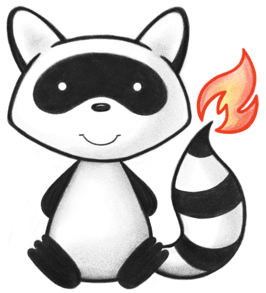
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A kind of specimen with associated set of requirements. 052 */ 053@ResourceDef(name="SpecimenDefinition", profile="http://hl7.org/fhir/StructureDefinition/SpecimenDefinition") 054public class SpecimenDefinition extends DomainResource { 055 056 public enum SpecimenContainedPreference { 057 /** 058 * This type of contained specimen is preferred to collect this kind of specimen. 059 */ 060 PREFERRED, 061 /** 062 * This type of conditioned specimen is an alternate. 063 */ 064 ALTERNATE, 065 /** 066 * added to help the parsers with the generic types 067 */ 068 NULL; 069 public static SpecimenContainedPreference fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("preferred".equals(codeString)) 073 return PREFERRED; 074 if ("alternate".equals(codeString)) 075 return ALTERNATE; 076 if (Configuration.isAcceptInvalidEnums()) 077 return null; 078 else 079 throw new FHIRException("Unknown SpecimenContainedPreference code '"+codeString+"'"); 080 } 081 public String toCode() { 082 switch (this) { 083 case PREFERRED: return "preferred"; 084 case ALTERNATE: return "alternate"; 085 case NULL: return null; 086 default: return "?"; 087 } 088 } 089 public String getSystem() { 090 switch (this) { 091 case PREFERRED: return "http://hl7.org/fhir/specimen-contained-preference"; 092 case ALTERNATE: return "http://hl7.org/fhir/specimen-contained-preference"; 093 case NULL: return null; 094 default: return "?"; 095 } 096 } 097 public String getDefinition() { 098 switch (this) { 099 case PREFERRED: return "This type of contained specimen is preferred to collect this kind of specimen."; 100 case ALTERNATE: return "This type of conditioned specimen is an alternate."; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDisplay() { 106 switch (this) { 107 case PREFERRED: return "Preferred"; 108 case ALTERNATE: return "Alternate"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 } 114 115 public static class SpecimenContainedPreferenceEnumFactory implements EnumFactory<SpecimenContainedPreference> { 116 public SpecimenContainedPreference fromCode(String codeString) throws IllegalArgumentException { 117 if (codeString == null || "".equals(codeString)) 118 if (codeString == null || "".equals(codeString)) 119 return null; 120 if ("preferred".equals(codeString)) 121 return SpecimenContainedPreference.PREFERRED; 122 if ("alternate".equals(codeString)) 123 return SpecimenContainedPreference.ALTERNATE; 124 throw new IllegalArgumentException("Unknown SpecimenContainedPreference code '"+codeString+"'"); 125 } 126 public Enumeration<SpecimenContainedPreference> fromType(PrimitiveType<?> code) throws FHIRException { 127 if (code == null) 128 return null; 129 if (code.isEmpty()) 130 return new Enumeration<SpecimenContainedPreference>(this, SpecimenContainedPreference.NULL, code); 131 String codeString = ((PrimitiveType) code).asStringValue(); 132 if (codeString == null || "".equals(codeString)) 133 return new Enumeration<SpecimenContainedPreference>(this, SpecimenContainedPreference.NULL, code); 134 if ("preferred".equals(codeString)) 135 return new Enumeration<SpecimenContainedPreference>(this, SpecimenContainedPreference.PREFERRED, code); 136 if ("alternate".equals(codeString)) 137 return new Enumeration<SpecimenContainedPreference>(this, SpecimenContainedPreference.ALTERNATE, code); 138 throw new FHIRException("Unknown SpecimenContainedPreference code '"+codeString+"'"); 139 } 140 public String toCode(SpecimenContainedPreference code) { 141 if (code == SpecimenContainedPreference.NULL) 142 return null; 143 if (code == SpecimenContainedPreference.PREFERRED) 144 return "preferred"; 145 if (code == SpecimenContainedPreference.ALTERNATE) 146 return "alternate"; 147 return "?"; 148 } 149 public String toSystem(SpecimenContainedPreference code) { 150 return code.getSystem(); 151 } 152 } 153 154 @Block() 155 public static class SpecimenDefinitionTypeTestedComponent extends BackboneElement implements IBaseBackboneElement { 156 /** 157 * Primary of secondary specimen. 158 */ 159 @Child(name = "isDerived", type = {BooleanType.class}, order=1, min=0, max=1, modifier=false, summary=false) 160 @Description(shortDefinition="Primary or secondary specimen", formalDefinition="Primary of secondary specimen." ) 161 protected BooleanType isDerived; 162 163 /** 164 * The kind of specimen conditioned for testing expected by lab. 165 */ 166 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 167 @Description(shortDefinition="Type of intended specimen", formalDefinition="The kind of specimen conditioned for testing expected by lab." ) 168 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v2-0487") 169 protected CodeableConcept type; 170 171 /** 172 * The preference for this type of conditioned specimen. 173 */ 174 @Child(name = "preference", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=false) 175 @Description(shortDefinition="preferred | alternate", formalDefinition="The preference for this type of conditioned specimen." ) 176 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/specimen-contained-preference") 177 protected Enumeration<SpecimenContainedPreference> preference; 178 179 /** 180 * The specimen's container. 181 */ 182 @Child(name = "container", type = {}, order=4, min=0, max=1, modifier=false, summary=false) 183 @Description(shortDefinition="The specimen's container", formalDefinition="The specimen's container." ) 184 protected SpecimenDefinitionTypeTestedContainerComponent container; 185 186 /** 187 * Requirements for delivery and special handling of this kind of conditioned specimen. 188 */ 189 @Child(name = "requirement", type = {MarkdownType.class}, order=5, min=0, max=1, modifier=false, summary=false) 190 @Description(shortDefinition="Requirements for specimen delivery and special handling", formalDefinition="Requirements for delivery and special handling of this kind of conditioned specimen." ) 191 protected MarkdownType requirement; 192 193 /** 194 * The usual time that a specimen of this kind is retained after the ordered tests are completed, for the purpose of additional testing. 195 */ 196 @Child(name = "retentionTime", type = {Duration.class}, order=6, min=0, max=1, modifier=false, summary=false) 197 @Description(shortDefinition="The usual time for retaining this kind of specimen", formalDefinition="The usual time that a specimen of this kind is retained after the ordered tests are completed, for the purpose of additional testing." ) 198 protected Duration retentionTime; 199 200 /** 201 * Specimen can be used by only one test or panel if the value is "true". 202 */ 203 @Child(name = "singleUse", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=false) 204 @Description(shortDefinition="Specimen for single use only", formalDefinition="Specimen can be used by only one test or panel if the value is \"true\"." ) 205 protected BooleanType singleUse; 206 207 /** 208 * Criterion for rejection of the specimen in its container by the laboratory. 209 */ 210 @Child(name = "rejectionCriterion", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 211 @Description(shortDefinition="Criterion specified for specimen rejection", formalDefinition="Criterion for rejection of the specimen in its container by the laboratory." ) 212 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/rejection-criteria") 213 protected List<CodeableConcept> rejectionCriterion; 214 215 /** 216 * Set of instructions for preservation/transport of the specimen at a defined temperature interval, prior the testing process. 217 */ 218 @Child(name = "handling", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 219 @Description(shortDefinition="Specimen handling before testing", formalDefinition="Set of instructions for preservation/transport of the specimen at a defined temperature interval, prior the testing process." ) 220 protected List<SpecimenDefinitionTypeTestedHandlingComponent> handling; 221 222 /** 223 * Where the specimen will be tested: e.g., lab, sector, device or any combination of these. 224 */ 225 @Child(name = "testingDestination", type = {CodeableConcept.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 226 @Description(shortDefinition="Where the specimen will be tested", formalDefinition="Where the specimen will be tested: e.g., lab, sector, device or any combination of these." ) 227 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/diagnostic-service-sections") 228 protected List<CodeableConcept> testingDestination; 229 230 private static final long serialVersionUID = -609761988L; 231 232 /** 233 * Constructor 234 */ 235 public SpecimenDefinitionTypeTestedComponent() { 236 super(); 237 } 238 239 /** 240 * Constructor 241 */ 242 public SpecimenDefinitionTypeTestedComponent(SpecimenContainedPreference preference) { 243 super(); 244 this.setPreference(preference); 245 } 246 247 /** 248 * @return {@link #isDerived} (Primary of secondary specimen.). This is the underlying object with id, value and extensions. The accessor "getIsDerived" gives direct access to the value 249 */ 250 public BooleanType getIsDerivedElement() { 251 if (this.isDerived == null) 252 if (Configuration.errorOnAutoCreate()) 253 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedComponent.isDerived"); 254 else if (Configuration.doAutoCreate()) 255 this.isDerived = new BooleanType(); // bb 256 return this.isDerived; 257 } 258 259 public boolean hasIsDerivedElement() { 260 return this.isDerived != null && !this.isDerived.isEmpty(); 261 } 262 263 public boolean hasIsDerived() { 264 return this.isDerived != null && !this.isDerived.isEmpty(); 265 } 266 267 /** 268 * @param value {@link #isDerived} (Primary of secondary specimen.). This is the underlying object with id, value and extensions. The accessor "getIsDerived" gives direct access to the value 269 */ 270 public SpecimenDefinitionTypeTestedComponent setIsDerivedElement(BooleanType value) { 271 this.isDerived = value; 272 return this; 273 } 274 275 /** 276 * @return Primary of secondary specimen. 277 */ 278 public boolean getIsDerived() { 279 return this.isDerived == null || this.isDerived.isEmpty() ? false : this.isDerived.getValue(); 280 } 281 282 /** 283 * @param value Primary of secondary specimen. 284 */ 285 public SpecimenDefinitionTypeTestedComponent setIsDerived(boolean value) { 286 if (this.isDerived == null) 287 this.isDerived = new BooleanType(); 288 this.isDerived.setValue(value); 289 return this; 290 } 291 292 /** 293 * @return {@link #type} (The kind of specimen conditioned for testing expected by lab.) 294 */ 295 public CodeableConcept getType() { 296 if (this.type == null) 297 if (Configuration.errorOnAutoCreate()) 298 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedComponent.type"); 299 else if (Configuration.doAutoCreate()) 300 this.type = new CodeableConcept(); // cc 301 return this.type; 302 } 303 304 public boolean hasType() { 305 return this.type != null && !this.type.isEmpty(); 306 } 307 308 /** 309 * @param value {@link #type} (The kind of specimen conditioned for testing expected by lab.) 310 */ 311 public SpecimenDefinitionTypeTestedComponent setType(CodeableConcept value) { 312 this.type = value; 313 return this; 314 } 315 316 /** 317 * @return {@link #preference} (The preference for this type of conditioned specimen.). This is the underlying object with id, value and extensions. The accessor "getPreference" gives direct access to the value 318 */ 319 public Enumeration<SpecimenContainedPreference> getPreferenceElement() { 320 if (this.preference == null) 321 if (Configuration.errorOnAutoCreate()) 322 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedComponent.preference"); 323 else if (Configuration.doAutoCreate()) 324 this.preference = new Enumeration<SpecimenContainedPreference>(new SpecimenContainedPreferenceEnumFactory()); // bb 325 return this.preference; 326 } 327 328 public boolean hasPreferenceElement() { 329 return this.preference != null && !this.preference.isEmpty(); 330 } 331 332 public boolean hasPreference() { 333 return this.preference != null && !this.preference.isEmpty(); 334 } 335 336 /** 337 * @param value {@link #preference} (The preference for this type of conditioned specimen.). This is the underlying object with id, value and extensions. The accessor "getPreference" gives direct access to the value 338 */ 339 public SpecimenDefinitionTypeTestedComponent setPreferenceElement(Enumeration<SpecimenContainedPreference> value) { 340 this.preference = value; 341 return this; 342 } 343 344 /** 345 * @return The preference for this type of conditioned specimen. 346 */ 347 public SpecimenContainedPreference getPreference() { 348 return this.preference == null ? null : this.preference.getValue(); 349 } 350 351 /** 352 * @param value The preference for this type of conditioned specimen. 353 */ 354 public SpecimenDefinitionTypeTestedComponent setPreference(SpecimenContainedPreference value) { 355 if (this.preference == null) 356 this.preference = new Enumeration<SpecimenContainedPreference>(new SpecimenContainedPreferenceEnumFactory()); 357 this.preference.setValue(value); 358 return this; 359 } 360 361 /** 362 * @return {@link #container} (The specimen's container.) 363 */ 364 public SpecimenDefinitionTypeTestedContainerComponent getContainer() { 365 if (this.container == null) 366 if (Configuration.errorOnAutoCreate()) 367 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedComponent.container"); 368 else if (Configuration.doAutoCreate()) 369 this.container = new SpecimenDefinitionTypeTestedContainerComponent(); // cc 370 return this.container; 371 } 372 373 public boolean hasContainer() { 374 return this.container != null && !this.container.isEmpty(); 375 } 376 377 /** 378 * @param value {@link #container} (The specimen's container.) 379 */ 380 public SpecimenDefinitionTypeTestedComponent setContainer(SpecimenDefinitionTypeTestedContainerComponent value) { 381 this.container = value; 382 return this; 383 } 384 385 /** 386 * @return {@link #requirement} (Requirements for delivery and special handling of this kind of conditioned specimen.). This is the underlying object with id, value and extensions. The accessor "getRequirement" gives direct access to the value 387 */ 388 public MarkdownType getRequirementElement() { 389 if (this.requirement == null) 390 if (Configuration.errorOnAutoCreate()) 391 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedComponent.requirement"); 392 else if (Configuration.doAutoCreate()) 393 this.requirement = new MarkdownType(); // bb 394 return this.requirement; 395 } 396 397 public boolean hasRequirementElement() { 398 return this.requirement != null && !this.requirement.isEmpty(); 399 } 400 401 public boolean hasRequirement() { 402 return this.requirement != null && !this.requirement.isEmpty(); 403 } 404 405 /** 406 * @param value {@link #requirement} (Requirements for delivery and special handling of this kind of conditioned specimen.). This is the underlying object with id, value and extensions. The accessor "getRequirement" gives direct access to the value 407 */ 408 public SpecimenDefinitionTypeTestedComponent setRequirementElement(MarkdownType value) { 409 this.requirement = value; 410 return this; 411 } 412 413 /** 414 * @return Requirements for delivery and special handling of this kind of conditioned specimen. 415 */ 416 public String getRequirement() { 417 return this.requirement == null ? null : this.requirement.getValue(); 418 } 419 420 /** 421 * @param value Requirements for delivery and special handling of this kind of conditioned specimen. 422 */ 423 public SpecimenDefinitionTypeTestedComponent setRequirement(String value) { 424 if (Utilities.noString(value)) 425 this.requirement = null; 426 else { 427 if (this.requirement == null) 428 this.requirement = new MarkdownType(); 429 this.requirement.setValue(value); 430 } 431 return this; 432 } 433 434 /** 435 * @return {@link #retentionTime} (The usual time that a specimen of this kind is retained after the ordered tests are completed, for the purpose of additional testing.) 436 */ 437 public Duration getRetentionTime() { 438 if (this.retentionTime == null) 439 if (Configuration.errorOnAutoCreate()) 440 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedComponent.retentionTime"); 441 else if (Configuration.doAutoCreate()) 442 this.retentionTime = new Duration(); // cc 443 return this.retentionTime; 444 } 445 446 public boolean hasRetentionTime() { 447 return this.retentionTime != null && !this.retentionTime.isEmpty(); 448 } 449 450 /** 451 * @param value {@link #retentionTime} (The usual time that a specimen of this kind is retained after the ordered tests are completed, for the purpose of additional testing.) 452 */ 453 public SpecimenDefinitionTypeTestedComponent setRetentionTime(Duration value) { 454 this.retentionTime = value; 455 return this; 456 } 457 458 /** 459 * @return {@link #singleUse} (Specimen can be used by only one test or panel if the value is "true".). This is the underlying object with id, value and extensions. The accessor "getSingleUse" gives direct access to the value 460 */ 461 public BooleanType getSingleUseElement() { 462 if (this.singleUse == null) 463 if (Configuration.errorOnAutoCreate()) 464 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedComponent.singleUse"); 465 else if (Configuration.doAutoCreate()) 466 this.singleUse = new BooleanType(); // bb 467 return this.singleUse; 468 } 469 470 public boolean hasSingleUseElement() { 471 return this.singleUse != null && !this.singleUse.isEmpty(); 472 } 473 474 public boolean hasSingleUse() { 475 return this.singleUse != null && !this.singleUse.isEmpty(); 476 } 477 478 /** 479 * @param value {@link #singleUse} (Specimen can be used by only one test or panel if the value is "true".). This is the underlying object with id, value and extensions. The accessor "getSingleUse" gives direct access to the value 480 */ 481 public SpecimenDefinitionTypeTestedComponent setSingleUseElement(BooleanType value) { 482 this.singleUse = value; 483 return this; 484 } 485 486 /** 487 * @return Specimen can be used by only one test or panel if the value is "true". 488 */ 489 public boolean getSingleUse() { 490 return this.singleUse == null || this.singleUse.isEmpty() ? false : this.singleUse.getValue(); 491 } 492 493 /** 494 * @param value Specimen can be used by only one test or panel if the value is "true". 495 */ 496 public SpecimenDefinitionTypeTestedComponent setSingleUse(boolean value) { 497 if (this.singleUse == null) 498 this.singleUse = new BooleanType(); 499 this.singleUse.setValue(value); 500 return this; 501 } 502 503 /** 504 * @return {@link #rejectionCriterion} (Criterion for rejection of the specimen in its container by the laboratory.) 505 */ 506 public List<CodeableConcept> getRejectionCriterion() { 507 if (this.rejectionCriterion == null) 508 this.rejectionCriterion = new ArrayList<CodeableConcept>(); 509 return this.rejectionCriterion; 510 } 511 512 /** 513 * @return Returns a reference to <code>this</code> for easy method chaining 514 */ 515 public SpecimenDefinitionTypeTestedComponent setRejectionCriterion(List<CodeableConcept> theRejectionCriterion) { 516 this.rejectionCriterion = theRejectionCriterion; 517 return this; 518 } 519 520 public boolean hasRejectionCriterion() { 521 if (this.rejectionCriterion == null) 522 return false; 523 for (CodeableConcept item : this.rejectionCriterion) 524 if (!item.isEmpty()) 525 return true; 526 return false; 527 } 528 529 public CodeableConcept addRejectionCriterion() { //3 530 CodeableConcept t = new CodeableConcept(); 531 if (this.rejectionCriterion == null) 532 this.rejectionCriterion = new ArrayList<CodeableConcept>(); 533 this.rejectionCriterion.add(t); 534 return t; 535 } 536 537 public SpecimenDefinitionTypeTestedComponent addRejectionCriterion(CodeableConcept t) { //3 538 if (t == null) 539 return this; 540 if (this.rejectionCriterion == null) 541 this.rejectionCriterion = new ArrayList<CodeableConcept>(); 542 this.rejectionCriterion.add(t); 543 return this; 544 } 545 546 /** 547 * @return The first repetition of repeating field {@link #rejectionCriterion}, creating it if it does not already exist {3} 548 */ 549 public CodeableConcept getRejectionCriterionFirstRep() { 550 if (getRejectionCriterion().isEmpty()) { 551 addRejectionCriterion(); 552 } 553 return getRejectionCriterion().get(0); 554 } 555 556 /** 557 * @return {@link #handling} (Set of instructions for preservation/transport of the specimen at a defined temperature interval, prior the testing process.) 558 */ 559 public List<SpecimenDefinitionTypeTestedHandlingComponent> getHandling() { 560 if (this.handling == null) 561 this.handling = new ArrayList<SpecimenDefinitionTypeTestedHandlingComponent>(); 562 return this.handling; 563 } 564 565 /** 566 * @return Returns a reference to <code>this</code> for easy method chaining 567 */ 568 public SpecimenDefinitionTypeTestedComponent setHandling(List<SpecimenDefinitionTypeTestedHandlingComponent> theHandling) { 569 this.handling = theHandling; 570 return this; 571 } 572 573 public boolean hasHandling() { 574 if (this.handling == null) 575 return false; 576 for (SpecimenDefinitionTypeTestedHandlingComponent item : this.handling) 577 if (!item.isEmpty()) 578 return true; 579 return false; 580 } 581 582 public SpecimenDefinitionTypeTestedHandlingComponent addHandling() { //3 583 SpecimenDefinitionTypeTestedHandlingComponent t = new SpecimenDefinitionTypeTestedHandlingComponent(); 584 if (this.handling == null) 585 this.handling = new ArrayList<SpecimenDefinitionTypeTestedHandlingComponent>(); 586 this.handling.add(t); 587 return t; 588 } 589 590 public SpecimenDefinitionTypeTestedComponent addHandling(SpecimenDefinitionTypeTestedHandlingComponent t) { //3 591 if (t == null) 592 return this; 593 if (this.handling == null) 594 this.handling = new ArrayList<SpecimenDefinitionTypeTestedHandlingComponent>(); 595 this.handling.add(t); 596 return this; 597 } 598 599 /** 600 * @return The first repetition of repeating field {@link #handling}, creating it if it does not already exist {3} 601 */ 602 public SpecimenDefinitionTypeTestedHandlingComponent getHandlingFirstRep() { 603 if (getHandling().isEmpty()) { 604 addHandling(); 605 } 606 return getHandling().get(0); 607 } 608 609 /** 610 * @return {@link #testingDestination} (Where the specimen will be tested: e.g., lab, sector, device or any combination of these.) 