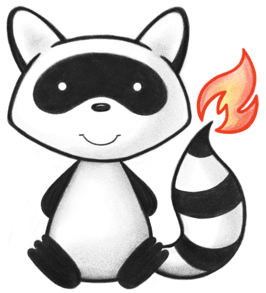
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types. 052 */ 053@ResourceDef(name="StructureDefinition", profile="http://hl7.org/fhir/StructureDefinition/StructureDefinition") 054public class StructureDefinition extends CanonicalResource { 055 056 public enum ExtensionContextType { 057 /** 058 * The context is all elements that match the FHIRPath query found in the expression. 059 */ 060 FHIRPATH, 061 /** 062 * The context is any element that has an ElementDefinition.id that matches that found in the expression. This includes ElementDefinition Ids that have slicing identifiers. The full path for the element is [url]#[elementid]. If there is no #, the Element id is one defined in the base specification. 063 */ 064 ELEMENT, 065 /** 066 * The context is a particular extension from a particular StructureDefinition, and the expression is just a uri that identifies the extension. 067 */ 068 EXTENSION, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static ExtensionContextType fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("fhirpath".equals(codeString)) 077 return FHIRPATH; 078 if ("element".equals(codeString)) 079 return ELEMENT; 080 if ("extension".equals(codeString)) 081 return EXTENSION; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown ExtensionContextType code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case FHIRPATH: return "fhirpath"; 090 case ELEMENT: return "element"; 091 case EXTENSION: return "extension"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case FHIRPATH: return "http://hl7.org/fhir/extension-context-type"; 099 case ELEMENT: return "http://hl7.org/fhir/extension-context-type"; 100 case EXTENSION: return "http://hl7.org/fhir/extension-context-type"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case FHIRPATH: return "The context is all elements that match the FHIRPath query found in the expression."; 108 case ELEMENT: return "The context is any element that has an ElementDefinition.id that matches that found in the expression. This includes ElementDefinition Ids that have slicing identifiers. The full path for the element is [url]#[elementid]. If there is no #, the Element id is one defined in the base specification."; 109 case EXTENSION: return "The context is a particular extension from a particular StructureDefinition, and the expression is just a uri that identifies the extension."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case FHIRPATH: return "FHIRPath"; 117 case ELEMENT: return "Element ID"; 118 case EXTENSION: return "Extension URL"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class ExtensionContextTypeEnumFactory implements EnumFactory<ExtensionContextType> { 126 public ExtensionContextType fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("fhirpath".equals(codeString)) 131 return ExtensionContextType.FHIRPATH; 132 if ("element".equals(codeString)) 133 return ExtensionContextType.ELEMENT; 134 if ("extension".equals(codeString)) 135 return ExtensionContextType.EXTENSION; 136 throw new IllegalArgumentException("Unknown ExtensionContextType code '"+codeString+"'"); 137 } 138 public Enumeration<ExtensionContextType> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<ExtensionContextType>(this, ExtensionContextType.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<ExtensionContextType>(this, ExtensionContextType.NULL, code); 146 if ("fhirpath".equals(codeString)) 147 return new Enumeration<ExtensionContextType>(this, ExtensionContextType.FHIRPATH, code); 148 if ("element".equals(codeString)) 149 return new Enumeration<ExtensionContextType>(this, ExtensionContextType.ELEMENT, code); 150 if ("extension".equals(codeString)) 151 return new Enumeration<ExtensionContextType>(this, ExtensionContextType.EXTENSION, code); 152 throw new FHIRException("Unknown ExtensionContextType code '"+codeString+"'"); 153 } 154 public String toCode(ExtensionContextType code) { 155 if (code == ExtensionContextType.NULL) 156 return null; 157 if (code == ExtensionContextType.FHIRPATH) 158 return "fhirpath"; 159 if (code == ExtensionContextType.ELEMENT) 160 return "element"; 161 if (code == ExtensionContextType.EXTENSION) 162 return "extension"; 163 return "?"; 164 } 165 public String toSystem(ExtensionContextType code) { 166 return code.getSystem(); 167 } 168 } 169 170 public enum StructureDefinitionKind { 171 /** 172 * A primitive type that has a value and an extension. These can be used throughout complex datatype, Resource and extension definitions. Only the base specification can define primitive types. 173 */ 174 PRIMITIVETYPE, 175 /** 176 * A complex structure that defines a set of data elements that is suitable for use in 'resources'. The base specification defines a number of complex types, and other specifications can define additional types. These structures do not have a maintained identity. 177 */ 178 COMPLEXTYPE, 179 /** 180 * A 'resource' - a directed acyclic graph of elements that aggregrates other types into an identifiable entity. The base FHIR resources are defined by the FHIR specification itself but other 'resources' can be defined in additional specifications (though these will not be recognized as 'resources' by the FHIR specification; i.e. they do not get end-points etc., or act as the targets of references in FHIR defined resources - though other specifications can treat them this way). 181 */ 182 RESOURCE, 183 /** 184 * A pattern or a template that is not intended to be a real resource or complex type. 185 */ 186 LOGICAL, 187 /** 188 * added to help the parsers with the generic types 189 */ 190 NULL; 191 public static StructureDefinitionKind fromCode(String codeString) throws FHIRException { 192 if (codeString == null || "".equals(codeString)) 193 return null; 194 if ("primitive-type".equals(codeString)) 195 return PRIMITIVETYPE; 196 if ("complex-type".equals(codeString)) 197 return COMPLEXTYPE; 198 if ("resource".equals(codeString)) 199 return RESOURCE; 200 if ("logical".equals(codeString)) 201 return LOGICAL; 202 if (Configuration.isAcceptInvalidEnums()) 203 return null; 204 else 205 throw new FHIRException("Unknown StructureDefinitionKind code '"+codeString+"'"); 206 } 207 public String toCode() { 208 switch (this) { 209 case PRIMITIVETYPE: return "primitive-type"; 210 case COMPLEXTYPE: return "complex-type"; 211 case RESOURCE: return "resource"; 212 case LOGICAL: return "logical"; 213 case NULL: return null; 214 default: return "?"; 215 } 216 } 217 public String getSystem() { 218 switch (this) { 219 case PRIMITIVETYPE: return "http://hl7.org/fhir/structure-definition-kind"; 220 case COMPLEXTYPE: return "http://hl7.org/fhir/structure-definition-kind"; 221 case RESOURCE: return "http://hl7.org/fhir/structure-definition-kind"; 222 case LOGICAL: return "http://hl7.org/fhir/structure-definition-kind"; 223 case NULL: return null; 224 default: return "?"; 225 } 226 } 227 public String getDefinition() { 228 switch (this) { 229 case PRIMITIVETYPE: return "A primitive type that has a value and an extension. These can be used throughout complex datatype, Resource and extension definitions. Only the base specification can define primitive types."; 230 case COMPLEXTYPE: return "A complex structure that defines a set of data elements that is suitable for use in 'resources'. The base specification defines a number of complex types, and other specifications can define additional types. These structures do not have a maintained identity."; 231 case RESOURCE: return "A 'resource' - a directed acyclic graph of elements that aggregrates other types into an identifiable entity. The base FHIR resources are defined by the FHIR specification itself but other 'resources' can be defined in additional specifications (though these will not be recognized as 'resources' by the FHIR specification; i.e. they do not get end-points etc., or act as the targets of references in FHIR defined resources - though other specifications can treat them this way)."; 232 case LOGICAL: return "A pattern or a template that is not intended to be a real resource or complex type."; 233 case NULL: return null; 234 default: return "?"; 235 } 236 } 237 public String getDisplay() { 238 switch (this) { 239 case PRIMITIVETYPE: return "Primitive Data Type"; 240 case COMPLEXTYPE: return "Complex Data Type"; 241 case RESOURCE: return "Resource"; 242 case LOGICAL: return "Logical"; 243 case NULL: return null; 244 default: return "?"; 245 } 246 } 247 } 248 249 public static class StructureDefinitionKindEnumFactory implements EnumFactory<StructureDefinitionKind> { 250 public StructureDefinitionKind fromCode(String codeString) throws IllegalArgumentException { 251 if (codeString == null || "".equals(codeString)) 252 if (codeString == null || "".equals(codeString)) 253 return null; 254 if ("primitive-type".equals(codeString)) 255 return StructureDefinitionKind.PRIMITIVETYPE; 256 if ("complex-type".equals(codeString)) 257 return StructureDefinitionKind.COMPLEXTYPE; 258 if ("resource".equals(codeString)) 259 return StructureDefinitionKind.RESOURCE; 260 if ("logical".equals(codeString)) 261 return StructureDefinitionKind.LOGICAL; 262 throw new IllegalArgumentException("Unknown StructureDefinitionKind code '"+codeString+"'"); 263 } 264 public Enumeration<StructureDefinitionKind> fromType(PrimitiveType<?> code) throws FHIRException { 265 if (code == null) 266 return null; 267 if (code.isEmpty()) 268 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.NULL, code); 269 String codeString = ((PrimitiveType) code).asStringValue(); 270 if (codeString == null || "".equals(codeString)) 271 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.NULL, code); 272 if ("primitive-type".equals(codeString)) 273 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.PRIMITIVETYPE, code); 274 if ("complex-type".equals(codeString)) 275 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.COMPLEXTYPE, code); 276 if ("resource".equals(codeString)) 277 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.RESOURCE, code); 278 if ("logical".equals(codeString)) 279 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.LOGICAL, code); 280 throw new FHIRException("Unknown StructureDefinitionKind code '"+codeString+"'"); 281 } 282 public String toCode(StructureDefinitionKind code) { 283 if (code == StructureDefinitionKind.NULL) 284 return null; 285 if (code == StructureDefinitionKind.PRIMITIVETYPE) 286 return "primitive-type"; 287 if (code == StructureDefinitionKind.COMPLEXTYPE) 288 return "complex-type"; 289 if (code == StructureDefinitionKind.RESOURCE) 290 return "resource"; 291 if (code == StructureDefinitionKind.LOGICAL) 292 return "logical"; 293 return "?"; 294 } 295 public String toSystem(StructureDefinitionKind code) { 296 return code.getSystem(); 297 } 298 } 299 300 public enum TypeDerivationRule { 301 /** 302 * This definition defines a new type that adds additional elements and optionally additional rules to the base type. 303 */ 304 SPECIALIZATION, 305 /** 306 * This definition adds additional rules to an existing concrete type. 307 */ 308 CONSTRAINT, 309 /** 310 * added to help the parsers with the generic types 311 */ 312 NULL; 313 public static TypeDerivationRule fromCode(String codeString) throws FHIRException { 314 if (codeString == null || "".equals(codeString)) 315 return null; 316 if ("specialization".equals(codeString)) 317 return SPECIALIZATION; 318 if ("constraint".equals(codeString)) 319 return CONSTRAINT; 320 if (Configuration.isAcceptInvalidEnums()) 321 return null; 322 else 323 throw new FHIRException("Unknown TypeDerivationRule code '"+codeString+"'"); 324 } 325 public String toCode() { 326 switch (this) { 327 case SPECIALIZATION: return "specialization"; 328 case CONSTRAINT: return "constraint"; 329 case NULL: return null; 330 default: return "?"; 331 } 332 } 333 public String getSystem() { 334 switch (this) { 335 case SPECIALIZATION: return "http://hl7.org/fhir/type-derivation-rule"; 336 case CONSTRAINT: return "http://hl7.org/fhir/type-derivation-rule"; 337 case NULL: return null; 338 default: return "?"; 339 } 340 } 341 public String getDefinition() { 342 switch (this) { 343 case SPECIALIZATION: return "This definition defines a new type that adds additional elements and optionally additional rules to the base type."; 344 case CONSTRAINT: return "This definition adds additional rules to an existing concrete type."; 345 case NULL: return null; 346 default: return "?"; 347 } 348 } 349 public String getDisplay() { 350 switch (this) { 351 case SPECIALIZATION: return "Specialization"; 352 case CONSTRAINT: return "Constraint"; 353 case NULL: return null; 354 default: return "?"; 355 } 356 } 357 } 358 359 public static class TypeDerivationRuleEnumFactory implements EnumFactory<TypeDerivationRule> { 360 public TypeDerivationRule fromCode(String codeString) throws IllegalArgumentException { 361 if (codeString == null || "".equals(codeString)) 362 if (codeString == null || "".equals(codeString)) 363 return null; 364 if ("specialization".equals(codeString)) 365 return TypeDerivationRule.SPECIALIZATION; 366 if ("constraint".equals(codeString)) 367 return TypeDerivationRule.CONSTRAINT; 368 throw new IllegalArgumentException("Unknown TypeDerivationRule code '"+codeString+"'"); 369 } 370 public Enumeration<TypeDerivationRule> fromType(PrimitiveType<?> code) throws FHIRException { 371 if (code == null) 372 return null; 373 if (code.isEmpty()) 374 return new Enumeration<TypeDerivationRule>(this, TypeDerivationRule.NULL, code); 375 String codeString = ((PrimitiveType) code).asStringValue(); 376 if (codeString == null || "".equals(codeString)) 377 return new Enumeration<TypeDerivationRule>(this, TypeDerivationRule.NULL, code); 378 if ("specialization".equals(codeString)) 379 return new Enumeration<TypeDerivationRule>(this, TypeDerivationRule.SPECIALIZATION, code); 380 if ("constraint".equals(codeString)) 381 return new Enumeration<TypeDerivationRule>(this, TypeDerivationRule.CONSTRAINT, code); 382 throw new FHIRException("Unknown TypeDerivationRule code '"+codeString+"'"); 383 } 384 public String toCode(TypeDerivationRule code) { 385 if (code == TypeDerivationRule.NULL) 386 return null; 387 if (code == TypeDerivationRule.SPECIALIZATION) 388 return "specialization"; 389 if (code == TypeDerivationRule.CONSTRAINT) 390 return "constraint"; 391 return "?"; 392 } 393 public String toSystem(TypeDerivationRule code) { 394 return code.getSystem(); 395 } 396 } 397 398 @Block() 399 public static class StructureDefinitionMappingComponent extends BackboneElement implements IBaseBackboneElement { 400 @Override 401 public String toString() { 402 return identity + "=" + uri + " (\""+name+"\")"; 403 } 404 405 /** 406 * An Internal id that is used to identify this mapping set when specific mappings are made. 407 */ 408 @Child(name = "identity", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=false) 409 @Description(shortDefinition="Internal id when this mapping is used", formalDefinition="An Internal id that is used to identify this mapping set when specific mappings are made." ) 410 protected IdType identity; 411 412 /** 413 * An absolute URI that identifies the specification that this mapping is expressed to. 414 */ 415 @Child(name = "uri", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 416 @Description(shortDefinition="Identifies what this mapping refers to", formalDefinition="An absolute URI that identifies the specification that this mapping is expressed to." ) 417 protected UriType uri; 418 419 /** 420 * A name for the specification that is being mapped to. 421 */ 422 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 423 @Description(shortDefinition="Names what this mapping refers to", formalDefinition="A name for the specification that is being mapped to." ) 424 protected StringType name; 425 426 /** 427 * Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage. 428 */ 429 @Child(name = "comment", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 430 @Description(shortDefinition="Versions, Issues, Scope limitations etc", formalDefinition="Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage." ) 431 protected StringType comment; 432 433 private static final long serialVersionUID = 9610265L; 434 435 /** 436 * Constructor 437 */ 438 public StructureDefinitionMappingComponent() { 439 super(); 440 } 441 442 /** 443 * Constructor 444 */ 445 public StructureDefinitionMappingComponent(String identity) { 446 super(); 447 this.setIdentity(identity); 448 } 449 450 /** 451 * @return {@link #identity} (An Internal id that is used to identify this mapping set when specific mappings are made.). This is the underlying object with id, value and extensions. The accessor "getIdentity" gives direct access to the value 452 */ 453 public IdType getIdentityElement() { 454 if (this.identity == null) 455 if (Configuration.errorOnAutoCreate()) 456 throw new Error("Attempt to auto-create StructureDefinitionMappingComponent.identity"); 457 else if (Configuration.doAutoCreate()) 458 this.identity = new IdType(); // bb 459 return this.identity; 460 } 461 462 public boolean hasIdentityElement() { 463 return this.identity != null && !this.identity.isEmpty(); 464 } 465 466 public boolean hasIdentity() { 467 return this.identity != null && !this.identity.isEmpty(); 468 } 469 470 /** 471 * @param value {@link #identity} (An Internal id that is used to identify this mapping set when specific mappings are made.). This is the underlying object with id, value and extensions. The accessor "getIdentity" gives direct access to the value 472 */ 473 public StructureDefinitionMappingComponent setIdentityElement(IdType value) { 474 this.identity = value; 475 return this; 476 } 477 478 /** 479 * @return An Internal id that is used to identify this mapping set when specific mappings are made. 480 */ 481 public String getIdentity() { 482 return this.identity == null ? null : this.identity.getValue(); 483 } 484 485 /** 486 * @param value An Internal id that is used to identify this mapping set when specific mappings are made. 487 */ 488 public StructureDefinitionMappingComponent setIdentity(String value) { 489 if (this.identity == null) 490 this.identity = new IdType(); 491 this.identity.setValue(value); 492 return this; 493 } 494 495 /** 496 * @return {@link #uri} (An absolute URI that identifies the specification that this mapping is expressed to.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 497 */ 498 public UriType getUriElement() { 499 if (this.uri == null) 500 if (Configuration.errorOnAutoCreate()) 501 throw new Error("Attempt to auto-create StructureDefinitionMappingComponent.uri"); 502 else if (Configuration.doAutoCreate()) 503 this.uri = new UriType(); // bb 504 return this.uri; 505 } 506 507 public boolean hasUriElement() { 508 return this.uri != null && !this.uri.isEmpty(); 509 } 510 511 public boolean hasUri() { 512 return this.uri != null && !this.uri.isEmpty(); 513 } 514 515 /** 516 * @param value {@link #uri} (An absolute URI that identifies the specification that this mapping is expressed to.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 517 */ 518 public StructureDefinitionMappingComponent setUriElement(UriType value) { 519 this.uri = value; 520 return this; 521 } 522 523 /** 524 * @return An absolute URI that identifies the specification that this mapping is expressed to. 525 */ 526 public String getUri() { 527 return this.uri == null ? null : this.uri.getValue(); 528 } 529 530 /** 531 * @param value An absolute URI that identifies the specification that this mapping is expressed to. 532 */ 533 public StructureDefinitionMappingComponent setUri(String value) { 534 if (Utilities.noString(value)) 535 this.uri = null; 536 else { 537 if (this.uri == null) 538 this.uri = new UriType(); 539 this.uri.setValue(value); 540 } 541 return this; 542 } 543 544 /** 545 * @return {@link #name} (A name for the specification that is being mapped to.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 546 */ 547 public StringType getNameElement() { 548 if (this.name == null) 549 if (Configuration.errorOnAutoCreate()) 550 throw new Error("Attempt to auto-create StructureDefinitionMappingComponent.name"); 551 else if (Configuration.doAutoCreate()) 552 this.name = new StringType(); // bb 553 return this.name; 554 } 555 556 public boolean hasNameElement() { 557 return this.name != null && !this.name.isEmpty(); 558 } 559 560 public boolean hasName() { 561 return this.name != null && !this.name.isEmpty(); 562 } 563 564 /** 565 * @param value {@link #name} (A name for the specification that is being mapped to.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 566 */ 567 public StructureDefinitionMappingComponent setNameElement(StringType value) { 568 this.name = value; 569 return this; 570 } 571 572 /** 573 * @return A name for the specification that is being mapped to. 574 */ 575 public String getName() { 576 return this.name == null ? null : this.name.getValue(); 577 } 578 579 /** 580 * @param value A name for the specification that is being mapped to. 581 */ 582 public StructureDefinitionMappingComponent setName(String value) { 583 if (Utilities.noString(value)) 584 this.name = null; 585 else { 586 if (this.name == null) 587 this.name = new StringType(); 588 this.name.setValue(value); 589 } 590 return this; 591 } 592 593 /** 594 * @return {@link #comment} (Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 595 */ 596 public StringType getCommentElement() { 597 if (this.comment == null) 598 if (Configuration.errorOnAutoCreate()) 599 throw new Error("Attempt to auto-create StructureDefinitionMappingComponent.comment"); 600 else if (Configuration.doAutoCreate()) 601 this.comment = new StringType(); // bb 602 return this.comment; 603 } 604 605 public boolean hasCommentElement() { 606 return this.comment != null && !this.comment.isEmpty(); 607 } 608 609 public boolean hasComment() { 610 return this.comment != null && !this.comment.isEmpty(); 611 } 612 613 /** 614 * @param value {@link #comment} (Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 615 */ 616 public StructureDefinitionMappingComponent setCommentElement(StringType value) { 617 this.comment = value; 618 return this; 619 } 620 621 /** 622 * @return Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage. 623 */ 624 public String getComment() { 625 return this.comment == null ? null : this.comment.getValue(); 626 } 627 628 /** 629 * @param value Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage. 630 */ 631 public StructureDefinitionMappingComponent setComment(String value) { 632 if (Utilities.noString(value)) 633 this.comment = null; 634 else { 635 if (this.comment == null) 636 this.comment = new StringType(); 637 this.comment.setValue(value); 638 } 639 return this; 640 } 641 642 protected void listChildren(List<Property> children) { 643 super.listChildren(children); 644 children.add(new Property("identity", "id", "An Internal id that is used to identify this mapping set when specific mappings are made.", 0, 1, identity)); 645 children.add(new Property("uri", "uri", "An absolute URI that identifies the specification that this mapping is expressed to.", 0, 1, uri)); 646 children.add(new Property("name", "string", "A name for the specification that is being mapped to.", 0, 1, name)); 647 children.add(new Property("comment", "string", "Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.", 0, 1, comment)); 648 } 649 650 @Override 651 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 652 switch (_hash) { 653 case -135761730: /*identity*/ return new Property("identity", "id", "An Internal id that is used to identify this mapping set when specific mappings are made.", 0, 1, identity); 654 case 116076: /*uri*/ return new Property("uri", "uri", "An absolute URI that identifies the specification that this mapping is expressed to.", 0, 1, uri); 655 case 3373707: /*name*/ return new Property("name", "string", "A name for the specification that is being mapped to.", 0, 1, name); 656 case 950398559: /*comment*/ return new Property("comment", "string", "Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.", 0, 1, comment); 657 default: return super.getNamedProperty(_hash, _name, _checkValid); 658 } 659 660 } 661 662 @Override 663 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 664 switch (hash) { 665 case -135761730: /*identity*/ return this.identity == null ? new Base[0] : new Base[] {this.identity}; // IdType 666 case 116076: /*uri*/ return this.uri == null ? new Base[0] : new Base[] {this.uri}; // UriType 667 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 668 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 669 default: return super.getProperty(hash, name, checkValid); 670 } 671 672 } 673 674 @Override 675 public Base setProperty(int hash, String name, Base value) throws FHIRException { 676 switch (hash) { 677 case -135761730: // identity 678 this.identity = TypeConvertor.castToId(value); // IdType 679 return value; 680 case 116076: // uri 681 this.uri = TypeConvertor.castToUri(value); // UriType 682 return value; 683 case 3373707: // name 684 this.name = TypeConvertor.castToString(value); // StringType 685 return value; 686 case 950398559: // comment 687 this.comment = TypeConvertor.castToString(value); // StringType 688 return value; 689 default: return super.setProperty(hash, name, value); 690 } 691 692 } 693 694 @Override 695 public Base setProperty(String name, Base value) throws FHIRException { 696 if (name.equals("identity")) { 697 this.identity = TypeConvertor.castToId(value); // IdType 698 } else if (name.equals("uri")) { 699 this.uri = TypeConvertor.castToUri(value); // UriType 700 } else if (name.equals("name")) { 701 this.name = TypeConvertor.castToString(value); // StringType 702 } else if (name.equals("comment")) { 703 this.comment = TypeConvertor.castToString(value); // StringType 704 } else 705 return super.setProperty(name, value); 706 return value; 707 } 708 709 @Override 710 public void removeChild(String name, Base value) throws FHIRException { 711 if (name.equals("identity")) { 712 this.identity = null; 713 } else if (name.equals("uri")) { 714 this.uri = null; 715 } else if (name.equals("name")) { 716 this.name = null; 717 } else if (name.equals("comment")) { 718 this.comment = null; 719 } else 720 super.removeChild(name, value); 721 722 } 723 724 @Override 725 public Base makeProperty(int hash, String name) throws FHIRException { 726 switch (hash) { 727 case -135761730: return getIdentityElement(); 728 case 116076: return getUriElement(); 729 case 3373707: return getNameElement(); 730 case 950398559: return getCommentElement(); 731 default: return super.makeProperty(hash, name); 732 } 733 734 } 735 736 @Override 737 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 738 switch (hash) { 739 case -135761730: /*identity*/ return new String[] {"id"}; 740 case 116076: /*uri*/ return new String[] {"uri"}; 741 case 3373707: /*name*/ return new String[] {"string"}; 742 case 950398559: /*comment*/ return new String[] {"string"}; 743 default: return super.getTypesForProperty(hash, name); 744 } 745 746 } 747 748 @Override 749 public Base addChild(String name) throws FHIRException { 750 if (name.equals("identity")) { 751 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.mapping.identity"); 752 } 753 else if (name.equals("uri")) { 754 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.mapping.uri"); 755 } 756 else if (name.equals("name")) { 757 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.mapping.name"); 758 } 759 else if (name.equals("comment")) { 760 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.mapping.comment"); 761 } 762 else 763 return super.addChild(name); 764 } 765 766 public StructureDefinitionMappingComponent copy() { 767 StructureDefinitionMappingComponent dst = new StructureDefinitionMappingComponent(); 768 copyValues(dst); 769 return dst; 770 } 771 772 public void copyValues(StructureDefinitionMappingComponent dst) { 773 super.copyValues(dst); 774 dst.identity = identity == null ? null : identity.copy(); 775 dst.uri = uri == null ? null : uri.copy(); 776 dst.name = name == null ? null : name.copy(); 777 dst.comment = comment == null ? null : comment.copy(); 778 } 779 780 @Override 781 public boolean equalsDeep(Base other_) { 782 if (!super.equalsDeep(other_)) 783 return false; 784 if (!(other_ instanceof StructureDefinitionMappingComponent)) 785 return false; 786 StructureDefinitionMappingComponent o = (StructureDefinitionMappingComponent) other_; 787 return compareDeep(identity, o.identity, true) && compareDeep(uri, o.uri, true) && compareDeep(name, o.name, true) 788 && compareDeep(comment, o.comment, true); 789 } 790 791 @Override 792 public boolean equalsShallow(Base other_) { 793 if (!super.equalsShallow(other_)) 794 return false; 795 if (!