && !this.title.isEmpty(); 930 } 931 932 /** 933 * @param value {@link #title} (A short, descriptive, user-friendly title for the search parameter.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 934 */ 935 public SearchParameter setTitleElement(StringType value) { 936 this.title = value; 937 return this; 938 } 939 940 /** 941 * @return A short, descriptive, user-friendly title for the search parameter. 942 */ 943 public String getTitle() { 944 return this.title == null ? null : this.title.getValue(); 945 } 946 947 /** 948 * @param value A short, descriptive, user-friendly title for the search parameter. 949 */ 950 public SearchParameter setTitle(String value) { 951 if (Utilities.noString(value)) 952 this.title = null; 953 else { 954 if (this.title == null) 955 this.title = new StringType(); 956 this.title.setValue(value); 957 } 958 return this; 959 } 960 961 /** 962 * @return {@link #derivedFrom} (Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.). This is the underlying object with id, value and extensions. The accessor "getDerivedFrom" gives direct access to the value 963 */ 964 public CanonicalType getDerivedFromElement() { 965 if (this.derivedFrom == null) 966 if (Configuration.errorOnAutoCreate()) 967 throw new Error("Attempt to auto-create SearchParameter.derivedFrom"); 968 else if (Configuration.doAutoCreate()) 969 this.derivedFrom = new CanonicalType(); // bb 970 return this.derivedFrom; 971 } 972 973 public boolean hasDerivedFromElement() { 974 return this.derivedFrom != null && !this.derivedFrom.isEmpty(); 975 } 976 977 public boolean hasDerivedFrom() { 978 return this.derivedFrom != null && !this.derivedFrom.isEmpty(); 979 } 980 981 /** 982 * @param value {@link #derivedFrom} (Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.). This is the underlying object with id, value and extensions. The accessor "getDerivedFrom" gives direct access to the value 983 */ 984 public SearchParameter setDerivedFromElement(CanonicalType value) { 985 this.derivedFrom = value; 986 return this; 987 } 988 989 /** 990 * @return Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter. 991 */ 992 public String getDerivedFrom() { 993 return this.derivedFrom == null ? null : this.derivedFrom.getValue(); 994 } 995 996 /** 997 * @param value Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter. 998 */ 999 public SearchParameter setDerivedFrom(String value) { 1000 if (Utilities.noString(value)) 1001 this.derivedFrom = null; 1002 else { 1003 if (this.derivedFrom == null) 1004 this.derivedFrom = new CanonicalType(); 1005 this.derivedFrom.setValue(value); 1006 } 1007 return this; 1008 } 1009 1010 /** 1011 * @return {@link #status} (The status of this search parameter. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1012 */ 1013 public Enumeration<PublicationStatus> getStatusElement() { 1014 if (this.status == null) 1015 if (Configuration.errorOnAutoCreate()) 1016 throw new Error("Attempt to auto-create SearchParameter.status"); 1017 else if (Configuration.doAutoCreate()) 1018 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1019 return this.status; 1020 } 1021 1022 public boolean hasStatusElement() { 1023 return this.status != null && !this.status.isEmpty(); 1024 } 1025 1026 public boolean hasStatus() { 1027 return this.status != null && !this.status.isEmpty(); 1028 } 1029 1030 /** 1031 * @param value {@link #status} (The status of this search parameter. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1032 */ 1033 public SearchParameter setStatusElement(Enumeration<PublicationStatus> value) { 1034 this.status = value; 1035 return this; 1036 } 1037 1038 /** 1039 * @return The status of this search parameter. Enables tracking the life-cycle of the content. 1040 */ 1041 public PublicationStatus getStatus() { 1042 return this.status == null ? null : this.status.getValue(); 1043 } 1044 1045 /** 1046 * @param value The status of this search parameter. Enables tracking the life-cycle of the content. 1047 */ 1048 public SearchParameter setStatus(PublicationStatus value) { 1049 if (this.status == null) 1050 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1051 this.status.setValue(value); 1052 return this; 1053 } 1054 1055 /** 1056 * @return {@link #experimental} (A Boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1057 */ 1058 public BooleanType getExperimentalElement() { 1059 if (this.experimental == null) 1060 if (Configuration.errorOnAutoCreate()) 1061 throw new Error("Attempt to auto-create SearchParameter.experimental"); 1062 else if (Configuration.doAutoCreate()) 1063 this.experimental = new BooleanType(); // bb 1064 return this.experimental; 1065 } 1066 1067 public boolean hasExperimentalElement() { 1068 return this.experimental != null && !this.experimental.isEmpty(); 1069 } 1070 1071 public boolean hasExperimental() { 1072 return this.experimental != null && !this.experimental.isEmpty(); 1073 } 1074 1075 /** 1076 * @param value {@link #experimental} (A Boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1077 */ 1078 public SearchParameter setExperimentalElement(BooleanType value) { 1079 this.experimental = value; 1080 return this; 1081 } 1082 1083 /** 1084 * @return A Boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1085 */ 1086 public boolean getExperimental() { 1087 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1088 } 1089 1090 /** 1091 * @param value A Boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1092 */ 1093 public SearchParameter setExperimental(boolean value) { 1094 if (this.experimental == null) 1095 this.experimental = new BooleanType(); 1096 this.experimental.setValue(value); 1097 return this; 1098 } 1099 1100 /** 1101 * @return {@link #date} (The date (and optionally time) when the search parameter was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1102 */ 1103 public DateTimeType getDateElement() { 1104 if (this.date == null) 1105 if (Configuration.errorOnAutoCreate()) 1106 throw new Error("Attempt to auto-create SearchParameter.date"); 1107 else if (Configuration.doAutoCreate()) 1108 this.date = new DateTimeType(); // bb 1109 return this.date; 1110 } 1111 1112 public boolean hasDateElement() { 1113 return this.date != null && !this.date.isEmpty(); 1114 } 1115 1116 public boolean hasDate() { 1117 return this.date != null && !this.date.isEmpty(); 1118 } 1119 1120 /** 1121 * @param value {@link #date} (The date (and optionally time) when the search parameter was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1122 */ 1123 public SearchParameter setDateElement(DateTimeType value) { 1124 this.date = value; 1125 return this; 1126 } 1127 1128 /** 1129 * @return The date (and optionally time) when the search parameter was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes. 1130 */ 1131 public Date getDate() { 1132 return this.date == null ? null : this.date.getValue(); 1133 } 1134 1135 /** 1136 * @param value The date (and optionally time) when the search parameter was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes. 1137 */ 1138 public SearchParameter setDate(Date value) { 1139 if (value == null) 1140 this.date = null; 1141 else { 1142 if (this.date == null) 1143 this.date = new DateTimeType(); 1144 this.date.setValue(value); 1145 } 1146 return this; 1147 } 1148 1149 /** 1150 * @return {@link #publisher} (The name of the organization or individual tresponsible for the release and ongoing maintenance of the search parameter.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1151 */ 1152 public StringType getPublisherElement() { 1153 if (this.publisher == null) 1154 if (Configuration.errorOnAutoCreate()) 1155 throw new Error("Attempt to auto-create SearchParameter.publisher"); 1156 else if (Configuration.doAutoCreate()) 1157 this.publisher = new StringType(); // bb 1158 return this.publisher; 1159 } 1160 1161 public boolean hasPublisherElement() { 1162 return this.publisher != null && !this.publisher.isEmpty(); 1163 } 1164 1165 public boolean hasPublisher() { 1166 return this.publisher != null && !this.publisher.isEmpty(); 1167 } 1168 1169 /** 1170 * @param value {@link #publisher} (The name of the organization or individual tresponsible for the release and ongoing maintenance of the search parameter.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1171 */ 1172 public SearchParameter setPublisherElement(StringType value) { 1173 this.publisher = value; 1174 return this; 1175 } 1176 1177 /** 1178 * @return The name of the organization or individual tresponsible for the release and ongoing maintenance of the search parameter. 1179 */ 1180 public String getPublisher() { 1181 return this.publisher == null ? null : this.publisher.getValue(); 1182 } 1183 1184 /** 1185 * @param value The name of the organization or individual tresponsible for the release and ongoing maintenance of the search parameter. 1186 */ 1187 public SearchParameter setPublisher(String value) { 1188 if (Utilities.noString(value)) 1189 this.publisher = null; 1190 else { 1191 if (this.publisher == null) 1192 this.publisher = new StringType(); 1193 this.publisher.setValue(value); 1194 } 1195 return this; 1196 } 1197 1198 /** 1199 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 1200 */ 1201 public List<ContactDetail> getContact() { 1202 if (this.contact == null) 1203 this.contact = new ArrayList<ContactDetail>(); 1204 return this.contact; 1205 } 1206 1207 /** 1208 * @return Returns a reference to <code>this</code> for easy method chaining 1209 */ 1210 public SearchParameter setContact(List<ContactDetail> theContact) { 1211 this.contact = theContact; 1212 return this; 1213 } 1214 1215 public boolean hasContact() { 1216 if (this.contact == null) 1217 return false; 1218 for (ContactDetail item : this.contact) 1219 if (!item.isEmpty()) 1220 return true; 1221 return false; 1222 } 1223 1224 public ContactDetail addContact() { //3 1225 ContactDetail t = new ContactDetail(); 1226 if (this.contact == null) 1227 this.contact = new ArrayList<ContactDetail>(); 1228 this.contact.add(t); 1229 return t; 1230 } 1231 1232 public SearchParameter addContact(ContactDetail t) { //3 1233 if (t == null) 1234 return this; 1235 if (this.contact == null) 1236 this.contact = new ArrayList<ContactDetail>(); 1237 this.contact.add(t); 1238 return this; 1239 } 1240 1241 /** 1242 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 1243 */ 1244 public ContactDetail getContactFirstRep() { 1245 if (getContact().isEmpty()) { 1246 addContact(); 1247 } 1248 return getContact().get(0); 1249 } 1250 1251 /** 1252 * @return {@link #description} (And how it used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1253 */ 1254 public MarkdownType getDescriptionElement() { 1255 if (this.description == null) 1256 if (Configuration.errorOnAutoCreate()) 1257 throw new Error("Attempt to auto-create SearchParameter.description"); 1258 else if (Configuration.doAutoCreate()) 1259 this.description = new MarkdownType(); // bb 1260 return this.description; 1261 } 1262 1263 public boolean hasDescriptionElement() { 1264 return this.description != null && !this.description.isEmpty(); 1265 } 1266 1267 public boolean hasDescription() { 1268 return this.description != null && !this.description.isEmpty(); 1269 } 1270 1271 /** 1272 * @param value {@link #description} (And how it used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1273 */ 1274 public SearchParameter setDescriptionElement(MarkdownType value) { 1275 this.description = value; 1276 return this; 1277 } 1278 1279 /** 1280 * @return And how it used. 1281 */ 1282 public String getDescription() { 1283 return this.description == null ? null : this.description.getValue(); 1284 } 1285 1286 /** 1287 * @param value And how it used. 1288 */ 1289 public SearchParameter setDescription(String value) { 1290 if (this.description == null) 1291 this.description = new MarkdownType(); 1292 this.description.setValue(value); 1293 return this; 1294 } 1295 1296 /** 1297 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate search parameter instances.) 1298 */ 1299 public List<UsageContext> getUseContext() { 1300 if (this.useContext == null) 1301 this.useContext = new ArrayList<UsageContext>(); 1302 return this.useContext; 1303 } 1304 1305 /** 1306 * @return Returns a reference to <code>this</code> for easy method chaining 1307 */ 1308 public SearchParameter setUseContext(List<UsageContext> theUseContext) { 1309 this.useContext = theUseContext; 1310 return this; 1311 } 1312 1313 public boolean hasUseContext() { 1314 if (this.useContext == null) 1315 return false; 1316 for (UsageContext item : this.useContext) 1317 if (!item.isEmpty()) 1318 return true; 1319 return false; 1320 } 1321 1322 public UsageContext addUseContext() { //3 1323 UsageContext t = new UsageContext(); 1324 if (this.useContext == null) 1325 this.useContext = new ArrayList<UsageContext>(); 1326 this.useContext.add(t); 1327 return t; 1328 } 1329 1330 public SearchParameter addUseContext(UsageContext t) { //3 1331 if (t == null) 1332 return this; 1333 if (this.useContext == null) 1334 this.useContext = new ArrayList<UsageContext>(); 1335 this.useContext.add(t); 1336 return this; 1337 } 1338 1339 /** 1340 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 1341 */ 1342 public UsageContext getUseContextFirstRep() { 1343 if (getUseContext().isEmpty()) { 1344 addUseContext(); 1345 } 1346 return getUseContext().get(0); 1347 } 1348 1349 /** 1350 * @return {@link #jurisdiction} (A legal or geographic region in which the search parameter is intended to be used.) 1351 */ 1352 public List<CodeableConcept> getJurisdiction() { 1353 if (this.jurisdiction == null) 1354 this.jurisdiction = new ArrayList<CodeableConcept>(); 1355 return this.jurisdiction; 1356 } 1357 1358 /** 1359 * @return Returns a reference to <code>this</code> for easy method chaining 1360 */ 1361 public SearchParameter setJurisdiction(List<CodeableConcept> theJurisdiction) { 1362 this.jurisdiction = theJurisdiction; 1363 return this; 1364 } 1365 1366 public boolean hasJurisdiction() { 1367 if (this.jurisdiction == null) 1368 return false; 1369 for (CodeableConcept item : this.jurisdiction) 1370 if (!item.isEmpty()) 1371 return true; 1372 return false; 1373 } 1374 1375 public CodeableConcept addJurisdiction() { //3 1376 CodeableConcept t = new CodeableConcept(); 1377 if (this.jurisdiction == null) 1378 this.jurisdiction = new ArrayList<CodeableConcept>(); 1379 this.jurisdiction.add(t); 1380 return t; 1381 } 1382 1383 public SearchParameter addJurisdiction(CodeableConcept t) { //3 1384 if (t == null) 1385 return this; 1386 if (this.jurisdiction == null) 1387 this.jurisdiction = new ArrayList<CodeableConcept>(); 1388 this.jurisdiction.add(t); 1389 return this; 1390 } 1391 1392 /** 1393 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 1394 */ 1395 public CodeableConcept getJurisdictionFirstRep() { 1396 if (getJurisdiction().isEmpty()) { 1397 addJurisdiction(); 1398 } 1399 return getJurisdiction().get(0); 1400 } 1401 1402 /** 1403 * @return {@link #purpose} (Explanation of why this search parameter is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1404 */ 1405 public MarkdownType getPurposeElement() { 1406 if (this.purpose == null) 1407 if (Configuration.errorOnAutoCreate()) 1408 throw new Error("Attempt to auto-create SearchParameter.purpose"); 1409 else if (Configuration.doAutoCreate()) 1410 this.purpose = new MarkdownType(); // bb 1411 return this.purpose; 1412 } 1413 1414 public boolean hasPurposeElement() { 1415 return this.purpose != null && !this.purpose.isEmpty(); 1416 } 1417 1418 public boolean hasPurpose() { 1419 return this.purpose != null && !this.purpose.isEmpty(); 1420 } 1421 1422 /** 1423 * @param value {@link #purpose} (Explanation of why this search parameter is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1424 */ 1425 public SearchParameter setPurposeElement(MarkdownType value) { 1426 this.purpose = value; 1427 return this; 1428 } 1429 1430 /** 1431 * @return Explanation of why this search parameter is needed and why it has been designed as it has. 1432 */ 1433 public String getPurpose() { 1434 return this.purpose == null ? null : this.purpose.getValue(); 1435 } 1436 1437 /** 1438 * @param value Explanation of why this search parameter is needed and why it has been designed as it has. 1439 */ 1440 public SearchParameter setPurpose(String value) { 1441 if (Utilities.noString(value)) 1442 this.purpose = null; 1443 else { 1444 if (this.purpose == null) 1445 this.purpose = new MarkdownType(); 1446 this.purpose.setValue(value); 1447 } 1448 return this; 1449 } 1450 1451 /** 1452 * @return {@link #copyright} (A copyright statement relating to the search parameter and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the search parameter.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1453 */ 1454 public MarkdownType getCopyrightElement() { 1455 if (this.copyright == null) 1456 if (Configuration.errorOnAutoCreate()) 1457 throw new Error("Attempt to auto-create SearchParameter.copyright"); 1458 else if (Configuration.doAutoCreate()) 1459 this.copyright = new MarkdownType(); // bb 1460 return this.copyright; 1461 } 1462 1463 public boolean hasCopyrightElement() { 1464 return this.copyright != null && !this.copyright.isEmpty(); 1465 } 1466 1467 public boolean hasCopyright() { 1468 return this.copyright != null && !this.copyright.isEmpty(); 1469 } 1470 1471 /** 1472 * @param value {@link #copyright} (A copyright statement relating to the search parameter and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the search parameter.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1473 */ 1474 public SearchParameter setCopyrightElement(MarkdownType value) { 1475 this.copyright = value; 1476 return this; 1477 } 1478 1479 /** 1480 * @return A copyright statement relating to the search parameter and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the search parameter. 1481 */ 1482 public String getCopyright() { 1483 return this.copyright == null ? null : this.copyright.getValue(); 1484 } 1485 1486 /** 1487 * @param value A copyright statement relating to the search parameter and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the search parameter. 