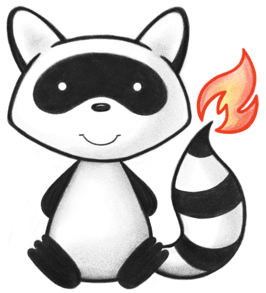
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Tue, May 4, 2021 07:17+1000 for FHIR v5.0.0-snapshot2 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.ChildOrder; 045import ca.uhn.fhir.model.api.annotation.DatatypeDef; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.Block; 048 049/** 050 * Base StructureDefinition for Statistic Type: A fact or piece of data from a study of a large quantity of numerical data. A mathematical or quantified characteristic of a group of observations. 051 */ 052@DatatypeDef(name="Statistic") 053public class Statistic extends BackboneType implements ICompositeType { 054 055 @Block() 056 public static class StatisticSampleSizeComponent extends Element implements IBaseDatatypeElement { 057 /** 058 * Human-readable summary of population sample size. 059 */ 060 @Child(name = "description", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 061 @Description(shortDefinition="Textual description of sample size for statistic", formalDefinition="Human-readable summary of population sample size." ) 062 protected StringType description; 063 064 /** 065 * Footnote or explanatory note about the sample size. 066 */ 067 @Child(name = "note", type = {Annotation.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 068 @Description(shortDefinition="Footnote or explanatory note about the sample size", formalDefinition="Footnote or explanatory note about the sample size." ) 069 protected List<Annotation> note; 070 071 /** 072 * Number of participants in the population. 073 */ 074 @Child(name = "numberOfStudies", type = {UnsignedIntType.class}, order=3, min=0, max=1, modifier=false, summary=true) 075 @Description(shortDefinition="Number of contributing studies", formalDefinition="Number of participants in the population." ) 076 protected UnsignedIntType numberOfStudies; 077 078 /** 079 * A human-readable string to clarify or explain concepts about the sample size. 080 */ 081 @Child(name = "numberOfParticipants", type = {UnsignedIntType.class}, order=4, min=0, max=1, modifier=false, summary=true) 082 @Description(shortDefinition="Cumulative number of participants", formalDefinition="A human-readable string to clarify or explain concepts about the sample size." ) 083 protected UnsignedIntType numberOfParticipants; 084 085 /** 086 * Number of participants with known results for measured variables. 087 */ 088 @Child(name = "knownDataCount", type = {UnsignedIntType.class}, order=5, min=0, max=1, modifier=false, summary=true) 089 @Description(shortDefinition="Number of participants with known results for measured variables", formalDefinition="Number of participants with known results for measured variables." ) 090 protected UnsignedIntType knownDataCount; 091 092 private static final long serialVersionUID = -1870635979L; 093 094 /** 095 * Constructor 096 */ 097 public StatisticSampleSizeComponent() { 098 super(); 099 } 100 101 /** 102 * @return {@link #description} (Human-readable summary of population sample size.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 103 */ 104 public StringType getDescriptionElement() { 105 if (this.description == null) 106 if (Configuration.errorOnAutoCreate()) 107 throw new Error("Attempt to auto-create StatisticSampleSizeComponent.description"); 108 else if (Configuration.doAutoCreate()) 109 this.description = new StringType(); // bb 110 return this.description; 111 } 112 113 public boolean hasDescriptionElement() { 114 return this.description != null && !this.description.isEmpty(); 115 } 116 117 public boolean hasDescription() { 118 return this.description != null && !this.description.isEmpty(); 119 } 120 121 /** 122 * @param value {@link #description} (Human-readable summary of population sample size.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 123 */ 124 public StatisticSampleSizeComponent setDescriptionElement(StringType value) { 125 this.description = value; 126 return this; 127 } 128 129 /** 130 * @return Human-readable summary of population sample size. 131 */ 132 public String getDescription() { 133 return this.description == null ? null : this.description.getValue(); 134 } 135 136 /** 137 * @param value Human-readable summary of population sample size. 138 */ 139 public StatisticSampleSizeComponent setDescription(String value) { 140 if (Utilities.noString(value)) 141 this.description = null; 142 else { 143 if (this.description == null) 144 this.description = new StringType(); 145 this.description.setValue(value); 146 } 147 return this; 148 } 149 150 /** 151 * @return {@link #note} (Footnote or explanatory note about the sample size.) 152 */ 153 public List<Annotation> getNote() { 154 if (this.note == null) 155 this.note = new ArrayList<Annotation>(); 156 return this.note; 157 } 158 159 /** 160 * @return Returns a reference to <code>this</code> for easy method chaining 161 */ 162 public StatisticSampleSizeComponent setNote(List<Annotation> theNote) { 163 this.note = theNote; 164 return this; 165 } 166 167 public boolean hasNote() { 168 if (this.note == null) 169 return false; 170 for (Annotation item : this.note) 171 if (!item.isEmpty()) 172 return true; 173 return false; 174 } 175 176 public Annotation addNote() { //3 177 Annotation t = new Annotation(); 178 if (this.note == null) 179 this.note = new ArrayList<Annotation>(); 180 this.note.add(t); 181 return t; 182 } 183 184 public StatisticSampleSizeComponent addNote(Annotation t) { //3 185 if (t == null) 186 return this; 187 if (this.note == null) 188 this.note = new ArrayList<Annotation>(); 189 this.note.add(t); 190 return this; 191 } 192 193 /** 194 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 195 */ 196 public Annotation getNoteFirstRep() { 197 if (getNote().isEmpty()) { 198 addNote(); 199 } 200 return getNote().get(0); 201 } 202 203 /** 204 * @return {@link #numberOfStudies} (Number of participants in the population.). This is the underlying object with id, value and extensions. The accessor "getNumberOfStudies" gives direct access to the value 205 */ 206 public UnsignedIntType getNumberOfStudiesElement() { 207 if (this.numberOfStudies == null) 208 if (Configuration.errorOnAutoCreate()) 209 throw new Error("Attempt to auto-create StatisticSampleSizeComponent.numberOfStudies"); 210 else if (Configuration.doAutoCreate()) 211 this.numberOfStudies = new UnsignedIntType(); // bb 212 return this.numberOfStudies; 213 } 214 215 public boolean hasNumberOfStudiesElement() { 216 return this.numberOfStudies != null && !this.numberOfStudies.isEmpty(); 217 } 218 219 public boolean hasNumberOfStudies() { 220 return this.numberOfStudies != null && !this.numberOfStudies.isEmpty(); 221 } 222 223 /** 224 * @param value {@link #numberOfStudies} (Number of participants in the population.). This is the underlying object with id, value and extensions. The accessor "getNumberOfStudies" gives direct access to the value 225 */ 226 public StatisticSampleSizeComponent setNumberOfStudiesElement(UnsignedIntType value) { 227 this.numberOfStudies = value; 228 return this; 229 } 230 231 /** 232 * @return Number of participants in the population. 233 */ 234 public int getNumberOfStudies() { 235 return this.numberOfStudies == null || this.numberOfStudies.isEmpty() ? 0 : this.numberOfStudies.getValue(); 236 } 237 238 /** 239 * @param value Number of participants in the population. 240 */ 241 public StatisticSampleSizeComponent setNumberOfStudies(int value) { 242 if (this.numberOfStudies == null) 243 this.numberOfStudies = new UnsignedIntType(); 244 this.numberOfStudies.setValue(value); 245 return this; 246 } 247 248 /** 249 * @return {@link #numberOfParticipants} (A human-readable string to clarify or explain concepts about the sample size.). This is the underlying object with id, value and extensions. The accessor "getNumberOfParticipants" gives direct access to the value 250 */ 251 public UnsignedIntType getNumberOfParticipantsElement() { 252 if (this.numberOfParticipants == null) 253 if (Configuration.errorOnAutoCreate()) 254 throw new Error("Attempt to auto-create StatisticSampleSizeComponent.numberOfParticipants"); 255 else if (Configuration.doAutoCreate()) 256 this.numberOfParticipants = new UnsignedIntType(); // bb 257 return this.numberOfParticipants; 258 } 259 260 public boolean hasNumberOfParticipantsElement() { 261 return this.numberOfParticipants != null && !this.numberOfParticipants.isEmpty(); 262 } 263 264 public boolean hasNumberOfParticipants() { 265 return this.numberOfParticipants != null && !this.numberOfParticipants.isEmpty(); 266 } 267 268 /** 269 * @param value {@link #numberOfParticipants} (A human-readable string to clarify or explain concepts about the sample size.). This is the underlying object with id, value and extensions. The accessor "getNumberOfParticipants" gives direct access to the value 270 */ 271 public StatisticSampleSizeComponent setNumberOfParticipantsElement(UnsignedIntType value) { 272 this.numberOfParticipants = value; 273 return this; 274 } 275 276 /** 277 * @return A human-readable string to clarify or explain concepts about the sample size. 278 */ 279 public int getNumberOfParticipants() { 280 return this.numberOfParticipants == null || this.numberOfParticipants.isEmpty() ? 0 : this.numberOfParticipants.getValue(); 281 } 282 283 /** 284 * @param value A human-readable string to clarify or explain concepts about the sample size. 285 */ 286 public StatisticSampleSizeComponent setNumberOfParticipants(int value) { 287 if (this.numberOfParticipants == null) 288 this.numberOfParticipants = new UnsignedIntType(); 289 this.numberOfParticipants.setValue(value); 290 return this; 291 } 292 293 /** 294 * @return {@link #knownDataCount} (Number of participants with known results for measured variables.). This is the underlying object with id, value and extensions. The accessor "getKnownDataCount" gives direct access to the value 295 */ 296 public UnsignedIntType getKnownDataCountElement() { 297 if (this.knownDataCount == null) 298 if (Configuration.errorOnAutoCreate()) 299 throw new Error("Attempt to auto-create StatisticSampleSizeComponent.knownDataCount"); 300 else if (Configuration.doAutoCreate()) 301 this.knownDataCount = new UnsignedIntType(); // bb 302 return this.knownDataCount; 303 } 304 305 public boolean hasKnownDataCountElement() { 306 return this.knownDataCount != null && !this.knownDataCount.isEmpty(); 307 } 308 309 public boolean hasKnownDataCount() { 310 return this.knownDataCount != null && !this.knownDataCount.isEmpty(); 311 } 312 313 /** 314 * @param value {@link #knownDataCount} (Number of participants with known results for measured variables.). This is the underlying object with id, value and extensions. The accessor "getKnownDataCount" gives direct access to the value 315 */ 316 public StatisticSampleSizeComponent setKnownDataCountElement(UnsignedIntType value) { 317 this.knownDataCount = value; 318 return this; 319 } 320 321 /** 322 * @return Number of participants with known results for measured variables. 323 */ 324 public int getKnownDataCount() { 325 return this.knownDataCount == null || this.knownDataCount.isEmpty() ? 0 : this.knownDataCount.getValue(); 326 } 327 328 /** 329 * @param value Number of participants with known results for measured variables. 330 */ 331 public StatisticSampleSizeComponent setKnownDataCount(int value) { 332 if (this.knownDataCount == null) 333 this.knownDataCount = new UnsignedIntType(); 334 this.knownDataCount.setValue(value); 335 return this; 336 } 337 338 protected void listChildren(List<Property> children) { 339 super.listChildren(children); 340 children.add(new Property("description", "string", "Human-readable summary of population sample size.", 0, 1, description)); 341 children.add(new Property("note", "Annotation", "Footnote or explanatory note about the sample size.", 0, java.lang.Integer.MAX_VALUE, note)); 342 children.add(new Property("numberOfStudies", "unsignedInt", "Number of participants in the population.", 0, 1, numberOfStudies)); 343 children.add(new Property("numberOfParticipants", "unsignedInt", "A human-readable string to clarify or explain concepts about the sample size.", 0, 1, numberOfParticipants)); 344 children.add(new Property("knownDataCount", "unsignedInt", "Number of participants with known results for measured variables.", 0, 1, knownDataCount)); 345 } 346 347 @Override 348 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 349 switch (_hash) { 350 case -1724546052: /*description*/ return new Property("description", "string", "Human-readable summary of population sample size.", 0, 1, description); 351 case 3387378: /*note*/ return new Property("note", "Annotation", "Footnote or explanatory note about the sample size.", 0, java.lang.Integer.MAX_VALUE, note); 352 case -177467129: /*numberOfStudies*/ return new Property("numberOfStudies", "unsignedInt", "Number of participants in the population.", 0, 1, numberOfStudies); 353 case 1799357120: /*numberOfParticipants*/ return new Property("numberOfParticipants", "unsignedInt", "A human-readable string to clarify or explain concepts about the sample size.", 0, 1, numberOfParticipants); 354 case -937344126: /*knownDataCount*/ return new Property("knownDataCount", "unsignedInt", "Number of participants with known results for measured variables.", 0, 1, knownDataCount); 355 default: return super.getNamedProperty(_hash, _name, _checkValid); 356 } 357 358 } 359 360 @Override 361 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 362 switch (hash) { 363 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 364 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 365 case -177467129: /*numberOfStudies*/ return this.numberOfStudies == null ? new Base[0] : new Base[] {this.numberOfStudies}; // UnsignedIntType 366 case 1799357120: /*numberOfParticipants*/ return this.numberOfParticipants == null ? new Base[0] : new Base[] {this.numberOfParticipants}; // UnsignedIntType 367 case -937344126: /*knownDataCount*/ return this.knownDataCount == null ? new Base[0] : new Base[] {this.knownDataCount}; // UnsignedIntType 368 default: return super.getProperty(hash, name, checkValid); 369 } 370 371 } 372 373 @Override 374 public Base setProperty(int hash, String name, Base value) throws FHIRException { 375 switch (hash) { 376 case -1724546052: // description 377 this.description = TypeConvertor.castToString(value); // StringType 378 return value; 379 case 3387378: // note 380 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 381 return value; 382 case -177467129: // numberOfStudies 383 this.numberOfStudies = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 384 return value; 385 case 1799357120: // numberOfParticipants 386 this.numberOfParticipants = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 387 return value; 388 case -937344126: // knownDataCount 389 this.knownDataCount = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 390 return value; 391 default: return super.setProperty(hash, name, value); 392 } 393 394 } 395 396 @Override 397 public Base setProperty(String name, Base value) throws FHIRException { 398 if (name.equals("description")) { 399 this.description = TypeConvertor.castToString(value); // StringType 400 } else if (name.equals("note")) { 401 this.getNote().add(TypeConvertor.castToAnnotation(value)); 402 } else if (name.equals("numberOfStudies")) { 403 this.numberOfStudies = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 404 } else if (name.equals("numberOfParticipants")) { 405 this.numberOfParticipants = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 406 } else if (name.equals("knownDataCount")) { 407 this.knownDataCount = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 408 } else 409 return super.setProperty(name, value); 410 return value; 411 } 412 413 @Override 414 public void removeChild(String name, Base value) throws FHIRException { 415 if (name.equals("description")) { 416 