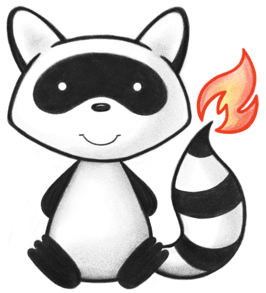
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A slot of time on a schedule that may be available for booking appointments. 052 */ 053@ResourceDef(name="Slot", profile="http://hl7.org/fhir/StructureDefinition/Slot") 054public class Slot extends DomainResource { 055 056 public enum SlotStatus { 057 /** 058 * Indicates that the time interval is busy because one or more events have been scheduled for that interval. 059 */ 060 BUSY, 061 /** 062 * Indicates that the time interval is free for scheduling. 063 */ 064 FREE, 065 /** 066 * Indicates that the time interval is busy and that the interval cannot be scheduled. 067 */ 068 BUSYUNAVAILABLE, 069 /** 070 * Indicates that the time interval is busy because one or more events have been tentatively scheduled for that interval. 071 */ 072 BUSYTENTATIVE, 073 /** 074 * This instance should not have been part of this patient's medical record. 075 */ 076 ENTEREDINERROR, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 public static SlotStatus fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("busy".equals(codeString)) 085 return BUSY; 086 if ("free".equals(codeString)) 087 return FREE; 088 if ("busy-unavailable".equals(codeString)) 089 return BUSYUNAVAILABLE; 090 if ("busy-tentative".equals(codeString)) 091 return BUSYTENTATIVE; 092 if ("entered-in-error".equals(codeString)) 093 return ENTEREDINERROR; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown SlotStatus code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case BUSY: return "busy"; 102 case FREE: return "free"; 103 case BUSYUNAVAILABLE: return "busy-unavailable"; 104 case BUSYTENTATIVE: return "busy-tentative"; 105 case ENTEREDINERROR: return "entered-in-error"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getSystem() { 111 switch (this) { 112 case BUSY: return "http://hl7.org/fhir/slotstatus"; 113 case FREE: return "http://hl7.org/fhir/slotstatus"; 114 case BUSYUNAVAILABLE: return "http://hl7.org/fhir/slotstatus"; 115 case BUSYTENTATIVE: return "http://hl7.org/fhir/slotstatus"; 116 case ENTEREDINERROR: return "http://hl7.org/fhir/slotstatus"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDefinition() { 122 switch (this) { 123 case BUSY: return "Indicates that the time interval is busy because one or more events have been scheduled for that interval."; 124 case FREE: return "Indicates that the time interval is free for scheduling."; 125 case BUSYUNAVAILABLE: return "Indicates that the time interval is busy and that the interval cannot be scheduled."; 126 case BUSYTENTATIVE: return "Indicates that the time interval is busy because one or more events have been tentatively scheduled for that interval."; 127 case ENTEREDINERROR: return "This instance should not have been part of this patient's medical record."; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case BUSY: return "Busy"; 135 case FREE: return "Free"; 136 case BUSYUNAVAILABLE: return "Busy (Unavailable)"; 137 case BUSYTENTATIVE: return "Busy (Tentative)"; 138 case ENTEREDINERROR: return "Entered in error"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 } 144 145 public static class SlotStatusEnumFactory implements EnumFactory<SlotStatus> { 146 public SlotStatus fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("busy".equals(codeString)) 151 return SlotStatus.BUSY; 152 if ("free".equals(codeString)) 153 return SlotStatus.FREE; 154 if ("busy-unavailable".equals(codeString)) 155 return SlotStatus.BUSYUNAVAILABLE; 156 if ("busy-tentative".equals(codeString)) 157 return SlotStatus.BUSYTENTATIVE; 158 if ("entered-in-error".equals(codeString)) 159 return SlotStatus.ENTEREDINERROR; 160 throw new IllegalArgumentException("Unknown SlotStatus code '"+codeString+"'"); 161 } 162 public Enumeration<SlotStatus> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<SlotStatus>(this, SlotStatus.NULL, code); 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<SlotStatus>(this, SlotStatus.NULL, code); 170 if ("busy".equals(codeString)) 171 return new Enumeration<SlotStatus>(this, SlotStatus.BUSY, code); 172 if ("free".equals(codeString)) 173 return new Enumeration<SlotStatus>(this, SlotStatus.FREE, code); 174 if ("busy-unavailable".equals(codeString)) 175 return new Enumeration<SlotStatus>(this, SlotStatus.BUSYUNAVAILABLE, code); 176 if ("busy-tentative".equals(codeString)) 177 return new Enumeration<SlotStatus>(this, SlotStatus.BUSYTENTATIVE, code); 178 if ("entered-in-error".equals(codeString)) 179 return new Enumeration<SlotStatus>(this, SlotStatus.ENTEREDINERROR, code); 180 throw new FHIRException("Unknown SlotStatus code '"+codeString+"'"); 181 } 182 public String toCode(SlotStatus code) { 183 if (code == SlotStatus.NULL) 184 return null; 185 if (code == SlotStatus.BUSY) 186 return "busy"; 187 if (code == SlotStatus.FREE) 188 return "free"; 189 if (code == SlotStatus.BUSYUNAVAILABLE) 190 return "busy-unavailable"; 191 if (code == SlotStatus.BUSYTENTATIVE) 192 return "busy-tentative"; 193 if (code == SlotStatus.ENTEREDINERROR) 194 return "entered-in-error"; 195 return "?"; 196 } 197 public String toSystem(SlotStatus code) { 198 return code.getSystem(); 199 } 200 } 201 202 /** 203 * External Ids for this item. 204 */ 205 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 206 @Description(shortDefinition="External Ids for this item", formalDefinition="External Ids for this item." ) 207 protected List<Identifier> identifier; 208 209 /** 210 * A broad categorization of the service that is to be performed during this appointment. 211 */ 212 @Child(name = "serviceCategory", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 213 @Description(shortDefinition="A broad categorization of the service that is to be performed during this appointment", formalDefinition="A broad categorization of the service that is to be performed during this appointment." ) 214 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-category") 215 protected List<CodeableConcept> serviceCategory; 216 217 /** 218 * The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the Schedule resource. 219 */ 220 @Child(name = "serviceType", type = {CodeableReference.