1488 */ 1489 public SearchParameter setCopyright(String value) { 1490 if (Utilities.noString(value)) 1491 this.copyright = null; 1492 else { 1493 if (this.copyright == null) 1494 this.copyright = new MarkdownType(); 1495 this.copyright.setValue(value); 1496 } 1497 return this; 1498 } 1499 1500 /** 1501 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 1502 */ 1503 public StringType getCopyrightLabelElement() { 1504 if (this.copyrightLabel == null) 1505 if (Configuration.errorOnAutoCreate()) 1506 throw new Error("Attempt to auto-create SearchParameter.copyrightLabel"); 1507 else if (Configuration.doAutoCreate()) 1508 this.copyrightLabel = new StringType(); // bb 1509 return this.copyrightLabel; 1510 } 1511 1512 public boolean hasCopyrightLabelElement() { 1513 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 1514 } 1515 1516 public boolean hasCopyrightLabel() { 1517 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 1518 } 1519 1520 /** 1521 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 1522 */ 1523 public SearchParameter setCopyrightLabelElement(StringType value) { 1524 this.copyrightLabel = value; 1525 return this; 1526 } 1527 1528 /** 1529 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1530 */ 1531 public String getCopyrightLabel() { 1532 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 1533 } 1534 1535 /** 1536 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1537 */ 1538 public SearchParameter setCopyrightLabel(String value) { 1539 if (Utilities.noString(value)) 1540 this.copyrightLabel = null; 1541 else { 1542 if (this.copyrightLabel == null) 1543 this.copyrightLabel = new StringType(); 1544 this.copyrightLabel.setValue(value); 1545 } 1546 return this; 1547 } 1548 1549 /** 1550 * @return {@link #code} (The label that is recommended to be used in the URL or the parameter name in a parameters resource for this search parameter. In some cases, servers may need to use a different CapabilityStatement searchParam.name to differentiate between multiple SearchParameters that happen to have the same code.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1551 */ 1552 public CodeType getCodeElement() { 1553 if (this.code == null) 1554 if (Configuration.errorOnAutoCreate()) 1555 throw new Error("Attempt to auto-create SearchParameter.code"); 1556 else if (Configuration.doAutoCreate()) 1557 this.code = new CodeType(); // bb 1558 return this.code; 1559 } 1560 1561 public boolean hasCodeElement() { 1562 return this.code != null && !this.code.isEmpty(); 1563 } 1564 1565 public boolean hasCode() { 1566 return this.code != null && !this.code.isEmpty(); 1567 } 1568 1569 /** 1570 * @param value {@link #code} (The label that is recommended to be used in the URL or the parameter name in a parameters resource for this search parameter. In some cases, servers may need to use a different CapabilityStatement searchParam.name to differentiate between multiple SearchParameters that happen to have the same code.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1571 */ 1572 public SearchParameter setCodeElement(CodeType value) { 1573 this.code = value; 1574 return this; 1575 } 1576 1577 /** 1578 * @return The label that is recommended to be used in the URL or the parameter name in a parameters resource for this search parameter. In some cases, servers may need to use a different CapabilityStatement searchParam.name to differentiate between multiple SearchParameters that happen to have the same code. 1579 */ 1580 public String getCode() { 1581 return this.code == null ? null : this.code.getValue(); 1582 } 1583 1584 /** 1585 * @param value The label that is recommended to be used in the URL or the parameter name in a parameters resource for this search parameter. In some cases, servers may need to use a different CapabilityStatement searchParam.name to differentiate between multiple SearchParameters that happen to have the same code. 1586 */ 1587 public SearchParameter setCode(String value) { 1588 if (this.code == null) 1589 this.code = new CodeType(); 1590 this.code.setValue(value); 1591 return this; 1592 } 1593 1594 /** 1595 * @return {@link #base} (The base resource type(s) that this search parameter can be used against.) 1596 */ 1597 public List<Enumeration<VersionIndependentResourceTypesAll>> getBase() { 1598 if (this.base == null) 1599 this.base = new ArrayList<Enumeration<VersionIndependentResourceTypesAll>>(); 1600 return this.base; 1601 } 1602 1603 /** 1604 * @return Returns a reference to <code>this</code> for easy method chaining 1605 */ 1606 public SearchParameter setBase(List<Enumeration<VersionIndependentResourceTypesAll>> theBase) { 1607 this.base = theBase; 1608 return this; 1609 } 1610 1611 public boolean hasBase() { 1612 if (this.base == null) 1613 return false; 1614 for (Enumeration<VersionIndependentResourceTypesAll> item : this.base) 1615 if (!item.isEmpty()) 1616 return true; 1617 return false; 1618 } 1619 1620 /** 1621 * @return {@link #base} (The base resource type(s) that this search parameter can be used against.) 1622 */ 1623 public Enumeration<VersionIndependentResourceTypesAll> addBaseElement() {//2 1624 Enumeration<VersionIndependentResourceTypesAll> t = new Enumeration<VersionIndependentResourceTypesAll>(new VersionIndependentResourceTypesAllEnumFactory()); 1625 if (this.base == null) 1626 this.base = new ArrayList<Enumeration<VersionIndependentResourceTypesAll>>(); 1627 this.base.add(t); 1628 return t; 1629 } 1630 1631 /** 1632 * @param value {@link #base} (The base resource type(s) that this search parameter can be used against.) 1633 */ 1634 public SearchParameter addBase(VersionIndependentResourceTypesAll value) { //1 1635 Enumeration<VersionIndependentResourceTypesAll> t = new Enumeration<VersionIndependentResourceTypesAll>(new VersionIndependentResourceTypesAllEnumFactory()); 1636 t.setValue(value); 1637 if (this.base == null) 1638 this.base = new ArrayList<Enumeration<VersionIndependentResourceTypesAll>>(); 1639 this.base.add(t); 1640 return this; 1641 } 1642 1643 /** 1644 * @param value {@link #base} (The base resource type(s) that this search parameter can be used against.) 1645 */ 1646 public boolean hasBase(VersionIndependentResourceTypesAll value) { 1647 if (this.base == null) 1648 return false; 1649 for (Enumeration<VersionIndependentResourceTypesAll> v : this.base) 1650 if (v.getValue().equals(value)) // code 1651 return true; 1652 return false; 1653 } 1654 1655 /** 1656 * @return {@link #type} (The type of value that a search parameter may contain, and how the content is interpreted.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1657 */ 1658 public Enumeration<SearchParamType> getTypeElement() { 1659 if (this.type == null) 1660 if (Configuration.errorOnAutoCreate()) 1661 throw new Error("Attempt to auto-create SearchParameter.type"); 1662 else if (Configuration.doAutoCreate()) 1663 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); // bb 1664 return this.type; 1665 } 1666 1667 public boolean hasTypeElement() { 1668 return this.type != null && !this.type.isEmpty(); 1669 } 1670 1671 public boolean hasType() { 1672 return this.type != null && !this.type.isEmpty(); 1673 } 1674 1675 /** 1676 * @param value {@link #type} (The type of value that a search parameter may contain, and how the content is interpreted.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1677 */ 1678 public SearchParameter setTypeElement(Enumeration<SearchParamType> value) { 1679 this.type = value; 1680 return this; 1681 } 1682 1683 /** 1684 * @return The type of value that a search parameter may contain, and how the content is interpreted. 1685 */ 1686 public SearchParamType getType() { 1687 return this.type == null ? null : this.type.getValue(); 1688 } 1689 1690 /** 1691 * @param value The type of value that a search parameter may contain, and how the content is interpreted. 1692 */ 1693 public SearchParameter setType(SearchParamType value) { 1694 if (this.type == null) 1695 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); 1696 this.type.setValue(value); 1697 return this; 1698 } 1699 1700 /** 1701 * @return {@link #expression} (A FHIRPath expression that returns a set of elements for the search parameter.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 1702 */ 1703 public StringType getExpressionElement() { 1704 if (this.expression == null) 1705 if (Configuration.errorOnAutoCreate()) 1706 throw new Error("Attempt to auto-create SearchParameter.expression"); 1707 else if (Configuration.doAutoCreate()) 1708 this.expression = new StringType(); // bb 1709 return this.expression; 1710 } 1711 1712 public boolean hasExpressionElement() { 1713 return this.expression != null && !this.expression.isEmpty(); 1714 } 1715 1716 public boolean hasExpression() { 1717 return this.expression != null && !this.expression.isEmpty(); 1718 } 1719 1720 /** 1721 * @param value {@link #expression} (A FHIRPath expression that returns a set of elements for the search parameter.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 1722 */ 1723 public SearchParameter setExpressionElement(StringType value) { 1724 this.expression = value; 1725 return this; 1726 } 1727 1728 /** 1729 * @return A FHIRPath expression that returns a set of elements for the search parameter. 1730 */ 1731 public String getExpression() { 1732 return this.expression == null ? null : this.expression.getValue(); 1733 } 1734 1735 /** 1736 * @param value A FHIRPath expression that returns a set of elements for the search parameter. 1737 */ 1738 public SearchParameter setExpression(String value) { 1739 if (Utilities.noString(value)) 1740 this.expression = null; 1741 else { 1742 if (this.expression == null) 1743 this.expression = new StringType(); 1744 this.expression.setValue(value); 1745 } 1746 return this; 1747 } 1748 1749 /** 1750 * @return {@link #processingMode} (How the search parameter relates to the set of elements returned by evaluating the expression query.). This is the underlying object with id, value and extensions. The accessor "getProcessingMode" gives direct access to the value 1751 */ 1752 public Enumeration<SearchProcessingModeType> getProcessingModeElement() { 1753 if (this.processingMode == null) 1754 if (Configuration.errorOnAutoCreate()) 1755 throw new Error("Attempt to auto-create SearchParameter.processingMode"); 1756 else if (Configuration.doAutoCreate()) 1757 this.processingMode = new Enumeration<SearchProcessingModeType>(new SearchProcessingModeTypeEnumFactory()); // bb 1758 return this.processingMode; 1759 } 1760 1761 public boolean hasProcessingModeElement() { 1762 return this.processingMode != null && !this.processingMode.isEmpty(); 1763 } 1764 1765 public boolean hasProcessingMode() { 1766 return this.processingMode != null && !this.processingMode.isEmpty(); 1767 } 1768 1769 /** 1770 * @param value {@link #processingMode} (How the search parameter relates to the set of elements returned by evaluating the expression query.). This is the underlying object with id, value and extensions. The accessor "getProcessingMode" gives direct access to the value 1771 */ 1772 public SearchParameter setProcessingModeElement(Enumeration<SearchProcessingModeType> value) { 1773 this.processingMode = value; 1774 return this; 1775 } 1776 1777 /** 1778 * @return How the search parameter relates to the set of elements returned by evaluating the expression query. 1779 */ 1780 public SearchProcessingModeType getProcessingMode() { 1781 return this.processingMode == null ? null : this.processingMode.getValue(); 1782 } 1783 1784 /** 1785 * @param value How the search parameter relates to the set of elements returned by evaluating the expression query. 1786 */ 1787 public SearchParameter setProcessingMode(SearchProcessingModeType value) { 1788 if (value == null) 1789 this.processingMode = null; 1790 else { 1791 if (this.processingMode == null) 1792 this.processingMode = new Enumeration<SearchProcessingModeType>(new SearchProcessingModeTypeEnumFactory()); 1793 this.processingMode.setValue(value); 1794 } 1795 return this; 1796 } 1797 1798 /** 1799 * @return {@link #constraint} (FHIRPath expression that defines/sets a complex constraint for when this SearchParameter is applicable.). This is the underlying object with id, value and extensions. The accessor "getConstraint" gives direct access to the value 1800 */ 1801 public StringType getConstraintElement() { 1802 if (this.constraint == null) 1803 if (Configuration.errorOnAutoCreate()) 1804 throw new Error("Attempt to auto-create SearchParameter.constraint"); 1805 else if (Configuration.doAutoCreate()) 1806 this.constraint = new StringType(); // bb 1807 return this.constraint; 1808 } 1809 1810 public boolean hasConstraintElement() { 1811 return this.constraint != null && !this.constraint.isEmpty(); 1812 } 1813 1814 public boolean hasConstraint() { 1815 return this.constraint != null && !this.constraint.isEmpty(); 1816 } 1817 1818 /** 1819 * @param value {@link #constraint} (FHIRPath expression that defines/sets a complex constraint for when this SearchParameter is applicable.). This is the underlying object with id, value and extensions. The accessor "getConstraint" gives direct access to the value 1820 */ 1821 public SearchParameter setConstraintElement(StringType value) { 1822 this.constraint = value; 1823 return this; 1824 } 1825 1826 /** 1827 * @return FHIRPath expression that defines/sets a complex constraint for when this SearchParameter is applicable. 1828 */ 1829 public String getConstraint() { 1830 return this.constraint == null ? null : this.constraint.getValue(); 1831 } 1832 1833 /** 1834 * @param value FHIRPath expression that defines/sets a complex constraint for when this SearchParameter is applicable. 1835 */ 1836 public SearchParameter setConstraint(String value) { 1837 if (Utilities.noString(value)) 1838 this.constraint = null; 1839 else { 1840 if (this.constraint == null) 1841 this.constraint = new StringType(); 1842 this.constraint.setValue(value); 1843 } 1844 return this; 1845 } 1846 1847 /** 1848 * @return {@link #target} (Types of resource (if a resource is referenced).) 1849 */ 1850 public List<Enumeration<VersionIndependentResourceTypesAll>> getTarget() { 1851 if (this.target == null) 1852 this.target = new ArrayList<Enumeration<VersionIndependentResourceTypesAll>>(); 1853 return this.target; 1854 } 1855 1856 /** 1857 * @return Returns a reference to <code>this</code> for easy method chaining 1858 */ 1859 public SearchParameter setTarget(List<Enumeration<VersionIndependentResourceTypesAll>> theTarget) { 1860 this.target = theTarget; 1861 return this; 1862 } 1863 1864 public boolean hasTarget() { 1865 if (this.target == null) 1866 return false; 1867 for (Enumeration<VersionIndependentResourceTypesAll> item : this.target) 1868 if (!item.isEmpty()) 1869 return true; 1870 return false; 1871 } 1872 1873 /** 1874 * @return {@link #target} (Types of resource (if a resource is referenced).) 1875 */ 1876 public Enumeration<VersionIndependentResourceTypesAll> addTargetElement() {//2 1877 Enumeration<VersionIndependentResourceTypesAll> t = new Enumeration<VersionIndependentResourceTypesAll>(new VersionIndependentResourceTypesAllEnumFactory()); 1878 if (this.target == null) 1879 this.target = new ArrayList<Enumeration<VersionIndependentResourceTypesAll>>(); 1880 this.target.add(t); 1881 return t; 1882 } 1883 1884 /** 1885 * @param value {@link #target} (Types of resource (if a resource is referenced).) 1886 */ 1887 public SearchParameter addTarget(VersionIndependentResourceTypesAll value) { //1 1888 Enumeration<VersionIndependentResourceTypesAll> t = new Enumeration<VersionIndependentResourceTypesAll>(new VersionIndependentResourceTypesAllEnumFactory()); 1889 t.setValue(value); 1890 if (this.target == null) 1891 this.target = new ArrayList<Enumeration<VersionIndependentResourceTypesAll>>(); 1892 this.target.add(t); 1893 return this; 1894 } 1895 1896 /** 1897 * @param value {@link #target} (Types of resource (if a resource is referenced).) 1898 */ 1899 public boolean hasTarget(VersionIndependentResourceTypesAll value) { 1900 if (this.target == null) 1901 return false; 1902 for (Enumeration<VersionIndependentResourceTypesAll> v : this.target) 1903 if (v.getValue().equals(value)) // code 1904 return true; 1905 return false; 1906 } 1907 1908 /** 1909 * @return {@link #multipleOr} (Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match.). This is the underlying object with id, value and extensions. The accessor "getMultipleOr" gives direct access to the value 1910 */ 1911 public BooleanType getMultipleOrElement() { 1912 if (this.multipleOr == null) 1913 if (Configuration.errorOnAutoCreate()) 1914 throw new Error("Attempt to auto-create SearchParameter.multipleOr"); 1915 else if (Configuration.doAutoCreate()) 1916 this.multipleOr = new BooleanType(); // bb 1917 return this.multipleOr; 1918 } 1919 1920 public boolean hasMultipleOrElement() { 1921 return this.multipleOr != null && !this.multipleOr.isEmpty(); 1922 } 1923 1924 public boolean hasMultipleOr() { 1925 return this.multipleOr != null && !this.multipleOr.isEmpty(); 1926 } 1927 1928 /** 1929 * @param value {@link #multipleOr} (Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match.). This is the underlying object with id, value and extensions. The accessor "getMultipleOr" gives direct access to the value 1930 */ 1931 public SearchParameter setMultipleOrElement(BooleanType value) { 1932 this.multipleOr = value; 1933 return this; 1934 } 1935 1936 /** 1937 * @return Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match. 1938 */ 1939 public boolean getMultipleOr() { 1940 return this.multipleOr == null || this.multipleOr.isEmpty() ? false : this.multipleOr.getValue(); 1941 } 1942 1943 /** 1944 * @param value Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match. 1945 */ 1946 public SearchParameter setMultipleOr(boolean value) { 1947 if (this.multipleOr == null) 1948 this.multipleOr = new BooleanType(); 1949 this.multipleOr.setValue(value); 1950 return this; 1951 } 1952 1953 /** 1954 * @return {@link #multipleAnd} (Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match.). This is the underlying object with id, value and extensions. The accessor "getMultipleAnd" gives direct access to the value 1955 */ 1956 public BooleanType getMultipleAndElement() { 1957 if (this.multipleAnd == null) 1958 if (Configuration.errorOnAutoCreate()) 1959 throw new Error("Attempt to auto-create SearchParameter.multipleAnd"); 1960 else if (Configuration.doAutoCreate()) 1961 this.multipleAnd = new BooleanType(); // bb 1962 return this.multipleAnd; 1963 } 1964 1965 public boolean hasMultipleAndElement() { 1966 return this.multipleAnd != null && !this.multipleAnd.isEmpty(); 1967 } 1968 1969 public boolean hasMultipleAnd() { 1970 return this.multipleAnd != null && !this.multipleAnd.isEmpty(); 1971 } 1972 1973 /** 1974 * @param value {@link #multipleAnd} (Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match.). This is the underlying object with id, value and extensions. The accessor "getMultipleAnd" gives direct access to the value 1975 */ 1976 public SearchParameter setMultipleAndElement(BooleanType value) { 1977 this.multipleAnd = value; 1978 return this; 1979 } 1980 1981 /** 1982 * @return Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match. 1983 */ 1984 public boolean getMultipleAnd() { 1985 return this.multipleAnd == null || this.multipleAnd.isEmpty() ? false : this.multipleAnd.getValue(); 1986 } 1987 1988 /** 1989 * @param value Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match. 1990 */ 1991 public SearchParameter setMultipleAnd(boolean value) { 1992 if (this.multipleAnd == null) 1993 this.multipleAnd = new BooleanType(); 1994 this.multipleAnd.setValue(value); 1995 return this; 1996 } 1997 1998 /** 1999 * @return {@link #comparator} (Comparators supported for the search parameter.) 