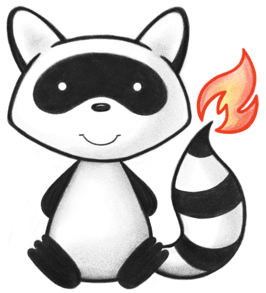
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Describes a stream of resource state changes identified by trigger criteria and annotated with labels useful to filter projections from this topic. 052 */ 053@ResourceDef(name="SubscriptionTopic", profile="http://hl7.org/fhir/StructureDefinition/SubscriptionTopic") 054public class SubscriptionTopic extends CanonicalResource { 055 056 public enum CriteriaNotExistsBehavior { 057 /** 058 * The requested conditional statement will pass if a matching state does not exist (e.g., previous state during create). 059 */ 060 TESTPASSES, 061 /** 062 * The requested conditional statement will fail if a matching state does not exist (e.g., previous state during create). 063 */ 064 TESTFAILS, 065 /** 066 * added to help the parsers with the generic types 067 */ 068 NULL; 069 public static CriteriaNotExistsBehavior fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("test-passes".equals(codeString)) 073 return TESTPASSES; 074 if ("test-fails".equals(codeString)) 075 return TESTFAILS; 076 if (Configuration.isAcceptInvalidEnums()) 077 return null; 078 else 079 throw new FHIRException("Unknown CriteriaNotExistsBehavior code '"+codeString+"'"); 080 } 081 public String toCode() { 082 switch (this) { 083 case TESTPASSES: return "test-passes"; 084 case TESTFAILS: return "test-fails"; 085 case NULL: return null; 086 default: return "?"; 087 } 088 } 089 public String getSystem() { 090 switch (this) { 091 case TESTPASSES: return "http://hl7.org/fhir/subscriptiontopic-cr-behavior"; 092 case TESTFAILS: return "http://hl7.org/fhir/subscriptiontopic-cr-behavior"; 093 case NULL: return null; 094 default: return "?"; 095 } 096 } 097 public String getDefinition() { 098 switch (this) { 099 case TESTPASSES: return "The requested conditional statement will pass if a matching state does not exist (e.g., previous state during create)."; 100 case TESTFAILS: return "The requested conditional statement will fail if a matching state does not exist (e.g., previous state during create)."; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDisplay() { 106 switch (this) { 107 case TESTPASSES: return "Test passes"; 108 case TESTFAILS: return "Test fails"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 } 114 115 public static class CriteriaNotExistsBehaviorEnumFactory implements EnumFactory<CriteriaNotExistsBehavior> { 116 public CriteriaNotExistsBehavior fromCode(String codeString) throws IllegalArgumentException { 117 if (codeString == null || "".equals(codeString)) 118 if (codeString == null || "".equals(codeString)) 119 return null; 120 if ("test-passes".equals(codeString)) 121 return CriteriaNotExistsBehavior.TESTPASSES; 122 if ("test-fails".equals(codeString)) 123 return CriteriaNotExistsBehavior.TESTFAILS; 124 throw new IllegalArgumentException("Unknown CriteriaNotExistsBehavior code '"+codeString+"'"); 125 } 126 public Enumeration<CriteriaNotExistsBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 127 if (code == null) 128 return null; 129 if (code.isEmpty()) 130 return new Enumeration<CriteriaNotExistsBehavior>(this, CriteriaNotExistsBehavior.NULL, code); 131 String codeString = ((PrimitiveType) code).asStringValue(); 132 if (codeString == null || "".equals(codeString)) 133 return new Enumeration<CriteriaNotExistsBehavior>(this, CriteriaNotExistsBehavior.NULL, code); 134 if ("test-passes".equals(codeString)) 135 return new Enumeration<CriteriaNotExistsBehavior>(this, CriteriaNotExistsBehavior.TESTPASSES, code); 136 if ("test-fails".equals(codeString)) 137 return new Enumeration<CriteriaNotExistsBehavior>(this, CriteriaNotExistsBehavior.TESTFAILS, code); 138 throw new FHIRException("Unknown CriteriaNotExistsBehavior code '"+codeString+"'"); 139 } 140 public String toCode(CriteriaNotExistsBehavior code) { 141 if (code == CriteriaNotExistsBehavior.NULL) 142 return null; 143 if (code == CriteriaNotExistsBehavior.TESTPASSES) 144 return "test-passes"; 145 if (code == CriteriaNotExistsBehavior.TESTFAILS) 146 return "test-fails"; 147 return "?"; 148 } 149 public String toSystem(CriteriaNotExistsBehavior code) { 150 return code.getSystem(); 151 } 152 } 153 154 public enum InteractionTrigger { 155 /** 156 * Create a new resource with a server assigned id. 157 */ 158 CREATE, 159 /** 160 * Update an existing resource by its id (or create it if it is new). 161 */ 162 UPDATE, 163 /** 164 * Delete a resource. 165 */ 166 DELETE, 167 /** 168 * added to help the parsers with the generic types 169 */ 170 NULL; 171 public static InteractionTrigger fromCode(String codeString) throws FHIRException { 172 if (codeString == null || "".equals(codeString)) 173 return null; 174 if ("create".equals(codeString)) 175 return CREATE; 176 if ("update".equals(codeString)) 177 return UPDATE; 178 if ("delete".equals(codeString)) 179 return DELETE; 180 if (Configuration.isAcceptInvalidEnums()) 181 return null; 182 else 183 throw new FHIRException("Unknown InteractionTrigger code '"+codeString+"'"); 184 } 185 public String toCode() { 186 switch (this) { 187 case CREATE: return "create"; 188 case UPDATE: return "update"; 189 case DELETE: return "delete"; 190 case NULL: return null; 191 default: return "?"; 192 } 193 } 194 public String getSystem() { 195 switch (this) { 196 case CREATE: return "http://hl7.org/fhir/restful-interaction"; 197 case UPDATE: return "http://hl7.org/fhir/restful-interaction"; 198 case DELETE: return "http://hl7.org/fhir/restful-interaction"; 199 case NULL: return null; 200 default: return "?"; 201 } 202 } 203 public String getDefinition() { 204 switch (this) { 205 case CREATE: return "Create a new resource with a server assigned id."; 206 case UPDATE: return "Update an existing resource by its id (or create it if it is new)."; 207 case DELETE: return "Delete a resource."; 208 case NULL: return null; 209 default: return "?"; 210 } 211 } 212 public String getDisplay() { 213 switch (this) { 214 case CREATE: return "create"; 215 case UPDATE: return "update"; 216 case DELETE: return "delete"; 217 case NULL: return null; 218 default: return "?"; 219 } 220 } 221 } 222 223 public static class InteractionTriggerEnumFactory implements EnumFactory<InteractionTrigger> { 224 public InteractionTrigger fromCode(String codeString) throws IllegalArgumentException { 225 if (codeString == null || "".equals(codeString)) 226 if (codeString == null || "".equals(codeString)) 227 return null; 228 if ("create".equals(codeString)) 229 return InteractionTrigger.CREATE; 230 if ("update".equals(codeString)) 231 return InteractionTrigger.UPDATE; 232 if ("delete".equals(codeString)) 233 return InteractionTrigger.DELETE; 234 throw new IllegalArgumentException("Unknown InteractionTrigger code '"+codeString+"'"); 235 } 236 public Enumeration<InteractionTrigger> fromType(PrimitiveType<?> code) throws FHIRException { 237 if (code == null) 238 return null; 239 if (code.isEmpty()) 240 return new Enumeration<InteractionTrigger>(this, InteractionTrigger.NULL, code); 241 String codeString = ((PrimitiveType) code).asStringValue(); 242 if (codeString == null || "".equals(codeString)) 243 return new Enumeration<InteractionTrigger>(this, InteractionTrigger.NULL, code); 244 if ("create".equals(codeString)) 245 return new Enumeration<InteractionTrigger>(this, InteractionTrigger.CREATE, code); 246 if ("update".equals(codeString)) 247 return new Enumeration<InteractionTrigger>(this, InteractionTrigger.UPDATE, code); 248 if ("delete".equals(codeString)) 249 return new Enumeration<InteractionTrigger>(this, InteractionTrigger.DELETE, code); 250 throw new FHIRException("Unknown InteractionTrigger code '"+codeString+"'"); 251 } 252 public String toCode(InteractionTrigger code) { 253 if (code == InteractionTrigger.NULL) 254 return null; 255 if (code == InteractionTrigger.CREATE) 256 return "create"; 257 if (code == InteractionTrigger.UPDATE) 258 return "update"; 259 if (code == InteractionTrigger.DELETE) 260 return "delete"; 261 return "?"; 262 } 263 public String toSystem(InteractionTrigger code) { 264 return code.getSystem(); 265 } 266 } 267 268 @Block() 269 public static class SubscriptionTopicResourceTriggerComponent extends BackboneElement implements IBaseBackboneElement { 270 /** 271 * The human readable description of this resource trigger for the SubscriptionTopic - for example, "An Encounter enters the 'in-progress' state". 272 */ 273 @Child(name = "description", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=true) 274 @Description(shortDefinition="Text representation of the resource trigger", formalDefinition="The human readable description of this resource trigger for the SubscriptionTopic - for example, \"An Encounter enters the 'in-progress' state\"." ) 275 protected MarkdownType description; 276 277 /** 278 * URL of the Resource that is the type used in this resource trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, "Patient" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href="elementdefinition-definitions.html#ElementDefinition.type.code">ElementDefinition.type.code</a>. 279 */ 280 @Child(name = "resource", type = {UriType.class}, order=2, min=1, max=1, modifier=false, summary=true) 281 @Description(shortDefinition="Data Type or Resource (reference to definition) for this trigger definition", formalDefinition="URL of the Resource that is the type used in this resource trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, \"Patient\" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href=\"elementdefinition-definitions.html#ElementDefinition.type.code\">ElementDefinition.type.code</a>." ) 282 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscription-types") 283 protected UriType resource; 284 285 /** 286 * The FHIR RESTful interaction which can be used to trigger a notification for the SubscriptionTopic. Multiple values are considered OR joined (e.g., CREATE or UPDATE). If not present, all supported interactions are assumed. 287 */ 288 @Child(name = "supportedInteraction", type = {CodeType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 289 @Description(shortDefinition="create | update | delete", formalDefinition="The FHIR RESTful interaction which can be used to trigger a notification for the SubscriptionTopic. Multiple values are considered OR joined (e.g., CREATE or UPDATE). If not present, all supported interactions are assumed." ) 290 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/interaction-trigger") 291 protected List<Enumeration<InteractionTrigger>> supportedInteraction; 292 293 /** 294 * The FHIR query based rules that the server should use to determine when to trigger a notification for this subscription topic. 295 */ 296 @Child(name = "queryCriteria", type = {}, order=4, min=0, max=1, modifier=false, summary=true) 297 @Description(shortDefinition="Query based trigger rule", formalDefinition="The FHIR query based rules that the server should use to determine when to trigger a notification for this subscription topic." ) 298 protected SubscriptionTopicResourceTriggerQueryCriteriaComponent queryCriteria; 299 300 /** 301 * The FHIRPath based rules that the server should use to determine when to trigger a notification for this topic. 302 */ 303 @Child(name = "fhirPathCriteria", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 304 @Description(shortDefinition="FHIRPath based trigger rule", formalDefinition="The FHIRPath based rules that the server should use to determine when to trigger a notification for this topic." ) 305 protected StringType fhirPathCriteria; 306 307 private static final long serialVersionUID = -1086940999L; 308 309 /** 310 * Constructor 311 */ 312 public SubscriptionTopicResourceTriggerComponent() { 313 super(); 314 } 315 316 /** 317 * Constructor 318 */ 319 public SubscriptionTopicResourceTriggerComponent(String resource) { 320 super(); 321 this.setResource(resource); 322 } 323 324 /** 325 * @return {@link #description} (The human readable description of this resource trigger for the SubscriptionTopic - for example, "An Encounter enters the 'in-progress' state".). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 326 */ 327 public MarkdownType getDescriptionElement() { 328 if (this.description == null) 329 if (Configuration.errorOnAutoCreate()) 330 throw new Error("Attempt to auto-create SubscriptionTopicResourceTriggerComponent.description"); 331 else if (Configuration.doAutoCreate()) 332 this.description = new MarkdownType(); // bb 333 return this.description; 334 } 335 336 public boolean hasDescriptionElement() { 337 return this.description != null && !this.description.isEmpty(); 338 } 339 340 public boolean hasDescription() { 341 return this.description != null && !this.description.isEmpty(); 342 } 343 344 /** 345 * @param value {@link #description} (The human readable description of this resource trigger for the SubscriptionTopic - for example, "An Encounter enters the 'in-progress' state".). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 346 */ 347 public SubscriptionTopicResourceTriggerComponent setDescriptionElement(MarkdownType value) { 348 this.description = value; 349 return this; 350 } 351 352 /** 353 * @return The human readable description of this resource trigger for the SubscriptionTopic - for example, "An Encounter enters the 'in-progress' state". 354 */ 355 public String getDescription() { 356 return this.description == null ? null : this.description.getValue(); 357 } 358 359 /** 360 * @param value The human readable description of this resource trigger for the SubscriptionTopic - for example, "An Encounter enters the 'in-progress' state". 361 */ 362 public SubscriptionTopicResourceTriggerComponent setDescription(String value) { 363 if (Utilities.noString(value)) 364 this.description = null; 365 else { 366 if (this.description == null) 367 this.description = new MarkdownType(); 368 this.description.setValue(value); 369 } 370 return this; 371 } 372 373 /** 374 * @return {@link #resource} (URL of the Resource that is the type used in this resource trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, "Patient" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href="elementdefinition-definitions.html#ElementDefinition.type.code">ElementDefinition.type.code</a>.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 375 */ 376 public UriType getResourceElement() { 377 if (this.resource == null) 378 if (Configuration.errorOnAutoCreate()) 379 throw new Error("Attempt to auto-create SubscriptionTopicResourceTriggerComponent.resource"); 380 else if (Configuration.doAutoCreate()) 381 this.resource = new UriType(); // bb 382 return this.resource; 383 } 384 385 public boolean hasResourceElement() { 386 return this.resource != null && !this.resource.isEmpty(); 387 } 388 389 public boolean hasResource() { 390 return this.resource != null && !this.resource.isEmpty(); 391 } 392 393 /** 394 * @param value {@link #resource} (URL of the Resource that is the type used in this resource trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, "Patient" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href="elementdefinition-definitions.html#ElementDefinition.type.code">ElementDefinition.type.code</a>.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 395 */ 396 public SubscriptionTopicResourceTriggerComponent setResourceElement(UriType value) { 397 this.resource = value; 398 return this; 399 } 400 401 /** 402 * @return URL of the Resource that is the type used in this resource trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, "Patient" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href="elementdefinition-definitions.html#ElementDefinition.type.code">ElementDefinition.type.code</a>. 403 */ 404 public String getResource() { 405 return this.resource == null ? null : this.resource.getValue(); 406 } 407 408 /** 409 * @param value URL of the Resource that is the type used in this resource trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, "Patient" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href="elementdefinition-definitions.html#ElementDefinition.type.code">ElementDefinition.type.code</a>. 410 */ 411 public SubscriptionTopicResourceTriggerComponent setResource(String value) { 412 if (this.resource == null) 413 this.resource = new UriType(); 414 this.resource.setValue(value); 415 return this; 416 } 417 418 /** 419 * @return {@link #supportedInteraction} (The FHIR RESTful interaction which can be used to trigger a notification for the SubscriptionTopic. Multiple values are considered OR joined (e.g., CREATE or UPDATE). If not present, all supported interactions are assumed.) 420 */ 421 public List<Enumeration<InteractionTrigger>> getSupportedInteraction() { 422 if (this.supportedInteraction == null) 423 this.supportedInteraction = new ArrayList<Enumeration<InteractionTrigger>>(); 424 return this.supportedInteraction; 425 } 426 427 /** 428 * @return Returns a reference to <code>this</code> for easy method chaining 429 */ 430 public SubscriptionTopicResourceTriggerComponent setSupportedInteraction(List<Enumeration<InteractionTrigger>> theSupportedInteraction) { 431 this.supportedInteraction = theSupportedInteraction; 432 return this; 433 } 434 435 public boolean hasSupportedInteraction() { 436 if (this.supportedInteraction == null) 437 return false; 438 for (Enumeration<InteractionTrigger> item : this.supportedInteraction) 439 if (!item.isEmpty()) 440 return true; 441 return false; 442 } 443 444 /** 445 * @return {@link #supportedInteraction} (The FHIR RESTful interaction which can be used to trigger a notification for the SubscriptionTopic. Multiple values are considered OR joined (e.g., CREATE or UPDATE). If not present, all supported interactions are assumed.) 446 */ 447 public Enumeration<InteractionTrigger> addSupportedInteractionElement() {//2 448 Enumeration<InteractionTrigger> t = new Enumeration<InteractionTrigger>(new InteractionTriggerEnumFactory()); 449 if (this.supportedInteraction == null) 450 this.supportedInteraction = new ArrayList<Enumeration<InteractionTrigger>>(); 451 this.supportedInteraction.add(t); 452 return t; 453 } 454 455 /** 456 * @param value {@link #supportedInteraction} (The FHIR RESTful interaction which can be used to trigger a notification for the SubscriptionTopic. Multiple values are considered OR joined (e.g., CREATE or UPDATE). If not present, all supported interactions are assumed.) 457 */ 458 public SubscriptionTopicResourceTriggerComponent addSupportedInteraction(InteractionTrigger value) { //1 459 Enumeration<InteractionTrigger> t = new Enumeration<InteractionTrigger>(new InteractionTriggerEnumFactory()); 460 t.setValue(value); 461 if (this.supportedInteraction == null) 462 this.supportedInteraction = new ArrayList<Enumeration<InteractionTrigger>>(); 463 this.supportedInteraction.add(t); 464 return this; 465 } 466 467 /** 468 * @param value {@link #supportedInteraction} (The FHIR RESTful interaction which can be used to trigger a notification for the SubscriptionTopic. Multiple values are considered OR joined (e.g., CREATE or UPDATE). If not present, all supported interactions are assumed.) 469 */ 470 public boolean hasSupportedInteraction(InteractionTrigger value) { 471 if (this.supportedInteraction == null) 472 return false; 473 for (Enumeration<InteractionTrigger> v : this.supportedInteraction) 474 if (v.getValue().equals(value)) // code 475 return true; 476 return false; 477 } 478 479 /** 480 * @return {@link #queryCriteria} (The FHIR query based rules that the server should use to determine when to trigger a notification for this subscription topic.) 481 */ 482 public SubscriptionTopicResourceTriggerQueryCriteriaComponent getQueryCriteria() { 483 if (this.queryCriteria == null) 484 if (Configuration.errorOnAutoCreate()) 485 throw new Error("Attempt to auto-create SubscriptionTopicResourceTriggerComponent.queryCriteria"); 486 else if (Configuration.doAutoCreate()) 487 this.queryCriteria = new SubscriptionTopicResourceTriggerQueryCriteriaComponent(); // cc 488 return this.queryCriteria; 489 } 490 491 public boolean hasQueryCriteria() { 492 return this.queryCriteria != null && !this.queryCriteria.isEmpty(); 493 } 494 495 /** 496 * @param value {@link #queryCriteria} (The FHIR query based rules that the server should use to determine when to trigger a notification for this subscription topic.) 497 */ 498 public SubscriptionTopicResourceTriggerComponent setQueryCriteria(SubscriptionTopicResourceTriggerQueryCriteriaComponent value) { 499 this.queryCriteria = value; 500 return this; 501 } 502 503 /** 504 * @return {@link #fhirPathCriteria} (The FHIRPath based rules that the server should use to determine when to trigger a notification for this topic.). This is the underlying object with id, value and extensions. The accessor "getFhirPathCriteria" gives direct access to the value 505 */ 506 public StringType getFhirPathCriteriaElement() { 507 if (this.fhirPathCriteria == null) 508 if (Configuration.errorOnAutoCreate()) 509 throw new Error("Attempt to auto-create SubscriptionTopicResourceTriggerComponent.fhirPathCriteria"); 510 else if (Configuration.doAutoCreate()) 511 this.fhirPathCriteria = new StringType(); // bb 512 return this.fhirPathCriteria; 513 } 514 515 public boolean hasFhirPathCriteriaElement() { 516 return this.fhirPathCriteria != null && !this.fhirPathCriteria.isEmpty(); 517 } 518 519 public boolean hasFhirPathCriteria() { 520 return this.fhirPathCriteria != null && !this.fhirPathCriteria.isEmpty(); 521 } 522 523 /** 524 * @param value {@link #fhirPathCriteria} (The FHIRPath based rules that the server should use to determine when to trigger a notification for this topic.). This is the underlying object with id, value and extensions. The accessor "getFhirPathCriteria" gives direct access to the value 525 */ 526 public SubscriptionTopicResourceTriggerComponent setFhirPathCriteriaElement(StringType value) { 527 this.fhirPathCriteria = value; 528 return this; 529 } 530 531 /** 532 * @return The FHIRPath based rules that the server should use to determine when to trigger a notification for this topic. 533 */ 534 public String getFhirPathCriteria() { 535 return this.fhirPathCriteria == null ? null : this.fhirPathCriteria.getValue(); 536 } 537 538 /** 539 * @param value The FHIRPath based rules that the server should use to determine when to trigger a notification for this topic. 540 */ 541 public SubscriptionTopicResourceTriggerComponent setFhirPathCriteria(String value) { 542 if (Utilities.noString(value)) 543 this.fhirPathCriteria = null; 544 else { 545 if (this.fhirPathCriteria == null) 546 this.fhirPathCriteria = new StringType(); 547 this.fhirPathCriteria.setValue(value); 548 } 549 return this; 550 } 551 552 protected void listChildren(List<Property> children) { 553 super.listChildren(children); 554 children.add(new Property("description", "markdown", "The human readable description of this resource trigger for the SubscriptionTopic - for example, \"An Encounter enters the 'in-progress' state\".", 0, 1, description)); 555 children.add(new Property("resource", "uri", "URL of the Resource that is the type used in this resource trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, \"Patient\" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href=\"elementdefinition-definitions.html#ElementDefinition.type.code\">ElementDefinition.type.code</a>.", 0, 1, resource)); 556 children.add(new Property("supportedInteraction", "code", "The FHIR RESTful interaction which can be used to trigger a notification for the SubscriptionTopic. Multiple values are considered OR joined (e.g., CREATE or UPDATE). If not present, all supported interactions are assumed.", 0, java.lang.Integer.MAX_VALUE, supportedInteraction)); 557 children.add(new Property("queryCriteria", "", "The FHIR query based rules that the server should use to determine when to trigger a notification for this subscription topic.", 0, 1, queryCriteria)); 558 children.add(new Property("fhirPathCriteria", "string", "The FHIRPath based rules that the server should use to determine when to trigger a notification for this topic.", 0, 1, fhirPathCriteria)); 559 } 560 561 @Override 562 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 563 switch (_hash) { 564 case -1724546052: /*description*/ return new Property("description", "markdown", "The human readable description of this resource trigger for the SubscriptionTopic - for example, \"An Encounter enters the 'in-progress' state\".", 0, 1, description); 565 case -341064690: /*resource*/ return new Property("resource", "uri", "URL of the Resource that is the type used in this resource trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, \"Patient\" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href=\"elementdefinition-definitions.html#ElementDefinition.type.code\">ElementDefinition.type.code</a>.", 0, 1, resource); 566 case 1838450820: /*supportedInteraction*/ return new Property("supportedInteraction", "code", "The FHIR RESTful interaction which can be used to trigger a notification for the SubscriptionTopic. Multiple values are considered OR joined (e.g., CREATE or UPDATE). If not present, all supported interactions are assumed.", 0, java.lang.Integer.MAX_VALUE, supportedInteraction); 567 case -545123257: /*queryCriteria*/ return new Property("queryCriteria", "", "The FHIR query based rules that the server should use to determine when to trigger a notification for this subscription topic.", 0, 1, queryCriteria); 568 case 1929785263: /*fhirPathCriteria*/ return new Property("fhirPathCriteria", "string", "The FHIRPath based rules that the server should use to determine when to trigger a notification for this topic.", 0, 1, fhirPathCriteria); 569 default: return super.getNamedProperty(_hash, _name, _checkValid); 570 } 571 572 } 573 574 @Override 575 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 576 switch (hash) { 577 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 578 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // UriType 579 case 1838450820: /*supportedInteraction*/ return this.supportedInteraction == null ? new Base[0] : this.supportedInteraction.toArray(new Base[this.supportedInteraction.size()]); // Enumeration<InteractionTrigger> 580 case -545123257: /*queryCriteria*/ return this.queryCriteria == null ? new Base[0] : new Base[] {this.queryCriteria}; // SubscriptionTopicResourceTriggerQueryCriteriaComponent 581 case 1929785263: /*fhirPathCriteria*/ return this.fhirPathCriteria == null ? new Base[0] : new Base[] {this.fhirPathCriteria}; // StringType 582 default: return super.getProperty(hash, name, checkValid); 583 } 584 585 } 586 587 @Override 588 public Base setProperty(int hash, String name, Base value) throws FHIRException { 589 switch (hash) { 590 case -1724546052: // description 591 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 592 return value; 593 case -341064690: // resource 594 this.resource = TypeConvertor.castToUri(value); // UriType 595 return value; 596 case 1838450820: // supportedInteraction 597 value = new InteractionTriggerEnumFactory().fromType(TypeConvertor.castToCode(value)); 598 this.getSupportedInteraction().add((Enumeration) value); // Enumeration<InteractionTrigger> 599 return value; 600 case -545123257: // queryCriteria 601 this.queryCriteria = (SubscriptionTopicResourceTriggerQueryCriteriaComponent) value; // SubscriptionTopicResourceTriggerQueryCriteriaComponent 602 return value; 603 case 1929785263: // fhirPathCriteria 604 this.fhirPathCriteria = TypeConvertor.castToString(value); // StringType 605 return value; 606 default: return super.setProperty(hash, name, value); 607 } 608 609 } 610 611 @Override 612 public Base setProperty(String name, Base value) throws FHIRException { 613 if (name.equals("description")) { 614 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 615 } else if (name.equals("resource")) { 616 this.resource = TypeConvertor.castToUri(value); // UriType 617 } else if (name.equals("supportedInteraction")) { 618 value = new InteractionTriggerEnumFactory().fromType(TypeConvertor.castToCode(value)); 619 this.getSupportedInteraction().add((Enumeration) value); 620 } else if (name.equals("queryCriteria")) { 621 this.queryCriteria = (SubscriptionTopicResourceTriggerQueryCriteriaComponent) value; // SubscriptionTopicResourceTriggerQueryCriteriaComponent 622 } else if (name.equals("fhirPathCriteria")) { 623 this.fhirPathCriteria = TypeConvertor.castToString(value); // StringType 624 } else 625 return super.setProperty(name, value); 626 return value; 627 } 628 629 @Override 630 public void removeChild(String name, Base value) throws FHIRException { 631 if (name.equals("description")) { 632 this.description = null; 633 } else if (name.equals("resource")) { 634 this.resource = null; 635 } else if (name.equals("supportedInteraction")) { 636 value = new InteractionTriggerEnumFactory().fromType(TypeConvertor.castToCode(value)); 637 this.getSupportedInteraction().remove((Enumeration) value); 638 } else if (name.equals("queryCriteria")) { 639 this.queryCriteria = (SubscriptionTopicResourceTriggerQueryCriteriaComponent) value; // SubscriptionTopicResourceTriggerQueryCriteriaComponent 640 } else if (name.equals("fhirPathCriteria")) { 641 this.fhirPathCriteria = null; 642 } else 643 super.removeChild(name, value); 644 645 } 646 647 @Override 648 public Base makeProperty(int hash, String name) throws FHIRException { 649 switch (hash) { 650 case -1724546052: return getDescriptionElement(); 651 case -341064690: return getResourceElement(); 652 case 1838450820: return addSupportedInteractionElement(); 653 case -545123257: return getQueryCriteria(); 654 case 1929785263: return getFhirPathCriteriaElement(); 655 default: return super.makeProperty(hash, name); 656 } 657 658 } 659 660 @Override 661 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 662 switch (hash) { 663 case -1724546052: /*description*/ return new String[] {"markdown"}; 664 case -341064690: /*resource*/ return new String[] {"uri"}; 665 case 1838450820: /*supportedInteraction*/ return new String[] {"code"}; 666 case -545123257: /*queryCriteria*/ return new String[] {}; 667 case 1929785263: /*fhirPathCriteria*/ return new String[] {"string"}; 668 default: return super.getTypesForProperty(hash, name); 669 } 670 671 } 672 673 @Override 674 public Base addChild(String name) throws FHIRException { 675 if (name.equals("description")) { 676 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.resourceTrigger.description"); 677 } 678 else if (name.equals("resource")) { 679 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.resourceTrigger.resource"); 680 } 681 else if (name.equals("supportedInteraction")) { 682 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.resourceTrigger.supportedInteraction"); 683 } 684 else if (name.equals("queryCriteria")) { 685 this.queryCriteria = new SubscriptionTopicResourceTriggerQueryCriteriaComponent(); 686 return this.queryCriteria; 687 } 688 else if (name.equals("fhirPathCriteria")) { 689 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.resourceTrigger.fhirPathCriteria"); 690 } 691 else 692 return super.addChild(name); 693 } 694 695 public SubscriptionTopicResourceTriggerComponent copy() { 696 SubscriptionTopicResourceTriggerComponent dst = new SubscriptionTopicResourceTriggerComponent(); 697 copyValues(dst); 698 return dst; 699 } 700 701 public void copyValues(SubscriptionTopicResourceTriggerComponent dst) { 702 super.copyValues(dst); 703 dst.description = description == null ? null : description.copy(); 704 dst.resource = resource == null ? null : resource.copy(); 705 if (supportedInteraction != null) { 706 dst.supportedInteraction = new ArrayList<Enumeration<InteractionTrigger>>(); 707 for (Enumeration<InteractionTrigger> i : supportedInteraction) 708 dst.supportedInteraction.add(i.copy()); 709 }; 710 dst.queryCriteria = queryCriteria == null ? null : queryCriteria.copy(); 711 dst.fhirPathCriteria = fhirPathCriteria == null ? null : fhirPathCriteria.copy(); 712 } 713 714 @Override 715 public boolean equalsDeep(Base other_) { 716 if (!super.equalsDeep(other_)) 717 return false; 718 if (!