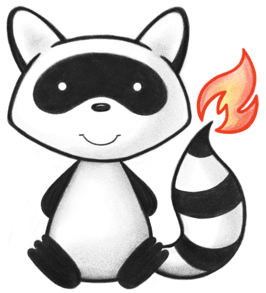
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Describes a stream of resource state changes identified by trigger criteria and annotated with labels useful to filter projections from this topic. 052 */ 053@ResourceDef(name="SubscriptionTopic", profile="http://hl7.org/fhir/StructureDefinition/SubscriptionTopic") 054public class SubscriptionTopic extends CanonicalResource { 055 056 public enum CriteriaNotExistsBehavior { 057 /** 058 * The requested conditional statement will pass if a matching state does not exist (e.g., previous state during create). 059 */ 060 TESTPASSES, 061 /** 062 * The requested conditional statement will fail if a matching state does not exist (e.g., previous state during create). 063 */ 064 TESTFAILS, 065 /** 066 * added to help the parsers with the generic types 067 */ 068 NULL; 069 public static CriteriaNotExistsBehavior fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("test-passes".equals(codeString)) 073 return TESTPASSES; 074 if ("test-fails".equals(codeString)) 075 return TESTFAILS; 076 if (Configuration.isAcceptInvalidEnums()) 077 return null; 078 else 079 throw new FHIRException("Unknown CriteriaNotExistsBehavior code '"+codeString+"'"); 080 } 081 public String toCode() { 082 switch (this) { 083 case TESTPASSES: return "test-passes"; 084 case TESTFAILS: return "test-fails"; 085 case NULL: return null; 086 default: return "?"; 087 } 088 } 089 public String getSystem() { 090 switch (this) { 091 case TESTPASSES: return "http://hl7.org/fhir/subscriptiontopic-cr-behavior"; 092 case TESTFAILS: return "http://hl7.org/fhir/subscriptiontopic-cr-behavior"; 093 case NULL: return null; 094 default: return "?"; 095 } 096 } 097 public String getDefinition() { 098 switch (this) { 099 case TESTPASSES: return "The requested conditional statement will pass if a matching state does not exist (e.g., previous state during create)."; 100 case TESTFAILS: return "The requested conditional statement will fail if a matching state does not exist (e.g., previous state during create)."; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDisplay() { 106 switch (this) { 107 case TESTPASSES: return "Test passes"; 108 case TESTFAILS: return "Test fails"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 } 114 115 public static class CriteriaNotExistsBehaviorEnumFactory implements EnumFactory<CriteriaNotExistsBehavior> { 116 public CriteriaNotExistsBehavior fromCode(String codeString) throws IllegalArgumentException { 117 if (codeString == null || "".equals(codeString)) 118 if (codeString == null || "".equals(codeString)) 119 return null; 120 if ("test-passes".equals(codeString)) 121 return CriteriaNotExistsBehavior.TESTPASSES; 122 if ("test-fails".equals(codeString)) 123 return CriteriaNotExistsBehavior.TESTFAILS; 124 throw new IllegalArgumentException("Unknown CriteriaNotExistsBehavior code '"+codeString+"'"); 125 } 126 public Enumeration<CriteriaNotExistsBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 127 if (code == null) 128 return null; 129 if (code.isEmpty()) 130 return new Enumeration<CriteriaNotExistsBehavior>(this, CriteriaNotExistsBehavior.NULL, code); 131 String codeString = ((PrimitiveType) code).asStringValue(); 132 if (codeString == null || "".equals(codeString)) 133 return new Enumeration<CriteriaNotExistsBehavior>(this, CriteriaNotExistsBehavior.NULL, code); 134 if ("test-passes".equals(codeString)) 135 return new Enumeration<CriteriaNotExistsBehavior>(this, CriteriaNotExistsBehavior.TESTPASSES, code); 136 if ("test-fails".equals(codeString)) 137 return new Enumeration<CriteriaNotExistsBehavior>(this, CriteriaNotExistsBehavior.TESTFAILS, code); 138 throw new FHIRException("Unknown CriteriaNotExistsBehavior code '"+codeString+"'"); 139 } 140 public String toCode(CriteriaNotExistsBehavior code) { 141 if (code == CriteriaNotExistsBehavior.TESTPASSES) 142 return "test-passes"; 143 if (code == CriteriaNotExistsBehavior.TESTFAILS) 144 return "test-fails"; 145 return "?"; 146 } 147 public String toSystem(CriteriaNotExistsBehavior code) { 148 return code.getSystem(); 149 } 150 } 151 152 public enum InteractionTrigger { 153 /** 154 * Create a new resource with a server assigned id. 155 */ 156 CREATE, 157 /** 158 * Update an existing resource by its id (or create it if it is new). 159 */ 160 UPDATE, 161 /** 162 * Delete a resource. 163 */ 164 DELETE, 165 /** 166 * added to help the parsers with the generic types 167 */ 168 NULL; 169 public static InteractionTrigger fromCode(String codeString) throws FHIRException { 170 if (codeString == null || "".equals(codeString)) 171 return null; 172 if ("create".equals(codeString)) 173 return CREATE; 174 if ("update".equals(codeString)) 175 return UPDATE; 176 if ("delete".equals(codeString)) 177 return DELETE; 178 if (Configuration.isAcceptInvalidEnums()) 179 return null; 180 else 181 throw new FHIRException("Unknown InteractionTrigger code '"+codeString+"'"); 182 } 183 public String toCode() { 184 switch (this) { 185 case CREATE: return "create"; 186 case UPDATE: return "update"; 187 case DELETE: return "delete"; 188 case NULL: return null; 189 default: return "?"; 190 } 191 } 192 public String getSystem() { 193 switch (this) { 194 case CREATE: return "http://hl7.org/fhir/restful-interaction"; 195 case UPDATE: return "http://hl7.org/fhir/restful-interaction"; 196 case DELETE: return "http://hl7.org/fhir/restful-interaction"; 197 case NULL: return null; 198 default: return "?"; 199 } 200 } 201 public String getDefinition() { 202 switch (this) { 203 case CREATE: return "Create a new resource with a server assigned id."; 204 case UPDATE: return "Update an existing resource by its id (or create it if it is new)."; 205 case DELETE: return "Delete a resource."; 206 case NULL: return null; 207 default: return "?"; 208 } 209 } 210 public String getDisplay() { 211 switch (this) { 212 case CREATE: return "create"; 213 case UPDATE: return "update"; 214 case DELETE: return "delete"; 215 case NULL: return null; 216 default: return "?"; 217 } 218 } 219 } 220 221 public static class InteractionTriggerEnumFactory implements EnumFactory<InteractionTrigger> { 222 public InteractionTrigger fromCode(String codeString) throws IllegalArgumentException { 223 if (codeString == null || "".equals(codeString)) 224 if (codeString == null || "".equals(codeString)) 225 return null; 226 if ("create".equals(codeString)) 227 return InteractionTrigger.CREATE; 228 if ("update".equals(codeString)) 229 return InteractionTrigger.UPDATE; 230 if ("delete".equals(codeString)) 231 return InteractionTrigger.DELETE; 232 throw new IllegalArgumentException("Unknown InteractionTrigger code '"+codeString+"'"); 233 } 234 public Enumeration<InteractionTrigger> fromType(PrimitiveType<?> code) throws FHIRException { 235 if (code == null) 236 return null; 237 if (code.isEmpty()) 238 return new Enumeration<InteractionTrigger>(this, InteractionTrigger.NULL, code); 239 String codeString = ((PrimitiveType) code).asStringValue(); 240 if (codeString == null || "".equals(codeString)) 241 return new Enumeration<InteractionTrigger>(this, InteractionTrigger.NULL, code); 242 if ("create".equals(codeString)) 243 return new Enumeration<InteractionTrigger>(this, InteractionTrigger.CREATE, code); 244 if ("update".equals(codeString)) 245 return new Enumeration<InteractionTrigger>(this, InteractionTrigger.UPDATE, code); 246 if ("delete".equals(codeString)) 247 return new Enumeration<InteractionTrigger>(this, InteractionTrigger.DELETE, code); 248 throw new FHIRException("Unknown InteractionTrigger code '"+codeString+"'"); 249 } 250 public String toCode(InteractionTrigger code) { 251 if (code == InteractionTrigger.CREATE) 252 return "create"; 253 if (code == InteractionTrigger.UPDATE) 254 return "update"; 255 if (code == InteractionTrigger.DELETE) 256 return "delete"; 257 return "?"; 258 } 259 public String toSystem(InteractionTrigger code) { 260 return code.getSystem(); 261 } 262 } 263 264 @Block() 265 public static class SubscriptionTopicResourceTriggerComponent extends BackboneElement implements IBaseBackboneElement { 266 /** 267 * The human readable description of this resource trigger for the SubscriptionTopic - for example, "An Encounter enters the 'in-progress' state". 268 */ 269 @Child(name = "description", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=true) 270 @Description(shortDefinition="Text representation of the resource trigger", formalDefinition="The human readable description of this resource trigger for the SubscriptionTopic - for example, \"An Encounter enters the 'in-progress' state\"." ) 271 protected MarkdownType description; 272 273 /** 274 * URL of the Resource that is the type used in this resource trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, "Patient" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href="elementdefinition-definitions.html#ElementDefinition.type.code">ElementDefinition.type.code</a>. 275 */ 276 @Child(name = "resource", type = {UriType.class}, order=2, min=1, max=1, modifier=false, summary=true) 277 @Description(shortDefinition="Data Type or Resource (reference to definition) for this trigger definition", formalDefinition="URL of the Resource that is the type used in this resource trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, \"Patient\" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href=\"elementdefinition-definitions.html#ElementDefinition.type.code\">ElementDefinition.type.code</a>." ) 278 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscription-types") 279 protected UriType resource; 280 281 /** 282 * The FHIR RESTful interaction which can be used to trigger a notification for the SubscriptionTopic. Multiple values are considered OR joined (e.g., CREATE or UPDATE). If not present, all supported interactions are assumed. 283 */ 284 @Child(name = "supportedInteraction", type = {CodeType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 285 @Description(shortDefinition="create | update | delete", formalDefinition="The FHIR RESTful interaction which can be used to trigger a notification for the SubscriptionTopic. Multiple values are considered OR joined (e.g., CREATE or UPDATE). If not present, all supported interactions are assumed." ) 286 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/interaction-trigger") 287 protected List<Enumeration<InteractionTrigger>> supportedInteraction; 288 289 /** 290 * The FHIR query based rules that the server should use to determine when to trigger a notification for this subscription topic. 291 */ 292 @Child(name = "queryCriteria", type = {}, order=4, min=0, max=1, modifier=false, summary=true) 293 @Description(shortDefinition="Query based trigger rule", formalDefinition="The FHIR query based rules that the server should use to determine when to trigger a notification for this subscription topic." ) 294 protected SubscriptionTopicResourceTriggerQueryCriteriaComponent queryCriteria; 295 296 /** 297 * The FHIRPath based rules that the server should use to determine when to trigger a notification for this topic. 298 */ 299 @Child(name = "fhirPathCriteria", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 300 @Description(shortDefinition="FHIRPath based trigger rule", formalDefinition="The FHIRPath based rules that the server should use to determine when to trigger a notification for this topic." ) 301 protected StringType fhirPathCriteria; 302 303 private static final long serialVersionUID = -1086940999L; 304 305 /** 306 * Constructor 307 */ 308 public SubscriptionTopicResourceTriggerComponent() { 309 super(); 310 } 311 312 /** 313 * Constructor 314 */ 315 public SubscriptionTopicResourceTriggerComponent(String resource) { 316 super(); 317 this.setResource(resource); 318 } 319 320 /** 321 * @return {@link #description} (The human readable description of this resource trigger for the SubscriptionTopic - for example, "An Encounter enters the 'in-progress' state".). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 322 */ 323 public MarkdownType getDescriptionElement() { 324 if (this.description == null) 325 if (Configuration.errorOnAutoCreate()) 326 throw new Error("Attempt to auto-create SubscriptionTopicResourceTriggerComponent.description"); 327 else if (Configuration.doAutoCreate()) 328 this.description = new MarkdownType(); // bb 329 return this.description; 330 } 331 332 public boolean hasDescriptionElement() { 333 return this.description != null && !this.description.isEmpty(); 334 } 335 336 public boolean hasDescription() { 337 return this.description != null && !this.description.isEmpty(); 338 } 339 340 /** 341 * @param value {@link #description} (The human readable description of this resource trigger for the SubscriptionTopic - for example, "An Encounter enters the 'in-progress' state".). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 342 */ 343 public SubscriptionTopicResourceTriggerComponent setDescriptionElement(MarkdownType value) { 344 this.description = value; 345 return this; 346 } 347 348 /** 349 * @return The human readable description of this resource trigger for the SubscriptionTopic - for example, "An Encounter enters the 'in-progress' state". 350 */ 351 public String getDescription() { 352 return this.description == null ? null : this.description.getValue(); 353 } 354 355 /** 356 * @param value The human readable description of this resource trigger for the SubscriptionTopic - for example, "An Encounter enters the 'in-progress' state". 357 */ 358 public SubscriptionTopicResourceTriggerComponent setDescription(String value) { 359 if (Utilities.noString(value)) 360 this.description = null; 361 else { 362 if (this.description == null) 363 this.description = new MarkdownType(); 364 this.description.setValue(value); 365 } 366 return this; 367 } 368 369 /** 370 * @return {@link #resource} (URL of the Resource that is the type used in this resource trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, "Patient" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href="elementdefinition-definitions.html#ElementDefinition.type.code">ElementDefinition.type.code</a>.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 371 */ 372 public UriType getResourceElement() { 373 if (this.resource == null) 374 if (Configuration.errorOnAutoCreate()) 375 throw new Error("Attempt to auto-create SubscriptionTopicResourceTriggerComponent.resource"); 376 else if (Configuration.doAutoCreate()) 377 this.resource = new UriType(); // bb 378 return this.resource; 379 } 380 381 public boolean hasResourceElement() { 382 return this.resource != null && !this.resource.isEmpty(); 383 } 384 385 public boolean hasResource() { 386 return this.resource != null && !this.resource.isEmpty(); 387 } 388 389 /** 390 * @param value {@link #resource} (URL of the Resource that is the type used in this resource trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, "Patient" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href="elementdefinition-definitions.html#ElementDefinition.type.code">ElementDefinition.type.code</a>.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 391 */ 392 public SubscriptionTopicResourceTriggerComponent setResourceElement(UriType value) { 393 this.resource = value; 394 return this; 395 } 396 397 /** 398 * @return URL of the Resource that is the type used in this resource trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, "Patient" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href="elementdefinition-definitions.html#ElementDefinition.type.code">ElementDefinition.type.code</a>. 399 */ 400 public String getResource() { 401 return this.resource == null ? null : this.resource.getValue(); 402 } 403 404 /** 405 * @param value URL of the Resource that is the type used in this resource trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, "Patient" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href="elementdefinition-definitions.html#ElementDefinition.type.code">ElementDefinition.type.code</a>. 406 */ 407 public SubscriptionTopicResourceTriggerComponent setResource(String value) { 408 if (this.resource == null) 409 this.resource = new UriType(); 410 this.resource.setValue(value); 411 return this; 412 } 413 414 /** 415 * @return {@link #supportedInteraction} (The FHIR RESTful interaction which can be used to trigger a notification for the SubscriptionTopic. Multiple values are considered OR joined (e.g., CREATE or UPDATE). If not present, all supported interactions are assumed.) 416 */ 417 public List<Enumeration<InteractionTrigger>> getSupportedInteraction() { 418 if (this.supportedInteraction == null) 419 this.supportedInteraction = new ArrayList<Enumeration<InteractionTrigger>>(); 420 return this.supportedInteraction; 421 } 422 423 /** 424 * @return Returns a reference to <code>this</code> for easy method chaining 425 */ 426 public SubscriptionTopicResourceTriggerComponent setSupportedInteraction(List<Enumeration<InteractionTrigger>> theSupportedInteraction) { 427 this.supportedInteraction = theSupportedInteraction; 428 return this; 429 } 430 431 public boolean hasSupportedInteraction() { 432 if (this.supportedInteraction == null) 433 return false; 434 for (Enumeration<InteractionTrigger> item : this.supportedInteraction) 435 if (!item.isEmpty()) 436 return true; 437 return false; 438 } 439 440 /** 441 * @return {@link #supportedInteraction} (The FHIR RESTful interaction which can be used to trigger a notification for the SubscriptionTopic. Multiple values are considered OR joined (e.g., CREATE or UPDATE). If not present, all supported interactions are assumed.) 442 */ 443 public Enumeration<InteractionTrigger> addSupportedInteractionElement() {//2 444 Enumeration<InteractionTrigger> t = new Enumeration<InteractionTrigger>(new InteractionTriggerEnumFactory()); 445 if (this.supportedInteraction == null) 446 this.supportedInteraction = new ArrayList<Enumeration<InteractionTrigger>>(); 447 this.supportedInteraction.add(t); 448 return t; 449 } 450 451 /** 452 * @param value {@link #supportedInteraction} (The FHIR RESTful interaction which can be used to trigger a notification for the SubscriptionTopic. Multiple values are considered OR joined (e.g., CREATE or UPDATE). If not present, all supported interactions are assumed.) 453 */ 454 public SubscriptionTopicResourceTriggerComponent addSupportedInteraction(InteractionTrigger value) { //1 455 Enumeration<InteractionTrigger> t = new Enumeration<InteractionTrigger>(new InteractionTriggerEnumFactory()); 456 t.setValue(value); 457 if (this.supportedInteraction == null) 458 this.supportedInteraction = new ArrayList<Enumeration<InteractionTrigger>>(); 459 this.supportedInteraction.add(t); 460 return this; 461 } 462 463 /** 464 * @param value {@link #supportedInteraction} (The FHIR RESTful interaction which can be used to trigger a notification for the SubscriptionTopic. Multiple values are considered OR joined (e.g., CREATE or UPDATE). If not present, all supported interactions are assumed.) 465 */ 466 public boolean hasSupportedInteraction(InteractionTrigger value) { 467 if (this.supportedInteraction == null) 468 return false; 469 for (Enumeration<InteractionTrigger> v : this.supportedInteraction) 470 if (v.getValue().equals(value)) // code 471 return true; 472 return false; 473 } 474 475 /** 476 * @return {@link #queryCriteria} (The FHIR query based rules that the server should use to determine when to trigger a notification for this subscription topic.) 477 */ 478 public SubscriptionTopicResourceTriggerQueryCriteriaComponent getQueryCriteria() { 479 if (this.queryCriteria == null) 480 if (Configuration.errorOnAutoCreate()) 481 throw new Error("Attempt to auto-create SubscriptionTopicResourceTriggerComponent.queryCriteria"); 482 else if (Configuration.doAutoCreate()) 483 this.queryCriteria = new SubscriptionTopicResourceTriggerQueryCriteriaComponent(); // cc 484 return this.queryCriteria; 485 } 486 487 public boolean hasQueryCriteria() { 488 return this.queryCriteria != null && !this.queryCriteria.isEmpty(); 489 } 490 491 /** 492 * @param value {@link #queryCriteria} (The FHIR query based rules that the server should use to determine when to trigger a notification for this subscription topic.) 493 */ 494 public SubscriptionTopicResourceTriggerComponent setQueryCriteria(SubscriptionTopicResourceTriggerQueryCriteriaComponent value) { 495 this.queryCriteria = value; 496 return this; 497 } 498 499 /** 500 * @return {@link #fhirPathCriteria} (The FHIRPath based rules that the server should use to determine when to trigger a notification for this topic.). This is the underlying object with id, value and extensions. The accessor "getFhirPathCriteria" gives direct access to the value 501 */ 502 public StringType getFhirPathCriteriaElement() { 503 if (this.fhirPathCriteria == null) 504 if (Configuration.errorOnAutoCreate()) 505 throw new Error("Attempt to auto-create SubscriptionTopicResourceTriggerComponent.fhirPathCriteria"); 506 else if (Configuration.doAutoCreate()) 507 this.fhirPathCriteria = new StringType(); // bb 508 return this.fhirPathCriteria; 509 } 510 511 public boolean hasFhirPathCriteriaElement() { 512 return this.fhirPathCriteria != null && !this.fhirPathCriteria.isEmpty(); 513 } 514 515 public boolean hasFhirPathCriteria() { 516 return this.fhirPathCriteria != null && !this.fhirPathCriteria.isEmpty(); 517 } 518 519 /** 520 * @param value {@link #fhirPathCriteria} (The FHIRPath based rules that the server should use to determine when to trigger a notification for this topic.). This is the underlying object with id, value and extensions. The accessor "getFhirPathCriteria" gives direct access to the value 521 */ 522 public SubscriptionTopicResourceTriggerComponent setFhirPathCriteriaElement(StringType value) { 523 this.fhirPathCriteria = value; 524 return this; 525 } 526 527 /** 528 * @return The FHIRPath based rules that the server should use to determine when to trigger a notification for this topic. 529 */ 530 public String getFhirPathCriteria() { 531 return this.fhirPathCriteria == null ? null : this.fhirPathCriteria.getValue(); 532 } 533 534 /** 535 * @param value The FHIRPath based rules that the server should use to determine when to trigger a notification for this topic. 536 */ 537 public SubscriptionTopicResourceTriggerComponent setFhirPathCriteria(String value) { 538 if (Utilities.noString(value)) 539 this.fhirPathCriteria = null; 540 else { 541 if (this.fhirPathCriteria == null) 542 this.fhirPathCriteria = new StringType(); 543 this.fhirPathCriteria.setValue(value); 544 } 545 return this; 546 } 547 548 protected void listChildren(List<Property> children) { 549 super.listChildren(children); 550 children.add(new Property("description", "markdown", "The human readable description of this resource trigger for the SubscriptionTopic - for example, \"An Encounter enters the 'in-progress' state\".", 0, 1, description)); 551 children.add(new Property("resource", "uri", "URL of the Resource that is the type used in this resource trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, \"Patient\" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href=\"elementdefinition-definitions.html#ElementDefinition.type.code\">ElementDefinition.type.code</a>.", 0, 1, resource)); 552 children.add(new Property("supportedInteraction", "code", "The FHIR RESTful interaction which can be used to trigger a notification for the SubscriptionTopic. Multiple values are considered OR joined (e.g., CREATE or UPDATE). If not present, all supported interactions are assumed.", 0, java.lang.Integer.MAX_VALUE, supportedInteraction)); 553 children.add(new Property("queryCriteria", "", "The FHIR query based rules that the server should use to determine when to trigger a notification for this subscription topic.", 0, 1, queryCriteria)); 554 children.add(new Property("fhirPathCriteria", "string", "The FHIRPath based rules that the server should use to determine when to trigger a notification for this topic.", 0, 1, fhirPathCriteria)); 555 } 556 557 @Override 558 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 559 switch (_hash) { 560 case -1724546052: /*description*/ return new Property("description", "markdown", "The human readable description of this resource trigger for the SubscriptionTopic - for example, \"An Encounter enters the 'in-progress' state\".", 0, 1, description); 561 case -341064690: /*resource*/ return new Property("resource", "uri", "URL of the Resource that is the type used in this resource trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, \"Patient\" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href=\"elementdefinition-definitions.html#ElementDefinition.type.code\">ElementDefinition.type.code</a>.", 0, 1, resource); 562 case 1838450820: /*supportedInteraction*/ return new Property("supportedInteraction", "code", "The FHIR RESTful interaction which can be used to trigger a notification for the SubscriptionTopic. Multiple values are considered OR joined (e.g., CREATE or UPDATE). If not present, all supported interactions are assumed.", 0, java.lang.Integer.MAX_VALUE, supportedInteraction); 563 case -545123257: /*queryCriteria*/ return new Property("queryCriteria", "", "The FHIR query based rules that the server should use to determine when to trigger a notification for this subscription topic.", 0, 1, queryCriteria); 564 case 1929785263: /*fhirPathCriteria*/ return new Property("fhirPathCriteria", "string", "The FHIRPath based rules that the server should use to determine when to trigger a notification for this topic.", 0, 1, fhirPathCriteria); 565 default: return super.getNamedProperty(_hash, _name, _checkValid); 566 } 567 568 } 569 570 @Override 571 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 572 switch (hash) { 573 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 574 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // UriType 575 case 1838450820: /*supportedInteraction*/ return this.supportedInteraction == null ? new Base[0] : this.supportedInteraction.toArray(new Base[this.supportedInteraction.size()]); // Enumeration<InteractionTrigger> 576 case -545123257: /*queryCriteria*/ return this.queryCriteria == null ? new Base[0] : new Base[] {this.queryCriteria}; // SubscriptionTopicResourceTriggerQueryCriteriaComponent 577 case 1929785263: /*fhirPathCriteria*/ return this.fhirPathCriteria == null ? new Base[0] : new Base[] {this.fhirPathCriteria}; // StringType 578 default: return super.getProperty(hash, name, checkValid); 579 } 580 581 } 582 583 @Override 584 public Base setProperty(int hash, String name, Base value) throws FHIRException { 585 switch (hash) { 586 case -1724546052: // description 587 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 588 return value; 589 case -341064690: // resource 590 this.resource = TypeConvertor.castToUri(value); // UriType 591 return value; 592 case 1838450820: // supportedInteraction 593 value = new InteractionTriggerEnumFactory().fromType(TypeConvertor.castToCode(value)); 594 this.getSupportedInteraction().add((Enumeration) value); // Enumeration<InteractionTrigger> 595 return value; 596 case -545123257: // queryCriteria 597 this.queryCriteria = (SubscriptionTopicResourceTriggerQueryCriteriaComponent) value; // SubscriptionTopicResourceTriggerQueryCriteriaComponent 598 return value; 599 case 1929785263: // fhirPathCriteria 600 this.fhirPathCriteria = TypeConvertor.castToString(value); // StringType 601 return value; 602 default: return super.setProperty(hash, name, value); 603 } 604 605 } 606 607 @Override 608 public Base setProperty(String name, Base value) throws FHIRException { 609 if (name.equals("description")) { 610 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 611 } else if (name.equals("resource")) { 612 this.resource = TypeConvertor.castToUri(value); // UriType 613 } else if (name.equals("supportedInteraction")) { 614 value = new InteractionTriggerEnumFactory().fromType(TypeConvertor.castToCode(value)); 615 this.getSupportedInteraction().add((Enumeration) value); 616 } else if (name.equals("queryCriteria")) { 617 this.queryCriteria = (SubscriptionTopicResourceTriggerQueryCriteriaComponent) value; // SubscriptionTopicResourceTriggerQueryCriteriaComponent 618 } else if (name.equals("fhirPathCriteria")) { 619 this.fhirPathCriteria = TypeConvertor.castToString(value); // StringType 620 } else 621 return super.setProperty(name, value); 622 return value; 623 } 624 625 @Override 626 public void removeChild(String name, Base value) throws FHIRException { 627 if (name.equals("description")) { 628 this.description = null; 629 } else if (name.equals("resource")) { 630 this.resource = null; 631 } else if (name.equals("supportedInteraction")) { 632 value = new InteractionTriggerEnumFactory().fromType(TypeConvertor.castToCode(value)); 633 this.getSupportedInteraction().remove((Enumeration) value); 634 } else if (name.equals("queryCriteria")) { 635 this.queryCriteria = (SubscriptionTopicResourceTriggerQueryCriteriaComponent) value; // SubscriptionTopicResourceTriggerQueryCriteriaComponent 636 } else if (name.equals("fhirPathCriteria")) { 637 this.fhirPathCriteria = null; 638 } else 639 super.removeChild(name, value); 640 641 } 642 643 @Override 644 public Base makeProperty(int hash, String name) throws FHIRException { 645 switch (hash) { 646 case -1724546052: return getDescriptionElement(); 647 case -341064690: return getResourceElement(); 648 case 1838450820: return addSupportedInteractionElement(); 649 case -545123257: return getQueryCriteria(); 650 case 1929785263: return getFhirPathCriteriaElement(); 651 default: return super.makeProperty(hash, name); 652 } 653 654 } 655 656 @Override 657 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 658 switch (hash) { 659 case -1724546052: /*description*/ return new String[] {"markdown"}; 660 case -341064690: /*resource*/ return new String[] {"uri"}; 661 case 1838450820: /*supportedInteraction*/ return new String[] {"code"}; 662 case -545123257: /*queryCriteria*/ return new String[] {}; 663 case 1929785263: /*fhirPathCriteria*/ return new String[] {"string"}; 664 default: return super.getTypesForProperty(hash, name); 665 } 666 667 } 668 669 @Override 670 public Base addChild(String name) throws FHIRException { 671 if (name.equals("description")) { 672 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.resourceTrigger.description"); 673 } 674 else if (name.equals("resource")) { 675 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.resourceTrigger.resource"); 676 } 677 else if (name.equals("supportedInteraction")) { 678 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.resourceTrigger.supportedInteraction"); 679 } 680 else if (name.equals("queryCriteria")) { 681 this.queryCriteria = new SubscriptionTopicResourceTriggerQueryCriteriaComponent(); 682 return this.queryCriteria; 683 } 684 else if (name.equals("fhirPathCriteria")) { 685 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.resourceTrigger.fhirPathCriteria"); 686 } 687 else 688 return super.addChild(name); 689 } 690 691 public SubscriptionTopicResourceTriggerComponent copy() { 692 SubscriptionTopicResourceTriggerComponent dst = new SubscriptionTopicResourceTriggerComponent(); 693 copyValues(dst); 694 return dst; 695 } 696 697 public void copyValues(SubscriptionTopicResourceTriggerComponent dst) { 698 super.copyValues(dst); 699 dst.description = description == null ? null : description.copy(); 700 dst.resource = resource == null ? null : resource.copy(); 701 if (supportedInteraction != null) { 702 dst.supportedInteraction = new ArrayList<Enumeration<InteractionTrigger>>(); 703 for (Enumeration<InteractionTrigger> i : supportedInteraction) 704 dst.supportedInteraction.add(i.copy()); 705 }; 706 dst.queryCriteria = queryCriteria == null ? null : queryCriteria.copy(); 707 dst.fhirPathCriteria = fhirPathCriteria == null ? null : fhirPathCriteria.copy(); 708 } 709 710 @Override 711 public boolean equalsDeep(Base other_) { 712 if (!super.equalsDeep(other_)) 713 return false; 714 if (!