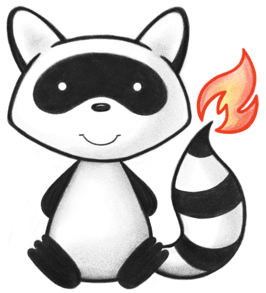
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The detailed description of a substance, typically at a level beyond what is used for prescribing. 052 */ 053@ResourceDef(name="SubstanceDefinition", profile="http://hl7.org/fhir/StructureDefinition/SubstanceDefinition") 054public class SubstanceDefinition extends DomainResource { 055 056 @Block() 057 public static class SubstanceDefinitionMoietyComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Role that the moiety is playing. 060 */ 061 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="Role that the moiety is playing", formalDefinition="Role that the moiety is playing." ) 063 protected CodeableConcept role; 064 065 /** 066 * Identifier by which this moiety substance is known. 067 */ 068 @Child(name = "identifier", type = {Identifier.class}, order=2, min=0, max=1, modifier=false, summary=true) 069 @Description(shortDefinition="Identifier by which this moiety substance is known", formalDefinition="Identifier by which this moiety substance is known." ) 070 protected Identifier identifier; 071 072 /** 073 * Textual name for this moiety substance. 074 */ 075 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 076 @Description(shortDefinition="Textual name for this moiety substance", formalDefinition="Textual name for this moiety substance." ) 077 protected StringType name; 078 079 /** 080 * Stereochemistry type. 081 */ 082 @Child(name = "stereochemistry", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 083 @Description(shortDefinition="Stereochemistry type", formalDefinition="Stereochemistry type." ) 084 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-stereochemistry") 085 protected CodeableConcept stereochemistry; 086 087 /** 088 * Optical activity type. 089 */ 090 @Child(name = "opticalActivity", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 091 @Description(shortDefinition="Optical activity type", formalDefinition="Optical activity type." ) 092 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-optical-activity") 093 protected CodeableConcept opticalActivity; 094 095 /** 096 * Molecular formula for this moiety of this substance, typically using the Hill system. 097 */ 098 @Child(name = "molecularFormula", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 099 @Description(shortDefinition="Molecular formula for this moiety (e.g. with the Hill system)", formalDefinition="Molecular formula for this moiety of this substance, typically using the Hill system." ) 100 protected StringType molecularFormula; 101 102 /** 103 * Quantitative value for this moiety. 104 */ 105 @Child(name = "amount", type = {Quantity.class, StringType.class}, order=7, min=0, max=1, modifier=false, summary=true) 106 @Description(shortDefinition="Quantitative value for this moiety", formalDefinition="Quantitative value for this moiety." ) 107 protected DataType amount; 108 109 /** 110 * The measurement type of the quantitative value. In capturing the actual relative amounts of substances or molecular fragments it may be necessary to indicate whether the amount refers to, for example, a mole ratio or weight ratio. 111 */ 112 @Child(name = "measurementType", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=true) 113 @Description(shortDefinition="The measurement type of the quantitative value", formalDefinition="The measurement type of the quantitative value. In capturing the actual relative amounts of substances or molecular fragments it may be necessary to indicate whether the amount refers to, for example, a mole ratio or weight ratio." ) 114 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-amount-type") 115 protected CodeableConcept measurementType; 116 117 private static final long serialVersionUID = 271962476L; 118 119 /** 120 * Constructor 121 */ 122 public SubstanceDefinitionMoietyComponent() { 123 super(); 124 } 125 126 /** 127 * @return {@link #role} (Role that the moiety is playing.) 128 */ 129 public CodeableConcept getRole() { 130 if (this.role == null) 131 if (Configuration.errorOnAutoCreate()) 132 throw new Error("Attempt to auto-create SubstanceDefinitionMoietyComponent.role"); 133 else if (Configuration.doAutoCreate()) 134 this.role = new CodeableConcept(); // cc 135 return this.role; 136 } 137 138 public boolean hasRole() { 139 return this.role != null && !this.role.isEmpty(); 140 } 141 142 /** 143 * @param value {@link #role} (Role that the moiety is playing.) 144 */ 145 public SubstanceDefinitionMoietyComponent setRole(CodeableConcept value) { 146 this.role = value; 147 return this; 148 } 149 150 /** 151 * @return {@link #identifier} (Identifier by which this moiety substance is known.) 152 */ 153 public Identifier getIdentifier() { 154 if (this.identifier == null) 155 if (Configuration.errorOnAutoCreate()) 156 throw new Error("Attempt to auto-create SubstanceDefinitionMoietyComponent.identifier"); 157 else if (Configuration.doAutoCreate()) 158 this.identifier = new Identifier(); // cc 159 return this.identifier; 160 } 161 162 public boolean hasIdentifier() { 163 return this.identifier != null && !this.identifier.isEmpty(); 164 } 165 166 /** 167 * @param value {@link #identifier} (Identifier by which this moiety substance is known.) 168 */ 169 public SubstanceDefinitionMoietyComponent setIdentifier(Identifier value) { 170 this.identifier = value; 171 return this; 172 } 173 174 /** 175 * @return {@link #name} (Textual name for this moiety substance.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 176 */ 177 public StringType getNameElement() { 178 if (this.name == null) 179 if (Configuration.errorOnAutoCreate()) 180 throw new Error("Attempt to auto-create SubstanceDefinitionMoietyComponent.name"); 181 else if (Configuration.doAutoCreate()) 182 this.name = new StringType(); // bb 183 return this.name; 184 } 185 186 public boolean hasNameElement() { 187 return this.name != null && !this.name.isEmpty(); 188 } 189 190 public boolean hasName() { 191 return this.name != null && !this.name.isEmpty(); 192 } 193 194 /** 195 * @param value {@link #name} (Textual name for this moiety substance.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 196 */ 197 public SubstanceDefinitionMoietyComponent setNameElement(StringType value) { 198 this.name = value; 199 return this; 200 } 201 202 /** 203 * @return Textual name for this moiety substance. 204 */ 205 public String getName() { 206 return this.name == null ? null : this.name.getValue(); 207 } 208 209 /** 210 * @param value Textual name for this moiety substance. 211 */ 212 public SubstanceDefinitionMoietyComponent setName(String value) { 213 if (Utilities.noString(value)) 214 this.name = null; 215 else { 216 if (this.name == null) 217 this.name = new StringType(); 218 this.name.setValue(value); 219 } 220 return this; 221 } 222 223 /** 224 * @return {@link #stereochemistry} (Stereochemistry type.) 225 */ 226 public CodeableConcept getStereochemistry() { 227 if (this.stereochemistry == null) 228 if (Configuration.errorOnAutoCreate()) 229 throw new Error("Attempt to auto-create SubstanceDefinitionMoietyComponent.stereochemistry"); 230 else if (Configuration.doAutoCreate()) 231 this.stereochemistry = new CodeableConcept(); // cc 232 return this.stereochemistry; 233 } 234 235 public boolean hasStereochemistry() { 236 return this.stereochemistry != null && !this.stereochemistry.isEmpty(); 237 } 238 239 /** 240 * @param value {@link #stereochemistry} (Stereochemistry type.) 241 */ 242 public SubstanceDefinitionMoietyComponent setStereochemistry(CodeableConcept value) { 243 this.stereochemistry = value; 244 return this; 245 } 246 247 /** 248 * @return {@link #opticalActivity} (Optical activity type.) 249 */ 250 public CodeableConcept getOpticalActivity() { 251 if (this.opticalActivity == null) 252 if (Configuration.errorOnAutoCreate()) 253 throw new Error("Attempt to auto-create SubstanceDefinitionMoietyComponent.opticalActivity"); 254 else if (Configuration.doAutoCreate()) 255 this.opticalActivity = new CodeableConcept(); // cc 256 return this.opticalActivity; 257 } 258 259 public boolean hasOpticalActivity() { 260 return this.opticalActivity != null && !this.opticalActivity.isEmpty(); 261 } 262 263 /** 264 * @param value {@link #opticalActivity} (Optical activity type.) 265 */ 266 public SubstanceDefinitionMoietyComponent setOpticalActivity(CodeableConcept value) { 267 this.opticalActivity = value; 268 return this; 269 } 270 271 /** 272 * @return {@link #molecularFormula} (Molecular formula for this moiety of this substance, typically using the Hill system.). This is the underlying object with id, value and extensions. The accessor "getMolecularFormula" gives direct access to the value 273 */ 274 public StringType getMolecularFormulaElement() { 275 if (this.molecularFormula == null) 276 if (Configuration.errorOnAutoCreate()) 277 throw new Error("Attempt to auto-create SubstanceDefinitionMoietyComponent.molecularFormula"); 278 else if (Configuration.doAutoCreate()) 279 this.molecularFormula = new StringType(); // bb 280 return this.molecularFormula; 281 } 282 283 public boolean hasMolecularFormulaElement() { 284 return this.molecularFormula != null && !this.molecularFormula.isEmpty(); 285 } 286 287 public boolean hasMolecularFormula() { 288 return this.molecularFormula != null && !this.molecularFormula.isEmpty(); 289 } 290 291 /** 292 * @param value {@link #molecularFormula} (Molecular formula for this moiety of this substance, typically using the Hill system.). This is the underlying object with id, value and extensions. The accessor "getMolecularFormula" gives direct access to the value 293 */ 294 public SubstanceDefinitionMoietyComponent setMolecularFormulaElement(StringType value) { 295 this.molecularFormula = value; 296 return this; 297 } 298 299 /** 300 * @return Molecular formula for this moiety of this substance, typically using the Hill system. 301 */ 302 public String getMolecularFormula() { 303 return this.molecularFormula == null ? null : this.molecularFormula.getValue(); 304 } 305 306 /** 307 * @param value Molecular formula for this moiety of this substance, typically using the Hill system. 308 */ 309 public SubstanceDefinitionMoietyComponent setMolecularFormula(String value) { 310 if (Utilities.noString(value)) 311 this.molecularFormula = null; 312 else { 313 if (this.molecularFormula == null) 314 this.molecularFormula = new StringType(); 315 this.molecularFormula.setValue(value); 316 } 317 return this; 318 } 319 320 /** 321 * @return {@link #amount} (Quantitative value for this moiety.) 322 */ 323 public DataType getAmount() { 324 return this.amount; 325 } 326 327 /** 328 * @return {@link #amount} (Quantitative value for this moiety.) 329 */ 330 public Quantity getAmountQuantity() throws FHIRException { 331 if (this.amount == null) 332 this.amount = new Quantity(); 333 if (!(this.amount instanceof Quantity)) 334 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.amount.getClass().getName()+" was encountered"); 335 return (Quantity) this.amount; 336 } 337 338 public boolean hasAmountQuantity() { 339 return this != null && this.amount instanceof Quantity; 340 } 341 342 /** 343 * @return {@link #amount} (Quantitative value for this moiety.) 344 */ 345 public StringType getAmountStringType() throws FHIRException { 346 if (this.amount == null) 347 this.amount = new StringType(); 348 if (!(this.amount instanceof StringType)) 349 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.amount.getClass().getName()+" was encountered"); 350 return (StringType) this.amount; 351 } 352 353 public boolean hasAmountStringType() { 354 return this != null && this.amount instanceof StringType; 355 } 356 357 public boolean hasAmount() { 358 return this.amount != null && !this.amount.isEmpty(); 359 } 360 361 /** 362 * @param value {@link #amount} (Quantitative value for this moiety.) 363 */ 364 public SubstanceDefinitionMoietyComponent setAmount(DataType value) { 365 if (value != null && !(value instanceof Quantity || value instanceof StringType)) 366 throw new FHIRException("Not the right type for SubstanceDefinition.moiety.amount[x]: "+value.fhirType()); 367 this.amount = value; 368 return this; 369 } 370 371 /** 372 * @return {@link #measurementType} (The measurement type of the quantitative value. In capturing the actual relative amounts of substances or molecular fragments it may be necessary to indicate whether the amount refers to, for example, a mole ratio or weight ratio.) 373 */ 374 public CodeableConcept getMeasurementType() { 375 if (this.measurementType == null) 376 if (Configuration.errorOnAutoCreate()) 377 throw new Error("Attempt to auto-create SubstanceDefinitionMoietyComponent.measurementType"); 378 else if (Configuration.doAutoCreate()) 379 this.measurementType = new CodeableConcept(); // cc 380 return this.measurementType; 381 } 382 383 public boolean hasMeasurementType() { 384 return this.measurementType != null && !this.measurementType.isEmpty(); 385 } 386 387 /** 388 * @param value {@link #measurementType} (The measurement type of the quantitative value. In capturing the actual relative amounts of substances or molecular fragments it may be necessary to indicate whether the amount refers to, for example, a mole ratio or weight ratio.) 389 */ 390 public SubstanceDefinitionMoietyComponent setMeasurementType(CodeableConcept value) { 391 this.measurementType = value; 392 return this; 393 } 394 395 protected void listChildren(List<Property> children) { 396 super.listChildren(children); 397 children.add(new Property("role", "CodeableConcept", "Role that the moiety is playing.", 0, 1, role)); 398 children.add(new Property("identifier", "Identifier", "Identifier by which this moiety substance is known.", 0, 1, identifier)); 399 children.add(new Property("name", "string", "Textual name for this moiety substance.", 0, 1, name)); 400 children.add(new Property("stereochemistry", "CodeableConcept", "Stereochemistry type.", 0, 1, stereochemistry)); 401 children.add(new Property("opticalActivity", "CodeableConcept", "Optical activity type.", 0, 1, opticalActivity)); 402 children.add(new Property("molecularFormula", "string", "Molecular formula for this moiety of this substance, typically using the Hill system.", 0, 1, molecularFormula)); 403 children.add(new Property("amount[x]", "Quantity|string", "Quantitative value for this moiety.", 0, 1, amount)); 404 children.add(new Property("measurementType", "CodeableConcept", "The measurement type of the quantitative value. In capturing the actual relative amounts of substances or molecular fragments it may be necessary to indicate whether the amount refers to, for example, a mole ratio or weight ratio.", 0, 1, measurementType)); 405 } 406 407 @Override 408 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 409 switch (_hash) { 410 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Role that the moiety is playing.", 0, 1, role); 411 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier by which this moiety substance is known.", 0, 1, identifier); 412 case 3373707: /*name*/ return new Property("name", "string", "Textual name for this moiety substance.", 0, 1, name); 413 case 263475116: /*stereochemistry*/ return new Property("stereochemistry", "CodeableConcept", "Stereochemistry type.", 0, 1, stereochemistry); 414 case 1420900135: /*opticalActivity*/ return new Property("opticalActivity", "CodeableConcept", "Optical activity type.", 0, 1, opticalActivity); 415 case 616660246: /*molecularFormula*/ return new Property("molecularFormula", "string", "Molecular formula for this moiety of this substance, typically using the Hill system.", 0, 1, molecularFormula); 416 case 646780200: /*amount[x]*/ return new Property("amount[x]", "Quantity|string", "Quantitative value for this moiety.", 0, 1, amount); 417 case -1413853096: /*amount*/ return new Property("amount[x]", "Quantity|string", "Quantitative value for this moiety.", 0, 1, amount); 418 case 1664303363: /*amountQuantity*/ return new Property("amount[x]", "Quantity", "Quantitative value for this moiety.", 0, 1, amount); 419 case 773651081: /*amountString*/ return new Property("amount[x]", "string", "Quantitative value for this moiety.", 0, 1, amount); 420 case 1670291734: /*measurementType*/ return new Property("measurementType", "CodeableConcept", "The measurement type of the quantitative value. In capturing the actual relative amounts of substances or molecular fragments it may be necessary to indicate whether the amount refers to, for example, a mole ratio or weight ratio.", 0, 1, measurementType); 421 default: return super.getNamedProperty(_hash, _name, _checkValid); 422 } 423 424 } 425 426 @Override 427 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 428 switch (hash) { 429 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 430 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 431 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 432 case 263475116: /*stereochemistry*/ return this.stereochemistry == null ? new Base[0] : new Base[] {this.stereochemistry}; // CodeableConcept 433 case 1420900135: /*opticalActivity*/ return this.opticalActivity == null ? new Base[0] : new Base[] {this.opticalActivity}; // CodeableConcept 434 case 616660246: /*molecularFormula*/ return this.molecularFormula == null ? new Base[0] : new Base[] {this.molecularFormula}; // StringType 435 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // DataType 436 case 1670291734: /*measurementType*/ return this.measurementType == null ? new Base[0] : new Base[] {this.measurementType}; // CodeableConcept 437 default: return super.getProperty(hash, name, checkValid); 438 } 439 440 } 441 442 @Override 443 public Base setProperty(int hash, String name, Base value) throws FHIRException { 444 switch (hash) { 445 case 3506294: // role 446 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 447 return value; 448 case -1618432855: // identifier 449 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 450 return value; 451 case 3373707: // name 452 this.name = TypeConvertor.castToString(value); // StringType 453 return value; 454 case 263475116: // stereochemistry 455 this.stereochemistry = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 456 return value; 457 case 1420900135: // opticalActivity 458 this.opticalActivity = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 459 return value; 460 case 616660246: // molecularFormula 461 this.molecularFormula = TypeConvertor.castToString(value); // StringType 462 return value; 463 case -1413853096: // amount 464 this.amount = TypeConvertor.castToType(value); // DataType 465 return value; 466 case 1670291734: // measurementType 467 this.measurementType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 468 return value; 469 default: return super.setProperty(hash, name, value); 470 } 471 472 } 473 474 @Override 475 public Base setProperty(String name, Base value) throws FHIRException { 476 if (name.equals("role")) { 477 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 478 } else if (name.equals("identifier")) { 479 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 480 } else if (name.equals("name")) { 481 this.name = TypeConvertor.castToString(value); // StringType 482 } else if (name.equals("stereochemistry")) { 483 this.stereochemistry = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 484 } else if (name.equals("opticalActivity")) { 485 this.opticalActivity = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 486 } else if (name.equals("molecularFormula")) { 487 this.molecularFormula = TypeConvertor.castToString(value); // StringType 488 } else if (name.equals("amount[x]")) { 489 this.amount = TypeConvertor.castToType(value); // DataType 490 } else if (name.equals("measurementType")) { 491 this.measurementType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 492 } else 493 return super.setProperty(name, value); 494 return value; 495 } 496 497 @Override 498 public void removeChild(String name, Base value) throws FHIRException { 499 if (name.equals("role")) { 500 this.role = null; 501 } else if (name.equals("identifier")) { 502 this.identifier = null; 503 } else if (name.equals("name")) { 504 this.name = null; 505 } else if (name.equals("stereochemistry")) { 506 this.stereochemistry = null; 507 } else if (name.equals("opticalActivity")) { 508 this.opticalActivity = null; 509 } else if (name.equals("molecularFormula")) { 510 this.molecularFormula = null; 511 } else if (name.equals("amount[x]")) { 512 this.amount = null; 513 } else if (name.equals("measurementType")) { 514 this.measurementType = null; 515 } else 516 super.removeChild(name, value); 517 518 } 519 520 @Override 521 public Base makeProperty(int hash, String name) throws FHIRException { 522 switch (hash) { 523 case 3506294: return getRole(); 524 case -1618432855: return getIdentifier(); 525 case 3373707: return getNameElement(); 526 case 263475116: return getStereochemistry(); 527 case 1420900135: return getOpticalActivity(); 528 case 616660246: return getMolecularFormulaElement(); 529 case 646780200: return getAmount(); 530 case -1413853096: return getAmount(); 531 case 1670291734: return getMeasurementType(); 532 default: return super.makeProperty(hash, name); 533 } 534 535 } 536 537 @Override 538 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 539 switch (hash) { 540 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 541 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 542 case 3373707: /*name*/ return new String[] {"string"}; 543 case 263475116: /*stereochemistry*/ return new String[] {"CodeableConcept"}; 544 case 1420900135: /*opticalActivity*/ return new String[] {"CodeableConcept"}; 545 case 616660246: /*molecularFormula*/ return new String[] {"string"}; 546 case -1413853096: /*amount*/ return new String[] {"Quantity", "string"}; 547 case 1670291734: /*measurementType*/ return new String[] {"CodeableConcept"}; 548 default: return super.getTypesForProperty(hash, name); 549 } 550 551 } 552 553 @Override 554 public Base addChild(String name) throws FHIRException { 555 if (name.equals("role")) { 556 this.role = new CodeableConcept(); 557 return this.role; 558 } 559 else if (name.equals("identifier")) { 560 this.identifier = new Identifier(); 561 return this.identifier; 562 } 563 else if (name.equals("name")) { 564 throw new FHIRException("Cannot call addChild on a singleton property SubstanceDefinition.moiety.name"); 565 } 566 else if (name.equals("stereochemistry")) { 567 this.stereochemistry = new CodeableConcept(); 568 return this.stereochemistry; 569 } 570 else if (name.equals("opticalActivity")) { 571 this.opticalActivity = new CodeableConcept(); 572 return this.opticalActivity; 573 } 574 else if (name.equals("molecularFormula")) { 575 throw new FHIRException("Cannot call addChild on a singleton property SubstanceDefinition.moiety.molecularFormula"); 576 } 577 else if (name.equals("amountQuantity")) { 578 this.amount = new Quantity(); 579 return this.amount; 580 } 581 else if (name.equals("amountString")) { 582 this.amount = new StringType(); 583 return this.amount; 584 } 585 else if (name.equals("measurementType")) { 586 this.measurementType = new CodeableConcept(); 587 return this.measurementType; 588 } 589 else 590 return super.addChild(name); 591 } 592 593 public SubstanceDefinitionMoietyComponent copy() { 594 SubstanceDefinitionMoietyComponent dst = new SubstanceDefinitionMoietyComponent(); 595 copyValues(dst); 596 return dst; 597 } 598 599 public void copyValues(SubstanceDefinitionMoietyComponent dst) { 600 super.copyValues(dst); 601 dst.role = role == null ? null : role.copy(); 602 dst.identifier = identifier == null ? null : identifier.copy(); 603 dst.name = name == null ? null : name.copy(); 604 dst.stereochemistry = stereochemistry == null ? null : stereochemistry.copy(); 605 dst.opticalActivity = opticalActivity == null ? null : opticalActivity.copy(); 606 dst.molecularFormula = molecularFormula == null ? null : molecularFormula.copy(); 607 dst.amount = amount == null ? null : amount.copy(); 608 dst.measurementType = measurementType == null ? null : measurementType.copy(); 609 } 610 611 @Override 612 public boolean equalsDeep(Base other_) { 613 if (!super.equalsDeep(other_)) 614 return false; 615 if (!