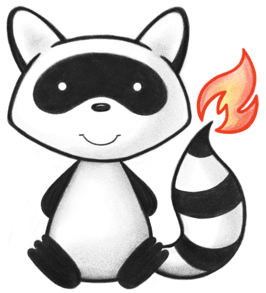
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The SubscriptionStatus resource describes the state of a Subscription during notifications. It is not persisted. 052 */ 053@ResourceDef(name="SubscriptionStatus", profile="http://hl7.org/fhir/StructureDefinition/SubscriptionStatus") 054public class SubscriptionStatus extends DomainResource { 055 056 public enum SubscriptionNotificationType { 057 /** 058 * The status was generated as part of the setup or verification of a communications channel. 059 */ 060 HANDSHAKE, 061 /** 062 * The status was generated to perform a heartbeat notification to the subscriber. 063 */ 064 HEARTBEAT, 065 /** 066 * The status was generated for an event to the subscriber. 067 */ 068 EVENTNOTIFICATION, 069 /** 070 * The status was generated in response to a status query/request. 071 */ 072 QUERYSTATUS, 073 /** 074 * The status was generated in response to an event query/request. 075 */ 076 QUERYEVENT, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 public static SubscriptionNotificationType fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("handshake".equals(codeString)) 085 return HANDSHAKE; 086 if ("heartbeat".equals(codeString)) 087 return HEARTBEAT; 088 if ("event-notification".equals(codeString)) 089 return EVENTNOTIFICATION; 090 if ("query-status".equals(codeString)) 091 return QUERYSTATUS; 092 if ("query-event".equals(codeString)) 093 return QUERYEVENT; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown SubscriptionNotificationType code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case HANDSHAKE: return "handshake"; 102 case HEARTBEAT: return "heartbeat"; 103 case EVENTNOTIFICATION: return "event-notification"; 104 case QUERYSTATUS: return "query-status"; 105 case QUERYEVENT: return "query-event"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getSystem() { 111 switch (this) { 112 case HANDSHAKE: return "http://hl7.org/fhir/subscription-notification-type"; 113 case HEARTBEAT: return "http://hl7.org/fhir/subscription-notification-type"; 114 case EVENTNOTIFICATION: return "http://hl7.org/fhir/subscription-notification-type"; 115 case QUERYSTATUS: return "http://hl7.org/fhir/subscription-notification-type"; 116 case QUERYEVENT: return "http://hl7.org/fhir/subscription-notification-type"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDefinition() { 122 switch (this) { 123 case HANDSHAKE: return "The status was generated as part of the setup or verification of a communications channel."; 124 case HEARTBEAT: return "The status was generated to perform a heartbeat notification to the subscriber."; 125 case EVENTNOTIFICATION: return "The status was generated for an event to the subscriber."; 126 case QUERYSTATUS: return "The status was generated in response to a status query/request."; 127 case QUERYEVENT: return "The status was generated in response to an event query/request."; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case HANDSHAKE: return "Handshake"; 135 case HEARTBEAT: return "Heartbeat"; 136 case EVENTNOTIFICATION: return "Event Notification"; 137 case QUERYSTATUS: return "Query Status"; 138 case QUERYEVENT: return "Query Event"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 } 144 145 public static class SubscriptionNotificationTypeEnumFactory implements EnumFactory<SubscriptionNotificationType> { 146 public SubscriptionNotificationType fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("handshake".equals(codeString)) 151 return SubscriptionNotificationType.HANDSHAKE; 152 if ("heartbeat".equals(codeString)) 153 return SubscriptionNotificationType.HEARTBEAT; 154 if ("event-notification".equals(codeString)) 155 return SubscriptionNotificationType.EVENTNOTIFICATION; 156 if ("query-status".equals(codeString)) 157 return SubscriptionNotificationType.QUERYSTATUS; 158 if ("query-event".equals(codeString)) 159 return SubscriptionNotificationType.QUERYEVENT; 160 throw new IllegalArgumentException("Unknown SubscriptionNotificationType code '"+codeString+"'"); 161 } 162 public Enumeration<SubscriptionNotificationType> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<SubscriptionNotificationType>(this, SubscriptionNotificationType.NULL, code); 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<SubscriptionNotificationType>(this, SubscriptionNotificationType.NULL, code); 170 if ("handshake".equals(codeString)) 171 return new Enumeration<SubscriptionNotificationType>(this, SubscriptionNotificationType.HANDSHAKE, code); 172 if ("heartbeat".equals(codeString)) 173 return new Enumeration<SubscriptionNotificationType>(this, SubscriptionNotificationType.HEARTBEAT, code); 174 if ("event-notification".equals(codeString)) 175 return new Enumeration<SubscriptionNotificationType>(this, SubscriptionNotificationType.EVENTNOTIFICATION, code); 176 if ("query-status".equals(codeString)) 177 return new Enumeration<SubscriptionNotificationType>(this, SubscriptionNotificationType.QUERYSTATUS, code); 178 if ("query-event".equals(codeString)) 179 return new Enumeration<SubscriptionNotificationType>(this, SubscriptionNotificationType.QUERYEVENT, code); 180 throw new FHIRException("Unknown SubscriptionNotificationType code '"+codeString+"'"); 181 } 182 public String toCode(SubscriptionNotificationType code) { 183 if (code == SubscriptionNotificationType.NULL) 184 return null; 185 if (code == SubscriptionNotificationType.HANDSHAKE) 186 return "handshake"; 187 if (code == SubscriptionNotificationType.HEARTBEAT) 188 return "heartbeat"; 189 if (code == SubscriptionNotificationType.EVENTNOTIFICATION) 190 return "event-notification"; 191 if (code == SubscriptionNotificationType.QUERYSTATUS) 192 return "query-status"; 193 if (code == SubscriptionNotificationType.QUERYEVENT) 194 return "query-event"; 195 return "?"; 196 } 197 public String toSystem(SubscriptionNotificationType code) { 198 return code.getSystem(); 199 } 200 } 201 202 @Block() 203 public static class SubscriptionStatusNotificationEventComponent extends BackboneElement implements IBaseBackboneElement { 204 /** 205 * Either the sequential number of this event in this subscription context or a relative event number for this notification. 206 */ 207 @Child(name = "eventNumber", type = {Integer64Type.class}, order=1, min=1, max=1, modifier=false, summary=false) 208 @Description(shortDefinition="Sequencing index of this event", formalDefinition="Either the sequential number of this event in this subscription context or a relative event number for this notification." ) 209 protected Integer64Type eventNumber; 210 211 /** 212 * The actual time this event occurred on the server. 