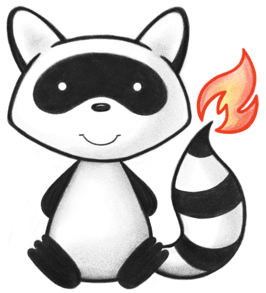
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050import org.hl7.fhir.r5.utils.structuremap.StructureMapUtilities; 051/** 052 * A Map of relationships between 2 structures that can be used to transform data. 053 */ 054@ResourceDef(name="StructureMap", profile="http://hl7.org/fhir/StructureDefinition/StructureMap") 055public class StructureMap extends CanonicalResource { 056 057 public enum StructureMapGroupTypeMode { 058 /** 059 * This group is a default mapping group for the specified types and for the primary source type. 060 */ 061 TYPES, 062 /** 063 * This group is a default mapping group for the specified types. 064 */ 065 TYPEANDTYPES, 066 /** 067 * added to help the parsers with the generic types 068 */ 069 NULL; 070 public static StructureMapGroupTypeMode fromCode(String codeString) throws FHIRException { 071 if (codeString == null || "".equals(codeString)) 072 return null; 073 if ("types".equals(codeString)) 074 return TYPES; 075 if ("type-and-types".equals(codeString)) 076 return TYPEANDTYPES; 077 if (Configuration.isAcceptInvalidEnums()) 078 return null; 079 else 080 throw new FHIRException("Unknown StructureMapGroupTypeMode code '"+codeString+"'"); 081 } 082 public String toCode() { 083 switch (this) { 084 case TYPES: return "types"; 085 case TYPEANDTYPES: return "type-and-types"; 086 case NULL: return null; 087 default: return "?"; 088 } 089 } 090 public String getSystem() { 091 switch (this) { 092 case TYPES: return "http://hl7.org/fhir/map-group-type-mode"; 093 case TYPEANDTYPES: return "http://hl7.org/fhir/map-group-type-mode"; 094 case NULL: return null; 095 default: return "?"; 096 } 097 } 098 public String getDefinition() { 099 switch (this) { 100 case TYPES: return "This group is a default mapping group for the specified types and for the primary source type."; 101 case TYPEANDTYPES: return "This group is a default mapping group for the specified types."; 102 case NULL: return null; 103 default: return "?"; 104 } 105 } 106 public String getDisplay() { 107 switch (this) { 108 case TYPES: return "Default for Type Combination"; 109 case TYPEANDTYPES: return "Default for type + combination"; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 } 115 116 public static class StructureMapGroupTypeModeEnumFactory implements EnumFactory<StructureMapGroupTypeMode> { 117 public StructureMapGroupTypeMode fromCode(String codeString) throws IllegalArgumentException { 118 if (codeString == null || "".equals(codeString)) 119 if (codeString == null || "".equals(codeString)) 120 return null; 121 if ("types".equals(codeString)) 122 return StructureMapGroupTypeMode.TYPES; 123 if ("type-and-types".equals(codeString)) 124 return StructureMapGroupTypeMode.TYPEANDTYPES; 125 throw new IllegalArgumentException("Unknown StructureMapGroupTypeMode code '"+codeString+"'"); 126 } 127 public Enumeration<StructureMapGroupTypeMode> fromType(PrimitiveType<?> code) throws FHIRException { 128 if (code == null) 129 return null; 130 if (code.isEmpty()) 131 return new Enumeration<StructureMapGroupTypeMode>(this, StructureMapGroupTypeMode.NULL, code); 132 String codeString = ((PrimitiveType) code).asStringValue(); 133 if (codeString == null || "".equals(codeString)) 134 return new Enumeration<StructureMapGroupTypeMode>(this, StructureMapGroupTypeMode.NULL, code); 135 if ("types".equals(codeString)) 136 return new Enumeration<StructureMapGroupTypeMode>(this, StructureMapGroupTypeMode.TYPES, code); 137 if ("type-and-types".equals(codeString)) 138 return new Enumeration<StructureMapGroupTypeMode>(this, StructureMapGroupTypeMode.TYPEANDTYPES, code); 139 throw new FHIRException("Unknown StructureMapGroupTypeMode code '"+codeString+"'"); 140 } 141 public String toCode(StructureMapGroupTypeMode code) { 142 if (code == StructureMapGroupTypeMode.TYPES) 143 return "types"; 144 if (code == StructureMapGroupTypeMode.TYPEANDTYPES) 145 return "type-and-types"; 146 return "?"; 147 } 148 public String toSystem(StructureMapGroupTypeMode code) { 149 return code.getSystem(); 150 } 151 } 152 153 public enum StructureMapInputMode { 154 /** 155 * Names an input instance used a source for mapping. 156 */ 157 SOURCE, 158 /** 159 * Names an instance that is being populated. 160 */ 161 TARGET, 162 /** 163 * added to help the parsers with the generic types 164 */ 165 NULL; 166 public static StructureMapInputMode fromCode(String codeString) throws FHIRException { 167 if (codeString == null || "".equals(codeString)) 168 return null; 169 if ("source".equals(codeString)) 170 return SOURCE; 171 if ("target".equals(codeString)) 172 return TARGET; 173 if (Configuration.isAcceptInvalidEnums()) 174 return null; 175 else 176 throw new FHIRException("Unknown StructureMapInputMode code '"+codeString+"'"); 177 } 178 public String toCode() { 179 switch (this) { 180 case SOURCE: return "source"; 181 case TARGET: return "target"; 182 case NULL: return null; 183 default: return "?"; 184 } 185 } 186 public String getSystem() { 187 switch (this) { 188 case SOURCE: return "http://hl7.org/fhir/map-input-mode"; 189 case TARGET: return "http://hl7.org/fhir/map-input-mode"; 190 case NULL: return null; 191 default: return "?"; 192 } 193 } 194 public String getDefinition() { 195 switch (this) { 196 case SOURCE: return "Names an input instance used a source for mapping."; 197 case TARGET: return "Names an instance that is being populated."; 198 case NULL: return null; 199 default: return "?"; 200 } 201 } 202 public String getDisplay() { 203 switch (this) { 204 case SOURCE: return "Source Instance"; 205 case TARGET: return "Target Instance"; 206 case NULL: return null; 207 default: return "?"; 208 } 209 } 210 } 211 212 public static class StructureMapInputModeEnumFactory implements EnumFactory<StructureMapInputMode> { 213 public StructureMapInputMode fromCode(String codeString) throws IllegalArgumentException { 214 if (codeString == null || "".equals(codeString)) 215 if (codeString == null || "".equals(codeString)) 216 return null; 217 if ("source".equals(codeString)) 218 return StructureMapInputMode.SOURCE; 219 if ("target".equals(codeString)) 220 return StructureMapInputMode.TARGET; 221 throw new IllegalArgumentException("Unknown StructureMapInputMode code '"+codeString+"'"); 222 } 223 public Enumeration<StructureMapInputMode> fromType(PrimitiveType<?> code) throws FHIRException { 224 if (code == null) 225 return null; 226 if (code.isEmpty()) 227 return new Enumeration<StructureMapInputMode>(this, StructureMapInputMode.NULL, code); 228 String codeString = ((PrimitiveType) code).asStringValue(); 229 if (codeString == null || "".equals(codeString)) 230 return new Enumeration<StructureMapInputMode>(this, StructureMapInputMode.NULL, code); 231 if ("source".equals(codeString)) 232 return new Enumeration<StructureMapInputMode>(this, StructureMapInputMode.SOURCE, code); 233 if ("target".equals(codeString)) 234 return new Enumeration<StructureMapInputMode>(this, StructureMapInputMode.TARGET, code); 235 throw new FHIRException("Unknown StructureMapInputMode code '"+codeString+"'"); 236 } 237 public String toCode(StructureMapInputMode code) { 238 if (code == StructureMapInputMode.SOURCE) 239 return "source"; 240 if (code == StructureMapInputMode.TARGET) 241 return "target"; 242 return "?"; 243 } 244 public String toSystem(StructureMapInputMode code) { 245 return code.getSystem(); 246 } 247 } 248 249 public enum StructureMapModelMode { 250 /** 251 * This structure describes an instance passed to the mapping engine that is used a source of data. 252 */ 253 SOURCE, 254 /** 255 * This structure describes an instance that the mapping engine may ask for that is used a source of data. 256 */ 257 QUERIED, 258 /** 259 * This structure describes an instance passed to the mapping engine that is used a target of data. 260 */ 261 TARGET, 262 /** 263 * This structure describes an instance that the mapping engine may ask to create that is used a target of data. 264 */ 265 PRODUCED, 266 /** 267 * added to help the parsers with the generic types 268 */ 269 NULL; 270 public static StructureMapModelMode fromCode(String codeString) throws FHIRException { 271 if (codeString == null || "".equals(codeString)) 272 return null; 273 if ("source".equals(codeString)) 274 return SOURCE; 275 if ("queried".equals(codeString)) 276 return QUERIED; 277 if ("target".equals(codeString)) 278 return TARGET; 279 if ("produced".equals(codeString)) 280 return PRODUCED; 281 if (Configuration.isAcceptInvalidEnums()) 282 return null; 283 else 284 throw new FHIRException("Unknown StructureMapModelMode code '"+codeString+"'"); 285 } 286 public String toCode() { 287 switch (this) { 288 case SOURCE: return "source"; 289 case QUERIED: return "queried"; 290 case TARGET: return "target"; 291 case PRODUCED: return "produced"; 292 case NULL: return null; 293 default: return "?"; 294 } 295 } 296 public String getSystem() { 297 switch (this) { 298 case SOURCE: return "http://hl7.org/fhir/map-model-mode"; 299 case QUERIED: return "http://hl7.org/fhir/map-model-mode"; 300 case TARGET: return "http://hl7.org/fhir/map-model-mode"; 301 case PRODUCED: return "http://hl7.org/fhir/map-model-mode"; 302 case NULL: return null; 303 default: return "?"; 304 } 305 } 306 public String getDefinition() { 307 switch (this) { 308 case SOURCE: return "This structure describes an instance passed to the mapping engine that is used a source of data."; 309 case QUERIED: return "This structure describes an instance that the mapping engine may ask for that is used a source of data."; 310 case TARGET: return "This structure describes an instance passed to the mapping engine that is used a target of data."; 311 case PRODUCED: return "This structure describes an instance that the mapping engine may ask to create that is used a target of data."; 312 case NULL: return null; 313 default: return "?"; 314 } 315 } 316 public String getDisplay() { 317 switch (this) { 318 case SOURCE: return "Source Structure Definition"; 319 case QUERIED: return "Queried Structure Definition"; 320 case TARGET: return "Target Structure Definition"; 321 case PRODUCED: return "Produced Structure Definition"; 322 case NULL: return null; 323 default: return "?"; 324 } 325 } 326 } 327 328 public static class StructureMapModelModeEnumFactory implements EnumFactory<StructureMapModelMode> { 329 public StructureMapModelMode fromCode(String codeString) throws IllegalArgumentException { 330 if (codeString == null || "".equals(codeString)) 331 if (codeString == null || "".equals(codeString)) 332 return null; 333 if ("source".equals(codeString)) 334 return StructureMapModelMode.SOURCE; 335 if ("queried".equals(codeString)) 336 return StructureMapModelMode.QUERIED; 337 if ("target".equals(codeString)) 338 return StructureMapModelMode.TARGET; 339 if ("produced".equals(codeString)) 340 return StructureMapModelMode.PRODUCED; 341 throw new IllegalArgumentException("Unknown StructureMapModelMode code '"+codeString+"'"); 342 } 343 public Enumeration<StructureMapModelMode> fromType(PrimitiveType<?> code) throws FHIRException { 344 if (code == null) 345 return null; 346 if (code.isEmpty()) 347 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.NULL, code); 348 String codeString = ((PrimitiveType) code).asStringValue(); 349 if (codeString == null || "".equals(codeString)) 350 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.NULL, code); 351 if ("source".equals(codeString)) 352 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.SOURCE, code); 353 if ("queried".equals(codeString)) 354 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.QUERIED, code); 355 if ("target".equals(codeString)) 356 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.TARGET, code); 357 if ("produced".equals(codeString)) 358 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.PRODUCED, code); 359 throw new FHIRException("Unknown StructureMapModelMode code '"+codeString+"'"); 360 } 361 public String toCode(StructureMapModelMode code) { 362 if (code == StructureMapModelMode.SOURCE) 363 return "source"; 364 if (code == StructureMapModelMode.QUERIED) 365 return "queried"; 366 if (code == StructureMapModelMode.TARGET) 367 return "target"; 368 if (code == StructureMapModelMode.PRODUCED) 369 return "produced"; 370 return "?"; 371 } 372 public String toSystem(StructureMapModelMode code) { 373 return code.getSystem(); 374 } 375 } 376 377 public enum StructureMapSourceListMode { 378 /** 379 * Only process this rule for the first in the list. 380 */ 381 FIRST, 382 /** 383 * Process this rule for all but the first. 384 */ 385 NOTFIRST, 386 /** 387 * Only process this rule for the last in the list. 388 */ 389 LAST, 390 /** 391 * Process this rule for all but the last. 392 */ 393 NOTLAST, 394 /** 395 * Only process this rule is there is only item. 396 */ 397 ONLYONE, 398 /** 399 * added to help the parsers with the generic types 400 */ 401 NULL; 402 public static StructureMapSourceListMode fromCode(String codeString) throws FHIRException { 403 if (codeString == null || "".equals(codeString)) 404 return null; 405 if ("first".equals(codeString)) 406 return FIRST; 407 if ("not_first".equals(codeString)) 408 return NOTFIRST; 409 if ("last".equals(codeString)) 410 return LAST; 411 if ("not_last".equals(codeString)) 412 return NOTLAST; 413 if ("only_one".equals(codeString)) 414 return ONLYONE; 415 if (Configuration.isAcceptInvalidEnums()) 416 return null; 417 else 418 throw new FHIRException("Unknown StructureMapSourceListMode code '"+codeString+"'"); 419 } 420 public String toCode() { 421 switch (this) { 422 case FIRST: return "first"; 423 case NOTFIRST: return "not_first"; 424 case LAST: return "last"; 425 case NOTLAST: return "not_last"; 426 case ONLYONE: return "only_one"; 427 case NULL: return null; 428 default: return "?"; 429 } 430 } 431 public String getSystem() { 432 switch (this) { 433 case FIRST: return "http://hl7.org/fhir/map-source-list-mode"; 434 case NOTFIRST: return "http://hl7.org/fhir/map-source-list-mode"; 435 case LAST: return "http://hl7.org/fhir/map-source-list-mode"; 436 case NOTLAST: return "http://hl7.org/fhir/map-source-list-mode"; 437 case ONLYONE: return "http://hl7.org/fhir/map-source-list-mode"; 438 case NULL: return null; 439 default: return "?"; 440 } 441 } 442 public String getDefinition() { 443 switch (this) { 444 case FIRST: return "Only process this rule for the first in the list."; 445 case NOTFIRST: return "Process this rule for all but the first."; 446 case LAST: return "Only process this rule for the last in the list."; 447 case NOTLAST: return "Process this rule for all but the last."; 448 case ONLYONE: return "Only process this rule is there is only item."; 449 case NULL: return null; 450 default: return "?"; 451 } 452 } 453 public String getDisplay() { 454 switch (this) { 455 case FIRST: return "First"; 456 case NOTFIRST: return "All but the first"; 457 case LAST: return "Last"; 458 case NOTLAST: return "All but the last"; 459 case ONLYONE: return "Enforce only one"; 460 case NULL: return null; 461 default: return "?"; 462 } 463 } 464 } 465 466 public static class StructureMapSourceListModeEnumFactory implements EnumFactory<StructureMapSourceListMode> { 467 public StructureMapSourceListMode fromCode(String codeString) throws IllegalArgumentException { 468 if (codeString == null || "".equals(codeString)) 469 if (codeString == null || "".equals(codeString)) 470 return null; 471 if ("first".equals(codeString)) 472 return StructureMapSourceListMode.FIRST; 473 if ("not_first".equals(codeString)) 474 return StructureMapSourceListMode.NOTFIRST; 475 if ("last".equals(codeString)) 476 return StructureMapSourceListMode.LAST; 477 if ("not_last".equals(codeString)) 478 return StructureMapSourceListMode.NOTLAST; 479 if ("only_one".equals(codeString)) 480 return StructureMapSourceListMode.ONLYONE; 481 throw new IllegalArgumentException("Unknown StructureMapSourceListMode code '"+codeString+"'"); 482 } 483 public Enumeration<StructureMapSourceListMode> fromType(PrimitiveType<?> code) throws FHIRException { 484 if (code == null) 485 return null; 486 if (code.isEmpty()) 487 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.NULL, code); 488 String codeString = ((PrimitiveType) code).asStringValue(); 489 if (codeString == null || "".equals(codeString)) 490 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.NULL, code); 491 if ("first".equals(codeString)) 492 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.FIRST, code); 493 if ("not_first".equals(codeString)) 494 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.NOTFIRST, code); 495 if ("last".equals(codeString)) 496 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.LAST, code); 497 if ("not_last".equals(codeString)) 498 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.NOTLAST, code); 499 if ("only_one".equals(codeString)) 500 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.ONLYONE, code); 501 throw new FHIRException("Unknown StructureMapSourceListMode code '"+codeString+"'"); 502 } 503 public String toCode(StructureMapSourceListMode code) { 504 if (code == StructureMapSourceListMode.FIRST) 505 return "first"; 506 if (code == StructureMapSourceListMode.NOTFIRST) 507 return "not_first"; 508 if (code == StructureMapSourceListMode.LAST) 509 return "last"; 510 if (code == StructureMapSourceListMode.NOTLAST) 511 return "not_last"; 512 if (code == StructureMapSourceListMode.ONLYONE) 513 return "only_one"; 514 return "?"; 515 } 516 public String toSystem(StructureMapSourceListMode code) { 517 return code.getSystem(); 518 } 519 } 520 521 public enum StructureMapTargetListMode { 522 /** 523 * when the target list is being assembled, the items for this rule go first. If more than one rule defines a first item (for a given instance of mapping) then this is an error. 524 */ 525 FIRST, 526 /** 527 * the target instance is shared with the target instances generated by another rule (up to the first common n items, then create new ones). 528 */ 529 SHARE, 530 /** 531 * when the target list is being assembled, the items for this rule go last. If more than one rule defines a last item (for a given instance of mapping) then this is an error. 532 */ 533 LAST, 534 /** 535 * the target instance is shared with a number of target instances generated by another rule (up to the first common n items, then create new ones). 536 */ 537 SINGLE, 538 /** 539 * added to help the parsers with the generic types 540 */ 541 NULL; 542 public static StructureMapTargetListMode fromCode(String codeString) throws FHIRException { 543 if (codeString == null || "".equals(codeString)) 544 return null; 545 if ("first".equals(codeString)) 546 return FIRST; 547 if ("share".equals(codeString)) 548 return SHARE; 549 if ("last".equals(codeString)) 550 return LAST; 551 if ("single".equals(codeString)) 552 return SINGLE; 553 if (Configuration.isAcceptInvalidEnums()) 554 return null; 555 else 556 throw new FHIRException("Unknown StructureMapTargetListMode code '"+codeString+"'"); 557 } 558 public String toCode() { 559 switch (this) { 560 case FIRST: return "first"; 561 case SHARE: return "share"; 562 case LAST: return "last"; 563 case SINGLE: return "single"; 564 case NULL: return null; 565 default: return "?"; 566 } 567 } 568 public String getSystem() { 569 switch (this) { 570 case FIRST: return "http://hl7.org/fhir/map-target-list-mode"; 571 case SHARE: return "http://hl7.org/fhir/map-target-list-mode"; 572 case LAST: return "http://hl7.org/fhir/map-target-list-mode"; 573 case SINGLE: return "http://hl7.org/fhir/map-target-list-mode"; 574 case NULL: return null; 575 default: return "?"; 576 } 577 } 578 public String getDefinition() { 579 switch (this) { 580 case FIRST: return "when the target list is being assembled, the items for this rule go first. If more than one rule defines a first item (for a given instance of mapping) then this is an error."; 581 case SHARE: return "the target instance is shared with the target instances generated by another rule (up to the first common n items, then create new ones)."; 582 case LAST: return "when the target list is being assembled, the items for this rule go last. If more than one rule defines a last item (for a given instance of mapping) then this is an error."; 583 case SINGLE: return "the target instance is shared with a number of target instances generated by another rule (up to the first common n items, then create new ones)."; 584 case NULL: return null; 585 default: return "?"; 586 } 587 } 588 public String getDisplay() { 589 switch (this) { 590 case FIRST: return "First"; 591 case SHARE: return "Share"; 592 case LAST: return "Last"; 593 case SINGLE: return "single"; 594 case NULL: return null; 595 default: return "?"; 596 } 597 } 598 } 599 600 public static class StructureMapTargetListModeEnumFactory implements EnumFactory<StructureMapTargetListMode> { 601 public StructureMapTargetListMode fromCode(String codeString) throws IllegalArgumentException { 602 if (codeString == null || "".equals(codeString)) 603 if (codeString == null || "".equals(codeString)) 604 return null; 605 if ("first".equals(codeString)) 606 return StructureMapTargetListMode.FIRST; 607 if ("share".equals(codeString)) 608 return StructureMapTargetListMode.SHARE; 609 if ("last".equals(codeString)) 610 return StructureMapTargetListMode.LAST; 611 if ("single".equals(codeString)) 612 return StructureMapTargetListMode.SINGLE; 613 throw new IllegalArgumentException("Unknown StructureMapTargetListMode code '"+codeString+"'"); 614 } 615 public Enumeration<StructureMapTargetListMode> fromType(PrimitiveType<?> code) throws FHIRException { 616 if (code == null) 617 return null; 618 if (code.isEmpty()) 619 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.NULL, code); 620 String codeString = ((PrimitiveType) code).asStringValue(); 621 if (codeString == null || "".equals(codeString)) 622 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.NULL, code); 623 if ("first".equals(codeString)) 624 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.FIRST, code); 625 if ("share".equals(codeString)) 626 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.SHARE, code); 627 if ("last".equals(codeString)) 628 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.LAST, code); 629 if ("single".equals(codeString)) 630 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.SINGLE, code); 631 throw new FHIRException("Unknown StructureMapTargetListMode code '"+codeString+"'"); 632 } 633 public String toCode(StructureMapTargetListMode code) { 634 if (code == StructureMapTargetListMode.FIRST) 635 return "first"; 636 if (code == StructureMapTargetListMode.SHARE) 637 return "share"; 638 if (code == StructureMapTargetListMode.LAST) 639 return "last"; 640 if (code == StructureMapTargetListMode.SINGLE) 641 return "single"; 642 return "?"; 643 } 644 public String toSystem(StructureMapTargetListMode code) { 645 return code.getSystem(); 646 } 647 } 648 649 public enum StructureMapTransform { 650 /** 651 * create(type : string) - type is passed through to the application on the standard API, and must be known by it. 652 */ 653 CREATE, 654 /** 655 * copy(source). 656 */ 657 COPY, 658 /** 659 * truncate(source, length) - source must be stringy type. 660 */ 661 TRUNCATE, 662 /** 663 * escape(source, fmt1, fmt2) - change source from one kind of escaping to another (plain, java, xml, json). note that this is for when the string itself is escaped. 664 */ 665 ESCAPE, 666 /** 667 * cast(source, type?) - cast (convert) source from one type to another. Target type can be left as implicit if there is one and only one target type known. The default namespace for the type is 'FHIR' (see [FHIRPath type specifiers](http://hl7.org/fhirpath/N1/#is-type-specifier)) 668 */ 669 CAST, 670 /** 671 * append(source...) - source is element or string. 672 */ 673 APPEND, 674 /** 675 * translate(source, uri_of_map) - use the translate operation. 676 */ 677 TRANSLATE, 678 /** 679 * reference(source : object) - return a string that references the provided tree properly. 680 */ 681 REFERENCE, 682 /** 683 * Perform a date operation. *Parameters to be documented*. 684 */ 685 DATEOP, 686 /** 687 * Generate a random UUID (in lowercase). No Parameters. 688 */ 689 UUID, 690 /** 691 * Return the appropriate string to put in a reference that refers to the resource provided as a parameter. 692 */ 693 POINTER, 694 /** 695 * Execute the supplied FHIRPath expression and use the value returned by that. 696 */ 697 EVALUATE, 698 /** 699 * Create a CodeableConcept. Parameters = (text) or (system. Code[, display]). 700 */ 701 CC, 702 /** 703 * Create a Coding. Parameters = (system. Code[, display]). 704 */ 705 C, 706 /** 707 * Create a quantity. Parameters = (text) or (value, unit, [system, code]) where text is the natural representation e.g. [comparator]value[space]unit. 708 */ 709 QTY, 710 /** 711 * Create an identifier. Parameters = (system, value[, type]) where type is a code from the identifier type value set. 712 */ 713 ID, 714 /** 715 * Create a contact details. Parameters = (value) or (system, value). If no system is provided, the system should be inferred from the content of the value. 716 */ 717 CP, 718 /** 719 * added to help the parsers with the generic types 720 */ 721 NULL; 722 public static StructureMapTransform fromCode(String codeString) throws FHIRException { 723 if (codeString == null || "".equals(codeString)) 724 return null; 725 if ("create".equals(codeString)) 726 return CREATE; 727 if ("copy".equals(codeString)) 728 return COPY; 729 if ("truncate".equals(codeString)) 730 return TRUNCATE; 731 if ("escape".equals(codeString)) 732 return ESCAPE; 733 if ("cast".equals(codeString)) 734 return CAST; 735 if ("append".equals(codeString)) 736 return APPEND; 737 if ("translate".equals(codeString)) 738 return TRANSLATE; 739 if ("reference".equals(codeString)) 740 return REFERENCE; 741 if ("dateOp".equals(codeString)) 742 return DATEOP; 743 if ("uuid".equals(codeString)) 744 return UUID; 745 if ("pointer".equals(codeString)) 746 return POINTER; 747 if ("evaluate".equals(codeString)) 748 return EVALUATE; 749 if ("cc".equals(codeString)) 750 return CC; 751 if ("c".equals(codeString)) 752 return C; 753 if ("qty".equals(codeString)) 754 return QTY; 755 if ("id".equals(codeString)) 756 return ID; 757 if ("cp".equals(codeString)) 758 return CP; 759 if (Configuration.isAcceptInvalidEnums()) 760 return null; 761 else 762 throw new FHIRException("Unknown StructureMapTransform code '"+codeString+"'"); 763 } 764 public String toCode() { 765 switch (this) { 766 case CREATE: return "create"; 767 case COPY: return "copy"; 768 case TRUNCATE: return "truncate"; 769 case ESCAPE: return "escape"; 770 case CAST: return "cast"; 771 case APPEND: return "append"; 772 case TRANSLATE: return "translate"; 773 case REFERENCE: return "reference"; 774 case DATEOP: return "dateOp"; 775 case UUID: return "uuid"; 776 case POINTER: return "pointer"; 777 case EVALUATE: return "evaluate"; 778 case CC: return "cc"; 779 case C: return "c"; 780 case QTY: return "qty"; 781 case ID: return "id"; 782 case CP: return "cp"; 783 case NULL: return null; 784 default: return "?"; 785 } 786 } 787 public String getSystem() { 788 switch (this) { 789 case CREATE: return "http://hl7.org/fhir/map-transform"; 790 case COPY: return "http://hl7.org/fhir/map-transform"; 791 case TRUNCATE: return "http://hl7.org/fhir/map-transform"; 792 case ESCAPE: return "http://hl7.org/fhir/map-transform"; 793 case CAST: return "http://hl7.org/fhir/map-transform"; 794 case APPEND: return "http://hl7.org/fhir/map-transform"; 795 case TRANSLATE: return "http://hl7.org/fhir/map-transform"; 796 case REFERENCE: return "http://hl7.org/fhir/map-transform"; 797 case DATEOP: return "http://hl7.org/fhir/map-transform"; 798 case UUID: return "http://hl7.org/fhir/map-transform"; 799 case POINTER: return "http://hl7.org/fhir/map-transform"; 800 case EVALUATE: return "http://hl7.org/fhir/map-transform"; 801 case CC: return "http://hl7.org/fhir/map-transform"; 802 case C: return "http://hl7.org/fhir/map-transform"; 803 case QTY: return "http://hl7.org/fhir/map-transform"; 804 case ID: return "http://hl7.org/fhir/map-transform"; 805 case CP: return "http://hl7.org/fhir/map-transform"; 806 case NULL: return null; 807 default: return "?"; 808 } 809 } 810 public String getDefinition() { 811 switch (this) { 812 case CREATE: return "create(type : string) - type is passed through to the application on the standard API, and must be known by it."; 813 case COPY: return "copy(source)."; 814 case TRUNCATE: return "truncate(source, length) - source must be stringy type."; 815 case ESCAPE: return "escape(source, fmt1, fmt2) - change source from one kind of escaping to another (plain, java, xml, json). note that this is for when the string itself is escaped."; 816 case CAST: return "cast(source, type?) - cast (convert) source from one type to another. Target type can be left as implicit if there is one and only one target type known. The default namespace for the type is 'FHIR' (see [FHIRPath type specifiers](http://hl7.org/fhirpath/N1/#is-type-specifier))"; 817 case APPEND: return "append(source...) - source is element or string."; 818 case TRANSLATE: return "translate(source, uri_of_map) - use the translate operation."; 819 case REFERENCE: return "reference(source : object) - return a string that references the provided tree properly."; 820 case DATEOP: return "Perform a date operation. *Parameters to be documented*."; 821 case UUID: return "Generate a random UUID (in lowercase). No Parameters."; 822 case POINTER: return "Return the appropriate string to put in a reference that refers to the resource provided as a parameter."; 823 case EVALUATE: return "Execute the supplied FHIRPath expression and use the value returned by that."; 824 case CC: return "Create a CodeableConcept. Parameters = (text) or (system. Code[, display])."; 825 case C: return "Create a Coding. Parameters = (system. Code[, display])."; 826 case QTY: return "Create a quantity. Parameters = (text) or (value, unit, [system, code]) where text is the natural representation e.g. [comparator]value[space]unit."; 827 case ID: return "Create an identifier. Parameters = (system, value[, type]) where type is a code from the identifier type value set."; 828 case CP: return "Create a contact details. Parameters = (value) or (system, value). If no system is provided, the system should be inferred from the content of the value."; 829 case NULL: return null; 830 default: return "?"; 831 } 832 } 833 public String getDisplay() { 834 switch (this) { 835 case CREATE: return "create"; 836 case COPY: return "copy"; 837 case TRUNCATE: return "truncate"; 838 case ESCAPE: return "escape"; 839 case CAST: return "cast"; 840 case APPEND: return "append"; 841 case TRANSLATE: return "translate"; 842 case REFERENCE: return "reference"; 843 case DATEOP: return "dateOp"; 844 case UUID: return "uuid"; 845 case POINTER: return "pointer"; 846 case EVALUATE: return "evaluate"; 847 case CC: return "cc"; 848 case C: return "c"; 849 case QTY: return "qty"; 850 case ID: return "id"; 851 case CP: return "cp"; 852 case NULL: return null; 853 default: return "?"; 854 } 855 } 856 } 857 858 public static class StructureMapTransformEnumFactory implements EnumFactory<StructureMapTransform> { 859 public StructureMapTransform fromCode(String codeString) throws IllegalArgumentException { 860 if (codeString == null || "".equals(codeString)) 861 if (codeString == null || "".equals(codeString)) 862 return null; 863 if ("create".equals(codeString)) 864 return StructureMapTransform.CREATE; 865 if ("copy".equals(codeString)) 866 return StructureMapTransform.COPY; 867 if ("truncate".equals(codeString)) 868 return StructureMapTransform.TRUNCATE; 869 if ("escape".equals(codeString)) 870 return StructureMapTransform.ESCAPE; 871 if ("cast".equals(codeString)) 872 return StructureMapTransform.CAST; 873 if ("append".equals(codeString)) 874 return StructureMapTransform.APPEND; 875 if ("translate".equals(codeString)) 876 return StructureMapTransform.TRANSLATE; 877 if ("reference".equals(codeString)) 878 return StructureMapTransform.REFERENCE; 879 if ("dateOp".equals(codeString)) 880 return StructureMapTransform.DATEOP; 881 if ("uuid".equals(codeString)) 882 return StructureMapTransform.UUID; 883 if ("pointer".equals(codeString)) 884 return StructureMapTransform.POINTER; 885 if ("evaluate".equals(codeString)) 886 return StructureMapTransform.EVALUATE; 887 if ("cc".equals(codeString)) 888 return StructureMapTransform.CC; 889 if ("c".equals(codeString)) 890 return StructureMapTransform.C; 891 if ("qty".equals(codeString)) 892 return StructureMapTransform.QTY; 893 if ("id".equals(codeString)) 894 return StructureMapTransform.ID; 895 if ("cp".equals(codeString)) 896 return StructureMapTransform.CP; 897 throw new IllegalArgumentException("Unknown StructureMapTransform code '"+codeString+"'"); 898 } 899 public Enumeration<StructureMapTransform> fromType(PrimitiveType<?> code) throws FHIRException { 900 if (code == null) 901 return null; 902 if (code.isEmpty()) 903 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.NULL, code); 904 String codeString = ((PrimitiveType) code).asStringValue(); 905 if (codeString == null || "".equals(codeString)) 906 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.NULL, code); 907 if ("create".equals(codeString)) 908 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.CREATE, code); 909 if ("copy".equals(codeString)) 910 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.COPY, code); 911 if ("truncate".equals(codeString)) 912 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.TRUNCATE, code); 913 if ("escape".equals(codeString)) 914 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.ESCAPE, code); 915 if ("cast".equals(codeString)) 916 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.CAST, code); 917 if ("append".equals(codeString)) 918 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.APPEND, code); 919 if ("translate".equals(codeString)) 920 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.TRANSLATE, code); 921 if ("reference".equals(codeString)) 922 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.REFERENCE, code); 923 if ("dateOp".equals(codeString)) 924 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.DATEOP, code); 925 if ("uuid".equals(codeString)) 926 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.UUID, code); 927 if ("pointer".equals(codeString)) 928 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.POINTER, code); 929 if ("evaluate".equals(codeString)) 930 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.EVALUATE, code); 931 if ("cc".equals(codeString)) 932 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.CC, code); 933 if ("c".equals(codeString)) 934 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.C, code); 935 if ("qty".equals(codeString)) 936 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.QTY, code); 937 if ("id".equals(codeString)) 938 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.ID, code); 939 if ("cp".equals(codeString)) 940 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.CP, code); 941 throw new FHIRException("Unknown StructureMapTransform code '"+codeString+"'"); 942 } 943 public String toCode(StructureMapTransform code) { 944 if (code == StructureMapTransform.CREATE) 945 return "create"; 946 if (code == StructureMapTransform.COPY) 947 return "copy"; 948 if (code == StructureMapTransform.TRUNCATE) 949 return "truncate"; 950 if (code == StructureMapTransform.ESCAPE) 951 return "escape"; 952 if (code == StructureMapTransform.CAST) 953 return "cast"; 954 if (code == StructureMapTransform.APPEND) 955 return "append"; 956 if (code == StructureMapTransform.TRANSLATE) 957 return "translate"; 958 if (code == StructureMapTransform.REFERENCE) 959 return "reference"; 960 if (code == StructureMapTransform.DATEOP) 961 return "dateOp"; 962 if (code == StructureMapTransform.UUID) 963 return "uuid"; 964 if (code == StructureMapTransform.POINTER) 965 return "pointer"; 966 if (code == StructureMapTransform.EVALUATE) 967 return "evaluate"; 968 if (code == StructureMapTransform.CC) 969 return "cc"; 970 if (code == StructureMapTransform.C) 971 return "c"; 972 if (code == StructureMapTransform.QTY) 973 return "qty"; 974 if (code == StructureMapTransform.ID) 975 return "id"; 976 if (code == StructureMapTransform.CP) 977 return "cp"; 978 return "?"; 979 } 980 public String toSystem(StructureMapTransform code) { 981 return code.getSystem(); 982 } 983 } 984 985 @Block() 986 public static class StructureMapStructureComponent extends BackboneElement implements IBaseBackboneElement { 987 /** 988 * The canonical reference to the structure. 989 */ 990 @Child(name = "url", type = {CanonicalType.class}, order=1, min=1, max=1, modifier=false, summary=true) 991 @Description(shortDefinition="Canonical reference to structure definition", formalDefinition="The canonical reference to the structure." ) 992 protected CanonicalType url; 993 994 /** 995 * How the referenced structure is used in this mapping. 996 */ 997 @Child(name = "mode", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 998 @Description(shortDefinition="source | queried | target | produced", formalDefinition="How the referenced structure is used in this mapping." ) 999 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/map-model-mode") 1000 protected Enumeration<StructureMapModelMode> mode; 1001 1002 /** 1003 * The name used for this type in the map. 1004 */ 1005 @Child(name = "alias", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1006 @Description(shortDefinition="Name for type in this map", formalDefinition="The name used for this type in the map." ) 1007 protected StringType alias; 1008 1009 /** 1010 * Documentation that describes how the structure is used in the mapping. 1011 */ 1012 @Child(name = "documentation", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1013 @Description(shortDefinition="Documentation on use of structure", formalDefinition="Documentation that describes how the structure is used in the mapping." ) 1014 protected StringType documentation; 1015 1016 private static final long serialVersionUID = 364750586L; 1017 1018 /** 1019 * Constructor 1020 */ 1021 public StructureMapStructureComponent() { 1022 super(); 1023 } 1024 1025 /** 1026 * Constructor 1027 */ 1028 public StructureMapStructureComponent(String url, StructureMapModelMode mode) { 1029 super(); 1030 this.setUrl(url); 1031 this.setMode(mode); 1032 } 1033 1034 /** 1035 * @return {@link #url} (The canonical reference to the structure.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1036 */ 1037 public CanonicalType getUrlElement() { 1038 if (this.url == null) 1039 if (Configuration.errorOnAutoCreate()) 1040 throw new Error("Attempt to auto-create StructureMapStructureComponent.url"); 1041 else if (Configuration.doAutoCreate()) 1042 this.url = new CanonicalType(); // bb 1043 return this.url; 1044 } 1045 1046 public boolean hasUrlElement() { 1047 return this.url != null && !this.url.isEmpty(); 1048 } 1049 1050 public boolean hasUrl() { 1051 return this.url != null && !this.url.isEmpty(); 1052 } 1053 1054 /** 1055 * @param value {@link #url} (The canonical reference to the structure.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1056 */ 1057 public StructureMapStructureComponent setUrlElement(CanonicalType value) { 1058 this.url = value; 1059 return this; 1060 } 1061 1062 /** 1063 * @return The canonical reference to the structure. 1064 */ 1065 public String getUrl() { 1066 return this.url == null ? null : this.url.getValue(); 1067 } 1068 1069 /** 1070 * @param value The canonical reference to the structure. 1071 */ 1072 public StructureMapStructureComponent setUrl(String value) { 1073 if (this.url == null) 1074 this.url = new CanonicalType(); 1075 this.url.setValue(value); 1076 return this; 1077 } 1078 1079 /** 1080 * @return {@link #mode} (How the referenced structure is used in this mapping.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1081 */ 1082 public Enumeration<StructureMapModelMode> getModeElement() { 1083 if (this.mode == null) 1084 if (Configuration.errorOnAutoCreate()) 1085 throw new Error("Attempt to auto-create StructureMapStructureComponent.mode"); 1086 else if (Configuration.doAutoCreate()) 1087 this.mode = new Enumeration<StructureMapModelMode>(new StructureMapModelModeEnumFactory()); // bb 1088 return this.mode; 1089 } 1090 1091 public boolean hasModeElement() { 1092 return this.mode != null && !this.mode.isEmpty(); 1093 } 1094 1095 public boolean hasMode() { 1096 return this.mode != null && !this.mode.isEmpty(); 1097 } 1098 1099 /** 1100 * @param value {@link #mode} (How the referenced structure is used in this mapping.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1101 */ 1102 public StructureMapStructureComponent setModeElement(Enumeration<StructureMapModelMode> value) { 1103 this.mode = value; 1104 return this; 1105 } 1106 1107 /** 1108 * @return How the referenced structure is used in this mapping. 1109 */ 1110 public StructureMapModelMode getMode() { 1111 return this.mode == null ? null : this.mode.getValue(); 1112 } 1113 1114 /** 1115 * @param value How the referenced structure is used in this mapping. 1116 */ 1117 public StructureMapStructureComponent setMode(StructureMapModelMode value) { 1118 if (this.mode == null) 1119 this.mode = new Enumeration<StructureMapModelMode>(new StructureMapModelModeEnumFactory()); 1120 this.mode.setValue(value); 1121 return this; 1122 } 1123 1124 /** 1125 * @return {@link #alias} (The name used for this type in the map.). This is the underlying object with id, value and extensions. The accessor "getAlias" gives direct access to the value 1126 */ 1127 public StringType getAliasElement() { 1128 if (this.alias == null) 1129 if (Configuration.errorOnAutoCreate()) 1130 throw new Error("Attempt to auto-create StructureMapStructureComponent.alias"); 1131 else if (Configuration.doAutoCreate()) 1132 this.alias = new StringType(); // bb 1133 return this.alias; 1134 } 1135 1136 public boolean hasAliasElement() { 1137 return this.alias != null && !this.alias.isEmpty(); 1138 } 1139 1140 public boolean hasAlias() { 1141 return this.alias != null && !this.alias.isEmpty(); 1142 } 1143 1144 /** 1145 * @param value {@link #alias} (The name used for this type in the map.). This is the underlying object with id, value and extensions. The accessor "getAlias" gives direct access to the value 1146 */ 1147 public StructureMapStructureComponent setAliasElement(StringType value) { 1148 this.alias = value; 1149 return this; 1150 } 1151 1152 /** 1153 * @return The name used for this type in the map. 1154 */ 1155 public String getAlias() { 1156 return this.alias == null ? null : this.alias.getValue(); 1157 } 1158 1159 /** 1160 * @param value The name used for this type in the map. 1161 */ 1162 public StructureMapStructureComponent setAlias(String value) { 1163 if (Utilities.noString(value)) 1164 this.alias = null; 1165 else { 1166 if (this.alias == null) 1167 this.alias = new StringType(); 1168 this.alias.setValue(value); 1169 } 1170 return this; 1171 } 1172 1173 /** 1174 * @return {@link #documentation} (Documentation that describes how the structure is used in the mapping.