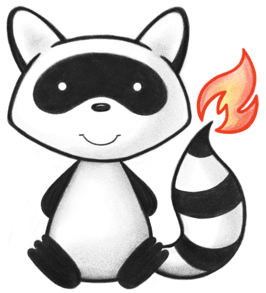
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A homogeneous material with a definite composition. 052 */ 053@ResourceDef(name="Substance", profile="http://hl7.org/fhir/StructureDefinition/Substance") 054public class Substance extends DomainResource { 055 056 public enum FHIRSubstanceStatus { 057 /** 058 * The substance is considered for use or reference. 059 */ 060 ACTIVE, 061 /** 062 * The substance is considered for reference, but not for use. 063 */ 064 INACTIVE, 065 /** 066 * The substance was entered in error. 067 */ 068 ENTEREDINERROR, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static FHIRSubstanceStatus fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("active".equals(codeString)) 077 return ACTIVE; 078 if ("inactive".equals(codeString)) 079 return INACTIVE; 080 if ("entered-in-error".equals(codeString)) 081 return ENTEREDINERROR; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown FHIRSubstanceStatus code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case ACTIVE: return "active"; 090 case INACTIVE: return "inactive"; 091 case ENTEREDINERROR: return "entered-in-error"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case ACTIVE: return "http://hl7.org/fhir/substance-status"; 099 case INACTIVE: return "http://hl7.org/fhir/substance-status"; 100 case ENTEREDINERROR: return "http://hl7.org/fhir/substance-status"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case ACTIVE: return "The substance is considered for use or reference."; 108 case INACTIVE: return "The substance is considered for reference, but not for use."; 109 case ENTEREDINERROR: return "The substance was entered in error."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case ACTIVE: return "Active"; 117 case INACTIVE: return "Inactive"; 118 case ENTEREDINERROR: return "Entered in Error"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class FHIRSubstanceStatusEnumFactory implements EnumFactory<FHIRSubstanceStatus> { 126 public FHIRSubstanceStatus fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("active".equals(codeString)) 131 return FHIRSubstanceStatus.ACTIVE; 132 if ("inactive".equals(codeString)) 133 return FHIRSubstanceStatus.INACTIVE; 134 if ("entered-in-error".equals(codeString)) 135 return FHIRSubstanceStatus.ENTEREDINERROR; 136 throw new IllegalArgumentException("Unknown FHIRSubstanceStatus code '"+codeString+"'"); 137 } 138 public Enumeration<FHIRSubstanceStatus> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<FHIRSubstanceStatus>(this, FHIRSubstanceStatus.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<FHIRSubstanceStatus>(this, FHIRSubstanceStatus.NULL, code); 146 if ("active".equals(codeString)) 147 return new Enumeration<FHIRSubstanceStatus>(this, FHIRSubstanceStatus.ACTIVE, code); 148 if ("inactive".equals(codeString)) 149 return new Enumeration<FHIRSubstanceStatus>(this, FHIRSubstanceStatus.INACTIVE, code); 150 if ("entered-in-error".equals(codeString)) 151 return new Enumeration<FHIRSubstanceStatus>(this, FHIRSubstanceStatus.ENTEREDINERROR, code); 152 throw new FHIRException("Unknown FHIRSubstanceStatus code '"+codeString+"'"); 153 } 154 public String toCode(FHIRSubstanceStatus code) { 155 if (code == FHIRSubstanceStatus.NULL) 156 return null; 157 if (code == FHIRSubstanceStatus.ACTIVE) 158 return "active"; 159 if (code == FHIRSubstanceStatus.INACTIVE) 160 return "inactive"; 161 if (code == FHIRSubstanceStatus.ENTEREDINERROR) 162 return "entered-in-error"; 163 return "?"; 164 } 165 public String toSystem(FHIRSubstanceStatus code) { 166 return code.getSystem(); 167 } 168 } 169 170 @Block() 171 public static class SubstanceIngredientComponent extends BackboneElement implements IBaseBackboneElement { 172 /** 173 * The amount of the ingredient in the substance - a concentration ratio. 174 */ 175 @Child(name = "quantity", type = {Ratio.class}, order=1, min=0, max=1, modifier=false, summary=true) 176 @Description(shortDefinition="Optional amount (concentration)", formalDefinition="The amount of the ingredient in the substance - a concentration ratio." ) 177 protected Ratio quantity; 178 179 /** 180 * Another substance that is a component of this substance. 181 */ 182 @Child(name = "substance", type = {CodeableConcept.class, Substance.class}, order=2, min=1, max=1, modifier=false, summary=true) 183 @Description(shortDefinition="A component of the substance", formalDefinition="Another substance that is a component of this substance." ) 184 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-code") 185 protected DataType substance; 186 187 private static final long serialVersionUID = 2068995180L; 188 189 /** 190 * Constructor 191 */ 192 public SubstanceIngredientComponent() { 193 super(); 194 } 195 196 /** 197 * Constructor 198 */ 199 public SubstanceIngredientComponent(DataType substance) { 200 super(); 201 this.setSubstance(substance); 202 } 203 204 /** 205 * @return {@link #quantity} (The amount of the ingredient in the substance - a concentration ratio.) 206 */ 207 public Ratio getQuantity() { 208 if (this.quantity == null) 209 if (Configuration.errorOnAutoCreate()) 210 throw new Error("Attempt to auto-create SubstanceIngredientComponent.quantity"); 211 else if (Configuration.doAutoCreate()) 212 this.quantity = new Ratio(); // cc 213 return this.quantity; 214 } 215 216 public boolean hasQuantity() { 217 return this.quantity != null && !this.quantity.isEmpty(); 218 } 219 220 /** 221 * @param value {@link #quantity} (The amount of the ingredient in the substance - a concentration ratio.) 222 */ 223 public SubstanceIngredientComponent setQuantity(Ratio value) { 224 this.quantity = value; 225 return this; 226 } 227 228 /** 229 * @return {@link #substance} (Another substance that is a component of this substance.) 230 */ 231 public DataType getSubstance() { 232 return this.substance; 233 } 234 235 /** 236 * @return {@link #substance} (Another substance that is a component of this substance.) 237 */ 238 public CodeableConcept getSubstanceCodeableConcept() throws FHIRException { 239 if (this.substance == null) 240 this.substance = new CodeableConcept(); 241 if (!