611 */ 612 public List<CodeableConcept> getTestingDestination() { 613 if (this.testingDestination == null) 614 this.testingDestination = new ArrayList<CodeableConcept>(); 615 return this.testingDestination; 616 } 617 618 /** 619 * @return Returns a reference to <code>this</code> for easy method chaining 620 */ 621 public SpecimenDefinitionTypeTestedComponent setTestingDestination(List<CodeableConcept> theTestingDestination) { 622 this.testingDestination = theTestingDestination; 623 return this; 624 } 625 626 public boolean hasTestingDestination() { 627 if (this.testingDestination == null) 628 return false; 629 for (CodeableConcept item : this.testingDestination) 630 if (!item.isEmpty()) 631 return true; 632 return false; 633 } 634 635 public CodeableConcept addTestingDestination() { //3 636 CodeableConcept t = new CodeableConcept(); 637 if (this.testingDestination == null) 638 this.testingDestination = new ArrayList<CodeableConcept>(); 639 this.testingDestination.add(t); 640 return t; 641 } 642 643 public SpecimenDefinitionTypeTestedComponent addTestingDestination(CodeableConcept t) { //3 644 if (t == null) 645 return this; 646 if (this.testingDestination == null) 647 this.testingDestination = new ArrayList<CodeableConcept>(); 648 this.testingDestination.add(t); 649 return this; 650 } 651 652 /** 653 * @return The first repetition of repeating field {@link #testingDestination}, creating it if it does not already exist {3} 654 */ 655 public CodeableConcept getTestingDestinationFirstRep() { 656 if (getTestingDestination().isEmpty()) { 657 addTestingDestination(); 658 } 659 return getTestingDestination().get(0); 660 } 661 662 protected void listChildren(List<Property> children) { 663 super.listChildren(children); 664 children.add(new Property("isDerived", "boolean", "Primary of secondary specimen.", 0, 1, isDerived)); 665 children.add(new Property("type", "CodeableConcept", "The kind of specimen conditioned for testing expected by lab.", 0, 1, type)); 666 children.add(new Property("preference", "code", "The preference for this type of conditioned specimen.", 0, 1, preference)); 667 children.add(new Property("container", "", "The specimen's container.", 0, 1, container)); 668 children.add(new Property("requirement", "markdown", "Requirements for delivery and special handling of this kind of conditioned specimen.", 0, 1, requirement)); 669 children.add(new Property("retentionTime", "Duration", "The usual time that a specimen of this kind is retained after the ordered tests are completed, for the purpose of additional testing.", 0, 1, retentionTime)); 670 children.add(new Property("singleUse", "boolean", "Specimen can be used by only one test or panel if the value is \"true\".", 0, 1, singleUse)); 671 children.add(new Property("rejectionCriterion", "CodeableConcept", "Criterion for rejection of the specimen in its container by the laboratory.", 0, java.lang.Integer.MAX_VALUE, rejectionCriterion)); 672 children.add(new Property("handling", "", "Set of instructions for preservation/transport of the specimen at a defined temperature interval, prior the testing process.", 0, java.lang.Integer.MAX_VALUE, handling)); 673 children.add(new Property("testingDestination", "CodeableConcept", "Where the specimen will be tested: e.g., lab, sector, device or any combination of these.", 0, java.lang.Integer.MAX_VALUE, testingDestination)); 674 } 675 676 @Override 677 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 678 switch (_hash) { 679 case 976346515: /*isDerived*/ return new Property("isDerived", "boolean", "Primary of secondary specimen.", 0, 1, isDerived); 680 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The kind of specimen conditioned for testing expected by lab.", 0, 1, type); 681 case -1459831589: /*preference*/ return new Property("preference", "code", "The preference for this type of conditioned specimen.", 0, 1, preference); 682 case -410956671: /*container*/ return new Property("container", "", "The specimen's container.", 0, 1, container); 683 case 363387971: /*requirement*/ return new Property("requirement", "markdown", "Requirements for delivery and special handling of this kind of conditioned specimen.", 0, 1, requirement); 684 case 1434969867: /*retentionTime*/ return new Property("retentionTime", "Duration", "The usual time that a specimen of this kind is retained after the ordered tests are completed, for the purpose of additional testing.", 0, 1, retentionTime); 685 case -1494547425: /*singleUse*/ return new Property("singleUse", "boolean", "Specimen can be used by only one test or panel if the value is \"true\".", 0, 1, singleUse); 686 case -553706344: /*rejectionCriterion*/ return new Property("rejectionCriterion", "CodeableConcept", "Criterion for rejection of the specimen in its container by the laboratory.", 0, java.lang.Integer.MAX_VALUE, rejectionCriterion); 687 case 2072805: /*handling*/ return new Property("handling", "", "Set of instructions for preservation/transport of the specimen at a defined temperature interval, prior the testing process.", 0, java.lang.Integer.MAX_VALUE, handling); 688 case 939511774: /*testingDestination*/ return new Property("testingDestination", "CodeableConcept", "Where the specimen will be tested: e.g., lab, sector, device or any combination of these.", 0, java.lang.Integer.MAX_VALUE, testingDestination); 689 default: return super.getNamedProperty(_hash, _name, _checkValid); 690 } 691 692 } 693 694 @Override 695 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 696 switch (hash) { 697 case 976346515: /*isDerived*/ return this.isDerived == null ? new Base[0] : new Base[] {this.isDerived}; // BooleanType 698 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 699 case -1459831589: /*preference*/ return this.preference == null ? new Base[0] : new Base[] {this.preference}; // Enumeration<SpecimenContainedPreference> 700 case -410956671: /*container*/ return this.container == null ? new Base[0] : new Base[] {this.container}; // SpecimenDefinitionTypeTestedContainerComponent 701 case 363387971: /*requirement*/ return this.requirement == null ? new Base[0] : new Base[] {this.requirement}; // MarkdownType 702 case 1434969867: /*retentionTime*/ return this.retentionTime == null ? new Base[0] : new Base[] {this.retentionTime}; // Duration 703 case -1494547425: /*singleUse*/ return this.singleUse == null ? new Base[0] : new Base[] {this.singleUse}; // BooleanType 704 case -553706344: /*rejectionCriterion*/ return this.rejectionCriterion == null ? new Base[0] : this.rejectionCriterion.toArray(new Base[this.rejectionCriterion.size()]); // CodeableConcept 705 case 2072805: /*handling*/ return this.handling == null ? new Base[0] : this.handling.toArray(new Base[this.handling.size()]); // SpecimenDefinitionTypeTestedHandlingComponent 706 case 939511774: /*testingDestination*/ return this.testingDestination == null ? new Base[0] : this.testingDestination.toArray(new Base[this.testingDestination.size()]); // CodeableConcept 707 default: return super.getProperty(hash, name, checkValid); 708 } 709 710 } 711 712 @Override 713 public Base setProperty(int hash, String name, Base value) throws FHIRException { 714 switch (hash) { 715 case 976346515: // isDerived 716 this.isDerived = TypeConvertor.castToBoolean(value); // BooleanType 717 return value; 718 case 3575610: // type 719 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 720 return value; 721 case -1459831589: // preference 722 value = new SpecimenContainedPreferenceEnumFactory().fromType(TypeConvertor.castToCode(value)); 723 this.preference = (Enumeration) value; // Enumeration<SpecimenContainedPreference> 724 return value; 725 case -410956671: // container 726 this.container = (SpecimenDefinitionTypeTestedContainerComponent) value; // SpecimenDefinitionTypeTestedContainerComponent 727 return value; 728 case 363387971: // requirement 729 this.requirement = TypeConvertor.castToMarkdown(value); // MarkdownType 730 return value; 731 case 1434969867: // retentionTime 732 this.retentionTime = TypeConvertor.castToDuration(value); // Duration 733 return value; 734 case -1494547425: // singleUse 735 this.singleUse = TypeConvertor.castToBoolean(value); // BooleanType 736 return value; 737 case -553706344: // rejectionCriterion 738 this.getRejectionCriterion().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 739 return value; 740 case 2072805: // handling 741 this.getHandling().add((SpecimenDefinitionTypeTestedHandlingComponent) value); // SpecimenDefinitionTypeTestedHandlingComponent 742 return value; 743 case 939511774: // testingDestination 744 this.getTestingDestination().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 745 return value; 746 default: return super.setProperty(hash, name, value); 747 } 748 749 } 750 751 @Override 752 public Base setProperty(String name, Base value) throws FHIRException { 753 if (name.equals("isDerived")) { 754 this.isDerived = TypeConvertor.castToBoolean(value); // BooleanType 755 } else if (name.equals("type")) { 756 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 757 } else if (name.equals("preference")) { 758 value = new SpecimenContainedPreferenceEnumFactory().fromType(TypeConvertor.castToCode(value)); 759 this.preference = (Enumeration) value; // Enumeration<SpecimenContainedPreference> 760 } else if (name.equals("container")) { 761 this.container = (SpecimenDefinitionTypeTestedContainerComponent) value; // SpecimenDefinitionTypeTestedContainerComponent 762 } else if (name.equals("requirement")) { 763 this.requirement = TypeConvertor.castToMarkdown(value); // MarkdownType 764 } else if (name.equals("retentionTime")) { 765 this.retentionTime = TypeConvertor.castToDuration(value); // Duration 766 } else if (name.equals("singleUse")) { 767 this.singleUse = TypeConvertor.castToBoolean(value); // BooleanType 768 } else if (name.equals("rejectionCriterion")) { 769 this.getRejectionCriterion().add(TypeConvertor.castToCodeableConcept(value)); 770 } else if (name.equals("handling")) { 771 this.getHandling().add((SpecimenDefinitionTypeTestedHandlingComponent) value); 772 } else if (name.equals("testingDestination")) { 773 this.getTestingDestination().add(TypeConvertor.castToCodeableConcept(value)); 774 } else 775 return super.setProperty(name, value); 776 return value; 777 } 778 779 @Override 780 public void removeChild(String name, Base value) throws FHIRException { 781 if (name.equals("isDerived")) { 782 this.isDerived = null; 783 } else if (name.equals("type")) { 784 this.type = null; 785 } else if (name.equals("preference")) { 786 value = new SpecimenContainedPreferenceEnumFactory().fromType(TypeConvertor.castToCode(value)); 787 this.preference = (Enumeration) value; // Enumeration<SpecimenContainedPreference> 788 } else if (name.equals("container")) { 789 this.container = (SpecimenDefinitionTypeTestedContainerComponent) value; // SpecimenDefinitionTypeTestedContainerComponent 790 } else if (name.equals("requirement")) { 791 this.requirement = null; 792 } else if (name.equals("retentionTime")) { 793 this.retentionTime = null; 794 } else if (name.equals("singleUse")) { 795 this.singleUse = null; 796 } else if (name.equals("rejectionCriterion")) { 797 this.getRejectionCriterion().remove(value); 798 } else if (name.equals("handling")) { 799 this.getHandling().remove((SpecimenDefinitionTypeTestedHandlingComponent) value); 800 } else if (name.equals("testingDestination")) { 801 this.getTestingDestination().remove(value); 802 } else 803 super.removeChild(name, value); 804 805 } 806 807 @Override 808 public Base makeProperty(int hash, String name) throws FHIRException { 809 switch (hash) { 810 case 976346515: return getIsDerivedElement(); 811 case 3575610: return getType(); 812 case -1459831589: return getPreferenceElement(); 813 case -410956671: return getContainer(); 814 case 363387971: return getRequirementElement(); 815 case 1434969867: return getRetentionTime(); 816 case -1494547425: return getSingleUseElement(); 817 case -553706344: return addRejectionCriterion(); 818 case 2072805: return addHandling(); 819 case 939511774: return addTestingDestination(); 820 default: return super.makeProperty(hash, name); 821 } 822 823 } 824 825 @Override 826 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 827 switch (hash) { 828 case 976346515: /*isDerived*/ return new String[] {"boolean"}; 829 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 830 case -1459831589: /*preference*/ return new String[] {"code"}; 831 case -410956671: /*container*/ return new String[] {}; 832 case 363387971: /*requirement*/ return new String[] {"markdown"}; 833 case 1434969867: /*retentionTime*/ return new String[] {"Duration"}; 834 case -1494547425: /*singleUse*/ return new String[] {"boolean"}; 835 case -553706344: /*rejectionCriterion*/ return new String[] {"CodeableConcept"}; 836 case 2072805: /*handling*/ return new String[] {}; 837 case 939511774: /*testingDestination*/ return new String[] {"CodeableConcept"}; 838 default: return super.getTypesForProperty(hash, name); 839 } 840 841 } 842 843 @Override 844 public Base addChild(String name) throws FHIRException { 845 if (name.equals("isDerived")) { 846 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.typeTested.isDerived"); 847 } 848 else if (name.equals("type")) { 849 this.type = new CodeableConcept(); 850 return this.type; 851 } 852 else if (name.equals("preference")) { 853 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.typeTested.preference"); 854 } 855 else if (name.equals("container")) { 856 this.container = new SpecimenDefinitionTypeTestedContainerComponent(); 857 return this.container; 858 } 859 else if (name.equals("requirement")) { 860 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.typeTested.requirement"); 861 } 862 else if (name.equals("retentionTime")) { 863 this.retentionTime = new Duration(); 864 return this.retentionTime; 865 } 866 else if (name.equals("singleUse")) { 867 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.typeTested.singleUse"); 868 } 869 else if (name.equals("rejectionCriterion")) { 870 return addRejectionCriterion(); 871 } 872 else if (name.equals("handling")) { 873 return addHandling(); 874 } 875 else if (name.equals("testingDestination")) { 876 return addTestingDestination(); 877 } 878 else 879 return super.addChild(name); 880 } 881 882 public SpecimenDefinitionTypeTestedComponent copy() { 883 SpecimenDefinitionTypeTestedComponent dst = new SpecimenDefinitionTypeTestedComponent(); 884 copyValues(dst); 885 return dst; 886 } 887 888 public void copyValues(SpecimenDefinitionTypeTestedComponent dst) { 889 super.copyValues(dst); 890 dst.isDerived = isDerived == null ? null : isDerived.copy(); 891 dst.type = type == null ? null : type.copy(); 892 dst.preference = preference == null ? null : preference.copy(); 893 dst.container = container == null ? null : container.copy(); 894 dst.requirement = requirement == null ? null : requirement.copy(); 895 dst.retentionTime = retentionTime == null ? null : retentionTime.copy(); 896 dst.singleUse = singleUse == null ? null : singleUse.copy(); 897 if (rejectionCriterion != null) { 898 dst.rejectionCriterion = new ArrayList<CodeableConcept>(); 899 for (CodeableConcept i : rejectionCriterion) 900 dst.rejectionCriterion.add(i.copy()); 901 }; 902 if (handling != null) { 903 dst.handling = new ArrayList<SpecimenDefinitionTypeTestedHandlingComponent>(); 904 for (SpecimenDefinitionTypeTestedHandlingComponent i : handling) 905 dst.handling.add(i.copy()); 906 }; 907 if (testingDestination != null) { 908 dst.testingDestination = new ArrayList<CodeableConcept>(); 909 for (CodeableConcept i : testingDestination) 910 dst.testingDestination.add(i.copy()); 911 }; 912 } 913 914 @Override 915 public boolean equalsDeep(Base other_) { 916 if (!super.equalsDeep(other_)) 917 return false; 918 if (!(other_ instanceof SpecimenDefinitionTypeTestedComponent)) 919 return false; 920 SpecimenDefinitionTypeTestedComponent o = (SpecimenDefinitionTypeTestedComponent) other_; 921 return compareDeep(isDerived, o.isDerived, true) && compareDeep(type, o.type, true) && compareDeep(preference, o.preference, true) 922 && compareDeep(container, o.container, true) && compareDeep(requirement, o.requirement, true) && compareDeep(retentionTime, o.retentionTime, true) 923 && compareDeep(singleUse, o.singleUse, true) && compareDeep(rejectionCriterion, o.rejectionCriterion, true) 924 && compareDeep(handling, o.handling, true) && compareDeep(testingDestination, o.testingDestination, true) 925 ; 926 } 927 928 @Override 929 public boolean equalsShallow(Base other_) { 930 if (!super.equalsShallow(other_)) 931 return false; 932 if (!(other_ instanceof SpecimenDefinitionTypeTestedComponent)) 933 return false; 934 SpecimenDefinitionTypeTestedComponent o = (SpecimenDefinitionTypeTestedComponent) other_; 935 return compareValues(isDerived, o.isDerived, true) && compareValues(preference, o.preference, true) 936 && compareValues(requirement, o.requirement, true) && compareValues(singleUse, o.singleUse, true); 937 } 938 939 public boolean isEmpty() { 940 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(isDerived, type, preference 941 , container, requirement, retentionTime, singleUse, rejectionCriterion, handling 942 , testingDestination); 943 } 944 945 public String fhirType() { 946 return "SpecimenDefinition.typeTested"; 947 948 } 949 950 } 951 952 @Block() 953 public static class SpecimenDefinitionTypeTestedContainerComponent extends BackboneElement implements IBaseBackboneElement { 954 /** 955 * The type of material of the container. 956 */ 957 @Child(name = "material", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 958 @Description(shortDefinition="The material type used for the container", formalDefinition="The type of material of the container." ) 959 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/container-material") 960 protected CodeableConcept material; 961 962 /** 963 * The type of container used to contain this kind of specimen. 964 */ 965 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 966 @Description(shortDefinition="Kind of container associated with the kind of specimen", formalDefinition="The type of container used to contain this kind of specimen." ) 967 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/specimen-container-type") 968 protected CodeableConcept type; 969 970 /** 971 * Color of container cap. 972 */ 973 @Child(name = "cap", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 974 @Description(shortDefinition="Color of container cap", formalDefinition="Color of container cap." ) 975 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/container-cap") 976 protected CodeableConcept cap; 977 978 /** 979 * The textual description of the kind of container. 980 */ 981 @Child(name = "description", type = {MarkdownType.class}, order=4, min=0, max=1, modifier=false, summary=false) 982 @Description(shortDefinition="The description of the kind of container", formalDefinition="The textual description of the kind of container." ) 983 protected MarkdownType description; 984 985 /** 986 * The capacity (volume or other measure) of this kind of container. 987 */ 988 @Child(name = "capacity", type = {Quantity.class}, order=5, min=0, max=1, modifier=false, summary=false) 989 @Description(shortDefinition="The capacity of this kind of container", formalDefinition="The capacity (volume or other measure) of this kind of container." ) 990 protected Quantity capacity; 991 992 /** 993 * The minimum volume to be conditioned in the container. 994 */ 995 @Child(name = "minimumVolume", type = {Quantity.class, StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 996 @Description(shortDefinition="Minimum volume", formalDefinition="The minimum volume to be conditioned in the container." ) 997 protected DataType minimumVolume; 998 999 /** 1000 * Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA. 1001 */ 1002 @Child(name = "additive", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1003 @Description(shortDefinition="Additive associated with container", formalDefinition="Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA." ) 1004 protected List<SpecimenDefinitionTypeTestedContainerAdditiveComponent> additive; 1005 1006 /** 1007 * Special processing that should be applied to the container for this kind of specimen. 1008 */ 1009 @Child(name = "preparation", type = {MarkdownType.class}, order=8, min=0, max=1, modifier=false, summary=false) 1010 @Description(shortDefinition="Special processing applied to the container for this specimen type", formalDefinition="Special processing that should be applied to the container for this kind of specimen." ) 1011 protected MarkdownType preparation; 1012 1013 private static final long serialVersionUID = -932984420L; 1014 1015 /** 1016 * Constructor 1017 */ 1018 public SpecimenDefinitionTypeTestedContainerComponent() { 1019 super(); 1020 } 1021 1022 /** 1023 * @return {@link #material} (The type of material of the container.) 1024 */ 1025 public CodeableConcept getMaterial() { 1026 if (this.material == null) 1027 if (Configuration.errorOnAutoCreate()) 1028 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedContainerComponent.material"); 1029 else if (Configuration.doAutoCreate()) 1030 this.material = new CodeableConcept(); // cc 1031 return this.material; 1032 } 1033 1034 public boolean hasMaterial() { 1035 return this.material != null && !this.material.isEmpty(); 1036 } 1037 1038 /** 1039 * @param value {@link #material} (The type of material of the container.) 1040 */ 1041 public SpecimenDefinitionTypeTestedContainerComponent setMaterial(CodeableConcept value) { 1042 this.material = value; 1043 return this; 1044 } 1045 1046 /** 1047 * @return {@link #type} (The type of container used to contain this kind of specimen.) 1048 */ 1049 public CodeableConcept getType() { 1050 if (this.type == null) 1051 if (Configuration.errorOnAutoCreate()) 1052 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedContainerComponent.type"); 1053 else if (Configuration.doAutoCreate()) 1054 this.type = new CodeableConcept(); // cc 1055 return this.type; 1056 } 1057 1058 public boolean hasType() { 1059 return this.type != null && !this.type.isEmpty(); 1060 } 1061 1062 /** 1063 * @param value {@link #type} (The type of container used to contain this kind of specimen.) 1064 */ 1065 public SpecimenDefinitionTypeTestedContainerComponent setType(CodeableConcept value) { 1066 this.type = value; 1067 return this; 1068 } 1069 1070 /** 1071 * @return {@link #cap} (Color of container cap.) 1072 */ 1073 public CodeableConcept getCap() { 1074 if (this.cap == null) 1075 if (Configuration.errorOnAutoCreate()) 1076 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedContainerComponent.cap"); 1077 else if (Configuration.doAutoCreate()) 1078 this.cap = new CodeableConcept(); // cc 1079 return this.cap; 1080 } 1081 1082 public boolean hasCap() { 1083 return this.cap != null && !this.cap.isEmpty(); 1084 } 1085 1086 /** 1087 * @param value {@link #cap} (Color of container cap.) 1088 */ 1089 public SpecimenDefinitionTypeTestedContainerComponent setCap(CodeableConcept value) { 1090 this.cap = value; 1091 return this; 1092 } 1093 1094 /** 1095 * @return {@link #description} (The textual description of the kind of container.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1096 */ 1097 public MarkdownType getDescriptionElement() { 1098 if (this.description == null) 1099 if (Configuration.errorOnAutoCreate()) 1100 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedContainerComponent.description"); 1101 else if (Configuration.doAutoCreate()) 1102 this.description = new MarkdownType(); // bb 1103 return this.description; 1104 } 1105 1106 public boolean hasDescriptionElement() { 1107 return this.description != null && !this.description.isEmpty(); 1108 } 1109 1110 public boolean hasDescription() { 1111 return this.description != null && !this.description.isEmpty(); 1112 } 1113 1114 /** 1115 * @param value {@link #description} (The textual description of the kind of container.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1116 */ 1117 public SpecimenDefinitionTypeTestedContainerComponent setDescriptionElement(MarkdownType value) { 1118 this.description = value; 1119 return this; 1120 } 1121 1122 /** 1123 * @return The textual description of the kind of container. 1124 */ 1125 public String getDescription() { 1126 return this.description == null ? null : this.description.getValue(); 1127 } 1128 1129 /** 1130 * @param value The textual description of the kind of container. 1131 */ 1132 public SpecimenDefinitionTypeTestedContainerComponent setDescription(String value) { 1133 if (Utilities.noString(value)) 1134 this.description = null; 1135 else { 1136 if (this.description == null) 1137 this.description = new MarkdownType(); 1138 this.description.setValue(value); 1139 } 1140 return this; 1141 } 1142 1143 /** 1144 * @return {@link #capacity} (The capacity (volume or other measure) of this kind of container.) 1145 */ 1146 public Quantity getCapacity() { 1147 if (this.capacity == null) 1148 if (Configuration.errorOnAutoCreate()) 1149 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedContainerComponent.capacity"); 1150 else if (Configuration.doAutoCreate()) 1151 this.capacity = new Quantity(); // cc 1152 return this.capacity; 1153 } 1154 1155 public boolean hasCapacity() { 1156 return this.capacity != null && !this.capacity.isEmpty(); 1157 } 1158 1159 /** 1160 * @param value {@link #capacity} (The capacity (volume or other measure) of this kind of container.) 1161 */ 1162 public SpecimenDefinitionTypeTestedContainerComponent setCapacity(Quantity value) { 1163 this.capacity = value; 1164 return this; 1165 } 1166 1167 /** 1168 * @return {@link #minimumVolume} (The minimum volume to be conditioned in the container.) 1169 */ 1170 public DataType getMinimumVolume() { 1171 return this.minimumVolume; 1172 } 1173 1174 /** 1175 * @return {@link #minimumVolume} (The minimum volume to be conditioned in the container.) 1176 */ 1177 public Quantity getMinimumVolumeQuantity() throws FHIRException { 1178 if (this.minimumVolume == null) 1179 this.minimumVolume = new Quantity(); 1180 if (!(this.minimumVolume instanceof Quantity)) 1181 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.minimumVolume.getClass().getName()+" was encountered"); 1182 return (Quantity) this.minimumVolume; 1183 } 1184 1185 public boolean hasMinimumVolumeQuantity() { 1186 return this != null && this.minimumVolume instanceof Quantity; 1187 } 1188 1189 /** 1190 * @return {@link #minimumVolume} (The minimum volume to be conditioned in the container.) 1191 */ 1192 public StringType getMinimumVolumeStringType() throws FHIRException { 1193 if (this.minimumVolume == null) 1194 this.minimumVolume = new StringType(); 1195 if (!(this.minimumVolume instanceof StringType)) 1196 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.minimumVolume.getClass().getName()+" was encountered"); 1197 return (StringType) this.minimumVolume; 1198 } 1199 1200 public boolean hasMinimumVolumeStringType() { 1201 return this != null && this.minimumVolume instanceof StringType; 1202 } 1203 1204 public boolean hasMinimumVolume() { 1205 return this.minimumVolume != null && !this.minimumVolume.isEmpty(); 1206 } 1207 1208 /** 1209 * @param value {@link #minimumVolume} (The minimum volume to be conditioned in the container.) 1210 */ 1211 public SpecimenDefinitionTypeTestedContainerComponent setMinimumVolume(DataType value) { 1212 if (value != null && !(value instanceof Quantity || value instanceof StringType)) 1213 throw new FHIRException("Not the right type for SpecimenDefinition.typeTested.container.minimumVolume[x]: "+value.fhirType()); 1214 this.minimumVolume = value; 1215 return this; 1216 } 1217 1218 /** 1219 * @return {@link #additive} (Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1220 */ 1221 public List<SpecimenDefinitionTypeTestedContainerAdditiveComponent> getAdditive() { 1222 if (this.additive == null) 1223 this.additive = new ArrayList<SpecimenDefinitionTypeTestedContainerAdditiveComponent>(); 1224 return this.additive; 1225 } 1226 1227 /** 1228 * @return Returns a reference to <code>this</code> for easy method chaining 1229 */ 1230 public SpecimenDefinitionTypeTestedContainerComponent setAdditive(List<SpecimenDefinitionTypeTestedContainerAdditiveComponent> theAdditive) { 1231 this.additive = theAdditive; 1232 return this; 1233 } 1234 1235 public boolean hasAdditive() { 1236 if (this.additive == null) 1237 return false; 1238 for (SpecimenDefinitionTypeTestedContainerAdditiveComponent item : this.additive) 1239 if (!item.isEmpty()) 1240 return true; 1241 return false; 1242 } 1243 1244 public SpecimenDefinitionTypeTestedContainerAdditiveComponent addAdditive() { //3 1245 SpecimenDefinitionTypeTestedContainerAdditiveComponent t = new SpecimenDefinitionTypeTestedContainerAdditiveComponent(); 1246 if (this.additive == null) 1247 this.additive = new ArrayList<SpecimenDefinitionTypeTestedContainerAdditiveComponent>(); 1248 this.additive.add(t); 1249 return t; 1250 } 1251 1252 public SpecimenDefinitionTypeTestedContainerComponent addAdditive(SpecimenDefinitionTypeTestedContainerAdditiveComponent t) { //3 1253 if (t == null) 1254 return this; 1255 if (this.additive == null) 1256 this.additive = new ArrayList<SpecimenDefinitionTypeTestedContainerAdditiveComponent>(); 1257 this.additive.add(t); 1258 return this; 1259 } 1260 1261 /** 1262 * @return The first repetition of repeating field {@link #additive}, creating it if it does not already exist {3} 1263 */ 1264 public SpecimenDefinitionTypeTestedContainerAdditiveComponent getAdditiveFirstRep() { 1265 if (getAdditive().isEmpty()) { 1266 addAdditive(); 1267 } 1268 return getAdditive().get(0); 1269 } 1270 1271 /** 1272 * @return {@link #preparation} (Special processing that should be applied to the container for this kind of specimen.). This is the underlying object with id, value and extensions. The accessor "getPreparation" gives direct access to the value 1273 */ 1274 public MarkdownType getPreparationElement() { 1275 if (this.preparation == null) 1276 if (Configuration.errorOnAutoCreate()) 1277 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedContainerComponent.preparation"); 1278 else if (Configuration.doAutoCreate()) 1279 this.preparation = new MarkdownType(); // bb 1280 return this.preparation; 1281 } 1282 1283 public boolean hasPreparationElement() { 1284 return this.preparation != null && !this.preparation.isEmpty(); 1285 } 1286 1287 public boolean hasPreparation() { 1288 return this.preparation != null && !this.preparation.isEmpty(); 1289 } 1290 1291 /** 1292 * @param value {@link #preparation} (Special processing that should be applied to the container for this kind of specimen.). This is the underlying object with id, value and extensions. The accessor "getPreparation" gives direct access to the value 1293 */ 1294 public SpecimenDefinitionTypeTestedContainerComponent setPreparationElement(MarkdownType value) { 1295 this.preparation = value; 1296 return this; 1297 } 1298 1299 /** 1300 * @return Special processing that should be applied to the container for this kind of specimen. 