2000 */ 2001 public List<Enumeration<SearchComparator>> getComparator() { 2002 if (this.comparator == null) 2003 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2004 return this.comparator; 2005 } 2006 2007 /** 2008 * @return Returns a reference to <code>this</code> for easy method chaining 2009 */ 2010 public SearchParameter setComparator(List<Enumeration<SearchComparator>> theComparator) { 2011 this.comparator = theComparator; 2012 return this; 2013 } 2014 2015 public boolean hasComparator() { 2016 if (this.comparator == null) 2017 return false; 2018 for (Enumeration<SearchComparator> item : this.comparator) 2019 if (!item.isEmpty()) 2020 return true; 2021 return false; 2022 } 2023 2024 /** 2025 * @return {@link #comparator} (Comparators supported for the search parameter.) 2026 */ 2027 public Enumeration<SearchComparator> addComparatorElement() {//2 2028 Enumeration<SearchComparator> t = new Enumeration<SearchComparator>(new SearchComparatorEnumFactory()); 2029 if (this.comparator == null) 2030 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2031 this.comparator.add(t); 2032 return t; 2033 } 2034 2035 /** 2036 * @param value {@link #comparator} (Comparators supported for the search parameter.) 2037 */ 2038 public SearchParameter addComparator(SearchComparator value) { //1 2039 Enumeration<SearchComparator> t = new Enumeration<SearchComparator>(new SearchComparatorEnumFactory()); 2040 t.setValue(value); 2041 if (this.comparator == null) 2042 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2043 this.comparator.add(t); 2044 return this; 2045 } 2046 2047 /** 2048 * @param value {@link #comparator} (Comparators supported for the search parameter.) 2049 */ 2050 public boolean hasComparator(SearchComparator value) { 2051 if (this.comparator == null) 2052 return false; 2053 for (Enumeration<SearchComparator> v : this.comparator) 2054 if (v.getValue().equals(value)) // code 2055 return true; 2056 return false; 2057 } 2058 2059 /** 2060 * @return {@link #modifier} (A modifier supported for the search parameter.) 2061 */ 2062 public List<Enumeration<SearchModifierCode>> getModifier() { 2063 if (this.modifier == null) 2064 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2065 return this.modifier; 2066 } 2067 2068 /** 2069 * @return Returns a reference to <code>this</code> for easy method chaining 2070 */ 2071 public SearchParameter setModifier(List<Enumeration<SearchModifierCode>> theModifier) { 2072 this.modifier = theModifier; 2073 return this; 2074 } 2075 2076 public boolean hasModifier() { 2077 if (this.modifier == null) 2078 return false; 2079 for (Enumeration<SearchModifierCode> item : this.modifier) 2080 if (!item.isEmpty()) 2081 return true; 2082 return false; 2083 } 2084 2085 /** 2086 * @return {@link #modifier} (A modifier supported for the search parameter.) 2087 */ 2088 public Enumeration<SearchModifierCode> addModifierElement() {//2 2089 Enumeration<SearchModifierCode> t = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 2090 if (this.modifier == null) 2091 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2092 this.modifier.add(t); 2093 return t; 2094 } 2095 2096 /** 2097 * @param value {@link #modifier} (A modifier supported for the search parameter.) 2098 */ 2099 public SearchParameter addModifier(SearchModifierCode value) { //1 2100 Enumeration<SearchModifierCode> t = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 2101 t.setValue(value); 2102 if (this.modifier == null) 2103 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2104 this.modifier.add(t); 2105 return this; 2106 } 2107 2108 /** 2109 * @param value {@link #modifier} (A modifier supported for the search parameter.) 2110 */ 2111 public boolean hasModifier(SearchModifierCode value) { 2112 if (this.modifier == null) 2113 return false; 2114 for (Enumeration<SearchModifierCode> v : this.modifier) 2115 if (v.getValue().equals(value)) // code 2116 return true; 2117 return false; 2118 } 2119 2120 /** 2121 * @return {@link #chain} (Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.) 2122 */ 2123 public List<StringType> getChain() { 2124 if (this.chain == null) 2125 this.chain = new ArrayList<StringType>(); 2126 return this.chain; 2127 } 2128 2129 /** 2130 * @return Returns a reference to <code>this</code> for easy method chaining 2131 */ 2132 public SearchParameter setChain(List<StringType> theChain) { 2133 this.chain = theChain; 2134 return this; 2135 } 2136 2137 public boolean hasChain() { 2138 if (this.chain == null) 2139 return false; 2140 for (StringType item : this.chain) 2141 if (!item.isEmpty()) 2142 return true; 2143 return false; 2144 } 2145 2146 /** 2147 * @return {@link #chain} (Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.) 2148 */ 2149 public StringType addChainElement() {//2 2150 StringType t = new StringType(); 2151 if (this.chain == null) 2152 this.chain = new ArrayList<StringType>(); 2153 this.chain.add(t); 2154 return t; 2155 } 2156 2157 /** 2158 * @param value {@link #chain} (Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.) 2159 */ 2160 public SearchParameter addChain(String value) { //1 2161 StringType t = new StringType(); 2162 t.setValue(value); 2163 if (this.chain == null) 2164 this.chain = new ArrayList<StringType>(); 2165 this.chain.add(t); 2166 return this; 2167 } 2168 2169 /** 2170 * @param value {@link #chain} (Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.) 2171 */ 2172 public boolean hasChain(String value) { 2173 if (this.chain == null) 2174 return false; 2175 for (StringType v : this.chain) 2176 if (v.getValue().equals(value)) // string 2177 return true; 2178 return false; 2179 } 2180 2181 /** 2182 * @return {@link #component} (Used to define the parts of a composite search parameter.) 2183 */ 2184 public List<SearchParameterComponentComponent> getComponent() { 2185 if (this.component == null) 2186 this.component = new ArrayList<SearchParameterComponentComponent>(); 2187 return this.component; 2188 } 2189 2190 /** 2191 * @return Returns a reference to <code>this</code> for easy method chaining 2192 */ 2193 public SearchParameter setComponent(List<SearchParameterComponentComponent> theComponent) { 2194 this.component = theComponent; 2195 return this; 2196 } 2197 2198 public boolean hasComponent() { 2199 if (this.component == null) 2200 return false; 2201 for (SearchParameterComponentComponent item : this.component) 2202 if (!item.isEmpty()) 2203 return true; 2204 return false; 2205 } 2206 2207 public SearchParameterComponentComponent addComponent() { //3 2208 SearchParameterComponentComponent t = new SearchParameterComponentComponent(); 2209 if (this.component == null) 2210 this.component = new ArrayList<SearchParameterComponentComponent>(); 2211 this.component.add(t); 2212 return t; 2213 } 2214 2215 public SearchParameter addComponent(SearchParameterComponentComponent t) { //3 2216 if (t == null) 2217 return this; 2218 if (this.component == null) 2219 this.component = new ArrayList<SearchParameterComponentComponent>(); 2220 this.component.add(t); 2221 return this; 2222 } 2223 2224 /** 2225 * @return The first repetition of repeating field {@link #component}, creating it if it does not already exist {3} 2226 */ 2227 public SearchParameterComponentComponent getComponentFirstRep() { 2228 if (getComponent().isEmpty()) { 2229 addComponent(); 2230 } 2231 return getComponent().get(0); 2232 } 2233 2234 protected void listChildren(List<Property> children) { 2235 super.listChildren(children); 2236 children.add(new Property("url", "uri", "An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this search parameter is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the search parameter is stored on different servers.", 0, 1, url)); 2237 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this search parameter when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2238 children.add(new Property("version", "string", "The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 2239 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 2240 children.add(new Property("name", "string", "A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 2241 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the search parameter.", 0, 1, title)); 2242 children.add(new Property("derivedFrom", "canonical(SearchParameter)", "Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.", 0, 1, derivedFrom)); 2243 children.add(new Property("status", "code", "The status of this search parameter. Enables tracking the life-cycle of the content.", 0, 1, status)); 2244 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 2245 children.add(new Property("date", "dateTime", "The date (and optionally time) when the search parameter was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.", 0, 1, date)); 2246 children.add(new Property("publisher", "string", "The name of the organization or individual tresponsible for the release and ongoing maintenance of the search parameter.", 0, 1, publisher)); 2247 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 2248 children.add(new Property("description", "markdown", "And how it used.", 0, 1, description)); 2249 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate search parameter instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 2250 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the search parameter is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 2251 children.add(new Property("purpose", "markdown", "Explanation of why this search parameter is needed and why it has been designed as it has.", 0, 1, purpose)); 2252 children.add(new Property("copyright", "markdown", "A copyright statement relating to the search parameter and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the search parameter.", 0, 1, copyright)); 2253 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 2254 children.add(new Property("code", "code", "The label that is recommended to be used in the URL or the parameter name in a parameters resource for this search parameter. In some cases, servers may need to use a different CapabilityStatement searchParam.name to differentiate between multiple SearchParameters that happen to have the same code.", 0, 1, code)); 2255 children.add(new Property("base", "code", "The base resource type(s) that this search parameter can be used against.", 0, java.lang.Integer.MAX_VALUE, base)); 2256 children.add(new Property("type", "code", "The type of value that a search parameter may contain, and how the content is interpreted.", 0, 1, type)); 2257 children.add(new Property("expression", "string", "A FHIRPath expression that returns a set of elements for the search parameter.", 0, 1, expression)); 2258 children.add(new Property("processingMode", "code", "How the search parameter relates to the set of elements returned by evaluating the expression query.", 0, 1, processingMode)); 2259 children.add(new Property("constraint", "string", "FHIRPath expression that defines/sets a complex constraint for when this SearchParameter is applicable.", 0, 1, constraint)); 2260 children.add(new Property("target", "code", "Types of resource (if a resource is referenced).", 0, java.lang.Integer.MAX_VALUE, target)); 2261 children.add(new Property("multipleOr", "boolean", "Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match.", 0, 1, multipleOr)); 2262 children.add(new Property("multipleAnd", "boolean", "Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match.", 0, 1, multipleAnd)); 2263 children.add(new Property("comparator", "code", "Comparators supported for the search parameter.", 0, java.lang.Integer.MAX_VALUE, comparator)); 2264 children.add(new Property("modifier", "code", "A modifier supported for the search parameter.", 0, java.lang.Integer.MAX_VALUE, modifier)); 2265 children.add(new Property("chain", "string", "Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.", 0, java.lang.Integer.MAX_VALUE, chain)); 2266 children.add(new Property("component", "", "Used to define the parts of a composite search parameter.", 0, java.lang.Integer.MAX_VALUE, component)); 2267 } 2268 2269 @Override 2270 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2271 switch (_hash) { 2272 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this search parameter is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the search parameter is stored on different servers.", 0, 1, url); 2273 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this search parameter when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 2274 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 2275 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2276 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2277 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2278 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2279 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 2280 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the search parameter.", 0, 1, title); 2281 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "canonical(SearchParameter)", "Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.", 0, 1, derivedFrom); 2282 case -892481550: /*status*/ return new Property("status", "code", "The status of this search parameter. Enables tracking the life-cycle of the content.", 0, 1, status); 2283 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 2284 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the search parameter was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.", 0, 1, date); 2285 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual tresponsible for the release and ongoing maintenance of the search parameter.", 0, 1, publisher); 2286 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 2287 case -1724546052: /*description*/ return new Property("description", "markdown", "And how it used.", 0, 1, description); 2288 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate search parameter instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 2289 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the search parameter is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 2290 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this search parameter is needed and why it has been designed as it has.", 0, 1, purpose); 2291 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the search parameter and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the search parameter.", 0, 1, copyright); 2292 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 2293 case 3059181: /*code*/ return new Property("code", "code", "The label that is recommended to be used in the URL or the parameter name in a parameters resource for this search parameter. In some cases, servers may need to use a different CapabilityStatement searchParam.name to differentiate between multiple SearchParameters that happen to have the same code.", 0, 1, code); 2294 case 3016401: /*base*/ return new Property("base", "code", "The base resource type(s) that this search parameter can be used against.", 0, java.lang.Integer.MAX_VALUE, base); 2295 case 3575610: /*type*/ return new Property("type", "code", "The type of value that a search parameter may contain, and how the content is interpreted.", 0, 1, type); 2296 case -1795452264: /*expression*/ return new Property("expression", "string", "A FHIRPath expression that returns a set of elements for the search parameter.", 0, 1, expression); 2297 case 195763030: /*processingMode*/ return new Property("processingMode", "code", "How the search parameter relates to the set of elements returned by evaluating the expression query.", 0, 1, processingMode); 2298 case -190376483: /*constraint*/ return new Property("constraint", "string", "FHIRPath expression that defines/sets a complex constraint for when this SearchParameter is applicable.", 0, 1, constraint); 2299 case -880905839: /*target*/ return new Property("target", "code", "Types of resource (if a resource is referenced).", 0, java.lang.Integer.MAX_VALUE, target); 2300 case 1265069075: /*multipleOr*/ return new Property("multipleOr", "boolean", "Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match.", 0, 1, multipleOr); 2301 case 562422183: /*multipleAnd*/ return new Property("multipleAnd", "boolean", "Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match.", 0, 1, multipleAnd); 2302 case -844673834: /*comparator*/ return new Property("comparator", "code", "Comparators supported for the search parameter.", 0, java.lang.Integer.MAX_VALUE, comparator); 2303 case -615513385: /*modifier*/ return new Property("modifier", "code", "A modifier supported for the search parameter.", 0, java.lang.Integer.MAX_VALUE, modifier); 2304 case 94623425: /*chain*/ return new Property("chain", "string", "Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.", 0, java.lang.Integer.MAX_VALUE, chain); 2305 case -1399907075: /*component*/ return new Property("component", "", "Used to define the parts of a composite search parameter.", 0, java.lang.Integer.MAX_VALUE, component); 2306 default: return super.getNamedProperty(_hash, _name, _checkValid); 2307 } 2308 2309 } 2310 2311 @Override 2312 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2313 switch (hash) { 2314 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2315 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2316 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2317 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 2318 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2319 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2320 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : new Base[] {this.derivedFrom}; // CanonicalType 2321 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2322 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 2323 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2324 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 2325 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2326 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2327 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2328 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2329 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 2330 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 2331 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 2332 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 2333 case 3016401: /*base*/ return this.base == null ? new Base[0] : this.base.toArray(new Base[this.base.size()]); // Enumeration<VersionIndependentResourceTypesAll> 2334 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<SearchParamType> 2335 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 2336 case 195763030: /*processingMode*/ return this.processingMode == null ? new Base[0] : new Base[] {this.processingMode}; // Enumeration<SearchProcessingModeType> 2337 case -190376483: /*constraint*/ return this.constraint == null ? new Base[0] : new Base[] {this.constraint}; // StringType 2338 case -880905839: /*target*/ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // Enumeration<VersionIndependentResourceTypesAll> 2339 case 1265069075: /*multipleOr*/ return this.multipleOr == null ? new Base[0] : new Base[] {this.multipleOr}; // BooleanType 2340 case 562422183: /*multipleAnd*/ return this.multipleAnd == null ? new Base[0] : new Base[] {this.multipleAnd}; // BooleanType 2341 case -844673834: /*comparator*/ return this.comparator == null ? new Base[0] : this.comparator.toArray(new Base[this.comparator.size()]); // Enumeration<SearchComparator> 2342 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // Enumeration<SearchModifierCode> 2343 case 94623425: /*chain*/ return this.chain == null ? new Base[0] : this.chain.toArray(new Base[this.chain.size()]); // StringType 2344 case -1399907075: /*component*/ return this.component == null ? new Base[0] : this.component.toArray(new Base[this.component.size()]); // SearchParameterComponentComponent 2345 default: return super.getProperty(hash, name, checkValid); 2346 } 2347 2348 } 2349 2350 @Override 2351 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2352 switch (hash) { 2353 case 116079: // url 2354 this.url = TypeConvertor.castToUri(value); // UriType 2355 return value; 2356 case -1618432855: // identifier 2357 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2358 return value; 2359 case 351608024: // version 2360 this.version = TypeConvertor.castToString(value); // StringType 2361 return value; 2362 case 1508158071: // versionAlgorithm 2363 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2364 return value; 2365 case 3373707: // name 2366 this.name = TypeConvertor.castToString(value); // StringType 2367 return value; 2368 case 110371416: // title 2369 this.title = TypeConvertor.castToString(value); // StringType 2370 return value; 2371 case 1077922663: // derivedFrom 2372 this.derivedFrom = TypeConvertor.castToCanonical(value); // CanonicalType 2373 return value; 2374 case -892481550: // status 2375 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2376 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2377 return value; 2378 case -404562712: // experimental 2379 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2380 return value; 2381 case 3076014: // date 2382 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2383 return value; 2384 case 1447404028: // publisher 2385 this.publisher = TypeConvertor.castToString(value); // StringType 2386 return value; 2387 case 951526432: // contact 2388 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2389 return value; 2390 case -1724546052: // description 2391 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2392 return value; 2393 case -669707736: // useContext 2394 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 2395 return value; 2396 case -507075711: // jurisdiction 2397 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2398 return value; 2399 case -220463842: // purpose 2400 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2401 return value; 2402 case 1522889671: // copyright 2403 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2404 return value; 2405 case 765157229: // copyrightLabel 2406 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2407 return value; 2408 case 3059181: // code 2409 this.code = TypeConvertor.castToCode(value); // CodeType 2410 return value; 2411 case 3016401: // base 2412 value = new VersionIndependentResourceTypesAllEnumFactory().fromType(TypeConvertor.castToCode(value)); 2413 this.getBase().add((Enumeration) value); // Enumeration<VersionIndependentResourceTypesAll> 2414 return value; 2415 case 3575610: // type 2416 value = new SearchParamTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2417 this.type = (Enumeration) value; // Enumeration<SearchParamType> 2418 return value; 2419 case -1795452264: // expression 2420 this.expression = TypeConvertor.castToString(value); // StringType 2421 return value; 2422 case 195763030: // processingMode 2423 value = new SearchProcessingModeTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2424 this.processingMode = (Enumeration) value; // Enumeration<SearchProcessingModeType> 2425 return value; 2426 case -190376483: // constraint 2427 this.constraint = TypeConvertor.castToString(value); // StringType 2428 return value; 2429 case -880905839: // target 2430 value = new VersionIndependentResourceTypesAllEnumFactory().fromType(TypeConvertor.castToCode(value)); 2431 this.getTarget().add((Enumeration) value); // Enumeration<VersionIndependentResourceTypesAll> 2432 return value; 2433 case 1265069075: // multipleOr 2434 this.multipleOr = TypeConvertor.castToBoolean(value); // BooleanType 2435 return value; 2436 case 562422183: // multipleAnd 2437 this.multipleAnd = TypeConvertor.castToBoolean(value); // BooleanType 2438 return value; 2439 case -844673834: // comparator 2440 value = new SearchComparatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 2441 this.getComparator().add((Enumeration) value); // Enumeration<SearchComparator> 2442 return value; 2443 case -615513385: // modifier 2444 value = new SearchModifierCodeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2445 this.getModifier().add((Enumeration) value); // Enumeration<SearchModifierCode> 2446 return value; 2447 case 94623425: // chain 2448 this.getChain().add(TypeConvertor.castToString(value)); // StringType 2449 return value; 2450 case -1399907075: // component 2451 this.getComponent().add((SearchParameterComponentComponent) value); // SearchParameterComponentComponent 2452 return value; 2453 default: return super.setProperty(hash, name, value); 2454 } 2455 2456 } 2457 2458 @Override 2459 public Base setProperty(String name, Base value) throws FHIRException { 2460 if (name.equals("url")) { 2461 this.url = TypeConvertor.castToUri(value); // UriType 2462 } else if (name.equals("identifier")) { 2463 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2464 } else if (name.equals("version")) { 2465 this.version = TypeConvertor.castToString(value); // StringType 2466 } else if (name.equals("versionAlgorithm[x]")) { 2467 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2468 } else if (name.equals("name")) { 2469 this.name = TypeConvertor.castToString(value); // StringType 2470 } else if (name.equals("title")) { 2471 this.title = TypeConvertor.castToString(value); // StringType 2472 } else if (name.equals("derivedFrom")) { 2473 this.derivedFrom = TypeConvertor.castToCanonical(value); // CanonicalType 2474 } else if (name.equals("status")) { 2475 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2476 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2477 } else if (name.equals("experimental")) { 2478 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2479 } else if (name.equals("date")) { 2480 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2481 } else if (name.equals("publisher")) { 2482 this.publisher = TypeConvertor.castToString(value); // StringType 2483 } else if (name.equals("contact")) { 2484 this.getContact().add(TypeConvertor.castToContactDetail(value)); 2485 } else if (name.equals("description")) { 2486 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2487 } else if (name.equals("useContext")) { 2488 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 2489 } else if (name.equals("jurisdiction")) { 2490 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 2491 } else if (name.equals("purpose")) { 2492 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2493 } else if (name.equals("copyright")) { 2494 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2495 } else if (name.equals("copyrightLabel")) { 2496 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2497 } else if (name.equals("code")) { 2498 this.code = TypeConvertor.castToCode(value); // CodeType 2499 } else if (name.equals("base")) { 2500 value = new VersionIndependentResourceTypesAllEnumFactory().fromType(TypeConvertor.castToCode(value)); 2501 this.getBase().add((Enumeration) value); 2502 } else if (name.equals("type")) { 2503 value = new SearchParamTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2504 this.type = (Enumeration) value; // Enumeration<SearchParamType> 2505 } else if (name.equals("expression")) { 2506 this.expression = TypeConvertor.castToString(value); // StringType 2507 } else if (name.equals("processingMode")) { 2508 value = new SearchProcessingModeTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2509 this.processingMode = (Enumeration) value; // Enumeration<SearchProcessingModeType> 2510 } else if (name.equals("constraint")) { 2511 this.constraint = TypeConvertor.castToString(value); // StringType 2512 } else if (name.equals("target")) { 2513 value = new VersionIndependentResourceTypesAllEnumFactory().fromType(TypeConvertor.castToCode(value)); 2514 this.getTarget().add((Enumeration) value); 2515 } else if (name.equals("multipleOr")) { 2516 this.multipleOr = TypeConvertor.castToBoolean(value); // BooleanType 2517 } else if (name.equals("multipleAnd")) { 2518 this.multipleAnd = TypeConvertor.castToBoolean(value); // BooleanType 2519 } else if (name.equals("comparator")) { 2520 value = new SearchComparatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 2521 this.getComparator().add((Enumeration) value); 2522 } else if (name.equals("modifier")) { 2523 value = new SearchModifierCodeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2524 this.getModifier().add((Enumeration) value); 2525 } else if (name.equals("chain")) { 2526 this.getChain().add(TypeConvertor.castToString(value)); 2527 } else if (name.equals("component")) { 2528 this.getComponent().add((SearchParameterComponentComponent) value); 2529 } else 2530 return super.setProperty(name, value); 2531 return value; 2532 } 2533 2534 @Override 2535 public Base makeProperty(int hash, String name) throws FHIRException { 2536 switch (hash) { 2537 case 116079: return getUrlElement(); 2538 case -1618432855: return addIdentifier(); 2539 case 351608024: return getVersionElement(); 2540 case -115699031: return getVersionAlgorithm(); 2541 case 1508158071: return getVersionAlgorithm(); 2542 case 3373707: return getNameElement(); 2543 case 110371416: return getTitleElement(); 2544 case 1077922663: return getDerivedFromElement(); 2545 case -892481550: return getStatusElement(); 2546 case -404562712: return getExperimentalElement(); 2547 case 3076014: return getDateElement(); 2548 case 1447404028: return getPublisherElement(); 2549 case 951526432: return addContact(); 2550 case -1724546052: return getDescriptionElement(); 2551 case -669707736: return addUseContext(); 2552 case -507075711: return addJurisdiction(); 2553 case -220463842: return getPurposeElement(); 2554 case 1522889671: return getCopyrightElement(); 2555 case 765157229: return getCopyrightLabelElement(); 2556 case 3059181: return getCodeElement(); 2557 case 3016401: return addBaseElement(); 2558 case 3575610: return getTypeElement(); 2559 case -1795452264: return getExpressionElement(); 2560 case 195763030: return getProcessingModeElement(); 2561 case -190376483: return getConstraintElement(); 2562 case -880905839: return addTargetElement(); 2563 case 1265069075: return getMultipleOrElement(); 2564 case 562422183: return getMultipleAndElement(); 2565 case -844673834: return addComparatorElement(); 2566 case -615513385: return addModifierElement(); 2567 case 94623425: return addChainElement(); 2568 case -1399907075: return addComponent(); 2569 default: return super.makeProperty(hash, name); 2570 } 2571 2572 } 2573 2574 @Override 2575 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2576 switch (hash) { 2577 case 116079: /*url*/ return new String[] {"uri"}; 2578 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2579 case 351608024: /*version*/ return new String[] {"string"}; 2580 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 2581 case 3373707: /*name*/ return new String[] {"string"}; 2582 case 110371416: /*title*/ return new String[] {"string"}; 2583 case 1077922663: /*derivedFrom*/ return new String[] {"canonical"}; 2584 case -892481550: /*status*/ return new String[] {"code"}; 2585 case -404562712: /*experimental*/ return new String[] {"boolean"}; 2586 case 3076014: /*date*/ return new String[] {"dateTime"}; 2587 case 1447404028: /*publisher*/ return new String[] {"string"}; 2588 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 2589 case -1724546052: /*description*/ return new String[] {"markdown"}; 2590 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 2591 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 2592 case -220463842: /*purpose*/ return new String[] {"markdown"}; 2593 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 2594 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 2595 case 3059181: /*code*/ return new String[] {"code"}; 2596 case 3016401: /*base*/ return new String[] {"code"}; 2597 case 3575610: /*type*/ return new String[] {"code"}; 2598 case -1795452264: /*expression*/ return new String[] {"string"}; 2599 case 195763030: /*processingMode*/ return new String[] {"code"}; 2600 case -190376483: /*constraint*/ return new String[] {"string"}; 2601 case -880905839: /*target*/ return new String[] {"code"}; 2602 case 1265069075: /*multipleOr*/ return new String[] {"boolean"}; 2603 case 562422183: /*multipleAnd*/ return new String[] {"boolean"}; 2604 case -844673834: /*comparator*/ return new String[] {"code"}; 2605 case -615513385: /*modifier*/ return new String[] {"code"}; 2606 case 94623425: /*chain*/ return new String[] {"string"}; 2607 case -1399907075: /*component*/ return new String[] {}; 2608 default: return super.getTypesForProperty(hash, name); 2609 } 2610 2611 } 2612 2613 @Override 2614 public Base addChild(String name) throws FHIRException { 2615 if (name.equals("url")) { 2616 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.url"); 2617 } 2618 else if (name.equals("identifier")) { 2619 return addIdentifier(); 2620 } 2621 else if (name.equals("version")) { 2622 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.version"); 2623 } 2624 else if (name.equals("versionAlgorithmString")) { 2625 this.versionAlgorithm = new StringType(); 2626 return this.versionAlgorithm; 2627 } 2628 else if (name.equals("versionAlgorithmCoding")) { 2629 this.versionAlgorithm = new Coding(); 2630 return this.versionAlgorithm; 2631 } 2632 else if (name.equals("name")) { 2633 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.name"); 2634 } 2635 else if (name.equals("title")) { 2636 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.title"); 2637 } 2638 else if (name.equals("derivedFrom")) { 2639 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.derivedFrom"); 2640 } 2641 else if (name.equals("status")) { 2642 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.status"); 2643 } 2644 else if (name.equals("experimental")) { 2645 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.experimental"); 2646 } 2647 else if (name.equals("date")) { 2648 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.date"); 2649 } 2650 else if (name.equals("publisher")) { 2651 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.publisher"); 2652 } 2653 else if (name.equals("contact")) { 2654 return addContact(); 2655 } 2656 else if (name.equals("description")) { 2657 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.description"); 2658 } 2659 else if (name.equals("useContext")) { 2660 return addUseContext(); 2661 } 2662 else if (name.equals("jurisdiction")) { 2663 return addJurisdiction(); 2664 } 2665 else if (name.equals("purpose")) { 2666 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.purpose"); 2667 } 2668 else if (name.equals("copyright")) { 2669 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.copyright"); 2670 } 2671 else if (name.equals("copyrightLabel")) { 2672 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.copyrightLabel"); 2673 } 2674 else if (name.equals("code")) { 2675 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.code"); 2676 } 2677 else if (name.equals("base")) { 2678 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.base"); 2679 } 2680 else if (name.equals("type")) { 2681 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.type"); 2682 } 2683 else if (name.equals("expression")) { 2684 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.expression"); 2685 } 2686 else if (name.equals("processingMode")) { 2687 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.processingMode"); 2688 } 2689 else if (name.equals("constraint")) { 2690 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.constraint"); 2691 } 2692 else if (name.equals("target")) { 2693 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.target"); 2694 } 2695 else if (name.equals("multipleOr")) { 2696 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.multipleOr"); 2697 } 2698 else if (name.equals("multipleAnd")) { 2699 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.multipleAnd"); 2700 } 2701 else if (name.equals("comparator")) { 2702 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.comparator"); 2703 } 2704 else if (name.equals("modifier")) { 2705 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.modifier"); 2706 } 2707 else if (name.equals("chain")) { 2708 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.chain"); 2709 } 2710 else if (name.equals("component")) { 2711 return addComponent(); 2712 } 2713 else 2714 return super.addChild(name); 2715 } 2716 2717 public String fhirType() { 2718 return "SearchParameter"; 2719 2720 } 2721 2722 public SearchParameter copy() { 2723 SearchParameter dst = new SearchParameter(); 2724 copyValues(dst); 2725 return dst; 2726 } 2727 2728 public void copyValues(SearchParameter dst) { 2729 super.copyValues(dst); 2730 dst.url = url == null ? null : url.copy(); 2731 if (identifier != null) { 2732 dst.identifier = new ArrayList<Identifier>(); 2733 for (Identifier i : identifier) 2734 dst.identifier.add(i.copy()); 2735 }; 2736 dst.version = version == null ? null : version.copy(); 2737 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 2738 dst.name = name == null ? null : name.copy(); 2739 dst.title = title == null ? null : title.copy(); 2740 dst.derivedFrom = derivedFrom == null ? null : derivedFrom.copy(); 2741 dst.status = status == null ? null : status.copy(); 2742 dst.experimental = experimental == null ? null : experimental.copy(); 2743 dst.date = date == null ? null : date.copy(); 2744 dst.publisher = publisher == null ? null : publisher.copy(); 2745 if (contact != null) { 2746 dst.contact = new ArrayList<ContactDetail>(); 2747 for (ContactDetail i : contact) 2748 dst.contact.add(i.copy()); 2749 }; 2750 dst.description = description == null ? null : description.copy(); 2751 if (useContext != null) { 2752 dst.useContext = new ArrayList<UsageContext>(); 2753 for (UsageContext i : useContext) 2754 dst.useContext.add(i.copy()); 2755 }; 2756 if (jurisdiction != null) { 2757 dst.jurisdiction = new ArrayList<CodeableConcept>(); 2758 for (CodeableConcept i : jurisdiction) 2759 dst.jurisdiction.add(i.copy()); 2760 }; 2761 dst.purpose = purpose == null ? null : purpose.copy(); 2762 dst.copyright = copyright == null ? null : copyright.copy(); 2763 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 2764 dst.code = code == null ? null : code.copy(); 2765 if (base != null) { 2766 dst.base = new ArrayList<Enumeration<VersionIndependentResourceTypesAll>>(); 2767 for (Enumeration<VersionIndependentResourceTypesAll> i : base) 2768 dst.base.add(i.copy()); 2769 }; 2770 dst.type = type == null ? null : type.copy(); 2771 dst.expression = expression == null ? null : expression.copy(); 2772 dst.processingMode = processingMode == null ? null : processingMode.copy(); 2773 dst.constraint = constraint == null ? null : constraint.copy(); 2774 if (target != null) { 2775 dst.target = new ArrayList<Enumeration<VersionIndependentResourceTypesAll>>(); 2776 for (Enumeration<VersionIndependentResourceTypesAll> i : target) 2777 dst.target.add(i.copy()); 2778 }; 2779 dst.multipleOr = multipleOr == null ? null : multipleOr.copy(); 2780 dst.multipleAnd = multipleAnd == null ? null : multipleAnd.copy(); 2781 if (comparator != null) { 2782 dst.