this.description = null; 417 } else if (name.equals("note")) { 418 this.getNote().remove(value); 419 } else if (name.equals("numberOfStudies")) { 420 this.numberOfStudies = null; 421 } else if (name.equals("numberOfParticipants")) { 422 this.numberOfParticipants = null; 423 } else if (name.equals("knownDataCount")) { 424 this.knownDataCount = null; 425 } else 426 super.removeChild(name, value); 427 428 } 429 430 @Override 431 public Base makeProperty(int hash, String name) throws FHIRException { 432 switch (hash) { 433 case -1724546052: return getDescriptionElement(); 434 case 3387378: return addNote(); 435 case -177467129: return getNumberOfStudiesElement(); 436 case 1799357120: return getNumberOfParticipantsElement(); 437 case -937344126: return getKnownDataCountElement(); 438 default: return super.makeProperty(hash, name); 439 } 440 441 } 442 443 @Override 444 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 445 switch (hash) { 446 case -1724546052: /*description*/ return new String[] {"string"}; 447 case 3387378: /*note*/ return new String[] {"Annotation"}; 448 case -177467129: /*numberOfStudies*/ return new String[] {"unsignedInt"}; 449 case 1799357120: /*numberOfParticipants*/ return new String[] {"unsignedInt"}; 450 case -937344126: /*knownDataCount*/ return new String[] {"unsignedInt"}; 451 default: return super.getTypesForProperty(hash, name); 452 } 453 454 } 455 456 @Override 457 public Base addChild(String name) throws FHIRException { 458 if (name.equals("description")) { 459 throw new FHIRException("Cannot call addChild on a singleton property Statistic.sampleSize.description"); 460 } 461 else if (name.equals("note")) { 462 return addNote(); 463 } 464 else if (name.equals("numberOfStudies")) { 465 throw new FHIRException("Cannot call addChild on a singleton property Statistic.sampleSize.numberOfStudies"); 466 } 467 else if (name.equals("numberOfParticipants")) { 468 throw new FHIRException("Cannot call addChild on a singleton property Statistic.sampleSize.numberOfParticipants"); 469 } 470 else if (name.equals("knownDataCount")) { 471 throw new FHIRException("Cannot call addChild on a singleton property Statistic.sampleSize.knownDataCount"); 472 } 473 else 474 return super.addChild(name); 475 } 476 477 public StatisticSampleSizeComponent copy() { 478 StatisticSampleSizeComponent dst = new StatisticSampleSizeComponent(); 479 copyValues(dst); 480 return dst; 481 } 482 483 public void copyValues(StatisticSampleSizeComponent dst) { 484 super.copyValues(dst); 485 dst.description = description == null ? null : description.copy(); 486 if (note != null) { 487 dst.note = new ArrayList<Annotation>(); 488 for (Annotation i : note) 489 dst.note.add(i.copy()); 490 }; 491 dst.numberOfStudies = numberOfStudies == null ? null : numberOfStudies.copy(); 492 dst.numberOfParticipants = numberOfParticipants == null ? null : numberOfParticipants.copy(); 493 dst.knownDataCount = knownDataCount == null ? null : knownDataCount.copy(); 494 } 495 496 @Override 497 public boolean equalsDeep(Base other_) { 498 if (!super.equalsDeep(other_)) 499 return false; 500 if (!(other_ instanceof StatisticSampleSizeComponent)) 501 return false; 502 StatisticSampleSizeComponent o = (StatisticSampleSizeComponent) other_; 503 return compareDeep(description, o.description, true) && compareDeep(note, o.note, true) && compareDeep(numberOfStudies, o.numberOfStudies, true) 504 && compareDeep(numberOfParticipants, o.numberOfParticipants, true) && compareDeep(knownDataCount, o.knownDataCount, true) 505 ; 506 } 507 508 @Override 509 public boolean equalsShallow(Base other_) { 510 if (!super.equalsShallow(other_)) 511 return false; 512 if (!(other_ instanceof StatisticSampleSizeComponent)) 513 return false; 514 StatisticSampleSizeComponent o = (StatisticSampleSizeComponent) other_; 515 return compareValues(description, o.description, true) && compareValues(numberOfStudies, o.numberOfStudies, true) 516 && compareValues(numberOfParticipants, o.numberOfParticipants, true) && compareValues(knownDataCount, o.knownDataCount, true) 517 ; 518 } 519 520 public boolean isEmpty() { 521 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, note, numberOfStudies 522 , numberOfParticipants, knownDataCount); 523 } 524 525 public String fhirType() { 526 return "Statistic.sampleSize"; 527 528 } 529 530 } 531 532 @Block() 533 public static class StatisticAttributeEstimateComponent extends Element implements IBaseDatatypeElement { 534 /** 535 * Human-readable summary of the estimate. 536 */ 537 @Child(name = "description", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 538 @Description(shortDefinition="Textual description of the attribute estimate", formalDefinition="Human-readable summary of the estimate." ) 539 protected StringType description; 540 541 /** 542 * Footnote or explanatory note about the estimate. 543 */ 544 @Child(name = "note", type = {Annotation.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 545 @Description(shortDefinition="Footnote or explanatory note about the estimate", formalDefinition="Footnote or explanatory note about the estimate." ) 546 protected List<Annotation> note; 547 548 /** 549 * The type of attribute estimate, eg confidence interval or p value. 550 */ 551 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 552 @Description(shortDefinition="The type of attribute estimate, eg confidence interval or p value", formalDefinition="The type of attribute estimate, eg confidence interval or p value." ) 553 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/attribute-estimate-type") 554 protected CodeableConcept type; 555 556 /** 557 * The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure. 558 */ 559 @Child(name = "quantity", type = {Quantity.class}, order=4, min=0, max=1, modifier=false, summary=true) 560 @Description(shortDefinition="The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure", formalDefinition="The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure." ) 561 protected Quantity quantity; 562 563 /** 564 * Use 95 for a 95% confidence interval. 565 */ 566 @Child(name = "level", type = {DecimalType.class}, order=5, min=0, max=1, modifier=false, summary=true) 567 @Description(shortDefinition="Level of confidence interval, eg 0.95 for 95% confidence interval", formalDefinition="Use 95 for a 95% confidence interval." ) 568 protected DecimalType level; 569 570 /** 571 * Lower bound of confidence interval. 572 */ 573 @Child(name = "range", type = {Range.class}, order=6, min=0, max=1, modifier=false, summary=true) 574 @Description(shortDefinition="Lower and upper bound values of the attribute estimate", formalDefinition="Lower bound of confidence interval." ) 575 protected Range range; 576 577 /** 578 * A nested attribute estimate; which is the attribute estimate of an attribute estimate. 579 */ 580 @Child(name = "attributeEstimate", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 581 @Description(shortDefinition="A nested attribute estimate; which is the attribute estimate of an attribute estimate", formalDefinition="A nested attribute estimate; which is the attribute estimate of an attribute estimate." ) 582 protected List<StatisticAttributeEstimateAttributeEstimateComponent> attributeEstimate; 583 584 private static final long serialVersionUID = 2062805621L; 585 586 /** 587 * Constructor 588 */ 589 public StatisticAttributeEstimateComponent() { 590 super(); 591 } 592 593 /** 594 * @return {@link #description} (Human-readable summary of the estimate.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 595 */ 596 public StringType getDescriptionElement() { 597 if (this.description == null) 598 if (Configuration.errorOnAutoCreate()) 599 throw new Error("Attempt to auto-create StatisticAttributeEstimateComponent.description"); 600 else if (Configuration.doAutoCreate()) 601 this.description = new StringType(); // bb 602 return this.description; 603 } 604 605 public boolean hasDescriptionElement() { 606 return this.description != null && !this.description.isEmpty(); 607 } 608 609 public boolean hasDescription() { 610 return this.description != null && !this.description.isEmpty(); 611 } 612 613 /** 614 * @param value {@link #description} (Human-readable summary of the estimate.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 615 */ 616 public StatisticAttributeEstimateComponent setDescriptionElement(StringType value) { 617 this.description = value; 618 return this; 619 } 620 621 /** 622 * @return Human-readable summary of the estimate. 623 */ 624 public String getDescription() { 625 return this.description == null ? null : this.description.getValue(); 626 } 627 628 /** 629 * @param value Human-readable summary of the estimate. 630 */ 631 public StatisticAttributeEstimateComponent setDescription(String value) { 632 if (Utilities.noString(value)) 633 this.description = null; 634 else { 635 if (this.description == null) 636 this.description = new StringType(); 637 this.description.setValue(value); 638 } 639 return this; 640 } 641 642 /** 643 * @return {@link #note} (Footnote or explanatory note about the estimate.) 644 */ 645 public List<Annotation> getNote() { 646 if (this.note == null) 647 this.note = new ArrayList<Annotation>(); 648 return this.note; 649 } 650 651 /** 652 * @return Returns a reference to <code>this</code> for easy method chaining 653 */ 654 public StatisticAttributeEstimateComponent setNote(List<Annotation> theNote) { 655 this.note = theNote; 656 return this; 657 } 658 659 public boolean hasNote() { 660 if (this.note == null) 661 return false; 662 for (Annotation item : this.note) 663 if (!item.isEmpty()) 664 return true; 665 return false; 666 } 667 668 public Annotation addNote() { //3 669 Annotation t = new Annotation(); 670 if (this.note == null) 671 this.note = new ArrayList<Annotation>(); 672 this.note.add(t); 673 return t; 674 } 675 676 public StatisticAttributeEstimateComponent addNote(Annotation t) { //3 677 if (t == null) 678 return this; 679 if (this.note == null) 680 this.note = new ArrayList<Annotation>(); 681 this.note.add(t); 682 return this; 683 } 684 685 /** 686 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 687 */ 688 public Annotation getNoteFirstRep() { 689 if (getNote().isEmpty()) { 690 addNote(); 691 } 692 return getNote().get(0); 693 } 694 695 /** 696 * @return {@link #type} (The type of attribute estimate, eg confidence interval or p value.) 697 */ 698 public CodeableConcept getType() { 699 if (this.type == null) 700 if (Configuration.errorOnAutoCreate()) 701 throw new Error("Attempt to auto-create StatisticAttributeEstimateComponent.type"); 702 else if (Configuration.doAutoCreate()) 703 this.type = new CodeableConcept(); // cc 704 return this.type; 705 } 706 707 public boolean hasType() { 708 return this.type != null && !this.type.isEmpty(); 709 } 710 711 /** 712 * @param value {@link #type} (The type of attribute estimate, eg confidence interval or p value.) 713 */ 714 public StatisticAttributeEstimateComponent setType(CodeableConcept value) { 715 this.type = value; 716 return this; 717 } 718 719 /** 720 * @return {@link #quantity} (The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure.) 721 */ 722 public Quantity getQuantity() { 723 if (this.quantity == null) 724 if (Configuration.errorOnAutoCreate()) 725 throw new Error("Attempt to auto-create StatisticAttributeEstimateComponent.quantity"); 726 else if (Configuration.doAutoCreate()) 727 this.quantity = new Quantity(); // cc 728 return this.quantity; 729 } 730 731 public boolean hasQuantity() { 732 return this.quantity != null && !this.quantity.isEmpty(); 733 } 734 735 /** 736 * @param value {@link #quantity} (The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure.) 737 */ 738 public StatisticAttributeEstimateComponent setQuantity(Quantity value) { 739 this.quantity = value; 740 return this; 741 } 742 743 /** 744 * @return {@link #level} (Use 95 for a 95% confidence interval.). This is the underlying object with id, value and extensions. The accessor "getLevel" gives direct access to the value 745 */ 746 public DecimalType getLevelElement() { 747 if (this.level == null) 748 if (Configuration.errorOnAutoCreate()) 749 throw new Error("Attempt to auto-create StatisticAttributeEstimateComponent.level"); 750 else if (Configuration.doAutoCreate()) 751 this.level = new DecimalType(); // bb 752 return this.level; 753 } 754 755 public boolean hasLevelElement() { 756 return this.level != null && !this.level.isEmpty(); 757 } 758 759 public boolean hasLevel() { 760 return this.level != null && !this.level.isEmpty(); 761 } 762 763 /** 764 * @param value {@link #level} (Use 95 for a 95% confidence interval.). This is the underlying object with id, value and extensions. The accessor "getLevel" gives direct access to the value 765 */ 766 public StatisticAttributeEstimateComponent setLevelElement(DecimalType value) { 767 this.level = value; 768 return this; 769 } 770 771 /** 772 * @return Use 95 for a 95% confidence interval. 773 */ 774 public BigDecimal getLevel() { 775 return this.level == null ? null : this.level.getValue(); 776 } 777 778 /** 779 * @param value Use 95 for a 95% confidence interval. 780 */ 781 public StatisticAttributeEstimateComponent setLevel(BigDecimal value) { 782 if (value == null) 783 this.level = null; 784 else { 785 if (this.level == null) 786 this.level = new DecimalType(); 787 this.level.setValue(value); 788 } 789 return this; 790 } 791 792 /** 793 * @param value Use 95 for a 95% confidence interval. 794 */ 795 public StatisticAttributeEstimateComponent setLevel(long value) { 796 this.level = new DecimalType(); 797 this.level.setValue(value); 798 return this; 799 } 800 801 /** 802 * @param value Use 95 for a 95% confidence interval. 803 */ 804 public StatisticAttributeEstimateComponent setLevel(double value) { 805 this.level = new DecimalType(); 806 this.level.setValue(value); 807 return this; 808 } 809 810 /** 811 * @return {@link #range} (Lower bound of confidence interval.) 812 */ 813 public Range getRange() { 814 if (this.range == null) 815 if (Configuration.errorOnAutoCreate()) 816 throw new Error("Attempt to auto-create StatisticAttributeEstimateComponent.range"); 817 else if (Configuration.doAutoCreate()) 818 this.range = new Range(); // cc 819 return this.range; 820 } 821 822 public boolean hasRange() { 823 return this.range != null && !this.range.isEmpty(); 824 } 825 826 /** 827 * @param value {@link #range} (Lower bound of confidence interval.) 828 */ 829 public StatisticAttributeEstimateComponent setRange(Range value) { 830 this.range = value; 831 return this; 832 } 833 834 /** 835 * @return {@link #attributeEstimate} (A nested attribute estimate; which is the attribute estimate of an attribute estimate.) 