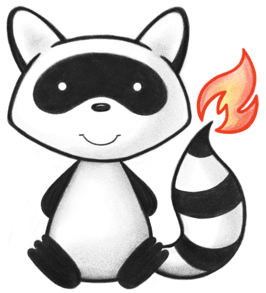
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * Signature Type: A signature along with supporting context. The signature may be a digital signature that is cryptographic in nature, or some other signature acceptable to the domain. This other signature may be as simple as a graphical image representing a hand-written signature, or a signature ceremony Different signature approaches have different utilities. 050 */ 051@DatatypeDef(name="Signature") 052public class Signature extends DataType implements ICompositeType { 053 054 /** 055 * An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document. 056 */ 057 @Child(name = "type", type = {Coding.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 058 @Description(shortDefinition="Indication of the reason the entity signed the object(s)", formalDefinition="An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document." ) 059 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/signature-type") 060 protected List<Coding> type; 061 062 /** 063 * When the digital signature was signed. 064 */ 065 @Child(name = "when", type = {InstantType.class}, order=1, min=0, max=1, modifier=false, summary=true) 066 @Description(shortDefinition="When the signature was created", formalDefinition="When the digital signature was signed." ) 067 protected InstantType when; 068 069 /** 070 * A reference to an application-usable description of the identity that signed (e.g. the signature used their private key). 071 */ 072 @Child(name = "who", type = {Practitioner.class, PractitionerRole.class, RelatedPerson.class, Patient.class, Device.class, Organization.class}, order=2, min=0, max=1, modifier=false, summary=true) 073 @Description(shortDefinition="Who signed", formalDefinition="A reference to an application-usable description of the identity that signed (e.g. the signature used their private key)." ) 074 protected Reference who; 075 076 /** 077 * A reference to an application-usable description of the identity that is represented by the signature. 078 */ 079 @Child(name = "onBehalfOf", type = {Practitioner.class, PractitionerRole.class, RelatedPerson.class, Patient.class, Device.class, Organization.class}, order=3, min=0, max=1, modifier=false, summary=true) 080 @Description(shortDefinition="The party represented", formalDefinition="A reference to an application-usable description of the identity that is represented by the signature." ) 081 protected Reference onBehalfOf; 082 083 /** 084 * A mime type that indicates the technical format of the target resources signed by the signature. 085 */ 086 @Child(name = "targetFormat", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=false) 087 @Description(shortDefinition="The technical format of the signed resources", formalDefinition="A mime type that indicates the technical format of the target resources signed by the signature." ) 088 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/mimetypes") 089 protected CodeType targetFormat; 090 091 /** 092 * A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jose for JWS, and image/* for a graphical image of a signature, etc. 093 */ 094 @Child(name = "sigFormat", type = {CodeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 095 @Description(shortDefinition="The technical format of the signature", formalDefinition="A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jose for JWS, and image/* for a graphical image of a signature, etc." ) 096 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/mimetypes") 097 protected CodeType sigFormat; 098 099 /** 100 * The base64 encoding of the Signature content. When signature is not recorded electronically this element would be empty. 101 */ 102 @Child(name = "data", type = {Base64BinaryType.class}, order=6, min=0, max=1, modifier=false, summary=false) 103 @Description(shortDefinition="The actual signature content (XML DigSig. JWS, picture, etc.)", formalDefinition="The base64 encoding of the Signature content. When signature is not recorded electronically this element would be empty." ) 104 protected Base64BinaryType data; 105 106 private static final long serialVersionUID = -986223243L; 107 108 /** 109 * Constructor 110 */ 111 public Signature() { 112 super(); 113 } 114 115 /** 116 * @return {@link #type} (An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document.) 