213 */ 214 @Child(name = "timestamp", type = {InstantType.class}, order=2, min=0, max=1, modifier=false, summary=false) 215 @Description(shortDefinition="The instant this event occurred", formalDefinition="The actual time this event occurred on the server." ) 216 protected InstantType timestamp; 217 218 /** 219 * The focus of this event. While this will usually be a reference to the focus resource of the event, it MAY contain a reference to a non-FHIR object. 220 */ 221 @Child(name = "focus", type = {Reference.class}, order=3, min=0, max=1, modifier=false, summary=false) 222 @Description(shortDefinition="Reference to the primary resource or information of this event", formalDefinition="The focus of this event. While this will usually be a reference to the focus resource of the event, it MAY contain a reference to a non-FHIR object." ) 223 protected Reference focus; 224 225 /** 226 * Additional context information for this event. Generally, this will contain references to additional resources included with the event (e.g., the Patient relevant to an Encounter), however it MAY refer to non-FHIR objects. 227 */ 228 @Child(name = "additionalContext", type = {Reference.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 229 @Description(shortDefinition="References related to the focus resource and/or context of this event", formalDefinition="Additional context information for this event. Generally, this will contain references to additional resources included with the event (e.g., the Patient relevant to an Encounter), however it MAY refer to non-FHIR objects." ) 230 protected List<Reference> additionalContext; 231 232 private static final long serialVersionUID = 855121922L; 233 234 /** 235 * Constructor 236 */ 237 public SubscriptionStatusNotificationEventComponent() { 238 super(); 239 } 240 241 /** 242 * Constructor 243 */ 244 public SubscriptionStatusNotificationEventComponent(long eventNumber) { 245 super(); 246 this.setEventNumber(eventNumber); 247 } 248 249 /** 250 * @return {@link #eventNumber} (Either the sequential number of this event in this subscription context or a relative event number for this notification.). This is the underlying object with id, value and extensions. The accessor "getEventNumber" gives direct access to the value 251 */ 252 public Integer64Type getEventNumberElement() { 253 if (this.eventNumber == null) 254 if (Configuration.errorOnAutoCreate()) 255 throw new Error("Attempt to auto-create SubscriptionStatusNotificationEventComponent.eventNumber"); 256 else if (Configuration.doAutoCreate()) 257 this.eventNumber = new Integer64Type(); // bb 258 return this.eventNumber; 259 } 260 261 public boolean hasEventNumberElement() { 262 return this.eventNumber != null && !this.eventNumber.isEmpty(); 263 } 264 265 public boolean hasEventNumber() { 266 return this.eventNumber != null && !this.eventNumber.isEmpty(); 267 } 268 269 /** 270 * @param value {@link #eventNumber} (Either the sequential number of this event in this subscription context or a relative event number for this notification.). This is the underlying object with id, value and extensions. The accessor "getEventNumber" gives direct access to the value 271 */ 272 public SubscriptionStatusNotificationEventComponent setEventNumberElement(Integer64Type value) { 273 this.eventNumber = value; 274 return this; 275 } 276 277 /** 278 * @return Either the sequential number of this event in this subscription context or a relative event number for this notification. 279 */ 280 public long getEventNumber() { 281 return this.eventNumber == null || this.eventNumber.isEmpty() ? 0 : this.eventNumber.getValue(); 282 } 283 284 /** 285 * @param value Either the sequential number of this event in this subscription context or a relative event number for this notification. 286 */ 287 public SubscriptionStatusNotificationEventComponent setEventNumber(long value) { 288 this.eventNumber = new Integer64Type(); 289 this.eventNumber.setValue(value); 290 return this; 291 } 292 293 /** 294 * @return {@link #timestamp} (The actual time this event occurred on the server.). This is the underlying object with id, value and extensions. The accessor "getTimestamp" gives direct access to the value 295 */ 296 public InstantType getTimestampElement() { 297 if (this.timestamp == null) 298 if (Configuration.errorOnAutoCreate()) 299 throw new Error("Attempt to auto-create SubscriptionStatusNotificationEventComponent.timestamp"); 300 else if (Configuration.doAutoCreate()) 301 this.timestamp = new InstantType(); // bb 302 return this.timestamp; 303 } 304 305 public boolean hasTimestampElement() { 306 return this.timestamp != null && !this.timestamp.isEmpty(); 307 } 308 309 public boolean hasTimestamp() { 310 return this.timestamp != null && !this.timestamp.isEmpty(); 311 } 312 313 /** 314 * @param value {@link #timestamp} (The actual time this event occurred on the server.). This is the underlying object with id, value and extensions. The accessor "getTimestamp" gives direct access to the value 315 */ 316 public SubscriptionStatusNotificationEventComponent setTimestampElement(InstantType value) { 317 this.timestamp = value; 318 return this; 319 } 320 321 /** 322 * @return The actual time this event occurred on the server. 323 */ 324 public Date getTimestamp() { 325 return this.timestamp == null ? null : this.timestamp.getValue(); 326 } 327 328 /** 329 * @param value The actual time this event occurred on the server. 330 */ 331 public SubscriptionStatusNotificationEventComponent setTimestamp(Date value) { 332 if (value == null) 333 this.timestamp = null; 334 else { 335 if (this.timestamp == null) 336 this.timestamp = new InstantType(); 337 this.timestamp.setValue(value); 338 } 339 return this; 340 } 341 342 /** 343 * @return {@link #focus} (The focus of this event. While this will usually be a reference to the focus resource of the event, it MAY contain a reference to a non-FHIR object.) 344 */ 345 public Reference getFocus() { 346 if (this.focus == null) 347 if (Configuration.errorOnAutoCreate()) 348 throw new Error("Attempt to auto-create SubscriptionStatusNotificationEventComponent.focus"); 349 else if (Configuration.doAutoCreate()) 350 this.focus = new Reference(); // cc 351 return this.focus; 352 } 353 354 public boolean hasFocus() { 355 return this.focus != null && !this.focus.isEmpty(); 356 } 357 358 /** 359 * @param value {@link #focus} (The focus of this event. While this will usually be a reference to the focus resource of the event, it MAY contain a reference to a non-FHIR object.) 