(other_ instanceof StructureDefinitionMappingComponent)) 796 return false; 797 StructureDefinitionMappingComponent o = (StructureDefinitionMappingComponent) other_; 798 return compareValues(identity, o.identity, true) && compareValues(uri, o.uri, true) && compareValues(name, o.name, true) 799 && compareValues(comment, o.comment, true); 800 } 801 802 public boolean isEmpty() { 803 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identity, uri, name, comment 804 ); 805 } 806 807 public String fhirType() { 808 return "StructureDefinition.mapping"; 809 810 } 811 812 } 813 814 @Block() 815 public static class StructureDefinitionContextComponent extends BackboneElement implements IBaseBackboneElement { 816 /** 817 * Defines how to interpret the expression that defines what the context of the extension is. 818 */ 819 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 820 @Description(shortDefinition="fhirpath | element | extension", formalDefinition="Defines how to interpret the expression that defines what the context of the extension is." ) 821 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/extension-context-type") 822 protected Enumeration<ExtensionContextType> type; 823 824 /** 825 * An expression that defines where an extension can be used in resources. 826 */ 827 @Child(name = "expression", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=true) 828 @Description(shortDefinition="Where the extension can be used in instances", formalDefinition="An expression that defines where an extension can be used in resources." ) 829 protected StringType expression; 830 831 private static final long serialVersionUID = 1958074856L; 832 833 /** 834 * Constructor 835 */ 836 public StructureDefinitionContextComponent() { 837 super(); 838 } 839 840 /** 841 * Constructor 842 */ 843 public StructureDefinitionContextComponent(ExtensionContextType type, String expression) { 844 super(); 845 this.setType(type); 846 this.setExpression(expression); 847 } 848 849 /** 850 * @return {@link #type} (Defines how to interpret the expression that defines what the context of the extension is.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 851 */ 852 public Enumeration<ExtensionContextType> getTypeElement() { 853 if (this.type == null) 854 if (Configuration.errorOnAutoCreate()) 855 throw new Error("Attempt to auto-create StructureDefinitionContextComponent.type"); 856 else if (Configuration.doAutoCreate()) 857 this.type = new Enumeration<ExtensionContextType>(new ExtensionContextTypeEnumFactory()); // bb 858 return this.type; 859 } 860 861 public boolean hasTypeElement() { 862 return this.type != null && !this.type.isEmpty(); 863 } 864 865 public boolean hasType() { 866 return this.type != null && !this.type.isEmpty(); 867 } 868 869 /** 870 * @param value {@link #type} (Defines how to interpret the expression that defines what the context of the extension is.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 871 */ 872 public StructureDefinitionContextComponent setTypeElement(Enumeration<ExtensionContextType> value) { 873 this.type = value; 874 return this; 875 } 876 877 /** 878 * @return Defines how to interpret the expression that defines what the context of the extension is. 879 */ 880 public ExtensionContextType getType() { 881 return this.type == null ? null : this.type.getValue(); 882 } 883 884 /** 885 * @param value Defines how to interpret the expression that defines what the context of the extension is. 886 */ 887 public StructureDefinitionContextComponent setType(ExtensionContextType value) { 888 if (this.type == null) 889 this.type = new Enumeration<ExtensionContextType>(new ExtensionContextTypeEnumFactory()); 890 this.type.setValue(value); 891 return this; 892 } 893 894 /** 895 * @return {@link #expression} (An expression that defines where an extension can be used in resources.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 896 */ 897 public StringType getExpressionElement() { 898 if (this.expression == null) 899 if (Configuration.errorOnAutoCreate()) 900 throw new Error("Attempt to auto-create StructureDefinitionContextComponent.expression"); 901 else if (Configuration.doAutoCreate()) 902 this.expression = new StringType(); // bb 903 return this.expression; 904 } 905 906 public boolean hasExpressionElement() { 907 return this.expression != null && !this.expression.isEmpty(); 908 } 909 910 public boolean hasExpression() { 911 return this.expression != null && !this.expression.isEmpty(); 912 } 913 914 /** 915 * @param value {@link #expression} (An expression that defines where an extension can be used in resources.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 916 */ 917 public StructureDefinitionContextComponent setExpressionElement(StringType value) { 918 this.expression = value; 919 return this; 920 } 921 922 /** 923 * @return An expression that defines where an extension can be used in resources. 924 */ 925 public String getExpression() { 926 return this.expression == null ? null : this.expression.getValue(); 927 } 928 929 /** 930 * @param value An expression that defines where an extension can be used in resources. 931 */ 932 public StructureDefinitionContextComponent setExpression(String value) { 933 if (this.expression == null) 934 this.expression = new StringType(); 935 this.expression.setValue(value); 936 return this; 937 } 938 939 protected void listChildren(List<Property> children) { 940 super.listChildren(children); 941 children.add(new Property("type", "code", "Defines how to interpret the expression that defines what the context of the extension is.", 0, 1, type)); 942 children.add(new Property("expression", "string", "An expression that defines where an extension can be used in resources.", 0, 1, expression)); 943 } 944 945 @Override 946 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 947 switch (_hash) { 948 case 3575610: /*type*/ return new Property("type", "code", "Defines how to interpret the expression that defines what the context of the extension is.", 0, 1, type); 949 case -1795452264: /*expression*/ return new Property("expression", "string", "An expression that defines where an extension can be used in resources.", 0, 1, expression); 950 default: return super.getNamedProperty(_hash, _name, _checkValid); 951 } 952 953 } 954 955 @Override 956 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 957 switch (hash) { 958 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ExtensionContextType> 959 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 960 default: return super.getProperty(hash, name, checkValid); 961 } 962 963 } 964 965 @Override 966 public Base setProperty(int hash, String name, Base value) throws FHIRException { 967 switch (hash) { 968 case 3575610: // type 969 value = new ExtensionContextTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 970 this.type = (Enumeration) value; // Enumeration<ExtensionContextType> 971 return value; 972 case -1795452264: // expression 973 this.expression = TypeConvertor.castToString(value); // StringType 974 return value; 975 default: return super.setProperty(hash, name, value); 976 } 977 978 } 979 980 @Override 981 public Base setProperty(String name, Base value) throws FHIRException { 982 if (name.equals("type")) { 983 value = new ExtensionContextTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 984 this.type = (Enumeration) value; // Enumeration<ExtensionContextType> 985 } else if (name.equals("expression")) { 986 this.expression = TypeConvertor.castToString(value); // StringType 987 } else 988 return super.setProperty(name, value); 989 return value; 990 } 991 992 @Override 993 public void removeChild(String name, Base value) throws FHIRException { 994 if (name.equals("type")) { 995 value = new ExtensionContextTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 996 this.type = (Enumeration) value; // Enumeration<ExtensionContextType> 997 } else if (name.equals("expression")) { 998 this.expression = null; 999 } else 1000 super.removeChild(name, value); 1001 1002 } 1003 1004 @Override 1005 public Base makeProperty(int hash, String name) throws FHIRException { 1006 switch (hash) { 1007 case 3575610: return getTypeElement(); 1008 case -1795452264: return getExpressionElement(); 1009 default: return super.makeProperty(hash, name); 1010 } 1011 1012 } 1013 1014 @Override 1015 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1016 switch (hash) { 1017 case 3575610: /*type*/ return new String[] {"code"}; 1018 case -1795452264: /*expression*/ return new String[] {"string"}; 1019 default: return super.getTypesForProperty(hash, name); 1020 } 1021 1022 } 1023 1024 @Override 1025 public Base addChild(String name) throws FHIRException { 1026 if (name.equals("type")) { 1027 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.context.type"); 1028 } 1029 else if (name.equals("expression")) { 1030 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.context.expression"); 1031 } 1032 else 1033 return super.addChild(name); 1034 } 1035 1036 public StructureDefinitionContextComponent copy() { 1037 StructureDefinitionContextComponent dst = new StructureDefinitionContextComponent(); 1038 copyValues(dst); 1039 return dst; 1040 } 1041 1042 public void copyValues(StructureDefinitionContextComponent dst) { 1043 super.copyValues(dst); 1044 dst.type = type == null ? null : type.copy(); 1045 dst.expression = expression == null ? null : expression.copy(); 1046 } 1047 1048 @Override 1049 public boolean equalsDeep(Base other_) { 1050 if (!super.equalsDeep(other_)) 1051 return false; 1052 if (!(other_ instanceof StructureDefinitionContextComponent)) 1053 return false; 1054 StructureDefinitionContextComponent o = (StructureDefinitionContextComponent) other_; 1055 return compareDeep(type, o.type, true) && compareDeep(expression, o.expression, true); 1056 } 1057 1058 @Override 1059 public boolean equalsShallow(Base other_) { 1060 if (!super.equalsShallow(other_)) 1061 return false; 1062 if (!(other_ instanceof StructureDefinitionContextComponent)) 1063 return false; 1064 StructureDefinitionContextComponent o = (StructureDefinitionContextComponent) other_; 1065 return compareValues(type, o.type, true) && compareValues(expression, o.expression, true); 1066 } 1067 1068 public boolean isEmpty() { 1069 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, expression); 1070 } 1071 1072 public String fhirType() { 1073 return "StructureDefinition.context"; 1074 1075 } 1076 1077 } 1078 1079 @Block() 1080 public static class StructureDefinitionSnapshotComponent extends BackboneElement implements IBaseBackboneElement { 1081 /** 1082 * Captures constraints on each element within the resource. 1083 */ 1084 @Child(name = "element", type = {ElementDefinition.class}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1085 @Description(shortDefinition="Definition of elements in the resource (if no StructureDefinition)", formalDefinition="Captures constraints on each element within the resource." ) 1086 protected List<ElementDefinition> element; 1087 1088 private static final long serialVersionUID = 53896641L; 1089 1090 /** 1091 * Constructor 1092 */ 1093 public StructureDefinitionSnapshotComponent() { 1094 super(); 1095 } 1096 1097 /** 1098 * Constructor 1099 */ 1100 public StructureDefinitionSnapshotComponent(ElementDefinition element) { 1101 super(); 1102 this.addElement(element); 1103 } 1104 1105 /** 1106 * @return {@link #element} (Captures constraints on each element within the resource.) 1107 */ 1108 public List<ElementDefinition> getElement() { 1109 if (this.element == null) 1110 this.element = new ArrayList<ElementDefinition>(); 1111 return this.element; 1112 } 1113 1114 /** 1115 * @return Returns a reference to <code>this</code> for easy method chaining 1116 */ 1117 public StructureDefinitionSnapshotComponent setElement(List<ElementDefinition> theElement) { 1118 this.element = theElement; 1119 return this; 1120 } 1121 1122 public boolean hasElement() { 1123 if (this.element == null) 1124 return false; 1125 for (ElementDefinition item : this.element) 1126 if (!item.isEmpty()) 1127 return true; 1128 return false; 1129 } 1130 1131 public ElementDefinition addElement() { //3 1132 ElementDefinition t = new ElementDefinition(); 1133 if (this.element == null) 1134 this.element = new ArrayList<ElementDefinition>(); 1135 this.element.add(t); 1136 return t; 1137 } 1138 1139 public StructureDefinitionSnapshotComponent addElement(ElementDefinition t) { //3 1140 if (t == null) 1141 return this; 1142 if (this.element == null) 1143 this.element = new ArrayList<ElementDefinition>(); 1144 this.element.add(t); 1145 return this; 1146 } 1147 1148 /** 1149 * @return The first repetition of repeating field {@link #element}, creating it if it does not already exist {3} 1150 */ 1151 public ElementDefinition getElementFirstRep() { 1152 if (getElement().isEmpty()) { 1153 addElement(); 1154 } 1155 return getElement().get(0); 1156 } 1157 1158 protected void listChildren(List<Property> children) { 1159 super.listChildren(children); 1160 children.add(new Property("element", "ElementDefinition", "Captures constraints on each element within the resource.", 0, java.lang.Integer.MAX_VALUE, element)); 1161 } 1162 1163 @Override 1164 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1165 switch (_hash) { 1166 case -1662836996: /*element*/ return new Property("element", "ElementDefinition", "Captures constraints on each element within the resource.", 0, java.lang.Integer.MAX_VALUE, element); 1167 default: return super.getNamedProperty(_hash, _name, _checkValid); 1168 } 1169 1170 } 1171 1172 @Override 1173 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1174 switch (hash) { 1175 case -1662836996: /*element*/ return this.element == null ? new Base[0] : this.element.toArray(new Base[this.element.size()]); // ElementDefinition 1176 default: return super.getProperty(hash, name, checkValid); 1177 } 1178 1179 } 1180 1181 @Override 1182 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1183 switch (hash) { 1184 case -1662836996: // element 1185 this.getElement().add(TypeConvertor.castToElementDefinition(value)); // ElementDefinition 1186 return value; 1187 default: return super.setProperty(hash, name, value); 1188 } 1189 1190 } 1191 1192 @Override 1193 public Base setProperty(String name, Base value) throws FHIRException { 1194 if (name.equals("element")) { 1195 this.getElement().add(TypeConvertor.castToElementDefinition(value)); 1196 } else 1197 return super.setProperty(name, value); 1198 return value; 1199 } 1200 1201 @Override 1202 public void removeChild(String name, Base value) throws FHIRException { 1203 if (name.equals("element")) { 1204 this.getElement().remove(value); 1205 } else 1206 super.removeChild(name, value); 1207 1208 } 1209 1210 @Override 1211 public Base makeProperty(int hash, String name) throws FHIRException { 1212 switch (hash) { 1213 case -1662836996: return addElement(); 1214 default: return super.makeProperty(hash, name); 1215 } 1216 1217 } 1218 1219 @Override 1220 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1221 switch (hash) { 1222 case -1662836996: /*element*/ return new String[] {"ElementDefinition"}; 1223 default: return super.getTypesForProperty(hash, name); 1224 } 1225 1226 } 1227 1228 @Override 1229 public Base addChild(String name) throws FHIRException { 1230 if (name.equals("element")) { 1231 return addElement(); 1232 } 1233 else 1234 return super.addChild(name); 1235 } 1236 1237 public StructureDefinitionSnapshotComponent copy() { 1238 StructureDefinitionSnapshotComponent dst = new StructureDefinitionSnapshotComponent(); 1239 copyValues(dst); 1240 return dst; 1241 } 1242 1243 public void copyValues(StructureDefinitionSnapshotComponent dst) { 1244 super.copyValues(dst); 1245 if (element != null) { 1246 dst.element = new ArrayList<ElementDefinition>(); 1247 for (ElementDefinition i : element) 1248 dst.element.add(i.copy()); 1249 }; 1250 } 1251 1252 @Override 1253 public boolean equalsDeep(Base other_) { 1254 if (!super.equalsDeep(other_)) 1255 return false; 1256 if (!(other_ instanceof StructureDefinitionSnapshotComponent)) 1257 return false; 1258 StructureDefinitionSnapshotComponent o = (StructureDefinitionSnapshotComponent) other_; 1259 return compareDeep(element, o.element, true); 1260 } 1261 1262 @Override 1263 public boolean equalsShallow(Base other_) { 1264 if (!super.equalsShallow(other_)) 1265 return false; 1266 if (!(other_ instanceof StructureDefinitionSnapshotComponent)) 1267 return false; 1268 StructureDefinitionSnapshotComponent o = (StructureDefinitionSnapshotComponent) other_; 1269 return true; 1270 } 1271 1272 public boolean isEmpty() { 1273 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(element); 1274 } 1275 1276 public String fhirType() { 1277 return "StructureDefinition.snapshot"; 1278 1279 } 1280 1281// added from java-adornments.txt: 1282 1283 public ElementDefinition getElementByPath(String path) { 1284 if (path == null) { 1285 return null; 1286 } 1287 for (ElementDefinition ed : getElement()) { 1288 if (path.equals(ed.getPath()) || (path+"[x]").equals(ed.getPath())) { 1289 return ed; 1290 } 1291 } 1292 return null; 1293 } 1294 1295 1296 public ElementDefinition getElementById(String id) { 1297 if (id == null) { 1298 return null; 1299 } 1300 for (ElementDefinition ed : getElement()) { 1301 if (id.equals(ed.getId())) { 1302 return ed; 1303 } 1304 } 1305 return null; 1306 } 1307 1308// end addition 1309 } 1310 1311 @Block() 1312 public static class StructureDefinitionDifferentialComponent extends BackboneElement implements IBaseBackboneElement { 1313 /** 1314 * Captures constraints on each element within the resource. 1315 */ 1316 @Child(name = "element", type = {ElementDefinition.class}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1317 @Description(shortDefinition="Definition of elements in the resource (if no StructureDefinition)", formalDefinition="Captures constraints on each element within the resource." ) 1318 protected List<ElementDefinition> element; 1319 1320 private static final long serialVersionUID = 53896641L; 1321 1322 /** 1323 * Constructor 1324 */ 1325 public StructureDefinitionDifferentialComponent() { 1326 super(); 1327 } 1328 1329 /** 1330 * Constructor 1331 */ 1332 public StructureDefinitionDifferentialComponent(ElementDefinition element) { 1333 super(); 1334 this.addElement(element); 1335 } 1336 1337 /** 1338 * @return {@link #element} (Captures constraints on each element within the resource.) 1339 */ 1340 public List<ElementDefinition> getElement() { 1341 if (this.element == null) 1342 this.element = new ArrayList<ElementDefinition>(); 1343 return this.element; 1344 } 1345 1346 /** 1347 * @return Returns a reference to <code>this</code> for easy method chaining 1348 */ 1349 public StructureDefinitionDifferentialComponent setElement(List<ElementDefinition> theElement) { 1350 this.element = theElement; 1351 return this; 1352 } 1353 1354 public boolean hasElement() { 1355 if (this.element == null) 1356 return false; 1357 for (ElementDefinition item : this.element) 1358 if (!item.isEmpty()) 1359 return true; 1360 return false; 1361 } 1362 1363 public ElementDefinition addElement() { //3 1364 ElementDefinition t = new ElementDefinition(); 1365 if (this.element == null) 1366 this.element = new ArrayList<ElementDefinition>(); 1367 this.element.add(t); 1368 return t; 1369 } 1370 1371 public StructureDefinitionDifferentialComponent addElement(ElementDefinition t) { //3 1372 if (t == null) 1373 return this; 1374 if (this.element == null) 1375 this.element = new ArrayList<ElementDefinition>(); 1376 this.element.add(t); 1377 return this; 1378 } 1379 1380 /** 1381 * @return The first repetition of repeating field {@link #element}, creating it if it does not already exist {3} 1382 */ 1383 public ElementDefinition getElementFirstRep() { 1384 if (getElement().isEmpty()) { 1385 addElement(); 1386 } 1387 return getElement().get(0); 1388 } 1389 1390 protected void listChildren(List<Property> children) { 1391 super.listChildren(children); 1392 children.add(new Property("element", "ElementDefinition", "Captures constraints on each element within the resource.", 0, java.lang.Integer.MAX_VALUE, element)); 1393 } 1394 1395 @Override 1396 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1397 switch (_hash) { 1398 case -1662836996: /*element*/ return new Property("element", "ElementDefinition", "Captures constraints on each element within the resource.", 0, java.lang.Integer.MAX_VALUE, element); 1399 default: return super.getNamedProperty(_hash, _name, _checkValid); 1400 } 1401 1402 } 1403 1404 @Override 1405 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1406 switch (hash) { 1407 case -1662836996: /*element*/ return this.element == null ? new Base[0] : this.element.toArray(new Base[this.element.size()]); // ElementDefinition 1408 default: return super.getProperty(hash, name, checkValid); 1409 } 1410 1411 } 1412 1413 @Override 1414 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1415 switch (hash) { 1416 case -1662836996: // element 1417 this.getElement().add(TypeConvertor.castToElementDefinition(value)); // ElementDefinition 1418 return value; 1419 default: return super.setProperty(hash, name, value); 1420 } 1421 1422 } 1423 1424 @Override 1425 public Base setProperty(String name, Base value) throws FHIRException { 1426 if (name.equals("element")) { 1427 this.getElement().add(TypeConvertor.castToElementDefinition(value)); 1428 } else 1429 return super.setProperty(name, value); 1430 return value; 1431 } 1432 1433 @Override 1434 public void removeChild(String name, Base value) throws FHIRException { 1435 if (name.equals("element")) { 1436 this.getElement().remove(value); 1437 } else 1438 super.removeChild(name, value); 1439 1440 } 1441 1442 @Override 1443 public Base makeProperty(int hash, String name) throws FHIRException { 1444 switch (hash) { 1445 case -1662836996: return addElement(); 1446 default: return super.makeProperty(hash, name); 1447 } 1448 1449 } 1450 1451 @Override 1452 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1453 switch (hash) { 1454 case -1662836996: /*element*/ return new String[] {"ElementDefinition"}; 1455 default: return super.getTypesForProperty(hash, name); 1456 } 1457 1458 } 1459 1460 @Override 1461 public Base addChild(String name) throws FHIRException { 1462 if (name.equals("element")) { 1463 return addElement(); 1464 } 1465 else 1466 return super.addChild(name); 1467 } 1468 1469 public StructureDefinitionDifferentialComponent copy() { 1470 StructureDefinitionDifferentialComponent dst = new StructureDefinitionDifferentialComponent(); 1471 copyValues(dst); 1472 return dst; 1473 } 1474 1475 public void copyValues(StructureDefinitionDifferentialComponent dst) { 1476 super.copyValues(dst); 1477 if (element != null) { 1478 dst.element = new ArrayList<ElementDefinition>(); 1479 for (ElementDefinition i : element) 1480 dst.element.add(i.copy()); 1481 }; 1482 } 1483 1484 @Override 1485 public boolean equalsDeep(Base other_) { 1486 if (!super.equalsDeep(other_)) 1487 return false; 1488 if (!(other_ instanceof StructureDefinitionDifferentialComponent)) 1489 return false; 1490 StructureDefinitionDifferentialComponent o = (StructureDefinitionDifferentialComponent) other_; 1491 return compareDeep(element, o.element, true); 1492 } 1493 1494 @Override 1495 public boolean equalsShallow(Base other_) { 1496 if (!super.equalsShallow(other_)) 1497 return false; 1498 if (!(other_ instanceof StructureDefinitionDifferentialComponent)) 1499 return false; 1500 StructureDefinitionDifferentialComponent o = (StructureDefinitionDifferentialComponent) other_; 1501 return true; 1502 } 1503 1504 public boolean isEmpty() { 1505 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(element); 1506 } 1507 1508 public String fhirType() { 1509 return "StructureDefinition.differential"; 1510 1511 } 1512 1513//added from java-adornments.txt: 1514 1515 public ElementDefinition getElementByPath(String path) { 1516 if (path == null) { 1517 return null; 1518 } 1519 for (ElementDefinition ed : getElement()) { 1520 if (path.equals(ed.getPath()) || (path+"[x]").equals(ed.getPath())) { 1521 return ed; 1522 } 1523 } 1524 return null; 1525 } 1526 1527 1528 public ElementDefinition getElementById(String id) { 1529 if (id == null) { 1530 return null; 1531 } 1532 for (ElementDefinition ed : getElement()) { 1533 if (id.equals(ed.getId())) { 1534 return ed; 1535 } 1536 } 1537 return null; 1538 } 1539 1540//end addition 1541 } 1542 1543 /** 1544 * An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure definition is stored on different servers. 1545 */ 1546 @Child(name = "url", type = {UriType.class}, order=0, min=1, max=1, modifier=false, summary=true) 1547 @Description(shortDefinition="Canonical identifier for this structure definition, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure definition is stored on different servers." ) 1548 protected UriType url; 1549 1550 /** 1551 * A formal identifier that is used to identify this structure definition when it is represented in other formats, or referenced in a specification, model, design or an instance. 1552 */ 1553 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1554 @Description(shortDefinition="Additional identifier for the structure definition", formalDefinition="A formal identifier that is used to identify this structure definition when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 1555 protected List<Identifier> identifier; 1556 1557 /** 1558 * The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. There is no expectation that versions can be placed in a lexicographical sequence, so authors are encouraged to populate the StructureDefinition.versionAlgorithm[x] element to enable comparisons. If there is no managed version available, authors can consider using ISO date/time syntax (e.g., '2023-01-01'). 1559 */ 1560 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1561 @Description(shortDefinition="Business version of the structure definition", formalDefinition="The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. There is no expectation that versions can be placed in a lexicographical sequence, so authors are encouraged to populate the StructureDefinition.versionAlgorithm[x] element to enable comparisons. If there is no managed version available, authors can consider using ISO date/time syntax (e.g., '2023-01-01')." ) 1562 protected StringType version; 1563 1564 /** 1565 * Indicates the mechanism used to compare versions to determine which is more current. 1566 */ 1567 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 1568 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 1569 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 1570 protected DataType versionAlgorithm; 1571 1572 /** 1573 * A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1574 */ 1575 @Child(name = "name", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=true) 1576 @Description(shortDefinition="Name for this structure definition (computer friendly)", formalDefinition="A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 1577 protected StringType name; 1578 1579 /** 1580 * A short, descriptive, user-friendly title for the structure definition. 1581 */ 1582 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1583 @Description(shortDefinition="Name for this structure definition (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the structure definition." ) 1584 protected StringType title; 1585 1586 /** 1587 * The status of this structure definition. Enables tracking the life-cycle of the content. 1588 */ 1589 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 1590 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this structure definition. Enables tracking the life-cycle of the content." ) 1591 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 1592 protected Enumeration<PublicationStatus> status; 1593 1594 /** 1595 * A Boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1596 */ 1597 @Child(name = "experimental", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1598 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 1599 protected BooleanType experimental; 1600 1601 /** 1602 * The date (and optionally time) when the structure definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes. 1603 */ 1604 @Child(name = "date", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1605 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the structure definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes." ) 1606 protected DateTimeType date; 1607 1608 /** 1609 * The name of the organization or individual responsible for the release and ongoing maintenance of the structure definition. 1610 */ 1611 @Child(name = "publisher", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1612 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the structure definition." ) 1613 protected StringType publisher; 1614 1615 /** 1616 * Contact details to assist a user in finding and communicating with the publisher. 1617 */ 1618 @Child(name = "contact", type = {ContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1619 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 1620 protected List<ContactDetail> contact; 1621 1622 /** 1623 * A free text natural language description of the structure definition from a consumer's perspective. 1624 */ 1625 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 1626 @Description(shortDefinition="Natural language description of the structure definition", formalDefinition="A free text natural language description of the structure definition from a consumer's perspective." ) 1627 protected MarkdownType description; 1628 1629 /** 1630 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure definition instances. 1631 */ 1632 @Child(name = "useContext", type = {UsageContext.