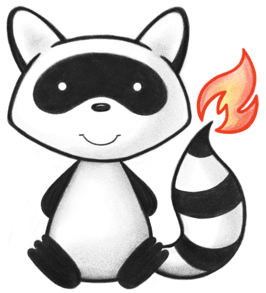
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A record of a request for service such as diagnostic investigations, treatments, or operations to be performed. 052 */ 053@ResourceDef(name="ServiceRequest", profile="http://hl7.org/fhir/StructureDefinition/ServiceRequest") 054public class ServiceRequest extends DomainResource { 055 056 @Block() 057 public static class ServiceRequestOrderDetailComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Indicates the context of the order details by reference. 060 */ 061 @Child(name = "parameterFocus", type = {CodeableReference.class}, order=1, min=0, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="The context of the order details by reference", formalDefinition="Indicates the context of the order details by reference." ) 063 protected CodeableReference parameterFocus; 064 065 /** 066 * The parameter details for the service being requested. 067 */ 068 @Child(name = "parameter", type = {}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 069 @Description(shortDefinition="The parameter details for the service being requested", formalDefinition="The parameter details for the service being requested." ) 070 protected List<ServiceRequestOrderDetailParameterComponent> parameter; 071 072 private static final long serialVersionUID = -404214439L; 073 074 /** 075 * Constructor 076 */ 077 public ServiceRequestOrderDetailComponent() { 078 super(); 079 } 080 081 /** 082 * Constructor 083 */ 084 public ServiceRequestOrderDetailComponent(ServiceRequestOrderDetailParameterComponent parameter) { 085 super(); 086 this.addParameter(parameter); 087 } 088 089 /** 090 * @return {@link #parameterFocus} (Indicates the context of the order details by reference.) 091 */ 092 public CodeableReference getParameterFocus() { 093 if (this.parameterFocus == null) 094 if (Configuration.errorOnAutoCreate()) 095 throw new Error("Attempt to auto-create ServiceRequestOrderDetailComponent.parameterFocus"); 096 else if (Configuration.doAutoCreate()) 097 this.parameterFocus = new CodeableReference(); // cc 098 return this.parameterFocus; 099 } 100 101 public boolean hasParameterFocus() { 102 return this.parameterFocus != null && !this.parameterFocus.isEmpty(); 103 } 104 105 /** 106 * @param value {@link #parameterFocus} (Indicates the context of the order details by reference.) 107 */ 108 public ServiceRequestOrderDetailComponent setParameterFocus(CodeableReference value) { 109 this.parameterFocus = value; 110 return this; 111 } 112 113 /** 114 * @return {@link #parameter} (The parameter details for the service being requested.) 115 */ 116 public List<ServiceRequestOrderDetailParameterComponent> getParameter() { 117 if (this.parameter == null) 118 this.parameter = new ArrayList<ServiceRequestOrderDetailParameterComponent>(); 119 return this.parameter; 120 } 121 122 /** 123 * @return Returns a reference to <code>this</code> for easy method chaining 124 */ 125 public ServiceRequestOrderDetailComponent setParameter(List<ServiceRequestOrderDetailParameterComponent> theParameter) { 126 this.parameter = theParameter; 127 return this; 128 } 129 130 public boolean hasParameter() { 131 if (this.parameter == null) 132 return false; 133 for (ServiceRequestOrderDetailParameterComponent item : this.parameter) 134 if (!item.isEmpty()) 135 return true; 136 return false; 137 } 138 139 public ServiceRequestOrderDetailParameterComponent addParameter() { //3 140 ServiceRequestOrderDetailParameterComponent t = new ServiceRequestOrderDetailParameterComponent(); 141 if (this.parameter == null) 142 this.parameter = new ArrayList<ServiceRequestOrderDetailParameterComponent>(); 143 this.parameter.add(t); 144 return t; 145 } 146 147 public ServiceRequestOrderDetailComponent addParameter(ServiceRequestOrderDetailParameterComponent t) { //3 148 if (t == null) 149 return this; 150 if (this.parameter == null) 151 this.parameter = new ArrayList<ServiceRequestOrderDetailParameterComponent>(); 152 this.parameter.add(t); 153 return this; 154 } 155 156 /** 157 * @return The first repetition of repeating field {@link #parameter}, creating it if it does not already exist {3} 158 */ 159 public ServiceRequestOrderDetailParameterComponent getParameterFirstRep() { 160 if (getParameter().isEmpty()) { 161 addParameter(); 162 } 163 return getParameter().get(0); 164 } 165 166 protected void listChildren(List<Property> children) { 167 super.listChildren(children); 168 children.add(new Property("parameterFocus", "CodeableReference(Device|DeviceDefinition|DeviceRequest|SupplyRequest|Medication|MedicationRequest|BiologicallyDerivedProduct|Substance)", "Indicates the context of the order details by reference.", 0, 1, parameterFocus)); 169 children.add(new Property("parameter", "", "The parameter details for the service being requested.", 0, java.lang.Integer.MAX_VALUE, parameter)); 170 } 171 172 @Override 173 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 174 switch (_hash) { 175 case 1110086319: /*parameterFocus*/ return new Property("parameterFocus", "CodeableReference(Device|DeviceDefinition|DeviceRequest|SupplyRequest|Medication|MedicationRequest|BiologicallyDerivedProduct|Substance)", "Indicates the context of the order details by reference.", 0, 1, parameterFocus); 176 case 1954460585: /*parameter*/ return new Property("parameter", "", "The parameter details for the service being requested.", 0, java.lang.Integer.MAX_VALUE, parameter); 177 default: return super.getNamedProperty(_hash, _name, _checkValid); 178 } 179 180 } 181 182 @Override 183 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 184 switch (hash) { 185 case 1110086319: /*parameterFocus*/ return this.parameterFocus == null ? new Base[0] : new Base[] {this.parameterFocus}; // CodeableReference 186 case 1954460585: /*parameter*/ return this.parameter == null ? new Base[0] : this.parameter.toArray(new Base[this.parameter.size()]); // ServiceRequestOrderDetailParameterComponent 187 default: return super.getProperty(hash, name, checkValid); 188 } 189 190 } 191 192 @Override 193 public Base setProperty(int hash, String name, Base value) throws FHIRException { 194 switch (hash) { 195 case 1110086319: // parameterFocus 196 this.parameterFocus = TypeConvertor.castToCodeableReference(value); // CodeableReference 197 return value; 198 case 1954460585: // parameter 199 this.getParameter().add((ServiceRequestOrderDetailParameterComponent) value); // ServiceRequestOrderDetailParameterComponent 200 return value; 201 default: return super.setProperty(hash, name, value); 202 } 203 204 } 205 206 @Override 207 public Base setProperty(String name, Base value) throws FHIRException { 208 if (name.equals("parameterFocus")) { 209 this.parameterFocus = TypeConvertor.castToCodeableReference(value); // CodeableReference 210 } else if (name.equals("parameter")) { 211 this.getParameter().add((ServiceRequestOrderDetailParameterComponent) value); 212 } else 213 return super.setProperty(name, value); 214 return value; 215 } 216 217 @Override 218 public void removeChild(String name, Base value) throws FHIRException { 219 if (name.equals("parameterFocus")) { 220 this.parameterFocus = null; 221 } else if (name.equals("parameter")) { 222 this.getParameter().remove((ServiceRequestOrderDetailParameterComponent) value); 223 } else 224 super.removeChild(name, value); 225 226 } 227 228 @Override 229 public Base makeProperty(int hash, String name) throws FHIRException { 230 switch (hash) { 231 case 1110086319: return getParameterFocus(); 232 case 1954460585: return addParameter(); 233 default: return super.makeProperty(hash, name); 234 } 235 236 } 237 238 @Override 239 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 240 switch (hash) { 241 case 1110086319: /*parameterFocus*/ return new String[] {"CodeableReference"}; 242 case 1954460585: /*parameter*/ return new String[] {}; 243 default: return super.getTypesForProperty(hash, name); 244 } 245 246 } 247 248 @Override 249 public Base addChild(String name) throws FHIRException { 250 if (name.equals("parameterFocus")) { 251 this.parameterFocus = new CodeableReference(); 252 return this.parameterFocus; 253 } 254 else if (name.equals("parameter")) { 255 return addParameter(); 256 } 257 else 258 return super.addChild(name); 259 } 260 261 public ServiceRequestOrderDetailComponent copy() { 262 ServiceRequestOrderDetailComponent dst = new ServiceRequestOrderDetailComponent(); 263 copyValues(dst); 264 return dst; 265 } 266 267 public void copyValues(ServiceRequestOrderDetailComponent dst) { 268 super.copyValues(dst); 269 dst.parameterFocus = parameterFocus == null ? null : parameterFocus.copy(); 270 if (parameter != null) { 271 dst.parameter = new ArrayList<ServiceRequestOrderDetailParameterComponent>(); 272 for (ServiceRequestOrderDetailParameterComponent i : parameter) 273 dst.parameter.add(i.copy()); 274 }; 275 } 276 277 @Override 278 public boolean equalsDeep(Base other_) { 279 if (!super.equalsDeep(other_)) 280 return false; 281 if (!(other_ instanceof ServiceRequestOrderDetailComponent)) 282 return false; 283 ServiceRequestOrderDetailComponent o = (ServiceRequestOrderDetailComponent) other_; 284 return compareDeep(parameterFocus, o.parameterFocus, true) && compareDeep(parameter, o.parameter, true) 285 ; 286 } 287 288 @Override 289 public boolean equalsShallow(Base other_) { 290 if (!super.equalsShallow(other_)) 291 return false; 292 if (!(other_ instanceof ServiceRequestOrderDetailComponent)) 293 return false; 294 ServiceRequestOrderDetailComponent o = (ServiceRequestOrderDetailComponent) other_; 295 return true; 296 } 297 298 public boolean isEmpty() { 299 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(parameterFocus, parameter 300 ); 301 } 302 303 public String fhirType() { 304 return "ServiceRequest.orderDetail"; 305 306 } 307 308 } 309 310 @Block() 311 public static class ServiceRequestOrderDetailParameterComponent extends BackboneElement implements IBaseBackboneElement { 312 /** 313 * A value representing the additional detail or instructions for the order (e.g., catheter insertion, body elevation, descriptive device configuration and/or setting instructions). 314 */ 315 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 316 @Description(shortDefinition="The detail of the order being requested", formalDefinition="A value representing the additional detail or instructions for the order (e.g., catheter insertion, body elevation, descriptive device configuration and/or setting instructions)." ) 317 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/servicerequest-orderdetail-parameter-code") 318 protected CodeableConcept code; 319 320 /** 321 * Indicates a value for the order detail. 322 */ 323 @Child(name = "value", type = {Quantity.class, Ratio.class, Range.class, BooleanType.class, CodeableConcept.class, StringType.class, Period.class}, order=2, min=1, max=1, modifier=false, summary=true) 324 @Description(shortDefinition="The value for the order detail", formalDefinition="Indicates a value for the order detail." ) 325 protected DataType value; 326 327 private static final long serialVersionUID = -1950789033L; 328 329 /** 330 * Constructor 331 */ 332 public ServiceRequestOrderDetailParameterComponent() { 333 super(); 334 } 335 336 /** 337 * Constructor 338 */ 339 public ServiceRequestOrderDetailParameterComponent(CodeableConcept code, DataType value) { 340 super(); 341 this.setCode(code); 342 this.setValue(value); 343 } 344 345 /** 346 * @return {@link #code} (A value representing the additional detail or instructions for the order (e.g., catheter insertion, body elevation, descriptive device configuration and/or setting instructions).) 347 */ 348 public CodeableConcept getCode() { 349 if (this.code == null) 350 if (Configuration.errorOnAutoCreate()) 351 throw new Error("Attempt to auto-create ServiceRequestOrderDetailParameterComponent.code"); 352 else if (Configuration.doAutoCreate()) 353 this.code = new CodeableConcept(); // cc 354 return this.code; 355 } 356 357 public boolean hasCode() { 358 return this.code != null && !this.code.isEmpty(); 359 } 360 361 /** 362 * @param value {@link #code} (A value representing the additional detail or instructions for the order (e.g., catheter insertion, body elevation, descriptive device configuration and/or setting instructions).) 363 */ 364 public ServiceRequestOrderDetailParameterComponent setCode(CodeableConcept value) { 365 this.code = value; 366 return this; 367 } 368 369 /** 370 * @return {@link #value} (Indicates a value for the order detail.) 371 */ 372 public DataType getValue() { 373 return this.value; 374 } 375 376 /** 377 * @return {@link #value} (Indicates a value for the order detail.) 378 */ 379 public Quantity getValueQuantity() throws FHIRException { 380 if (this.value == null) 381 this.value = new Quantity(); 382 if (!(this.value instanceof Quantity)) 383 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 384 return (Quantity) this.value; 385 } 386 387 public boolean hasValueQuantity() { 388 return this != null && this.value instanceof Quantity; 389 } 390 391 /** 392 * @return {@link #value} (Indicates a value for the order detail.) 393 */ 394 public Ratio getValueRatio() throws FHIRException { 395 if (this.value == null) 396 this.value = new Ratio(); 397 if (!(this.value instanceof Ratio)) 398 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.value.getClass().getName()+" was encountered"); 399 return (Ratio) this.value; 400 } 401 402 public boolean hasValueRatio() { 403 return this != null && this.value instanceof Ratio; 404 } 405 406 /** 407 * @return {@link #value} (Indicates a value for the order detail.) 408 */ 409 public Range getValueRange() throws FHIRException { 410 if (this.value == null) 411 this.value = new Range(); 412 if (!(this.value instanceof Range)) 413 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 414 return (Range) this.value; 415 } 416 417 public boolean hasValueRange() { 418 return this != null && this.value instanceof Range; 419 } 420 421 /** 422 * @return {@link #value} (Indicates a value for the order detail.) 423 */ 424 public BooleanType getValueBooleanType() throws FHIRException { 425 if (this.value == null) 426 this.value = new BooleanType(); 427 if (!(this.value instanceof BooleanType)) 428 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 429 return (BooleanType) this.value; 430 } 431 432 public boolean hasValueBooleanType() { 433 return this != null && this.value instanceof BooleanType; 434 } 435 436 /** 437 * @return {@link #value} (Indicates a value for the order detail.) 438 */ 439 public CodeableConcept getValueCodeableConcept() throws FHIRException { 440 if (this.value == null) 441 this.value = new CodeableConcept(); 442 if (!(this.value instanceof CodeableConcept)) 443 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 444 return (CodeableConcept) this.value; 445 } 446 447 public boolean hasValueCodeableConcept() { 448 return this != null && this.value instanceof CodeableConcept; 449 } 450 451 /** 452 * @return {@link #value} (Indicates a value for the order detail.) 453 */ 454 public StringType getValueStringType() throws FHIRException { 455 if (this.value == null) 456 this.value = new StringType(); 457 if (!(this.value instanceof StringType)) 458 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 459 return (StringType) this.value; 460 } 461 462 public boolean hasValueStringType() { 463 return this != null && this.value instanceof StringType; 464 } 465 466 /** 467 * @return {@link #value} (Indicates a value for the order detail.) 468 */ 469 public Period getValuePeriod() throws FHIRException { 470 if (this.value == null) 471 this.value = new Period(); 472 if (!(this.value instanceof Period)) 473 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.value.getClass().getName()+" was encountered"); 474 return (Period) this.value; 475 } 476 477 public boolean hasValuePeriod() { 478 return this != null && this.value instanceof Period; 479 } 480 481 public boolean hasValue() { 482 return this.value != null && !this.value.isEmpty(); 483 } 484 485 /** 486 * @param value {@link #value} (Indicates a value for the order detail.) 487 */ 488 public ServiceRequestOrderDetailParameterComponent setValue(DataType value) { 489 if (value != null && !(value instanceof Quantity || value instanceof Ratio || value instanceof Range || value instanceof BooleanType || value instanceof CodeableConcept || value instanceof StringType || value instanceof Period)) 490 throw new FHIRException("Not the right type for ServiceRequest.orderDetail.parameter.value[x]: "+value.fhirType()); 491 this.value = value; 492 return this; 493 } 494 495 protected void listChildren(List<Property> children) { 496 super.listChildren(children); 497 children.add(new Property("code", "CodeableConcept", "A value representing the additional detail or instructions for the order (e.g., catheter insertion, body elevation, descriptive device configuration and/or setting instructions).", 0, 1, code)); 498 children.add(new Property("value[x]", "Quantity|Ratio|Range|boolean|CodeableConcept|string|Period", "Indicates a value for the order detail.", 0, 1, value)); 499 } 500 501 @Override 502 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 503 switch (_hash) { 504 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A value representing the additional detail or instructions for the order (e.g., catheter insertion, body elevation, descriptive device configuration and/or setting instructions).", 0, 1, code); 505 case -1410166417: /*value[x]*/ return new Property("value[x]", "Quantity|Ratio|Range|boolean|CodeableConcept|string|Period", "Indicates a value for the order detail.", 0, 1, value); 506 case 111972721: /*value*/ return new Property("value[x]", "Quantity|Ratio|Range|boolean|CodeableConcept|string|Period", "Indicates a value for the order detail.", 0, 1, value); 507 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Indicates a value for the order detail.", 0, 1, value); 508 case 2030767386: /*valueRatio*/ return new Property("value[x]", "Ratio", "Indicates a value for the order detail.", 0, 1, value); 509 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "Indicates a value for the order detail.", 0, 1, value); 510 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "Indicates a value for the order detail.", 0, 1, value); 511 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "Indicates a value for the order detail.", 0, 1, value); 512 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "Indicates a value for the order detail.", 0, 1, value); 513 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "Period", "Indicates a value for the order detail.", 0, 1, value); 514 default: return super.getNamedProperty(_hash, _name, _checkValid); 515 } 516 517 } 518 519 @Override 520 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 521 switch (hash) { 522 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 523 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 524 default: return super.getProperty(hash, name, checkValid); 525 } 526 527 } 528 529 @Override 530 public Base setProperty(int hash, String name, Base value) throws FHIRException { 531 switch (hash) { 532 case 3059181: // code 533 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 534 return value; 535 case 111972721: // value 536 this.value = TypeConvertor.castToType(value); // DataType 537 return value; 538 default: return super.setProperty(hash, name, value); 539 } 540 541 } 542 543 @Override 544 public Base setProperty(String name, Base value) throws FHIRException { 545 if (name.equals("code")) { 546 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 547 } else if (name.equals("value[x]")) { 548 this.value = TypeConvertor.castToType(value); // DataType 549 } else 550 return super.setProperty(name, value); 551 return value; 552 } 553 554 @Override 555 public void removeChild(String name, Base value) throws FHIRException { 556 if (name.equals("code")) { 557 this.code = null; 558 } else if (name.equals("value[x]")) { 559 this.value = null; 560 } else 561 super.removeChild(name, value); 562 563 } 564 565 @Override 566 public Base makeProperty(int hash, String name) throws FHIRException { 567 switch (hash) { 568 case 3059181: return getCode(); 569 case -1410166417: return getValue(); 570 case 111972721: return getValue(); 571 default: return super.makeProperty(hash, name); 572 } 573 574 } 575 576 @Override 577 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 578 switch (hash) { 579 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 580 case 111972721: /*value*/ return new String[] {"Quantity", "Ratio", "Range", "boolean", "CodeableConcept", "string", "Period"}; 581 default: return super.getTypesForProperty(hash, name); 582 } 583 584 } 585 586 @Override 587 public Base addChild(String name) throws FHIRException { 588 if (name.equals("code")) { 589 this.code = new CodeableConcept(); 590 return this.code; 591 } 592 else if (name.equals("valueQuantity")) { 593 this.value = new Quantity(); 594 return this.value; 595 } 596 else if (name.equals("valueRatio")) { 597 this.value = new Ratio(); 598 return this.value; 599 } 600 else if (name.equals("valueRange")) { 601 this.value = new Range(); 602 return this.value; 603 } 604 else if (name.equals("valueBoolean")) { 605 this.value = new BooleanType(); 606 return this.value; 607 } 608 else if (name.equals("valueCodeableConcept")) { 609 this.value = new CodeableConcept(); 610 return this.value; 611 } 612 else if (name.equals("valueString")) { 613 this.value = new StringType(); 614 return this.value; 615 } 616 else if (name.equals("valuePeriod")) { 617 this.value = new Period(); 618 return this.value; 619 } 620 else 621 return super.addChild(name); 622 } 623 624 public ServiceRequestOrderDetailParameterComponent copy() { 625 ServiceRequestOrderDetailParameterComponent dst = new ServiceRequestOrderDetailParameterComponent(); 626 copyValues(dst); 627 return dst; 628 } 629 630 public void copyValues(ServiceRequestOrderDetailParameterComponent dst) { 631 super.copyValues(dst); 632 dst.code = code == null ? null : code.copy(); 633 dst.value = value == null ? null : value.copy(); 634 } 635 636 @Override 637 public boolean equalsDeep(Base other_) { 638 if (!super.equalsDeep(other_)) 639 return false; 640 if (!(other_ instanceof ServiceRequestOrderDetailParameterComponent)) 641 return false; 642 ServiceRequestOrderDetailParameterComponent o = (ServiceRequestOrderDetailParameterComponent) other_; 643 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 644 } 645 646 @Override 647 public boolean equalsShallow(Base other_) { 648 if (!super.equalsShallow(other_)) 649 return false; 650 if (!(other_ instanceof ServiceRequestOrderDetailParameterComponent)) 651 return false; 652 ServiceRequestOrderDetailParameterComponent o = (ServiceRequestOrderDetailParameterComponent) other_; 653 return true; 654 } 655 656 public boolean isEmpty() { 657 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value); 658 } 659 660 public String fhirType() { 661 return "ServiceRequest.orderDetail.parameter"; 662 663 } 664 665 } 666 667 @Block() 668 public static class ServiceRequestPatientInstructionComponent extends BackboneElement implements IBaseBackboneElement { 669 /** 670 * Instructions in terms that are understood by the patient or consumer. 671 */ 672 @Child(name = "instruction", type = {MarkdownType.class, DocumentReference.class}, order=1, min=0, max=1, modifier=false, summary=true) 673 @Description(shortDefinition="Patient or consumer-oriented instructions", formalDefinition="Instructions in terms that are understood by the patient or consumer." ) 674 protected DataType instruction; 675 676 private static final long serialVersionUID = 474568404L; 677 678 /** 679 * Constructor 680 */ 681 public ServiceRequestPatientInstructionComponent() { 682 super(); 683 } 684 685 /** 686 * @return {@link #instruction} (Instructions in terms that are understood by the patient or consumer.) 