117 */ 118 public List<Coding> getType() { 119 if (this.type == null) 120 this.type = new ArrayList<Coding>(); 121 return this.type; 122 } 123 124 /** 125 * @return Returns a reference to <code>this</code> for easy method chaining 126 */ 127 public Signature setType(List<Coding> theType) { 128 this.type = theType; 129 return this; 130 } 131 132 public boolean hasType() { 133 if (this.type == null) 134 return false; 135 for (Coding item : this.type) 136 if (!item.isEmpty()) 137 return true; 138 return false; 139 } 140 141 public Coding addType() { //3 142 Coding t = new Coding(); 143 if (this.type == null) 144 this.type = new ArrayList<Coding>(); 145 this.type.add(t); 146 return t; 147 } 148 149 public Signature addType(Coding t) { //3 150 if (t == null) 151 return this; 152 if (this.type == null) 153 this.type = new ArrayList<Coding>(); 154 this.type.add(t); 155 return this; 156 } 157 158 /** 159 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 160 */ 161 public Coding getTypeFirstRep() { 162 if (getType().isEmpty()) { 163 addType(); 164 } 165 return getType().get(0); 166 } 167 168 /** 169 * @return {@link #when} (When the digital signature was signed.). This is the underlying object with id, value and extensions. The accessor "getWhen" gives direct access to the value 170 */ 171 public InstantType getWhenElement() { 172 if (this.when == null) 173 if (Configuration.errorOnAutoCreate()) 174 throw new Error("Attempt to auto-create Signature.when"); 175 else if (Configuration.doAutoCreate()) 176 this.when = new InstantType(); // bb 177 return this.when; 178 } 179 180 public boolean hasWhenElement() { 181 return this.when != null && !this.when.isEmpty(); 182 } 183 184 public boolean hasWhen() { 185 return this.when != null && !this.when.isEmpty(); 186 } 187 188 /** 189 * @param value {@link #when} (When the digital signature was signed.). This is the underlying object with id, value and extensions. The accessor "getWhen" gives direct access to the value 190 */ 191 public Signature setWhenElement(InstantType value) { 192 this.when = value; 193 return this; 194 } 195 196 /** 197 * @return When the digital signature was signed. 198 */ 199 public Date getWhen() { 200 return this.when == null ? null : this.when.getValue(); 201 } 202 203 /** 204 * @param value When the digital signature was signed. 205 */ 206 public Signature setWhen(Date value) { 207 if (value == null) 208 this.when = null; 209 else { 210 if (this.when == null) 211 this.when = new InstantType(); 212 this.when.setValue(value); 213 } 214 return this; 215 } 216 217 /** 218 * @return {@link #who} (A reference to an application-usable description of the identity that signed (e.g. the signature used their private key).) 219 */ 220 public Reference getWho() { 221 if (this.who == null) 222 if (Configuration.errorOnAutoCreate()) 223 throw new Error("Attempt to auto-create Signature.who"); 224 else if (Configuration.doAutoCreate()) 225 this.who = new Reference(); // cc 226 return this.who; 227 } 228 229 public boolean hasWho() { 230 return this.who != null && !this.who.isEmpty(); 231 } 232 233 /** 234 * @param value {@link #who} (A reference to an application-usable description of the identity that signed (e.g. the signature used their private key).) 235 */ 236 public Signature setWho(Reference value) { 237 this.who = value; 238 return this; 239 } 240 241 /** 242 * @return {@link #onBehalfOf} (A reference to an application-usable description of the identity that is represented by the signature.) 243 */ 244 public Reference getOnBehalfOf() { 245 if (this.onBehalfOf == null) 246 if (Configuration.errorOnAutoCreate()) 247 throw new Error("Attempt to auto-create Signature.onBehalfOf"); 248 else if (Configuration.doAutoCreate()) 249 this.onBehalfOf = new Reference(); // cc 250 return this.onBehalfOf; 251 } 252 253 public boolean hasOnBehalfOf() { 254 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 255 } 256 257 /** 258 * @param value {@link #onBehalfOf} (A reference to an application-usable description of the identity that is represented by the signature.) 