(this.substance instanceof CodeableConcept)) 242 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.substance.getClass().getName()+" was encountered"); 243 return (CodeableConcept) this.substance; 244 } 245 246 public boolean hasSubstanceCodeableConcept() { 247 return this.substance instanceof CodeableConcept; 248 } 249 250 /** 251 * @return {@link #substance} (Another substance that is a component of this substance.) 252 */ 253 public Reference getSubstanceReference() throws FHIRException { 254 if (this.substance == null) 255 this.substance = new Reference(); 256 if (!(this.substance instanceof Reference)) 257 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.substance.getClass().getName()+" was encountered"); 258 return (Reference) this.substance; 259 } 260 261 public boolean hasSubstanceReference() { 262 return this.substance instanceof Reference; 263 } 264 265 public boolean hasSubstance() { 266 return this.substance != null && !this.substance.isEmpty(); 267 } 268 269 /** 270 * @param value {@link #substance} (Another substance that is a component of this substance.) 271 */ 272 public SubstanceIngredientComponent setSubstance(DataType value) { 273 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 274 throw new FHIRException("Not the right type for Substance.ingredient.substance[x]: "+value.fhirType()); 275 this.substance = value; 276 return this; 277 } 278 279 protected void listChildren(List<Property> children) { 280 super.listChildren(children); 281 children.add(new Property("quantity", "Ratio", "The amount of the ingredient in the substance - a concentration ratio.", 0, 1, quantity)); 282 children.add(new Property("substance[x]", "CodeableConcept|Reference(Substance)", "Another substance that is a component of this substance.", 0, 1, substance)); 283 } 284 285 @Override 286 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 287 switch (_hash) { 288 case -1285004149: /*quantity*/ return new Property("quantity", "Ratio", "The amount of the ingredient in the substance - a concentration ratio.", 0, 1, quantity); 289 case 2127194384: /*substance[x]*/ return new Property("substance[x]", "CodeableConcept|Reference(Substance)", "Another substance that is a component of this substance.", 0, 1, substance); 290 case 530040176: /*substance*/ return new Property("substance[x]", "CodeableConcept|Reference(Substance)", "Another substance that is a component of this substance.", 0, 1, substance); 291 case -1974119407: /*substanceCodeableConcept*/ return new Property("substance[x]", "CodeableConcept", "Another substance that is a component of this substance.", 0, 1, substance); 292 case 516208571: /*substanceReference*/ return new Property("substance[x]", "Reference(Substance)", "Another substance that is a component of this substance.", 0, 1, substance); 293 default: return super.getNamedProperty(_hash, _name, _checkValid); 294 } 295 296 } 297 298 @Override 299 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 300 switch (hash) { 301 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Ratio 302 case 530040176: /*substance*/ return this.substance == null ? new Base[0] : new Base[] {this.substance}; // DataType 303 default: return super.getProperty(hash, name, checkValid); 304 } 305 306 } 307 308 @Override 309 public Base setProperty(int hash, String name, Base value) throws FHIRException { 310 switch (hash) { 311 case -1285004149: // quantity 312 this.quantity = TypeConvertor.castToRatio(value); // Ratio 313 return value; 314 case 530040176: // substance 315 this.substance = TypeConvertor.castToType(value); // DataType 316 return value; 317 default: return super.setProperty(hash, name, value); 318 } 319 320 } 321 322 @Override 323 public Base setProperty(String name, Base value) throws FHIRException { 324 if (name.equals("quantity")) { 325 this.quantity = TypeConvertor.castToRatio(value); // Ratio 326 } else if (name.equals("substance[x]")) { 327 this.substance = TypeConvertor.castToType(value); // DataType 328 } else 329 return super.setProperty(name, value); 330 return value; 331 } 332 333 @Override 334 public void removeChild(String name, Base value) throws FHIRException { 335 if (name.equals("quantity")) { 336 this.quantity = null; 337 } else if (name.equals("substance[x]")) { 338 this.substance = null; 339 } else 340 super.removeChild(name, value); 341 342 } 343 344 @Override 345 public Base makeProperty(int hash, String name) throws FHIRException { 346 switch (hash) { 347 case -1285004149: return getQuantity(); 348 case 2127194384: return getSubstance(); 349 case 530040176: return getSubstance(); 350 default: return super.makeProperty(hash, name); 351 } 352 353 } 354 355 @Override 356 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 357 switch (hash) { 358 case -1285004149: /*quantity*/ return new String[] {"Ratio"}; 359 case 530040176: /*substance*/ return new String[] {"CodeableConcept", "Reference"}; 360 default: return super.getTypesForProperty(hash, name); 361 } 362 363 } 364 365 @Override 366 public Base addChild(String name) throws FHIRException { 367 if (name.equals("quantity")) { 368 this.quantity = new Ratio(); 369 return this.quantity; 370 } 371 else if (name.equals("substanceCodeableConcept")) { 372 this.substance = new CodeableConcept(); 373 return this.substance; 374 } 375 else if (name.equals("substanceReference")) { 376 this.substance = new Reference(); 377 return this.substance; 378 } 379 else 380 return super.addChild(name); 381 } 382 383 public SubstanceIngredientComponent copy() { 384 SubstanceIngredientComponent dst = new SubstanceIngredientComponent(); 385 copyValues(dst); 386 return dst; 387 } 388 389 public void copyValues(SubstanceIngredientComponent dst) { 390 super.copyValues(dst); 391 dst.quantity = quantity == null ? null : quantity.copy(); 392 dst.substance = substance == null ? null : substance.copy(); 393 } 394 395 @Override 396 public boolean equalsDeep(Base other_) { 397 if (!super.equalsDeep(other_)) 398 return false; 399 if (!(other_ instanceof SubstanceIngredientComponent)) 400 return false; 401 SubstanceIngredientComponent o = (SubstanceIngredientComponent) other_; 402 return compareDeep(quantity, o.quantity, true) && compareDeep(substance, o.substance, true); 403 } 404 405 @Override 406 public boolean equalsShallow(Base other_) { 407 if (!super.equalsShallow(other_)) 408 return false; 409 if (!