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1633 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure definition instances." ) 1634 protected List<UsageContext> useContext; 1635 1636 /** 1637 * A legal or geographic region in which the structure definition is intended to be used. 1638 */ 1639 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1640 @Description(shortDefinition="Intended jurisdiction for structure definition (if applicable)", formalDefinition="A legal or geographic region in which the structure definition is intended to be used." ) 1641 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 1642 protected List<CodeableConcept> jurisdiction; 1643 1644 /** 1645 * Explanation of why this structure definition is needed and why it has been designed as it has. 1646 */ 1647 @Child(name = "purpose", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 1648 @Description(shortDefinition="Why this structure definition is defined", formalDefinition="Explanation of why this structure definition is needed and why it has been designed as it has." ) 1649 protected MarkdownType purpose; 1650 1651 /** 1652 * A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element. 1653 */ 1654 @Child(name = "copyright", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 1655 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element." ) 1656 protected MarkdownType copyright; 1657 1658 /** 1659 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1660 */ 1661 @Child(name = "copyrightLabel", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 1662 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 1663 protected StringType copyrightLabel; 1664 1665 /** 1666 * (DEPRECATED) A set of key words or terms from external terminologies that may be used to assist with indexing and searching of templates nby describing the use of this structure definition, or the content it describes. 1667 */ 1668 @Child(name = "keyword", type = {Coding.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1669 @Description(shortDefinition="Assist with indexing and finding", formalDefinition="(DEPRECATED) A set of key words or terms from external terminologies that may be used to assist with indexing and searching of templates nby describing the use of this structure definition, or the content it describes." ) 1670 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/definition-use") 1671 protected List<Coding> keyword; 1672 1673 /** 1674 * The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version. 1675 */ 1676 @Child(name = "fhirVersion", type = {CodeType.class}, order=18, min=0, max=1, modifier=false, summary=true) 1677 @Description(shortDefinition="FHIR Version this StructureDefinition targets", formalDefinition="The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version." ) 1678 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/FHIR-version") 1679 protected Enumeration<FHIRVersion> fhirVersion; 1680 1681 /** 1682 * An external specification that the content is mapped to. 1683 */ 1684 @Child(name = "mapping", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1685 @Description(shortDefinition="External specification that the content is mapped to", formalDefinition="An external specification that the content is mapped to." ) 1686 protected List<StructureDefinitionMappingComponent> mapping; 1687 1688 /** 1689 * Defines the kind of structure that this definition is describing. 1690 */ 1691 @Child(name = "kind", type = {CodeType.class}, order=20, min=1, max=1, modifier=false, summary=true) 1692 @Description(shortDefinition="primitive-type | complex-type | resource | logical", formalDefinition="Defines the kind of structure that this definition is describing." ) 1693 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/structure-definition-kind") 1694 protected Enumeration<StructureDefinitionKind> kind; 1695 1696 /** 1697 * Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems. 1698 */ 1699 @Child(name = "abstract", type = {BooleanType.class}, order=21, min=1, max=1, modifier=false, summary=true) 1700 @Description(shortDefinition="Whether the structure is abstract", formalDefinition="Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems." ) 1701 protected BooleanType abstract_; 1702 1703 /** 1704 * Identifies the types of resource or data type elements to which the extension can be applied. For more guidance on using the 'context' element, see the [defining extensions page](defining-extensions.html#context). 1705 */ 1706 @Child(name = "context", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1707 @Description(shortDefinition="If an extension, where it can be used in instances", formalDefinition="Identifies the types of resource or data type elements to which the extension can be applied. For more guidance on using the 'context' element, see the [defining extensions page](defining-extensions.html#context)." ) 1708 protected List<StructureDefinitionContextComponent> context; 1709 1710 /** 1711 * A set of rules as FHIRPath Invariants about when the extension can be used (e.g. co-occurrence variants for the extension). All the rules must be true. 1712 */ 1713 @Child(name = "contextInvariant", type = {StringType.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1714 @Description(shortDefinition="FHIRPath invariants - when the extension can be used", formalDefinition="A set of rules as FHIRPath Invariants about when the extension can be used (e.g. co-occurrence variants for the extension). All the rules must be true." ) 1715 protected List<StringType> contextInvariant; 1716 1717 /** 1718 * The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models, where they are required. 1719 */ 1720 @Child(name = "type", type = {UriType.class}, order=24, min=1, max=1, modifier=false, summary=true) 1721 @Description(shortDefinition="Type defined or constrained by this structure", formalDefinition="The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models, where they are required." ) 1722 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fhir-types") 1723 protected UriType type; 1724 1725 /** 1726 * An absolute URI that is the base structure from which this type is derived, either by specialization or constraint. 1727 */ 1728 @Child(name = "baseDefinition", type = {CanonicalType.class}, order=25, min=0, max=1, modifier=false, summary=true) 1729 @Description(shortDefinition="Definition that this type is constrained/specialized from", formalDefinition="An absolute URI that is the base structure from which this type is derived, either by specialization or constraint." ) 1730 protected CanonicalType baseDefinition; 1731 1732 /** 1733 * How the type relates to the baseDefinition. 1734 */ 1735 @Child(name = "derivation", type = {CodeType.class}, order=26, min=0, max=1, modifier=false, summary=true) 1736 @Description(shortDefinition="specialization | constraint - How relates to base definition", formalDefinition="How the type relates to the baseDefinition." ) 1737 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/type-derivation-rule") 1738 protected Enumeration<TypeDerivationRule> derivation; 1739 1740 /** 1741 * A snapshot view is expressed in a standalone form that can be used and interpreted without considering the base StructureDefinition. 1742 */ 1743 @Child(name = "snapshot", type = {}, order=27, min=0, max=1, modifier=false, summary=false) 1744 @Description(shortDefinition="Snapshot view of the structure", formalDefinition="A snapshot view is expressed in a standalone form that can be used and interpreted without considering the base StructureDefinition." ) 1745 protected StructureDefinitionSnapshotComponent snapshot; 1746 1747 /** 1748 * A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies. 1749 */ 1750 @Child(name = "differential", type = {}, order=28, min=0, max=1, modifier=false, summary=false) 1751 @Description(shortDefinition="Differential view of the structure", formalDefinition="A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies." ) 1752 protected StructureDefinitionDifferentialComponent differential; 1753 1754 private static final long serialVersionUID = -1127285723L; 1755 1756 /** 1757 * Constructor 1758 */ 1759 public StructureDefinition() { 1760 super(); 1761 } 1762 1763 /** 1764 * Constructor 1765 */ 1766 public StructureDefinition(String url, String name, PublicationStatus status, StructureDefinitionKind kind, boolean abstract_, String type) { 1767 super(); 1768 this.setUrl(url); 1769 this.setName(name); 1770 this.setStatus(status); 1771 this.setKind(kind); 1772 this.setAbstract(abstract_); 1773 this.setType(type); 1774 } 1775 1776 /** 1777 * @return {@link #url} (An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1778 */ 1779 public UriType getUrlElement() { 1780 if (this.url == null) 1781 if (Configuration.errorOnAutoCreate()) 1782 throw new Error("Attempt to auto-create StructureDefinition.url"); 1783 else if (Configuration.doAutoCreate()) 1784 this.url = new UriType(); // bb 1785 return this.url; 1786 } 1787 1788 public boolean hasUrlElement() { 1789 return this.url != null && !this.url.isEmpty(); 1790 } 1791 1792 public boolean hasUrl() { 1793 return this.url != null && !this.url.isEmpty(); 1794 } 1795 1796 /** 1797 * @param value {@link #url} (An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1798 */ 1799 public StructureDefinition setUrlElement(UriType value) { 1800 this.url = value; 1801 return this; 1802 } 1803 1804 /** 1805 * @return An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure definition is stored on different servers. 1806 */ 1807 public String getUrl() { 1808 return this.url == null ? null : this.url.getValue(); 1809 } 1810 1811 /** 1812 * @param value An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure definition is stored on different servers. 1813 */ 1814 public StructureDefinition setUrl(String value) { 1815 if (this.url == null) 1816 this.url = new UriType(); 1817 this.url.setValue(value); 1818 return this; 1819 } 1820 1821 /** 1822 * @return {@link #identifier} (A formal identifier that is used to identify this structure definition when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1823 */ 1824 public List<Identifier> getIdentifier() { 1825 if (this.identifier == null) 1826 this.identifier = new ArrayList<Identifier>(); 1827 return this.identifier; 1828 } 1829 1830 /** 1831 * @return Returns a reference to <code>this</code> for easy method chaining 1832 */ 1833 public StructureDefinition setIdentifier(List<Identifier> theIdentifier) { 1834 this.identifier = theIdentifier; 1835 return this; 1836 } 1837 1838 public boolean hasIdentifier() { 1839 if (this.identifier == null) 1840 return false; 1841 for (Identifier item : this.identifier) 1842 if (!item.isEmpty()) 1843 return true; 1844 return false; 1845 } 1846 1847 public Identifier addIdentifier() { //3 1848 Identifier t = new Identifier(); 1849 if (this.identifier == null) 1850 this.identifier = new ArrayList<Identifier>(); 1851 this.identifier.add(t); 1852 return t; 1853 } 1854 1855 public StructureDefinition addIdentifier(Identifier t) { //3 1856 if (t == null) 1857 return this; 1858 if (this.identifier == null) 1859 this.identifier = new ArrayList<Identifier>(); 1860 this.identifier.add(t); 1861 return this; 1862 } 1863 1864 /** 1865 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1866 */ 1867 public Identifier getIdentifierFirstRep() { 1868 if (getIdentifier().isEmpty()) { 1869 addIdentifier(); 1870 } 1871 return getIdentifier().get(0); 1872 } 1873 1874 /** 1875 * @return {@link #version} (The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. There is no expectation that versions can be placed in a lexicographical sequence, so authors are encouraged to populate the StructureDefinition.versionAlgorithm[x] element to enable comparisons. If there is no managed version available, authors can consider using ISO date/time syntax (e.g., '2023-01-01').). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1876 */ 1877 public StringType getVersionElement() { 1878 if (this.version == null) 1879 if (Configuration.errorOnAutoCreate()) 1880 throw new Error("Attempt to auto-create StructureDefinition.version"); 1881 else if (Configuration.doAutoCreate()) 1882 this.version = new StringType(); // bb 1883 return this.version; 1884 } 1885 1886 public boolean hasVersionElement() { 1887 return this.version != null && !this.version.isEmpty(); 1888 } 1889 1890 public boolean hasVersion() { 1891 return this.version != null && !this.version.isEmpty(); 1892 } 1893 1894 /** 1895 * @param value {@link #version} (The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. There is no expectation that versions can be placed in a lexicographical sequence, so authors are encouraged to populate the StructureDefinition.versionAlgorithm[x] element to enable comparisons. If there is no managed version available, authors can consider using ISO date/time syntax (e.g., '2023-01-01').). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1896 */ 1897 public StructureDefinition setVersionElement(StringType value) { 1898 this.version = value; 1899 return this; 1900 } 1901 1902 /** 1903 * @return The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. There is no expectation that versions can be placed in a lexicographical sequence, so authors are encouraged to populate the StructureDefinition.versionAlgorithm[x] element to enable comparisons. If there is no managed version available, authors can consider using ISO date/time syntax (e.g., '2023-01-01'). 1904 */ 1905 public String getVersion() { 1906 return this.version == null ? null : this.version.getValue(); 1907 } 1908 1909 /** 1910 * @param value The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. There is no expectation that versions can be placed in a lexicographical sequence, so authors are encouraged to populate the StructureDefinition.versionAlgorithm[x] element to enable comparisons. If there is no managed version available, authors can consider using ISO date/time syntax (e.g., '2023-01-01'). 1911 */ 1912 public StructureDefinition setVersion(String value) { 1913 if (Utilities.noString(value)) 1914 this.version = null; 1915 else { 1916 if (this.version == null) 1917 this.version = new StringType(); 1918 this.version.setValue(value); 1919 } 1920 return this; 1921 } 1922 1923 /** 1924 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1925 */ 1926 public DataType getVersionAlgorithm() { 1927 return this.versionAlgorithm; 1928 } 1929 1930 /** 1931 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1932 */ 1933 public StringType getVersionAlgorithmStringType() throws FHIRException { 1934 if (this.versionAlgorithm == null) 1935 this.versionAlgorithm = new StringType(); 1936 if (!(this.versionAlgorithm instanceof StringType)) 1937 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1938 return (StringType) this.versionAlgorithm; 1939 } 1940 1941 public boolean hasVersionAlgorithmStringType() { 1942 return this != null && this.versionAlgorithm instanceof StringType; 1943 } 1944 1945 /** 1946 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1947 */ 1948 public Coding getVersionAlgorithmCoding() throws FHIRException { 1949 if (this.versionAlgorithm == null) 1950 this.versionAlgorithm = new Coding(); 1951 if (!(this.versionAlgorithm instanceof Coding)) 1952 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1953 return (Coding) this.versionAlgorithm; 1954 } 1955 1956 public boolean hasVersionAlgorithmCoding() { 1957 return this != null && this.versionAlgorithm instanceof Coding; 1958 } 1959 1960 public boolean hasVersionAlgorithm() { 1961 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 1962 } 1963 1964 /** 1965 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1966 */ 1967 public StructureDefinition setVersionAlgorithm(DataType value) { 1968 if (value != null && !(value instanceof StringType || value instanceof Coding)) 1969 throw new FHIRException("Not the right type for StructureDefinition.versionAlgorithm[x]: "+value.fhirType()); 1970 this.versionAlgorithm = value; 1971 return this; 1972 } 1973 1974 /** 1975 * @return {@link #name} (A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1976 */ 1977 public StringType getNameElement() { 1978 if (this.name == null) 1979 if (Configuration.errorOnAutoCreate()) 1980 throw new Error("Attempt to auto-create StructureDefinition.name"); 1981 else if (Configuration.doAutoCreate()) 1982 this.name = new StringType(); // bb 1983 return this.name; 1984 } 1985 1986 public boolean hasNameElement() { 1987 return this.name != null && !this.name.isEmpty(); 1988 } 1989 1990 public boolean hasName() { 1991 return this.name != null && !this.name.isEmpty(); 1992 } 1993 1994 /** 1995 * @param value {@link #name} (A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1996 */ 1997 public StructureDefinition setNameElement(StringType value) { 1998 this.name = value; 1999 return this; 2000 } 2001 2002 /** 2003 * @return A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2004 */ 2005 public String getName() { 2006 return this.name == null ? null : this.name.getValue(); 2007 } 2008 2009 /** 2010 * @param value A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2011 */ 2012 public StructureDefinition setName(String value) { 2013 if (this.name == null) 2014 this.name = new StringType(); 2015 this.name.setValue(value); 2016 return this; 2017 } 2018 2019 /** 2020 * @return {@link #title} (A short, descriptive, user-friendly title for the structure definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2021 */ 2022 public StringType getTitleElement() { 2023 if (this.title == null) 2024 if (Configuration.errorOnAutoCreate()) 2025 throw new Error("Attempt to auto-create StructureDefinition.title"); 2026 else if (Configuration.doAutoCreate()) 2027 this.title = new StringType(); // bb 2028 return this.title; 2029 } 2030 2031 public boolean hasTitleElement() { 2032 return this.title != null && !this.title.isEmpty(); 2033 } 2034 2035 public boolean hasTitle() { 2036 return this.title != null && !this.title.isEmpty(); 2037 } 2038 2039 /** 2040 * @param value {@link #title} (A short, descriptive, user-friendly title for the structure definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2041 */ 2042 public StructureDefinition setTitleElement(StringType value) { 2043 this.title = value; 2044 return this; 2045 } 2046 2047 /** 2048 * @return A short, descriptive, user-friendly title for the structure definition. 2049 */ 2050 public String getTitle() { 2051 return this.title == null ? null : this.title.getValue(); 2052 } 2053 2054 /** 2055 * @param value A short, descriptive, user-friendly title for the structure definition. 2056 */ 2057 public StructureDefinition setTitle(String value) { 2058 if (Utilities.noString(value)) 2059 this.title = null; 2060 else { 2061 if (this.title == null) 2062 this.title = new StringType(); 2063 this.title.setValue(value); 2064 } 2065 return this; 2066 } 2067 2068 /** 2069 * @return {@link #status} (The status of this structure definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2070 */ 2071 public Enumeration<PublicationStatus> getStatusElement() { 2072 if (this.status == null) 2073 if (Configuration.errorOnAutoCreate()) 2074 throw new Error("Attempt to auto-create StructureDefinition.status"); 2075 else if (Configuration.doAutoCreate()) 2076 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2077 return this.status; 2078 } 2079 2080 public boolean hasStatusElement() { 2081 return this.status != null && !this.status.isEmpty(); 2082 } 2083 2084 public boolean hasStatus() { 2085 return this.status != null && !this.status.isEmpty(); 2086 } 2087 2088 /** 2089 * @param value {@link #status} (The status of this structure definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2090 */ 2091 public StructureDefinition setStatusElement(Enumeration<PublicationStatus> value) { 2092 this.status = value; 2093 return this; 2094 } 2095 2096 /** 2097 * @return The status of this structure definition. Enables tracking the life-cycle of the content. 2098 */ 2099 public PublicationStatus getStatus() { 2100 return this.status == null ? null : this.status.getValue(); 2101 } 2102 2103 /** 2104 * @param value The status of this structure definition. Enables tracking the life-cycle of the content. 2105 */ 2106 public StructureDefinition setStatus(PublicationStatus value) { 2107 if (this.status == null) 2108 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2109 this.status.setValue(value); 2110 return this; 2111 } 2112 2113 /** 2114 * @return {@link #experimental} (A Boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2115 */ 2116 public BooleanType getExperimentalElement() { 2117 if (this.experimental == null) 2118 if (Configuration.errorOnAutoCreate()) 2119 throw new Error("Attempt to auto-create StructureDefinition.experimental"); 2120 else if (Configuration.doAutoCreate()) 2121 this.experimental = new BooleanType(); // bb 2122 return this.experimental; 2123 } 2124 2125 public boolean hasExperimentalElement() { 2126 return this.experimental != null && !this.experimental.isEmpty(); 2127 } 2128 2129 public boolean hasExperimental() { 2130 return this.experimental != null && !this.experimental.isEmpty(); 2131 } 2132 2133 /** 2134 * @param value {@link #experimental} (A Boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2135 */ 2136 public StructureDefinition setExperimentalElement(BooleanType value) { 2137 this.experimental = value; 2138 return this; 2139 } 2140 2141 /** 2142 * @return A Boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 2143 */ 2144 public boolean getExperimental() { 2145 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2146 } 2147 2148 /** 2149 * @param value A Boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 2150 */ 2151 public StructureDefinition setExperimental(boolean value) { 2152 if (this.experimental == null) 2153 this.experimental = new BooleanType(); 2154 this.experimental.setValue(value); 2155 return this; 2156 } 2157 2158 /** 2159 * @return {@link #date} (The date (and optionally time) when the structure definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2160 */ 2161 public DateTimeType getDateElement() { 2162 if (this.date == null) 2163 if (Configuration.errorOnAutoCreate()) 2164 throw new Error("Attempt to auto-create StructureDefinition.date"); 2165 else if (Configuration.doAutoCreate()) 2166 this.date = new DateTimeType(); // bb 2167 return this.date; 2168 } 2169 2170 public boolean hasDateElement() { 2171 return this.date != null && !this.date.isEmpty(); 2172 } 2173 2174 public boolean hasDate() { 2175 return this.date != null && !this.date.isEmpty(); 2176 } 2177 2178 /** 2179 * @param value {@link #date} (The date (and optionally time) when the structure definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2180 */ 2181 public StructureDefinition setDateElement(DateTimeType value) { 2182 this.date = value; 2183 return this; 2184 } 2185 2186 /** 2187 * @return The date (and optionally time) when the structure definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes. 2188 */ 2189 public Date getDate() { 2190 return this.date == null ? null : this.date.getValue(); 2191 } 2192 2193 /** 2194 * @param value The date (and optionally time) when the structure definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes. 2195 */ 2196 public StructureDefinition setDate(Date value) { 2197 if (value == null) 2198 this.date = null; 2199 else { 2200 if (this.date == null) 2201 this.date = new DateTimeType(); 2202 this.date.setValue(value); 2203 } 2204 return this; 2205 } 2206 2207 /** 2208 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the structure definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2209 */ 2210 public StringType getPublisherElement() { 2211 if (this.publisher == null) 2212 if (Configuration.errorOnAutoCreate()) 2213 throw new Error("Attempt to auto-create StructureDefinition.publisher"); 2214 else if (Configuration.doAutoCreate()) 2215 this.publisher = new StringType(); // bb 2216 return this.publisher; 2217 } 2218 2219 public boolean hasPublisherElement() { 2220 return this.publisher != null && !this.publisher.isEmpty(); 2221 } 2222 2223 public boolean hasPublisher() { 2224 return this.publisher != null && !this.publisher.isEmpty(); 2225 } 2226 2227 /** 2228 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the structure definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2229 */ 2230 public StructureDefinition setPublisherElement(StringType value) { 2231 this.publisher = value; 2232 return this; 2233 } 2234 2235 /** 2236 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the structure definition. 2237 */ 2238 public String getPublisher() { 2239 return this.publisher == null ? null : this.publisher.getValue(); 2240 } 2241 2242 /** 2243 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the structure definition. 2244 */ 2245 public StructureDefinition setPublisher(String value) { 2246 if (Utilities.noString(value)) 2247 this.publisher = null; 2248 else { 2249 if (this.publisher == null) 2250 this.publisher = new StringType(); 2251 this.publisher.setValue(value); 2252 } 2253 return this; 2254 } 2255 2256 /** 2257 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 2258 */ 2259 public List<ContactDetail> getContact() { 2260 if (this.contact == null) 2261 this.contact = new ArrayList<ContactDetail>(); 2262 return this.contact; 2263 } 2264 2265 /** 2266 * @return Returns a reference to <code>this</code> for easy method chaining 2267 */ 2268 public StructureDefinition setContact(List<ContactDetail> theContact) { 2269 this.contact = theContact; 2270 return this; 2271 } 2272 2273 public boolean hasContact() { 2274 if (this.contact == null) 2275 return false; 2276 for (ContactDetail item : this.contact) 2277 if (!item.isEmpty()) 2278 return true; 2279 return false; 2280 } 2281 2282 public ContactDetail addContact() { //3 2283 ContactDetail t = new ContactDetail(); 2284 if (this.contact == null) 2285 this.contact = new ArrayList<ContactDetail>(); 2286 this.contact.add(t); 2287 return t; 2288 } 2289 2290 public StructureDefinition addContact(ContactDetail t) { //3 2291 if (t == null) 2292 return this; 2293 if (this.contact == null) 2294 this.contact = new ArrayList<ContactDetail>(); 2295 this.contact.add(t); 2296 return this; 2297 } 2298 2299 /** 2300 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 2301 */ 2302 public ContactDetail getContactFirstRep() { 2303 if (getContact().isEmpty()) { 2304 addContact(); 2305 } 2306 return getContact().get(0); 2307 } 2308 2309 /** 2310 * @return {@link #description} (A free text natural language description of the structure definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2311 */ 2312 public MarkdownType getDescriptionElement() { 2313 if (this.description == null) 2314 if (Configuration.errorOnAutoCreate()) 2315 throw new Error("Attempt to auto-create StructureDefinition.description"); 2316 else if (Configuration.doAutoCreate()) 2317 this.description = new MarkdownType(); // bb 2318 return this.description; 2319 } 2320 2321 public boolean hasDescriptionElement() { 2322 return this.description != null && !this.description.isEmpty(); 2323 } 2324 2325 public boolean hasDescription() { 2326 return this.description != null && !this.description.isEmpty(); 2327 } 2328 2329 /** 2330 * @param value {@link #description} (A free text natural language description of the structure definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2331 */ 2332 public StructureDefinition setDescriptionElement(MarkdownType value) { 2333 this.description = value; 2334 return this; 2335 } 2336 2337 /** 2338 * @return A free text natural language description of the structure definition from a consumer's perspective. 2339 */ 2340 public String getDescription() { 2341 return this.description == null ? null : this.description.getValue(); 2342 } 2343 2344 /** 2345 * @param value A free text natural language description of the structure definition from a consumer's perspective. 2346 */ 2347 public StructureDefinition setDescription(String value) { 2348 if (Utilities.noString(value)) 2349 this.description = null; 2350 else { 2351 if (this.description == null) 2352 this.description = new MarkdownType(); 2353 this.description.setValue(value); 2354 } 2355 return this; 2356 } 2357 2358 /** 2359 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure definition instances.) 