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 221 @Description(shortDefinition="The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the Schedule resource", formalDefinition="The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the Schedule resource." ) 222 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-type") 223 protected List<CodeableReference> serviceType; 224 225 /** 226 * The specialty of a practitioner that would be required to perform the service requested in this appointment. 227 */ 228 @Child(name = "specialty", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 229 @Description(shortDefinition="The specialty of a practitioner that would be required to perform the service requested in this appointment", formalDefinition="The specialty of a practitioner that would be required to perform the service requested in this appointment." ) 230 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/c80-practice-codes") 231 protected List<CodeableConcept> specialty; 232 233 /** 234 * The style of appointment or patient that may be booked in the slot (not service type). 235 */ 236 @Child(name = "appointmentType", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 237 @Description(shortDefinition="The style of appointment or patient that may be booked in the slot (not service type)", formalDefinition="The style of appointment or patient that may be booked in the slot (not service type)." ) 238 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v2-0276") 239 protected List<CodeableConcept> appointmentType; 240 241 /** 242 * The schedule resource that this slot defines an interval of status information. 243 */ 244 @Child(name = "schedule", type = {Schedule.class}, order=5, min=1, max=1, modifier=false, summary=true) 245 @Description(shortDefinition="The schedule resource that this slot defines an interval of status information", formalDefinition="The schedule resource that this slot defines an interval of status information." ) 246 protected Reference schedule; 247 248 /** 249 * busy | free | busy-unavailable | busy-tentative | entered-in-error. 250 */ 251 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=false, summary=true) 252 @Description(shortDefinition="busy | free | busy-unavailable | busy-tentative | entered-in-error", formalDefinition="busy | free | busy-unavailable | busy-tentative | entered-in-error." ) 253 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/slotstatus") 254 protected Enumeration<SlotStatus> status; 255 256 /** 257 * Date/Time that the slot is to begin. 258 */ 259 @Child(name = "start", type = {InstantType.class}, order=7, min=1, max=1, modifier=false, summary=true) 260 @Description(shortDefinition="Date/Time that the slot is to begin", formalDefinition="Date/Time that the slot is to begin." ) 261 protected InstantType start; 262 263 /** 264 * Date/Time that the slot is to conclude. 265 */ 266 @Child(name = "end", type = {InstantType.class}, order=8, min=1, max=1, modifier=false, summary=true) 267 @Description(shortDefinition="Date/Time that the slot is to conclude", formalDefinition="Date/Time that the slot is to conclude." ) 268 protected InstantType end; 269 270 /** 271 * This slot has already been overbooked, appointments are unlikely to be accepted for this time. 272 */ 273 @Child(name = "overbooked", type = {BooleanType.class}, order=9, min=0, max=1, modifier=false, summary=false) 274 @Description(shortDefinition="This slot has already been overbooked, appointments are unlikely to be accepted for this time", formalDefinition="This slot has already been overbooked, appointments are unlikely to be accepted for this time." ) 275 protected BooleanType overbooked; 276 277 /** 278 * Comments on the slot to describe any extended information. Such as custom constraints on the slot. 279 */ 280 @Child(name = "comment", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=false) 281 @Description(shortDefinition="Comments on the slot to describe any extended information. Such as custom constraints on the slot", formalDefinition="Comments on the slot to describe any extended information. Such as custom constraints on the slot." ) 282 protected StringType comment; 283 284 private static final long serialVersionUID = 2060370370L; 285 286 /** 287 * Constructor 288 */ 289 public Slot() { 290 super(); 291 } 292 293 /** 294 * Constructor 295 */ 296 public Slot(Reference schedule, SlotStatus status, Date start, Date end) { 297 super(); 298 this.setSchedule(schedule); 299 this.setStatus(status); 300 this.setStart(start); 301 this.setEnd(end); 302 } 303 304 /** 305 * @return {@link #identifier} (External Ids for this item.) 306 */ 307 public List<Identifier> getIdentifier() { 308 if (this.identifier == null) 309 this.identifier = new ArrayList<Identifier>(); 310 return this.identifier; 311 } 312 313 /** 314 * @return Returns a reference to <code>this</code> for easy method chaining 315 */ 316 public Slot setIdentifier(List<Identifier> theIdentifier) { 317 this.identifier = theIdentifier; 318 return this; 319 } 320 321 public boolean hasIdentifier() { 322 if (this.identifier == null) 323 return false; 324 for (Identifier item : this.identifier) 325 if (!item.isEmpty()) 326 return true; 327 return false; 328 } 329 330 public Identifier addIdentifier() { //3 331 Identifier t = new Identifier(); 332 if (this.identifier == null) 333 this.identifier = new ArrayList<Identifier>(); 334 this.identifier.add(t); 335 return t; 336 } 337 338 public Slot addIdentifier(Identifier t) { //3 339 if (t == null) 340 return this; 341 if (this.identifier == null) 342 this.identifier = new ArrayList<Identifier>(); 343 this.identifier.add(t); 344 return this; 345 } 346 347 /** 348 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 349 */ 350 public Identifier getIdentifierFirstRep() { 351 if (getIdentifier().isEmpty()) { 352 addIdentifier(); 353 } 354 return getIdentifier().get(0); 355 } 356 357 /** 358 * @return {@link #serviceCategory} (A broad categorization of the service that is to be performed during this appointment.) 