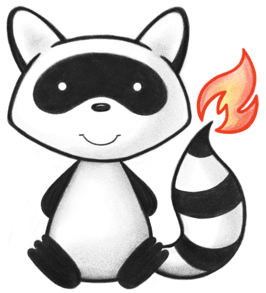
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050import org.hl7.fhir.r5.utils.structuremap.StructureMapUtilities; 051/** 052 * A Map of relationships between 2 structures that can be used to transform data. 053 */ 054@ResourceDef(name="StructureMap", profile="http://hl7.org/fhir/StructureDefinition/StructureMap") 055public class StructureMap extends CanonicalResource { 056 057 public enum StructureMapGroupTypeMode { 058 /** 059 * This group is a default mapping group for the specified types and for the primary source type. 060 */ 061 TYPES, 062 /** 063 * This group is a default mapping group for the specified types. 064 */ 065 TYPEANDTYPES, 066 /** 067 * added to help the parsers with the generic types 068 */ 069 NULL; 070 public static StructureMapGroupTypeMode fromCode(String codeString) throws FHIRException { 071 if (codeString == null || "".equals(codeString)) 072 return null; 073 if ("types".equals(codeString)) 074 return TYPES; 075 if ("type-and-types".equals(codeString)) 076 return TYPEANDTYPES; 077 if (Configuration.isAcceptInvalidEnums()) 078 return null; 079 else 080 throw new FHIRException("Unknown StructureMapGroupTypeMode code '"+codeString+"'"); 081 } 082 public String toCode() { 083 switch (this) { 084 case TYPES: return "types"; 085 case TYPEANDTYPES: return "type-and-types"; 086 case NULL: return null; 087 default: return "?"; 088 } 089 } 090 public String getSystem() { 091 switch (this) { 092 case TYPES: return "http://hl7.org/fhir/map-group-type-mode"; 093 case TYPEANDTYPES: return "http://hl7.org/fhir/map-group-type-mode"; 094 case NULL: return null; 095 default: return "?"; 096 } 097 } 098 public String getDefinition() { 099 switch (this) { 100 case TYPES: return "This group is a default mapping group for the specified types and for the primary source type."; 101 case TYPEANDTYPES: return "This group is a default mapping group for the specified types."; 102 case NULL: return null; 103 default: return "?"; 104 } 105 } 106 public String getDisplay() { 107 switch (this) { 108 case TYPES: return "Default for Type Combination"; 109 case TYPEANDTYPES: return "Default for type + combination"; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 } 115 116 public static class StructureMapGroupTypeModeEnumFactory implements EnumFactory<StructureMapGroupTypeMode> { 117 public StructureMapGroupTypeMode fromCode(String codeString) throws IllegalArgumentException { 118 if (codeString == null || "".equals(codeString)) 119 if (codeString == null || "".equals(codeString)) 120 return null; 121 if ("types".equals(codeString)) 122 return StructureMapGroupTypeMode.TYPES; 123 if ("type-and-types".equals(codeString)) 124 return StructureMapGroupTypeMode.TYPEANDTYPES; 125 throw new IllegalArgumentException("Unknown StructureMapGroupTypeMode code '"+codeString+"'"); 126 } 127 public Enumeration<StructureMapGroupTypeMode> fromType(PrimitiveType<?> code) throws FHIRException { 128 if (code == null) 129 return null; 130 if (code.isEmpty()) 131 return new Enumeration<StructureMapGroupTypeMode>(this, StructureMapGroupTypeMode.NULL, code); 132 String codeString = ((PrimitiveType) code).asStringValue(); 133 if (codeString == null || "".equals(codeString)) 134 return new Enumeration<StructureMapGroupTypeMode>(this, StructureMapGroupTypeMode.NULL, code); 135 if ("types".equals(codeString)) 136 return new Enumeration<StructureMapGroupTypeMode>(this, StructureMapGroupTypeMode.TYPES, code); 137 if ("type-and-types".equals(codeString)) 138 return new Enumeration<StructureMapGroupTypeMode>(this, StructureMapGroupTypeMode.TYPEANDTYPES, code); 139 throw new FHIRException("Unknown StructureMapGroupTypeMode code '"+codeString+"'"); 140 } 141 public String toCode(StructureMapGroupTypeMode code) { 142 if (code == StructureMapGroupTypeMode.NULL) 143 return null; 144 if (code == StructureMapGroupTypeMode.TYPES) 145 return "types"; 146 if (code == StructureMapGroupTypeMode.TYPEANDTYPES) 147 return "type-and-types"; 148 return "?"; 149 } 150 public String toSystem(StructureMapGroupTypeMode code) { 151 return code.getSystem(); 152 } 153 } 154 155 public enum StructureMapInputMode { 156 /** 157 * Names an input instance used a source for mapping. 158 */ 159 SOURCE, 160 /** 161 * Names an instance that is being populated. 162 */ 163 TARGET, 164 /** 165 * added to help the parsers with the generic types 166 */ 167 NULL; 168 public static StructureMapInputMode fromCode(String codeString) throws FHIRException { 169 if (codeString == null || "".equals(codeString)) 170 return null; 171 if ("source".equals(codeString)) 172 return SOURCE; 173 if ("target".equals(codeString)) 174 return TARGET; 175 if (Configuration.isAcceptInvalidEnums()) 176 return null; 177 else 178 throw new FHIRException("Unknown StructureMapInputMode code '"+codeString+"'"); 179 } 180 public String toCode() { 181 switch (this) { 182 case SOURCE: return "source"; 183 case TARGET: return "target"; 184 case NULL: return null; 185 default: return "?"; 186 } 187 } 188 public String getSystem() { 189 switch (this) { 190 case SOURCE: return "http://hl7.org/fhir/map-input-mode"; 191 case TARGET: return "http://hl7.org/fhir/map-input-mode"; 192 case NULL: return null; 193 default: return "?"; 194 } 195 } 196 public String getDefinition() { 197 switch (this) { 198 case SOURCE: return "Names an input instance used a source for mapping."; 199 case TARGET: return "Names an instance that is being populated."; 200 case NULL: return null; 201 default: return "?"; 202 } 203 } 204 public String getDisplay() { 205 switch (this) { 206 case SOURCE: return "Source Instance"; 207 case TARGET: return "Target Instance"; 208 case NULL: return null; 209 default: return "?"; 210 } 211 } 212 } 213 214 public static class StructureMapInputModeEnumFactory implements EnumFactory<StructureMapInputMode> { 215 public StructureMapInputMode fromCode(String codeString) throws IllegalArgumentException { 216 if (codeString == null || "".equals(codeString)) 217 if (codeString == null || "".equals(codeString)) 218 return null; 219 if ("source".equals(codeString)) 220 return StructureMapInputMode.SOURCE; 221 if ("target".equals(codeString)) 222 return StructureMapInputMode.TARGET; 223 throw new IllegalArgumentException("Unknown StructureMapInputMode code '"+codeString+"'"); 224 } 225 public Enumeration<StructureMapInputMode> fromType(PrimitiveType<?> code) throws FHIRException { 226 if (code == null) 227 return null; 228 if (code.isEmpty()) 229 return new Enumeration<StructureMapInputMode>(this, StructureMapInputMode.NULL, code); 230 String codeString = ((PrimitiveType) code).asStringValue(); 231 if (codeString == null || "".equals(codeString)) 232 return new Enumeration<StructureMapInputMode>(this, StructureMapInputMode.NULL, code); 233 if ("source".equals(codeString)) 234 return new Enumeration<StructureMapInputMode>(this, StructureMapInputMode.SOURCE, code); 235 if ("target".equals(codeString)) 236 return new Enumeration<StructureMapInputMode>(this, StructureMapInputMode.TARGET, code); 237 throw new FHIRException("Unknown StructureMapInputMode code '"+codeString+"'"); 238 } 239 public String toCode(StructureMapInputMode code) { 240 if (code == StructureMapInputMode.NULL) 241 return null; 242 if (code == StructureMapInputMode.SOURCE) 243 return "source"; 244 if (code == StructureMapInputMode.TARGET) 245 return "target"; 246 return "?"; 247 } 248 public String toSystem(StructureMapInputMode code) { 249 return code.getSystem(); 250 } 251 } 252 253 public enum StructureMapModelMode { 254 /** 255 * This structure describes an instance passed to the mapping engine that is used a source of data. 256 */ 257 SOURCE, 258 /** 259 * This structure describes an instance that the mapping engine may ask for that is used a source of data. 260 */ 261 QUERIED, 262 /** 263 * This structure describes an instance passed to the mapping engine that is used a target of data. 264 */ 265 TARGET, 266 /** 267 * This structure describes an instance that the mapping engine may ask to create that is used a target of data. 268 */ 269 PRODUCED, 270 /** 271 * added to help the parsers with the generic types 272 */ 273 NULL; 274 public static StructureMapModelMode fromCode(String codeString) throws FHIRException { 275 if (codeString == null || "".equals(codeString)) 276 return null; 277 if ("source".equals(codeString)) 278 return SOURCE; 279 if ("queried".equals(codeString)) 280 return QUERIED; 281 if ("target".equals(codeString)) 282 return TARGET; 283 if ("produced".equals(codeString)) 284 return PRODUCED; 285 if (Configuration.isAcceptInvalidEnums()) 286 return null; 287 else 288 throw new FHIRException("Unknown StructureMapModelMode code '"+codeString+"'"); 289 } 290 public String toCode() { 291 switch (this) { 292 case SOURCE: return "source"; 293 case QUERIED: return "queried"; 294 case TARGET: return "target"; 295 case PRODUCED: return "produced"; 296 case NULL: return null; 297 default: return "?"; 298 } 299 } 300 public String getSystem() { 301 switch (this) { 302 case SOURCE: return "http://hl7.org/fhir/map-model-mode"; 303 case QUERIED: return "http://hl7.org/fhir/map-model-mode"; 304 case TARGET: return "http://hl7.org/fhir/map-model-mode"; 305 case PRODUCED: return "http://hl7.org/fhir/map-model-mode"; 306 case NULL: return null; 307 default: return "?"; 308 } 309 } 310 public String getDefinition() { 311 switch (this) { 312 case SOURCE: return "This structure describes an instance passed to the mapping engine that is used a source of data."; 313 case QUERIED: return "This structure describes an instance that the mapping engine may ask for that is used a source of data."; 314 case TARGET: return "This structure describes an instance passed to the mapping engine that is used a target of data."; 315 case PRODUCED: return "This structure describes an instance that the mapping engine may ask to create that is used a target of data."; 316 case NULL: return null; 317 default: return "?"; 318 } 319 } 320 public String getDisplay() { 321 switch (this) { 322 case SOURCE: return "Source Structure Definition"; 323 case QUERIED: return "Queried Structure Definition"; 324 case TARGET: return "Target Structure Definition"; 325 case PRODUCED: return "Produced Structure Definition"; 326 case NULL: return null; 327 default: return "?"; 328 } 329 } 330 } 331 332 public static class StructureMapModelModeEnumFactory implements EnumFactory<StructureMapModelMode> { 333 public StructureMapModelMode fromCode(String codeString) throws IllegalArgumentException { 334 if (codeString == null || "".equals(codeString)) 335 if (codeString == null || "".equals(codeString)) 336 return null; 337 if ("source".equals(codeString)) 338 return StructureMapModelMode.SOURCE; 339 if ("queried".equals(codeString)) 340 return StructureMapModelMode.QUERIED; 341 if ("target".equals(codeString)) 342 return StructureMapModelMode.TARGET; 343 if ("produced".equals(codeString)) 344 return StructureMapModelMode.PRODUCED; 345 throw new IllegalArgumentException("Unknown StructureMapModelMode code '"+codeString+"'"); 346 } 347 public Enumeration<StructureMapModelMode> fromType(PrimitiveType<?> code) throws FHIRException { 348 if (code == null) 349 return null; 350 if (code.isEmpty()) 351 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.NULL, code); 352 String codeString = ((PrimitiveType) code).asStringValue(); 353 if (codeString == null || "".equals(codeString)) 354 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.NULL, code); 355 if ("source".equals(codeString)) 356 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.SOURCE, code); 357 if ("queried".equals(codeString)) 358 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.QUERIED, code); 359 if ("target".equals(codeString)) 360 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.TARGET, code); 361 if ("produced".equals(codeString)) 362 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.PRODUCED, code); 363 throw new FHIRException("Unknown StructureMapModelMode code '"+codeString+"'"); 364 } 365 public String toCode(StructureMapModelMode code) { 366 if (code == StructureMapModelMode.NULL) 367 return null; 368 if (code == StructureMapModelMode.SOURCE) 369 return "source"; 370 if (code == StructureMapModelMode.QUERIED) 371 return "queried"; 372 if (code == StructureMapModelMode.TARGET) 373 return "target"; 374 if (code == StructureMapModelMode.PRODUCED) 375 return "produced"; 376 return "?"; 377 } 378 public String toSystem(StructureMapModelMode code) { 379 return code.getSystem(); 380 } 381 } 382 383 public enum StructureMapSourceListMode { 384 /** 385 * Only process this rule for the first in the list. 386 */ 387 FIRST, 388 /** 389 * Process this rule for all but the first. 390 */ 391 NOTFIRST, 392 /** 393 * Only process this rule for the last in the list. 394 */ 395 LAST, 396 /** 397 * Process this rule for all but the last. 398 */ 399 NOTLAST, 400 /** 401 * Only process this rule is there is only item. 402 */ 403 ONLYONE, 404 /** 405 * added to help the parsers with the generic types 406 */ 407 NULL; 408 public static StructureMapSourceListMode fromCode(String codeString) throws FHIRException { 409 if (codeString == null || "".equals(codeString)) 410 return null; 411 if ("first".equals(codeString)) 412 return FIRST; 413 if ("not_first".equals(codeString)) 414 return NOTFIRST; 415 if ("last".equals(codeString)) 416 return LAST; 417 if ("not_last".equals(codeString)) 418 return NOTLAST; 419 if ("only_one".equals(codeString)) 420 return ONLYONE; 421 if (Configuration.isAcceptInvalidEnums()) 422 return null; 423 else 424 throw new FHIRException("Unknown StructureMapSourceListMode code '"+codeString+"'"); 425 } 426 public String toCode() { 427 switch (this) { 428 case FIRST: return "first"; 429 case NOTFIRST: return "not_first"; 430 case LAST: return "last"; 431 case NOTLAST: return "not_last"; 432 case ONLYONE: return "only_one"; 433 case NULL: return null; 434 default: return "?"; 435 } 436 } 437 public String getSystem() { 438 switch (this) { 439 case FIRST: return "http://hl7.org/fhir/map-source-list-mode"; 440 case NOTFIRST: return "http://hl7.org/fhir/map-source-list-mode"; 441 case LAST: return "http://hl7.org/fhir/map-source-list-mode"; 442 case NOTLAST: return "http://hl7.org/fhir/map-source-list-mode"; 443 case ONLYONE: return "http://hl7.org/fhir/map-source-list-mode"; 444 case NULL: return null; 445 default: return "?"; 446 } 447 } 448 public String getDefinition() { 449 switch (this) { 450 case FIRST: return "Only process this rule for the first in the list."; 451 case NOTFIRST: return "Process this rule for all but the first."; 452 case LAST: return "Only process this rule for the last in the list."; 453 case NOTLAST: return "Process this rule for all but the last."; 454 case ONLYONE: return "Only process this rule is there is only item."; 455 case NULL: return null; 456 default: return "?"; 457 } 458 } 459 public String getDisplay() { 460 switch (this) { 461 case FIRST: return "First"; 462 case NOTFIRST: return "All but the first"; 463 case LAST: return "Last"; 464 case NOTLAST: return "All but the last"; 465 case ONLYONE: return "Enforce only one"; 466 case NULL: return null; 467 default: return "?"; 468 } 469 } 470 } 471 472 public static class StructureMapSourceListModeEnumFactory implements EnumFactory<StructureMapSourceListMode> { 473 public StructureMapSourceListMode fromCode(String codeString) throws IllegalArgumentException { 474 if (codeString == null || "".equals(codeString)) 475 if (codeString == null || "".equals(codeString)) 476 return null; 477 if ("first".equals(codeString)) 478 return StructureMapSourceListMode.FIRST; 479 if ("not_first".equals(codeString)) 480 return StructureMapSourceListMode.NOTFIRST; 481 if ("last".equals(codeString)) 482 return StructureMapSourceListMode.LAST; 483 if ("not_last".equals(codeString)) 484 return StructureMapSourceListMode.NOTLAST; 485 if ("only_one".equals(codeString)) 486 return StructureMapSourceListMode.ONLYONE; 487 throw new IllegalArgumentException("Unknown StructureMapSourceListMode code '"+codeString+"'"); 488 } 489 public Enumeration<StructureMapSourceListMode> fromType(PrimitiveType<?> code) throws FHIRException { 490 if (code == null) 491 return null; 492 if (code.isEmpty()) 493 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.NULL, code); 494 String codeString = ((PrimitiveType) code).asStringValue(); 495 if (codeString == null || "".equals(codeString)) 496 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.NULL, code); 497 if ("first".equals(codeString)) 498 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.FIRST, code); 499 if ("not_first".equals(codeString)) 500 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.NOTFIRST, code); 501 if ("last".equals(codeString)) 502 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.LAST, code); 503 if ("not_last".equals(codeString)) 504 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.NOTLAST, code); 505 if ("only_one".equals(codeString)) 506 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.ONLYONE, code); 507 throw new FHIRException("Unknown StructureMapSourceListMode code '"+codeString+"'"); 508 } 509 public String toCode(StructureMapSourceListMode code) { 510 if (code == StructureMapSourceListMode.NULL) 511 return null; 512 if (code == StructureMapSourceListMode.FIRST) 513 return "first"; 514 if (code == StructureMapSourceListMode.NOTFIRST) 515 return "not_first"; 516 if (code == StructureMapSourceListMode.LAST) 517 return "last"; 518 if (code == StructureMapSourceListMode.NOTLAST) 519 return "not_last"; 520 if (code == StructureMapSourceListMode.ONLYONE) 521 return "only_one"; 522 return "?"; 523 } 524 public String toSystem(StructureMapSourceListMode code) { 525 return code.getSystem(); 526 } 527 } 528 529 public enum StructureMapTargetListMode { 530 /** 531 * when the target list is being assembled, the items for this rule go first. If more than one rule defines a first item (for a given instance of mapping) then this is an error. 532 */ 533 FIRST, 534 /** 535 * the target instance is shared with the target instances generated by another rule (up to the first common n items, then create new ones). 536 */ 537 SHARE, 538 /** 539 * when the target list is being assembled, the items for this rule go last. If more than one rule defines a last item (for a given instance of mapping) then this is an error. 540 */ 541 LAST, 542 /** 543 * the target instance is shared with a number of target instances generated by another rule (up to the first common n items, then create new ones). 544 */ 545 SINGLE, 546 /** 547 * added to help the parsers with the generic types 548 */ 549 NULL; 550 public static StructureMapTargetListMode fromCode(String codeString) throws FHIRException { 551 if (codeString == null || "".equals(codeString)) 552 return null; 553 if ("first".equals(codeString)) 554 return FIRST; 555 if ("share".equals(codeString)) 556 return SHARE; 557 if ("last".equals(codeString)) 558 return LAST; 559 if ("single".equals(codeString)) 560 return SINGLE; 561 if (Configuration.isAcceptInvalidEnums()) 562 return null; 563 else 564 throw new FHIRException("Unknown StructureMapTargetListMode code '"+codeString+"'"); 565 } 566 public String toCode() { 567 switch (this) { 568 case FIRST: return "first"; 569 case SHARE: return "share"; 570 case LAST: return "last"; 571 case SINGLE: return "single"; 572 case NULL: return null; 573 default: return "?"; 574 } 575 } 576 public String getSystem() { 577 switch (this) { 578 case FIRST: return "http://hl7.org/fhir/map-target-list-mode"; 579 case SHARE: return "http://hl7.org/fhir/map-target-list-mode"; 580 case LAST: return "http://hl7.org/fhir/map-target-list-mode"; 581 case SINGLE: return "http://hl7.org/fhir/map-target-list-mode"; 582 case NULL: return null; 583 default: return "?"; 584 } 585 } 586 public String getDefinition() { 587 switch (this) { 588 case FIRST: return "when the target list is being assembled, the items for this rule go first. If more than one rule defines a first item (for a given instance of mapping) then this is an error."; 589 case SHARE: return "the target instance is shared with the target instances generated by another rule (up to the first common n items, then create new ones)."; 590 case LAST: return "when the target list is being assembled, the items for this rule go last. If more than one rule defines a last item (for a given instance of mapping) then this is an error."; 591 case SINGLE: return "the target instance is shared with a number of target instances generated by another rule (up to the first common n items, then create new ones)."; 592 case NULL: return null; 593 default: return "?"; 594 } 595 } 596 public String getDisplay() { 597 switch (this) { 598 case FIRST: return "First"; 599 case SHARE: return "Share"; 600 case LAST: return "Last"; 601 case SINGLE: return "single"; 602 case NULL: return null; 603 default: return "?"; 604 } 605 } 606 } 607 608 public static class StructureMapTargetListModeEnumFactory implements EnumFactory<StructureMapTargetListMode> { 609 public StructureMapTargetListMode fromCode(String codeString) throws IllegalArgumentException { 610 if (codeString == null || "".equals(codeString)) 611 if (codeString == null || "".equals(codeString)) 612 return null; 613 if ("first".equals(codeString)) 614 return StructureMapTargetListMode.FIRST; 615 if ("share".equals(codeString)) 616 return StructureMapTargetListMode.SHARE; 617 if ("last".equals(codeString)) 618 return StructureMapTargetListMode.LAST; 619 if ("single".equals(codeString)) 620 return StructureMapTargetListMode.SINGLE; 621 throw new IllegalArgumentException("Unknown StructureMapTargetListMode code '"+codeString+"'"); 622 } 623 public Enumeration<StructureMapTargetListMode> fromType(PrimitiveType<?> code) throws FHIRException { 624 if (code == null) 625 return null; 626 if (code.isEmpty()) 627 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.NULL, code); 628 String codeString = ((PrimitiveType) code).asStringValue(); 629 if (codeString == null || "".equals(codeString)) 630 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.NULL, code); 631 if ("first".equals(codeString)) 632 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.FIRST, code); 633 if ("share".equals(codeString)) 634 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.SHARE, code); 635 if ("last".equals(codeString)) 636 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.LAST, code); 637 if ("single".equals(codeString)) 638 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.SINGLE, code); 639 throw new FHIRException("Unknown StructureMapTargetListMode code '"+codeString+"'"); 640 } 641 public String toCode(StructureMapTargetListMode code) { 642 if (code == StructureMapTargetListMode.NULL) 643 return null; 644 if (code == StructureMapTargetListMode.FIRST) 645 return "first"; 646 if (code == StructureMapTargetListMode.SHARE) 647 return "share"; 648 if (code == StructureMapTargetListMode.LAST) 649 return "last"; 650 if (code == StructureMapTargetListMode.SINGLE) 651 return "single"; 652 return "?"; 653 } 654 public String toSystem(StructureMapTargetListMode code) { 655 return code.getSystem(); 656 } 657 } 658 659 public enum StructureMapTransform { 660 /** 661 * create(type : string) - type is passed through to the application on the standard API, and must be known by it. 662 */ 663 CREATE, 664 /** 665 * copy(source). 666 */ 667 COPY, 668 /** 669 * truncate(source, length) - source must be stringy type. 670 */ 671 TRUNCATE, 672 /** 673 * escape(source, fmt1, fmt2) - change source from one kind of escaping to another (plain, java, xml, json). note that this is for when the string itself is escaped. 674 */ 675 ESCAPE, 676 /** 677 * cast(source, type?) - cast (convert) source from one type to another. Target type can be left as implicit if there is one and only one target type known. The default namespace for the type is 'FHIR' (see [FHIRPath type specifiers](http://hl7.org/fhirpath/N1/#is-type-specifier)) 678 */ 679 CAST, 680 /** 681 * append(source...) - source is element or string. 682 */ 683 APPEND, 684 /** 685 * translate(source, uri_of_map) - use the translate operation. 686 */ 687 TRANSLATE, 688 /** 689 * reference(source : object) - return a string that references the provided tree properly. 690 */ 691 REFERENCE, 692 /** 693 * Perform a date operation. *Parameters to be documented*. 694 */ 695 DATEOP, 696 /** 697 * Generate a random UUID (in lowercase). No Parameters. 698 */ 699 UUID, 700 /** 701 * Return the appropriate string to put in a reference that refers to the resource provided as a parameter. 702 */ 703 POINTER, 704 /** 705 * Execute the supplied FHIRPath expression and use the value returned by that. 706 */ 707 EVALUATE, 708 /** 709 * Create a CodeableConcept. Parameters = (text) or (system. Code[, display]). 710 */ 711 CC, 712 /** 713 * Create a Coding. Parameters = (system. Code[, display]). 714 */ 715 C, 716 /** 717 * Create a quantity. Parameters = (text) or (value, unit, [system, code]) where text is the natural representation e.g. [comparator]value[space]unit. 718 */ 719 QTY, 720 /** 721 * Create an identifier. Parameters = (system, value[, type]) where type is a code from the identifier type value set. 722 */ 723 ID, 724 /** 725 * Create a contact details. Parameters = (value) or (system, value). If no system is provided, the system should be inferred from the content of the value. 726 */ 727 CP, 728 /** 729 * added to help the parsers with the generic types 730 */ 731 NULL; 732 public static StructureMapTransform fromCode(String codeString) throws FHIRException { 733 if (codeString == null || "".equals(codeString)) 734 return null; 735 if ("create".equals(codeString)) 736 return CREATE; 737 if ("copy".equals(codeString)) 738 return COPY; 739 if ("truncate".equals(codeString)) 740 return TRUNCATE; 741 if ("escape".equals(codeString)) 742 return ESCAPE; 743 if ("cast".equals(codeString)) 744 return CAST; 745 if ("append".equals(codeString)) 746 return APPEND; 747 if ("translate".equals(codeString)) 748 return TRANSLATE; 749 if ("reference".equals(codeString)) 750 return REFERENCE; 751 if ("dateOp".equals(codeString)) 752 return DATEOP; 753 if ("uuid".equals(codeString)) 754 return UUID; 755 if ("pointer".equals(codeString)) 756 return POINTER; 757 if ("evaluate".equals(codeString)) 758 return EVALUATE; 759 if ("cc".equals(codeString)) 760 return CC; 761 if ("c".equals(codeString)) 762 return C; 763 if ("qty".equals(codeString)) 764 return QTY; 765 if ("id".equals(codeString)) 766 return ID; 767 if ("cp".equals(codeString)) 768 return CP; 769 if (Configuration.isAcceptInvalidEnums()) 770 return null; 771 else 772 throw new FHIRException("Unknown StructureMapTransform code '"+codeString+"'"); 773 } 774 public String toCode() { 775 switch (this) { 776 case CREATE: return "create"; 777 case COPY: return "copy"; 778 case TRUNCATE: return "truncate"; 779 case ESCAPE: return "escape"; 780 case CAST: return "cast"; 781 case APPEND: return "append"; 782 case TRANSLATE: return "translate"; 783 case REFERENCE: return "reference"; 784 case DATEOP: return "dateOp"; 785 case UUID: return "uuid"; 786 case POINTER: return "pointer"; 787 case EVALUATE: return "evaluate"; 788 case CC: return "cc"; 789 case C: return "c"; 790 case QTY: return "qty"; 791 case ID: return "id"; 792 case CP: return "cp"; 793 case NULL: return null; 794 default: return "?"; 795 } 796 } 797 public String getSystem() { 798 switch (this) { 799 case CREATE: return "http://hl7.org/fhir/map-transform"; 800 case COPY: return "http://hl7.org/fhir/map-transform"; 801 case TRUNCATE: return "http://hl7.org/fhir/map-transform"; 802 case ESCAPE: return "http://hl7.org/fhir/map-transform"; 803 case CAST: return "http://hl7.org/fhir/map-transform"; 804 case APPEND: return "http://hl7.org/fhir/map-transform"; 805 case TRANSLATE: return "http://hl7.org/fhir/map-transform"; 806 case REFERENCE: return "http://hl7.org/fhir/map-transform"; 807 case DATEOP: return "http://hl7.org/fhir/map-transform"; 808 case UUID: return "http://hl7.org/fhir/map-transform"; 809 case POINTER: return "http://hl7.org/fhir/map-transform"; 810 case EVALUATE: return "http://hl7.org/fhir/map-transform"; 811 case CC: return "http://hl7.org/fhir/map-transform"; 812 case C: return "http://hl7.org/fhir/map-transform"; 813 case QTY: return "http://hl7.org/fhir/map-transform"; 814 case ID: return "http://hl7.org/fhir/map-transform"; 815 case CP: return "http://hl7.org/fhir/map-transform"; 816 case NULL: return null; 817 default: return "?"; 818 } 819 } 820 public String getDefinition() { 821 switch (this) { 822 case CREATE: return "create(type : string) - type is passed through to the application on the standard API, and must be known by it."; 823 case COPY: return "copy(source)."; 824 case TRUNCATE: return "truncate(source, length) - source must be stringy type."; 825 case ESCAPE: return "escape(source, fmt1, fmt2) - change source from one kind of escaping to another (plain, java, xml, json). note that this is for when the string itself is escaped."; 826 case CAST: return "cast(source, type?) - cast (convert) source from one type to another. Target type can be left as implicit if there is one and only one target type known. The default namespace for the type is 'FHIR' (see [FHIRPath type specifiers](http://hl7.org/fhirpath/N1/#is-type-specifier))"; 827 case APPEND: return "append(source...) - source is element or string."; 828 case TRANSLATE: return "translate(source, uri_of_map) - use the translate operation."; 829 case REFERENCE: return "reference(source : object) - return a string that references the provided tree properly."; 830 case DATEOP: return "Perform a date operation. *Parameters to be documented*."; 831 case UUID: return "Generate a random UUID (in lowercase). No Parameters."; 832 case POINTER: return "Return the appropriate string to put in a reference that refers to the resource provided as a parameter."; 833 case EVALUATE: return "Execute the supplied FHIRPath expression and use the value returned by that."; 834 case CC: return "Create a CodeableConcept. Parameters = (text) or (system. Code[, display])."; 835 case C: return "Create a Coding. Parameters = (system. Code[, display])."; 836 case QTY: return "Create a quantity. Parameters = (text) or (value, unit, [system, code]) where text is the natural representation e.g. [comparator]value[space]unit."; 837 case ID: return "Create an identifier. Parameters = (system, value[, type]) where type is a code from the identifier type value set."; 838 case CP: return "Create a contact details. Parameters = (value) or (system, value). If no system is provided, the system should be inferred from the content of the value."; 839 case NULL: return null; 840 default: return "?"; 841 } 842 } 843 public String getDisplay() { 844 switch (this) { 845 case CREATE: return "create"; 846 case COPY: return "copy"; 847 case TRUNCATE: return "truncate"; 848 case ESCAPE: return "escape"; 849 case CAST: return "cast"; 850 case APPEND: return "append"; 851 case TRANSLATE: return "translate"; 852 case REFERENCE: return "reference"; 853 case DATEOP: return "dateOp"; 854 case UUID: return "uuid"; 855 case POINTER: return "pointer"; 856 case EVALUATE: return "evaluate"; 857 case CC: return "cc"; 858 case C: return "c"; 859 case QTY: return "qty"; 860 case ID: return "id"; 861 case CP: return "cp"; 862 case NULL: return null; 863 default: return "?"; 864 } 865 } 866 } 867 868 public static class StructureMapTransformEnumFactory implements EnumFactory<StructureMapTransform> { 869 public StructureMapTransform fromCode(String codeString) throws IllegalArgumentException { 870 if (codeString == null || "".equals(codeString)) 871 if (codeString == null || "".equals(codeString)) 872 return null; 873 if ("create".equals(codeString)) 874 return StructureMapTransform.CREATE; 875 if ("copy".equals(codeString)) 876 return StructureMapTransform.COPY; 877 if ("truncate".equals(codeString)) 878 return StructureMapTransform.TRUNCATE; 879 if ("escape".equals(codeString)) 880 return StructureMapTransform.ESCAPE; 881 if ("cast".equals(codeString)) 882 return StructureMapTransform.CAST; 883 if ("append".equals(codeString)) 884 return StructureMapTransform.APPEND; 885 if ("translate".equals(codeString)) 886 return StructureMapTransform.TRANSLATE; 887 if ("reference".equals(codeString)) 888 return StructureMapTransform.REFERENCE; 889 if ("dateOp".equals(codeString)) 890 return StructureMapTransform.DATEOP; 891 if ("uuid".equals(codeString)) 892 return StructureMapTransform.UUID; 893 if ("pointer".equals(codeString)) 894 return StructureMapTransform.POINTER; 895 if ("evaluate".equals(codeString)) 896 return StructureMapTransform.EVALUATE; 897 if ("cc".equals(codeString)) 898 return StructureMapTransform.CC; 899 if ("c".equals(codeString)) 900 return StructureMapTransform.C; 901 if ("qty".equals(codeString)) 902 return StructureMapTransform.QTY; 903 if ("id".equals(codeString)) 904 return StructureMapTransform.ID; 905 if ("cp".equals(codeString)) 906 return StructureMapTransform.CP; 907 throw new IllegalArgumentException("Unknown StructureMapTransform code '"+codeString+"'"); 908 } 909 public Enumeration<StructureMapTransform> fromType(PrimitiveType<?> code) throws FHIRException { 910 if (code == null) 911 return null; 912 if (code.isEmpty()) 913 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.NULL, code); 914 String codeString = ((PrimitiveType) code).asStringValue(); 915 if (codeString == null || "".equals(codeString)) 916 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.NULL, code); 917 if ("create".equals(codeString)) 918 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.CREATE, code); 919 if ("copy".equals(codeString)) 920 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.COPY, code); 921 if ("truncate".equals(codeString)) 922 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.TRUNCATE, code); 923 if ("escape".equals(codeString)) 924 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.ESCAPE, code); 925 if ("cast".equals(codeString)) 926 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.CAST, code); 927 if ("append".equals(codeString)) 928 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.APPEND, code); 929 if ("translate".equals(codeString)) 930 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.TRANSLATE, code); 931 if ("reference".equals(codeString)) 932 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.REFERENCE, code); 933 if ("dateOp".equals(codeString)) 934 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.DATEOP, code); 935 if ("uuid".equals(codeString)) 936 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.UUID, code); 937 if ("pointer".equals(codeString)) 938 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.POINTER, code); 939 if ("evaluate".equals(codeString)) 940 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.EVALUATE, code); 941 if ("cc".equals(codeString)) 942 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.CC, code); 943 if ("c".equals(codeString)) 944 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.C, code); 945 if ("qty".equals(codeString)) 946 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.QTY, code); 947 if ("id".equals(codeString)) 948 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.ID, code); 949 if ("cp".equals(codeString)) 950 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.CP, code); 951 throw new FHIRException("Unknown StructureMapTransform code '"+codeString+"'"); 952 } 953 public String toCode(StructureMapTransform code) { 954 if (code == StructureMapTransform.NULL) 955 return null; 956 if (code == StructureMapTransform.CREATE) 957 return "create"; 958 if (code == StructureMapTransform.COPY) 959 return "copy"; 960 if (code == StructureMapTransform.TRUNCATE) 961 return "truncate"; 962 if (code == StructureMapTransform.ESCAPE) 963 return "escape"; 964 if (code == StructureMapTransform.CAST) 965 return "cast"; 966 if (code == StructureMapTransform.APPEND) 967 return "append"; 968 if (code == StructureMapTransform.TRANSLATE) 969 return "translate"; 970 if (code == StructureMapTransform.REFERENCE) 971 return "reference"; 972 if (code == StructureMapTransform.DATEOP) 973 return "dateOp"; 974 if (code == StructureMapTransform.UUID) 975 return "uuid"; 976 if (code == StructureMapTransform.POINTER) 977 return "pointer"; 978 if (code == StructureMapTransform.EVALUATE) 979 return "evaluate"; 980 if (code == StructureMapTransform.CC) 981 return "cc"; 982 if (code == StructureMapTransform.C) 983 return "c"; 984 if (code == StructureMapTransform.QTY) 985 return "qty"; 986 if (code == StructureMapTransform.ID) 987 return "id"; 988 if (code == StructureMapTransform.CP) 989 return "cp"; 990 return "?"; 991 } 992 public String toSystem(StructureMapTransform code) { 993 return code.getSystem(); 994 } 995 } 996 997 @Block() 998 public static class StructureMapStructureComponent extends BackboneElement implements IBaseBackboneElement { 999 /** 1000 * The canonical reference to the structure. 1001 */ 1002 @Child(name = "url", type = {CanonicalType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1003 @Description(shortDefinition="Canonical reference to structure definition", formalDefinition="The canonical reference to the structure." ) 1004 protected CanonicalType url; 1005 1006 /** 1007 * How the referenced structure is used in this mapping. 1008 */ 1009 @Child(name = "mode", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1010 @Description(shortDefinition="source | queried | target | produced", formalDefinition="How the referenced structure is used in this mapping." ) 1011 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/map-model-mode") 1012 protected Enumeration<StructureMapModelMode> mode; 1013 1014 /** 1015 * The name used for this type in the map. 1016 */ 1017 @Child(name = "alias", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1018 @Description(shortDefinition="Name for type in this map", formalDefinition="The name used for this type in the map." ) 1019 protected StringType alias; 1020 1021 /** 1022 * Documentation that describes how the structure is used in the mapping. 1023 */ 1024 @Child(name = "documentation", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1025 @Description(shortDefinition="Documentation on use of structure", formalDefinition="Documentation that describes how the structure is used in the mapping." ) 1026 protected StringType documentation; 1027 1028 private static final long serialVersionUID = 364750586L; 1029 1030 /** 1031 * Constructor 1032 */ 1033 public StructureMapStructureComponent() { 1034 super(); 1035 } 1036 1037 /** 1038 * Constructor 1039 */ 1040 public StructureMapStructureComponent(String url, StructureMapModelMode mode) { 1041 super(); 1042 this.setUrl(url); 1043 this.setMode(mode); 1044 } 1045 1046 /** 1047 * @return {@link #url} (The canonical reference to the structure.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1048 */ 1049 public CanonicalType getUrlElement() { 1050 if (this.url == null) 1051 if (Configuration.errorOnAutoCreate()) 1052 throw new Error("Attempt to auto-create StructureMapStructureComponent.url"); 1053 else if (Configuration.doAutoCreate()) 1054 this.url = new CanonicalType(); // bb 1055 return this.url; 1056 } 1057 1058 public boolean hasUrlElement() { 1059 return this.url != null && !this.url.isEmpty(); 1060 } 1061 1062 public boolean hasUrl() { 1063 return this.url != null && !this.url.isEmpty(); 1064 } 1065 1066 /** 1067 * @param value {@link #url} (The canonical reference to the structure.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1068 */ 1069 public StructureMapStructureComponent setUrlElement(CanonicalType value) { 1070 this.url = value; 1071 return this; 1072 } 1073 1074 /** 1075 * @return The canonical reference to the structure. 1076 */ 1077 public String getUrl() { 1078 return this.url == null ? null : this.url.getValue(); 1079 } 1080 1081 /** 1082 * @param value The canonical reference to the structure. 1083 */ 1084 public StructureMapStructureComponent setUrl(String value) { 1085 if (this.url == null) 1086 this.url = new CanonicalType(); 1087 this.url.setValue(value); 1088 return this; 1089 } 1090 1091 /** 1092 * @return {@link #mode} (How the referenced structure is used in this mapping.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1093 */ 1094 public Enumeration<StructureMapModelMode> getModeElement() { 1095 if (this.mode == null) 1096 if (Configuration.errorOnAutoCreate()) 1097 throw new Error("Attempt to auto-create StructureMapStructureComponent.mode"); 1098 else if (Configuration.doAutoCreate()) 1099 this.mode = new Enumeration<StructureMapModelMode>(new StructureMapModelModeEnumFactory()); // bb 1100 return this.mode; 1101 } 1102 1103 public boolean hasModeElement() { 1104 return this.mode != null && !this.mode.isEmpty(); 1105 } 1106 1107 public boolean hasMode() { 1108 return this.mode != null && !this.mode.isEmpty(); 1109 } 1110 1111 /** 1112 * @param value {@link #mode} (How the referenced structure is used in this mapping.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1113 */ 1114 public StructureMapStructureComponent setModeElement(Enumeration<StructureMapModelMode> value) { 1115 this.mode = value; 1116 return this; 1117 } 1118 1119 /** 1120 * @return How the referenced structure is used in this mapping. 1121 */ 1122 public StructureMapModelMode getMode() { 1123 return this.mode == null ? null : this.mode.getValue(); 1124 } 1125 1126 /** 1127 * @param value How the referenced structure is used in this mapping. 1128 */ 1129 public StructureMapStructureComponent setMode(StructureMapModelMode value) { 1130 if (this.mode == null) 1131 this.mode = new Enumeration<StructureMapModelMode>(new StructureMapModelModeEnumFactory()); 1132 this.mode.setValue(value); 1133 return this; 1134 } 1135 1136 /** 1137 * @return {@link #alias} (The name used for this type in the map.). This is the underlying object with id, value and extensions. The accessor "getAlias" gives direct access to the value 1138 */ 1139 public StringType getAliasElement() { 1140 if (this.alias == null) 1141 if (Configuration.errorOnAutoCreate()) 1142 throw new Error("Attempt to auto-create StructureMapStructureComponent.alias"); 1143 else if (Configuration.doAutoCreate()) 1144 this.alias = new StringType(); // bb 1145 return this.alias; 1146 } 1147 1148 public boolean hasAliasElement() { 1149 return this.alias != null && !this.alias.isEmpty(); 1150 } 1151 1152 public boolean hasAlias() { 1153 return this.alias != null && !this.alias.isEmpty(); 1154 } 1155 1156 /** 1157 * @param value {@link #alias} (The name used for this type in the map.). This is the underlying object with id, value and extensions. The accessor "getAlias" gives direct access to the value 1158 */ 1159 public StructureMapStructureComponent setAliasElement(StringType value) { 1160 this.alias = value; 1161 return this; 1162 } 1163 1164 /** 1165 * @return The name used for this type in the map. 1166 */ 1167 public String getAlias() { 1168 return this.alias == null ? null : this.alias.getValue(); 1169 } 1170 1171 /** 1172 * @param value The name used for this type in the map. 1173 */ 1174 public StructureMapStructureComponent setAlias(String value) { 1175 if (Utilities.noString(value)) 1176 this.alias = null; 1177 else { 1178 if (this.alias == null) 1179 this.alias = new StringType(); 1180 this.alias.setValue(value); 1181 } 1182 return this; 1183 } 1184 1185 /** 1186 * @return {@link #documentation} (Documentation that describes how the structure is used in the mapping.