2360 */ 2361 public List<UsageContext> getUseContext() { 2362 if (this.useContext == null) 2363 this.useContext = new ArrayList<UsageContext>(); 2364 return this.useContext; 2365 } 2366 2367 /** 2368 * @return Returns a reference to <code>this</code> for easy method chaining 2369 */ 2370 public StructureDefinition setUseContext(List<UsageContext> theUseContext) { 2371 this.useContext = theUseContext; 2372 return this; 2373 } 2374 2375 public boolean hasUseContext() { 2376 if (this.useContext == null) 2377 return false; 2378 for (UsageContext item : this.useContext) 2379 if (!item.isEmpty()) 2380 return true; 2381 return false; 2382 } 2383 2384 public UsageContext addUseContext() { //3 2385 UsageContext t = new UsageContext(); 2386 if (this.useContext == null) 2387 this.useContext = new ArrayList<UsageContext>(); 2388 this.useContext.add(t); 2389 return t; 2390 } 2391 2392 public StructureDefinition addUseContext(UsageContext t) { //3 2393 if (t == null) 2394 return this; 2395 if (this.useContext == null) 2396 this.useContext = new ArrayList<UsageContext>(); 2397 this.useContext.add(t); 2398 return this; 2399 } 2400 2401 /** 2402 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 2403 */ 2404 public UsageContext getUseContextFirstRep() { 2405 if (getUseContext().isEmpty()) { 2406 addUseContext(); 2407 } 2408 return getUseContext().get(0); 2409 } 2410 2411 /** 2412 * @return {@link #jurisdiction} (A legal or geographic region in which the structure definition is intended to be used.) 2413 */ 2414 public List<CodeableConcept> getJurisdiction() { 2415 if (this.jurisdiction == null) 2416 this.jurisdiction = new ArrayList<CodeableConcept>(); 2417 return this.jurisdiction; 2418 } 2419 2420 /** 2421 * @return Returns a reference to <code>this</code> for easy method chaining 2422 */ 2423 public StructureDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 2424 this.jurisdiction = theJurisdiction; 2425 return this; 2426 } 2427 2428 public boolean hasJurisdiction() { 2429 if (this.jurisdiction == null) 2430 return false; 2431 for (CodeableConcept item : this.jurisdiction) 2432 if (!item.isEmpty()) 2433 return true; 2434 return false; 2435 } 2436 2437 public CodeableConcept addJurisdiction() { //3 2438 CodeableConcept t = new CodeableConcept(); 2439 if (this.jurisdiction == null) 2440 this.jurisdiction = new ArrayList<CodeableConcept>(); 2441 this.jurisdiction.add(t); 2442 return t; 2443 } 2444 2445 public StructureDefinition addJurisdiction(CodeableConcept t) { //3 2446 if (t == null) 2447 return this; 2448 if (this.jurisdiction == null) 2449 this.jurisdiction = new ArrayList<CodeableConcept>(); 2450 this.jurisdiction.add(t); 2451 return this; 2452 } 2453 2454 /** 2455 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 2456 */ 2457 public CodeableConcept getJurisdictionFirstRep() { 2458 if (getJurisdiction().isEmpty()) { 2459 addJurisdiction(); 2460 } 2461 return getJurisdiction().get(0); 2462 } 2463 2464 /** 2465 * @return {@link #purpose} (Explanation of why this structure definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 2466 */ 2467 public MarkdownType getPurposeElement() { 2468 if (this.purpose == null) 2469 if (Configuration.errorOnAutoCreate()) 2470 throw new Error("Attempt to auto-create StructureDefinition.purpose"); 2471 else if (Configuration.doAutoCreate()) 2472 this.purpose = new MarkdownType(); // bb 2473 return this.purpose; 2474 } 2475 2476 public boolean hasPurposeElement() { 2477 return this.purpose != null && !this.purpose.isEmpty(); 2478 } 2479 2480 public boolean hasPurpose() { 2481 return this.purpose != null && !this.purpose.isEmpty(); 2482 } 2483 2484 /** 2485 * @param value {@link #purpose} (Explanation of why this structure definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 2486 */ 2487 public StructureDefinition setPurposeElement(MarkdownType value) { 2488 this.purpose = value; 2489 return this; 2490 } 2491 2492 /** 2493 * @return Explanation of why this structure definition is needed and why it has been designed as it has. 2494 */ 2495 public String getPurpose() { 2496 return this.purpose == null ? null : this.purpose.getValue(); 2497 } 2498 2499 /** 2500 * @param value Explanation of why this structure definition is needed and why it has been designed as it has. 2501 */ 2502 public StructureDefinition setPurpose(String value) { 2503 if (Utilities.noString(value)) 2504 this.purpose = null; 2505 else { 2506 if (this.purpose == null) 2507 this.purpose = new MarkdownType(); 2508 this.purpose.setValue(value); 2509 } 2510 return this; 2511 } 2512 2513 /** 2514 * @return {@link #copyright} (A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 2515 */ 2516 public MarkdownType getCopyrightElement() { 2517 if (this.copyright == null) 2518 if (Configuration.errorOnAutoCreate()) 2519 throw new Error("Attempt to auto-create StructureDefinition.copyright"); 2520 else if (Configuration.doAutoCreate()) 2521 this.copyright = new MarkdownType(); // bb 2522 return this.copyright; 2523 } 2524 2525 public boolean hasCopyrightElement() { 2526 return this.copyright != null && !this.copyright.isEmpty(); 2527 } 2528 2529 public boolean hasCopyright() { 2530 return this.copyright != null && !this.copyright.isEmpty(); 2531 } 2532 2533 /** 2534 * @param value {@link #copyright} (A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 2535 */ 2536 public StructureDefinition setCopyrightElement(MarkdownType value) { 2537 this.copyright = value; 2538 return this; 2539 } 2540 2541 /** 2542 * @return A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element. 2543 */ 2544 public String getCopyright() { 2545 return this.copyright == null ? null : this.copyright.getValue(); 2546 } 2547 2548 /** 2549 * @param value A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element. 2550 */ 2551 public StructureDefinition setCopyright(String value) { 2552 if (Utilities.noString(value)) 2553 this.copyright = null; 2554 else { 2555 if (this.copyright == null) 2556 this.copyright = new MarkdownType(); 2557 this.copyright.setValue(value); 2558 } 2559 return this; 2560 } 2561 2562 /** 2563 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2564 */ 2565 public StringType getCopyrightLabelElement() { 2566 if (this.copyrightLabel == null) 2567 if (Configuration.errorOnAutoCreate()) 2568 throw new Error("Attempt to auto-create StructureDefinition.copyrightLabel"); 2569 else if (Configuration.doAutoCreate()) 2570 this.copyrightLabel = new StringType(); // bb 2571 return this.copyrightLabel; 2572 } 2573 2574 public boolean hasCopyrightLabelElement() { 2575 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2576 } 2577 2578 public boolean hasCopyrightLabel() { 2579 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2580 } 2581 2582 /** 2583 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2584 */ 2585 public StructureDefinition setCopyrightLabelElement(StringType value) { 2586 this.copyrightLabel = value; 2587 return this; 2588 } 2589 2590 /** 2591 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2592 */ 2593 public String getCopyrightLabel() { 2594 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 2595 } 2596 2597 /** 2598 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2599 */ 2600 public StructureDefinition setCopyrightLabel(String value) { 2601 if (Utilities.noString(value)) 2602 this.copyrightLabel = null; 2603 else { 2604 if (this.copyrightLabel == null) 2605 this.copyrightLabel = new StringType(); 2606 this.copyrightLabel.setValue(value); 2607 } 2608 return this; 2609 } 2610 2611 /** 2612 * @return {@link #keyword} ((DEPRECATED) A set of key words or terms from external terminologies that may be used to assist with indexing and searching of templates nby describing the use of this structure definition, or the content it describes.) 2613 */ 2614 public List<Coding> getKeyword() { 2615 if (this.keyword == null) 2616 this.keyword = new ArrayList<Coding>(); 2617 return this.keyword; 2618 } 2619 2620 /** 2621 * @return Returns a reference to <code>this</code> for easy method chaining 2622 */ 2623 public StructureDefinition setKeyword(List<Coding> theKeyword) { 2624 this.keyword = theKeyword; 2625 return this; 2626 } 2627 2628 public boolean hasKeyword() { 2629 if (this.keyword == null) 2630 return false; 2631 for (Coding item : this.keyword) 2632 if (!item.isEmpty()) 2633 return true; 2634 return false; 2635 } 2636 2637 public Coding addKeyword() { //3 2638 Coding t = new Coding(); 2639 if (this.keyword == null) 2640 this.keyword = new ArrayList<Coding>(); 2641 this.keyword.add(t); 2642 return t; 2643 } 2644 2645 public StructureDefinition addKeyword(Coding t) { //3 2646 if (t == null) 2647 return this; 2648 if (this.keyword == null) 2649 this.keyword = new ArrayList<Coding>(); 2650 this.keyword.add(t); 2651 return this; 2652 } 2653 2654 /** 2655 * @return The first repetition of repeating field {@link #keyword}, creating it if it does not already exist {3} 2656 */ 2657 public Coding getKeywordFirstRep() { 2658 if (getKeyword().isEmpty()) { 2659 addKeyword(); 2660 } 2661 return getKeyword().get(0); 2662 } 2663 2664 /** 2665 * @return {@link #fhirVersion} (The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version.). This is the underlying object with id, value and extensions. The accessor "getFhirVersion" gives direct access to the value 2666 */ 2667 public Enumeration<FHIRVersion> getFhirVersionElement() { 2668 if (this.fhirVersion == null) 2669 if (Configuration.errorOnAutoCreate()) 2670 throw new Error("Attempt to auto-create StructureDefinition.fhirVersion"); 2671 else if (Configuration.doAutoCreate()) 2672 this.fhirVersion = new Enumeration<FHIRVersion>(new FHIRVersionEnumFactory()); // bb 2673 return this.fhirVersion; 2674 } 2675 2676 public boolean hasFhirVersionElement() { 2677 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 2678 } 2679 2680 public boolean hasFhirVersion() { 2681 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 2682 } 2683 2684 /** 2685 * @param value {@link #fhirVersion} (The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version.). This is the underlying object with id, value and extensions. The accessor "getFhirVersion" gives direct access to the value 2686 */ 2687 public StructureDefinition setFhirVersionElement(Enumeration<FHIRVersion> value) { 2688 this.fhirVersion = value; 2689 return this; 2690 } 2691 2692 /** 2693 * @return The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version. 2694 */ 2695 public FHIRVersion getFhirVersion() { 2696 return this.fhirVersion == null ? null : this.fhirVersion.getValue(); 2697 } 2698 2699 /** 2700 * @param value The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version. 2701 */ 2702 public StructureDefinition setFhirVersion(FHIRVersion value) { 2703 if (value == null) 2704 this.fhirVersion = null; 2705 else { 2706 if (this.fhirVersion == null) 2707 this.fhirVersion = new Enumeration<FHIRVersion>(new FHIRVersionEnumFactory()); 2708 this.fhirVersion.setValue(value); 2709 } 2710 return this; 2711 } 2712 2713 /** 2714 * @return {@link #mapping} (An external specification that the content is mapped to.) 2715 */ 2716 public List<StructureDefinitionMappingComponent> getMapping() { 2717 if (this.mapping == null) 2718 this.mapping = new ArrayList<StructureDefinitionMappingComponent>(); 2719 return this.mapping; 2720 } 2721 2722 /** 2723 * @return Returns a reference to <code>this</code> for easy method chaining 2724 */ 2725 public StructureDefinition setMapping(List<StructureDefinitionMappingComponent> theMapping) { 2726 this.mapping = theMapping; 2727 return this; 2728 } 2729 2730 public boolean hasMapping() { 2731 if (this.mapping == null) 2732 return false; 2733 for (StructureDefinitionMappingComponent item : this.mapping) 2734 if (!item.isEmpty()) 2735 return true; 2736 return false; 2737 } 2738 2739 public StructureDefinitionMappingComponent addMapping() { //3 2740 StructureDefinitionMappingComponent t = new StructureDefinitionMappingComponent(); 2741 if (this.mapping == null) 2742 this.mapping = new ArrayList<StructureDefinitionMappingComponent>(); 2743 this.mapping.add(t); 2744 return t; 2745 } 2746 2747 public StructureDefinition addMapping(StructureDefinitionMappingComponent t) { //3 2748 if (t == null) 2749 return this; 2750 if (this.mapping == null) 2751 this.mapping = new ArrayList<StructureDefinitionMappingComponent>(); 2752 this.mapping.add(t); 2753 return this; 2754 } 2755 2756 /** 2757 * @return The first repetition of repeating field {@link #mapping}, creating it if it does not already exist {3} 2758 */ 2759 public StructureDefinitionMappingComponent getMappingFirstRep() { 2760 if (getMapping().isEmpty()) { 2761 addMapping(); 2762 } 2763 return getMapping().get(0); 2764 } 2765 2766 /** 2767 * @return {@link #kind} (Defines the kind of structure that this definition is describing.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 2768 */ 2769 public Enumeration<StructureDefinitionKind> getKindElement() { 2770 if (this.kind == null) 2771 if (Configuration.errorOnAutoCreate()) 2772 throw new Error("Attempt to auto-create StructureDefinition.kind"); 2773 else if (Configuration.doAutoCreate()) 2774 this.kind = new Enumeration<StructureDefinitionKind>(new StructureDefinitionKindEnumFactory()); // bb 2775 return this.kind; 2776 } 2777 2778 public boolean hasKindElement() { 2779 return this.kind != null && !this.kind.isEmpty(); 2780 } 2781 2782 public boolean hasKind() { 2783 return this.kind != null && !this.kind.isEmpty(); 2784 } 2785 2786 /** 2787 * @param value {@link #kind} (Defines the kind of structure that this definition is describing.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 2788 */ 2789 public StructureDefinition setKindElement(Enumeration<StructureDefinitionKind> value) { 2790 this.kind = value; 2791 return this; 2792 } 2793 2794 /** 2795 * @return Defines the kind of structure that this definition is describing. 2796 */ 2797 public StructureDefinitionKind getKind() { 2798 return this.kind == null ? null : this.kind.getValue(); 2799 } 2800 2801 /** 2802 * @param value Defines the kind of structure that this definition is describing. 2803 */ 2804 public StructureDefinition setKind(StructureDefinitionKind value) { 2805 if (this.kind == null) 2806 this.kind = new Enumeration<StructureDefinitionKind>(new StructureDefinitionKindEnumFactory()); 2807 this.kind.setValue(value); 2808 return this; 2809 } 2810 2811 /** 2812 * @return {@link #abstract_} (Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems.). This is the underlying object with id, value and extensions. The accessor "getAbstract" gives direct access to the value 2813 */ 2814 public BooleanType getAbstractElement() { 2815 if (this.abstract_ == null) 2816 if (Configuration.errorOnAutoCreate()) 2817 throw new Error("Attempt to auto-create StructureDefinition.abstract_"); 2818 else if (Configuration.doAutoCreate()) 2819 this.abstract_ = new BooleanType(); // bb 2820 return this.abstract_; 2821 } 2822 2823 public boolean hasAbstractElement() { 2824 return this.abstract_ != null && !this.abstract_.isEmpty(); 2825 } 2826 2827 public boolean hasAbstract() { 2828 return this.abstract_ != null && !this.abstract_.isEmpty(); 2829 } 2830 2831 /** 2832 * @param value {@link #abstract_} (Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems.). This is the underlying object with id, value and extensions. The accessor "getAbstract" gives direct access to the value 2833 */ 2834 public StructureDefinition setAbstractElement(BooleanType value) { 2835 this.abstract_ = value; 2836 return this; 2837 } 2838 2839 /** 2840 * @return Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems. 2841 */ 2842 public boolean getAbstract() { 2843 return this.abstract_ == null || this.abstract_.isEmpty() ? false : this.abstract_.getValue(); 2844 } 2845 2846 /** 2847 * @param value Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems. 2848 */ 2849 public StructureDefinition setAbstract(boolean value) { 2850 if (this.abstract_ == null) 2851 this.abstract_ = new BooleanType(); 2852 this.abstract_.setValue(value); 2853 return this; 2854 } 2855 2856 /** 2857 * @return {@link #context} (Identifies the types of resource or data type elements to which the extension can be applied. For more guidance on using the 'context' element, see the [defining extensions page](defining-extensions.html#context).) 2858 */ 2859 public List<StructureDefinitionContextComponent> getContext() { 2860 if (this.context == null) 2861 this.context = new ArrayList<StructureDefinitionContextComponent>(); 2862 return this.context; 2863 } 2864 2865 /** 2866 * @return Returns a reference to <code>this</code> for easy method chaining 2867 */ 2868 public StructureDefinition setContext(List<StructureDefinitionContextComponent> theContext) { 2869 this.context = theContext; 2870 return this; 2871 } 2872 2873 public boolean hasContext() { 2874 if (this.context == null) 2875 return false; 2876 for (StructureDefinitionContextComponent item : this.context) 2877 if (!item.isEmpty()) 2878 return true; 2879 return false; 2880 } 2881 2882 public StructureDefinitionContextComponent addContext() { //3 2883 StructureDefinitionContextComponent t = new StructureDefinitionContextComponent(); 2884 if (this.context == null) 2885 this.context = new ArrayList<StructureDefinitionContextComponent>(); 2886 this.context.add(t); 2887 return t; 2888 } 2889 2890 public StructureDefinition addContext(StructureDefinitionContextComponent t) { //3 2891 if (t == null) 2892 return this; 2893 if (this.context == null) 2894 this.context = new ArrayList<StructureDefinitionContextComponent>(); 2895 this.context.add(t); 2896 return this; 2897 } 2898 2899 /** 2900 * @return The first repetition of repeating field {@link #context}, creating it if it does not already exist {3} 2901 */ 2902 public StructureDefinitionContextComponent getContextFirstRep() { 2903 if (getContext().isEmpty()) { 2904 addContext(); 2905 } 2906 return getContext().get(0); 2907 } 2908 2909 /** 2910 * @return {@link #contextInvariant} (A set of rules as FHIRPath Invariants about when the extension can be used (e.g. co-occurrence variants for the extension). All the rules must be true.) 2911 */ 2912 public List<StringType> getContextInvariant() { 2913 if (this.contextInvariant == null) 2914 this.contextInvariant = new ArrayList<StringType>(); 2915 return this.contextInvariant; 2916 } 2917 2918 /** 2919 * @return Returns a reference to <code>this</code> for easy method chaining 2920 */ 2921 public StructureDefinition setContextInvariant(List<StringType> theContextInvariant) { 2922 this.contextInvariant = theContextInvariant; 2923 return this; 2924 } 2925 2926 public boolean hasContextInvariant() { 2927 if (this.contextInvariant == null) 2928 return false; 2929 for (StringType item : this.contextInvariant) 2930 if (!item.isEmpty()) 2931 return true; 2932 return false; 2933 } 2934 2935 /** 2936 * @return {@link #contextInvariant} (A set of rules as FHIRPath Invariants about when the extension can be used (e.g. co-occurrence variants for the extension). All the rules must be true.) 2937 */ 2938 public StringType addContextInvariantElement() {//2 2939 StringType t = new StringType(); 2940 if (this.contextInvariant == null) 2941 this.contextInvariant = new ArrayList<StringType>(); 2942 this.contextInvariant.add(t); 2943 return t; 2944 } 2945 2946 /** 2947 * @param value {@link #contextInvariant} (A set of rules as FHIRPath Invariants about when the extension can be used (e.g. co-occurrence variants for the extension). All the rules must be true.) 2948 */ 2949 public StructureDefinition addContextInvariant(String value) { //1 2950 StringType t = new StringType(); 2951 t.setValue(value); 2952 if (this.contextInvariant == null) 2953 this.contextInvariant = new ArrayList<StringType>(); 2954 this.contextInvariant.add(t); 2955 return this; 2956 } 2957 2958 /** 2959 * @param value {@link #contextInvariant} (A set of rules as FHIRPath Invariants about when the extension can be used (e.g. co-occurrence variants for the extension). All the rules must be true.) 2960 */ 2961 public boolean hasContextInvariant(String value) { 2962 if (this.contextInvariant == null) 2963 return false; 2964 for (StringType v : this.contextInvariant) 2965 if (v.getValue().equals(value)) // string 2966 return true; 2967 return false; 2968 } 2969 2970 /** 2971 * @return {@link #type} (The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models, where they are required.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2972 */ 2973 public UriType getTypeElement() { 2974 if (this.type == null) 2975 if (Configuration.errorOnAutoCreate()) 2976 throw new Error("Attempt to auto-create StructureDefinition.type"); 2977 else if (Configuration.doAutoCreate()) 2978 this.type = new UriType(); // bb 2979 return this.type; 2980 } 2981 2982 public boolean hasTypeElement() { 2983 return this.type != null && !this.type.isEmpty(); 2984 } 2985 2986 public boolean hasType() { 2987 return this.type != null && !this.type.isEmpty(); 2988 } 2989 2990 /** 2991 * @param value {@link #type} (The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models, where they are required.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2992 */ 2993 public StructureDefinition setTypeElement(UriType value) { 2994 this.type = value; 2995 return this; 2996 } 2997 2998 /** 2999 * @return The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models, where they are required. 3000 */ 3001 public String getType() { 3002 return this.type == null ? null : this.type.getValue(); 3003 } 3004 3005 /** 3006 * @param value The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models, where they are required. 3007 */ 3008 public StructureDefinition setType(String value) { 3009 if (this.type == null) 3010 this.type = new UriType(); 3011 this.type.setValue(value); 3012 return this; 3013 } 3014 3015 /** 3016 * @return {@link #baseDefinition} (An absolute URI that is the base structure from which this type is derived, either by specialization or constraint.). This is the underlying object with id, value and extensions. The accessor "getBaseDefinition" gives direct access to the value 3017 */ 3018 public CanonicalType getBaseDefinitionElement() { 3019 if (this.baseDefinition == null) 3020 if (Configuration.errorOnAutoCreate()) 3021 throw new Error("Attempt to auto-create StructureDefinition.baseDefinition"); 3022 else if (Configuration.doAutoCreate()) 3023 this.baseDefinition = new CanonicalType(); // bb 3024 return this.baseDefinition; 3025 } 3026 3027 public boolean hasBaseDefinitionElement() { 3028 return this.baseDefinition != null && !this.baseDefinition.isEmpty(); 3029 } 3030 3031 public boolean hasBaseDefinition() { 3032 return this.baseDefinition != null && !this.baseDefinition.isEmpty(); 3033 } 3034 3035 /** 3036 * @param value {@link #baseDefinition} (An absolute URI that is the base structure from which this type is derived, either by specialization or constraint.). This is the underlying object with id, value and extensions. The accessor "getBaseDefinition" gives direct access to the value 3037 */ 3038 public StructureDefinition setBaseDefinitionElement(CanonicalType value) { 3039 this.baseDefinition = value; 3040 return this; 3041 } 3042 3043 /** 3044 * @return An absolute URI that is the base structure from which this type is derived, either by specialization or constraint. 3045 */ 3046 public String getBaseDefinition() { 3047 return this.baseDefinition == null ? null : this.baseDefinition.getValue(); 3048 } 3049 3050 /** 3051 * @param value An absolute URI that is the base structure from which this type is derived, either by specialization or constraint. 3052 */ 3053 public StructureDefinition setBaseDefinition(String value) { 3054 if (Utilities.noString(value)) 3055 this.baseDefinition = null; 3056 else { 3057 if (this.baseDefinition == null) 3058 this.baseDefinition = new CanonicalType(); 3059 this.baseDefinition.setValue(value); 3060 } 3061 return this; 3062 } 3063 3064 /** 3065 * @return {@link #derivation} (How the type relates to the baseDefinition.). This is the underlying object with id, value and extensions. The accessor "getDerivation" gives direct access to the value 3066 */ 3067 public Enumeration<TypeDerivationRule> getDerivationElement() { 3068 if (this.derivation == null) 3069 if (Configuration.errorOnAutoCreate()) 3070 throw new Error("Attempt to auto-create StructureDefinition.derivation"); 3071 else if (Configuration.doAutoCreate()) 3072 this.derivation = new Enumeration<TypeDerivationRule>(new TypeDerivationRuleEnumFactory()); // bb 3073 return this.derivation; 3074 } 3075 3076 public boolean hasDerivationElement() { 3077 return this.derivation != null && !this.derivation.isEmpty(); 3078 } 3079 3080 public boolean hasDerivation() { 3081 return this.derivation != null && !this.derivation.isEmpty(); 3082 } 3083 3084 /** 3085 * @param value {@link #derivation} (How the type relates to the baseDefinition.). This is the underlying object with id, value and extensions. The accessor "getDerivation" gives direct access to the value 3086 */ 3087 public StructureDefinition setDerivationElement(Enumeration<TypeDerivationRule> value) { 3088 this.derivation = value; 3089 return this; 3090 } 3091 3092 /** 3093 * @return How the type relates to the baseDefinition. 3094 */ 3095 public TypeDerivationRule getDerivation() { 3096 return this.derivation == null ? null : this.derivation.getValue(); 3097 } 3098 3099 /** 3100 * @param value How the type relates to the baseDefinition. 3101 */ 3102 public StructureDefinition setDerivation(TypeDerivationRule value) { 3103 if (value == null) 3104 this.derivation = null; 3105 else { 3106 if (this.derivation == null) 3107 this.derivation = new Enumeration<TypeDerivationRule>(new TypeDerivationRuleEnumFactory()); 3108 this.derivation.setValue(value); 3109 } 3110 return this; 3111 } 3112 3113 /** 3114 * @return {@link #snapshot} (A snapshot view is expressed in a standalone form that can be used and interpreted without considering the base StructureDefinition.) 3115 */ 3116 public StructureDefinitionSnapshotComponent getSnapshot() { 3117 if (this.snapshot == null) 3118 if (Configuration.errorOnAutoCreate()) 3119 throw new Error("Attempt to auto-create StructureDefinition.snapshot"); 3120 else if (Configuration.doAutoCreate()) 3121 this.snapshot = new StructureDefinitionSnapshotComponent(); // cc 3122 return this.snapshot; 3123 } 3124 3125 public boolean hasSnapshot() { 3126 return this.snapshot != null && !this.snapshot.isEmpty(); 3127 } 3128 3129 /** 3130 * @param value {@link #snapshot} (A snapshot view is expressed in a standalone form that can be used and interpreted without considering the base StructureDefinition.) 