359 */ 360 public List<CodeableConcept> getServiceCategory() { 361 if (this.serviceCategory == null) 362 this.serviceCategory = new ArrayList<CodeableConcept>(); 363 return this.serviceCategory; 364 } 365 366 /** 367 * @return Returns a reference to <code>this</code> for easy method chaining 368 */ 369 public Slot setServiceCategory(List<CodeableConcept> theServiceCategory) { 370 this.serviceCategory = theServiceCategory; 371 return this; 372 } 373 374 public boolean hasServiceCategory() { 375 if (this.serviceCategory == null) 376 return false; 377 for (CodeableConcept item : this.serviceCategory) 378 if (!item.isEmpty()) 379 return true; 380 return false; 381 } 382 383 public CodeableConcept addServiceCategory() { //3 384 CodeableConcept t = new CodeableConcept(); 385 if (this.serviceCategory == null) 386 this.serviceCategory = new ArrayList<CodeableConcept>(); 387 this.serviceCategory.add(t); 388 return t; 389 } 390 391 public Slot addServiceCategory(CodeableConcept t) { //3 392 if (t == null) 393 return this; 394 if (this.serviceCategory == null) 395 this.serviceCategory = new ArrayList<CodeableConcept>(); 396 this.serviceCategory.add(t); 397 return this; 398 } 399 400 /** 401 * @return The first repetition of repeating field {@link #serviceCategory}, creating it if it does not already exist {3} 402 */ 403 public CodeableConcept getServiceCategoryFirstRep() { 404 if (getServiceCategory().isEmpty()) { 405 addServiceCategory(); 406 } 407 return getServiceCategory().get(0); 408 } 409 410 /** 411 * @return {@link #serviceType} (The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the Schedule resource.) 412 */ 413 public List<CodeableReference> getServiceType() { 414 if (this.serviceType == null) 415 this.serviceType = new ArrayList<CodeableReference>(); 416 return this.serviceType; 417 } 418 419 /** 420 * @return Returns a reference to <code>this</code> for easy method chaining 421 */ 422 public Slot setServiceType(List<CodeableReference> theServiceType) { 423 this.serviceType = theServiceType; 424 return this; 425 } 426 427 public boolean hasServiceType() { 428 if (this.serviceType == null) 429 return false; 430 for (CodeableReference item : this.serviceType) 431 if (!item.isEmpty()) 432 return true; 433 return false; 434 } 435 436 public CodeableReference addServiceType() { //3 437 CodeableReference t = new CodeableReference(); 438 if (this.serviceType == null) 439 this.serviceType = new ArrayList<CodeableReference>(); 440 this.serviceType.add(t); 441 return t; 442 } 443 444 public Slot addServiceType(CodeableReference t) { //3 445 if (t == null) 446 return this; 447 if (this.serviceType == null) 448 this.serviceType = new ArrayList<CodeableReference>(); 449 this.serviceType.add(t); 450 return this; 451 } 452 453 /** 454 * @return The first repetition of repeating field {@link #serviceType}, creating it if it does not already exist {3} 455 */ 456 public CodeableReference getServiceTypeFirstRep() { 457 if (getServiceType().isEmpty()) { 458 addServiceType(); 459 } 460 return getServiceType().get(0); 461 } 462 463 /** 464 * @return {@link #specialty} (The specialty of a practitioner that would be required to perform the service requested in this appointment.) 465 */ 466 public List<CodeableConcept> getSpecialty() { 467 if (this.specialty == null) 468 this.specialty = new ArrayList<CodeableConcept>(); 469 return this.specialty; 470 } 471 472 /** 473 * @return Returns a reference to <code>this</code> for easy method chaining 474 */ 475 public Slot setSpecialty(List<CodeableConcept> theSpecialty) { 476 this.specialty = theSpecialty; 477 return this; 478 } 479 480 public boolean hasSpecialty() { 481 if (this.specialty == null) 482 return false; 483 for (CodeableConcept item : this.specialty) 484 if (!item.isEmpty()) 485 return true; 486 return false; 487 } 488 489 public CodeableConcept addSpecialty() { //3 490 CodeableConcept t = new CodeableConcept(); 491 if (this.specialty == null) 492 this.specialty = new ArrayList<CodeableConcept>(); 493 this.specialty.add(t); 494 return t; 495 } 496 497 public Slot addSpecialty(CodeableConcept t) { //3 498 if (t == null) 499 return this; 500 if (this.specialty == null) 501 this.specialty = new ArrayList<CodeableConcept>(); 502 this.specialty.add(t); 503 return this; 504 } 505 506 /** 507 * @return The first repetition of repeating field {@link #specialty}, creating it if it does not already exist {3} 508 */ 509 public CodeableConcept getSpecialtyFirstRep() { 510 if (getSpecialty().isEmpty()) { 511 addSpecialty(); 512 } 513 return getSpecialty().get(0); 514 } 515 516 /** 517 * @return {@link #appointmentType} (The style of appointment or patient that may be booked in the slot (not service type).) 518 */ 519 public List<CodeableConcept> getAppointmentType() { 520 if (this.appointmentType == null) 521 this.appointmentType = new ArrayList<CodeableConcept>(); 522 return this.appointmentType; 523 } 524 525 /** 526 * @return Returns a reference to <code>this</code> for easy method chaining 527 */ 528 public Slot setAppointmentType(List<CodeableConcept> theAppointmentType) { 529 this.appointmentType = theAppointmentType; 530 return this; 531 } 532 533 public boolean hasAppointmentType() { 534 if (this.appointmentType == null) 535 return false; 536 for (CodeableConcept item : this.appointmentType) 537 if (!item.isEmpty()) 538 return true; 539 return false; 540 } 541 542 public CodeableConcept addAppointmentType() { //3 543 CodeableConcept t = new CodeableConcept(); 544 if (this.appointmentType == null) 545 this.appointmentType = new ArrayList<CodeableConcept>(); 546 this.appointmentType.add(t); 547 return t; 548 } 549 550 public Slot addAppointmentType(CodeableConcept t) { //3 551 if (t == null) 552 return this; 553 if (this.appointmentType == null) 554 this.appointmentType = new ArrayList<CodeableConcept>(); 555 this.appointmentType.add(t); 556 return this; 557 } 558 559 /** 560 * @return The first repetition of repeating field {@link #appointmentType}, creating it if it does not already exist {3} 561 */ 562 public CodeableConcept getAppointmentTypeFirstRep() { 563 if (getAppointmentType().isEmpty()) { 564 addAppointmentType(); 565 } 566 return getAppointmentType().get(0); 567 } 568 569 /** 570 * @return {@link #schedule} (The schedule resource that this slot defines an interval of status information.) 571 */ 572 public Reference getSchedule() { 573 if (this.schedule == null) 574 if (Configuration.errorOnAutoCreate()) 575 throw new Error("Attempt to auto-create Slot.schedule"); 576 else if (Configuration.doAutoCreate()) 577 this.schedule = new Reference(); // cc 578 return this.schedule; 579 } 580 581 public boolean hasSchedule() { 582 return this.schedule != null && !this.schedule.isEmpty(); 583 } 584 585 /** 586 * @param value {@link #schedule} (The schedule resource that this slot defines an interval of status information.) 