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 1187 */ 1188 public StringType getDocumentationElement() { 1189 if (this.documentation == null) 1190 if (Configuration.errorOnAutoCreate()) 1191 throw new Error("Attempt to auto-create StructureMapStructureComponent.documentation"); 1192 else if (Configuration.doAutoCreate()) 1193 this.documentation = new StringType(); // bb 1194 return this.documentation; 1195 } 1196 1197 public boolean hasDocumentationElement() { 1198 return this.documentation != null && !this.documentation.isEmpty(); 1199 } 1200 1201 public boolean hasDocumentation() { 1202 return this.documentation != null && !this.documentation.isEmpty(); 1203 } 1204 1205 /** 1206 * @param value {@link #documentation} (Documentation that describes how the structure is used in the mapping.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 1207 */ 1208 public StructureMapStructureComponent setDocumentationElement(StringType value) { 1209 this.documentation = value; 1210 return this; 1211 } 1212 1213 /** 1214 * @return Documentation that describes how the structure is used in the mapping. 1215 */ 1216 public String getDocumentation() { 1217 return this.documentation == null ? null : this.documentation.getValue(); 1218 } 1219 1220 /** 1221 * @param value Documentation that describes how the structure is used in the mapping. 1222 */ 1223 public StructureMapStructureComponent setDocumentation(String value) { 1224 if (Utilities.noString(value)) 1225 this.documentation = null; 1226 else { 1227 if (this.documentation == null) 1228 this.documentation = new StringType(); 1229 this.documentation.setValue(value); 1230 } 1231 return this; 1232 } 1233 1234 protected void listChildren(List<Property> children) { 1235 super.listChildren(children); 1236 children.add(new Property("url", "canonical(StructureDefinition)", "The canonical reference to the structure.", 0, 1, url)); 1237 children.add(new Property("mode", "code", "How the referenced structure is used in this mapping.", 0, 1, mode)); 1238 children.add(new Property("alias", "string", "The name used for this type in the map.", 0, 1, alias)); 1239 children.add(new Property("documentation", "string", "Documentation that describes how the structure is used in the mapping.", 0, 1, documentation)); 1240 } 1241 1242 @Override 1243 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1244 switch (_hash) { 1245 case 116079: /*url*/ return new Property("url", "canonical(StructureDefinition)", "The canonical reference to the structure.", 0, 1, url); 1246 case 3357091: /*mode*/ return new Property("mode", "code", "How the referenced structure is used in this mapping.", 0, 1, mode); 1247 case 92902992: /*alias*/ return new Property("alias", "string", "The name used for this type in the map.", 0, 1, alias); 1248 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Documentation that describes how the structure is used in the mapping.", 0, 1, documentation); 1249 default: return super.getNamedProperty(_hash, _name, _checkValid); 1250 } 1251 1252 } 1253 1254 @Override 1255 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1256 switch (hash) { 1257 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // CanonicalType 1258 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<StructureMapModelMode> 1259 case 92902992: /*alias*/ return this.alias == null ? new Base[0] : new Base[] {this.alias}; // StringType 1260 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 1261 default: return super.getProperty(hash, name, checkValid); 1262 } 1263 1264 } 1265 1266 @Override 1267 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1268 switch (hash) { 1269 case 116079: // url 1270 this.url = TypeConvertor.castToCanonical(value); // CanonicalType 1271 return value; 1272 case 3357091: // mode 1273 value = new StructureMapModelModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1274 this.mode = (Enumeration) value; // Enumeration<StructureMapModelMode> 1275 return value; 1276 case 92902992: // alias 1277 this.alias = TypeConvertor.castToString(value); // StringType 1278 return value; 1279 case 1587405498: // documentation 1280 this.documentation = TypeConvertor.castToString(value); // StringType 1281 return value; 1282 default: return super.setProperty(hash, name, value); 1283 } 1284 1285 } 1286 1287 @Override 1288 public Base setProperty(String name, Base value) throws FHIRException { 1289 if (name.equals("url")) { 1290 this.url = TypeConvertor.castToCanonical(value); // CanonicalType 1291 } else if (name.equals("mode")) { 1292 value = new StructureMapModelModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1293 this.mode = (Enumeration) value; // Enumeration<StructureMapModelMode> 1294 } else if (name.equals("alias")) { 1295 this.alias = TypeConvertor.castToString(value); // StringType 1296 } else if (name.equals("documentation")) { 1297 this.documentation = TypeConvertor.castToString(value); // StringType 1298 } else 1299 return super.setProperty(name, value); 1300 return value; 1301 } 1302 1303 @Override 1304 public void removeChild(String name, Base value) throws FHIRException { 1305 if (name.equals("url")) { 1306 this.url = null; 1307 } else if (name.equals("mode")) { 1308 value = new StructureMapModelModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1309 this.mode = (Enumeration) value; // Enumeration<StructureMapModelMode> 1310 } else if (name.equals("alias")) { 1311 this.alias = null; 1312 } else if (name.equals("documentation")) { 1313 this.documentation = null; 1314 } else 1315 super.removeChild(name, value); 1316 1317 } 1318 1319 @Override 1320 public Base makeProperty(int hash, String name) throws FHIRException { 1321 switch (hash) { 1322 case 116079: return getUrlElement(); 1323 case 3357091: return getModeElement(); 1324 case 92902992: return getAliasElement(); 1325 case 1587405498: return getDocumentationElement(); 1326 default: return super.makeProperty(hash, name); 1327 } 1328 1329 } 1330 1331 @Override 1332 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1333 switch (hash) { 1334 case 116079: /*url*/ return new String[] {"canonical"}; 1335 case 3357091: /*mode*/ return new String[] {"code"}; 1336 case 92902992: /*alias*/ return new String[] {"string"}; 1337 case 1587405498: /*documentation*/ return new String[] {"string"}; 1338 default: return super.getTypesForProperty(hash, name); 1339 } 1340 1341 } 1342 1343 @Override 1344 public Base addChild(String name) throws FHIRException { 1345 if (name.equals("url")) { 1346 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.structure.url"); 1347 } 1348 else if (name.equals("mode")) { 1349 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.structure.mode"); 1350 } 1351 else if (name.equals("alias")) { 1352 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.structure.alias"); 1353 } 1354 else if (name.equals("documentation")) { 1355 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.structure.documentation"); 1356 } 1357 else 1358 return super.addChild(name); 1359 } 1360 1361 public StructureMapStructureComponent copy() { 1362 StructureMapStructureComponent dst = new StructureMapStructureComponent(); 1363 copyValues(dst); 1364 return dst; 1365 } 1366 1367 public void copyValues(StructureMapStructureComponent dst) { 1368 super.copyValues(dst); 1369 dst.url = url == null ? null : url.copy(); 1370 dst.mode = mode == null ? null : mode.copy(); 1371 dst.alias = alias == null ? null : alias.copy(); 1372 dst.documentation = documentation == null ? null : documentation.copy(); 1373 } 1374 1375 @Override 1376 public boolean equalsDeep(Base other_) { 1377 if (!super.equalsDeep(other_)) 1378 return false; 1379 if (!(other_ instanceof StructureMapStructureComponent)) 1380 return false; 1381 StructureMapStructureComponent o = (StructureMapStructureComponent) other_; 1382 return compareDeep(url, o.url, true) && compareDeep(mode, o.mode, true) && compareDeep(alias, o.alias, true) 1383 && compareDeep(documentation, o.documentation, true); 1384 } 1385 1386 @Override 1387 public boolean equalsShallow(Base other_) { 1388 if (!super.equalsShallow(other_)) 1389 return false; 1390 if (!(other_ instanceof StructureMapStructureComponent)) 1391 return false; 1392 StructureMapStructureComponent o = (StructureMapStructureComponent) other_; 1393 return compareValues(url, o.url, true) && compareValues(mode, o.mode, true) && compareValues(alias, o.alias, true) 1394 && compareValues(documentation, o.documentation, true); 1395 } 1396 1397 public boolean isEmpty() { 1398 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, mode, alias, documentation 1399 ); 1400 } 1401 1402 public String fhirType() { 1403 return "StructureMap.structure"; 1404 1405 } 1406 1407 } 1408 1409 @Block() 1410 public static class StructureMapConstComponent extends BackboneElement implements IBaseBackboneElement { 1411 /** 1412 * Other maps used by this map (canonical URLs). 1413 */ 1414 @Child(name = "name", type = {IdType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1415 @Description(shortDefinition="Constant name", formalDefinition="Other maps used by this map (canonical URLs)." ) 1416 protected IdType name; 1417 1418 /** 1419 * A FHIRPath expression that is the value of this variable. 1420 */ 1421 @Child(name = "value", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1422 @Description(shortDefinition="FHIRPath exression - value of the constant", formalDefinition="A FHIRPath expression that is the value of this variable." ) 1423 protected StringType value; 1424 1425 private static final long serialVersionUID = 19170614L; 1426 1427 /** 1428 * Constructor 1429 */ 1430 public StructureMapConstComponent() { 1431 super(); 1432 } 1433 1434 /** 1435 * @return {@link #name} (Other maps used by this map (canonical URLs).). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1436 */ 1437 public IdType getNameElement() { 1438 if (this.name == null) 1439 if (Configuration.errorOnAutoCreate()) 1440 throw new Error("Attempt to auto-create StructureMapConstComponent.name"); 1441 else if (Configuration.doAutoCreate()) 1442 this.name = new IdType(); // bb 1443 return this.name; 1444 } 1445 1446 public boolean hasNameElement() { 1447 return this.name != null && !this.name.isEmpty(); 1448 } 1449 1450 public boolean hasName() { 1451 return this.name != null && !this.name.isEmpty(); 1452 } 1453 1454 /** 1455 * @param value {@link #name} (Other maps used by this map (canonical URLs).). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1456 */ 1457 public StructureMapConstComponent setNameElement(IdType value) { 1458 this.name = value; 1459 return this; 1460 } 1461 1462 /** 1463 * @return Other maps used by this map (canonical URLs). 1464 */ 1465 public String getName() { 1466 return this.name == null ? null : this.name.getValue(); 1467 } 1468 1469 /** 1470 * @param value Other maps used by this map (canonical URLs). 1471 */ 1472 public StructureMapConstComponent setName(String value) { 1473 if (Utilities.noString(value)) 1474 this.name = null; 1475 else { 1476 if (this.name == null) 1477 this.name = new IdType(); 1478 this.name.setValue(value); 1479 } 1480 return this; 1481 } 1482 1483 /** 1484 * @return {@link #value} (A FHIRPath expression that is the value of this variable.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 1485 */ 1486 public StringType getValueElement() { 1487 if (this.value == null) 1488 if (Configuration.errorOnAutoCreate()) 1489 throw new Error("Attempt to auto-create StructureMapConstComponent.value"); 1490 else if (Configuration.doAutoCreate()) 1491 this.value = new StringType(); // bb 1492 return this.value; 1493 } 1494 1495 public boolean hasValueElement() { 1496 return this.value != null && !this.value.isEmpty(); 1497 } 1498 1499 public boolean hasValue() { 1500 return this.value != null && !this.value.isEmpty(); 1501 } 1502 1503 /** 1504 * @param value {@link #value} (A FHIRPath expression that is the value of this variable.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 1505 */ 1506 public StructureMapConstComponent setValueElement(StringType value) { 1507 this.value = value; 1508 return this; 1509 } 1510 1511 /** 1512 * @return A FHIRPath expression that is the value of this variable. 1513 */ 1514 public String getValue() { 1515 return this.value == null ? null : this.value.getValue(); 1516 } 1517 1518 /** 1519 * @param value A FHIRPath expression that is the value of this variable. 1520 */ 1521 public StructureMapConstComponent setValue(String value) { 1522 if (Utilities.noString(value)) 1523 this.value = null; 1524 else { 1525 if (this.value == null) 1526 this.value = new StringType(); 1527 this.value.setValue(value); 1528 } 1529 return this; 1530 } 1531 1532 protected void listChildren(List<Property> children) { 1533 super.listChildren(children); 1534 children.add(new Property("name", "id", "Other maps used by this map (canonical URLs).", 0, 1, name)); 1535 children.add(new Property("value", "string", "A FHIRPath expression that is the value of this variable.", 0, 1, value)); 1536 } 1537 1538 @Override 1539 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1540 switch (_hash) { 1541 case 3373707: /*name*/ return new Property("name", "id", "Other maps used by this map (canonical URLs).", 0, 1, name); 1542 case 111972721: /*value*/ return new Property("value", "string", "A FHIRPath expression that is the value of this variable.", 0, 1, value); 1543 default: return super.getNamedProperty(_hash, _name, _checkValid); 1544 } 1545 1546 } 1547 1548 @Override 1549 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1550 switch (hash) { 1551 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // IdType 1552 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 1553 default: return super.getProperty(hash, name, checkValid); 1554 } 1555 1556 } 1557 1558 @Override 1559 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1560 switch (hash) { 1561 case 3373707: // name 1562 this.name = TypeConvertor.castToId(value); // IdType 1563 return value; 1564 case 111972721: // value 1565 this.value = TypeConvertor.castToString(value); // StringType 1566 return value; 1567 default: return super.setProperty(hash, name, value); 1568 } 1569 1570 } 1571 1572 @Override 1573 public Base setProperty(String name, Base value) throws FHIRException { 1574 if (name.equals("name")) { 1575 this.name = TypeConvertor.castToId(value); // IdType 1576 } else if (name.equals("value")) { 1577 this.value = TypeConvertor.castToString(value); // StringType 1578 } else 1579 return super.setProperty(name, value); 1580 return value; 1581 } 1582 1583 @Override 1584 public void removeChild(String name, Base value) throws FHIRException { 1585 if (name.equals("name")) { 1586 this.name = null; 1587 } else if (name.equals("value")) { 1588 this.value = null; 1589 } else 1590 super.removeChild(name, value); 1591 1592 } 1593 1594 @Override 1595 public Base makeProperty(int hash, String name) throws FHIRException { 1596 switch (hash) { 1597 case 3373707: return getNameElement(); 1598 case 111972721: return getValueElement(); 1599 default: return super.makeProperty(hash, name); 1600 } 1601 1602 } 1603 1604 @Override 1605 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1606 switch (hash) { 1607 case 3373707: /*name*/ return new String[] {"id"}; 1608 case 111972721: /*value*/ return new String[] {"string"}; 1609 default: return super.getTypesForProperty(hash, name); 1610 } 1611 1612 } 1613 1614 @Override 1615 public Base addChild(String name) throws FHIRException { 1616 if (name.equals("name")) { 1617 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.const.name"); 1618 } 1619 else if (name.equals("value")) { 1620 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.const.value"); 1621 } 1622 else 1623 return super.addChild(name); 1624 } 1625 1626 public StructureMapConstComponent copy() { 1627 StructureMapConstComponent dst = new StructureMapConstComponent(); 1628 copyValues(dst); 1629 return dst; 1630 } 1631 1632 public void copyValues(StructureMapConstComponent dst) { 1633 super.copyValues(dst); 1634 dst.name = name == null ? null : name.copy(); 1635 dst.value = value == null ? null : value.copy(); 1636 } 1637 1638 @Override 1639 public boolean equalsDeep(Base other_) { 1640 if (!super.equalsDeep(other_)) 1641 return false; 1642 if (!(other_ instanceof StructureMapConstComponent)) 1643 return false; 1644 StructureMapConstComponent o = (StructureMapConstComponent) other_; 1645 return compareDeep(name, o.name, true) && compareDeep(value, o.value, true); 1646 } 1647 1648 @Override 1649 public boolean equalsShallow(Base other_) { 1650 if (!super.equalsShallow(other_)) 1651 return false; 1652 if (!(other_ instanceof StructureMapConstComponent)) 1653 return false; 1654 StructureMapConstComponent o = (StructureMapConstComponent) other_; 1655 return compareValues(name, o.name, true) && compareValues(value, o.value, true); 1656 } 1657 1658 public boolean isEmpty() { 1659 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, value); 1660 } 1661 1662 public String fhirType() { 1663 return "StructureMap.const"; 1664 1665 } 1666 1667 } 1668 1669 @Block() 1670 public static class StructureMapGroupComponent extends BackboneElement implements IBaseBackboneElement { 1671 /** 1672 * A unique name for the group for the convenience of human readers. 1673 */ 1674 @Child(name = "name", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1675 @Description(shortDefinition="Human-readable label", formalDefinition="A unique name for the group for the convenience of human readers." ) 1676 protected IdType name; 1677 1678 /** 1679 * Another group that this group adds rules to. 1680 */ 1681 @Child(name = "extends", type = {IdType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1682 @Description(shortDefinition="Another group that this group adds rules to", formalDefinition="Another group that this group adds rules to." ) 1683 protected IdType extends_; 1684 1685 /** 1686 * If this is the default rule set to apply for the source type or this combination of types. 1687 */ 1688 @Child(name = "typeMode", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1689 @Description(shortDefinition="types | type-and-types", formalDefinition="If this is the default rule set to apply for the source type or this combination of types." ) 1690 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/map-group-type-mode") 1691 protected Enumeration<StructureMapGroupTypeMode> typeMode; 1692 1693 /** 1694 * Additional supporting documentation that explains the purpose of the group and the types of mappings within it. 1695 */ 1696 @Child(name = "documentation", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1697 @Description(shortDefinition="Additional description/explanation for group", formalDefinition="Additional supporting documentation that explains the purpose of the group and the types of mappings within it." ) 1698 protected StringType documentation; 1699 1700 /** 1701 * A name assigned to an instance of data. The instance must be provided when the mapping is invoked. 1702 */ 1703 @Child(name = "input", type = {}, order=5, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1704 @Description(shortDefinition="Named instance provided when invoking the map", formalDefinition="A name assigned to an instance of data. The instance must be provided when the mapping is invoked." ) 1705 protected List<StructureMapGroupInputComponent> input; 1706 1707 /** 1708 * Transform Rule from source to target. 1709 */ 1710 @Child(name = "rule", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1711 @Description(shortDefinition="Transform Rule from source to target", formalDefinition="Transform Rule from source to target." ) 1712 protected List<StructureMapGroupRuleComponent> rule; 1713 1714 private static final long serialVersionUID = -1474595081L; 1715 1716 /** 1717 * Constructor 1718 */ 1719 public StructureMapGroupComponent() { 1720 super(); 1721 } 1722 1723 /** 1724 * Constructor 1725 */ 1726 public StructureMapGroupComponent(String name, StructureMapGroupInputComponent input) { 1727 super(); 1728 this.setName(name); 1729 this.addInput(input); 1730 } 1731 1732 /** 1733 * @return {@link #name} (A unique name for the group for the convenience of human readers.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1734 */ 1735 public IdType getNameElement() { 1736 if (this.name == null) 1737 if (Configuration.errorOnAutoCreate()) 1738 throw new Error("Attempt to auto-create StructureMapGroupComponent.name"); 1739 else if (Configuration.doAutoCreate()) 1740 this.name = new IdType(); // bb 1741 return this.name; 1742 } 1743 1744 public boolean hasNameElement() { 1745 return this.name != null && !this.name.isEmpty(); 1746 } 1747 1748 public boolean hasName() { 1749 return this.name != null && !this.name.isEmpty(); 1750 } 1751 1752 /** 1753 * @param value {@link #name} (A unique name for the group for the convenience of human readers.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1754 */ 1755 public StructureMapGroupComponent setNameElement(IdType value) { 1756 this.name = value; 1757 return this; 1758 } 1759 1760 /** 1761 * @return A unique name for the group for the convenience of human readers. 1762 */ 1763 public String getName() { 1764 return this.name == null ? null : this.name.getValue(); 1765 } 1766 1767 /** 1768 * @param value A unique name for the group for the convenience of human readers. 1769 */ 1770 public StructureMapGroupComponent setName(String value) { 1771 if (this.name == null) 1772 this.name = new IdType(); 1773 this.name.setValue(value); 1774 return this; 1775 } 1776 1777 /** 1778 * @return {@link #extends_} (Another group that this group adds rules to.). This is the underlying object with id, value and extensions. The accessor "getExtends" gives direct access to the value 1779 */ 1780 public IdType getExtendsElement() { 1781 if (this.extends_ == null) 1782 if (Configuration.errorOnAutoCreate()) 1783 throw new Error("Attempt to auto-create StructureMapGroupComponent.extends_"); 1784 else if (Configuration.doAutoCreate()) 1785 this.extends_ = new IdType(); // bb 1786 return this.extends_; 1787 } 1788 1789 public boolean hasExtendsElement() { 1790 return this.extends_ != null && !this.extends_.isEmpty(); 1791 } 1792 1793 public boolean hasExtends() { 1794 return this.extends_ != null && !this.extends_.isEmpty(); 1795 } 1796 1797 /** 1798 * @param value {@link #extends_} (Another group that this group adds rules to.). This is the underlying object with id, value and extensions. The accessor "getExtends" gives direct access to the value 1799 */ 1800 public StructureMapGroupComponent setExtendsElement(IdType value) { 1801 this.extends_ = value; 1802 return this; 1803 } 1804 1805 /** 1806 * @return Another group that this group adds rules to. 1807 */ 1808 public String getExtends() { 1809 return this.extends_ == null ? null : this.extends_.getValue(); 1810 } 1811 1812 /** 1813 * @param value Another group that this group adds rules to. 1814 */ 1815 public StructureMapGroupComponent setExtends(String value) { 1816 if (Utilities.noString(value)) 1817 this.extends_ = null; 1818 else { 1819 if (this.extends_ == null) 1820 this.extends_ = new IdType(); 1821 this.extends_.setValue(value); 1822 } 1823 return this; 1824 } 1825 1826 /** 1827 * @return {@link #typeMode} (If this is the default rule set to apply for the source type or this combination of types.). This is the underlying object with id, value and extensions. The accessor "getTypeMode" gives direct access to the value 1828 */ 1829 public Enumeration<StructureMapGroupTypeMode> getTypeModeElement() { 1830 if (this.typeMode == null) 1831 if (Configuration.errorOnAutoCreate()) 1832 throw new Error("Attempt to auto-create StructureMapGroupComponent.typeMode"); 1833 else if (Configuration.doAutoCreate()) 1834 this.typeMode = new Enumeration<StructureMapGroupTypeMode>(new StructureMapGroupTypeModeEnumFactory()); // bb 1835 return this.typeMode; 1836 } 1837 1838 public boolean hasTypeModeElement() { 1839 return this.typeMode != null && !this.typeMode.isEmpty(); 1840 } 1841 1842 public boolean hasTypeMode() { 1843 return this.typeMode != null && !this.typeMode.isEmpty(); 1844 } 1845 1846 /** 1847 * @param value {@link #typeMode} (If this is the default rule set to apply for the source type or this combination of types.). This is the underlying object with id, value and extensions. The accessor "getTypeMode" gives direct access to the value 1848 */ 1849 public StructureMapGroupComponent setTypeModeElement(Enumeration<StructureMapGroupTypeMode> value) { 1850 this.typeMode = value; 1851 return this; 1852 } 1853 1854 /** 1855 * @return If this is the default rule set to apply for the source type or this combination of types. 1856 */ 1857 public StructureMapGroupTypeMode getTypeMode() { 1858 return this.typeMode == null ? null : this.typeMode.getValue(); 1859 } 1860 1861 /** 1862 * @param value If this is the default rule set to apply for the source type or this combination of types. 1863 */ 1864 public StructureMapGroupComponent setTypeMode(StructureMapGroupTypeMode value) { 1865 if (value == null) 1866 this.typeMode = null; 1867 else { 1868 if (this.typeMode == null) 1869 this.typeMode = new Enumeration<StructureMapGroupTypeMode>(new StructureMapGroupTypeModeEnumFactory()); 1870 this.typeMode.setValue(value); 1871 } 1872 return this; 1873 } 1874 1875 /** 1876 * @return {@link #documentation} (Additional supporting documentation that explains the purpose of the group and the types of mappings within it.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 1877 */ 1878 public StringType getDocumentationElement() { 1879 if (this.documentation == null) 1880 if (Configuration.errorOnAutoCreate()) 1881 throw new Error("Attempt to auto-create StructureMapGroupComponent.documentation"); 1882 else if (Configuration.doAutoCreate()) 1883 this.documentation = new StringType(); // bb 1884 return this.documentation; 1885 } 1886 1887 public boolean hasDocumentationElement() { 1888 return this.documentation != null && !this.documentation.isEmpty(); 1889 } 1890 1891 public boolean hasDocumentation() { 1892 return this.documentation != null && !this.documentation.isEmpty(); 1893 } 1894 1895 /** 1896 * @param value {@link #documentation} (Additional supporting documentation that explains the purpose of the group and the types of mappings within it.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 1897 */ 1898 public StructureMapGroupComponent setDocumentationElement(StringType value) { 1899 this.documentation = value; 1900 return this; 1901 } 1902 1903 /** 1904 * @return Additional supporting documentation that explains the purpose of the group and the types of mappings within it. 1905 */ 1906 public String getDocumentation() { 1907 return this.documentation == null ? null : this.documentation.getValue(); 1908 } 1909 1910 /** 1911 * @param value Additional supporting documentation that explains the purpose of the group and the types of mappings within it. 1912 */ 1913 public StructureMapGroupComponent setDocumentation(String value) { 1914 if (Utilities.noString(value)) 1915 this.documentation = null; 1916 else { 1917 if (this.documentation == null) 1918 this.documentation = new StringType(); 1919 this.documentation.setValue(value); 1920 } 1921 return this; 1922 } 1923 1924 /** 1925 * @return {@link #input} (A name assigned to an instance of data. The instance must be provided when the mapping is invoked.) 1926 */ 1927 public List<StructureMapGroupInputComponent> getInput() { 1928 if (this.input == null) 1929 this.input = new ArrayList<StructureMapGroupInputComponent>(); 1930 return this.input; 1931 } 1932 1933 /** 1934 * @return Returns a reference to <code>this</code> for easy method chaining 1935 */ 1936 public StructureMapGroupComponent setInput(List<StructureMapGroupInputComponent> theInput) { 1937 this.input = theInput; 1938 return this; 1939 } 1940 1941 public boolean hasInput() { 1942 if (this.input == null) 1943 return false; 1944 for (StructureMapGroupInputComponent item : this.input) 1945 if (!item.isEmpty()) 1946 return true; 1947 return false; 1948 } 1949 1950 public StructureMapGroupInputComponent addInput() { //3 1951 StructureMapGroupInputComponent t = new StructureMapGroupInputComponent(); 1952 if (this.input == null) 1953 this.input = new ArrayList<StructureMapGroupInputComponent>(); 1954 this.input.add(t); 1955 return t; 1956 } 1957 1958 public StructureMapGroupComponent addInput(StructureMapGroupInputComponent t) { //3 1959 if (t == null) 1960 return this; 1961 if (this.input == null) 1962 this.input = new ArrayList<StructureMapGroupInputComponent>(); 1963 this.input.add(t); 1964 return this; 1965 } 1966 1967 /** 1968 * @return The first repetition of repeating field {@link #input}, creating it if it does not already exist {3} 1969 */ 1970 public StructureMapGroupInputComponent getInputFirstRep() { 1971 if (getInput().isEmpty()) { 1972 addInput(); 1973 } 1974 return getInput().get(0); 1975 } 1976 1977 /** 1978 * @return {@link #rule} (Transform Rule from source to target.) 1979 */ 1980 public List<StructureMapGroupRuleComponent> getRule() { 1981 if (this.rule == null) 1982 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 1983 return this.rule; 1984 } 1985 1986 /** 1987 * @return Returns a reference to <code>this</code> for easy method chaining 1988 */ 1989 public StructureMapGroupComponent setRule(List<StructureMapGroupRuleComponent> theRule) { 1990 this.rule = theRule; 1991 return this; 1992 } 1993 1994 public boolean hasRule() { 1995 if (this.rule == null) 1996 return false; 1997 for (StructureMapGroupRuleComponent item : this.rule) 1998 if (!item.isEmpty()) 1999 return true; 2000 return false; 2001 } 2002 2003 public StructureMapGroupRuleComponent addRule() { //3 2004 StructureMapGroupRuleComponent t = new StructureMapGroupRuleComponent(); 2005 if (this.rule == null) 2006 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 2007 this.rule.add(t); 2008 return t; 2009 } 2010 2011 public StructureMapGroupComponent addRule(StructureMapGroupRuleComponent t) { //3 2012 if (t == null) 2013 return this; 2014 if (this.rule == null) 2015 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 2016 this.rule.add(t); 2017 return this; 2018 } 2019 2020 /** 2021 * @return The first repetition of repeating field {@link #rule}, creating it if it does not already exist {3} 2022 */ 2023 public StructureMapGroupRuleComponent getRuleFirstRep() { 2024 if (getRule().isEmpty()) { 2025 addRule(); 2026 } 2027 return getRule().get(0); 2028 } 2029 2030 protected void listChildren(List<Property> children) { 2031 super.listChildren(children); 2032 children.add(new Property("name", "id", "A unique name for the group for the convenience of human readers.", 0, 1, name)); 2033 children.add(new Property("extends", "id", "Another group that this group adds rules to.", 0, 1, extends_)); 2034 children.add(new Property("typeMode", "code", "If this is the default rule set to apply for the source type or this combination of types.", 0, 1, typeMode)); 2035 children.add(new Property("documentation", "string", "Additional supporting documentation that explains the purpose of the group and the types of mappings within it.", 0, 1, documentation)); 2036 children.add(new Property("input", "", "A name assigned to an instance of data. The instance must be provided when the mapping is invoked.", 0, java.lang.Integer.MAX_VALUE, input)); 2037 children.add(new Property("rule", "", "Transform Rule from source to target.", 0, java.lang.Integer.MAX_VALUE, rule)); 2038 } 2039 2040 @Override 2041 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2042 switch (_hash) { 2043 case 3373707: /*name*/ return new Property("name", "id", "A unique name for the group for the convenience of human readers.", 0, 1, name); 2044 case -1305664359: /*extends*/ return new Property("extends", "id", "Another group that this group adds rules to.", 0, 1, extends_); 2045 case -676524035: /*typeMode*/ return new Property("typeMode", "code", "If this is the default rule set to apply for the source type or this combination of types.", 0, 1, typeMode); 2046 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Additional supporting documentation that explains the purpose of the group and the types of mappings within it.", 0, 1, documentation); 2047 case 100358090: /*input*/ return new Property("input", "", "A name assigned to an instance of data. The instance must be provided when the mapping is invoked.", 0, java.lang.Integer.MAX_VALUE, input); 2048 case 3512060: /*rule*/ return new Property("rule", "", "Transform Rule from source to target.", 0, java.lang.Integer.MAX_VALUE, rule); 2049 default: return super.getNamedProperty(_hash, _name, _checkValid); 2050 } 2051 2052 } 2053 2054 @Override 2055 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2056 switch (hash) { 2057 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // IdType 2058 case -1305664359: /*extends*/ return this.extends_ == null ? new Base[0] : new Base[] {this.extends_}; // IdType 2059 case -676524035: /*typeMode*/ return this.typeMode == null ? new Base[0] : new Base[] {this.typeMode}; // Enumeration<StructureMapGroupTypeMode> 2060 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 2061 case 100358090: /*input*/ return this.input == null ? new Base[0] : this.input.toArray(new Base[this.input.size()]); // StructureMapGroupInputComponent 2062 case 3512060: /*rule*/ return this.rule == null ? new Base[0] : this.rule.toArray(new Base[this.rule.size()]); // StructureMapGroupRuleComponent 2063 default: return super.getProperty(hash, name, checkValid); 2064 } 2065 2066 } 2067 2068 @Override 2069 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2070 switch (hash) { 2071 case 3373707: // name 2072 this.name = TypeConvertor.castToId(value); // IdType 2073 return value; 2074 case -1305664359: // extends 2075 this.extends_ = TypeConvertor.castToId(value); // IdType 2076 return value; 2077 case -676524035: // typeMode 2078 value = new StructureMapGroupTypeModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2079 this.typeMode = (Enumeration) value; // Enumeration<StructureMapGroupTypeMode> 2080 return value; 2081 case 1587405498: // documentation 2082 this.documentation = TypeConvertor.castToString(value); // StringType 2083 return value; 2084 case 100358090: // input 2085 this.getInput().add((StructureMapGroupInputComponent) value); // StructureMapGroupInputComponent 2086 return value; 2087 case 3512060: // rule 2088 this.getRule().add((StructureMapGroupRuleComponent) value); // StructureMapGroupRuleComponent 2089 return value; 2090 default: return super.setProperty(hash, name, value); 2091 } 2092 2093 } 2094 2095 @Override 2096 public Base setProperty(String name, Base value) throws FHIRException { 2097 if (name.equals("name")) { 2098 this.name = TypeConvertor.castToId(value); // IdType 2099 } else if (name.equals("extends")) { 2100 this.extends_ = TypeConvertor.castToId(value); // IdType 2101 } else if (name.equals("typeMode")) { 2102 value = new StructureMapGroupTypeModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2103 this.typeMode = (Enumeration) value; // Enumeration<StructureMapGroupTypeMode> 2104 } else if (name.equals("documentation")) { 2105 this.documentation = TypeConvertor.castToString(value); // StringType 2106 } else if (name.equals("input")) { 2107 this.getInput().add((StructureMapGroupInputComponent) value); 2108 } else if (name.equals("rule")) { 2109 this.getRule().add((StructureMapGroupRuleComponent) value); 2110 } else 2111 return super.setProperty(name, value); 2112 return value; 2113 } 2114 2115 @Override 2116 public void removeChild(String name, Base value) throws FHIRException { 2117 if (name.equals("name")) { 2118 this.name = null; 2119 } else if (name.equals("extends")) { 2120 this.extends_ = null; 2121 } else if (name.equals("typeMode")) { 2122 value = new StructureMapGroupTypeModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2123 this.typeMode = (Enumeration) value; // Enumeration<StructureMapGroupTypeMode> 2124 } else if (name.equals("documentation")) { 2125 this.documentation = null; 2126 } else if (name.equals("input")) { 2127 this.getInput().remove((StructureMapGroupInputComponent) value); 2128 } else if (name.equals("rule")) { 2129 this.getRule().remove((StructureMapGroupRuleComponent) value); 2130 } else 2131 super.removeChild(name, value); 2132 2133 } 2134 2135 @Override 2136 public Base makeProperty(int hash, String name) throws FHIRException { 2137 switch (hash) { 2138 case 3373707: return getNameElement(); 2139 case -1305664359: return getExtendsElement(); 2140 case -676524035: return getTypeModeElement(); 2141 case 1587405498: return getDocumentationElement(); 2142 case 100358090: return addInput(); 2143 case 3512060: return addRule(); 2144 default: return super.makeProperty(hash, name); 2145 } 2146 2147 } 2148 2149 @Override 2150 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2151 switch (hash) { 2152 case 3373707: /*name*/ return new String[] {"id"}; 2153 case -1305664359: /*extends*/ return new String[] {"id"}; 2154 case -676524035: /*typeMode*/ return new String[] {"code"}; 2155 case 1587405498: /*documentation*/ return new String[] {"string"}; 2156 case 100358090: /*input*/ return new String[] {}; 2157 case 3512060: /*rule*/ return new String[] {}; 2158 default: return super.getTypesForProperty(hash, name); 2159 } 2160 2161 } 2162 2163 @Override 2164 public Base addChild(String name) throws FHIRException { 2165 if (name.equals("name")) { 2166 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.name"); 2167 } 2168 else if (name.equals("extends")) { 2169 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.extends"); 2170 } 2171 else if (name.equals("typeMode")) { 2172 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.typeMode"); 2173 } 2174 else if (name.equals("documentation")) { 2175 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.documentation"); 2176 } 2177 else if (name.equals("input")) { 2178 return addInput(); 2179 } 2180 else if (name.equals("rule")) { 2181 return addRule(); 2182 } 2183 else 2184 return super.addChild(name); 2185 } 2186 2187 public StructureMapGroupComponent copy() { 2188 StructureMapGroupComponent dst = new StructureMapGroupComponent(); 2189 copyValues(dst); 2190 return dst; 2191 } 2192 2193 public void copyValues(StructureMapGroupComponent dst) { 2194 super.copyValues(dst); 2195 dst.name = name == null ? null : name.copy(); 2196 dst.extends_ = extends_ == null ? null : extends_.copy(); 2197 dst.typeMode = typeMode == null ? null : typeMode.copy(); 2198 dst.documentation = documentation == null ? null : documentation.copy(); 2199 if (input != null) { 2200 dst.input = new ArrayList<StructureMapGroupInputComponent>(); 2201 for (StructureMapGroupInputComponent i : input) 2202 dst.input.add(i.copy()); 2203 }; 2204 if (rule != null) { 2205 dst.rule = new ArrayList<StructureMapGroupRuleComponent>(); 2206 for (StructureMapGroupRuleComponent i : rule) 2207 dst.rule.add(i.copy()); 2208 }; 2209 } 2210 2211 @Override 2212 public boolean equalsDeep(Base other_) { 2213 if (!super.equalsDeep(other_)) 2214 return false; 2215 if (!(other_ instanceof StructureMapGroupComponent)) 2216 return false; 2217 StructureMapGroupComponent o = (StructureMapGroupComponent) other_; 2218 return compareDeep(name, o.name, true) && compareDeep(extends_, o.extends_, true) && compareDeep(typeMode, o.typeMode, true) 2219 && compareDeep(documentation, o.documentation, true) && compareDeep(input, o.input, true) && compareDeep(rule, o.rule, true) 2220 ; 2221 } 2222 2223 @Override 2224 public boolean equalsShallow(Base other_) { 2225 if (!super.equalsShallow(other_)) 2226 return false; 2227 if (!