1301 */ 1302 public String getPreparation() { 1303 return this.preparation == null ? null : this.preparation.getValue(); 1304 } 1305 1306 /** 1307 * @param value Special processing that should be applied to the container for this kind of specimen. 1308 */ 1309 public SpecimenDefinitionTypeTestedContainerComponent setPreparation(String value) { 1310 if (Utilities.noString(value)) 1311 this.preparation = null; 1312 else { 1313 if (this.preparation == null) 1314 this.preparation = new MarkdownType(); 1315 this.preparation.setValue(value); 1316 } 1317 return this; 1318 } 1319 1320 protected void listChildren(List<Property> children) { 1321 super.listChildren(children); 1322 children.add(new Property("material", "CodeableConcept", "The type of material of the container.", 0, 1, material)); 1323 children.add(new Property("type", "CodeableConcept", "The type of container used to contain this kind of specimen.", 0, 1, type)); 1324 children.add(new Property("cap", "CodeableConcept", "Color of container cap.", 0, 1, cap)); 1325 children.add(new Property("description", "markdown", "The textual description of the kind of container.", 0, 1, description)); 1326 children.add(new Property("capacity", "Quantity", "The capacity (volume or other measure) of this kind of container.", 0, 1, capacity)); 1327 children.add(new Property("minimumVolume[x]", "Quantity|string", "The minimum volume to be conditioned in the container.", 0, 1, minimumVolume)); 1328 children.add(new Property("additive", "", "Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, java.lang.Integer.MAX_VALUE, additive)); 1329 children.add(new Property("preparation", "markdown", "Special processing that should be applied to the container for this kind of specimen.", 0, 1, preparation)); 1330 } 1331 1332 @Override 1333 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1334 switch (_hash) { 1335 case 299066663: /*material*/ return new Property("material", "CodeableConcept", "The type of material of the container.", 0, 1, material); 1336 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of container used to contain this kind of specimen.", 0, 1, type); 1337 case 98258: /*cap*/ return new Property("cap", "CodeableConcept", "Color of container cap.", 0, 1, cap); 1338 case -1724546052: /*description*/ return new Property("description", "markdown", "The textual description of the kind of container.", 0, 1, description); 1339 case -67824454: /*capacity*/ return new Property("capacity", "Quantity", "The capacity (volume or other measure) of this kind of container.", 0, 1, capacity); 1340 case 371830456: /*minimumVolume[x]*/ return new Property("minimumVolume[x]", "Quantity|string", "The minimum volume to be conditioned in the container.", 0, 1, minimumVolume); 1341 case -1674665784: /*minimumVolume*/ return new Property("minimumVolume[x]", "Quantity|string", "The minimum volume to be conditioned in the container.", 0, 1, minimumVolume); 1342 case -532143757: /*minimumVolumeQuantity*/ return new Property("minimumVolume[x]", "Quantity", "The minimum volume to be conditioned in the container.", 0, 1, minimumVolume); 1343 case 248461049: /*minimumVolumeString*/ return new Property("minimumVolume[x]", "string", "The minimum volume to be conditioned in the container.", 0, 1, minimumVolume); 1344 case -1226589236: /*additive*/ return new Property("additive", "", "Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, java.lang.Integer.MAX_VALUE, additive); 1345 case -1315428713: /*preparation*/ return new Property("preparation", "markdown", "Special processing that should be applied to the container for this kind of specimen.", 0, 1, preparation); 1346 default: return super.getNamedProperty(_hash, _name, _checkValid); 1347 } 1348 1349 } 1350 1351 @Override 1352 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1353 switch (hash) { 1354 case 299066663: /*material*/ return this.material == null ? new Base[0] : new Base[] {this.material}; // CodeableConcept 1355 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1356 case 98258: /*cap*/ return this.cap == null ? new Base[0] : new Base[] {this.cap}; // CodeableConcept 1357 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1358 case -67824454: /*capacity*/ return this.capacity == null ? new Base[0] : new Base[] {this.capacity}; // Quantity 1359 case -1674665784: /*minimumVolume*/ return this.minimumVolume == null ? new Base[0] : new Base[] {this.minimumVolume}; // DataType 1360 case -1226589236: /*additive*/ return this.additive == null ? new Base[0] : this.additive.toArray(new Base[this.additive.size()]); // SpecimenDefinitionTypeTestedContainerAdditiveComponent 1361 case -1315428713: /*preparation*/ return this.preparation == null ? new Base[0] : new Base[] {this.preparation}; // MarkdownType 1362 default: return super.getProperty(hash, name, checkValid); 1363 } 1364 1365 } 1366 1367 @Override 1368 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1369 switch (hash) { 1370 case 299066663: // material 1371 this.material = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1372 return value; 1373 case 3575610: // type 1374 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1375 return value; 1376 case 98258: // cap 1377 this.cap = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1378 return value; 1379 case -1724546052: // description 1380 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1381 return value; 1382 case -67824454: // capacity 1383 this.capacity = TypeConvertor.castToQuantity(value); // Quantity 1384 return value; 1385 case -1674665784: // minimumVolume 1386 this.minimumVolume = TypeConvertor.castToType(value); // DataType 1387 return value; 1388 case -1226589236: // additive 1389 this.getAdditive().add((SpecimenDefinitionTypeTestedContainerAdditiveComponent) value); // SpecimenDefinitionTypeTestedContainerAdditiveComponent 1390 return value; 1391 case -1315428713: // preparation 1392 this.preparation = TypeConvertor.castToMarkdown(value); // MarkdownType 1393 return value; 1394 default: return super.setProperty(hash, name, value); 1395 } 1396 1397 } 1398 1399 @Override 1400 public Base setProperty(String name, Base value) throws FHIRException { 1401 if (name.equals("material")) { 1402 this.material = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1403 } else if (name.equals("type")) { 1404 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1405 } else if (name.equals("cap")) { 1406 this.cap = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1407 } else if (name.equals("description")) { 1408 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1409 } else if (name.equals("capacity")) { 1410 this.capacity = TypeConvertor.castToQuantity(value); // Quantity 1411 } else if (name.equals("minimumVolume[x]")) { 1412 this.minimumVolume = TypeConvertor.castToType(value); // DataType 1413 } else if (name.equals("additive")) { 1414 this.getAdditive().add((SpecimenDefinitionTypeTestedContainerAdditiveComponent) value); 1415 } else if (name.equals("preparation")) { 1416 this.preparation = TypeConvertor.castToMarkdown(value); // MarkdownType 1417 } else 1418 return super.setProperty(name, value); 1419 return value; 1420 } 1421 1422 @Override 1423 public void removeChild(String name, Base value) throws FHIRException { 1424 if (name.equals("material")) { 1425 this.material = null; 1426 } else if (name.equals("type")) { 1427 this.type = null; 1428 } else if (name.equals("cap")) { 1429 this.cap = null; 1430 } else if (name.equals("description")) { 1431 this.description = null; 1432 } else if (name.equals("capacity")) { 1433 this.capacity = null; 1434 } else if (name.equals("minimumVolume[x]")) { 1435 this.minimumVolume = null; 1436 } else if (name.equals("additive")) { 1437 this.getAdditive().remove((SpecimenDefinitionTypeTestedContainerAdditiveComponent) value); 1438 } else if (name.equals("preparation")) { 1439 this.preparation = null; 1440 } else 1441 super.removeChild(name, value); 1442 1443 } 1444 1445 @Override 1446 public Base makeProperty(int hash, String name) throws FHIRException { 1447 switch (hash) { 1448 case 299066663: return getMaterial(); 1449 case 3575610: return getType(); 1450 case 98258: return getCap(); 1451 case -1724546052: return getDescriptionElement(); 1452 case -67824454: return getCapacity(); 1453 case 371830456: return getMinimumVolume(); 1454 case -1674665784: return getMinimumVolume(); 1455 case -1226589236: return addAdditive(); 1456 case -1315428713: return getPreparationElement(); 1457 default: return super.makeProperty(hash, name); 1458 } 1459 1460 } 1461 1462 @Override 1463 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1464 switch (hash) { 1465 case 299066663: /*material*/ return new String[] {"CodeableConcept"}; 1466 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1467 case 98258: /*cap*/ return new String[] {"CodeableConcept"}; 1468 case -1724546052: /*description*/ return new String[] {"markdown"}; 1469 case -67824454: /*capacity*/ return new String[] {"Quantity"}; 1470 case -1674665784: /*minimumVolume*/ return new String[] {"Quantity", "string"}; 1471 case -1226589236: /*additive*/ return new String[] {}; 1472 case -1315428713: /*preparation*/ return new String[] {"markdown"}; 1473 default: return super.getTypesForProperty(hash, name); 1474 } 1475 1476 } 1477 1478 @Override 1479 public Base addChild(String name) throws FHIRException { 1480 if (name.equals("material")) { 1481 this.material = new CodeableConcept(); 1482 return this.material; 1483 } 1484 else if (name.equals("type")) { 1485 this.type = new CodeableConcept(); 1486 return this.type; 1487 } 1488 else if (name.equals("cap")) { 1489 this.cap = new CodeableConcept(); 1490 return this.cap; 1491 } 1492 else if (name.equals("description")) { 1493 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.typeTested.container.description"); 1494 } 1495 else if (name.equals("capacity")) { 1496 this.capacity = new Quantity(); 1497 return this.capacity; 1498 } 1499 else if (name.equals("minimumVolumeQuantity")) { 1500 this.minimumVolume = new Quantity(); 1501 return this.minimumVolume; 1502 } 1503 else if (name.equals("minimumVolumeString")) { 1504 this.minimumVolume = new StringType(); 1505 return this.minimumVolume; 1506 } 1507 else if (name.equals("additive")) { 1508 return addAdditive(); 1509 } 1510 else if (name.equals("preparation")) { 1511 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.typeTested.container.preparation"); 1512 } 1513 else 1514 return super.addChild(name); 1515 } 1516 1517 public SpecimenDefinitionTypeTestedContainerComponent copy() { 1518 SpecimenDefinitionTypeTestedContainerComponent dst = new SpecimenDefinitionTypeTestedContainerComponent(); 1519 copyValues(dst); 1520 return dst; 1521 } 1522 1523 public void copyValues(SpecimenDefinitionTypeTestedContainerComponent dst) { 1524 super.copyValues(dst); 1525 dst.material = material == null ? null : material.copy(); 1526 dst.type = type == null ? null : type.copy(); 1527 dst.cap = cap == null ? null : cap.copy(); 1528 dst.description = description == null ? null : description.copy(); 1529 dst.capacity = capacity == null ? null : capacity.copy(); 1530 dst.minimumVolume = minimumVolume == null ? null : minimumVolume.copy(); 1531 if (additive != null) { 1532 dst.additive = new ArrayList<SpecimenDefinitionTypeTestedContainerAdditiveComponent>(); 1533 for (SpecimenDefinitionTypeTestedContainerAdditiveComponent i : additive) 1534 dst.additive.add(i.copy()); 1535 }; 1536 dst.preparation = preparation == null ? null : preparation.copy(); 1537 } 1538 1539 @Override 1540 public boolean equalsDeep(Base other_) { 1541 if (!super.equalsDeep(other_)) 1542 return false; 1543 if (!(other_ instanceof SpecimenDefinitionTypeTestedContainerComponent)) 1544 return false; 1545 SpecimenDefinitionTypeTestedContainerComponent o = (SpecimenDefinitionTypeTestedContainerComponent) other_; 1546 return compareDeep(material, o.material, true) && compareDeep(type, o.type, true) && compareDeep(cap, o.cap, true) 1547 && compareDeep(description, o.description, true) && compareDeep(capacity, o.capacity, true) && compareDeep(minimumVolume, o.minimumVolume, true) 1548 && compareDeep(additive, o.additive, true) && compareDeep(preparation, o.preparation, true); 1549 } 1550 1551 @Override 1552 public boolean equalsShallow(Base other_) { 1553 if (!super.equalsShallow(other_)) 1554 return false; 1555 if (!(other_ instanceof SpecimenDefinitionTypeTestedContainerComponent)) 1556 return false; 1557 SpecimenDefinitionTypeTestedContainerComponent o = (SpecimenDefinitionTypeTestedContainerComponent) other_; 1558 return compareValues(description, o.description, true) && compareValues(preparation, o.preparation, true) 1559 ; 1560 } 1561 1562 public boolean isEmpty() { 1563 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(material, type, cap, description 1564 , capacity, minimumVolume, additive, preparation); 1565 } 1566 1567 public String fhirType() { 1568 return "SpecimenDefinition.typeTested.container"; 1569 1570 } 1571 1572 } 1573 1574 @Block() 1575 public static class SpecimenDefinitionTypeTestedContainerAdditiveComponent extends BackboneElement implements IBaseBackboneElement { 1576 /** 1577 * Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA. 1578 */ 1579 @Child(name = "additive", type = {CodeableConcept.class, SubstanceDefinition.class}, order=1, min=1, max=1, modifier=false, summary=false) 1580 @Description(shortDefinition="Additive associated with container", formalDefinition="Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA." ) 1581 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v2-0371") 1582 protected DataType additive; 1583 1584 private static final long serialVersionUID = 201856258L; 1585 1586 /** 1587 * Constructor 1588 */ 1589 public SpecimenDefinitionTypeTestedContainerAdditiveComponent() { 1590 super(); 1591 } 1592 1593 /** 1594 * Constructor 1595 */ 1596 public SpecimenDefinitionTypeTestedContainerAdditiveComponent(DataType additive) { 1597 super(); 1598 this.setAdditive(additive); 1599 } 1600 1601 /** 1602 * @return {@link #additive} (Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1603 */ 1604 public DataType getAdditive() { 1605 return this.additive; 1606 } 1607 1608 /** 1609 * @return {@link #additive} (Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1610 */ 1611 public CodeableConcept getAdditiveCodeableConcept() throws FHIRException { 1612 if (this.additive == null) 1613 this.additive = new CodeableConcept(); 1614 if (!(this.additive instanceof CodeableConcept)) 1615 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.additive.getClass().getName()+" was encountered"); 1616 return (CodeableConcept) this.additive; 1617 } 1618 1619 public boolean hasAdditiveCodeableConcept() { 1620 return this != null && this.additive instanceof CodeableConcept; 1621 } 1622 1623 /** 1624 * @return {@link #additive} (Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1625 */ 1626 public Reference getAdditiveReference() throws FHIRException { 1627 if (this.additive == null) 1628 this.additive = new Reference(); 1629 if (!(this.additive instanceof Reference)) 1630 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.additive.getClass().getName()+" was encountered"); 1631 return (Reference) this.additive; 1632 } 1633 1634 public boolean hasAdditiveReference() { 1635 return this != null && this.additive instanceof Reference; 1636 } 1637 1638 public boolean hasAdditive() { 1639 return this.additive != null && !this.additive.isEmpty(); 1640 } 1641 1642 /** 1643 * @param value {@link #additive} (Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1644 */ 1645 public SpecimenDefinitionTypeTestedContainerAdditiveComponent setAdditive(DataType value) { 1646 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1647 throw new FHIRException("Not the right type for SpecimenDefinition.typeTested.container.additive.additive[x]: "+value.fhirType()); 1648 this.additive = value; 1649 return this; 1650 } 1651 1652 protected void listChildren(List<Property> children) { 1653 super.listChildren(children); 1654 children.add(new Property("additive[x]", "CodeableConcept|Reference(SubstanceDefinition)", "Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1, additive)); 1655 } 1656 1657 @Override 1658 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1659 switch (_hash) { 1660 case 261915956: /*additive[x]*/ return new Property("additive[x]", "CodeableConcept|Reference(SubstanceDefinition)", "Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1, additive); 1661 case -1226589236: /*additive*/ return new Property("additive[x]", "CodeableConcept|Reference(SubstanceDefinition)", "Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1, additive); 1662 case 1330272821: /*additiveCodeableConcept*/ return new Property("additive[x]", "CodeableConcept", "Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1, additive); 1663 case -386783009: /*additiveReference*/ return new Property("additive[x]", "Reference(SubstanceDefinition)", "Substance introduced in the kind of container to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1, additive); 1664 default: return super.getNamedProperty(_hash, _name, _checkValid); 1665 } 1666 1667 } 1668 1669 @Override 1670 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1671 switch (hash) { 1672 case -1226589236: /*additive*/ return this.additive == null ? new Base[0] : new Base[] {this.additive}; // DataType 1673 default: return super.getProperty(hash, name, checkValid); 1674 } 1675 1676 } 1677 1678 @Override 1679 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1680 switch (hash) { 1681 case -1226589236: // additive 1682 this.additive = TypeConvertor.castToType(value); // DataType 1683 return value; 1684 default: return super.setProperty(hash, name, value); 1685 } 1686 1687 } 1688 1689 @Override 1690 public Base setProperty(String name, Base value) throws FHIRException { 1691 if (name.equals("additive[x]")) { 1692 this.additive = TypeConvertor.castToType(value); // DataType 1693 } else 1694 return super.setProperty(name, value); 1695 return value; 1696 } 1697 1698 @Override 1699 public void removeChild(String name, Base value) throws FHIRException { 1700 if (name.equals("additive[x]")) { 1701 this.additive = null; 1702 } else 1703 super.removeChild(name, value); 1704 1705 } 1706 1707 @Override 1708 public Base makeProperty(int hash, String name) throws FHIRException { 1709 switch (hash) { 1710 case 261915956: return getAdditive(); 1711 case -1226589236: return getAdditive(); 1712 default: return super.makeProperty(hash, name); 1713 } 1714 1715 } 1716 1717 @Override 1718 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1719 switch (hash) { 1720 case -1226589236: /*additive*/ return new String[] {"CodeableConcept", "Reference"}; 1721 default: return super.getTypesForProperty(hash, name); 1722 } 1723 1724 } 1725 1726 @Override 1727 public Base addChild(String name) throws FHIRException { 1728 if (name.equals("additiveCodeableConcept")) { 1729 this.additive = new CodeableConcept(); 1730 return this.additive; 1731 } 1732 else if (name.equals("additiveReference")) { 1733 this.additive = new Reference(); 1734 return this.additive; 1735 } 1736 else 1737 return super.addChild(name); 1738 } 1739 1740 public SpecimenDefinitionTypeTestedContainerAdditiveComponent copy() { 1741 SpecimenDefinitionTypeTestedContainerAdditiveComponent dst = new SpecimenDefinitionTypeTestedContainerAdditiveComponent(); 1742 copyValues(dst); 1743 return dst; 1744 } 1745 1746 public void copyValues(SpecimenDefinitionTypeTestedContainerAdditiveComponent dst) { 1747 super.copyValues(dst); 1748 dst.additive = additive == null ? null : additive.copy(); 1749 } 1750 1751 @Override 1752 public boolean equalsDeep(Base other_) { 1753 if (!super.equalsDeep(other_)) 1754 return false; 1755 if (!(other_ instanceof SpecimenDefinitionTypeTestedContainerAdditiveComponent)) 1756 return false; 1757 SpecimenDefinitionTypeTestedContainerAdditiveComponent o = (SpecimenDefinitionTypeTestedContainerAdditiveComponent) other_; 1758 return compareDeep(additive, o.additive, true); 1759 } 1760 1761 @Override 1762 public boolean equalsShallow(Base other_) { 1763 if (!super.equalsShallow(other_)) 1764 return false; 1765 if (!(other_ instanceof SpecimenDefinitionTypeTestedContainerAdditiveComponent)) 1766 return false; 1767 SpecimenDefinitionTypeTestedContainerAdditiveComponent o = (SpecimenDefinitionTypeTestedContainerAdditiveComponent) other_; 1768 return true; 1769 } 1770 1771 public boolean isEmpty() { 1772 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(additive); 1773 } 1774 1775 public String fhirType() { 1776 return "SpecimenDefinition.typeTested.container.additive"; 1777 1778 } 1779 1780 } 1781 1782 @Block() 1783 public static class SpecimenDefinitionTypeTestedHandlingComponent extends BackboneElement implements IBaseBackboneElement { 1784 /** 1785 * It qualifies the interval of temperature, which characterizes an occurrence of handling. Conditions that are not related to temperature may be handled in the instruction element. 1786 */ 1787 @Child(name = "temperatureQualifier", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1788 @Description(shortDefinition="Qualifies the interval of temperature", formalDefinition="It qualifies the interval of temperature, which characterizes an occurrence of handling. Conditions that are not related to temperature may be handled in the instruction element." ) 1789 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/handling-condition") 1790 protected CodeableConcept temperatureQualifier; 1791 1792 /** 1793 * The temperature interval for this set of handling instructions. 1794 */ 1795 @Child(name = "temperatureRange", type = {Range.class}, order=2, min=0, max=1, modifier=false, summary=false) 1796 @Description(shortDefinition="Temperature range for these handling instructions", formalDefinition="The temperature interval for this set of handling instructions." ) 1797 protected Range temperatureRange; 1798 1799 /** 1800 * The maximum time interval of preservation of the specimen with these conditions. 1801 */ 1802 @Child(name = "maxDuration", type = {Duration.class}, order=3, min=0, max=1, modifier=false, summary=false) 1803 @Description(shortDefinition="Maximum preservation time", formalDefinition="The maximum time interval of preservation of the specimen with these conditions." ) 1804 protected Duration maxDuration; 1805 1806 /** 1807 * Additional textual instructions for the preservation or transport of the specimen. For instance, 'Protect from light exposure'. 1808 */ 1809 @Child(name = "instruction", type = {MarkdownType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1810 @Description(shortDefinition="Preservation instruction", formalDefinition="Additional textual instructions for the preservation or transport of the specimen. For instance, 'Protect from light exposure'." ) 1811 protected MarkdownType instruction; 1812 1813 private static final long serialVersionUID = 113016318L; 1814 1815 /** 1816 * Constructor 1817 */ 1818 public SpecimenDefinitionTypeTestedHandlingComponent() { 1819 super(); 1820 } 1821 1822 /** 1823 * @return {@link #temperatureQualifier} (It qualifies the interval of temperature, which characterizes an occurrence of handling. Conditions that are not related to temperature may be handled in the instruction element.) 1824 */ 1825 public CodeableConcept getTemperatureQualifier() { 1826 if (this.temperatureQualifier == null) 1827 if (Configuration.errorOnAutoCreate()) 1828 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedHandlingComponent.temperatureQualifier"); 1829 else if (Configuration.doAutoCreate()) 1830 this.temperatureQualifier = new CodeableConcept(); // cc 1831 return this.temperatureQualifier; 1832 } 1833 1834 public boolean hasTemperatureQualifier() { 1835 return this.temperatureQualifier != null && !this.temperatureQualifier.isEmpty(); 1836 } 1837 1838 /** 1839 * @param value {@link #temperatureQualifier} (It qualifies the interval of temperature, which characterizes an occurrence of handling. Conditions that are not related to temperature may be handled in the instruction element.) 1840 */ 1841 public SpecimenDefinitionTypeTestedHandlingComponent setTemperatureQualifier(CodeableConcept value) { 1842 this.temperatureQualifier = value; 1843 return this; 1844 } 1845 1846 /** 1847 * @return {@link #temperatureRange} (The temperature interval for this set of handling instructions.) 1848 */ 1849 public Range getTemperatureRange() { 1850 if (this.temperatureRange == null) 1851 if (Configuration.errorOnAutoCreate()) 1852 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedHandlingComponent.temperatureRange"); 1853 else if (Configuration.doAutoCreate()) 1854 this.temperatureRange = new Range(); // cc 1855 return this.temperatureRange; 1856 } 1857 1858 public boolean hasTemperatureRange() { 1859 return this.temperatureRange != null && !this.temperatureRange.isEmpty(); 1860 } 1861 1862 /** 1863 * @param value {@link #temperatureRange} (The temperature interval for this set of handling instructions.) 