687 */ 688 public DataType getInstruction() { 689 return this.instruction; 690 } 691 692 /** 693 * @return {@link #instruction} (Instructions in terms that are understood by the patient or consumer.) 694 */ 695 public MarkdownType getInstructionMarkdownType() throws FHIRException { 696 if (this.instruction == null) 697 this.instruction = new MarkdownType(); 698 if (!(this.instruction instanceof MarkdownType)) 699 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.instruction.getClass().getName()+" was encountered"); 700 return (MarkdownType) this.instruction; 701 } 702 703 public boolean hasInstructionMarkdownType() { 704 return this != null && this.instruction instanceof MarkdownType; 705 } 706 707 /** 708 * @return {@link #instruction} (Instructions in terms that are understood by the patient or consumer.) 709 */ 710 public Reference getInstructionReference() throws FHIRException { 711 if (this.instruction == null) 712 this.instruction = new Reference(); 713 if (!(this.instruction instanceof Reference)) 714 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.instruction.getClass().getName()+" was encountered"); 715 return (Reference) this.instruction; 716 } 717 718 public boolean hasInstructionReference() { 719 return this != null && this.instruction instanceof Reference; 720 } 721 722 public boolean hasInstruction() { 723 return this.instruction != null && !this.instruction.isEmpty(); 724 } 725 726 /** 727 * @param value {@link #instruction} (Instructions in terms that are understood by the patient or consumer.) 728 */ 729 public ServiceRequestPatientInstructionComponent setInstruction(DataType value) { 730 if (value != null && !(value instanceof MarkdownType || value instanceof Reference)) 731 throw new FHIRException("Not the right type for ServiceRequest.patientInstruction.instruction[x]: "+value.fhirType()); 732 this.instruction = value; 733 return this; 734 } 735 736 protected void listChildren(List<Property> children) { 737 super.listChildren(children); 738 children.add(new Property("instruction[x]", "markdown|Reference(DocumentReference)", "Instructions in terms that are understood by the patient or consumer.", 0, 1, instruction)); 739 } 740 741 @Override 742 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 743 switch (_hash) { 744 case 1989248306: /*instruction[x]*/ return new Property("instruction[x]", "markdown|Reference(DocumentReference)", "Instructions in terms that are understood by the patient or consumer.", 0, 1, instruction); 745 case 301526158: /*instruction*/ return new Property("instruction[x]", "markdown|Reference(DocumentReference)", "Instructions in terms that are understood by the patient or consumer.", 0, 1, instruction); 746 case 13950877: /*instructionMarkdown*/ return new Property("instruction[x]", "markdown", "Instructions in terms that are understood by the patient or consumer.", 0, 1, instruction); 747 case 442151517: /*instructionReference*/ return new Property("instruction[x]", "Reference(DocumentReference)", "Instructions in terms that are understood by the patient or consumer.", 0, 1, instruction); 748 default: return super.getNamedProperty(_hash, _name, _checkValid); 749 } 750 751 } 752 753 @Override 754 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 755 switch (hash) { 756 case 301526158: /*instruction*/ return this.instruction == null ? new Base[0] : new Base[] {this.instruction}; // DataType 757 default: return super.getProperty(hash, name, checkValid); 758 } 759 760 } 761 762 @Override 763 public Base setProperty(int hash, String name, Base value) throws FHIRException { 764 switch (hash) { 765 case 301526158: // instruction 766 this.instruction = TypeConvertor.castToType(value); // DataType 767 return value; 768 default: return super.setProperty(hash, name, value); 769 } 770 771 } 772 773 @Override 774 public Base setProperty(String name, Base value) throws FHIRException { 775 if (name.equals("instruction[x]")) { 776 this.instruction = TypeConvertor.castToType(value); // DataType 777 } else 778 return super.setProperty(name, value); 779 return value; 780 } 781 782 @Override 783 public void removeChild(String name, Base value) throws FHIRException { 784 if (name.equals("instruction[x]")) { 785 this.instruction = null; 786 } else 787 super.removeChild(name, value); 788 789 } 790 791 @Override 792 public Base makeProperty(int hash, String name) throws FHIRException { 793 switch (hash) { 794 case 1989248306: return getInstruction(); 795 case 301526158: return getInstruction(); 796 default: return super.makeProperty(hash, name); 797 } 798 799 } 800 801 @Override 802 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 803 switch (hash) { 804 case 301526158: /*instruction*/ return new String[] {"markdown", "Reference"}; 805 default: return super.getTypesForProperty(hash, name); 806 } 807 808 } 809 810 @Override 811 public Base addChild(String name) throws FHIRException { 812 if (name.equals("instructionMarkdown")) { 813 this.instruction = new MarkdownType(); 814 return this.instruction; 815 } 816 else if (name.equals("instructionReference")) { 817 this.instruction = new Reference(); 818 return this.instruction; 819 } 820 else 821 return super.addChild(name); 822 } 823 824 public ServiceRequestPatientInstructionComponent copy() { 825 ServiceRequestPatientInstructionComponent dst = new ServiceRequestPatientInstructionComponent(); 826 copyValues(dst); 827 return dst; 828 } 829 830 public void copyValues(ServiceRequestPatientInstructionComponent dst) { 831 super.copyValues(dst); 832 dst.instruction = instruction == null ? null : instruction.copy(); 833 } 834 835 @Override 836 public boolean equalsDeep(Base other_) { 837 if (!super.equalsDeep(other_)) 838 return false; 839 if (!(other_ instanceof ServiceRequestPatientInstructionComponent)) 840 return false; 841 ServiceRequestPatientInstructionComponent o = (ServiceRequestPatientInstructionComponent) other_; 842 return compareDeep(instruction, o.instruction, true); 843 } 844 845 @Override 846 public boolean equalsShallow(Base other_) { 847 if (!super.equalsShallow(other_)) 848 return false; 849 if (!(other_ instanceof ServiceRequestPatientInstructionComponent)) 850 return false; 851 ServiceRequestPatientInstructionComponent o = (ServiceRequestPatientInstructionComponent) other_; 852 return true; 853 } 854 855 public boolean isEmpty() { 856 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(instruction); 857 } 858 859 public String fhirType() { 860 return "ServiceRequest.patientInstruction"; 861 862 } 863 864 } 865 866 /** 867 * Identifiers assigned to this order instance by the orderer and/or the receiver and/or order fulfiller. 868 */ 869 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 870 @Description(shortDefinition="Identifiers assigned to this order", formalDefinition="Identifiers assigned to this order instance by the orderer and/or the receiver and/or order fulfiller." ) 871 protected List<Identifier> identifier; 872 873 /** 874 * The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest. 875 */ 876 @Child(name = "instantiatesCanonical", type = {CanonicalType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 877 @Description(shortDefinition="Instantiates FHIR protocol or definition", formalDefinition="The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest." ) 878 protected List<CanonicalType> instantiatesCanonical; 879 880 /** 881 * The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest. 882 */ 883 @Child(name = "instantiatesUri", type = {UriType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 884 @Description(shortDefinition="Instantiates external protocol or definition", formalDefinition="The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest." ) 885 protected List<UriType> instantiatesUri; 886 887 /** 888 * Plan/proposal/order fulfilled by this request. 889 */ 890 @Child(name = "basedOn", type = {CarePlan.class, ServiceRequest.class, MedicationRequest.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 891 @Description(shortDefinition="What request fulfills", formalDefinition="Plan/proposal/order fulfilled by this request." ) 892 protected List<Reference> basedOn; 893 894 /** 895 * The request takes the place of the referenced completed or terminated request(s). 896 */ 897 @Child(name = "replaces", type = {ServiceRequest.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 898 @Description(shortDefinition="What request replaces", formalDefinition="The request takes the place of the referenced completed or terminated request(s)." ) 899 protected List<Reference> replaces; 900 901 /** 902 * A shared identifier common to all service requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier. 903 */ 904 @Child(name = "requisition", type = {Identifier.class}, order=5, min=0, max=1, modifier=false, summary=true) 905 @Description(shortDefinition="Composite Request ID", formalDefinition="A shared identifier common to all service requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier." ) 906 protected Identifier requisition; 907 908 /** 909 * The status of the order. 910 */ 911 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 912 @Description(shortDefinition="draft | active | on-hold | revoked | completed | entered-in-error | unknown", formalDefinition="The status of the order." ) 913 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-status") 914 protected Enumeration<RequestStatus> status; 915 916 /** 917 * Whether the request is a proposal, plan, an original order or a reflex order. 918 */ 919 @Child(name = "intent", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 920 @Description(shortDefinition="proposal | plan | directive | order +", formalDefinition="Whether the request is a proposal, plan, an original order or a reflex order." ) 921 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-intent") 922 protected Enumeration<RequestIntent> intent; 923 924 /** 925 * A code that classifies the service for searching, sorting and display purposes (e.g. "Surgical Procedure"). 926 */ 927 @Child(name = "category", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 928 @Description(shortDefinition="Classification of service", formalDefinition="A code that classifies the service for searching, sorting and display purposes (e.g. \"Surgical Procedure\")." ) 929 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/servicerequest-category") 930 protected List<CodeableConcept> category; 931 932 /** 933 * Indicates how quickly the ServiceRequest should be addressed with respect to other requests. 934 */ 935 @Child(name = "priority", type = {CodeType.class}, order=9, min=0, max=1, modifier=false, summary=true) 936 @Description(shortDefinition="routine | urgent | asap | stat", formalDefinition="Indicates how quickly the ServiceRequest should be addressed with respect to other requests." ) 937 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 938 protected Enumeration<RequestPriority> priority; 939 940 /** 941 * Set this to true if the record is saying that the service/procedure should NOT be performed. 942 */ 943 @Child(name = "doNotPerform", type = {BooleanType.class}, order=10, min=0, max=1, modifier=true, summary=true) 944 @Description(shortDefinition="True if service/procedure should not be performed", formalDefinition="Set this to true if the record is saying that the service/procedure should NOT be performed." ) 945 protected BooleanType doNotPerform; 946 947 /** 948 * A code or reference that identifies a particular service (i.e., procedure, diagnostic investigation, or panel of investigations) that have been requested. 949 */ 950 @Child(name = "code", type = {CodeableReference.class}, order=11, min=0, max=1, modifier=false, summary=true) 951 @Description(shortDefinition="What is being requested/ordered", formalDefinition="A code or reference that identifies a particular service (i.e., procedure, diagnostic investigation, or panel of investigations) that have been requested." ) 952 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-code") 953 protected CodeableReference code; 954 955 /** 956 * Additional details and instructions about the how the services are to be delivered. For example, and order for a urinary catheter may have an order detail for an external or indwelling catheter, or an order for a bandage may require additional instructions specifying how the bandage should be applied. 957 */ 958 @Child(name = "orderDetail", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 959 @Description(shortDefinition="Additional order information", formalDefinition="Additional details and instructions about the how the services are to be delivered. For example, and order for a urinary catheter may have an order detail for an external or indwelling catheter, or an order for a bandage may require additional instructions specifying how the bandage should be applied." ) 960 protected List<ServiceRequestOrderDetailComponent> orderDetail; 961 962 /** 963 * An amount of service being requested which can be a quantity ( for example $1,500 home modification), a ratio ( for example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per fraction). 964 */ 965 @Child(name = "quantity", type = {Quantity.class, Ratio.class, Range.class}, order=13, min=0, max=1, modifier=false, summary=true) 966 @Description(shortDefinition="Service amount", formalDefinition="An amount of service being requested which can be a quantity ( for example $1,500 home modification), a ratio ( for example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per fraction)." ) 967 protected DataType quantity; 968 969 /** 970 * On whom or what the service is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans). 971 */ 972 @Child(name = "subject", type = {Patient.class, Group.class, Location.class, Device.class}, order=14, min=1, max=1, modifier=false, summary=true) 973 @Description(shortDefinition="Individual or Entity the service is ordered for", formalDefinition="On whom or what the service is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans)." ) 974 protected Reference subject; 975 976 /** 977 * The actual focus of a service request when it is not the subject of record representing something or someone associated with the subject such as a spouse, parent, fetus, or donor. The focus of a service request could also be an existing condition, an intervention, the subject's diet, another service request on the subject, or a body structure such as tumor or implanted device. 978 */ 979 @Child(name = "focus", type = {Reference.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 980 @Description(shortDefinition="What the service request is about, when it is not about the subject of record", formalDefinition="The actual focus of a service request when it is not the subject of record representing something or someone associated with the subject such as a spouse, parent, fetus, or donor. The focus of a service request could also be an existing condition, an intervention, the subject's diet, another service request on the subject, or a body structure such as tumor or implanted device." ) 981 protected List<Reference> focus; 982 983 /** 984 * An encounter that provides additional information about the healthcare context in which this request is made. 985 */ 986 @Child(name = "encounter", type = {Encounter.class}, order=16, min=0, max=1, modifier=false, summary=true) 987 @Description(shortDefinition="Encounter in which the request was created", formalDefinition="An encounter that provides additional information about the healthcare context in which this request is made." ) 988 protected Reference encounter; 989 990 /** 991 * The date/time at which the requested service should occur. 992 */ 993 @Child(name = "occurrence", type = {DateTimeType.class, Period.class, Timing.class}, order=17, min=0, max=1, modifier=false, summary=true) 994 @Description(shortDefinition="When service should occur", formalDefinition="The date/time at which the requested service should occur." ) 995 protected DataType occurrence; 996 997 /** 998 * If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example "pain", "on flare-up", etc. 999 */ 1000 @Child(name = "asNeeded", type = {BooleanType.class, CodeableConcept.class}, order=18, min=0, max=1, modifier=false, summary=true) 1001 @Description(shortDefinition="Preconditions for service", formalDefinition="If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc." ) 1002 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-as-needed-reason") 1003 protected DataType asNeeded; 1004 1005 /** 1006 * When the request transitioned to being actionable. 1007 */ 1008 @Child(name = "authoredOn", type = {DateTimeType.class}, order=19, min=0, max=1, modifier=false, summary=true) 1009 @Description(shortDefinition="Date request signed", formalDefinition="When the request transitioned to being actionable." ) 1010 protected DateTimeType authoredOn; 1011 1012 /** 1013 * The individual who initiated the request and has responsibility for its activation. 1014 */ 1015 @Child(name = "requester", type = {Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, RelatedPerson.class, Device.class}, order=20, min=0, max=1, modifier=false, summary=true) 1016 @Description(shortDefinition="Who/what is requesting service", formalDefinition="The individual who initiated the request and has responsibility for its activation." ) 1017 protected Reference requester; 1018 1019 /** 1020 * Desired type of performer for doing the requested service. 1021 */ 1022 @Child(name = "performerType", type = {CodeableConcept.class}, order=21, min=0, max=1, modifier=false, summary=true) 1023 @Description(shortDefinition="Performer role", formalDefinition="Desired type of performer for doing the requested service." ) 1024 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participant-role") 1025 protected CodeableConcept performerType; 1026 1027 /** 1028 * The desired performer for doing the requested service. For example, the surgeon, dermatopathologist, endoscopist, etc. 1029 */ 1030 @Child(name = "performer", type = {Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, HealthcareService.class, Patient.class, Device.class, RelatedPerson.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1031 @Description(shortDefinition="Requested performer", formalDefinition="The desired performer for doing the requested service. For example, the surgeon, dermatopathologist, endoscopist, etc." ) 1032 protected List<Reference> performer; 1033 1034 /** 1035 * The preferred location(s) where the procedure should actually happen in coded or free text form. E.g. at home or nursing day care center. 1036 */ 1037 @Child(name = "location", type = {CodeableReference.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1038 @Description(shortDefinition="Requested location", formalDefinition="The preferred location(s) where the procedure should actually happen in coded or free text form. E.g. at home or nursing day care center." ) 1039 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-ServiceDeliveryLocationRoleType") 1040 protected List<CodeableReference> location; 1041 1042 /** 1043 * An explanation or justification for why this service is being requested in coded or textual form. This is often for billing purposes. May relate to the resources referred to in `supportingInfo`. 1044 */ 1045 @Child(name = "reason", type = {CodeableReference.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1046 @Description(shortDefinition="Explanation/Justification for procedure or service", formalDefinition="An explanation or justification for why this service is being requested in coded or textual form. This is often for billing purposes. May relate to the resources referred to in `supportingInfo`." ) 1047 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-reason") 1048 protected List<CodeableReference> reason; 1049 1050 /** 1051 * Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be needed for delivering the requested service. 1052 */ 1053 @Child(name = "insurance", type = {Coverage.class, ClaimResponse.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1054 @Description(shortDefinition="Associated insurance coverage", formalDefinition="Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be needed for delivering the requested service." ) 1055 protected List<Reference> insurance; 1056 1057 /** 1058 * Additional clinical information about the patient or specimen that may influence the services or their interpretations. This information includes diagnosis, clinical findings and other observations. In laboratory ordering these are typically referred to as "ask at order entry questions (AOEs)". This includes observations explicitly requested by the producer (filler) to provide context or supporting information needed to complete the order. For example, reporting the amount of inspired oxygen for blood gas measurements. 1059 */ 1060 @Child(name = "supportingInfo", type = {CodeableReference.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1061 @Description(shortDefinition="Additional clinical information", formalDefinition="Additional clinical information about the patient or specimen that may influence the services or their interpretations. This information includes diagnosis, clinical findings and other observations. In laboratory ordering these are typically referred to as \"ask at order entry questions (AOEs)\". This includes observations explicitly requested by the producer (filler) to provide context or supporting information needed to complete the order. For example, reporting the amount of inspired oxygen for blood gas measurements." ) 1062 protected List<CodeableReference> supportingInfo; 1063 1064 /** 1065 * One or more specimens that the laboratory procedure will use. 1066 */ 1067 @Child(name = "specimen", type = {Specimen.class}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1068 @Description(shortDefinition="Procedure Samples", formalDefinition="One or more specimens that the laboratory procedure will use." ) 1069 protected List<Reference> specimen; 1070 1071 /** 1072 * Anatomic location where the procedure should be performed. This is the target site. 1073 */ 1074 @Child(name = "bodySite", type = {CodeableConcept.class}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1075 @Description(shortDefinition="Coded location on Body", formalDefinition="Anatomic location where the procedure should be performed. This is the target site." ) 1076 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 1077 protected List<CodeableConcept> bodySite; 1078 1079 /** 1080 * Anatomic location where the procedure should be performed. This is the target site. 1081 */ 1082 @Child(name = "bodyStructure", type = {BodyStructure.class}, order=29, min=0, max=1, modifier=false, summary=true) 1083 @Description(shortDefinition="BodyStructure-based location on the body", formalDefinition="Anatomic location where the procedure should be performed. This is the target site." ) 1084 protected Reference bodyStructure; 1085 1086 /** 1087 * Any other notes and comments made about the service request. For example, internal billing notes. 1088 */ 1089 @Child(name = "note", type = {Annotation.class}, order=30, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1090 @Description(shortDefinition="Comments", formalDefinition="Any other notes and comments made about the service request. For example, internal billing notes." ) 1091 protected List<Annotation> note; 1092 1093 /** 1094 * Instructions in terms that are understood by the patient or consumer. 1095 */ 1096 @Child(name = "patientInstruction", type = {}, order=31, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1097 @Description(shortDefinition="Patient or consumer-oriented instructions", formalDefinition="Instructions in terms that are understood by the patient or consumer." ) 1098 protected List<ServiceRequestPatientInstructionComponent> patientInstruction; 1099 1100 /** 1101 * Key events in the history of the request. 