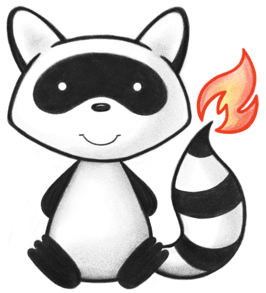
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A sample to be used for analysis. 052 */ 053@ResourceDef(name="Specimen", profile="http://hl7.org/fhir/StructureDefinition/Specimen") 054public class Specimen extends DomainResource { 055 056 public enum SpecimenCombined { 057 /** 058 * The specimen is in a group. 059 */ 060 GROUPED, 061 /** 062 * The specimen is pooled. 063 */ 064 POOLED, 065 /** 066 * added to help the parsers with the generic types 067 */ 068 NULL; 069 public static SpecimenCombined fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("grouped".equals(codeString)) 073 return GROUPED; 074 if ("pooled".equals(codeString)) 075 return POOLED; 076 if (Configuration.isAcceptInvalidEnums()) 077 return null; 078 else 079 throw new FHIRException("Unknown SpecimenCombined code '"+codeString+"'"); 080 } 081 public String toCode() { 082 switch (this) { 083 case GROUPED: return "grouped"; 084 case POOLED: return "pooled"; 085 case NULL: return null; 086 default: return "?"; 087 } 088 } 089 public String getSystem() { 090 switch (this) { 091 case GROUPED: return "http://hl7.org/fhir/specimen-combined"; 092 case POOLED: return "http://hl7.org/fhir/specimen-combined"; 093 case NULL: return null; 094 default: return "?"; 095 } 096 } 097 public String getDefinition() { 098 switch (this) { 099 case GROUPED: return "The specimen is in a group."; 100 case POOLED: return "The specimen is pooled."; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDisplay() { 106 switch (this) { 107 case GROUPED: return "Grouped"; 108 case POOLED: return "Pooled"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 } 114 115 public static class SpecimenCombinedEnumFactory implements EnumFactory<SpecimenCombined> { 116 public SpecimenCombined fromCode(String codeString) throws IllegalArgumentException { 117 if (codeString == null || "".equals(codeString)) 118 if (codeString == null || "".equals(codeString)) 119 return null; 120 if ("grouped".equals(codeString)) 121 return SpecimenCombined.GROUPED; 122 if ("pooled".equals(codeString)) 123 return SpecimenCombined.POOLED; 124 throw new IllegalArgumentException("Unknown SpecimenCombined code '"+codeString+"'"); 125 } 126 public Enumeration<SpecimenCombined> fromType(PrimitiveType<?> code) throws FHIRException { 127 if (code == null) 128 return null; 129 if (code.isEmpty()) 130 return new Enumeration<SpecimenCombined>(this, SpecimenCombined.NULL, code); 131 String codeString = ((PrimitiveType) code).asStringValue(); 132 if (codeString == null || "".equals(codeString)) 133 return new Enumeration<SpecimenCombined>(this, SpecimenCombined.NULL, code); 134 if ("grouped".equals(codeString)) 135 return new Enumeration<SpecimenCombined>(this, SpecimenCombined.GROUPED, code); 136 if ("pooled".equals(codeString)) 137 return new Enumeration<SpecimenCombined>(this, SpecimenCombined.POOLED, code); 138 throw new FHIRException("Unknown SpecimenCombined code '"+codeString+"'"); 139 } 140 public String toCode(SpecimenCombined code) { 141 if (code == SpecimenCombined.NULL) 142 return null; 143 if (code == SpecimenCombined.GROUPED) 144 return "grouped"; 145 if (code == SpecimenCombined.POOLED) 146 return "pooled"; 147 return "?"; 148 } 149 public String toSystem(SpecimenCombined code) { 150 return code.getSystem(); 151 } 152 } 153 154 public enum SpecimenStatus { 155 /** 156 * The physical specimen is present and in good condition. 157 */ 158 AVAILABLE, 159 /** 160 * There is no physical specimen because it is either lost, destroyed or consumed. 161 */ 162 UNAVAILABLE, 163 /** 164 * The specimen cannot be used because of a quality issue such as a broken container, contamination, or too old. 165 */ 166 UNSATISFACTORY, 167 /** 168 * The specimen was entered in error and therefore nullified. 169 */ 170 ENTEREDINERROR, 171 /** 172 * added to help the parsers with the generic types 173 */ 174 NULL; 175 public static SpecimenStatus fromCode(String codeString) throws FHIRException { 176 if (codeString == null || "".equals(codeString)) 177 return null; 178 if ("available".equals(codeString)) 179 return AVAILABLE; 180 if ("unavailable".equals(codeString)) 181 return UNAVAILABLE; 182 if ("unsatisfactory".equals(codeString)) 183 return UNSATISFACTORY; 184 if ("entered-in-error".equals(codeString)) 185 return ENTEREDINERROR; 186 if (Configuration.isAcceptInvalidEnums()) 187 return null; 188 else 189 throw new FHIRException("Unknown SpecimenStatus code '"+codeString+"'"); 190 } 191 public String toCode() { 192 switch (this) { 193 case AVAILABLE: return "available"; 194 case UNAVAILABLE: return "unavailable"; 195 case UNSATISFACTORY: return "unsatisfactory"; 196 case ENTEREDINERROR: return "entered-in-error"; 197 case NULL: return null; 198 default: return "?"; 199 } 200 } 201 public String getSystem() { 202 switch (this) { 203 case AVAILABLE: return "http://hl7.org/fhir/specimen-status"; 204 case UNAVAILABLE: return "http://hl7.org/fhir/specimen-status"; 205 case UNSATISFACTORY: return "http://hl7.org/fhir/specimen-status"; 206 case ENTEREDINERROR: return "http://hl7.org/fhir/specimen-status"; 207 case NULL: return null; 208 default: return "?"; 209 } 210 } 211 public String getDefinition() { 212 switch (this) { 213 case AVAILABLE: return "The physical specimen is present and in good condition."; 214 case UNAVAILABLE: return "There is no physical specimen because it is either lost, destroyed or consumed."; 215 case UNSATISFACTORY: return "The specimen cannot be used because of a quality issue such as a broken container, contamination, or too old."; 216 case ENTEREDINERROR: return "The specimen was entered in error and therefore nullified."; 217 case NULL: return null; 218 default: return "?"; 219 } 220 } 221 public String getDisplay() { 222 switch (this) { 223 case AVAILABLE: return "Available"; 224 case UNAVAILABLE: return "Unavailable"; 225 case UNSATISFACTORY: return "Unsatisfactory"; 226 case ENTEREDINERROR: return "Entered in Error"; 227 case NULL: return null; 228 default: return "?"; 229 } 230 } 231 } 232 233 public static class SpecimenStatusEnumFactory implements EnumFactory<SpecimenStatus> { 234 public SpecimenStatus fromCode(String codeString) throws IllegalArgumentException { 235 if (codeString == null || "".equals(codeString)) 236 if (codeString == null || "".equals(codeString)) 237 return null; 238 if ("available".equals(codeString)) 239 return SpecimenStatus.AVAILABLE; 240 if ("unavailable".equals(codeString)) 241 return SpecimenStatus.UNAVAILABLE; 242 if ("unsatisfactory".equals(codeString)) 243 return SpecimenStatus.UNSATISFACTORY; 244 if ("entered-in-error".equals(codeString)) 245 return SpecimenStatus.ENTEREDINERROR; 246 throw new IllegalArgumentException("Unknown SpecimenStatus code '"+codeString+"'"); 247 } 248 public Enumeration<SpecimenStatus> fromType(PrimitiveType<?> code) throws FHIRException { 249 if (code == null) 250 return null; 251 if (code.isEmpty()) 252 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.NULL, code); 253 String codeString = ((PrimitiveType) code).asStringValue(); 254 if (codeString == null || "".equals(codeString)) 255 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.NULL, code); 256 if ("available".equals(codeString)) 257 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.AVAILABLE, code); 258 if ("unavailable".equals(codeString)) 259 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.UNAVAILABLE, code); 260 if ("unsatisfactory".equals(codeString)) 261 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.UNSATISFACTORY, code); 262 if ("entered-in-error".equals(codeString)) 263 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.ENTEREDINERROR, code); 264 throw new FHIRException("Unknown SpecimenStatus code '"+codeString+"'"); 265 } 266 public String toCode(SpecimenStatus code) { 267 if (code == SpecimenStatus.NULL) 268 return null; 269 if (code == SpecimenStatus.AVAILABLE) 270 return "available"; 271 if (code == SpecimenStatus.UNAVAILABLE) 272 return "unavailable"; 273 if (code == SpecimenStatus.UNSATISFACTORY) 274 return "unsatisfactory"; 275 if (code == SpecimenStatus.ENTEREDINERROR) 276 return "entered-in-error"; 277 return "?"; 278 } 279 public String toSystem(SpecimenStatus code) { 280 return code.getSystem(); 281 } 282 } 283 284 @Block() 285 public static class SpecimenFeatureComponent extends BackboneElement implements IBaseBackboneElement { 286 /** 287 * The landmark or feature being highlighted. 288 */ 289 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 290 @Description(shortDefinition="Highlighted feature", formalDefinition="The landmark or feature being highlighted." ) 291 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 292 protected CodeableConcept type; 293 294 /** 295 * Description of the feature of the specimen. 296 */ 297 @Child(name = "description", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 298 @Description(shortDefinition="Information about the feature", formalDefinition="Description of the feature of the specimen." ) 299 protected StringType description; 300 301 private static final long serialVersionUID = 1762224562L; 302 303 /** 304 * Constructor 305 */ 306 public SpecimenFeatureComponent() { 307 super(); 308 } 309 310 /** 311 * Constructor 312 */ 313 public SpecimenFeatureComponent(CodeableConcept type, String description) { 314 super(); 315 this.setType(type); 316 this.setDescription(description); 317 } 318 319 /** 320 * @return {@link #type} (The landmark or feature being highlighted.) 321 */ 322 public CodeableConcept getType() { 323 if (this.type == null) 324 if (Configuration.errorOnAutoCreate()) 325 throw new Error("Attempt to auto-create SpecimenFeatureComponent.type"); 326 else if (Configuration.doAutoCreate()) 327 this.type = new CodeableConcept(); // cc 328 return this.type; 329 } 330 331 public boolean hasType() { 332 return this.type != null && !this.type.isEmpty(); 333 } 334 335 /** 336 * @param value {@link #type} (The landmark or feature being highlighted.) 337 */ 338 public SpecimenFeatureComponent setType(CodeableConcept value) { 339 this.type = value; 340 return this; 341 } 342 343 /** 344 * @return {@link #description} (Description of the feature of the specimen.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 345 */ 346 public StringType getDescriptionElement() { 347 if (this.description == null) 348 if (Configuration.errorOnAutoCreate()) 349 throw new Error("Attempt to auto-create SpecimenFeatureComponent.description"); 350 else if (Configuration.doAutoCreate()) 351 this.description = new StringType(); // bb 352 return this.description; 353 } 354 355 public boolean hasDescriptionElement() { 356 return this.description != null && !this.description.isEmpty(); 357 } 358 359 public boolean hasDescription() { 360 return this.description != null && !this.description.isEmpty(); 361 } 362 363 /** 364 * @param value {@link #description} (Description of the feature of the specimen.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 365 */ 366 public SpecimenFeatureComponent setDescriptionElement(StringType value) { 367 this.description = value; 368 return this; 369 } 370 371 /** 372 * @return Description of the feature of the specimen. 373 */ 374 public String getDescription() { 375 return this.description == null ? null : this.description.getValue(); 376 } 377 378 /** 379 * @param value Description of the feature of the specimen. 380 */ 381 public SpecimenFeatureComponent setDescription(String value) { 382 if (this.description == null) 383 this.description = new StringType(); 384 this.description.setValue(value); 385 return this; 386 } 387 388 protected void listChildren(List<Property> children) { 389 super.listChildren(children); 390 children.add(new Property("type", "CodeableConcept", "The landmark or feature being highlighted.", 0, 1, type)); 391 children.add(new Property("description", "string", "Description of the feature of the specimen.", 0, 1, description)); 392 } 393 394 @Override 395 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 396 switch (_hash) { 397 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The landmark or feature being highlighted.", 0, 1, type); 398 case -1724546052: /*description*/ return new Property("description", "string", "Description of the feature of the specimen.", 0, 1, description); 399 default: return super.getNamedProperty(_hash, _name, _checkValid); 400 } 401 402 } 403 404 @Override 405 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 406 switch (hash) { 407 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 408 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 409 default: return super.getProperty(hash, name, checkValid); 410 } 411 412 } 413 414 @Override 415 public Base setProperty(int hash, String name, Base value) throws FHIRException { 416 switch (hash) { 417 case 3575610: // type 418 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 419 return value; 420 case -1724546052: // description 421 this.description = TypeConvertor.castToString(value); // StringType 422 return value; 423 default: return super.setProperty(hash, name, value); 424 } 425 426 } 427 428 @Override 429 public Base setProperty(String name, Base value) throws FHIRException { 430 if (name.equals("type")) { 431 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 432 } else if (name.equals("description")) { 433 this.description = TypeConvertor.castToString(value); // StringType 434 } else 435 return super.setProperty(name, value); 436 return value; 437 } 438 439 @Override 440 public void removeChild(String name, Base value) throws FHIRException { 441 if (name.equals("type")) { 442 this.type = null; 443 } else if (name.equals("description")) { 444 this.description = null; 445 } else 446 super.removeChild(name, value); 447 448 } 449 450 @Override 451 public Base makeProperty(int hash, String name) throws FHIRException { 452 switch (hash) { 453 case 3575610: return getType(); 454 case -1724546052: return getDescriptionElement(); 455 default: return super.makeProperty(hash, name); 456 } 457 458 } 459 460 @Override 461 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 462 switch (hash) { 463 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 464 case -1724546052: /*description*/ return new String[] {"string"}; 465 default: return super.getTypesForProperty(hash, name); 466 } 467 468 } 469 470 @Override 471 public Base addChild(String name) throws FHIRException { 472 if (name.equals("type")) { 473 this.type = new CodeableConcept(); 474 return this.type; 475 } 476 else if (name.equals("description")) { 477 throw new FHIRException("Cannot call addChild on a singleton property Specimen.feature.description"); 478 } 479 else 480 return super.addChild(name); 481 } 482 483 public SpecimenFeatureComponent copy() { 484 SpecimenFeatureComponent dst = new SpecimenFeatureComponent(); 485 copyValues(dst); 486 return dst; 487 } 488 489 public void copyValues(SpecimenFeatureComponent dst) { 490 super.copyValues(dst); 491 dst.type = type == null ? null : type.copy(); 492 dst.description = description == null ? null : description.copy(); 493 } 494 495 @Override 496 public boolean equalsDeep(Base other_) { 497 if (!super.equalsDeep(other_)) 498 return false; 499 if (!(other_ instanceof SpecimenFeatureComponent)) 500 return false; 501 SpecimenFeatureComponent o = (SpecimenFeatureComponent) other_; 502 return compareDeep(type, o.type, true) && compareDeep(description, o.description, true); 503 } 504 505 @Override 506 public boolean equalsShallow(Base other_) { 507 if (!super.equalsShallow(other_)) 508 return false; 509 if (!(other_ instanceof SpecimenFeatureComponent)) 510 return false; 511 SpecimenFeatureComponent o = (SpecimenFeatureComponent) other_; 512 return compareValues(description, o.description, true); 513 } 514 515 public boolean isEmpty() { 516 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, description); 517 } 518 519 public String fhirType() { 520 return "Specimen.feature"; 521 522 } 523 524 } 525 526 @Block() 527 public static class SpecimenCollectionComponent extends BackboneElement implements IBaseBackboneElement { 528 /** 529 * Person who collected the specimen. 530 */ 531 @Child(name = "collector", type = {Practitioner.class, PractitionerRole.class, Patient.class, RelatedPerson.class}, order=1, min=0, max=1, modifier=false, summary=true) 532 @Description(shortDefinition="Who collected the specimen", formalDefinition="Person who collected the specimen." ) 533 protected Reference collector; 534 535 /** 536 * Time when specimen was collected from subject - the physiologically relevant time. 537 */ 538 @Child(name = "collected", type = {DateTimeType.class, Period.class}, order=2, min=0, max=1, modifier=false, summary=true) 539 @Description(shortDefinition="Collection time", formalDefinition="Time when specimen was collected from subject - the physiologically relevant time." ) 540 protected DataType collected; 541 542 /** 543 * The span of time over which the collection of a specimen occurred. 544 */ 545 @Child(name = "duration", type = {Duration.class}, order=3, min=0, max=1, modifier=false, summary=true) 546 @Description(shortDefinition="How long it took to collect specimen", formalDefinition="The span of time over which the collection of a specimen occurred." ) 547 protected Duration duration; 548 549 /** 550 * The quantity of specimen collected; for instance the volume of a blood sample, or the physical measurement of an anatomic pathology sample. 551 */ 552 @Child(name = "quantity", type = {Quantity.class}, order=4, min=0, max=1, modifier=false, summary=false) 553 @Description(shortDefinition="The quantity of specimen collected", formalDefinition="The quantity of specimen collected; for instance the volume of a blood sample, or the physical measurement of an anatomic pathology sample." ) 554 protected Quantity quantity; 555 556 /** 557 * A coded value specifying the technique that is used to perform the procedure. 558 */ 559 @Child(name = "method", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 560 @Description(shortDefinition="Technique used to perform collection", formalDefinition="A coded value specifying the technique that is used to perform the procedure." ) 561 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/specimen-collection-method") 562 protected CodeableConcept method; 563 564 /** 565 * A coded value specifying the technique that is used to perform the procedure. 566 */ 567 @Child(name = "device", type = {CodeableReference.class}, order=6, min=0, max=1, modifier=false, summary=false) 568 @Description(shortDefinition="Device used to perform collection", formalDefinition="A coded value specifying the technique that is used to perform the procedure." ) 569 protected CodeableReference device; 570 571 /** 572 * The procedure event during which the specimen was collected (e.g. the surgery leading to the collection of a pathology sample). 573 */ 574 @Child(name = "procedure", type = {Procedure.class}, order=7, min=0, max=1, modifier=false, summary=false) 575 @Description(shortDefinition="The procedure that collects the specimen", formalDefinition="The procedure event during which the specimen was collected (e.g. the surgery leading to the collection of a pathology sample)." ) 576 protected Reference procedure; 577 578 /** 579 * Anatomical location from which the specimen was collected (if subject is a patient). This is the target site. This element is not used for environmental specimens. 