836 */ 837 public List<StatisticAttributeEstimateAttributeEstimateComponent> getAttributeEstimate() { 838 if (this.attributeEstimate == null) 839 this.attributeEstimate = new ArrayList<StatisticAttributeEstimateAttributeEstimateComponent>(); 840 return this.attributeEstimate; 841 } 842 843 /** 844 * @return Returns a reference to <code>this</code> for easy method chaining 845 */ 846 public StatisticAttributeEstimateComponent setAttributeEstimate(List<StatisticAttributeEstimateAttributeEstimateComponent> theAttributeEstimate) { 847 this.attributeEstimate = theAttributeEstimate; 848 return this; 849 } 850 851 public boolean hasAttributeEstimate() { 852 if (this.attributeEstimate == null) 853 return false; 854 for (StatisticAttributeEstimateAttributeEstimateComponent item : this.attributeEstimate) 855 if (!item.isEmpty()) 856 return true; 857 return false; 858 } 859 860 public StatisticAttributeEstimateAttributeEstimateComponent addAttributeEstimate() { //3 861 StatisticAttributeEstimateAttributeEstimateComponent t = new StatisticAttributeEstimateAttributeEstimateComponent(); 862 if (this.attributeEstimate == null) 863 this.attributeEstimate = new ArrayList<StatisticAttributeEstimateAttributeEstimateComponent>(); 864 this.attributeEstimate.add(t); 865 return t; 866 } 867 868 public StatisticAttributeEstimateComponent addAttributeEstimate(StatisticAttributeEstimateAttributeEstimateComponent t) { //3 869 if (t == null) 870 return this; 871 if (this.attributeEstimate == null) 872 this.attributeEstimate = new ArrayList<StatisticAttributeEstimateAttributeEstimateComponent>(); 873 this.attributeEstimate.add(t); 874 return this; 875 } 876 877 /** 878 * @return The first repetition of repeating field {@link #attributeEstimate}, creating it if it does not already exist {3} 879 */ 880 public StatisticAttributeEstimateAttributeEstimateComponent getAttributeEstimateFirstRep() { 881 if (getAttributeEstimate().isEmpty()) { 882 addAttributeEstimate(); 883 } 884 return getAttributeEstimate().get(0); 885 } 886 887 protected void listChildren(List<Property> children) { 888 super.listChildren(children); 889 children.add(new Property("description", "string", "Human-readable summary of the estimate.", 0, 1, description)); 890 children.add(new Property("note", "Annotation", "Footnote or explanatory note about the estimate.", 0, java.lang.Integer.MAX_VALUE, note)); 891 children.add(new Property("type", "CodeableConcept", "The type of attribute estimate, eg confidence interval or p value.", 0, 1, type)); 892 children.add(new Property("quantity", "Quantity", "The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure.", 0, 1, quantity)); 893 children.add(new Property("level", "decimal", "Use 95 for a 95% confidence interval.", 0, 1, level)); 894 children.add(new Property("range", "Range", "Lower bound of confidence interval.", 0, 1, range)); 895 children.add(new Property("attributeEstimate", "", "A nested attribute estimate; which is the attribute estimate of an attribute estimate.", 0, java.lang.Integer.MAX_VALUE, attributeEstimate)); 896 } 897 898 @Override 899 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 900 switch (_hash) { 901 case -1724546052: /*description*/ return new Property("description", "string", "Human-readable summary of the estimate.", 0, 1, description); 902 case 3387378: /*note*/ return new Property("note", "Annotation", "Footnote or explanatory note about the estimate.", 0, java.lang.Integer.MAX_VALUE, note); 903 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of attribute estimate, eg confidence interval or p value.", 0, 1, type); 904 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure.", 0, 1, quantity); 905 case 102865796: /*level*/ return new Property("level", "decimal", "Use 95 for a 95% confidence interval.", 0, 1, level); 906 case 108280125: /*range*/ return new Property("range", "Range", "Lower bound of confidence interval.", 0, 1, range); 907 case -1539581980: /*attributeEstimate*/ return new Property("attributeEstimate", "", "A nested attribute estimate; which is the attribute estimate of an attribute estimate.", 0, java.lang.Integer.MAX_VALUE, attributeEstimate); 908 default: return super.getNamedProperty(_hash, _name, _checkValid); 909 } 910 911 } 912 913 @Override 914 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 915 switch (hash) { 916 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 917 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 918 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 919 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 920 case 102865796: /*level*/ return this.level == null ? new Base[0] : new Base[] {this.level}; // DecimalType 921 case 108280125: /*range*/ return this.range == null ? new Base[0] : new Base[] {this.range}; // Range 922 case -1539581980: /*attributeEstimate*/ return this.attributeEstimate == null ? new Base[0] : this.attributeEstimate.toArray(new Base[this.attributeEstimate.size()]); // StatisticAttributeEstimateAttributeEstimateComponent 923 default: return super.getProperty(hash, name, checkValid); 924 } 925 926 } 927 928 @Override 929 public Base setProperty(int hash, String name, Base value) throws FHIRException { 930 switch (hash) { 931 case -1724546052: // description 932 this.description = TypeConvertor.castToString(value); // StringType 933 return value; 934 case 3387378: // note 935 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 936 return value; 937 case 3575610: // type 938 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 939 return value; 940 case -1285004149: // quantity 941 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 942 return value; 943 case 102865796: // level 944 this.level = TypeConvertor.castToDecimal(value); // DecimalType 945 return value; 946 case 108280125: // range 947 this.range = TypeConvertor.castToRange(value); // Range 948 return value; 949 case -1539581980: // attributeEstimate 950 this.getAttributeEstimate().add((StatisticAttributeEstimateAttributeEstimateComponent) value); // StatisticAttributeEstimateAttributeEstimateComponent 951 return value; 952 default: return super.setProperty(hash, name, value); 953 } 954 955 } 956 957 @Override 958 public Base setProperty(String name, Base value) throws FHIRException { 959 if (name.equals("description")) { 960 this.description = TypeConvertor.castToString(value); // StringType 961 } else if (name.equals("note")) { 962 this.getNote().add(TypeConvertor.castToAnnotation(value)); 963 } else if (name.equals("type")) { 964 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 965 } else if (name.equals("quantity")) { 966 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 967 } else if (name.equals("level")) { 968 this.level = TypeConvertor.castToDecimal(value); // DecimalType 969 } else if (name.equals("range")) { 970 this.range = TypeConvertor.castToRange(value); // Range 971 } else if (name.equals("attributeEstimate")) { 972 this.getAttributeEstimate().add((StatisticAttributeEstimateAttributeEstimateComponent) value); 973 } else 974 return super.setProperty(name, value); 975 return value; 976 } 977 978 @Override 979 public void removeChild(String name, Base value) throws FHIRException { 980 if (name.equals("description")) { 981 this.description = null; 982 } else if (name.equals("note")) { 983 this.getNote().remove(value); 984 } else if (name.equals("type")) { 985 this.type = null; 986 } else if (name.equals("quantity")) { 987 this.quantity = null; 988 } else if (name.equals("level")) { 989 this.level = null; 990 } else if (name.equals("range")) { 991 this.range = null; 992 } else if (name.equals("attributeEstimate")) { 993 this.getAttributeEstimate().remove((StatisticAttributeEstimateAttributeEstimateComponent) value); 994 } else 995 super.removeChild(name, value); 996 997 } 998 999 @Override 1000 public Base makeProperty(int hash, String name) throws FHIRException { 1001 switch (hash) { 1002 case -1724546052: return getDescriptionElement(); 1003 case 3387378: return addNote(); 1004 case 3575610: return getType(); 1005 case -1285004149: return getQuantity(); 1006 case 102865796: return getLevelElement(); 1007 case 108280125: return getRange(); 1008 case -1539581980: return addAttributeEstimate(); 1009 default: return super.makeProperty(hash, name); 1010 } 1011 1012 } 1013 1014 @Override 1015 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1016 switch (hash) { 1017 case -1724546052: /*description*/ return new String[] {"string"}; 1018 case 3387378: /*note*/ return new String[] {"Annotation"}; 1019 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1020 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 1021 case 102865796: /*level*/ return new String[] {"decimal"}; 1022 case 108280125: /*range*/ return new String[] {"Range"}; 1023 case -1539581980: /*attributeEstimate*/ return new String[] {}; 1024 default: return super.getTypesForProperty(hash, name); 1025 } 1026 1027 } 1028 1029 @Override 1030 public Base addChild(String name) throws FHIRException { 1031 if (name.equals("description")) { 1032 throw new FHIRException("Cannot call addChild on a singleton property Statistic.attributeEstimate.description"); 1033 } 1034 else if (name.equals("note")) { 1035 return addNote(); 1036 } 1037 else if (name.equals("type")) { 1038 this.type = new CodeableConcept(); 1039 return this.type; 1040 } 1041 else if (name.equals("quantity")) { 1042 this.quantity = new Quantity(); 1043 return this.quantity; 1044 } 1045 else if (name.equals("level")) { 1046 throw new FHIRException("Cannot call addChild on a singleton property Statistic.attributeEstimate.level"); 1047 } 1048 else if (name.equals("range")) { 1049 this.range = new Range(); 1050 return this.range; 1051 } 1052 else if (name.equals("attributeEstimate")) { 1053 return addAttributeEstimate(); 1054 } 1055 else 1056 return super.addChild(name); 1057 } 1058 1059 public StatisticAttributeEstimateComponent copy() { 1060 StatisticAttributeEstimateComponent dst = new StatisticAttributeEstimateComponent(); 1061 copyValues(dst); 1062 return dst; 1063 } 1064 1065 public void copyValues(StatisticAttributeEstimateComponent dst) { 1066 super.copyValues(dst); 1067 dst.description = description == null ? null : description.copy(); 1068 if (note != null) { 1069 dst.note = new ArrayList<Annotation>(); 1070 for (Annotation i : note) 1071 dst.note.add(i.copy()); 1072 }; 1073 dst.type = type == null ? null : type.copy(); 1074 dst.quantity = quantity == null ? null : quantity.copy(); 1075 dst.level = level == null ? null : level.copy(); 1076 dst.range = range == null ? null : range.copy(); 1077 if (attributeEstimate != null) { 1078 dst.attributeEstimate = new ArrayList<StatisticAttributeEstimateAttributeEstimateComponent>(); 1079 for (StatisticAttributeEstimateAttributeEstimateComponent i : attributeEstimate) 1080 dst.attributeEstimate.add(i.copy()); 1081 }; 1082 } 1083 1084 @Override 1085 public boolean equalsDeep(Base other_) { 1086 if (!super.equalsDeep(other_)) 1087 return false; 1088 if (!(other_ instanceof StatisticAttributeEstimateComponent)) 1089 return false; 1090 StatisticAttributeEstimateComponent o = (StatisticAttributeEstimateComponent) other_; 1091 return compareDeep(description, o.description, true) && compareDeep(note, o.note, true) && compareDeep(type, o.type, true) 1092 && compareDeep(quantity, o.quantity, true) && compareDeep(level, o.level, true) && compareDeep(range, o.range, true) 1093 && compareDeep(attributeEstimate, o.attributeEstimate, true); 1094 } 1095 1096 @Override 1097 public boolean equalsShallow(Base other_) { 1098 if (!super.equalsShallow(other_)) 1099 return false; 1100 if (!(other_ instanceof StatisticAttributeEstimateComponent)) 1101 return false; 1102 StatisticAttributeEstimateComponent o = (StatisticAttributeEstimateComponent) other_; 1103 return compareValues(description, o.description, true) && compareValues(level, o.level, true); 1104 } 1105 1106 public boolean isEmpty() { 1107 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, note, type 1108 , quantity, level, range, attributeEstimate); 1109 } 1110 1111 public String fhirType() { 1112 return "Statistic.attributeEstimate"; 1113 1114 } 1115 1116 } 1117 1118 @Block() 1119 public static class StatisticAttributeEstimateAttributeEstimateComponent extends Element implements IBaseDatatypeElement { 1120 /** 1121 * Human-readable summary of the estimate. 1122 */ 1123 @Child(name = "description", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1124 @Description(shortDefinition="Textual description of the attribute estimate", formalDefinition="Human-readable summary of the estimate." ) 1125 protected StringType description; 1126 1127 /** 1128 * Footnote or explanatory note about the estimate. 1129 */ 1130 @Child(name = "note", type = {Annotation.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1131 @Description(shortDefinition="Footnote or explanatory note about the estimate", formalDefinition="Footnote or explanatory note about the estimate." ) 1132 protected List<Annotation> note; 1133 1134 /** 1135 * The type of attribute estimate, eg confidence interval or p value. 1136 */ 1137 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 1138 @Description(shortDefinition="The type of attribute estimate, eg confidence interval or p value", formalDefinition="The type of attribute estimate, eg confidence interval or p value." ) 1139 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/attribute-estimate-type") 1140 protected CodeableConcept type; 1141 1142 /** 1143 * The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure. 1144 */ 1145 @Child(name = "quantity", type = {Quantity.class}, order=4, min=0, max=1, modifier=false, summary=true) 1146 @Description(shortDefinition="The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure", formalDefinition="The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure." ) 1147 protected Quantity quantity; 1148 1149 /** 1150 * Use 95 for a 95% confidence interval. 1151 */ 1152 @Child(name = "level", type = {DecimalType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1153 @Description(shortDefinition="Level of confidence interval, eg 0.95 for 95% confidence interval", formalDefinition="Use 95 for a 95% confidence interval." ) 1154 protected DecimalType level; 1155 1156 /** 1157 * Lower bound of confidence interval. 1158 */ 1159 @Child(name = "range", type = {Range.class}, order=6, min=0, max=1, modifier=false, summary=true) 1160 @Description(shortDefinition="Lower and upper bound values of the attribute estimate", formalDefinition="Lower bound of confidence interval." ) 1161 protected Range range; 1162 1163 private static final long serialVersionUID = 1873606362L; 1164 1165 /** 1166 * Constructor 1167 */ 1168 public StatisticAttributeEstimateAttributeEstimateComponent() { 1169 super(); 1170 } 1171 1172 /** 1173 * @return {@link #description} (Human-readable summary of the estimate.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1174 */ 1175 public StringType getDescriptionElement() { 1176 if (this.description == null) 1177 if (Configuration.errorOnAutoCreate()) 1178 throw new Error("Attempt to auto-create StatisticAttributeEstimateAttributeEstimateComponent.description"); 1179 else if (Configuration.doAutoCreate()) 1180 this.description = new StringType(); // bb 1181 return this.description; 1182 } 1183 1184 public boolean hasDescriptionElement() { 1185 return this.description != null && !this.description.isEmpty(); 1186 } 1187 1188 public boolean hasDescription() { 1189 return this.description != null && !this.description.isEmpty(); 1190 } 1191 1192 /** 1193 * @param value {@link #description} (Human-readable summary of the estimate.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1194 */ 1195 public StatisticAttributeEstimateAttributeEstimateComponent setDescriptionElement(StringType value) { 1196 this.description = value; 1197 return this; 1198 } 1199 1200 /** 1201 * @return Human-readable summary of the estimate. 1202 */ 1203 public String getDescription() { 1204 return this.description == null ? null : this.description.getValue(); 1205 } 1206 1207 /** 1208 * @param value Human-readable summary of the estimate. 1209 */ 1210 public StatisticAttributeEstimateAttributeEstimateComponent setDescription(String value) { 1211 if (Utilities.noString(value)) 1212 this.description = null; 1213 else { 1214 if (this.description == null) 1215 this.description = new StringType(); 1216 this.description.setValue(value); 1217 } 1218 return this; 1219 } 1220 1221 /** 1222 * @return {@link #note} (Footnote or explanatory note about the estimate.) 