1102 */ 1103 @Child(name = "relevantHistory", type = {Provenance.class}, order=32, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1104 @Description(shortDefinition="Request provenance", formalDefinition="Key events in the history of the request." ) 1105 protected List<Reference> relevantHistory; 1106 1107 private static final long serialVersionUID = -1785672942L; 1108 1109 /** 1110 * Constructor 1111 */ 1112 public ServiceRequest() { 1113 super(); 1114 } 1115 1116 /** 1117 * Constructor 1118 */ 1119 public ServiceRequest(RequestStatus status, RequestIntent intent, Reference subject) { 1120 super(); 1121 this.setStatus(status); 1122 this.setIntent(intent); 1123 this.setSubject(subject); 1124 } 1125 1126 /** 1127 * @return {@link #identifier} (Identifiers assigned to this order instance by the orderer and/or the receiver and/or order fulfiller.) 1128 */ 1129 public List<Identifier> getIdentifier() { 1130 if (this.identifier == null) 1131 this.identifier = new ArrayList<Identifier>(); 1132 return this.identifier; 1133 } 1134 1135 /** 1136 * @return Returns a reference to <code>this</code> for easy method chaining 1137 */ 1138 public ServiceRequest setIdentifier(List<Identifier> theIdentifier) { 1139 this.identifier = theIdentifier; 1140 return this; 1141 } 1142 1143 public boolean hasIdentifier() { 1144 if (this.identifier == null) 1145 return false; 1146 for (Identifier item : this.identifier) 1147 if (!item.isEmpty()) 1148 return true; 1149 return false; 1150 } 1151 1152 public Identifier addIdentifier() { //3 1153 Identifier t = new Identifier(); 1154 if (this.identifier == null) 1155 this.identifier = new ArrayList<Identifier>(); 1156 this.identifier.add(t); 1157 return t; 1158 } 1159 1160 public ServiceRequest addIdentifier(Identifier t) { //3 1161 if (t == null) 1162 return this; 1163 if (this.identifier == null) 1164 this.identifier = new ArrayList<Identifier>(); 1165 this.identifier.add(t); 1166 return this; 1167 } 1168 1169 /** 1170 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1171 */ 1172 public Identifier getIdentifierFirstRep() { 1173 if (getIdentifier().isEmpty()) { 1174 addIdentifier(); 1175 } 1176 return getIdentifier().get(0); 1177 } 1178 1179 /** 1180 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest.) 1181 */ 1182 public List<CanonicalType> getInstantiatesCanonical() { 1183 if (this.instantiatesCanonical == null) 1184 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1185 return this.instantiatesCanonical; 1186 } 1187 1188 /** 1189 * @return Returns a reference to <code>this</code> for easy method chaining 1190 */ 1191 public ServiceRequest setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 1192 this.instantiatesCanonical = theInstantiatesCanonical; 1193 return this; 1194 } 1195 1196 public boolean hasInstantiatesCanonical() { 1197 if (this.instantiatesCanonical == null) 1198 return false; 1199 for (CanonicalType item : this.instantiatesCanonical) 1200 if (!item.isEmpty()) 1201 return true; 1202 return false; 1203 } 1204 1205 /** 1206 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest.) 1207 */ 1208 public CanonicalType addInstantiatesCanonicalElement() {//2 1209 CanonicalType t = new CanonicalType(); 1210 if (this.instantiatesCanonical == null) 1211 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1212 this.instantiatesCanonical.add(t); 1213 return t; 1214 } 1215 1216 /** 1217 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest.) 1218 */ 1219 public ServiceRequest addInstantiatesCanonical(String value) { //1 1220 CanonicalType t = new CanonicalType(); 1221 t.setValue(value); 1222 if (this.instantiatesCanonical == null) 1223 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1224 this.instantiatesCanonical.add(t); 1225 return this; 1226 } 1227 1228 /** 1229 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest.) 1230 */ 1231 public boolean hasInstantiatesCanonical(String value) { 1232 if (this.instantiatesCanonical == null) 1233 return false; 1234 for (CanonicalType v : this.instantiatesCanonical) 1235 if (v.getValue().equals(value)) // canonical 1236 return true; 1237 return false; 1238 } 1239 1240 /** 1241 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest.) 1242 */ 1243 public List<UriType> getInstantiatesUri() { 1244 if (this.instantiatesUri == null) 1245 this.instantiatesUri = new ArrayList<UriType>(); 1246 return this.instantiatesUri; 1247 } 1248 1249 /** 1250 * @return Returns a reference to <code>this</code> for easy method chaining 1251 */ 1252 public ServiceRequest setInstantiatesUri(List<UriType> theInstantiatesUri) { 1253 this.instantiatesUri = theInstantiatesUri; 1254 return this; 1255 } 1256 1257 public boolean hasInstantiatesUri() { 1258 if (this.instantiatesUri == null) 1259 return false; 1260 for (UriType item : this.instantiatesUri) 1261 if (!item.isEmpty()) 1262 return true; 1263 return false; 1264 } 1265 1266 /** 1267 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest.) 1268 */ 1269 public UriType addInstantiatesUriElement() {//2 1270 UriType t = new UriType(); 1271 if (this.instantiatesUri == null) 1272 this.instantiatesUri = new ArrayList<UriType>(); 1273 this.instantiatesUri.add(t); 1274 return t; 1275 } 1276 1277 /** 1278 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest.) 1279 */ 1280 public ServiceRequest addInstantiatesUri(String value) { //1 1281 UriType t = new UriType(); 1282 t.setValue(value); 1283 if (this.instantiatesUri == null) 1284 this.instantiatesUri = new ArrayList<UriType>(); 1285 this.instantiatesUri.add(t); 1286 return this; 1287 } 1288 1289 /** 1290 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest.) 1291 */ 1292 public boolean hasInstantiatesUri(String value) { 1293 if (this.instantiatesUri == null) 1294 return false; 1295 for (UriType v : this.instantiatesUri) 1296 if (v.getValue().equals(value)) // uri 1297 return true; 1298 return false; 1299 } 1300 1301 /** 1302 * @return {@link #basedOn} (Plan/proposal/order fulfilled by this request.) 1303 */ 1304 public List<Reference> getBasedOn() { 1305 if (this.basedOn == null) 1306 this.basedOn = new ArrayList<Reference>(); 1307 return this.basedOn; 1308 } 1309 1310 /** 1311 * @return Returns a reference to <code>this</code> for easy method chaining 1312 */ 1313 public ServiceRequest setBasedOn(List<Reference> theBasedOn) { 1314 this.basedOn = theBasedOn; 1315 return this; 1316 } 1317 1318 public boolean hasBasedOn() { 1319 if (this.basedOn == null) 1320 return false; 1321 for (Reference item : this.basedOn) 1322 if (!item.isEmpty()) 1323 return true; 1324 return false; 1325 } 1326 1327 public Reference addBasedOn() { //3 1328 Reference t = new Reference(); 1329 if (this.basedOn == null) 1330 this.basedOn = new ArrayList<Reference>(); 1331 this.basedOn.add(t); 1332 return t; 1333 } 1334 1335 public ServiceRequest addBasedOn(Reference t) { //3 1336 if (t == null) 1337 return this; 1338 if (this.basedOn == null) 1339 this.basedOn = new ArrayList<Reference>(); 1340 this.basedOn.add(t); 1341 return this; 1342 } 1343 1344 /** 1345 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 1346 */ 1347 public Reference getBasedOnFirstRep() { 1348 if (getBasedOn().isEmpty()) { 1349 addBasedOn(); 1350 } 1351 return getBasedOn().get(0); 1352 } 1353 1354 /** 1355 * @return {@link #replaces} (The request takes the place of the referenced completed or terminated request(s).) 1356 */ 1357 public List<Reference> getReplaces() { 1358 if (this.replaces == null) 1359 this.replaces = new ArrayList<Reference>(); 1360 return this.replaces; 1361 } 1362 1363 /** 1364 * @return Returns a reference to <code>this</code> for easy method chaining 1365 */ 1366 public ServiceRequest setReplaces(List<Reference> theReplaces) { 1367 this.replaces = theReplaces; 1368 return this; 1369 } 1370 1371 public boolean hasReplaces() { 1372 if (this.replaces == null) 1373 return false; 1374 for (Reference item : this.replaces) 1375 if (!item.isEmpty()) 1376 return true; 1377 return false; 1378 } 1379 1380 public Reference addReplaces() { //3 1381 Reference t = new Reference(); 1382 if (this.replaces == null) 1383 this.replaces = new ArrayList<Reference>(); 1384 this.replaces.add(t); 1385 return t; 1386 } 1387 1388 public ServiceRequest addReplaces(Reference t) { //3 1389 if (t == null) 1390 return this; 1391 if (this.replaces == null) 1392 this.replaces = new ArrayList<Reference>(); 1393 this.replaces.add(t); 1394 return this; 1395 } 1396 1397 /** 1398 * @return The first repetition of repeating field {@link #replaces}, creating it if it does not already exist {3} 1399 */ 1400 public Reference getReplacesFirstRep() { 1401 if (getReplaces().isEmpty()) { 1402 addReplaces(); 1403 } 1404 return getReplaces().get(0); 1405 } 1406 1407 /** 1408 * @return {@link #requisition} (A shared identifier common to all service requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier.) 1409 */ 1410 public Identifier getRequisition() { 1411 if (this.requisition == null) 1412 if (Configuration.errorOnAutoCreate()) 1413 throw new Error("Attempt to auto-create ServiceRequest.requisition"); 1414 else if (Configuration.doAutoCreate()) 1415 this.requisition = new Identifier(); // cc 1416 return this.requisition; 1417 } 1418 1419 public boolean hasRequisition() { 1420 return this.requisition != null && !this.requisition.isEmpty(); 1421 } 1422 1423 /** 1424 * @param value {@link #requisition} (A shared identifier common to all service requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier.) 1425 */ 1426 public ServiceRequest setRequisition(Identifier value) { 1427 this.requisition = value; 1428 return this; 1429 } 1430 1431 /** 1432 * @return {@link #status} (The status of the order.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1433 */ 1434 public Enumeration<RequestStatus> getStatusElement() { 1435 if (this.status == null) 1436 if (Configuration.errorOnAutoCreate()) 1437 throw new Error("Attempt to auto-create ServiceRequest.status"); 1438 else if (Configuration.doAutoCreate()) 1439 this.status = new Enumeration<RequestStatus>(new RequestStatusEnumFactory()); // bb 1440 return this.status; 1441 } 1442 1443 public boolean hasStatusElement() { 1444 return this.status != null && !this.status.isEmpty(); 1445 } 1446 1447 public boolean hasStatus() { 1448 return this.status != null && !this.status.isEmpty(); 1449 } 1450 1451 /** 1452 * @param value {@link #status} (The status of the order.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1453 */ 1454 public ServiceRequest setStatusElement(Enumeration<RequestStatus> value) { 1455 this.status = value; 1456 return this; 1457 } 1458 1459 /** 1460 * @return The status of the order. 1461 */ 1462 public RequestStatus getStatus() { 1463 return this.status == null ? null : this.status.getValue(); 1464 } 1465 1466 /** 1467 * @param value The status of the order. 1468 */ 1469 public ServiceRequest setStatus(RequestStatus value) { 1470 if (this.status == null) 1471 this.status = new Enumeration<RequestStatus>(new RequestStatusEnumFactory()); 1472 this.status.setValue(value); 1473 return this; 1474 } 1475 1476 /** 1477 * @return {@link #intent} (Whether the request is a proposal, plan, an original order or a reflex order.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 1478 */ 1479 public Enumeration<RequestIntent> getIntentElement() { 1480 if (this.intent == null) 1481 if (Configuration.errorOnAutoCreate()) 1482 throw new Error("Attempt to auto-create ServiceRequest.intent"); 1483 else if (Configuration.doAutoCreate()) 1484 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); // bb 1485 return this.intent; 1486 } 1487 1488 public boolean hasIntentElement() { 1489 return this.intent != null && !this.intent.isEmpty(); 1490 } 1491 1492 public boolean hasIntent() { 1493 return this.intent != null && !this.intent.isEmpty(); 1494 } 1495 1496 /** 1497 * @param value {@link #intent} (Whether the request is a proposal, plan, an original order or a reflex order.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 1498 */ 1499 public ServiceRequest setIntentElement(Enumeration<RequestIntent> value) { 1500 this.intent = value; 1501 return this; 1502 } 1503 1504 /** 1505 * @return Whether the request is a proposal, plan, an original order or a reflex order. 1506 */ 1507 public RequestIntent getIntent() { 1508 return this.intent == null ? null : this.intent.getValue(); 1509 } 1510 1511 /** 1512 * @param value Whether the request is a proposal, plan, an original order or a reflex order. 1513 */ 1514 public ServiceRequest setIntent(RequestIntent value) { 1515 if (this.intent == null) 1516 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); 1517 this.intent.setValue(value); 1518 return this; 1519 } 1520 1521 /** 1522 * @return {@link #category} (A code that classifies the service for searching, sorting and display purposes (e.g. "Surgical Procedure").) 1523 */ 1524 public List<CodeableConcept> getCategory() { 1525 if (this.category == null) 1526 this.category = new ArrayList<CodeableConcept>(); 1527 return this.category; 1528 } 1529 1530 /** 1531 * @return Returns a reference to <code>this</code> for easy method chaining 1532 */ 1533 public ServiceRequest setCategory(List<CodeableConcept> theCategory) { 1534 this.category = theCategory; 1535 return this; 1536 } 1537 1538 public boolean hasCategory() { 1539 if (this.category == null) 1540 return false; 1541 for (CodeableConcept item : this.category) 1542 if (!item.isEmpty()) 1543 return true; 1544 return false; 1545 } 1546 1547 public CodeableConcept addCategory() { //3 1548 CodeableConcept t = new CodeableConcept(); 1549 if (this.category == null) 1550 this.category = new ArrayList<CodeableConcept>(); 1551 this.category.add(t); 1552 return t; 1553 } 1554 1555 public ServiceRequest addCategory(CodeableConcept t) { //3 1556 if (t == null) 1557 return this; 1558 if (this.category == null) 1559 this.category = new ArrayList<CodeableConcept>(); 1560 this.category.add(t); 1561 return this; 1562 } 1563 1564 /** 1565 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 1566 */ 1567 public CodeableConcept getCategoryFirstRep() { 1568 if (getCategory().isEmpty()) { 1569 addCategory(); 1570 } 1571 return getCategory().get(0); 1572 } 1573 1574 /** 1575 * @return {@link #priority} (Indicates how quickly the ServiceRequest should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 1576 */ 1577 public Enumeration<RequestPriority> getPriorityElement() { 1578 if (this.priority == null) 1579 if (Configuration.errorOnAutoCreate()) 1580 throw new Error("Attempt to auto-create ServiceRequest.priority"); 1581 else if (Configuration.doAutoCreate()) 1582 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 1583 return this.priority; 1584 } 1585 1586 public boolean hasPriorityElement() { 1587 return this.priority != null && !this.priority.isEmpty(); 1588 } 1589 1590 public boolean hasPriority() { 1591 return this.priority != null && !this.priority.isEmpty(); 1592 } 1593 1594 /** 1595 * @param value {@link #priority} (Indicates how quickly the ServiceRequest should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 1596 */ 1597 public ServiceRequest setPriorityElement(Enumeration<RequestPriority> value) { 1598 this.priority = value; 1599 return this; 1600 } 1601 1602 /** 1603 * @return Indicates how quickly the ServiceRequest should be addressed with respect to other requests. 1604 */ 1605 public RequestPriority getPriority() { 1606 return this.priority == null ? null : this.priority.getValue(); 1607 } 1608 1609 /** 1610 * @param value Indicates how quickly the ServiceRequest should be addressed with respect to other requests. 1611 */ 1612 public ServiceRequest setPriority(RequestPriority value) { 1613 if (value == null) 1614 this.priority = null; 1615 else { 1616 if (this.priority == null) 1617 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 1618 this.priority.setValue(value); 1619 } 1620 return this; 1621 } 1622 1623 /** 1624 * @return {@link #doNotPerform} (Set this to true if the record is saying that the service/procedure should NOT be performed.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 1625 */ 1626 public BooleanType getDoNotPerformElement() { 1627 if (this.doNotPerform == null) 1628 if (Configuration.errorOnAutoCreate()) 1629 throw new Error("Attempt to auto-create ServiceRequest.doNotPerform"); 1630 else if (Configuration.doAutoCreate()) 1631 this.doNotPerform = new BooleanType(); // bb 1632 return this.doNotPerform; 1633 } 1634 1635 public boolean hasDoNotPerformElement() { 1636 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 1637 } 1638 1639 public boolean hasDoNotPerform() { 1640 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 1641 } 1642 1643 /** 1644 * @param value {@link #doNotPerform} (Set this to true if the record is saying that the service/procedure should NOT be performed.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 1645 */ 1646 public ServiceRequest setDoNotPerformElement(BooleanType value) { 1647 this.doNotPerform = value; 1648 return this; 1649 } 1650 1651 /** 1652 * @return Set this to true if the record is saying that the service/procedure should NOT be performed. 1653 */ 1654 public boolean getDoNotPerform() { 1655 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 1656 } 1657 1658 /** 1659 * @param value Set this to true if the record is saying that the service/procedure should NOT be performed. 1660 */ 1661 public ServiceRequest setDoNotPerform(boolean value) { 1662 if (this.doNotPerform == null) 1663 this.doNotPerform = new BooleanType(); 1664 this.doNotPerform.setValue(value); 1665 return this; 1666 } 1667 1668 /** 1669 * @return {@link #code} (A code or reference that identifies a particular service (i.e., procedure, diagnostic investigation, or panel of investigations) that have been requested.) 1670 */ 1671 public CodeableReference getCode() { 1672 if (this.code == null) 1673 if (Configuration.errorOnAutoCreate()) 1674 throw new Error("Attempt to auto-create ServiceRequest.code"); 1675 else if (Configuration.doAutoCreate()) 1676 this.code = new CodeableReference(); // cc 1677 return this.code; 1678 } 1679 1680 public boolean hasCode() { 1681 return this.code != null && !this.code.isEmpty(); 1682 } 1683 1684 /** 1685 * @param value {@link #code} (A code or reference that identifies a particular service (i.e., procedure, diagnostic investigation, or panel of investigations) that have been requested.) 1686 */ 1687 public ServiceRequest setCode(CodeableReference value) { 1688 this.code = value; 1689 return this; 1690 } 1691 1692 /** 1693 * @return {@link #orderDetail} (Additional details and instructions about the how the services are to be delivered. For example, and order for a urinary catheter may have an order detail for an external or indwelling catheter, or an order for a bandage may require additional instructions specifying how the bandage should be applied.) 1694 */ 1695 public List<ServiceRequestOrderDetailComponent> getOrderDetail() { 1696 if (this.orderDetail == null) 1697 this.orderDetail = new ArrayList<ServiceRequestOrderDetailComponent>(); 1698 return this.orderDetail; 1699 } 1700 1701 /** 1702 * @return Returns a reference to <code>this</code> for easy method chaining 1703 */ 1704 public ServiceRequest setOrderDetail(List<ServiceRequestOrderDetailComponent> theOrderDetail) { 1705 this.orderDetail = theOrderDetail; 1706 return this; 1707 } 1708 1709 public boolean hasOrderDetail() { 1710 if (this.orderDetail == null) 1711 return false; 1712 for (ServiceRequestOrderDetailComponent item : this.orderDetail) 1713 if (!item.isEmpty()) 1714 return true; 1715 return false; 1716 } 1717 1718 public ServiceRequestOrderDetailComponent addOrderDetail() { //3 1719 ServiceRequestOrderDetailComponent t = new ServiceRequestOrderDetailComponent(); 1720 if (this.orderDetail == null) 1721 this.orderDetail = new ArrayList<ServiceRequestOrderDetailComponent>(); 1722 this.orderDetail.add(t); 1723 return t; 1724 } 1725 1726 public ServiceRequest addOrderDetail(ServiceRequestOrderDetailComponent t) { //3 1727 if (t == null) 1728 return this; 1729 if (this.orderDetail == null) 1730 this.orderDetail = new ArrayList<ServiceRequestOrderDetailComponent>(); 1731 this.orderDetail.add(t); 1732 return this; 1733 } 1734 1735 /** 1736 * @return The first repetition of repeating field {@link #orderDetail}, creating it if it does not already exist {3} 1737 */ 1738 public ServiceRequestOrderDetailComponent getOrderDetailFirstRep() { 1739 if (getOrderDetail().isEmpty()) { 1740 addOrderDetail(); 1741 } 1742 return getOrderDetail().get(0); 1743 } 1744 1745 /** 1746 * @return {@link #quantity} (An amount of service being requested which can be a quantity ( for example $1,500 home modification), a ratio ( for example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per fraction).) 1747 */ 1748 public DataType getQuantity() { 1749 return this.quantity; 1750 } 1751 1752 /** 1753 * @return {@link #quantity} (An amount of service being requested which can be a quantity ( for example $1,500 home modification), a ratio ( for example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per fraction).) 1754 */ 1755 public Quantity getQuantityQuantity() throws FHIRException { 1756 if (this.quantity == null) 1757 this.quantity = new Quantity(); 1758 if (!(this.quantity instanceof Quantity)) 1759 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.quantity.getClass().getName()+" was encountered"); 1760 return (Quantity) this.quantity; 1761 } 1762 1763 public boolean hasQuantityQuantity() { 1764 return this != null && this.quantity instanceof Quantity; 1765 } 1766 1767 /** 1768 * @return {@link #quantity} (An amount of service being requested which can be a quantity ( for example $1,500 home modification), a ratio ( for example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per fraction).) 1769 */ 1770 public Ratio getQuantityRatio() throws FHIRException { 1771 if (this.quantity == null) 1772 this.quantity = new Ratio(); 1773 if (!(this.quantity instanceof Ratio)) 1774 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.quantity.getClass().getName()+" was encountered"); 1775 return (Ratio) this.quantity; 1776 } 1777 1778 public boolean hasQuantityRatio() { 1779 return this != null && this.quantity instanceof Ratio; 1780 } 1781 1782 /** 1783 * @return {@link #quantity} (An amount of service being requested which can be a quantity ( for example $1,500 home modification), a ratio ( for example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per fraction).) 1784 */ 1785 public Range getQuantityRange() throws FHIRException { 1786 if (this.quantity == null) 1787 this.quantity = new Range(); 1788 if (!(this.quantity instanceof Range)) 1789 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.quantity.getClass().getName()+" was encountered"); 1790 return (Range) this.quantity; 1791 } 1792 1793 public boolean hasQuantityRange() { 1794 return this != null && this.quantity instanceof Range; 1795 } 1796 1797 public boolean hasQuantity() { 1798 return this.quantity != null && !this.quantity.isEmpty(); 1799 } 1800 1801 /** 1802 * @param value {@link #quantity} (An amount of service being requested which can be a quantity ( for example $1,500 home modification), a ratio ( for example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per fraction).) 1803 */ 1804 public ServiceRequest setQuantity(DataType value) { 1805 if (value != null && !(value instanceof Quantity || value instanceof Ratio || value instanceof Range)) 1806 throw new FHIRException("Not the right type for ServiceRequest.quantity[x]: "+value.fhirType()); 1807 this.quantity = value; 1808 return this; 1809 } 1810 1811 /** 1812 * @return {@link #subject} (On whom or what the service is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).) 