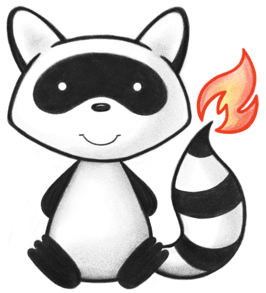
001package org.hl7.fhir.r5.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, \ 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this \ 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, \ 016 this list of conditions and the following disclaimer in the documentation \ 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 031 POSSIBILITY OF SUCH DAMAGE. 032 */ 033 034// Generated on Mon, May 11, 2020 09:58+1000 for FHIR v5.0.0-snapshot2 035 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039import org.hl7.fhir.utilities.Utilities; 040import org.hl7.fhir.r5.model.Enumerations.*; 041import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 042import org.hl7.fhir.exceptions.FHIRException; 043import org.hl7.fhir.instance.model.api.ICompositeType; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.DatatypeDef; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Base StructureDefinition for SubstanceAmount Type: Chemical substances are a single substance type whose primary defining element is the molecular structure. Chemical substances shall be defined on the basis of their complete covalent molecular structure; the presence of a salt (counter-ion) and/or solvates (water, alcohols) is also captured. Purity, grade, physical form or particle size are not taken into account in the definition of a chemical substance or in the assignment of a Substance ID. 052 */ 053@DatatypeDef(name="SubstanceAmount") 054public class SubstanceAmount extends BackboneType implements ICompositeType { 055 056 @Block() 057 public static class SubstanceAmountReferenceRangeComponent extends Element implements IBaseDatatypeElement { 058 /** 059 * Lower limit possible or expected. 060 */ 061 @Child(name = "lowLimit", type = {Quantity.class}, order=1, min=0, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="Lower limit possible or expected", formalDefinition="Lower limit possible or expected." ) 063 protected Quantity lowLimit; 064 065 /** 066 * Upper limit possible or expected. 067 */ 068 @Child(name = "highLimit", type = {Quantity.class}, order=2, min=0, max=1, modifier=false, summary=true) 069 @Description(shortDefinition="Upper limit possible or expected", formalDefinition="Upper limit possible or expected." ) 070 protected Quantity highLimit; 071 072 private static final long serialVersionUID = -193230412L; 073 074 /** 075 * Constructor 076 */ 077 public SubstanceAmountReferenceRangeComponent() { 078 super(); 079 } 080 081 /** 082 * @return {@link #lowLimit} (Lower limit possible or expected.) 083 */ 084 public Quantity getLowLimit() { 085 if (this.lowLimit == null) 086 if (Configuration.errorOnAutoCreate()) 087 throw new Error("Attempt to auto-create SubstanceAmountReferenceRangeComponent.lowLimit"); 088 else if (Configuration.doAutoCreate()) 089 this.lowLimit = new Quantity(); // cc 090 return this.lowLimit; 091 } 092 093 public boolean hasLowLimit() { 094 return this.lowLimit != null && !this.lowLimit.isEmpty(); 095 } 096 097 /** 098 * @param value {@link #lowLimit} (Lower limit possible or expected.) 099 */ 100 public SubstanceAmountReferenceRangeComponent setLowLimit(Quantity value) { 101 this.lowLimit = value; 102 return this; 103 } 104 105 /** 106 * @return {@link #highLimit} (Upper limit possible or expected.) 107 */ 108 public Quantity getHighLimit() { 109 if (this.highLimit == null) 110 if (Configuration.errorOnAutoCreate()) 111 throw new Error("Attempt to auto-create SubstanceAmountReferenceRangeComponent.highLimit"); 112 else if (Configuration.doAutoCreate()) 113 this.highLimit = new Quantity(); // cc 114 return this.highLimit; 115 } 116 117 public boolean hasHighLimit() { 118 return this.highLimit != null && !this.highLimit.isEmpty(); 119 } 120 121 /** 122 * @param value {@link #highLimit} (Upper limit possible or expected.) 