(other_ instanceof SubscriptionTopicResourceTriggerComponent)) 719 return false; 720 SubscriptionTopicResourceTriggerComponent o = (SubscriptionTopicResourceTriggerComponent) other_; 721 return compareDeep(description, o.description, true) && compareDeep(resource, o.resource, true) 722 && compareDeep(supportedInteraction, o.supportedInteraction, true) && compareDeep(queryCriteria, o.queryCriteria, true) 723 && compareDeep(fhirPathCriteria, o.fhirPathCriteria, true); 724 } 725 726 @Override 727 public boolean equalsShallow(Base other_) { 728 if (!super.equalsShallow(other_)) 729 return false; 730 if (!(other_ instanceof SubscriptionTopicResourceTriggerComponent)) 731 return false; 732 SubscriptionTopicResourceTriggerComponent o = (SubscriptionTopicResourceTriggerComponent) other_; 733 return compareValues(description, o.description, true) && compareValues(resource, o.resource, true) 734 && compareValues(supportedInteraction, o.supportedInteraction, true) && compareValues(fhirPathCriteria, o.fhirPathCriteria, true) 735 ; 736 } 737 738 public boolean isEmpty() { 739 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, resource, supportedInteraction 740 , queryCriteria, fhirPathCriteria); 741 } 742 743 public String fhirType() { 744 return "SubscriptionTopic.resourceTrigger"; 745 746 } 747 748 } 749 750 @Block() 751 public static class SubscriptionTopicResourceTriggerQueryCriteriaComponent extends BackboneElement implements IBaseBackboneElement { 752 /** 753 * The FHIR query based rules are applied to the previous resource state (e.g., state before an update). 754 */ 755 @Child(name = "previous", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 756 @Description(shortDefinition="Rule applied to previous resource state", formalDefinition="The FHIR query based rules are applied to the previous resource state (e.g., state before an update)." ) 757 protected StringType previous; 758 759 /** 760 * For `create` interactions, should the `previous` criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `create` interactions is unspecified (server discretion). 761 */ 762 @Child(name = "resultForCreate", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 763 @Description(shortDefinition="test-passes | test-fails", formalDefinition="For `create` interactions, should the `previous` criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `create` interactions is unspecified (server discretion)." ) 764 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscriptiontopic-cr-behavior") 765 protected Enumeration<CriteriaNotExistsBehavior> resultForCreate; 766 767 /** 768 * The FHIR query based rules are applied to the current resource state (e.g., state after an update). 769 */ 770 @Child(name = "current", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 771 @Description(shortDefinition="Rule applied to current resource state", formalDefinition="The FHIR query based rules are applied to the current resource state (e.g., state after an update)." ) 772 protected StringType current; 773 774 /** 775 * For 'delete' interactions, should the 'current' query criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `delete` interactions is unspecified (server discretion). 776 */ 777 @Child(name = "resultForDelete", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 778 @Description(shortDefinition="test-passes | test-fails", formalDefinition="For 'delete' interactions, should the 'current' query criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `delete` interactions is unspecified (server discretion)." ) 779 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscriptiontopic-cr-behavior") 780 protected Enumeration<CriteriaNotExistsBehavior> resultForDelete; 781 782 /** 783 * If set to `true`, both the `current` and `previous` query criteria must evaluate `true` to trigger a notification for this topic. If set to `false` or not present, a notification for this topic will be triggered if either the `current` or `previous` tests evaluate to `true`. 784 */ 785 @Child(name = "requireBoth", type = {BooleanType.class}, order=5, min=0, max=1, modifier=false, summary=true) 786 @Description(shortDefinition="Both must be true flag", formalDefinition="If set to `true`, both the `current` and `previous` query criteria must evaluate `true` to trigger a notification for this topic. If set to `false` or not present, a notification for this topic will be triggered if either the `current` or `previous` tests evaluate to `true`." ) 787 protected BooleanType requireBoth; 788 789 private static final long serialVersionUID = -291746067L; 790 791 /** 792 * Constructor 793 */ 794 public SubscriptionTopicResourceTriggerQueryCriteriaComponent() { 795 super(); 796 } 797 798 /** 799 * @return {@link #previous} (The FHIR query based rules are applied to the previous resource state (e.g., state before an update).). This is the underlying object with id, value and extensions. The accessor "getPrevious" gives direct access to the value 800 */ 801 public StringType getPreviousElement() { 802 if (this.previous == null) 803 if (Configuration.errorOnAutoCreate()) 804 throw new Error("Attempt to auto-create SubscriptionTopicResourceTriggerQueryCriteriaComponent.previous"); 805 else if (Configuration.doAutoCreate()) 806 this.previous = new StringType(); // bb 807 return this.previous; 808 } 809 810 public boolean hasPreviousElement() { 811 return this.previous != null && !this.previous.isEmpty(); 812 } 813 814 public boolean hasPrevious() { 815 return this.previous != null && !this.previous.isEmpty(); 816 } 817 818 /** 819 * @param value {@link #previous} (The FHIR query based rules are applied to the previous resource state (e.g., state before an update).). This is the underlying object with id, value and extensions. The accessor "getPrevious" gives direct access to the value 820 */ 821 public SubscriptionTopicResourceTriggerQueryCriteriaComponent setPreviousElement(StringType value) { 822 this.previous = value; 823 return this; 824 } 825 826 /** 827 * @return The FHIR query based rules are applied to the previous resource state (e.g., state before an update). 828 */ 829 public String getPrevious() { 830 return this.previous == null ? null : this.previous.getValue(); 831 } 832 833 /** 834 * @param value The FHIR query based rules are applied to the previous resource state (e.g., state before an update). 835 */ 836 public SubscriptionTopicResourceTriggerQueryCriteriaComponent setPrevious(String value) { 837 if (Utilities.noString(value)) 838 this.previous = null; 839 else { 840 if (this.previous == null) 841 this.previous = new StringType(); 842 this.previous.setValue(value); 843 } 844 return this; 845 } 846 847 /** 848 * @return {@link #resultForCreate} (For `create` interactions, should the `previous` criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `create` interactions is unspecified (server discretion).). This is the underlying object with id, value and extensions. The accessor "getResultForCreate" gives direct access to the value 849 */ 850 public Enumeration<CriteriaNotExistsBehavior> getResultForCreateElement() { 851 if (this.resultForCreate == null) 852 if (Configuration.errorOnAutoCreate()) 853 throw new Error("Attempt to auto-create SubscriptionTopicResourceTriggerQueryCriteriaComponent.resultForCreate"); 854 else if (Configuration.doAutoCreate()) 855 this.resultForCreate = new Enumeration<CriteriaNotExistsBehavior>(new CriteriaNotExistsBehaviorEnumFactory()); // bb 856 return this.resultForCreate; 857 } 858 859 public boolean hasResultForCreateElement() { 860 return this.resultForCreate != null && !this.resultForCreate.isEmpty(); 861 } 862 863 public boolean hasResultForCreate() { 864 return this.resultForCreate != null && !this.resultForCreate.isEmpty(); 865 } 866 867 /** 868 * @param value {@link #resultForCreate} (For `create` interactions, should the `previous` criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `create` interactions is unspecified (server discretion).). This is the underlying object with id, value and extensions. The accessor "getResultForCreate" gives direct access to the value 869 */ 870 public SubscriptionTopicResourceTriggerQueryCriteriaComponent setResultForCreateElement(Enumeration<CriteriaNotExistsBehavior> value) { 871 this.resultForCreate = value; 872 return this; 873 } 874 875 /** 876 * @return For `create` interactions, should the `previous` criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `create` interactions is unspecified (server discretion). 877 */ 878 public CriteriaNotExistsBehavior getResultForCreate() { 879 return this.resultForCreate == null ? null : this.resultForCreate.getValue(); 880 } 881 882 /** 883 * @param value For `create` interactions, should the `previous` criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `create` interactions is unspecified (server discretion). 884 */ 885 public SubscriptionTopicResourceTriggerQueryCriteriaComponent setResultForCreate(CriteriaNotExistsBehavior value) { 886 if (value == null) 887 this.resultForCreate = null; 888 else { 889 if (this.resultForCreate == null) 890 this.resultForCreate = new Enumeration<CriteriaNotExistsBehavior>(new CriteriaNotExistsBehaviorEnumFactory()); 891 this.resultForCreate.setValue(value); 892 } 893 return this; 894 } 895 896 /** 897 * @return {@link #current} (The FHIR query based rules are applied to the current resource state (e.g., state after an update).). This is the underlying object with id, value and extensions. The accessor "getCurrent" gives direct access to the value 898 */ 899 public StringType getCurrentElement() { 900 if (this.current == null) 901 if (Configuration.errorOnAutoCreate()) 902 throw new Error("Attempt to auto-create SubscriptionTopicResourceTriggerQueryCriteriaComponent.current"); 903 else if (Configuration.doAutoCreate()) 904 this.current = new StringType(); // bb 905 return this.current; 906 } 907 908 public boolean hasCurrentElement() { 909 return this.current != null && !this.current.isEmpty(); 910 } 911 912 public boolean hasCurrent() { 913 return this.current != null && !this.current.isEmpty(); 914 } 915 916 /** 917 * @param value {@link #current} (The FHIR query based rules are applied to the current resource state (e.g., state after an update).). This is the underlying object with id, value and extensions. The accessor "getCurrent" gives direct access to the value 918 */ 919 public SubscriptionTopicResourceTriggerQueryCriteriaComponent setCurrentElement(StringType value) { 920 this.current = value; 921 return this; 922 } 923 924 /** 925 * @return The FHIR query based rules are applied to the current resource state (e.g., state after an update). 926 */ 927 public String getCurrent() { 928 return this.current == null ? null : this.current.getValue(); 929 } 930 931 /** 932 * @param value The FHIR query based rules are applied to the current resource state (e.g., state after an update). 933 */ 934 public SubscriptionTopicResourceTriggerQueryCriteriaComponent setCurrent(String value) { 935 if (Utilities.noString(value)) 936 this.current = null; 937 else { 938 if (this.current == null) 939 this.current = new StringType(); 940 this.current.setValue(value); 941 } 942 return this; 943 } 944 945 /** 946 * @return {@link #resultForDelete} (For 'delete' interactions, should the 'current' query criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `delete` interactions is unspecified (server discretion).). This is the underlying object with id, value and extensions. The accessor "getResultForDelete" gives direct access to the value 947 */ 948 public Enumeration<CriteriaNotExistsBehavior> getResultForDeleteElement() { 949 if (this.resultForDelete == null) 950 if (Configuration.errorOnAutoCreate()) 951 throw new Error("Attempt to auto-create SubscriptionTopicResourceTriggerQueryCriteriaComponent.resultForDelete"); 952 else if (Configuration.doAutoCreate()) 953 this.resultForDelete = new Enumeration<CriteriaNotExistsBehavior>(new CriteriaNotExistsBehaviorEnumFactory()); // bb 954 return this.resultForDelete; 955 } 956 957 public boolean hasResultForDeleteElement() { 958 return this.resultForDelete != null && !this.resultForDelete.isEmpty(); 959 } 960 961 public boolean hasResultForDelete() { 962 return this.resultForDelete != null && !this.resultForDelete.isEmpty(); 963 } 964 965 /** 966 * @param value {@link #resultForDelete} (For 'delete' interactions, should the 'current' query criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `delete` interactions is unspecified (server discretion).). This is the underlying object with id, value and extensions. The accessor "getResultForDelete" gives direct access to the value 967 */ 968 public SubscriptionTopicResourceTriggerQueryCriteriaComponent setResultForDeleteElement(Enumeration<CriteriaNotExistsBehavior> value) { 969 this.resultForDelete = value; 970 return this; 971 } 972 973 /** 974 * @return For 'delete' interactions, should the 'current' query criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `delete` interactions is unspecified (server discretion). 975 */ 976 public CriteriaNotExistsBehavior getResultForDelete() { 977 return this.resultForDelete == null ? null : this.resultForDelete.getValue(); 978 } 979 980 /** 981 * @param value For 'delete' interactions, should the 'current' query criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `delete` interactions is unspecified (server discretion). 982 */ 983 public SubscriptionTopicResourceTriggerQueryCriteriaComponent setResultForDelete(CriteriaNotExistsBehavior value) { 984 if (value == null) 985 this.resultForDelete = null; 986 else { 987 if (this.resultForDelete == null) 988 this.resultForDelete = new Enumeration<CriteriaNotExistsBehavior>(new CriteriaNotExistsBehaviorEnumFactory()); 989 this.resultForDelete.setValue(value); 990 } 991 return this; 992 } 993 994 /** 995 * @return {@link #requireBoth} (If set to `true`, both the `current` and `previous` query criteria must evaluate `true` to trigger a notification for this topic. If set to `false` or not present, a notification for this topic will be triggered if either the `current` or `previous` tests evaluate to `true`.). This is the underlying object with id, value and extensions. The accessor "getRequireBoth" gives direct access to the value 996 */ 997 public BooleanType getRequireBothElement() { 998 if (this.requireBoth == null) 999 if (Configuration.errorOnAutoCreate()) 1000 throw new Error("Attempt to auto-create SubscriptionTopicResourceTriggerQueryCriteriaComponent.requireBoth"); 1001 else if (Configuration.doAutoCreate()) 1002 this.requireBoth = new BooleanType(); // bb 1003 return this.requireBoth; 1004 } 1005 1006 public boolean hasRequireBothElement() { 1007 return this.requireBoth != null && !this.requireBoth.isEmpty(); 1008 } 1009 1010 public boolean hasRequireBoth() { 1011 return this.requireBoth != null && !this.requireBoth.isEmpty(); 1012 } 1013 1014 /** 1015 * @param value {@link #requireBoth} (If set to `true`, both the `current` and `previous` query criteria must evaluate `true` to trigger a notification for this topic. If set to `false` or not present, a notification for this topic will be triggered if either the `current` or `previous` tests evaluate to `true`.). This is the underlying object with id, value and extensions. The accessor "getRequireBoth" gives direct access to the value 1016 */ 1017 public SubscriptionTopicResourceTriggerQueryCriteriaComponent setRequireBothElement(BooleanType value) { 1018 this.requireBoth = value; 1019 return this; 1020 } 1021 1022 /** 1023 * @return If set to `true`, both the `current` and `previous` query criteria must evaluate `true` to trigger a notification for this topic. If set to `false` or not present, a notification for this topic will be triggered if either the `current` or `previous` tests evaluate to `true`. 1024 */ 1025 public boolean getRequireBoth() { 1026 return this.requireBoth == null || this.requireBoth.isEmpty() ? false : this.requireBoth.getValue(); 1027 } 1028 1029 /** 1030 * @param value If set to `true`, both the `current` and `previous` query criteria must evaluate `true` to trigger a notification for this topic. If set to `false` or not present, a notification for this topic will be triggered if either the `current` or `previous` tests evaluate to `true`. 1031 */ 1032 public SubscriptionTopicResourceTriggerQueryCriteriaComponent setRequireBoth(boolean value) { 1033 if (this.requireBoth == null) 1034 this.requireBoth = new BooleanType(); 1035 this.requireBoth.setValue(value); 1036 return this; 1037 } 1038 1039 protected void listChildren(List<Property> children) { 1040 super.listChildren(children); 1041 children.add(new Property("previous", "string", "The FHIR query based rules are applied to the previous resource state (e.g., state before an update).", 0, 1, previous)); 1042 children.add(new Property("resultForCreate", "code", "For `create` interactions, should the `previous` criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `create` interactions is unspecified (server discretion).", 0, 1, resultForCreate)); 1043 children.add(new Property("current", "string", "The FHIR query based rules are applied to the current resource state (e.g., state after an update).", 0, 1, current)); 1044 children.add(new Property("resultForDelete", "code", "For 'delete' interactions, should the 'current' query criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `delete` interactions is unspecified (server discretion).", 0, 1, resultForDelete)); 1045 children.add(new Property("requireBoth", "boolean", "If set to `true`, both the `current` and `previous` query criteria must evaluate `true` to trigger a notification for this topic. If set to `false` or not present, a notification for this topic will be triggered if either the `current` or `previous` tests evaluate to `true`.", 0, 1, requireBoth)); 1046 } 1047 1048 @Override 1049 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1050 switch (_hash) { 1051 case -1273775369: /*previous*/ return new Property("previous", "string", "The FHIR query based rules are applied to the previous resource state (e.g., state before an update).", 0, 1, previous); 1052 case -407976056: /*resultForCreate*/ return new Property("resultForCreate", "code", "For `create` interactions, should the `previous` criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `create` interactions is unspecified (server discretion).", 0, 1, resultForCreate); 1053 case 1126940025: /*current*/ return new Property("current", "string", "The FHIR query based rules are applied to the current resource state (e.g., state after an update).", 0, 1, current); 1054 case -391140297: /*resultForDelete*/ return new Property("resultForDelete", "code", "For 'delete' interactions, should the 'current' query criteria count as an automatic pass or an automatic fail. If not present, the testing behavior during `delete` interactions is unspecified (server discretion).", 0, 1, resultForDelete); 1055 case 362116742: /*requireBoth*/ return new Property("requireBoth", "boolean", "If set to `true`, both the `current` and `previous` query criteria must evaluate `true` to trigger a notification for this topic. If set to `false` or not present, a notification for this topic will be triggered if either the `current` or `previous` tests evaluate to `true`.", 0, 1, requireBoth); 1056 default: return super.getNamedProperty(_hash, _name, _checkValid); 1057 } 1058 1059 } 1060 1061 @Override 1062 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1063 switch (hash) { 1064 case -1273775369: /*previous*/ return this.previous == null ? new Base[0] : new Base[] {this.previous}; // StringType 1065 case -407976056: /*resultForCreate*/ return this.resultForCreate == null ? new Base[0] : new Base[] {this.resultForCreate}; // Enumeration<CriteriaNotExistsBehavior> 1066 case 1126940025: /*current*/ return this.current == null ? new Base[0] : new Base[] {this.current}; // StringType 1067 case -391140297: /*resultForDelete*/ return this.resultForDelete == null ? new Base[0] : new Base[] {this.resultForDelete}; // Enumeration<CriteriaNotExistsBehavior> 1068 case 362116742: /*requireBoth*/ return this.requireBoth == null ? new Base[0] : new Base[] {this.requireBoth}; // BooleanType 1069 default: return super.getProperty(hash, name, checkValid); 1070 } 1071 1072 } 1073 1074 @Override 1075 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1076 switch (hash) { 1077 case -1273775369: // previous 1078 this.previous = TypeConvertor.castToString(value); // StringType 1079 return value; 1080 case -407976056: // resultForCreate 1081 value = new CriteriaNotExistsBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1082 this.resultForCreate = (Enumeration) value; // Enumeration<CriteriaNotExistsBehavior> 1083 return value; 1084 case 1126940025: // current 1085 this.current = TypeConvertor.castToString(value); // StringType 1086 return value; 1087 case -391140297: // resultForDelete 1088 value = new CriteriaNotExistsBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1089 this.resultForDelete = (Enumeration) value; // Enumeration<CriteriaNotExistsBehavior> 1090 return value; 1091 case 362116742: // requireBoth 1092 this.requireBoth = TypeConvertor.castToBoolean(value); // BooleanType 1093 return value; 1094 default: return super.setProperty(hash, name, value); 1095 } 1096 1097 } 1098 1099 @Override 1100 public Base setProperty(String name, Base value) throws FHIRException { 1101 if (name.equals("previous")) { 1102 this.previous = TypeConvertor.castToString(value); // StringType 1103 } else if (name.equals("resultForCreate")) { 1104 value = new CriteriaNotExistsBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1105 this.resultForCreate = (Enumeration) value; // Enumeration<CriteriaNotExistsBehavior> 1106 } else if (name.equals("current")) { 1107 this.current = TypeConvertor.castToString(value); // StringType 1108 } else if (name.equals("resultForDelete")) { 1109 value = new CriteriaNotExistsBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1110 this.resultForDelete = (Enumeration) value; // Enumeration<CriteriaNotExistsBehavior> 1111 } else if (name.equals("requireBoth")) { 1112 this.requireBoth = TypeConvertor.castToBoolean(value); // BooleanType 1113 } else 1114 return super.setProperty(name, value); 1115 return value; 1116 } 1117 1118 @Override 1119 public void removeChild(String name, Base value) throws FHIRException { 1120 if (name.equals("previous")) { 1121 this.previous = null; 1122 } else if (name.equals("resultForCreate")) { 1123 value = new CriteriaNotExistsBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1124 this.resultForCreate = (Enumeration) value; // Enumeration<CriteriaNotExistsBehavior> 1125 } else if (name.equals("current")) { 1126 this.current = null; 1127 } else if (name.equals("resultForDelete")) { 1128 value = new CriteriaNotExistsBehaviorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1129 this.resultForDelete = (Enumeration) value; // Enumeration<CriteriaNotExistsBehavior> 1130 } else if (name.equals("requireBoth")) { 1131 this.requireBoth = null; 1132 } else 1133 super.removeChild(name, value); 1134 1135 } 1136 1137 @Override 1138 public Base makeProperty(int hash, String name) throws FHIRException { 1139 switch (hash) { 1140 case -1273775369: return getPreviousElement(); 1141 case -407976056: return getResultForCreateElement(); 1142 case 1126940025: return getCurrentElement(); 1143 case -391140297: return getResultForDeleteElement(); 1144 case 362116742: return getRequireBothElement(); 1145 default: return super.makeProperty(hash, name); 1146 } 1147 1148 } 1149 1150 @Override 1151 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1152 switch (hash) { 1153 case -1273775369: /*previous*/ return new String[] {"string"}; 1154 case -407976056: /*resultForCreate*/ return new String[] {"code"}; 1155 case 1126940025: /*current*/ return new String[] {"string"}; 1156 case -391140297: /*resultForDelete*/ return new String[] {"code"}; 1157 case 362116742: /*requireBoth*/ return new String[] {"boolean"}; 1158 default: return super.getTypesForProperty(hash, name); 1159 } 1160 1161 } 1162 1163 @Override 1164 public Base addChild(String name) throws FHIRException { 1165 if (name.equals("previous")) { 1166 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.resourceTrigger.queryCriteria.previous"); 1167 } 1168 else if (name.equals("resultForCreate")) { 1169 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.resourceTrigger.queryCriteria.resultForCreate"); 1170 } 1171 else if (name.equals("current")) { 1172 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.resourceTrigger.queryCriteria.current"); 1173 } 1174 else if (name.equals("resultForDelete")) { 1175 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.resourceTrigger.queryCriteria.resultForDelete"); 1176 } 1177 else if (name.equals("requireBoth")) { 1178 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.resourceTrigger.queryCriteria.requireBoth"); 1179 } 1180 else 1181 return super.addChild(name); 1182 } 1183 1184 public SubscriptionTopicResourceTriggerQueryCriteriaComponent copy() { 1185 SubscriptionTopicResourceTriggerQueryCriteriaComponent dst = new SubscriptionTopicResourceTriggerQueryCriteriaComponent(); 1186 copyValues(dst); 1187 return dst; 1188 } 1189 1190 public void copyValues(SubscriptionTopicResourceTriggerQueryCriteriaComponent dst) { 1191 super.copyValues(dst); 1192 dst.previous = previous == null ? null : previous.copy(); 1193 dst.resultForCreate = resultForCreate == null ? null : resultForCreate.copy(); 1194 dst.current = current == null ? null : current.copy(); 1195 dst.resultForDelete = resultForDelete == null ? null : resultForDelete.copy(); 1196 dst.requireBoth = requireBoth == null ? null : requireBoth.copy(); 1197 } 1198 1199 @Override 1200 public boolean equalsDeep(Base other_) { 1201 if (!super.equalsDeep(other_)) 1202 return false; 1203 if (!(other_ instanceof SubscriptionTopicResourceTriggerQueryCriteriaComponent)) 1204 return false; 1205 SubscriptionTopicResourceTriggerQueryCriteriaComponent o = (SubscriptionTopicResourceTriggerQueryCriteriaComponent) other_; 1206 return compareDeep(previous, o.previous, true) && compareDeep(resultForCreate, o.resultForCreate, true) 1207 && compareDeep(current, o.current, true) && compareDeep(resultForDelete, o.resultForDelete, true) 1208 && compareDeep(requireBoth, o.requireBoth, true); 1209 } 1210 1211 @Override 1212 public boolean equalsShallow(Base other_) { 1213 if (!super.equalsShallow(other_)) 1214 return false; 1215 if (!(other_ instanceof SubscriptionTopicResourceTriggerQueryCriteriaComponent)) 1216 return false; 1217 SubscriptionTopicResourceTriggerQueryCriteriaComponent o = (SubscriptionTopicResourceTriggerQueryCriteriaComponent) other_; 1218 return compareValues(previous, o.previous, true) && compareValues(resultForCreate, o.resultForCreate, true) 1219 && compareValues(current, o.current, true) && compareValues(resultForDelete, o.resultForDelete, true) 1220 && compareValues(requireBoth, o.requireBoth, true); 1221 } 1222 1223 public boolean isEmpty() { 1224 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(previous, resultForCreate 1225 , current, resultForDelete, requireBoth); 1226 } 1227 1228 public String fhirType() { 1229 return "SubscriptionTopic.resourceTrigger.queryCriteria"; 1230 1231 } 1232 1233 } 1234 1235 @Block() 1236 public static class SubscriptionTopicEventTriggerComponent extends BackboneElement implements IBaseBackboneElement { 1237 /** 1238 * The human readable description of an event to trigger a notification for the SubscriptionTopic - for example, "Patient Admission, as defined in HL7v2 via message ADT^A01". Multiple values are considered OR joined (e.g., matching any single event listed). 1239 */ 1240 @Child(name = "description", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1241 @Description(shortDefinition="Text representation of the event trigger", formalDefinition="The human readable description of an event to trigger a notification for the SubscriptionTopic - for example, \"Patient Admission, as defined in HL7v2 via message ADT^A01\". Multiple values are considered OR joined (e.g., matching any single event listed)." ) 1242 protected MarkdownType description; 1243 1244 /** 1245 * A well-defined event which can be used to trigger notifications from the SubscriptionTopic. 