1223 */ 1224 public List<Annotation> getNote() { 1225 if (this.note == null) 1226 this.note = new ArrayList<Annotation>(); 1227 return this.note; 1228 } 1229 1230 /** 1231 * @return Returns a reference to <code>this</code> for easy method chaining 1232 */ 1233 public StatisticAttributeEstimateAttributeEstimateComponent setNote(List<Annotation> theNote) { 1234 this.note = theNote; 1235 return this; 1236 } 1237 1238 public boolean hasNote() { 1239 if (this.note == null) 1240 return false; 1241 for (Annotation item : this.note) 1242 if (!item.isEmpty()) 1243 return true; 1244 return false; 1245 } 1246 1247 public Annotation addNote() { //3 1248 Annotation t = new Annotation(); 1249 if (this.note == null) 1250 this.note = new ArrayList<Annotation>(); 1251 this.note.add(t); 1252 return t; 1253 } 1254 1255 public StatisticAttributeEstimateAttributeEstimateComponent addNote(Annotation t) { //3 1256 if (t == null) 1257 return this; 1258 if (this.note == null) 1259 this.note = new ArrayList<Annotation>(); 1260 this.note.add(t); 1261 return this; 1262 } 1263 1264 /** 1265 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1266 */ 1267 public Annotation getNoteFirstRep() { 1268 if (getNote().isEmpty()) { 1269 addNote(); 1270 } 1271 return getNote().get(0); 1272 } 1273 1274 /** 1275 * @return {@link #type} (The type of attribute estimate, eg confidence interval or p value.) 1276 */ 1277 public CodeableConcept getType() { 1278 if (this.type == null) 1279 if (Configuration.errorOnAutoCreate()) 1280 throw new Error("Attempt to auto-create StatisticAttributeEstimateAttributeEstimateComponent.type"); 1281 else if (Configuration.doAutoCreate()) 1282 this.type = new CodeableConcept(); // cc 1283 return this.type; 1284 } 1285 1286 public boolean hasType() { 1287 return this.type != null && !this.type.isEmpty(); 1288 } 1289 1290 /** 1291 * @param value {@link #type} (The type of attribute estimate, eg confidence interval or p value.) 1292 */ 1293 public StatisticAttributeEstimateAttributeEstimateComponent setType(CodeableConcept value) { 1294 this.type = value; 1295 return this; 1296 } 1297 1298 /** 1299 * @return {@link #quantity} (The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure.) 1300 */ 1301 public Quantity getQuantity() { 1302 if (this.quantity == null) 1303 if (Configuration.errorOnAutoCreate()) 1304 throw new Error("Attempt to auto-create StatisticAttributeEstimateAttributeEstimateComponent.quantity"); 1305 else if (Configuration.doAutoCreate()) 1306 this.quantity = new Quantity(); // cc 1307 return this.quantity; 1308 } 1309 1310 public boolean hasQuantity() { 1311 return this.quantity != null && !this.quantity.isEmpty(); 1312 } 1313 1314 /** 1315 * @param value {@link #quantity} (The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure.) 1316 */ 1317 public StatisticAttributeEstimateAttributeEstimateComponent setQuantity(Quantity value) { 1318 this.quantity = value; 1319 return this; 1320 } 1321 1322 /** 1323 * @return {@link #level} (Use 95 for a 95% confidence interval.). This is the underlying object with id, value and extensions. The accessor "getLevel" gives direct access to the value 1324 */ 1325 public DecimalType getLevelElement() { 1326 if (this.level == null) 1327 if (Configuration.errorOnAutoCreate()) 1328 throw new Error("Attempt to auto-create StatisticAttributeEstimateAttributeEstimateComponent.level"); 1329 else if (Configuration.doAutoCreate()) 1330 this.level = new DecimalType(); // bb 1331 return this.level; 1332 } 1333 1334 public boolean hasLevelElement() { 1335 return this.level != null && !this.level.isEmpty(); 1336 } 1337 1338 public boolean hasLevel() { 1339 return this.level != null && !this.level.isEmpty(); 1340 } 1341 1342 /** 1343 * @param value {@link #level} (Use 95 for a 95% confidence interval.). This is the underlying object with id, value and extensions. The accessor "getLevel" gives direct access to the value 1344 */ 1345 public StatisticAttributeEstimateAttributeEstimateComponent setLevelElement(DecimalType value) { 1346 this.level = value; 1347 return this; 1348 } 1349 1350 /** 1351 * @return Use 95 for a 95% confidence interval. 1352 */ 1353 public BigDecimal getLevel() { 1354 return this.level == null ? null : this.level.getValue(); 1355 } 1356 1357 /** 1358 * @param value Use 95 for a 95% confidence interval. 1359 */ 1360 public StatisticAttributeEstimateAttributeEstimateComponent setLevel(BigDecimal value) { 1361 if (value == null) 1362 this.level = null; 1363 else { 1364 if (this.level == null) 1365 this.level = new DecimalType(); 1366 this.level.setValue(value); 1367 } 1368 return this; 1369 } 1370 1371 /** 1372 * @param value Use 95 for a 95% confidence interval. 1373 */ 1374 public StatisticAttributeEstimateAttributeEstimateComponent setLevel(long value) { 1375 this.level = new DecimalType(); 1376 this.level.setValue(value); 1377 return this; 1378 } 1379 1380 /** 1381 * @param value Use 95 for a 95% confidence interval. 1382 */ 1383 public StatisticAttributeEstimateAttributeEstimateComponent setLevel(double value) { 1384 this.level = new DecimalType(); 1385 this.level.setValue(value); 1386 return this; 1387 } 1388 1389 /** 1390 * @return {@link #range} (Lower bound of confidence interval.) 1391 */ 1392 public Range getRange() { 1393 if (this.range == null) 1394 if (Configuration.errorOnAutoCreate()) 1395 throw new Error("Attempt to auto-create StatisticAttributeEstimateAttributeEstimateComponent.range"); 1396 else if (Configuration.doAutoCreate()) 1397 this.range = new Range(); // cc 1398 return this.range; 1399 } 1400 1401 public boolean hasRange() { 1402 return this.range != null && !this.range.isEmpty(); 1403 } 1404 1405 /** 1406 * @param value {@link #range} (Lower bound of confidence interval.) 1407 */ 1408 public StatisticAttributeEstimateAttributeEstimateComponent setRange(Range value) { 1409 this.range = value; 1410 return this; 1411 } 1412 1413 protected void listChildren(List<Property> children) { 1414 super.listChildren(children); 1415 children.add(new Property("description", "string", "Human-readable summary of the estimate.", 0, 1, description)); 1416 children.add(new Property("note", "Annotation", "Footnote or explanatory note about the estimate.", 0, java.lang.Integer.MAX_VALUE, note)); 1417 children.add(new Property("type", "CodeableConcept", "The type of attribute estimate, eg confidence interval or p value.", 0, 1, type)); 1418 children.add(new Property("quantity", "Quantity", "The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure.", 0, 1, quantity)); 1419 children.add(new Property("level", "decimal", "Use 95 for a 95% confidence interval.", 0, 1, level)); 1420 children.add(new Property("range", "Range", "Lower bound of confidence interval.", 0, 1, range)); 1421 } 1422 1423 @Override 1424 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1425 switch (_hash) { 1426 case -1724546052: /*description*/ return new Property("description", "string", "Human-readable summary of the estimate.", 0, 1, description); 1427 case 3387378: /*note*/ return new Property("note", "Annotation", "Footnote or explanatory note about the estimate.", 0, java.lang.Integer.MAX_VALUE, note); 1428 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of attribute estimate, eg confidence interval or p value.", 0, 1, type); 1429 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The singular quantity of the attribute estimate, for attribute estimates represented as single values; also used to report unit of measure.", 0, 1, quantity); 1430 case 102865796: /*level*/ return new Property("level", "decimal", "Use 95 for a 95% confidence interval.", 0, 1, level); 1431 case 108280125: /*range*/ return new Property("range", "Range", "Lower bound of confidence interval.", 0, 1, range); 1432 default: return super.getNamedProperty(_hash, _name, _checkValid); 1433 } 1434 1435 } 1436 1437 @Override 1438 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1439 switch (hash) { 1440 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1441 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1442 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1443 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 1444 case 102865796: /*level*/ return this.level == null ? new Base[0] : new Base[] {this.level}; // DecimalType 1445 case 108280125: /*range*/ return this.range == null ? new Base[0] : new Base[] {this.range}; // Range 1446 default: return super.getProperty(hash, name, checkValid); 1447 } 1448 1449 } 1450 1451 @Override 1452 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1453 switch (hash) { 1454 case -1724546052: // description 1455 this.description = TypeConvertor.castToString(value); // StringType 1456 return value; 1457 case 3387378: // note 1458 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1459 return value; 1460 case 3575610: // type 1461 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1462 return value; 1463 case -1285004149: // quantity 1464 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1465 return value; 1466 case 102865796: // level 1467 this.level = TypeConvertor.castToDecimal(value); // DecimalType 1468 return value; 1469 case 108280125: // range 1470 this.range = TypeConvertor.castToRange(value); // Range 1471 return value; 1472 default: return super.setProperty(hash, name, value); 1473 } 1474 1475 } 1476 1477 @Override 1478 public Base setProperty(String name, Base value) throws FHIRException { 1479 if (name.equals("description")) { 1480 this.description = TypeConvertor.castToString(value); // StringType 1481 } else if (name.equals("note")) { 1482 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1483 } else if (name.equals("type")) { 1484 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1485 } else if (name.equals("quantity")) { 1486 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1487 } else if (name.equals("level")) { 1488 this.level = TypeConvertor.castToDecimal(value); // DecimalType 1489 } else if (name.equals("range")) { 1490 this.range = TypeConvertor.castToRange(value); // Range 1491 } else 1492 return super.setProperty(name, value); 1493 return value; 1494 } 1495 1496 @Override 1497 public void removeChild(String name, Base value) throws FHIRException { 1498 if (name.equals("description")) { 1499 this.description = null; 1500 } else if (name.equals("note")) { 1501 this.getNote().remove(value); 1502 } else if (name.equals("type")) { 1503 this.type = null; 1504 } else if (name.equals("quantity")) { 1505 this.quantity = null; 1506 } else if (name.equals("level")) { 1507 this.level = null; 1508 } else if (name.equals("range")) { 1509 this.range = null; 1510 } else 1511 super.removeChild(name, value); 1512 1513 } 1514 1515 @Override 1516 public Base makeProperty(int hash, String name) throws FHIRException { 1517 switch (hash) { 1518 case -1724546052: return getDescriptionElement(); 1519 case 3387378: return addNote(); 1520 case 3575610: return getType(); 1521 case -1285004149: return getQuantity(); 1522 case 102865796: return getLevelElement(); 1523 case 108280125: return getRange(); 1524 default: return super.makeProperty(hash, name); 1525 } 1526 1527 } 1528 1529 @Override 1530 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1531 switch (hash) { 1532 case -1724546052: /*description*/ return new String[] {"string"}; 1533 case 3387378: /*note*/ return new String[] {"Annotation"}; 1534 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1535 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 1536 case 102865796: /*level*/ return new String[] {"decimal"}; 1537 case 108280125: /*range*/ return new String[] {"Range"}; 1538 default: return super.getTypesForProperty(hash, name); 1539 } 1540 1541 } 1542 1543 @Override 1544 public Base addChild(String name) throws FHIRException { 1545 if (name.equals("description")) { 1546 throw new FHIRException("Cannot call addChild on a singleton property Statistic.attributeEstimate.attributeEstimate.description"); 1547 } 1548 else if (name.equals("note")) { 1549 return addNote(); 1550 } 1551 else if (name.equals("type")) { 1552 this.type = new CodeableConcept(); 1553 return this.type; 1554 } 1555 else if (name.equals("quantity")) { 1556 this.quantity = new Quantity(); 1557 return this.quantity; 1558 } 1559 else if (name.equals("level")) { 1560 throw new FHIRException("Cannot call addChild on a singleton property Statistic.attributeEstimate.attributeEstimate.level"); 1561 } 1562 else if (name.equals("range")) { 1563 this.range = new Range(); 1564 return this.range; 1565 } 1566 else 1567 return super.addChild(name); 1568 } 1569 1570 public StatisticAttributeEstimateAttributeEstimateComponent copy() { 1571 StatisticAttributeEstimateAttributeEstimateComponent dst = new StatisticAttributeEstimateAttributeEstimateComponent(); 1572 copyValues(dst); 1573 return dst; 1574 } 1575 1576 public void copyValues(StatisticAttributeEstimateAttributeEstimateComponent dst) { 1577 super.copyValues(dst); 1578 dst.description = description == null ? null : description.copy(); 1579 if (note != null) { 1580 dst.note = new ArrayList<Annotation>(); 1581 for (Annotation i : note) 1582 dst.note.add(i.copy()); 1583 }; 1584 dst.type = type == null ? null : type.copy(); 1585 dst.quantity = quantity == null ? null : quantity.copy(); 1586 dst.level = level == null ? null : level.copy(); 1587 dst.range = range == null ? null : range.copy(); 1588 } 1589 1590 @Override 1591 public boolean equalsDeep(Base other_) { 1592 if (!super.equalsDeep(other_)) 1593 return false; 1594 if (!(other_ instanceof StatisticAttributeEstimateAttributeEstimateComponent)) 1595 return false; 1596 StatisticAttributeEstimateAttributeEstimateComponent o = (StatisticAttributeEstimateAttributeEstimateComponent) other_; 1597 return compareDeep(description, o.description, true) && compareDeep(note, o.note, true) && compareDeep(type, o.type, true) 1598 && compareDeep(quantity, o.quantity, true) && compareDeep(level, o.level, true) && compareDeep(range, o.range, true) 1599 ; 1600 } 1601 1602 @Override 1603 public boolean equalsShallow(Base other_) { 1604 if (!super.equalsShallow(other_)) 1605 return false; 1606 if (!(other_ instanceof StatisticAttributeEstimateAttributeEstimateComponent)) 1607 return false; 1608 StatisticAttributeEstimateAttributeEstimateComponent o = (StatisticAttributeEstimateAttributeEstimateComponent) other_; 1609 return compareValues(description, o.description, true) && compareValues(level, o.level, true); 1610 } 1611 1612 public boolean isEmpty() { 1613 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, note, type 1614 , quantity, level, range); 1615 } 1616 1617 public String fhirType() { 1618 return "Statistic.attributeEstimate.attributeEstimate"; 1619 1620 } 1621 1622 } 1623 1624 @Block() 1625 public static class StatisticModelCharacteristicComponent extends Element implements IBaseDatatypeElement { 1626 /** 1627 * Description of a component of the method to generate the statistic. 1628 */ 1629 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1630 @Description(shortDefinition="Model specification", formalDefinition="Description of a component of the method to generate the statistic." ) 1631 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/statistic-model-code") 1632 protected CodeableConcept code; 1633 1634 /** 1635 * Further specification of the quantified value of the component of the method to generate the statistic. 1636 */ 1637 @Child(name = "value", type = {Quantity.class}, order=2, min=0, max=1, modifier=false, summary=true) 1638 @Description(shortDefinition="Numerical value to complete model specification", formalDefinition="Further specification of the quantified value of the component of the method to generate the statistic." ) 1639 protected Quantity value; 1640 1641 /** 1642 * A variable adjusted for in the adjusted analysis. 1643 */ 1644 @Child(name = "variable", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1645 @Description(shortDefinition="A variable adjusted for in the adjusted analysis", formalDefinition="A variable adjusted for in the adjusted analysis." ) 1646 protected List<StatisticModelCharacteristicVariableComponent> variable; 1647 1648 private static final long serialVersionUID = 1539071113L; 1649 1650 /** 1651 * Constructor 1652 */ 1653 public StatisticModelCharacteristicComponent() { 1654 super(); 1655 } 1656 1657 /** 1658 * Constructor 1659 */ 1660 public StatisticModelCharacteristicComponent(CodeableConcept code) { 1661 super(); 1662 this.setCode(code); 1663 } 1664 1665 /** 1666 * @return {@link #code} (Description of a component of the method to generate the statistic.) 