123 */ 124 public SubstanceAmountReferenceRangeComponent setHighLimit(Quantity value) { 125 this.highLimit = value; 126 return this; 127 } 128 129 protected void listChildren(List<Property> children) { 130 super.listChildren(children); 131 children.add(new Property("lowLimit", "Quantity", "Lower limit possible or expected.", 0, 1, lowLimit)); 132 children.add(new Property("highLimit", "Quantity", "Upper limit possible or expected.", 0, 1, highLimit)); 133 } 134 135 @Override 136 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 137 switch (_hash) { 138 case -1841058617: /*lowLimit*/ return new Property("lowLimit", "Quantity", "Lower limit possible or expected.", 0, 1, lowLimit); 139 case -710757575: /*highLimit*/ return new Property("highLimit", "Quantity", "Upper limit possible or expected.", 0, 1, highLimit); 140 default: return super.getNamedProperty(_hash, _name, _checkValid); 141 } 142 143 } 144 145 @Override 146 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 147 switch (hash) { 148 case -1841058617: /*lowLimit*/ return this.lowLimit == null ? new Base[0] : new Base[] {this.lowLimit}; // Quantity 149 case -710757575: /*highLimit*/ return this.highLimit == null ? new Base[0] : new Base[] {this.highLimit}; // Quantity 150 default: return super.getProperty(hash, name, checkValid); 151 } 152 153 } 154 155 @Override 156 public Base setProperty(int hash, String name, Base value) throws FHIRException { 157 switch (hash) { 158 case -1841058617: // lowLimit 159 this.lowLimit = TypeConvertor.castToQuantity(value); // Quantity 160 return value; 161 case -710757575: // highLimit 162 this.highLimit = TypeConvertor.castToQuantity(value); // Quantity 163 return value; 164 default: return super.setProperty(hash, name, value); 165 } 166 167 } 168 169 @Override 170 public Base setProperty(String name, Base value) throws FHIRException { 171 if (name.equals("lowLimit")) { 172 this.lowLimit = TypeConvertor.castToQuantity(value); // Quantity 173 } else if (name.equals("highLimit")) { 174 this.highLimit = TypeConvertor.castToQuantity(value); // Quantity 175 } else 176 return super.setProperty(name, value); 177 return value; 178 } 179 180 @Override 181 public void removeChild(String name, Base value) throws FHIRException { 182 if (name.equals("lowLimit")) { 183 this.lowLimit = null; 184 } else if (name.equals("highLimit")) { 185 this.highLimit = null; 186 } else 187 super.removeChild(name, value); 188 189 } 190 191 @Override 192 public Base makeProperty(int hash, String name) throws FHIRException { 193 switch (hash) { 194 case -1841058617: return getLowLimit(); 195 case -710757575: return getHighLimit(); 196 default: return super.makeProperty(hash, name); 197 } 198 199 } 200 201 @Override 202 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 203 switch (hash) { 204 case -1841058617: /*lowLimit*/ return new String[] {"Quantity"}; 205 case -710757575: /*highLimit*/ return new String[] {"Quantity"}; 206 default: return super.getTypesForProperty(hash, name); 207 } 208 209 } 210 211 @Override 212 public Base addChild(String name) throws FHIRException { 213 if (name.equals("lowLimit")) { 214 this.lowLimit = new Quantity(); 215 return this.lowLimit; 216 } 217 else if (name.equals("highLimit")) { 218 this.highLimit = new Quantity(); 219 return this.highLimit; 220 } 221 else 222 return super.addChild(name); 223 } 224 225 public SubstanceAmountReferenceRangeComponent copy() { 226 SubstanceAmountReferenceRangeComponent dst = new SubstanceAmountReferenceRangeComponent(); 227 copyValues(dst); 228 return dst; 229 } 230 231 public void copyValues(SubstanceAmountReferenceRangeComponent dst) { 232 super.copyValues(dst); 233 dst.lowLimit = lowLimit == null ? null : lowLimit.copy(); 234 dst.highLimit = highLimit == null ? null : highLimit.copy(); 235 } 236 237 @Override 238 public boolean equalsDeep(Base other_) { 239 if (!super.equalsDeep(other_)) 240 return false; 241 if (!(other_ instanceof SubstanceAmountReferenceRangeComponent)) 242 return false; 243 SubstanceAmountReferenceRangeComponent o = (SubstanceAmountReferenceRangeComponent) other_; 244 return compareDeep(lowLimit, o.lowLimit, true) && compareDeep(highLimit, o.highLimit, true); 245 } 246 247 @Override 248 public boolean equalsShallow(Base other_) { 249 if (!super.equalsShallow(other_)) 250 return false; 251 if (!(other_ instanceof SubstanceAmountReferenceRangeComponent)) 252 return false; 253 SubstanceAmountReferenceRangeComponent o = (SubstanceAmountReferenceRangeComponent) other_; 254 return true; 255 } 256 257 public boolean isEmpty() { 258 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(lowLimit, highLimit); 259 } 260 261 public String fhirType() { 262 return "SubstanceAmount.referenceRange"; 263 264 } 265 266 } 267 268 /** 269 * Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field. 