1246 */ 1247 @Child(name = "event", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=true) 1248 @Description(shortDefinition="Event which can trigger a notification from the SubscriptionTopic", formalDefinition="A well-defined event which can be used to trigger notifications from the SubscriptionTopic." ) 1249 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v2-0003") 1250 protected CodeableConcept event; 1251 1252 /** 1253 * URL of the Resource that is the focus type used in this event trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, "Patient" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href="elementdefinition-definitions.html#ElementDefinition.type.code">ElementDefinition.type.code</a>. 1254 */ 1255 @Child(name = "resource", type = {UriType.class}, order=3, min=1, max=1, modifier=false, summary=true) 1256 @Description(shortDefinition="Data Type or Resource (reference to definition) for this trigger definition", formalDefinition="URL of the Resource that is the focus type used in this event trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, \"Patient\" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href=\"elementdefinition-definitions.html#ElementDefinition.type.code\">ElementDefinition.type.code</a>." ) 1257 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscription-types") 1258 protected UriType resource; 1259 1260 private static final long serialVersionUID = 1818872110L; 1261 1262 /** 1263 * Constructor 1264 */ 1265 public SubscriptionTopicEventTriggerComponent() { 1266 super(); 1267 } 1268 1269 /** 1270 * Constructor 1271 */ 1272 public SubscriptionTopicEventTriggerComponent(CodeableConcept event, String resource) { 1273 super(); 1274 this.setEvent(event); 1275 this.setResource(resource); 1276 } 1277 1278 /** 1279 * @return {@link #description} (The human readable description of an event to trigger a notification for the SubscriptionTopic - for example, "Patient Admission, as defined in HL7v2 via message ADT^A01". Multiple values are considered OR joined (e.g., matching any single event listed).). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1280 */ 1281 public MarkdownType getDescriptionElement() { 1282 if (this.description == null) 1283 if (Configuration.errorOnAutoCreate()) 1284 throw new Error("Attempt to auto-create SubscriptionTopicEventTriggerComponent.description"); 1285 else if (Configuration.doAutoCreate()) 1286 this.description = new MarkdownType(); // bb 1287 return this.description; 1288 } 1289 1290 public boolean hasDescriptionElement() { 1291 return this.description != null && !this.description.isEmpty(); 1292 } 1293 1294 public boolean hasDescription() { 1295 return this.description != null && !this.description.isEmpty(); 1296 } 1297 1298 /** 1299 * @param value {@link #description} (The human readable description of an event to trigger a notification for the SubscriptionTopic - for example, "Patient Admission, as defined in HL7v2 via message ADT^A01". Multiple values are considered OR joined (e.g., matching any single event listed).). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1300 */ 1301 public SubscriptionTopicEventTriggerComponent setDescriptionElement(MarkdownType value) { 1302 this.description = value; 1303 return this; 1304 } 1305 1306 /** 1307 * @return The human readable description of an event to trigger a notification for the SubscriptionTopic - for example, "Patient Admission, as defined in HL7v2 via message ADT^A01". Multiple values are considered OR joined (e.g., matching any single event listed). 1308 */ 1309 public String getDescription() { 1310 return this.description == null ? null : this.description.getValue(); 1311 } 1312 1313 /** 1314 * @param value The human readable description of an event to trigger a notification for the SubscriptionTopic - for example, "Patient Admission, as defined in HL7v2 via message ADT^A01". Multiple values are considered OR joined (e.g., matching any single event listed). 1315 */ 1316 public SubscriptionTopicEventTriggerComponent setDescription(String value) { 1317 if (Utilities.noString(value)) 1318 this.description = null; 1319 else { 1320 if (this.description == null) 1321 this.description = new MarkdownType(); 1322 this.description.setValue(value); 1323 } 1324 return this; 1325 } 1326 1327 /** 1328 * @return {@link #event} (A well-defined event which can be used to trigger notifications from the SubscriptionTopic.) 1329 */ 1330 public CodeableConcept getEvent() { 1331 if (this.event == null) 1332 if (Configuration.errorOnAutoCreate()) 1333 throw new Error("Attempt to auto-create SubscriptionTopicEventTriggerComponent.event"); 1334 else if (Configuration.doAutoCreate()) 1335 this.event = new CodeableConcept(); // cc 1336 return this.event; 1337 } 1338 1339 public boolean hasEvent() { 1340 return this.event != null && !this.event.isEmpty(); 1341 } 1342 1343 /** 1344 * @param value {@link #event} (A well-defined event which can be used to trigger notifications from the SubscriptionTopic.) 1345 */ 1346 public SubscriptionTopicEventTriggerComponent setEvent(CodeableConcept value) { 1347 this.event = value; 1348 return this; 1349 } 1350 1351 /** 1352 * @return {@link #resource} (URL of the Resource that is the focus type used in this event trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, "Patient" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href="elementdefinition-definitions.html#ElementDefinition.type.code">ElementDefinition.type.code</a>.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 1353 */ 1354 public UriType getResourceElement() { 1355 if (this.resource == null) 1356 if (Configuration.errorOnAutoCreate()) 1357 throw new Error("Attempt to auto-create SubscriptionTopicEventTriggerComponent.resource"); 1358 else if (Configuration.doAutoCreate()) 1359 this.resource = new UriType(); // bb 1360 return this.resource; 1361 } 1362 1363 public boolean hasResourceElement() { 1364 return this.resource != null && !this.resource.isEmpty(); 1365 } 1366 1367 public boolean hasResource() { 1368 return this.resource != null && !this.resource.isEmpty(); 1369 } 1370 1371 /** 1372 * @param value {@link #resource} (URL of the Resource that is the focus type used in this event trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, "Patient" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href="elementdefinition-definitions.html#ElementDefinition.type.code">ElementDefinition.type.code</a>.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 1373 */ 1374 public SubscriptionTopicEventTriggerComponent setResourceElement(UriType value) { 1375 this.resource = value; 1376 return this; 1377 } 1378 1379 /** 1380 * @return URL of the Resource that is the focus type used in this event trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, "Patient" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href="elementdefinition-definitions.html#ElementDefinition.type.code">ElementDefinition.type.code</a>. 1381 */ 1382 public String getResource() { 1383 return this.resource == null ? null : this.resource.getValue(); 1384 } 1385 1386 /** 1387 * @param value URL of the Resource that is the focus type used in this event trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, "Patient" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href="elementdefinition-definitions.html#ElementDefinition.type.code">ElementDefinition.type.code</a>. 1388 */ 1389 public SubscriptionTopicEventTriggerComponent setResource(String value) { 1390 if (this.resource == null) 1391 this.resource = new UriType(); 1392 this.resource.setValue(value); 1393 return this; 1394 } 1395 1396 protected void listChildren(List<Property> children) { 1397 super.listChildren(children); 1398 children.add(new Property("description", "markdown", "The human readable description of an event to trigger a notification for the SubscriptionTopic - for example, \"Patient Admission, as defined in HL7v2 via message ADT^A01\". Multiple values are considered OR joined (e.g., matching any single event listed).", 0, 1, description)); 1399 children.add(new Property("event", "CodeableConcept", "A well-defined event which can be used to trigger notifications from the SubscriptionTopic.", 0, 1, event)); 1400 children.add(new Property("resource", "uri", "URL of the Resource that is the focus type used in this event trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, \"Patient\" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href=\"elementdefinition-definitions.html#ElementDefinition.type.code\">ElementDefinition.type.code</a>.", 0, 1, resource)); 1401 } 1402 1403 @Override 1404 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1405 switch (_hash) { 1406 case -1724546052: /*description*/ return new Property("description", "markdown", "The human readable description of an event to trigger a notification for the SubscriptionTopic - for example, \"Patient Admission, as defined in HL7v2 via message ADT^A01\". Multiple values are considered OR joined (e.g., matching any single event listed).", 0, 1, description); 1407 case 96891546: /*event*/ return new Property("event", "CodeableConcept", "A well-defined event which can be used to trigger notifications from the SubscriptionTopic.", 0, 1, event); 1408 case -341064690: /*resource*/ return new Property("resource", "uri", "URL of the Resource that is the focus type used in this event trigger. Relative URLs are relative to the StructureDefinition root of the implemented FHIR version (e.g., http://hl7.org/fhir/StructureDefinition). For example, \"Patient\" maps to http://hl7.org/fhir/StructureDefinition/Patient. For more information, see <a href=\"elementdefinition-definitions.html#ElementDefinition.type.code\">ElementDefinition.type.code</a>.", 0, 1, resource); 1409 default: return super.getNamedProperty(_hash, _name, _checkValid); 1410 } 1411 1412 } 1413 1414 @Override 1415 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1416 switch (hash) { 1417 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1418 case 96891546: /*event*/ return this.event == null ? new Base[0] : new Base[] {this.event}; // CodeableConcept 1419 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // UriType 1420 default: return super.getProperty(hash, name, checkValid); 1421 } 1422 1423 } 1424 1425 @Override 1426 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1427 switch (hash) { 1428 case -1724546052: // description 1429 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1430 return value; 1431 case 96891546: // event 1432 this.event = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1433 return value; 1434 case -341064690: // resource 1435 this.resource = TypeConvertor.castToUri(value); // UriType 1436 return value; 1437 default: return super.setProperty(hash, name, value); 1438 } 1439 1440 } 1441 1442 @Override 1443 public Base setProperty(String name, Base value) throws FHIRException { 1444 if (name.equals("description")) { 1445 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1446 } else if (name.equals("event")) { 1447 this.event = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1448 } else if (name.equals("resource")) { 1449 this.resource = TypeConvertor.castToUri(value); // UriType 1450 } else 1451 return super.setProperty(name, value); 1452 return value; 1453 } 1454 1455 @Override 1456 public void removeChild(String name, Base value) throws FHIRException { 1457 if (name.equals("description")) { 1458 this.description = null; 1459 } else if (name.equals("event")) { 1460 this.event = null; 1461 } else if (name.equals("resource")) { 1462 this.resource = null; 1463 } else 1464 super.removeChild(name, value); 1465 1466 } 1467 1468 @Override 1469 public Base makeProperty(int hash, String name) throws FHIRException { 1470 switch (hash) { 1471 case -1724546052: return getDescriptionElement(); 1472 case 96891546: return getEvent(); 1473 case -341064690: return getResourceElement(); 1474 default: return super.makeProperty(hash, name); 1475 } 1476 1477 } 1478 1479 @Override 1480 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1481 switch (hash) { 1482 case -1724546052: /*description*/ return new String[] {"markdown"}; 1483 case 96891546: /*event*/ return new String[] {"CodeableConcept"}; 1484 case -341064690: /*resource*/ return new String[] {"uri"}; 1485 default: return super.getTypesForProperty(hash, name); 1486 } 1487 1488 } 1489 1490 @Override 1491 public Base addChild(String name) throws FHIRException { 1492 if (name.equals("description")) { 1493 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.eventTrigger.description"); 1494 } 1495 else if (name.equals("event")) { 1496 this.event = new CodeableConcept(); 1497 return this.event; 1498 } 1499 else if (name.equals("resource")) { 1500 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.eventTrigger.resource"); 1501 } 1502 else 1503 return super.addChild(name); 1504 } 1505 1506 public SubscriptionTopicEventTriggerComponent copy() { 1507 SubscriptionTopicEventTriggerComponent dst = new SubscriptionTopicEventTriggerComponent(); 1508 copyValues(dst); 1509 return dst; 1510 } 1511 1512 public void copyValues(SubscriptionTopicEventTriggerComponent dst) { 1513 super.copyValues(dst); 1514 dst.description = description == null ? null : description.copy(); 1515 dst.event = event == null ? null : event.copy(); 1516 dst.resource = resource == null ? null : resource.copy(); 1517 } 1518 1519 @Override 1520 public boolean equalsDeep(Base other_) { 1521 if (!super.equalsDeep(other_)) 1522 return false; 1523 if (!(other_ instanceof SubscriptionTopicEventTriggerComponent)) 1524 return false; 1525 SubscriptionTopicEventTriggerComponent o = (SubscriptionTopicEventTriggerComponent) other_; 1526 return compareDeep(description, o.description, true) && compareDeep(event, o.event, true) && compareDeep(resource, o.resource, true) 1527 ; 1528 } 1529 1530 @Override 1531 public boolean equalsShallow(Base other_) { 1532 if (!super.equalsShallow(other_)) 1533 return false; 1534 if (!(other_ instanceof SubscriptionTopicEventTriggerComponent)) 1535 return false; 1536 SubscriptionTopicEventTriggerComponent o = (SubscriptionTopicEventTriggerComponent) other_; 1537 return compareValues(description, o.description, true) && compareValues(resource, o.resource, true) 1538 ; 1539 } 1540 1541 public boolean isEmpty() { 1542 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, event, resource 1543 ); 1544 } 1545 1546 public String fhirType() { 1547 return "SubscriptionTopic.eventTrigger"; 1548 1549 } 1550 1551 } 1552 1553 @Block() 1554 public static class SubscriptionTopicCanFilterByComponent extends BackboneElement implements IBaseBackboneElement { 1555 /** 1556 * Description of how this filtering parameter is intended to be used. 1557 */ 1558 @Child(name = "description", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1559 @Description(shortDefinition="Description of this filter parameter", formalDefinition="Description of how this filtering parameter is intended to be used." ) 1560 protected MarkdownType description; 1561 1562 /** 1563 * URL of the Resource that is the type used in this filter. This is the "focus" of the topic (or one of them if there are more than one). It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present. 1564 */ 1565 @Child(name = "resource", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1566 @Description(shortDefinition="URL of the triggering Resource that this filter applies to", formalDefinition="URL of the Resource that is the type used in this filter. This is the \"focus\" of the topic (or one of them if there are more than one). It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present." ) 1567 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscription-types") 1568 protected UriType resource; 1569 1570 /** 1571 * Either the canonical URL to a search parameter (like "http://hl7.org/fhir/SearchParameter/encounter-patient") or topic-defined parameter (like "hub.event") which is a label for the filter. 1572 */ 1573 @Child(name = "filterParameter", type = {StringType.class}, order=3, min=1, max=1, modifier=false, summary=true) 1574 @Description(shortDefinition="Human-readable and computation-friendly name for a filter parameter usable by subscriptions on this topic, via Subscription.filterBy.filterParameter", formalDefinition="Either the canonical URL to a search parameter (like \"http://hl7.org/fhir/SearchParameter/encounter-patient\") or topic-defined parameter (like \"hub.event\") which is a label for the filter." ) 1575 protected StringType filterParameter; 1576 1577 /** 1578 * Either the canonical URL to a search parameter (like "http://hl7.org/fhir/SearchParameter/encounter-patient") or the officially-defined URI for a shared filter concept (like "http://example.org/concepts/shared-common-event"). 1579 */ 1580 @Child(name = "filterDefinition", type = {UriType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1581 @Description(shortDefinition="Canonical URL for a filterParameter definition", formalDefinition="Either the canonical URL to a search parameter (like \"http://hl7.org/fhir/SearchParameter/encounter-patient\") or the officially-defined URI for a shared filter concept (like \"http://example.org/concepts/shared-common-event\")." ) 1582 protected UriType filterDefinition; 1583 1584 /** 1585 * Comparators allowed for the filter parameter. 1586 */ 1587 @Child(name = "comparator", type = {CodeType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1588 @Description(shortDefinition="eq | ne | gt | lt | ge | le | sa | eb | ap", formalDefinition="Comparators allowed for the filter parameter." ) 1589 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-comparator") 1590 protected List<Enumeration<SearchComparator>> comparator; 1591 1592 /** 1593 * Modifiers allowed for the filter parameter. 1594 */ 1595 @Child(name = "modifier", type = {CodeType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1596 @Description(shortDefinition="missing | exact | contains | not | text | in | not-in | below | above | type | identifier | of-type | code-text | text-advanced | iterate", formalDefinition="Modifiers allowed for the filter parameter." ) 1597 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/search-modifier-code") 1598 protected List<Enumeration<SearchModifierCode>> modifier; 1599 1600 private static final long serialVersionUID = -1521606527L; 1601 1602 /** 1603 * Constructor 1604 */ 1605 public SubscriptionTopicCanFilterByComponent() { 1606 super(); 1607 } 1608 1609 /** 1610 * Constructor 1611 */ 1612 public SubscriptionTopicCanFilterByComponent(String filterParameter) { 1613 super(); 1614 this.setFilterParameter(filterParameter); 1615 } 1616 1617 /** 1618 * @return {@link #description} (Description of how this filtering parameter is intended to be used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1619 */ 1620 public MarkdownType getDescriptionElement() { 1621 if (this.description == null) 1622 if (Configuration.errorOnAutoCreate()) 1623 throw new Error("Attempt to auto-create SubscriptionTopicCanFilterByComponent.description"); 1624 else if (Configuration.doAutoCreate()) 1625 this.description = new MarkdownType(); // bb 1626 return this.description; 1627 } 1628 1629 public boolean hasDescriptionElement() { 1630 return this.description != null && !this.description.isEmpty(); 1631 } 1632 1633 public boolean hasDescription() { 1634 return this.description != null && !this.description.isEmpty(); 1635 } 1636 1637 /** 1638 * @param value {@link #description} (Description of how this filtering parameter is intended to be used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1639 */ 1640 public SubscriptionTopicCanFilterByComponent setDescriptionElement(MarkdownType value) { 1641 this.description = value; 1642 return this; 1643 } 1644 1645 /** 1646 * @return Description of how this filtering parameter is intended to be used. 1647 */ 1648 public String getDescription() { 1649 return this.description == null ? null : this.description.getValue(); 1650 } 1651 1652 /** 1653 * @param value Description of how this filtering parameter is intended to be used. 1654 */ 1655 public SubscriptionTopicCanFilterByComponent setDescription(String value) { 1656 if (Utilities.noString(value)) 1657 this.description = null; 1658 else { 1659 if (this.description == null) 1660 this.description = new MarkdownType(); 1661 this.description.setValue(value); 1662 } 1663 return this; 1664 } 1665 1666 /** 1667 * @return {@link #resource} (URL of the Resource that is the type used in this filter. This is the "focus" of the topic (or one of them if there are more than one). It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 1668 */ 1669 public UriType getResourceElement() { 1670 if (this.resource == null) 1671 if (Configuration.errorOnAutoCreate()) 1672 throw new Error("Attempt to auto-create SubscriptionTopicCanFilterByComponent.resource"); 1673 else if (Configuration.doAutoCreate()) 1674 this.resource = new UriType(); // bb 1675 return this.resource; 1676 } 1677 1678 public boolean hasResourceElement() { 1679 return this.resource != null && !this.resource.isEmpty(); 1680 } 1681 1682 public boolean hasResource() { 1683 return this.resource != null && !this.resource.isEmpty(); 1684 } 1685 1686 /** 1687 * @param value {@link #resource} (URL of the Resource that is the type used in this filter. This is the "focus" of the topic (or one of them if there are more than one). It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 1688 */ 1689 public SubscriptionTopicCanFilterByComponent setResourceElement(UriType value) { 1690 this.resource = value; 1691 return this; 1692 } 1693 1694 /** 1695 * @return URL of the Resource that is the type used in this filter. This is the "focus" of the topic (or one of them if there are more than one). It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present. 1696 */ 1697 public String getResource() { 1698 return this.resource == null ? null : this.resource.getValue(); 1699 } 1700 1701 /** 1702 * @param value URL of the Resource that is the type used in this filter. This is the "focus" of the topic (or one of them if there are more than one). It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present. 1703 */ 1704 public SubscriptionTopicCanFilterByComponent setResource(String value) { 1705 if (Utilities.noString(value)) 1706 this.resource = null; 1707 else { 1708 if (this.resource == null) 1709 this.resource = new UriType(); 1710 this.resource.setValue(value); 1711 } 1712 return this; 1713 } 1714 1715 /** 1716 * @return {@link #filterParameter} (Either the canonical URL to a search parameter (like "http://hl7.org/fhir/SearchParameter/encounter-patient") or topic-defined parameter (like "hub.event") which is a label for the filter.). This is the underlying object with id, value and extensions. The accessor "getFilterParameter" gives direct access to the value 1717 */ 1718 public StringType getFilterParameterElement() { 1719 if (this.filterParameter == null) 1720 if (Configuration.errorOnAutoCreate()) 1721 throw new Error("Attempt to auto-create SubscriptionTopicCanFilterByComponent.filterParameter"); 1722 else if (Configuration.doAutoCreate()) 1723 this.filterParameter = new StringType(); // bb 1724 return this.filterParameter; 1725 } 1726 1727 public boolean hasFilterParameterElement() { 1728 return this.filterParameter != null && !this.filterParameter.isEmpty(); 1729 } 1730 1731 public boolean hasFilterParameter() { 1732 return this.filterParameter != null && !this.filterParameter.isEmpty(); 1733 } 1734 1735 /** 1736 * @param value {@link #filterParameter} (Either the canonical URL to a search parameter (like "http://hl7.org/fhir/SearchParameter/encounter-patient") or topic-defined parameter (like "hub.event") which is a label for the filter.). This is the underlying object with id, value and extensions. The accessor "getFilterParameter" gives direct access to the value 1737 */ 1738 public SubscriptionTopicCanFilterByComponent setFilterParameterElement(StringType value) { 1739 this.filterParameter = value; 1740 return this; 1741 } 1742 1743 /** 1744 * @return Either the canonical URL to a search parameter (like "http://hl7.org/fhir/SearchParameter/encounter-patient") or topic-defined parameter (like "hub.event") which is a label for the filter. 1745 */ 1746 public String getFilterParameter() { 1747 return this.filterParameter == null ? null : this.filterParameter.getValue(); 1748 } 1749 1750 /** 1751 * @param value Either the canonical URL to a search parameter (like "http://hl7.org/fhir/SearchParameter/encounter-patient") or topic-defined parameter (like "hub.event") which is a label for the filter. 1752 */ 1753 public SubscriptionTopicCanFilterByComponent setFilterParameter(String value) { 1754 if (this.filterParameter == null) 1755 this.filterParameter = new StringType(); 1756 this.filterParameter.setValue(value); 1757 return this; 1758 } 1759 1760 /** 1761 * @return {@link #filterDefinition} (Either the canonical URL to a search parameter (like "http://hl7.org/fhir/SearchParameter/encounter-patient") or the officially-defined URI for a shared filter concept (like "http://example.org/concepts/shared-common-event").). This is the underlying object with id, value and extensions. The accessor "getFilterDefinition" gives direct access to the value 1762 */ 1763 public UriType getFilterDefinitionElement() { 1764 if (this.filterDefinition == null) 1765 if (Configuration.errorOnAutoCreate()) 1766 throw new Error("Attempt to auto-create SubscriptionTopicCanFilterByComponent.filterDefinition"); 1767 else if (Configuration.doAutoCreate()) 1768 this.filterDefinition = new UriType(); // bb 1769 return this.filterDefinition; 1770 } 1771 1772 public boolean hasFilterDefinitionElement() { 1773 return this.filterDefinition != null && !this.filterDefinition.isEmpty(); 1774 } 1775 1776 public boolean hasFilterDefinition() { 1777 return this.filterDefinition != null && !this.filterDefinition.isEmpty(); 1778 } 1779 1780 /** 1781 * @param value {@link #filterDefinition} (Either the canonical URL to a search parameter (like "http://hl7.org/fhir/SearchParameter/encounter-patient") or the officially-defined URI for a shared filter concept (like "http://example.org/concepts/shared-common-event").). This is the underlying object with id, value and extensions. The accessor "getFilterDefinition" gives direct access to the value 1782 */ 1783 public SubscriptionTopicCanFilterByComponent setFilterDefinitionElement(UriType value) { 1784 this.filterDefinition = value; 1785 return this; 1786 } 1787 1788 /** 1789 * @return Either the canonical URL to a search parameter (like "http://hl7.org/fhir/SearchParameter/encounter-patient") or the officially-defined URI for a shared filter concept (like "http://example.org/concepts/shared-common-event"). 1790 */ 1791 public String getFilterDefinition() { 1792 return this.filterDefinition == null ? null : this.filterDefinition.getValue(); 1793 } 1794 1795 /** 1796 * @param value Either the canonical URL to a search parameter (like "http://hl7.org/fhir/SearchParameter/encounter-patient") or the officially-defined URI for a shared filter concept (like "http://example.org/concepts/shared-common-event"). 1797 */ 1798 public SubscriptionTopicCanFilterByComponent setFilterDefinition(String value) { 1799 if (Utilities.noString(value)) 1800 this.filterDefinition = null; 1801 else { 1802 if (this.filterDefinition == null) 1803 this.filterDefinition = new UriType(); 1804 this.filterDefinition.setValue(value); 1805 } 1806 return this; 1807 } 1808 1809 /** 1810 * @return {@link #comparator} (Comparators allowed for the filter parameter.) 1811 */ 1812 public List<Enumeration<SearchComparator>> getComparator() { 1813 if (this.comparator == null) 1814 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 1815 return this.comparator; 1816 } 1817 1818 /** 1819 * @return Returns a reference to <code>this</code> for easy method chaining 1820 */ 1821 public SubscriptionTopicCanFilterByComponent setComparator(List<Enumeration<SearchComparator>> theComparator) { 1822 this.comparator = theComparator; 1823 return this; 1824 } 1825 1826 public boolean hasComparator() { 1827 if (this.comparator == null) 1828 return false; 1829 for (Enumeration<SearchComparator> item : this.comparator) 1830 if (!item.isEmpty()) 1831 return true; 1832 return false; 1833 } 1834 1835 /** 1836 * @return {@link #comparator} (Comparators allowed for the filter parameter.) 1837 */ 1838 public Enumeration<SearchComparator> addComparatorElement() {//2 1839 Enumeration<SearchComparator> t = new Enumeration<SearchComparator>(new SearchComparatorEnumFactory()); 1840 if (this.comparator == null) 1841 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 1842 this.comparator.add(t); 1843 return t; 1844 } 1845 1846 /** 1847 * @param value {@link #comparator} (Comparators allowed for the filter parameter.) 1848 */ 1849 public SubscriptionTopicCanFilterByComponent addComparator(SearchComparator value) { //1 1850 Enumeration<SearchComparator> t = new Enumeration<SearchComparator>(new SearchComparatorEnumFactory()); 1851 t.setValue(value); 1852 if (this.comparator == null) 1853 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 1854 this.comparator.add(t); 1855 return this; 1856 } 1857 1858 /** 1859 * @param value {@link #comparator} (Comparators allowed for the filter parameter.) 1860 */ 1861 public boolean hasComparator(SearchComparator value) { 1862 if (this.comparator == null) 1863 return false; 1864 for (Enumeration<SearchComparator> v : this.comparator) 1865 if (v.getValue().equals(value)) // code 1866 return true; 1867 return false; 1868 } 1869 1870 /** 1871 * @return {@link #modifier} (Modifiers allowed for the filter parameter.) 1872 */ 1873 public List<Enumeration<SearchModifierCode>> getModifier() { 1874 if (this.modifier == null) 1875 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 1876 return this.modifier; 1877 } 1878 1879 /** 1880 * @return Returns a reference to <code>this</code> for easy method chaining 1881 */ 1882 public SubscriptionTopicCanFilterByComponent setModifier(List<Enumeration<SearchModifierCode>> theModifier) { 1883 this.modifier = theModifier; 1884 return this; 1885 } 1886 1887 public boolean hasModifier() { 1888 if (this.modifier == null) 1889 return false; 1890 for (Enumeration<SearchModifierCode> item : this.modifier) 1891 if (!item.isEmpty()) 1892 return true; 1893 return false; 1894 } 1895 1896 /** 1897 * @return {@link #modifier} (Modifiers allowed for the filter parameter.) 