1864 */ 1865 public SpecimenDefinitionTypeTestedHandlingComponent setTemperatureRange(Range value) { 1866 this.temperatureRange = value; 1867 return this; 1868 } 1869 1870 /** 1871 * @return {@link #maxDuration} (The maximum time interval of preservation of the specimen with these conditions.) 1872 */ 1873 public Duration getMaxDuration() { 1874 if (this.maxDuration == null) 1875 if (Configuration.errorOnAutoCreate()) 1876 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedHandlingComponent.maxDuration"); 1877 else if (Configuration.doAutoCreate()) 1878 this.maxDuration = new Duration(); // cc 1879 return this.maxDuration; 1880 } 1881 1882 public boolean hasMaxDuration() { 1883 return this.maxDuration != null && !this.maxDuration.isEmpty(); 1884 } 1885 1886 /** 1887 * @param value {@link #maxDuration} (The maximum time interval of preservation of the specimen with these conditions.) 1888 */ 1889 public SpecimenDefinitionTypeTestedHandlingComponent setMaxDuration(Duration value) { 1890 this.maxDuration = value; 1891 return this; 1892 } 1893 1894 /** 1895 * @return {@link #instruction} (Additional textual instructions for the preservation or transport of the specimen. For instance, 'Protect from light exposure'.). This is the underlying object with id, value and extensions. The accessor "getInstruction" gives direct access to the value 1896 */ 1897 public MarkdownType getInstructionElement() { 1898 if (this.instruction == null) 1899 if (Configuration.errorOnAutoCreate()) 1900 throw new Error("Attempt to auto-create SpecimenDefinitionTypeTestedHandlingComponent.instruction"); 1901 else if (Configuration.doAutoCreate()) 1902 this.instruction = new MarkdownType(); // bb 1903 return this.instruction; 1904 } 1905 1906 public boolean hasInstructionElement() { 1907 return this.instruction != null && !this.instruction.isEmpty(); 1908 } 1909 1910 public boolean hasInstruction() { 1911 return this.instruction != null && !this.instruction.isEmpty(); 1912 } 1913 1914 /** 1915 * @param value {@link #instruction} (Additional textual instructions for the preservation or transport of the specimen. For instance, 'Protect from light exposure'.). This is the underlying object with id, value and extensions. The accessor "getInstruction" gives direct access to the value 1916 */ 1917 public SpecimenDefinitionTypeTestedHandlingComponent setInstructionElement(MarkdownType value) { 1918 this.instruction = value; 1919 return this; 1920 } 1921 1922 /** 1923 * @return Additional textual instructions for the preservation or transport of the specimen. For instance, 'Protect from light exposure'. 1924 */ 1925 public String getInstruction() { 1926 return this.instruction == null ? null : this.instruction.getValue(); 1927 } 1928 1929 /** 1930 * @param value Additional textual instructions for the preservation or transport of the specimen. For instance, 'Protect from light exposure'. 1931 */ 1932 public SpecimenDefinitionTypeTestedHandlingComponent setInstruction(String value) { 1933 if (Utilities.noString(value)) 1934 this.instruction = null; 1935 else { 1936 if (this.instruction == null) 1937 this.instruction = new MarkdownType(); 1938 this.instruction.setValue(value); 1939 } 1940 return this; 1941 } 1942 1943 protected void listChildren(List<Property> children) { 1944 super.listChildren(children); 1945 children.add(new Property("temperatureQualifier", "CodeableConcept", "It qualifies the interval of temperature, which characterizes an occurrence of handling. Conditions that are not related to temperature may be handled in the instruction element.", 0, 1, temperatureQualifier)); 1946 children.add(new Property("temperatureRange", "Range", "The temperature interval for this set of handling instructions.", 0, 1, temperatureRange)); 1947 children.add(new Property("maxDuration", "Duration", "The maximum time interval of preservation of the specimen with these conditions.", 0, 1, maxDuration)); 1948 children.add(new Property("instruction", "markdown", "Additional textual instructions for the preservation or transport of the specimen. For instance, 'Protect from light exposure'.", 0, 1, instruction)); 1949 } 1950 1951 @Override 1952 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1953 switch (_hash) { 1954 case 548941206: /*temperatureQualifier*/ return new Property("temperatureQualifier", "CodeableConcept", "It qualifies the interval of temperature, which characterizes an occurrence of handling. Conditions that are not related to temperature may be handled in the instruction element.", 0, 1, temperatureQualifier); 1955 case -39203799: /*temperatureRange*/ return new Property("temperatureRange", "Range", "The temperature interval for this set of handling instructions.", 0, 1, temperatureRange); 1956 case 40284952: /*maxDuration*/ return new Property("maxDuration", "Duration", "The maximum time interval of preservation of the specimen with these conditions.", 0, 1, maxDuration); 1957 case 301526158: /*instruction*/ return new Property("instruction", "markdown", "Additional textual instructions for the preservation or transport of the specimen. For instance, 'Protect from light exposure'.", 0, 1, instruction); 1958 default: return super.getNamedProperty(_hash, _name, _checkValid); 1959 } 1960 1961 } 1962 1963 @Override 1964 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1965 switch (hash) { 1966 case 548941206: /*temperatureQualifier*/ return this.temperatureQualifier == null ? new Base[0] : new Base[] {this.temperatureQualifier}; // CodeableConcept 1967 case -39203799: /*temperatureRange*/ return this.temperatureRange == null ? new Base[0] : new Base[] {this.temperatureRange}; // Range 1968 case 40284952: /*maxDuration*/ return this.maxDuration == null ? new Base[0] : new Base[] {this.maxDuration}; // Duration 1969 case 301526158: /*instruction*/ return this.instruction == null ? new Base[0] : new Base[] {this.instruction}; // MarkdownType 1970 default: return super.getProperty(hash, name, checkValid); 1971 } 1972 1973 } 1974 1975 @Override 1976 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1977 switch (hash) { 1978 case 548941206: // temperatureQualifier 1979 this.temperatureQualifier = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1980 return value; 1981 case -39203799: // temperatureRange 1982 this.temperatureRange = TypeConvertor.castToRange(value); // Range 1983 return value; 1984 case 40284952: // maxDuration 1985 this.maxDuration = TypeConvertor.castToDuration(value); // Duration 1986 return value; 1987 case 301526158: // instruction 1988 this.instruction = TypeConvertor.castToMarkdown(value); // MarkdownType 1989 return value; 1990 default: return super.setProperty(hash, name, value); 1991 } 1992 1993 } 1994 1995 @Override 1996 public Base setProperty(String name, Base value) throws FHIRException { 1997 if (name.equals("temperatureQualifier")) { 1998 this.temperatureQualifier = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1999 } else if (name.equals("temperatureRange")) { 2000 this.temperatureRange = TypeConvertor.castToRange(value); // Range 2001 } else if (name.equals("maxDuration")) { 2002 this.maxDuration = TypeConvertor.castToDuration(value); // Duration 2003 } else if (name.equals("instruction")) { 2004 this.instruction = TypeConvertor.castToMarkdown(value); // MarkdownType 2005 } else 2006 return super.setProperty(name, value); 2007 return value; 2008 } 2009 2010 @Override 2011 public void removeChild(String name, Base value) throws FHIRException { 2012 if (name.equals("temperatureQualifier")) { 2013 this.temperatureQualifier = null; 2014 } else if (name.equals("temperatureRange")) { 2015 this.temperatureRange = null; 2016 } else if (name.equals("maxDuration")) { 2017 this.maxDuration = null; 2018 } else if (name.equals("instruction")) { 2019 this.instruction = null; 2020 } else 2021 super.removeChild(name, value); 2022 2023 } 2024 2025 @Override 2026 public Base makeProperty(int hash, String name) throws FHIRException { 2027 switch (hash) { 2028 case 548941206: return getTemperatureQualifier(); 2029 case -39203799: return getTemperatureRange(); 2030 case 40284952: return getMaxDuration(); 2031 case 301526158: return getInstructionElement(); 2032 default: return super.makeProperty(hash, name); 2033 } 2034 2035 } 2036 2037 @Override 2038 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2039 switch (hash) { 2040 case 548941206: /*temperatureQualifier*/ return new String[] {"CodeableConcept"}; 2041 case -39203799: /*temperatureRange*/ return new String[] {"Range"}; 2042 case 40284952: /*maxDuration*/ return new String[] {"Duration"}; 2043 case 301526158: /*instruction*/ return new String[] {"markdown"}; 2044 default: return super.getTypesForProperty(hash, name); 2045 } 2046 2047 } 2048 2049 @Override 2050 public Base addChild(String name) throws FHIRException { 2051 if (name.equals("temperatureQualifier")) { 2052 this.temperatureQualifier = new CodeableConcept(); 2053 return this.temperatureQualifier; 2054 } 2055 else if (name.equals("temperatureRange")) { 2056 this.temperatureRange = new Range(); 2057 return this.temperatureRange; 2058 } 2059 else if (name.equals("maxDuration")) { 2060 this.maxDuration = new Duration(); 2061 return this.maxDuration; 2062 } 2063 else if (name.equals("instruction")) { 2064 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.typeTested.handling.instruction"); 2065 } 2066 else 2067 return super.addChild(name); 2068 } 2069 2070 public SpecimenDefinitionTypeTestedHandlingComponent copy() { 2071 SpecimenDefinitionTypeTestedHandlingComponent dst = new SpecimenDefinitionTypeTestedHandlingComponent(); 2072 copyValues(dst); 2073 return dst; 2074 } 2075 2076 public void copyValues(SpecimenDefinitionTypeTestedHandlingComponent dst) { 2077 super.copyValues(dst); 2078 dst.temperatureQualifier = temperatureQualifier == null ? null : temperatureQualifier.copy(); 2079 dst.temperatureRange = temperatureRange == null ? null : temperatureRange.copy(); 2080 dst.maxDuration = maxDuration == null ? null : maxDuration.copy(); 2081 dst.instruction = instruction == null ? null : instruction.copy(); 2082 } 2083 2084 @Override 2085 public boolean equalsDeep(Base other_) { 2086 if (!super.equalsDeep(other_)) 2087 return false; 2088 if (!(other_ instanceof SpecimenDefinitionTypeTestedHandlingComponent)) 2089 return false; 2090 SpecimenDefinitionTypeTestedHandlingComponent o = (SpecimenDefinitionTypeTestedHandlingComponent) other_; 2091 return compareDeep(temperatureQualifier, o.temperatureQualifier, true) && compareDeep(temperatureRange, o.temperatureRange, true) 2092 && compareDeep(maxDuration, o.maxDuration, true) && compareDeep(instruction, o.instruction, true) 2093 ; 2094 } 2095 2096 @Override 2097 public boolean equalsShallow(Base other_) { 2098 if (!super.equalsShallow(other_)) 2099 return false; 2100 if (!(other_ instanceof SpecimenDefinitionTypeTestedHandlingComponent)) 2101 return false; 2102 SpecimenDefinitionTypeTestedHandlingComponent o = (SpecimenDefinitionTypeTestedHandlingComponent) other_; 2103 return compareValues(instruction, o.instruction, true); 2104 } 2105 2106 public boolean isEmpty() { 2107 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(temperatureQualifier, temperatureRange 2108 , maxDuration, instruction); 2109 } 2110 2111 public String fhirType() { 2112 return "SpecimenDefinition.typeTested.handling"; 2113 2114 } 2115 2116 } 2117 2118 /** 2119 * An absolute URL that is used to identify this SpecimenDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this SpecimenDefinition is (or will be) published. The URL SHOULD include the major version of the SpecimenDefinition. For more information see Technical and Business Versions. 2120 */ 2121 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 2122 @Description(shortDefinition="Logical canonical URL to reference this SpecimenDefinition (globally unique)", formalDefinition="An absolute URL that is used to identify this SpecimenDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this SpecimenDefinition is (or will be) published. The URL SHOULD include the major version of the SpecimenDefinition. For more information see Technical and Business Versions." ) 2123 protected UriType url; 2124 2125 /** 2126 * A business identifier assigned to this SpecimenDefinition. 2127 */ 2128 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 2129 @Description(shortDefinition="Business identifier", formalDefinition="A business identifier assigned to this SpecimenDefinition." ) 2130 protected Identifier identifier; 2131 2132 /** 2133 * The identifier that is used to identify this version of the SpecimenDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the SpecimenDefinition author and is not expected to be globally unique. 2134 */ 2135 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2136 @Description(shortDefinition="Business version of the SpecimenDefinition", formalDefinition="The identifier that is used to identify this version of the SpecimenDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the SpecimenDefinition author and is not expected to be globally unique." ) 2137 protected StringType version; 2138 2139 /** 2140 * Indicates the mechanism used to compare versions to determine which is more current. 2141 */ 2142 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 2143 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 2144 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 2145 protected DataType versionAlgorithm; 2146 2147 /** 2148 * A natural language name identifying the specimen definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2149 */ 2150 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 2151 @Description(shortDefinition="Name for this specimen definition (computer friendly)", formalDefinition="A natural language name identifying the specimen definition. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 2152 protected StringType name; 2153 2154 /** 2155 * A short, descriptive, user-friendly title for the SpecimenDefinition. 2156 */ 2157 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 2158 @Description(shortDefinition="Name for this SpecimenDefinition (Human friendly)", formalDefinition="A short, descriptive, user-friendly title for the SpecimenDefinition." ) 2159 protected StringType title; 2160 2161 /** 2162 * The canonical URL pointing to another FHIR-defined SpecimenDefinition that is adhered to in whole or in part by this definition. 2163 */ 2164 @Child(name = "derivedFromCanonical", type = {CanonicalType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2165 @Description(shortDefinition="Based on FHIR definition of another SpecimenDefinition", formalDefinition="The canonical URL pointing to another FHIR-defined SpecimenDefinition that is adhered to in whole or in part by this definition." ) 2166 protected List<CanonicalType> derivedFromCanonical; 2167 2168 /** 2169 * The URL pointing to an externally-defined type of specimen, guideline or other definition that is adhered to in whole or in part by this definition. 2170 */ 2171 @Child(name = "derivedFromUri", type = {UriType.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2172 @Description(shortDefinition="Based on external definition", formalDefinition="The URL pointing to an externally-defined type of specimen, guideline or other definition that is adhered to in whole or in part by this definition." ) 2173 protected List<UriType> derivedFromUri; 2174 2175 /** 2176 * The current state of theSpecimenDefinition. 2177 */ 2178 @Child(name = "status", type = {CodeType.class}, order=8, min=1, max=1, modifier=true, summary=true) 2179 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The current state of theSpecimenDefinition." ) 2180 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 2181 protected Enumeration<PublicationStatus> status; 2182 2183 /** 2184 * A flag to indicate that this SpecimenDefinition is not authored for genuine usage. 2185 */ 2186 @Child(name = "experimental", type = {BooleanType.class}, order=9, min=0, max=1, modifier=false, summary=true) 2187 @Description(shortDefinition="If this SpecimenDefinition is not for real usage", formalDefinition="A flag to indicate that this SpecimenDefinition is not authored for genuine usage." ) 2188 protected BooleanType experimental; 2189 2190 /** 2191 * A code or group definition that describes the intended subject from which this kind of specimen is to be collected. 2192 */ 2193 @Child(name = "subject", type = {CodeableConcept.class, Group.class}, order=10, min=0, max=1, modifier=false, summary=true) 2194 @Description(shortDefinition="Type of subject for specimen collection", formalDefinition="A code or group definition that describes the intended subject from which this kind of specimen is to be collected." ) 2195 protected DataType subject; 2196 2197 /** 2198 * For draft definitions, indicates the date of initial creation. For active definitions, represents the date of activation. For withdrawn definitions, indicates the date of withdrawal. 2199 */ 2200 @Child(name = "date", type = {DateTimeType.class}, order=11, min=0, max=1, modifier=false, summary=true) 2201 @Description(shortDefinition="Date status first applied", formalDefinition="For draft definitions, indicates the date of initial creation. For active definitions, represents the date of activation. For withdrawn definitions, indicates the date of withdrawal." ) 2202 protected DateTimeType date; 2203 2204 /** 2205 * Helps establish the "authority/credibility" of the SpecimenDefinition. May also allow for contact. 2206 */ 2207 @Child(name = "publisher", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=true) 2208 @Description(shortDefinition="The name of the individual or organization that published the SpecimenDefinition", formalDefinition="Helps establish the \"authority/credibility\" of the SpecimenDefinition. May also allow for contact." ) 2209 protected StringType publisher; 2210 2211 /** 2212 * Contact details to assist a user in finding and communicating with the publisher. 2213 */ 2214 @Child(name = "contact", type = {ContactDetail.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2215 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 2216 protected List<ContactDetail> contact; 2217 2218 /** 2219 * A free text natural language description of the SpecimenDefinition from the consumer's perspective. 2220 */ 2221 @Child(name = "description", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 2222 @Description(shortDefinition="Natural language description of the SpecimenDefinition", formalDefinition="A free text natural language description of the SpecimenDefinition from the consumer's perspective." ) 2223 protected MarkdownType description; 2224 2225 /** 2226 * The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of specimen definitions. 2227 */ 2228 @Child(name = "useContext", type = {UsageContext.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2229 @Description(shortDefinition="Content intends to support these contexts", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of specimen definitions." ) 2230 protected List<UsageContext> useContext; 2231 2232 /** 2233 * A jurisdiction in which the SpecimenDefinition is intended to be used. 2234 */ 2235 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2236 @Description(shortDefinition="Intended jurisdiction for this SpecimenDefinition (if applicable)", formalDefinition="A jurisdiction in which the SpecimenDefinition is intended to be used." ) 2237 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 2238 protected List<CodeableConcept> jurisdiction; 2239 2240 /** 2241 * Explains why this SpecimeDefinition is needed and why it has been designed as it has. 2242 */ 2243 @Child(name = "purpose", type = {MarkdownType.class}, order=17, min=0, max=1, modifier=false, summary=false) 2244 @Description(shortDefinition="Why this SpecimenDefinition is defined", formalDefinition="Explains why this SpecimeDefinition is needed and why it has been designed as it has." ) 2245 protected MarkdownType purpose; 2246 2247 /** 2248 * Copyright statement relating to the SpecimenDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SpecimenDefinition. 2249 */ 2250 @Child(name = "copyright", type = {MarkdownType.class}, order=18, min=0, max=1, modifier=false, summary=false) 2251 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="Copyright statement relating to the SpecimenDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SpecimenDefinition." ) 2252 protected MarkdownType copyright; 2253 2254 /** 2255 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2256 */ 2257 @Child(name = "copyrightLabel", type = {StringType.class}, order=19, min=0, max=1, modifier=false, summary=false) 2258 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 2259 protected StringType copyrightLabel; 2260 2261 /** 2262 * The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage. 2263 */ 2264 @Child(name = "approvalDate", type = {DateType.class}, order=20, min=0, max=1, modifier=false, summary=false) 2265 @Description(shortDefinition="When SpecimenDefinition was approved by publisher", formalDefinition="The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 2266 protected DateType approvalDate; 2267 2268 /** 2269 * The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date. 2270 */ 2271 @Child(name = "lastReviewDate", type = {DateType.class}, order=21, min=0, max=1, modifier=false, summary=false) 2272 @Description(shortDefinition="The date on which the asset content was last reviewed by the publisher", formalDefinition="The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date." ) 2273 protected DateType lastReviewDate; 2274 2275 /** 2276 * The period during which the SpecimenDefinition content was or is planned to be effective. 2277 */ 2278 @Child(name = "effectivePeriod", type = {Period.class}, order=22, min=0, max=1, modifier=false, summary=true) 2279 @Description(shortDefinition="The effective date range for the SpecimenDefinition", formalDefinition="The period during which the SpecimenDefinition content was or is planned to be effective." ) 2280 protected Period effectivePeriod; 2281 2282 /** 2283 * The kind of material to be collected. 2284 */ 2285 @Child(name = "typeCollected", type = {CodeableConcept.class}, order=23, min=0, max=1, modifier=false, summary=true) 2286 @Description(shortDefinition="Kind of material to collect", formalDefinition="The kind of material to be collected." ) 2287 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v2-0487") 2288 protected CodeableConcept typeCollected; 2289 2290 /** 2291 * Preparation of the patient for specimen collection. 2292 */ 2293 @Child(name = "patientPreparation", type = {CodeableConcept.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2294 @Description(shortDefinition="Patient preparation for collection", formalDefinition="Preparation of the patient for specimen collection." ) 2295 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/prepare-patient-prior-specimen-collection") 2296 protected List<CodeableConcept> patientPreparation; 2297 2298 /** 2299 * Time aspect of specimen collection (duration or offset). 2300 */ 2301 @Child(name = "timeAspect", type = {StringType.class}, order=25, min=0, max=1, modifier=false, summary=true) 2302 @Description(shortDefinition="Time aspect for collection", formalDefinition="Time aspect of specimen collection (duration or offset)." ) 2303 protected StringType timeAspect; 2304 2305 /** 2306 * The action to be performed for collecting the specimen. 2307 */ 2308 @Child(name = "collection", type = {CodeableConcept.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2309 @Description(shortDefinition="Specimen collection procedure", formalDefinition="The action to be performed for collecting the specimen." ) 2310 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/specimen-collection") 2311 protected List<CodeableConcept> collection; 2312 2313 /** 2314 * Specimen conditioned in a container as expected by the testing laboratory. 2315 */ 2316 @Child(name = "typeTested", type = {}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2317 @Description(shortDefinition="Specimen in container intended for testing by lab", formalDefinition="Specimen conditioned in a container as expected by the testing laboratory." ) 2318 protected List<SpecimenDefinitionTypeTestedComponent> typeTested; 2319 2320 private static final long serialVersionUID = 1698015458L; 2321 2322 /** 2323 * Constructor 2324 */ 2325 public SpecimenDefinition() { 2326 super(); 2327 } 2328 2329 /** 2330 * Constructor 2331 */ 2332 public SpecimenDefinition(PublicationStatus status) { 2333 super(); 2334 this.setStatus(status); 2335 } 2336 2337 /** 2338 * @return {@link #url} (An absolute URL that is used to identify this SpecimenDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this SpecimenDefinition is (or will be) published. The URL SHOULD include the major version of the SpecimenDefinition. For more information see Technical and Business Versions.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2339 */ 2340 public UriType getUrlElement() { 2341 if (this.url == null) 2342 if (Configuration.errorOnAutoCreate()) 2343 throw new Error("Attempt to auto-create SpecimenDefinition.url"); 2344 else if (Configuration.doAutoCreate()) 2345 this.url = new UriType(); // bb 2346 return this.url; 2347 } 2348 2349 public boolean hasUrlElement() { 2350 return this.url != null && !this.url.isEmpty(); 2351 } 2352 2353 public boolean hasUrl() { 2354 return this.url != null && !this.url.isEmpty(); 2355 } 2356 2357 /** 2358 * @param value {@link #url} (An absolute URL that is used to identify this SpecimenDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this SpecimenDefinition is (or will be) published. The URL SHOULD include the major version of the SpecimenDefinition. For more information see Technical and Business Versions.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2359 */ 2360 public SpecimenDefinition setUrlElement(UriType value) { 2361 this.url = value; 2362 return this; 2363 } 2364 2365 /** 2366 * @return An absolute URL that is used to identify this SpecimenDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this SpecimenDefinition is (or will be) published. The URL SHOULD include the major version of the SpecimenDefinition. For more information see Technical and Business Versions. 