(other_ instanceof SubstanceDefinitionMoietyComponent)) 616 return false; 617 SubstanceDefinitionMoietyComponent o = (SubstanceDefinitionMoietyComponent) other_; 618 return compareDeep(role, o.role, true) && compareDeep(identifier, o.identifier, true) && compareDeep(name, o.name, true) 619 && compareDeep(stereochemistry, o.stereochemistry, true) && compareDeep(opticalActivity, o.opticalActivity, true) 620 && compareDeep(molecularFormula, o.molecularFormula, true) && compareDeep(amount, o.amount, true) 621 && compareDeep(measurementType, o.measurementType, true); 622 } 623 624 @Override 625 public boolean equalsShallow(Base other_) { 626 if (!super.equalsShallow(other_)) 627 return false; 628 if (!(other_ instanceof SubstanceDefinitionMoietyComponent)) 629 return false; 630 SubstanceDefinitionMoietyComponent o = (SubstanceDefinitionMoietyComponent) other_; 631 return compareValues(name, o.name, true) && compareValues(molecularFormula, o.molecularFormula, true) 632 ; 633 } 634 635 public boolean isEmpty() { 636 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, identifier, name, stereochemistry 637 , opticalActivity, molecularFormula, amount, measurementType); 638 } 639 640 public String fhirType() { 641 return "SubstanceDefinition.moiety"; 642 643 } 644 645 } 646 647 @Block() 648 public static class SubstanceDefinitionCharacterizationComponent extends BackboneElement implements IBaseBackboneElement { 649 /** 650 * The method used to elucidate the characterization of the drug substance. Example: HPLC. 651 */ 652 @Child(name = "technique", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 653 @Description(shortDefinition="The method used to find the characterization e.g. HPLC", formalDefinition="The method used to elucidate the characterization of the drug substance. Example: HPLC." ) 654 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-structure-technique") 655 protected CodeableConcept technique; 656 657 /** 658 * Describes the nature of the chemical entity and explains, for instance, whether this is a base or a salt form. 659 */ 660 @Child(name = "form", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 661 @Description(shortDefinition="Describes the nature of the chemical entity and explains, for instance, whether this is a base or a salt form", formalDefinition="Describes the nature of the chemical entity and explains, for instance, whether this is a base or a salt form." ) 662 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-form") 663 protected CodeableConcept form; 664 665 /** 666 * The description or justification in support of the interpretation of the data file. 667 */ 668 @Child(name = "description", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=true) 669 @Description(shortDefinition="The description or justification in support of the interpretation of the data file", formalDefinition="The description or justification in support of the interpretation of the data file." ) 670 protected MarkdownType description; 671 672 /** 673 * The data produced by the analytical instrument or a pictorial representation of that data. Examples: a JCAMP, JDX, or ADX file, or a chromatogram or spectrum analysis. 674 */ 675 @Child(name = "file", type = {Attachment.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 676 @Description(shortDefinition="The data produced by the analytical instrument or a pictorial representation of that data. Examples: a JCAMP, JDX, or ADX file, or a chromatogram or spectrum analysis", formalDefinition="The data produced by the analytical instrument or a pictorial representation of that data. Examples: a JCAMP, JDX, or ADX file, or a chromatogram or spectrum analysis." ) 677 protected List<Attachment> file; 678 679 private static final long serialVersionUID = -1561571166L; 680 681 /** 682 * Constructor 683 */ 684 public SubstanceDefinitionCharacterizationComponent() { 685 super(); 686 } 687 688 /** 689 * @return {@link #technique} (The method used to elucidate the characterization of the drug substance. Example: HPLC.) 690 */ 691 public CodeableConcept getTechnique() { 692 if (this.technique == null) 693 if (Configuration.errorOnAutoCreate()) 694 throw new Error("Attempt to auto-create SubstanceDefinitionCharacterizationComponent.technique"); 695 else if (Configuration.doAutoCreate()) 696 this.technique = new CodeableConcept(); // cc 697 return this.technique; 698 } 699 700 public boolean hasTechnique() { 701 return this.technique != null && !this.technique.isEmpty(); 702 } 703 704 /** 705 * @param value {@link #technique} (The method used to elucidate the characterization of the drug substance. Example: HPLC.) 706 */ 707 public SubstanceDefinitionCharacterizationComponent setTechnique(CodeableConcept value) { 708 this.technique = value; 709 return this; 710 } 711 712 /** 713 * @return {@link #form} (Describes the nature of the chemical entity and explains, for instance, whether this is a base or a salt form.) 714 */ 715 public CodeableConcept getForm() { 716 if (this.form == null) 717 if (Configuration.errorOnAutoCreate()) 718 throw new Error("Attempt to auto-create SubstanceDefinitionCharacterizationComponent.form"); 719 else if (Configuration.doAutoCreate()) 720 this.form = new CodeableConcept(); // cc 721 return this.form; 722 } 723 724 public boolean hasForm() { 725 return this.form != null && !this.form.isEmpty(); 726 } 727 728 /** 729 * @param value {@link #form} (Describes the nature of the chemical entity and explains, for instance, whether this is a base or a salt form.) 730 */ 731 public SubstanceDefinitionCharacterizationComponent setForm(CodeableConcept value) { 732 this.form = value; 733 return this; 734 } 735 736 /** 737 * @return {@link #description} (The description or justification in support of the interpretation of the data file.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 738 */ 739 public MarkdownType getDescriptionElement() { 740 if (this.description == null) 741 if (Configuration.errorOnAutoCreate()) 742 throw new Error("Attempt to auto-create SubstanceDefinitionCharacterizationComponent.description"); 743 else if (Configuration.doAutoCreate()) 744 this.description = new MarkdownType(); // bb 745 return this.description; 746 } 747 748 public boolean hasDescriptionElement() { 749 return this.description != null && !this.description.isEmpty(); 750 } 751 752 public boolean hasDescription() { 753 return this.description != null && !this.description.isEmpty(); 754 } 755 756 /** 757 * @param value {@link #description} (The description or justification in support of the interpretation of the data file.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 758 */ 759 public SubstanceDefinitionCharacterizationComponent setDescriptionElement(MarkdownType value) { 760 this.description = value; 761 return this; 762 } 763 764 /** 765 * @return The description or justification in support of the interpretation of the data file. 766 */ 767 public String getDescription() { 768 return this.description == null ? null : this.description.getValue(); 769 } 770 771 /** 772 * @param value The description or justification in support of the interpretation of the data file. 773 */ 774 public SubstanceDefinitionCharacterizationComponent setDescription(String value) { 775 if (Utilities.noString(value)) 776 this.description = null; 777 else { 778 if (this.description == null) 779 this.description = new MarkdownType(); 780 this.description.setValue(value); 781 } 782 return this; 783 } 784 785 /** 786 * @return {@link #file} (The data produced by the analytical instrument or a pictorial representation of that data. Examples: a JCAMP, JDX, or ADX file, or a chromatogram or spectrum analysis.) 787 */ 788 public List<Attachment> getFile() { 789 if (this.file == null) 790 this.file = new ArrayList<Attachment>(); 791 return this.file; 792 } 793 794 /** 795 * @return Returns a reference to <code>this</code> for easy method chaining 796 */ 797 public SubstanceDefinitionCharacterizationComponent setFile(List<Attachment> theFile) { 798 this.file = theFile; 799 return this; 800 } 801 802 public boolean hasFile() { 803 if (this.file == null) 804 return false; 805 for (Attachment item : this.file) 806 if (!item.isEmpty()) 807 return true; 808 return false; 809 } 810 811 public Attachment addFile() { //3 812 Attachment t = new Attachment(); 813 if (this.file == null) 814 this.file = new ArrayList<Attachment>(); 815 this.file.add(t); 816 return t; 817 } 818 819 public SubstanceDefinitionCharacterizationComponent addFile(Attachment t) { //3 820 if (t == null) 821 return this; 822 if (this.file == null) 823 this.file = new ArrayList<Attachment>(); 824 this.file.add(t); 825 return this; 826 } 827 828 /** 829 * @return The first repetition of repeating field {@link #file}, creating it if it does not already exist {3} 830 */ 831 public Attachment getFileFirstRep() { 832 if (getFile().isEmpty()) { 833 addFile(); 834 } 835 return getFile().get(0); 836 } 837 838 protected void listChildren(List<Property> children) { 839 super.listChildren(children); 840 children.add(new Property("technique", "CodeableConcept", "The method used to elucidate the characterization of the drug substance. Example: HPLC.", 0, 1, technique)); 841 children.add(new Property("form", "CodeableConcept", "Describes the nature of the chemical entity and explains, for instance, whether this is a base or a salt form.", 0, 1, form)); 842 children.add(new Property("description", "markdown", "The description or justification in support of the interpretation of the data file.", 0, 1, description)); 843 children.add(new Property("file", "Attachment", "The data produced by the analytical instrument or a pictorial representation of that data. Examples: a JCAMP, JDX, or ADX file, or a chromatogram or spectrum analysis.", 0, java.lang.Integer.MAX_VALUE, file)); 844 } 845 846 @Override 847 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 848 switch (_hash) { 849 case 1469675088: /*technique*/ return new Property("technique", "CodeableConcept", "The method used to elucidate the characterization of the drug substance. Example: HPLC.", 0, 1, technique); 850 case 3148996: /*form*/ return new Property("form", "CodeableConcept", "Describes the nature of the chemical entity and explains, for instance, whether this is a base or a salt form.", 0, 1, form); 851 case -1724546052: /*description*/ return new Property("description", "markdown", "The description or justification in support of the interpretation of the data file.", 0, 1, description); 852 case 3143036: /*file*/ return new Property("file", "Attachment", "The data produced by the analytical instrument or a pictorial representation of that data. Examples: a JCAMP, JDX, or ADX file, or a chromatogram or spectrum analysis.", 0, java.lang.Integer.MAX_VALUE, file); 853 default: return super.getNamedProperty(_hash, _name, _checkValid); 854 } 855 856 } 857 858 @Override 859 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 860 switch (hash) { 861 case 1469675088: /*technique*/ return this.technique == null ? new Base[0] : new Base[] {this.technique}; // CodeableConcept 862 case 3148996: /*form*/ return this.form == null ? new Base[0] : new Base[] {this.form}; // CodeableConcept 863 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 864 case 3143036: /*file*/ return this.file == null ? new Base[0] : this.file.toArray(new Base[this.file.size()]); // Attachment 865 default: return super.getProperty(hash, name, checkValid); 866 } 867 868 } 869 870 @Override 871 public Base setProperty(int hash, String name, Base value) throws FHIRException { 872 switch (hash) { 873 case 1469675088: // technique 874 this.technique = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 875 return value; 876 case 3148996: // form 877 this.form = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 878 return value; 879 case -1724546052: // description 880 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 881 return value; 882 case 3143036: // file 883 this.getFile().add(TypeConvertor.castToAttachment(value)); // Attachment 884 return value; 885 default: return super.setProperty(hash, name, value); 886 } 887 888 } 889 890 @Override 891 public Base setProperty(String name, Base value) throws FHIRException { 892 if (name.equals("technique")) { 893 this.technique = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 894 } else if (name.equals("form")) { 895 this.form = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 896 } else if (name.equals("description")) { 897 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 898 } else if (name.equals("file")) { 899 this.getFile().add(TypeConvertor.castToAttachment(value)); 900 } else 901 return super.setProperty(name, value); 902 return value; 903 } 904 905 @Override 906 public void removeChild(String name, Base value) throws FHIRException { 907 if (name.equals("technique")) { 908 this.technique = null; 909 } else if (name.equals("form")) { 910 this.form = null; 911 } else if (name.equals("description")) { 912 this.description = null; 913 } else if (name.equals("file")) { 914 this.getFile().remove(value); 915 } else 916 super.removeChild(name, value); 917 918 } 919 920 @Override 921 public Base makeProperty(int hash, String name) throws FHIRException { 922 switch (hash) { 923 case 1469675088: return getTechnique(); 924 case 3148996: return getForm(); 925 case -1724546052: return getDescriptionElement(); 926 case 3143036: return addFile(); 927 default: return super.makeProperty(hash, name); 928 } 929 930 } 931 932 @Override 933 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 934 switch (hash) { 935 case 1469675088: /*technique*/ return new String[] {"CodeableConcept"}; 936 case 3148996: /*form*/ return new String[] {"CodeableConcept"}; 937 case -1724546052: /*description*/ return new String[] {"markdown"}; 938 case 3143036: /*file*/ return new String[] {"Attachment"}; 939 default: return super.getTypesForProperty(hash, name); 940 } 941 942 } 943 944 @Override 945 public Base addChild(String name) throws FHIRException { 946 if (name.equals("technique")) { 947 this.technique = new CodeableConcept(); 948 return this.technique; 949 } 950 else if (name.equals("form")) { 951 this.form = new CodeableConcept(); 952 return this.form; 953 } 954 else if (name.equals("description")) { 955 throw new FHIRException("Cannot call addChild on a singleton property SubstanceDefinition.characterization.description"); 956 } 957 else if (name.equals("file")) { 958 return addFile(); 959 } 960 else 961 return super.addChild(name); 962 } 963 964 public SubstanceDefinitionCharacterizationComponent copy() { 965 SubstanceDefinitionCharacterizationComponent dst = new SubstanceDefinitionCharacterizationComponent(); 966 copyValues(dst); 967 return dst; 968 } 969 970 public void copyValues(SubstanceDefinitionCharacterizationComponent dst) { 971 super.copyValues(dst); 972 dst.technique = technique == null ? null : technique.copy(); 973 dst.form = form == null ? null : form.copy(); 974 dst.description = description == null ? null : description.copy(); 975 if (file != null) { 976 dst.file = new ArrayList<Attachment>(); 977 for (Attachment i : file) 978 dst.file.add(i.copy()); 979 }; 980 } 981 982 @Override 983 public boolean equalsDeep(Base other_) { 984 if (!super.equalsDeep(other_)) 985 return false; 986 if (!(other_ instanceof SubstanceDefinitionCharacterizationComponent)) 987 return false; 988 SubstanceDefinitionCharacterizationComponent o = (SubstanceDefinitionCharacterizationComponent) other_; 989 return compareDeep(technique, o.technique, true) && compareDeep(form, o.form, true) && compareDeep(description, o.description, true) 990 && compareDeep(file, o.file, true); 991 } 992 993 @Override 994 public boolean equalsShallow(Base other_) { 995 if (!super.equalsShallow(other_)) 996 return false; 997 if (!(other_ instanceof SubstanceDefinitionCharacterizationComponent)) 998 return false; 999 SubstanceDefinitionCharacterizationComponent o = (SubstanceDefinitionCharacterizationComponent) other_; 1000 return compareValues(description, o.description, true); 1001 } 1002 1003 public boolean isEmpty() { 1004 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(technique, form, description 1005 , file); 1006 } 1007 1008 public String fhirType() { 1009 return "SubstanceDefinition.characterization"; 1010 1011 } 1012 1013 } 1014 1015 @Block() 1016 public static class SubstanceDefinitionPropertyComponent extends BackboneElement implements IBaseBackboneElement { 1017 /** 1018 * A code expressing the type of property. 1019 */ 1020 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 1021 @Description(shortDefinition="A code expressing the type of property", formalDefinition="A code expressing the type of property." ) 1022 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/product-characteristic-codes") 1023 protected CodeableConcept type; 1024 1025 /** 1026 * A value for the property. 1027 */ 1028 @Child(name = "value", type = {CodeableConcept.class, Quantity.class, DateType.class, BooleanType.class, Attachment.class}, order=2, min=0, max=1, modifier=false, summary=true) 1029 @Description(shortDefinition="A value for the property", formalDefinition="A value for the property." ) 1030 protected DataType value; 1031 1032 private static final long serialVersionUID = -1659186716L; 1033 1034 /** 1035 * Constructor 1036 */ 1037 public SubstanceDefinitionPropertyComponent() { 1038 super(); 1039 } 1040 1041 /** 1042 * Constructor 1043 */ 1044 public SubstanceDefinitionPropertyComponent(CodeableConcept type) { 1045 super(); 1046 this.setType(type); 1047 } 1048 1049 /** 1050 * @return {@link #type} (A code expressing the type of property.) 1051 */ 1052 public CodeableConcept getType() { 1053 if (this.type == null) 1054 if (Configuration.errorOnAutoCreate()) 1055 throw new Error("Attempt to auto-create SubstanceDefinitionPropertyComponent.type"); 1056 else if (Configuration.doAutoCreate()) 1057 this.type = new CodeableConcept(); // cc 1058 return this.type; 1059 } 1060 1061 public boolean hasType() { 1062 return this.type != null && !this.type.isEmpty(); 1063 } 1064 1065 /** 1066 * @param value {@link #type} (A code expressing the type of property.) 1067 */ 1068 public SubstanceDefinitionPropertyComponent setType(CodeableConcept value) { 1069 this.type = value; 1070 return this; 1071 } 1072 1073 /** 1074 * @return {@link #value} (A value for the property.) 1075 */ 1076 public DataType getValue() { 1077 return this.value; 1078 } 1079 1080 /** 1081 * @return {@link #value} (A value for the property.) 1082 */ 1083 public CodeableConcept getValueCodeableConcept() throws FHIRException { 1084 if (this.value == null) 1085 this.value = new CodeableConcept(); 1086 if (!(this.value instanceof CodeableConcept)) 1087 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 1088 return (CodeableConcept) this.value; 1089 } 1090 1091 public boolean hasValueCodeableConcept() { 1092 return this != null && this.value instanceof CodeableConcept; 1093 } 1094 1095 /** 1096 * @return {@link #value} (A value for the property.) 1097 */ 1098 public Quantity getValueQuantity() throws FHIRException { 1099 if (this.value == null) 1100 this.value = new Quantity(); 1101 if (!(this.value instanceof Quantity)) 1102 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1103 return (Quantity) this.value; 1104 } 1105 1106 public boolean hasValueQuantity() { 1107 return this != null && this.value instanceof Quantity; 1108 } 1109 1110 /** 1111 * @return {@link #value} (A value for the property.) 1112 */ 1113 public DateType getValueDateType() throws FHIRException { 1114 if (this.value == null) 1115 this.value = new DateType(); 1116 if (!(this.value instanceof DateType)) 1117 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 1118 return (DateType) this.value; 1119 } 1120 1121 public boolean hasValueDateType() { 1122 return this != null && this.value instanceof DateType; 1123 } 1124 1125 /** 1126 * @return {@link #value} (A value for the property.) 1127 */ 1128 public BooleanType getValueBooleanType() throws FHIRException { 1129 if (this.value == null) 1130 this.value = new BooleanType(); 1131 if (!(this.value instanceof BooleanType)) 1132 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 1133 return (BooleanType) this.value; 1134 } 1135 1136 public boolean hasValueBooleanType() { 1137 return this != null && this.value instanceof BooleanType; 1138 } 1139 1140 /** 1141 * @return {@link #value} (A value for the property.) 1142 */ 1143 public Attachment getValueAttachment() throws FHIRException { 1144 if (this.value == null) 1145 this.value = new Attachment(); 1146 if (!(this.value instanceof Attachment)) 1147 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 1148 return (Attachment) this.value; 1149 } 1150 1151 public boolean hasValueAttachment() { 1152 return this != null && this.value instanceof Attachment; 1153 } 1154 1155 public boolean hasValue() { 1156 return this.value != null && !this.value.isEmpty(); 1157 } 1158 1159 /** 1160 * @param value {@link #value} (A value for the property.) 1161 */ 1162 public SubstanceDefinitionPropertyComponent setValue(DataType value) { 1163 if (value != null && !