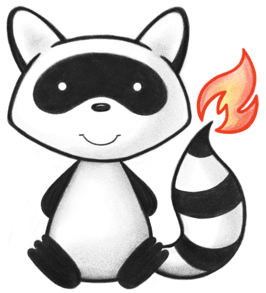
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The subscription resource describes a particular client's request to be notified about a SubscriptionTopic. 052 */ 053@ResourceDef(name="Subscription", profile="http://hl7.org/fhir/StructureDefinition/Subscription") 054public class Subscription extends DomainResource { 055 056 public enum SubscriptionPayloadContent { 057 /** 058 * No resource content is transacted in the notification payload. 059 */ 060 EMPTY, 061 /** 062 * Only the resource id is transacted in the notification payload. 063 */ 064 IDONLY, 065 /** 066 * The entire resource is transacted in the notification payload. 067 */ 068 FULLRESOURCE, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static SubscriptionPayloadContent fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("empty".equals(codeString)) 077 return EMPTY; 078 if ("id-only".equals(codeString)) 079 return IDONLY; 080 if ("full-resource".equals(codeString)) 081 return FULLRESOURCE; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown SubscriptionPayloadContent code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case EMPTY: return "empty"; 090 case IDONLY: return "id-only"; 091 case FULLRESOURCE: return "full-resource"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case EMPTY: return "http://hl7.org/fhir/subscription-payload-content"; 099 case IDONLY: return "http://hl7.org/fhir/subscription-payload-content"; 100 case FULLRESOURCE: return "http://hl7.org/fhir/subscription-payload-content"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case EMPTY: return "No resource content is transacted in the notification payload."; 108 case IDONLY: return "Only the resource id is transacted in the notification payload."; 109 case FULLRESOURCE: return "The entire resource is transacted in the notification payload."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case EMPTY: return "Empty"; 117 case IDONLY: return "Id-only"; 118 case FULLRESOURCE: return "Full-resource"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class SubscriptionPayloadContentEnumFactory implements EnumFactory<SubscriptionPayloadContent> { 126 public SubscriptionPayloadContent fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("empty".equals(codeString)) 131 return SubscriptionPayloadContent.EMPTY; 132 if ("id-only".equals(codeString)) 133 return SubscriptionPayloadContent.IDONLY; 134 if ("full-resource".equals(codeString)) 135 return SubscriptionPayloadContent.FULLRESOURCE; 136 throw new IllegalArgumentException("Unknown SubscriptionPayloadContent code '"+codeString+"'"); 137 } 138 public Enumeration<SubscriptionPayloadContent> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<SubscriptionPayloadContent>(this, SubscriptionPayloadContent.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<SubscriptionPayloadContent>(this, SubscriptionPayloadContent.NULL, code); 146 if ("empty".equals(codeString)) 147 return new Enumeration<SubscriptionPayloadContent>(this, SubscriptionPayloadContent.EMPTY, code); 148 if ("id-only".equals(codeString)) 149 return new Enumeration<SubscriptionPayloadContent>(this, SubscriptionPayloadContent.IDONLY, code); 150 if ("full-resource".equals(codeString)) 151 return new Enumeration<SubscriptionPayloadContent>(this, SubscriptionPayloadContent.FULLRESOURCE, code); 152 throw new FHIRException("Unknown SubscriptionPayloadContent code '"+codeString+"'"); 153 } 154 public String toCode(SubscriptionPayloadContent code) { 155 if (code == SubscriptionPayloadContent.NULL) 156 return null; 157 if (code == SubscriptionPayloadContent.EMPTY) 158 return "empty"; 159 if (code == SubscriptionPayloadContent.IDONLY) 160 return "id-only"; 161 if (code == SubscriptionPayloadContent.FULLRESOURCE) 162 return "full-resource"; 163 return "?"; 164 } 165 public String toSystem(SubscriptionPayloadContent code) { 166 return code.getSystem(); 167 } 168 } 169 170 @Block() 171 public static class SubscriptionFilterByComponent extends BackboneElement implements IBaseBackboneElement { 172 /** 173 * A resource listed in the `SubscriptionTopic` this `Subscription` references (`SubscriptionTopic.canFilterBy.resource`). This element can be used to differentiate filters for topics that include more than one resource type. 174 */ 175 @Child(name = "resourceType", type = {UriType.class}, order=1, min=0, max=1, modifier=false, summary=true) 176 @Description(shortDefinition="Allowed Resource (reference to definition) for this Subscription filter", formalDefinition="A resource listed in the `SubscriptionTopic` this `Subscription` references (`SubscriptionTopic.canFilterBy.resource`). This element can be used to differentiate filters for topics that include more than one resource type." ) 177 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscription-types") 178 protected UriType resourceType; 179 180 /** 181 * The filter as defined in the `SubscriptionTopic.canFilterBy.filterParameter` element. 182 */ 183 @Child(name = "filterParameter", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=true) 184 @Description(shortDefinition="Filter label defined in SubscriptionTopic", formalDefinition="The filter as defined in the `SubscriptionTopic.canFilterBy.filterParameter` element." ) 185 protected StringType filterParameter; 186 187 /** 188 * Comparator applied to this filter parameter. 189 */ 190 @Child(name = "comparator", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 191 @Description(shortDefinition="eq | ne | gt | lt | ge | le | sa | eb | ap", formalDefinition="Comparator applied to this filter parameter." ) 192 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-comparator") 193 protected Enumeration<SearchComparator> comparator; 194 195 /** 196 * Modifier applied to this filter parameter. 197 */ 198 @Child(name = "modifier", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=false) 199 @Description(shortDefinition="missing | exact | contains | not | text | in | not-in | below | above | type | identifier | of-type | code-text | text-advanced | iterate", formalDefinition="Modifier applied to this filter parameter." ) 200 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-modifier-code") 201 protected Enumeration<SearchModifierCode> modifier; 202 203 /** 204 * The literal value or resource path as is legal in search - for example, `Patient/123` or `le1950`. 205 */ 206 @Child(name = "value", type = {StringType.class}, order=5, min=1, max=1, modifier=false, summary=true) 207 @Description(shortDefinition="Literal value or resource path", formalDefinition="The literal value or resource path as is legal in search - for example, `Patient/123` or `le1950`." ) 208 protected StringType value; 209 210 private static final long serialVersionUID = 765605139L; 211 212 /** 213 * Constructor 214 */ 215 public SubscriptionFilterByComponent() { 216 super(); 217 } 218 219 /** 220 * Constructor 221 */ 222 public SubscriptionFilterByComponent(String filterParameter, String value) { 223 super(); 224 this.setFilterParameter(filterParameter); 225 this.setValue(value); 226 } 227 228 /** 229 * @return {@link #resourceType} (A resource listed in the `SubscriptionTopic` this `Subscription` references (`SubscriptionTopic.canFilterBy.resource`). This element can be used to differentiate filters for topics that include more than one resource type.). This is the underlying object with id, value and extensions. The accessor "getResourceType" gives direct access to the value 230 */ 231 public UriType getResourceTypeElement() { 232 if (this.resourceType == null) 233 if (Configuration.errorOnAutoCreate()) 234 throw new Error("Attempt to auto-create SubscriptionFilterByComponent.resourceType"); 235 else if (Configuration.doAutoCreate()) 236 this.resourceType = new UriType(); // bb 237 return this.resourceType; 238 } 239 240 public boolean hasResourceTypeElement() { 241 return this.resourceType != null && !this.resourceType.isEmpty(); 242 } 243 244 public boolean hasResourceType() { 245 return this.resourceType != null && !this.resourceType.isEmpty(); 246 } 247 248 /** 249 * @param value {@link #resourceType} (A resource listed in the `SubscriptionTopic` this `Subscription` references (`SubscriptionTopic.canFilterBy.resource`). This element can be used to differentiate filters for topics that include more than one resource type.). This is the underlying object with id, value and extensions. The accessor "getResourceType" gives direct access to the value 250 */ 251 public SubscriptionFilterByComponent setResourceTypeElement(UriType value) { 252 this.resourceType = value; 253 return this; 254 } 255 256 /** 257 * @return A resource listed in the `SubscriptionTopic` this `Subscription` references (`SubscriptionTopic.canFilterBy.resource`). This element can be used to differentiate filters for topics that include more than one resource type. 258 */ 259 public String getResourceType() { 260 return this.resourceType == null ? null : this.resourceType.getValue(); 261 } 262 263 /** 264 * @param value A resource listed in the `SubscriptionTopic` this `Subscription` references (`SubscriptionTopic.canFilterBy.resource`). This element can be used to differentiate filters for topics that include more than one resource type. 265 */ 266 public SubscriptionFilterByComponent setResourceType(String value) { 267 if (Utilities.noString(value)) 268 this.resourceType = null; 269 else { 270 if (this.resourceType == null) 271 this.resourceType = new UriType(); 272 this.resourceType.setValue(value); 273 } 274 return this; 275 } 276 277 /** 278 * @return {@link #filterParameter} (The filter as defined in the `SubscriptionTopic.canFilterBy.filterParameter` element.). This is the underlying object with id, value and extensions. The accessor "getFilterParameter" gives direct access to the value 279 */ 280 public StringType getFilterParameterElement() { 281 if (this.filterParameter == null) 282 if (Configuration.errorOnAutoCreate()) 283 throw new Error("Attempt to auto-create SubscriptionFilterByComponent.filterParameter"); 284 else if (Configuration.doAutoCreate()) 285 this.filterParameter = new StringType(); // bb 286 return this.filterParameter; 287 } 288 289 public boolean hasFilterParameterElement() { 290 return this.filterParameter != null && !this.filterParameter.isEmpty(); 291 } 292 293 public boolean hasFilterParameter() { 294 return this.filterParameter != null && !this.filterParameter.isEmpty(); 295 } 296 297 /** 298 * @param value {@link #filterParameter} (The filter as defined in the `SubscriptionTopic.canFilterBy.filterParameter` element.). This is the underlying object with id, value and extensions. The accessor "getFilterParameter" gives direct access to the value 299 */ 300 public SubscriptionFilterByComponent setFilterParameterElement(StringType value) { 301 this.filterParameter = value; 302 return this; 303 } 304 305 /** 306 * @return The filter as defined in the `SubscriptionTopic.canFilterBy.filterParameter` element. 307 */ 308 public String getFilterParameter() { 309 return this.filterParameter == null ? null : this.filterParameter.getValue(); 310 } 311 312 /** 313 * @param value The filter as defined in the `SubscriptionTopic.canFilterBy.filterParameter` element. 314 */ 315 public SubscriptionFilterByComponent setFilterParameter(String value) { 316 if (this.filterParameter == null) 317 this.filterParameter = new StringType(); 318 this.filterParameter.setValue(value); 319 return this; 320 } 321 322 /** 323 * @return {@link #comparator} (Comparator applied to this filter parameter.). This is the underlying object with id, value and extensions. The accessor "getComparator" gives direct access to the value 324 */ 325 public Enumeration<SearchComparator> getComparatorElement() { 326 if (this.comparator == null) 327 if (Configuration.errorOnAutoCreate()) 328 throw new Error("Attempt to auto-create SubscriptionFilterByComponent.comparator"); 329 else if (Configuration.doAutoCreate()) 330 this.comparator = new Enumeration<SearchComparator>(new SearchComparatorEnumFactory()); // bb 331 return this.comparator; 332 } 333 334 public boolean hasComparatorElement() { 335 return this.comparator != null && !this.comparator.isEmpty(); 336 } 337 338 public boolean hasComparator() { 339 return this.comparator != null && !this.comparator.isEmpty(); 340 } 341 342 /** 343 * @param value {@link #comparator} (Comparator applied to this filter parameter.). This is the underlying object with id, value and extensions. The accessor "getComparator" gives direct access to the value 344 */ 345 public SubscriptionFilterByComponent setComparatorElement(Enumeration<SearchComparator> value) { 346 this.comparator = value; 347 return this; 348 } 349 350 /** 351 * @return Comparator applied to this filter parameter. 352 */ 353 public SearchComparator getComparator() { 354 return this.comparator == null ? null : this.comparator.getValue(); 355 } 356 357 /** 358 * @param value Comparator applied to this filter parameter. 359 */ 360 public SubscriptionFilterByComponent setComparator(SearchComparator value) { 361 if (value == null) 362 this.comparator = null; 363 else { 364 if (this.comparator == null) 365 this.comparator = new Enumeration<SearchComparator>(new SearchComparatorEnumFactory()); 366 this.comparator.setValue(value); 367 } 368 return this; 369 } 370 371 /** 372 * @return {@link #modifier} (Modifier applied to this filter parameter.). This is the underlying object with id, value and extensions. The accessor "getModifier" gives direct access to the value 373 */ 374 public Enumeration<SearchModifierCode> getModifierElement() { 375 if (this.modifier == null) 376 if (Configuration.errorOnAutoCreate()) 377 throw new Error("Attempt to auto-create SubscriptionFilterByComponent.modifier"); 378 else if (Configuration.doAutoCreate()) 379 this.modifier = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); // bb 380 return this.modifier; 381 } 382 383 public boolean hasModifierElement() { 384 return this.modifier != null && !this.modifier.isEmpty(); 385 } 386 387 public boolean hasModifier() { 388 return this.modifier != null && !this.modifier.isEmpty(); 389 } 390 391 /** 392 * @param value {@link #modifier} (Modifier applied to this filter parameter.). This is the underlying object with id, value and extensions. The accessor "getModifier" gives direct access to the value 393 */ 394 public SubscriptionFilterByComponent setModifierElement(Enumeration<SearchModifierCode> value) { 395 this.modifier = value; 396 return this; 397 } 398 399 /** 400 * @return Modifier applied to this filter parameter. 