259 */ 260 public Signature setOnBehalfOf(Reference value) { 261 this.onBehalfOf = value; 262 return this; 263 } 264 265 /** 266 * @return {@link #targetFormat} (A mime type that indicates the technical format of the target resources signed by the signature.). This is the underlying object with id, value and extensions. The accessor "getTargetFormat" gives direct access to the value 267 */ 268 public CodeType getTargetFormatElement() { 269 if (this.targetFormat == null) 270 if (Configuration.errorOnAutoCreate()) 271 throw new Error("Attempt to auto-create Signature.targetFormat"); 272 else if (Configuration.doAutoCreate()) 273 this.targetFormat = new CodeType(); // bb 274 return this.targetFormat; 275 } 276 277 public boolean hasTargetFormatElement() { 278 return this.targetFormat != null && !this.targetFormat.isEmpty(); 279 } 280 281 public boolean hasTargetFormat() { 282 return this.targetFormat != null && !this.targetFormat.isEmpty(); 283 } 284 285 /** 286 * @param value {@link #targetFormat} (A mime type that indicates the technical format of the target resources signed by the signature.). This is the underlying object with id, value and extensions. The accessor "getTargetFormat" gives direct access to the value 287 */ 288 public Signature setTargetFormatElement(CodeType value) { 289 this.targetFormat = value; 290 return this; 291 } 292 293 /** 294 * @return A mime type that indicates the technical format of the target resources signed by the signature. 295 */ 296 public String getTargetFormat() { 297 return this.targetFormat == null ? null : this.targetFormat.getValue(); 298 } 299 300 /** 301 * @param value A mime type that indicates the technical format of the target resources signed by the signature. 302 */ 303 public Signature setTargetFormat(String value) { 304 if (Utilities.noString(value)) 305 this.targetFormat = null; 306 else { 307 if (this.targetFormat == null) 308 this.targetFormat = new CodeType(); 309 this.targetFormat.setValue(value); 310 } 311 return this; 312 } 313 314 /** 315 * @return {@link #sigFormat} (A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jose for JWS, and image/* for a graphical image of a signature, etc.). This is the underlying object with id, value and extensions. The accessor "getSigFormat" gives direct access to the value 316 */ 317 public CodeType getSigFormatElement() { 318 if (this.sigFormat == null) 319 if (Configuration.errorOnAutoCreate()) 320 throw new Error("Attempt to auto-create Signature.sigFormat"); 321 else if (Configuration.doAutoCreate()) 322 this.sigFormat = new CodeType(); // bb 323 return this.sigFormat; 324 } 325 326 public boolean hasSigFormatElement() { 327 return this.sigFormat != null && !this.sigFormat.isEmpty(); 328 } 329 330 public boolean hasSigFormat() { 331 return this.sigFormat != null && !this.sigFormat.isEmpty(); 332 } 333 334 /** 335 * @param value {@link #sigFormat} (A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jose for JWS, and image/* for a graphical image of a signature, etc.). This is the underlying object with id, value and extensions. The accessor "getSigFormat" gives direct access to the value 336 */ 337 public Signature setSigFormatElement(CodeType value) { 338 this.sigFormat = value; 339 return this; 340 } 341 342 /** 343 * @return A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jose for JWS, and image/* for a graphical image of a signature, etc. 344 */ 345 public String getSigFormat() { 346 return this.sigFormat == null ? null : this.sigFormat.getValue(); 347 } 348 349 /** 350 * @param value A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jose for JWS, and image/* for a graphical image of a signature, etc. 351 */ 352 public Signature setSigFormat(String value) { 353 if (Utilities.noString(value)) 354 this.sigFormat = null; 355 else { 356 if (this.sigFormat == null) 357 this.sigFormat = new CodeType(); 358 