2367 */ 2368 public String getUrl() { 2369 return this.url == null ? null : this.url.getValue(); 2370 } 2371 2372 /** 2373 * @param value An absolute URL that is used to identify this SpecimenDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this SpecimenDefinition is (or will be) published. The URL SHOULD include the major version of the SpecimenDefinition. For more information see Technical and Business Versions. 2374 */ 2375 public SpecimenDefinition setUrl(String value) { 2376 if (Utilities.noString(value)) 2377 this.url = null; 2378 else { 2379 if (this.url == null) 2380 this.url = new UriType(); 2381 this.url.setValue(value); 2382 } 2383 return this; 2384 } 2385 2386 /** 2387 * @return {@link #identifier} (A business identifier assigned to this SpecimenDefinition.) 2388 */ 2389 public Identifier getIdentifier() { 2390 if (this.identifier == null) 2391 if (Configuration.errorOnAutoCreate()) 2392 throw new Error("Attempt to auto-create SpecimenDefinition.identifier"); 2393 else if (Configuration.doAutoCreate()) 2394 this.identifier = new Identifier(); // cc 2395 return this.identifier; 2396 } 2397 2398 public boolean hasIdentifier() { 2399 return this.identifier != null && !this.identifier.isEmpty(); 2400 } 2401 2402 /** 2403 * @param value {@link #identifier} (A business identifier assigned to this SpecimenDefinition.) 2404 */ 2405 public SpecimenDefinition setIdentifier(Identifier value) { 2406 this.identifier = value; 2407 return this; 2408 } 2409 2410 /** 2411 * @return {@link #version} (The identifier that is used to identify this version of the SpecimenDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the SpecimenDefinition author and is not expected to be globally unique.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2412 */ 2413 public StringType getVersionElement() { 2414 if (this.version == null) 2415 if (Configuration.errorOnAutoCreate()) 2416 throw new Error("Attempt to auto-create SpecimenDefinition.version"); 2417 else if (Configuration.doAutoCreate()) 2418 this.version = new StringType(); // bb 2419 return this.version; 2420 } 2421 2422 public boolean hasVersionElement() { 2423 return this.version != null && !this.version.isEmpty(); 2424 } 2425 2426 public boolean hasVersion() { 2427 return this.version != null && !this.version.isEmpty(); 2428 } 2429 2430 /** 2431 * @param value {@link #version} (The identifier that is used to identify this version of the SpecimenDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the SpecimenDefinition author and is not expected to be globally unique.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2432 */ 2433 public SpecimenDefinition setVersionElement(StringType value) { 2434 this.version = value; 2435 return this; 2436 } 2437 2438 /** 2439 * @return The identifier that is used to identify this version of the SpecimenDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the SpecimenDefinition author and is not expected to be globally unique. 2440 */ 2441 public String getVersion() { 2442 return this.version == null ? null : this.version.getValue(); 2443 } 2444 2445 /** 2446 * @param value The identifier that is used to identify this version of the SpecimenDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the SpecimenDefinition author and is not expected to be globally unique. 2447 */ 2448 public SpecimenDefinition setVersion(String value) { 2449 if (Utilities.noString(value)) 2450 this.version = null; 2451 else { 2452 if (this.version == null) 2453 this.version = new StringType(); 2454 this.version.setValue(value); 2455 } 2456 return this; 2457 } 2458 2459 /** 2460 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2461 */ 2462 public DataType getVersionAlgorithm() { 2463 return this.versionAlgorithm; 2464 } 2465 2466 /** 2467 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2468 */ 2469 public StringType getVersionAlgorithmStringType() throws FHIRException { 2470 if (this.versionAlgorithm == null) 2471 this.versionAlgorithm = new StringType(); 2472 if (!(this.versionAlgorithm instanceof StringType)) 2473 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 2474 return (StringType) this.versionAlgorithm; 2475 } 2476 2477 public boolean hasVersionAlgorithmStringType() { 2478 return this != null && this.versionAlgorithm instanceof StringType; 2479 } 2480 2481 /** 2482 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2483 */ 2484 public Coding getVersionAlgorithmCoding() throws FHIRException { 2485 if (this.versionAlgorithm == null) 2486 this.versionAlgorithm = new Coding(); 2487 if (!(this.versionAlgorithm instanceof Coding)) 2488 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 2489 return (Coding) this.versionAlgorithm; 2490 } 2491 2492 public boolean hasVersionAlgorithmCoding() { 2493 return this != null && this.versionAlgorithm instanceof Coding; 2494 } 2495 2496 public boolean hasVersionAlgorithm() { 2497 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 2498 } 2499 2500 /** 2501 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2502 */ 2503 public SpecimenDefinition setVersionAlgorithm(DataType value) { 2504 if (value != null && !(value instanceof StringType || value instanceof Coding)) 2505 throw new FHIRException("Not the right type for SpecimenDefinition.versionAlgorithm[x]: "+value.fhirType()); 2506 this.versionAlgorithm = value; 2507 return this; 2508 } 2509 2510 /** 2511 * @return {@link #name} (A natural language name identifying the specimen definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2512 */ 2513 public StringType getNameElement() { 2514 if (this.name == null) 2515 if (Configuration.errorOnAutoCreate()) 2516 throw new Error("Attempt to auto-create SpecimenDefinition.name"); 2517 else if (Configuration.doAutoCreate()) 2518 this.name = new StringType(); // bb 2519 return this.name; 2520 } 2521 2522 public boolean hasNameElement() { 2523 return this.name != null && !this.name.isEmpty(); 2524 } 2525 2526 public boolean hasName() { 2527 return this.name != null && !this.name.isEmpty(); 2528 } 2529 2530 /** 2531 * @param value {@link #name} (A natural language name identifying the specimen definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2532 */ 2533 public SpecimenDefinition setNameElement(StringType value) { 2534 this.name = value; 2535 return this; 2536 } 2537 2538 /** 2539 * @return A natural language name identifying the specimen definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2540 */ 2541 public String getName() { 2542 return this.name == null ? null : this.name.getValue(); 2543 } 2544 2545 /** 2546 * @param value A natural language name identifying the specimen definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2547 */ 2548 public SpecimenDefinition setName(String value) { 2549 if (Utilities.noString(value)) 2550 this.name = null; 2551 else { 2552 if (this.name == null) 2553 this.name = new StringType(); 2554 this.name.setValue(value); 2555 } 2556 return this; 2557 } 2558 2559 /** 2560 * @return {@link #title} (A short, descriptive, user-friendly title for the SpecimenDefinition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2561 */ 2562 public StringType getTitleElement() { 2563 if (this.title == null) 2564 if (Configuration.errorOnAutoCreate()) 2565 throw new Error("Attempt to auto-create SpecimenDefinition.title"); 2566 else if (Configuration.doAutoCreate()) 2567 this.title = new StringType(); // bb 2568 return this.title; 2569 } 2570 2571 public boolean hasTitleElement() { 2572 return this.title != null && !this.title.isEmpty(); 2573 } 2574 2575 public boolean hasTitle() { 2576 return this.title != null && !this.title.isEmpty(); 2577 } 2578 2579 /** 2580 * @param value {@link #title} (A short, descriptive, user-friendly title for the SpecimenDefinition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2581 */ 2582 public SpecimenDefinition setTitleElement(StringType value) { 2583 this.title = value; 2584 return this; 2585 } 2586 2587 /** 2588 * @return A short, descriptive, user-friendly title for the SpecimenDefinition. 2589 */ 2590 public String getTitle() { 2591 return this.title == null ? null : this.title.getValue(); 2592 } 2593 2594 /** 2595 * @param value A short, descriptive, user-friendly title for the SpecimenDefinition. 2596 */ 2597 public SpecimenDefinition setTitle(String value) { 2598 if (Utilities.noString(value)) 2599 this.title = null; 2600 else { 2601 if (this.title == null) 2602 this.title = new StringType(); 2603 this.title.setValue(value); 2604 } 2605 return this; 2606 } 2607 2608 /** 2609 * @return {@link #derivedFromCanonical} (The canonical URL pointing to another FHIR-defined SpecimenDefinition that is adhered to in whole or in part by this definition.) 2610 */ 2611 public List<CanonicalType> getDerivedFromCanonical() { 2612 if (this.derivedFromCanonical == null) 2613 this.derivedFromCanonical = new ArrayList<CanonicalType>(); 2614 return this.derivedFromCanonical; 2615 } 2616 2617 /** 2618 * @return Returns a reference to <code>this</code> for easy method chaining 2619 */ 2620 public SpecimenDefinition setDerivedFromCanonical(List<CanonicalType> theDerivedFromCanonical) { 2621 this.derivedFromCanonical = theDerivedFromCanonical; 2622 return this; 2623 } 2624 2625 public boolean hasDerivedFromCanonical() { 2626 if (this.derivedFromCanonical == null) 2627 return false; 2628 for (CanonicalType item : this.derivedFromCanonical) 2629 if (!item.isEmpty()) 2630 return true; 2631 return false; 2632 } 2633 2634 /** 2635 * @return {@link #derivedFromCanonical} (The canonical URL pointing to another FHIR-defined SpecimenDefinition that is adhered to in whole or in part by this definition.) 2636 */ 2637 public CanonicalType addDerivedFromCanonicalElement() {//2 2638 CanonicalType t = new CanonicalType(); 2639 if (this.derivedFromCanonical == null) 2640 this.derivedFromCanonical = new ArrayList<CanonicalType>(); 2641 this.derivedFromCanonical.add(t); 2642 return t; 2643 } 2644 2645 /** 2646 * @param value {@link #derivedFromCanonical} (The canonical URL pointing to another FHIR-defined SpecimenDefinition that is adhered to in whole or in part by this definition.) 2647 */ 2648 public SpecimenDefinition addDerivedFromCanonical(String value) { //1 2649 CanonicalType t = new CanonicalType(); 2650 t.setValue(value); 2651 if (this.derivedFromCanonical == null) 2652 this.derivedFromCanonical = new ArrayList<CanonicalType>(); 2653 this.derivedFromCanonical.add(t); 2654 return this; 2655 } 2656 2657 /** 2658 * @param value {@link #derivedFromCanonical} (The canonical URL pointing to another FHIR-defined SpecimenDefinition that is adhered to in whole or in part by this definition.) 2659 */ 2660 public boolean hasDerivedFromCanonical(String value) { 2661 if (this.derivedFromCanonical == null) 2662 return false; 2663 for (CanonicalType v : this.derivedFromCanonical) 2664 if (v.getValue().equals(value)) // canonical 2665 return true; 2666 return false; 2667 } 2668 2669 /** 2670 * @return {@link #derivedFromUri} (The URL pointing to an externally-defined type of specimen, guideline or other definition that is adhered to in whole or in part by this definition.) 2671 */ 2672 public List<UriType> getDerivedFromUri() { 2673 if (this.derivedFromUri == null) 2674 this.derivedFromUri = new ArrayList<UriType>(); 2675 return this.derivedFromUri; 2676 } 2677 2678 /** 2679 * @return Returns a reference to <code>this</code> for easy method chaining 2680 */ 2681 public SpecimenDefinition setDerivedFromUri(List<UriType> theDerivedFromUri) { 2682 this.derivedFromUri = theDerivedFromUri; 2683 return this; 2684 } 2685 2686 public boolean hasDerivedFromUri() { 2687 if (this.derivedFromUri == null) 2688 return false; 2689 for (UriType item : this.derivedFromUri) 2690 if (!item.isEmpty()) 2691 return true; 2692 return false; 2693 } 2694 2695 /** 2696 * @return {@link #derivedFromUri} (The URL pointing to an externally-defined type of specimen, guideline or other definition that is adhered to in whole or in part by this definition.) 2697 */ 2698 public UriType addDerivedFromUriElement() {//2 2699 UriType t = new UriType(); 2700 if (this.derivedFromUri == null) 2701 this.derivedFromUri = new ArrayList<UriType>(); 2702 this.derivedFromUri.add(t); 2703 return t; 2704 } 2705 2706 /** 2707 * @param value {@link #derivedFromUri} (The URL pointing to an externally-defined type of specimen, guideline or other definition that is adhered to in whole or in part by this definition.) 2708 */ 2709 public SpecimenDefinition addDerivedFromUri(String value) { //1 2710 UriType t = new UriType(); 2711 t.setValue(value); 2712 if (this.derivedFromUri == null) 2713 this.derivedFromUri = new ArrayList<UriType>(); 2714 this.derivedFromUri.add(t); 2715 return this; 2716 } 2717 2718 /** 2719 * @param value {@link #derivedFromUri} (The URL pointing to an externally-defined type of specimen, guideline or other definition that is adhered to in whole or in part by this definition.) 2720 */ 2721 public boolean hasDerivedFromUri(String value) { 2722 if (this.derivedFromUri == null) 2723 return false; 2724 for (UriType v : this.derivedFromUri) 2725 if (v.getValue().equals(value)) // uri 2726 return true; 2727 return false; 2728 } 2729 2730 /** 2731 * @return {@link #status} (The current state of theSpecimenDefinition.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2732 */ 2733 public Enumeration<PublicationStatus> getStatusElement() { 2734 if (this.status == null) 2735 if (Configuration.errorOnAutoCreate()) 2736 throw new Error("Attempt to auto-create SpecimenDefinition.status"); 2737 else if (Configuration.doAutoCreate()) 2738 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2739 return this.status; 2740 } 2741 2742 public boolean hasStatusElement() { 2743 return this.status != null && !this.status.isEmpty(); 2744 } 2745 2746 public boolean hasStatus() { 2747 return this.status != null && !this.status.isEmpty(); 2748 } 2749 2750 /** 2751 * @param value {@link #status} (The current state of theSpecimenDefinition.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2752 */ 2753 public SpecimenDefinition setStatusElement(Enumeration<PublicationStatus> value) { 2754 this.status = value; 2755 return this; 2756 } 2757 2758 /** 2759 * @return The current state of theSpecimenDefinition. 2760 */ 2761 public PublicationStatus getStatus() { 2762 return this.status == null ? null : this.status.getValue(); 2763 } 2764 2765 /** 2766 * @param value The current state of theSpecimenDefinition. 2767 */ 2768 public SpecimenDefinition setStatus(PublicationStatus value) { 2769 if (this.status == null) 2770 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2771 this.status.setValue(value); 2772 return this; 2773 } 2774 2775 /** 2776 * @return {@link #experimental} (A flag to indicate that this SpecimenDefinition is not authored for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2777 */ 2778 public BooleanType getExperimentalElement() { 2779 if (this.experimental == null) 2780 if (Configuration.errorOnAutoCreate()) 2781 throw new Error("Attempt to auto-create SpecimenDefinition.experimental"); 2782 else if (Configuration.doAutoCreate()) 2783 this.experimental = new BooleanType(); // bb 2784 return this.experimental; 2785 } 2786 2787 public boolean hasExperimentalElement() { 2788 return this.experimental != null && !this.experimental.isEmpty(); 2789 } 2790 2791 public boolean hasExperimental() { 2792 return this.experimental != null && !this.experimental.isEmpty(); 2793 } 2794 2795 /** 2796 * @param value {@link #experimental} (A flag to indicate that this SpecimenDefinition is not authored for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2797 */ 2798 public SpecimenDefinition setExperimentalElement(BooleanType value) { 2799 this.experimental = value; 2800 return this; 2801 } 2802 2803 /** 2804 * @return A flag to indicate that this SpecimenDefinition is not authored for genuine usage. 2805 */ 2806 public boolean getExperimental() { 2807 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2808 } 2809 2810 /** 2811 * @param value A flag to indicate that this SpecimenDefinition is not authored for genuine usage. 2812 */ 2813 public SpecimenDefinition setExperimental(boolean value) { 2814 if (this.experimental == null) 2815 this.experimental = new BooleanType(); 2816 this.experimental.setValue(value); 2817 return this; 2818 } 2819 2820 /** 2821 * @return {@link #subject} (A code or group definition that describes the intended subject from which this kind of specimen is to be collected.) 2822 */ 2823 public DataType getSubject() { 2824 return this.subject; 2825 } 2826 2827 /** 2828 * @return {@link #subject} (A code or group definition that describes the intended subject from which this kind of specimen is to be collected.) 2829 */ 2830 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 2831 if (this.subject == null) 2832 this.subject = new CodeableConcept(); 2833 if (!(this.subject instanceof CodeableConcept)) 2834 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.subject.getClass().getName()+" was encountered"); 2835 return (CodeableConcept) this.subject; 2836 } 2837 2838 public boolean hasSubjectCodeableConcept() { 2839 return this != null && this.subject instanceof CodeableConcept; 2840 } 2841 2842 /** 2843 * @return {@link #subject} (A code or group definition that describes the intended subject from which this kind of specimen is to be collected.) 2844 */ 2845 public Reference getSubjectReference() throws FHIRException { 2846 if (this.subject == null) 2847 this.subject = new Reference(); 2848 if (!(this.subject instanceof Reference)) 2849 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.subject.getClass().getName()+" was encountered"); 2850 return (Reference) this.subject; 2851 } 2852 2853 public boolean hasSubjectReference() { 2854 return this != null && this.subject instanceof Reference; 2855 } 2856 2857 public boolean hasSubject() { 2858 return this.subject != null && !this.subject.isEmpty(); 2859 } 2860 2861 /** 2862 * @param value {@link #subject} (A code or group definition that describes the intended subject from which this kind of specimen is to be collected.) 2863 */ 2864 public SpecimenDefinition setSubject(DataType value) { 2865 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2866 throw new FHIRException("Not the right type for SpecimenDefinition.subject[x]: "+value.fhirType()); 2867 this.subject = value; 2868 return this; 2869 } 2870 2871 /** 2872 * @return {@link #date} (For draft definitions, indicates the date of initial creation. For active definitions, represents the date of activation. For withdrawn definitions, indicates the date of withdrawal.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2873 */ 2874 public DateTimeType getDateElement() { 2875 if (this.date == null) 2876 if (Configuration.errorOnAutoCreate()) 2877 throw new Error("Attempt to auto-create SpecimenDefinition.date"); 2878 else if (Configuration.doAutoCreate()) 2879 this.date = new DateTimeType(); // bb 2880 return this.date; 2881 } 2882 2883 public boolean hasDateElement() { 2884 return this.date != null && !this.date.isEmpty(); 2885 } 2886 2887 public boolean hasDate() { 2888 return this.date != null && !this.date.isEmpty(); 2889 } 2890 2891 /** 2892 * @param value {@link #date} (For draft definitions, indicates the date of initial creation. For active definitions, represents the date of activation. For withdrawn definitions, indicates the date of withdrawal.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2893 */ 2894 public SpecimenDefinition setDateElement(DateTimeType value) { 2895 this.date = value; 2896 return this; 2897 } 2898 2899 /** 2900 * @return For draft definitions, indicates the date of initial creation. For active definitions, represents the date of activation. For withdrawn definitions, indicates the date of withdrawal. 2901 */ 2902 public Date getDate() { 2903 return this.date == null ? null : this.date.getValue(); 2904 } 2905 2906 /** 2907 * @param value For draft definitions, indicates the date of initial creation. For active definitions, represents the date of activation. For withdrawn definitions, indicates the date of withdrawal. 2908 */ 2909 public SpecimenDefinition setDate(Date value) { 2910 if (value == null) 2911 this.date = null; 2912 else { 2913 if (this.date == null) 2914 this.date = new DateTimeType(); 2915 this.date.setValue(value); 2916 } 2917 return this; 2918 } 2919 2920 /** 2921 * @return {@link #publisher} (Helps establish the "authority/credibility" of the SpecimenDefinition. May also allow for contact.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2922 */ 2923 public StringType getPublisherElement() { 2924 if (this.publisher == null) 2925 if (Configuration.errorOnAutoCreate()) 2926 throw new Error("Attempt to auto-create SpecimenDefinition.publisher"); 2927 else if (Configuration.doAutoCreate()) 2928 this.publisher = new StringType(); // bb 2929 return this.publisher; 2930 } 2931 2932 public boolean hasPublisherElement() { 2933 return this.publisher != null && !this.publisher.isEmpty(); 2934 } 2935 2936 public boolean hasPublisher() { 2937 return this.publisher != null && !this.publisher.isEmpty(); 2938 } 2939 2940 /** 2941 * @param value {@link #publisher} (Helps establish the "authority/credibility" of the SpecimenDefinition. May also allow for contact.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2942 */ 2943 public SpecimenDefinition setPublisherElement(StringType value) { 2944 this.publisher = value; 2945 return this; 2946 } 2947 2948 /** 2949 * @return Helps establish the "authority/credibility" of the SpecimenDefinition. May also allow for contact. 2950 */ 2951 public String getPublisher() { 2952 return this.publisher == null ? null : this.publisher.getValue(); 2953 } 2954 2955 /** 2956 * @param value Helps establish the "authority/credibility" of the SpecimenDefinition. May also allow for contact. 2957 */ 2958 public SpecimenDefinition setPublisher(String value) { 2959 if (Utilities.noString(value)) 2960 this.publisher = null; 2961 else { 2962 if (this.publisher == null) 2963 this.publisher = new StringType(); 2964 this.publisher.setValue(value); 2965 } 2966 return this; 2967 } 2968 2969 /** 2970 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 2971 */ 2972 public List<ContactDetail> getContact() { 2973 if (this.contact == null) 2974 this.contact = new ArrayList<ContactDetail>(); 2975 return this.contact; 2976 } 2977 2978 /** 2979 * @return Returns a reference to <code>this</code> for easy method chaining 2980 */ 2981 public SpecimenDefinition setContact(List<ContactDetail> theContact) { 2982 this.contact = theContact; 2983 return this; 2984 } 2985 2986 public boolean hasContact() { 2987 if (this.contact == null) 2988 return false; 2989 for (ContactDetail item : this.contact) 2990 if (!item.isEmpty()) 2991 return true; 2992 return false; 2993 } 2994 2995 public ContactDetail addContact() { //3 2996 ContactDetail t = new ContactDetail(); 2997 if (this.contact == null) 2998 this.contact = new ArrayList<ContactDetail>(); 2999 this.contact.add(t); 3000 return t; 3001 } 3002 3003 public SpecimenDefinition addContact(ContactDetail t) { //3 3004 if (t == null) 3005 return this; 3006 if (this.contact == null) 3007 this.contact = new ArrayList<ContactDetail>(); 3008 this.contact.add(t); 3009 return this; 3010 } 3011 3012 /** 3013 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 3014 */ 3015 public ContactDetail getContactFirstRep() { 3016 if (getContact().isEmpty()) { 3017 addContact(); 3018 } 3019 return getContact().get(0); 3020 } 3021 3022 /** 3023 * @return {@link #description} (A free text natural language description of the SpecimenDefinition from the consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3024 */ 3025 public MarkdownType getDescriptionElement() { 3026 if (this.description == null) 3027 if (Configuration.errorOnAutoCreate()) 3028 throw new Error("Attempt to auto-create SpecimenDefinition.description"); 3029 else if (Configuration.doAutoCreate()) 3030 this.description = new MarkdownType(); // bb 3031 return this.description; 3032 } 3033 3034 public boolean hasDescriptionElement() { 3035 return this.description != null && !this.description.isEmpty(); 3036 } 3037 3038 public boolean hasDescription() { 3039 return this.description != null && !this.description.isEmpty(); 3040 } 3041 3042 /** 3043 * @param value {@link #description} (A free text natural language description of the SpecimenDefinition from the consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3044 */ 3045 public SpecimenDefinition setDescriptionElement(MarkdownType value) { 3046 this.description = value; 3047 return this; 3048 } 3049 3050 /** 3051 * @return A free text natural language description of the SpecimenDefinition from the consumer's perspective. 3052 */ 3053 public String getDescription() { 3054 return this.description == null ? null : this.description.getValue(); 3055 } 3056 3057 /** 3058 * @param value A free text natural language description of the SpecimenDefinition from the consumer's perspective. 3059 */ 3060 public SpecimenDefinition setDescription(String value) { 3061 if (Utilities.noString(value)) 3062 this.description = null; 3063 else { 3064 if (this.description == null) 3065 this.description = new MarkdownType(); 3066 this.description.setValue(value); 3067 } 3068 return this; 3069 } 3070 3071 /** 3072 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of specimen definitions.) 