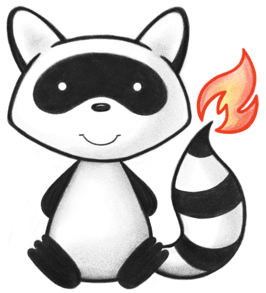
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A search parameter that defines a named search item that can be used to search/filter on a resource. 052 */ 053@ResourceDef(name="SearchParameter", profile="http://hl7.org/fhir/StructureDefinition/SearchParameter") 054public class SearchParameter extends CanonicalResource { 055 056 public enum SearchProcessingModeType { 057 /** 058 * The search parameter is derived directly from the selected nodes based on the type definitions. 059 */ 060 NORMAL, 061 /** 062 * The search parameter is derived by a phonetic transform from the selected nodes. 063 */ 064 PHONETIC, 065 /** 066 * The interpretation of the xpath statement is unknown (and can't be automated). 067 */ 068 OTHER, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static SearchProcessingModeType fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("normal".equals(codeString)) 077 return NORMAL; 078 if ("phonetic".equals(codeString)) 079 return PHONETIC; 080 if ("other".equals(codeString)) 081 return OTHER; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown SearchProcessingModeType code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case NORMAL: return "normal"; 090 case PHONETIC: return "phonetic"; 091 case OTHER: return "other"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case NORMAL: return "http://hl7.org/fhir/search-processingmode"; 099 case PHONETIC: return "http://hl7.org/fhir/search-processingmode"; 100 case OTHER: return "http://hl7.org/fhir/search-processingmode"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case NORMAL: return "The search parameter is derived directly from the selected nodes based on the type definitions."; 108 case PHONETIC: return "The search parameter is derived by a phonetic transform from the selected nodes."; 109 case OTHER: return "The interpretation of the xpath statement is unknown (and can't be automated)."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case NORMAL: return "Normal"; 117 case PHONETIC: return "Phonetic"; 118 case OTHER: return "Other"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class SearchProcessingModeTypeEnumFactory implements EnumFactory<SearchProcessingModeType> { 126 public SearchProcessingModeType fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("normal".equals(codeString)) 131 return SearchProcessingModeType.NORMAL; 132 if ("phonetic".equals(codeString)) 133 return SearchProcessingModeType.PHONETIC; 134 if ("other".equals(codeString)) 135 return SearchProcessingModeType.OTHER; 136 throw new IllegalArgumentException("Unknown SearchProcessingModeType code '"+codeString+"'"); 137 } 138 public Enumeration<SearchProcessingModeType> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<SearchProcessingModeType>(this, SearchProcessingModeType.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<SearchProcessingModeType>(this, SearchProcessingModeType.NULL, code); 146 if ("normal".equals(codeString)) 147 return new Enumeration<SearchProcessingModeType>(this, SearchProcessingModeType.NORMAL, code); 148 if ("phonetic".equals(codeString)) 149 return new Enumeration<SearchProcessingModeType>(this, SearchProcessingModeType.PHONETIC, code); 150 if ("other".equals(codeString)) 151 return new Enumeration<SearchProcessingModeType>(this, SearchProcessingModeType.OTHER, code); 152 throw new FHIRException("Unknown SearchProcessingModeType code '"+codeString+"'"); 153 } 154 public String toCode(SearchProcessingModeType code) { 155 if (code == SearchProcessingModeType.NULL) 156 return null; 157 if (code == SearchProcessingModeType.NORMAL) 158 return "normal"; 159 if (code == SearchProcessingModeType.PHONETIC) 160 return "phonetic"; 161 if (code == SearchProcessingModeType.OTHER) 162 return "other"; 163 return "?"; 164 } 165 public String toSystem(SearchProcessingModeType code) { 166 return code.getSystem(); 167 } 168 } 169 170 @Block() 171 public static class SearchParameterComponentComponent extends BackboneElement implements IBaseBackboneElement { 172 /** 173 * The definition of the search parameter that describes this part. 174 */ 175 @Child(name = "definition", type = {CanonicalType.class}, order=1, min=1, max=1, modifier=false, summary=false) 176 @Description(shortDefinition="Defines how the part works", formalDefinition="The definition of the search parameter that describes this part." ) 177 protected CanonicalType definition; 178 179 /** 180 * A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression. 181 */ 182 @Child(name = "expression", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 183 @Description(shortDefinition="Subexpression relative to main expression", formalDefinition="A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression." ) 184 protected StringType expression; 185 186 private static final long serialVersionUID = -1469435618L; 187 188 /** 189 * Constructor 190 */ 191 public SearchParameterComponentComponent() { 192 super(); 193 } 194 195 /** 196 * Constructor 197 */ 198 public SearchParameterComponentComponent(String definition, String expression) { 199 super(); 200 this.setDefinition(definition); 201 this.setExpression(expression); 202 } 203 204 /** 205 * @return {@link #definition} (The definition of the search parameter that describes this part.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 206 */ 207 public CanonicalType getDefinitionElement() { 208 if (this.definition == null) 209 if (Configuration.errorOnAutoCreate()) 210 throw new Error("Attempt to auto-create SearchParameterComponentComponent.definition"); 211 else if (Configuration.doAutoCreate()) 212 this.definition = new CanonicalType(); // bb 213 return this.definition; 214 } 215 216 public boolean hasDefinitionElement() { 217 return this.definition != null && !this.definition.isEmpty(); 218 } 219 220 public boolean hasDefinition() { 221 return this.definition != null && !this.definition.isEmpty(); 222 } 223 224 /** 225 * @param value {@link #definition} (The definition of the search parameter that describes this part.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 226 */ 227 public SearchParameterComponentComponent setDefinitionElement(CanonicalType value) { 228 this.definition = value; 229 return this; 230 } 231 232 /** 233 * @return The definition of the search parameter that describes this part. 234 */ 235 public String getDefinition() { 236 return this.definition == null ? null : this.definition.getValue(); 237 } 238 239 /** 240 * @param value The definition of the search parameter that describes this part. 241 */ 242 public SearchParameterComponentComponent setDefinition(String value) { 243 if (this.definition == null) 244 this.definition = new CanonicalType(); 245 this.definition.setValue(value); 246 return this; 247 } 248 249 /** 250 * @return {@link #expression} (A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 251 */ 252 public StringType getExpressionElement() { 253 if (this.expression == null) 254 if (Configuration.errorOnAutoCreate()) 255 throw new Error("Attempt to auto-create SearchParameterComponentComponent.expression"); 256 else if (Configuration.doAutoCreate()) 257 this.expression = new StringType(); // bb 258 return this.expression; 259 } 260 261 public boolean hasExpressionElement() { 262 return this.expression != null && !this.expression.isEmpty(); 263 } 264 265 public boolean hasExpression() { 266 return this.expression != null && !this.expression.isEmpty(); 267 } 268 269 /** 270 * @param value {@link #expression} (A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 271 */ 272 public SearchParameterComponentComponent setExpressionElement(StringType value) { 273 this.expression = value; 274 return this; 275 } 276 277 /** 278 * @return A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression. 279 */ 280 public String getExpression() { 281 return this.expression == null ? null : this.expression.getValue(); 282 } 283 284 /** 285 * @param value A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression. 286 */ 287 public SearchParameterComponentComponent setExpression(String value) { 288 if (this.expression == null) 289 this.expression = new StringType(); 290 this.expression.setValue(value); 291 return this; 292 } 293 294 protected void listChildren(List<Property> children) { 295 super.listChildren(children); 296 children.add(new Property("definition", "canonical(SearchParameter)", "The definition of the search parameter that describes this part.", 0, 1, definition)); 297 children.add(new Property("expression", "string", "A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.", 0, 1, expression)); 298 } 299 300 @Override 301 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 302 switch (_hash) { 303 case -1014418093: /*definition*/ return new Property("definition", "canonical(SearchParameter)", "The definition of the search parameter that describes this part.", 0, 1, definition); 304 case -1795452264: /*expression*/ return new Property("expression", "string", "A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.", 0, 1, expression); 305 default: return super.getNamedProperty(_hash, _name, _checkValid); 306 } 307 308 } 309 310 @Override 311 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 312 switch (hash) { 313 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // CanonicalType 314 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 315 default: return super.getProperty(hash, name, checkValid); 316 } 317 318 } 319 320 @Override 321 public Base setProperty(int hash, String name, Base value) throws FHIRException { 322 switch (hash) { 323 case -1014418093: // definition 324 this.definition = TypeConvertor.castToCanonical(value); // CanonicalType 325 return value; 326 case -1795452264: // expression 327 this.expression = TypeConvertor.castToString(value); // StringType 328 return value; 329 default: return super.setProperty(hash, name, value); 330 } 331 332 } 333 334 @Override 335 public Base setProperty(String name, Base value) throws FHIRException { 336 if (name.equals("definition")) { 337 this.definition = TypeConvertor.castToCanonical(value); // CanonicalType 338 } else if (name.equals("expression")) { 339 this.expression = TypeConvertor.castToString(value); // StringType 340 } else 341 return super.setProperty(name, value); 342 return value; 343 } 344 345 @Override 346 public void removeChild(String name, Base value) throws FHIRException { 347 if (name.equals("definition")) { 348 this.definition = null; 349 } else if (name.equals("expression")) { 350 this.expression = null; 351 } else 352 super.removeChild(name, value); 353 354 } 355 356 @Override 357 public Base makeProperty(int hash, String name) throws FHIRException { 358 switch (hash) { 359 case -1014418093: return getDefinitionElement(); 360 case -1795452264: return getExpressionElement(); 361 default: return super.makeProperty(hash, name); 362 } 363 364 } 365 366 @Override 367 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 368 switch (hash) { 369 case -1014418093: /*definition*/ return new String[] {"canonical"}; 370 case -1795452264: /*expression*/ return new String[] {"string"}; 371 default: return super.getTypesForProperty(hash, name); 372 } 373 374 } 375 376 @Override 377 public Base addChild(String name) throws FHIRException { 378 if (name.equals("definition")) { 379 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.component.definition"); 380 } 381 else if (name.equals("expression")) { 382 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.component.expression"); 383 } 384 else 385 return super.addChild(name); 386 } 387 388 public SearchParameterComponentComponent copy() { 389 SearchParameterComponentComponent dst = new SearchParameterComponentComponent(); 390 copyValues(dst); 391 return dst; 392 } 393 394 public void copyValues(SearchParameterComponentComponent dst) { 395 super.copyValues(dst); 396 dst.definition = definition == null ? null : definition.copy(); 397 dst.expression = expression == null ? null : expression.copy(); 398 } 399 400 @Override 401 public boolean equalsDeep(Base other_) { 402 if (!super.equalsDeep(other_)) 403 return false; 404 if (!(other_ instanceof SearchParameterComponentComponent)) 405 return false; 406 SearchParameterComponentComponent o = (SearchParameterComponentComponent) other_; 407 return compareDeep(definition, o.definition, true) && compareDeep(expression, o.expression, true) 408 ; 409 } 410 411 @Override 412 public boolean equalsShallow(Base other_) { 413 if (!super.equalsShallow(other_)) 414 return false; 415 if (!(other_ instanceof SearchParameterComponentComponent)) 416 return false; 417 SearchParameterComponentComponent o = (SearchParameterComponentComponent) other_; 418 return compareValues(definition, o.definition, true) && compareValues(expression, o.expression, true) 419 ; 420 } 421 422 public boolean isEmpty() { 423 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(definition, expression); 424 } 425 426 public String fhirType() { 427 return "SearchParameter.component"; 428 429 } 430 431 } 432 433 /** 434 * An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this search parameter is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the search parameter is stored on different servers. 435 */ 436 @Child(name = "url", type = {UriType.class}, order=0, min=1, max=1, modifier=false, summary=true) 437 @Description(shortDefinition="Canonical identifier for this search parameter, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this search parameter is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the search parameter is stored on different servers." ) 438 protected UriType url; 439 440 /** 441 * A formal identifier that is used to identify this search parameter when it is represented in other formats, or referenced in a specification, model, design or an instance. 442 */ 443 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 444 @Description(shortDefinition="Additional identifier for the search parameter (business identifier)", formalDefinition="A formal identifier that is used to identify this search parameter when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 445 protected List<Identifier> identifier; 446 447 /** 448 * The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 449 */ 450 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 451 @Description(shortDefinition="Business version of the search parameter", formalDefinition="The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 452 protected StringType version; 453 454 /** 455 * Indicates the mechanism used to compare versions to determine which is more current. 456 */ 457 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 458 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 459 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 460 protected DataType versionAlgorithm; 461 462 /** 463 * A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation. 464 */ 465 @Child(name = "name", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=true) 466 @Description(shortDefinition="Name for this search parameter (computer friendly)", formalDefinition="A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 467 protected StringType name; 468 469 /** 470 * A short, descriptive, user-friendly title for the search parameter. 471 */ 472 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 473 @Description(shortDefinition="Name for this search parameter (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the search parameter." ) 474 protected StringType title; 475 476 /** 477 * Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter. 478 */ 479 @Child(name = "derivedFrom", type = {CanonicalType.class}, order=6, min=0, max=1, modifier=false, summary=false) 480 @Description(shortDefinition="Original definition for the search parameter", formalDefinition="Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter." ) 481 protected CanonicalType derivedFrom; 482 483 /** 484 * The status of this search parameter. Enables tracking the life-cycle of the content. 485 */ 486 @Child(name = "status", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 487 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this search parameter. Enables tracking the life-cycle of the content." ) 488 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 489 protected Enumeration<PublicationStatus> status; 490 491 /** 492 * A Boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 493 */ 494 @Child(name = "experimental", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=true) 495 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 496 protected BooleanType experimental; 497 498 /** 499 * The date (and optionally time) when the search parameter was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes. 500 */ 501 @Child(name = "date", type = {DateTimeType.class}, order=9, min=0, max=1, modifier=false, summary=true) 502 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the search parameter was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes." ) 503 protected DateTimeType date; 504 505 /** 506 * The name of the organization or individual tresponsible for the release and ongoing maintenance of the search parameter. 507 */ 508 @Child(name = "publisher", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 509 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual tresponsible for the release and ongoing maintenance of the search parameter." ) 510 protected StringType publisher; 511 512 /** 513 * Contact details to assist a user in finding and communicating with the publisher. 514 */ 515 @Child(name = "contact", type = {ContactDetail.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 516 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 517 protected List<ContactDetail> contact; 518 519 /** 520 * And how it used. 521 */ 522 @Child(name = "description", type = {MarkdownType.class}, order=12, min=1, max=1, modifier=false, summary=true) 523 @Description(shortDefinition="Natural language description of the search parameter", formalDefinition="And how it used." ) 524 protected MarkdownType description; 525 526 /** 527 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate search parameter instances. 528 */ 529 @Child(name = "useContext", type = {UsageContext.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 530 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate search parameter instances." ) 531 protected List<UsageContext> useContext; 532 533 /** 534 * A legal or geographic region in which the search parameter is intended to be used. 535 */ 536 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 537 @Description(shortDefinition="Intended jurisdiction for search parameter (if applicable)", formalDefinition="A legal or geographic region in which the search parameter is intended to be used." ) 538 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 539 protected List<CodeableConcept> jurisdiction; 540 541 /** 542 * Explanation of why this search parameter is needed and why it has been designed as it has. 543 */ 544 @Child(name = "purpose", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 545 @Description(shortDefinition="Why this search parameter is defined", formalDefinition="Explanation of why this search parameter is needed and why it has been designed as it has." ) 546 protected MarkdownType purpose; 547 548 /** 549 * A copyright statement relating to the search parameter and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the search parameter. 550 */ 551 @Child(name = "copyright", type = {MarkdownType.class}, order=16, min=0, max=1, modifier=false, summary=false) 552 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the search parameter and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the search parameter." ) 553 protected MarkdownType copyright; 554 555 /** 556 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 557 */ 558 @Child(name = "copyrightLabel", type = {StringType.class}, order=17, min=0, max=1, modifier=false, summary=false) 559 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 560 protected StringType copyrightLabel; 561 562 /** 563 * The label that is recommended to be used in the URL or the parameter name in a parameters resource for this search parameter. In some cases, servers may need to use a different CapabilityStatement searchParam.name to differentiate between multiple SearchParameters that happen to have the same code. 564 */ 565 @Child(name = "code", type = {CodeType.class}, order=18, min=1, max=1, modifier=false, summary=true) 566 @Description(shortDefinition="Recommended name for parameter in search url", formalDefinition="The label that is recommended to be used in the URL or the parameter name in a parameters resource for this search parameter. In some cases, servers may need to use a different CapabilityStatement searchParam.name to differentiate between multiple SearchParameters that happen to have the same code." ) 567 protected CodeType code; 568 569 /** 570 * The base resource type(s) that this search parameter can be used against. 571 */ 572 @Child(name = "base", type = {CodeType.class}, order=19, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 573 @Description(shortDefinition="The resource type(s) this search parameter applies to", formalDefinition="The base resource type(s) that this search parameter can be used against." ) 574 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-independent-all-resource-types") 575 protected List<Enumeration<VersionIndependentResourceTypesAll>> base; 576 577 /** 578 * The type of value that a search parameter may contain, and how the content is interpreted. 579 */ 580 @Child(name = "type", type = {CodeType.class}, order=20, min=1, max=1, modifier=false, summary=true) 581 @Description(shortDefinition="number | date | string | token | reference | composite | quantity | uri | special", formalDefinition="The type of value that a search parameter may contain, and how the content is interpreted." ) 582 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-param-type") 583 protected Enumeration<SearchParamType> type; 584 585 /** 586 * A FHIRPath expression that returns a set of elements for the search parameter. 587 */ 588 @Child(name = "expression", type = {StringType.class}, order=21, min=0, max=1, modifier=false, summary=false) 589 @Description(shortDefinition="FHIRPath expression that extracts the values", formalDefinition="A FHIRPath expression that returns a set of elements for the search parameter." ) 590 protected StringType expression; 591 592 /** 593 * How the search parameter relates to the set of elements returned by evaluating the expression query. 594 */ 595 @Child(name = "processingMode", type = {CodeType.class}, order=22, min=0, max=1, modifier=false, summary=false) 596 @Description(shortDefinition="normal | phonetic | other", formalDefinition="How the search parameter relates to the set of elements returned by evaluating the expression query." ) 597 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-processingmode") 598 protected Enumeration<SearchProcessingModeType> processingMode; 599 600 /** 601 * FHIRPath expression that defines/sets a complex constraint for when this SearchParameter is applicable. 602 */ 603 @Child(name = "constraint", type = {StringType.class}, order=23, min=0, max=1, modifier=false, summary=false) 604 @Description(shortDefinition="FHIRPath expression that constraints the usage of this SearchParamete", formalDefinition="FHIRPath expression that defines/sets a complex constraint for when this SearchParameter is applicable." ) 605 protected StringType constraint; 606 607 /** 608 * Types of resource (if a resource is referenced). 609 */ 610 @Child(name = "target", type = {CodeType.