270 */ 271 @Child(name = "amount", type = {Quantity.class, Range.class, StringType.class}, order=0, min=0, max=1, modifier=false, summary=true) 272 @Description(shortDefinition="Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field", formalDefinition="Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field." ) 273 protected DataType amount; 274 275 /** 276 * Most elements that require a quantitative value will also have a field called amount type. Amount type should always be specified because the actual value of the amount is often dependent on it. EXAMPLE: In capturing the actual relative amounts of substances or molecular fragments it is essential to indicate whether the amount refers to a mole ratio or weight ratio. For any given element an effort should be made to use same the amount type for all related definitional elements. 277 */ 278 @Child(name = "amountType", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 279 @Description(shortDefinition="Most elements that require a quantitative value will also have a field called amount type. Amount type should always be specified because the actual value of the amount is often dependent on it. EXAMPLE: In capturing the actual relative amounts of substances or molecular fragments it is essential to indicate whether the amount refers to a mole ratio or weight ratio. For any given element an effort should be made to use same the amount type for all related definitional elements", formalDefinition="Most elements that require a quantitative value will also have a field called amount type. Amount type should always be specified because the actual value of the amount is often dependent on it. EXAMPLE: In capturing the actual relative amounts of substances or molecular fragments it is essential to indicate whether the amount refers to a mole ratio or weight ratio. For any given element an effort should be made to use same the amount type for all related definitional elements." ) 280 protected CodeableConcept amountType; 281 282 /** 283 * A textual comment on a numeric value. 284 */ 285 @Child(name = "amountText", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 286 @Description(shortDefinition="A textual comment on a numeric value", formalDefinition="A textual comment on a numeric value." ) 287 protected StringType amountText; 288 289 /** 290 * Reference range of possible or expected values. 291 */ 292 @Child(name = "referenceRange", type = {}, order=3, min=0, max=1, modifier=false, summary=true) 293 @Description(shortDefinition="Reference range of possible or expected values", formalDefinition="Reference range of possible or expected values." ) 294 protected SubstanceAmountReferenceRangeComponent referenceRange; 295 296 private static final long serialVersionUID = 585120586L; 297 298 /** 299 * Constructor 300 */ 301 public SubstanceAmount() { 302 super(); 303 } 304 305 /** 306 * @return {@link #amount} (Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.) 307 */ 308 public DataType getAmount() { 309 return this.amount; 310 } 311 312 /** 313 * @return {@link #amount} (Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.) 314 */ 315 public Quantity getAmountQuantity() throws FHIRException { 316 if (this.amount == null) 317 this.amount = new Quantity(); 318 if (!(this.amount instanceof Quantity)) 319 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.amount.getClass().getName()+" was encountered"); 320 return (Quantity) this.amount; 321 } 322 323 public boolean hasAmountQuantity() { 324 return this.amount instanceof Quantity; 325 } 326 327 /** 328 * @return {@link #amount} (Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.) 329 */ 330 public Range getAmountRange() throws FHIRException { 331 if (this.amount == null) 332 this.amount = new Range(); 333 if (!(this.amount instanceof Range)) 334 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.amount.getClass().getName()+" was encountered"); 335 return (Range) this.amount; 336 } 337 338 public boolean hasAmountRange() { 339 return this.amount instanceof Range; 340 } 341 342 /** 343 * @return {@link #amount} (Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.) 344 */ 345 public StringType getAmountStringType() throws FHIRException { 346 if (this.amount == null) 347 this.amount = new StringType(); 348 if (!(this.amount instanceof StringType)) 349 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.amount.getClass().getName()+" was encountered"); 350 return (StringType) this.amount; 351 } 352 353 public boolean hasAmountStringType() { 354 return this.amount instanceof StringType; 355 } 356 357 public boolean hasAmount() { 358 return this.amount != null && !this.amount.isEmpty(); 359 } 360 361 /** 362 * @param value {@link #amount} (Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.) 