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 1175 */ 1176 public StringType getDocumentationElement() { 1177 if (this.documentation == null) 1178 if (Configuration.errorOnAutoCreate()) 1179 throw new Error("Attempt to auto-create StructureMapStructureComponent.documentation"); 1180 else if (Configuration.doAutoCreate()) 1181 this.documentation = new StringType(); // bb 1182 return this.documentation; 1183 } 1184 1185 public boolean hasDocumentationElement() { 1186 return this.documentation != null && !this.documentation.isEmpty(); 1187 } 1188 1189 public boolean hasDocumentation() { 1190 return this.documentation != null && !this.documentation.isEmpty(); 1191 } 1192 1193 /** 1194 * @param value {@link #documentation} (Documentation that describes how the structure is used in the mapping.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 1195 */ 1196 public StructureMapStructureComponent setDocumentationElement(StringType value) { 1197 this.documentation = value; 1198 return this; 1199 } 1200 1201 /** 1202 * @return Documentation that describes how the structure is used in the mapping. 1203 */ 1204 public String getDocumentation() { 1205 return this.documentation == null ? null : this.documentation.getValue(); 1206 } 1207 1208 /** 1209 * @param value Documentation that describes how the structure is used in the mapping. 1210 */ 1211 public StructureMapStructureComponent setDocumentation(String value) { 1212 if (Utilities.noString(value)) 1213 this.documentation = null; 1214 else { 1215 if (this.documentation == null) 1216 this.documentation = new StringType(); 1217 this.documentation.setValue(value); 1218 } 1219 return this; 1220 } 1221 1222 protected void listChildren(List<Property> children) { 1223 super.listChildren(children); 1224 children.add(new Property("url", "canonical(StructureDefinition)", "The canonical reference to the structure.", 0, 1, url)); 1225 children.add(new Property("mode", "code", "How the referenced structure is used in this mapping.", 0, 1, mode)); 1226 children.add(new Property("alias", "string", "The name used for this type in the map.", 0, 1, alias)); 1227 children.add(new Property("documentation", "string", "Documentation that describes how the structure is used in the mapping.", 0, 1, documentation)); 1228 } 1229 1230 @Override 1231 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1232 switch (_hash) { 1233 case 116079: /*url*/ return new Property("url", "canonical(StructureDefinition)", "The canonical reference to the structure.", 0, 1, url); 1234 case 3357091: /*mode*/ return new Property("mode", "code", "How the referenced structure is used in this mapping.", 0, 1, mode); 1235 case 92902992: /*alias*/ return new Property("alias", "string", "The name used for this type in the map.", 0, 1, alias); 1236 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Documentation that describes how the structure is used in the mapping.", 0, 1, documentation); 1237 default: return super.getNamedProperty(_hash, _name, _checkValid); 1238 } 1239 1240 } 1241 1242 @Override 1243 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1244 switch (hash) { 1245 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // CanonicalType 1246 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<StructureMapModelMode> 1247 case 92902992: /*alias*/ return this.alias == null ? new Base[0] : new Base[] {this.alias}; // StringType 1248 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 1249 default: return super.getProperty(hash, name, checkValid); 1250 } 1251 1252 } 1253 1254 @Override 1255 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1256 switch (hash) { 1257 case 116079: // url 1258 this.url = TypeConvertor.castToCanonical(value); // CanonicalType 1259 return value; 1260 case 3357091: // mode 1261 value = new StructureMapModelModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1262 this.mode = (Enumeration) value; // Enumeration<StructureMapModelMode> 1263 return value; 1264 case 92902992: // alias 1265 this.alias = TypeConvertor.castToString(value); // StringType 1266 return value; 1267 case 1587405498: // documentation 1268 this.documentation = TypeConvertor.castToString(value); // StringType 1269 return value; 1270 default: return super.setProperty(hash, name, value); 1271 } 1272 1273 } 1274 1275 @Override 1276 public Base setProperty(String name, Base value) throws FHIRException { 1277 if (name.equals("url")) { 1278 this.url = TypeConvertor.castToCanonical(value); // CanonicalType 1279 } else if (name.equals("mode")) { 1280 value = new StructureMapModelModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1281 this.mode = (Enumeration) value; // Enumeration<StructureMapModelMode> 1282 } else if (name.equals("alias")) { 1283 this.alias = TypeConvertor.castToString(value); // StringType 1284 } else if (name.equals("documentation")) { 1285 this.documentation = TypeConvertor.castToString(value); // StringType 1286 } else 1287 return super.setProperty(name, value); 1288 return value; 1289 } 1290 1291 @Override 1292 public void removeChild(String name, Base value) throws FHIRException { 1293 if (name.equals("url")) { 1294 this.url = null; 1295 } else if (name.equals("mode")) { 1296 value = new StructureMapModelModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1297 this.mode = (Enumeration) value; // Enumeration<StructureMapModelMode> 1298 } else if (name.equals("alias")) { 1299 this.alias = null; 1300 } else if (name.equals("documentation")) { 1301 this.documentation = null; 1302 } else 1303 super.removeChild(name, value); 1304 1305 } 1306 1307 @Override 1308 public Base makeProperty(int hash, String name) throws FHIRException { 1309 switch (hash) { 1310 case 116079: return getUrlElement(); 1311 case 3357091: return getModeElement(); 1312 case 92902992: return getAliasElement(); 1313 case 1587405498: return getDocumentationElement(); 1314 default: return super.makeProperty(hash, name); 1315 } 1316 1317 } 1318 1319 @Override 1320 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1321 switch (hash) { 1322 case 116079: /*url*/ return new String[] {"canonical"}; 1323 case 3357091: /*mode*/ return new String[] {"code"}; 1324 case 92902992: /*alias*/ return new String[] {"string"}; 1325 case 1587405498: /*documentation*/ return new String[] {"string"}; 1326 default: return super.getTypesForProperty(hash, name); 1327 } 1328 1329 } 1330 1331 @Override 1332 public Base addChild(String name) throws FHIRException { 1333 if (name.equals("url")) { 1334 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.structure.url"); 1335 } 1336 else if (name.equals("mode")) { 1337 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.structure.mode"); 1338 } 1339 else if (name.equals("alias")) { 1340 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.structure.alias"); 1341 } 1342 else if (name.equals("documentation")) { 1343 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.structure.documentation"); 1344 } 1345 else 1346 return super.addChild(name); 1347 } 1348 1349 public StructureMapStructureComponent copy() { 1350 StructureMapStructureComponent dst = new StructureMapStructureComponent(); 1351 copyValues(dst); 1352 return dst; 1353 } 1354 1355 public void copyValues(StructureMapStructureComponent dst) { 1356 super.copyValues(dst); 1357 dst.url = url == null ? null : url.copy(); 1358 dst.mode = mode == null ? null : mode.copy(); 1359 dst.alias = alias == null ? null : alias.copy(); 1360 dst.documentation = documentation == null ? null : documentation.copy(); 1361 } 1362 1363 @Override 1364 public boolean equalsDeep(Base other_) { 1365 if (!super.equalsDeep(other_)) 1366 return false; 1367 if (!(other_ instanceof StructureMapStructureComponent)) 1368 return false; 1369 StructureMapStructureComponent o = (StructureMapStructureComponent) other_; 1370 return compareDeep(url, o.url, true) && compareDeep(mode, o.mode, true) && compareDeep(alias, o.alias, true) 1371 && compareDeep(documentation, o.documentation, true); 1372 } 1373 1374 @Override 1375 public boolean equalsShallow(Base other_) { 1376 if (!super.equalsShallow(other_)) 1377 return false; 1378 if (!(other_ instanceof StructureMapStructureComponent)) 1379 return false; 1380 StructureMapStructureComponent o = (StructureMapStructureComponent) other_; 1381 return compareValues(url, o.url, true) && compareValues(mode, o.mode, true) && compareValues(alias, o.alias, true) 1382 && compareValues(documentation, o.documentation, true); 1383 } 1384 1385 public boolean isEmpty() { 1386 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, mode, alias, documentation 1387 ); 1388 } 1389 1390 public String fhirType() { 1391 return "StructureMap.structure"; 1392 1393 } 1394 1395 } 1396 1397 @Block() 1398 public static class StructureMapConstComponent extends BackboneElement implements IBaseBackboneElement { 1399 /** 1400 * Other maps used by this map (canonical URLs). 1401 */ 1402 @Child(name = "name", type = {IdType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1403 @Description(shortDefinition="Constant name", formalDefinition="Other maps used by this map (canonical URLs)." ) 1404 protected IdType name; 1405 1406 /** 1407 * A FHIRPath expression that is the value of this variable. 1408 */ 1409 @Child(name = "value", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1410 @Description(shortDefinition="FHIRPath exression - value of the constant", formalDefinition="A FHIRPath expression that is the value of this variable." ) 1411 protected StringType value; 1412 1413 private static final long serialVersionUID = 19170614L; 1414 1415 /** 1416 * Constructor 1417 */ 1418 public StructureMapConstComponent() { 1419 super(); 1420 } 1421 1422 /** 1423 * @return {@link #name} (Other maps used by this map (canonical URLs).). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1424 */ 1425 public IdType getNameElement() { 1426 if (this.name == null) 1427 if (Configuration.errorOnAutoCreate()) 1428 throw new Error("Attempt to auto-create StructureMapConstComponent.name"); 1429 else if (Configuration.doAutoCreate()) 1430 this.name = new IdType(); // bb 1431 return this.name; 1432 } 1433 1434 public boolean hasNameElement() { 1435 return this.name != null && !this.name.isEmpty(); 1436 } 1437 1438 public boolean hasName() { 1439 return this.name != null && !this.name.isEmpty(); 1440 } 1441 1442 /** 1443 * @param value {@link #name} (Other maps used by this map (canonical URLs).). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1444 */ 1445 public StructureMapConstComponent setNameElement(IdType value) { 1446 this.name = value; 1447 return this; 1448 } 1449 1450 /** 1451 * @return Other maps used by this map (canonical URLs). 1452 */ 1453 public String getName() { 1454 return this.name == null ? null : this.name.getValue(); 1455 } 1456 1457 /** 1458 * @param value Other maps used by this map (canonical URLs). 1459 */ 1460 public StructureMapConstComponent setName(String value) { 1461 if (Utilities.noString(value)) 1462 this.name = null; 1463 else { 1464 if (this.name == null) 1465 this.name = new IdType(); 1466 this.name.setValue(value); 1467 } 1468 return this; 1469 } 1470 1471 /** 1472 * @return {@link #value} (A FHIRPath expression that is the value of this variable.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 1473 */ 1474 public StringType getValueElement() { 1475 if (this.value == null) 1476 if (Configuration.errorOnAutoCreate()) 1477 throw new Error("Attempt to auto-create StructureMapConstComponent.value"); 1478 else if (Configuration.doAutoCreate()) 1479 this.value = new StringType(); // bb 1480 return this.value; 1481 } 1482 1483 public boolean hasValueElement() { 1484 return this.value != null && !this.value.isEmpty(); 1485 } 1486 1487 public boolean hasValue() { 1488 return this.value != null && !this.value.isEmpty(); 1489 } 1490 1491 /** 1492 * @param value {@link #value} (A FHIRPath expression that is the value of this variable.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 1493 */ 1494 public StructureMapConstComponent setValueElement(StringType value) { 1495 this.value = value; 1496 return this; 1497 } 1498 1499 /** 1500 * @return A FHIRPath expression that is the value of this variable. 1501 */ 1502 public String getValue() { 1503 return this.value == null ? null : this.value.getValue(); 1504 } 1505 1506 /** 1507 * @param value A FHIRPath expression that is the value of this variable. 1508 */ 1509 public StructureMapConstComponent setValue(String value) { 1510 if (Utilities.noString(value)) 1511 this.value = null; 1512 else { 1513 if (this.value == null) 1514 this.value = new StringType(); 1515 this.value.setValue(value); 1516 } 1517 return this; 1518 } 1519 1520 protected void listChildren(List<Property> children) { 1521 super.listChildren(children); 1522 children.add(new Property("name", "id", "Other maps used by this map (canonical URLs).", 0, 1, name)); 1523 children.add(new Property("value", "string", "A FHIRPath expression that is the value of this variable.", 0, 1, value)); 1524 } 1525 1526 @Override 1527 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1528 switch (_hash) { 1529 case 3373707: /*name*/ return new Property("name", "id", "Other maps used by this map (canonical URLs).", 0, 1, name); 1530 case 111972721: /*value*/ return new Property("value", "string", "A FHIRPath expression that is the value of this variable.", 0, 1, value); 1531 default: return super.getNamedProperty(_hash, _name, _checkValid); 1532 } 1533 1534 } 1535 1536 @Override 1537 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1538 switch (hash) { 1539 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // IdType 1540 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 1541 default: return super.getProperty(hash, name, checkValid); 1542 } 1543 1544 } 1545 1546 @Override 1547 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1548 switch (hash) { 1549 case 3373707: // name 1550 this.name = TypeConvertor.castToId(value); // IdType 1551 return value; 1552 case 111972721: // value 1553 this.value = TypeConvertor.castToString(value); // StringType 1554 return value; 1555 default: return super.setProperty(hash, name, value); 1556 } 1557 1558 } 1559 1560 @Override 1561 public Base setProperty(String name, Base value) throws FHIRException { 1562 if (name.equals("name")) { 1563 this.name = TypeConvertor.castToId(value); // IdType 1564 } else if (name.equals("value")) { 1565 this.value = TypeConvertor.castToString(value); // StringType 1566 } else 1567 return super.setProperty(name, value); 1568 return value; 1569 } 1570 1571 @Override 1572 public void removeChild(String name, Base value) throws FHIRException { 1573 if (name.equals("name")) { 1574 this.name = null; 1575 } else if (name.equals("value")) { 1576 this.value = null; 1577 } else 1578 super.removeChild(name, value); 1579 1580 } 1581 1582 @Override 1583 public Base makeProperty(int hash, String name) throws FHIRException { 1584 switch (hash) { 1585 case 3373707: return getNameElement(); 1586 case 111972721: return getValueElement(); 1587 default: return super.makeProperty(hash, name); 1588 } 1589 1590 } 1591 1592 @Override 1593 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1594 switch (hash) { 1595 case 3373707: /*name*/ return new String[] {"id"}; 1596 case 111972721: /*value*/ return new String[] {"string"}; 1597 default: return super.getTypesForProperty(hash, name); 1598 } 1599 1600 } 1601 1602 @Override 1603 public Base addChild(String name) throws FHIRException { 1604 if (name.equals("name")) { 1605 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.const.name"); 1606 } 1607 else if (name.equals("value")) { 1608 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.const.value"); 1609 } 1610 else 1611 return super.addChild(name); 1612 } 1613 1614 public StructureMapConstComponent copy() { 1615 StructureMapConstComponent dst = new StructureMapConstComponent(); 1616 copyValues(dst); 1617 return dst; 1618 } 1619 1620 public void copyValues(StructureMapConstComponent dst) { 1621 super.copyValues(dst); 1622 dst.name = name == null ? null : name.copy(); 1623 dst.value = value == null ? null : value.copy(); 1624 } 1625 1626 @Override 1627 public boolean equalsDeep(Base other_) { 1628 if (!super.equalsDeep(other_)) 1629 return false; 1630 if (!(other_ instanceof StructureMapConstComponent)) 1631 return false; 1632 StructureMapConstComponent o = (StructureMapConstComponent) other_; 1633 return compareDeep(name, o.name, true) && compareDeep(value, o.value, true); 1634 } 1635 1636 @Override 1637 public boolean equalsShallow(Base other_) { 1638 if (!super.equalsShallow(other_)) 1639 return false; 1640 if (!(other_ instanceof StructureMapConstComponent)) 1641 return false; 1642 StructureMapConstComponent o = (StructureMapConstComponent) other_; 1643 return compareValues(name, o.name, true) && compareValues(value, o.value, true); 1644 } 1645 1646 public boolean isEmpty() { 1647 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, value); 1648 } 1649 1650 public String fhirType() { 1651 return "StructureMap.const"; 1652 1653 } 1654 1655 } 1656 1657 @Block() 1658 public static class StructureMapGroupComponent extends BackboneElement implements IBaseBackboneElement { 1659 /** 1660 * A unique name for the group for the convenience of human readers. 1661 */ 1662 @Child(name = "name", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1663 @Description(shortDefinition="Human-readable label", formalDefinition="A unique name for the group for the convenience of human readers." ) 1664 protected IdType name; 1665 1666 /** 1667 * Another group that this group adds rules to. 1668 */ 1669 @Child(name = "extends", type = {IdType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1670 @Description(shortDefinition="Another group that this group adds rules to", formalDefinition="Another group that this group adds rules to." ) 1671 protected IdType extends_; 1672 1673 /** 1674 * If this is the default rule set to apply for the source type or this combination of types. 1675 */ 1676 @Child(name = "typeMode", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1677 @Description(shortDefinition="types | type-and-types", formalDefinition="If this is the default rule set to apply for the source type or this combination of types." ) 1678 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/map-group-type-mode") 1679 protected Enumeration<StructureMapGroupTypeMode> typeMode; 1680 1681 /** 1682 * Additional supporting documentation that explains the purpose of the group and the types of mappings within it. 1683 */ 1684 @Child(name = "documentation", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1685 @Description(shortDefinition="Additional description/explanation for group", formalDefinition="Additional supporting documentation that explains the purpose of the group and the types of mappings within it." ) 1686 protected StringType documentation; 1687 1688 /** 1689 * A name assigned to an instance of data. The instance must be provided when the mapping is invoked. 1690 */ 1691 @Child(name = "input", type = {}, order=5, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1692 @Description(shortDefinition="Named instance provided when invoking the map", formalDefinition="A name assigned to an instance of data. The instance must be provided when the mapping is invoked." ) 1693 protected List<StructureMapGroupInputComponent> input; 1694 1695 /** 1696 * Transform Rule from source to target. 1697 */ 1698 @Child(name = "rule", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1699 @Description(shortDefinition="Transform Rule from source to target", formalDefinition="Transform Rule from source to target." ) 1700 protected List<StructureMapGroupRuleComponent> rule; 1701 1702 private static final long serialVersionUID = -1474595081L; 1703 1704 /** 1705 * Constructor 1706 */ 1707 public StructureMapGroupComponent() { 1708 super(); 1709 } 1710 1711 /** 1712 * Constructor 1713 */ 1714 public StructureMapGroupComponent(String name, StructureMapGroupInputComponent input) { 1715 super(); 1716 this.setName(name); 1717 this.addInput(input); 1718 } 1719 1720 /** 1721 * @return {@link #name} (A unique name for the group for the convenience of human readers.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1722 */ 1723 public IdType getNameElement() { 1724 if (this.name == null) 1725 if (Configuration.errorOnAutoCreate()) 1726 throw new Error("Attempt to auto-create StructureMapGroupComponent.name"); 1727 else if (Configuration.doAutoCreate()) 1728 this.name = new IdType(); // bb 1729 return this.name; 1730 } 1731 1732 public boolean hasNameElement() { 1733 return this.name != null && !this.name.isEmpty(); 1734 } 1735 1736 public boolean hasName() { 1737 return this.name != null && !this.name.isEmpty(); 1738 } 1739 1740 /** 1741 * @param value {@link #name} (A unique name for the group for the convenience of human readers.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1742 */ 1743 public StructureMapGroupComponent setNameElement(IdType value) { 1744 this.name = value; 1745 return this; 1746 } 1747 1748 /** 1749 * @return A unique name for the group for the convenience of human readers. 1750 */ 1751 public String getName() { 1752 return this.name == null ? null : this.name.getValue(); 1753 } 1754 1755 /** 1756 * @param value A unique name for the group for the convenience of human readers. 1757 */ 1758 public StructureMapGroupComponent setName(String value) { 1759 if (this.name == null) 1760 this.name = new IdType(); 1761 this.name.setValue(value); 1762 return this; 1763 } 1764 1765 /** 1766 * @return {@link #extends_} (Another group that this group adds rules to.). This is the underlying object with id, value and extensions. The accessor "getExtends" gives direct access to the value 1767 */ 1768 public IdType getExtendsElement() { 1769 if (this.extends_ == null) 1770 if (Configuration.errorOnAutoCreate()) 1771 throw new Error("Attempt to auto-create StructureMapGroupComponent.extends_"); 1772 else if (Configuration.doAutoCreate()) 1773 this.extends_ = new IdType(); // bb 1774 return this.extends_; 1775 } 1776 1777 public boolean hasExtendsElement() { 1778 return this.extends_ != null && !this.extends_.isEmpty(); 1779 } 1780 1781 public boolean hasExtends() { 1782 return this.extends_ != null && !this.extends_.isEmpty(); 1783 } 1784 1785 /** 1786 * @param value {@link #extends_} (Another group that this group adds rules to.). This is the underlying object with id, value and extensions. The accessor "getExtends" gives direct access to the value 1787 */ 1788 public StructureMapGroupComponent setExtendsElement(IdType value) { 1789 this.extends_ = value; 1790 return this; 1791 } 1792 1793 /** 1794 * @return Another group that this group adds rules to. 1795 */ 1796 public String getExtends() { 1797 return this.extends_ == null ? null : this.extends_.getValue(); 1798 } 1799 1800 /** 1801 * @param value Another group that this group adds rules to. 1802 */ 1803 public StructureMapGroupComponent setExtends(String value) { 1804 if (Utilities.noString(value)) 1805 this.extends_ = null; 1806 else { 1807 if (this.extends_ == null) 1808 this.extends_ = new IdType(); 1809 this.extends_.setValue(value); 1810 } 1811 return this; 1812 } 1813 1814 /** 1815 * @return {@link #typeMode} (If this is the default rule set to apply for the source type or this combination of types.). This is the underlying object with id, value and extensions. The accessor "getTypeMode" gives direct access to the value 1816 */ 1817 public Enumeration<StructureMapGroupTypeMode> getTypeModeElement() { 1818 if (this.typeMode == null) 1819 if (Configuration.errorOnAutoCreate()) 1820 throw new Error("Attempt to auto-create StructureMapGroupComponent.typeMode"); 1821 else if (Configuration.doAutoCreate()) 1822 this.typeMode = new Enumeration<StructureMapGroupTypeMode>(new StructureMapGroupTypeModeEnumFactory()); // bb 1823 return this.typeMode; 1824 } 1825 1826 public boolean hasTypeModeElement() { 1827 return this.typeMode != null && !this.typeMode.isEmpty(); 1828 } 1829 1830 public boolean hasTypeMode() { 1831 return this.typeMode != null && !this.typeMode.isEmpty(); 1832 } 1833 1834 /** 1835 * @param value {@link #typeMode} (If this is the default rule set to apply for the source type or this combination of types.). This is the underlying object with id, value and extensions. The accessor "getTypeMode" gives direct access to the value 1836 */ 1837 public StructureMapGroupComponent setTypeModeElement(Enumeration<StructureMapGroupTypeMode> value) { 1838 this.typeMode = value; 1839 return this; 1840 } 1841 1842 /** 1843 * @return If this is the default rule set to apply for the source type or this combination of types. 1844 */ 1845 public StructureMapGroupTypeMode getTypeMode() { 1846 return this.typeMode == null ? null : this.typeMode.getValue(); 1847 } 1848 1849 /** 1850 * @param value If this is the default rule set to apply for the source type or this combination of types. 1851 */ 1852 public StructureMapGroupComponent setTypeMode(StructureMapGroupTypeMode value) { 1853 if (value == null) 1854 this.typeMode = null; 1855 else { 1856 if (this.typeMode == null) 1857 this.typeMode = new Enumeration<StructureMapGroupTypeMode>(new StructureMapGroupTypeModeEnumFactory()); 1858 this.typeMode.setValue(value); 1859 } 1860 return this; 1861 } 1862 1863 /** 1864 * @return {@link #documentation} (Additional supporting documentation that explains the purpose of the group and the types of mappings within it.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 1865 */ 1866 public StringType getDocumentationElement() { 1867 if (this.documentation == null) 1868 if (Configuration.errorOnAutoCreate()) 1869 throw new Error("Attempt to auto-create StructureMapGroupComponent.documentation"); 1870 else if (Configuration.doAutoCreate()) 1871 this.documentation = new StringType(); // bb 1872 return this.documentation; 1873 } 1874 1875 public boolean hasDocumentationElement() { 1876 return this.documentation != null && !this.documentation.isEmpty(); 1877 } 1878 1879 public boolean hasDocumentation() { 1880 return this.documentation != null && !this.documentation.isEmpty(); 1881 } 1882 1883 /** 1884 * @param value {@link #documentation} (Additional supporting documentation that explains the purpose of the group and the types of mappings within it.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 1885 */ 1886 public StructureMapGroupComponent setDocumentationElement(StringType value) { 1887 this.documentation = value; 1888 return this; 1889 } 1890 1891 /** 1892 * @return Additional supporting documentation that explains the purpose of the group and the types of mappings within it. 1893 */ 1894 public String getDocumentation() { 1895 return this.documentation == null ? null : this.documentation.getValue(); 1896 } 1897 1898 /** 1899 * @param value Additional supporting documentation that explains the purpose of the group and the types of mappings within it. 1900 */ 1901 public StructureMapGroupComponent setDocumentation(String value) { 1902 if (Utilities.noString(value)) 1903 this.documentation = null; 1904 else { 1905 if (this.documentation == null) 1906 this.documentation = new StringType(); 1907 this.documentation.setValue(value); 1908 } 1909 return this; 1910 } 1911 1912 /** 1913 * @return {@link #input} (A name assigned to an instance of data. The instance must be provided when the mapping is invoked.) 1914 */ 1915 public List<StructureMapGroupInputComponent> getInput() { 1916 if (this.input == null) 1917 this.input = new ArrayList<StructureMapGroupInputComponent>(); 1918 return this.input; 1919 } 1920 1921 /** 1922 * @return Returns a reference to <code>this</code> for easy method chaining 1923 */ 1924 public StructureMapGroupComponent setInput(List<StructureMapGroupInputComponent> theInput) { 1925 this.input = theInput; 1926 return this; 1927 } 1928 1929 public boolean hasInput() { 1930 if (this.input == null) 1931 return false; 1932 for (StructureMapGroupInputComponent item : this.input) 1933 if (!item.isEmpty()) 1934 return true; 1935 return false; 1936 } 1937 1938 public StructureMapGroupInputComponent addInput() { //3 1939 StructureMapGroupInputComponent t = new StructureMapGroupInputComponent(); 1940 if (this.input == null) 1941 this.input = new ArrayList<StructureMapGroupInputComponent>(); 1942 this.input.add(t); 1943 return t; 1944 } 1945 1946 public StructureMapGroupComponent addInput(StructureMapGroupInputComponent t) { //3 1947 if (t == null) 1948 return this; 1949 if (this.input == null) 1950 this.input = new ArrayList<StructureMapGroupInputComponent>(); 1951 this.input.add(t); 1952 return this; 1953 } 1954 1955 /** 1956 * @return The first repetition of repeating field {@link #input}, creating it if it does not already exist {3} 1957 */ 1958 public StructureMapGroupInputComponent getInputFirstRep() { 1959 if (getInput().isEmpty()) { 1960 addInput(); 1961 } 1962 return getInput().get(0); 1963 } 1964 1965 /** 1966 * @return {@link #rule} (Transform Rule from source to target.) 1967 */ 1968 public List<StructureMapGroupRuleComponent> getRule() { 1969 if (this.rule == null) 1970 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 1971 return this.rule; 1972 } 1973 1974 /** 1975 * @return Returns a reference to <code>this</code> for easy method chaining 1976 */ 1977 public StructureMapGroupComponent setRule(List<StructureMapGroupRuleComponent> theRule) { 1978 this.rule = theRule; 1979 return this; 1980 } 1981 1982 public boolean hasRule() { 1983 if (this.rule == null) 1984 return false; 1985 for (StructureMapGroupRuleComponent item : this.rule) 1986 if (!item.isEmpty()) 1987 return true; 1988 return false; 1989 } 1990 1991 public StructureMapGroupRuleComponent addRule() { //3 1992 StructureMapGroupRuleComponent t = new StructureMapGroupRuleComponent(); 1993 if (this.rule == null) 1994 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 1995 this.rule.add(t); 1996 return t; 1997 } 1998 1999 public StructureMapGroupComponent addRule(StructureMapGroupRuleComponent t) { //3 2000 if (t == null) 2001 return this; 2002 if (this.rule == null) 2003 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 2004 this.rule.add(t); 2005 return this; 2006 } 2007 2008 /** 2009 * @return The first repetition of repeating field {@link #rule}, creating it if it does not already exist {3} 2010 */ 2011 public StructureMapGroupRuleComponent getRuleFirstRep() { 2012 if (getRule().isEmpty()) { 2013 addRule(); 2014 } 2015 return getRule().get(0); 2016 } 2017 2018 protected void listChildren(List<Property> children) { 2019 super.listChildren(children); 2020 children.add(new Property("name", "id", "A unique name for the group for the convenience of human readers.", 0, 1, name)); 2021 children.add(new Property("extends", "id", "Another group that this group adds rules to.", 0, 1, extends_)); 2022 children.add(new Property("typeMode", "code", "If this is the default rule set to apply for the source type or this combination of types.", 0, 1, typeMode)); 2023 children.add(new Property("documentation", "string", "Additional supporting documentation that explains the purpose of the group and the types of mappings within it.", 0, 1, documentation)); 2024 children.add(new Property("input", "", "A name assigned to an instance of data. The instance must be provided when the mapping is invoked.", 0, java.lang.Integer.MAX_VALUE, input)); 2025 children.add(new Property("rule", "", "Transform Rule from source to target.", 0, java.lang.Integer.MAX_VALUE, rule)); 2026 } 2027 2028 @Override 2029 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2030 switch (_hash) { 2031 case 3373707: /*name*/ return new Property("name", "id", "A unique name for the group for the convenience of human readers.", 0, 1, name); 2032 case -1305664359: /*extends*/ return new Property("extends", "id", "Another group that this group adds rules to.", 0, 1, extends_); 2033 case -676524035: /*typeMode*/ return new Property("typeMode", "code", "If this is the default rule set to apply for the source type or this combination of types.", 0, 1, typeMode); 2034 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Additional supporting documentation that explains the purpose of the group and the types of mappings within it.", 0, 1, documentation); 2035 case 100358090: /*input*/ return new Property("input", "", "A name assigned to an instance of data. The instance must be provided when the mapping is invoked.", 0, java.lang.Integer.MAX_VALUE, input); 2036 case 3512060: /*rule*/ return new Property("rule", "", "Transform Rule from source to target.", 0, java.lang.Integer.MAX_VALUE, rule); 2037 default: return super.getNamedProperty(_hash, _name, _checkValid); 2038 } 2039 2040 } 2041 2042 @Override 2043 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2044 switch (hash) { 2045 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // IdType 2046 case -1305664359: /*extends*/ return this.extends_ == null ? new Base[0] : new Base[] {this.extends_}; // IdType 2047 case -676524035: /*typeMode*/ return this.typeMode == null ? new Base[0] : new Base[] {this.typeMode}; // Enumeration<StructureMapGroupTypeMode> 2048 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 2049 case 100358090: /*input*/ return this.input == null ? new Base[0] : this.input.toArray(new Base[this.input.size()]); // StructureMapGroupInputComponent 2050 case 3512060: /*rule*/ return this.rule == null ? new Base[0] : this.rule.toArray(new Base[this.rule.size()]); // StructureMapGroupRuleComponent 2051 default: return super.getProperty(hash, name, checkValid); 2052 } 2053 2054 } 2055 2056 @Override 2057 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2058 switch (hash) { 2059 case 3373707: // name 2060 this.name = TypeConvertor.castToId(value); // IdType 2061 return value; 2062 case -1305664359: // extends 2063 this.extends_ = TypeConvertor.castToId(value); // IdType 2064 return value; 2065 case -676524035: // typeMode 2066 value = new StructureMapGroupTypeModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2067 this.typeMode = (Enumeration) value; // Enumeration<StructureMapGroupTypeMode> 2068 return value; 2069 case 1587405498: // documentation 2070 this.documentation = TypeConvertor.castToString(value); // StringType 2071 return value; 2072 case 100358090: // input 2073 this.getInput().add((StructureMapGroupInputComponent) value); // StructureMapGroupInputComponent 2074 return value; 2075 case 3512060: // rule 2076 this.getRule().add((StructureMapGroupRuleComponent) value); // StructureMapGroupRuleComponent 2077 return value; 2078 default: return super.setProperty(hash, name, value); 2079 } 2080 2081 } 2082 2083 @Override 2084 public Base setProperty(String name, Base value) throws FHIRException { 2085 if (name.equals("name")) { 2086 this.name = TypeConvertor.castToId(value); // IdType 2087 } else if (name.equals("extends")) { 2088 this.extends_ = TypeConvertor.castToId(value); // IdType 2089 } else if (name.equals("typeMode")) { 2090 value = new StructureMapGroupTypeModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2091 this.typeMode = (Enumeration) value; // Enumeration<StructureMapGroupTypeMode> 2092 } else if (name.equals("documentation")) { 2093 this.documentation = TypeConvertor.castToString(value); // StringType 2094 } else if (name.equals("input")) { 2095 this.getInput().add((StructureMapGroupInputComponent) value); 2096 } else if (name.equals("rule")) { 2097 this.getRule().add((StructureMapGroupRuleComponent) value); 2098 } else 2099 return super.setProperty(name, value); 2100 return value; 2101 } 2102 2103 @Override 2104 public void removeChild(String name, Base value) throws FHIRException { 2105 if (name.equals("name")) { 2106 this.name = null; 2107 } else if (name.equals("extends")) { 2108 this.extends_ = null; 2109 } else if (name.equals("typeMode")) { 2110 value = new StructureMapGroupTypeModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2111 this.typeMode = (Enumeration) value; // Enumeration<StructureMapGroupTypeMode> 2112 } else if (name.equals("documentation")) { 2113 this.documentation = null; 2114 } else if (name.equals("input")) { 2115 this.getInput().remove((StructureMapGroupInputComponent) value); 2116 } else if (name.equals("rule")) { 2117 this.getRule().remove((StructureMapGroupRuleComponent) value); 2118 } else 2119 super.removeChild(name, value); 2120 2121 } 2122 2123 @Override 2124 public Base makeProperty(int hash, String name) throws FHIRException { 2125 switch (hash) { 2126 case 3373707: return getNameElement(); 2127 case -1305664359: return getExtendsElement(); 2128 case -676524035: return getTypeModeElement(); 2129 case 1587405498: return getDocumentationElement(); 2130 case 100358090: return addInput(); 2131 case 3512060: return addRule(); 2132 default: return super.makeProperty(hash, name); 2133 } 2134 2135 } 2136 2137 @Override 2138 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2139 switch (hash) { 2140 case 3373707: /*name*/ return new String[] {"id"}; 2141 case -1305664359: /*extends*/ return new String[] {"id"}; 2142 case -676524035: /*typeMode*/ return new String[] {"code"}; 2143 case 1587405498: /*documentation*/ return new String[] {"string"}; 2144 case 100358090: /*input*/ return new String[] {}; 2145 case 3512060: /*rule*/ return new String[] {}; 2146 default: return super.getTypesForProperty(hash, name); 2147 } 2148 2149 } 2150 2151 @Override 2152 public Base addChild(String name) throws FHIRException { 2153 if (name.equals("name")) { 2154 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.name"); 2155 } 2156 else if (name.equals("extends")) { 2157 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.extends"); 2158 } 2159 else if (name.equals("typeMode")) { 2160 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.typeMode"); 2161 } 2162 else if (name.equals("documentation")) { 2163 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.documentation"); 2164 } 2165 else if (name.equals("input")) { 2166 return addInput(); 2167 } 2168 else if (name.equals("rule")) { 2169 return addRule(); 2170 } 2171 else 2172 return super.addChild(name); 2173 } 2174 2175 public StructureMapGroupComponent copy() { 2176 StructureMapGroupComponent dst = new StructureMapGroupComponent(); 2177 copyValues(dst); 2178 return dst; 2179 } 2180 2181 public void copyValues(StructureMapGroupComponent dst) { 2182 super.copyValues(dst); 2183 dst.name = name == null ? null : name.copy(); 2184 dst.extends_ = extends_ == null ? null : extends_.copy(); 2185 dst.typeMode = typeMode == null ? null : typeMode.copy(); 2186 dst.documentation = documentation == null ? null : documentation.copy(); 2187 if (input != null) { 2188 dst.input = new ArrayList<StructureMapGroupInputComponent>(); 2189 for (StructureMapGroupInputComponent i : input) 2190 dst.input.add(i.copy()); 2191 }; 2192 if (rule != null) { 2193 dst.rule = new ArrayList<StructureMapGroupRuleComponent>(); 2194 for (StructureMapGroupRuleComponent i : rule) 2195 dst.rule.add(i.copy()); 2196 }; 2197 } 2198 2199 @Override 2200 public boolean equalsDeep(Base other_) { 2201 if (!super.equalsDeep(other_)) 2202 return false; 2203 if (!(other_ instanceof StructureMapGroupComponent)) 2204 return false; 2205 StructureMapGroupComponent o = (StructureMapGroupComponent) other_; 2206 return compareDeep(name, o.name, true) && compareDeep(extends_, o.extends_, true) && compareDeep(typeMode, o.typeMode, true) 2207 && compareDeep(documentation, o.documentation, true) && compareDeep(input, o.input, true) && compareDeep(rule, o.rule, true) 2208 ; 2209 } 2210 2211 @Override 2212 public boolean equalsShallow(Base other_) { 2213 if (!super.equalsShallow(other_)) 2214 return false; 2215 if (!