3073 */ 3074 public List<UsageContext> getUseContext() { 3075 if (this.useContext == null) 3076 this.useContext = new ArrayList<UsageContext>(); 3077 return this.useContext; 3078 } 3079 3080 /** 3081 * @return Returns a reference to <code>this</code> for easy method chaining 3082 */ 3083 public SpecimenDefinition setUseContext(List<UsageContext> theUseContext) { 3084 this.useContext = theUseContext; 3085 return this; 3086 } 3087 3088 public boolean hasUseContext() { 3089 if (this.useContext == null) 3090 return false; 3091 for (UsageContext item : this.useContext) 3092 if (!item.isEmpty()) 3093 return true; 3094 return false; 3095 } 3096 3097 public UsageContext addUseContext() { //3 3098 UsageContext t = new UsageContext(); 3099 if (this.useContext == null) 3100 this.useContext = new ArrayList<UsageContext>(); 3101 this.useContext.add(t); 3102 return t; 3103 } 3104 3105 public SpecimenDefinition addUseContext(UsageContext t) { //3 3106 if (t == null) 3107 return this; 3108 if (this.useContext == null) 3109 this.useContext = new ArrayList<UsageContext>(); 3110 this.useContext.add(t); 3111 return this; 3112 } 3113 3114 /** 3115 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 3116 */ 3117 public UsageContext getUseContextFirstRep() { 3118 if (getUseContext().isEmpty()) { 3119 addUseContext(); 3120 } 3121 return getUseContext().get(0); 3122 } 3123 3124 /** 3125 * @return {@link #jurisdiction} (A jurisdiction in which the SpecimenDefinition is intended to be used.) 3126 */ 3127 public List<CodeableConcept> getJurisdiction() { 3128 if (this.jurisdiction == null) 3129 this.jurisdiction = new ArrayList<CodeableConcept>(); 3130 return this.jurisdiction; 3131 } 3132 3133 /** 3134 * @return Returns a reference to <code>this</code> for easy method chaining 3135 */ 3136 public SpecimenDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 3137 this.jurisdiction = theJurisdiction; 3138 return this; 3139 } 3140 3141 public boolean hasJurisdiction() { 3142 if (this.jurisdiction == null) 3143 return false; 3144 for (CodeableConcept item : this.jurisdiction) 3145 if (!item.isEmpty()) 3146 return true; 3147 return false; 3148 } 3149 3150 public CodeableConcept addJurisdiction() { //3 3151 CodeableConcept t = new CodeableConcept(); 3152 if (this.jurisdiction == null) 3153 this.jurisdiction = new ArrayList<CodeableConcept>(); 3154 this.jurisdiction.add(t); 3155 return t; 3156 } 3157 3158 public SpecimenDefinition addJurisdiction(CodeableConcept t) { //3 3159 if (t == null) 3160 return this; 3161 if (this.jurisdiction == null) 3162 this.jurisdiction = new ArrayList<CodeableConcept>(); 3163 this.jurisdiction.add(t); 3164 return this; 3165 } 3166 3167 /** 3168 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 3169 */ 3170 public CodeableConcept getJurisdictionFirstRep() { 3171 if (getJurisdiction().isEmpty()) { 3172 addJurisdiction(); 3173 } 3174 return getJurisdiction().get(0); 3175 } 3176 3177 /** 3178 * @return {@link #purpose} (Explains why this SpecimeDefinition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3179 */ 3180 public MarkdownType getPurposeElement() { 3181 if (this.purpose == null) 3182 if (Configuration.errorOnAutoCreate()) 3183 throw new Error("Attempt to auto-create SpecimenDefinition.purpose"); 3184 else if (Configuration.doAutoCreate()) 3185 this.purpose = new MarkdownType(); // bb 3186 return this.purpose; 3187 } 3188 3189 public boolean hasPurposeElement() { 3190 return this.purpose != null && !this.purpose.isEmpty(); 3191 } 3192 3193 public boolean hasPurpose() { 3194 return this.purpose != null && !this.purpose.isEmpty(); 3195 } 3196 3197 /** 3198 * @param value {@link #purpose} (Explains why this SpecimeDefinition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3199 */ 3200 public SpecimenDefinition setPurposeElement(MarkdownType value) { 3201 this.purpose = value; 3202 return this; 3203 } 3204 3205 /** 3206 * @return Explains why this SpecimeDefinition is needed and why it has been designed as it has. 3207 */ 3208 public String getPurpose() { 3209 return this.purpose == null ? null : this.purpose.getValue(); 3210 } 3211 3212 /** 3213 * @param value Explains why this SpecimeDefinition is needed and why it has been designed as it has. 3214 */ 3215 public SpecimenDefinition setPurpose(String value) { 3216 if (Utilities.noString(value)) 3217 this.purpose = null; 3218 else { 3219 if (this.purpose == null) 3220 this.purpose = new MarkdownType(); 3221 this.purpose.setValue(value); 3222 } 3223 return this; 3224 } 3225 3226 /** 3227 * @return {@link #copyright} (Copyright statement relating to the SpecimenDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SpecimenDefinition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3228 */ 3229 public MarkdownType getCopyrightElement() { 3230 if (this.copyright == null) 3231 if (Configuration.errorOnAutoCreate()) 3232 throw new Error("Attempt to auto-create SpecimenDefinition.copyright"); 3233 else if (Configuration.doAutoCreate()) 3234 this.copyright = new MarkdownType(); // bb 3235 return this.copyright; 3236 } 3237 3238 public boolean hasCopyrightElement() { 3239 return this.copyright != null && !this.copyright.isEmpty(); 3240 } 3241 3242 public boolean hasCopyright() { 3243 return this.copyright != null && !this.copyright.isEmpty(); 3244 } 3245 3246 /** 3247 * @param value {@link #copyright} (Copyright statement relating to the SpecimenDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SpecimenDefinition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3248 */ 3249 public SpecimenDefinition setCopyrightElement(MarkdownType value) { 3250 this.copyright = value; 3251 return this; 3252 } 3253 3254 /** 3255 * @return Copyright statement relating to the SpecimenDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SpecimenDefinition. 3256 */ 3257 public String getCopyright() { 3258 return this.copyright == null ? null : this.copyright.getValue(); 3259 } 3260 3261 /** 3262 * @param value Copyright statement relating to the SpecimenDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SpecimenDefinition. 3263 */ 3264 public SpecimenDefinition setCopyright(String value) { 3265 if (Utilities.noString(value)) 3266 this.copyright = null; 3267 else { 3268 if (this.copyright == null) 3269 this.copyright = new MarkdownType(); 3270 this.copyright.setValue(value); 3271 } 3272 return this; 3273 } 3274 3275 /** 3276 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 3277 */ 3278 public StringType getCopyrightLabelElement() { 3279 if (this.copyrightLabel == null) 3280 if (Configuration.errorOnAutoCreate()) 3281 throw new Error("Attempt to auto-create SpecimenDefinition.copyrightLabel"); 3282 else if (Configuration.doAutoCreate()) 3283 this.copyrightLabel = new StringType(); // bb 3284 return this.copyrightLabel; 3285 } 3286 3287 public boolean hasCopyrightLabelElement() { 3288 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 3289 } 3290 3291 public boolean hasCopyrightLabel() { 3292 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 3293 } 3294 3295 /** 3296 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 3297 */ 3298 public SpecimenDefinition setCopyrightLabelElement(StringType value) { 3299 this.copyrightLabel = value; 3300 return this; 3301 } 3302 3303 /** 3304 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3305 */ 3306 public String getCopyrightLabel() { 3307 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 3308 } 3309 3310 /** 3311 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3312 */ 3313 public SpecimenDefinition setCopyrightLabel(String value) { 3314 if (Utilities.noString(value)) 3315 this.copyrightLabel = null; 3316 else { 3317 if (this.copyrightLabel == null) 3318 this.copyrightLabel = new StringType(); 3319 this.copyrightLabel.setValue(value); 3320 } 3321 return this; 3322 } 3323 3324 /** 3325 * @return {@link #approvalDate} (The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 3326 */ 3327 public DateType getApprovalDateElement() { 3328 if (this.approvalDate == null) 3329 if (Configuration.errorOnAutoCreate()) 3330 throw new Error("Attempt to auto-create SpecimenDefinition.approvalDate"); 3331 else if (Configuration.doAutoCreate()) 3332 this.approvalDate = new DateType(); // bb 3333 return this.approvalDate; 3334 } 3335 3336 public boolean hasApprovalDateElement() { 3337 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3338 } 3339 3340 public boolean hasApprovalDate() { 3341 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3342 } 3343 3344 /** 3345 * @param value {@link #approvalDate} (The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 3346 */ 3347 public SpecimenDefinition setApprovalDateElement(DateType value) { 3348 this.approvalDate = value; 3349 return this; 3350 } 3351 3352 /** 3353 * @return The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3354 */ 3355 public Date getApprovalDate() { 3356 return this.approvalDate == null ? null : this.approvalDate.getValue(); 3357 } 3358 3359 /** 3360 * @param value The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3361 */ 3362 public SpecimenDefinition setApprovalDate(Date value) { 3363 if (value == null) 3364 this.approvalDate = null; 3365 else { 3366 if (this.approvalDate == null) 3367 this.approvalDate = new DateType(); 3368 this.approvalDate.setValue(value); 3369 } 3370 return this; 3371 } 3372 3373 /** 3374 * @return {@link #lastReviewDate} (The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 3375 */ 3376 public DateType getLastReviewDateElement() { 3377 if (this.lastReviewDate == null) 3378 if (Configuration.errorOnAutoCreate()) 3379 throw new Error("Attempt to auto-create SpecimenDefinition.lastReviewDate"); 3380 else if (Configuration.doAutoCreate()) 3381 this.lastReviewDate = new DateType(); // bb 3382 return this.lastReviewDate; 3383 } 3384 3385 public boolean hasLastReviewDateElement() { 3386 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3387 } 3388 3389 public boolean hasLastReviewDate() { 3390 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3391 } 3392 3393 /** 3394 * @param value {@link #lastReviewDate} (The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 3395 */ 3396 public SpecimenDefinition setLastReviewDateElement(DateType value) { 3397 this.lastReviewDate = value; 3398 return this; 3399 } 3400 3401 /** 3402 * @return The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date. 3403 */ 3404 public Date getLastReviewDate() { 3405 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 3406 } 3407 3408 /** 3409 * @param value The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date. 3410 */ 3411 public SpecimenDefinition setLastReviewDate(Date value) { 3412 if (value == null) 3413 this.lastReviewDate = null; 3414 else { 3415 if (this.lastReviewDate == null) 3416 this.lastReviewDate = new DateType(); 3417 this.lastReviewDate.setValue(value); 3418 } 3419 return this; 3420 } 3421 3422 /** 3423 * @return {@link #effectivePeriod} (The period during which the SpecimenDefinition content was or is planned to be effective.) 3424 */ 3425 public Period getEffectivePeriod() { 3426 if (this.effectivePeriod == null) 3427 if (Configuration.errorOnAutoCreate()) 3428 throw new Error("Attempt to auto-create SpecimenDefinition.effectivePeriod"); 3429 else if (Configuration.doAutoCreate()) 3430 this.effectivePeriod = new Period(); // cc 3431 return this.effectivePeriod; 3432 } 3433 3434 public boolean hasEffectivePeriod() { 3435 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 3436 } 3437 3438 /** 3439 * @param value {@link #effectivePeriod} (The period during which the SpecimenDefinition content was or is planned to be effective.) 3440 */ 3441 public SpecimenDefinition setEffectivePeriod(Period value) { 3442 this.effectivePeriod = value; 3443 return this; 3444 } 3445 3446 /** 3447 * @return {@link #typeCollected} (The kind of material to be collected.) 3448 */ 3449 public CodeableConcept getTypeCollected() { 3450 if (this.typeCollected == null) 3451 if (Configuration.errorOnAutoCreate()) 3452 throw new Error("Attempt to auto-create SpecimenDefinition.typeCollected"); 3453 else if (Configuration.doAutoCreate()) 3454 this.typeCollected = new CodeableConcept(); // cc 3455 return this.typeCollected; 3456 } 3457 3458 public boolean hasTypeCollected() { 3459 return this.typeCollected != null && !this.typeCollected.isEmpty(); 3460 } 3461 3462 /** 3463 * @param value {@link #typeCollected} (The kind of material to be collected.) 3464 */ 3465 public SpecimenDefinition setTypeCollected(CodeableConcept value) { 3466 this.typeCollected = value; 3467 return this; 3468 } 3469 3470 /** 3471 * @return {@link #patientPreparation} (Preparation of the patient for specimen collection.) 3472 */ 3473 public List<CodeableConcept> getPatientPreparation() { 3474 if (this.patientPreparation == null) 3475 this.patientPreparation = new ArrayList<CodeableConcept>(); 3476 return this.patientPreparation; 3477 } 3478 3479 /** 3480 * @return Returns a reference to <code>this</code> for easy method chaining 3481 */ 3482 public SpecimenDefinition setPatientPreparation(List<CodeableConcept> thePatientPreparation) { 3483 this.patientPreparation = thePatientPreparation; 3484 return this; 3485 } 3486 3487 public boolean hasPatientPreparation() { 3488 if (this.patientPreparation == null) 3489 return false; 3490 for (CodeableConcept item : this.patientPreparation) 3491 if (!item.isEmpty()) 3492 return true; 3493 return false; 3494 } 3495 3496 public CodeableConcept addPatientPreparation() { //3 3497 CodeableConcept t = new CodeableConcept(); 3498 if (this.patientPreparation == null) 3499 this.patientPreparation = new ArrayList<CodeableConcept>(); 3500 this.patientPreparation.add(t); 3501 return t; 3502 } 3503 3504 public SpecimenDefinition addPatientPreparation(CodeableConcept t) { //3 3505 if (t == null) 3506 return this; 3507 if (this.patientPreparation == null) 3508 this.patientPreparation = new ArrayList<CodeableConcept>(); 3509 this.patientPreparation.add(t); 3510 return this; 3511 } 3512 3513 /** 3514 * @return The first repetition of repeating field {@link #patientPreparation}, creating it if it does not already exist {3} 3515 */ 3516 public CodeableConcept getPatientPreparationFirstRep() { 3517 if (getPatientPreparation().isEmpty()) { 3518 addPatientPreparation(); 3519 } 3520 return getPatientPreparation().get(0); 3521 } 3522 3523 /** 3524 * @return {@link #timeAspect} (Time aspect of specimen collection (duration or offset).). This is the underlying object with id, value and extensions. The accessor "getTimeAspect" gives direct access to the value 3525 */ 3526 public StringType getTimeAspectElement() { 3527 if (this.timeAspect == null) 3528 if (Configuration.errorOnAutoCreate()) 3529 throw new Error("Attempt to auto-create SpecimenDefinition.timeAspect"); 3530 else if (Configuration.doAutoCreate()) 3531 this.timeAspect = new StringType(); // bb 3532 return this.timeAspect; 3533 } 3534 3535 public boolean hasTimeAspectElement() { 3536 return this.timeAspect != null && !this.timeAspect.isEmpty(); 3537 } 3538 3539 public boolean hasTimeAspect() { 3540 return this.timeAspect != null && !this.timeAspect.isEmpty(); 3541 } 3542 3543 /** 3544 * @param value {@link #timeAspect} (Time aspect of specimen collection (duration or offset).). This is the underlying object with id, value and extensions. The accessor "getTimeAspect" gives direct access to the value 3545 */ 3546 public SpecimenDefinition setTimeAspectElement(StringType value) { 3547 this.timeAspect = value; 3548 return this; 3549 } 3550 3551 /** 3552 * @return Time aspect of specimen collection (duration or offset). 3553 */ 3554 public String getTimeAspect() { 3555 return this.timeAspect == null ? null : this.timeAspect.getValue(); 3556 } 3557 3558 /** 3559 * @param value Time aspect of specimen collection (duration or offset). 3560 */ 3561 public SpecimenDefinition setTimeAspect(String value) { 3562 if (Utilities.noString(value)) 3563 this.timeAspect = null; 3564 else { 3565 if (this.timeAspect == null) 3566 this.timeAspect = new StringType(); 3567 this.timeAspect.setValue(value); 3568 } 3569 return this; 3570 } 3571 3572 /** 3573 * @return {@link #collection} (The action to be performed for collecting the specimen.) 3574 */ 3575 public List<CodeableConcept> getCollection() { 3576 if (this.collection == null) 3577 this.collection = new ArrayList<CodeableConcept>(); 3578 return this.collection; 3579 } 3580 3581 /** 3582 * @return Returns a reference to <code>this</code> for easy method chaining 3583 */ 3584 public SpecimenDefinition setCollection(List<CodeableConcept> theCollection) { 3585 this.collection = theCollection; 3586 return this; 3587 } 3588 3589 public boolean hasCollection() { 3590 if (this.collection == null) 3591 return false; 3592 for (CodeableConcept item : this.collection) 3593 if (!item.isEmpty()) 3594 return true; 3595 return false; 3596 } 3597 3598 public CodeableConcept addCollection() { //3 3599 CodeableConcept t = new CodeableConcept(); 3600 if (this.collection == null) 3601 this.collection = new ArrayList<CodeableConcept>(); 3602 this.collection.add(t); 3603 return t; 3604 } 3605 3606 public SpecimenDefinition addCollection(CodeableConcept t) { //3 3607 if (t == null) 3608 return this; 3609 if (this.collection == null) 3610 this.collection = new ArrayList<CodeableConcept>(); 3611 this.collection.add(t); 3612 return this; 3613 } 3614 3615 /** 3616 * @return The first repetition of repeating field {@link #collection}, creating it if it does not already exist {3} 3617 */ 3618 public CodeableConcept getCollectionFirstRep() { 3619 if (getCollection().isEmpty()) { 3620 addCollection(); 3621 } 3622 return getCollection().get(0); 3623 } 3624 3625 /** 3626 * @return {@link #typeTested} (Specimen conditioned in a container as expected by the testing laboratory.) 3627 */ 3628 public List<SpecimenDefinitionTypeTestedComponent> getTypeTested() { 3629 if (this.typeTested == null) 3630 this.typeTested = new ArrayList<SpecimenDefinitionTypeTestedComponent>(); 3631 return this.typeTested; 3632 } 3633 3634 /** 3635 * @return Returns a reference to <code>this</code> for easy method chaining 3636 */ 3637 public SpecimenDefinition setTypeTested(List<SpecimenDefinitionTypeTestedComponent> theTypeTested) { 3638 this.typeTested = theTypeTested; 3639 return this; 3640 } 3641 3642 public boolean hasTypeTested() { 3643 if (this.typeTested == null) 3644 return false; 3645 for (SpecimenDefinitionTypeTestedComponent item : this.typeTested) 3646 if (!item.isEmpty()) 3647 return true; 3648 return false; 3649 } 3650 3651 public SpecimenDefinitionTypeTestedComponent addTypeTested() { //3 3652 SpecimenDefinitionTypeTestedComponent t = new SpecimenDefinitionTypeTestedComponent(); 3653 if (this.typeTested == null) 3654 this.typeTested = new ArrayList<SpecimenDefinitionTypeTestedComponent>(); 3655 this.typeTested.add(t); 3656 return t; 3657 } 3658 3659 public SpecimenDefinition addTypeTested(SpecimenDefinitionTypeTestedComponent t) { //3 3660 if (t == null) 3661 return this; 3662 if (this.typeTested == null) 3663 this.typeTested = new ArrayList<SpecimenDefinitionTypeTestedComponent>(); 3664 this.typeTested.add(t); 3665 return this; 3666 } 3667 3668 /** 3669 * @return The first repetition of repeating field {@link #typeTested}, creating it if it does not already exist {3} 3670 */ 3671 public SpecimenDefinitionTypeTestedComponent getTypeTestedFirstRep() { 3672 if (getTypeTested().isEmpty()) { 3673 addTypeTested(); 3674 } 3675 return getTypeTested().get(0); 3676 } 3677 3678 protected void listChildren(List<Property> children) { 3679 super.listChildren(children); 3680 children.add(new Property("url", "uri", "An absolute URL that is used to identify this SpecimenDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this SpecimenDefinition is (or will be) published. The URL SHOULD include the major version of the SpecimenDefinition. For more information see Technical and Business Versions.", 0, 1, url)); 3681 children.add(new Property("identifier", "Identifier", "A business identifier assigned to this SpecimenDefinition.", 0, 1, identifier)); 3682 children.add(new Property("version", "string", "The identifier that is used to identify this version of the SpecimenDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the SpecimenDefinition author and is not expected to be globally unique.", 0, 1, version)); 3683 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 3684 children.add(new Property("name", "string", "A natural language name identifying the specimen definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 3685 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the SpecimenDefinition.", 0, 1, title)); 3686 children.add(new Property("derivedFromCanonical", "canonical(SpecimenDefinition)", "The canonical URL pointing to another FHIR-defined SpecimenDefinition that is adhered to in whole or in part by this definition.", 0, java.lang.Integer.MAX_VALUE, derivedFromCanonical)); 3687 children.add(new Property("derivedFromUri", "uri", "The URL pointing to an externally-defined type of specimen, guideline or other definition that is adhered to in whole or in part by this definition.", 0, java.lang.Integer.MAX_VALUE, derivedFromUri)); 3688 children.add(new Property("status", "code", "The current state of theSpecimenDefinition.", 0, 1, status)); 3689 children.add(new Property("experimental", "boolean", "A flag to indicate that this SpecimenDefinition is not authored for genuine usage.", 0, 1, experimental)); 3690 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group)", "A code or group definition that describes the intended subject from which this kind of specimen is to be collected.", 0, 1, subject)); 3691 children.add(new Property("date", "dateTime", "For draft definitions, indicates the date of initial creation. For active definitions, represents the date of activation. For withdrawn definitions, indicates the date of withdrawal.", 0, 1, date)); 3692 children.add(new Property("publisher", "string", "Helps establish the \"authority/credibility\" of the SpecimenDefinition. May also allow for contact.", 0, 1, publisher)); 3693 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 3694 children.add(new Property("description", "markdown", "A free text natural language description of the SpecimenDefinition from the consumer's perspective.", 0, 1, description)); 3695 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of specimen definitions.", 0, java.lang.Integer.MAX_VALUE, useContext)); 3696 children.add(new Property("jurisdiction", "CodeableConcept", "A jurisdiction in which the SpecimenDefinition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 3697 children.add(new Property("purpose", "markdown", "Explains why this SpecimeDefinition is needed and why it has been designed as it has.", 0, 1, purpose)); 3698 children.add(new Property("copyright", "markdown", "Copyright statement relating to the SpecimenDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SpecimenDefinition.", 0, 1, copyright)); 3699 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 3700 children.add(new Property("approvalDate", "date", "The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 3701 children.add(new Property("lastReviewDate", "date", "The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.", 0, 1, lastReviewDate)); 3702 children.add(new Property("effectivePeriod", "Period", "The period during which the SpecimenDefinition content was or is planned to be effective.", 0, 1, effectivePeriod)); 3703 children.add(new Property("typeCollected", "CodeableConcept", "The kind of material to be collected.", 0, 1, typeCollected)); 3704 children.add(new Property("patientPreparation", "CodeableConcept", "Preparation of the patient for specimen collection.", 0, java.lang.Integer.MAX_VALUE, patientPreparation)); 3705 children.add(new Property("timeAspect", "string", "Time aspect of specimen collection (duration or offset).", 0, 1, timeAspect)); 3706 children.add(new Property("collection", "CodeableConcept", "The action to be performed for collecting the specimen.", 0, java.lang.Integer.MAX_VALUE, collection)); 3707 children.add(new Property("typeTested", "", "Specimen conditioned in a container as expected by the testing laboratory.", 0, java.lang.Integer.MAX_VALUE, typeTested)); 3708 } 3709 3710 @Override 3711 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3712 switch (_hash) { 3713 case 116079: /*url*/ return new Property("url", "uri", "An absolute URL that is used to identify this SpecimenDefinition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this SpecimenDefinition is (or will be) published. The URL SHOULD include the major version of the SpecimenDefinition. For more information see Technical and Business Versions.", 0, 1, url); 3714 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A business identifier assigned to this SpecimenDefinition.", 0, 1, identifier); 3715 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the SpecimenDefinition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the SpecimenDefinition author and is not expected to be globally unique.", 0, 1, version); 3716 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3717 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3718 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3719 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3720 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the specimen definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 3721 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the SpecimenDefinition.", 0, 1, title); 3722 case -978133683: /*derivedFromCanonical*/ return new Property("derivedFromCanonical", "canonical(SpecimenDefinition)", "The canonical URL pointing to another FHIR-defined SpecimenDefinition that is adhered to in whole or in part by this definition.", 0, java.lang.Integer.MAX_VALUE, derivedFromCanonical); 3723 case -1076333435: /*derivedFromUri*/ return new Property("derivedFromUri", "uri", "The URL pointing to an externally-defined type of specimen, guideline or other definition that is adhered to in whole or in part by this definition.", 0, java.lang.Integer.MAX_VALUE, derivedFromUri); 3724 case -892481550: /*status*/ return new Property("status", "code", "The current state of theSpecimenDefinition.", 0, 1, status); 3725 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A flag to indicate that this SpecimenDefinition is not authored for genuine usage.", 0, 1, experimental); 3726 case -573640748: /*subject[x]*/ return new Property("subject[x]", "CodeableConcept|Reference(Group)", "A code or group definition that describes the intended subject from which this kind of specimen is to be collected.", 0, 1, subject); 3727 case -1867885268: /*subject*/ return new Property("subject[x]", "CodeableConcept|Reference(Group)", "A code or group definition that describes the intended subject from which this