580 */ 581 @Child(name = "bodySite", type = {CodeableReference.class}, order=8, min=0, max=1, modifier=false, summary=false) 582 @Description(shortDefinition="Anatomical collection site", formalDefinition="Anatomical location from which the specimen was collected (if subject is a patient). This is the target site. This element is not used for environmental specimens." ) 583 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 584 protected CodeableReference bodySite; 585 586 /** 587 * Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection. 588 */ 589 @Child(name = "fastingStatus", type = {CodeableConcept.class, Duration.class}, order=9, min=0, max=1, modifier=false, summary=true) 590 @Description(shortDefinition="Whether or how long patient abstained from food and/or drink", formalDefinition="Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection." ) 591 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v2-0916") 592 protected DataType fastingStatus; 593 594 private static final long serialVersionUID = 953983070L; 595 596 /** 597 * Constructor 598 */ 599 public SpecimenCollectionComponent() { 600 super(); 601 } 602 603 /** 604 * @return {@link #collector} (Person who collected the specimen.) 605 */ 606 public Reference getCollector() { 607 if (this.collector == null) 608 if (Configuration.errorOnAutoCreate()) 609 throw new Error("Attempt to auto-create SpecimenCollectionComponent.collector"); 610 else if (Configuration.doAutoCreate()) 611 this.collector = new Reference(); // cc 612 return this.collector; 613 } 614 615 public boolean hasCollector() { 616 return this.collector != null && !this.collector.isEmpty(); 617 } 618 619 /** 620 * @param value {@link #collector} (Person who collected the specimen.) 621 */ 622 public SpecimenCollectionComponent setCollector(Reference value) { 623 this.collector = value; 624 return this; 625 } 626 627 /** 628 * @return {@link #collected} (Time when specimen was collected from subject - the physiologically relevant time.) 629 */ 630 public DataType getCollected() { 631 return this.collected; 632 } 633 634 /** 635 * @return {@link #collected} (Time when specimen was collected from subject - the physiologically relevant time.) 636 */ 637 public DateTimeType getCollectedDateTimeType() throws FHIRException { 638 if (this.collected == null) 639 this.collected = new DateTimeType(); 640 if (!(this.collected instanceof DateTimeType)) 641 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.collected.getClass().getName()+" was encountered"); 642 return (DateTimeType) this.collected; 643 } 644 645 public boolean hasCollectedDateTimeType() { 646 return this.collected instanceof DateTimeType; 647 } 648 649 /** 650 * @return {@link #collected} (Time when specimen was collected from subject - the physiologically relevant time.) 651 */ 652 public Period getCollectedPeriod() throws FHIRException { 653 if (this.collected == null) 654 this.collected = new Period(); 655 if (!(this.collected instanceof Period)) 656 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.collected.getClass().getName()+" was encountered"); 657 return (Period) this.collected; 658 } 659 660 public boolean hasCollectedPeriod() { 661 return this.collected instanceof Period; 662 } 663 664 public boolean hasCollected() { 665 return this.collected != null && !this.collected.isEmpty(); 666 } 667 668 /** 669 * @param value {@link #collected} (Time when specimen was collected from subject - the physiologically relevant time.) 670 */ 671 public SpecimenCollectionComponent setCollected(DataType value) { 672 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 673 throw new FHIRException("Not the right type for Specimen.collection.collected[x]: "+value.fhirType()); 674 this.collected = value; 675 return this; 676 } 677 678 /** 679 * @return {@link #duration} (The span of time over which the collection of a specimen occurred.) 680 */ 681 public Duration getDuration() { 682 if (this.duration == null) 683 if (Configuration.errorOnAutoCreate()) 684 throw new Error("Attempt to auto-create SpecimenCollectionComponent.duration"); 685 else if (Configuration.doAutoCreate()) 686 this.duration = new Duration(); // cc 687 return this.duration; 688 } 689 690 public boolean hasDuration() { 691 return this.duration != null && !this.duration.isEmpty(); 692 } 693 694 /** 695 * @param value {@link #duration} (The span of time over which the collection of a specimen occurred.) 696 */ 697 public SpecimenCollectionComponent setDuration(Duration value) { 698 this.duration = value; 699 return this; 700 } 701 702 /** 703 * @return {@link #quantity} (The quantity of specimen collected; for instance the volume of a blood sample, or the physical measurement of an anatomic pathology sample.) 704 */ 705 public Quantity getQuantity() { 706 if (this.quantity == null) 707 if (Configuration.errorOnAutoCreate()) 708 throw new Error("Attempt to auto-create SpecimenCollectionComponent.quantity"); 709 else if (Configuration.doAutoCreate()) 710 this.quantity = new Quantity(); // cc 711 return this.quantity; 712 } 713 714 public boolean hasQuantity() { 715 return this.quantity != null && !this.quantity.isEmpty(); 716 } 717 718 /** 719 * @param value {@link #quantity} (The quantity of specimen collected; for instance the volume of a blood sample, or the physical measurement of an anatomic pathology sample.) 720 */ 721 public SpecimenCollectionComponent setQuantity(Quantity value) { 722 this.quantity = value; 723 return this; 724 } 725 726 /** 727 * @return {@link #method} (A coded value specifying the technique that is used to perform the procedure.) 728 */ 729 public CodeableConcept getMethod() { 730 if (this.method == null) 731 if (Configuration.errorOnAutoCreate()) 732 throw new Error("Attempt to auto-create SpecimenCollectionComponent.method"); 733 else if (Configuration.doAutoCreate()) 734 this.method = new CodeableConcept(); // cc 735 return this.method; 736 } 737 738 public boolean hasMethod() { 739 return this.method != null && !this.method.isEmpty(); 740 } 741 742 /** 743 * @param value {@link #method} (A coded value specifying the technique that is used to perform the procedure.) 744 */ 745 public SpecimenCollectionComponent setMethod(CodeableConcept value) { 746 this.method = value; 747 return this; 748 } 749 750 /** 751 * @return {@link #device} (A coded value specifying the technique that is used to perform the procedure.) 752 */ 753 public CodeableReference getDevice() { 754 if (this.device == null) 755 if (Configuration.errorOnAutoCreate()) 756 throw new Error("Attempt to auto-create SpecimenCollectionComponent.device"); 757 else if (Configuration.doAutoCreate()) 758 this.device = new CodeableReference(); // cc 759 return this.device; 760 } 761 762 public boolean hasDevice() { 763 return this.device != null && !this.device.isEmpty(); 764 } 765 766 /** 767 * @param value {@link #device} (A coded value specifying the technique that is used to perform the procedure.) 768 */ 769 public SpecimenCollectionComponent setDevice(CodeableReference value) { 770 this.device = value; 771 return this; 772 } 773 774 /** 775 * @return {@link #procedure} (The procedure event during which the specimen was collected (e.g. the surgery leading to the collection of a pathology sample).) 776 */ 777 public Reference getProcedure() { 778 if (this.procedure == null) 779 if (Configuration.errorOnAutoCreate()) 780 throw new Error("Attempt to auto-create SpecimenCollectionComponent.procedure"); 781 else if (Configuration.doAutoCreate()) 782 this.procedure = new Reference(); // cc 783 return this.procedure; 784 } 785 786 public boolean hasProcedure() { 787 return this.procedure != null && !this.procedure.isEmpty(); 788 } 789 790 /** 791 * @param value {@link #procedure} (The procedure event during which the specimen was collected (e.g. the surgery leading to the collection of a pathology sample).) 792 */ 793 public SpecimenCollectionComponent setProcedure(Reference value) { 794 this.procedure = value; 795 return this; 796 } 797 798 /** 799 * @return {@link #bodySite} (Anatomical location from which the specimen was collected (if subject is a patient). This is the target site. This element is not used for environmental specimens.) 800 */ 801 public CodeableReference getBodySite() { 802 if (this.bodySite == null) 803 if (Configuration.errorOnAutoCreate()) 804 throw new Error("Attempt to auto-create SpecimenCollectionComponent.bodySite"); 805 else if (Configuration.doAutoCreate()) 806 this.bodySite = new CodeableReference(); // cc 807 return this.bodySite; 808 } 809 810 public boolean hasBodySite() { 811 return this.bodySite != null && !this.bodySite.isEmpty(); 812 } 813 814 /** 815 * @param value {@link #bodySite} (Anatomical location from which the specimen was collected (if subject is a patient). This is the target site. This element is not used for environmental specimens.) 816 */ 817 public SpecimenCollectionComponent setBodySite(CodeableReference value) { 818 this.bodySite = value; 819 return this; 820 } 821 822 /** 823 * @return {@link #fastingStatus} (Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.) 824 */ 825 public DataType getFastingStatus() { 826 return this.fastingStatus; 827 } 828 829 /** 830 * @return {@link #fastingStatus} (Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.) 831 */ 832 public CodeableConcept getFastingStatusCodeableConcept() throws FHIRException { 833 if (this.fastingStatus == null) 834 this.fastingStatus = new CodeableConcept(); 835 if (!(this.fastingStatus instanceof CodeableConcept)) 836 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.fastingStatus.getClass().getName()+" was encountered"); 837 return (CodeableConcept) this.fastingStatus; 838 } 839 840 public boolean hasFastingStatusCodeableConcept() { 841 return this.fastingStatus instanceof CodeableConcept; 842 } 843 844 /** 845 * @return {@link #fastingStatus} (Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.) 846 */ 847 public Duration getFastingStatusDuration() throws FHIRException { 848 if (this.fastingStatus == null) 849 this.fastingStatus = new Duration(); 850 if (!(this.fastingStatus instanceof Duration)) 851 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.fastingStatus.getClass().getName()+" was encountered"); 852 return (Duration) this.fastingStatus; 853 } 854 855 public boolean hasFastingStatusDuration() { 856 return this.fastingStatus instanceof Duration; 857 } 858 859 public boolean hasFastingStatus() { 860 return this.fastingStatus != null && !this.fastingStatus.isEmpty(); 861 } 862 863 /** 864 * @param value {@link #fastingStatus} (Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.) 865 */ 866 public SpecimenCollectionComponent setFastingStatus(DataType value) { 867 if (value != null && !(value instanceof CodeableConcept || value instanceof Duration)) 868 throw new FHIRException("Not the right type for Specimen.collection.fastingStatus[x]: "+value.fhirType()); 869 this.fastingStatus = value; 870 return this; 871 } 872 873 protected void listChildren(List<Property> children) { 874 super.listChildren(children); 875 children.add(new Property("collector", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson)", "Person who collected the specimen.", 0, 1, collector)); 876 children.add(new Property("collected[x]", "dateTime|Period", "Time when specimen was collected from subject - the physiologically relevant time.", 0, 1, collected)); 877 children.add(new Property("duration", "Duration", "The span of time over which the collection of a specimen occurred.", 0, 1, duration)); 878 children.add(new Property("quantity", "Quantity", "The quantity of specimen collected; for instance the volume of a blood sample, or the physical measurement of an anatomic pathology sample.", 0, 1, quantity)); 879 children.add(new Property("method", "CodeableConcept", "A coded value specifying the technique that is used to perform the procedure.", 0, 1, method)); 880 children.add(new Property("device", "CodeableReference(Device)", "A coded value specifying the technique that is used to perform the procedure.", 0, 1, device)); 881 children.add(new Property("procedure", "Reference(Procedure)", "The procedure event during which the specimen was collected (e.g. the surgery leading to the collection of a pathology sample).", 0, 1, procedure)); 882 children.add(new Property("bodySite", "CodeableReference(BodyStructure)", "Anatomical location from which the specimen was collected (if subject is a patient). This is the target site. This element is not used for environmental specimens.", 0, 1, bodySite)); 883 children.add(new Property("fastingStatus[x]", "CodeableConcept|Duration", "Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.", 0, 1, fastingStatus)); 884 } 885 886 @Override 887 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 888 switch (_hash) { 889 case 1883491469: /*collector*/ return new Property("collector", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson)", "Person who collected the specimen.", 0, 1, collector); 890 case 1632037015: /*collected[x]*/ return new Property("collected[x]", "dateTime|Period", "Time when specimen was collected from subject - the physiologically relevant time.", 0, 1, collected); 891 case 1883491145: /*collected*/ return new Property("collected[x]", "dateTime|Period", "Time when specimen was collected from subject - the physiologically relevant time.", 0, 1, collected); 892 case 2005009924: /*collectedDateTime*/ return new Property("collected[x]", "dateTime", "Time when specimen was collected from subject - the physiologically relevant time.", 0, 1, collected); 893 case 653185642: /*collectedPeriod*/ return new Property("collected[x]", "Period", "Time when specimen was collected from subject - the physiologically relevant time.", 0, 1, collected); 894 case -1992012396: /*duration*/ return new Property("duration", "Duration", "The span of time over which the collection of a specimen occurred.", 0, 1, duration); 895 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The quantity of specimen collected; for instance the volume of a blood sample, or the physical measurement of an anatomic pathology sample.", 0, 1, quantity); 896 case -1077554975: /*method*/ return new Property("method", "CodeableConcept", "A coded value specifying the technique that is used to perform the procedure.", 0, 1, method); 897 case -1335157162: /*device*/ return new Property("device", "CodeableReference(Device)", "A coded value specifying the technique that is used to perform the procedure.", 0, 1, device); 898 case -1095204141: /*procedure*/ return new Property("procedure", "Reference(Procedure)", "The procedure event during which the specimen was collected (e.g. the surgery leading to the collection of a pathology sample).", 0, 1, procedure); 899 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableReference(BodyStructure)", "Anatomical location from which the specimen was collected (if subject is a patient). This is the target site. This element is not used for environmental specimens.", 0, 1, bodySite); 900 case -570577944: /*fastingStatus[x]*/ return new Property("fastingStatus[x]", "CodeableConcept|Duration", "Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.", 0, 1, fastingStatus); 901 case -701550184: /*fastingStatus*/ return new Property("fastingStatus[x]", "CodeableConcept|Duration", "Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.", 0, 1, fastingStatus); 902 case -1153232151: /*fastingStatusCodeableConcept*/ return new Property("fastingStatus[x]", "CodeableConcept", "Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.", 0, 1, fastingStatus); 903 case -433140916: /*fastingStatusDuration*/ return new Property("fastingStatus[x]", "Duration", "Abstinence or reduction from some or all food, drink, or both, for a period of time prior to sample collection.", 0, 1, fastingStatus); 904 default: return super.getNamedProperty(_hash, _name, _checkValid); 905 } 906 907 } 908 909 @Override 910 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 911 switch (hash) { 912 case 1883491469: /*collector*/ return this.collector == null ? new Base[0] : new Base[] {this.collector}; // Reference 913 case 1883491145: /*collected*/ return this.collected == null ? new Base[0] : new Base[] {this.collected}; // DataType 914 case -1992012396: /*duration*/ return this.duration == null ? new Base[0] : new Base[] {this.duration}; // Duration 915 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 916 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // CodeableConcept 917 case -1335157162: /*device*/ return this.device == null ? new Base[0] : new Base[] {this.device}; // CodeableReference 918 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : new Base[] {this.procedure}; // Reference 919 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : new Base[] {this.bodySite}; // CodeableReference 920 case -701550184: /*fastingStatus*/ return this.fastingStatus == null ? new Base[0] : new Base[] {this.fastingStatus}; // DataType 921 default: return super.getProperty(hash, name, checkValid); 922 } 923 924 } 925 926 @Override 927 public Base setProperty(int hash, String name, Base value) throws FHIRException { 928 switch (hash) { 929 case 1883491469: // collector 930 this.collector = TypeConvertor.castToReference(value); // Reference 931 return value; 932 case 1883491145: // collected 933 this.collected = TypeConvertor.castToType(value); // DataType 934 return value; 935 case -1992012396: // duration 936 this.duration = TypeConvertor.castToDuration(value); // Duration 937 return value; 938 case -1285004149: // quantity 939 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 940 return value; 941 case -1077554975: // method 942 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 943 return value; 944 case -1335157162: // device 945 this.device = TypeConvertor.castToCodeableReference(value); // CodeableReference 946 return value; 947 case -1095204141: // procedure 948 this.procedure = TypeConvertor.castToReference(value); // Reference 949 return value; 950 case 1702620169: // bodySite 951 this.bodySite = TypeConvertor.castToCodeableReference(value); // CodeableReference 952 return value; 953 case -701550184: // fastingStatus 954 this.fastingStatus = TypeConvertor.castToType(value); // DataType 955 return value; 956 default: return super.setProperty(hash, name, value); 957 } 958 959 } 960 961 @Override 962 public Base setProperty(String name, Base value) throws FHIRException { 963 if (name.equals("collector")) { 964 this.collector = TypeConvertor.castToReference(value); // Reference 965 } else if (name.equals("collected[x]")) { 966 this.collected = TypeConvertor.castToType(value); // DataType 967 } else if (name.equals("duration")) { 968 this.duration = TypeConvertor.castToDuration(value); // Duration 969 } else if (name.equals("quantity")) { 970 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 971 } else if (name.equals("method")) { 972 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 973 } else if (name.equals("device")) { 974 this.device = TypeConvertor.castToCodeableReference(value); // CodeableReference 975 } else if (name.equals("procedure")) { 976 this.procedure = TypeConvertor.castToReference(value); // Reference 977 } else if (name.equals("bodySite")) { 978 this.bodySite = TypeConvertor.castToCodeableReference(value); // CodeableReference 979 } else if (name.equals("fastingStatus[x]")) { 980 this.fastingStatus = TypeConvertor.castToType(value); // DataType 981 } else 982 return super.setProperty(name, value); 983 return value; 984 } 985 986 @Override 987 public void removeChild(String name, Base value) throws FHIRException { 988 if (name.equals("collector")) { 989 this.collector = null; 990 } else if (name.equals("collected[x]")) { 991 this.collected = null; 992 } else if (name.equals("duration")) { 993 this.duration = null; 994 } else if (name.equals("quantity")) { 995 this.quantity = null; 996 } else if (name.equals("method")) { 997 this.method = null; 998 } else if (name.equals("device")) { 999 this.device = null; 1000 } else if (name.equals("procedure")) { 1001 this.procedure = null; 1002 } else if (name.equals("bodySite")) { 1003 this.bodySite = null; 1004 } else if (name.equals("fastingStatus[x]")) { 1005 this.fastingStatus = null; 1006 } else 1007 super.removeChild(name, value); 1008 1009 } 1010 1011 @Override 1012 public Base makeProperty(int hash, String name) throws FHIRException { 1013 switch (hash) { 1014 case 1883491469: return getCollector(); 1015 case 1632037015: return getCollected(); 1016 case 1883491145: return getCollected(); 1017 case -1992012396: return getDuration(); 1018 case -1285004149: return getQuantity(); 1019 case -1077554975: return getMethod(); 1020 case -1335157162: return getDevice(); 1021 case -1095204141: return getProcedure(); 1022 case 1702620169: return getBodySite(); 1023 case -570577944: return getFastingStatus(); 1024 case -701550184: return getFastingStatus(); 1025 default: return super.makeProperty(hash, name); 1026 } 1027 1028 } 1029 1030 @Override 1031 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1032 switch (hash) { 1033 case 1883491469: /*collector*/ return new String[] {"Reference"}; 1034 case 1883491145: /*collected*/ return new String[] {"dateTime", "Period"}; 1035 case -1992012396: /*duration*/ return new String[] {"Duration"}; 1036 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 1037 case -1077554975: /*method*/ return new String[] {"CodeableConcept"}; 1038 case -1335157162: /*device*/ return new String[] {"CodeableReference"}; 1039 case -1095204141: /*procedure*/ return new String[] {"Reference"}; 1040 case 1702620169: /*bodySite*/ return new String[] {"CodeableReference"}; 1041 case -701550184: /*fastingStatus*/ return new String[] {"CodeableConcept", "Duration"}; 1042 default: return super.getTypesForProperty(hash, name); 1043 } 1044 1045 } 1046 1047 @Override 1048 public Base addChild(String name) throws FHIRException { 1049 if (name.equals("collector")) { 1050 this.collector = new Reference(); 1051 return this.collector; 1052 } 1053 else if (name.equals("collectedDateTime")) { 1054 this.collected = new DateTimeType(); 1055 return this.collected; 1056 } 1057 else if (name.equals("collectedPeriod")) { 1058 this.collected = new Period(); 1059 return this.collected; 1060 } 1061 else if (name.equals("duration")) { 1062 this.duration = new Duration(); 1063 return this.duration; 1064 } 1065 else if (name.equals("quantity")) { 1066 this.quantity = new Quantity(); 1067 return this.quantity; 1068 } 1069 else if (name.equals("method")) { 1070 this.method = new CodeableConcept(); 1071 return this.method; 1072 } 1073 else if (name.equals("device")) { 1074 this.device = new CodeableReference(); 1075 return this.device; 1076 } 1077 else if (name.equals("procedure")) { 1078 this.procedure = new Reference(); 1079 return this.procedure; 1080 } 1081 else if (name.equals("bodySite")) { 1082 this.bodySite = new CodeableReference(); 1083 return this.bodySite; 1084 } 1085 else if (name.equals("fastingStatusCodeableConcept")) { 1086 this.fastingStatus = new CodeableConcept(); 1087 return this.fastingStatus; 1088 } 1089 else if (name.equals("fastingStatusDuration")) { 1090 this.fastingStatus = new Duration(); 1091 return this.fastingStatus; 1092 } 1093 else 1094 return super.addChild(name); 1095 } 1096 1097 public SpecimenCollectionComponent copy() { 1098 SpecimenCollectionComponent dst = new SpecimenCollectionComponent(); 1099 copyValues(dst); 1100 return dst; 1101 } 1102 1103 public void copyValues(SpecimenCollectionComponent dst) { 1104 super.copyValues(dst); 1105 dst.collector = collector == null ? null : collector.copy(); 1106 dst.collected = collected == null ? null : collected.copy(); 1107 dst.duration = duration == null ? null : duration.copy(); 1108 dst.quantity = quantity == null ? null : quantity.copy(); 1109 dst.method = method == null ? null : method.copy(); 1110 dst.device = device == null ? null : device.copy(); 1111 dst.procedure = procedure == null ? null : procedure.copy(); 1112 dst.bodySite = bodySite == null ? null : bodySite.copy(); 1113 dst.fastingStatus = fastingStatus == null ? null : fastingStatus.copy(); 1114 } 1115 1116 @Override 1117 public boolean equalsDeep(Base other_) { 1118 if (!super.equalsDeep(other_)) 1119 return false; 1120 if (!(other_ instanceof SpecimenCollectionComponent)) 1121 return false; 1122 SpecimenCollectionComponent o = (SpecimenCollectionComponent) other_; 1123 return compareDeep(collector, o.collector, true) && compareDeep(collected, o.collected, true) && compareDeep(duration, o.duration, true) 1124 && compareDeep(quantity, o.quantity, true) && compareDeep(method, o.method, true) && compareDeep(device, o.device, true) 1125 && compareDeep(procedure, o.procedure, true) && compareDeep(bodySite, o.bodySite, true) && compareDeep(fastingStatus, o.fastingStatus, true) 1126 ; 1127 } 1128 1129 @Override 1130 public boolean equalsShallow(Base other_) { 1131 if (!super.equalsShallow(other_)) 1132 return false; 1133 if (!(other_ instanceof SpecimenCollectionComponent)) 1134 return false; 1135 SpecimenCollectionComponent o = (SpecimenCollectionComponent) other_; 1136 return true; 1137 } 1138 1139 public boolean isEmpty() { 1140 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(collector, collected, duration 1141 , quantity, method, device, procedure, bodySite, fastingStatus); 1142 } 1143 1144 public String fhirType() { 1145 return "Specimen.collection"; 1146 1147 } 1148 1149 } 1150 1151 @Block() 1152 public static class SpecimenProcessingComponent extends BackboneElement implements IBaseBackboneElement { 1153 /** 1154 * Textual description of procedure. 1155 */ 1156 @Child(name = "description", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1157 @Description(shortDefinition="Textual description of procedure", formalDefinition="Textual description of procedure." ) 1158 protected StringType description; 1159 1160 /** 1161 * A coded value specifying the method used to process the specimen. 1162 */ 1163 @Child(name = "method", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1164 @Description(shortDefinition="Indicates the treatment step applied to the specimen", formalDefinition="A coded value specifying the method used to process the specimen." ) 1165 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/specimen-processing-method") 1166 protected CodeableConcept method; 1167 1168 /** 1169 * Material used in the processing step. 1170 */ 1171 @Child(name = "additive", type = {Substance.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1172 @Description(shortDefinition="Material used in the processing step", formalDefinition="Material used in the processing step." ) 1173 protected List<Reference> additive; 1174 1175 /** 1176 * A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin. 1177 */ 1178 @Child(name = "time", type = {DateTimeType.class, Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 1179 @Description(shortDefinition="Date and time of specimen processing", formalDefinition="A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin." ) 1180 protected DataType time; 1181 1182 private static final long serialVersionUID = -329044827L; 1183 1184 /** 1185 * Constructor 1186 */ 1187 public SpecimenProcessingComponent() { 1188 super(); 1189 } 1190 1191 /** 1192 * @return {@link #description} (Textual description of procedure.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1193 */ 1194 public StringType getDescriptionElement() { 1195 if (this.description == null) 1196 if (Configuration.errorOnAutoCreate()) 1197 throw new Error("Attempt to auto-create SpecimenProcessingComponent.description"); 1198 else if (Configuration.doAutoCreate()) 1199 this.description = new StringType(); // bb 1200 return this.description; 1201 } 1202 1203 public boolean hasDescriptionElement() { 1204 return this.description != null && !this.description.isEmpty(); 1205 } 1206 1207 public boolean hasDescription() { 1208 return this.description != null && !this.description.isEmpty(); 1209 } 1210 1211 /** 1212 * @param value {@link #description} (Textual description of procedure.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1213 */ 1214 public SpecimenProcessingComponent setDescriptionElement(StringType value) { 1215 this.description = value; 1216 return this; 1217 } 1218 1219 /** 1220 * @return Textual description of procedure. 1221 */ 1222 public String getDescription() { 1223 return this.description == null ? null : this.description.getValue(); 1224 } 1225 1226 /** 1227 * @param value Textual description of procedure. 1228 */ 1229 public SpecimenProcessingComponent setDescription(String value) { 1230 if (Utilities.noString(value)) 1231 this.description = null; 1232 else { 1233 if (this.description == null) 1234 this.description = new StringType(); 1235 this.description.setValue(value); 1236 } 1237 return this; 1238 } 1239 1240 /** 1241 * @return {@link #method} (A coded value specifying the method used to process the specimen.) 1242 */ 1243 public CodeableConcept getMethod() { 1244 if (this.method == null) 1245 if (Configuration.errorOnAutoCreate()) 1246 throw new Error("Attempt to auto-create SpecimenProcessingComponent.method"); 1247 else if (Configuration.doAutoCreate()) 1248 this.method = new CodeableConcept(); // cc 1249 return this.method; 1250 } 1251 1252 public boolean hasMethod() { 1253 return this.method != null && !this.method.isEmpty(); 1254 } 1255 1256 /** 1257 * @param value {@link #method} (A coded value specifying the method used to process the specimen.) 1258 */ 1259 public SpecimenProcessingComponent setMethod(CodeableConcept value) { 1260 this.method = value; 1261 return this; 1262 } 1263 1264 /** 1265 * @return {@link #additive} (Material used in the processing step.) 1266 */ 1267 public List<Reference> getAdditive() { 1268 if (this.additive == null) 1269 this.additive = new ArrayList<Reference>(); 1270 return this.additive; 1271 } 1272 1273 /** 1274 * @return Returns a reference to <code>this</code> for easy method chaining 1275 */ 1276 public SpecimenProcessingComponent setAdditive(List<Reference> theAdditive) { 1277 this.additive = theAdditive; 1278 return this; 1279 } 1280 1281 public boolean hasAdditive() { 1282 if (this.additive == null) 1283 return false; 1284 for (Reference item : this.additive) 1285 if (!item.isEmpty()) 1286 return true; 1287 return false; 1288 } 1289 1290 public Reference addAdditive() { //3 1291 Reference t = new Reference(); 1292 if (this.additive == null) 1293 this.additive = new ArrayList<Reference>(); 1294 this.additive.add(t); 1295 return t; 1296 } 1297 1298 public SpecimenProcessingComponent addAdditive(Reference t) { //3 1299 if (t == null) 1300 return this; 1301 if (this.additive == null) 1302 this.additive = new ArrayList<Reference>(); 1303 this.additive.add(t); 1304 return this; 1305 } 1306 1307 /** 1308 * @return The first repetition of repeating field {@link #additive}, creating it if it does not already exist {3} 1309 */ 1310 public Reference getAdditiveFirstRep() { 1311 if (getAdditive().isEmpty()) { 1312 addAdditive(); 1313 } 1314 return getAdditive().get(0); 1315 } 1316 1317 /** 1318 * @return {@link #time} (A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.) 1319 */ 1320 public DataType getTime() { 1321 return this.time; 1322 } 1323 1324 /** 1325 * @return {@link #time} (A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.) 1326 */ 1327 public DateTimeType getTimeDateTimeType() throws FHIRException { 1328 if (this.time == null) 1329 this.time = new DateTimeType(); 1330 if (!(this.time instanceof DateTimeType)) 1331 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.time.getClass().getName()+" was encountered"); 1332 return (DateTimeType) this.time; 1333 } 1334 1335 public boolean hasTimeDateTimeType() { 1336 return this.time instanceof DateTimeType; 1337 } 1338 1339 /** 1340 * @return {@link #time} (A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.) 1341 */ 1342 public Period getTimePeriod() throws FHIRException { 1343 if (this.time == null) 1344 this.time = new Period(); 1345 if (!(this.time instanceof Period)) 1346 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.time.getClass().getName()+" was encountered"); 1347 return (Period) this.time; 1348 } 1349 1350 public boolean hasTimePeriod() { 1351 return this.time instanceof Period; 1352 } 1353 1354 public boolean hasTime() { 1355 return this.time != null && !this.time.isEmpty(); 1356 } 1357 1358 /** 1359 * @param value {@link #time} (A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.) 1360 */ 1361 public SpecimenProcessingComponent setTime(DataType value) { 1362 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1363 throw new FHIRException("Not the right type for Specimen.processing.time[x]: "+value.fhirType()); 1364 this.time = value; 1365 return this; 1366 } 1367 1368 protected void listChildren(List<Property> children) { 1369 super.listChildren(children); 1370 children.add(new Property("description", "string", "Textual description of procedure.", 0, 1, description)); 1371 children.add(new Property("method", "CodeableConcept", "A coded value specifying the method used to process the specimen.", 0, 1, method)); 1372 children.add(new Property("additive", "Reference(Substance)", "Material used in the processing step.", 0, java.lang.Integer.MAX_VALUE, additive)); 1373 children.add(new Property("time[x]", "dateTime|Period", "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.", 0, 1, time)); 1374 } 1375 1376 @Override 1377 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1378 switch (_hash) { 1379 case -1724546052: /*description*/ return new Property("description", "string", "Textual description of procedure.", 0, 1, description); 1380 case -1077554975: /*method*/ return new Property("method", "CodeableConcept", "A coded value specifying the method used to process the specimen.", 0, 1, method); 1381 case -1226589236: /*additive*/ return new Property("additive", "Reference(Substance)", "Material used in the processing step.", 0, java.lang.Integer.MAX_VALUE, additive); 1382 case -1313930605: /*time[x]*/ return new Property("time[x]", "dateTime|Period", "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.", 0, 1, time); 1383 case 3560141: /*time*/ return new Property("time[x]", "dateTime|Period", "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.", 0, 1, time); 1384 case 2135345544: /*timeDateTime*/ return new Property("time[x]", "dateTime", "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.", 0, 1, time); 1385 case 693544686: /*timePeriod*/ return new Property("time[x]", "Period", "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.", 0, 1, time); 1386 default: return super.getNamedProperty(_hash, _name, _checkValid); 1387 } 1388 1389 } 1390 1391 @Override 1392 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1393 switch (hash) { 1394 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1395 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // CodeableConcept 1396 case -1226589236: /*additive*/ return this.additive == null ? new Base[0] : this.additive.toArray(new Base[this.additive.size()]); // Reference 1397 case 3560141: /*time*/ return this.time == null ? new Base[0] : new Base[] {this.time}; // DataType 1398 default: return super.getProperty(hash, name, checkValid); 1399 } 1400 1401 } 1402 1403 @Override 1404 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1405 switch (hash) { 1406 case -1724546052: // description 1407 this.description = TypeConvertor.castToString(value); // StringType 1408 return value; 1409 case -1077554975: // method 1410 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1411 return value; 1412 case -1226589236: // additive 1413 this.getAdditive().add(TypeConvertor.castToReference(value)); // Reference 1414 return value; 1415 case 3560141: // time 1416 this.time = TypeConvertor.castToType(value); // DataType 1417 return value; 1418 default: return super.setProperty(hash, name, value); 1419 } 1420 1421 } 1422 1423 @Override 1424 public Base setProperty(String name, Base value) throws FHIRException { 1425 if (name.equals("description")) { 1426 this.description = TypeConvertor.castToString(value); // StringType 1427 } else if (name.equals("method")) { 1428 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1429 } else if (name.equals("additive")) { 1430 this.getAdditive().add(TypeConvertor.castToReference(value)); 1431 } else if (name.equals("time[x]")) { 1432 this.time = TypeConvertor.castToType(value); // DataType 1433 } else 1434 return super.setProperty(name, value); 1435 return value; 1436 } 1437 1438 @Override 1439 public void removeChild(String name, Base value) throws FHIRException { 1440 if (name.equals("description")) { 1441 this.description = null; 1442 } else if (name.equals("method")) { 1443 this.method = null; 1444 } else if (name.equals("additive")) { 1445 this.getAdditive().remove(value); 1446 } else if (name.equals("time[x]")) { 1447 this.time = null; 1448 } else 1449 super.removeChild(name, value); 1450 1451 } 1452 1453 @Override 1454 public Base makeProperty(int hash, String name) throws FHIRException { 1455 switch (hash) { 1456 case -1724546052: return getDescriptionElement(); 1457 case -1077554975: return getMethod(); 1458 case -1226589236: return addAdditive(); 1459 case -1313930605: return getTime(); 1460 case 3560141: return getTime(); 1461 default: return super.makeProperty(hash, name); 1462 } 1463 1464 } 1465 1466 @Override 1467 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1468 switch (hash) { 1469 case -1724546052: /*description*/ return new String[] {"string"}; 1470 case -1077554975: /*method*/ return new String[] {"CodeableConcept"}; 1471 case -1226589236: /*additive*/ return new String[] {"Reference"}; 1472 case 3560141: /*time*/ return new String[] {"dateTime", "Period"}; 1473 default: return super.getTypesForProperty(hash, name); 1474 } 1475 1476 } 1477 1478 @Override 1479 public Base addChild(String name) throws FHIRException { 1480 if (name.equals("description")) { 1481 throw new FHIRException("Cannot call addChild on a singleton property Specimen.processing.description"); 1482 } 1483 else if (name.equals("method")) { 1484 this.method = new CodeableConcept(); 1485 return this.method; 1486 } 1487 else if (name.equals("additive")) { 1488 return addAdditive(); 1489 } 1490 else if (name.equals("timeDateTime")) { 1491 this.time = new DateTimeType(); 1492 return this.time; 1493 } 1494 else if (name.equals("timePeriod")) { 1495 this.time = new Period(); 1496 return this.time; 1497 } 1498 else 1499 return super.addChild(name); 1500 } 1501 1502 public SpecimenProcessingComponent copy() { 1503 SpecimenProcessingComponent dst = new SpecimenProcessingComponent(); 1504 copyValues(dst); 1505 return dst; 1506 } 1507 1508 public void copyValues(SpecimenProcessingComponent dst) { 1509 super.copyValues(dst); 1510 dst.description = description == null ? null : description.copy(); 1511 dst.method = method == null ? null : method.copy(); 1512 if (additive != null) { 1513 dst.additive = new ArrayList<Reference>(); 1514 for (Reference i : additive) 1515 dst.additive.add(i.copy()); 1516 }; 1517 dst.time = time == null ? null : time.copy(); 1518 } 1519 1520 @Override 1521 public boolean equalsDeep(Base other_) { 1522 if (!super.equalsDeep(other_)) 1523 return false; 1524 if (!