401 */ 402 public SearchModifierCode getModifier() { 403 return this.modifier == null ? null : this.modifier.getValue(); 404 } 405 406 /** 407 * @param value Modifier applied to this filter parameter. 408 */ 409 public SubscriptionFilterByComponent setModifier(SearchModifierCode value) { 410 if (value == null) 411 this.modifier = null; 412 else { 413 if (this.modifier == null) 414 this.modifier = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 415 this.modifier.setValue(value); 416 } 417 return this; 418 } 419 420 /** 421 * @return {@link #value} (The literal value or resource path as is legal in search - for example, `Patient/123` or `le1950`.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 422 */ 423 public StringType getValueElement() { 424 if (this.value == null) 425 if (Configuration.errorOnAutoCreate()) 426 throw new Error("Attempt to auto-create SubscriptionFilterByComponent.value"); 427 else if (Configuration.doAutoCreate()) 428 this.value = new StringType(); // bb 429 return this.value; 430 } 431 432 public boolean hasValueElement() { 433 return this.value != null && !this.value.isEmpty(); 434 } 435 436 public boolean hasValue() { 437 return this.value != null && !this.value.isEmpty(); 438 } 439 440 /** 441 * @param value {@link #value} (The literal value or resource path as is legal in search - for example, `Patient/123` or `le1950`.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 442 */ 443 public SubscriptionFilterByComponent setValueElement(StringType value) { 444 this.value = value; 445 return this; 446 } 447 448 /** 449 * @return The literal value or resource path as is legal in search - for example, `Patient/123` or `le1950`. 450 */ 451 public String getValue() { 452 return this.value == null ? null : this.value.getValue(); 453 } 454 455 /** 456 * @param value The literal value or resource path as is legal in search - for example, `Patient/123` or `le1950`. 457 */ 458 public SubscriptionFilterByComponent setValue(String value) { 459 if (this.value == null) 460 this.value = new StringType(); 461 this.value.setValue(value); 462 return this; 463 } 464 465 protected void listChildren(List<Property> children) { 466 super.listChildren(children); 467 children.add(new Property("resourceType", "uri", "A resource listed in the `SubscriptionTopic` this `Subscription` references (`SubscriptionTopic.canFilterBy.resource`). This element can be used to differentiate filters for topics that include more than one resource type.", 0, 1, resourceType)); 468 children.add(new Property("filterParameter", "string", "The filter as defined in the `SubscriptionTopic.canFilterBy.filterParameter` element.", 0, 1, filterParameter)); 469 children.add(new Property("comparator", "code", "Comparator applied to this filter parameter.", 0, 1, comparator)); 470 children.add(new Property("modifier", "code", "Modifier applied to this filter parameter.", 0, 1, modifier)); 471 children.add(new Property("value", "string", "The literal value or resource path as is legal in search - for example, `Patient/123` or `le1950`.", 0, 1, value)); 472 } 473 474 @Override 475 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 476 switch (_hash) { 477 case -384364440: /*resourceType*/ return new Property("resourceType", "uri", "A resource listed in the `SubscriptionTopic` this `Subscription` references (`SubscriptionTopic.canFilterBy.resource`). This element can be used to differentiate filters for topics that include more than one resource type.", 0, 1, resourceType); 478 case 618257: /*filterParameter*/ return new Property("filterParameter", "string", "The filter as defined in the `SubscriptionTopic.canFilterBy.filterParameter` element.", 0, 1, filterParameter); 479 case -844673834: /*comparator*/ return new Property("comparator", "code", "Comparator applied to this filter parameter.", 0, 1, comparator); 480 case -615513385: /*modifier*/ return new Property("modifier", "code", "Modifier applied to this filter parameter.", 0, 1, modifier); 481 case 111972721: /*value*/ return new Property("value", "string", "The literal value or resource path as is legal in search - for example, `Patient/123` or `le1950`.", 0, 1, value); 482 default: return super.getNamedProperty(_hash, _name, _checkValid); 483 } 484 485 } 486 487 @Override 488 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 489 switch (hash) { 490 case -384364440: /*resourceType*/ return this.resourceType == null ? new Base[0] : new Base[] {this.resourceType}; // UriType 491 case 618257: /*filterParameter*/ return this.filterParameter == null ? new Base[0] : new Base[] {this.filterParameter}; // StringType 492 case -844673834: /*comparator*/ return this.comparator == null ? new Base[0] : new Base[] {this.comparator}; // Enumeration<SearchComparator> 493 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : new Base[] {this.modifier}; // Enumeration<SearchModifierCode> 494 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 495 default: return super.getProperty(hash, name, checkValid); 496 } 497 498 } 499 500 @Override 501 public Base setProperty(int hash, String name, Base value) throws FHIRException { 502 switch (hash) { 503 case -384364440: // resourceType 504 this.resourceType = TypeConvertor.castToUri(value); // UriType 505 return value; 506 case 618257: // filterParameter 507 this.filterParameter = TypeConvertor.castToString(value); // StringType 508 return value; 509 case -844673834: // comparator 510 value = new SearchComparatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 511 this.comparator = (Enumeration) value; // Enumeration<SearchComparator> 512 return value; 513 case -615513385: // modifier 514 value = new SearchModifierCodeEnumFactory().fromType(TypeConvertor.castToCode(value)); 515 this.modifier = (Enumeration) value; // Enumeration<SearchModifierCode> 516 return value; 517 case 111972721: // value 518 this.value = TypeConvertor.castToString(value); // StringType 519 return value; 520 default: return super.setProperty(hash, name, value); 521 } 522 523 } 524 525 @Override 526 public Base setProperty(String name, Base value) throws FHIRException { 527 if (name.equals("resourceType")) { 528 this.resourceType = TypeConvertor.castToUri(value); // UriType 529 } else if (name.equals("filterParameter")) { 530 this.filterParameter = TypeConvertor.castToString(value); // StringType 531 } else if (name.equals("comparator")) { 532 value = new SearchComparatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 533 this.comparator = (Enumeration) value; // Enumeration<SearchComparator> 534 } else if (name.equals("modifier")) { 535 value = new SearchModifierCodeEnumFactory().fromType(TypeConvertor.castToCode(value)); 536 this.modifier = (Enumeration) value; // Enumeration<SearchModifierCode> 537 } else if (name.equals("value")) { 538 this.value = TypeConvertor.castToString(value); // StringType 539 } else 540 return super.setProperty(name, value); 541 return value; 542 } 543 544 @Override 545 public void removeChild(String name, Base value) throws FHIRException { 546 if (name.equals("resourceType")) { 547 this.resourceType = null; 548 } else if (name.equals("filterParameter")) { 549 this.filterParameter = null; 550 } else if (name.equals("comparator")) { 551 value = new SearchComparatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 552 this.comparator = (Enumeration) value; // Enumeration<SearchComparator> 553 } else if (name.equals("modifier")) { 554 value = new SearchModifierCodeEnumFactory().fromType(TypeConvertor.castToCode(value)); 555 this.modifier = (Enumeration) value; // Enumeration<SearchModifierCode> 556 } else if (name.equals("value")) { 557 this.value = null; 558 } else 559 super.removeChild(name, value); 560 561 } 562 563 @Override 564 public Base makeProperty(int hash, String name) throws FHIRException { 565 switch (hash) { 566 case -384364440: return getResourceTypeElement(); 567 case 618257: return getFilterParameterElement(); 568 case -844673834: return getComparatorElement(); 569 case -615513385: return getModifierElement(); 570 case 111972721: return getValueElement(); 571 default: return super.makeProperty(hash, name); 572 } 573 574 } 575 576 @Override 577 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 578 switch (hash) { 579 case -384364440: /*resourceType*/ return new String[] {"uri"}; 580 case 618257: /*filterParameter*/ return new String[] {"string"}; 581 case -844673834: /*comparator*/ return new String[] {"code"}; 582 case -615513385: /*modifier*/ return new String[] {"code"}; 583 case 111972721: /*value*/ return new String[] {"string"}; 584 default: return super.getTypesForProperty(hash, name); 585 } 586 587 } 588 589 @Override 590 public Base addChild(String name) throws FHIRException { 591 if (name.equals("resourceType")) { 592 throw new FHIRException("Cannot call addChild on a singleton property Subscription.filterBy.resourceType"); 593 } 594 else if (name.equals("filterParameter")) { 595 throw new FHIRException("Cannot call addChild on a singleton property Subscription.filterBy.filterParameter"); 596 } 597 else if (name.equals("comparator")) { 598 throw new FHIRException("Cannot call addChild on a singleton property Subscription.filterBy.comparator"); 599 } 600 else if (name.equals("modifier")) { 601 throw new FHIRException("Cannot call addChild on a singleton property Subscription.filterBy.modifier"); 602 } 603 else if (name.equals("value")) { 604 throw new FHIRException("Cannot call addChild on a singleton property Subscription.filterBy.value"); 605 } 606 else 607 return super.addChild(name); 608 } 609 610 public SubscriptionFilterByComponent copy() { 611 SubscriptionFilterByComponent dst = new SubscriptionFilterByComponent(); 612 copyValues(dst); 613 return dst; 614 } 615 616 public void copyValues(SubscriptionFilterByComponent dst) { 617 super.copyValues(dst); 618 dst.resourceType = resourceType == null ? null : resourceType.copy(); 619 dst.filterParameter = filterParameter == null ? null : filterParameter.copy(); 620 dst.comparator = comparator == null ? null : comparator.copy(); 621 dst.modifier = modifier == null ? null : modifier.copy(); 622 dst.value = value == null ? null : value.copy(); 623 } 624 625 @Override 626 public boolean equalsDeep(Base other_) { 627 if (!super.equalsDeep(other_)) 628 return false; 629 if (!(other_ instanceof SubscriptionFilterByComponent)) 630 return false; 631 SubscriptionFilterByComponent o = (SubscriptionFilterByComponent) other_; 632 return compareDeep(resourceType, o.resourceType, true) && compareDeep(filterParameter, o.filterParameter, true) 633 && compareDeep(comparator, o.comparator, true) && compareDeep(modifier, o.modifier, true) && compareDeep(value, o.value, true) 634 ; 635 } 636 637 @Override 638 public boolean equalsShallow(Base other_) { 639 if (!super.equalsShallow(other_)) 640 return false; 641 if (!(other_ instanceof SubscriptionFilterByComponent)) 642 return false; 643 SubscriptionFilterByComponent o = (SubscriptionFilterByComponent) other_; 644 return compareValues(resourceType, o.resourceType, true) && compareValues(filterParameter, o.filterParameter, true) 645 && compareValues(comparator, o.comparator, true) && compareValues(modifier, o.modifier, true) && compareValues(value, o.value, true) 646 ; 647 } 648 649 public boolean isEmpty() { 650 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(resourceType, filterParameter 651 , comparator, modifier, value); 652 } 653 654 public String fhirType() { 655 return "Subscription.filterBy"; 656 657 } 658 659 } 660 661 @Block() 662 public static class SubscriptionParameterComponent extends BackboneElement implements IBaseBackboneElement { 663 /** 664 * Parameter name for information passed to the channel for notifications, for example in the case of a REST hook wanting to pass through an authorization header, the name would be Authorization. 665 */ 666 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 667 @Description(shortDefinition="Name (key) of the parameter", formalDefinition="Parameter name for information passed to the channel for notifications, for example in the case of a REST hook wanting to pass through an authorization header, the name would be Authorization." ) 668 protected StringType name; 669 670 /** 671 * Parameter value for information passed to the channel for notifications, for example in the case of a REST hook wanting to pass through an authorization header, the value would be `Bearer 0193...`. 672 */ 673 @Child(name = "value", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 674 @Description(shortDefinition="Value of the parameter to use or pass through", formalDefinition="Parameter value for information passed to the channel for notifications, for example in the case of a REST hook wanting to pass through an authorization header, the value would be `Bearer 0193...