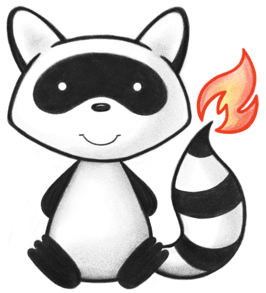
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.r5.utils.ToolingExtensions; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.Block; 050 051/** 052 * A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types. 053 */ 054@ResourceDef(name="StructureDefinition", profile="http://hl7.org/fhir/StructureDefinition/StructureDefinition") 055public class StructureDefinition extends CanonicalResource { 056 057 public enum ExtensionContextType { 058 /** 059 * The context is all elements that match the FHIRPath query found in the expression. 060 */ 061 FHIRPATH, 062 /** 063 * The context is any element that has an ElementDefinition.id that matches that found in the expression. This includes ElementDefinition Ids that have slicing identifiers. The full path for the element is [url]#[elementid]. If there is no #, the Element id is one defined in the base specification. 064 */ 065 ELEMENT, 066 /** 067 * The context is a particular extension from a particular StructureDefinition, and the expression is just a uri that identifies the extension. 068 */ 069 EXTENSION, 070 /** 071 * added to help the parsers with the generic types 072 */ 073 NULL; 074 public static ExtensionContextType fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("fhirpath".equals(codeString)) 078 return FHIRPATH; 079 if ("element".equals(codeString)) 080 return ELEMENT; 081 if ("extension".equals(codeString)) 082 return EXTENSION; 083 if (Configuration.isAcceptInvalidEnums()) 084 return null; 085 else 086 throw new FHIRException("Unknown ExtensionContextType code '"+codeString+"'"); 087 } 088 public String toCode() { 089 switch (this) { 090 case FHIRPATH: return "fhirpath"; 091 case ELEMENT: return "element"; 092 case EXTENSION: return "extension"; 093 case NULL: return null; 094 default: return "?"; 095 } 096 } 097 public String getSystem() { 098 switch (this) { 099 case FHIRPATH: return "http://hl7.org/fhir/extension-context-type"; 100 case ELEMENT: return "http://hl7.org/fhir/extension-context-type"; 101 case EXTENSION: return "http://hl7.org/fhir/extension-context-type"; 102 case NULL: return null; 103 default: return "?"; 104 } 105 } 106 public String getDefinition() { 107 switch (this) { 108 case FHIRPATH: return "The context is all elements that match the FHIRPath query found in the expression."; 109 case ELEMENT: return "The context is any element that has an ElementDefinition.id that matches that found in the expression. This includes ElementDefinition Ids that have slicing identifiers. The full path for the element is [url]#[elementid]. If there is no #, the Element id is one defined in the base specification."; 110 case EXTENSION: return "The context is a particular extension from a particular StructureDefinition, and the expression is just a uri that identifies the extension."; 111 case NULL: return null; 112 default: return "?"; 113 } 114 } 115 public String getDisplay() { 116 switch (this) { 117 case FHIRPATH: return "FHIRPath"; 118 case ELEMENT: return "Element ID"; 119 case EXTENSION: return "Extension URL"; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 } 125 126 public static class ExtensionContextTypeEnumFactory implements EnumFactory<ExtensionContextType> { 127 public ExtensionContextType fromCode(String codeString) throws IllegalArgumentException { 128 if (codeString == null || "".equals(codeString)) 129 if (codeString == null || "".equals(codeString)) 130 return null; 131 if ("fhirpath".equals(codeString)) 132 return ExtensionContextType.FHIRPATH; 133 if ("element".equals(codeString)) 134 return ExtensionContextType.ELEMENT; 135 if ("extension".equals(codeString)) 136 return ExtensionContextType.EXTENSION; 137 throw new IllegalArgumentException("Unknown ExtensionContextType code '"+codeString+"'"); 138 } 139 public Enumeration<ExtensionContextType> fromType(PrimitiveType<?> code) throws FHIRException { 140 if (code == null) 141 return null; 142 if (code.isEmpty()) 143 return new Enumeration<ExtensionContextType>(this, ExtensionContextType.NULL, code); 144 String codeString = ((PrimitiveType) code).asStringValue(); 145 if (codeString == null || "".equals(codeString)) 146 return new Enumeration<ExtensionContextType>(this, ExtensionContextType.NULL, code); 147 if ("fhirpath".equals(codeString)) 148 return new Enumeration<ExtensionContextType>(this, ExtensionContextType.FHIRPATH, code); 149 if ("element".equals(codeString)) 150 return new Enumeration<ExtensionContextType>(this, ExtensionContextType.ELEMENT, code); 151 if ("extension".equals(codeString)) 152 return new Enumeration<ExtensionContextType>(this, ExtensionContextType.EXTENSION, code); 153 throw new FHIRException("Unknown ExtensionContextType code '"+codeString+"'"); 154 } 155 public String toCode(ExtensionContextType code) { 156 if (code == ExtensionContextType.NULL) 157 return null; 158 if (code == ExtensionContextType.FHIRPATH) 159 return "fhirpath"; 160 if (code == ExtensionContextType.ELEMENT) 161 return "element"; 162 if (code == ExtensionContextType.EXTENSION) 163 return "extension"; 164 return "?"; 165 } 166 public String toSystem(ExtensionContextType code) { 167 return code.getSystem(); 168 } 169 } 170 171 public enum StructureDefinitionKind { 172 /** 173 * A primitive type that has a value and an extension. These can be used throughout complex datatype, Resource and extension definitions. Only the base specification can define primitive types. 174 */ 175 PRIMITIVETYPE, 176 /** 177 * A complex structure that defines a set of data elements that is suitable for use in 'resources'. The base specification defines a number of complex types, and other specifications can define additional types. These structures do not have a maintained identity. 178 */ 179 COMPLEXTYPE, 180 /** 181 * A 'resource' - a directed acyclic graph of elements that aggregrates other types into an identifiable entity. The base FHIR resources are defined by the FHIR specification itself but other 'resources' can be defined in additional specifications (though these will not be recognized as 'resources' by the FHIR specification; i.e. they do not get end-points etc., or act as the targets of references in FHIR defined resources - though other specifications can treat them this way). 182 */ 183 RESOURCE, 184 /** 185 * A pattern or a template that is not intended to be a real resource or complex type. 186 */ 187 LOGICAL, 188 /** 189 * added to help the parsers with the generic types 190 */ 191 NULL; 192 public static StructureDefinitionKind fromCode(String codeString) throws FHIRException { 193 if (codeString == null || "".equals(codeString)) 194 return null; 195 if ("primitive-type".equals(codeString)) 196 return PRIMITIVETYPE; 197 if ("complex-type".equals(codeString)) 198 return COMPLEXTYPE; 199 if ("resource".equals(codeString)) 200 return RESOURCE; 201 if ("logical".equals(codeString)) 202 return LOGICAL; 203 if (Configuration.isAcceptInvalidEnums()) 204 return null; 205 else 206 throw new FHIRException("Unknown StructureDefinitionKind code '"+codeString+"'"); 207 } 208 public String toCode() { 209 switch (this) { 210 case PRIMITIVETYPE: return "primitive-type"; 211 case COMPLEXTYPE: return "complex-type"; 212 case RESOURCE: return "resource"; 213 case LOGICAL: return "logical"; 214 case NULL: return null; 215 default: return "?"; 216 } 217 } 218 public String getSystem() { 219 switch (this) { 220 case PRIMITIVETYPE: return "http://hl7.org/fhir/structure-definition-kind"; 221 case COMPLEXTYPE: return "http://hl7.org/fhir/structure-definition-kind"; 222 case RESOURCE: return "http://hl7.org/fhir/structure-definition-kind"; 223 case LOGICAL: return "http://hl7.org/fhir/structure-definition-kind"; 224 case NULL: return null; 225 default: return "?"; 226 } 227 } 228 public String getDefinition() { 229 switch (this) { 230 case PRIMITIVETYPE: return "A primitive type that has a value and an extension. These can be used throughout complex datatype, Resource and extension definitions. Only the base specification can define primitive types."; 231 case COMPLEXTYPE: return "A complex structure that defines a set of data elements that is suitable for use in 'resources'. The base specification defines a number of complex types, and other specifications can define additional types. These structures do not have a maintained identity."; 232 case RESOURCE: return "A 'resource' - a directed acyclic graph of elements that aggregrates other types into an identifiable entity. The base FHIR resources are defined by the FHIR specification itself but other 'resources' can be defined in additional specifications (though these will not be recognized as 'resources' by the FHIR specification; i.e. they do not get end-points etc., or act as the targets of references in FHIR defined resources - though other specifications can treat them this way)."; 233 case LOGICAL: return "A pattern or a template that is not intended to be a real resource or complex type."; 234 case NULL: return null; 235 default: return "?"; 236 } 237 } 238 public String getDisplay() { 239 switch (this) { 240 case PRIMITIVETYPE: return "Primitive Data Type"; 241 case COMPLEXTYPE: return "Complex Data Type"; 242 case RESOURCE: return "Resource"; 243 case LOGICAL: return "Logical"; 244 case NULL: return null; 245 default: return "?"; 246 } 247 } 248 } 249 250 public static class StructureDefinitionKindEnumFactory implements EnumFactory<StructureDefinitionKind> { 251 public StructureDefinitionKind fromCode(String codeString) throws IllegalArgumentException { 252 if (codeString == null || "".equals(codeString)) 253 if (codeString == null || "".equals(codeString)) 254 return null; 255 if ("primitive-type".equals(codeString)) 256 return StructureDefinitionKind.PRIMITIVETYPE; 257 if ("complex-type".equals(codeString)) 258 return StructureDefinitionKind.COMPLEXTYPE; 259 if ("resource".equals(codeString)) 260 return StructureDefinitionKind.RESOURCE; 261 if ("logical".equals(codeString)) 262 return StructureDefinitionKind.LOGICAL; 263 throw new IllegalArgumentException("Unknown StructureDefinitionKind code '"+codeString+"'"); 264 } 265 public Enumeration<StructureDefinitionKind> fromType(PrimitiveType<?> code) throws FHIRException { 266 if (code == null) 267 return null; 268 if (code.isEmpty()) 269 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.NULL, code); 270 String codeString = ((PrimitiveType) code).asStringValue(); 271 if (codeString == null || "".equals(codeString)) 272 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.NULL, code); 273 if ("primitive-type".equals(codeString)) 274 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.PRIMITIVETYPE, code); 275 if ("complex-type".equals(codeString)) 276 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.COMPLEXTYPE, code); 277 if ("resource".equals(codeString)) 278 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.RESOURCE, code); 279 if ("logical".equals(codeString)) 280 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.LOGICAL, code); 281 throw new FHIRException("Unknown StructureDefinitionKind code '"+codeString+"'"); 282 } 283 public String toCode(StructureDefinitionKind code) { 284 if (code == StructureDefinitionKind.NULL) 285 return null; 286 if (code == StructureDefinitionKind.PRIMITIVETYPE) 287 return "primitive-type"; 288 if (code == StructureDefinitionKind.COMPLEXTYPE) 289 return "complex-type"; 290 if (code == StructureDefinitionKind.RESOURCE) 291 return "resource"; 292 if (code == StructureDefinitionKind.LOGICAL) 293 return "logical"; 294 return "?"; 295 } 296 public String toSystem(StructureDefinitionKind code) { 297 return code.getSystem(); 298 } 299 } 300 301 public enum TypeDerivationRule { 302 /** 303 * This definition defines a new type that adds additional elements and optionally additional rules to the base type. 304 */ 305 SPECIALIZATION, 306 /** 307 * This definition adds additional rules to an existing concrete type. 308 */ 309 CONSTRAINT, 310 /** 311 * added to help the parsers with the generic types 312 */ 313 NULL; 314 public static TypeDerivationRule fromCode(String codeString) throws FHIRException { 315 if (codeString == null || "".equals(codeString)) 316 return null; 317 if ("specialization".equals(codeString)) 318 return SPECIALIZATION; 319 if ("constraint".equals(codeString)) 320 return CONSTRAINT; 321 if (Configuration.isAcceptInvalidEnums()) 322 return null; 323 else 324 throw new FHIRException("Unknown TypeDerivationRule code '"+codeString+"'"); 325 } 326 public String toCode() { 327 switch (this) { 328 case SPECIALIZATION: return "specialization"; 329 case CONSTRAINT: return "constraint"; 330 case NULL: return null; 331 default: return "?"; 332 } 333 } 334 public String getSystem() { 335 switch (this) { 336 case SPECIALIZATION: return "http://hl7.org/fhir/type-derivation-rule"; 337 case CONSTRAINT: return "http://hl7.org/fhir/type-derivation-rule"; 338 case NULL: return null; 339 default: return "?"; 340 } 341 } 342 public String getDefinition() { 343 switch (this) { 344 case SPECIALIZATION: return "This definition defines a new type that adds additional elements and optionally additional rules to the base type."; 345 case CONSTRAINT: return "This definition adds additional rules to an existing concrete type."; 346 case NULL: return null; 347 default: return "?"; 348 } 349 } 350 public String getDisplay() { 351 switch (this) { 352 case SPECIALIZATION: return "Specialization"; 353 case CONSTRAINT: return "Constraint"; 354 case NULL: return null; 355 default: return "?"; 356 } 357 } 358 } 359 360 public static class TypeDerivationRuleEnumFactory implements EnumFactory<TypeDerivationRule> { 361 public TypeDerivationRule fromCode(String codeString) throws IllegalArgumentException { 362 if (codeString == null || "".equals(codeString)) 363 if (codeString == null || "".equals(codeString)) 364 return null; 365 if ("specialization".equals(codeString)) 366 return TypeDerivationRule.SPECIALIZATION; 367 if ("constraint".equals(codeString)) 368 return TypeDerivationRule.CONSTRAINT; 369 throw new IllegalArgumentException("Unknown TypeDerivationRule code '"+codeString+"'"); 370 } 371 public Enumeration<TypeDerivationRule> fromType(PrimitiveType<?> code) throws FHIRException { 372 if (code == null) 373 return null; 374 if (code.isEmpty()) 375 return new Enumeration<TypeDerivationRule>(this, TypeDerivationRule.NULL, code); 376 String codeString = ((PrimitiveType) code).asStringValue(); 377 if (codeString == null || "".equals(codeString)) 378 return new Enumeration<TypeDerivationRule>(this, TypeDerivationRule.NULL, code); 379 if ("specialization".equals(codeString)) 380 return new Enumeration<TypeDerivationRule>(this, TypeDerivationRule.SPECIALIZATION, code); 381 if ("constraint".equals(codeString)) 382 return new Enumeration<TypeDerivationRule>(this, TypeDerivationRule.CONSTRAINT, code); 383 throw new FHIRException("Unknown TypeDerivationRule code '"+codeString+"'"); 384 } 385 public String toCode(TypeDerivationRule code) { 386 if (code == TypeDerivationRule.NULL) 387 return null; 388 if (code == TypeDerivationRule.SPECIALIZATION) 389 return "specialization"; 390 if (code == TypeDerivationRule.CONSTRAINT) 391 return "constraint"; 392 return "?"; 393 } 394 public String toSystem(TypeDerivationRule code) { 395 return code.getSystem(); 396 } 397 } 398 399 @Block() 400 public static class StructureDefinitionMappingComponent extends BackboneElement implements IBaseBackboneElement { 401 @Override 402 public String toString() { 403 return identity + "=" + uri + " (\""+name+"\")"; 404 } 405 406 /** 407 * An Internal id that is used to identify this mapping set when specific mappings are made. 408 */ 409 @Child(name = "identity", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=false) 410 @Description(shortDefinition="Internal id when this mapping is used", formalDefinition="An Internal id that is used to identify this mapping set when specific mappings are made." ) 411 protected IdType identity; 412 413 /** 414 * An absolute URI that identifies the specification that this mapping is expressed to. 415 */ 416 @Child(name = "uri", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 417 @Description(shortDefinition="Identifies what this mapping refers to", formalDefinition="An absolute URI that identifies the specification that this mapping is expressed to." ) 418 protected UriType uri; 419 420 /** 421 * A name for the specification that is being mapped to. 422 */ 423 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 424 @Description(shortDefinition="Names what this mapping refers to", formalDefinition="A name for the specification that is being mapped to." ) 425 protected StringType name; 426 427 /** 428 * Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage. 429 */ 430 @Child(name = "comment", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 431 @Description(shortDefinition="Versions, Issues, Scope limitations etc", formalDefinition="Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage." ) 432 protected StringType comment; 433 434 private static final long serialVersionUID = 9610265L; 435 436 /** 437 * Constructor 438 */ 439 public StructureDefinitionMappingComponent() { 440 super(); 441 } 442 443 /** 444 * Constructor 445 */ 446 public StructureDefinitionMappingComponent(String identity) { 447 super(); 448 this.setIdentity(identity); 449 } 450 451 /** 452 * @return {@link #identity} (An Internal id that is used to identify this mapping set when specific mappings are made.). This is the underlying object with id, value and extensions. The accessor "getIdentity" gives direct access to the value 453 */ 454 public IdType getIdentityElement() { 455 if (this.identity == null) 456 if (Configuration.errorOnAutoCreate()) 457 throw new Error("Attempt to auto-create StructureDefinitionMappingComponent.identity"); 458 else if (Configuration.doAutoCreate()) 459 this.identity = new IdType(); // bb 460 return this.identity; 461 } 462 463 public boolean hasIdentityElement() { 464 return this.identity != null && !this.identity.isEmpty(); 465 } 466 467 public boolean hasIdentity() { 468 return this.identity != null && !this.identity.isEmpty(); 469 } 470 471 /** 472 * @param value {@link #identity} (An Internal id that is used to identify this mapping set when specific mappings are made.). This is the underlying object with id, value and extensions. The accessor "getIdentity" gives direct access to the value 473 */ 474 public StructureDefinitionMappingComponent setIdentityElement(IdType value) { 475 this.identity = value; 476 return this; 477 } 478 479 /** 480 * @return An Internal id that is used to identify this mapping set when specific mappings are made. 481 */ 482 public String getIdentity() { 483 return this.identity == null ? null : this.identity.getValue(); 484 } 485 486 /** 487 * @param value An Internal id that is used to identify this mapping set when specific mappings are made. 488 */ 489 public StructureDefinitionMappingComponent setIdentity(String value) { 490 if (this.identity == null) 491 this.identity = new IdType(); 492 this.identity.setValue(value); 493 return this; 494 } 495 496 /** 497 * @return {@link #uri} (An absolute URI that identifies the specification that this mapping is expressed to.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 498 */ 499 public UriType getUriElement() { 500 if (this.uri == null) 501 if (Configuration.errorOnAutoCreate()) 502 throw new Error("Attempt to auto-create StructureDefinitionMappingComponent.uri"); 503 else if (Configuration.doAutoCreate()) 504 this.uri = new UriType(); // bb 505 return this.uri; 506 } 507 508 public boolean hasUriElement() { 509 return this.uri != null && !this.uri.isEmpty(); 510 } 511 512 public boolean hasUri() { 513 return this.uri != null && !this.uri.isEmpty(); 514 } 515 516 /** 517 * @param value {@link #uri} (An absolute URI that identifies the specification that this mapping is expressed to.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 518 */ 519 public StructureDefinitionMappingComponent setUriElement(UriType value) { 520 this.uri = value; 521 return this; 522 } 523 524 /** 525 * @return An absolute URI that identifies the specification that this mapping is expressed to. 526 */ 527 public String getUri() { 528 return this.uri == null ? null : this.uri.getValue(); 529 } 530 531 /** 532 * @param value An absolute URI that identifies the specification that this mapping is expressed to. 533 */ 534 public StructureDefinitionMappingComponent setUri(String value) { 535 if (Utilities.noString(value)) 536 this.uri = null; 537 else { 538 if (this.uri == null) 539 this.uri = new UriType(); 540 this.uri.setValue(value); 541 } 542 return this; 543 } 544 545 /** 546 * @return {@link #name} (A name for the specification that is being mapped to.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 547 */ 548 public StringType getNameElement() { 549 if (this.name == null) 550 if (Configuration.errorOnAutoCreate()) 551 throw new Error("Attempt to auto-create StructureDefinitionMappingComponent.name"); 552 else if (Configuration.doAutoCreate()) 553 this.name = new StringType(); // bb 554 return this.name; 555 } 556 557 public boolean hasNameElement() { 558 return this.name != null && !this.name.isEmpty(); 559 } 560 561 public boolean hasName() { 562 return this.name != null && !this.name.isEmpty(); 563 } 564 565 /** 566 * @param value {@link #name} (A name for the specification that is being mapped to.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 567 */ 568 public StructureDefinitionMappingComponent setNameElement(StringType value) { 569 this.name = value; 570 return this; 571 } 572 573 /** 574 * @return A name for the specification that is being mapped to. 575 */ 576 public String getName() { 577 return this.name == null ? null : this.name.getValue(); 578 } 579 580 /** 581 * @param value A name for the specification that is being mapped to. 582 */ 583 public StructureDefinitionMappingComponent setName(String value) { 584 if (Utilities.noString(value)) 585 this.name = null; 586 else { 587 if (this.name == null) 588 this.name = new StringType(); 589 this.name.setValue(value); 590 } 591 return this; 592 } 593 594 /** 595 * @return {@link #comment} (Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 596 */ 597 public StringType getCommentElement() { 598 if (this.comment == null) 599 if (Configuration.errorOnAutoCreate()) 600 throw new Error("Attempt to auto-create StructureDefinitionMappingComponent.comment"); 601 else if (Configuration.doAutoCreate()) 602 this.comment = new StringType(); // bb 603 return this.comment; 604 } 605 606 public boolean hasCommentElement() { 607 return this.comment != null && !this.comment.isEmpty(); 608 } 609 610 public boolean hasComment() { 611 return this.comment != null && !this.comment.isEmpty(); 612 } 613 614 /** 615 * @param value {@link #comment} (Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 616 */ 617 public StructureDefinitionMappingComponent setCommentElement(StringType value) { 618 this.comment = value; 619 return this; 620 } 621 622 /** 623 * @return Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage. 624 */ 625 public String getComment() { 626 return this.comment == null ? null : this.comment.getValue(); 627 } 628 629 /** 630 * @param value Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage. 631 */ 632 public StructureDefinitionMappingComponent setComment(String value) { 633 if (Utilities.noString(value)) 634 this.comment = null; 635 else { 636 if (this.comment == null) 637 this.comment = new StringType(); 638 this.comment.setValue(value); 639 } 640 return this; 641 } 642 643 protected void listChildren(List<Property> children) { 644 super.listChildren(children); 645 children.add(new Property("identity", "id", "An Internal id that is used to identify this mapping set when specific mappings are made.", 0, 1, identity)); 646 children.add(new Property("uri", "uri", "An absolute URI that identifies the specification that this mapping is expressed to.", 0, 1, uri)); 647 children.add(new Property("name", "string", "A name for the specification that is being mapped to.", 0, 1, name)); 648 children.add(new Property("comment", "string", "Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.", 0, 1, comment)); 649 } 650 651 @Override 652 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 653 switch (_hash) { 654 case -135761730: /*identity*/ return new Property("identity", "id", "An Internal id that is used to identify this mapping set when specific mappings are made.", 0, 1, identity); 655 case 116076: /*uri*/ return new Property("uri", "uri", "An absolute URI that identifies the specification that this mapping is expressed to.", 0, 1, uri); 656 case 3373707: /*name*/ return new Property("name", "string", "A name for the specification that is being mapped to.", 0, 1, name); 657 case 950398559: /*comment*/ return new Property("comment", "string", "Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.", 0, 1, comment); 658 default: return super.getNamedProperty(_hash, _name, _checkValid); 659 } 660 661 } 662 663 @Override 664 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 665 switch (hash) { 666 case -135761730: /*identity*/ return this.identity == null ? new Base[0] : new Base[] {this.identity}; // IdType 667 case 116076: /*uri*/ return this.uri == null ? new Base[0] : new Base[] {this.uri}; // UriType 668 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 669 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 670 default: return super.getProperty(hash, name, checkValid); 671 } 672 673 } 674 675 @Override 676 public Base setProperty(int hash, String name, Base value) throws FHIRException { 677 switch (hash) { 678 case -135761730: // identity 679 this.identity = TypeConvertor.castToId(value); // IdType 680 return value; 681 case 116076: // uri 682 this.uri = TypeConvertor.castToUri(value); // UriType 683 return value; 684 case 3373707: // name 685 this.name = TypeConvertor.castToString(value); // StringType 686 return value; 687 case 950398559: // comment 688 this.comment = TypeConvertor.castToString(value); // StringType 689 return value; 690 default: return super.setProperty(hash, name, value); 691 } 692 693 } 694 695 @Override 696 public Base setProperty(String name, Base value) throws FHIRException { 697 if (name.equals("identity")) { 698 this.identity = TypeConvertor.castToId(value); // IdType 699 } else if (name.equals("uri")) { 700 this.uri = TypeConvertor.castToUri(value); // UriType 701 } else if (name.equals("name")) { 702 this.name = TypeConvertor.castToString(value); // StringType 703 } else if (name.equals("comment")) { 704 this.comment = TypeConvertor.castToString(value); // StringType 705 } else 706 return super.setProperty(name, value); 707 return value; 708 } 709 710 @Override 711 public void removeChild(String name, Base value) throws FHIRException { 712 if (name.equals("identity")) { 713 this.identity = null; 714 } else if (name.equals("uri")) { 715 this.uri = null; 716 } else if (name.equals("name")) { 717 this.name = null; 718 } else if (name.equals("comment")) { 719 this.comment = null; 720 } else 721 super.removeChild(name, value); 722 723 } 724 725 @Override 726 public Base makeProperty(int hash, String name) throws FHIRException { 727 switch (hash) { 728 case -135761730: return getIdentityElement(); 729 case 116076: return getUriElement(); 730 case 3373707: return getNameElement(); 731 case 950398559: return getCommentElement(); 732 default: return super.makeProperty(hash, name); 733 } 734 735 } 736 737 @Override 738 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 739 switch (hash) { 740 case -135761730: /*identity*/ return new String[] {"id"}; 741 case 116076: /*uri*/ return new String[] {"uri"}; 742 case 3373707: /*name*/ return new String[] {"string"}; 743 case 950398559: /*comment*/ return new String[] {"string"}; 744 default: return super.getTypesForProperty(hash, name); 745 } 746 747 } 748 749 @Override 750 public Base addChild(String name) throws FHIRException { 751 if (name.equals("identity")) { 752 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.mapping.identity"); 753 } 754 else if (name.equals("uri")) { 755 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.mapping.uri"); 756 } 757 else if (name.equals("name")) { 758 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.mapping.name"); 759 } 760 else if (name.equals("comment")) { 761 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.mapping.comment"); 762 } 763 else 764 return super.addChild(name); 765 } 766 767 public StructureDefinitionMappingComponent copy() { 768 StructureDefinitionMappingComponent dst = new StructureDefinitionMappingComponent(); 769 copyValues(dst); 770 return dst; 771 } 772 773 public void copyValues(StructureDefinitionMappingComponent dst) { 774 super.copyValues(dst); 775 dst.identity = identity == null ? null : identity.copy(); 776 dst.uri = uri == null ? null : uri.copy(); 777 dst.name = name == null ? null : name.copy(); 778 dst.comment = comment == null ? null : comment.copy(); 779 } 780 781 @Override 782 public boolean equalsDeep(Base other_) { 783 if (!super.equalsDeep(other_)) 784 return false; 785 if (!(other_ instanceof StructureDefinitionMappingComponent)) 786 return false; 787 StructureDefinitionMappingComponent o = (StructureDefinitionMappingComponent) other_; 788 return compareDeep(identity, o.identity, true) && compareDeep(uri, o.uri, true) && compareDeep(name, o.name, true) 789 && compareDeep(comment, o.comment, true); 790 } 791 792 @Override 793 public boolean equalsShallow(Base other_) { 794 if (!super.equalsShallow(other_)) 795 return false; 796 if (!