587 */ 588 public Slot setSchedule(Reference value) { 589 this.schedule = value; 590 return this; 591 } 592 593 /** 594 * @return {@link #status} (busy | free | busy-unavailable | busy-tentative | entered-in-error.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 595 */ 596 public Enumeration<SlotStatus> getStatusElement() { 597 if (this.status == null) 598 if (Configuration.errorOnAutoCreate()) 599 throw new Error("Attempt to auto-create Slot.status"); 600 else if (Configuration.doAutoCreate()) 601 this.status = new Enumeration<SlotStatus>(new SlotStatusEnumFactory()); // bb 602 return this.status; 603 } 604 605 public boolean hasStatusElement() { 606 return this.status != null && !this.status.isEmpty(); 607 } 608 609 public boolean hasStatus() { 610 return this.status != null && !this.status.isEmpty(); 611 } 612 613 /** 614 * @param value {@link #status} (busy | free | busy-unavailable | busy-tentative | entered-in-error.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 615 */ 616 public Slot setStatusElement(Enumeration<SlotStatus> value) { 617 this.status = value; 618 return this; 619 } 620 621 /** 622 * @return busy | free | busy-unavailable | busy-tentative | entered-in-error. 623 */ 624 public SlotStatus getStatus() { 625 return this.status == null ? null : this.status.getValue(); 626 } 627 628 /** 629 * @param value busy | free | busy-unavailable | busy-tentative | entered-in-error. 630 */ 631 public Slot setStatus(SlotStatus value) { 632 if (this.status == null) 633 this.status = new Enumeration<SlotStatus>(new SlotStatusEnumFactory()); 634 this.status.setValue(value); 635 return this; 636 } 637 638 /** 639 * @return {@link #start} (Date/Time that the slot is to begin.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 640 */ 641 public InstantType getStartElement() { 642 if (this.start == null) 643 if (Configuration.errorOnAutoCreate()) 644 throw new Error("Attempt to auto-create Slot.start"); 645 else if (Configuration.doAutoCreate()) 646 this.start = new InstantType(); // bb 647 return this.start; 648 } 649 650 public boolean hasStartElement() { 651 return this.start != null && !this.start.isEmpty(); 652 } 653 654 public boolean hasStart() { 655 return this.start != null && !this.start.isEmpty(); 656 } 657 658 /** 659 * @param value {@link #start} (Date/Time that the slot is to begin.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 660 */ 661 public Slot setStartElement(InstantType value) { 662 this.start = value; 663 return this; 664 } 665 666 /** 667 * @return Date/Time that the slot is to begin. 668 */ 669 public Date getStart() { 670 return this.start == null ? null : this.start.getValue(); 671 } 672 673 /** 674 * @param value Date/Time that the slot is to begin. 675 */ 676 public Slot setStart(Date value) { 677 if (this.start == null) 678 this.start = new InstantType(); 679 this.start.setValue(value); 680 return this; 681 } 682 683 /** 684 * @return {@link #end} (Date/Time that the slot is to conclude.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 685 */ 686 public InstantType getEndElement() { 687 if (this.end == null) 688 if (Configuration.errorOnAutoCreate()) 689 throw new Error("Attempt to auto-create Slot.end"); 690 else if (Configuration.doAutoCreate()) 691 this.end = new InstantType(); // bb 692 return this.end; 693 } 694 695 public boolean hasEndElement() { 696 return this.end != null && !this.end.isEmpty(); 697 } 698 699 public boolean hasEnd() { 700 return this.end != null && !this.end.isEmpty(); 701 } 702 703 /** 704 * @param value {@link #end} (Date/Time that the slot is to conclude.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 705 */ 706 public Slot setEndElement(InstantType value) { 707 this.end = value; 708 return this; 709 } 710 711 /** 712 * @return Date/Time that the slot is to conclude. 713 */ 714 public Date getEnd() { 715 return this.end == null ? null : this.end.getValue(); 716 } 717 718 /** 719 * @param value Date/Time that the slot is to conclude. 720 */ 721 public Slot setEnd(Date value) { 722 if (this.end == null) 723 this.end = new InstantType(); 724 this.end.setValue(value); 725 return this; 726 } 727 728 /** 729 * @return {@link #overbooked} (This slot has already been overbooked, appointments are unlikely to be accepted for this time.). This is the underlying object with id, value and extensions. The accessor "getOverbooked" gives direct access to the value 730 */ 731 public BooleanType getOverbookedElement() { 732 if (this.overbooked == null) 733 if (Configuration.errorOnAutoCreate()) 734 throw new Error("Attempt to auto-create Slot.overbooked"); 735 else if (Configuration.doAutoCreate()) 736 this.overbooked = new BooleanType(); // bb 737 return this.overbooked; 738 } 739 740 public boolean hasOverbookedElement() { 741 return this.overbooked != null && !this.overbooked.isEmpty(); 742 } 743 744 public boolean hasOverbooked() { 745 return this.overbooked != null && !this.overbooked.isEmpty(); 746 } 747 748 /** 749 * @param value {@link #overbooked} (This slot has already been overbooked, appointments are unlikely to be accepted for this time.). This is the underlying object with id, value and extensions. The accessor "getOverbooked" gives direct access to the value 750 */ 751 public Slot setOverbookedElement(BooleanType value) { 752 this.overbooked = value; 753 return this; 754 } 755 756 /** 757 * @return This slot has already been overbooked, appointments are unlikely to be accepted for this time. 758 */ 759 public boolean getOverbooked() { 760 return this.overbooked == null || this.overbooked.isEmpty() ? false : this.overbooked.getValue(); 761 } 762 763 /** 764 * @param value This slot has already been overbooked, appointments are unlikely to be accepted for this time. 765 */ 766 public Slot setOverbooked(boolean value) { 767 if (this.overbooked == null) 768 this.overbooked = new BooleanType(); 769 this.overbooked.setValue(value); 770 return this; 771 } 772 773 /** 774 * @return {@link #comment} (Comments on the slot to describe any extended information. Such as custom constraints on the slot.