`." ) 675 protected StringType value; 676 677 private static final long serialVersionUID = 395259392L; 678 679 /** 680 * Constructor 681 */ 682 public SubscriptionParameterComponent() { 683 super(); 684 } 685 686 /** 687 * Constructor 688 */ 689 public SubscriptionParameterComponent(String name, String value) { 690 super(); 691 this.setName(name); 692 this.setValue(value); 693 } 694 695 /** 696 * @return {@link #name} (Parameter name for information passed to the channel for notifications, for example in the case of a REST hook wanting to pass through an authorization header, the name would be Authorization.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 697 */ 698 public StringType getNameElement() { 699 if (this.name == null) 700 if (Configuration.errorOnAutoCreate()) 701 throw new Error("Attempt to auto-create SubscriptionParameterComponent.name"); 702 else if (Configuration.doAutoCreate()) 703 this.name = new StringType(); // bb 704 return this.name; 705 } 706 707 public boolean hasNameElement() { 708 return this.name != null && !this.name.isEmpty(); 709 } 710 711 public boolean hasName() { 712 return this.name != null && !this.name.isEmpty(); 713 } 714 715 /** 716 * @param value {@link #name} (Parameter name for information passed to the channel for notifications, for example in the case of a REST hook wanting to pass through an authorization header, the name would be Authorization.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 717 */ 718 public SubscriptionParameterComponent setNameElement(StringType value) { 719 this.name = value; 720 return this; 721 } 722 723 /** 724 * @return Parameter name for information passed to the channel for notifications, for example in the case of a REST hook wanting to pass through an authorization header, the name would be Authorization. 725 */ 726 public String getName() { 727 return this.name == null ? null : this.name.getValue(); 728 } 729 730 /** 731 * @param value Parameter name for information passed to the channel for notifications, for example in the case of a REST hook wanting to pass through an authorization header, the name would be Authorization. 732 */ 733 public SubscriptionParameterComponent setName(String value) { 734 if (this.name == null) 735 this.name = new StringType(); 736 this.name.setValue(value); 737 return this; 738 } 739 740 /** 741 * @return {@link #value} (Parameter value for information passed to the channel for notifications, for example in the case of a REST hook wanting to pass through an authorization header, the value would be `Bearer 0193...`.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 742 */ 743 public StringType getValueElement() { 744 if (this.value == null) 745 if (Configuration.errorOnAutoCreate()) 746 throw new Error("Attempt to auto-create SubscriptionParameterComponent.value"); 747 else if (Configuration.doAutoCreate()) 748 this.value = new StringType(); // bb 749 return this.value; 750 } 751 752 public boolean hasValueElement() { 753 return this.value != null && !this.value.isEmpty(); 754 } 755 756 public boolean hasValue() { 757 return this.value != null && !this.value.isEmpty(); 758 } 759 760 /** 761 * @param value {@link #value} (Parameter value for information passed to the channel for notifications, for example in the case of a REST hook wanting to pass through an authorization header, the value would be `Bearer 0193...`.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 762 */ 763 public SubscriptionParameterComponent setValueElement(StringType value) { 764 this.value = value; 765 return this; 766 } 767 768 /** 769 * @return Parameter value for information passed to the channel for notifications, for example in the case of a REST hook wanting to pass through an authorization header, the value would be `Bearer 0193...`. 770 */ 771 public String getValue() { 772 return this.value == null ? null : this.value.getValue(); 773 } 774 775 /** 776 * @param value Parameter value for information passed to the channel for notifications, for example in the case of a REST hook wanting to pass through an authorization header, the value would be `Bearer 0193...`. 777 */ 778 public SubscriptionParameterComponent setValue(String value) { 779 if (this.value == null) 780 this.value = new StringType(); 781 this.value.setValue(value); 782 return this; 783 } 784 785 protected void listChildren(List<Property> children) { 786 super.listChildren(children); 787 children.add(new Property("name", "string", "Parameter name for information passed to the channel for notifications, for example in the case of a REST hook wanting to pass through an authorization header, the name would be Authorization.", 0, 1, name)); 788 children.add(new Property("value", "string", "Parameter value for information passed to the channel for notifications, for example in the case of a REST hook wanting to pass through an authorization header, the value would be `Bearer 0193...`.", 0, 1, value)); 789 } 790 791 @Override 792 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 793 switch (_hash) { 794 case 3373707: /*name*/ return new Property("name", "string", "Parameter name for information passed to the channel for notifications, for example in the case of a REST hook wanting to pass through an authorization header, the name would be Authorization.", 0, 1, name); 795 case 111972721: /*value*/ return new Property("value", "string", "Parameter value for information passed to the channel for notifications, for example in the case of a REST hook wanting to pass through an authorization header, the value would be `Bearer 0193...`.", 0, 1, value); 796 default: return super.getNamedProperty(_hash, _name, _checkValid); 797 } 798 799 } 800 801 @Override 802 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 803 switch (hash) { 804 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 805 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 806 default: return super.getProperty(hash, name, checkValid); 807 } 808 809 } 810 811 @Override 812 public Base setProperty(int hash, String name, Base value) throws FHIRException { 813 switch (hash) { 814 case 3373707: // name 815 this.name = TypeConvertor.castToString(value); // StringType 816 return value; 817 case 111972721: // value 818 this.value = TypeConvertor.castToString(value); // StringType 819 return value; 820 default: return super.setProperty(hash, name, value); 821 } 822 823 } 824 825 @Override 826 public Base setProperty(String name, Base value) throws FHIRException { 827 if (name.equals("name")) { 828 this.name = TypeConvertor.castToString(value); // StringType 829 } else if (name.equals("value")) { 830 this.value = TypeConvertor.castToString(value); // StringType 831 } else 832 return super.setProperty(name, value); 833 return value; 834 } 835 836 @Override 837 public void removeChild(String name, Base value) throws FHIRException { 838 if (name.equals("name")) { 839 this.name = null; 840 } else if (name.equals("value")) { 841 this.value = null; 842 } else 843 super.removeChild(name, value); 844 845 } 846 847 @Override 848 public Base makeProperty(int hash, String name) throws FHIRException { 849 switch (hash) { 850 case 3373707: return getNameElement(); 851 case 111972721: return getValueElement(); 852 default: return super.makeProperty(hash, name); 853 } 854 855 } 856 857 @Override 858 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 859 switch (hash) { 860 case 3373707: /*name*/ return new String[] {"string"}; 861 case 111972721: /*value*/ return new String[] {"string"}; 862 default: return super.getTypesForProperty(hash, name); 863 } 864 865 } 866 867 @Override 868 public Base addChild(String name) throws FHIRException { 869 if (name.equals("name")) { 870 throw new FHIRException("Cannot call addChild on a singleton property Subscription.parameter.name"); 871 } 872 else if (name.equals("value")) { 873 throw new FHIRException("Cannot call addChild on a singleton property Subscription.parameter.value"); 874 } 875 else 876 return super.addChild(name); 877 } 878 879 public SubscriptionParameterComponent copy() { 880 SubscriptionParameterComponent dst = new SubscriptionParameterComponent(); 881 copyValues(dst); 882 return dst; 883 } 884 885 public void copyValues(SubscriptionParameterComponent dst) { 886 super.copyValues(dst); 887 dst.name = name == null ? null : name.copy(); 888 dst.value = value == null ? null : value.copy(); 889 } 890 891 @Override 892 public boolean equalsDeep(Base other_) { 893 if (!super.equalsDeep(other_)) 894 return false; 895 if (!(other_ instanceof SubscriptionParameterComponent)) 896 return false; 897 SubscriptionParameterComponent o = (SubscriptionParameterComponent) other_; 898 return compareDeep(name, o.name, true) && compareDeep(value, o.value, true); 899 } 900 901 @Override 902 public boolean equalsShallow(Base other_) { 903 if (!super.equalsShallow(other_)) 904 return false; 905 if (!(other_ instanceof SubscriptionParameterComponent)) 906 return false; 907 SubscriptionParameterComponent o = (SubscriptionParameterComponent) other_; 908 return compareValues(name, o.name, true) && compareValues(value, o.value, true); 909 } 910 911 public boolean isEmpty() { 912 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, value); 913 } 914 915 public String fhirType() { 916 return "Subscription.parameter"; 917 918 } 919 920 } 921 922 /** 923 * A formal identifier that is used to identify this code system when it is represented in other formats, or referenced in a specification, model, design or an instance. 924 */ 925 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 926 @Description(shortDefinition="Additional identifiers (business identifier)", formalDefinition="A formal identifier that is used to identify this code system when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 927 protected List<Identifier> identifier; 928 929 /** 930 * A natural language name identifying the subscription. 931 */ 932 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 933 @Description(shortDefinition="Human readable name for this subscription", formalDefinition="A natural language name identifying the subscription." ) 934 protected StringType name; 935 936 /** 937 * The status of the subscription, which marks the server state for managing the subscription. 938 */ 939 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 940 @Description(shortDefinition="requested | active | error | off | entered-in-error", formalDefinition="The status of the subscription, which marks the server state for managing the subscription." ) 941 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscription-status") 942 protected Enumeration<SubscriptionStatusCodes> status; 943 944 /** 945 * The reference to the subscription topic to be notified about. 946 */ 947 @Child(name = "topic", type = {CanonicalType.class}, order=3, min=1, max=1, modifier=false, summary=true) 948 @Description(shortDefinition="Reference to the subscription topic being subscribed to", formalDefinition="The reference to the subscription topic to be notified about." ) 949 protected CanonicalType topic; 950 951 /** 952 * Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting. 953 */ 954 @Child(name = "contact", type = {ContactPoint.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 955 @Description(shortDefinition="Contact details for source (e.g. troubleshooting)", formalDefinition="Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting." ) 956 protected List<ContactPoint> contact; 957 958 /** 959 * The time for the server to turn the subscription off. 960 */ 961 @Child(name = "end", type = {InstantType.class}, order=5, min=0, max=1, modifier=false, summary=true) 962 @Description(shortDefinition="When to automatically delete the subscription", formalDefinition="The time for the server to turn the subscription off." ) 963 protected InstantType end; 964 965 /** 966 * Entity with authorization to make subsequent revisions to the Subscription and also determines what data the subscription is authorized to disclose. 967 */ 968 @Child(name = "managingEntity", type = {CareTeam.class, HealthcareService.class, Organization.class, RelatedPerson.class, Patient.class, Practitioner.class, PractitionerRole.class}, order=6, min=0, max=1, modifier=false, summary=true) 969 @Description(shortDefinition="Entity responsible for Subscription changes", formalDefinition="Entity with authorization to make subsequent revisions to the Subscription and also determines what data the subscription is authorized to disclose." ) 970 protected Reference managingEntity; 971 972 /** 973 * A description of why this subscription is defined. 974 */ 975 @Child(name = "reason", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=true) 976 @Description(shortDefinition="Description of why this subscription was created", formalDefinition="A description of why this subscription is defined." ) 977 protected StringType reason; 978 979 /** 980 * The filter properties to be applied to narrow the subscription topic stream. When multiple filters are applied, evaluates to true if all the conditions applicable to that resource are met; otherwise it returns false (i.e., logical AND). 981 */ 982 @Child(name = "filterBy", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 983 @Description(shortDefinition="Criteria for narrowing the subscription topic stream", formalDefinition="The filter properties to be applied to narrow the subscription topic stream. When multiple filters are applied, evaluates to true if all the conditions applicable to that resource are met; otherwise it returns false (i.e., logical AND)." ) 984 protected List<SubscriptionFilterByComponent> filterBy; 985 986 /** 987 * The type of channel to send notifications on. 988 */ 989 @Child(name = "channelType", type = {Coding.class}, order=9, min=1, max=1, modifier=false, summary=true) 990 @Description(shortDefinition="Channel type for notifications", formalDefinition="The type of channel to send notifications on." ) 991 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscription-channel-type") 992 protected Coding channelType; 993 994 /** 995 * The url that describes the actual end-point to send notifications to. 996 */ 997 @Child(name = "endpoint", type = {UrlType.class}, order=10, min=0, max=1, modifier=false, summary=true) 998 @Description(shortDefinition="Where the channel points to", formalDefinition="The url that describes the actual end-point to send notifications to." ) 999 protected UrlType endpoint; 1000 1001 /** 1002 * Channel-dependent information to send as part of the notification (e.g., HTTP Headers). 