(value instanceof CodeableConcept || value instanceof Quantity || value instanceof DateType || value instanceof BooleanType || value instanceof Attachment)) 1164 throw new FHIRException("Not the right type for SubstanceDefinition.property.value[x]: "+value.fhirType()); 1165 this.value = value; 1166 return this; 1167 } 1168 1169 protected void listChildren(List<Property> children) { 1170 super.listChildren(children); 1171 children.add(new Property("type", "CodeableConcept", "A code expressing the type of property.", 0, 1, type)); 1172 children.add(new Property("value[x]", "CodeableConcept|Quantity|date|boolean|Attachment", "A value for the property.", 0, 1, value)); 1173 } 1174 1175 @Override 1176 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1177 switch (_hash) { 1178 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A code expressing the type of property.", 0, 1, type); 1179 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|Quantity|date|boolean|Attachment", "A value for the property.", 0, 1, value); 1180 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|Quantity|date|boolean|Attachment", "A value for the property.", 0, 1, value); 1181 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "A value for the property.", 0, 1, value); 1182 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "A value for the property.", 0, 1, value); 1183 case -766192449: /*valueDate*/ return new Property("value[x]", "date", "A value for the property.", 0, 1, value); 1184 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "A value for the property.", 0, 1, value); 1185 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "A value for the property.", 0, 1, value); 1186 default: return super.getNamedProperty(_hash, _name, _checkValid); 1187 } 1188 1189 } 1190 1191 @Override 1192 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1193 switch (hash) { 1194 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1195 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1196 default: return super.getProperty(hash, name, checkValid); 1197 } 1198 1199 } 1200 1201 @Override 1202 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1203 switch (hash) { 1204 case 3575610: // type 1205 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1206 return value; 1207 case 111972721: // value 1208 this.value = TypeConvertor.castToType(value); // DataType 1209 return value; 1210 default: return super.setProperty(hash, name, value); 1211 } 1212 1213 } 1214 1215 @Override 1216 public Base setProperty(String name, Base value) throws FHIRException { 1217 if (name.equals("type")) { 1218 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1219 } else if (name.equals("value[x]")) { 1220 this.value = TypeConvertor.castToType(value); // DataType 1221 } else 1222 return super.setProperty(name, value); 1223 return value; 1224 } 1225 1226 @Override 1227 public void removeChild(String name, Base value) throws FHIRException { 1228 if (name.equals("type")) { 1229 this.type = null; 1230 } else if (name.equals("value[x]")) { 1231 this.value = null; 1232 } else 1233 super.removeChild(name, value); 1234 1235 } 1236 1237 @Override 1238 public Base makeProperty(int hash, String name) throws FHIRException { 1239 switch (hash) { 1240 case 3575610: return getType(); 1241 case -1410166417: return getValue(); 1242 case 111972721: return getValue(); 1243 default: return super.makeProperty(hash, name); 1244 } 1245 1246 } 1247 1248 @Override 1249 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1250 switch (hash) { 1251 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1252 case 111972721: /*value*/ return new String[] {"CodeableConcept", "Quantity", "date", "boolean", "Attachment"}; 1253 default: return super.getTypesForProperty(hash, name); 1254 } 1255 1256 } 1257 1258 @Override 1259 public Base addChild(String name) throws FHIRException { 1260 if (name.equals("type")) { 1261 this.type = new CodeableConcept(); 1262 return this.type; 1263 } 1264 else if (name.equals("valueCodeableConcept")) { 1265 this.value = new CodeableConcept(); 1266 return this.value; 1267 } 1268 else if (name.equals("valueQuantity")) { 1269 this.value = new Quantity(); 1270 return this.value; 1271 } 1272 else if (name.equals("valueDate")) { 1273 this.value = new DateType(); 1274 return this.value; 1275 } 1276 else if (name.equals("valueBoolean")) { 1277 this.value = new BooleanType(); 1278 return this.value; 1279 } 1280 else if (name.equals("valueAttachment")) { 1281 this.value = new Attachment(); 1282 return this.value; 1283 } 1284 else 1285 return super.addChild(name); 1286 } 1287 1288 public SubstanceDefinitionPropertyComponent copy() { 1289 SubstanceDefinitionPropertyComponent dst = new SubstanceDefinitionPropertyComponent(); 1290 copyValues(dst); 1291 return dst; 1292 } 1293 1294 public void copyValues(SubstanceDefinitionPropertyComponent dst) { 1295 super.copyValues(dst); 1296 dst.type = type == null ? null : type.copy(); 1297 dst.value = value == null ? null : value.copy(); 1298 } 1299 1300 @Override 1301 public boolean equalsDeep(Base other_) { 1302 if (!super.equalsDeep(other_)) 1303 return false; 1304 if (!(other_ instanceof SubstanceDefinitionPropertyComponent)) 1305 return false; 1306 SubstanceDefinitionPropertyComponent o = (SubstanceDefinitionPropertyComponent) other_; 1307 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 1308 } 1309 1310 @Override 1311 public boolean equalsShallow(Base other_) { 1312 if (!super.equalsShallow(other_)) 1313 return false; 1314 if (!(other_ instanceof SubstanceDefinitionPropertyComponent)) 1315 return false; 1316 SubstanceDefinitionPropertyComponent o = (SubstanceDefinitionPropertyComponent) other_; 1317 return true; 1318 } 1319 1320 public boolean isEmpty() { 1321 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 1322 } 1323 1324 public String fhirType() { 1325 return "SubstanceDefinition.property"; 1326 1327 } 1328 1329 } 1330 1331 @Block() 1332 public static class SubstanceDefinitionMolecularWeightComponent extends BackboneElement implements IBaseBackboneElement { 1333 /** 1334 * The method by which the molecular weight was determined. 1335 */ 1336 @Child(name = "method", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 1337 @Description(shortDefinition="The method by which the weight was determined", formalDefinition="The method by which the molecular weight was determined." ) 1338 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-weight-method") 1339 protected CodeableConcept method; 1340 1341 /** 1342 * Type of molecular weight such as exact, average (also known as. number average), weight average. 1343 */ 1344 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 1345 @Description(shortDefinition="Type of molecular weight e.g. exact, average, weight average", formalDefinition="Type of molecular weight such as exact, average (also known as. number average), weight average." ) 1346 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-weight-type") 1347 protected CodeableConcept type; 1348 1349 /** 1350 * Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field. 1351 */ 1352 @Child(name = "amount", type = {Quantity.class}, order=3, min=1, max=1, modifier=false, summary=true) 1353 @Description(shortDefinition="Used to capture quantitative values for a variety of elements", formalDefinition="Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field." ) 1354 protected Quantity amount; 1355 1356 private static final long serialVersionUID = 805939780L; 1357 1358 /** 1359 * Constructor 1360 */ 1361 public SubstanceDefinitionMolecularWeightComponent() { 1362 super(); 1363 } 1364 1365 /** 1366 * Constructor 1367 */ 1368 public SubstanceDefinitionMolecularWeightComponent(Quantity amount) { 1369 super(); 1370 this.setAmount(amount); 1371 } 1372 1373 /** 1374 * @return {@link #method} (The method by which the molecular weight was determined.) 1375 */ 1376 public CodeableConcept getMethod() { 1377 if (this.method == null) 1378 if (Configuration.errorOnAutoCreate()) 1379 throw new Error("Attempt to auto-create SubstanceDefinitionMolecularWeightComponent.method"); 1380 else if (Configuration.doAutoCreate()) 1381 this.method = new CodeableConcept(); // cc 1382 return this.method; 1383 } 1384 1385 public boolean hasMethod() { 1386 return this.method != null && !this.method.isEmpty(); 1387 } 1388 1389 /** 1390 * @param value {@link #method} (The method by which the molecular weight was determined.) 1391 */ 1392 public SubstanceDefinitionMolecularWeightComponent setMethod(CodeableConcept value) { 1393 this.method = value; 1394 return this; 1395 } 1396 1397 /** 1398 * @return {@link #type} (Type of molecular weight such as exact, average (also known as. number average), weight average.) 1399 */ 1400 public CodeableConcept getType() { 1401 if (this.type == null) 1402 if (Configuration.errorOnAutoCreate()) 1403 throw new Error("Attempt to auto-create SubstanceDefinitionMolecularWeightComponent.type"); 1404 else if (Configuration.doAutoCreate()) 1405 this.type = new CodeableConcept(); // cc 1406 return this.type; 1407 } 1408 1409 public boolean hasType() { 1410 return this.type != null && !this.type.isEmpty(); 1411 } 1412 1413 /** 1414 * @param value {@link #type} (Type of molecular weight such as exact, average (also known as. number average), weight average.) 1415 */ 1416 public SubstanceDefinitionMolecularWeightComponent setType(CodeableConcept value) { 1417 this.type = value; 1418 return this; 1419 } 1420 1421 /** 1422 * @return {@link #amount} (Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.) 1423 */ 1424 public Quantity getAmount() { 1425 if (this.amount == null) 1426 if (Configuration.errorOnAutoCreate()) 1427 throw new Error("Attempt to auto-create SubstanceDefinitionMolecularWeightComponent.amount"); 1428 else if (Configuration.doAutoCreate()) 1429 this.amount = new Quantity(); // cc 1430 return this.amount; 1431 } 1432 1433 public boolean hasAmount() { 1434 return this.amount != null && !this.amount.isEmpty(); 1435 } 1436 1437 /** 1438 * @param value {@link #amount} (Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.) 1439 */ 1440 public SubstanceDefinitionMolecularWeightComponent setAmount(Quantity value) { 1441 this.amount = value; 1442 return this; 1443 } 1444 1445 protected void listChildren(List<Property> children) { 1446 super.listChildren(children); 1447 children.add(new Property("method", "CodeableConcept", "The method by which the molecular weight was determined.", 0, 1, method)); 1448 children.add(new Property("type", "CodeableConcept", "Type of molecular weight such as exact, average (also known as. number average), weight average.", 0, 1, type)); 1449 children.add(new Property("amount", "Quantity", "Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.", 0, 1, amount)); 1450 } 1451 1452 @Override 1453 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1454 switch (_hash) { 1455 case -1077554975: /*method*/ return new Property("method", "CodeableConcept", "The method by which the molecular weight was determined.", 0, 1, method); 1456 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of molecular weight such as exact, average (also known as. number average), weight average.", 0, 1, type); 1457 case -1413853096: /*amount*/ return new Property("amount", "Quantity", "Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.", 0, 1, amount); 1458 default: return super.getNamedProperty(_hash, _name, _checkValid); 1459 } 1460 1461 } 1462 1463 @Override 1464 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1465 switch (hash) { 1466 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // CodeableConcept 1467 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1468 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Quantity 1469 default: return super.getProperty(hash, name, checkValid); 1470 } 1471 1472 } 1473 1474 @Override 1475 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1476 switch (hash) { 1477 case -1077554975: // method 1478 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1479 return value; 1480 case 3575610: // type 1481 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1482 return value; 1483 case -1413853096: // amount 1484 this.amount = TypeConvertor.castToQuantity(value); // Quantity 1485 return value; 1486 default: return super.setProperty(hash, name, value); 1487 } 1488 1489 } 1490 1491 @Override 1492 public Base setProperty(String name, Base value) throws FHIRException { 1493 if (name.equals("method")) { 1494 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1495 } else if (name.equals("type")) { 1496 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1497 } else if (name.equals("amount")) { 1498 this.amount = TypeConvertor.castToQuantity(value); // Quantity 1499 } else 1500 return super.setProperty(name, value); 1501 return value; 1502 } 1503 1504 @Override 1505 public void removeChild(String name, Base value) throws FHIRException { 1506 if (name.equals("method")) { 1507 this.method = null; 1508 } else if (name.equals("type")) { 1509 this.type = null; 1510 } else if (name.equals("amount")) { 1511 this.amount = null; 1512 } else 1513 super.removeChild(name, value); 1514 1515 } 1516 1517 @Override 1518 public Base makeProperty(int hash, String name) throws FHIRException { 1519 switch (hash) { 1520 case -1077554975: return getMethod(); 1521 case 3575610: return getType(); 1522 case -1413853096: return getAmount(); 1523 default: return super.makeProperty(hash, name); 1524 } 1525 1526 } 1527 1528 @Override 1529 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1530 switch (hash) { 1531 case -1077554975: /*method*/ return new String[] {"CodeableConcept"}; 1532 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1533 case -1413853096: /*amount*/ return new String[] {"Quantity"}; 1534 default: return super.getTypesForProperty(hash, name); 1535 } 1536 1537 } 1538 1539 @Override 1540 public Base addChild(String name) throws FHIRException { 1541 if (name.equals("method")) { 1542 this.method = new CodeableConcept(); 1543 return this.method; 1544 } 1545 else if (name.equals("type")) { 1546 this.type = new CodeableConcept(); 1547 return this.type; 1548 } 1549 else if (name.equals("amount")) { 1550 this.amount = new Quantity(); 1551 return this.amount; 1552 } 1553 else 1554 return super.addChild(name); 1555 } 1556 1557 public SubstanceDefinitionMolecularWeightComponent copy() { 1558 SubstanceDefinitionMolecularWeightComponent dst = new SubstanceDefinitionMolecularWeightComponent(); 1559 copyValues(dst); 1560 return dst; 1561 } 1562 1563 public void copyValues(SubstanceDefinitionMolecularWeightComponent dst) { 1564 super.copyValues(dst); 1565 dst.method = method == null ? null : method.copy(); 1566 dst.type = type == null ? null : type.copy(); 1567 dst.amount = amount == null ? null : amount.copy(); 1568 } 1569 1570 @Override 1571 public boolean equalsDeep(Base other_) { 1572 if (!super.equalsDeep(other_)) 1573 return false; 1574 if (!(other_ instanceof SubstanceDefinitionMolecularWeightComponent)) 1575 return false; 1576 SubstanceDefinitionMolecularWeightComponent o = (SubstanceDefinitionMolecularWeightComponent) other_; 1577 return compareDeep(method, o.method, true) && compareDeep(type, o.type, true) && compareDeep(amount, o.amount, true) 1578 ; 1579 } 1580 1581 @Override 1582 public boolean equalsShallow(Base other_) { 1583 if (!super.equalsShallow(other_)) 1584 return false; 1585 if (!(other_ instanceof SubstanceDefinitionMolecularWeightComponent)) 1586 return false; 1587 SubstanceDefinitionMolecularWeightComponent o = (SubstanceDefinitionMolecularWeightComponent) other_; 1588 return true; 1589 } 1590 1591 public boolean isEmpty() { 1592 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(method, type, amount); 1593 } 1594 1595 public String fhirType() { 1596 return "SubstanceDefinition.molecularWeight"; 1597 1598 } 1599 1600 } 1601 1602 @Block() 1603 public static class SubstanceDefinitionStructureComponent extends BackboneElement implements IBaseBackboneElement { 1604 /** 1605 * Stereochemistry type. 1606 */ 1607 @Child(name = "stereochemistry", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 1608 @Description(shortDefinition="Stereochemistry type", formalDefinition="Stereochemistry type." ) 1609 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-stereochemistry") 1610 protected CodeableConcept stereochemistry; 1611 1612 /** 1613 * Optical activity type. 1614 */ 1615 @Child(name = "opticalActivity", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 1616 @Description(shortDefinition="Optical activity type", formalDefinition="Optical activity type." ) 1617 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-optical-activity") 1618 protected CodeableConcept opticalActivity; 1619 1620 /** 1621 * An expression which states the number and type of atoms present in a molecule of a substance. 1622 */ 1623 @Child(name = "molecularFormula", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1624 @Description(shortDefinition="An expression which states the number and type of atoms present in a molecule of a substance", formalDefinition="An expression which states the number and type of atoms present in a molecule of a substance." ) 1625 protected StringType molecularFormula; 1626 1627 /** 1628 * Specified per moiety according to the Hill system, i.e. first C, then H, then alphabetical, each moiety separated by a dot. 1629 */ 1630 @Child(name = "molecularFormulaByMoiety", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1631 @Description(shortDefinition="Specified per moiety according to the Hill system", formalDefinition="Specified per moiety according to the Hill system, i.e. first C, then H, then alphabetical, each moiety separated by a dot." ) 1632 protected StringType molecularFormulaByMoiety; 1633 1634 /** 1635 * The molecular weight or weight range (for proteins, polymers or nucleic acids). 1636 */ 1637 @Child(name = "molecularWeight", type = {SubstanceDefinitionMolecularWeightComponent.class}, order=5, min=0, max=1, modifier=false, summary=true) 1638 @Description(shortDefinition="The molecular weight or weight range", formalDefinition="The molecular weight or weight range (for proteins, polymers or nucleic acids)." ) 1639 protected SubstanceDefinitionMolecularWeightComponent molecularWeight; 1640 1641 /** 1642 * The method used to elucidate the structure of the drug substance. Examples: X-ray, NMR, Peptide mapping, Ligand binding assay. 1643 */ 1644 @Child(name = "technique", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1645 @Description(shortDefinition="The method used to find the structure e.g. X-ray, NMR", formalDefinition="The method used to elucidate the structure of the drug substance. Examples: X-ray, NMR, Peptide mapping, Ligand binding assay." ) 1646 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-structure-technique") 1647 protected List<CodeableConcept> technique; 1648 1649 /** 1650 * The source of information about the structure. 1651 */ 1652 @Child(name = "sourceDocument", type = {DocumentReference.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1653 @Description(shortDefinition="Source of information for the structure", formalDefinition="The source of information about the structure." ) 1654 protected List<Reference> sourceDocument; 1655 1656 /** 1657 * A depiction of the structure of the substance. 1658 */ 1659 @Child(name = "representation", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1660 @Description(shortDefinition="A depiction of the structure of the substance", formalDefinition="A depiction of the structure of the substance." ) 1661 protected List<SubstanceDefinitionStructureRepresentationComponent> representation; 1662 1663 private static final long serialVersionUID = -2137814144L; 1664 1665 /** 1666 * Constructor 1667 */ 1668 public SubstanceDefinitionStructureComponent() { 1669 super(); 1670 } 1671 1672 /** 1673 * @return {@link #stereochemistry} (Stereochemistry type.) 1674 */ 1675 public CodeableConcept getStereochemistry() { 1676 if (this.stereochemistry == null) 1677 if (Configuration.errorOnAutoCreate()) 1678 throw new Error("Attempt to auto-create SubstanceDefinitionStructureComponent.stereochemistry"); 1679 else if (Configuration.doAutoCreate()) 1680 this.stereochemistry = new CodeableConcept(); // cc 1681 return this.stereochemistry; 1682 } 1683 1684 public boolean hasStereochemistry() { 1685 return this.stereochemistry != null && !this.stereochemistry.isEmpty(); 1686 } 1687 1688 /** 1689 * @param value {@link #stereochemistry} (Stereochemistry type.) 1690 */ 1691 public SubstanceDefinitionStructureComponent setStereochemistry(CodeableConcept value) { 1692 this.stereochemistry = value; 1693 return this; 1694 } 1695 1696 /** 1697 * @return {@link #opticalActivity} (Optical activity type.) 1698 */ 1699 public CodeableConcept getOpticalActivity() { 1700 if (this.opticalActivity == null) 1701 if (Configuration.errorOnAutoCreate()) 1702 throw new Error("Attempt to auto-create SubstanceDefinitionStructureComponent.opticalActivity"); 1703 else if (Configuration.doAutoCreate()) 1704 this.opticalActivity = new CodeableConcept(); // cc 1705 return this.opticalActivity; 1706 } 1707 1708 public boolean hasOpticalActivity() { 1709 return this.opticalActivity != null && !this.opticalActivity.isEmpty(); 1710 } 1711 1712 /** 1713 * @param value {@link #opticalActivity} (Optical activity type.) 1714 */ 1715 public SubstanceDefinitionStructureComponent setOpticalActivity(CodeableConcept value) { 1716 this.opticalActivity = value; 1717 return this; 1718 } 1719 1720 /** 1721 * @return {@link #molecularFormula} (An expression which states the number and type of atoms present in a molecule of a substance.). This is the underlying object with id, value and extensions. The accessor "getMolecularFormula" gives direct access to the value 1722 */ 1723 public StringType getMolecularFormulaElement() { 1724 if (this.molecularFormula == null) 1725 if (Configuration.errorOnAutoCreate()) 1726 throw new Error("Attempt to auto-create SubstanceDefinitionStructureComponent.molecularFormula"); 1727 else if (Configuration.doAutoCreate()) 1728 this.molecularFormula = new StringType(); // bb 1729 return this.molecularFormula; 1730 } 1731 1732 public boolean hasMolecularFormulaElement() { 1733 return this.molecularFormula != null && !this.molecularFormula.isEmpty(); 1734 } 1735 1736 public boolean hasMolecularFormula() { 1737 return this.molecularFormula != null && !this.molecularFormula.isEmpty(); 1738 } 1739 1740 /** 1741 * @param value {@link #molecularFormula} (An expression which states the number and type of atoms present in a molecule of a substance.). This is the underlying object with id, value and extensions. The accessor "getMolecularFormula" gives direct access to the value 1742 */ 1743 public SubstanceDefinitionStructureComponent setMolecularFormulaElement(StringType value) { 1744 this.molecularFormula = value; 1745 return this; 1746 } 1747 1748 /** 1749 * @return An expression which states the number and type of atoms present in a molecule of a substance. 1750 */ 1751 public String getMolecularFormula() { 1752 return this.molecularFormula == null ? null : this.molecularFormula.getValue(); 1753 } 1754 1755 /** 1756 * @param value An expression which states the number and type of atoms present in a molecule of a substance. 