(other_ instanceof SubstanceIngredientComponent)) 410 return false; 411 SubstanceIngredientComponent o = (SubstanceIngredientComponent) other_; 412 return true; 413 } 414 415 public boolean isEmpty() { 416 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(quantity, substance); 417 } 418 419 public String fhirType() { 420 return "Substance.ingredient"; 421 422 } 423 424 } 425 426 /** 427 * Unique identifier for the substance. For an instance, an identifier associated with the package/container (usually a label affixed directly). 428 */ 429 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 430 @Description(shortDefinition="Unique identifier", formalDefinition="Unique identifier for the substance. For an instance, an identifier associated with the package/container (usually a label affixed directly)." ) 431 protected List<Identifier> identifier; 432 433 /** 434 * A boolean to indicate if this an instance of a substance or a kind of one (a definition). 435 */ 436 @Child(name = "instance", type = {BooleanType.class}, order=1, min=1, max=1, modifier=true, summary=true) 437 @Description(shortDefinition="Is this an instance of a substance or a kind of one", formalDefinition="A boolean to indicate if this an instance of a substance or a kind of one (a definition)." ) 438 protected BooleanType instance; 439 440 /** 441 * A code to indicate if the substance is actively used. 442 */ 443 @Child(name = "status", type = {CodeType.class}, order=2, min=0, max=1, modifier=true, summary=true) 444 @Description(shortDefinition="active | inactive | entered-in-error", formalDefinition="A code to indicate if the substance is actively used." ) 445 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-status") 446 protected Enumeration<FHIRSubstanceStatus> status; 447 448 /** 449 * A code that classifies the general type of substance. This is used for searching, sorting and display purposes. 450 */ 451 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 452 @Description(shortDefinition="What class/type of substance this is", formalDefinition="A code that classifies the general type of substance. This is used for searching, sorting and display purposes." ) 453 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-category") 454 protected List<CodeableConcept> category; 455 456 /** 457 * A code (or set of codes) that identify this substance. 458 */ 459 @Child(name = "code", type = {CodeableReference.class}, order=4, min=1, max=1, modifier=false, summary=true) 460 @Description(shortDefinition="What substance this is", formalDefinition="A code (or set of codes) that identify this substance." ) 461 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-code") 462 protected CodeableReference code; 463 464 /** 465 * A description of the substance - its appearance, handling requirements, and other usage notes. 466 */ 467 @Child(name = "description", type = {MarkdownType.class}, order=5, min=0, max=1, modifier=false, summary=true) 468 @Description(shortDefinition="Textual description of the substance, comments", formalDefinition="A description of the substance - its appearance, handling requirements, and other usage notes." ) 469 protected MarkdownType description; 470 471 /** 472 * When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry. 473 */ 474 @Child(name = "expiry", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 475 @Description(shortDefinition="When no longer valid to use", formalDefinition="When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry." ) 476 protected DateTimeType expiry; 477 478 /** 479 * The amount of the substance. 480 */ 481 @Child(name = "quantity", type = {Quantity.class}, order=7, min=0, max=1, modifier=false, summary=true) 482 @Description(shortDefinition="Amount of substance in the package", formalDefinition="The amount of the substance." ) 483 protected Quantity quantity; 484 485 /** 486 * A substance can be composed of other substances. 487 */ 488 @Child(name = "ingredient", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 489 @Description(shortDefinition="Composition information about the substance", formalDefinition="A substance can be composed of other substances." ) 490 protected List<SubstanceIngredientComponent> ingredient; 491 492 private static final long serialVersionUID = -3119765L; 493 494 /** 495 * Constructor 496 */ 497 public Substance() { 498 super(); 499 } 500 501 /** 502 * Constructor 503 */ 504 public Substance(boolean instance, CodeableReference code) { 505 super(); 506 this.setInstance(instance); 507 this.setCode(code); 508 } 509 510 /** 511 * @return {@link #identifier} (Unique identifier for the substance. For an instance, an identifier associated with the package/container (usually a label affixed directly).) 512 */ 513 public List<Identifier> getIdentifier() { 514 if (this.identifier == null) 515 this.identifier = new ArrayList<Identifier>(); 516 return this.identifier; 517 } 518 519 /** 520 * @return Returns a reference to <code>this</code> for easy method chaining 521 */ 522 public Substance setIdentifier(List<Identifier> theIdentifier) { 523 this.identifier = theIdentifier; 524 return this; 525 } 526 527 public boolean hasIdentifier() { 528 if (this.identifier == null) 529 return false; 530 for (Identifier item : this.identifier) 531 if (!item.isEmpty()) 532 return true; 533 return false; 534 } 535 536 public Identifier addIdentifier() { //3 537 Identifier t = new Identifier(); 538 if (this.identifier == null) 539 this.identifier = new ArrayList<Identifier>(); 540 this.identifier.add(t); 541 return t; 542 } 543 544 public Substance addIdentifier(Identifier t) { //3 545 if (t == null) 546 return this; 547 if (this.identifier == null) 548 this.identifier = new ArrayList<Identifier>(); 549 this.identifier.add(t); 550 return this; 551 } 552 553 /** 554 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 555 */ 556 public Identifier getIdentifierFirstRep() { 557 if (getIdentifier().isEmpty()) { 558 addIdentifier(); 559 } 560 return getIdentifier().get(0); 561 } 562 563 /** 564 * @return {@link #instance} (A boolean to indicate if this an instance of a substance or a kind of one (a definition).). This is the underlying object with