(other_ instanceof SpecimenProcessingComponent)) 1525 return false; 1526 SpecimenProcessingComponent o = (SpecimenProcessingComponent) other_; 1527 return compareDeep(description, o.description, true) && compareDeep(method, o.method, true) && compareDeep(additive, o.additive, true) 1528 && compareDeep(time, o.time, true); 1529 } 1530 1531 @Override 1532 public boolean equalsShallow(Base other_) { 1533 if (!super.equalsShallow(other_)) 1534 return false; 1535 if (!(other_ instanceof SpecimenProcessingComponent)) 1536 return false; 1537 SpecimenProcessingComponent o = (SpecimenProcessingComponent) other_; 1538 return compareValues(description, o.description, true); 1539 } 1540 1541 public boolean isEmpty() { 1542 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, method, additive 1543 , time); 1544 } 1545 1546 public String fhirType() { 1547 return "Specimen.processing"; 1548 1549 } 1550 1551 } 1552 1553 @Block() 1554 public static class SpecimenContainerComponent extends BackboneElement implements IBaseBackboneElement { 1555 /** 1556 * The device resource for the the container holding the specimen. If the container is in a holder then the referenced device will point to a parent device. 1557 */ 1558 @Child(name = "device", type = {Device.class}, order=1, min=1, max=1, modifier=false, summary=false) 1559 @Description(shortDefinition="Device resource for the container", formalDefinition="The device resource for the the container holding the specimen. If the container is in a holder then the referenced device will point to a parent device." ) 1560 protected Reference device; 1561 1562 /** 1563 * The location of the container holding the specimen. 1564 */ 1565 @Child(name = "location", type = {Location.class}, order=2, min=0, max=1, modifier=false, summary=false) 1566 @Description(shortDefinition="Where the container is", formalDefinition="The location of the container holding the specimen." ) 1567 protected Reference location; 1568 1569 /** 1570 * The quantity of specimen in the container; may be volume, dimensions, or other appropriate measurements, depending on the specimen type. 1571 */ 1572 @Child(name = "specimenQuantity", type = {Quantity.class}, order=3, min=0, max=1, modifier=false, summary=false) 1573 @Description(shortDefinition="Quantity of specimen within container", formalDefinition="The quantity of specimen in the container; may be volume, dimensions, or other appropriate measurements, depending on the specimen type." ) 1574 protected Quantity specimenQuantity; 1575 1576 private static final long serialVersionUID = 1973387427L; 1577 1578 /** 1579 * Constructor 1580 */ 1581 public SpecimenContainerComponent() { 1582 super(); 1583 } 1584 1585 /** 1586 * Constructor 1587 */ 1588 public SpecimenContainerComponent(Reference device) { 1589 super(); 1590 this.setDevice(device); 1591 } 1592 1593 /** 1594 * @return {@link #device} (The device resource for the the container holding the specimen. If the container is in a holder then the referenced device will point to a parent device.) 1595 */ 1596 public Reference getDevice() { 1597 if (this.device == null) 1598 if (Configuration.errorOnAutoCreate()) 1599 throw new Error("Attempt to auto-create SpecimenContainerComponent.device"); 1600 else if (Configuration.doAutoCreate()) 1601 this.device = new Reference(); // cc 1602 return this.device; 1603 } 1604 1605 public boolean hasDevice() { 1606 return this.device != null && !this.device.isEmpty(); 1607 } 1608 1609 /** 1610 * @param value {@link #device} (The device resource for the the container holding the specimen. If the container is in a holder then the referenced device will point to a parent device.) 1611 */ 1612 public SpecimenContainerComponent setDevice(Reference value) { 1613 this.device = value; 1614 return this; 1615 } 1616 1617 /** 1618 * @return {@link #location} (The location of the container holding the specimen.) 1619 */ 1620 public Reference getLocation() { 1621 if (this.location == null) 1622 if (Configuration.errorOnAutoCreate()) 1623 throw new Error("Attempt to auto-create SpecimenContainerComponent.location"); 1624 else if (Configuration.doAutoCreate()) 1625 this.location = new Reference(); // cc 1626 return this.location; 1627 } 1628 1629 public boolean hasLocation() { 1630 return this.location != null && !this.location.isEmpty(); 1631 } 1632 1633 /** 1634 * @param value {@link #location} (The location of the container holding the specimen.) 1635 */ 1636 public SpecimenContainerComponent setLocation(Reference value) { 1637 this.location = value; 1638 return this; 1639 } 1640 1641 /** 1642 * @return {@link #specimenQuantity} (The quantity of specimen in the container; may be volume, dimensions, or other appropriate measurements, depending on the specimen type.) 1643 */ 1644 public Quantity getSpecimenQuantity() { 1645 if (this.specimenQuantity == null) 1646 if (Configuration.errorOnAutoCreate()) 1647 throw new Error("Attempt to auto-create SpecimenContainerComponent.specimenQuantity"); 1648 else if (Configuration.doAutoCreate()) 1649 this.specimenQuantity = new Quantity(); // cc 1650 return this.specimenQuantity; 1651 } 1652 1653 public boolean hasSpecimenQuantity() { 1654 return this.specimenQuantity != null && !this.specimenQuantity.isEmpty(); 1655 } 1656 1657 /** 1658 * @param value {@link #specimenQuantity} (The quantity of specimen in the container; may be volume, dimensions, or other appropriate measurements, depending on the specimen type.) 1659 */ 1660 public SpecimenContainerComponent setSpecimenQuantity(Quantity value) { 1661 this.specimenQuantity = value; 1662 return this; 1663 } 1664 1665 protected void listChildren(List<Property> children) { 1666 super.listChildren(children); 1667 children.add(new Property("device", "Reference(Device)", "The device resource for the the container holding the specimen. If the container is in a holder then the referenced device will point to a parent device.", 0, 1, device)); 1668 children.add(new Property("location", "Reference(Location)", "The location of the container holding the specimen.", 0, 1, location)); 1669 children.add(new Property("specimenQuantity", "Quantity", "The quantity of specimen in the container; may be volume, dimensions, or other appropriate measurements, depending on the specimen type.", 0, 1, specimenQuantity)); 1670 } 1671 1672 @Override 1673 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1674 switch (_hash) { 1675 case -1335157162: /*device*/ return new Property("device", "Reference(Device)", "The device resource for the the container holding the specimen. If the container is in a holder then the referenced device will point to a parent device.", 0, 1, device); 1676 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The location of the container holding the specimen.", 0, 1, location); 1677 case 1485980595: /*specimenQuantity*/ return new Property("specimenQuantity", "Quantity", "The quantity of specimen in the container; may be volume, dimensions, or other appropriate measurements, depending on the specimen type.", 0, 1, specimenQuantity); 1678 default: return super.getNamedProperty(_hash, _name, _checkValid); 1679 } 1680 1681 } 1682 1683 @Override 1684 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1685 switch (hash) { 1686 case -1335157162: /*device*/ return this.device == null ? new Base[0] : new Base[] {this.device}; // Reference 1687 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 1688 case 1485980595: /*specimenQuantity*/ return this.specimenQuantity == null ? new Base[0] : new Base[] {this.specimenQuantity}; // Quantity 1689 default: return super.getProperty(hash, name, checkValid); 1690 } 1691 1692 } 1693 1694 @Override 1695 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1696 switch (hash) { 1697 case -1335157162: // device 1698 this.device = TypeConvertor.castToReference(value); // Reference 1699 return value; 1700 case 1901043637: // location 1701 this.location = TypeConvertor.castToReference(value); // Reference 1702 return value; 1703 case 1485980595: // specimenQuantity 1704 this.specimenQuantity = TypeConvertor.castToQuantity(value); // Quantity 1705 return value; 1706 default: return super.setProperty(hash, name, value); 1707 } 1708 1709 } 1710 1711 @Override 1712 public Base setProperty(String name, Base value) throws FHIRException { 1713 if (name.equals("device")) { 1714 this.device = TypeConvertor.castToReference(value); // Reference 1715 } else if (name.equals("location")) { 1716 this.location = TypeConvertor.castToReference(value); // Reference 1717 } else if (name.equals("specimenQuantity")) { 1718 this.specimenQuantity = TypeConvertor.castToQuantity(value); // Quantity 1719 } else 1720 return super.setProperty(name, value); 1721 return value; 1722 } 1723 1724 @Override 1725 public void removeChild(String name, Base value) throws FHIRException { 1726 if (name.equals("device")) { 1727 this.device = null; 1728 } else if (name.equals("location")) { 1729 this.location = null; 1730 } else if (name.equals("specimenQuantity")) { 1731 this.specimenQuantity = null; 1732 } else 1733 super.removeChild(name, value); 1734 1735 } 1736 1737 @Override 1738 public Base makeProperty(int hash, String name) throws FHIRException { 1739 switch (hash) { 1740 case -1335157162: return getDevice(); 1741 case 1901043637: return getLocation(); 1742 case 1485980595: return getSpecimenQuantity(); 1743 default: return super.makeProperty(hash, name); 1744 } 1745 1746 } 1747 1748 @Override 1749 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1750 switch (hash) { 1751 case -1335157162: /*device*/ return new String[] {"Reference"}; 1752 case 1901043637: /*location*/ return new String[] {"Reference"}; 1753 case 1485980595: /*specimenQuantity*/ return new String[] {"Quantity"}; 1754 default: return super.getTypesForProperty(hash, name); 1755 } 1756 1757 } 1758 1759 @Override 1760 public Base addChild(String name) throws FHIRException { 1761 if (name.equals("device")) { 1762 this.device = new Reference(); 1763 return this.device; 1764 } 1765 else if (name.equals("location")) { 1766 this.location = new Reference(); 1767 return this.location; 1768 } 1769 else if (name.equals("specimenQuantity")) { 1770 this.specimenQuantity = new Quantity(); 1771 return this.specimenQuantity; 1772 } 1773 else 1774 return super.addChild(name); 1775 } 1776 1777 public SpecimenContainerComponent copy() { 1778 SpecimenContainerComponent dst = new SpecimenContainerComponent(); 1779 copyValues(dst); 1780 return dst; 1781 } 1782 1783 public void copyValues(SpecimenContainerComponent dst) { 1784 super.copyValues(dst); 1785 dst.device = device == null ? null : device.copy(); 1786 dst.location = location == null ? null : location.copy(); 1787 dst.specimenQuantity = specimenQuantity == null ? null : specimenQuantity.copy(); 1788 } 1789 1790 @Override 1791 public boolean equalsDeep(Base other_) { 1792 if (!super.equalsDeep(other_)) 1793 return false; 1794 if (!(other_ instanceof SpecimenContainerComponent)) 1795 return false; 1796 SpecimenContainerComponent o = (SpecimenContainerComponent) other_; 1797 return compareDeep(device, o.device, true) && compareDeep(location, o.location, true) && compareDeep(specimenQuantity, o.specimenQuantity, true) 1798 ; 1799 } 1800 1801 @Override 1802 public boolean equalsShallow(Base other_) { 1803 if (!super.equalsShallow(other_)) 1804 return false; 1805 if (!(other_ instanceof SpecimenContainerComponent)) 1806 return false; 1807 SpecimenContainerComponent o = (SpecimenContainerComponent) other_; 1808 return true; 1809 } 1810 1811 public boolean isEmpty() { 1812 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(device, location, specimenQuantity 1813 ); 1814 } 1815 1816 public String fhirType() { 1817 return "Specimen.container"; 1818 1819 } 1820 1821 } 1822 1823 /** 1824 * Id for specimen. 1825 */ 1826 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1827 @Description(shortDefinition="External Identifier", formalDefinition="Id for specimen." ) 1828 protected List<Identifier> identifier; 1829 1830 /** 1831 * The identifier assigned by the lab when accessioning specimen(s). This is not necessarily the same as the specimen identifier, depending on local lab procedures. 1832 */ 1833 @Child(name = "accessionIdentifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 1834 @Description(shortDefinition="Identifier assigned by the lab", formalDefinition="The identifier assigned by the lab when accessioning specimen(s). This is not necessarily the same as the specimen identifier, depending on local lab procedures." ) 1835 protected Identifier accessionIdentifier; 1836 1837 /** 1838 * The availability of the specimen. 1839 */ 1840 @Child(name = "status", type = {CodeType.class}, order=2, min=0, max=1, modifier=true, summary=true) 1841 @Description(shortDefinition="available | unavailable | unsatisfactory | entered-in-error", formalDefinition="The availability of the specimen." ) 1842 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/specimen-status") 1843 protected Enumeration<SpecimenStatus> status; 1844 1845 /** 1846 * The kind of material that forms the specimen. 1847 */ 1848 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 1849 @Description(shortDefinition="Kind of material that forms the specimen", formalDefinition="The kind of material that forms the specimen." ) 1850 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v2-0487") 1851 protected CodeableConcept type; 1852 1853 /** 1854 * Where the specimen came from. This may be from patient(s), from a location (e.g., the source of an environmental sample), or a sampling of a substance, a biologically-derived product, or a device. 1855 */ 1856 @Child(name = "subject", type = {Patient.class, Group.class, Device.class, BiologicallyDerivedProduct.class, Substance.class, Location.class}, order=4, min=0, max=1, modifier=false, summary=true) 1857 @Description(shortDefinition="Where the specimen came from. This may be from patient(s), from a location (e.g., the source of an environmental sample), or a sampling of a substance, a biologically-derived product, or a device", formalDefinition="Where the specimen came from. This may be from patient(s), from a location (e.g., the source of an environmental sample), or a sampling of a substance, a biologically-derived product, or a device." ) 1858 protected Reference subject; 1859 1860 /** 1861 * Time when specimen is received by the testing laboratory for processing or testing. 1862 */ 1863 @Child(name = "receivedTime", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1864 @Description(shortDefinition="The time when specimen is received by the testing laboratory", formalDefinition="Time when specimen is received by the testing laboratory for processing or testing." ) 1865 protected DateTimeType receivedTime; 1866 1867 /** 1868 * Reference to the parent (source) specimen which is used when the specimen was either derived from or a component of another specimen. 1869 */ 1870 @Child(name = "parent", type = {Specimen.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1871 @Description(shortDefinition="Specimen from which this specimen originated", formalDefinition="Reference to the parent (source) specimen which is used when the specimen was either derived from or a component of another specimen." ) 1872 protected List<Reference> parent; 1873 1874 /** 1875 * Details concerning a service request that required a specimen to be collected. 1876 */ 1877 @Child(name = "request", type = {ServiceRequest.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1878 @Description(shortDefinition="Why the specimen was collected", formalDefinition="Details concerning a service request that required a specimen to be collected." ) 1879 protected List<Reference> request; 1880 1881 /** 1882 * This element signifies if the specimen is part of a group or pooled. 1883 */ 1884 @Child(name = "combined", type = {CodeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1885 @Description(shortDefinition="grouped | pooled", formalDefinition="This element signifies if the specimen is part of a group or pooled." ) 1886 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/specimen-combined") 1887 protected Enumeration<SpecimenCombined> combined; 1888 1889 /** 1890 * The role or reason for the specimen in the testing workflow. 1891 */ 1892 @Child(name = "role", type = {CodeableConcept.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1893 @Description(shortDefinition="The role the specimen serves", formalDefinition="The role or reason for the specimen in the testing workflow." ) 1894 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/specimen-role") 1895 protected List<CodeableConcept> role; 1896 1897 /** 1898 * A physical feature or landmark on a specimen, highlighted for context by the collector of the specimen (e.g. surgeon), that identifies the type of feature as well as its meaning (e.g. the red ink indicating the resection margin of the right lobe of the excised prostate tissue or wire loop at radiologically suspected tumor location). 1899 */ 1900 @Child(name = "feature", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1901 @Description(shortDefinition="The physical feature of a specimen", formalDefinition="A physical feature or landmark on a specimen, highlighted for context by the collector of the specimen (e.g. surgeon), that identifies the type of feature as well as its meaning (e.g. the red ink indicating the resection margin of the right lobe of the excised prostate tissue or wire loop at radiologically suspected tumor location)." ) 1902 protected List<SpecimenFeatureComponent> feature; 1903 1904 /** 1905 * Details concerning the specimen collection. 1906 */ 1907 @Child(name = "collection", type = {}, order=11, min=0, max=1, modifier=false, summary=false) 1908 @Description(shortDefinition="Collection details", formalDefinition="Details concerning the specimen collection." ) 1909 protected SpecimenCollectionComponent collection; 1910 1911 /** 1912 * Details concerning processing and processing steps for the specimen. 1913 */ 1914 @Child(name = "processing", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1915 @Description(shortDefinition="Processing and processing step details", formalDefinition="Details concerning processing and processing steps for the specimen." ) 1916 protected List<SpecimenProcessingComponent> processing; 1917 1918 /** 1919 * The container holding the specimen. The recursive nature of containers; i.e. blood in tube in tray in rack is not addressed here. 1920 */ 1921 @Child(name = "container", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1922 @Description(shortDefinition="Direct container of specimen (tube/slide, etc.)", formalDefinition="The container holding the specimen. The recursive nature of containers; i.e. blood in tube in tray in rack is not addressed here." ) 1923 protected List<SpecimenContainerComponent> container; 1924 1925 /** 1926 * A mode or state of being that describes the nature of the specimen. 