360 */ 361 public SubscriptionStatusNotificationEventComponent setFocus(Reference value) { 362 this.focus = value; 363 return this; 364 } 365 366 /** 367 * @return {@link #additionalContext} (Additional context information for this event. Generally, this will contain references to additional resources included with the event (e.g., the Patient relevant to an Encounter), however it MAY refer to non-FHIR objects.) 368 */ 369 public List<Reference> getAdditionalContext() { 370 if (this.additionalContext == null) 371 this.additionalContext = new ArrayList<Reference>(); 372 return this.additionalContext; 373 } 374 375 /** 376 * @return Returns a reference to <code>this</code> for easy method chaining 377 */ 378 public SubscriptionStatusNotificationEventComponent setAdditionalContext(List<Reference> theAdditionalContext) { 379 this.additionalContext = theAdditionalContext; 380 return this; 381 } 382 383 public boolean hasAdditionalContext() { 384 if (this.additionalContext == null) 385 return false; 386 for (Reference item : this.additionalContext) 387 if (!item.isEmpty()) 388 return true; 389 return false; 390 } 391 392 public Reference addAdditionalContext() { //3 393 Reference t = new Reference(); 394 if (this.additionalContext == null) 395 this.additionalContext = new ArrayList<Reference>(); 396 this.additionalContext.add(t); 397 return t; 398 } 399 400 public SubscriptionStatusNotificationEventComponent addAdditionalContext(Reference t) { //3 401 if (t == null) 402 return this; 403 if (this.additionalContext == null) 404 this.additionalContext = new ArrayList<Reference>(); 405 this.additionalContext.add(t); 406 return this; 407 } 408 409 /** 410 * @return The first repetition of repeating field {@link #additionalContext}, creating it if it does not already exist {3} 411 */ 412 public Reference getAdditionalContextFirstRep() { 413 if (getAdditionalContext().isEmpty()) { 414 addAdditionalContext(); 415 } 416 return getAdditionalContext().get(0); 417 } 418 419 protected void listChildren(List<Property> children) { 420 super.listChildren(children); 421 children.add(new Property("eventNumber", "integer64", "Either the sequential number of this event in this subscription context or a relative event number for this notification.", 0, 1, eventNumber)); 422 children.add(new Property("timestamp", "instant", "The actual time this event occurred on the server.", 0, 1, timestamp)); 423 children.add(new Property("focus", "Reference(Any)", "The focus of this event. While this will usually be a reference to the focus resource of the event, it MAY contain a reference to a non-FHIR object.", 0, 1, focus)); 424 children.add(new Property("additionalContext", "Reference(Any)", "Additional context information for this event. Generally, this will contain references to additional resources included with the event (e.g., the Patient relevant to an Encounter), however it MAY refer to non-FHIR objects.", 0, java.lang.Integer.MAX_VALUE, additionalContext)); 425 } 426 427 @Override 428 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 429 switch (_hash) { 430 case -35234173: /*eventNumber*/ return new Property("eventNumber", "integer64", "Either the sequential number of this event in this subscription context or a relative event number for this notification.", 0, 1, eventNumber); 431 case 55126294: /*timestamp*/ return new Property("timestamp", "instant", "The actual time this event occurred on the server.", 0, 1, timestamp); 432 case 97604824: /*focus*/ return new Property("focus", "Reference(Any)", "The focus of this event. While this will usually be a reference to the focus resource of the event, it MAY contain a reference to a non-FHIR object.", 0, 1, focus); 433 case -908743800: /*additionalContext*/ return new Property("additionalContext", "Reference(Any)", "Additional context information for this event. Generally, this will contain references to additional resources included with the event (e.g., the Patient relevant to an Encounter), however it MAY refer to non-FHIR objects.", 0, java.lang.Integer.MAX_VALUE, additionalContext); 434 default: return super.getNamedProperty(_hash, _name, _checkValid); 435 } 436 437 } 438 439 @Override 440 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 441 switch (hash) { 442 case -35234173: /*eventNumber*/ return this.eventNumber == null ? new Base[0] : new Base[] {this.eventNumber}; // Integer64Type 443 case 55126294: /*timestamp*/ return this.timestamp == null ? new Base[0] : new Base[] {this.timestamp}; // InstantType 444 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : new Base[] {this.focus}; // Reference 445 case -908743800: /*additionalContext*/ return this.additionalContext == null ? new Base[0] : this.additionalContext.toArray(new Base[this.additionalContext.size()]); // Reference 446 default: return super.getProperty(hash, name, checkValid); 447 } 448 449 } 450 451 @Override 452 public Base setProperty(int hash, String name, Base value) throws FHIRException { 453 switch (hash) { 454 case -35234173: // eventNumber 455 this.eventNumber = TypeConvertor.castToInteger64(value); // Integer64Type 456 return value; 457 case 55126294: // timestamp 458 this.timestamp = TypeConvertor.castToInstant(value); // InstantType 459 return value; 460 case 97604824: // focus 461 this.focus = TypeConvertor.castToReference(value); // Reference 462 return value; 463 case -908743800: // additionalContext 464 this.getAdditionalContext().add(TypeConvertor.castToReference(value)); // Reference 465 return value; 466 default: return super.setProperty(hash, name, value); 467 } 468 469 } 470 471 @Override 472 public Base setProperty(String name, Base value) throws FHIRException { 473 if (name.equals("eventNumber")) { 474 this.eventNumber = TypeConvertor.castToInteger64(value); // Integer64Type 475 } else if (name.equals("timestamp")) { 476 this.timestamp = TypeConvertor.castToInstant(value); // InstantType 477 } else if (name.equals("focus")) { 478 this.focus = TypeConvertor.castToReference(value); // Reference 479 } else if (name.equals("additionalContext")) { 480 this.getAdditionalContext().add(TypeConvertor.castToReference(value)); 481 } else 482 return super.setProperty(name, value); 483 return value; 484 } 485 486 @Override 487 public void removeChild(String name, Base value) throws FHIRException { 488 if (name.equals("eventNumber")) { 489 this.eventNumber = null; 490 } else if (name.equals("timestamp")) { 491 this.timestamp = null; 492 } else if (name.equals("focus")) { 