1003 */ 1004 @Child(name = "parameter", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1005 @Description(shortDefinition="Channel type", formalDefinition="Channel-dependent information to send as part of the notification (e.g., HTTP Headers)." ) 1006 protected List<SubscriptionParameterComponent> parameter; 1007 1008 /** 1009 * If present, a 'heartbeat' notification (keep-alive) is sent via this channel with an interval period equal to this elements integer value in seconds. If not present, a heartbeat notification is not sent. 1010 */ 1011 @Child(name = "heartbeatPeriod", type = {UnsignedIntType.class}, order=12, min=0, max=1, modifier=false, summary=true) 1012 @Description(shortDefinition="Interval in seconds to send 'heartbeat' notification", formalDefinition="If present, a 'heartbeat' notification (keep-alive) is sent via this channel with an interval period equal to this elements integer value in seconds. If not present, a heartbeat notification is not sent." ) 1013 protected UnsignedIntType heartbeatPeriod; 1014 1015 /** 1016 * If present, the maximum amount of time a server will allow before failing a notification attempt. 1017 */ 1018 @Child(name = "timeout", type = {UnsignedIntType.class}, order=13, min=0, max=1, modifier=false, summary=true) 1019 @Description(shortDefinition="Timeout in seconds to attempt notification delivery", formalDefinition="If present, the maximum amount of time a server will allow before failing a notification attempt." ) 1020 protected UnsignedIntType timeout; 1021 1022 /** 1023 * The MIME type to send the payload in - e.g., `application/fhir+xml` or `application/fhir+json`. Note that: 1024 1025* clients may request notifications in a specific FHIR version by using the [FHIR Version Parameter](http.html#version-parameter) - e.g., `application/fhir+json; fhirVersion=4.0`. 1026 1027* additional MIME types can be allowed by channels - e.g., `text/plain` and `text/html` are defined by the Email channel. 1028 */ 1029 @Child(name = "contentType", type = {CodeType.class}, order=14, min=0, max=1, modifier=false, summary=true) 1030 @Description(shortDefinition="MIME type to send, or omit for no payload", formalDefinition="The MIME type to send the payload in - e.g., `application/fhir+xml` or `application/fhir+json`. Note that:\n\n* clients may request notifications in a specific FHIR version by using the [FHIR Version Parameter](http.html#version-parameter) - e.g., `application/fhir+json; fhirVersion=4.0`.\n\n* additional MIME types can be allowed by channels - e.g., `text/plain` and `text/html` are defined by the Email channel." ) 1031 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/mimetypes") 1032 protected CodeType contentType; 1033 1034 /** 1035 * How much of the resource content to deliver in the notification payload. The choices are an empty payload, only the resource id, or the full resource content. 1036 */ 1037 @Child(name = "content", type = {CodeType.class}, order=15, min=0, max=1, modifier=false, summary=true) 1038 @Description(shortDefinition="empty | id-only | full-resource", formalDefinition="How much of the resource content to deliver in the notification payload. The choices are an empty payload, only the resource id, or the full resource content." ) 1039 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscription-payload-content") 1040 protected Enumeration<SubscriptionPayloadContent> content; 1041 1042 /** 1043 * If present, the maximum number of events that will be included in a notification bundle. Note that this is not a strict limit on the number of entries in a bundle, as dependent resources can be included. 1044 */ 1045 @Child(name = "maxCount", type = {PositiveIntType.class}, order=16, min=0, max=1, modifier=false, summary=true) 1046 @Description(shortDefinition="Maximum number of events that can be combined in a single notification", formalDefinition="If present, the maximum number of events that will be included in a notification bundle. Note that this is not a strict limit on the number of entries in a bundle, as dependent resources can be included." ) 1047 protected PositiveIntType maxCount; 1048 1049 private static final long serialVersionUID = 1396960420L; 1050 1051 /** 1052 * Constructor 1053 */ 1054 public Subscription() { 1055 super(); 1056 } 1057 1058 /** 1059 * Constructor 1060 */ 1061 public Subscription(SubscriptionStatusCodes status, String topic, Coding channelType) { 1062 super(); 1063 this.setStatus(status); 1064 this.setTopic(topic); 1065 this.setChannelType(channelType); 1066 } 1067 1068 /** 1069 * @return {@link #identifier} (A formal identifier that is used to identify this code system when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1070 */ 1071 public List<Identifier> getIdentifier() { 1072 if (this.identifier == null) 1073 this.identifier = new ArrayList<Identifier>(); 1074 return this.identifier; 1075 } 1076 1077 /** 1078 * @return Returns a reference to <code>this</code> for easy method chaining 1079 */ 1080 public Subscription setIdentifier(List<Identifier> theIdentifier) { 1081 this.identifier = theIdentifier; 1082 return this; 1083 } 1084 1085 public boolean hasIdentifier() { 1086 if (this.identifier == null) 1087 return false; 1088 for (Identifier item : this.identifier) 1089 if (!item.isEmpty()) 1090 return true; 1091 return false; 1092 } 1093 1094 public Identifier addIdentifier() { //3 1095 Identifier t = new Identifier(); 1096 if (this.identifier == null) 1097 this.identifier = new ArrayList<Identifier>(); 1098 this.identifier.add(t); 1099 return t; 1100 } 1101 1102 public Subscription addIdentifier(Identifier t) { //3 1103 if (t == null) 1104 return this; 1105 if (this.identifier == null) 1106 this.identifier = new ArrayList<Identifier>(); 1107 this.identifier.add(t); 1108 return this; 1109 } 1110 1111 /** 1112 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1113 */ 1114 public Identifier getIdentifierFirstRep() { 1115 if (getIdentifier().isEmpty()) { 1116 addIdentifier(); 1117 } 1118 return getIdentifier().get(0); 1119 } 1120 1121 /** 1122 * @return {@link #name} (A natural language name identifying the subscription.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1123 */ 1124 public StringType getNameElement() { 1125 if (this.name == null) 1126 if (Configuration.errorOnAutoCreate()) 1127 throw new Error("Attempt to auto-create Subscription.name"); 1128 else if (Configuration.doAutoCreate()) 1129 this.name = new StringType(); // bb 1130 return this.name; 1131 } 1132 1133 public boolean hasNameElement() { 1134 return this.name != null && !this.name.isEmpty(); 1135 } 1136 1137 public boolean hasName() { 1138 return this.name != null && !this.name.isEmpty(); 1139 } 1140 1141 /** 1142 * @param value {@link #name} (A natural language name identifying the subscription.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1143 */ 1144 public Subscription setNameElement(StringType value) { 1145 this.name = value; 1146 return this; 1147 } 1148 1149 /** 1150 * @return A natural language name identifying the subscription. 1151 */ 1152 public String getName() { 1153 return this.name == null ? null : this.name.getValue(); 1154 } 1155 1156 /** 1157 * @param value A natural language name identifying the subscription. 1158 */ 1159 public Subscription setName(String value) { 1160 if (Utilities.noString(value)) 1161 this.name = null; 1162 else { 1163 if (this.name == null) 1164 this.name = new StringType(); 1165 this.name.setValue(value); 1166 } 1167 return this; 1168 } 1169 1170 /** 1171 * @return {@link #status} (The status of the subscription, which marks the server state for managing the subscription.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1172 */ 1173 public Enumeration<SubscriptionStatusCodes> getStatusElement() { 1174 if (this.status == null) 1175 if (Configuration.errorOnAutoCreate()) 1176 throw new Error("Attempt to auto-create Subscription.status"); 1177 else if (Configuration.doAutoCreate()) 1178 this.status = new Enumeration<SubscriptionStatusCodes>(new SubscriptionStatusCodesEnumFactory()); // bb 1179 return this.status; 1180 } 1181 1182 public boolean hasStatusElement() { 1183 return this.status != null && !this.status.isEmpty(); 1184 } 1185 1186 public boolean hasStatus() { 1187 return this.status != null && !this.status.isEmpty(); 1188 } 1189 1190 /** 1191 * @param value {@link #status} (The status of the subscription, which marks the server state for managing the subscription.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1192 */ 1193 public Subscription setStatusElement(Enumeration<SubscriptionStatusCodes> value) { 1194 this.status = value; 1195 return this; 1196 } 1197 1198 /** 1199 * @return The status of the subscription, which marks the server state for managing the subscription. 1200 */ 1201 public SubscriptionStatusCodes getStatus() { 1202 return this.status == null ? null : this.status.getValue(); 1203 } 1204 1205 /** 1206 * @param value The status of the subscription, which marks the server state for managing the subscription. 1207 */ 1208 public Subscription setStatus(SubscriptionStatusCodes value) { 1209 if (this.status == null) 1210 this.status = new Enumeration<SubscriptionStatusCodes>(new SubscriptionStatusCodesEnumFactory()); 1211 this.status.setValue(value); 1212 return this; 1213 } 1214 1215 /** 1216 * @return {@link #topic} (The reference to the subscription topic to be notified about.). This is the underlying object with id, value and extensions. The accessor "getTopic" gives direct access to the value 1217 */ 1218 public CanonicalType getTopicElement() { 1219 if (this.topic == null) 1220 if (Configuration.errorOnAutoCreate()) 1221 throw new Error("Attempt to auto-create Subscription.topic"); 1222 else if (Configuration.doAutoCreate()) 1223 this.topic = new CanonicalType(); // bb 1224 return this.topic; 1225 } 1226 1227 public boolean hasTopicElement() { 1228 return this.topic != null && !this.topic.isEmpty(); 1229 } 1230 1231 public boolean hasTopic() { 1232 return this.topic != null && !this.topic.isEmpty(); 1233 } 1234 1235 /** 1236 * @param value {@link #topic} (The reference to the subscription topic to be notified about.). This is the underlying object with id, value and extensions. The accessor "getTopic" gives direct access to the value 1237 */ 1238 public Subscription setTopicElement(CanonicalType value) { 1239 this.topic = value; 1240 return this; 1241 } 1242 1243 /** 1244 * @return The reference to the subscription topic to be notified about. 1245 */ 1246 public String getTopic() { 1247 return this.topic == null ? null : this.topic.getValue(); 1248 } 1249 1250 /** 1251 * @param value The reference to the subscription topic to be notified about. 1252 */ 1253 public Subscription setTopic(String value) { 1254 if (this.topic == null) 1255 this.topic = new CanonicalType(); 1256 this.topic.setValue(value); 1257 return this; 1258 } 1259 1260 /** 1261 * @return {@link #contact} (Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting.) 1262 */ 1263 public List<ContactPoint> getContact() { 1264 if (this.contact == null) 1265 this.contact = new ArrayList<ContactPoint>(); 1266 return this.contact; 1267 } 1268 1269 /** 1270 * @return Returns a reference to <code>this</code> for easy method chaining 1271 */ 1272 public Subscription setContact(List<ContactPoint> theContact) { 1273 this.contact = theContact; 1274 return this; 1275 } 1276 1277 public boolean hasContact() { 1278 if (this.contact == null) 1279 return false; 1280 for (ContactPoint item : this.contact) 1281 if (!item.isEmpty()) 1282 return true; 1283 return false; 1284 } 1285 1286 public ContactPoint addContact() { //3 1287 ContactPoint t = new ContactPoint(); 1288 if (this.contact == null) 1289 this.contact = new ArrayList<ContactPoint>(); 1290 this.contact.add(t); 1291 return t; 1292 } 1293 1294 public Subscription addContact(ContactPoint t) { //3 1295 if (t == null) 1296 return this; 1297 if (this.contact == null) 1298 this.contact = new ArrayList<ContactPoint>(); 1299 this.contact.add(t); 1300 return this; 1301 } 1302 1303 /** 1304 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 1305 */ 1306 public ContactPoint getContactFirstRep() { 1307 if (getContact().isEmpty()) { 1308 addContact(); 1309 } 1310 return getContact().get(0); 1311 } 1312 1313 /** 1314 * @return {@link #end} (The time for the server to turn the subscription off.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 1315 */ 1316 public InstantType getEndElement() { 1317 if (this.end == null) 1318 if (Configuration.errorOnAutoCreate()) 1319 throw new Error("Attempt to auto-create Subscription.end"); 1320 else if (Configuration.doAutoCreate()) 1321 this.end = new InstantType(); // bb 1322 return this.end; 1323 } 1324 1325 public boolean hasEndElement() { 1326 return this.end != null && !this.end.isEmpty(); 1327 } 1328 1329 public boolean hasEnd() { 1330 return this.end != null && !this.end.isEmpty(); 1331 } 1332 1333 /** 1334 * @param value {@link #end} (The time for the server to turn the subscription off.