1927 */ 1928 @Child(name = "condition", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1929 @Description(shortDefinition="State of the specimen", formalDefinition="A mode or state of being that describes the nature of the specimen." ) 1930 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v2-0493") 1931 protected List<CodeableConcept> condition; 1932 1933 /** 1934 * To communicate any details or issues about the specimen or during the specimen collection. (for example: broken vial, sent with patient, frozen). 1935 */ 1936 @Child(name = "note", type = {Annotation.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1937 @Description(shortDefinition="Comments", formalDefinition="To communicate any details or issues about the specimen or during the specimen collection. (for example: broken vial, sent with patient, frozen)." ) 1938 protected List<Annotation> note; 1939 1940 private static final long serialVersionUID = -445425000L; 1941 1942 /** 1943 * Constructor 1944 */ 1945 public Specimen() { 1946 super(); 1947 } 1948 1949 /** 1950 * @return {@link #identifier} (Id for specimen.) 1951 */ 1952 public List<Identifier> getIdentifier() { 1953 if (this.identifier == null) 1954 this.identifier = new ArrayList<Identifier>(); 1955 return this.identifier; 1956 } 1957 1958 /** 1959 * @return Returns a reference to <code>this</code> for easy method chaining 1960 */ 1961 public Specimen setIdentifier(List<Identifier> theIdentifier) { 1962 this.identifier = theIdentifier; 1963 return this; 1964 } 1965 1966 public boolean hasIdentifier() { 1967 if (this.identifier == null) 1968 return false; 1969 for (Identifier item : this.identifier) 1970 if (!item.isEmpty()) 1971 return true; 1972 return false; 1973 } 1974 1975 public Identifier addIdentifier() { //3 1976 Identifier t = new Identifier(); 1977 if (this.identifier == null) 1978 this.identifier = new ArrayList<Identifier>(); 1979 this.identifier.add(t); 1980 return t; 1981 } 1982 1983 public Specimen addIdentifier(Identifier t) { //3 1984 if (t == null) 1985 return this; 1986 if (this.identifier == null) 1987 this.identifier = new ArrayList<Identifier>(); 1988 this.identifier.add(t); 1989 return this; 1990 } 1991 1992 /** 1993 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1994 */ 1995 public Identifier getIdentifierFirstRep() { 1996 if (getIdentifier().isEmpty()) { 1997 addIdentifier(); 1998 } 1999 return getIdentifier().get(0); 2000 } 2001 2002 /** 2003 * @return {@link #accessionIdentifier} (The identifier assigned by the lab when accessioning specimen(s). This is not necessarily the same as the specimen identifier, depending on local lab procedures.) 2004 */ 2005 public Identifier getAccessionIdentifier() { 2006 if (this.accessionIdentifier == null) 2007 if (Configuration.errorOnAutoCreate()) 2008 throw new Error("Attempt to auto-create Specimen.accessionIdentifier"); 2009 else if (Configuration.doAutoCreate()) 2010 this.accessionIdentifier = new Identifier(); // cc 2011 return this.accessionIdentifier; 2012 } 2013 2014 public boolean hasAccessionIdentifier() { 2015 return this.accessionIdentifier != null && !this.accessionIdentifier.isEmpty(); 2016 } 2017 2018 /** 2019 * @param value {@link #accessionIdentifier} (The identifier assigned by the lab when accessioning specimen(s). This is not necessarily the same as the specimen identifier, depending on local lab procedures.) 2020 */ 2021 public Specimen setAccessionIdentifier(Identifier value) { 2022 this.accessionIdentifier = value; 2023 return this; 2024 } 2025 2026 /** 2027 * @return {@link #status} (The availability of the specimen.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2028 */ 2029 public Enumeration<SpecimenStatus> getStatusElement() { 2030 if (this.status == null) 2031 if (Configuration.errorOnAutoCreate()) 2032 throw new Error("Attempt to auto-create Specimen.status"); 2033 else if (Configuration.doAutoCreate()) 2034 this.status = new Enumeration<SpecimenStatus>(new SpecimenStatusEnumFactory()); // bb 2035 return this.status; 2036 } 2037 2038 public boolean hasStatusElement() { 2039 return this.status != null && !this.status.isEmpty(); 2040 } 2041 2042 public boolean hasStatus() { 2043 return this.status != null && !this.status.isEmpty(); 2044 } 2045 2046 /** 2047 * @param value {@link #status} (The availability of the specimen.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2048 */ 2049 public Specimen setStatusElement(Enumeration<SpecimenStatus> value) { 2050 this.status = value; 2051 return this; 2052 } 2053 2054 /** 2055 * @return The availability of the specimen. 2056 */ 2057 public SpecimenStatus getStatus() { 2058 return this.status == null ? null : this.status.getValue(); 2059 } 2060 2061 /** 2062 * @param value The availability of the specimen. 2063 */ 2064 public Specimen setStatus(SpecimenStatus value) { 2065 if (value == null) 2066 this.status = null; 2067 else { 2068 if (this.status == null) 2069 this.status = new Enumeration<SpecimenStatus>(new SpecimenStatusEnumFactory()); 2070 this.status.setValue(value); 2071 } 2072 return this; 2073 } 2074 2075 /** 2076 * @return {@link #type} (The kind of material that forms the specimen.) 2077 */ 2078 public CodeableConcept getType() { 2079 if (this.type == null) 2080 if (Configuration.errorOnAutoCreate()) 2081 throw new Error("Attempt to auto-create Specimen.type"); 2082 else if (Configuration.doAutoCreate()) 2083 this.type = new CodeableConcept(); // cc 2084 return this.type; 2085 } 2086 2087 public boolean hasType() { 2088 return this.type != null && !this.type.isEmpty(); 2089 } 2090 2091 /** 2092 * @param value {@link #type} (The kind of material that forms the specimen.) 2093 */ 2094 public Specimen setType(CodeableConcept value) { 2095 this.type = value; 2096 return this; 2097 } 2098 2099 /** 2100 * @return {@link #subject} (Where the specimen came from. This may be from patient(s), from a location (e.g., the source of an environmental sample), or a sampling of a substance, a biologically-derived product, or a device.) 2101 */ 2102 public Reference getSubject() { 2103 if (this.subject == null) 2104 if (Configuration.errorOnAutoCreate()) 2105 throw new Error("Attempt to auto-create Specimen.subject"); 2106 else if (Configuration.doAutoCreate()) 2107 this.subject = new Reference(); // cc 2108 return this.subject; 2109 } 2110 2111 public boolean hasSubject() { 2112 return this.subject != null && !this.subject.isEmpty(); 2113 } 2114 2115 /** 2116 * @param value {@link #subject} (Where the specimen came from. This may be from patient(s), from a location (e.g., the source of an environmental sample), or a sampling of a substance, a biologically-derived product, or a device.) 2117 */ 2118 public Specimen setSubject(Reference value) { 2119 this.subject = value; 2120 return this; 2121 } 2122 2123 /** 2124 * @return {@link #receivedTime} (Time when specimen is received by the testing laboratory for processing or testing.). This is the underlying object with id, value and extensions. The accessor "getReceivedTime" gives direct access to the value 2125 */ 2126 public DateTimeType getReceivedTimeElement() { 2127 if (this.receivedTime == null) 2128 if (Configuration.errorOnAutoCreate()) 2129 throw new Error("Attempt to auto-create Specimen.receivedTime"); 2130 else if (Configuration.doAutoCreate()) 2131 this.receivedTime = new DateTimeType(); // bb 2132 return this.receivedTime; 2133 } 2134 2135 public boolean hasReceivedTimeElement() { 2136 return this.receivedTime != null && !this.receivedTime.isEmpty(); 2137 } 2138 2139 public boolean hasReceivedTime() { 2140 return this.receivedTime != null && !this.receivedTime.isEmpty(); 2141 } 2142 2143 /** 2144 * @param value {@link #receivedTime} (Time when specimen is received by the testing laboratory for processing or testing.). This is the underlying object with id, value and extensions. The accessor "getReceivedTime" gives direct access to the value 2145 */ 2146 public Specimen setReceivedTimeElement(DateTimeType value) { 2147 this.receivedTime = value; 2148 return this; 2149 } 2150 2151 /** 2152 * @return Time when specimen is received by the testing laboratory for processing or testing. 2153 */ 2154 public Date getReceivedTime() { 2155 return this.receivedTime == null ? null : this.receivedTime.getValue(); 2156 } 2157 2158 /** 2159 * @param value Time when specimen is received by the testing laboratory for processing or testing. 2160 */ 2161 public Specimen setReceivedTime(Date value) { 2162 if (value == null) 2163 this.receivedTime = null; 2164 else { 2165 if (this.receivedTime == null) 2166 this.receivedTime = new DateTimeType(); 2167 this.receivedTime.setValue(value); 2168 } 2169 return this; 2170 } 2171 2172 /** 2173 * @return {@link #parent} (Reference to the parent (source) specimen which is used when the specimen was either derived from or a component of another specimen.) 2174 */ 2175 public List<Reference> getParent() { 2176 if (this.parent == null) 2177 this.parent = new ArrayList<Reference>(); 2178 return this.parent; 2179 } 2180 2181 /** 2182 * @return Returns a reference to <code>this</code> for easy method chaining 2183 */ 2184 public Specimen setParent(List<Reference> theParent) { 2185 this.parent = theParent; 2186 return this; 2187 } 2188 2189 public boolean hasParent() { 2190 if (this.parent == null) 2191 return false; 2192 for (Reference item : this.parent) 2193 if (!item.isEmpty()) 2194 return true; 2195 return false; 2196 } 2197 2198 public Reference addParent() { //3 2199 Reference t = new Reference(); 2200 if (this.parent == null) 2201 this.parent = new ArrayList<Reference>(); 2202 this.parent.add(t); 2203 return t; 2204 } 2205 2206 public Specimen addParent(Reference t) { //3 2207 if (t == null) 2208 return this; 2209 if (this.parent == null) 2210 this.parent = new ArrayList<Reference>(); 2211 this.parent.add(t); 2212 return this; 2213 } 2214 2215 /** 2216 * @return The first repetition of repeating field {@link #parent}, creating it if it does not already exist {3} 2217 */ 2218 public Reference getParentFirstRep() { 2219 if (getParent().isEmpty()) { 2220 addParent(); 2221 } 2222 return getParent().get(0); 2223 } 2224 2225 /** 2226 * @return {@link #request} (Details concerning a service request that required a specimen to be collected.) 2227 */ 2228 public List<Reference> getRequest() { 2229 if (this.request == null) 2230 this.request = new ArrayList<Reference>(); 2231 return this.request; 2232 } 2233 2234 /** 2235 * @return Returns a reference to <code>this</code> for easy method chaining 2236 */ 2237 public Specimen setRequest(List<Reference> theRequest) { 2238 this.request = theRequest; 2239 return this; 2240 } 2241 2242 public boolean hasRequest() { 2243 if (this.request == null) 2244 return false; 2245 for (Reference item : this.request) 2246 if (!item.isEmpty()) 2247 return true; 2248 return false; 2249 } 2250 2251 public Reference addRequest() { //3 2252 Reference t = new Reference(); 2253 if (this.request == null) 2254 this.request = new ArrayList<Reference>(); 2255 this.request.add(t); 2256 return t; 2257 } 2258 2259 public Specimen addRequest(Reference t) { //3 2260 if (t == null) 2261 return this; 2262 if (this.request == null) 2263 this.request = new ArrayList<Reference>(); 2264 this.request.add(t); 2265 return this; 2266 } 2267 2268 /** 2269 * @return The first repetition of repeating field {@link #request}, creating it if it does not already exist {3} 2270 */ 2271 public Reference getRequestFirstRep() { 2272 if (getRequest().isEmpty()) { 2273 addRequest(); 2274 } 2275 return getRequest().get(0); 2276 } 2277 2278 /** 2279 * @return {@link #combined} (This element signifies if the specimen is part of a group or pooled.). This is the underlying object with id, value and extensions. The accessor "getCombined" gives direct access to the value 2280 */ 2281 public Enumeration<SpecimenCombined> getCombinedElement() { 2282 if (this.combined == null) 2283 if (Configuration.errorOnAutoCreate()) 2284 throw new Error("Attempt to auto-create Specimen.combined"); 2285 else if (Configuration.doAutoCreate()) 2286 this.combined = new Enumeration<SpecimenCombined>(new SpecimenCombinedEnumFactory()); // bb 2287 return this.combined; 2288 } 2289 2290 public boolean hasCombinedElement() { 2291 return this.combined != null && !this.combined.isEmpty(); 2292 } 2293 2294 public boolean hasCombined() { 2295 return this.combined != null && !this.combined.isEmpty(); 2296 } 2297 2298 /** 2299 * @param value {@link #combined} (This element signifies if the specimen is part of a group or pooled.). This is the underlying object with id, value and extensions. The accessor "getCombined" gives direct access to the value 2300 */ 2301 public Specimen setCombinedElement(Enumeration<SpecimenCombined> value) { 2302 this.combined = value; 2303 return this; 2304 } 2305 2306 /** 2307 * @return This element signifies if the specimen is part of a group or pooled. 2308 */ 2309 public SpecimenCombined getCombined() { 2310 return this.combined == null ? null : this.combined.getValue(); 2311 } 2312 2313 /** 2314 * @param value This element signifies if the specimen is part of a group or pooled. 2315 */ 2316 public Specimen setCombined(SpecimenCombined value) { 2317 if (value == null) 2318 this.combined = null; 2319 else { 2320 if (this.combined == null) 2321 this.combined = new Enumeration<SpecimenCombined>(new SpecimenCombinedEnumFactory()); 2322 this.combined.setValue(value); 2323 } 2324 return this; 2325 } 2326 2327 /** 2328 * @return {@link #role} (The role or reason for the specimen in the testing workflow.) 2329 */ 2330 public List<CodeableConcept> getRole() { 2331 if (this.role == null) 2332 this.role = new ArrayList<CodeableConcept>(); 2333 return this.role; 2334 } 2335 2336 /** 2337 * @return Returns a reference to <code>this</code> for easy method chaining 2338 */ 2339 public Specimen setRole(List<CodeableConcept> theRole) { 2340 this.role = theRole; 2341 return this; 2342 } 2343 2344 public boolean hasRole() { 2345 if (this.role == null) 2346 return false; 2347 for (CodeableConcept item : this.role) 2348 if (!item.isEmpty()) 2349 return true; 2350 return false; 2351 } 2352 2353 public CodeableConcept addRole() { //3 2354 CodeableConcept t = new CodeableConcept(); 2355 if (this.role == null) 2356 this.role = new ArrayList<CodeableConcept>(); 2357 this.role.add(t); 2358 return t; 2359 } 2360 2361 public Specimen addRole(CodeableConcept t) { //3 2362 if (t == null) 2363 return this; 2364 if (this.role == null) 2365 this.role = new ArrayList<CodeableConcept>(); 2366 this.role.add(t); 2367 return this; 2368 } 2369 2370 /** 2371 * @return The first repetition of repeating field {@link #role}, creating it if it does not already exist {3} 2372 */ 2373 public CodeableConcept getRoleFirstRep() { 2374 if (getRole().isEmpty()) { 2375 addRole(); 2376 } 2377 return getRole().get(0); 2378 } 2379 2380 /** 2381 * @return {@link #feature} (A physical feature or landmark on a specimen, highlighted for context by the collector of the specimen (e.g. surgeon), that identifies the type of feature as well as its meaning (e.g. the red ink indicating the resection margin of the right lobe of the excised prostate tissue or wire loop at radiologically suspected tumor location).) 2382 */ 2383 public List<SpecimenFeatureComponent> getFeature() { 2384 if (this.feature == null) 2385 this.feature = new ArrayList<SpecimenFeatureComponent>(); 2386 return this.feature; 2387 } 2388 2389 /** 2390 * @return Returns a reference to <code>this</code> for easy method chaining 2391 */ 2392 public Specimen setFeature(List<SpecimenFeatureComponent> theFeature) { 2393 this.feature = theFeature; 2394 return this; 2395 } 2396 2397 public boolean hasFeature() { 2398 if (this.feature == null) 2399 return false; 2400 for (SpecimenFeatureComponent item : this.feature) 2401 if (!item.isEmpty()) 2402 return true; 2403 return false; 2404 } 2405 2406 public SpecimenFeatureComponent addFeature() { //3 2407 SpecimenFeatureComponent t = new SpecimenFeatureComponent(); 2408 if (this.feature == null) 2409 this.feature = new ArrayList<SpecimenFeatureComponent>(); 2410 this.feature.add(t); 2411 return t; 2412 } 2413 2414 public Specimen addFeature(SpecimenFeatureComponent t) { //3 2415 if (t == null) 2416 return this; 2417 if (this.feature == null) 2418 this.feature = new ArrayList<SpecimenFeatureComponent>(); 2419 this.feature.add(t); 2420 return this; 2421 } 2422 2423 /** 2424 * @return The first repetition of repeating field {@link #feature}, creating it if it does not already exist {3} 2425 */ 2426 public SpecimenFeatureComponent getFeatureFirstRep() { 2427 if (getFeature().isEmpty()) { 2428 addFeature(); 2429 } 2430 return getFeature().get(0); 2431 } 2432 2433 /** 2434 * @return {@link #collection} (Details concerning the specimen collection.) 2435 */ 2436 public SpecimenCollectionComponent getCollection() { 2437 if (this.collection == null) 2438 if (Configuration.errorOnAutoCreate()) 2439 throw new Error("Attempt to auto-create Specimen.collection"); 2440 else if (Configuration.doAutoCreate()) 2441 this.collection = new SpecimenCollectionComponent(); // cc 2442 return this.collection; 2443 } 2444 2445 public boolean hasCollection() { 2446 return this.collection != null && !this.collection.isEmpty(); 2447 } 2448 2449 /** 2450 * @param value {@link #collection} (Details concerning the specimen collection.) 2451 */ 2452 public Specimen setCollection(SpecimenCollectionComponent value) { 2453 this.collection = value; 2454 return this; 2455 } 2456 2457 /** 2458 * @return {@link #processing} (Details concerning processing and processing steps for the specimen.) 2459 */ 2460 public List<SpecimenProcessingComponent> getProcessing() { 2461 if (this.processing == null) 2462 this.processing = new ArrayList<SpecimenProcessingComponent>(); 2463 return this.processing; 2464 } 2465 2466 /** 2467 * @return Returns a reference to <code>this</code> for easy method chaining 2468 */ 2469 public Specimen setProcessing(List<SpecimenProcessingComponent> theProcessing) { 2470 this.processing = theProcessing; 2471 return this; 2472 } 2473 2474 public boolean hasProcessing() { 2475 if (this.processing == null) 2476 return false; 2477 for (SpecimenProcessingComponent item : this.processing) 2478 if (!item.isEmpty()) 2479 return true; 2480 return false; 2481 } 2482 2483 public SpecimenProcessingComponent addProcessing() { //3 2484 SpecimenProcessingComponent t = new SpecimenProcessingComponent(); 2485 if (this.processing == null) 2486 this.processing = new ArrayList<SpecimenProcessingComponent>(); 2487 this.processing.add(t); 2488 return t; 2489 } 2490 2491 public Specimen addProcessing(SpecimenProcessingComponent t) { //3 2492 if (t == null) 2493 return this; 2494 if (this.processing == null) 2495 this.processing = new ArrayList<SpecimenProcessingComponent>(); 2496 this.processing.add(t); 2497 return this; 2498 } 2499 2500 /** 2501 * @return The first repetition of repeating field {@link #processing}, creating it if it does not already exist {3} 2502 */ 2503 public SpecimenProcessingComponent getProcessingFirstRep() { 2504 if (getProcessing().isEmpty()) { 2505 addProcessing(); 2506 } 2507 return getProcessing().get(0); 2508 } 2509 2510 /** 2511 * @return {@link #container} (The container holding the specimen. The recursive nature of containers; i.e. blood in tube in tray in rack is not addressed here.) 2512 */ 2513 public List<SpecimenContainerComponent> getContainer() { 2514 if (this.container == null) 2515 this.container = new ArrayList<SpecimenContainerComponent>(); 2516 return this.container; 2517 } 2518 2519 /** 2520 * @return Returns a reference to <code>this</code> for easy method chaining 2521 */ 2522 public Specimen setContainer(List<SpecimenContainerComponent> theContainer) { 2523 this.container = theContainer; 2524 return this; 2525 } 2526 2527 public boolean hasContainer() { 2528 if (this.container == null) 2529 return false; 2530 for (SpecimenContainerComponent item : this.container) 2531 if (!item.isEmpty()) 2532 return true; 2533 return false; 2534 } 2535 2536 public SpecimenContainerComponent addContainer() { //3 2537 SpecimenContainerComponent t = new SpecimenContainerComponent(); 2538 if (this.container == null) 2539 this.container = new ArrayList<SpecimenContainerComponent>(); 2540 this.container.add(t); 2541 return t; 2542 } 2543 2544 public Specimen addContainer(SpecimenContainerComponent t) { //3 2545 if (t == null) 2546 return this; 2547 if (this.container == null) 2548 this.container = new ArrayList<SpecimenContainerComponent>(); 2549 this.container.add(t); 2550 return this; 2551 } 2552 2553 /** 2554 * @return The first repetition of repeating field {@link #container}, creating it if it does not already exist {3} 2555 */ 2556 public SpecimenContainerComponent getContainerFirstRep() { 2557 if (getContainer().isEmpty()) { 2558 addContainer(); 2559 } 2560 return getContainer().get(0); 2561 } 2562 2563 /** 2564 * @return {@link #condition} (A mode or state of being that describes the nature of the specimen.) 