this.sigFormat.setValue(value); 359 } 360 return this; 361 } 362 363 /** 364 * @return {@link #data} (The base64 encoding of the Signature content. When signature is not recorded electronically this element would be empty.). This is the underlying object with id, value and extensions. The accessor "getData" gives direct access to the value 365 */ 366 public Base64BinaryType getDataElement() { 367 if (this.data == null) 368 if (Configuration.errorOnAutoCreate()) 369 throw new Error("Attempt to auto-create Signature.data"); 370 else if (Configuration.doAutoCreate()) 371 this.data = new Base64BinaryType(); // bb 372 return this.data; 373 } 374 375 public boolean hasDataElement() { 376 return this.data != null && !this.data.isEmpty(); 377 } 378 379 public boolean hasData() { 380 return this.data != null && !this.data.isEmpty(); 381 } 382 383 /** 384 * @param value {@link #data} (The base64 encoding of the Signature content. When signature is not recorded electronically this element would be empty.). This is the underlying object with id, value and extensions. The accessor "getData" gives direct access to the value 385 */ 386 public Signature setDataElement(Base64BinaryType value) { 387 this.data = value; 388 return this; 389 } 390 391 /** 392 * @return The base64 encoding of the Signature content. When signature is not recorded electronically this element would be empty. 393 */ 394 public byte[] getData() { 395 return this.data == null ? null : this.data.getValue(); 396 } 397 398 /** 399 * @param value The base64 encoding of the Signature content. When signature is not recorded electronically this element would be empty. 400 */ 401 public Signature setData(byte[] value) { 402 if (value == null) 403 this.data = null; 404 else { 405 if (this.data == null) 406 this.data = new Base64BinaryType(); 407 this.data.setValue(value); 408 } 409 return this; 410 } 411 412 protected void listChildren(List<Property> children) { 413 super.listChildren(children); 414 children.add(new Property("type", "Coding", "An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document.", 0, java.lang.Integer.MAX_VALUE, type)); 415 children.add(new Property("when", "instant", "When the digital signature was signed.", 0, 1, when)); 416 children.add(new Property("who", "Reference(Practitioner|PractitionerRole|RelatedPerson|Patient|Device|Organization)", "A reference to an application-usable description of the identity that signed (e.g. the signature used their private key).", 0, 1, who)); 417 children.add(new Property("onBehalfOf", "Reference(Practitioner|PractitionerRole|RelatedPerson|Patient|Device|Organization)", "A reference to an application-usable description of the identity that is represented by the signature.", 0, 1, onBehalfOf)); 418 children.add(new Property("targetFormat", "code", "A mime type that indicates the technical format of the target resources signed by the signature.", 0, 1, targetFormat)); 419 children.add(new Property("sigFormat", "code", "A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jose for JWS, and image/* for a graphical image of a signature, etc.", 0, 1, sigFormat)); 420 children.add(new Property("data", "base64Binary", "The base64 encoding of the Signature content. When signature is not recorded electronically this element would be empty.", 0, 1, data)); 421 } 422 423 @Override 424 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 425 switch (_hash) { 426 case 3575610: /*type*/ return new Property("type", "Coding", "An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document.", 0, java.lang.Integer.MAX_VALUE, type); 427 case 3648314: /*when*/ return new Property("when", "instant", "When the digital signature was signed.", 0, 1, when); 428 case 117694: /*who*/ return new Property("who", "Reference(Practitioner|PractitionerRole|RelatedPerson|Patient|Device|Organization)", "A reference to an application-usable description of the identity that signed (e.g. the signature used their private key).", 0, 1, who); 429 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf", "Reference(Practitioner|PractitionerRole|RelatedPerson|Patient|Device|Organization)", "A