1667 */ 1668 public CodeableConcept getCode() { 1669 if (this.code == null) 1670 if (Configuration.errorOnAutoCreate()) 1671 throw new Error("Attempt to auto-create StatisticModelCharacteristicComponent.code"); 1672 else if (Configuration.doAutoCreate()) 1673 this.code = new CodeableConcept(); // cc 1674 return this.code; 1675 } 1676 1677 public boolean hasCode() { 1678 return this.code != null && !this.code.isEmpty(); 1679 } 1680 1681 /** 1682 * @param value {@link #code} (Description of a component of the method to generate the statistic.) 1683 */ 1684 public StatisticModelCharacteristicComponent setCode(CodeableConcept value) { 1685 this.code = value; 1686 return this; 1687 } 1688 1689 /** 1690 * @return {@link #value} (Further specification of the quantified value of the component of the method to generate the statistic.) 1691 */ 1692 public Quantity getValue() { 1693 if (this.value == null) 1694 if (Configuration.errorOnAutoCreate()) 1695 throw new Error("Attempt to auto-create StatisticModelCharacteristicComponent.value"); 1696 else if (Configuration.doAutoCreate()) 1697 this.value = new Quantity(); // cc 1698 return this.value; 1699 } 1700 1701 public boolean hasValue() { 1702 return this.value != null && !this.value.isEmpty(); 1703 } 1704 1705 /** 1706 * @param value {@link #value} (Further specification of the quantified value of the component of the method to generate the statistic.) 1707 */ 1708 public StatisticModelCharacteristicComponent setValue(Quantity value) { 1709 this.value = value; 1710 return this; 1711 } 1712 1713 /** 1714 * @return {@link #variable} (A variable adjusted for in the adjusted analysis.) 1715 */ 1716 public List<StatisticModelCharacteristicVariableComponent> getVariable() { 1717 if (this.variable == null) 1718 this.variable = new ArrayList<StatisticModelCharacteristicVariableComponent>(); 1719 return this.variable; 1720 } 1721 1722 /** 1723 * @return Returns a reference to <code>this</code> for easy method chaining 1724 */ 1725 public StatisticModelCharacteristicComponent setVariable(List<StatisticModelCharacteristicVariableComponent> theVariable) { 1726 this.variable = theVariable; 1727 return this; 1728 } 1729 1730 public boolean hasVariable() { 1731 if (this.variable == null) 1732 return false; 1733 for (StatisticModelCharacteristicVariableComponent item : this.variable) 1734 if (!item.isEmpty()) 1735 return true; 1736 return false; 1737 } 1738 1739 public StatisticModelCharacteristicVariableComponent addVariable() { //3 1740 StatisticModelCharacteristicVariableComponent t = new StatisticModelCharacteristicVariableComponent(); 1741 if (this.variable == null) 1742 this.variable = new ArrayList<StatisticModelCharacteristicVariableComponent>(); 1743 this.variable.add(t); 1744 return t; 1745 } 1746 1747 public StatisticModelCharacteristicComponent addVariable(StatisticModelCharacteristicVariableComponent t) { //3 1748 if (t == null) 1749 return this; 1750 if (this.variable == null) 1751 this.variable = new ArrayList<StatisticModelCharacteristicVariableComponent>(); 1752 this.variable.add(t); 1753 return this; 1754 } 1755 1756 /** 1757 * @return The first repetition of repeating field {@link #variable}, creating it if it does not already exist {3} 1758 */ 1759 public StatisticModelCharacteristicVariableComponent getVariableFirstRep() { 1760 if (getVariable().isEmpty()) { 1761 addVariable(); 1762 } 1763 return getVariable().get(0); 1764 } 1765 1766 protected void listChildren(List<Property> children) { 1767 super.listChildren(children); 1768 children.add(new Property("code", "CodeableConcept", "Description of a component of the method to generate the statistic.", 0, 1, code)); 1769 children.add(new Property("value", "Quantity", "Further specification of the quantified value of the component of the method to generate the statistic.", 0, 1, value)); 1770 children.add(new Property("variable", "", "A variable adjusted for in the adjusted analysis.", 0, java.lang.Integer.MAX_VALUE, variable)); 1771 } 1772 1773 @Override 1774 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1775 switch (_hash) { 1776 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Description of a component of the method to generate the statistic.", 0, 1, code); 1777 case 111972721: /*value*/ return new Property("value", "Quantity", "Further specification of the quantified value of the component of the method to generate the statistic.", 0, 1, value); 1778 case -1249586564: /*variable*/ return new Property("variable", "", "A variable adjusted for in the adjusted analysis.", 0, java.lang.Integer.MAX_VALUE, variable); 1779 default: return super.getNamedProperty(_hash, _name, _checkValid); 1780 } 1781 1782 } 1783 1784 @Override 1785 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1786 switch (hash) { 1787 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1788 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Quantity 1789 case -1249586564: /*variable*/ return this.variable == null ? new Base[0] : this.variable.toArray(new Base[this.variable.size()]); // StatisticModelCharacteristicVariableComponent 1790 default: return super.getProperty(hash, name, checkValid); 1791 } 1792 1793 } 1794 1795 @Override 1796 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1797 switch (hash) { 1798 case 3059181: // code 1799 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1800 return value; 1801 case 111972721: // value 1802 this.value = TypeConvertor.castToQuantity(value); // Quantity 1803 return value; 1804 case -1249586564: // variable 1805 this.getVariable().add((StatisticModelCharacteristicVariableComponent) value); // StatisticModelCharacteristicVariableComponent 1806 return value; 1807 default: return super.setProperty(hash, name, value); 1808 } 1809 1810 } 1811 1812 @Override 1813 public Base setProperty(String name, Base value) throws FHIRException { 1814 if (name.equals("code")) { 1815 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1816 } else if (name.equals("value")) { 1817 this.value = TypeConvertor.castToQuantity(value); // Quantity 1818 } else if (name.equals("variable")) { 1819 this.getVariable().add((StatisticModelCharacteristicVariableComponent) value); 1820 } else 1821 return super.setProperty(name, value); 1822 return value; 1823 } 1824 1825 @Override 1826 public void removeChild(String name, Base value) throws FHIRException { 1827 if (name.equals("code")) { 1828 this.code = null; 1829 } else if (name.equals("value")) { 1830 this.value = null; 1831 } else if (name.equals("variable")) { 1832 this.getVariable().remove((StatisticModelCharacteristicVariableComponent) value); 1833 } else 1834 super.removeChild(name, value); 1835 1836 } 1837 1838 @Override 1839 public Base makeProperty(int hash, String name) throws FHIRException { 1840 switch (hash) { 1841 case 3059181: return getCode(); 1842 case 111972721: return getValue(); 1843 case -1249586564: return addVariable(); 1844 default: return super.makeProperty(hash, name); 1845 } 1846 1847 } 1848 1849 @Override 1850 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1851 switch (hash) { 1852 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1853 case 111972721: /*value*/ return new String[] {"Quantity"}; 1854 case -1249586564: /*variable*/ return new String[] {}; 1855 default: return super.getTypesForProperty(hash, name); 1856 } 1857 1858 } 1859 1860 @Override 1861 public Base addChild(String name) throws FHIRException { 1862 if (name.equals("code")) { 1863 this.code = new CodeableConcept(); 1864 return this.code; 1865 } 1866 else if (name.equals("value")) { 1867 this.value = new Quantity(); 1868 return this.value; 1869 } 1870 else if (name.equals("variable")) { 1871 return addVariable(); 1872 } 1873 else 1874 return super.addChild(name); 1875 } 1876 1877 public StatisticModelCharacteristicComponent copy() { 1878 StatisticModelCharacteristicComponent dst = new StatisticModelCharacteristicComponent(); 1879 copyValues(dst); 1880 return dst; 1881 } 1882 1883 public void copyValues(StatisticModelCharacteristicComponent dst) { 1884 super.copyValues(dst); 1885 dst.code = code == null ? null : code.copy(); 1886 dst.value = value == null ? null : value.copy(); 1887 if (variable != null) { 1888 dst.variable = new ArrayList<StatisticModelCharacteristicVariableComponent>(); 1889 for (StatisticModelCharacteristicVariableComponent i : variable) 1890 dst.variable.add(i.copy()); 1891 }; 1892 } 1893 1894 @Override 1895 public boolean equalsDeep(Base other_) { 1896 if (!super.equalsDeep(other_)) 1897 return false; 1898 if (!(other_ instanceof StatisticModelCharacteristicComponent)) 1899 return false; 1900 StatisticModelCharacteristicComponent o = (StatisticModelCharacteristicComponent) other_; 1901 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true) && compareDeep(variable, o.variable, true) 1902 ; 1903 } 1904 1905 @Override 1906 public boolean equalsShallow(Base other_) { 1907 if (!super.equalsShallow(other_)) 1908 return false; 1909 if (!(other_ instanceof StatisticModelCharacteristicComponent)) 1910 return false; 1911 StatisticModelCharacteristicComponent o = (StatisticModelCharacteristicComponent) other_; 1912 return true; 1913 } 1914 1915 public boolean isEmpty() { 1916 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value, variable); 1917 } 1918 1919 public String fhirType() { 1920 return "Statistic.modelCharacteristic"; 1921 1922 } 1923 1924 } 1925 1926 @Block() 1927 public static class StatisticModelCharacteristicVariableComponent extends Element implements IBaseDatatypeElement { 1928 /** 1929 * Description of the variable. 1930 */ 1931 @Child(name = "variableDefinition", type = {Group.class, EvidenceVariable.class}, order=1, min=1, max=1, modifier=false, summary=true) 1932 @Description(shortDefinition="Description of the variable", formalDefinition="Description of the variable." ) 1933 protected Reference variableDefinition; 1934 1935 /** 1936 * How the variable is classified for use in adjusted analysis. 1937 */ 1938 @Child(name = "handling", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1939 @Description(shortDefinition="continuous | dichotomous | ordinal | polychotomous", formalDefinition="How the variable is classified for use in adjusted analysis." ) 1940 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/variable-handling") 1941 protected Enumeration<EvidenceVariableHandling> handling; 1942 1943 /** 1944 * Description for grouping of ordinal or polychotomous variables. 1945 */ 1946 @Child(name = "valueCategory", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1947 @Description(shortDefinition="Description for grouping of ordinal or polychotomous variables", formalDefinition="Description for grouping of ordinal or polychotomous variables." ) 1948 protected List<CodeableConcept> valueCategory; 1949 1950 /** 1951 * Discrete value for grouping of ordinal or polychotomous variables. 1952 */ 1953 @Child(name = "valueQuantity", type = {Quantity.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1954 @Description(shortDefinition="Discrete value for grouping of ordinal or polychotomous variables", formalDefinition="Discrete value for grouping of ordinal or polychotomous variables." ) 1955 protected List<Quantity> valueQuantity; 1956 1957 /** 1958 * Range of values for grouping of ordinal or polychotomous variables. 1959 */ 1960 @Child(name = "valueRange", type = {Range.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1961 @Description(shortDefinition="Range of values for grouping of ordinal or polychotomous variables", formalDefinition="Range of values for grouping of ordinal or polychotomous variables." ) 1962 protected List<Range> valueRange; 1963 1964 private static final long serialVersionUID = 1516174900L; 1965 1966 /** 1967 * Constructor 1968 */ 1969 public StatisticModelCharacteristicVariableComponent() { 1970 super(); 1971 } 1972 1973 /** 1974 * Constructor 1975 */ 1976 public StatisticModelCharacteristicVariableComponent(Reference variableDefinition) { 1977 super(); 1978 this.setVariableDefinition(variableDefinition); 1979 } 1980 1981 /** 1982 * @return {@link #variableDefinition} (Description of the variable.) 1983 */ 1984 public Reference getVariableDefinition() { 1985 if (this.variableDefinition == null) 1986 if (Configuration.errorOnAutoCreate()) 1987 throw new Error("Attempt to auto-create StatisticModelCharacteristicVariableComponent.variableDefinition"); 1988 else if (Configuration.doAutoCreate()) 1989 this.variableDefinition = new Reference(); // cc 1990 return this.variableDefinition; 1991 } 1992 1993 public boolean hasVariableDefinition() { 1994 return this.variableDefinition != null && !this.variableDefinition.isEmpty(); 1995 } 1996 1997 /** 1998 * @param value {@link #variableDefinition} (Description of the variable.) 1999 */ 2000 public StatisticModelCharacteristicVariableComponent setVariableDefinition(Reference value) { 2001 this.variableDefinition = value; 2002 return this; 2003 } 2004 2005 /** 2006 * @return {@link #handling} (How the variable is classified for use in adjusted analysis.). This is the underlying object with id, value and extensions. The accessor "getHandling" gives direct access to the value 2007 */ 2008 public Enumeration<EvidenceVariableHandling> getHandlingElement() { 2009 if (this.handling == null) 2010 if (Configuration.errorOnAutoCreate()) 2011 throw new Error("Attempt to auto-create StatisticModelCharacteristicVariableComponent.handling"); 2012 else if (Configuration.doAutoCreate()) 2013 this.handling = new Enumeration<EvidenceVariableHandling>(new EvidenceVariableHandlingEnumFactory()); // bb 2014 return this.handling; 2015 } 2016 2017 public boolean hasHandlingElement() { 2018 return this.handling != null && !this.handling.isEmpty(); 2019 } 2020 2021 public boolean hasHandling() { 2022 return this.handling != null && !this.handling.isEmpty(); 2023 } 2024 2025 /** 2026 * @param value {@link #handling} (How the variable is classified for use in adjusted analysis.). This is the underlying object with id, value and extensions. The accessor "getHandling" gives direct access to the value 2027 */ 2028 public StatisticModelCharacteristicVariableComponent setHandlingElement(Enumeration<EvidenceVariableHandling> value) { 2029 this.handling = value; 2030 return this; 2031 } 2032 2033 /** 2034 * @return How the variable is classified for use in adjusted analysis. 2035 */ 2036 public EvidenceVariableHandling getHandling() { 2037 return this.handling == null ? null : this.handling.getValue(); 2038 } 2039 2040 /** 2041 * @param value How the variable is classified for use in adjusted analysis. 2042 */ 2043 public StatisticModelCharacteristicVariableComponent setHandling(EvidenceVariableHandling value) { 2044 if (value == null) 2045 this.handling = null; 2046 else { 2047 if (this.handling == null) 2048 this.handling = new Enumeration<EvidenceVariableHandling>(new EvidenceVariableHandlingEnumFactory()); 2049 this.handling.setValue(value); 2050 } 2051 return this; 2052 } 2053 2054 /** 2055 * @return {@link #valueCategory} (Description for grouping of ordinal or polychotomous variables.) 