(other_ instanceof StructureDefinitionMappingComponent)) 797 return false; 798 StructureDefinitionMappingComponent o = (StructureDefinitionMappingComponent) other_; 799 return compareValues(identity, o.identity, true) && compareValues(uri, o.uri, true) && compareValues(name, o.name, true) 800 && compareValues(comment, o.comment, true); 801 } 802 803 public boolean isEmpty() { 804 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identity, uri, name, comment 805 ); 806 } 807 808 public String fhirType() { 809 return "StructureDefinition.mapping"; 810 811 } 812 813 } 814 815 @Block() 816 public static class StructureDefinitionContextComponent extends BackboneElement implements IBaseBackboneElement { 817 /** 818 * Defines how to interpret the expression that defines what the context of the extension is. 819 */ 820 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 821 @Description(shortDefinition="fhirpath | element | extension", formalDefinition="Defines how to interpret the expression that defines what the context of the extension is." ) 822 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/extension-context-type") 823 protected Enumeration<ExtensionContextType> type; 824 825 /** 826 * An expression that defines where an extension can be used in resources. 827 */ 828 @Child(name = "expression", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=true) 829 @Description(shortDefinition="Where the extension can be used in instances", formalDefinition="An expression that defines where an extension can be used in resources." ) 830 protected StringType expression; 831 832 private static final long serialVersionUID = 1958074856L; 833 834 /** 835 * Constructor 836 */ 837 public StructureDefinitionContextComponent() { 838 super(); 839 } 840 841 /** 842 * Constructor 843 */ 844 public StructureDefinitionContextComponent(ExtensionContextType type, String expression) { 845 super(); 846 this.setType(type); 847 this.setExpression(expression); 848 } 849 850 /** 851 * @return {@link #type} (Defines how to interpret the expression that defines what the context of the extension is.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 852 */ 853 public Enumeration<ExtensionContextType> getTypeElement() { 854 if (this.type == null) 855 if (Configuration.errorOnAutoCreate()) 856 throw new Error("Attempt to auto-create StructureDefinitionContextComponent.type"); 857 else if (Configuration.doAutoCreate()) 858 this.type = new Enumeration<ExtensionContextType>(new ExtensionContextTypeEnumFactory()); // bb 859 return this.type; 860 } 861 862 public boolean hasTypeElement() { 863 return this.type != null && !this.type.isEmpty(); 864 } 865 866 public boolean hasType() { 867 return this.type != null && !this.type.isEmpty(); 868 } 869 870 /** 871 * @param value {@link #type} (Defines how to interpret the expression that defines what the context of the extension is.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 872 */ 873 public StructureDefinitionContextComponent setTypeElement(Enumeration<ExtensionContextType> value) { 874 this.type = value; 875 return this; 876 } 877 878 /** 879 * @return Defines how to interpret the expression that defines what the context of the extension is. 880 */ 881 public ExtensionContextType getType() { 882 return this.type == null ? null : this.type.getValue(); 883 } 884 885 /** 886 * @param value Defines how to interpret the expression that defines what the context of the extension is. 887 */ 888 public StructureDefinitionContextComponent setType(ExtensionContextType value) { 889 if (this.type == null) 890 this.type = new Enumeration<ExtensionContextType>(new ExtensionContextTypeEnumFactory()); 891 this.type.setValue(value); 892 return this; 893 } 894 895 /** 896 * @return {@link #expression} (An expression that defines where an extension can be used in resources.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 897 */ 898 public StringType getExpressionElement() { 899 if (this.expression == null) 900 if (Configuration.errorOnAutoCreate()) 901 throw new Error("Attempt to auto-create StructureDefinitionContextComponent.expression"); 902 else if (Configuration.doAutoCreate()) 903 this.expression = new StringType(); // bb 904 return this.expression; 905 } 906 907 public boolean hasExpressionElement() { 908 return this.expression != null && !this.expression.isEmpty(); 909 } 910 911 public boolean hasExpression() { 912 return this.expression != null && !this.expression.isEmpty(); 913 } 914 915 /** 916 * @param value {@link #expression} (An expression that defines where an extension can be used in resources.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 917 */ 918 public StructureDefinitionContextComponent setExpressionElement(StringType value) { 919 this.expression = value; 920 return this; 921 } 922 923 /** 924 * @return An expression that defines where an extension can be used in resources. 925 */ 926 public String getExpression() { 927 return this.expression == null ? null : this.expression.getValue(); 928 } 929 930 /** 931 * @param value An expression that defines where an extension can be used in resources. 932 */ 933 public StructureDefinitionContextComponent setExpression(String value) { 934 if (this.expression == null) 935 this.expression = new StringType(); 936 this.expression.setValue(value); 937 return this; 938 } 939 940 protected void listChildren(List<Property> children) { 941 super.listChildren(children); 942 children.add(new Property("type", "code", "Defines how to interpret the expression that defines what the context of the extension is.", 0, 1, type)); 943 children.add(new Property("expression", "string", "An expression that defines where an extension can be used in resources.", 0, 1, expression)); 944 } 945 946 @Override 947 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 948 switch (_hash) { 949 case 3575610: /*type*/ return new Property("type", "code", "Defines how to interpret the expression that defines what the context of the extension is.", 0, 1, type); 950 case -1795452264: /*expression*/ return new Property("expression", "string", "An expression that defines where an extension can be used in resources.", 0, 1, expression); 951 default: return super.getNamedProperty(_hash, _name, _checkValid); 952 } 953 954 } 955 956 @Override 957 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 958 switch (hash) { 959 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ExtensionContextType> 960 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 961 default: return super.getProperty(hash, name, checkValid); 962 } 963 964 } 965 966 @Override 967 public Base setProperty(int hash, String name, Base value) throws FHIRException { 968 switch (hash) { 969 case 3575610: // type 970 value = new ExtensionContextTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 971 this.type = (Enumeration) value; // Enumeration<ExtensionContextType> 972 return value; 973 case -1795452264: // expression 974 this.expression = TypeConvertor.castToString(value); // StringType 975 return value; 976 default: return super.setProperty(hash, name, value); 977 } 978 979 } 980 981 @Override 982 public Base setProperty(String name, Base value) throws FHIRException { 983 if (name.equals("type")) { 984 value = new ExtensionContextTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 985 this.type = (Enumeration) value; // Enumeration<ExtensionContextType> 986 } else if (name.equals("expression")) { 987 this.expression = TypeConvertor.castToString(value); // StringType 988 } else 989 return super.setProperty(name, value); 990 return value; 991 } 992 993 @Override 994 public void removeChild(String name, Base value) throws FHIRException { 995 if (name.equals("type")) { 996 value = new ExtensionContextTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 997 this.type = (Enumeration) value; // Enumeration<ExtensionContextType> 998 } else if (name.equals("expression")) { 999 this.expression = null; 1000 } else 1001 super.removeChild(name, value); 1002 1003 } 1004 1005 @Override 1006 public Base makeProperty(int hash, String name) throws FHIRException { 1007 switch (hash) { 1008 case 3575610: return getTypeElement(); 1009 case -1795452264: return getExpressionElement(); 1010 default: return super.makeProperty(hash, name); 1011 } 1012 1013 } 1014 1015 @Override 1016 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1017 switch (hash) { 1018 case 3575610: /*type*/ return new String[] {"code"}; 1019 case -1795452264: /*expression*/ return new String[] {"string"}; 1020 default: return super.getTypesForProperty(hash, name); 1021 } 1022 1023 } 1024 1025 @Override 1026 public Base addChild(String name) throws FHIRException { 1027 if (name.equals("type")) { 1028 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.context.type"); 1029 } 1030 else if (name.equals("expression")) { 1031 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.context.expression"); 1032 } 1033 else 1034 return super.addChild(name); 1035 } 1036 1037 public StructureDefinitionContextComponent copy() { 1038 StructureDefinitionContextComponent dst = new StructureDefinitionContextComponent(); 1039 copyValues(dst); 1040 return dst; 1041 } 1042 1043 public void copyValues(StructureDefinitionContextComponent dst) { 1044 super.copyValues(dst); 1045 dst.type = type == null ? null : type.copy(); 1046 dst.expression = expression == null ? null : expression.copy(); 1047 } 1048 1049 @Override 1050 public boolean equalsDeep(Base other_) { 1051 if (!super.equalsDeep(other_)) 1052 return false; 1053 if (!(other_ instanceof StructureDefinitionContextComponent)) 1054 return false; 1055 StructureDefinitionContextComponent o = (StructureDefinitionContextComponent) other_; 1056 return compareDeep(type, o.type, true) && compareDeep(expression, o.expression, true); 1057 } 1058 1059 @Override 1060 public boolean equalsShallow(Base other_) { 1061 if (!super.equalsShallow(other_)) 1062 return false; 1063 if (!(other_ instanceof StructureDefinitionContextComponent)) 1064 return false; 1065 StructureDefinitionContextComponent o = (StructureDefinitionContextComponent) other_; 1066 return compareValues(type, o.type, true) && compareValues(expression, o.expression, true); 1067 } 1068 1069 public boolean isEmpty() { 1070 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, expression); 1071 } 1072 1073 public String fhirType() { 1074 return "StructureDefinition.context"; 1075 1076 } 1077 1078 } 1079 1080 @Block() 1081 public static class StructureDefinitionSnapshotComponent extends BackboneElement implements IBaseBackboneElement { 1082 /** 1083 * Captures constraints on each element within the resource. 1084 */ 1085 @Child(name = "element", type = {ElementDefinition.class}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1086 @Description(shortDefinition="Definition of elements in the resource (if no StructureDefinition)", formalDefinition="Captures constraints on each element within the resource." ) 1087 protected List<ElementDefinition> element; 1088 1089 private static final long serialVersionUID = 53896641L; 1090 1091 /** 1092 * Constructor 1093 */ 1094 public StructureDefinitionSnapshotComponent() { 1095 super(); 1096 } 1097 1098 /** 1099 * Constructor 1100 */ 1101 public StructureDefinitionSnapshotComponent(ElementDefinition element) { 1102 super(); 1103 this.addElement(element); 1104 } 1105 1106 /** 1107 * @return {@link #element} (Captures constraints on each element within the resource.) 1108 */ 1109 public List<ElementDefinition> getElement() { 1110 if (this.element == null) 1111 this.element = new ArrayList<ElementDefinition>(); 1112 return this.element; 1113 } 1114 1115 /** 1116 * @return Returns a reference to <code>this</code> for easy method chaining 1117 */ 1118 public StructureDefinitionSnapshotComponent setElement(List<ElementDefinition> theElement) { 1119 this.element = theElement; 1120 return this; 1121 } 1122 1123 public boolean hasElement() { 1124 if (this.element == null) 1125 return false; 1126 for (ElementDefinition item : this.element) 1127 if (!item.isEmpty()) 1128 return true; 1129 return false; 1130 } 1131 1132 public ElementDefinition addElement() { //3 1133 ElementDefinition t = new ElementDefinition(); 1134 if (this.element == null) 1135 this.element = new ArrayList<ElementDefinition>(); 1136 this.element.add(t); 1137 return t; 1138 } 1139 1140 public StructureDefinitionSnapshotComponent addElement(ElementDefinition t) { //3 1141 if (t == null) 1142 return this; 1143 if (this.element == null) 1144 this.element = new ArrayList<ElementDefinition>(); 1145 this.element.add(t); 1146 return this; 1147 } 1148 1149 /** 1150 * @return The first repetition of repeating field {@link #element}, creating it if it does not already exist {3} 1151 */ 1152 public ElementDefinition getElementFirstRep() { 1153 if (getElement().isEmpty()) { 1154 addElement(); 1155 } 1156 return getElement().get(0); 1157 } 1158 1159 protected void listChildren(List<Property> children) { 1160 super.listChildren(children); 1161 children.add(new Property("element", "ElementDefinition", "Captures constraints on each element within the resource.", 0, java.lang.Integer.MAX_VALUE, element)); 1162 } 1163 1164 @Override 1165 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1166 switch (_hash) { 1167 case -1662836996: /*element*/ return new Property("element", "ElementDefinition", "Captures constraints on each element within the resource.", 0, java.lang.Integer.MAX_VALUE, element); 1168 default: return super.getNamedProperty(_hash, _name, _checkValid); 1169 } 1170 1171 } 1172 1173 @Override 1174 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1175 switch (hash) { 1176 case -1662836996: /*element*/ return this.element == null ? new Base[0] : this.element.toArray(new Base[this.element.size()]); // ElementDefinition 1177 default: return super.getProperty(hash, name, checkValid); 1178 } 1179 1180 } 1181 1182 @Override 1183 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1184 switch (hash) { 1185 case -1662836996: // element 1186 this.getElement().add(TypeConvertor.castToElementDefinition(value)); // ElementDefinition 1187 return value; 1188 default: return super.setProperty(hash, name, value); 1189 } 1190 1191 } 1192 1193 @Override 1194 public Base setProperty(String name, Base value) throws FHIRException { 1195 if (name.equals("element")) { 1196 this.getElement().add(TypeConvertor.castToElementDefinition(value)); 1197 } else 1198 return super.setProperty(name, value); 1199 return value; 1200 } 1201 1202 @Override 1203 public void removeChild(String name, Base value) throws FHIRException { 1204 if (name.equals("element")) { 1205 this.getElement().remove(value); 1206 } else 1207 super.removeChild(name, value); 1208 1209 } 1210 1211 @Override 1212 public Base makeProperty(int hash, String name) throws FHIRException { 1213 switch (hash) { 1214 case -1662836996: return addElement(); 1215 default: return super.makeProperty(hash, name); 1216 } 1217 1218 } 1219 1220 @Override 1221 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1222 switch (hash) { 1223 case -1662836996: /*element*/ return new String[] {"ElementDefinition"}; 1224 default: return super.getTypesForProperty(hash, name); 1225 } 1226 1227 } 1228 1229 @Override 1230 public Base addChild(String name) throws FHIRException { 1231 if (name.equals("element")) { 1232 return addElement(); 1233 } 1234 else 1235 return super.addChild(name); 1236 } 1237 1238 public StructureDefinitionSnapshotComponent copy() { 1239 StructureDefinitionSnapshotComponent dst = new StructureDefinitionSnapshotComponent(); 1240 copyValues(dst); 1241 return dst; 1242 } 1243 1244 public void copyValues(StructureDefinitionSnapshotComponent dst) { 1245 super.copyValues(dst); 1246 if (element != null) { 1247 dst.element = new ArrayList<ElementDefinition>(); 1248 for (ElementDefinition i : element) 1249 dst.element.add(i.copy()); 1250 }; 1251 } 1252 1253 @Override 1254 public boolean equalsDeep(Base other_) { 1255 if (!super.equalsDeep(other_)) 1256 return false; 1257 if (!(other_ instanceof StructureDefinitionSnapshotComponent)) 1258 return false; 1259 StructureDefinitionSnapshotComponent o = (StructureDefinitionSnapshotComponent) other_; 1260 return compareDeep(element, o.element, true); 1261 } 1262 1263 @Override 1264 public boolean equalsShallow(Base other_) { 1265 if (!super.equalsShallow(other_)) 1266 return false; 1267 if (!(other_ instanceof StructureDefinitionSnapshotComponent)) 1268 return false; 1269 StructureDefinitionSnapshotComponent o = (StructureDefinitionSnapshotComponent) other_; 1270 return true; 1271 } 1272 1273 public boolean isEmpty() { 1274 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(element); 1275 } 1276 1277 public String fhirType() { 1278 return "StructureDefinition.snapshot"; 1279 1280 } 1281 1282// added from java-adornments.txt: 1283 1284 public ElementDefinition getElementByPath(String path) { 1285 if (path == null) { 1286 return null; 1287 } 1288 for (ElementDefinition ed : getElement()) { 1289 if (path.equals(ed.getPath()) || (path+"[x]").equals(ed.getPath())) { 1290 return ed; 1291 } 1292 } 1293 return null; 1294 } 1295 1296 1297 public ElementDefinition getElementById(String id) { 1298 if (id == null) { 1299 return null; 1300 } 1301 for (ElementDefinition ed : getElement()) { 1302 if (id.equals(ed.getId())) { 1303 return ed; 1304 } 1305 } 1306 return null; 1307 } 1308 1309// end addition 1310 } 1311 1312 @Block() 1313 public static class StructureDefinitionDifferentialComponent extends BackboneElement implements IBaseBackboneElement { 1314 /** 1315 * Captures constraints on each element within the resource. 1316 */ 1317 @Child(name = "element", type = {ElementDefinition.class}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1318 @Description(shortDefinition="Definition of elements in the resource (if no StructureDefinition)", formalDefinition="Captures constraints on each element within the resource." ) 1319 protected List<ElementDefinition> element; 1320 1321 private static final long serialVersionUID = 53896641L; 1322 1323 /** 1324 * Constructor 1325 */ 1326 public StructureDefinitionDifferentialComponent() { 1327 super(); 1328 } 1329 1330 /** 1331 * Constructor 1332 */ 1333 public StructureDefinitionDifferentialComponent(ElementDefinition element) { 1334 super(); 1335 this.addElement(element); 1336 } 1337 1338 /** 1339 * @return {@link #element} (Captures constraints on each element within the resource.) 1340 */ 1341 public List<ElementDefinition> getElement() { 1342 if (this.element == null) 1343 this.element = new ArrayList<ElementDefinition>(); 1344 return this.element; 1345 } 1346 1347 /** 1348 * @return Returns a reference to <code>this</code> for easy method chaining 1349 */ 1350 public StructureDefinitionDifferentialComponent setElement(List<ElementDefinition> theElement) { 1351 this.element = theElement; 1352 return this; 1353 } 1354 1355 public boolean hasElement() { 1356 if (this.element == null) 1357 return false; 1358 for (ElementDefinition item : this.element) 1359 if (!item.isEmpty()) 1360 return true; 1361 return false; 1362 } 1363 1364 public ElementDefinition addElement() { //3 1365 ElementDefinition t = new ElementDefinition(); 1366 if (this.element == null) 1367 this.element = new ArrayList<ElementDefinition>(); 1368 this.element.add(t); 1369 return t; 1370 } 1371 1372 public StructureDefinitionDifferentialComponent addElement(ElementDefinition t) { //3 1373 if (t == null) 1374 return this; 1375 if (this.element == null) 1376 this.element = new ArrayList<ElementDefinition>(); 1377 this.element.add(t); 1378 return this; 1379 } 1380 1381 /** 1382 * @return The first repetition of repeating field {@link #element}, creating it if it does not already exist {3} 1383 */ 1384 public ElementDefinition getElementFirstRep() { 1385 if (getElement().isEmpty()) { 1386 addElement(); 1387 } 1388 return getElement().get(0); 1389 } 1390 1391 protected void listChildren(List<Property> children) { 1392 super.listChildren(children); 1393 children.add(new Property("element", "ElementDefinition", "Captures constraints on each element within the resource.", 0, java.lang.Integer.MAX_VALUE, element)); 1394 } 1395 1396 @Override 1397 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1398 switch (_hash) { 1399 case -1662836996: /*element*/ return new Property("element", "ElementDefinition", "Captures constraints on each element within the resource.", 0, java.lang.Integer.MAX_VALUE, element); 1400 default: return super.getNamedProperty(_hash, _name, _checkValid); 1401 } 1402 1403 } 1404 1405 @Override 1406 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1407 switch (hash) { 1408 case -1662836996: /*element*/ return this.element == null ? new Base[0] : this.element.toArray(new Base[this.element.size()]); // ElementDefinition 1409 default: return super.getProperty(hash, name, checkValid); 1410 } 1411 1412 } 1413 1414 @Override 1415 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1416 switch (hash) { 1417 case -1662836996: // element 1418 this.getElement().add(TypeConvertor.castToElementDefinition(value)); // ElementDefinition 1419 return value; 1420 default: return super.setProperty(hash, name, value); 1421 } 1422 1423 } 1424 1425 @Override 1426 public Base setProperty(String name, Base value) throws FHIRException { 1427 if (name.equals("element")) { 1428 this.getElement().add(TypeConvertor.castToElementDefinition(value)); 1429 } else 1430 return super.setProperty(name, value); 1431 return value; 1432 } 1433 1434 @Override 1435 public void removeChild(String name, Base value) throws FHIRException { 1436 if (name.equals("element")) { 1437 this.getElement().remove(value); 1438 } else 1439 super.removeChild(name, value); 1440 1441 } 1442 1443 @Override 1444 public Base makeProperty(int hash, String name) throws FHIRException { 1445 switch (hash) { 1446 case -1662836996: return addElement(); 1447 default: return super.makeProperty(hash, name); 1448 } 1449 1450 } 1451 1452 @Override 1453 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1454 switch (hash) { 1455 case -1662836996: /*element*/ return new String[] {"ElementDefinition"}; 1456 default: return super.getTypesForProperty(hash, name); 1457 } 1458 1459 } 1460 1461 @Override 1462 public Base addChild(String name) throws FHIRException { 1463 if (name.equals("element")) { 1464 return addElement(); 1465 } 1466 else 1467 return super.addChild(name); 1468 } 1469 1470 public StructureDefinitionDifferentialComponent copy() { 1471 StructureDefinitionDifferentialComponent dst = new StructureDefinitionDifferentialComponent(); 1472 copyValues(dst); 1473 return dst; 1474 } 1475 1476 public void copyValues(StructureDefinitionDifferentialComponent dst) { 1477 super.copyValues(dst); 1478 if (element != null) { 1479 dst.element = new ArrayList<ElementDefinition>(); 1480 for (ElementDefinition i : element) 1481 dst.element.add(i.copy()); 1482 }; 1483 } 1484 1485 @Override 1486 public boolean equalsDeep(Base other_) { 1487 if (!super.equalsDeep(other_)) 1488 return false; 1489 if (!(other_ instanceof StructureDefinitionDifferentialComponent)) 1490 return false; 1491 StructureDefinitionDifferentialComponent o = (StructureDefinitionDifferentialComponent) other_; 1492 return compareDeep(element, o.element, true); 1493 } 1494 1495 @Override 1496 public boolean equalsShallow(Base other_) { 1497 if (!super.equalsShallow(other_)) 1498 return false; 1499 if (!(other_ instanceof StructureDefinitionDifferentialComponent)) 1500 return false; 1501 StructureDefinitionDifferentialComponent o = (StructureDefinitionDifferentialComponent) other_; 1502 return true; 1503 } 1504 1505 public boolean isEmpty() { 1506 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(element); 1507 } 1508 1509 public String fhirType() { 1510 return "StructureDefinition.differential"; 1511 1512 } 1513 1514//added from java-adornments.txt: 1515 1516 public ElementDefinition getElementByPath(String path) { 1517 if (path == null) { 1518 return null; 1519 } 1520 for (ElementDefinition ed : getElement()) { 1521 if (path.equals(ed.getPath()) || (path+"[x]").equals(ed.getPath())) { 1522 return ed; 1523 } 1524 } 1525 return null; 1526 } 1527 1528 1529 public ElementDefinition getElementById(String id) { 1530 if (id == null) { 1531 return null; 1532 } 1533 for (ElementDefinition ed : getElement()) { 1534 if (id.equals(ed.getId())) { 1535 return ed; 1536 } 1537 } 1538 return null; 1539 } 1540 1541//end addition 1542 } 1543 1544 /** 1545 * An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure definition is stored on different servers. 1546 */ 1547 @Child(name = "url", type = {UriType.class}, order=0, min=1, max=1, modifier=false, summary=true) 1548 @Description(shortDefinition="Canonical identifier for this structure definition, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure definition is stored on different servers." ) 1549 protected UriType url; 1550 1551 /** 1552 * A formal identifier that is used to identify this structure definition when it is represented in other formats, or referenced in a specification, model, design or an instance. 1553 */ 1554 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1555 @Description(shortDefinition="Additional identifier for the structure definition", formalDefinition="A formal identifier that is used to identify this structure definition when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 1556 protected List<Identifier> identifier; 1557 1558 /** 1559 * The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. There is no expectation that versions can be placed in a lexicographical sequence, so authors are encouraged to populate the StructureDefinition.versionAlgorithm[x] element to enable comparisons. If there is no managed version available, authors can consider using ISO date/time syntax (e.g., '2023-01-01'). 1560 */ 1561 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1562 @Description(shortDefinition="Business version of the structure definition", formalDefinition="The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. There is no expectation that versions can be placed in a lexicographical sequence, so authors are encouraged to populate the StructureDefinition.versionAlgorithm[x] element to enable comparisons. If there is no managed version available, authors can consider using ISO date/time syntax (e.g., '2023-01-01')." ) 1563 protected StringType version; 1564 1565 /** 1566 * Indicates the mechanism used to compare versions to determine which is more current. 1567 */ 1568 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 1569 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 1570 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 1571 protected DataType versionAlgorithm; 1572 1573 /** 1574 * A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1575 */ 1576 @Child(name = "name", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=true) 1577 @Description(shortDefinition="Name for this structure definition (computer friendly)", formalDefinition="A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 1578 protected StringType name; 1579 1580 /** 1581 * A short, descriptive, user-friendly title for the structure definition. 1582 */ 1583 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1584 @Description(shortDefinition="Name for this structure definition (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the structure definition." ) 1585 protected StringType title; 1586 1587 /** 1588 * The status of this structure definition. Enables tracking the life-cycle of the content. 1589 */ 1590 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 1591 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this structure definition. Enables tracking the life-cycle of the content." ) 1592 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 1593 protected Enumeration<PublicationStatus> status; 1594 1595 /** 1596 * A Boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 1597 */ 1598 @Child(name = "experimental", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1599 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 1600 protected BooleanType experimental; 1601 1602 /** 1603 * The date (and optionally time) when the structure definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes. 1604 */ 1605 @Child(name = "date", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1606 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the structure definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes." ) 1607 protected DateTimeType date; 1608 1609 /** 1610 * The name of the organization or individual responsible for the release and ongoing maintenance of the structure definition. 1611 */ 1612 @Child(name = "publisher", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1613 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the structure definition." ) 1614 protected StringType publisher; 1615 1616 /** 1617 * Contact details to assist a user in finding and communicating with the publisher. 1618 */ 1619 @Child(name = "contact", type = {ContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1620 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 1621 protected List<ContactDetail> contact; 1622 1623 /** 1624 * A free text natural language description of the structure definition from a consumer's perspective. 