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 775 */ 776 public StringType getCommentElement() { 777 if (this.comment == null) 778 if (Configuration.errorOnAutoCreate()) 779 throw new Error("Attempt to auto-create Slot.comment"); 780 else if (Configuration.doAutoCreate()) 781 this.comment = new StringType(); // bb 782 return this.comment; 783 } 784 785 public boolean hasCommentElement() { 786 return this.comment != null && !this.comment.isEmpty(); 787 } 788 789 public boolean hasComment() { 790 return this.comment != null && !this.comment.isEmpty(); 791 } 792 793 /** 794 * @param value {@link #comment} (Comments on the slot to describe any extended information. Such as custom constraints on the slot.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 795 */ 796 public Slot setCommentElement(StringType value) { 797 this.comment = value; 798 return this; 799 } 800 801 /** 802 * @return Comments on the slot to describe any extended information. Such as custom constraints on the slot. 803 */ 804 public String getComment() { 805 return this.comment == null ? null : this.comment.getValue(); 806 } 807 808 /** 809 * @param value Comments on the slot to describe any extended information. Such as custom constraints on the slot. 810 */ 811 public Slot setComment(String value) { 812 if (Utilities.noString(value)) 813 this.comment = null; 814 else { 815 if (this.comment == null) 816 this.comment = new StringType(); 817 this.comment.setValue(value); 818 } 819 return this; 820 } 821 822 protected void listChildren(List<Property> children) { 823 super.listChildren(children); 824 children.add(new Property("identifier", "Identifier", "External Ids for this item.", 0, java.lang.Integer.MAX_VALUE, identifier)); 825 children.add(new Property("serviceCategory", "CodeableConcept", "A broad categorization of the service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, serviceCategory)); 826 children.add(new Property("serviceType", "CodeableReference(HealthcareService)", "The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the Schedule resource.", 0, java.lang.Integer.MAX_VALUE, serviceType)); 827 children.add(new Property("specialty", "CodeableConcept", "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 0, java.lang.Integer.MAX_VALUE, specialty)); 828 children.add(new Property("appointmentType", "CodeableConcept", "The style of appointment or patient that may be booked in the slot (not service type).", 0, java.lang.Integer.MAX_VALUE, appointmentType)); 829 children.add(new Property("schedule", "Reference(Schedule)", "The schedule resource that this slot defines an interval of status information.", 0, 1, schedule)); 830 children.add(new Property("status", "code", "busy | free | busy-unavailable | busy-tentative | entered-in-error.", 0, 1, status)); 831 children.add(new Property("start", "instant", "Date/Time that the slot is to begin.", 0, 1, start)); 832 children.add(new Property("end", "instant", "Date/Time that the slot is to conclude.", 0, 1, end)); 833 children.add(new Property("overbooked", "boolean", "This slot has already been overbooked, appointments are unlikely to be accepted for this time.", 0, 1, overbooked)); 834 children.add(new Property("comment", "string", "Comments on the slot to describe any extended information. Such as custom constraints on the slot.", 0, 1, comment)); 835 } 836 837 @Override 838 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 839 switch (_hash) { 840 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "External Ids for this item.", 0, java.lang.Integer.MAX_VALUE, identifier); 841 case 1281188563: /*serviceCategory*/ return new Property("serviceCategory", "CodeableConcept", "A broad categorization of the service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, serviceCategory); 842 case -1928370289: /*serviceType*/ return new Property("serviceType", "CodeableReference(HealthcareService)", "The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the Schedule resource.", 0, java.lang.Integer.MAX_VALUE, serviceType); 843 case -1694759682: /*specialty*/ return new Property("specialty", "CodeableConcept", "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 0, java.lang.Integer.MAX_VALUE, specialty); 844 case -1596426375: /*appointmentType*/ return new Property("appointmentType", "CodeableConcept", "The style of appointment or patient that may be booked in the slot (not service type).", 0, java.lang.Integer.MAX_VALUE, appointmentType); 845 case -697920873: /*schedule*/ return new Property("schedule", "Reference(Schedule)", "The schedule resource that this slot defines an interval of status information.", 0, 1, schedule); 846 case -892481550: /*status*/ return new Property("status", "code", "busy | free | busy-unavailable | busy-tentative | entered-in-error.", 0, 1, status); 847 case 109757538: /*start*/ return new Property("start", "instant", "Date/Time that the slot is to begin.", 0, 1, start); 848 case 100571: /*end*/ return new Property("end", "instant", "Date/Time that the slot is to conclude.", 0, 1, end); 849 case 2068545308: /*overbooked*/ return new Property("overbooked", "boolean", "This slot has already been overbooked, appointments are unlikely to be accepted for this time.", 0, 1, overbooked); 850 case 950398559: /*comment*/ return new Property("comment", "string", "Comments on the slot to describe any extended information. Such as custom constraints on the slot.", 0, 1, comment); 851 default: return super.getNamedProperty(_hash, _name, _checkValid); 852 } 853 854 } 855 856 @Override 857 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 858 switch (hash) { 859 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 860 case 1281188563: /*serviceCategory*/ return this.serviceCategory == null ? new Base[0] : this.serviceCategory.toArray(new Base[this.serviceCategory.size()]); // CodeableConcept 861 case -1928370289: /*serviceType*/ return this.serviceType == null ? new Base[0] : this.serviceType.toArray(new Base[this.serviceType.size()]); // CodeableReference 862 case -1694759682: /*specialty*/ return this.specialty == null ? new Base[0] : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 863 case -1596426375: /*appointmentType*/ return this.appointmentType == null ? new Base[0] : this.appointmentType.toArray(new Base[this.appointmentType.size()]); // CodeableConcept 864 case -697920873: /*schedule*/ return this.schedule == null ? new Base[0] : new Base[] {this.schedule}; // Reference 865 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<SlotStatus> 866 case 109757538: /*start*/ return this.start == null ? new Base[0] : new Base[] {this.start}; // InstantType 867 case 100571: /*end*/ return this.end == null ? new Base[0] : new Base[] {this.end}; // InstantType 868 case 2068545308: /*overbooked*/ return this.overbooked == null ? new Base[0] : new Base[] {this.overbooked}; // BooleanType 869 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 870 default: return super.getProperty(hash, name, checkValid); 871 } 872 873 } 874 875 @Override 876 public Base setProperty(int hash, String name, Base value) throws FHIRException { 877 switch (hash) { 878 case -1618432855: // identifier 879 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 880 return value; 881 case 1281188563: // serviceCategory 882 this.getServiceCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 883 return value; 884 case -1928370289: // serviceType 885 this.getServiceType().