kind of specimen is to be collected.", 0, 1, subject); 3728 case -1257122603: /*subjectCodeableConcept*/ return new Property("subject[x]", "CodeableConcept", "A code or group definition that describes the intended subject from which this kind of specimen is to be collected.", 0, 1, subject); 3729 case 772938623: /*subjectReference*/ return new Property("subject[x]", "Reference(Group)", "A code or group definition that describes the intended subject from which this kind of specimen is to be collected.", 0, 1, subject); 3730 case 3076014: /*date*/ return new Property("date", "dateTime", "For draft definitions, indicates the date of initial creation. For active definitions, represents the date of activation. For withdrawn definitions, indicates the date of withdrawal.", 0, 1, date); 3731 case 1447404028: /*publisher*/ return new Property("publisher", "string", "Helps establish the \"authority/credibility\" of the SpecimenDefinition. May also allow for contact.", 0, 1, publisher); 3732 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 3733 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the SpecimenDefinition from the consumer's perspective.", 0, 1, description); 3734 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of specimen definitions.", 0, java.lang.Integer.MAX_VALUE, useContext); 3735 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A jurisdiction in which the SpecimenDefinition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 3736 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explains why this SpecimeDefinition is needed and why it has been designed as it has.", 0, 1, purpose); 3737 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "Copyright statement relating to the SpecimenDefinition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SpecimenDefinition.", 0, 1, copyright); 3738 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 3739 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 3740 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.", 0, 1, lastReviewDate); 3741 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the SpecimenDefinition content was or is planned to be effective.", 0, 1, effectivePeriod); 3742 case 588504367: /*typeCollected*/ return new Property("typeCollected", "CodeableConcept", "The kind of material to be collected.", 0, 1, typeCollected); 3743 case -879411630: /*patientPreparation*/ return new Property("patientPreparation", "CodeableConcept", "Preparation of the patient for specimen collection.", 0, java.lang.Integer.MAX_VALUE, patientPreparation); 3744 case 276972933: /*timeAspect*/ return new Property("timeAspect", "string", "Time aspect of specimen collection (duration or offset).", 0, 1, timeAspect); 3745 case -1741312354: /*collection*/ return new Property("collection", "CodeableConcept", "The action to be performed for collecting the specimen.", 0, java.lang.Integer.MAX_VALUE, collection); 3746 case -1407902581: /*typeTested*/ return new Property("typeTested", "", "Specimen conditioned in a container as expected by the testing laboratory.", 0, java.lang.Integer.MAX_VALUE, typeTested); 3747 default: return super.getNamedProperty(_hash, _name, _checkValid); 3748 } 3749 3750 } 3751 3752 @Override 3753 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3754 switch (hash) { 3755 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 3756 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 3757 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 3758 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 3759 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3760 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 3761 case -978133683: /*derivedFromCanonical*/ return this.derivedFromCanonical == null ? new Base[0] : this.derivedFromCanonical.toArray(new Base[this.derivedFromCanonical.size()]); // CanonicalType 3762 case -1076333435: /*derivedFromUri*/ return this.derivedFromUri == null ? new Base[0] : this.derivedFromUri.toArray(new Base[this.derivedFromUri.size()]); // UriType 3763 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 3764 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 3765 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // DataType 3766 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 3767 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 3768 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3769 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3770 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3771 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3772 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 3773 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 3774 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 3775 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 3776 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 3777 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 3778 case 588504367: /*typeCollected*/ return this.typeCollected == null ? new Base[0] : new Base[] {this.typeCollected}; // CodeableConcept 3779 case -879411630: /*patientPreparation*/ return this.patientPreparation == null ? new Base[0] : this.patientPreparation.toArray(new Base[this.patientPreparation.size()]); // CodeableConcept 3780 case 276972933: /*timeAspect*/ return this.timeAspect == null ? new Base[0] : new Base[] {this.timeAspect}; // StringType 3781 case -1741312354: /*collection*/ return this.collection == null ? new Base[0] : this.collection.toArray(new Base[this.collection.size()]); // CodeableConcept 3782 case -1407902581: /*typeTested*/ return this.typeTested == null ? new Base[0] : this.typeTested.toArray(new Base[this.typeTested.size()]); // SpecimenDefinitionTypeTestedComponent 3783 default: return super.getProperty(hash, name, checkValid); 3784 } 3785 3786 } 3787 3788 @Override 3789 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3790 switch (hash) { 3791 case 116079: // url 3792 this.url = TypeConvertor.castToUri(value); // UriType 3793 return value; 3794 case -1618432855: // identifier 3795 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 3796 return value; 3797 case 351608024: // version 3798 this.version = TypeConvertor.castToString(value); // StringType 3799 return value; 3800 case 1508158071: // versionAlgorithm 3801 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 3802 return value; 3803 case 3373707: // name 3804 this.name = TypeConvertor.castToString(value); // StringType 3805 return value; 3806 case 110371416: // title 3807 this.title = TypeConvertor.castToString(value); // StringType 3808 return value; 3809 case -978133683: // derivedFromCanonical 3810 this.getDerivedFromCanonical().add(TypeConvertor.castToCanonical(value)); // CanonicalType 3811 return value; 3812 case -1076333435: // derivedFromUri 3813 this.getDerivedFromUri().add(TypeConvertor.castToUri(value)); // UriType 3814 return value; 3815 case -892481550: // status 3816 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3817 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3818 return value; 3819 case -404562712: // experimental 3820 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 3821 return value; 3822 case -1867885268: // subject 3823 this.subject = TypeConvertor.castToType(value); // DataType 3824 return value; 3825 case 3076014: // date 3826 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 3827 return value; 3828 case 1447404028: // publisher 3829 this.publisher = TypeConvertor.castToString(value); // StringType 3830 return value; 3831 case 951526432: // contact 3832 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 3833 return value; 3834 case -1724546052: // description 3835 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3836 return value; 3837 case -669707736: // useContext 3838 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 3839 return value; 3840 case -507075711: // jurisdiction 3841 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3842 return value; 3843 case -220463842: // purpose 3844 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 3845 return value; 3846 case 1522889671: // copyright 3847 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 3848 return value; 3849 case 765157229: // copyrightLabel 3850 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 3851 return value; 3852 case 223539345: // approvalDate 3853 this.approvalDate = TypeConvertor.castToDate(value); // DateType 3854 return value; 3855 case -1687512484: // lastReviewDate 3856 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 3857 return value; 3858 case -403934648: // effectivePeriod 3859 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 3860 return value; 3861 case 588504367: // typeCollected 3862 this.typeCollected = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3863 return value; 3864 case -879411630: // patientPreparation 3865 this.getPatientPreparation().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3866 return value; 3867 case 276972933: // timeAspect 3868 this.timeAspect = TypeConvertor.castToString(value); // StringType 3869 return value; 3870 case -1741312354: // collection 3871 this.getCollection().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3872 return value; 3873 case -1407902581: // typeTested 3874 this.getTypeTested().add((SpecimenDefinitionTypeTestedComponent) value); // SpecimenDefinitionTypeTestedComponent 3875 return value; 3876 default: return super.setProperty(hash, name, value); 3877 } 3878 3879 } 3880 3881 @Override 3882 public Base setProperty(String name, Base value) throws FHIRException { 3883 if (name.equals("url")) { 3884 this.url = TypeConvertor.castToUri(value); // UriType 3885 } else if (name.equals("identifier")) { 3886 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 3887 } else if (name.equals("version")) { 3888 this.version = TypeConvertor.castToString(value); // StringType 3889 } else if (name.equals("versionAlgorithm[x]")) { 3890 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 3891 } else if (name.equals("name")) { 3892 this.name = TypeConvertor.castToString(value); // StringType 3893 } else if (name.equals("title")) { 3894 this.title = TypeConvertor.castToString(value); // StringType 3895 } else if (name.equals("derivedFromCanonical")) { 3896 this.getDerivedFromCanonical().add(TypeConvertor.castToCanonical(value)); 3897 } else if (name.equals("derivedFromUri")) { 3898 this.getDerivedFromUri().add(TypeConvertor.castToUri(value)); 3899 } else if (name.equals("status")) { 3900 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3901 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3902 } else if (name.equals("experimental")) { 3903 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 3904 } else if (name.equals("subject[x]")) { 3905 this.subject = TypeConvertor.castToType(value); // DataType 3906 } else if (name.equals("date")) { 3907 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 3908 } else if (name.equals("publisher")) { 3909 this.publisher = TypeConvertor.castToString(value); // StringType 3910 } else if (name.equals("contact")) { 3911 this.getContact().add(TypeConvertor.castToContactDetail(value)); 3912 } else if (name.equals("description")) { 3913 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3914 } else if (name.equals("useContext")) { 3915 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 3916 } else if (name.equals("jurisdiction")) { 3917 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 3918 } else if (name.equals("purpose")) { 3919 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 3920 } else if (name.equals("copyright")) { 3921 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 3922 } else if (name.equals("copyrightLabel")) { 3923 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 3924 } else if (name.equals("approvalDate")) { 3925 this.approvalDate = TypeConvertor.castToDate(value); // DateType 3926 } else if (name.equals("lastReviewDate")) { 3927 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 3928 } else if (name.equals("effectivePeriod")) { 3929 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 3930 } else if (name.equals("typeCollected")) { 3931 this.typeCollected = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3932 } else if (name.equals("patientPreparation")) { 3933 this.getPatientPreparation().add(TypeConvertor.castToCodeableConcept(value)); 3934 } else if (name.equals("timeAspect")) { 3935 this.timeAspect = TypeConvertor.castToString(value); // StringType 3936 } else if (name.equals("collection")) { 3937 this.getCollection().add(TypeConvertor.castToCodeableConcept(value)); 3938 } else if (name.equals("typeTested")) { 3939 this.getTypeTested().add((SpecimenDefinitionTypeTestedComponent) value); 3940 } else 3941 return super.setProperty(name, value); 3942 return value; 3943 } 3944 3945 @Override 3946 public void removeChild(String name, Base value) throws FHIRException { 3947 if (name.equals("url")) { 3948 this.url = null; 3949 } else if (name.equals("identifier")) { 3950 this.identifier = null; 3951 } else if (name.equals("version")) { 3952 this.version = null; 3953 } else if (name.equals("versionAlgorithm[x]")) { 3954 this.versionAlgorithm = null; 3955 } else if (name.equals("name")) { 3956 this.name = null; 3957 } else if (name.equals("title")) { 3958 this.title = null; 3959 } else if (name.equals("derivedFromCanonical")) { 3960 this.getDerivedFromCanonical().remove(value); 3961 } else if (name.equals("derivedFromUri")) { 3962 this.getDerivedFromUri().remove(value); 3963 } else if (name.equals("status")) { 3964 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3965 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3966 } else if (name.equals("experimental")) { 3967 this.experimental = null; 3968 } else if (name.equals("subject[x]")) { 3969 this.subject = null; 3970 } else if (name.equals("date")) { 3971 this.date = null; 3972 } else if (name.equals("publisher")) { 3973 this.publisher = null; 3974 } else if (name.equals("contact")) { 3975 this.getContact().remove(value); 3976 } else if (name.equals("description")) { 3977 this.description = null; 3978 } else if (name.equals("useContext")) { 3979 this.getUseContext().remove(value); 3980 } else if (name.equals("jurisdiction")) { 3981 this.getJurisdiction().remove(value); 3982 } else if (name.equals("purpose")) { 3983 this.purpose = null; 3984 } else if (name.equals("copyright")) { 3985 this.copyright = null; 3986 } else if (name.equals("copyrightLabel")) { 3987 this.copyrightLabel = null; 3988 } else if (name.equals("approvalDate")) { 3989 this.approvalDate = null; 3990 } else if (name.equals("lastReviewDate")) { 3991 this.lastReviewDate = null; 3992 } else if (name.equals("effectivePeriod")) { 3993 this.effectivePeriod = null; 3994 } else if (name.equals("typeCollected")) { 3995 this.typeCollected = null; 3996 } else if (name.equals("patientPreparation")) { 3997 this.getPatientPreparation().remove(value); 3998 } else if (name.equals("timeAspect")) { 3999 this.timeAspect = null; 4000 } else if (name.equals("collection")) { 4001 this.getCollection().remove(value); 4002 } else if (name.equals("typeTested")) { 4003 this.getTypeTested().remove((SpecimenDefinitionTypeTestedComponent) value); 4004 } else 4005 super.removeChild(name, value); 4006 4007 } 4008 4009 @Override 4010 public Base makeProperty(int hash, String name) throws FHIRException { 4011 switch (hash) { 4012 case 116079: return getUrlElement(); 4013 case -1618432855: return getIdentifier(); 4014 case 351608024: return getVersionElement(); 4015 case -115699031: return getVersionAlgorithm(); 4016 case 1508158071: return getVersionAlgorithm(); 4017 case 3373707: return getNameElement(); 4018 case 110371416: return getTitleElement(); 4019 case -978133683: return addDerivedFromCanonicalElement(); 4020 case -1076333435: return addDerivedFromUriElement(); 4021 case -892481550: return getStatusElement(); 4022 case -404562712: return getExperimentalElement(); 4023 case -573640748: return getSubject(); 4024 case -1867885268: return getSubject(); 4025 case 3076014: return getDateElement(); 4026 case 1447404028: return getPublisherElement(); 4027 case 951526432: return addContact(); 4028 case -1724546052: return getDescriptionElement(); 4029 case -669707736: return addUseContext(); 4030 case -507075711: return addJurisdiction(); 4031 case -220463842: return getPurposeElement(); 4032 case 1522889671: return getCopyrightElement(); 4033 case 765157229: return getCopyrightLabelElement(); 4034 case 223539345: return getApprovalDateElement(); 4035 case -1687512484: return getLastReviewDateElement(); 4036 case -403934648: return getEffectivePeriod(); 4037 case 588504367: return getTypeCollected(); 4038 case -879411630: return addPatientPreparation(); 4039 case 276972933: return getTimeAspectElement(); 4040 case -1741312354: return addCollection(); 4041 case -1407902581: return addTypeTested(); 4042 default: return super.makeProperty(hash, name); 4043 } 4044 4045 } 4046 4047 @Override 4048 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4049 switch (hash) { 4050 case 116079: /*url*/ return new String[] {"uri"}; 4051 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4052 case 351608024: /*version*/ return new String[] {"string"}; 4053 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 4054 case 3373707: /*name*/ return new String[] {"string"}; 4055 case 110371416: /*title*/ return new String[] {"string"}; 4056 case -978133683: /*derivedFromCanonical*/ return new String[] {"canonical"}; 4057 case -1076333435: /*derivedFromUri*/ return new String[] {"uri"}; 4058 case -892481550: /*status*/ return new String[] {"code"}; 4059 case -404562712: /*experimental*/ return new String[] {"boolean"}; 4060 case -1867885268: /*subject*/ return new String[] {"CodeableConcept", "Reference"}; 4061 case 3076014: /*date*/ return new String[] {"dateTime"}; 4062 case 1447404028: /*publisher*/ return new String[] {"string"}; 4063 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 4064 case -1724546052: /*description*/ return new String[] {"markdown"}; 4065 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 4066 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 4067 case -220463842: /*purpose*/ return new String[] {"markdown"}; 4068 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 4069 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 4070 case 223539345: /*approvalDate*/ return new String[] {"date"}; 4071 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 4072 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 4073 case 588504367: /*typeCollected*/ return new String[] {"CodeableConcept"}; 4074 case -879411630: /*patientPreparation*/ return new String[] {"CodeableConcept"}; 4075 case 276972933: /*timeAspect*/ return new String[] {"string"}; 4076 case -1741312354: /*collection*/ return new String[] {"CodeableConcept"}; 4077 case -1407902581: /*typeTested*/ return new String[] {}; 4078 default: return super.getTypesForProperty(hash, name); 4079 } 4080 4081 } 4082 4083 @Override 4084 public Base addChild(String name) throws FHIRException { 4085 if (name.equals("url")) { 4086 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.url"); 4087 } 4088 else if (name.equals("identifier")) { 4089 this.identifier = new Identifier(); 4090 return this.identifier; 4091 } 4092 else if (name.equals("version")) { 4093 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.version"); 4094 } 4095 else if (name.equals("versionAlgorithmString")) { 4096 this.versionAlgorithm = new StringType(); 4097 return this.versionAlgorithm; 4098 } 4099 else if (name.equals("versionAlgorithmCoding")) { 4100 this.versionAlgorithm = new Coding(); 4101 return this.versionAlgorithm; 4102 } 4103 else if (name.equals("name")) { 4104 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.name"); 4105 } 4106 else if (name.equals("title")) { 4107 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.title"); 4108 } 4109 else if (name.equals("derivedFromCanonical")) { 4110 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.derivedFromCanonical"); 4111 } 4112 else if (name.equals("derivedFromUri")) { 4113 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.derivedFromUri"); 4114 } 4115 else if (name.equals("status")) { 4116 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.status"); 4117 } 4118 else if (name.equals("experimental")) { 4119 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.experimental"); 4120 } 4121 else if (name.equals("subjectCodeableConcept")) { 4122 this.subject = new CodeableConcept(); 4123 return this.subject; 4124 } 4125 else if (name.equals("subjectReference")) { 4126 this.subject = new Reference(); 4127 return this.subject; 4128 } 4129 else if (name.equals("date")) { 4130 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.date"); 4131 } 4132 else if (name.equals("publisher")) { 4133 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.publisher"); 4134 } 4135 else if (name.equals("contact")) { 4136 return addContact(); 4137 } 4138 else if (name.equals("description")) { 4139 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.description"); 4140 } 4141 else if (name.equals("useContext")) { 4142 return addUseContext(); 4143 } 4144 else if (name.equals("jurisdiction")) { 4145 return addJurisdiction(); 4146 } 4147 else if (name.equals("purpose")) { 4148 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.purpose"); 4149 } 4150 else if (name.equals("copyright")) { 4151 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.copyright"); 4152 } 4153 else if (name.equals("copyrightLabel")) { 4154 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.copyrightLabel"); 4155 } 4156 else if (name.equals("approvalDate")) { 4157 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.approvalDate"); 4158 } 4159 else if (name.equals("lastReviewDate")) { 4160 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.lastReviewDate"); 4161 } 4162 else if (name.equals("effectivePeriod")) { 4163 this.effectivePeriod = new Period(); 4164 return this.effectivePeriod; 4165 } 4166 else if (name.equals("typeCollected")) { 4167 this.typeCollected = new CodeableConcept(); 4168 return this.typeCollected; 4169 } 4170 else if (name.equals("patientPreparation")) { 4171 return addPatientPreparation(); 4172 } 4173 else if (name.equals("timeAspect")) { 4174 throw new FHIRException("Cannot call addChild on a singleton property SpecimenDefinition.timeAspect"); 4175 } 4176 else if (name.equals("collection")) { 4177 return addCollection(); 4178 } 4179 else if (name.equals("typeTested")) { 4180 return addTypeTested(); 4181 } 4182 else 4183 return super.addChild(name); 4184 } 4185 4186 public String fhirType() { 4187 return "SpecimenDefinition"; 4188 4189 } 4190 4191 public SpecimenDefinition copy() { 4192 SpecimenDefinition dst = new SpecimenDefinition(); 4193 copyValues(dst); 4194 return dst; 4195 } 4196 4197 public void copyValues(SpecimenDefinition dst) { 4198 super.copyValues(dst); 4199 dst.url = url == null ? null : url.copy(); 4200 dst.identifier = identifier == null ? null : identifier.copy(); 4201 dst.version = version == null ? null : version.copy(); 4202 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 4203 dst.name = name == null ? null : name.copy(); 4204 dst.title = title == null ? null : title.copy(); 4205 if (derivedFromCanonical != null) { 4206 dst.derivedFromCanonical = new ArrayList<CanonicalType>(); 4207 for (CanonicalType i : derivedFromCanonical) 4208 dst.derivedFromCanonical.add(i.copy()); 4209 }; 4210 if (derivedFromUri != null) { 4211 dst.derivedFromUri = new ArrayList<UriType>(); 4212 for (UriType i : derivedFromUri) 4213 dst.derivedFromUri.add(i.copy()); 4214 }; 4215 dst.status = status == null ? null : status.copy(); 4216 dst.experimental = experimental == null ? null : experimental.copy(); 4217 dst.subject = subject == null ? null : subject.copy(); 4218 dst.date = date == null ? null : date.copy(); 4219 dst.publisher = publisher == null ? null : publisher.copy(); 4220 if (contact != null) { 4221 dst.contact = new ArrayList<ContactDetail>(); 4222 for (ContactDetail i : contact) 4223 dst.contact.add(i.copy()); 4224 }; 4225 dst.description = description == null ? null : description.copy(); 4226 if (useContext != null) { 4227 dst.useContext = new ArrayList<UsageContext>(); 4228 for (UsageContext i : useContext) 4229 dst.useContext.add(i.copy()); 4230 }; 4231 if (jurisdiction != null) { 4232 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4233 for (CodeableConcept i : jurisdiction) 4234 dst.jurisdiction.add(i.copy()); 4235 }; 4236 dst.purpose = purpose == null ? null : purpose.copy(); 4237 dst.copyright = copyright == null ? null : copyright.copy(); 4238 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 4239 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 4240 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 4241 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 4242 dst.typeCollected = typeCollected == null ? null : typeCollected.copy(); 4243 if (patientPreparation != null) { 4244 dst.patientPreparation = new ArrayList<CodeableConcept>(); 4245 for (CodeableConcept i : patientPreparation) 4246 dst.patientPreparation.add(i.copy()); 4247 }; 4248 dst.timeAspect = timeAspect == null ? null : timeAspect.copy(); 4249 if (collection != null) { 4250 dst.collection = new ArrayList<CodeableConcept>(); 4251 for (CodeableConcept i : collection) 4252 dst.collection.add(i.copy()); 4253 }; 4254 if (typeTested != null) { 4255 dst.typeTested = new ArrayList<SpecimenDefinitionTypeTestedComponent>(); 4256 for (SpecimenDefinitionTypeTestedComponent i : typeTested) 4257 dst.typeTested.add(i.copy()); 4258 }; 4259 } 4260 4261 protected SpecimenDefinition typedCopy() { 4262 return copy(); 4263 } 4264 4265 @Override 4266 public boolean equalsDeep(Base other_) { 4267 if (!super.equalsDeep(other_)) 4268 return false; 4269 if (!