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 611 @Description(shortDefinition="Types of resource (if a resource reference)", formalDefinition="Types of resource (if a resource is referenced)." ) 612 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-independent-all-resource-types") 613 protected List<Enumeration<VersionIndependentResourceTypesAll>> target; 614 615 /** 616 * Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match. 617 */ 618 @Child(name = "multipleOr", type = {BooleanType.class}, order=25, min=0, max=1, modifier=false, summary=false) 619 @Description(shortDefinition="Allow multiple values per parameter (or)", formalDefinition="Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match." ) 620 protected BooleanType multipleOr; 621 622 /** 623 * Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match. 624 */ 625 @Child(name = "multipleAnd", type = {BooleanType.class}, order=26, min=0, max=1, modifier=false, summary=false) 626 @Description(shortDefinition="Allow multiple parameters (and)", formalDefinition="Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match." ) 627 protected BooleanType multipleAnd; 628 629 /** 630 * Comparators supported for the search parameter. 631 */ 632 @Child(name = "comparator", type = {CodeType.class}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 633 @Description(shortDefinition="eq | ne | gt | lt | ge | le | sa | eb | ap", formalDefinition="Comparators supported for the search parameter." ) 634 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-comparator") 635 protected List<Enumeration<SearchComparator>> comparator; 636 637 /** 638 * A modifier supported for the search parameter. 639 */ 640 @Child(name = "modifier", type = {CodeType.class}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 641 @Description(shortDefinition="missing | exact | contains | not | text | in | not-in | below | above | type | identifier | of-type | code-text | text-advanced | iterate", formalDefinition="A modifier supported for the search parameter." ) 642 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-modifier-code") 643 protected List<Enumeration<SearchModifierCode>> modifier; 644 645 /** 646 * Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type. 647 */ 648 @Child(name = "chain", type = {StringType.class}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 649 @Description(shortDefinition="Chained names supported", formalDefinition="Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type." ) 650 protected List<StringType> chain; 651 652 /** 653 * Used to define the parts of a composite search parameter. 654 */ 655 @Child(name = "component", type = {}, order=30, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 656 @Description(shortDefinition="For Composite resources to define the parts", formalDefinition="Used to define the parts of a composite search parameter." ) 657 protected List<SearchParameterComponentComponent> component; 658 659 private static final long serialVersionUID = -327665794L; 660 661 /** 662 * Constructor 663 */ 664 public SearchParameter() { 665 super(); 666 } 667 668 /** 669 * Constructor 670 */ 671 public SearchParameter(String url, String name, PublicationStatus status, String description, String code, VersionIndependentResourceTypesAll base, SearchParamType type) { 672 super(); 673 this.setUrl(url); 674 this.setName(name); 675 this.setStatus(status); 676 this.setDescription(description); 677 this.setCode(code); 678 this.addBase(base); 679 this.setType(type); 680 } 681 682 /** 683 * @return {@link #url} (An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this search parameter is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the search parameter is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 684 */ 685 public UriType getUrlElement() { 686 if (this.url == null) 687 if (Configuration.errorOnAutoCreate()) 688 throw new Error("Attempt to auto-create SearchParameter.url"); 689 else if (Configuration.doAutoCreate()) 690 this.url = new UriType(); // bb 691 return this.url; 692 } 693 694 public boolean hasUrlElement() { 695 return this.url != null && !this.url.isEmpty(); 696 } 697 698 public boolean hasUrl() { 699 return this.url != null && !this.url.isEmpty(); 700 } 701 702 /** 703 * @param value {@link #url} (An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this search parameter is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the search parameter is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 704 */ 705 public SearchParameter setUrlElement(UriType value) { 706 this.url = value; 707 return this; 708 } 709 710 /** 711 * @return An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this search parameter is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the search parameter is stored on different servers. 712 */ 713 public String getUrl() { 714 return this.url == null ? null : this.url.getValue(); 715 } 716 717 /** 718 * @param value An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this search parameter is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the search parameter is stored on different servers. 719 */ 720 public SearchParameter setUrl(String value) { 721 if (this.url == null) 722 this.url = new UriType(); 723 this.url.setValue(value); 724 return this; 725 } 726 727 /** 728 * @return {@link #identifier} (A formal identifier that is used to identify this search parameter when it is represented in other formats, or referenced in a specification, model, design or an instance.) 729 */ 730 public List<Identifier> getIdentifier() { 731 if (this.identifier == null) 732 this.identifier = new ArrayList<Identifier>(); 733 return this.identifier; 734 } 735 736 /** 737 * @return Returns a reference to <code>this</code> for easy method chaining 738 */ 739 public SearchParameter setIdentifier(List<Identifier> theIdentifier) { 740 this.identifier = theIdentifier; 741 return this; 742 } 743 744 public boolean hasIdentifier() { 745 if (this.identifier == null) 746 return false; 747 for (Identifier item : this.identifier) 748 if (!item.isEmpty()) 749 return true; 750 return false; 751 } 752 753 public Identifier addIdentifier() { //3 754 Identifier t = new Identifier(); 755 if (this.identifier == null) 756 this.identifier = new ArrayList<Identifier>(); 757 this.identifier.add(t); 758 return t; 759 } 760 761 public SearchParameter addIdentifier(Identifier t) { //3 762 if (t == null) 763 return this; 764 if (this.identifier == null) 765 this.identifier = new ArrayList<Identifier>(); 766 this.identifier.add(t); 767 return this; 768 } 769 770 /** 771 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 772 */ 773 public Identifier getIdentifierFirstRep() { 774 if (getIdentifier().isEmpty()) { 775 addIdentifier(); 776 } 777 return getIdentifier().get(0); 778 } 779 780 /** 781 * @return {@link #version} (The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 782 */ 783 public StringType getVersionElement() { 784 if (this.version == null) 785 if (Configuration.errorOnAutoCreate()) 786 throw new Error("Attempt to auto-create SearchParameter.version"); 787 else if (Configuration.doAutoCreate()) 788 this.version = new StringType(); // bb 789 return this.version; 790 } 791 792 public boolean hasVersionElement() { 793 return this.version != null && !this.version.isEmpty(); 794 } 795 796 public boolean hasVersion() { 797 return this.version != null && !this.version.isEmpty(); 798 } 799 800 /** 801 * @param value {@link #version} (The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 802 */ 803 public SearchParameter setVersionElement(StringType value) { 804 this.version = value; 805 return this; 806 } 807 808 /** 809 * @return The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 810 */ 811 public String getVersion() { 812 return this.version == null ? null : this.version.getValue(); 813 } 814 815 /** 816 * @param value The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 817 */ 818 public SearchParameter setVersion(String value) { 819 if (Utilities.noString(value)) 820 this.version = null; 821 else { 822 if (this.version == null) 823 this.version = new StringType(); 824 this.version.setValue(value); 825 } 826 return this; 827 } 828 829 /** 830 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 831 */ 832 public DataType getVersionAlgorithm() { 833 return this.versionAlgorithm; 834 } 835 836 /** 837 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 838 */ 839 public StringType getVersionAlgorithmStringType() throws FHIRException { 840 if (this.versionAlgorithm == null) 841 this.versionAlgorithm = new StringType(); 842 if (!(this.versionAlgorithm instanceof StringType)) 843 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 844 return (StringType) this.versionAlgorithm; 845 } 846 847 public boolean hasVersionAlgorithmStringType() { 848 return this != null && this.versionAlgorithm instanceof StringType; 849 } 850 851 /** 852 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 853 */ 854 public Coding getVersionAlgorithmCoding() throws FHIRException { 855 if (this.versionAlgorithm == null) 856 this.versionAlgorithm = new Coding(); 857 if (!(this.versionAlgorithm instanceof Coding)) 858 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 859 return (Coding) this.versionAlgorithm; 860 } 861 862 public boolean hasVersionAlgorithmCoding() { 863 return this != null && this.versionAlgorithm instanceof Coding; 864 } 865 866 public boolean hasVersionAlgorithm() { 867 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 868 } 869 870 /** 871 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 872 */ 873 public SearchParameter setVersionAlgorithm(DataType value) { 874 if (value != null && !(value instanceof StringType || value instanceof Coding)) 875 throw new FHIRException("Not the right type for SearchParameter.versionAlgorithm[x]: "+value.fhirType()); 876 this.versionAlgorithm = value; 877 return this; 878 } 879 880 /** 881 * @return {@link #name} (A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 882 */ 883 public StringType getNameElement() { 884 if (this.name == null) 885 if (Configuration.errorOnAutoCreate()) 886 throw new Error("Attempt to auto-create SearchParameter.name"); 887 else if (Configuration.doAutoCreate()) 888 this.name = new StringType(); // bb 889 return this.name; 890 } 891 892 public boolean hasNameElement() { 893 return this.name != null && !this.name.isEmpty(); 894 } 895 896 public boolean hasName() { 897 return this.name != null && !this.name.isEmpty(); 898 } 899 900 /** 901 * @param value {@link #name} (A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 902 */ 903 public SearchParameter setNameElement(StringType value) { 904 this.name = value; 905 return this; 906 } 907 908 /** 909 * @return A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation. 910 */ 911 public String getName() { 912 return this.name == null ? null : this.name.getValue(); 913 } 914 915 /** 916 * @param value A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation. 917 */ 918 public SearchParameter setName(String value) { 919 if (this.name == null) 920 this.name = new StringType(); 921 this.name.setValue(value); 922 return this; 923 } 924 925 /** 926 * @return {@link #title} (A short, descriptive, user-friendly title for the search parameter.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 927 */ 928 public StringType getTitleElement() { 929 if (this.title == null) 930 if (Configuration.errorOnAutoCreate()) 931 throw new Error("Attempt to auto-create SearchParameter.title"); 932 else if (Configuration.doAutoCreate()) 933 this.title = new StringType(); // bb 934 return this.title; 935 } 936 937 public boolean hasTitleElement() { 938 return this.title != null && !this.title.isEmpty(); 939 } 940 941 public boolean hasTitle() { 942 return this.title != null && !this.title.isEmpty(); 943 } 944 945 /** 946 * @param value {@link #title} (A short, descriptive, user-friendly title for the search parameter.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 947 */ 948 public SearchParameter setTitleElement(StringType value) { 949 this.title = value; 950 return this; 951 } 952 953 /** 954 * @return A short, descriptive, user-friendly title for the search parameter. 955 */ 956 public String getTitle() { 957 return this.title == null ? null : this.title.getValue(); 958 } 959 960 /** 961 * @param value A short, descriptive, user-friendly title for the search parameter. 962 */ 963 public SearchParameter setTitle(String value) { 964 if (Utilities.noString(value)) 965 this.title = null; 966 else { 967 if (this.title == null) 968 this.title = new StringType(); 969 this.title.setValue(value); 970 } 971 return this; 972 } 973 974 /** 975 * @return {@link #derivedFrom} (Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.). This is the underlying object with id, value and extensions. The accessor "getDerivedFrom" gives direct access to the value 976 */ 977 public CanonicalType getDerivedFromElement() { 978 if (this.derivedFrom == null) 979 if (Configuration.errorOnAutoCreate()) 980 throw new Error("Attempt to auto-create SearchParameter.derivedFrom"); 981 else if (Configuration.doAutoCreate()) 982 this.derivedFrom = new CanonicalType(); // bb 983 return this.derivedFrom; 984 } 985 986 public boolean hasDerivedFromElement() { 987 return this.derivedFrom != null && !this.derivedFrom.isEmpty(); 988 } 989 990 public boolean hasDerivedFrom() { 991 return this.derivedFrom != null && !this.derivedFrom.isEmpty(); 992 } 993 994 /** 995 * @param value {@link #derivedFrom} (Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.). This is the underlying object with id, value and extensions. The accessor "getDerivedFrom" gives direct access to the value 996 */ 997 public SearchParameter setDerivedFromElement(CanonicalType value) { 998 this.derivedFrom = value; 999 return this; 1000 } 1001 1002 /** 1003 * @return Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter. 1004 */ 1005 public String getDerivedFrom() { 1006 return this.derivedFrom == null ? null : this.derivedFrom.getValue(); 1007 } 1008 1009 /** 1010 * @param value Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter. 1011 */ 1012 public SearchParameter setDerivedFrom(String value) { 1013 if (Utilities.noString(value)) 1014 this.derivedFrom = null; 1015 else { 1016 if (this.derivedFrom == null) 1017 this.derivedFrom = new CanonicalType(); 1018 this.derivedFrom.setValue(value); 1019 } 1020 return this; 1021 } 1022 1023 /** 1024 * @return {@link #status} (The status of this search parameter. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1025 */ 1026 public Enumeration<PublicationStatus> getStatusElement() { 1027 if (this.status == null) 1028 if (Configuration.errorOnAutoCreate()) 1029 throw new Error("Attempt to auto-create SearchParameter.status"); 1030 else if (Configuration.doAutoCreate()) 1031 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1032 return this.status; 1033 } 1034 1035 public boolean hasStatusElement() { 1036 return this.status != null && !this.status.isEmpty(); 1037 } 1038 1039 public boolean hasStatus() { 1040 return this.status != null && !this.status.isEmpty(); 1041 } 1042 1043 /** 1044 * @param value {@link #status} (The status of this search parameter. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1045 */ 1046 public SearchParameter setStatusElement(Enumeration<PublicationStatus> value) { 1047 this.status = value; 1048 return this; 1049 } 1050 1051 /** 1052 * @return The status of this search parameter. Enables tracking the life-cycle of the content. 1053 */ 1054 public PublicationStatus getStatus() { 1055 return this.status == null ? null : this.status.getValue(); 1056 } 1057 1058 /** 1059 * @param value The status of this search parameter. Enables tracking the life-cycle of the content. 1060 */ 1061 public SearchParameter setStatus(PublicationStatus value) { 1062 if (this.status == null) 1063 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1064 this.status.setValue(value); 1065 return this; 1066 } 1067 1068 /** 1069 * @return {@link #experimental} (A Boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1070 */ 1071 public BooleanType getExperimentalElement() { 1072 if (this.experimental == null) 1073 if (Configuration.errorOnAutoCreate()) 1074 throw new Error("Attempt to auto-create SearchParameter.experimental"); 1075 else if (Configuration.doAutoCreate()) 1076 this.experimental = new BooleanType(); // bb 1077 return this.experimental; 1078 } 1079 1080 public boolean hasExperimentalElement() { 1081 return this.experimental != null && !this.experimental.isEmpty(); 1082 } 1083 1084 public boolean hasExperimental() { 1085 return this.experimental != null && !this.experimental.isEmpty(); 1086 } 1087 1088 /** 1089 * @param value {@link #experimental} (A Boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1090 */ 1091 public SearchParameter setExperimentalElement(BooleanType value) { 1092 this.experimental = value; 1093 return this; 1094 } 1095 1096 /** 1097 * @return A Boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1098 */ 1099 public boolean getExperimental() { 1100 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1101 } 1102 1103 /** 1104 * @param value A Boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1105 */ 1106 public SearchParameter setExperimental(boolean value) { 1107 if (this.experimental == null) 1108 this.experimental = new BooleanType(); 1109 this.experimental.setValue(value); 1110 return this; 1111 } 1112 1113 /** 1114 * @return {@link #date} (The date (and optionally time) when the search parameter was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1115 */ 1116 public DateTimeType getDateElement() { 1117 if (this.date == null) 1118 if (Configuration.errorOnAutoCreate()) 1119 throw new Error("Attempt to auto-create SearchParameter.date"); 1120 else if (Configuration.doAutoCreate()) 1121 this.date = new DateTimeType(); // bb 1122 return this.date; 1123 } 1124 1125 public boolean hasDateElement() { 1126 return this.date != null && !this.date.isEmpty(); 1127 } 1128 1129 public boolean hasDate() { 1130 return this.date != null && !this.date.isEmpty(); 1131 } 1132 1133 /** 1134 * @param value {@link #date} (The date (and optionally time) when the search parameter was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1135 */ 1136 public SearchParameter setDateElement(DateTimeType value) { 1137 this.date = value; 1138 return this; 1139 } 1140 1141 /** 1142 * @return The date (and optionally time) when the search parameter was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes. 1143 */ 1144 public Date getDate() { 1145 return this.date == null ? null : this.date.getValue(); 1146 } 1147 1148 /** 1149 * @param value The date (and optionally time) when the search parameter was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes. 1150 */ 1151 public SearchParameter setDate(Date value) { 1152 if (value == null) 1153 this.date = null; 1154 else { 1155 if (this.date == null) 1156 this.date = new DateTimeType(); 1157 this.date.setValue(value); 1158 } 1159 return this; 1160 } 1161 1162 /** 1163 * @return {@link #publisher} (The name of the organization or individual tresponsible for the release and ongoing maintenance of the search parameter.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1164 */ 1165 public StringType getPublisherElement() { 1166 if (this.publisher == null) 1167 if (Configuration.errorOnAutoCreate()) 1168 throw new Error("Attempt to auto-create SearchParameter.publisher"); 1169 else if (Configuration.doAutoCreate()) 1170 this.publisher = new StringType(); // bb 1171 return this.publisher; 1172 } 1173 1174 public boolean hasPublisherElement() { 1175 return this.publisher != null && !this.publisher.isEmpty(); 1176 } 1177 1178 public boolean hasPublisher() { 1179 return this.publisher != null && !this.publisher.isEmpty(); 1180 } 1181 1182 /** 1183 * @param value {@link #publisher} (The name of the organization or individual tresponsible for the release and ongoing maintenance of the search parameter.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1184 */ 1185 public SearchParameter setPublisherElement(StringType value) { 1186 this.publisher = value; 1187 return this; 1188 } 1189 1190 /** 1191 * @return The name of the organization or individual tresponsible for the release and ongoing maintenance of the search parameter. 1192 */ 1193 public String getPublisher() { 1194 return this.publisher == null ? null : this.publisher.getValue(); 1195 } 1196 1197 /** 1198 * @param value The name of the organization or individual tresponsible for the release and ongoing maintenance of the search parameter. 1199 */ 1200 public SearchParameter setPublisher(String value) { 1201 if (Utilities.noString(value)) 1202 this.publisher = null; 1203 else { 1204 if (this.publisher == null) 1205 this.publisher = new StringType(); 1206 this.publisher.setValue(value); 1207 } 1208 return this; 1209 } 1210 1211 /** 1212 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 1213 */ 1214 public List<ContactDetail> getContact() { 1215 if (this.contact == null) 1216 this.contact = new ArrayList<ContactDetail>(); 1217 return this.contact; 1218 } 1219 1220 /** 1221 * @return Returns a reference to <code>this</code> for easy method chaining 1222 */ 1223 public SearchParameter setContact(List<ContactDetail> theContact) { 1224 this.contact = theContact; 1225 return this; 1226 } 1227 1228 public boolean hasContact() { 1229 if (this.contact == null) 1230 return false; 1231 for (ContactDetail item : this.contact) 1232 if (!item.isEmpty()) 1233 return true; 1234 return false; 1235 } 1236 1237 public ContactDetail addContact() { //3 1238 ContactDetail t = new ContactDetail(); 1239 if (this.contact == null) 1240 this.contact = new ArrayList<ContactDetail>(); 1241 this.contact.add(t); 1242 return t; 1243 } 1244 1245 public SearchParameter addContact(ContactDetail t) { //3 1246 if (t == null) 1247 return this; 1248 if (this.contact == null) 1249 this.contact = new ArrayList<ContactDetail>(); 1250 this.contact.add(t); 1251 return this; 1252 } 1253 1254 /** 1255 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 1256 */ 1257 public ContactDetail getContactFirstRep() { 1258 if (getContact().isEmpty()) { 1259 addContact(); 1260 } 1261 return getContact().get(0); 1262 } 1263 1264 /** 1265 * @return {@link #description} (And how it used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1266 */ 1267 public MarkdownType getDescriptionElement() { 1268 if (this.description == null) 1269 if (Configuration.errorOnAutoCreate()) 1270 throw new Error("Attempt to auto-create SearchParameter.description"); 1271 else if (Configuration.doAutoCreate()) 1272 this.description = new MarkdownType(); // bb 1273 return this.description; 1274 } 1275 1276 public boolean hasDescriptionElement() { 1277 return this.description != null && !this.description.isEmpty(); 1278 } 1279 1280 public boolean hasDescription() { 1281 return this.description != null && !this.description.isEmpty(); 1282 } 1283 1284 /** 1285 * @param value {@link #description} (And how it used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1286 */ 1287 public SearchParameter setDescriptionElement(MarkdownType value) { 1288 this.description = value; 1289 return this; 1290 } 1291 1292 /** 1293 * @return And how it used. 1294 */ 1295 public String getDescription() { 1296 return this.description == null ? null : this.description.getValue(); 1297 } 1298 1299 /** 1300 * @param value And how it used. 1301 */ 1302 public SearchParameter setDescription(String value) { 1303 if (this.description == null) 1304 this.description = new MarkdownType(); 1305 this.description.setValue(value); 1306 return this; 1307 } 1308 1309 /** 1310 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate search parameter instances.) 