363 */ 364 public SubstanceAmount setAmount(DataType value) { 365 if (value != null && !(value instanceof Quantity || value instanceof Range || value instanceof StringType)) 366 throw new FHIRException("Not the right type for SubstanceAmount.amount[x]: "+value.fhirType()); 367 this.amount = value; 368 return this; 369 } 370 371 /** 372 * @return {@link #amountType} (Most elements that require a quantitative value will also have a field called amount type. Amount type should always be specified because the actual value of the amount is often dependent on it. EXAMPLE: In capturing the actual relative amounts of substances or molecular fragments it is essential to indicate whether the amount refers to a mole ratio or weight ratio. For any given element an effort should be made to use same the amount type for all related definitional elements.) 373 */ 374 public CodeableConcept getAmountType() { 375 if (this.amountType == null) 376 if (Configuration.errorOnAutoCreate()) 377 throw new Error("Attempt to auto-create SubstanceAmount.amountType"); 378 else if (Configuration.doAutoCreate()) 379 this.amountType = new CodeableConcept(); // cc 380 return this.amountType; 381 } 382 383 public boolean hasAmountType() { 384 return this.amountType != null && !this.amountType.isEmpty(); 385 } 386 387 /** 388 * @param value {@link #amountType} (Most elements that require a quantitative value will also have a field called amount type. Amount type should always be specified because the actual value of the amount is often dependent on it. EXAMPLE: In capturing the actual relative amounts of substances or molecular fragments it is essential to indicate whether the amount refers to a mole ratio or weight ratio. For any given element an effort should be made to use same the amount type for all related definitional elements.) 389 */ 390 public SubstanceAmount setAmountType(CodeableConcept value) { 391 this.amountType = value; 392 return this; 393 } 394 395 /** 396 * @return {@link #amountText} (A textual comment on a numeric value.). This is the underlying object with id, value and extensions. The accessor "getAmountText" gives direct access to the value 397 */ 398 public StringType getAmountTextElement() { 399 if (this.amountText == null) 400 if (Configuration.errorOnAutoCreate()) 401 throw new Error("Attempt to auto-create SubstanceAmount.amountText"); 402 else if (Configuration.doAutoCreate()) 403 this.amountText = new StringType(); // bb 404 return this.amountText; 405 } 406 407 public boolean hasAmountTextElement() { 408 return this.amountText != null && !this.amountText.isEmpty(); 409 } 410 411 public boolean hasAmountText() { 412 return this.amountText != null && !this.amountText.isEmpty(); 413 } 414 415 /** 416 * @param value {@link #amountText} (A textual comment on a numeric value.). This is the underlying object with id, value and extensions. The accessor "getAmountText" gives direct access to the value 417 */ 418 public SubstanceAmount setAmountTextElement(StringType value) { 419 this.amountText = value; 420 return this; 421 } 422 423 /** 424 * @return A textual comment on a numeric value. 425 */ 426 public String getAmountText() { 427 return this.amountText == null ? null : this.amountText.getValue(); 428 } 429 430 /** 431 * @param value A textual comment on a numeric value. 432 */ 433 public SubstanceAmount setAmountText(String value) { 434 if (Utilities.noString(value)) 435 this.amountText = null; 436 else { 437 if (this.amountText == null) 438 this.amountText = new StringType(); 439 this.amountText.setValue(value); 440 } 441 return this; 442 } 443 444 /** 445 * @return {@link #referenceRange} (Reference range of possible or expected values.) 446 */ 447 public SubstanceAmountReferenceRangeComponent getReferenceRange() { 448 if (this.referenceRange == null) 449 if (Configuration.errorOnAutoCreate()) 450 throw new Error("Attempt to auto-create SubstanceAmount.referenceRange"); 451 else if (Configuration.doAutoCreate()) 452 this.referenceRange = new SubstanceAmountReferenceRangeComponent(); // cc 453 return this.referenceRange; 454 } 455 456 public boolean hasReferenceRange() { 457 return this.referenceRange != null && !this.referenceRange.isEmpty(); 458 } 459 460 /** 461 * @param value {@link #referenceRange} (Reference range of possible or expected values.) 