1813 */ 1814 public Reference getSubject() { 1815 if (this.subject == null) 1816 if (Configuration.errorOnAutoCreate()) 1817 throw new Error("Attempt to auto-create ServiceRequest.subject"); 1818 else if (Configuration.doAutoCreate()) 1819 this.subject = new Reference(); // cc 1820 return this.subject; 1821 } 1822 1823 public boolean hasSubject() { 1824 return this.subject != null && !this.subject.isEmpty(); 1825 } 1826 1827 /** 1828 * @param value {@link #subject} (On whom or what the service is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).) 1829 */ 1830 public ServiceRequest setSubject(Reference value) { 1831 this.subject = value; 1832 return this; 1833 } 1834 1835 /** 1836 * @return {@link #focus} (The actual focus of a service request when it is not the subject of record representing something or someone associated with the subject such as a spouse, parent, fetus, or donor. The focus of a service request could also be an existing condition, an intervention, the subject's diet, another service request on the subject, or a body structure such as tumor or implanted device.) 1837 */ 1838 public List<Reference> getFocus() { 1839 if (this.focus == null) 1840 this.focus = new ArrayList<Reference>(); 1841 return this.focus; 1842 } 1843 1844 /** 1845 * @return Returns a reference to <code>this</code> for easy method chaining 1846 */ 1847 public ServiceRequest setFocus(List<Reference> theFocus) { 1848 this.focus = theFocus; 1849 return this; 1850 } 1851 1852 public boolean hasFocus() { 1853 if (this.focus == null) 1854 return false; 1855 for (Reference item : this.focus) 1856 if (!item.isEmpty()) 1857 return true; 1858 return false; 1859 } 1860 1861 public Reference addFocus() { //3 1862 Reference t = new Reference(); 1863 if (this.focus == null) 1864 this.focus = new ArrayList<Reference>(); 1865 this.focus.add(t); 1866 return t; 1867 } 1868 1869 public ServiceRequest addFocus(Reference t) { //3 1870 if (t == null) 1871 return this; 1872 if (this.focus == null) 1873 this.focus = new ArrayList<Reference>(); 1874 this.focus.add(t); 1875 return this; 1876 } 1877 1878 /** 1879 * @return The first repetition of repeating field {@link #focus}, creating it if it does not already exist {3} 1880 */ 1881 public Reference getFocusFirstRep() { 1882 if (getFocus().isEmpty()) { 1883 addFocus(); 1884 } 1885 return getFocus().get(0); 1886 } 1887 1888 /** 1889 * @return {@link #encounter} (An encounter that provides additional information about the healthcare context in which this request is made.) 1890 */ 1891 public Reference getEncounter() { 1892 if (this.encounter == null) 1893 if (Configuration.errorOnAutoCreate()) 1894 throw new Error("Attempt to auto-create ServiceRequest.encounter"); 1895 else if (Configuration.doAutoCreate()) 1896 this.encounter = new Reference(); // cc 1897 return this.encounter; 1898 } 1899 1900 public boolean hasEncounter() { 1901 return this.encounter != null && !this.encounter.isEmpty(); 1902 } 1903 1904 /** 1905 * @param value {@link #encounter} (An encounter that provides additional information about the healthcare context in which this request is made.) 1906 */ 1907 public ServiceRequest setEncounter(Reference value) { 1908 this.encounter = value; 1909 return this; 1910 } 1911 1912 /** 1913 * @return {@link #occurrence} (The date/time at which the requested service should occur.) 1914 */ 1915 public DataType getOccurrence() { 1916 return this.occurrence; 1917 } 1918 1919 /** 1920 * @return {@link #occurrence} (The date/time at which the requested service should occur.) 1921 */ 1922 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1923 if (this.occurrence == null) 1924 this.occurrence = new DateTimeType(); 1925 if (!(this.occurrence instanceof DateTimeType)) 1926 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1927 return (DateTimeType) this.occurrence; 1928 } 1929 1930 public boolean hasOccurrenceDateTimeType() { 1931 return this != null && this.occurrence instanceof DateTimeType; 1932 } 1933 1934 /** 1935 * @return {@link #occurrence} (The date/time at which the requested service should occur.) 1936 */ 1937 public Period getOccurrencePeriod() throws FHIRException { 1938 if (this.occurrence == null) 1939 this.occurrence = new Period(); 1940 if (!(this.occurrence instanceof Period)) 1941 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1942 return (Period) this.occurrence; 1943 } 1944 1945 public boolean hasOccurrencePeriod() { 1946 return this != null && this.occurrence instanceof Period; 1947 } 1948 1949 /** 1950 * @return {@link #occurrence} (The date/time at which the requested service should occur.) 1951 */ 1952 public Timing getOccurrenceTiming() throws FHIRException { 1953 if (this.occurrence == null) 1954 this.occurrence = new Timing(); 1955 if (!(this.occurrence instanceof Timing)) 1956 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1957 return (Timing) this.occurrence; 1958 } 1959 1960 public boolean hasOccurrenceTiming() { 1961 return this != null && this.occurrence instanceof Timing; 1962 } 1963 1964 public boolean hasOccurrence() { 1965 return this.occurrence != null && !this.occurrence.isEmpty(); 1966 } 1967 1968 /** 1969 * @param value {@link #occurrence} (The date/time at which the requested service should occur.) 1970 */ 1971 public ServiceRequest setOccurrence(DataType value) { 1972 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 1973 throw new FHIRException("Not the right type for ServiceRequest.occurrence[x]: "+value.fhirType()); 1974 this.occurrence = value; 1975 return this; 1976 } 1977 1978 /** 1979 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example "pain", "on flare-up", etc.) 1980 */ 1981 public DataType getAsNeeded() { 1982 return this.asNeeded; 1983 } 1984 1985 /** 1986 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example "pain", "on flare-up", etc.) 1987 */ 1988 public BooleanType getAsNeededBooleanType() throws FHIRException { 1989 if (this.asNeeded == null) 1990 this.asNeeded = new BooleanType(); 1991 if (!(this.asNeeded instanceof BooleanType)) 1992 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.asNeeded.getClass().getName()+" was encountered"); 1993 return (BooleanType) this.asNeeded; 1994 } 1995 1996 public boolean hasAsNeededBooleanType() { 1997 return this != null && this.asNeeded instanceof BooleanType; 1998 } 1999 2000 /** 2001 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example "pain", "on flare-up", etc.) 2002 */ 2003 public CodeableConcept getAsNeededCodeableConcept() throws FHIRException { 2004 if (this.asNeeded == null) 2005 this.asNeeded = new CodeableConcept(); 2006 if (!(this.asNeeded instanceof CodeableConcept)) 2007 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.asNeeded.getClass().getName()+" was encountered"); 2008 return (CodeableConcept) this.asNeeded; 2009 } 2010 2011 public boolean hasAsNeededCodeableConcept() { 2012 return this != null && this.asNeeded instanceof CodeableConcept; 2013 } 2014 2015 public boolean hasAsNeeded() { 2016 return this.asNeeded != null && !this.asNeeded.isEmpty(); 2017 } 2018 2019 /** 2020 * @param value {@link #asNeeded} (If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example "pain", "on flare-up", etc.) 2021 */ 2022 public ServiceRequest setAsNeeded(DataType value) { 2023 if (value != null && !(value instanceof BooleanType || value instanceof CodeableConcept)) 2024 throw new FHIRException("Not the right type for ServiceRequest.asNeeded[x]: "+value.fhirType()); 2025 this.asNeeded = value; 2026 return this; 2027 } 2028 2029 /** 2030 * @return {@link #authoredOn} (When the request transitioned to being actionable.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 2031 */ 2032 public DateTimeType getAuthoredOnElement() { 2033 if (this.authoredOn == null) 2034 if (Configuration.errorOnAutoCreate()) 2035 throw new Error("Attempt to auto-create ServiceRequest.authoredOn"); 2036 else if (Configuration.doAutoCreate()) 2037 this.authoredOn = new DateTimeType(); // bb 2038 return this.authoredOn; 2039 } 2040 2041 public boolean hasAuthoredOnElement() { 2042 return this.authoredOn != null && !this.authoredOn.isEmpty(); 2043 } 2044 2045 public boolean hasAuthoredOn() { 2046 return this.authoredOn != null && !this.authoredOn.isEmpty(); 2047 } 2048 2049 /** 2050 * @param value {@link #authoredOn} (When the request transitioned to being actionable.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 2051 */ 2052 public ServiceRequest setAuthoredOnElement(DateTimeType value) { 2053 this.authoredOn = value; 2054 return this; 2055 } 2056 2057 /** 2058 * @return When the request transitioned to being actionable. 2059 */ 2060 public Date getAuthoredOn() { 2061 return this.authoredOn == null ? null : this.authoredOn.getValue(); 2062 } 2063 2064 /** 2065 * @param value When the request transitioned to being actionable. 2066 */ 2067 public ServiceRequest setAuthoredOn(Date value) { 2068 if (value == null) 2069 this.authoredOn = null; 2070 else { 2071 if (this.authoredOn == null) 2072 this.authoredOn = new DateTimeType(); 2073 this.authoredOn.setValue(value); 2074 } 2075 return this; 2076 } 2077 2078 /** 2079 * @return {@link #requester} (The individual who initiated the request and has responsibility for its activation.) 2080 */ 2081 public Reference getRequester() { 2082 if (this.requester == null) 2083 if (Configuration.errorOnAutoCreate()) 2084 throw new Error("Attempt to auto-create ServiceRequest.requester"); 2085 else if (Configuration.doAutoCreate()) 2086 this.requester = new Reference(); // cc 2087 return this.requester; 2088 } 2089 2090 public boolean hasRequester() { 2091 return this.requester != null && !this.requester.isEmpty(); 2092 } 2093 2094 /** 2095 * @param value {@link #requester} (The individual who initiated the request and has responsibility for its activation.) 2096 */ 2097 public ServiceRequest setRequester(Reference value) { 2098 this.requester = value; 2099 return this; 2100 } 2101 2102 /** 2103 * @return {@link #performerType} (Desired type of performer for doing the requested service.) 2104 */ 2105 public CodeableConcept getPerformerType() { 2106 if (this.performerType == null) 2107 if (Configuration.errorOnAutoCreate()) 2108 throw new Error("Attempt to auto-create ServiceRequest.performerType"); 2109 else if (Configuration.doAutoCreate()) 2110 this.performerType = new CodeableConcept(); // cc 2111 return this.performerType; 2112 } 2113 2114 public boolean hasPerformerType() { 2115 return this.performerType != null && !this.performerType.isEmpty(); 2116 } 2117 2118 /** 2119 * @param value {@link #performerType} (Desired type of performer for doing the requested service.) 2120 */ 2121 public ServiceRequest setPerformerType(CodeableConcept value) { 2122 this.performerType = value; 2123 return this; 2124 } 2125 2126 /** 2127 * @return {@link #performer} (The desired performer for doing the requested service. For example, the surgeon, dermatopathologist, endoscopist, etc.) 2128 */ 2129 public List<Reference> getPerformer() { 2130 if (this.performer == null) 2131 this.performer = new ArrayList<Reference>(); 2132 return this.performer; 2133 } 2134 2135 /** 2136 * @return Returns a reference to <code>this</code> for easy method chaining 2137 */ 2138 public ServiceRequest setPerformer(List<Reference> thePerformer) { 2139 this.performer = thePerformer; 2140 return this; 2141 } 2142 2143 public boolean hasPerformer() { 2144 if (this.performer == null) 2145 return false; 2146 for (Reference item : this.performer) 2147 if (!item.isEmpty()) 2148 return true; 2149 return false; 2150 } 2151 2152 public Reference addPerformer() { //3 2153 Reference t = new Reference(); 2154 if (this.performer == null) 2155 this.performer = new ArrayList<Reference>(); 2156 this.performer.add(t); 2157 return t; 2158 } 2159 2160 public ServiceRequest addPerformer(Reference t) { //3 2161 if (t == null) 2162 return this; 2163 if (this.performer == null) 2164 this.performer = new ArrayList<Reference>(); 2165 this.performer.add(t); 2166 return this; 2167 } 2168 2169 /** 2170 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist {3} 2171 */ 2172 public Reference getPerformerFirstRep() { 2173 if (getPerformer().isEmpty()) { 2174 addPerformer(); 2175 } 2176 return getPerformer().get(0); 2177 } 2178 2179 /** 2180 * @return {@link #location} (The preferred location(s) where the procedure should actually happen in coded or free text form. E.g. at home or nursing day care center.) 2181 */ 2182 public List<CodeableReference> getLocation() { 2183 if (this.location == null) 2184 this.location = new ArrayList<CodeableReference>(); 2185 return this.location; 2186 } 2187 2188 /** 2189 * @return Returns a reference to <code>this</code> for easy method chaining 2190 */ 2191 public ServiceRequest setLocation(List<CodeableReference> theLocation) { 2192 this.location = theLocation; 2193 return this; 2194 } 2195 2196 public boolean hasLocation() { 2197 if (this.location == null) 2198 return false; 2199 for (CodeableReference item : this.location) 2200 if (!item.isEmpty()) 2201 return true; 2202 return false; 2203 } 2204 2205 public CodeableReference addLocation() { //3 2206 CodeableReference t = new CodeableReference(); 2207 if (this.location == null) 2208 this.location = new ArrayList<CodeableReference>(); 2209 this.location.add(t); 2210 return t; 2211 } 2212 2213 public ServiceRequest addLocation(CodeableReference t) { //3 2214 if (t == null) 2215 return this; 2216 if (this.location == null) 2217 this.location = new ArrayList<CodeableReference>(); 2218 this.location.add(t); 2219 return this; 2220 } 2221 2222 /** 2223 * @return The first repetition of repeating field {@link #location}, creating it if it does not already exist {3} 2224 */ 2225 public CodeableReference getLocationFirstRep() { 2226 if (getLocation().isEmpty()) { 2227 addLocation(); 2228 } 2229 return getLocation().get(0); 2230 } 2231 2232 /** 2233 * @return {@link #reason} (An explanation or justification for why this service is being requested in coded or textual form. This is often for billing purposes. May relate to the resources referred to in `supportingInfo`.) 2234 */ 2235 public List<CodeableReference> getReason() { 2236 if (this.reason == null) 2237 this.reason = new ArrayList<CodeableReference>(); 2238 return this.reason; 2239 } 2240 2241 /** 2242 * @return Returns a reference to <code>this</code> for easy method chaining 2243 */ 2244 public ServiceRequest setReason(List<CodeableReference> theReason) { 2245 this.reason = theReason; 2246 return this; 2247 } 2248 2249 public boolean hasReason() { 2250 if (this.reason == null) 2251 return false; 2252 for (CodeableReference item : this.reason) 2253 if (!item.isEmpty()) 2254 return true; 2255 return false; 2256 } 2257 2258 public CodeableReference addReason() { //3 2259 CodeableReference t = new CodeableReference(); 2260 if (this.reason == null) 2261 this.reason = new ArrayList<CodeableReference>(); 2262 this.reason.add(t); 2263 return t; 2264 } 2265 2266 public ServiceRequest addReason(CodeableReference t) { //3 2267 if (t == null) 2268 return this; 2269 if (this.reason == null) 2270 this.reason = new ArrayList<CodeableReference>(); 2271 this.reason.add(t); 2272 return this; 2273 } 2274 2275 /** 2276 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 2277 */ 2278 public CodeableReference getReasonFirstRep() { 2279 if (getReason().isEmpty()) { 2280 addReason(); 2281 } 2282 return getReason().get(0); 2283 } 2284 2285 /** 2286 * @return {@link #insurance} (Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be needed for delivering the requested service.) 2287 */ 2288 public List<Reference> getInsurance() { 2289 if (this.insurance == null) 2290 this.insurance = new ArrayList<Reference>(); 2291 return this.insurance; 2292 } 2293 2294 /** 2295 * @return Returns a reference to <code>this</code> for easy method chaining 2296 */ 2297 public ServiceRequest setInsurance(List<Reference> theInsurance) { 2298 this.insurance = theInsurance; 2299 return this; 2300 } 2301 2302 public boolean hasInsurance() { 2303 if (this.insurance == null) 2304 return false; 2305 for (Reference item : this.insurance) 2306 if (!item.isEmpty()) 2307 return true; 2308 return false; 2309 } 2310 2311 public Reference addInsurance() { //3 2312 Reference t = new Reference(); 2313 if (this.insurance == null) 2314 this.insurance = new ArrayList<Reference>(); 2315 this.insurance.add(t); 2316 return t; 2317 } 2318 2319 public ServiceRequest addInsurance(Reference t) { //3 2320 if (t == null) 2321 return this; 2322 if (this.insurance == null) 2323 this.insurance = new ArrayList<Reference>(); 2324 this.insurance.add(t); 2325 return this; 2326 } 2327 2328 /** 2329 * @return The first repetition of repeating field {@link #insurance}, creating it if it does not already exist {3} 2330 */ 2331 public Reference getInsuranceFirstRep() { 2332 if (getInsurance().isEmpty()) { 2333 addInsurance(); 2334 } 2335 return getInsurance().get(0); 2336 } 2337 2338 /** 2339 * @return {@link #supportingInfo} (Additional clinical information about the patient or specimen that may influence the services or their interpretations. This information includes diagnosis, clinical findings and other observations. In laboratory ordering these are typically referred to as "ask at order entry questions (AOEs)". This includes observations explicitly requested by the producer (filler) to provide context or supporting information needed to complete the order. For example, reporting the amount of inspired oxygen for blood gas measurements.) 2340 */ 2341 public List<CodeableReference> getSupportingInfo() { 2342 if (this.supportingInfo == null) 2343 this.supportingInfo = new ArrayList<CodeableReference>(); 2344 return this.supportingInfo; 2345 } 2346 2347 /** 2348 * @return Returns a reference to <code>this</code> for easy method chaining 2349 */ 2350 public ServiceRequest setSupportingInfo(List<CodeableReference> theSupportingInfo) { 2351 this.supportingInfo = theSupportingInfo; 2352 return this; 2353 } 2354 2355 public boolean hasSupportingInfo() { 2356 if (this.supportingInfo == null) 2357 return false; 2358 for (CodeableReference item : this.supportingInfo) 2359 if (!item.isEmpty()) 2360 return true; 2361 return false; 2362 } 2363 2364 public CodeableReference addSupportingInfo() { //3 2365 CodeableReference t = new CodeableReference(); 2366 if (this.supportingInfo == null) 2367 this.supportingInfo = new ArrayList<CodeableReference>(); 2368 this.supportingInfo.add(t); 2369 return t; 2370 } 2371 2372 public ServiceRequest addSupportingInfo(CodeableReference t) { //3 2373 if (t == null) 2374 return this; 2375 if (this.supportingInfo == null) 2376 this.supportingInfo = new ArrayList<CodeableReference>(); 2377 this.supportingInfo.add(t); 2378 return this; 2379 } 2380 2381 /** 2382 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist {3} 2383 */ 2384 public CodeableReference getSupportingInfoFirstRep() { 2385 if (getSupportingInfo().isEmpty()) { 2386 addSupportingInfo(); 2387 } 2388 return getSupportingInfo().get(0); 2389 } 2390 2391 /** 2392 * @return {@link #specimen} (One or more specimens that the laboratory procedure will use.) 2393 */ 2394 public List<Reference> getSpecimen() { 2395 if (this.specimen == null) 2396 this.specimen = new ArrayList<Reference>(); 2397 return this.specimen; 2398 } 2399 2400 /** 2401 * @return Returns a reference to <code>this</code> for easy method chaining 2402 */ 2403 public ServiceRequest setSpecimen(List<Reference> theSpecimen) { 2404 this.specimen = theSpecimen; 2405 return this; 2406 } 2407 2408 public boolean hasSpecimen() { 2409 if (this.specimen == null) 2410 return false; 2411 for (Reference item : this.specimen) 2412 if (!item.isEmpty()) 2413 return true; 2414 return false; 2415 } 2416 2417 public Reference addSpecimen() { //3 2418 Reference t = new Reference(); 2419 if (this.specimen == null) 2420 this.specimen = new ArrayList<Reference>(); 2421 this.specimen.add(t); 2422 return t; 2423 } 2424 2425 public ServiceRequest addSpecimen(Reference t) { //3 2426 if (t == null) 2427 return this; 2428 if (this.specimen == null) 2429 this.specimen = new ArrayList<Reference>(); 2430 this.specimen.add(t); 2431 return this; 2432 } 2433 2434 /** 2435 * @return The first repetition of repeating field {@link #specimen}, creating it if it does not already exist {3} 2436 */ 2437 public Reference getSpecimenFirstRep() { 2438 if (getSpecimen().isEmpty()) { 2439 addSpecimen(); 2440 } 2441 return getSpecimen().get(0); 2442 } 2443 2444 /** 2445 * @return {@link #bodySite} (Anatomic location where the procedure should be performed. This is the target site.) 2446 */ 2447 public List<CodeableConcept> getBodySite() { 2448 if (this.bodySite == null) 2449 this.bodySite = new ArrayList<CodeableConcept>(); 2450 return this.bodySite; 2451 } 2452 2453 /** 2454 * @return Returns a reference to <code>this</code> for easy method chaining 2455 */ 2456 public ServiceRequest setBodySite(List<CodeableConcept> theBodySite) { 2457 this.bodySite = theBodySite; 2458 return this; 2459 } 2460 2461 public boolean hasBodySite() { 2462 if (this.bodySite == null) 2463 return false; 2464 for (CodeableConcept item : this.bodySite) 2465 if (!item.isEmpty()) 2466 return true; 2467 return false; 2468 } 2469 2470 public CodeableConcept addBodySite() { //3 2471 CodeableConcept t = new CodeableConcept(); 2472 if (this.bodySite == null) 2473 this.bodySite = new ArrayList<CodeableConcept>(); 2474 this.bodySite.add(t); 2475 return t; 2476 } 2477 2478 public ServiceRequest addBodySite(CodeableConcept t) { //3 2479 if (t == null) 2480 return this; 2481 if (this.bodySite == null) 2482 this.bodySite = new ArrayList<CodeableConcept>(); 2483 this.bodySite.add(t); 2484 return this; 2485 } 2486 2487 /** 2488 * @return The first repetition of repeating field {@link #bodySite}, creating it if it does not already exist {3} 2489 */ 2490 public CodeableConcept getBodySiteFirstRep() { 2491 if (getBodySite().isEmpty()) { 2492 addBodySite(); 2493 } 2494 return getBodySite().get(0); 2495 } 2496 2497 /** 2498 * @return {@link #bodyStructure} (Anatomic location where the procedure should be performed. This is the target site.) 2499 */ 2500 public Reference getBodyStructure() { 2501 if (this.bodyStructure == null) 2502 if (Configuration.errorOnAutoCreate()) 2503 throw new Error("Attempt to auto-create ServiceRequest.bodyStructure"); 2504 else if (Configuration.doAutoCreate()) 2505 this.bodyStructure = new Reference(); // cc 2506 return this.bodyStructure; 2507 } 2508 2509 public boolean hasBodyStructure() { 2510 return this.bodyStructure != null && !this.bodyStructure.isEmpty(); 2511 } 2512 2513 /** 2514 * @param value {@link #bodyStructure} (Anatomic location where the procedure should be performed. This is the target site.) 2515 */ 2516 public ServiceRequest setBodyStructure(Reference value) { 2517 this.bodyStructure = value; 2518 return this; 2519 } 2520 2521 /** 2522 * @return {@link #note} (Any other notes and comments made about the service request. For example, internal billing notes.) 2523 */ 2524 public List<Annotation> getNote() { 2525 if (this.note == null) 2526 this.note = new ArrayList<Annotation>(); 2527 return this.note; 2528 } 2529 2530 /** 2531 * @return Returns a reference to <code>this</code> for easy method chaining 2532 */ 2533 public ServiceRequest setNote(List<Annotation> theNote) { 2534 this.note = theNote; 2535 return this; 2536 } 2537 2538 public boolean hasNote() { 2539 if (this.note == null) 2540 return false; 2541 for (Annotation item : this.note) 2542 if (!item.isEmpty()) 2543 return true; 2544 return false; 2545 } 2546 2547 public Annotation addNote() { //3 2548 Annotation t = new Annotation(); 2549 if (this.note == null) 2550 this.note = new ArrayList<Annotation>(); 2551 this.note.add(t); 2552 return t; 2553 } 2554 2555 public ServiceRequest addNote(Annotation t) { //3 2556 if (t == null) 2557 return this; 2558 if (this.note == null) 2559 this.note = new ArrayList<Annotation>(); 2560 this.note.add(t); 2561 return this; 2562 } 2563 2564 /** 2565 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 2566 */ 2567 public Annotation getNoteFirstRep() { 2568 if (getNote().isEmpty()) { 2569 addNote(); 2570 } 2571 return getNote().get(0); 2572 } 2573 2574 /** 2575 * @return {@link #patientInstruction} (Instructions in terms that are understood by the patient or consumer.) 