id, value and extensions. The accessor "getInstance" gives direct access to the value 565 */ 566 public BooleanType getInstanceElement() { 567 if (this.instance == null) 568 if (Configuration.errorOnAutoCreate()) 569 throw new Error("Attempt to auto-create Substance.instance"); 570 else if (Configuration.doAutoCreate()) 571 this.instance = new BooleanType(); // bb 572 return this.instance; 573 } 574 575 public boolean hasInstanceElement() { 576 return this.instance != null && !this.instance.isEmpty(); 577 } 578 579 public boolean hasInstance() { 580 return this.instance != null && !this.instance.isEmpty(); 581 } 582 583 /** 584 * @param value {@link #instance} (A boolean to indicate if this an instance of a substance or a kind of one (a definition).). This is the underlying object with id, value and extensions. The accessor "getInstance" gives direct access to the value 585 */ 586 public Substance setInstanceElement(BooleanType value) { 587 this.instance = value; 588 return this; 589 } 590 591 /** 592 * @return A boolean to indicate if this an instance of a substance or a kind of one (a definition). 593 */ 594 public boolean getInstance() { 595 return this.instance == null || this.instance.isEmpty() ? false : this.instance.getValue(); 596 } 597 598 /** 599 * @param value A boolean to indicate if this an instance of a substance or a kind of one (a definition). 600 */ 601 public Substance setInstance(boolean value) { 602 if (this.instance == null) 603 this.instance = new BooleanType(); 604 this.instance.setValue(value); 605 return this; 606 } 607 608 /** 609 * @return {@link #status} (A code to indicate if the substance is actively used.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 610 */ 611 public Enumeration<FHIRSubstanceStatus> getStatusElement() { 612 if (this.status == null) 613 if (Configuration.errorOnAutoCreate()) 614 throw new Error("Attempt to auto-create Substance.status"); 615 else if (Configuration.doAutoCreate()) 616 this.status = new Enumeration<FHIRSubstanceStatus>(new FHIRSubstanceStatusEnumFactory()); // bb 617 return this.status; 618 } 619 620 public boolean hasStatusElement() { 621 return this.status != null && !this.status.isEmpty(); 622 } 623 624 public boolean hasStatus() { 625 return this.status != null && !this.status.isEmpty(); 626 } 627 628 /** 629 * @param value {@link #status} (A code to indicate if the substance is actively used.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 630 */ 631 public Substance setStatusElement(Enumeration<FHIRSubstanceStatus> value) { 632 this.status = value; 633 return this; 634 } 635 636 /** 637 * @return A code to indicate if the substance is actively used. 638 */ 639 public FHIRSubstanceStatus getStatus() { 640 return this.status == null ? null : this.status.getValue(); 641 } 642 643 /** 644 * @param value A code to indicate if the substance is actively used. 645 */ 646 public Substance setStatus(FHIRSubstanceStatus value) { 647 if (value == null) 648 this.status = null; 649 else { 650 if (this.status == null) 651 this.status = new Enumeration<FHIRSubstanceStatus>(new FHIRSubstanceStatusEnumFactory()); 652 this.status.setValue(value); 653 } 654 return this; 655 } 656 657 /** 658 * @return {@link #category} (A code that classifies the general type of substance. This is used for searching, sorting and display purposes.) 659 */ 660 public List<CodeableConcept> getCategory() { 661 if (this.category == null) 662 this.category = new ArrayList<CodeableConcept>(); 663 return this.category; 664 } 665 666 /** 667 * @return Returns a reference to <code>this</code> for easy method chaining 668 */ 669 public Substance setCategory(List<CodeableConcept> theCategory) { 670 this.category = theCategory; 671 return this; 672 } 673 674 public boolean hasCategory() { 675 if (this.category == null) 676 return false; 677 for (CodeableConcept item : this.category) 678 if (!item.isEmpty()) 679 return true; 680 return false; 681 } 682 683 public CodeableConcept addCategory() { //3 684 CodeableConcept t = new CodeableConcept(); 685 if (this.category == null) 686 this.category = new ArrayList<CodeableConcept>(); 687 this.category.add(t); 688 return t; 689 } 690 691 public Substance addCategory(CodeableConcept t) { //3 692 if (t == null) 693 return this; 694 if (this.category == null) 695 this.category = new ArrayList<CodeableConcept>(); 696 this.category.add(t); 697 return this; 698 } 699 700 /** 701 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 702 */ 703 public CodeableConcept getCategoryFirstRep() { 704 if (getCategory().isEmpty()) { 705 addCategory(); 706 } 707 return getCategory().get(0); 708 } 709 710 /** 711 * @return {@link #code} (A code (or set of codes) that identify this substance.) 712 */ 713 public CodeableReference getCode() { 714 if (this.code == null) 715 if (Configuration.errorOnAutoCreate()) 716 throw new Error("Attempt to auto-create Substance.code"); 717 else if (Configuration.doAutoCreate()) 718 this.code = new CodeableReference(); // cc 719 return this.code; 720 } 721 722 public boolean hasCode() { 723 return this.code != null && !this.code.isEmpty(); 724 } 725 726 /** 727 * @param value {@link #code} (A code (or set of codes) that identify this substance.) 728 */ 729 public Substance setCode(CodeableReference value) { 730 this.code = value; 731 return this; 732 } 733 734 /** 735 * @return {@link #description} (A description of the substance - its appearance, handling requirements, and other usage notes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 736 */ 737 public MarkdownType getDescriptionElement() { 738 if (this.description == null) 739 if (Configuration.errorOnAutoCreate()) 740 throw new Error("Attempt to auto-create Substance.description"); 741 else if (Configuration.doAutoCreate()) 742 this.description = new MarkdownType(); // bb 743 return this.description; 744 } 745 746 public boolean hasDescriptionElement() { 747 return this.description != null && !this.description.isEmpty(); 748 } 749 750 public boolean hasDescription() { 751 return this.description != null && !this.description.isEmpty(); 752 } 753 754 /** 755 * @param value {@link #description} (A description of the substance - its appearance, handling requirements, and other usage notes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 756 */ 757 public Substance setDescriptionElement(MarkdownType value) { 758 this.description = value; 759 return this; 760 } 761 762 /** 763 * @return A description of the substance - its appearance, handling requirements, and other usage notes. 