3131 */ 3132 public StructureDefinition setSnapshot(StructureDefinitionSnapshotComponent value) { 3133 this.snapshot = value; 3134 return this; 3135 } 3136 3137 /** 3138 * @return {@link #differential} (A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies.) 3139 */ 3140 public StructureDefinitionDifferentialComponent getDifferential() { 3141 if (this.differential == null) 3142 if (Configuration.errorOnAutoCreate()) 3143 throw new Error("Attempt to auto-create StructureDefinition.differential"); 3144 else if (Configuration.doAutoCreate()) 3145 this.differential = new StructureDefinitionDifferentialComponent(); // cc 3146 return this.differential; 3147 } 3148 3149 public boolean hasDifferential() { 3150 return this.differential != null && !this.differential.isEmpty(); 3151 } 3152 3153 /** 3154 * @param value {@link #differential} (A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies.) 3155 */ 3156 public StructureDefinition setDifferential(StructureDefinitionDifferentialComponent value) { 3157 this.differential = value; 3158 return this; 3159 } 3160 3161 protected void listChildren(List<Property> children) { 3162 super.listChildren(children); 3163 children.add(new Property("url", "uri", "An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure definition is stored on different servers.", 0, 1, url)); 3164 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this structure definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3165 children.add(new Property("version", "string", "The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. There is no expectation that versions can be placed in a lexicographical sequence, so authors are encouraged to populate the StructureDefinition.versionAlgorithm[x] element to enable comparisons. If there is no managed version available, authors can consider using ISO date/time syntax (e.g., '2023-01-01').", 0, 1, version)); 3166 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 3167 children.add(new Property("name", "string", "A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 3168 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the structure definition.", 0, 1, title)); 3169 children.add(new Property("status", "code", "The status of this structure definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 3170 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 3171 children.add(new Property("date", "dateTime", "The date (and optionally time) when the structure definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes.", 0, 1, date)); 3172 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the structure definition.", 0, 1, publisher)); 3173 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 3174 children.add(new Property("description", "markdown", "A free text natural language description of the structure definition from a consumer's perspective.", 0, 1, description)); 3175 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 3176 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the structure definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 3177 children.add(new Property("purpose", "markdown", "Explanation of why this structure definition is needed and why it has been designed as it has.", 0, 1, purpose)); 3178 children.add(new Property("copyright", "markdown", "A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element.", 0, 1, copyright)); 3179 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 3180 children.add(new Property("keyword", "Coding", "(DEPRECATED) A set of key words or terms from external terminologies that may be used to assist with indexing and searching of templates nby describing the use of this structure definition, or the content it describes.", 0, java.lang.Integer.MAX_VALUE, keyword)); 3181 children.add(new Property("fhirVersion", "code", "The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version.", 0, 1, fhirVersion)); 3182 children.add(new Property("mapping", "", "An external specification that the content is mapped to.", 0, java.lang.Integer.MAX_VALUE, mapping)); 3183 children.add(new Property("kind", "code", "Defines the kind of structure that this definition is describing.", 0, 1, kind)); 3184 children.add(new Property("abstract", "boolean", "Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems.", 0, 1, abstract_)); 3185 children.add(new Property("context", "", "Identifies the types of resource or data type elements to which the extension can be applied. For more guidance on using the 'context' element, see the [defining extensions page](defining-extensions.html#context).", 0, java.lang.Integer.MAX_VALUE, context)); 3186 children.add(new Property("contextInvariant", "string", "A set of rules as FHIRPath Invariants about when the extension can be used (e.g. co-occurrence variants for the extension). All the rules must be true.", 0, java.lang.Integer.MAX_VALUE, contextInvariant)); 3187 children.add(new Property("type", "uri", "The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models, where they are required.", 0, 1, type)); 3188 children.add(new Property("baseDefinition", "canonical(StructureDefinition)", "An absolute URI that is the base structure from which this type is derived, either by specialization or constraint.", 0, 1, baseDefinition)); 3189 children.add(new Property("derivation", "code", "How the type relates to the baseDefinition.", 0, 1, derivation)); 3190 children.add(new Property("snapshot", "", "A snapshot view is expressed in a standalone form that can be used and interpreted without considering the base StructureDefinition.", 0, 1, snapshot)); 3191 children.add(new Property("differential", "", "A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies.", 0, 1, differential)); 3192 } 3193 3194 @Override 3195 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3196 switch (_hash) { 3197 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure definition is stored on different servers.", 0, 1, url); 3198 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this structure definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 3199 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. There is no expectation that versions can be placed in a lexicographical sequence, so authors are encouraged to populate the StructureDefinition.versionAlgorithm[x] element to enable comparisons. If there is no managed version available, authors can consider using ISO date/time syntax (e.g., '2023-01-01').", 0, 1, version); 3200 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3201 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3202 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3203 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3204 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 3205 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the structure definition.", 0, 1, title); 3206 case -892481550: /*status*/ return new Property("status", "code", "The status of this structure definition. Enables tracking the life-cycle of the content.", 0, 1, status); 3207 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 3208 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the structure definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes.", 0, 1, date); 3209 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the structure definition.", 0, 1, publisher); 3210 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 3211 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the structure definition from a consumer's perspective.", 0, 1, description); 3212 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 3213 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the structure definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 3214 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this structure definition is needed and why it has been designed as it has.", 0, 1, purpose); 3215 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element.", 0, 1, copyright); 3216 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 3217 case -814408215: /*keyword*/ return new Property("keyword", "Coding", "(DEPRECATED) A set of key words or terms from external terminologies that may be used to assist with indexing and searching of templates nby describing the use of this structure definition, or the content it describes.", 0, java.lang.Integer.MAX_VALUE, keyword); 3218 case 461006061: /*fhirVersion*/ return new Property("fhirVersion", "code", "The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version.", 0, 1, fhirVersion); 3219 case 837556430: /*mapping*/ return new Property("mapping", "", "An external specification that the content is mapped to.", 0, java.lang.Integer.MAX_VALUE, mapping); 3220 case 3292052: /*kind*/ return new Property("kind", "code", "Defines the kind of structure that this definition is describing.", 0, 1, kind); 3221 case 1732898850: /*abstract*/ return new Property("abstract", "boolean", "Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems.", 0, 1, abstract_); 3222 case 951530927: /*context*/ return new Property("context", "", "Identifies the types of resource or data type elements to which the extension can be applied. For more guidance on using the 'context' element, see the [defining extensions page](defining-extensions.html#context).", 0, java.lang.Integer.MAX_VALUE, context); 3223 case -802505007: /*contextInvariant*/ return new Property("contextInvariant", "string", "A set of rules as FHIRPath Invariants about when the extension can be used (e.g. co-occurrence variants for the extension). All the rules must be true.", 0, java.lang.Integer.MAX_VALUE, contextInvariant); 3224 case 3575610: /*type*/ return new Property("type", "uri", "The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models, where they are required.", 0, 1, type); 3225 case 1139771140: /*baseDefinition*/ return new Property("baseDefinition", "canonical(StructureDefinition)", "An absolute URI that is the base structure from which this type is derived, either by specialization or constraint.", 0, 1, baseDefinition); 3226 case -1353885513: /*derivation*/ return new Property("derivation", "code", "How the type relates to the baseDefinition.", 0, 1, derivation); 3227 case 284874180: /*snapshot*/ return new Property("snapshot", "", "A snapshot view is expressed in a standalone form that can be used and interpreted without considering the base StructureDefinition.", 0, 1, snapshot); 3228 case -1196150917: /*differential*/ return new Property("differential", "", "A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies.", 0, 1, differential); 3229 default: return super.getNamedProperty(_hash, _name, _checkValid); 3230 } 3231 3232 } 3233 3234 @Override 3235 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3236 switch (hash) { 3237 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 3238 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3239 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 3240 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 3241 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3242 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 3243 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 3244 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 3245 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 3246 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 3247 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3248 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3249 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3250 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3251 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 3252 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 3253 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 3254 case -814408215: /*keyword*/ return this.keyword == null ? new Base[0] : this.keyword.toArray(new Base[this.keyword.size()]); // Coding 3255 case 461006061: /*fhirVersion*/ return this.fhirVersion == null ? new Base[0] : new Base[] {this.fhirVersion}; // Enumeration<FHIRVersion> 3256 case 837556430: /*mapping*/ return this.mapping == null ? new Base[0] : this.mapping.toArray(new Base[this.mapping.size()]); // StructureDefinitionMappingComponent 3257 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // Enumeration<StructureDefinitionKind> 3258 case 1732898850: /*abstract*/ return this.abstract_ == null ? new Base[0] : new Base[] {this.abstract_}; // BooleanType 3259 case 951530927: /*context*/ return this.context == null ? new Base[0] : this.context.toArray(new Base[this.context.size()]); // StructureDefinitionContextComponent 3260 case -802505007: /*contextInvariant*/ return this.contextInvariant == null ? new Base[0] : this.contextInvariant.toArray(new Base[this.contextInvariant.size()]); // StringType 3261 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // UriType 3262 case 1139771140: /*baseDefinition*/ return this.baseDefinition == null ? new Base[0] : new Base[] {this.baseDefinition}; // CanonicalType 3263 case -1353885513: /*derivation*/ return this.derivation == null ? new Base[0] : new Base[] {this.derivation}; // Enumeration<TypeDerivationRule> 3264 case 284874180: /*snapshot*/ return this.snapshot == null ? new Base[0] : new Base[] {this.snapshot}; // StructureDefinitionSnapshotComponent 3265 case -1196150917: /*differential*/ return this.differential == null ? new Base[0] : new Base[] {this.differential}; // StructureDefinitionDifferentialComponent 3266 default: return super.getProperty(hash, name, checkValid); 3267 } 3268 3269 } 3270 3271 @Override 3272 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3273 switch (hash) { 3274 case 116079: // url 3275 this.url = TypeConvertor.castToUri(value); // UriType 3276 return value; 3277 case -1618432855: // identifier 3278 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3279 return value; 3280 case 351608024: // version 3281 this.version = TypeConvertor.castToString(value); // StringType 3282 return value; 3283 case 1508158071: // versionAlgorithm 3284 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 3285 return value; 3286 case 3373707: // name 3287 this.name = TypeConvertor.castToString(value); // StringType 3288 return value; 3289 case 110371416: // title 3290 this.title = TypeConvertor.castToString(value); // StringType 3291 return value; 3292 case -892481550: // status 3293 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3294 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3295 return value; 3296 case -404562712: // experimental 3297 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 3298 return value; 3299 case 3076014: // date 3300 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 3301 return value; 3302 case 1447404028: // publisher 3303 this.publisher = TypeConvertor.castToString(value); // StringType 3304 return value; 3305 case 951526432: // contact 3306 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 3307 return value; 3308 case -1724546052: // description 3309 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3310 return value; 3311 case -669707736: // useContext 3312 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 3313 return value; 3314 case -507075711: // jurisdiction 3315 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3316 return value; 3317 case -220463842: // purpose 3318 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 3319 return value; 3320 case 1522889671: // copyright 3321 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 3322 return value; 3323 case 765157229: // copyrightLabel 3324 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 3325 return value; 3326 case -814408215: // keyword 3327 this.getKeyword().add(TypeConvertor.castToCoding(value)); // Coding 3328 return value; 3329 case 461006061: // fhirVersion 3330 value = new FHIRVersionEnumFactory().fromType(TypeConvertor.castToCode(value)); 3331 this.fhirVersion = (Enumeration) value; // Enumeration<FHIRVersion> 3332 return value; 3333 case 837556430: // mapping 3334 this.getMapping().add((StructureDefinitionMappingComponent) value); // StructureDefinitionMappingComponent 3335 return value; 3336 case 3292052: // kind 3337 value = new StructureDefinitionKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 3338 this.kind = (Enumeration) value; // Enumeration<StructureDefinitionKind> 3339 return value; 3340 case 1732898850: // abstract 3341 this.abstract_ = TypeConvertor.castToBoolean(value); // BooleanType 3342 return value; 3343 case 951530927: // context 3344 this.getContext().add((StructureDefinitionContextComponent) value); // StructureDefinitionContextComponent 3345 return value; 3346 case -802505007: // contextInvariant 3347 this.getContextInvariant().add(TypeConvertor.castToString(value)); // StringType 3348 return value; 3349 case 3575610: // type 3350 this.type = TypeConvertor.castToUri(value); // UriType 3351 return value; 3352 case 1139771140: // baseDefinition 3353 this.baseDefinition = TypeConvertor.castToCanonical(value); // CanonicalType 3354 return value; 3355 case -1353885513: // derivation 3356 value = new TypeDerivationRuleEnumFactory().fromType(TypeConvertor.castToCode(value)); 3357 this.derivation = (Enumeration) value; // Enumeration<TypeDerivationRule> 3358 return value; 3359 case 284874180: // snapshot 3360 this.snapshot = (StructureDefinitionSnapshotComponent) value; // StructureDefinitionSnapshotComponent 3361 return value; 3362 case -1196150917: // differential 3363 this.differential = (StructureDefinitionDifferentialComponent) value; // StructureDefinitionDifferentialComponent 3364 return value; 3365 default: return super.setProperty(hash, name, value); 3366 } 3367 3368 } 3369 3370 @Override 3371 public Base setProperty(String name, Base value) throws FHIRException { 3372 if (name.equals("url")) { 3373 this.url = TypeConvertor.castToUri(value); // UriType 3374 } else if (name.equals("identifier")) { 3375 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3376 } else if (name.equals("version")) { 3377 this.version = TypeConvertor.castToString(value); // StringType 3378 } else if (name.equals("versionAlgorithm[x]")) { 3379 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 3380 } else if (name.equals("name")) { 3381 this.name = TypeConvertor.castToString(value); // StringType 3382 } else if (name.equals("title")) { 3383 this.title = TypeConvertor.castToString(value); // StringType 3384 } else if (name.equals("status")) { 3385 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3386 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3387 } else if (name.equals("experimental")) { 3388 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 3389 } else if (name.equals("date")) { 3390 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 3391 } else if (name.equals("publisher")) { 3392 this.publisher = TypeConvertor.castToString(value); // StringType 3393 } else if (name.equals("contact")) { 3394 this.getContact().add(TypeConvertor.castToContactDetail(value)); 3395 } else if (name.equals("description")) { 3396 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3397 } else if (name.equals("useContext")) { 3398 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 3399 } else if (name.equals("jurisdiction")) { 3400 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 3401 } else if (name.equals("purpose")) { 3402 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 3403 } else if (name.equals("copyright")) { 3404 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 3405 } else if (name.equals("copyrightLabel")) { 3406 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 3407 } else if (name.equals("keyword")) { 3408 this.getKeyword().add(TypeConvertor.castToCoding(value)); 3409 } else if (name.equals("fhirVersion")) { 3410 value = new FHIRVersionEnumFactory().fromType(TypeConvertor.castToCode(value)); 3411 this.fhirVersion = (Enumeration) value; // Enumeration<FHIRVersion> 3412 } else if (name.equals("mapping")) { 3413 this.getMapping().add((StructureDefinitionMappingComponent) value); 3414 } else if (name.equals("kind")) { 3415 value = new StructureDefinitionKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 3416 this.kind = (Enumeration) value; // Enumeration<StructureDefinitionKind> 3417 } else if (name.equals("abstract")) { 3418 this.abstract_ = TypeConvertor.castToBoolean(value); // BooleanType 3419 } else if (name.equals("context")) { 3420 this.getContext().add((StructureDefinitionContextComponent) value); 3421 } else if (name.equals("contextInvariant")) { 3422 this.getContextInvariant().add(TypeConvertor.castToString(value)); 3423 } else if (name.equals("type")) { 3424 this.type = TypeConvertor.castToUri(value); // UriType 3425 } else if (name.equals("baseDefinition")) { 3426 this.baseDefinition = TypeConvertor.castToCanonical(value); // CanonicalType 3427 } else if (name.equals("derivation")) { 3428 value = new TypeDerivationRuleEnumFactory().fromType(TypeConvertor.castToCode(value)); 3429 this.derivation = (Enumeration) value; // Enumeration<TypeDerivationRule> 3430 } else if (name.equals("snapshot")) { 3431 this.snapshot = (StructureDefinitionSnapshotComponent) value; // StructureDefinitionSnapshotComponent 3432 } else if (name.equals("differential")) { 3433 this.differential = (StructureDefinitionDifferentialComponent) value; // StructureDefinitionDifferentialComponent 3434 } else 3435 return super.setProperty(name, value); 3436 return value; 3437 } 3438 3439 @Override 3440 public void removeChild(String name, Base value) throws FHIRException { 3441 if (name.equals("url")) { 3442 this.url = null; 3443 } else if (name.equals("identifier")) { 3444 this.getIdentifier().remove(value); 3445 } else if (name.equals("version")) { 3446 this.version = null; 3447 } else if (name.equals("versionAlgorithm[x]")) { 3448 this.versionAlgorithm = null; 3449 } else if (name.equals("name")) { 3450 this.name = null; 3451 } else if (name.equals("title")) { 3452 this.title = null; 3453 } else if (name.equals("status")) { 3454 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3455 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3456 } else if (name.equals("experimental")) { 3457 this.experimental = null; 3458 } else if (name.equals("date")) { 3459 this.date = null; 3460 } else if (name.equals("publisher")) { 3461 this.publisher = null; 3462 } else if (name.equals("contact")) { 3463 this.getContact().remove(value); 3464 } else if (name.equals("description")) { 3465 this.description = null; 3466 } else if (name.equals("useContext")) { 3467 this.getUseContext().remove(value); 3468 } else if (name.equals("jurisdiction")) { 3469 this.getJurisdiction().remove(value); 3470 } else if (name.equals("purpose")) { 3471 this.purpose = null; 3472 } else if (name.equals("copyright")) { 3473 this.copyright = null; 3474 } else if (name.equals("copyrightLabel")) { 3475 this.copyrightLabel = null; 3476 } else if (name.equals("keyword")) { 3477 this.getKeyword().remove(value); 3478 } else if (name.equals("fhirVersion")) { 3479 value = new FHIRVersionEnumFactory().fromType(TypeConvertor.castToCode(value)); 3480 this.fhirVersion = (Enumeration) value; // Enumeration<FHIRVersion> 3481 } else if (name.equals("mapping")) { 3482 this.getMapping().remove((StructureDefinitionMappingComponent) value); 3483 } else if (name.equals("kind")) { 3484 value = new StructureDefinitionKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 3485 this.kind = (Enumeration) value; // Enumeration<StructureDefinitionKind> 3486 } else if (name.equals("abstract")) { 3487 this.abstract_ = null; 3488 } else if (name.equals("context")) { 3489 this.getContext().remove((StructureDefinitionContextComponent) value); 3490 } else if (name.equals("contextInvariant")) { 3491 this.getContextInvariant().remove(value); 3492 } else if (name.equals("type")) { 3493 this.type = null; 3494 } else if (name.equals("baseDefinition")) { 3495 this.baseDefinition = null; 3496 } else if (name.equals("derivation")) { 3497 value = new TypeDerivationRuleEnumFactory().fromType(TypeConvertor.castToCode(value)); 3498 this.derivation = (Enumeration) value; // Enumeration<TypeDerivationRule> 3499 } else if (name.equals("snapshot")) { 3500 this.snapshot = (StructureDefinitionSnapshotComponent) value; // StructureDefinitionSnapshotComponent 3501 } else if (name.equals("differential")) { 3502 this.differential = (StructureDefinitionDifferentialComponent) value; // StructureDefinitionDifferentialComponent 3503 } else 3504 super.removeChild(name, value); 3505 3506 } 3507 3508 @Override 3509 public Base makeProperty(int hash, String name) throws FHIRException { 3510 switch (hash) { 3511 case 116079: return getUrlElement(); 3512 case -1618432855: return addIdentifier(); 3513 case 351608024: return getVersionElement(); 3514 case -115699031: return getVersionAlgorithm(); 3515 case 1508158071: return getVersionAlgorithm(); 3516 case 3373707: return getNameElement(); 3517 case 110371416: return getTitleElement(); 3518 case -892481550: return getStatusElement(); 3519 case -404562712: return getExperimentalElement(); 3520 case 3076014: return getDateElement(); 3521 case 1447404028: return getPublisherElement(); 3522 case 951526432: return addContact(); 3523 case -1724546052: return getDescriptionElement(); 3524 case -669707736: return addUseContext(); 3525 case -507075711: return addJurisdiction(); 3526 case -220463842: return getPurposeElement(); 3527 case 1522889671: return getCopyrightElement(); 3528 case 765157229: return getCopyrightLabelElement(); 3529 case -814408215: return addKeyword(); 3530 case 461006061: return getFhirVersionElement(); 3531 case 837556430: return addMapping(); 3532 case 3292052: return getKindElement(); 3533 case 1732898850: return getAbstractElement(); 3534 case 951530927: return addContext(); 3535 case -802505007: return addContextInvariantElement(); 3536 case 3575610: return getTypeElement(); 3537 case 1139771140: return getBaseDefinitionElement(); 3538 case -1353885513: return getDerivationElement(); 3539 case 284874180: return getSnapshot(); 3540 case -1196150917: return getDifferential(); 3541 default: return super.makeProperty(hash, name); 3542 } 3543 3544 } 3545 3546 @Override 3547 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3548 switch (hash) { 3549 case 116079: /*url*/ return new String[] {"uri"}; 3550 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3551 case 351608024: /*version*/ return new String[] {"string"}; 3552 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 3553 case 3373707: /*name*/ return new String[] {"string"}; 3554 case 110371416: /*title*/ return new String[] {"string"}; 3555 case -892481550: /*status*/ return new String[] {"code"}; 3556 case -404562712: /*experimental*/ return new String[] {"boolean"}; 3557 case 3076014: /*date*/ return new String[] {"dateTime"}; 3558 case 1447404028: /*publisher*/ return new String[] {"string"}; 3559 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 3560 case -1724546052: /*description*/ return new String[] {"markdown"}; 3561 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 3562 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 3563 case -220463842: /*purpose*/ return new String[] {"markdown"}; 3564 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 3565 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 3566 case -814408215: /*keyword*/ return new String[] {"Coding"}; 3567 case 461006061: /*fhirVersion*/ return new String[] {"code"}; 3568 case 837556430: /*mapping*/ return new String[] {}; 3569 case 3292052: /*kind*/ return new String[] {"code"}; 3570 case 1732898850: /*abstract*/ return new String[] {"boolean"}; 3571 case 951530927: /*context*/ return new String[] {}; 3572 case -802505007: /*contextInvariant*/ return new String[] {"string"}; 3573 case 3575610: /*type*/ return new String[] {"uri"}; 3574 case 1139771140: /*baseDefinition*/ return new String[] {"canonical"}; 3575 case -1353885513: /*derivation*/ return new String[] {"code"}; 3576 case 284874180: /*snapshot*/ return new String[] {}; 3577 case -1196150917: /*differential*/ return new String[] {}; 3578 default: return super.getTypesForProperty(hash, name); 3579 } 3580 3581 } 3582 3583 @Override 3584 public Base addChild(String name) throws FHIRException { 3585 if (name.equals("url")) { 3586 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.url"); 3587 } 3588 else if (name.equals("identifier")) { 3589 return addIdentifier(); 3590 } 3591 else if (name.equals("version")) { 3592 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.version"); 3593 } 3594 else if (name.equals("versionAlgorithmString")) { 3595 this.versionAlgorithm = new StringType(); 3596 return this.versionAlgorithm; 3597 } 3598 else if (name.equals("versionAlgorithmCoding")) { 3599 this.versionAlgorithm = new Coding(); 3600 return this.versionAlgorithm; 3601 } 3602 else if (name.equals("name")) { 3603 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.name"); 3604 } 3605 else if (name.equals("title")) { 3606 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.title"); 3607 } 3608 else if (name.equals("status")) { 3609 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.status"); 3610 } 3611 else if (name.equals("experimental")) { 3612 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.experimental"); 3613 } 3614 else if (name.equals("date")) { 3615 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.date"); 3616 } 3617 else if (name.equals("publisher")) { 3618 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.publisher"); 3619 } 3620 else if (name.equals("contact")) { 3621 return addContact(); 3622 } 3623 else if (name.equals("description")) { 3624 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.description"); 3625 } 3626 else if (name.equals("useContext")) { 3627 return addUseContext(); 3628 } 3629 else if (name.equals("jurisdiction")) { 3630 return addJurisdiction(); 3631 } 3632 else if (name.equals("purpose")) { 3633 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.purpose"); 3634 } 3635 else if (name.equals("copyright")) { 3636 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.copyright"); 3637 } 3638 else if (name.equals("copyrightLabel")) { 3639 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.copyrightLabel"); 3640 } 3641 else if (name.equals("keyword")) { 3642 return addKeyword(); 3643 } 3644 else if (name.equals("fhirVersion")) { 3645 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.fhirVersion"); 3646 } 3647 else if (name.equals("mapping")) { 3648 return addMapping(); 3649 } 3650 else if (name.equals("kind")) { 3651 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.kind"); 3652 } 3653 else if (name.equals("abstract")) { 3654 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.abstract"); 3655 } 3656 else if (name.equals("context")) { 3657 return addContext(); 3658 } 3659 else if (name.equals("contextInvariant")) { 3660 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.contextInvariant"); 3661 } 3662 else if (name.equals("type")) { 3663 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.type"); 3664 } 3665 else if (name.equals("baseDefinition")) { 3666 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.baseDefinition"); 3667 } 3668 else if (name.equals("derivation")) { 3669 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.derivation"); 3670 } 3671 else if (name.equals("snapshot")) { 3672 this.snapshot = new StructureDefinitionSnapshotComponent(); 3673 return this.snapshot; 3674 } 3675 else if (name.equals("differential")) { 3676 this.differential = new StructureDefinitionDifferentialComponent(); 3677 return this.differential; 3678 } 3679 else 3680 return super.addChild(name); 3681 } 3682 3683 public String fhirType() { 3684 return "StructureDefinition"; 3685 3686 } 3687 3688 public StructureDefinition copy() { 3689 StructureDefinition dst = new StructureDefinition(); 3690 copyValues(dst); 3691 return dst; 3692 } 3693 3694 public void copyValues(StructureDefinition dst) { 3695 super.copyValues(dst); 3696 dst.url = url == null ? null : url.copy(); 3697 if (identifier != null) { 3698 dst.identifier = new ArrayList<Identifier>(); 3699 for (Identifier i : identifier) 3700 dst.identifier.add(i.copy()); 3701 }; 3702 dst.version = version == null ? null : version.copy(); 3703 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 3704 dst.name = name == null ? null : name.copy(); 3705 dst.title = title == null ? null : title.copy(); 3706 dst.status = status == null ? null : status.copy(); 3707 dst.experimental = experimental == null ? null : experimental.copy(); 3708 dst.date = date == null ? null : date.copy(); 3709 dst.publisher = publisher == null ? null : publisher.copy(); 3710 if (contact != null) { 3711 dst.contact = new ArrayList<ContactDetail>(); 3712 for (ContactDetail i : contact) 3713 dst.contact.add(i.copy()); 3714 }; 3715 dst.description = description == null ? null : description.copy(); 3716 if (useContext != null) { 3717 dst.useContext = new ArrayList<UsageContext>(); 3718 for (UsageContext i : useContext) 3719 dst.useContext.add(i.copy()); 3720 }; 3721 if (jurisdiction != null) { 3722 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3723 for (CodeableConcept i : jurisdiction) 3724 dst.jurisdiction.add(i.copy()); 3725 }; 3726 dst.purpose = purpose == null ? null : purpose.copy(); 3727 dst.copyright = copyright == null ? null : copyright.copy(); 3728 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 3729 if (keyword != null) { 3730 dst.keyword = new ArrayList<Coding>(); 3731 for (Coding i : keyword) 3732 dst.keyword.add(i.copy()); 3733 }; 3734 dst.fhirVersion = fhirVersion == null ? null : fhirVersion.copy(); 3735 if (mapping != null) { 3736 dst.mapping = new ArrayList<StructureDefinitionMappingComponent>(); 3737 for (StructureDefinitionMappingComponent i : mapping) 3738 dst.mapping.add(i.copy()); 3739 }; 3740 dst.kind = kind == null ? null : kind.copy(); 3741 dst.abstract_ = abstract_ == null ? null : abstract_.copy(); 3742 if (context != null) { 3743 dst.context = new ArrayList<StructureDefinitionContextComponent>(); 3744 for (StructureDefinitionContextComponent i : context) 3745 dst.context.add(i.copy()); 3746 }; 3747 if (contextInvariant != null) { 3748 dst.contextInvariant = new ArrayList<StringType>(); 3749 for (StringType i : contextInvariant) 3750 dst.contextInvariant.add(i.copy()); 3751 }; 3752 dst.type = type == null ? null : type.copy(); 3753 dst.baseDefinition = baseDefinition == null ? null : baseDefinition.copy(); 3754 dst.derivation = derivation == null ? null : derivation.copy(); 3755 dst.snapshot = snapshot == null ? null : snapshot.copy(); 3756 dst.differential = differential == null ? null : differential.copy(); 3757 } 3758 3759 protected StructureDefinition typedCopy() { 3760 return copy(); 3761 } 3762 3763 @Override 3764 public boolean equalsDeep(Base other_) { 3765 if (!super.equalsDeep(other_)) 3766 return false; 3767 if (!