1898 */ 1899 public Enumeration<SearchModifierCode> addModifierElement() {//2 1900 Enumeration<SearchModifierCode> t = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 1901 if (this.modifier == null) 1902 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 1903 this.modifier.add(t); 1904 return t; 1905 } 1906 1907 /** 1908 * @param value {@link #modifier} (Modifiers allowed for the filter parameter.) 1909 */ 1910 public SubscriptionTopicCanFilterByComponent addModifier(SearchModifierCode value) { //1 1911 Enumeration<SearchModifierCode> t = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 1912 t.setValue(value); 1913 if (this.modifier == null) 1914 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 1915 this.modifier.add(t); 1916 return this; 1917 } 1918 1919 /** 1920 * @param value {@link #modifier} (Modifiers allowed for the filter parameter.) 1921 */ 1922 public boolean hasModifier(SearchModifierCode value) { 1923 if (this.modifier == null) 1924 return false; 1925 for (Enumeration<SearchModifierCode> v : this.modifier) 1926 if (v.getValue().equals(value)) // code 1927 return true; 1928 return false; 1929 } 1930 1931 protected void listChildren(List<Property> children) { 1932 super.listChildren(children); 1933 children.add(new Property("description", "markdown", "Description of how this filtering parameter is intended to be used.", 0, 1, description)); 1934 children.add(new Property("resource", "uri", "URL of the Resource that is the type used in this filter. This is the \"focus\" of the topic (or one of them if there are more than one). It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present.", 0, 1, resource)); 1935 children.add(new Property("filterParameter", "string", "Either the canonical URL to a search parameter (like \"http://hl7.org/fhir/SearchParameter/encounter-patient\") or topic-defined parameter (like \"hub.event\") which is a label for the filter.", 0, 1, filterParameter)); 1936 children.add(new Property("filterDefinition", "uri", "Either the canonical URL to a search parameter (like \"http://hl7.org/fhir/SearchParameter/encounter-patient\") or the officially-defined URI for a shared filter concept (like \"http://example.org/concepts/shared-common-event\").", 0, 1, filterDefinition)); 1937 children.add(new Property("comparator", "code", "Comparators allowed for the filter parameter.", 0, java.lang.Integer.MAX_VALUE, comparator)); 1938 children.add(new Property("modifier", "code", "Modifiers allowed for the filter parameter.", 0, java.lang.Integer.MAX_VALUE, modifier)); 1939 } 1940 1941 @Override 1942 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1943 switch (_hash) { 1944 case -1724546052: /*description*/ return new Property("description", "markdown", "Description of how this filtering parameter is intended to be used.", 0, 1, description); 1945 case -341064690: /*resource*/ return new Property("resource", "uri", "URL of the Resource that is the type used in this filter. This is the \"focus\" of the topic (or one of them if there are more than one). It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present.", 0, 1, resource); 1946 case 618257: /*filterParameter*/ return new Property("filterParameter", "string", "Either the canonical URL to a search parameter (like \"http://hl7.org/fhir/SearchParameter/encounter-patient\") or topic-defined parameter (like \"hub.event\") which is a label for the filter.", 0, 1, filterParameter); 1947 case -1453988117: /*filterDefinition*/ return new Property("filterDefinition", "uri", "Either the canonical URL to a search parameter (like \"http://hl7.org/fhir/SearchParameter/encounter-patient\") or the officially-defined URI for a shared filter concept (like \"http://example.org/concepts/shared-common-event\").", 0, 1, filterDefinition); 1948 case -844673834: /*comparator*/ return new Property("comparator", "code", "Comparators allowed for the filter parameter.", 0, java.lang.Integer.MAX_VALUE, comparator); 1949 case -615513385: /*modifier*/ return new Property("modifier", "code", "Modifiers allowed for the filter parameter.", 0, java.lang.Integer.MAX_VALUE, modifier); 1950 default: return super.getNamedProperty(_hash, _name, _checkValid); 1951 } 1952 1953 } 1954 1955 @Override 1956 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1957 switch (hash) { 1958 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1959 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // UriType 1960 case 618257: /*filterParameter*/ return this.filterParameter == null ? new Base[0] : new Base[] {this.filterParameter}; // StringType 1961 case -1453988117: /*filterDefinition*/ return this.filterDefinition == null ? new Base[0] : new Base[] {this.filterDefinition}; // UriType 1962 case -844673834: /*comparator*/ return this.comparator == null ? new Base[0] : this.comparator.toArray(new Base[this.comparator.size()]); // Enumeration<SearchComparator> 1963 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // Enumeration<SearchModifierCode> 1964 default: return super.getProperty(hash, name, checkValid); 1965 } 1966 1967 } 1968 1969 @Override 1970 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1971 switch (hash) { 1972 case -1724546052: // description 1973 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1974 return value; 1975 case -341064690: // resource 1976 this.resource = TypeConvertor.castToUri(value); // UriType 1977 return value; 1978 case 618257: // filterParameter 1979 this.filterParameter = TypeConvertor.castToString(value); // StringType 1980 return value; 1981 case -1453988117: // filterDefinition 1982 this.filterDefinition = TypeConvertor.castToUri(value); // UriType 1983 return value; 1984 case -844673834: // comparator 1985 value = new SearchComparatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 1986 this.getComparator().add((Enumeration) value); // Enumeration<SearchComparator> 1987 return value; 1988 case -615513385: // modifier 1989 value = new SearchModifierCodeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1990 this.getModifier().add((Enumeration) value); // Enumeration<SearchModifierCode> 1991 return value; 1992 default: return super.setProperty(hash, name, value); 1993 } 1994 1995 } 1996 1997 @Override 1998 public Base setProperty(String name, Base value) throws FHIRException { 1999 if (name.equals("description")) { 2000 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2001 } else if (name.equals("resource")) { 2002 this.resource = TypeConvertor.castToUri(value); // UriType 2003 } else if (name.equals("filterParameter")) { 2004 this.filterParameter = TypeConvertor.castToString(value); // StringType 2005 } else if (name.equals("filterDefinition")) { 2006 this.filterDefinition = TypeConvertor.castToUri(value); // UriType 2007 } else if (name.equals("comparator")) { 2008 value = new SearchComparatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 2009 this.getComparator().add((Enumeration) value); 2010 } else if (name.equals("modifier")) { 2011 value = new SearchModifierCodeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2012 this.getModifier().add((Enumeration) value); 2013 } else 2014 return super.setProperty(name, value); 2015 return value; 2016 } 2017 2018 @Override 2019 public void removeChild(String name, Base value) throws FHIRException { 2020 if (name.equals("description")) { 2021 this.description = null; 2022 } else if (name.equals("resource")) { 2023 this.resource = null; 2024 } else if (name.equals("filterParameter")) { 2025 this.filterParameter = null; 2026 } else if (name.equals("filterDefinition")) { 2027 this.filterDefinition = null; 2028 } else if (name.equals("comparator")) { 2029 value = new SearchComparatorEnumFactory().fromType(TypeConvertor.castToCode(value)); 2030 this.getComparator().remove((Enumeration) value); 2031 } else if (name.equals("modifier")) { 2032 value = new SearchModifierCodeEnumFactory().fromType(TypeConvertor.castToCode(value)); 2033 this.getModifier().remove((Enumeration) value); 2034 } else 2035 super.removeChild(name, value); 2036 2037 } 2038 2039 @Override 2040 public Base makeProperty(int hash, String name) throws FHIRException { 2041 switch (hash) { 2042 case -1724546052: return getDescriptionElement(); 2043 case -341064690: return getResourceElement(); 2044 case 618257: return getFilterParameterElement(); 2045 case -1453988117: return getFilterDefinitionElement(); 2046 case -844673834: return addComparatorElement(); 2047 case -615513385: return addModifierElement(); 2048 default: return super.makeProperty(hash, name); 2049 } 2050 2051 } 2052 2053 @Override 2054 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2055 switch (hash) { 2056 case -1724546052: /*description*/ return new String[] {"markdown"}; 2057 case -341064690: /*resource*/ return new String[] {"uri"}; 2058 case 618257: /*filterParameter*/ return new String[] {"string"}; 2059 case -1453988117: /*filterDefinition*/ return new String[] {"uri"}; 2060 case -844673834: /*comparator*/ return new String[] {"code"}; 2061 case -615513385: /*modifier*/ return new String[] {"code"}; 2062 default: return super.getTypesForProperty(hash, name); 2063 } 2064 2065 } 2066 2067 @Override 2068 public Base addChild(String name) throws FHIRException { 2069 if (name.equals("description")) { 2070 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.canFilterBy.description"); 2071 } 2072 else if (name.equals("resource")) { 2073 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.canFilterBy.resource"); 2074 } 2075 else if (name.equals("filterParameter")) { 2076 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.canFilterBy.filterParameter"); 2077 } 2078 else if (name.equals("filterDefinition")) { 2079 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.canFilterBy.filterDefinition"); 2080 } 2081 else if (name.equals("comparator")) { 2082 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.canFilterBy.comparator"); 2083 } 2084 else if (name.equals("modifier")) { 2085 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.canFilterBy.modifier"); 2086 } 2087 else 2088 return super.addChild(name); 2089 } 2090 2091 public SubscriptionTopicCanFilterByComponent copy() { 2092 SubscriptionTopicCanFilterByComponent dst = new SubscriptionTopicCanFilterByComponent(); 2093 copyValues(dst); 2094 return dst; 2095 } 2096 2097 public void copyValues(SubscriptionTopicCanFilterByComponent dst) { 2098 super.copyValues(dst); 2099 dst.description = description == null ? null : description.copy(); 2100 dst.resource = resource == null ? null : resource.copy(); 2101 dst.filterParameter = filterParameter == null ? null : filterParameter.copy(); 2102 dst.filterDefinition = filterDefinition == null ? null : filterDefinition.copy(); 2103 if (comparator != null) { 2104 dst.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2105 for (Enumeration<SearchComparator> i : comparator) 2106 dst.comparator.add(i.copy()); 2107 }; 2108 if (modifier != null) { 2109 dst.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2110 for (Enumeration<SearchModifierCode> i : modifier) 2111 dst.modifier.add(i.copy()); 2112 }; 2113 } 2114 2115 @Override 2116 public boolean equalsDeep(Base other_) { 2117 if (!super.equalsDeep(other_)) 2118 return false; 2119 if (!(other_ instanceof SubscriptionTopicCanFilterByComponent)) 2120 return false; 2121 SubscriptionTopicCanFilterByComponent o = (SubscriptionTopicCanFilterByComponent) other_; 2122 return compareDeep(description, o.description, true) && compareDeep(resource, o.resource, true) 2123 && compareDeep(filterParameter, o.filterParameter, true) && compareDeep(filterDefinition, o.filterDefinition, true) 2124 && compareDeep(comparator, o.comparator, true) && compareDeep(modifier, o.modifier, true); 2125 } 2126 2127 @Override 2128 public boolean equalsShallow(Base other_) { 2129 if (!super.equalsShallow(other_)) 2130 return false; 2131 if (!(other_ instanceof SubscriptionTopicCanFilterByComponent)) 2132 return false; 2133 SubscriptionTopicCanFilterByComponent o = (SubscriptionTopicCanFilterByComponent) other_; 2134 return compareValues(description, o.description, true) && compareValues(resource, o.resource, true) 2135 && compareValues(filterParameter, o.filterParameter, true) && compareValues(filterDefinition, o.filterDefinition, true) 2136 && compareValues(comparator, o.comparator, true) && compareValues(modifier, o.modifier, true); 2137 } 2138 2139 public boolean isEmpty() { 2140 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, resource, filterParameter 2141 , filterDefinition, comparator, modifier); 2142 } 2143 2144 public String fhirType() { 2145 return "SubscriptionTopic.canFilterBy"; 2146 2147 } 2148 2149 } 2150 2151 @Block() 2152 public static class SubscriptionTopicNotificationShapeComponent extends BackboneElement implements IBaseBackboneElement { 2153 /** 2154 * URL of the Resource that is the type used in this shape. This is the 'focus' resource of the topic (or one of them if there are more than one) and the root resource for this shape definition. It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present. 2155 */ 2156 @Child(name = "resource", type = {UriType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2157 @Description(shortDefinition="URL of the Resource that is the focus (main) resource in a notification shape", formalDefinition="URL of the Resource that is the type used in this shape. This is the 'focus' resource of the topic (or one of them if there are more than one) and the root resource for this shape definition. It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present." ) 2158 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscription-types") 2159 protected UriType resource; 2160 2161 /** 2162 * Search-style _include directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them. 2163 */ 2164 @Child(name = "include", type = {StringType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2165 @Description(shortDefinition="Include directives, rooted in the resource for this shape", formalDefinition="Search-style _include directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them." ) 2166 protected List<StringType> include; 2167 2168 /** 2169 * Search-style _revinclude directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them. 2170 */ 2171 @Child(name = "revInclude", type = {StringType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2172 @Description(shortDefinition="Reverse include directives, rooted in the resource for this shape", formalDefinition="Search-style _revinclude directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them." ) 2173 protected List<StringType> revInclude; 2174 2175 private static final long serialVersionUID = -1718592091L; 2176 2177 /** 2178 * Constructor 2179 */ 2180 public SubscriptionTopicNotificationShapeComponent() { 2181 super(); 2182 } 2183 2184 /** 2185 * Constructor 2186 */ 2187 public SubscriptionTopicNotificationShapeComponent(String resource) { 2188 super(); 2189 this.setResource(resource); 2190 } 2191 2192 /** 2193 * @return {@link #resource} (URL of the Resource that is the type used in this shape. This is the 'focus' resource of the topic (or one of them if there are more than one) and the root resource for this shape definition. It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 2194 */ 2195 public UriType getResourceElement() { 2196 if (this.resource == null) 2197 if (Configuration.errorOnAutoCreate()) 2198 throw new Error("Attempt to auto-create SubscriptionTopicNotificationShapeComponent.resource"); 2199 else if (Configuration.doAutoCreate()) 2200 this.resource = new UriType(); // bb 2201 return this.resource; 2202 } 2203 2204 public boolean hasResourceElement() { 2205 return this.resource != null && !this.resource.isEmpty(); 2206 } 2207 2208 public boolean hasResource() { 2209 return this.resource != null && !this.resource.isEmpty(); 2210 } 2211 2212 /** 2213 * @param value {@link #resource} (URL of the Resource that is the type used in this shape. This is the 'focus' resource of the topic (or one of them if there are more than one) and the root resource for this shape definition. It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 2214 */ 2215 public SubscriptionTopicNotificationShapeComponent setResourceElement(UriType value) { 2216 this.resource = value; 2217 return this; 2218 } 2219 2220 /** 2221 * @return URL of the Resource that is the type used in this shape. This is the 'focus' resource of the topic (or one of them if there are more than one) and the root resource for this shape definition. It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present. 2222 */ 2223 public String getResource() { 2224 return this.resource == null ? null : this.resource.getValue(); 2225 } 2226 2227 /** 2228 * @param value URL of the Resource that is the type used in this shape. This is the 'focus' resource of the topic (or one of them if there are more than one) and the root resource for this shape definition. It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present. 2229 */ 2230 public SubscriptionTopicNotificationShapeComponent setResource(String value) { 2231 if (this.resource == null) 2232 this.resource = new UriType(); 2233 this.resource.setValue(value); 2234 return this; 2235 } 2236 2237 /** 2238 * @return {@link #include} (Search-style _include directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.) 2239 */ 2240 public List<StringType> getInclude() { 2241 if (this.include == null) 2242 this.include = new ArrayList<StringType>(); 2243 return this.include; 2244 } 2245 2246 /** 2247 * @return Returns a reference to <code>this</code> for easy method chaining 2248 */ 2249 public SubscriptionTopicNotificationShapeComponent setInclude(List<StringType> theInclude) { 2250 this.include = theInclude; 2251 return this; 2252 } 2253 2254 public boolean hasInclude() { 2255 if (this.include == null) 2256 return false; 2257 for (StringType item : this.include) 2258 if (!item.isEmpty()) 2259 return true; 2260 return false; 2261 } 2262 2263 /** 2264 * @return {@link #include} (Search-style _include directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.) 2265 */ 2266 public StringType addIncludeElement() {//2 2267 StringType t = new StringType(); 2268 if (this.include == null) 2269 this.include = new ArrayList<StringType>(); 2270 this.include.add(t); 2271 return t; 2272 } 2273 2274 /** 2275 * @param value {@link #include} (Search-style _include directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.) 2276 */ 2277 public SubscriptionTopicNotificationShapeComponent addInclude(String value) { //1 2278 StringType t = new StringType(); 2279 t.setValue(value); 2280 if (this.include == null) 2281 this.include = new ArrayList<StringType>(); 2282 this.include.add(t); 2283 return this; 2284 } 2285 2286 /** 2287 * @param value {@link #include} (Search-style _include directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.) 2288 */ 2289 public boolean hasInclude(String value) { 2290 if (this.include == null) 2291 return false; 2292 for (StringType v : this.include) 2293 if (v.getValue().equals(value)) // string 2294 return true; 2295 return false; 2296 } 2297 2298 /** 2299 * @return {@link #revInclude} (Search-style _revinclude directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.) 2300 */ 2301 public List<StringType> getRevInclude() { 2302 if (this.revInclude == null) 2303 this.revInclude = new ArrayList<StringType>(); 2304 return this.revInclude; 2305 } 2306 2307 /** 2308 * @return Returns a reference to <code>this</code> for easy method chaining 2309 */ 2310 public SubscriptionTopicNotificationShapeComponent setRevInclude(List<StringType> theRevInclude) { 2311 this.revInclude = theRevInclude; 2312 return this; 2313 } 2314 2315 public boolean hasRevInclude() { 2316 if (this.revInclude == null) 2317 return false; 2318 for (StringType item : this.revInclude) 2319 if (!item.isEmpty()) 2320 return true; 2321 return false; 2322 } 2323 2324 /** 2325 * @return {@link #revInclude} (Search-style _revinclude directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.) 2326 */ 2327 public StringType addRevIncludeElement() {//2 2328 StringType t = new StringType(); 2329 if (this.revInclude == null) 2330 this.revInclude = new ArrayList<StringType>(); 2331 this.revInclude.add(t); 2332 return t; 2333 } 2334 2335 /** 2336 * @param value {@link #revInclude} (Search-style _revinclude directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.) 2337 */ 2338 public SubscriptionTopicNotificationShapeComponent addRevInclude(String value) { //1 2339 StringType t = new StringType(); 2340 t.setValue(value); 2341 if (this.revInclude == null) 2342 this.revInclude = new ArrayList<StringType>(); 2343 this.revInclude.add(t); 2344 return this; 2345 } 2346 2347 /** 2348 * @param value {@link #revInclude} (Search-style _revinclude directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.) 2349 */ 2350 public boolean hasRevInclude(String value) { 2351 if (this.revInclude == null) 2352 return false; 2353 for (StringType v : this.revInclude) 2354 if (v.getValue().equals(value)) // string 2355 return true; 2356 return false; 2357 } 2358 2359 protected void listChildren(List<Property> children) { 2360 super.listChildren(children); 2361 children.add(new Property("resource", "uri", "URL of the Resource that is the type used in this shape. This is the 'focus' resource of the topic (or one of them if there are more than one) and the root resource for this shape definition. It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present.", 0, 1, resource)); 2362 children.add(new Property("include", "string", "Search-style _include directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.", 0, java.lang.Integer.MAX_VALUE, include)); 2363 children.add(new Property("revInclude", "string", "Search-style _revinclude directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.", 0, java.lang.Integer.MAX_VALUE, revInclude)); 2364 } 2365 2366 @Override 2367 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2368 switch (_hash) { 2369 case -341064690: /*resource*/ return new Property("resource", "uri", "URL of the Resource that is the type used in this shape. This is the 'focus' resource of the topic (or one of them if there are more than one) and the root resource for this shape definition. It will be the same, a generality, or a specificity of SubscriptionTopic.resourceTrigger.resource or SubscriptionTopic.eventTrigger.resource when they are present.", 0, 1, resource); 2370 case 1942574248: /*include*/ return new Property("include", "string", "Search-style _include directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.", 0, java.lang.Integer.MAX_VALUE, include); 2371 case 8439429: /*revInclude*/ return new Property("revInclude", "string", "Search-style _revinclude directives, rooted in the resource for this shape. Servers SHOULD include resources listed here, if they exist and the user is authorized to receive them. Clients SHOULD be prepared to receive these additional resources, but SHALL function properly without them.", 0, java.lang.Integer.MAX_VALUE, revInclude); 2372 default: return super.getNamedProperty(_hash, _name, _checkValid); 2373 } 2374 2375 } 2376 2377 @Override 2378 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2379 switch (hash) { 2380 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // UriType 2381 case 1942574248: /*include*/ return this.include == null ? new Base[0] : this.include.toArray(new Base[this.include.size()]); // StringType 2382 case 8439429: /*revInclude*/ return this.revInclude == null ? new Base[0] : this.revInclude.toArray(new Base[this.revInclude.size()]); // StringType 2383 default: return super.getProperty(hash, name, checkValid); 2384 } 2385 2386 } 2387 2388 @Override 2389 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2390 switch (hash) { 2391 case -341064690: // resource 2392 this.resource = TypeConvertor.castToUri(value); // UriType 2393 return value; 2394 case 1942574248: // include 2395 this.getInclude().add(TypeConvertor.castToString(value)); // StringType 2396 return value; 2397 case 8439429: // revInclude 2398 this.getRevInclude().add(TypeConvertor.castToString(value)); // StringType 2399 return value; 2400 default: return super.setProperty(hash, name, value); 2401 } 2402 2403 } 2404 2405 @Override 2406 public Base setProperty(String name, Base value) throws FHIRException { 2407 if (name.equals("resource")) { 2408 this.resource = TypeConvertor.castToUri(value); // UriType 2409 } else if (name.equals("include")) { 2410 this.getInclude().add(TypeConvertor.castToString(value)); 2411 } else if (name.equals("revInclude")) { 2412 this.getRevInclude().add(TypeConvertor.castToString(value)); 2413 } else 2414 return super.setProperty(name, value); 2415 return value; 2416 } 2417 2418 @Override 2419 public void removeChild(String name, Base value) throws FHIRException { 2420 if (name.equals("resource")) { 2421 this.resource = null; 2422 } else if (name.equals("include")) { 2423 this.getInclude().remove(value); 2424 } else if (name.equals("revInclude")) { 2425 this.getRevInclude().remove(value); 2426 } else 2427 super.removeChild(name, value); 2428 2429 } 2430 2431 @Override 2432 public Base makeProperty(int hash, String name) throws FHIRException { 2433 switch (hash) { 2434 case -341064690: return getResourceElement(); 2435 case 1942574248: return addIncludeElement(); 2436 case 8439429: return addRevIncludeElement(); 2437 default: return super.makeProperty(hash, name); 2438 } 2439 2440 } 2441 2442 @Override 2443 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2444 switch (hash) { 2445 case -341064690: /*resource*/ return new String[] {"uri"}; 2446 case 1942574248: /*include*/ return new String[] {"string"}; 2447 case 8439429: /*revInclude*/ return new String[] {"string"}; 2448 default: return super.getTypesForProperty(hash, name); 2449 } 2450 2451 } 2452 2453 @Override 2454 public Base addChild(String name) throws FHIRException { 2455 if (name.equals("resource")) { 2456 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.notificationShape.resource"); 2457 } 2458 else if (name.equals("include")) { 2459 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.notificationShape.include"); 2460 } 2461 else if (name.equals("revInclude")) { 2462 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.notificationShape.revInclude"); 2463 } 2464 else 2465 return super.addChild(name); 2466 } 2467 2468 public SubscriptionTopicNotificationShapeComponent copy() { 2469 SubscriptionTopicNotificationShapeComponent dst = new SubscriptionTopicNotificationShapeComponent(); 2470 copyValues(dst); 2471 return dst; 2472 } 2473 2474 public void copyValues(SubscriptionTopicNotificationShapeComponent dst) { 2475 super.copyValues(dst); 2476 dst.resource = resource == null ? null : resource.copy(); 2477 if (include != null) { 2478 dst.include = new ArrayList<StringType>(); 2479 for (StringType i : include) 2480 dst.include.add(i.copy()); 2481 }; 2482 if (revInclude != null) { 2483 dst.revInclude = new ArrayList<StringType>(); 2484 for (StringType i : revInclude) 2485 dst.revInclude.add(i.copy()); 2486 }; 2487 } 2488 2489 @Override 2490 public boolean equalsDeep(Base other_) { 2491 if (!super.equalsDeep(other_)) 2492 return false; 2493 if (!(other_ instanceof SubscriptionTopicNotificationShapeComponent)) 2494 return false; 2495 SubscriptionTopicNotificationShapeComponent o = (SubscriptionTopicNotificationShapeComponent) other_; 2496 return compareDeep(resource, o.resource, true) && compareDeep(include, o.include, true) && compareDeep(revInclude, o.revInclude, true) 2497 ; 2498 } 2499 2500 @Override 2501 public boolean equalsShallow(Base other_) { 2502 if (!super.equalsShallow(other_)) 2503 return false; 2504 if (!(other_ instanceof SubscriptionTopicNotificationShapeComponent)) 2505 return false; 2506 SubscriptionTopicNotificationShapeComponent o = (SubscriptionTopicNotificationShapeComponent) other_; 2507 return compareValues(resource, o.resource, true) && compareValues(include, o.include, true) && compareValues(revInclude, o.revInclude, true) 2508 ; 2509 } 2510 2511 public boolean isEmpty() { 2512 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(resource, include, revInclude 2513 ); 2514 } 2515 2516 public String fhirType() { 2517 return "SubscriptionTopic.notificationShape"; 2518 2519 } 2520 2521 } 2522 2523 /** 2524 * An absolute URI that is used to identify this subscription topic when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this subscription topic is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the subscription topic is stored on different servers. 2525 */ 2526 @Child(name = "url", type = {UriType.class}, order=0, min=1, max=1, modifier=false, summary=true) 2527 @Description(shortDefinition="Canonical identifier for this subscription topic, represented as an absolute URI (globally unique)", formalDefinition="An absolute URI that is used to identify this subscription topic when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this subscription topic is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the subscription topic is stored on different servers." ) 2528 protected UriType url; 2529 2530 /** 2531 * Business identifiers assigned to this subscription topic by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server. 2532 */ 2533 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2534 @Description(shortDefinition="Business identifier for subscription topic", formalDefinition="Business identifiers assigned to this subscription topic by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server." ) 2535 protected List<Identifier> identifier; 2536 2537 /** 2538 * The identifier that is used to identify this version of the subscription topic when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Topic author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable. 2539 */ 2540 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2541 @Description(shortDefinition="Business version of the subscription topic", formalDefinition="The identifier that is used to identify this version of the subscription topic when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Topic author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable." ) 2542 protected StringType version; 2543 2544 /** 2545 * Indicates the mechanism used to compare versions to determine which is more current. 