1757 */ 1758 public SubstanceDefinitionStructureComponent setMolecularFormula(String value) { 1759 if (Utilities.noString(value)) 1760 this.molecularFormula = null; 1761 else { 1762 if (this.molecularFormula == null) 1763 this.molecularFormula = new StringType(); 1764 this.molecularFormula.setValue(value); 1765 } 1766 return this; 1767 } 1768 1769 /** 1770 * @return {@link #molecularFormulaByMoiety} (Specified per moiety according to the Hill system, i.e. first C, then H, then alphabetical, each moiety separated by a dot.). This is the underlying object with id, value and extensions. The accessor "getMolecularFormulaByMoiety" gives direct access to the value 1771 */ 1772 public StringType getMolecularFormulaByMoietyElement() { 1773 if (this.molecularFormulaByMoiety == null) 1774 if (Configuration.errorOnAutoCreate()) 1775 throw new Error("Attempt to auto-create SubstanceDefinitionStructureComponent.molecularFormulaByMoiety"); 1776 else if (Configuration.doAutoCreate()) 1777 this.molecularFormulaByMoiety = new StringType(); // bb 1778 return this.molecularFormulaByMoiety; 1779 } 1780 1781 public boolean hasMolecularFormulaByMoietyElement() { 1782 return this.molecularFormulaByMoiety != null && !this.molecularFormulaByMoiety.isEmpty(); 1783 } 1784 1785 public boolean hasMolecularFormulaByMoiety() { 1786 return this.molecularFormulaByMoiety != null && !this.molecularFormulaByMoiety.isEmpty(); 1787 } 1788 1789 /** 1790 * @param value {@link #molecularFormulaByMoiety} (Specified per moiety according to the Hill system, i.e. first C, then H, then alphabetical, each moiety separated by a dot.). This is the underlying object with id, value and extensions. The accessor "getMolecularFormulaByMoiety" gives direct access to the value 1791 */ 1792 public SubstanceDefinitionStructureComponent setMolecularFormulaByMoietyElement(StringType value) { 1793 this.molecularFormulaByMoiety = value; 1794 return this; 1795 } 1796 1797 /** 1798 * @return Specified per moiety according to the Hill system, i.e. first C, then H, then alphabetical, each moiety separated by a dot. 1799 */ 1800 public String getMolecularFormulaByMoiety() { 1801 return this.molecularFormulaByMoiety == null ? null : this.molecularFormulaByMoiety.getValue(); 1802 } 1803 1804 /** 1805 * @param value Specified per moiety according to the Hill system, i.e. first C, then H, then alphabetical, each moiety separated by a dot. 1806 */ 1807 public SubstanceDefinitionStructureComponent setMolecularFormulaByMoiety(String value) { 1808 if (Utilities.noString(value)) 1809 this.molecularFormulaByMoiety = null; 1810 else { 1811 if (this.molecularFormulaByMoiety == null) 1812 this.molecularFormulaByMoiety = new StringType(); 1813 this.molecularFormulaByMoiety.setValue(value); 1814 } 1815 return this; 1816 } 1817 1818 /** 1819 * @return {@link #molecularWeight} (The molecular weight or weight range (for proteins, polymers or nucleic acids).) 1820 */ 1821 public SubstanceDefinitionMolecularWeightComponent getMolecularWeight() { 1822 if (this.molecularWeight == null) 1823 if (Configuration.errorOnAutoCreate()) 1824 throw new Error("Attempt to auto-create SubstanceDefinitionStructureComponent.molecularWeight"); 1825 else if (Configuration.doAutoCreate()) 1826 this.molecularWeight = new SubstanceDefinitionMolecularWeightComponent(); // cc 1827 return this.molecularWeight; 1828 } 1829 1830 public boolean hasMolecularWeight() { 1831 return this.molecularWeight != null && !this.molecularWeight.isEmpty(); 1832 } 1833 1834 /** 1835 * @param value {@link #molecularWeight} (The molecular weight or weight range (for proteins, polymers or nucleic acids).) 1836 */ 1837 public SubstanceDefinitionStructureComponent setMolecularWeight(SubstanceDefinitionMolecularWeightComponent value) { 1838 this.molecularWeight = value; 1839 return this; 1840 } 1841 1842 /** 1843 * @return {@link #technique} (The method used to elucidate the structure of the drug substance. Examples: X-ray, NMR, Peptide mapping, Ligand binding assay.) 1844 */ 1845 public List<CodeableConcept> getTechnique() { 1846 if (this.technique == null) 1847 this.technique = new ArrayList<CodeableConcept>(); 1848 return this.technique; 1849 } 1850 1851 /** 1852 * @return Returns a reference to <code>this</code> for easy method chaining 1853 */ 1854 public SubstanceDefinitionStructureComponent setTechnique(List<CodeableConcept> theTechnique) { 1855 this.technique = theTechnique; 1856 return this; 1857 } 1858 1859 public boolean hasTechnique() { 1860 if (this.technique == null) 1861 return false; 1862 for (CodeableConcept item : this.technique) 1863 if (!item.isEmpty()) 1864 return true; 1865 return false; 1866 } 1867 1868 public CodeableConcept addTechnique() { //3 1869 CodeableConcept t = new CodeableConcept(); 1870 if (this.technique == null) 1871 this.technique = new ArrayList<CodeableConcept>(); 1872 this.technique.add(t); 1873 return t; 1874 } 1875 1876 public SubstanceDefinitionStructureComponent addTechnique(CodeableConcept t) { //3 1877 if (t == null) 1878 return this; 1879 if (this.technique == null) 1880 this.technique = new ArrayList<CodeableConcept>(); 1881 this.technique.add(t); 1882 return this; 1883 } 1884 1885 /** 1886 * @return The first repetition of repeating field {@link #technique}, creating it if it does not already exist {3} 1887 */ 1888 public CodeableConcept getTechniqueFirstRep() { 1889 if (getTechnique().isEmpty()) { 1890 addTechnique(); 1891 } 1892 return getTechnique().get(0); 1893 } 1894 1895 /** 1896 * @return {@link #sourceDocument} (The source of information about the structure.) 1897 */ 1898 public List<Reference> getSourceDocument() { 1899 if (this.sourceDocument == null) 1900 this.sourceDocument = new ArrayList<Reference>(); 1901 return this.sourceDocument; 1902 } 1903 1904 /** 1905 * @return Returns a reference to <code>this</code> for easy method chaining 1906 */ 1907 public SubstanceDefinitionStructureComponent setSourceDocument(List<Reference> theSourceDocument) { 1908 this.sourceDocument = theSourceDocument; 1909 return this; 1910 } 1911 1912 public boolean hasSourceDocument() { 1913 if (this.sourceDocument == null) 1914 return false; 1915 for (Reference item : this.sourceDocument) 1916 if (!item.isEmpty()) 1917 return true; 1918 return false; 1919 } 1920 1921 public Reference addSourceDocument() { //3 1922 Reference t = new Reference(); 1923 if (this.sourceDocument == null) 1924 this.sourceDocument = new ArrayList<Reference>(); 1925 this.sourceDocument.add(t); 1926 return t; 1927 } 1928 1929 public SubstanceDefinitionStructureComponent addSourceDocument(Reference t) { //3 1930 if (t == null) 1931 return this; 1932 if (this.sourceDocument == null) 1933 this.sourceDocument = new ArrayList<Reference>(); 1934 this.sourceDocument.add(t); 1935 return this; 1936 } 1937 1938 /** 1939 * @return The first repetition of repeating field {@link #sourceDocument}, creating it if it does not already exist {3} 1940 */ 1941 public Reference getSourceDocumentFirstRep() { 1942 if (getSourceDocument().isEmpty()) { 1943 addSourceDocument(); 1944 } 1945 return getSourceDocument().get(0); 1946 } 1947 1948 /** 1949 * @return {@link #representation} (A depiction of the structure of the substance.) 1950 */ 1951 public List<SubstanceDefinitionStructureRepresentationComponent> getRepresentation() { 1952 if (this.representation == null) 1953 this.representation = new ArrayList<SubstanceDefinitionStructureRepresentationComponent>(); 1954 return this.representation; 1955 } 1956 1957 /** 1958 * @return Returns a reference to <code>this</code> for easy method chaining 1959 */ 1960 public SubstanceDefinitionStructureComponent setRepresentation(List<SubstanceDefinitionStructureRepresentationComponent> theRepresentation) { 1961 this.representation = theRepresentation; 1962 return this; 1963 } 1964 1965 public boolean hasRepresentation() { 1966 if (this.representation == null) 1967 return false; 1968 for (SubstanceDefinitionStructureRepresentationComponent item : this.representation) 1969 if (!item.isEmpty()) 1970 return true; 1971 return false; 1972 } 1973 1974 public SubstanceDefinitionStructureRepresentationComponent addRepresentation() { //3 1975 SubstanceDefinitionStructureRepresentationComponent t = new SubstanceDefinitionStructureRepresentationComponent(); 1976 if (this.representation == null) 1977 this.representation = new ArrayList<SubstanceDefinitionStructureRepresentationComponent>(); 1978 this.representation.add(t); 1979 return t; 1980 } 1981 1982 public SubstanceDefinitionStructureComponent addRepresentation(SubstanceDefinitionStructureRepresentationComponent t) { //3 1983 if (t == null) 1984 return this; 1985 if (this.representation == null) 1986 this.representation = new ArrayList<SubstanceDefinitionStructureRepresentationComponent>(); 1987 this.representation.add(t); 1988 return this; 1989 } 1990 1991 /** 1992 * @return The first repetition of repeating field {@link #representation}, creating it if it does not already exist {3} 1993 */ 1994 public SubstanceDefinitionStructureRepresentationComponent getRepresentationFirstRep() { 1995 if (getRepresentation().isEmpty()) { 1996 addRepresentation(); 1997 } 1998 return getRepresentation().get(0); 1999 } 2000 2001 protected void listChildren(List<Property> children) { 2002 super.listChildren(children); 2003 children.add(new Property("stereochemistry", "CodeableConcept", "Stereochemistry type.", 0, 1, stereochemistry)); 2004 children.add(new Property("opticalActivity", "CodeableConcept", "Optical activity type.", 0, 1, opticalActivity)); 2005 children.add(new Property("molecularFormula", "string", "An expression which states the number and type of atoms present in a molecule of a substance.", 0, 1, molecularFormula)); 2006 children.add(new Property("molecularFormulaByMoiety", "string", "Specified per moiety according to the Hill system, i.e. first C, then H, then alphabetical, each moiety separated by a dot.", 0, 1, molecularFormulaByMoiety)); 2007 children.add(new Property("molecularWeight", "@SubstanceDefinition.molecularWeight", "The molecular weight or weight range (for proteins, polymers or nucleic acids).", 0, 1, molecularWeight)); 2008 children.add(new Property("technique", "CodeableConcept", "The method used to elucidate the structure of the drug substance. Examples: X-ray, NMR, Peptide mapping, Ligand binding assay.", 0, java.lang.Integer.MAX_VALUE, technique)); 2009 children.add(new Property("sourceDocument", "Reference(DocumentReference)", "The source of information about the structure.", 0, java.lang.Integer.MAX_VALUE, sourceDocument)); 2010 children.add(new Property("representation", "", "A depiction of the structure of the substance.", 0, java.lang.Integer.MAX_VALUE, representation)); 2011 } 2012 2013 @Override 2014 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2015 switch (_hash) { 2016 case 263475116: /*stereochemistry*/ return new Property("stereochemistry", "CodeableConcept", "Stereochemistry type.", 0, 1, stereochemistry); 2017 case 1420900135: /*opticalActivity*/ return new Property("opticalActivity", "CodeableConcept", "Optical activity type.", 0, 1, opticalActivity); 2018 case 616660246: /*molecularFormula*/ return new Property("molecularFormula", "string", "An expression which states the number and type of atoms present in a molecule of a substance.", 0, 1, molecularFormula); 2019 case 1315452848: /*molecularFormulaByMoiety*/ return new Property("molecularFormulaByMoiety", "string", "Specified per moiety according to the Hill system, i.e. first C, then H, then alphabetical, each moiety separated by a dot.", 0, 1, molecularFormulaByMoiety); 2020 case 635625672: /*molecularWeight*/ return new Property("molecularWeight", "@SubstanceDefinition.molecularWeight", "The molecular weight or weight range (for proteins, polymers or nucleic acids).", 0, 1, molecularWeight); 2021 case 1469675088: /*technique*/ return new Property("technique", "CodeableConcept", "The method used to elucidate the structure of the drug substance. Examples: X-ray, NMR, Peptide mapping, Ligand binding assay.", 0, java.lang.Integer.MAX_VALUE, technique); 2022 case -501788074: /*sourceDocument*/ return new Property("sourceDocument", "Reference(DocumentReference)", "The source of information about the structure.", 0, java.lang.Integer.MAX_VALUE, sourceDocument); 2023 case -671065907: /*representation*/ return new Property("representation", "", "A depiction of the structure of the substance.", 0, java.lang.Integer.MAX_VALUE, representation); 2024 default: return super.getNamedProperty(_hash, _name, _checkValid); 2025 } 2026 2027 } 2028 2029 @Override 2030 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2031 switch (hash) { 2032 case 263475116: /*stereochemistry*/ return this.stereochemistry == null ? new Base[0] : new Base[] {this.stereochemistry}; // CodeableConcept 2033 case 1420900135: /*opticalActivity*/ return this.opticalActivity == null ? new Base[0] : new Base[] {this.opticalActivity}; // CodeableConcept 2034 case 616660246: /*molecularFormula*/ return this.molecularFormula == null ? new Base[0] : new Base[] {this.molecularFormula}; // StringType 2035 case 1315452848: /*molecularFormulaByMoiety*/ return this.molecularFormulaByMoiety == null ? new Base[0] : new Base[] {this.molecularFormulaByMoiety}; // StringType 2036 case 635625672: /*molecularWeight*/ return this.molecularWeight == null ? new Base[0] : new Base[] {this.molecularWeight}; // SubstanceDefinitionMolecularWeightComponent 2037 case 1469675088: /*technique*/ return this.technique == null ? new Base[0] : this.technique.toArray(new Base[this.technique.size()]); // CodeableConcept 2038 case -501788074: /*sourceDocument*/ return this.sourceDocument == null ? new Base[0] : this.sourceDocument.toArray(new Base[this.sourceDocument.size()]); // Reference 2039 case -671065907: /*representation*/ return this.representation == null ? new Base[0] : this.representation.toArray(new Base[this.representation.size()]); // SubstanceDefinitionStructureRepresentationComponent 2040 default: return super.getProperty(hash, name, checkValid); 2041 } 2042 2043 } 2044 2045 @Override 2046 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2047 switch (hash) { 2048 case 263475116: // stereochemistry 2049 this.stereochemistry = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2050 return value; 2051 case 1420900135: // opticalActivity 2052 this.opticalActivity = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2053 return value; 2054 case 616660246: // molecularFormula 2055 this.molecularFormula = TypeConvertor.castToString(value); // StringType 2056 return value; 2057 case 1315452848: // molecularFormulaByMoiety 2058 this.molecularFormulaByMoiety = TypeConvertor.castToString(value); // StringType 2059 return value; 2060 case 635625672: // molecularWeight 2061 this.molecularWeight = (SubstanceDefinitionMolecularWeightComponent) value; // SubstanceDefinitionMolecularWeightComponent 2062 return value; 2063 case 1469675088: // technique 2064 this.getTechnique().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2065 return value; 2066 case -501788074: // sourceDocument 2067 this.getSourceDocument().add(TypeConvertor.castToReference(value)); // Reference 2068 return value; 2069 case -671065907: // representation 2070 this.getRepresentation().add((SubstanceDefinitionStructureRepresentationComponent) value); // SubstanceDefinitionStructureRepresentationComponent 2071 return value; 2072 default: return super.setProperty(hash, name, value); 2073 } 2074 2075 } 2076 2077 @Override 2078 public Base setProperty(String name, Base value) throws FHIRException { 2079 if (name.equals("stereochemistry")) { 2080 this.stereochemistry = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2081 } else if (name.equals("opticalActivity")) { 2082 this.opticalActivity = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2083 } else if (name.equals("molecularFormula")) { 2084 this.molecularFormula = TypeConvertor.castToString(value); // StringType 2085 } else if (name.equals("molecularFormulaByMoiety")) { 2086 this.molecularFormulaByMoiety = TypeConvertor.castToString(value); // StringType 2087 } else if (name.equals("molecularWeight")) { 2088 this.molecularWeight = (SubstanceDefinitionMolecularWeightComponent) value; // SubstanceDefinitionMolecularWeightComponent 2089 } else if (name.equals("technique")) { 2090 this.getTechnique().add(TypeConvertor.castToCodeableConcept(value)); 2091 } else if (name.equals("sourceDocument")) { 2092 this.getSourceDocument().add(TypeConvertor.castToReference(value)); 2093 } else if (name.equals("representation")) { 2094 this.getRepresentation().add((SubstanceDefinitionStructureRepresentationComponent) value); 2095 } else 2096 return super.setProperty(name, value); 2097 return value; 2098 } 2099 2100 @Override 2101 public void removeChild(String name, Base value) throws FHIRException { 2102 if (name.equals("stereochemistry")) { 2103 this.stereochemistry = null; 2104 } else if (name.equals("opticalActivity")) { 2105 this.opticalActivity = null; 2106 } else if (name.equals("molecularFormula")) { 2107 this.molecularFormula = null; 2108 } else if (name.equals("molecularFormulaByMoiety")) { 2109 this.molecularFormulaByMoiety = null; 2110 } else if (name.equals("molecularWeight")) { 2111 this.molecularWeight = (SubstanceDefinitionMolecularWeightComponent) value; // SubstanceDefinitionMolecularWeightComponent 2112 } else if (name.equals("technique")) { 2113 this.getTechnique().remove(value); 2114 } else if (name.equals("sourceDocument")) { 2115 this.getSourceDocument().remove(value); 2116 } else if (name.equals("representation")) { 2117 this.getRepresentation().remove((SubstanceDefinitionStructureRepresentationComponent) value); 2118 } else 2119 super.removeChild(name, value); 2120 2121 } 2122 2123 @Override 2124 public Base makeProperty(int hash, String name) throws FHIRException { 2125 switch (hash) { 2126 case 263475116: return getStereochemistry(); 2127 case 1420900135: return getOpticalActivity(); 2128 case 616660246: return getMolecularFormulaElement(); 2129 case 1315452848: return getMolecularFormulaByMoietyElement(); 2130 case 635625672: return getMolecularWeight(); 2131 case 1469675088: return addTechnique(); 2132 case -501788074: return addSourceDocument(); 2133 case -671065907: return addRepresentation(); 2134 default: return super.makeProperty(hash, name); 2135 } 2136 2137 } 2138 2139 @Override 2140 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2141 switch (hash) { 2142 case 263475116: /*stereochemistry*/ return new String[] {"CodeableConcept"}; 2143 case 1420900135: /*opticalActivity*/ return new String[] {"CodeableConcept"}; 2144 case 616660246: /*molecularFormula*/ return new String[] {"string"}; 2145 case 1315452848: /*molecularFormulaByMoiety*/ return new String[] {"string"}; 2146 case 635625672: /*molecularWeight*/ return new String[] {"@SubstanceDefinition.molecularWeight"}; 2147 case 1469675088: /*technique*/ return new String[] {"CodeableConcept"}; 2148 case -501788074: /*sourceDocument*/ return new String[] {"Reference"}; 2149 case -671065907: /*representation*/ return new String[] {}; 2150 default: return super.getTypesForProperty(hash, name); 2151 } 2152 2153 } 2154 2155 @Override 2156 public Base addChild(String name) throws FHIRException { 2157 if (name.equals("stereochemistry")) { 2158 this.stereochemistry = new CodeableConcept(); 2159 return this.stereochemistry; 2160 } 2161 else if (name.equals("opticalActivity")) { 2162 this.opticalActivity = new CodeableConcept(); 2163 return this.opticalActivity; 2164 } 2165 else if (name.equals("molecularFormula")) { 2166 throw new FHIRException("Cannot call addChild on a singleton property SubstanceDefinition.structure.molecularFormula"); 2167 } 2168 else if (name.equals("molecularFormulaByMoiety")) { 2169 throw new FHIRException("Cannot call addChild on a singleton property SubstanceDefinition.structure.molecularFormulaByMoiety"); 2170 } 2171 else if (name.equals("molecularWeight")) { 2172 this.molecularWeight = new SubstanceDefinitionMolecularWeightComponent(); 2173 return this.molecularWeight; 2174 } 2175 else if (name.equals("technique")) { 2176 return addTechnique(); 2177 } 2178 else if (name.equals("sourceDocument")) { 2179 return addSourceDocument(); 2180 } 2181 else if (name.equals("representation")) { 2182 return addRepresentation(); 2183 } 2184 else 2185 return super.addChild(name); 2186 } 2187 2188 public SubstanceDefinitionStructureComponent copy() { 2189 SubstanceDefinitionStructureComponent dst = new SubstanceDefinitionStructureComponent(); 2190 copyValues(dst); 2191 return dst; 2192 } 2193 2194 public void copyValues(SubstanceDefinitionStructureComponent dst) { 2195 super.copyValues(dst); 2196 dst.stereochemistry = stereochemistry == null ? null : stereochemistry.copy(); 2197 dst.opticalActivity = opticalActivity == null ? null : opticalActivity.copy(); 2198 dst.molecularFormula = molecularFormula == null ? null : molecularFormula.copy(); 2199 dst.molecularFormulaByMoiety = molecularFormulaByMoiety == null ? null : molecularFormulaByMoiety.copy(); 2200 dst.molecularWeight = molecularWeight == null ? null : molecularWeight.copy(); 2201 if (technique != null) { 2202 dst.technique = new ArrayList<CodeableConcept>(); 2203 for (CodeableConcept i : technique) 2204 dst.technique.add(i.copy()); 2205 }; 2206 if (sourceDocument != null) { 2207 dst.sourceDocument = new ArrayList<Reference>(); 2208 for (Reference i : sourceDocument) 2209 dst.sourceDocument.add(i.copy()); 2210 }; 2211 if (representation != null) { 2212 dst.representation = new ArrayList<SubstanceDefinitionStructureRepresentationComponent>(); 2213 for (SubstanceDefinitionStructureRepresentationComponent i : representation) 2214 dst.representation.add(i.copy()); 2215 }; 2216 } 2217 2218 @Override 2219 public boolean equalsDeep(Base other_) { 2220 if (!super.equalsDeep(other_)) 2221 return false; 2222 if (!(other_ instanceof SubstanceDefinitionStructureComponent)) 2223 return false; 2224 SubstanceDefinitionStructureComponent o = (SubstanceDefinitionStructureComponent) other_; 2225 return compareDeep(stereochemistry, o.stereochemistry, true) && compareDeep(opticalActivity, o.opticalActivity, true) 2226 && compareDeep(molecularFormula, o.molecularFormula, true) && compareDeep(molecularFormulaByMoiety, o.molecularFormulaByMoiety, true) 2227 && compareDeep(molecularWeight, o.molecularWeight, true) && compareDeep(technique, o.technique, true) 2228 && compareDeep(sourceDocument, o.sourceDocument, true) && compareDeep(representation, o.representation, true) 2229 ; 2230 } 2231 2232 @Override 2233 public boolean equalsShallow(Base other_) { 2234 if (!super.equalsShallow(other_)) 2235 return false; 2236 if (!(other_ instanceof SubstanceDefinitionStructureComponent)) 2237 return false; 2238 SubstanceDefinitionStructureComponent o = (SubstanceDefinitionStructureComponent) other_; 2239 return compareValues(molecularFormula, o.molecularFormula, true) && compareValues(molecularFormulaByMoiety, o.molecularFormulaByMoiety, true) 2240 ; 2241 } 2242 2243 public boolean isEmpty() { 2244 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(stereochemistry, opticalActivity 2245 , molecularFormula, molecularFormulaByMoiety, molecularWeight, technique, sourceDocument 2246 , representation); 2247 } 2248 2249 public String fhirType() { 2250 return "SubstanceDefinition.structure"; 2251 2252 } 2253 2254 } 2255 2256 @Block() 2257 public static class SubstanceDefinitionStructureRepresentationComponent extends BackboneElement implements IBaseBackboneElement { 2258 /** 2259 * The kind of structural representation (e.g. full, partial). 