(other_ instanceof StructureMapGroupComponent)) 2216 return false; 2217 StructureMapGroupComponent o = (StructureMapGroupComponent) other_; 2218 return compareValues(name, o.name, true) && compareValues(extends_, o.extends_, true) && compareValues(typeMode, o.typeMode, true) 2219 && compareValues(documentation, o.documentation, true); 2220 } 2221 2222 public boolean isEmpty() { 2223 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, extends_, typeMode 2224 , documentation, input, rule); 2225 } 2226 2227 public String fhirType() { 2228 return "StructureMap.group"; 2229 2230 } 2231 2232// added from java-adornments.txt: 2233public String toString() { 2234 return StructureMapUtilities.groupToString(this); 2235 } 2236// end addition 2237 } 2238 2239 @Block() 2240 public static class StructureMapGroupInputComponent extends BackboneElement implements IBaseBackboneElement { 2241 /** 2242 * Name for this instance of data. 2243 */ 2244 @Child(name = "name", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2245 @Description(shortDefinition="Name for this instance of data", formalDefinition="Name for this instance of data." ) 2246 protected IdType name; 2247 2248 /** 2249 * Type for this instance of data. 2250 */ 2251 @Child(name = "type", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2252 @Description(shortDefinition="Type for this instance of data", formalDefinition="Type for this instance of data." ) 2253 protected StringType type; 2254 2255 /** 2256 * Mode for this instance of data. 2257 */ 2258 @Child(name = "mode", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=true) 2259 @Description(shortDefinition="source | target", formalDefinition="Mode for this instance of data." ) 2260 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/map-input-mode") 2261 protected Enumeration<StructureMapInputMode> mode; 2262 2263 /** 2264 * Documentation for this instance of data. 2265 */ 2266 @Child(name = "documentation", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 2267 @Description(shortDefinition="Documentation for this instance of data", formalDefinition="Documentation for this instance of data." ) 2268 protected StringType documentation; 2269 2270 private static final long serialVersionUID = -25050724L; 2271 2272 /** 2273 * Constructor 2274 */ 2275 public StructureMapGroupInputComponent() { 2276 super(); 2277 } 2278 2279 /** 2280 * Constructor 2281 */ 2282 public StructureMapGroupInputComponent(String name, StructureMapInputMode mode) { 2283 super(); 2284 this.setName(name); 2285 this.setMode(mode); 2286 } 2287 2288 /** 2289 * @return {@link #name} (Name for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2290 */ 2291 public IdType getNameElement() { 2292 if (this.name == null) 2293 if (Configuration.errorOnAutoCreate()) 2294 throw new Error("Attempt to auto-create StructureMapGroupInputComponent.name"); 2295 else if (Configuration.doAutoCreate()) 2296 this.name = new IdType(); // bb 2297 return this.name; 2298 } 2299 2300 public boolean hasNameElement() { 2301 return this.name != null && !this.name.isEmpty(); 2302 } 2303 2304 public boolean hasName() { 2305 return this.name != null && !this.name.isEmpty(); 2306 } 2307 2308 /** 2309 * @param value {@link #name} (Name for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2310 */ 2311 public StructureMapGroupInputComponent setNameElement(IdType value) { 2312 this.name = value; 2313 return this; 2314 } 2315 2316 /** 2317 * @return Name for this instance of data. 2318 */ 2319 public String getName() { 2320 return this.name == null ? null : this.name.getValue(); 2321 } 2322 2323 /** 2324 * @param value Name for this instance of data. 2325 */ 2326 public StructureMapGroupInputComponent setName(String value) { 2327 if (this.name == null) 2328 this.name = new IdType(); 2329 this.name.setValue(value); 2330 return this; 2331 } 2332 2333 /** 2334 * @return {@link #type} (Type for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2335 */ 2336 public StringType getTypeElement() { 2337 if (this.type == null) 2338 if (Configuration.errorOnAutoCreate()) 2339 throw new Error("Attempt to auto-create StructureMapGroupInputComponent.type"); 2340 else if (Configuration.doAutoCreate()) 2341 this.type = new StringType(); // bb 2342 return this.type; 2343 } 2344 2345 public boolean hasTypeElement() { 2346 return this.type != null && !this.type.isEmpty(); 2347 } 2348 2349 public boolean hasType() { 2350 return this.type != null && !this.type.isEmpty(); 2351 } 2352 2353 /** 2354 * @param value {@link #type} (Type for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2355 */ 2356 public StructureMapGroupInputComponent setTypeElement(StringType value) { 2357 this.type = value; 2358 return this; 2359 } 2360 2361 /** 2362 * @return Type for this instance of data. 2363 */ 2364 public String getType() { 2365 return this.type == null ? null : this.type.getValue(); 2366 } 2367 2368 /** 2369 * @param value Type for this instance of data. 2370 */ 2371 public StructureMapGroupInputComponent setType(String value) { 2372 if (Utilities.noString(value)) 2373 this.type = null; 2374 else { 2375 if (this.type == null) 2376 this.type = new StringType(); 2377 this.type.setValue(value); 2378 } 2379 return this; 2380 } 2381 2382 /** 2383 * @return {@link #mode} (Mode for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 2384 */ 2385 public Enumeration<StructureMapInputMode> getModeElement() { 2386 if (this.mode == null) 2387 if (Configuration.errorOnAutoCreate()) 2388 throw new Error("Attempt to auto-create StructureMapGroupInputComponent.mode"); 2389 else if (Configuration.doAutoCreate()) 2390 this.mode = new Enumeration<StructureMapInputMode>(new StructureMapInputModeEnumFactory()); // bb 2391 return this.mode; 2392 } 2393 2394 public boolean hasModeElement() { 2395 return this.mode != null && !this.mode.isEmpty(); 2396 } 2397 2398 public boolean hasMode() { 2399 return this.mode != null && !this.mode.isEmpty(); 2400 } 2401 2402 /** 2403 * @param value {@link #mode} (Mode for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 2404 */ 2405 public StructureMapGroupInputComponent setModeElement(Enumeration<StructureMapInputMode> value) { 2406 this.mode = value; 2407 return this; 2408 } 2409 2410 /** 2411 * @return Mode for this instance of data. 2412 */ 2413 public StructureMapInputMode getMode() { 2414 return this.mode == null ? null : this.mode.getValue(); 2415 } 2416 2417 /** 2418 * @param value Mode for this instance of data. 2419 */ 2420 public StructureMapGroupInputComponent setMode(StructureMapInputMode value) { 2421 if (this.mode == null) 2422 this.mode = new Enumeration<StructureMapInputMode>(new StructureMapInputModeEnumFactory()); 2423 this.mode.setValue(value); 2424 return this; 2425 } 2426 2427 /** 2428 * @return {@link #documentation} (Documentation for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 2429 */ 2430 public StringType getDocumentationElement() { 2431 if (this.documentation == null) 2432 if (Configuration.errorOnAutoCreate()) 2433 throw new Error("Attempt to auto-create StructureMapGroupInputComponent.documentation"); 2434 else if (Configuration.doAutoCreate()) 2435 this.documentation = new StringType(); // bb 2436 return this.documentation; 2437 } 2438 2439 public boolean hasDocumentationElement() { 2440 return this.documentation != null && !this.documentation.isEmpty(); 2441 } 2442 2443 public boolean hasDocumentation() { 2444 return this.documentation != null && !this.documentation.isEmpty(); 2445 } 2446 2447 /** 2448 * @param value {@link #documentation} (Documentation for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 2449 */ 2450 public StructureMapGroupInputComponent setDocumentationElement(StringType value) { 2451 this.documentation = value; 2452 return this; 2453 } 2454 2455 /** 2456 * @return Documentation for this instance of data. 2457 */ 2458 public String getDocumentation() { 2459 return this.documentation == null ? null : this.documentation.getValue(); 2460 } 2461 2462 /** 2463 * @param value Documentation for this instance of data. 2464 */ 2465 public StructureMapGroupInputComponent setDocumentation(String value) { 2466 if (Utilities.noString(value)) 2467 this.documentation = null; 2468 else { 2469 if (this.documentation == null) 2470 this.documentation = new StringType(); 2471 this.documentation.setValue(value); 2472 } 2473 return this; 2474 } 2475 2476 protected void listChildren(List<Property> children) { 2477 super.listChildren(children); 2478 children.add(new Property("name", "id", "Name for this instance of data.", 0, 1, name)); 2479 children.add(new Property("type", "string", "Type for this instance of data.", 0, 1, type)); 2480 children.add(new Property("mode", "code", "Mode for this instance of data.", 0, 1, mode)); 2481 children.add(new Property("documentation", "string", "Documentation for this instance of data.", 0, 1, documentation)); 2482 } 2483 2484 @Override 2485 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2486 switch (_hash) { 2487 case 3373707: /*name*/ return new Property("name", "id", "Name for this instance of data.", 0, 1, name); 2488 case 3575610: /*type*/ return new Property("type", "string", "Type for this instance of data.", 0, 1, type); 2489 case 3357091: /*mode*/ return new Property("mode", "code", "Mode for this instance of data.", 0, 1, mode); 2490 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Documentation for this instance of data.", 0, 1, documentation); 2491 default: return super.getNamedProperty(_hash, _name, _checkValid); 2492 } 2493 2494 } 2495 2496 @Override 2497 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2498 switch (hash) { 2499 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // IdType 2500 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // StringType 2501 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<StructureMapInputMode> 2502 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 2503 default: return super.getProperty(hash, name, checkValid); 2504 } 2505 2506 } 2507 2508 @Override 2509 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2510 switch (hash) { 2511 case 3373707: // name 2512 this.name = TypeConvertor.castToId(value); // IdType 2513 return value; 2514 case 3575610: // type 2515 this.type = TypeConvertor.castToString(value); // StringType 2516 return value; 2517 case 3357091: // mode 2518 value = new StructureMapInputModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2519 this.mode = (Enumeration) value; // Enumeration<StructureMapInputMode> 2520 return value; 2521 case 1587405498: // documentation 2522 this.documentation = TypeConvertor.castToString(value); // StringType 2523 return value; 2524 default: return super.setProperty(hash, name, value); 2525 } 2526 2527 } 2528 2529 @Override 2530 public Base setProperty(String name, Base value) throws FHIRException { 2531 if (name.equals("name")) { 2532 this.name = TypeConvertor.castToId(value); // IdType 2533 } else if (name.equals("type")) { 2534 this.type = TypeConvertor.castToString(value); // StringType 2535 } else if (name.equals("mode")) { 2536 value = new StructureMapInputModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2537 this.mode = (Enumeration) value; // Enumeration<StructureMapInputMode> 2538 } else if (name.equals("documentation")) { 2539 this.documentation = TypeConvertor.castToString(value); // StringType 2540 } else 2541 return super.setProperty(name, value); 2542 return value; 2543 } 2544 2545 @Override 2546 public void removeChild(String name, Base value) throws FHIRException { 2547 if (name.equals("name")) { 2548 this.name = null; 2549 } else if (name.equals("type")) { 2550 this.type = null; 2551 } else if (name.equals("mode")) { 2552 value = new StructureMapInputModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2553 this.mode = (Enumeration) value; // Enumeration<StructureMapInputMode> 2554 } else if (name.equals("documentation")) { 2555 this.documentation = null; 2556 } else 2557 super.removeChild(name, value); 2558 2559 } 2560 2561 @Override 2562 public Base makeProperty(int hash, String name) throws FHIRException { 2563 switch (hash) { 2564 case 3373707: return getNameElement(); 2565 case 3575610: return getTypeElement(); 2566 case 3357091: return getModeElement(); 2567 case 1587405498: return getDocumentationElement(); 2568 default: return super.makeProperty(hash, name); 2569 } 2570 2571 } 2572 2573 @Override 2574 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2575 switch (hash) { 2576 case 3373707: /*name*/ return new String[] {"id"}; 2577 case 3575610: /*type*/ return new String[] {"string"}; 2578 case 3357091: /*mode*/ return new String[] {"code"}; 2579 case 1587405498: /*documentation*/ return new String[] {"string"}; 2580 default: return super.getTypesForProperty(hash, name); 2581 } 2582 2583 } 2584 2585 @Override 2586 public Base addChild(String name) throws FHIRException { 2587 if (name.equals("name")) { 2588 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.input.name"); 2589 } 2590 else if (name.equals("type")) { 2591 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.input.type"); 2592 } 2593 else if (name.equals("mode")) { 2594 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.input.mode"); 2595 } 2596 else if (name.equals("documentation")) { 2597 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.input.documentation"); 2598 } 2599 else 2600 return super.addChild(name); 2601 } 2602 2603 public StructureMapGroupInputComponent copy() { 2604 StructureMapGroupInputComponent dst = new StructureMapGroupInputComponent(); 2605 copyValues(dst); 2606 return dst; 2607 } 2608 2609 public void copyValues(StructureMapGroupInputComponent dst) { 2610 super.copyValues(dst); 2611 dst.name = name == null ? null : name.copy(); 2612 dst.type = type == null ? null : type.copy(); 2613 dst.mode = mode == null ? null : mode.copy(); 2614 dst.documentation = documentation == null ? null : documentation.copy(); 2615 } 2616 2617 @Override 2618 public boolean equalsDeep(Base other_) { 2619 if (!super.equalsDeep(other_)) 2620 return false; 2621 if (!(other_ instanceof StructureMapGroupInputComponent)) 2622 return false; 2623 StructureMapGroupInputComponent o = (StructureMapGroupInputComponent) other_; 2624 return compareDeep(name, o.name, true) && compareDeep(type, o.type, true) && compareDeep(mode, o.mode, true) 2625 && compareDeep(documentation, o.documentation, true); 2626 } 2627 2628 @Override 2629 public boolean equalsShallow(Base other_) { 2630 if (!super.equalsShallow(other_)) 2631 return false; 2632 if (!(other_ instanceof StructureMapGroupInputComponent)) 2633 return false; 2634 StructureMapGroupInputComponent o = (StructureMapGroupInputComponent) other_; 2635 return compareValues(name, o.name, true) && compareValues(type, o.type, true) && compareValues(mode, o.mode, true) 2636 && compareValues(documentation, o.documentation, true); 2637 } 2638 2639 public boolean isEmpty() { 2640 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, type, mode, documentation 2641 ); 2642 } 2643 2644 public String fhirType() { 2645 return "StructureMap.group.input"; 2646 2647 } 2648 2649 } 2650 2651 @Block() 2652 public static class StructureMapGroupRuleComponent extends BackboneElement implements IBaseBackboneElement { 2653 /** 2654 * Name of the rule for internal references. 2655 */ 2656 @Child(name = "name", type = {IdType.class}, order=1, min=0, max=1, modifier=false, summary=true) 2657 @Description(shortDefinition="Name of the rule for internal references", formalDefinition="Name of the rule for internal references." ) 2658 protected IdType name; 2659 2660 /** 2661 * Source inputs to the mapping. 2662 */ 2663 @Child(name = "source", type = {}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2664 @Description(shortDefinition="Source inputs to the mapping", formalDefinition="Source inputs to the mapping." ) 2665 protected List<StructureMapGroupRuleSourceComponent> source; 2666 2667 /** 2668 * Content to create because of this mapping rule. 2669 */ 2670 @Child(name = "target", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2671 @Description(shortDefinition="Content to create because of this mapping rule", formalDefinition="Content to create because of this mapping rule." ) 2672 protected List<StructureMapGroupRuleTargetComponent> target; 2673 2674 /** 2675 * Rules contained in this rule. 2676 */ 2677 @Child(name = "rule", type = {StructureMapGroupRuleComponent.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2678 @Description(shortDefinition="Rules contained in this rule", formalDefinition="Rules contained in this rule." ) 2679 protected List<StructureMapGroupRuleComponent> rule; 2680 2681 /** 2682 * Which other rules to apply in the context of this rule. 2683 */ 2684 @Child(name = "dependent", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2685 @Description(shortDefinition="Which other rules to apply in the context of this rule", formalDefinition="Which other rules to apply in the context of this rule." ) 2686 protected List<StructureMapGroupRuleDependentComponent> dependent; 2687 2688 /** 2689 * Documentation for this instance of data. 2690 */ 2691 @Child(name = "documentation", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 2692 @Description(shortDefinition="Documentation for this instance of data", formalDefinition="Documentation for this instance of data." ) 2693 protected StringType documentation; 2694 2695 private static final long serialVersionUID = 773925517L; 2696 2697 /** 2698 * Constructor 2699 */ 2700 public StructureMapGroupRuleComponent() { 2701 super(); 2702 } 2703 2704 /** 2705 * Constructor 2706 */ 2707 public StructureMapGroupRuleComponent(StructureMapGroupRuleSourceComponent source) { 2708 super(); 2709 this.addSource(source); 2710 } 2711 2712 /** 2713 * @return {@link #name} (Name of the rule for internal references.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2714 */ 2715 public IdType getNameElement() { 2716 if (this.name == null) 2717 if (Configuration.errorOnAutoCreate()) 2718 throw new Error("Attempt to auto-create StructureMapGroupRuleComponent.name"); 2719 else if (Configuration.doAutoCreate()) 2720 this.name = new IdType(); // bb 2721 return this.name; 2722 } 2723 2724 public boolean hasNameElement() { 2725 return this.name != null && !this.name.isEmpty(); 2726 } 2727 2728 public boolean hasName() { 2729 return this.name != null && !this.name.isEmpty(); 2730 } 2731 2732 /** 2733 * @param value {@link #name} (Name of the rule for internal references.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2734 */ 2735 public StructureMapGroupRuleComponent setNameElement(IdType value) { 2736 this.name = value; 2737 return this; 2738 } 2739 2740 /** 2741 * @return Name of the rule for internal references. 2742 */ 2743 public String getName() { 2744 return this.name == null ? null : this.name.getValue(); 2745 } 2746 2747 /** 2748 * @param value Name of the rule for internal references. 2749 */ 2750 public StructureMapGroupRuleComponent setName(String value) { 2751 if (Utilities.noString(value)) 2752 this.name = null; 2753 else { 2754 if (this.name == null) 2755 this.name = new IdType(); 2756 this.name.setValue(value); 2757 } 2758 return this; 2759 } 2760 2761 /** 2762 * @return {@link #source} (Source inputs to the mapping.) 2763 */ 2764 public List<StructureMapGroupRuleSourceComponent> getSource() { 2765 if (this.source == null) 2766 this.source = new ArrayList<StructureMapGroupRuleSourceComponent>(); 2767 return this.source; 2768 } 2769 2770 /** 2771 * @return Returns a reference to <code>this</code> for easy method chaining 2772 */ 2773 public StructureMapGroupRuleComponent setSource(List<StructureMapGroupRuleSourceComponent> theSource) { 2774 this.source = theSource; 2775 return this; 2776 } 2777 2778 public boolean hasSource() { 2779 if (this.source == null) 2780 return false; 2781 for (StructureMapGroupRuleSourceComponent item : this.source) 2782 if (!item.isEmpty()) 2783 return true; 2784 return false; 2785 } 2786 2787 public StructureMapGroupRuleSourceComponent addSource() { //3 2788 StructureMapGroupRuleSourceComponent t = new StructureMapGroupRuleSourceComponent(); 2789 if (this.source == null) 2790 this.source = new ArrayList<StructureMapGroupRuleSourceComponent>(); 2791 this.source.add(t); 2792 return t; 2793 } 2794 2795 public StructureMapGroupRuleComponent addSource(StructureMapGroupRuleSourceComponent t) { //3 2796 if (t == null) 2797 return this; 2798 if (this.source == null) 2799 this.source = new ArrayList<StructureMapGroupRuleSourceComponent>(); 2800 this.source.add(t); 2801 return this; 2802 } 2803 2804 /** 2805 * @return The first repetition of repeating field {@link #source}, creating it if it does not already exist {3} 2806 */ 2807 public StructureMapGroupRuleSourceComponent getSourceFirstRep() { 2808 if (getSource().isEmpty()) { 2809 addSource(); 2810 } 2811 return getSource().get(0); 2812 } 2813 2814 /** 2815 * @return {@link #target} (Content to create because of this mapping rule.) 2816 */ 2817 public List<StructureMapGroupRuleTargetComponent> getTarget() { 2818 if (this.target == null) 2819 this.target = new ArrayList<StructureMapGroupRuleTargetComponent>(); 2820 return this.target; 2821 } 2822 2823 /** 2824 * @return Returns a reference to <code>this</code> for easy method chaining 2825 */ 2826 public StructureMapGroupRuleComponent setTarget(List<StructureMapGroupRuleTargetComponent> theTarget) { 2827 this.target = theTarget; 2828 return this; 2829 } 2830 2831 public boolean hasTarget() { 2832 if (this.target == null) 2833 return false; 2834 for (StructureMapGroupRuleTargetComponent item : this.target) 2835 if (!item.isEmpty()) 2836 return true; 2837 return false; 2838 } 2839 2840 public StructureMapGroupRuleTargetComponent addTarget() { //3 2841 StructureMapGroupRuleTargetComponent t = new StructureMapGroupRuleTargetComponent(); 2842 if (this.target == null) 2843 this.target = new ArrayList<StructureMapGroupRuleTargetComponent>(); 2844 this.target.add(t); 2845 return t; 2846 } 2847 2848 public StructureMapGroupRuleComponent addTarget(StructureMapGroupRuleTargetComponent t) { //3 2849 if (t == null) 2850 return this; 2851 if (this.target == null) 2852 this.target = new ArrayList<StructureMapGroupRuleTargetComponent>(); 2853 this.target.add(t); 2854 return this; 2855 } 2856 2857 /** 2858 * @return The first repetition of repeating field {@link #target}, creating it if it does not already exist {3} 2859 */ 2860 public StructureMapGroupRuleTargetComponent getTargetFirstRep() { 2861 if (getTarget().isEmpty()) { 2862 addTarget(); 2863 } 2864 return getTarget().get(0); 2865 } 2866 2867 /** 2868 * @return {@link #rule} (Rules contained in this rule.) 2869 */ 2870 public List<StructureMapGroupRuleComponent> getRule() { 2871 if (this.rule == null) 2872 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 2873 return this.rule; 2874 } 2875 2876 /** 2877 * @return Returns a reference to <code>this</code> for easy method chaining 2878 */ 2879 public StructureMapGroupRuleComponent setRule(List<StructureMapGroupRuleComponent> theRule) { 2880 this.rule = theRule; 2881 return this; 2882 } 2883 2884 public boolean hasRule() { 2885 if (this.rule == null) 2886 return false; 2887 for (StructureMapGroupRuleComponent item : this.rule) 2888 if (!item.isEmpty()) 2889 return true; 2890 return false; 2891 } 2892 2893 public StructureMapGroupRuleComponent addRule() { //3 2894 StructureMapGroupRuleComponent t = new StructureMapGroupRuleComponent(); 2895 if (this.rule == null) 2896 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 2897 this.rule.add(t); 2898 return t; 2899 } 2900 2901 public StructureMapGroupRuleComponent addRule(StructureMapGroupRuleComponent t) { //3 2902 if (t == null) 2903 return this; 2904 if (this.rule == null) 2905 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 2906 this.rule.add(t); 2907 return this; 2908 } 2909 2910 /** 2911 * @return The first repetition of repeating field {@link #rule}, creating it if it does not already exist {3} 2912 */ 2913 public StructureMapGroupRuleComponent getRuleFirstRep() { 2914 if (getRule().isEmpty()) { 2915 addRule(); 2916 } 2917 return getRule().get(0); 2918 } 2919 2920 /** 2921 * @return {@link #dependent} (Which other rules to apply in the context of this rule.) 2922 */ 2923 public List<StructureMapGroupRuleDependentComponent> getDependent() { 2924 if (this.dependent == null) 2925 this.dependent = new ArrayList<StructureMapGroupRuleDependentComponent>(); 2926 return this.dependent; 2927 } 2928 2929 /** 2930 * @return Returns a reference to <code>this</code> for easy method chaining 2931 */ 2932 public StructureMapGroupRuleComponent setDependent(List<StructureMapGroupRuleDependentComponent> theDependent) { 2933 this.dependent = theDependent; 2934 return this; 2935 } 2936 2937 public boolean hasDependent() { 2938 if (this.dependent == null) 2939 return false; 2940 for (StructureMapGroupRuleDependentComponent item : this.dependent) 2941 if (!item.isEmpty()) 2942 return true; 2943 return false; 2944 } 2945 2946 public StructureMapGroupRuleDependentComponent addDependent() { //3 2947 StructureMapGroupRuleDependentComponent t = new StructureMapGroupRuleDependentComponent(); 2948 if (this.dependent == null) 2949 this.dependent = new ArrayList<StructureMapGroupRuleDependentComponent>(); 2950 this.dependent.add(t); 2951 return t; 2952 } 2953 2954 public StructureMapGroupRuleComponent addDependent(StructureMapGroupRuleDependentComponent t) { //3 2955 if (t == null) 2956 return this; 2957 if (this.dependent == null) 2958 this.dependent = new ArrayList<StructureMapGroupRuleDependentComponent>(); 2959 this.dependent.add(t); 2960 return this; 2961 } 2962 2963 /** 2964 * @return The first repetition of repeating field {@link #dependent}, creating it if it does not already exist {3} 2965 */ 2966 public StructureMapGroupRuleDependentComponent getDependentFirstRep() { 2967 if (getDependent().isEmpty()) { 2968 addDependent(); 2969 } 2970 return getDependent().get(0); 2971 } 2972 2973 /** 2974 * @return {@link #documentation} (Documentation for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 2975 */ 2976 public StringType getDocumentationElement() { 2977 if (this.documentation == null) 2978 if (Configuration.errorOnAutoCreate()) 2979 throw new Error("Attempt to auto-create StructureMapGroupRuleComponent.documentation"); 2980 else if (Configuration.doAutoCreate()) 2981 this.documentation = new StringType(); // bb 2982 return this.documentation; 2983 } 2984 2985 public boolean hasDocumentationElement() { 2986 return this.documentation != null && !this.documentation.isEmpty(); 2987 } 2988 2989 public boolean hasDocumentation() { 2990 return this.documentation != null && !this.documentation.isEmpty(); 2991 } 2992 2993 /** 2994 * @param value {@link #documentation} (Documentation for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 2995 */ 2996 public StructureMapGroupRuleComponent setDocumentationElement(StringType value) { 2997 this.documentation = value; 2998 return this; 2999 } 3000 3001 /** 3002 * @return Documentation for this instance of data. 3003 */ 3004 public String getDocumentation() { 3005 return this.documentation == null ? null : this.documentation.getValue(); 3006 } 3007 3008 /** 3009 * @param value Documentation for this instance of data. 3010 */ 3011 public StructureMapGroupRuleComponent setDocumentation(String value) { 3012 if (Utilities.noString(value)) 3013 this.documentation = null; 3014 else { 3015 if (this.documentation == null) 3016 this.documentation = new StringType(); 3017 this.documentation.setValue(value); 3018 } 3019 return this; 3020 } 3021 3022 protected void listChildren(List<Property> children) { 3023 super.listChildren(children); 3024 children.add(new Property("name", "id", "Name of the rule for internal references.", 0, 1, name)); 3025 children.add(new Property("source", "", "Source inputs to the mapping.", 0, java.lang.Integer.MAX_VALUE, source)); 3026 children.add(new Property("target", "", "Content to create because of this mapping rule.", 0, java.lang.Integer.MAX_VALUE, target)); 3027 children.add(new Property("rule", "@StructureMap.group.rule", "Rules contained in this rule.", 0, java.lang.Integer.MAX_VALUE, rule)); 3028 children.add(new Property("dependent", "", "Which other rules to apply in the context of this rule.", 0, java.lang.Integer.MAX_VALUE, dependent)); 3029 children.add(new Property("documentation", "string", "Documentation for this instance of data.", 0, 1, documentation)); 3030 } 3031 3032 @Override 3033 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3034 switch (_hash) { 3035 case 3373707: /*name*/ return new Property("name", "id", "Name of the rule for internal references.", 0, 1, name); 3036 case -896505829: /*source*/ return new Property("source", "", "Source inputs to the mapping.", 0, java.lang.Integer.MAX_VALUE, source); 3037 case -880905839: /*target*/ return new Property("target", "", "Content to create because of this mapping rule.", 0, java.lang.Integer.MAX_VALUE, target); 3038 case 3512060: /*rule*/ return new Property("rule", "@StructureMap.group.rule", "Rules contained in this rule.", 0, java.lang.Integer.MAX_VALUE, rule); 3039 case -1109226753: /*dependent*/ return new Property("dependent", "", "Which other rules to apply in the context of this rule.", 0, java.lang.Integer.MAX_VALUE, dependent); 3040 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Documentation for this instance of data.", 0, 1, documentation); 3041 default: return super.getNamedProperty(_hash, _name, _checkValid); 3042 } 3043 3044 } 3045 3046 @Override 3047 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3048 switch (hash) { 3049 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // IdType 3050 case -896505829: /*source*/ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // StructureMapGroupRuleSourceComponent 3051 case -880905839: /*target*/ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // StructureMapGroupRuleTargetComponent 3052 case 3512060: /*rule*/ return this.rule == null ? new Base[0] : this.rule.toArray(new Base[this.rule.size()]); // StructureMapGroupRuleComponent 3053 case -1109226753: /*dependent*/ return this.dependent == null ? new Base[0] : this.dependent.toArray(new Base[this.dependent.size()]); // StructureMapGroupRuleDependentComponent 3054 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 3055 default: return super.getProperty(hash, name, checkValid); 3056 } 3057 3058 } 3059 3060 @Override 3061 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3062 switch (hash) { 3063 case 3373707: // name 3064 this.name = TypeConvertor.castToId(value); // IdType 3065 return value; 3066 case -896505829: // source 3067 this.getSource().add((StructureMapGroupRuleSourceComponent) value); // StructureMapGroupRuleSourceComponent 3068 return value; 3069 case -880905839: // target 3070 this.getTarget().add((StructureMapGroupRuleTargetComponent) value); // StructureMapGroupRuleTargetComponent 3071 return value; 3072 case 3512060: // rule 3073 this.getRule().add((StructureMapGroupRuleComponent) value); // StructureMapGroupRuleComponent 3074 return value; 3075 case -1109226753: // dependent 3076 this.getDependent().add((StructureMapGroupRuleDependentComponent) value); // StructureMapGroupRuleDependentComponent 3077 return value; 3078 case 1587405498: // documentation 3079 this.documentation = TypeConvertor.castToString(value); // StringType 3080 return value; 3081 default: return super.setProperty(hash, name, value); 3082 } 3083 3084 } 3085 3086 @Override 3087 public Base setProperty(String name, Base value) throws FHIRException { 3088 if (name.equals("name")) { 3089 this.name = TypeConvertor.castToId(value); // IdType 3090 } else if (name.equals("source")) { 3091 this.getSource().add((StructureMapGroupRuleSourceComponent) value); 3092 } else if (name.equals("target")) { 3093 this.getTarget().add((StructureMapGroupRuleTargetComponent) value); 3094 } else if (name.equals("rule")) { 3095 this.getRule().add((StructureMapGroupRuleComponent) value); 3096 } else if (name.equals("dependent")) { 3097 this.getDependent().add((StructureMapGroupRuleDependentComponent) value); 3098 } else if (name.equals("documentation")) { 3099 this.documentation = TypeConvertor.castToString(value); // StringType 3100 } else 3101 return super.setProperty(name, value); 3102 return value; 3103 } 3104 3105 @Override 3106 public void removeChild(String name, Base value) throws FHIRException { 3107 if (name.equals("name")) { 3108 this.name = null; 3109 } else if (name.equals("source")) { 3110 this.getSource().remove((StructureMapGroupRuleSourceComponent) value); 3111 } else if (name.equals("target")) { 3112 this.getTarget().remove((StructureMapGroupRuleTargetComponent) value); 3113 } else if (name.equals("rule")) { 3114 this.getRule().remove((StructureMapGroupRuleComponent) value); 3115 } else if (name.equals("dependent")) { 3116 this.getDependent().remove((StructureMapGroupRuleDependentComponent) value); 3117 } else if (name.equals("documentation")) { 3118 this.documentation = null; 3119 } else 3120 super.removeChild(name, value); 3121 3122 } 3123 3124 @Override 3125 public Base makeProperty(int hash, String name) throws FHIRException { 3126 switch (hash) { 3127 case 3373707: return getNameElement(); 3128 case -896505829: return addSource(); 3129 case -880905839: return addTarget(); 3130 case 3512060: return addRule(); 3131 case -1109226753: return addDependent(); 3132 case 1587405498: return getDocumentationElement(); 3133 default: return super.makeProperty(hash, name); 3134 } 3135 3136 } 3137 3138 @Override 3139 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3140 switch (hash) { 3141 case 3373707: /*name*/ return new String[] {"id"}; 3142 case -896505829: /*source*/ return new String[] {}; 3143 case -880905839: /*target*/ return new String[] {}; 3144 case 3512060: /*rule*/ return new String[] {"@StructureMap.group.rule"}; 3145 case -1109226753: /*dependent*/ return new String[] {}; 3146 case 1587405498: /*documentation*/ return new String[] {"string"}; 3147 default: return super.getTypesForProperty(hash, name); 3148 } 3149 3150 } 3151 3152 @Override 3153 public Base addChild(String name) throws FHIRException { 3154 if (name.equals("name")) { 3155 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.name"); 3156 } 3157 else if (name.equals("source")) { 3158 return addSource(); 3159 } 3160 else if (name.equals("target")) { 3161 return addTarget(); 3162 } 3163 else if (name.equals("rule")) { 3164 return addRule(); 3165 } 3166 else if (name.equals("dependent")) { 3167 return addDependent(); 3168 } 3169 else if (name.equals("documentation")) { 3170 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.documentation"); 3171 } 3172 else 3173 return super.addChild(name); 3174 } 3175 3176 public StructureMapGroupRuleComponent copy() { 3177 StructureMapGroupRuleComponent dst = new StructureMapGroupRuleComponent(); 3178 copyValues(dst); 3179 return dst; 3180 } 3181 3182 public void copyValues(StructureMapGroupRuleComponent dst) { 3183 super.copyValues(dst); 3184 dst.name = name == null ? null : name.copy(); 3185 if (source != null) { 3186 dst.source = new ArrayList<StructureMapGroupRuleSourceComponent>(); 3187 for (StructureMapGroupRuleSourceComponent i : source) 3188 dst.source.add(i.copy()); 3189 }; 3190 if (target != null) { 3191 dst.target = new ArrayList<StructureMapGroupRuleTargetComponent>(); 3192 for (StructureMapGroupRuleTargetComponent i : target) 3193 dst.target.add(i.copy()); 3194 }; 3195 if (rule != null) { 3196 dst.rule = new ArrayList<StructureMapGroupRuleComponent>(); 3197 for (StructureMapGroupRuleComponent i : rule) 3198 dst.rule.add(i.copy()); 3199 }; 3200 if (dependent != null) { 3201 dst.dependent = new ArrayList<StructureMapGroupRuleDependentComponent>(); 3202 for (StructureMapGroupRuleDependentComponent i : dependent) 3203 dst.dependent.add(i.copy()); 3204 }; 3205 dst.documentation = documentation == null ? null : documentation.copy(); 3206 } 3207 3208 @Override 3209 public boolean equalsDeep(Base other_) { 3210 if (!super.equalsDeep(other_)) 3211 return false; 3212 if (!(other_ instanceof StructureMapGroupRuleComponent)) 3213 return false; 3214 StructureMapGroupRuleComponent o = (StructureMapGroupRuleComponent) other_; 3215 return compareDeep(name, o.name, true) && compareDeep(source, o.source, true) && compareDeep(target, o.target, true) 3216 && compareDeep(rule, o.rule, true) && compareDeep(dependent, o.dependent, true) && compareDeep(documentation, o.documentation, true) 3217 ; 3218 } 3219 3220 @Override 3221 public boolean equalsShallow(Base other_) { 3222 if (!super.equalsShallow(other_)) 3223 return false; 3224 if (!(other_ instanceof StructureMapGroupRuleComponent)) 3225 return false; 3226 StructureMapGroupRuleComponent o = (StructureMapGroupRuleComponent) other_; 3227 return compareValues(name, o.name, true) && compareValues(documentation, o.documentation, true); 3228 } 3229 3230 public boolean isEmpty() { 3231 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, source, target, rule 3232 , dependent, documentation); 3233 } 3234 3235 public String fhirType() { 3236 return "StructureMap.group.rule"; 3237 3238 } 3239 3240// added from java-adornments.txt: 3241public String toString() { 3242 return StructureMapUtilities.ruleToString(this); 3243 } 3244// end addition 3245 } 3246 3247 @Block() 3248 public static class StructureMapGroupRuleSourceComponent extends BackboneElement implements IBaseBackboneElement { 3249 /** 3250 * Type or variable this rule applies to. 3251 */ 3252 @Child(name = "context", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 3253 @Description(shortDefinition="Type or variable this rule applies to", formalDefinition="Type or variable this rule applies to." ) 3254 protected IdType context; 3255 3256 /** 3257 * Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content. 3258 */ 3259 @Child(name = "min", type = {IntegerType.class}, order=2, min=0, max=1, modifier=false, summary=true) 3260 @Description(shortDefinition="Specified minimum cardinality", formalDefinition="Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content." ) 3261 protected IntegerType min; 3262 3263 /** 3264 * Specified maximum cardinality for the element - a number or a "*". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value). 3265 */ 3266 @Child(name = "max", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 3267 @Description(shortDefinition="Specified maximum cardinality (number or *)", formalDefinition="Specified maximum cardinality for the element - a number or a \"*\". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value)." ) 3268 protected StringType max; 3269 3270 /** 3271 * Specified type for the element. This works as a condition on the mapping - use for polymorphic elements. 3272 */ 3273 @Child(name = "type", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 3274 @Description(shortDefinition="Rule only applies if source has this type", formalDefinition="Specified type for the element. This works as a condition on the mapping - use for polymorphic elements." ) 3275 protected StringType type; 3276 3277 /** 3278 * A value to use if there is no existing value in the source object. 3279 */ 3280 @Child(name = "defaultValue", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 3281 @Description(shortDefinition="Default value if no value exists", formalDefinition="A value to use if there is no existing value in the source object." ) 3282 protected StringType defaultValue; 3283 3284 /** 3285 * Optional field for this source. 3286 */ 3287 @Child(name = "element", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 3288 @Description(shortDefinition="Optional field for this source", formalDefinition="Optional field for this source." ) 3289 protected StringType element; 3290 3291 /** 3292 * How to handle the list mode for this element. 3293 */ 3294 @Child(name = "listMode", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 3295 @Description(shortDefinition="first | not_first | last | not_last | only_one", formalDefinition="How to handle the list mode for this element." ) 3296 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/map-source-list-mode") 3297 protected Enumeration<StructureMapSourceListMode> listMode; 3298 3299 /** 3300 * Named context for field, if a field is specified. 3301 */ 3302 @Child(name = "variable", type = {IdType.class}, order=8, min=0, max=1, modifier=false, summary=true) 3303 @Description(shortDefinition="Named context for field, if a field is specified", formalDefinition="Named context for field, if a field is specified." ) 3304 protected IdType variable; 3305 3306 /** 3307 * FHIRPath expression - must be true or the rule does not apply. 3308 */ 3309 @Child(name = "condition", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 3310 @Description(shortDefinition="FHIRPath expression - must be true or the rule does not apply", formalDefinition="FHIRPath expression - must be true or the rule does not apply." ) 3311 protected StringType condition; 3312 3313 /** 3314 * FHIRPath expression - must be true or the mapping engine throws an error instead of completing. 3315 */ 3316 @Child(name = "check", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 3317 @Description(shortDefinition="FHIRPath expression - must be true or the mapping engine throws an error instead of completing", formalDefinition="FHIRPath expression - must be true or the mapping engine throws an error instead of completing." ) 3318 protected StringType check; 3319 3320 /** 3321 * A FHIRPath expression which specifies a message to put in the transform log when content matching the source rule is found. 3322 */ 3323 @Child(name = "logMessage", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=true) 3324 @Description(shortDefinition="Message to put in log if source exists (FHIRPath)", formalDefinition="A FHIRPath expression which specifies a message to put in the transform log when content matching the source rule is found." ) 3325 protected StringType logMessage; 3326 3327 private static final long serialVersionUID = 214178119L; 3328 3329 /** 3330 * Constructor 3331 */ 3332 public StructureMapGroupRuleSourceComponent() { 3333 super(); 3334 } 3335 3336 /** 3337 * Constructor 3338 */ 3339 public StructureMapGroupRuleSourceComponent(String context) { 3340 super(); 3341 this.setContext(context); 3342 } 3343 3344 /** 3345 * @return {@link #context} (Type or variable this rule applies to.). This is the underlying object with id, value and extensions. The accessor "getContext" gives direct access to the value 3346 */ 3347 public IdType getContextElement() { 3348 if (this.context == null) 3349 if (Configuration.errorOnAutoCreate()) 3350 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.context"); 3351 else if (Configuration.doAutoCreate()) 3352 this.context = new IdType(); // bb 3353 return this.context; 3354 } 3355 3356 public boolean hasContextElement() { 3357 return this.context != null && !this.context.isEmpty(); 3358 } 3359 3360 public boolean hasContext() { 3361 return this.context != null && !this.context.isEmpty(); 3362 } 3363 3364 /** 3365 * @param value {@link #context} (Type or variable this rule applies to.). This is the underlying object with id, value and extensions. The accessor "getContext" gives direct access to the value 3366 */ 3367 public StructureMapGroupRuleSourceComponent setContextElement(IdType value) { 3368 this.context = value; 3369 return this; 3370 } 3371 3372 /** 3373 * @return Type or variable this rule applies to. 3374 */ 3375 public String getContext() { 3376 return this.context == null ? null : this.context.getValue(); 3377 } 3378 3379 /** 3380 * @param value Type or variable this rule applies to. 3381 */ 3382 public StructureMapGroupRuleSourceComponent setContext(String value) { 3383 if (this.context == null) 3384 this.context = new IdType(); 3385 this.context.setValue(value); 3386 return this; 3387 } 3388 3389 /** 3390 * @return {@link #min} (Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 3391 */ 3392 public IntegerType getMinElement() { 3393 if (this.min == null) 3394 if (Configuration.errorOnAutoCreate()) 3395 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.min"); 3396 else if (Configuration.doAutoCreate()) 3397 this.min = new IntegerType(); // bb 3398 return this.min; 3399 } 3400 3401 public boolean hasMinElement() { 3402 return this.min != null && !this.min.isEmpty(); 3403 } 3404 3405 public boolean hasMin() { 3406 return this.min != null && !this.min.isEmpty(); 3407 } 3408 3409 /** 3410 * @param value {@link #min} (Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 3411 */ 3412 public StructureMapGroupRuleSourceComponent setMinElement(IntegerType value) { 3413 this.min = value; 3414 return this; 3415 } 3416 3417 /** 3418 * @return Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content. 3419 */ 3420 public int getMin() { 3421 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 3422 } 3423 3424 /** 3425 * @param value Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content. 3426 */ 3427 public StructureMapGroupRuleSourceComponent setMin(int value) { 3428 if (this.min == null) 3429 this.min = new IntegerType(); 3430 this.min.setValue(value); 3431 return this; 3432 } 3433 3434 /** 3435 * @return {@link #max} (Specified maximum cardinality for the element - a number or a "*". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value).). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 3436 */ 3437 public StringType getMaxElement() { 3438 if (this.max == null) 3439 if (Configuration.errorOnAutoCreate()) 3440 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.max"); 3441 else if (Configuration.doAutoCreate()) 3442 this.max = new StringType(); // bb 3443 return this.max; 3444 } 3445 3446 public boolean hasMaxElement() { 3447 return this.max != null && !this.max.isEmpty(); 3448 } 3449 3450 public boolean hasMax() { 3451 return this.max != null && !this.max.isEmpty(); 3452 } 3453 3454 /** 3455 * @param value {@link #max} (Specified maximum cardinality for the element - a number or a "*". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value).). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 3456 */ 3457 public StructureMapGroupRuleSourceComponent setMaxElement(StringType value) { 3458 this.max = value; 3459 return this; 3460 } 3461 3462 /** 3463 * @return Specified maximum cardinality for the element - a number or a "*". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value). 3464 */ 3465 public String getMax() { 3466 return this.max == null ? null : this.max.getValue(); 3467 } 3468 3469 /** 3470 * @param value Specified maximum cardinality for the element - a number or a "*". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value). 3471 */ 3472 public StructureMapGroupRuleSourceComponent setMax(String value) { 3473 if (Utilities.noString(value)) 3474 this.max = null; 3475 else { 3476 if (this.max == null) 3477 this.max = new StringType(); 3478 this.max.setValue(value); 3479 } 3480 return this; 3481 } 3482 3483 /** 3484 * @return {@link #type} (Specified type for the element. This works as a condition on the mapping - use for polymorphic elements.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3485 */ 3486 public StringType getTypeElement() { 3487 if (this.type == null) 3488 if (Configuration.errorOnAutoCreate()) 3489 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.type"); 3490 else if (Configuration.doAutoCreate()) 3491 this.type = new StringType(); // bb 3492 return this.type; 3493 } 3494 3495 public boolean hasTypeElement() { 3496 return this.type != null && !this.type.isEmpty(); 3497 } 3498 3499 public boolean hasType() { 3500 return this.type != null && !this.type.isEmpty(); 3501 } 3502 3503 /** 3504 * @param value {@link #type} (Specified type for the element. This works as a condition on the mapping - use for polymorphic elements.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3505 */ 3506 public StructureMapGroupRuleSourceComponent setTypeElement(StringType value) { 3507 this.type = value; 3508 return this; 3509 } 3510 3511 /** 3512 * @return Specified type for the element. This works as a condition on the mapping - use for polymorphic elements. 3513 */ 3514 public String getType() { 3515 return this.type == null ? null : this.type.getValue(); 3516 } 3517 3518 /** 3519 * @param value Specified type for the element. This works as a condition on the mapping - use for polymorphic elements. 3520 */ 3521 public StructureMapGroupRuleSourceComponent setType(String value) { 3522 if (Utilities.noString(value)) 3523 this.type = null; 3524 else { 3525 if (this.type == null) 3526 this.type = new StringType(); 3527 this.type.setValue(value); 3528 } 3529 return this; 3530 } 3531 3532 /** 3533 * @return {@link #defaultValue} (A value to use if there is no existing value in the source object.). This is the underlying object with id, value and extensions. The accessor "getDefaultValue" gives direct access to the value 3534 */ 3535 public StringType getDefaultValueElement() { 3536 if (this.defaultValue == null) 3537 if (Configuration.errorOnAutoCreate()) 3538 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.defaultValue"); 3539 else if (Configuration.doAutoCreate()) 3540 this.defaultValue = new StringType(); // bb 3541 return this.defaultValue; 3542 } 3543 3544 public boolean hasDefaultValueElement() { 3545 return this.defaultValue != null && !this.defaultValue.isEmpty(); 3546 } 3547 3548 public boolean hasDefaultValue() { 3549 return this.defaultValue != null && !this.defaultValue.isEmpty(); 3550 } 3551 3552 /** 3553 * @param value {@link #defaultValue} (A value to use if there is no existing value in the source object.). This is the underlying object with id, value and extensions. The accessor "getDefaultValue" gives direct access to the value 3554 */ 3555 public StructureMapGroupRuleSourceComponent setDefaultValueElement(StringType value) { 3556 this.defaultValue = value; 3557 return this; 3558 } 3559 3560 /** 3561 * @return A value to use if there is no existing value in the source object. 3562 */ 3563 public String getDefaultValue() { 3564 return this.defaultValue == null ? null : this.defaultValue.getValue(); 3565 } 3566 3567 /** 3568 * @param value A value to use if there is no existing value in the source object. 3569 */ 3570 public StructureMapGroupRuleSourceComponent setDefaultValue(String value) { 3571 if (Utilities.noString(value)) 3572 this.defaultValue = null; 3573 else { 3574 if (this.defaultValue == null) 3575 this.defaultValue = new StringType(); 3576 this.defaultValue.setValue(value); 3577 } 3578 return this; 3579 } 3580 3581 /** 3582 * @return {@link #element} (Optional field for this source.). This is the underlying object with id, value and extensions. The accessor "getElement" gives direct access to the value 3583 */ 3584 public StringType getElementElement() { 3585 if (this.element == null) 3586 if (Configuration.errorOnAutoCreate()) 3587 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.element"); 3588 else if (Configuration.doAutoCreate()) 3589 this.element = new StringType(); // bb 3590 return this.element; 3591 } 3592 3593 public boolean hasElementElement() { 3594 return this.element != null && !this.element.isEmpty(); 3595 } 3596 3597 public boolean hasElement() { 3598 return this.element != null && !this.element.isEmpty(); 3599 } 3600 3601 /** 3602 * @param value {@link #element} (Optional field for this source.). This is the underlying object with id, value and extensions. The accessor "getElement" gives direct access to the value 3603 */ 3604 public StructureMapGroupRuleSourceComponent setElementElement(StringType value) { 3605 this.element = value; 3606 return this; 3607 } 3608 3609 /** 3610 * @return Optional field for this source. 3611 */ 3612 public String getElement() { 3613 return this.element == null ? null : this.element.getValue(); 3614 } 3615 3616 /** 3617 * @param value Optional field for this source. 3618 */ 3619 public StructureMapGroupRuleSourceComponent setElement(String value) { 3620 if (Utilities.noString(value)) 3621 this.element = null; 3622 else { 3623 if (this.element == null) 3624 this.element = new StringType(); 3625 this.element.setValue(value); 3626 } 3627 return this; 3628 } 3629 3630 /** 3631 * @return {@link #listMode} (How to handle the list mode for this element.). This is the underlying object with id, value and extensions. The accessor "getListMode" gives direct access to the value 3632 */ 3633 public Enumeration<StructureMapSourceListMode> getListModeElement() { 3634 if (this.listMode == null) 3635 if (Configuration.errorOnAutoCreate()) 3636 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.listMode"); 3637 else if (Configuration.doAutoCreate()) 3638 this.listMode = new Enumeration<StructureMapSourceListMode>(new StructureMapSourceListModeEnumFactory()); // bb 3639 return this.listMode; 3640 } 3641 3642 public boolean hasListModeElement() { 3643 return this.listMode != null && !this.listMode.isEmpty(); 3644 } 3645 3646 public boolean hasListMode() { 3647 return this.listMode != null && !this.listMode.isEmpty(); 3648 } 3649 3650 /** 3651 * @param value {@link #listMode} (How to handle the list mode for this element.). This is the underlying object with id, value and extensions. The accessor "getListMode" gives direct access to the value 3652 */ 3653 public StructureMapGroupRuleSourceComponent setListModeElement(Enumeration<StructureMapSourceListMode> value) { 3654 this.listMode = value; 3655 return this; 3656 } 3657 3658 /** 3659 * @return How to handle the list mode for this element. 3660 */ 3661 public StructureMapSourceListMode getListMode() { 3662 return this.listMode == null ? null : this.listMode.getValue(); 3663 } 3664 3665 /** 3666 * @param value How to handle the list mode for this element. 3667 */ 3668 public StructureMapGroupRuleSourceComponent setListMode(StructureMapSourceListMode value) { 3669 if (value == null) 3670 this.listMode = null; 3671 else { 3672 if (this.listMode == null) 3673 this.listMode = new Enumeration<StructureMapSourceListMode>(new StructureMapSourceListModeEnumFactory()); 3674 this.listMode.setValue(value); 3675 } 3676 return this; 3677 } 3678 3679 /** 3680 * @return {@link #variable} (Named context for field, if a field is specified.). This is the underlying object with id, value and extensions. The accessor "getVariable" gives direct access to the value 3681 */ 3682 public IdType getVariableElement() { 3683 if (this.variable == null) 3684 if (Configuration.errorOnAutoCreate()) 3685 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.variable"); 3686 else if (Configuration.doAutoCreate()) 3687 this.variable = new IdType(); // bb 3688 return this.variable; 3689 } 3690 3691 public boolean hasVariableElement() { 3692 return this.variable != null && !this.variable.isEmpty(); 3693 } 3694 3695 public boolean hasVariable() { 3696 return this.variable != null && !this.variable.isEmpty(); 3697 } 3698 3699 /** 3700 * @param value {@link #variable} (Named context for field, if a field is specified.). This is the underlying object with id, value and extensions. The accessor "getVariable" gives direct access to the value 3701 */ 3702 public StructureMapGroupRuleSourceComponent setVariableElement(IdType value) { 3703 this.variable = value; 3704 return this; 3705 } 3706 3707 /** 3708 * @return Named context for field, if a field is specified. 3709 */ 3710 public String getVariable() { 3711 return this.variable == null ? null : this.variable.getValue(); 3712 } 3713 3714 /** 3715 * @param value Named context for field, if a field is specified. 3716 */ 3717 public StructureMapGroupRuleSourceComponent setVariable(String value) { 3718 if (Utilities.noString(value)) 3719 this.variable = null; 3720 else { 3721 if (this.variable == null) 3722 this.variable = new IdType(); 3723 this.variable.setValue(value); 3724 } 3725 return this; 3726 } 3727 3728 /** 3729 * @return {@link #condition} (FHIRPath expression - must be true or the rule does not apply.). This is the underlying object with id, value and extensions. The accessor "getCondition" gives direct access to the value 3730 */ 3731 public StringType getConditionElement() { 3732 if (this.condition == null) 3733 if (Configuration.errorOnAutoCreate()) 3734 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.condition"); 3735 else if (Configuration.doAutoCreate()) 3736 this.condition = new StringType(); // bb 3737 return this.condition; 3738 } 3739 3740 public boolean hasConditionElement() { 3741 return this.condition != null && !this.condition.isEmpty(); 3742 } 3743 3744 public boolean hasCondition() { 3745 return this.condition != null && !this.condition.isEmpty(); 3746 } 3747 3748 /** 3749 * @param value {@link #condition} (FHIRPath expression - must be true or the rule does not apply.). This is the underlying object with id, value and extensions. The accessor "getCondition" gives direct access to the value 3750 */ 3751 public StructureMapGroupRuleSourceComponent setConditionElement(StringType value) { 3752 this.condition = value; 3753 return this; 3754 } 3755 3756 /** 3757 * @return FHIRPath expression - must be true or the rule does not apply. 3758 */ 3759 public String getCondition() { 3760 return this.condition == null ? null : this.condition.getValue(); 3761 } 3762 3763 /** 3764 * @param value FHIRPath expression - must be true or the rule does not apply. 3765 */ 3766 public StructureMapGroupRuleSourceComponent setCondition(String value) { 3767 if (Utilities.noString(value)) 3768 this.condition = null; 3769 else { 3770 if (this.condition == null) 3771 this.condition = new StringType(); 3772 this.condition.setValue(value); 3773 } 3774 return this; 3775 } 3776 3777 /** 3778 * @return {@link #check} (FHIRPath expression - must be true or the mapping engine throws an error instead of completing.). This is the underlying object with id, value and extensions. The accessor "getCheck" gives direct access to the value 3779 */ 3780 public StringType getCheckElement() { 3781 if (this.check == null) 3782 if (Configuration.errorOnAutoCreate()) 3783 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.check"); 3784 else if (Configuration.doAutoCreate()) 3785 this.check = new StringType(); // bb 3786 return this.check; 3787 } 3788 3789 public boolean hasCheckElement() { 3790 return this.check != null && !this.check.isEmpty(); 3791 } 3792 3793 public boolean hasCheck() { 3794 return this.check != null && !this.check.isEmpty(); 3795 } 3796 3797 /** 3798 * @param value {@link #check} (FHIRPath expression - must be true or the mapping engine throws an error instead of completing.). This is the underlying object with id, value and extensions. The accessor "getCheck" gives direct access to the value 3799 */ 3800 public StructureMapGroupRuleSourceComponent setCheckElement(StringType value) { 3801 this.check = value; 3802 return this; 3803 } 3804 3805 /** 3806 * @return FHIRPath expression - must be true or the mapping engine throws an error instead of completing. 3807 */ 3808 public String getCheck() { 3809 return this.check == null ? null : this.check.getValue(); 3810 } 3811 3812 /** 3813 * @param value FHIRPath expression - must be true or the mapping engine throws an error instead of completing. 3814 */ 3815 public StructureMapGroupRuleSourceComponent setCheck(String value) { 3816 if (Utilities.noString(value)) 3817 this.check = null; 3818 else { 3819 if (this.check == null) 3820 this.check = new StringType(); 3821 this.check.setValue(value); 3822 } 3823 return this; 3824 } 3825 3826 /** 3827 * @return {@link #logMessage} (A FHIRPath expression which specifies a message to put in the transform log when content matching the source rule is found.). This is the underlying object with id, value and extensions. The accessor "getLogMessage" gives direct access to the value 3828 */ 3829 public StringType getLogMessageElement() { 3830 if (this.logMessage == null) 3831 if (Configuration.errorOnAutoCreate()) 3832 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.logMessage"); 3833 else if (Configuration.doAutoCreate()) 3834 this.logMessage = new StringType(); // bb 3835 return this.logMessage; 3836 } 3837 3838 public boolean hasLogMessageElement() { 3839 return this.logMessage != null && !this.logMessage.isEmpty(); 3840 } 3841 3842 public boolean hasLogMessage() { 3843 return this.logMessage != null && !this.logMessage.isEmpty(); 3844 } 3845 3846 /** 3847 * @param value {@link #logMessage} (A FHIRPath expression which specifies a message to put in the transform log when content matching the source rule is found.). This is the underlying object with id, value and extensions. The accessor "getLogMessage" gives direct access to the value 3848 */ 3849 public StructureMapGroupRuleSourceComponent setLogMessageElement(StringType value) { 3850 this.logMessage = value; 3851 return this; 3852 } 3853 3854 /** 3855 * @return A FHIRPath expression which specifies a message to put in the transform log when content matching the source rule is found. 3856 */ 3857 public String getLogMessage() { 3858 return this.logMessage == null ? null : this.logMessage.getValue(); 3859 } 3860 3861 /** 3862 * @param value A FHIRPath expression which specifies a message to put in the transform log when content matching the source rule is found. 3863 */ 3864 public StructureMapGroupRuleSourceComponent setLogMessage(String value) { 3865 if (Utilities.noString(value)) 3866 this.logMessage = null; 3867 else { 3868 if (this.logMessage == null) 3869 this.logMessage = new StringType(); 3870 this.logMessage.setValue(value); 3871 } 3872 return this; 3873 } 3874 3875 protected void listChildren(List<Property> children) { 3876 super.listChildren(children); 3877 children.add(new Property("context", "id", "Type or variable this rule applies to.", 0, 1, context)); 3878 children.add(new Property("min", "integer", "Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content.", 0, 1, min)); 3879 children.add(new Property("max", "string", "Specified maximum cardinality for the element - a number or a \"*\". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value).", 0, 1, max)); 3880 children.add(new Property("type", "string", "Specified type for the element. This works as a condition on the mapping - use for polymorphic elements.", 0, 1, type)); 3881 children.add(new Property("defaultValue", "string", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue)); 3882 children.add(new Property("element", "string", "Optional field for this source.", 0, 1, element)); 3883 children.add(new Property("listMode", "code", "How to handle the list mode for this element.", 0, 1, listMode)); 3884 children.add(new Property("variable", "id", "Named context for field, if a field is specified.", 0, 1, variable)); 3885 children.add(new Property("condition", "string", "FHIRPath expression - must be true or the rule does not apply.", 0, 1, condition)); 3886 children.add(new Property("check", "string", "FHIRPath expression - must be true or the mapping engine throws an error instead of completing.", 0, 1, check)); 3887 children.add(new Property("logMessage", "string", "A FHIRPath expression which specifies a message to put in the transform log when content matching the source rule is found.", 0, 1, logMessage)); 3888 } 3889 3890 @Override 3891 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3892 switch (_hash) { 3893 case 951530927: /*context*/ return new Property("context", "id", "Type or variable this rule applies to.", 0, 1, context); 3894 case 108114: /*min*/ return new Property("min", "integer", "Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content.", 0, 1, min); 3895 case 107876: /*max*/ return new Property("max", "string", "Specified maximum cardinality for the element - a number or a \"*\". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value).", 0, 1, max); 3896 case 3575610: /*type*/ return new Property("type", "string", "Specified type for the element. This works as a condition on the mapping - use for polymorphic elements.", 0, 1, type); 3897 case -659125328: /*defaultValue*/ return new Property("defaultValue", "string", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3898 case -1662836996: /*element*/ return new Property("element", "string", "Optional field for this source.", 0, 1, element); 3899 case 1345445729: /*listMode*/ return new Property("listMode", "code", "How to handle the list mode for this element.", 0, 1, listMode); 3900 case -1249586564: /*variable*/ return new Property("variable", "id", "Named context for field, if a field is specified.", 0, 1, variable); 3901 case -861311717: /*condition*/ return new Property("condition", "string", "FHIRPath expression - must be true or the rule does not apply.", 0, 1, condition); 3902 case 94627080: /*check*/ return new Property("check", "string", "FHIRPath expression - must be true or the mapping engine throws an error instead of completing.", 0, 1, check); 3903 case -1067155421: /*logMessage*/ return new Property("logMessage", "string", "A FHIRPath expression which specifies a message to put in the transform log when content matching the source rule is found.", 0, 1, logMessage); 3904 default: return super.getNamedProperty(_hash, _name, _checkValid); 3905 } 3906 3907 } 3908 3909 @Override 3910 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3911 switch (hash) { 3912 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // IdType 3913 case 108114: /*min*/ return this.min == null ? new Base[0] : new Base[] {this.min}; // IntegerType 3914 case 107876: /*max*/ return this.max == null ? new Base[0] : new Base[] {this.max}; // StringType 3915 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // StringType 3916 case -659125328: /*defaultValue*/ return this.defaultValue == null ? new Base[0] : new Base[] {this.defaultValue}; // StringType 3917 case -1662836996: /*element*/ return this.element == null ? new Base[0] : new Base[] {this.element}; // StringType 3918 case 1345445729: /*listMode*/ return this.listMode == null ? new Base[0] : new Base[] {this.listMode}; // Enumeration<StructureMapSourceListMode> 3919 case -1249586564: /*variable*/ return this.variable == null ? new Base[0] : new Base[] {this.variable}; // IdType 3920 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : new Base[] {this.condition}; // StringType 3921 case 94627080: /*check*/ return this.check == null ? new Base[0] : new Base[] {this.check}; // StringType 3922 case -1067155421: /*logMessage*/ return this.logMessage == null ? new Base[0] : new Base[] {this.logMessage}; // StringType 3923 default: return super.getProperty(hash, name, checkValid); 3924 } 3925 3926 } 3927 3928 @Override 3929 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3930 switch (hash) { 3931 case 951530927: // context 3932 this.context = TypeConvertor.castToId(value); // IdType 3933 return value; 3934 case 108114: // min 3935 this.min = TypeConvertor.castToInteger(value); // IntegerType 3936 return value; 3937 case 107876: // max 3938 this.max = TypeConvertor.castToString(value); // StringType 3939 return value; 3940 case 3575610: // type 3941 this.type = TypeConvertor.castToString(value); // StringType 3942 return value; 3943 case -659125328: // defaultValue 3944 this.defaultValue = TypeConvertor.castToString(value); // StringType 3945 return value; 3946 case -1662836996: // element 3947 this.element = TypeConvertor.castToString(value); // StringType 3948 return value; 3949 case 1345445729: // listMode 3950 value = new StructureMapSourceListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3951 this.listMode = (Enumeration) value; // Enumeration<StructureMapSourceListMode> 3952 return value; 3953 case -1249586564: // variable 3954 this.variable = TypeConvertor.castToId(value); // IdType 3955 return value; 3956 case -861311717: // condition 3957 this.condition = TypeConvertor.castToString(value); // StringType 3958 return value; 3959 case 94627080: // check 3960 this.check = TypeConvertor.castToString(value); // StringType 3961 return value; 3962 case -1067155421: // logMessage 3963 this.logMessage = TypeConvertor.castToString(value); // StringType 3964 return value; 3965 default: return super.setProperty(hash, name, value); 3966 } 3967 3968 } 3969 3970 @Override 3971 public Base setProperty(String name, Base value) throws FHIRException { 3972 if (name.equals("context")) { 3973 this.context = TypeConvertor.castToId(value); // IdType 3974 } else if (name.equals("min")) { 3975 this.min = TypeConvertor.castToInteger(value); // IntegerType 3976 } else if (name.equals("max")) { 3977 this.max = TypeConvertor.castToString(value); // StringType 3978 } else if (name.equals("type")) { 3979 this.type = TypeConvertor.castToString(value); // StringType 3980 } else if (name.equals("defaultValue")) { 3981 this.defaultValue = TypeConvertor.castToString(value); // StringType 3982 } else if (name.equals("element")) { 3983 this.element = TypeConvertor.castToString(value); // StringType 3984 } else if (name.equals("listMode")) { 3985 value = new StructureMapSourceListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3986 this.listMode = (Enumeration) value; // Enumeration<StructureMapSourceListMode> 3987 } else if (name.equals("variable")) { 3988 this.variable = TypeConvertor.castToId(value); // IdType 3989 } else if (name.equals("condition")) { 3990 this.condition = TypeConvertor.castToString(value); // StringType 3991 } else if (name.equals("check")) { 3992 this.check = TypeConvertor.castToString(value); // StringType 3993 } else if (name.equals("logMessage")) { 3994 this.logMessage = TypeConvertor.castToString(value); // StringType 3995 } else 3996 return super.setProperty(name, value); 3997 return value; 3998 } 3999 4000 @Override 4001 public void removeChild(String name, Base value) throws FHIRException { 4002 if (name.equals("context")) { 4003 this.context = null; 4004 } else if (name.equals("min")) { 4005 this.min = null; 4006 } else if (name.equals("max")) { 4007 this.max = null; 4008 } else if (name.equals("type")) { 4009 this.type = null; 4010 } else if (name.equals("defaultValue")) { 4011 this.defaultValue = null; 4012 } else if (name.equals("element")) { 4013 this.element = null; 4014 } else if (name.equals("listMode")) { 4015 value = new StructureMapSourceListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4016 this.listMode = (Enumeration) value; // Enumeration<StructureMapSourceListMode> 4017 } else if (name.equals("variable")) { 4018 this.variable = null; 4019 } else if (name.equals("condition")) { 4020 this.condition = null; 4021 } else if (name.equals("check")) { 4022 this.check = null; 4023 } else if (name.equals("logMessage")) { 4024 this.logMessage = null; 4025 } else 4026 super.removeChild(name, value); 4027 4028 } 4029 4030 @Override 4031 public Base makeProperty(int hash, String name) throws FHIRException { 4032 switch (hash) { 4033 case 951530927: return getContextElement(); 4034 case 108114: return getMinElement(); 4035 case 107876: return getMaxElement(); 4036 case 3575610: return getTypeElement(); 4037 case -659125328: return getDefaultValueElement(); 4038 case -1662836996: return getElementElement(); 4039 case 1345445729: return getListModeElement(); 4040 case -1249586564: return getVariableElement(); 4041 case -861311717: return getConditionElement(); 4042 case 94627080: return getCheckElement(); 4043 case -1067155421: return getLogMessageElement(); 4044 default: return super.makeProperty(hash, name); 4045 } 4046 4047 } 4048 4049 @Override 4050 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4051 switch (hash) { 4052 case 951530927: /*context*/ return new String[] {"id"}; 4053 case 108114: /*min*/ return new String[] {"integer"}; 4054 case 107876: /*max*/ return new String[] {"string"}; 4055 case 3575610: /*type*/ return new String[] {"string"}; 4056 case -659125328: /*defaultValue*/ return new String[] {"string"}; 4057 case -1662836996: /*element*/ return new String[] {"string"}; 4058 case 1345445729: /*listMode*/ return new String[] {"code"}; 4059 case -1249586564: /*variable*/ return new String[] {"id"}; 4060 case -861311717: /*condition*/ return new String[] {"string"}; 4061 case 94627080: /*check*/ return new String[] {"string"}; 4062 case -1067155421: /*logMessage*/ return new String[] {"string"}; 4063 default: return super.getTypesForProperty(hash, name); 4064 } 4065 4066 } 4067 4068 @Override 4069 public Base addChild(String name) throws FHIRException { 4070 if (name.equals("context")) { 4071 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.context"); 4072 } 4073 else if (name.equals("min")) { 4074 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.min"); 4075 } 4076 else if (name.equals("max")) { 4077 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.max"); 4078 } 4079 else if (name.equals("type")) { 4080 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.type"); 4081 } 4082 else if (name.equals("defaultValue")) { 4083 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.defaultValue"); 4084 } 4085 else if (name.equals("element")) { 4086 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.element"); 4087 } 4088 else if (name.equals("listMode")) { 4089 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.listMode"); 4090 } 4091 else if (name.equals("variable")) { 4092 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.variable"); 4093 } 4094 else if (name.equals("condition")) { 4095 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.condition"); 4096 } 4097 else if (name.equals("check")) { 4098 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.check"); 4099 } 4100 else if (name.equals("logMessage")) { 4101 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.logMessage"); 4102 } 4103 else 4104 return super.addChild(name); 4105 } 4106 4107 public StructureMapGroupRuleSourceComponent copy() { 4108 StructureMapGroupRuleSourceComponent dst = new StructureMapGroupRuleSourceComponent(); 4109 copyValues(dst); 4110 return dst; 4111 } 4112 4113 public void copyValues(StructureMapGroupRuleSourceComponent dst) { 4114 super.copyValues(dst); 4115 dst.context = context == null ? null : context.copy(); 4116 dst.min = min == null ? null : min.copy(); 4117 dst.max = max == null ? null : max.copy(); 4118 dst.type = type == null ? null : type.copy(); 4119 dst.defaultValue = defaultValue == null ? null : defaultValue.copy(); 4120 dst.element = element == null ? null : element.copy(); 4121 dst.listMode = listMode == null ? null : listMode.copy(); 4122 dst.variable = variable == null ? null : variable.copy(); 4123 dst.condition = condition == null ? null : condition.copy(); 4124 dst.check = check == null ? null : check.copy(); 4125 dst.logMessage = logMessage == null ? null : logMessage.copy(); 4126 } 4127 4128 @Override 4129 public boolean equalsDeep(Base other_) { 4130 if (!super.equalsDeep(other_)) 4131 return false; 4132 if (!(other_ instanceof StructureMapGroupRuleSourceComponent)) 4133 return false; 4134 StructureMapGroupRuleSourceComponent o = (StructureMapGroupRuleSourceComponent) other_; 4135 return compareDeep(context, o.context, true) && compareDeep(min, o.min, true) && compareDeep(max, o.max, true) 4136 && compareDeep(type, o.type, true) && compareDeep(defaultValue, o.defaultValue, true) && compareDeep(element, o.element, true) 4137 && compareDeep(listMode, o.listMode, true) && compareDeep(variable, o.variable, true) && compareDeep(condition, o.condition, true) 4138 && compareDeep(check, o.check, true) && compareDeep(logMessage, o.logMessage, true); 4139 } 4140 4141 @Override 4142 public boolean equalsShallow(Base other_) { 4143 if (!super.equalsShallow(other_)) 4144 return false; 4145 if (!(other_ instanceof StructureMapGroupRuleSourceComponent)) 4146 return false; 4147 StructureMapGroupRuleSourceComponent o = (StructureMapGroupRuleSourceComponent) other_; 4148 return compareValues(context, o.context, true) && compareValues(min, o.min, true) && compareValues(max, o.max, true) 4149 && compareValues(type, o.type, true) && compareValues(defaultValue, o.defaultValue, true) && compareValues(element, o.element, true) 4150 && compareValues(listMode, o.listMode, true) && compareValues(variable, o.variable, true) && compareValues(condition, o.condition, true) 4151 && compareValues(check, o.check, true) && compareValues(logMessage, o.logMessage, true); 4152 } 4153 4154 public boolean isEmpty() { 4155 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(context, min, max, type 4156 , defaultValue, element, listMode, variable, condition, check, logMessage); 4157 } 4158 4159 public String fhirType() { 4160 return "StructureMap.group.rule.source"; 4161 4162 } 4163 4164// added from java-adornments.txt: 4165public String toString() { 4166 return StructureMapUtilities.sourceToString(this); 4167 } 4168// end addition 4169 } 4170 4171 @Block() 4172 public static class StructureMapGroupRuleTargetComponent extends BackboneElement implements IBaseBackboneElement { 4173 /** 4174 * Variable this rule applies to. 4175 */ 4176 @Child(name = "context", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 4177 @Description(shortDefinition="Variable this rule applies to", formalDefinition="Variable this rule applies to." ) 4178 protected StringType context; 4179 4180 /** 4181 * Field to create in the context. 4182 */ 4183 @Child(name = "element", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 4184 @Description(shortDefinition="Field to create in the context", formalDefinition="Field to create in the context." ) 4185 protected StringType element; 4186 4187 /** 4188 * Named context for field, if desired, and a field is specified. 4189 */ 4190 @Child(name = "variable", type = {IdType.class}, order=3, min=0, max=1, modifier=false, summary=true) 4191 @Description(shortDefinition="Named context for field, if desired, and a field is specified", formalDefinition="Named context for field, if desired, and a field is specified." ) 4192 protected IdType variable; 4193 4194 /** 4195 * If field is a list, how to manage the list. 4196 */ 4197 @Child(name = "listMode", type = {CodeType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4198 @Description(shortDefinition="first | share | last | single", formalDefinition="If field is a list, how to manage the list." ) 4199 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/map-target-list-mode") 4200 protected List<Enumeration<StructureMapTargetListMode>> listMode; 4201 4202 /** 4203 * Internal rule reference for shared list items. 4204 */ 4205 @Child(name = "listRuleId", type = {IdType.class}, order=5, min=0, max=1, modifier=false, summary=true) 4206 @Description(shortDefinition="Internal rule reference for shared list items", formalDefinition="Internal rule reference for shared list items." ) 4207 protected IdType listRuleId; 4208 4209 /** 4210 * How the data is copied / created. 4211 */ 4212 @Child(name = "transform", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 4213 @Description(shortDefinition="create | copy +", formalDefinition="How the data is copied / created." ) 4214 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/map-transform") 4215 protected Enumeration<StructureMapTransform> transform; 4216 4217 /** 4218 * Parameters to the transform. 4219 */ 4220 @Child(name = "parameter", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4221 @Description(shortDefinition="Parameters to the transform", formalDefinition="Parameters to the transform." ) 4222 protected List<StructureMapGroupRuleTargetParameterComponent> parameter; 4223 4224 private static final long serialVersionUID = 1565495071L; 4225 4226 /** 4227 * Constructor 4228 */ 4229 public StructureMapGroupRuleTargetComponent() { 4230 super(); 4231 } 4232 4233 /** 4234 * @return {@link #context} (Variable this rule applies to.). This is the underlying object with id, value and extensions. The accessor "getContext" gives direct access to the value 4235 */ 4236 public StringType getContextElement() { 4237 if (this.context == null) 4238 if (Configuration.errorOnAutoCreate()) 4239 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.context"); 4240 else if (Configuration.doAutoCreate()) 4241 this.context = new StringType(); // bb 4242 return this.context; 4243 } 4244 4245 public boolean hasContextElement() { 4246 return this.context != null && !this.context.isEmpty(); 4247 } 4248 4249 public boolean hasContext() { 4250 return this.context != null && !this.context.isEmpty(); 4251 } 4252 4253 /** 4254 * @param value {@link #context} (Variable this rule applies to.). This is the underlying object with id, value and extensions. The accessor "getContext" gives direct access to the value 4255 */ 4256 public StructureMapGroupRuleTargetComponent setContextElement(StringType value) { 4257 this.context = value; 4258 return this; 4259 } 4260 4261 /** 4262 * @return Variable this rule applies to. 4263 */ 4264 public String getContext() { 4265 return this.context == null ? null : this.context.getValue(); 4266 } 4267 4268 /** 4269 * @param value Variable this rule applies to. 4270 */ 4271 public StructureMapGroupRuleTargetComponent setContext(String value) { 4272 if (Utilities.noString(value)) 4273 this.context = null; 4274 else { 4275 if (this.context == null) 4276 this.context = new StringType(); 4277 this.context.setValue(value); 4278 } 4279 return this; 4280 } 4281 4282 /** 4283 * @return {@link #element} (Field to create in the context.). This is the underlying object with id, value and extensions. The accessor "getElement" gives direct access to the value 4284 */ 4285 public StringType getElementElement() { 4286 if (this.element == null) 4287 if (Configuration.errorOnAutoCreate()) 4288 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.element"); 4289 else if (Configuration.doAutoCreate()) 4290 this.element = new StringType(); // bb 4291 return this.element; 4292 } 4293 4294 public boolean hasElementElement() { 4295 return this.element != null && !this.element.isEmpty(); 4296 } 4297 4298 public boolean hasElement() { 4299 return this.element != null && !this.element.isEmpty(); 4300 } 4301 4302 /** 4303 * @param value {@link #element} (Field to create in the context.). This is the underlying object with id, value and extensions. The accessor "getElement" gives direct access to the value 4304 */ 4305 public StructureMapGroupRuleTargetComponent setElementElement(StringType value) { 4306 this.element = value; 4307 return this; 4308 } 4309 4310 /** 4311 * @return Field to create in the context. 4312 */ 4313 public String getElement() { 4314 return this.element == null ? null : this.element.getValue(); 4315 } 4316 4317 /** 4318 * @param value Field to create in the context. 4319 */ 4320 public StructureMapGroupRuleTargetComponent setElement(String value) { 4321 if (Utilities.noString(value)) 4322 this.element = null; 4323 else { 4324 if (this.element == null) 4325 this.element = new StringType(); 4326 this.element.setValue(value); 4327 } 4328 return this; 4329 } 4330 4331 /** 4332 * @return {@link #variable} (Named context for field, if desired, and a field is specified.). This is the underlying object with id, value and extensions. The accessor "getVariable" gives direct access to the value 4333 */ 4334 public IdType getVariableElement() { 4335 if (this.variable == null) 4336 if (Configuration.errorOnAutoCreate()) 4337 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.variable"); 4338 else if (Configuration.doAutoCreate()) 4339 this.variable = new IdType(); // bb 4340 return this.variable; 4341 } 4342 4343 public boolean hasVariableElement() { 4344 return this.variable != null && !this.variable.isEmpty(); 4345 } 4346 4347 public boolean hasVariable() { 4348 return this.variable != null && !this.variable.isEmpty(); 4349 } 4350 4351 /** 4352 * @param value {@link #variable} (Named context for field, if desired, and a field is specified.). This is the underlying object with id, value and extensions. The accessor "getVariable" gives direct access to the value 4353 */ 4354 public StructureMapGroupRuleTargetComponent setVariableElement(IdType value) { 4355 this.variable = value; 4356 return this; 4357 } 4358 4359 /** 4360 * @return Named context for field, if desired, and a field is specified. 4361 */ 4362 public String getVariable() { 4363 return this.variable == null ? null : this.variable.getValue(); 4364 } 4365 4366 /** 4367 * @param value Named context for field, if desired, and a field is specified. 4368 */ 4369 public StructureMapGroupRuleTargetComponent setVariable(String value) { 4370 if (Utilities.noString(value)) 4371 this.variable = null; 4372 else { 4373 if (this.variable == null) 4374 this.variable = new IdType(); 4375 this.variable.setValue(value); 4376 } 4377 return this; 4378 } 4379 4380 /** 4381 * @return {@link #listMode} (If field is a list, how to manage the list.) 4382 */ 4383 public List<Enumeration<StructureMapTargetListMode>> getListMode() { 4384 if (this.listMode == null) 4385 this.listMode = new ArrayList<Enumeration<StructureMapTargetListMode>>(); 4386 return this.listMode; 4387 } 4388 4389 /** 4390 * @return Returns a reference to <code>this</code> for easy method chaining 4391 */ 4392 public StructureMapGroupRuleTargetComponent setListMode(List<Enumeration<StructureMapTargetListMode>> theListMode) { 4393 this.listMode = theListMode; 4394 return this; 4395 } 4396 4397 public boolean hasListMode() { 4398 if (this.listMode == null) 4399 return false; 4400 for (Enumeration<StructureMapTargetListMode> item : this.listMode) 4401 if (!item.isEmpty()) 4402 return true; 4403 return false; 4404 } 4405 4406 /** 4407 * @return {@link #listMode} (If field is a list, how to manage the list.) 4408 */ 4409 public Enumeration<StructureMapTargetListMode> addListModeElement() {//2 4410 Enumeration<StructureMapTargetListMode> t = new Enumeration<StructureMapTargetListMode>(new StructureMapTargetListModeEnumFactory()); 4411 if (this.listMode == null) 4412 this.listMode = new ArrayList<Enumeration<StructureMapTargetListMode>>(); 4413 this.listMode.add(t); 4414 return t; 4415 } 4416 4417 /** 4418 * @param value {@link #listMode} (If field is a list, how to manage the list.) 4419 */ 4420 public StructureMapGroupRuleTargetComponent addListMode(StructureMapTargetListMode value) { //1 4421 Enumeration<StructureMapTargetListMode> t = new Enumeration<StructureMapTargetListMode>(new StructureMapTargetListModeEnumFactory()); 4422 t.setValue(value); 4423 if (this.listMode == null) 4424 this.listMode = new ArrayList<Enumeration<StructureMapTargetListMode>>(); 4425 this.listMode.add(t); 4426 return this; 4427 } 4428 4429 /** 4430 * @param value {@link #listMode} (If field is a list, how to manage the list.) 4431 */ 4432 public boolean hasListMode(StructureMapTargetListMode value) { 4433 if (this.listMode == null) 4434 return false; 4435 for (Enumeration<StructureMapTargetListMode> v : this.listMode) 4436 if (v.getValue().equals(value)) // code 4437 return true; 4438 return false; 4439 } 4440 4441 /** 4442 * @return {@link #listRuleId} (Internal rule reference for shared list items.). This is the underlying object with id, value and extensions. The accessor "getListRuleId" gives direct access to the value 4443 */ 4444 public IdType getListRuleIdElement() { 4445 if (this.listRuleId == null) 4446 if (Configuration.errorOnAutoCreate()) 4447 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.listRuleId"); 4448 else if (Configuration.doAutoCreate()) 4449 this.listRuleId = new IdType(); // bb 4450 return this.listRuleId; 4451 } 4452 4453 public boolean hasListRuleIdElement() { 4454 return this.listRuleId != null && !this.listRuleId.isEmpty(); 4455 } 4456 4457 public boolean hasListRuleId() { 4458 return this.listRuleId != null && !this.listRuleId.isEmpty(); 4459 } 4460 4461 /** 4462 * @param value {@link #listRuleId} (Internal rule reference for shared list items.). This is the underlying object with id, value and extensions. The accessor "getListRuleId" gives direct access to the value 4463 */ 4464 public StructureMapGroupRuleTargetComponent setListRuleIdElement(IdType value) { 4465 this.listRuleId = value; 4466 return this; 4467 } 4468 4469 /** 4470 * @return Internal rule reference for shared list items. 4471 */ 4472 public String getListRuleId() { 4473 return this.listRuleId == null ? null : this.listRuleId.getValue(); 4474 } 4475 4476 /** 4477 * @param value Internal rule reference for shared list items. 