reference to an application-usable description of the identity that is represented by the signature.", 0, 1, onBehalfOf); 430 case -917363480: /*targetFormat*/ return new Property("targetFormat", "code", "A mime type that indicates the technical format of the target resources signed by the signature.", 0, 1, targetFormat); 431 case -58720216: /*sigFormat*/ return new Property("sigFormat", "code", "A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jose for JWS, and image/* for a graphical image of a signature, etc.", 0, 1, sigFormat); 432 case 3076010: /*data*/ return new Property("data", "base64Binary", "The base64 encoding of the Signature content. When signature is not recorded electronically this element would be empty.", 0, 1, data); 433 default: return super.getNamedProperty(_hash, _name, _checkValid); 434 } 435 436 } 437 438 @Override 439 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 440 switch (hash) { 441 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // Coding 442 case 3648314: /*when*/ return this.when == null ? new Base[0] : new Base[] {this.when}; // InstantType 443 case 117694: /*who*/ return this.who == null ? new Base[0] : new Base[] {this.who}; // Reference 444 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Reference 445 case -917363480: /*targetFormat*/ return this.targetFormat == null ? new Base[0] : new Base[] {this.targetFormat}; // CodeType 446 case -58720216: /*sigFormat*/ return this.sigFormat == null ? new Base[0] : new Base[] {this.sigFormat}; // CodeType 447 case 3076010: /*data*/ return this.data == null ? new Base[0] : new Base[] {this.data}; // Base64BinaryType 448 default: return super.getProperty(hash, name, checkValid); 449 } 450 451 } 452 453 @Override 454 public Base setProperty(int hash, String name, Base value) throws FHIRException { 455 switch (hash) { 456 case 3575610: // type 457 this.getType().add(TypeConvertor.castToCoding(value)); // Coding 458 return value; 459 case 3648314: // when 460 this.when = TypeConvertor.castToInstant(value); // InstantType 461 return value; 462 case 117694: // who 463 this.who = TypeConvertor.castToReference(value); // Reference 464 return value; 465 case -14402964: // onBehalfOf 466 this.onBehalfOf = TypeConvertor.castToReference(value); // Reference 467 return value; 468 case -917363480: // targetFormat 469 this.targetFormat = TypeConvertor.castToCode(value); // CodeType 470 return value; 471 case -58720216: // sigFormat 472 this.sigFormat = TypeConvertor.castToCode(value); // CodeType 473 return value; 474 case 3076010: // data 475 this.data = TypeConvertor.castToBase64Binary(value); // Base64BinaryType 476 return value; 477 default: return super.setProperty(hash, name, value); 478 } 479 480 } 481 482 @Override 483 public Base setProperty(String name, Base value) throws FHIRException { 484 if (name.equals("type")) { 485 this.getType().add(TypeConvertor.castToCoding(value)); 486 } else if (name.equals("when")) { 487 this.when = TypeConvertor.castToInstant(value); // InstantType 488 } else if (name.equals("who")) { 489 this.who = TypeConvertor.castToReference(value); // Reference 490 } else if (name.equals("onBehalfOf")) { 491 this.onBehalfOf = TypeConvertor.castToReference(value); // Reference 492 } else if (name.equals("targetFormat")) { 493 this.targetFormat = TypeConvertor.castToCode(value); // CodeType 494 } else if (name.equals("sigFormat")) { 495 this.sigFormat = TypeConvertor.castToCode(value); // CodeType 496 } else if (name.equals("data")) { 497 this.data = TypeConvertor.castToBase64Binary(value); // Base64BinaryType 498 } else 499 return super.setProperty(name, value); 500 return value; 501 } 502 503 @Override 504 public void removeChild(String name, Base value) throws FHIRException { 505 if (name.equals("type")) { 506 this.getType().remove(value); 507 } else if (name.equals("when")) { 508 this.when = null; 509 } else if (name.equals("who")) { 510 this.who = null; 511 } else if (name.equals("onBehalfOf")) { 512 this.onBehalfOf = null; 513 } else if (name.equals("targetFormat")) { 514 this.targetFormat = null; 515 } else if (name.equals("sigFormat")) { 