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 886 return value; 887 case -1694759682: // specialty 888 this.getSpecialty().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 889 return value; 890 case -1596426375: // appointmentType 891 this.getAppointmentType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 892 return value; 893 case -697920873: // schedule 894 this.schedule = TypeConvertor.castToReference(value); // Reference 895 return value; 896 case -892481550: // status 897 value = new SlotStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 898 this.status = (Enumeration) value; // Enumeration<SlotStatus> 899 return value; 900 case 109757538: // start 901 this.start = TypeConvertor.castToInstant(value); // InstantType 902 return value; 903 case 100571: // end 904 this.end = TypeConvertor.castToInstant(value); // InstantType 905 return value; 906 case 2068545308: // overbooked 907 this.overbooked = TypeConvertor.castToBoolean(value); // BooleanType 908 return value; 909 case 950398559: // comment 910 this.comment = TypeConvertor.castToString(value); // StringType 911 return value; 912 default: return super.setProperty(hash, name, value); 913 } 914 915 } 916 917 @Override 918 public Base setProperty(String name, Base value) throws FHIRException { 919 if (name.equals("identifier")) { 920 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 921 } else if (name.equals("serviceCategory")) { 922 this.getServiceCategory().add(TypeConvertor.castToCodeableConcept(value)); 923 } else if (name.equals("serviceType")) { 924 this.getServiceType().add(TypeConvertor.castToCodeableReference(value)); 925 } else if (name.equals("specialty")) { 926 this.getSpecialty().add(TypeConvertor.castToCodeableConcept(value)); 927 } else if (name.equals("appointmentType")) { 928 this.getAppointmentType().add(TypeConvertor.castToCodeableConcept(value)); 929 } else if (name.equals("schedule")) { 930 this.schedule = TypeConvertor.castToReference(value); // Reference 931 } else if (name.equals("status")) { 932 value = new SlotStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 933 this.status = (Enumeration) value; // Enumeration<SlotStatus> 934 } else if (name.equals("start")) { 935 this.start = TypeConvertor.castToInstant(value); // InstantType 936 } else if (name.equals("end")) { 937 this.end = TypeConvertor.castToInstant(value); // InstantType 938 } else if (name.equals("overbooked")) { 939 this.overbooked = TypeConvertor.castToBoolean(value); // BooleanType 940 } else if (name.equals("comment")) { 941 this.comment = TypeConvertor.castToString(value); // StringType 942 } else 943 return super.setProperty(name, value); 944 return value; 945 } 946 947 @Override 948 public void removeChild(String name, Base value) throws FHIRException { 949 if (name.equals("identifier")) { 950 this.getIdentifier().remove(value); 951 } else if (name.equals("serviceCategory")) { 952 this.getServiceCategory().remove(value); 953 } else if (name.equals("serviceType")) { 954 this.getServiceType().remove(value); 955 } else if (name.equals("specialty")) { 956 this.getSpecialty().remove(value); 957 } else if (name.equals("appointmentType")) { 958 this.getAppointmentType().remove(value); 959 } else if (name.equals("schedule")) { 960 this.schedule = null; 961 } else if (name.equals("status")) { 962 value = new SlotStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 963 this.status = (Enumeration) value; // Enumeration<SlotStatus> 964 } else if (name.equals("start")) { 965 this.start = null; 966 } else if (name.equals("end")) { 967 this.end = null; 968 } else if (name.equals("overbooked")) { 969 this.overbooked = null; 970 } else if (name.equals("comment")) { 971 this.comment = null; 972 } else 973 super.removeChild(name, value); 974 975 } 976 977 @Override 978 public Base makeProperty(int hash, String name) throws FHIRException { 979 switch (hash) { 980 case -1618432855: return addIdentifier(); 981 case 1281188563: return addServiceCategory(); 982 case -1928370289: return addServiceType(); 983 case -1694759682: return addSpecialty(); 984 case -1596426375: return addAppointmentType(); 985 case -697920873: return getSchedule(); 986 case -892481550: return getStatusElement(); 987 case 109757538: return getStartElement(); 988 case 100571: return getEndElement(); 989 case 2068545308: return getOverbookedElement(); 990 case 950398559: return getCommentElement(); 991 default: return super.makeProperty(hash, name); 992 } 993 994 } 995 996 @Override 997 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 998 switch (hash) { 999 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1000 case 1281188563: /*serviceCategory*/ return new String[] {"CodeableConcept"}; 1001 case -1928370289: /*serviceType*/ return new String[] {"CodeableReference"}; 1002 case -1694759682: /*specialty*/ return new String[] {"CodeableConcept"}; 1003 case -1596426375: /*appointmentType*/ return new String[] {"CodeableConcept"}; 1004 case -697920873: /*schedule*/ return new String[] {"Reference"}; 1005 case -892481550: /*status*/ return new String[] {"code"}; 1006 case 109757538: /*start*/ return new String[] {"instant"}; 1007 case 100571: /*end*/ return new String[] {"instant"}; 1008 case 2068545308: /*overbooked*/ return new String[] {"boolean"}; 1009 case 950398559: /*comment*/ return new String[] {"string"}; 1010 default: return super.getTypesForProperty(hash, name); 1011 } 1012 1013 } 1014 1015 @Override 1016 public Base addChild(String name) throws FHIRException { 1017 if (name.equals("identifier")) { 1018 return addIdentifier(); 1019 } 1020 else if (name.equals("serviceCategory")) { 1021 return addServiceCategory(); 