2546 */ 2547 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 2548 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 2549 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 2550 protected DataType versionAlgorithm; 2551 2552 /** 2553 * A natural language name identifying the subscription topic This name should be usable as an identifier for the module by machine processing applications such as code generation. 2554 */ 2555 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 2556 @Description(shortDefinition="Name for this subscription topic (computer friendly)", formalDefinition="A natural language name identifying the subscription topic This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 2557 protected StringType name; 2558 2559 /** 2560 * A short, descriptive, user-friendly title for the subscription topic. For example, "admission". 2561 */ 2562 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 2563 @Description(shortDefinition="Name for this subscription topic (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the subscription topic. For example, \"admission\"." ) 2564 protected StringType title; 2565 2566 /** 2567 * The canonical URL pointing to another FHIR-defined SubscriptionTopic that is adhered to in whole or in part by this SubscriptionTopic. 2568 */ 2569 @Child(name = "derivedFrom", type = {CanonicalType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2570 @Description(shortDefinition="Based on FHIR protocol or definition", formalDefinition="The canonical URL pointing to another FHIR-defined SubscriptionTopic that is adhered to in whole or in part by this SubscriptionTopic." ) 2571 protected List<CanonicalType> derivedFrom; 2572 2573 /** 2574 * The current state of the SubscriptionTopic. 2575 */ 2576 @Child(name = "status", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 2577 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The current state of the SubscriptionTopic." ) 2578 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 2579 protected Enumeration<PublicationStatus> status; 2580 2581 /** 2582 * A flag to indicate that this TopSubscriptionTopicic is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 2583 */ 2584 @Child(name = "experimental", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=true) 2585 @Description(shortDefinition="If for testing purposes, not real usage", formalDefinition="A flag to indicate that this TopSubscriptionTopicic is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage." ) 2586 protected BooleanType experimental; 2587 2588 /** 2589 * The date (and optionally time) when the subscription topic was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the subscription topic changes. 2590 */ 2591 @Child(name = "date", type = {DateTimeType.class}, order=9, min=0, max=1, modifier=false, summary=true) 2592 @Description(shortDefinition="Date status first applied", formalDefinition="The date (and optionally time) when the subscription topic was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the subscription topic changes." ) 2593 protected DateTimeType date; 2594 2595 /** 2596 * Helps establish the "authority/credibility" of the SubscriptionTopic. May also allow for contact. 2597 */ 2598 @Child(name = "publisher", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 2599 @Description(shortDefinition="The name of the individual or organization that published the SubscriptionTopic", formalDefinition="Helps establish the \"authority/credibility\" of the SubscriptionTopic. May also allow for contact." ) 2600 protected StringType publisher; 2601 2602 /** 2603 * Contact details to assist a user in finding and communicating with the publisher. 2604 */ 2605 @Child(name = "contact", type = {ContactDetail.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2606 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 2607 protected List<ContactDetail> contact; 2608 2609 /** 2610 * A free text natural language description of the Topic from the consumer's perspective. 2611 */ 2612 @Child(name = "description", type = {MarkdownType.class}, order=12, min=0, max=1, modifier=false, summary=false) 2613 @Description(shortDefinition="Natural language description of the SubscriptionTopic", formalDefinition="A free text natural language description of the Topic from the consumer's perspective." ) 2614 protected MarkdownType description; 2615 2616 /** 2617 * The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of code system definitions. 2618 */ 2619 @Child(name = "useContext", type = {UsageContext.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2620 @Description(shortDefinition="Content intends to support these contexts", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of code system definitions." ) 2621 protected List<UsageContext> useContext; 2622 2623 /** 2624 * A jurisdiction in which the Topic is intended to be used. 2625 */ 2626 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2627 @Description(shortDefinition="Intended jurisdiction of the SubscriptionTopic (if applicable)", formalDefinition="A jurisdiction in which the Topic is intended to be used." ) 2628 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 2629 protected List<CodeableConcept> jurisdiction; 2630 2631 /** 2632 * Explains why this Topic is needed and why it has been designed as it has. 2633 */ 2634 @Child(name = "purpose", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 2635 @Description(shortDefinition="Why this SubscriptionTopic is defined", formalDefinition="Explains why this Topic is needed and why it has been designed as it has." ) 2636 protected MarkdownType purpose; 2637 2638 /** 2639 * A copyright statement relating to the SubscriptionTopic and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SubscriptionTopic. 2640 */ 2641 @Child(name = "copyright", type = {MarkdownType.class}, order=16, min=0, max=1, modifier=false, summary=false) 2642 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the SubscriptionTopic and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SubscriptionTopic." ) 2643 protected MarkdownType copyright; 2644 2645 /** 2646 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2647 */ 2648 @Child(name = "copyrightLabel", type = {StringType.class}, order=17, min=0, max=1, modifier=false, summary=false) 2649 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 2650 protected StringType copyrightLabel; 2651 2652 /** 2653 * The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage. 2654 */ 2655 @Child(name = "approvalDate", type = {DateType.class}, order=18, min=0, max=1, modifier=false, summary=false) 2656 @Description(shortDefinition="When SubscriptionTopic is/was approved by publisher", formalDefinition="The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 2657 protected DateType approvalDate; 2658 2659 /** 2660 * The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date. 2661 */ 2662 @Child(name = "lastReviewDate", type = {DateType.class}, order=19, min=0, max=1, modifier=false, summary=false) 2663 @Description(shortDefinition="Date the Subscription Topic was last reviewed by the publisher", formalDefinition="The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date." ) 2664 protected DateType lastReviewDate; 2665 2666 /** 2667 * The period during which the SubscriptionTopic content was or is planned to be effective. 2668 */ 2669 @Child(name = "effectivePeriod", type = {Period.class}, order=20, min=0, max=1, modifier=false, summary=true) 2670 @Description(shortDefinition="The effective date range for the SubscriptionTopic", formalDefinition="The period during which the SubscriptionTopic content was or is planned to be effective." ) 2671 protected Period effectivePeriod; 2672 2673 /** 2674 * A definition of a resource-based event that triggers a notification based on the SubscriptionTopic. The criteria may be just a human readable description and/or a full FHIR search string or FHIRPath expression. Multiple triggers are considered OR joined (e.g., a resource update matching ANY of the definitions will trigger a notification). 2675 */ 2676 @Child(name = "resourceTrigger", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2677 @Description(shortDefinition="Definition of a resource-based trigger for the subscription topic", formalDefinition="A definition of a resource-based event that triggers a notification based on the SubscriptionTopic. The criteria may be just a human readable description and/or a full FHIR search string or FHIRPath expression. Multiple triggers are considered OR joined (e.g., a resource update matching ANY of the definitions will trigger a notification)." ) 2678 protected List<SubscriptionTopicResourceTriggerComponent> resourceTrigger; 2679 2680 /** 2681 * Event definition which can be used to trigger the SubscriptionTopic. 2682 */ 2683 @Child(name = "eventTrigger", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2684 @Description(shortDefinition="Event definitions the SubscriptionTopic", formalDefinition="Event definition which can be used to trigger the SubscriptionTopic." ) 2685 protected List<SubscriptionTopicEventTriggerComponent> eventTrigger; 2686 2687 /** 2688 * List of properties by which Subscriptions on the SubscriptionTopic can be filtered. May be defined Search Parameters (e.g., Encounter.patient) or parameters defined within this SubscriptionTopic context (e.g., hub.event). 2689 */ 2690 @Child(name = "canFilterBy", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2691 @Description(shortDefinition="Properties by which a Subscription can filter notifications from the SubscriptionTopic", formalDefinition="List of properties by which Subscriptions on the SubscriptionTopic can be filtered. May be defined Search Parameters (e.g., Encounter.patient) or parameters defined within this SubscriptionTopic context (e.g., hub.event)." ) 2692 protected List<SubscriptionTopicCanFilterByComponent> canFilterBy; 2693 2694 /** 2695 * List of properties to describe the shape (e.g., resources) included in notifications from this Subscription Topic. 2696 */ 2697 @Child(name = "notificationShape", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2698 @Description(shortDefinition="Properties for describing the shape of notifications generated by this topic", formalDefinition="List of properties to describe the shape (e.g., resources) included in notifications from this Subscription Topic." ) 2699 protected List<SubscriptionTopicNotificationShapeComponent> notificationShape; 2700 2701 private static final long serialVersionUID = -194061063L; 2702 2703 /** 2704 * Constructor 2705 */ 2706 public SubscriptionTopic() { 2707 super(); 2708 } 2709 2710 /** 2711 * Constructor 2712 */ 2713 public SubscriptionTopic(String url, PublicationStatus status) { 2714 super(); 2715 this.setUrl(url); 2716 this.setStatus(status); 2717 } 2718 2719 /** 2720 * @return {@link #url} (An absolute URI that is used to identify this subscription topic when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this subscription topic is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the subscription topic is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2721 */ 2722 public UriType getUrlElement() { 2723 if (this.url == null) 2724 if (Configuration.errorOnAutoCreate()) 2725 throw new Error("Attempt to auto-create SubscriptionTopic.url"); 2726 else if (Configuration.doAutoCreate()) 2727 this.url = new UriType(); // bb 2728 return this.url; 2729 } 2730 2731 public boolean hasUrlElement() { 2732 return this.url != null && !this.url.isEmpty(); 2733 } 2734 2735 public boolean hasUrl() { 2736 return this.url != null && !this.url.isEmpty(); 2737 } 2738 2739 /** 2740 * @param value {@link #url} (An absolute URI that is used to identify this subscription topic when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this subscription topic is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the subscription topic is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2741 */ 2742 public SubscriptionTopic setUrlElement(UriType value) { 2743 this.url = value; 2744 return this; 2745 } 2746 2747 /** 2748 * @return An absolute URI that is used to identify this subscription topic when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this subscription topic is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the subscription topic is stored on different servers. 2749 */ 2750 public String getUrl() { 2751 return this.url == null ? null : this.url.getValue(); 2752 } 2753 2754 /** 2755 * @param value An absolute URI that is used to identify this subscription topic when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this subscription topic is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the subscription topic is stored on different servers. 2756 */ 2757 public SubscriptionTopic setUrl(String value) { 2758 if (this.url == null) 2759 this.url = new UriType(); 2760 this.url.setValue(value); 2761 return this; 2762 } 2763 2764 /** 2765 * @return {@link #identifier} (Business identifiers assigned to this subscription topic by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.) 2766 */ 2767 public List<Identifier> getIdentifier() { 2768 if (this.identifier == null) 2769 this.identifier = new ArrayList<Identifier>(); 2770 return this.identifier; 2771 } 2772 2773 /** 2774 * @return Returns a reference to <code>this</code> for easy method chaining 2775 */ 2776 public SubscriptionTopic setIdentifier(List<Identifier> theIdentifier) { 2777 this.identifier = theIdentifier; 2778 return this; 2779 } 2780 2781 public boolean hasIdentifier() { 2782 if (this.identifier == null) 2783 return false; 2784 for (Identifier item : this.identifier) 2785 if (!item.isEmpty()) 2786 return true; 2787 return false; 2788 } 2789 2790 public Identifier addIdentifier() { //3 2791 Identifier t = new Identifier(); 2792 if (this.identifier == null) 2793 this.identifier = new ArrayList<Identifier>(); 2794 this.identifier.add(t); 2795 return t; 2796 } 2797 2798 public SubscriptionTopic addIdentifier(Identifier t) { //3 2799 if (t == null) 2800 return this; 2801 if (this.identifier == null) 2802 this.identifier = new ArrayList<Identifier>(); 2803 this.identifier.add(t); 2804 return this; 2805 } 2806 2807 /** 2808 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2809 */ 2810 public Identifier getIdentifierFirstRep() { 2811 if (getIdentifier().isEmpty()) { 2812 addIdentifier(); 2813 } 2814 return getIdentifier().get(0); 2815 } 2816 2817 /** 2818 * @return {@link #version} (The identifier that is used to identify this version of the subscription topic when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Topic author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2819 */ 2820 public StringType getVersionElement() { 2821 if (this.version == null) 2822 if (Configuration.errorOnAutoCreate()) 2823 throw new Error("Attempt to auto-create SubscriptionTopic.version"); 2824 else if (Configuration.doAutoCreate()) 2825 this.version = new StringType(); // bb 2826 return this.version; 2827 } 2828 2829 public boolean hasVersionElement() { 2830 return this.version != null && !this.version.isEmpty(); 2831 } 2832 2833 public boolean hasVersion() { 2834 return this.version != null && !this.version.isEmpty(); 2835 } 2836 2837 /** 2838 * @param value {@link #version} (The identifier that is used to identify this version of the subscription topic when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Topic author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2839 */ 2840 public SubscriptionTopic setVersionElement(StringType value) { 2841 this.version = value; 2842 return this; 2843 } 2844 2845 /** 2846 * @return The identifier that is used to identify this version of the subscription topic when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Topic author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable. 2847 */ 2848 public String getVersion() { 2849 return this.version == null ? null : this.version.getValue(); 2850 } 2851 2852 /** 2853 * @param value The identifier that is used to identify this version of the subscription topic when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Topic author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable. 2854 */ 2855 public SubscriptionTopic setVersion(String value) { 2856 if (Utilities.noString(value)) 2857 this.version = null; 2858 else { 2859 if (this.version == null) 2860 this.version = new StringType(); 2861 this.version.setValue(value); 2862 } 2863 return this; 2864 } 2865 2866 /** 2867 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2868 */ 2869 public DataType getVersionAlgorithm() { 2870 return this.versionAlgorithm; 2871 } 2872 2873 /** 2874 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2875 */ 2876 public StringType getVersionAlgorithmStringType() throws FHIRException { 2877 if (this.versionAlgorithm == null) 2878 this.versionAlgorithm = new StringType(); 2879 if (!(this.versionAlgorithm instanceof StringType)) 2880 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 2881 return (StringType) this.versionAlgorithm; 2882 } 2883 2884 public boolean hasVersionAlgorithmStringType() { 2885 return this.versionAlgorithm instanceof StringType; 2886 } 2887 2888 /** 2889 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2890 */ 2891 public Coding getVersionAlgorithmCoding() throws FHIRException { 2892 if (this.versionAlgorithm == null) 2893 this.versionAlgorithm = new Coding(); 2894 if (!(this.versionAlgorithm instanceof Coding)) 2895 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 2896 return (Coding) this.versionAlgorithm; 2897 } 2898 2899 public boolean hasVersionAlgorithmCoding() { 2900 return this.versionAlgorithm instanceof Coding; 2901 } 2902 2903 public boolean hasVersionAlgorithm() { 2904 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 2905 } 2906 2907 /** 2908 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2909 */ 2910 public SubscriptionTopic setVersionAlgorithm(DataType value) { 2911 if (value != null && !(value instanceof StringType || value instanceof Coding)) 2912 throw new FHIRException("Not the right type for SubscriptionTopic.versionAlgorithm[x]: "+value.fhirType()); 2913 this.versionAlgorithm = value; 2914 return this; 2915 } 2916 2917 /** 2918 * @return {@link #name} (A natural language name identifying the subscription topic This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2919 */ 2920 public StringType getNameElement() { 2921 if (this.name == null) 2922 if (Configuration.errorOnAutoCreate()) 2923 throw new Error("Attempt to auto-create SubscriptionTopic.name"); 2924 else if (Configuration.doAutoCreate()) 2925 this.name = new StringType(); // bb 2926 return this.name; 2927 } 2928 2929 public boolean hasNameElement() { 2930 return this.name != null && !this.name.isEmpty(); 2931 } 2932 2933 public boolean hasName() { 2934 return this.name != null && !this.name.isEmpty(); 2935 } 2936 2937 /** 2938 * @param value {@link #name} (A natural language name identifying the subscription topic This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2939 */ 2940 public SubscriptionTopic setNameElement(StringType value) { 2941 this.name = value; 2942 return this; 2943 } 2944 2945 /** 2946 * @return A natural language name identifying the subscription topic This name should be usable as an identifier for the module by machine processing applications such as code generation. 2947 */ 2948 public String getName() { 2949 return this.name == null ? null : this.name.getValue(); 2950 } 2951 2952 /** 2953 * @param value A natural language name identifying the subscription topic This name should be usable as an identifier for the module by machine processing applications such as code generation. 2954 */ 2955 public SubscriptionTopic setName(String value) { 2956 if (Utilities.noString(value)) 2957 this.name = null; 2958 else { 2959 if (this.name == null) 2960 this.name = new StringType(); 2961 this.name.setValue(value); 2962 } 2963 return this; 2964 } 2965 2966 /** 2967 * @return {@link #title} (A short, descriptive, user-friendly title for the subscription topic. For example, "admission".). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2968 */ 2969 public StringType getTitleElement() { 2970 if (this.title == null) 2971 if (Configuration.errorOnAutoCreate()) 2972 throw new Error("Attempt to auto-create SubscriptionTopic.title"); 2973 else if (Configuration.doAutoCreate()) 2974 this.title = new StringType(); // bb 2975 return this.title; 2976 } 2977 2978 public boolean hasTitleElement() { 2979 return this.title != null && !this.title.isEmpty(); 2980 } 2981 2982 public boolean hasTitle() { 2983 return this.title != null && !this.title.isEmpty(); 2984 } 2985 2986 /** 2987 * @param value {@link #title} (A short, descriptive, user-friendly title for the subscription topic. For example, "admission".). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2988 */ 2989 public SubscriptionTopic setTitleElement(StringType value) { 2990 this.title = value; 2991 return this; 2992 } 2993 2994 /** 2995 * @return A short, descriptive, user-friendly title for the subscription topic. For example, "admission". 2996 */ 2997 public String getTitle() { 2998 return this.title == null ? null : this.title.getValue(); 2999 } 3000 3001 /** 3002 * @param value A short, descriptive, user-friendly title for the subscription topic. For example, "admission". 3003 */ 3004 public SubscriptionTopic setTitle(String value) { 3005 if (Utilities.noString(value)) 3006 this.title = null; 3007 else { 3008 if (this.title == null) 3009 this.title = new StringType(); 3010 this.title.setValue(value); 3011 } 3012 return this; 3013 } 3014 3015 /** 3016 * @return {@link #derivedFrom} (The canonical URL pointing to another FHIR-defined SubscriptionTopic that is adhered to in whole or in part by this SubscriptionTopic.) 3017 */ 3018 public List<CanonicalType> getDerivedFrom() { 3019 if (this.derivedFrom == null) 3020 this.derivedFrom = new ArrayList<CanonicalType>(); 3021 return this.derivedFrom; 3022 } 3023 3024 /** 3025 * @return Returns a reference to <code>this</code> for easy method chaining 3026 */ 3027 public SubscriptionTopic setDerivedFrom(List<CanonicalType> theDerivedFrom) { 3028 this.derivedFrom = theDerivedFrom; 3029 return this; 3030 } 3031 3032 public boolean hasDerivedFrom() { 3033 if (this.derivedFrom == null) 3034 return false; 3035 for (CanonicalType item : this.derivedFrom) 3036 if (!item.isEmpty()) 3037 return true; 3038 return false; 3039 } 3040 3041 /** 3042 * @return {@link #derivedFrom} (The canonical URL pointing to another FHIR-defined SubscriptionTopic that is adhered to in whole or in part by this SubscriptionTopic.) 3043 */ 3044 public CanonicalType addDerivedFromElement() {//2 3045 CanonicalType t = new CanonicalType(); 3046 if (this.derivedFrom == null) 3047 this.derivedFrom = new ArrayList<CanonicalType>(); 3048 this.derivedFrom.add(t); 3049 return t; 3050 } 3051 3052 /** 3053 * @param value {@link #derivedFrom} (The canonical URL pointing to another FHIR-defined SubscriptionTopic that is adhered to in whole or in part by this SubscriptionTopic.) 3054 */ 3055 public SubscriptionTopic addDerivedFrom(String value) { //1 3056 CanonicalType t = new CanonicalType(); 3057 t.setValue(value); 3058 if (this.derivedFrom == null) 3059 this.derivedFrom = new ArrayList<CanonicalType>(); 3060 this.derivedFrom.add(t); 3061 return this; 3062 } 3063 3064 /** 3065 * @param value {@link #derivedFrom} (The canonical URL pointing to another FHIR-defined SubscriptionTopic that is adhered to in whole or in part by this SubscriptionTopic.) 3066 */ 3067 public boolean hasDerivedFrom(String value) { 3068 if (this.derivedFrom == null) 3069 return false; 3070 for (CanonicalType v : this.derivedFrom) 3071 if (v.getValue().equals(value)) // canonical 3072 return true; 3073 return false; 3074 } 3075 3076 /** 3077 * @return {@link #status} (The current state of the SubscriptionTopic.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3078 */ 3079 public Enumeration<PublicationStatus> getStatusElement() { 3080 if (this.status == null) 3081 if (Configuration.errorOnAutoCreate()) 3082 throw new Error("Attempt to auto-create SubscriptionTopic.status"); 3083 else if (Configuration.doAutoCreate()) 3084 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 3085 return this.status; 3086 } 3087 3088 public boolean hasStatusElement() { 3089 return this.status != null && !this.status.isEmpty(); 3090 } 3091 3092 public boolean hasStatus() { 3093 return this.status != null && !this.status.isEmpty(); 3094 } 3095 3096 /** 3097 * @param value {@link #status} (The current state of the SubscriptionTopic.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3098 */ 3099 public SubscriptionTopic setStatusElement(Enumeration<PublicationStatus> value) { 3100 this.status = value; 3101 return this; 3102 } 3103 3104 /** 3105 * @return The current state of the SubscriptionTopic. 3106 */ 3107 public PublicationStatus getStatus() { 3108 return this.status == null ? null : this.status.getValue(); 3109 } 3110 3111 /** 3112 * @param value The current state of the SubscriptionTopic. 3113 */ 3114 public SubscriptionTopic setStatus(PublicationStatus value) { 3115 if (this.status == null) 3116 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 3117 this.status.setValue(value); 3118 return this; 3119 } 3120 3121 /** 3122 * @return {@link #experimental} (A flag to indicate that this TopSubscriptionTopicic is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3123 */ 3124 public BooleanType getExperimentalElement() { 3125 if (this.experimental == null) 3126 if (Configuration.errorOnAutoCreate()) 3127 throw new Error("Attempt to auto-create SubscriptionTopic.experimental"); 3128 else if (Configuration.doAutoCreate()) 3129 this.experimental = new BooleanType(); // bb 3130 return this.experimental; 3131 } 3132 3133 public boolean hasExperimentalElement() { 3134 return this.experimental != null && !this.experimental.isEmpty(); 3135 } 3136 3137 public boolean hasExperimental() { 3138 return this.experimental != null && !this.experimental.isEmpty(); 3139 } 3140 3141 /** 3142 * @param value {@link #experimental} (A flag to indicate that this TopSubscriptionTopicic is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3143 */ 3144 public SubscriptionTopic setExperimentalElement(BooleanType value) { 3145 this.experimental = value; 3146 return this; 3147 } 3148 3149 /** 3150 * @return A flag to indicate that this TopSubscriptionTopicic is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 3151 */ 3152 public boolean getExperimental() { 3153 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 3154 } 3155 3156 /** 3157 * @param value A flag to indicate that this TopSubscriptionTopicic is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 3158 */ 3159 public SubscriptionTopic setExperimental(boolean value) { 3160 if (this.experimental == null) 3161 this.experimental = new BooleanType(); 3162 this.experimental.setValue(value); 3163 return this; 3164 } 3165 3166 /** 3167 * @return {@link #date} (The date (and optionally time) when the subscription topic was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the subscription topic changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3168 */ 3169 public DateTimeType getDateElement() { 3170 if (this.date == null) 3171 if (Configuration.errorOnAutoCreate()) 3172 throw new Error("Attempt to auto-create SubscriptionTopic.date"); 3173 else if (Configuration.doAutoCreate()) 3174 this.date = new DateTimeType(); // bb 3175 return this.date; 3176 } 3177 3178 public boolean hasDateElement() { 3179 return this.date != null && !this.date.isEmpty(); 3180 } 3181 3182 public boolean hasDate() { 3183 return this.date != null && !this.date.isEmpty(); 3184 } 3185 3186 /** 3187 * @param value {@link #date} (The date (and optionally time) when the subscription topic was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the subscription topic changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3188 */ 3189 public SubscriptionTopic setDateElement(DateTimeType value) { 3190 this.date = value; 3191 return this; 3192 } 3193 3194 /** 3195 * @return The date (and optionally time) when the subscription topic was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the subscription topic changes. 3196 */ 3197 public Date getDate() { 3198 return this.date == null ? null : this.date.getValue(); 3199 } 3200 3201 /** 3202 * @param value The date (and optionally time) when the subscription topic was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the subscription topic changes. 3203 */ 3204 public SubscriptionTopic setDate(Date value) { 3205 if (value == null) 3206 this.date = null; 3207 else { 3208 if (this.date == null) 3209 this.date = new DateTimeType(); 3210 this.date.setValue(value); 3211 } 3212 return this; 3213 } 3214 3215 /** 3216 * @return {@link #publisher} (Helps establish the "authority/credibility" of the SubscriptionTopic. May also allow for contact.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3217 */ 3218 public StringType getPublisherElement() { 3219 if (this.publisher == null) 3220 if (Configuration.errorOnAutoCreate()) 3221 throw new Error("Attempt to auto-create SubscriptionTopic.publisher"); 3222 else if (Configuration.doAutoCreate()) 3223 this.publisher = new StringType(); // bb 3224 return this.publisher; 3225 } 3226 3227 public boolean hasPublisherElement() { 3228 return this.publisher != null && !this.publisher.isEmpty(); 3229 } 3230 3231 public boolean hasPublisher() { 3232 return this.publisher != null && !this.publisher.isEmpty(); 3233 } 3234 3235 /** 3236 * @param value {@link #publisher} (Helps establish the "authority/credibility" of the SubscriptionTopic. May also allow for contact.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3237 */ 3238 public SubscriptionTopic setPublisherElement(StringType value) { 3239 this.publisher = value; 3240 return this; 3241 } 3242 3243 /** 3244 * @return Helps establish the "authority/credibility" of the SubscriptionTopic. May also allow for contact. 