493 this.focus = null; 494 } else if (name.equals("additionalContext")) { 495 this.getAdditionalContext().remove(value); 496 } else 497 super.removeChild(name, value); 498 499 } 500 501 @Override 502 public Base makeProperty(int hash, String name) throws FHIRException { 503 switch (hash) { 504 case -35234173: return getEventNumberElement(); 505 case 55126294: return getTimestampElement(); 506 case 97604824: return getFocus(); 507 case -908743800: return addAdditionalContext(); 508 default: return super.makeProperty(hash, name); 509 } 510 511 } 512 513 @Override 514 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 515 switch (hash) { 516 case -35234173: /*eventNumber*/ return new String[] {"integer64"}; 517 case 55126294: /*timestamp*/ return new String[] {"instant"}; 518 case 97604824: /*focus*/ return new String[] {"Reference"}; 519 case -908743800: /*additionalContext*/ return new String[] {"Reference"}; 520 default: return super.getTypesForProperty(hash, name); 521 } 522 523 } 524 525 @Override 526 public Base addChild(String name) throws FHIRException { 527 if (name.equals("eventNumber")) { 528 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionStatus.notificationEvent.eventNumber"); 529 } 530 else if (name.equals("timestamp")) { 531 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionStatus.notificationEvent.timestamp"); 532 } 533 else if (name.equals("focus")) { 534 this.focus = new Reference(); 535 return this.focus; 536 } 537 else if (name.equals("additionalContext")) { 538 return addAdditionalContext(); 539 } 540 else 541 return super.addChild(name); 542 } 543 544 public SubscriptionStatusNotificationEventComponent copy() { 545 SubscriptionStatusNotificationEventComponent dst = new SubscriptionStatusNotificationEventComponent(); 546 copyValues(dst); 547 return dst; 548 } 549 550 public void copyValues(SubscriptionStatusNotificationEventComponent dst) { 551 super.copyValues(dst); 552 dst.eventNumber = eventNumber == null ? null : eventNumber.copy(); 553 dst.timestamp = timestamp == null ? null : timestamp.copy(); 554 dst.focus = focus == null ? null : focus.copy(); 555 if (additionalContext != null) { 556 dst.additionalContext = new ArrayList<Reference>(); 557 for (Reference i : additionalContext) 558 dst.additionalContext.add(i.copy()); 559 }; 560 } 561 562 @Override 563 public boolean equalsDeep(Base other_) { 564 if (!super.equalsDeep(other_)) 565 return false; 566 if (!(other_ instanceof SubscriptionStatusNotificationEventComponent)) 567 return false; 568 SubscriptionStatusNotificationEventComponent o = (SubscriptionStatusNotificationEventComponent) other_; 569 return compareDeep(eventNumber, o.eventNumber, true) && compareDeep(timestamp, o.timestamp, true) 570 && compareDeep(focus, o.focus, true) && compareDeep(additionalContext, o.additionalContext, true) 571 ; 572 } 573 574 @Override 575 public boolean equalsShallow(Base other_) { 576 if (!super.equalsShallow(other_)) 577 return false; 578 if (!(other_ instanceof SubscriptionStatusNotificationEventComponent)) 579 return false; 580 SubscriptionStatusNotificationEventComponent o = (SubscriptionStatusNotificationEventComponent) other_; 581 return compareValues(eventNumber, o.eventNumber, true) && compareValues(timestamp, o.timestamp, true) 582 ; 583 } 584 585 public boolean isEmpty() { 586 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(eventNumber, timestamp, focus 587 , additionalContext); 588 } 589 590 public String fhirType() { 591 return "SubscriptionStatus.notificationEvent"; 592 593 } 594 595 } 596 597 /** 598 * The status of the subscription, which marks the server state for managing the subscription. 599 */ 600 @Child(name = "status", type = {CodeType.class}, order=0, min=0, max=1, modifier=false, summary=true) 601 @Description(shortDefinition="requested | active | error | off | entered-in-error", formalDefinition="The status of the subscription, which marks the server state for managing the subscription." ) 602 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscription-status") 603 protected Enumeration<SubscriptionStatusCodes> status; 604 605 /** 606 * The type of event being conveyed with this notification. 607 */ 608 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 609 @Description(shortDefinition="handshake | heartbeat | event-notification | query-status | query-event", formalDefinition="The type of event being conveyed with this notification." ) 610 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscription-notification-type") 611 protected Enumeration<SubscriptionNotificationType> type; 612 613 /** 614 * The total number of actual events which have been generated since the Subscription was created (inclusive of this notification) - regardless of how many have been successfully communicated. This number is NOT incremented for handshake and heartbeat notifications. 615 */ 616 @Child(name = "eventsSinceSubscriptionStart", type = {Integer64Type.class}, order=2, min=0, max=1, modifier=false, summary=true) 617 @Description(shortDefinition="Events since the Subscription was created", formalDefinition="The total number of actual events which have been generated since the Subscription was created (inclusive of this notification) - regardless of how many have been successfully communicated. This number is NOT incremented for handshake and heartbeat notifications." ) 618 protected Integer64Type eventsSinceSubscriptionStart; 619 620 /** 621 * Detailed information about events relevant to this subscription notification. 622 */ 623 @Child(name = "notificationEvent", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 624 @Description(shortDefinition="Detailed information about any events relevant to this notification", formalDefinition="Detailed information about events relevant to this subscription notification." ) 625 protected List<SubscriptionStatusNotificationEventComponent> notificationEvent; 626 627 /** 628 * The reference to the Subscription which generated this notification. 629 */ 630 @Child(name = "subscription", type = {Subscription.class}, order=4, min=1, max=1, modifier=false, summary=true) 631 @Description(shortDefinition="Reference to the Subscription responsible for this notification", formalDefinition="The reference to the Subscription which generated this notification." ) 632 protected Reference subscription; 633 634 /** 635 * The reference to the SubscriptionTopic for the Subscription which generated this notification. 