(other_ instanceof StructureDefinition)) 3768 return false; 3769 StructureDefinition o = (StructureDefinition) other_; 3770 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 3771 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 3772 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 3773 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 3774 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 3775 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 3776 && compareDeep(keyword, o.keyword, true) && compareDeep(fhirVersion, o.fhirVersion, true) && compareDeep(mapping, o.mapping, true) 3777 && compareDeep(kind, o.kind, true) && compareDeep(abstract_, o.abstract_, true) && compareDeep(context, o.context, true) 3778 && compareDeep(contextInvariant, o.contextInvariant, true) && compareDeep(type, o.type, true) && compareDeep(baseDefinition, o.baseDefinition, true) 3779 && compareDeep(derivation, o.derivation, true) && compareDeep(snapshot, o.snapshot, true) && compareDeep(differential, o.differential, true) 3780 ; 3781 } 3782 3783 @Override 3784 public boolean equalsShallow(Base other_) { 3785 if (!super.equalsShallow(other_)) 3786 return false; 3787 if (!(other_ instanceof StructureDefinition)) 3788 return false; 3789 StructureDefinition o = (StructureDefinition) other_; 3790 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 3791 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 3792 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 3793 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 3794 && compareValues(fhirVersion, o.fhirVersion, true) && compareValues(kind, o.kind, true) && compareValues(abstract_, o.abstract_, true) 3795 && compareValues(contextInvariant, o.contextInvariant, true) && compareValues(type, o.type, true) && compareValues(baseDefinition, o.baseDefinition, true) 3796 && compareValues(derivation, o.derivation, true); 3797 } 3798 3799 public boolean isEmpty() { 3800 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 3801 , versionAlgorithm, name, title, status, experimental, date, publisher, contact 3802 , description, useContext, jurisdiction, purpose, copyright, copyrightLabel, keyword 3803 , fhirVersion, mapping, kind, abstract_, context, contextInvariant, type, baseDefinition 3804 , derivation, snapshot, differential); 3805 } 3806 3807 @Override 3808 public ResourceType getResourceType() { 3809 return ResourceType.StructureDefinition; 3810 } 3811 3812 /** 3813 * Search parameter: <b>context-quantity</b> 3814 * <p> 3815 * Description: <b>Multiple Resources: 3816 3817* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 3818* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 3819* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 3820* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 3821* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 3822* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 3823* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 3824* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 3825* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 3826* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 3827* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 3828* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 3829* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 3830* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 3831* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 3832* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 3833* [Library](library.html): A quantity- or range-valued use context assigned to the library 3834* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 3835* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 3836* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 3837* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 3838* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 3839* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 3840* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 3841* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 3842* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 3843* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 3844* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 3845* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 3846* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 3847</b><br> 3848 * Type: <b>quantity</b><br> 3849 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 3850 * </p> 3851 */ 3852 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 3853 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 3854 /** 3855 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 3856 * <p> 3857 * Description: <b>Multiple Resources: 3858 3859* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 3860* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 3861* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 3862* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 3863* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 3864* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 3865* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 3866* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 3867* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 3868* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 3869* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 3870* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 3871* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 3872* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 3873* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 3874* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 3875* [Library](library.html): A quantity- or range-valued use context assigned to the library 3876* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 3877* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 3878* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 3879* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 3880* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 3881* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 3882* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 3883* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 3884* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 3885* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 3886* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 3887* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 3888* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 3889</b><br> 3890 * Type: <b>quantity</b><br> 3891 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 3892 * </p> 3893 */ 3894 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 3895 3896 /** 3897 * Search parameter: <b>context-type-quantity</b> 3898 * <p> 3899 * Description: <b>Multiple Resources: 3900 3901* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 3902* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 3903* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 3904* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 3905* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 3906* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 3907* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 3908* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 3909* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 3910* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 3911* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 3912* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 3913* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 3914* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 3915* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 3916* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 3917* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 3918* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 3919* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 3920* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 3921* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 3922* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 3923* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 3924* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 3925* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 3926* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 3927* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 3928* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 3929* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3930* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3931</b><br> 3932 * Type: <b>composite</b><br> 3933 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3934 * </p> 3935 */ 3936 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 3937 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 3938 /** 3939 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 3940 * <p> 3941 * Description: <b>Multiple Resources: 3942 3943* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 3944* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 3945* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 3946* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 3947* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 3948* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 3949* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 3950* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 3951* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 3952* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 3953* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 3954* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 3955* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 3956* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 3957* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 3958* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 3959* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 3960* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 3961* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 3962* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 3963* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 3964* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 3965* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 3966* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 3967* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 3968* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 3969* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 3970* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 3971* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3972* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3973</b><br> 3974 * Type: <b>composite</b><br> 3975 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3976 * </p> 3977 */ 3978 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 3979 3980 /** 3981 * Search parameter: <b>context-type-value</b> 3982 * <p> 3983 * Description: <b>Multiple Resources: 3984 3985* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3986* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3987* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3988* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3989* [Citation](citation.html): A use context type and value assigned to the citation 3990* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3991* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3992* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3993* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3994* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3995* [Evidence](evidence.html): A use context type and value assigned to the evidence 3996* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3997* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3998* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3999* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 4000* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 4001* [Library](library.html): A use context type and value assigned to the library 4002* [Measure](measure.html): A use context type and value assigned to the measure 4003* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 4004* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 4005* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 4006* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 4007* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 4008* [Requirements](requirements.html): A use context type and value assigned to the requirements 4009* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 4010* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 4011* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 4012* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 4013* [TestScript](testscript.html): A use context type and value assigned to the test script 4014* [ValueSet](valueset.html): A use context type and value assigned to the value set 4015</b><br> 4016 * Type: <b>composite</b><br> 4017 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4018 * </p> 4019 */ 4020 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 4021 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 4022 /** 4023 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 4024 * <p> 4025 * Description: <b>Multiple Resources: 4026 4027* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 4028* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 4029* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 4030* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 4031* [Citation](citation.html): A use context type and value assigned to the citation 4032* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 4033* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 4034* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 4035* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 4036* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 4037* [Evidence](evidence.html): A use context type and value assigned to the evidence 4038* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 4039* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 4040* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 4041* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 4042* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 4043* [Library](library.html): A use context type and value assigned to the library 4044* [Measure](measure.html): A use context type and value assigned to the measure 4045* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 4046* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 4047* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 4048* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 4049* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 4050* [Requirements](requirements.html): A use context type and value assigned to the requirements 4051* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 4052* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 4053* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 4054* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 4055* [TestScript](testscript.html): A use context type and value assigned to the test script 4056* [ValueSet](valueset.html): A use context type and value assigned to the value set 4057</b><br> 4058 * Type: <b>composite</b><br> 4059 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4060 * </p> 4061 */ 4062 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 4063 4064 /** 4065 * Search parameter: <b>context-type</b> 4066 * <p> 4067 * Description: <b>Multiple Resources: 4068 4069* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 4070* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 4071* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 4072* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 4073* [Citation](citation.html): A type of use context assigned to the citation 4074* [CodeSystem](codesystem.html): A type of use context assigned to the code system 4075* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 4076* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 4077* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 4078* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 4079* [Evidence](evidence.html): A type of use context assigned to the evidence 4080* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 4081* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 4082* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 4083* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 4084* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 4085* [Library](library.html): A type of use context assigned to the library 4086* [Measure](measure.html): A type of use context assigned to the measure 4087* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 4088* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 4089* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 4090* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 4091* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 4092* [Requirements](requirements.html): A type of use context assigned to the requirements 4093* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 4094* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 4095* [StructureMap](structuremap.html): A type of use context assigned to the structure map 4096* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 4097* [TestScript](testscript.html): A type of use context assigned to the test script 4098* [ValueSet](valueset.html): A type of use context assigned to the value set 4099</b><br> 4100 * Type: <b>token</b><br> 4101 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 4102 * </p> 4103 */ 4104 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 4105 public static final String SP_CONTEXT_TYPE = "context-type"; 4106 /** 4107 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 4108 * <p> 4109 * Description: <b>Multiple Resources: 4110 4111* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 4112* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 4113* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 4114* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 4115* [Citation](citation.html): A type of use context assigned to the citation 4116* [CodeSystem](codesystem.html): A type of use context assigned to the code system 4117* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 4118* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 4119* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 4120* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 4121* [Evidence](evidence.html): A type of use context assigned to the evidence 4122* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 4123* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 4124* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 4125* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 4126* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 4127* [Library](library.html): A type of use context assigned to the library 4128* [Measure](measure.html): A type of use context assigned to the measure 4129* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 4130* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 4131* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 4132* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 4133* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 4134* [Requirements](requirements.html): A type of use context assigned to the requirements 4135* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 4136* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 4137* [StructureMap](structuremap.html): A type of use context assigned to the structure map 4138* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 4139* [TestScript](testscript.html): A type of use context assigned to the test script 4140* [ValueSet](valueset.html): A type of use context assigned to the value set 4141</b><br> 4142 * Type: <b>token</b><br> 4143 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 4144 * </p> 4145 */ 4146 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 4147 4148 /** 4149 * Search parameter: <b>context</b> 4150 * <p> 4151 * Description: <b>Multiple Resources: 4152 4153* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 4154* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 4155* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 4156* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 4157* [Citation](citation.html): A use context assigned to the citation 4158* [CodeSystem](codesystem.html): A use context assigned to the code system 4159* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 4160* [ConceptMap](conceptmap.html): A use context assigned to the concept map 4161* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 4162* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 4163* [Evidence](evidence.html): A use context assigned to the evidence 4164* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 4165* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 4166* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 4167* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 4168* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 4169* [Library](library.html): A use context assigned to the library 4170* [Measure](measure.html): A use context assigned to the measure 4171* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 4172* [NamingSystem](namingsystem.html): A use context assigned to the naming system 4173* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 4174* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 4175* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 4176* [Requirements](requirements.html): A use context assigned to the requirements 4177* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 4178* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 4179* [StructureMap](structuremap.html): A use context assigned to the structure map 4180* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 4181* [TestScript](testscript.html): A use context assigned to the test script 4182* [ValueSet](valueset.html): A use context assigned to the value set 4183</b><br> 4184 * Type: <b>token</b><br> 4185 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 4186 * </p> 4187 */ 4188 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 4189 public static final String SP_CONTEXT = "context"; 4190 /** 4191 * <b>Fluent Client</b> search parameter constant for <b>context</b> 4192 * <p> 4193 * Description: <b>Multiple Resources: 4194 4195* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 4196* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 4197* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 4198* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 4199* [Citation](citation.html): A use context assigned to the citation 4200* [CodeSystem](codesystem.html): A use context assigned to the code system 4201* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 4202* [ConceptMap](conceptmap.html): A use context assigned to the concept map 4203* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 4204* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 4205* [Evidence](evidence.html): A use context assigned to the evidence 4206* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 4207* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 4208* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 4209* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 4210* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 4211* [Library](library.html): A use context assigned to the library 4212* [Measure](measure.html): A use context assigned to the measure 4213* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 4214* [NamingSystem](namingsystem.html): A use context assigned to the naming system 4215* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 4216* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 4217* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 4218* [Requirements](requirements.html): A use context assigned to the requirements 4219* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 4220* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 4221* [StructureMap](structuremap.html): A use context assigned to the structure map 4222* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 4223* [TestScript](testscript.html): A use context assigned to the test script 4224* [ValueSet](valueset.html): A use context assigned to the value set 4225</b><br> 4226 * Type: <b>token</b><br> 4227 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 4228 * </p> 4229 */ 4230 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 4231 4232 /** 4233 * Search parameter: <b>date</b> 4234 * <p> 4235 * Description: <b>Multiple Resources: 4236 4237* [ActivityDefinition](activitydefinition.html): The activity definition publication date 4238* [ActorDefinition](actordefinition.html): The Actor Definition publication date 4239* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 4240* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 4241* [Citation](citation.html): The citation publication date 4242* [CodeSystem](codesystem.html): The code system publication date 4243* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 4244* [ConceptMap](conceptmap.html): The concept map publication date 4245* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 4246* [EventDefinition](eventdefinition.html): The event definition publication date 4247* [Evidence](evidence.html): The evidence publication date 4248* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 4249* [ExampleScenario](examplescenario.html): The example scenario publication date 4250* [GraphDefinition](graphdefinition.html): The graph definition publication date 4251* [ImplementationGuide](implementationguide.html): The implementation guide publication date 4252* [Library](library.html): The library publication date 4253* [Measure](measure.html): The measure publication date 4254* [MessageDefinition](messagedefinition.html): The message definition publication date 4255* [NamingSystem](namingsystem.html): The naming system publication date 4256* [OperationDefinition](operationdefinition.html): The operation definition publication date 4257* [PlanDefinition](plandefinition.html): The plan definition publication date 4258* [Questionnaire](questionnaire.html): The questionnaire publication date 4259* [Requirements](requirements.html): The requirements publication date 4260* [SearchParameter](searchparameter.html): The search parameter publication date 4261* [StructureDefinition](structuredefinition.html): The structure definition publication date 4262* [StructureMap](structuremap.html): The structure map publication date 4263* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 4264* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 4265* [TestScript](testscript.html): The test script publication date 4266* [ValueSet](valueset.html): The value set publication date 4267</b><br> 4268 * Type: <b>date</b><br> 4269 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 4270 * </p> 4271 */ 4272 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 4273 public static final String SP_DATE = "date"; 4274 /** 4275 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4276 * <p> 4277 * Description: <b>Multiple Resources: 4278 4279* [ActivityDefinition](activitydefinition.html): The activity definition publication date 4280* [ActorDefinition](actordefinition.html): The Actor Definition publication date 4281* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 