2056 */ 2057 public List<CodeableConcept> getValueCategory() { 2058 if (this.valueCategory == null) 2059 this.valueCategory = new ArrayList<CodeableConcept>(); 2060 return this.valueCategory; 2061 } 2062 2063 /** 2064 * @return Returns a reference to <code>this</code> for easy method chaining 2065 */ 2066 public StatisticModelCharacteristicVariableComponent setValueCategory(List<CodeableConcept> theValueCategory) { 2067 this.valueCategory = theValueCategory; 2068 return this; 2069 } 2070 2071 public boolean hasValueCategory() { 2072 if (this.valueCategory == null) 2073 return false; 2074 for (CodeableConcept item : this.valueCategory) 2075 if (!item.isEmpty()) 2076 return true; 2077 return false; 2078 } 2079 2080 public CodeableConcept addValueCategory() { //3 2081 CodeableConcept t = new CodeableConcept(); 2082 if (this.valueCategory == null) 2083 this.valueCategory = new ArrayList<CodeableConcept>(); 2084 this.valueCategory.add(t); 2085 return t; 2086 } 2087 2088 public StatisticModelCharacteristicVariableComponent addValueCategory(CodeableConcept t) { //3 2089 if (t == null) 2090 return this; 2091 if (this.valueCategory == null) 2092 this.valueCategory = new ArrayList<CodeableConcept>(); 2093 this.valueCategory.add(t); 2094 return this; 2095 } 2096 2097 /** 2098 * @return The first repetition of repeating field {@link #valueCategory}, creating it if it does not already exist {3} 2099 */ 2100 public CodeableConcept getValueCategoryFirstRep() { 2101 if (getValueCategory().isEmpty()) { 2102 addValueCategory(); 2103 } 2104 return getValueCategory().get(0); 2105 } 2106 2107 /** 2108 * @return {@link #valueQuantity} (Discrete value for grouping of ordinal or polychotomous variables.) 2109 */ 2110 public List<Quantity> getValueQuantity() { 2111 if (this.valueQuantity == null) 2112 this.valueQuantity = new ArrayList<Quantity>(); 2113 return this.valueQuantity; 2114 } 2115 2116 /** 2117 * @return Returns a reference to <code>this</code> for easy method chaining 2118 */ 2119 public StatisticModelCharacteristicVariableComponent setValueQuantity(List<Quantity> theValueQuantity) { 2120 this.valueQuantity = theValueQuantity; 2121 return this; 2122 } 2123 2124 public boolean hasValueQuantity() { 2125 if (this.valueQuantity == null) 2126 return false; 2127 for (Quantity item : this.valueQuantity) 2128 if (!item.isEmpty()) 2129 return true; 2130 return false; 2131 } 2132 2133 public Quantity addValueQuantity() { //3 2134 Quantity t = new Quantity(); 2135 if (this.valueQuantity == null) 2136 this.valueQuantity = new ArrayList<Quantity>(); 2137 this.valueQuantity.add(t); 2138 return t; 2139 } 2140 2141 public StatisticModelCharacteristicVariableComponent addValueQuantity(Quantity t) { //3 2142 if (t == null) 2143 return this; 2144 if (this.valueQuantity == null) 2145 this.valueQuantity = new ArrayList<Quantity>(); 2146 this.valueQuantity.add(t); 2147 return this; 2148 } 2149 2150 /** 2151 * @return The first repetition of repeating field {@link #valueQuantity}, creating it if it does not already exist {3} 2152 */ 2153 public Quantity getValueQuantityFirstRep() { 2154 if (getValueQuantity().isEmpty()) { 2155 addValueQuantity(); 2156 } 2157 return getValueQuantity().get(0); 2158 } 2159 2160 /** 2161 * @return {@link #valueRange} (Range of values for grouping of ordinal or polychotomous variables.) 2162 */ 2163 public List<Range> getValueRange() { 2164 if (this.valueRange == null) 2165 this.valueRange = new ArrayList<Range>(); 2166 return this.valueRange; 2167 } 2168 2169 /** 2170 * @return Returns a reference to <code>this</code> for easy method chaining 2171 */ 2172 public StatisticModelCharacteristicVariableComponent setValueRange(List<Range> theValueRange) { 2173 this.valueRange = theValueRange; 2174 return this; 2175 } 2176 2177 public boolean hasValueRange() { 2178 if (this.valueRange == null) 2179 return false; 2180 for (Range item : this.valueRange) 2181 if (!item.isEmpty()) 2182 return true; 2183 return false; 2184 } 2185 2186 public Range addValueRange() { //3 2187 Range t = new Range(); 2188 if (this.valueRange == null) 2189 this.valueRange = new ArrayList<Range>(); 2190 this.valueRange.add(t); 2191 return t; 2192 } 2193 2194 public StatisticModelCharacteristicVariableComponent addValueRange(Range t) { //3 2195 if (t == null) 2196 return this; 2197 if (this.valueRange == null) 2198 this.valueRange = new ArrayList<Range>(); 2199 this.valueRange.add(t); 2200 return this; 2201 } 2202 2203 /** 2204 * @return The first repetition of repeating field {@link #valueRange}, creating it if it does not already exist {3} 2205 */ 2206 public Range getValueRangeFirstRep() { 2207 if (getValueRange().isEmpty()) { 2208 addValueRange(); 2209 } 2210 return getValueRange().get(0); 2211 } 2212 2213 protected void listChildren(List<Property> children) { 2214 super.listChildren(children); 2215 children.add(new Property("variableDefinition", "Reference(Group|EvidenceVariable)", "Description of the variable.", 0, 1, variableDefinition)); 2216 children.add(new Property("handling", "code", "How the variable is classified for use in adjusted analysis.", 0, 1, handling)); 2217 children.add(new Property("valueCategory", "CodeableConcept", "Description for grouping of ordinal or polychotomous variables.", 0, java.lang.Integer.MAX_VALUE, valueCategory)); 2218 children.add(new Property("valueQuantity", "Quantity", "Discrete value for grouping of ordinal or polychotomous variables.", 0, java.lang.Integer.MAX_VALUE, valueQuantity)); 2219 children.add(new Property("valueRange", "Range", "Range of values for grouping of ordinal or polychotomous variables.", 0, java.lang.Integer.MAX_VALUE, valueRange)); 2220 } 2221 2222 @Override 2223 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2224 switch (_hash) { 2225 case -1807222545: /*variableDefinition*/ return new Property("variableDefinition", "Reference(Group|EvidenceVariable)", "Description of the variable.", 0, 1, variableDefinition); 2226 case 2072805: /*handling*/ return new Property("handling", "code", "How the variable is classified for use in adjusted analysis.", 0, 1, handling); 2227 case -694308465: /*valueCategory*/ return new Property("valueCategory", "CodeableConcept", "Description for grouping of ordinal or polychotomous variables.", 0, java.lang.Integer.MAX_VALUE, valueCategory); 2228 case -2029823716: /*valueQuantity*/ return new Property("valueQuantity", "Quantity", "Discrete value for grouping of ordinal or polychotomous variables.", 0, java.lang.Integer.MAX_VALUE, valueQuantity); 2229 case 2030761548: /*valueRange*/ return new Property("valueRange", "Range", "Range of values for grouping of ordinal or polychotomous variables.", 0, java.lang.Integer.MAX_VALUE, valueRange); 2230 default: return super.getNamedProperty(_hash, _name, _checkValid); 2231 } 2232 2233 } 2234 2235 @Override 2236 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2237 switch (hash) { 2238 case -1807222545: /*variableDefinition*/ return this.variableDefinition == null ? new Base[0] : new Base[] {this.variableDefinition}; // Reference 2239 case 2072805: /*handling*/ return this.handling == null ? new Base[0] : new Base[] {this.handling}; // Enumeration<EvidenceVariableHandling> 2240 case -694308465: /*valueCategory*/ return this.valueCategory == null ? new Base[0] : this.valueCategory.toArray(new Base[this.valueCategory.size()]); // CodeableConcept 2241 case -2029823716: /*valueQuantity*/ return this.valueQuantity == null ? new Base[0] : this.valueQuantity.toArray(new Base[this.valueQuantity.size()]); // Quantity 2242 case 2030761548: /*valueRange*/ return this.valueRange == null ? new Base[0] : this.valueRange.toArray(new Base[this.valueRange.size()]); // Range 2243 default: return super.getProperty(hash, name, checkValid); 2244 } 2245 2246 } 2247 2248 @Override 2249 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2250 switch (hash) { 2251 case -1807222545: // variableDefinition 2252 this.variableDefinition = TypeConvertor.castToReference(value); // Reference 2253 return value; 2254 case 2072805: // handling 2255 value = new EvidenceVariableHandlingEnumFactory().fromType(TypeConvertor.castToCode(value)); 2256 this.handling = (Enumeration) value; // Enumeration<EvidenceVariableHandling> 2257 return value; 2258 case -694308465: // valueCategory 2259 this.getValueCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2260 return value; 2261 case -2029823716: // valueQuantity 2262 this.getValueQuantity().add(TypeConvertor.castToQuantity(value)); // Quantity 2263 return value; 2264 case 2030761548: // valueRange 2265 this.getValueRange().add(TypeConvertor.castToRange(value)); // Range 2266 return value; 2267 default: return super.setProperty(hash, name, value); 2268 } 2269 2270 } 2271 2272 @Override 2273 public Base setProperty(String name, Base value) throws FHIRException { 2274 if (name.equals("variableDefinition")) { 2275 this.variableDefinition = TypeConvertor.castToReference(value); // Reference 2276 } else if (name.equals("handling")) { 2277 value = new EvidenceVariableHandlingEnumFactory().fromType(TypeConvertor.castToCode(value)); 2278 this.handling = (Enumeration) value; // Enumeration<EvidenceVariableHandling> 2279 } else if (name.equals("valueCategory")) { 2280 this.getValueCategory().add(TypeConvertor.castToCodeableConcept(value)); 2281 } else if (name.equals("valueQuantity")) { 2282 this.getValueQuantity().add(TypeConvertor.castToQuantity(value)); 2283 } else if (name.equals("valueRange")) { 2284 this.getValueRange().add(TypeConvertor.castToRange(value)); 2285 } else 2286 return super.setProperty(name, value); 2287 return value; 2288 } 2289 2290 @Override 2291 public void removeChild(String name, Base value) throws FHIRException { 2292 if (name.equals("variableDefinition")) { 2293 this.variableDefinition = null; 2294 } else if (name.equals("handling")) { 2295 value = new EvidenceVariableHandlingEnumFactory().fromType(TypeConvertor.castToCode(value)); 2296 this.handling = (Enumeration) value; // Enumeration<EvidenceVariableHandling> 2297 } else if (name.equals("valueCategory")) { 2298 this.getValueCategory().remove(value); 2299 } else if (name.equals("valueQuantity")) { 2300 this.getValueQuantity().remove(value); 2301 } else if (name.equals("valueRange")) { 2302 this.getValueRange().remove(value); 2303 } else 2304 super.removeChild(name, value); 2305 2306 } 2307 2308 @Override 2309 public Base makeProperty(int hash, String name) throws FHIRException { 2310 switch (hash) { 2311 case -1807222545: return getVariableDefinition(); 2312 case 2072805: return getHandlingElement(); 2313 case -694308465: return addValueCategory(); 2314 case -2029823716: return addValueQuantity(); 2315 case 2030761548: return addValueRange(); 2316 default: return super.makeProperty(hash, name); 2317 } 2318 2319 } 2320 2321 @Override 2322 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2323 switch (hash) { 2324 case -1807222545: /*variableDefinition*/ return new String[] {"Reference"}; 2325 case 2072805: /*handling*/ return new String[] {"code"}; 2326 case -694308465: /*valueCategory*/ return new String[] {"CodeableConcept"}; 2327 case -2029823716: /*valueQuantity*/ return new String[] {"Quantity"}; 2328 case 2030761548: /*valueRange*/ return new String[] {"Range"}; 2329 default: return super.getTypesForProperty(hash, name); 2330 } 2331 2332 } 2333 2334 @Override 2335 public Base addChild(String name) throws FHIRException { 2336 if (name.equals("variableDefinition")) { 2337 this.variableDefinition = new Reference(); 2338 return this.variableDefinition; 2339 } 2340 else if (name.equals("handling")) { 2341 throw new FHIRException("Cannot call addChild on a singleton property Statistic.modelCharacteristic.variable.handling"); 2342 } 2343 else if (name.equals("valueCategory")) { 2344 return addValueCategory(); 2345 } 2346 else if (name.equals("valueQuantity")) { 2347 return addValueQuantity(); 2348 } 2349 else if (name.equals("valueRange")) { 2350 return addValueRange(); 2351 } 2352 else 2353 return super.addChild(name); 2354 } 2355 2356 public StatisticModelCharacteristicVariableComponent copy() { 2357 StatisticModelCharacteristicVariableComponent dst = new StatisticModelCharacteristicVariableComponent(); 2358 copyValues(dst); 2359 return dst; 2360 } 2361 2362 public void copyValues(StatisticModelCharacteristicVariableComponent dst) { 2363 super.copyValues(dst); 2364 dst.variableDefinition = variableDefinition == null ? null : variableDefinition.copy(); 2365 dst.handling = handling == null ? null : handling.copy(); 2366 if (valueCategory != null) { 2367 dst.valueCategory = new ArrayList<CodeableConcept>(); 2368 for (CodeableConcept i : valueCategory) 2369 dst.valueCategory.add(i.copy()); 2370 }; 2371 if (valueQuantity != null) { 2372 dst.valueQuantity = new ArrayList<Quantity>(); 2373 for (Quantity i : valueQuantity) 2374 dst.valueQuantity.add(i.copy()); 2375 }; 2376 if (valueRange != null) { 2377 dst.valueRange = new ArrayList<Range>(); 2378 for (Range i : valueRange) 2379 dst.valueRange.add(i.copy()); 2380 }; 2381 } 2382 2383 @Override 2384 public boolean equalsDeep(Base other_) { 2385 if (!super.equalsDeep(other_)) 2386 return false; 2387 if (!(other_ instanceof StatisticModelCharacteristicVariableComponent)) 2388 return false; 2389 StatisticModelCharacteristicVariableComponent o = (StatisticModelCharacteristicVariableComponent) other_; 2390 return compareDeep(variableDefinition, o.variableDefinition, true) && compareDeep(handling, o.handling, true) 2391 && compareDeep(valueCategory, o.valueCategory, true) && compareDeep(valueQuantity, o.valueQuantity, true) 2392 && compareDeep(valueRange, o.valueRange, true); 2393 } 2394 2395 @Override 2396 public boolean equalsShallow(Base other_) { 2397 if (!super.equalsShallow(other_)) 2398 return false; 2399 if (!(other_ instanceof StatisticModelCharacteristicVariableComponent)) 2400 return false; 2401 StatisticModelCharacteristicVariableComponent o = (StatisticModelCharacteristicVariableComponent) other_; 2402 return compareValues(handling, o.handling, true); 2403 } 2404 2405 public boolean isEmpty() { 2406 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(variableDefinition, handling 2407 , valueCategory, valueQuantity, valueRange); 2408 } 2409 2410 public String fhirType() { 2411 return "Statistic.modelCharacteristic.variable"; 2412 2413 } 2414 2415 } 2416 2417 /** 2418 * A description of the content value of the statistic. 2419 */ 2420 @Child(name = "description", type = {StringType.class}, order=0, min=0, max=1, modifier=false, summary=true) 2421 @Description(shortDefinition="Description of content", formalDefinition="A description of the content value of the statistic." ) 2422 protected StringType description; 2423 2424 /** 2425 * Footnotes and/or explanatory notes. 2426 */ 2427 @Child(name = "note", type = {Annotation.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2428 @Description(shortDefinition="Footnotes and/or explanatory notes", formalDefinition="Footnotes and/or explanatory notes." ) 2429 protected List<Annotation> note; 2430 2431 /** 2432 * Type of statistic, eg relative risk. 2433 */ 2434 @Child(name = "statisticType", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 2435 @Description(shortDefinition="Type of statistic, eg relative risk", formalDefinition="Type of statistic, eg relative risk." ) 2436 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/statistic-type") 2437 protected CodeableConcept statisticType; 2438 2439 /** 2440 * When the measured variable is handled categorically, the category element is used to define which category the statistic is reporting. 2441 */ 2442 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 2443 @Description(shortDefinition="Associated category for categorical variable\"", formalDefinition="When the measured variable is handled categorically, the category element is used to define which category the statistic is reporting." ) 2444 protected CodeableConcept category; 2445 2446 /** 2447 * Statistic value. 2448 */ 2449 @Child(name = "quantity", type = {Quantity.class}, order=4, min=0, max=1, modifier=false, summary=true) 2450 @Description(shortDefinition="Statistic value", formalDefinition="Statistic value." ) 2451 protected Quantity quantity; 2452 2453 /** 2454 * The number of events associated with the statistic, where the unit of analysis is different from numberAffected, sampleSize.knownDataCount and sampleSize.numberOfParticipants. 