2260 */ 2261 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 2262 @Description(shortDefinition="The kind of structural representation (e.g. full, partial)", formalDefinition="The kind of structural representation (e.g. full, partial)." ) 2263 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-representation-type") 2264 protected CodeableConcept type; 2265 2266 /** 2267 * The structural representation as a text string in a standard format. 2268 */ 2269 @Child(name = "representation", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2270 @Description(shortDefinition="The structural representation as a text string in a standard format", formalDefinition="The structural representation as a text string in a standard format." ) 2271 protected StringType representation; 2272 2273 /** 2274 * The format of the representation e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF. The logical content type rather than the physical file format of a document. 2275 */ 2276 @Child(name = "format", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 2277 @Description(shortDefinition="The format of the representation e.g. InChI, SMILES, MOLFILE (note: not the physical file format)", formalDefinition="The format of the representation e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF. The logical content type rather than the physical file format of a document." ) 2278 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-representation-format") 2279 protected CodeableConcept format; 2280 2281 /** 2282 * An attached file with the structural representation e.g. a molecular structure graphic of the substance, a JCAMP or AnIML file. 2283 */ 2284 @Child(name = "document", type = {DocumentReference.class}, order=4, min=0, max=1, modifier=false, summary=true) 2285 @Description(shortDefinition="An attachment with the structural representation e.g. a structure graphic or AnIML file", formalDefinition="An attached file with the structural representation e.g. a molecular structure graphic of the substance, a JCAMP or AnIML file." ) 2286 protected Reference document; 2287 2288 private static final long serialVersionUID = 138704347L; 2289 2290 /** 2291 * Constructor 2292 */ 2293 public SubstanceDefinitionStructureRepresentationComponent() { 2294 super(); 2295 } 2296 2297 /** 2298 * @return {@link #type} (The kind of structural representation (e.g. full, partial).) 2299 */ 2300 public CodeableConcept getType() { 2301 if (this.type == null) 2302 if (Configuration.errorOnAutoCreate()) 2303 throw new Error("Attempt to auto-create SubstanceDefinitionStructureRepresentationComponent.type"); 2304 else if (Configuration.doAutoCreate()) 2305 this.type = new CodeableConcept(); // cc 2306 return this.type; 2307 } 2308 2309 public boolean hasType() { 2310 return this.type != null && !this.type.isEmpty(); 2311 } 2312 2313 /** 2314 * @param value {@link #type} (The kind of structural representation (e.g. full, partial).) 2315 */ 2316 public SubstanceDefinitionStructureRepresentationComponent setType(CodeableConcept value) { 2317 this.type = value; 2318 return this; 2319 } 2320 2321 /** 2322 * @return {@link #representation} (The structural representation as a text string in a standard format.). This is the underlying object with id, value and extensions. The accessor "getRepresentation" gives direct access to the value 2323 */ 2324 public StringType getRepresentationElement() { 2325 if (this.representation == null) 2326 if (Configuration.errorOnAutoCreate()) 2327 throw new Error("Attempt to auto-create SubstanceDefinitionStructureRepresentationComponent.representation"); 2328 else if (Configuration.doAutoCreate()) 2329 this.representation = new StringType(); // bb 2330 return this.representation; 2331 } 2332 2333 public boolean hasRepresentationElement() { 2334 return this.representation != null && !this.representation.isEmpty(); 2335 } 2336 2337 public boolean hasRepresentation() { 2338 return this.representation != null && !this.representation.isEmpty(); 2339 } 2340 2341 /** 2342 * @param value {@link #representation} (The structural representation as a text string in a standard format.). This is the underlying object with id, value and extensions. The accessor "getRepresentation" gives direct access to the value 2343 */ 2344 public SubstanceDefinitionStructureRepresentationComponent setRepresentationElement(StringType value) { 2345 this.representation = value; 2346 return this; 2347 } 2348 2349 /** 2350 * @return The structural representation as a text string in a standard format. 2351 */ 2352 public String getRepresentation() { 2353 return this.representation == null ? null : this.representation.getValue(); 2354 } 2355 2356 /** 2357 * @param value The structural representation as a text string in a standard format. 2358 */ 2359 public SubstanceDefinitionStructureRepresentationComponent setRepresentation(String value) { 2360 if (Utilities.noString(value)) 2361 this.representation = null; 2362 else { 2363 if (this.representation == null) 2364 this.representation = new StringType(); 2365 this.representation.setValue(value); 2366 } 2367 return this; 2368 } 2369 2370 /** 2371 * @return {@link #format} (The format of the representation e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF. The logical content type rather than the physical file format of a document.) 2372 */ 2373 public CodeableConcept getFormat() { 2374 if (this.format == null) 2375 if (Configuration.errorOnAutoCreate()) 2376 throw new Error("Attempt to auto-create SubstanceDefinitionStructureRepresentationComponent.format"); 2377 else if (Configuration.doAutoCreate()) 2378 this.format = new CodeableConcept(); // cc 2379 return this.format; 2380 } 2381 2382 public boolean hasFormat() { 2383 return this.format != null && !this.format.isEmpty(); 2384 } 2385 2386 /** 2387 * @param value {@link #format} (The format of the representation e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF. The logical content type rather than the physical file format of a document.) 2388 */ 2389 public SubstanceDefinitionStructureRepresentationComponent setFormat(CodeableConcept value) { 2390 this.format = value; 2391 return this; 2392 } 2393 2394 /** 2395 * @return {@link #document} (An attached file with the structural representation e.g. a molecular structure graphic of the substance, a JCAMP or AnIML file.) 2396 */ 2397 public Reference getDocument() { 2398 if (this.document == null) 2399 if (Configuration.errorOnAutoCreate()) 2400 throw new Error("Attempt to auto-create SubstanceDefinitionStructureRepresentationComponent.document"); 2401 else if (Configuration.doAutoCreate()) 2402 this.document = new Reference(); // cc 2403 return this.document; 2404 } 2405 2406 public boolean hasDocument() { 2407 return this.document != null && !this.document.isEmpty(); 2408 } 2409 2410 /** 2411 * @param value {@link #document} (An attached file with the structural representation e.g. a molecular structure graphic of the substance, a JCAMP or AnIML file.) 2412 */ 2413 public SubstanceDefinitionStructureRepresentationComponent setDocument(Reference value) { 2414 this.document = value; 2415 return this; 2416 } 2417 2418 protected void listChildren(List<Property> children) { 2419 super.listChildren(children); 2420 children.add(new Property("type", "CodeableConcept", "The kind of structural representation (e.g. full, partial).", 0, 1, type)); 2421 children.add(new Property("representation", "string", "The structural representation as a text string in a standard format.", 0, 1, representation)); 2422 children.add(new Property("format", "CodeableConcept", "The format of the representation e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF. The logical content type rather than the physical file format of a document.", 0, 1, format)); 2423 children.add(new Property("document", "Reference(DocumentReference)", "An attached file with the structural representation e.g. a molecular structure graphic of the substance, a JCAMP or AnIML file.", 0, 1, document)); 2424 } 2425 2426 @Override 2427 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2428 switch (_hash) { 2429 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The kind of structural representation (e.g. full, partial).", 0, 1, type); 2430 case -671065907: /*representation*/ return new Property("representation", "string", "The structural representation as a text string in a standard format.", 0, 1, representation); 2431 case -1268779017: /*format*/ return new Property("format", "CodeableConcept", "The format of the representation e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF. The logical content type rather than the physical file format of a document.", 0, 1, format); 2432 case 861720859: /*document*/ return new Property("document", "Reference(DocumentReference)", "An attached file with the structural representation e.g. a molecular structure graphic of the substance, a JCAMP or AnIML file.", 0, 1, document); 2433 default: return super.getNamedProperty(_hash, _name, _checkValid); 2434 } 2435 2436 } 2437 2438 @Override 2439 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2440 switch (hash) { 2441 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2442 case -671065907: /*representation*/ return this.representation == null ? new Base[0] : new Base[] {this.representation}; // StringType 2443 case -1268779017: /*format*/ return this.format == null ? new Base[0] : new Base[] {this.format}; // CodeableConcept 2444 case 861720859: /*document*/ return this.document == null ? new Base[0] : new Base[] {this.document}; // Reference 2445 default: return super.getProperty(hash, name, checkValid); 2446 } 2447 2448 } 2449 2450 @Override 2451 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2452 switch (hash) { 2453 case 3575610: // type 2454 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2455 return value; 2456 case -671065907: // representation 2457 this.representation = TypeConvertor.castToString(value); // StringType 2458 return value; 2459 case -1268779017: // format 2460 this.format = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2461 return value; 2462 case 861720859: // document 2463 this.document = TypeConvertor.castToReference(value); // Reference 2464 return value; 2465 default: return super.setProperty(hash, name, value); 2466 } 2467 2468 } 2469 2470 @Override 2471 public Base setProperty(String name, Base value) throws FHIRException { 2472 if (name.equals("type")) { 2473 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2474 } else if (name.equals("representation")) { 2475 this.representation = TypeConvertor.castToString(value); // StringType 2476 } else if (name.equals("format")) { 2477 this.format = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2478 } else if (name.equals("document")) { 2479 this.document = TypeConvertor.castToReference(value); // Reference 2480 } else 2481 return super.setProperty(name, value); 2482 return value; 2483 } 2484 2485 @Override 2486 public void removeChild(String name, Base value) throws FHIRException { 2487 if (name.equals("type")) { 2488 this.type = null; 2489 } else if (name.equals("representation")) { 2490 this.representation = null; 2491 } else if (name.equals("format")) { 2492 this.format = null; 2493 } else if (name.equals("document")) { 2494 this.document = null; 2495 } else 2496 super.removeChild(name, value); 2497 2498 } 2499 2500 @Override 2501 public Base makeProperty(int hash, String name) throws FHIRException { 2502 switch (hash) { 2503 case 3575610: return getType(); 2504 case -671065907: return getRepresentationElement(); 2505 case -1268779017: return getFormat(); 2506 case 861720859: return getDocument(); 2507 default: return super.makeProperty(hash, name); 2508 } 2509 2510 } 2511 2512 @Override 2513 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2514 switch (hash) { 2515 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2516 case -671065907: /*representation*/ return new String[] {"string"}; 2517 case -1268779017: /*format*/ return new String[] {"CodeableConcept"}; 2518 case 861720859: /*document*/ return new String[] {"Reference"}; 2519 default: return super.getTypesForProperty(hash, name); 2520 } 2521 2522 } 2523 2524 @Override 2525 public Base addChild(String name) throws FHIRException { 2526 if (name.equals("type")) { 2527 this.type = new CodeableConcept(); 2528 return this.type; 2529 } 2530 else if (name.equals("representation")) { 2531 throw new FHIRException("Cannot call addChild on a singleton property SubstanceDefinition.structure.representation.representation"); 2532 } 2533 else if (name.equals("format")) { 2534 this.format = new CodeableConcept(); 2535 return this.format; 2536 } 2537 else if (name.equals("document")) { 2538 this.document = new Reference(); 2539 return this.document; 2540 } 2541 else 2542 return super.addChild(name); 2543 } 2544 2545 public SubstanceDefinitionStructureRepresentationComponent copy() { 2546 SubstanceDefinitionStructureRepresentationComponent dst = new SubstanceDefinitionStructureRepresentationComponent(); 2547 copyValues(dst); 2548 return dst; 2549 } 2550 2551 public void copyValues(SubstanceDefinitionStructureRepresentationComponent dst) { 2552 super.copyValues(dst); 2553 dst.type = type == null ? null : type.copy(); 2554 dst.representation = representation == null ? null : representation.copy(); 2555 dst.format = format == null ? null : format.copy(); 2556 dst.document = document == null ? null : document.copy(); 2557 } 2558 2559 @Override 2560 public boolean equalsDeep(Base other_) { 2561 if (!super.equalsDeep(other_)) 2562 return false; 2563 if (!(other_ instanceof SubstanceDefinitionStructureRepresentationComponent)) 2564 return false; 2565 SubstanceDefinitionStructureRepresentationComponent o = (SubstanceDefinitionStructureRepresentationComponent) other_; 2566 return compareDeep(type, o.type, true) && compareDeep(representation, o.representation, true) && compareDeep(format, o.format, true) 2567 && compareDeep(document, o.document, true); 2568 } 2569 2570 @Override 2571 public boolean equalsShallow(Base other_) { 2572 if (!super.equalsShallow(other_)) 2573 return false; 2574 if (!(other_ instanceof SubstanceDefinitionStructureRepresentationComponent)) 2575 return false; 2576 SubstanceDefinitionStructureRepresentationComponent o = (SubstanceDefinitionStructureRepresentationComponent) other_; 2577 return compareValues(representation, o.representation, true); 2578 } 2579 2580 public boolean isEmpty() { 2581 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, representation, format 2582 , document); 2583 } 2584 2585 public String fhirType() { 2586 return "SubstanceDefinition.structure.representation"; 2587 2588 } 2589 2590 } 2591 2592 @Block() 2593 public static class SubstanceDefinitionCodeComponent extends BackboneElement implements IBaseBackboneElement { 2594 /** 2595 * The specific code. 2596 */ 2597 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 2598 @Description(shortDefinition="The specific code", formalDefinition="The specific code." ) 2599 protected CodeableConcept code; 2600 2601 /** 2602 * Status of the code assignment, for example 'provisional', 'approved'. 2603 */ 2604 @Child(name = "status", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 2605 @Description(shortDefinition="Status of the code assignment, for example 'provisional', 'approved'", formalDefinition="Status of the code assignment, for example 'provisional', 'approved'." ) 2606 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 2607 protected CodeableConcept status; 2608 2609 /** 2610 * The date at which the code status was changed as part of the terminology maintenance. 2611 */ 2612 @Child(name = "statusDate", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 2613 @Description(shortDefinition="The date at which the code status was changed", formalDefinition="The date at which the code status was changed as part of the terminology maintenance." ) 2614 protected DateTimeType statusDate; 2615 2616 /** 2617 * Any comment can be provided in this field, if necessary. 2618 */ 2619 @Child(name = "note", type = {Annotation.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2620 @Description(shortDefinition="Any comment can be provided in this field", formalDefinition="Any comment can be provided in this field, if necessary." ) 2621 protected List<Annotation> note; 2622 2623 /** 2624 * Supporting literature. 2625 */ 2626 @Child(name = "source", type = {DocumentReference.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2627 @Description(shortDefinition="Supporting literature", formalDefinition="Supporting literature." ) 2628 protected List<Reference> source; 2629 2630 private static final long serialVersionUID = 1140562105L; 2631 2632 /** 2633 * Constructor 2634 */ 2635 public SubstanceDefinitionCodeComponent() { 2636 super(); 2637 } 2638 2639 /** 2640 * @return {@link #code} (The specific code.) 2641 */ 2642 public CodeableConcept getCode() { 2643 if (this.code == null) 2644 if (Configuration.errorOnAutoCreate()) 2645 throw new Error("Attempt to auto-create SubstanceDefinitionCodeComponent.code"); 2646 else if (Configuration.doAutoCreate()) 2647 this.code = new CodeableConcept(); // cc 2648 return this.code; 2649 } 2650 2651 public boolean hasCode() { 2652 return this.code != null && !this.code.isEmpty(); 2653 } 2654 2655 /** 2656 * @param value {@link #code} (The specific code.) 2657 */ 2658 public SubstanceDefinitionCodeComponent setCode(CodeableConcept value) { 2659 this.code = value; 2660 return this; 2661 } 2662 2663 /** 2664 * @return {@link #status} (Status of the code assignment, for example 'provisional', 'approved'.) 2665 */ 2666 public CodeableConcept getStatus() { 2667 if (this.status == null) 2668 if (Configuration.errorOnAutoCreate()) 2669 throw new Error("Attempt to auto-create SubstanceDefinitionCodeComponent.status"); 2670 else if (Configuration.doAutoCreate()) 2671 this.status = new CodeableConcept(); // cc 2672 return this.status; 2673 } 2674 2675 public boolean hasStatus() { 2676 return this.status != null && !this.status.isEmpty(); 2677 } 2678 2679 /** 2680 * @param value {@link #status} (Status of the code assignment, for example 'provisional', 'approved'.) 2681 */ 2682 public SubstanceDefinitionCodeComponent setStatus(CodeableConcept value) { 2683 this.status = value; 2684 return this; 2685 } 2686 2687 /** 2688 * @return {@link #statusDate} (The date at which the code status was changed as part of the terminology maintenance.). This is the underlying object with id, value and extensions. The accessor "getStatusDate" gives direct access to the value 2689 */ 2690 public DateTimeType getStatusDateElement() { 2691 if (this.statusDate == null) 2692 if (Configuration.errorOnAutoCreate()) 2693 throw new Error("Attempt to auto-create SubstanceDefinitionCodeComponent.statusDate"); 2694 else if (Configuration.doAutoCreate()) 2695 this.statusDate = new DateTimeType(); // bb 2696 return this.statusDate; 2697 } 2698 2699 public boolean hasStatusDateElement() { 2700 return this.statusDate != null && !this.statusDate.isEmpty(); 2701 } 2702 2703 public boolean hasStatusDate() { 2704 return this.statusDate != null && !this.statusDate.isEmpty(); 2705 } 2706 2707 /** 2708 * @param value {@link #statusDate} (The date at which the code status was changed as part of the terminology maintenance.). This is the underlying object with id, value and extensions. The accessor "getStatusDate" gives direct access to the value 2709 */ 2710 public SubstanceDefinitionCodeComponent setStatusDateElement(DateTimeType value) { 2711 this.statusDate = value; 2712 return this; 2713 } 2714 2715 /** 2716 * @return The date at which the code status was changed as part of the terminology maintenance. 2717 */ 2718 public Date getStatusDate() { 2719 return this.statusDate == null ? null : this.statusDate.getValue(); 2720 } 2721 2722 /** 2723 * @param value The date at which the code status was changed as part of the terminology maintenance. 2724 */ 2725 public SubstanceDefinitionCodeComponent setStatusDate(Date value) { 2726 if (value == null) 2727 this.statusDate = null; 2728 else { 2729 if (this.statusDate == null) 2730 this.statusDate = new DateTimeType(); 2731 this.statusDate.setValue(value); 2732 } 2733 return this; 2734 } 2735 2736 /** 2737 * @return {@link #note} (Any comment can be provided in this field, if necessary.) 2738 */ 2739 public List<Annotation> getNote() { 2740 if (this.note == null) 2741 this.note = new ArrayList<Annotation>(); 2742 return this.note; 2743 } 2744 2745 /** 2746 * @return Returns a reference to <code>this</code> for easy method chaining 2747 */ 2748 public SubstanceDefinitionCodeComponent setNote(List<Annotation> theNote) { 2749 this.note = theNote; 2750 return this; 2751 } 2752 2753 public boolean hasNote() { 2754 if (this.note == null) 2755 return false; 2756 for (Annotation item : this.note) 2757 if (!item.isEmpty()) 2758 return true; 2759 return false; 2760 } 2761 2762 public Annotation addNote() { //3 2763 Annotation t = new Annotation(); 2764 if (this.note == null) 2765 this.note = new ArrayList<Annotation>(); 2766 this.note.add(t); 2767 return t; 2768 } 2769 2770 public SubstanceDefinitionCodeComponent addNote(Annotation t) { //3 2771 if (t == null) 2772 return this; 2773 if (this.note == null) 2774 this.note = new ArrayList<Annotation>(); 2775 this.note.add(t); 2776 return this; 2777 } 2778 2779 /** 2780 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 2781 */ 2782 public Annotation getNoteFirstRep() { 2783 if (getNote().isEmpty()) { 2784 addNote(); 2785 } 2786 return getNote().get(0); 2787 } 2788 2789 /** 2790 * @return {@link #source} (Supporting literature.) 