2576 */ 2577 public List<ServiceRequestPatientInstructionComponent> getPatientInstruction() { 2578 if (this.patientInstruction == null) 2579 this.patientInstruction = new ArrayList<ServiceRequestPatientInstructionComponent>(); 2580 return this.patientInstruction; 2581 } 2582 2583 /** 2584 * @return Returns a reference to <code>this</code> for easy method chaining 2585 */ 2586 public ServiceRequest setPatientInstruction(List<ServiceRequestPatientInstructionComponent> thePatientInstruction) { 2587 this.patientInstruction = thePatientInstruction; 2588 return this; 2589 } 2590 2591 public boolean hasPatientInstruction() { 2592 if (this.patientInstruction == null) 2593 return false; 2594 for (ServiceRequestPatientInstructionComponent item : this.patientInstruction) 2595 if (!item.isEmpty()) 2596 return true; 2597 return false; 2598 } 2599 2600 public ServiceRequestPatientInstructionComponent addPatientInstruction() { //3 2601 ServiceRequestPatientInstructionComponent t = new ServiceRequestPatientInstructionComponent(); 2602 if (this.patientInstruction == null) 2603 this.patientInstruction = new ArrayList<ServiceRequestPatientInstructionComponent>(); 2604 this.patientInstruction.add(t); 2605 return t; 2606 } 2607 2608 public ServiceRequest addPatientInstruction(ServiceRequestPatientInstructionComponent t) { //3 2609 if (t == null) 2610 return this; 2611 if (this.patientInstruction == null) 2612 this.patientInstruction = new ArrayList<ServiceRequestPatientInstructionComponent>(); 2613 this.patientInstruction.add(t); 2614 return this; 2615 } 2616 2617 /** 2618 * @return The first repetition of repeating field {@link #patientInstruction}, creating it if it does not already exist {3} 2619 */ 2620 public ServiceRequestPatientInstructionComponent getPatientInstructionFirstRep() { 2621 if (getPatientInstruction().isEmpty()) { 2622 addPatientInstruction(); 2623 } 2624 return getPatientInstruction().get(0); 2625 } 2626 2627 /** 2628 * @return {@link #relevantHistory} (Key events in the history of the request.) 2629 */ 2630 public List<Reference> getRelevantHistory() { 2631 if (this.relevantHistory == null) 2632 this.relevantHistory = new ArrayList<Reference>(); 2633 return this.relevantHistory; 2634 } 2635 2636 /** 2637 * @return Returns a reference to <code>this</code> for easy method chaining 2638 */ 2639 public ServiceRequest setRelevantHistory(List<Reference> theRelevantHistory) { 2640 this.relevantHistory = theRelevantHistory; 2641 return this; 2642 } 2643 2644 public boolean hasRelevantHistory() { 2645 if (this.relevantHistory == null) 2646 return false; 2647 for (Reference item : this.relevantHistory) 2648 if (!item.isEmpty()) 2649 return true; 2650 return false; 2651 } 2652 2653 public Reference addRelevantHistory() { //3 2654 Reference t = new Reference(); 2655 if (this.relevantHistory == null) 2656 this.relevantHistory = new ArrayList<Reference>(); 2657 this.relevantHistory.add(t); 2658 return t; 2659 } 2660 2661 public ServiceRequest addRelevantHistory(Reference t) { //3 2662 if (t == null) 2663 return this; 2664 if (this.relevantHistory == null) 2665 this.relevantHistory = new ArrayList<Reference>(); 2666 this.relevantHistory.add(t); 2667 return this; 2668 } 2669 2670 /** 2671 * @return The first repetition of repeating field {@link #relevantHistory}, creating it if it does not already exist {3} 2672 */ 2673 public Reference getRelevantHistoryFirstRep() { 2674 if (getRelevantHistory().isEmpty()) { 2675 addRelevantHistory(); 2676 } 2677 return getRelevantHistory().get(0); 2678 } 2679 2680 protected void listChildren(List<Property> children) { 2681 super.listChildren(children); 2682 children.add(new Property("identifier", "Identifier", "Identifiers assigned to this order instance by the orderer and/or the receiver and/or order fulfiller.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2683 children.add(new Property("instantiatesCanonical", "canonical(ActivityDefinition|PlanDefinition)", "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest.", 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 2684 children.add(new Property("instantiatesUri", "uri", "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest.", 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 2685 children.add(new Property("basedOn", "Reference(CarePlan|ServiceRequest|MedicationRequest)", "Plan/proposal/order fulfilled by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 2686 children.add(new Property("replaces", "Reference(ServiceRequest)", "The request takes the place of the referenced completed or terminated request(s).", 0, java.lang.Integer.MAX_VALUE, replaces)); 2687 children.add(new Property("requisition", "Identifier", "A shared identifier common to all service requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier.", 0, 1, requisition)); 2688 children.add(new Property("status", "code", "The status of the order.", 0, 1, status)); 2689 children.add(new Property("intent", "code", "Whether the request is a proposal, plan, an original order or a reflex order.", 0, 1, intent)); 2690 children.add(new Property("category", "CodeableConcept", "A code that classifies the service for searching, sorting and display purposes (e.g. \"Surgical Procedure\").", 0, java.lang.Integer.MAX_VALUE, category)); 2691 children.add(new Property("priority", "code", "Indicates how quickly the ServiceRequest should be addressed with respect to other requests.", 0, 1, priority)); 2692 children.add(new Property("doNotPerform", "boolean", "Set this to true if the record is saying that the service/procedure should NOT be performed.", 0, 1, doNotPerform)); 2693 children.add(new Property("code", "CodeableReference(ActivityDefinition|PlanDefinition)", "A code or reference that identifies a particular service (i.e., procedure, diagnostic investigation, or panel of investigations) that have been requested.", 0, 1, code)); 2694 children.add(new Property("orderDetail", "", "Additional details and instructions about the how the services are to be delivered. For example, and order for a urinary catheter may have an order detail for an external or indwelling catheter, or an order for a bandage may require additional instructions specifying how the bandage should be applied.", 0, java.lang.Integer.MAX_VALUE, orderDetail)); 2695 children.add(new Property("quantity[x]", "Quantity|Ratio|Range", "An amount of service being requested which can be a quantity ( for example $1,500 home modification), a ratio ( for example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per fraction).", 0, 1, quantity)); 2696 children.add(new Property("subject", "Reference(Patient|Group|Location|Device)", "On whom or what the service is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).", 0, 1, subject)); 2697 children.add(new Property("focus", "Reference(Any)", "The actual focus of a service request when it is not the subject of record representing something or someone associated with the subject such as a spouse, parent, fetus, or donor. The focus of a service request could also be an existing condition, an intervention, the subject's diet, another service request on the subject, or a body structure such as tumor or implanted device.", 0, java.lang.Integer.MAX_VALUE, focus)); 2698 children.add(new Property("encounter", "Reference(Encounter)", "An encounter that provides additional information about the healthcare context in which this request is made.", 0, 1, encounter)); 2699 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "The date/time at which the requested service should occur.", 0, 1, occurrence)); 2700 children.add(new Property("asNeeded[x]", "boolean|CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded)); 2701 children.add(new Property("authoredOn", "dateTime", "When the request transitioned to being actionable.", 0, 1, authoredOn)); 2702 children.add(new Property("requester", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", "The individual who initiated the request and has responsibility for its activation.", 0, 1, requester)); 2703 children.add(new Property("performerType", "CodeableConcept", "Desired type of performer for doing the requested service.", 0, 1, performerType)); 2704 children.add(new Property("performer", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|HealthcareService|Patient|Device|RelatedPerson)", "The desired performer for doing the requested service. For example, the surgeon, dermatopathologist, endoscopist, etc.", 0, java.lang.Integer.MAX_VALUE, performer)); 2705 children.add(new Property("location", "CodeableReference(Location)", "The preferred location(s) where the procedure should actually happen in coded or free text form. E.g. at home or nursing day care center.", 0, java.lang.Integer.MAX_VALUE, location)); 2706 children.add(new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport|DocumentReference|DetectedIssue)", "An explanation or justification for why this service is being requested in coded or textual form. This is often for billing purposes. May relate to the resources referred to in `supportingInfo`.", 0, java.lang.Integer.MAX_VALUE, reason)); 2707 children.add(new Property("insurance", "Reference(Coverage|ClaimResponse)", "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be needed for delivering the requested service.", 0, java.lang.Integer.MAX_VALUE, insurance)); 2708 children.add(new Property("supportingInfo", "CodeableReference(Any)", "Additional clinical information about the patient or specimen that may influence the services or their interpretations. This information includes diagnosis, clinical findings and other observations. In laboratory ordering these are typically referred to as \"ask at order entry questions (AOEs)\". This includes observations explicitly requested by the producer (filler) to provide context or supporting information needed to complete the order. For example, reporting the amount of inspired oxygen for blood gas measurements.", 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 2709 children.add(new Property("specimen", "Reference(Specimen)", "One or more specimens that the laboratory procedure will use.", 0, java.lang.Integer.MAX_VALUE, specimen)); 2710 children.add(new Property("bodySite", "CodeableConcept", "Anatomic location where the procedure should be performed. This is the target site.", 0, java.lang.Integer.MAX_VALUE, bodySite)); 2711 children.add(new Property("bodyStructure", "Reference(BodyStructure)", "Anatomic location where the procedure should be performed. This is the target site.", 0, 1, bodyStructure)); 2712 children.add(new Property("note", "Annotation", "Any other notes and comments made about the service request. For example, internal billing notes.", 0, java.lang.Integer.MAX_VALUE, note)); 2713 children.add(new Property("patientInstruction", "", "Instructions in terms that are understood by the patient or consumer.", 0, java.lang.Integer.MAX_VALUE, patientInstruction)); 2714 children.add(new Property("relevantHistory", "Reference(Provenance)", "Key events in the history of the request.", 0, java.lang.Integer.MAX_VALUE, relevantHistory)); 2715 } 2716 2717 @Override 2718 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2719 switch (_hash) { 2720 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers assigned to this order instance by the orderer and/or the receiver and/or order fulfiller.", 0, java.lang.Integer.MAX_VALUE, identifier); 2721 case 8911915: /*instantiatesCanonical*/ return new Property("instantiatesCanonical", "canonical(ActivityDefinition|PlanDefinition)", "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest.", 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 2722 case -1926393373: /*instantiatesUri*/ return new Property("instantiatesUri", "uri", "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest.", 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 2723 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|ServiceRequest|MedicationRequest)", "Plan/proposal/order fulfilled by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn); 2724 case -430332865: /*replaces*/ return new Property("replaces", "Reference(ServiceRequest)", "The request takes the place of the referenced completed or terminated request(s).", 0, java.lang.Integer.MAX_VALUE, replaces); 2725 case 395923612: /*requisition*/ return new Property("requisition", "Identifier", "A shared identifier common to all service requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier.", 0, 1, requisition); 2726 case -892481550: /*status*/ return new Property("status", "code", "The status of the order.", 0, 1, status); 2727 case -1183762788: /*intent*/ return new Property("intent", "code", "Whether the request is a proposal, plan, an original order or a reflex order.", 0, 1, intent); 2728 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code that classifies the service for searching, sorting and display purposes (e.g. \"Surgical Procedure\").", 0, java.lang.Integer.MAX_VALUE, category); 2729 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly the ServiceRequest should be addressed with respect to other requests.", 0, 1, priority); 2730 case -1788508167: /*doNotPerform*/ return new Property("doNotPerform", "boolean", "Set this to true if the record is saying that the service/procedure should NOT be performed.", 0, 1, doNotPerform); 2731 case 3059181: /*code*/ return new Property("code", "CodeableReference(ActivityDefinition|PlanDefinition)", "A code or reference that identifies a particular service (i.e., procedure, diagnostic investigation, or panel of investigations) that have been requested.", 0, 1, code); 2732 case 1187338559: /*orderDetail*/ return new Property("orderDetail", "", "Additional details and instructions about the how the services are to be delivered. For example, and order for a urinary catheter may have an order detail for an external or indwelling catheter, or an order for a bandage may require additional instructions specifying how the bandage should be applied.", 0, java.lang.Integer.MAX_VALUE, orderDetail); 2733 case -515002347: /*quantity[x]*/ return new Property("quantity[x]", "Quantity|Ratio|Range", "An amount of service being requested which can be a quantity ( for example $1,500 home modification), a ratio ( for example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per fraction).", 0, 1, quantity); 2734 case -1285004149: /*quantity*/ return new Property("quantity[x]", "Quantity|Ratio|Range", "An amount of service being requested which can be a quantity ( for example $1,500 home modification), a ratio ( for example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per fraction).", 0, 1, quantity); 2735 case -1087409610: /*quantityQuantity*/ return new Property("quantity[x]", "Quantity", "An amount of service being requested which can be a quantity ( for example $1,500 home modification), a ratio ( for example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per fraction).", 0, 1, quantity); 2736 case -1004987840: /*quantityRatio*/ return new Property("quantity[x]", "Ratio", "An amount of service being requested which can be a quantity ( for example $1,500 home modification), a ratio ( for example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per fraction).", 0, 1, quantity); 2737 case -1004993678: /*quantityRange*/ return new Property("quantity[x]", "Range", "An amount of service being requested which can be a quantity ( for example $1,500 home modification), a ratio ( for example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per fraction).", 0, 1, quantity); 2738 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Location|Device)", "On whom or what the service is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).", 0, 1, subject); 2739 case 97604824: /*focus*/ return new Property("focus", "Reference(Any)", "The actual focus of a service request when it is not the subject of record representing something or someone associated with the subject such as a spouse, parent, fetus, or donor. The focus of a service request could also be an existing condition, an intervention, the subject's diet, another service request on the subject, or a body structure such as tumor or implanted device.", 0, java.lang.Integer.MAX_VALUE, focus); 2740 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "An encounter that provides additional information about the healthcare context in which this request is made.", 0, 1, encounter); 2741 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date/time at which the requested service should occur.", 0, 1, occurrence); 2742 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date/time at which the requested service should occur.", 0, 1, occurrence); 2743 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime", "The date/time at which the requested service should occur.", 0, 1, occurrence); 2744 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "Period", "The date/time at which the requested service should occur.", 0, 1, occurrence); 2745 case 1515218299: /*occurrenceTiming*/ return new Property("occurrence[x]", "Timing", "The date/time at which the requested service should occur.", 0, 1, occurrence); 2746 case -544329575: /*asNeeded[x]*/ return new Property("asNeeded[x]", "boolean|CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded); 2747 case -1432923513: /*asNeeded*/ return new Property("asNeeded[x]", "boolean|CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded); 2748 case -591717471: /*asNeededBoolean*/ return new Property("asNeeded[x]", "boolean", "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded); 2749 case 1556420122: /*asNeededCodeableConcept*/ return new Property("asNeeded[x]", "CodeableConcept", "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.", 0, 1, asNeeded); 2750 case -1500852503: /*authoredOn*/ return new Property("authoredOn", "dateTime", "When the request transitioned to being actionable.", 0, 1, authoredOn); 2751 case 693933948: /*requester*/ return new Property("requester", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", "The individual who initiated the request and has responsibility for its activation.", 0, 1, requester); 2752 case -901444568: /*performerType*/ return new Property("performerType", "CodeableConcept", "Desired type of performer for doing the requested service.", 0, 1, performerType); 2753 case 481140686: /*performer*/ return new Property("performer", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|HealthcareService|Patient|Device|RelatedPerson)", "The desired performer for doing the requested service. For example, the surgeon, dermatopathologist, endoscopist, etc.", 0, java.lang.Integer.MAX_VALUE, performer); 2754 case 1901043637: /*location*/ return new Property("location", "CodeableReference(Location)", "The preferred location(s) where the procedure should actually happen in coded or free text form. E.g. at home or nursing day care center.", 0, java.lang.Integer.MAX_VALUE, location); 2755 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport|DocumentReference|DetectedIssue)", "An explanation or justification for why this service is being requested in coded or textual form. This is often for billing purposes. May relate to the resources referred to in `supportingInfo`.", 0, java.lang.Integer.MAX_VALUE, reason); 2756 case 73049818: /*insurance*/ return new Property("insurance", "Reference(Coverage|ClaimResponse)", "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be needed for delivering the requested service.", 0, java.lang.Integer.MAX_VALUE, insurance); 2757 case 1922406657: /*supportingInfo*/ return new Property("supportingInfo", "CodeableReference(Any)", "Additional clinical information about the patient or specimen that may influence the services or their interpretations. This information includes diagnosis, clinical findings and other observations. In laboratory ordering these are typically referred to as \"ask at order entry questions (AOEs)\". This includes observations explicitly requested by the producer (filler) to provide context or supporting information needed to complete the order. For example, reporting the amount of inspired oxygen for blood gas measurements.", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 2758 case -2132868344: /*specimen*/ return new Property("specimen", "Reference(Specimen)", "One or more specimens that the laboratory procedure will use.", 0, java.lang.Integer.MAX_VALUE, specimen); 2759 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "Anatomic location where the procedure should be performed. This is the target site.", 0, java.lang.Integer.MAX_VALUE, bodySite); 2760 case -1001731599: /*bodyStructure*/ return new Property("bodyStructure", "Reference(BodyStructure)", "Anatomic location where the procedure should be performed. This is the target site.", 0, 1, bodyStructure); 2761 case 3387378: /*note*/ return new Property("note", "Annotation", "Any other notes and comments made about the service request. For example, internal billing notes.", 0, java.lang.Integer.MAX_VALUE, note); 2762 case 737543241: /*patientInstruction*/ return new Property("patientInstruction", "", "Instructions in terms that are understood by the patient or consumer.", 0, java.lang.Integer.MAX_VALUE, patientInstruction); 2763 case 1538891575: /*relevantHistory*/ return new Property("relevantHistory", "Reference(Provenance)", "Key events in the history of the request.", 0, java.lang.Integer.MAX_VALUE, relevantHistory); 2764 default: return super.getNamedProperty(_hash, _name, _checkValid); 2765 } 2766 2767 } 2768 2769 @Override 2770 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2771 switch (hash) { 2772 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2773 case 8911915: /*instantiatesCanonical*/ return this.instantiatesCanonical == null ? new Base[0] : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 2774 case -1926393373: /*instantiatesUri*/ return this.instantiatesUri == null ? new Base[0] : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 2775 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2776 case -430332865: /*replaces*/ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 2777 case 395923612: /*requisition*/ return this.requisition == null ? new Base[0] : new Base[] {this.requisition}; // Identifier 2778 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<RequestStatus> 2779 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // Enumeration<RequestIntent> 2780 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2781 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<RequestPriority> 2782 case -1788508167: /*doNotPerform*/ return this.doNotPerform == null ? new Base[0] : new Base[] {this.doNotPerform}; // BooleanType 2783 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableReference 2784 case 1187338559: /*orderDetail*/ return this.orderDetail == null ? new Base[0] : this.orderDetail.toArray(new Base[this.orderDetail.size()]); // ServiceRequestOrderDetailComponent 2785 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // DataType 2786 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2787 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // Reference 2788 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 2789 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // DataType 2790 case -1432923513: /*asNeeded*/ return this.asNeeded == null ? new Base[0] : new Base[] {this.asNeeded}; // DataType 2791 case -1500852503: /*authoredOn*/ return this.authoredOn == null ? new Base[0] : new Base[] {this.authoredOn}; // DateTimeType 2792 case 693933948: /*requester*/ return this.requester == null ? new Base[0] : new Base[] {this.requester}; // Reference 2793 case -901444568: /*performerType*/ return this.performerType == null ? new Base[0] : new Base[] {this.performerType}; // CodeableConcept 2794 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // Reference 2795 case 1901043637: /*location*/ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // CodeableReference 2796 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 2797 case 73049818: /*insurance*/ return this.insurance == null ? new Base[0] : this.insurance.toArray(new Base[this.insurance.size()]); // Reference 2798 case 1922406657: /*supportingInfo*/ return this.supportingInfo == null ? new Base[0] : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // CodeableReference 2799 case -2132868344: /*specimen*/ return this.specimen == null ? new Base[0] : this.specimen.toArray(new Base[this.specimen.size()]); // Reference 2800 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // CodeableConcept 2801 case -1001731599: /*bodyStructure*/ return this.bodyStructure == null ? new Base[0] : new Base[] {this.bodyStructure}; // Reference 2802 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2803 case 737543241: /*patientInstruction*/ return this.patientInstruction == null ? new Base[0] : this.patientInstruction.toArray(new Base[this.patientInstruction.size()]); // ServiceRequestPatientInstructionComponent 2804 case 1538891575: /*relevantHistory*/ return this.relevantHistory == null ? new Base[0] : this.relevantHistory.toArray(new Base[this.relevantHistory.size()]); // Reference 2805 default: return super.getProperty(hash, name, checkValid); 2806 } 2807 2808 } 2809 2810 @Override 2811 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2812 switch (hash) { 2813 case -1618432855: // identifier 2814 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2815 return value; 2816 case 8911915: // instantiatesCanonical 2817 this.getInstantiatesCanonical().