4478 */ 4479 public StructureMapGroupRuleTargetComponent setListRuleId(String value) { 4480 if (Utilities.noString(value)) 4481 this.listRuleId = null; 4482 else { 4483 if (this.listRuleId == null) 4484 this.listRuleId = new IdType(); 4485 this.listRuleId.setValue(value); 4486 } 4487 return this; 4488 } 4489 4490 /** 4491 * @return {@link #transform} (How the data is copied / created.). This is the underlying object with id, value and extensions. The accessor "getTransform" gives direct access to the value 4492 */ 4493 public Enumeration<StructureMapTransform> getTransformElement() { 4494 if (this.transform == null) 4495 if (Configuration.errorOnAutoCreate()) 4496 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.transform"); 4497 else if (Configuration.doAutoCreate()) 4498 this.transform = new Enumeration<StructureMapTransform>(new StructureMapTransformEnumFactory()); // bb 4499 return this.transform; 4500 } 4501 4502 public boolean hasTransformElement() { 4503 return this.transform != null && !this.transform.isEmpty(); 4504 } 4505 4506 public boolean hasTransform() { 4507 return this.transform != null && !this.transform.isEmpty(); 4508 } 4509 4510 /** 4511 * @param value {@link #transform} (How the data is copied / created.). This is the underlying object with id, value and extensions. The accessor "getTransform" gives direct access to the value 4512 */ 4513 public StructureMapGroupRuleTargetComponent setTransformElement(Enumeration<StructureMapTransform> value) { 4514 this.transform = value; 4515 return this; 4516 } 4517 4518 /** 4519 * @return How the data is copied / created. 4520 */ 4521 public StructureMapTransform getTransform() { 4522 return this.transform == null ? null : this.transform.getValue(); 4523 } 4524 4525 /** 4526 * @param value How the data is copied / created. 4527 */ 4528 public StructureMapGroupRuleTargetComponent setTransform(StructureMapTransform value) { 4529 if (value == null) 4530 this.transform = null; 4531 else { 4532 if (this.transform == null) 4533 this.transform = new Enumeration<StructureMapTransform>(new StructureMapTransformEnumFactory()); 4534 this.transform.setValue(value); 4535 } 4536 return this; 4537 } 4538 4539 /** 4540 * @return {@link #parameter} (Parameters to the transform.) 4541 */ 4542 public List<StructureMapGroupRuleTargetParameterComponent> getParameter() { 4543 if (this.parameter == null) 4544 this.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 4545 return this.parameter; 4546 } 4547 4548 /** 4549 * @return Returns a reference to <code>this</code> for easy method chaining 4550 */ 4551 public StructureMapGroupRuleTargetComponent setParameter(List<StructureMapGroupRuleTargetParameterComponent> theParameter) { 4552 this.parameter = theParameter; 4553 return this; 4554 } 4555 4556 public boolean hasParameter() { 4557 if (this.parameter == null) 4558 return false; 4559 for (StructureMapGroupRuleTargetParameterComponent item : this.parameter) 4560 if (!item.isEmpty()) 4561 return true; 4562 return false; 4563 } 4564 4565 public StructureMapGroupRuleTargetParameterComponent addParameter() { //3 4566 StructureMapGroupRuleTargetParameterComponent t = new StructureMapGroupRuleTargetParameterComponent(); 4567 if (this.parameter == null) 4568 this.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 4569 this.parameter.add(t); 4570 return t; 4571 } 4572 4573 public StructureMapGroupRuleTargetComponent addParameter(StructureMapGroupRuleTargetParameterComponent t) { //3 4574 if (t == null) 4575 return this; 4576 if (this.parameter == null) 4577 this.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 4578 this.parameter.add(t); 4579 return this; 4580 } 4581 4582 /** 4583 * @return The first repetition of repeating field {@link #parameter}, creating it if it does not already exist {3} 4584 */ 4585 public StructureMapGroupRuleTargetParameterComponent getParameterFirstRep() { 4586 if (getParameter().isEmpty()) { 4587 addParameter(); 4588 } 4589 return getParameter().get(0); 4590 } 4591 4592 protected void listChildren(List<Property> children) { 4593 super.listChildren(children); 4594 children.add(new Property("context", "string", "Variable this rule applies to.", 0, 1, context)); 4595 children.add(new Property("element", "string", "Field to create in the context.", 0, 1, element)); 4596 children.add(new Property("variable", "id", "Named context for field, if desired, and a field is specified.", 0, 1, variable)); 4597 children.add(new Property("listMode", "code", "If field is a list, how to manage the list.", 0, java.lang.Integer.MAX_VALUE, listMode)); 4598 children.add(new Property("listRuleId", "id", "Internal rule reference for shared list items.", 0, 1, listRuleId)); 4599 children.add(new Property("transform", "code", "How the data is copied / created.", 0, 1, transform)); 4600 children.add(new Property("parameter", "", "Parameters to the transform.", 0, java.lang.Integer.MAX_VALUE, parameter)); 4601 } 4602 4603 @Override 4604 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4605 switch (_hash) { 4606 case 951530927: /*context*/ return new Property("context", "string", "Variable this rule applies to.", 0, 1, context); 4607 case -1662836996: /*element*/ return new Property("element", "string", "Field to create in the context.", 0, 1, element); 4608 case -1249586564: /*variable*/ return new Property("variable", "id", "Named context for field, if desired, and a field is specified.", 0, 1, variable); 4609 case 1345445729: /*listMode*/ return new Property("listMode", "code", "If field is a list, how to manage the list.", 0, java.lang.Integer.MAX_VALUE, listMode); 4610 case 337117045: /*listRuleId*/ return new Property("listRuleId", "id", "Internal rule reference for shared list items.", 0, 1, listRuleId); 4611 case 1052666732: /*transform*/ return new Property("transform", "code", "How the data is copied / created.", 0, 1, transform); 4612 case 1954460585: /*parameter*/ return new Property("parameter", "", "Parameters to the transform.", 0, java.lang.Integer.MAX_VALUE, parameter); 4613 default: return super.getNamedProperty(_hash, _name, _checkValid); 4614 } 4615 4616 } 4617 4618 @Override 4619 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4620 switch (hash) { 4621 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // StringType 4622 case -1662836996: /*element*/ return this.element == null ? new Base[0] : new Base[] {this.element}; // StringType 4623 case -1249586564: /*variable*/ return this.variable == null ? new Base[0] : new Base[] {this.variable}; // IdType 4624 case 1345445729: /*listMode*/ return this.listMode == null ? new Base[0] : this.listMode.toArray(new Base[this.listMode.size()]); // Enumeration<StructureMapTargetListMode> 4625 case 337117045: /*listRuleId*/ return this.listRuleId == null ? new Base[0] : new Base[] {this.listRuleId}; // IdType 4626 case 1052666732: /*transform*/ return this.transform == null ? new Base[0] : new Base[] {this.transform}; // Enumeration<StructureMapTransform> 4627 case 1954460585: /*parameter*/ return this.parameter == null ? new Base[0] : this.parameter.toArray(new Base[this.parameter.size()]); // StructureMapGroupRuleTargetParameterComponent 4628 default: return super.getProperty(hash, name, checkValid); 4629 } 4630 4631 } 4632 4633 @Override 4634 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4635 switch (hash) { 4636 case 951530927: // context 4637 this.context = TypeConvertor.castToString(value); // StringType 4638 return value; 4639 case -1662836996: // element 4640 this.element = TypeConvertor.castToString(value); // StringType 4641 return value; 4642 case -1249586564: // variable 4643 this.variable = TypeConvertor.castToId(value); // IdType 4644 return value; 4645 case 1345445729: // listMode 4646 value = new StructureMapTargetListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4647 this.getListMode().add((Enumeration) value); // Enumeration<StructureMapTargetListMode> 4648 return value; 4649 case 337117045: // listRuleId 4650 this.listRuleId = TypeConvertor.castToId(value); // IdType 4651 return value; 4652 case 1052666732: // transform 4653 value = new StructureMapTransformEnumFactory().fromType(TypeConvertor.castToCode(value)); 4654 this.transform = (Enumeration) value; // Enumeration<StructureMapTransform> 4655 return value; 4656 case 1954460585: // parameter 4657 this.getParameter().add((StructureMapGroupRuleTargetParameterComponent) value); // StructureMapGroupRuleTargetParameterComponent 4658 return value; 4659 default: return super.setProperty(hash, name, value); 4660 } 4661 4662 } 4663 4664 @Override 4665 public Base setProperty(String name, Base value) throws FHIRException { 4666 if (name.equals("context")) { 4667 this.context = TypeConvertor.castToString(value); // StringType 4668 } else if (name.equals("element")) { 4669 this.element = TypeConvertor.castToString(value); // StringType 4670 } else if (name.equals("variable")) { 4671 this.variable = TypeConvertor.castToId(value); // IdType 4672 } else if (name.equals("listMode")) { 4673 value = new StructureMapTargetListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4674 this.getListMode().add((Enumeration) value); 4675 } else if (name.equals("listRuleId")) { 4676 this.listRuleId = TypeConvertor.castToId(value); // IdType 4677 } else if (name.equals("transform")) { 4678 value = new StructureMapTransformEnumFactory().fromType(TypeConvertor.castToCode(value)); 4679 this.transform = (Enumeration) value; // Enumeration<StructureMapTransform> 4680 } else if (name.equals("parameter")) { 4681 this.getParameter().add((StructureMapGroupRuleTargetParameterComponent) value); 4682 } else 4683 return super.setProperty(name, value); 4684 return value; 4685 } 4686 4687 @Override 4688 public void removeChild(String name, Base value) throws FHIRException { 4689 if (name.equals("context")) { 4690 this.context = null; 4691 } else if (name.equals("element")) { 4692 this.element = null; 4693 } else if (name.equals("variable")) { 4694 this.variable = null; 4695 } else if (name.equals("listMode")) { 4696 value = new StructureMapTargetListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4697 this.getListMode().remove((Enumeration) value); 4698 } else if (name.equals("listRuleId")) { 4699 this.listRuleId = null; 4700 } else if (name.equals("transform")) { 4701 value = new StructureMapTransformEnumFactory().fromType(TypeConvertor.castToCode(value)); 4702 this.transform = (Enumeration) value; // Enumeration<StructureMapTransform> 4703 } else if (name.equals("parameter")) { 4704 this.getParameter().remove((StructureMapGroupRuleTargetParameterComponent) value); 4705 } else 4706 super.removeChild(name, value); 4707 4708 } 4709 4710 @Override 4711 public Base makeProperty(int hash, String name) throws FHIRException { 4712 switch (hash) { 4713 case 951530927: return getContextElement(); 4714 case -1662836996: return getElementElement(); 4715 case -1249586564: return getVariableElement(); 4716 case 1345445729: return addListModeElement(); 4717 case 337117045: return getListRuleIdElement(); 4718 case 1052666732: return getTransformElement(); 4719 case 1954460585: return addParameter(); 4720 default: return super.makeProperty(hash, name); 4721 } 4722 4723 } 4724 4725 @Override 4726 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4727 switch (hash) { 4728 case 951530927: /*context*/ return new String[] {"string"}; 4729 case -1662836996: /*element*/ return new String[] {"string"}; 4730 case -1249586564: /*variable*/ return new String[] {"id"}; 4731 case 1345445729: /*listMode*/ return new String[] {"code"}; 4732 case 337117045: /*listRuleId*/ return new String[] {"id"}; 4733 case 1052666732: /*transform*/ return new String[] {"code"}; 4734 case 1954460585: /*parameter*/ return new String[] {}; 4735 default: return super.getTypesForProperty(hash, name); 4736 } 4737 4738 } 4739 4740 @Override 4741 public Base addChild(String name) throws FHIRException { 4742 if (name.equals("context")) { 4743 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.target.context"); 4744 } 4745 else if (name.equals("element")) { 4746 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.target.element"); 4747 } 4748 else if (name.equals("variable")) { 4749 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.target.variable"); 4750 } 4751 else if (name.equals("listMode")) { 4752 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.target.listMode"); 4753 } 4754 else if (name.equals("listRuleId")) { 4755 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.target.listRuleId"); 4756 } 4757 else if (name.equals("transform")) { 4758 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.target.transform"); 4759 } 4760 else if (name.equals("parameter")) { 4761 return addParameter(); 4762 } 4763 else 4764 return super.addChild(name); 4765 } 4766 4767 public StructureMapGroupRuleTargetComponent copy() { 4768 StructureMapGroupRuleTargetComponent dst = new StructureMapGroupRuleTargetComponent(); 4769 copyValues(dst); 4770 return dst; 4771 } 4772 4773 public void copyValues(StructureMapGroupRuleTargetComponent dst) { 4774 super.copyValues(dst); 4775 dst.context = context == null ? null : context.copy(); 4776 dst.element = element == null ? null : element.copy(); 4777 dst.variable = variable == null ? null : variable.copy(); 4778 if (listMode != null) { 4779 dst.listMode = new ArrayList<Enumeration<StructureMapTargetListMode>>(); 4780 for (Enumeration<StructureMapTargetListMode> i : listMode) 4781 dst.listMode.add(i.copy()); 4782 }; 4783 dst.listRuleId = listRuleId == null ? null : listRuleId.copy(); 4784 dst.transform = transform == null ? null : transform.copy(); 4785 if (parameter != null) { 4786 dst.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 4787 for (StructureMapGroupRuleTargetParameterComponent i : parameter) 4788 dst.parameter.add(i.copy()); 4789 }; 4790 } 4791 4792 @Override 4793 public boolean equalsDeep(Base other_) { 4794 if (!super.equalsDeep(other_)) 4795 return false; 4796 if (!(other_ instanceof StructureMapGroupRuleTargetComponent)) 4797 return false; 4798 StructureMapGroupRuleTargetComponent o = (StructureMapGroupRuleTargetComponent) other_; 4799 return compareDeep(context, o.context, true) && compareDeep(element, o.element, true) && compareDeep(variable, o.variable, true) 4800 && compareDeep(listMode, o.listMode, true) && compareDeep(listRuleId, o.listRuleId, true) && compareDeep(transform, o.transform, true) 4801 && compareDeep(parameter, o.parameter, true); 4802 } 4803 4804 @Override 4805 public boolean equalsShallow(Base other_) { 4806 if (!super.equalsShallow(other_)) 4807 return false; 4808 if (!(other_ instanceof StructureMapGroupRuleTargetComponent)) 4809 return false; 4810 StructureMapGroupRuleTargetComponent o = (StructureMapGroupRuleTargetComponent) other_; 4811 return compareValues(context, o.context, true) && compareValues(element, o.element, true) && compareValues(variable, o.variable, true) 4812 && compareValues(listMode, o.listMode, true) && compareValues(listRuleId, o.listRuleId, true) && compareValues(transform, o.transform, true) 4813 ; 4814 } 4815 4816 public boolean isEmpty() { 4817 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(context, element, variable 4818 , listMode, listRuleId, transform, parameter); 4819 } 4820 4821 public String fhirType() { 4822 return "StructureMap.group.rule.target"; 4823 4824 } 4825 4826// added from java-adornments.txt: 4827public String toString() { 4828 return StructureMapUtilities.targetToString(this); 4829 } 4830// end addition 4831 } 4832 4833 @Block() 4834 public static class StructureMapGroupRuleTargetParameterComponent extends BackboneElement implements IBaseBackboneElement { 4835 /** 4836 * Parameter value - variable or literal. 4837 */ 4838 @Child(name = "value", type = {IdType.class, StringType.class, BooleanType.class, IntegerType.class, DecimalType.class, DateType.class, TimeType.class, DateTimeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 4839 @Description(shortDefinition="Parameter value - variable or literal", formalDefinition="Parameter value - variable or literal." ) 4840 protected DataType value; 4841 4842 private static final long serialVersionUID = -1135414639L; 4843 4844 /** 4845 * Constructor 4846 */ 4847 public StructureMapGroupRuleTargetParameterComponent() { 4848 super(); 4849 } 4850 4851 /** 4852 * Constructor 4853 */ 4854 public StructureMapGroupRuleTargetParameterComponent(DataType value) { 4855 super(); 4856 this.setValue(value); 4857 } 4858 4859 /** 4860 * @return {@link #value} (Parameter value - variable or literal.) 4861 */ 4862 public DataType getValue() { 4863 return this.value; 4864 } 4865 4866 /** 4867 * @return {@link #value} (Parameter value - variable or literal.) 4868 */ 4869 public IdType getValueIdType() throws FHIRException { 4870 if (this.value == null) 4871 this.value = new IdType(); 4872 if (!(this.value instanceof IdType)) 4873 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.value.getClass().getName()+" was encountered"); 4874 return (IdType) this.value; 4875 } 4876 4877 public boolean hasValueIdType() { 4878 return this != null && this.value instanceof IdType; 4879 } 4880 4881 /** 4882 * @return {@link #value} (Parameter value - variable or literal.) 4883 */ 4884 public StringType getValueStringType() throws FHIRException { 4885 if (this.value == null) 4886 this.value = new StringType(); 4887 if (!(this.value instanceof StringType)) 4888 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 4889 return (StringType) this.value; 4890 } 4891 4892 public boolean hasValueStringType() { 4893 return this != null && this.value instanceof StringType; 4894 } 4895 4896 /** 4897 * @return {@link #value} (Parameter value - variable or literal.) 4898 */ 4899 public BooleanType getValueBooleanType() throws FHIRException { 4900 if (this.value == null) 4901 this.value = new BooleanType(); 4902 if (!(this.value instanceof BooleanType)) 4903 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 4904 return (BooleanType) this.value; 4905 } 4906 4907 public boolean hasValueBooleanType() { 4908 return this != null && this.value instanceof BooleanType; 4909 } 4910 4911 /** 4912 * @return {@link #value} (Parameter value - variable or literal.) 4913 */ 4914 public IntegerType getValueIntegerType() throws FHIRException { 4915 if (this.value == null) 4916 this.value = new IntegerType(); 4917 if (!(this.value instanceof IntegerType)) 4918 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 4919 return (IntegerType) this.value; 4920 } 4921 4922 public boolean hasValueIntegerType() { 4923 return this != null && this.value instanceof IntegerType; 4924 } 4925 4926 /** 4927 * @return {@link #value} (Parameter value - variable or literal.) 4928 */ 4929 public DecimalType getValueDecimalType() throws FHIRException { 4930 if (this.value == null) 4931 this.value = new DecimalType(); 4932 if (!(this.value instanceof DecimalType)) 4933 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 4934 return (DecimalType) this.value; 4935 } 4936 4937 public boolean hasValueDecimalType() { 4938 return this != null && this.value instanceof DecimalType; 4939 } 4940 4941 /** 4942 * @return {@link #value} (Parameter value - variable or literal.) 4943 */ 4944 public DateType getValueDateType() throws FHIRException { 4945 if (this.value == null) 4946 this.value = new DateType(); 4947 if (!(this.value instanceof DateType)) 4948 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 4949 return (DateType) this.value; 4950 } 4951 4952 public boolean hasValueDateType() { 4953 return this != null && this.value instanceof DateType; 4954 } 4955 4956 /** 4957 * @return {@link #value} (Parameter value - variable or literal.) 4958 */ 4959 public TimeType getValueTimeType() throws FHIRException { 4960 if (this.value == null) 4961 this.value = new TimeType(); 4962 if (!(this.value instanceof TimeType)) 4963 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 4964 return (TimeType) this.value; 4965 } 4966 4967 public boolean hasValueTimeType() { 4968 return this != null && this.value instanceof TimeType; 4969 } 4970 4971 /** 4972 * @return {@link #value} (Parameter value - variable or literal.) 4973 */ 4974 public DateTimeType getValueDateTimeType() throws FHIRException { 4975 if (this.value == null) 4976 this.value = new DateTimeType(); 4977 if (!(this.value instanceof DateTimeType)) 4978 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 4979 return (DateTimeType) this.value; 4980 } 4981 4982 public boolean hasValueDateTimeType() { 4983 return this != null && this.value instanceof DateTimeType; 4984 } 4985 4986 public boolean hasValue() { 4987 return this.value != null && !this.value.isEmpty(); 4988 } 4989 4990 /** 4991 * @param value {@link #value} (Parameter value - variable or literal.) 4992 */ 4993 public StructureMapGroupRuleTargetParameterComponent setValue(DataType value) { 4994 if (value != null && !(value instanceof IdType || value instanceof StringType || value instanceof BooleanType || value instanceof IntegerType || value instanceof DecimalType || value instanceof DateType || value instanceof TimeType || value instanceof DateTimeType)) 4995 throw new FHIRException("Not the right type for StructureMap.group.rule.target.parameter.value[x]: "+value.fhirType()); 4996 this.value = value; 4997 return this; 4998 } 4999 5000 protected void listChildren(List<Property> children) { 5001 super.listChildren(children); 5002 children.add(new Property("value[x]", "id|string|boolean|integer|decimal|date|time|dateTime", "Parameter value - variable or literal.", 0, 1, value)); 5003 } 5004 5005 @Override 5006 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5007 switch (_hash) { 5008 case -1410166417: /*value[x]*/ return new Property("value[x]", "id|string|boolean|integer|decimal|date|time|dateTime", "Parameter value - variable or literal.", 0, 1, value); 5009 case 111972721: /*value*/ return new Property("value[x]", "id|string|boolean|integer|decimal|date|time|dateTime", "Parameter value - variable or literal.", 0, 1, value); 5010 case 231604844: /*valueId*/ return new Property("value[x]", "id", "Parameter value - variable or literal.", 0, 1, value); 5011 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "Parameter value - variable or literal.", 0, 1, value); 5012 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "Parameter value - variable or literal.", 0, 1, value); 5013 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "Parameter value - variable or literal.", 0, 1, value); 5014 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "Parameter value - variable or literal.", 0, 1, value); 5015 case -766192449: /*valueDate*/ return new Property("value[x]", "date", "Parameter value - variable or literal.", 0, 1, value); 5016 case -765708322: /*valueTime*/ return new Property("value[x]", "time", "Parameter value - variable or literal.", 0, 1, value); 5017 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "Parameter value - variable or literal.", 0, 1, value); 5018 default: return super.getNamedProperty(_hash, _name, _checkValid); 5019 } 5020 5021 } 5022 5023 @Override 5024 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5025 switch (hash) { 5026 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 5027 default: return super.getProperty(hash, name, checkValid); 5028 } 5029 5030 } 5031 5032 @Override 5033 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5034 switch (hash) { 5035 case 111972721: // value 5036 this.value = TypeConvertor.castToType(value); // DataType 5037 return value; 5038 default: return super.setProperty(hash, name, value); 5039 } 5040 5041 } 5042 5043 @Override 5044 public Base setProperty(String name, Base value) throws FHIRException { 5045 if (name.equals("value[x]")) { 5046 this.value = TypeConvertor.castToType(value); // DataType 5047 } else 5048 return super.setProperty(name, value); 5049 return value; 5050 } 5051 5052 @Override 5053 public void removeChild(String name, Base value) throws FHIRException { 5054 if (name.equals("value[x]")) { 5055 this.value = null; 5056 } else 5057 super.removeChild(name, value); 5058 5059 } 5060 5061 @Override 5062 public Base makeProperty(int hash, String name) throws FHIRException { 5063 switch (hash) { 5064 case -1410166417: return getValue(); 5065 case 111972721: return getValue(); 5066 default: return super.makeProperty(hash, name); 5067 } 5068 5069 } 5070 5071 @Override 5072 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5073 switch (hash) { 5074 case 111972721: /*value*/ return new String[] {"id", "string", "boolean", "integer", "decimal", "date", "time", "dateTime"}; 5075 default: return super.getTypesForProperty(hash, name); 5076 } 5077 5078 } 5079 5080 @Override 5081 public Base addChild(String name) throws FHIRException { 5082 if (name.equals("valueId")) { 5083 this.value = new IdType(); 5084 return this.value; 5085 } 5086 else if (name.equals("valueString")) { 5087 this.value = new StringType(); 5088 return this.value; 5089 } 5090 else if (name.equals("valueBoolean")) { 5091 this.value = new BooleanType(); 5092 return this.value; 5093 } 5094 else if (name.equals("valueInteger")) { 5095 this.value = new IntegerType(); 5096 return this.value; 5097 } 5098 else if (name.equals("valueDecimal")) { 5099 this.value = new DecimalType(); 5100 return this.value; 5101 } 5102 else if (name.equals("valueDate")) { 5103 this.value = new DateType(); 5104 return this.value; 5105 } 5106 else if (name.equals("valueTime")) { 5107 this.value = new TimeType(); 5108 return this.value; 5109 } 5110 else if (name.equals("valueDateTime")) { 5111 this.value = new DateTimeType(); 5112 return this.value; 5113 } 5114 else 5115 return super.addChild(name); 5116 } 5117 5118 public StructureMapGroupRuleTargetParameterComponent copy() { 5119 StructureMapGroupRuleTargetParameterComponent dst = new StructureMapGroupRuleTargetParameterComponent(); 5120 copyValues(dst); 5121 return dst; 5122 } 5123 5124 public void copyValues(StructureMapGroupRuleTargetParameterComponent dst) { 5125 super.copyValues(dst); 5126 dst.value = value == null ? null : value.copy(); 5127 } 5128 5129 @Override 5130 public boolean equalsDeep(Base other_) { 5131 if (!super.equalsDeep(other_)) 5132 return false; 5133 if (!(other_ instanceof StructureMapGroupRuleTargetParameterComponent)) 5134 return false; 5135 StructureMapGroupRuleTargetParameterComponent o = (StructureMapGroupRuleTargetParameterComponent) other_; 5136 return compareDeep(value, o.value, true); 5137 } 5138 5139 @Override 5140 public boolean equalsShallow(Base other_) { 5141 if (!super.equalsShallow(other_)) 5142 return false; 5143 if (!(other_ instanceof StructureMapGroupRuleTargetParameterComponent)) 5144 return false; 5145 StructureMapGroupRuleTargetParameterComponent o = (StructureMapGroupRuleTargetParameterComponent) other_; 5146 return true; 5147 } 5148 5149 public boolean isEmpty() { 5150 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value); 5151 } 5152 5153 public String fhirType() { 5154 return "StructureMap.group.rule.target.parameter"; 5155 5156 } 5157 5158// added from java-adornments.txt: 5159public String toString() { 5160 return value == null ? "null!" : value.toString(); 5161 } 5162// end addition 5163 } 5164 5165 @Block() 5166 public static class StructureMapGroupRuleDependentComponent extends BackboneElement implements IBaseBackboneElement { 5167 /** 5168 * Name of a rule or group to apply. 5169 */ 5170 @Child(name = "name", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 5171 @Description(shortDefinition="Name of a rule or group to apply", formalDefinition="Name of a rule or group to apply." ) 5172 protected IdType name; 5173 5174 /** 5175 * Parameter to pass to the rule or group. 5176 */ 5177 @Child(name = "parameter", type = {StructureMapGroupRuleTargetParameterComponent.class}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5178 @Description(shortDefinition="Parameter to pass to the rule or group", formalDefinition="Parameter to pass to the rule or group." ) 5179 protected List<StructureMapGroupRuleTargetParameterComponent> parameter; 5180 5181 private static final long serialVersionUID = -290346576L; 5182 5183 /** 5184 * Constructor 5185 */ 5186 public StructureMapGroupRuleDependentComponent() { 5187 super(); 5188 } 5189 5190 /** 5191 * Constructor 5192 */ 5193 public StructureMapGroupRuleDependentComponent(String name, StructureMapGroupRuleTargetParameterComponent parameter) { 5194 super(); 5195 this.setName(name); 5196 this.addParameter(parameter); 5197 } 5198 5199 /** 5200 * @return {@link #name} (Name of a rule or group to apply.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5201 */ 5202 public IdType getNameElement() { 5203 if (this.name == null) 5204 if (Configuration.errorOnAutoCreate()) 5205 throw new Error("Attempt to auto-create StructureMapGroupRuleDependentComponent.name"); 5206 else if (Configuration.doAutoCreate()) 5207 this.name = new IdType(); // bb 5208 return this.name; 5209 } 5210 5211 public boolean hasNameElement() { 5212 return this.name != null && !this.name.isEmpty(); 5213 } 5214 5215 public boolean hasName() { 5216 return this.name != null && !this.name.isEmpty(); 5217 } 5218 5219 /** 5220 * @param value {@link #name} (Name of a rule or group to apply.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5221 */ 5222 public StructureMapGroupRuleDependentComponent setNameElement(IdType value) { 5223 this.name = value; 5224 return this; 5225 } 5226 5227 /** 5228 * @return Name of a rule or group to apply. 5229 */ 5230 public String getName() { 5231 return this.name == null ? null : this.name.getValue(); 5232 } 5233 5234 /** 5235 * @param value Name of a rule or group to apply. 5236 */ 5237 public StructureMapGroupRuleDependentComponent setName(String value) { 5238 if (this.name == null) 5239 this.name = new IdType(); 5240 this.name.setValue(value); 5241 return this; 5242 } 5243 5244 /** 5245 * @return {@link #parameter} (Parameter to pass to the rule or group.) 5246 */ 5247 public List<StructureMapGroupRuleTargetParameterComponent> getParameter() { 5248 if (this.parameter == null) 5249 this.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 5250 return this.parameter; 5251 } 5252 5253 /** 5254 * @return Returns a reference to <code>this</code> for easy method chaining 5255 */ 5256 public StructureMapGroupRuleDependentComponent setParameter(List<StructureMapGroupRuleTargetParameterComponent> theParameter) { 5257 this.parameter = theParameter; 5258 return this; 5259 } 5260 5261 public boolean hasParameter() { 5262 if (this.parameter == null) 5263 return false; 5264 for (StructureMapGroupRuleTargetParameterComponent item : this.parameter) 5265 if (!item.isEmpty()) 5266 return true; 5267 return false; 5268 } 5269 5270 public StructureMapGroupRuleTargetParameterComponent addParameter() { //3 5271 StructureMapGroupRuleTargetParameterComponent t = new StructureMapGroupRuleTargetParameterComponent(); 5272 if (this.parameter == null) 5273 this.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 5274 this.parameter.add(t); 5275 return t; 5276 } 5277 5278 public StructureMapGroupRuleDependentComponent addParameter(StructureMapGroupRuleTargetParameterComponent t) { //3 5279 if (t == null) 5280 return this; 5281 if (this.parameter == null) 5282 this.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 5283 this.parameter.add(t); 5284 return this; 5285 } 5286 5287 /** 5288 * @return The first repetition of repeating field {@link #parameter}, creating it if it does not already exist {3} 5289 */ 5290 public StructureMapGroupRuleTargetParameterComponent getParameterFirstRep() { 5291 if (getParameter().isEmpty()) { 5292 addParameter(); 5293 } 5294 return getParameter().get(0); 5295 } 5296 5297 protected void listChildren(List<Property> children) { 5298 super.listChildren(children); 5299 children.add(new Property("name", "id", "Name of a rule or group to apply.", 0, 1, name)); 5300 children.add(new Property("parameter", "@StructureMap.group.rule.target.parameter", "Parameter to pass to the rule or group.", 0, java.lang.Integer.MAX_VALUE, parameter)); 5301 } 5302 5303 @Override 5304 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5305 switch (_hash) { 5306 case 3373707: /*name*/ return new Property("name", "id", "Name of a rule or group to apply.", 0, 1, name); 5307 case 1954460585: /*parameter*/ return new Property("parameter", "@StructureMap.group.rule.target.parameter", "Parameter to pass to the rule or group.", 0, java.lang.Integer.MAX_VALUE, parameter); 5308 default: return super.getNamedProperty(_hash, _name, _checkValid); 5309 } 5310 5311 } 5312 5313 @Override 5314 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5315 switch (hash) { 5316 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // IdType 5317 case 1954460585: /*parameter*/ return this.parameter == null ? new Base[0] : this.parameter.toArray(new Base[this.parameter.size()]); // StructureMapGroupRuleTargetParameterComponent 5318 default: return super.getProperty(hash, name, checkValid); 5319 } 5320 5321 } 5322 5323 @Override 5324 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5325 switch (hash) { 5326 case 3373707: // name 5327 this.name = TypeConvertor.castToId(value); // IdType 5328 return value; 5329 case 1954460585: // parameter 5330 this.getParameter().add((StructureMapGroupRuleTargetParameterComponent) value); // StructureMapGroupRuleTargetParameterComponent 5331 return value; 5332 default: return super.setProperty(hash, name, value); 5333 } 5334 5335 } 5336 5337 @Override 5338 public Base setProperty(String name, Base value) throws FHIRException { 5339 if (name.equals("name")) { 5340 this.name = TypeConvertor.castToId(value); // IdType 5341 } else if (name.equals("parameter")) { 5342 this.getParameter().add((StructureMapGroupRuleTargetParameterComponent) value); 5343 } else 5344 return super.setProperty(name, value); 5345 return value; 5346 } 5347 5348 @Override 5349 public void removeChild(String name, Base value) throws FHIRException { 5350 if (name.equals("name")) { 5351 this.name = null; 5352 } else if (name.equals("parameter")) { 5353 this.getParameter().remove((StructureMapGroupRuleTargetParameterComponent) value); 5354 } else 5355 super.removeChild(name, value); 5356 5357 } 5358 5359 @Override 5360 public Base makeProperty(int hash, String name) throws FHIRException { 5361 switch (hash) { 5362 case 3373707: return getNameElement(); 5363 case 1954460585: return addParameter(); 5364 default: return super.makeProperty(hash, name); 5365 } 5366 5367 } 5368 5369 @Override 5370 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5371 switch (hash) { 5372 case 3373707: /*name*/ return new String[] {"id"}; 5373 case 1954460585: /*parameter*/ return new String[] {"@StructureMap.group.rule.target.parameter"}; 5374 default: return super.getTypesForProperty(hash, name); 5375 } 5376 5377 } 5378 5379 @Override 5380 public Base addChild(String name) throws FHIRException { 5381 if (name.equals("name")) { 5382 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.dependent.name"); 5383 } 5384 else if (name.equals("parameter")) { 5385 return addParameter(); 5386 } 5387 else 5388 return super.addChild(name); 5389 } 5390 5391 public StructureMapGroupRuleDependentComponent copy() { 5392 StructureMapGroupRuleDependentComponent dst = new StructureMapGroupRuleDependentComponent(); 5393 copyValues(dst); 5394 return dst; 5395 } 5396 5397 public void copyValues(StructureMapGroupRuleDependentComponent dst) { 5398 super.copyValues(dst); 5399 dst.name = name == null ? null : name.copy(); 5400 if (parameter != null) { 5401 dst.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 5402 for (StructureMapGroupRuleTargetParameterComponent i : parameter) 5403 dst.parameter.add(i.copy()); 5404 }; 5405 } 5406 5407 @Override 5408 public boolean equalsDeep(Base other_) { 5409 if (!super.equalsDeep(other_)) 5410 return false; 5411 if (!(other_ instanceof StructureMapGroupRuleDependentComponent)) 5412 return false; 5413 StructureMapGroupRuleDependentComponent o = (StructureMapGroupRuleDependentComponent) other_; 5414 return compareDeep(name, o.name, true) && compareDeep(parameter, o.parameter, true); 5415 } 5416 5417 @Override 5418 public boolean equalsShallow(Base other_) { 5419 if (!super.equalsShallow(other_)) 5420 return false; 5421 if (!(other_ instanceof StructureMapGroupRuleDependentComponent)) 5422 return false; 5423 StructureMapGroupRuleDependentComponent o = (StructureMapGroupRuleDependentComponent) other_; 5424 return compareValues(name, o.name, true); 5425 } 5426 5427 public boolean isEmpty() { 5428 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, parameter); 5429 } 5430 5431 public String fhirType() { 5432 return "StructureMap.group.rule.dependent"; 5433 5434 } 5435 5436 } 5437 5438 /** 5439 * An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure map is stored on different servers. 5440 */ 5441 @Child(name = "url", type = {UriType.class}, order=0, min=1, max=1, modifier=false, summary=true) 5442 @Description(shortDefinition="Canonical identifier for this structure map, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure map is stored on different servers." ) 5443 protected UriType url; 5444 5445 /** 5446 * A formal identifier that is used to identify this structure map when it is represented in other formats, or referenced in a specification, model, design or an instance. 5447 */ 5448 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5449 @Description(shortDefinition="Additional identifier for the structure map", formalDefinition="A formal identifier that is used to identify this structure map when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 5450 protected List<Identifier> identifier; 5451 5452 /** 5453 * The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 5454 */ 5455 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 5456 @Description(shortDefinition="Business version of the structure map", formalDefinition="The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 5457 protected StringType version; 5458 5459 /** 5460 * Indicates the mechanism used to compare versions to determine which is more current. 5461 */ 5462 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 5463 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 5464 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 5465 protected DataType versionAlgorithm; 5466 5467 /** 5468 * A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation. 5469 */ 5470 @Child(name = "name", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=true) 5471 @Description(shortDefinition="Name for this structure map (computer friendly)", formalDefinition="A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 5472 protected StringType name; 5473 5474 /** 5475 * A short, descriptive, user-friendly title for the structure map. 5476 */ 5477 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 5478 @Description(shortDefinition="Name for this structure map (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the structure map." ) 5479 protected StringType title; 5480 5481 /** 5482 * The status of this structure map. Enables tracking the life-cycle of the content. 5483 */ 5484 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 5485 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this structure map. Enables tracking the life-cycle of the content." ) 5486 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 5487 protected Enumeration<PublicationStatus> status; 5488 5489 /** 5490 * A Boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 5491 */ 5492 @Child(name = "experimental", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 5493 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 5494 protected BooleanType experimental; 5495 5496 /** 5497 * The date (and optionally time) when the structure map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes. 5498 */ 5499 @Child(name = "date", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 5500 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the structure map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes." ) 5501 protected DateTimeType date; 5502 5503 /** 5504 * The name of the organization or individual responsible for the release and ongoing maintenance of the structure map. 5505 */ 5506 @Child(name = "publisher", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 5507 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the structure map." ) 5508 protected StringType publisher; 5509 5510 /** 5511 * Contact details to assist a user in finding and communicating with the publisher. 5512 */ 5513 @Child(name = "contact", type = {ContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5514 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 5515 protected List<ContactDetail> contact; 5516 5517 /** 5518 * A free text natural language description of the structure map from a consumer's perspective. 5519 */ 5520 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 5521 @Description(shortDefinition="Natural language description of the structure map", formalDefinition="A free text natural language description of the structure map from a consumer's perspective." ) 5522 protected MarkdownType description; 5523 5524 /** 5525 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure map instances. 5526 */ 5527 @Child(name = "useContext", type = {UsageContext.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5528 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure map instances." ) 5529 protected List<UsageContext> useContext; 5530 5531 /** 5532 * A legal or geographic region in which the structure map is intended to be used. 5533 */ 5534 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5535 @Description(shortDefinition="Intended jurisdiction for structure map (if applicable)", formalDefinition="A legal or geographic region in which the structure map is intended to be used." ) 5536 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 5537 protected List<CodeableConcept> jurisdiction; 5538 5539 /** 5540 * Explanation of why this structure map is needed and why it has been designed as it has. 5541 */ 5542 @Child(name = "purpose", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 5543 @Description(shortDefinition="Why this structure map is defined", formalDefinition="Explanation of why this structure map is needed and why it has been designed as it has." ) 5544 protected MarkdownType purpose; 5545 5546 /** 5547 * A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map. 5548 */ 5549 @Child(name = "copyright", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 5550 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map." ) 5551 protected MarkdownType copyright; 5552 5553 /** 5554 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 5555 */ 5556 @Child(name = "copyrightLabel", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 5557 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 5558 protected StringType copyrightLabel; 5559 5560 /** 5561 * A structure definition used by this map. The structure definition may describe instances that are converted, or the instances that are produced. 5562 */ 5563 @Child(name = "structure", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5564 @Description(shortDefinition="Structure Definition used by this map", formalDefinition="A structure definition used by this map. The structure definition may describe instances that are converted, or the instances that are produced." ) 5565 protected List<StructureMapStructureComponent> structure; 5566 5567 /** 5568 * Other maps used by this map (canonical URLs). 