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 1335 */ 1336 public Subscription setEndElement(InstantType value) { 1337 this.end = value; 1338 return this; 1339 } 1340 1341 /** 1342 * @return The time for the server to turn the subscription off. 1343 */ 1344 public Date getEnd() { 1345 return this.end == null ? null : this.end.getValue(); 1346 } 1347 1348 /** 1349 * @param value The time for the server to turn the subscription off. 1350 */ 1351 public Subscription setEnd(Date value) { 1352 if (value == null) 1353 this.end = null; 1354 else { 1355 if (this.end == null) 1356 this.end = new InstantType(); 1357 this.end.setValue(value); 1358 } 1359 return this; 1360 } 1361 1362 /** 1363 * @return {@link #managingEntity} (Entity with authorization to make subsequent revisions to the Subscription and also determines what data the subscription is authorized to disclose.) 1364 */ 1365 public Reference getManagingEntity() { 1366 if (this.managingEntity == null) 1367 if (Configuration.errorOnAutoCreate()) 1368 throw new Error("Attempt to auto-create Subscription.managingEntity"); 1369 else if (Configuration.doAutoCreate()) 1370 this.managingEntity = new Reference(); // cc 1371 return this.managingEntity; 1372 } 1373 1374 public boolean hasManagingEntity() { 1375 return this.managingEntity != null && !this.managingEntity.isEmpty(); 1376 } 1377 1378 /** 1379 * @param value {@link #managingEntity} (Entity with authorization to make subsequent revisions to the Subscription and also determines what data the subscription is authorized to disclose.) 1380 */ 1381 public Subscription setManagingEntity(Reference value) { 1382 this.managingEntity = value; 1383 return this; 1384 } 1385 1386 /** 1387 * @return {@link #reason} (A description of why this subscription is defined.). This is the underlying object with id, value and extensions. The accessor "getReason" gives direct access to the value 1388 */ 1389 public StringType getReasonElement() { 1390 if (this.reason == null) 1391 if (Configuration.errorOnAutoCreate()) 1392 throw new Error("Attempt to auto-create Subscription.reason"); 1393 else if (Configuration.doAutoCreate()) 1394 this.reason = new StringType(); // bb 1395 return this.reason; 1396 } 1397 1398 public boolean hasReasonElement() { 1399 return this.reason != null && !this.reason.isEmpty(); 1400 } 1401 1402 public boolean hasReason() { 1403 return this.reason != null && !this.reason.isEmpty(); 1404 } 1405 1406 /** 1407 * @param value {@link #reason} (A description of why this subscription is defined.). This is the underlying object with id, value and extensions. The accessor "getReason" gives direct access to the value 1408 */ 1409 public Subscription setReasonElement(StringType value) { 1410 this.reason = value; 1411 return this; 1412 } 1413 1414 /** 1415 * @return A description of why this subscription is defined. 1416 */ 1417 public String getReason() { 1418 return this.reason == null ? null : this.reason.getValue(); 1419 } 1420 1421 /** 1422 * @param value A description of why this subscription is defined. 1423 */ 1424 public Subscription setReason(String value) { 1425 if (Utilities.noString(value)) 1426 this.reason = null; 1427 else { 1428 if (this.reason == null) 1429 this.reason = new StringType(); 1430 this.reason.setValue(value); 1431 } 1432 return this; 1433 } 1434 1435 /** 1436 * @return {@link #filterBy} (The filter properties to be applied to narrow the subscription topic stream. When multiple filters are applied, evaluates to true if all the conditions applicable to that resource are met; otherwise it returns false (i.e., logical AND).) 1437 */ 1438 public List<SubscriptionFilterByComponent> getFilterBy() { 1439 if (this.filterBy == null) 1440 this.filterBy = new ArrayList<SubscriptionFilterByComponent>(); 1441 return this.filterBy; 1442 } 1443 1444 /** 1445 * @return Returns a reference to <code>this</code> for easy method chaining 1446 */ 1447 public Subscription setFilterBy(List<SubscriptionFilterByComponent> theFilterBy) { 1448 this.filterBy = theFilterBy; 1449 return this; 1450 } 1451 1452 public boolean hasFilterBy() { 1453 if (this.filterBy == null) 1454 return false; 1455 for (SubscriptionFilterByComponent item : this.filterBy) 1456 if (!item.isEmpty()) 1457 return true; 1458 return false; 1459 } 1460 1461 public SubscriptionFilterByComponent addFilterBy() { //3 1462 SubscriptionFilterByComponent t = new SubscriptionFilterByComponent(); 1463 if (this.filterBy == null) 1464 this.filterBy = new ArrayList<SubscriptionFilterByComponent>(); 1465 this.filterBy.add(t); 1466 return t; 1467 } 1468 1469 public Subscription addFilterBy(SubscriptionFilterByComponent t) { //3 1470 if (t == null) 1471 return this; 1472 if (this.filterBy == null) 1473 this.filterBy = new ArrayList<SubscriptionFilterByComponent>(); 1474 this.filterBy.add(t); 1475 return this; 1476 } 1477 1478 /** 1479 * @return The first repetition of repeating field {@link #filterBy}, creating it if it does not already exist {3} 1480 */ 1481 public SubscriptionFilterByComponent getFilterByFirstRep() { 1482 if (getFilterBy().isEmpty()) { 1483 addFilterBy(); 1484 } 1485 return getFilterBy().get(0); 1486 } 1487 1488 /** 1489 * @return {@link #channelType} (The type of channel to send notifications on.) 1490 */ 1491 public Coding getChannelType() { 1492 if (this.channelType == null) 1493 if (Configuration.errorOnAutoCreate()) 1494 throw new Error("Attempt to auto-create Subscription.channelType"); 1495 else if (Configuration.doAutoCreate()) 1496 this.channelType = new Coding(); // cc 1497 return this.channelType; 1498 } 1499 1500 public boolean hasChannelType() { 1501 return this.channelType != null && !this.channelType.isEmpty(); 1502 } 1503 1504 /** 1505 * @param value {@link #channelType} (The type of channel to send notifications on.) 1506 */ 1507 public Subscription setChannelType(Coding value) { 1508 this.channelType = value; 1509 return this; 1510 } 1511 1512 /** 1513 * @return {@link #endpoint} (The url that describes the actual end-point to send notifications to.). This is the underlying object with id, value and extensions. The accessor "getEndpoint" gives direct access to the value 1514 */ 1515 public UrlType getEndpointElement() { 1516 if (this.endpoint == null) 1517 if (Configuration.errorOnAutoCreate()) 1518 throw new Error("Attempt to auto-create Subscription.endpoint"); 1519 else if (Configuration.doAutoCreate()) 1520 this.endpoint = new UrlType(); // bb 1521 return this.endpoint; 1522 } 1523 1524 public boolean hasEndpointElement() { 1525 return this.endpoint != null && !this.endpoint.isEmpty(); 1526 } 1527 1528 public boolean hasEndpoint() { 1529 return this.endpoint != null && !this.endpoint.isEmpty(); 1530 } 1531 1532 /** 1533 * @param value {@link #endpoint} (The url that describes the actual end-point to send notifications to.). This is the underlying object with id, value and extensions. The accessor "getEndpoint" gives direct access to the value 1534 */ 1535 public Subscription setEndpointElement(UrlType value) { 1536 this.endpoint = value; 1537 return this; 1538 } 1539 1540 /** 1541 * @return The url that describes the actual end-point to send notifications to. 1542 */ 1543 public String getEndpoint() { 1544 return this.endpoint == null ? null : this.endpoint.getValue(); 1545 } 1546 1547 /** 1548 * @param value The url that describes the actual end-point to send notifications to. 1549 */ 1550 public Subscription setEndpoint(String value) { 1551 if (Utilities.noString(value)) 1552 this.endpoint = null; 1553 else { 1554 if (this.endpoint == null) 1555 this.endpoint = new UrlType(); 1556 this.endpoint.setValue(value); 1557 } 1558 return this; 1559 } 1560 1561 /** 1562 * @return {@link #parameter} (Channel-dependent information to send as part of the notification (e.g., HTTP Headers).) 1563 */ 1564 public List<SubscriptionParameterComponent> getParameter() { 1565 if (this.parameter == null) 1566 this.parameter = new ArrayList<SubscriptionParameterComponent>(); 1567 return this.parameter; 1568 } 1569 1570 /** 1571 * @return Returns a reference to <code>this</code> for easy method chaining 1572 */ 1573 public Subscription setParameter(List<SubscriptionParameterComponent> theParameter) { 1574 this.parameter = theParameter; 1575 return this; 1576 } 1577 1578 public boolean hasParameter() { 1579 if (this.parameter == null) 1580 return false; 1581 for (SubscriptionParameterComponent item : this.parameter) 1582 if (!item.isEmpty()) 1583 return true; 1584 return false; 1585 } 1586 1587 public SubscriptionParameterComponent addParameter() { //3 1588 SubscriptionParameterComponent t = new SubscriptionParameterComponent(); 1589 if (this.parameter == null) 1590 this.parameter = new ArrayList<SubscriptionParameterComponent>(); 1591 this.parameter.add(t); 1592 return t; 1593 } 1594 1595 public Subscription addParameter(SubscriptionParameterComponent t) { //3 1596 if (t == null) 1597 return this; 1598 if (this.parameter == null) 1599 this.parameter = new ArrayList<SubscriptionParameterComponent>(); 1600 this.parameter.add(t); 1601 return this; 1602 } 1603 1604 /** 1605 * @return The first repetition of repeating field {@link #parameter}, creating it if it does not already exist {3} 1606 */ 1607 public SubscriptionParameterComponent getParameterFirstRep() { 1608 if (getParameter().isEmpty()) { 1609 addParameter(); 1610 } 1611 return getParameter().get(0); 1612 } 1613 1614 /** 1615 * @return {@link #heartbeatPeriod} (If present, a 'heartbeat' notification (keep-alive) is sent via this channel with an interval period equal to this elements integer value in seconds. If not present, a heartbeat notification is not sent.). This is the underlying object with id, value and extensions. The accessor "getHeartbeatPeriod" gives direct access to the value 1616 */ 1617 public UnsignedIntType getHeartbeatPeriodElement() { 1618 if (this.heartbeatPeriod == null) 1619 if (Configuration.errorOnAutoCreate()) 1620 throw new Error("Attempt to auto-create Subscription.heartbeatPeriod"); 1621 else if (Configuration.doAutoCreate()) 1622 this.heartbeatPeriod = new UnsignedIntType(); // bb 1623 return this.heartbeatPeriod; 1624 } 1625 1626 public boolean hasHeartbeatPeriodElement() { 1627 return this.heartbeatPeriod != null && !this.heartbeatPeriod.isEmpty(); 1628 } 1629 1630 public boolean hasHeartbeatPeriod() { 1631 return this.heartbeatPeriod != null && !this.heartbeatPeriod.isEmpty(); 1632 } 1633 1634 /** 1635 * @param value {@link #heartbeatPeriod} (If present, a 'heartbeat' notification (keep-alive) is sent via this channel with an interval period equal to this elements integer value in seconds. If not present, a heartbeat notification is not sent.). This is the underlying object with id, value and extensions. The accessor "getHeartbeatPeriod" gives direct access to the value 1636 */ 1637 public Subscription setHeartbeatPeriodElement(UnsignedIntType value) { 1638 this.heartbeatPeriod = value; 1639 return this; 1640 } 1641 1642 /** 1643 * @return If present, a 'heartbeat' notification (keep-alive) is sent via this channel with an interval period equal to this elements integer value in seconds. If not present, a heartbeat notification is not sent. 1644 */ 1645 public int getHeartbeatPeriod() { 1646 return this.heartbeatPeriod == null || this.heartbeatPeriod.isEmpty() ? 0 : this.heartbeatPeriod.getValue(); 1647 } 1648 1649 /** 1650 * @param value If present, a 'heartbeat' notification (keep-alive) is sent via this channel with an interval period equal to this elements integer value in seconds. If not present, a heartbeat notification is not sent. 1651 */ 1652 public Subscription setHeartbeatPeriod(int value) { 1653 if (this.heartbeatPeriod == null) 1654 this.heartbeatPeriod = new UnsignedIntType(); 1655 this.heartbeatPeriod.setValue(value); 1656 return this; 1657 } 1658 1659 /** 1660 * @return {@link #timeout} (If present, the maximum amount of time a server will allow before failing a notification attempt.). This is the underlying object with id, value and extensions. The accessor "getTimeout" gives direct access to the value 1661 */ 1662 public UnsignedIntType getTimeoutElement() { 1663 if (this.timeout == null) 1664 if (Configuration.errorOnAutoCreate()) 1665 throw new Error("Attempt to auto-create Subscription.timeout"); 1666 else if (Configuration.doAutoCreate()) 1667 this.timeout = new UnsignedIntType(); // bb 1668 return this.timeout; 1669 } 1670 1671 public boolean hasTimeoutElement() { 1672 return this.timeout != null && !this.timeout.isEmpty(); 1673 } 1674 1675 public boolean hasTimeout() { 1676 return this.timeout != null && !this.timeout.isEmpty(); 1677 } 1678 1679 /** 1680 * @param value {@link #timeout} (If present, the maximum amount of time a server will allow before failing a notification attempt.). This is the underlying object with id, value and extensions. The accessor "getTimeout" gives direct access to the value 1681 */ 1682 public Subscription setTimeoutElement(UnsignedIntType value) { 1683 this.timeout = value; 1684 return this; 1685 } 1686 1687 /** 1688 * @return If present, the maximum amount of time a server will allow before failing a notification attempt. 1689 */ 1690 public int getTimeout() { 1691 return this.timeout == null || this.timeout.isEmpty() ? 0 : this.timeout.getValue(); 1692 } 1693 1694 /** 1695 * @param value If present, the maximum amount of time a server will allow before failing a notification attempt. 1696 */ 1697 public Subscription setTimeout(int value) { 1698 if (this.timeout == null) 1699 this.timeout = new UnsignedIntType(); 1700 this.timeout.setValue(value); 1701 return this; 1702 } 1703 1704 /** 1705 * @return {@link #contentType} (The MIME type to send the payload in - e.g., `application/fhir+xml` or `application/fhir+json`. Note that: 1706 1707* clients may request notifications in a specific FHIR version by using the [FHIR Version Parameter](http.html#version-parameter) - e.g., `application/fhir+json; fhirVersion=4.0`. 