1022 } 1023 else if (name.equals("serviceType")) { 1024 return addServiceType(); 1025 } 1026 else if (name.equals("specialty")) { 1027 return addSpecialty(); 1028 } 1029 else if (name.equals("appointmentType")) { 1030 return addAppointmentType(); 1031 } 1032 else if (name.equals("schedule")) { 1033 this.schedule = new Reference(); 1034 return this.schedule; 1035 } 1036 else if (name.equals("status")) { 1037 throw new FHIRException("Cannot call addChild on a singleton property Slot.status"); 1038 } 1039 else if (name.equals("start")) { 1040 throw new FHIRException("Cannot call addChild on a singleton property Slot.start"); 1041 } 1042 else if (name.equals("end")) { 1043 throw new FHIRException("Cannot call addChild on a singleton property Slot.end"); 1044 } 1045 else if (name.equals("overbooked")) { 1046 throw new FHIRException("Cannot call addChild on a singleton property Slot.overbooked"); 1047 } 1048 else if (name.equals("comment")) { 1049 throw new FHIRException("Cannot call addChild on a singleton property Slot.comment"); 1050 } 1051 else 1052 return super.addChild(name); 1053 } 1054 1055 public String fhirType() { 1056 return "Slot"; 1057 1058 } 1059 1060 public Slot copy() { 1061 Slot dst = new Slot(); 1062 copyValues(dst); 1063 return dst; 1064 } 1065 1066 public void copyValues(Slot dst) { 1067 super.copyValues(dst); 1068 if (identifier != null) { 1069 dst.identifier = new ArrayList<Identifier>(); 1070 for (Identifier i : identifier) 1071 dst.identifier.add(i.copy()); 1072 }; 1073 if (serviceCategory != null) { 1074 dst.serviceCategory = new ArrayList<CodeableConcept>(); 1075 for (CodeableConcept i : serviceCategory) 1076 dst.serviceCategory.add(i.copy()); 1077 }; 1078 if (serviceType != null) { 1079 dst.serviceType = new ArrayList<CodeableReference>(); 1080 for (CodeableReference i : serviceType) 1081 dst.serviceType.add(i.copy()); 1082 }; 1083 if (specialty != null) { 1084 dst.specialty = new ArrayList<CodeableConcept>(); 1085 for (CodeableConcept i : specialty) 1086 dst.specialty.add(i.copy()); 1087 }; 1088 if (appointmentType != null) { 1089 dst.appointmentType = new ArrayList<CodeableConcept>(); 1090 for (CodeableConcept i : appointmentType) 1091 dst.appointmentType.add(i.copy()); 1092 }; 1093 dst.schedule = schedule == null ? null : schedule.copy(); 1094 dst.status = status == null ? null : status.copy(); 1095 dst.start = start == null ? null : start.copy(); 1096 dst.end = end == null ? null : end.copy(); 1097 dst.overbooked = overbooked == null ? null : overbooked.copy(); 1098 dst.comment = comment == null ? null : comment.copy(); 1099 } 1100 1101 protected Slot typedCopy() { 1102 return copy(); 1103 } 1104 1105 @Override 1106 public boolean equalsDeep(Base other_) { 1107 if (!super.equalsDeep(other_)) 1108 return false; 1109 if (!(other_ instanceof Slot)) 1110 return false; 1111 Slot o = (Slot) other_; 1112 return compareDeep(identifier, o.identifier, true) && compareDeep(serviceCategory, o.serviceCategory, true) 1113 && compareDeep(serviceType, o.serviceType, true) && compareDeep(specialty, o.specialty, true) && compareDeep(appointmentType, o.appointmentType, true) 1114 && compareDeep(schedule, o.schedule, true) && compareDeep(status, o.status, true) && compareDeep(start, o.start, true) 1115 && compareDeep(end, o.end, true) && compareDeep(overbooked, o.overbooked, true) && compareDeep(comment, o.comment, true) 1116 ; 1117 } 1118 1119 @Override 1120 public boolean equalsShallow(Base other_) { 1121 if (!super.equalsShallow(other_)) 1122 return false; 1123 if (!(other_ instanceof Slot)) 1124 return false; 1125 Slot o = (Slot) other_; 1126 return compareValues(status, o.status, true) && compareValues(start, o.start, true) && compareValues(end, o.end, true) 1127 && compareValues(overbooked, o.overbooked, true) && compareValues(comment, o.comment, true); 1128 } 1129 1130 public boolean isEmpty() { 1131 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, serviceCategory 1132 , serviceType, specialty, appointmentType, schedule, status, start, end, overbooked 1133 , comment); 1134 } 1135 1136 @Override 1137 public ResourceType getResourceType() { 1138 return ResourceType.Slot; 1139 } 1140 1141 /** 1142 * Search parameter: <b>appointment-type</b> 1143 * <p> 1144 * Description: <b>The style of appointment or patient that may be booked in the slot (not service type)</b><br> 1145 * Type: <b>token</b><br> 1146 * Path: <b>Slot.appointmentType</b><br> 1147 * </p> 1148 */ 1149 @SearchParamDefinition(name="appointment-type", path="Slot.appointmentType", description="The style of appointment or patient that may be booked in the slot (not service type)", type="token" ) 1150 public static final String SP_APPOINTMENT_TYPE = "appointment-type"; 1151 /** 1152 * <b>Fluent Client</b> search parameter constant for <b>appointment-type</b> 1153 * <p> 1154 * Description: <b>The style of appointment or patient that may be booked in the slot (not service type)</b><br> 1155 * Type: <b>token</b><br> 1156 * Path: <b>Slot.appointmentType</b><br> 1157 * </p> 1158 */ 1159 public static final ca.uhn.fhir.rest.gclient.TokenClientParam APPOINTMENT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_APPOINTMENT_TYPE); 1160 1161 /** 1162 * Search parameter: <b>identifier</b> 1163 * <p> 1164 * Description: <b>A Slot Identifier</b><br> 1165 * Type: <b>token</b><br> 1166 * Path: <b>Slot.identifier</b><br> 1167 * </p> 1168 */ 1169 @SearchParamDefinition(name="identifier", path="Slot.identifier", description="A Slot Identifier", type="token" ) 1170 public static final String SP_IDENTIFIER = "identifier"; 1171 /** 1172 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1173 * <p> 1174 * Description: <b>A Slot Identifier</b><br> 1175 * Type: <b>token</b><br> 1176 * Path: <b>Slot.identifier</b><br> 1177 * </p> 1178 */ 1179 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1180 1181 /** 1182 * Search parameter: <b>schedule</b> 1183 * <p> 1184 * Description: <b>The Schedule Resource that we are seeking a slot within</b><br> 1185 * Type: <b>reference</b><br> 1186 * Path: <b>Slot.schedule</b><br> 1187 * </p> 1188 */ 1189 @SearchParamDefinition(name="schedule", path="Slot.schedule", description="The Schedule Resource that we are seeking a slot within", type="reference", target={Schedule.class } ) 1190 public static final String SP_SCHEDULE = "schedule"; 1191 /** 1192 * <b>Fluent Client</b> search parameter constant for <b>schedule</b> 1193 * <p> 1194 * Description: <b>The Schedule Resource that we are seeking a slot within</b><br> 1195 * Type: <b>reference</b><br> 1196 * Path: <b>Slot.schedule</b><br> 1197 * </p> 1198 */ 1199 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SCHEDULE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SCHEDULE); 1200 1201/** 1202 * Constant for fluent queries to be used to add include statements. Specifies 1203 * the path value of "<b>Slot:schedule</b>". 