3245 */ 3246 public String getPublisher() { 3247 return this.publisher == null ? null : this.publisher.getValue(); 3248 } 3249 3250 /** 3251 * @param value Helps establish the "authority/credibility" of the SubscriptionTopic. May also allow for contact. 3252 */ 3253 public SubscriptionTopic setPublisher(String value) { 3254 if (Utilities.noString(value)) 3255 this.publisher = null; 3256 else { 3257 if (this.publisher == null) 3258 this.publisher = new StringType(); 3259 this.publisher.setValue(value); 3260 } 3261 return this; 3262 } 3263 3264 /** 3265 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 3266 */ 3267 public List<ContactDetail> getContact() { 3268 if (this.contact == null) 3269 this.contact = new ArrayList<ContactDetail>(); 3270 return this.contact; 3271 } 3272 3273 /** 3274 * @return Returns a reference to <code>this</code> for easy method chaining 3275 */ 3276 public SubscriptionTopic setContact(List<ContactDetail> theContact) { 3277 this.contact = theContact; 3278 return this; 3279 } 3280 3281 public boolean hasContact() { 3282 if (this.contact == null) 3283 return false; 3284 for (ContactDetail item : this.contact) 3285 if (!item.isEmpty()) 3286 return true; 3287 return false; 3288 } 3289 3290 public ContactDetail addContact() { //3 3291 ContactDetail t = new ContactDetail(); 3292 if (this.contact == null) 3293 this.contact = new ArrayList<ContactDetail>(); 3294 this.contact.add(t); 3295 return t; 3296 } 3297 3298 public SubscriptionTopic addContact(ContactDetail t) { //3 3299 if (t == null) 3300 return this; 3301 if (this.contact == null) 3302 this.contact = new ArrayList<ContactDetail>(); 3303 this.contact.add(t); 3304 return this; 3305 } 3306 3307 /** 3308 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 3309 */ 3310 public ContactDetail getContactFirstRep() { 3311 if (getContact().isEmpty()) { 3312 addContact(); 3313 } 3314 return getContact().get(0); 3315 } 3316 3317 /** 3318 * @return {@link #description} (A free text natural language description of the Topic from the consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3319 */ 3320 public MarkdownType getDescriptionElement() { 3321 if (this.description == null) 3322 if (Configuration.errorOnAutoCreate()) 3323 throw new Error("Attempt to auto-create SubscriptionTopic.description"); 3324 else if (Configuration.doAutoCreate()) 3325 this.description = new MarkdownType(); // bb 3326 return this.description; 3327 } 3328 3329 public boolean hasDescriptionElement() { 3330 return this.description != null && !this.description.isEmpty(); 3331 } 3332 3333 public boolean hasDescription() { 3334 return this.description != null && !this.description.isEmpty(); 3335 } 3336 3337 /** 3338 * @param value {@link #description} (A free text natural language description of the Topic from the consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3339 */ 3340 public SubscriptionTopic setDescriptionElement(MarkdownType value) { 3341 this.description = value; 3342 return this; 3343 } 3344 3345 /** 3346 * @return A free text natural language description of the Topic from the consumer's perspective. 3347 */ 3348 public String getDescription() { 3349 return this.description == null ? null : this.description.getValue(); 3350 } 3351 3352 /** 3353 * @param value A free text natural language description of the Topic from the consumer's perspective. 3354 */ 3355 public SubscriptionTopic setDescription(String value) { 3356 if (Utilities.noString(value)) 3357 this.description = null; 3358 else { 3359 if (this.description == null) 3360 this.description = new MarkdownType(); 3361 this.description.setValue(value); 3362 } 3363 return this; 3364 } 3365 3366 /** 3367 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of code system definitions.) 3368 */ 3369 public List<UsageContext> getUseContext() { 3370 if (this.useContext == null) 3371 this.useContext = new ArrayList<UsageContext>(); 3372 return this.useContext; 3373 } 3374 3375 /** 3376 * @return Returns a reference to <code>this</code> for easy method chaining 3377 */ 3378 public SubscriptionTopic setUseContext(List<UsageContext> theUseContext) { 3379 this.useContext = theUseContext; 3380 return this; 3381 } 3382 3383 public boolean hasUseContext() { 3384 if (this.useContext == null) 3385 return false; 3386 for (UsageContext item : this.useContext) 3387 if (!item.isEmpty()) 3388 return true; 3389 return false; 3390 } 3391 3392 public UsageContext addUseContext() { //3 3393 UsageContext t = new UsageContext(); 3394 if (this.useContext == null) 3395 this.useContext = new ArrayList<UsageContext>(); 3396 this.useContext.add(t); 3397 return t; 3398 } 3399 3400 public SubscriptionTopic addUseContext(UsageContext t) { //3 3401 if (t == null) 3402 return this; 3403 if (this.useContext == null) 3404 this.useContext = new ArrayList<UsageContext>(); 3405 this.useContext.add(t); 3406 return this; 3407 } 3408 3409 /** 3410 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 3411 */ 3412 public UsageContext getUseContextFirstRep() { 3413 if (getUseContext().isEmpty()) { 3414 addUseContext(); 3415 } 3416 return getUseContext().get(0); 3417 } 3418 3419 /** 3420 * @return {@link #jurisdiction} (A jurisdiction in which the Topic is intended to be used.) 3421 */ 3422 public List<CodeableConcept> getJurisdiction() { 3423 if (this.jurisdiction == null) 3424 this.jurisdiction = new ArrayList<CodeableConcept>(); 3425 return this.jurisdiction; 3426 } 3427 3428 /** 3429 * @return Returns a reference to <code>this</code> for easy method chaining 3430 */ 3431 public SubscriptionTopic setJurisdiction(List<CodeableConcept> theJurisdiction) { 3432 this.jurisdiction = theJurisdiction; 3433 return this; 3434 } 3435 3436 public boolean hasJurisdiction() { 3437 if (this.jurisdiction == null) 3438 return false; 3439 for (CodeableConcept item : this.jurisdiction) 3440 if (!item.isEmpty()) 3441 return true; 3442 return false; 3443 } 3444 3445 public CodeableConcept addJurisdiction() { //3 3446 CodeableConcept t = new CodeableConcept(); 3447 if (this.jurisdiction == null) 3448 this.jurisdiction = new ArrayList<CodeableConcept>(); 3449 this.jurisdiction.add(t); 3450 return t; 3451 } 3452 3453 public SubscriptionTopic addJurisdiction(CodeableConcept t) { //3 3454 if (t == null) 3455 return this; 3456 if (this.jurisdiction == null) 3457 this.jurisdiction = new ArrayList<CodeableConcept>(); 3458 this.jurisdiction.add(t); 3459 return this; 3460 } 3461 3462 /** 3463 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 3464 */ 3465 public CodeableConcept getJurisdictionFirstRep() { 3466 if (getJurisdiction().isEmpty()) { 3467 addJurisdiction(); 3468 } 3469 return getJurisdiction().get(0); 3470 } 3471 3472 /** 3473 * @return {@link #purpose} (Explains why this Topic is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3474 */ 3475 public MarkdownType getPurposeElement() { 3476 if (this.purpose == null) 3477 if (Configuration.errorOnAutoCreate()) 3478 throw new Error("Attempt to auto-create SubscriptionTopic.purpose"); 3479 else if (Configuration.doAutoCreate()) 3480 this.purpose = new MarkdownType(); // bb 3481 return this.purpose; 3482 } 3483 3484 public boolean hasPurposeElement() { 3485 return this.purpose != null && !this.purpose.isEmpty(); 3486 } 3487 3488 public boolean hasPurpose() { 3489 return this.purpose != null && !this.purpose.isEmpty(); 3490 } 3491 3492 /** 3493 * @param value {@link #purpose} (Explains why this Topic is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3494 */ 3495 public SubscriptionTopic setPurposeElement(MarkdownType value) { 3496 this.purpose = value; 3497 return this; 3498 } 3499 3500 /** 3501 * @return Explains why this Topic is needed and why it has been designed as it has. 3502 */ 3503 public String getPurpose() { 3504 return this.purpose == null ? null : this.purpose.getValue(); 3505 } 3506 3507 /** 3508 * @param value Explains why this Topic is needed and why it has been designed as it has. 3509 */ 3510 public SubscriptionTopic setPurpose(String value) { 3511 if (Utilities.noString(value)) 3512 this.purpose = null; 3513 else { 3514 if (this.purpose == null) 3515 this.purpose = new MarkdownType(); 3516 this.purpose.setValue(value); 3517 } 3518 return this; 3519 } 3520 3521 /** 3522 * @return {@link #copyright} (A copyright statement relating to the SubscriptionTopic and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SubscriptionTopic.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3523 */ 3524 public MarkdownType getCopyrightElement() { 3525 if (this.copyright == null) 3526 if (Configuration.errorOnAutoCreate()) 3527 throw new Error("Attempt to auto-create SubscriptionTopic.copyright"); 3528 else if (Configuration.doAutoCreate()) 3529 this.copyright = new MarkdownType(); // bb 3530 return this.copyright; 3531 } 3532 3533 public boolean hasCopyrightElement() { 3534 return this.copyright != null && !this.copyright.isEmpty(); 3535 } 3536 3537 public boolean hasCopyright() { 3538 return this.copyright != null && !this.copyright.isEmpty(); 3539 } 3540 3541 /** 3542 * @param value {@link #copyright} (A copyright statement relating to the SubscriptionTopic and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SubscriptionTopic.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3543 */ 3544 public SubscriptionTopic setCopyrightElement(MarkdownType value) { 3545 this.copyright = value; 3546 return this; 3547 } 3548 3549 /** 3550 * @return A copyright statement relating to the SubscriptionTopic and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SubscriptionTopic. 3551 */ 3552 public String getCopyright() { 3553 return this.copyright == null ? null : this.copyright.getValue(); 3554 } 3555 3556 /** 3557 * @param value A copyright statement relating to the SubscriptionTopic and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SubscriptionTopic. 3558 */ 3559 public SubscriptionTopic setCopyright(String value) { 3560 if (Utilities.noString(value)) 3561 this.copyright = null; 3562 else { 3563 if (this.copyright == null) 3564 this.copyright = new MarkdownType(); 3565 this.copyright.setValue(value); 3566 } 3567 return this; 3568 } 3569 3570 /** 3571 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 3572 */ 3573 public StringType getCopyrightLabelElement() { 3574 if (this.copyrightLabel == null) 3575 if (Configuration.errorOnAutoCreate()) 3576 throw new Error("Attempt to auto-create SubscriptionTopic.copyrightLabel"); 3577 else if (Configuration.doAutoCreate()) 3578 this.copyrightLabel = new StringType(); // bb 3579 return this.copyrightLabel; 3580 } 3581 3582 public boolean hasCopyrightLabelElement() { 3583 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 3584 } 3585 3586 public boolean hasCopyrightLabel() { 3587 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 3588 } 3589 3590 /** 3591 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 3592 */ 3593 public SubscriptionTopic setCopyrightLabelElement(StringType value) { 3594 this.copyrightLabel = value; 3595 return this; 3596 } 3597 3598 /** 3599 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3600 */ 3601 public String getCopyrightLabel() { 3602 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 3603 } 3604 3605 /** 3606 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3607 */ 3608 public SubscriptionTopic setCopyrightLabel(String value) { 3609 if (Utilities.noString(value)) 3610 this.copyrightLabel = null; 3611 else { 3612 if (this.copyrightLabel == null) 3613 this.copyrightLabel = new StringType(); 3614 this.copyrightLabel.setValue(value); 3615 } 3616 return this; 3617 } 3618 3619 /** 3620 * @return {@link #approvalDate} (The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 3621 */ 3622 public DateType getApprovalDateElement() { 3623 if (this.approvalDate == null) 3624 if (Configuration.errorOnAutoCreate()) 3625 throw new Error("Attempt to auto-create SubscriptionTopic.approvalDate"); 3626 else if (Configuration.doAutoCreate()) 3627 this.approvalDate = new DateType(); // bb 3628 return this.approvalDate; 3629 } 3630 3631 public boolean hasApprovalDateElement() { 3632 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3633 } 3634 3635 public boolean hasApprovalDate() { 3636 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3637 } 3638 3639 /** 3640 * @param value {@link #approvalDate} (The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 3641 */ 3642 public SubscriptionTopic setApprovalDateElement(DateType value) { 3643 this.approvalDate = value; 3644 return this; 3645 } 3646 3647 /** 3648 * @return The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3649 */ 3650 public Date getApprovalDate() { 3651 return this.approvalDate == null ? null : this.approvalDate.getValue(); 3652 } 3653 3654 /** 3655 * @param value The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3656 */ 3657 public SubscriptionTopic setApprovalDate(Date value) { 3658 if (value == null) 3659 this.approvalDate = null; 3660 else { 3661 if (this.approvalDate == null) 3662 this.approvalDate = new DateType(); 3663 this.approvalDate.setValue(value); 3664 } 3665 return this; 3666 } 3667 3668 /** 3669 * @return {@link #lastReviewDate} (The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 3670 */ 3671 public DateType getLastReviewDateElement() { 3672 if (this.lastReviewDate == null) 3673 if (Configuration.errorOnAutoCreate()) 3674 throw new Error("Attempt to auto-create SubscriptionTopic.lastReviewDate"); 3675 else if (Configuration.doAutoCreate()) 3676 this.lastReviewDate = new DateType(); // bb 3677 return this.lastReviewDate; 3678 } 3679 3680 public boolean hasLastReviewDateElement() { 3681 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3682 } 3683 3684 public boolean hasLastReviewDate() { 3685 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3686 } 3687 3688 /** 3689 * @param value {@link #lastReviewDate} (The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 3690 */ 3691 public SubscriptionTopic setLastReviewDateElement(DateType value) { 3692 this.lastReviewDate = value; 3693 return this; 3694 } 3695 3696 /** 3697 * @return The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date. 3698 */ 3699 public Date getLastReviewDate() { 3700 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 3701 } 3702 3703 /** 3704 * @param value The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date. 3705 */ 3706 public SubscriptionTopic setLastReviewDate(Date value) { 3707 if (value == null) 3708 this.lastReviewDate = null; 3709 else { 3710 if (this.lastReviewDate == null) 3711 this.lastReviewDate = new DateType(); 3712 this.lastReviewDate.setValue(value); 3713 } 3714 return this; 3715 } 3716 3717 /** 3718 * @return {@link #effectivePeriod} (The period during which the SubscriptionTopic content was or is planned to be effective.) 3719 */ 3720 public Period getEffectivePeriod() { 3721 if (this.effectivePeriod == null) 3722 if (Configuration.errorOnAutoCreate()) 3723 throw new Error("Attempt to auto-create SubscriptionTopic.effectivePeriod"); 3724 else if (Configuration.doAutoCreate()) 3725 this.effectivePeriod = new Period(); // cc 3726 return this.effectivePeriod; 3727 } 3728 3729 public boolean hasEffectivePeriod() { 3730 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 3731 } 3732 3733 /** 3734 * @param value {@link #effectivePeriod} (The period during which the SubscriptionTopic content was or is planned to be effective.) 3735 */ 3736 public SubscriptionTopic setEffectivePeriod(Period value) { 3737 this.effectivePeriod = value; 3738 return this; 3739 } 3740 3741 /** 3742 * @return {@link #resourceTrigger} (A definition of a resource-based event that triggers a notification based on the SubscriptionTopic. The criteria may be just a human readable description and/or a full FHIR search string or FHIRPath expression. Multiple triggers are considered OR joined (e.g., a resource update matching ANY of the definitions will trigger a notification).) 3743 */ 3744 public List<SubscriptionTopicResourceTriggerComponent> getResourceTrigger() { 3745 if (this.resourceTrigger == null) 3746 this.resourceTrigger = new ArrayList<SubscriptionTopicResourceTriggerComponent>(); 3747 return this.resourceTrigger; 3748 } 3749 3750 /** 3751 * @return Returns a reference to <code>this</code> for easy method chaining 3752 */ 3753 public SubscriptionTopic setResourceTrigger(List<SubscriptionTopicResourceTriggerComponent> theResourceTrigger) { 3754 this.resourceTrigger = theResourceTrigger; 3755 return this; 3756 } 3757 3758 public boolean hasResourceTrigger() { 3759 if (this.resourceTrigger == null) 3760 return false; 3761 for (SubscriptionTopicResourceTriggerComponent item : this.resourceTrigger) 3762 if (!item.isEmpty()) 3763 return true; 3764 return false; 3765 } 3766 3767 public SubscriptionTopicResourceTriggerComponent addResourceTrigger() { //3 3768 SubscriptionTopicResourceTriggerComponent t = new SubscriptionTopicResourceTriggerComponent(); 3769 if (this.resourceTrigger == null) 3770 this.resourceTrigger = new ArrayList<SubscriptionTopicResourceTriggerComponent>(); 3771 this.resourceTrigger.add(t); 3772 return t; 3773 } 3774 3775 public SubscriptionTopic addResourceTrigger(SubscriptionTopicResourceTriggerComponent t) { //3 3776 if (t == null) 3777 return this; 3778 if (this.resourceTrigger == null) 3779 this.resourceTrigger = new ArrayList<SubscriptionTopicResourceTriggerComponent>(); 3780 this.resourceTrigger.add(t); 3781 return this; 3782 } 3783 3784 /** 3785 * @return The first repetition of repeating field {@link #resourceTrigger}, creating it if it does not already exist {3} 3786 */ 3787 public SubscriptionTopicResourceTriggerComponent getResourceTriggerFirstRep() { 3788 if (getResourceTrigger().isEmpty()) { 3789 addResourceTrigger(); 3790 } 3791 return getResourceTrigger().get(0); 3792 } 3793 3794 /** 3795 * @return {@link #eventTrigger} (Event definition which can be used to trigger the SubscriptionTopic.) 3796 */ 3797 public List<SubscriptionTopicEventTriggerComponent> getEventTrigger() { 3798 if (this.eventTrigger == null) 3799 this.eventTrigger = new ArrayList<SubscriptionTopicEventTriggerComponent>(); 3800 return this.eventTrigger; 3801 } 3802 3803 /** 3804 * @return Returns a reference to <code>this</code> for easy method chaining 3805 */ 3806 public SubscriptionTopic setEventTrigger(List<SubscriptionTopicEventTriggerComponent> theEventTrigger) { 3807 this.eventTrigger = theEventTrigger; 3808 return this; 3809 } 3810 3811 public boolean hasEventTrigger() { 3812 if (this.eventTrigger == null) 3813 return false; 3814 for (SubscriptionTopicEventTriggerComponent item : this.eventTrigger) 3815 if (!item.isEmpty()) 3816 return true; 3817 return false; 3818 } 3819 3820 public SubscriptionTopicEventTriggerComponent addEventTrigger() { //3 3821 SubscriptionTopicEventTriggerComponent t = new SubscriptionTopicEventTriggerComponent(); 3822 if (this.eventTrigger == null) 3823 this.eventTrigger = new ArrayList<SubscriptionTopicEventTriggerComponent>(); 3824 this.eventTrigger.add(t); 3825 return t; 3826 } 3827 3828 public SubscriptionTopic addEventTrigger(SubscriptionTopicEventTriggerComponent t) { //3 3829 if (t == null) 3830 return this; 3831 if (this.eventTrigger == null) 3832 this.eventTrigger = new ArrayList<SubscriptionTopicEventTriggerComponent>(); 3833 this.eventTrigger.add(t); 3834 return this; 3835 } 3836 3837 /** 3838 * @return The first repetition of repeating field {@link #eventTrigger}, creating it if it does not already exist {3} 3839 */ 3840 public SubscriptionTopicEventTriggerComponent getEventTriggerFirstRep() { 3841 if (getEventTrigger().isEmpty()) { 3842 addEventTrigger(); 3843 } 3844 return getEventTrigger().get(0); 3845 } 3846 3847 /** 3848 * @return {@link #canFilterBy} (List of properties by which Subscriptions on the SubscriptionTopic can be filtered. May be defined Search Parameters (e.g., Encounter.patient) or parameters defined within this SubscriptionTopic context (e.g., hub.event).) 3849 */ 3850 public List<SubscriptionTopicCanFilterByComponent> getCanFilterBy() { 3851 if (this.canFilterBy == null) 3852 this.canFilterBy = new ArrayList<SubscriptionTopicCanFilterByComponent>(); 3853 return this.canFilterBy; 3854 } 3855 3856 /** 3857 * @return Returns a reference to <code>this</code> for easy method chaining 3858 */ 3859 public SubscriptionTopic setCanFilterBy(List<SubscriptionTopicCanFilterByComponent> theCanFilterBy) { 3860 this.canFilterBy = theCanFilterBy; 3861 return this; 3862 } 3863 3864 public boolean hasCanFilterBy() { 3865 if (this.canFilterBy == null) 3866 return false; 3867 for (SubscriptionTopicCanFilterByComponent item : this.canFilterBy) 3868 if (!item.isEmpty()) 3869 return true; 3870 return false; 3871 } 3872 3873 public SubscriptionTopicCanFilterByComponent addCanFilterBy() { //3 3874 SubscriptionTopicCanFilterByComponent t = new SubscriptionTopicCanFilterByComponent(); 3875 if (this.canFilterBy == null) 3876 this.canFilterBy = new ArrayList<SubscriptionTopicCanFilterByComponent>(); 3877 this.canFilterBy.add(t); 3878 return t; 3879 } 3880 3881 public SubscriptionTopic addCanFilterBy(SubscriptionTopicCanFilterByComponent t) { //3 3882 if (t == null) 3883 return this; 3884 if (this.canFilterBy == null) 3885 this.canFilterBy = new ArrayList<SubscriptionTopicCanFilterByComponent>(); 3886 this.canFilterBy.add(t); 3887 return this; 3888 } 3889 3890 /** 3891 * @return The first repetition of repeating field {@link #canFilterBy}, creating it if it does not already exist {3} 3892 */ 3893 public SubscriptionTopicCanFilterByComponent getCanFilterByFirstRep() { 3894 if (getCanFilterBy().isEmpty()) { 3895 addCanFilterBy(); 3896 } 3897 return getCanFilterBy().get(0); 3898 } 3899 3900 /** 3901 * @return {@link #notificationShape} (List of properties to describe the shape (e.g., resources) included in notifications from this Subscription Topic.) 3902 */ 3903 public List<SubscriptionTopicNotificationShapeComponent> getNotificationShape() { 3904 if (this.notificationShape == null) 3905 this.notificationShape = new ArrayList<SubscriptionTopicNotificationShapeComponent>(); 3906 return this.notificationShape; 3907 } 3908 3909 /** 3910 * @return Returns a reference to <code>this</code> for easy method chaining 3911 */ 3912 public SubscriptionTopic setNotificationShape(List<SubscriptionTopicNotificationShapeComponent> theNotificationShape) { 3913 this.notificationShape = theNotificationShape; 3914 return this; 3915 } 3916 3917 public boolean hasNotificationShape() { 3918 if (this.notificationShape == null) 3919 return false; 3920 for (SubscriptionTopicNotificationShapeComponent item : this.notificationShape) 3921 if (!item.isEmpty()) 3922 return true; 3923 return false; 3924 } 3925 3926 public SubscriptionTopicNotificationShapeComponent addNotificationShape() { //3 3927 SubscriptionTopicNotificationShapeComponent t = new SubscriptionTopicNotificationShapeComponent(); 3928 if (this.notificationShape == null) 3929 this.notificationShape = new ArrayList<SubscriptionTopicNotificationShapeComponent>(); 3930 this.notificationShape.add(t); 3931 return t; 3932 } 3933 3934 public SubscriptionTopic addNotificationShape(SubscriptionTopicNotificationShapeComponent t) { //3 3935 if (t == null) 3936 return this; 3937 if (this.notificationShape == null) 3938 this.notificationShape = new ArrayList<SubscriptionTopicNotificationShapeComponent>(); 3939 this.notificationShape.add(t); 3940 return this; 3941 } 3942 3943 /** 3944 * @return The first repetition of repeating field {@link #notificationShape}, creating it if it does not already exist {3} 3945 */ 3946 public SubscriptionTopicNotificationShapeComponent getNotificationShapeFirstRep() { 3947 if (getNotificationShape().isEmpty()) { 3948 addNotificationShape(); 3949 } 3950 return getNotificationShape().get(0); 3951 } 3952 3953 protected void listChildren(List<Property> children) { 3954 super.listChildren(children); 3955 children.add(new Property("url", "uri", "An absolute URI that is used to identify this subscription topic when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this subscription topic is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the subscription topic is stored on different servers.", 0, 1, url)); 3956 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this subscription topic by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3957 children.add(new Property("version", "string", "The identifier that is used to identify this version of the subscription topic when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Topic author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable.", 0, 1, version)); 3958 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 3959 children.add(new Property("name", "string", "A natural language name identifying the subscription topic This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 3960 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the subscription topic. For example, \"admission\".", 0, 1, title)); 3961 children.add(new Property("derivedFrom", "canonical(SubscriptionTopic)", "The canonical URL pointing to another FHIR-defined SubscriptionTopic that is adhered to in whole or in part by this SubscriptionTopic.", 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 3962 children.add(new Property("status", "code", "The current state of the SubscriptionTopic.", 0, 1, status)); 3963 children.add(new Property("experimental", "boolean", "A flag to indicate that this TopSubscriptionTopicic is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 3964 children.add(new Property("date", "dateTime", "The date (and optionally time) when the subscription topic was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the subscription topic changes.", 0, 1, date)); 3965 children.add(new Property("publisher", "string", "Helps establish the \"authority/credibility\" of the SubscriptionTopic. May also allow for contact.", 0, 1, publisher)); 3966 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 3967 children.add(new Property("description", "markdown", "A free text natural language description of the Topic from the consumer's perspective.", 0, 1, description)); 3968 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of code system definitions.", 0, java.lang.Integer.MAX_VALUE, useContext)); 3969 children.add(new Property("jurisdiction", "CodeableConcept", "A jurisdiction in which the Topic is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 3970 children.add(new Property("purpose", "markdown", "Explains why this Topic is needed and why it has been designed as it has.", 0, 1, purpose)); 3971 children.add(new Property("copyright", "markdown", "A copyright statement relating to the SubscriptionTopic and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SubscriptionTopic.", 0, 1, copyright)); 3972 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 3973 children.add(new Property("approvalDate", "date", "The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 3974 children.add(new Property("lastReviewDate", "date", "The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.", 0, 1, lastReviewDate)); 3975 children.add(new Property("effectivePeriod", "Period", "The period during which the SubscriptionTopic content was or is planned to be effective.", 0, 1, effectivePeriod)); 3976 children.add(new Property("resourceTrigger", "", "A definition of a resource-based event that triggers a notification based on the SubscriptionTopic. The criteria may be just a human readable description and/or a full FHIR search string or FHIRPath expression. Multiple triggers are considered OR joined (e.g., a resource update matching ANY of the definitions will trigger a notification).", 0, java.lang.Integer.MAX_VALUE, resourceTrigger)); 3977 children.add(new Property("eventTrigger", "", "Event definition which can be used to trigger the SubscriptionTopic.", 0, java.lang.Integer.MAX_VALUE, eventTrigger)); 3978 children.add(new Property("canFilterBy", "", "List of properties by which Subscriptions on the SubscriptionTopic can be filtered. May be defined Search Parameters (e.g., Encounter.patient) or parameters defined within this SubscriptionTopic context (e.g., hub.event).", 0, java.lang.Integer.MAX_VALUE, canFilterBy)); 3979 children.add(new Property("notificationShape", "", "List of properties to describe the shape (e.g., resources) included in notifications from this Subscription Topic.", 0, java.lang.Integer.MAX_VALUE, notificationShape)); 3980 } 3981 3982 @Override 3983 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3984 switch (_hash) { 3985 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this subscription topic when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this subscription topic is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the subscription topic is stored on different servers.", 0, 1, url); 3986 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this subscription topic by the performer and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 3987 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the subscription topic when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the Topic author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions are orderable.", 0, 1, version); 3988 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3989 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3990 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3991 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3992 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the subscription topic This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 3993 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the subscription topic. For example, \"admission\".", 0, 1, title); 3994 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "canonical(SubscriptionTopic)", "The canonical URL pointing to another FHIR-defined SubscriptionTopic that is adhered to in whole or in part by this SubscriptionTopic.", 0, java.lang.Integer.MAX_VALUE, derivedFrom); 3995 case -892481550: /*status*/ return new Property("status", "code", "The current state of the SubscriptionTopic.", 0, 1, status); 3996 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A flag to indicate that this TopSubscriptionTopicic is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 3997 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the