(other_ instanceof StructureMapGroupComponent)) 2228 return false; 2229 StructureMapGroupComponent o = (StructureMapGroupComponent) other_; 2230 return compareValues(name, o.name, true) && compareValues(extends_, o.extends_, true) && compareValues(typeMode, o.typeMode, true) 2231 && compareValues(documentation, o.documentation, true); 2232 } 2233 2234 public boolean isEmpty() { 2235 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, extends_, typeMode 2236 , documentation, input, rule); 2237 } 2238 2239 public String fhirType() { 2240 return "StructureMap.group"; 2241 2242 } 2243 2244// added from java-adornments.txt: 2245public String toString() { 2246 return StructureMapUtilities.groupToString(this); 2247 } 2248// end addition 2249 } 2250 2251 @Block() 2252 public static class StructureMapGroupInputComponent extends BackboneElement implements IBaseBackboneElement { 2253 /** 2254 * Name for this instance of data. 2255 */ 2256 @Child(name = "name", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2257 @Description(shortDefinition="Name for this instance of data", formalDefinition="Name for this instance of data." ) 2258 protected IdType name; 2259 2260 /** 2261 * Type for this instance of data. 2262 */ 2263 @Child(name = "type", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2264 @Description(shortDefinition="Type for this instance of data", formalDefinition="Type for this instance of data." ) 2265 protected StringType type; 2266 2267 /** 2268 * Mode for this instance of data. 2269 */ 2270 @Child(name = "mode", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=true) 2271 @Description(shortDefinition="source | target", formalDefinition="Mode for this instance of data." ) 2272 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/map-input-mode") 2273 protected Enumeration<StructureMapInputMode> mode; 2274 2275 /** 2276 * Documentation for this instance of data. 2277 */ 2278 @Child(name = "documentation", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 2279 @Description(shortDefinition="Documentation for this instance of data", formalDefinition="Documentation for this instance of data." ) 2280 protected StringType documentation; 2281 2282 private static final long serialVersionUID = -25050724L; 2283 2284 /** 2285 * Constructor 2286 */ 2287 public StructureMapGroupInputComponent() { 2288 super(); 2289 } 2290 2291 /** 2292 * Constructor 2293 */ 2294 public StructureMapGroupInputComponent(String name, StructureMapInputMode mode) { 2295 super(); 2296 this.setName(name); 2297 this.setMode(mode); 2298 } 2299 2300 /** 2301 * @return {@link #name} (Name for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2302 */ 2303 public IdType getNameElement() { 2304 if (this.name == null) 2305 if (Configuration.errorOnAutoCreate()) 2306 throw new Error("Attempt to auto-create StructureMapGroupInputComponent.name"); 2307 else if (Configuration.doAutoCreate()) 2308 this.name = new IdType(); // bb 2309 return this.name; 2310 } 2311 2312 public boolean hasNameElement() { 2313 return this.name != null && !this.name.isEmpty(); 2314 } 2315 2316 public boolean hasName() { 2317 return this.name != null && !this.name.isEmpty(); 2318 } 2319 2320 /** 2321 * @param value {@link #name} (Name for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2322 */ 2323 public StructureMapGroupInputComponent setNameElement(IdType value) { 2324 this.name = value; 2325 return this; 2326 } 2327 2328 /** 2329 * @return Name for this instance of data. 2330 */ 2331 public String getName() { 2332 return this.name == null ? null : this.name.getValue(); 2333 } 2334 2335 /** 2336 * @param value Name for this instance of data. 2337 */ 2338 public StructureMapGroupInputComponent setName(String value) { 2339 if (this.name == null) 2340 this.name = new IdType(); 2341 this.name.setValue(value); 2342 return this; 2343 } 2344 2345 /** 2346 * @return {@link #type} (Type for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2347 */ 2348 public StringType getTypeElement() { 2349 if (this.type == null) 2350 if (Configuration.errorOnAutoCreate()) 2351 throw new Error("Attempt to auto-create StructureMapGroupInputComponent.type"); 2352 else if (Configuration.doAutoCreate()) 2353 this.type = new StringType(); // bb 2354 return this.type; 2355 } 2356 2357 public boolean hasTypeElement() { 2358 return this.type != null && !this.type.isEmpty(); 2359 } 2360 2361 public boolean hasType() { 2362 return this.type != null && !this.type.isEmpty(); 2363 } 2364 2365 /** 2366 * @param value {@link #type} (Type for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2367 */ 2368 public StructureMapGroupInputComponent setTypeElement(StringType value) { 2369 this.type = value; 2370 return this; 2371 } 2372 2373 /** 2374 * @return Type for this instance of data. 2375 */ 2376 public String getType() { 2377 return this.type == null ? null : this.type.getValue(); 2378 } 2379 2380 /** 2381 * @param value Type for this instance of data. 2382 */ 2383 public StructureMapGroupInputComponent setType(String value) { 2384 if (Utilities.noString(value)) 2385 this.type = null; 2386 else { 2387 if (this.type == null) 2388 this.type = new StringType(); 2389 this.type.setValue(value); 2390 } 2391 return this; 2392 } 2393 2394 /** 2395 * @return {@link #mode} (Mode for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 2396 */ 2397 public Enumeration<StructureMapInputMode> getModeElement() { 2398 if (this.mode == null) 2399 if (Configuration.errorOnAutoCreate()) 2400 throw new Error("Attempt to auto-create StructureMapGroupInputComponent.mode"); 2401 else if (Configuration.doAutoCreate()) 2402 this.mode = new Enumeration<StructureMapInputMode>(new StructureMapInputModeEnumFactory()); // bb 2403 return this.mode; 2404 } 2405 2406 public boolean hasModeElement() { 2407 return this.mode != null && !this.mode.isEmpty(); 2408 } 2409 2410 public boolean hasMode() { 2411 return this.mode != null && !this.mode.isEmpty(); 2412 } 2413 2414 /** 2415 * @param value {@link #mode} (Mode for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 2416 */ 2417 public StructureMapGroupInputComponent setModeElement(Enumeration<StructureMapInputMode> value) { 2418 this.mode = value; 2419 return this; 2420 } 2421 2422 /** 2423 * @return Mode for this instance of data. 2424 */ 2425 public StructureMapInputMode getMode() { 2426 return this.mode == null ? null : this.mode.getValue(); 2427 } 2428 2429 /** 2430 * @param value Mode for this instance of data. 2431 */ 2432 public StructureMapGroupInputComponent setMode(StructureMapInputMode value) { 2433 if (this.mode == null) 2434 this.mode = new Enumeration<StructureMapInputMode>(new StructureMapInputModeEnumFactory()); 2435 this.mode.setValue(value); 2436 return this; 2437 } 2438 2439 /** 2440 * @return {@link #documentation} (Documentation for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 2441 */ 2442 public StringType getDocumentationElement() { 2443 if (this.documentation == null) 2444 if (Configuration.errorOnAutoCreate()) 2445 throw new Error("Attempt to auto-create StructureMapGroupInputComponent.documentation"); 2446 else if (Configuration.doAutoCreate()) 2447 this.documentation = new StringType(); // bb 2448 return this.documentation; 2449 } 2450 2451 public boolean hasDocumentationElement() { 2452 return this.documentation != null && !this.documentation.isEmpty(); 2453 } 2454 2455 public boolean hasDocumentation() { 2456 return this.documentation != null && !this.documentation.isEmpty(); 2457 } 2458 2459 /** 2460 * @param value {@link #documentation} (Documentation for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 2461 */ 2462 public StructureMapGroupInputComponent setDocumentationElement(StringType value) { 2463 this.documentation = value; 2464 return this; 2465 } 2466 2467 /** 2468 * @return Documentation for this instance of data. 2469 */ 2470 public String getDocumentation() { 2471 return this.documentation == null ? null : this.documentation.getValue(); 2472 } 2473 2474 /** 2475 * @param value Documentation for this instance of data. 2476 */ 2477 public StructureMapGroupInputComponent setDocumentation(String value) { 2478 if (Utilities.noString(value)) 2479 this.documentation = null; 2480 else { 2481 if (this.documentation == null) 2482 this.documentation = new StringType(); 2483 this.documentation.setValue(value); 2484 } 2485 return this; 2486 } 2487 2488 protected void listChildren(List<Property> children) { 2489 super.listChildren(children); 2490 children.add(new Property("name", "id", "Name for this instance of data.", 0, 1, name)); 2491 children.add(new Property("type", "string", "Type for this instance of data.", 0, 1, type)); 2492 children.add(new Property("mode", "code", "Mode for this instance of data.", 0, 1, mode)); 2493 children.add(new Property("documentation", "string", "Documentation for this instance of data.", 0, 1, documentation)); 2494 } 2495 2496 @Override 2497 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2498 switch (_hash) { 2499 case 3373707: /*name*/ return new Property("name", "id", "Name for this instance of data.", 0, 1, name); 2500 case 3575610: /*type*/ return new Property("type", "string", "Type for this instance of data.", 0, 1, type); 2501 case 3357091: /*mode*/ return new Property("mode", "code", "Mode for this instance of data.", 0, 1, mode); 2502 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Documentation for this instance of data.", 0, 1, documentation); 2503 default: return super.getNamedProperty(_hash, _name, _checkValid); 2504 } 2505 2506 } 2507 2508 @Override 2509 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2510 switch (hash) { 2511 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // IdType 2512 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // StringType 2513 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<StructureMapInputMode> 2514 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 2515 default: return super.getProperty(hash, name, checkValid); 2516 } 2517 2518 } 2519 2520 @Override 2521 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2522 switch (hash) { 2523 case 3373707: // name 2524 this.name = TypeConvertor.castToId(value); // IdType 2525 return value; 2526 case 3575610: // type 2527 this.type = TypeConvertor.castToString(value); // StringType 2528 return value; 2529 case 3357091: // mode 2530 value = new StructureMapInputModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2531 this.mode = (Enumeration) value; // Enumeration<StructureMapInputMode> 2532 return value; 2533 case 1587405498: // documentation 2534 this.documentation = TypeConvertor.castToString(value); // StringType 2535 return value; 2536 default: return super.setProperty(hash, name, value); 2537 } 2538 2539 } 2540 2541 @Override 2542 public Base setProperty(String name, Base value) throws FHIRException { 2543 if (name.equals("name")) { 2544 this.name = TypeConvertor.castToId(value); // IdType 2545 } else if (name.equals("type")) { 2546 this.type = TypeConvertor.castToString(value); // StringType 2547 } else if (name.equals("mode")) { 2548 value = new StructureMapInputModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2549 this.mode = (Enumeration) value; // Enumeration<StructureMapInputMode> 2550 } else if (name.equals("documentation")) { 2551 this.documentation = TypeConvertor.castToString(value); // StringType 2552 } else 2553 return super.setProperty(name, value); 2554 return value; 2555 } 2556 2557 @Override 2558 public void removeChild(String name, Base value) throws FHIRException { 2559 if (name.equals("name")) { 2560 this.name = null; 2561 } else if (name.equals("type")) { 2562 this.type = null; 2563 } else if (name.equals("mode")) { 2564 value = new StructureMapInputModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2565 this.mode = (Enumeration) value; // Enumeration<StructureMapInputMode> 2566 } else if (name.equals("documentation")) { 2567 this.documentation = null; 2568 } else 2569 super.removeChild(name, value); 2570 2571 } 2572 2573 @Override 2574 public Base makeProperty(int hash, String name) throws FHIRException { 2575 switch (hash) { 2576 case 3373707: return getNameElement(); 2577 case 3575610: return getTypeElement(); 2578 case 3357091: return getModeElement(); 2579 case 1587405498: return getDocumentationElement(); 2580 default: return super.makeProperty(hash, name); 2581 } 2582 2583 } 2584 2585 @Override 2586 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2587 switch (hash) { 2588 case 3373707: /*name*/ return new String[] {"id"}; 2589 case 3575610: /*type*/ return new String[] {"string"}; 2590 case 3357091: /*mode*/ return new String[] {"code"}; 2591 case 1587405498: /*documentation*/ return new String[] {"string"}; 2592 default: return super.getTypesForProperty(hash, name); 2593 } 2594 2595 } 2596 2597 @Override 2598 public Base addChild(String name) throws FHIRException { 2599 if (name.equals("name")) { 2600 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.input.name"); 2601 } 2602 else if (name.equals("type")) { 2603 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.input.type"); 2604 } 2605 else if (name.equals("mode")) { 2606 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.input.mode"); 2607 } 2608 else if (name.equals("documentation")) { 2609 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.input.documentation"); 2610 } 2611 else 2612 return super.addChild(name); 2613 } 2614 2615 public StructureMapGroupInputComponent copy() { 2616 StructureMapGroupInputComponent dst = new StructureMapGroupInputComponent(); 2617 copyValues(dst); 2618 return dst; 2619 } 2620 2621 public void copyValues(StructureMapGroupInputComponent dst) { 2622 super.copyValues(dst); 2623 dst.name = name == null ? null : name.copy(); 2624 dst.type = type == null ? null : type.copy(); 2625 dst.mode = mode == null ? null : mode.copy(); 2626 dst.documentation = documentation == null ? null : documentation.copy(); 2627 } 2628 2629 @Override 2630 public boolean equalsDeep(Base other_) { 2631 if (!super.equalsDeep(other_)) 2632 return false; 2633 if (!(other_ instanceof StructureMapGroupInputComponent)) 2634 return false; 2635 StructureMapGroupInputComponent o = (StructureMapGroupInputComponent) other_; 2636 return compareDeep(name, o.name, true) && compareDeep(type, o.type, true) && compareDeep(mode, o.mode, true) 2637 && compareDeep(documentation, o.documentation, true); 2638 } 2639 2640 @Override 2641 public boolean equalsShallow(Base other_) { 2642 if (!super.equalsShallow(other_)) 2643 return false; 2644 if (!(other_ instanceof StructureMapGroupInputComponent)) 2645 return false; 2646 StructureMapGroupInputComponent o = (StructureMapGroupInputComponent) other_; 2647 return compareValues(name, o.name, true) && compareValues(type, o.type, true) && compareValues(mode, o.mode, true) 2648 && compareValues(documentation, o.documentation, true); 2649 } 2650 2651 public boolean isEmpty() { 2652 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, type, mode, documentation 2653 ); 2654 } 2655 2656 public String fhirType() { 2657 return "StructureMap.group.input"; 2658 2659 } 2660 2661 } 2662 2663 @Block() 2664 public static class StructureMapGroupRuleComponent extends BackboneElement implements IBaseBackboneElement { 2665 /** 2666 * Name of the rule for internal references. 2667 */ 2668 @Child(name = "name", type = {IdType.class}, order=1, min=0, max=1, modifier=false, summary=true) 2669 @Description(shortDefinition="Name of the rule for internal references", formalDefinition="Name of the rule for internal references." ) 2670 protected IdType name; 2671 2672 /** 2673 * Source inputs to the mapping. 2674 */ 2675 @Child(name = "source", type = {}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2676 @Description(shortDefinition="Source inputs to the mapping", formalDefinition="Source inputs to the mapping." ) 2677 protected List<StructureMapGroupRuleSourceComponent> source; 2678 2679 /** 2680 * Content to create because of this mapping rule. 2681 */ 2682 @Child(name = "target", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2683 @Description(shortDefinition="Content to create because of this mapping rule", formalDefinition="Content to create because of this mapping rule." ) 2684 protected List<StructureMapGroupRuleTargetComponent> target; 2685 2686 /** 2687 * Rules contained in this rule. 2688 */ 2689 @Child(name = "rule", type = {StructureMapGroupRuleComponent.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2690 @Description(shortDefinition="Rules contained in this rule", formalDefinition="Rules contained in this rule." ) 2691 protected List<StructureMapGroupRuleComponent> rule; 2692 2693 /** 2694 * Which other rules to apply in the context of this rule. 2695 */ 2696 @Child(name = "dependent", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2697 @Description(shortDefinition="Which other rules to apply in the context of this rule", formalDefinition="Which other rules to apply in the context of this rule." ) 2698 protected List<StructureMapGroupRuleDependentComponent> dependent; 2699 2700 /** 2701 * Documentation for this instance of data. 2702 */ 2703 @Child(name = "documentation", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 2704 @Description(shortDefinition="Documentation for this instance of data", formalDefinition="Documentation for this instance of data." ) 2705 protected StringType documentation; 2706 2707 private static final long serialVersionUID = 773925517L; 2708 2709 /** 2710 * Constructor 2711 */ 2712 public StructureMapGroupRuleComponent() { 2713 super(); 2714 } 2715 2716 /** 2717 * Constructor 2718 */ 2719 public StructureMapGroupRuleComponent(StructureMapGroupRuleSourceComponent source) { 2720 super(); 2721 this.addSource(source); 2722 } 2723 2724 /** 2725 * @return {@link #name} (Name of the rule for internal references.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2726 */ 2727 public IdType getNameElement() { 2728 if (this.name == null) 2729 if (Configuration.errorOnAutoCreate()) 2730 throw new Error("Attempt to auto-create StructureMapGroupRuleComponent.name"); 2731 else if (Configuration.doAutoCreate()) 2732 this.name = new IdType(); // bb 2733 return this.name; 2734 } 2735 2736 public boolean hasNameElement() { 2737 return this.name != null && !this.name.isEmpty(); 2738 } 2739 2740 public boolean hasName() { 2741 return this.name != null && !this.name.isEmpty(); 2742 } 2743 2744 /** 2745 * @param value {@link #name} (Name of the rule for internal references.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2746 */ 2747 public StructureMapGroupRuleComponent setNameElement(IdType value) { 2748 this.name = value; 2749 return this; 2750 } 2751 2752 /** 2753 * @return Name of the rule for internal references. 2754 */ 2755 public String getName() { 2756 return this.name == null ? null : this.name.getValue(); 2757 } 2758 2759 /** 2760 * @param value Name of the rule for internal references. 2761 */ 2762 public StructureMapGroupRuleComponent setName(String value) { 2763 if (Utilities.noString(value)) 2764 this.name = null; 2765 else { 2766 if (this.name == null) 2767 this.name = new IdType(); 2768 this.name.setValue(value); 2769 } 2770 return this; 2771 } 2772 2773 /** 2774 * @return {@link #source} (Source inputs to the mapping.) 2775 */ 2776 public List<StructureMapGroupRuleSourceComponent> getSource() { 2777 if (this.source == null) 2778 this.source = new ArrayList<StructureMapGroupRuleSourceComponent>(); 2779 return this.source; 2780 } 2781 2782 /** 2783 * @return Returns a reference to <code>this</code> for easy method chaining 2784 */ 2785 public StructureMapGroupRuleComponent setSource(List<StructureMapGroupRuleSourceComponent> theSource) { 2786 this.source = theSource; 2787 return this; 2788 } 2789 2790 public boolean hasSource() { 2791 if (this.source == null) 2792 return false; 2793 for (StructureMapGroupRuleSourceComponent item : this.source) 2794 if (!item.isEmpty()) 2795 return true; 2796 return false; 2797 } 2798 2799 public StructureMapGroupRuleSourceComponent addSource() { //3 2800 StructureMapGroupRuleSourceComponent t = new StructureMapGroupRuleSourceComponent(); 2801 if (this.source == null) 2802 this.source = new ArrayList<StructureMapGroupRuleSourceComponent>(); 2803 this.source.add(t); 2804 return t; 2805 } 2806 2807 public StructureMapGroupRuleComponent addSource(StructureMapGroupRuleSourceComponent t) { //3 2808 if (t == null) 2809 return this; 2810 if (this.source == null) 2811 this.source = new ArrayList<StructureMapGroupRuleSourceComponent>(); 2812 this.source.add(t); 2813 return this; 2814 } 2815 2816 /** 2817 * @return The first repetition of repeating field {@link #source}, creating it if it does not already exist {3} 2818 */ 2819 public StructureMapGroupRuleSourceComponent getSourceFirstRep() { 2820 if (getSource().isEmpty()) { 2821 addSource(); 2822 } 2823 return getSource().get(0); 2824 } 2825 2826 /** 2827 * @return {@link #target} (Content to create because of this mapping rule.) 2828 */ 2829 public List<StructureMapGroupRuleTargetComponent> getTarget() { 2830 if (this.target == null) 2831 this.target = new ArrayList<StructureMapGroupRuleTargetComponent>(); 2832 return this.target; 2833 } 2834 2835 /** 2836 * @return Returns a reference to <code>this</code> for easy method chaining 2837 */ 2838 public StructureMapGroupRuleComponent setTarget(List<StructureMapGroupRuleTargetComponent> theTarget) { 2839 this.target = theTarget; 2840 return this; 2841 } 2842 2843 public boolean hasTarget() { 2844 if (this.target == null) 2845 return false; 2846 for (StructureMapGroupRuleTargetComponent item : this.target) 2847 if (!item.isEmpty()) 2848 return true; 2849 return false; 2850 } 2851 2852 public StructureMapGroupRuleTargetComponent addTarget() { //3 2853 StructureMapGroupRuleTargetComponent t = new StructureMapGroupRuleTargetComponent(); 2854 if (this.target == null) 2855 this.target = new ArrayList<StructureMapGroupRuleTargetComponent>(); 2856 this.target.add(t); 2857 return t; 2858 } 2859 2860 public StructureMapGroupRuleComponent addTarget(StructureMapGroupRuleTargetComponent t) { //3 2861 if (t == null) 2862 return this; 2863 if (this.target == null) 2864 this.target = new ArrayList<StructureMapGroupRuleTargetComponent>(); 2865 this.target.add(t); 2866 return this; 2867 } 2868 2869 /** 2870 * @return The first repetition of repeating field {@link #target}, creating it if it does not already exist {3} 2871 */ 2872 public StructureMapGroupRuleTargetComponent getTargetFirstRep() { 2873 if (getTarget().isEmpty()) { 2874 addTarget(); 2875 } 2876 return getTarget().get(0); 2877 } 2878 2879 /** 2880 * @return {@link #rule} (Rules contained in this rule.) 2881 */ 2882 public List<StructureMapGroupRuleComponent> getRule() { 2883 if (this.rule == null) 2884 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 2885 return this.rule; 2886 } 2887 2888 /** 2889 * @return Returns a reference to <code>this</code> for easy method chaining 2890 */ 2891 public StructureMapGroupRuleComponent setRule(List<StructureMapGroupRuleComponent> theRule) { 2892 this.rule = theRule; 2893 return this; 2894 } 2895 2896 public boolean hasRule() { 2897 if (this.rule == null) 2898 return false; 2899 for (StructureMapGroupRuleComponent item : this.rule) 2900 if (!item.isEmpty()) 2901 return true; 2902 return false; 2903 } 2904 2905 public StructureMapGroupRuleComponent addRule() { //3 2906 StructureMapGroupRuleComponent t = new StructureMapGroupRuleComponent(); 2907 if (this.rule == null) 2908 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 2909 this.rule.add(t); 2910 return t; 2911 } 2912 2913 public StructureMapGroupRuleComponent addRule(StructureMapGroupRuleComponent t) { //3 2914 if (t == null) 2915 return this; 2916 if (this.rule == null) 2917 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 2918 this.rule.add(t); 2919 return this; 2920 } 2921 2922 /** 2923 * @return The first repetition of repeating field {@link #rule}, creating it if it does not already exist {3} 2924 */ 2925 public StructureMapGroupRuleComponent getRuleFirstRep() { 2926 if (getRule().isEmpty()) { 2927 addRule(); 2928 } 2929 return getRule().get(0); 2930 } 2931 2932 /** 2933 * @return {@link #dependent} (Which other rules to apply in the context of this rule.) 2934 */ 2935 public List<StructureMapGroupRuleDependentComponent> getDependent() { 2936 if (this.dependent == null) 2937 this.dependent = new ArrayList<StructureMapGroupRuleDependentComponent>(); 2938 return this.dependent; 2939 } 2940 2941 /** 2942 * @return Returns a reference to <code>this</code> for easy method chaining 2943 */ 2944 public StructureMapGroupRuleComponent setDependent(List<StructureMapGroupRuleDependentComponent> theDependent) { 2945 this.dependent = theDependent; 2946 return this; 2947 } 2948 2949 public boolean hasDependent() { 2950 if (this.dependent == null) 2951 return false; 2952 for (StructureMapGroupRuleDependentComponent item : this.dependent) 2953 if (!item.isEmpty()) 2954 return true; 2955 return false; 2956 } 2957 2958 public StructureMapGroupRuleDependentComponent addDependent() { //3 2959 StructureMapGroupRuleDependentComponent t = new StructureMapGroupRuleDependentComponent(); 2960 if (this.dependent == null) 2961 this.dependent = new ArrayList<StructureMapGroupRuleDependentComponent>(); 2962 this.dependent.add(t); 2963 return t; 2964 } 2965 2966 public StructureMapGroupRuleComponent addDependent(StructureMapGroupRuleDependentComponent t) { //3 2967 if (t == null) 2968 return this; 2969 if (this.dependent == null) 2970 this.dependent = new ArrayList<StructureMapGroupRuleDependentComponent>(); 2971 this.dependent.add(t); 2972 return this; 2973 } 2974 2975 /** 2976 * @return The first repetition of repeating field {@link #dependent}, creating it if it does not already exist {3} 2977 */ 2978 public StructureMapGroupRuleDependentComponent getDependentFirstRep() { 2979 if (getDependent().isEmpty()) { 2980 addDependent(); 2981 } 2982 return getDependent().get(0); 2983 } 2984 2985 /** 2986 * @return {@link #documentation} (Documentation for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 2987 */ 2988 public StringType getDocumentationElement() { 2989 if (this.documentation == null) 2990 if (Configuration.errorOnAutoCreate()) 2991 throw new Error("Attempt to auto-create StructureMapGroupRuleComponent.documentation"); 2992 else if (Configuration.doAutoCreate()) 2993 this.documentation = new StringType(); // bb 2994 return this.documentation; 2995 } 2996 2997 public boolean hasDocumentationElement() { 2998 return this.documentation != null && !this.documentation.isEmpty(); 2999 } 3000 3001 public boolean hasDocumentation() { 3002 return this.documentation != null && !this.documentation.isEmpty(); 3003 } 3004 3005 /** 3006 * @param value {@link #documentation} (Documentation for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 3007 */ 3008 public StructureMapGroupRuleComponent setDocumentationElement(StringType value) { 3009 this.documentation = value; 3010 return this; 3011 } 3012 3013 /** 3014 * @return Documentation for this instance of data. 3015 */ 3016 public String getDocumentation() { 3017 return this.documentation == null ? null : this.documentation.getValue(); 3018 } 3019 3020 /** 3021 * @param value Documentation for this instance of data. 3022 */ 3023 public StructureMapGroupRuleComponent setDocumentation(String value) { 3024 if (Utilities.noString(value)) 3025 this.documentation = null; 3026 else { 3027 if (this.documentation == null) 3028 this.documentation = new StringType(); 3029 this.documentation.setValue(value); 3030 } 3031 return this; 3032 } 3033 3034 protected void listChildren(List<Property> children) { 3035 super.listChildren(children); 3036 children.add(new Property("name", "id", "Name of the rule for internal references.", 0, 1, name)); 3037 children.add(new Property("source", "", "Source inputs to the mapping.", 0, java.lang.Integer.MAX_VALUE, source)); 3038 children.add(new Property("target", "", "Content to create because of this mapping rule.", 0, java.lang.Integer.MAX_VALUE, target)); 3039 children.add(new Property("rule", "@StructureMap.group.rule", "Rules contained in this rule.", 0, java.lang.Integer.MAX_VALUE, rule)); 3040 children.add(new Property("dependent", "", "Which other rules to apply in the context of this rule.", 0, java.lang.Integer.MAX_VALUE, dependent)); 3041 children.add(new Property("documentation", "string", "Documentation for this instance of data.", 0, 1, documentation)); 3042 } 3043 3044 @Override 3045 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3046 switch (_hash) { 3047 case 3373707: /*name*/ return new Property("name", "id", "Name of the rule for internal references.", 0, 1, name); 3048 case -896505829: /*source*/ return new Property("source", "", "Source inputs to the mapping.", 0, java.lang.Integer.MAX_VALUE, source); 3049 case -880905839: /*target*/ return new Property("target", "", "Content to create because of this mapping rule.", 0, java.lang.Integer.MAX_VALUE, target); 3050 case 3512060: /*rule*/ return new Property("rule", "@StructureMap.group.rule", "Rules contained in this rule.", 0, java.lang.Integer.MAX_VALUE, rule); 3051 case -1109226753: /*dependent*/ return new Property("dependent", "", "Which other rules to apply in the context of this rule.", 0, java.lang.Integer.MAX_VALUE, dependent); 3052 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Documentation for this instance of data.", 0, 1, documentation); 3053 default: return super.getNamedProperty(_hash, _name, _checkValid); 3054 } 3055 3056 } 3057 3058 @Override 3059 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3060 switch (hash) { 3061 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // IdType 3062 case -896505829: /*source*/ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // StructureMapGroupRuleSourceComponent 3063 case -880905839: /*target*/ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // StructureMapGroupRuleTargetComponent 3064 case 3512060: /*rule*/ return this.rule == null ? new Base[0] : this.rule.toArray(new Base[this.rule.size()]); // StructureMapGroupRuleComponent 3065 case -1109226753: /*dependent*/ return this.dependent == null ? new Base[0] : this.dependent.toArray(new Base[this.dependent.size()]); // StructureMapGroupRuleDependentComponent 3066 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 3067 default: return super.getProperty(hash, name, checkValid); 3068 } 3069 3070 } 3071 3072 @Override 3073 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3074 switch (hash) { 3075 case 3373707: // name 3076 this.name = TypeConvertor.castToId(value); // IdType 3077 return value; 3078 case -896505829: // source 3079 this.getSource().add((StructureMapGroupRuleSourceComponent) value); // StructureMapGroupRuleSourceComponent 3080 return value; 3081 case -880905839: // target 3082 this.getTarget().add((StructureMapGroupRuleTargetComponent) value); // StructureMapGroupRuleTargetComponent 3083 return value; 3084 case 3512060: // rule 3085 this.getRule().add((StructureMapGroupRuleComponent) value); // StructureMapGroupRuleComponent 3086 return value; 3087 case -1109226753: // dependent 3088 this.getDependent().add((StructureMapGroupRuleDependentComponent) value); // StructureMapGroupRuleDependentComponent 3089 return value; 3090 case 1587405498: // documentation 3091 this.documentation = TypeConvertor.castToString(value); // StringType 3092 return value; 3093 default: return super.setProperty(hash, name, value); 3094 } 3095 3096 } 3097 3098 @Override 3099 public Base setProperty(String name, Base value) throws FHIRException { 3100 if (name.equals("name")) { 3101 this.name = TypeConvertor.castToId(value); // IdType 3102 } else if (name.equals("source")) { 3103 this.getSource().add((StructureMapGroupRuleSourceComponent) value); 3104 } else if (name.equals("target")) { 3105 this.getTarget().add((StructureMapGroupRuleTargetComponent) value); 3106 } else if (name.equals("rule")) { 3107 this.getRule().add((StructureMapGroupRuleComponent) value); 3108 } else if (name.equals("dependent")) { 3109 this.getDependent().add((StructureMapGroupRuleDependentComponent) value); 3110 } else if (name.equals("documentation")) { 3111 this.documentation = TypeConvertor.castToString(value); // StringType 3112 } else 3113 return super.setProperty(name, value); 3114 return value; 3115 } 3116 3117 @Override 3118 public void removeChild(String name, Base value) throws FHIRException { 3119 if (name.equals("name")) { 3120 this.name = null; 3121 } else if (name.equals("source")) { 3122 this.getSource().remove((StructureMapGroupRuleSourceComponent) value); 3123 } else if (name.equals("target")) { 3124 this.getTarget().remove((StructureMapGroupRuleTargetComponent) value); 3125 } else if (name.equals("rule")) { 3126 this.getRule().remove((StructureMapGroupRuleComponent) value); 3127 } else if (name.equals("dependent")) { 3128 this.getDependent().remove((StructureMapGroupRuleDependentComponent) value); 3129 } else if (name.equals("documentation")) { 3130 this.documentation = null; 3131 } else 3132 super.removeChild(name, value); 3133 3134 } 3135 3136 @Override 3137 public Base makeProperty(int hash, String name) throws FHIRException { 3138 switch (hash) { 3139 case 3373707: return getNameElement(); 3140 case -896505829: return addSource(); 3141 case -880905839: return addTarget(); 3142 case 3512060: return addRule(); 3143 case -1109226753: return addDependent(); 3144 case 1587405498: return getDocumentationElement(); 3145 default: return super.makeProperty(hash, name); 3146 } 3147 3148 } 3149 3150 @Override 3151 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3152 switch (hash) { 3153 case 3373707: /*name*/ return new String[] {"id"}; 3154 case -896505829: /*source*/ return new String[] {}; 3155 case -880905839: /*target*/ return new String[] {}; 3156 case 3512060: /*rule*/ return new String[] {"@StructureMap.group.rule"}; 3157 case -1109226753: /*dependent*/ return new String[] {}; 3158 case 1587405498: /*documentation*/ return new String[] {"string"}; 3159 default: return super.getTypesForProperty(hash, name); 3160 } 3161 3162 } 3163 3164 @Override 3165 public Base addChild(String name) throws FHIRException { 3166 if (name.equals("name")) { 3167 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.name"); 3168 } 3169 else if (name.equals("source")) { 3170 return addSource(); 3171 } 3172 else if (name.equals("target")) { 3173 return addTarget(); 3174 } 3175 else if (name.equals("rule")) { 3176 return addRule(); 3177 } 3178 else if (name.equals("dependent")) { 3179 return addDependent(); 3180 } 3181 else if (name.equals("documentation")) { 3182 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.documentation"); 3183 } 3184 else 3185 return super.addChild(name); 3186 } 3187 3188 public StructureMapGroupRuleComponent copy() { 3189 StructureMapGroupRuleComponent dst = new StructureMapGroupRuleComponent(); 3190 copyValues(dst); 3191 return dst; 3192 } 3193 3194 public void copyValues(StructureMapGroupRuleComponent dst) { 3195 super.copyValues(dst); 3196 dst.name = name == null ? null : name.copy(); 3197 if (source != null) { 3198 dst.source = new ArrayList<StructureMapGroupRuleSourceComponent>(); 3199 for (StructureMapGroupRuleSourceComponent i : source) 3200 dst.source.add(i.copy()); 3201 }; 3202 if (target != null) { 3203 dst.target = new ArrayList<StructureMapGroupRuleTargetComponent>(); 3204 for (StructureMapGroupRuleTargetComponent i : target) 3205 dst.target.add(i.copy()); 3206 }; 3207 if (rule != null) { 3208 dst.rule = new ArrayList<StructureMapGroupRuleComponent>(); 3209 for (StructureMapGroupRuleComponent i : rule) 3210 dst.rule.add(i.copy()); 3211 }; 3212 if (dependent != null) { 3213 dst.dependent = new ArrayList<StructureMapGroupRuleDependentComponent>(); 3214 for (StructureMapGroupRuleDependentComponent i : dependent) 3215 dst.dependent.add(i.copy()); 3216 }; 3217 dst.documentation = documentation == null ? null : documentation.copy(); 3218 } 3219 3220 @Override 3221 public boolean equalsDeep(Base other_) { 3222 if (!super.equalsDeep(other_)) 3223 return false; 3224 if (!(other_ instanceof StructureMapGroupRuleComponent)) 3225 return false; 3226 StructureMapGroupRuleComponent o = (StructureMapGroupRuleComponent) other_; 3227 return compareDeep(name, o.name, true) && compareDeep(source, o.source, true) && compareDeep(target, o.target, true) 3228 && compareDeep(rule, o.rule, true) && compareDeep(dependent, o.dependent, true) && compareDeep(documentation, o.documentation, true) 3229 ; 3230 } 3231 3232 @Override 3233 public boolean equalsShallow(Base other_) { 3234 if (!super.equalsShallow(other_)) 3235 return false; 3236 if (!(other_ instanceof StructureMapGroupRuleComponent)) 3237 return false; 3238 StructureMapGroupRuleComponent o = (StructureMapGroupRuleComponent) other_; 3239 return compareValues(name, o.name, true) && compareValues(documentation, o.documentation, true); 3240 } 3241 3242 public boolean isEmpty() { 3243 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, source, target, rule 3244 , dependent, documentation); 3245 } 3246 3247 public String fhirType() { 3248 return "StructureMap.group.rule"; 3249 3250 } 3251 3252// added from java-adornments.txt: 3253public String toString() { 3254 return StructureMapUtilities.ruleToString(this); 3255 } 3256// end addition 3257 } 3258 3259 @Block() 3260 public static class StructureMapGroupRuleSourceComponent extends BackboneElement implements IBaseBackboneElement { 3261 /** 3262 * Type or variable this rule applies to. 3263 */ 3264 @Child(name = "context", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 3265 @Description(shortDefinition="Type or variable this rule applies to", formalDefinition="Type or variable this rule applies to." ) 3266 protected IdType context; 3267 3268 /** 3269 * Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content. 3270 */ 3271 @Child(name = "min", type = {IntegerType.class}, order=2, min=0, max=1, modifier=false, summary=true) 3272 @Description(shortDefinition="Specified minimum cardinality", formalDefinition="Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content." ) 3273 protected IntegerType min; 3274 3275 /** 3276 * Specified maximum cardinality for the element - a number or a "*". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value). 3277 */ 3278 @Child(name = "max", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 3279 @Description(shortDefinition="Specified maximum cardinality (number or *)", formalDefinition="Specified maximum cardinality for the element - a number or a \"*\". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value)." ) 3280 protected StringType max; 3281 3282 /** 3283 * Specified type for the element. This works as a condition on the mapping - use for polymorphic elements. 3284 */ 3285 @Child(name = "type", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 3286 @Description(shortDefinition="Rule only applies if source has this type", formalDefinition="Specified type for the element. This works as a condition on the mapping - use for polymorphic elements." ) 3287 protected StringType type; 3288 3289 /** 3290 * A value to use if there is no existing value in the source object. 3291 */ 3292 @Child(name = "defaultValue", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 3293 @Description(shortDefinition="Default value if no value exists", formalDefinition="A value to use if there is no existing value in the source object." ) 3294 protected StringType defaultValue; 3295 3296 /** 3297 * Optional field for this source. 3298 */ 3299 @Child(name = "element", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 3300 @Description(shortDefinition="Optional field for this source", formalDefinition="Optional field for this source." ) 3301 protected StringType element; 3302 3303 /** 3304 * How to handle the list mode for this element. 3305 */ 3306 @Child(name = "listMode", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 3307 @Description(shortDefinition="first | not_first | last | not_last | only_one", formalDefinition="How to handle the list mode for this element." ) 3308 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/map-source-list-mode") 3309 protected Enumeration<StructureMapSourceListMode> listMode; 3310 3311 /** 3312 * Named context for field, if a field is specified. 3313 */ 3314 @Child(name = "variable", type = {IdType.class}, order=8, min=0, max=1, modifier=false, summary=true) 3315 @Description(shortDefinition="Named context for field, if a field is specified", formalDefinition="Named context for field, if a field is specified." ) 3316 protected IdType variable; 3317 3318 /** 3319 * FHIRPath expression - must be true or the rule does not apply. 3320 */ 3321 @Child(name = "condition", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 3322 @Description(shortDefinition="FHIRPath expression - must be true or the rule does not apply", formalDefinition="FHIRPath expression - must be true or the rule does not apply." ) 3323 protected StringType condition; 3324 3325 /** 3326 * FHIRPath expression - must be true or the mapping engine throws an error instead of completing. 3327 */ 3328 @Child(name = "check", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 3329 @Description(shortDefinition="FHIRPath expression - must be true or the mapping engine throws an error instead of completing", formalDefinition="FHIRPath expression - must be true or the mapping engine throws an error instead of completing." ) 3330 protected StringType check; 3331 3332 /** 3333 * A FHIRPath expression which specifies a message to put in the transform log when content matching the source rule is found. 3334 */ 3335 @Child(name = "logMessage", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=true) 3336 @Description(shortDefinition="Message to put in log if source exists (FHIRPath)", formalDefinition="A FHIRPath expression which specifies a message to put in the transform log when content matching the source rule is found." ) 3337 protected StringType logMessage; 3338 3339 private static final long serialVersionUID = 214178119L; 3340 3341 /** 3342 * Constructor 3343 */ 3344 public StructureMapGroupRuleSourceComponent() { 3345 super(); 3346 } 3347 3348 /** 3349 * Constructor 3350 */ 3351 public StructureMapGroupRuleSourceComponent(String context) { 3352 super(); 3353 this.setContext(context); 3354 } 3355 3356 /** 3357 * @return {@link #context} (Type or variable this rule applies to.). This is the underlying object with id, value and extensions. The accessor "getContext" gives direct access to the value 3358 */ 3359 public IdType getContextElement() { 3360 if (this.context == null) 3361 if (Configuration.errorOnAutoCreate()) 3362 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.context"); 3363 else if (Configuration.doAutoCreate()) 3364 this.context = new IdType(); // bb 3365 return this.context; 3366 } 3367 3368 public boolean hasContextElement() { 3369 return this.context != null && !this.context.isEmpty(); 3370 } 3371 3372 public boolean hasContext() { 3373 return this.context != null && !this.context.isEmpty(); 3374 } 3375 3376 /** 3377 * @param value {@link #context} (Type or variable this rule applies to.). This is the underlying object with id, value and extensions. The accessor "getContext" gives direct access to the value 3378 */ 3379 public StructureMapGroupRuleSourceComponent setContextElement(IdType value) { 3380 this.context = value; 3381 return this; 3382 } 3383 3384 /** 3385 * @return Type or variable this rule applies to. 3386 */ 3387 public String getContext() { 3388 return this.context == null ? null : this.context.getValue(); 3389 } 3390 3391 /** 3392 * @param value Type or variable this rule applies to. 3393 */ 3394 public StructureMapGroupRuleSourceComponent setContext(String value) { 3395 if (this.context == null) 3396 this.context = new IdType(); 3397 this.context.setValue(value); 3398 return this; 3399 } 3400 3401 /** 3402 * @return {@link #min} (Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 3403 */ 3404 public IntegerType getMinElement() { 3405 if (this.min == null) 3406 if (Configuration.errorOnAutoCreate()) 3407 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.min"); 3408 else if (Configuration.doAutoCreate()) 3409 this.min = new IntegerType(); // bb 3410 return this.min; 3411 } 3412 3413 public boolean hasMinElement() { 3414 return this.min != null && !this.min.isEmpty(); 3415 } 3416 3417 public boolean hasMin() { 3418 return this.min != null && !this.min.isEmpty(); 3419 } 3420 3421 /** 3422 * @param value {@link #min} (Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 3423 */ 3424 public StructureMapGroupRuleSourceComponent setMinElement(IntegerType value) { 3425 this.min = value; 3426 return this; 3427 } 3428 3429 /** 3430 * @return Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content. 3431 */ 3432 public int getMin() { 3433 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 3434 } 3435 3436 /** 3437 * @param value Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content. 3438 */ 3439 public StructureMapGroupRuleSourceComponent setMin(int value) { 3440 if (this.min == null) 3441 this.min = new IntegerType(); 3442 this.min.setValue(value); 3443 return this; 3444 } 3445 3446 /** 3447 * @return {@link #max} (Specified maximum cardinality for the element - a number or a "*". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value).). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 3448 */ 3449 public StringType getMaxElement() { 3450 if (this.max == null) 3451 if (Configuration.errorOnAutoCreate()) 3452 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.max"); 3453 else if (Configuration.doAutoCreate()) 3454 this.max = new StringType(); // bb 3455 return this.max; 3456 } 3457 3458 public boolean hasMaxElement() { 3459 return this.max != null && !this.max.isEmpty(); 3460 } 3461 3462 public boolean hasMax() { 3463 return this.max != null && !this.max.isEmpty(); 3464 } 3465 3466 /** 3467 * @param value {@link #max} (Specified maximum cardinality for the element - a number or a "*". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value).). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 3468 */ 3469 public StructureMapGroupRuleSourceComponent setMaxElement(StringType value) { 3470 this.max = value; 3471 return this; 3472 } 3473 3474 /** 3475 * @return Specified maximum cardinality for the element - a number or a "*". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value). 3476 */ 3477 public String getMax() { 3478 return this.max == null ? null : this.max.getValue(); 3479 } 3480 3481 /** 3482 * @param value Specified maximum cardinality for the element - a number or a "*". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value). 3483 */ 3484 public StructureMapGroupRuleSourceComponent setMax(String value) { 3485 if (Utilities.noString(value)) 3486 this.max = null; 3487 else { 3488 if (this.max == null) 3489 this.max = new StringType(); 3490 this.max.setValue(value); 3491 } 3492 return this; 3493 } 3494 3495 /** 3496 * @return {@link #type} (Specified type for the element. This works as a condition on the mapping - use for polymorphic elements.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3497 */ 3498 public StringType getTypeElement() { 3499 if (this.type == null) 3500 if (Configuration.errorOnAutoCreate()) 3501 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.type"); 3502 else if (Configuration.doAutoCreate()) 3503 this.type = new StringType(); // bb 3504 return this.type; 3505 } 3506 3507 public boolean hasTypeElement() { 3508 return this.type != null && !this.type.isEmpty(); 3509 } 3510 3511 public boolean hasType() { 3512 return this.type != null && !this.type.isEmpty(); 3513 } 3514 3515 /** 3516 * @param value {@link #type} (Specified type for the element. This works as a condition on the mapping - use for polymorphic elements.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3517 */ 3518 public StructureMapGroupRuleSourceComponent setTypeElement(StringType value) { 3519 this.type = value; 3520 return this; 3521 } 3522 3523 /** 3524 * @return Specified type for the element. This works as a condition on the mapping - use for polymorphic elements. 3525 */ 3526 public String getType() { 3527 return this.type == null ? null : this.type.getValue(); 3528 } 3529 3530 /** 3531 * @param value Specified type for the element. This works as a condition on the mapping - use for polymorphic elements. 3532 */ 3533 public StructureMapGroupRuleSourceComponent setType(String value) { 3534 if (Utilities.noString(value)) 3535 this.type = null; 3536 else { 3537 if (this.type == null) 3538 this.type = new StringType(); 3539 this.type.setValue(value); 3540 } 3541 return this; 3542 } 3543 3544 /** 3545 * @return {@link #defaultValue} (A value to use if there is no existing value in the source object.). This is the underlying object with id, value and extensions. The accessor "getDefaultValue" gives direct access to the value 3546 */ 3547 public StringType getDefaultValueElement() { 3548 if (this.defaultValue == null) 3549 if (Configuration.errorOnAutoCreate()) 3550 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.defaultValue"); 3551 else if (Configuration.doAutoCreate()) 3552 this.defaultValue = new StringType(); // bb 3553 return this.defaultValue; 3554 } 3555 3556 public boolean hasDefaultValueElement() { 3557 return this.defaultValue != null && !this.defaultValue.isEmpty(); 3558 } 3559 3560 public boolean hasDefaultValue() { 3561 return this.defaultValue != null && !this.defaultValue.isEmpty(); 3562 } 3563 3564 /** 3565 * @param value {@link #defaultValue} (A value to use if there is no existing value in the source object.). This is the underlying object with id, value and extensions. The accessor "getDefaultValue" gives direct access to the value 3566 */ 3567 public StructureMapGroupRuleSourceComponent setDefaultValueElement(StringType value) { 3568 this.defaultValue = value; 3569 return this; 3570 } 3571 3572 /** 3573 * @return A value to use if there is no existing value in the source object. 3574 */ 3575 public String getDefaultValue() { 3576 return this.defaultValue == null ? null : this.defaultValue.getValue(); 3577 } 3578 3579 /** 3580 * @param value A value to use if there is no existing value in the source object. 3581 */ 3582 public StructureMapGroupRuleSourceComponent setDefaultValue(String value) { 3583 if (Utilities.noString(value)) 3584 this.defaultValue = null; 3585 else { 3586 if (this.defaultValue == null) 3587 this.defaultValue = new StringType(); 3588 this.defaultValue.setValue(value); 3589 } 3590 return this; 3591 } 3592 3593 /** 3594 * @return {@link #element} (Optional field for this source.). This is the underlying object with id, value and extensions. The accessor "getElement" gives direct access to the value 3595 */ 3596 public StringType getElementElement() { 3597 if (this.element == null) 3598 if (Configuration.errorOnAutoCreate()) 3599 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.element"); 3600 else if (Configuration.doAutoCreate()) 3601 this.element = new StringType(); // bb 3602 return this.element; 3603 } 3604 3605 public boolean hasElementElement() { 3606 return this.element != null && !this.element.isEmpty(); 3607 } 3608 3609 public boolean hasElement() { 3610 return this.element != null && !this.element.isEmpty(); 3611 } 3612 3613 /** 3614 * @param value {@link #element} (Optional field for this source.). This is the underlying object with id, value and extensions. The accessor "getElement" gives direct access to the value 3615 */ 3616 public StructureMapGroupRuleSourceComponent setElementElement(StringType value) { 3617 this.element = value; 3618 return this; 3619 } 3620 3621 /** 3622 * @return Optional field for this source. 3623 */ 3624 public String getElement() { 3625 return this.element == null ? null : this.element.getValue(); 3626 } 3627 3628 /** 3629 * @param value Optional field for this source. 3630 */ 3631 public StructureMapGroupRuleSourceComponent setElement(String value) { 3632 if (Utilities.noString(value)) 3633 this.element = null; 3634 else { 3635 if (this.element == null) 3636 this.element = new StringType(); 3637 this.element.setValue(value); 3638 } 3639 return this; 3640 } 3641 3642 /** 3643 * @return {@link #listMode} (How to handle the list mode for this element.). This is the underlying object with id, value and extensions. The accessor "getListMode" gives direct access to the value 3644 */ 3645 public Enumeration<StructureMapSourceListMode> getListModeElement() { 3646 if (this.listMode == null) 3647 if (Configuration.errorOnAutoCreate()) 3648 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.listMode"); 3649 else if (Configuration.doAutoCreate()) 3650 this.listMode = new Enumeration<StructureMapSourceListMode>(new StructureMapSourceListModeEnumFactory()); // bb 3651 return this.listMode; 3652 } 3653 3654 public boolean hasListModeElement() { 3655 return this.listMode != null && !this.listMode.isEmpty(); 3656 } 3657 3658 public boolean hasListMode() { 3659 return this.listMode != null && !this.listMode.isEmpty(); 3660 } 3661 3662 /** 3663 * @param value {@link #listMode} (How to handle the list mode for this element.). This is the underlying object with id, value and extensions. The accessor "getListMode" gives direct access to the value 3664 */ 3665 public StructureMapGroupRuleSourceComponent setListModeElement(Enumeration<StructureMapSourceListMode> value) { 3666 this.listMode = value; 3667 return this; 3668 } 3669 3670 /** 3671 * @return How to handle the list mode for this element. 3672 */ 3673 public StructureMapSourceListMode getListMode() { 3674 return this.listMode == null ? null : this.listMode.getValue(); 3675 } 3676 3677 /** 3678 * @param value How to handle the list mode for this element. 3679 */ 3680 public StructureMapGroupRuleSourceComponent setListMode(StructureMapSourceListMode value) { 3681 if (value == null) 3682 this.listMode = null; 3683 else { 3684 if (this.listMode == null) 3685 this.listMode = new Enumeration<StructureMapSourceListMode>(new StructureMapSourceListModeEnumFactory()); 3686 this.listMode.setValue(value); 3687 } 3688 return this; 3689 } 3690 3691 /** 3692 * @return {@link #variable} (Named context for field, if a field is specified.). This is the underlying object with id, value and extensions. The accessor "getVariable" gives direct access to the value 3693 */ 3694 public IdType getVariableElement() { 3695 if (this.variable == null) 3696 if (Configuration.errorOnAutoCreate()) 3697 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.variable"); 3698 else if (Configuration.doAutoCreate()) 3699 this.variable = new IdType(); // bb 3700 return this.variable; 3701 } 3702 3703 public boolean hasVariableElement() { 3704 return this.variable != null && !this.variable.isEmpty(); 3705 } 3706 3707 public boolean hasVariable() { 3708 return this.variable != null && !this.variable.isEmpty(); 3709 } 3710 3711 /** 3712 * @param value {@link #variable} (Named context for field, if a field is specified.). This is the underlying object with id, value and extensions. The accessor "getVariable" gives direct access to the value 3713 */ 3714 public StructureMapGroupRuleSourceComponent setVariableElement(IdType value) { 3715 this.variable = value; 3716 return this; 3717 } 3718 3719 /** 3720 * @return Named context for field, if a field is specified. 3721 */ 3722 public String getVariable() { 3723 return this.variable == null ? null : this.variable.getValue(); 3724 } 3725 3726 /** 3727 * @param value Named context for field, if a field is specified. 3728 */ 3729 public StructureMapGroupRuleSourceComponent setVariable(String value) { 3730 if (Utilities.noString(value)) 3731 this.variable = null; 3732 else { 3733 if (this.variable == null) 3734 this.variable = new IdType(); 3735 this.variable.setValue(value); 3736 } 3737 return this; 3738 } 3739 3740 /** 3741 * @return {@link #condition} (FHIRPath expression - must be true or the rule does not apply.). This is the underlying object with id, value and extensions. The accessor "getCondition" gives direct access to the value 3742 */ 3743 public StringType getConditionElement() { 3744 if (this.condition == null) 3745 if (Configuration.errorOnAutoCreate()) 3746 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.condition"); 3747 else if (Configuration.doAutoCreate()) 3748 this.condition = new StringType(); // bb 3749 return this.condition; 3750 } 3751 3752 public boolean hasConditionElement() { 3753 return this.condition != null && !this.condition.isEmpty(); 3754 } 3755 3756 public boolean hasCondition() { 3757 return this.condition != null && !this.condition.isEmpty(); 3758 } 3759 3760 /** 3761 * @param value {@link #condition} (FHIRPath expression - must be true or the rule does not apply.). This is the underlying object with id, value and extensions. The accessor "getCondition" gives direct access to the value 3762 */ 3763 public StructureMapGroupRuleSourceComponent setConditionElement(StringType value) { 3764 this.condition = value; 3765 return this; 3766 } 3767 3768 /** 3769 * @return FHIRPath expression - must be true or the rule does not apply. 3770 */ 3771 public String getCondition() { 3772 return this.condition == null ? null : this.condition.getValue(); 3773 } 3774 3775 /** 3776 * @param value FHIRPath expression - must be true or the rule does not apply. 3777 */ 3778 public StructureMapGroupRuleSourceComponent setCondition(String value) { 3779 if (Utilities.noString(value)) 3780 this.condition = null; 3781 else { 3782 if (this.condition == null) 3783 this.condition = new StringType(); 3784 this.condition.setValue(value); 3785 } 3786 return this; 3787 } 3788 3789 /** 3790 * @return {@link #check} (FHIRPath expression - must be true or the mapping engine throws an error instead of completing.). This is the underlying object with id, value and extensions. The accessor "getCheck" gives direct access to the value 3791 */ 3792 public StringType getCheckElement() { 3793 if (this.check == null) 3794 if (Configuration.errorOnAutoCreate()) 3795 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.check"); 3796 else if (Configuration.doAutoCreate()) 3797 this.check = new StringType(); // bb 3798 return this.check; 3799 } 3800 3801 public boolean hasCheckElement() { 3802 return this.check != null && !this.check.isEmpty(); 3803 } 3804 3805 public boolean hasCheck() { 3806 return this.check != null && !this.check.isEmpty(); 3807 } 3808 3809 /** 3810 * @param value {@link #check} (FHIRPath expression - must be true or the mapping engine throws an error instead of completing.). This is the underlying object with id, value and extensions. The accessor "getCheck" gives direct access to the value 3811 */ 3812 public StructureMapGroupRuleSourceComponent setCheckElement(StringType value) { 3813 this.check = value; 3814 return this; 3815 } 3816 3817 /** 3818 * @return FHIRPath expression - must be true or the mapping engine throws an error instead of completing. 3819 */ 3820 public String getCheck() { 3821 return this.check == null ? null : this.check.getValue(); 3822 } 3823 3824 /** 3825 * @param value FHIRPath expression - must be true or the mapping engine throws an error instead of completing. 3826 */ 3827 public StructureMapGroupRuleSourceComponent setCheck(String value) { 3828 if (Utilities.noString(value)) 3829 this.check = null; 3830 else { 3831 if (this.check == null) 3832 this.check = new StringType(); 3833 this.check.setValue(value); 3834 } 3835 return this; 3836 } 3837 3838 /** 3839 * @return {@link #logMessage} (A FHIRPath expression which specifies a message to put in the transform log when content matching the source rule is found.). This is the underlying object with id, value and extensions. The accessor "getLogMessage" gives direct access to the value 3840 */ 3841 public StringType getLogMessageElement() { 3842 if (this.logMessage == null) 3843 if (Configuration.errorOnAutoCreate()) 3844 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.logMessage"); 3845 else if (Configuration.doAutoCreate()) 3846 this.logMessage = new StringType(); // bb 3847 return this.logMessage; 3848 } 3849 3850 public boolean hasLogMessageElement() { 3851 return this.logMessage != null && !this.logMessage.isEmpty(); 3852 } 3853 3854 public boolean hasLogMessage() { 3855 return this.logMessage != null && !this.logMessage.isEmpty(); 3856 } 3857 3858 /** 3859 * @param value {@link #logMessage} (A FHIRPath expression which specifies a message to put in the transform log when content matching the source rule is found.). This is the underlying object with id, value and extensions. The accessor "getLogMessage" gives direct access to the value 3860 */ 3861 public StructureMapGroupRuleSourceComponent setLogMessageElement(StringType value) { 3862 this.logMessage = value; 3863 return this; 3864 } 3865 3866 /** 3867 * @return A FHIRPath expression which specifies a message to put in the transform log when content matching the source rule is found. 3868 */ 3869 public String getLogMessage() { 3870 return this.logMessage == null ? null : this.logMessage.getValue(); 3871 } 3872 3873 /** 3874 * @param value A FHIRPath expression which specifies a message to put in the transform log when content matching the source rule is found. 3875 */ 3876 public StructureMapGroupRuleSourceComponent setLogMessage(String value) { 3877 if (Utilities.noString(value)) 3878 this.logMessage = null; 3879 else { 3880 if (this.logMessage == null) 3881 this.logMessage = new StringType(); 3882 this.logMessage.setValue(value); 3883 } 3884 return this; 3885 } 3886 3887 protected void listChildren(List<Property> children) { 3888 super.listChildren(children); 3889 children.add(new Property("context", "id", "Type or variable this rule applies to.", 0, 1, context)); 3890 children.add(new Property("min", "integer", "Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content.", 0, 1, min)); 3891 children.add(new Property("max", "string", "Specified maximum cardinality for the element - a number or a \"*\". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value).", 0, 1, max)); 3892 children.add(new Property("type", "string", "Specified type for the element. This works as a condition on the mapping - use for polymorphic elements.", 0, 1, type)); 3893 children.add(new Property("defaultValue", "string", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue)); 3894 children.add(new Property("element", "string", "Optional field for this source.", 0, 1, element)); 3895 children.add(new Property("listMode", "code", "How to handle the list mode for this element.", 0, 1, listMode)); 3896 children.add(new Property("variable", "id", "Named context for field, if a field is specified.", 0, 1, variable)); 3897 children.add(new Property("condition", "string", "FHIRPath expression - must be true or the rule does not apply.", 0, 1, condition)); 3898 children.add(new Property("check", "string", "FHIRPath expression - must be true or the mapping engine throws an error instead of completing.", 0, 1, check)); 3899 children.add(new Property("logMessage", "string", "A FHIRPath expression which specifies a message to put in the transform log when content matching the source rule is found.", 0, 1, logMessage)); 3900 } 3901 3902 @Override 3903 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3904 switch (_hash) { 3905 case 951530927: /*context*/ return new Property("context", "id", "Type or variable this rule applies to.", 0, 1, context); 3906 case 108114: /*min*/ return new Property("min", "integer", "Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content.", 0, 1, min); 3907 case 107876: /*max*/ return new Property("max", "string", "Specified maximum cardinality for the element - a number or a \"*\". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value).", 0, 1, max); 3908 case 3575610: /*type*/ return new Property("type", "string", "Specified type for the element. This works as a condition on the mapping - use for polymorphic elements.", 0, 1, type); 3909 case -659125328: /*defaultValue*/ return new Property("defaultValue", "string", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3910 case -1662836996: /*element*/ return new Property("element", "string", "Optional field for this source.", 0, 1, element); 3911 case 1345445729: /*listMode*/ return new Property("listMode", "code", "How to handle the list mode for this element.", 0, 1, listMode); 3912 case -1249586564: /*variable*/ return new Property("variable", "id", "Named context for field, if a field is specified.", 0, 1, variable); 3913 case -861311717: /*condition*/ return new Property("condition", "string", "FHIRPath expression - must be true or the rule does not apply.", 0, 1, condition); 3914 case 94627080: /*check*/ return new Property("check", "string", "FHIRPath expression - must be true or the mapping engine throws an error instead of completing.", 0, 1, check); 3915 case -1067155421: /*logMessage*/ return new Property("logMessage", "string", "A FHIRPath expression which specifies a message to put in the transform log when content matching the source rule is found.", 0, 1, logMessage); 3916 default: return super.getNamedProperty(_hash, _name, _checkValid); 3917 } 3918 3919 } 3920 3921 @Override 3922 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3923 switch (hash) { 3924 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // IdType 3925 case 108114: /*min*/ return this.min == null ? new Base[0] : new Base[] {this.min}; // IntegerType 3926 case 107876: /*max*/ return this.max == null ? new Base[0] : new Base[] {this.max}; // StringType 3927 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // StringType 3928 case -659125328: /*defaultValue*/ return this.defaultValue == null ? new Base[0] : new Base[] {this.defaultValue}; // StringType 3929 case -1662836996: /*element*/ return this.element == null ? new Base[0] : new Base[] {this.element}; // StringType 3930 case 1345445729: /*listMode*/ return this.listMode == null ? new Base[0] : new Base[] {this.listMode}; // Enumeration<StructureMapSourceListMode> 3931 case -1249586564: /*variable*/ return this.variable == null ? new Base[0] : new Base[] {this.variable}; // IdType 3932 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : new Base[] {this.condition}; // StringType 3933 case 94627080: /*check*/ return this.check == null ? new Base[0] : new Base[] {this.check}; // StringType 3934 case -1067155421: /*logMessage*/ return this.logMessage == null ? new Base[0] : new Base[] {this.logMessage}; // StringType 3935 default: return super.getProperty(hash, name, checkValid); 3936 } 3937 3938 } 3939 3940 @Override 3941 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3942 switch (hash) { 3943 case 951530927: // context 3944 this.context = TypeConvertor.castToId(value); // IdType 3945 return value; 3946 case 108114: // min 3947 this.min = TypeConvertor.castToInteger(value); // IntegerType 3948 return value; 3949 case 107876: // max 3950 this.max = TypeConvertor.castToString(value); // StringType 3951 return value; 3952 case 3575610: // type 3953 this.type = TypeConvertor.castToString(value); // StringType 3954 return value; 3955 case -659125328: // defaultValue 3956 this.defaultValue = TypeConvertor.castToString(value); // StringType 3957 return value; 3958 case -1662836996: // element 3959 this.element = TypeConvertor.castToString(value); // StringType 3960 return value; 3961 case 1345445729: // listMode 3962 value = new StructureMapSourceListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3963 this.listMode = (Enumeration) value; // Enumeration<StructureMapSourceListMode> 3964 return value; 3965 case -1249586564: // variable 3966 this.variable = TypeConvertor.castToId(value); // IdType 3967 return value; 3968 case -861311717: // condition 3969 this.condition = TypeConvertor.castToString(value); // StringType 3970 return value; 3971 case 94627080: // check 3972 this.check = TypeConvertor.castToString(value); // StringType 3973 return value; 3974 case -1067155421: // logMessage 3975 this.logMessage = TypeConvertor.castToString(value); // StringType 3976 return value; 3977 default: return super.setProperty(hash, name, value); 3978 } 3979 3980 } 3981 3982 @Override 3983 public Base setProperty(String name, Base value) throws FHIRException { 3984 if (name.equals("context")) { 3985 this.context = TypeConvertor.castToId(value); // IdType 3986 } else if (name.equals("min")) { 3987 this.min = TypeConvertor.castToInteger(value); // IntegerType 3988 } else if (name.equals("max")) { 3989 this.max = TypeConvertor.castToString(value); // StringType 3990 } else if (name.equals("type")) { 3991 this.type = TypeConvertor.castToString(value); // StringType 3992 } else if (name.equals("defaultValue")) { 3993 this.defaultValue = TypeConvertor.castToString(value); // StringType 3994 } else if (name.equals("element")) { 3995 this.element = TypeConvertor.castToString(value); // StringType 3996 } else if (name.equals("listMode")) { 3997 value = new StructureMapSourceListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 3998 this.listMode = (Enumeration) value; // Enumeration<StructureMapSourceListMode> 3999 } else if (name.equals("variable")) { 4000 this.variable = TypeConvertor.castToId(value); // IdType 4001 } else if (name.equals("condition")) { 4002 this.condition = TypeConvertor.castToString(value); // StringType 4003 } else if (name.equals("check")) { 4004 this.check = TypeConvertor.castToString(value); // StringType 4005 } else if (name.equals("logMessage")) { 4006 this.logMessage = TypeConvertor.castToString(value); // StringType 4007 } else 4008 return super.setProperty(name, value); 4009 return value; 4010 } 4011 4012 @Override 4013 public void removeChild(String name, Base value) throws FHIRException { 4014 if (name.equals("context")) { 4015 this.context = null; 4016 } else if (name.equals("min")) { 4017 this.min = null; 4018 } else if (name.equals("max")) { 4019 this.max = null; 4020 } else if (name.equals("type")) { 4021 this.type = null; 4022 } else if (name.equals("defaultValue")) { 4023 this.defaultValue = null; 4024 } else if (name.equals("element")) { 4025 this.element = null; 4026 } else if (name.equals("listMode")) { 4027 value = new StructureMapSourceListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4028 this.listMode = (Enumeration) value; // Enumeration<StructureMapSourceListMode> 4029 } else if (name.equals("variable")) { 4030 this.variable = null; 4031 } else if (name.equals("condition")) { 4032 this.condition = null; 4033 } else if (name.equals("check")) { 4034 this.check = null; 4035 } else if (name.equals("logMessage")) { 4036 this.logMessage = null; 4037 } else 4038 super.removeChild(name, value); 4039 4040 } 4041 4042 @Override 4043 public Base makeProperty(int hash, String name) throws FHIRException { 4044 switch (hash) { 4045 case 951530927: return getContextElement(); 4046 case 108114: return getMinElement(); 4047 case 107876: return getMaxElement(); 4048 case 3575610: return getTypeElement(); 4049 case -659125328: return getDefaultValueElement(); 4050 case -1662836996: return getElementElement(); 4051 case 1345445729: return getListModeElement(); 4052 case -1249586564: return getVariableElement(); 4053 case -861311717: return getConditionElement(); 4054 case 94627080: return getCheckElement(); 4055 case -1067155421: return getLogMessageElement(); 4056 default: return super.makeProperty(hash, name); 4057 } 4058 4059 } 4060 4061 @Override 4062 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4063 switch (hash) { 4064 case 951530927: /*context*/ return new String[] {"id"}; 4065 case 108114: /*min*/ return new String[] {"integer"}; 4066 case 107876: /*max*/ return new String[] {"string"}; 4067 case 3575610: /*type*/ return new String[] {"string"}; 4068 case -659125328: /*defaultValue*/ return new String[] {"string"}; 4069 case -1662836996: /*element*/ return new String[] {"string"}; 4070 case 1345445729: /*listMode*/ return new String[] {"code"}; 4071 case -1249586564: /*variable*/ return new String[] {"id"}; 4072 case -861311717: /*condition*/ return new String[] {"string"}; 4073 case 94627080: /*check*/ return new String[] {"string"}; 4074 case -1067155421: /*logMessage*/ return new String[] {"string"}; 4075 default: return super.getTypesForProperty(hash, name); 4076 } 4077 4078 } 4079 4080 @Override 4081 public Base addChild(String name) throws FHIRException { 4082 if (name.equals("context")) { 4083 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.context"); 4084 } 4085 else if (name.equals("min")) { 4086 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.min"); 4087 } 4088 else if (name.equals("max")) { 4089 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.max"); 4090 } 4091 else if (name.equals("type")) { 4092 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.type"); 4093 } 4094 else if (name.equals("defaultValue")) { 4095 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.defaultValue"); 4096 } 4097 else if (name.equals("element")) { 4098 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.element"); 4099 } 4100 else if (name.equals("listMode")) { 4101 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.listMode"); 4102 } 4103 else if (name.equals("variable")) { 4104 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.variable"); 4105 } 4106 else if (name.equals("condition")) { 4107 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.condition"); 4108 } 4109 else if (name.equals("check")) { 4110 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.check"); 4111 } 4112 else if (name.equals("logMessage")) { 4113 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.source.logMessage"); 4114 } 4115 else 4116 return super.addChild(name); 4117 } 4118 4119 public StructureMapGroupRuleSourceComponent copy() { 4120 StructureMapGroupRuleSourceComponent dst = new StructureMapGroupRuleSourceComponent(); 4121 copyValues(dst); 4122 return dst; 4123 } 4124 4125 public void copyValues(StructureMapGroupRuleSourceComponent dst) { 4126 super.copyValues(dst); 4127 dst.context = context == null ? null : context.copy(); 4128 dst.min = min == null ? null : min.copy(); 4129 dst.max = max == null ? null : max.copy(); 4130 dst.type = type == null ? null : type.copy(); 4131 dst.defaultValue = defaultValue == null ? null : defaultValue.copy(); 4132 dst.element = element == null ? null : element.copy(); 4133 dst.listMode = listMode == null ? null : listMode.copy(); 4134 dst.variable = variable == null ? null : variable.copy(); 4135 dst.condition = condition == null ? null : condition.copy(); 4136 dst.check = check == null ? null : check.copy(); 4137 dst.logMessage = logMessage == null ? null : logMessage.copy(); 4138 } 4139 4140 @Override 4141 public boolean equalsDeep(Base other_) { 4142 if (!super.equalsDeep(other_)) 4143 return false; 4144 if (!(other_ instanceof StructureMapGroupRuleSourceComponent)) 4145 return false; 4146 StructureMapGroupRuleSourceComponent o = (StructureMapGroupRuleSourceComponent) other_; 4147 return compareDeep(context, o.context, true) && compareDeep(min, o.min, true) && compareDeep(max, o.max, true) 4148 && compareDeep(type, o.type, true) && compareDeep(defaultValue, o.defaultValue, true) && compareDeep(element, o.element, true) 4149 && compareDeep(listMode, o.listMode, true) && compareDeep(variable, o.variable, true) && compareDeep(condition, o.condition, true) 4150 && compareDeep(check, o.check, true) && compareDeep(logMessage, o.logMessage, true); 4151 } 4152 4153 @Override 4154 public boolean equalsShallow(Base other_) { 4155 if (!super.equalsShallow(other_)) 4156 return false; 4157 if (!(other_ instanceof StructureMapGroupRuleSourceComponent)) 4158 return false; 4159 StructureMapGroupRuleSourceComponent o = (StructureMapGroupRuleSourceComponent) other_; 4160 return compareValues(context, o.context, true) && compareValues(min, o.min, true) && compareValues(max, o.max, true) 4161 && compareValues(type, o.type, true) && compareValues(defaultValue, o.defaultValue, true) && compareValues(element, o.element, true) 4162 && compareValues(listMode, o.listMode, true) && compareValues(variable, o.variable, true) && compareValues(condition, o.condition, true) 4163 && compareValues(check, o.check, true) && compareValues(logMessage, o.logMessage, true); 4164 } 4165 4166 public boolean isEmpty() { 4167 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(context, min, max, type 4168 , defaultValue, element, listMode, variable, condition, check, logMessage); 4169 } 4170 4171 public String fhirType() { 4172 return "StructureMap.group.rule.source"; 4173 4174 } 4175 4176// added from java-adornments.txt: 4177public String toString() { 4178 return StructureMapUtilities.sourceToString(this); 4179 } 4180// end addition 4181 } 4182 4183 @Block() 4184 public static class StructureMapGroupRuleTargetComponent extends BackboneElement implements IBaseBackboneElement { 4185 /** 4186 * Variable this rule applies to. 4187 */ 4188 @Child(name = "context", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 4189 @Description(shortDefinition="Variable this rule applies to", formalDefinition="Variable this rule applies to." ) 4190 protected StringType context; 4191 4192 /** 4193 * Field to create in the context. 4194 */ 4195 @Child(name = "element", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 4196 @Description(shortDefinition="Field to create in the context", formalDefinition="Field to create in the context." ) 4197 protected StringType element; 4198 4199 /** 4200 * Named context for field, if desired, and a field is specified. 4201 */ 4202 @Child(name = "variable", type = {IdType.class}, order=3, min=0, max=1, modifier=false, summary=true) 4203 @Description(shortDefinition="Named context for field, if desired, and a field is specified", formalDefinition="Named context for field, if desired, and a field is specified." ) 4204 protected IdType variable; 4205 4206 /** 4207 * If field is a list, how to manage the list. 4208 */ 4209 @Child(name = "listMode", type = {CodeType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4210 @Description(shortDefinition="first | share | last | single", formalDefinition="If field is a list, how to manage the list." ) 4211 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/map-target-list-mode") 4212 protected List<Enumeration<StructureMapTargetListMode>> listMode; 4213 4214 /** 4215 * Internal rule reference for shared list items. 4216 */ 4217 @Child(name = "listRuleId", type = {IdType.class}, order=5, min=0, max=1, modifier=false, summary=true) 4218 @Description(shortDefinition="Internal rule reference for shared list items", formalDefinition="Internal rule reference for shared list items." ) 4219 protected IdType listRuleId; 4220 4221 /** 4222 * How the data is copied / created. 4223 */ 4224 @Child(name = "transform", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 4225 @Description(shortDefinition="create | copy +", formalDefinition="How the data is copied / created." ) 4226 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/map-transform") 4227 protected Enumeration<StructureMapTransform> transform; 4228 4229 /** 4230 * Parameters to the transform. 4231 */ 4232 @Child(name = "parameter", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4233 @Description(shortDefinition="Parameters to the transform", formalDefinition="Parameters to the transform." ) 4234 protected List<StructureMapGroupRuleTargetParameterComponent> parameter; 4235 4236 private static final long serialVersionUID = 1565495071L; 4237 4238 /** 4239 * Constructor 4240 */ 4241 public StructureMapGroupRuleTargetComponent() { 4242 super(); 4243 } 4244 4245 /** 4246 * @return {@link #context} (Variable this rule applies to.). This is the underlying object with id, value and extensions. The accessor "getContext" gives direct access to the value 4247 */ 4248 public StringType getContextElement() { 4249 if (this.context == null) 4250 if (Configuration.errorOnAutoCreate()) 4251 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.context"); 4252 else if (Configuration.doAutoCreate()) 4253 this.context = new StringType(); // bb 4254 return this.context; 4255 } 4256 4257 public boolean hasContextElement() { 4258 return this.context != null && !this.context.isEmpty(); 4259 } 4260 4261 public boolean hasContext() { 4262 return this.context != null && !this.context.isEmpty(); 4263 } 4264 4265 /** 4266 * @param value {@link #context} (Variable this rule applies to.). This is the underlying object with id, value and extensions. The accessor "getContext" gives direct access to the value 4267 */ 4268 public StructureMapGroupRuleTargetComponent setContextElement(StringType value) { 4269 this.context = value; 4270 return this; 4271 } 4272 4273 /** 4274 * @return Variable this rule applies to. 4275 */ 4276 public String getContext() { 4277 return this.context == null ? null : this.context.getValue(); 4278 } 4279 4280 /** 4281 * @param value Variable this rule applies to. 4282 */ 4283 public StructureMapGroupRuleTargetComponent setContext(String value) { 4284 if (Utilities.noString(value)) 4285 this.context = null; 4286 else { 4287 if (this.context == null) 4288 this.context = new StringType(); 4289 this.context.setValue(value); 4290 } 4291 return this; 4292 } 4293 4294 /** 4295 * @return {@link #element} (Field to create in the context.). This is the underlying object with id, value and extensions. The accessor "getElement" gives direct access to the value 4296 */ 4297 public StringType getElementElement() { 4298 if (this.element == null) 4299 if (Configuration.errorOnAutoCreate()) 4300 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.element"); 4301 else if (Configuration.doAutoCreate()) 4302 this.element = new StringType(); // bb 4303 return this.element; 4304 } 4305 4306 public boolean hasElementElement() { 4307 return this.element != null && !this.element.isEmpty(); 4308 } 4309 4310 public boolean hasElement() { 4311 return this.element != null && !this.element.isEmpty(); 4312 } 4313 4314 /** 4315 * @param value {@link #element} (Field to create in the context.). This is the underlying object with id, value and extensions. The accessor "getElement" gives direct access to the value 4316 */ 4317 public StructureMapGroupRuleTargetComponent setElementElement(StringType value) { 4318 this.element = value; 4319 return this; 4320 } 4321 4322 /** 4323 * @return Field to create in the context. 4324 */ 4325 public String getElement() { 4326 return this.element == null ? null : this.element.getValue(); 4327 } 4328 4329 /** 4330 * @param value Field to create in the context. 4331 */ 4332 public StructureMapGroupRuleTargetComponent setElement(String value) { 4333 if (Utilities.noString(value)) 4334 this.element = null; 4335 else { 4336 if (this.element == null) 4337 this.element = new StringType(); 4338 this.element.setValue(value); 4339 } 4340 return this; 4341 } 4342 4343 /** 4344 * @return {@link #variable} (Named context for field, if desired, and a field is specified.). This is the underlying object with id, value and extensions. The accessor "getVariable" gives direct access to the value 4345 */ 4346 public IdType getVariableElement() { 4347 if (this.variable == null) 4348 if (Configuration.errorOnAutoCreate()) 4349 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.variable"); 4350 else if (Configuration.doAutoCreate()) 4351 this.variable = new IdType(); // bb 4352 return this.variable; 4353 } 4354 4355 public boolean hasVariableElement() { 4356 return this.variable != null && !this.variable.isEmpty(); 4357 } 4358 4359 public boolean hasVariable() { 4360 return this.variable != null && !this.variable.isEmpty(); 4361 } 4362 4363 /** 4364 * @param value {@link #variable} (Named context for field, if desired, and a field is specified.). This is the underlying object with id, value and extensions. The accessor "getVariable" gives direct access to the value 4365 */ 4366 public StructureMapGroupRuleTargetComponent setVariableElement(IdType value) { 4367 this.variable = value; 4368 return this; 4369 } 4370 4371 /** 4372 * @return Named context for field, if desired, and a field is specified. 4373 */ 4374 public String getVariable() { 4375 return this.variable == null ? null : this.variable.getValue(); 4376 } 4377 4378 /** 4379 * @param value Named context for field, if desired, and a field is specified. 4380 */ 4381 public StructureMapGroupRuleTargetComponent setVariable(String value) { 4382 if (Utilities.noString(value)) 4383 this.variable = null; 4384 else { 4385 if (this.variable == null) 4386 this.variable = new IdType(); 4387 this.variable.setValue(value); 4388 } 4389 return this; 4390 } 4391 4392 /** 4393 * @return {@link #listMode} (If field is a list, how to manage the list.) 4394 */ 4395 public List<Enumeration<StructureMapTargetListMode>> getListMode() { 4396 if (this.listMode == null) 4397 this.listMode = new ArrayList<Enumeration<StructureMapTargetListMode>>(); 4398 return this.listMode; 4399 } 4400 4401 /** 4402 * @return Returns a reference to <code>this</code> for easy method chaining 4403 */ 4404 public StructureMapGroupRuleTargetComponent setListMode(List<Enumeration<StructureMapTargetListMode>> theListMode) { 4405 this.listMode = theListMode; 4406 return this; 4407 } 4408 4409 public boolean hasListMode() { 4410 if (this.listMode == null) 4411 return false; 4412 for (Enumeration<StructureMapTargetListMode> item : this.listMode) 4413 if (!item.isEmpty()) 4414 return true; 4415 return false; 4416 } 4417 4418 /** 4419 * @return {@link #listMode} (If field is a list, how to manage the list.) 4420 */ 4421 public Enumeration<StructureMapTargetListMode> addListModeElement() {//2 4422 Enumeration<StructureMapTargetListMode> t = new Enumeration<StructureMapTargetListMode>(new StructureMapTargetListModeEnumFactory()); 4423 if (this.listMode == null) 4424 this.listMode = new ArrayList<Enumeration<StructureMapTargetListMode>>(); 4425 this.listMode.add(t); 4426 return t; 4427 } 4428 4429 /** 4430 * @param value {@link #listMode} (If field is a list, how to manage the list.) 4431 */ 4432 public StructureMapGroupRuleTargetComponent addListMode(StructureMapTargetListMode value) { //1 4433 Enumeration<StructureMapTargetListMode> t = new Enumeration<StructureMapTargetListMode>(new StructureMapTargetListModeEnumFactory()); 4434 t.setValue(value); 4435 if (this.listMode == null) 4436 this.listMode = new ArrayList<Enumeration<StructureMapTargetListMode>>(); 4437 this.listMode.add(t); 4438 return this; 4439 } 4440 4441 /** 4442 * @param value {@link #listMode} (If field is a list, how to manage the list.) 4443 */ 4444 public boolean hasListMode(StructureMapTargetListMode value) { 4445 if (this.listMode == null) 4446 return false; 4447 for (Enumeration<StructureMapTargetListMode> v : this.listMode) 4448 if (v.getValue().equals(value)) // code 4449 return true; 4450 return false; 4451 } 4452 4453 /** 4454 * @return {@link #listRuleId} (Internal rule reference for shared list items.). This is the underlying object with id, value and extensions. The accessor "getListRuleId" gives direct access to the value 4455 */ 4456 public IdType getListRuleIdElement() { 4457 if (this.listRuleId == null) 4458 if (Configuration.errorOnAutoCreate()) 4459 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.listRuleId"); 4460 else if (Configuration.doAutoCreate()) 4461 this.listRuleId = new IdType(); // bb 4462 return this.listRuleId; 4463 } 4464 4465 public boolean hasListRuleIdElement() { 4466 return this.listRuleId != null && !this.listRuleId.isEmpty(); 4467 } 4468 4469 public boolean hasListRuleId() { 4470 return this.listRuleId != null && !this.listRuleId.isEmpty(); 4471 } 4472 4473 /** 4474 * @param value {@link #listRuleId} (Internal rule reference for shared list items.). This is the underlying object with id, value and extensions. The accessor "getListRuleId" gives direct access to the value 4475 */ 4476 public StructureMapGroupRuleTargetComponent setListRuleIdElement(IdType value) { 4477 this.listRuleId = value; 4478 return this; 4479 } 4480 4481 /** 4482 * @return Internal rule reference for shared list items. 4483 */ 4484 public String getListRuleId() { 4485 return this.listRuleId == null ? null : this.listRuleId.getValue(); 4486 } 4487 4488 /** 4489 * @param value Internal rule reference for shared list items. 