1625 */ 1626 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 1627 @Description(shortDefinition="Natural language description of the structure definition", formalDefinition="A free text natural language description of the structure definition from a consumer's perspective." ) 1628 protected MarkdownType description; 1629 1630 /** 1631 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure definition instances. 1632 */ 1633 @Child(name = "useContext", type = {UsageContext.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1634 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure definition instances." ) 1635 protected List<UsageContext> useContext; 1636 1637 /** 1638 * A legal or geographic region in which the structure definition is intended to be used. 1639 */ 1640 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1641 @Description(shortDefinition="Intended jurisdiction for structure definition (if applicable)", formalDefinition="A legal or geographic region in which the structure definition is intended to be used." ) 1642 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 1643 protected List<CodeableConcept> jurisdiction; 1644 1645 /** 1646 * Explanation of why this structure definition is needed and why it has been designed as it has. 1647 */ 1648 @Child(name = "purpose", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 1649 @Description(shortDefinition="Why this structure definition is defined", formalDefinition="Explanation of why this structure definition is needed and why it has been designed as it has." ) 1650 protected MarkdownType purpose; 1651 1652 /** 1653 * A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element. 1654 */ 1655 @Child(name = "copyright", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 1656 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element." ) 1657 protected MarkdownType copyright; 1658 1659 /** 1660 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1661 */ 1662 @Child(name = "copyrightLabel", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 1663 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 1664 protected StringType copyrightLabel; 1665 1666 /** 1667 * (DEPRECATED) A set of key words or terms from external terminologies that may be used to assist with indexing and searching of templates nby describing the use of this structure definition, or the content it describes. 1668 */ 1669 @Child(name = "keyword", type = {Coding.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1670 @Description(shortDefinition="Assist with indexing and finding", formalDefinition="(DEPRECATED) A set of key words or terms from external terminologies that may be used to assist with indexing and searching of templates nby describing the use of this structure definition, or the content it describes." ) 1671 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/definition-use") 1672 protected List<Coding> keyword; 1673 1674 /** 1675 * The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version. 1676 */ 1677 @Child(name = "fhirVersion", type = {CodeType.class}, order=18, min=0, max=1, modifier=false, summary=true) 1678 @Description(shortDefinition="FHIR Version this StructureDefinition targets", formalDefinition="The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version." ) 1679 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/FHIR-version") 1680 protected Enumeration<FHIRVersion> fhirVersion; 1681 1682 /** 1683 * An external specification that the content is mapped to. 1684 */ 1685 @Child(name = "mapping", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1686 @Description(shortDefinition="External specification that the content is mapped to", formalDefinition="An external specification that the content is mapped to." ) 1687 protected List<StructureDefinitionMappingComponent> mapping; 1688 1689 /** 1690 * Defines the kind of structure that this definition is describing. 1691 */ 1692 @Child(name = "kind", type = {CodeType.class}, order=20, min=1, max=1, modifier=false, summary=true) 1693 @Description(shortDefinition="primitive-type | complex-type | resource | logical", formalDefinition="Defines the kind of structure that this definition is describing." ) 1694 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/structure-definition-kind") 1695 protected Enumeration<StructureDefinitionKind> kind; 1696 1697 /** 1698 * Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems. 1699 */ 1700 @Child(name = "abstract", type = {BooleanType.class}, order=21, min=1, max=1, modifier=false, summary=true) 1701 @Description(shortDefinition="Whether the structure is abstract", formalDefinition="Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems." ) 1702 protected BooleanType abstract_; 1703 1704 /** 1705 * Identifies the types of resource or data type elements to which the extension can be applied. For more guidance on using the 'context' element, see the [defining extensions page](defining-extensions.html#context). 1706 */ 1707 @Child(name = "context", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1708 @Description(shortDefinition="If an extension, where it can be used in instances", formalDefinition="Identifies the types of resource or data type elements to which the extension can be applied. For more guidance on using the 'context' element, see the [defining extensions page](defining-extensions.html#context)." ) 1709 protected List<StructureDefinitionContextComponent> context; 1710 1711 /** 1712 * A set of rules as FHIRPath Invariants about when the extension can be used (e.g. co-occurrence variants for the extension). All the rules must be true. 1713 */ 1714 @Child(name = "contextInvariant", type = {StringType.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1715 @Description(shortDefinition="FHIRPath invariants - when the extension can be used", formalDefinition="A set of rules as FHIRPath Invariants about when the extension can be used (e.g. co-occurrence variants for the extension). All the rules must be true." ) 1716 protected List<StringType> contextInvariant; 1717 1718 /** 1719 * The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models, where they are required. 1720 */ 1721 @Child(name = "type", type = {UriType.class}, order=24, min=1, max=1, modifier=false, summary=true) 1722 @Description(shortDefinition="Type defined or constrained by this structure", formalDefinition="The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models, where they are required." ) 1723 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fhir-types") 1724 protected UriType type; 1725 1726 /** 1727 * An absolute URI that is the base structure from which this type is derived, either by specialization or constraint. 1728 */ 1729 @Child(name = "baseDefinition", type = {CanonicalType.class}, order=25, min=0, max=1, modifier=false, summary=true) 1730 @Description(shortDefinition="Definition that this type is constrained/specialized from", formalDefinition="An absolute URI that is the base structure from which this type is derived, either by specialization or constraint." ) 1731 protected CanonicalType baseDefinition; 1732 1733 /** 1734 * How the type relates to the baseDefinition. 1735 */ 1736 @Child(name = "derivation", type = {CodeType.class}, order=26, min=0, max=1, modifier=false, summary=true) 1737 @Description(shortDefinition="specialization | constraint - How relates to base definition", formalDefinition="How the type relates to the baseDefinition." ) 1738 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/type-derivation-rule") 1739 protected Enumeration<TypeDerivationRule> derivation; 1740 1741 /** 1742 * A snapshot view is expressed in a standalone form that can be used and interpreted without considering the base StructureDefinition. 1743 */ 1744 @Child(name = "snapshot", type = {}, order=27, min=0, max=1, modifier=false, summary=false) 1745 @Description(shortDefinition="Snapshot view of the structure", formalDefinition="A snapshot view is expressed in a standalone form that can be used and interpreted without considering the base StructureDefinition." ) 1746 protected StructureDefinitionSnapshotComponent snapshot; 1747 1748 /** 1749 * A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies. 1750 */ 1751 @Child(name = "differential", type = {}, order=28, min=0, max=1, modifier=false, summary=false) 1752 @Description(shortDefinition="Differential view of the structure", formalDefinition="A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies." ) 1753 protected StructureDefinitionDifferentialComponent differential; 1754 1755 private static final long serialVersionUID = -1127285723L; 1756 1757 /** 1758 * Constructor 1759 */ 1760 public StructureDefinition() { 1761 super(); 1762 } 1763 1764 /** 1765 * Constructor 1766 */ 1767 public StructureDefinition(String url, String name, PublicationStatus status, StructureDefinitionKind kind, boolean abstract_, String type) { 1768 super(); 1769 this.setUrl(url); 1770 this.setName(name); 1771 this.setStatus(status); 1772 this.setKind(kind); 1773 this.setAbstract(abstract_); 1774 this.setType(type); 1775 } 1776 1777 /** 1778 * @return {@link #url} (An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1779 */ 1780 public UriType getUrlElement() { 1781 if (this.url == null) 1782 if (Configuration.errorOnAutoCreate()) 1783 throw new Error("Attempt to auto-create StructureDefinition.url"); 1784 else if (Configuration.doAutoCreate()) 1785 this.url = new UriType(); // bb 1786 return this.url; 1787 } 1788 1789 public boolean hasUrlElement() { 1790 return this.url != null && !this.url.isEmpty(); 1791 } 1792 1793 public boolean hasUrl() { 1794 return this.url != null && !this.url.isEmpty(); 1795 } 1796 1797 /** 1798 * @param value {@link #url} (An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1799 */ 1800 public StructureDefinition setUrlElement(UriType value) { 1801 this.url = value; 1802 return this; 1803 } 1804 1805 /** 1806 * @return An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure definition is stored on different servers. 1807 */ 1808 public String getUrl() { 1809 return this.url == null ? null : this.url.getValue(); 1810 } 1811 1812 /** 1813 * @param value An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure definition is stored on different servers. 1814 */ 1815 public StructureDefinition setUrl(String value) { 1816 if (this.url == null) 1817 this.url = new UriType(); 1818 this.url.setValue(value); 1819 return this; 1820 } 1821 1822 /** 1823 * @return {@link #identifier} (A formal identifier that is used to identify this structure definition when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1824 */ 1825 public List<Identifier> getIdentifier() { 1826 if (this.identifier == null) 1827 this.identifier = new ArrayList<Identifier>(); 1828 return this.identifier; 1829 } 1830 1831 /** 1832 * @return Returns a reference to <code>this</code> for easy method chaining 1833 */ 1834 public StructureDefinition setIdentifier(List<Identifier> theIdentifier) { 1835 this.identifier = theIdentifier; 1836 return this; 1837 } 1838 1839 public boolean hasIdentifier() { 1840 if (this.identifier == null) 1841 return false; 1842 for (Identifier item : this.identifier) 1843 if (!item.isEmpty()) 1844 return true; 1845 return false; 1846 } 1847 1848 public Identifier addIdentifier() { //3 1849 Identifier t = new Identifier(); 1850 if (this.identifier == null) 1851 this.identifier = new ArrayList<Identifier>(); 1852 this.identifier.add(t); 1853 return t; 1854 } 1855 1856 public StructureDefinition addIdentifier(Identifier t) { //3 1857 if (t == null) 1858 return this; 1859 if (this.identifier == null) 1860 this.identifier = new ArrayList<Identifier>(); 1861 this.identifier.add(t); 1862 return this; 1863 } 1864 1865 /** 1866 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1867 */ 1868 public Identifier getIdentifierFirstRep() { 1869 if (getIdentifier().isEmpty()) { 1870 addIdentifier(); 1871 } 1872 return getIdentifier().get(0); 1873 } 1874 1875 /** 1876 * @return {@link #version} (The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. There is no expectation that versions can be placed in a lexicographical sequence, so authors are encouraged to populate the StructureDefinition.versionAlgorithm[x] element to enable comparisons. If there is no managed version available, authors can consider using ISO date/time syntax (e.g., '2023-01-01').). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1877 */ 1878 public StringType getVersionElement() { 1879 if (this.version == null) 1880 if (Configuration.errorOnAutoCreate()) 1881 throw new Error("Attempt to auto-create StructureDefinition.version"); 1882 else if (Configuration.doAutoCreate()) 1883 this.version = new StringType(); // bb 1884 return this.version; 1885 } 1886 1887 public boolean hasVersionElement() { 1888 return this.version != null && !this.version.isEmpty(); 1889 } 1890 1891 public boolean hasVersion() { 1892 return this.version != null && !this.version.isEmpty(); 1893 } 1894 1895 /** 1896 * @param value {@link #version} (The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. There is no expectation that versions can be placed in a lexicographical sequence, so authors are encouraged to populate the StructureDefinition.versionAlgorithm[x] element to enable comparisons. If there is no managed version available, authors can consider using ISO date/time syntax (e.g., '2023-01-01').). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1897 */ 1898 public StructureDefinition setVersionElement(StringType value) { 1899 this.version = value; 1900 return this; 1901 } 1902 1903 /** 1904 * @return The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. There is no expectation that versions can be placed in a lexicographical sequence, so authors are encouraged to populate the StructureDefinition.versionAlgorithm[x] element to enable comparisons. If there is no managed version available, authors can consider using ISO date/time syntax (e.g., '2023-01-01'). 1905 */ 1906 public String getVersion() { 1907 return this.version == null ? null : this.version.getValue(); 1908 } 1909 1910 /** 1911 * @param value The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. There is no expectation that versions can be placed in a lexicographical sequence, so authors are encouraged to populate the StructureDefinition.versionAlgorithm[x] element to enable comparisons. If there is no managed version available, authors can consider using ISO date/time syntax (e.g., '2023-01-01'). 1912 */ 1913 public StructureDefinition setVersion(String value) { 1914 if (Utilities.noString(value)) 1915 this.version = null; 1916 else { 1917 if (this.version == null) 1918 this.version = new StringType(); 1919 this.version.setValue(value); 1920 } 1921 return this; 1922 } 1923 1924 /** 1925 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1926 */ 1927 public DataType getVersionAlgorithm() { 1928 return this.versionAlgorithm; 1929 } 1930 1931 /** 1932 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1933 */ 1934 public StringType getVersionAlgorithmStringType() throws FHIRException { 1935 if (this.versionAlgorithm == null) 1936 this.versionAlgorithm = new StringType(); 1937 if (!(this.versionAlgorithm instanceof StringType)) 1938 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1939 return (StringType) this.versionAlgorithm; 1940 } 1941 1942 public boolean hasVersionAlgorithmStringType() { 1943 return this.versionAlgorithm instanceof StringType; 1944 } 1945 1946 /** 1947 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1948 */ 1949 public Coding getVersionAlgorithmCoding() throws FHIRException { 1950 if (this.versionAlgorithm == null) 1951 this.versionAlgorithm = new Coding(); 1952 if (!(this.versionAlgorithm instanceof Coding)) 1953 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 1954 return (Coding) this.versionAlgorithm; 1955 } 1956 1957 public boolean hasVersionAlgorithmCoding() { 1958 return this.versionAlgorithm instanceof Coding; 1959 } 1960 1961 public boolean hasVersionAlgorithm() { 1962 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 1963 } 1964 1965 /** 1966 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 1967 */ 1968 public StructureDefinition setVersionAlgorithm(DataType value) { 1969 if (value != null && !(value instanceof StringType || value instanceof Coding)) 1970 throw new FHIRException("Not the right type for StructureDefinition.versionAlgorithm[x]: "+value.fhirType()); 1971 this.versionAlgorithm = value; 1972 return this; 1973 } 1974 1975 /** 1976 * @return {@link #name} (A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1977 */ 1978 public StringType getNameElement() { 1979 if (this.name == null) 1980 if (Configuration.errorOnAutoCreate()) 1981 throw new Error("Attempt to auto-create StructureDefinition.name"); 1982 else if (Configuration.doAutoCreate()) 1983 this.name = new StringType(); // bb 1984 return this.name; 1985 } 1986 1987 public boolean hasNameElement() { 1988 return this.name != null && !this.name.isEmpty(); 1989 } 1990 1991 public boolean hasName() { 1992 return this.name != null && !this.name.isEmpty(); 1993 } 1994 1995 /** 1996 * @param value {@link #name} (A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1997 */ 1998 public StructureDefinition setNameElement(StringType value) { 1999 this.name = value; 2000 return this; 2001 } 2002 2003 /** 2004 * @return A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2005 */ 2006 public String getName() { 2007 return this.name == null ? null : this.name.getValue(); 2008 } 2009 2010 /** 2011 * @param value A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2012 */ 2013 public StructureDefinition setName(String value) { 2014 if (this.name == null) 2015 this.name = new StringType(); 2016 this.name.setValue(value); 2017 return this; 2018 } 2019 2020 /** 2021 * @return {@link #title} (A short, descriptive, user-friendly title for the structure definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2022 */ 2023 public StringType getTitleElement() { 2024 if (this.title == null) 2025 if (Configuration.errorOnAutoCreate()) 2026 throw new Error("Attempt to auto-create StructureDefinition.title"); 2027 else if (Configuration.doAutoCreate()) 2028 this.title = new StringType(); // bb 2029 return this.title; 2030 } 2031 2032 public boolean hasTitleElement() { 2033 return this.title != null && !this.title.isEmpty(); 2034 } 2035 2036 public boolean hasTitle() { 2037 return this.title != null && !this.title.isEmpty(); 2038 } 2039 2040 /** 2041 * @param value {@link #title} (A short, descriptive, user-friendly title for the structure definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2042 */ 2043 public StructureDefinition setTitleElement(StringType value) { 2044 this.title = value; 2045 return this; 2046 } 2047 2048 /** 2049 * @return A short, descriptive, user-friendly title for the structure definition. 2050 */ 2051 public String getTitle() { 2052 return this.title == null ? null : this.title.getValue(); 2053 } 2054 2055 /** 2056 * @param value A short, descriptive, user-friendly title for the structure definition. 2057 */ 2058 public StructureDefinition setTitle(String value) { 2059 if (Utilities.noString(value)) 2060 this.title = null; 2061 else { 2062 if (this.title == null) 2063 this.title = new StringType(); 2064 this.title.setValue(value); 2065 } 2066 return this; 2067 } 2068 2069 /** 2070 * @return {@link #status} (The status of this structure definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2071 */ 2072 public Enumeration<PublicationStatus> getStatusElement() { 2073 if (this.status == null) 2074 if (Configuration.errorOnAutoCreate()) 2075 throw new Error("Attempt to auto-create StructureDefinition.status"); 2076 else if (Configuration.doAutoCreate()) 2077 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2078 return this.status; 2079 } 2080 2081 public boolean hasStatusElement() { 2082 return this.status != null && !this.status.isEmpty(); 2083 } 2084 2085 public boolean hasStatus() { 2086 return this.status != null && !this.status.isEmpty(); 2087 } 2088 2089 /** 2090 * @param value {@link #status} (The status of this structure definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2091 */ 2092 public StructureDefinition setStatusElement(Enumeration<PublicationStatus> value) { 2093 this.status = value; 2094 return this; 2095 } 2096 2097 /** 2098 * @return The status of this structure definition. Enables tracking the life-cycle of the content. 2099 */ 2100 public PublicationStatus getStatus() { 2101 return this.status == null ? null : this.status.getValue(); 2102 } 2103 2104 /** 2105 * @param value The status of this structure definition. Enables tracking the life-cycle of the content. 2106 */ 2107 public StructureDefinition setStatus(PublicationStatus value) { 2108 if (this.status == null) 2109 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2110 this.status.setValue(value); 2111 return this; 2112 } 2113 2114 /** 2115 * @return {@link #experimental} (A Boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2116 */ 2117 public BooleanType getExperimentalElement() { 2118 if (this.experimental == null) 2119 if (Configuration.errorOnAutoCreate()) 2120 throw new Error("Attempt to auto-create StructureDefinition.experimental"); 2121 else if (Configuration.doAutoCreate()) 2122 this.experimental = new BooleanType(); // bb 2123 return this.experimental; 2124 } 2125 2126 public boolean hasExperimentalElement() { 2127 return this.experimental != null && !this.experimental.isEmpty(); 2128 } 2129 2130 public boolean hasExperimental() { 2131 return this.experimental != null && !this.experimental.isEmpty(); 2132 } 2133 2134 /** 2135 * @param value {@link #experimental} (A Boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2136 */ 2137 public StructureDefinition setExperimentalElement(BooleanType value) { 2138 this.experimental = value; 2139 return this; 2140 } 2141 2142 /** 2143 * @return A Boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 2144 */ 2145 public boolean getExperimental() { 2146 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2147 } 2148 2149 /** 2150 * @param value A Boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 2151 */ 2152 public StructureDefinition setExperimental(boolean value) { 2153 if (this.experimental == null) 2154 this.experimental = new BooleanType(); 2155 this.experimental.setValue(value); 2156 return this; 2157 } 2158 2159 /** 2160 * @return {@link #date} (The date (and optionally time) when the structure definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2161 */ 2162 public DateTimeType getDateElement() { 2163 if (this.date == null) 2164 if (Configuration.errorOnAutoCreate()) 2165 throw new Error("Attempt to auto-create StructureDefinition.date"); 2166 else if (Configuration.doAutoCreate()) 2167 this.date = new DateTimeType(); // bb 2168 return this.date; 2169 } 2170 2171 public boolean hasDateElement() { 2172 return this.date != null && !this.date.isEmpty(); 2173 } 2174 2175 public boolean hasDate() { 2176 return this.date != null && !this.date.isEmpty(); 2177 } 2178 2179 /** 2180 * @param value {@link #date} (The date (and optionally time) when the structure definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2181 */ 2182 public StructureDefinition setDateElement(DateTimeType value) { 2183 this.date = value; 2184 return this; 2185 } 2186 2187 /** 2188 * @return The date (and optionally time) when the structure definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes. 2189 */ 2190 public Date getDate() { 2191 return this.date == null ? null : this.date.getValue(); 2192 } 2193 2194 /** 2195 * @param value The date (and optionally time) when the structure definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes. 2196 */ 2197 public StructureDefinition setDate(Date value) { 2198 if (value == null) 2199 this.date = null; 2200 else { 2201 if (this.date == null) 2202 this.date = new DateTimeType(); 2203 this.date.setValue(value); 2204 } 2205 return this; 2206 } 2207 2208 /** 2209 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the structure definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2210 */ 2211 public StringType getPublisherElement() { 2212 if (this.publisher == null) 2213 if (Configuration.errorOnAutoCreate()) 2214 throw new Error("Attempt to auto-create StructureDefinition.publisher"); 2215 else if (Configuration.doAutoCreate()) 2216 this.publisher = new StringType(); // bb 2217 return this.publisher; 2218 } 2219 2220 public boolean hasPublisherElement() { 2221 return this.publisher != null && !this.publisher.isEmpty(); 2222 } 2223 2224 public boolean hasPublisher() { 2225 return this.publisher != null && !this.publisher.isEmpty(); 2226 } 2227 2228 /** 2229 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the structure definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2230 */ 2231 public StructureDefinition setPublisherElement(StringType value) { 2232 this.publisher = value; 2233 return this; 2234 } 2235 2236 /** 2237 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the structure definition. 2238 */ 2239 public String getPublisher() { 2240 return this.publisher == null ? null : this.publisher.getValue(); 2241 } 2242 2243 /** 2244 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the structure definition. 2245 */ 2246 public StructureDefinition setPublisher(String value) { 2247 if (Utilities.noString(value)) 2248 this.publisher = null; 2249 else { 2250 if (this.publisher == null) 2251 this.publisher = new StringType(); 2252 this.publisher.setValue(value); 2253 } 2254 return this; 2255 } 2256 2257 /** 2258 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 2259 */ 2260 public List<ContactDetail> getContact() { 2261 if (this.contact == null) 2262 this.contact = new ArrayList<ContactDetail>(); 2263 return this.contact; 2264 } 2265 2266 /** 2267 * @return Returns a reference to <code>this</code> for easy method chaining 2268 */ 2269 public StructureDefinition setContact(List<ContactDetail> theContact) { 2270 this.contact = theContact; 2271 return this; 2272 } 2273 2274 public boolean hasContact() { 2275 if (this.contact == null) 2276 return false; 2277 for (ContactDetail item : this.contact) 2278 if (!item.isEmpty()) 2279 return true; 2280 return false; 2281 } 2282 2283 public ContactDetail addContact() { //3 2284 ContactDetail t = new ContactDetail(); 2285 if (this.contact == null) 2286 this.contact = new ArrayList<ContactDetail>(); 2287 this.contact.add(t); 2288 return t; 2289 } 2290 2291 public StructureDefinition addContact(ContactDetail t) { //3 2292 if (t == null) 2293 return this; 2294 if (this.contact == null) 2295 this.contact = new ArrayList<ContactDetail>(); 2296 this.contact.add(t); 2297 return this; 2298 } 2299 2300 /** 2301 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 2302 */ 2303 public ContactDetail getContactFirstRep() { 2304 if (getContact().isEmpty()) { 2305 addContact(); 2306 } 2307 return getContact().get(0); 2308 } 2309 2310 /** 2311 * @return {@link #description} (A free text natural language description of the structure definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2312 */ 2313 public MarkdownType getDescriptionElement() { 2314 if (this.description == null) 2315 if (Configuration.errorOnAutoCreate()) 2316 throw new Error("Attempt to auto-create StructureDefinition.description"); 2317 else if (Configuration.doAutoCreate()) 2318 this.description = new MarkdownType(); // bb 2319 return this.description; 2320 } 2321 2322 public boolean hasDescriptionElement() { 2323 return this.description != null && !this.description.isEmpty(); 2324 } 2325 2326 public boolean hasDescription() { 2327 return this.description != null && !this.description.isEmpty(); 2328 } 2329 2330 /** 2331 * @param value {@link #description} (A free text natural language description of the structure definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2332 */ 2333 public StructureDefinition setDescriptionElement(MarkdownType value) { 2334 this.description = value; 2335 return this; 2336 } 2337 2338 /** 2339 * @return A free text natural language description of the structure definition from a consumer's perspective. 2340 */ 2341 public String getDescription() { 2342 return this.description == null ? null : this.description.getValue(); 2343 } 2344 2345 /** 2346 * @param value A free text natural language description of the structure definition from a consumer's perspective. 2347 */ 2348 public StructureDefinition setDescription(String value) { 2349 if (Utilities.noString(value)) 2350 this.description = null; 2351 else { 2352 if (this.description == null) 2353 this.description = new MarkdownType(); 2354 this.description.setValue(value); 2355 } 2356 return this; 2357 } 2358 2359 /** 2360 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure definition instances.) 