2565 */ 2566 public List<CodeableConcept> getCondition() { 2567 if (this.condition == null) 2568 this.condition = new ArrayList<CodeableConcept>(); 2569 return this.condition; 2570 } 2571 2572 /** 2573 * @return Returns a reference to <code>this</code> for easy method chaining 2574 */ 2575 public Specimen setCondition(List<CodeableConcept> theCondition) { 2576 this.condition = theCondition; 2577 return this; 2578 } 2579 2580 public boolean hasCondition() { 2581 if (this.condition == null) 2582 return false; 2583 for (CodeableConcept item : this.condition) 2584 if (!item.isEmpty()) 2585 return true; 2586 return false; 2587 } 2588 2589 public CodeableConcept addCondition() { //3 2590 CodeableConcept t = new CodeableConcept(); 2591 if (this.condition == null) 2592 this.condition = new ArrayList<CodeableConcept>(); 2593 this.condition.add(t); 2594 return t; 2595 } 2596 2597 public Specimen addCondition(CodeableConcept t) { //3 2598 if (t == null) 2599 return this; 2600 if (this.condition == null) 2601 this.condition = new ArrayList<CodeableConcept>(); 2602 this.condition.add(t); 2603 return this; 2604 } 2605 2606 /** 2607 * @return The first repetition of repeating field {@link #condition}, creating it if it does not already exist {3} 2608 */ 2609 public CodeableConcept getConditionFirstRep() { 2610 if (getCondition().isEmpty()) { 2611 addCondition(); 2612 } 2613 return getCondition().get(0); 2614 } 2615 2616 /** 2617 * @return {@link #note} (To communicate any details or issues about the specimen or during the specimen collection. (for example: broken vial, sent with patient, frozen).) 2618 */ 2619 public List<Annotation> getNote() { 2620 if (this.note == null) 2621 this.note = new ArrayList<Annotation>(); 2622 return this.note; 2623 } 2624 2625 /** 2626 * @return Returns a reference to <code>this</code> for easy method chaining 2627 */ 2628 public Specimen setNote(List<Annotation> theNote) { 2629 this.note = theNote; 2630 return this; 2631 } 2632 2633 public boolean hasNote() { 2634 if (this.note == null) 2635 return false; 2636 for (Annotation item : this.note) 2637 if (!item.isEmpty()) 2638 return true; 2639 return false; 2640 } 2641 2642 public Annotation addNote() { //3 2643 Annotation t = new Annotation(); 2644 if (this.note == null) 2645 this.note = new ArrayList<Annotation>(); 2646 this.note.add(t); 2647 return t; 2648 } 2649 2650 public Specimen addNote(Annotation t) { //3 2651 if (t == null) 2652 return this; 2653 if (this.note == null) 2654 this.note = new ArrayList<Annotation>(); 2655 this.note.add(t); 2656 return this; 2657 } 2658 2659 /** 2660 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 2661 */ 2662 public Annotation getNoteFirstRep() { 2663 if (getNote().isEmpty()) { 2664 addNote(); 2665 } 2666 return getNote().get(0); 2667 } 2668 2669 protected void listChildren(List<Property> children) { 2670 super.listChildren(children); 2671 children.add(new Property("identifier", "Identifier", "Id for specimen.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2672 children.add(new Property("accessionIdentifier", "Identifier", "The identifier assigned by the lab when accessioning specimen(s). This is not necessarily the same as the specimen identifier, depending on local lab procedures.", 0, 1, accessionIdentifier)); 2673 children.add(new Property("status", "code", "The availability of the specimen.", 0, 1, status)); 2674 children.add(new Property("type", "CodeableConcept", "The kind of material that forms the specimen.", 0, 1, type)); 2675 children.add(new Property("subject", "Reference(Patient|Group|Device|BiologicallyDerivedProduct|Substance|Location)", "Where the specimen came from. This may be from patient(s), from a location (e.g., the source of an environmental sample), or a sampling of a substance, a biologically-derived product, or a device.", 0, 1, subject)); 2676 children.add(new Property("receivedTime", "dateTime", "Time when specimen is received by the testing laboratory for processing or testing.", 0, 1, receivedTime)); 2677 children.add(new Property("parent", "Reference(Specimen)", "Reference to the parent (source) specimen which is used when the specimen was either derived from or a component of another specimen.", 0, java.lang.Integer.MAX_VALUE, parent)); 2678 children.add(new Property("request", "Reference(ServiceRequest)", "Details concerning a service request that required a specimen to be collected.", 0, java.lang.Integer.MAX_VALUE, request)); 2679 children.add(new Property("combined", "code", "This element signifies if the specimen is part of a group or pooled.", 0, 1, combined)); 2680 children.add(new Property("role", "CodeableConcept", "The role or reason for the specimen in the testing workflow.", 0, java.lang.Integer.MAX_VALUE, role)); 2681 children.add(new Property("feature", "", "A physical feature or landmark on a specimen, highlighted for context by the collector of the specimen (e.g. surgeon), that identifies the type of feature as well as its meaning (e.g. the red ink indicating the resection margin of the right lobe of the excised prostate tissue or wire loop at radiologically suspected tumor location).", 0, java.lang.Integer.MAX_VALUE, feature)); 2682 children.add(new Property("collection", "", "Details concerning the specimen collection.", 0, 1, collection)); 2683 children.add(new Property("processing", "", "Details concerning processing and processing steps for the specimen.", 0, java.lang.Integer.MAX_VALUE, processing)); 2684 children.add(new Property("container", "", "The container holding the specimen. The recursive nature of containers; i.e. blood in tube in tray in rack is not addressed here.", 0, java.lang.Integer.MAX_VALUE, container)); 2685 children.add(new Property("condition", "CodeableConcept", "A mode or state of being that describes the nature of the specimen.", 0, java.lang.Integer.MAX_VALUE, condition)); 2686 children.add(new Property("note", "Annotation", "To communicate any details or issues about the specimen or during the specimen collection. (for example: broken vial, sent with patient, frozen).", 0, java.lang.Integer.MAX_VALUE, note)); 2687 } 2688 2689 @Override 2690 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2691 switch (_hash) { 2692 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Id for specimen.", 0, java.lang.Integer.MAX_VALUE, identifier); 2693 case 818734061: /*accessionIdentifier*/ return new Property("accessionIdentifier", "Identifier", "The identifier assigned by the lab when accessioning specimen(s). This is not necessarily the same as the specimen identifier, depending on local lab procedures.", 0, 1, accessionIdentifier); 2694 case -892481550: /*status*/ return new Property("status", "code", "The availability of the specimen.", 0, 1, status); 2695 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The kind of material that forms the specimen.", 0, 1, type); 2696 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Device|BiologicallyDerivedProduct|Substance|Location)", "Where the specimen came from. This may be from patient(s), from a location (e.g., the source of an environmental sample), or a sampling of a substance, a biologically-derived product, or a device.", 0, 1, subject); 2697 case -767961010: /*receivedTime*/ return new Property("receivedTime", "dateTime", "Time when specimen is received by the testing laboratory for processing or testing.", 0, 1, receivedTime); 2698 case -995424086: /*parent*/ return new Property("parent", "Reference(Specimen)", "Reference to the parent (source) specimen which is used when the specimen was either derived from or a component of another specimen.", 0, java.lang.Integer.MAX_VALUE, parent); 2699 case 1095692943: /*request*/ return new Property("request", "Reference(ServiceRequest)", "Details concerning a service request that required a specimen to be collected.", 0, java.lang.Integer.MAX_VALUE, request); 2700 case -612455675: /*combined*/ return new Property("combined", "code", "This element signifies if the specimen is part of a group or pooled.", 0, 1, combined); 2701 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The role or reason for the specimen in the testing workflow.", 0, java.lang.Integer.MAX_VALUE, role); 2702 case -979207434: /*feature*/ return new Property("feature", "", "A physical feature or landmark on a specimen, highlighted for context by the collector of the specimen (e.g. surgeon), that identifies the type of feature as well as its meaning (e.g. the red ink indicating the resection margin of the right lobe of the excised prostate tissue or wire loop at radiologically suspected tumor location).", 0, java.lang.Integer.MAX_VALUE, feature); 2703 case -1741312354: /*collection*/ return new Property("collection", "", "Details concerning the specimen collection.", 0, 1, collection); 2704 case 422194963: /*processing*/ return new Property("processing", "", "Details concerning processing and processing steps for the specimen.", 0, java.lang.Integer.MAX_VALUE, processing); 2705 case -410956671: /*container*/ return new Property("container", "", "The container holding the specimen. The recursive nature of containers; i.e. blood in tube in tray in rack is not addressed here.", 0, java.lang.Integer.MAX_VALUE, container); 2706 case -861311717: /*condition*/ return new Property("condition", "CodeableConcept", "A mode or state of being that describes the nature of the specimen.", 0, java.lang.Integer.MAX_VALUE, condition); 2707 case 3387378: /*note*/ return new Property("note", "Annotation", "To communicate any details or issues about the specimen or during the specimen collection. (for example: broken vial, sent with patient, frozen).", 0, java.lang.Integer.MAX_VALUE, note); 2708 default: return super.getNamedProperty(_hash, _name, _checkValid); 2709 } 2710 2711 } 2712 2713 @Override 2714 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2715 switch (hash) { 2716 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2717 case 818734061: /*accessionIdentifier*/ return this.accessionIdentifier == null ? new Base[0] : new Base[] {this.accessionIdentifier}; // Identifier 2718 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<SpecimenStatus> 2719 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2720 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2721 case -767961010: /*receivedTime*/ return this.receivedTime == null ? new Base[0] : new Base[] {this.receivedTime}; // DateTimeType 2722 case -995424086: /*parent*/ return this.parent == null ? new Base[0] : this.parent.toArray(new Base[this.parent.size()]); // Reference 2723 case 1095692943: /*request*/ return this.request == null ? new Base[0] : this.request.toArray(new Base[this.request.size()]); // Reference 2724 case -612455675: /*combined*/ return this.combined == null ? new Base[0] : new Base[] {this.combined}; // Enumeration<SpecimenCombined> 2725 case 3506294: /*role*/ return this.role == null ? new Base[0] : this.role.toArray(new Base[this.role.size()]); // CodeableConcept 2726 case -979207434: /*feature*/ return this.feature == null ? new Base[0] : this.feature.toArray(new Base[this.feature.size()]); // SpecimenFeatureComponent 2727 case -1741312354: /*collection*/ return this.collection == null ? new Base[0] : new Base[] {this.collection}; // SpecimenCollectionComponent 2728 case 422194963: /*processing*/ return this.processing == null ? new Base[0] : this.processing.toArray(new Base[this.processing.size()]); // SpecimenProcessingComponent 2729 case -410956671: /*container*/ return this.container == null ? new Base[0] : this.container.toArray(new Base[this.container.size()]); // SpecimenContainerComponent 2730 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : this.condition.toArray(new Base[this.condition.size()]); // CodeableConcept 2731 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2732 default: return super.getProperty(hash, name, checkValid); 2733 } 2734 2735 } 2736 2737 @Override 2738 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2739 switch (hash) { 2740 case -1618432855: // identifier 2741 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2742 return value; 2743 case 818734061: // accessionIdentifier 2744 this.accessionIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 2745 return value; 2746 case -892481550: // status 2747 value = new SpecimenStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2748 this.status = (Enumeration) value; // Enumeration<SpecimenStatus> 2749 return value; 2750 case 3575610: // type 2751 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2752 return value; 2753 case -1867885268: // subject 2754 this.subject = TypeConvertor.castToReference(value); // Reference 2755 return value; 2756 case -767961010: // receivedTime 2757 this.receivedTime = TypeConvertor.castToDateTime(value); // DateTimeType 2758 return value; 2759 case -995424086: // parent 2760 this.getParent().add(TypeConvertor.castToReference(value)); // Reference 2761 return value; 2762 case 1095692943: // request 2763 this.getRequest().add(TypeConvertor.castToReference(value)); // Reference 2764 return value; 2765 case -612455675: // combined 2766 value = new SpecimenCombinedEnumFactory().fromType(TypeConvertor.castToCode(value)); 2767 this.combined = (Enumeration) value; // Enumeration<SpecimenCombined> 2768 return value; 2769 case 3506294: // role 2770 this.getRole().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2771 return value; 2772 case -979207434: // feature 2773 this.getFeature().add((SpecimenFeatureComponent) value); // SpecimenFeatureComponent 2774 return value; 2775 case -1741312354: // collection 2776 this.collection = (SpecimenCollectionComponent) value; // SpecimenCollectionComponent 2777 return value; 2778 case 422194963: // processing 2779 this.getProcessing().add((SpecimenProcessingComponent) value); // SpecimenProcessingComponent 2780 return value; 2781 case -410956671: // container 2782 this.getContainer().add((SpecimenContainerComponent) value); // SpecimenContainerComponent 2783 return value; 2784 case -861311717: // condition 2785 this.getCondition().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2786 return value; 2787 case 3387378: // note 2788 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 2789 return value; 2790 default: return super.setProperty(hash, name, value); 2791 } 2792 2793 } 2794 2795 @Override 2796 public Base setProperty(String name, Base value) throws FHIRException { 2797 if (name.equals("identifier")) { 2798 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2799 } else if (name.equals("accessionIdentifier")) { 2800 this.accessionIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 2801 } else if (name.equals("status")) { 2802 value = new SpecimenStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2803 this.status = (Enumeration) value; // Enumeration<SpecimenStatus> 2804 } else if (name.equals("type")) { 2805 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2806 } else if (name.equals("subject")) { 2807 this.subject = TypeConvertor.castToReference(value); // Reference 2808 } else if (name.equals("receivedTime")) { 2809 this.receivedTime = TypeConvertor.castToDateTime(value); // DateTimeType 2810 } else if (name.equals("parent")) { 2811 this.getParent().add(TypeConvertor.castToReference(value)); 2812 } else if (name.equals("request")) { 2813 this.getRequest().add(TypeConvertor.castToReference(value)); 2814 } else if (name.equals("combined")) { 2815 value = new SpecimenCombinedEnumFactory().fromType(TypeConvertor.castToCode(value)); 2816 this.combined = (Enumeration) value; // Enumeration<SpecimenCombined> 2817 } else if (name.equals("role")) { 2818 this.getRole().add(TypeConvertor.castToCodeableConcept(value)); 2819 } else if (name.equals("feature")) { 2820 this.getFeature().add((SpecimenFeatureComponent) value); 2821 } else if (name.equals("collection")) { 2822 this.collection = (SpecimenCollectionComponent) value; // SpecimenCollectionComponent 2823 } else if (name.equals("processing")) { 2824 this.getProcessing().add((SpecimenProcessingComponent) value); 2825 } else if (name.equals("container")) { 2826 this.getContainer().add((SpecimenContainerComponent) value); 2827 } else if (name.equals("condition")) { 2828 this.getCondition().add(TypeConvertor.castToCodeableConcept(value)); 2829 } else if (name.equals("note")) { 2830 this.getNote().add(TypeConvertor.castToAnnotation(value)); 2831 } else 2832 return super.setProperty(name, value); 2833 return value; 2834 } 2835 2836 @Override 2837 public void removeChild(String name, Base value) throws FHIRException { 2838 if (name.equals("identifier")) { 2839 this.getIdentifier().remove(value); 2840 } else if (name.equals("accessionIdentifier")) { 2841 this.accessionIdentifier = null; 2842 } else if (name.equals("status")) { 2843 value = new SpecimenStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2844 this.status = (Enumeration) value; // Enumeration<SpecimenStatus> 2845 } else if (name.equals("type")) { 2846 this.type = null; 2847 } else if (name.equals("subject")) { 2848 this.subject = null; 2849 } else if (name.equals("receivedTime")) { 2850 this.receivedTime = null; 2851 } else if (name.equals("parent")) { 2852 this.getParent().remove(value); 2853 } else if (name.equals("request")) { 2854 this.getRequest().remove(value); 2855 } else if (name.equals("combined")) { 2856 value = new SpecimenCombinedEnumFactory().fromType(TypeConvertor.castToCode(value)); 2857 this.combined = (Enumeration) value; // Enumeration<SpecimenCombined> 2858 } else if (name.equals("role")) { 2859 this.getRole().remove(value); 2860 } else if (name.equals("feature")) { 2861 this.getFeature().remove((SpecimenFeatureComponent) value); 2862 } else if (name.equals("collection")) { 2863 this.collection = (SpecimenCollectionComponent) value; // SpecimenCollectionComponent 2864 } else if (name.equals("processing")) { 2865 this.getProcessing().remove((SpecimenProcessingComponent) value); 2866 } else if (name.equals("container")) { 2867 this.getContainer().remove((SpecimenContainerComponent) value); 2868 } else if (name.equals("condition")) { 2869 this.getCondition().remove(value); 2870 } else if (name.equals("note")) { 2871 this.getNote().remove(value); 2872 } else 2873 super.removeChild(name, value); 2874 2875 } 2876 2877 @Override 2878 public Base makeProperty(int hash, String name) throws FHIRException { 2879 switch (hash) { 2880 case -1618432855: return addIdentifier(); 2881 case 818734061: return getAccessionIdentifier(); 2882 case -892481550: return getStatusElement(); 2883 case 3575610: return getType(); 2884 case -1867885268: return getSubject(); 2885 case -767961010: return getReceivedTimeElement(); 2886 case -995424086: return addParent(); 2887 case 1095692943: return addRequest(); 2888 case -612455675: return getCombinedElement(); 2889 case 3506294: return addRole(); 2890 case -979207434: return addFeature(); 2891 case -1741312354: return getCollection(); 2892 case 422194963: return addProcessing(); 2893 case -410956671: return addContainer(); 2894 case -861311717: return addCondition(); 2895 case 3387378: return addNote(); 2896 default: return super.makeProperty(hash, name); 2897 } 2898 2899 } 2900 2901 @Override 2902 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2903 switch (hash) { 2904 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2905 case 818734061: /*accessionIdentifier*/ return new String[] {"Identifier"}; 2906 case -892481550: /*status*/ return new String[] {"code"}; 2907 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2908 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2909 case -767961010: /*receivedTime*/ return new String[] {"dateTime"}; 2910 case -995424086: /*parent*/ return new String[] {"Reference"}; 2911 case 1095692943: /*request*/ return new String[] {"Reference"}; 2912 case -612455675: /*combined*/ return new String[] {"code"}; 2913 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 2914 case -979207434: /*feature*/ return new String[] {}; 2915 case -1741312354: /*collection*/ return new String[] {}; 2916 case 422194963: /*processing*/ return new String[] {}; 2917 case -410956671: /*container*/ return new String[] {}; 2918 case -861311717: /*condition*/ return new String[] {"CodeableConcept"}; 2919 case 3387378: /*note*/ return new String[] {"Annotation"}; 2920 default: return super.getTypesForProperty(hash, name); 2921 } 2922 2923 } 2924 2925 @Override 2926 public Base addChild(String name) throws FHIRException { 2927 if (name.equals("identifier")) { 2928 return addIdentifier(); 2929 } 2930 else if (name.equals("accessionIdentifier")) { 2931 this.accessionIdentifier = new Identifier(); 2932 return this.accessionIdentifier; 2933 } 2934 else if (name.equals("status")) { 2935 throw new FHIRException("Cannot call addChild on a singleton property Specimen.status"); 2936 } 2937 else if (name.equals("type")) { 2938 this.type = new CodeableConcept(); 2939 return this.type; 2940 } 2941 else if (name.equals("subject")) { 2942 this.subject = new Reference(); 2943 return this.subject; 2944 } 2945 else if (name.equals("receivedTime")) { 2946 throw new FHIRException("Cannot call addChild on a singleton property Specimen.receivedTime"); 2947 } 2948 else if (name.equals("parent")) { 2949 return addParent(); 2950 } 2951 else if (name.equals("request")) { 2952 return addRequest(); 2953 } 2954 else if (name.equals("combined")) { 2955 throw new FHIRException("Cannot call addChild on a singleton property Specimen.combined"); 2956 } 2957 else if (name.equals("role")) { 2958 return addRole(); 2959 } 2960 else if (name.equals("feature")) { 2961 return addFeature(); 2962 } 2963 else if (name.equals("collection")) { 2964 this.collection = new SpecimenCollectionComponent(); 2965 return this.collection; 2966 } 2967 else if (name.equals("processing")) { 2968 return addProcessing(); 2969 } 2970 else if (name.equals("container")) { 2971 return addContainer(); 2972 } 2973 else if (name.equals("condition")) { 2974 return addCondition(); 2975 } 2976 else if (name.equals("note")) { 2977 return addNote(); 2978 } 2979 else 2980 return super.addChild(name); 2981 } 2982 2983 public String fhirType() { 2984 return "Specimen"; 2985 2986 } 2987 2988 public Specimen copy() { 2989 Specimen dst = new Specimen(); 2990 copyValues(dst); 2991 return dst; 2992 } 2993 2994 public void copyValues(Specimen dst) { 2995 super.copyValues(dst); 2996 if (identifier != null) { 2997 dst.identifier = new ArrayList<Identifier>(); 2998 for (Identifier i : identifier) 2999 dst.identifier.add(i.copy()); 3000 }; 3001 dst.accessionIdentifier = accessionIdentifier == null ? null : accessionIdentifier.copy(); 3002 dst.status = status == null ? null : status.copy(); 3003 dst.type = type == null ? null : type.copy(); 3004 dst.subject = subject == null ? null : subject.copy(); 3005 dst.receivedTime = receivedTime == null ? null : receivedTime.copy(); 3006 if (parent != null) { 3007 dst.parent = new ArrayList<Reference>(); 3008 for (Reference i : parent) 3009 dst.parent.add(i.copy()); 3010 }; 3011 if (request != null) { 3012 dst.request = new ArrayList<Reference>(); 3013 for (Reference i : request) 3014 dst.request.add(i.copy()); 3015 }; 3016 dst.combined = combined == null ? null : combined.copy(); 3017 if (role != null) { 3018 dst.role = new ArrayList<CodeableConcept>(); 3019 for (CodeableConcept i : role) 3020 dst.role.add(i.copy()); 3021 }; 3022 if (feature != null) { 3023 dst.feature = new ArrayList<SpecimenFeatureComponent>(); 3024 for (SpecimenFeatureComponent i : feature) 3025 dst.feature.add(i.copy()); 3026 }; 3027 dst.collection = collection == null ? null : collection.copy(); 3028 if (processing != null) { 3029 dst.processing = new ArrayList<SpecimenProcessingComponent>(); 3030 for (SpecimenProcessingComponent i : processing) 3031 dst.processing.add(i.copy()); 3032 }; 3033 if (container != null) { 3034 dst.container = new ArrayList<SpecimenContainerComponent>(); 3035 for (SpecimenContainerComponent i : container) 3036 dst.container.add(i.copy()); 3037 }; 3038 if (condition != null) { 3039 dst.condition = new ArrayList<CodeableConcept>(); 3040 for (CodeableConcept i : condition) 3041 dst.condition.add(i.copy()); 3042 }; 3043 if (note != null) { 3044 dst.note = new ArrayList<Annotation>(); 3045 for (Annotation i : note) 3046 dst.note.add(i.copy()); 3047 }; 3048 } 3049 3050 protected Specimen typedCopy() { 3051 return copy(); 3052 } 3053 3054 @Override 3055 public boolean equalsDeep(Base other_) { 3056 if (!super.equalsDeep(other_)) 3057 return false; 3058 if (!(other_ instanceof Specimen)) 3059 return false; 3060 Specimen o = (Specimen) other_; 3061 return compareDeep(identifier, o.identifier, true) && compareDeep(accessionIdentifier, o.accessionIdentifier, true) 3062 && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) && compareDeep(subject, o.subject, true) 3063 && compareDeep(receivedTime, o.receivedTime, true) && compareDeep(parent, o.parent, true) && compareDeep(request, o.request, true) 3064 && compareDeep(combined, o.combined, true) && compareDeep(role, o.role, true) && compareDeep(feature, o.feature, true) 3065 && compareDeep(collection, o.collection, true) && compareDeep(processing, o.processing, true) && compareDeep(container, o.container, true) 3066 && compareDeep(condition, o.condition, true) && compareDeep(note, o.note, true); 3067 } 3068 3069 @Override 3070 public boolean equalsShallow(Base other_) { 3071 if (!super.equalsShallow(other_)) 3072 return false; 3073 if (!(other_ instanceof Specimen)) 3074 return false; 3075 Specimen o = (Specimen) other_; 3076 return compareValues(status, o.status, true) && compareValues(receivedTime, o.receivedTime, true) && compareValues(combined, o.combined, true) 3077 ; 3078 } 3079 3080 public boolean isEmpty() { 3081 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, accessionIdentifier 3082 , status, type, subject, receivedTime, parent, request, combined, role, feature 3083 , collection, processing, container, condition, note); 3084 } 3085 3086 @Override 3087 public ResourceType getResourceType() { 3088 return ResourceType.Specimen; 3089 } 3090 3091 /** 3092 * Search parameter: <b>accession</b> 3093 * <p> 3094 * Description: <b>The accession number associated with the specimen</b><br> 3095 * Type: <b>token</b><br> 3096 * Path: <b>Specimen.accessionIdentifier</b><br> 3097 * </p> 3098 */ 3099 @SearchParamDefinition(name="accession", path="Specimen.accessionIdentifier", description="The accession number associated with the specimen", type="token" ) 3100 public static final String SP_ACCESSION = "accession"; 3101 /** 3102 * <b>Fluent Client</b> search parameter constant for <b>accession</b> 3103 * <p> 3104 * Description: <b>The accession number associated with the specimen</b><br> 3105 * Type: <b>token</b><br> 3106 * Path: <b>Specimen.accessionIdentifier</b><br> 3107 * </p> 3108 */ 3109 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACCESSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACCESSION); 3110 3111 /** 3112 * Search parameter: <b>bodysite</b> 3113 * <p> 3114 * Description: <b>Reference to a resource (by instance)</b><br> 3115 * Type: <b>reference</b><br> 3116 * Path: <b>Specimen.collection.bodySite.reference</b><br> 3117 * </p> 3118 */ 3119 @SearchParamDefinition(name="bodysite", path="Specimen.collection.bodySite.reference", description="Reference to a resource (by instance)", type="reference", target={BodyStructure.class } ) 3120 public static final String SP_BODYSITE = "bodysite"; 3121 /** 3122 * <b>Fluent Client</b> search parameter constant for <b>bodysite</b> 3123 * <p> 3124 * Description: <b>Reference to a resource (by instance)</b><br> 3125 * Type: <b>reference</b><br> 3126 * Path: <b>Specimen.collection.bodySite.reference</b><br> 3127 * </p> 3128 */ 3129 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BODYSITE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BODYSITE); 3130 3131/** 3132 * Constant for fluent queries to be used to add include statements. Specifies 3133 * the path value of "<b>Specimen:bodysite</b>". 3134 */ 3135 public static final ca.uhn.fhir.model.api.Include INCLUDE_BODYSITE = new ca.uhn.fhir.model.api.Include("Specimen:bodysite").toLocked(); 3136 3137 /** 3138 * Search parameter: <b>collected</b> 3139 * <p> 3140 * Description: <b>The date the specimen was collected</b><br> 3141 * Type: <b>date</b><br> 3142 * Path: <b>Specimen.collection.collected.ofType(dateTime) | Specimen.collection.collected.ofType(Period)</b><br> 3143 * </p> 3144 */ 3145 @SearchParamDefinition(name="collected", path="Specimen.collection.collected.ofType(dateTime) | Specimen.collection.collected.ofType(Period)", description="The date the specimen was collected", type="date" ) 3146 public static final String SP_COLLECTED = "collected"; 3147 /** 3148 * <b>Fluent Client</b> search parameter constant for <b>collected</b> 3149 * <p> 3150 * Description: <b>The date the specimen was collected</b><br> 3151 * Type: <b>date</b><br> 3152 * Path: <b>Specimen.collection.collected.ofType(dateTime) | Specimen.collection.collected.ofType(Period)</b><br> 3153 * </p> 3154 */ 3155 public static final ca.uhn.fhir.rest.gclient.DateClientParam COLLECTED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_COLLECTED); 3156 3157 /** 3158 * Search parameter: <b>collector</b> 3159 * <p> 3160 * Description: <b>Who collected the specimen</b><br> 3161 * Type: <b>reference</b><br> 3162 * Path: <b>Specimen.collection.collector</b><br> 3163 * </p> 3164 */ 3165 @SearchParamDefinition(name="collector", path="Specimen.collection.collector", description="Who collected the specimen", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 3166 public static final String SP_COLLECTOR = "collector"; 3167 /** 3168 * <b>Fluent Client</b> search parameter constant for <b>collector</b> 3169 * <p> 3170 * Description: <b>Who collected the specimen</b><br> 3171 * Type: <b>reference</b><br> 3172 * Path: <b>Specimen.collection.collector</b><br> 3173 * </p> 3174 */ 3175 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COLLECTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COLLECTOR); 3176 3177/** 3178 * Constant for fluent queries to be used to add include statements. Specifies 3179 * the path value of "<b>Specimen:collector</b>". 3180 */ 3181 public static final ca.uhn.fhir.model.api.Include INCLUDE_COLLECTOR = new ca.uhn.fhir.model.api.Include("Specimen:collector").toLocked(); 3182 3183 /** 3184 * Search parameter: <b>container-device</b> 3185 * <p> 3186 * Description: <b>The unique identifier associated with the specimen container</b><br> 3187 * Type: <b>reference</b><br> 3188 * Path: <b>Specimen.container.device.where(resolve() is Device)</b><br> 3189 * </p> 3190 */ 3191 @SearchParamDefinition(name="container-device", path="Specimen.container.device.where(resolve() is Device)", description="The unique identifier associated with the specimen container", type="reference", target={Device.class } ) 3192 public static final String SP_CONTAINER_DEVICE = "container-device"; 3193 /** 3194 * <b>Fluent Client</b> search parameter constant for <b>container-device</b> 3195 * <p> 3196 * Description: <b>The unique identifier associated with the specimen container</b><br> 3197 * Type: <b>reference</b><br> 3198 * Path: <b>Specimen.container.device.where(resolve() is Device)</b><br> 3199 * </p> 3200 */ 3201 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTAINER_DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTAINER_DEVICE); 3202 3203/** 3204 * Constant for fluent queries to be used to add include statements. Specifies 3205 * the path value of "<b>Specimen:container-device</b>". 3206 */ 3207 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTAINER_DEVICE = new ca.uhn.fhir.model.api.Include("Specimen:container-device").toLocked(); 3208 3209 /** 3210 * Search parameter: <b>parent</b> 3211 * <p> 3212 * Description: <b>The parent of the specimen</b><br> 3213 * Type: <b>reference</b><br> 3214 * Path: <b>Specimen.parent</b><br> 3215 * </p> 3216 */ 3217 @SearchParamDefinition(name="parent", path="Specimen.parent", description="The parent of the specimen", type="reference", target={Specimen.class } ) 3218 public static final String SP_PARENT = "parent"; 3219 /** 3220 * <b>Fluent Client</b> search parameter constant for <b>parent</b> 3221 * <p> 3222 * Description: <b>The parent of the specimen</b><br> 3223 * Type: <b>reference</b><br> 3224 * Path: <b>Specimen.parent</b><br> 3225 * </p> 3226 */ 3227 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARENT); 3228 3229/** 3230 * Constant for fluent queries to be used to add include statements. Specifies 3231 * the path value of "<b>Specimen:parent</b>". 3232 */ 3233 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARENT = new ca.uhn.fhir.model.api.Include("Specimen:parent").toLocked(); 3234 3235 /** 3236 * Search parameter: <b>procedure</b> 3237 * <p> 3238 * Description: <b>The procedure that collected the specimen</b><br> 3239 * Type: <b>reference</b><br> 3240 * Path: <b>Specimen.collection.procedure</b><br> 3241 * </p> 3242 */ 3243 @SearchParamDefinition(name="procedure", path="Specimen.collection.procedure", description="The procedure that collected the specimen", type="reference", target={Procedure.class } ) 3244 public static final String SP_PROCEDURE = "procedure"; 3245 /** 3246 * <b>Fluent Client</b> search parameter constant for <b>procedure</b> 3247 * <p> 3248 * Description: <b>The procedure that collected the specimen</b><br> 3249 * Type: <b>reference</b><br> 3250 * Path: <b>Specimen.collection.procedure</b><br> 3251 * </p> 3252 */ 3253 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROCEDURE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROCEDURE); 3254 3255/** 3256 * Constant for fluent queries to be used to add include statements. Specifies 3257 * the path value of "<b>Specimen:procedure</b>". 3258 */ 3259 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROCEDURE = new ca.uhn.fhir.model.api.Include("Specimen:procedure").toLocked(); 3260 3261 /** 3262 * Search parameter: <b>status</b> 3263 * <p> 3264 * Description: <b>available | unavailable | unsatisfactory | entered-in-error</b><br> 3265 * Type: <b>token</b><br> 3266 * Path: <b>Specimen.status</b><br> 3267 * </p> 3268 */ 3269 @SearchParamDefinition(name="status", path="Specimen.status", description="available | unavailable | unsatisfactory | entered-in-error", type="token" ) 3270 public static final String SP_STATUS = "status"; 3271 /** 3272 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3273 * <p> 3274 * Description: <b>available | unavailable | unsatisfactory | entered-in-error</b><br> 3275 * Type: <b>token</b><br> 3276 * Path: <b>Specimen.status</b><br> 3277 * </p> 3278 */ 3279 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3280 3281 /** 3282 * Search parameter: <b>subject</b> 3283 * <p> 3284 * Description: <b>The subject of the specimen</b><br> 3285 * Type: <b>reference</b><br> 3286 * Path: <b>Specimen.subject</b><br> 3287 * </p> 3288 */ 3289 @SearchParamDefinition(name="subject", path="Specimen.subject", description="The subject of the specimen", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={BiologicallyDerivedProduct.class, Device.class, Group.class, Location.class, Patient.class, Substance.class } ) 3290 public static final String SP_SUBJECT = "subject"; 3291 /** 3292 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3293 * <p> 3294 * Description: <b>The subject of the specimen</b><br> 3295 * Type: <b>reference</b><br> 3296 * Path: <b>Specimen.subject</b><br> 3297 * </p> 3298 */ 3299 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 3300 3301/** 3302 * Constant for fluent queries to be used to add include statements. Specifies 3303 * the path value of "<b>Specimen:subject</b>". 