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2783 for (Enumeration<SearchComparator> i : comparator) 2784 dst.comparator.add(i.copy()); 2785 }; 2786 if (modifier != null) { 2787 dst.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2788 for (Enumeration<SearchModifierCode> i : modifier) 2789 dst.modifier.add(i.copy()); 2790 }; 2791 if (chain != null) { 2792 dst.chain = new ArrayList<StringType>(); 2793 for (StringType i : chain) 2794 dst.chain.add(i.copy()); 2795 }; 2796 if (component != null) { 2797 dst.component = new ArrayList<SearchParameterComponentComponent>(); 2798 for (SearchParameterComponentComponent i : component) 2799 dst.component.add(i.copy()); 2800 }; 2801 } 2802 2803 protected SearchParameter typedCopy() { 2804 return copy(); 2805 } 2806 2807 @Override 2808 public boolean equalsDeep(Base other_) { 2809 if (!super.equalsDeep(other_)) 2810 return false; 2811 if (!(other_ instanceof SearchParameter)) 2812 return false; 2813 SearchParameter o = (SearchParameter) other_; 2814 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 2815 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 2816 && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 2817 && compareDeep(date, o.date, true) && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 2818 && compareDeep(description, o.description, true) && compareDeep(useContext, o.useContext, true) 2819 && compareDeep(jurisdiction, o.jurisdiction, true) && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 2820 && compareDeep(copyrightLabel, o.copyrightLabel, true) && compareDeep(code, o.code, true) && compareDeep(base, o.base, true) 2821 && compareDeep(type, o.type, true) && compareDeep(expression, o.expression, true) && compareDeep(processingMode, o.processingMode, true) 2822 && compareDeep(constraint, o.constraint, true) && compareDeep(target, o.target, true) && compareDeep(multipleOr, o.multipleOr, true) 2823 && compareDeep(multipleAnd, o.multipleAnd, true) && compareDeep(comparator, o.comparator, true) 2824 && compareDeep(modifier, o.modifier, true) && compareDeep(chain, o.chain, true) && compareDeep(component, o.component, true) 2825 ; 2826 } 2827 2828 @Override 2829 public boolean equalsShallow(Base other_) { 2830 if (!super.equalsShallow(other_)) 2831 return false; 2832 if (!(other_ instanceof SearchParameter)) 2833 return false; 2834 SearchParameter o = (SearchParameter) other_; 2835 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 2836 && compareValues(title, o.title, true) && compareValues(derivedFrom, o.derivedFrom, true) && compareValues(status, o.status, true) 2837 && compareValues(experimental, o.experimental, true) && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) 2838 && compareValues(description, o.description, true) && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) 2839 && compareValues(copyrightLabel, o.copyrightLabel, true) && compareValues(code, o.code, true) && compareValues(base, o.base, true) 2840 && compareValues(type, o.type, true) && compareValues(expression, o.expression, true) && compareValues(processingMode, o.processingMode, true) 2841 && compareValues(constraint, o.constraint, true) && compareValues(target, o.target, true) && compareValues(multipleOr, o.multipleOr, true) 2842 && compareValues(multipleAnd, o.multipleAnd, true) && compareValues(comparator, o.comparator, true) 2843 && compareValues(modifier, o.modifier, true) && compareValues(chain, o.chain, true); 2844 } 2845 2846 public boolean isEmpty() { 2847 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 2848 , versionAlgorithm, name, title, derivedFrom, status, experimental, date, publisher 2849 , contact, description, useContext, jurisdiction, purpose, copyright, copyrightLabel 2850 , code, base, type, expression, processingMode, constraint, target, multipleOr 2851 , multipleAnd, comparator, modifier, chain, component); 2852 } 2853 2854 @Override 2855 public ResourceType getResourceType() { 2856 return ResourceType.SearchParameter; 2857 } 2858 2859 /** 2860 * Search parameter: <b>context-quantity</b> 2861 * <p> 2862 * Description: <b>Multiple Resources: 2863 2864* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 2865* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 2866* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 2867* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 2868* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 2869* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 2870* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 2871* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 2872* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 2873* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 2874* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 2875* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 2876* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 2877* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 2878* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 2879* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 2880* [Library](library.html): A quantity- or range-valued use context assigned to the library 2881* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 2882* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 2883* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 2884* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 2885* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 2886* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 2887* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 2888* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 2889* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 2890* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 2891* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 2892* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 2893* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 2894</b><br> 2895 * Type: <b>quantity</b><br> 2896 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 2897 * </p> 2898 */ 2899 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 2900 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 2901 /** 2902 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 2903 * <p> 2904 * Description: <b>Multiple Resources: 2905 2906* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 2907* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 2908* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 2909* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 2910* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 2911* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 2912* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 2913* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 2914* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 2915* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 2916* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 2917* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 2918* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 2919* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 2920* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 2921* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 2922* [Library](library.html): A quantity- or range-valued use context assigned to the library 2923* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 2924* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 2925* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 2926* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 2927* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 2928* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 2929* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 2930* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 2931* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 2932* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 2933* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 2934* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 2935* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 2936</b><br> 2937 * Type: <b>quantity</b><br> 2938 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 2939 * </p> 2940 */ 2941 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 2942 2943 /** 2944 * Search parameter: <b>context-type-quantity</b> 2945 * <p> 2946 * Description: <b>Multiple Resources: 2947 2948* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 2949* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 2950* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 2951* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 2952* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 2953* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 2954* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 2955* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 2956* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 2957* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 2958* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 2959* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 2960* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 2961* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 2962* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 2963* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 2964* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 2965* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 2966* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 2967* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 2968* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 2969* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 2970* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 2971* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 2972* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 2973* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 2974* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 2975* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 2976* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 2977* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 2978</b><br> 2979 * Type: <b>composite</b><br> 2980 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2981 * </p> 2982 */ 2983 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 2984 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 2985 /** 2986 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 2987 * <p> 2988 * Description: <b>Multiple Resources: 2989 2990* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 2991* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 2992* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 2993* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 2994* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 2995* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 2996* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 2997* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 2998* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 2999* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 3000* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 3001* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 3002* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 3003* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 3004* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 3005* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 3006* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 3007* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 3008* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 3009* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 3010* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 3011* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 3012* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 3013* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 3014* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 3015* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 3016* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 3017* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 3018* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3019* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3020</b><br> 3021 * Type: <b>composite</b><br> 3022 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3023 * </p> 3024 */ 3025 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 3026 3027 /** 3028 * Search parameter: <b>context-type-value</b> 3029 * <p> 3030 * Description: <b>Multiple Resources: 3031 3032* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3033* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3034* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3035* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3036* [Citation](citation.html): A use context type and value assigned to the citation 3037* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3038* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3039* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3040* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3041* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3042* [Evidence](evidence.html): A use context type and value assigned to the evidence 3043* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3044* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3045* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3046* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 3047* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 3048* [Library](library.html): A use context type and value assigned to the library 3049* [Measure](measure.html): A use context type and value assigned to the measure 3050* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 3051* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 3052* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 3053* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 3054* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 3055* [Requirements](requirements.html): A use context type and value assigned to the requirements 3056* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3057* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3058* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3059* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3060* [TestScript](testscript.html): A use context type and value assigned to the test script 3061* [ValueSet](valueset.html): A use context type and value assigned to the value set 3062</b><br> 3063 * Type: <b>composite</b><br> 3064 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3065 * </p> 3066 */ 3067 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 3068 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3069 /** 3070 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3071 * <p> 3072 * Description: <b>Multiple Resources: 3073 3074* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3075* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3076* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3077* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3078* [Citation](citation.html): A use context type and value assigned to the citation 3079* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3080* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3081* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3082* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3083* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3084* [Evidence](evidence.html): A use context type and value assigned to the evidence 3085* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3086* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3087* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3088* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 3089* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 3090* [Library](library.html): A use context type and value assigned to the library 3091* [Measure](measure.html): A use context type and value assigned to the measure 3092* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 3093* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 3094* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 3095* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 3096* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 3097* [Requirements](requirements.html): A use context type and value assigned to the requirements 3098* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3099* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3100* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3101* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3102* [TestScript](testscript.html): A use context type and value assigned to the test script 3103* [ValueSet](valueset.html): A use context type and value assigned to the value set 3104</b><br> 3105 * Type: <b>composite</b><br> 3106 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3107 * </p> 3108 */ 3109 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 3110 3111 /** 3112 * Search parameter: <b>context-type</b> 3113 * <p> 3114 * Description: <b>Multiple Resources: 3115 3116* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3117* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3118* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3119* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3120* [Citation](citation.html): A type of use context assigned to the citation 3121* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3122* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3123* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3124* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3125* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3126* [Evidence](evidence.html): A type of use context assigned