2791 */ 2792 public List<Reference> getSource() { 2793 if (this.source == null) 2794 this.source = new ArrayList<Reference>(); 2795 return this.source; 2796 } 2797 2798 /** 2799 * @return Returns a reference to <code>this</code> for easy method chaining 2800 */ 2801 public SubstanceDefinitionCodeComponent setSource(List<Reference> theSource) { 2802 this.source = theSource; 2803 return this; 2804 } 2805 2806 public boolean hasSource() { 2807 if (this.source == null) 2808 return false; 2809 for (Reference item : this.source) 2810 if (!item.isEmpty()) 2811 return true; 2812 return false; 2813 } 2814 2815 public Reference addSource() { //3 2816 Reference t = new Reference(); 2817 if (this.source == null) 2818 this.source = new ArrayList<Reference>(); 2819 this.source.add(t); 2820 return t; 2821 } 2822 2823 public SubstanceDefinitionCodeComponent addSource(Reference t) { //3 2824 if (t == null) 2825 return this; 2826 if (this.source == null) 2827 this.source = new ArrayList<Reference>(); 2828 this.source.add(t); 2829 return this; 2830 } 2831 2832 /** 2833 * @return The first repetition of repeating field {@link #source}, creating it if it does not already exist {3} 2834 */ 2835 public Reference getSourceFirstRep() { 2836 if (getSource().isEmpty()) { 2837 addSource(); 2838 } 2839 return getSource().get(0); 2840 } 2841 2842 protected void listChildren(List<Property> children) { 2843 super.listChildren(children); 2844 children.add(new Property("code", "CodeableConcept", "The specific code.", 0, 1, code)); 2845 children.add(new Property("status", "CodeableConcept", "Status of the code assignment, for example 'provisional', 'approved'.", 0, 1, status)); 2846 children.add(new Property("statusDate", "dateTime", "The date at which the code status was changed as part of the terminology maintenance.", 0, 1, statusDate)); 2847 children.add(new Property("note", "Annotation", "Any comment can be provided in this field, if necessary.", 0, java.lang.Integer.MAX_VALUE, note)); 2848 children.add(new Property("source", "Reference(DocumentReference)", "Supporting literature.", 0, java.lang.Integer.MAX_VALUE, source)); 2849 } 2850 2851 @Override 2852 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2853 switch (_hash) { 2854 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The specific code.", 0, 1, code); 2855 case -892481550: /*status*/ return new Property("status", "CodeableConcept", "Status of the code assignment, for example 'provisional', 'approved'.", 0, 1, status); 2856 case 247524032: /*statusDate*/ return new Property("statusDate", "dateTime", "The date at which the code status was changed as part of the terminology maintenance.", 0, 1, statusDate); 2857 case 3387378: /*note*/ return new Property("note", "Annotation", "Any comment can be provided in this field, if necessary.", 0, java.lang.Integer.MAX_VALUE, note); 2858 case -896505829: /*source*/ return new Property("source", "Reference(DocumentReference)", "Supporting literature.", 0, java.lang.Integer.MAX_VALUE, source); 2859 default: return super.getNamedProperty(_hash, _name, _checkValid); 2860 } 2861 2862 } 2863 2864 @Override 2865 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2866 switch (hash) { 2867 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2868 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // CodeableConcept 2869 case 247524032: /*statusDate*/ return this.statusDate == null ? new Base[0] : new Base[] {this.statusDate}; // DateTimeType 2870 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2871 case -896505829: /*source*/ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 2872 default: return super.getProperty(hash, name, checkValid); 2873 } 2874 2875 } 2876 2877 @Override 2878 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2879 switch (hash) { 2880 case 3059181: // code 2881 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2882 return value; 2883 case -892481550: // status 2884 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2885 return value; 2886 case 247524032: // statusDate 2887 this.statusDate = TypeConvertor.castToDateTime(value); // DateTimeType 2888 return value; 2889 case 3387378: // note 2890 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 2891 return value; 2892 case -896505829: // source 2893 this.getSource().add(TypeConvertor.castToReference(value)); // Reference 2894 return value; 2895 default: return super.setProperty(hash, name, value); 2896 } 2897 2898 } 2899 2900 @Override 2901 public Base setProperty(String name, Base value) throws FHIRException { 2902 if (name.equals("code")) { 2903 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2904 } else if (name.equals("status")) { 2905 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2906 } else if (name.equals("statusDate")) { 2907 this.statusDate = TypeConvertor.castToDateTime(value); // DateTimeType 2908 } else if (name.equals("note")) { 2909 this.getNote().add(TypeConvertor.castToAnnotation(value)); 2910 } else if (name.equals("source")) { 2911 this.getSource().add(TypeConvertor.castToReference(value)); 2912 } else 2913 return super.setProperty(name, value); 2914 return value; 2915 } 2916 2917 @Override 2918 public void removeChild(String name, Base value) throws FHIRException { 2919 if (name.equals("code")) { 2920 this.code = null; 2921 } else if (name.equals("status")) { 2922 this.status = null; 2923 } else if (name.equals("statusDate")) { 2924 this.statusDate = null; 2925 } else if (name.equals("note")) { 2926 this.getNote().remove(value); 2927 } else if (name.equals("source")) { 2928 this.getSource().remove(value); 2929 } else 2930 super.removeChild(name, value); 2931 2932 } 2933 2934 @Override 2935 public Base makeProperty(int hash, String name) throws FHIRException { 2936 switch (hash) { 2937 case 3059181: return getCode(); 2938 case -892481550: return getStatus(); 2939 case 247524032: return getStatusDateElement(); 2940 case 3387378: return addNote(); 2941 case -896505829: return addSource(); 2942 default: return super.makeProperty(hash, name); 2943 } 2944 2945 } 2946 2947 @Override 2948 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2949 switch (hash) { 2950 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2951 case -892481550: /*status*/ return new String[] {"CodeableConcept"}; 2952 case 247524032: /*statusDate*/ return new String[] {"dateTime"}; 2953 case 3387378: /*note*/ return new String[] {"Annotation"}; 2954 case -896505829: /*source*/ return new String[] {"Reference"}; 2955 default: return super.getTypesForProperty(hash, name); 2956 } 2957 2958 } 2959 2960 @Override 2961 public Base addChild(String name) throws FHIRException { 2962 if (name.equals("code")) { 2963 this.code = new CodeableConcept(); 2964 return this.code; 2965 } 2966 else if (name.equals("status")) { 2967 this.status = new CodeableConcept(); 2968 return this.status; 2969 } 2970 else if (name.equals("statusDate")) { 2971 throw new FHIRException("Cannot call addChild on a singleton property SubstanceDefinition.code.statusDate"); 2972 } 2973 else if (name.equals("note")) { 2974 return addNote(); 2975 } 2976 else if (name.equals("source")) { 2977 return addSource(); 2978 } 2979 else 2980 return super.addChild(name); 2981 } 2982 2983 public SubstanceDefinitionCodeComponent copy() { 2984 SubstanceDefinitionCodeComponent dst = new SubstanceDefinitionCodeComponent(); 2985 copyValues(dst); 2986 return dst; 2987 } 2988 2989 public void copyValues(SubstanceDefinitionCodeComponent dst) { 2990 super.copyValues(dst); 2991 dst.code = code == null ? null : code.copy(); 2992 dst.status = status == null ? null : status.copy(); 2993 dst.statusDate = statusDate == null ? null : statusDate.copy(); 2994 if (note != null) { 2995 dst.note = new ArrayList<Annotation>(); 2996 for (Annotation i : note) 2997 dst.note.add(i.copy()); 2998 }; 2999 if (source != null) { 3000 dst.source = new ArrayList<Reference>(); 3001 for (Reference i : source) 3002 dst.source.add(i.copy()); 3003 }; 3004 } 3005 3006 @Override 3007 public boolean equalsDeep(Base other_) { 3008 if (!super.equalsDeep(other_)) 3009 return false; 3010 if (!(other_ instanceof SubstanceDefinitionCodeComponent)) 3011 return false; 3012 SubstanceDefinitionCodeComponent o = (SubstanceDefinitionCodeComponent) other_; 3013 return compareDeep(code, o.code, true) && compareDeep(status, o.status, true) && compareDeep(statusDate, o.statusDate, true) 3014 && compareDeep(note, o.note, true) && compareDeep(source, o.source, true); 3015 } 3016 3017 @Override 3018 public boolean equalsShallow(Base other_) { 3019 if (!super.equalsShallow(other_)) 3020 return false; 3021 if (!(other_ instanceof SubstanceDefinitionCodeComponent)) 3022 return false; 3023 SubstanceDefinitionCodeComponent o = (SubstanceDefinitionCodeComponent) other_; 3024 return compareValues(statusDate, o.statusDate, true); 3025 } 3026 3027 public boolean isEmpty() { 3028 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, status, statusDate 3029 , note, source); 3030 } 3031 3032 public String fhirType() { 3033 return "SubstanceDefinition.code"; 3034 3035 } 3036 3037 } 3038 3039 @Block() 3040 public static class SubstanceDefinitionNameComponent extends BackboneElement implements IBaseBackboneElement { 3041 /** 3042 * The actual name. 3043 */ 3044 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 3045 @Description(shortDefinition="The actual name", formalDefinition="The actual name." ) 3046 protected StringType name; 3047 3048 /** 3049 * Name type, for example 'systematic', 'scientific, 'brand'. 3050 */ 3051 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 3052 @Description(shortDefinition="Name type e.g. 'systematic', 'scientific, 'brand'", formalDefinition="Name type, for example 'systematic', 'scientific, 'brand'." ) 3053 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-name-type") 3054 protected CodeableConcept type; 3055 3056 /** 3057 * The status of the name, for example 'current', 'proposed'. 3058 */ 3059 @Child(name = "status", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 3060 @Description(shortDefinition="The status of the name e.g. 'current', 'proposed'", formalDefinition="The status of the name, for example 'current', 'proposed'." ) 3061 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 3062 protected CodeableConcept status; 3063 3064 /** 3065 * If this is the preferred name for this substance. 3066 */ 3067 @Child(name = "preferred", type = {BooleanType.class}, order=4, min=0, max=1, modifier=false, summary=true) 3068 @Description(shortDefinition="If this is the preferred name for this substance", formalDefinition="If this is the preferred name for this substance." ) 3069 protected BooleanType preferred; 3070 3071 /** 3072 * Human language that the name is written in. 3073 */ 3074 @Child(name = "language", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3075 @Description(shortDefinition="Human language that the name is written in", formalDefinition="Human language that the name is written in." ) 3076 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/all-languages") 3077 protected List<CodeableConcept> language; 3078 3079 /** 3080 * The use context of this name for example if there is a different name a drug active ingredient as opposed to a food colour additive. 3081 */ 3082 @Child(name = "domain", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3083 @Description(shortDefinition="The use context of this name e.g. as an active ingredient or as a food colour additive", formalDefinition="The use context of this name for example if there is a different name a drug active ingredient as opposed to a food colour additive." ) 3084 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-name-domain") 3085 protected List<CodeableConcept> domain; 3086 3087 /** 3088 * The jurisdiction where this name applies. 3089 */ 3090 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3091 @Description(shortDefinition="The jurisdiction where this name applies", formalDefinition="The jurisdiction where this name applies." ) 3092 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 3093 protected List<CodeableConcept> jurisdiction; 3094 3095 /** 3096 * A synonym of this particular name, by which the substance is also known. 3097 */ 3098 @Child(name = "synonym", type = {SubstanceDefinitionNameComponent.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3099 @Description(shortDefinition="A synonym of this particular name, by which the substance is also known", formalDefinition="A synonym of this particular name, by which the substance is also known." ) 3100 protected List<SubstanceDefinitionNameComponent> synonym; 3101 3102 /** 3103 * A translation for this name into another human language. 3104 */ 3105 @Child(name = "translation", type = {SubstanceDefinitionNameComponent.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3106 @Description(shortDefinition="A translation for this name into another human language", formalDefinition="A translation for this name into another human language." ) 3107 protected List<SubstanceDefinitionNameComponent> translation; 3108 3109 /** 3110 * Details of the official nature of this name. 3111 */ 3112 @Child(name = "official", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3113 @Description(shortDefinition="Details of the official nature of this name", formalDefinition="Details of the official nature of this name." ) 3114 protected List<SubstanceDefinitionNameOfficialComponent> official; 3115 3116 /** 3117 * Supporting literature. 3118 */ 3119 @Child(name = "source", type = {DocumentReference.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3120 @Description(shortDefinition="Supporting literature", formalDefinition="Supporting literature." ) 3121 protected List<Reference> source; 3122 3123 private static final long serialVersionUID = -1184238780L; 3124 3125 /** 3126 * Constructor 3127 */ 3128 public SubstanceDefinitionNameComponent() { 3129 super(); 3130 } 3131 3132 /** 3133 * Constructor 3134 */ 3135 public SubstanceDefinitionNameComponent(String name) { 3136 super(); 3137 this.setName(name); 3138 } 3139 3140 /** 3141 * @return {@link #name} (The actual name.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3142 */ 3143 public StringType getNameElement() { 3144 if (this.name == null) 3145 if (Configuration.errorOnAutoCreate()) 3146 throw new Error("Attempt to auto-create SubstanceDefinitionNameComponent.name"); 3147 else if (Configuration.doAutoCreate()) 3148 this.name = new StringType(); // bb 3149 return this.name; 3150 } 3151 3152 public boolean hasNameElement() { 3153 return this.name != null && !this.name.isEmpty(); 3154 } 3155 3156 public boolean hasName() { 3157 return this.name != null && !this.name.isEmpty(); 3158 } 3159 3160 /** 3161 * @param value {@link #name} (The actual name.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3162 */ 3163 public SubstanceDefinitionNameComponent setNameElement(StringType value) { 3164 this.name = value; 3165 return this; 3166 } 3167 3168 /** 3169 * @return The actual name. 3170 */ 3171 public String getName() { 3172 return this.name == null ? null : this.name.getValue(); 3173 } 3174 3175 /** 3176 * @param value The actual name. 3177 */ 3178 public SubstanceDefinitionNameComponent setName(String value) { 3179 if (this.name == null) 3180 this.name = new StringType(); 3181 this.name.setValue(value); 3182 return this; 3183 } 3184 3185 /** 3186 * @return {@link #type} (Name type, for example 'systematic', 'scientific, 'brand'.) 3187 */ 3188 public CodeableConcept getType() { 3189 if (this.type == null) 3190 if (Configuration.errorOnAutoCreate()) 3191 throw new Error("Attempt to auto-create SubstanceDefinitionNameComponent.type"); 3192 else if (Configuration.doAutoCreate()) 3193 this.type = new CodeableConcept(); // cc 3194 return this.type; 3195 } 3196 3197 public boolean hasType() { 3198 return this.type != null && !this.type.isEmpty(); 3199 } 3200 3201 /** 3202 * @param value {@link #type} (Name type, for example 'systematic', 'scientific, 'brand'.) 3203 */ 3204 public SubstanceDefinitionNameComponent setType(CodeableConcept value) { 3205 this.type = value; 3206 return this; 3207 } 3208 3209 /** 3210 * @return {@link #status} (The status of the name, for example 'current', 'proposed'.) 3211 */ 3212 public CodeableConcept getStatus() { 3213 if (this.status == null) 3214 if (Configuration.errorOnAutoCreate()) 3215 throw new Error("Attempt to auto-create SubstanceDefinitionNameComponent.status"); 3216 else if (Configuration.doAutoCreate()) 3217 this.status = new CodeableConcept(); // cc 3218 return this.status; 3219 } 3220 3221 public boolean hasStatus() { 3222 return this.status != null && !this.status.isEmpty(); 3223 } 3224 3225 /** 3226 * @param value {@link #status} (The status of the name, for example 'current', 'proposed'.) 3227 */ 3228 public SubstanceDefinitionNameComponent setStatus(CodeableConcept value) { 3229 this.status = value; 3230 return this; 3231 } 3232 3233 /** 3234 * @return {@link #preferred} (If this is the preferred name for this substance.). This is the underlying object with id, value and extensions. The accessor "getPreferred" gives direct access to the value 3235 */ 3236 public BooleanType getPreferredElement() { 3237 if (this.preferred == null) 3238 if (Configuration.errorOnAutoCreate()) 3239 throw new Error("Attempt to auto-create SubstanceDefinitionNameComponent.preferred"); 3240 else if (Configuration.doAutoCreate()) 3241 this.preferred = new BooleanType(); // bb 3242 return this.preferred; 3243 } 3244 3245 public boolean hasPreferredElement() { 3246 return this.preferred != null && !this.preferred.isEmpty(); 3247 } 3248 3249 public boolean hasPreferred() { 3250 return this.preferred != null && !this.preferred.isEmpty(); 3251 } 3252 3253 /** 3254 * @param value {@link #preferred} (If this is the preferred name for this substance.). This is the underlying object with id, value and extensions. The accessor "getPreferred" gives direct access to the value 3255 */ 3256 public SubstanceDefinitionNameComponent setPreferredElement(BooleanType value) { 3257 this.preferred = value; 3258 return this; 3259 } 3260 3261 /** 3262 * @return If this is the preferred name for this substance. 3263 */ 3264 public boolean getPreferred() { 3265 return this.preferred == null || this.preferred.isEmpty() ? false : this.preferred.getValue(); 3266 } 3267 3268 /** 3269 * @param value If this is the preferred name for this substance. 3270 */ 3271 public SubstanceDefinitionNameComponent setPreferred(boolean value) { 3272 if (this.preferred == null) 3273 this.preferred = new BooleanType(); 3274 this.preferred.setValue(value); 3275 return this; 3276 } 3277 3278 /** 3279 * @return {@link #language} (Human language that the name is written in.) 3280 */ 3281 public List<CodeableConcept> getLanguage() { 3282 if (this.language == null) 3283 this.language = new ArrayList<CodeableConcept>(); 3284 return this.language; 3285 } 3286 3287 /** 3288 * @return Returns a reference to <code>this</code> for easy method chaining 3289 */ 3290 public SubstanceDefinitionNameComponent setLanguage(List<CodeableConcept> theLanguage) { 3291 this.language = theLanguage; 3292 return this; 3293 } 3294 3295 public boolean hasLanguage() { 3296 if (this.language == null) 3297 return false; 3298 for (CodeableConcept item : this.language) 3299 if (!item.isEmpty()) 3300 return true; 3301 return false; 3302 } 3303 3304 public CodeableConcept addLanguage() { //3 3305 CodeableConcept t = new CodeableConcept(); 3306 if (this.language == null) 3307 this.language = new ArrayList<CodeableConcept>(); 3308 this.language.add(t); 3309 return t; 3310 } 3311 3312 public SubstanceDefinitionNameComponent addLanguage(CodeableConcept t) { //3 3313 if (t == null) 3314 return this; 3315 if (this.language == null) 3316 this.language = new ArrayList<CodeableConcept>(); 3317 this.language.add(t); 3318 return this; 3319 } 3320 3321 /** 3322 * @return The first repetition of repeating field {@link #language}, creating it if it does not already exist {3} 3323 */ 3324 public CodeableConcept getLanguageFirstRep() { 3325 if (getLanguage().isEmpty()) { 3326 addLanguage(); 3327 } 3328 return getLanguage().get(0); 3329 } 3330 3331 /** 3332 * @return {@link #domain} (The use context of this name for example if there is a different name a drug active ingredient as opposed to a food colour additive.) 3333 */ 3334 public List<CodeableConcept> getDomain() { 3335 if (this.domain == null) 3336 this.domain = new ArrayList<CodeableConcept>(); 3337 return this.domain; 3338 } 3339 3340 /** 3341 * @return Returns a reference to <code>this</code> for easy method chaining 3342 */ 3343 public SubstanceDefinitionNameComponent setDomain(List<CodeableConcept> theDomain) { 3344 this.domain = theDomain; 3345 return this; 3346 } 3347 3348 public boolean hasDomain() { 3349 if (this.domain == null) 3350 return false; 3351 for (CodeableConcept item : this.domain) 3352 if (!item.isEmpty()) 3353 return true; 3354 return false; 3355 } 3356 3357 public CodeableConcept addDomain() { //3 3358 CodeableConcept t = new CodeableConcept(); 3359 if (this.domain == null) 3360 this.domain = new ArrayList<CodeableConcept>(); 3361 this.domain.add(t); 3362 return t; 3363 } 3364 3365 public SubstanceDefinitionNameComponent addDomain(CodeableConcept t) { //3 3366 if (t == null) 3367 return this; 3368 if (this.domain == null) 3369 this.domain = new ArrayList<CodeableConcept>(); 3370 this.domain.add(t); 3371 return this; 3372 } 3373 3374 /** 3375 * @return The first repetition of repeating field {@link #domain}, creating it if it does not already exist {3} 3376 */ 3377 public CodeableConcept getDomainFirstRep() { 3378 if (getDomain().isEmpty()) { 3379 addDomain(); 3380 } 3381 return getDomain().get(0); 3382 } 3383 3384 /** 3385 * @return {@link #jurisdiction} (The jurisdiction where this name applies.) 3386 */ 3387 public List<CodeableConcept> getJurisdiction() { 3388 if (this.jurisdiction == null) 3389 this.jurisdiction = new ArrayList<CodeableConcept>(); 3390 return this.jurisdiction; 3391 } 3392 3393 /** 3394 * @return Returns a reference to <code>this</code> for easy method chaining 3395 */ 3396 public SubstanceDefinitionNameComponent setJurisdiction(List<CodeableConcept> theJurisdiction) { 3397 this.jurisdiction = theJurisdiction; 3398 return this; 3399 } 3400 3401 public boolean hasJurisdiction() { 3402 if (this.jurisdiction == null) 3403 return false; 3404 for (CodeableConcept item : this.jurisdiction) 3405 if (!item.isEmpty()) 3406 return true; 3407 return false; 3408 } 3409 3410 public CodeableConcept addJurisdiction() { //3 3411 CodeableConcept t = new CodeableConcept(); 3412 if (this.jurisdiction == null) 3413 this.jurisdiction = new ArrayList<CodeableConcept>(); 3414 this.jurisdiction.add(t); 3415 return t; 3416 } 3417 3418 public SubstanceDefinitionNameComponent addJurisdiction(CodeableConcept t) { //3 3419 if (t == null) 3420 return this; 3421 if (this.jurisdiction == null) 3422 this.jurisdiction = new ArrayList<CodeableConcept>(); 3423 this.jurisdiction.add(t); 3424 return this; 3425 } 3426 3427 /** 3428 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 3429 */ 3430 public CodeableConcept getJurisdictionFirstRep() { 3431 if (getJurisdiction().isEmpty()) { 3432 addJurisdiction(); 3433 } 3434 return getJurisdiction().get(0); 3435 } 3436 3437 /** 3438 * @return {@link #synonym} (A synonym of this particular name, by which the substance is also known.) 