(other_ instanceof SpecimenDefinition)) 4270 return false; 4271 SpecimenDefinition o = (SpecimenDefinition) other_; 4272 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 4273 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 4274 && compareDeep(derivedFromCanonical, o.derivedFromCanonical, true) && compareDeep(derivedFromUri, o.derivedFromUri, true) 4275 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(subject, o.subject, true) 4276 && compareDeep(date, o.date, true) && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 4277 && compareDeep(description, o.description, true) && compareDeep(useContext, o.useContext, true) 4278 && compareDeep(jurisdiction, o.jurisdiction, true) && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 4279 && compareDeep(copyrightLabel, o.copyrightLabel, true) && compareDeep(approvalDate, o.approvalDate, true) 4280 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 4281 && compareDeep(typeCollected, o.typeCollected, true) && compareDeep(patientPreparation, o.patientPreparation, true) 4282 && compareDeep(timeAspect, o.timeAspect, true) && compareDeep(collection, o.collection, true) && compareDeep(typeTested, o.typeTested, true) 4283 ; 4284 } 4285 4286 @Override 4287 public boolean equalsShallow(Base other_) { 4288 if (!super.equalsShallow(other_)) 4289 return false; 4290 if (!(other_ instanceof SpecimenDefinition)) 4291 return false; 4292 SpecimenDefinition o = (SpecimenDefinition) other_; 4293 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 4294 && compareValues(title, o.title, true) && compareValues(derivedFromCanonical, o.derivedFromCanonical, true) 4295 && compareValues(derivedFromUri, o.derivedFromUri, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 4296 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 4297 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 4298 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 4299 && compareValues(timeAspect, o.timeAspect, true); 4300 } 4301 4302 public boolean isEmpty() { 4303 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 4304 , versionAlgorithm, name, title, derivedFromCanonical, derivedFromUri, status, experimental 4305 , subject, date, publisher, contact, description, useContext, jurisdiction, purpose 4306 , copyright, copyrightLabel, approvalDate, lastReviewDate, effectivePeriod, typeCollected 4307 , patientPreparation, timeAspect, collection, typeTested); 4308 } 4309 4310 @Override 4311 public ResourceType getResourceType() { 4312 return ResourceType.SpecimenDefinition; 4313 } 4314 4315 /** 4316 * Search parameter: <b>identifier</b> 4317 * <p> 4318 * Description: <b>Multiple Resources: 4319 4320* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4321* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4322* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4323* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4324* [Citation](citation.html): External identifier for the citation 4325* [CodeSystem](codesystem.html): External identifier for the code system 4326* [ConceptMap](conceptmap.html): External identifier for the concept map 4327* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4328* [EventDefinition](eventdefinition.html): External identifier for the event definition 4329* [Evidence](evidence.html): External identifier for the evidence 4330* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4331* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4332* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4333* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4334* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4335* [Library](library.html): External identifier for the library 4336* [Measure](measure.html): External identifier for the measure 4337* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4338* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4339* [NamingSystem](namingsystem.html): External identifier for the naming system 4340* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4341* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4342* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4343* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4344* [Requirements](requirements.html): External identifier for the requirements 4345* [SearchParameter](searchparameter.html): External identifier for the search parameter 4346* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4347* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4348* [StructureMap](structuremap.html): External identifier for the structure map 4349* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4350* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4351* [TestPlan](testplan.html): An identifier for the test plan 4352* [TestScript](testscript.html): External identifier for the test script 4353* [ValueSet](valueset.html): External identifier for the value set 4354</b><br> 4355 * Type: <b>token</b><br> 4356 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4357 * </p> 4358 */ 4359 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 4360 public static final String SP_IDENTIFIER = "identifier"; 4361 /** 4362 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4363 * <p> 4364 * Description: <b>Multiple Resources: 4365 4366* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4367* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4368* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4369* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4370* [Citation](citation.html): External identifier for the citation 4371* [CodeSystem](codesystem.html): External identifier for the code system 4372* [ConceptMap](conceptmap.html): External identifier for the concept map 4373* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4374* [EventDefinition](eventdefinition.html): External identifier for the event definition 4375* [Evidence](evidence.html): External identifier for the evidence 4376* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4377* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4378* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4379* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4380* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4381* [Library](library.html): External identifier for the library 4382* [Measure](measure.html): External identifier for the measure 4383* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4384* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4385* [NamingSystem](namingsystem.html): External identifier for the naming system 4386* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4387* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4388* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4389* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4390* [Requirements](requirements.html): External identifier for the requirements 4391* [SearchParameter](searchparameter.html): External identifier for the search parameter 4392* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4393* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4394* [StructureMap](structuremap.html): External identifier for the structure map 4395* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4396* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4397* [TestPlan](testplan.html): An identifier for the test plan 4398* [TestScript](testscript.html): External identifier for the test script 4399* [ValueSet](valueset.html): External identifier for the value set 4400</b><br> 4401 * Type: <b>token</b><br> 4402 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4403 * </p> 4404 */ 4405 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4406 4407 /** 4408 * Search parameter: <b>status</b> 4409 * <p> 4410 * Description: <b>Multiple Resources: 4411 4412* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4413* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4414* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4415* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4416* [Citation](citation.html): The current status of the citation 4417* [CodeSystem](codesystem.html): The current status of the code system 4418* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4419* [ConceptMap](conceptmap.html): The current status of the concept map 4420* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4421* [EventDefinition](eventdefinition.html): The current status of the event definition 4422* [Evidence](evidence.html): The current status of the evidence 4423* [EvidenceReport](evidencereport.html): The current status of the evidence report 4424* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4425* [ExampleScenario](examplescenario.html): The current status of the example scenario 4426* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4427* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4428* [Library](library.html): The current status of the library 4429* [Measure](measure.html): The current status of the measure 4430* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4431* [MessageDefinition](messagedefinition.html): The current status of the message definition 4432* [NamingSystem](namingsystem.html): The current status of the naming system 4433* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4434* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4435* [PlanDefinition](plandefinition.html): The current status of the plan definition 4436* [Questionnaire](questionnaire.html): The current status of the questionnaire 4437* [Requirements](requirements.html): The current status of the requirements 4438* [SearchParameter](searchparameter.html): The current status of the search parameter 4439* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4440* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4441* [StructureMap](structuremap.html): The current status of the structure map 4442* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4443* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4444* [TestPlan](testplan.html): The current status of the test plan 4445* [TestScript](testscript.html): The current status of the test script 4446* [ValueSet](valueset.html): The current status of the value set 4447</b><br> 4448 * Type: <b>token</b><br> 4449 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4450 * </p> 4451 */ 4452 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 4453 public static final String SP_STATUS = "status"; 4454 /** 4455 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4456 * <p> 4457 * Description: <b>Multiple Resources: 4458 4459* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4460* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4461* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4462* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4463* [Citation](citation.html): The current status of the citation 4464* [CodeSystem](codesystem.html): The current status of the code system 4465* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4466* [ConceptMap](conceptmap.html): The current status of the concept map 4467* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4468* [EventDefinition](eventdefinition.html): The current status of the event definition 4469* [Evidence](evidence.html): The current status of the evidence 4470* [EvidenceReport](evidencereport.html): The current status of the evidence report 4471* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4472* [ExampleScenario](examplescenario.html): The current status of the example scenario 4473* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4474* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4475* [Library](library.html): The current status of the library 4476* [Measure](measure.html): The current status of the measure 4477* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4478* [MessageDefinition](messagedefinition.html): The current status of the message definition 4479* [NamingSystem](namingsystem.html): The current status of the naming system 4480* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4481* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4482* [PlanDefinition](plandefinition.html): The current status of the plan definition 4483* [Questionnaire](questionnaire.html): The current status of the questionnaire 4484* [Requirements](requirements.html): The current status of the requirements 4485* [SearchParameter](searchparameter.html): The current status of the search parameter 4486* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4487* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4488* [StructureMap](structuremap.html): The current status of the structure map 4489* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4490* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4491* [TestPlan](testplan.html): The current status of the test plan 4492* [TestScript](testscript.html): The current status of the test script 4493* [ValueSet](valueset.html): The current status of the value set 4494</b><br> 4495 * Type: <b>token</b><br> 4496 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4497 * </p> 4498 */ 4499 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4500 4501 /** 4502 * Search parameter: <b>title</b> 4503 * <p> 4504 * Description: <b>Multiple Resources: 4505 4506* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 4507* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 4508* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 4509* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 4510* [Citation](citation.html): The human-friendly name of the citation 4511* [CodeSystem](codesystem.html): The human-friendly name of the code system 4512* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 4513* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 4514* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 4515* [Evidence](evidence.html): The human-friendly name of the evidence 4516* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 4517* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 4518* [Library](library.html): The human-friendly name of the library 4519* [Measure](measure.html): The human-friendly name of the measure 4520* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 4521* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 4522* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 4523* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 4524* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 4525* [Requirements](requirements.html): The human-friendly name of the requirements 4526* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 4527* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 4528* [StructureMap](structuremap.html): The human-friendly name of the structure map 4529* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 4530* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 4531* [TestScript](testscript.html): The human-friendly name of the test script 4532* [ValueSet](valueset.html): The human-friendly name of the value set 4533</b><br> 4534 * Type: <b>string</b><br> 4535 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 4536 * </p> 4537 */ 4538 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 4539 public static final String SP_TITLE = "title"; 4540 /** 4541 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4542 * <p> 4543 * Description: <b>Multiple Resources: 4544 4545* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 4546* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 4547* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 4548* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 4549* [Citation](citation.html): The human-friendly name of the citation 4550* [CodeSystem](codesystem.html): The human-friendly name of the code system 4551* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 4552* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 4553* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 4554* [Evidence](evidence.html): The human-friendly name of the evidence 4555* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 4556* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 4557* [Library](library.html): The human-friendly name of the library 4558* [Measure](measure.html): The human-friendly name of the measure 4559* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 4560* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 4561* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 4562* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 4563* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 4564* [Requirements](requirements.html): The human-friendly name of the requirements 4565* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 4566* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 4567* [StructureMap](structuremap.html): The human-friendly name of the structure map 4568* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 4569* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 4570* [TestScript](testscript.html): The human-friendly name of the test script 4571* [ValueSet](valueset.html): The human-friendly name of the value set 4572</b><br> 4573 * Type: <b>string</b><br> 4574 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 4575 * </p> 4576 */ 4577 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 4578 4579 /** 4580 * Search parameter: <b>url</b> 4581 * <p> 4582 * Description: <b>Multiple Resources: 4583 4584* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4585* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4586* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4587* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4588* [Citation](citation.html): The uri that identifies the citation 4589* [CodeSystem](codesystem.html): The uri that identifies the code system 4590* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4591* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4592* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4593* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4594* [Evidence](evidence.html): The uri that identifies the evidence 4595* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4596* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4597* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4598* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4599* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4600* [Library](library.html): The uri that identifies the library 4601* [Measure](measure.html): The uri that identifies the measure 4602* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4603* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4604* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4605* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4606* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4607* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4608* [Requirements](requirements.html): The uri that identifies the requirements 4609* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4610* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4611* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4612* [StructureMap](structuremap.html): The uri that identifies the structure map 4613* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4614* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4615* [TestPlan](testplan.html): The uri that identifies the test plan 4616* [TestScript](testscript.html): The uri that identifies the test script 4617* [ValueSet](valueset.html): The uri that identifies the value set 4618</b><br> 4619 * Type: <b>uri</b><br> 4620 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4621 * </p> 4622 */ 4623 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 4624 public static final String SP_URL = "url"; 4625 /** 4626 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4627 * <p> 4628 * Description: <b>Multiple Resources: 4629 4630* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4631* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4632* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4633* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4634* [Citation](citation.html): The uri that identifies the citation 4635* [CodeSystem](codesystem.html): The uri that identifies the code system 4636* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4637* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4638* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4639* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4640* [Evidence](evidence.html): The uri that identifies the evidence 4641* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4642* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4643* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4644* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4645* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4646* [Library](library.html): The uri that identifies the library 4647* [Measure](measure.html): The uri that identifies the measure 4648* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4649* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4650* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4651* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4652* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4653* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4654* [Requirements](requirements.html): The uri that identifies the requirements 4655* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4656* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4657* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4658* [StructureMap](structuremap.html): The uri that identifies the structure map 4659* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4660* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4661* [TestPlan](testplan.html): The uri that identifies the test plan 4662* [TestScript](testscript.html): The uri that identifies the test script 4663* [ValueSet](valueset.html): The uri that identifies the value set 4664</b><br> 4665 * Type: <b>uri</b><br> 4666 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4667 * </p> 4668 */ 4669 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4670 4671 /** 4672 * Search parameter: <b>container</b> 4673 * <p> 4674 * Description: <b>The type of specimen conditioned in container expected by the lab</b><br> 4675 * Type: <b>token</b><br> 4676 * Path: <b>SpecimenDefinition.typeTested.container.type</b><br> 4677 * </p> 4678 */ 4679 @SearchParamDefinition(name="container", path="SpecimenDefinition.typeTested.container.type", description="The type of specimen conditioned in container expected by the lab", type="token" ) 4680 public static final String SP_CONTAINER = "container"; 4681 /** 4682 * <b>Fluent Client</b> search parameter constant for <b>container</b> 4683 * <p> 4684 * Description: <b>The type of specimen conditioned in container expected by the lab</b><br> 4685 * Type: <b>token</b><br> 4686 * Path: <b>SpecimenDefinition.typeTested.container.type</b><br> 4687 * </p> 4688 */ 4689 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTAINER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTAINER); 4690 4691 /** 4692 * Search parameter: <b>experimental</b> 4693 * <p> 4694 * Description: <b>Not for genuine usage (true)</b><br> 4695 * Type: <b>token</b><br> 4696 * Path: <b>SpecimenDefinition.experimental</b><br> 4697 * </p> 4698 */ 4699 @SearchParamDefinition(name="experimental", path="SpecimenDefinition.experimental", description="Not for genuine usage (true)", type="token" ) 4700 public static final String SP_EXPERIMENTAL = "experimental"; 4701 /** 4702 * <b>Fluent Client</b> search parameter constant for <b>experimental</b> 4703 * <p> 4704 * Description: <b>Not for genuine usage (true)</b><br> 4705 * Type: <b>token</b><br> 4706 * Path: <b>SpecimenDefinition.experimental</b><br> 4707 * </p> 4708 */ 4709 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXPERIMENTAL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EXPERIMENTAL); 4710 4711 /** 4712 * Search parameter: <b>is-derived</b> 4713 * <p> 4714 * Description: <b>Primary specimen (false) or derived specimen (true)</b><br> 4715 * Type: <b>token</b><br> 4716 * Path: <b>SpecimenDefinition.typeTested.isDerived</b><br> 4717 * </p> 4718 */ 4719 @SearchParamDefinition(name="is-derived", path="SpecimenDefinition.typeTested.isDerived", description="Primary specimen (false) or derived specimen (true)", type="token" ) 4720 public static final String SP_IS_DERIVED = "is-derived"; 4721 /** 4722 * <b>Fluent Client</b> search parameter constant for <b>is-derived</b> 4723 * <p> 4724 * Description: <b>Primary specimen (false) or derived specimen (true)</b><br> 4725 * Type: <b>token</b><br> 4726 * Path: <b>SpecimenDefinition.typeTested.isDerived</b><br> 4727 * </p> 4728 */ 4729 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IS_DERIVED = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IS_DERIVED); 4730 4731 /** 4732 * Search parameter: <b>type-tested</b> 4733 * <p> 4734 * Description: <b>The type of specimen conditioned for testing</b><br> 4735 * Type: <b>token</b><br> 4736 * Path: <b>SpecimenDefinition.typeTested.type</b><br> 4737 * </p> 4738 */ 4739 @SearchParamDefinition(name="type-tested", path="SpecimenDefinition.typeTested.type", description="The type of specimen conditioned for testing", type="token" ) 4740 public static final String SP_TYPE_TESTED = "type-tested"; 4741 /** 4742 * <b>Fluent Client</b> search parameter constant for <b>type-tested</b> 4743 * <p> 4744 * Description: <b>The type of specimen conditioned for testing</b><br> 4745 * Type: <b>token</b><br> 4746 * Path: <b>SpecimenDefinition.typeTested.type</b><br> 4747 * </p> 4748 */ 4749 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE_TESTED = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE_TESTED); 4750 4751 /** 4752 * Search parameter: <b>type</b> 4753 * <p> 4754 * Description: <b>The type of collected specimen</b><br> 4755 * Type: <b>token</b><br> 4756 * Path: <b>SpecimenDefinition.typeCollected</b><br> 4757 * </p> 4758 */ 4759 @SearchParamDefinition(name="type", path="SpecimenDefinition.typeCollected", description="The type of collected specimen", type="token" ) 4760 public static final String SP_TYPE = "type"; 4761 /** 4762 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4763 * <p> 4764 * Description: <b>The type of collected specimen</b><br> 4765 * Type: <b>token</b><br> 4766 * Path: <b>SpecimenDefinition.typeCollected</b><br> 4767 * </p> 4768 */ 4769 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 4770 4771 4772} 4773