to the evidence 3127* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3128* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3129* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3130* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3131* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3132* [Library](library.html): A type of use context assigned to the library 3133* [Measure](measure.html): A type of use context assigned to the measure 3134* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3135* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3136* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3137* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3138* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3139* [Requirements](requirements.html): A type of use context assigned to the requirements 3140* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3141* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3142* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3143* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3144* [TestScript](testscript.html): A type of use context assigned to the test script 3145* [ValueSet](valueset.html): A type of use context assigned to the value set 3146</b><br> 3147 * Type: <b>token</b><br> 3148 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3149 * </p> 3150 */ 3151 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 3152 public static final String SP_CONTEXT_TYPE = "context-type"; 3153 /** 3154 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3155 * <p> 3156 * Description: <b>Multiple Resources: 3157 3158* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3159* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3160* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3161* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3162* [Citation](citation.html): A type of use context assigned to the citation 3163* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3164* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3165* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3166* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3167* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3168* [Evidence](evidence.html): A type of use context assigned to the evidence 3169* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3170* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3171* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3172* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3173* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3174* [Library](library.html): A type of use context assigned to the library 3175* [Measure](measure.html): A type of use context assigned to the measure 3176* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3177* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3178* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3179* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3180* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3181* [Requirements](requirements.html): A type of use context assigned to the requirements 3182* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3183* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3184* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3185* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3186* [TestScript](testscript.html): A type of use context assigned to the test script 3187* [ValueSet](valueset.html): A type of use context assigned to the value set 3188</b><br> 3189 * Type: <b>token</b><br> 3190 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3191 * </p> 3192 */ 3193 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 3194 3195 /** 3196 * Search parameter: <b>context</b> 3197 * <p> 3198 * Description: <b>Multiple Resources: 3199 3200* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3201* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3202* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3203* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3204* [Citation](citation.html): A use context assigned to the citation 3205* [CodeSystem](codesystem.html): A use context assigned to the code system 3206* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3207* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3208* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3209* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3210* [Evidence](evidence.html): A use context assigned to the evidence 3211* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3212* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3213* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3214* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3215* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3216* [Library](library.html): A use context assigned to the library 3217* [Measure](measure.html): A use context assigned to the measure 3218* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3219* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3220* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3221* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3222* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3223* [Requirements](requirements.html): A use context assigned to the requirements 3224* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3225* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3226* [StructureMap](structuremap.html): A use context assigned to the structure map 3227* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3228* [TestScript](testscript.html): A use context assigned to the test script 3229* [ValueSet](valueset.html): A use context assigned to the value set 3230</b><br> 3231 * Type: <b>token</b><br> 3232 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3233 * </p> 3234 */ 3235 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 3236 public static final String SP_CONTEXT = "context"; 3237 /** 3238 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3239 * <p> 3240 * Description: <b>Multiple Resources: 3241 3242* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3243* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3244* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3245* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3246* [Citation](citation.html): A use context assigned to the citation 3247* [CodeSystem](codesystem.html): A use context assigned to the code system 3248* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3249* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3250* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3251* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3252* [Evidence](evidence.html): A use context assigned to the evidence 3253* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3254* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3255* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3256* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3257* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3258* [Library](library.html): A use context assigned to the library 3259* [Measure](measure.html): A use context assigned to the measure 3260* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3261* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3262* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3263* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3264* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3265* [Requirements](requirements.html): A use context assigned to the requirements 3266* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3267* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3268* [StructureMap](structuremap.html): A use context assigned to the structure map 3269* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3270* [TestScript](testscript.html): A use context assigned to the test script 3271* [ValueSet](valueset.html): A use context assigned to the value set 3272</b><br> 3273 * Type: <b>token</b><br> 3274 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3275 * </p> 3276 */ 3277 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 3278 3279 /** 3280 * Search parameter: <b>date</b> 3281 * <p> 3282 * Description: <b>Multiple Resources: 3283 3284* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3285* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3286* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3287* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3288* [Citation](citation.html): The citation publication date 3289* [CodeSystem](codesystem.html): The code system publication date 3290* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3291* [ConceptMap](conceptmap.html): The concept map publication date 3292* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3293* [EventDefinition](eventdefinition.html): The event definition publication date 3294* [Evidence](evidence.html): The evidence publication date 3295* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3296* [ExampleScenario](examplescenario.html): The example scenario publication date 3297* [GraphDefinition](graphdefinition.html): The graph definition publication date 3298* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3299* [Library](library.html): The library publication date 3300* [Measure](measure.html): The measure publication date 3301* [MessageDefinition](messagedefinition.html): The message definition publication date 3302* [NamingSystem](namingsystem.html): The naming system publication date 3303* [OperationDefinition](operationdefinition.html): The operation definition publication date 3304* [PlanDefinition](plandefinition.html): The plan definition publication date 3305* [Questionnaire](questionnaire.html): The questionnaire publication date 3306* [Requirements](requirements.html): The requirements publication date 3307* [SearchParameter](searchparameter.html): The search parameter publication date 3308* [StructureDefinition](structuredefinition.html): The structure definition publication date 3309* [StructureMap](structuremap.html): The structure map publication date 3310* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3311* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3312* [TestScript](testscript.html): The test script publication date 3313* [ValueSet](valueset.html): The value set publication date 3314</b><br> 3315 * Type: <b>date</b><br> 3316 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3317 * </p> 3318 */ 3319 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 3320 public static final String SP_DATE = "date"; 3321 /** 3322 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3323 * <p> 3324 * Description: <b>Multiple Resources: 3325 3326* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3327* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3328* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3329* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3330* [Citation](citation.html): The citation publication date 3331* [CodeSystem](codesystem.html): The code system publication date 3332* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3333* [ConceptMap](conceptmap.html): The concept map publication date 3334* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3335* [EventDefinition](eventdefinition.html): The event definition publication date 3336* [Evidence](evidence.html): The evidence publication date 3337* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3338* [ExampleScenario](examplescenario.html): The example scenario publication date 3339* [GraphDefinition](graphdefinition.html): The graph definition publication date 3340* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3341* [Library](library.html): The library publication date 3342* [Measure](measure.html): The measure publication date 3343* [MessageDefinition](messagedefinition.html): The message definition publication date 3344* [NamingSystem](namingsystem.html): The naming system publication date 3345* [OperationDefinition](operationdefinition.html): The operation definition publication date 3346* [PlanDefinition](plandefinition.html): The plan definition publication date 3347* [Questionnaire](questionnaire.html): The questionnaire publication date 3348* [Requirements](requirements.html): The requirements publication date 3349* [SearchParameter](searchparameter.html): The search parameter publication date 3350* [StructureDefinition](structuredefinition.html): The structure definition publication date 3351* [StructureMap](structuremap.html): The structure map publication date 3352* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3353* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3354* [TestScript](testscript.html): The test script publication date 3355* [ValueSet](valueset.html): The value set publication date 3356</b><br> 3357 * Type: <b>date</b><br> 3358 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3359 * </p> 3360 */ 3361 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3362 3363 /** 3364 * Search parameter: <b>description</b> 3365 * <p> 3366 * Description: <b>Multiple Resources: 3367 3368* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3369* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3370* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3371* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3372* [Citation](citation.html): The description of the citation 3373* [CodeSystem](codesystem.html): The description of the code system 3374* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3375* [ConceptMap](conceptmap.html): The description of the concept map 3376* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3377* [EventDefinition](eventdefinition.html): The description of the event definition 3378* [Evidence](evidence.html): The description of the evidence 3379* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3380* [GraphDefinition](graphdefinition.html): The description of the graph definition 3381* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3382* [Library](library.html): The description of the library 3383* [Measure](measure.html): The description of the measure 3384* [MessageDefinition](messagedefinition.html): The description of the message definition 3385* [NamingSystem](namingsystem.html): The description of the naming system 3386* [OperationDefinition](operationdefinition.html): The description of the operation definition 3387* [PlanDefinition](plandefinition.html): The description of the plan definition 3388* [Questionnaire](questionnaire.html): The description of the questionnaire 3389* [Requirements](requirements.html): The description of the requirements 3390* [SearchParameter](searchparameter.html): The description of the search parameter 3391* [StructureDefinition](structuredefinition.html): The description of the structure definition 3392* [StructureMap](structuremap.html): The description of the structure map 3393* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3394* [TestScript](testscript.html): The description of the test script 3395* [ValueSet](valueset.html): The description of the value set 3396</b><br> 3397 * Type: <b>string</b><br> 3398 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3399 * </p> 3400 */ 3401 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 3402 public static final String SP_DESCRIPTION = "description"; 3403 /** 3404 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3405 * <p> 3406 * Description: <b>Multiple Resources: 3407 3408* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3409* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3410* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3411* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3412* [Citation](citation.html): The description of the citation 3413* [CodeSystem](codesystem.html): The description of the code system 3414* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3415* [ConceptMap](conceptmap.html): The description of the concept map 3416* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3417* [EventDefinition](eventdefinition.html): The description of the event definition 3418* [Evidence](evidence.html): The description of the evidence 3419* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3420* [GraphDefinition](graphdefinition.html): The description of the graph definition 3421* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3422* [Library](library.html): The description of the library 3423* [Measure](measure.html): The description of the measure 3424* [MessageDefinition](messagedefinition.html): The description of the message definition 3425* [NamingSystem](namingsystem.html): The description of the naming system 3426* [OperationDefinition](operationdefinition.html): The description of the operation definition 3427* [PlanDefinition](plandefinition.html): The description of the plan definition 3428* [Questionnaire](questionnaire.html): The description of the questionnaire 3429* [Requirements](requirements.html): The description of the requirements 3430* [SearchParameter](searchparameter.html): The description of the search parameter 3431* [StructureDefinition](structuredefinition.html): The description of the structure definition 3432* [StructureMap](structuremap.html): The description of the structure map 3433* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3434* [TestScript](testscript.html): The description of the test script 3435* [ValueSet](valueset.html): The description of the value set 3436</b><br> 3437 * Type: <b>string</b><br> 3438 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3439 * </p> 3440 */ 3441 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 3442 3443 /** 3444 * Search parameter: <b>identifier</b> 3445 * <p> 3446 * Description: <b>Multiple Resources: 3447 3448* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3449* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3450* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3451* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3452* [Citation](citation.html): External identifier for the citation 3453* [CodeSystem](codesystem.html): External identifier for the code system 3454* [ConceptMap](conceptmap.html): External identifier for the concept map 3455* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3456* [EventDefinition](eventdefinition.html): External identifier for the event definition 3457* [Evidence](evidence.html): External identifier for the evidence 3458* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3459* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3460* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3461* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3462* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3463* [Library](library.html): External identifier for the library 3464* [Measure](measure.html): External identifier for the measure 3465* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3466* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3467* [NamingSystem](namingsystem.html): External