636 */ 637 @Child(name = "topic", type = {CanonicalType.class}, order=5, min=0, max=1, modifier=false, summary=true) 638 @Description(shortDefinition="Reference to the SubscriptionTopic this notification relates to", formalDefinition="The reference to the SubscriptionTopic for the Subscription which generated this notification." ) 639 protected CanonicalType topic; 640 641 /** 642 * A record of errors that occurred when the server processed a notification. 643 */ 644 @Child(name = "error", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 645 @Description(shortDefinition="List of errors on the subscription", formalDefinition="A record of errors that occurred when the server processed a notification." ) 646 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscription-error") 647 protected List<CodeableConcept> error; 648 649 private static final long serialVersionUID = -285503955L; 650 651 /** 652 * Constructor 653 */ 654 public SubscriptionStatus() { 655 super(); 656 } 657 658 /** 659 * Constructor 660 */ 661 public SubscriptionStatus(SubscriptionNotificationType type, Reference subscription) { 662 super(); 663 this.setType(type); 664 this.setSubscription(subscription); 665 } 666 667 /** 668 * @return {@link #status} (The status of the subscription, which marks the server state for managing the subscription.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 669 */ 670 public Enumeration<SubscriptionStatusCodes> getStatusElement() { 671 if (this.status == null) 672 if (Configuration.errorOnAutoCreate()) 673 throw new Error("Attempt to auto-create SubscriptionStatus.status"); 674 else if (Configuration.doAutoCreate()) 675 this.status = new Enumeration<SubscriptionStatusCodes>(new SubscriptionStatusCodesEnumFactory()); // bb 676 return this.status; 677 } 678 679 public boolean hasStatusElement() { 680 return this.status != null && !this.status.isEmpty(); 681 } 682 683 public boolean hasStatus() { 684 return this.status != null && !this.status.isEmpty(); 685 } 686 687 /** 688 * @param value {@link #status} (The status of the subscription, which marks the server state for managing the subscription.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 689 */ 690 public SubscriptionStatus setStatusElement(Enumeration<SubscriptionStatusCodes> value) { 691 this.status = value; 692 return this; 693 } 694 695 /** 696 * @return The status of the subscription, which marks the server state for managing the subscription. 697 */ 698 public SubscriptionStatusCodes getStatus() { 699 return this.status == null ? null : this.status.getValue(); 700 } 701 702 /** 703 * @param value The status of the subscription, which marks the server state for managing the subscription. 704 */ 705 public SubscriptionStatus setStatus(SubscriptionStatusCodes value) { 706 if (value == null) 707 this.status = null; 708 else { 709 if (this.status == null) 710 this.status = new Enumeration<SubscriptionStatusCodes>(new SubscriptionStatusCodesEnumFactory()); 711 this.status.setValue(value); 712 } 713 return this; 714 } 715 716 /** 717 * @return {@link #type} (The type of event being conveyed with this notification.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 718 */ 719 public Enumeration<SubscriptionNotificationType> getTypeElement() { 720 if (this.type == null) 721 if (Configuration.errorOnAutoCreate()) 722 throw new Error("Attempt to auto-create SubscriptionStatus.type"); 723 else if (Configuration.doAutoCreate()) 724 this.type = new Enumeration<SubscriptionNotificationType>(new SubscriptionNotificationTypeEnumFactory()); // bb 725 return this.type; 726 } 727 728 public boolean hasTypeElement() { 729 return this.type != null && !this.type.isEmpty(); 730 } 731 732 public boolean hasType() { 733 return this.type != null && !this.type.isEmpty(); 734 } 735 736 /** 737 * @param value {@link #type} (The type of event being conveyed with this notification.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 738 */ 739 public SubscriptionStatus setTypeElement(Enumeration<SubscriptionNotificationType> value) { 740 this.type = value; 741 return this; 742 } 743 744 /** 745 * @return The type of event being conveyed with this notification. 746 */ 747 public SubscriptionNotificationType getType() { 748 return this.type == null ? null : this.type.getValue(); 749 } 750 751 /** 752 * @param value The type of event being conveyed with this notification. 753 */ 754 public SubscriptionStatus setType(SubscriptionNotificationType value) { 755 if (this.type == null) 756 this.type = new Enumeration<SubscriptionNotificationType>(new SubscriptionNotificationTypeEnumFactory()); 757 this.type.setValue(value); 758 return this; 759 } 760 761 /** 762 * @return {@link #eventsSinceSubscriptionStart} (The total number of actual events which have been generated since the Subscription was created (inclusive of this notification) - regardless of how many have been successfully communicated. This number is NOT incremented for handshake and heartbeat notifications.). This is the underlying object with id, value and extensions. The accessor "getEventsSinceSubscriptionStart" gives direct access to the value 763 */ 764 public Integer64Type getEventsSinceSubscriptionStartElement() { 765 if (this.eventsSinceSubscriptionStart == null) 766 if (Configuration.errorOnAutoCreate()) 767 throw new Error("Attempt to auto-create SubscriptionStatus.eventsSinceSubscriptionStart"); 768 else if (Configuration.doAutoCreate()) 769 this.eventsSinceSubscriptionStart = new Integer64Type(); // bb 770 return this.eventsSinceSubscriptionStart; 771 } 772 773 public boolean hasEventsSinceSubscriptionStartElement() { 774 return this.eventsSinceSubscriptionStart != null && !this.eventsSinceSubscriptionStart.isEmpty(); 775 } 776 777 public boolean hasEventsSinceSubscriptionStart() { 778 return this.eventsSinceSubscriptionStart != null && !this.eventsSinceSubscriptionStart.isEmpty(); 779 } 780 781 /** 782 * @param value {@link #eventsSinceSubscriptionStart} (The total number of actual events which have been generated since the Subscription was created (inclusive of this notification) - regardless of how many have been successfully communicated. This number is NOT incremented for handshake and heartbeat notifications.). This is the underlying object with id, value and extensions. The accessor "getEventsSinceSubscriptionStart" gives direct access to the value 783 */ 784 public SubscriptionStatus setEventsSinceSubscriptionStartElement(Integer64Type value) { 785 this.eventsSinceSubscriptionStart = value; 786 return this; 787 } 788 789 /** 790 * @return The total number of actual events which have been generated since the Subscription was created (inclusive of this notification) - regardless of how many have been successfully communicated. This number is NOT incremented for handshake and heartbeat notifications. 