2361 */ 2362 public List<UsageContext> getUseContext() { 2363 if (this.useContext == null) 2364 this.useContext = new ArrayList<UsageContext>(); 2365 return this.useContext; 2366 } 2367 2368 /** 2369 * @return Returns a reference to <code>this</code> for easy method chaining 2370 */ 2371 public StructureDefinition setUseContext(List<UsageContext> theUseContext) { 2372 this.useContext = theUseContext; 2373 return this; 2374 } 2375 2376 public boolean hasUseContext() { 2377 if (this.useContext == null) 2378 return false; 2379 for (UsageContext item : this.useContext) 2380 if (!item.isEmpty()) 2381 return true; 2382 return false; 2383 } 2384 2385 public UsageContext addUseContext() { //3 2386 UsageContext t = new UsageContext(); 2387 if (this.useContext == null) 2388 this.useContext = new ArrayList<UsageContext>(); 2389 this.useContext.add(t); 2390 return t; 2391 } 2392 2393 public StructureDefinition addUseContext(UsageContext t) { //3 2394 if (t == null) 2395 return this; 2396 if (this.useContext == null) 2397 this.useContext = new ArrayList<UsageContext>(); 2398 this.useContext.add(t); 2399 return this; 2400 } 2401 2402 /** 2403 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 2404 */ 2405 public UsageContext getUseContextFirstRep() { 2406 if (getUseContext().isEmpty()) { 2407 addUseContext(); 2408 } 2409 return getUseContext().get(0); 2410 } 2411 2412 /** 2413 * @return {@link #jurisdiction} (A legal or geographic region in which the structure definition is intended to be used.) 2414 */ 2415 public List<CodeableConcept> getJurisdiction() { 2416 if (this.jurisdiction == null) 2417 this.jurisdiction = new ArrayList<CodeableConcept>(); 2418 return this.jurisdiction; 2419 } 2420 2421 /** 2422 * @return Returns a reference to <code>this</code> for easy method chaining 2423 */ 2424 public StructureDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 2425 this.jurisdiction = theJurisdiction; 2426 return this; 2427 } 2428 2429 public boolean hasJurisdiction() { 2430 if (this.jurisdiction == null) 2431 return false; 2432 for (CodeableConcept item : this.jurisdiction) 2433 if (!item.isEmpty()) 2434 return true; 2435 return false; 2436 } 2437 2438 public CodeableConcept addJurisdiction() { //3 2439 CodeableConcept t = new CodeableConcept(); 2440 if (this.jurisdiction == null) 2441 this.jurisdiction = new ArrayList<CodeableConcept>(); 2442 this.jurisdiction.add(t); 2443 return t; 2444 } 2445 2446 public StructureDefinition addJurisdiction(CodeableConcept t) { //3 2447 if (t == null) 2448 return this; 2449 if (this.jurisdiction == null) 2450 this.jurisdiction = new ArrayList<CodeableConcept>(); 2451 this.jurisdiction.add(t); 2452 return this; 2453 } 2454 2455 /** 2456 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 2457 */ 2458 public CodeableConcept getJurisdictionFirstRep() { 2459 if (getJurisdiction().isEmpty()) { 2460 addJurisdiction(); 2461 } 2462 return getJurisdiction().get(0); 2463 } 2464 2465 /** 2466 * @return {@link #purpose} (Explanation of why this structure definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 2467 */ 2468 public MarkdownType getPurposeElement() { 2469 if (this.purpose == null) 2470 if (Configuration.errorOnAutoCreate()) 2471 throw new Error("Attempt to auto-create StructureDefinition.purpose"); 2472 else if (Configuration.doAutoCreate()) 2473 this.purpose = new MarkdownType(); // bb 2474 return this.purpose; 2475 } 2476 2477 public boolean hasPurposeElement() { 2478 return this.purpose != null && !this.purpose.isEmpty(); 2479 } 2480 2481 public boolean hasPurpose() { 2482 return this.purpose != null && !this.purpose.isEmpty(); 2483 } 2484 2485 /** 2486 * @param value {@link #purpose} (Explanation of why this structure definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 2487 */ 2488 public StructureDefinition setPurposeElement(MarkdownType value) { 2489 this.purpose = value; 2490 return this; 2491 } 2492 2493 /** 2494 * @return Explanation of why this structure definition is needed and why it has been designed as it has. 2495 */ 2496 public String getPurpose() { 2497 return this.purpose == null ? null : this.purpose.getValue(); 2498 } 2499 2500 /** 2501 * @param value Explanation of why this structure definition is needed and why it has been designed as it has. 2502 */ 2503 public StructureDefinition setPurpose(String value) { 2504 if (Utilities.noString(value)) 2505 this.purpose = null; 2506 else { 2507 if (this.purpose == null) 2508 this.purpose = new MarkdownType(); 2509 this.purpose.setValue(value); 2510 } 2511 return this; 2512 } 2513 2514 /** 2515 * @return {@link #copyright} (A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 2516 */ 2517 public MarkdownType getCopyrightElement() { 2518 if (this.copyright == null) 2519 if (Configuration.errorOnAutoCreate()) 2520 throw new Error("Attempt to auto-create StructureDefinition.copyright"); 2521 else if (Configuration.doAutoCreate()) 2522 this.copyright = new MarkdownType(); // bb 2523 return this.copyright; 2524 } 2525 2526 public boolean hasCopyrightElement() { 2527 return this.copyright != null && !this.copyright.isEmpty(); 2528 } 2529 2530 public boolean hasCopyright() { 2531 return this.copyright != null && !this.copyright.isEmpty(); 2532 } 2533 2534 /** 2535 * @param value {@link #copyright} (A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 2536 */ 2537 public StructureDefinition setCopyrightElement(MarkdownType value) { 2538 this.copyright = value; 2539 return this; 2540 } 2541 2542 /** 2543 * @return A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element. 2544 */ 2545 public String getCopyright() { 2546 return this.copyright == null ? null : this.copyright.getValue(); 2547 } 2548 2549 /** 2550 * @param value A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element. 2551 */ 2552 public StructureDefinition setCopyright(String value) { 2553 if (Utilities.noString(value)) 2554 this.copyright = null; 2555 else { 2556 if (this.copyright == null) 2557 this.copyright = new MarkdownType(); 2558 this.copyright.setValue(value); 2559 } 2560 return this; 2561 } 2562 2563 /** 2564 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2565 */ 2566 public StringType getCopyrightLabelElement() { 2567 if (this.copyrightLabel == null) 2568 if (Configuration.errorOnAutoCreate()) 2569 throw new Error("Attempt to auto-create StructureDefinition.copyrightLabel"); 2570 else if (Configuration.doAutoCreate()) 2571 this.copyrightLabel = new StringType(); // bb 2572 return this.copyrightLabel; 2573 } 2574 2575 public boolean hasCopyrightLabelElement() { 2576 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2577 } 2578 2579 public boolean hasCopyrightLabel() { 2580 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2581 } 2582 2583 /** 2584 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2585 */ 2586 public StructureDefinition setCopyrightLabelElement(StringType value) { 2587 this.copyrightLabel = value; 2588 return this; 2589 } 2590 2591 /** 2592 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2593 */ 2594 public String getCopyrightLabel() { 2595 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 2596 } 2597 2598 /** 2599 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2600 */ 2601 public StructureDefinition setCopyrightLabel(String value) { 2602 if (Utilities.noString(value)) 2603 this.copyrightLabel = null; 2604 else { 2605 if (this.copyrightLabel == null) 2606 this.copyrightLabel = new StringType(); 2607 this.copyrightLabel.setValue(value); 2608 } 2609 return this; 2610 } 2611 2612 /** 2613 * @return {@link #keyword} ((DEPRECATED) A set of key words or terms from external terminologies that may be used to assist with indexing and searching of templates nby describing the use of this structure definition, or the content it describes.) 2614 */ 2615 public List<Coding> getKeyword() { 2616 if (this.keyword == null) 2617 this.keyword = new ArrayList<Coding>(); 2618 return this.keyword; 2619 } 2620 2621 /** 2622 * @return Returns a reference to <code>this</code> for easy method chaining 2623 */ 2624 public StructureDefinition setKeyword(List<Coding> theKeyword) { 2625 this.keyword = theKeyword; 2626 return this; 2627 } 2628 2629 public boolean hasKeyword() { 2630 if (this.keyword == null) 2631 return false; 2632 for (Coding item : this.keyword) 2633 if (!item.isEmpty()) 2634 return true; 2635 return false; 2636 } 2637 2638 public Coding addKeyword() { //3 2639 Coding t = new Coding(); 2640 if (this.keyword == null) 2641 this.keyword = new ArrayList<Coding>(); 2642 this.keyword.add(t); 2643 return t; 2644 } 2645 2646 public StructureDefinition addKeyword(Coding t) { //3 2647 if (t == null) 2648 return this; 2649 if (this.keyword == null) 2650 this.keyword = new ArrayList<Coding>(); 2651 this.keyword.add(t); 2652 return this; 2653 } 2654 2655 /** 2656 * @return The first repetition of repeating field {@link #keyword}, creating it if it does not already exist {3} 2657 */ 2658 public Coding getKeywordFirstRep() { 2659 if (getKeyword().isEmpty()) { 2660 addKeyword(); 2661 } 2662 return getKeyword().get(0); 2663 } 2664 2665 /** 2666 * @return {@link #fhirVersion} (The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version.). This is the underlying object with id, value and extensions. The accessor "getFhirVersion" gives direct access to the value 2667 */ 2668 public Enumeration<FHIRVersion> getFhirVersionElement() { 2669 if (this.fhirVersion == null) 2670 if (Configuration.errorOnAutoCreate()) 2671 throw new Error("Attempt to auto-create StructureDefinition.fhirVersion"); 2672 else if (Configuration.doAutoCreate()) 2673 this.fhirVersion = new Enumeration<FHIRVersion>(new FHIRVersionEnumFactory()); // bb 2674 return this.fhirVersion; 2675 } 2676 2677 public boolean hasFhirVersionElement() { 2678 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 2679 } 2680 2681 public boolean hasFhirVersion() { 2682 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 2683 } 2684 2685 /** 2686 * @param value {@link #fhirVersion} (The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version.). This is the underlying object with id, value and extensions. The accessor "getFhirVersion" gives direct access to the value 2687 */ 2688 public StructureDefinition setFhirVersionElement(Enumeration<FHIRVersion> value) { 2689 this.fhirVersion = value; 2690 return this; 2691 } 2692 2693 /** 2694 * @return The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version. 2695 */ 2696 public FHIRVersion getFhirVersion() { 2697 return this.fhirVersion == null ? null : this.fhirVersion.getValue(); 2698 } 2699 2700 /** 2701 * @param value The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version. 2702 */ 2703 public StructureDefinition setFhirVersion(FHIRVersion value) { 2704 if (value == null) 2705 this.fhirVersion = null; 2706 else { 2707 if (this.fhirVersion == null) 2708 this.fhirVersion = new Enumeration<FHIRVersion>(new FHIRVersionEnumFactory()); 2709 this.fhirVersion.setValue(value); 2710 } 2711 return this; 2712 } 2713 2714 /** 2715 * @return {@link #mapping} (An external specification that the content is mapped to.) 2716 */ 2717 public List<StructureDefinitionMappingComponent> getMapping() { 2718 if (this.mapping == null) 2719 this.mapping = new ArrayList<StructureDefinitionMappingComponent>(); 2720 return this.mapping; 2721 } 2722 2723 /** 2724 * @return Returns a reference to <code>this</code> for easy method chaining 2725 */ 2726 public StructureDefinition setMapping(List<StructureDefinitionMappingComponent> theMapping) { 2727 this.mapping = theMapping; 2728 return this; 2729 } 2730 2731 public boolean hasMapping() { 2732 if (this.mapping == null) 2733 return false; 2734 for (StructureDefinitionMappingComponent item : this.mapping) 2735 if (!item.isEmpty()) 2736 return true; 2737 return false; 2738 } 2739 2740 public StructureDefinitionMappingComponent addMapping() { //3 2741 StructureDefinitionMappingComponent t = new StructureDefinitionMappingComponent(); 2742 if (this.mapping == null) 2743 this.mapping = new ArrayList<StructureDefinitionMappingComponent>(); 2744 this.mapping.add(t); 2745 return t; 2746 } 2747 2748 public StructureDefinition addMapping(StructureDefinitionMappingComponent t) { //3 2749 if (t == null) 2750 return this; 2751 if (this.mapping == null) 2752 this.mapping = new ArrayList<StructureDefinitionMappingComponent>(); 2753 this.mapping.add(t); 2754 return this; 2755 } 2756 2757 /** 2758 * @return The first repetition of repeating field {@link #mapping}, creating it if it does not already exist {3} 2759 */ 2760 public StructureDefinitionMappingComponent getMappingFirstRep() { 2761 if (getMapping().isEmpty()) { 2762 addMapping(); 2763 } 2764 return getMapping().get(0); 2765 } 2766 2767 /** 2768 * @return {@link #kind} (Defines the kind of structure that this definition is describing.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 2769 */ 2770 public Enumeration<StructureDefinitionKind> getKindElement() { 2771 if (this.kind == null) 2772 if (Configuration.errorOnAutoCreate()) 2773 throw new Error("Attempt to auto-create StructureDefinition.kind"); 2774 else if (Configuration.doAutoCreate()) 2775 this.kind = new Enumeration<StructureDefinitionKind>(new StructureDefinitionKindEnumFactory()); // bb 2776 return this.kind; 2777 } 2778 2779 public boolean hasKindElement() { 2780 return this.kind != null && !this.kind.isEmpty(); 2781 } 2782 2783 public boolean hasKind() { 2784 return this.kind != null && !this.kind.isEmpty(); 2785 } 2786 2787 /** 2788 * @param value {@link #kind} (Defines the kind of structure that this definition is describing.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 2789 */ 2790 public StructureDefinition setKindElement(Enumeration<StructureDefinitionKind> value) { 2791 this.kind = value; 2792 return this; 2793 } 2794 2795 /** 2796 * @return Defines the kind of structure that this definition is describing. 2797 */ 2798 public StructureDefinitionKind getKind() { 2799 return this.kind == null ? null : this.kind.getValue(); 2800 } 2801 2802 /** 2803 * @param value Defines the kind of structure that this definition is describing. 2804 */ 2805 public StructureDefinition setKind(StructureDefinitionKind value) { 2806 if (this.kind == null) 2807 this.kind = new Enumeration<StructureDefinitionKind>(new StructureDefinitionKindEnumFactory()); 2808 this.kind.setValue(value); 2809 return this; 2810 } 2811 2812 /** 2813 * @return {@link #abstract_} (Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems.). This is the underlying object with id, value and extensions. The accessor "getAbstract" gives direct access to the value 2814 */ 2815 public BooleanType getAbstractElement() { 2816 if (this.abstract_ == null) 2817 if (Configuration.errorOnAutoCreate()) 2818 throw new Error("Attempt to auto-create StructureDefinition.abstract_"); 2819 else if (Configuration.doAutoCreate()) 2820 this.abstract_ = new BooleanType(); // bb 2821 return this.abstract_; 2822 } 2823 2824 public boolean hasAbstractElement() { 2825 return this.abstract_ != null && !this.abstract_.isEmpty(); 2826 } 2827 2828 public boolean hasAbstract() { 2829 return this.abstract_ != null && !this.abstract_.isEmpty(); 2830 } 2831 2832 /** 2833 * @param value {@link #abstract_} (Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems.). This is the underlying object with id, value and extensions. The accessor "getAbstract" gives direct access to the value 2834 */ 2835 public StructureDefinition setAbstractElement(BooleanType value) { 2836 this.abstract_ = value; 2837 return this; 2838 } 2839 2840 /** 2841 * @return Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems. 2842 */ 2843 public boolean getAbstract() { 2844 return this.abstract_ == null || this.abstract_.isEmpty() ? false : this.abstract_.getValue(); 2845 } 2846 2847 /** 2848 * @param value Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems. 2849 */ 2850 public StructureDefinition setAbstract(boolean value) { 2851 if (this.abstract_ == null) 2852 this.abstract_ = new BooleanType(); 2853 this.abstract_.setValue(value); 2854 return this; 2855 } 2856 2857 /** 2858 * @return {@link #context} (Identifies the types of resource or data type elements to which the extension can be applied. For more guidance on using the 'context' element, see the [defining extensions page](defining-extensions.html#context).) 2859 */ 2860 public List<StructureDefinitionContextComponent> getContext() { 2861 if (this.context == null) 2862 this.context = new ArrayList<StructureDefinitionContextComponent>(); 2863 return this.context; 2864 } 2865 2866 /** 2867 * @return Returns a reference to <code>this</code> for easy method chaining 2868 */ 2869 public StructureDefinition setContext(List<StructureDefinitionContextComponent> theContext) { 2870 this.context = theContext; 2871 return this; 2872 } 2873 2874 public boolean hasContext() { 2875 if (this.context == null) 2876 return false; 2877 for (StructureDefinitionContextComponent item : this.context) 2878 if (!item.isEmpty()) 2879 return true; 2880 return false; 2881 } 2882 2883 public StructureDefinitionContextComponent addContext() { //3 2884 StructureDefinitionContextComponent t = new StructureDefinitionContextComponent(); 2885 if (this.context == null) 2886 this.context = new ArrayList<StructureDefinitionContextComponent>(); 2887 this.context.add(t); 2888 return t; 2889 } 2890 2891 public StructureDefinition addContext(StructureDefinitionContextComponent t) { //3 2892 if (t == null) 2893 return this; 2894 if (this.context == null) 2895 this.context = new ArrayList<StructureDefinitionContextComponent>(); 2896 this.context.add(t); 2897 return this; 2898 } 2899 2900 /** 2901 * @return The first repetition of repeating field {@link #context}, creating it if it does not already exist {3} 2902 */ 2903 public StructureDefinitionContextComponent getContextFirstRep() { 2904 if (getContext().isEmpty()) { 2905 addContext(); 2906 } 2907 return getContext().get(0); 2908 } 2909 2910 /** 2911 * @return {@link #contextInvariant} (A set of rules as FHIRPath Invariants about when the extension can be used (e.g. co-occurrence variants for the extension). All the rules must be true.) 2912 */ 2913 public List<StringType> getContextInvariant() { 2914 if (this.contextInvariant == null) 2915 this.contextInvariant = new ArrayList<StringType>(); 2916 return this.contextInvariant; 2917 } 2918 2919 /** 2920 * @return Returns a reference to <code>this</code> for easy method chaining 2921 */ 2922 public StructureDefinition setContextInvariant(List<StringType> theContextInvariant) { 2923 this.contextInvariant = theContextInvariant; 2924 return this; 2925 } 2926 2927 public boolean hasContextInvariant() { 2928 if (this.contextInvariant == null) 2929 return false; 2930 for (StringType item : this.contextInvariant) 2931 if (!item.isEmpty()) 2932 return true; 2933 return false; 2934 } 2935 2936 /** 2937 * @return {@link #contextInvariant} (A set of rules as FHIRPath Invariants about when the extension can be used (e.g. co-occurrence variants for the extension). All the rules must be true.) 2938 */ 2939 public StringType addContextInvariantElement() {//2 2940 StringType t = new StringType(); 2941 if (this.contextInvariant == null) 2942 this.contextInvariant = new ArrayList<StringType>(); 2943 this.contextInvariant.add(t); 2944 return t; 2945 } 2946 2947 /** 2948 * @param value {@link #contextInvariant} (A set of rules as FHIRPath Invariants about when the extension can be used (e.g. co-occurrence variants for the extension). All the rules must be true.) 2949 */ 2950 public StructureDefinition addContextInvariant(String value) { //1 2951 StringType t = new StringType(); 2952 t.setValue(value); 2953 if (this.contextInvariant == null) 2954 this.contextInvariant = new ArrayList<StringType>(); 2955 this.contextInvariant.add(t); 2956 return this; 2957 } 2958 2959 /** 2960 * @param value {@link #contextInvariant} (A set of rules as FHIRPath Invariants about when the extension can be used (e.g. co-occurrence variants for the extension). All the rules must be true.) 2961 */ 2962 public boolean hasContextInvariant(String value) { 2963 if (this.contextInvariant == null) 2964 return false; 2965 for (StringType v : this.contextInvariant) 2966 if (v.getValue().equals(value)) // string 2967 return true; 2968 return false; 2969 } 2970 2971 /** 2972 * @return {@link #type} (The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models, where they are required.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2973 */ 2974 public UriType getTypeElement() { 2975 if (this.type == null) 2976 if (Configuration.errorOnAutoCreate()) 2977 throw new Error("Attempt to auto-create StructureDefinition.type"); 2978 else if (Configuration.doAutoCreate()) 2979 this.type = new UriType(); // bb 2980 return this.type; 2981 } 2982 2983 public boolean hasTypeElement() { 2984 return this.type != null && !this.type.isEmpty(); 2985 } 2986 2987 public boolean hasType() { 2988 return this.type != null && !this.type.isEmpty(); 2989 } 2990 2991 /** 2992 * @param value {@link #type} (The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models, where they are required.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2993 */ 2994 public StructureDefinition setTypeElement(UriType value) { 2995 this.type = value; 2996 return this; 2997 } 2998 2999 /** 3000 * @return The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models, where they are required. 3001 */ 3002 public String getType() { 3003 return this.type == null ? null : this.type.getValue(); 3004 } 3005 3006 /** 3007 * @param value The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models, where they are required. 3008 */ 3009 public StructureDefinition setType(String value) { 3010 if (this.type == null) 3011 this.type = new UriType(); 3012 this.type.setValue(value); 3013 return this; 3014 } 3015 3016 /** 3017 * @return {@link #baseDefinition} (An absolute URI that is the base structure from which this type is derived, either by specialization or constraint.). This is the underlying object with id, value and extensions. The accessor "getBaseDefinition" gives direct access to the value 3018 */ 3019 public CanonicalType getBaseDefinitionElement() { 3020 if (this.baseDefinition == null) 3021 if (Configuration.errorOnAutoCreate()) 3022 throw new Error("Attempt to auto-create StructureDefinition.baseDefinition"); 3023 else if (Configuration.doAutoCreate()) 3024 this.baseDefinition = new CanonicalType(); // bb 3025 return this.baseDefinition; 3026 } 3027 3028 public boolean hasBaseDefinitionElement() { 3029 return this.baseDefinition != null && !this.baseDefinition.isEmpty(); 3030 } 3031 3032 public boolean hasBaseDefinition() { 3033 return this.baseDefinition != null && !this.baseDefinition.isEmpty(); 3034 } 3035 3036 /** 3037 * @param value {@link #baseDefinition} (An absolute URI that is the base structure from which this type is derived, either by specialization or constraint.). This is the underlying object with id, value and extensions. The accessor "getBaseDefinition" gives direct access to the value 3038 */ 3039 public StructureDefinition setBaseDefinitionElement(CanonicalType value) { 3040 this.baseDefinition = value; 3041 return this; 3042 } 3043 3044 /** 3045 * @return An absolute URI that is the base structure from which this type is derived, either by specialization or constraint. 3046 */ 3047 public String getBaseDefinition() { 3048 return this.baseDefinition == null ? null : this.baseDefinition.getValue(); 3049 } 3050 3051 /** 3052 * @param value An absolute URI that is the base structure from which this type is derived, either by specialization or constraint. 3053 */ 3054 public StructureDefinition setBaseDefinition(String value) { 3055 if (Utilities.noString(value)) 3056 this.baseDefinition = null; 3057 else { 3058 if (this.baseDefinition == null) 3059 this.baseDefinition = new CanonicalType(); 3060 this.baseDefinition.setValue(value); 3061 } 3062 return this; 3063 } 3064 3065 /** 3066 * @return {@link #derivation} (How the type relates to the baseDefinition.). This is the underlying object with id, value and extensions. The accessor "getDerivation" gives direct access to the value 3067 */ 3068 public Enumeration<TypeDerivationRule> getDerivationElement() { 3069 if (this.derivation == null) 3070 if (Configuration.errorOnAutoCreate()) 3071 throw new Error("Attempt to auto-create StructureDefinition.derivation"); 3072 else if (Configuration.doAutoCreate()) 3073 this.derivation = new Enumeration<TypeDerivationRule>(new TypeDerivationRuleEnumFactory()); // bb 3074 return this.derivation; 3075 } 3076 3077 public boolean hasDerivationElement() { 3078 return this.derivation != null && !this.derivation.isEmpty(); 3079 } 3080 3081 public boolean hasDerivation() { 3082 return this.derivation != null && !this.derivation.isEmpty(); 3083 } 3084 3085 /** 3086 * @param value {@link #derivation} (How the type relates to the baseDefinition.). This is the underlying object with id, value and extensions. The accessor "getDerivation" gives direct access to the value 3087 */ 3088 public StructureDefinition setDerivationElement(Enumeration<TypeDerivationRule> value) { 3089 this.derivation = value; 3090 return this; 3091 } 3092 3093 /** 3094 * @return How the type relates to the baseDefinition. 3095 */ 3096 public TypeDerivationRule getDerivation() { 3097 return this.derivation == null ? null : this.derivation.getValue(); 3098 } 3099 3100 /** 3101 * @param value How the type relates to the baseDefinition. 3102 */ 3103 public StructureDefinition setDerivation(TypeDerivationRule value) { 3104 if (value == null) 3105 this.derivation = null; 3106 else { 3107 if (this.derivation == null) 3108 this.derivation = new Enumeration<TypeDerivationRule>(new TypeDerivationRuleEnumFactory()); 3109 this.derivation.setValue(value); 3110 } 3111 return this; 3112 } 3113 3114 /** 3115 * @return {@link #snapshot} (A snapshot view is expressed in a standalone form that can be used and interpreted without considering the base StructureDefinition.) 3116 */ 3117 public StructureDefinitionSnapshotComponent getSnapshot() { 3118 if (this.snapshot == null) 3119 if (Configuration.errorOnAutoCreate()) 3120 throw new Error("Attempt to auto-create StructureDefinition.snapshot"); 3121 else if (Configuration.doAutoCreate()) 3122 this.snapshot = new StructureDefinitionSnapshotComponent(); // cc 3123 return this.snapshot; 3124 } 3125 3126 public boolean hasSnapshot() { 3127 return this.snapshot != null && !this.snapshot.isEmpty(); 3128 } 3129 3130 /** 3131 * @param value {@link #snapshot} (A snapshot view is expressed in a standalone form that can be used and interpreted without considering the base StructureDefinition.) 