516 this.sigFormat = null; 517 } else if (name.equals("data")) { 518 this.data = null; 519 } else 520 super.removeChild(name, value); 521 522 } 523 524 @Override 525 public Base makeProperty(int hash, String name) throws FHIRException { 526 switch (hash) { 527 case 3575610: return addType(); 528 case 3648314: return getWhenElement(); 529 case 117694: return getWho(); 530 case -14402964: return getOnBehalfOf(); 531 case -917363480: return getTargetFormatElement(); 532 case -58720216: return getSigFormatElement(); 533 case 3076010: return getDataElement(); 534 default: return super.makeProperty(hash, name); 535 } 536 537 } 538 539 @Override 540 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 541 switch (hash) { 542 case 3575610: /*type*/ return new String[] {"Coding"}; 543 case 3648314: /*when*/ return new String[] {"instant"}; 544 case 117694: /*who*/ return new String[] {"Reference"}; 545 case -14402964: /*onBehalfOf*/ return new String[] {"Reference"}; 546 case -917363480: /*targetFormat*/ return new String[] {"code"}; 547 case -58720216: /*sigFormat*/ return new String[] {"code"}; 548 case 3076010: /*data*/ return new String[] {"base64Binary"}; 549 default: return super.getTypesForProperty(hash, name); 550 } 551 552 } 553 554 @Override 555 public Base addChild(String name) throws FHIRException { 556 if (name.equals("type")) { 557 return addType(); 558 } 559 else if (name.equals("when")) { 560 throw new FHIRException("Cannot call addChild on a singleton property Signature.when"); 561 } 562 else if (name.equals("who")) { 563 this.who = new Reference(); 564 return this.who; 565 } 566 else if (name.equals("onBehalfOf")) { 567 this.onBehalfOf = new Reference(); 568 return this.onBehalfOf; 569 } 570 else if (name.equals("targetFormat")) { 571 throw new FHIRException("Cannot call addChild on a singleton property Signature.targetFormat"); 572 } 573 else if (name.equals("sigFormat")) { 574 throw new FHIRException("Cannot call addChild on a singleton property Signature.sigFormat"); 575 } 576 else if (name.equals("data")) { 577 throw new FHIRException("Cannot call addChild on a singleton property Signature.data"); 578 } 579 else 580 return super.addChild(name); 581 } 582 583 public String fhirType() { 584 return "Signature"; 585 586 } 587 588 public Signature copy() { 589 Signature dst = new Signature(); 590 copyValues(dst); 591 return dst; 592 } 593 594 public void copyValues(Signature dst) { 595 super.copyValues(dst); 596 if (type != null) { 597 dst.type = new ArrayList<Coding>(); 598 for (Coding i : type) 599 dst.type.add(i.copy()); 600 }; 601 dst.when = when == null ? null : when.copy(); 602 dst.who = who == null ? null : who.copy(); 603 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 604 dst.targetFormat = targetFormat == null ? null : targetFormat.copy(); 605 dst.sigFormat = sigFormat == null ? null : sigFormat.copy(); 606 dst.data = data == null ? null : data.copy(); 607 } 608 609 protected Signature typedCopy() { 610 return copy(); 611 } 612 613 @Override 614 public boolean equalsDeep(Base other_) { 615 if (!super.equalsDeep(other_)) 616 return false; 617 if (!(other_ instanceof Signature)) 618 return false; 619 Signature o = (Signature) other_; 620 return compareDeep(type, o.type, true) && compareDeep(when, o.when, true) && compareDeep(who, o.who, true) 621 && compareDeep(onBehalfOf, o.onBehalfOf, true) && compareDeep(targetFormat, o.targetFormat, true) 622 && compareDeep(sigFormat, o.sigFormat, true) && compareDeep(data, o.data, true); 623 } 624 625 @Override 626 public boolean equalsShallow(Base other_) { 627 if (!super.equalsShallow(other_)) 628 return false; 629 if (!(other_ instanceof Signature)) 630 return false; 631 Signature o = (Signature) other_; 632 return compareValues(when, o.when, true) && compareValues(targetFormat, o.targetFormat, true) && compareValues(sigFormat, o.sigFormat, true) 633 && compareValues(data, o.data, true); 634 } 635 636 public boolean isEmpty() { 637 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, when, who, onBehalfOf 638 , targetFormat, sigFormat, data); 639 } 640 641 642} 643