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2818 return value; 2819 case -1926393373: // instantiatesUri 2820 this.getInstantiatesUri().add(TypeConvertor.castToUri(value)); // UriType 2821 return value; 2822 case -332612366: // basedOn 2823 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 2824 return value; 2825 case -430332865: // replaces 2826 this.getReplaces().add(TypeConvertor.castToReference(value)); // Reference 2827 return value; 2828 case 395923612: // requisition 2829 this.requisition = TypeConvertor.castToIdentifier(value); // Identifier 2830 return value; 2831 case -892481550: // status 2832 value = new RequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2833 this.status = (Enumeration) value; // Enumeration<RequestStatus> 2834 return value; 2835 case -1183762788: // intent 2836 value = new RequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 2837 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 2838 return value; 2839 case 50511102: // category 2840 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2841 return value; 2842 case -1165461084: // priority 2843 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 2844 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 2845 return value; 2846 case -1788508167: // doNotPerform 2847 this.doNotPerform = TypeConvertor.castToBoolean(value); // BooleanType 2848 return value; 2849 case 3059181: // code 2850 this.code = TypeConvertor.castToCodeableReference(value); // CodeableReference 2851 return value; 2852 case 1187338559: // orderDetail 2853 this.getOrderDetail().add((ServiceRequestOrderDetailComponent) value); // ServiceRequestOrderDetailComponent 2854 return value; 2855 case -1285004149: // quantity 2856 this.quantity = TypeConvertor.castToType(value); // DataType 2857 return value; 2858 case -1867885268: // subject 2859 this.subject = TypeConvertor.castToReference(value); // Reference 2860 return value; 2861 case 97604824: // focus 2862 this.getFocus().add(TypeConvertor.castToReference(value)); // Reference 2863 return value; 2864 case 1524132147: // encounter 2865 this.encounter = TypeConvertor.castToReference(value); // Reference 2866 return value; 2867 case 1687874001: // occurrence 2868 this.occurrence = TypeConvertor.castToType(value); // DataType 2869 return value; 2870 case -1432923513: // asNeeded 2871 this.asNeeded = TypeConvertor.castToType(value); // DataType 2872 return value; 2873 case -1500852503: // authoredOn 2874 this.authoredOn = TypeConvertor.castToDateTime(value); // DateTimeType 2875 return value; 2876 case 693933948: // requester 2877 this.requester = TypeConvertor.castToReference(value); // Reference 2878 return value; 2879 case -901444568: // performerType 2880 this.performerType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2881 return value; 2882 case 481140686: // performer 2883 this.getPerformer().add(TypeConvertor.castToReference(value)); // Reference 2884 return value; 2885 case 1901043637: // location 2886 this.getLocation().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 2887 return value; 2888 case -934964668: // reason 2889 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 2890 return value; 2891 case 73049818: // insurance 2892 this.getInsurance().add(TypeConvertor.castToReference(value)); // Reference 2893 return value; 2894 case 1922406657: // supportingInfo 2895 this.getSupportingInfo().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 2896 return value; 2897 case -2132868344: // specimen 2898 this.getSpecimen().add(TypeConvertor.castToReference(value)); // Reference 2899 return value; 2900 case 1702620169: // bodySite 2901 this.getBodySite().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2902 return value; 2903 case -1001731599: // bodyStructure 2904 this.bodyStructure = TypeConvertor.castToReference(value); // Reference 2905 return value; 2906 case 3387378: // note 2907 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 2908 return value; 2909 case 737543241: // patientInstruction 2910 this.getPatientInstruction().add((ServiceRequestPatientInstructionComponent) value); // ServiceRequestPatientInstructionComponent 2911 return value; 2912 case 1538891575: // relevantHistory 2913 this.getRelevantHistory().add(TypeConvertor.castToReference(value)); // Reference 2914 return value; 2915 default: return super.setProperty(hash, name, value); 2916 } 2917 2918 } 2919 2920 @Override 2921 public Base setProperty(String name, Base value) throws FHIRException { 2922 if (name.equals("identifier")) { 2923 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2924 } else if (name.equals("instantiatesCanonical")) { 2925 this.getInstantiatesCanonical().add(TypeConvertor.castToCanonical(value)); 2926 } else if (name.equals("instantiatesUri")) { 2927 this.getInstantiatesUri().add(TypeConvertor.castToUri(value)); 2928 } else if (name.equals("basedOn")) { 2929 this.getBasedOn().add(TypeConvertor.castToReference(value)); 2930 } else if (name.equals("replaces")) { 2931 this.getReplaces().add(TypeConvertor.castToReference(value)); 2932 } else if (name.equals("requisition")) { 2933 this.requisition = TypeConvertor.castToIdentifier(value); // Identifier 2934 } else if (name.equals("status")) { 2935 value = new RequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2936 this.status = (Enumeration) value; // Enumeration<RequestStatus> 2937 } else if (name.equals("intent")) { 2938 value = new RequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 2939 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 2940 } else if (name.equals("category")) { 2941 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 2942 } else if (name.equals("priority")) { 2943 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 2944 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 2945 } else if (name.equals("doNotPerform")) { 2946 this.doNotPerform = TypeConvertor.castToBoolean(value); // BooleanType 2947 } else if (name.equals("code")) { 2948 this.code = TypeConvertor.castToCodeableReference(value); // CodeableReference 2949 } else if (name.equals("orderDetail")) { 2950 this.getOrderDetail().add((ServiceRequestOrderDetailComponent) value); 2951 } else if (name.equals("quantity[x]")) { 2952 this.quantity = TypeConvertor.castToType(value); // DataType 2953 } else if (name.equals("subject")) { 2954 this.subject = TypeConvertor.castToReference(value); // Reference 2955 } else if (name.equals("focus")) { 2956 this.getFocus().add(TypeConvertor.castToReference(value)); 2957 } else if (name.equals("encounter")) { 2958 this.encounter = TypeConvertor.castToReference(value); // Reference 2959 } else if (name.equals("occurrence[x]")) { 2960 this.occurrence = TypeConvertor.castToType(value); // DataType 2961 } else if (name.equals("asNeeded[x]")) { 2962 this.asNeeded = TypeConvertor.castToType(value); // DataType 2963 } else if (name.equals("authoredOn")) { 2964 this.authoredOn = TypeConvertor.castToDateTime(value); // DateTimeType 2965 } else if (name.equals("requester")) { 2966 this.requester = TypeConvertor.castToReference(value); // Reference 2967 } else if (name.equals("performerType")) { 2968 this.performerType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2969 } else if (name.equals("performer")) { 2970 this.getPerformer().add(TypeConvertor.castToReference(value)); 2971 } else if (name.equals("location")) { 2972 this.getLocation().add(TypeConvertor.castToCodeableReference(value)); 2973 } else if (name.equals("reason")) { 2974 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 2975 } else if (name.equals("insurance")) { 2976 this.getInsurance().add(TypeConvertor.castToReference(value)); 2977 } else if (name.equals("supportingInfo")) { 2978 this.getSupportingInfo().add(TypeConvertor.castToCodeableReference(value)); 2979 } else if (name.equals("specimen")) { 2980 this.getSpecimen().add(TypeConvertor.castToReference(value)); 2981 } else if (name.equals("bodySite")) { 2982 this.getBodySite().add(TypeConvertor.castToCodeableConcept(value)); 2983 } else if (name.equals("bodyStructure")) { 2984 this.bodyStructure = TypeConvertor.castToReference(value); // Reference 2985 } else if (name.equals("note")) { 2986 this.getNote().add(TypeConvertor.castToAnnotation(value)); 2987 } else if (name.equals("patientInstruction")) { 2988 this.getPatientInstruction().add((ServiceRequestPatientInstructionComponent) value); 2989 } else if (name.equals("relevantHistory")) { 2990 this.getRelevantHistory().add(TypeConvertor.castToReference(value)); 2991 } else 2992 return super.setProperty(name, value); 2993 return value; 2994 } 2995 2996 @Override 2997 public void removeChild(String name, Base value) throws FHIRException { 2998 if (name.equals("identifier")) { 2999 this.getIdentifier().remove(value); 3000 } else if (name.equals("instantiatesCanonical")) { 3001 this.getInstantiatesCanonical().remove(value); 3002 } else if (name.equals("instantiatesUri")) { 3003 this.getInstantiatesUri().remove(value); 3004 } else if (name.equals("basedOn")) { 3005 this.getBasedOn().remove(value); 3006 } else if (name.equals("replaces")) { 3007 this.getReplaces().remove(value); 3008 } else if (name.equals("requisition")) { 3009 this.requisition = null; 3010 } else if (name.equals("status")) { 3011 value = new RequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3012 this.status = (Enumeration) value; // Enumeration<RequestStatus> 3013 } else if (name.equals("intent")) { 3014 value = new RequestIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 3015 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 3016 } else if (name.equals("category")) { 3017 this.getCategory().remove(value); 3018 } else if (name.equals("priority")) { 3019 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 3020 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 3021 } else if (name.equals("doNotPerform")) { 3022 this.doNotPerform = null; 3023 } else if (name.equals("code")) { 3024 this.code = null; 3025 } else if (name.equals("orderDetail")) { 3026 this.getOrderDetail().remove((ServiceRequestOrderDetailComponent) value); 3027 } else if (name.equals("quantity[x]")) { 3028 this.quantity = null; 3029 } else if (name.equals("subject")) { 3030 this.subject = null; 3031 } else if (name.equals("focus")) { 3032 this.getFocus().remove(value); 3033 } else if (name.equals("encounter")) { 3034 this.encounter = null; 3035 } else if (name.equals("occurrence[x]")) { 3036 this.occurrence = null; 3037 } else if (name.equals("asNeeded[x]")) { 3038 this.asNeeded = null; 3039 } else if (name.equals("authoredOn")) { 3040 this.authoredOn = null; 3041 } else if (name.equals("requester")) { 3042 this.requester = null; 3043 } else if (name.equals("performerType")) { 3044 this.performerType = null; 3045 } else if (name.equals("performer")) { 3046 this.getPerformer().remove(value); 3047 } else if (name.equals("location")) { 3048 this.getLocation().remove(value); 3049 } else if (name.equals("reason")) { 3050 this.getReason().remove(value); 3051 } else if (name.equals("insurance")) { 3052 this.getInsurance().remove(value); 3053 } else if (name.equals("supportingInfo")) { 3054 this.getSupportingInfo().remove(value); 3055 } else if (name.equals("specimen")) { 3056 this.getSpecimen().remove(value); 3057 } else if (name.equals("bodySite")) { 3058 this.getBodySite().remove(value); 3059 } else if (name.equals("bodyStructure")) { 3060 this.bodyStructure = null; 3061 } else if (name.equals("note")) { 3062 this.getNote().remove(value); 3063 } else if (name.equals("patientInstruction")) { 3064 this.getPatientInstruction().remove((ServiceRequestPatientInstructionComponent) value); 3065 } else if (name.equals("relevantHistory")) { 3066 this.getRelevantHistory().remove(value); 3067 } else 3068 super.removeChild(name, value); 3069 3070 } 3071 3072 @Override 3073 public Base makeProperty(int hash, String name) throws FHIRException { 3074 switch (hash) { 3075 case -1618432855: return addIdentifier(); 3076 case 8911915: return addInstantiatesCanonicalElement(); 3077 case -1926393373: return addInstantiatesUriElement(); 3078 case -332612366: return addBasedOn(); 3079 case -430332865: return addReplaces(); 3080 case 395923612: return getRequisition(); 3081 case -892481550: return getStatusElement(); 3082 case -1183762788: return getIntentElement(); 3083 case 50511102: return addCategory(); 3084 case -1165461084: return getPriorityElement(); 3085 case -1788508167: return getDoNotPerformElement(); 3086 case 3059181: return getCode(); 3087 case 1187338559: return addOrderDetail(); 3088 case -515002347: return getQuantity(); 3089 case -1285004149: return getQuantity(); 3090 case -1867885268: return getSubject(); 3091 case 97604824: return addFocus(); 3092 case 1524132147: return getEncounter(); 3093 case -2022646513: return getOccurrence(); 3094 case 1687874001: return getOccurrence(); 3095 case -544329575: return getAsNeeded(); 3096 case -1432923513: return getAsNeeded(); 3097 case -1500852503: return getAuthoredOnElement(); 3098 case 693933948: return getRequester(); 3099 case -901444568: return getPerformerType(); 3100 case 481140686: return addPerformer(); 3101 case 1901043637: return addLocation(); 3102 case -934964668: return addReason(); 3103 case 73049818: return addInsurance(); 3104 case 1922406657: return addSupportingInfo(); 3105 case -2132868344: return addSpecimen(); 3106 case 1702620169: return addBodySite(); 3107 case -1001731599: return getBodyStructure(); 3108 case 3387378: return addNote(); 3109 case 737543241: return addPatientInstruction(); 3110 case 1538891575: return addRelevantHistory(); 3111 default: return super.makeProperty(hash, name); 3112 } 3113 3114 } 3115 3116 @Override 3117 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3118 switch (hash) { 3119 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3120 case 8911915: /*instantiatesCanonical*/ return new String[] {"canonical"}; 3121 case -1926393373: /*instantiatesUri*/ return new String[] {"uri"}; 3122 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 3123 case -430332865: /*replaces*/ return new String[] {"Reference"}; 3124 case 395923612: /*requisition*/ return new String[] {"Identifier"}; 3125 case -892481550: /*status*/ return new String[] {"code"}; 3126 case -1183762788: /*intent*/ return new String[] {"code"}; 3127 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3128 case -1165461084: /*priority*/ return new String[] {"code"}; 3129 case -1788508167: /*doNotPerform*/ return new String[] {"boolean"}; 3130 case 3059181: /*code*/ return new String[] {"CodeableReference"}; 3131 case 1187338559: /*orderDetail*/ return new String[] {}; 3132 case -1285004149: /*quantity*/ return new String[] {"Quantity", "Ratio", "Range"}; 3133 case -1867885268: /*subject*/ return new String[] {"Reference"}; 3134 case 97604824: /*focus*/ return new String[] {"Reference"}; 3135 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 3136 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period", "Timing"}; 3137 case -1432923513: /*asNeeded*/ return new String[] {"boolean", "CodeableConcept"}; 3138 case -1500852503: /*authoredOn*/ return new String[] {"dateTime"}; 3139 case 693933948: /*requester*/ return new String[] {"Reference"}; 3140 case -901444568: /*performerType*/ return new String[] {"CodeableConcept"}; 3141 case 481140686: /*performer*/ return new String[] {"Reference"}; 3142 case 1901043637: /*location*/ return new String[] {"CodeableReference"}; 3143 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 3144 case 73049818: /*insurance*/ return new String[] {"Reference"}; 3145 case 1922406657: /*supportingInfo*/ return new String[] {"CodeableReference"}; 3146 case -2132868344: /*specimen*/ return new String[] {"Reference"}; 3147 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 3148 case -1001731599: /*bodyStructure*/ return new String[] {"Reference"}; 3149 case 3387378: /*note*/ return new String[] {"Annotation"}; 3150 case 737543241: /*patientInstruction*/ return new String[] {}; 3151 case 1538891575: /*relevantHistory*/ return new String[] {"Reference"}; 3152 default: return super.getTypesForProperty(hash, name); 3153 } 3154 3155 } 3156 3157 @Override 3158 public Base addChild(String name) throws FHIRException { 3159 if (name.equals("identifier")) { 3160 return addIdentifier(); 3161 } 3162 else if (name.equals("instantiatesCanonical")) { 3163 throw new FHIRException("Cannot call addChild on a singleton property ServiceRequest.instantiatesCanonical"); 3164 } 3165 else if (name.equals("instantiatesUri")) { 3166 throw new FHIRException("Cannot call addChild on a singleton property ServiceRequest.instantiatesUri"); 3167 } 3168 else if (name.equals("basedOn")) { 3169 return addBasedOn(); 3170 } 3171 else if (name.equals("replaces")) { 3172 return addReplaces(); 3173 } 3174 else if (name.equals("requisition")) { 3175 this.requisition = new Identifier(); 3176 return this.requisition; 3177 } 3178 else if (name.equals("status")) { 3179 throw new FHIRException("Cannot call addChild on a singleton property ServiceRequest.status"); 3180 } 3181 else if (name.equals("intent")) { 3182 throw new FHIRException("Cannot call addChild on a singleton property ServiceRequest.intent"); 3183 } 3184 else if (name.equals("category")) { 3185 return addCategory(); 3186 } 3187 else if (name.equals("priority")) { 3188 throw new FHIRException("Cannot call addChild on a singleton property ServiceRequest.priority"); 3189 } 3190 else if (name.equals("doNotPerform")) { 3191 throw new FHIRException("Cannot call addChild on a singleton property ServiceRequest.doNotPerform"); 3192 } 3193 else if (name.equals("code")) { 3194 this.code = new CodeableReference(); 3195 return this.code; 3196 } 3197 else if (name.equals("orderDetail")) { 3198 return addOrderDetail(); 3199 } 3200 else if (name.equals("quantityQuantity")) { 3201 this.quantity = new Quantity(); 3202 return this.quantity; 3203 } 3204 else if (name.equals("quantityRatio")) { 3205 this.quantity = new Ratio(); 3206 return this.quantity; 3207 } 3208 else if (name.equals("quantityRange")) { 3209 this.quantity = new Range(); 3210 return this.quantity; 3211 } 3212 else if (name.equals("subject")) { 3213 this.subject = new Reference(); 3214 return this.subject; 3215 } 3216 else if (name.equals("focus")) { 3217 return addFocus(); 3218 } 3219 else if (name.equals("encounter")) { 3220 this.encounter = new Reference(); 3221 return this.encounter; 3222 } 3223 else if (name.equals("occurrenceDateTime")) { 3224 this.occurrence = new DateTimeType(); 3225 return this.occurrence; 3226 } 3227 else if (name.equals("occurrencePeriod")) { 3228 this.occurrence = new Period(); 3229 return this.occurrence; 3230 } 3231 else if (name.equals("occurrenceTiming")) { 3232 this.occurrence = new Timing(); 3233 return this.occurrence; 3234 } 3235 else if (name.equals("asNeededBoolean")) { 3236 this.asNeeded = new BooleanType(); 3237 return this.asNeeded; 3238 } 3239 else if (name.equals("asNeededCodeableConcept")) { 3240 this.asNeeded = new CodeableConcept(); 3241 return this.asNeeded; 3242 } 3243 else if (name.equals("authoredOn")) { 3244 throw new FHIRException("Cannot call addChild on a singleton property ServiceRequest.authoredOn"); 3245 } 3246 else if (name.equals("requester")) { 3247 this.requester = new Reference(); 3248 return this.requester; 3249 } 3250 else if (name.equals("performerType")) { 3251 this.performerType = new CodeableConcept(); 3252 return this.performerType; 3253 } 3254 else if (name.equals("performer")) { 3255 return addPerformer(); 3256 } 3257 else if (name.equals("location")) { 3258 return addLocation(); 3259 } 3260 else if (name.equals("reason")) { 3261 return addReason(); 3262 } 3263 else if (name.equals("insurance")) { 3264 return addInsurance(); 3265 } 3266 else if (name.equals("supportingInfo")) { 3267 return addSupportingInfo(); 3268 } 3269 else if (name.equals("specimen")) { 3270 return addSpecimen(); 3271 } 3272 else if (name.equals("bodySite")) { 3273 return addBodySite(); 3274 } 3275 else if (name.equals("bodyStructure")) { 3276 this.bodyStructure = new Reference(); 3277 return this.bodyStructure; 3278 } 3279 else if (name.equals("note")) { 3280 return addNote(); 3281 } 3282 else if (name.equals("patientInstruction")) { 3283 return addPatientInstruction(); 3284 } 3285 else if (name.equals("relevantHistory")) { 3286 return addRelevantHistory(); 3287 } 3288 else 3289 return super.addChild(name); 3290 } 3291 3292 public String fhirType() { 3293 return "ServiceRequest"; 3294 3295 } 3296 3297 public ServiceRequest copy() { 3298 ServiceRequest dst = new ServiceRequest(); 3299 copyValues(dst); 3300 return dst; 3301 } 3302 3303 public void copyValues(ServiceRequest dst) { 3304 super.copyValues(dst); 3305 if (identifier != null) { 3306 dst.identifier = new ArrayList<Identifier>(); 3307 for (Identifier i : identifier) 3308 dst.identifier.add(i.copy()); 3309 }; 3310 if (instantiatesCanonical != null) { 3311 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 3312 for (CanonicalType i : instantiatesCanonical) 3313 dst.instantiatesCanonical.add(i.copy()); 3314 }; 3315 if (instantiatesUri != null) { 3316 dst.instantiatesUri = new ArrayList<UriType>(); 3317 for (UriType i : instantiatesUri) 3318 dst.instantiatesUri.add(i.copy()); 3319 }; 3320 if (basedOn != null) { 3321 dst.basedOn = new ArrayList<Reference>(); 3322 for (Reference i : basedOn) 3323 dst.basedOn.add(i.copy()); 3324 }; 3325 if (replaces != null) { 3326 dst.replaces = new ArrayList<Reference>(); 3327 for (Reference i : replaces) 3328 dst.replaces.add(i.copy()); 3329 }; 3330 dst.requisition = requisition == null ? null : requisition.copy(); 3331 dst.status = status == null ? null : status.copy(); 3332 dst.intent = intent == null ? null : intent.copy(); 3333 if (category != null) { 3334 dst.category = new ArrayList<CodeableConcept>(); 3335 for (CodeableConcept i : category) 3336 dst.category.add(i.copy()); 3337 }; 3338 dst.priority = priority == null ? null : priority.copy(); 3339 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 3340 dst.code = code == null ? null : code.copy(); 3341 if (orderDetail != null) { 3342 dst.orderDetail = new ArrayList<ServiceRequestOrderDetailComponent>(); 3343 for (ServiceRequestOrderDetailComponent i : orderDetail) 3344 dst.orderDetail.add(i.copy()); 3345 }; 3346 dst.quantity = quantity == null ? null : quantity.copy(); 3347 dst.subject = subject == null ? null : subject.copy(); 3348 if (focus != null) { 3349 dst.focus = new ArrayList<Reference>(); 3350 for (Reference i : focus) 3351 dst.focus.add(i.copy()); 3352 }; 3353 dst.encounter = encounter == null ? null : encounter.copy(); 3354 dst.occurrence = occurrence == null ? null : occurrence.copy(); 3355 dst.asNeeded = asNeeded == null ? null : asNeeded.copy(); 3356 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 3357 dst.requester = requester == null ? null : requester.copy(); 3358 dst.performerType = performerType == null ? null : performerType.copy(); 3359 if (performer != null) { 3360 dst.performer = new ArrayList<Reference>(); 3361 for (Reference i : performer) 3362 dst.performer.add(i.copy()); 3363 }; 3364 if (location != null) { 3365 dst.location = new ArrayList<CodeableReference>(); 3366 for (CodeableReference i : location) 3367 dst.location.add(i.copy()); 3368 }; 3369 if (reason != null) { 3370 dst.reason = new ArrayList<CodeableReference>(); 3371 for (CodeableReference i : reason) 3372 dst.reason.add(i.copy()); 3373 }; 3374 if (insurance != null) { 3375 dst.insurance = new ArrayList<Reference>(); 3376 for (Reference i : insurance) 3377 dst.insurance.add(i.copy()); 3378 }; 3379 if (supportingInfo != null) { 3380 dst.supportingInfo = new ArrayList<CodeableReference>(); 3381 for (CodeableReference i : supportingInfo) 3382 dst.supportingInfo.add(i.copy()); 3383 }; 3384 if (specimen != null) { 3385 dst.specimen = new ArrayList<Reference>(); 3386 for (Reference i : specimen) 3387 dst.specimen.add(i.copy()); 3388 }; 3389 if (bodySite != null) { 3390 dst.bodySite = new ArrayList<CodeableConcept>(); 3391 for (CodeableConcept i : bodySite) 3392 dst.bodySite.add(i.copy()); 3393 }; 3394 dst.bodyStructure = bodyStructure == null ? null : bodyStructure.copy(); 3395 if (note != null) { 3396 dst.note = new ArrayList<Annotation>(); 3397 for (Annotation i : note) 3398 dst.note.add(i.copy()); 3399 }; 3400 if (patientInstruction != null) { 3401 dst.patientInstruction = new ArrayList<ServiceRequestPatientInstructionComponent>(); 3402 for (ServiceRequestPatientInstructionComponent i : patientInstruction) 3403 dst.patientInstruction.add(i.copy()); 3404 }; 3405 if (relevantHistory != null) { 3406 dst.relevantHistory = new ArrayList<Reference>(); 3407 for (Reference i : relevantHistory) 3408 dst.relevantHistory.add(i.copy()); 3409 }; 3410 } 3411 3412 protected ServiceRequest typedCopy() { 3413 return copy(); 3414 } 3415 3416 @Override 3417 public boolean equalsDeep(Base other_) { 3418 if (!super.equalsDeep(other_)) 3419 return false; 3420 if (!