3132 */ 3133 public StructureDefinition setSnapshot(StructureDefinitionSnapshotComponent value) { 3134 this.snapshot = value; 3135 return this; 3136 } 3137 3138 /** 3139 * @return {@link #differential} (A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies.) 3140 */ 3141 public StructureDefinitionDifferentialComponent getDifferential() { 3142 if (this.differential == null) 3143 if (Configuration.errorOnAutoCreate()) 3144 throw new Error("Attempt to auto-create StructureDefinition.differential"); 3145 else if (Configuration.doAutoCreate()) 3146 this.differential = new StructureDefinitionDifferentialComponent(); // cc 3147 return this.differential; 3148 } 3149 3150 public boolean hasDifferential() { 3151 return this.differential != null && !this.differential.isEmpty(); 3152 } 3153 3154 /** 3155 * @param value {@link #differential} (A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies.) 3156 */ 3157 public StructureDefinition setDifferential(StructureDefinitionDifferentialComponent value) { 3158 this.differential = value; 3159 return this; 3160 } 3161 3162 protected void listChildren(List<Property> children) { 3163 super.listChildren(children); 3164 children.add(new Property("url", "uri", "An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure definition is stored on different servers.", 0, 1, url)); 3165 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this structure definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3166 children.add(new Property("version", "string", "The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. There is no expectation that versions can be placed in a lexicographical sequence, so authors are encouraged to populate the StructureDefinition.versionAlgorithm[x] element to enable comparisons. If there is no managed version available, authors can consider using ISO date/time syntax (e.g., '2023-01-01').", 0, 1, version)); 3167 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 3168 children.add(new Property("name", "string", "A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 3169 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the structure definition.", 0, 1, title)); 3170 children.add(new Property("status", "code", "The status of this structure definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 3171 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 3172 children.add(new Property("date", "dateTime", "The date (and optionally time) when the structure definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes.", 0, 1, date)); 3173 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the structure definition.", 0, 1, publisher)); 3174 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 3175 children.add(new Property("description", "markdown", "A free text natural language description of the structure definition from a consumer's perspective.", 0, 1, description)); 3176 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 3177 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the structure definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 3178 children.add(new Property("purpose", "markdown", "Explanation of why this structure definition is needed and why it has been designed as it has.", 0, 1, purpose)); 3179 children.add(new Property("copyright", "markdown", "A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element.", 0, 1, copyright)); 3180 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 3181 children.add(new Property("keyword", "Coding", "(DEPRECATED) A set of key words or terms from external terminologies that may be used to assist with indexing and searching of templates nby describing the use of this structure definition, or the content it describes.", 0, java.lang.Integer.MAX_VALUE, keyword)); 3182 children.add(new Property("fhirVersion", "code", "The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version.", 0, 1, fhirVersion)); 3183 children.add(new Property("mapping", "", "An external specification that the content is mapped to.", 0, java.lang.Integer.MAX_VALUE, mapping)); 3184 children.add(new Property("kind", "code", "Defines the kind of structure that this definition is describing.", 0, 1, kind)); 3185 children.add(new Property("abstract", "boolean", "Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems.", 0, 1, abstract_)); 3186 children.add(new Property("context", "", "Identifies the types of resource or data type elements to which the extension can be applied. For more guidance on using the 'context' element, see the [defining extensions page](defining-extensions.html#context).", 0, java.lang.Integer.MAX_VALUE, context)); 3187 children.add(new Property("contextInvariant", "string", "A set of rules as FHIRPath Invariants about when the extension can be used (e.g. co-occurrence variants for the extension). All the rules must be true.", 0, java.lang.Integer.MAX_VALUE, contextInvariant)); 3188 children.add(new Property("type", "uri", "The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models, where they are required.", 0, 1, type)); 3189 children.add(new Property("baseDefinition", "canonical(StructureDefinition)", "An absolute URI that is the base structure from which this type is derived, either by specialization or constraint.", 0, 1, baseDefinition)); 3190 children.add(new Property("derivation", "code", "How the type relates to the baseDefinition.", 0, 1, derivation)); 3191 children.add(new Property("snapshot", "", "A snapshot view is expressed in a standalone form that can be used and interpreted without considering the base StructureDefinition.", 0, 1, snapshot)); 3192 children.add(new Property("differential", "", "A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies.", 0, 1, differential)); 3193 } 3194 3195 @Override 3196 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3197 switch (_hash) { 3198 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure definition is stored on different servers.", 0, 1, url); 3199 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this structure definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 3200 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. There is no expectation that versions can be placed in a lexicographical sequence, so authors are encouraged to populate the StructureDefinition.versionAlgorithm[x] element to enable comparisons. If there is no managed version available, authors can consider using ISO date/time syntax (e.g., '2023-01-01').", 0, 1, version); 3201 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3202 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3203 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3204 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3205 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 3206 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the structure definition.", 0, 1, title); 3207 case -892481550: /*status*/ return new Property("status", "code", "The status of this structure definition. Enables tracking the life-cycle of the content.", 0, 1, status); 3208 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 3209 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the structure definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes.", 0, 1, date); 3210 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the structure definition.", 0, 1, publisher); 3211 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 3212 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the structure definition from a consumer's perspective.", 0, 1, description); 3213 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 3214 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the structure definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 3215 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this structure definition is needed and why it has been designed as it has.", 0, 1, purpose); 3216 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element.", 0, 1, copyright); 3217 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 3218 case -814408215: /*keyword*/ return new Property("keyword", "Coding", "(DEPRECATED) A set of key words or terms from external terminologies that may be used to assist with indexing and searching of templates nby describing the use of this structure definition, or the content it describes.", 0, java.lang.Integer.MAX_VALUE, keyword); 3219 case 461006061: /*fhirVersion*/ return new Property("fhirVersion", "code", "The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version.", 0, 1, fhirVersion); 3220 case 837556430: /*mapping*/ return new Property("mapping", "", "An external specification that the content is mapped to.", 0, java.lang.Integer.MAX_VALUE, mapping); 3221 case 3292052: /*kind*/ return new Property("kind", "code", "Defines the kind of structure that this definition is describing.", 0, 1, kind); 3222 case 1732898850: /*abstract*/ return new Property("abstract", "boolean", "Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems.", 0, 1, abstract_); 3223 case 951530927: /*context*/ return new Property("context", "", "Identifies the types of resource or data type elements to which the extension can be applied. For more guidance on using the 'context' element, see the [defining extensions page](defining-extensions.html#context).", 0, java.lang.Integer.MAX_VALUE, context); 3224 case -802505007: /*contextInvariant*/ return new Property("contextInvariant", "string", "A set of rules as FHIRPath Invariants about when the extension can be used (e.g. co-occurrence variants for the extension). All the rules must be true.", 0, java.lang.Integer.MAX_VALUE, contextInvariant); 3225 case 3575610: /*type*/ return new Property("type", "uri", "The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models, where they are required.", 0, 1, type); 3226 case 1139771140: /*baseDefinition*/ return new Property("baseDefinition", "canonical(StructureDefinition)", "An absolute URI that is the base structure from which this type is derived, either by specialization or constraint.", 0, 1, baseDefinition); 3227 case -1353885513: /*derivation*/ return new Property("derivation", "code", "How the type relates to the baseDefinition.", 0, 1, derivation); 3228 case 284874180: /*snapshot*/ return new Property("snapshot", "", "A snapshot view is expressed in a standalone form that can be used and interpreted without considering the base StructureDefinition.", 0, 1, snapshot); 3229 case -1196150917: /*differential*/ return new Property("differential", "", "A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies.", 0, 1, differential); 3230 default: return super.getNamedProperty(_hash, _name, _checkValid); 3231 } 3232 3233 } 3234 3235 @Override 3236 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3237 switch (hash) { 3238 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 3239 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3240 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 3241 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 3242 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3243 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 3244 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 3245 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 3246 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 3247 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 3248 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3249 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3250 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3251 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3252 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 3253 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 3254 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 3255 case -814408215: /*keyword*/ return this.keyword == null ? new Base[0] : this.keyword.toArray(new Base[this.keyword.size()]); // Coding 3256 case 461006061: /*fhirVersion*/ return this.fhirVersion == null ? new Base[0] : new Base[] {this.fhirVersion}; // Enumeration<FHIRVersion> 3257 case 837556430: /*mapping*/ return this.mapping == null ? new Base[0] : this.mapping.toArray(new Base[this.mapping.size()]); // StructureDefinitionMappingComponent 3258 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // Enumeration<StructureDefinitionKind> 3259 case 1732898850: /*abstract*/ return this.abstract_ == null ? new Base[0] : new Base[] {this.abstract_}; // BooleanType 3260 case 951530927: /*context*/ return this.context == null ? new Base[0] : this.context.toArray(new Base[this.context.size()]); // StructureDefinitionContextComponent 3261 case -802505007: /*contextInvariant*/ return this.contextInvariant == null ? new Base[0] : this.contextInvariant.toArray(new Base[this.contextInvariant.size()]); // StringType 3262 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // UriType 3263 case 1139771140: /*baseDefinition*/ return this.baseDefinition == null ? new Base[0] : new Base[] {this.baseDefinition}; // CanonicalType 3264 case -1353885513: /*derivation*/ return this.derivation == null ? new Base[0] : new Base[] {this.derivation}; // Enumeration<TypeDerivationRule> 3265 case 284874180: /*snapshot*/ return this.snapshot == null ? new Base[0] : new Base[] {this.snapshot}; // StructureDefinitionSnapshotComponent 3266 case -1196150917: /*differential*/ return this.differential == null ? new Base[0] : new Base[] {this.differential}; // StructureDefinitionDifferentialComponent 3267 default: return super.getProperty(hash, name, checkValid); 3268 } 3269 3270 } 3271 3272 @Override 3273 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3274 switch (hash) { 3275 case 116079: // url 3276 this.url = TypeConvertor.castToUri(value); // UriType 3277 return value; 3278 case -1618432855: // identifier 3279 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3280 return value; 3281 case 351608024: // version 3282 this.version = TypeConvertor.castToString(value); // StringType 3283 return value; 3284 case 1508158071: // versionAlgorithm 3285 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 3286 return value; 3287 case 3373707: // name 3288 this.name = TypeConvertor.castToString(value); // StringType 3289 return value; 3290 case 110371416: // title 3291 this.title = TypeConvertor.castToString(value); // StringType 3292 return value; 3293 case -892481550: // status 3294 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3295 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3296 return value; 3297 case -404562712: // experimental 3298 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 3299 return value; 3300 case 3076014: // date 3301 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 3302 return value; 3303 case 1447404028: // publisher 3304 this.publisher = TypeConvertor.castToString(value); // StringType 3305 return value; 3306 case 951526432: // contact 3307 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 3308 return value; 3309 case -1724546052: // description 3310 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3311 return value; 3312 case -669707736: // useContext 3313 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 3314 return value; 3315 case -507075711: // jurisdiction 3316 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3317 return value; 3318 case -220463842: // purpose 3319 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 3320 return value; 3321 case 1522889671: // copyright 3322 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 3323 return value; 3324 case 765157229: // copyrightLabel 3325 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 3326 return value; 3327 case -814408215: // keyword 3328 this.getKeyword().add(TypeConvertor.castToCoding(value)); // Coding 3329 return value; 3330 case 461006061: // fhirVersion 3331 value = new FHIRVersionEnumFactory().fromType(TypeConvertor.castToCode(value)); 3332 this.fhirVersion = (Enumeration) value; // Enumeration<FHIRVersion> 3333 return value; 3334 case 837556430: // mapping 3335 this.getMapping().add((StructureDefinitionMappingComponent) value); // StructureDefinitionMappingComponent 3336 return value; 3337 case 3292052: // kind 3338 value = new StructureDefinitionKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 3339 this.kind = (Enumeration) value; // Enumeration<StructureDefinitionKind> 3340 return value; 3341 case 1732898850: // abstract 3342 this.abstract_ = TypeConvertor.castToBoolean(value); // BooleanType 3343 return value; 3344 case 951530927: // context 3345 this.getContext().add((StructureDefinitionContextComponent) value); // StructureDefinitionContextComponent 3346 return value; 3347 case -802505007: // contextInvariant 3348 this.getContextInvariant().add(TypeConvertor.castToString(value)); // StringType 3349 return value; 3350 case 3575610: // type 3351 this.type = TypeConvertor.castToUri(value); // UriType 3352 return value; 3353 case 1139771140: // baseDefinition 3354 this.baseDefinition = TypeConvertor.castToCanonical(value); // CanonicalType 3355 return value; 3356 case -1353885513: // derivation 3357 value = new TypeDerivationRuleEnumFactory().fromType(TypeConvertor.castToCode(value)); 3358 this.derivation = (Enumeration) value; // Enumeration<TypeDerivationRule> 3359 return value; 3360 case 284874180: // snapshot 3361 this.snapshot = (StructureDefinitionSnapshotComponent) value; // StructureDefinitionSnapshotComponent 3362 return value; 3363 case -1196150917: // differential 3364 this.differential = (StructureDefinitionDifferentialComponent) value; // StructureDefinitionDifferentialComponent 3365 return value; 3366 default: return super.setProperty(hash, name, value); 3367 } 3368 3369 } 3370 3371 @Override 3372 public Base setProperty(String name, Base value) throws FHIRException { 3373 if (name.equals("url")) { 3374 this.url = TypeConvertor.castToUri(value); // UriType 3375 } else if (name.equals("identifier")) { 3376 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3377 } else if (name.equals("version")) { 3378 this.version = TypeConvertor.castToString(value); // StringType 3379 } else if (name.equals("versionAlgorithm[x]")) { 3380 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 3381 } else if (name.equals("name")) { 3382 this.name = TypeConvertor.castToString(value); // StringType 3383 } else if (name.equals("title")) { 3384 this.title = TypeConvertor.castToString(value); // StringType 3385 } else if (name.equals("status")) { 3386 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3387 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3388 } else if (name.equals("experimental")) { 3389 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 3390 } else if (name.equals("date")) { 3391 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 3392 } else if (name.equals("publisher")) { 3393 this.publisher = TypeConvertor.castToString(value); // StringType 3394 } else if (name.equals("contact")) { 3395 this.getContact().add(TypeConvertor.castToContactDetail(value)); 3396 } else if (name.equals("description")) { 3397 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3398 } else if (name.equals("useContext")) { 3399 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 3400 } else if (name.equals("jurisdiction")) { 3401 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 3402 } else if (name.equals("purpose")) { 3403 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 3404 } else if (name.equals("copyright")) { 3405 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 3406 } else if (name.equals("copyrightLabel")) { 3407 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 3408 } else if (name.equals("keyword")) { 3409 this.getKeyword().add(TypeConvertor.castToCoding(value)); 3410 } else if (name.equals("fhirVersion")) { 3411 value = new FHIRVersionEnumFactory().fromType(TypeConvertor.castToCode(value)); 3412 this.fhirVersion = (Enumeration) value; // Enumeration<FHIRVersion> 3413 } else if (name.equals("mapping")) { 3414 this.getMapping().add((StructureDefinitionMappingComponent) value); 3415 } else if (name.equals("kind")) { 3416 value = new StructureDefinitionKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 3417 this.kind = (Enumeration) value; // Enumeration<StructureDefinitionKind> 3418 } else if (name.equals("abstract")) { 3419 this.abstract_ = TypeConvertor.castToBoolean(value); // BooleanType 3420 } else if (name.equals("context")) { 3421 this.getContext().add((StructureDefinitionContextComponent) value); 3422 } else if (name.equals("contextInvariant")) { 3423 this.getContextInvariant().add(TypeConvertor.castToString(value)); 3424 } else if (name.equals("type")) { 3425 this.type = TypeConvertor.castToUri(value); // UriType 3426 } else if (name.equals("baseDefinition")) { 3427 this.baseDefinition = TypeConvertor.castToCanonical(value); // CanonicalType 3428 } else if (name.equals("derivation")) { 3429 value = new TypeDerivationRuleEnumFactory().fromType(TypeConvertor.castToCode(value)); 3430 this.derivation = (Enumeration) value; // Enumeration<TypeDerivationRule> 3431 } else if (name.equals("snapshot")) { 3432 this.snapshot = (StructureDefinitionSnapshotComponent) value; // StructureDefinitionSnapshotComponent 3433 } else if (name.equals("differential")) { 3434 this.differential = (StructureDefinitionDifferentialComponent) value; // StructureDefinitionDifferentialComponent 3435 } else 3436 return super.setProperty(name, value); 3437 return value; 3438 } 3439 3440 @Override 3441 public void removeChild(String name, Base value) throws FHIRException { 3442 if (name.equals("url")) { 3443 this.url = null; 3444 } else if (name.equals("identifier")) { 3445 this.getIdentifier().remove(value); 3446 } else if (name.equals("version")) { 3447 this.version = null; 3448 } else if (name.equals("versionAlgorithm[x]")) { 3449 this.versionAlgorithm = null; 3450 } else if (name.equals("name")) { 3451 this.name = null; 3452 } else if (name.equals("title")) { 3453 this.title = null; 3454 } else if (name.equals("status")) { 3455 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3456 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3457 } else if (name.equals("experimental")) { 3458 this.experimental = null; 3459 } else if (name.equals("date")) { 3460 this.date = null; 3461 } else if (name.equals("publisher")) { 3462 this.publisher = null; 3463 } else if (name.equals("contact")) { 3464 this.getContact().remove(value); 3465 } else if (name.equals("description")) { 3466 this.description = null; 3467 } else if (name.equals("useContext")) { 3468 this.getUseContext().remove(value); 3469 } else if (name.equals("jurisdiction")) { 3470 this.getJurisdiction().remove(value); 3471 } else if (name.equals("purpose")) { 3472 this.purpose = null; 3473 } else if (name.equals("copyright")) { 3474 this.copyright = null; 3475 } else if (name.equals("copyrightLabel")) { 3476 this.copyrightLabel = null; 3477 } else if (name.equals("keyword")) { 3478 this.getKeyword().remove(value); 3479 } else if (name.equals("fhirVersion")) { 3480 value = new FHIRVersionEnumFactory().fromType(TypeConvertor.castToCode(value)); 3481 this.fhirVersion = (Enumeration) value; // Enumeration<FHIRVersion> 3482 } else if (name.equals("mapping")) { 3483 this.getMapping().remove((StructureDefinitionMappingComponent) value); 3484 } else if (name.equals("kind")) { 3485 value = new StructureDefinitionKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 3486 this.kind = (Enumeration) value; // Enumeration<StructureDefinitionKind> 3487 } else if (name.equals("abstract")) { 3488 this.abstract_ = null; 3489 } else if (name.equals("context")) { 3490 this.getContext().remove((StructureDefinitionContextComponent) value); 3491 } else if (name.equals("contextInvariant")) { 3492 this.getContextInvariant().remove(value); 3493 } else if (name.equals("type")) { 3494 this.type = null; 3495 } else if (name.equals("baseDefinition")) { 3496 this.baseDefinition = null; 3497 } else if (name.equals("derivation")) { 3498 value = new TypeDerivationRuleEnumFactory().fromType(TypeConvertor.castToCode(value)); 3499 this.derivation = (Enumeration) value; // Enumeration<TypeDerivationRule> 3500 } else if (name.equals("snapshot")) { 3501 this.snapshot = (StructureDefinitionSnapshotComponent) value; // StructureDefinitionSnapshotComponent 3502 } else if (name.equals("differential")) { 3503 this.differential = (StructureDefinitionDifferentialComponent) value; // StructureDefinitionDifferentialComponent 3504 } else 3505 super.removeChild(name, value); 3506 3507 } 3508 3509 @Override 3510 public Base makeProperty(int hash, String name) throws FHIRException { 3511 switch (hash) { 3512 case 116079: return getUrlElement(); 3513 case -1618432855: return addIdentifier(); 3514 case 351608024: return getVersionElement(); 3515 case -115699031: return getVersionAlgorithm(); 3516 case 1508158071: return getVersionAlgorithm(); 3517 case 3373707: return getNameElement(); 3518 case 110371416: return getTitleElement(); 3519 case -892481550: return getStatusElement(); 3520 case -404562712: return getExperimentalElement(); 3521 case 3076014: return getDateElement(); 3522 case 1447404028: return getPublisherElement(); 3523 case 951526432: return addContact(); 3524 case -1724546052: return getDescriptionElement(); 3525 case -669707736: return addUseContext(); 3526 case -507075711: return addJurisdiction(); 3527 case -220463842: return getPurposeElement(); 3528 case 1522889671: return getCopyrightElement(); 3529 case 765157229: return getCopyrightLabelElement(); 3530 case -814408215: return addKeyword(); 3531 case 461006061: return getFhirVersionElement(); 3532 case 837556430: return addMapping(); 3533 case 3292052: return getKindElement(); 3534 case 1732898850: return getAbstractElement(); 3535 case 951530927: return addContext(); 3536 case -802505007: return addContextInvariantElement(); 3537 case 3575610: return getTypeElement(); 3538 case 1139771140: return getBaseDefinitionElement(); 3539 case -1353885513: return getDerivationElement(); 3540 case 284874180: return getSnapshot(); 3541 case -1196150917: return getDifferential(); 3542 default: return super.makeProperty(hash, name); 3543 } 3544 3545 } 3546 3547 @Override 3548 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3549 switch (hash) { 3550 case 116079: /*url*/ return new String[] {"uri"}; 3551 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3552 case 351608024: /*version*/ return new String[] {"string"}; 3553 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 3554 case 3373707: /*name*/ return new String[] {"string"}; 3555 case 110371416: /*title*/ return new String[] {"string"}; 3556 case -892481550: /*status*/ return new String[] {"code"}; 3557 case -404562712: /*experimental*/ return new String[] {"boolean"}; 3558 case 3076014: /*date*/ return new String[] {"dateTime"}; 3559 case 1447404028: /*publisher*/ return new String[] {"string"}; 3560 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 3561 case -1724546052: /*description*/ return new String[] {"markdown"}; 3562 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 3563 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 3564 case -220463842: /*purpose*/ return new String[] {"markdown"}; 3565 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 3566 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 3567 case -814408215: /*keyword*/ return new String[] {"Coding"}; 3568 case 461006061: /*fhirVersion*/ return new String[] {"code"}; 3569 case 837556430: /*mapping*/ return new String[] {}; 3570 case 3292052: /*kind*/ return new String[] {"code"}; 3571 case 1732898850: /*abstract*/ return new String[] {"boolean"}; 3572 case 951530927: /*context*/ return new String[] {}; 3573 case -802505007: /*contextInvariant*/ return new String[] {"string"}; 3574 case 3575610: /*type*/ return new String[] {"uri"}; 3575 case 1139771140: /*baseDefinition*/ return new String[] {"canonical"}; 3576 case -1353885513: /*derivation*/ return new String[] {"code"}; 3577 case 284874180: /*snapshot*/ return new String[] {}; 3578 case -1196150917: /*differential*/ return new String[] {}; 3579 default: return super.getTypesForProperty(hash, name); 3580 } 3581 3582 } 3583 3584 @Override 3585 public Base addChild(String name) throws FHIRException { 3586 if (name.equals("url")) { 3587 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.url"); 3588 } 3589 else if (name.equals("identifier")) { 3590 return addIdentifier(); 3591 } 3592 else if (name.equals("version")) { 3593 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.version"); 3594 } 3595 else if (name.equals("versionAlgorithmString")) { 3596 this.versionAlgorithm = new StringType(); 3597 return this.versionAlgorithm; 3598 } 3599 else if (name.equals("versionAlgorithmCoding")) { 3600 this.versionAlgorithm = new Coding(); 3601 return this.versionAlgorithm; 3602 } 3603 else if (name.equals("name")) { 3604 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.name"); 3605 } 3606 else if (name.equals("title")) { 3607 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.title"); 3608 } 3609 else if (name.equals("status")) { 3610 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.status"); 3611 } 3612 else if (name.equals("experimental")) { 3613 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.experimental"); 3614 } 3615 else if (name.equals("date")) { 3616 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.date"); 3617 } 3618 else if (name.equals("publisher")) { 3619 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.publisher"); 3620 } 3621 else if (name.equals("contact")) { 3622 return addContact(); 3623 } 3624 else if (name.equals("description")) { 3625 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.description"); 3626 } 3627 else if (name.equals("useContext")) { 3628 return addUseContext(); 3629 } 3630 else if (name.equals("jurisdiction")) { 3631 return addJurisdiction(); 3632 } 3633 else if (name.equals("purpose")) { 3634 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.purpose"); 3635 } 3636 else if (name.equals("copyright")) { 3637 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.copyright"); 3638 } 3639 else if (name.equals("copyrightLabel")) { 3640 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.copyrightLabel"); 3641 } 3642 else if (name.equals("keyword")) { 3643 return addKeyword(); 3644 } 3645 else if (name.equals("fhirVersion")) { 3646 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.fhirVersion"); 3647 } 3648 else if (name.equals("mapping")) { 3649 return addMapping(); 3650 } 3651 else if (name.equals("kind")) { 3652 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.kind"); 3653 } 3654 else if (name.equals("abstract")) { 3655 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.abstract"); 3656 } 3657 else if (name.equals("context")) { 3658 return addContext(); 3659 } 3660 else if (name.equals("contextInvariant")) { 3661 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.contextInvariant"); 3662 } 3663 else if (name.equals("type")) { 3664 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.type"); 3665 } 3666 else if (name.equals("baseDefinition")) { 3667 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.baseDefinition"); 3668 } 3669 else if (name.equals("derivation")) { 3670 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.derivation"); 3671 } 3672 else if (name.equals("snapshot")) { 3673 this.snapshot = new StructureDefinitionSnapshotComponent(); 3674 return this.snapshot; 3675 } 3676 else if (name.equals("differential")) { 3677 this.differential = new StructureDefinitionDifferentialComponent(); 3678 return this.differential; 3679 } 3680 else 3681 return super.addChild(name); 3682 } 3683 3684 public String fhirType() { 3685 return "StructureDefinition"; 3686 3687 } 3688 3689 public StructureDefinition copy() { 3690 StructureDefinition dst = new StructureDefinition(); 3691 copyValues(dst); 3692 return dst; 3693 } 3694 3695 public void copyValues(StructureDefinition dst) { 3696 super.copyValues(dst); 3697 dst.url = url == null ? null : url.copy(); 3698 if (identifier != null) { 3699 dst.identifier = new ArrayList<Identifier>(); 3700 for (Identifier i : identifier) 3701 dst.identifier.add(i.copy()); 3702 }; 3703 dst.version = version == null ? null : version.copy(); 3704 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 3705 dst.name = name == null ? null : name.copy(); 3706 dst.title = title == null ? null : title.copy(); 3707 dst.status = status == null ? null : status.copy(); 3708 dst.experimental = experimental == null ? null : experimental.copy(); 3709 dst.date = date == null ? null : date.copy(); 3710 dst.publisher = publisher == null ? null : publisher.copy(); 3711 if (contact != null) { 3712 dst.contact = new ArrayList<ContactDetail>(); 3713 for (ContactDetail i : contact) 3714 dst.contact.add(i.copy()); 3715 }; 3716 dst.description = description == null ? null : description.copy(); 3717 if (useContext != null) { 3718 dst.useContext = new ArrayList<UsageContext>(); 3719 for (UsageContext i : useContext) 3720 dst.useContext.add(i.copy()); 3721 }; 3722 if (jurisdiction != null) { 3723 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3724 for (CodeableConcept i : jurisdiction) 3725 dst.jurisdiction.add(i.copy()); 3726 }; 3727 dst.purpose = purpose == null ? null : purpose.copy(); 3728 dst.copyright = copyright == null ? null : copyright.copy(); 3729 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 3730 if (keyword != null) { 3731 dst.keyword = new ArrayList<Coding>(); 3732 for (Coding i : keyword) 3733 dst.keyword.add(i.copy()); 3734 }; 3735 dst.fhirVersion = fhirVersion == null ? null : fhirVersion.copy(); 3736 if (mapping != null) { 3737 dst.mapping = new ArrayList<StructureDefinitionMappingComponent>(); 3738 for (StructureDefinitionMappingComponent i : mapping) 3739 dst.mapping.add(i.copy()); 3740 }; 3741 dst.kind = kind == null ? null : kind.copy(); 3742 dst.abstract_ = abstract_ == null ? null : abstract_.copy(); 3743 if (context != null) { 3744 dst.context = new ArrayList<StructureDefinitionContextComponent>(); 3745 for (StructureDefinitionContextComponent i : context) 3746 dst.context.add(i.copy()); 3747 }; 3748 if (contextInvariant != null) { 3749 dst.contextInvariant = new ArrayList<StringType>(); 3750 for (StringType i : contextInvariant) 3751 dst.contextInvariant.add(i.copy()); 3752 }; 3753 dst.type = type == null ? null : type.copy(); 3754 dst.baseDefinition = baseDefinition == null ? null : baseDefinition.copy(); 3755 dst.derivation = derivation == null ? null : derivation.copy(); 3756 dst.snapshot = snapshot == null ? null : snapshot.copy(); 3757 dst.differential = differential == null ? null : differential.copy(); 3758 } 3759 3760 protected StructureDefinition typedCopy() { 3761 return copy(); 3762 } 3763 3764 @Override 3765 public boolean equalsDeep(Base other_) { 3766 if (!super.equalsDeep(other_)) 3767 return false; 3768 if (!