subscription topic was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the subscription topic changes.", 0, 1, date); 3998 case 1447404028: /*publisher*/ return new Property("publisher", "string", "Helps establish the \"authority/credibility\" of the SubscriptionTopic. May also allow for contact.", 0, 1, publisher); 3999 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 4000 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the Topic from the consumer's perspective.", 0, 1, description); 4001 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching of code system definitions.", 0, java.lang.Integer.MAX_VALUE, useContext); 4002 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A jurisdiction in which the Topic is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 4003 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explains why this Topic is needed and why it has been designed as it has.", 0, 1, purpose); 4004 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the SubscriptionTopic and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the SubscriptionTopic.", 0, 1, copyright); 4005 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 4006 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the asset content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 4007 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the asset content was last reviewed. Review happens periodically after that, but doesn't change the original approval date.", 0, 1, lastReviewDate); 4008 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the SubscriptionTopic content was or is planned to be effective.", 0, 1, effectivePeriod); 4009 case -424927798: /*resourceTrigger*/ return new Property("resourceTrigger", "", "A definition of a resource-based event that triggers a notification based on the SubscriptionTopic. The criteria may be just a human readable description and/or a full FHIR search string or FHIRPath expression. Multiple triggers are considered OR joined (e.g., a resource update matching ANY of the definitions will trigger a notification).", 0, java.lang.Integer.MAX_VALUE, resourceTrigger); 4010 case -151635522: /*eventTrigger*/ return new Property("eventTrigger", "", "Event definition which can be used to trigger the SubscriptionTopic.", 0, java.lang.Integer.MAX_VALUE, eventTrigger); 4011 case -1299519009: /*canFilterBy*/ return new Property("canFilterBy", "", "List of properties by which Subscriptions on the SubscriptionTopic can be filtered. May be defined Search Parameters (e.g., Encounter.patient) or parameters defined within this SubscriptionTopic context (e.g., hub.event).", 0, java.lang.Integer.MAX_VALUE, canFilterBy); 4012 case -1583369866: /*notificationShape*/ return new Property("notificationShape", "", "List of properties to describe the shape (e.g., resources) included in notifications from this Subscription Topic.", 0, java.lang.Integer.MAX_VALUE, notificationShape); 4013 default: return super.getNamedProperty(_hash, _name, _checkValid); 4014 } 4015 4016 } 4017 4018 @Override 4019 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4020 switch (hash) { 4021 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 4022 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4023 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 4024 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 4025 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 4026 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 4027 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // CanonicalType 4028 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 4029 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 4030 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 4031 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 4032 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 4033 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 4034 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 4035 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 4036 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 4037 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 4038 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 4039 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 4040 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 4041 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 4042 case -424927798: /*resourceTrigger*/ return this.resourceTrigger == null ? new Base[0] : this.resourceTrigger.toArray(new Base[this.resourceTrigger.size()]); // SubscriptionTopicResourceTriggerComponent 4043 case -151635522: /*eventTrigger*/ return this.eventTrigger == null ? new Base[0] : this.eventTrigger.toArray(new Base[this.eventTrigger.size()]); // SubscriptionTopicEventTriggerComponent 4044 case -1299519009: /*canFilterBy*/ return this.canFilterBy == null ? new Base[0] : this.canFilterBy.toArray(new Base[this.canFilterBy.size()]); // SubscriptionTopicCanFilterByComponent 4045 case -1583369866: /*notificationShape*/ return this.notificationShape == null ? new Base[0] : this.notificationShape.toArray(new Base[this.notificationShape.size()]); // SubscriptionTopicNotificationShapeComponent 4046 default: return super.getProperty(hash, name, checkValid); 4047 } 4048 4049 } 4050 4051 @Override 4052 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4053 switch (hash) { 4054 case 116079: // url 4055 this.url = TypeConvertor.castToUri(value); // UriType 4056 return value; 4057 case -1618432855: // identifier 4058 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 4059 return value; 4060 case 351608024: // version 4061 this.version = TypeConvertor.castToString(value); // StringType 4062 return value; 4063 case 1508158071: // versionAlgorithm 4064 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 4065 return value; 4066 case 3373707: // name 4067 this.name = TypeConvertor.castToString(value); // StringType 4068 return value; 4069 case 110371416: // title 4070 this.title = TypeConvertor.castToString(value); // StringType 4071 return value; 4072 case 1077922663: // derivedFrom 4073 this.getDerivedFrom().add(TypeConvertor.castToCanonical(value)); // CanonicalType 4074 return value; 4075 case -892481550: // status 4076 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4077 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4078 return value; 4079 case -404562712: // experimental 4080 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 4081 return value; 4082 case 3076014: // date 4083 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4084 return value; 4085 case 1447404028: // publisher 4086 this.publisher = TypeConvertor.castToString(value); // StringType 4087 return value; 4088 case 951526432: // contact 4089 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4090 return value; 4091 case -1724546052: // description 4092 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4093 return value; 4094 case -669707736: // useContext 4095 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 4096 return value; 4097 case -507075711: // jurisdiction 4098 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4099 return value; 4100 case -220463842: // purpose 4101 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 4102 return value; 4103 case 1522889671: // copyright 4104 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 4105 return value; 4106 case 765157229: // copyrightLabel 4107 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 4108 return value; 4109 case 223539345: // approvalDate 4110 this.approvalDate = TypeConvertor.castToDate(value); // DateType 4111 return value; 4112 case -1687512484: // lastReviewDate 4113 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 4114 return value; 4115 case -403934648: // effectivePeriod 4116 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 4117 return value; 4118 case -424927798: // resourceTrigger 4119 this.getResourceTrigger().add((SubscriptionTopicResourceTriggerComponent) value); // SubscriptionTopicResourceTriggerComponent 4120 return value; 4121 case -151635522: // eventTrigger 4122 this.getEventTrigger().add((SubscriptionTopicEventTriggerComponent) value); // SubscriptionTopicEventTriggerComponent 4123 return value; 4124 case -1299519009: // canFilterBy 4125 this.getCanFilterBy().add((SubscriptionTopicCanFilterByComponent) value); // SubscriptionTopicCanFilterByComponent 4126 return value; 4127 case -1583369866: // notificationShape 4128 this.getNotificationShape().add((SubscriptionTopicNotificationShapeComponent) value); // SubscriptionTopicNotificationShapeComponent 4129 return value; 4130 default: return super.setProperty(hash, name, value); 4131 } 4132 4133 } 4134 4135 @Override 4136 public Base setProperty(String name, Base value) throws FHIRException { 4137 if (name.equals("url")) { 4138 this.url = TypeConvertor.castToUri(value); // UriType 4139 } else if (name.equals("identifier")) { 4140 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 4141 } else if (name.equals("version")) { 4142 this.version = TypeConvertor.castToString(value); // StringType 4143 } else if (name.equals("versionAlgorithm[x]")) { 4144 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 4145 } else if (name.equals("name")) { 4146 this.name = TypeConvertor.castToString(value); // StringType 4147 } else if (name.equals("title")) { 4148 this.title = TypeConvertor.castToString(value); // StringType 4149 } else if (name.equals("derivedFrom")) { 4150 this.getDerivedFrom().add(TypeConvertor.castToCanonical(value)); 4151 } else if (name.equals("status")) { 4152 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4153 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4154 } else if (name.equals("experimental")) { 4155 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 4156 } else if (name.equals("date")) { 4157 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4158 } else if (name.equals("publisher")) { 4159 this.publisher = TypeConvertor.castToString(value); // StringType 4160 } else if (name.equals("contact")) { 4161 this.getContact().add(TypeConvertor.castToContactDetail(value)); 4162 } else if (name.equals("description")) { 4163 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4164 } else if (name.equals("useContext")) { 4165 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 4166 } else if (name.equals("jurisdiction")) { 4167 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 4168 } else if (name.equals("purpose")) { 4169 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 4170 } else if (name.equals("copyright")) { 4171 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 4172 } else if (name.equals("copyrightLabel")) { 4173 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 4174 } else if (name.equals("approvalDate")) { 4175 this.approvalDate = TypeConvertor.castToDate(value); // DateType 4176 } else if (name.equals("lastReviewDate")) { 4177 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 4178 } else if (name.equals("effectivePeriod")) { 4179 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 4180 } else if (name.equals("resourceTrigger")) { 4181 this.getResourceTrigger().add((SubscriptionTopicResourceTriggerComponent) value); 4182 } else if (name.equals("eventTrigger")) { 4183 this.getEventTrigger().add((SubscriptionTopicEventTriggerComponent) value); 4184 } else if (name.equals("canFilterBy")) { 4185 this.getCanFilterBy().add((SubscriptionTopicCanFilterByComponent) value); 4186 } else if (name.equals("notificationShape")) { 4187 this.getNotificationShape().add((SubscriptionTopicNotificationShapeComponent) value); 4188 } else 4189 return super.setProperty(name, value); 4190 return value; 4191 } 4192 4193 @Override 4194 public void removeChild(String name, Base value) throws FHIRException { 4195 if (name.equals("url")) { 4196 this.url = null; 4197 } else if (name.equals("identifier")) { 4198 this.getIdentifier().remove(value); 4199 } else if (name.equals("version")) { 4200 this.version = null; 4201 } else if (name.equals("versionAlgorithm[x]")) { 4202 this.versionAlgorithm = null; 4203 } else if (name.equals("name")) { 4204 this.name = null; 4205 } else if (name.equals("title")) { 4206 this.title = null; 4207 } else if (name.equals("derivedFrom")) { 4208 this.getDerivedFrom().remove(value); 4209 } else if (name.equals("status")) { 4210 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4211 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4212 } else if (name.equals("experimental")) { 4213 this.experimental = null; 4214 } else if (name.equals("date")) { 4215 this.date = null; 4216 } else if (name.equals("publisher")) { 4217 this.publisher = null; 4218 } else if (name.equals("contact")) { 4219 this.getContact().remove(value); 4220 } else if (name.equals("description")) { 4221 this.description = null; 4222 } else if (name.equals("useContext")) { 4223 this.getUseContext().remove(value); 4224 } else if (name.equals("jurisdiction")) { 4225 this.getJurisdiction().remove(value); 4226 } else if (name.equals("purpose")) { 4227 this.purpose = null; 4228 } else if (name.equals("copyright")) { 4229 this.copyright = null; 4230 } else if (name.equals("copyrightLabel")) { 4231 this.copyrightLabel = null; 4232 } else if (name.equals("approvalDate")) { 4233 this.approvalDate = null; 4234 } else if (name.equals("lastReviewDate")) { 4235 this.lastReviewDate = null; 4236 } else if (name.equals("effectivePeriod")) { 4237 this.effectivePeriod = null; 4238 } else if (name.equals("resourceTrigger")) { 4239 this.getResourceTrigger().remove((SubscriptionTopicResourceTriggerComponent) value); 4240 } else if (name.equals("eventTrigger")) { 4241 this.getEventTrigger().remove((SubscriptionTopicEventTriggerComponent) value); 4242 } else if (name.equals("canFilterBy")) { 4243 this.getCanFilterBy().remove((SubscriptionTopicCanFilterByComponent) value); 4244 } else if (name.equals("notificationShape")) { 4245 this.getNotificationShape().remove((SubscriptionTopicNotificationShapeComponent) value); 4246 } else 4247 super.removeChild(name, value); 4248 4249 } 4250 4251 @Override 4252 public Base makeProperty(int hash, String name) throws FHIRException { 4253 switch (hash) { 4254 case 116079: return getUrlElement(); 4255 case -1618432855: return addIdentifier(); 4256 case 351608024: return getVersionElement(); 4257 case -115699031: return getVersionAlgorithm(); 4258 case 1508158071: return getVersionAlgorithm(); 4259 case 3373707: return getNameElement(); 4260 case 110371416: return getTitleElement(); 4261 case 1077922663: return addDerivedFromElement(); 4262 case -892481550: return getStatusElement(); 4263 case -404562712: return getExperimentalElement(); 4264 case 3076014: return getDateElement(); 4265 case 1447404028: return getPublisherElement(); 4266 case 951526432: return addContact(); 4267 case -1724546052: return getDescriptionElement(); 4268 case -669707736: return addUseContext(); 4269 case -507075711: return addJurisdiction(); 4270 case -220463842: return getPurposeElement(); 4271 case 1522889671: return getCopyrightElement(); 4272 case 765157229: return getCopyrightLabelElement(); 4273 case 223539345: return getApprovalDateElement(); 4274 case -1687512484: return getLastReviewDateElement(); 4275 case -403934648: return getEffectivePeriod(); 4276 case -424927798: return addResourceTrigger(); 4277 case -151635522: return addEventTrigger(); 4278 case -1299519009: return addCanFilterBy(); 4279 case -1583369866: return addNotificationShape(); 4280 default: return super.makeProperty(hash, name); 4281 } 4282 4283 } 4284 4285 @Override 4286 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4287 switch (hash) { 4288 case 116079: /*url*/ return new String[] {"uri"}; 4289 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4290 case 351608024: /*version*/ return new String[] {"string"}; 4291 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 4292 case 3373707: /*name*/ return new String[] {"string"}; 4293 case 110371416: /*title*/ return new String[] {"string"}; 4294 case 1077922663: /*derivedFrom*/ return new String[] {"canonical"}; 4295 case -892481550: /*status*/ return new String[] {"code"}; 4296 case -404562712: /*experimental*/ return new String[] {"boolean"}; 4297 case 3076014: /*date*/ return new String[] {"dateTime"}; 4298 case 1447404028: /*publisher*/ return new String[] {"string"}; 4299 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 4300 case -1724546052: /*description*/ return new String[] {"markdown"}; 4301 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 4302 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 4303 case -220463842: /*purpose*/ return new String[] {"markdown"}; 4304 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 4305 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 4306 case 223539345: /*approvalDate*/ return new String[] {"date"}; 4307 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 4308 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 4309 case -424927798: /*resourceTrigger*/ return new String[] {}; 4310 case -151635522: /*eventTrigger*/ return new String[] {}; 4311 case -1299519009: /*canFilterBy*/ return new String[] {}; 4312 case -1583369866: /*notificationShape*/ return new String[] {}; 4313 default: return super.getTypesForProperty(hash, name); 4314 } 4315 4316 } 4317 4318 @Override 4319 public Base addChild(String name) throws FHIRException { 4320 if (name.equals("url")) { 4321 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.url"); 4322 } 4323 else if (name.equals("identifier")) { 4324 return addIdentifier(); 4325 } 4326 else if (name.equals("version")) { 4327 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.version"); 4328 } 4329 else if (name.equals("versionAlgorithmString")) { 4330 this.versionAlgorithm = new StringType(); 4331 return this.versionAlgorithm; 4332 } 4333 else if (name.equals("versionAlgorithmCoding")) { 4334 this.versionAlgorithm = new Coding(); 4335 return this.versionAlgorithm; 4336 } 4337 else if (name.equals("name")) { 4338 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.name"); 4339 } 4340 else if (name.equals("title")) { 4341 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.title"); 4342 } 4343 else if (name.equals("derivedFrom")) { 4344 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.derivedFrom"); 4345 } 4346 else if (name.equals("status")) { 4347 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.status"); 4348 } 4349 else if (name.equals("experimental")) { 4350 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.experimental"); 4351 } 4352 else if (name.equals("date")) { 4353 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.date"); 4354 } 4355 else if (name.equals("publisher")) { 4356 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.publisher"); 4357 } 4358 else if (name.equals("contact")) { 4359 return addContact(); 4360 } 4361 else if (name.equals("description")) { 4362 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.description"); 4363 } 4364 else if (name.equals("useContext")) { 4365 return addUseContext(); 4366 } 4367 else if (name.equals("jurisdiction")) { 4368 return addJurisdiction(); 4369 } 4370 else if (name.equals("purpose")) { 4371 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.purpose"); 4372 } 4373 else if (name.equals("copyright")) { 4374 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.copyright"); 4375 } 4376 else if (name.equals("copyrightLabel")) { 4377 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.copyrightLabel"); 4378 } 4379 else if (name.equals("approvalDate")) { 4380 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.approvalDate"); 4381 } 4382 else if (name.equals("lastReviewDate")) { 4383 throw new FHIRException("Cannot call addChild on a singleton property SubscriptionTopic.lastReviewDate"); 4384 } 4385 else if (name.equals("effectivePeriod")) { 4386 this.effectivePeriod = new Period(); 4387 return this.effectivePeriod; 4388 } 4389 else if (name.equals("resourceTrigger")) { 4390 return addResourceTrigger(); 4391 } 4392 else if (name.equals("eventTrigger")) { 4393 return addEventTrigger(); 4394 } 4395 else if (name.equals("canFilterBy")) { 4396 return addCanFilterBy(); 4397 } 4398 else if (name.equals("notificationShape")) { 4399 return addNotificationShape(); 4400 } 4401 else 4402 return super.addChild(name); 4403 } 4404 4405 public String fhirType() { 4406 return "SubscriptionTopic"; 4407 4408 } 4409 4410 public SubscriptionTopic copy() { 4411 SubscriptionTopic dst = new SubscriptionTopic(); 4412 copyValues(dst); 4413 return dst; 4414 } 4415 4416 public void copyValues(SubscriptionTopic dst) { 4417 super.copyValues(dst); 4418 dst.url = url == null ? null : url.copy(); 4419 if (identifier != null) { 4420 dst.identifier = new ArrayList<Identifier>(); 4421 for (Identifier i : identifier) 4422 dst.identifier.add(i.copy()); 4423 }; 4424 dst.version = version == null ? null : version.copy(); 4425 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 4426 dst.name = name == null ? null : name.copy(); 4427 dst.title = title == null ? null : title.copy(); 4428 if (derivedFrom != null) { 4429 dst.derivedFrom = new ArrayList<CanonicalType>(); 4430 for (CanonicalType i : derivedFrom) 4431 dst.derivedFrom.add(i.copy()); 4432 }; 4433 dst.status = status == null ? null : status.copy(); 4434 dst.experimental = experimental == null ? null : experimental.copy(); 4435 dst.date = date == null ? null : date.copy(); 4436 dst.publisher = publisher == null ? null : publisher.copy(); 4437 if (contact != null) { 4438 dst.contact = new ArrayList<ContactDetail>(); 4439 for (ContactDetail i : contact) 4440 dst.contact.add(i.copy()); 4441 }; 4442 dst.description = description == null ? null : description.copy(); 4443 if (useContext != null) { 4444 dst.useContext = new ArrayList<UsageContext>(); 4445 for (UsageContext i : useContext) 4446 dst.useContext.add(i.copy()); 4447 }; 4448 if (jurisdiction != null) { 4449 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4450 for (CodeableConcept i : jurisdiction) 4451 dst.jurisdiction.add(i.copy()); 4452 }; 4453 dst.purpose = purpose == null ? null : purpose.copy(); 4454 dst.copyright = copyright == null ? null : copyright.copy(); 4455 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 4456 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 4457 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 4458 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 4459 if (resourceTrigger != null) { 4460 dst.resourceTrigger = new ArrayList<SubscriptionTopicResourceTriggerComponent>(); 4461 for (SubscriptionTopicResourceTriggerComponent i : resourceTrigger) 4462 dst.resourceTrigger.add(i.copy()); 4463 }; 4464 if (eventTrigger != null) { 4465 dst.eventTrigger = new ArrayList<SubscriptionTopicEventTriggerComponent>(); 4466 for (SubscriptionTopicEventTriggerComponent i : eventTrigger) 4467 dst.eventTrigger.add(i.copy()); 4468 }; 4469 if (canFilterBy != null) { 4470 dst.canFilterBy = new ArrayList<SubscriptionTopicCanFilterByComponent>(); 4471 for (SubscriptionTopicCanFilterByComponent i : canFilterBy) 4472 dst.canFilterBy.add(i.copy()); 4473 }; 4474 if (notificationShape != null) { 4475 dst.notificationShape = new ArrayList<SubscriptionTopicNotificationShapeComponent>(); 4476 for (SubscriptionTopicNotificationShapeComponent i : notificationShape) 4477 dst.notificationShape.add(i.copy()); 4478 }; 4479 } 4480 4481 protected SubscriptionTopic typedCopy() { 4482 return copy(); 4483 } 4484 4485 @Override 4486 public boolean equalsDeep(Base other_) { 4487 if (!super.equalsDeep(other_)) 4488 return false; 4489 if (!(other_ instanceof SubscriptionTopic)) 4490 return false; 4491 SubscriptionTopic o = (SubscriptionTopic) other_; 4492 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 4493 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 4494 && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 4495 && compareDeep(date, o.date, true) && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 4496 && compareDeep(description, o.description, true) && compareDeep(useContext, o.useContext, true) 4497 && compareDeep(jurisdiction, o.jurisdiction, true) && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 4498 && compareDeep(copyrightLabel, o.copyrightLabel, true) && compareDeep(approvalDate, o.approvalDate, true) 4499 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 4500 && compareDeep(resourceTrigger, o.resourceTrigger, true) && compareDeep(eventTrigger, o.eventTrigger, true) 4501 && compareDeep(canFilterBy, o.canFilterBy, true) && compareDeep(notificationShape, o.notificationShape, true) 4502 ; 4503 } 4504 4505 @Override 4506 public boolean equalsShallow(Base other_) { 4507 if (!super.equalsShallow(other_)) 4508 return false; 4509 if (!(other_ instanceof SubscriptionTopic)) 4510 return false; 4511 SubscriptionTopic o = (SubscriptionTopic) other_; 4512 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 4513 && compareValues(title, o.title, true) && compareValues(derivedFrom, o.derivedFrom, true) && compareValues(status, o.status, true) 4514 && compareValues(experimental, o.experimental, true) && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) 4515 && compareValues(description, o.description, true) && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) 4516 && compareValues(copyrightLabel, o.copyrightLabel, true) && compareValues(approvalDate, o.approvalDate, true) 4517 && compareValues(lastReviewDate, o.lastReviewDate, true); 4518 } 4519 4520 public boolean isEmpty() { 4521 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 4522 , versionAlgorithm, name, title, derivedFrom, status, experimental, date, publisher 4523 , contact, description, useContext, jurisdiction, purpose, copyright, copyrightLabel 4524 , approvalDate, lastReviewDate, effectivePeriod, resourceTrigger, eventTrigger, canFilterBy 4525 , notificationShape); 4526 } 4527 4528 @Override 4529 public ResourceType getResourceType() { 4530 return ResourceType.SubscriptionTopic; 4531 } 4532 4533 /** 4534 * Search parameter: <b>date</b> 4535 * <p> 4536 * Description: <b>Multiple Resources: 4537 4538* [ActivityDefinition](activitydefinition.html): The activity definition publication date 4539* [ActorDefinition](actordefinition.html): The Actor Definition publication date 4540* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 4541* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 4542* [Citation](citation.html): The citation publication date 4543* [CodeSystem](codesystem.html): The code system publication date 4544* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 4545* [ConceptMap](conceptmap.html): The concept map publication date 4546* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 4547* [EventDefinition](eventdefinition.html): The event definition publication date 4548* [Evidence](evidence.html): The evidence publication date 4549* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 4550* [ExampleScenario](examplescenario.html): The example scenario publication date 4551* [GraphDefinition](graphdefinition.html): The graph definition publication date 4552* [ImplementationGuide](implementationguide.html): The implementation guide publication date 4553* [Library](library.html): The library publication date 4554* [Measure](measure.html): The measure publication date 4555* [MessageDefinition](messagedefinition.html): The message definition publication date 4556* [NamingSystem](namingsystem.html): The naming system publication date 4557* [OperationDefinition](operationdefinition.html): The operation definition publication date 4558* [PlanDefinition](plandefinition.html): The plan definition publication date 4559* [Questionnaire](questionnaire.html): The questionnaire publication date 4560* [Requirements](requirements.html): The requirements publication date 4561* [SearchParameter](searchparameter.html): The search parameter publication date 4562* [StructureDefinition](structuredefinition.html): The structure definition publication date 4563* [StructureMap](structuremap.html): The structure map publication date 4564* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 4565* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 4566* [TestScript](testscript.html): The test script publication date 4567* [ValueSet](valueset.html): The value set publication date 4568</b><br> 4569 * Type: <b>date</b><br> 4570 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 4571 * </p> 4572 */ 4573 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 4574 public static final String SP_DATE = "date"; 4575 /** 4576 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4577 * <p> 4578 * Description: <b>Multiple Resources: 4579 4580* [ActivityDefinition](activitydefinition.html): The activity definition publication date 4581* [ActorDefinition](actordefinition.html): The Actor Definition publication date 4582* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 4583* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 4584* [Citation](citation.html): The citation publication date 4585* [CodeSystem](codesystem.html): The code system publication date 4586* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 4587* [ConceptMap](conceptmap.html): The concept map publication date 4588* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 4589* [EventDefinition](eventdefinition.html): The event definition publication date 4590* [Evidence](evidence.html): The evidence publication date 4591* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 4592* [ExampleScenario](examplescenario.html): The example scenario publication date 4593* [GraphDefinition](graphdefinition.html): The graph definition publication date 4594* [ImplementationGuide](implementationguide.html): The implementation guide publication date 4595* [Library](library.html): The library publication date 4596* [Measure](measure.html): The measure publication date 4597* [MessageDefinition](messagedefinition.html): The message definition publication date 4598* [NamingSystem](namingsystem.html): The naming system publication date 4599* [OperationDefinition](operationdefinition.html): The operation definition publication date 4600* [PlanDefinition](plandefinition.html): The plan definition publication date 4601* [Questionnaire](questionnaire.html): The questionnaire