4490 */ 4491 public StructureMapGroupRuleTargetComponent setListRuleId(String value) { 4492 if (Utilities.noString(value)) 4493 this.listRuleId = null; 4494 else { 4495 if (this.listRuleId == null) 4496 this.listRuleId = new IdType(); 4497 this.listRuleId.setValue(value); 4498 } 4499 return this; 4500 } 4501 4502 /** 4503 * @return {@link #transform} (How the data is copied / created.). This is the underlying object with id, value and extensions. The accessor "getTransform" gives direct access to the value 4504 */ 4505 public Enumeration<StructureMapTransform> getTransformElement() { 4506 if (this.transform == null) 4507 if (Configuration.errorOnAutoCreate()) 4508 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.transform"); 4509 else if (Configuration.doAutoCreate()) 4510 this.transform = new Enumeration<StructureMapTransform>(new StructureMapTransformEnumFactory()); // bb 4511 return this.transform; 4512 } 4513 4514 public boolean hasTransformElement() { 4515 return this.transform != null && !this.transform.isEmpty(); 4516 } 4517 4518 public boolean hasTransform() { 4519 return this.transform != null && !this.transform.isEmpty(); 4520 } 4521 4522 /** 4523 * @param value {@link #transform} (How the data is copied / created.). This is the underlying object with id, value and extensions. The accessor "getTransform" gives direct access to the value 4524 */ 4525 public StructureMapGroupRuleTargetComponent setTransformElement(Enumeration<StructureMapTransform> value) { 4526 this.transform = value; 4527 return this; 4528 } 4529 4530 /** 4531 * @return How the data is copied / created. 4532 */ 4533 public StructureMapTransform getTransform() { 4534 return this.transform == null ? null : this.transform.getValue(); 4535 } 4536 4537 /** 4538 * @param value How the data is copied / created. 4539 */ 4540 public StructureMapGroupRuleTargetComponent setTransform(StructureMapTransform value) { 4541 if (value == null) 4542 this.transform = null; 4543 else { 4544 if (this.transform == null) 4545 this.transform = new Enumeration<StructureMapTransform>(new StructureMapTransformEnumFactory()); 4546 this.transform.setValue(value); 4547 } 4548 return this; 4549 } 4550 4551 /** 4552 * @return {@link #parameter} (Parameters to the transform.) 4553 */ 4554 public List<StructureMapGroupRuleTargetParameterComponent> getParameter() { 4555 if (this.parameter == null) 4556 this.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 4557 return this.parameter; 4558 } 4559 4560 /** 4561 * @return Returns a reference to <code>this</code> for easy method chaining 4562 */ 4563 public StructureMapGroupRuleTargetComponent setParameter(List<StructureMapGroupRuleTargetParameterComponent> theParameter) { 4564 this.parameter = theParameter; 4565 return this; 4566 } 4567 4568 public boolean hasParameter() { 4569 if (this.parameter == null) 4570 return false; 4571 for (StructureMapGroupRuleTargetParameterComponent item : this.parameter) 4572 if (!item.isEmpty()) 4573 return true; 4574 return false; 4575 } 4576 4577 public StructureMapGroupRuleTargetParameterComponent addParameter() { //3 4578 StructureMapGroupRuleTargetParameterComponent t = new StructureMapGroupRuleTargetParameterComponent(); 4579 if (this.parameter == null) 4580 this.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 4581 this.parameter.add(t); 4582 return t; 4583 } 4584 4585 public StructureMapGroupRuleTargetComponent addParameter(StructureMapGroupRuleTargetParameterComponent t) { //3 4586 if (t == null) 4587 return this; 4588 if (this.parameter == null) 4589 this.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 4590 this.parameter.add(t); 4591 return this; 4592 } 4593 4594 /** 4595 * @return The first repetition of repeating field {@link #parameter}, creating it if it does not already exist {3} 4596 */ 4597 public StructureMapGroupRuleTargetParameterComponent getParameterFirstRep() { 4598 if (getParameter().isEmpty()) { 4599 addParameter(); 4600 } 4601 return getParameter().get(0); 4602 } 4603 4604 protected void listChildren(List<Property> children) { 4605 super.listChildren(children); 4606 children.add(new Property("context", "string", "Variable this rule applies to.", 0, 1, context)); 4607 children.add(new Property("element", "string", "Field to create in the context.", 0, 1, element)); 4608 children.add(new Property("variable", "id", "Named context for field, if desired, and a field is specified.", 0, 1, variable)); 4609 children.add(new Property("listMode", "code", "If field is a list, how to manage the list.", 0, java.lang.Integer.MAX_VALUE, listMode)); 4610 children.add(new Property("listRuleId", "id", "Internal rule reference for shared list items.", 0, 1, listRuleId)); 4611 children.add(new Property("transform", "code", "How the data is copied / created.", 0, 1, transform)); 4612 children.add(new Property("parameter", "", "Parameters to the transform.", 0, java.lang.Integer.MAX_VALUE, parameter)); 4613 } 4614 4615 @Override 4616 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4617 switch (_hash) { 4618 case 951530927: /*context*/ return new Property("context", "string", "Variable this rule applies to.", 0, 1, context); 4619 case -1662836996: /*element*/ return new Property("element", "string", "Field to create in the context.", 0, 1, element); 4620 case -1249586564: /*variable*/ return new Property("variable", "id", "Named context for field, if desired, and a field is specified.", 0, 1, variable); 4621 case 1345445729: /*listMode*/ return new Property("listMode", "code", "If field is a list, how to manage the list.", 0, java.lang.Integer.MAX_VALUE, listMode); 4622 case 337117045: /*listRuleId*/ return new Property("listRuleId", "id", "Internal rule reference for shared list items.", 0, 1, listRuleId); 4623 case 1052666732: /*transform*/ return new Property("transform", "code", "How the data is copied / created.", 0, 1, transform); 4624 case 1954460585: /*parameter*/ return new Property("parameter", "", "Parameters to the transform.", 0, java.lang.Integer.MAX_VALUE, parameter); 4625 default: return super.getNamedProperty(_hash, _name, _checkValid); 4626 } 4627 4628 } 4629 4630 @Override 4631 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4632 switch (hash) { 4633 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // StringType 4634 case -1662836996: /*element*/ return this.element == null ? new Base[0] : new Base[] {this.element}; // StringType 4635 case -1249586564: /*variable*/ return this.variable == null ? new Base[0] : new Base[] {this.variable}; // IdType 4636 case 1345445729: /*listMode*/ return this.listMode == null ? new Base[0] : this.listMode.toArray(new Base[this.listMode.size()]); // Enumeration<StructureMapTargetListMode> 4637 case 337117045: /*listRuleId*/ return this.listRuleId == null ? new Base[0] : new Base[] {this.listRuleId}; // IdType 4638 case 1052666732: /*transform*/ return this.transform == null ? new Base[0] : new Base[] {this.transform}; // Enumeration<StructureMapTransform> 4639 case 1954460585: /*parameter*/ return this.parameter == null ? new Base[0] : this.parameter.toArray(new Base[this.parameter.size()]); // StructureMapGroupRuleTargetParameterComponent 4640 default: return super.getProperty(hash, name, checkValid); 4641 } 4642 4643 } 4644 4645 @Override 4646 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4647 switch (hash) { 4648 case 951530927: // context 4649 this.context = TypeConvertor.castToString(value); // StringType 4650 return value; 4651 case -1662836996: // element 4652 this.element = TypeConvertor.castToString(value); // StringType 4653 return value; 4654 case -1249586564: // variable 4655 this.variable = TypeConvertor.castToId(value); // IdType 4656 return value; 4657 case 1345445729: // listMode 4658 value = new StructureMapTargetListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4659 this.getListMode().add((Enumeration) value); // Enumeration<StructureMapTargetListMode> 4660 return value; 4661 case 337117045: // listRuleId 4662 this.listRuleId = TypeConvertor.castToId(value); // IdType 4663 return value; 4664 case 1052666732: // transform 4665 value = new StructureMapTransformEnumFactory().fromType(TypeConvertor.castToCode(value)); 4666 this.transform = (Enumeration) value; // Enumeration<StructureMapTransform> 4667 return value; 4668 case 1954460585: // parameter 4669 this.getParameter().add((StructureMapGroupRuleTargetParameterComponent) value); // StructureMapGroupRuleTargetParameterComponent 4670 return value; 4671 default: return super.setProperty(hash, name, value); 4672 } 4673 4674 } 4675 4676 @Override 4677 public Base setProperty(String name, Base value) throws FHIRException { 4678 if (name.equals("context")) { 4679 this.context = TypeConvertor.castToString(value); // StringType 4680 } else if (name.equals("element")) { 4681 this.element = TypeConvertor.castToString(value); // StringType 4682 } else if (name.equals("variable")) { 4683 this.variable = TypeConvertor.castToId(value); // IdType 4684 } else if (name.equals("listMode")) { 4685 value = new StructureMapTargetListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4686 this.getListMode().add((Enumeration) value); 4687 } else if (name.equals("listRuleId")) { 4688 this.listRuleId = TypeConvertor.castToId(value); // IdType 4689 } else if (name.equals("transform")) { 4690 value = new StructureMapTransformEnumFactory().fromType(TypeConvertor.castToCode(value)); 4691 this.transform = (Enumeration) value; // Enumeration<StructureMapTransform> 4692 } else if (name.equals("parameter")) { 4693 this.getParameter().add((StructureMapGroupRuleTargetParameterComponent) value); 4694 } else 4695 return super.setProperty(name, value); 4696 return value; 4697 } 4698 4699 @Override 4700 public void removeChild(String name, Base value) throws FHIRException { 4701 if (name.equals("context")) { 4702 this.context = null; 4703 } else if (name.equals("element")) { 4704 this.element = null; 4705 } else if (name.equals("variable")) { 4706 this.variable = null; 4707 } else if (name.equals("listMode")) { 4708 value = new StructureMapTargetListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4709 this.getListMode().remove((Enumeration) value); 4710 } else if (name.equals("listRuleId")) { 4711 this.listRuleId = null; 4712 } else if (name.equals("transform")) { 4713 value = new StructureMapTransformEnumFactory().fromType(TypeConvertor.castToCode(value)); 4714 this.transform = (Enumeration) value; // Enumeration<StructureMapTransform> 4715 } else if (name.equals("parameter")) { 4716 this.getParameter().remove((StructureMapGroupRuleTargetParameterComponent) value); 4717 } else 4718 super.removeChild(name, value); 4719 4720 } 4721 4722 @Override 4723 public Base makeProperty(int hash, String name) throws FHIRException { 4724 switch (hash) { 4725 case 951530927: return getContextElement(); 4726 case -1662836996: return getElementElement(); 4727 case -1249586564: return getVariableElement(); 4728 case 1345445729: return addListModeElement(); 4729 case 337117045: return getListRuleIdElement(); 4730 case 1052666732: return getTransformElement(); 4731 case 1954460585: return addParameter(); 4732 default: return super.makeProperty(hash, name); 4733 } 4734 4735 } 4736 4737 @Override 4738 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4739 switch (hash) { 4740 case 951530927: /*context*/ return new String[] {"string"}; 4741 case -1662836996: /*element*/ return new String[] {"string"}; 4742 case -1249586564: /*variable*/ return new String[] {"id"}; 4743 case 1345445729: /*listMode*/ return new String[] {"code"}; 4744 case 337117045: /*listRuleId*/ return new String[] {"id"}; 4745 case 1052666732: /*transform*/ return new String[] {"code"}; 4746 case 1954460585: /*parameter*/ return new String[] {}; 4747 default: return super.getTypesForProperty(hash, name); 4748 } 4749 4750 } 4751 4752 @Override 4753 public Base addChild(String name) throws FHIRException { 4754 if (name.equals("context")) { 4755 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.target.context"); 4756 } 4757 else if (name.equals("element")) { 4758 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.target.element"); 4759 } 4760 else if (name.equals("variable")) { 4761 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.target.variable"); 4762 } 4763 else if (name.equals("listMode")) { 4764 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.target.listMode"); 4765 } 4766 else if (name.equals("listRuleId")) { 4767 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.target.listRuleId"); 4768 } 4769 else if (name.equals("transform")) { 4770 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.target.transform"); 4771 } 4772 else if (name.equals("parameter")) { 4773 return addParameter(); 4774 } 4775 else 4776 return super.addChild(name); 4777 } 4778 4779 public StructureMapGroupRuleTargetComponent copy() { 4780 StructureMapGroupRuleTargetComponent dst = new StructureMapGroupRuleTargetComponent(); 4781 copyValues(dst); 4782 return dst; 4783 } 4784 4785 public void copyValues(StructureMapGroupRuleTargetComponent dst) { 4786 super.copyValues(dst); 4787 dst.context = context == null ? null : context.copy(); 4788 dst.element = element == null ? null : element.copy(); 4789 dst.variable = variable == null ? null : variable.copy(); 4790 if (listMode != null) { 4791 dst.listMode = new ArrayList<Enumeration<StructureMapTargetListMode>>(); 4792 for (Enumeration<StructureMapTargetListMode> i : listMode) 4793 dst.listMode.add(i.copy()); 4794 }; 4795 dst.listRuleId = listRuleId == null ? null : listRuleId.copy(); 4796 dst.transform = transform == null ? null : transform.copy(); 4797 if (parameter != null) { 4798 dst.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 4799 for (StructureMapGroupRuleTargetParameterComponent i : parameter) 4800 dst.parameter.add(i.copy()); 4801 }; 4802 } 4803 4804 @Override 4805 public boolean equalsDeep(Base other_) { 4806 if (!super.equalsDeep(other_)) 4807 return false; 4808 if (!(other_ instanceof StructureMapGroupRuleTargetComponent)) 4809 return false; 4810 StructureMapGroupRuleTargetComponent o = (StructureMapGroupRuleTargetComponent) other_; 4811 return compareDeep(context, o.context, true) && compareDeep(element, o.element, true) && compareDeep(variable, o.variable, true) 4812 && compareDeep(listMode, o.listMode, true) && compareDeep(listRuleId, o.listRuleId, true) && compareDeep(transform, o.transform, true) 4813 && compareDeep(parameter, o.parameter, true); 4814 } 4815 4816 @Override 4817 public boolean equalsShallow(Base other_) { 4818 if (!super.equalsShallow(other_)) 4819 return false; 4820 if (!(other_ instanceof StructureMapGroupRuleTargetComponent)) 4821 return false; 4822 StructureMapGroupRuleTargetComponent o = (StructureMapGroupRuleTargetComponent) other_; 4823 return compareValues(context, o.context, true) && compareValues(element, o.element, true) && compareValues(variable, o.variable, true) 4824 && compareValues(listMode, o.listMode, true) && compareValues(listRuleId, o.listRuleId, true) && compareValues(transform, o.transform, true) 4825 ; 4826 } 4827 4828 public boolean isEmpty() { 4829 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(context, element, variable 4830 , listMode, listRuleId, transform, parameter); 4831 } 4832 4833 public String fhirType() { 4834 return "StructureMap.group.rule.target"; 4835 4836 } 4837 4838// added from java-adornments.txt: 4839public String toString() { 4840 return StructureMapUtilities.targetToString(this); 4841 } 4842// end addition 4843 } 4844 4845 @Block() 4846 public static class StructureMapGroupRuleTargetParameterComponent extends BackboneElement implements IBaseBackboneElement { 4847 /** 4848 * Parameter value - variable or literal. 4849 */ 4850 @Child(name = "value", type = {IdType.class, StringType.class, BooleanType.class, IntegerType.class, DecimalType.class, DateType.class, TimeType.class, DateTimeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 4851 @Description(shortDefinition="Parameter value - variable or literal", formalDefinition="Parameter value - variable or literal." ) 4852 protected DataType value; 4853 4854 private static final long serialVersionUID = -1135414639L; 4855 4856 /** 4857 * Constructor 4858 */ 4859 public StructureMapGroupRuleTargetParameterComponent() { 4860 super(); 4861 } 4862 4863 /** 4864 * Constructor 4865 */ 4866 public StructureMapGroupRuleTargetParameterComponent(DataType value) { 4867 super(); 4868 this.setValue(value); 4869 } 4870 4871 /** 4872 * @return {@link #value} (Parameter value - variable or literal.) 4873 */ 4874 public DataType getValue() { 4875 return this.value; 4876 } 4877 4878 /** 4879 * @return {@link #value} (Parameter value - variable or literal.) 4880 */ 4881 public IdType getValueIdType() throws FHIRException { 4882 if (this.value == null) 4883 this.value = new IdType(); 4884 if (!(this.value instanceof IdType)) 4885 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.value.getClass().getName()+" was encountered"); 4886 return (IdType) this.value; 4887 } 4888 4889 public boolean hasValueIdType() { 4890 return this.value instanceof IdType; 4891 } 4892 4893 /** 4894 * @return {@link #value} (Parameter value - variable or literal.) 4895 */ 4896 public StringType getValueStringType() throws FHIRException { 4897 if (this.value == null) 4898 this.value = new StringType(); 4899 if (!(this.value instanceof StringType)) 4900 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 4901 return (StringType) this.value; 4902 } 4903 4904 public boolean hasValueStringType() { 4905 return this.value instanceof StringType; 4906 } 4907 4908 /** 4909 * @return {@link #value} (Parameter value - variable or literal.) 4910 */ 4911 public BooleanType getValueBooleanType() throws FHIRException { 4912 if (this.value == null) 4913 this.value = new BooleanType(); 4914 if (!(this.value instanceof BooleanType)) 4915 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 4916 return (BooleanType) this.value; 4917 } 4918 4919 public boolean hasValueBooleanType() { 4920 return this.value instanceof BooleanType; 4921 } 4922 4923 /** 4924 * @return {@link #value} (Parameter value - variable or literal.) 4925 */ 4926 public IntegerType getValueIntegerType() throws FHIRException { 4927 if (this.value == null) 4928 this.value = new IntegerType(); 4929 if (!(this.value instanceof IntegerType)) 4930 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 4931 return (IntegerType) this.value; 4932 } 4933 4934 public boolean hasValueIntegerType() { 4935 return this.value instanceof IntegerType; 4936 } 4937 4938 /** 4939 * @return {@link #value} (Parameter value - variable or literal.) 4940 */ 4941 public DecimalType getValueDecimalType() throws FHIRException { 4942 if (this.value == null) 4943 this.value = new DecimalType(); 4944 if (!(this.value instanceof DecimalType)) 4945 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 4946 return (DecimalType) this.value; 4947 } 4948 4949 public boolean hasValueDecimalType() { 4950 return this.value instanceof DecimalType; 4951 } 4952 4953 /** 4954 * @return {@link #value} (Parameter value - variable or literal.) 4955 */ 4956 public DateType getValueDateType() throws FHIRException { 4957 if (this.value == null) 4958 this.value = new DateType(); 4959 if (!(this.value instanceof DateType)) 4960 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 4961 return (DateType) this.value; 4962 } 4963 4964 public boolean hasValueDateType() { 4965 return this.value instanceof DateType; 4966 } 4967 4968 /** 4969 * @return {@link #value} (Parameter value - variable or literal.) 4970 */ 4971 public TimeType getValueTimeType() throws FHIRException { 4972 if (this.value == null) 4973 this.value = new TimeType(); 4974 if (!(this.value instanceof TimeType)) 4975 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 4976 return (TimeType) this.value; 4977 } 4978 4979 public boolean hasValueTimeType() { 4980 return this.value instanceof TimeType; 4981 } 4982 4983 /** 4984 * @return {@link #value} (Parameter value - variable or literal.) 4985 */ 4986 public DateTimeType getValueDateTimeType() throws FHIRException { 4987 if (this.value == null) 4988 this.value = new DateTimeType(); 4989 if (!(this.value instanceof DateTimeType)) 4990 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 4991 return (DateTimeType) this.value; 4992 } 4993 4994 public boolean hasValueDateTimeType() { 4995 return this.value instanceof DateTimeType; 4996 } 4997 4998 public boolean hasValue() { 4999 return this.value != null && !this.value.isEmpty(); 5000 } 5001 5002 /** 5003 * @param value {@link #value} (Parameter value - variable or literal.) 5004 */ 5005 public StructureMapGroupRuleTargetParameterComponent setValue(DataType value) { 5006 if (value != null && !(value instanceof IdType || value instanceof StringType || value instanceof BooleanType || value instanceof IntegerType || value instanceof DecimalType || value instanceof DateType || value instanceof TimeType || value instanceof DateTimeType)) 5007 throw new FHIRException("Not the right type for StructureMap.group.rule.target.parameter.value[x]: "+value.fhirType()); 5008 this.value = value; 5009 return this; 5010 } 5011 5012 protected void listChildren(List<Property> children) { 5013 super.listChildren(children); 5014 children.add(new Property("value[x]", "id|string|boolean|integer|decimal|date|time|dateTime", "Parameter value - variable or literal.", 0, 1, value)); 5015 } 5016 5017 @Override 5018 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5019 switch (_hash) { 5020 case -1410166417: /*value[x]*/ return new Property("value[x]", "id|string|boolean|integer|decimal|date|time|dateTime", "Parameter value - variable or literal.", 0, 1, value); 5021 case 111972721: /*value*/ return new Property("value[x]", "id|string|boolean|integer|decimal|date|time|dateTime", "Parameter value - variable or literal.", 0, 1, value); 5022 case 231604844: /*valueId*/ return new Property("value[x]", "id", "Parameter value - variable or literal.", 0, 1, value); 5023 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "Parameter value - variable or literal.", 0, 1, value); 5024 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "Parameter value - variable or literal.", 0, 1, value); 5025 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "Parameter value - variable or literal.", 0, 1, value); 5026 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "Parameter value - variable or literal.", 0, 1, value); 5027 case -766192449: /*valueDate*/ return new Property("value[x]", "date", "Parameter value - variable or literal.", 0, 1, value); 5028 case -765708322: /*valueTime*/ return new Property("value[x]", "time", "Parameter value - variable or literal.", 0, 1, value); 5029 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "Parameter value - variable or literal.", 0, 1, value); 5030 default: return super.getNamedProperty(_hash, _name, _checkValid); 5031 } 5032 5033 } 5034 5035 @Override 5036 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5037 switch (hash) { 5038 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 5039 default: return super.getProperty(hash, name, checkValid); 5040 } 5041 5042 } 5043 5044 @Override 5045 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5046 switch (hash) { 5047 case 111972721: // value 5048 this.value = TypeConvertor.castToType(value); // DataType 5049 return value; 5050 default: return super.setProperty(hash, name, value); 5051 } 5052 5053 } 5054 5055 @Override 5056 public Base setProperty(String name, Base value) throws FHIRException { 5057 if (name.equals("value[x]")) { 5058 this.value = TypeConvertor.castToType(value); // DataType 5059 } else 5060 return super.setProperty(name, value); 5061 return value; 5062 } 5063 5064 @Override 5065 public void removeChild(String name, Base value) throws FHIRException { 5066 if (name.equals("value[x]")) { 5067 this.value = null; 5068 } else 5069 super.removeChild(name, value); 5070 5071 } 5072 5073 @Override 5074 public Base makeProperty(int hash, String name) throws FHIRException { 5075 switch (hash) { 5076 case -1410166417: return getValue(); 5077 case 111972721: return getValue(); 5078 default: return super.makeProperty(hash, name); 5079 } 5080 5081 } 5082 5083 @Override 5084 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5085 switch (hash) { 5086 case 111972721: /*value*/ return new String[] {"id", "string", "boolean", "integer", "decimal", "date", "time", "dateTime"}; 5087 default: return super.getTypesForProperty(hash, name); 5088 } 5089 5090 } 5091 5092 @Override 5093 public Base addChild(String name) throws FHIRException { 5094 if (name.equals("valueId")) { 5095 this.value = new IdType(); 5096 return this.value; 5097 } 5098 else if (name.equals("valueString")) { 5099 this.value = new StringType(); 5100 return this.value; 5101 } 5102 else if (name.equals("valueBoolean")) { 5103 this.value = new BooleanType(); 5104 return this.value; 5105 } 5106 else if (name.equals("valueInteger")) { 5107 this.value = new IntegerType(); 5108 return this.value; 5109 } 5110 else if (name.equals("valueDecimal")) { 5111 this.value = new DecimalType(); 5112 return this.value; 5113 } 5114 else if (name.equals("valueDate")) { 5115 this.value = new DateType(); 5116 return this.value; 5117 } 5118 else if (name.equals("valueTime")) { 5119 this.value = new TimeType(); 5120 return this.value; 5121 } 5122 else if (name.equals("valueDateTime")) { 5123 this.value = new DateTimeType(); 5124 return this.value; 5125 } 5126 else 5127 return super.addChild(name); 5128 } 5129 5130 public StructureMapGroupRuleTargetParameterComponent copy() { 5131 StructureMapGroupRuleTargetParameterComponent dst = new StructureMapGroupRuleTargetParameterComponent(); 5132 copyValues(dst); 5133 return dst; 5134 } 5135 5136 public void copyValues(StructureMapGroupRuleTargetParameterComponent dst) { 5137 super.copyValues(dst); 5138 dst.value = value == null ? null : value.copy(); 5139 } 5140 5141 @Override 5142 public boolean equalsDeep(Base other_) { 5143 if (!super.equalsDeep(other_)) 5144 return false; 5145 if (!(other_ instanceof StructureMapGroupRuleTargetParameterComponent)) 5146 return false; 5147 StructureMapGroupRuleTargetParameterComponent o = (StructureMapGroupRuleTargetParameterComponent) other_; 5148 return compareDeep(value, o.value, true); 5149 } 5150 5151 @Override 5152 public boolean equalsShallow(Base other_) { 5153 if (!super.equalsShallow(other_)) 5154 return false; 5155 if (!(other_ instanceof StructureMapGroupRuleTargetParameterComponent)) 5156 return false; 5157 StructureMapGroupRuleTargetParameterComponent o = (StructureMapGroupRuleTargetParameterComponent) other_; 5158 return true; 5159 } 5160 5161 public boolean isEmpty() { 5162 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value); 5163 } 5164 5165 public String fhirType() { 5166 return "StructureMap.group.rule.target.parameter"; 5167 5168 } 5169 5170// added from java-adornments.txt: 5171public String toString() { 5172 return value == null ? "null!" : value.toString(); 5173 } 5174// end addition 5175 } 5176 5177 @Block() 5178 public static class StructureMapGroupRuleDependentComponent extends BackboneElement implements IBaseBackboneElement { 5179 /** 5180 * Name of a rule or group to apply. 5181 */ 5182 @Child(name = "name", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 5183 @Description(shortDefinition="Name of a rule or group to apply", formalDefinition="Name of a rule or group to apply." ) 5184 protected IdType name; 5185 5186 /** 5187 * Parameter to pass to the rule or group. 5188 */ 5189 @Child(name = "parameter", type = {StructureMapGroupRuleTargetParameterComponent.class}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5190 @Description(shortDefinition="Parameter to pass to the rule or group", formalDefinition="Parameter to pass to the rule or group." ) 5191 protected List<StructureMapGroupRuleTargetParameterComponent> parameter; 5192 5193 private static final long serialVersionUID = -290346576L; 5194 5195 /** 5196 * Constructor 5197 */ 5198 public StructureMapGroupRuleDependentComponent() { 5199 super(); 5200 } 5201 5202 /** 5203 * Constructor 5204 */ 5205 public StructureMapGroupRuleDependentComponent(String name, StructureMapGroupRuleTargetParameterComponent parameter) { 5206 super(); 5207 this.setName(name); 5208 this.addParameter(parameter); 5209 } 5210 5211 /** 5212 * @return {@link #name} (Name of a rule or group to apply.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5213 */ 5214 public IdType getNameElement() { 5215 if (this.name == null) 5216 if (Configuration.errorOnAutoCreate()) 5217 throw new Error("Attempt to auto-create StructureMapGroupRuleDependentComponent.name"); 5218 else if (Configuration.doAutoCreate()) 5219 this.name = new IdType(); // bb 5220 return this.name; 5221 } 5222 5223 public boolean hasNameElement() { 5224 return this.name != null && !this.name.isEmpty(); 5225 } 5226 5227 public boolean hasName() { 5228 return this.name != null && !this.name.isEmpty(); 5229 } 5230 5231 /** 5232 * @param value {@link #name} (Name of a rule or group to apply.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5233 */ 5234 public StructureMapGroupRuleDependentComponent setNameElement(IdType value) { 5235 this.name = value; 5236 return this; 5237 } 5238 5239 /** 5240 * @return Name of a rule or group to apply. 5241 */ 5242 public String getName() { 5243 return this.name == null ? null : this.name.getValue(); 5244 } 5245 5246 /** 5247 * @param value Name of a rule or group to apply. 5248 */ 5249 public StructureMapGroupRuleDependentComponent setName(String value) { 5250 if (this.name == null) 5251 this.name = new IdType(); 5252 this.name.setValue(value); 5253 return this; 5254 } 5255 5256 /** 5257 * @return {@link #parameter} (Parameter to pass to the rule or group.) 5258 */ 5259 public List<StructureMapGroupRuleTargetParameterComponent> getParameter() { 5260 if (this.parameter == null) 5261 this.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 5262 return this.parameter; 5263 } 5264 5265 /** 5266 * @return Returns a reference to <code>this</code> for easy method chaining 5267 */ 5268 public StructureMapGroupRuleDependentComponent setParameter(List<StructureMapGroupRuleTargetParameterComponent> theParameter) { 5269 this.parameter = theParameter; 5270 return this; 5271 } 5272 5273 public boolean hasParameter() { 5274 if (this.parameter == null) 5275 return false; 5276 for (StructureMapGroupRuleTargetParameterComponent item : this.parameter) 5277 if (!item.isEmpty()) 5278 return true; 5279 return false; 5280 } 5281 5282 public StructureMapGroupRuleTargetParameterComponent addParameter() { //3 5283 StructureMapGroupRuleTargetParameterComponent t = new StructureMapGroupRuleTargetParameterComponent(); 5284 if (this.parameter == null) 5285 this.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 5286 this.parameter.add(t); 5287 return t; 5288 } 5289 5290 public StructureMapGroupRuleDependentComponent addParameter(StructureMapGroupRuleTargetParameterComponent t) { //3 5291 if (t == null) 5292 return this; 5293 if (this.parameter == null) 5294 this.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 5295 this.parameter.add(t); 5296 return this; 5297 } 5298 5299 /** 5300 * @return The first repetition of repeating field {@link #parameter}, creating it if it does not already exist {3} 5301 */ 5302 public StructureMapGroupRuleTargetParameterComponent getParameterFirstRep() { 5303 if (getParameter().isEmpty()) { 5304 addParameter(); 5305 } 5306 return getParameter().get(0); 5307 } 5308 5309 protected void listChildren(List<Property> children) { 5310 super.listChildren(children); 5311 children.add(new Property("name", "id", "Name of a rule or group to apply.", 0, 1, name)); 5312 children.add(new Property("parameter", "@StructureMap.group.rule.target.parameter", "Parameter to pass to the rule or group.", 0, java.lang.Integer.MAX_VALUE, parameter)); 5313 } 5314 5315 @Override 5316 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5317 switch (_hash) { 5318 case 3373707: /*name*/ return new Property("name", "id", "Name of a rule or group to apply.", 0, 1, name); 5319 case 1954460585: /*parameter*/ return new Property("parameter", "@StructureMap.group.rule.target.parameter", "Parameter to pass to the rule or group.", 0, java.lang.Integer.MAX_VALUE, parameter); 5320 default: return super.getNamedProperty(_hash, _name, _checkValid); 5321 } 5322 5323 } 5324 5325 @Override 5326 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5327 switch (hash) { 5328 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // IdType 5329 case 1954460585: /*parameter*/ return this.parameter == null ? new Base[0] : this.parameter.toArray(new Base[this.parameter.size()]); // StructureMapGroupRuleTargetParameterComponent 5330 default: return super.getProperty(hash, name, checkValid); 5331 } 5332 5333 } 5334 5335 @Override 5336 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5337 switch (hash) { 5338 case 3373707: // name 5339 this.name = TypeConvertor.castToId(value); // IdType 5340 return value; 5341 case 1954460585: // parameter 5342 this.getParameter().add((StructureMapGroupRuleTargetParameterComponent) value); // StructureMapGroupRuleTargetParameterComponent 5343 return value; 5344 default: return super.setProperty(hash, name, value); 5345 } 5346 5347 } 5348 5349 @Override 5350 public Base setProperty(String name, Base value) throws FHIRException { 5351 if (name.equals("name")) { 5352 this.name = TypeConvertor.castToId(value); // IdType 5353 } else if (name.equals("parameter")) { 5354 this.getParameter().add((StructureMapGroupRuleTargetParameterComponent) value); 5355 } else 5356 return super.setProperty(name, value); 5357 return value; 5358 } 5359 5360 @Override 5361 public void removeChild(String name, Base value) throws FHIRException { 5362 if (name.equals("name")) { 5363 this.name = null; 5364 } else if (name.equals("parameter")) { 5365 this.getParameter().remove((StructureMapGroupRuleTargetParameterComponent) value); 5366 } else 5367 super.removeChild(name, value); 5368 5369 } 5370 5371 @Override 5372 public Base makeProperty(int hash, String name) throws FHIRException { 5373 switch (hash) { 5374 case 3373707: return getNameElement(); 5375 case 1954460585: return addParameter(); 5376 default: return super.makeProperty(hash, name); 5377 } 5378 5379 } 5380 5381 @Override 5382 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5383 switch (hash) { 5384 case 3373707: /*name*/ return new String[] {"id"}; 5385 case 1954460585: /*parameter*/ return new String[] {"@StructureMap.group.rule.target.parameter"}; 5386 default: return super.getTypesForProperty(hash, name); 5387 } 5388 5389 } 5390 5391 @Override 5392 public Base addChild(String name) throws FHIRException { 5393 if (name.equals("name")) { 5394 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.group.rule.dependent.name"); 5395 } 5396 else if (name.equals("parameter")) { 5397 return addParameter(); 5398 } 5399 else 5400 return super.addChild(name); 5401 } 5402 5403 public StructureMapGroupRuleDependentComponent copy() { 5404 StructureMapGroupRuleDependentComponent dst = new StructureMapGroupRuleDependentComponent(); 5405 copyValues(dst); 5406 return dst; 5407 } 5408 5409 public void copyValues(StructureMapGroupRuleDependentComponent dst) { 5410 super.copyValues(dst); 5411 dst.name = name == null ? null : name.copy(); 5412 if (parameter != null) { 5413 dst.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 5414 for (StructureMapGroupRuleTargetParameterComponent i : parameter) 5415 dst.parameter.add(i.copy()); 5416 }; 5417 } 5418 5419 @Override 5420 public boolean equalsDeep(Base other_) { 5421 if (!super.equalsDeep(other_)) 5422 return false; 5423 if (!(other_ instanceof StructureMapGroupRuleDependentComponent)) 5424 return false; 5425 StructureMapGroupRuleDependentComponent o = (StructureMapGroupRuleDependentComponent) other_; 5426 return compareDeep(name, o.name, true) && compareDeep(parameter, o.parameter, true); 5427 } 5428 5429 @Override 5430 public boolean equalsShallow(Base other_) { 5431 if (!super.equalsShallow(other_)) 5432 return false; 5433 if (!(other_ instanceof StructureMapGroupRuleDependentComponent)) 5434 return false; 5435 StructureMapGroupRuleDependentComponent o = (StructureMapGroupRuleDependentComponent) other_; 5436 return compareValues(name, o.name, true); 5437 } 5438 5439 public boolean isEmpty() { 5440 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, parameter); 5441 } 5442 5443 public String fhirType() { 5444 return "StructureMap.group.rule.dependent"; 5445 5446 } 5447 5448 } 5449 5450 /** 5451 * An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure map is stored on different servers. 5452 */ 5453 @Child(name = "url", type = {UriType.class}, order=0, min=1, max=1, modifier=false, summary=true) 5454 @Description(shortDefinition="Canonical identifier for this structure map, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure map is stored on different servers." ) 5455 protected UriType url; 5456 5457 /** 5458 * A formal identifier that is used to identify this structure map when it is represented in other formats, or referenced in a specification, model, design or an instance. 5459 */ 5460 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5461 @Description(shortDefinition="Additional identifier for the structure map", formalDefinition="A formal identifier that is used to identify this structure map when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 5462 protected List<Identifier> identifier; 5463 5464 /** 5465 * The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 5466 */ 5467 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 5468 @Description(shortDefinition="Business version of the structure map", formalDefinition="The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 5469 protected StringType version; 5470 5471 /** 5472 * Indicates the mechanism used to compare versions to determine which is more current. 5473 */ 5474 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 5475 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 5476 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 5477 protected DataType versionAlgorithm; 5478 5479 /** 5480 * A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation. 5481 */ 5482 @Child(name = "name", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=true) 5483 @Description(shortDefinition="Name for this structure map (computer friendly)", formalDefinition="A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 5484 protected StringType name; 5485 5486 /** 5487 * A short, descriptive, user-friendly title for the structure map. 5488 */ 5489 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 5490 @Description(shortDefinition="Name for this structure map (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the structure map." ) 5491 protected StringType title; 5492 5493 /** 5494 * The status of this structure map. Enables tracking the life-cycle of the content. 5495 */ 5496 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 5497 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this structure map. Enables tracking the life-cycle of the content." ) 5498 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 5499 protected Enumeration<PublicationStatus> status; 5500 5501 /** 5502 * A Boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 5503 */ 5504 @Child(name = "experimental", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 5505 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 5506 protected BooleanType experimental; 5507 5508 /** 5509 * The date (and optionally time) when the structure map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes. 5510 */ 5511 @Child(name = "date", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 5512 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the structure map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes." ) 5513 protected DateTimeType date; 5514 5515 /** 5516 * The name of the organization or individual responsible for the release and ongoing maintenance of the structure map. 5517 */ 5518 @Child(name = "publisher", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 5519 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the structure map." ) 5520 protected StringType publisher; 5521 5522 /** 5523 * Contact details to assist a user in finding and communicating with the publisher. 5524 */ 5525 @Child(name = "contact", type = {ContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5526 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 5527 protected List<ContactDetail> contact; 5528 5529 /** 5530 * A free text natural language description of the structure map from a consumer's perspective. 5531 */ 5532 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 5533 @Description(shortDefinition="Natural language description of the structure map", formalDefinition="A free text natural language description of the structure map from a consumer's perspective." ) 5534 protected MarkdownType description; 5535 5536 /** 5537 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure map instances. 5538 */ 5539 @Child(name = "useContext", type = {UsageContext.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5540 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure map instances." ) 5541 protected List<UsageContext> useContext; 5542 5543 /** 5544 * A legal or geographic region in which the structure map is intended to be used. 5545 */ 5546 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5547 @Description(shortDefinition="Intended jurisdiction for structure map (if applicable)", formalDefinition="A legal or geographic region in which the structure map is intended to be used." ) 5548 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 5549 protected List<CodeableConcept> jurisdiction; 5550 5551 /** 5552 * Explanation of why this structure map is needed and why it has been designed as it has. 5553 */ 5554 @Child(name = "purpose", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 5555 @Description(shortDefinition="Why this structure map is defined", formalDefinition="Explanation of why this structure map is needed and why it has been designed as it has." ) 5556 protected MarkdownType purpose; 5557 5558 /** 5559 * A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map. 5560 */ 5561 @Child(name = "copyright", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 5562 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map." ) 5563 protected MarkdownType copyright; 5564 5565 /** 5566 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 5567 */ 5568 @Child(name = "copyrightLabel", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 5569 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 5570 protected StringType copyrightLabel; 5571 5572 /** 5573 * A structure definition used by this map. The structure definition may describe instances that are converted, or the instances that are produced. 