(other_ instanceof StructureDefinition)) 3769 return false; 3770 StructureDefinition o = (StructureDefinition) other_; 3771 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 3772 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 3773 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 3774 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 3775 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 3776 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 3777 && compareDeep(keyword, o.keyword, true) && compareDeep(fhirVersion, o.fhirVersion, true) && compareDeep(mapping, o.mapping, true) 3778 && compareDeep(kind, o.kind, true) && compareDeep(abstract_, o.abstract_, true) && compareDeep(context, o.context, true) 3779 && compareDeep(contextInvariant, o.contextInvariant, true) && compareDeep(type, o.type, true) && compareDeep(baseDefinition, o.baseDefinition, true) 3780 && compareDeep(derivation, o.derivation, true) && compareDeep(snapshot, o.snapshot, true) && compareDeep(differential, o.differential, true) 3781 ; 3782 } 3783 3784 @Override 3785 public boolean equalsShallow(Base other_) { 3786 if (!super.equalsShallow(other_)) 3787 return false; 3788 if (!(other_ instanceof StructureDefinition)) 3789 return false; 3790 StructureDefinition o = (StructureDefinition) other_; 3791 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 3792 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 3793 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 3794 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 3795 && compareValues(fhirVersion, o.fhirVersion, true) && compareValues(kind, o.kind, true) && compareValues(abstract_, o.abstract_, true) 3796 && compareValues(contextInvariant, o.contextInvariant, true) && compareValues(type, o.type, true) && compareValues(baseDefinition, o.baseDefinition, true) 3797 && compareValues(derivation, o.derivation, true); 3798 } 3799 3800 public boolean isEmpty() { 3801 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 3802 , versionAlgorithm, name, title, status, experimental, date, publisher, contact 3803 , description, useContext, jurisdiction, purpose, copyright, copyrightLabel, keyword 3804 , fhirVersion, mapping, kind, abstract_, context, contextInvariant, type, baseDefinition 3805 , derivation, snapshot, differential); 3806 } 3807 3808 @Override 3809 public ResourceType getResourceType() { 3810 return ResourceType.StructureDefinition; 3811 } 3812 3813 /** 3814 * Search parameter: <b>context-quantity</b> 3815 * <p> 3816 * Description: <b>Multiple Resources: 3817 3818* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 3819* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 3820* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 3821* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 3822* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 3823* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 3824* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 3825* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 3826* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 3827* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 3828* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 3829* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 3830* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 3831* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 3832* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 3833* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 3834* [Library](library.html): A quantity- or range-valued use context assigned to the library 3835* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 3836* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 3837* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 3838* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 3839* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 3840* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 3841* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 3842* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 3843* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 3844* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 3845* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 3846* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 3847* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 3848</b><br> 3849 * Type: <b>quantity</b><br> 3850 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 3851 * </p> 3852 */ 3853 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 3854 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 3855 /** 3856 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 3857 * <p> 3858 * Description: <b>Multiple Resources: 3859 3860* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 3861* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 3862* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 3863* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 3864* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 3865* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 3866* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 3867* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 3868* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 3869* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 3870* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 3871* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 3872* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 3873* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 3874* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 3875* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 3876* [Library](library.html): A quantity- or range-valued use context assigned to the library 3877* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 3878* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 3879* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 3880* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 3881* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 3882* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 3883* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 3884* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 3885* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 3886* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 3887* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 3888* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 3889* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 3890</b><br> 3891 * Type: <b>quantity</b><br> 3892 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 3893 * </p> 3894 */ 3895 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 3896 3897 /** 3898 * Search parameter: <b>context-type-quantity</b> 3899 * <p> 3900 * Description: <b>Multiple Resources: 3901 3902* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 3903* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 3904* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 3905* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 3906* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 3907* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 3908* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 3909* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 3910* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 3911* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 3912* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 3913* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 3914* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 3915* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 3916* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 3917* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 3918* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 3919* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 3920* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 3921* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 3922* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 3923* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 3924* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 3925* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 3926* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 3927* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 3928* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 3929* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 3930* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3931* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3932</b><br> 3933 * Type: <b>composite</b><br> 3934 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3935 * </p> 3936 */ 3937 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 3938 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 3939 /** 3940 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 3941 * <p> 3942 * Description: <b>Multiple Resources: 3943 3944* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 3945* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 3946* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 3947* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 3948* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 3949* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 3950* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 3951* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 3952* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 3953* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 3954* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 3955* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 3956* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 3957* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 3958* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 3959* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 3960* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 3961* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 3962* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 3963* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 3964* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 3965* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 3966* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 3967* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 3968* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 3969* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 3970* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 3971* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 3972* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3973* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3974</b><br> 3975 * Type: <b>composite</b><br> 3976 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3977 * </p> 3978 */ 3979 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 3980 3981 /** 3982 * Search parameter: <b>context-type-value</b> 3983 * <p> 3984 * Description: <b>Multiple Resources: 3985 3986* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3987* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3988* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3989* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3990* [Citation](citation.html): A use context type and value assigned to the citation 3991* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3992* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3993* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3994* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3995* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3996* [Evidence](evidence.html): A use context type and value assigned to the evidence 3997* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3998* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3999* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 4000* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 4001* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 4002* [Library](library.html): A use context type and value assigned to the library 4003* [Measure](measure.html): A use context type and value assigned to the measure 4004* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 4005* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 4006* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 4007* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 4008* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 4009* [Requirements](requirements.html): A use context type and value assigned to the requirements 4010* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 4011* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 4012* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 4013* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 4014* [TestScript](testscript.html): A use context type and value assigned to the test script 4015* [ValueSet](valueset.html): A use context type and value assigned to the value set 4016</b><br> 4017 * Type: <b>composite</b><br> 4018 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4019 * </p> 4020 */ 4021 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 4022 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 4023 /** 4024 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 4025 * <p> 4026 * Description: <b>Multiple Resources: 4027 4028* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 4029* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 4030* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 4031* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 4032* [Citation](citation.html): A use context type and value assigned to the citation 4033* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 4034* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 4035* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 4036* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 4037* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 4038* [Evidence](evidence.html): A use context type and value assigned to the evidence 4039* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 4040* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 4041* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 4042* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 4043* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 4044* [Library](library.html): A use context type and value assigned to the library 4045* [Measure](measure.html): A use context type and value assigned to the measure 4046* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 4047* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 4048* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 4049* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 4050* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 4051* [Requirements](requirements.html): A use context type and value assigned to the requirements 4052* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 4053* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 4054* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 4055* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 4056* [TestScript](testscript.html): A use context type and value assigned to the test script 4057* [ValueSet](valueset.html): A use context type and value assigned to the value set 4058</b><br> 4059 * Type: <b>composite</b><br> 4060 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 4061 * </p> 4062 */ 4063 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 4064 4065 /** 4066 * Search parameter: <b>context-type</b> 4067 * <p> 4068 * Description: <b>Multiple Resources: 4069 4070* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 4071* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 4072* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 4073* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 4074* [Citation](citation.html): A type of use context assigned to the citation 4075* [CodeSystem](codesystem.html): A type of use context assigned to the code system 4076* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 4077* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 4078* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 4079* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 4080* [Evidence](evidence.html): A type of use context assigned to the evidence 4081* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 4082* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 4083* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 4084* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 4085* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 4086* [Library](library.html): A type of use context assigned to the library 4087* [Measure](measure.html): A type of use context assigned to the measure 4088* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 4089* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 4090* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 4091* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 4092* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 4093* [Requirements](requirements.html): A type of use context assigned to the requirements 4094* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 4095* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 4096* [StructureMap](structuremap.html): A type of use context assigned to the structure map 4097* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 4098* [TestScript](testscript.html): A type of use context assigned to the test script 4099* [ValueSet](valueset.html): A type of use context assigned to the value set 4100</b><br> 4101 * Type: <b>token</b><br> 4102 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 4103 * </p> 4104 */ 4105 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 4106 public static final String SP_CONTEXT_TYPE = "context-type"; 4107 /** 4108 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 4109 * <p> 4110 * Description: <b>Multiple Resources: 4111 4112* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 4113* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 4114* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 4115* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 4116* [Citation](citation.html): A type of use context assigned to the citation 4117* [CodeSystem](codesystem.html): A type of use context assigned to the code system 4118* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 4119* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 4120* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 4121* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 4122* [Evidence](evidence.html): A type of use context assigned to the evidence 4123* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 4124* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 4125* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 4126* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 4127* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 4128* [Library](library.html): A type of use context assigned to the library 4129* [Measure](measure.html): A type of use context assigned to the measure 4130* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 4131* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 4132* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 4133* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 4134* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 4135* [Requirements](requirements.html): A type of use context assigned to the requirements 4136* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 4137* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 4138* [StructureMap](structuremap.html): A type of use context assigned to the structure map 4139* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 4140* [TestScript](testscript.html): A type of use context assigned to the test script 4141* [ValueSet](valueset.html): A type of use context assigned to the value set 4142</b><br> 4143 * Type: <b>token</b><br> 4144 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 4145 * </p> 4146 */ 4147 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 4148 4149 /** 4150 * Search parameter: <b>context</b> 4151 * <p> 4152 * Description: <b>Multiple Resources: 4153 4154* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 4155* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 4156* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 4157* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 4158* [Citation](citation.html): A use context assigned to the citation 4159* [CodeSystem](codesystem.html): A use context assigned to the code system 4160* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 4161* [ConceptMap](conceptmap.html): A use context assigned to the concept map 4162* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 4163* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 4164* [Evidence](evidence.html): A use context assigned to the evidence 4165* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 4166* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 4167* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 4168* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 4169* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 4170* [Library](library.html): A use context assigned to the library 4171* [Measure](measure.html): A use context assigned to the measure 4172* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 4173* [NamingSystem](namingsystem.html): A use context assigned to the naming system 4174* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 4175* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 4176* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 4177* [Requirements](requirements.html): A use context assigned to the requirements 4178* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 4179* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 4180* [StructureMap](structuremap.html): A use context assigned to the structure map 4181* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 4182* [TestScript](testscript.html): A use context assigned to the test script 4183* [ValueSet](valueset.html): A use context assigned to the value set 4184</b><br> 4185 * Type: <b>token</b><br> 4186 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 4187 * </p> 4188 */ 4189 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 4190 public static final String SP_CONTEXT = "context"; 4191 /** 4192 * <b>Fluent Client</b> search parameter constant for <b>context</b> 4193 * <p> 4194 * Description: <b>Multiple Resources: 4195 4196* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 4197* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 4198* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 4199* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 4200* [Citation](citation.html): A use context assigned to the citation 4201* [CodeSystem](codesystem.html): A use context assigned to the code system 4202* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 4203* [ConceptMap](conceptmap.html): A use context assigned to the concept map 4204* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 4205* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 4206* [Evidence](evidence.html): A use context assigned to the evidence 4207* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 4208* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 4209* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 4210* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 4211* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 4212* [Library](library.html): A use context assigned to the library 4213* [Measure](measure.html): A use context assigned to the measure 4214* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 4215* [NamingSystem](namingsystem.html): A use context assigned to the naming system 4216* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 4217* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 4218* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 4219* [Requirements](requirements.html): A use context assigned to the requirements 4220* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 4221* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 4222* [StructureMap](structuremap.html): A use context assigned to the structure map 4223* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 4224* [TestScript](testscript.html): A use context assigned to the test script 4225* [ValueSet](valueset.html): A use context assigned to the value set 4226</b><br> 4227 * Type: <b>token</b><br> 4228 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 4229 * </p> 4230 */ 4231 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 4232 4233 /** 4234 * Search parameter: <b>date</b> 4235 * <p> 4236 * Description: <b>Multiple Resources: 4237 4238* [ActivityDefinition](activitydefinition.html): The activity definition publication date 4239* [ActorDefinition](actordefinition.html): The Actor Definition publication date 4240* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 4241* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 4242* [Citation](citation.html): The citation publication date 4243* [CodeSystem](codesystem.html): The code system publication date 4244* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 4245* [ConceptMap](conceptmap.html): The concept map publication date 4246* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 4247* [EventDefinition](eventdefinition.html): The event definition publication date 4248* [Evidence](evidence.html): The evidence publication date 4249* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 4250* [ExampleScenario](examplescenario.html): The example scenario publication date 4251* [GraphDefinition](graphdefinition.html): The graph definition publication date 4252* [ImplementationGuide](implementationguide.html): The implementation guide publication date 4253* [Library](library.html): The library publication date 4254* [Measure](measure.html): The measure publication date 4255* [MessageDefinition](messagedefinition.html): The message definition publication date 4256* [NamingSystem](namingsystem.html): The naming system publication date 4257* [OperationDefinition](operationdefinition.html): The operation definition publication date 4258* [PlanDefinition](plandefinition.html): The plan definition publication date 4259* [Questionnaire](questionnaire.html): The questionnaire publication date 4260* [Requirements](requirements.html): The requirements publication date 4261* [SearchParameter](searchparameter.html): The search parameter publication date 4262* [StructureDefinition](structuredefinition.html): The structure definition publication date 4263* [StructureMap](structuremap.html): The structure map publication date 4264* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 4265* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 4266* [TestScript](testscript.html): The test script publication date 4267* [ValueSet](valueset.html): The value set publication date 4268</b><br> 4269 * Type: <b>date</b><br> 4270 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 4271 * </p> 4272 */ 4273 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 4274 public static final String SP_DATE = "date"; 4275 /** 4276 