5569 */ 5570 @Child(name = "import", type = {CanonicalType.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5571 @Description(shortDefinition="Other maps used by this map (canonical URLs)", formalDefinition="Other maps used by this map (canonical URLs)." ) 5572 protected List<CanonicalType> import_; 5573 5574 /** 5575 * Definition of a constant value used in the map rules. 5576 */ 5577 @Child(name = "const", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5578 @Description(shortDefinition="Definition of the constant value used in the map rules", formalDefinition="Definition of a constant value used in the map rules." ) 5579 protected List<StructureMapConstComponent> const_; 5580 5581 /** 5582 * Organizes the mapping into managable chunks for human review/ease of maintenance. 5583 */ 5584 @Child(name = "group", type = {}, order=20, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5585 @Description(shortDefinition="Named sections for reader convenience", formalDefinition="Organizes the mapping into managable chunks for human review/ease of maintenance." ) 5586 protected List<StructureMapGroupComponent> group; 5587 5588 private static final long serialVersionUID = -1875777589L; 5589 5590 /** 5591 * Constructor 5592 */ 5593 public StructureMap() { 5594 super(); 5595 } 5596 5597 /** 5598 * Constructor 5599 */ 5600 public StructureMap(String url, String name, PublicationStatus status, StructureMapGroupComponent group) { 5601 super(); 5602 this.setUrl(url); 5603 this.setName(name); 5604 this.setStatus(status); 5605 this.addGroup(group); 5606 } 5607 5608 /** 5609 * @return {@link #url} (An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure map is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 5610 */ 5611 public UriType getUrlElement() { 5612 if (this.url == null) 5613 if (Configuration.errorOnAutoCreate()) 5614 throw new Error("Attempt to auto-create StructureMap.url"); 5615 else if (Configuration.doAutoCreate()) 5616 this.url = new UriType(); // bb 5617 return this.url; 5618 } 5619 5620 public boolean hasUrlElement() { 5621 return this.url != null && !this.url.isEmpty(); 5622 } 5623 5624 public boolean hasUrl() { 5625 return this.url != null && !this.url.isEmpty(); 5626 } 5627 5628 /** 5629 * @param value {@link #url} (An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure map is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 5630 */ 5631 public StructureMap setUrlElement(UriType value) { 5632 this.url = value; 5633 return this; 5634 } 5635 5636 /** 5637 * @return An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure map is stored on different servers. 5638 */ 5639 public String getUrl() { 5640 return this.url == null ? null : this.url.getValue(); 5641 } 5642 5643 /** 5644 * @param value An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure map is stored on different servers. 5645 */ 5646 public StructureMap setUrl(String value) { 5647 if (this.url == null) 5648 this.url = new UriType(); 5649 this.url.setValue(value); 5650 return this; 5651 } 5652 5653 /** 5654 * @return {@link #identifier} (A formal identifier that is used to identify this structure map when it is represented in other formats, or referenced in a specification, model, design or an instance.) 5655 */ 5656 public List<Identifier> getIdentifier() { 5657 if (this.identifier == null) 5658 this.identifier = new ArrayList<Identifier>(); 5659 return this.identifier; 5660 } 5661 5662 /** 5663 * @return Returns a reference to <code>this</code> for easy method chaining 5664 */ 5665 public StructureMap setIdentifier(List<Identifier> theIdentifier) { 5666 this.identifier = theIdentifier; 5667 return this; 5668 } 5669 5670 public boolean hasIdentifier() { 5671 if (this.identifier == null) 5672 return false; 5673 for (Identifier item : this.identifier) 5674 if (!item.isEmpty()) 5675 return true; 5676 return false; 5677 } 5678 5679 public Identifier addIdentifier() { //3 5680 Identifier t = new Identifier(); 5681 if (this.identifier == null) 5682 this.identifier = new ArrayList<Identifier>(); 5683 this.identifier.add(t); 5684 return t; 5685 } 5686 5687 public StructureMap addIdentifier(Identifier t) { //3 5688 if (t == null) 5689 return this; 5690 if (this.identifier == null) 5691 this.identifier = new ArrayList<Identifier>(); 5692 this.identifier.add(t); 5693 return this; 5694 } 5695 5696 /** 5697 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 5698 */ 5699 public Identifier getIdentifierFirstRep() { 5700 if (getIdentifier().isEmpty()) { 5701 addIdentifier(); 5702 } 5703 return getIdentifier().get(0); 5704 } 5705 5706 /** 5707 * @return {@link #version} (The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 5708 */ 5709 public StringType getVersionElement() { 5710 if (this.version == null) 5711 if (Configuration.errorOnAutoCreate()) 5712 throw new Error("Attempt to auto-create StructureMap.version"); 5713 else if (Configuration.doAutoCreate()) 5714 this.version = new StringType(); // bb 5715 return this.version; 5716 } 5717 5718 public boolean hasVersionElement() { 5719 return this.version != null && !this.version.isEmpty(); 5720 } 5721 5722 public boolean hasVersion() { 5723 return this.version != null && !this.version.isEmpty(); 5724 } 5725 5726 /** 5727 * @param value {@link #version} (The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 5728 */ 5729 public StructureMap setVersionElement(StringType value) { 5730 this.version = value; 5731 return this; 5732 } 5733 5734 /** 5735 * @return The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 5736 */ 5737 public String getVersion() { 5738 return this.version == null ? null : this.version.getValue(); 5739 } 5740 5741 /** 5742 * @param value The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 5743 */ 5744 public StructureMap setVersion(String value) { 5745 if (Utilities.noString(value)) 5746 this.version = null; 5747 else { 5748 if (this.version == null) 5749 this.version = new StringType(); 5750 this.version.setValue(value); 5751 } 5752 return this; 5753 } 5754 5755 /** 5756 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 5757 */ 5758 public DataType getVersionAlgorithm() { 5759 return this.versionAlgorithm; 5760 } 5761 5762 /** 5763 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 5764 */ 5765 public StringType getVersionAlgorithmStringType() throws FHIRException { 5766 if (this.versionAlgorithm == null) 5767 this.versionAlgorithm = new StringType(); 5768 if (!(this.versionAlgorithm instanceof StringType)) 5769 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 5770 return (StringType) this.versionAlgorithm; 5771 } 5772 5773 public boolean hasVersionAlgorithmStringType() { 5774 return this != null && this.versionAlgorithm instanceof StringType; 5775 } 5776 5777 /** 5778 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 5779 */ 5780 public Coding getVersionAlgorithmCoding() throws FHIRException { 5781 if (this.versionAlgorithm == null) 5782 this.versionAlgorithm = new Coding(); 5783 if (!(this.versionAlgorithm instanceof Coding)) 5784 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 5785 return (Coding) this.versionAlgorithm; 5786 } 5787 5788 public boolean hasVersionAlgorithmCoding() { 5789 return this != null && this.versionAlgorithm instanceof Coding; 5790 } 5791 5792 public boolean hasVersionAlgorithm() { 5793 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 5794 } 5795 5796 /** 5797 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 5798 */ 5799 public StructureMap setVersionAlgorithm(DataType value) { 5800 if (value != null && !(value instanceof StringType || value instanceof Coding)) 5801 throw new FHIRException("Not the right type for StructureMap.versionAlgorithm[x]: "+value.fhirType()); 5802 this.versionAlgorithm = value; 5803 return this; 5804 } 5805 5806 /** 5807 * @return {@link #name} (A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5808 */ 5809 public StringType getNameElement() { 5810 if (this.name == null) 5811 if (Configuration.errorOnAutoCreate()) 5812 throw new Error("Attempt to auto-create StructureMap.name"); 5813 else if (Configuration.doAutoCreate()) 5814 this.name = new StringType(); // bb 5815 return this.name; 5816 } 5817 5818 public boolean hasNameElement() { 5819 return this.name != null && !this.name.isEmpty(); 5820 } 5821 5822 public boolean hasName() { 5823 return this.name != null && !this.name.isEmpty(); 5824 } 5825 5826 /** 5827 * @param value {@link #name} (A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5828 */ 5829 public StructureMap setNameElement(StringType value) { 5830 this.name = value; 5831 return this; 5832 } 5833 5834 /** 5835 * @return A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation. 5836 */ 5837 public String getName() { 5838 return this.name == null ? null : this.name.getValue(); 5839 } 5840 5841 /** 5842 * @param value A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation. 5843 */ 5844 public StructureMap setName(String value) { 5845 if (this.name == null) 5846 this.name = new StringType(); 5847 this.name.setValue(value); 5848 return this; 5849 } 5850 5851 /** 5852 * @return {@link #title} (A short, descriptive, user-friendly title for the structure map.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 5853 */ 5854 public StringType getTitleElement() { 5855 if (this.title == null) 5856 if (Configuration.errorOnAutoCreate()) 5857 throw new Error("Attempt to auto-create StructureMap.title"); 5858 else if (Configuration.doAutoCreate()) 5859 this.title = new StringType(); // bb 5860 return this.title; 5861 } 5862 5863 public boolean hasTitleElement() { 5864 return this.title != null && !this.title.isEmpty(); 5865 } 5866 5867 public boolean hasTitle() { 5868 return this.title != null && !this.title.isEmpty(); 5869 } 5870 5871 /** 5872 * @param value {@link #title} (A short, descriptive, user-friendly title for the structure map.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 5873 */ 5874 public StructureMap setTitleElement(StringType value) { 5875 this.title = value; 5876 return this; 5877 } 5878 5879 /** 5880 * @return A short, descriptive, user-friendly title for the structure map. 5881 */ 5882 public String getTitle() { 5883 return this.title == null ? null : this.title.getValue(); 5884 } 5885 5886 /** 5887 * @param value A short, descriptive, user-friendly title for the structure map. 5888 */ 5889 public StructureMap setTitle(String value) { 5890 if (Utilities.noString(value)) 5891 this.title = null; 5892 else { 5893 if (this.title == null) 5894 this.title = new StringType(); 5895 this.title.setValue(value); 5896 } 5897 return this; 5898 } 5899 5900 /** 5901 * @return {@link #status} (The status of this structure map. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 5902 */ 5903 public Enumeration<PublicationStatus> getStatusElement() { 5904 if (this.status == null) 5905 if (Configuration.errorOnAutoCreate()) 5906 throw new Error("Attempt to auto-create StructureMap.status"); 5907 else if (Configuration.doAutoCreate()) 5908 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 5909 return this.status; 5910 } 5911 5912 public boolean hasStatusElement() { 5913 return this.status != null && !this.status.isEmpty(); 5914 } 5915 5916 public boolean hasStatus() { 5917 return this.status != null && !this.status.isEmpty(); 5918 } 5919 5920 /** 5921 * @param value {@link #status} (The status of this structure map. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 5922 */ 5923 public StructureMap setStatusElement(Enumeration<PublicationStatus> value) { 5924 this.status = value; 5925 return this; 5926 } 5927 5928 /** 5929 * @return The status of this structure map. Enables tracking the life-cycle of the content. 5930 */ 5931 public PublicationStatus getStatus() { 5932 return this.status == null ? null : this.status.getValue(); 5933 } 5934 5935 /** 5936 * @param value The status of this structure map. Enables tracking the life-cycle of the content. 5937 */ 5938 public StructureMap setStatus(PublicationStatus value) { 5939 if (this.status == null) 5940 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 5941 this.status.setValue(value); 5942 return this; 5943 } 5944 5945 /** 5946 * @return {@link #experimental} (A Boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 5947 */ 5948 public BooleanType getExperimentalElement() { 5949 if (this.experimental == null) 5950 if (Configuration.errorOnAutoCreate()) 5951 throw new Error("Attempt to auto-create StructureMap.experimental"); 5952 else if (Configuration.doAutoCreate()) 5953 this.experimental = new BooleanType(); // bb 5954 return this.experimental; 5955 } 5956 5957 public boolean hasExperimentalElement() { 5958 return this.experimental != null && !this.experimental.isEmpty(); 5959 } 5960 5961 public boolean hasExperimental() { 5962 return this.experimental != null && !this.experimental.isEmpty(); 5963 } 5964 5965 /** 5966 * @param value {@link #experimental} (A Boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 5967 */ 5968 public StructureMap setExperimentalElement(BooleanType value) { 5969 this.experimental = value; 5970 return this; 5971 } 5972 5973 /** 5974 * @return A Boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 5975 */ 5976 public boolean getExperimental() { 5977 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 5978 } 5979 5980 /** 5981 * @param value A Boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 5982 */ 5983 public StructureMap setExperimental(boolean value) { 5984 if (this.experimental == null) 5985 this.experimental = new BooleanType(); 5986 this.experimental.setValue(value); 5987 return this; 5988 } 5989 5990 /** 5991 * @return {@link #date} (The date (and optionally time) when the structure map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 5992 */ 5993 public DateTimeType getDateElement() { 5994 if (this.date == null) 5995 if (Configuration.errorOnAutoCreate()) 5996 throw new Error("Attempt to auto-create StructureMap.date"); 5997 else if (Configuration.doAutoCreate()) 5998 this.date = new DateTimeType(); // bb 5999 return this.date; 6000 } 6001 6002 public boolean hasDateElement() { 6003 return this.date != null && !this.date.isEmpty(); 6004 } 6005 6006 public boolean hasDate() { 6007 return this.date != null && !this.date.isEmpty(); 6008 } 6009 6010 /** 6011 * @param value {@link #date} (The date (and optionally time) when the structure map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 6012 */ 6013 public StructureMap setDateElement(DateTimeType value) { 6014 this.date = value; 6015 return this; 6016 } 6017 6018 /** 6019 * @return The date (and optionally time) when the structure map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes. 6020 */ 6021 public Date getDate() { 6022 return this.date == null ? null : this.date.getValue(); 6023 } 6024 6025 /** 6026 * @param value The date (and optionally time) when the structure map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes. 6027 */ 6028 public StructureMap setDate(Date value) { 6029 if (value == null) 6030 this.date = null; 6031 else { 6032 if (this.date == null) 6033 this.date = new DateTimeType(); 6034 this.date.setValue(value); 6035 } 6036 return this; 6037 } 6038 6039 /** 6040 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the structure map.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 6041 */ 6042 public StringType getPublisherElement() { 6043 if (this.publisher == null) 6044 if (Configuration.errorOnAutoCreate()) 6045 throw new Error("Attempt to auto-create StructureMap.publisher"); 6046 else if (Configuration.doAutoCreate()) 6047 this.publisher = new StringType(); // bb 6048 return this.publisher; 6049 } 6050 6051 public boolean hasPublisherElement() { 6052 return this.publisher != null && !this.publisher.isEmpty(); 6053 } 6054 6055 public boolean hasPublisher() { 6056 return this.publisher != null && !this.publisher.isEmpty(); 6057 } 6058 6059 /** 6060 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the structure map.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 6061 */ 6062 public StructureMap setPublisherElement(StringType value) { 6063 this.publisher = value; 6064 return this; 6065 } 6066 6067 /** 6068 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the structure map. 6069 */ 6070 public String getPublisher() { 6071 return this.publisher == null ? null : this.publisher.getValue(); 6072 } 6073 6074 /** 6075 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the structure map. 6076 */ 6077 public StructureMap setPublisher(String value) { 6078 if (Utilities.noString(value)) 6079 this.publisher = null; 6080 else { 6081 if (this.publisher == null) 6082 this.publisher = new StringType(); 6083 this.publisher.setValue(value); 6084 } 6085 return this; 6086 } 6087 6088 /** 6089 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 6090 */ 6091 public List<ContactDetail> getContact() { 6092 if (this.contact == null) 6093 this.contact = new ArrayList<ContactDetail>(); 6094 return this.contact; 6095 } 6096 6097 /** 6098 * @return Returns a reference to <code>this</code> for easy method chaining 6099 */ 6100 public StructureMap setContact(List<ContactDetail> theContact) { 6101 this.contact = theContact; 6102 return this; 6103 } 6104 6105 public boolean hasContact() { 6106 if (this.contact == null) 6107 return false; 6108 for (ContactDetail item : this.contact) 6109 if (!item.isEmpty()) 6110 return true; 6111 return false; 6112 } 6113 6114 public ContactDetail addContact() { //3 6115 ContactDetail t = new ContactDetail(); 6116 if (this.contact == null) 6117 this.contact = new ArrayList<ContactDetail>(); 6118 this.contact.add(t); 6119 return t; 6120 } 6121 6122 public StructureMap addContact(ContactDetail t) { //3 6123 if (t == null) 6124 return this; 6125 if (this.contact == null) 6126 this.contact = new ArrayList<ContactDetail>(); 6127 this.contact.add(t); 6128 return this; 6129 } 6130 6131 /** 6132 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 6133 */ 6134 public ContactDetail getContactFirstRep() { 6135 if (getContact().isEmpty()) { 6136 addContact(); 6137 } 6138 return getContact().get(0); 6139 } 6140 6141 /** 6142 * @return {@link #description} (A free text natural language description of the structure map from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 6143 */ 6144 public MarkdownType getDescriptionElement() { 6145 if (this.description == null) 6146 if (Configuration.errorOnAutoCreate()) 6147 throw new Error("Attempt to auto-create StructureMap.description"); 6148 else if (Configuration.doAutoCreate()) 6149 this.description = new MarkdownType(); // bb 6150 return this.description; 6151 } 6152 6153 public boolean hasDescriptionElement() { 6154 return this.description != null && !this.description.isEmpty(); 6155 } 6156 6157 public boolean hasDescription() { 6158 return this.description != null && !this.description.isEmpty(); 6159 } 6160 6161 /** 6162 * @param value {@link #description} (A free text natural language description of the structure map from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 6163 */ 6164 public StructureMap setDescriptionElement(MarkdownType value) { 6165 this.description = value; 6166 return this; 6167 } 6168 6169 /** 6170 * @return A free text natural language description of the structure map from a consumer's perspective. 6171 */ 6172 public String getDescription() { 6173 return this.description == null ? null : this.description.getValue(); 6174 } 6175 6176 /** 6177 * @param value A free text natural language description of the structure map from a consumer's perspective. 6178 */ 6179 public StructureMap setDescription(String value) { 6180 if (Utilities.noString(value)) 6181 this.description = null; 6182 else { 6183 if (this.description == null) 6184 this.description = new MarkdownType(); 6185 this.description.setValue(value); 6186 } 6187 return this; 6188 } 6189 6190 /** 6191 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure map instances.) 6192 */ 6193 public List<UsageContext> getUseContext() { 6194 if (this.useContext == null) 6195 this.useContext = new ArrayList<UsageContext>(); 6196 return this.useContext; 6197 } 6198 6199 /** 6200 * @return Returns a reference to <code>this</code> for easy method chaining 6201 */ 6202 public StructureMap setUseContext(List<UsageContext> theUseContext) { 6203 this.useContext = theUseContext; 6204 return this; 6205 } 6206 6207 public boolean hasUseContext() { 6208 if (this.useContext == null) 6209 return false; 6210 for (UsageContext item : this.useContext) 6211 if (!item.isEmpty()) 6212 return true; 6213 return false; 6214 } 6215 6216 public UsageContext addUseContext() { //3 6217 UsageContext t = new UsageContext(); 6218 if (this.useContext == null) 6219 this.useContext = new ArrayList<UsageContext>(); 6220 this.useContext.add(t); 6221 return t; 6222 } 6223 6224 public StructureMap addUseContext(UsageContext t) { //3 6225 if (t == null) 6226 return this; 6227 if (this.useContext == null) 6228 this.useContext = new ArrayList<UsageContext>(); 6229 this.useContext.add(t); 6230 return this; 6231 } 6232 6233 /** 6234 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 6235 */ 6236 public UsageContext getUseContextFirstRep() { 6237 if (getUseContext().isEmpty()) { 6238 addUseContext(); 6239 } 6240 return getUseContext().get(0); 6241 } 6242 6243 /** 6244 * @return {@link #jurisdiction} (A legal or geographic region in which the structure map is intended to be used.) 6245 */ 6246 public List<CodeableConcept> getJurisdiction() { 6247 if (this.jurisdiction == null) 6248 this.jurisdiction = new ArrayList<CodeableConcept>(); 6249 return this.jurisdiction; 6250 } 6251 6252 /** 6253 * @return Returns a reference to <code>this</code> for easy method chaining 6254 */ 6255 public StructureMap setJurisdiction(List<CodeableConcept> theJurisdiction) { 6256 this.jurisdiction = theJurisdiction; 6257 return this; 6258 } 6259 6260 public boolean hasJurisdiction() { 6261 if (this.jurisdiction == null) 6262 return false; 6263 for (CodeableConcept item : this.jurisdiction) 6264 if (!item.isEmpty()) 6265 return true; 6266 return false; 6267 } 6268 6269 public CodeableConcept addJurisdiction() { //3 6270 CodeableConcept t = new CodeableConcept(); 6271 if (this.jurisdiction == null) 6272 this.jurisdiction = new ArrayList<CodeableConcept>(); 6273 this.jurisdiction.add(t); 6274 return t; 6275 } 6276 6277 public StructureMap addJurisdiction(CodeableConcept t) { //3 6278 if (t == null) 6279 return this; 6280 if (this.jurisdiction == null) 6281 this.jurisdiction = new ArrayList<CodeableConcept>(); 6282 this.jurisdiction.add(t); 6283 return this; 6284 } 6285 6286 /** 6287 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 6288 */ 6289 public CodeableConcept getJurisdictionFirstRep() { 6290 if (getJurisdiction().isEmpty()) { 6291 addJurisdiction(); 6292 } 6293 return getJurisdiction().get(0); 6294 } 6295 6296 /** 6297 * @return {@link #purpose} (Explanation of why this structure map is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 6298 */ 6299 public MarkdownType getPurposeElement() { 6300 if (this.purpose == null) 6301 if (Configuration.errorOnAutoCreate()) 6302 throw new Error("Attempt to auto-create StructureMap.purpose"); 6303 else if (Configuration.doAutoCreate()) 6304 this.purpose = new MarkdownType(); // bb 6305 return this.purpose; 6306 } 6307 6308 public boolean hasPurposeElement() { 6309 return this.purpose != null && !this.purpose.isEmpty(); 6310 } 6311 6312 public boolean hasPurpose() { 6313 return this.purpose != null && !this.purpose.isEmpty(); 6314 } 6315 6316 /** 6317 * @param value {@link #purpose} (Explanation of why this structure map is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 6318 */ 6319 public StructureMap setPurposeElement(MarkdownType value) { 6320 this.purpose = value; 6321 return this; 6322 } 6323 6324 /** 6325 * @return Explanation of why this structure map is needed and why it has been designed as it has. 6326 */ 6327 public String getPurpose() { 6328 return this.purpose == null ? null : this.purpose.getValue(); 6329 } 6330 6331 /** 6332 * @param value Explanation of why this structure map is needed and why it has been designed as it has. 6333 */ 6334 public StructureMap setPurpose(String value) { 6335 if (Utilities.noString(value)) 6336 this.purpose = null; 6337 else { 6338 if (this.purpose == null) 6339 this.purpose = new MarkdownType(); 6340 this.purpose.setValue(value); 6341 } 6342 return this; 6343 } 6344 6345 /** 6346 * @return {@link #copyright} (A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 6347 */ 6348 public MarkdownType getCopyrightElement() { 6349 if (this.copyright == null) 6350 if (Configuration.errorOnAutoCreate()) 6351 throw new Error("Attempt to auto-create StructureMap.copyright"); 6352 else if (Configuration.doAutoCreate()) 6353 this.copyright = new MarkdownType(); // bb 6354 return this.copyright; 6355 } 6356 6357 public boolean hasCopyrightElement() { 6358 return this.copyright != null && !this.copyright.isEmpty(); 6359 } 6360 6361 public boolean hasCopyright() { 6362 return this.copyright != null && !this.copyright.isEmpty(); 6363 } 6364 6365 /** 6366 * @param value {@link #copyright} (A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 6367 */ 6368 public StructureMap setCopyrightElement(MarkdownType value) { 6369 this.copyright = value; 6370 return this; 6371 } 6372 6373 /** 6374 * @return A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map. 6375 */ 6376 public String getCopyright() { 6377 return this.copyright == null ? null : this.copyright.getValue(); 6378 } 6379 6380 /** 6381 * @param value A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map. 6382 */ 6383 public StructureMap setCopyright(String value) { 6384 if (Utilities.noString(value)) 6385 this.copyright = null; 6386 else { 6387 if (this.copyright == null) 6388 this.copyright = new MarkdownType(); 6389 this.copyright.setValue(value); 6390 } 6391 return this; 6392 } 6393 6394 /** 6395 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 6396 */ 6397 public StringType getCopyrightLabelElement() { 6398 if (this.copyrightLabel == null) 6399 if (Configuration.errorOnAutoCreate()) 6400 throw new Error("Attempt to auto-create StructureMap.copyrightLabel"); 6401 else if (Configuration.doAutoCreate()) 6402 this.copyrightLabel = new StringType(); // bb 6403 return this.copyrightLabel; 6404 } 6405 6406 public boolean hasCopyrightLabelElement() { 6407 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 6408 } 6409 6410 public boolean hasCopyrightLabel() { 6411 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 6412 } 6413 6414 /** 6415 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 6416 */ 6417 public StructureMap setCopyrightLabelElement(StringType value) { 6418 this.copyrightLabel = value; 6419 return this; 6420 } 6421 6422 /** 6423 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 6424 */ 6425 public String getCopyrightLabel() { 6426 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 6427 } 6428 6429 /** 6430 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 6431 */ 6432 public StructureMap setCopyrightLabel(String value) { 6433 if (Utilities.noString(value)) 6434 this.copyrightLabel = null; 6435 else { 6436 if (this.copyrightLabel == null) 6437 this.copyrightLabel = new StringType(); 6438 this.copyrightLabel.setValue(value); 6439 } 6440 return this; 6441 } 6442 6443 /** 6444 * @return {@link #structure} (A structure definition used by this map. The structure definition may describe instances that are converted, or the instances that are produced.) 6445 */ 6446 public List<StructureMapStructureComponent> getStructure() { 6447 if (this.structure == null) 6448 this.structure = new ArrayList<StructureMapStructureComponent>(); 6449 return this.structure; 6450 } 6451 6452 /** 6453 * @return Returns a reference to <code>this</code> for easy method chaining 6454 */ 6455 public StructureMap setStructure(List<StructureMapStructureComponent> theStructure) { 6456 this.structure = theStructure; 6457 return this; 6458 } 6459 6460 public boolean hasStructure() { 6461 if (this.structure == null) 6462 return false; 6463 for (StructureMapStructureComponent item : this.structure) 6464 if (!item.isEmpty()) 6465 return true; 6466 return false; 6467 } 6468 6469 public StructureMapStructureComponent addStructure() { //3 6470 StructureMapStructureComponent t = new StructureMapStructureComponent(); 6471 if (this.structure == null) 6472 this.structure = new ArrayList<StructureMapStructureComponent>(); 6473 this.structure.add(t); 6474 return t; 6475 } 6476 6477 public StructureMap addStructure(StructureMapStructureComponent t) { //3 6478 if (t == null) 6479 return this; 6480 if (this.structure == null) 6481 this.structure = new ArrayList<StructureMapStructureComponent>(); 6482 this.structure.add(t); 6483 return this; 6484 } 6485 6486 /** 6487 * @return The first repetition of repeating field {@link #structure}, creating it if it does not already exist {3} 6488 */ 6489 public StructureMapStructureComponent getStructureFirstRep() { 6490 if (getStructure().isEmpty()) { 6491 addStructure(); 6492 } 6493 return getStructure().get(0); 6494 } 6495 6496 /** 6497 * @return {@link #import_} (Other maps used by this map (canonical URLs).) 6498 */ 6499 public List<CanonicalType> getImport() { 6500 if (this.import_ == null) 6501 this.import_ = new ArrayList<CanonicalType>(); 6502 return this.import_; 6503 } 6504 6505 /** 6506 * @return Returns a reference to <code>this</code> for easy method chaining 6507 */ 6508 public StructureMap setImport(List<CanonicalType> theImport) { 6509 this.import_ = theImport; 6510 return this; 6511 } 6512 6513 public boolean hasImport() { 6514 if (this.import_ == null) 6515 return false; 6516 for (CanonicalType item : this.import_) 6517 if (!item.isEmpty()) 6518 return true; 6519 return false; 6520 } 6521 6522 /** 6523 * @return {@link #import_} (Other maps used by this map (canonical URLs).) 6524 */ 6525 public CanonicalType addImportElement() {//2 6526 CanonicalType t = new CanonicalType(); 6527 if (this.import_ == null) 6528 this.import_ = new ArrayList<CanonicalType>(); 6529 this.import_.add(t); 6530 return t; 6531 } 6532 6533 /** 6534 * @param value {@link #import_} (Other maps used by this map (canonical URLs).) 6535 */ 6536 public StructureMap addImport(String value) { //1 6537 CanonicalType t = new CanonicalType(); 6538 t.setValue(value); 6539 if (this.import_ == null) 6540 this.import_ = new ArrayList<CanonicalType>(); 6541 this.import_.add(t); 6542 return this; 6543 } 6544 6545 /** 6546 * @param value {@link #import_} (Other maps used by this map (canonical URLs).) 6547 */ 6548 public boolean hasImport(String value) { 6549 if (this.import_ == null) 6550 return false; 6551 for (CanonicalType v : this.import_) 6552 if (v.getValue().equals(value)) // canonical 6553 return true; 6554 return false; 6555 } 6556 6557 /** 6558 * @return {@link #const_} (Definition of a constant value used in the map rules.) 6559 */ 6560 public List<StructureMapConstComponent> getConst() { 6561 if (this.const_ == null) 6562 this.const_ = new ArrayList<StructureMapConstComponent>(); 6563 return this.const_; 6564 } 6565 6566 /** 6567 * @return Returns a reference to <code>this</code> for easy method chaining 6568 */ 6569 public StructureMap setConst(List<StructureMapConstComponent> theConst) { 6570 this.const_ = theConst; 6571 return this; 6572 } 6573 6574 public boolean hasConst() { 6575 if (this.const_ == null) 6576 return false; 6577 for (StructureMapConstComponent item : this.const_) 6578 if (!item.isEmpty()) 6579 return true; 6580 return false; 6581 } 6582 6583 public StructureMapConstComponent addConst() { //3 6584 StructureMapConstComponent t = new StructureMapConstComponent(); 6585 if (this.const_ == null) 6586 this.const_ = new ArrayList<StructureMapConstComponent>(); 6587 this.const_.add(t); 6588 return t; 6589 } 6590 6591 public StructureMap addConst(StructureMapConstComponent t) { //3 6592 if (t == null) 6593 return this; 6594 if (this.const_ == null) 6595 this.const_ = new ArrayList<StructureMapConstComponent>(); 6596 this.const_.add(t); 6597 return this; 6598 } 6599 6600 /** 6601 * @return The first repetition of repeating field {@link #const_}, creating it if it does not already exist {3} 6602 */ 6603 public StructureMapConstComponent getConstFirstRep() { 6604 if (getConst().isEmpty()) { 6605 addConst(); 6606 } 6607 return getConst().get(0); 6608 } 6609 6610 /** 6611 * @return {@link #group} (Organizes the mapping into managable chunks for human review/ease of maintenance.) 6612 */ 6613 public List<StructureMapGroupComponent> getGroup() { 6614 if (this.group == null) 6615 this.group = new ArrayList<StructureMapGroupComponent>(); 6616 return this.group; 6617 } 6618 6619 /** 6620 * @return Returns a reference to <code>this</code> for easy method chaining 6621 */ 6622 public StructureMap setGroup(List<StructureMapGroupComponent> theGroup) { 6623 this.group = theGroup; 6624 return this; 6625 } 6626 6627 public boolean hasGroup() { 6628 if (this.group == null) 6629 return false; 6630 for (StructureMapGroupComponent item : this.group) 6631 if (!item.isEmpty()) 6632 return true; 6633 return false; 6634 } 6635 6636 public StructureMapGroupComponent addGroup() { //3 6637 StructureMapGroupComponent t = new StructureMapGroupComponent(); 6638 if (this.group == null) 6639 this.group = new ArrayList<StructureMapGroupComponent>(); 6640 this.group.add(t); 6641 return t; 6642 } 6643 6644 public StructureMap addGroup(StructureMapGroupComponent t) { //3 6645 if (t == null) 6646 return this; 6647 if (this.group == null) 6648 this.group = new ArrayList<StructureMapGroupComponent>(); 6649 this.group.add(t); 6650 return this; 6651 } 6652 6653 /** 6654 * @return The first repetition of repeating field {@link #group}, creating it if it does not already exist {3} 6655 */ 6656 public StructureMapGroupComponent getGroupFirstRep() { 6657 if (getGroup().isEmpty()) { 6658 addGroup(); 6659 } 6660 return getGroup().get(0); 6661 } 6662 6663 protected void listChildren(List<Property> children) { 6664 super.listChildren(children); 6665 children.add(new Property("url", "uri", "An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure map is stored on different servers.", 0, 1, url)); 6666 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this structure map when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 6667 children.add(new Property("version", "string", "The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 6668 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 6669 children.add(new Property("name", "string", "A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 6670 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the structure map.", 0, 1, title)); 6671 children.add(new Property("status", "code", "The status of this structure map. Enables tracking the life-cycle of the content.", 0, 1, status)); 6672 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 6673 children.add(new Property("date", "dateTime", "The date (and optionally time) when the structure map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes.", 0, 1, date)); 6674 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the structure map.", 0, 1, publisher)); 6675 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 6676 children.add(new Property("description", "markdown", "A free text natural language description of the structure map from a consumer's perspective.", 0, 1, description)); 6677 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure map instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 6678 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the structure map is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 6679 children.add(new Property("purpose", "markdown", "Explanation of why this structure map is needed and why it has been designed as it has.", 0, 1, purpose)); 6680 children.add(new Property("copyright", "markdown", "A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map.", 0, 1, copyright)); 6681 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 6682 children.add(new Property("structure", "", "A structure definition used by this map. The structure definition may describe instances that are converted, or the instances that are produced.", 0, java.lang.Integer.MAX_VALUE, structure)); 6683 children.add(new Property("import", "canonical(StructureMap)", "Other maps used by this map (canonical URLs).", 0, java.lang.Integer.MAX_VALUE, import_)); 6684 children.add(new Property("const", "", "Definition of a constant value used in the map rules.", 0, java.lang.Integer.MAX_VALUE, const_)); 6685 children.add(new Property("group", "", "Organizes the mapping into managable chunks for human review/ease of maintenance.", 0, java.lang.Integer.MAX_VALUE, group)); 6686 } 6687 6688 @Override 6689 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6690 switch (_hash) { 6691 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure map is stored on different servers.", 0, 1, url); 6692 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this structure map when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 6693 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 6694 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 6695 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 6696 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 6697 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 6698 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 6699 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the structure map.", 0, 1, title); 6700 case -892481550: /*status*/ return new Property("status", "code", "The status of this structure map. Enables tracking the life-cycle of the content.", 0, 1, status); 6701 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 6702 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the structure map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes.", 0, 1, date); 6703 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the structure map.", 0, 1, publisher); 6704 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 6705 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the structure map from a consumer's perspective.", 0, 1, description); 6706 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure map instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 6707 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the structure map is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 6708 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this structure map is needed and why it has been designed as it has.", 0, 1, purpose); 6709 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map.", 0, 1, copyright); 6710 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 6711 case 144518515: /*structure*/ return new Property("structure", "", "A structure definition used by this map. The structure definition may describe instances that are converted, or the instances that are produced.", 0, java.lang.Integer.MAX_VALUE, structure); 6712 case -1184795739: /*import*/ return new Property("import", "canonical(StructureMap)", "Other maps used by this map (canonical URLs).", 0, java.lang.Integer.MAX_VALUE, import_); 6713 case 94844771: /*const*/ return new Property("const", "", "Definition of a constant value used in the map rules.", 0, java.lang.Integer.MAX_VALUE, const_); 6714 case 98629247: /*group*/ return new Property("group", "", "Organizes the mapping into managable chunks for human review/ease of maintenance.", 0, java.lang.Integer.MAX_VALUE, group); 6715 default: return super.getNamedProperty(_hash, _name, _checkValid); 6716 } 6717 6718 } 6719 6720 @Override 6721 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6722 switch (hash) { 6723 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 6724 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 6725 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 6726 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 6727 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 6728 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 6729 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 6730 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 6731 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 6732 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 6733 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 6734 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 6735 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 6736 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 6737 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 6738 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 6739 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 6740 case 144518515: /*structure*/ return this.structure == null ? new Base[0] : this.structure.toArray(new Base[this.structure.size()]); // StructureMapStructureComponent 6741 case -1184795739: /*import*/ return this.import_ == null ? new Base[0] : this.import_.toArray(new Base[this.import_.size()]); // CanonicalType 6742 case 94844771: /*const*/ return this.const_ == null ? new Base[0] : this.const_.toArray(new Base[this.const_.size()]); // StructureMapConstComponent 6743 case 98629247: /*group*/ return this.group == null ? new Base[0] : this.group.toArray(new Base[this.group.size()]); // StructureMapGroupComponent 6744 default: return super.getProperty(hash, name, checkValid); 6745 } 6746 6747 } 6748 6749 @Override 6750 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6751 switch (hash) { 6752 case 116079: // url 6753 this.url = TypeConvertor.castToUri(value); // UriType 6754 return value; 6755 case -1618432855: // identifier 6756 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 6757 return value; 6758 case 351608024: // version 6759 this.version = TypeConvertor.castToString(value); // StringType 6760 return value; 6761 case 1508158071: // versionAlgorithm 6762 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 6763 return value; 6764 case 3373707: // name 6765 this.name = TypeConvertor.castToString(value); // StringType 6766 return value; 6767 case 110371416: // title 6768 this.title = TypeConvertor.castToString(value); // StringType 6769 return value; 6770 case -892481550: // status 6771 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 6772 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 6773 return value; 6774 case -404562712: // experimental 6775 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 6776 return value; 6777 case 3076014: // date 6778 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 6779 return value; 6780 case 1447404028: // publisher 6781 this.publisher = TypeConvertor.castToString(value); // StringType 6782 