791 */ 792 public long getEventsSinceSubscriptionStart() { 793 return this.eventsSinceSubscriptionStart == null || this.eventsSinceSubscriptionStart.isEmpty() ? 0 : this.eventsSinceSubscriptionStart.getValue(); 794 } 795 796 /** 797 * @param value The total number of actual events which have been generated since the Subscription was created (inclusive of this notification) - regardless of how many have been successfully communicated. This number is NOT incremented for handshake and heartbeat notifications. 798 */ 799 public SubscriptionStatus setEventsSinceSubscriptionStart(long value) { 800 this.eventsSinceSubscriptionStart = new Integer64Type(); 801 this.eventsSinceSubscriptionStart.setValue(value); 802 return this; 803 } 804 805 /** 806 * @return {@link #notificationEvent} (Detailed information about events relevant to this subscription notification.) 807 */ 808 public List<SubscriptionStatusNotificationEventComponent> getNotificationEvent() { 809 if (this.notificationEvent == null) 810 this.notificationEvent = new ArrayList<SubscriptionStatusNotificationEventComponent>(); 811 return this.notificationEvent; 812 } 813 814 /** 815 * @return Returns a reference to <code>this</code> for easy method chaining 816 */ 817 public SubscriptionStatus setNotificationEvent(List<SubscriptionStatusNotificationEventComponent> theNotificationEvent) { 818 this.notificationEvent = theNotificationEvent; 819 return this; 820 } 821 822 public boolean hasNotificationEvent() { 823 if (this.notificationEvent == null) 824 return false; 825 for (SubscriptionStatusNotificationEventComponent item : this.notificationEvent) 826 if (!item.isEmpty()) 827 return true; 828 return false; 829 } 830 831 public SubscriptionStatusNotificationEventComponent addNotificationEvent() { //3 832 SubscriptionStatusNotificationEventComponent t = new SubscriptionStatusNotificationEventComponent(); 833 if (this.notificationEvent == null) 834 this.notificationEvent = new ArrayList<SubscriptionStatusNotificationEventComponent>(); 835 this.notificationEvent.add(t); 836 return t; 837 } 838 839 public SubscriptionStatus addNotificationEvent(SubscriptionStatusNotificationEventComponent t) { //3 840 if (t == null) 841 return this; 842 if (this.notificationEvent == null) 843 this.notificationEvent = new ArrayList<SubscriptionStatusNotificationEventComponent>(); 844 this.notificationEvent.add(t); 845 return this; 846 } 847 848 /** 849 * @return The first repetition of repeating field {@link #notificationEvent}, creating it if it does not already exist {3} 850 */ 851 public SubscriptionStatusNotificationEventComponent getNotificationEventFirstRep() { 852 if (getNotificationEvent().isEmpty()) { 853 addNotificationEvent(); 854 } 855 return getNotificationEvent().get(0); 856 } 857 858 /** 859 * @return {@link #subscription} (The reference to the Subscription which generated this notification.) 860 */ 861 public Reference getSubscription() { 862 if (this.subscription == null) 863 if (Configuration.errorOnAutoCreate()) 864 throw new Error("Attempt to auto-create SubscriptionStatus.subscription"); 865 else if (Configuration.doAutoCreate()) 866 this.subscription = new Reference(); // cc 867 return this.subscription; 868 } 869 870 public boolean hasSubscription() { 871 return this.subscription != null && !this.subscription.isEmpty(); 872 } 873 874 /** 875 * @param value {@link #subscription} (The reference to the Subscription which generated this notification.) 876 */ 877 public SubscriptionStatus setSubscription(Reference value) { 878 this.subscription = value; 879 return this; 880 } 881 882 /** 883 * @return {@link #topic} (The reference to the SubscriptionTopic for the Subscription which generated this notification.). This is the underlying object with id, value and extensions. The accessor "getTopic" gives direct access to the value 884 */ 885 public CanonicalType getTopicElement() { 886 if (this.topic == null) 887 if (Configuration.errorOnAutoCreate()) 888 throw new Error("Attempt to auto-create SubscriptionStatus.topic"); 889 else if (Configuration.doAutoCreate()) 890 this.topic = new CanonicalType(); // bb 891 return this.topic; 892 } 893 894 public boolean hasTopicElement() { 895 return this.topic != null && !this.topic.isEmpty(); 896 } 897 898 public boolean hasTopic() { 899 return this.topic != null && !this.topic.isEmpty(); 900 } 901 902 /** 903 * @param value {@link #topic} (The reference to the SubscriptionTopic for the Subscription which generated this notification.). This is the underlying object with id, value and extensions. The accessor "getTopic" gives direct access to the value 904 */ 905 public SubscriptionStatus setTopicElement(CanonicalType value) { 906 this.topic = value; 907 return this; 908 } 909 910 /** 911 * @return The reference to the SubscriptionTopic for the Subscription which generated this notification. 912 */ 913 public String getTopic() { 914 return this.topic == null ? null : this.topic.getValue(); 915 } 916 917 /** 918 * @param value The reference to the SubscriptionTopic for the Subscription which generated this notification. 919 */ 920 public SubscriptionStatus setTopic(String value) { 921 if (Utilities.noString(value)) 922 this.topic = null; 923 else { 924 if (this.topic == null) 925 this.topic = new CanonicalType(); 926 this.topic.setValue(value); 927 } 928 return this; 929 } 930 931 /** 932 * @return {@link #error} (A record of errors that occurred when the server processed a notification.) 