(other_ instanceof ServiceRequest)) 3421 return false; 3422 ServiceRequest o = (ServiceRequest) other_; 3423 return compareDeep(identifier, o.identifier, true) && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 3424 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(basedOn, o.basedOn, true) 3425 && compareDeep(replaces, o.replaces, true) && compareDeep(requisition, o.requisition, true) && compareDeep(status, o.status, true) 3426 && compareDeep(intent, o.intent, true) && compareDeep(category, o.category, true) && compareDeep(priority, o.priority, true) 3427 && compareDeep(doNotPerform, o.doNotPerform, true) && compareDeep(code, o.code, true) && compareDeep(orderDetail, o.orderDetail, true) 3428 && compareDeep(quantity, o.quantity, true) && compareDeep(subject, o.subject, true) && compareDeep(focus, o.focus, true) 3429 && compareDeep(encounter, o.encounter, true) && compareDeep(occurrence, o.occurrence, true) && compareDeep(asNeeded, o.asNeeded, true) 3430 && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(requester, o.requester, true) && compareDeep(performerType, o.performerType, true) 3431 && compareDeep(performer, o.performer, true) && compareDeep(location, o.location, true) && compareDeep(reason, o.reason, true) 3432 && compareDeep(insurance, o.insurance, true) && compareDeep(supportingInfo, o.supportingInfo, true) 3433 && compareDeep(specimen, o.specimen, true) && compareDeep(bodySite, o.bodySite, true) && compareDeep(bodyStructure, o.bodyStructure, true) 3434 && compareDeep(note, o.note, true) && compareDeep(patientInstruction, o.patientInstruction, true) 3435 && compareDeep(relevantHistory, o.relevantHistory, true); 3436 } 3437 3438 @Override 3439 public boolean equalsShallow(Base other_) { 3440 if (!super.equalsShallow(other_)) 3441 return false; 3442 if (!(other_ instanceof ServiceRequest)) 3443 return false; 3444 ServiceRequest o = (ServiceRequest) other_; 3445 return compareValues(instantiatesCanonical, o.instantiatesCanonical, true) && compareValues(instantiatesUri, o.instantiatesUri, true) 3446 && compareValues(status, o.status, true) && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) 3447 && compareValues(doNotPerform, o.doNotPerform, true) && compareValues(authoredOn, o.authoredOn, true) 3448 ; 3449 } 3450 3451 public boolean isEmpty() { 3452 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical 3453 , instantiatesUri, basedOn, replaces, requisition, status, intent, category, priority 3454 , doNotPerform, code, orderDetail, quantity, subject, focus, encounter, occurrence 3455 , asNeeded, authoredOn, requester, performerType, performer, location, reason 3456 , insurance, supportingInfo, specimen, bodySite, bodyStructure, note, patientInstruction 3457 , relevantHistory); 3458 } 3459 3460 @Override 3461 public ResourceType getResourceType() { 3462 return ResourceType.ServiceRequest; 3463 } 3464 3465 /** 3466 * Search parameter: <b>authored</b> 3467 * <p> 3468 * Description: <b>Date request signed</b><br> 3469 * Type: <b>date</b><br> 3470 * Path: <b>ServiceRequest.authoredOn</b><br> 3471 * </p> 3472 */ 3473 @SearchParamDefinition(name="authored", path="ServiceRequest.authoredOn", description="Date request signed", type="date" ) 3474 public static final String SP_AUTHORED = "authored"; 3475 /** 3476 * <b>Fluent Client</b> search parameter constant for <b>authored</b> 3477 * <p> 3478 * Description: <b>Date request signed</b><br> 3479 * Type: <b>date</b><br> 3480 * Path: <b>ServiceRequest.authoredOn</b><br> 3481 * </p> 3482 */ 3483 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHORED); 3484 3485 /** 3486 * Search parameter: <b>based-on</b> 3487 * <p> 3488 * Description: <b>What request fulfills</b><br> 3489 * Type: <b>reference</b><br> 3490 * Path: <b>ServiceRequest.basedOn</b><br> 3491 * </p> 3492 */ 3493 @SearchParamDefinition(name="based-on", path="ServiceRequest.basedOn", description="What request fulfills", type="reference", target={CarePlan.class, MedicationRequest.class, ServiceRequest.class } ) 3494 public static final String SP_BASED_ON = "based-on"; 3495 /** 3496 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 3497 * <p> 3498 * Description: <b>What request fulfills</b><br> 3499 * Type: <b>reference</b><br> 3500 * Path: <b>ServiceRequest.basedOn</b><br> 3501 * </p> 3502 */ 3503 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 3504 3505/** 3506 * Constant for fluent queries to be used to add include statements. Specifies 3507 * the path value of "<b>ServiceRequest:based-on</b>". 3508 */ 3509 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("ServiceRequest:based-on").toLocked(); 3510 3511 /** 3512 * Search parameter: <b>body-site</b> 3513 * <p> 3514 * Description: <b>Where procedure is going to be done</b><br> 3515 * Type: <b>token</b><br> 3516 * Path: <b>ServiceRequest.bodySite</b><br> 3517 * </p> 3518 */ 3519 @SearchParamDefinition(name="body-site", path="ServiceRequest.bodySite", description="Where procedure is going to be done", type="token" ) 3520 public static final String SP_BODY_SITE = "body-site"; 3521 /** 3522 * <b>Fluent Client</b> search parameter constant for <b>body-site</b> 3523 * <p> 3524 * Description: <b>Where procedure is going to be done</b><br> 3525 * Type: <b>token</b><br> 3526 * Path: <b>ServiceRequest.bodySite</b><br> 3527 * </p> 3528 */ 3529 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BODY_SITE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BODY_SITE); 3530 3531 /** 3532 * Search parameter: <b>body-structure</b> 3533 * <p> 3534 * Description: <b>Body structure Where procedure is going to be done</b><br> 3535 * Type: <b>reference</b><br> 3536 * Path: <b>ServiceRequest.bodyStructure</b><br> 3537 * </p> 3538 */ 3539 @SearchParamDefinition(name="body-structure", path="ServiceRequest.bodyStructure", description="Body structure Where procedure is going to be done", type="reference", target={BodyStructure.class } ) 3540 public static final String SP_BODY_STRUCTURE = "body-structure"; 3541 /** 3542 * <b>Fluent Client</b> search parameter constant for <b>body-structure</b> 3543 * <p> 3544 * Description: <b>Body structure Where procedure is going to be done</b><br> 3545 * Type: <b>reference</b><br> 3546 * Path: <b>ServiceRequest.bodyStructure</b><br> 3547 * </p> 3548 */ 3549 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BODY_STRUCTURE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BODY_STRUCTURE); 3550 3551/** 3552 * Constant for fluent queries to be used to add include statements. Specifies 3553 * the path value of "<b>ServiceRequest:body-structure</b>". 3554 */ 3555 public static final ca.uhn.fhir.model.api.Include INCLUDE_BODY_STRUCTURE = new ca.uhn.fhir.model.api.Include("ServiceRequest:body-structure").toLocked(); 3556 3557 /** 3558 * Search parameter: <b>category</b> 3559 * <p> 3560 * Description: <b>Classification of service</b><br> 3561 * Type: <b>token</b><br> 3562 * Path: <b>ServiceRequest.category</b><br> 3563 * </p> 3564 */ 3565 @SearchParamDefinition(name="category", path="ServiceRequest.category", description="Classification of service", type="token" ) 3566 public static final String SP_CATEGORY = "category"; 3567 /** 3568 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3569 * <p> 3570 * Description: <b>Classification of service</b><br> 3571 * Type: <b>token</b><br> 3572 * Path: <b>ServiceRequest.category</b><br> 3573 * </p> 3574 */ 3575 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 3576 3577 /** 3578 * Search parameter: <b>code-concept</b> 3579 * <p> 3580 * Description: <b>What is being requested/ordered</b><br> 3581 * Type: <b>token</b><br> 3582 * Path: <b>ServiceRequest.code.concept</b><br> 3583 * </p> 3584 */ 3585 @SearchParamDefinition(name="code-concept", path="ServiceRequest.code.concept", description="What is being requested/ordered", type="token" ) 3586 public static final String SP_CODE_CONCEPT = "code-concept"; 3587 /** 3588 * <b>Fluent Client</b> search parameter constant for <b>code-concept</b> 3589 * <p> 3590 * Description: <b>What is being requested/ordered</b><br> 3591 * Type: <b>token</b><br> 3592 * Path: <b>ServiceRequest.code.concept</b><br> 3593 * </p> 3594 */ 3595 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE_CONCEPT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE_CONCEPT); 3596 3597 /** 3598 * Search parameter: <b>code-reference</b> 3599 * <p> 3600 * Description: <b>What is being requested/ordered</b><br> 3601 * Type: <b>reference</b><br> 3602 * Path: <b>ServiceRequest.code.reference</b><br> 3603 * </p> 3604 */ 3605 @SearchParamDefinition(name="code-reference", path="ServiceRequest.code.reference", description="What is being requested/ordered", type="reference", target={ActivityDefinition.class, PlanDefinition.class } ) 3606 public static final String SP_CODE_REFERENCE = "code-reference"; 3607 /** 3608 * <b>Fluent Client</b> search parameter constant for <b>code-reference</b> 3609 * <p> 3610 * Description: <b>What is being requested/ordered</b><br> 3611 * Type: <b>reference</b><br> 3612 * Path: <b>ServiceRequest.code.reference</b><br> 3613 * </p> 3614 */ 3615 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CODE_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CODE_REFERENCE); 3616 3617/** 3618 * Constant for fluent queries to be used to add include statements. Specifies 3619 * the path value of "<b>ServiceRequest:code-reference</b>". 3620 */ 3621 public static final ca.uhn.fhir.model.api.Include INCLUDE_CODE_REFERENCE = new ca.uhn.fhir.model.api.Include("ServiceRequest:code-reference").toLocked(); 3622 3623 /** 3624 * Search parameter: <b>instantiates-canonical</b> 3625 * <p> 3626 * Description: <b>Instantiates FHIR protocol or definition</b><br> 3627 * Type: <b>reference</b><br> 3628 * Path: <b>ServiceRequest.instantiatesCanonical</b><br> 3629 * </p> 3630 */ 3631 @SearchParamDefinition(name="instantiates-canonical", path="ServiceRequest.instantiatesCanonical", description="Instantiates FHIR protocol or definition", type="reference", target={ActivityDefinition.class, PlanDefinition.class } ) 3632 public static final String SP_INSTANTIATES_CANONICAL = "instantiates-canonical"; 3633 /** 3634 * <b>Fluent Client</b> search parameter constant for <b>instantiates-canonical</b> 3635 * <p> 3636 * Description: <b>Instantiates FHIR protocol or definition</b><br> 3637 * Type: <b>reference</b><br> 3638 * Path: <b>ServiceRequest.instantiatesCanonical</b><br> 3639 * </p> 3640 */ 3641 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSTANTIATES_CANONICAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INSTANTIATES_CANONICAL); 3642 3643/** 3644 * Constant for fluent queries to be used to add include statements. Specifies 3645 * the path value of "<b>ServiceRequest:instantiates-canonical</b>". 3646 */ 3647 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSTANTIATES_CANONICAL = new ca.uhn.fhir.model.api.Include("ServiceRequest:instantiates-canonical").toLocked(); 3648 3649 /** 3650 * Search parameter: <b>instantiates-uri</b> 3651 * <p> 3652 * Description: <b>Instantiates external protocol or definition</b><br> 3653 * Type: <b>uri</b><br> 3654 * Path: <b>ServiceRequest.instantiatesUri</b><br> 3655 * </p> 3656 */ 3657 @SearchParamDefinition(name="instantiates-uri", path="ServiceRequest.instantiatesUri", description="Instantiates external protocol or definition", type="uri" ) 3658 public static final String SP_INSTANTIATES_URI = "instantiates-uri"; 3659 /** 3660 * <b>Fluent Client</b> search parameter constant for <b>instantiates-uri</b> 3661 * <p> 3662 * Description: <b>Instantiates external protocol or definition</b><br> 3663 * Type: <b>uri</b><br> 3664 * Path: <b>ServiceRequest.instantiatesUri</b><br> 3665 * </p> 3666 */ 3667 public static final ca.uhn.fhir.rest.gclient.UriClientParam INSTANTIATES_URI = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_INSTANTIATES_URI); 3668 3669 /** 3670 * Search parameter: <b>intent</b> 3671 * <p> 3672 * Description: <b>proposal | plan | directive | order +</b><br> 3673 * Type: <b>token</b><br> 3674 * Path: <b>ServiceRequest.intent</b><br> 3675 * </p> 3676 */ 3677 @SearchParamDefinition(name="intent", path="ServiceRequest.intent", description="proposal | plan | directive | order +", type="token" ) 3678 public static final String SP_INTENT = "intent"; 3679 /** 3680 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 3681 * <p> 3682 * Description: <b>proposal | plan | directive | order +</b><br> 3683 * Type: <b>token</b><br> 3684 * Path: <b>ServiceRequest.intent</b><br> 3685 * </p> 3686 */ 3687 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INTENT); 3688 3689 /** 3690 * Search parameter: <b>occurrence</b> 3691 * <p> 3692 * Description: <b>When service should occur</b><br> 3693 * Type: <b>date</b><br> 3694 * Path: <b>ServiceRequest.occurrence.ofType(dateTime) | ServiceRequest.occurrence.ofType(Period) | ServiceRequest.occurrence.ofType(Timing)</b><br> 3695 * </p> 3696 */ 3697 @SearchParamDefinition(name="occurrence", path="ServiceRequest.occurrence.ofType(dateTime) | ServiceRequest.occurrence.ofType(Period) | ServiceRequest.occurrence.ofType(Timing)", description="When service should occur", type="date" ) 3698 public static final String SP_OCCURRENCE = "occurrence"; 3699 /** 3700 * <b>Fluent Client</b> search parameter constant for <b>occurrence</b> 3701 * <p> 3702 * Description: <b>When service should occur</b><br> 3703 * Type: <b>date</b><br> 3704 * Path: <b>ServiceRequest.occurrence.ofType(dateTime) | ServiceRequest.occurrence.ofType(Period) | ServiceRequest.occurrence.ofType(Timing)</b><br> 3705 * </p> 3706 */ 3707 public static final ca.uhn.fhir.rest.gclient.DateClientParam OCCURRENCE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_OCCURRENCE); 3708 3709 /** 3710 * Search parameter: <b>performer-type</b> 3711 * <p> 3712 * Description: <b>Performer role</b><br> 3713 * Type: <b>token</b><br> 3714 * Path: <b>ServiceRequest.performerType</b><br> 3715 * </p> 3716 */ 3717 @SearchParamDefinition(name="performer-type", path="ServiceRequest.performerType", description="Performer role", type="token" ) 3718 public static final String SP_PERFORMER_TYPE = "performer-type"; 3719 /** 3720 * <b>Fluent Client</b> search parameter constant for <b>performer-type</b> 3721 * <p> 3722 * Description: <b>Performer role</b><br> 3723 * Type: <b>token</b><br> 3724 * Path: <b>ServiceRequest.performerType</b><br> 3725 * </p> 3726 */ 3727 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PERFORMER_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PERFORMER_TYPE); 3728 3729 /** 3730 * Search parameter: <b>performer</b> 3731 * <p> 3732 * Description: <b>Requested performer</b><br> 3733 * Type: <b>reference</b><br> 3734 * Path: <b>ServiceRequest.performer</b><br> 3735 * </p> 3736 */ 3737 @SearchParamDefinition(name="performer", path="ServiceRequest.performer", description="Requested performer", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={CareTeam.class, Device.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 3738 public static final String SP_PERFORMER = "performer"; 3739 /** 3740 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 3741 * <p> 3742 * Description: <b>Requested performer</b><br> 3743 * Type: <b>reference</b><br> 3744 * Path: <b>ServiceRequest.performer</b><br> 3745 * </p> 3746 */ 3747 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 3748 3749/** 3750 * Constant for fluent queries to be used to add include statements. Specifies 3751 * the path value of "<b>ServiceRequest:performer</b>". 3752 */ 3753 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("ServiceRequest:performer").toLocked(); 3754 3755 /** 3756 * Search parameter: <b>priority</b> 3757 * <p> 3758 * Description: <b>routine | urgent | asap | stat</b><br> 3759 * Type: <b>token</b><br> 3760 * Path: <b>ServiceRequest.priority</b><br> 3761 * </p> 3762 */ 3763 @SearchParamDefinition(name="priority", path="ServiceRequest.priority", description="routine | urgent | asap | stat", type="token" ) 3764 public static final String SP_PRIORITY = "priority"; 3765 /** 3766 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 3767 * <p> 3768 * Description: <b>routine | urgent | asap | stat</b><br> 3769 * Type: <b>token</b><br> 3770 * Path: <b>ServiceRequest.priority</b><br> 3771 * </p> 3772 */ 3773 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRIORITY); 3774 3775 /** 3776 * Search parameter: <b>replaces</b> 3777 * <p> 3778 * Description: <b>What request replaces</b><br> 3779 * Type: <b>reference</b><br> 3780 * Path: <b>ServiceRequest.replaces</b><br> 3781 * </p> 3782 */ 3783 @SearchParamDefinition(name="replaces", path="ServiceRequest.replaces", description="What request replaces", type="reference", target={ServiceRequest.class } ) 3784 public static final String SP_REPLACES = "replaces"; 3785 /** 3786 * <b>Fluent Client</b> search parameter constant for <b>replaces</b> 3787 * <p> 3788 * Description: <b>What request replaces</b><br> 3789 * Type: <b>reference</b><br> 3790 * Path: <b>ServiceRequest.replaces</b><br> 3791 * </p> 3792 */ 3793 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REPLACES = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REPLACES); 3794 3795/** 3796 * Constant for fluent queries to be used to add include statements. Specifies 3797 * the path value of "<b>ServiceRequest:replaces</b>". 3798 */ 3799 public static final ca.uhn.fhir.model.api.Include INCLUDE_REPLACES = new ca.uhn.fhir.model.api.Include("ServiceRequest:replaces").toLocked(); 3800 3801 /** 3802 * Search parameter: <b>requester</b> 3803 * <p> 3804 * Description: <b>Who/what is requesting service</b><br> 3805 * Type: <b>reference</b><br> 3806 * Path: <b>ServiceRequest.requester</b><br> 3807 * </p> 3808 */ 3809 @SearchParamDefinition(name="requester", path="ServiceRequest.requester", description="Who/what is requesting service", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 3810 public static final String SP_REQUESTER = "requester"; 3811 /** 3812 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 3813 * <p> 3814 * Description: <b>Who/what is requesting service</b><br> 3815 * Type: <b>reference</b><br> 3816 * Path: <b>ServiceRequest.requester</b><br> 3817 * </p> 3818 */ 3819 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTER); 3820 3821/** 3822 * Constant for fluent queries to be used to add include statements. Specifies 3823 * the path value of "<b>ServiceRequest:requester</b>". 3824 */ 3825 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include("ServiceRequest:requester").toLocked(); 3826 3827 /** 3828 * Search parameter: <b>requisition</b> 3829 * <p> 3830 * Description: <b>Composite Request ID</b><br> 3831 * Type: <b>token</b><br> 3832 * Path: <b>ServiceRequest.requisition</b><br> 3833 * </p> 3834 */ 3835 @SearchParamDefinition(name="requisition", path="ServiceRequest.requisition", description="Composite Request ID", type="token" ) 3836 public static final String SP_REQUISITION = "requisition"; 3837 /** 3838 * <b>Fluent Client</b> search parameter constant for <b>requisition</b> 3839 * <p> 3840 * Description: <b>Composite Request ID</b><br> 3841 * Type: <b>token</b><br> 3842 * Path: <b>ServiceRequest.requisition</b><br> 3843 * </p> 3844 */ 3845 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REQUISITION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REQUISITION); 3846 3847 /** 3848 * Search parameter: <b>specimen</b> 3849 * <p> 3850 * Description: <b>Specimen to be tested</b><br> 3851 * Type: <b>reference</b><br> 3852 * Path: <b>ServiceRequest.specimen</b><br> 3853 * </p> 3854 */ 3855 @SearchParamDefinition(name="specimen", path="ServiceRequest.specimen", description="Specimen to be tested", type="reference", target={Specimen.class } ) 3856 public static final String SP_SPECIMEN = "specimen"; 3857 /** 3858 * <b>Fluent Client</b> search parameter constant for <b>specimen</b> 3859 * <p> 3860 * Description: <b>Specimen to be tested</b><br> 3861 * Type: <b>reference</b><br> 3862 * Path: <b>ServiceRequest.specimen</b><br> 3863 * </p> 3864 */ 3865 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SPECIMEN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SPECIMEN); 3866 3867/** 3868 * Constant for fluent queries to be used to add include statements. Specifies 3869 * the path value of "<b>ServiceRequest:specimen</b>". 3870 */ 3871 public static final ca.uhn.fhir.model.api.Include INCLUDE_SPECIMEN = new ca.uhn.fhir.model.api.Include("ServiceRequest:specimen").toLocked(); 3872 3873 /** 3874 * Search parameter: <b>status</b> 3875 * <p> 3876 * Description: <b>draft | active | on-hold | revoked | completed | entered-in-error | unknown</b><br> 3877 * Type: <b>token</b><br> 3878 * Path: <b>ServiceRequest.status</b><br> 3879 * </p> 3880 */ 3881 @SearchParamDefinition(name="status", path="ServiceRequest.status", description="draft | active | on-hold | revoked | completed | entered-in-error | unknown", type="token" ) 3882 public static final String SP_STATUS = "status"; 3883 /** 3884 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3885 * <p> 3886 * Description: <b>draft | active | on-hold | revoked | completed | entered-in-error | unknown</b><br> 3887 * Type: <b>token</b><br> 3888 * Path: <b>ServiceRequest.status</b><br> 3889 * </p> 3890 */ 3891 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3892 3893 /** 3894 * Search parameter: <b>subject</b> 3895 * <p> 3896 * Description: <b>Search by subject</b><br> 3897 * Type: <b>reference</b><br> 3898 * Path: <b>ServiceRequest.subject</b><br> 3899 * </p> 3900 */ 3901 @SearchParamDefinition(name="subject", path="ServiceRequest.subject", description="Search by subject", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Device.class, Group.class, Location.class, Patient.class } ) 3902 public static final String SP_SUBJECT = "subject"; 3903 /** 3904 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3905 * <p> 3906 * Description: <b>Search by subject</b><br> 3907 * Type: <b>reference</b><br> 3908 * Path: <b>ServiceRequest.subject</b><br> 3909 * </p> 3910 */ 3911 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 3912 3913/** 3914 * Constant for fluent queries to be used to add include statements. Specifies 3915 * the path value of "<b>ServiceRequest:subject</b>". 