4282* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 4283* [Citation](citation.html): The citation publication date 4284* [CodeSystem](codesystem.html): The code system publication date 4285* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 4286* [ConceptMap](conceptmap.html): The concept map publication date 4287* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 4288* [EventDefinition](eventdefinition.html): The event definition publication date 4289* [Evidence](evidence.html): The evidence publication date 4290* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 4291* [ExampleScenario](examplescenario.html): The example scenario publication date 4292* [GraphDefinition](graphdefinition.html): The graph definition publication date 4293* [ImplementationGuide](implementationguide.html): The implementation guide publication date 4294* [Library](library.html): The library publication date 4295* [Measure](measure.html): The measure publication date 4296* [MessageDefinition](messagedefinition.html): The message definition publication date 4297* [NamingSystem](namingsystem.html): The naming system publication date 4298* [OperationDefinition](operationdefinition.html): The operation definition publication date 4299* [PlanDefinition](plandefinition.html): The plan definition publication date 4300* [Questionnaire](questionnaire.html): The questionnaire publication date 4301* [Requirements](requirements.html): The requirements publication date 4302* [SearchParameter](searchparameter.html): The search parameter publication date 4303* [StructureDefinition](structuredefinition.html): The structure definition publication date 4304* [StructureMap](structuremap.html): The structure map publication date 4305* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 4306* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 4307* [TestScript](testscript.html): The test script publication date 4308* [ValueSet](valueset.html): The value set publication date 4309</b><br> 4310 * Type: <b>date</b><br> 4311 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 4312 * </p> 4313 */ 4314 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4315 4316 /** 4317 * Search parameter: <b>description</b> 4318 * <p> 4319 * Description: <b>Multiple Resources: 4320 4321* [ActivityDefinition](activitydefinition.html): The description of the activity definition 4322* [ActorDefinition](actordefinition.html): The description of the Actor Definition 4323* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 4324* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 4325* [Citation](citation.html): The description of the citation 4326* [CodeSystem](codesystem.html): The description of the code system 4327* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 4328* [ConceptMap](conceptmap.html): The description of the concept map 4329* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 4330* [EventDefinition](eventdefinition.html): The description of the event definition 4331* [Evidence](evidence.html): The description of the evidence 4332* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 4333* [GraphDefinition](graphdefinition.html): The description of the graph definition 4334* [ImplementationGuide](implementationguide.html): The description of the implementation guide 4335* [Library](library.html): The description of the library 4336* [Measure](measure.html): The description of the measure 4337* [MessageDefinition](messagedefinition.html): The description of the message definition 4338* [NamingSystem](namingsystem.html): The description of the naming system 4339* [OperationDefinition](operationdefinition.html): The description of the operation definition 4340* [PlanDefinition](plandefinition.html): The description of the plan definition 4341* [Questionnaire](questionnaire.html): The description of the questionnaire 4342* [Requirements](requirements.html): The description of the requirements 4343* [SearchParameter](searchparameter.html): The description of the search parameter 4344* [StructureDefinition](structuredefinition.html): The description of the structure definition 4345* [StructureMap](structuremap.html): The description of the structure map 4346* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 4347* [TestScript](testscript.html): The description of the test script 4348* [ValueSet](valueset.html): The description of the value set 4349</b><br> 4350 * Type: <b>string</b><br> 4351 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 4352 * </p> 4353 */ 4354 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 4355 public static final String SP_DESCRIPTION = "description"; 4356 /** 4357 * <b>Fluent Client</b> search parameter constant for <b>description</b> 4358 * <p> 4359 * Description: <b>Multiple Resources: 4360 4361* [ActivityDefinition](activitydefinition.html): The description of the activity definition 4362* [ActorDefinition](actordefinition.html): The description of the Actor Definition 4363* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 4364* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 4365* [Citation](citation.html): The description of the citation 4366* [CodeSystem](codesystem.html): The description of the code system 4367* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 4368* [ConceptMap](conceptmap.html): The description of the concept map 4369* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 4370* [EventDefinition](eventdefinition.html): The description of the event definition 4371* [Evidence](evidence.html): The description of the evidence 4372* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 4373* [GraphDefinition](graphdefinition.html): The description of the graph definition 4374* [ImplementationGuide](implementationguide.html): The description of the implementation guide 4375* [Library](library.html): The description of the library 4376* [Measure](measure.html): The description of the measure 4377* [MessageDefinition](messagedefinition.html): The description of the message definition 4378* [NamingSystem](namingsystem.html): The description of the naming system 4379* [OperationDefinition](operationdefinition.html): The description of the operation definition 4380* [PlanDefinition](plandefinition.html): The description of the plan definition 4381* [Questionnaire](questionnaire.html): The description of the questionnaire 4382* [Requirements](requirements.html): The description of the requirements 4383* [SearchParameter](searchparameter.html): The description of the search parameter 4384* [StructureDefinition](structuredefinition.html): The description of the structure definition 4385* [StructureMap](structuremap.html): The description of the structure map 4386* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 4387* [TestScript](testscript.html): The description of the test script 4388* [ValueSet](valueset.html): The description of the value set 4389</b><br> 4390 * Type: <b>string</b><br> 4391 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 4392 * </p> 4393 */ 4394 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 4395 4396 /** 4397 * Search parameter: <b>identifier</b> 4398 * <p> 4399 * Description: <b>Multiple Resources: 4400 4401* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4402* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4403* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4404* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4405* [Citation](citation.html): External identifier for the citation 4406* [CodeSystem](codesystem.html): External identifier for the code system 4407* [ConceptMap](conceptmap.html): External identifier for the concept map 4408* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4409* [EventDefinition](eventdefinition.html): External identifier for the event definition 4410* [Evidence](evidence.html): External identifier for the evidence 4411* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4412* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4413* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4414* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4415* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4416* [Library](library.html): External identifier for the library 4417* [Measure](measure.html): External identifier for the measure 4418* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4419* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4420* [NamingSystem](namingsystem.html): External identifier for the naming system 4421* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4422* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4423* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4424* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4425* [Requirements](requirements.html): External identifier for the requirements 4426* [SearchParameter](searchparameter.html): External identifier for the search parameter 4427* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4428* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4429* [StructureMap](structuremap.html): External identifier for the structure map 4430* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4431* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4432* [TestPlan](testplan.html): An identifier for the test plan 4433* [TestScript](testscript.html): External identifier for the test script 4434* [ValueSet](valueset.html): External identifier for the value set 4435</b><br> 4436 * Type: <b>token</b><br> 4437 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4438 * </p> 4439 */ 4440 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 4441 public static final String SP_IDENTIFIER = "identifier"; 4442 /** 4443 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4444 * <p> 4445 * Description: <b>Multiple Resources: 4446 4447* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4448* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4449* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4450* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4451* [Citation](citation.html): External identifier for the citation 4452* [CodeSystem](codesystem.html): External identifier for the code system 4453* [ConceptMap](conceptmap.html): External identifier for the concept map 4454* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4455* [EventDefinition](eventdefinition.html): External identifier for the event definition 4456* [Evidence](evidence.html): External identifier for the evidence 4457* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4458* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4459* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4460* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4461* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4462* [Library](library.html): External identifier for the library 4463* [Measure](measure.html): External identifier for the measure 4464* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4465* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4466* [NamingSystem](namingsystem.html): External identifier for the naming system 4467* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4468* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4469* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4470* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4471* [Requirements](requirements.html): External identifier for the requirements 4472* [SearchParameter](searchparameter.html): External identifier for the search parameter 4473* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4474* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4475* [StructureMap](structuremap.html): External identifier for the structure map 4476* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4477* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4478* [TestPlan](testplan.html): An identifier for the test plan 4479* [TestScript](testscript.html): External identifier for the test script 4480* [ValueSet](valueset.html): External identifier for the value set 4481</b><br> 4482 * Type: <b>token</b><br> 4483 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4484 * </p> 4485 */ 4486 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4487 4488 /** 4489 * Search parameter: <b>jurisdiction</b> 4490 * <p> 4491 * Description: <b>Multiple Resources: 4492 4493* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 4494* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 4495* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 4496* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 4497* [Citation](citation.html): Intended jurisdiction for the citation 4498* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 4499* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 4500* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 4501* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 4502* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 4503* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 4504* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 4505* [Library](library.html): Intended jurisdiction for the library 4506* [Measure](measure.html): Intended jurisdiction for the measure 4507* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 4508* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 4509* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 4510* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 4511* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 4512* [Requirements](requirements.html): Intended jurisdiction for the requirements 4513* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 4514* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 4515* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 4516* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 4517* [TestScript](testscript.html): Intended jurisdiction for the test script 4518* [ValueSet](valueset.html): Intended jurisdiction for the value set 4519</b><br> 4520 * Type: <b>token</b><br> 4521 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 4522 * </p> 4523 */ 4524 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 4525 public static final String SP_JURISDICTION = "jurisdiction"; 4526 /** 4527 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 4528 * <p> 4529 * Description: <b>Multiple Resources: 4530 4531* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 4532* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 4533* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 4534* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 4535* [Citation](citation.html): Intended jurisdiction for the citation 4536* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 4537* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 4538* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 4539* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 4540* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 4541* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 4542* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 4543* [Library](library.html): Intended jurisdiction for the library 4544* [Measure](measure.html): Intended jurisdiction for the measure 4545* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 4546* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 4547* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 4548* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 4549* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 4550* [Requirements](requirements.html): Intended jurisdiction for the requirements 4551* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 4552* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 4553* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 4554* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 4555* [TestScript](testscript.html): Intended jurisdiction for the test script 4556* [ValueSet](valueset.html): Intended jurisdiction for the value set 4557</b><br> 4558 * Type: <b>token</b><br> 4559 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 4560 * </p> 4561 */ 4562 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 4563 4564 /** 4565 * Search parameter: <b>name</b> 4566 * <p> 4567 * Description: <b>Multiple Resources: 4568 4569* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 4570* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 4571* [Citation](citation.html): Computationally friendly name of the citation 4572* [CodeSystem](codesystem.html): Computationally friendly name of the code system 4573* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 4574* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 4575* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 4576* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 4577* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 4578* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 4579* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 4580* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 4581* [Library](library.html): Computationally friendly name of the library 4582* [Measure](measure.html): Computationally friendly name of the measure 4583* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 4584* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 4585* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 4586* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 4587* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 4588* [Requirements](requirements.html): Computationally friendly name of the requirements 4589* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 4590* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 4591* [StructureMap](structuremap.html): Computationally friendly name of the structure map 4592* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 4593* [TestScript](testscript.html): Computationally friendly name of the test script 4594* [ValueSet](valueset.html): Computationally friendly name of the value set 4595</b><br> 4596 * Type: <b>string</b><br> 4597 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 4598 * </p> 4599 */ 4600 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 4601 public static final String SP_NAME = "name"; 4602 /** 4603 * <b>Fluent Client</b> search parameter constant for <b>name</b> 4604 * <p> 4605 * Description: <b>Multiple Resources: 4606 4607* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 4608* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 4609* [Citation](citation.html): Computationally friendly name of the citation 4610* [CodeSystem](codesystem.html): Computationally friendly name of the code system 4611* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 4612* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 4613* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 4614* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 4615* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 4616* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 4617* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 4618* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 4619* [Library](library.html): Computationally friendly name of the library 4620* [Measure](measure.html): Computationally friendly name of the measure 4621* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 4622* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 4623* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 4624* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 4625* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 4626* [Requirements](requirements.html): Computationally friendly name of the requirements 4627* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 4628* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 4629* [StructureMap](structuremap.html): Computationally friendly name of the structure map 4630* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 4631* [TestScript](testscript.html): Computationally friendly name of the test script 4632* [ValueSet](valueset.html): Computationally friendly name of the value set 4633</b><br> 4634 * Type: <b>string</b><br> 4635 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 4636 * </p> 4637 */ 4638 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 4639 4640 /** 4641 * Search parameter: <b>publisher</b> 4642 * <p> 4643 * Description: <b>Multiple Resources: 4644 4645* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 4646* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 4647* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 4648* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 4649* [Citation](citation.html): Name of the publisher of the citation 4650* [CodeSystem](codesystem.html): Name of the publisher of the code system 4651* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 4652* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 4653* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 4654* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 4655* [Evidence](evidence.html): Name of the publisher of the evidence 4656* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 4657* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 4658* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 4659* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 4660* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 4661* [Library](library.html): Name of the publisher of the library 4662* [Measure](measure.html): Name of the publisher of the measure 4663* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 4664* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 4665* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 4666* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 4667* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 4668* [Requirements](requirements.html): Name of the publisher of the requirements 4669* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 4670* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 4671* [StructureMap](structuremap.html): Name of the publisher of the structure map 4672* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 4673* [TestScript](testscript.html): Name of the publisher of the test script 4674* [ValueSet](valueset.html): Name of the publisher of the value set 4675</b><br> 4676 * Type: <b>string</b><br> 4677 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 4678 * </p> 4679 */ 4680 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 4681 public static final String SP_PUBLISHER = "publisher"; 4682 /** 4683 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 4684 * <p> 4685 * Description: <b>Multiple Resources: 4686 4687* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 4688* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 4689* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 4690* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 4691* [Citation](citation.html): Name of the publisher of the citation 4692* [CodeSystem](codesystem.html): Name of the publisher of the code system 4693* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 4694* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 4695* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 4696* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 4697* [Evidence](evidence.html): Name of the publisher of the evidence 4698* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 4699* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 4700* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 4701* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 4702* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 4703* [Library](library.html): Name of the publisher of the library 4704* [Measure](measure.html): Name of the publisher of the measure 4705* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 4706* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 4707* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 4708* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 4709* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 4710* [Requirements](requirements.html): Name of the publisher of the requirements 4711* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 4712* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 4713* [StructureMap](structuremap.html): Name of the publisher of the structure map 4714* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 4715* [TestScript](testscript.html): Name of the publisher of the test script 4716* [ValueSet](valueset.html): Name of the publisher of the value set 4717</b><br> 4718 * Type: <b>string</b><br> 4719 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 4720 * </p> 4721 */ 4722 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 4723 4724 /** 4725 * Search parameter: <b>status</b> 4726 * <p> 4727 * Description: <b>Multiple Resources: 4728 4729* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4730* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4731* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4732* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4733* [Citation](citation.html): The current status of the citation 4734* [CodeSystem](codesystem.html): The current status of the code system 4735* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4736* [ConceptMap](conceptmap.html): The current status of the concept map 4737* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4738* [EventDefinition](eventdefinition.html): The current status of the event definition 4739* [Evidence](evidence.html): The current status of the evidence 4740* [EvidenceReport](evidencereport.html): The current status of the evidence report 4741* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4742* [ExampleScenario](examplescenario.html): The current status of the example scenario 4743* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4744* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4745* [Library](library.html): The current status of the library 4746* [Measure](measure.html): The current status of the measure 4747* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4748* [MessageDefinition](messagedefinition.html): The current status of the message definition 4749* [NamingSystem](namingsystem.html): The current status of the naming system 4750* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4751* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4752* [PlanDefinition](plandefinition.html): The current status of the plan definition 4753* [Questionnaire](questionnaire.html): The current status of the questionnaire 4754* [Requirements](requirements.html): The current status of the requirements 4755* [SearchParameter](searchparameter.html): The current status of the search parameter 4756* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4757* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4758* [StructureMap](structuremap.html): The current status of the structure map 4759* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4760* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4761* [TestPlan](testplan.html): The current status of the test plan 4762* [TestScript](testscript.html): The current status of the test script 4763* [ValueSet](valueset.html): The current status of the value set 4764</b><br> 4765 * Type: <b>token</b><br> 4766 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4767 * </p> 4768 */ 4769 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 4770 public static final String SP_STATUS = "status"; 4771 /** 4772 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4773 * <p> 4774 * Description: <b>Multiple Resources: 4775 4776* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4777* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4778* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4779* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4780* [Citation](citation.html): The current status of the citation 4781* [CodeSystem](codesystem.html): The current status of the code system 4782* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4783* [ConceptMap](conceptmap.html): The current status of the concept map 4784* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4785* [EventDefinition](eventdefinition.html): The current status of the event definition 4786* [Evidence](evidence.html): The current status of the evidence 4787* [EvidenceReport](evidencereport.html): The current status of the evidence report 4788* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4789* [ExampleScenario](examplescenario.html): The current status of the example scenario 4790* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4791* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4792* [Library](library.html): The current status of the library 4793* [Measure](measure.html): The current status of the measure 4794* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4795* [MessageDefinition](messagedefinition.html): The current status of the message definition 4796* [NamingSystem](namingsystem.html): The current status of the naming system 4797* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4798* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4799* [PlanDefinition](plandefinition.html): The current status of the plan definition 4800* [Questionnaire](questionnaire.html): The current status of the questionnaire 4801* [Requirements](requirements.html): The current status of the requirements 