933 */ 934 public List<CodeableConcept> getError() { 935 if (this.error == null) 936 this.error = new ArrayList<CodeableConcept>(); 937 return this.error; 938 } 939 940 /** 941 * @return Returns a reference to <code>this</code> for easy method chaining 942 */ 943 public SubscriptionStatus setError(List<CodeableConcept> theError) { 944 this.error = theError; 945 return this; 946 } 947 948 public boolean hasError() { 949 if (this.error == null) 950 return false; 951 for (CodeableConcept item : this.error) 952 if (!item.isEmpty()) 953 return true; 954 return false; 955 } 956 957 public CodeableConcept addError() { //3 958 CodeableConcept t = new CodeableConcept(); 959 if (this.error == null) 960 this.error = new ArrayList<CodeableConcept>(); 961 this.error.add(t); 962 return t; 963 } 964 965 public SubscriptionStatus addError(CodeableConcept t) { //3 966 if (t == null) 967 return this; 968 if (this.error == null) 969 this.error = new ArrayList<CodeableConcept>(); 970 this.error.add(t); 971 return this; 972 } 973 974 /** 975 * @return The first repetition of repeating field {@link #error}, creating it if it does not already exist {3} 976 */ 977 public CodeableConcept getErrorFirstRep() { 978 if (getError().isEmpty()) { 979 addError(); 980 } 981 return getError().get(0); 982 } 983 984 protected void listChildren(List<Property> children) { 985 super.listChildren(children); 986 children.add(new Property("status", "code", "The status of the subscription, which marks the server state for managing the subscription.", 0, 1, status)); 987 children.add(new Property("type", "code", "The type of event being conveyed with this notification.", 0, 1, type)); 988 children.add(new Property("eventsSinceSubscriptionStart", "integer64", "The total number of actual events which have been generated since the Subscription was created (inclusive of this notification) - regardless of how many have been successfully communicated. This number is NOT incremented for handshake and heartbeat notifications.", 0, 1, eventsSinceSubscriptionStart)); 989 children.add(new Property("notificationEvent", "", "Detailed information about events relevant to this subscription notification.", 0, java.lang.Integer.MAX_VALUE, notificationEvent)); 990 children.add(new Property("subscription", "Reference(Subscription)", "The reference to the Subscription which generated this notification.", 0, 1, subscription)); 991 children.add(new Property("topic", "canonical(SubscriptionTopic)", "The reference to the SubscriptionTopic for the Subscription which generated this notification.", 0, 1, topic)); 992 children.add(new Property("error", "CodeableConcept", "A record of errors that occurred when the server processed a notification.", 0, java.lang.Integer.MAX_VALUE, error)); 993 } 994 995 @Override 996 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 997 switch (_hash) { 998 case -892481550: /*status*/ return new Property("status", "code", "The status of the subscription, which marks the server state for managing the subscription.", 0, 1, status); 999 case 3575610: /*type*/ return new Property("type", "code", "The type of event being conveyed with this notification.", 0, 1, type); 1000 case 304566692: /*eventsSinceSubscriptionStart*/ return new Property("eventsSinceSubscriptionStart", "integer64", "The total number of actual events which have been generated since the Subscription was created (inclusive of this notification) - regardless of how many have been successfully communicated. This number is NOT incremented for handshake and heartbeat notifications.", 0, 1, eventsSinceSubscriptionStart); 1001 case -1595878289: /*notificationEvent*/ return new Property("notificationEvent", "", "Detailed information about events relevant to this subscription notification.", 0, java.lang.Integer.MAX_VALUE, notificationEvent); 1002 case 341203229: /*subscription*/ return new Property("subscription", "Reference(Subscription)", "The reference to the Subscription which generated this notification.", 0, 1, subscription); 1003 case 110546223: /*topic*/ return new Property("topic", "canonical(SubscriptionTopic)", "The reference to the SubscriptionTopic for the Subscription which generated this notification.", 0, 1, topic); 1004 case 96784904: /*error*/ return new Property("error", "CodeableConcept", "A record of errors that occurred when the server processed a notification.", 0, java.lang.Integer.MAX_VALUE, error); 1005 default: return super.getNamedProperty(_hash, _name, _checkValid); 1006 } 1007 1008 } 1009 1010 @Override 1011 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1012 switch (hash) { 1013 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<SubscriptionStatusCodes> 1014 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<SubscriptionNotificationType> 1015 case 304566692: /*eventsSinceSubscriptionStart*/ return this.eventsSinceSubscriptionStart == null ? new Base[0] : new Base[] {this.eventsSinceSubscriptionStart}; // Integer64Type 1016 case -1595878289: /*notificationEvent*/ return this.notificationEvent == null ? new Base[0] : this.notificationEvent.toArray(new Base[this.notificationEvent.size()]); // SubscriptionStatusNotificationEventComponent 1017 case 341203229: /*subscription*/ return this.subscription == null ? new Base[0] : new Base[] {this.subscription}; // Reference 1018 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : new Base[] {this.topic}; // CanonicalType 1019 case 96784904: /*error*/ return this.error == null ? new Base[0] : this.error.toArray(new Base[this.error.size()]); // CodeableConcept 1020 default: return super.getProperty(hash, name, checkValid); 1021 } 1022 1023 } 1024 1025 @Override 1026 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1027 switch (hash) { 1028 case -892481550: // status 1029 value = new SubscriptionStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1030 this.status = (Enumeration) value; // Enumeration<SubscriptionStatusCodes> 1031 return value; 1032 case 3575610: // type 1033 value = new SubscriptionNotificationTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1034 this.type = (Enumeration) value; // Enumeration<SubscriptionNotificationType> 1035 return value; 1036 case 304566692: // eventsSinceSubscriptionStart 1037 this.eventsSinceSubscriptionStart = TypeConvertor.castToInteger64(value); // Integer64Type 1038 return value; 1039 case -1595878289: // notificationEvent 1040 this.getNotificationEvent().add((SubscriptionStatusNotificationEventComponent) value); // SubscriptionStatusNotificationEventComponent 1041 return value; 1042 case 341203229: // subscription 1043 this.subscription = TypeConvertor.castToReference(value); // Reference 1044 return value; 1045 case 110546223: // topic 1046 this.topic = TypeConvertor.castToCanonical(value); // CanonicalType 1047 return value; 1048 case 96784904: // error 1049 this.getError().