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4277 * <p> 4278 * Description: <b>Multiple Resources: 4279 4280* [ActivityDefinition](activitydefinition.html): The activity definition publication date 4281* [ActorDefinition](actordefinition.html): The Actor Definition publication date 4282* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 4283* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 4284* [Citation](citation.html): The citation publication date 4285* [CodeSystem](codesystem.html): The code system publication date 4286* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 4287* [ConceptMap](conceptmap.html): The concept map publication date 4288* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 4289* [EventDefinition](eventdefinition.html): The event definition publication date 4290* [Evidence](evidence.html): The evidence publication date 4291* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 4292* [ExampleScenario](examplescenario.html): The example scenario publication date 4293* [GraphDefinition](graphdefinition.html): The graph definition publication date 4294* [ImplementationGuide](implementationguide.html): The implementation guide publication date 4295* [Library](library.html): The library publication date 4296* [Measure](measure.html): The measure publication date 4297* [MessageDefinition](messagedefinition.html): The message definition publication date 4298* [NamingSystem](namingsystem.html): The naming system publication date 4299* [OperationDefinition](operationdefinition.html): The operation definition publication date 4300* [PlanDefinition](plandefinition.html): The plan definition publication date 4301* [Questionnaire](questionnaire.html): The questionnaire publication date 4302* [Requirements](requirements.html): The requirements publication date 4303* [SearchParameter](searchparameter.html): The search parameter publication date 4304* [StructureDefinition](structuredefinition.html): The structure definition publication date 4305* [StructureMap](structuremap.html): The structure map publication date 4306* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 4307* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 4308* [TestScript](testscript.html): The test script publication date 4309* [ValueSet](valueset.html): The value set publication date 4310</b><br> 4311 * Type: <b>date</b><br> 4312 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 4313 * </p> 4314 */ 4315 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4316 4317 /** 4318 * Search parameter: <b>description</b> 4319 * <p> 4320 * Description: <b>Multiple Resources: 4321 4322* [ActivityDefinition](activitydefinition.html): The description of the activity definition 4323* [ActorDefinition](actordefinition.html): The description of the Actor Definition 4324* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 4325* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 4326* [Citation](citation.html): The description of the citation 4327* [CodeSystem](codesystem.html): The description of the code system 4328* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 4329* [ConceptMap](conceptmap.html): The description of the concept map 4330* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 4331* [EventDefinition](eventdefinition.html): The description of the event definition 4332* [Evidence](evidence.html): The description of the evidence 4333* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 4334* [GraphDefinition](graphdefinition.html): The description of the graph definition 4335* [ImplementationGuide](implementationguide.html): The description of the implementation guide 4336* [Library](library.html): The description of the library 4337* [Measure](measure.html): The description of the measure 4338* [MessageDefinition](messagedefinition.html): The description of the message definition 4339* [NamingSystem](namingsystem.html): The description of the naming system 4340* [OperationDefinition](operationdefinition.html): The description of the operation definition 4341* [PlanDefinition](plandefinition.html): The description of the plan definition 4342* [Questionnaire](questionnaire.html): The description of the questionnaire 4343* [Requirements](requirements.html): The description of the requirements 4344* [SearchParameter](searchparameter.html): The description of the search parameter 4345* [StructureDefinition](structuredefinition.html): The description of the structure definition 4346* [StructureMap](structuremap.html): The description of the structure map 4347* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 4348* [TestScript](testscript.html): The description of the test script 4349* [ValueSet](valueset.html): The description of the value set 4350</b><br> 4351 * Type: <b>string</b><br> 4352 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 4353 * </p> 4354 */ 4355 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 4356 public static final String SP_DESCRIPTION = "description"; 4357 /** 4358 * <b>Fluent Client</b> search parameter constant for <b>description</b> 4359 * <p> 4360 * Description: <b>Multiple Resources: 4361 4362* [ActivityDefinition](activitydefinition.html): The description of the activity definition 4363* [ActorDefinition](actordefinition.html): The description of the Actor Definition 4364* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 4365* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 4366* [Citation](citation.html): The description of the citation 4367* [CodeSystem](codesystem.html): The description of the code system 4368* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 4369* [ConceptMap](conceptmap.html): The description of the concept map 4370* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 4371* [EventDefinition](eventdefinition.html): The description of the event definition 4372* [Evidence](evidence.html): The description of the evidence 4373* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 4374* [GraphDefinition](graphdefinition.html): The description of the graph definition 4375* [ImplementationGuide](implementationguide.html): The description of the implementation guide 4376* [Library](library.html): The description of the library 4377* [Measure](measure.html): The description of the measure 4378* [MessageDefinition](messagedefinition.html): The description of the message definition 4379* [NamingSystem](namingsystem.html): The description of the naming system 4380* [OperationDefinition](operationdefinition.html): The description of the operation definition 4381* [PlanDefinition](plandefinition.html): The description of the plan definition 4382* [Questionnaire](questionnaire.html): The description of the questionnaire 4383* [Requirements](requirements.html): The description of the requirements 4384* [SearchParameter](searchparameter.html): The description of the search parameter 4385* [StructureDefinition](structuredefinition.html): The description of the structure definition 4386* [StructureMap](structuremap.html): The description of the structure map 4387* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 4388* [TestScript](testscript.html): The description of the test script 4389* [ValueSet](valueset.html): The description of the value set 4390</b><br> 4391 * Type: <b>string</b><br> 4392 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 4393 * </p> 4394 */ 4395 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 4396 4397 /** 4398 * Search parameter: <b>identifier</b> 4399 * <p> 4400 * Description: <b>Multiple Resources: 4401 4402* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4403* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4404* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4405* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4406* [Citation](citation.html): External identifier for the citation 4407* [CodeSystem](codesystem.html): External identifier for the code system 4408* [ConceptMap](conceptmap.html): External identifier for the concept map 4409* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4410* [EventDefinition](eventdefinition.html): External identifier for the event definition 4411* [Evidence](evidence.html): External identifier for the evidence 4412* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4413* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4414* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4415* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4416* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4417* [Library](library.html): External identifier for the library 4418* [Measure](measure.html): External identifier for the measure 4419* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4420* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4421* [NamingSystem](namingsystem.html): External identifier for the naming system 4422* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4423* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4424* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4425* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4426* [Requirements](requirements.html): External identifier for the requirements 4427* [SearchParameter](searchparameter.html): External identifier for the search parameter 4428* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4429* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4430* [StructureMap](structuremap.html): External identifier for the structure map 4431* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4432* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4433* [TestPlan](testplan.html): An identifier for the test plan 4434* [TestScript](testscript.html): External identifier for the test script 4435* [ValueSet](valueset.html): External identifier for the value set 4436</b><br> 4437 * Type: <b>token</b><br> 4438 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4439 * </p> 4440 */ 4441 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 4442 public static final String SP_IDENTIFIER = "identifier"; 4443 /** 4444 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4445 * <p> 4446 * Description: <b>Multiple Resources: 4447 4448* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4449* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4450* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4451* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4452* [Citation](citation.html): External identifier for the citation 4453* [CodeSystem](codesystem.html): External identifier for the code system 4454* [ConceptMap](conceptmap.html): External identifier for the concept map 4455* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4456* [EventDefinition](eventdefinition.html): External identifier for the event definition 4457* [Evidence](evidence.html): External identifier for the evidence 4458* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4459* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4460* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4461* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4462* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4463* [Library](library.html): External identifier for the library 4464* [Measure](measure.html): External identifier for the measure 4465* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4466* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4467* [NamingSystem](namingsystem.html): External identifier for the naming system 4468* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4469* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4470* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4471* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4472* [Requirements](requirements.html): External identifier for the requirements 4473* [SearchParameter](searchparameter.html): External identifier for the search parameter 4474* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4475* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4476* [StructureMap](structuremap.html): External identifier for the structure map 4477* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4478* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4479* [TestPlan](testplan.html): An identifier for the test plan 4480* [TestScript](testscript.html): External identifier for the test script 4481* [ValueSet](valueset.html): External identifier for the value set 4482</b><br> 4483 * Type: <b>token</b><br> 4484 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4485 * </p> 4486 */ 4487 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4488 4489 /** 4490 * Search parameter: <b>jurisdiction</b> 4491 * <p> 4492 * Description: <b>Multiple Resources: 4493 4494* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 4495* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 4496* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 4497* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 4498* [Citation](citation.html): Intended jurisdiction for the citation 4499* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 4500* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 4501* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 4502* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 4503* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 4504* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 4505* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 4506* [Library](library.html): Intended jurisdiction for the library 4507* [Measure](measure.html): Intended jurisdiction for the measure 4508* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 4509* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 4510* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 4511* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 4512* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 4513* [Requirements](requirements.html): Intended jurisdiction for the requirements 4514* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 4515* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 4516* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 4517* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 4518* [TestScript](testscript.html): Intended jurisdiction for the test script 4519* [ValueSet](valueset.html): Intended jurisdiction for the value set 4520</b><br> 4521 * Type: <b>token</b><br> 4522 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 4523 * </p> 4524 */ 4525 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 4526 public static final String SP_JURISDICTION = "jurisdiction"; 4527 /** 4528 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 4529 * <p> 4530 * Description: <b>Multiple Resources: 4531 4532* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 4533* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 4534* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 4535* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 4536* [Citation](citation.html): Intended jurisdiction for the citation 4537* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 4538* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 4539* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 4540* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 4541* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 4542* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 4543* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 4544* [Library](library.html): Intended jurisdiction for the library 4545* [Measure](measure.html): Intended jurisdiction for the measure 4546* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 4547* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 4548* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 4549* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 4550* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 4551* [Requirements](requirements.html): Intended jurisdiction for the requirements 4552* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 4553* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 4554* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 4555* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 4556* [TestScript](testscript.html): Intended jurisdiction for the test script 4557* [ValueSet](valueset.html): Intended jurisdiction for the value set 4558</b><br> 4559 * Type: <b>token</b><br> 4560 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 4561 * </p> 4562 */ 4563 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 4564 4565 /** 4566 * Search parameter: <b>name</b> 4567 * <p> 4568 * Description: <b>Multiple Resources: 4569 4570* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 4571* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 4572* [Citation](citation.html): Computationally friendly name of the citation 4573* [CodeSystem](codesystem.html): Computationally friendly name of the code system 4574* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 4575* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 4576* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 4577* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 4578* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 4579* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 4580* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 4581* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 4582* [Library](library.html): Computationally friendly name of the library 4583* [Measure](measure.html): Computationally friendly name of the measure 4584* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 4585* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 4586* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 4587* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 4588* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 4589* [Requirements](requirements.html): Computationally friendly name of the requirements 4590* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 4591* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 4592* [StructureMap](structuremap.html): Computationally friendly name of the structure map 4593* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 4594* [TestScript](testscript.html): Computationally friendly name of the test script 4595* [ValueSet](valueset.html): Computationally friendly name of the value set 4596</b><br> 4597 * Type: <b>string</b><br> 4598 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 4599 * </p> 4600 */ 4601 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 4602 public static final String SP_NAME = "name"; 4603 /** 4604 * <b>Fluent Client</b> search parameter constant for <b>name</b> 4605 * <p> 4606 * Description: <b>Multiple Resources: 4607 4608* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 4609* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 4610* [Citation](citation.html): Computationally friendly name of the citation 4611* [CodeSystem](codesystem.html): Computationally friendly name of the code system 4612* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 4613* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 4614* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 4615* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 4616* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 4617* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 4618* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 4619* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 4620* [Library](library.html): Computationally friendly name of the library 4621* [Measure](measure.html): Computationally friendly name of the measure 4622* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 4623* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 4624* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 4625* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 4626* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 4627* [Requirements](requirements.html): Computationally friendly name of the requirements 4628* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 4629* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 4630* [StructureMap](structuremap.html): Computationally friendly name of the structure map 4631* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 4632* [TestScript](testscript.html): Computationally friendly name of the test script 4633* [ValueSet](valueset.html): Computationally friendly name of the value set 4634</b><br> 4635 * Type: <b>string</b><br> 4636 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 4637 * </p> 4638 */ 4639 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 4640 4641 /** 4642 * Search parameter: <b>publisher</b> 4643 * <p> 4644 * Description: <b>Multiple Resources: 4645 4646* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 4647* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 4648* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 4649* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 4650* [Citation](citation.html): Name of the publisher of the citation 4651* [CodeSystem](codesystem.html): Name of the publisher of the code system 4652* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 4653* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 4654* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 4655* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 4656* [Evidence](evidence.html): Name of the publisher of the evidence 4657* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 4658* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 4659* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 4660* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 4661* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 4662* [Library](library.html): Name of the publisher of the library 4663* [Measure](measure.html): Name of the publisher of the measure 4664* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 4665* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 4666* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 4667* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 4668* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 4669* [Requirements](requirements.html): Name of the publisher of the requirements 4670* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 4671* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 4672* [StructureMap](structuremap.html): Name of the publisher of the structure map 4673* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 4674* [TestScript](testscript.html): Name of the publisher of the test script 4675* [ValueSet](valueset.html): Name of the publisher of the value set 4676</b><br> 4677 * Type: <b>string</b><br> 4678 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 4679 * </p> 4680 */ 4681 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 4682 public static final String SP_PUBLISHER = "publisher"; 4683 /** 4684 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 4685 * <p> 4686 * Description: <b>Multiple Resources: 4687 4688* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 4689* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 4690* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 4691* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 4692* [Citation](citation.html): Name of the publisher of the citation 4693* [CodeSystem](codesystem.html): Name of the publisher of the code system 4694* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 4695* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 4696* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 4697* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 4698* [Evidence](evidence.html): Name of the publisher of the evidence 4699* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 4700* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 4701* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 4702* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 4703* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 4704* [Library](library.html): Name of the publisher of the library 4705* [Measure](measure.html): Name of the publisher of the measure 4706* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 4707* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 4708* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 4709* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 4710* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 4711* [Requirements](requirements.html): Name of the publisher of the requirements 4712* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 4713* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 4714* [StructureMap](structuremap.html): Name of the publisher of the structure map 4715* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 4716* [TestScript](testscript.html): Name of the publisher of the test script 4717* [ValueSet](valueset.html): Name of the publisher of the value set 4718</b><br> 4719 * Type: <b>string</b><br> 4720 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 4721 * </p> 4722 */ 4723 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 4724 4725 /** 4726 * Search parameter: <b>status</b> 4727 * <p> 4728 * Description: <b>Multiple Resources: 4729 4730* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4731* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4732* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4733* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4734* [Citation](citation.html): The current status of the citation 4735* [CodeSystem](codesystem.html): The current status of the code system 4736* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4737* [ConceptMap](conceptmap.html): The current status of the concept map 4738* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4739* [EventDefinition](eventdefinition.html): The current status of the event definition 4740* [Evidence](evidence.html): The current status of the evidence 4741* [EvidenceReport](evidencereport.html): The current status of the evidence report 4742* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4743* [ExampleScenario](examplescenario.html): The current status of the example scenario 4744* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4745* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4746* [Library](library.html): The current status of the library 4747* [Measure](measure.html): The current status of the measure 4748* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4749* [MessageDefinition](messagedefinition.html): The current status of the message definition 4750* [NamingSystem](namingsystem.html): The current status of the naming system 4751* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4752* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4753* [PlanDefinition](plandefinition.html): The current status of the plan definition 4754* [Questionnaire](questionnaire.html): The current status of the questionnaire 4755* [Requirements](requirements.html): The current status of the requirements 4756* [SearchParameter](searchparameter.html): The current status of the search parameter 4757* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4758* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4759* [StructureMap](structuremap.html): The current status of the structure map 4760* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4761* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4762* [TestPlan](testplan.html): The current status of the test plan 4763* [TestScript](testscript.html): The current status of the test script 4764* [ValueSet](valueset.html): The current status of the value set 4765</b><br> 4766 * Type: <b>token</b><br> 4767 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4768 * </p> 4769 */ 4770 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 4771 public static final String SP_STATUS = "status"; 4772 /** 4773 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4774 * <p> 4775 * Description: <b>Multiple Resources: 4776 4777* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4778* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4779* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4780* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4781* [Citation](citation.html): The current status of the citation 4782* [CodeSystem](codesystem.html): The current status of the code system 4783* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4784* [ConceptMap](conceptmap.html): The current status of the concept map 4785* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4786* [EventDefinition](eventdefinition.html): The current status of the event definition 4787* [Evidence](evidence.html): The current status of the evidence 4788* [EvidenceReport](evidencereport.html): The current status of the evidence report 4789* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4790* [ExampleScenario](examplescenario.html): The current status of the example scenario 4791* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4792* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4793* [Library](library.html): The current status of the library 4794* [Measure](measure.html): The current status of the measure 4795* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4796* [MessageDefinition](messagedefinition.html): The current status of the message definition 4797* [NamingSystem](namingsystem.html): The current status of the naming system 4798* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4799* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4800* [PlanDefinition](plandefinition.html): The