3439 */ 3440 public List<SubstanceDefinitionNameComponent> getSynonym() { 3441 if (this.synonym == null) 3442 this.synonym = new ArrayList<SubstanceDefinitionNameComponent>(); 3443 return this.synonym; 3444 } 3445 3446 /** 3447 * @return Returns a reference to <code>this</code> for easy method chaining 3448 */ 3449 public SubstanceDefinitionNameComponent setSynonym(List<SubstanceDefinitionNameComponent> theSynonym) { 3450 this.synonym = theSynonym; 3451 return this; 3452 } 3453 3454 public boolean hasSynonym() { 3455 if (this.synonym == null) 3456 return false; 3457 for (SubstanceDefinitionNameComponent item : this.synonym) 3458 if (!item.isEmpty()) 3459 return true; 3460 return false; 3461 } 3462 3463 public SubstanceDefinitionNameComponent addSynonym() { //3 3464 SubstanceDefinitionNameComponent t = new SubstanceDefinitionNameComponent(); 3465 if (this.synonym == null) 3466 this.synonym = new ArrayList<SubstanceDefinitionNameComponent>(); 3467 this.synonym.add(t); 3468 return t; 3469 } 3470 3471 public SubstanceDefinitionNameComponent addSynonym(SubstanceDefinitionNameComponent t) { //3 3472 if (t == null) 3473 return this; 3474 if (this.synonym == null) 3475 this.synonym = new ArrayList<SubstanceDefinitionNameComponent>(); 3476 this.synonym.add(t); 3477 return this; 3478 } 3479 3480 /** 3481 * @return The first repetition of repeating field {@link #synonym}, creating it if it does not already exist {3} 3482 */ 3483 public SubstanceDefinitionNameComponent getSynonymFirstRep() { 3484 if (getSynonym().isEmpty()) { 3485 addSynonym(); 3486 } 3487 return getSynonym().get(0); 3488 } 3489 3490 /** 3491 * @return {@link #translation} (A translation for this name into another human language.) 3492 */ 3493 public List<SubstanceDefinitionNameComponent> getTranslation() { 3494 if (this.translation == null) 3495 this.translation = new ArrayList<SubstanceDefinitionNameComponent>(); 3496 return this.translation; 3497 } 3498 3499 /** 3500 * @return Returns a reference to <code>this</code> for easy method chaining 3501 */ 3502 public SubstanceDefinitionNameComponent setTranslation(List<SubstanceDefinitionNameComponent> theTranslation) { 3503 this.translation = theTranslation; 3504 return this; 3505 } 3506 3507 public boolean hasTranslation() { 3508 if (this.translation == null) 3509 return false; 3510 for (SubstanceDefinitionNameComponent item : this.translation) 3511 if (!item.isEmpty()) 3512 return true; 3513 return false; 3514 } 3515 3516 public SubstanceDefinitionNameComponent addTranslation() { //3 3517 SubstanceDefinitionNameComponent t = new SubstanceDefinitionNameComponent(); 3518 if (this.translation == null) 3519 this.translation = new ArrayList<SubstanceDefinitionNameComponent>(); 3520 this.translation.add(t); 3521 return t; 3522 } 3523 3524 public SubstanceDefinitionNameComponent addTranslation(SubstanceDefinitionNameComponent t) { //3 3525 if (t == null) 3526 return this; 3527 if (this.translation == null) 3528 this.translation = new ArrayList<SubstanceDefinitionNameComponent>(); 3529 this.translation.add(t); 3530 return this; 3531 } 3532 3533 /** 3534 * @return The first repetition of repeating field {@link #translation}, creating it if it does not already exist {3} 3535 */ 3536 public SubstanceDefinitionNameComponent getTranslationFirstRep() { 3537 if (getTranslation().isEmpty()) { 3538 addTranslation(); 3539 } 3540 return getTranslation().get(0); 3541 } 3542 3543 /** 3544 * @return {@link #official} (Details of the official nature of this name.) 3545 */ 3546 public List<SubstanceDefinitionNameOfficialComponent> getOfficial() { 3547 if (this.official == null) 3548 this.official = new ArrayList<SubstanceDefinitionNameOfficialComponent>(); 3549 return this.official; 3550 } 3551 3552 /** 3553 * @return Returns a reference to <code>this</code> for easy method chaining 3554 */ 3555 public SubstanceDefinitionNameComponent setOfficial(List<SubstanceDefinitionNameOfficialComponent> theOfficial) { 3556 this.official = theOfficial; 3557 return this; 3558 } 3559 3560 public boolean hasOfficial() { 3561 if (this.official == null) 3562 return false; 3563 for (SubstanceDefinitionNameOfficialComponent item : this.official) 3564 if (!item.isEmpty()) 3565 return true; 3566 return false; 3567 } 3568 3569 public SubstanceDefinitionNameOfficialComponent addOfficial() { //3 3570 SubstanceDefinitionNameOfficialComponent t = new SubstanceDefinitionNameOfficialComponent(); 3571 if (this.official == null) 3572 this.official = new ArrayList<SubstanceDefinitionNameOfficialComponent>(); 3573 this.official.add(t); 3574 return t; 3575 } 3576 3577 public SubstanceDefinitionNameComponent addOfficial(SubstanceDefinitionNameOfficialComponent t) { //3 3578 if (t == null) 3579 return this; 3580 if (this.official == null) 3581 this.official = new ArrayList<SubstanceDefinitionNameOfficialComponent>(); 3582 this.official.add(t); 3583 return this; 3584 } 3585 3586 /** 3587 * @return The first repetition of repeating field {@link #official}, creating it if it does not already exist {3} 3588 */ 3589 public SubstanceDefinitionNameOfficialComponent getOfficialFirstRep() { 3590 if (getOfficial().isEmpty()) { 3591 addOfficial(); 3592 } 3593 return getOfficial().get(0); 3594 } 3595 3596 /** 3597 * @return {@link #source} (Supporting literature.) 3598 */ 3599 public List<Reference> getSource() { 3600 if (this.source == null) 3601 this.source = new ArrayList<Reference>(); 3602 return this.source; 3603 } 3604 3605 /** 3606 * @return Returns a reference to <code>this</code> for easy method chaining 3607 */ 3608 public SubstanceDefinitionNameComponent setSource(List<Reference> theSource) { 3609 this.source = theSource; 3610 return this; 3611 } 3612 3613 public boolean hasSource() { 3614 if (this.source == null) 3615 return false; 3616 for (Reference item : this.source) 3617 if (!item.isEmpty()) 3618 return true; 3619 return false; 3620 } 3621 3622 public Reference addSource() { //3 3623 Reference t = new Reference(); 3624 if (this.source == null) 3625 this.source = new ArrayList<Reference>(); 3626 this.source.add(t); 3627 return t; 3628 } 3629 3630 public SubstanceDefinitionNameComponent addSource(Reference t) { //3 3631 if (t == null) 3632 return this; 3633 if (this.source == null) 3634 this.source = new ArrayList<Reference>(); 3635 this.source.add(t); 3636 return this; 3637 } 3638 3639 /** 3640 * @return The first repetition of repeating field {@link #source}, creating it if it does not already exist {3} 3641 */ 3642 public Reference getSourceFirstRep() { 3643 if (getSource().isEmpty()) { 3644 addSource(); 3645 } 3646 return getSource().get(0); 3647 } 3648 3649 protected void listChildren(List<Property> children) { 3650 super.listChildren(children); 3651 children.add(new Property("name", "string", "The actual name.", 0, 1, name)); 3652 children.add(new Property("type", "CodeableConcept", "Name type, for example 'systematic', 'scientific, 'brand'.", 0, 1, type)); 3653 children.add(new Property("status", "CodeableConcept", "The status of the name, for example 'current', 'proposed'.", 0, 1, status)); 3654 children.add(new Property("preferred", "boolean", "If this is the preferred name for this substance.", 0, 1, preferred)); 3655 children.add(new Property("language", "CodeableConcept", "Human language that the name is written in.", 0, java.lang.Integer.MAX_VALUE, language)); 3656 children.add(new Property("domain", "CodeableConcept", "The use context of this name for example if there is a different name a drug active ingredient as opposed to a food colour additive.", 0, java.lang.Integer.MAX_VALUE, domain)); 3657 children.add(new Property("jurisdiction", "CodeableConcept", "The jurisdiction where this name applies.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 3658 children.add(new Property("synonym", "@SubstanceDefinition.name", "A synonym of this particular name, by which the substance is also known.", 0, java.lang.Integer.MAX_VALUE, synonym)); 3659 children.add(new Property("translation", "@SubstanceDefinition.name", "A translation for this name into another human language.", 0, java.lang.Integer.MAX_VALUE, translation)); 3660 children.add(new Property("official", "", "Details of the official nature of this name.", 0, java.lang.Integer.MAX_VALUE, official)); 3661 children.add(new Property("source", "Reference(DocumentReference)", "Supporting literature.", 0, java.lang.Integer.MAX_VALUE, source)); 3662 } 3663 3664 @Override 3665 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3666 switch (_hash) { 3667 case 3373707: /*name*/ return new Property("name", "string", "The actual name.", 0, 1, name); 3668 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Name type, for example 'systematic', 'scientific, 'brand'.", 0, 1, type); 3669 case -892481550: /*status*/ return new Property("status", "CodeableConcept", "The status of the name, for example 'current', 'proposed'.", 0, 1, status); 3670 case -1294005119: /*preferred*/ return new Property("preferred", "boolean", "If this is the preferred name for this substance.", 0, 1, preferred); 3671 case -1613589672: /*language*/ return new Property("language", "CodeableConcept", "Human language that the name is written in.", 0, java.lang.Integer.MAX_VALUE, language); 3672 case -1326197564: /*domain*/ return new Property("domain", "CodeableConcept", "The use context of this name for example if there is a different name a drug active ingredient as opposed to a food colour additive.", 0, java.lang.Integer.MAX_VALUE, domain); 3673 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "The jurisdiction where this name applies.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 3674 case -1742128133: /*synonym*/ return new Property("synonym", "@SubstanceDefinition.name", "A synonym of this particular name, by which the substance is also known.", 0, java.lang.Integer.MAX_VALUE, synonym); 3675 case -1840647503: /*translation*/ return new Property("translation", "@SubstanceDefinition.name", "A translation for this name into another human language.", 0, java.lang.Integer.MAX_VALUE, translation); 3676 case -765289749: /*official*/ return new Property("official", "", "Details of the official nature of this name.", 0, java.lang.Integer.MAX_VALUE, official); 3677 case -896505829: /*source*/ return new Property("source", "Reference(DocumentReference)", "Supporting literature.", 0, java.lang.Integer.MAX_VALUE, source); 3678 default: return super.getNamedProperty(_hash, _name, _checkValid); 3679 } 3680 3681 } 3682 3683 @Override 3684 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3685 switch (hash) { 3686 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3687 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3688 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // CodeableConcept 3689 case -1294005119: /*preferred*/ return this.preferred == null ? new Base[0] : new Base[] {this.preferred}; // BooleanType 3690 case -1613589672: /*language*/ return this.language == null ? new Base[0] : this.language.toArray(new Base[this.language.size()]); // CodeableConcept 3691 case -1326197564: /*domain*/ return this.domain == null ? new Base[0] : this.domain.toArray(new Base[this.domain.size()]); // CodeableConcept 3692 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3693 case -1742128133: /*synonym*/ return this.synonym == null ? new Base[0] : this.synonym.toArray(new Base[this.synonym.size()]); // SubstanceDefinitionNameComponent 3694 case -1840647503: /*translation*/ return this.translation == null ? new Base[0] : this.translation.toArray(new Base[this.translation.size()]); // SubstanceDefinitionNameComponent 3695 case -765289749: /*official*/ return this.official == null ? new Base[0] : this.official.toArray(new Base[this.official.size()]); // SubstanceDefinitionNameOfficialComponent 3696 case -896505829: /*source*/ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 3697 default: return super.getProperty(hash, name, checkValid); 3698 } 3699 3700 } 3701 3702 @Override 3703 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3704 switch (hash) { 3705 case 3373707: // name 3706 this.name = TypeConvertor.castToString(value); // StringType 3707 return value; 3708 case 3575610: // type 3709 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3710 return value; 3711 case -892481550: // status 3712 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3713 return value; 3714 case -1294005119: // preferred 3715 this.preferred = TypeConvertor.castToBoolean(value); // BooleanType 3716 return value; 3717 case -1613589672: // language 3718 this.getLanguage().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3719 return value; 3720 case -1326197564: // domain 3721 this.getDomain().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3722 return value; 3723 case -507075711: // jurisdiction 3724 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3725 return value; 3726 case -1742128133: // synonym 3727 this.getSynonym().add((SubstanceDefinitionNameComponent) value); // SubstanceDefinitionNameComponent 3728 return value; 3729 case -1840647503: // translation 3730 this.getTranslation().add((SubstanceDefinitionNameComponent) value); // SubstanceDefinitionNameComponent 3731 return value; 3732 case -765289749: // official 3733 this.getOfficial().add((SubstanceDefinitionNameOfficialComponent) value); // SubstanceDefinitionNameOfficialComponent 3734 return value; 3735 case -896505829: // source 3736 this.getSource().add(TypeConvertor.castToReference(value)); // Reference 3737 return value; 3738 default: return super.setProperty(hash, name, value); 3739 } 3740 3741 } 3742 3743 @Override 3744 public Base setProperty(String name, Base value) throws FHIRException { 3745 if (name.equals("name")) { 3746 this.name = TypeConvertor.castToString(value); // StringType 3747 } else if (name.equals("type")) { 3748 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3749 } else if (name.equals("status")) { 3750 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3751 } else if (name.equals("preferred")) { 3752 this.preferred = TypeConvertor.castToBoolean(value); // BooleanType 3753 } else if (name.equals("language")) { 3754 this.getLanguage().add(TypeConvertor.castToCodeableConcept(value)); 3755 } else if (name.equals("domain")) { 3756 this.getDomain().add(TypeConvertor.castToCodeableConcept(value)); 3757 } else if (name.equals("jurisdiction")) { 3758 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 3759 } else if (name.equals("synonym")) { 3760 this.getSynonym().add((SubstanceDefinitionNameComponent) value); 3761 } else if (name.equals("translation")) { 3762 this.getTranslation().add((SubstanceDefinitionNameComponent) value); 3763 } else if (name.equals("official")) { 3764 this.getOfficial().add((SubstanceDefinitionNameOfficialComponent) value); 3765 } else if (name.equals("source")) { 3766 this.getSource().add(TypeConvertor.castToReference(value)); 3767 } else 3768 return super.setProperty(name, value); 3769 return value; 3770 } 3771 3772 @Override 3773 public void removeChild(String name, Base value) throws FHIRException { 3774 if (name.equals("name")) { 3775 this.name = null; 3776 } else if (name.equals("type")) { 3777 this.type = null; 3778 } else if (name.equals("status")) { 3779 this.status = null; 3780 } else if (name.equals("preferred")) { 3781 this.preferred = null; 3782 } else if (name.equals("language")) { 3783 this.getLanguage().remove(value); 3784 } else if (name.equals("domain")) { 3785 this.getDomain().remove(value); 3786 } else if (name.equals("jurisdiction")) { 3787 this.getJurisdiction().remove(value); 3788 } else if (name.equals("synonym")) { 3789 this.getSynonym().remove((SubstanceDefinitionNameComponent) value); 3790 } else if (name.equals("translation")) { 3791 this.getTranslation().remove((SubstanceDefinitionNameComponent) value); 3792 } else if (name.equals("official")) { 3793 this.getOfficial().remove((SubstanceDefinitionNameOfficialComponent) value); 3794 } else if (name.equals("source")) { 3795 this.getSource().remove(value); 3796 } else 3797 super.removeChild(name, value); 3798 3799 } 3800 3801 @Override 3802 public Base makeProperty(int hash, String name) throws FHIRException { 3803 switch (hash) { 3804 case 3373707: return getNameElement(); 3805 case 3575610: return getType(); 3806 case -892481550: return getStatus(); 3807 case -1294005119: return getPreferredElement(); 3808 case -1613589672: return addLanguage(); 3809 case -1326197564: return addDomain(); 3810 case -507075711: return addJurisdiction(); 3811 case -1742128133: return addSynonym(); 3812 case -1840647503: return addTranslation(); 3813 case -765289749: return addOfficial(); 3814 case -896505829: return addSource(); 3815 default: return super.makeProperty(hash, name); 3816 } 3817 3818 } 3819 3820 @Override 3821 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3822 switch (hash) { 3823 case 3373707: /*name*/ return new String[] {"string"}; 3824 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3825 case -892481550: /*status*/ return new String[] {"CodeableConcept"}; 3826 case -1294005119: /*preferred*/ return new String[] {"boolean"}; 3827 case -1613589672: /*language*/ return new String[] {"CodeableConcept"}; 3828 case -1326197564: /*domain*/ return new String[] {"CodeableConcept"}; 3829 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 3830 case -1742128133: /*synonym*/ return new String[] {"@SubstanceDefinition.name"}; 3831 case -1840647503: /*translation*/ return new String[] {"@SubstanceDefinition.name"}; 3832 case -765289749: /*official*/ return new String[] {}; 3833 case -896505829: /*source*/ return new String[] {"Reference"}; 3834 default: return super.getTypesForProperty(hash, name); 3835 } 3836 3837 } 3838 3839 @Override 3840 public Base addChild(String name) throws FHIRException { 3841 if (name.equals("name")) { 3842 throw new FHIRException("Cannot call addChild on a singleton property SubstanceDefinition.name.name"); 3843 } 3844 else if (name.equals("type")) { 3845 this.type = new CodeableConcept(); 3846 return this.type; 3847 } 3848 else if (name.equals("status")) { 3849 this.status = new CodeableConcept(); 3850 return this.status; 3851 } 3852 else if (name.equals("preferred")) { 3853 throw new FHIRException("Cannot call addChild on a singleton property SubstanceDefinition.name.preferred"); 3854 } 3855 else if (name.equals("language")) { 3856 return addLanguage(); 3857 } 3858 else if (name.equals("domain")) { 3859 return addDomain(); 3860 } 3861 else if (name.equals("jurisdiction")) { 3862 return addJurisdiction(); 3863 } 3864 else if (name.equals("synonym")) { 3865 return addSynonym(); 3866 } 3867 else if (name.equals("translation")) { 3868 return addTranslation(); 3869 } 3870 else if (name.equals("official")) { 3871 return addOfficial(); 3872 } 3873 else if (name.equals("source")) { 3874 return addSource(); 3875 } 3876 else 3877 return super.addChild(name); 3878 } 3879 3880 public SubstanceDefinitionNameComponent copy() { 3881 SubstanceDefinitionNameComponent dst = new SubstanceDefinitionNameComponent(); 3882 copyValues(dst); 3883 return dst; 3884 } 3885 3886 public void copyValues(SubstanceDefinitionNameComponent dst) { 3887 super.copyValues(dst); 3888 dst.name = name == null ? null : name.copy(); 3889 dst.type = type == null ? null : type.copy(); 3890 dst.status = status == null ? null : status.copy(); 3891 dst.preferred = preferred == null ? null : preferred.copy(); 3892 if (language != null) { 3893 dst.language = new ArrayList<CodeableConcept>(); 3894 for (CodeableConcept i : language) 3895 dst.language.add(i.copy()); 3896 }; 3897 if (domain != null) { 3898 dst.domain = new ArrayList<CodeableConcept>(); 3899 for (CodeableConcept i : domain) 3900 dst.domain.add(i.copy()); 3901 }; 3902 if (jurisdiction != null) { 3903 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3904 for (CodeableConcept i : jurisdiction) 3905 dst.jurisdiction.add(i.copy()); 3906 }; 3907 if (synonym != null) { 3908 dst.synonym = new ArrayList<SubstanceDefinitionNameComponent>(); 3909 for (SubstanceDefinitionNameComponent i : synonym) 3910 dst.synonym.add(i.copy()); 3911 }; 3912 if (translation != null) { 3913 dst.translation = new ArrayList<SubstanceDefinitionNameComponent>(); 3914 for (SubstanceDefinitionNameComponent i : translation) 3915 dst.translation.add(i.copy()); 3916 }; 3917 if (official != null) { 3918 dst.official = new ArrayList<SubstanceDefinitionNameOfficialComponent>(); 3919 for (SubstanceDefinitionNameOfficialComponent i : official) 3920 dst.official.add(i.copy()); 3921 }; 3922 if (source != null) { 3923 dst.source = new ArrayList<Reference>(); 3924 for (Reference i : source) 3925 dst.source.add(i.copy()); 3926 }; 3927 } 3928 3929 @Override 3930 public boolean equalsDeep(Base other_) { 3931 if (!super.equalsDeep(other_)) 3932 return false; 3933 if (!(other_ instanceof SubstanceDefinitionNameComponent)) 3934 return false; 3935 SubstanceDefinitionNameComponent o = (SubstanceDefinitionNameComponent) other_; 3936 return compareDeep(name, o.name, true) && compareDeep(type, o.type, true) && compareDeep(status, o.status, true) 3937 && compareDeep(preferred, o.preferred, true) && compareDeep(language, o.language, true) && compareDeep(domain, o.domain, true) 3938 && compareDeep(jurisdiction, o.jurisdiction, true) && compareDeep(synonym, o.synonym, true) && compareDeep(translation, o.translation, true) 3939 && compareDeep(official, o.official, true) && compareDeep(source, o.source, true); 3940 } 3941 3942 @Override 3943 public boolean equalsShallow(Base other_) { 3944 if (!super.equalsShallow(other_)) 3945 return false; 3946 if (!(other_ instanceof SubstanceDefinitionNameComponent)) 3947 return false; 3948 SubstanceDefinitionNameComponent o = (SubstanceDefinitionNameComponent) other_; 3949 return compareValues(name, o.name, true) && compareValues(preferred, o.preferred, true); 3950 } 3951 3952 public boolean isEmpty() { 3953 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, type, status, preferred 3954 , language, domain, jurisdiction, synonym, translation, official, source); 3955 } 3956 3957 public String fhirType() { 3958 return "SubstanceDefinition.name"; 3959 3960 } 3961 3962 } 3963 3964 @Block() 3965 public static class SubstanceDefinitionNameOfficialComponent extends BackboneElement implements IBaseBackboneElement { 3966 /** 3967 * Which authority uses this official name. 3968 */ 3969 @Child(name = "authority", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 3970 @Description(shortDefinition="Which authority uses this official name", formalDefinition="Which authority uses this official name." ) 3971 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-name-authority") 3972 protected CodeableConcept authority; 3973 3974 /** 3975 * The status of the official name, for example 'draft', 'active', 'retired'. 3976 */ 3977 @Child(name = "status", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 3978 @Description(shortDefinition="The status of the official name, for example 'draft', 'active'", formalDefinition="The status of the official name, for example 'draft', 'active', 'retired'." ) 3979 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 3980 protected CodeableConcept status; 3981 3982 /** 3983 * Date of the official name change. 