identifier for the naming system 3468* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3469* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3470* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3471* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3472* [Requirements](requirements.html): External identifier for the requirements 3473* [SearchParameter](searchparameter.html): External identifier for the search parameter 3474* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3475* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3476* [StructureMap](structuremap.html): External identifier for the structure map 3477* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3478* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3479* [TestPlan](testplan.html): An identifier for the test plan 3480* [TestScript](testscript.html): External identifier for the test script 3481* [ValueSet](valueset.html): External identifier for the value set 3482</b><br> 3483 * Type: <b>token</b><br> 3484 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3485 * </p> 3486 */ 3487 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 3488 public static final String SP_IDENTIFIER = "identifier"; 3489 /** 3490 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3491 * <p> 3492 * Description: <b>Multiple Resources: 3493 3494* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3495* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3496* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3497* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3498* [Citation](citation.html): External identifier for the citation 3499* [CodeSystem](codesystem.html): External identifier for the code system 3500* [ConceptMap](conceptmap.html): External identifier for the concept map 3501* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3502* [EventDefinition](eventdefinition.html): External identifier for the event definition 3503* [Evidence](evidence.html): External identifier for the evidence 3504* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3505* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3506* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3507* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3508* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3509* [Library](library.html): External identifier for the library 3510* [Measure](measure.html): External identifier for the measure 3511* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3512* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3513* [NamingSystem](namingsystem.html): External identifier for the naming system 3514* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3515* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3516* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3517* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3518* [Requirements](requirements.html): External identifier for the requirements 3519* [SearchParameter](searchparameter.html): External identifier for the search parameter 3520* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3521* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3522* [StructureMap](structuremap.html): External identifier for the structure map 3523* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3524* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3525* [TestPlan](testplan.html): An identifier for the test plan 3526* [TestScript](testscript.html): External identifier for the test script 3527* [ValueSet](valueset.html): External identifier for the value set 3528</b><br> 3529 * Type: <b>token</b><br> 3530 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3531 * </p> 3532 */ 3533 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3534 3535 /** 3536 * Search parameter: <b>jurisdiction</b> 3537 * <p> 3538 * Description: <b>Multiple Resources: 3539 3540* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3541* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3542* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3543* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3544* [Citation](citation.html): Intended jurisdiction for the citation 3545* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3546* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3547* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3548* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3549* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3550* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3551* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3552* [Library](library.html): Intended jurisdiction for the library 3553* [Measure](measure.html): Intended jurisdiction for the measure 3554* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3555* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3556* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3557* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3558* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3559* [Requirements](requirements.html): Intended jurisdiction for the requirements 3560* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3561* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3562* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3563* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3564* [TestScript](testscript.html): Intended jurisdiction for the test script 3565* [ValueSet](valueset.html): Intended jurisdiction for the value set 3566</b><br> 3567 * Type: <b>token</b><br> 3568 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3569 * </p> 3570 */ 3571 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 3572 public static final String SP_JURISDICTION = "jurisdiction"; 3573 /** 3574 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3575 * <p> 3576 * Description: <b>Multiple Resources: 3577 3578* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3579* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3580* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3581* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3582* [Citation](citation.html): Intended jurisdiction for the citation 3583* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3584* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3585* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3586* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3587* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3588* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3589* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3590* [Library](library.html): Intended jurisdiction for the library 3591* [Measure](measure.html): Intended jurisdiction for the measure 3592* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3593* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3594* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3595* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3596* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3597* [Requirements](requirements.html): Intended jurisdiction for the requirements 3598* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3599* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3600* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3601* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3602* [TestScript](testscript.html): Intended jurisdiction for the test script 3603* [ValueSet](valueset.html): Intended jurisdiction for the value set 3604</b><br> 3605 * Type: <b>token</b><br> 3606 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3607 * </p> 3608 */ 3609 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 3610 3611 /** 3612 * Search parameter: <b>name</b> 3613 * <p> 3614 * Description: <b>Multiple Resources: 3615 3616* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 3617* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 3618* [Citation](citation.html): Computationally friendly name of the citation 3619* [CodeSystem](codesystem.html): Computationally friendly name of the code system 3620* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 3621* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 3622* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 3623* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 3624* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 3625* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 3626* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 3627* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 3628* [Library](library.html): Computationally friendly name of the library 3629* [Measure](measure.html): Computationally friendly name of the measure 3630* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 3631* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 3632* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 3633* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 3634* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 3635* [Requirements](requirements.html): Computationally friendly name of the requirements 3636* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 3637* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 3638* [StructureMap](structuremap.html): Computationally friendly name of the structure map 3639* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 3640* [TestScript](testscript.html): Computationally friendly name of the test script 3641* [ValueSet](valueset.html): Computationally friendly name of the value set 3642</b><br> 3643 * Type: <b>string</b><br> 3644 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 3645 * </p> 3646 */ 3647 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 3648 public static final String SP_NAME = "name"; 3649 /** 3650 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3651 * <p> 3652 * Description: <b>Multiple Resources: 3653 3654* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 3655* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 3656* [Citation](citation.html): Computationally friendly name of the citation 3657* [CodeSystem](codesystem.html): Computationally friendly name of the code system 3658* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 3659* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 3660* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 3661* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 3662* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 3663* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 3664* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 3665* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 3666* [Library](library.html): Computationally friendly name of the library 3667* [Measure](measure.html): Computationally friendly name of the measure 3668* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 3669* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 3670* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 3671* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 3672* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 3673* [Requirements](requirements.html): Computationally friendly name of the requirements 3674* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 3675* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 3676* [StructureMap](structuremap.html): Computationally friendly name of the structure map 3677* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 3678* [TestScript](testscript.html): Computationally friendly name of the test script 3679* [ValueSet](valueset.html): Computationally friendly name of the value set 3680</b><br> 3681 * Type: <b>string</b><br> 3682 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 3683 * </p> 3684 */ 3685 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 3686 3687 /** 3688 * Search parameter: <b>publisher</b> 3689 * <p> 3690 * Description: <b>Multiple Resources: 3691 3692* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 3693* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 3694* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 3695* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 3696* [Citation](citation.html): Name of the publisher of the citation 3697* [CodeSystem](codesystem.html): Name of the publisher of the code system 3698* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 3699* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 3700* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 3701* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 3702* [Evidence](evidence.html): Name of the publisher of the evidence 3703* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 3704* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 3705* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 3706* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 3707* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 3708* [Library](library.html): Name of the publisher of the library 3709* [Measure](measure.html): Name of the publisher of the measure 3710* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 3711* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 3712* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 3713* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 3714* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 3715* [Requirements](requirements.html): Name of the publisher of the requirements 3716* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 3717* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 3718* [StructureMap](structuremap.html): Name of the publisher of the structure map 3719* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 3720* [TestScript](testscript.html): Name of the publisher of the test script 3721* [ValueSet](valueset.html): Name of the publisher of the value set 3722</b><br> 3723 * Type: <b>string</b><br> 3724 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 3725 * </p> 3726 */ 3727 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 3728 public static final String SP_PUBLISHER = "publisher"; 3729 /** 3730 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3731 * <p> 3732 * Description: <b>Multiple Resources: 3733 3734* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 3735* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 3736* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 3737* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 3738* [Citation](citation.html): Name of the publisher of the citation 3739* [CodeSystem](codesystem.html): Name of the publisher of the code system 3740* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 3741* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 3742* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 3743* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 3744* [Evidence](evidence.html): Name of the publisher of the evidence 3745* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 3746* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 3747* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 3748* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 3749* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 3750* [Library](library.html): Name of the publisher of the library 3751* [Measure](measure.html): Name of the publisher of the measure 3752* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 3753* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 3754* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 3755* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 3756* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 3757* [Requirements](requirements.html): Name of the publisher of the requirements 3758* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 3759* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 3760* [StructureMap](structuremap.html): Name of the publisher of the structure map 3761* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 3762* [TestScript](testscript.html): Name of the publisher of the test script 3763* [ValueSet](valueset.html): Name of the publisher of the value set 3764</b><br> 3765 * Type: <b>string</b><br> 3766 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 3767 * </p> 3768 */ 3769 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 3770 3771 /** 3772 * Search parameter: <b>status</b> 3773 * <p> 3774 * Description: <b>Multiple Resources: 3775 3776* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 3777* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 3778* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 3779* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 3780* [Citation](citation.html): The current status of the citation 3781* [CodeSystem](codesystem.html): The current status of the code system 3782* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 3783* [ConceptMap](conceptmap.html): The current status of the concept map 3784* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 3785* [EventDefinition](eventdefinition.html): The current status of the event definition 3786* [Evidence](evidence.html): The current status of the evidence 3787* [EvidenceReport](evidencereport.html): The current status of the evidence report 3788* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 3789* [ExampleScenario](examplescenario.html): The current status of the example scenario 3790* [GraphDefinition](graphdefinition.html): The current status of the graph definition 3791* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 3792* [Library](library.html): The current status of the library 3793* [Measure](measure.html): The current status of the measure 3794* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 3795* [MessageDefinition](messagedefinition.html): The current status of the message definition 3796* [NamingSystem](namingsystem.html): The current status of the naming system 3797* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 3798* [OperationDefinition](operationdefinition.html): The current status of the operation definition 3799* [PlanDefinition](plandefinition.html): The current status of the plan definition 3800* [Questionnaire](questionnaire.html): The current status of the questionnaire 3801* [Requirements](requirements.html): The current status of the requirements 3802* [SearchParameter](searchparameter.html): The current status of the search parameter 3803* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 3804* [StructureDefinition](structuredefinition.html): The current status of the structure definition 3805* [StructureMap](structuremap.html): The current status of the structure map 3806* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 3807* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 3808* [TestPlan](testplan.html): The current status of the test plan 3809* [TestScript](testscript.html): The current status of the test script 3810* [ValueSet](valueset.html): The current status of the value set 3811</b><br> 3812 * Type: <b>token</b><br> 3813 