(other_ instanceof SubscriptionTopicResourceTriggerComponent)) 715 return false; 716 SubscriptionTopicResourceTriggerComponent o = (SubscriptionTopicResourceTriggerComponent) other_; 717 return compareDeep(description, o.description, true) && compareDeep(resource, o.resource, true) 718 && compareDeep(supportedInteraction, o.supportedInteraction, true) && compareDeep(queryCriteria, o.queryCriteria, true) 719 && compareDeep(fhirPathCriteria, o.fhirPathCriteria, true); 720 } 721 722 @Override 723 public boolean equalsShallow(Base other_) { 724 if (!super.equalsShallow(other_)) 725 return false; 726 if (!(other_ instanceof SubscriptionTopicResourceTriggerComponent)) 727 return false; 728 SubscriptionTopicResourceTriggerComponent o = (SubscriptionTopicResourceTriggerComponent) other_; 729 return compareValues(description, o.description, true) && compareValues(resource, o.resource, true) 730 && compareValues(supportedInteraction, o.supportedInteraction, true) && compareValues(fhirPathCriteria, o.fhirPathCriteria, true) 731 ; 732 } 733 734 public boolean isEmpty() { 735 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, resource, supportedInteraction 736 , queryCriteria, fhirPathCriteria); 737 } 738 739 public String fhirType() { 740 return "SubscriptionTopic.resourceTrigger"; 741 742 } 743 744 } 745 746 @Block() 747 public static class SubscriptionTopicResourceTriggerQueryCriteriaComponent extends BackboneElement implements IBaseBackboneElement { 748 /** 749 * The FHIR query based rules are applied to the previous resource state (e.g., state before an update). 750 */ 751 @Child(name = "previous", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 752 @Description(shortDefinition="Rule applied to previous resource state", formalDefinition="The FHIR query based rules are applied to the previous resource state (e.g., state before an update)." ) 753 protected StringType previous; 754 755 /** 756 * For `create` interactions, should the `previous` criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `create` interactions is unspecified (server discretion). 757 */ 758 @Child(name = "resultForCreate", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 759 @Description(shortDefinition="test-passes | test-fails", formalDefinition="For `create` interactions, should the `previous` criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `create` interactions is unspecified (server discretion)." ) 760 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscriptiontopic-cr-behavior") 761 protected Enumeration<CriteriaNotExistsBehavior> resultForCreate; 762 763 /** 764 * The FHIR query based rules are applied to the current resource state (e.g., state after an update). 765 */ 766 @Child(name = "current", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 767 @Description(shortDefinition="Rule applied to current resource state", formalDefinition="The FHIR query based rules are applied to the current resource state (e.g., state after an update)." ) 768 protected StringType current; 769 770 /** 771 * For 'delete' interactions, should the 'current' query criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `delete` interactions is unspecified (server discretion). 772 */ 773 @Child(name = "resultForDelete", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 774 @Description(shortDefinition="test-passes | test-fails", formalDefinition="For 'delete' interactions, should the 'current' query criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `delete` interactions is unspecified (server discretion)." ) 775 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscriptiontopic-cr-behavior") 776 protected Enumeration<CriteriaNotExistsBehavior> resultForDelete; 777 778 /** 779 * If set to `true`, both the `current` and `previous` query criteria must evaluate `true` to trigger a notification for this topic. If set to `false` or not present, a notification for this topic will be triggered if either the `current` or `previous` tests evaluate to `true`. 780 */ 781 @Child(name = "requireBoth", type = {BooleanType.class}, order=5, min=0, max=1, modifier=false, summary=true) 782 @Description(shortDefinition="Both must be true flag", formalDefinition="If set to `true`, both the `current` and `previous` query criteria must evaluate `true` to trigger a notification for this topic. If set to `false` or not present, a notification for this topic will be triggered if either the `current` or `previous` tests evaluate to `true`." ) 783 protected BooleanType requireBoth; 784 785 private static final long serialVersionUID = -291746067L; 786 787 /** 788 * Constructor 789 */ 790 public SubscriptionTopicResourceTriggerQueryCriteriaComponent() { 791 super(); 792 } 793 794 /** 795 * @return {@link #previous} (The FHIR query based rules are applied to the previous resource state (e.g., state before an update).). This is the underlying object with id, value and extensions. The accessor "getPrevious" gives direct access to the value 796 */ 797 public StringType getPreviousElement() { 798 if (this.previous == null) 799 if (Configuration.errorOnAutoCreate()) 800 throw new Error("Attempt to auto-create SubscriptionTopicResourceTriggerQueryCriteriaComponent.previous"); 801 else if (Configuration.doAutoCreate()) 802 this.previous = new StringType(); // bb 803 return this.previous; 804 } 805 806 public boolean hasPreviousElement() { 807 return this.previous != null && !this.previous.isEmpty(); 808 } 809 810 public boolean hasPrevious() { 811 return this.previous != null && !this.previous.isEmpty(); 812 } 813 814 /** 815 * @param value {@link #previous} (The FHIR query based rules are applied to the previous resource state (e.g., state before an update).). This is the underlying object with id, value and extensions. The accessor "getPrevious" gives direct access to the value 816 */ 817 public SubscriptionTopicResourceTriggerQueryCriteriaComponent setPreviousElement(StringType value) { 818 this.previous = value; 819 return this; 820 } 821 822 /** 823 * @return The FHIR query based rules are applied to the previous resource state (e.g., state before an update). 824 */ 825 public String getPrevious() { 826 return this.previous == null ? null : this.previous.getValue(); 827 } 828 829 /** 830 * @param value The FHIR query based rules are applied to the previous resource state (e.g., state before an update). 831 */ 832 public SubscriptionTopicResourceTriggerQueryCriteriaComponent setPrevious(String value) { 833 if (Utilities.noString(value)) 834 this.previous = null; 835 else { 836 if (this.previous == null) 837 this.previous = new StringType(); 838 this.previous.setValue(value); 839 } 840 return this; 841 } 842 843 /** 844 * @return {@link #resultForCreate} (For `create` interactions, should the `previous` criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `create` interactions is unspecified (server discretion).). This is the underlying object with id, value and extensions. The accessor "getResultForCreate" gives direct access to the value 845 */ 846 public Enumeration<CriteriaNotExistsBehavior> getResultForCreateElement() { 847 if (this.resultForCreate == null) 848 if (Configuration.errorOnAutoCreate()) 849 throw new Error("Attempt to auto-create SubscriptionTopicResourceTriggerQueryCriteriaComponent.resultForCreate"); 850 else if (Configuration.doAutoCreate()) 851 this.resultForCreate = new Enumeration<CriteriaNotExistsBehavior>(new CriteriaNotExistsBehaviorEnumFactory()); // bb 852 return this.resultForCreate; 853 } 854 855 public boolean hasResultForCreateElement() { 856 return this.resultForCreate != null && !this.resultForCreate.isEmpty(); 857 } 858 859 public boolean hasResultForCreate() { 860 return this.resultForCreate != null && !this.resultForCreate.isEmpty(); 861 } 862 863 /** 864 * @param value {@link #resultForCreate} (For `create` interactions, should the `previous` criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `create` interactions is unspecified (server discretion).). This is the underlying object with id, value and extensions. The accessor "getResultForCreate" gives direct access to the value 865 */ 866 public SubscriptionTopicResourceTriggerQueryCriteriaComponent setResultForCreateElement(Enumeration<CriteriaNotExistsBehavior> value) { 867 this.resultForCreate = value; 868 return this; 869 } 870 871 /** 872 * @return For `create` interactions, should the `previous` criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `create` interactions is unspecified (server discretion). 873 */ 874 public CriteriaNotExistsBehavior getResultForCreate() { 875 return this.resultForCreate == null ? null : this.resultForCreate.getValue(); 876 } 877 878 /** 879 * @param value For `create` interactions, should the `previous` criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `create` interactions is unspecified (server discretion). 880 */ 881 public SubscriptionTopicResourceTriggerQueryCriteriaComponent setResultForCreate(CriteriaNotExistsBehavior value) { 882 if (value == null) 883 this.resultForCreate = null; 884 else { 885 if (this.resultForCreate == null) 886 this.resultForCreate = new Enumeration<CriteriaNotExistsBehavior>(new CriteriaNotExistsBehaviorEnumFactory()); 887 this.resultForCreate.setValue(value); 888 } 889 return this; 890 } 891 892 /** 893 * @return {@link #current} (The FHIR query based rules are applied to the current resource state (e.g., state after an update).). This is the underlying object with id, value and extensions. The accessor "getCurrent" gives direct access to the value 894 */ 895 public StringType getCurrentElement() { 896 if (this.current == null) 897 if (Configuration.errorOnAutoCreate()) 898 throw new Error("Attempt to auto-create SubscriptionTopicResourceTriggerQueryCriteriaComponent.current"); 899 else if (Configuration.doAutoCreate()) 900 this.current = new StringType(); // bb 901 return this.current; 902 } 903 904 public boolean hasCurrentElement() { 905 return this.current != null && !this.current.isEmpty(); 906 } 907 908 public boolean hasCurrent() { 909 return this.current != null && !this.current.isEmpty(); 910 } 911 912 /** 913 * @param value {@link #current} (The FHIR query based rules are applied to the current resource state (e.g., state after an update).). This is the underlying object with id, value and extensions. The accessor "getCurrent" gives direct access to the value 914 */ 915 public SubscriptionTopicResourceTriggerQueryCriteriaComponent setCurrentElement(StringType value) { 916 this.current = value; 917 return this; 918 } 919 920 /** 921 * @return The FHIR query based rules are applied to the current resource state (e.g., state after an update). 922 */ 923 public String getCurrent() { 924 return this.current == null ? null : this.current.getValue(); 925 } 926 927 /** 928 * @param value The FHIR query based rules are applied to the current resource state (e.g., state after an update). 929 */ 930 public SubscriptionTopicResourceTriggerQueryCriteriaComponent setCurrent(String value) { 931 if (Utilities.noString(value)) 932 this.current = null; 933 else { 934 if (this.current == null) 935 this.current = new StringType(); 936 this.current.setValue(value); 937 } 938 return this; 939 } 940 941 /** 942 * @return {@link #resultForDelete} (For 'delete' interactions, should the 'current' query criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `delete` interactions is unspecified (server discretion).). This is the underlying object with id, value and extensions. The accessor "getResultForDelete" gives direct access to the value 943 */ 944 public Enumeration<CriteriaNotExistsBehavior> getResultForDeleteElement() { 945 if (this.resultForDelete == null) 946 if (Configuration.errorOnAutoCreate()) 947 throw new Error("Attempt to auto-create SubscriptionTopicResourceTriggerQueryCriteriaComponent.resultForDelete"); 948 else if (Configuration.doAutoCreate()) 949 this.resultForDelete = new Enumeration<CriteriaNotExistsBehavior>(new CriteriaNotExistsBehaviorEnumFactory()); // bb 950 return this.resultForDelete; 951 } 952 953 public boolean hasResultForDeleteElement() { 954 return this.resultForDelete != null && !this.resultForDelete.isEmpty(); 955 } 956 957 public boolean hasResultForDelete() { 958 return this.resultForDelete != null && !this.resultForDelete.isEmpty(); 959 } 960 961 /** 962 * @param value {@link #resultForDelete} (For 'delete' interactions, should the 'current' query criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `delete` interactions is unspecified (server discretion).). This is the underlying object with id, value and extensions. The accessor "getResultForDelete" gives direct access to the value 963 */ 964 public SubscriptionTopicResourceTriggerQueryCriteriaComponent setResultForDeleteElement(Enumeration<CriteriaNotExistsBehavior> value) { 965 this.resultForDelete = value; 966 return this; 967 } 968 969 /** 970 * @return For 'delete' interactions, should the 'current' query criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `delete` interactions is unspecified (server discretion). 971 */ 972 public CriteriaNotExistsBehavior getResultForDelete() { 973 return this.resultForDelete == null ? null : this.resultForDelete.getValue(); 974 } 975 976 /** 977 * @param value For 'delete' interactions, should the 'current' query criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `delete` interactions is unspecified (server discretion). 978 */ 979 public SubscriptionTopicResourceTriggerQueryCriteriaComponent setResultForDelete(CriteriaNotExistsBehavior value) { 980 if (value == null) 981 this.resultForDelete = null; 982 else { 983 if (this.resultForDelete == null) 984 this.resultForDelete = new Enumeration<CriteriaNotExistsBehavior>(new CriteriaNotExistsBehaviorEnumFactory()); 985 this.resultForDelete.setValue(value); 986 } 987 return this; 988 } 989 990 /** 991 * @return {@link #requireBoth} (If set to `true`, both the `current` and `previous` query criteria must evaluate `true` to trigger a notification for this topic. If set to `false` or not present, a notification for this topic will be triggered if either the `current` or `previous` tests evaluate to `true`.). This is the underlying object with id, value and extensions. The accessor "getRequireBoth" gives direct access to the value 992 */ 993 public BooleanType getRequireBothElement() { 994 if (this.requireBoth == null) 995 if (Configuration.errorOnAutoCreate()) 996 throw new Error("Attempt to auto-create SubscriptionTopicResourceTriggerQueryCriteriaComponent.requireBoth"); 997 else if (Configuration.doAutoCreate()) 998 this.requireBoth = new BooleanType(); // bb 999 return this.requireBoth; 1000 } 1001 1002 public boolean hasRequireBothElement() { 1003 return this.requireBoth != null && !this.requireBoth.isEmpty(); 1004 } 1005 1006 public boolean hasRequireBoth() { 1007 return this.requireBoth != null && !this.requireBoth.isEmpty(); 1008 } 1009 1010 /** 1011 * @param value {@link #requireBoth} (If set to `true`, both the `current` and `previous` query criteria must evaluate `true` to trigger a notification for this topic. If set to `false` or not present, a notification for this topic will be triggered if either the `current` or `previous` tests evaluate to `true`.). This is the underlying object with id, value and extensions. The accessor "getRequireBoth" gives direct access to the value 1012 */ 1013 public SubscriptionTopicResourceTriggerQueryCriteriaComponent setRequireBothElement(BooleanType value) { 1014 this.requireBoth = value; 1015 return this; 1016 } 1017 1018 /** 1019 * @return If set to `true`, both the `current` and `previous` query criteria must evaluate `true` to trigger a notification for this topic. If set to `false` or not present, a notification for this topic will be triggered if either the `current` or `previous` tests evaluate to `true`. 1020 */ 1021 public boolean getRequireBoth() { 1022 return this.requireBoth == null || this.requireBoth.isEmpty() ? false : this.requireBoth.getValue(); 1023 } 1024 1025 /** 1026 * @param value If set to `true`, both the `current` and `previous` query criteria must evaluate `true` to trigger a notification for this topic. If set to `false` or not present, a notification for this topic will be triggered if either the `current` or `previous` tests evaluate to `true`. 1027 */ 1028 public SubscriptionTopicResourceTriggerQueryCriteriaComponent setRequireBoth(boolean value) { 1029 if (this.requireBoth == null) 1030 this.requireBoth = new BooleanType(); 1031 this.requireBoth.setValue(value); 1032 return this; 1033 } 1034 1035 protected void listChildren(List<Property> children) { 1036 super.listChildren(children); 1037 children.add(new Property("previous", "string", "The FHIR query based rules are applied to the previous resource state (e.g., state before an update).", 0, 1, previous)); 1038 children.add(new Property("resultForCreate", "code", "For `create` interactions, should the `previous` criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `create` interactions is unspecified (server discretion).", 0, 1, resultForCreate)); 1039 children.add(new Property("current", "string", "The FHIR query based rules are applied to the current resource state (e.g., state after an update).", 0, 1, current)); 1040 children.add(new Property("resultForDelete", "code", "For 'delete' interactions, should the 'current' query criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `delete` interactions is unspecified (server discretion).", 0, 1, resultForDelete)); 1041 children.add(new Property("requireBoth", "boolean", "If set to `true`, both the `current` and `previous` query criteria must evaluate `true` to trigger a notification for this topic. If set to `false` or not present, a notification for this topic will be triggered if either the `current` or `previous` tests evaluate to `true`.", 0, 1, requireBoth)); 1042 } 1043 1044 @Override 1045 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1046 switch (_hash) { 1047 case -1273775369: /*previous*/ return new Property("previous", "string", "The FHIR query based rules are applied to the previous resource state (e.g., state before an update).", 0, 1, previous); 1048 case -407976056: /*resultForCreate*/ return new Property("resultForCreate", "code", "For `create` interactions, should the `previous` criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `create` interactions is unspecified (server discretion).", 0, 1, resultForCreate); 1049 case 1126940025: /*current*/ return new Property("current", "string", "The FHIR query based rules are applied to the current resource state (e.g., state after an update).", 0, 1, current); 1050 case -391140297: /*resultForDelete*/ return new Property("resultForDelete", "code", "For 'delete' interactions, should the 'current' query criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `delete` interactions is unspecified (server discretion).", 0, 1, resultForDelete); 1051 case 362116742: /*requireBoth*/ return new Property("requireBoth", "boolean", "If set to `true`, both the `current` and `previous` query criteria must evaluate `true` to trigger a notification for this topic. If set to `false` or not present, a notification for this topic will be triggered if either the `current` or `previous` tests evaluate to `true`.", 0, 1, requireBoth); 1052 default: return super.getNamedProperty(_hash, _name, _checkValid); 1053 } 1054 1055 } 1056 1057 @Override 1058 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1059 switch (hash) { 1060 case -1273775369: /*previous*/ return this.previous == null ? new Base[0] : new Base[] {this.previous}; // StringType 1061 case -407976056: /*resultForCreate*/ return this.resultForCreate == null ? new Base[0] : new Base[] {this.resultForCreate}; // Enumeration<CriteriaNotExistsBehavior> 1062 case 1126940025: /*current*/ return this.current == null ? new Base[0] : new Base[] {this.current}; // StringType 1063 case -391140297: /*resultForDelete*/ return this.resultForDelete == null ? new Base[0] : new Base[] {this.resultForDelete}; // Enumeration<CriteriaNotExistsBehavior> 1064 case 362116742: /*requireBoth*/ return this.requireBoth == null ? new Base[0] : new Base[] {this.requireBoth}; // BooleanType 1065 default: return super.getProperty(hash, name, checkValid); 1066 } 1067 1068 } 1069 1070 @Override 1071 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1072 switch (hash) { 1073 case -1273775369: // previous 1074 this.previous = TypeConvertor.castToString(value); // StringType 1075 return value; 1076 case -407976056: // resultForCreate 1077 value = new CriteriaNotExistsBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1078 this.resultForCreate = (Enumeration) value; // Enumeration<CriteriaNotExistsBehavior> 1079 return value; 1080 case 1126940025: // current 1081 this.current = TypeConvertor.castToString(value); // StringType 1082 return value; 1083 case -391140297: // resultForDelete 1084 value = new CriteriaNotExistsBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1085 this.resultForDelete = (Enumeration) value; // Enumeration<CriteriaNotExistsBehavior> 1086 return value; 1087 case 362116742: // requireBoth 1088 this.requireBoth = TypeConvertor.castToBoolean(value); // BooleanType 1089 return value; 1090 default: return super.setProperty(hash, name, value); 1091 } 1092 1093 } 1094 1095 @Override 1096 public Base setProperty(String name, Base value) throws FHIRException { 1097 if (name.equals("previous")) { 1098 this.previous = TypeConvertor.castToString(value); // StringType 1099 } else if (name.equals("resultForCreate")) { 1100 value = new CriteriaNotExistsBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1101 this.resultForCreate = (Enumeration) value; // Enumeration<CriteriaNotExistsBehavior> 1102 } else if (name.equals("current")) { 1103 this.current = TypeConvertor.castToString(value); // StringType 1104 } else if (name.equals("resultForDelete")) { 1105 value = new CriteriaNotExistsBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1106 this.resultForDelete = (Enumeration) value; // Enumeration<CriteriaNotExistsBehavior> 1107 } else if (name.equals("requireBoth")) { 1108 this.requireBoth = TypeConvertor.castToBoolean(value); // BooleanType 1109 } else 1110 return super.setProperty(name, value); 1111 return value; 1112 } 1113 1114 @Override 1115 public void removeChild(String name, Base value) throws FHIRException { 1116 if (name.equals("previous")) { 1117 this.previous = null; 1118 } else if (name.equals("resultForCreate")) { 1119 value = new CriteriaNotExistsBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1120 this.resultForCreate = (Enumeration) value; // Enumeration<CriteriaNotExistsBehavior> 1121 } else if (name.equals("current")) { 1122 this.current = null; 1123 } else if (name.equals("resultForDelete")) { 1124 value = new CriteriaNotExistsBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1125 this.resultForDelete = (Enumeration) value; // Enumeration<CriteriaNotExistsBehavior> 1126 } else if (name.equals("requireBoth")) { 1127 this.requireBoth = null; 1128 } else 1129 super.removeChild(name, value); 1130 1131 } 1132 1133 @Override 1134 public Base makeProperty(int hash, String name) throws FHIRException { 1135 switch (hash) { 1136 case -1273775369: return getPreviousElement(); 1137 case -407976056: return getResultForCreateElement(); 1138 case 1126940025: return getCurrentElement(); 1139 case -391140297: return getResultForDeleteElement(); 1140 case 362116742: return getRequireBothElement(); 1141 default: return super.makeProperty(hash, name); 1142 } 1143 1144 } 1145 1146 @Override 1147 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1148 switch (hash) { 1149 case -1273775369: /*previous*/ return new String[] {"string"}; 1150 case -407976056: /*resultForCreate*/ return new String[] {"code"}; 1151 case 1126940025: /*current*/ return new String[] {"string"}; 1152 case -391140297: /*resultForDelete*/ return new String[] {"code"}; 1153 case 362116742: /*requireBoth*/ return new String[] {"boolean"}; 1154 default: return super.getTypesForProperty(hash, name); 1155 } 1156 1157 } 1158 1159 @Override 1160 public Base addChild(String name) throws FHIRException { 1161 if (name.equals("previous")) { 1162 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.resourceTrigger.queryCriteria.previous"); 1163 } 1164 else if (name.equals("resultForCreate")) { 1165 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.resourceTrigger.queryCriteria.resultForCreate"); 1166 } 1167 else if (name.equals("current")) { 1168 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.resourceTrigger.queryCriteria.current"); 1169 } 1170 else if (name.equals("resultForDelete")) { 1171 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.resourceTrigger.queryCriteria.resultForDelete"); 1172 } 1173 else if (name.equals("requireBoth")) { 1174 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.resourceTrigger.queryCriteria.requireBoth"); 1175 } 1176 else 1177 return super.addChild(name); 1178 } 1179 1180 public SubscriptionTopicResourceTriggerQueryCriteriaComponent copy() { 1181 SubscriptionTopicResourceTriggerQueryCriteriaComponent dst = new SubscriptionTopicResourceTriggerQueryCriteriaComponent(); 1182 copyValues(dst); 1183 return dst; 1184 } 1185 1186 public void copyValues(SubscriptionTopicResourceTriggerQueryCriteriaComponent dst) { 1187 super.copyValues(dst); 1188 dst.previous = previous == null ? null : previous.copy(); 1189 dst.resultForCreate = resultForCreate == null ? null : resultForCreate.copy(); 1190 dst.current = current == null ? null : current.copy(); 1191 dst.resultForDelete = resultForDelete == null ? null : resultForDelete.copy(); 1192 dst.requireBoth = requireBoth == null ? null : requireBoth.copy(); 1193 } 1194 1195 @Override 1196 public boolean equalsDeep(Base other_) { 1197 if (!super.equalsDeep(other_)) 1198 return false; 1199 if (!(other_ instanceof SubscriptionTopicResourceTriggerQueryCriteriaComponent)) 1200 return false; 1201 SubscriptionTopicResourceTriggerQueryCriteriaComponent o = (SubscriptionTopicResourceTriggerQueryCriteriaComponent) other_; 1202 return compareDeep(previous, o.previous, true) && compareDeep(resultForCreate, o.resultForCreate, true) 1203 && compareDeep(current, o.current, true) && compareDeep(resultForDelete, o.resultForDelete, true) 1204 && compareDeep(requireBoth, o.requireBoth, true); 1205 } 1206 1207 @Override 1208 public boolean equalsShallow(Base other_) { 1209 if (!super.equalsShallow(other_)) 1210 return false; 1211 if (!(other_ instanceof SubscriptionTopicResourceTriggerQueryCriteriaComponent)) 1212 return false; 1213 SubscriptionTopicResourceTriggerQueryCriteriaComponent o = (SubscriptionTopicResourceTriggerQueryCriteriaComponent) other_; 1214 return compareValues(previous, o.previous, true) && compareValues(resultForCreate, o.resultForCreate, true) 1215 && compareValues(current, o.current, true) && compareValues(resultForDelete, o.resultForDelete, true) 1216 && compareValues(requireBoth, o.requireBoth, true); 1217 } 1218 1219 public boolean isEmpty() { 1220 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(previous, resultForCreate 1221 , current, resultForDelete, requireBoth); 1222 } 1223 1224 public String fhirType() { 1225 return "SubscriptionTopic.resourceTrigger.queryCriteria"; 1226 1227 } 1228 1229 } 1230 1231 @Block() 1232 public static class SubscriptionTopicEventTriggerComponent extends BackboneElement implements IBaseBackboneElement { 1233 /** 1234 * The human readable description of an event to trigger a notification for the SubscriptionTopic - for example, "Patient Admission, as defined in HL7v2 via message ADT^A01". Multiple values are considered OR joined (e.g., matching any single event listed). 1235 */ 1236 @Child(name = "description", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1237 @Description(shortDefinition="Text representation of the event trigger", formalDefinition="The human readable description of an event to trigger a notification for the SubscriptionTopic - for example, \"Patient Admission, as defined in HL7v2 via message ADT^A01\". Multiple values are considered OR joined (e.g., matching any single event listed)." ) 1238 protected MarkdownType description; 1239 1240 /** 1241 * A well-defined event which can be used to trigger notifications from the SubscriptionTopic. 