462 */ 463 public SubstanceAmount setReferenceRange(SubstanceAmountReferenceRangeComponent value) { 464 this.referenceRange = value; 465 return this; 466 } 467 468 protected void listChildren(List<Property> children) { 469 super.listChildren(children); 470 children.add(new Property("amount[x]", "Quantity|Range|string", "Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.", 0, 1, amount)); 471 children.add(new Property("amountType", "CodeableConcept", "Most elements that require a quantitative value will also have a field called amount type. Amount type should always be specified because the actual value of the amount is often dependent on it. EXAMPLE: In capturing the actual relative amounts of substances or molecular fragments it is essential to indicate whether the amount refers to a mole ratio or weight ratio. For any given element an effort should be made to use same the amount type for all related definitional elements.", 0, 1, amountType)); 472 children.add(new Property("amountText", "string", "A textual comment on a numeric value.", 0, 1, amountText)); 473 children.add(new Property("referenceRange", "", "Reference range of possible or expected values.", 0, 1, referenceRange)); 474 } 475 476 @Override 477 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 478 switch (_hash) { 479 case 646780200: /*amount[x]*/ return new Property("amount[x]", "Quantity|Range|string", "Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.", 0, 1, amount); 480 case -1413853096: /*amount*/ return new Property("amount[x]", "Quantity|Range|string", "Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.", 0, 1, amount); 481 case 1664303363: /*amountQuantity*/ return new Property("amount[x]", "Quantity", "Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.", 0, 1, amount); 482 case -1223462971: /*amountRange*/ return new Property("amount[x]", "Range", "Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.", 0, 1, amount); 483 case 773651081: /*amountString*/ return new Property("amount[x]", "string", "Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.", 0, 1, amount); 484 case -1424857166: /*amountType*/ return new Property("amountType", "CodeableConcept", "Most elements that require a quantitative value will also have a field called amount type. Amount type should always be specified because the actual value of the amount is often dependent on it. EXAMPLE: In capturing the actual relative amounts of substances or molecular fragments it is essential to indicate whether the amount refers to a mole ratio or weight ratio. For any given element an effort should be made to use same the amount type for all related definitional elements.", 0, 1, amountType); 485 case -1424876123: /*amountText*/ return new Property("amountText", "string", "A textual comment on a numeric value.", 0, 1, amountText); 486 case -1912545102: /*referenceRange*/ return new Property("referenceRange", "", "Reference range of possible or expected values.", 0, 1, referenceRange); 487 default: return super.getNamedProperty(_hash, _name, _checkValid); 488 } 489 490 } 491 492 @Override 493 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 494 switch (hash) { 495 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // DataType 496 case -1424857166: /*amountType*/ return this.amountType == null ? new Base[0] : new Base[] {this.amountType}; // CodeableConcept 497 case -1424876123: /*amountText*/ return this.amountText == null ? new Base[0] : new Base[] {this.amountText}; // StringType 498 case -1912545102: /*referenceRange*/ return this.referenceRange == null ? new Base[0] : new Base[] {this.referenceRange}; // SubstanceAmountReferenceRangeComponent 499 default: return super.getProperty(hash, name, checkValid); 500 } 501 502 } 503 504 @Override 505 public Base setProperty(int hash, String name, Base value) throws FHIRException { 506 switch (hash) { 507 case -1413853096: // amount 508 this.amount = TypeConvertor.castToType(value); // DataType 509 return value; 510 case -1424857166: // amountType 511 this.amountType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 512 return value; 513 case -1424876123: // amountText 514 this.amountText = TypeConvertor.castToString(value); // StringType 515 return value; 516 case -1912545102: // referenceRange 517 this.referenceRange = (SubstanceAmountReferenceRangeComponent) value; // SubstanceAmountReferenceRangeComponent 518 return value; 519 default: return super.setProperty(hash, name, value); 520 } 521 522 } 523 524 @Override 525 