5574 */ 5575 @Child(name = "structure", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5576 @Description(shortDefinition="Structure Definition used by this map", formalDefinition="A structure definition used by this map. The structure definition may describe instances that are converted, or the instances that are produced." ) 5577 protected List<StructureMapStructureComponent> structure; 5578 5579 /** 5580 * Other maps used by this map (canonical URLs). 5581 */ 5582 @Child(name = "import", type = {CanonicalType.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5583 @Description(shortDefinition="Other maps used by this map (canonical URLs)", formalDefinition="Other maps used by this map (canonical URLs)." ) 5584 protected List<CanonicalType> import_; 5585 5586 /** 5587 * Definition of a constant value used in the map rules. 5588 */ 5589 @Child(name = "const", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5590 @Description(shortDefinition="Definition of the constant value used in the map rules", formalDefinition="Definition of a constant value used in the map rules." ) 5591 protected List<StructureMapConstComponent> const_; 5592 5593 /** 5594 * Organizes the mapping into managable chunks for human review/ease of maintenance. 5595 */ 5596 @Child(name = "group", type = {}, order=20, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5597 @Description(shortDefinition="Named sections for reader convenience", formalDefinition="Organizes the mapping into managable chunks for human review/ease of maintenance." ) 5598 protected List<StructureMapGroupComponent> group; 5599 5600 private static final long serialVersionUID = -1875777589L; 5601 5602 /** 5603 * Constructor 5604 */ 5605 public StructureMap() { 5606 super(); 5607 } 5608 5609 /** 5610 * Constructor 5611 */ 5612 public StructureMap(String url, String name, PublicationStatus status, StructureMapGroupComponent group) { 5613 super(); 5614 this.setUrl(url); 5615 this.setName(name); 5616 this.setStatus(status); 5617 this.addGroup(group); 5618 } 5619 5620 /** 5621 * @return {@link #url} (An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure map is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 5622 */ 5623 public UriType getUrlElement() { 5624 if (this.url == null) 5625 if (Configuration.errorOnAutoCreate()) 5626 throw new Error("Attempt to auto-create StructureMap.url"); 5627 else if (Configuration.doAutoCreate()) 5628 this.url = new UriType(); // bb 5629 return this.url; 5630 } 5631 5632 public boolean hasUrlElement() { 5633 return this.url != null && !this.url.isEmpty(); 5634 } 5635 5636 public boolean hasUrl() { 5637 return this.url != null && !this.url.isEmpty(); 5638 } 5639 5640 /** 5641 * @param value {@link #url} (An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure map is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 5642 */ 5643 public StructureMap setUrlElement(UriType value) { 5644 this.url = value; 5645 return this; 5646 } 5647 5648 /** 5649 * @return An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure map is stored on different servers. 5650 */ 5651 public String getUrl() { 5652 return this.url == null ? null : this.url.getValue(); 5653 } 5654 5655 /** 5656 * @param value An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure map is stored on different servers. 5657 */ 5658 public StructureMap setUrl(String value) { 5659 if (this.url == null) 5660 this.url = new UriType(); 5661 this.url.setValue(value); 5662 return this; 5663 } 5664 5665 /** 5666 * @return {@link #identifier} (A formal identifier that is used to identify this structure map when it is represented in other formats, or referenced in a specification, model, design or an instance.) 5667 */ 5668 public List<Identifier> getIdentifier() { 5669 if (this.identifier == null) 5670 this.identifier = new ArrayList<Identifier>(); 5671 return this.identifier; 5672 } 5673 5674 /** 5675 * @return Returns a reference to <code>this</code> for easy method chaining 5676 */ 5677 public StructureMap setIdentifier(List<Identifier> theIdentifier) { 5678 this.identifier = theIdentifier; 5679 return this; 5680 } 5681 5682 public boolean hasIdentifier() { 5683 if (this.identifier == null) 5684 return false; 5685 for (Identifier item : this.identifier) 5686 if (!item.isEmpty()) 5687 return true; 5688 return false; 5689 } 5690 5691 public Identifier addIdentifier() { //3 5692 Identifier t = new Identifier(); 5693 if (this.identifier == null) 5694 this.identifier = new ArrayList<Identifier>(); 5695 this.identifier.add(t); 5696 return t; 5697 } 5698 5699 public StructureMap addIdentifier(Identifier t) { //3 5700 if (t == null) 5701 return this; 5702 if (this.identifier == null) 5703 this.identifier = new ArrayList<Identifier>(); 5704 this.identifier.add(t); 5705 return this; 5706 } 5707 5708 /** 5709 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 5710 */ 5711 public Identifier getIdentifierFirstRep() { 5712 if (getIdentifier().isEmpty()) { 5713 addIdentifier(); 5714 } 5715 return getIdentifier().get(0); 5716 } 5717 5718 /** 5719 * @return {@link #version} (The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 5720 */ 5721 public StringType getVersionElement() { 5722 if (this.version == null) 5723 if (Configuration.errorOnAutoCreate()) 5724 throw new Error("Attempt to auto-create StructureMap.version"); 5725 else if (Configuration.doAutoCreate()) 5726 this.version = new StringType(); // bb 5727 return this.version; 5728 } 5729 5730 public boolean hasVersionElement() { 5731 return this.version != null && !this.version.isEmpty(); 5732 } 5733 5734 public boolean hasVersion() { 5735 return this.version != null && !this.version.isEmpty(); 5736 } 5737 5738 /** 5739 * @param value {@link #version} (The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 5740 */ 5741 public StructureMap setVersionElement(StringType value) { 5742 this.version = value; 5743 return this; 5744 } 5745 5746 /** 5747 * @return The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 5748 */ 5749 public String getVersion() { 5750 return this.version == null ? null : this.version.getValue(); 5751 } 5752 5753 /** 5754 * @param value The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 5755 */ 5756 public StructureMap setVersion(String value) { 5757 if (Utilities.noString(value)) 5758 this.version = null; 5759 else { 5760 if (this.version == null) 5761 this.version = new StringType(); 5762 this.version.setValue(value); 5763 } 5764 return this; 5765 } 5766 5767 /** 5768 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 5769 */ 5770 public DataType getVersionAlgorithm() { 5771 return this.versionAlgorithm; 5772 } 5773 5774 /** 5775 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 5776 */ 5777 public StringType getVersionAlgorithmStringType() throws FHIRException { 5778 if (this.versionAlgorithm == null) 5779 this.versionAlgorithm = new StringType(); 5780 if (!(this.versionAlgorithm instanceof StringType)) 5781 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 5782 return (StringType) this.versionAlgorithm; 5783 } 5784 5785 public boolean hasVersionAlgorithmStringType() { 5786 return this.versionAlgorithm instanceof StringType; 5787 } 5788 5789 /** 5790 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 5791 */ 5792 public Coding getVersionAlgorithmCoding() throws FHIRException { 5793 if (this.versionAlgorithm == null) 5794 this.versionAlgorithm = new Coding(); 5795 if (!(this.versionAlgorithm instanceof Coding)) 5796 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 5797 return (Coding) this.versionAlgorithm; 5798 } 5799 5800 public boolean hasVersionAlgorithmCoding() { 5801 return this.versionAlgorithm instanceof Coding; 5802 } 5803 5804 public boolean hasVersionAlgorithm() { 5805 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 5806 } 5807 5808 /** 5809 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 5810 */ 5811 public StructureMap setVersionAlgorithm(DataType value) { 5812 if (value != null && !(value instanceof StringType || value instanceof Coding)) 5813 throw new FHIRException("Not the right type for StructureMap.versionAlgorithm[x]: "+value.fhirType()); 5814 this.versionAlgorithm = value; 5815 return this; 5816 } 5817 5818 /** 5819 * @return {@link #name} (A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5820 */ 5821 public StringType getNameElement() { 5822 if (this.name == null) 5823 if (Configuration.errorOnAutoCreate()) 5824 throw new Error("Attempt to auto-create StructureMap.name"); 5825 else if (Configuration.doAutoCreate()) 5826 this.name = new StringType(); // bb 5827 return this.name; 5828 } 5829 5830 public boolean hasNameElement() { 5831 return this.name != null && !this.name.isEmpty(); 5832 } 5833 5834 public boolean hasName() { 5835 return this.name != null && !this.name.isEmpty(); 5836 } 5837 5838 /** 5839 * @param value {@link #name} (A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5840 */ 5841 public StructureMap setNameElement(StringType value) { 5842 this.name = value; 5843 return this; 5844 } 5845 5846 /** 5847 * @return A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation. 5848 */ 5849 public String getName() { 5850 return this.name == null ? null : this.name.getValue(); 5851 } 5852 5853 /** 5854 * @param value A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation. 5855 */ 5856 public StructureMap setName(String value) { 5857 if (this.name == null) 5858 this.name = new StringType(); 5859 this.name.setValue(value); 5860 return this; 5861 } 5862 5863 /** 5864 * @return {@link #title} (A short, descriptive, user-friendly title for the structure map.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 5865 */ 5866 public StringType getTitleElement() { 5867 if (this.title == null) 5868 if (Configuration.errorOnAutoCreate()) 5869 throw new Error("Attempt to auto-create StructureMap.title"); 5870 else if (Configuration.doAutoCreate()) 5871 this.title = new StringType(); // bb 5872 return this.title; 5873 } 5874 5875 public boolean hasTitleElement() { 5876 return this.title != null && !this.title.isEmpty(); 5877 } 5878 5879 public boolean hasTitle() { 5880 return this.title != null && !this.title.isEmpty(); 5881 } 5882 5883 /** 5884 * @param value {@link #title} (A short, descriptive, user-friendly title for the structure map.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 5885 */ 5886 public StructureMap setTitleElement(StringType value) { 5887 this.title = value; 5888 return this; 5889 } 5890 5891 /** 5892 * @return A short, descriptive, user-friendly title for the structure map. 5893 */ 5894 public String getTitle() { 5895 return this.title == null ? null : this.title.getValue(); 5896 } 5897 5898 /** 5899 * @param value A short, descriptive, user-friendly title for the structure map. 5900 */ 5901 public StructureMap setTitle(String value) { 5902 if (Utilities.noString(value)) 5903 this.title = null; 5904 else { 5905 if (this.title == null) 5906 this.title = new StringType(); 5907 this.title.setValue(value); 5908 } 5909 return this; 5910 } 5911 5912 /** 5913 * @return {@link #status} (The status of this structure map. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 5914 */ 5915 public Enumeration<PublicationStatus> getStatusElement() { 5916 if (this.status == null) 5917 if (Configuration.errorOnAutoCreate()) 5918 throw new Error("Attempt to auto-create StructureMap.status"); 5919 else if (Configuration.doAutoCreate()) 5920 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 5921 return this.status; 5922 } 5923 5924 public boolean hasStatusElement() { 5925 return this.status != null && !this.status.isEmpty(); 5926 } 5927 5928 public boolean hasStatus() { 5929 return this.status != null && !this.status.isEmpty(); 5930 } 5931 5932 /** 5933 * @param value {@link #status} (The status of this structure map. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 5934 */ 5935 public StructureMap setStatusElement(Enumeration<PublicationStatus> value) { 5936 this.status = value; 5937 return this; 5938 } 5939 5940 /** 5941 * @return The status of this structure map. Enables tracking the life-cycle of the content. 5942 */ 5943 public PublicationStatus getStatus() { 5944 return this.status == null ? null : this.status.getValue(); 5945 } 5946 5947 /** 5948 * @param value The status of this structure map. Enables tracking the life-cycle of the content. 5949 */ 5950 public StructureMap setStatus(PublicationStatus value) { 5951 if (this.status == null) 5952 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 5953 this.status.setValue(value); 5954 return this; 5955 } 5956 5957 /** 5958 * @return {@link #experimental} (A Boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 5959 */ 5960 public BooleanType getExperimentalElement() { 5961 if (this.experimental == null) 5962 if (Configuration.errorOnAutoCreate()) 5963 throw new Error("Attempt to auto-create StructureMap.experimental"); 5964 else if (Configuration.doAutoCreate()) 5965 this.experimental = new BooleanType(); // bb 5966 return this.experimental; 5967 } 5968 5969 public boolean hasExperimentalElement() { 5970 return this.experimental != null && !this.experimental.isEmpty(); 5971 } 5972 5973 public boolean hasExperimental() { 5974 return this.experimental != null && !this.experimental.isEmpty(); 5975 } 5976 5977 /** 5978 * @param value {@link #experimental} (A Boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 5979 */ 5980 public StructureMap setExperimentalElement(BooleanType value) { 5981 this.experimental = value; 5982 return this; 5983 } 5984 5985 /** 5986 * @return A Boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 5987 */ 5988 public boolean getExperimental() { 5989 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 5990 } 5991 5992 /** 5993 * @param value A Boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 5994 */ 5995 public StructureMap setExperimental(boolean value) { 5996 if (this.experimental == null) 5997 this.experimental = new BooleanType(); 5998 this.experimental.setValue(value); 5999 return this; 6000 } 6001 6002 /** 6003 * @return {@link #date} (The date (and optionally time) when the structure map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 6004 */ 6005 public DateTimeType getDateElement() { 6006 if (this.date == null) 6007 if (Configuration.errorOnAutoCreate()) 6008 throw new Error("Attempt to auto-create StructureMap.date"); 6009 else if (Configuration.doAutoCreate()) 6010 this.date = new DateTimeType(); // bb 6011 return this.date; 6012 } 6013 6014 public boolean hasDateElement() { 6015 return this.date != null && !this.date.isEmpty(); 6016 } 6017 6018 public boolean hasDate() { 6019 return this.date != null && !this.date.isEmpty(); 6020 } 6021 6022 /** 6023 * @param value {@link #date} (The date (and optionally time) when the structure map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 6024 */ 6025 public StructureMap setDateElement(DateTimeType value) { 6026 this.date = value; 6027 return this; 6028 } 6029 6030 /** 6031 * @return The date (and optionally time) when the structure map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes. 6032 */ 6033 public Date getDate() { 6034 return this.date == null ? null : this.date.getValue(); 6035 } 6036 6037 /** 6038 * @param value The date (and optionally time) when the structure map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes. 6039 */ 6040 public StructureMap setDate(Date value) { 6041 if (value == null) 6042 this.date = null; 6043 else { 6044 if (this.date == null) 6045 this.date = new DateTimeType(); 6046 this.date.setValue(value); 6047 } 6048 return this; 6049 } 6050 6051 /** 6052 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the structure map.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 6053 */ 6054 public StringType getPublisherElement() { 6055 if (this.publisher == null) 6056 if (Configuration.errorOnAutoCreate()) 6057 throw new Error("Attempt to auto-create StructureMap.publisher"); 6058 else if (Configuration.doAutoCreate()) 6059 this.publisher = new StringType(); // bb 6060 return this.publisher; 6061 } 6062 6063 public boolean hasPublisherElement() { 6064 return this.publisher != null && !this.publisher.isEmpty(); 6065 } 6066 6067 public boolean hasPublisher() { 6068 return this.publisher != null && !this.publisher.isEmpty(); 6069 } 6070 6071 /** 6072 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the structure map.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 6073 */ 6074 public StructureMap setPublisherElement(StringType value) { 6075 this.publisher = value; 6076 return this; 6077 } 6078 6079 /** 6080 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the structure map. 6081 */ 6082 public String getPublisher() { 6083 return this.publisher == null ? null : this.publisher.getValue(); 6084 } 6085 6086 /** 6087 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the structure map. 6088 */ 6089 public StructureMap setPublisher(String value) { 6090 if (Utilities.noString(value)) 6091 this.publisher = null; 6092 else { 6093 if (this.publisher == null) 6094 this.publisher = new StringType(); 6095 this.publisher.setValue(value); 6096 } 6097 return this; 6098 } 6099 6100 /** 6101 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 6102 */ 6103 public List<ContactDetail> getContact() { 6104 if (this.contact == null) 6105 this.contact = new ArrayList<ContactDetail>(); 6106 return this.contact; 6107 } 6108 6109 /** 6110 * @return Returns a reference to <code>this</code> for easy method chaining 6111 */ 6112 public StructureMap setContact(List<ContactDetail> theContact) { 6113 this.contact = theContact; 6114 return this; 6115 } 6116 6117 public boolean hasContact() { 6118 if (this.contact == null) 6119 return false; 6120 for (ContactDetail item : this.contact) 6121 if (!item.isEmpty()) 6122 return true; 6123 return false; 6124 } 6125 6126 public ContactDetail addContact() { //3 6127 ContactDetail t = new ContactDetail(); 6128 if (this.contact == null) 6129 this.contact = new ArrayList<ContactDetail>(); 6130 this.contact.add(t); 6131 return t; 6132 } 6133 6134 public StructureMap addContact(ContactDetail t) { //3 6135 if (t == null) 6136 return this; 6137 if (this.contact == null) 6138 this.contact = new ArrayList<ContactDetail>(); 6139 this.contact.add(t); 6140 return this; 6141 } 6142 6143 /** 6144 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 6145 */ 6146 public ContactDetail getContactFirstRep() { 6147 if (getContact().isEmpty()) { 6148 addContact(); 6149 } 6150 return getContact().get(0); 6151 } 6152 6153 /** 6154 * @return {@link #description} (A free text natural language description of the structure map from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 6155 */ 6156 public MarkdownType getDescriptionElement() { 6157 if (this.description == null) 6158 if (Configuration.errorOnAutoCreate()) 6159 throw new Error("Attempt to auto-create StructureMap.description"); 6160 else if (Configuration.doAutoCreate()) 6161 this.description = new MarkdownType(); // bb 6162 return this.description; 6163 } 6164 6165 public boolean hasDescriptionElement() { 6166 return this.description != null && !this.description.isEmpty(); 6167 } 6168 6169 public boolean hasDescription() { 6170 return this.description != null && !this.description.isEmpty(); 6171 } 6172 6173 /** 6174 * @param value {@link #description} (A free text natural language description of the structure map from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 6175 */ 6176 public StructureMap setDescriptionElement(MarkdownType value) { 6177 this.description = value; 6178 return this; 6179 } 6180 6181 /** 6182 * @return A free text natural language description of the structure map from a consumer's perspective. 6183 */ 6184 public String getDescription() { 6185 return this.description == null ? null : this.description.getValue(); 6186 } 6187 6188 /** 6189 * @param value A free text natural language description of the structure map from a consumer's perspective. 6190 */ 6191 public StructureMap setDescription(String value) { 6192 if (Utilities.noString(value)) 6193 this.description = null; 6194 else { 6195 if (this.description == null) 6196 this.description = new MarkdownType(); 6197 this.description.setValue(value); 6198 } 6199 return this; 6200 } 6201 6202 /** 6203 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure map instances.) 6204 */ 6205 public List<UsageContext> getUseContext() { 6206 if (this.useContext == null) 6207 this.useContext = new ArrayList<UsageContext>(); 6208 return this.useContext; 6209 } 6210 6211 /** 6212 * @return Returns a reference to <code>this</code> for easy method chaining 6213 */ 6214 public StructureMap setUseContext(List<UsageContext> theUseContext) { 6215 this.useContext = theUseContext; 6216 return this; 6217 } 6218 6219 public boolean hasUseContext() { 6220 if (this.useContext == null) 6221 return false; 6222 for (UsageContext item : this.useContext) 6223 if (!item.isEmpty()) 6224 return true; 6225 return false; 6226 } 6227 6228 public UsageContext addUseContext() { //3 6229 UsageContext t = new UsageContext(); 6230 if (this.useContext == null) 6231 this.useContext = new ArrayList<UsageContext>(); 6232 this.useContext.add(t); 6233 return t; 6234 } 6235 6236 public StructureMap addUseContext(UsageContext t) { //3 6237 if (t == null) 6238 return this; 6239 if (this.useContext == null) 6240 this.useContext = new ArrayList<UsageContext>(); 6241 this.useContext.add(t); 6242 return this; 6243 } 6244 6245 /** 6246 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 6247 */ 6248 public UsageContext getUseContextFirstRep() { 6249 if (getUseContext().isEmpty()) { 6250 addUseContext(); 6251 } 6252 return getUseContext().get(0); 6253 } 6254 6255 /** 6256 * @return {@link #jurisdiction} (A legal or geographic region in which the structure map is intended to be used.) 6257 */ 6258 public List<CodeableConcept> getJurisdiction() { 6259 if (this.jurisdiction == null) 6260 this.jurisdiction = new ArrayList<CodeableConcept>(); 6261 return this.jurisdiction; 6262 } 6263 6264 /** 6265 * @return Returns a reference to <code>this</code> for easy method chaining 6266 */ 6267 public StructureMap setJurisdiction(List<CodeableConcept> theJurisdiction) { 6268 this.jurisdiction = theJurisdiction; 6269 return this; 6270 } 6271 6272 public boolean hasJurisdiction() { 6273 if (this.jurisdiction == null) 6274 return false; 6275 for (CodeableConcept item : this.jurisdiction) 6276 if (!item.isEmpty()) 6277 return true; 6278 return false; 6279 } 6280 6281 public CodeableConcept addJurisdiction() { //3 6282 CodeableConcept t = new CodeableConcept(); 6283 if (this.jurisdiction == null) 6284 this.jurisdiction = new ArrayList<CodeableConcept>(); 6285 this.jurisdiction.add(t); 6286 return t; 6287 } 6288 6289 public StructureMap addJurisdiction(CodeableConcept t) { //3 6290 if (t == null) 6291 return this; 6292 if (this.jurisdiction == null) 6293 this.jurisdiction = new ArrayList<CodeableConcept>(); 6294 this.jurisdiction.add(t); 6295 return this; 6296 } 6297 6298 /** 6299 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 6300 */ 6301 public CodeableConcept getJurisdictionFirstRep() { 6302 if (getJurisdiction().isEmpty()) { 6303 addJurisdiction(); 6304 } 6305 return getJurisdiction().get(0); 6306 } 6307 6308 /** 6309 * @return {@link #purpose} (Explanation of why this structure map is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 6310 */ 6311 public MarkdownType getPurposeElement() { 6312 if (this.purpose == null) 6313 if (Configuration.errorOnAutoCreate()) 6314 throw new Error("Attempt to auto-create StructureMap.purpose"); 6315 else if (Configuration.doAutoCreate()) 6316 this.purpose = new MarkdownType(); // bb 6317 return this.purpose; 6318 } 6319 6320 public boolean hasPurposeElement() { 6321 return this.purpose != null && !this.purpose.isEmpty(); 6322 } 6323 6324 public boolean hasPurpose() { 6325 return this.purpose != null && !this.purpose.isEmpty(); 6326 } 6327 6328 /** 6329 * @param value {@link #purpose} (Explanation of why this structure map is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 6330 */ 6331 public StructureMap setPurposeElement(MarkdownType value) { 6332 this.purpose = value; 6333 return this; 6334 } 6335 6336 /** 6337 * @return Explanation of why this structure map is needed and why it has been designed as it has. 6338 */ 6339 public String getPurpose() { 6340 return this.purpose == null ? null : this.purpose.getValue(); 6341 } 6342 6343 /** 6344 * @param value Explanation of why this structure map is needed and why it has been designed as it has. 6345 */ 6346 public StructureMap setPurpose(String value) { 6347 if (Utilities.noString(value)) 6348 this.purpose = null; 6349 else { 6350 if (this.purpose == null) 6351 this.purpose = new MarkdownType(); 6352 this.purpose.setValue(value); 6353 } 6354 return this; 6355 } 6356 6357 /** 6358 * @return {@link #copyright} (A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 6359 */ 6360 public MarkdownType getCopyrightElement() { 6361 if (this.copyright == null) 6362 if (Configuration.errorOnAutoCreate()) 6363 throw new Error("Attempt to auto-create StructureMap.copyright"); 6364 else if (Configuration.doAutoCreate()) 6365 this.copyright = new MarkdownType(); // bb 6366 return this.copyright; 6367 } 6368 6369 public boolean hasCopyrightElement() { 6370 return this.copyright != null && !this.copyright.isEmpty(); 6371 } 6372 6373 public boolean hasCopyright() { 6374 return this.copyright != null && !this.copyright.isEmpty(); 6375 } 6376 6377 /** 6378 * @param value {@link #copyright} (A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 6379 */ 6380 public StructureMap setCopyrightElement(MarkdownType value) { 6381 this.copyright = value; 6382 return this; 6383 } 6384 6385 /** 6386 * @return A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map. 6387 */ 6388 public String getCopyright() { 6389 return this.copyright == null ? null : this.copyright.getValue(); 6390 } 6391 6392 /** 6393 * @param value A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map. 6394 */ 6395 public StructureMap setCopyright(String value) { 6396 if (Utilities.noString(value)) 6397 this.copyright = null; 6398 else { 6399 if (this.copyright == null) 6400 this.copyright = new MarkdownType(); 6401 this.copyright.setValue(value); 6402 } 6403 return this; 6404 } 6405 6406 /** 6407 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 6408 */ 6409 public StringType getCopyrightLabelElement() { 6410 if (this.copyrightLabel == null) 6411 if (Configuration.errorOnAutoCreate()) 6412 throw new Error("Attempt to auto-create StructureMap.copyrightLabel"); 6413 else if (Configuration.doAutoCreate()) 6414 this.copyrightLabel = new StringType(); // bb 6415 return this.copyrightLabel; 6416 } 6417 6418 public boolean hasCopyrightLabelElement() { 6419 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 6420 } 6421 6422 public boolean hasCopyrightLabel() { 6423 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 6424 } 6425 6426 /** 6427 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 6428 */ 6429 public StructureMap setCopyrightLabelElement(StringType value) { 6430 this.copyrightLabel = value; 6431 return this; 6432 } 6433 6434 /** 6435 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 6436 */ 6437 public String getCopyrightLabel() { 6438 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 6439 } 6440 6441 /** 6442 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 6443 */ 6444 public StructureMap setCopyrightLabel(String value) { 6445 if (Utilities.noString(value)) 6446 this.copyrightLabel = null; 6447 else { 6448 if (this.copyrightLabel == null) 6449 this.copyrightLabel = new StringType(); 6450 this.copyrightLabel.setValue(value); 6451 } 6452 return this; 6453 } 6454 6455 /** 6456 * @return {@link #structure} (A structure definition used by this map. The structure definition may describe instances that are converted, or the instances that are produced.) 6457 */ 6458 public List<StructureMapStructureComponent> getStructure() { 6459 if (this.structure == null) 6460 this.structure = new ArrayList<StructureMapStructureComponent>(); 6461 return this.structure; 6462 } 6463 6464 /** 6465 * @return Returns a reference to <code>this</code> for easy method chaining 6466 */ 6467 public StructureMap setStructure(List<StructureMapStructureComponent> theStructure) { 6468 this.structure = theStructure; 6469 return this; 6470 } 6471 6472 public boolean hasStructure() { 6473 if (this.structure == null) 6474 return false; 6475 for (StructureMapStructureComponent item : this.structure) 6476 if (!item.isEmpty()) 6477 return true; 6478 return false; 6479 } 6480 6481 public StructureMapStructureComponent addStructure() { //3 6482 StructureMapStructureComponent t = new StructureMapStructureComponent(); 6483 if (this.structure == null) 6484 this.structure = new ArrayList<StructureMapStructureComponent>(); 6485 this.structure.add(t); 6486 return t; 6487 } 6488 6489 public StructureMap addStructure(StructureMapStructureComponent t) { //3 6490 if (t == null) 6491 return this; 6492 if (this.structure == null) 6493 this.structure = new ArrayList<StructureMapStructureComponent>(); 6494 this.structure.add(t); 6495 return this; 6496 } 6497 6498 /** 6499 * @return The first repetition of repeating field {@link #structure}, creating it if it does not already exist {3} 6500 */ 6501 public StructureMapStructureComponent getStructureFirstRep() { 6502 if (getStructure().isEmpty()) { 6503 addStructure(); 6504 } 6505 return getStructure().get(0); 6506 } 6507 6508 /** 6509 * @return {@link #import_} (Other maps used by this map (canonical URLs).) 6510 */ 6511 public List<CanonicalType> getImport() { 6512 if (this.import_ == null) 6513 this.import_ = new ArrayList<CanonicalType>(); 6514 return this.import_; 6515 } 6516 6517 /** 6518 * @return Returns a reference to <code>this</code> for easy method chaining 6519 */ 6520 public StructureMap setImport(List<CanonicalType> theImport) { 6521 this.import_ = theImport; 6522 return this; 6523 } 6524 6525 public boolean hasImport() { 6526 if (this.import_ == null) 6527 return false; 6528 for (CanonicalType item : this.import_) 6529 if (!item.isEmpty()) 6530 return true; 6531 return false; 6532 } 6533 6534 /** 6535 * @return {@link #import_} (Other maps used by this map (canonical URLs).) 6536 */ 6537 public CanonicalType addImportElement() {//2 6538 CanonicalType t = new CanonicalType(); 6539 if (this.import_ == null) 6540 this.import_ = new ArrayList<CanonicalType>(); 6541 this.import_.add(t); 6542 return t; 6543 } 6544 6545 /** 6546 * @param value {@link #import_} (Other maps used by this map (canonical URLs).) 6547 */ 6548 public StructureMap addImport(String value) { //1 6549 CanonicalType t = new CanonicalType(); 6550 t.setValue(value); 6551 if (this.import_ == null) 6552 this.import_ = new ArrayList<CanonicalType>(); 6553 this.import_.add(t); 6554 return this; 6555 } 6556 6557 /** 6558 * @param value {@link #import_} (Other maps used by this map (canonical URLs).) 6559 */ 6560 public boolean hasImport(String value) { 6561 if (this.import_ == null) 6562 return false; 6563 for (CanonicalType v : this.import_) 6564 if (v.getValue().equals(value)) // canonical 6565 return true; 6566 return false; 6567 } 6568 6569 /** 6570 * @return {@link #const_} (Definition of a constant value used in the map rules.) 6571 */ 6572 public List<StructureMapConstComponent> getConst() { 6573 if (this.const_ == null) 6574 this.const_ = new ArrayList<StructureMapConstComponent>(); 6575 return this.const_; 6576 } 6577 6578 /** 6579 * @return Returns a reference to <code>this</code> for easy method chaining 6580 */ 6581 public StructureMap setConst(List<StructureMapConstComponent> theConst) { 6582 this.const_ = theConst; 6583 return this; 6584 } 6585 6586 public boolean hasConst() { 6587 if (this.const_ == null) 6588 return false; 6589 for (StructureMapConstComponent item : this.const_) 6590 if (!item.isEmpty()) 6591 return true; 6592 return false; 6593 } 6594 6595 public StructureMapConstComponent addConst() { //3 6596 StructureMapConstComponent t = new StructureMapConstComponent(); 6597 if (this.const_ == null) 6598 this.const_ = new ArrayList<StructureMapConstComponent>(); 6599 this.const_.add(t); 6600 return t; 6601 } 6602 6603 public StructureMap addConst(StructureMapConstComponent t) { //3 6604 if (t == null) 6605 return this; 6606 if (this.const_ == null) 6607 this.const_ = new ArrayList<StructureMapConstComponent>(); 6608 this.const_.add(t); 6609 return this; 6610 } 6611 6612 /** 6613 * @return The first repetition of repeating field {@link #const_}, creating it if it does not already exist {3} 6614 */ 6615 public StructureMapConstComponent getConstFirstRep() { 6616 if (getConst().isEmpty()) { 6617 addConst(); 6618 } 6619 return getConst().get(0); 6620 } 6621 6622 /** 6623 * @return {@link #group} (Organizes the mapping into managable chunks for human review/ease of maintenance.) 6624 */ 6625 public List<StructureMapGroupComponent> getGroup() { 6626 if (this.group == null) 6627 this.group = new ArrayList<StructureMapGroupComponent>(); 6628 return this.group; 6629 } 6630 6631 /** 6632 * @return Returns a reference to <code>this</code> for easy method chaining 6633 */ 6634 public StructureMap setGroup(List<StructureMapGroupComponent> theGroup) { 6635 this.group = theGroup; 6636 return this; 6637 } 6638 6639 public boolean hasGroup() { 6640 if (this.group == null) 6641 return false; 6642 for (StructureMapGroupComponent item : this.group) 6643 if (!item.isEmpty()) 6644 return true; 6645 return false; 6646 } 6647 6648 public StructureMapGroupComponent addGroup() { //3 6649 StructureMapGroupComponent t = new StructureMapGroupComponent(); 6650 if (this.group == null) 6651 this.group = new ArrayList<StructureMapGroupComponent>(); 6652 this.group.add(t); 6653 return t; 6654 } 6655 6656 public StructureMap addGroup(StructureMapGroupComponent t) { //3 6657 if (t == null) 6658 return this; 6659 if (this.group == null) 6660 this.group = new ArrayList<StructureMapGroupComponent>(); 6661 this.group.add(t); 6662 return this; 6663 } 6664 6665 /** 6666 * @return The first repetition of repeating field {@link #group}, creating it if it does not already exist {3} 6667 */ 6668 public StructureMapGroupComponent getGroupFirstRep() { 6669 if (getGroup().isEmpty()) { 6670 addGroup(); 6671 } 6672 return getGroup().get(0); 6673 } 6674 6675 protected void listChildren(List<Property> children) { 6676 super.listChildren(children); 6677 children.add(new Property("url", "uri", "An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure map is stored on different servers.", 0, 1, url)); 6678 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this structure map when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 6679 children.add(new Property("version", "string", "The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 6680 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 6681 children.add(new Property("name", "string", "A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 6682 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the structure map.", 0, 1, title)); 6683 children.add(new Property("status", "code", "The status of this structure map. Enables tracking the life-cycle of the content.", 0, 1, status)); 6684 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 6685 children.add(new Property("date", "dateTime", "The date (and optionally time) when the structure map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes.", 0, 1, date)); 6686 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the structure map.", 0, 1, publisher)); 6687 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 6688 children.add(new Property("description", "markdown", "A free text natural language description of the structure map from a consumer's perspective.", 0, 1, description)); 6689 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure map instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 6690 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the structure map is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 6691 children.add(new Property("purpose", "markdown", "Explanation of why this structure map is needed and why it has been designed as it has.", 0, 1, purpose)); 6692 children.add(new Property("copyright", "markdown", "A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map.", 0, 1, copyright)); 6693 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 6694 children.add(new Property("structure", "", "A structure definition used by this map. The structure definition may describe instances that are converted, or the instances that are produced.", 0, java.lang.Integer.MAX_VALUE, structure)); 6695 children.add(new Property("import", "canonical(StructureMap)", "Other maps used by this map (canonical URLs).", 0, java.lang.Integer.MAX_VALUE, import_)); 6696 children.add(new Property("const", "", "Definition of a constant value used in the map rules.", 0, java.lang.Integer.MAX_VALUE, const_)); 6697 children.add(new Property("group", "", "Organizes the mapping into managable chunks for human review/ease of maintenance.", 0, java.lang.Integer.MAX_VALUE, group)); 6698 } 6699 6700 @Override 6701 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6702 switch (_hash) { 6703 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this structure map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure map is stored on different servers.", 0, 1, url); 6704 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this structure map when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 6705 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 6706 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 6707 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 6708 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 6709 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 6710 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 6711 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the structure map.", 0, 1, title); 6712 case -892481550: /*status*/ return new Property("status", "code", "The status of this structure map. Enables tracking the life-cycle of the content.", 0, 1, status); 6713 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 6714 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the structure map was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes.", 0, 1, date); 6715 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the structure map.", 0, 1, publisher); 6716 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 6717 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the structure map from a consumer's perspective.", 0, 1, description); 6718 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure map instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 6719 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the structure map is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 6720 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this structure map is needed and why it has been designed as it has.", 0, 1, purpose); 6721 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map.", 0, 1, copyright); 6722 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 6723 case 144518515: /*structure*/ return new Property("structure", "", "A structure definition used by this map. The structure definition may describe instances that are converted, or the instances that are produced.", 0, java.lang.Integer.MAX_VALUE, structure); 6724 case -1184795739: /*import*/ return new Property("import", "canonical(StructureMap)", "Other maps used by this map (canonical URLs).", 0, java.lang.Integer.MAX_VALUE, import_); 6725 case 94844771: /*const*/ return new Property("const", "", "Definition of a constant value used in the map rules.", 0, java.lang.Integer.MAX_VALUE, const_); 6726 case 98629247: /*group*/ return new Property("group", "", "Organizes the mapping into managable chunks for human review/ease of maintenance.", 0, java.lang.Integer.MAX_VALUE, group); 6727 default: return super.getNamedProperty(_hash, _name, _checkValid); 6728 } 6729 6730 } 6731 6732 @Override 6733 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6734 switch (hash) { 6735 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 6736 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 6737 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 6738 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 6739 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 6740 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 6741 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 6742 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 6743 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 6744 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 6745 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 6746 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 6747 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 6748 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 6749 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 6750 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 6751 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 6752 case 144518515: /*structure*/ return this.structure == null ? new Base[0] : this.structure.toArray(new Base[this.structure.size()]); // StructureMapStructureComponent 6753 case -1184795739: /*import*/ return this.import_ == null ? new Base[0] : this.import_.toArray(new Base[this.import_.size()]); // CanonicalType 6754 case 94844771: /*const*/ return this.const_ == null ? new Base[0] : this.const_.toArray(new Base[this.const_.size()]); // StructureMapConstComponent 6755 case 98629247: /*group*/ return this.group == null ? new Base[0] : this.group.toArray(new Base[this.group.size()]); // StructureMapGroupComponent 6756 default: return super.getProperty(hash, name, checkValid); 6757 } 6758 6759 } 6760 6761 @Override 6762 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6763 switch (hash) { 6764 case 116079: // url 6765 this.url = TypeConvertor.castToUri(value); // UriType 6766 return value; 6767 case -1618432855: // identifier 6768 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 6769 return value; 6770 case 351608024: // version 6771 this.version = TypeConvertor.castToString(value); // StringType 6772 return value; 6773 case 1508158071: // versionAlgorithm 6774 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 6775 return value; 6776 case 3373707: // name 6777 this.name = TypeConvertor.castToString(value); // StringType 6778 return value; 6779 case 110371416: // title 6780 this.title = TypeConvertor.castToString(value); // StringType 6781 return value; 6782 case -892481550: // status 6783 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 6784 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 6785 return value; 6786 case -404562712: // experimental 6787 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 6788 return value; 6789 case 3076014: // date 6790 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 6791 return value; 6792 case 1447404028: // publisher 