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1050 return value; 1051 default: return super.setProperty(hash, name, value); 1052 } 1053 1054 } 1055 1056 @Override 1057 public Base setProperty(String name, Base value) throws FHIRException { 1058 if (name.equals("status")) { 1059 value = new SubscriptionStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1060 this.status = (Enumeration) value; // Enumeration<SubscriptionStatusCodes> 1061 } else if (name.equals("type")) { 1062 value = new SubscriptionNotificationTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1063 this.type = (Enumeration) value; // Enumeration<SubscriptionNotificationType> 1064 } else if (name.equals("eventsSinceSubscriptionStart")) { 1065 this.eventsSinceSubscriptionStart = TypeConvertor.castToInteger64(value); // Integer64Type 1066 } else if (name.equals("notificationEvent")) { 1067 this.getNotificationEvent().add((SubscriptionStatusNotificationEventComponent) value); 1068 } else if (name.equals("subscription")) { 1069 this.subscription = TypeConvertor.castToReference(value); // Reference 1070 } else if (name.equals("topic")) { 1071 this.topic = TypeConvertor.castToCanonical(value); // CanonicalType 1072 } else if (name.equals("error")) { 1073 this.getError().add(TypeConvertor.castToCodeableConcept(value)); 1074 } else 1075 return super.setProperty(name, value); 1076 return value; 1077 } 1078 1079 @Override 1080 public void removeChild(String name, Base value) throws FHIRException { 1081 if (name.equals("status")) { 1082 value = new SubscriptionStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1083 this.status = (Enumeration) value; // Enumeration<SubscriptionStatusCodes> 1084 } else if (name.equals("type")) { 1085 value = new SubscriptionNotificationTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1086 this.type = (Enumeration) value; // Enumeration<SubscriptionNotificationType> 1087 } else if (name.equals("eventsSinceSubscriptionStart")) { 1088 this.eventsSinceSubscriptionStart = null; 1089 } else if (name.equals("notificationEvent")) { 1090 this.getNotificationEvent().remove((SubscriptionStatusNotificationEventComponent) value); 1091 } else if (name.equals("subscription")) { 1092 this.subscription = null; 1093 } else if (name.equals("topic")) { 1094 this.topic = null; 1095 } else if (name.equals("error")) { 1096 this.getError().remove(value); 1097 } else 1098 super.removeChild(name, value); 1099 1100 } 1101 1102 @Override 1103 public Base makeProperty(int hash, String name) throws FHIRException { 1104 switch (hash) { 1105 case -892481550: return getStatusElement(); 1106 case 3575610: return getTypeElement(); 1107 case 304566692: return getEventsSinceSubscriptionStartElement(); 1108 case -1595878289: return addNotificationEvent(); 1109 case 341203229: return getSubscription(); 1110 case 110546223: return getTopicElement(); 1111 case 96784904: return addError(); 1112 default: return super.makeProperty(hash, name); 1113 } 1114 1115 } 1116 1117 @Override 1118 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1119 switch (hash) { 1120 case -892481550: /*status*/ return new String[] {"code"}; 1121 case 3575610: /*type*/ return new String[] {"code"}; 1122 case 304566692: /*eventsSinceSubscriptionStart*/ return new String[] {"integer64"}; 1123 case -1595878289: /*notificationEvent*/ return new String[] {}; 1124 case 341203229: /*subscription*/ return new String[] {"Reference"}; 1125 case 110546223: /*topic*/ return new String[] {"canonical"}; 1126 case 96784904: /*error*/ return new String[] {"CodeableConcept"}; 1127 default: return super.getTypesForProperty(hash, name); 1128 } 1129 1130 } 1131 1132 @Override 1133 public Base addChild(String name) throws FHIRException { 1134 if (name.equals("status")) { 1135 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionStatus.status"); 1136 } 1137 else if (name.equals("type")) { 1138 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionStatus.type"); 1139 } 1140 else if (name.equals("eventsSinceSubscriptionStart")) { 1141 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionStatus.eventsSinceSubscriptionStart"); 1142 } 1143 else if (name.equals("notificationEvent")) { 1144 return addNotificationEvent(); 1145 } 1146 else if (name.equals("subscription")) { 1147 this.subscription = new Reference(); 1148 return this.subscription; 1149 } 1150 else if (name.equals("topic")) { 1151 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionStatus.topic"); 1152 } 1153 else if (name.equals("error")) { 1154 return addError(); 1155 } 1156 else 1157 return super.addChild(name); 1158 } 1159 1160 public String fhirType() { 1161 return "SubscriptionStatus"; 1162 1163 } 1164 1165 public SubscriptionStatus copy() { 1166 SubscriptionStatus dst = new SubscriptionStatus(); 1167 copyValues(dst); 1168 return dst; 1169 } 1170 1171 public void copyValues(SubscriptionStatus dst) { 1172 super.copyValues(dst); 1173 dst.status = status == null ? null : status.copy(); 1174 dst.type = type == null ? null : type.copy(); 1175 dst.eventsSinceSubscriptionStart = eventsSinceSubscriptionStart == null ? null : eventsSinceSubscriptionStart.copy(); 1176 if (notificationEvent != null) { 1177 dst.notificationEvent = new ArrayList<SubscriptionStatusNotificationEventComponent>(); 1178 for (SubscriptionStatusNotificationEventComponent i : notificationEvent) 1179 dst.notificationEvent.add(i.copy()); 1180 }; 1181 dst.subscription = subscription == null ? null : subscription.copy(); 1182 dst.topic = topic == null ? null : topic.copy(); 1183 if (error != null) { 1184 dst.error = new ArrayList<CodeableConcept>(); 1185 for (CodeableConcept i : error) 1186 dst.error.add(i.copy()); 1187 }; 1188 } 1189 1190 protected SubscriptionStatus typedCopy() { 1191 return copy(); 1192 } 1193 1194 @Override 1195 public boolean equalsDeep(Base other_) { 1196 if (!super.equalsDeep(other_)) 1197 return false; 1198 if (!(other_ instanceof SubscriptionStatus)) 1199 return false; 1200 SubscriptionStatus o = (SubscriptionStatus) other_; 1201 return compareDeep(status, o.status, true) && compareDeep(type, o.type, true) && compareDeep(eventsSinceSubscriptionStart, o.eventsSinceSubscriptionStart, true) 1202 && compareDeep(notificationEvent, o.notificationEvent, true) && compareDeep(subscription, o.subscription, true) 1203 && compareDeep(topic, o.topic, true) && compareDeep(error, o.error, true); 1204 } 1205 1206 @Override 1207 public boolean equalsShallow(Base other_) { 1208 if (!super.equalsShallow(other_)) 1209 return false; 1210 if (!(other_ instanceof SubscriptionStatus)) 1211 return false; 1212 SubscriptionStatus o = (SubscriptionStatus) other_; 1213 return compareValues(status, o.status, true) && compareValues(type, o.type, true) && compareValues(eventsSinceSubscriptionStart, o.eventsSinceSubscriptionStart, true) 1214 && compareValues(topic, o.topic, true); 1215 } 1216 1217 public boolean isEmpty() { 1218 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(status, type, eventsSinceSubscriptionStart 1219 , notificationEvent, subscription, topic, error); 1220 } 1221 1222 @Override 1223 public ResourceType getResourceType() { 1224 return ResourceType.SubscriptionStatus; 1225 } 1226 1227 1228} 1229