publication date 4602* [Requirements](requirements.html): The requirements publication date 4603* [SearchParameter](searchparameter.html): The search parameter publication date 4604* [StructureDefinition](structuredefinition.html): The structure definition publication date 4605* [StructureMap](structuremap.html): The structure map publication date 4606* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 4607* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 4608* [TestScript](testscript.html): The test script publication date 4609* [ValueSet](valueset.html): The value set publication date 4610</b><br> 4611 * Type: <b>date</b><br> 4612 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 4613 * </p> 4614 */ 4615 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4616 4617 /** 4618 * Search parameter: <b>identifier</b> 4619 * <p> 4620 * Description: <b>Multiple Resources: 4621 4622* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4623* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4624* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4625* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4626* [Citation](citation.html): External identifier for the citation 4627* [CodeSystem](codesystem.html): External identifier for the code system 4628* [ConceptMap](conceptmap.html): External identifier for the concept map 4629* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4630* [EventDefinition](eventdefinition.html): External identifier for the event definition 4631* [Evidence](evidence.html): External identifier for the evidence 4632* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4633* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4634* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4635* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4636* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4637* [Library](library.html): External identifier for the library 4638* [Measure](measure.html): External identifier for the measure 4639* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4640* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4641* [NamingSystem](namingsystem.html): External identifier for the naming system 4642* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4643* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4644* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4645* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4646* [Requirements](requirements.html): External identifier for the requirements 4647* [SearchParameter](searchparameter.html): External identifier for the search parameter 4648* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4649* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4650* [StructureMap](structuremap.html): External identifier for the structure map 4651* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4652* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4653* [TestPlan](testplan.html): An identifier for the test plan 4654* [TestScript](testscript.html): External identifier for the test script 4655* [ValueSet](valueset.html): External identifier for the value set 4656</b><br> 4657 * Type: <b>token</b><br> 4658 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4659 * </p> 4660 */ 4661 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 4662 public static final String SP_IDENTIFIER = "identifier"; 4663 /** 4664 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4665 * <p> 4666 * Description: <b>Multiple Resources: 4667 4668* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4669* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4670* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4671* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4672* [Citation](citation.html): External identifier for the citation 4673* [CodeSystem](codesystem.html): External identifier for the code system 4674* [ConceptMap](conceptmap.html): External identifier for the concept map 4675* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4676* [EventDefinition](eventdefinition.html): External identifier for the event definition 4677* [Evidence](evidence.html): External identifier for the evidence 4678* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4679* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4680* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4681* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4682* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4683* [Library](library.html): External identifier for the library 4684* [Measure](measure.html): External identifier for the measure 4685* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4686* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4687* [NamingSystem](namingsystem.html): External identifier for the naming system 4688* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4689* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4690* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4691* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4692* [Requirements](requirements.html): External identifier for the requirements 4693* [SearchParameter](searchparameter.html): External identifier for the search parameter 4694* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4695* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4696* [StructureMap](structuremap.html): External identifier for the structure map 4697* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4698* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4699* [TestPlan](testplan.html): An identifier for the test plan 4700* [TestScript](testscript.html): External identifier for the test script 4701* [ValueSet](valueset.html): External identifier for the value set 4702</b><br> 4703 * Type: <b>token</b><br> 4704 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4705 * </p> 4706 */ 4707 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4708 4709 /** 4710 * Search parameter: <b>status</b> 4711 * <p> 4712 * Description: <b>Multiple Resources: 4713 4714* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4715* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4716* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4717* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4718* [Citation](citation.html): The current status of the citation 4719* [CodeSystem](codesystem.html): The current status of the code system 4720* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4721* [ConceptMap](conceptmap.html): The current status of the concept map 4722* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4723* [EventDefinition](eventdefinition.html): The current status of the event definition 4724* [Evidence](evidence.html): The current status of the evidence 4725* [EvidenceReport](evidencereport.html): The current status of the evidence report 4726* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4727* [ExampleScenario](examplescenario.html): The current status of the example scenario 4728* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4729* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4730* [Library](library.html): The current status of the library 4731* [Measure](measure.html): The current status of the measure 4732* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4733* [MessageDefinition](messagedefinition.html): The current status of the message definition 4734* [NamingSystem](namingsystem.html): The current status of the naming system 4735* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4736* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4737* [PlanDefinition](plandefinition.html): The current status of the plan definition 4738* [Questionnaire](questionnaire.html): The current status of the questionnaire 4739* [Requirements](requirements.html): The current status of the requirements 4740* [SearchParameter](searchparameter.html): The current status of the search parameter 4741* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4742* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4743* [StructureMap](structuremap.html): The current status of the structure map 4744* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4745* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4746* [TestPlan](testplan.html): The current status of the test plan 4747* [TestScript](testscript.html): The current status of the test script 4748* [ValueSet](valueset.html): The current status of the value set 4749</b><br> 4750 * Type: <b>token</b><br> 4751 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4752 * </p> 4753 */ 4754 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 4755 public static final String SP_STATUS = "status"; 4756 /** 4757 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4758 * <p> 4759 * Description: <b>Multiple Resources: 4760 4761* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4762* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4763* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4764* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4765* [Citation](citation.html): The current status of the citation 4766* [CodeSystem](codesystem.html): The current status of the code system 4767* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4768* [ConceptMap](conceptmap.html): The current status of the concept map 4769* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4770* [EventDefinition](eventdefinition.html): The current status of the event definition 4771* [Evidence](evidence.html): The current status of the evidence 4772* [EvidenceReport](evidencereport.html): The current status of the evidence report 4773* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4774* [ExampleScenario](examplescenario.html): The current status of the example scenario 4775* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4776* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4777* [Library](library.html): The current status of the library 4778* [Measure](measure.html): The current status of the measure 4779* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4780* [MessageDefinition](messagedefinition.html): The current status of the message definition 4781* [NamingSystem](namingsystem.html): The current status of the naming system 4782* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4783* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4784* [PlanDefinition](plandefinition.html): The current status of the plan definition 4785* [Questionnaire](questionnaire.html): The current status of the questionnaire 4786* [Requirements](requirements.html): The current status of the requirements 4787* [SearchParameter](searchparameter.html): The current status of the search parameter 4788* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4789* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4790* [StructureMap](structuremap.html): The current status of the structure map 4791* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4792* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4793* [TestPlan](testplan.html): The current status of the test plan 4794* [TestScript](testscript.html): The current status of the test script 4795* [ValueSet](valueset.html): The current status of the value set 4796</b><br> 4797 * Type: <b>token</b><br> 4798 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4799 * </p> 4800 */ 4801 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4802 4803 /** 4804 * Search parameter: <b>title</b> 4805 * <p> 4806 * Description: <b>Multiple Resources: 4807 4808* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 4809* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 4810* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 4811* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 4812* [Citation](citation.html): The human-friendly name of the citation 4813* [CodeSystem](codesystem.html): The human-friendly name of the code system 4814* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 4815* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 4816* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 4817* [Evidence](evidence.html): The human-friendly name of the evidence 4818* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 4819* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 4820* [Library](library.html): The human-friendly name of the library 4821* [Measure](measure.html): The human-friendly name of the measure 4822* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 4823* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 4824* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 4825* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 4826* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 4827* [Requirements](requirements.html): The human-friendly name of the requirements 4828* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 4829* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 4830* [StructureMap](structuremap.html): The human-friendly name of the structure map 4831* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 4832* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 4833* [TestScript](testscript.html): The human-friendly name of the test script 4834* [ValueSet](valueset.html): The human-friendly name of the value set 4835</b><br> 4836 * Type: <b>string</b><br> 4837 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 4838 * </p> 4839 */ 4840 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 4841 public static final String SP_TITLE = "title"; 4842 /** 4843 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4844 * <p> 4845 * Description: <b>Multiple Resources: 4846 4847* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 4848* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 4849* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 4850* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 4851* [Citation](citation.html): The human-friendly name of the citation 4852* [CodeSystem](codesystem.html): The human-friendly name of the code system 4853* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 4854* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 4855* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 4856* [Evidence](evidence.html): The human-friendly name of the evidence 4857* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 4858* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 4859* [Library](library.html): The human-friendly name of the library 4860* [Measure](measure.html): The human-friendly name of the measure 4861* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 4862* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 4863* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 4864* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 4865* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 4866* [Requirements](requirements.html): The human-friendly name of the requirements 4867* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 4868* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 4869* [StructureMap](structuremap.html): The human-friendly name of the structure map 4870* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 4871* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 4872* [TestScript](testscript.html): The human-friendly name of the test script 4873* [ValueSet](valueset.html): The human-friendly name of the value set 4874</b><br> 4875 * Type: <b>string</b><br> 4876 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 4877 * </p> 4878 */ 4879 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 4880 4881 /** 4882 * Search parameter: <b>url</b> 4883 * <p> 4884 * Description: <b>Multiple Resources: 4885 4886* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4887* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4888* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4889* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4890* [Citation](citation.html): The uri that identifies the citation 4891* [CodeSystem](codesystem.html): The uri that identifies the code system 4892* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4893* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4894* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4895* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4896* [Evidence](evidence.html): The uri that identifies the evidence 4897* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4898* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4899* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4900* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4901* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4902* [Library](library.html): The uri that identifies the library 4903* [Measure](measure.html): The uri that identifies the measure 4904* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4905* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4906* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4907* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4908* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4909* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4910* [Requirements](requirements.html): The uri that identifies the requirements 4911* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4912* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4913* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4914* [StructureMap](structuremap.html): The uri that identifies the structure map 4915* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4916* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4917* [TestPlan](testplan.html): The uri that identifies the test plan 4918* [TestScript](testscript.html): The uri that identifies the test script 4919* [ValueSet](valueset.html): The uri that identifies the value set 4920</b><br> 4921 * Type: <b>uri</b><br> 4922 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4923 * </p> 4924 */ 4925 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 4926 public static final String SP_URL = "url"; 4927 /** 4928 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4929 * <p> 4930 * Description: <b>Multiple Resources: 4931 4932* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4933* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4934* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4935* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4936* [Citation](citation.html): The uri that identifies the citation 4937* [CodeSystem](codesystem.html): The uri that identifies the code system 4938* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4939* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4940* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4941* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4942* [Evidence](evidence.html): The uri that identifies the evidence 4943* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4944* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4945* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4946* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4947* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4948* [Library](library.html): The uri that identifies the library 4949* [Measure](measure.html): The uri that identifies the measure 4950* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4951* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4952* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4953* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4954* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4955* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4956* [Requirements](requirements.html): The uri that identifies the requirements 4957* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4958* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4959* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4960* [StructureMap](structuremap.html): The uri that identifies the structure map 4961* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4962* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4963* [TestPlan](testplan.html): The uri that identifies the test plan 4964* [TestScript](testscript.html): The uri that identifies the test script 4965* [ValueSet](valueset.html): The uri that identifies the value set 4966</b><br> 4967 * Type: <b>uri</b><br> 4968 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4969 * </p> 4970 */ 4971 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4972 4973 /** 4974 * Search parameter: <b>version</b> 4975 * <p> 4976 * Description: <b>Multiple Resources: 4977 4978* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4979* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4980* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4981* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4982* [Citation](citation.html): The business version of the citation 4983* [CodeSystem](codesystem.html): The business version of the code system 4984* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 4985* [ConceptMap](conceptmap.html): The business version of the concept map 4986* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 4987* [EventDefinition](eventdefinition.html): The business version of the event definition 4988* [Evidence](evidence.html): The business version of the evidence 4989* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 4990* [ExampleScenario](examplescenario.html): The business version of the example scenario 4991* [GraphDefinition](graphdefinition.html): The business version of the graph definition 4992* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 4993* [Library](library.html): The business version of the library 4994* [Measure](measure.html): The business version of the measure 4995* [MessageDefinition](messagedefinition.html): The business version of the message definition 4996* [NamingSystem](namingsystem.html): The business version of the naming system 4997* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4998* [PlanDefinition](plandefinition.html): The business version of the plan definition 4999* [Questionnaire](questionnaire.html): The business version of the questionnaire 5000* [Requirements](requirements.html): The business version of the requirements 5001* [SearchParameter](searchparameter.html): The business version of the search parameter 5002* [StructureDefinition](structuredefinition.html): The business version of the structure definition 5003* [StructureMap](structuremap.html): The business version of the structure map 5004* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 5005* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 5006* [TestScript](testscript.html): The business version of the test script 5007* [ValueSet](valueset.html): The business version of the value set 5008</b><br> 5009 * Type: <b>token</b><br> 5010 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 5011 * </p> 5012 */ 5013 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 5014 public static final String SP_VERSION = "version"; 5015 /** 5016 * <b>Fluent Client</b> search parameter constant for <b>version</b> 5017 * <p> 5018 * Description: <b>Multiple Resources: 5019 5020* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 5021* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 5022* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 5023* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 5024* [Citation](citation.html): The business version of the citation 5025* [CodeSystem](codesystem.html): The business version of the code system 5026* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 5027* [ConceptMap](conceptmap.html): The business version of the concept map 5028* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 5029* [EventDefinition](eventdefinition.html): The business version of the event definition 5030* [Evidence](evidence.html): The business version of the evidence 5031* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 5032* [ExampleScenario](examplescenario.html): The business version of the example scenario 5033* [GraphDefinition](graphdefinition.html): The business version of the graph definition 5034* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 5035* [Library](library.html): The business version of the library 5036* [Measure](measure.html): The business version of the measure 5037* [MessageDefinition](messagedefinition.html): The business version of the message definition 5038* [NamingSystem](namingsystem.html): The business version of the naming system 5039* [OperationDefinition](operationdefinition.html): The business version of the operation definition 5040* [PlanDefinition](plandefinition.html): The business version of the plan definition 5041* [Questionnaire](questionnaire.html): The business version of the questionnaire 5042* [Requirements](requirements.html): The business version of the requirements 5043* [SearchParameter](searchparameter.html): The business version of the search parameter 5044* [StructureDefinition](structuredefinition.html): The business version of the structure definition 5045* [StructureMap](structuremap.html): The business version of the structure map 5046* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 5047* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 5048* [TestScript](testscript.html): The business version of the test script 5049* [ValueSet](valueset.html): The business version of the value set 5050</b><br> 5051 * Type: <b>token</b><br> 5052 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 5053 * </p> 5054 */ 5055 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 5056 5057 /** 5058 * Search parameter: <b>derived-or-self</b> 5059 * <p> 5060 * Description: <b>A server defined search that matches either the url or derivedFrom</b><br> 5061 * Type: <b>uri</b><br> 5062 * Path: <b>SubscriptionTopic.url | SubscriptionTopic.derivedFrom</b><br> 5063 * </p> 5064 */ 5065 @SearchParamDefinition(name="derived-or-self", path="SubscriptionTopic.url | SubscriptionTopic.derivedFrom", description="A server defined search that matches either the url or derivedFrom", type="uri" ) 5066 public static final String SP_DERIVED_OR_SELF = "derived-or-self"; 5067 /** 5068 * <b>Fluent Client</b> search parameter constant for <b>derived-or-self</b> 5069 * <p> 5070 * Description: <b>A server defined search that matches either the url or derivedFrom</b><br> 5071 * Type: <b>uri</b><br> 5072 * Path: <b>SubscriptionTopic.url | SubscriptionTopic.derivedFrom</b><br> 5073 * </p> 5074 */ 5075 public static final ca.uhn.fhir.rest.gclient.UriClientParam DERIVED_OR_SELF = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_DERIVED_OR_SELF); 5076 5077 /** 5078 * Search parameter: <b>effective</b> 5079 * <p> 5080 * Description: <b>Effective period</b><br> 5081 * Type: <b>date</b><br> 5082 * Path: <b>SubscriptionTopic.effectivePeriod</b><br> 5083 * </p> 5084 */ 5085 @SearchParamDefinition(name="effective", path="SubscriptionTopic.effectivePeriod", description="Effective period", type="date" ) 5086 public static final String SP_EFFECTIVE = "effective"; 5087 /** 5088 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 5089 * <p> 5090 * Description: <b>Effective period</b><br> 5091 * Type: <b>date</b><br> 5092 * Path: <b>SubscriptionTopic.effectivePeriod</b><br> 5093 * </p> 5094 */ 5095 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 5096 5097 /** 5098 * Search parameter: <b>event</b> 5099 * <p> 5100 * Description: <b>Event trigger</b><br> 5101 * Type: <b>token</b><br> 5102 * Path: <b>SubscriptionTopic.eventTrigger.event</b><br> 5103 * </p> 5104 */ 5105 @SearchParamDefinition(name="event", path="SubscriptionTopic.eventTrigger.event", description="Event trigger", type="token" ) 5106 public static final String SP_EVENT = "event"; 5107 /** 5108 * <b>Fluent Client</b> search parameter constant for <b>event</b> 5109 * <p> 5110 * Description: <b>Event trigger</b><br> 5111 * Type: <b>token</b><br> 5112 * Path: <b>SubscriptionTopic.eventTrigger.event</b><br> 5113 * </p> 5114 */ 5115 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EVENT); 5116 5117 /** 5118 * Search parameter: <b>resource</b> 5119 * <p> 5120 * Description: <b>Allowed resource for this definition</b><br> 5121 * Type: <b>uri</b><br> 5122 * Path: <b>SubscriptionTopic.resourceTrigger.resource | SubscriptionTopic.eventTrigger.resource | SubscriptionTopic.canFilterBy.resource | SubscriptionTopic.notificationShape.resource</b><br> 5123 * </p> 5124 */ 5125 @SearchParamDefinition(name="resource", path="SubscriptionTopic.resourceTrigger.resource | SubscriptionTopic.eventTrigger.resource | SubscriptionTopic.canFilterBy.resource | SubscriptionTopic.notificationShape.resource", description="Allowed resource for this definition", type="uri" ) 5126 public static final String SP_RESOURCE = "resource"; 5127 /** 5128 * <b>Fluent Client</b> search parameter constant for <b>resource</b> 5129 * <p> 5130 * Description: <b>Allowed resource for this definition</b><br> 5131 * Type: <b>uri</b><br> 5132 * Path: <b>SubscriptionTopic.resourceTrigger.resource | SubscriptionTopic.eventTrigger.resource | SubscriptionTopic.canFilterBy.resource | SubscriptionTopic.notificationShape.resource</b><br> 5133 * </p> 5134 */ 5135 public static final ca.uhn.fhir.rest.gclient.UriClientParam RESOURCE = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_RESOURCE); 5136 5137 /** 5138 * Search parameter: <b>trigger-description</b> 5139 * <p> 5140 * Description: <b>Text representation of the trigger</b><br> 5141 * Type: <b>string</b><br> 5142 * Path: <b>SubscriptionTopic.resourceTrigger.description</b><br> 5143 * </p> 5144 */ 5145 @SearchParamDefinition(name="trigger-description", path="SubscriptionTopic.resourceTrigger.description", description="Text representation of the trigger", type="string" ) 5146 public static final String SP_TRIGGER_DESCRIPTION = "trigger-description"; 5147 /** 5148 * <b>Fluent Client</b> search parameter constant for <b>trigger-description</b> 5149 * <p> 5150 * Description: <b>Text representation of the trigger</b><br> 5151 * Type: <b>string</b><br> 5152 * Path: <b>SubscriptionTopic.resourceTrigger.description</b><br> 5153 * </p> 5154 */ 5155 public static final ca.uhn.fhir.rest.gclient.StringClientParam TRIGGER_DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TRIGGER_DESCRIPTION); 5156 5157 5158} 5159