1242 */ 1243 @Child(name = "event", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=true) 1244 @Description(shortDefinition="Event which can trigger a notification from the SubscriptionTopic", formalDefinition="A well-defined event which can be used to trigger notifications from the SubscriptionTopic." ) 1245 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v2-0003") 1246 protected CodeableConcept event; 1247 1248 /** 1249 * URL of the Resource that is the focus type used in this event trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, "Patient" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href="elementdefinition-definitions.html#ElementDefinition.type.code">ElementDefinition.type.code</a>. 1250 */ 1251 @Child(name = "resource", type = {UriType.class}, order=3, min=1, max=1, modifier=false, summary=true) 1252 @Description(shortDefinition="Data Type or Resource (reference to definition) for this trigger definition", formalDefinition="URL of the Resource that is the focus type used in this event trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, \"Patient\" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href=\"elementdefinition-definitions.html#ElementDefinition.type.code\">ElementDefinition.type.code</a>." ) 1253 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscription-types") 1254 protected UriType resource; 1255 1256 private static final long serialVersionUID = 1818872110L; 1257 1258 /** 1259 * Constructor 1260 */ 1261 public SubscriptionTopicEventTriggerComponent() { 1262 super(); 1263 } 1264 1265 /** 1266 * Constructor 1267 */ 1268 public SubscriptionTopicEventTriggerComponent(CodeableConcept event, String resource) { 1269 super(); 1270 this.setEvent(event); 1271 this.setResource(resource); 1272 } 1273 1274 /** 1275 * @return {@link #description} (The human readable description of an event to trigger a notification for the SubscriptionTopic - for example, "Patient Admission, as defined in HL7v2 via message ADT^A01". Multiple values are considered OR joined (e.g., matching any single event listed).). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1276 */ 1277 public MarkdownType getDescriptionElement() { 1278 if (this.description == null) 1279 if (Configuration.errorOnAutoCreate()) 1280 throw new Error("Attempt to auto-create SubscriptionTopicEventTriggerComponent.description"); 1281 else if (Configuration.doAutoCreate()) 1282 this.description = new MarkdownType(); // bb 1283 return this.description; 1284 } 1285 1286 public boolean hasDescriptionElement() { 1287 return this.description != null && !this.description.isEmpty(); 1288 } 1289 1290 public boolean hasDescription() { 1291 return this.description != null && !this.description.isEmpty(); 1292 } 1293 1294 /** 1295 * @param value {@link #description} (The human readable description of an event to trigger a notification for the SubscriptionTopic - for example, "Patient Admission, as defined in HL7v2 via message ADT^A01". Multiple values are considered OR joined (e.g., matching any single event listed).). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1296 */ 1297 public SubscriptionTopicEventTriggerComponent setDescriptionElement(MarkdownType value) { 1298 this.description = value; 1299 return this; 1300 } 1301 1302 /** 1303 * @return The human readable description of an event to trigger a notification for the SubscriptionTopic - for example, "Patient Admission, as defined in HL7v2 via message ADT^A01". Multiple values are considered OR joined (e.g., matching any single event listed). 1304 */ 1305 public String getDescription() { 1306 return this.description == null ? null : this.description.getValue(); 1307 } 1308 1309 /** 1310 * @param value The human readable description of an event to trigger a notification for the SubscriptionTopic - for example, "Patient Admission, as defined in HL7v2 via message ADT^A01". Multiple values are considered OR joined (e.g., matching any single event listed). 1311 */ 1312 public SubscriptionTopicEventTriggerComponent setDescription(String value) { 1313 if (Utilities.noString(value)) 1314 this.description = null; 1315 else { 1316 if (this.description == null) 1317 this.description = new MarkdownType(); 1318 this.description.setValue(value); 1319 } 1320 return this; 1321 } 1322 1323 /** 1324 * @return {@link #event} (A well-defined event which can be used to trigger notifications from the SubscriptionTopic.) 1325 */ 1326 public CodeableConcept getEvent() { 1327 if (this.event == null) 1328 if (Configuration.errorOnAutoCreate()) 1329 throw new Error("Attempt to auto-create SubscriptionTopicEventTriggerComponent.event"); 1330 else if (Configuration.doAutoCreate()) 1331 this.event = new CodeableConcept(); // cc 1332 return this.event; 1333 } 1334 1335 public boolean hasEvent() { 1336 return this.event != null && !this.event.isEmpty(); 1337 } 1338 1339 /** 1340 * @param value {@link #event} (A well-defined event which can be used to trigger notifications from the SubscriptionTopic.) 1341 */ 1342 public SubscriptionTopicEventTriggerComponent setEvent(CodeableConcept value) { 1343 this.event = value; 1344 return this; 1345 } 1346 1347 /** 1348 * @return {@link #resource} (URL of the Resource that is the focus type used in this event trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, "Patient" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href="elementdefinition-definitions.html#ElementDefinition.type.code">ElementDefinition.type.code</a>.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 1349 */ 1350 public UriType getResourceElement() { 1351 if (this.resource == null) 1352 if (Configuration.errorOnAutoCreate()) 1353 throw new Error("Attempt to auto-create SubscriptionTopicEventTriggerComponent.resource"); 1354 else if (Configuration.doAutoCreate()) 1355 this.resource = new UriType(); // bb 1356 return this.resource; 1357 } 1358 1359 public boolean hasResourceElement() { 1360 return this.resource != null && !this.resource.isEmpty(); 1361 } 1362 1363 public boolean hasResource() { 1364 return this.resource != null && !this.resource.isEmpty(); 1365 } 1366 1367 /** 1368 * @param value {@link #resource} (URL of the Resource that is the focus type used in this event trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, "Patient" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href="elementdefinition-definitions.html#ElementDefinition.type.code">ElementDefinition.type.code</a>.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 1369 */ 1370 public SubscriptionTopicEventTriggerComponent setResourceElement(UriType value) { 1371 this.resource = value; 1372 return this; 1373 } 1374 1375 /** 1376 * @return URL of the Resource that is the focus type used in this event trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, "Patient" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href="elementdefinition-definitions.html#ElementDefinition.type.code">ElementDefinition.type.code</a>. 1377 */ 1378 public String getResource() { 1379 return this.resource == null ? null : this.resource.getValue(); 1380 } 1381 1382 /** 1383 * @param value URL of the Resource that is the focus type used in this event trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, "Patient" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href="elementdefinition-definitions.html#ElementDefinition.type.code">ElementDefinition.type.code</a>. 1384 */ 1385 public SubscriptionTopicEventTriggerComponent setResource(String value) { 1386 if (this.resource == null) 1387 this.resource = new UriType(); 1388 this.resource.setValue(value); 1389 return this; 1390 } 1391 1392 protected void listChildren(List<Property> children) { 1393 super.listChildren(children); 1394 children.add(new Property("description", "markdown", "The human readable description of an event to trigger a notification for the SubscriptionTopic - for example, \"Patient Admission, as defined in HL7v2 via message ADT^A01\". Multiple values are considered OR joined (e.g., matching any single event listed).", 0, 1, description)); 1395 children.add(new Property("event", "CodeableConcept", "A well-defined event which can be used to trigger notifications from the SubscriptionTopic.", 0, 1, event)); 1396 children.add(new Property("resource", "uri", "URL of the Resource that is the focus type used in this event trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, \"Patient\" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href=\"elementdefinition-definitions.html#ElementDefinition.type.code\">ElementDefinition.type.code</a>.", 0, 1, resource)); 1397 } 1398 1399 @Override 1400 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1401 switch (_hash) { 1402 case -1724546052: /*description*/ return new Property("description", "markdown", "The human readable description of an event to trigger a notification for the SubscriptionTopic - for example, \"Patient Admission, as defined in HL7v2 via message ADT^A01\". Multiple values are considered OR joined (e.g., matching any single event listed).", 0, 1, description); 1403 case 96891546: /*event*/ return new Property("event", "CodeableConcept", "A well-defined event which can be used to trigger notifications from the SubscriptionTopic.", 0, 1, event); 1404 case -341064690: /*resource*/ return new Property("resource", "uri", "URL of the Resource that is the focus type used in this event trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, \"Patient\" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href=\"elementdefinition-definitions.html#ElementDefinition.type.code\">ElementDefinition.type.code</a>.", 0, 1, resource); 1405 default: return super.getNamedProperty(_hash, _name, _checkValid); 1406 } 1407 1408 } 1409 1410 @Override 1411 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1412 switch (hash) { 1413 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1414 case 96891546: /*event*/ return this.event == null ? new Base[0] : new Base[] {this.event}; // CodeableConcept 1415 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // UriType 1416 default: return super.getProperty(hash, name, checkValid); 1417 } 1418 1419 } 1420 1421 @Override 1422 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1423 switch (hash) { 1424 case -1724546052: // description 1425 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1426 return value; 1427 case 96891546: // event 1428 this.event = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1429 return value; 1430 case -341064690: // resource 1431 this.resource = TypeConvertor.castToUri(value); // UriType 1432 return value; 1433 default: return super.setProperty(hash, name, value); 1434 } 1435 1436 } 1437 1438 @Override 1439 public Base setProperty(String name, Base value) throws FHIRException { 1440 if (name.equals("description")) { 1441 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1442 } else if (name.equals("event")) { 1443 this.event = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1444 } else if (name.equals("resource")) { 1445 this.resource = TypeConvertor.castToUri(value); // UriType 1446 } else 1447 return super.setProperty(name, value); 1448 return value; 1449 } 1450 1451 @Override 1452 public void removeChild(String name, Base value) throws FHIRException { 1453 if (name.equals("description")) { 1454 this.description = null; 1455 } else if (name.equals("event")) { 1456 this.event = null; 1457 } else if (name.equals("resource")) { 1458 this.resource = null; 1459 } else 1460 super.removeChild(name, value); 1461 1462 } 1463 1464 @Override 1465 public Base makeProperty(int hash, String name) throws FHIRException { 1466 switch (hash) { 1467 case -1724546052: return getDescriptionElement(); 1468 case 96891546: return getEvent(); 1469 case -341064690: return getResourceElement(); 1470 default: return super.makeProperty(hash, name); 1471 } 1472 1473 } 1474 1475 @Override 1476 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1477 switch (hash) { 1478 case -1724546052: /*description*/ return new String[] {"markdown"}; 1479 case 96891546: /*event*/ return new String[] {"CodeableConcept"}; 1480 case -341064690: /*resource*/ return new String[] {"uri"}; 1481 default: return super.getTypesForProperty(hash, name); 1482 } 1483 1484 } 1485 1486 @Override 1487 public Base addChild(String name) throws FHIRException { 1488 if (name.equals("description")) { 1489 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.eventTrigger.description"); 1490 } 1491 else if (name.equals("event")) { 1492 this.event = new CodeableConcept(); 1493 return this.event; 1494 } 1495 else if (name.equals("resource")) { 1496 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.eventTrigger.resource"); 1497 } 1498 else 1499 return super.addChild(name); 1500 } 1501 1502 public SubscriptionTopicEventTriggerComponent copy() { 1503 SubscriptionTopicEventTriggerComponent dst = new SubscriptionTopicEventTriggerComponent(); 1504 copyValues(dst); 1505 return dst; 1506 } 1507 1508 public void copyValues(SubscriptionTopicEventTriggerComponent dst) { 1509 super.copyValues(dst); 1510 dst.description = description == null ? null : description.copy(); 1511 dst.event = event == null ? null : event.copy(); 1512 dst.resource = resource == null ? null : resource.copy(); 1513 } 1514 1515 @Override 1516 public boolean equalsDeep(Base other_) { 1517 if (!super.equalsDeep(other_)) 1518 return false; 1519 if (!(other_ instanceof SubscriptionTopicEventTriggerComponent)) 1520 return false; 1521 SubscriptionTopicEventTriggerComponent o = (SubscriptionTopicEventTriggerComponent) other_; 1522 return compareDeep(description, o.description, true) && compareDeep(event, o.event, true) && compareDeep(resource, o.resource, true) 1523 ; 1524 } 1525 1526 @Override 1527 public boolean equalsShallow(Base other_) { 1528 if (!super.equalsShallow(other_)) 1529 return false; 1530 if (!(other_ instanceof SubscriptionTopicEventTriggerComponent)) 1531 return false; 1532 SubscriptionTopicEventTriggerComponent o = (SubscriptionTopicEventTriggerComponent) other_; 1533 return compareValues(description, o.description, true) && compareValues(resource, o.resource, true) 1534 ; 1535 } 1536 1537 public boolean isEmpty() { 1538 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, event, resource 1539 ); 1540 } 1541 1542 public String fhirType() { 1543 return "SubscriptionTopic.eventTrigger"; 1544 1545 } 1546 1547 } 1548 1549 @Block() 1550 public static class SubscriptionTopicCanFilterByComponent extends BackboneElement implements IBaseBackboneElement { 1551 /** 1552 * Description of how this filtering parameter is intended to be used. 1553 */ 1554 @Child(name = "description", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1555 @Description(shortDefinition="Description of this filter parameter", formalDefinition="Description of how this filtering parameter is intended to be used." ) 1556 protected MarkdownType description; 1557 1558 /** 1559 * URL of the Resource that is the type used in this filter. This is the "focus" of the topic (or one of them if there are more than one). It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present. 1560 */ 1561 @Child(name = "resource", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1562 @Description(shortDefinition="URL of the triggering Resource that this filter applies to", formalDefinition="URL of the Resource that is the type used in this filter. This is the \"focus\" of the topic (or one of them if there are more than one). It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present." ) 1563 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscription-types") 1564 protected UriType resource; 1565 1566 /** 1567 * Either the canonical URL to a search parameter (like "http://hl7.org/fhir/SearchParameter/encounter-patient") or topic-defined parameter (like "hub.event") which is a label for the filter. 1568 */ 1569 @Child(name = "filterParameter", type = {StringType.class}, order=3, min=1, max=1, modifier=false, summary=true) 1570 @Description(shortDefinition="Human-readable and computation-friendly name for a filter parameter usable by subscriptions on this topic, via Subscription.filterBy.filterParameter", formalDefinition="Either the canonical URL to a search parameter (like \"http://hl7.org/fhir/SearchParameter/encounter-patient\") or topic-defined parameter (like \"hub.event\") which is a label for the filter." ) 1571 protected StringType filterParameter; 1572 1573 /** 1574 * Either the canonical URL to a search parameter (like "http://hl7.org/fhir/SearchParameter/encounter-patient") or the officially-defined URI for a shared filter concept (like "http://example.org/concepts/shared-common-event"). 1575 */ 1576 @Child(name = "filterDefinition", type = {UriType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1577 @Description(shortDefinition="Canonical URL for a filterParameter definition", formalDefinition="Either the canonical URL to a search parameter (like \"http://hl7.org/fhir/SearchParameter/encounter-patient\") or the officially-defined URI for a shared filter concept (like \"http://example.org/concepts/shared-common-event\")." ) 1578 protected UriType filterDefinition; 1579 1580 /** 1581 * Comparators allowed for the filter parameter. 1582 */ 1583 @Child(name = "comparator", type = {CodeType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1584 @Description(shortDefinition="eq | ne | gt | lt | ge | le | sa | eb | ap", formalDefinition="Comparators allowed for the filter parameter." ) 1585 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-comparator") 1586 protected List<Enumeration<SearchComparator>> comparator; 1587 1588 /** 1589 * Modifiers allowed for the filter parameter. 1590 */ 1591 @Child(name = "modifier", type = {CodeType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1592 @Description(shortDefinition="missing | exact | contains | not | text | in | not-in | below | above | type | identifier | of-type | code-text | text-advanced | iterate", formalDefinition="Modifiers allowed for the filter parameter." ) 1593 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-modifier-code") 1594 protected List<Enumeration<SearchModifierCode>> modifier; 1595 1596 private static final long serialVersionUID = -1521606527L; 1597 1598 /** 1599 * Constructor 1600 */ 1601 public SubscriptionTopicCanFilterByComponent() { 1602 super(); 1603 } 1604 1605 /** 1606 * Constructor 1607 */ 1608 public SubscriptionTopicCanFilterByComponent(String filterParameter) { 1609 super(); 1610 this.setFilterParameter(filterParameter); 1611 } 1612 1613 /** 1614 * @return {@link #description} (Description of how this filtering parameter is intended to be used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1615 */ 1616 public MarkdownType getDescriptionElement() { 1617 if (this.description == null) 1618 if (Configuration.errorOnAutoCreate()) 1619 throw new Error("Attempt to auto-create SubscriptionTopicCanFilterByComponent.description"); 1620 else if (Configuration.doAutoCreate()) 1621 this.description = new MarkdownType(); // bb 1622 return this.description; 1623 } 1624 1625 public boolean hasDescriptionElement() { 1626 return this.description != null && !this.description.isEmpty(); 1627 } 1628 1629 public boolean hasDescription() { 1630 return this.description != null && !this.description.isEmpty(); 1631 } 1632 1633 /** 1634 * @param value {@link #description} (Description of how this filtering parameter is intended to be used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1635 */ 1636 public SubscriptionTopicCanFilterByComponent setDescriptionElement(MarkdownType value) { 1637 this.description = value; 1638 return this; 1639 } 1640 1641 /** 1642 * @return Description of how this filtering parameter is intended to be used. 1643 */ 1644 public String getDescription() { 1645 return this.description == null ? null : this.description.getValue(); 1646 } 1647 1648 /** 1649 * @param value Description of how this filtering parameter is intended to be used. 1650 */ 1651 public SubscriptionTopicCanFilterByComponent setDescription(String value) { 1652 if (Utilities.noString(value)) 1653 this.description = null; 1654 else { 1655 if (this.description == null) 1656 this.description = new MarkdownType(); 1657 this.description.setValue(value); 1658 } 1659 return this; 1660 } 1661 1662 /** 1663 * @return {@link #resource} (URL of the Resource that is the type used in this filter. This is the "focus" of the topic (or one of them if there are more than one). It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 1664 */ 1665 public UriType getResourceElement() { 1666 if (this.resource == null) 1667 if (Configuration.errorOnAutoCreate()) 1668 throw new Error("Attempt to auto-create SubscriptionTopicCanFilterByComponent.resource"); 1669 else if (Configuration.doAutoCreate()) 1670 this.resource = new UriType(); // bb 1671 return this.resource; 1672 } 1673 1674 public boolean hasResourceElement() { 1675 return this.resource != null && !this.resource.isEmpty(); 1676 } 1677 1678 public boolean hasResource() { 1679 return this.resource != null && !this.resource.isEmpty(); 1680 } 1681 1682 /** 1683 * @param value {@link #resource} (URL of the Resource that is the type used in this filter. This is the "focus" of the topic (or one of them if there are more than one). It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 1684 */ 1685 public SubscriptionTopicCanFilterByComponent setResourceElement(UriType value) { 1686 this.resource = value; 1687 return this; 1688 } 1689 1690 /** 1691 * @return URL of the Resource that is the type used in this filter. This is the "focus" of the topic (or one of them if there are more than one). It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present. 1692 */ 1693 public String getResource() { 1694 return this.resource == null ? null : this.resource.getValue(); 1695 } 1696 1697 /** 1698 * @param value URL of the Resource that is the type used in this filter. This is the "focus" of the topic (or one of them if there are more than one). It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present. 1699 */ 1700 public SubscriptionTopicCanFilterByComponent setResource(String value) { 1701 if (Utilities.noString(value)) 1702 this.resource = null; 1703 else { 1704 if (this.resource == null) 1705 this.resource = new UriType(); 1706 this.resource.setValue(value); 1707 } 1708 return this; 1709 } 1710 1711 /** 1712 * @return {@link #filterParameter} (Either the canonical URL to a search parameter (like "http://hl7.org/fhir/SearchParameter/encounter-patient") or topic-defined parameter (like "hub.event") which is a label for the filter.). This is the underlying object with id, value and extensions. The accessor "getFilterParameter" gives direct access to the value 1713 */ 1714 public StringType getFilterParameterElement() { 1715 if (this.filterParameter == null) 1716 if (Configuration.errorOnAutoCreate()) 1717 throw new Error("Attempt to auto-create SubscriptionTopicCanFilterByComponent.filterParameter"); 1718 else if (Configuration.doAutoCreate()) 1719 this.filterParameter = new StringType(); // bb 1720 return this.filterParameter; 1721 } 1722 1723 public boolean hasFilterParameterElement() { 1724 return this.filterParameter != null && !this.filterParameter.isEmpty(); 1725 } 1726 1727 public boolean hasFilterParameter() { 1728 return this.filterParameter != null && !this.filterParameter.isEmpty(); 1729 } 1730 1731 /** 1732 * @param value {@link #filterParameter} (Either the canonical URL to a search parameter (like "http://hl7.org/fhir/SearchParameter/encounter-patient") or topic-defined parameter (like "hub.event") which is a label for the filter.). This is the underlying object with id, value and extensions. The accessor "getFilterParameter" gives direct access to the value 1733 */ 1734 public SubscriptionTopicCanFilterByComponent setFilterParameterElement(StringType value) { 1735 this.filterParameter = value; 1736 return this; 1737 } 1738 1739 /** 1740 * @return Either the canonical URL to a search parameter (like "http://hl7.org/fhir/SearchParameter/encounter-patient") or topic-defined parameter (like "hub.event") which is a label for the filter. 1741 */ 1742 public String getFilterParameter() { 1743 return this.filterParameter == null ? null : this.filterParameter.getValue(); 1744 } 1745 1746 /** 1747 * @param value Either the canonical URL to a search parameter (like "http://hl7.org/fhir/SearchParameter/encounter-patient") or topic-defined parameter (like "hub.event") which is a label for the filter. 1748 */ 1749 public SubscriptionTopicCanFilterByComponent setFilterParameter(String value) { 1750 if (this.filterParameter == null) 1751 this.filterParameter = new StringType(); 1752 this.filterParameter.setValue(value); 1753 return this; 1754 } 1755 1756 /** 1757 * @return {@link #filterDefinition} (Either the canonical URL to a search parameter (like "http://hl7.org/fhir/SearchParameter/encounter-patient") or the officially-defined URI for a shared filter concept (like "http://example.org/concepts/shared-common-event").). This is the underlying object with id, value and extensions. The accessor "getFilterDefinition" gives direct access to the value 1758 */ 1759 public UriType getFilterDefinitionElement() { 1760 if (this.filterDefinition == null) 1761 if (Configuration.errorOnAutoCreate()) 1762 throw new Error("Attempt to auto-create SubscriptionTopicCanFilterByComponent.filterDefinition"); 1763 else if (Configuration.doAutoCreate()) 1764 this.filterDefinition = new UriType(); // bb 1765 return this.filterDefinition; 1766 } 1767 1768 public boolean hasFilterDefinitionElement() { 1769 return this.filterDefinition != null && !this.filterDefinition.isEmpty(); 1770 } 1771 1772 public boolean hasFilterDefinition() { 1773 return this.filterDefinition != null && !this.filterDefinition.isEmpty(); 1774 } 1775 1776 /** 1777 * @param value {@link #filterDefinition} (Either the canonical URL to a search parameter (like "http://hl7.org/fhir/SearchParameter/encounter-patient") or the officially-defined URI for a shared filter concept (like "http://example.org/concepts/shared-common-event").). This is the underlying object with id, value and extensions. The accessor "getFilterDefinition" gives direct access to the value 1778 */ 1779 public SubscriptionTopicCanFilterByComponent setFilterDefinitionElement(UriType value) { 1780 this.filterDefinition = value; 1781 return this; 1782 } 1783 1784 /** 1785 * @return Either the canonical URL to a search parameter (like "http://hl7.org/fhir/SearchParameter/encounter-patient") or the officially-defined URI for a shared filter concept (like "http://example.org/concepts/shared-common-event"). 1786 */ 1787 public String getFilterDefinition() { 1788 return this.filterDefinition == null ? null : this.filterDefinition.getValue(); 1789 } 1790 1791 /** 1792 * @param value Either the canonical URL to a search parameter (like "http://hl7.org/fhir/SearchParameter/encounter-patient") or the officially-defined URI for a shared filter concept (like "http://example.org/concepts/shared-common-event"). 1793 */ 1794 public SubscriptionTopicCanFilterByComponent setFilterDefinition(String value) { 1795 if (Utilities.noString(value)) 1796 this.filterDefinition = null; 1797 else { 1798 if (this.filterDefinition == null) 1799 this.filterDefinition = new UriType(); 1800 this.filterDefinition.setValue(value); 1801 } 1802 return this; 1803 } 1804 1805 /** 1806 * @return {@link #comparator} (Comparators allowed for the filter parameter.) 1807 */ 1808 public List<Enumeration<SearchComparator>> getComparator() { 1809 if (this.comparator == null) 1810 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 1811 return this.comparator; 1812 } 1813 1814 /** 1815 * @return Returns a reference to <code>this</code> for easy method chaining 1816 */ 1817 public SubscriptionTopicCanFilterByComponent setComparator(List<Enumeration<SearchComparator>> theComparator) { 1818 this.comparator = theComparator; 1819 return this; 1820 } 1821 1822 public boolean hasComparator() { 1823 if (this.comparator == null) 1824 return false; 1825 for (Enumeration<SearchComparator> item : this.comparator) 1826 if (!item.isEmpty()) 1827 return true; 1828 return false; 1829 } 1830 1831 /** 1832 * @return {@link #comparator} (Comparators allowed for the filter parameter.) 1833 */ 1834 public Enumeration<SearchComparator> addComparatorElement() {//2 1835 Enumeration<SearchComparator> t = new Enumeration<SearchComparator>(new SearchComparatorEnumFactory()); 1836 if (this.comparator == null) 1837 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 1838 this.comparator.add(t); 1839 return t; 1840 } 1841 1842 /** 1843 * @param value {@link #comparator} (Comparators allowed for the filter parameter.) 1844 */ 1845 public SubscriptionTopicCanFilterByComponent addComparator(SearchComparator value) { //1 1846 Enumeration<SearchComparator> t = new Enumeration<SearchComparator>(new SearchComparatorEnumFactory()); 1847 t.setValue(value); 1848 if (this.comparator == null) 1849 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 1850 this.comparator.add(t); 1851 return this; 1852 } 1853 1854 /** 1855 * @param value {@link #comparator} (Comparators allowed for the filter parameter.) 1856 */ 1857 public boolean hasComparator(SearchComparator value) { 1858 if (this.comparator == null) 1859 return false; 1860 for (Enumeration<SearchComparator> v : this.comparator) 1861 if (v.getValue().equals(value)) // code 1862 return true; 1863 return false; 1864 } 1865 1866 /** 1867 * @return {@link #modifier} (Modifiers allowed for the filter parameter.) 1868 */ 1869 public List<Enumeration<SearchModifierCode>> getModifier() { 1870 if (this.modifier == null) 1871 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 1872 return this.modifier; 1873 } 1874 1875 /** 1876 * @return Returns a reference to <code>this</code> for easy method chaining 1877 */ 1878 public SubscriptionTopicCanFilterByComponent setModifier(List<Enumeration<SearchModifierCode>> theModifier) { 1879 this.modifier = theModifier; 1880 return this; 1881 } 1882 1883 public boolean hasModifier() { 1884 if (this.modifier == null) 1885 return false; 1886 for (Enumeration<SearchModifierCode> item : this.modifier) 1887 if (!item.isEmpty()) 1888 return true; 1889 return false; 1890 } 1891 1892 /** 1893 * @return {@link #modifier} (Modifiers allowed for the filter parameter.) 