6793 this.publisher = TypeConvertor.castToString(value); // StringType 6794 return value; 6795 case 951526432: // contact 6796 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 6797 return value; 6798 case -1724546052: // description 6799 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 6800 return value; 6801 case -669707736: // useContext 6802 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 6803 return value; 6804 case -507075711: // jurisdiction 6805 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 6806 return value; 6807 case -220463842: // purpose 6808 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 6809 return value; 6810 case 1522889671: // copyright 6811 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 6812 return value; 6813 case 765157229: // copyrightLabel 6814 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 6815 return value; 6816 case 144518515: // structure 6817 this.getStructure().add((StructureMapStructureComponent) value); // StructureMapStructureComponent 6818 return value; 6819 case -1184795739: // import 6820 this.getImport().add(TypeConvertor.castToCanonical(value)); // CanonicalType 6821 return value; 6822 case 94844771: // const 6823 this.getConst().add((StructureMapConstComponent) value); // StructureMapConstComponent 6824 return value; 6825 case 98629247: // group 6826 this.getGroup().add((StructureMapGroupComponent) value); // StructureMapGroupComponent 6827 return value; 6828 default: return super.setProperty(hash, name, value); 6829 } 6830 6831 } 6832 6833 @Override 6834 public Base setProperty(String name, Base value) throws FHIRException { 6835 if (name.equals("url")) { 6836 this.url = TypeConvertor.castToUri(value); // UriType 6837 } else if (name.equals("identifier")) { 6838 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 6839 } else if (name.equals("version")) { 6840 this.version = TypeConvertor.castToString(value); // StringType 6841 } else if (name.equals("versionAlgorithm[x]")) { 6842 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 6843 } else if (name.equals("name")) { 6844 this.name = TypeConvertor.castToString(value); // StringType 6845 } else if (name.equals("title")) { 6846 this.title = TypeConvertor.castToString(value); // StringType 6847 } else if (name.equals("status")) { 6848 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 6849 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 6850 } else if (name.equals("experimental")) { 6851 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 6852 } else if (name.equals("date")) { 6853 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 6854 } else if (name.equals("publisher")) { 6855 this.publisher = TypeConvertor.castToString(value); // StringType 6856 } else if (name.equals("contact")) { 6857 this.getContact().add(TypeConvertor.castToContactDetail(value)); 6858 } else if (name.equals("description")) { 6859 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 6860 } else if (name.equals("useContext")) { 6861 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 6862 } else if (name.equals("jurisdiction")) { 6863 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 6864 } else if (name.equals("purpose")) { 6865 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 6866 } else if (name.equals("copyright")) { 6867 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 6868 } else if (name.equals("copyrightLabel")) { 6869 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 6870 } else if (name.equals("structure")) { 6871 this.getStructure().add((StructureMapStructureComponent) value); 6872 } else if (name.equals("import")) { 6873 this.getImport().add(TypeConvertor.castToCanonical(value)); 6874 } else if (name.equals("const")) { 6875 this.getConst().add((StructureMapConstComponent) value); 6876 } else if (name.equals("group")) { 6877 this.getGroup().add((StructureMapGroupComponent) value); 6878 } else 6879 return super.setProperty(name, value); 6880 return value; 6881 } 6882 6883 @Override 6884 public void removeChild(String name, Base value) throws FHIRException { 6885 if (name.equals("url")) { 6886 this.url = null; 6887 } else if (name.equals("identifier")) { 6888 this.getIdentifier().remove(value); 6889 } else if (name.equals("version")) { 6890 this.version = null; 6891 } else if (name.equals("versionAlgorithm[x]")) { 6892 this.versionAlgorithm = null; 6893 } else if (name.equals("name")) { 6894 this.name = null; 6895 } else if (name.equals("title")) { 6896 this.title = null; 6897 } else if (name.equals("status")) { 6898 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 6899 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 6900 } else if (name.equals("experimental")) { 6901 this.experimental = null; 6902 } else if (name.equals("date")) { 6903 this.date = null; 6904 } else if (name.equals("publisher")) { 6905 this.publisher = null; 6906 } else if (name.equals("contact")) { 6907 this.getContact().remove(value); 6908 } else if (name.equals("description")) { 6909 this.description = null; 6910 } else if (name.equals("useContext")) { 6911 this.getUseContext().remove(value); 6912 } else if (name.equals("jurisdiction")) { 6913 this.getJurisdiction().remove(value); 6914 } else if (name.equals("purpose")) { 6915 this.purpose = null; 6916 } else if (name.equals("copyright")) { 6917 this.copyright = null; 6918 } else if (name.equals("copyrightLabel")) { 6919 this.copyrightLabel = null; 6920 } else if (name.equals("structure")) { 6921 this.getStructure().remove((StructureMapStructureComponent) value); 6922 } else if (name.equals("import")) { 6923 this.getImport().remove(value); 6924 } else if (name.equals("const")) { 6925 this.getConst().remove((StructureMapConstComponent) value); 6926 } else if (name.equals("group")) { 6927 this.getGroup().remove((StructureMapGroupComponent) value); 6928 } else 6929 super.removeChild(name, value); 6930 6931 } 6932 6933 @Override 6934 public Base makeProperty(int hash, String name) throws FHIRException { 6935 switch (hash) { 6936 case 116079: return getUrlElement(); 6937 case -1618432855: return addIdentifier(); 6938 case 351608024: return getVersionElement(); 6939 case -115699031: return getVersionAlgorithm(); 6940 case 1508158071: return getVersionAlgorithm(); 6941 case 3373707: return getNameElement(); 6942 case 110371416: return getTitleElement(); 6943 case -892481550: return getStatusElement(); 6944 case -404562712: return getExperimentalElement(); 6945 case 3076014: return getDateElement(); 6946 case 1447404028: return getPublisherElement(); 6947 case 951526432: return addContact(); 6948 case -1724546052: return getDescriptionElement(); 6949 case -669707736: return addUseContext(); 6950 case -507075711: return addJurisdiction(); 6951 case -220463842: return getPurposeElement(); 6952 case 1522889671: return getCopyrightElement(); 6953 case 765157229: return getCopyrightLabelElement(); 6954 case 144518515: return addStructure(); 6955 case -1184795739: return addImportElement(); 6956 case 94844771: return addConst(); 6957 case 98629247: return addGroup(); 6958 default: return super.makeProperty(hash, name); 6959 } 6960 6961 } 6962 6963 @Override 6964 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6965 switch (hash) { 6966 case 116079: /*url*/ return new String[] {"uri"}; 6967 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 6968 case 351608024: /*version*/ return new String[] {"string"}; 6969 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 6970 case 3373707: /*name*/ return new String[] {"string"}; 6971 case 110371416: /*title*/ return new String[] {"string"}; 6972 case -892481550: /*status*/ return new String[] {"code"}; 6973 case -404562712: /*experimental*/ return new String[] {"boolean"}; 6974 case 3076014: /*date*/ return new String[] {"dateTime"}; 6975 case 1447404028: /*publisher*/ return new String[] {"string"}; 6976 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 6977 case -1724546052: /*description*/ return new String[] {"markdown"}; 6978 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 6979 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 6980 case -220463842: /*purpose*/ return new String[] {"markdown"}; 6981 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 6982 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 6983 case 144518515: /*structure*/ return new String[] {}; 6984 case -1184795739: /*import*/ return new String[] {"canonical"}; 6985 case 94844771: /*const*/ return new String[] {}; 6986 case 98629247: /*group*/ return new String[] {}; 6987 default: return super.getTypesForProperty(hash, name); 6988 } 6989 6990 } 6991 6992 @Override 6993 public Base addChild(String name) throws FHIRException { 6994 if (name.equals("url")) { 6995 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.url"); 6996 } 6997 else if (name.equals("identifier")) { 6998 return addIdentifier(); 6999 } 7000 else if (name.equals("version")) { 7001 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.version"); 7002 } 7003 else if (name.equals("versionAlgorithmString")) { 7004 this.versionAlgorithm = new StringType(); 7005 return this.versionAlgorithm; 7006 } 7007 else if (name.equals("versionAlgorithmCoding")) { 7008 this.versionAlgorithm = new Coding(); 7009 return this.versionAlgorithm; 7010 } 7011 else if (name.equals("name")) { 7012 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.name"); 7013 } 7014 else if (name.equals("title")) { 7015 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.title"); 7016 } 7017 else if (name.equals("status")) { 7018 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.status"); 7019 } 7020 else if (name.equals("experimental")) { 7021 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.experimental"); 7022 } 7023 else if (name.equals("date")) { 7024 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.date"); 7025 } 7026 else if (name.equals("publisher")) { 7027 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.publisher"); 7028 } 7029 else if (name.equals("contact")) { 7030 return addContact(); 7031 } 7032 else if (name.equals("description")) { 7033 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.description"); 7034 } 7035 else if (name.equals("useContext")) { 7036 return addUseContext(); 7037 } 7038 else if (name.equals("jurisdiction")) { 7039 return addJurisdiction(); 7040 } 7041 else if (name.equals("purpose")) { 7042 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.purpose"); 7043 } 7044 else if (name.equals("copyright")) { 7045 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.copyright"); 7046 } 7047 else if (name.equals("copyrightLabel")) { 7048 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.copyrightLabel"); 7049 } 7050 else if (name.equals("structure")) { 7051 return addStructure(); 7052 } 7053 else if (name.equals("import")) { 7054 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.import"); 7055 } 7056 else if (name.equals("const")) { 7057 return addConst(); 7058 } 7059 else if (name.equals("group")) { 7060 return addGroup(); 7061 } 7062 else 7063 return super.addChild(name); 7064 } 7065 7066 public String fhirType() { 7067 return "StructureMap"; 7068 7069 } 7070 7071 public StructureMap copy() { 7072 StructureMap dst = new StructureMap(); 7073 copyValues(dst); 7074 return dst; 7075 } 7076 7077 public void copyValues(StructureMap dst) { 7078 super.copyValues(dst); 7079 dst.url = url == null ? null : url.copy(); 7080 if (identifier != null) { 7081 dst.identifier = new ArrayList<Identifier>(); 7082 for (Identifier i : identifier) 7083 dst.identifier.add(i.copy()); 7084 }; 7085 dst.version = version == null ? null : version.copy(); 7086 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 7087 dst.name = name == null ? null : name.copy(); 7088 dst.title = title == null ? null : title.copy(); 7089 dst.status = status == null ? null : status.copy(); 7090 dst.experimental = experimental == null ? null : experimental.copy(); 7091 dst.date = date == null ? null : date.copy(); 7092 dst.publisher = publisher == null ? null : publisher.copy(); 7093 if (contact != null) { 7094 dst.contact = new ArrayList<ContactDetail>(); 7095 for (ContactDetail i : contact) 7096 dst.contact.add(i.copy()); 7097 }; 7098 dst.description = description == null ? null : description.copy(); 7099 if (useContext != null) { 7100 dst.useContext = new ArrayList<UsageContext>(); 7101 for (UsageContext i : useContext) 7102 dst.useContext.add(i.copy()); 7103 }; 7104 if (jurisdiction != null) { 7105 dst.jurisdiction = new ArrayList<CodeableConcept>(); 7106 for (CodeableConcept i : jurisdiction) 7107 dst.jurisdiction.add(i.copy()); 7108 }; 7109 dst.purpose = purpose == null ? null : purpose.copy(); 7110 dst.copyright = copyright == null ? null : copyright.copy(); 7111 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 7112 if (structure != null) { 7113 dst.structure = new ArrayList<StructureMapStructureComponent>(); 7114 for (StructureMapStructureComponent i : structure) 7115 dst.structure.add(i.copy()); 7116 }; 7117 if (import_ != null) { 7118 dst.import_ = new ArrayList<CanonicalType>(); 7119 for (CanonicalType i : import_) 7120 dst.import_.add(i.copy()); 7121 }; 7122 if (const_ != null) { 7123 dst.const_ = new ArrayList<StructureMapConstComponent>(); 7124 for (StructureMapConstComponent i : const_) 7125 dst.const_.add(i.copy()); 7126 }; 7127 if (group != null) { 7128 dst.group = new ArrayList<StructureMapGroupComponent>(); 7129 for (StructureMapGroupComponent i : group) 7130 dst.group.add(i.copy()); 7131 }; 7132 } 7133 7134 protected StructureMap typedCopy() { 7135 return copy(); 7136 } 7137 7138 @Override 7139 public boolean equalsDeep(Base other_) { 7140 if (!super.equalsDeep(other_)) 7141 return false; 7142 if (!(other_ instanceof StructureMap)) 7143 return false; 7144 StructureMap o = (StructureMap) other_; 7145 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 7146 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 7147 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 7148 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 7149 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 7150 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 7151 && compareDeep(structure, o.structure, true) && compareDeep(import_, o.import_, true) && compareDeep(const_, o.const_, true) 7152 && compareDeep(group, o.group, true); 7153 } 7154 7155 @Override 7156 public boolean equalsShallow(Base other_) { 7157 if (!super.equalsShallow(other_)) 7158 return false; 7159 if (!(other_ instanceof StructureMap)) 7160 return false; 7161 StructureMap o = (StructureMap) other_; 7162 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 7163 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 7164 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 7165 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 7166 && compareValues(import_, o.import_, true); 7167 } 7168 7169 public boolean isEmpty() { 7170 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 7171 , versionAlgorithm, name, title, status, experimental, date, publisher, contact 7172 , description, useContext, jurisdiction, purpose, copyright, copyrightLabel, structure 7173 , import_, const_, group); 7174 } 7175 7176 @Override 7177 public ResourceType getResourceType() { 7178 return ResourceType.StructureMap; 7179 } 7180 7181 /** 7182 * Search parameter: <b>context-quantity</b> 7183 * <p> 7184 * Description: <b>Multiple Resources: 7185 7186* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 7187* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 7188* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 7189* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 7190* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 7191* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 7192* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 7193* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 7194* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 7195* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 7196* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 7197* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 7198* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 7199* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 7200* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 7201* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 7202* [Library](library.html): A quantity- or range-valued use context assigned to the library 7203* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 7204* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 7205* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 7206* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 7207* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 7208* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 7209* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 7210* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 7211* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 7212* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 7213* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 7214* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 7215* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 7216</b><br> 7217 * Type: <b>quantity</b><br> 7218 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 7219 * </p> 7220 */ 7221 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 7222 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 7223 /** 7224 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 7225 * <p> 7226 * Description: <b>Multiple Resources: 7227 7228* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 7229* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 7230* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 7231* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 7232* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 7233* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 7234* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 7235* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 7236* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 7237* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 7238* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 7239* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 7240* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 7241* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 7242* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 7243* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 7244* [Library](library.html): A quantity- or range-valued use context assigned to the library 7245* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 7246* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 7247* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 7248* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 7249* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 7250* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 7251* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 7252* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 7253* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 7254* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 7255* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 7256* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 7257* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 7258</b><br> 7259 * Type: <b>quantity</b><br> 7260 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 7261 * </p> 7262 */ 7263 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 7264 7265 /** 7266 * Search parameter: <b>context-type-quantity</b> 7267 * <p> 7268 * Description: <b>Multiple Resources: 7269 7270* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 7271* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 7272* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 7273* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 7274* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 7275* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 7276* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 7277* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 7278* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 7279* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 7280* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 7281* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 7282* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 7283* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 7284* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 7285* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 7286* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 7287* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 7288* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 7289* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 7290* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 7291* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 7292* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 7293* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 7294* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 7295* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 7296* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 7297* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 7298* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 7299* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 7300</b><br> 7301 * Type: <b>composite</b><br> 7302 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 7303 * </p> 7304 */ 7305 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 7306 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 7307 /** 7308 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 7309 * <p> 7310 * Description: <b>Multiple Resources: 7311 7312* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 7313* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 7314* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 7315* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 7316* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 7317* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 7318* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 7319* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 7320* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 7321* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 7322* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 7323* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 7324* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 7325* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 7326* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 7327* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 7328* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 7329* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 7330* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 7331* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 7332* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 7333* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 7334* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 7335* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 7336* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 7337* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 7338* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 7339* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 7340* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 7341* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 7342</b><br> 7343 * Type: <b>composite</b><br> 7344 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 7345 * </p> 7346 */ 7347 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 7348 7349 /** 7350 * Search parameter: <b>context-type-value</b> 7351 * <p> 7352 * Description: <b>Multiple Resources: 7353 7354* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 7355* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 7356* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 7357* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 7358* [Citation](citation.html): A use context type and value assigned to the citation 7359* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 7360* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 7361* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 7362* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 7363* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 7364* [Evidence](evidence.html): A use context type and value assigned to the evidence 7365* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 7366* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 7367* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 7368* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 7369* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 7370* [Library](library.html): A use context type and value assigned to the library 7371* [Measure](measure.html): A use context type and value assigned to the measure 7372* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 7373* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 7374* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 7375* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 7376* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 7377* [Requirements](requirements.html): A use context type and value assigned to the requirements 7378* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 7379* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 7380* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 7381* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 7382* [TestScript](testscript.html): A use context type and value assigned to the test script 7383* [ValueSet](valueset.html): A use context type and value assigned to the value set 7384</b><br> 7385 * Type: <b>composite</b><br> 7386 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 7387 * </p> 7388 */ 7389 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 7390 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 7391 /** 7392 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 7393 * <p> 7394 * Description: <b>Multiple Resources: 7395 7396* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 7397* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 7398* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 7399* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 7400* [Citation](citation.html): A use context type and value assigned to the citation 7401* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 7402* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 7403* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 7404* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 7405* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 7406* [Evidence](evidence.html): A use context type and value assigned to the evidence 7407* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 7408* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 7409* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 7410* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 7411* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 7412* [Library](library.html): A use context type and value assigned to the library 7413* [Measure](measure.html): A use context type and value assigned to the measure 7414* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 7415* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 7416* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 7417* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 7418* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 7419* [Requirements](requirements.html): A use context type and value assigned to the requirements 7420* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 7421* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 7422* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 7423* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 7424* [TestScript](testscript.html): A use context type and value assigned to the test script 7425* [ValueSet](valueset.html): A use context type and value assigned to the value set 7426</b><br> 7427 * Type: <b>composite</b><br> 7428 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 7429 * </p> 7430 */ 7431 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 7432 7433 /** 7434 * Search parameter: <b>context-type</b> 7435 * <p> 7436 * Description: <b>Multiple Resources: 7437 7438* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 7439* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 7440* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 7441* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 7442* [Citation](citation.html): A type of use context assigned to the citation 7443* [CodeSystem](codesystem.html): A type of use context assigned to the code system 7444* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 7445* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 7446* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 7447* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 7448* [Evidence](evidence.html): A type of use context assigned to the evidence 7449* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 7450* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 7451* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 7452* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 7453* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 7454* [Library](library.html): A type of use context assigned to the library 7455* [Measure](measure.html): A type of use context assigned to the measure 7456* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 7457* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 7458* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 7459* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 7460* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 7461* [Requirements](requirements.html): A type of use context assigned to the requirements 7462* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 7463* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 7464* [StructureMap](structuremap.html): A type of use context assigned to the structure map 7465* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 7466* [TestScript](testscript.html): A type of use context assigned to the test script 7467* [ValueSet](valueset.html): A type of use context assigned to the value set 7468</b><br> 7469 * Type: <b>token</b><br> 7470 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 7471 * </p> 7472 */ 7473 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 7474 public static final String SP_CONTEXT_TYPE = "context-type"; 7475 /** 7476 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 7477 * <p> 7478 * Description: <b>Multiple Resources: 7479 7480* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 7481* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 7482* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 7483* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 7484* [Citation](citation.html): A type of use context assigned to the citation 7485* [CodeSystem](codesystem.html): A type of use context assigned to the code system 7486* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 7487* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 7488* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 7489* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 7490* [Evidence](evidence.html): A type of use context assigned to the evidence 7491* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 7492* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 7493* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 7494* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 7495* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 7496* [Library](library.html): A type of use context assigned to the library 7497* [Measure](measure.html): A type of use context assigned to the measure 7498* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 7499* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 7500* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 7501* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 7502* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 7503* [Requirements](requirements.html): A type of use context assigned to the requirements 7504* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 7505* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 7506* [StructureMap](structuremap.html): A type of use context assigned to the structure map 7507* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 7508* [TestScript](testscript.html): A type of use context assigned to the test script 7509* [ValueSet](valueset.html): A type of use context assigned to the value set 7510</b><br> 7511 * Type: <b>token</b><br> 7512 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 7513 * </p> 7514 */ 7515 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 7516 7517 /** 7518 * Search parameter: <b>context</b> 7519 * <p> 7520 * Description: <b>Multiple Resources: 7521 7522* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 7523* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 7524* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 7525* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 7526* [Citation](citation.html): A use context assigned to the citation 7527* [CodeSystem](codesystem.html): A use context assigned to the code system 7528* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 7529* [ConceptMap](conceptmap.html): A use context assigned to the concept map 7530* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 7531* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 7532* [Evidence](evidence.html): A use context assigned to the evidence 7533* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 7534* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 7535* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 7536* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 7537* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 7538* [Library](library.html): A use context assigned to the library 7539* [Measure](measure.html): A use context assigned to the measure 7540* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 7541* [NamingSystem](namingsystem.html): A use context assigned to the naming system 7542* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 7543* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 7544* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 7545* [Requirements](requirements.html): A use context assigned to the requirements 7546* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 7547* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 7548* [StructureMap](structuremap.html): A use context assigned to the structure map 7549* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 7550* [TestScript](testscript.html): A use context assigned to the test script 7551* [ValueSet](valueset.html): A use context assigned to the value set 7552</b><br> 7553 * Type: <b>token</b><br> 7554 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 7555 * </p> 7556 */ 7557 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 7558 public static final String SP_CONTEXT = "context"; 7559 /** 7560 * <b>Fluent Client</b> search parameter constant for <b>context</b> 7561 * <p> 7562 * Description: <b>Multiple Resources: 7563 7564* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 7565* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 7566* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 7567* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 7568* [Citation](citation.html): A use context assigned to the citation 7569* [CodeSystem](codesystem.html): A use context assigned to the code system 7570* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 7571* [ConceptMap](conceptmap.html): A use context assigned to the concept map 7572* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 7573* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 7574* [Evidence](evidence.html): A use context assigned to the evidence 7575* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 7576* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 7577* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 7578* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 7579* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 7580* [Library](library.html): A use context assigned to the library 7581* [Measure](measure.html): A use context assigned to the measure 7582* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 7583* [NamingSystem](namingsystem.html): A use context assigned to the naming system 7584* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 7585* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 7586* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 7587* [Requirements](requirements.html): A use context assigned to the requirements 7588* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 7589* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 7590* [StructureMap](structuremap.html): A use context assigned to the structure map 7591* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 7592* [TestScript](testscript.html): A use context assigned to the test script 7593* [ValueSet](valueset.html): A use context assigned to the value set 7594</b><br> 7595 * Type: <b>token</b><br> 7596 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 7597 * </p> 7598 */ 7599 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 7600 7601 /** 7602 * Search parameter: <b>date</b> 7603 * <p> 7604 * Description: <b>Multiple Resources: 7605 7606* [ActivityDefinition](activitydefinition.html): The activity definition publication date 7607* [ActorDefinition](actordefinition.html): The Actor Definition publication date 7608* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 7609* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 7610* [Citation](citation.html): The citation publication date 7611* [CodeSystem](codesystem.html): The code system publication date 7612* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 7613* [ConceptMap](conceptmap.html): The concept map publication date 7614* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 7615* [EventDefinition](eventdefinition.html): The event definition publication date 7616* [Evidence](evidence.html): The evidence publication date 7617* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 7618* [ExampleScenario](examplescenario.html): The example scenario publication date 7619* [GraphDefinition](graphdefinition.html): The graph definition publication date 7620* [ImplementationGuide](implementationguide.html): The implementation guide publication date 7621* [Library](library.html): The library publication date 7622* [Measure](measure.html): The measure publication date 7623* [MessageDefinition](messagedefinition.html): The message definition publication date 7624* [NamingSystem](namingsystem.html): The naming system publication date 7625* [OperationDefinition](operationdefinition.html): The operation definition publication date 7626* [PlanDefinition](plandefinition.html): The plan definition publication date 7627* [Questionnaire](questionnaire.html): The questionnaire publication date 7628* [Requirements](requirements.html): The requirements publication date 7629* [SearchParameter](searchparameter.html): The search parameter publication date 7630* [StructureDefinition](structuredefinition.html): The structure definition publication date 7631* [StructureMap](structuremap.html): The structure map publication date 7632* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 7633* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 7634* [TestScript](testscript.html): The test script publication date 7635* [ValueSet](valueset.html): The value set publication date 7636</b><br> 7637 * Type: <b>date</b><br> 7638 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 7639 * </p> 7640 */ 7641 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 7642 public static final String SP_DATE = "date"; 7643 /** 7644 * <b>Fluent Client</b> search parameter constant for <b>date</b> 7645 * <p> 7646 * Description: <b>Multiple Resources: 7647 7648* [ActivityDefinition](activitydefinition.html): The activity definition publication date 7649* [ActorDefinition](actordefinition.html): The Actor Definition publication date 7650* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 7651* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 7652* [Citation](citation.html): The citation publication date 7653* [CodeSystem](codesystem.html): The code system publication date 7654* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 7655* [ConceptMap](conceptmap.html): The concept map publication date 7656* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 7657* [EventDefinition](eventdefinition.html): The event definition publication date 7658* [Evidence](evidence.html): The evidence publication date 7659* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 7660* [ExampleScenario](examplescenario.html): The example scenario publication date 7661* [GraphDefinition](graphdefinition.html): The graph definition publication date 7662* [ImplementationGuide](implementationguide.html): The implementation guide publication date 7663* [Library](library.html): The library publication date 7664* [Measure](measure.html): The measure publication date 7665* [MessageDefinition](messagedefinition.html): The message definition publication date 7666* [NamingSystem](namingsystem.html): The naming system publication date 7667* [OperationDefinition](operationdefinition.html): The operation definition publication date 7668* [PlanDefinition](plandefinition.html): The plan definition publication date 7669* [Questionnaire](questionnaire.html): The questionnaire publication date 7670* [Requirements](requirements.html): The requirements publication date 7671* [SearchParameter](searchparameter.html): The search parameter publication date 7672* [StructureDefinition](structuredefinition.html): The structure definition publication date 7673* [StructureMap](structuremap.html): The structure map publication date 7674* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 7675* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 7676* [TestScript](testscript.html): The test script publication date 7677* [ValueSet](valueset.html): The value set publication date 7678</b><br> 7679 * Type: <b>date</b><br> 7680 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 7681 * </p> 7682 */ 7683 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 7684 7685 /** 7686 * Search parameter: <b>description</b> 7687 * <p> 7688 * Description: <b>Multiple Resources: 7689 7690* [ActivityDefinition](activitydefinition.html): The description of the activity definition 