1311 */ 1312 public List<UsageContext> getUseContext() { 1313 if (this.useContext == null) 1314 this.useContext = new ArrayList<UsageContext>(); 1315 return this.useContext; 1316 } 1317 1318 /** 1319 * @return Returns a reference to <code>this</code> for easy method chaining 1320 */ 1321 public SearchParameter setUseContext(List<UsageContext> theUseContext) { 1322 this.useContext = theUseContext; 1323 return this; 1324 } 1325 1326 public boolean hasUseContext() { 1327 if (this.useContext == null) 1328 return false; 1329 for (UsageContext item : this.useContext) 1330 if (!item.isEmpty()) 1331 return true; 1332 return false; 1333 } 1334 1335 public UsageContext addUseContext() { //3 1336 UsageContext t = new UsageContext(); 1337 if (this.useContext == null) 1338 this.useContext = new ArrayList<UsageContext>(); 1339 this.useContext.add(t); 1340 return t; 1341 } 1342 1343 public SearchParameter addUseContext(UsageContext t) { //3 1344 if (t == null) 1345 return this; 1346 if (this.useContext == null) 1347 this.useContext = new ArrayList<UsageContext>(); 1348 this.useContext.add(t); 1349 return this; 1350 } 1351 1352 /** 1353 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 1354 */ 1355 public UsageContext getUseContextFirstRep() { 1356 if (getUseContext().isEmpty()) { 1357 addUseContext(); 1358 } 1359 return getUseContext().get(0); 1360 } 1361 1362 /** 1363 * @return {@link #jurisdiction} (A legal or geographic region in which the search parameter is intended to be used.) 1364 */ 1365 public List<CodeableConcept> getJurisdiction() { 1366 if (this.jurisdiction == null) 1367 this.jurisdiction = new ArrayList<CodeableConcept>(); 1368 return this.jurisdiction; 1369 } 1370 1371 /** 1372 * @return Returns a reference to <code>this</code> for easy method chaining 1373 */ 1374 public SearchParameter setJurisdiction(List<CodeableConcept> theJurisdiction) { 1375 this.jurisdiction = theJurisdiction; 1376 return this; 1377 } 1378 1379 public boolean hasJurisdiction() { 1380 if (this.jurisdiction == null) 1381 return false; 1382 for (CodeableConcept item : this.jurisdiction) 1383 if (!item.isEmpty()) 1384 return true; 1385 return false; 1386 } 1387 1388 public CodeableConcept addJurisdiction() { //3 1389 CodeableConcept t = new CodeableConcept(); 1390 if (this.jurisdiction == null) 1391 this.jurisdiction = new ArrayList<CodeableConcept>(); 1392 this.jurisdiction.add(t); 1393 return t; 1394 } 1395 1396 public SearchParameter addJurisdiction(CodeableConcept t) { //3 1397 if (t == null) 1398 return this; 1399 if (this.jurisdiction == null) 1400 this.jurisdiction = new ArrayList<CodeableConcept>(); 1401 this.jurisdiction.add(t); 1402 return this; 1403 } 1404 1405 /** 1406 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 1407 */ 1408 public CodeableConcept getJurisdictionFirstRep() { 1409 if (getJurisdiction().isEmpty()) { 1410 addJurisdiction(); 1411 } 1412 return getJurisdiction().get(0); 1413 } 1414 1415 /** 1416 * @return {@link #purpose} (Explanation of why this search parameter is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1417 */ 1418 public MarkdownType getPurposeElement() { 1419 if (this.purpose == null) 1420 if (Configuration.errorOnAutoCreate()) 1421 throw new Error("Attempt to auto-create SearchParameter.purpose"); 1422 else if (Configuration.doAutoCreate()) 1423 this.purpose = new MarkdownType(); // bb 1424 return this.purpose; 1425 } 1426 1427 public boolean hasPurposeElement() { 1428 return this.purpose != null && !this.purpose.isEmpty(); 1429 } 1430 1431 public boolean hasPurpose() { 1432 return this.purpose != null && !this.purpose.isEmpty(); 1433 } 1434 1435 /** 1436 * @param value {@link #purpose} (Explanation of why this search parameter is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1437 */ 1438 public SearchParameter setPurposeElement(MarkdownType value) { 1439 this.purpose = value; 1440 return this; 1441 } 1442 1443 /** 1444 * @return Explanation of why this search parameter is needed and why it has been designed as it has. 1445 */ 1446 public String getPurpose() { 1447 return this.purpose == null ? null : this.purpose.getValue(); 1448 } 1449 1450 /** 1451 * @param value Explanation of why this search parameter is needed and why it has been designed as it has. 1452 */ 1453 public SearchParameter setPurpose(String value) { 1454 if (Utilities.noString(value)) 1455 this.purpose = null; 1456 else { 1457 if (this.purpose == null) 1458 this.purpose = new MarkdownType(); 1459 this.purpose.setValue(value); 1460 } 1461 return this; 1462 } 1463 1464 /** 1465 * @return {@link #copyright} (A copyright statement relating to the search parameter and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the search parameter.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1466 */ 1467 public MarkdownType getCopyrightElement() { 1468 if (this.copyright == null) 1469 if (Configuration.errorOnAutoCreate()) 1470 throw new Error("Attempt to auto-create SearchParameter.copyright"); 1471 else if (Configuration.doAutoCreate()) 1472 this.copyright = new MarkdownType(); // bb 1473 return this.copyright; 1474 } 1475 1476 public boolean hasCopyrightElement() { 1477 return this.copyright != null && !this.copyright.isEmpty(); 1478 } 1479 1480 public boolean hasCopyright() { 1481 return this.copyright != null && !this.copyright.isEmpty(); 1482 } 1483 1484 /** 1485 * @param value {@link #copyright} (A copyright statement relating to the search parameter and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the search parameter.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1486 */ 1487 public SearchParameter setCopyrightElement(MarkdownType value) { 1488 this.copyright = value; 1489 return this; 1490 } 1491 1492 /** 1493 * @return A copyright statement relating to the search parameter and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the search parameter. 1494 */ 1495 public String getCopyright() { 1496 return this.copyright == null ? null : this.copyright.getValue(); 1497 } 1498 1499 /** 1500 * @param value A copyright statement relating to the search parameter and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the search parameter. 1501 */ 1502 public SearchParameter setCopyright(String value) { 1503 if (Utilities.noString(value)) 1504 this.copyright = null; 1505 else { 1506 if (this.copyright == null) 1507 this.copyright = new MarkdownType(); 1508 this.copyright.setValue(value); 1509 } 1510 return this; 1511 } 1512 1513 /** 1514 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 1515 */ 1516 public StringType getCopyrightLabelElement() { 1517 if (this.copyrightLabel == null) 1518 if (Configuration.errorOnAutoCreate()) 1519 throw new Error("Attempt to auto-create SearchParameter.copyrightLabel"); 1520 else if (Configuration.doAutoCreate()) 1521 this.copyrightLabel = new StringType(); // bb 1522 return this.copyrightLabel; 1523 } 1524 1525 public boolean hasCopyrightLabelElement() { 1526 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 1527 } 1528 1529 public boolean hasCopyrightLabel() { 1530 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 1531 } 1532 1533 /** 1534 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 1535 */ 1536 public SearchParameter setCopyrightLabelElement(StringType value) { 1537 this.copyrightLabel = value; 1538 return this; 1539 } 1540 1541 /** 1542 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1543 */ 1544 public String getCopyrightLabel() { 1545 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 1546 } 1547 1548 /** 1549 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1550 */ 1551 public SearchParameter setCopyrightLabel(String value) { 1552 if (Utilities.noString(value)) 1553 this.copyrightLabel = null; 1554 else { 1555 if (this.copyrightLabel == null) 1556 this.copyrightLabel = new StringType(); 1557 this.copyrightLabel.setValue(value); 1558 } 1559 return this; 1560 } 1561 1562 /** 1563 * @return {@link #code} (The label that is recommended to be used in the URL or the parameter name in a parameters resource for this search parameter. In some cases, servers may need to use a different CapabilityStatement searchParam.name to differentiate between multiple SearchParameters that happen to have the same code.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1564 */ 1565 public CodeType getCodeElement() { 1566 if (this.code == null) 1567 if (Configuration.errorOnAutoCreate()) 1568 throw new Error("Attempt to auto-create SearchParameter.code"); 1569 else if (Configuration.doAutoCreate()) 1570 this.code = new CodeType(); // bb 1571 return this.code; 1572 } 1573 1574 public boolean hasCodeElement() { 1575 return this.code != null && !this.code.isEmpty(); 1576 } 1577 1578 public boolean hasCode() { 1579 return this.code != null && !this.code.isEmpty(); 1580 } 1581 1582 /** 1583 * @param value {@link #code} (The label that is recommended to be used in the URL or the parameter name in a parameters resource for this search parameter. In some cases, servers may need to use a different CapabilityStatement searchParam.name to differentiate between multiple SearchParameters that happen to have the same code.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1584 */ 1585 public SearchParameter setCodeElement(CodeType value) { 1586 this.code = value; 1587 return this; 1588 } 1589 1590 /** 1591 * @return The label that is recommended to be used in the URL or the parameter name in a parameters resource for this search parameter. In some cases, servers may need to use a different CapabilityStatement searchParam.name to differentiate between multiple SearchParameters that happen to have the same code. 1592 */ 1593 public String getCode() { 1594 return this.code == null ? null : this.code.getValue(); 1595 } 1596 1597 /** 1598 * @param value The label that is recommended to be used in the URL or the parameter name in a parameters resource for this search parameter. In some cases, servers may need to use a different CapabilityStatement searchParam.name to differentiate between multiple SearchParameters that happen to have the same code. 1599 */ 1600 public SearchParameter setCode(String value) { 1601 if (this.code == null) 1602 this.code = new CodeType(); 1603 this.code.setValue(value); 1604 return this; 1605 } 1606 1607 /** 1608 * @return {@link #base} (The base resource type(s) that this search parameter can be used against.) 1609 */ 1610 public List<Enumeration<VersionIndependentResourceTypesAll>> getBase() { 1611 if (this.base == null) 1612 this.base = new ArrayList<Enumeration<VersionIndependentResourceTypesAll>>(); 1613 return this.base; 1614 } 1615 1616 /** 1617 * @return Returns a reference to <code>this</code> for easy method chaining 1618 */ 1619 public SearchParameter setBase(List<Enumeration<VersionIndependentResourceTypesAll>> theBase) { 1620 this.base = theBase; 1621 return this; 1622 } 1623 1624 public boolean hasBase() { 1625 if (this.base == null) 1626 return false; 1627 for (Enumeration<VersionIndependentResourceTypesAll> item : this.base) 1628 if (!item.isEmpty()) 1629 return true; 1630 return false; 1631 } 1632 1633 /** 1634 * @return {@link #base} (The base resource type(s) that this search parameter can be used against.) 1635 */ 1636 public Enumeration<VersionIndependentResourceTypesAll> addBaseElement() {//2 1637 Enumeration<VersionIndependentResourceTypesAll> t = new Enumeration<VersionIndependentResourceTypesAll>(new VersionIndependentResourceTypesAllEnumFactory()); 1638 if (this.base == null) 1639 this.base = new ArrayList<Enumeration<VersionIndependentResourceTypesAll>>(); 1640 this.base.add(t); 1641 return t; 1642 } 1643 1644 /** 1645 * @param value {@link #base} (The base resource type(s) that this search parameter can be used against.) 1646 */ 1647 public SearchParameter addBase(VersionIndependentResourceTypesAll value) { //1 1648 Enumeration<VersionIndependentResourceTypesAll> t = new Enumeration<VersionIndependentResourceTypesAll>(new VersionIndependentResourceTypesAllEnumFactory()); 1649 t.setValue(value); 1650 if (this.base == null) 1651 this.base = new ArrayList<Enumeration<VersionIndependentResourceTypesAll>>(); 1652 this.base.add(t); 1653 return this; 1654 } 1655 1656 /** 1657 * @param value {@link #base} (The base resource type(s) that this search parameter can be used against.) 1658 */ 1659 public boolean hasBase(VersionIndependentResourceTypesAll value) { 1660 if (this.base == null) 1661 return false; 1662 for (Enumeration<VersionIndependentResourceTypesAll> v : this.base) 1663 if (v.getValue().equals(value)) // code 1664 return true; 1665 return false; 1666 } 1667 1668 /** 1669 * @return {@link #type} (The type of value that a search parameter may contain, and how the content is interpreted.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1670 */ 1671 public Enumeration<SearchParamType> getTypeElement() { 1672 if (this.type == null) 1673 if (Configuration.errorOnAutoCreate()) 1674 throw new Error("Attempt to auto-create SearchParameter.type"); 1675 else if (Configuration.doAutoCreate()) 1676 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); // bb 1677 return this.type; 1678 } 1679 1680 public boolean hasTypeElement() { 1681 return this.type != null && !this.type.isEmpty(); 1682 } 1683 1684 public boolean hasType() { 1685 return this.type != null && !this.type.isEmpty(); 1686 } 1687 1688 /** 1689 * @param value {@link #type} (The type of value that a search parameter may contain, and how the content is interpreted.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1690 */ 1691 public SearchParameter setTypeElement(Enumeration<SearchParamType> value) { 1692 this.type = value; 1693 return this; 1694 } 1695 1696 /** 1697 * @return The type of value that a search parameter may contain, and how the content is interpreted. 1698 */ 1699 public SearchParamType getType() { 1700 return this.type == null ? null : this.type.getValue(); 1701 } 1702 1703 /** 1704 * @param value The type of value that a search parameter may contain, and how the content is interpreted. 1705 */ 1706 public SearchParameter setType(SearchParamType value) { 1707 if (this.type == null) 1708 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); 1709 this.type.setValue(value); 1710 return this; 1711 } 1712 1713 /** 1714 * @return {@link #expression} (A FHIRPath expression that returns a set of elements for the search parameter.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 1715 */ 1716 public StringType getExpressionElement() { 1717 if (this.expression == null) 1718 if (Configuration.errorOnAutoCreate()) 1719 throw new Error("Attempt to auto-create SearchParameter.expression"); 1720 else if (Configuration.doAutoCreate()) 1721 this.expression = new StringType(); // bb 1722 return this.expression; 1723 } 1724 1725 public boolean hasExpressionElement() { 1726 return this.expression != null && !this.expression.isEmpty(); 1727 } 1728 1729 public boolean hasExpression() { 1730 return this.expression != null && !this.expression.isEmpty(); 1731 } 1732 1733 /** 1734 * @param value {@link #expression} (A FHIRPath expression that returns a set of elements for the search parameter.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 1735 */ 1736 public SearchParameter setExpressionElement(StringType value) { 1737 this.expression = value; 1738 return this; 1739 } 1740 1741 /** 1742 * @return A FHIRPath expression that returns a set of elements for the search parameter. 1743 */ 1744 public String getExpression() { 1745 return this.expression == null ? null : this.expression.getValue(); 1746 } 1747 1748 /** 1749 * @param value A FHIRPath expression that returns a set of elements for the search parameter. 1750 */ 1751 public SearchParameter setExpression(String value) { 1752 if (Utilities.noString(value)) 1753 this.expression = null; 1754 else { 1755 if (this.expression == null) 1756 this.expression = new StringType(); 1757 this.expression.setValue(value); 1758 } 1759 return this; 1760 } 1761 1762 /** 1763 * @return {@link #processingMode} (How the search parameter relates to the set of elements returned by evaluating the expression query.). This is the underlying object with id, value and extensions. The accessor "getProcessingMode" gives direct access to the value 1764 */ 1765 public Enumeration<SearchProcessingModeType> getProcessingModeElement() { 1766 if (this.processingMode == null) 1767 if (Configuration.errorOnAutoCreate()) 1768 throw new Error("Attempt to auto-create SearchParameter.processingMode"); 1769 else if (Configuration.doAutoCreate()) 1770 this.processingMode = new Enumeration<SearchProcessingModeType>(new SearchProcessingModeTypeEnumFactory()); // bb 1771 return this.processingMode; 1772 } 1773 1774 public boolean hasProcessingModeElement() { 1775 return this.processingMode != null && !this.processingMode.isEmpty(); 1776 } 1777 1778 public boolean hasProcessingMode() { 1779 return this.processingMode != null && !this.processingMode.isEmpty(); 1780 } 1781 1782 /** 1783 * @param value {@link #processingMode} (How the search parameter relates to the set of elements returned by evaluating the expression query.). This is the underlying object with id, value and extensions. The accessor "getProcessingMode" gives direct access to the value 1784 */ 1785 public SearchParameter setProcessingModeElement(Enumeration<SearchProcessingModeType> value) { 1786 this.processingMode = value; 1787 return this; 1788 } 1789 1790 /** 1791 * @return How the search parameter relates to the set of elements returned by evaluating the expression query. 1792 */ 1793 public SearchProcessingModeType getProcessingMode() { 1794 return this.processingMode == null ? null : this.processingMode.getValue(); 1795 } 1796 1797 /** 1798 * @param value How the search parameter relates to the set of elements returned by evaluating the expression query. 1799 */ 1800 public SearchParameter setProcessingMode(SearchProcessingModeType value) { 1801 if (value == null) 1802 this.processingMode = null; 1803 else { 1804 if (this.processingMode == null) 1805 this.processingMode = new Enumeration<SearchProcessingModeType>(new SearchProcessingModeTypeEnumFactory()); 1806 this.processingMode.setValue(value); 1807 } 1808 return this; 1809 } 1810 1811 /** 1812 * @return {@link #constraint} (FHIRPath expression that defines/sets a complex constraint for when this SearchParameter is applicable.). This is the underlying object with id, value and extensions. The accessor "getConstraint" gives direct access to the value 1813 */ 1814 public StringType getConstraintElement() { 1815 if (this.constraint == null) 1816 if (Configuration.errorOnAutoCreate()) 1817 throw new Error("Attempt to auto-create SearchParameter.constraint"); 1818 else if (Configuration.doAutoCreate()) 1819 this.constraint = new StringType(); // bb 1820 return this.constraint; 1821 } 1822 1823 public boolean hasConstraintElement() { 1824 return this.constraint != null && !this.constraint.isEmpty(); 1825 } 1826 1827 public boolean hasConstraint() { 1828 return this.constraint != null && !this.constraint.isEmpty(); 1829 } 1830 1831 /** 1832 * @param value {@link #constraint} (FHIRPath expression that defines/sets a complex constraint for when this SearchParameter is applicable.). This is the underlying object with id, value and extensions. The accessor "getConstraint" gives direct access to the value 1833 */ 1834 public SearchParameter setConstraintElement(StringType value) { 1835 this.constraint = value; 1836 return this; 1837 } 1838 1839 /** 1840 * @return FHIRPath expression that defines/sets a complex constraint for when this SearchParameter is applicable. 1841 */ 1842 public String getConstraint() { 1843 return this.constraint == null ? null : this.constraint.getValue(); 1844 } 1845 1846 /** 1847 * @param value FHIRPath expression that defines/sets a complex constraint for when this SearchParameter is applicable. 1848 */ 1849 public SearchParameter setConstraint(String value) { 1850 if (Utilities.noString(value)) 1851 this.constraint = null; 1852 else { 1853 if (this.constraint == null) 1854 this.constraint = new StringType(); 1855 this.constraint.setValue(value); 1856 } 1857 return this; 1858 } 1859 1860 /** 1861 * @return {@link #target} (Types of resource (if a resource is referenced).) 1862 */ 1863 public List<Enumeration<VersionIndependentResourceTypesAll>> getTarget() { 1864 if (this.target == null) 1865 this.target = new ArrayList<Enumeration<VersionIndependentResourceTypesAll>>(); 1866 return this.target; 1867 } 1868 1869 /** 1870 * @return Returns a reference to <code>this</code> for easy method chaining 1871 */ 1872 public SearchParameter setTarget(List<Enumeration<VersionIndependentResourceTypesAll>> theTarget) { 1873 this.target = theTarget; 1874 return this; 1875 } 1876 1877 public boolean hasTarget() { 1878 if (this.target == null) 1879 return false; 1880 for (Enumeration<VersionIndependentResourceTypesAll> item : this.target) 1881 if (!item.isEmpty()) 1882 return true; 1883 return false; 1884 } 1885 1886 /** 1887 * @return {@link #target} (Types of resource (if a resource is referenced).) 1888 */ 1889 public Enumeration<VersionIndependentResourceTypesAll> addTargetElement() {//2 1890 Enumeration<VersionIndependentResourceTypesAll> t = new Enumeration<VersionIndependentResourceTypesAll>(new VersionIndependentResourceTypesAllEnumFactory()); 1891 if (this.target == null) 1892 this.target = new ArrayList<Enumeration<VersionIndependentResourceTypesAll>>(); 1893 this.target.add(t); 1894 return t; 1895 } 1896 1897 /** 1898 * @param value {@link #target} (Types of resource (if a resource is referenced).) 1899 */ 1900 public SearchParameter addTarget(VersionIndependentResourceTypesAll value) { //1 1901 Enumeration<VersionIndependentResourceTypesAll> t = new Enumeration<VersionIndependentResourceTypesAll>(new VersionIndependentResourceTypesAllEnumFactory()); 1902 t.setValue(value); 1903 if (this.target == null) 1904 this.target = new ArrayList<Enumeration<VersionIndependentResourceTypesAll>>(); 1905 this.target.add(t); 1906 return this; 1907 } 1908 1909 /** 1910 * @param value {@link #target} (Types of resource (if a resource is referenced).) 1911 */ 1912 public boolean hasTarget(VersionIndependentResourceTypesAll value) { 1913 if (this.target == null) 1914 return false; 1915 for (Enumeration<VersionIndependentResourceTypesAll> v : this.target) 1916 if (v.getValue().equals(value)) // code 1917 return true; 1918 return false; 1919 } 1920 1921 /** 1922 * @return {@link #multipleOr} (Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match.). This is the underlying object with id, value and extensions. The accessor "getMultipleOr" gives direct access to the value 1923 */ 1924 public BooleanType getMultipleOrElement() { 1925 if (this.multipleOr == null) 1926 if (Configuration.errorOnAutoCreate()) 1927 throw new Error("Attempt to auto-create SearchParameter.multipleOr"); 1928 else if (Configuration.doAutoCreate()) 1929 this.multipleOr = new BooleanType(); // bb 1930 return this.multipleOr; 1931 } 1932 1933 public boolean hasMultipleOrElement() { 1934 return this.multipleOr != null && !this.multipleOr.isEmpty(); 1935 } 1936 1937 public boolean hasMultipleOr() { 1938 return this.multipleOr != null && !this.multipleOr.isEmpty(); 1939 } 1940 1941 /** 1942 * @param value {@link #multipleOr} (Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match.). This is the underlying object with id, value and extensions. The accessor "getMultipleOr" gives direct access to the value 1943 */ 1944 public SearchParameter setMultipleOrElement(BooleanType value) { 1945 this.multipleOr = value; 1946 return this; 1947 } 1948 1949 /** 1950 * @return Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match. 1951 */ 1952 public boolean getMultipleOr() { 1953 return this.multipleOr == null || this.multipleOr.isEmpty() ? false : this.multipleOr.getValue(); 1954 } 1955 1956 /** 1957 * @param value Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match. 