1708 1709* additional MIME types can be allowed by channels - e.g., `text/plain` and `text/html` are defined by the Email channel.). This is the underlying object with id, value and extensions. The accessor "getContentType" gives direct access to the value 1710 */ 1711 public CodeType getContentTypeElement() { 1712 if (this.contentType == null) 1713 if (Configuration.errorOnAutoCreate()) 1714 throw new Error("Attempt to auto-create Subscription.contentType"); 1715 else if (Configuration.doAutoCreate()) 1716 this.contentType = new CodeType(); // bb 1717 return this.contentType; 1718 } 1719 1720 public boolean hasContentTypeElement() { 1721 return this.contentType != null && !this.contentType.isEmpty(); 1722 } 1723 1724 public boolean hasContentType() { 1725 return this.contentType != null && !this.contentType.isEmpty(); 1726 } 1727 1728 /** 1729 * @param value {@link #contentType} (The MIME type to send the payload in - e.g., `application/fhir+xml` or `application/fhir+json`. Note that: 1730 1731* clients may request notifications in a specific FHIR version by using the [FHIR Version Parameter](http.html#version-parameter) - e.g., `application/fhir+json; fhirVersion=4.0`. 1732 1733* additional MIME types can be allowed by channels - e.g., `text/plain` and `text/html` are defined by the Email channel.). This is the underlying object with id, value and extensions. The accessor "getContentType" gives direct access to the value 1734 */ 1735 public Subscription setContentTypeElement(CodeType value) { 1736 this.contentType = value; 1737 return this; 1738 } 1739 1740 /** 1741 * @return The MIME type to send the payload in - e.g., `application/fhir+xml` or `application/fhir+json`. Note that: 1742 1743* clients may request notifications in a specific FHIR version by using the [FHIR Version Parameter](http.html#version-parameter) - e.g., `application/fhir+json; fhirVersion=4.0`. 1744 1745* additional MIME types can be allowed by channels - e.g., `text/plain` and `text/html` are defined by the Email channel. 1746 */ 1747 public String getContentType() { 1748 return this.contentType == null ? null : this.contentType.getValue(); 1749 } 1750 1751 /** 1752 * @param value The MIME type to send the payload in - e.g., `application/fhir+xml` or `application/fhir+json`. Note that: 1753 1754* clients may request notifications in a specific FHIR version by using the [FHIR Version Parameter](http.html#version-parameter) - e.g., `application/fhir+json; fhirVersion=4.0`. 1755 1756* additional MIME types can be allowed by channels - e.g., `text/plain` and `text/html` are defined by the Email channel. 1757 */ 1758 public Subscription setContentType(String value) { 1759 if (Utilities.noString(value)) 1760 this.contentType = null; 1761 else { 1762 if (this.contentType == null) 1763 this.contentType = new CodeType(); 1764 this.contentType.setValue(value); 1765 } 1766 return this; 1767 } 1768 1769 /** 1770 * @return {@link #content} (How much of the resource content to deliver in the notification payload. The choices are an empty payload, only the resource id, or the full resource content.). This is the underlying object with id, value and extensions. The accessor "getContent" gives direct access to the value 1771 */ 1772 public Enumeration<SubscriptionPayloadContent> getContentElement() { 1773 if (this.content == null) 1774 if (Configuration.errorOnAutoCreate()) 1775 throw new Error("Attempt to auto-create Subscription.content"); 1776 else if (Configuration.doAutoCreate()) 1777 this.content = new Enumeration<SubscriptionPayloadContent>(new SubscriptionPayloadContentEnumFactory()); // bb 1778 return this.content; 1779 } 1780 1781 public boolean hasContentElement() { 1782 return this.content != null && !this.content.isEmpty(); 1783 } 1784 1785 public boolean hasContent() { 1786 return this.content != null && !this.content.isEmpty(); 1787 } 1788 1789 /** 1790 * @param value {@link #content} (How much of the resource content to deliver in the notification payload. The choices are an empty payload, only the resource id, or the full resource content.). This is the underlying object with id, value and extensions. The accessor "getContent" gives direct access to the value 1791 */ 1792 public Subscription setContentElement(Enumeration<SubscriptionPayloadContent> value) { 1793 this.content = value; 1794 return this; 1795 } 1796 1797 /** 1798 * @return How much of the resource content to deliver in the notification payload. The choices are an empty payload, only the resource id, or the full resource content. 1799 */ 1800 public SubscriptionPayloadContent getContent() { 1801 return this.content == null ? null : this.content.getValue(); 1802 } 1803 1804 /** 1805 * @param value How much of the resource content to deliver in the notification payload. The choices are an empty payload, only the resource id, or the full resource content. 1806 */ 1807 public Subscription setContent(SubscriptionPayloadContent value) { 1808 if (value == null) 1809 this.content = null; 1810 else { 1811 if (this.content == null) 1812 this.content = new Enumeration<SubscriptionPayloadContent>(new SubscriptionPayloadContentEnumFactory()); 1813 this.content.setValue(value); 1814 } 1815 return this; 1816 } 1817 1818 /** 1819 * @return {@link #maxCount} (If present, the maximum number of events that will be included in a notification bundle. Note that this is not a strict limit on the number of entries in a bundle, as dependent resources can be included.). This is the underlying object with id, value and extensions. The accessor "getMaxCount" gives direct access to the value 1820 */ 1821 public PositiveIntType getMaxCountElement() { 1822 if (this.maxCount == null) 1823 if (Configuration.errorOnAutoCreate()) 1824 throw new Error("Attempt to auto-create Subscription.maxCount"); 1825 else if (Configuration.doAutoCreate()) 1826 this.maxCount = new PositiveIntType(); // bb 1827 return this.maxCount; 1828 } 1829 1830 public boolean hasMaxCountElement() { 1831 return this.maxCount != null && !this.maxCount.isEmpty(); 1832 } 1833 1834 public boolean hasMaxCount() { 1835 return this.maxCount != null && !this.maxCount.isEmpty(); 1836 } 1837 1838 /** 1839 * @param value {@link #maxCount} (If present, the maximum number of events that will be included in a notification bundle. Note that this is not a strict limit on the number of entries in a bundle, as dependent resources can be included.). This is the underlying object with id, value and extensions. The accessor "getMaxCount" gives direct access to the value 1840 */ 1841 public Subscription setMaxCountElement(PositiveIntType value) { 1842 this.maxCount = value; 1843 return this; 1844 } 1845 1846 /** 1847 * @return If present, the maximum number of events that will be included in a notification bundle. Note that this is not a strict limit on the number of entries in a bundle, as dependent resources can be included. 1848 */ 1849 public int getMaxCount() { 1850 return this.maxCount == null || this.maxCount.isEmpty() ? 0 : this.maxCount.getValue(); 1851 } 1852 1853 /** 1854 * @param value If present, the maximum number of events that will be included in a notification bundle. Note that this is not a strict limit on the number of entries in a bundle, as dependent resources can be included. 1855 */ 1856 public Subscription setMaxCount(int value) { 1857 if (this.maxCount == null) 1858 this.maxCount = new PositiveIntType(); 1859 this.maxCount.setValue(value); 1860 return this; 1861 } 1862 1863 protected void listChildren(List<Property> children) { 1864 super.listChildren(children); 1865 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this code system when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1866 children.add(new Property("name", "string", "A natural language name identifying the subscription.", 0, 1, name)); 1867 children.add(new Property("status", "code", "The status of the subscription, which marks the server state for managing the subscription.", 0, 1, status)); 1868 children.add(new Property("topic", "canonical(SubscriptionTopic)", "The reference to the subscription topic to be notified about.", 0, 1, topic)); 1869 children.add(new Property("contact", "ContactPoint", "Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting.", 0, java.lang.Integer.MAX_VALUE, contact)); 1870 children.add(new Property("end", "instant", "The time for the server to turn the subscription off.", 0, 1, end)); 1871 children.add(new Property("managingEntity", "Reference(CareTeam|HealthcareService|Organization|RelatedPerson|Patient|Practitioner|PractitionerRole)", "Entity with authorization to make subsequent revisions to the Subscription and also determines what data the subscription is authorized to disclose.", 0, 1, managingEntity)); 1872 children.add(new Property("reason", "string", "A description of why this subscription is defined.", 0, 1, reason)); 1873 children.add(new Property("filterBy", "", "The filter properties to be applied to narrow the subscription topic stream. When multiple filters are applied, evaluates to true if all the conditions applicable to that resource are met; otherwise it returns false (i.e., logical AND).", 0, java.lang.Integer.MAX_VALUE, filterBy)); 1874 children.add(new Property("channelType", "Coding", "The type of channel to send notifications on.", 0, 1, channelType)); 1875 children.add(new Property("endpoint", "url", "The url that describes the actual end-point to send notifications to.", 0, 1, endpoint)); 1876 children.add(new Property("parameter", "", "Channel-dependent information to send as part of the notification (e.g., HTTP Headers).", 0, java.lang.Integer.MAX_VALUE, parameter)); 1877 children.add(new Property("heartbeatPeriod", "unsignedInt", "If present, a 'heartbeat' notification (keep-alive) is sent via this channel with an interval period equal to this elements integer value in seconds. If not present, a heartbeat notification is not sent.", 0, 1, heartbeatPeriod)); 1878 children.add(new Property("timeout", "unsignedInt", "If present, the maximum amount of time a server will allow before failing a notification attempt.", 0, 1, timeout)); 1879 children.add(new Property("contentType", "code", "The MIME type to send the payload in - e.g., `application/fhir+xml` or `application/fhir+json`. Note that:\n\n* clients may request notifications in a specific FHIR version by using the [FHIR Version Parameter](http.html#version-parameter) - e.g., `application/fhir+json; fhirVersion=4.0`.\n\n* additional MIME types can be allowed by channels - e.g., `text/plain` and `text/html` are defined by the Email channel.", 0, 1, contentType)); 1880 children.add(new Property("content", "code", "How much of the resource content to deliver in the notification payload. The choices are an empty payload, only the resource id, or the full resource content.", 0, 1, content)); 1881 children.add(new Property("maxCount", "positiveInt", "If present, the maximum number of events that will be included in a notification bundle. Note that this is not a strict limit on the number of entries in a bundle, as dependent resources can be included.", 0, 1, maxCount)); 1882 } 1883 1884 @Override 1885 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1886 switch (_hash) { 1887 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this code system when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 1888 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the subscription.", 0, 1, name); 1889 case -892481550: /*status*/ return new Property("status", "code", "The status of the subscription, which marks the server state for managing the subscription.", 0, 1, status); 1890 case 110546223: /*topic*/ return new Property("topic", "canonical(SubscriptionTopic)", "The reference to the subscription topic to be notified about.", 0, 1, topic); 1891 case 951526432: /*contact*/ return new Property("contact", "ContactPoint", "Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting.", 0, java.lang.Integer.MAX_VALUE, contact); 1892 case 100571: /*end*/ return new Property("end", "instant", "The time for the server to turn the subscription off.", 0, 1, end); 1893 case -988474523: /*managingEntity*/ return new Property("managingEntity", "Reference(CareTeam|HealthcareService|Organization|RelatedPerson|Patient|Practitioner|PractitionerRole)", "Entity with authorization to make subsequent revisions to the Subscription and also determines what data the subscription is authorized to disclose.", 0, 1, managingEntity); 1894 case -934964668: /*reason*/ return new Property("reason", "string", "A description of why this subscription is defined.", 0, 1, reason); 1895 case -721168913: /*filterBy*/ return new Property("filterBy", "", "The filter properties to be applied to narrow the subscription topic stream. When multiple filters are applied, evaluates to true if all the conditions applicable to that resource are met; otherwise it returns false (i.e., logical AND).", 0, java.lang.Integer.MAX_VALUE, filterBy); 1896 case 274155229: /*channelType*/ return new Property("channelType", "Coding", "The type of channel to send notifications on.", 0, 1, channelType); 1897 case 1741102485: /*endpoint*/ return new Property("endpoint", "url", "The url that describes the actual end-point to send notifications to.", 0, 1, endpoint); 1898 case 1954460585: /*parameter*/ return new Property("parameter", "", "Channel-dependent information to send as part of the notification (e.g., HTTP Headers).", 0, java.lang.Integer.MAX_VALUE, parameter); 1899 case -938465827: /*heartbeatPeriod*/ return new Property("heartbeatPeriod", "unsignedInt", "If present, a 'heartbeat' notification (keep-alive) is sent via this channel with an interval period equal to this elements integer value in seconds. If not present, a heartbeat notification is not sent.", 0, 1, heartbeatPeriod); 1900 case -1313911455: /*timeout*/ return new Property("timeout", "unsignedInt", "If present, the maximum amount of time a server will allow before failing a notification attempt.", 0, 1, timeout); 1901 case -389131437: /*contentType*/ return new Property("contentType", "code", "The MIME type to send the payload in - e.g., `application/fhir+xml` or `application/fhir+json`. Note that:\n\n* clients may request notifications in a specific FHIR version by using the [FHIR Version Parameter](http.html#version-parameter) - e.g., `application/fhir+json; fhirVersion=4.0`.