1894 */ 1895 public Enumeration<SearchModifierCode> addModifierElement() {//2 1896 Enumeration<SearchModifierCode> t = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 1897 if (this.modifier == null) 1898 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 1899 this.modifier.add(t); 1900 return t; 1901 } 1902 1903 /** 1904 * @param value {@link #modifier} (Modifiers allowed for the filter parameter.) 1905 */ 1906 public SubscriptionTopicCanFilterByComponent addModifier(SearchModifierCode value) { //1 1907 Enumeration<SearchModifierCode> t = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 1908 t.setValue(value); 1909 if (this.modifier == null) 1910 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 1911 this.modifier.add(t); 1912 return this; 1913 } 1914 1915 /** 1916 * @param value {@link #modifier} (Modifiers allowed for the filter parameter.) 1917 */ 1918 public boolean hasModifier(SearchModifierCode value) { 1919 if (this.modifier == null) 1920 return false; 1921 for (Enumeration<SearchModifierCode> v : this.modifier) 1922 if (v.getValue().equals(value)) // code 1923 return true; 1924 return false; 1925 } 1926 1927 protected void listChildren(List<Property> children) { 1928 super.listChildren(children); 1929 children.add(new Property("description", "markdown", "Description of how this filtering parameter is intended to be used.", 0, 1, description)); 1930 children.add(new Property("resource", "uri", "URL of the Resource that is the type used in this filter. This is the \"focus\" of the topic (or one of them if there are more than one). It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present.", 0, 1, resource)); 1931 children.add(new Property("filterParameter", "string", "Either the canonical URL to a search parameter (like \"http://hl7.org/fhir/SearchParameter/encounter-patient\") or topic-defined parameter (like \"hub.event\") which is a label for the filter.", 0, 1, filterParameter)); 1932 children.add(new Property("filterDefinition", "uri", "Either the canonical URL to a search parameter (like \"http://hl7.org/fhir/SearchParameter/encounter-patient\") or the officially-defined URI for a shared filter concept (like \"http://example.org/concepts/shared-common-event\").", 0, 1, filterDefinition)); 1933 children.add(new Property("comparator", "code", "Comparators allowed for the filter parameter.", 0, java.lang.Integer.MAX_VALUE, comparator)); 1934 children.add(new Property("modifier", "code", "Modifiers allowed for the filter parameter.", 0, java.lang.Integer.MAX_VALUE, modifier)); 1935 } 1936 1937 @Override 1938 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1939 switch (_hash) { 1940 case -1724546052: /*description*/ return new Property("description", "markdown", "Description of how this filtering parameter is intended to be used.", 0, 1, description); 1941 case -341064690: /*resource*/ return new Property("resource", "uri", "URL of the Resource that is the type used in this filter. This is the \"focus\" of the topic (or one of them if there are more than one). It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present.", 0, 1, resource); 1942 case 618257: /*filterParameter*/ return new Property("filterParameter", "string", "Either the canonical URL to a search parameter (like \"http://hl7.org/fhir/SearchParameter/encounter-patient\") or topic-defined parameter (like \"hub.event\") which is a label for the filter.", 0, 1, filterParameter); 1943 case -1453988117: /*filterDefinition*/ return new Property("filterDefinition", "uri", "Either the canonical URL to a search parameter (like \"http://hl7.org/fhir/SearchParameter/encounter-patient\") or the officially-defined URI for a shared filter concept (like \"http://example.org/concepts/shared-common-event\").", 0, 1, filterDefinition); 1944 case -844673834: /*comparator*/ return new Property("comparator", "code", "Comparators allowed for the filter parameter.", 0, java.lang.Integer.MAX_VALUE, comparator); 1945 case -615513385: /*modifier*/ return new Property("modifier", "code", "Modifiers allowed for the filter parameter.", 0, java.lang.Integer.MAX_VALUE, modifier); 1946 default: return super.getNamedProperty(_hash, _name, _checkValid); 1947 } 1948 1949 } 1950 1951 @Override 1952 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1953 switch (hash) { 1954 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1955 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // UriType 1956 case 618257: /*filterParameter*/ return this.filterParameter == null ? new Base[0] : new Base[] {this.filterParameter}; // StringType 1957 case -1453988117: /*filterDefinition*/ return this.filterDefinition == null ? new Base[0] : new Base[] {this.filterDefinition}; // UriType 1958 case -844673834: /*comparator*/ return this.comparator == null ? new Base[0] : this.comparator.toArray(new Base[this.comparator.size()]); // Enumeration<SearchComparator> 1959 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // Enumeration<SearchModifierCode> 1960 default: return super.getProperty(hash, name, checkValid); 1961 } 1962 1963 } 1964 1965 @Override 1966 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1967 switch (hash) { 1968 case -1724546052: // description 1969 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1970 return value; 1971 case -341064690: // resource 1972 this.resource = TypeConvertor.castToUri(value); // UriType 1973 return value; 1974 case 618257: // filterParameter 1975 this.filterParameter = TypeConvertor.castToString(value); // StringType 1976 return value; 1977 case -1453988117: // filterDefinition 1978 this.filterDefinition = TypeConvertor.castToUri(value); // UriType 1979 return value; 1980 case -844673834: // comparator 1981 value = new SearchComparatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1982 this.getComparator().add((Enumeration) value); // Enumeration<SearchComparator> 1983 return value; 1984 case -615513385: // modifier 1985 value = new SearchModifierCodeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1986 this.getModifier().add((Enumeration) value); // Enumeration<SearchModifierCode> 1987 return value; 1988 default: return super.setProperty(hash, name, value); 1989 } 1990 1991 } 1992 1993 @Override 1994 public Base setProperty(String name, Base value) throws FHIRException { 1995 if (name.equals("description")) { 1996 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1997 } else if (name.equals("resource")) { 1998 this.resource = TypeConvertor.castToUri(value); // UriType 1999 } else if (name.equals("filterParameter")) { 2000 this.filterParameter = TypeConvertor.castToString(value); // StringType 2001 } else if (name.equals("filterDefinition")) { 2002 this.filterDefinition = TypeConvertor.castToUri(value); // UriType 2003 } else if (name.equals("comparator")) { 2004 value = new SearchComparatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 2005 this.getComparator().add((Enumeration) value); 2006 } else if (name.equals("modifier")) { 2007 value = new SearchModifierCodeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2008 this.getModifier().add((Enumeration) value); 2009 } else 2010 return super.setProperty(name, value); 2011 return value; 2012 } 2013 2014 @Override 2015 public void removeChild(String name, Base value) throws FHIRException { 2016 if (name.equals("description")) { 2017 this.description = null; 2018 } else if (name.equals("resource")) { 2019 this.resource = null; 2020 } else if (name.equals("filterParameter")) { 2021 this.filterParameter = null; 2022 } else if (name.equals("filterDefinition")) { 2023 this.filterDefinition = null; 2024 } else if (name.equals("comparator")) { 2025 value = new SearchComparatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 2026 this.getComparator().remove((Enumeration) value); 2027 } else if (name.equals("modifier")) { 2028 value = new SearchModifierCodeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2029 this.getModifier().remove((Enumeration) value); 2030 } else 2031 super.removeChild(name, value); 2032 2033 } 2034 2035 @Override 2036 public Base makeProperty(int hash, String name) throws FHIRException { 2037 switch (hash) { 2038 case -1724546052: return getDescriptionElement(); 2039 case -341064690: return getResourceElement(); 2040 case 618257: return getFilterParameterElement(); 2041 case -1453988117: return getFilterDefinitionElement(); 2042 case -844673834: return addComparatorElement(); 2043 case -615513385: return addModifierElement(); 2044 default: return super.makeProperty(hash, name); 2045 } 2046 2047 } 2048 2049 @Override 2050 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2051 switch (hash) { 2052 case -1724546052: /*description*/ return new String[] {"markdown"}; 2053 case -341064690: /*resource*/ return new String[] {"uri"}; 2054 case 618257: /*filterParameter*/ return new String[] {"string"}; 2055 case -1453988117: /*filterDefinition*/ return new String[] {"uri"}; 2056 case -844673834: /*comparator*/ return new String[] {"code"}; 2057 case -615513385: /*modifier*/ return new String[] {"code"}; 2058 default: return super.getTypesForProperty(hash, name); 2059 } 2060 2061 } 2062 2063 @Override 2064 public Base addChild(String name) throws FHIRException { 2065 if (name.equals("description")) { 2066 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.canFilterBy.description"); 2067 } 2068 else if (name.equals("resource")) { 2069 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.canFilterBy.resource"); 2070 } 2071 else if (name.equals("filterParameter")) { 2072 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.canFilterBy.filterParameter"); 2073 } 2074 else if (name.equals("filterDefinition")) { 2075 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.canFilterBy.filterDefinition"); 2076 } 2077 else if (name.equals("comparator")) { 2078 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.canFilterBy.comparator"); 2079 } 2080 else if (name.equals("modifier")) { 2081 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.canFilterBy.modifier"); 2082 } 2083 else 2084 return super.addChild(name); 2085 } 2086 2087 public SubscriptionTopicCanFilterByComponent copy() { 2088 SubscriptionTopicCanFilterByComponent dst = new SubscriptionTopicCanFilterByComponent(); 2089 copyValues(dst); 2090 return dst; 2091 } 2092 2093 public void copyValues(SubscriptionTopicCanFilterByComponent dst) { 2094 super.copyValues(dst); 2095 dst.description = description == null ? null : description.copy(); 2096 dst.resource = resource == null ? null : resource.copy(); 2097 dst.filterParameter = filterParameter == null ? null : filterParameter.copy(); 2098 dst.filterDefinition = filterDefinition == null ? null : filterDefinition.copy(); 2099 if (comparator != null) { 2100 dst.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2101 for (Enumeration<SearchComparator> i : comparator) 2102 dst.comparator.add(i.copy()); 2103 }; 2104 if (modifier != null) { 2105 dst.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2106 for (Enumeration<SearchModifierCode> i : modifier) 2107 dst.modifier.add(i.copy()); 2108 }; 2109 } 2110 2111 @Override 2112 public boolean equalsDeep(Base other_) { 2113 if (!super.equalsDeep(other_)) 2114 return false; 2115 if (!(other_ instanceof SubscriptionTopicCanFilterByComponent)) 2116 return false; 2117 SubscriptionTopicCanFilterByComponent o = (SubscriptionTopicCanFilterByComponent) other_; 2118 return compareDeep(description, o.description, true) && compareDeep(resource, o.resource, true) 2119 && compareDeep(filterParameter, o.filterParameter, true) && compareDeep(filterDefinition, o.filterDefinition, true) 2120 && compareDeep(comparator, o.comparator, true) && compareDeep(modifier, o.modifier, true); 2121 } 2122 2123 @Override 2124 public boolean equalsShallow(Base other_) { 2125 if (!super.equalsShallow(other_)) 2126 return false; 2127 if (!(other_ instanceof SubscriptionTopicCanFilterByComponent)) 2128 return false; 2129 SubscriptionTopicCanFilterByComponent o = (SubscriptionTopicCanFilterByComponent) other_; 2130 return compareValues(description, o.description, true) && compareValues(resource, o.resource, true) 2131 && compareValues(filterParameter, o.filterParameter, true) && compareValues(filterDefinition, o.filterDefinition, true) 2132 && compareValues(comparator, o.comparator, true) && compareValues(modifier, o.modifier, true); 2133 } 2134 2135 public boolean isEmpty() { 2136 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, resource, filterParameter 2137 , filterDefinition, comparator, modifier); 2138 } 2139 2140 public String fhirType() { 2141 return "SubscriptionTopic.canFilterBy"; 2142 2143 } 2144 2145 } 2146 2147 @Block() 2148 public static class SubscriptionTopicNotificationShapeComponent extends BackboneElement implements IBaseBackboneElement { 2149 /** 2150 * URL of the Resource that is the type used in this shape. This is the 'focus' resource of the topic (or one of them if there are more than one) and the root resource for this shape definition. It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present. 2151 */ 2152 @Child(name = "resource", type = {UriType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2153 @Description(shortDefinition="URL of the Resource that is the focus (main) resource in a notification shape", formalDefinition="URL of the Resource that is the type used in this shape. This is the 'focus' resource of the topic (or one of them if there are more than one) and the root resource for this shape definition. It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present." ) 2154 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscription-types") 2155 protected UriType resource; 2156 2157 /** 2158 * Search-style _include directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them. 2159 */ 2160 @Child(name = "include", type = {StringType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2161 @Description(shortDefinition="Include directives, rooted in the resource for this shape", formalDefinition="Search-style _include directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them." ) 2162 protected List<StringType> include; 2163 2164 /** 2165 * Search-style _revinclude directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them. 2166 */ 2167 @Child(name = "revInclude", type = {StringType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2168 @Description(shortDefinition="Reverse include directives, rooted in the resource for this shape", formalDefinition="Search-style _revinclude directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them." ) 2169 protected List<StringType> revInclude; 2170 2171 private static final long serialVersionUID = -1718592091L; 2172 2173 /** 2174 * Constructor 2175 */ 2176 public SubscriptionTopicNotificationShapeComponent() { 2177 super(); 2178 } 2179 2180 /** 2181 * Constructor 2182 */ 2183 public SubscriptionTopicNotificationShapeComponent(String resource) { 2184 super(); 2185 this.setResource(resource); 2186 } 2187 2188 /** 2189 * @return {@link #resource} (URL of the Resource that is the type used in this shape. This is the 'focus' resource of the topic (or one of them if there are more than one) and the root resource for this shape definition. It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 2190 */ 2191 public UriType getResourceElement() { 2192 if (this.resource == null) 2193 if (Configuration.errorOnAutoCreate()) 2194 throw new Error("Attempt to auto-create SubscriptionTopicNotificationShapeComponent.resource"); 2195 else if (Configuration.doAutoCreate()) 2196 this.resource = new UriType(); // bb 2197 return this.resource; 2198 } 2199 2200 public boolean hasResourceElement() { 2201 return this.resource != null && !this.resource.isEmpty(); 2202 } 2203 2204 public boolean hasResource() { 2205 return this.resource != null && !this.resource.isEmpty(); 2206 } 2207 2208 /** 2209 * @param value {@link #resource} (URL of the Resource that is the type used in this shape. This is the 'focus' resource of the topic (or one of them if there are more than one) and the root resource for this shape definition. It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 2210 */ 2211 public SubscriptionTopicNotificationShapeComponent setResourceElement(UriType value) { 2212 this.resource = value; 2213 return this; 2214 } 2215 2216 /** 2217 * @return URL of the Resource that is the type used in this shape. This is the 'focus' resource of the topic (or one of them if there are more than one) and the root resource for this shape definition. It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present. 2218 */ 2219 public String getResource() { 2220 return this.resource == null ? null : this.resource.getValue(); 2221 } 2222 2223 /** 2224 * @param value URL of the Resource that is the type used in this shape. This is the 'focus' resource of the topic (or one of them if there are more than one) and the root resource for this shape definition. It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present. 2225 */ 2226 public SubscriptionTopicNotificationShapeComponent setResource(String value) { 2227 if (this.resource == null) 2228 this.resource = new UriType(); 2229 this.resource.setValue(value); 2230 return this; 2231 } 2232 2233 /** 2234 * @return {@link #include} (Search-style _include directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.) 2235 */ 2236 public List<StringType> getInclude() { 2237 if (this.include == null) 2238 this.include = new ArrayList<StringType>(); 2239 return this.include; 2240 } 2241 2242 /** 2243 * @return Returns a reference to <code>this</code> for easy method chaining 2244 */ 2245 public SubscriptionTopicNotificationShapeComponent setInclude(List<StringType> theInclude) { 2246 this.include = theInclude; 2247 return this; 2248 } 2249 2250 public boolean hasInclude() { 2251 if (this.include == null) 2252 return false; 2253 for (StringType item : this.include) 2254 if (!item.isEmpty()) 2255 return true; 2256 return false; 2257 } 2258 2259 /** 2260 * @return {@link #include} (Search-style _include directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.) 2261 */ 2262 public StringType addIncludeElement() {//2 2263 StringType t = new StringType(); 2264 if (this.include == null) 2265 this.include = new ArrayList<StringType>(); 2266 this.include.add(t); 2267 return t; 2268 } 2269 2270 /** 2271 * @param value {@link #include} (Search-style _include directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.) 2272 */ 2273 public SubscriptionTopicNotificationShapeComponent addInclude(String value) { //1 2274 StringType t = new StringType(); 2275 t.setValue(value); 2276 if (this.include == null) 2277 this.include = new ArrayList<StringType>(); 2278 this.include.add(t); 2279 return this; 2280 } 2281 2282 /** 2283 * @param value {@link #include} (Search-style _include directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.) 2284 */ 2285 public boolean hasInclude(String value) { 2286 if (this.include == null) 2287 return false; 2288 for (StringType v : this.include) 2289 if (v.getValue().equals(value)) // string 2290 return true; 2291 return false; 2292 } 2293 2294 /** 2295 * @return {@link #revInclude} (Search-style _revinclude directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.) 2296 */ 2297 public List<StringType> getRevInclude() { 2298 if (this.revInclude == null) 2299 this.revInclude = new ArrayList<StringType>(); 2300 return this.revInclude; 2301 } 2302 2303 /** 2304 * @return Returns a reference to <code>this</code> for easy method chaining 2305 */ 2306 public SubscriptionTopicNotificationShapeComponent setRevInclude(List<StringType> theRevInclude) { 2307 this.revInclude = theRevInclude; 2308 return this; 2309 } 2310 2311 public boolean hasRevInclude() { 2312 if (this.revInclude == null) 2313 return false; 2314 for (StringType item : this.revInclude) 2315 if (!item.isEmpty()) 2316 return true; 2317 return false; 2318 } 2319 2320 /** 2321 * @return {@link #revInclude} (Search-style _revinclude directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.) 2322 */ 2323 public StringType addRevIncludeElement() {//2 2324 StringType t = new StringType(); 2325 if (this.revInclude == null) 2326 this.revInclude = new ArrayList<StringType>(); 2327 this.revInclude.add(t); 2328 return t; 2329 } 2330 2331 /** 2332 * @param value {@link #revInclude} (Search-style _revinclude directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.) 2333 */ 2334 public SubscriptionTopicNotificationShapeComponent addRevInclude(String value) { //1 2335 StringType t = new StringType(); 2336 t.setValue(value); 2337 if (this.revInclude == null) 2338 this.revInclude = new ArrayList<StringType>(); 2339 this.revInclude.add(t); 2340 return this; 2341 } 2342 2343 /** 2344 * @param value {@link #revInclude} (Search-style _revinclude directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.) 2345 */ 2346 public boolean hasRevInclude(String value) { 2347 if (this.revInclude == null) 2348 return false; 2349 for (StringType v : this.revInclude) 2350 if (v.getValue().equals(value)) // string 2351 return true; 2352 return false; 2353 } 2354 2355 protected void listChildren(List<Property> children) { 2356 super.listChildren(children); 2357 children.add(new Property("resource", "uri", "URL of the Resource that is the type used in this shape. This is the 'focus' resource of the topic (or one of them if there are more than one) and the root resource for this shape definition. It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present.", 0, 1, resource)); 2358 children.add(new Property("include", "string", "Search-style _include directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.", 0, java.lang.Integer.MAX_VALUE, include)); 2359 children.add(new Property("revInclude", "string", "Search-style _revinclude directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.", 0, java.lang.Integer.MAX_VALUE, revInclude)); 2360 } 2361 2362 @Override 2363 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2364 switch (_hash) { 2365 case -341064690: /*resource*/ return new Property("resource", "uri", "URL of the Resource that is the type used in this shape. This is the 'focus' resource of the topic (or one of them if there are more than one) and the root resource for this shape definition. It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present.", 0, 1, resource); 2366 case 1942574248: /*include*/ return new Property("include", "string", "Search-style _include directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.", 0, java.lang.Integer.MAX_VALUE, include); 2367 case 8439429: /*revInclude*/ return new Property("revInclude", "string", "Search-style _revinclude directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.", 0, java.lang.Integer.MAX_VALUE, revInclude); 2368 default: return super.getNamedProperty(_hash, _name, _checkValid); 2369 } 2370 2371 } 2372 2373 @Override 2374 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2375 switch (hash) { 2376 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // UriType 2377 case 1942574248: /*include*/ return this.include == null ? new Base[0] : this.include.toArray(new Base[this.include.size()]); // StringType 2378 case 8439429: /*revInclude*/ return this.revInclude == null ? new Base[0] : this.revInclude.toArray(new Base[this.revInclude.size()]); // StringType 2379 default: return super.getProperty(hash, name, checkValid); 2380 } 2381 2382 } 2383 2384 @Override 2385 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2386 switch (hash) { 2387 case -341064690: // resource 2388 this.resource = TypeConvertor.castToUri(value); // UriType 2389 return value; 2390 case 1942574248: // include 2391 this.getInclude().add(TypeConvertor.castToString(value)); // StringType 2392 return value; 2393 case 8439429: // revInclude 2394 this.getRevInclude().add(TypeConvertor.castToString(value)); // StringType 2395 return value; 2396 default: return super.setProperty(hash, name, value); 2397 } 2398 2399 } 2400 2401 @Override 2402 public Base setProperty(String name, Base value) throws FHIRException { 2403 if (name.equals("resource")) { 2404 this.resource = TypeConvertor.castToUri(value); // UriType 2405 } else if (name.equals("include")) { 2406 this.getInclude().add(TypeConvertor.castToString(value)); 2407 } else if (name.equals("revInclude")) { 2408 this.getRevInclude().add(TypeConvertor.castToString(value)); 2409 } else 2410 return super.setProperty(name, value); 2411 return value; 2412 } 2413 2414 @Override 2415 public void removeChild(String name, Base value) throws FHIRException { 2416 if (name.equals("resource")) { 2417 this.resource = null; 2418 } else if (name.equals("include")) { 2419 this.getInclude().remove(value); 2420 } else if (name.equals("revInclude")) { 2421 this.getRevInclude().remove(value); 2422 } else 2423 super.removeChild(name, value); 2424 2425 } 2426 2427 @Override 2428 public Base makeProperty(int hash, String name) throws FHIRException { 2429 switch (hash) { 2430 case -341064690: return getResourceElement(); 2431 case 1942574248: return addIncludeElement(); 2432 case 8439429: return addRevIncludeElement(); 2433 default: return super.makeProperty(hash, name); 2434 } 2435 2436 } 2437 2438 @Override 2439 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2440 switch (hash) { 2441 case -341064690: /*resource*/ return new String[] {"uri"}; 2442 case 1942574248: /*include*/ return new String[] {"string"}; 2443 case 8439429: /*revInclude*/ return new String[] {"string"}; 2444 default: return super.getTypesForProperty(hash, name); 2445 } 2446 2447 } 2448 2449 @Override 2450 public Base addChild(String name) throws FHIRException { 2451 if (name.equals("resource")) { 2452 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.notificationShape.resource"); 2453 } 2454 else if (name.equals("include")) { 2455 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.notificationShape.include"); 2456 } 2457 else if (name.equals("revInclude")) { 2458 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.notificationShape.revInclude"); 2459 } 2460 else 2461 return super.addChild(name); 2462 } 2463 2464 public SubscriptionTopicNotificationShapeComponent copy() { 2465 SubscriptionTopicNotificationShapeComponent dst = new SubscriptionTopicNotificationShapeComponent(); 2466 copyValues(dst); 2467 return dst; 2468 } 2469 2470 public void copyValues(SubscriptionTopicNotificationShapeComponent dst) { 2471 super.copyValues(dst); 2472 dst.resource = resource == null ? null : resource.copy(); 2473 if (include != null) { 2474 dst.include = new ArrayList<StringType>(); 2475 for (StringType i : include) 2476 dst.include.add(i.copy()); 2477 }; 2478 if (revInclude != null) { 2479 dst.revInclude = new ArrayList<StringType>(); 2480 for (StringType i : revInclude) 2481 dst.revInclude.add(i.copy()); 2482 }; 2483 } 2484 2485 @Override 2486 public boolean equalsDeep(Base other_) { 2487 if (!super.equalsDeep(other_)) 2488 return false; 2489 if (!(other_ instanceof SubscriptionTopicNotificationShapeComponent)) 2490 return false; 2491 SubscriptionTopicNotificationShapeComponent o = (SubscriptionTopicNotificationShapeComponent) other_; 2492 return compareDeep(resource, o.resource, true) && compareDeep(include, o.include, true) && compareDeep(revInclude, o.revInclude, true) 2493 ; 2494 } 2495 2496 @Override 2497 public boolean equalsShallow(Base other_) { 2498 if (!super.equalsShallow(other_)) 2499 return false; 2500 if (!(other_ instanceof SubscriptionTopicNotificationShapeComponent)) 2501 return false; 2502 SubscriptionTopicNotificationShapeComponent o = (SubscriptionTopicNotificationShapeComponent) other_; 2503 return compareValues(resource, o.resource, true) && compareValues(include, o.include, true) && compareValues(revInclude, o.revInclude, true) 2504 ; 2505 } 2506 2507 public boolean isEmpty() { 2508 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(resource, include, revInclude 2509 ); 2510 } 2511 2512 public String fhirType() { 2513 return "SubscriptionTopic.notificationShape"; 2514 2515 } 2516 2517 } 2518 2519 /** 2520 * An absolute URI that is used to identify this subscription topic when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this subscription topic is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the subscription topic is stored on different servers. 2521 */ 2522 @Child(name = "url", type = {UriType.class}, order=0, min=1, max=1, modifier=false, summary=true) 2523 @Description(shortDefinition="Canonical identifier for this subscription topic, represented as an absolute URI (globally unique)", formalDefinition="An absolute URI that is used to identify this subscription topic when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this subscription topic is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the subscription topic is stored on different servers." ) 2524 protected UriType url; 2525 2526 /** 2527 * Business identifiers assigned to this subscription topic by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server. 2528 */ 2529 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2530 @Description(shortDefinition="Business identifier for subscription topic", formalDefinition="Business identifiers assigned to this subscription topic by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server." ) 2531 protected List<Identifier> identifier; 2532 2533 /** 2534 * The identifier that is used to identify this version of the subscription topic when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Topic author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable. 2535 */ 2536 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2537 @Description(shortDefinition="Business version of the subscription topic", formalDefinition="The identifier that is used to identify this version of the subscription topic when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Topic author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable." ) 2538 protected StringType version; 2539 2540 /** 2541 * Indicates the mechanism used to compare versions to determine which is more current. 