1958 */ 1959 public SearchParameter setMultipleOr(boolean value) { 1960 if (this.multipleOr == null) 1961 this.multipleOr = new BooleanType(); 1962 this.multipleOr.setValue(value); 1963 return this; 1964 } 1965 1966 /** 1967 * @return {@link #multipleAnd} (Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match.). This is the underlying object with id, value and extensions. The accessor "getMultipleAnd" gives direct access to the value 1968 */ 1969 public BooleanType getMultipleAndElement() { 1970 if (this.multipleAnd == null) 1971 if (Configuration.errorOnAutoCreate()) 1972 throw new Error("Attempt to auto-create SearchParameter.multipleAnd"); 1973 else if (Configuration.doAutoCreate()) 1974 this.multipleAnd = new BooleanType(); // bb 1975 return this.multipleAnd; 1976 } 1977 1978 public boolean hasMultipleAndElement() { 1979 return this.multipleAnd != null && !this.multipleAnd.isEmpty(); 1980 } 1981 1982 public boolean hasMultipleAnd() { 1983 return this.multipleAnd != null && !this.multipleAnd.isEmpty(); 1984 } 1985 1986 /** 1987 * @param value {@link #multipleAnd} (Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match.). This is the underlying object with id, value and extensions. The accessor "getMultipleAnd" gives direct access to the value 1988 */ 1989 public SearchParameter setMultipleAndElement(BooleanType value) { 1990 this.multipleAnd = value; 1991 return this; 1992 } 1993 1994 /** 1995 * @return Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match. 1996 */ 1997 public boolean getMultipleAnd() { 1998 return this.multipleAnd == null || this.multipleAnd.isEmpty() ? false : this.multipleAnd.getValue(); 1999 } 2000 2001 /** 2002 * @param value Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match. 2003 */ 2004 public SearchParameter setMultipleAnd(boolean value) { 2005 if (this.multipleAnd == null) 2006 this.multipleAnd = new BooleanType(); 2007 this.multipleAnd.setValue(value); 2008 return this; 2009 } 2010 2011 /** 2012 * @return {@link #comparator} (Comparators supported for the search parameter.) 2013 */ 2014 public List<Enumeration<SearchComparator>> getComparator() { 2015 if (this.comparator == null) 2016 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2017 return this.comparator; 2018 } 2019 2020 /** 2021 * @return Returns a reference to <code>this</code> for easy method chaining 2022 */ 2023 public SearchParameter setComparator(List<Enumeration<SearchComparator>> theComparator) { 2024 this.comparator = theComparator; 2025 return this; 2026 } 2027 2028 public boolean hasComparator() { 2029 if (this.comparator == null) 2030 return false; 2031 for (Enumeration<SearchComparator> item : this.comparator) 2032 if (!item.isEmpty()) 2033 return true; 2034 return false; 2035 } 2036 2037 /** 2038 * @return {@link #comparator} (Comparators supported for the search parameter.) 2039 */ 2040 public Enumeration<SearchComparator> addComparatorElement() {//2 2041 Enumeration<SearchComparator> t = new Enumeration<SearchComparator>(new SearchComparatorEnumFactory()); 2042 if (this.comparator == null) 2043 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2044 this.comparator.add(t); 2045 return t; 2046 } 2047 2048 /** 2049 * @param value {@link #comparator} (Comparators supported for the search parameter.) 2050 */ 2051 public SearchParameter addComparator(SearchComparator value) { //1 2052 Enumeration<SearchComparator> t = new Enumeration<SearchComparator>(new SearchComparatorEnumFactory()); 2053 t.setValue(value); 2054 if (this.comparator == null) 2055 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2056 this.comparator.add(t); 2057 return this; 2058 } 2059 2060 /** 2061 * @param value {@link #comparator} (Comparators supported for the search parameter.) 2062 */ 2063 public boolean hasComparator(SearchComparator value) { 2064 if (this.comparator == null) 2065 return false; 2066 for (Enumeration<SearchComparator> v : this.comparator) 2067 if (v.getValue().equals(value)) // code 2068 return true; 2069 return false; 2070 } 2071 2072 /** 2073 * @return {@link #modifier} (A modifier supported for the search parameter.) 2074 */ 2075 public List<Enumeration<SearchModifierCode>> getModifier() { 2076 if (this.modifier == null) 2077 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2078 return this.modifier; 2079 } 2080 2081 /** 2082 * @return Returns a reference to <code>this</code> for easy method chaining 2083 */ 2084 public SearchParameter setModifier(List<Enumeration<SearchModifierCode>> theModifier) { 2085 this.modifier = theModifier; 2086 return this; 2087 } 2088 2089 public boolean hasModifier() { 2090 if (this.modifier == null) 2091 return false; 2092 for (Enumeration<SearchModifierCode> item : this.modifier) 2093 if (!item.isEmpty()) 2094 return true; 2095 return false; 2096 } 2097 2098 /** 2099 * @return {@link #modifier} (A modifier supported for the search parameter.) 2100 */ 2101 public Enumeration<SearchModifierCode> addModifierElement() {//2 2102 Enumeration<SearchModifierCode> t = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 2103 if (this.modifier == null) 2104 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2105 this.modifier.add(t); 2106 return t; 2107 } 2108 2109 /** 2110 * @param value {@link #modifier} (A modifier supported for the search parameter.) 2111 */ 2112 public SearchParameter addModifier(SearchModifierCode value) { //1 2113 Enumeration<SearchModifierCode> t = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 2114 t.setValue(value); 2115 if (this.modifier == null) 2116 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2117 this.modifier.add(t); 2118 return this; 2119 } 2120 2121 /** 2122 * @param value {@link #modifier} (A modifier supported for the search parameter.) 2123 */ 2124 public boolean hasModifier(SearchModifierCode value) { 2125 if (this.modifier == null) 2126 return false; 2127 for (Enumeration<SearchModifierCode> v : this.modifier) 2128 if (v.getValue().equals(value)) // code 2129 return true; 2130 return false; 2131 } 2132 2133 /** 2134 * @return {@link #chain} (Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.) 2135 */ 2136 public List<StringType> getChain() { 2137 if (this.chain == null) 2138 this.chain = new ArrayList<StringType>(); 2139 return this.chain; 2140 } 2141 2142 /** 2143 * @return Returns a reference to <code>this</code> for easy method chaining 2144 */ 2145 public SearchParameter setChain(List<StringType> theChain) { 2146 this.chain = theChain; 2147 return this; 2148 } 2149 2150 public boolean hasChain() { 2151 if (this.chain == null) 2152 return false; 2153 for (StringType item : this.chain) 2154 if (!item.isEmpty()) 2155 return true; 2156 return false; 2157 } 2158 2159 /** 2160 * @return {@link #chain} (Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.) 2161 */ 2162 public StringType addChainElement() {//2 2163 StringType t = new StringType(); 2164 if (this.chain == null) 2165 this.chain = new ArrayList<StringType>(); 2166 this.chain.add(t); 2167 return t; 2168 } 2169 2170 /** 2171 * @param value {@link #chain} (Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.) 2172 */ 2173 public SearchParameter addChain(String value) { //1 2174 StringType t = new StringType(); 2175 t.setValue(value); 2176 if (this.chain == null) 2177 this.chain = new ArrayList<StringType>(); 2178 this.chain.add(t); 2179 return this; 2180 } 2181 2182 /** 2183 * @param value {@link #chain} (Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.) 2184 */ 2185 public boolean hasChain(String value) { 2186 if (this.chain == null) 2187 return false; 2188 for (StringType v : this.chain) 2189 if (v.getValue().equals(value)) // string 2190 return true; 2191 return false; 2192 } 2193 2194 /** 2195 * @return {@link #component} (Used to define the parts of a composite search parameter.) 2196 */ 2197 public List<SearchParameterComponentComponent> getComponent() { 2198 if (this.component == null) 2199 this.component = new ArrayList<SearchParameterComponentComponent>(); 2200 return this.component; 2201 } 2202 2203 /** 2204 * @return Returns a reference to <code>this</code> for easy method chaining 2205 */ 2206 public SearchParameter setComponent(List<SearchParameterComponentComponent> theComponent) { 2207 this.component = theComponent; 2208 return this; 2209 } 2210 2211 public boolean hasComponent() { 2212 if (this.component == null) 2213 return false; 2214 for (SearchParameterComponentComponent item : this.component) 2215 if (!item.isEmpty()) 2216 return true; 2217 return false; 2218 } 2219 2220 public SearchParameterComponentComponent addComponent() { //3 2221 SearchParameterComponentComponent t = new SearchParameterComponentComponent(); 2222 if (this.component == null) 2223 this.component = new ArrayList<SearchParameterComponentComponent>(); 2224 this.component.add(t); 2225 return t; 2226 } 2227 2228 public SearchParameter addComponent(SearchParameterComponentComponent t) { //3 2229 if (t == null) 2230 return this; 2231 if (this.component == null) 2232 this.component = new ArrayList<SearchParameterComponentComponent>(); 2233 this.component.add(t); 2234 return this; 2235 } 2236 2237 /** 2238 * @return The first repetition of repeating field {@link #component}, creating it if it does not already exist {3} 2239 */ 2240 public SearchParameterComponentComponent getComponentFirstRep() { 2241 if (getComponent().isEmpty()) { 2242 addComponent(); 2243 } 2244 return getComponent().get(0); 2245 } 2246 2247 protected void listChildren(List<Property> children) { 2248 super.listChildren(children); 2249 children.add(new Property("url", "uri", "An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this search parameter is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the search parameter is stored on different servers.", 0, 1, url)); 2250 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this search parameter when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2251 children.add(new Property("version", "string", "The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 2252 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 2253 children.add(new Property("name", "string", "A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 2254 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the search parameter.", 0, 1, title)); 2255 children.add(new Property("derivedFrom", "canonical(SearchParameter)", "Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.", 0, 1, derivedFrom)); 2256 children.add(new Property("status", "code", "The status of this search parameter. Enables tracking the life-cycle of the content.", 0, 1, status)); 2257 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 2258 children.add(new Property("date", "dateTime", "The date (and optionally time) when the search parameter was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.", 0, 1, date)); 2259 children.add(new Property("publisher", "string", "The name of the organization or individual tresponsible for the release and ongoing maintenance of the search parameter.", 0, 1, publisher)); 2260 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 2261 children.add(new Property("description", "markdown", "And how it used.", 0, 1, description)); 2262 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate search parameter instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 2263 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the search parameter is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 2264 children.add(new Property("purpose", "markdown", "Explanation of why this search parameter is needed and why it has been designed as it has.", 0, 1, purpose)); 2265 children.add(new Property("copyright", "markdown", "A copyright statement relating to the search parameter and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the search parameter.", 0, 1, copyright)); 2266 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 2267 children.add(new Property("code", "code", "The label that is recommended to be used in the URL or the parameter name in a parameters resource for this search parameter. In some cases, servers may need to use a different CapabilityStatement searchParam.name to differentiate between multiple SearchParameters that happen to have the same code.", 0, 1, code)); 2268 children.add(new Property("base", "code", "The base resource type(s) that this search parameter can be used against.", 0, java.lang.Integer.MAX_VALUE, base)); 2269 children.add(new Property("type", "code", "The type of value that a search parameter may contain, and how the content is interpreted.", 0, 1, type)); 2270 children.add(new Property("expression", "string", "A FHIRPath expression that returns a set of elements for the search parameter.", 0, 1, expression)); 2271 children.add(new Property("processingMode", "code", "How the search parameter relates to the set of elements returned by evaluating the expression query.", 0, 1, processingMode)); 2272 children.add(new Property("constraint", "string", "FHIRPath expression that defines/sets a complex constraint for when this SearchParameter is applicable.", 0, 1, constraint)); 2273 children.add(new Property("target", "code", "Types of resource (if a resource is referenced).", 0, java.lang.Integer.MAX_VALUE, target)); 2274 children.add(new Property("multipleOr", "boolean", "Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match.", 0, 1, multipleOr)); 2275 children.add(new Property("multipleAnd", "boolean", "Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match.", 0, 1, multipleAnd)); 2276 children.add(new Property("comparator", "code", "Comparators supported for the search parameter.", 0, java.lang.Integer.MAX_VALUE, comparator)); 2277 children.add(new Property("modifier", "code", "A modifier supported for the search parameter.", 0, java.lang.Integer.MAX_VALUE, modifier)); 2278 children.add(new Property("chain", "string", "Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.", 0, java.lang.Integer.MAX_VALUE, chain)); 2279 children.add(new Property("component", "", "Used to define the parts of a composite search parameter.", 0, java.lang.Integer.MAX_VALUE, component)); 2280 } 2281 2282 @Override 2283 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2284 switch (_hash) { 2285 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this search parameter is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the search parameter is stored on different servers.", 0, 1, url); 2286 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this search parameter when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 2287 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 2288 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2289 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2290 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2291 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 2292 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 2293 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the search parameter.", 0, 1, title); 2294 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "canonical(SearchParameter)", "Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.", 0, 1, derivedFrom); 2295 case -892481550: /*status*/ return new Property("status", "code", "The status of this search parameter. Enables tracking the life-cycle of the content.", 0, 1, status); 2296 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 2297 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the search parameter was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.", 0, 1, date); 2298 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual tresponsible for the release and ongoing maintenance of the search parameter.", 0, 1, publisher); 2299 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 2300 case -1724546052: /*description*/ return new Property("description", "markdown", "And how it used.", 0, 1, description); 2301 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate search parameter instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 2302 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the search parameter is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 2303 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this search parameter is needed and why it has been designed as it has.", 0, 1, purpose); 2304 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the search parameter and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the search parameter.", 0, 1, copyright); 2305 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 2306 case 3059181: /*code*/ return new Property("code", "code", "The label that is recommended to be used in the URL or the parameter name in a parameters resource for this search parameter. In some cases, servers may need to use a different CapabilityStatement searchParam.name to differentiate between multiple SearchParameters that happen to have the same code.", 0, 1, code); 2307 case 3016401: /*base*/ return new Property("base", "code", "The base resource type(s) that this search parameter can be used against.", 0, java.lang.Integer.MAX_VALUE, base); 2308 case 3575610: /*type*/ return new Property("type", "code", "The type of value that a search parameter may contain, and how the content is interpreted.", 0, 1, type); 2309 case -1795452264: /*expression*/ return new Property("expression", "string", "A FHIRPath expression that returns a set of elements for the search parameter.", 0, 1, expression); 2310 case 195763030: /*processingMode*/ return new Property("processingMode", "code", "How the search parameter relates to the set of elements returned by evaluating the expression query.", 0, 1, processingMode); 2311 case -190376483: /*constraint*/ return new Property("constraint", "string", "FHIRPath expression that defines/sets a complex constraint for when this SearchParameter is applicable.", 0, 1, constraint); 2312 case -880905839: /*target*/ return new Property("target", "code", "Types of resource (if a resource is referenced).", 0, java.lang.Integer.MAX_VALUE, target); 2313 case 1265069075: /*multipleOr*/ return new Property("multipleOr", "boolean", "Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match.", 0, 1, multipleOr); 2314 case 562422183: /*multipleAnd*/ return new Property("multipleAnd", "boolean", "Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match.", 0, 1, multipleAnd); 2315 case -844673834: /*comparator*/ return new Property("comparator", "code", "Comparators supported for the search parameter.", 0, java.lang.Integer.MAX_VALUE, comparator); 2316 case -615513385: /*modifier*/ return new Property("modifier", "code", "A modifier supported for the search parameter.", 0, java.lang.Integer.MAX_VALUE, modifier); 2317 case 94623425: /*chain*/ return new Property("chain", "string", "Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.", 0, java.lang.Integer.MAX_VALUE, chain); 2318 case -1399907075: /*component*/ return new Property("component", "", "Used to define the parts of a composite search parameter.", 0, java.lang.Integer.MAX_VALUE, component); 2319 default: return super.getNamedProperty(_hash, _name, _checkValid); 2320 } 2321 2322 } 2323 2324 @Override 2325 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2326 switch (hash) { 2327 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2328 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2329 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2330 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 2331 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2332 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2333 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : new Base[] {this.derivedFrom}; // CanonicalType 2334 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2335 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 2336 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2337 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 2338 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2339 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2340 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2341 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2342 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 2343 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 2344 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 2345 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 2346 case 3016401: /*base*/ return this.base == null ? new Base[0] : this.base.toArray(new Base[this.base.size()]); // Enumeration<VersionIndependentResourceTypesAll> 2347 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<SearchParamType> 2348 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 2349 case 195763030: /*processingMode*/ return this.processingMode == null ? new Base[0] : new Base[] {this.processingMode}; // Enumeration<SearchProcessingModeType> 2350 case -190376483: /*constraint*/ return this.constraint == null ? new Base[0] : new Base[] {this.constraint}; // StringType 2351 case -880905839: /*target*/ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // Enumeration<VersionIndependentResourceTypesAll> 2352 case 1265069075: /*multipleOr*/ return this.multipleOr == null ? new Base[0] : new Base[] {this.multipleOr}; // BooleanType 2353 case 562422183: /*multipleAnd*/ return this.multipleAnd == null ? new Base[0] : new Base[] {this.multipleAnd}; // BooleanType 2354 case -844673834: /*comparator*/ return this.comparator == null ? new Base[0] : this.comparator.toArray(new Base[this.comparator.size()]); // Enumeration<SearchComparator> 2355 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // Enumeration<SearchModifierCode> 2356 case 94623425: /*chain*/ return this.chain == null ? new Base[0] : this.chain.toArray(new Base[this.chain.size()]); // StringType 2357 case -1399907075: /*component*/ return this.component == null ? new Base[0] : this.component.toArray(new Base[this.component.size()]); // SearchParameterComponentComponent 2358 default: return super.getProperty(hash, name, checkValid); 2359 } 2360 2361 } 2362 2363 @Override 2364 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2365 switch (hash) { 2366 case 116079: // url 2367 this.url = TypeConvertor.castToUri(value); // UriType 2368 return value; 2369 case -1618432855: // identifier 2370 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2371 return value; 2372 case 351608024: // version 2373 this.version = TypeConvertor.castToString(value); // StringType 2374 return value; 2375 case 1508158071: // versionAlgorithm 2376 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2377 return value; 2378 case 3373707: // name 2379 this.name = TypeConvertor.castToString(value); // StringType 2380 return value; 2381 case 110371416: // title 2382 this.title = TypeConvertor.castToString(value); // StringType 2383 return value; 2384 case 1077922663: // derivedFrom 2385 this.derivedFrom = TypeConvertor.castToCanonical(value); // CanonicalType 2386 return value; 2387 case -892481550: // status 2388 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2389 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2390 return value; 2391 case -404562712: // experimental 2392 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2393 return value; 2394 case 3076014: // date 2395 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2396 return value; 2397 case 1447404028: // publisher 2398 this.publisher = TypeConvertor.castToString(value); // StringType 2399 return value; 2400 case 951526432: // contact 2401 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2402 return value; 2403 case -1724546052: // description 2404 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2405 return value; 2406 case -669707736: // useContext 2407 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 2408 return value; 2409 case -507075711: // jurisdiction 2410 