return value; 6783 case 951526432: // contact 6784 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 6785 return value; 6786 case -1724546052: // description 6787 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 6788 return value; 6789 case -669707736: // useContext 6790 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 6791 return value; 6792 case -507075711: // jurisdiction 6793 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 6794 return value; 6795 case -220463842: // purpose 6796 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 6797 return value; 6798 case 1522889671: // copyright 6799 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 6800 return value; 6801 case 765157229: // copyrightLabel 6802 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 6803 return value; 6804 case 144518515: // structure 6805 this.getStructure().add((StructureMapStructureComponent) value); // StructureMapStructureComponent 6806 return value; 6807 case -1184795739: // import 6808 this.getImport().add(TypeConvertor.castToCanonical(value)); // CanonicalType 6809 return value; 6810 case 94844771: // const 6811 this.getConst().add((StructureMapConstComponent) value); // StructureMapConstComponent 6812 return value; 6813 case 98629247: // group 6814 this.getGroup().add((StructureMapGroupComponent) value); // StructureMapGroupComponent 6815 return value; 6816 default: return super.setProperty(hash, name, value); 6817 } 6818 6819 } 6820 6821 @Override 6822 public Base setProperty(String name, Base value) throws FHIRException { 6823 if (name.equals("url")) { 6824 this.url = TypeConvertor.castToUri(value); // UriType 6825 } else if (name.equals("identifier")) { 6826 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 6827 } else if (name.equals("version")) { 6828 this.version = TypeConvertor.castToString(value); // StringType 6829 } else if (name.equals("versionAlgorithm[x]")) { 6830 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 6831 } else if (name.equals("name")) { 6832 this.name = TypeConvertor.castToString(value); // StringType 6833 } else if (name.equals("title")) { 6834 this.title = TypeConvertor.castToString(value); // StringType 6835 } else if (name.equals("status")) { 6836 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 6837 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 6838 } else if (name.equals("experimental")) { 6839 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 6840 } else if (name.equals("date")) { 6841 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 6842 } else if (name.equals("publisher")) { 6843 this.publisher = TypeConvertor.castToString(value); // StringType 6844 } else if (name.equals("contact")) { 6845 this.getContact().add(TypeConvertor.castToContactDetail(value)); 6846 } else if (name.equals("description")) { 6847 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 6848 } else if (name.equals("useContext")) { 6849 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 6850 } else if (name.equals("jurisdiction")) { 6851 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 6852 } else if (name.equals("purpose")) { 6853 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 6854 } else if (name.equals("copyright")) { 6855 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 6856 } else if (name.equals("copyrightLabel")) { 6857 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 6858 } else if (name.equals("structure")) { 6859 this.getStructure().add((StructureMapStructureComponent) value); 6860 } else if (name.equals("import")) { 6861 this.getImport().add(TypeConvertor.castToCanonical(value)); 6862 } else if (name.equals("const")) { 6863 this.getConst().add((StructureMapConstComponent) value); 6864 } else if (name.equals("group")) { 6865 this.getGroup().add((StructureMapGroupComponent) value); 6866 } else 6867 return super.setProperty(name, value); 6868 return value; 6869 } 6870 6871 @Override 6872 public void removeChild(String name, Base value) throws FHIRException { 6873 if (name.equals("url")) { 6874 this.url = null; 6875 } else if (name.equals("identifier")) { 6876 this.getIdentifier().remove(value); 6877 } else if (name.equals("version")) { 6878 this.version = null; 6879 } else if (name.equals("versionAlgorithm[x]")) { 6880 this.versionAlgorithm = null; 6881 } else if (name.equals("name")) { 6882 this.name = null; 6883 } else if (name.equals("title")) { 6884 this.title = null; 6885 } else if (name.equals("status")) { 6886 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 6887 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 6888 } else if (name.equals("experimental")) { 6889 this.experimental = null; 6890 } else if (name.equals("date")) { 6891 this.date = null; 6892 } else if (name.equals("publisher")) { 6893 this.publisher = null; 6894 } else if (name.equals("contact")) { 6895 this.getContact().remove(value); 6896 } else if (name.equals("description")) { 6897 this.description = null; 6898 } else if (name.equals("useContext")) { 6899 this.getUseContext().remove(value); 6900 } else if (name.equals("jurisdiction")) { 6901 this.getJurisdiction().remove(value); 6902 } else if (name.equals("purpose")) { 6903 this.purpose = null; 6904 } else if (name.equals("copyright")) { 6905 this.copyright = null; 6906 } else if (name.equals("copyrightLabel")) { 6907 this.copyrightLabel = null; 6908 } else if (name.equals("structure")) { 6909 this.getStructure().remove((StructureMapStructureComponent) value); 6910 } else if (name.equals("import")) { 6911 this.getImport().remove(value); 6912 } else if (name.equals("const")) { 6913 this.getConst().remove((StructureMapConstComponent) value); 6914 } else if (name.equals("group")) { 6915 this.getGroup().remove((StructureMapGroupComponent) value); 6916 } else 6917 super.removeChild(name, value); 6918 6919 } 6920 6921 @Override 6922 public Base makeProperty(int hash, String name) throws FHIRException { 6923 switch (hash) { 6924 case 116079: return getUrlElement(); 6925 case -1618432855: return addIdentifier(); 6926 case 351608024: return getVersionElement(); 6927 case -115699031: return getVersionAlgorithm(); 6928 case 1508158071: return getVersionAlgorithm(); 6929 case 3373707: return getNameElement(); 6930 case 110371416: return getTitleElement(); 6931 case -892481550: return getStatusElement(); 6932 case -404562712: return getExperimentalElement(); 6933 case 3076014: return getDateElement(); 6934 case 1447404028: return getPublisherElement(); 6935 case 951526432: return addContact(); 6936 case -1724546052: return getDescriptionElement(); 6937 case -669707736: return addUseContext(); 6938 case -507075711: return addJurisdiction(); 6939 case -220463842: return getPurposeElement(); 6940 case 1522889671: return getCopyrightElement(); 6941 case 765157229: return getCopyrightLabelElement(); 6942 case 144518515: return addStructure(); 6943 case -1184795739: return addImportElement(); 6944 case 94844771: return addConst(); 6945 case 98629247: return addGroup(); 6946 default: return super.makeProperty(hash, name); 6947 } 6948 6949 } 6950 6951 @Override 6952 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6953 switch (hash) { 6954 case 116079: /*url*/ return new String[] {"uri"}; 6955 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 6956 case 351608024: /*version*/ return new String[] {"string"}; 6957 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 6958 case 3373707: /*name*/ return new String[] {"string"}; 6959 case 110371416: /*title*/ return new String[] {"string"}; 6960 case -892481550: /*status*/ return new String[] {"code"}; 6961 case -404562712: /*experimental*/ return new String[] {"boolean"}; 6962 case 3076014: /*date*/ return new String[] {"dateTime"}; 6963 case 1447404028: /*publisher*/ return new String[] {"string"}; 6964 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 6965 case -1724546052: /*description*/ return new String[] {"markdown"}; 6966 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 6967 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 6968 case -220463842: /*purpose*/ return new String[] {"markdown"}; 6969 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 6970 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 6971 case 144518515: /*structure*/ return new String[] {}; 6972 case -1184795739: /*import*/ return new String[] {"canonical"}; 6973 case 94844771: /*const*/ return new String[] {}; 6974 case 98629247: /*group*/ return new String[] {}; 6975 default: return super.getTypesForProperty(hash, name); 6976 } 6977 6978 } 6979 6980 @Override 6981 public Base addChild(String name) throws FHIRException { 6982 if (name.equals("url")) { 6983 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.url"); 6984 } 6985 else if (name.equals("identifier")) { 6986 return addIdentifier(); 6987 } 6988 else if (name.equals("version")) { 6989 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.version"); 6990 } 6991 else if (name.equals("versionAlgorithmString")) { 6992 this.versionAlgorithm = new StringType(); 6993 return this.versionAlgorithm; 6994 } 6995 else if (name.equals("versionAlgorithmCoding")) { 6996 this.versionAlgorithm = new Coding(); 6997 return this.versionAlgorithm; 6998 } 6999 else if (name.equals("name")) { 7000 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.name"); 7001 } 7002 else if (name.equals("title")) { 7003 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.title"); 7004 } 7005 else if (name.equals("status")) { 7006 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.status"); 7007 } 7008 else if (name.equals("experimental")) { 7009 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.experimental"); 7010 } 7011 else if (name.equals("date")) { 7012 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.date"); 7013 } 7014 else if (name.equals("publisher")) { 7015 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.publisher"); 7016 } 7017 else if (name.equals("contact")) { 7018 return addContact(); 7019 } 7020 else if (name.equals("description")) { 7021 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.description"); 7022 } 7023 else if (name.equals("useContext")) { 7024 return addUseContext(); 7025 } 7026 else if (name.equals("jurisdiction")) { 7027 return addJurisdiction(); 7028 } 7029 else if (name.equals("purpose")) { 7030 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.purpose"); 7031 } 7032 else if (name.equals("copyright")) { 7033 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.copyright"); 7034 } 7035 else if (name.equals("copyrightLabel")) { 7036 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.copyrightLabel"); 7037 } 7038 else if (name.equals("structure")) { 7039 return addStructure(); 7040 } 7041 else if (name.equals("import")) { 7042 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.import"); 7043 } 7044 else if (name.equals("const")) { 7045 return addConst(); 7046 } 7047 else if (name.equals("group")) { 7048 return addGroup(); 7049 } 7050 else 7051 return super.addChild(name); 7052 } 7053 7054 public String fhirType() { 7055 return "StructureMap"; 7056 7057 } 7058 7059 public StructureMap copy() { 7060 StructureMap dst = new StructureMap(); 7061 copyValues(dst); 7062 return dst; 7063 } 7064 7065 public void copyValues(StructureMap dst) { 7066 super.copyValues(dst); 7067 dst.url = url == null ? null : url.copy(); 7068 if (identifier != null) { 7069 dst.identifier = new ArrayList<Identifier>(); 7070 for (Identifier i : identifier) 7071 dst.identifier.add(i.copy()); 7072 }; 7073 dst.version = version == null ? null : version.copy(); 7074 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 7075 dst.name = name == null ? null : name.copy(); 7076 dst.title = title == null ? null : title.copy(); 7077 dst.status = status == null ? null : status.copy(); 7078 dst.experimental = experimental == null ? null : experimental.copy(); 7079 dst.date = date == null ? null : date.copy(); 7080 dst.publisher = publisher == null ? null : publisher.copy(); 7081 if (contact != null) { 7082 dst.contact = new ArrayList<ContactDetail>(); 7083 for (ContactDetail i : contact) 7084 dst.contact.add(i.copy()); 7085 }; 7086 dst.description = description == null ? null : description.copy(); 7087 if (useContext != null) { 7088 dst.useContext = new ArrayList<UsageContext>(); 7089 for (UsageContext i : useContext) 7090 dst.useContext.add(i.copy()); 7091 }; 7092 if (jurisdiction != null) { 7093 dst.jurisdiction = new ArrayList<CodeableConcept>(); 7094 for (CodeableConcept i : jurisdiction) 7095 dst.jurisdiction.add(i.copy()); 7096 }; 7097 dst.purpose = purpose == null ? null : purpose.copy(); 7098 dst.copyright = copyright == null ? null : copyright.copy(); 7099 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 7100 if (structure != null) { 7101 dst.structure = new ArrayList<StructureMapStructureComponent>(); 7102 for (StructureMapStructureComponent i : structure) 7103 dst.structure.add(i.copy()); 7104 }; 7105 if (import_ != null) { 7106 dst.import_ = new ArrayList<CanonicalType>(); 7107 for (CanonicalType i : import_) 7108 dst.import_.add(i.copy()); 7109 }; 7110 if (const_ != null) { 7111 dst.const_ = new ArrayList<StructureMapConstComponent>(); 7112 for (StructureMapConstComponent i : const_) 7113 dst.const_.add(i.copy()); 7114 }; 7115 if (group != null) { 7116 dst.group = new ArrayList<StructureMapGroupComponent>(); 7117 for (StructureMapGroupComponent i : group) 7118 dst.group.add(i.copy()); 7119 }; 7120 } 7121 7122 protected StructureMap typedCopy() { 7123 return copy(); 7124 } 7125 7126 @Override 7127 public boolean equalsDeep(Base other_) { 7128 if (!super.equalsDeep(other_)) 7129 return false; 7130 if (!(other_ instanceof StructureMap)) 7131 return false; 7132 StructureMap o = (StructureMap) other_; 7133 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 7134 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 7135 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 7136 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 7137 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 7138 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 7139 && compareDeep(structure, o.structure, true) && compareDeep(import_, o.import_, true) && compareDeep(const_, o.const_, true) 7140 && compareDeep(group, o.group, true); 7141 } 7142 7143 @Override 7144 public boolean equalsShallow(Base other_) { 7145 if (!super.equalsShallow(other_)) 7146 return false; 7147 if (!(other_ instanceof StructureMap)) 7148 return false; 7149 StructureMap o = (StructureMap) other_; 7150 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 7151 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 7152 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 7153 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 7154 && compareValues(import_, o.import_, true); 7155 } 7156 7157 public boolean isEmpty() { 7158 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 7159 , versionAlgorithm, name, title, status, experimental, date, publisher, contact 7160 , description, useContext, jurisdiction, purpose, copyright, copyrightLabel, structure 7161 , import_, const_, group); 7162 } 7163 7164 @Override 7165 public ResourceType getResourceType() { 7166 return ResourceType.StructureMap; 7167 } 7168 7169 /** 7170 * Search parameter: <b>context-quantity</b> 7171 * <p> 7172 * Description: <b>Multiple Resources: 7173 7174* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 7175* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 7176* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 7177* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 7178* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 7179* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 7180* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 7181* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 7182* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 7183* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 7184* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 7185* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 7186* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 7187* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 7188* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 7189* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 7190* [Library](library.html): A quantity- or range-valued use context assigned to the library 7191* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 7192* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 7193* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 7194* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 7195* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 7196* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 7197* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 7198* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 7199* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 7200* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 7201* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 7202* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 7203* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 7204</b><br> 7205 * Type: <b>quantity</b><br> 7206 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 7207 * </p> 7208 */ 7209 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 7210 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 7211 /** 7212 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 7213 * <p> 7214 * Description: <b>Multiple Resources: 7215 7216* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 7217* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 7218* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 7219* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 7220* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 7221* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 7222* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 7223* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 7224* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 7225* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 7226* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 7227* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 7228* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 7229* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 7230* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 7231* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 7232* [Library](library.html): A quantity- or range-valued use context assigned to the library 7233* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 7234* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 7235* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 7236* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 7237* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 7238* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 7239* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 7240* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 7241* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 7242* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 7243* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 7244* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 7245* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 7246</b><br> 7247 * Type: <b>quantity</b><br> 7248 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 7249 * </p> 7250 */ 7251 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 7252 7253 /** 7254 * Search parameter: <b>context-type-quantity</b> 7255 * <p> 7256 * Description: <b>Multiple Resources: 7257 7258* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 7259* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 7260* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 7261* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 7262* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 7263* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 7264* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 7265* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 7266* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 7267* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 7268* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 7269* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 7270* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 7271* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 7272* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 7273* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 7274* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 7275* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 7276* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 7277* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 7278* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 7279* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 7280* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 7281* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 7282* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 7283* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 7284* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 7285* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 7286* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 7287* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 7288</b><br> 7289 * Type: <b>composite</b><br> 7290 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 7291 * </p> 7292 */ 7293 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 7294 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 7295 /** 7296 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 7297 * <p> 7298 * Description: <b>Multiple Resources: 7299 7300* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 7301* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 7302* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 7303* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 7304* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 7305* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 7306* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 7307* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 7308* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 7309* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 7310* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 7311* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 7312* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 7313* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 7314* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 7315* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 7316* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 7317* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 7318* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 7319* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 7320* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 7321* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 7322* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 7323* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 7324* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 7325* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 7326* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 7327* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 7328* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 7329* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 7330</b><br> 7331 * Type: <b>composite</b><br> 7332 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 7333 * </p> 7334 */ 7335 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 7336 7337 /** 7338 * Search parameter: <b>context-type-value</b> 7339 * <p> 7340 * Description: <b>Multiple Resources: 7341 7342* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 7343* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 7344* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 7345* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 7346* [Citation](citation.html): A use context type and value assigned to the citation 7347* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 7348* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 7349* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 7350* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 7351* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 7352* [Evidence](evidence.html): A use context type and value assigned to the evidence 7353* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 7354* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 7355* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 7356* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 7357* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 7358* [Library](library.html): A use context type and value assigned to the library 7359* [Measure](measure.html): A use context type and value assigned to the measure 7360* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 7361* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 7362* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 7363* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 7364* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 7365* [Requirements](requirements.html): A use context type and value assigned to the requirements 7366* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 7367* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 7368* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 7369* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 7370* [TestScript](testscript.html): A use context type and value assigned to the test script 7371* [ValueSet](valueset.html): A use context type and value assigned to the value set 7372</b><br> 7373 * Type: <b>composite</b><br> 7374 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 7375 * </p> 7376 */ 7377 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 7378 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 7379 /** 7380 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 7381 * <p> 7382 * Description: <b>Multiple Resources: 7383 7384* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 7385* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 7386* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 7387* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 7388* [Citation](citation.html): A use context type and value assigned to the citation 7389* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 7390* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 7391* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 7392* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 7393* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 7394* [Evidence](evidence.html): A use context type and value assigned to the evidence 7395* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 7396* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 7397* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 7398* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 7399* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 7400* [Library](library.html): A use context type and value assigned to the library 7401* [Measure](measure.html): A use context type and value assigned to the measure 7402* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 7403* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 7404* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 7405* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 7406* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 7407* [Requirements](requirements.html): A use context type and value assigned to the requirements 7408* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 7409* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 7410* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 7411* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 7412* [TestScript](testscript.html): A use context type and value assigned to the test script 7413* [ValueSet](valueset.html): A use context type and value assigned to the value set 7414</b><br> 7415 * Type: <b>composite</b><br> 7416 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 7417 * </p> 7418 */ 7419 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 7420 7421 /** 7422 * Search parameter: <b>context-type</b> 7423 * <p> 7424 * Description: <b>Multiple Resources: 7425 7426* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 7427* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 7428* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 7429* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 7430* [Citation](citation.html): A type of use context assigned to the citation 7431* [CodeSystem](codesystem.html): A type of use context assigned to the code system 7432* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 7433* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 7434* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 7435* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 7436* [Evidence](evidence.html): A type of use context assigned to the evidence 7437* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 7438* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 7439* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 7440* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 7441* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 7442* [Library](library.html): A type of use context assigned to the library 7443* [Measure](measure.html): A type of use context assigned to the measure 7444* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 7445* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 7446* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 7447* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 7448* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 7449* [Requirements](requirements.html): A type of use context assigned to the requirements 7450* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 7451* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 7452* [StructureMap](structuremap.html): A type of use context assigned to the structure map 7453* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 7454* [TestScript](testscript.html): A type of use context assigned to the test script 7455* [ValueSet](valueset.html): A type of use context assigned to the value set 7456</b><br> 7457 * Type: <b>token</b><br> 7458 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 7459 * </p> 7460 */ 7461 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 7462 public static final String SP_CONTEXT_TYPE = "context-type"; 7463 /** 7464 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 7465 * <p> 7466 * Description: <b>Multiple Resources: 7467 7468* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 7469* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 7470* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 7471* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 7472* [Citation](citation.html): A type of use context assigned to the citation 7473* [CodeSystem](codesystem.html): A type of use context assigned to the code system 7474* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 7475* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 7476* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 7477* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 7478* [Evidence](evidence.html): A type of use context assigned to the evidence 7479* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 7480* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 7481* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 7482* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 7483* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 7484* [Library](library.html): A type of use context assigned to the library 7485* [Measure](measure.html): A type of use context assigned to the measure 7486* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 7487* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 7488* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 7489* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 7490* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 7491* [Requirements](requirements.html): A type of use context assigned to the requirements 7492* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 7493* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 7494* [StructureMap](structuremap.html): A type of use context assigned to the structure map 7495* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 7496* [TestScript](testscript.html): A type of use context assigned to the test script 7497* [ValueSet](valueset.html): A type of use context assigned to the value set 7498</b><br> 7499 * Type: <b>token</b><br> 7500 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 7501 * </p> 7502 */ 7503 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 7504 7505 /** 7506 * Search parameter: <b>context</b> 7507 * <p> 7508 * Description: <b>Multiple Resources: 7509 7510* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 7511* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 7512* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 7513* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 7514* [Citation](citation.html): A use context assigned to the citation 7515* [CodeSystem](codesystem.html): A use context assigned to the code system 7516* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 7517* [ConceptMap](conceptmap.html): A use context assigned to the concept map 7518* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 7519* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 7520* [Evidence](evidence.html): A use context assigned to the evidence 7521* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 7522* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 7523* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 7524* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 7525* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 7526* [Library](library.html): A use context assigned to the library 7527* [Measure](measure.html): A use context assigned to the measure 7528* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 7529* [NamingSystem](namingsystem.html): A use context assigned to the naming system 7530* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 7531* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 7532* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 7533* [Requirements](requirements.html): A use context assigned to the requirements 7534* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 7535* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 7536* [StructureMap](structuremap.html): A use context assigned to the structure map 7537* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 7538* [TestScript](testscript.html): A use context assigned to the test script 7539* [ValueSet](valueset.html): A use context assigned to the value set 7540</b><br> 7541 * Type: <b>token</b><br> 7542 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 7543 * </p> 7544 */ 7545 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 7546 public static final String SP_CONTEXT = "context"; 7547 /** 7548 * <b>Fluent Client</b> search parameter constant for <b>context</b> 7549 * <p> 7550 * Description: <b>Multiple Resources: 7551 7552* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 7553* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 7554* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 7555* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 7556* [Citation](citation.html): A use context assigned to the citation 7557* [CodeSystem](codesystem.html): A use context assigned to the code system 7558* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 7559* [ConceptMap](conceptmap.html): A use context assigned to the concept map 7560* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 7561* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 7562* [Evidence](evidence.html): A use context assigned to the evidence 7563* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 7564* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 7565* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 7566* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 7567* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 7568* [Library](library.html): A use context assigned to the library 7569* [Measure](measure.html): A use context assigned to the measure 7570* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 7571* [NamingSystem](namingsystem.html): A use context assigned to the naming system 7572* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 7573* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 7574* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 7575* [Requirements](requirements.html): A use context assigned to the requirements 7576* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 7577* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 7578* [StructureMap](structuremap.html): A use context assigned to the structure map 7579* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 7580* [TestScript](testscript.html): A use context assigned to the test script 7581* [ValueSet](valueset.html): A use context assigned to the value set 7582</b><br> 7583 * Type: <b>token</b><br> 7584 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 7585 * </p> 7586 */ 7587 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 7588 7589 /** 7590 * Search parameter: <b>date</b> 7591 * <p> 7592 * Description: <b>Multiple Resources: 7593 7594* [ActivityDefinition](activitydefinition.html): The activity definition publication date 7595* [ActorDefinition](actordefinition.html): The Actor Definition publication date 7596* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 7597* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 7598* [Citation](citation.html): The citation publication date 7599* [CodeSystem](codesystem.html): The code system publication date 7600* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 7601* [ConceptMap](conceptmap.html): The concept map publication date 7602* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 7603* [EventDefinition](eventdefinition.html): The event definition publication date 7604* [Evidence](evidence.html): The evidence publication date 7605* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 7606* [ExampleScenario](examplescenario.html): The example scenario publication date 7607* [GraphDefinition](graphdefinition.html): The graph definition publication date 7608* [ImplementationGuide](implementationguide.html): The implementation guide publication date 7609* [Library](library.html): The library publication date 7610* [Measure](measure.html): The measure publication date 7611* [MessageDefinition](messagedefinition.html): The message definition publication date 7612* [NamingSystem](namingsystem.html): The naming system publication date 7613* [OperationDefinition](operationdefinition.html): The operation definition publication date 7614* [PlanDefinition](plandefinition.html): The plan definition publication date 7615* [Questionnaire](questionnaire.html): The questionnaire publication date 7616* [Requirements](requirements.html): The requirements publication date 7617* [SearchParameter](searchparameter.html): The search parameter publication date 7618* [StructureDefinition](structuredefinition.html): The structure definition publication date 7619* [StructureMap](structuremap.html): The structure map publication date 7620* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 7621* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 7622* [TestScript](testscript.html): The test script publication date 7623* [ValueSet](valueset.html): The value set publication date 7624</b><br> 7625 * Type: <b>date</b><br> 7626 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 7627 * </p> 7628 */ 7629 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 7630 public static final String SP_DATE = "date"; 7631 /** 7632 * <b>Fluent Client</b> search parameter constant for <b>date</b> 7633 * <p> 7634 * Description: <b>Multiple Resources: 7635 7636* [ActivityDefinition](activitydefinition.html): The activity definition publication date 7637* [ActorDefinition](actordefinition.html): The Actor Definition publication date 7638* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 7639* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 7640* [Citation](citation.html): The citation publication date 7641* [CodeSystem](codesystem.html): The code system publication date 7642* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 7643* [ConceptMap](conceptmap.html): The concept map publication date 7644* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 7645* [EventDefinition](eventdefinition.html): The event definition publication date 7646* [Evidence](evidence.html): The evidence publication date 7647* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 7648* [ExampleScenario](examplescenario.html): The example scenario publication date 7649* [GraphDefinition](graphdefinition.html): The graph definition publication date 7650* [ImplementationGuide](implementationguide.html): The implementation guide publication date 7651* [Library](library.html): The library publication date 7652* [Measure](measure.html): The measure publication date 7653* [MessageDefinition](messagedefinition.html): The message definition publication date 7654* [NamingSystem](namingsystem.html): The naming system publication date 7655* [OperationDefinition](operationdefinition.html): The operation definition publication date 7656* [PlanDefinition](plandefinition.html): The plan definition publication date 7657* [Questionnaire](questionnaire.html): The questionnaire publication date 7658* [Requirements](requirements.html): The requirements publication date 7659* [SearchParameter](searchparameter.html): The search parameter publication date 7660* [StructureDefinition](structuredefinition.html): The structure definition publication date 7661* [StructureMap](structuremap.html): The structure map publication date 7662* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 7663* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 7664* [TestScript](testscript.html): The test script publication date 7665* [ValueSet](valueset.html): The value set publication date 7666</b><br> 7667 * Type: <b>date</b><br> 7668 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 7669 * </p> 7670 */ 7671 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 7672 7673 /** 7674 * Search parameter: <b>description</b> 7675 * <p> 7676 * Description: <b>Multiple Resources: 7677 7678* [ActivityDefinition](activitydefinition.html): The description of the activity definition 7679* [ActorDefinition](actordefinition.html): The description