2455 */ 2456 @Child(name = "numberOfEvents", type = {UnsignedIntType.class}, order=5, min=0, max=1, modifier=false, summary=true) 2457 @Description(shortDefinition="The number of events associated with the statistic", formalDefinition="The number of events associated with the statistic, where the unit of analysis is different from numberAffected, sampleSize.knownDataCount and sampleSize.numberOfParticipants." ) 2458 protected UnsignedIntType numberOfEvents; 2459 2460 /** 2461 * The number of participants affected where the unit of analysis is the same as sampleSize.knownDataCount and sampleSize.numberOfParticipants. 2462 */ 2463 @Child(name = "numberAffected", type = {UnsignedIntType.class}, order=6, min=0, max=1, modifier=false, summary=true) 2464 @Description(shortDefinition="The number of participants affected", formalDefinition="The number of participants affected where the unit of analysis is the same as sampleSize.knownDataCount and sampleSize.numberOfParticipants." ) 2465 protected UnsignedIntType numberAffected; 2466 2467 /** 2468 * Number of samples in the statistic. 2469 */ 2470 @Child(name = "sampleSize", type = {}, order=7, min=0, max=1, modifier=false, summary=true) 2471 @Description(shortDefinition="Number of samples in the statistic", formalDefinition="Number of samples in the statistic." ) 2472 protected StatisticSampleSizeComponent sampleSize; 2473 2474 /** 2475 * A statistical attribute of the statistic such as a measure of heterogeneity. 2476 */ 2477 @Child(name = "attributeEstimate", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2478 @Description(shortDefinition="An attribute of the Statistic", formalDefinition="A statistical attribute of the statistic such as a measure of heterogeneity." ) 2479 protected List<StatisticAttributeEstimateComponent> attributeEstimate; 2480 2481 /** 2482 * A component of the method to generate the statistic. 2483 */ 2484 @Child(name = "modelCharacteristic", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2485 @Description(shortDefinition="Model characteristic", formalDefinition="A component of the method to generate the statistic." ) 2486 protected List<StatisticModelCharacteristicComponent> modelCharacteristic; 2487 2488 private static final long serialVersionUID = -1861373489L; 2489 2490 /** 2491 * Constructor 2492 */ 2493 public Statistic() { 2494 super(); 2495 } 2496 2497 /** 2498 * @return {@link #description} (A description of the content value of the statistic.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2499 */ 2500 public StringType getDescriptionElement() { 2501 if (this.description == null) 2502 if (Configuration.errorOnAutoCreate()) 2503 throw new Error("Attempt to auto-create Statistic.description"); 2504 else if (Configuration.doAutoCreate()) 2505 this.description = new StringType(); // bb 2506 return this.description; 2507 } 2508 2509 public boolean hasDescriptionElement() { 2510 return this.description != null && !this.description.isEmpty(); 2511 } 2512 2513 public boolean hasDescription() { 2514 return this.description != null && !this.description.isEmpty(); 2515 } 2516 2517 /** 2518 * @param value {@link #description} (A description of the content value of the statistic.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2519 */ 2520 public Statistic setDescriptionElement(StringType value) { 2521 this.description = value; 2522 return this; 2523 } 2524 2525 /** 2526 * @return A description of the content value of the statistic. 2527 */ 2528 public String getDescription() { 2529 return this.description == null ? null : this.description.getValue(); 2530 } 2531 2532 /** 2533 * @param value A description of the content value of the statistic. 2534 */ 2535 public Statistic setDescription(String value) { 2536 if (Utilities.noString(value)) 2537 this.description = null; 2538 else { 2539 if (this.description == null) 2540 this.description = new StringType(); 2541 this.description.setValue(value); 2542 } 2543 return this; 2544 } 2545 2546 /** 2547 * @return {@link #note} (Footnotes and/or explanatory notes.) 2548 */ 2549 public List<Annotation> getNote() { 2550 if (this.note == null) 2551 this.note = new ArrayList<Annotation>(); 2552 return this.note; 2553 } 2554 2555 /** 2556 * @return Returns a reference to <code>this</code> for easy method chaining 2557 */ 2558 public Statistic setNote(List<Annotation> theNote) { 2559 this.note = theNote; 2560 return this; 2561 } 2562 2563 public boolean hasNote() { 2564 if (this.note == null) 2565 return false; 2566 for (Annotation item : this.note) 2567 if (!item.isEmpty()) 2568 return true; 2569 return false; 2570 } 2571 2572 public Annotation addNote() { //3 2573 Annotation t = new Annotation(); 2574 if (this.note == null) 2575 this.note = new ArrayList<Annotation>(); 2576 this.note.add(t); 2577 return t; 2578 } 2579 2580 public Statistic addNote(Annotation t) { //3 2581 if (t == null) 2582 return this; 2583 if (this.note == null) 2584 this.note = new ArrayList<Annotation>(); 2585 this.note.add(t); 2586 return this; 2587 } 2588 2589 /** 2590 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 2591 */ 2592 public Annotation getNoteFirstRep() { 2593 if (getNote().isEmpty()) { 2594 addNote(); 2595 } 2596 return getNote().get(0); 2597 } 2598 2599 /** 2600 * @return {@link #statisticType} (Type of statistic, eg relative risk.) 2601 */ 2602 public CodeableConcept getStatisticType() { 2603 if (this.statisticType == null) 2604 if (Configuration.errorOnAutoCreate()) 2605 throw new Error("Attempt to auto-create Statistic.statisticType"); 2606 else if (Configuration.doAutoCreate()) 2607 this.statisticType = new CodeableConcept(); // cc 2608 return this.statisticType; 2609 } 2610 2611 public boolean hasStatisticType() { 2612 return this.statisticType != null && !this.statisticType.isEmpty(); 2613 } 2614 2615 /** 2616 * @param value {@link #statisticType} (Type of statistic, eg relative risk.) 2617 */ 2618 public Statistic setStatisticType(CodeableConcept value) { 2619 this.statisticType = value; 2620 return this; 2621 } 2622 2623 /** 2624 * @return {@link #category} (When the measured variable is handled categorically, the category element is used to define which category the statistic is reporting.) 2625 */ 2626 public CodeableConcept getCategory() { 2627 if (this.category == null) 2628 if (Configuration.errorOnAutoCreate()) 2629 throw new Error("Attempt to auto-create Statistic.category"); 2630 else if (Configuration.doAutoCreate()) 2631 this.category = new CodeableConcept(); // cc 2632 return this.category; 2633 } 2634 2635 public boolean hasCategory() { 2636 return this.category != null && !this.category.isEmpty(); 2637 } 2638 2639 /** 2640 * @param value {@link #category} (When the measured variable is handled categorically, the category element is used to define which category the statistic is reporting.) 2641 */ 2642 public Statistic setCategory(CodeableConcept value) { 2643 this.category = value; 2644 return this; 2645 } 2646 2647 /** 2648 * @return {@link #quantity} (Statistic value.) 2649 */ 2650 public Quantity getQuantity() { 2651 if (this.quantity == null) 2652 if (Configuration.errorOnAutoCreate()) 2653 throw new Error("Attempt to auto-create Statistic.quantity"); 2654 else if (Configuration.doAutoCreate()) 2655 this.quantity = new Quantity(); // cc 2656 return this.quantity; 2657 } 2658 2659 public boolean hasQuantity() { 2660 return this.quantity != null && !this.quantity.isEmpty(); 2661 } 2662 2663 /** 2664 * @param value {@link #quantity} (Statistic value.) 2665 */ 2666 public Statistic setQuantity(Quantity value) { 2667 this.quantity = value; 2668 return this; 2669 } 2670 2671 /** 2672 * @return {@link #numberOfEvents} (The number of events associated with the statistic, where the unit of analysis is different from numberAffected, sampleSize.knownDataCount and sampleSize.numberOfParticipants.). This is the underlying object with id, value and extensions. The accessor "getNumberOfEvents" gives direct access to the value 2673 */ 2674 public UnsignedIntType getNumberOfEventsElement() { 2675 if (this.numberOfEvents == null) 2676 if (Configuration.errorOnAutoCreate()) 2677 throw new Error("Attempt to auto-create Statistic.numberOfEvents"); 2678 else if (Configuration.doAutoCreate()) 2679 this.numberOfEvents = new UnsignedIntType(); // bb 2680 return this.numberOfEvents; 2681 } 2682 2683 public boolean hasNumberOfEventsElement() { 2684 return this.numberOfEvents != null && !this.numberOfEvents.isEmpty(); 2685 } 2686 2687 public boolean hasNumberOfEvents() { 2688 return this.numberOfEvents != null && !this.numberOfEvents.isEmpty(); 2689 } 2690 2691 /** 2692 * @param value {@link #numberOfEvents} (The number of events associated with the statistic, where the unit of analysis is different from numberAffected, sampleSize.knownDataCount and sampleSize.numberOfParticipants.). This is the underlying object with id, value and extensions. The accessor "getNumberOfEvents" gives direct access to the value 2693 */ 2694 public Statistic setNumberOfEventsElement(UnsignedIntType value) { 2695 this.numberOfEvents = value; 2696 return this; 2697 } 2698 2699 /** 2700 * @return The number of events associated with the statistic, where the unit of analysis is different from numberAffected, sampleSize.knownDataCount and sampleSize.numberOfParticipants. 2701 */ 2702 public int getNumberOfEvents() { 2703 return this.numberOfEvents == null || this.numberOfEvents.isEmpty() ? 0 : this.numberOfEvents.getValue(); 2704 } 2705 2706 /** 2707 * @param value The number of events associated with the statistic, where the unit of analysis is different from numberAffected, sampleSize.knownDataCount and sampleSize.numberOfParticipants. 2708 */ 2709 public Statistic setNumberOfEvents(int value) { 2710 if (this.numberOfEvents == null) 2711 this.numberOfEvents = new UnsignedIntType(); 2712 this.numberOfEvents.setValue(value); 2713 return this; 2714 } 2715 2716 /** 2717 * @return {@link #numberAffected} (The number of participants affected where the unit of analysis is the same as sampleSize.knownDataCount and sampleSize.numberOfParticipants.). This is the underlying object with id, value and extensions. The accessor "getNumberAffected" gives direct access to the value 2718 */ 2719 public UnsignedIntType getNumberAffectedElement() { 2720 if (this.numberAffected == null) 2721 if (Configuration.errorOnAutoCreate()) 2722 throw new Error("Attempt to auto-create Statistic.numberAffected"); 2723 else if (Configuration.doAutoCreate()) 2724 this.numberAffected = new UnsignedIntType(); // bb 2725 return this.numberAffected; 2726 } 2727 2728 public boolean hasNumberAffectedElement() { 2729 return this.numberAffected != null && !this.numberAffected.isEmpty(); 2730 } 2731 2732 public boolean hasNumberAffected() { 2733 return this.numberAffected != null && !this.numberAffected.isEmpty(); 2734 } 2735 2736 /** 2737 * @param value {@link #numberAffected} (The number of participants affected where the unit of analysis is the same as sampleSize.knownDataCount and sampleSize.numberOfParticipants.). This is the underlying object with id, value and extensions. The accessor "getNumberAffected" gives direct access to the value 2738 */ 2739 public Statistic setNumberAffectedElement(UnsignedIntType value) { 2740 this.numberAffected = value; 2741 return this; 2742 } 2743 2744 /** 2745 * @return The number of participants affected where the unit of analysis is the same as sampleSize.knownDataCount and sampleSize.numberOfParticipants. 2746 */ 2747 public int getNumberAffected() { 2748 return this.numberAffected == null || this.numberAffected.isEmpty() ? 0 : this.numberAffected.getValue(); 2749 } 2750 2751 /** 2752 * @param value The number of participants affected where the unit of analysis is the same as sampleSize.knownDataCount and sampleSize.numberOfParticipants. 2753 */ 2754 public Statistic setNumberAffected(int value) { 2755 if (this.numberAffected == null) 2756 this.numberAffected = new UnsignedIntType(); 2757 this.numberAffected.setValue(value); 2758 return this; 2759 } 2760 2761 /** 2762 * @return {@link #sampleSize} (Number of samples in the statistic.) 2763 */ 2764 public StatisticSampleSizeComponent getSampleSize() { 2765 if (this.sampleSize == null) 2766 if (Configuration.errorOnAutoCreate()) 2767 throw new Error("Attempt to auto-create Statistic.sampleSize"); 2768 else if (Configuration.doAutoCreate()) 2769 this.sampleSize = new StatisticSampleSizeComponent(); // cc 2770 return this.sampleSize; 2771 } 2772 2773 public boolean hasSampleSize() { 2774 return this.sampleSize != null && !this.sampleSize.isEmpty(); 2775 } 2776 2777 /** 2778 * @param value {@link #sampleSize} (Number of samples in the statistic.) 2779 */ 2780 public Statistic setSampleSize(StatisticSampleSizeComponent value) { 2781 this.sampleSize = value; 2782 return this; 2783 } 2784 2785 /** 2786 * @return {@link #attributeEstimate} (A statistical attribute of the statistic such as a measure of heterogeneity.) 2787 */ 2788 public List<StatisticAttributeEstimateComponent> getAttributeEstimate() { 2789 if (this.attributeEstimate == null) 2790 this.attributeEstimate = new ArrayList<StatisticAttributeEstimateComponent>(); 2791 return this.attributeEstimate; 2792 } 2793 2794 /** 2795 * @return Returns a reference to <code>this</code> for easy method chaining 2796 */ 2797 public Statistic setAttributeEstimate(List<StatisticAttributeEstimateComponent> theAttributeEstimate) { 2798 this.attributeEstimate = theAttributeEstimate; 2799 return this; 2800 } 2801 2802 public boolean hasAttributeEstimate() { 2803 if (this.attributeEstimate == null) 2804 return false; 2805 for (StatisticAttributeEstimateComponent item : this.attributeEstimate) 2806 if (!item.isEmpty()) 2807 return true; 2808 return false; 2809 } 2810 2811 public StatisticAttributeEstimateComponent addAttributeEstimate() { //3 2812 StatisticAttributeEstimateComponent t = new StatisticAttributeEstimateComponent(); 2813 if (this.attributeEstimate == null) 2814 this.attributeEstimate = new ArrayList<StatisticAttributeEstimateComponent>(); 2815 this.attributeEstimate.add(t); 2816 return t; 2817 } 2818 2819 public Statistic addAttributeEstimate(StatisticAttributeEstimateComponent t) { //3 2820 if (t == null) 2821 return this; 2822 if (this.attributeEstimate == null) 2823 this.attributeEstimate = new ArrayList<StatisticAttributeEstimateComponent>(); 2824 this.attributeEstimate.add(t); 2825 return this; 2826 } 2827 2828 /** 2829 * @return The first repetition of repeating field {@link #attributeEstimate}, creating it if it does not already exist {3} 2830 */ 2831 public StatisticAttributeEstimateComponent getAttributeEstimateFirstRep() { 2832 if (getAttributeEstimate().isEmpty()) { 2833 addAttributeEstimate(); 2834 } 2835 return getAttributeEstimate().get(0); 2836 } 2837 2838 /** 2839 * @return {@link #modelCharacteristic} (A component of the method to generate the statistic.) 