764 */ 765 public String getDescription() { 766 return this.description == null ? null : this.description.getValue(); 767 } 768 769 /** 770 * @param value A description of the substance - its appearance, handling requirements, and other usage notes. 771 */ 772 public Substance setDescription(String value) { 773 if (Utilities.noString(value)) 774 this.description = null; 775 else { 776 if (this.description == null) 777 this.description = new MarkdownType(); 778 this.description.setValue(value); 779 } 780 return this; 781 } 782 783 /** 784 * @return {@link #expiry} (When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry.). This is the underlying object with id, value and extensions. The accessor "getExpiry" gives direct access to the value 785 */ 786 public DateTimeType getExpiryElement() { 787 if (this.expiry == null) 788 if (Configuration.errorOnAutoCreate()) 789 throw new Error("Attempt to auto-create Substance.expiry"); 790 else if (Configuration.doAutoCreate()) 791 this.expiry = new DateTimeType(); // bb 792 return this.expiry; 793 } 794 795 public boolean hasExpiryElement() { 796 return this.expiry != null && !this.expiry.isEmpty(); 797 } 798 799 public boolean hasExpiry() { 800 return this.expiry != null && !this.expiry.isEmpty(); 801 } 802 803 /** 804 * @param value {@link #expiry} (When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry.). This is the underlying object with id, value and extensions. The accessor "getExpiry" gives direct access to the value 805 */ 806 public Substance setExpiryElement(DateTimeType value) { 807 this.expiry = value; 808 return this; 809 } 810 811 /** 812 * @return When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry. 813 */ 814 public Date getExpiry() { 815 return this.expiry == null ? null : this.expiry.getValue(); 816 } 817 818 /** 819 * @param value When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry. 820 */ 821 public Substance setExpiry(Date value) { 822 if (value == null) 823 this.expiry = null; 824 else { 825 if (this.expiry == null) 826 this.expiry = new DateTimeType(); 827 this.expiry.setValue(value); 828 } 829 return this; 830 } 831 832 /** 833 * @return {@link #quantity} (The amount of the substance.) 834 */ 835 public Quantity getQuantity() { 836 if (this.quantity == null) 837 if (Configuration.errorOnAutoCreate()) 838 throw new Error("Attempt to auto-create Substance.quantity"); 839 else if (Configuration.doAutoCreate()) 840 this.quantity = new Quantity(); // cc 841 return this.quantity; 842 } 843 844 public boolean hasQuantity() { 845 return this.quantity != null && !this.quantity.isEmpty(); 846 } 847 848 /** 849 * @param value {@link #quantity} (The amount of the substance.) 850 */ 851 public Substance setQuantity(Quantity value) { 852 this.quantity = value; 853 return this; 854 } 855 856 /** 857 * @return {@link #ingredient} (A substance can be composed of other substances.) 858 */ 859 public List<SubstanceIngredientComponent> getIngredient() { 860 if (this.ingredient == null) 861 this.ingredient = new ArrayList<SubstanceIngredientComponent>(); 862 return this.ingredient; 863 } 864 865 /** 866 * @return Returns a reference to <code>this</code> for easy method chaining 867 */ 868 public Substance setIngredient(List<SubstanceIngredientComponent> theIngredient) { 869 this.ingredient = theIngredient; 870 return this; 871 } 872 873 public boolean hasIngredient() { 874 if (this.ingredient == null) 875 return false; 876 for (SubstanceIngredientComponent item : this.ingredient) 877 if (!item.isEmpty()) 878 return true; 879 return false; 880 } 881 882 public SubstanceIngredientComponent addIngredient() { //3 883 SubstanceIngredientComponent t = new SubstanceIngredientComponent(); 884 if (this.ingredient == null) 885 this.ingredient = new ArrayList<SubstanceIngredientComponent>(); 886 this.ingredient.add(t); 887 return t; 888 } 889 890 public Substance addIngredient(SubstanceIngredientComponent t) { //3 891 if (t == null) 892 return this; 893 if (this.ingredient == null) 894 this.ingredient = new ArrayList<SubstanceIngredientComponent>(); 895 this.ingredient.add(t); 896 return this; 897 } 898 899 /** 900 * @return The first repetition of repeating field {@link #ingredient}, creating it if it does not already exist {3} 901 */ 902 public SubstanceIngredientComponent getIngredientFirstRep() { 903 if (getIngredient().isEmpty()) { 904 addIngredient(); 905 } 906 return getIngredient().get(0); 907 } 908 909 protected void listChildren(List<Property> children) { 910 super.listChildren(children); 911 children.add(new Property("identifier", "Identifier", "Unique identifier for the substance. For an instance, an identifier associated with the package/container (usually a label affixed directly).", 0, java.lang.Integer.MAX_VALUE, identifier)); 912 children.add(new Property("instance", "boolean", "A boolean to indicate if this an instance of a substance or a kind of one (a definition).", 0, 1, instance)); 913 children.add(new Property("status", "code", "A code to indicate if the substance is actively used.", 0, 1, status)); 914 children.add(new Property("category", "CodeableConcept", "A code that classifies the general type of substance. This is used for searching, sorting and display purposes.", 0, java.lang.Integer.MAX_VALUE, category)); 915 children.add(new Property("code", "CodeableReference(SubstanceDefinition)", "A code (or set of codes) that identify this substance.", 0, 1, code)); 916 children.add(new Property("description", "markdown", "A description of the substance - its appearance, handling requirements, and other usage notes.", 0, 1, description)); 917 children.add(new Property("expiry", "dateTime", "When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry.", 0, 1, expiry)); 918 children.add(new Property("quantity", "Quantity", "The amount of the substance.", 0, 1, quantity)); 919 children.add(new Property("ingredient", "", "A substance can be composed