of the Actor Definition 7680* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 7681* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 7682* [Citation](citation.html): The description of the citation 7683* [CodeSystem](codesystem.html): The description of the code system 7684* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 7685* [ConceptMap](conceptmap.html): The description of the concept map 7686* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 7687* [EventDefinition](eventdefinition.html): The description of the event definition 7688* [Evidence](evidence.html): The description of the evidence 7689* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 7690* [GraphDefinition](graphdefinition.html): The description of the graph definition 7691* [ImplementationGuide](implementationguide.html): The description of the implementation guide 7692* [Library](library.html): The description of the library 7693* [Measure](measure.html): The description of the measure 7694* [MessageDefinition](messagedefinition.html): The description of the message definition 7695* [NamingSystem](namingsystem.html): The description of the naming system 7696* [OperationDefinition](operationdefinition.html): The description of the operation definition 7697* [PlanDefinition](plandefinition.html): The description of the plan definition 7698* [Questionnaire](questionnaire.html): The description of the questionnaire 7699* [Requirements](requirements.html): The description of the requirements 7700* [SearchParameter](searchparameter.html): The description of the search parameter 7701* [StructureDefinition](structuredefinition.html): The description of the structure definition 7702* [StructureMap](structuremap.html): The description of the structure map 7703* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 7704* [TestScript](testscript.html): The description of the test script 7705* [ValueSet](valueset.html): The description of the value set 7706</b><br> 7707 * Type: <b>string</b><br> 7708 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 7709 * </p> 7710 */ 7711 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 7712 public static final String SP_DESCRIPTION = "description"; 7713 /** 7714 * <b>Fluent Client</b> search parameter constant for <b>description</b> 7715 * <p> 7716 * Description: <b>Multiple Resources: 7717 7718* [ActivityDefinition](activitydefinition.html): The description of the activity definition 7719* [ActorDefinition](actordefinition.html): The description of the Actor Definition 7720* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 7721* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 7722* [Citation](citation.html): The description of the citation 7723* [CodeSystem](codesystem.html): The description of the code system 7724* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 7725* [ConceptMap](conceptmap.html): The description of the concept map 7726* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 7727* [EventDefinition](eventdefinition.html): The description of the event definition 7728* [Evidence](evidence.html): The description of the evidence 7729* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 7730* [GraphDefinition](graphdefinition.html): The description of the graph definition 7731* [ImplementationGuide](implementationguide.html): The description of the implementation guide 7732* [Library](library.html): The description of the library 7733* [Measure](measure.html): The description of the measure 7734* [MessageDefinition](messagedefinition.html): The description of the message definition 7735* [NamingSystem](namingsystem.html): The description of the naming system 7736* [OperationDefinition](operationdefinition.html): The description of the operation definition 7737* [PlanDefinition](plandefinition.html): The description of the plan definition 7738* [Questionnaire](questionnaire.html): The description of the questionnaire 7739* [Requirements](requirements.html): The description of the requirements 7740* [SearchParameter](searchparameter.html): The description of the search parameter 7741* [StructureDefinition](structuredefinition.html): The description of the structure definition 7742* [StructureMap](structuremap.html): The description of the structure map 7743* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 7744* [TestScript](testscript.html): The description of the test script 7745* [ValueSet](valueset.html): The description of the value set 7746</b><br> 7747 * Type: <b>string</b><br> 7748 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 7749 * </p> 7750 */ 7751 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 7752 7753 /** 7754 * Search parameter: <b>identifier</b> 7755 * <p> 7756 * Description: <b>Multiple Resources: 7757 7758* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 7759* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 7760* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 7761* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 7762* [Citation](citation.html): External identifier for the citation 7763* [CodeSystem](codesystem.html): External identifier for the code system 7764* [ConceptMap](conceptmap.html): External identifier for the concept map 7765* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 7766* [EventDefinition](eventdefinition.html): External identifier for the event definition 7767* [Evidence](evidence.html): External identifier for the evidence 7768* [EvidenceReport](evidencereport.html): External identifier for the evidence report 7769* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 7770* [ExampleScenario](examplescenario.html): External identifier for the example scenario 7771* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 7772* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 7773* [Library](library.html): External identifier for the library 7774* [Measure](measure.html): External identifier for the measure 7775* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 7776* [MessageDefinition](messagedefinition.html): External identifier for the message definition 7777* [NamingSystem](namingsystem.html): External identifier for the naming system 7778* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 7779* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 7780* [PlanDefinition](plandefinition.html): External identifier for the plan definition 7781* [Questionnaire](questionnaire.html): External identifier for the questionnaire 7782* [Requirements](requirements.html): External identifier for the requirements 7783* [SearchParameter](searchparameter.html): External identifier for the search parameter 7784* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 7785* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 7786* [StructureMap](structuremap.html): External identifier for the structure map 7787* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 7788* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 7789* [TestPlan](testplan.html): An identifier for the test plan 7790* [TestScript](testscript.html): External identifier for the test script 7791* [ValueSet](valueset.html): External identifier for the value set 7792</b><br> 7793 * Type: <b>token</b><br> 7794 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 7795 * </p> 7796 */ 7797 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 7798 public static final String SP_IDENTIFIER = "identifier"; 7799 /** 7800 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 7801 * <p> 7802 * Description: <b>Multiple Resources: 7803 7804* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 7805* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 7806* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 7807* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 7808* [Citation](citation.html): External identifier for the citation 7809* [CodeSystem](codesystem.html): External identifier for the code system 7810* [ConceptMap](conceptmap.html): External identifier for the concept map 7811* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 7812* [EventDefinition](eventdefinition.html): External identifier for the event definition 7813* [Evidence](evidence.html): External identifier for the evidence 7814* [EvidenceReport](evidencereport.html): External identifier for the evidence report 7815* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 7816* [ExampleScenario](examplescenario.html): External identifier for the example scenario 7817* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 7818* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 7819* [Library](library.html): External identifier for the library 7820* [Measure](measure.html): External identifier for the measure 7821* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 7822* [MessageDefinition](messagedefinition.html): External identifier for the message definition 7823* [NamingSystem](namingsystem.html): External identifier for the naming system 7824* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 7825* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 7826* [PlanDefinition](plandefinition.html): External identifier for the plan definition 7827* [Questionnaire](questionnaire.html): External identifier for the questionnaire 7828* [Requirements](requirements.html): External identifier for the requirements 7829* [SearchParameter](searchparameter.html): External identifier for the search parameter 7830* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 7831* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 7832* [StructureMap](structuremap.html): External identifier for the structure map 7833* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 7834* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 7835* [TestPlan](testplan.html): An identifier for the test plan 7836* [TestScript](testscript.html): External identifier for the test script 7837* [ValueSet](valueset.html): External identifier for the value set 7838</b><br> 7839 * Type: <b>token</b><br> 7840 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 7841 * </p> 7842 */ 7843 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 7844 7845 /** 7846 * Search parameter: <b>jurisdiction</b> 7847 * <p> 7848 * Description: <b>Multiple Resources: 7849 7850* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 7851* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 7852* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 7853* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 7854* [Citation](citation.html): Intended jurisdiction for the citation 7855* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 7856* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 7857* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 7858* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 7859* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 7860* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 7861* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 7862* [Library](library.html): Intended jurisdiction for the library 7863* [Measure](measure.html): Intended jurisdiction for the measure 7864* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 7865* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 7866* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 7867* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 7868* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 7869* [Requirements](requirements.html): Intended jurisdiction for the requirements 7870* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 7871* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 7872* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 7873* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 7874* [TestScript](testscript.html): Intended jurisdiction for the test script 7875* [ValueSet](valueset.html): Intended jurisdiction for the value set 7876</b><br> 7877 * Type: <b>token</b><br> 7878 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 7879 * </p> 7880 */ 7881 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 7882 public static final String SP_JURISDICTION = "jurisdiction"; 7883 /** 7884 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 7885 * <p> 7886 * Description: <b>Multiple Resources: 7887 7888* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 7889* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 7890* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 7891* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 7892* [Citation](citation.html): Intended jurisdiction for the citation 7893* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 7894* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 7895* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 7896* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 7897* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 7898* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 7899* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 7900* [Library](library.html): Intended jurisdiction for the library 7901* [Measure](measure.html): Intended jurisdiction for the measure 7902* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 7903* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 7904* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 7905* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 7906* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 7907* [Requirements](requirements.html): Intended jurisdiction for the requirements 7908* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 7909* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 7910* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 7911* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 7912* [TestScript](testscript.html): Intended jurisdiction for the test script 7913* [ValueSet](valueset.html): Intended jurisdiction for the value set 7914</b><br> 7915 * Type: <b>token</b><br> 7916 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 7917 * </p> 7918 */ 7919 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 7920 7921 /** 7922 * Search parameter: <b>name</b> 7923 * <p> 7924 * Description: <b>Multiple Resources: 7925 7926* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 7927* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 7928* [Citation](citation.html): Computationally friendly name of the citation 7929* [CodeSystem](codesystem.html): Computationally friendly name of the code system 7930* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 7931* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 7932* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 7933* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 7934* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 7935* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 7936* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 7937* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 7938* [Library](library.html): Computationally friendly name of the library 7939* [Measure](measure.html): Computationally friendly name of the measure 7940* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 7941* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 7942* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 7943* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 7944* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 7945* [Requirements](requirements.html): Computationally friendly name of the requirements 7946* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 7947* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 7948* [StructureMap](structuremap.html): Computationally friendly name of the structure map 7949* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 7950* [TestScript](testscript.html): Computationally friendly name of the test script 7951* [ValueSet](valueset.html): Computationally friendly name of the value set 7952</b><br> 7953 * Type: <b>string</b><br> 7954 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 7955 * </p> 7956 */ 7957 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 7958 public static final String SP_NAME = "name"; 7959 /** 7960 * <b>Fluent Client</b> search parameter constant for <b>name</b> 7961 * <p> 7962 * Description: <b>Multiple Resources: 7963 7964* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 7965* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 7966* [Citation](citation.html): Computationally friendly name of the citation 7967* [CodeSystem](codesystem.html): Computationally friendly name of the code system 7968* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 7969* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 7970* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 7971* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 7972* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 7973* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 7974* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 7975* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 7976* [Library](library.html): Computationally friendly name of the library 7977* [Measure](measure.html): Computationally friendly name of the measure 7978* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 7979* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 7980* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 7981* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 7982* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 7983* [Requirements](requirements.html): Computationally friendly name of the requirements 7984* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 7985* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 7986* [StructureMap](structuremap.html): Computationally friendly name of the structure map 7987* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 7988* [TestScript](testscript.html): Computationally friendly name of the test script 7989* [ValueSet](valueset.html): Computationally friendly name of the value set 7990</b><br> 7991 * Type: <b>string</b><br> 7992 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 7993 * </p> 7994 */ 7995 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 7996 7997 /** 7998 * Search parameter: <b>publisher</b> 7999 * <p> 8000 * Description: <b>Multiple Resources: 8001 8002* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 8003* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 8004* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 8005* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 8006* [Citation](citation.html): Name of the publisher of the citation 8007* [CodeSystem](codesystem.html): Name of the publisher of the code system 8008* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 8009* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 8010* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 8011* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 8012* [Evidence](evidence.html): Name of the publisher of the evidence 8013* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 8014* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 8015* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 8016* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 8017* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 8018* [Library](library.html): Name of the publisher of the library 8019* [Measure](measure.html): Name of the publisher of the measure 8020* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 8021* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 8022* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 8023* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 8024* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 8025* [Requirements](requirements.html): Name of the publisher of the requirements 8026* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 8027* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 8028* [StructureMap](structuremap.html): Name of the publisher of the structure map 8029* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 8030* [TestScript](testscript.html): Name of the publisher of the test script 8031* [ValueSet](valueset.html): Name of the publisher of the value set 8032</b><br> 8033 * Type: <b>string</b><br> 8034 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 8035 * </p> 8036 */ 8037 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 8038 public static final String SP_PUBLISHER = "publisher"; 8039 /** 8040 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 8041 * <p> 8042 * Description: <b>Multiple Resources: 8043 8044* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 8045* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 8046* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 8047* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 8048* [Citation](citation.html): Name of the publisher of the citation 8049* [CodeSystem](codesystem.html): Name of the publisher of the code system 8050* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 8051* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 8052* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 8053* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 8054* [Evidence](evidence.html): Name of the publisher of the evidence 8055* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 8056* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 8057* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 8058* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 8059* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 8060* [Library](library.html): Name of the publisher of the library 8061* [Measure](measure.html): Name of the publisher of the measure 8062* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 8063* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 8064* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 8065* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 8066* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 8067* [Requirements](requirements.html): Name of the publisher of the requirements 8068* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 8069* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 8070* [StructureMap](structuremap.html): Name of the publisher of the structure map 8071* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 8072* [TestScript](testscript.html): Name of the publisher of the test script 8073* [ValueSet](valueset.html): Name of the publisher of the value set 8074</b><br> 8075 * Type: <b>string</b><br> 8076 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 8077 * </p> 8078 */ 8079 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 8080 8081 /** 8082 * Search parameter: <b>status</b> 8083 * <p> 8084 * Description: <b>Multiple Resources: 8085 8086* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 8087* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 8088* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 8089* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 8090* [Citation](citation.html): The current status of the citation 8091* [CodeSystem](codesystem.html): The current status of the code system 8092* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 8093* [ConceptMap](conceptmap.html): The current status of the concept map 8094* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 8095* [EventDefinition](eventdefinition.html): The current status of the event definition 8096* [Evidence](evidence.html): The current status of the evidence 8097* [EvidenceReport](evidencereport.html): The current status of the evidence report 8098* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 8099* [ExampleScenario](examplescenario.html): The current status of the example scenario 8100* [GraphDefinition](graphdefinition.html): The current status of the graph definition 8101* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 8102* [Library](library.html): The current status of the library 8103* [Measure](measure.html): The current status of the measure 8104* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 8105* [MessageDefinition](messagedefinition.html): The current status of the message definition 8106* [NamingSystem](namingsystem.html): The current status of the naming system 8107* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 8108* [OperationDefinition](operationdefinition.html): The current status of the operation definition 8109* [PlanDefinition](plandefinition.html): The current status of the plan definition 8110* [Questionnaire](questionnaire.html): The current status of the questionnaire 8111* [Requirements](requirements.html): The current status of the requirements 8112* [SearchParameter](searchparameter.html): The current status of the search parameter 8113* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 8114* [StructureDefinition](structuredefinition.html): The current status of the structure definition 8115* [StructureMap](structuremap.html): The current status of the structure map 8116* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 8117* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 8118* [TestPlan](testplan.html): The current status of the test plan 8119* [TestScript](testscript.html): The current status of the test script 8120* [ValueSet](valueset.html): The current status of the value set 8121</b><br> 8122 * Type: <b>token</b><br> 8123 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 8124 * </p> 8125 */ 8126 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 8127 public static final String SP_STATUS = "status"; 8128 /** 8129 * <b>Fluent Client</b> search parameter constant for <b>status</b> 8130 * <p> 8131 * Description: <b>Multiple Resources: 8132 8133* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 8134* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 8135* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 8136* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 8137* [Citation](citation.html): The current status of the citation 8138* [CodeSystem](codesystem.html): The current status of the code system 8139* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 8140* [ConceptMap](conceptmap.html): The current status of the concept map 8141* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 8142* [EventDefinition](eventdefinition.html): The current status of the event definition 8143* [Evidence](evidence.html): The current status of the evidence 8144* [EvidenceReport](evidencereport.html): The current status of the evidence report 8145* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 8146* [ExampleScenario](examplescenario.html): The current status of the example scenario 8147* [GraphDefinition](graphdefinition.html): The current status of the graph definition 8148* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 8149* [Library](library.html): The current status of the library 8150* [Measure](measure.html): The current status of the measure 8151* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 8152* [MessageDefinition](messagedefinition.html): The current status of the message definition 8153* [NamingSystem](namingsystem.html): The current status of the naming system 8154* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 8155* [OperationDefinition](operationdefinition.html): The current status of the operation definition 8156* [PlanDefinition](plandefinition.html): The current status of the plan definition 8157* [Questionnaire](questionnaire.html): The current status of the questionnaire 8158* [Requirements](requirements.html): The current status of the requirements 8159* [SearchParameter](searchparameter.html): The current status of the search parameter 8160* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 8161* [StructureDefinition](structuredefinition.html): The current status of the structure definition 8162* [StructureMap](structuremap.html): The current status of the structure map 8163* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 8164* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 8165* [TestPlan](testplan.html): The current status of the test plan 8166* [TestScript](testscript.html): The current status of the test script 8167* [ValueSet](valueset.html): The current status of the value set 8168</b><br> 8169 * Type: <b>token</b><br> 8170 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 8171 * </p> 8172 */ 8173 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 8174 8175 /** 8176 * Search parameter: <b>title</b> 8177 * <p> 8178 * Description: <b>Multiple Resources: 8179 8180* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 8181* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 8182* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 8183* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 8184* [Citation](citation.html): The human-friendly name of the citation 8185* [CodeSystem](codesystem.html): The human-friendly name of the code system 8186* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 8187* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 8188* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 8189* [Evidence](evidence.html): The human-friendly name of the evidence 8190* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 8191* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 8192* [Library](library.html): The human-friendly name of the library 8193* [Measure](measure.html): The human-friendly name of the measure 8194* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 8195* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 8196* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 8197* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 8198* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 8199* [Requirements](requirements.html): The human-friendly name of the requirements 8200* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 8201* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 8202* [StructureMap](structuremap.html): The human-friendly name of the structure map 8203* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 8204* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 8205* [TestScript](testscript.html): The human-friendly name of the test script 8206* [ValueSet](valueset.html): The human-friendly name of the value set 8207</b><br> 8208 * Type: <b>string</b><br> 8209 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 8210 * </p> 8211 */ 8212 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 8213 public static final String SP_TITLE = "title"; 8214 /** 8215 * <b>Fluent Client</b> search parameter constant for <b>title</b> 8216 * <p> 8217 * Description: <b>Multiple Resources: 8218 8219* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 8220* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 8221* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 8222* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 8223* [Citation](citation.html): The human-friendly name of the citation 8224* [CodeSystem](codesystem.html): The human-friendly name of the code system 8225* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 8226* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 8227* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 8228* [Evidence](evidence.html): The human-friendly name of the evidence 8229* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 8230* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 8231* [Library](library.html): The human-friendly name of the library 8232* [Measure](measure.html): The human-friendly name of the measure 8233* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 8234* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 8235* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 8236* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 8237* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 8238* [Requirements](requirements.html): The human-friendly name of the requirements 8239* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 8240* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 8241* [StructureMap](structuremap.html): The human-friendly name of the structure map 8242* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 8243* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 8244* [TestScript](testscript.html): The human-friendly name of the test script 8245* [ValueSet](valueset.html): The human-friendly name of the value set 8246</b><br> 8247 * Type: <b>string</b><br> 8248 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 8249 * </p> 8250 */ 8251 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 8252 8253 /** 8254 * Search parameter: <b>url</b> 8255 * <p> 8256 * Description: <b>Multiple Resources: 8257 8258* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 8259* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 8260* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 8261* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 8262* [Citation](citation.html): The uri that identifies the citation 8263* [CodeSystem](codesystem.html): The uri that identifies the code system 8264* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 8265* [ConceptMap](conceptmap.html): The URI that identifies the concept map 8266* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 8267* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 8268* [Evidence](evidence.html): The uri that identifies the evidence 8269* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 8270* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 8271* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 8272* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 8273* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 8274* [Library](library.html): The uri that identifies the library 8275* [Measure](measure.html): The uri that identifies the measure 8276* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 8277* [NamingSystem](namingsystem.html): The uri that identifies the naming system 8278* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 8279* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 8280* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 8281* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 8282* [Requirements](requirements.html): The uri that identifies the requirements 8283* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 8284* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 8285* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 8286* [StructureMap](structuremap.html): The uri that identifies the structure map 8287* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 8288* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 8289* [TestPlan](testplan.html): The uri that identifies the test plan 8290* [TestScript](testscript.html): The uri that identifies the test script 8291* [ValueSet](valueset.html): The uri that identifies the value set 8292</b><br> 8293 * Type: <b>uri</b><br> 8294 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 8295 * </p> 8296 */ 8297 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 8298 public static final String SP_URL = "url"; 8299 /** 8300 * <b>Fluent Client</b> search parameter constant for <b>url</b> 8301 * <p> 8302 * Description: <b>Multiple Resources: 8303 8304* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 8305* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 8306* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 8307* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 8308* [Citation](citation.html): The uri that identifies the citation 8309* [CodeSystem](codesystem.html): The uri that identifies the code system 8310* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 8311* [ConceptMap](conceptmap.html): The URI that identifies the concept map 8312* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 8313* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 8314* [Evidence](evidence.html): The uri that identifies the evidence 8315* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 8316* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 8317* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 8318* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 8319* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 8320* [Library](library.html): The uri that identifies the library 8321* [Measure](measure.html): The uri that identifies the measure 8322* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 8323* [NamingSystem](namingsystem.html): The uri that identifies the naming system 8324* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 8325* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 8326* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 8327* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 8328* [Requirements](requirements.html): The uri that identifies the requirements 8329* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 8330* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 8331* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 8332* [StructureMap](structuremap.html): The uri that identifies the structure map 8333* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 8334* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 8335* [TestPlan](testplan.html): The uri that identifies the test plan 8336* [TestScript](testscript.html): The uri that identifies the test script 8337* [ValueSet](valueset.html): The uri that identifies the value set 8338</b><br> 8339 * Type: <b>uri</b><br> 8340 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 8341 * </p> 8342 */ 8343 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 8344 8345 /** 8346 * Search parameter: <b>version</b> 8347 * <p> 8348 * Description: <b>Multiple Resources: 8349 8350* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 8351* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 8352* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 8353* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 8354* [Citation](citation.html): The business version of the citation 8355* [CodeSystem](codesystem.html): The business version of the code system 8356* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 8357* [ConceptMap](conceptmap.html): The business version of the concept map 8358* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 8359* [EventDefinition](eventdefinition.html): The business version of the event definition 8360* [Evidence](evidence.html): The business version of the evidence 8361* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 8362* [ExampleScenario](examplescenario.html): The business version of the example scenario 8363* [GraphDefinition](graphdefinition.html): The business version of the graph definition 8364* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 8365* [Library](library.html): The business version of the library 8366* [Measure](measure.html): The business version of the measure 8367* [MessageDefinition](messagedefinition.html): The business version of the message definition 8368* [NamingSystem](namingsystem.html): The business version of the naming system 8369* [OperationDefinition](operationdefinition.html): The business version of the operation definition 8370* [PlanDefinition](plandefinition.html): The business version of the plan definition 8371* [Questionnaire](questionnaire.html): The business version of the questionnaire 8372* [Requirements](requirements.html): The business version of the requirements 8373* [SearchParameter](searchparameter.html): The business version of the search parameter 8374* [StructureDefinition](structuredefinition.html): The business version of the structure definition 8375* [StructureMap](structuremap.html): The business version of the structure map 8376* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 8377* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 8378* [TestScript](testscript.html): The business version of the test script 8379* [ValueSet](valueset.html): The business version of the value set 8380</b><br> 8381 * Type: <b>token</b><br> 8382 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 8383 * </p> 8384 */ 8385 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 8386 public static final String SP_VERSION = "version"; 8387 /** 8388 * <b>Fluent Client</b> search parameter constant for <b>version</b> 8389 * <p> 8390 * Description: <b>Multiple Resources: 8391 8392* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 8393* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 8394* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 8395* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 8396* [Citation](citation.html): The business version of the citation 8397* [CodeSystem](codesystem.html): The business version of the code system 8398* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 8399* [ConceptMap](conceptmap.html): The business version of the concept map 8400* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 8401* [EventDefinition](eventdefinition.html): The business version of the event definition 8402* [Evidence](evidence.html): The business version of the evidence 8403* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 8404* [ExampleScenario](examplescenario.html): The business version of the example scenario 8405* [GraphDefinition](graphdefinition.html): The business version of the graph definition 8406* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 8407* [Library](library.html): The business version of the library 8408* [Measure](measure.html): The business version of the measure 8409* [MessageDefinition](messagedefinition.html): The business version of the message definition 8410* [NamingSystem](namingsystem.html): The business version of the naming system 8411* [OperationDefinition](operationdefinition.html): The business version of the operation definition 8412* [PlanDefinition](plandefinition.html): The business version of the plan definition 8413* [Questionnaire](questionnaire.html): The business version of the questionnaire 8414* [Requirements](requirements.html): The business version of the requirements 8415* [SearchParameter](searchparameter.html): The business version of the search parameter 8416* [StructureDefinition](structuredefinition.html): The business version of the structure definition 8417* [StructureMap](structuremap.html): The business version of the structure map 8418* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 8419* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 8420* [TestScript](testscript.html): The business version of the test script 8421* [ValueSet](valueset.html): The business version of the value set 8422</b><br> 8423 * Type: <b>token</b><br> 8424 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 8425 * </p> 8426 */ 8427 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 8428 8429// Manual code (from Configuration.txt): 8430public String toString() { 8431 return StructureMapUtilities.render(this); 8432 } 8433// end addition 8434 8435} 8436