\n\n* additional MIME types can be allowed by channels - e.g., `text/plain` and `text/html` are defined by the Email channel.", 0, 1, contentType); 1902 case 951530617: /*content*/ return new Property("content", "code", "How much of the resource content to deliver in the notification payload. The choices are an empty payload, only the resource id, or the full resource content.", 0, 1, content); 1903 case 382106123: /*maxCount*/ return new Property("maxCount", "positiveInt", "If present, the maximum number of events that will be included in a notification bundle. Note that this is not a strict limit on the number of entries in a bundle, as dependent resources can be included.", 0, 1, maxCount); 1904 default: return super.getNamedProperty(_hash, _name, _checkValid); 1905 } 1906 1907 } 1908 1909 @Override 1910 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1911 switch (hash) { 1912 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1913 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1914 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<SubscriptionStatusCodes> 1915 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : new Base[] {this.topic}; // CanonicalType 1916 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactPoint 1917 case 100571: /*end*/ return this.end == null ? new Base[0] : new Base[] {this.end}; // InstantType 1918 case -988474523: /*managingEntity*/ return this.managingEntity == null ? new Base[0] : new Base[] {this.managingEntity}; // Reference 1919 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // StringType 1920 case -721168913: /*filterBy*/ return this.filterBy == null ? new Base[0] : this.filterBy.toArray(new Base[this.filterBy.size()]); // SubscriptionFilterByComponent 1921 case 274155229: /*channelType*/ return this.channelType == null ? new Base[0] : new Base[] {this.channelType}; // Coding 1922 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : new Base[] {this.endpoint}; // UrlType 1923 case 1954460585: /*parameter*/ return this.parameter == null ? new Base[0] : this.parameter.toArray(new Base[this.parameter.size()]); // SubscriptionParameterComponent 1924 case -938465827: /*heartbeatPeriod*/ return this.heartbeatPeriod == null ? new Base[0] : new Base[] {this.heartbeatPeriod}; // UnsignedIntType 1925 case -1313911455: /*timeout*/ return this.timeout == null ? new Base[0] : new Base[] {this.timeout}; // UnsignedIntType 1926 case -389131437: /*contentType*/ return this.contentType == null ? new Base[0] : new Base[] {this.contentType}; // CodeType 1927 case 951530617: /*content*/ return this.content == null ? new Base[0] : new Base[] {this.content}; // Enumeration<SubscriptionPayloadContent> 1928 case 382106123: /*maxCount*/ return this.maxCount == null ? new Base[0] : new Base[] {this.maxCount}; // PositiveIntType 1929 default: return super.getProperty(hash, name, checkValid); 1930 } 1931 1932 } 1933 1934 @Override 1935 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1936 switch (hash) { 1937 case -1618432855: // identifier 1938 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1939 return value; 1940 case 3373707: // name 1941 this.name = TypeConvertor.castToString(value); // StringType 1942 return value; 1943 case -892481550: // status 1944 value = new SubscriptionStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1945 this.status = (Enumeration) value; // Enumeration<SubscriptionStatusCodes> 1946 return value; 1947 case 110546223: // topic 1948 this.topic = TypeConvertor.castToCanonical(value); // CanonicalType 1949 return value; 1950 case 951526432: // contact 1951 this.getContact().add(TypeConvertor.castToContactPoint(value)); // ContactPoint 1952 return value; 1953 case 100571: // end 1954 this.end = TypeConvertor.castToInstant(value); // InstantType 1955 return value; 1956 case -988474523: // managingEntity 1957 this.managingEntity = TypeConvertor.castToReference(value); // Reference 1958 return value; 1959 case -934964668: // reason 1960 this.reason = TypeConvertor.castToString(value); // StringType 1961 return value; 1962 case -721168913: // filterBy 1963 this.getFilterBy().add((SubscriptionFilterByComponent) value); // SubscriptionFilterByComponent 1964 return value; 1965 case 274155229: // channelType 1966 this.channelType = TypeConvertor.castToCoding(value); // Coding 1967 return value; 1968 case 1741102485: // endpoint 1969 this.endpoint = TypeConvertor.castToUrl(value); // UrlType 1970 return value; 1971 case 1954460585: // parameter 1972 this.getParameter().add((SubscriptionParameterComponent) value); // SubscriptionParameterComponent 1973 return value; 1974 case -938465827: // heartbeatPeriod 1975 this.heartbeatPeriod = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1976 return value; 1977 case -1313911455: // timeout 1978 this.timeout = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1979 return value; 1980 case -389131437: // contentType 1981 this.contentType = TypeConvertor.castToCode(value); // CodeType 1982 return value; 1983 case 951530617: // content 1984 value = new SubscriptionPayloadContentEnumFactory().fromType(TypeConvertor.castToCode(value)); 1985 this.content = (Enumeration) value; // Enumeration<SubscriptionPayloadContent> 1986 return value; 1987 case 382106123: // maxCount 1988 this.maxCount = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1989 return value; 1990 default: return super.setProperty(hash, name, value); 1991 } 1992 1993 } 1994 1995 @Override 1996 public Base setProperty(String name, Base value) throws FHIRException { 1997 if (name.equals("identifier")) { 1998 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1999 } else if (name.equals("name")) { 2000 this.name = TypeConvertor.castToString(value); // StringType 2001 } else if (name.equals("status")) { 2002 value = new SubscriptionStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2003 this.status = (Enumeration) value; // Enumeration<SubscriptionStatusCodes> 2004 } else if (name.equals("topic")) { 2005 this.topic = TypeConvertor.castToCanonical(value); // CanonicalType 2006 } else if (name.equals("contact")) { 2007 this.getContact().add(TypeConvertor.castToContactPoint(value)); 2008 } else if (name.equals("end")) { 2009 this.end = TypeConvertor.castToInstant(value); // InstantType 2010 } else if (name.equals("managingEntity")) { 2011 this.managingEntity = TypeConvertor.castToReference(value); // Reference 2012 } else if (name.equals("reason")) { 2013 this.reason = TypeConvertor.castToString(value); // StringType 2014 } else if (name.equals("filterBy")) { 2015 this.getFilterBy().add((SubscriptionFilterByComponent) value); 2016 } else if (name.equals("channelType")) { 2017 this.channelType = TypeConvertor.castToCoding(value); // Coding 2018 } else if (name.equals("endpoint")) { 2019 this.endpoint = TypeConvertor.castToUrl(value); // UrlType 2020 } else if (name.equals("parameter")) { 2021 this.getParameter().add((SubscriptionParameterComponent) value); 2022 } else if (name.equals("heartbeatPeriod")) { 2023 this.heartbeatPeriod = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 2024 } else if (name.equals("timeout")) { 2025 this.timeout = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 2026 } else if (name.equals("contentType")) { 2027 this.contentType = TypeConvertor.castToCode(value); // CodeType 2028 } else if (name.equals("content")) { 2029 value = new SubscriptionPayloadContentEnumFactory().fromType(TypeConvertor.castToCode(value)); 2030 this.content = (Enumeration) value; // Enumeration<SubscriptionPayloadContent> 2031 } else if (name.equals("maxCount")) { 2032 this.maxCount = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2033 } else 2034 return super.setProperty(name, value); 2035 return value; 2036 } 2037 2038 @Override 2039 public void removeChild(String name, Base value) throws FHIRException { 2040 if (name.equals("identifier")) { 2041 this.getIdentifier().remove(value); 2042 } else if (name.equals("name")) { 2043 this.name = null; 2044 } else if (name.equals("status")) { 2045 value = new SubscriptionStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2046 this.status = (Enumeration) value; // Enumeration<SubscriptionStatusCodes> 2047 } else if (name.equals("topic")) { 2048 this.topic = null; 2049 } else if (name.equals("contact")) { 2050 this.getContact().remove(value); 2051 } else if (name.equals("end")) { 2052 this.end = null; 2053 } else if (name.equals("managingEntity")) { 2054 this.managingEntity = null; 2055 } else if (name.equals("reason")) { 2056 this.reason = null; 2057 } else if (name.equals("filterBy")) { 2058 this.getFilterBy().remove((SubscriptionFilterByComponent) value); 2059 } else if (name.equals("channelType")) { 2060 this.channelType = null; 2061 } else if (name.equals("endpoint")) { 2062 this.endpoint = null; 2063 } else if (name.equals("parameter")) { 2064 this.getParameter().remove((SubscriptionParameterComponent) value); 2065 } else if (name.equals("heartbeatPeriod")) { 2066 this.heartbeatPeriod = null; 2067 } else if (name.equals("timeout")) { 2068 this.timeout = null; 2069 } else if (name.equals("contentType")) { 2070 this.contentType = null; 2071 } else if (name.equals("content")) { 2072 value = new SubscriptionPayloadContentEnumFactory().fromType(TypeConvertor.castToCode(value)); 2073 this.content = (Enumeration) value; // Enumeration<SubscriptionPayloadContent> 2074 } else if (name.equals("maxCount")) { 2075 this.maxCount = null; 2076 } else 2077 super.removeChild(name, value); 2078 2079 } 2080 2081 @Override 2082 public Base makeProperty(int hash, String name) throws FHIRException { 2083 switch (hash) { 2084 case -1618432855: return addIdentifier(); 2085 case 3373707: return getNameElement(); 2086 case -892481550: return getStatusElement(); 2087 case 110546223: return getTopicElement(); 2088 case 951526432: return addContact(); 2089 case 100571: return getEndElement(); 2090 case -988474523: return getManagingEntity(); 2091 case -934964668: return getReasonElement(); 2092 case -721168913: return addFilterBy(); 2093 case 274155229: return getChannelType(); 2094 case 1741102485: return getEndpointElement(); 2095 case 1954460585: return addParameter(); 2096 case -938465827: return getHeartbeatPeriodElement(); 2097 case -1313911455: return getTimeoutElement(); 2098 case -389131437: return getContentTypeElement(); 2099 case 951530617: return getContentElement(); 2100 case 382106123: return getMaxCountElement(); 2101 default: return super.makeProperty(hash, name); 2102 } 2103 2104 } 2105 2106 @Override 2107 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2108 switch (hash) { 2109 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2110 case 3373707: /*name*/ return new String[] {"string"}; 2111 case -892481550: /*status*/ return new String[] {"code"}; 2112 case 110546223: /*topic*/ return new String[] {"canonical"}; 2113 case 951526432: /*contact*/ return new String[] {"ContactPoint"}; 2114 case 100571: /*end*/ return new String[] {"instant"}; 2115 case -988474523: /*managingEntity*/ return new String[] {"Reference"}; 2116 case -934964668: /*reason*/ return new String[] {"string"}; 2117 case -721168913: /*filterBy*/ return new String[] {}; 2118 case 274155229: /*channelType*/ return new String[] {"Coding"}; 2119 case 1741102485: /*endpoint*/ return new String[] {"url"}; 2120 case 1954460585: /*parameter*/ return new String[] {}; 2121 case -938465827: /*heartbeatPeriod*/ return new String[] {"unsignedInt"}; 2122 case -1313911455: /*timeout*/ return new String[] {"unsignedInt"}; 2123 case -389131437: /*contentType*/ return new String[] {"code"}; 2124 case 951530617: /*content*/ return new String[] {"code"}; 2125 case 382106123: /*maxCount*/ return new String[] {"positiveInt"}; 2126 default: return super.getTypesForProperty(hash, name); 2127 } 2128 2129 } 2130 2131 @Override 2132 public Base addChild(String name) throws FHIRException { 2133 if (name.equals("identifier")) { 2134 return addIdentifier(); 2135 } 2136 else if (name.equals("name")) { 2137 throw new FHIRException("Cannot call addChild on a singleton property Subscription.name"); 2138 } 2139 else if (name.equals("status")) { 2140 throw new FHIRException("Cannot call addChild on a singleton property Subscription.status"); 2141 } 2142 else if (name.equals("topic")) { 2143 throw new FHIRException("Cannot call addChild on a singleton property Subscription.topic"); 2144 } 2145 else if (name.equals("contact")) { 2146 return addContact(); 2147 } 2148 else if (name.equals("end")) { 2149 throw new FHIRException("Cannot call addChild on a singleton property Subscription.end"); 2150 } 2151 else if (name.equals("managingEntity")) { 2152 this.managingEntity = new Reference(); 2153 return this.managingEntity; 2154 } 2155 else if (name.equals("reason")) { 2156 throw new FHIRException("Cannot call addChild on a singleton property Subscription.reason"); 2157 } 2158 else if (name.equals("filterBy")) { 2159 return addFilterBy(); 2160 } 2161 else if (name.equals("channelType")) { 2162 this.channelType = new Coding(); 2163 return this.channelType; 2164 } 2165 else if (name.equals("endpoint")) { 2166 throw new FHIRException("Cannot call addChild on a singleton property Subscription.endpoint"); 2167 } 2168 else if (name.equals("parameter")) { 