public Base setProperty(String name, Base value) throws FHIRException { 526 if (name.equals("amount[x]")) { 527 this.amount = TypeConvertor.castToType(value); // DataType 528 } else if (name.equals("amountType")) { 529 this.amountType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 530 } else if (name.equals("amountText")) { 531 this.amountText = TypeConvertor.castToString(value); // StringType 532 } else if (name.equals("referenceRange")) { 533 this.referenceRange = (SubstanceAmountReferenceRangeComponent) value; // SubstanceAmountReferenceRangeComponent 534 } else 535 return super.setProperty(name, value); 536 return value; 537 } 538 539 @Override 540 public void removeChild(String name, Base value) throws FHIRException { 541 if (name.equals("amount[x]")) { 542 this.amount = null; 543 } else if (name.equals("amountType")) { 544 this.amountType = null; 545 } else if (name.equals("amountText")) { 546 this.amountText = null; 547 } else if (name.equals("referenceRange")) { 548 this.referenceRange = (SubstanceAmountReferenceRangeComponent) value; // SubstanceAmountReferenceRangeComponent 549 } else 550 super.removeChild(name, value); 551 552 } 553 554 @Override 555 public Base makeProperty(int hash, String name) throws FHIRException { 556 switch (hash) { 557 case 646780200: return getAmount(); 558 case -1413853096: return getAmount(); 559 case -1424857166: return getAmountType(); 560 case -1424876123: return getAmountTextElement(); 561 case -1912545102: return getReferenceRange(); 562 default: return super.makeProperty(hash, name); 563 } 564 565 } 566 567 @Override 568 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 569 switch (hash) { 570 case -1413853096: /*amount*/ return new String[] {"Quantity", "Range", "string"}; 571 case -1424857166: /*amountType*/ return new String[] {"CodeableConcept"}; 572 case -1424876123: /*amountText*/ return new String[] {"string"}; 573 case -1912545102: /*referenceRange*/ return new String[] {}; 574 default: return super.getTypesForProperty(hash, name); 575 } 576 577 } 578 579 @Override 580 public Base addChild(String name) throws FHIRException { 581 if (name.equals("amountQuantity")) { 582 this.amount = new Quantity(); 583 return this.amount; 584 } 585 else if (name.equals("amountRange")) { 586 this.amount = new Range(); 587 return this.amount; 588 } 589 else if (name.equals("amountString")) { 590 this.amount = new StringType(); 591 return this.amount; 592 } 593 else if (name.equals("amountType")) { 594 this.amountType = new CodeableConcept(); 595 return this.amountType; 596 } 597 else if (name.equals("amountText")) { 598 throw new FHIRException("Cannot call addChild on a singleton property SubstanceAmount.amountText"); 599 } 600 else if (name.equals("referenceRange")) { 601 this.referenceRange = new SubstanceAmountReferenceRangeComponent(); 602 return this.referenceRange; 603 } 604 else 605 return super.addChild(name); 606 } 607 608 public String fhirType() { 609 return "SubstanceAmount"; 610 611 } 612 613 public SubstanceAmount copy() { 614 SubstanceAmount dst = new SubstanceAmount(); 615 copyValues(dst); 616 return dst; 617 } 618 619 public void copyValues(SubstanceAmount dst) { 620 super.copyValues(dst); 621 dst.amount = amount == null ? null : amount.copy(); 622 dst.amountType = amountType == null ? null : amountType.copy(); 623 dst.amountText = amountText == null ? null : amountText.copy(); 624 dst.referenceRange = referenceRange == null ? null : referenceRange.copy(); 625 } 626 627 protected SubstanceAmount typedCopy() { 628 return copy(); 629 } 630 631 @Override 632 public boolean equalsDeep(Base other_) { 633 if (!super.equalsDeep(other_)) 634 return false; 635 if (!(other_ instanceof SubstanceAmount)) 636 return false; 637 SubstanceAmount o = (SubstanceAmount) other_; 638 return compareDeep(amount, o.amount, true) && compareDeep(amountType, o.amountType, true) && compareDeep(amountText, o.amountText, true) 639 && compareDeep(referenceRange, o.referenceRange, true); 640 } 641 642 @Override 643 public boolean equalsShallow(Base other_) { 644 if (!super.equalsShallow(other_)) 645 return false; 646 if (!(other_ instanceof SubstanceAmount)) 647 return false; 648 SubstanceAmount o = (SubstanceAmount) other_; 649 return compareValues(amountText, o.amountText, true); 650 } 651 652 public boolean isEmpty() { 653 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(amount, amountType, amountText 654 , referenceRange); 655 } 656 657 658}