2542 */ 2543 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 2544 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 2545 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 2546 protected DataType versionAlgorithm; 2547 2548 /** 2549 * A natural language name identifying the subscription topic This name should be usable as an identifier for the module by machine processing applications such as code generation. 2550 */ 2551 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 2552 @Description(shortDefinition="Name for this subscription topic (computer friendly)", formalDefinition="A natural language name identifying the subscription topic This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 2553 protected StringType name; 2554 2555 /** 2556 * A short, descriptive, user-friendly title for the subscription topic. For example, "admission". 2557 */ 2558 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 2559 @Description(shortDefinition="Name for this subscription topic (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the subscription topic. For example, \"admission\"." ) 2560 protected StringType title; 2561 2562 /** 2563 * The canonical URL pointing to another FHIR-defined SubscriptionTopic that is adhered to in whole or in part by this SubscriptionTopic. 2564 */ 2565 @Child(name = "derivedFrom", type = {CanonicalType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2566 @Description(shortDefinition="Based on FHIR protocol or definition", formalDefinition="The canonical URL pointing to another FHIR-defined SubscriptionTopic that is adhered to in whole or in part by this SubscriptionTopic." ) 2567 protected List<CanonicalType> derivedFrom; 2568 2569 /** 2570 * The current state of the SubscriptionTopic. 2571 */ 2572 @Child(name = "status", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 2573 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The current state of the SubscriptionTopic." ) 2574 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 2575 protected Enumeration<PublicationStatus> status; 2576 2577 /** 2578 * A flag to indicate that this TopSubscriptionTopicic is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 2579 */ 2580 @Child(name = "experimental", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=true) 2581 @Description(shortDefinition="If for testing purposes, not real usage", formalDefinition="A flag to indicate that this TopSubscriptionTopicic is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage." ) 2582 protected BooleanType experimental; 2583 2584 /** 2585 * The date (and optionally time) when the subscription topic was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the subscription topic changes. 2586 */ 2587 @Child(name = "date", type = {DateTimeType.class}, order=9, min=0, max=1, modifier=false, summary=true) 2588 @Description(shortDefinition="Date status first applied", formalDefinition="The date (and optionally time) when the subscription topic was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the subscription topic changes." ) 2589 protected DateTimeType date; 2590 2591 /** 2592 * Helps establish the "authority/credibility" of the SubscriptionTopic. May also allow for contact. 2593 */ 2594 @Child(name = "publisher", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 2595 @Description(shortDefinition="The name of the individual or organization that published the SubscriptionTopic", formalDefinition="Helps establish the \"authority/credibility\" of the SubscriptionTopic. May also allow for contact." ) 2596 protected StringType publisher; 2597 2598 /** 2599 * Contact details to assist a user in finding and communicating with the publisher. 2600 */ 2601 @Child(name = "contact", type = {ContactDetail.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2602 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 2603 protected List<ContactDetail> contact; 2604 2605 /** 2606 * A free text natural language description of the Topic from the consumer's perspective. 2607 */ 2608 @Child(name = "description", type = {MarkdownType.class}, order=12, min=0, max=1, modifier=false, summary=false) 2609 @Description(shortDefinition="Natural language description of the SubscriptionTopic", formalDefinition="A free text natural language description of the Topic from the consumer's perspective." ) 2610 protected MarkdownType description; 2611 2612 /** 2613 * The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of code system definitions. 2614 */ 2615 @Child(name = "useContext", type = {UsageContext.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2616 @Description(shortDefinition="Content intends to support these contexts", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of code system definitions." ) 2617 protected List<UsageContext> useContext; 2618 2619 /** 2620 * A jurisdiction in which the Topic is intended to be used. 2621 */ 2622 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2623 @Description(shortDefinition="Intended jurisdiction of the SubscriptionTopic (if applicable)", formalDefinition="A jurisdiction in which the Topic is intended to be used." ) 2624 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 2625 protected List<CodeableConcept> jurisdiction; 2626 2627 /** 2628 * Explains why this Topic is needed and why it has been designed as it has. 2629 */ 2630 @Child(name = "purpose", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 2631 @Description(shortDefinition="Why this SubscriptionTopic is defined", formalDefinition="Explains why this Topic is needed and why it has been designed as it has." ) 2632 protected MarkdownType purpose; 2633 2634 /** 2635 * A copyright statement relating to the SubscriptionTopic and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SubscriptionTopic. 2636 */ 2637 @Child(name = "copyright", type = {MarkdownType.class}, order=16, min=0, max=1, modifier=false, summary=false) 2638 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the SubscriptionTopic and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SubscriptionTopic." ) 2639 protected MarkdownType copyright; 2640 2641 /** 2642 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2643 */ 2644 @Child(name = "copyrightLabel", type = {StringType.class}, order=17, min=0, max=1, modifier=false, summary=false) 2645 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 2646 protected StringType copyrightLabel; 2647 2648 /** 2649 * The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage. 2650 */ 2651 @Child(name = "approvalDate", type = {DateType.class}, order=18, min=0, max=1, modifier=false, summary=false) 2652 @Description(shortDefinition="When SubscriptionTopic is/was approved by publisher", formalDefinition="The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 2653 protected DateType approvalDate; 2654 2655 /** 2656 * The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date. 2657 */ 2658 @Child(name = "lastReviewDate", type = {DateType.class}, order=19, min=0, max=1, modifier=false, summary=false) 2659 @Description(shortDefinition="Date the Subscription Topic was last reviewed by the publisher", formalDefinition="The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date." ) 2660 protected DateType lastReviewDate; 2661 2662 /** 2663 * The period during which the SubscriptionTopic content was or is planned to be effective. 2664 */ 2665 @Child(name = "effectivePeriod", type = {Period.class}, order=20, min=0, max=1, modifier=false, summary=true) 2666 @Description(shortDefinition="The effective date range for the SubscriptionTopic", formalDefinition="The period during which the SubscriptionTopic content was or is planned to be effective." ) 2667 protected Period effectivePeriod; 2668 2669 /** 2670 * A definition of a resource-based event that triggers a notification based on the SubscriptionTopic. The criteria may be just a human readable description and/or a full FHIR search string or FHIRPath expression. Multiple triggers are considered OR joined (e.g., a resource update matching ANY of the definitions will trigger a notification). 2671 */ 2672 @Child(name = "resourceTrigger", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2673 @Description(shortDefinition="Definition of a resource-based trigger for the subscription topic", formalDefinition="A definition of a resource-based event that triggers a notification based on the SubscriptionTopic. The criteria may be just a human readable description and/or a full FHIR search string or FHIRPath expression. Multiple triggers are considered OR joined (e.g., a resource update matching ANY of the definitions will trigger a notification)." ) 2674 protected List<SubscriptionTopicResourceTriggerComponent> resourceTrigger; 2675 2676 /** 2677 * Event definition which can be used to trigger the SubscriptionTopic. 2678 */ 2679 @Child(name = "eventTrigger", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2680 @Description(shortDefinition="Event definitions the SubscriptionTopic", formalDefinition="Event definition which can be used to trigger the SubscriptionTopic." ) 2681 protected List<SubscriptionTopicEventTriggerComponent> eventTrigger; 2682 2683 /** 2684 * List of properties by which Subscriptions on the SubscriptionTopic can be filtered. May be defined Search Parameters (e.g., Encounter.patient) or parameters defined within this SubscriptionTopic context (e.g., hub.event). 2685 */ 2686 @Child(name = "canFilterBy", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2687 @Description(shortDefinition="Properties by which a Subscription can filter notifications from the SubscriptionTopic", formalDefinition="List of properties by which Subscriptions on the SubscriptionTopic can be filtered. May be defined Search Parameters (e.g., Encounter.patient) or parameters defined within this SubscriptionTopic context (e.g., hub.event)." ) 2688 protected List<SubscriptionTopicCanFilterByComponent> canFilterBy; 2689 2690 /** 2691 * List of properties to describe the shape (e.g., resources) included in notifications from this Subscription Topic. 2692 */ 2693 @Child(name = "notificationShape", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2694 @Description(shortDefinition="Properties for describing the shape of notifications generated by this topic", formalDefinition="List of properties to describe the shape (e.g., resources) included in notifications from this Subscription Topic." ) 2695 protected List<SubscriptionTopicNotificationShapeComponent> notificationShape; 2696 2697 private static final long serialVersionUID = -194061063L; 2698 2699 /** 2700 * Constructor 2701 */ 2702 public SubscriptionTopic() { 2703 super(); 2704 } 2705 2706 /** 2707 * Constructor 2708 */ 2709 public SubscriptionTopic(String url, PublicationStatus status) { 2710 super(); 2711 this.setUrl(url); 2712 this.setStatus(status); 2713 } 2714 2715 /** 2716 * @return {@link #url} (An absolute URI that is used to identify this subscription topic when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this subscription topic is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the subscription topic is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2717 */ 2718 public UriType getUrlElement() { 2719 if (this.url == null) 2720 if (Configuration.errorOnAutoCreate()) 2721 throw new Error("Attempt to auto-create SubscriptionTopic.url"); 2722 else if (Configuration.doAutoCreate()) 2723 this.url = new UriType(); // bb 2724 return this.url; 2725 } 2726 2727 public boolean hasUrlElement() { 2728 return this.url != null && !this.url.isEmpty(); 2729 } 2730 2731 public boolean hasUrl() { 2732 return this.url != null && !this.url.isEmpty(); 2733 } 2734 2735 /** 2736 * @param value {@link #url} (An absolute URI that is used to identify this subscription topic when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this subscription topic is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the subscription topic is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2737 */ 2738 public SubscriptionTopic setUrlElement(UriType value) { 2739 this.url = value; 2740 return this; 2741 } 2742 2743 /** 2744 * @return An absolute URI that is used to identify this subscription topic when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this subscription topic is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the subscription topic is stored on different servers. 2745 */ 2746 public String getUrl() { 2747 return this.url == null ? null : this.url.getValue(); 2748 } 2749 2750 /** 2751 * @param value An absolute URI that is used to identify this subscription topic when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this subscription topic is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the subscription topic is stored on different servers. 2752 */ 2753 public SubscriptionTopic setUrl(String value) { 2754 if (this.url == null) 2755 this.url = new UriType(); 2756 this.url.setValue(value); 2757 return this; 2758 } 2759 2760 /** 2761 * @return {@link #identifier} (Business identifiers assigned to this subscription topic by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.) 2762 */ 2763 public List<Identifier> getIdentifier() { 2764 if (this.identifier == null) 2765 this.identifier = new ArrayList<Identifier>(); 2766 return this.identifier; 2767 } 2768 2769 /** 2770 * @return Returns a reference to <code>this</code> for easy method chaining 2771 */ 2772 public SubscriptionTopic setIdentifier(List<Identifier> theIdentifier) { 2773 this.identifier = theIdentifier; 2774 return this; 2775 } 2776 2777 public boolean hasIdentifier() { 2778 if (this.identifier == null) 2779 return false; 2780 for (Identifier item : this.identifier) 2781 if (!item.isEmpty()) 2782 return true; 2783 return false; 2784 } 2785 2786 public Identifier addIdentifier() { //3 2787 Identifier t = new Identifier(); 2788 if (this.identifier == null) 2789 this.identifier = new ArrayList<Identifier>(); 2790 this.identifier.add(t); 2791 return t; 2792 } 2793 2794 public SubscriptionTopic addIdentifier(Identifier t) { //3 2795 if (t == null) 2796 return this; 2797 if (this.identifier == null) 2798 this.identifier = new ArrayList<Identifier>(); 2799 this.identifier.add(t); 2800 return this; 2801 } 2802 2803 /** 2804 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2805 */ 2806 public Identifier getIdentifierFirstRep() { 2807 if (getIdentifier().isEmpty()) { 2808 addIdentifier(); 2809 } 2810 return getIdentifier().get(0); 2811 } 2812 2813 /** 2814 * @return {@link #version} (The identifier that is used to identify this version of the subscription topic when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Topic author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2815 */ 2816 public StringType getVersionElement() { 2817 if (this.version == null) 2818 if (Configuration.errorOnAutoCreate()) 2819 throw new Error("Attempt to auto-create SubscriptionTopic.version"); 2820 else if (Configuration.doAutoCreate()) 2821 this.version = new StringType(); // bb 2822 return this.version; 2823 } 2824 2825 public boolean hasVersionElement() { 2826 return this.version != null && !this.version.isEmpty(); 2827 } 2828 2829 public boolean hasVersion() { 2830 return this.version != null && !this.version.isEmpty(); 2831 } 2832 2833 /** 2834 * @param value {@link #version} (The identifier that is used to identify this version of the subscription topic when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Topic author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2835 */ 2836 public SubscriptionTopic setVersionElement(StringType value) { 2837 this.version = value; 2838 return this; 2839 } 2840 2841 /** 2842 * @return The identifier that is used to identify this version of the subscription topic when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Topic author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable. 2843 */ 2844 public String getVersion() { 2845 return this.version == null ? null : this.version.getValue(); 2846 } 2847 2848 /** 2849 * @param value The identifier that is used to identify this version of the subscription topic when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Topic author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable. 2850 */ 2851 public SubscriptionTopic setVersion(String value) { 2852 if (Utilities.noString(value)) 2853 this.version = null; 2854 else { 2855 if (this.version == null) 2856 this.version = new StringType(); 2857 this.version.setValue(value); 2858 } 2859 return this; 2860 } 2861 2862 /** 2863 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2864 */ 2865 public DataType getVersionAlgorithm() { 2866 return this.versionAlgorithm; 2867 } 2868 2869 /** 2870 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2871 */ 2872 public StringType getVersionAlgorithmStringType() throws FHIRException { 2873 if (this.versionAlgorithm == null) 2874 this.versionAlgorithm = new StringType(); 2875 if (!(this.versionAlgorithm instanceof StringType)) 2876 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 2877 return (StringType) this.versionAlgorithm; 2878 } 2879 2880 public boolean hasVersionAlgorithmStringType() { 2881 return this != null && this.versionAlgorithm instanceof StringType; 2882 } 2883 2884 /** 2885 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2886 */ 2887 public Coding getVersionAlgorithmCoding() throws FHIRException { 2888 if (this.versionAlgorithm == null) 2889 this.versionAlgorithm = new Coding(); 2890 if (!(this.versionAlgorithm instanceof Coding)) 2891 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 2892 return (Coding) this.versionAlgorithm; 2893 } 2894 2895 public boolean hasVersionAlgorithmCoding() { 2896 return this != null && this.versionAlgorithm instanceof Coding; 2897 } 2898 2899 public boolean hasVersionAlgorithm() { 2900 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 2901 } 2902 2903 /** 2904 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2905 */ 2906 public SubscriptionTopic setVersionAlgorithm(DataType value) { 2907 if (value != null && !(value instanceof StringType || value instanceof Coding)) 2908 throw new FHIRException("Not the right type for SubscriptionTopic.versionAlgorithm[x]: "+value.fhirType()); 2909 this.versionAlgorithm = value; 2910 return this; 2911 } 2912 2913 /** 2914 * @return {@link #name} (A natural language name identifying the subscription topic This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2915 */ 2916 public StringType getNameElement() { 2917 if (this.name == null) 2918 if (Configuration.errorOnAutoCreate()) 2919 throw new Error("Attempt to auto-create SubscriptionTopic.name"); 2920 else if (Configuration.doAutoCreate()) 2921 this.name = new StringType(); // bb 2922 return this.name; 2923 } 2924 2925 public boolean hasNameElement() { 2926 return this.name != null && !this.name.isEmpty(); 2927 } 2928 2929 public boolean hasName() { 2930 return this.name != null && !this.name.isEmpty(); 2931 } 2932 2933 /** 2934 * @param value {@link #name} (A natural language name identifying the subscription topic This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2935 */ 2936 public SubscriptionTopic setNameElement(StringType value) { 2937 this.name = value; 2938 return this; 2939 } 2940 2941 /** 2942 * @return A natural language name identifying the subscription topic This name should be usable as an identifier for the module by machine processing applications such as code generation. 2943 */ 2944 public String getName() { 2945 return this.name == null ? null : this.name.getValue(); 2946 } 2947 2948 /** 2949 * @param value A natural language name identifying the subscription topic This name should be usable as an identifier for the module by machine processing applications such as code generation. 2950 */ 2951 public SubscriptionTopic setName(String value) { 2952 if (Utilities.noString(value)) 2953 this.name = null; 2954 else { 2955 if (this.name == null) 2956 this.name = new StringType(); 2957 this.name.setValue(value); 2958 } 2959 return this; 2960 } 2961 2962 /** 2963 * @return {@link #title} (A short, descriptive, user-friendly title for the subscription topic. For example, "admission".). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2964 */ 2965 public StringType getTitleElement() { 2966 if (this.title == null) 2967 if (Configuration.errorOnAutoCreate()) 2968 throw new Error("Attempt to auto-create SubscriptionTopic.title"); 2969 else if (Configuration.doAutoCreate()) 2970 this.title = new StringType(); // bb 2971 return this.title; 2972 } 2973 2974 public boolean hasTitleElement() { 2975 return this.title != null && !this.title.isEmpty(); 2976 } 2977 2978 public boolean hasTitle() { 2979 return this.title != null && !this.title.isEmpty(); 2980 } 2981 2982 /** 2983 * @param value {@link #title} (A short, descriptive, user-friendly title for the subscription topic. For example, "admission".). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2984 */ 2985 public SubscriptionTopic setTitleElement(StringType value) { 2986 this.title = value; 2987 return this; 2988 } 2989 2990 /** 2991 * @return A short, descriptive, user-friendly title for the subscription topic. For example, "admission". 2992 */ 2993 public String getTitle() { 2994 return this.title == null ? null : this.title.getValue(); 2995 } 2996 2997 /** 2998 * @param value A short, descriptive, user-friendly title for the subscription topic. For example, "admission". 2999 */ 3000 public SubscriptionTopic setTitle(String value) { 3001 if (Utilities.noString(value)) 3002 this.title = null; 3003 else { 3004 if (this.title == null) 3005 this.title = new StringType(); 3006 this.title.setValue(value); 3007 } 3008 return this; 3009 } 3010 3011 /** 3012 * @return {@link #derivedFrom} (The canonical URL pointing to another FHIR-defined SubscriptionTopic that is adhered to in whole or in part by this SubscriptionTopic.) 3013 */ 3014 public List<CanonicalType> getDerivedFrom() { 3015 if (this.derivedFrom == null) 3016 this.derivedFrom = new ArrayList<CanonicalType>(); 3017 return this.derivedFrom; 3018 } 3019 3020 /** 3021 * @return Returns a reference to <code>this</code> for easy method chaining 3022 */ 3023 public SubscriptionTopic setDerivedFrom(List<CanonicalType> theDerivedFrom) { 3024 this.derivedFrom = theDerivedFrom; 3025 return this; 3026 } 3027 3028 public boolean hasDerivedFrom() { 3029 if (this.derivedFrom == null) 3030 return false; 3031 for (CanonicalType item : this.derivedFrom) 3032 if (!item.isEmpty()) 3033 return true; 3034 return false; 3035 } 3036 3037 /** 3038 * @return {@link #derivedFrom} (The canonical URL pointing to another FHIR-defined SubscriptionTopic that is adhered to in whole or in part by this SubscriptionTopic.) 3039 */ 3040 public CanonicalType addDerivedFromElement() {//2 3041 CanonicalType t = new CanonicalType(); 3042 if (this.derivedFrom == null) 3043 this.derivedFrom = new ArrayList<CanonicalType>(); 3044 this.derivedFrom.add(t); 3045 return t; 3046 } 3047 3048 /** 3049 * @param value {@link #derivedFrom} (The canonical URL pointing to another FHIR-defined SubscriptionTopic that is adhered to in whole or in part by this SubscriptionTopic.) 3050 */ 3051 public SubscriptionTopic addDerivedFrom(String value) { //1 3052 CanonicalType t = new CanonicalType(); 3053 t.setValue(value); 3054 if (this.derivedFrom == null) 3055 this.derivedFrom = new ArrayList<CanonicalType>(); 3056 this.derivedFrom.add(t); 3057 return this; 3058 } 3059 3060 /** 3061 * @param value {@link #derivedFrom} (The canonical URL pointing to another FHIR-defined SubscriptionTopic that is adhered to in whole or in part by this SubscriptionTopic.) 3062 */ 3063 public boolean hasDerivedFrom(String value) { 3064 if (this.derivedFrom == null) 3065 return false; 3066 for (CanonicalType v : this.derivedFrom) 3067 if (v.getValue().equals(value)) // canonical 3068 return true; 3069 return false; 3070 } 3071 3072 /** 3073 * @return {@link #status} (The current state of the SubscriptionTopic.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3074 */ 3075 public Enumeration<PublicationStatus> getStatusElement() { 3076 if (this.status == null) 3077 if (Configuration.errorOnAutoCreate()) 3078 throw new Error("Attempt to auto-create SubscriptionTopic.status"); 3079 else if (Configuration.doAutoCreate()) 3080 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 3081 return this.status; 3082 } 3083 3084 public boolean hasStatusElement() { 3085 return this.status != null && !this.status.isEmpty(); 3086 } 3087 3088 public boolean hasStatus() { 3089 return this.status != null && !this.status.isEmpty(); 3090 } 3091 3092 /** 3093 * @param value {@link #status} (The current state of the SubscriptionTopic.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3094 */ 3095 public SubscriptionTopic setStatusElement(Enumeration<PublicationStatus> value) { 3096 this.status = value; 3097 return this; 3098 } 3099 3100 /** 3101 * @return The current state of the SubscriptionTopic. 3102 */ 3103 public PublicationStatus getStatus() { 3104 return this.status == null ? null : this.status.getValue(); 3105 } 3106 3107 /** 3108 * @param value The current state of the SubscriptionTopic. 3109 */ 3110 public SubscriptionTopic setStatus(PublicationStatus value) { 3111 if (this.status == null) 3112 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 3113 this.status.setValue(value); 3114 return this; 3115 } 3116 3117 /** 3118 * @return {@link #experimental} (A flag to indicate that this TopSubscriptionTopicic is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3119 */ 3120 public BooleanType getExperimentalElement() { 3121 if (this.experimental == null) 3122 if (Configuration.errorOnAutoCreate()) 3123 throw new Error("Attempt to auto-create SubscriptionTopic.experimental"); 3124 else if (Configuration.doAutoCreate()) 3125 this.experimental = new BooleanType(); // bb 3126 return this.experimental; 3127 } 3128 3129 public boolean hasExperimentalElement() { 3130 return this.experimental != null && !this.experimental.isEmpty(); 3131 } 3132 3133 public boolean hasExperimental() { 3134 return this.experimental != null && !this.experimental.isEmpty(); 3135 } 3136 3137 /** 3138 * @param value {@link #experimental} (A flag to indicate that this TopSubscriptionTopicic is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3139 */ 3140 public SubscriptionTopic setExperimentalElement(BooleanType value) { 3141 this.experimental = value; 3142 return this; 3143 } 3144 3145 /** 3146 * @return A flag to indicate that this TopSubscriptionTopicic is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 3147 */ 3148 public boolean getExperimental() { 3149 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 3150 } 3151 3152 /** 3153 * @param value A flag to indicate that this TopSubscriptionTopicic is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 3154 */ 3155 public SubscriptionTopic setExperimental(boolean value) { 3156 if (this.experimental == null) 3157 this.experimental = new BooleanType(); 3158 this.experimental.setValue(value); 3159 return this; 3160 } 3161 3162 /** 3163 * @return {@link #date} (The date (and optionally time) when the subscription topic was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the subscription topic changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3164 */ 3165 public DateTimeType getDateElement() { 3166 if (this.date == null) 3167 if (Configuration.errorOnAutoCreate()) 3168 throw new Error("Attempt to auto-create SubscriptionTopic.date"); 3169 else if (Configuration.doAutoCreate()) 3170 this.date = new DateTimeType(); // bb 3171 return this.date; 3172 } 3173 3174 public boolean hasDateElement() { 3175 return this.date != null && !this.date.isEmpty(); 3176 } 3177 3178 public boolean hasDate() { 3179 return this.date != null && !this.date.isEmpty(); 3180 } 3181 3182 /** 3183 * @param value {@link #date} (The date (and optionally time) when the subscription topic was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the subscription topic changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3184 */ 3185 public SubscriptionTopic setDateElement(DateTimeType value) { 3186 this.date = value; 3187 return this; 3188 } 3189 3190 /** 3191 * @return The date (and optionally time) when the subscription topic was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the subscription topic changes. 3192 */ 3193 public Date getDate() { 3194 return this.date == null ? null : this.date.getValue(); 3195 } 3196 3197 /** 3198 * @param value The date (and optionally time) when the subscription topic was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the subscription topic changes. 3199 */ 3200 public SubscriptionTopic setDate(Date value) { 3201 if (value == null) 3202 this.date = null; 3203 else { 3204 if (this.date == null) 3205 this.date = new DateTimeType(); 3206 this.date.setValue(value); 3207 } 3208 return this; 3209 } 3210 3211 /** 3212 * @return {@link #publisher} (Helps establish the "authority/credibility" of the SubscriptionTopic. May also allow for contact.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3213 */ 3214 public StringType getPublisherElement() { 3215 if (this.publisher == null) 3216 if (Configuration.errorOnAutoCreate()) 3217 throw new Error("Attempt to auto-create SubscriptionTopic.publisher"); 3218 else if (Configuration.doAutoCreate()) 3219 this.publisher = new StringType(); // bb 3220 return this.publisher; 3221 } 3222 3223 public boolean hasPublisherElement() { 3224 return this.publisher != null && !this.publisher.isEmpty(); 3225 } 3226 3227 public boolean hasPublisher() { 3228 return this.publisher != null && !this.publisher.isEmpty(); 3229 } 3230 3231 /** 3232 * @param value {@link #publisher} (Helps establish the "authority/credibility" of the SubscriptionTopic. May also allow for contact.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3233 */ 3234 public SubscriptionTopic setPublisherElement(StringType value) { 3235 this.publisher = value; 3236 return this; 3237 } 3238 3239 /** 3240 * @return Helps establish the "authority/credibility" of the SubscriptionTopic. May also allow for contact. 