7691* [ActorDefinition](actordefinition.html): The description of the Actor Definition 7692* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 7693* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 7694* [Citation](citation.html): The description of the citation 7695* [CodeSystem](codesystem.html): The description of the code system 7696* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 7697* [ConceptMap](conceptmap.html): The description of the concept map 7698* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 7699* [EventDefinition](eventdefinition.html): The description of the event definition 7700* [Evidence](evidence.html): The description of the evidence 7701* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 7702* [GraphDefinition](graphdefinition.html): The description of the graph definition 7703* [ImplementationGuide](implementationguide.html): The description of the implementation guide 7704* [Library](library.html): The description of the library 7705* [Measure](measure.html): The description of the measure 7706* [MessageDefinition](messagedefinition.html): The description of the message definition 7707* [NamingSystem](namingsystem.html): The description of the naming system 7708* [OperationDefinition](operationdefinition.html): The description of the operation definition 7709* [PlanDefinition](plandefinition.html): The description of the plan definition 7710* [Questionnaire](questionnaire.html): The description of the questionnaire 7711* [Requirements](requirements.html): The description of the requirements 7712* [SearchParameter](searchparameter.html): The description of the search parameter 7713* [StructureDefinition](structuredefinition.html): The description of the structure definition 7714* [StructureMap](structuremap.html): The description of the structure map 7715* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 7716* [TestScript](testscript.html): The description of the test script 7717* [ValueSet](valueset.html): The description of the value set 7718</b><br> 7719 * Type: <b>string</b><br> 7720 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 7721 * </p> 7722 */ 7723 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 7724 public static final String SP_DESCRIPTION = "description"; 7725 /** 7726 * <b>Fluent Client</b> search parameter constant for <b>description</b> 7727 * <p> 7728 * Description: <b>Multiple Resources: 7729 7730* [ActivityDefinition](activitydefinition.html): The description of the activity definition 7731* [ActorDefinition](actordefinition.html): The description of the Actor Definition 7732* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 7733* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 7734* [Citation](citation.html): The description of the citation 7735* [CodeSystem](codesystem.html): The description of the code system 7736* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 7737* [ConceptMap](conceptmap.html): The description of the concept map 7738* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 7739* [EventDefinition](eventdefinition.html): The description of the event definition 7740* [Evidence](evidence.html): The description of the evidence 7741* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 7742* [GraphDefinition](graphdefinition.html): The description of the graph definition 7743* [ImplementationGuide](implementationguide.html): The description of the implementation guide 7744* [Library](library.html): The description of the library 7745* [Measure](measure.html): The description of the measure 7746* [MessageDefinition](messagedefinition.html): The description of the message definition 7747* [NamingSystem](namingsystem.html): The description of the naming system 7748* [OperationDefinition](operationdefinition.html): The description of the operation definition 7749* [PlanDefinition](plandefinition.html): The description of the plan definition 7750* [Questionnaire](questionnaire.html): The description of the questionnaire 7751* [Requirements](requirements.html): The description of the requirements 7752* [SearchParameter](searchparameter.html): The description of the search parameter 7753* [StructureDefinition](structuredefinition.html): The description of the structure definition 7754* [StructureMap](structuremap.html): The description of the structure map 7755* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 7756* [TestScript](testscript.html): The description of the test script 7757* [ValueSet](valueset.html): The description of the value set 7758</b><br> 7759 * Type: <b>string</b><br> 7760 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 7761 * </p> 7762 */ 7763 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 7764 7765 /** 7766 * Search parameter: <b>identifier</b> 7767 * <p> 7768 * Description: <b>Multiple Resources: 7769 7770* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 7771* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 7772* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 7773* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 7774* [Citation](citation.html): External identifier for the citation 7775* [CodeSystem](codesystem.html): External identifier for the code system 7776* [ConceptMap](conceptmap.html): External identifier for the concept map 7777* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 7778* [EventDefinition](eventdefinition.html): External identifier for the event definition 7779* [Evidence](evidence.html): External identifier for the evidence 7780* [EvidenceReport](evidencereport.html): External identifier for the evidence report 7781* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 7782* [ExampleScenario](examplescenario.html): External identifier for the example scenario 7783* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 7784* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 7785* [Library](library.html): External identifier for the library 7786* [Measure](measure.html): External identifier for the measure 7787* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 7788* [MessageDefinition](messagedefinition.html): External identifier for the message definition 7789* [NamingSystem](namingsystem.html): External identifier for the naming system 7790* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 7791* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 7792* [PlanDefinition](plandefinition.html): External identifier for the plan definition 7793* [Questionnaire](questionnaire.html): External identifier for the questionnaire 7794* [Requirements](requirements.html): External identifier for the requirements 7795* [SearchParameter](searchparameter.html): External identifier for the search parameter 7796* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 7797* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 7798* [StructureMap](structuremap.html): External identifier for the structure map 7799* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 7800* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 7801* [TestPlan](testplan.html): An identifier for the test plan 7802* [TestScript](testscript.html): External identifier for the test script 7803* [ValueSet](valueset.html): External identifier for the value set 7804</b><br> 7805 * Type: <b>token</b><br> 7806 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 7807 * </p> 7808 */ 7809 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 7810 public static final String SP_IDENTIFIER = "identifier"; 7811 /** 7812 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 7813 * <p> 7814 * Description: <b>Multiple Resources: 7815 7816* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 7817* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 7818* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 7819* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 7820* [Citation](citation.html): External identifier for the citation 7821* [CodeSystem](codesystem.html): External identifier for the code system 7822* [ConceptMap](conceptmap.html): External identifier for the concept map 7823* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 7824* [EventDefinition](eventdefinition.html): External identifier for the event definition 7825* [Evidence](evidence.html): External identifier for the evidence 7826* [EvidenceReport](evidencereport.html): External identifier for the evidence report 7827* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 7828* [ExampleScenario](examplescenario.html): External identifier for the example scenario 7829* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 7830* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 7831* [Library](library.html): External identifier for the library 7832* [Measure](measure.html): External identifier for the measure 7833* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 7834* [MessageDefinition](messagedefinition.html): External identifier for the message definition 7835* [NamingSystem](namingsystem.html): External identifier for the naming system 7836* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 7837* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 7838* [PlanDefinition](plandefinition.html): External identifier for the plan definition 7839* [Questionnaire](questionnaire.html): External identifier for the questionnaire 7840* [Requirements](requirements.html): External identifier for the requirements 7841* [SearchParameter](searchparameter.html): External identifier for the search parameter 7842* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 7843* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 7844* [StructureMap](structuremap.html): External identifier for the structure map 7845* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 7846* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 7847* [TestPlan](testplan.html): An identifier for the test plan 7848* [TestScript](testscript.html): External identifier for the test script 7849* [ValueSet](valueset.html): External identifier for the value set 7850</b><br> 7851 * Type: <b>token</b><br> 7852 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 7853 * </p> 7854 */ 7855 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 7856 7857 /** 7858 * Search parameter: <b>jurisdiction</b> 7859 * <p> 7860 * Description: <b>Multiple Resources: 7861 7862* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 7863* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 7864* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 7865* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 7866* [Citation](citation.html): Intended jurisdiction for the citation 7867* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 7868* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 7869* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 7870* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 7871* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 7872* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 7873* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 7874* [Library](library.html): Intended jurisdiction for the library 7875* [Measure](measure.html): Intended jurisdiction for the measure 7876* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 7877* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 7878* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 7879* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 7880* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 7881* [Requirements](requirements.html): Intended jurisdiction for the requirements 7882* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 7883* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 7884* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 7885* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 7886* [TestScript](testscript.html): Intended jurisdiction for the test script 7887* [ValueSet](valueset.html): Intended jurisdiction for the value set 7888</b><br> 7889 * Type: <b>token</b><br> 7890 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 7891 * </p> 7892 */ 7893 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 7894 public static final String SP_JURISDICTION = "jurisdiction"; 7895 /** 7896 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 7897 * <p> 7898 * Description: <b>Multiple Resources: 7899 7900* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 7901* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 7902* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 7903* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 7904* [Citation](citation.html): Intended jurisdiction for the citation 7905* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 7906* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 7907* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 7908* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 7909* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 7910* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 7911* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 7912* [Library](library.html): Intended jurisdiction for the library 7913* [Measure](measure.html): Intended jurisdiction for the measure 7914* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 7915* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 7916* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 7917* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 7918* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 7919* [Requirements](requirements.html): Intended jurisdiction for the requirements 7920* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 7921* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 7922* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 7923* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 7924* [TestScript](testscript.html): Intended jurisdiction for the test script 7925* [ValueSet](valueset.html): Intended jurisdiction for the value set 7926</b><br> 7927 * Type: <b>token</b><br> 7928 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 7929 * </p> 7930 */ 7931 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 7932 7933 /** 7934 * Search parameter: <b>name</b> 7935 * <p> 7936 * Description: <b>Multiple Resources: 7937 7938* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 7939* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 7940* [Citation](citation.html): Computationally friendly name of the citation 7941* [CodeSystem](codesystem.html): Computationally friendly name of the code system 7942* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 7943* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 7944* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 7945* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 7946* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 7947* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 7948* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 7949* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 7950* [Library](library.html): Computationally friendly name of the library 7951* [Measure](measure.html): Computationally friendly name of the measure 7952* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 7953* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 7954* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 7955* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 7956* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 7957* [Requirements](requirements.html): Computationally friendly name of the requirements 7958* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 7959* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 7960* [StructureMap](structuremap.html): Computationally friendly name of the structure map 7961* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 7962* [TestScript](testscript.html): Computationally friendly name of the test script 7963* [ValueSet](valueset.html): Computationally friendly name of the value set 7964</b><br> 7965 * Type: <b>string</b><br> 7966 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 7967 * </p> 7968 */ 7969 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 7970 public static final String SP_NAME = "name"; 7971 /** 7972 * <b>Fluent Client</b> search parameter constant for <b>name</b> 7973 * <p> 7974 * Description: <b>Multiple Resources: 7975 7976* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 7977* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 7978* [Citation](citation.html): Computationally friendly name of the citation 7979* [CodeSystem](codesystem.html): Computationally friendly name of the code system 7980* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 7981* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 7982* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 7983* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 7984* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 7985* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 7986* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 7987* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 7988* [Library](library.html): Computationally friendly name of the library 7989* [Measure](measure.html): Computationally friendly name of the measure 7990* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 7991* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 7992* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 7993* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 7994* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 7995* [Requirements](requirements.html): Computationally friendly name of the requirements 7996* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 7997* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 7998* [StructureMap](structuremap.html): Computationally friendly name of the structure map 7999* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 8000* [TestScript](testscript.html): Computationally friendly name of the test script 8001* [ValueSet](valueset.html): Computationally friendly name of the value set 8002</b><br> 8003 * Type: <b>string</b><br> 8004 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 8005 * </p> 8006 */ 8007 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 8008 8009 /** 8010 * Search parameter: <b>publisher</b> 8011 * <p> 8012 * Description: <b>Multiple Resources: 8013 8014* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 8015* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 8016* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 8017* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 8018* [Citation](citation.html): Name of the publisher of the citation 8019* [CodeSystem](codesystem.html): Name of the publisher of the code system 8020* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 8021* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 8022* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 8023* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 8024* [Evidence](evidence.html): Name of the publisher of the evidence 8025* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 8026* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 8027* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 8028* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 8029* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 8030* [Library](library.html): Name of the publisher of the library 8031* [Measure](measure.html): Name of the publisher of the measure 8032* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 8033* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 8034* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 8035* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 8036* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 8037* [Requirements](requirements.html): Name of the publisher of the requirements 8038* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 8039* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 8040* [StructureMap](structuremap.html): Name of the publisher of the structure map 8041* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 8042* [TestScript](testscript.html): Name of the publisher of the test script 8043* [ValueSet](valueset.html): Name of the publisher of the value set 8044</b><br> 8045 * Type: <b>string</b><br> 8046 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 8047 * </p> 8048 */ 8049 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 8050 public static final String SP_PUBLISHER = "publisher"; 8051 /** 8052 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 8053 * <p> 8054 * Description: <b>Multiple Resources: 8055 8056* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 8057* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 8058* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 8059* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 8060* [Citation](citation.html): Name of the publisher of the citation 8061* [CodeSystem](codesystem.html): Name of the publisher of the code system 8062* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 8063* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 8064* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 8065* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 8066* [Evidence](evidence.html): Name of the publisher of the evidence 8067* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 8068* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 8069* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 8070* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 8071* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 8072* [Library](library.html): Name of the publisher of the library 8073* [Measure](measure.html): Name of the publisher of the measure 8074* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 8075* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 8076* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 8077* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 8078* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 8079* [Requirements](requirements.html): Name of the publisher of the requirements 8080* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 8081* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 8082* [StructureMap](structuremap.html): Name of the publisher of the structure map 8083* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 8084* [TestScript](testscript.html): Name of the publisher of the test script 8085* [ValueSet](valueset.html): Name of the publisher of the value set 8086</b><br> 8087 * Type: <b>string</b><br> 8088 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 8089 * </p> 8090 */ 8091 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 8092 8093 /** 8094 * Search parameter: <b>status</b> 8095 * <p> 8096 * Description: <b>Multiple Resources: 8097 8098* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 8099* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 8100* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 8101* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 8102* [Citation](citation.html): The current status of the citation 8103* [CodeSystem](codesystem.html): The current status of the code system 8104* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 8105* [ConceptMap](conceptmap.html): The current status of the concept map 8106* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 8107* [EventDefinition](eventdefinition.html): The current status of the event definition 8108* [Evidence](evidence.html): The current status of the evidence 8109* [EvidenceReport](evidencereport.html): The current status of the evidence report 8110* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 8111* [ExampleScenario](examplescenario.html): The current status of the example scenario 8112* [GraphDefinition](graphdefinition.html): The current status of the graph definition 8113* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 8114* [Library](library.html): The current status of the library 8115* [Measure](measure.html): The current status of the measure 8116* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 8117* [MessageDefinition](messagedefinition.html): The current status of the message definition 8118* [NamingSystem](namingsystem.html): The current status of the naming system 8119* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 8120* [OperationDefinition](operationdefinition.html): The current status of the operation definition 8121* [PlanDefinition](plandefinition.html): The current status of the plan definition 8122* [Questionnaire](questionnaire.html): The current status of the questionnaire 8123* [Requirements](requirements.html): The current status of the requirements 8124* [SearchParameter](searchparameter.html): The current status of the search parameter 8125* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 8126* [StructureDefinition](structuredefinition.html): The current status of the structure definition 8127* [StructureMap](structuremap.html): The current status of the structure map 8128* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 8129* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 8130* [TestPlan](testplan.html): The current status of the test plan 8131* [TestScript](testscript.html): The current status of the test script 8132* [ValueSet](valueset.html): The current status of the value set 8133</b><br> 8134 * Type: <b>token</b><br> 8135 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 8136 * </p> 8137 */ 8138 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 8139 public static final String SP_STATUS = "status"; 8140 /** 8141 * <b>Fluent Client</b> search parameter constant for <b>status</b> 8142 * <p> 8143 * Description: <b>Multiple Resources: 8144 8145* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 8146* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 8147* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 8148* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 8149* [Citation](citation.html): The current status of the citation 8150* [CodeSystem](codesystem.html): The current status of the code system 8151* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 8152* [ConceptMap](conceptmap.html): The current status of the concept map 8153* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 8154* [EventDefinition](eventdefinition.html): The current status of the event definition 8155* [Evidence](evidence.html): The current status of the evidence 8156* [EvidenceReport](evidencereport.html): The current status of the evidence report 8157* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 8158* [ExampleScenario](examplescenario.html): The current status of the example scenario 8159* [GraphDefinition](graphdefinition.html): The current status of the graph definition 8160* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 8161* [Library](library.html): The current status of the library 8162* [Measure](measure.html): The current status of the measure 8163* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 8164* [MessageDefinition](messagedefinition.html): The current status of the message definition 8165* [NamingSystem](namingsystem.html): The current status of the naming system 8166* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 8167* [OperationDefinition](operationdefinition.html): The current status of the operation definition 8168* [PlanDefinition](plandefinition.html): The current status of the plan definition 8169* [Questionnaire](questionnaire.html): The current status of the questionnaire 8170* [Requirements](requirements.html): The current status of the requirements 8171* [SearchParameter](searchparameter.html): The current status of the search parameter 8172* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 8173* [StructureDefinition](structuredefinition.html): The current status of the structure definition 8174* [StructureMap](structuremap.html): The current status of the structure map 8175* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 8176* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 8177* [TestPlan](testplan.html): The current status of the test plan 8178* [TestScript](testscript.html): The current status of the test script 8179* [ValueSet](valueset.html): The current status of the value set 8180</b><br> 8181 * Type: <b>token</b><br> 8182 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 8183 * </p> 8184 */ 8185 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 8186 8187 /** 8188 * Search parameter: <b>title</b> 8189 * <p> 8190 * Description: <b>Multiple Resources: 8191 8192* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 8193* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 8194* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 8195* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 8196* [Citation](citation.html): The human-friendly name of the citation 8197* [CodeSystem](codesystem.html): The human-friendly name of the code system 8198* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 8199* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 8200* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 8201* [Evidence](evidence.html): The human-friendly name of the evidence 8202* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 8203* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 8204* [Library](library.html): The human-friendly name of the library 8205* [Measure](measure.html): The human-friendly name of the measure 8206* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 8207* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 8208* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 8209* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 8210* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 8211* [Requirements](requirements.html): The human-friendly name of the requirements 8212* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 8213* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 8214* [StructureMap](structuremap.html): The human-friendly name of the structure map 8215* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 8216* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 8217* [TestScript](testscript.html): The human-friendly name of the test script 8218* [ValueSet](valueset.html): The human-friendly name of the value set 8219</b><br> 8220 * Type: <b>string</b><br> 8221 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 8222 * </p> 8223 */ 8224 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 8225 public static final String SP_TITLE = "title"; 8226 /** 8227 * <b>Fluent Client</b> search parameter constant for <b>title</b> 8228 * <p> 8229 * Description: <b>Multiple Resources: 8230 8231* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 8232* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 8233* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 8234* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 8235* [Citation](citation.html): The human-friendly name of the citation 8236* [CodeSystem](codesystem.html): The human-friendly name of the code system 8237* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 8238* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 8239* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 8240* [Evidence](evidence.html): The human-friendly name of the evidence 8241* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 8242* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 8243* [Library](library.html): The human-friendly name of the library 8244* [Measure](measure.html): The human-friendly name of the measure 8245* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 8246* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 8247* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 8248* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 8249* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 8250* [Requirements](requirements.html): The human-friendly name of the requirements 8251* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 8252* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 8253* [StructureMap](structuremap.html): The human-friendly name of the structure map 8254* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 8255* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 8256* [TestScript](testscript.html): The human-friendly name of the test script 8257* [ValueSet](valueset.html): The human-friendly name of the value set 8258</b><br> 8259 * Type: <b>string</b><br> 8260 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 8261 * </p> 8262 */ 8263 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 8264 8265 /** 8266 * Search parameter: <b>url</b> 8267 * <p> 8268 * Description: <b>Multiple Resources: 8269 8270* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 8271* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 8272* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 8273* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 8274* [Citation](citation.html): The uri that identifies the citation 8275* [CodeSystem](codesystem.html): The uri that identifies the code system 8276* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 8277* [ConceptMap](conceptmap.html): The URI that identifies the concept map 8278* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 8279* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 8280* [Evidence](evidence.html): The uri that identifies the evidence 8281* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 8282* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 8283* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 8284* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 8285* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 8286* [Library](library.html): The uri that identifies the library 8287* [Measure](measure.html): The uri that identifies the measure 8288* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 8289* [NamingSystem](namingsystem.html): The uri that identifies the naming system 8290* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 8291* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 8292* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 8293* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 8294* [Requirements](requirements.html): The uri that identifies the requirements 8295* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 8296* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 8297* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 8298* [StructureMap](structuremap.html): The uri that identifies the structure map 8299* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 8300* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 8301* [TestPlan](testplan.html): The uri that identifies the test plan 8302* [TestScript](testscript.html): The uri that identifies the test script 8303* [ValueSet](valueset.html): The uri that identifies the value set 8304</b><br> 8305 * Type: <b>uri</b><br> 8306 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 8307 * </p> 8308 */ 8309 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 8310 public static final String SP_URL = "url"; 8311 /** 8312 * <b>Fluent Client</b> search parameter constant for <b>url</b> 8313 * <p> 8314 * Description: <b>Multiple Resources: 8315 8316* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 8317* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 8318* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 8319* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 8320* [Citation](citation.html): The uri that identifies the citation 8321* [CodeSystem](codesystem.html): The uri that identifies the code system 8322* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 8323* [ConceptMap](conceptmap.html): The URI that identifies the concept map 8324* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 8325* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 8326* [Evidence](evidence.html): The uri that identifies the evidence 8327* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 8328* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 8329* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 8330* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 8331* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 8332* [Library](library.html): The uri that identifies the library 8333* [Measure](measure.html): The uri that identifies the measure 8334* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 8335* [NamingSystem](namingsystem.html): The uri that identifies the naming system 8336* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 8337* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 8338* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 8339* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 8340* [Requirements](requirements.html): The uri that identifies the requirements 8341* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 8342* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 8343* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 8344* [StructureMap](structuremap.html): The uri that identifies the structure map 8345* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 8346* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 8347* [TestPlan](testplan.html): The uri that identifies the test plan 8348* [TestScript](testscript.html): The uri that identifies the test script 8349* [ValueSet](valueset.html): The uri that identifies the value set 8350</b><br> 8351 * Type: <b>uri</b><br> 8352 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 8353 * </p> 8354 */ 8355 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 8356 8357 /** 8358 * Search parameter: <b>version</b> 8359 * <p> 8360 * Description: <b>Multiple Resources: 8361 8362* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 8363* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 8364* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 8365* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 8366* [Citation](citation.html): The business version of the citation 8367* [CodeSystem](codesystem.html): The business version of the code system 8368* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 8369* [ConceptMap](conceptmap.html): The business version of the concept map 8370* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 8371* [EventDefinition](eventdefinition.html): The business version of the event definition 8372* [Evidence](evidence.html): The business version of the evidence 8373* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 8374* [ExampleScenario](examplescenario.html): The business version of the example scenario 8375* [GraphDefinition](graphdefinition.html): The business version of the graph definition 8376* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 8377* [Library](library.html): The business version of the library 8378* [Measure](measure.html): The business version of the measure 8379* [MessageDefinition](messagedefinition.html): The business version of the message definition 8380* [NamingSystem](namingsystem.html): The business version of the naming system 8381* [OperationDefinition](operationdefinition.html): The business version of the operation definition 8382* [PlanDefinition](plandefinition.html): The business version of the plan definition 8383* [Questionnaire](questionnaire.html): The business version of the questionnaire 8384* [Requirements](requirements.html): The business version of the requirements 8385* [SearchParameter](searchparameter.html): The business version of the search parameter 8386* [StructureDefinition](structuredefinition.html): The business version of the structure definition 8387* [StructureMap](structuremap.html): The business version of the structure map 8388* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 8389* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 8390* [TestScript](testscript.html): The business version of the test script 8391* [ValueSet](valueset.html): The business version of the value set 8392</b><br> 8393 * Type: <b>token</b><br> 8394 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 8395 * </p> 8396 */ 8397 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 8398 public static final String SP_VERSION = "version"; 8399 /** 8400 * <b>Fluent Client</b> search parameter constant for <b>version</b> 8401 * <p> 8402 * Description: <b>Multiple Resources: 8403 8404* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 8405* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 8406* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 8407* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 8408* [Citation](citation.html): The business version of the citation 8409* [CodeSystem](codesystem.html): The business version of the code system 8410* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 8411* [ConceptMap](conceptmap.html): The business version of the concept map 8412* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 8413* [EventDefinition](eventdefinition.html): The business version of the event definition 8414* [Evidence](evidence.html): The business version of the evidence 8415* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 8416* [ExampleScenario](examplescenario.html): The business version of the example scenario 8417* [GraphDefinition](graphdefinition.html): The business version of the graph definition 8418* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 8419* [Library](library.html): The business version of the library 8420* [Measure](measure.html): The business version of the measure 8421* [MessageDefinition](messagedefinition.html): The business version of the message definition 8422* [NamingSystem](namingsystem.html): The business version of the naming system 8423* [OperationDefinition](operationdefinition.html): The business version of the operation definition 8424* [PlanDefinition](plandefinition.html): The business version of the plan definition 8425* [Questionnaire](questionnaire.html): The business version of the questionnaire 8426* [Requirements](requirements.html): The business version of the requirements 8427* [SearchParameter](searchparameter.html): The business version of the search parameter 8428* [StructureDefinition](structuredefinition.html): The business version of the structure definition 8429* [StructureMap](structuremap.html): The business version of the structure map 8430* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 8431* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 8432* [TestScript](testscript.html): The business version of the test script 8433* [ValueSet](valueset.html): The business version of the value set 8434</b><br> 8435 * Type: <b>token</b><br> 8436 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 8437 * </p> 8438 */ 8439 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 8440 8441// Manual code (from Configuration.txt): 8442public String toString() { 8443 return StructureMapUtilities.render(this); 8444 } 8445// end addition 8446 8447} 8448