of other substances.", 0, java.lang.Integer.MAX_VALUE, ingredient)); 920 } 921 922 @Override 923 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 924 switch (_hash) { 925 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique identifier for the substance. For an instance, an identifier associated with the package/container (usually a label affixed directly).", 0, java.lang.Integer.MAX_VALUE, identifier); 926 case 555127957: /*instance*/ return new Property("instance", "boolean", "A boolean to indicate if this an instance of a substance or a kind of one (a definition).", 0, 1, instance); 927 case -892481550: /*status*/ return new Property("status", "code", "A code to indicate if the substance is actively used.", 0, 1, status); 928 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code that classifies the general type of substance. This is used for searching, sorting and display purposes.", 0, java.lang.Integer.MAX_VALUE, category); 929 case 3059181: /*code*/ return new Property("code", "CodeableReference(SubstanceDefinition)", "A code (or set of codes) that identify this substance.", 0, 1, code); 930 case -1724546052: /*description*/ return new Property("description", "markdown", "A description of the substance - its appearance, handling requirements, and other usage notes.", 0, 1, description); 931 case -1289159373: /*expiry*/ return new Property("expiry", "dateTime", "When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry.", 0, 1, expiry); 932 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The amount of the substance.", 0, 1, quantity); 933 case -206409263: /*ingredient*/ return new Property("ingredient", "", "A substance can be composed of other substances.", 0, java.lang.Integer.MAX_VALUE, ingredient); 934 default: return super.getNamedProperty(_hash, _name, _checkValid); 935 } 936 937 } 938 939 @Override 940 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 941 switch (hash) { 942 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 943 case 555127957: /*instance*/ return this.instance == null ? new Base[0] : new Base[] {this.instance}; // BooleanType 944 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FHIRSubstanceStatus> 945 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 946 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableReference 947 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 948 case -1289159373: /*expiry*/ return this.expiry == null ? new Base[0] : new Base[] {this.expiry}; // DateTimeType 949 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 950 case -206409263: /*ingredient*/ return this.ingredient == null ? new Base[0] : this.ingredient.toArray(new Base[this.ingredient.size()]); // SubstanceIngredientComponent 951 default: return super.getProperty(hash, name, checkValid); 952 } 953 954 } 955 956 @Override 957 public Base setProperty(int hash, String name, Base value) throws FHIRException { 958 switch (hash) { 959 case -1618432855: // identifier 960 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 961 return value; 962 case 555127957: // instance 963 this.instance = TypeConvertor.castToBoolean(value); // BooleanType 964 return value; 965 case -892481550: // status 966 value = new FHIRSubstanceStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 967 this.status = (Enumeration) value; // Enumeration<FHIRSubstanceStatus> 968 return value; 969 case 50511102: // category 970 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 971 return value; 972 case 3059181: // code 973 this.code = TypeConvertor.castToCodeableReference(value); // CodeableReference 974 return value; 975 case -1724546052: // description 976 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 977 return value; 978 case -1289159373: // expiry 979 this.expiry = TypeConvertor.castToDateTime(value); // DateTimeType 980 return value; 981 case -1285004149: // quantity 982 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 983 return value; 984 case -206409263: // ingredient 985 this.getIngredient().add((SubstanceIngredientComponent) value); // SubstanceIngredientComponent 986 return value; 987 default: return super.setProperty(hash, name, value); 988 } 989 990 } 991 992 @Override 993 public Base setProperty(String name, Base value) throws FHIRException { 994 if (name.equals("identifier")) { 995 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 996 } else if (name.equals("instance")) { 997 this.instance = TypeConvertor.castToBoolean(value); // BooleanType 998 } else if (name.equals("status")) { 999 value = new FHIRSubstanceStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1000 this.status = (Enumeration) value; // Enumeration<FHIRSubstanceStatus> 1001 } else if (name.equals("category")) { 1002 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 1003 } else if (name.equals("code")) { 1004 this.code = TypeConvertor.castToCodeableReference(value); // CodeableReference 1005 } else if (name.equals("description")) { 1006 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1007 } else if (name.equals("expiry")) { 1008 this.expiry = TypeConvertor.castToDateTime(value); // DateTimeType 1009 } else if (name.equals("quantity")) { 1010 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1011 } else if (name.equals("ingredient")) { 1012 this.getIngredient().add((SubstanceIngredientComponent) value); 1013 } else 1014 return super.setProperty(name, value); 1015 return value; 1016 } 1017 1018 @Override 1019 public void removeChild(String name, Base value) throws FHIRException { 1020 if (name.equals("identifier")) { 1021 this.getIdentifier().remove(value); 1022 } else if (name.equals("instance")) { 1023 this.instance = null; 1024 } else if (name.equals("status")) { 1025 value = new FHIRSubstanceStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1026 this.status = (Enumeration) value; // Enumeration<FHIRSubstanceStatus> 1027 } else if (name.equals("category")) { 1028 this.getCategory().remove(value); 1029 } else if (name.equals("code")) { 1030 this.code = null; 1031 } else if (name.equals("description")) { 1032 this.description = null; 1033 } else if (name.equals("expiry")) { 1034 this.expiry = null; 1035 } else if (name.equals("quantity")) { 1036 this.quantity = null; 1037 } else