3304 */ 3305 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Specimen:subject").toLocked(); 3306 3307 /** 3308 * Search parameter: <b>identifier</b> 3309 * <p> 3310 * Description: <b>Multiple Resources: 3311 3312* [Account](account.html): Account number 3313* [AdverseEvent](adverseevent.html): Business identifier for the event 3314* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3315* [Appointment](appointment.html): An Identifier of the Appointment 3316* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3317* [Basic](basic.html): Business identifier 3318* [BodyStructure](bodystructure.html): Bodystructure identifier 3319* [CarePlan](careplan.html): External Ids for this plan 3320* [CareTeam](careteam.html): External Ids for this team 3321* [ChargeItem](chargeitem.html): Business Identifier for item 3322* [Claim](claim.html): The primary identifier of the financial resource 3323* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3324* [ClinicalImpression](clinicalimpression.html): Business identifier 3325* [Communication](communication.html): Unique identifier 3326* [CommunicationRequest](communicationrequest.html): Unique identifier 3327* [Composition](composition.html): Version-independent identifier for the Composition 3328* [Condition](condition.html): A unique identifier of the condition record 3329* [Consent](consent.html): Identifier for this record (external references) 3330* [Contract](contract.html): The identity of the contract 3331* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3332* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3333* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3334* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3335* [DeviceRequest](devicerequest.html): Business identifier for request/order 3336* [DeviceUsage](deviceusage.html): Search by identifier 3337* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3338* [DocumentReference](documentreference.html): Identifier of the attachment binary 3339* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3340* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3341* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3342* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3343* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3344* [Flag](flag.html): Business identifier 3345* [Goal](goal.html): External Ids for this goal 3346* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3347* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3348* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3349* [Immunization](immunization.html): Business identifier 3350* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3351* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3352* [Invoice](invoice.html): Business Identifier for item 3353* [List](list.html): Business identifier 3354* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3355* [Medication](medication.html): Returns medications with this external identifier 3356* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3357* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3358* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3359* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3360* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3361* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3362* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3363* [Observation](observation.html): The unique id for a particular observation 3364* [Person](person.html): A person Identifier 3365* [Procedure](procedure.html): A unique identifier for a procedure 3366* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3367* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3368* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3369* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3370* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3371* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3372* [Specimen](specimen.html): The unique identifier associated with the specimen 3373* [SupplyDelivery](supplydelivery.html): External identifier 3374* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3375* [Task](task.html): Search for a task instance by its business identifier 3376* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3377</b><br> 3378 * Type: <b>token</b><br> 3379 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3380 * </p> 3381 */ 3382 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 3383 public static final String SP_IDENTIFIER = "identifier"; 3384 /** 3385 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3386 * <p> 3387 * Description: <b>Multiple Resources: 3388 3389* [Account](account.html): Account number 3390* [AdverseEvent](adverseevent.html): Business identifier for the event 3391* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3392* [Appointment](appointment.html): An Identifier of the Appointment 3393* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3394* [Basic](basic.html): Business identifier 3395* [BodyStructure](bodystructure.html): Bodystructure identifier 3396* [CarePlan](careplan.html): External Ids for this plan 3397* [CareTeam](careteam.html): External Ids for this team 3398* [ChargeItem](chargeitem.html): Business Identifier for item 3399* [Claim](claim.html): The primary identifier of the financial resource 3400* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3401* [ClinicalImpression](clinicalimpression.html): Business identifier 3402* [Communication](communication.html): Unique identifier 3403* [CommunicationRequest](communicationrequest.html): Unique identifier 3404* [Composition](composition.html): Version-independent identifier for the Composition 3405* [Condition](condition.html): A unique identifier of the condition record 3406* [Consent](consent.html): Identifier for this record (external references) 3407* [Contract](contract.html): The identity of the contract 3408* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3409* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3410* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3411* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3412* [DeviceRequest](devicerequest.html): Business identifier for request/order 3413* [DeviceUsage](deviceusage.html): Search by identifier 3414* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3415* [DocumentReference](documentreference.html): Identifier of the attachment binary 3416* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3417* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3418* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3419* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3420* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3421* [Flag](flag.html): Business identifier 3422* [Goal](goal.html): External Ids for this goal 3423* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3424* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3425* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3426* [Immunization](immunization.html): Business identifier 3427* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3428* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3429* [Invoice](invoice.html): Business Identifier for item 3430* [List](list.html): Business identifier 3431* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3432* [Medication](medication.html): Returns medications with this external identifier 3433* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3434* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3435* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3436* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3437* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3438* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3439* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3440* [Observation](observation.html): The unique id for a particular observation 3441* [Person](person.html): A person Identifier 3442* [Procedure](procedure.html): A unique identifier for a procedure 3443* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3444* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3445* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3446* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3447* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3448* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3449* [Specimen](specimen.html): The unique identifier associated with the specimen 3450* [SupplyDelivery](supplydelivery.html): External identifier 3451* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3452* [Task](task.html): Search for a task instance by its business identifier 3453* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3454</b><br> 3455 * Type: <b>token</b><br> 3456 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3457 * </p> 3458 */ 3459 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3460 3461 /** 3462 * Search parameter: <b>patient</b> 3463 * <p> 3464 * Description: <b>Multiple Resources: 3465 3466* [Account](account.html): The entity that caused the expenses 3467* [AdverseEvent](adverseevent.html): Subject impacted by event 3468* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3469* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3470* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3471* [AuditEvent](auditevent.html): Where the activity involved patient data 3472* [Basic](basic.html): Identifies the focus of this resource 3473* [BodyStructure](bodystructure.html): Who this is about 3474* [CarePlan](careplan.html): Who the care plan is for 3475* [CareTeam](careteam.html): Who care team is for 3476* [ChargeItem](chargeitem.html): Individual service was done for/to 3477* [Claim](claim.html): Patient receiving the products or services 3478* [ClaimResponse](claimresponse.html): The subject of care 3479* [ClinicalImpression](clinicalimpression.html): Patient assessed 3480* [Communication](communication.html): Focus of message 3481* [CommunicationRequest](communicationrequest.html): Focus of message 3482* [Composition](composition.html): Who and/or what the composition is about 3483* [Condition](condition.html): Who has the condition? 3484* [Consent](consent.html): Who the consent applies to 3485* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3486* [Coverage](coverage.html): Retrieve coverages for a patient 3487* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3488* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3489* [DetectedIssue](detectedissue.html): Associated patient 3490* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3491* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3492* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3493* [DocumentReference](documentreference.html): Who/what is the subject of the document 3494* [Encounter](encounter.html): The patient present at the encounter 3495* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3496* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3497* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3498* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3499* [Flag](flag.html): The identity of a subject to list flags for 3500* [Goal](goal.html): Who this goal is intended for 3501* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3502* [ImagingSelection](imagingselection.html): Who the study is about 3503* [ImagingStudy](imagingstudy.html): Who the study is about 3504* [Immunization](immunization.html): The patient for the vaccination record 3505* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3506* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3507* [Invoice](invoice.html): Recipient(s) of goods and services 3508* [List](list.html): If all resources have the same subject 3509* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3510* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3511* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3512* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3513* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3514* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3515* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3516* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3517* [Observation](observation.html): The subject that the observation is about (if patient) 3518* [Person](person.html): The Person links to this Patient 3519* [Procedure](procedure.html): Search by subject - a patient 3520* [Provenance](provenance.html): Where the activity involved patient data 3521* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3522* [RelatedPerson](relatedperson.html): The patient this related person is related to 3523* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3524* [ResearchSubject](researchsubject.html): Who or what is part of study 3525* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3526* [ServiceRequest](servicerequest.html): Search by subject - a patient 3527* [Specimen](specimen.html): The patient the specimen comes from 3528* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3529* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3530* [Task](task.html): Search by patient 3531* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3532</b><br> 3533 * Type: <b>reference</b><br> 3534 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3535 * </p> 3536 */ 3537 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 3538 public static final String SP_PATIENT = "patient"; 3539 /** 3540 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3541 * <p> 3542 * Description: <b>Multiple Resources: 3543 3544* [Account](account.html): The entity that caused the expenses 3545* [AdverseEvent](adverseevent.html): Subject impacted by event 3546* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3547* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3548* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3549* [AuditEvent](auditevent.html): Where the activity involved patient data 3550* [Basic](basic.html): Identifies the focus of this resource 3551* [BodyStructure](bodystructure.html): Who this is about 3552* [CarePlan](careplan.html): Who the care plan is for 3553* [CareTeam](careteam.html): Who care team is for 3554* [ChargeItem](chargeitem.html): Individual service was done for/to 3555* [Claim](claim.html): Patient receiving the products or services 3556* [ClaimResponse](claimresponse.html): The subject of care 3557* [ClinicalImpression](clinicalimpression.html): Patient assessed 3558* [Communication](communication.html): Focus of message 3559* [CommunicationRequest](communicationrequest.html): Focus of message 3560* [Composition](composition.html): Who and/or what the composition is about 3561* [Condition](condition.html): Who has the condition? 3562* [Consent](consent.html): Who the consent applies to 3563* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3564* [Coverage](coverage.html): Retrieve coverages for a patient 3565* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3566* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3567* [DetectedIssue](detectedissue.html): Associated patient 3568* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3569* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3570* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3571* [DocumentReference](documentreference.html): Who/what is the subject of the document 3572* [Encounter](encounter.html): The patient present at the encounter 3573* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3574* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3575* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3576* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3577* [Flag](flag.html): The identity of a subject to list flags for 3578* [Goal](goal.html): Who this goal is intended for 3579* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3580* [ImagingSelection](imagingselection.html): Who the study is about 3581* [ImagingStudy](imagingstudy.html): Who the study is about 3582* [Immunization](immunization.html): The patient for the vaccination record 3583* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3584* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3585* [Invoice](invoice.html): Recipient(s) of goods and services 3586* [List](list.html): If all resources have the same subject 3587* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3588* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3589* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3590* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3591* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3592* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3593* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3594* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3595* [Observation](observation.html): The subject that the observation is about (if patient) 3596* [Person](person.html): The Person links to this Patient 3597* [Procedure](procedure.html): Search by subject - a patient 3598* [Provenance](provenance.html): Where the activity involved patient data 3599* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3600* [RelatedPerson](relatedperson.html): The patient this related person is related to 3601* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3602* [ResearchSubject](researchsubject.html): Who or what is part of study 3603* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3604* [ServiceRequest](servicerequest.html): Search by subject - a patient 3605* [Specimen](specimen.html): The patient the specimen comes from 3606* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3607* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3608* [Task](task.html): Search by patient 3609* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3610</b><br> 3611 * Type: <b>reference</b><br> 3612 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3613 * </p> 3614 */ 3615 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3616 3617/** 3618 * Constant for fluent queries to be used to add include statements. Specifies 3619 * the path value of "<b>Specimen:patient</b>". 3620 */ 3621 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Specimen:patient").toLocked(); 3622 3623 /** 3624 * Search parameter: <b>type</b> 3625 * <p> 3626 * Description: <b>Multiple Resources: 3627 3628* [Account](account.html): E.g. patient, expense, depreciation 3629* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 3630* [Composition](composition.html): Kind of composition (LOINC if possible) 3631* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 3632* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 3633* [Encounter](encounter.html): Specific type of encounter 3634* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 3635* [Invoice](invoice.html): Type of Invoice 3636* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 3637* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 3638* [Specimen](specimen.html): The specimen type 3639</b><br> 3640 * Type: <b>token</b><br> 3641 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 3642 * </p> 3643 */ 3644 @SearchParamDefinition(name="type", path="Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type", description="Multiple Resources: \r\n\r\n* [Account](account.html): E.g. patient, expense, depreciation\r\n* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known)\r\n* [Composition](composition.html): Kind of composition (LOINC if possible)\r\n* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation)\r\n* [DocumentReference](documentreference.html): Kind of document (LOINC if possible)\r\n* [Encounter](encounter.html): Specific type of encounter\r\n* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management\r\n* [Invoice](invoice.html): Type of Invoice\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type\r\n* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence\r\n* [Specimen](specimen.html): The specimen type\r\n", type="token" ) 3645 public static final String SP_TYPE = "type"; 3646 /** 3647 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3648 * <p> 3649 * Description: <b>Multiple Resources: 3650 3651* [Account](account.html): E.g. patient, expense, depreciation 3652* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 3653* [Composition](composition.html): Kind of composition (LOINC if possible) 3654* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 3655* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 3656* [Encounter](encounter.html): Specific type of encounter 3657* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 3658* [Invoice](invoice.html): Type of Invoice 3659* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 3660* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 3661* [Specimen](specimen.html): The specimen type 3662</b><br> 3663 * Type: <b>token</b><br> 3664 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 3665 * </p> 3666 */ 3667 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 3668 3669 3670} 3671