2169 return addParameter(); 2170 } 2171 else if (name.equals("heartbeatPeriod")) { 2172 throw new FHIRException("Cannot call addChild on a singleton property Subscription.heartbeatPeriod"); 2173 } 2174 else if (name.equals("timeout")) { 2175 throw new FHIRException("Cannot call addChild on a singleton property Subscription.timeout"); 2176 } 2177 else if (name.equals("contentType")) { 2178 throw new FHIRException("Cannot call addChild on a singleton property Subscription.contentType"); 2179 } 2180 else if (name.equals("content")) { 2181 throw new FHIRException("Cannot call addChild on a singleton property Subscription.content"); 2182 } 2183 else if (name.equals("maxCount")) { 2184 throw new FHIRException("Cannot call addChild on a singleton property Subscription.maxCount"); 2185 } 2186 else 2187 return super.addChild(name); 2188 } 2189 2190 public String fhirType() { 2191 return "Subscription"; 2192 2193 } 2194 2195 public Subscription copy() { 2196 Subscription dst = new Subscription(); 2197 copyValues(dst); 2198 return dst; 2199 } 2200 2201 public void copyValues(Subscription dst) { 2202 super.copyValues(dst); 2203 if (identifier != null) { 2204 dst.identifier = new ArrayList<Identifier>(); 2205 for (Identifier i : identifier) 2206 dst.identifier.add(i.copy()); 2207 }; 2208 dst.name = name == null ? null : name.copy(); 2209 dst.status = status == null ? null : status.copy(); 2210 dst.topic = topic == null ? null : topic.copy(); 2211 if (contact != null) { 2212 dst.contact = new ArrayList<ContactPoint>(); 2213 for (ContactPoint i : contact) 2214 dst.contact.add(i.copy()); 2215 }; 2216 dst.end = end == null ? null : end.copy(); 2217 dst.managingEntity = managingEntity == null ? null : managingEntity.copy(); 2218 dst.reason = reason == null ? null : reason.copy(); 2219 if (filterBy != null) { 2220 dst.filterBy = new ArrayList<SubscriptionFilterByComponent>(); 2221 for (SubscriptionFilterByComponent i : filterBy) 2222 dst.filterBy.add(i.copy()); 2223 }; 2224 dst.channelType = channelType == null ? null : channelType.copy(); 2225 dst.endpoint = endpoint == null ? null : endpoint.copy(); 2226 if (parameter != null) { 2227 dst.parameter = new ArrayList<SubscriptionParameterComponent>(); 2228 for (SubscriptionParameterComponent i : parameter) 2229 dst.parameter.add(i.copy()); 2230 }; 2231 dst.heartbeatPeriod = heartbeatPeriod == null ? null : heartbeatPeriod.copy(); 2232 dst.timeout = timeout == null ? null : timeout.copy(); 2233 dst.contentType = contentType == null ? null : contentType.copy(); 2234 dst.content = content == null ? null : content.copy(); 2235 dst.maxCount = maxCount == null ? null : maxCount.copy(); 2236 } 2237 2238 protected Subscription typedCopy() { 2239 return copy(); 2240 } 2241 2242 @Override 2243 public boolean equalsDeep(Base other_) { 2244 if (!super.equalsDeep(other_)) 2245 return false; 2246 if (!(other_ instanceof Subscription)) 2247 return false; 2248 Subscription o = (Subscription) other_; 2249 return compareDeep(identifier, o.identifier, true) && compareDeep(name, o.name, true) && compareDeep(status, o.status, true) 2250 && compareDeep(topic, o.topic, true) && compareDeep(contact, o.contact, true) && compareDeep(end, o.end, true) 2251 && compareDeep(managingEntity, o.managingEntity, true) && compareDeep(reason, o.reason, true) && compareDeep(filterBy, o.filterBy, true) 2252 && compareDeep(channelType, o.channelType, true) && compareDeep(endpoint, o.endpoint, true) && compareDeep(parameter, o.parameter, true) 2253 && compareDeep(heartbeatPeriod, o.heartbeatPeriod, true) && compareDeep(timeout, o.timeout, true) 2254 && compareDeep(contentType, o.contentType, true) && compareDeep(content, o.content, true) && compareDeep(maxCount, o.maxCount, true) 2255 ; 2256 } 2257 2258 @Override 2259 public boolean equalsShallow(Base other_) { 2260 if (!super.equalsShallow(other_)) 2261 return false; 2262 if (!(other_ instanceof Subscription)) 2263 return false; 2264 Subscription o = (Subscription) other_; 2265 return compareValues(name, o.name, true) && compareValues(status, o.status, true) && compareValues(topic, o.topic, true) 2266 && compareValues(end, o.end, true) && compareValues(reason, o.reason, true) && compareValues(endpoint, o.endpoint, true) 2267 && compareValues(heartbeatPeriod, o.heartbeatPeriod, true) && compareValues(timeout, o.timeout, true) 2268 && compareValues(contentType, o.contentType, true) && compareValues(content, o.content, true) && compareValues(maxCount, o.maxCount, true) 2269 ; 2270 } 2271 2272 public boolean isEmpty() { 2273 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, name, status 2274 , topic, contact, end, managingEntity, reason, filterBy, channelType, endpoint 2275 , parameter, heartbeatPeriod, timeout, contentType, content, maxCount); 2276 } 2277 2278 @Override 2279 public ResourceType getResourceType() { 2280 return ResourceType.Subscription; 2281 } 2282 2283 /** 2284 * Search parameter: <b>contact</b> 2285 * <p> 2286 * Description: <b>Contact details for the subscription</b><br> 2287 * Type: <b>token</b><br> 2288 * Path: <b>Subscription.contact</b><br> 2289 * </p> 2290 */ 2291 @SearchParamDefinition(name="contact", path="Subscription.contact", description="Contact details for the subscription", type="token" ) 2292 public static final String SP_CONTACT = "contact"; 2293 /** 2294 * <b>Fluent Client</b> search parameter constant for <b>contact</b> 2295 * <p> 2296 * Description: <b>Contact details for the subscription</b><br> 2297 * Type: <b>token</b><br> 2298 * Path: <b>Subscription.contact</b><br> 2299 * </p> 2300 */ 2301 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTACT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTACT); 2302 2303 /** 2304 * Search parameter: <b>content-level</b> 2305 * <p> 2306 * Description: <b>Content level included in notifications</b><br> 2307 * Type: <b>token</b><br> 2308 * Path: <b>Subscription.content</b><br> 2309 * </p> 2310 */ 2311 @SearchParamDefinition(name="content-level", path="Subscription.content", description="Content level included in notifications", type="token" ) 2312 public static final String SP_CONTENT_LEVEL = "content-level"; 2313 /** 2314 * <b>Fluent Client</b> search parameter constant for <b>content-level</b> 2315 * <p> 2316 * Description: <b>Content level included in notifications</b><br> 2317 * Type: <b>token</b><br> 2318 * Path: <b>Subscription.content</b><br> 2319 * </p> 2320 */ 2321 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTENT_LEVEL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTENT_LEVEL); 2322 2323 /** 2324 * Search parameter: <b>filter-value</b> 2325 * <p> 2326 * Description: <b>Filter value used to narrow notifications</b><br> 2327 * Type: <b>string</b><br> 2328 * Path: <b>Subscription.filterBy.value</b><br> 2329 * </p> 2330 */ 2331 @SearchParamDefinition(name="filter-value", path="Subscription.filterBy.value", description="Filter value used to narrow notifications", type="string" ) 2332 public static final String SP_FILTER_VALUE = "filter-value"; 2333 /** 2334 * <b>Fluent Client</b> search parameter constant for <b>filter-value</b> 2335 * <p> 2336 * Description: <b>Filter value used to narrow notifications</b><br> 2337 * Type: <b>string</b><br> 2338 * Path: <b>Subscription.filterBy.value</b><br> 2339 * </p> 2340 */ 2341 public static final ca.uhn.fhir.rest.gclient.StringClientParam FILTER_VALUE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_FILTER_VALUE); 2342 2343 /** 2344 * Search parameter: <b>identifier</b> 2345 * <p> 2346 * Description: <b>A subscription identifier</b><br> 2347 * Type: <b>token</b><br> 2348 * Path: <b>Subscription.identifier</b><br> 2349 * </p> 2350 */ 2351 @SearchParamDefinition(name="identifier", path="Subscription.identifier", description="A subscription identifier", type="token" ) 2352 public static final String SP_IDENTIFIER = "identifier"; 2353 /** 2354 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2355 * <p> 2356 * Description: <b>A subscription identifier</b><br> 2357 * Type: <b>token</b><br> 2358 * Path: <b>Subscription.identifier</b><br> 2359 * </p> 2360 */ 2361 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2362 2363 /** 2364 * Search parameter: <b>name</b> 2365 * <p> 2366 * Description: <b>A human-readable name</b><br> 2367 * Type: <b>string</b><br> 2368 * Path: <b>Subscription.name</b><br> 2369 * </p> 2370 */ 2371 @SearchParamDefinition(name="name", path="Subscription.name", description="A human-readable name", type="string" ) 2372 public static final String SP_NAME = "name"; 2373 /** 2374 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2375 * <p> 2376 * Description: <b>A human-readable name</b><br> 2377 * Type: <b>string</b><br> 2378 * Path: <b>Subscription.name</b><br> 2379 * </p> 2380 */ 2381 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 2382 2383 /** 2384 * Search parameter: <b>owner</b> 2385 * <p> 2386 * Description: <b>The managing entity</b><br> 2387 * Type: <b>reference</b><br> 2388 * Path: <b>Subscription.managingEntity</b><br> 2389 * </p> 2390 */ 2391 @SearchParamDefinition(name="owner", path="Subscription.managingEntity", description="The managing entity", type="reference", target={CareTeam.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2392 public static final String SP_OWNER = "owner"; 2393 /** 2394 * <b>Fluent Client</b> search parameter constant for <b>owner</b> 2395 * <p> 2396 * Description: <b>The managing entity</b><br> 2397 * Type: <b>reference</b><br> 2398 * Path: <b>Subscription.managingEntity</b><br> 2399 * </p> 2400 */ 2401 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OWNER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_OWNER); 2402 2403/** 2404 * Constant for fluent queries to be used to add include statements. Specifies 2405 * the path value of "<b>Subscription:owner</b>". 2406 */ 2407 public static final ca.uhn.fhir.model.api.Include INCLUDE_OWNER = new ca.uhn.fhir.model.api.Include("Subscription:owner").toLocked(); 2408 2409 /** 2410 * Search parameter: <b>payload</b> 2411 * <p> 2412 * Description: <b>The mime-type of notifications</b><br> 2413 * Type: <b>token</b><br> 2414 * Path: <b>Subscription.contentType</b><br> 2415 * </p> 2416 */ 2417 @SearchParamDefinition(name="payload", path="Subscription.contentType", description="The mime-type of notifications", type="token" ) 2418 public static final String SP_PAYLOAD = "payload"; 2419 /** 2420 * <b>Fluent Client</b> search parameter constant for <b>payload</b> 2421 * <p> 2422 * Description: <b>The mime-type of notifications</b><br> 2423 * Type: <b>token</b><br> 2424 * Path: <b>Subscription.contentType</b><br> 2425 * </p> 2426 */ 2427 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PAYLOAD = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PAYLOAD); 2428 2429 /** 2430 * Search parameter: <b>status</b> 2431 * <p> 2432 * Description: <b>The current state of the subscription</b><br> 2433 * Type: <b>token</b><br> 2434 * Path: <b>Subscription.status</b><br> 2435 * </p> 2436 */ 2437 @SearchParamDefinition(name="status", path="Subscription.status", description="The current state of the subscription", type="token" ) 2438 public static final String SP_STATUS = "status"; 2439 /** 2440 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2441 * <p> 2442 * Description: <b>The current state of the subscription</b><br> 2443 * Type: <b>token</b><br> 2444 * Path: <b>Subscription.status</b><br> 2445 * </p> 2446 */ 2447 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2448 2449 /** 2450 * Search parameter: <b>topic</b> 2451 * <p> 2452 * Description: <b>The canonical topic url that triggers notifications</b><br> 2453 * Type: <b>uri</b><br> 2454 * Path: <b>Subscription.topic</b><br> 2455 * </p> 2456 */ 2457 @SearchParamDefinition(name="topic", path="Subscription.topic", description="The canonical topic url that triggers notifications", type="uri" ) 2458 public static final String SP_TOPIC = "topic"; 2459 /** 2460 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 2461 * <p> 2462 * Description: <b>The canonical topic url that triggers notifications</b><br> 2463 * Type: <b>uri</b><br> 2464 * Path: <b>Subscription.topic</b><br> 2465 * </p> 2466 */ 2467 public static final ca.uhn.fhir.rest.gclient.UriClientParam TOPIC = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_TOPIC); 2468 2469 /** 2470 * Search parameter: <b>type</b> 2471 * <p> 2472 * Description: <b>The type of channel for the sent notifications</b><br> 2473 * Type: <b>token</b><br> 2474 * Path: <b>Subscription.channelType</b><br> 2475 * </p> 2476 */ 2477 @SearchParamDefinition(name="type", path="Subscription.channelType", description="The type of channel for the sent notifications", type="token" ) 2478 public static final String SP_TYPE = "type"; 2479 /** 2480 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2481 * <p> 2482 * Description: <b>The type of channel for the sent notifications</b><br> 2483 * Type: <b>token</b><br> 2484 * Path: <b>Subscription.channelType</b><br> 2485 * </p> 2486 */ 2487 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 2488 2489 /** 2490 * Search parameter: <b>url</b> 2491 * <p> 2492 * Description: <b>The uri that will receive the notifications</b><br> 2493 * Type: <b>uri</b><br> 2494 * Path: <b>Subscription.endpoint</b><br> 2495 * </p> 2496 */ 2497 @SearchParamDefinition(name="url", path="Subscription.endpoint", description="The uri that will receive the notifications", type="uri" ) 2498 public static final String SP_URL = "url"; 2499 /** 2500 * <b>Fluent Client</b> search parameter constant for <b>url</b> 2501 * <p> 2502 * Description: <b>The uri that will receive the notifications</b><br> 2503 * Type: <b>uri</b><br> 2504 * Path: <b>Subscription.endpoint</b><br> 2505 * </p> 2506 */ 2507 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 2508 2509 2510} 2511