if (name.equals("ingredient")) { 1038 this.getIngredient().remove((SubstanceIngredientComponent) value); 1039 } else 1040 super.removeChild(name, value); 1041 1042 } 1043 1044 @Override 1045 public Base makeProperty(int hash, String name) throws FHIRException { 1046 switch (hash) { 1047 case -1618432855: return addIdentifier(); 1048 case 555127957: return getInstanceElement(); 1049 case -892481550: return getStatusElement(); 1050 case 50511102: return addCategory(); 1051 case 3059181: return getCode(); 1052 case -1724546052: return getDescriptionElement(); 1053 case -1289159373: return getExpiryElement(); 1054 case -1285004149: return getQuantity(); 1055 case -206409263: return addIngredient(); 1056 default: return super.makeProperty(hash, name); 1057 } 1058 1059 } 1060 1061 @Override 1062 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1063 switch (hash) { 1064 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1065 case 555127957: /*instance*/ return new String[] {"boolean"}; 1066 case -892481550: /*status*/ return new String[] {"code"}; 1067 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1068 case 3059181: /*code*/ return new String[] {"CodeableReference"}; 1069 case -1724546052: /*description*/ return new String[] {"markdown"}; 1070 case -1289159373: /*expiry*/ return new String[] {"dateTime"}; 1071 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 1072 case -206409263: /*ingredient*/ return new String[] {}; 1073 default: return super.getTypesForProperty(hash, name); 1074 } 1075 1076 } 1077 1078 @Override 1079 public Base addChild(String name) throws FHIRException { 1080 if (name.equals("identifier")) { 1081 return addIdentifier(); 1082 } 1083 else if (name.equals("instance")) { 1084 throw new FHIRException("Cannot call addChild on a singleton property Substance.instance"); 1085 } 1086 else if (name.equals("status")) { 1087 throw new FHIRException("Cannot call addChild on a singleton property Substance.status"); 1088 } 1089 else if (name.equals("category")) { 1090 return addCategory(); 1091 } 1092 else if (name.equals("code")) { 1093 this.code = new CodeableReference(); 1094 return this.code; 1095 } 1096 else if (name.equals("description")) { 1097 throw new FHIRException("Cannot call addChild on a singleton property Substance.description"); 1098 } 1099 else if (name.equals("expiry")) { 1100 throw new FHIRException("Cannot call addChild on a singleton property Substance.expiry"); 1101 } 1102 else if (name.equals("quantity")) { 1103 this.quantity = new Quantity(); 1104 return this.quantity; 1105 } 1106 else if (name.equals("ingredient")) { 1107 return addIngredient(); 1108 } 1109 else 1110 return super.addChild(name); 1111 } 1112 1113 public String fhirType() { 1114 return "Substance"; 1115 1116 } 1117 1118 public Substance copy() { 1119 Substance dst = new Substance(); 1120 copyValues(dst); 1121 return dst; 1122 } 1123 1124 public void copyValues(Substance dst) { 1125 super.copyValues(dst); 1126 if (identifier != null) { 1127 dst.identifier = new ArrayList<Identifier>(); 1128 for (Identifier i : identifier) 1129 dst.identifier.add(i.copy()); 1130 }; 1131 dst.instance = instance == null ? null : instance.copy(); 1132 dst.status = status == null ? null : status.copy(); 1133 if (category != null) { 1134 dst.category = new ArrayList<CodeableConcept>(); 1135 for (CodeableConcept i : category) 1136 dst.category.add(i.copy()); 1137 }; 1138 dst.code = code == null ? null : code.copy(); 1139 dst.description = description == null ? null : description.copy(); 1140 dst.expiry = expiry == null ? null : expiry.copy(); 1141 dst.quantity = quantity == null ? null : quantity.copy(); 1142 if (ingredient != null) { 1143 dst.ingredient = new ArrayList<SubstanceIngredientComponent>(); 1144 for (SubstanceIngredientComponent i : ingredient) 1145 dst.ingredient.add(i.copy()); 1146 }; 1147 } 1148 1149 protected Substance typedCopy() { 1150 return copy(); 1151 } 1152 1153 @Override 1154 public boolean equalsDeep(Base other_) { 1155 if (!super.equalsDeep(other_)) 1156 return false; 1157 if (!(other_ instanceof Substance)) 1158 return false; 1159 Substance o = (Substance) other_; 1160 return compareDeep(identifier, o.identifier, true) && compareDeep(instance, o.instance, true) && compareDeep(status, o.status, true) 1161 && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) && compareDeep(description, o.description, true) 1162 && compareDeep(expiry, o.expiry, true) && compareDeep(quantity, o.quantity, true) && compareDeep(ingredient, o.ingredient, true) 1163 ; 1164 } 1165 1166 @Override 1167 public boolean equalsShallow(Base other_) { 1168 if (!super.equalsShallow(other_)) 1169 return false; 1170 if (!(other_ instanceof Substance)) 1171 return false; 1172 Substance o = (Substance) other_; 1173 return compareValues(instance, o.instance, true) && compareValues(status, o.status, true) && compareValues(description, o.description, true) 1174 && compareValues(expiry, o.expiry, true); 1175 } 1176 1177 public boolean isEmpty() { 1178 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instance, status 1179 , category, code, description, expiry, quantity, ingredient); 1180 } 1181 1182 @Override 1183 public ResourceType getResourceType() { 1184 return ResourceType.Substance; 1185 } 1186 1187 /** 1188 * Search parameter: <b>category</b> 1189 * <p> 1190 * Description: <b>The category of the substance</b><br> 1191 * Type: <b>token</b><br> 1192 * Path: <b>Substance.category</b><br> 1193 * </p> 1194 */ 1195 @SearchParamDefinition(name="category", path="Substance.category", description="The category of the substance", type="token" ) 1196 public static final String SP_CATEGORY = "category"; 1197 /** 1198 * <b>Fluent Client</b> search parameter constant for <b>category</b> 1199 * <p> 1200 * Description: <b>The category of the substance</b><br> 1201 * Type: <b>token</b><br> 1202 * Path: <b>Substance.category</b><br> 1203 * </p> 1204 */ 1205 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 1206 1207 /** 1208 * Search parameter: <b>code-reference</b> 1209 * <p> 1210 * Description: <b>A reference to the defining substance</b><br> 1211 * Type: <b>reference</b><br> 1212 * Path: <b>Substance.code.reference</b><br> 1213 * </p> 1214 */ 1215 @SearchParamDefinition(name="code-reference", path="Substance.code.reference", description="A reference to the defining substance", type="reference", target={SubstanceDefinition.class } ) 1216 public static final String SP_CODE_REFERENCE = "code-reference"; 1217 /** 1218 * <b>Fluent Client</b> search parameter constant for <b>code-reference</b> 1219 * <p> 1220 * Description: <b>A reference to the defining substance</b><br> 1221 * Type: <b>reference</b><br> 1222 * Path: <b>Substance.code.reference</b><br> 1223 * </p> 1224 */ 1225 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CODE_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CODE_REFERENCE); 1226 1227/** 1228 * Constant for fluent queries to be used to add include statements. Specifies 1229 * the path value of "<b>Substance:code-reference</b>". 