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2411 return value; 2412 case -220463842: // purpose 2413 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2414 return value; 2415 case 1522889671: // copyright 2416 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2417 return value; 2418 case 765157229: // copyrightLabel 2419 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2420 return value; 2421 case 3059181: // code 2422 this.code = TypeConvertor.castToCode(value); // CodeType 2423 return value; 2424 case 3016401: // base 2425 value = new VersionIndependentResourceTypesAllEnumFactory().fromType(TypeConvertor.castToCode(value)); 2426 this.getBase().add((Enumeration) value); // Enumeration<VersionIndependentResourceTypesAll> 2427 return value; 2428 case 3575610: // type 2429 value = new SearchParamTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2430 this.type = (Enumeration) value; // Enumeration<SearchParamType> 2431 return value; 2432 case -1795452264: // expression 2433 this.expression = TypeConvertor.castToString(value); // StringType 2434 return value; 2435 case 195763030: // processingMode 2436 value = new SearchProcessingModeTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2437 this.processingMode = (Enumeration) value; // Enumeration<SearchProcessingModeType> 2438 return value; 2439 case -190376483: // constraint 2440 this.constraint = TypeConvertor.castToString(value); // StringType 2441 return value; 2442 case -880905839: // target 2443 value = new VersionIndependentResourceTypesAllEnumFactory().fromType(TypeConvertor.castToCode(value)); 2444 this.getTarget().add((Enumeration) value); // Enumeration<VersionIndependentResourceTypesAll> 2445 return value; 2446 case 1265069075: // multipleOr 2447 this.multipleOr = TypeConvertor.castToBoolean(value); // BooleanType 2448 return value; 2449 case 562422183: // multipleAnd 2450 this.multipleAnd = TypeConvertor.castToBoolean(value); // BooleanType 2451 return value; 2452 case -844673834: // comparator 2453 value = new SearchComparatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 2454 this.getComparator().add((Enumeration) value); // Enumeration<SearchComparator> 2455 return value; 2456 case -615513385: // modifier 2457 value = new SearchModifierCodeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2458 this.getModifier().add((Enumeration) value); // Enumeration<SearchModifierCode> 2459 return value; 2460 case 94623425: // chain 2461 this.getChain().add(TypeConvertor.castToString(value)); // StringType 2462 return value; 2463 case -1399907075: // component 2464 this.getComponent().add((SearchParameterComponentComponent) value); // SearchParameterComponentComponent 2465 return value; 2466 default: return super.setProperty(hash, name, value); 2467 } 2468 2469 } 2470 2471 @Override 2472 public Base setProperty(String name, Base value) throws FHIRException { 2473 if (name.equals("url")) { 2474 this.url = TypeConvertor.castToUri(value); // UriType 2475 } else if (name.equals("identifier")) { 2476 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2477 } else if (name.equals("version")) { 2478 this.version = TypeConvertor.castToString(value); // StringType 2479 } else if (name.equals("versionAlgorithm[x]")) { 2480 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2481 } else if (name.equals("name")) { 2482 this.name = TypeConvertor.castToString(value); // StringType 2483 } else if (name.equals("title")) { 2484 this.title = TypeConvertor.castToString(value); // StringType 2485 } else if (name.equals("derivedFrom")) { 2486 this.derivedFrom = TypeConvertor.castToCanonical(value); // CanonicalType 2487 } else if (name.equals("status")) { 2488 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2489 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2490 } else if (name.equals("experimental")) { 2491 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2492 } else if (name.equals("date")) { 2493 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2494 } else if (name.equals("publisher")) { 2495 this.publisher = TypeConvertor.castToString(value); // StringType 2496 } else if (name.equals("contact")) { 2497 this.getContact().add(TypeConvertor.castToContactDetail(value)); 2498 } else if (name.equals("description")) { 2499 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2500 } else if (name.equals("useContext")) { 2501 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 2502 } else if (name.equals("jurisdiction")) { 2503 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 2504 } else if (name.equals("purpose")) { 2505 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2506 } else if (name.equals("copyright")) { 2507 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2508 } else if (name.equals("copyrightLabel")) { 2509 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2510 } else if (name.equals("code")) { 2511 this.code = TypeConvertor.castToCode(value); // CodeType 2512 } else if (name.equals("base")) { 2513 value = new VersionIndependentResourceTypesAllEnumFactory().fromType(TypeConvertor.castToCode(value)); 2514 this.getBase().add((Enumeration) value); 2515 } else if (name.equals("type")) { 2516 value = new SearchParamTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2517 this.type = (Enumeration) value; // Enumeration<SearchParamType> 2518 } else if (name.equals("expression")) { 2519 this.expression = TypeConvertor.castToString(value); // StringType 2520 } else if (name.equals("processingMode")) { 2521 value = new SearchProcessingModeTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2522 this.processingMode = (Enumeration) value; // Enumeration<SearchProcessingModeType> 2523 } else if (name.equals("constraint")) { 2524 this.constraint = TypeConvertor.castToString(value); // StringType 2525 } else if (name.equals("target")) { 2526 value = new VersionIndependentResourceTypesAllEnumFactory().fromType(TypeConvertor.castToCode(value)); 2527 this.getTarget().add((Enumeration) value); 2528 } else if (name.equals("multipleOr")) { 2529 this.multipleOr = TypeConvertor.castToBoolean(value); // BooleanType 2530 } else if (name.equals("multipleAnd")) { 2531 this.multipleAnd = TypeConvertor.castToBoolean(value); // BooleanType 2532 } else if (name.equals("comparator")) { 2533 value = new SearchComparatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 2534 this.getComparator().add((Enumeration) value); 2535 } else if (name.equals("modifier")) { 2536 value = new SearchModifierCodeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2537 this.getModifier().add((Enumeration) value); 2538 } else if (name.equals("chain")) { 2539 this.getChain().add(TypeConvertor.castToString(value)); 2540 } else if (name.equals("component")) { 2541 this.getComponent().add((SearchParameterComponentComponent) value); 2542 } else 2543 return super.setProperty(name, value); 2544 return value; 2545 } 2546 2547 @Override 2548 public void removeChild(String name, Base value) throws FHIRException { 2549 if (name.equals("url")) { 2550 this.url = null; 2551 } else if (name.equals("identifier")) { 2552 this.getIdentifier().remove(value); 2553 } else if (name.equals("version")) { 2554 this.version = null; 2555 } else if (name.equals("versionAlgorithm[x]")) { 2556 this.versionAlgorithm = null; 2557 } else if (name.equals("name")) { 2558 this.name = null; 2559 } else if (name.equals("title")) { 2560 this.title = null; 2561 } else if (name.equals("derivedFrom")) { 2562 this.derivedFrom = null; 2563 } else if (name.equals("status")) { 2564 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2565 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2566 } else if (name.equals("experimental")) { 2567 this.experimental = null; 2568 } else if (name.equals("date")) { 2569 this.date = null; 2570 } else if (name.equals("publisher")) { 2571 this.publisher = null; 2572 } else if (name.equals("contact")) { 2573 this.getContact().remove(value); 2574 } else if (name.equals("description")) { 2575 this.description = null; 2576 } else if (name.equals("useContext")) { 2577 this.getUseContext().remove(value); 2578 } else if (name.equals("jurisdiction")) { 2579 this.getJurisdiction().remove(value); 2580 } else if (name.equals("purpose")) { 2581 this.purpose = null; 2582 } else if (name.equals("copyright")) { 2583 this.copyright = null; 2584 } else if (name.equals("copyrightLabel")) { 2585 this.copyrightLabel = null; 2586 } else if (name.equals("code")) { 2587 this.code = null; 2588 } else if (name.equals("base")) { 2589 value = new VersionIndependentResourceTypesAllEnumFactory().fromType(TypeConvertor.castToCode(value)); 2590 this.getBase().remove((Enumeration) value); 2591 } else if (name.equals("type")) { 2592 value = new SearchParamTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2593 this.type = (Enumeration) value; // Enumeration<SearchParamType> 2594 } else if (name.equals("expression")) { 2595 this.expression = null; 2596 } else if (name.equals("processingMode")) { 2597 value = new SearchProcessingModeTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2598 this.processingMode = (Enumeration) value; // Enumeration<SearchProcessingModeType> 2599 } else if (name.equals("constraint")) { 2600 this.constraint = null; 2601 } else if (name.equals("target")) { 2602 value = new VersionIndependentResourceTypesAllEnumFactory().fromType(TypeConvertor.castToCode(value)); 2603 this.getTarget().remove((Enumeration) value); 2604 } else if (name.equals("multipleOr")) { 2605 this.multipleOr = null; 2606 } else if (name.equals("multipleAnd")) { 2607 this.multipleAnd = null; 2608 } else if (name.equals("comparator")) { 2609 value = new SearchComparatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 2610 this.getComparator().remove((Enumeration) value); 2611 } else if (name.equals("modifier")) { 2612 value = new SearchModifierCodeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2613 this.getModifier().remove((Enumeration) value); 2614 } else if (name.equals("chain")) { 2615 this.getChain().remove(value); 2616 } else if (name.equals("component")) { 2617 this.getComponent().remove((SearchParameterComponentComponent) value); 2618 } else 2619 super.removeChild(name, value); 2620 2621 } 2622 2623 @Override 2624 public Base makeProperty(int hash, String name) throws FHIRException { 2625 switch (hash) { 2626 case 116079: return getUrlElement(); 2627 case -1618432855: return addIdentifier(); 2628 case 351608024: return getVersionElement(); 2629 case -115699031: return getVersionAlgorithm(); 2630 case 1508158071: return getVersionAlgorithm(); 2631 case 3373707: return getNameElement(); 2632 case 110371416: return getTitleElement(); 2633 case 1077922663: return getDerivedFromElement(); 2634 case -892481550: return getStatusElement(); 2635 case -404562712: return getExperimentalElement(); 2636 case 3076014: return getDateElement(); 2637 case 1447404028: return getPublisherElement(); 2638 case 951526432: return addContact(); 2639 case -1724546052: return getDescriptionElement(); 2640 case -669707736: return addUseContext(); 2641 case -507075711: return addJurisdiction(); 2642 case -220463842: return getPurposeElement(); 2643 case 1522889671: return getCopyrightElement(); 2644 case 765157229: return getCopyrightLabelElement(); 2645 case 3059181: return getCodeElement(); 2646 case 3016401: return addBaseElement(); 2647 case 3575610: return getTypeElement(); 2648 case -1795452264: return getExpressionElement(); 2649 case 195763030: return getProcessingModeElement(); 2650 case -190376483: return getConstraintElement(); 2651 case -880905839: return addTargetElement(); 2652 case 1265069075: return getMultipleOrElement(); 2653 case 562422183: return getMultipleAndElement(); 2654 case -844673834: return addComparatorElement(); 2655 case -615513385: return addModifierElement(); 2656 case 94623425: return addChainElement(); 2657 case -1399907075: return addComponent(); 2658 default: return super.makeProperty(hash, name); 2659 } 2660 2661 } 2662 2663 @Override 2664 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2665 switch (hash) { 2666 case 116079: /*url*/ return new String[] {"uri"}; 2667 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2668 case 351608024: /*version*/ return new String[] {"string"}; 2669 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 2670 case 3373707: /*name*/ return new String[] {"string"}; 2671 case 110371416: /*title*/ return new String[] {"string"}; 2672 case 1077922663: /*derivedFrom*/ return new String[] {"canonical"}; 2673 case -892481550: /*status*/ return new String[] {"code"}; 2674 case -404562712: /*experimental*/ return new String[] {"boolean"}; 2675 case 3076014: /*date*/ return new String[] {"dateTime"}; 2676 case 1447404028: /*publisher*/ return new String[] {"string"}; 2677 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 2678 case -1724546052: /*description*/ return new String[] {"markdown"}; 2679 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 2680 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 2681 case -220463842: /*purpose*/ return new String[] {"markdown"}; 2682 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 2683 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 2684 case 3059181: /*code*/ return new String[] {"code"}; 2685 case 3016401: /*base*/ return new String[] {"code"}; 2686 case 3575610: /*type*/ return new String[] {"code"}; 2687 case -1795452264: /*expression*/ return new String[] {"string"}; 2688 case 195763030: /*processingMode*/ return new String[] {"code"}; 2689 case -190376483: /*constraint*/ return new String[] {"string"}; 2690 case -880905839: /*target*/ return new String[] {"code"}; 2691 case 1265069075: /*multipleOr*/ return new String[] {"boolean"}; 2692 case 562422183: /*multipleAnd*/ return new String[] {"boolean"}; 2693 case -844673834: /*comparator*/ return new String[] {"code"}; 2694 case -615513385: /*modifier*/ return new String[] {"code"}; 2695 case 94623425: /*chain*/ return new String[] {"string"}; 2696 case -1399907075: /*component*/ return new String[] {}; 2697 default: return super.getTypesForProperty(hash, name); 2698 } 2699 2700 } 2701 2702 @Override 2703 public Base addChild(String name) throws FHIRException { 2704 if (name.equals("url")) { 2705 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.url"); 2706 } 2707 else if (name.equals("identifier")) { 2708 return addIdentifier(); 2709 } 2710 else if (name.equals("version")) { 2711 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.version"); 2712 } 2713 else if (name.equals("versionAlgorithmString")) { 2714 this.versionAlgorithm = new StringType(); 2715 return this.versionAlgorithm; 2716 } 2717 else if (name.equals("versionAlgorithmCoding")) { 2718 this.versionAlgorithm = new Coding(); 2719 return this.versionAlgorithm; 2720 } 2721 else if (name.equals("name")) { 2722 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.name"); 2723 } 2724 else if (name.equals("title")) { 2725 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.title"); 2726 } 2727 else if (name.equals("derivedFrom")) { 2728 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.derivedFrom"); 2729 } 2730 else if (name.equals("status")) { 2731 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.status"); 2732 } 2733 else if (name.equals("experimental")) { 2734 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.experimental"); 2735 } 2736 else if (name.equals("date")) { 2737 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.date"); 2738 } 2739 else if (name.equals("publisher")) { 2740 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.publisher"); 2741 } 2742 else if (name.equals("contact")) { 2743 return addContact(); 2744 } 2745 else if (name.equals("description")) { 2746 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.description"); 2747 } 2748 else if (name.equals("useContext")) { 2749 return addUseContext(); 2750 } 2751 else if (name.equals("jurisdiction")) { 2752 return addJurisdiction(); 2753 } 2754 else if (name.equals("purpose")) { 2755 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.purpose"); 2756 } 2757 else if (name.equals("copyright")) { 2758 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.copyright"); 2759 } 2760 else if (name.equals("copyrightLabel")) { 2761 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.copyrightLabel"); 2762 } 2763 else if (name.equals("code")) { 2764 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.code"); 2765 } 2766 else if (name.equals("base")) { 2767 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.base"); 2768 } 2769 else if (name.equals("type")) { 2770 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.type"); 2771 } 2772 else if (name.equals("expression")) { 2773 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.expression"); 2774 } 2775 else if (name.equals("processingMode")) { 2776 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.processingMode"); 2777 } 2778 else if (name.equals("constraint")) { 2779 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.constraint"); 2780 } 2781 else if (name.equals("target")) { 2782 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.target"); 2783 } 2784 else if (name.equals("multipleOr")) { 2785 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.multipleOr"); 2786 } 2787 else if (name.equals("multipleAnd")) { 2788 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.multipleAnd"); 2789 } 2790 else if (name.equals("comparator")) { 2791 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.comparator"); 2792 } 2793 else if (name.equals("modifier")) { 2794 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.modifier"); 2795 } 2796 else if (name.equals("chain")) { 2797 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.chain"); 2798 } 2799 else if (name.equals("component")) { 2800 return addComponent(); 2801 } 2802 else 2803 return super.addChild(name); 2804 } 2805 2806 public String fhirType() { 2807 return "SearchParameter"; 2808 2809 } 2810 2811 public SearchParameter copy() { 2812 SearchParameter dst = new SearchParameter(); 2813 copyValues(dst); 2814 return dst; 2815 } 2816 2817 public void copyValues(SearchParameter dst) { 2818 super.copyValues(dst); 2819 dst.url = url == null ? null : url.copy(); 2820 if (identifier != null) { 2821 dst.identifier = new ArrayList<Identifier>(); 2822 for (Identifier i : identifier) 2823 dst.identifier.add(i.copy()); 2824 }; 2825 dst.version = version == null ? null : version.copy(); 2826 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 2827 dst.name = name == null ? null : name.copy(); 2828 dst.title = title == null ? null : title.copy(); 2829 dst.derivedFrom = derivedFrom == null ? null : derivedFrom.copy(); 2830 dst.status = status == null ? null : status.copy(); 2831 dst.experimental = experimental == null ? null : experimental.copy(); 2832 dst.date = date == null ? null : date.copy(); 2833 dst.publisher = publisher == null ? null : publisher.copy(); 2834 if (contact != null) { 2835 dst.contact = new ArrayList<ContactDetail>(); 2836 for (ContactDetail i : contact) 2837 dst.contact.add(i.copy()); 2838 }; 2839 dst.description = description == null ? null : description.copy(); 2840 if (useContext != null) { 2841 dst.useContext = new ArrayList<UsageContext>(); 2842 for (UsageContext i : useContext) 2843 dst.useContext.add(i.copy()); 2844 }; 2845 if (jurisdiction != null) { 2846 dst.jurisdiction = new ArrayList<CodeableConcept>(); 2847 for (CodeableConcept i : jurisdiction) 2848 dst.jurisdiction.add(i.copy()); 2849 }; 2850 dst.purpose = purpose == null ? null : purpose.copy(); 2851 dst.copyright = copyright == null ? null : copyright.copy(); 2852 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 2853 dst.code = code == null ? null : code.copy(); 2854 if (base != null) { 2855 dst.base = new ArrayList<Enumeration<VersionIndependentResourceTypesAll>>(); 2856 for (Enumeration<VersionIndependentResourceTypesAll> i : base) 2857 dst.base.add(i.copy()); 2858 }; 2859 dst.type = type == null ? null : type.copy(); 2860 dst.expression = expression == null ? null : expression.copy(); 2861 dst.processingMode = processingMode == null ? null : processingMode.copy(); 2862 dst.constraint = constraint == null ? null : constraint.copy(); 2863 if (target != null) { 2864 dst.target = new ArrayList<Enumeration<VersionIndependentResourceTypesAll>>(); 2865 for (Enumeration<VersionIndependentResourceTypesAll> i : target) 2866 dst.target.add(i.copy()); 2867 }; 2868 dst.multipleOr = multipleOr == null ? null : multipleOr.copy(); 2869 dst.multipleAnd = multipleAnd == null ? null : multipleAnd.copy(); 2870 if (comparator != null) { 2871 dst.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2872 for (Enumeration<SearchComparator> i : comparator) 2873 dst.comparator.add(i.copy()); 2874 }; 2875 if (modifier != null) { 2876 dst.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2877 for (Enumeration<SearchModifierCode> i : modifier) 2878 dst.modifier.add(i.copy()); 2879 }; 2880 if (chain != null) { 2881 dst.chain = new ArrayList<StringType>(); 2882 for (StringType i : chain) 2883 dst.chain.add(i.copy()); 2884 }; 2885 if (component != null) { 2886 dst.component = new ArrayList<SearchParameterComponentComponent>(); 2887 for (SearchParameterComponentComponent i : component) 2888 dst.component.add(i.copy()); 2889 }; 2890 } 2891 2892 protected SearchParameter typedCopy() { 2893 return copy(); 2894 } 2895 2896 @Override 2897 public boolean equalsDeep(Base other_) { 2898 if (!super.equalsDeep(other_)) 2899 return false; 2900 if (!(other_ instanceof SearchParameter)) 2901 return false; 2902 SearchParameter o = (SearchParameter) other_; 2903 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 2904 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 2905 && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 2906 && compareDeep(date, o.date, true) && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 2907 && compareDeep(description, o.description, true) && compareDeep(useContext, o.useContext, true) 2908 && compareDeep(jurisdiction, o.jurisdiction, true) && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 2909 && compareDeep(copyrightLabel, o.copyrightLabel, true) && compareDeep(code, o.code, true) && compareDeep(base, o.base, true) 2910 && compareDeep(type, o.type, true) && compareDeep(expression, o.expression, true) && compareDeep(processingMode, o.processingMode, true) 2911 && compareDeep(constraint, o.constraint, true) && compareDeep(target, o.target, true) && compareDeep(multipleOr, o.multipleOr, true) 2912 && compareDeep(multipleAnd, o.multipleAnd, true) && compareDeep(comparator, o.comparator, true) 2913 && compareDeep(modifier, o.modifier, true) && compareDeep(chain, o.chain, true) && compareDeep(component, o.component, true) 2914 ; 2915 } 2916 2917 @Override 2918 public boolean equalsShallow(Base other_) { 2919 if (!super.equalsShallow(other_)) 2920 return false; 2921 if (!