3241 */ 3242 public String getPublisher() { 3243 return this.publisher == null ? null : this.publisher.getValue(); 3244 } 3245 3246 /** 3247 * @param value Helps establish the "authority/credibility" of the SubscriptionTopic. May also allow for contact. 3248 */ 3249 public SubscriptionTopic setPublisher(String value) { 3250 if (Utilities.noString(value)) 3251 this.publisher = null; 3252 else { 3253 if (this.publisher == null) 3254 this.publisher = new StringType(); 3255 this.publisher.setValue(value); 3256 } 3257 return this; 3258 } 3259 3260 /** 3261 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 3262 */ 3263 public List<ContactDetail> getContact() { 3264 if (this.contact == null) 3265 this.contact = new ArrayList<ContactDetail>(); 3266 return this.contact; 3267 } 3268 3269 /** 3270 * @return Returns a reference to <code>this</code> for easy method chaining 3271 */ 3272 public SubscriptionTopic setContact(List<ContactDetail> theContact) { 3273 this.contact = theContact; 3274 return this; 3275 } 3276 3277 public boolean hasContact() { 3278 if (this.contact == null) 3279 return false; 3280 for (ContactDetail item : this.contact) 3281 if (!item.isEmpty()) 3282 return true; 3283 return false; 3284 } 3285 3286 public ContactDetail addContact() { //3 3287 ContactDetail t = new ContactDetail(); 3288 if (this.contact == null) 3289 this.contact = new ArrayList<ContactDetail>(); 3290 this.contact.add(t); 3291 return t; 3292 } 3293 3294 public SubscriptionTopic addContact(ContactDetail t) { //3 3295 if (t == null) 3296 return this; 3297 if (this.contact == null) 3298 this.contact = new ArrayList<ContactDetail>(); 3299 this.contact.add(t); 3300 return this; 3301 } 3302 3303 /** 3304 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 3305 */ 3306 public ContactDetail getContactFirstRep() { 3307 if (getContact().isEmpty()) { 3308 addContact(); 3309 } 3310 return getContact().get(0); 3311 } 3312 3313 /** 3314 * @return {@link #description} (A free text natural language description of the Topic from the consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3315 */ 3316 public MarkdownType getDescriptionElement() { 3317 if (this.description == null) 3318 if (Configuration.errorOnAutoCreate()) 3319 throw new Error("Attempt to auto-create SubscriptionTopic.description"); 3320 else if (Configuration.doAutoCreate()) 3321 this.description = new MarkdownType(); // bb 3322 return this.description; 3323 } 3324 3325 public boolean hasDescriptionElement() { 3326 return this.description != null && !this.description.isEmpty(); 3327 } 3328 3329 public boolean hasDescription() { 3330 return this.description != null && !this.description.isEmpty(); 3331 } 3332 3333 /** 3334 * @param value {@link #description} (A free text natural language description of the Topic from the consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3335 */ 3336 public SubscriptionTopic setDescriptionElement(MarkdownType value) { 3337 this.description = value; 3338 return this; 3339 } 3340 3341 /** 3342 * @return A free text natural language description of the Topic from the consumer's perspective. 3343 */ 3344 public String getDescription() { 3345 return this.description == null ? null : this.description.getValue(); 3346 } 3347 3348 /** 3349 * @param value A free text natural language description of the Topic from the consumer's perspective. 3350 */ 3351 public SubscriptionTopic setDescription(String value) { 3352 if (Utilities.noString(value)) 3353 this.description = null; 3354 else { 3355 if (this.description == null) 3356 this.description = new MarkdownType(); 3357 this.description.setValue(value); 3358 } 3359 return this; 3360 } 3361 3362 /** 3363 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of code system definitions.) 3364 */ 3365 public List<UsageContext> getUseContext() { 3366 if (this.useContext == null) 3367 this.useContext = new ArrayList<UsageContext>(); 3368 return this.useContext; 3369 } 3370 3371 /** 3372 * @return Returns a reference to <code>this</code> for easy method chaining 3373 */ 3374 public SubscriptionTopic setUseContext(List<UsageContext> theUseContext) { 3375 this.useContext = theUseContext; 3376 return this; 3377 } 3378 3379 public boolean hasUseContext() { 3380 if (this.useContext == null) 3381 return false; 3382 for (UsageContext item : this.useContext) 3383 if (!item.isEmpty()) 3384 return true; 3385 return false; 3386 } 3387 3388 public UsageContext addUseContext() { //3 3389 UsageContext t = new UsageContext(); 3390 if (this.useContext == null) 3391 this.useContext = new ArrayList<UsageContext>(); 3392 this.useContext.add(t); 3393 return t; 3394 } 3395 3396 public SubscriptionTopic addUseContext(UsageContext t) { //3 3397 if (t == null) 3398 return this; 3399 if (this.useContext == null) 3400 this.useContext = new ArrayList<UsageContext>(); 3401 this.useContext.add(t); 3402 return this; 3403 } 3404 3405 /** 3406 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 3407 */ 3408 public UsageContext getUseContextFirstRep() { 3409 if (getUseContext().isEmpty()) { 3410 addUseContext(); 3411 } 3412 return getUseContext().get(0); 3413 } 3414 3415 /** 3416 * @return {@link #jurisdiction} (A jurisdiction in which the Topic is intended to be used.) 3417 */ 3418 public List<CodeableConcept> getJurisdiction() { 3419 if (this.jurisdiction == null) 3420 this.jurisdiction = new ArrayList<CodeableConcept>(); 3421 return this.jurisdiction; 3422 } 3423 3424 /** 3425 * @return Returns a reference to <code>this</code> for easy method chaining 3426 */ 3427 public SubscriptionTopic setJurisdiction(List<CodeableConcept> theJurisdiction) { 3428 this.jurisdiction = theJurisdiction; 3429 return this; 3430 } 3431 3432 public boolean hasJurisdiction() { 3433 if (this.jurisdiction == null) 3434 return false; 3435 for (CodeableConcept item : this.jurisdiction) 3436 if (!item.isEmpty()) 3437 return true; 3438 return false; 3439 } 3440 3441 public CodeableConcept addJurisdiction() { //3 3442 CodeableConcept t = new CodeableConcept(); 3443 if (this.jurisdiction == null) 3444 this.jurisdiction = new ArrayList<CodeableConcept>(); 3445 this.jurisdiction.add(t); 3446 return t; 3447 } 3448 3449 public SubscriptionTopic addJurisdiction(CodeableConcept t) { //3 3450 if (t == null) 3451 return this; 3452 if (this.jurisdiction == null) 3453 this.jurisdiction = new ArrayList<CodeableConcept>(); 3454 this.jurisdiction.add(t); 3455 return this; 3456 } 3457 3458 /** 3459 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 3460 */ 3461 public CodeableConcept getJurisdictionFirstRep() { 3462 if (getJurisdiction().isEmpty()) { 3463 addJurisdiction(); 3464 } 3465 return getJurisdiction().get(0); 3466 } 3467 3468 /** 3469 * @return {@link #purpose} (Explains why this Topic is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3470 */ 3471 public MarkdownType getPurposeElement() { 3472 if (this.purpose == null) 3473 if (Configuration.errorOnAutoCreate()) 3474 throw new Error("Attempt to auto-create SubscriptionTopic.purpose"); 3475 else if (Configuration.doAutoCreate()) 3476 this.purpose = new MarkdownType(); // bb 3477 return this.purpose; 3478 } 3479 3480 public boolean hasPurposeElement() { 3481 return this.purpose != null && !this.purpose.isEmpty(); 3482 } 3483 3484 public boolean hasPurpose() { 3485 return this.purpose != null && !this.purpose.isEmpty(); 3486 } 3487 3488 /** 3489 * @param value {@link #purpose} (Explains why this Topic is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3490 */ 3491 public SubscriptionTopic setPurposeElement(MarkdownType value) { 3492 this.purpose = value; 3493 return this; 3494 } 3495 3496 /** 3497 * @return Explains why this Topic is needed and why it has been designed as it has. 3498 */ 3499 public String getPurpose() { 3500 return this.purpose == null ? null : this.purpose.getValue(); 3501 } 3502 3503 /** 3504 * @param value Explains why this Topic is needed and why it has been designed as it has. 3505 */ 3506 public SubscriptionTopic setPurpose(String value) { 3507 if (Utilities.noString(value)) 3508 this.purpose = null; 3509 else { 3510 if (this.purpose == null) 3511 this.purpose = new MarkdownType(); 3512 this.purpose.setValue(value); 3513 } 3514 return this; 3515 } 3516 3517 /** 3518 * @return {@link #copyright} (A copyright statement relating to the SubscriptionTopic and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SubscriptionTopic.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3519 */ 3520 public MarkdownType getCopyrightElement() { 3521 if (this.copyright == null) 3522 if (Configuration.errorOnAutoCreate()) 3523 throw new Error("Attempt to auto-create SubscriptionTopic.copyright"); 3524 else if (Configuration.doAutoCreate()) 3525 this.copyright = new MarkdownType(); // bb 3526 return this.copyright; 3527 } 3528 3529 public boolean hasCopyrightElement() { 3530 return this.copyright != null && !this.copyright.isEmpty(); 3531 } 3532 3533 public boolean hasCopyright() { 3534 return this.copyright != null && !this.copyright.isEmpty(); 3535 } 3536 3537 /** 3538 * @param value {@link #copyright} (A copyright statement relating to the SubscriptionTopic and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SubscriptionTopic.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3539 */ 3540 public SubscriptionTopic setCopyrightElement(MarkdownType value) { 3541 this.copyright = value; 3542 return this; 3543 } 3544 3545 /** 3546 * @return A copyright statement relating to the SubscriptionTopic and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SubscriptionTopic. 3547 */ 3548 public String getCopyright() { 3549 return this.copyright == null ? null : this.copyright.getValue(); 3550 } 3551 3552 /** 3553 * @param value A copyright statement relating to the SubscriptionTopic and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SubscriptionTopic. 3554 */ 3555 public SubscriptionTopic setCopyright(String value) { 3556 if (Utilities.noString(value)) 3557 this.copyright = null; 3558 else { 3559 if (this.copyright == null) 3560 this.copyright = new MarkdownType(); 3561 this.copyright.setValue(value); 3562 } 3563 return this; 3564 } 3565 3566 /** 3567 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 3568 */ 3569 public StringType getCopyrightLabelElement() { 3570 if (this.copyrightLabel == null) 3571 if (Configuration.errorOnAutoCreate()) 3572 throw new Error("Attempt to auto-create SubscriptionTopic.copyrightLabel"); 3573 else if (Configuration.doAutoCreate()) 3574 this.copyrightLabel = new StringType(); // bb 3575 return this.copyrightLabel; 3576 } 3577 3578 public boolean hasCopyrightLabelElement() { 3579 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 3580 } 3581 3582 public boolean hasCopyrightLabel() { 3583 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 3584 } 3585 3586 /** 3587 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 3588 */ 3589 public SubscriptionTopic setCopyrightLabelElement(StringType value) { 3590 this.copyrightLabel = value; 3591 return this; 3592 } 3593 3594 /** 3595 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3596 */ 3597 public String getCopyrightLabel() { 3598 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 3599 } 3600 3601 /** 3602 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3603 */ 3604 public SubscriptionTopic setCopyrightLabel(String value) { 3605 if (Utilities.noString(value)) 3606 this.copyrightLabel = null; 3607 else { 3608 if (this.copyrightLabel == null) 3609 this.copyrightLabel = new StringType(); 3610 this.copyrightLabel.setValue(value); 3611 } 3612 return this; 3613 } 3614 3615 /** 3616 * @return {@link #approvalDate} (The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 3617 */ 3618 public DateType getApprovalDateElement() { 3619 if (this.approvalDate == null) 3620 if (Configuration.errorOnAutoCreate()) 3621 throw new Error("Attempt to auto-create SubscriptionTopic.approvalDate"); 3622 else if (Configuration.doAutoCreate()) 3623 this.approvalDate = new DateType(); // bb 3624 return this.approvalDate; 3625 } 3626 3627 public boolean hasApprovalDateElement() { 3628 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3629 } 3630 3631 public boolean hasApprovalDate() { 3632 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3633 } 3634 3635 /** 3636 * @param value {@link #approvalDate} (The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 3637 */ 3638 public SubscriptionTopic setApprovalDateElement(DateType value) { 3639 this.approvalDate = value; 3640 return this; 3641 } 3642 3643 /** 3644 * @return The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3645 */ 3646 public Date getApprovalDate() { 3647 return this.approvalDate == null ? null : this.approvalDate.getValue(); 3648 } 3649 3650 /** 3651 * @param value The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3652 */ 3653 public SubscriptionTopic setApprovalDate(Date value) { 3654 if (value == null) 3655 this.approvalDate = null; 3656 else { 3657 if (this.approvalDate == null) 3658 this.approvalDate = new DateType(); 3659 this.approvalDate.setValue(value); 3660 } 3661 return this; 3662 } 3663 3664 /** 3665 * @return {@link #lastReviewDate} (The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 3666 */ 3667 public DateType getLastReviewDateElement() { 3668 if (this.lastReviewDate == null) 3669 if (Configuration.errorOnAutoCreate()) 3670 throw new Error("Attempt to auto-create SubscriptionTopic.lastReviewDate"); 3671 else if (Configuration.doAutoCreate()) 3672 this.lastReviewDate = new DateType(); // bb 3673 return this.lastReviewDate; 3674 } 3675 3676 public boolean hasLastReviewDateElement() { 3677 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3678 } 3679 3680 public boolean hasLastReviewDate() { 3681 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3682 } 3683 3684 /** 3685 * @param value {@link #lastReviewDate} (The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 3686 */ 3687 public SubscriptionTopic setLastReviewDateElement(DateType value) { 3688 this.lastReviewDate = value; 3689 return this; 3690 } 3691 3692 /** 3693 * @return The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date. 3694 */ 3695 public Date getLastReviewDate() { 3696 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 3697 } 3698 3699 /** 3700 * @param value The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date. 3701 */ 3702 public SubscriptionTopic setLastReviewDate(Date value) { 3703 if (value == null) 3704 this.lastReviewDate = null; 3705 else { 3706 if (this.lastReviewDate == null) 3707 this.lastReviewDate = new DateType(); 3708 this.lastReviewDate.setValue(value); 3709 } 3710 return this; 3711 } 3712 3713 /** 3714 * @return {@link #effectivePeriod} (The period during which the SubscriptionTopic content was or is planned to be effective.) 3715 */ 3716 public Period getEffectivePeriod() { 3717 if (this.effectivePeriod == null) 3718 if (Configuration.errorOnAutoCreate()) 3719 throw new Error("Attempt to auto-create SubscriptionTopic.effectivePeriod"); 3720 else if (Configuration.doAutoCreate()) 3721 this.effectivePeriod = new Period(); // cc 3722 return this.effectivePeriod; 3723 } 3724 3725 public boolean hasEffectivePeriod() { 3726 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 3727 } 3728 3729 /** 3730 * @param value {@link #effectivePeriod} (The period during which the SubscriptionTopic content was or is planned to be effective.) 3731 */ 3732 public SubscriptionTopic setEffectivePeriod(Period value) { 3733 this.effectivePeriod = value; 3734 return this; 3735 } 3736 3737 /** 3738 * @return {@link #resourceTrigger} (A definition of a resource-based event that triggers a notification based on the SubscriptionTopic. The criteria may be just a human readable description and/or a full FHIR search string or FHIRPath expression. Multiple triggers are considered OR joined (e.g., a resource update matching ANY of the definitions will trigger a notification).) 3739 */ 3740 public List<SubscriptionTopicResourceTriggerComponent> getResourceTrigger() { 3741 if (this.resourceTrigger == null) 3742 this.resourceTrigger = new ArrayList<SubscriptionTopicResourceTriggerComponent>(); 3743 return this.resourceTrigger; 3744 } 3745 3746 /** 3747 * @return Returns a reference to <code>this</code> for easy method chaining 3748 */ 3749 public SubscriptionTopic setResourceTrigger(List<SubscriptionTopicResourceTriggerComponent> theResourceTrigger) { 3750 this.resourceTrigger = theResourceTrigger; 3751 return this; 3752 } 3753 3754 public boolean hasResourceTrigger() { 3755 if (this.resourceTrigger == null) 3756 return false; 3757 for (SubscriptionTopicResourceTriggerComponent item : this.resourceTrigger) 3758 if (!item.isEmpty()) 3759 return true; 3760 return false; 3761 } 3762 3763 public SubscriptionTopicResourceTriggerComponent addResourceTrigger() { //3 3764 SubscriptionTopicResourceTriggerComponent t = new SubscriptionTopicResourceTriggerComponent(); 3765 if (this.resourceTrigger == null) 3766 this.resourceTrigger = new ArrayList<SubscriptionTopicResourceTriggerComponent>(); 3767 this.resourceTrigger.add(t); 3768 return t; 3769 } 3770 3771 public SubscriptionTopic addResourceTrigger(SubscriptionTopicResourceTriggerComponent t) { //3 3772 if (t == null) 3773 return this; 3774 if (this.resourceTrigger == null) 3775 this.resourceTrigger = new ArrayList<SubscriptionTopicResourceTriggerComponent>(); 3776 this.resourceTrigger.add(t); 3777 return this; 3778 } 3779 3780 /** 3781 * @return The first repetition of repeating field {@link #resourceTrigger}, creating it if it does not already exist {3} 3782 */ 3783 public SubscriptionTopicResourceTriggerComponent getResourceTriggerFirstRep() { 3784 if (getResourceTrigger().isEmpty()) { 3785 addResourceTrigger(); 3786 } 3787 return getResourceTrigger().get(0); 3788 } 3789 3790 /** 3791 * @return {@link #eventTrigger} (Event definition which can be used to trigger the SubscriptionTopic.) 3792 */ 3793 public List<SubscriptionTopicEventTriggerComponent> getEventTrigger() { 3794 if (this.eventTrigger == null) 3795 this.eventTrigger = new ArrayList<SubscriptionTopicEventTriggerComponent>(); 3796 return this.eventTrigger; 3797 } 3798 3799 /** 3800 * @return Returns a reference to <code>this</code> for easy method chaining 3801 */ 3802 public SubscriptionTopic setEventTrigger(List<SubscriptionTopicEventTriggerComponent> theEventTrigger) { 3803 this.eventTrigger = theEventTrigger; 3804 return this; 3805 } 3806 3807 public boolean hasEventTrigger() { 3808 if (this.eventTrigger == null) 3809 return false; 3810 for (SubscriptionTopicEventTriggerComponent item : this.eventTrigger) 3811 if (!item.isEmpty()) 3812 return true; 3813 return false; 3814 } 3815 3816 public SubscriptionTopicEventTriggerComponent addEventTrigger() { //3 3817 SubscriptionTopicEventTriggerComponent t = new SubscriptionTopicEventTriggerComponent(); 3818 if (this.eventTrigger == null) 3819 this.eventTrigger = new ArrayList<SubscriptionTopicEventTriggerComponent>(); 3820 this.eventTrigger.add(t); 3821 return t; 3822 } 3823 3824 public SubscriptionTopic addEventTrigger(SubscriptionTopicEventTriggerComponent t) { //3 3825 if (t == null) 3826 return this; 3827 if (this.eventTrigger == null) 3828 this.eventTrigger = new ArrayList<SubscriptionTopicEventTriggerComponent>(); 3829 this.eventTrigger.add(t); 3830 return this; 3831 } 3832 3833 /** 3834 * @return The first repetition of repeating field {@link #eventTrigger}, creating it if it does not already exist {3} 3835 */ 3836 public SubscriptionTopicEventTriggerComponent getEventTriggerFirstRep() { 3837 if (getEventTrigger().isEmpty()) { 3838 addEventTrigger(); 3839 } 3840 return getEventTrigger().get(0); 3841 } 3842 3843 /** 3844 * @return {@link #canFilterBy} (List of properties by which Subscriptions on the SubscriptionTopic can be filtered. May be defined Search Parameters (e.g., Encounter.patient) or parameters defined within this SubscriptionTopic context (e.g., hub.event).) 3845 */ 3846 public List<SubscriptionTopicCanFilterByComponent> getCanFilterBy() { 3847 if (this.canFilterBy == null) 3848 this.canFilterBy = new ArrayList<SubscriptionTopicCanFilterByComponent>(); 3849 return this.canFilterBy; 3850 } 3851 3852 /** 3853 * @return Returns a reference to <code>this</code> for easy method chaining 3854 */ 3855 public SubscriptionTopic setCanFilterBy(List<SubscriptionTopicCanFilterByComponent> theCanFilterBy) { 3856 this.canFilterBy = theCanFilterBy; 3857 return this; 3858 } 3859 3860 public boolean hasCanFilterBy() { 3861 if (this.canFilterBy == null) 3862 return false; 3863 for (SubscriptionTopicCanFilterByComponent item : this.canFilterBy) 3864 if (!item.isEmpty()) 3865 return true; 3866 return false; 3867 } 3868 3869 public SubscriptionTopicCanFilterByComponent addCanFilterBy() { //3 3870 SubscriptionTopicCanFilterByComponent t = new SubscriptionTopicCanFilterByComponent(); 3871 if (this.canFilterBy == null) 3872 this.canFilterBy = new ArrayList<SubscriptionTopicCanFilterByComponent>(); 3873 this.canFilterBy.add(t); 3874 return t; 3875 } 3876 3877 public SubscriptionTopic addCanFilterBy(SubscriptionTopicCanFilterByComponent t) { //3 3878 if (t == null) 3879 return this; 3880 if (this.canFilterBy == null) 3881 this.canFilterBy = new ArrayList<SubscriptionTopicCanFilterByComponent>(); 3882 this.canFilterBy.add(t); 3883 return this; 3884 } 3885 3886 /** 3887 * @return The first repetition of repeating field {@link #canFilterBy}, creating it if it does not already exist {3} 3888 */ 3889 public SubscriptionTopicCanFilterByComponent getCanFilterByFirstRep() { 3890 if (getCanFilterBy().isEmpty()) { 3891 addCanFilterBy(); 3892 } 3893 return getCanFilterBy().get(0); 3894 } 3895 3896 /** 3897 * @return {@link #notificationShape} (List of properties to describe the shape (e.g., resources) included in notifications from this Subscription Topic.) 3898 */ 3899 public List<SubscriptionTopicNotificationShapeComponent> getNotificationShape() { 3900 if (this.notificationShape == null) 3901 this.notificationShape = new ArrayList<SubscriptionTopicNotificationShapeComponent>(); 3902 return this.notificationShape; 3903 } 3904 3905 /** 3906 * @return Returns a reference to <code>this</code> for easy method chaining 3907 */ 3908 public SubscriptionTopic setNotificationShape(List<SubscriptionTopicNotificationShapeComponent> theNotificationShape) { 3909 this.notificationShape = theNotificationShape; 3910 return this; 3911 } 3912 3913 public boolean hasNotificationShape() { 3914 if (this.notificationShape == null) 3915 return false; 3916 for (SubscriptionTopicNotificationShapeComponent item : this.notificationShape) 3917 if (!item.isEmpty()) 3918 return true; 3919 return false; 3920 } 3921 3922 public SubscriptionTopicNotificationShapeComponent addNotificationShape() { //3 3923 SubscriptionTopicNotificationShapeComponent t = new SubscriptionTopicNotificationShapeComponent(); 3924 if (this.notificationShape == null) 3925 this.notificationShape = new ArrayList<SubscriptionTopicNotificationShapeComponent>(); 3926 this.notificationShape.add(t); 3927 return t; 3928 } 3929 3930 public SubscriptionTopic addNotificationShape(SubscriptionTopicNotificationShapeComponent t) { //3 3931 if (t == null) 3932 return this; 3933 if (this.notificationShape == null) 3934 this.notificationShape = new ArrayList<SubscriptionTopicNotificationShapeComponent>(); 3935 this.notificationShape.add(t); 3936 return this; 3937 } 3938 3939 /** 3940 * @return The first repetition of repeating field {@link #notificationShape}, creating it if it does not already exist {3} 3941 */ 3942 public SubscriptionTopicNotificationShapeComponent getNotificationShapeFirstRep() { 3943 if (getNotificationShape().isEmpty()) { 3944 addNotificationShape(); 3945 } 3946 return getNotificationShape().get(0); 3947 } 3948 3949 protected void listChildren(List<Property> children) { 3950 super.listChildren(children); 3951 children.add(new Property("url", "uri", "An absolute URI that is used to identify this subscription topic when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this subscription topic is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the subscription topic is stored on different servers.", 0, 1, url)); 3952 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this subscription topic by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3953 children.add(new Property("version", "string", "The identifier that is used to identify this version of the subscription topic when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Topic author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable.", 0, 1, version)); 3954 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 3955 children.add(new Property("name", "string", "A natural language name identifying the subscription topic This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 3956 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the subscription topic. For example, \"admission\".", 0, 1, title)); 3957 children.add(new Property("derivedFrom", "canonical(SubscriptionTopic)", "The canonical URL pointing to another FHIR-defined SubscriptionTopic that is adhered to in whole or in part by this SubscriptionTopic.", 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 3958 children.add(new Property("status", "code", "The current state of the SubscriptionTopic.", 0, 1, status)); 3959 children.add(new Property("experimental", "boolean", "A flag to indicate that this TopSubscriptionTopicic is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 3960 children.add(new Property("date", "dateTime", "The date (and optionally time) when the subscription topic was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the subscription topic changes.", 0, 1, date)); 3961 children.add(new Property("publisher", "string", "Helps establish the \"authority/credibility\" of the SubscriptionTopic. May also allow for contact.", 0, 1, publisher)); 3962 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 3963 children.add(new Property("description", "markdown", "A free text natural language description of the Topic from the consumer's perspective.", 0, 1, description)); 3964 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of code system definitions.", 0, java.lang.Integer.MAX_VALUE, useContext)); 3965 children.add(new Property("jurisdiction", "CodeableConcept", "A jurisdiction in which the Topic is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 3966 children.add(new Property("purpose", "markdown", "Explains why this Topic is needed and why it has been designed as it has.", 0, 1, purpose)); 3967 children.add(new Property("copyright", "markdown", "A copyright statement relating to the SubscriptionTopic and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SubscriptionTopic.", 0, 1, copyright)); 3968 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 3969 children.add(new Property("approvalDate", "date", "The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 3970 children.add(new Property("lastReviewDate", "date", "The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.", 0, 1, lastReviewDate)); 3971 children.add(new Property("effectivePeriod", "Period", "The period during which the SubscriptionTopic content was or is planned to be effective.", 0, 1, effectivePeriod)); 3972 children.add(new Property("resourceTrigger", "", "A definition of a resource-based event that triggers a notification based on the SubscriptionTopic. The criteria may be just a human readable description and/or a full FHIR search string or FHIRPath expression. Multiple triggers are considered OR joined (e.g., a resource update matching ANY of the definitions will trigger a notification).", 0, java.lang.Integer.MAX_VALUE, resourceTrigger)); 3973 children.add(new Property("eventTrigger", "", "Event definition which can be used to trigger the SubscriptionTopic.", 0, java.lang.Integer.MAX_VALUE, eventTrigger)); 3974 children.add(new Property("canFilterBy", "", "List of properties by which Subscriptions on the SubscriptionTopic can be filtered. May be defined Search Parameters (e.g., Encounter.patient) or parameters defined within this SubscriptionTopic context (e.g., hub.event).", 0, java.lang.Integer.MAX_VALUE, canFilterBy)); 3975 children.add(new Property("notificationShape", "", "List of properties to describe the shape (e.g., resources) included in notifications from this Subscription Topic.", 0, java.lang.Integer.MAX_VALUE, notificationShape)); 3976 } 3977 3978 @Override 3979 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3980 switch (_hash) { 3981 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this subscription topic when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this subscription topic is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the subscription topic is stored on different servers.", 0, 1, url); 3982 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this subscription topic by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 3983 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the subscription topic when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Topic author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable.", 0, 1, version); 3984 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3985 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3986 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3987 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3988 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the subscription topic This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 3989 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the subscription topic. For example, \"admission\".", 0, 1, title); 3990 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "canonical(SubscriptionTopic)", "The canonical URL pointing to another FHIR-defined SubscriptionTopic that is adhered to in whole or in part by this SubscriptionTopic.", 0, java.lang.Integer.MAX_VALUE, derivedFrom); 3991 case -892481550: /*status*/ return new Property("status", "code", "The current state of the SubscriptionTopic.", 0, 1, status); 3992 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A flag to indicate that this TopSubscriptionTopicic is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 3993 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the