current status of the plan definition 4801* [Questionnaire](questionnaire.html): The current status of the questionnaire 4802* [Requirements](requirements.html): The current status of the requirements 4803* [SearchParameter](searchparameter.html): The current status of the search parameter 4804* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4805* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4806* [StructureMap](structuremap.html): The current status of the structure map 4807* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4808* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4809* [TestPlan](testplan.html): The current status of the test plan 4810* [TestScript](testscript.html): The current status of the test script 4811* [ValueSet](valueset.html): The current status of the value set 4812</b><br> 4813 * Type: <b>token</b><br> 4814 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4815 * </p> 4816 */ 4817 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4818 4819 /** 4820 * Search parameter: <b>title</b> 4821 * <p> 4822 * Description: <b>Multiple Resources: 4823 4824* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 4825* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 4826* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 4827* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 4828* [Citation](citation.html): The human-friendly name of the citation 4829* [CodeSystem](codesystem.html): The human-friendly name of the code system 4830* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 4831* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 4832* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 4833* [Evidence](evidence.html): The human-friendly name of the evidence 4834* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 4835* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 4836* [Library](library.html): The human-friendly name of the library 4837* [Measure](measure.html): The human-friendly name of the measure 4838* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 4839* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 4840* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 4841* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 4842* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 4843* [Requirements](requirements.html): The human-friendly name of the requirements 4844* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 4845* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 4846* [StructureMap](structuremap.html): The human-friendly name of the structure map 4847* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 4848* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 4849* [TestScript](testscript.html): The human-friendly name of the test script 4850* [ValueSet](valueset.html): The human-friendly name of the value set 4851</b><br> 4852 * Type: <b>string</b><br> 4853 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 4854 * </p> 4855 */ 4856 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 4857 public static final String SP_TITLE = "title"; 4858 /** 4859 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4860 * <p> 4861 * Description: <b>Multiple Resources: 4862 4863* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 4864* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 4865* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 4866* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 4867* [Citation](citation.html): The human-friendly name of the citation 4868* [CodeSystem](codesystem.html): The human-friendly name of the code system 4869* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 4870* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 4871* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 4872* [Evidence](evidence.html): The human-friendly name of the evidence 4873* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 4874* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 4875* [Library](library.html): The human-friendly name of the library 4876* [Measure](measure.html): The human-friendly name of the measure 4877* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 4878* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 4879* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 4880* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 4881* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 4882* [Requirements](requirements.html): The human-friendly name of the requirements 4883* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 4884* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 4885* [StructureMap](structuremap.html): The human-friendly name of the structure map 4886* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 4887* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 4888* [TestScript](testscript.html): The human-friendly name of the test script 4889* [ValueSet](valueset.html): The human-friendly name of the value set 4890</b><br> 4891 * Type: <b>string</b><br> 4892 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 4893 * </p> 4894 */ 4895 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 4896 4897 /** 4898 * Search parameter: <b>url</b> 4899 * <p> 4900 * Description: <b>Multiple Resources: 4901 4902* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4903* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4904* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4905* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4906* [Citation](citation.html): The uri that identifies the citation 4907* [CodeSystem](codesystem.html): The uri that identifies the code system 4908* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4909* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4910* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4911* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4912* [Evidence](evidence.html): The uri that identifies the evidence 4913* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4914* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4915* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4916* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4917* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4918* [Library](library.html): The uri that identifies the library 4919* [Measure](measure.html): The uri that identifies the measure 4920* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4921* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4922* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4923* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4924* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4925* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4926* [Requirements](requirements.html): The uri that identifies the requirements 4927* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4928* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4929* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4930* [StructureMap](structuremap.html): The uri that identifies the structure map 4931* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4932* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4933* [TestPlan](testplan.html): The uri that identifies the test plan 4934* [TestScript](testscript.html): The uri that identifies the test script 4935* [ValueSet](valueset.html): The uri that identifies the value set 4936</b><br> 4937 * Type: <b>uri</b><br> 4938 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4939 * </p> 4940 */ 4941 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 4942 public static final String SP_URL = "url"; 4943 /** 4944 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4945 * <p> 4946 * Description: <b>Multiple Resources: 4947 4948* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4949* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4950* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4951* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4952* [Citation](citation.html): The uri that identifies the citation 4953* [CodeSystem](codesystem.html): The uri that identifies the code system 4954* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4955* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4956* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4957* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4958* [Evidence](evidence.html): The uri that identifies the evidence 4959* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4960* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4961* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4962* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4963* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4964* [Library](library.html): The uri that identifies the library 4965* [Measure](measure.html): The uri that identifies the measure 4966* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4967* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4968* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4969* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4970* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4971* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4972* [Requirements](requirements.html): The uri that identifies the requirements 4973* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4974* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4975* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4976* [StructureMap](structuremap.html): The uri that identifies the structure map 4977* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4978* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4979* [TestPlan](testplan.html): The uri that identifies the test plan 4980* [TestScript](testscript.html): The uri that identifies the test script 4981* [ValueSet](valueset.html): The uri that identifies the value set 4982</b><br> 4983 * Type: <b>uri</b><br> 4984 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4985 * </p> 4986 */ 4987 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4988 4989 /** 4990 * Search parameter: <b>version</b> 4991 * <p> 4992 * Description: <b>Multiple Resources: 4993 4994* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4995* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4996* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4997* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4998* [Citation](citation.html): The business version of the citation 4999* [CodeSystem](codesystem.html): The business version of the code system 5000* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 5001* [ConceptMap](conceptmap.html): The business version of the concept map 5002* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 5003* [EventDefinition](eventdefinition.html): The business version of the event definition 5004* [Evidence](evidence.html): The business version of the evidence 5005* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 5006* [ExampleScenario](examplescenario.html): The business version of the example scenario 5007* [GraphDefinition](graphdefinition.html): The business version of the graph definition 5008* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 5009* [Library](library.html): The business version of the library 5010* [Measure](measure.html): The business version of the measure 5011* [MessageDefinition](messagedefinition.html): The business version of the message definition 5012* [NamingSystem](namingsystem.html): The business version of the naming system 5013* [OperationDefinition](operationdefinition.html): The business version of the operation definition 5014* [PlanDefinition](plandefinition.html): The business version of the plan definition 5015* [Questionnaire](questionnaire.html): The business version of the questionnaire 5016* [Requirements](requirements.html): The business version of the requirements 5017* [SearchParameter](searchparameter.html): The business version of the search parameter 5018* [StructureDefinition](structuredefinition.html): The business version of the structure definition 5019* [StructureMap](structuremap.html): The business version of the structure map 5020* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 5021* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 5022* [TestScript](testscript.html): The business version of the test script 5023* [ValueSet](valueset.html): The business version of the value set 5024</b><br> 5025 * Type: <b>token</b><br> 5026 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 5027 * </p> 5028 */ 5029 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 5030 public static final String SP_VERSION = "version"; 5031 /** 5032 * <b>Fluent Client</b> search parameter constant for <b>version</b> 5033 * <p> 5034 * Description: <b>Multiple Resources: 5035 5036* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 5037* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 5038* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 5039* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 5040* [Citation](citation.html): The business version of the citation 5041* [CodeSystem](codesystem.html): The business version of the code system 5042* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 5043* [ConceptMap](conceptmap.html): The business version of the concept map 5044* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 5045* [EventDefinition](eventdefinition.html): The business version of the event definition 5046* [Evidence](evidence.html): The business version of the evidence 5047* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 5048* [ExampleScenario](examplescenario.html): The business version of the example scenario 5049* [GraphDefinition](graphdefinition.html): The business version of the graph definition 5050* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 5051* [Library](library.html): The business version of the library 5052* [Measure](measure.html): The business version of the measure 5053* [MessageDefinition](messagedefinition.html): The business version of the message definition 5054* [NamingSystem](namingsystem.html): The business version of the naming system 5055* [OperationDefinition](operationdefinition.html): The business version of the operation definition 5056* [PlanDefinition](plandefinition.html): The business version of the plan definition 5057* [Questionnaire](questionnaire.html): The business version of the questionnaire 5058* [Requirements](requirements.html): The business version of the requirements 5059* [SearchParameter](searchparameter.html): The business version of the search parameter 5060* [StructureDefinition](structuredefinition.html): The business version of the structure definition 5061* [StructureMap](structuremap.html): The business version of the structure map 5062* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 5063* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 5064* [TestScript](testscript.html): The business version of the test script 5065* [ValueSet](valueset.html): The business version of the value set 5066</b><br> 5067 * Type: <b>token</b><br> 5068 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 5069 * </p> 5070 */ 5071 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 5072 5073 /** 5074 * Search parameter: <b>abstract</b> 5075 * <p> 5076 * Description: <b>Whether the structure is abstract</b><br> 5077 * Type: <b>token</b><br> 5078 * Path: <b>StructureDefinition.abstract</b><br> 5079 * </p> 5080 */ 5081 @SearchParamDefinition(name="abstract", path="StructureDefinition.abstract", description="Whether the structure is abstract", type="token" ) 5082 public static final String SP_ABSTRACT = "abstract"; 5083 /** 5084 * <b>Fluent Client</b> search parameter constant for <b>abstract</b> 5085 * <p> 5086 * Description: <b>Whether the structure is abstract</b><br> 5087 * Type: <b>token</b><br> 5088 * Path: <b>StructureDefinition.abstract</b><br> 5089 * </p> 5090 */ 5091 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ABSTRACT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ABSTRACT); 5092 5093 /** 5094 * Search parameter: <b>base-path</b> 5095 * <p> 5096 * Description: <b>Path that identifies the base element</b><br> 5097 * Type: <b>token</b><br> 5098 * Path: <b>StructureDefinition.snapshot.element.base.path | StructureDefinition.differential.element.base.path</b><br> 5099 * </p> 5100 */ 5101 @SearchParamDefinition(name="base-path", path="StructureDefinition.snapshot.element.base.path | StructureDefinition.differential.element.base.path", description="Path that identifies the base element", type="token" ) 5102 public static final String SP_BASE_PATH = "base-path"; 5103 /** 5104 * <b>Fluent Client</b> search parameter constant for <b>base-path</b> 5105 * <p> 5106 * Description: <b>Path that identifies the base element</b><br> 5107 * Type: <b>token</b><br> 5108 * Path: <b>StructureDefinition.snapshot.element.base.path | StructureDefinition.differential.element.base.path</b><br> 5109 * </p> 5110 */ 5111 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BASE_PATH = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BASE_PATH); 5112 5113 /** 5114 * Search parameter: <b>base</b> 5115 * <p> 5116 * Description: <b>Definition that this type is constrained/specialized from</b><br> 5117 * Type: <b>reference</b><br> 5118 * Path: <b>StructureDefinition.baseDefinition</b><br> 5119 * </p> 5120 */ 5121 @SearchParamDefinition(name="base", path="StructureDefinition.baseDefinition", description="Definition that this type is constrained/specialized from", type="reference", target={StructureDefinition.class } ) 5122 public static final String SP_BASE = "base"; 5123 /** 5124 * <b>Fluent Client</b> search parameter constant for <b>base</b> 5125 * <p> 5126 * Description: <b>Definition that this type is constrained/specialized from</b><br> 5127 * Type: <b>reference</b><br> 5128 * Path: <b>StructureDefinition.baseDefinition</b><br> 5129 * </p> 5130 */ 5131 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASE); 5132 5133/** 5134 * Constant for fluent queries to be used to add include statements. Specifies 5135 * the path value of "<b>StructureDefinition:base</b>". 5136 */ 5137 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASE = new ca.uhn.fhir.model.api.Include("StructureDefinition:base").toLocked(); 5138 5139 /** 5140 * Search parameter: <b>derivation</b> 5141 * <p> 5142 * Description: <b>specialization | constraint - How relates to base definition</b><br> 5143 * Type: <b>token</b><br> 5144 * Path: <b>StructureDefinition.derivation</b><br> 5145 * </p> 5146 */ 5147 @SearchParamDefinition(name="derivation", path="StructureDefinition.derivation", description="specialization | constraint - How relates to base definition", type="token" ) 5148 public static final String SP_DERIVATION = "derivation"; 5149 /** 5150 * <b>Fluent Client</b> search parameter constant for <b>derivation</b> 5151 * <p> 5152 * Description: <b>specialization | constraint - How relates to base definition</b><br> 5153 * Type: <b>token</b><br> 5154 * Path: <b>StructureDefinition.derivation</b><br> 5155 * </p> 5156 */ 5157 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DERIVATION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DERIVATION); 5158 5159 /** 5160 * Search parameter: <b>experimental</b> 5161 * <p> 5162 * Description: <b>For testing purposes, not real usage</b><br> 5163 * Type: <b>token</b><br> 5164 * Path: <b>StructureDefinition.experimental</b><br> 5165 * </p> 5166 */ 5167 @SearchParamDefinition(name="experimental", path="StructureDefinition.experimental", description="For testing purposes, not real usage", type="token" ) 5168 public static final String SP_EXPERIMENTAL = "experimental"; 5169 /** 5170 * <b>Fluent Client</b> search parameter constant for <b>experimental</b> 5171 * <p> 5172 * Description: <b>For testing purposes, not real usage</b><br> 5173 * Type: <b>token</b><br> 5174 * Path: <b>StructureDefinition.experimental</b><br> 5175 * </p> 5176 */ 5177 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXPERIMENTAL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EXPERIMENTAL); 5178 5179 /** 5180 * Search parameter: <b>ext-context-expression</b> 5181 * <p> 5182 * Description: <b>An expression of extension context assigned to the structure definition</b><br> 5183 * Type: <b>token</b><br> 5184 * Path: <b>StructureDefinition.context.expression</b><br> 5185 * </p> 5186 */ 5187 @SearchParamDefinition(name="ext-context-expression", path="StructureDefinition.context.expression", description="An expression of extension context assigned to the structure definition", type="token" ) 5188 public static final String SP_EXT_CONTEXT_EXPRESSION = "ext-context-expression"; 5189 /** 5190 * <b>Fluent Client</b> search parameter constant for <b>ext-context-expression</b> 5191 * <p> 5192 * Description: <b>An expression of extension context assigned to the structure definition</b><br> 5193 * Type: <b>token</b><br> 5194 * Path: <b>StructureDefinition.context.expression</b><br> 5195 * </p> 5196 */ 5197 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXT_CONTEXT_EXPRESSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EXT_CONTEXT_EXPRESSION); 5198 5199 /** 5200 * Search parameter: <b>ext-context-type</b> 5201 * <p> 5202 * Description: <b>A type of extension context assigned to the structure definition</b><br> 5203 * Type: <b>token</b><br> 5204 * Path: <b>StructureDefinition.context.type</b><br> 5205 * </p> 5206 */ 5207 @SearchParamDefinition(name="ext-context-type", path="StructureDefinition.context.type", description="A type of extension context assigned to the structure definition", type="token" ) 5208 public static final String SP_EXT_CONTEXT_TYPE = "ext-context-type"; 5209 /** 5210 * <b>Fluent Client</b> search parameter constant for <b>ext-context-type</b> 5211 * <p> 5212 * Description: <b>A type of extension context assigned to the structure definition</b><br> 5213 * Type: <b>token</b><br> 5214 * Path: <b>StructureDefinition.context.type</b><br> 5215 * </p> 5216 */ 5217 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXT_CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EXT_CONTEXT_TYPE); 5218 5219 /** 5220 * Search parameter: <b>ext-context</b> 5221 * <p> 5222 * Description: <b>An extension context assigned to the structure definition</b><br> 5223 * Type: <b>composite</b><br> 5224 * Path: <b>StructureDefinition.context</b><br> 5225 * </p> 5226 */ 5227 @SearchParamDefinition(name="ext-context", path="StructureDefinition.context", description="An extension context assigned to the structure definition", type="composite", compositeOf={"ext-context-type", "ext-context-expression"} ) 5228 public static final String SP_EXT_CONTEXT = "ext-context"; 5229 /** 5230 * <b>Fluent Client</b> search parameter constant for <b>ext-context</b> 5231 * <p> 5232 * Description: <b>An extension context assigned to the structure definition</b><br> 5233 * Type: <b>composite</b><br> 5234 * Path: <b>StructureDefinition.context</b><br> 5235 * </p> 5236 */ 5237 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> EXT_CONTEXT = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_EXT_CONTEXT); 5238 5239 /** 5240 * Search parameter: <b>keyword</b> 5241 * <p> 5242 * Description: <b>A code for the StructureDefinition</b><br> 5243 * Type: <b>token</b><br> 5244 * Path: <b>StructureDefinition.keyword</b><br> 5245 * </p> 5246 */ 5247 @SearchParamDefinition(name="keyword", path="StructureDefinition.keyword", description="A code for the StructureDefinition", type="token" ) 5248 public static final String SP_KEYWORD = "keyword"; 5249 /** 5250 * <b>Fluent Client</b> search parameter constant for <b>keyword</b> 5251 * <p> 5252 * Description: <b>A code for the StructureDefinition</b><br> 5253 * Type: <b>token</b><br> 5254 * Path: <b>StructureDefinition.keyword</b><br> 5255 * </p> 5256 */ 5257 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KEYWORD = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_KEYWORD); 5258 5259 /** 5260 * Search parameter: <b>kind</b> 5261 * <p> 5262 * Description: <b>primitive-type | complex-type | resource | logical</b><br> 5263 * Type: <b>token</b><br> 5264 * Path: <b>StructureDefinition.kind</b><br> 5265 * </p> 5266 */ 5267 @SearchParamDefinition(name="kind", path="StructureDefinition.kind", description="primitive-type | complex-type | resource | logical", type="token" ) 5268 public static final String SP_KIND = "kind"; 5269 /** 5270 * <b>Fluent Client</b> search parameter constant for <b>kind</b> 5271 * <p> 5272 * Description: <b>primitive-type | complex-type | resource | logical</b><br> 5273 * Type: <b>token</b><br> 5274 * Path: <b>StructureDefinition.kind</b><br> 5275 * </p> 5276 */ 5277 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KIND = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_KIND); 5278 5279 /** 5280 * Search parameter: <b>path</b> 5281 * <p> 5282 * Description: <b>A path that is constrained in the StructureDefinition</b><br> 5283 * Type: <b>token</b><br> 5284 * Path: <b>StructureDefinition.snapshot.element.path | StructureDefinition.differential.element.path</b><br> 5285 * </p> 5286 */ 5287 @SearchParamDefinition(name="path", path="StructureDefinition.snapshot.element.path | StructureDefinition.differential.element.path", description="A path that is constrained in the StructureDefinition", type="token" ) 5288 public static final String SP_PATH = "path"; 5289 /** 5290 * <b>Fluent Client</b> search parameter constant for <b>path</b> 5291 * <p> 5292 * Description: <b>A path that is constrained in the StructureDefinition</b><br> 5293 * Type: <b>token</b><br> 5294 * Path: <b>StructureDefinition.snapshot.element.path | StructureDefinition.differential.element.path</b><br> 5295 * </p> 5296 */ 5297 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PATH = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PATH); 5298 5299 /** 5300 * Search parameter: <b>type</b> 5301 * <p> 5302 * Description: <b>Type defined or constrained by this structure</b><br> 5303 * Type: <b>uri</b><br> 5304 * Path: <b>StructureDefinition.type</b><br> 5305 * </p> 5306 */ 5307 @SearchParamDefinition(name="type", path="StructureDefinition.type", description="Type defined or constrained by this structure", type="uri" ) 5308 public static final String SP_TYPE = "type"; 5309 /** 5310 * <b>Fluent Client</b> search parameter constant for <b>type</b> 5311 * <p> 5312 * Description: <b>Type defined or constrained by this structure</b><br> 5313 * Type: <b>uri</b><br> 5314 * Path: <b>StructureDefinition.type</b><br> 5315 * </p> 5316 */ 5317 public static final ca.uhn.fhir.rest.gclient.UriClientParam TYPE = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_TYPE); 5318 5319 /** 5320 * Search parameter: <b>valueset</b> 5321 * <p> 5322 * Description: <b>A vocabulary binding reference</b><br> 5323 * Type: <b>reference</b><br> 5324 * Path: <b>StructureDefinition.snapshot.element.binding.valueSet</b><br> 5325 * </p> 5326 */ 5327 @SearchParamDefinition(name="valueset", path="StructureDefinition.snapshot.element.binding.valueSet", description="A vocabulary binding reference", type="reference", target={ValueSet.class } ) 5328 public static final String SP_VALUESET = "valueset"; 5329 /** 5330 * <b>Fluent Client</b> search parameter constant for <b>valueset</b> 5331 * <p> 5332 * Description: <b>A vocabulary binding reference</b><br> 5333 * Type: <b>reference</b><br> 5334 * Path: <b>StructureDefinition.snapshot.element.binding.valueSet</b><br> 5335 * </p> 5336 */ 5337 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam VALUESET = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_VALUESET); 5338 5339/** 5340 * Constant for fluent queries to be used to add include statements. Specifies 5341 * the path value of "<b>StructureDefinition:valueset</b>". 5342 */ 5343 public static final ca.uhn.fhir.model.api.Include INCLUDE_VALUESET = new ca.uhn.fhir.model.api.Include("StructureDefinition:valueset").toLocked(); 5344 5345// Manual code (from Configuration.txt): 5346 5347 5348 public String getTypeName() { 5349 String t = getType(); 5350 return StructureDefinitionKind.LOGICAL.equals(getKind()) && t.contains("/") ? t.substring(t.lastIndexOf("/")+1) : t; 5351 } 5352 5353 public String getTypeTail() { 5354 if (getType().contains("/")) { 5355 return getType().substring(getType().lastIndexOf("/")+1); 5356 } else { 5357 return getType(); 5358 } 5359 } 5360 5361 private boolean generatedSnapshot; 5362 private boolean generatingSnapshot; 5363 5364 private List<String> baseDefinitions; 5365 5366 public boolean isGeneratedSnapshot() { 5367 return generatedSnapshot; 5368 } 5369 5370 public void setGeneratedSnapshot(boolean generatedSnapshot) { 5371 this.generatedSnapshot = generatedSnapshot; 5372 } 5373 5374 public boolean isGeneratingSnapshot() { 5375 return generatingSnapshot; 5376 } 5377 5378 public void setGeneratingSnapshot(boolean generatingSnapshot) { 5379 this.generatingSnapshot = generatingSnapshot; 5380 } 5381 5382 public List<String> getBaseDefinitions() { 5383 if (baseDefinitions == null) { 5384 baseDefinitions = new ArrayList<>(); 5385 baseDefinitions.add(getBaseDefinition()); 5386 for (Extension ex : getExtensionsByUrl(ToolingExtensions.EXT_ADDITIONAL_BASE)) { 5387 if (ex.hasValue() && ex.getValue().hasPrimitiveValue()) { 5388 baseDefinitions.add(ex.getValue().primitiveValue()); 5389 } 5390 } 5391 } 5392 return baseDefinitions; 5393 } 5394 5395// end addition 5396 5397} 5398