(other_ instanceof SearchParameter)) 2922 return false; 2923 SearchParameter o = (SearchParameter) other_; 2924 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 2925 && compareValues(title, o.title, true) && compareValues(derivedFrom, o.derivedFrom, true) && compareValues(status, o.status, true) 2926 && compareValues(experimental, o.experimental, true) && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) 2927 && compareValues(description, o.description, true) && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) 2928 && compareValues(copyrightLabel, o.copyrightLabel, true) && compareValues(code, o.code, true) && compareValues(base, o.base, true) 2929 && compareValues(type, o.type, true) && compareValues(expression, o.expression, true) && compareValues(processingMode, o.processingMode, true) 2930 && compareValues(constraint, o.constraint, true) && compareValues(target, o.target, true) && compareValues(multipleOr, o.multipleOr, true) 2931 && compareValues(multipleAnd, o.multipleAnd, true) && compareValues(comparator, o.comparator, true) 2932 && compareValues(modifier, o.modifier, true) && compareValues(chain, o.chain, true); 2933 } 2934 2935 public boolean isEmpty() { 2936 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 2937 , versionAlgorithm, name, title, derivedFrom, status, experimental, date, publisher 2938 , contact, description, useContext, jurisdiction, purpose, copyright, copyrightLabel 2939 , code, base, type, expression, processingMode, constraint, target, multipleOr 2940 , multipleAnd, comparator, modifier, chain, component); 2941 } 2942 2943 @Override 2944 public ResourceType getResourceType() { 2945 return ResourceType.SearchParameter; 2946 } 2947 2948 /** 2949 * Search parameter: <b>context-quantity</b> 2950 * <p> 2951 * Description: <b>Multiple Resources: 2952 2953* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 2954* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 2955* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 2956* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 2957* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 2958* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 2959* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 2960* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 2961* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 2962* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 2963* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 2964* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 2965* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 2966* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 2967* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 2968* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 2969* [Library](library.html): A quantity- or range-valued use context assigned to the library 2970* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 2971* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 2972* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 2973* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 2974* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 2975* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 2976* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 2977* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 2978* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 2979* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 2980* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 2981* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 2982* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 2983</b><br> 2984 * Type: <b>quantity</b><br> 2985 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 2986 * </p> 2987 */ 2988 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 2989 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 2990 /** 2991 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 2992 * <p> 2993 * Description: <b>Multiple Resources: 2994 2995* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 2996* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 2997* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 2998* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 2999* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 3000* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 3001* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 3002* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 3003* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 3004* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 3005* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 3006* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 3007* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 3008* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 3009* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 3010* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 3011* [Library](library.html): A quantity- or range-valued use context assigned to the library 3012* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 3013* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 3014* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 3015* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 3016* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 3017* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 3018* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 3019* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 3020* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 3021* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 3022* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 3023* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 3024* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 3025</b><br> 3026 * Type: <b>quantity</b><br> 3027 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 3028 * </p> 3029 */ 3030 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 3031 3032 /** 3033 * Search parameter: <b>context-type-quantity</b> 3034 * <p> 3035 * Description: <b>Multiple Resources: 3036 3037* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 3038* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 3039* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 3040* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 3041* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 3042* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 3043* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 3044* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 3045* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 3046* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 3047* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 3048* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 3049* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 3050* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 3051* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 3052* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 3053* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 3054* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 3055* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 3056* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 3057* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 3058* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 3059* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 3060* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 3061* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 3062* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 3063* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 3064* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 3065* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3066* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3067</b><br> 3068 * Type: <b>composite</b><br> 3069 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3070 * </p> 3071 */ 3072 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 3073 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 3074 /** 3075 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 3076 * <p> 3077 * Description: <b>Multiple Resources: 3078 3079* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 3080* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 3081* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 3082* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 3083* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 3084* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 3085* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 3086* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 3087* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 3088* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 3089* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 3090* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 3091* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 3092* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 3093* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 3094* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 3095* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 3096* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 3097* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 3098* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 3099* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 3100* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 3101* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 3102* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 3103* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 3104* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 3105* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 3106* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 3107* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3108* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3109</b><br> 3110 * Type: <b>composite</b><br> 3111 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3112 * </p> 3113 */ 3114 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 3115 3116 /** 3117 * Search parameter: <b>context-type-value</b> 3118 * <p> 3119 * Description: <b>Multiple Resources: 3120 3121* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3122* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3123* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3124* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3125* [Citation](citation.html): A use context type and value assigned to the citation 3126* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3127* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3128* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3129* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3130* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3131* [Evidence](evidence.html): A use context type and value assigned to the evidence 3132* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3133* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3134* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3135* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 3136* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 3137* [Library](library.html): A use context type and value assigned to the library 3138* [Measure](measure.html): A use context type and value assigned to the measure 3139* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 3140* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 3141* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 3142* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 3143* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 3144* [Requirements](requirements.html): A use context type and value assigned to the requirements 3145* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3146* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3147* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3148* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3149* [TestScript](testscript.html): A use context type and value assigned to the test script 3150* [ValueSet](valueset.html): A use context type and value assigned to the value set 3151</b><br> 3152 * Type: <b>composite</b><br> 3153 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3154 * </p> 3155 */ 3156 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 3157 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3158 /** 3159 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3160 * <p> 3161 * Description: <b>Multiple Resources: 3162 3163* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3164* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3165* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3166* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3167* [Citation](citation.html): A use context type and value assigned to the citation 3168* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3169* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3170* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3171* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3172* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3173* [Evidence](evidence.html): A use context type and value assigned to the evidence 3174* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3175* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3176* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3177* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 3178* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 3179* [Library](library.html): A use context type and value assigned to the library 3180* [Measure](measure.html): A use context type and value assigned to the measure 3181* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 3182* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 3183* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 3184* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 3185* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 3186* [Requirements](requirements.html): A use context type and value assigned to the requirements 3187* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3188* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3189* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3190* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3191* [TestScript](testscript.html): A use context type and value assigned to the test script 3192* [ValueSet](valueset.html): A use context type and value assigned to the value set 3193</b><br> 3194 * Type: <b>composite</b><br> 3195 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3196 * </p> 3197 */ 3198 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 3199 3200 /** 3201 * Search parameter: <b>context-type</b> 3202 * <p> 3203 * Description: <b>Multiple Resources: 3204 3205* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3206* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3207* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3208* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3209* [Citation](citation.html): A type of use context assigned to the citation 3210* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3211* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3212* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3213* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3214* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3215* [Evidence](evidence.html): A type of use context assigned to the evidence 3216* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3217* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3218* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3219* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3220* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3221* [Library](library.html): A type of use context assigned to the library 3222* [Measure](measure.html): A type of use context assigned to the measure 3223* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3224* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3225* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3226* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3227* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3228* [Requirements](requirements.html): A type of use context assigned to the requirements 3229* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3230* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3231* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3232* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3233* [TestScript](testscript.html): A type of use context assigned to the test script 3234* [ValueSet](valueset.html): A type of use context assigned to the value set 3235</b><br> 3236 * Type: <b>token</b><br> 3237 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3238 * </p> 3239 */ 3240 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 3241 public static final String SP_CONTEXT_TYPE = "context-type"; 3242 /** 3243 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3244 * <p> 3245 * Description: <b>Multiple Resources: 3246 3247* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3248* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3249* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3250* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3251* [Citation](citation.html): A type of use context assigned to the citation 3252* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3253* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3254* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3255* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3256* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3257* [Evidence](evidence.html): A type of use context assigned to the evidence 3258* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3259* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3260* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3261* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3262* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3263* [Library](library.html): A type of use context assigned to the library 3264* [Measure](measure.html): A type of use context assigned to the measure 3265* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3266* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3267* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3268* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3269* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3270* [Requirements](requirements.html): A type of use context assigned to the requirements 3271* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3272* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3273* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3274* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3275* [TestScript](testscript.html): A type of use context assigned to the test script 3276* [ValueSet](valueset.html): A type of use context assigned to the value set 3277</b><br> 3278 * Type: <b>token</b><br> 3279 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3280 * </p> 3281 */ 3282 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 3283 3284 /** 3285 * Search parameter: <b>context</b> 3286 * <p> 3287 * Description: <b>Multiple Resources: 3288 3289* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3290* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3291* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3292* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3293* [Citation](citation.html): A use context assigned to the citation 3294* [CodeSystem](codesystem.html): A use context assigned to the code system 3295* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3296* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3297* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3298* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3299* [Evidence](evidence.html): A use context assigned to the evidence 3300* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3301* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3302* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3303* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3304* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3305* [Library](library.html): A use context assigned to the library 3306* [Measure](measure.html): A use context assigned to the measure 3307* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3308* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3309* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3310* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3311* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3312* [Requirements](requirements.html): A use context assigned to the requirements 3313* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3314* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3315* [StructureMap](structuremap.html): A use context assigned to the structure map 3316* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3317* [TestScript](testscript.html): A use context assigned to the test script 3318* [ValueSet](valueset.html): A use context assigned to the value set 3319</b><br> 3320 * Type: <b>token</b><br> 3321 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3322 * </p> 3323 */ 3324 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 3325 public static final String SP_CONTEXT = "context"; 3326 /** 3327 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3328 * <p> 3329 * Description: <b>Multiple Resources: 3330 3331* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3332* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3333* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3334* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3335* [Citation](citation.html): A use context assigned to the citation 3336* [CodeSystem](codesystem.html): A use context assigned to the code system 3337* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3338* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3339* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3340* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3341* [Evidence](evidence.html): A use context assigned to the evidence 3342* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3343* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3344* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3345* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3346* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3347* [Library](library.html): A use context assigned to the library 3348* [Measure](measure.html): A use context assigned to the measure 3349* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3350* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3351* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3352* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3353* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3354* [Requirements](requirements.html): A use context assigned to the requirements 3355* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3356* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3357* [StructureMap](structuremap.html): A use context assigned to the structure map 3358* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3359* [TestScript](testscript.html): A use context assigned to the test script 3360* [ValueSet](valueset.html): A use context assigned to the value set 3361</b><br> 3362 * Type: <b>token</b><br> 3363 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3364 * </p> 3365 */ 3366 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 3367 3368 /** 3369 * Search parameter: <b>date</b> 3370 * <p> 3371 * Description: <b>Multiple Resources: 3372 3373* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3374* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3375* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3376* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3377* [Citation](citation.html): The citation publication date 3378* [CodeSystem](codesystem.html): The code system publication date 3379* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3380* [ConceptMap](conceptmap.html): The concept map publication date 3381* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3382* [EventDefinition](eventdefinition.html): The event definition publication date 3383* [Evidence](evidence.html): The evidence publication date 3384* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3385* [ExampleScenario](examplescenario.html): The example scenario publication date 3386* [GraphDefinition](graphdefinition.html): The graph definition publication date 