4802* [SearchParameter](searchparameter.html): The current status of the search parameter 4803* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4804* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4805* [StructureMap](structuremap.html): The current status of the structure map 4806* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4807* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4808* [TestPlan](testplan.html): The current status of the test plan 4809* [TestScript](testscript.html): The current status of the test script 4810* [ValueSet](valueset.html): The current status of the value set 4811</b><br> 4812 * Type: <b>token</b><br> 4813 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4814 * </p> 4815 */ 4816 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4817 4818 /** 4819 * Search parameter: <b>title</b> 4820 * <p> 4821 * Description: <b>Multiple Resources: 4822 4823* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 4824* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 4825* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 4826* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 4827* [Citation](citation.html): The human-friendly name of the citation 4828* [CodeSystem](codesystem.html): The human-friendly name of the code system 4829* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 4830* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 4831* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 4832* [Evidence](evidence.html): The human-friendly name of the evidence 4833* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 4834* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 4835* [Library](library.html): The human-friendly name of the library 4836* [Measure](measure.html): The human-friendly name of the measure 4837* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 4838* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 4839* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 4840* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 4841* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 4842* [Requirements](requirements.html): The human-friendly name of the requirements 4843* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 4844* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 4845* [StructureMap](structuremap.html): The human-friendly name of the structure map 4846* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 4847* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 4848* [TestScript](testscript.html): The human-friendly name of the test script 4849* [ValueSet](valueset.html): The human-friendly name of the value set 4850</b><br> 4851 * Type: <b>string</b><br> 4852 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 4853 * </p> 4854 */ 4855 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 4856 public static final String SP_TITLE = "title"; 4857 /** 4858 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4859 * <p> 4860 * Description: <b>Multiple Resources: 4861 4862* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 4863* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 4864* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 4865* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 4866* [Citation](citation.html): The human-friendly name of the citation 4867* [CodeSystem](codesystem.html): The human-friendly name of the code system 4868* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 4869* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 4870* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 4871* [Evidence](evidence.html): The human-friendly name of the evidence 4872* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 4873* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 4874* [Library](library.html): The human-friendly name of the library 4875* [Measure](measure.html): The human-friendly name of the measure 4876* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 4877* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 4878* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 4879* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 4880* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 4881* [Requirements](requirements.html): The human-friendly name of the requirements 4882* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 4883* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 4884* [StructureMap](structuremap.html): The human-friendly name of the structure map 4885* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 4886* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 4887* [TestScript](testscript.html): The human-friendly name of the test script 4888* [ValueSet](valueset.html): The human-friendly name of the value set 4889</b><br> 4890 * Type: <b>string</b><br> 4891 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 4892 * </p> 4893 */ 4894 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 4895 4896 /** 4897 * Search parameter: <b>url</b> 4898 * <p> 4899 * Description: <b>Multiple Resources: 4900 4901* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4902* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4903* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4904* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4905* [Citation](citation.html): The uri that identifies the citation 4906* [CodeSystem](codesystem.html): The uri that identifies the code system 4907* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4908* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4909* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4910* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4911* [Evidence](evidence.html): The uri that identifies the evidence 4912* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4913* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4914* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4915* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4916* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4917* [Library](library.html): The uri that identifies the library 4918* [Measure](measure.html): The uri that identifies the measure 4919* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4920* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4921* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4922* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4923* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4924* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4925* [Requirements](requirements.html): The uri that identifies the requirements 4926* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4927* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4928* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4929* [StructureMap](structuremap.html): The uri that identifies the structure map 4930* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4931* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4932* [TestPlan](testplan.html): The uri that identifies the test plan 4933* [TestScript](testscript.html): The uri that identifies the test script 4934* [ValueSet](valueset.html): The uri that identifies the value set 4935</b><br> 4936 * Type: <b>uri</b><br> 4937 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4938 * </p> 4939 */ 4940 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 4941 public static final String SP_URL = "url"; 4942 /** 4943 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4944 * <p> 4945 * Description: <b>Multiple Resources: 4946 4947* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4948* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4949* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4950* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4951* [Citation](citation.html): The uri that identifies the citation 4952* [CodeSystem](codesystem.html): The uri that identifies the code system 4953* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4954* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4955* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4956* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4957* [Evidence](evidence.html): The uri that identifies the evidence 4958* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4959* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4960* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4961* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4962* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4963* [Library](library.html): The uri that identifies the library 4964* [Measure](measure.html): The uri that identifies the measure 4965* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4966* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4967* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4968* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4969* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4970* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4971* [Requirements](requirements.html): The uri that identifies the requirements 4972* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4973* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4974* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4975* [StructureMap](structuremap.html): The uri that identifies the structure map 4976* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4977* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4978* [TestPlan](testplan.html): The uri that identifies the test plan 4979* [TestScript](testscript.html): The uri that identifies the test script 4980* [ValueSet](valueset.html): The uri that identifies the value set 4981</b><br> 4982 * Type: <b>uri</b><br> 4983 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4984 * </p> 4985 */ 4986 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4987 4988 /** 4989 * Search parameter: <b>version</b> 4990 * <p> 4991 * Description: <b>Multiple Resources: 4992 4993* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4994* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4995* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4996* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4997* [Citation](citation.html): The business version of the citation 4998* [CodeSystem](codesystem.html): The business version of the code system 4999* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 5000* [ConceptMap](conceptmap.html): The business version of the concept map 5001* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 5002* [EventDefinition](eventdefinition.html): The business version of the event definition 5003* [Evidence](evidence.html): The business version of the evidence 5004* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 5005* [ExampleScenario](examplescenario.html): The business version of the example scenario 5006* [GraphDefinition](graphdefinition.html): The business version of the graph definition 5007* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 5008* [Library](library.html): The business version of the library 5009* [Measure](measure.html): The business version of the measure 5010* [MessageDefinition](messagedefinition.html): The business version of the message definition 5011* [NamingSystem](namingsystem.html): The business version of the naming system 5012* [OperationDefinition](operationdefinition.html): The business version of the operation definition 5013* [PlanDefinition](plandefinition.html): The business version of the plan definition 5014* [Questionnaire](questionnaire.html): The business version of the questionnaire 5015* [Requirements](requirements.html): The business version of the requirements 5016* [SearchParameter](searchparameter.html): The business version of the search parameter 5017* [StructureDefinition](structuredefinition.html): The business version of the structure definition 5018* [StructureMap](structuremap.html): The business version of the structure map 5019* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 5020* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 5021* [TestScript](testscript.html): The business version of the test script 5022* [ValueSet](valueset.html): The business version of the value set 5023</b><br> 5024 * Type: <b>token</b><br> 5025 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 5026 * </p> 5027 */ 5028 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 5029 public static final String SP_VERSION = "version"; 5030 /** 5031 * <b>Fluent Client</b> search parameter constant for <b>version</b> 5032 * <p> 5033 * Description: <b>Multiple Resources: 5034 5035* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 5036* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 5037* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 5038* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 5039* [Citation](citation.html): The business version of the citation 5040* [CodeSystem](codesystem.html): The business version of the code system 5041* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 5042* [ConceptMap](conceptmap.html): The business version of the concept map 5043* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 5044* [EventDefinition](eventdefinition.html): The business version of the event definition 5045* [Evidence](evidence.html): The business version of the evidence 5046* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 5047* [ExampleScenario](examplescenario.html): The business version of the example scenario 5048* [GraphDefinition](graphdefinition.html): The business version of the graph definition 5049* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 5050* [Library](library.html): The business version of the library 5051* [Measure](measure.html): The business version of the measure 5052* [MessageDefinition](messagedefinition.html): The business version of the message definition 5053* [NamingSystem](namingsystem.html): The business version of the naming system 5054* [OperationDefinition](operationdefinition.html): The business version of the operation definition 5055* [PlanDefinition](plandefinition.html): The business version of the plan definition 5056* [Questionnaire](questionnaire.html): The business version of the questionnaire 5057* [Requirements](requirements.html): The business version of the requirements 5058* [SearchParameter](searchparameter.html): The business version of the search parameter 5059* [StructureDefinition](structuredefinition.html): The business version of the structure definition 5060* [StructureMap](structuremap.html): The business version of the structure map 5061* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 5062* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 5063* [TestScript](testscript.html): The business version of the test script 5064* [ValueSet](valueset.html): The business version of the value set 5065</b><br> 5066 * Type: <b>token</b><br> 5067 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 5068 * </p> 5069 */ 5070 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 5071 5072 /** 5073 * Search parameter: <b>abstract</b> 5074 * <p> 5075 * Description: <b>Whether the structure is abstract</b><br> 5076 * Type: <b>token</b><br> 5077 * Path: <b>StructureDefinition.abstract</b><br> 5078 * </p> 5079 */ 5080 @SearchParamDefinition(name="abstract", path="StructureDefinition.abstract", description="Whether the structure is abstract", type="token" ) 5081 public static final String SP_ABSTRACT = "abstract"; 5082 /** 5083 * <b>Fluent Client</b> search parameter constant for <b>abstract</b> 5084 * <p> 5085 * Description: <b>Whether the structure is abstract</b><br> 5086 * Type: <b>token</b><br> 5087 * Path: <b>StructureDefinition.abstract</b><br> 5088 * </p> 5089 */ 5090 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ABSTRACT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ABSTRACT); 5091 5092 /** 5093 * Search parameter: <b>base-path</b> 5094 * <p> 5095 * Description: <b>Path that identifies the base element</b><br> 5096 * Type: <b>token</b><br> 5097 * Path: <b>StructureDefinition.snapshot.element.base.path | StructureDefinition.differential.element.base.path</b><br> 5098 * </p> 5099 */ 5100 @SearchParamDefinition(name="base-path", path="StructureDefinition.snapshot.element.base.path | StructureDefinition.differential.element.base.path", description="Path that identifies the base element", type="token" ) 5101 public static final String SP_BASE_PATH = "base-path"; 5102 /** 5103 * <b>Fluent Client</b> search parameter constant for <b>base-path</b> 5104 * <p> 5105 * Description: <b>Path that identifies the base element</b><br> 5106 * Type: <b>token</b><br> 5107 * Path: <b>StructureDefinition.snapshot.element.base.path | StructureDefinition.differential.element.base.path</b><br> 5108 * </p> 5109 */ 5110 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BASE_PATH = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BASE_PATH); 5111 5112 /** 5113 * Search parameter: <b>base</b> 5114 * <p> 5115 * Description: <b>Definition that this type is constrained/specialized from</b><br> 5116 * Type: <b>reference</b><br> 5117 * Path: <b>StructureDefinition.baseDefinition</b><br> 5118 * </p> 5119 */ 5120 @SearchParamDefinition(name="base", path="StructureDefinition.baseDefinition", description="Definition that this type is constrained/specialized from", type="reference", target={StructureDefinition.class } ) 5121 public static final String SP_BASE = "base"; 5122 /** 5123 * <b>Fluent Client</b> search parameter constant for <b>base</b> 5124 * <p> 5125 * Description: <b>Definition that this type is constrained/specialized from</b><br> 5126 * Type: <b>reference</b><br> 5127 * Path: <b>StructureDefinition.baseDefinition</b><br> 5128 * </p> 5129 */ 5130 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASE); 5131 5132/** 5133 * Constant for fluent queries to be used to add include statements. Specifies 5134 * the path value of "<b>StructureDefinition:base</b>". 5135 */ 5136 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASE = new ca.uhn.fhir.model.api.Include("StructureDefinition:base").toLocked(); 5137 5138 /** 5139 * Search parameter: <b>derivation</b> 5140 * <p> 5141 * Description: <b>specialization | constraint - How relates to base definition</b><br> 5142 * Type: <b>token</b><br> 5143 * Path: <b>StructureDefinition.derivation</b><br> 5144 * </p> 5145 */ 5146 @SearchParamDefinition(name="derivation", path="StructureDefinition.derivation", description="specialization | constraint - How relates to base definition", type="token" ) 5147 public static final String SP_DERIVATION = "derivation"; 5148 /** 5149 * <b>Fluent Client</b> search parameter constant for <b>derivation</b> 5150 * <p> 5151 * Description: <b>specialization | constraint - How relates to base definition</b><br> 5152 * Type: <b>token</b><br> 5153 * Path: <b>StructureDefinition.derivation</b><br> 5154 * </p> 5155 */ 5156 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DERIVATION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DERIVATION); 5157 5158 /** 5159 * Search parameter: <b>experimental</b> 5160 * <p> 5161 * Description: <b>For testing purposes, not real usage</b><br> 5162 * Type: <b>token</b><br> 5163 * Path: <b>StructureDefinition.experimental</b><br> 5164 * </p> 5165 */ 5166 @SearchParamDefinition(name="experimental", path="StructureDefinition.experimental", description="For testing purposes, not real usage", type="token" ) 5167 public static final String SP_EXPERIMENTAL = "experimental"; 5168 /** 5169 * <b>Fluent Client</b> search parameter constant for <b>experimental</b> 5170 * <p> 5171 * Description: <b>For testing purposes, not real usage</b><br> 5172 * Type: <b>token</b><br> 5173 * Path: <b>StructureDefinition.experimental</b><br> 5174 * </p> 5175 */ 5176 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXPERIMENTAL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EXPERIMENTAL); 5177 5178 /** 5179 * Search parameter: <b>ext-context-expression</b> 5180 * <p> 5181 * Description: <b>An expression of extension context assigned to the structure definition</b><br> 5182 * Type: <b>token</b><br> 5183 * Path: <b>StructureDefinition.context.expression</b><br> 5184 * </p> 5185 */ 5186 @SearchParamDefinition(name="ext-context-expression", path="StructureDefinition.context.expression", description="An expression of extension context assigned to the structure definition", type="token" ) 5187 public static final String SP_EXT_CONTEXT_EXPRESSION = "ext-context-expression"; 5188 /** 5189 * <b>Fluent Client</b> search parameter constant for <b>ext-context-expression</b> 5190 * <p> 5191 * Description: <b>An expression of extension context assigned to the structure definition</b><br> 5192 * Type: <b>token</b><br> 5193 * Path: <b>StructureDefinition.context.expression</b><br> 5194 * </p> 5195 */ 5196 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXT_CONTEXT_EXPRESSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EXT_CONTEXT_EXPRESSION); 5197 5198 /** 5199 * Search parameter: <b>ext-context-type</b> 5200 * <p> 5201 * Description: <b>A type of extension context assigned to the structure definition</b><br> 5202 * Type: <b>token</b><br> 5203 * Path: <b>StructureDefinition.context.type</b><br> 5204 * </p> 5205 */ 5206 @SearchParamDefinition(name="ext-context-type", path="StructureDefinition.context.type", description="A type of extension context assigned to the structure definition", type="token" ) 5207 public static final String SP_EXT_CONTEXT_TYPE = "ext-context-type"; 5208 /** 5209 * <b>Fluent Client</b> search parameter constant for <b>ext-context-type</b> 5210 * <p> 5211 * Description: <b>A type of extension context assigned to the structure definition</b><br> 5212 * Type: <b>token</b><br> 5213 * Path: <b>StructureDefinition.context.type</b><br> 5214 * </p> 5215 */ 5216 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXT_CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EXT_CONTEXT_TYPE); 5217 5218 /** 5219 * Search parameter: <b>ext-context</b> 5220 * <p> 5221 * Description: <b>An extension context assigned to the structure definition</b><br> 5222 * Type: <b>composite</b><br> 5223 * Path: <b>StructureDefinition.context</b><br> 5224 * </p> 5225 */ 5226 @SearchParamDefinition(name="ext-context", path="StructureDefinition.context", description="An extension context assigned to the structure definition", type="composite", compositeOf={"ext-context-type", "ext-context-expression"} ) 5227 public static final String SP_EXT_CONTEXT = "ext-context"; 5228 /** 5229 * <b>Fluent Client</b> search parameter constant for <b>ext-context</b> 5230 * <p> 5231 * Description: <b>An extension context assigned to the structure definition</b><br> 5232 * Type: <b>composite</b><br> 5233 * Path: <b>StructureDefinition.context</b><br> 5234 * </p> 5235 */ 5236 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> EXT_CONTEXT = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_EXT_CONTEXT); 5237 5238 /** 5239 * Search parameter: <b>keyword</b> 5240 * <p> 5241 * Description: <b>A code for the StructureDefinition</b><br> 5242 * Type: <b>token</b><br> 5243 * Path: <b>StructureDefinition.keyword</b><br> 5244 * </p> 5245 */ 5246 @SearchParamDefinition(name="keyword", path="StructureDefinition.keyword", description="A code for the StructureDefinition", type="token" ) 5247 public static final String SP_KEYWORD = "keyword"; 5248 /** 5249 * <b>Fluent Client</b> search parameter constant for <b>keyword</b> 5250 * <p> 5251 * Description: <b>A code for the StructureDefinition</b><br> 5252 * Type: <b>token</b><br> 5253 * Path: <b>StructureDefinition.keyword</b><br> 5254 * </p> 5255 */ 5256 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KEYWORD = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_KEYWORD); 5257 5258 /** 5259 * Search parameter: <b>kind</b> 5260 * <p> 5261 * Description: <b>primitive-type | complex-type | resource | logical</b><br> 5262 * Type: <b>token</b><br> 5263 * Path: <b>StructureDefinition.kind</b><br> 5264 * </p> 5265 */ 5266 @SearchParamDefinition(name="kind", path="StructureDefinition.kind", description="primitive-type | complex-type | resource | logical", type="token" ) 5267 public static final String SP_KIND = "kind"; 5268 /** 5269 * <b>Fluent Client</b> search parameter constant for <b>kind</b> 5270 * <p> 5271 * Description: <b>primitive-type | complex-type | resource | logical</b><br> 5272 * Type: <b>token</b><br> 5273 * Path: <b>StructureDefinition.kind</b><br> 5274 * </p> 5275 */ 5276 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KIND = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_KIND); 5277 5278 /** 5279 * Search parameter: <b>path</b> 5280 * <p> 5281 * Description: <b>A path that is constrained in the StructureDefinition</b><br> 5282 * Type: <b>token</b><br> 5283 * Path: <b>StructureDefinition.snapshot.element.path | StructureDefinition.differential.element.path</b><br> 5284 * </p> 5285 */ 5286 @SearchParamDefinition(name="path", path="StructureDefinition.snapshot.element.path | StructureDefinition.differential.element.path", description="A path that is constrained in the StructureDefinition", type="token" ) 5287 public static final String SP_PATH = "path"; 5288 /** 5289 * <b>Fluent Client</b> search parameter constant for <b>path</b> 5290 * <p> 5291 * Description: <b>A path that is constrained in the StructureDefinition</b><br> 5292 * Type: <b>token</b><br> 5293 * Path: <b>StructureDefinition.snapshot.element.path | StructureDefinition.differential.element.path</b><br> 5294 * </p> 5295 */ 5296 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PATH = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PATH); 5297 5298 /** 5299 * Search parameter: <b>type</b> 5300 * <p> 5301 * Description: <b>Type defined or constrained by this structure</b><br> 5302 * Type: <b>uri</b><br> 5303 * Path: <b>StructureDefinition.type</b><br> 5304 * </p> 5305 */ 5306 @SearchParamDefinition(name="type", path="StructureDefinition.type", description="Type defined or constrained by this structure", type="uri" ) 5307 public static final String SP_TYPE = "type"; 5308 /** 5309 * <b>Fluent Client</b> search parameter constant for <b>type</b> 5310 * <p> 5311 * Description: <b>Type defined or constrained by this structure</b><br> 5312 * Type: <b>uri</b><br> 5313 * Path: <b>StructureDefinition.type</b><br> 5314 * </p> 5315 */ 5316 public static final ca.uhn.fhir.rest.gclient.UriClientParam TYPE = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_TYPE); 5317 5318 /** 5319 * Search parameter: <b>valueset</b> 5320 * <p> 5321 * Description: <b>A vocabulary binding reference</b><br> 5322 * Type: <b>reference</b><br> 5323 * Path: <b>StructureDefinition.snapshot.element.binding.valueSet</b><br> 5324 * </p> 5325 */ 5326 @SearchParamDefinition(name="valueset", path="StructureDefinition.snapshot.element.binding.valueSet", description="A vocabulary binding reference", type="reference", target={ValueSet.class } ) 5327 public static final String SP_VALUESET = "valueset"; 5328 /** 5329 * <b>Fluent Client</b> search parameter constant for <b>valueset</b> 5330 * <p> 5331 * Description: <b>A vocabulary binding reference</b><br> 5332 * Type: <b>reference</b><br> 5333 * Path: <b>StructureDefinition.snapshot.element.binding.valueSet</b><br> 5334 * </p> 5335 */ 5336 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam VALUESET = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_VALUESET); 5337 5338/** 5339 * Constant for fluent queries to be used to add include statements. Specifies 5340 * the path value of "<b>StructureDefinition:valueset</b>". 5341 */ 5342 public static final ca.uhn.fhir.model.api.Include INCLUDE_VALUESET = new ca.uhn.fhir.model.api.Include("StructureDefinition:valueset").toLocked(); 5343 5344// Manual code (from Configuration.txt): 5345public String describeType() { 5346 if ("Extension".equals(getType())) 5347 return "Extension" ; 5348 switch (getKind()) { 5349 case COMPLEXTYPE: return getDerivation() == TypeDerivationRule.CONSTRAINT ? "DataType Constraint" : "DataType" ; 5350 case LOGICAL: return getDerivation() == TypeDerivationRule.CONSTRAINT ? "Logical Model" : "Logical Model Profile"; 5351 case PRIMITIVETYPE: return getDerivation() == TypeDerivationRule.CONSTRAINT ? "PrimitiveType Constraint" : "PrimitiveType"; 5352 case RESOURCE: return getDerivation() == TypeDerivationRule.CONSTRAINT ? "Resource Profile" : "Resource"; 5353 default: 5354 return "Definition"; 5355 } 5356 } 5357 5358 5359 public String getTypeName() { 5360 String t = getType(); 5361 return StructureDefinitionKind.LOGICAL.equals(getKind()) && t.contains("/") ? t.substring(t.lastIndexOf("/")+1) : t; 5362 } 5363 5364 public String getTypeTail() { 5365 if (getType().contains("/")) { 5366 return getType().substring(getType().lastIndexOf("/")+1); 5367 } else { 5368 return getType(); 5369 } 5370 } 5371 5372 private boolean generatedSnapshot; 5373 private boolean generatingSnapshot; 5374 5375 public boolean isGeneratedSnapshot() { 5376 return generatedSnapshot; 5377 } 5378 5379 public void setGeneratedSnapshot(boolean generatedSnapshot) { 5380 this.generatedSnapshot = generatedSnapshot; 5381 } 5382 5383 public boolean isGeneratingSnapshot() { 5384 return generatingSnapshot; 5385 } 5386 5387 public void setGeneratingSnapshot(boolean generatingSnapshot) { 5388 this.generatingSnapshot = generatingSnapshot; 5389 } 5390 5391// end addition 5392 5393} 5394