1230 */ 1231 public static final ca.uhn.fhir.model.api.Include INCLUDE_CODE_REFERENCE = new ca.uhn.fhir.model.api.Include("Substance:code-reference").toLocked(); 1232 1233 /** 1234 * Search parameter: <b>code</b> 1235 * <p> 1236 * Description: <b>The code of the substance or ingredient</b><br> 1237 * Type: <b>token</b><br> 1238 * Path: <b>Substance.code.concept | (Substance.ingredient.substance.ofType(CodeableConcept))</b><br> 1239 * </p> 1240 */ 1241 @SearchParamDefinition(name="code", path="Substance.code.concept | (Substance.ingredient.substance.ofType(CodeableConcept))", description="The code of the substance or ingredient", type="token" ) 1242 public static final String SP_CODE = "code"; 1243 /** 1244 * <b>Fluent Client</b> search parameter constant for <b>code</b> 1245 * <p> 1246 * Description: <b>The code of the substance or ingredient</b><br> 1247 * Type: <b>token</b><br> 1248 * Path: <b>Substance.code.concept | (Substance.ingredient.substance.ofType(CodeableConcept))</b><br> 1249 * </p> 1250 */ 1251 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 1252 1253 /** 1254 * Search parameter: <b>expiry</b> 1255 * <p> 1256 * Description: <b>Expiry date of package or container of substance</b><br> 1257 * Type: <b>date</b><br> 1258 * Path: <b>Substance.expiry</b><br> 1259 * </p> 1260 */ 1261 @SearchParamDefinition(name="expiry", path="Substance.expiry", description="Expiry date of package or container of substance", type="date" ) 1262 public static final String SP_EXPIRY = "expiry"; 1263 /** 1264 * <b>Fluent Client</b> search parameter constant for <b>expiry</b> 1265 * <p> 1266 * Description: <b>Expiry date of package or container of substance</b><br> 1267 * Type: <b>date</b><br> 1268 * Path: <b>Substance.expiry</b><br> 1269 * </p> 1270 */ 1271 public static final ca.uhn.fhir.rest.gclient.DateClientParam EXPIRY = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EXPIRY); 1272 1273 /** 1274 * Search parameter: <b>identifier</b> 1275 * <p> 1276 * Description: <b>Unique identifier for the substance</b><br> 1277 * Type: <b>token</b><br> 1278 * Path: <b>Substance.identifier</b><br> 1279 * </p> 1280 */ 1281 @SearchParamDefinition(name="identifier", path="Substance.identifier", description="Unique identifier for the substance", type="token" ) 1282 public static final String SP_IDENTIFIER = "identifier"; 1283 /** 1284 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1285 * <p> 1286 * Description: <b>Unique identifier for the substance</b><br> 1287 * Type: <b>token</b><br> 1288 * Path: <b>Substance.identifier</b><br> 1289 * </p> 1290 */ 1291 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1292 1293 /** 1294 * Search parameter: <b>quantity</b> 1295 * <p> 1296 * Description: <b>Amount of substance in the package</b><br> 1297 * Type: <b>quantity</b><br> 1298 * Path: <b>Substance.quantity</b><br> 1299 * </p> 1300 */ 1301 @SearchParamDefinition(name="quantity", path="Substance.quantity", description="Amount of substance in the package", type="quantity" ) 1302 public static final String SP_QUANTITY = "quantity"; 1303 /** 1304 * <b>Fluent Client</b> search parameter constant for <b>quantity</b> 1305 * <p> 1306 * Description: <b>Amount of substance in the package</b><br> 1307 * Type: <b>quantity</b><br> 1308 * Path: <b>Substance.quantity</b><br> 1309 * </p> 1310 */ 1311 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_QUANTITY); 1312 1313 /** 1314 * Search parameter: <b>status</b> 1315 * <p> 1316 * Description: <b>active | inactive | entered-in-error</b><br> 1317 * Type: <b>token</b><br> 1318 * Path: <b>Substance.status</b><br> 1319 * </p> 1320 */ 1321 @SearchParamDefinition(name="status", path="Substance.status", description="active | inactive | entered-in-error", type="token" ) 1322 public static final String SP_STATUS = "status"; 1323 /** 1324 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1325 * <p> 1326 * Description: <b>active | inactive | entered-in-error</b><br> 1327 * Type: <b>token</b><br> 1328 * Path: <b>Substance.status</b><br> 1329 * </p> 1330 */ 1331 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1332 1333 /** 1334 * Search parameter: <b>substance-reference</b> 1335 * <p> 1336 * Description: <b>A component of the substance</b><br> 1337 * Type: <b>reference</b><br> 1338 * Path: <b>(Substance.ingredient.substance.ofType(Reference))</b><br> 1339 * </p> 1340 */ 1341 @SearchParamDefinition(name="substance-reference", path="(Substance.ingredient.substance.ofType(Reference))", description="A component of the substance", type="reference", target={Substance.class } ) 1342 public static final String SP_SUBSTANCE_REFERENCE = "substance-reference"; 1343 /** 1344 * <b>Fluent Client</b> search parameter constant for <b>substance-reference</b> 1345 * <p> 1346 * Description: <b>A component of the substance</b><br> 1347 * Type: <b>reference</b><br> 1348 * Path: <b>(Substance.ingredient.substance.ofType(Reference))</b><br> 1349 * </p> 1350 */ 1351 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBSTANCE_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBSTANCE_REFERENCE); 1352 1353/** 1354 * Constant for fluent queries to be used to add include statements. Specifies 1355 * the path value of "<b>Substance:substance-reference</b>". 1356 */ 1357 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBSTANCE_REFERENCE = new ca.uhn.fhir.model.api.Include("Substance:substance-reference").toLocked(); 1358 1359 1360} 1361