subscription topic was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the subscription topic changes.", 0, 1, date); 3994 case 1447404028: /*publisher*/ return new Property("publisher", "string", "Helps establish the \"authority/credibility\" of the SubscriptionTopic. May also allow for contact.", 0, 1, publisher); 3995 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 3996 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the Topic from the consumer's perspective.", 0, 1, description); 3997 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of code system definitions.", 0, java.lang.Integer.MAX_VALUE, useContext); 3998 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A jurisdiction in which the Topic is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 3999 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explains why this Topic is needed and why it has been designed as it has.", 0, 1, purpose); 4000 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the SubscriptionTopic and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SubscriptionTopic.", 0, 1, copyright); 4001 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 4002 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 4003 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.", 0, 1, lastReviewDate); 4004 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the SubscriptionTopic content was or is planned to be effective.", 0, 1, effectivePeriod); 4005 case -424927798: /*resourceTrigger*/ return new Property("resourceTrigger", "", "A definition of a resource-based event that triggers a notification based on the SubscriptionTopic. The criteria may be just a human readable description and/or a full FHIR search string or FHIRPath expression. Multiple triggers are considered OR joined (e.g., a resource update matching ANY of the definitions will trigger a notification).", 0, java.lang.Integer.MAX_VALUE, resourceTrigger); 4006 case -151635522: /*eventTrigger*/ return new Property("eventTrigger", "", "Event definition which can be used to trigger the SubscriptionTopic.", 0, java.lang.Integer.MAX_VALUE, eventTrigger); 4007 case -1299519009: /*canFilterBy*/ return new Property("canFilterBy", "", "List of properties by which Subscriptions on the SubscriptionTopic can be filtered. May be defined Search Parameters (e.g., Encounter.patient) or parameters defined within this SubscriptionTopic context (e.g., hub.event).", 0, java.lang.Integer.MAX_VALUE, canFilterBy); 4008 case -1583369866: /*notificationShape*/ return new Property("notificationShape", "", "List of properties to describe the shape (e.g., resources) included in notifications from this Subscription Topic.", 0, java.lang.Integer.MAX_VALUE, notificationShape); 4009 default: return super.getNamedProperty(_hash, _name, _checkValid); 4010 } 4011 4012 } 4013 4014 @Override 4015 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4016 switch (hash) { 4017 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 4018 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4019 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 4020 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 4021 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 4022 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 4023 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // CanonicalType 4024 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 4025 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 4026 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 4027 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 4028 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 4029 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 4030 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 4031 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 4032 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 4033 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 4034 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 4035 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 4036 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 4037 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 4038 case -424927798: /*resourceTrigger*/ return this.resourceTrigger == null ? new Base[0] : this.resourceTrigger.toArray(new Base[this.resourceTrigger.size()]); // SubscriptionTopicResourceTriggerComponent 4039 case -151635522: /*eventTrigger*/ return this.eventTrigger == null ? new Base[0] : this.eventTrigger.toArray(new Base[this.eventTrigger.size()]); // SubscriptionTopicEventTriggerComponent 4040 case -1299519009: /*canFilterBy*/ return this.canFilterBy == null ? new Base[0] : this.canFilterBy.toArray(new Base[this.canFilterBy.size()]); // SubscriptionTopicCanFilterByComponent 4041 case -1583369866: /*notificationShape*/ return this.notificationShape == null ? new Base[0] : this.notificationShape.toArray(new Base[this.notificationShape.size()]); // SubscriptionTopicNotificationShapeComponent 4042 default: return super.getProperty(hash, name, checkValid); 4043 } 4044 4045 } 4046 4047 @Override 4048 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4049 switch (hash) { 4050 case 116079: // url 4051 this.url = TypeConvertor.castToUri(value); // UriType 4052 return value; 4053 case -1618432855: // identifier 4054 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 4055 return value; 4056 case 351608024: // version 4057 this.version = TypeConvertor.castToString(value); // StringType 4058 return value; 4059 case 1508158071: // versionAlgorithm 4060 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 4061 return value; 4062 case 3373707: // name 4063 this.name = TypeConvertor.castToString(value); // StringType 4064 return value; 4065 case 110371416: // title 4066 this.title = TypeConvertor.castToString(value); // StringType 4067 return value; 4068 case 1077922663: // derivedFrom 4069 this.getDerivedFrom().add(TypeConvertor.castToCanonical(value)); // CanonicalType 4070 return value; 4071 case -892481550: // status 4072 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4073 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4074 return value; 4075 case -404562712: // experimental 4076 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 4077 return value; 4078 case 3076014: // date 4079 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4080 return value; 4081 case 1447404028: // publisher 4082 this.publisher = TypeConvertor.castToString(value); // StringType 4083 return value; 4084 case 951526432: // contact 4085 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4086 return value; 4087 case -1724546052: // description 4088 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4089 return value; 4090 case -669707736: // useContext 4091 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 4092 return value; 4093 case -507075711: // jurisdiction 4094 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4095 return value; 4096 case -220463842: // purpose 4097 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 4098 return value; 4099 case 1522889671: // copyright 4100 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 4101 return value; 4102 case 765157229: // copyrightLabel 4103 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 4104 return value; 4105 case 223539345: // approvalDate 4106 this.approvalDate = TypeConvertor.castToDate(value); // DateType 4107 return value; 4108 case -1687512484: // lastReviewDate 4109 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 4110 return value; 4111 case -403934648: // effectivePeriod 4112 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 4113 return value; 4114 case -424927798: // resourceTrigger 4115 this.getResourceTrigger().add((SubscriptionTopicResourceTriggerComponent) value); // SubscriptionTopicResourceTriggerComponent 4116 return value; 4117 case -151635522: // eventTrigger 4118 this.getEventTrigger().add((SubscriptionTopicEventTriggerComponent) value); // SubscriptionTopicEventTriggerComponent 4119 return value; 4120 case -1299519009: // canFilterBy 4121 this.getCanFilterBy().add((SubscriptionTopicCanFilterByComponent) value); // SubscriptionTopicCanFilterByComponent 4122 return value; 4123 case -1583369866: // notificationShape 4124 this.getNotificationShape().add((SubscriptionTopicNotificationShapeComponent) value); // SubscriptionTopicNotificationShapeComponent 4125 return value; 4126 default: return super.setProperty(hash, name, value); 4127 } 4128 4129 } 4130 4131 @Override 4132 public Base setProperty(String name, Base value) throws FHIRException { 4133 if (name.equals("url")) { 4134 this.url = TypeConvertor.castToUri(value); // UriType 4135 } else if (name.equals("identifier")) { 4136 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 4137 } else if (name.equals("version")) { 4138 this.version = TypeConvertor.castToString(value); // StringType 4139 } else if (name.equals("versionAlgorithm[x]")) { 4140 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 4141 } else if (name.equals("name")) { 4142 this.name = TypeConvertor.castToString(value); // StringType 4143 } else if (name.equals("title")) { 4144 this.title = TypeConvertor.castToString(value); // StringType 4145 } else if (name.equals("derivedFrom")) { 4146 this.getDerivedFrom().add(TypeConvertor.castToCanonical(value)); 4147 } else if (name.equals("status")) { 4148 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4149 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4150 } else if (name.equals("experimental")) { 4151 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 4152 } else if (name.equals("date")) { 4153 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4154 } else if (name.equals("publisher")) { 4155 this.publisher = TypeConvertor.castToString(value); // StringType 4156 } else if (name.equals("contact")) { 4157 this.getContact().add(TypeConvertor.castToContactDetail(value)); 4158 } else if (name.equals("description")) { 4159 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4160 } else if (name.equals("useContext")) { 4161 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 4162 } else if (name.equals("jurisdiction")) { 4163 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 4164 } else if (name.equals("purpose")) { 4165 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 4166 } else if (name.equals("copyright")) { 4167 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 4168 } else if (name.equals("copyrightLabel")) { 4169 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 4170 } else if (name.equals("approvalDate")) { 4171 this.approvalDate = TypeConvertor.castToDate(value); // DateType 4172 } else if (name.equals("lastReviewDate")) { 4173 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 4174 } else if (name.equals("effectivePeriod")) { 4175 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 4176 } else if (name.equals("resourceTrigger")) { 4177 this.getResourceTrigger().add((SubscriptionTopicResourceTriggerComponent) value); 4178 } else if (name.equals("eventTrigger")) { 4179 this.getEventTrigger().add((SubscriptionTopicEventTriggerComponent) value); 4180 } else if (name.equals("canFilterBy")) { 4181 this.getCanFilterBy().add((SubscriptionTopicCanFilterByComponent) value); 4182 } else if (name.equals("notificationShape")) { 4183 this.getNotificationShape().add((SubscriptionTopicNotificationShapeComponent) value); 4184 } else 4185 return super.setProperty(name, value); 4186 return value; 4187 } 4188 4189 @Override 4190 public void removeChild(String name, Base value) throws FHIRException { 4191 if (name.equals("url")) { 4192 this.url = null; 4193 } else if (name.equals("identifier")) { 4194 this.getIdentifier().remove(value); 4195 } else if (name.equals("version")) { 4196 this.version = null; 4197 } else if (name.equals("versionAlgorithm[x]")) { 4198 this.versionAlgorithm = null; 4199 } else if (name.equals("name")) { 4200 this.name = null; 4201 } else if (name.equals("title")) { 4202 this.title = null; 4203 } else if (name.equals("derivedFrom")) { 4204 this.getDerivedFrom().remove(value); 4205 } else if (name.equals("status")) { 4206 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4207 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4208 } else if (name.equals("experimental")) { 4209 this.experimental = null; 4210 } else if (name.equals("date")) { 4211 this.date = null; 4212 } else if (name.equals("publisher")) { 4213 this.publisher = null; 4214 } else if (name.equals("contact")) { 4215 this.getContact().remove(value); 4216 } else if (name.equals("description")) { 4217 this.description = null; 4218 } else if (name.equals("useContext")) { 4219 this.getUseContext().remove(value); 4220 } else if (name.equals("jurisdiction")) { 4221 this.getJurisdiction().remove(value); 4222 } else if (name.equals("purpose")) { 4223 this.purpose = null; 4224 } else if (name.equals("copyright")) { 4225 this.copyright = null; 4226 } else if (name.equals("copyrightLabel")) { 4227 this.copyrightLabel = null; 4228 } else if (name.equals("approvalDate")) { 4229 this.approvalDate = null; 4230 } else if (name.equals("lastReviewDate")) { 4231 this.lastReviewDate = null; 4232 } else if (name.equals("effectivePeriod")) { 4233 this.effectivePeriod = null; 4234 } else if (name.equals("resourceTrigger")) { 4235 this.getResourceTrigger().remove((SubscriptionTopicResourceTriggerComponent) value); 4236 } else if (name.equals("eventTrigger")) { 4237 this.getEventTrigger().remove((SubscriptionTopicEventTriggerComponent) value); 4238 } else if (name.equals("canFilterBy")) { 4239 this.getCanFilterBy().remove((SubscriptionTopicCanFilterByComponent) value); 4240 } else if (name.equals("notificationShape")) { 4241 this.getNotificationShape().remove((SubscriptionTopicNotificationShapeComponent) value); 4242 } else 4243 super.removeChild(name, value); 4244 4245 } 4246 4247 @Override 4248 public Base makeProperty(int hash, String name) throws FHIRException { 4249 switch (hash) { 4250 case 116079: return getUrlElement(); 4251 case -1618432855: return addIdentifier(); 4252 case 351608024: return getVersionElement(); 4253 case -115699031: return getVersionAlgorithm(); 4254 case 1508158071: return getVersionAlgorithm(); 4255 case 3373707: return getNameElement(); 4256 case 110371416: return getTitleElement(); 4257 case 1077922663: return addDerivedFromElement(); 4258 case -892481550: return getStatusElement(); 4259 case -404562712: return getExperimentalElement(); 4260 case 3076014: return getDateElement(); 4261 case 1447404028: return getPublisherElement(); 4262 case 951526432: return addContact(); 4263 case -1724546052: return getDescriptionElement(); 4264 case -669707736: return addUseContext(); 4265 case -507075711: return addJurisdiction(); 4266 case -220463842: return getPurposeElement(); 4267 case 1522889671: return getCopyrightElement(); 4268 case 765157229: return getCopyrightLabelElement(); 4269 case 223539345: return getApprovalDateElement(); 4270 case -1687512484: return getLastReviewDateElement(); 4271 case -403934648: return getEffectivePeriod(); 4272 case -424927798: return addResourceTrigger(); 4273 case -151635522: return addEventTrigger(); 4274 case -1299519009: return addCanFilterBy(); 4275 case -1583369866: return addNotificationShape(); 4276 default: return super.makeProperty(hash, name); 4277 } 4278 4279 } 4280 4281 @Override 4282 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4283 switch (hash) { 4284 case 116079: /*url*/ return new String[] {"uri"}; 4285 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4286 case 351608024: /*version*/ return new String[] {"string"}; 4287 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 4288 case 3373707: /*name*/ return new String[] {"string"}; 4289 case 110371416: /*title*/ return new String[] {"string"}; 4290 case 1077922663: /*derivedFrom*/ return new String[] {"canonical"}; 4291 case -892481550: /*status*/ return new String[] {"code"}; 4292 case -404562712: /*experimental*/ return new String[] {"boolean"}; 4293 case 3076014: /*date*/ return new String[] {"dateTime"}; 4294 case 1447404028: /*publisher*/ return new String[] {"string"}; 4295 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 4296 case -1724546052: /*description*/ return new String[] {"markdown"}; 4297 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 4298 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 4299 case -220463842: /*purpose*/ return new String[] {"markdown"}; 4300 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 4301 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 4302 case 223539345: /*approvalDate*/ return new String[] {"date"}; 4303 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 4304 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 4305 case -424927798: /*resourceTrigger*/ return new String[] {}; 4306 case -151635522: /*eventTrigger*/ return new String[] {}; 4307 case -1299519009: /*canFilterBy*/ return new String[] {}; 4308 case -1583369866: /*notificationShape*/ return new String[] {}; 4309 default: return super.getTypesForProperty(hash, name); 4310 } 4311 4312 } 4313 4314 @Override 4315 public Base addChild(String name) throws FHIRException { 4316 if (name.equals("url")) { 4317 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.url"); 4318 } 4319 else if (name.equals("identifier")) { 4320 return addIdentifier(); 4321 } 4322 else if (name.equals("version")) { 4323 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.version"); 4324 } 4325 else if (name.equals("versionAlgorithmString")) { 4326 this.versionAlgorithm = new StringType(); 4327 return this.versionAlgorithm; 4328 } 4329 else if (name.equals("versionAlgorithmCoding")) { 4330 this.versionAlgorithm = new Coding(); 4331 return this.versionAlgorithm; 4332 } 4333 else if (name.equals("name")) { 4334 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.name"); 4335 } 4336 else if (name.equals("title")) { 4337 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.title"); 4338 } 4339 else if (name.equals("derivedFrom")) { 4340 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.derivedFrom"); 4341 } 4342 else if (name.equals("status")) { 4343 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.status"); 4344 } 4345 else if (name.equals("experimental")) { 4346 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.experimental"); 4347 } 4348 else if (name.equals("date")) { 4349 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.date"); 4350 } 4351 else if (name.equals("publisher")) { 4352 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.publisher"); 4353 } 4354 else if (name.equals("contact")) { 4355 return addContact(); 4356 } 4357 else if (name.equals("description")) { 4358 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.description"); 4359 } 4360 else if (name.equals("useContext")) { 4361 return addUseContext(); 4362 } 4363 else if (name.equals("jurisdiction")) { 4364 return addJurisdiction(); 4365 } 4366 else if (name.equals("purpose")) { 4367 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.purpose"); 4368 } 4369 else if (name.equals("copyright")) { 4370 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.copyright"); 4371 } 4372 else if (name.equals("copyrightLabel")) { 4373 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.copyrightLabel"); 4374 } 4375 else if (name.equals("approvalDate")) { 4376 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.approvalDate"); 4377 } 4378 else if (name.equals("lastReviewDate")) { 4379 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.lastReviewDate"); 4380 } 4381 else if (name.equals("effectivePeriod")) { 4382 this.effectivePeriod = new Period(); 4383 return this.effectivePeriod; 4384 } 4385 else if (name.equals("resourceTrigger")) { 4386 return addResourceTrigger(); 4387 } 4388 else if (name.equals("eventTrigger")) { 4389 return addEventTrigger(); 4390 } 4391 else if (name.equals("canFilterBy")) { 4392 return addCanFilterBy(); 4393 } 4394 else if (name.equals("notificationShape")) { 4395 return addNotificationShape(); 4396 } 4397 else 4398 return super.addChild(name); 4399 } 4400 4401 public String fhirType() { 4402 return "SubscriptionTopic"; 4403 4404 } 4405 4406 public SubscriptionTopic copy() { 4407 SubscriptionTopic dst = new SubscriptionTopic(); 4408 copyValues(dst); 4409 return dst; 4410 } 4411 4412 public void copyValues(SubscriptionTopic dst) { 4413 super.copyValues(dst); 4414 dst.url = url == null ? null : url.copy(); 4415 if (identifier != null) { 4416 dst.identifier = new ArrayList<Identifier>(); 4417 for (Identifier i : identifier) 4418 dst.identifier.add(i.copy()); 4419 }; 4420 dst.version = version == null ? null : version.copy(); 4421 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 4422 dst.name = name == null ? null : name.copy(); 4423 dst.title = title == null ? null : title.copy(); 4424 if (derivedFrom != null) { 4425 dst.derivedFrom = new ArrayList<CanonicalType>(); 4426 for (CanonicalType i : derivedFrom) 4427 dst.derivedFrom.add(i.copy()); 4428 }; 4429 dst.status = status == null ? null : status.copy(); 4430 dst.experimental = experimental == null ? null : experimental.copy(); 4431 dst.date = date == null ? null : date.copy(); 4432 dst.publisher = publisher == null ? null : publisher.copy(); 4433 if (contact != null) { 4434 dst.contact = new ArrayList<ContactDetail>(); 4435 for (ContactDetail i : contact) 4436 dst.contact.add(i.copy()); 4437 }; 4438 dst.description = description == null ? null : description.copy(); 4439 if (useContext != null) { 4440 dst.useContext = new ArrayList<UsageContext>(); 4441 for (UsageContext i : useContext) 4442 dst.useContext.add(i.copy()); 4443 }; 4444 if (jurisdiction != null) { 4445 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4446 for (CodeableConcept i : jurisdiction) 4447 dst.jurisdiction.add(i.copy()); 4448 }; 4449 dst.purpose = purpose == null ? null : purpose.copy(); 4450 dst.copyright = copyright == null ? null : copyright.copy(); 4451 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 4452 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 4453 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 4454 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 4455 if (resourceTrigger != null) { 4456 dst.resourceTrigger = new ArrayList<SubscriptionTopicResourceTriggerComponent>(); 4457 for (SubscriptionTopicResourceTriggerComponent i : resourceTrigger) 4458 dst.resourceTrigger.add(i.copy()); 4459 }; 4460 if (eventTrigger != null) { 4461 dst.eventTrigger = new ArrayList<SubscriptionTopicEventTriggerComponent>(); 4462 for (SubscriptionTopicEventTriggerComponent i : eventTrigger) 4463 dst.eventTrigger.add(i.copy()); 4464 }; 4465 if (canFilterBy != null) { 4466 dst.canFilterBy = new ArrayList<SubscriptionTopicCanFilterByComponent>(); 4467 for (SubscriptionTopicCanFilterByComponent i : canFilterBy) 4468 dst.canFilterBy.add(i.copy()); 4469 }; 4470 if (notificationShape != null) { 4471 dst.notificationShape = new ArrayList<SubscriptionTopicNotificationShapeComponent>(); 4472 for (SubscriptionTopicNotificationShapeComponent i : notificationShape) 4473 dst.notificationShape.add(i.copy()); 4474 }; 4475 } 4476 4477 protected SubscriptionTopic typedCopy() { 4478 return copy(); 4479 } 4480 4481 @Override 4482 public boolean equalsDeep(Base other_) { 4483 if (!super.equalsDeep(other_)) 4484 return false; 4485 if (!(other_ instanceof SubscriptionTopic)) 4486 return false; 4487 SubscriptionTopic o = (SubscriptionTopic) other_; 4488 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 4489 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 4490 && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 4491 && compareDeep(date, o.date, true) && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 4492 && compareDeep(description, o.description, true) && compareDeep(useContext, o.useContext, true) 4493 && compareDeep(jurisdiction, o.jurisdiction, true) && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 4494 && compareDeep(copyrightLabel, o.copyrightLabel, true) && compareDeep(approvalDate, o.approvalDate, true) 4495 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 4496 && compareDeep(resourceTrigger, o.resourceTrigger, true) && compareDeep(eventTrigger, o.eventTrigger, true) 4497 && compareDeep(canFilterBy, o.canFilterBy, true) && compareDeep(notificationShape, o.notificationShape, true) 4498 ; 4499 } 4500 4501 @Override 4502 public boolean equalsShallow(Base other_) { 4503 if (!super.equalsShallow(other_)) 4504 return false; 4505 if (!(other_ instanceof SubscriptionTopic)) 4506 return false; 4507 SubscriptionTopic o = (SubscriptionTopic) other_; 4508 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 4509 && compareValues(title, o.title, true) && compareValues(derivedFrom, o.derivedFrom, true) && compareValues(status, o.status, true) 4510 && compareValues(experimental, o.experimental, true) && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) 4511 && compareValues(description, o.description, true) && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) 4512 && compareValues(copyrightLabel, o.copyrightLabel, true) && compareValues(approvalDate, o.approvalDate, true) 4513 && compareValues(lastReviewDate, o.lastReviewDate, true); 4514 } 4515 4516 public boolean isEmpty() { 4517 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 4518 , versionAlgorithm, name, title, derivedFrom, status, experimental, date, publisher 4519 , contact, description, useContext, jurisdiction, purpose, copyright, copyrightLabel 4520 , approvalDate, lastReviewDate, effectivePeriod, resourceTrigger, eventTrigger, canFilterBy 4521 , notificationShape); 4522 } 4523 4524 @Override 4525 public ResourceType getResourceType() { 4526 return ResourceType.SubscriptionTopic; 4527 } 4528 4529 /** 4530 * Search parameter: <b>date</b> 4531 * <p> 4532 * Description: <b>Multiple Resources: 4533 4534* [ActivityDefinition](activitydefinition.html): The activity definition publication date 4535* [ActorDefinition](actordefinition.html): The Actor Definition publication date 4536* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 4537* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 4538* [Citation](citation.html): The citation publication date 4539* [CodeSystem](codesystem.html): The code system publication date 4540* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 4541* [ConceptMap](conceptmap.html): The concept map publication date 4542* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 4543* [EventDefinition](eventdefinition.html): The event definition publication date 4544* [Evidence](evidence.html): The evidence publication date 4545* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 4546* [ExampleScenario](examplescenario.html): The example scenario publication date 4547* [GraphDefinition](graphdefinition.html): The graph definition publication date 4548* [ImplementationGuide](implementationguide.html): The implementation guide publication date 4549* [Library](library.html): The library publication date 4550* [Measure](measure.html): The measure publication date 4551* [MessageDefinition](messagedefinition.html): The message definition publication date 4552* [NamingSystem](namingsystem.html): The naming system publication date 4553* [OperationDefinition](operationdefinition.html): The operation definition publication date 4554* [PlanDefinition](plandefinition.html): The plan definition publication date 4555* [Questionnaire](questionnaire.html): The questionnaire publication date 4556* [Requirements](requirements.html): The requirements publication date 4557* [SearchParameter](searchparameter.html): The search parameter publication date 4558* [StructureDefinition](structuredefinition.html): The structure definition publication date 4559* [StructureMap](structuremap.html): The structure map publication date 4560* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 4561* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 4562* [TestScript](testscript.html): The test script publication date 4563* [ValueSet](valueset.html): The value set publication date 4564</b><br> 4565 * Type: <b>date</b><br> 4566 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 4567 * </p> 4568 */ 4569 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 4570 public static final String SP_DATE = "date"; 4571 /** 4572 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4573 * <p> 4574 * Description: <b>Multiple Resources: 4575 4576* [ActivityDefinition](activitydefinition.html): The activity definition publication date 4577* [ActorDefinition](actordefinition.html): The Actor Definition publication date 4578* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 4579* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 4580* [Citation](citation.html): The citation publication date 4581* [CodeSystem](codesystem.html): The code system publication date 4582* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 4583* [ConceptMap](conceptmap.html): The concept map publication date 4584* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 4585* [EventDefinition](eventdefinition.html): The event definition publication date 4586* [Evidence](evidence.html): The evidence publication date 4587* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 4588* [ExampleScenario](examplescenario.html): The example scenario publication date 4589* [GraphDefinition](graphdefinition.html): The graph definition publication date 4590* [ImplementationGuide](implementationguide.html): The implementation guide publication date 4591* [Library](library.html): The library publication date 4592* [Measure](measure.html): The measure publication date 4593* [MessageDefinition](messagedefinition.html): The message definition publication date 4594* [NamingSystem](namingsystem.html): The naming system publication date 4595* [OperationDefinition](operationdefinition.html): The operation definition publication date 4596* [PlanDefinition](plandefinition.html): The plan definition publication date 4597* [Questionnaire](questionnaire.html): The questionnaire publication date 4598* [Requirements](requirements.html): The requirements publication date 