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 3814 * </p> 3815 */ 3816 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 3817 public static final String SP_STATUS = "status"; 3818 /** 3819 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3820 * <p> 3821 * Description: <b>Multiple Resources: 3822 3823* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 3824* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 3825* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 3826* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 3827* [Citation](citation.html): The current status of the citation 3828* [CodeSystem](codesystem.html): The current status of the code system 3829* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 3830* [ConceptMap](conceptmap.html): The current status of the concept map 3831* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 3832* [EventDefinition](eventdefinition.html): The current status of the event definition 3833* [Evidence](evidence.html): The current status of the evidence 3834* [EvidenceReport](evidencereport.html): The current status of the evidence report 3835* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 3836* [ExampleScenario](examplescenario.html): The current status of the example scenario 3837* [GraphDefinition](graphdefinition.html): The current status of the graph definition 3838* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 3839* [Library](library.html): The current status of the library 3840* [Measure](measure.html): The current status of the measure 3841* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 3842* [MessageDefinition](messagedefinition.html): The current status of the message definition 3843* [NamingSystem](namingsystem.html): The current status of the naming system 3844* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 3845* [OperationDefinition](operationdefinition.html): The current status of the operation definition 3846* [PlanDefinition](plandefinition.html): The current status of the plan definition 3847* [Questionnaire](questionnaire.html): The current status of the questionnaire 3848* [Requirements](requirements.html): The current status of the requirements 3849* [SearchParameter](searchparameter.html): The current status of the search parameter 3850* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 3851* [StructureDefinition](structuredefinition.html): The current status of the structure definition 3852* [StructureMap](structuremap.html): The current status of the structure map 3853* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 3854* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 3855* [TestPlan](testplan.html): The current status of the test plan 3856* [TestScript](testscript.html): The current status of the test script 3857* [ValueSet](valueset.html): The current status of the value set 3858</b><br> 3859 * Type: <b>token</b><br> 3860 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 3861 * </p> 3862 */ 3863 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3864 3865 /** 3866 * Search parameter: <b>url</b> 3867 * <p> 3868 * Description: <b>Multiple Resources: 3869 3870* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 3871* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 3872* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 3873* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 3874* [Citation](citation.html): The uri that identifies the citation 3875* [CodeSystem](codesystem.html): The uri that identifies the code system 3876* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 3877* [ConceptMap](conceptmap.html): The URI that identifies the concept map 3878* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 3879* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 3880* [Evidence](evidence.html): The uri that identifies the evidence 3881* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 3882* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 3883* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 3884* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 3885* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 3886* [Library](library.html): The uri that identifies the library 3887* [Measure](measure.html): The uri that identifies the measure 3888* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 3889* [NamingSystem](namingsystem.html): The uri that identifies the naming system 3890* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 3891* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 3892* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 3893* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 3894* [Requirements](requirements.html): The uri that identifies the requirements 3895* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 3896* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 3897* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 3898* [StructureMap](structuremap.html): The uri that identifies the structure map 3899* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 3900* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 3901* [TestPlan](testplan.html): The uri that identifies the test plan 3902* [TestScript](testscript.html): The uri that identifies the test script 3903* [ValueSet](valueset.html): The uri that identifies the value set 3904</b><br> 3905 * Type: <b>uri</b><br> 3906 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 3907 * </p> 3908 */ 3909 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 3910 public static final String SP_URL = "url"; 3911 /** 3912 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3913 * <p> 3914 * Description: <b>Multiple Resources: 3915 3916* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 3917* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 3918* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 3919* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 3920* [Citation](citation.html): The uri that identifies the citation 3921* [CodeSystem](codesystem.html): The uri that identifies the code system 3922* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 3923* [ConceptMap](conceptmap.html): The URI that identifies the concept map 3924* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 3925* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 3926* [Evidence](evidence.html): The uri that identifies the evidence 3927* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 3928* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 3929* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 3930* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 3931* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 3932* [Library](library.html): The uri that identifies the library 3933* [Measure](measure.html): The uri that identifies the measure 3934* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 3935* [NamingSystem](namingsystem.html): The uri that identifies the naming system 3936* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 3937* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 3938* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 3939* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 3940* [Requirements](requirements.html): The uri that identifies the requirements 3941* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 3942* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 3943* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 3944* [StructureMap](structuremap.html): The uri that identifies the structure map 3945* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 3946* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 3947* [TestPlan](testplan.html): The uri that identifies the test plan 3948* [TestScript](testscript.html): The uri that identifies the test script 3949* [ValueSet](valueset.html): The uri that identifies the value set 3950</b><br> 3951 * Type: <b>uri</b><br> 3952 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 3953 * </p> 3954 */ 3955 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3956 3957 /** 3958 * Search parameter: <b>version</b> 3959 * <p> 3960 * Description: <b>Multiple Resources: 3961 3962* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 3963* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 3964* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 3965* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 3966* [Citation](citation.html): The business version of the citation 3967* [CodeSystem](codesystem.html): The business version of the code system 3968* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 3969* [ConceptMap](conceptmap.html): The business version of the concept map 3970* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 3971* [EventDefinition](eventdefinition.html): The business version of the event definition 3972* [Evidence](evidence.html): The business version of the evidence 3973* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 3974* [ExampleScenario](examplescenario.html): The business version of the example scenario 3975* [GraphDefinition](graphdefinition.html): The business version of the graph definition 3976* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 3977* [Library](library.html): The business version of the library 3978* [Measure](measure.html): The business version of the measure 3979* [MessageDefinition](messagedefinition.html): The business version of the message definition 3980* [NamingSystem](namingsystem.html): The business version of the naming system 3981* [OperationDefinition](operationdefinition.html): The business version of the operation definition 3982* [PlanDefinition](plandefinition.html): The business version of the plan definition 3983* [Questionnaire](questionnaire.html): The business version of the questionnaire 3984* [Requirements](requirements.html): The business version of the requirements 3985* [SearchParameter](searchparameter.html): The business version of the search parameter 3986* [StructureDefinition](structuredefinition.html): The business version of the structure definition 3987* [StructureMap](structuremap.html): The business version of the structure map 3988* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 3989* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 3990* [TestScript](testscript.html): The business version of the test script 3991* [ValueSet](valueset.html): The business version of the value set 3992</b><br> 3993 * Type: <b>token</b><br> 3994 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 3995 * </p> 3996 */ 3997 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 3998 public static final String SP_VERSION = "version"; 3999 /** 4000 * <b>Fluent Client</b> search parameter constant for <b>version</b> 4001 * <p> 4002 * Description: <b>Multiple Resources: 4003 4004* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4005* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4006* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4007* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4008* [Citation](citation.html): The business version of the citation 4009* [CodeSystem](codesystem.html): The business version of the code system 4010* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 4011* [ConceptMap](conceptmap.html): The business version of the concept map 4012* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 4013* [EventDefinition](eventdefinition.html): The business version of the event definition 4014* [Evidence](evidence.html): The business version of the evidence 4015* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 4016* [ExampleScenario](examplescenario.html): The business version of the example scenario 4017* [GraphDefinition](graphdefinition.html): The business version of the graph definition 4018* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 4019* [Library](library.html): The business version of the library 4020* [Measure](measure.html): The business version of the measure 4021* [MessageDefinition](messagedefinition.html): The business version of the message definition 4022* [NamingSystem](namingsystem.html): The business version of the naming system 4023* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4024* [PlanDefinition](plandefinition.html): The business version of the plan definition 4025* [Questionnaire](questionnaire.html): The business version of the questionnaire 4026* [Requirements](requirements.html): The business version of the requirements 4027* [SearchParameter](searchparameter.html): The business version of the search parameter 4028* [StructureDefinition](structuredefinition.html): The business version of the structure definition 4029* [StructureMap](structuremap.html): The business version of the structure map 4030* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 4031* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 4032* [TestScript](testscript.html): The business version of the test script 4033* [ValueSet](valueset.html): The business version of the value set 4034</b><br> 4035 * Type: <b>token</b><br> 4036 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 4037 * </p> 4038 */ 4039 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 4040 4041 /** 4042 * Search parameter: <b>base</b> 4043 * <p> 4044 * Description: <b>The resource type(s) this search parameter applies to</b><br> 4045 * Type: <b>token</b><br> 4046 * Path: <b>SearchParameter.base</b><br> 4047 * </p> 4048 */ 4049 @SearchParamDefinition(name="base", path="SearchParameter.base", description="The resource type(s) this search parameter applies to", type="token" ) 4050 public static final String SP_BASE = "base"; 4051 /** 4052 * <b>Fluent Client</b> search parameter constant for <b>base</b> 4053 * <p> 4054 * Description: <b>The resource type(s) this search parameter applies to</b><br> 4055 * Type: <b>token</b><br> 4056 * Path: <b>SearchParameter.base</b><br> 4057 * </p> 4058 */ 4059 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BASE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BASE); 4060 4061 /** 4062 * Search parameter: <b>code</b> 4063 * <p> 4064 * Description: <b>Code used in URL</b><br> 4065 * Type: <b>token</b><br> 4066 * Path: <b>SearchParameter.code</b><br> 4067 * </p> 4068 */ 4069 @SearchParamDefinition(name="code", path="SearchParameter.code", description="Code used in URL", type="token" ) 4070 public static final String SP_CODE = "code"; 4071 /** 4072 * <b>Fluent Client</b> search parameter constant for <b>code</b> 4073 * <p> 4074 * Description: <b>Code used in URL</b><br> 4075 * Type: <b>token</b><br> 4076 * Path: <b>SearchParameter.code</b><br> 4077 * </p> 4078 */ 4079 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 4080 4081 /** 4082 * Search parameter: <b>component</b> 4083 * <p> 4084 * Description: <b>Defines how the part works</b><br> 4085 * Type: <b>reference</b><br> 4086 * Path: <b>SearchParameter.component.definition</b><br> 4087 * </p> 4088 */ 4089 @SearchParamDefinition(name="component", path="SearchParameter.component.definition", description="Defines how the part works", type="reference", target={SearchParameter.class } ) 4090 public static final String SP_COMPONENT = "component"; 4091 /** 4092 * <b>Fluent Client</b> search parameter constant for <b>component</b> 4093 * <p> 4094 * Description: <b>Defines how the part works</b><br> 4095 * Type: <b>reference</b><br> 4096 * Path: <b>SearchParameter.component.definition</b><br> 4097 * </p> 4098 */ 4099 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPONENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COMPONENT); 4100 4101/** 4102 * Constant for fluent queries to be used to add include statements. Specifies 4103 * the path value of "<b>SearchParameter:component</b>". 4104 */ 4105 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPONENT = new ca.uhn.fhir.model.api.Include("SearchParameter:component").toLocked(); 4106 4107 /** 4108 * Search parameter: <b>derived-from</b> 4109 * <p> 4110 * Description: <b>Original definition for the search parameter</b><br> 4111 * Type: <b>reference</b><br> 4112 * Path: <b>SearchParameter.derivedFrom</b><br> 4113 * </p> 4114 */ 4115 @SearchParamDefinition(name="derived-from", path="SearchParameter.derivedFrom", description="Original definition for the search parameter", type="reference", target={SearchParameter.class } ) 4116 public static final String SP_DERIVED_FROM = "derived-from"; 4117 /** 4118 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 4119 * <p> 4120 * Description: <b>Original definition for the search parameter</b><br> 4121 * Type: <b>reference</b><br> 4122 * Path: <b>SearchParameter.derivedFrom</b><br> 4123 * </p> 4124 */ 4125 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 4126 4127/** 4128 * Constant for fluent queries to be used to add include statements. Specifies 4129 * the path value of "<b>SearchParameter:derived-from</b>". 4130 */ 4131 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("SearchParameter:derived-from").toLocked(); 4132 4133 /** 4134 * Search parameter: <b>target</b> 4135 * <p> 4136 * Description: <b>Types of resource (if a resource reference)</b><br> 4137 * Type: <b>token</b><br> 4138 * Path: <b>SearchParameter.target</b><br> 4139 * </p> 4140 */ 4141 @SearchParamDefinition(name="target", path="SearchParameter.target", description="Types of resource (if a resource reference)", type="token" ) 4142 public static final String SP_TARGET = "target"; 4143 /** 4144 * <b>Fluent Client</b> search parameter constant for <b>target</b> 4145 * <p> 4146 * Description: <b>Types of resource (if a resource reference)</b><br> 4147 * Type: <b>token</b><br> 4148 * Path: <b>SearchParameter.target</b><br> 4149 * </p> 4150 */ 4151 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TARGET); 4152 4153 /** 4154 * Search parameter: <b>type</b> 4155 * <p> 4156 * Description: <b>number | date | string | token | reference | composite | quantity | uri | special</b><br> 4157 * Type: <b>token</b><br> 4158 * Path: <b>SearchParameter.type</b><br> 4159 * </p> 4160 */ 4161 @SearchParamDefinition(name="type", path="SearchParameter.type", description="number | date | string | token | reference | composite | quantity | uri | special", type="token" ) 4162 public static final String SP_TYPE = "type"; 4163 /** 4164 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4165 * <p> 4166 * Description: <b>number | date | string | token | reference | composite | quantity | uri | special</b><br> 4167 * Type: <b>token</b><br> 4168 * Path: <b>SearchParameter.type</b><br> 4169 * </p> 4170 */ 4171 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 4172 4173// Manual code (from Configuration.txt): 4174 public boolean supportsCopyright() { 4175 return true; 4176 } 4177 4178 4179 public boolean hasBase(String value) { 4180 if (this.base == null) 4181 return false; 4182 for (Enumeration<VersionIndependentResourceTypesAll> v : this.base) 4183 if (v.getCode().equals(value)) // code 4184 return true; 4185 return false; 4186 } 4187 4188// end addition 4189 4190} 4191