3916 */ 3917 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("ServiceRequest:subject").toLocked(); 3918 3919 /** 3920 * Search parameter: <b>encounter</b> 3921 * <p> 3922 * Description: <b>Multiple Resources: 3923 3924* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 3925* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 3926* [ChargeItem](chargeitem.html): Encounter associated with event 3927* [Claim](claim.html): Encounters associated with a billed line item 3928* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 3929* [Communication](communication.html): The Encounter during which this Communication was created 3930* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 3931* [Composition](composition.html): Context of the Composition 3932* [Condition](condition.html): The Encounter during which this Condition was created 3933* [DeviceRequest](devicerequest.html): Encounter during which request was created 3934* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 3935* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 3936* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 3937* [Flag](flag.html): Alert relevant during encounter 3938* [ImagingStudy](imagingstudy.html): The context of the study 3939* [List](list.html): Context in which list created 3940* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 3941* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 3942* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 3943* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 3944* [Observation](observation.html): Encounter related to the observation 3945* [Procedure](procedure.html): The Encounter during which this Procedure was created 3946* [Provenance](provenance.html): Encounter related to the Provenance 3947* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 3948* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 3949* [RiskAssessment](riskassessment.html): Where was assessment performed? 3950* [ServiceRequest](servicerequest.html): An encounter in which this request is made 3951* [Task](task.html): Search by encounter 3952* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 3953</b><br> 3954 * Type: <b>reference</b><br> 3955 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 3956 * </p> 3957 */ 3958 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 3959 public static final String SP_ENCOUNTER = "encounter"; 3960 /** 3961 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3962 * <p> 3963 * Description: <b>Multiple Resources: 3964 3965* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 3966* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 3967* [ChargeItem](chargeitem.html): Encounter associated with event 3968* [Claim](claim.html): Encounters associated with a billed line item 3969* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 3970* [Communication](communication.html): The Encounter during which this Communication was created 3971* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 3972* [Composition](composition.html): Context of the Composition 3973* [Condition](condition.html): The Encounter during which this Condition was created 3974* [DeviceRequest](devicerequest.html): Encounter during which request was created 3975* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 3976* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 3977* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 3978* [Flag](flag.html): Alert relevant during encounter 3979* [ImagingStudy](imagingstudy.html): The context of the study 3980* [List](list.html): Context in which list created 3981* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 3982* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 3983* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 3984* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 3985* [Observation](observation.html): Encounter related to the observation 3986* [Procedure](procedure.html): The Encounter during which this Procedure was created 3987* [Provenance](provenance.html): Encounter related to the Provenance 3988* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 3989* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 3990* [RiskAssessment](riskassessment.html): Where was assessment performed? 3991* [ServiceRequest](servicerequest.html): An encounter in which this request is made 3992* [Task](task.html): Search by encounter 3993* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 3994</b><br> 3995 * Type: <b>reference</b><br> 3996 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 3997 * </p> 3998 */ 3999 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 4000 4001/** 4002 * Constant for fluent queries to be used to add include statements. Specifies 4003 * the path value of "<b>ServiceRequest:encounter</b>". 4004 */ 4005 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("ServiceRequest:encounter").toLocked(); 4006 4007 /** 4008 * Search parameter: <b>identifier</b> 4009 * <p> 4010 * Description: <b>Multiple Resources: 4011 4012* [Account](account.html): Account number 4013* [AdverseEvent](adverseevent.html): Business identifier for the event 4014* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4015* [Appointment](appointment.html): An Identifier of the Appointment 4016* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4017* [Basic](basic.html): Business identifier 4018* [BodyStructure](bodystructure.html): Bodystructure identifier 4019* [CarePlan](careplan.html): External Ids for this plan 4020* [CareTeam](careteam.html): External Ids for this team 4021* [ChargeItem](chargeitem.html): Business Identifier for item 4022* [Claim](claim.html): The primary identifier of the financial resource 4023* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4024* [ClinicalImpression](clinicalimpression.html): Business identifier 4025* [Communication](communication.html): Unique identifier 4026* [CommunicationRequest](communicationrequest.html): Unique identifier 4027* [Composition](composition.html): Version-independent identifier for the Composition 4028* [Condition](condition.html): A unique identifier of the condition record 4029* [Consent](consent.html): Identifier for this record (external references) 4030* [Contract](contract.html): The identity of the contract 4031* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4032* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4033* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4034* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4035* [DeviceRequest](devicerequest.html): Business identifier for request/order 4036* [DeviceUsage](deviceusage.html): Search by identifier 4037* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4038* [DocumentReference](documentreference.html): Identifier of the attachment binary 4039* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4040* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4041* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4042* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4043* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4044* [Flag](flag.html): Business identifier 4045* [Goal](goal.html): External Ids for this goal 4046* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4047* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4048* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4049* [Immunization](immunization.html): Business identifier 4050* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4051* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4052* [Invoice](invoice.html): Business Identifier for item 4053* [List](list.html): Business identifier 4054* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4055* [Medication](medication.html): Returns medications with this external identifier 4056* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4057* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4058* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4059* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4060* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4061* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4062* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4063* [Observation](observation.html): The unique id for a particular observation 4064* [Person](person.html): A person Identifier 4065* [Procedure](procedure.html): A unique identifier for a procedure 4066* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4067* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4068* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4069* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4070* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4071* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4072* [Specimen](specimen.html): The unique identifier associated with the specimen 4073* [SupplyDelivery](supplydelivery.html): External identifier 4074* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4075* [Task](task.html): Search for a task instance by its business identifier 4076* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4077</b><br> 4078 * Type: <b>token</b><br> 4079 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4080 * </p> 4081 */ 4082 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 4083 public static final String SP_IDENTIFIER = "identifier"; 4084 /** 4085 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4086 * <p> 4087 * Description: <b>Multiple Resources: 4088 4089* [Account](account.html): Account number 4090* [AdverseEvent](adverseevent.html): Business identifier for the event 4091* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4092* [Appointment](appointment.html): An Identifier of the Appointment 4093* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4094* [Basic](basic.html): Business identifier 4095* [BodyStructure](bodystructure.html): Bodystructure identifier 4096* [CarePlan](careplan.html): External Ids for this plan 4097* [CareTeam](careteam.html): External Ids for this team 4098* [ChargeItem](chargeitem.html): Business Identifier for item 4099* [Claim](claim.html): The primary identifier of the financial resource 4100* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4101* [ClinicalImpression](clinicalimpression.html): Business identifier 4102* [Communication](communication.html): Unique identifier 4103* [CommunicationRequest](communicationrequest.html): Unique identifier 4104* [Composition](composition.html): Version-independent identifier for the Composition 4105* [Condition](condition.html): A unique identifier of the condition record 4106* [Consent](consent.html): Identifier for this record (external references) 4107* [Contract](contract.html): The identity of the contract 4108* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4109* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4110* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4111* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4112* [DeviceRequest](devicerequest.html): Business identifier for request/order 4113* [DeviceUsage](deviceusage.html): Search by identifier 4114* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4115* [DocumentReference](documentreference.html): Identifier of the attachment binary 4116* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4117* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4118* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4119* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4120* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4121* [Flag](flag.html): Business identifier 4122* [Goal](goal.html): External Ids for this goal 4123* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4124* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4125* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4126* [Immunization](immunization.html): Business identifier 4127* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4128* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4129* [Invoice](invoice.html): Business Identifier for item 4130* [List](list.html): Business identifier 4131* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4132* [Medication](medication.html): Returns medications with this external identifier 4133* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4134* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4135* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4136* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4137* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4138* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4139* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4140* [Observation](observation.html): The unique id for a particular observation 4141* [Person](person.html): A person Identifier 4142* [Procedure](procedure.html): A unique identifier for a procedure 4143* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4144* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4145* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4146* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4147* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4148* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4149* [Specimen](specimen.html): The unique identifier associated with the specimen 4150* [SupplyDelivery](supplydelivery.html): External identifier 4151* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4152* [Task](task.html): Search for a task instance by its business identifier 4153* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4154</b><br> 4155 * Type: <b>token</b><br> 4156 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4157 * </p> 4158 */ 4159 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4160 4161 /** 4162 * Search parameter: <b>patient</b> 4163 * <p> 4164 * Description: <b>Multiple Resources: 4165 4166* [Account](account.html): The entity that caused the expenses 4167* [AdverseEvent](adverseevent.html): Subject impacted by event 4168* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4169* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4170* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4171* [AuditEvent](auditevent.html): Where the activity involved patient data 4172* [Basic](basic.html): Identifies the focus of this resource 4173* [BodyStructure](bodystructure.html): Who this is about 4174* [CarePlan](careplan.html): Who the care plan is for 4175* [CareTeam](careteam.html): Who care team is for 4176* [ChargeItem](chargeitem.html): Individual service was done for/to 4177* [Claim](claim.html): Patient receiving the products or services 4178* [ClaimResponse](claimresponse.html): The subject of care 4179* [ClinicalImpression](clinicalimpression.html): Patient assessed 4180* [Communication](communication.html): Focus of message 4181* [CommunicationRequest](communicationrequest.html): Focus of message 4182* [Composition](composition.html): Who and/or what the composition is about 4183* [Condition](condition.html): Who has the condition? 4184* [Consent](consent.html): Who the consent applies to 4185* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4186* [Coverage](coverage.html): Retrieve coverages for a patient 4187* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4188* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4189* [DetectedIssue](detectedissue.html): Associated patient 4190* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4191* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4192* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4193* [DocumentReference](documentreference.html): Who/what is the subject of the document 4194* [Encounter](encounter.html): The patient present at the encounter 4195* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4196* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4197* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4198* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4199* [Flag](flag.html): The identity of a subject to list flags for 4200* [Goal](goal.html): Who this goal is intended for 4201* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4202* [ImagingSelection](imagingselection.html): Who the study is about 4203* [ImagingStudy](imagingstudy.html): Who the study is about 4204* [Immunization](immunization.html): The patient for the vaccination record 4205* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4206* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4207* [Invoice](invoice.html): Recipient(s) of goods and services 4208* [List](list.html): If all resources have the same subject 4209* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4210* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4211* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4212* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4213* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4214* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4215* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4216* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4217* [Observation](observation.html): The subject that the observation is about (if patient) 4218* [Person](person.html): The Person links to this Patient 4219* [Procedure](procedure.html): Search by subject - a patient 4220* [Provenance](provenance.html): Where the activity involved patient data 4221* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4222* [RelatedPerson](relatedperson.html): The patient this related person is related to 4223* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4224* [ResearchSubject](researchsubject.html): Who or what is part of study 4225* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4226* [ServiceRequest](servicerequest.html): Search by subject - a patient 4227* [Specimen](specimen.html): The patient the specimen comes from 4228* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4229* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4230* [Task](task.html): Search by patient 4231* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4232</b><br> 4233 * Type: <b>reference</b><br> 4234 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4235 * </p> 4236 */ 4237 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 4238 public static final String SP_PATIENT = "patient"; 4239 /** 4240 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4241 * <p> 4242 * Description: <b>Multiple Resources: 4243 4244* [Account](account.html): The entity that caused the expenses 4245* [AdverseEvent](adverseevent.html): Subject impacted by event 4246* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4247* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4248* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4249* [AuditEvent](auditevent.html): Where the activity involved patient data 4250* [Basic](basic.html): Identifies the focus of this resource 4251* [BodyStructure](bodystructure.html): Who this is about 4252* [CarePlan](careplan.html): Who the care plan is for 4253* [CareTeam](careteam.html): Who care team is for 4254* [ChargeItem](chargeitem.html): Individual service was done for/to 4255* [Claim](claim.html): Patient receiving the products or services 4256* [ClaimResponse](claimresponse.html): The subject of care 4257* [ClinicalImpression](clinicalimpression.html): Patient assessed 4258* [Communication](communication.html): Focus of message 4259* [CommunicationRequest](communicationrequest.html): Focus of message 4260* [Composition](composition.html): Who and/or what the composition is about 4261* [Condition](condition.html): Who has the condition? 4262* [Consent](consent.html): Who the consent applies to 4263* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4264* [Coverage](coverage.html): Retrieve coverages for a patient 4265* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4266* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4267* [DetectedIssue](detectedissue.html): Associated patient 4268* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4269* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4270* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4271* [DocumentReference](documentreference.html): Who/what is the subject of the document 4272* [Encounter](encounter.html): The patient present at the encounter 4273* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4274* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4275* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4276* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4277* [Flag](flag.html): The identity of a subject to list flags for 4278* [Goal](goal.html): Who this goal is intended for 4279* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4280* [ImagingSelection](imagingselection.html): Who the study is about 4281* [ImagingStudy](imagingstudy.html): Who the study is about 4282* [Immunization](immunization.html): The patient for the vaccination record 4283* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4284* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4285* [Invoice](invoice.html): Recipient(s) of goods and services 4286* [List](list.html): If all resources have the same subject 4287* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4288* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4289* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4290* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4291* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4292* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4293* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4294* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4295* [Observation](observation.html): The subject that the observation is about (if patient) 4296* [Person](person.html): The Person links to this Patient 4297* [Procedure](procedure.html): Search by subject - a patient 4298* [Provenance](provenance.html): Where the activity involved patient data 4299* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4300* [RelatedPerson](relatedperson.html): The patient this related person is related to 4301* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4302* [ResearchSubject](researchsubject.html): Who or what is part of study 4303* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4304* [ServiceRequest](servicerequest.html): Search by subject - a patient 4305* [Specimen](specimen.html): The patient the specimen comes from 4306* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4307* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4308* [Task](task.html): Search by patient 4309* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4310</b><br> 4311 * Type: <b>reference</b><br> 4312 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4313 * </p> 4314 */ 4315 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 4316 4317/** 4318 * Constant for fluent queries to be used to add include statements. Specifies 4319 * the path value of "<b>ServiceRequest:patient</b>". 4320 */ 4321 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ServiceRequest:patient").toLocked(); 4322 4323 4324} 4325