4599* [SearchParameter](searchparameter.html): The search parameter publication date 4600* [StructureDefinition](structuredefinition.html): The structure definition publication date 4601* [StructureMap](structuremap.html): The structure map publication date 4602* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 4603* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 4604* [TestScript](testscript.html): The test script publication date 4605* [ValueSet](valueset.html): The value set publication date 4606</b><br> 4607 * Type: <b>date</b><br> 4608 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 4609 * </p> 4610 */ 4611 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4612 4613 /** 4614 * Search parameter: <b>identifier</b> 4615 * <p> 4616 * Description: <b>Multiple Resources: 4617 4618* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4619* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4620* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4621* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4622* [Citation](citation.html): External identifier for the citation 4623* [CodeSystem](codesystem.html): External identifier for the code system 4624* [ConceptMap](conceptmap.html): External identifier for the concept map 4625* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4626* [EventDefinition](eventdefinition.html): External identifier for the event definition 4627* [Evidence](evidence.html): External identifier for the evidence 4628* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4629* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4630* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4631* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4632* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4633* [Library](library.html): External identifier for the library 4634* [Measure](measure.html): External identifier for the measure 4635* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4636* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4637* [NamingSystem](namingsystem.html): External identifier for the naming system 4638* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4639* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4640* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4641* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4642* [Requirements](requirements.html): External identifier for the requirements 4643* [SearchParameter](searchparameter.html): External identifier for the search parameter 4644* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4645* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4646* [StructureMap](structuremap.html): External identifier for the structure map 4647* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4648* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4649* [TestPlan](testplan.html): An identifier for the test plan 4650* [TestScript](testscript.html): External identifier for the test script 4651* [ValueSet](valueset.html): External identifier for the value set 4652</b><br> 4653 * Type: <b>token</b><br> 4654 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4655 * </p> 4656 */ 4657 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 4658 public static final String SP_IDENTIFIER = "identifier"; 4659 /** 4660 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4661 * <p> 4662 * Description: <b>Multiple Resources: 4663 4664* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4665* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4666* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4667* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4668* [Citation](citation.html): External identifier for the citation 4669* [CodeSystem](codesystem.html): External identifier for the code system 4670* [ConceptMap](conceptmap.html): External identifier for the concept map 4671* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4672* [EventDefinition](eventdefinition.html): External identifier for the event definition 4673* [Evidence](evidence.html): External identifier for the evidence 4674* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4675* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4676* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4677* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4678* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4679* [Library](library.html): External identifier for the library 4680* [Measure](measure.html): External identifier for the measure 4681* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4682* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4683* [NamingSystem](namingsystem.html): External identifier for the naming system 4684* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4685* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4686* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4687* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4688* [Requirements](requirements.html): External identifier for the requirements 4689* [SearchParameter](searchparameter.html): External identifier for the search parameter 4690* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4691* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4692* [StructureMap](structuremap.html): External identifier for the structure map 4693* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4694* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4695* [TestPlan](testplan.html): An identifier for the test plan 4696* [TestScript](testscript.html): External identifier for the test script 4697* [ValueSet](valueset.html): External identifier for the value set 4698</b><br> 4699 * Type: <b>token</b><br> 4700 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4701 * </p> 4702 */ 4703 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4704 4705 /** 4706 * Search parameter: <b>status</b> 4707 * <p> 4708 * Description: <b>Multiple Resources: 4709 4710* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4711* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4712* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4713* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4714* [Citation](citation.html): The current status of the citation 4715* [CodeSystem](codesystem.html): The current status of the code system 4716* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4717* [ConceptMap](conceptmap.html): The current status of the concept map 4718* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4719* [EventDefinition](eventdefinition.html): The current status of the event definition 4720* [Evidence](evidence.html): The current status of the evidence 4721* [EvidenceReport](evidencereport.html): The current status of the evidence report 4722* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4723* [ExampleScenario](examplescenario.html): The current status of the example scenario 4724* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4725* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4726* [Library](library.html): The current status of the library 4727* [Measure](measure.html): The current status of the measure 4728* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4729* [MessageDefinition](messagedefinition.html): The current status of the message definition 4730* [NamingSystem](namingsystem.html): The current status of the naming system 4731* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4732* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4733* [PlanDefinition](plandefinition.html): The current status of the plan definition 4734* [Questionnaire](questionnaire.html): The current status of the questionnaire 4735* [Requirements](requirements.html): The current status of the requirements 4736* [SearchParameter](searchparameter.html): The current status of the search parameter 4737* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4738* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4739* [StructureMap](structuremap.html): The current status of the structure map 4740* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4741* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4742* [TestPlan](testplan.html): The current status of the test plan 4743* [TestScript](testscript.html): The current status of the test script 4744* [ValueSet](valueset.html): The current status of the value set 4745</b><br> 4746 * Type: <b>token</b><br> 4747 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4748 * </p> 4749 */ 4750 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 4751 public static final String SP_STATUS = "status"; 4752 /** 4753 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4754 * <p> 4755 * Description: <b>Multiple Resources: 4756 4757* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4758* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4759* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4760* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4761* [Citation](citation.html): The current status of the citation 4762* [CodeSystem](codesystem.html): The current status of the code system 4763* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4764* [ConceptMap](conceptmap.html): The current status of the concept map 4765* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4766* [EventDefinition](eventdefinition.html): The current status of the event definition 4767* [Evidence](evidence.html): The current status of the evidence 4768* [EvidenceReport](evidencereport.html): The current status of the evidence report 4769* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4770* [ExampleScenario](examplescenario.html): The current status of the example scenario 4771* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4772* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4773* [Library](library.html): The current status of the library 4774* [Measure](measure.html): The current status of the measure 4775* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4776* [MessageDefinition](messagedefinition.html): The current status of the message definition 4777* [NamingSystem](namingsystem.html): The current status of the naming system 4778* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4779* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4780* [PlanDefinition](plandefinition.html): The current status of the plan definition 4781* [Questionnaire](questionnaire.html): The current status of the questionnaire 4782* [Requirements](requirements.html): The current status of the requirements 4783* [SearchParameter](searchparameter.html): The current status of the search parameter 4784* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4785* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4786* [StructureMap](structuremap.html): The current status of the structure map 4787* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4788* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4789* [TestPlan](testplan.html): The current status of the test plan 4790* [TestScript](testscript.html): The current status of the test script 4791* [ValueSet](valueset.html): The current status of the value set 4792</b><br> 4793 * Type: <b>token</b><br> 4794 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4795 * </p> 4796 */ 4797 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4798 4799 /** 4800 * Search parameter: <b>title</b> 4801 * <p> 4802 * Description: <b>Multiple Resources: 4803 4804* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 4805* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 4806* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 4807* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 4808* [Citation](citation.html): The human-friendly name of the citation 4809* [CodeSystem](codesystem.html): The human-friendly name of the code system 4810* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 4811* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 4812* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 4813* [Evidence](evidence.html): The human-friendly name of the evidence 4814* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 4815* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 4816* [Library](library.html): The human-friendly name of the library 4817* [Measure](measure.html): The human-friendly name of the measure 4818* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 4819* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 4820* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 4821* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 4822* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 4823* [Requirements](requirements.html): The human-friendly name of the requirements 4824* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 4825* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 4826* [StructureMap](structuremap.html): The human-friendly name of the structure map 4827* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 4828* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 4829* [TestScript](testscript.html): The human-friendly name of the test script 4830* [ValueSet](valueset.html): The human-friendly name of the value set 4831</b><br> 4832 * Type: <b>string</b><br> 4833 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 4834 * </p> 4835 */ 4836 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 4837 public static final String SP_TITLE = "title"; 4838 /** 4839 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4840 * <p> 4841 * Description: <b>Multiple Resources: 4842 4843* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 4844* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 4845* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 4846* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 4847* [Citation](citation.html): The human-friendly name of the citation 4848* [CodeSystem](codesystem.html): The human-friendly name of the code system 4849* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 4850* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 4851* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 4852* [Evidence](evidence.html): The human-friendly name of the evidence 4853* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 4854* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 4855* [Library](library.html): The human-friendly name of the library 4856* [Measure](measure.html): The human-friendly name of the measure 4857* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 4858* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 4859* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 4860* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 4861* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 4862* [Requirements](requirements.html): The human-friendly name of the requirements 4863* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 4864* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 4865* [StructureMap](structuremap.html): The human-friendly name of the structure map 4866* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 4867* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 4868* [TestScript](testscript.html): The human-friendly name of the test script 4869* [ValueSet](valueset.html): The human-friendly name of the value set 4870</b><br> 4871 * Type: <b>string</b><br> 4872 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 4873 * </p> 4874 */ 4875 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 4876 4877 /** 4878 * Search parameter: <b>url</b> 4879 * <p> 4880 * Description: <b>Multiple Resources: 4881 4882* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4883* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4884* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4885* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4886* [Citation](citation.html): The uri that identifies the citation 4887* [CodeSystem](codesystem.html): The uri that identifies the code system 4888* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4889* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4890* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4891* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4892* [Evidence](evidence.html): The uri that identifies the evidence 4893* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4894* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4895* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4896* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4897* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4898* [Library](library.html): The uri that identifies the library 4899* [Measure](measure.html): The uri that identifies the measure 4900* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4901* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4902* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4903* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4904* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4905* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4906* [Requirements](requirements.html): The uri that identifies the requirements 4907* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4908* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4909* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4910* [StructureMap](structuremap.html): The uri that identifies the structure map 4911* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4912* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4913* [TestPlan](testplan.html): The uri that identifies the test plan 4914* [TestScript](testscript.html): The uri that identifies the test script 4915* [ValueSet](valueset.html): The uri that identifies the value set 4916</b><br> 4917 * Type: <b>uri</b><br> 4918 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4919 * </p> 4920 */ 4921 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 4922 public static final String SP_URL = "url"; 4923 /** 4924 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4925 * <p> 4926 * Description: <b>Multiple Resources: 4927 4928* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4929* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4930* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4931* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4932* [Citation](citation.html): The uri that identifies the citation 4933* [CodeSystem](codesystem.html): The uri that identifies the code system 4934* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4935* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4936* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4937* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4938* [Evidence](evidence.html): The uri that identifies the evidence 4939* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4940* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4941* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4942* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4943* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4944* [Library](library.html): The uri that identifies the library 4945* [Measure](measure.html): The uri that identifies the measure 4946* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4947* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4948* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4949* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4950* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4951* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4952* [Requirements](requirements.html): The uri that identifies the requirements 4953* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4954* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4955* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4956* [StructureMap](structuremap.html): The uri that identifies the structure map 4957* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4958* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4959* [TestPlan](testplan.html): The uri that identifies the test plan 4960* [TestScript](testscript.html): The uri that identifies the test script 4961* [ValueSet](valueset.html): The uri that identifies the value set 4962</b><br> 4963 * Type: <b>uri</b><br> 4964 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4965 * </p> 4966 */ 4967 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4968 4969 /** 4970 * Search parameter: <b>version</b> 4971 * <p> 4972 * Description: <b>Multiple Resources: 4973 4974* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4975* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4976* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4977* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4978* [Citation](citation.html): The business version of the citation 4979* [CodeSystem](codesystem.html): The business version of the code system 4980* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 4981* [ConceptMap](conceptmap.html): The business version of the concept map 4982* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 4983* [EventDefinition](eventdefinition.html): The business version of the event definition 4984* [Evidence](evidence.html): The business version of the evidence 4985* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 4986* [ExampleScenario](examplescenario.html): The business version of the example scenario 4987* [GraphDefinition](graphdefinition.html): The business version of the graph definition 4988* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 4989* [Library](library.html): The business version of the library 4990* [Measure](measure.html): The business version of the measure 4991* [MessageDefinition](messagedefinition.html): The business version of the message definition 4992* [NamingSystem](namingsystem.html): The business version of the naming system 4993* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4994* [PlanDefinition](plandefinition.html): The business version of the plan definition 4995* [Questionnaire](questionnaire.html): The business version of the questionnaire 4996* [Requirements](requirements.html): The business version of the requirements 4997* [SearchParameter](searchparameter.html): The business version of the search parameter 4998* [StructureDefinition](structuredefinition.html): The business version of the structure definition 4999* [StructureMap](structuremap.html): The business version of the structure map 5000* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 5001* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 5002* [TestScript](testscript.html): The business version of the test script 5003* [ValueSet](valueset.html): The business version of the value set 5004</b><br> 5005 * Type: <b>token</b><br> 5006 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 5007 * </p> 5008 */ 5009 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 5010 public static final String SP_VERSION = "version"; 5011 /** 5012 * <b>Fluent Client</b> search parameter constant for <b>version</b> 5013 * <p> 5014 * Description: <b>Multiple Resources: 5015 5016* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 5017* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 5018* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 5019* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 5020* [Citation](citation.html): The business version of the citation 5021* [CodeSystem](codesystem.html): The business version of the code system 5022* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 5023* [ConceptMap](conceptmap.html): The business version of the concept map 5024* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 5025* [EventDefinition](eventdefinition.html): The business version of the event definition 5026* [Evidence](evidence.html): The business version of the evidence 5027* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 5028* [ExampleScenario](examplescenario.html): The business version of the example scenario 5029* [GraphDefinition](graphdefinition.html): The business version of the graph definition 5030* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 5031* [Library](library.html): The business version of the library 5032* [Measure](measure.html): The business version of the measure 5033* [MessageDefinition](messagedefinition.html): The business version of the message definition 5034* [NamingSystem](namingsystem.html): The business version of the naming system 5035* [OperationDefinition](operationdefinition.html): The business version of the operation definition 5036* [PlanDefinition](plandefinition.html): The business version of the plan definition 5037* [Questionnaire](questionnaire.html): The business version of the questionnaire 5038* [Requirements](requirements.html): The business version of the requirements 5039* [SearchParameter](searchparameter.html): The business version of the search parameter 5040* [StructureDefinition](structuredefinition.html): The business version of the structure definition 5041* [StructureMap](structuremap.html): The business version of the structure map 5042* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 5043* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 5044* [TestScript](testscript.html): The business version of the test script 5045* [ValueSet](valueset.html): The business version of the value set 5046</b><br> 5047 * Type: <b>token</b><br> 5048 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 5049 * </p> 5050 */ 5051 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 5052 5053 /** 5054 * Search parameter: <b>derived-or-self</b> 5055 * <p> 5056 * Description: <b>A server defined search that matches either the url or derivedFrom</b><br> 5057 * Type: <b>uri</b><br> 5058 * Path: <b>SubscriptionTopic.url | SubscriptionTopic.derivedFrom</b><br> 5059 * </p> 5060 */ 5061 @SearchParamDefinition(name="derived-or-self", path="SubscriptionTopic.url | SubscriptionTopic.derivedFrom", description="A server defined search that matches either the url or derivedFrom", type="uri" ) 5062 public static final String SP_DERIVED_OR_SELF = "derived-or-self"; 5063 /** 5064 * <b>Fluent Client</b> search parameter constant for <b>derived-or-self</b> 5065 * <p> 5066 * Description: <b>A server defined search that matches either the url or derivedFrom</b><br> 5067 * Type: <b>uri</b><br> 5068 * Path: <b>SubscriptionTopic.url | SubscriptionTopic.derivedFrom</b><br> 5069 * </p> 5070 */ 5071 public static final ca.uhn.fhir.rest.gclient.UriClientParam DERIVED_OR_SELF = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_DERIVED_OR_SELF); 5072 5073 /** 5074 * Search parameter: <b>effective</b> 5075 * <p> 5076 * Description: <b>Effective period</b><br> 5077 * Type: <b>date</b><br> 5078 * Path: <b>SubscriptionTopic.effectivePeriod</b><br> 5079 * </p> 5080 */ 5081 @SearchParamDefinition(name="effective", path="SubscriptionTopic.effectivePeriod", description="Effective period", type="date" ) 5082 public static final String SP_EFFECTIVE = "effective"; 5083 /** 5084 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 5085 * <p> 5086 * Description: <b>Effective period</b><br> 5087 * Type: <b>date</b><br> 5088 * Path: <b>SubscriptionTopic.effectivePeriod</b><br> 5089 * </p> 5090 */ 5091 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 5092 5093 /** 5094 * Search parameter: <b>event</b> 5095 * <p> 5096 * Description: <b>Event trigger</b><br> 5097 * Type: <b>token</b><br> 5098 * Path: <b>SubscriptionTopic.eventTrigger.event</b><br> 5099 * </p> 5100 */ 5101 @SearchParamDefinition(name="event", path="SubscriptionTopic.eventTrigger.event", description="Event trigger", type="token" ) 5102 public static final String SP_EVENT = "event"; 5103 /** 5104 * <b>Fluent Client</b> search parameter constant for <b>event</b> 5105 * <p> 5106 * Description: <b>Event trigger</b><br> 5107 * Type: <b>token</b><br> 5108 * Path: <b>SubscriptionTopic.eventTrigger.event</b><br> 5109 * </p> 5110 */ 5111 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EVENT); 5112 5113 /** 5114 * Search parameter: <b>resource</b> 5115 * <p> 5116 * Description: <b>Allowed resource for this definition</b><br> 5117 * Type: <b>uri</b><br> 5118 * Path: <b>SubscriptionTopic.resourceTrigger.resource | SubscriptionTopic.eventTrigger.resource | SubscriptionTopic.canFilterBy.resource | SubscriptionTopic.notificationShape.resource</b><br> 5119 * </p> 5120 */ 5121 @SearchParamDefinition(name="resource", path="SubscriptionTopic.resourceTrigger.resource | SubscriptionTopic.eventTrigger.resource | SubscriptionTopic.canFilterBy.resource | SubscriptionTopic.notificationShape.resource", description="Allowed resource for this definition", type="uri" ) 5122 public static final String SP_RESOURCE = "resource"; 5123 /** 5124 * <b>Fluent Client</b> search parameter constant for <b>resource</b> 5125 * <p> 5126 * Description: <b>Allowed resource for this definition</b><br> 5127 * Type: <b>uri</b><br> 5128 * Path: <b>SubscriptionTopic.resourceTrigger.resource | SubscriptionTopic.eventTrigger.resource | SubscriptionTopic.canFilterBy.resource | SubscriptionTopic.notificationShape.resource</b><br> 5129 * </p> 5130 */ 5131 public static final ca.uhn.fhir.rest.gclient.UriClientParam RESOURCE = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_RESOURCE); 5132 5133 /** 5134 * Search parameter: <b>trigger-description</b> 5135 * <p> 5136 * Description: <b>Text representation of the trigger</b><br> 5137 * Type: <b>string</b><br> 5138 * Path: <b>SubscriptionTopic.resourceTrigger.description</b><br> 5139 * </p> 5140 */ 5141 @SearchParamDefinition(name="trigger-description", path="SubscriptionTopic.resourceTrigger.description", description="Text representation of the trigger", type="string" ) 5142 public static final String SP_TRIGGER_DESCRIPTION = "trigger-description"; 5143 /** 5144 * <b>Fluent Client</b> search parameter constant for <b>trigger-description</b> 5145 * <p> 5146 * Description: <b>Text representation of the trigger</b><br> 5147 * Type: <b>string</b><br> 5148 * Path: <b>SubscriptionTopic.resourceTrigger.description</b><br> 5149 * </p> 5150 */ 5151 public static final ca.uhn.fhir.rest.gclient.StringClientParam TRIGGER_DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TRIGGER_DESCRIPTION); 5152 5153 5154} 5155