3387* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3388* [Library](library.html): The library publication date 3389* [Measure](measure.html): The measure publication date 3390* [MessageDefinition](messagedefinition.html): The message definition publication date 3391* [NamingSystem](namingsystem.html): The naming system publication date 3392* [OperationDefinition](operationdefinition.html): The operation definition publication date 3393* [PlanDefinition](plandefinition.html): The plan definition publication date 3394* [Questionnaire](questionnaire.html): The questionnaire publication date 3395* [Requirements](requirements.html): The requirements publication date 3396* [SearchParameter](searchparameter.html): The search parameter publication date 3397* [StructureDefinition](structuredefinition.html): The structure definition publication date 3398* [StructureMap](structuremap.html): The structure map publication date 3399* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3400* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3401* [TestScript](testscript.html): The test script publication date 3402* [ValueSet](valueset.html): The value set publication date 3403</b><br> 3404 * Type: <b>date</b><br> 3405 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3406 * </p> 3407 */ 3408 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 3409 public static final String SP_DATE = "date"; 3410 /** 3411 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3412 * <p> 3413 * Description: <b>Multiple Resources: 3414 3415* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3416* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3417* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3418* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3419* [Citation](citation.html): The citation publication date 3420* [CodeSystem](codesystem.html): The code system publication date 3421* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3422* [ConceptMap](conceptmap.html): The concept map publication date 3423* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3424* [EventDefinition](eventdefinition.html): The event definition publication date 3425* [Evidence](evidence.html): The evidence publication date 3426* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3427* [ExampleScenario](examplescenario.html): The example scenario publication date 3428* [GraphDefinition](graphdefinition.html): The graph definition publication date 3429* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3430* [Library](library.html): The library publication date 3431* [Measure](measure.html): The measure publication date 3432* [MessageDefinition](messagedefinition.html): The message definition publication date 3433* [NamingSystem](namingsystem.html): The naming system publication date 3434* [OperationDefinition](operationdefinition.html): The operation definition publication date 3435* [PlanDefinition](plandefinition.html): The plan definition publication date 3436* [Questionnaire](questionnaire.html): The questionnaire publication date 3437* [Requirements](requirements.html): The requirements publication date 3438* [SearchParameter](searchparameter.html): The search parameter publication date 3439* [StructureDefinition](structuredefinition.html): The structure definition publication date 3440* [StructureMap](structuremap.html): The structure map publication date 3441* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3442* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3443* [TestScript](testscript.html): The test script publication date 3444* [ValueSet](valueset.html): The value set publication date 3445</b><br> 3446 * Type: <b>date</b><br> 3447 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3448 * </p> 3449 */ 3450 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3451 3452 /** 3453 * Search parameter: <b>description</b> 3454 * <p> 3455 * Description: <b>Multiple Resources: 3456 3457* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3458* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3459* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3460* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3461* [Citation](citation.html): The description of the citation 3462* [CodeSystem](codesystem.html): The description of the code system 3463* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3464* [ConceptMap](conceptmap.html): The description of the concept map 3465* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3466* [EventDefinition](eventdefinition.html): The description of the event definition 3467* [Evidence](evidence.html): The description of the evidence 3468* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3469* [GraphDefinition](graphdefinition.html): The description of the graph definition 3470* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3471* [Library](library.html): The description of the library 3472* [Measure](measure.html): The description of the measure 3473* [MessageDefinition](messagedefinition.html): The description of the message definition 3474* [NamingSystem](namingsystem.html): The description of the naming system 3475* [OperationDefinition](operationdefinition.html): The description of the operation definition 3476* [PlanDefinition](plandefinition.html): The description of the plan definition 3477* [Questionnaire](questionnaire.html): The description of the questionnaire 3478* [Requirements](requirements.html): The description of the requirements 3479* [SearchParameter](searchparameter.html): The description of the search parameter 3480* [StructureDefinition](structuredefinition.html): The description of the structure definition 3481* [StructureMap](structuremap.html): The description of the structure map 3482* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3483* [TestScript](testscript.html): The description of the test script 3484* [ValueSet](valueset.html): The description of the value set 3485</b><br> 3486 * Type: <b>string</b><br> 3487 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3488 * </p> 3489 */ 3490 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 3491 public static final String SP_DESCRIPTION = "description"; 3492 /** 3493 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3494 * <p> 3495 * Description: <b>Multiple Resources: 3496 3497* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3498* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3499* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3500* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3501* [Citation](citation.html): The description of the citation 3502* [CodeSystem](codesystem.html): The description of the code system 3503* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3504* [ConceptMap](conceptmap.html): The description of the concept map 3505* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3506* [EventDefinition](eventdefinition.html): The description of the event definition 3507* [Evidence](evidence.html): The description of the evidence 3508* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3509* [GraphDefinition](graphdefinition.html): The description of the graph definition 3510* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3511* [Library](library.html): The description of the library 3512* [Measure](measure.html): The description of the measure 3513* [MessageDefinition](messagedefinition.html): The description of the message definition 3514* [NamingSystem](namingsystem.html): The description of the naming system 3515* [OperationDefinition](operationdefinition.html): The description of the operation definition 3516* [PlanDefinition](plandefinition.html): The description of the plan definition 3517* [Questionnaire](questionnaire.html): The description of the questionnaire 3518* [Requirements](requirements.html): The description of the requirements 3519* [SearchParameter](searchparameter.html): The description of the search parameter 3520* [StructureDefinition](structuredefinition.html): The description of the structure definition 3521* [StructureMap](structuremap.html): The description of the structure map 3522* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3523* [TestScript](testscript.html): The description of the test script 3524* [ValueSet](valueset.html): The description of the value set 3525</b><br> 3526 * Type: <b>string</b><br> 3527 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3528 * </p> 3529 */ 3530 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 3531 3532 /** 3533 * Search parameter: <b>identifier</b> 3534 * <p> 3535 * Description: <b>Multiple Resources: 3536 3537* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3538* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3539* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3540* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3541* [Citation](citation.html): External identifier for the citation 3542* [CodeSystem](codesystem.html): External identifier for the code system 3543* [ConceptMap](conceptmap.html): External identifier for the concept map 3544* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3545* [EventDefinition](eventdefinition.html): External identifier for the event definition 3546* [Evidence](evidence.html): External identifier for the evidence 3547* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3548* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3549* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3550* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3551* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3552* [Library](library.html): External identifier for the library 3553* [Measure](measure.html): External identifier for the measure 3554* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3555* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3556* [NamingSystem](namingsystem.html): External identifier for the naming system 3557* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3558* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3559* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3560* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3561* [Requirements](requirements.html): External identifier for the requirements 3562* [SearchParameter](searchparameter.html): External identifier for the search parameter 3563* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3564* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3565* [StructureMap](structuremap.html): External identifier for the structure map 3566* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3567* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3568* [TestPlan](testplan.html): An identifier for the test plan 3569* [TestScript](testscript.html): External identifier for the test script 3570* [ValueSet](valueset.html): External identifier for the value set 3571</b><br> 3572 * Type: <b>token</b><br> 3573 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3574 * </p> 3575 */ 3576 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 3577 public static final String SP_IDENTIFIER = "identifier"; 3578 /** 3579 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3580 * <p> 3581 * Description: <b>Multiple Resources: 3582 3583* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3584* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3585* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3586* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3587* [Citation](citation.html): External identifier for the citation 3588* [CodeSystem](codesystem.html): External identifier for the code system 3589* [ConceptMap](conceptmap.html): External identifier for the concept map 3590* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3591* [EventDefinition](eventdefinition.html): External identifier for the event definition 3592* [Evidence](evidence.html): External identifier for the evidence 3593* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3594* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3595* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3596* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3597* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3598* [Library](library.html): External identifier for the library 3599* [Measure](measure.html): External identifier for the measure 3600* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3601* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3602* [NamingSystem](namingsystem.html): External identifier for the naming system 3603* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3604* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3605* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3606* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3607* [Requirements](requirements.html): External identifier for the requirements 3608* [SearchParameter](searchparameter.html): External identifier for the search parameter 3609* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3610* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3611* [StructureMap](structuremap.html): External identifier for the structure map 3612* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3613* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3614* [TestPlan](testplan.html): An identifier for the test plan 3615* [TestScript](testscript.html): External identifier for the test script 3616* [ValueSet](valueset.html): External identifier for the value set 3617</b><br> 3618 * Type: <b>token</b><br> 3619 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3620 * </p> 3621 */ 3622 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3623 3624 /** 3625 * Search parameter: <b>jurisdiction</b> 3626 * <p> 3627 * Description: <b>Multiple Resources: 3628 3629* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3630* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3631* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3632* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3633* [Citation](citation.html): Intended jurisdiction for the citation 3634* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3635* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3636* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3637* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3638* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3639* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3640* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3641* [Library](library.html): Intended jurisdiction for the library 3642* [Measure](measure.html): Intended jurisdiction for the measure 3643* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3644* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3645* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3646* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3647* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3648* [Requirements](requirements.html): Intended jurisdiction for the requirements 3649* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3650* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3651* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3652* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3653* [TestScript](testscript.html): Intended jurisdiction for the test script 3654* [ValueSet](valueset.html): Intended jurisdiction for the value set 3655</b><br> 3656 * Type: <b>token</b><br> 3657 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3658 * </p> 3659 */ 3660 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 3661 public static final String SP_JURISDICTION = "jurisdiction"; 3662 /** 3663 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3664 * <p> 3665 * Description: <b>Multiple Resources: 3666 3667* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3668* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3669* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3670* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3671* [Citation](citation.html): Intended jurisdiction for the citation 3672* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3673* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3674* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3675* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3676* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3677* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3678* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3679* [Library](library.html): Intended jurisdiction for the library 3680* [Measure](measure.html): Intended jurisdiction for the measure 3681* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3682* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3683* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3684* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3685* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3686* [Requirements](requirements.html): Intended jurisdiction for the requirements 3687* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3688* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3689* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3690* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3691* [TestScript](testscript.html): Intended jurisdiction for the test script 3692* [ValueSet](valueset.html): Intended jurisdiction for the value set 3693</b><br> 3694 * Type: <b>token</b><br> 3695 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3696 * </p> 3697 */ 3698 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 3699 3700 /** 3701 * Search parameter: <b>name</b> 3702 * <p> 3703 * Description: <b>Multiple Resources: 3704 3705* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 3706* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 3707* [Citation](citation.html): Computationally friendly name of the citation 3708* [CodeSystem](codesystem.html): Computationally friendly name of the code system 3709* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 3710* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 3711* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 3712* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 3713* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 3714* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 3715* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 3716* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 3717* [Library](library.html): Computationally friendly name of the library 3718* [Measure](measure.html): Computationally friendly name of the measure 3719* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 3720* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 3721* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 3722* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 3723* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 3724* [Requirements](requirements.html): Computationally friendly name of the requirements 3725* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 3726* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 3727* [StructureMap](structuremap.html): Computationally friendly name of the structure map 3728* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 3729* [TestScript](testscript.html): Computationally friendly name of the test script 3730* [ValueSet](valueset.html): Computationally friendly name of the value set 3731</b><br> 3732 * Type: <b>string</b><br> 3733 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 3734 * </p> 3735 */ 3736 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 3737 public static final String SP_NAME = "name"; 3738 /** 3739 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3740 * <p> 3741 * Description: <b>Multiple Resources: 3742 3743* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 3744* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 3745* [Citation](citation.html): Computationally friendly name of the citation 3746* [CodeSystem](codesystem.html): Computationally friendly name of the code system 3747* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 3748* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 3749* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 3750* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 3751* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 3752* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 3753* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 3754* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 3755* [Library](library.html): Computationally friendly name of the library 3756* [Measure](measure.html): Computationally friendly name of the measure 3757* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 3758* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 3759* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 3760* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 3761* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 3762* [Requirements](requirements.html): Computationally friendly name of the requirements 3763* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 3764* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 3765* [StructureMap](structuremap.html): Computationally friendly name of the structure map 3766* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 3767* [TestScript](testscript.html): Computationally friendly name of the test script 3768* [ValueSet](valueset.html): Computationally friendly name of the value set 3769</b><br> 3770 * Type: <b>string</b><br> 3771 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 3772 * </p> 3773 */ 3774 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 3775 3776 /** 3777 * Search parameter: <b>publisher</b> 3778 * <p> 3779 * Description: <b>Multiple Resources: 3780 3781* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 3782* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 3783* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 3784* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 3785* [Citation](citation.html): Name of the publisher of the citation 3786* [CodeSystem](codesystem.html): Name of the publisher of the code system 3787* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 3788* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 3789* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 3790* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 3791* [Evidence](evidence.html): Name of the publisher of the evidence 3792* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 3793* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 3794* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 3795* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 3796* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 3797* [Library](library.html): Name of the publisher of the library 3798* [Measure](measure.html): Name of the publisher of the measure 3799* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 3800* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 3801* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 3802* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 3803* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 3804* [Requirements](requirements.html): Name of the publisher of the requirements 3805* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 3806* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 3807* [StructureMap](structuremap.html): Name of the publisher of the structure map 3808* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 3809* [TestScript](testscript.html): Name of the publisher of the test script 3810* [ValueSet](valueset.html): Name of the publisher of the value set 3811</b><br> 3812 * Type: <b>string</b><br> 3813 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 3814 * </p> 3815 */ 3816 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 3817 public static final String SP_PUBLISHER = "publisher"; 3818 /** 3819 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3820 * <p> 3821 * Description: <b>Multiple Resources: 3822 3823* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 3824* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 3825* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 3826* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 3827* [Citation](citation.html): Name of the publisher of the citation 3828* [CodeSystem](codesystem.html): Name of the publisher of the code system 3829* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 3830* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 3831* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 3832* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 3833* [Evidence](evidence.html): Name of the publisher of the evidence 3834* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 3835* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 3836* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 3837* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 3838* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 3839* [Library](library.html): Name of the publisher of the library 3840* [Measure](measure.html): Name of the publisher of the measure 3841* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 3842* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 3843* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 3844* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 3845* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 3846* [Requirements](requirements.html): Name of the publisher of the requirements 3847* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 3848* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 3849* [StructureMap](structuremap.html): Name of the publisher of the structure map 3850* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 3851* [TestScript](testscript.html): Name of the publisher of the test script 3852* [ValueSet](valueset.html): Name of the publisher of the value set 3853</b><br> 3854 * Type: <b>string</b><br> 3855 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 3856 * </p> 3857 */ 3858 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 3859 3860 /** 3861 * Search parameter: <b>status</b> 3862 * <p> 3863 * Description: <b>Multiple Resources: 3864 3865* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 3866* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 3867* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 3868* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 3869* [Citation](citation.html): The current status of the citation 3870* [CodeSystem](codesystem.html): The current status of the code system 3871* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 3872* [ConceptMap](conceptmap.html): The current status of the concept map 3873* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 3874* [EventDefinition](eventdefinition.html): The current status of the event definition 3875* [Evidence](evidence.html): The current status of the evidence 3876* [EvidenceReport](evidencereport.html): The current status of the evidence report 3877* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 3878* [ExampleScenario](examplescenario.html): The current status of the example scenario 3879* [GraphDefinition](graphdefinition.html): The current status of the graph definition 3880* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 3881* [Library](library.html): The current status of the library 3882* [Measure](measure.html): The current status of the measure 3883* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 3884* [MessageDefinition](messagedefinition.html): The current status of the message definition 3885* [NamingSystem](namingsystem.html): The current status of the naming system 3886* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 3887* [OperationDefinition](operationdefinition.html): The current status of the operation definition 3888* [PlanDefinition](plandefinition.html): The current status of the plan definition 3889* [Questionnaire](questionnaire.html): The current status of the questionnaire 3890* [Requirements](requirements.html): The current status of the requirements 3891* [SearchParameter](searchparameter.html): The current status of the search parameter 3892* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 3893* [StructureDefinition](structuredefinition.html): The current status of the structure definition 3894* [StructureMap](structuremap.html): The current status of the structure map 3895* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 3896* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 3897* [TestPlan](testplan.html): The current status of the test plan 3898* [TestScript](testscript.html): The current status of the test script 3899* [ValueSet](valueset.html): The current status of the value set 3900</b><br> 3901 * Type: <b>token</b><br> 3902 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 3903 * </p> 3904 */ 3905 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 3906 public static final String SP_STATUS = "status"; 3907 /** 3908 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3909 * <p> 3910 * Description: <b>Multiple Resources: 3911 3912* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 3913* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 3914* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 3915* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 3916* [Citation](citation.html): The current status of the citation 3917* [CodeSystem](codesystem.html): The current status of the code system 3918* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 3919* [ConceptMap](conceptmap.html): The current status of the concept map 3920* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 3921* [EventDefinition](eventdefinition.html): The current status of the event definition 3922* [Evidence](evidence.html): The current status of the evidence 3923* [EvidenceReport](evidencereport.html): The current status of the evidence report 3924* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 3925* [ExampleScenario](examplescenario.html): The current status of the example scenario 3926* [GraphDefinition](graphdefinition.html): The current status of the graph definition 3927* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 3928* [Library](library.html): The current status of the library 3929* [Measure](measure.html): The current status of the measure 3930* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 3931* [MessageDefinition](messagedefinition.html): The current status of the message definition 3932* [NamingSystem](namingsystem.html): The current status of the naming system 3933* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 3934* [OperationDefinition](operationdefinition.html): The current status of the operation definition 3935* [PlanDefinition](plandefinition.html): The current status of the plan definition 3936* [Questionnaire](questionnaire.html): The current status of the questionnaire 3937* [Requirements](requirements.html): The current status of the requirements 3938* [SearchParameter](searchparameter.html): The current status of the search parameter 3939* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 3940* [StructureDefinition](structuredefinition.html): The current status of the structure definition 3941* [StructureMap](structuremap.html): The current status of the structure map 3942* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 3943* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 3944* [TestPlan](testplan.html): The current status of the test plan 3945* [TestScript](testscript.html): The current status of the test script 3946* [ValueSet](valueset.html): The current status of the value set 3947</b><br> 3948 * Type: <b>token</b><br> 3949 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 3950 * </p> 3951 */ 3952 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3953 3954 /** 3955 * Search parameter: <b>url</b> 3956 * <p> 3957 * Description: <b>Multiple Resources: 3958 3959* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 3960* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 3961* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 3962* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 3963* [Citation](citation.html): The uri that identifies the citation 3964* [CodeSystem](codesystem.html): The uri that identifies the code system 3965* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 3966* [ConceptMap](conceptmap.html): The URI that identifies the concept map 3967* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 3968* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 3969* [Evidence](evidence.html): The uri that identifies the evidence 3970* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 3971* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 3972* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 3973* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 3974* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 3975* [Library](library.html): The uri that identifies the library 3976* [Measure](measure.html): The uri that identifies the measure 3977* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 3978* [NamingSystem](namingsystem.html): The uri that identifies the naming system 3979* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 3980* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 3981* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 3982* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 3983* [Requirements](requirements.html): The uri that identifies the requirements 3984* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 3985* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 3986* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 3987* [StructureMap](structuremap.html): The uri that identifies the structure map 3988* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 3989* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 3990* [TestPlan](testplan.html): The uri that identifies the test plan 3991* [TestScript](testscript.html): The uri that identifies the test script 3992* [ValueSet](valueset.html): The uri that identifies the value set 3993</b><br> 3994 * Type: <b>uri</b><br> 3995 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 3996 * </p> 3997 */ 3998 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 3999 public static final String SP_URL = "url"; 4000 /** 4001 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4002 * <p> 4003 * Description: <b>Multiple Resources: 4004 4005* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4006* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4007* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4008* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4009* [Citation](citation.html): The uri that identifies the citation 4010* [CodeSystem](codesystem.html): The uri that identifies the code system 4011* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4012* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4013* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4014* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4015* [Evidence](evidence.html): The uri that identifies the evidence 4016* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4017* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4018* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4019* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4020* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4021* [Library](library.html): The uri that identifies the library 4022* [Measure](measure.html): The uri that identifies the measure 4023* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4024* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4025* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4026* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4027* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4028* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4029* [Requirements](requirements.html): The uri that identifies the requirements 4030* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4031* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4032* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4033* [StructureMap](structuremap.html): The uri that identifies the structure map 4034* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4035* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4036* [TestPlan](testplan.html): The uri that identifies the test plan 4037* [TestScript](testscript.html): The uri that identifies the test script 4038* [ValueSet](valueset.html): The uri that identifies the value set 4039</b><br> 4040 * Type: <b>uri</b><br> 4041 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4042 * </p> 4043 */ 4044 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4045 4046 /** 4047 * Search parameter: <b>version</b> 4048 * <p> 4049 * Description: <b>Multiple Resources: 4050 4051* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4052* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4053* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4054* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4055* [Citation](citation.html): The business version of the citation 4056* [CodeSystem](codesystem.html): The business version of the code system 4057* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 4058* [ConceptMap](conceptmap.html): The business version of the concept map 4059* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 4060* [EventDefinition](eventdefinition.html): The business version of the event definition 4061* [Evidence](evidence.html): The business version of the evidence 4062* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 4063* [ExampleScenario](examplescenario.html): The business version of the example scenario 4064* [GraphDefinition](graphdefinition.html): The business version of the graph definition 4065* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 4066* [Library](library.html): The business version of the library 4067* [Measure](measure.html): The business version of the measure 4068* [MessageDefinition](messagedefinition.html): The business version of the message definition 4069* [NamingSystem](namingsystem.html): The business version of the naming system 4070* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4071* [PlanDefinition](plandefinition.html): The business version of the plan definition 4072* [Questionnaire](questionnaire.html): The business version of the questionnaire 4073* [Requirements](requirements.html): The business version of the requirements 4074* [SearchParameter](searchparameter.html): The business version of the search parameter 4075* [StructureDefinition](structuredefinition.html): The business version of the structure definition 4076* [StructureMap](structuremap.html): The business version of the structure map 4077* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 4078* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 4079* [TestScript](testscript.html): The business version of the test script 4080* [ValueSet](valueset.html): The business version of the value set 4081</b><br> 4082 * Type: <b>token</b><br> 4083 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 4084 * </p> 4085 */ 4086 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 4087 public static final String SP_VERSION = "version"; 4088 /** 4089 * <b>Fluent Client</b> search parameter constant for <b>version</b> 4090 * <p> 4091 * Description: <b>Multiple Resources: 4092 4093* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4094* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4095* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4096* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4097* [Citation](citation.html): The business version of the citation 4098* [CodeSystem](codesystem.html): The business version of the code system 4099* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 4100* [ConceptMap](conceptmap.html): The business version of the concept map 4101* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 4102* [EventDefinition](eventdefinition.html): The business version of the event definition 4103* [Evidence](evidence.html): The business version of the evidence 4104* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 4105* [ExampleScenario](examplescenario.html): The business version of the example scenario 4106* [GraphDefinition](graphdefinition.html): The business version of the graph definition 4107* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 4108* [Library](library.html): The business version of the library 4109* [Measure](measure.html): The business version of the measure 4110* [MessageDefinition](messagedefinition.html): The business version of the message definition 4111* [NamingSystem](namingsystem.html): The business version of the naming system 4112* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4113* [PlanDefinition](plandefinition.html): The business version of the plan definition 4114* [Questionnaire](questionnaire.html): The business version of the questionnaire 4115* [Requirements](requirements.html): The business version of the requirements 4116* [SearchParameter](searchparameter.html): The business version of the search parameter 4117* [StructureDefinition](structuredefinition.html): The business version of the structure definition 4118* [StructureMap](structuremap.html): The business version of the structure map 4119* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 4120* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 4121* [TestScript](testscript.html): The business version of the test script 4122* [ValueSet](valueset.html): The business version of the value set 4123</b><br> 4124 * Type: <b>token</b><br> 4125 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 4126 * </p> 4127 */ 4128 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 4129 4130 /** 4131 * Search parameter: <b>base</b> 4132 * <p> 4133 * Description: <b>The resource type(s) this search parameter applies to</b><br> 4134 * Type: <b>token</b><br> 4135 * Path: <b>SearchParameter.base</b><br> 4136 * </p> 4137 */ 4138 @SearchParamDefinition(name="base", path="SearchParameter.base", description="The resource type(s) this search parameter applies to", type="token" ) 4139 public static final String SP_BASE = "base"; 4140 /** 4141 * <b>Fluent Client</b> search parameter constant for <b>base</b> 4142 * <p> 4143 * Description: <b>The resource type(s) this search parameter applies to</b><br> 4144 * Type: <b>token</b><br> 4145 * Path: <b>SearchParameter.base</b><br> 4146 * </p> 4147 */ 4148 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BASE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BASE); 4149 4150 /** 4151 * Search parameter: <b>code</b> 4152 * <p> 4153 * Description: <b>Code used in URL</b><br> 4154 * Type: <b>token</b><br> 4155 * Path: <b>SearchParameter.code</b><br> 4156 * </p> 4157 */ 4158 @SearchParamDefinition(name="code", path="SearchParameter.code", description="Code used in URL", type="token" ) 4159 public static final String SP_CODE = "code"; 4160 /** 4161 * <b>Fluent Client</b> search parameter constant for <b>code</b> 4162 * <p> 4163 * Description: <b>Code used in URL</b><br> 4164 * Type: <b>token</b><br> 4165 * Path: <b>SearchParameter.code</b><br> 4166 * </p> 4167 */ 4168 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 4169 4170 /** 4171 * Search parameter: <b>component</b> 4172 * <p> 4173 * Description: <b>Defines how the part works</b><br> 4174 * Type: <b>reference</b><br> 4175 * Path: <b>SearchParameter.component.definition</b><br> 4176 * </p> 4177 */ 4178 @SearchParamDefinition(name="component", path="SearchParameter.component.definition", description="Defines how the part works", type="reference", target={SearchParameter.class } ) 4179 public static final String SP_COMPONENT = "component"; 4180 /** 4181 * <b>Fluent Client</b> search parameter constant for <b>component</b> 4182 * <p> 4183 * Description: <b>Defines how the part works</b><br> 4184 * Type: <b>reference</b><br> 4185 * Path: <b>SearchParameter.component.definition</b><br> 4186 * </p> 4187 */ 4188 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPONENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COMPONENT); 4189 4190/** 4191 * Constant for fluent queries to be used to add include statements. Specifies 4192 * the path value of "<b>SearchParameter:component</b>". 4193 */ 4194 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPONENT = new ca.uhn.fhir.model.api.Include("SearchParameter:component").toLocked(); 4195 4196 /** 4197 * Search parameter: <b>derived-from</b> 4198 * <p> 4199 * Description: <b>Original definition for the search parameter</b><br> 4200 * Type: <b>reference</b><br> 4201 * Path: <b>SearchParameter.derivedFrom</b><br> 4202 * </p> 4203 */ 4204 @SearchParamDefinition(name="derived-from", path="SearchParameter.derivedFrom", description="Original definition for the search parameter", type="reference", target={SearchParameter.class } ) 4205 public static final String SP_DERIVED_FROM = "derived-from"; 4206 /** 4207 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 4208 * <p> 4209 * Description: <b>Original definition for the search parameter</b><br> 4210 * Type: <b>reference</b><br> 4211 * Path: <b>SearchParameter.derivedFrom</b><br> 4212 * </p> 4213 */ 4214 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 4215 4216/** 4217 * Constant for fluent queries to be used to add include statements. Specifies 4218 * the path value of "<b>SearchParameter:derived-from</b>". 4219 */ 4220 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("SearchParameter:derived-from").toLocked(); 4221 4222 /** 4223 * Search parameter: <b>target</b> 4224 * <p> 4225 * Description: <b>Types of resource (if a resource reference)</b><br> 4226 * Type: <b>token</b><br> 4227 * Path: <b>SearchParameter.target</b><br> 4228 * </p> 4229 */ 4230 @SearchParamDefinition(name="target", path="SearchParameter.target", description="Types of resource (if a resource reference)", type="token" ) 4231 public static final String SP_TARGET = "target"; 4232 /** 4233 * <b>Fluent Client</b> search parameter constant for <b>target</b> 4234 * <p> 4235 * Description: <b>Types of resource (if a resource reference)</b><br> 4236 * Type: <b>token</b><br> 4237 * Path: <b>SearchParameter.target</b><br> 4238 * </p> 4239 */ 4240 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TARGET); 4241 4242 /** 4243 * Search parameter: <b>type</b> 4244 * <p> 4245 * Description: <b>number | date | string | token | reference | composite | quantity | uri | special</b><br> 4246 * Type: <b>token</b><br> 4247 * Path: <b>SearchParameter.type</b><br> 4248 * </p> 4249 */ 4250 @SearchParamDefinition(name="type", path="SearchParameter.type", description="number | date | string | token | reference | composite | quantity | uri | special", type="token" ) 4251 public static final String SP_TYPE = "type"; 4252 /** 4253 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4254 * <p> 4255 * Description: <b>number | date | string | token | reference | composite | quantity | uri | special</b><br> 4256 * Type: <b>token</b><br> 4257 * Path: <b>SearchParameter.type</b><br> 4258 * </p> 4259 */ 4260 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 4261 4262// Manual code (from Configuration.txt): 4263 public boolean supportsCopyright() { 4264 return true; 4265 } 4266 4267 4268 public boolean hasBase(String value) { 4269 if (this.base == null) 4270 return false; 4271 for (Enumeration<VersionIndependentResourceTypesAll> v : this.base) 4272 if (v.getCode().equals(value)) // code 4273 return true; 4274 return false; 4275 } 4276 4277// end addition 4278 4279} 4280