1204 */ 1205 public static final ca.uhn.fhir.model.api.Include INCLUDE_SCHEDULE = new ca.uhn.fhir.model.api.Include("Slot:schedule").toLocked(); 1206 1207 /** 1208 * Search parameter: <b>service-category</b> 1209 * <p> 1210 * Description: <b>A broad categorization of the service that is to be performed during this appointment</b><br> 1211 * Type: <b>token</b><br> 1212 * Path: <b>Slot.serviceCategory</b><br> 1213 * </p> 1214 */ 1215 @SearchParamDefinition(name="service-category", path="Slot.serviceCategory", description="A broad categorization of the service that is to be performed during this appointment", type="token" ) 1216 public static final String SP_SERVICE_CATEGORY = "service-category"; 1217 /** 1218 * <b>Fluent Client</b> search parameter constant for <b>service-category</b> 1219 * <p> 1220 * Description: <b>A broad categorization of the service that is to be performed during this appointment</b><br> 1221 * Type: <b>token</b><br> 1222 * Path: <b>Slot.serviceCategory</b><br> 1223 * </p> 1224 */ 1225 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SERVICE_CATEGORY); 1226 1227 /** 1228 * Search parameter: <b>service-type-reference</b> 1229 * <p> 1230 * Description: <b>The type (by HealthcareService) of appointments that can be booked into the slot</b><br> 1231 * Type: <b>reference</b><br> 1232 * Path: <b>Slot.serviceType.reference</b><br> 1233 * </p> 1234 */ 1235 @SearchParamDefinition(name="service-type-reference", path="Slot.serviceType.reference", description="The type (by HealthcareService) of appointments that can be booked into the slot", type="reference", target={HealthcareService.class } ) 1236 public static final String SP_SERVICE_TYPE_REFERENCE = "service-type-reference"; 1237 /** 1238 * <b>Fluent Client</b> search parameter constant for <b>service-type-reference</b> 1239 * <p> 1240 * Description: <b>The type (by HealthcareService) of appointments that can be booked into the slot</b><br> 1241 * Type: <b>reference</b><br> 1242 * Path: <b>Slot.serviceType.reference</b><br> 1243 * </p> 1244 */ 1245 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SERVICE_TYPE_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SERVICE_TYPE_REFERENCE); 1246 1247/** 1248 * Constant for fluent queries to be used to add include statements. Specifies 1249 * the path value of "<b>Slot:service-type-reference</b>". 1250 */ 1251 public static final ca.uhn.fhir.model.api.Include INCLUDE_SERVICE_TYPE_REFERENCE = new ca.uhn.fhir.model.api.Include("Slot:service-type-reference").toLocked(); 1252 1253 /** 1254 * Search parameter: <b>service-type</b> 1255 * <p> 1256 * Description: <b>The type (by coding) of appointments that can be booked into the slot</b><br> 1257 * Type: <b>token</b><br> 1258 * Path: <b>Slot.serviceType.concept</b><br> 1259 * </p> 1260 */ 1261 @SearchParamDefinition(name="service-type", path="Slot.serviceType.concept", description="The type (by coding) of appointments that can be booked into the slot", type="token" ) 1262 public static final String SP_SERVICE_TYPE = "service-type"; 1263 /** 1264 * <b>Fluent Client</b> search parameter constant for <b>service-type</b> 1265 * <p> 1266 * Description: <b>The type (by coding) of appointments that can be booked into the slot</b><br> 1267 * Type: <b>token</b><br> 1268 * Path: <b>Slot.serviceType.concept</b><br> 1269 * </p> 1270 */ 1271 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SERVICE_TYPE); 1272 1273 /** 1274 * Search parameter: <b>specialty</b> 1275 * <p> 1276 * Description: <b>The specialty of a practitioner that would be required to perform the service requested in this appointment</b><br> 1277 * Type: <b>token</b><br> 1278 * Path: <b>Slot.specialty</b><br> 1279 * </p> 1280 */ 1281 @SearchParamDefinition(name="specialty", path="Slot.specialty", description="The specialty of a practitioner that would be required to perform the service requested in this appointment", type="token" ) 1282 public static final String SP_SPECIALTY = "specialty"; 1283 /** 1284 * <b>Fluent Client</b> search parameter constant for <b>specialty</b> 1285 * <p> 1286 * Description: <b>The specialty of a practitioner that would be required to perform the service requested in this appointment</b><br> 1287 * Type: <b>token</b><br> 1288 * Path: <b>Slot.specialty</b><br> 1289 * </p> 1290 */ 1291 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIALTY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SPECIALTY); 1292 1293 /** 1294 * Search parameter: <b>start</b> 1295 * <p> 1296 * Description: <b>Appointment date/time.</b><br> 1297 * Type: <b>date</b><br> 1298 * Path: <b>Slot.start</b><br> 1299 * </p> 1300 */ 1301 @SearchParamDefinition(name="start", path="Slot.start", description="Appointment date/time.", type="date" ) 1302 public static final String SP_START = "start"; 1303 /** 1304 * <b>Fluent Client</b> search parameter constant for <b>start</b> 1305 * <p> 1306 * Description: <b>Appointment date/time.</b><br> 1307 * Type: <b>date</b><br> 1308 * Path: <b>Slot.start</b><br> 1309 * </p> 1310 */ 1311 public static final ca.uhn.fhir.rest.gclient.DateClientParam START = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_START); 1312 1313 /** 1314 * Search parameter: <b>status</b> 1315 * <p> 1316 * Description: <b>The free/busy status of the appointment</b><br> 1317 * Type: <b>token</b><br> 1318 * Path: <b>Slot.status</b><br> 1319 * </p> 1320 */ 1321 @SearchParamDefinition(name="status", path="Slot.status", description="The free/busy status of the appointment", type="token" ) 1322 public static final String SP_STATUS = "status"; 1323 /** 1324 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1325 * <p> 1326 * Description: <b>The free/busy status of the appointment</b><br> 1327 * Type: <b>token</b><br> 1328 * Path: <b>Slot.status</b><br> 1329 * </p> 1330 */ 1331 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1332 1333 1334} 1335