2840 */ 2841 public List<StatisticModelCharacteristicComponent> getModelCharacteristic() { 2842 if (this.modelCharacteristic == null) 2843 this.modelCharacteristic = new ArrayList<StatisticModelCharacteristicComponent>(); 2844 return this.modelCharacteristic; 2845 } 2846 2847 /** 2848 * @return Returns a reference to <code>this</code> for easy method chaining 2849 */ 2850 public Statistic setModelCharacteristic(List<StatisticModelCharacteristicComponent> theModelCharacteristic) { 2851 this.modelCharacteristic = theModelCharacteristic; 2852 return this; 2853 } 2854 2855 public boolean hasModelCharacteristic() { 2856 if (this.modelCharacteristic == null) 2857 return false; 2858 for (StatisticModelCharacteristicComponent item : this.modelCharacteristic) 2859 if (!item.isEmpty()) 2860 return true; 2861 return false; 2862 } 2863 2864 public StatisticModelCharacteristicComponent addModelCharacteristic() { //3 2865 StatisticModelCharacteristicComponent t = new StatisticModelCharacteristicComponent(); 2866 if (this.modelCharacteristic == null) 2867 this.modelCharacteristic = new ArrayList<StatisticModelCharacteristicComponent>(); 2868 this.modelCharacteristic.add(t); 2869 return t; 2870 } 2871 2872 public Statistic addModelCharacteristic(StatisticModelCharacteristicComponent t) { //3 2873 if (t == null) 2874 return this; 2875 if (this.modelCharacteristic == null) 2876 this.modelCharacteristic = new ArrayList<StatisticModelCharacteristicComponent>(); 2877 this.modelCharacteristic.add(t); 2878 return this; 2879 } 2880 2881 /** 2882 * @return The first repetition of repeating field {@link #modelCharacteristic}, creating it if it does not already exist {3} 2883 */ 2884 public StatisticModelCharacteristicComponent getModelCharacteristicFirstRep() { 2885 if (getModelCharacteristic().isEmpty()) { 2886 addModelCharacteristic(); 2887 } 2888 return getModelCharacteristic().get(0); 2889 } 2890 2891 protected void listChildren(List<Property> children) { 2892 super.listChildren(children); 2893 children.add(new Property("description", "string", "A description of the content value of the statistic.", 0, 1, description)); 2894 children.add(new Property("note", "Annotation", "Footnotes and/or explanatory notes.", 0, java.lang.Integer.MAX_VALUE, note)); 2895 children.add(new Property("statisticType", "CodeableConcept", "Type of statistic, eg relative risk.", 0, 1, statisticType)); 2896 children.add(new Property("category", "CodeableConcept", "When the measured variable is handled categorically, the category element is used to define which category the statistic is reporting.", 0, 1, category)); 2897 children.add(new Property("quantity", "Quantity", "Statistic value.", 0, 1, quantity)); 2898 children.add(new Property("numberOfEvents", "unsignedInt", "The number of events associated with the statistic, where the unit of analysis is different from numberAffected, sampleSize.knownDataCount and sampleSize.numberOfParticipants.", 0, 1, numberOfEvents)); 2899 children.add(new Property("numberAffected", "unsignedInt", "The number of participants affected where the unit of analysis is the same as sampleSize.knownDataCount and sampleSize.numberOfParticipants.", 0, 1, numberAffected)); 2900 children.add(new Property("sampleSize", "", "Number of samples in the statistic.", 0, 1, sampleSize)); 2901 children.add(new Property("attributeEstimate", "", "A statistical attribute of the statistic such as a measure of heterogeneity.", 0, java.lang.Integer.MAX_VALUE, attributeEstimate)); 2902 children.add(new Property("modelCharacteristic", "", "A component of the method to generate the statistic.", 0, java.lang.Integer.MAX_VALUE, modelCharacteristic)); 2903 } 2904 2905 @Override 2906 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2907 switch (_hash) { 2908 case -1724546052: /*description*/ return new Property("description", "string", "A description of the content value of the statistic.", 0, 1, description); 2909 case 3387378: /*note*/ return new Property("note", "Annotation", "Footnotes and/or explanatory notes.", 0, java.lang.Integer.MAX_VALUE, note); 2910 case -392342358: /*statisticType*/ return new Property("statisticType", "CodeableConcept", "Type of statistic, eg relative risk.", 0, 1, statisticType); 2911 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "When the measured variable is handled categorically, the category element is used to define which category the statistic is reporting.", 0, 1, category); 2912 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "Statistic value.", 0, 1, quantity); 2913 case 1534510137: /*numberOfEvents*/ return new Property("numberOfEvents", "unsignedInt", "The number of events associated with the statistic, where the unit of analysis is different from numberAffected, sampleSize.knownDataCount and sampleSize.numberOfParticipants.", 0, 1, numberOfEvents); 2914 case -460990243: /*numberAffected*/ return new Property("numberAffected", "unsignedInt", "The number of participants affected where the unit of analysis is the same as sampleSize.knownDataCount and sampleSize.numberOfParticipants.", 0, 1, numberAffected); 2915 case 143123659: /*sampleSize*/ return new Property("sampleSize", "", "Number of samples in the statistic.", 0, 1, sampleSize); 2916 case -1539581980: /*attributeEstimate*/ return new Property("attributeEstimate", "", "A statistical attribute of the statistic such as a measure of heterogeneity.", 0, java.lang.Integer.MAX_VALUE, attributeEstimate); 2917 case 274795812: /*modelCharacteristic*/ return new Property("modelCharacteristic", "", "A component of the method to generate the statistic.", 0, java.lang.Integer.MAX_VALUE, modelCharacteristic); 2918 default: return super.getNamedProperty(_hash, _name, _checkValid); 2919 } 2920 2921 } 2922 2923 @Override 2924 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2925 switch (hash) { 2926 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 2927 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2928 case -392342358: /*statisticType*/ return this.statisticType == null ? new Base[0] : new Base[] {this.statisticType}; // CodeableConcept 2929 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 2930 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 2931 case 1534510137: /*numberOfEvents*/ return this.numberOfEvents == null ? new Base[0] : new Base[] {this.numberOfEvents}; // UnsignedIntType 2932 case -460990243: /*numberAffected*/ return this.numberAffected == null ? new Base[0] : new Base[] {this.numberAffected}; // UnsignedIntType 2933 case 143123659: /*sampleSize*/ return this.sampleSize == null ? new Base[0] : new Base[] {this.sampleSize}; // StatisticSampleSizeComponent 2934 case -1539581980: /*attributeEstimate*/ return this.attributeEstimate == null ? new Base[0] : this.attributeEstimate.toArray(new Base[this.attributeEstimate.size()]); // StatisticAttributeEstimateComponent 2935 case 274795812: /*modelCharacteristic*/ return this.modelCharacteristic == null ? new Base[0] : this.modelCharacteristic.toArray(new Base[this.modelCharacteristic.size()]); // StatisticModelCharacteristicComponent 2936 default: return super.getProperty(hash, name, checkValid); 2937 } 2938 2939 } 2940 2941 @Override 2942 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2943 switch (hash) { 2944 case -1724546052: // description 2945 this.description = TypeConvertor.castToString(value); // StringType 2946 return value; 2947 case 3387378: // note 2948 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 2949 return value; 2950 case -392342358: // statisticType 2951 this.statisticType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2952 return value; 2953 case 50511102: // category 2954 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2955 return value; 2956 case -1285004149: // quantity 2957 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 2958 return value; 2959 case 1534510137: // numberOfEvents 2960 this.numberOfEvents = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 2961 return value; 2962 case -460990243: // numberAffected 2963 this.numberAffected = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 2964 return value; 2965 case 143123659: // sampleSize 2966 this.sampleSize = (StatisticSampleSizeComponent) value; // StatisticSampleSizeComponent 2967 return value; 2968 case -1539581980: // attributeEstimate 2969 this.getAttributeEstimate().add((StatisticAttributeEstimateComponent) value); // StatisticAttributeEstimateComponent 2970 return value; 2971 case 274795812: // modelCharacteristic 2972 this.getModelCharacteristic().add((StatisticModelCharacteristicComponent) value); // StatisticModelCharacteristicComponent 2973 return value; 2974 default: return super.setProperty(hash, name, value); 2975 } 2976 2977 } 2978 2979 @Override 2980 public Base setProperty(String name, Base value) throws FHIRException { 2981 if (name.equals("description")) { 2982 this.description = TypeConvertor.castToString(value); // StringType 2983 } else if (name.equals("note")) { 2984 this.getNote().add(TypeConvertor.castToAnnotation(value)); 2985 } else if (name.equals("statisticType")) { 2986 this.statisticType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2987 } else if (name.equals("category")) { 2988 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2989 } else if (name.equals("quantity")) { 2990 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 2991 } else if (name.equals("numberOfEvents")) { 2992 this.numberOfEvents = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 2993 } else if (name.equals("numberAffected")) { 2994 this.numberAffected = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 2995 } else if (name.equals("sampleSize")) { 2996 this.sampleSize = (StatisticSampleSizeComponent) value; // StatisticSampleSizeComponent 2997 } else if (name.equals("attributeEstimate")) { 2998 this.getAttributeEstimate().add((StatisticAttributeEstimateComponent) value); 2999 } else if (name.equals("modelCharacteristic")) { 3000 this.getModelCharacteristic().add((StatisticModelCharacteristicComponent) value); 3001 } else 3002 return super.setProperty(name, value); 3003 return value; 3004 } 3005 3006 @Override 3007 public void removeChild(String name, Base value) throws FHIRException { 3008 if (name.equals("description")) { 3009 this.description = null; 3010 } else if (name.equals("note")) { 3011 this.getNote().remove(value); 3012 } else if (name.equals("statisticType")) { 3013 this.statisticType = null; 3014 } else if (name.equals("category")) { 3015 this.category = null; 3016 } else if (name.equals("quantity")) { 3017 this.quantity = null; 3018 } else if (name.equals("numberOfEvents")) { 3019 this.numberOfEvents = null; 3020 } else if (name.equals("numberAffected")) { 3021 this.numberAffected = null; 3022 } else if (name.equals("sampleSize")) { 3023 this.sampleSize = (StatisticSampleSizeComponent) value; // StatisticSampleSizeComponent 3024 } else if (name.equals("attributeEstimate")) { 3025 this.getAttributeEstimate().remove((StatisticAttributeEstimateComponent) value); 3026 } else if (name.equals("modelCharacteristic")) { 3027 this.getModelCharacteristic().remove((StatisticModelCharacteristicComponent) value); 3028 } else 3029 super.removeChild(name, value); 3030 3031 } 3032 3033 @Override 3034 public Base makeProperty(int hash, String name) throws FHIRException { 3035 switch (hash) { 3036 case -1724546052: return getDescriptionElement(); 3037 case 3387378: return addNote(); 3038 case -392342358: return getStatisticType(); 3039 case 50511102: return getCategory(); 3040 case -1285004149: return getQuantity(); 3041 case 1534510137: return getNumberOfEventsElement(); 3042 case -460990243: return getNumberAffectedElement(); 3043 case 143123659: return getSampleSize(); 3044 case -1539581980: return addAttributeEstimate(); 3045 case 274795812: return addModelCharacteristic(); 3046 default: return super.makeProperty(hash, name); 3047 } 3048 3049 } 3050 3051 @Override 3052 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3053 switch (hash) { 3054 case -1724546052: /*description*/ return new String[] {"string"}; 3055 case 3387378: /*note*/ return new String[] {"Annotation"}; 3056 case -392342358: /*statisticType*/ return new String[] {"CodeableConcept"}; 3057 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3058 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 3059 case 1534510137: /*numberOfEvents*/ return new String[] {"unsignedInt"}; 3060 case -460990243: /*numberAffected*/ return new String[] {"unsignedInt"}; 3061 case 143123659: /*sampleSize*/ return new String[] {}; 3062 case -1539581980: /*attributeEstimate*/ return new String[] {}; 3063 case 274795812: /*modelCharacteristic*/ return new String[] {}; 3064 default: return super.getTypesForProperty(hash, name); 3065 } 3066 3067 } 3068 3069 @Override 3070 public Base addChild(String name) throws FHIRException { 3071 if (name.equals("description")) { 3072 throw new FHIRException("Cannot call addChild on a singleton property Statistic.description"); 3073 } 3074 else if (name.equals("note")) { 3075 return addNote(); 3076 } 3077 else if (name.equals("statisticType")) { 3078 this.statisticType = new CodeableConcept(); 3079 return this.statisticType; 3080 } 3081 else if (name.equals("category")) { 3082 this.category = new CodeableConcept(); 3083 return this.category; 3084 } 3085 else if (name.equals("quantity")) { 3086 this.quantity = new Quantity(); 3087 return this.quantity; 3088 } 3089 else if (name.equals("numberOfEvents")) { 3090 throw new FHIRException("Cannot call addChild on a singleton property Statistic.numberOfEvents"); 3091 } 3092 else if (name.equals("numberAffected")) { 3093 throw new FHIRException("Cannot call addChild on a singleton property Statistic.numberAffected"); 3094 } 3095 else if (name.equals("sampleSize")) { 3096 this.sampleSize = new StatisticSampleSizeComponent(); 3097 return this.sampleSize; 3098 } 3099 else if (name.equals("attributeEstimate")) { 3100 return addAttributeEstimate(); 3101 } 3102 else if (name.equals("modelCharacteristic")) { 3103 return addModelCharacteristic(); 3104 } 3105 else 3106 return super.addChild(name); 3107 } 3108 3109 public String fhirType() { 3110 return "Statistic"; 3111 3112 } 3113 3114 public Statistic copy() { 3115 Statistic dst = new Statistic(); 3116 copyValues(dst); 3117 return dst; 3118 } 3119 3120 public void copyValues(Statistic dst) { 3121 super.copyValues(dst); 3122 dst.description = description == null ? null : description.copy(); 3123 if (note != null) { 3124 dst.note = new ArrayList<Annotation>(); 3125 for (Annotation i : note) 3126 dst.note.add(i.copy()); 3127 }; 3128 dst.statisticType = statisticType == null ? null : statisticType.copy(); 3129 dst.category = category == null ? null : category.copy(); 3130 dst.quantity = quantity == null ? null : quantity.copy(); 3131 dst.numberOfEvents = numberOfEvents == null ? null : numberOfEvents.copy(); 3132 dst.numberAffected = numberAffected == null ? null : numberAffected.copy(); 3133 dst.sampleSize = sampleSize == null ? null : sampleSize.copy(); 3134 if (attributeEstimate != null) { 3135 dst.attributeEstimate = new ArrayList<StatisticAttributeEstimateComponent>(); 3136 for (StatisticAttributeEstimateComponent i : attributeEstimate) 3137 dst.attributeEstimate.add(i.copy()); 3138 }; 3139 if (modelCharacteristic != null) { 3140 dst.modelCharacteristic = new ArrayList<StatisticModelCharacteristicComponent>(); 3141 for (StatisticModelCharacteristicComponent i : modelCharacteristic) 3142 dst.modelCharacteristic.add(i.copy()); 3143 }; 3144 } 3145 3146 protected Statistic typedCopy() { 3147 return copy(); 3148 } 3149 3150 @Override 3151 public boolean equalsDeep(Base other_) { 3152 if (!super.equalsDeep(other_)) 3153 return false; 3154 if (!(other_ instanceof Statistic)) 3155 return false; 3156 Statistic o = (Statistic) other_; 3157 return compareDeep(description, o.description, true) && compareDeep(note, o.note, true) && compareDeep(statisticType, o.statisticType, true) 3158 && compareDeep(category, o.category, true) && compareDeep(quantity, o.quantity, true) && compareDeep(numberOfEvents, o.numberOfEvents, true) 3159 && compareDeep(numberAffected, o.numberAffected, true) && compareDeep(sampleSize, o.sampleSize, true) 3160 && compareDeep(attributeEstimate, o.attributeEstimate, true) && compareDeep(modelCharacteristic, o.modelCharacteristic, true) 3161 ; 3162 } 3163 3164 @Override 3165 public boolean equalsShallow(Base other_) { 3166 if (!super.equalsShallow(other_)) 3167 return false; 3168 if (!(other_ instanceof Statistic)) 3169 return false; 3170 Statistic o = (Statistic) other_; 3171 return compareValues(description, o.description, true) && compareValues(numberOfEvents, o.numberOfEvents, true) 3172 && compareValues(numberAffected, o.numberAffected, true); 3173 } 3174 3175 public boolean isEmpty() { 3176 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, note, statisticType 3177 , category, quantity, numberOfEvents, numberAffected, sampleSize, attributeEstimate 3178 , modelCharacteristic); 3179 } 3180 3181 3182} 3183