3984 */ 3985 @Child(name = "date", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 3986 @Description(shortDefinition="Date of official name change", formalDefinition="Date of the official name change." ) 3987 protected DateTimeType date; 3988 3989 private static final long serialVersionUID = -2040011008L; 3990 3991 /** 3992 * Constructor 3993 */ 3994 public SubstanceDefinitionNameOfficialComponent() { 3995 super(); 3996 } 3997 3998 /** 3999 * @return {@link #authority} (Which authority uses this official name.) 4000 */ 4001 public CodeableConcept getAuthority() { 4002 if (this.authority == null) 4003 if (Configuration.errorOnAutoCreate()) 4004 throw new Error("Attempt to auto-create SubstanceDefinitionNameOfficialComponent.authority"); 4005 else if (Configuration.doAutoCreate()) 4006 this.authority = new CodeableConcept(); // cc 4007 return this.authority; 4008 } 4009 4010 public boolean hasAuthority() { 4011 return this.authority != null && !this.authority.isEmpty(); 4012 } 4013 4014 /** 4015 * @param value {@link #authority} (Which authority uses this official name.) 4016 */ 4017 public SubstanceDefinitionNameOfficialComponent setAuthority(CodeableConcept value) { 4018 this.authority = value; 4019 return this; 4020 } 4021 4022 /** 4023 * @return {@link #status} (The status of the official name, for example 'draft', 'active', 'retired'.) 4024 */ 4025 public CodeableConcept getStatus() { 4026 if (this.status == null) 4027 if (Configuration.errorOnAutoCreate()) 4028 throw new Error("Attempt to auto-create SubstanceDefinitionNameOfficialComponent.status"); 4029 else if (Configuration.doAutoCreate()) 4030 this.status = new CodeableConcept(); // cc 4031 return this.status; 4032 } 4033 4034 public boolean hasStatus() { 4035 return this.status != null && !this.status.isEmpty(); 4036 } 4037 4038 /** 4039 * @param value {@link #status} (The status of the official name, for example 'draft', 'active', 'retired'.) 4040 */ 4041 public SubstanceDefinitionNameOfficialComponent setStatus(CodeableConcept value) { 4042 this.status = value; 4043 return this; 4044 } 4045 4046 /** 4047 * @return {@link #date} (Date of the official name change.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 4048 */ 4049 public DateTimeType getDateElement() { 4050 if (this.date == null) 4051 if (Configuration.errorOnAutoCreate()) 4052 throw new Error("Attempt to auto-create SubstanceDefinitionNameOfficialComponent.date"); 4053 else if (Configuration.doAutoCreate()) 4054 this.date = new DateTimeType(); // bb 4055 return this.date; 4056 } 4057 4058 public boolean hasDateElement() { 4059 return this.date != null && !this.date.isEmpty(); 4060 } 4061 4062 public boolean hasDate() { 4063 return this.date != null && !this.date.isEmpty(); 4064 } 4065 4066 /** 4067 * @param value {@link #date} (Date of the official name change.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 4068 */ 4069 public SubstanceDefinitionNameOfficialComponent setDateElement(DateTimeType value) { 4070 this.date = value; 4071 return this; 4072 } 4073 4074 /** 4075 * @return Date of the official name change. 4076 */ 4077 public Date getDate() { 4078 return this.date == null ? null : this.date.getValue(); 4079 } 4080 4081 /** 4082 * @param value Date of the official name change. 4083 */ 4084 public SubstanceDefinitionNameOfficialComponent setDate(Date value) { 4085 if (value == null) 4086 this.date = null; 4087 else { 4088 if (this.date == null) 4089 this.date = new DateTimeType(); 4090 this.date.setValue(value); 4091 } 4092 return this; 4093 } 4094 4095 protected void listChildren(List<Property> children) { 4096 super.listChildren(children); 4097 children.add(new Property("authority", "CodeableConcept", "Which authority uses this official name.", 0, 1, authority)); 4098 children.add(new Property("status", "CodeableConcept", "The status of the official name, for example 'draft', 'active', 'retired'.", 0, 1, status)); 4099 children.add(new Property("date", "dateTime", "Date of the official name change.", 0, 1, date)); 4100 } 4101 4102 @Override 4103 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4104 switch (_hash) { 4105 case 1475610435: /*authority*/ return new Property("authority", "CodeableConcept", "Which authority uses this official name.", 0, 1, authority); 4106 case -892481550: /*status*/ return new Property("status", "CodeableConcept", "The status of the official name, for example 'draft', 'active', 'retired'.", 0, 1, status); 4107 case 3076014: /*date*/ return new Property("date", "dateTime", "Date of the official name change.", 0, 1, date); 4108 default: return super.getNamedProperty(_hash, _name, _checkValid); 4109 } 4110 4111 } 4112 4113 @Override 4114 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4115 switch (hash) { 4116 case 1475610435: /*authority*/ return this.authority == null ? new Base[0] : new Base[] {this.authority}; // CodeableConcept 4117 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // CodeableConcept 4118 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 4119 default: return super.getProperty(hash, name, checkValid); 4120 } 4121 4122 } 4123 4124 @Override 4125 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4126 switch (hash) { 4127 case 1475610435: // authority 4128 this.authority = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4129 return value; 4130 case -892481550: // status 4131 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4132 return value; 4133 case 3076014: // date 4134 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4135 return value; 4136 default: return super.setProperty(hash, name, value); 4137 } 4138 4139 } 4140 4141 @Override 4142 public Base setProperty(String name, Base value) throws FHIRException { 4143 if (name.equals("authority")) { 4144 this.authority = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4145 } else if (name.equals("status")) { 4146 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4147 } else if (name.equals("date")) { 4148 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4149 } else 4150 return super.setProperty(name, value); 4151 return value; 4152 } 4153 4154 @Override 4155 public void removeChild(String name, Base value) throws FHIRException { 4156 if (name.equals("authority")) { 4157 this.authority = null; 4158 } else if (name.equals("status")) { 4159 this.status = null; 4160 } else if (name.equals("date")) { 4161 this.date = null; 4162 } else 4163 super.removeChild(name, value); 4164 4165 } 4166 4167 @Override 4168 public Base makeProperty(int hash, String name) throws FHIRException { 4169 switch (hash) { 4170 case 1475610435: return getAuthority(); 4171 case -892481550: return getStatus(); 4172 case 3076014: return getDateElement(); 4173 default: return super.makeProperty(hash, name); 4174 } 4175 4176 } 4177 4178 @Override 4179 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4180 switch (hash) { 4181 case 1475610435: /*authority*/ return new String[] {"CodeableConcept"}; 4182 case -892481550: /*status*/ return new String[] {"CodeableConcept"}; 4183 case 3076014: /*date*/ return new String[] {"dateTime"}; 4184 default: return super.getTypesForProperty(hash, name); 4185 } 4186 4187 } 4188 4189 @Override 4190 public Base addChild(String name) throws FHIRException { 4191 if (name.equals("authority")) { 4192 this.authority = new CodeableConcept(); 4193 return this.authority; 4194 } 4195 else if (name.equals("status")) { 4196 this.status = new CodeableConcept(); 4197 return this.status; 4198 } 4199 else if (name.equals("date")) { 4200 throw new FHIRException("Cannot call addChild on a singleton property SubstanceDefinition.name.official.date"); 4201 } 4202 else 4203 return super.addChild(name); 4204 } 4205 4206 public SubstanceDefinitionNameOfficialComponent copy() { 4207 SubstanceDefinitionNameOfficialComponent dst = new SubstanceDefinitionNameOfficialComponent(); 4208 copyValues(dst); 4209 return dst; 4210 } 4211 4212 public void copyValues(SubstanceDefinitionNameOfficialComponent dst) { 4213 super.copyValues(dst); 4214 dst.authority = authority == null ? null : authority.copy(); 4215 dst.status = status == null ? null : status.copy(); 4216 dst.date = date == null ? null : date.copy(); 4217 } 4218 4219 @Override 4220 public boolean equalsDeep(Base other_) { 4221 if (!super.equalsDeep(other_)) 4222 return false; 4223 if (!(other_ instanceof SubstanceDefinitionNameOfficialComponent)) 4224 return false; 4225 SubstanceDefinitionNameOfficialComponent o = (SubstanceDefinitionNameOfficialComponent) other_; 4226 return compareDeep(authority, o.authority, true) && compareDeep(status, o.status, true) && compareDeep(date, o.date, true) 4227 ; 4228 } 4229 4230 @Override 4231 public boolean equalsShallow(Base other_) { 4232 if (!super.equalsShallow(other_)) 4233 return false; 4234 if (!(other_ instanceof SubstanceDefinitionNameOfficialComponent)) 4235 return false; 4236 SubstanceDefinitionNameOfficialComponent o = (SubstanceDefinitionNameOfficialComponent) other_; 4237 return compareValues(date, o.date, true); 4238 } 4239 4240 public boolean isEmpty() { 4241 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(authority, status, date 4242 ); 4243 } 4244 4245 public String fhirType() { 4246 return "SubstanceDefinition.name.official"; 4247 4248 } 4249 4250 } 4251 4252 @Block() 4253 public static class SubstanceDefinitionRelationshipComponent extends BackboneElement implements IBaseBackboneElement { 4254 /** 4255 * A pointer to another substance, as a resource or just a representational code. 4256 */ 4257 @Child(name = "substanceDefinition", type = {SubstanceDefinition.class, CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 4258 @Description(shortDefinition="A pointer to another substance, as a resource or a representational code", formalDefinition="A pointer to another substance, as a resource or just a representational code." ) 4259 protected DataType substanceDefinition; 4260 4261 /** 4262 * For example "salt to parent", "active moiety", "starting material", "polymorph", "impurity of". 4263 */ 4264 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=true) 4265 @Description(shortDefinition="For example \"salt to parent\", \"active moiety\"", formalDefinition="For example \"salt to parent\", \"active moiety\", \"starting material\", \"polymorph\", \"impurity of\"." ) 4266 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-relationship-type") 4267 protected CodeableConcept type; 4268 4269 /** 4270 * For example where an enzyme strongly bonds with a particular substance, this is a defining relationship for that enzyme, out of several possible substance relationships. 4271 */ 4272 @Child(name = "isDefining", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=true) 4273 @Description(shortDefinition="For example where an enzyme strongly bonds with a particular substance, this is a defining relationship for that enzyme, out of several possible relationships", formalDefinition="For example where an enzyme strongly bonds with a particular substance, this is a defining relationship for that enzyme, out of several possible substance relationships." ) 4274 protected BooleanType isDefining; 4275 4276 /** 4277 * A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other. 4278 */ 4279 @Child(name = "amount", type = {Quantity.class, Ratio.class, StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 4280 @Description(shortDefinition="A numeric factor for the relationship, e.g. that a substance salt has some percentage of active substance in relation to some other", formalDefinition="A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other." ) 4281 protected DataType amount; 4282 4283 /** 4284 * For use when the numeric has an uncertain range. 4285 */ 4286 @Child(name = "ratioHighLimitAmount", type = {Ratio.class}, order=5, min=0, max=1, modifier=false, summary=true) 4287 @Description(shortDefinition="For use when the numeric has an uncertain range", formalDefinition="For use when the numeric has an uncertain range." ) 4288 protected Ratio ratioHighLimitAmount; 4289 4290 /** 4291 * An operator for the amount, for example "average", "approximately", "less than". 4292 */ 4293 @Child(name = "comparator", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=true) 4294 @Description(shortDefinition="An operator for the amount, for example \"average\", \"approximately\", \"less than\"", formalDefinition="An operator for the amount, for example \"average\", \"approximately\", \"less than\"." ) 4295 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-amount-type") 4296 protected CodeableConcept comparator; 4297 4298 /** 4299 * Supporting literature. 4300 */ 4301 @Child(name = "source", type = {DocumentReference.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4302 @Description(shortDefinition="Supporting literature", formalDefinition="Supporting literature." ) 4303 protected List<Reference> source; 4304 4305 private static final long serialVersionUID = 192222632L; 4306 4307 /** 4308 * Constructor 4309 */ 4310 public SubstanceDefinitionRelationshipComponent() { 4311 super(); 4312 } 4313 4314 /** 4315 * Constructor 4316 */ 4317 public SubstanceDefinitionRelationshipComponent(CodeableConcept type) { 4318 super(); 4319 this.setType(type); 4320 } 4321 4322 /** 4323 * @return {@link #substanceDefinition} (A pointer to another substance, as a resource or just a representational code.) 4324 */ 4325 public DataType getSubstanceDefinition() { 4326 return this.substanceDefinition; 4327 } 4328 4329 /** 4330 * @return {@link #substanceDefinition} (A pointer to another substance, as a resource or just a representational code.) 4331 */ 4332 public Reference getSubstanceDefinitionReference() throws FHIRException { 4333 if (this.substanceDefinition == null) 4334 this.substanceDefinition = new Reference(); 4335 if (!(this.substanceDefinition instanceof Reference)) 4336 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.substanceDefinition.getClass().getName()+" was encountered"); 4337 return (Reference) this.substanceDefinition; 4338 } 4339 4340 public boolean hasSubstanceDefinitionReference() { 4341 return this != null && this.substanceDefinition instanceof Reference; 4342 } 4343 4344 /** 4345 * @return {@link #substanceDefinition} (A pointer to another substance, as a resource or just a representational code.) 4346 */ 4347 public CodeableConcept getSubstanceDefinitionCodeableConcept() throws FHIRException { 4348 if (this.substanceDefinition == null) 4349 this.substanceDefinition = new CodeableConcept(); 4350 if (!(this.substanceDefinition instanceof CodeableConcept)) 4351 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.substanceDefinition.getClass().getName()+" was encountered"); 4352 return (CodeableConcept) this.substanceDefinition; 4353 } 4354 4355 public boolean hasSubstanceDefinitionCodeableConcept() { 4356 return this != null && this.substanceDefinition instanceof CodeableConcept; 4357 } 4358 4359 public boolean hasSubstanceDefinition() { 4360 return this.substanceDefinition != null && !this.substanceDefinition.isEmpty(); 4361 } 4362 4363 /** 4364 * @param value {@link #substanceDefinition} (A pointer to another substance, as a resource or just a representational code.) 4365 */ 4366 public SubstanceDefinitionRelationshipComponent setSubstanceDefinition(DataType value) { 4367 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 4368 throw new FHIRException("Not the right type for SubstanceDefinition.relationship.substanceDefinition[x]: "+value.fhirType()); 4369 this.substanceDefinition = value; 4370 return this; 4371 } 4372 4373 /** 4374 * @return {@link #type} (For example "salt to parent", "active moiety", "starting material", "polymorph", "impurity of".) 4375 */ 4376 public CodeableConcept getType() { 4377 if (this.type == null) 4378 if (Configuration.errorOnAutoCreate()) 4379 throw new Error("Attempt to auto-create SubstanceDefinitionRelationshipComponent.type"); 4380 else if (Configuration.doAutoCreate()) 4381 this.type = new CodeableConcept(); // cc 4382 return this.type; 4383 } 4384 4385 public boolean hasType() { 4386 return this.type != null && !this.type.isEmpty(); 4387 } 4388 4389 /** 4390 * @param value {@link #type} (For example "salt to parent", "active moiety", "starting material", "polymorph", "impurity of".) 4391 */ 4392 public SubstanceDefinitionRelationshipComponent setType(CodeableConcept value) { 4393 this.type = value; 4394 return this; 4395 } 4396 4397 /** 4398 * @return {@link #isDefining} (For example where an enzyme strongly bonds with a particular substance, this is a defining relationship for that enzyme, out of several possible substance relationships.). This is the underlying object with id, value and extensions. The accessor "getIsDefining" gives direct access to the value 4399 */ 4400 public BooleanType getIsDefiningElement() { 4401 if (this.isDefining == null) 4402 if (Configuration.errorOnAutoCreate()) 4403 throw new Error("Attempt to auto-create SubstanceDefinitionRelationshipComponent.isDefining"); 4404 else if (Configuration.doAutoCreate()) 4405 this.isDefining = new BooleanType(); // bb 4406 return this.isDefining; 4407 } 4408 4409 public boolean hasIsDefiningElement() { 4410 return this.isDefining != null && !this.isDefining.isEmpty(); 4411 } 4412 4413 public boolean hasIsDefining() { 4414 return this.isDefining != null && !this.isDefining.isEmpty(); 4415 } 4416 4417 /** 4418 * @param value {@link #isDefining} (For example where an enzyme strongly bonds with a particular substance, this is a defining relationship for that enzyme, out of several possible substance relationships.). This is the underlying object with id, value and extensions. The accessor "getIsDefining" gives direct access to the value 4419 */ 4420 public SubstanceDefinitionRelationshipComponent setIsDefiningElement(BooleanType value) { 4421 this.isDefining = value; 4422 return this; 4423 } 4424 4425 /** 4426 * @return For example where an enzyme strongly bonds with a particular substance, this is a defining relationship for that enzyme, out of several possible substance relationships. 4427 */ 4428 public boolean getIsDefining() { 4429 return this.isDefining == null || this.isDefining.isEmpty() ? false : this.isDefining.getValue(); 4430 } 4431 4432 /** 4433 * @param value For example where an enzyme strongly bonds with a particular substance, this is a defining relationship for that enzyme, out of several possible substance relationships. 4434 */ 4435 public SubstanceDefinitionRelationshipComponent setIsDefining(boolean value) { 4436 if (this.isDefining == null) 4437 this.isDefining = new BooleanType(); 4438 this.isDefining.setValue(value); 4439 return this; 4440 } 4441 4442 /** 4443 * @return {@link #amount} (A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other.) 4444 */ 4445 public DataType getAmount() { 4446 return this.amount; 4447 } 4448 4449 /** 4450 * @return {@link #amount} (A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other.) 4451 */ 4452 public Quantity getAmountQuantity() throws FHIRException { 4453 if (this.amount == null) 4454 this.amount = new Quantity(); 4455 if (!(this.amount instanceof Quantity)) 4456 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.amount.getClass().getName()+" was encountered"); 4457 return (Quantity) this.amount; 4458 } 4459 4460 public boolean hasAmountQuantity() { 4461 return this != null && this.amount instanceof Quantity; 4462 } 4463 4464 /** 4465 * @return {@link #amount} (A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other.) 4466 */ 4467 public Ratio getAmountRatio() throws FHIRException { 4468 if (this.amount == null) 4469 this.amount = new Ratio(); 4470 if (!(this.amount instanceof Ratio)) 4471 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.amount.getClass().getName()+" was encountered"); 4472 return (Ratio) this.amount; 4473 } 4474 4475 public boolean hasAmountRatio() { 4476 return this != null && this.amount instanceof Ratio; 4477 } 4478 4479 /** 4480 * @return {@link #amount} (A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other.) 4481 */ 4482 public StringType getAmountStringType() throws FHIRException { 4483 if (this.amount == null) 4484 this.amount = new StringType(); 4485 if (!(this.amount instanceof StringType)) 4486 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.amount.getClass().getName()+" was encountered"); 4487 return (StringType) this.amount; 4488 } 4489 4490 public boolean hasAmountStringType() { 4491 return this != null && this.amount instanceof StringType; 4492 } 4493 4494 public boolean hasAmount() { 4495 return this.amount != null && !this.amount.isEmpty(); 4496 } 4497 4498 /** 4499 * @param value {@link #amount} (A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other.) 4500 */ 4501 public SubstanceDefinitionRelationshipComponent setAmount(DataType value) { 4502 if (value != null && !(value instanceof Quantity || value instanceof Ratio || value instanceof StringType)) 4503 throw new FHIRException("Not the right type for SubstanceDefinition.relationship.amount[x]: "+value.fhirType()); 4504 this.amount = value; 4505 return this; 4506 } 4507 4508 /** 4509 * @return {@link #ratioHighLimitAmount} (For use when the numeric has an uncertain range.) 4510 */ 4511 public Ratio getRatioHighLimitAmount() { 4512 if (this.ratioHighLimitAmount == null) 4513 if (Configuration.errorOnAutoCreate()) 4514 throw new Error("Attempt to auto-create SubstanceDefinitionRelationshipComponent.ratioHighLimitAmount"); 4515 else if (Configuration.doAutoCreate()) 4516 this.ratioHighLimitAmount = new Ratio(); // cc 4517 return this.ratioHighLimitAmount; 4518 } 4519 4520 public boolean hasRatioHighLimitAmount() { 4521 return this.ratioHighLimitAmount != null && !this.ratioHighLimitAmount.isEmpty(); 4522 } 4523 4524 /** 4525 * @param value {@link #ratioHighLimitAmount} (For use when the numeric has an uncertain range.) 4526 */ 4527 public SubstanceDefinitionRelationshipComponent setRatioHighLimitAmount(Ratio value) { 4528 this.ratioHighLimitAmount = value; 4529 return this; 4530 } 4531 4532 /** 4533 * @return {@link #comparator} (An operator for the amount, for example "average", "approximately", "less than".) 4534 */ 4535 public CodeableConcept getComparator() { 4536 if (this.comparator == null) 4537 if (Configuration.errorOnAutoCreate()) 4538 throw new Error("Attempt to auto-create SubstanceDefinitionRelationshipComponent.comparator"); 4539 else if (Configuration.doAutoCreate()) 4540 this.comparator = new CodeableConcept(); // cc 4541 return this.comparator; 4542 } 4543 4544 public boolean hasComparator() { 4545 return this.comparator != null && !this.comparator.isEmpty(); 4546 } 4547 4548 /** 4549 * @param value {@link #comparator} (An operator for the amount, for example "average", "approximately", "less than".) 4550 */ 4551 public SubstanceDefinitionRelationshipComponent setComparator(CodeableConcept value) { 4552 this.comparator = value; 4553 return this; 4554 } 4555 4556 /** 4557 * @return {@link #source} (Supporting literature.) 4558 */ 4559 public List<Reference> getSource() { 4560 if (this.source == null) 4561 this.source = new ArrayList<Reference>(); 4562 return this.source; 4563 } 4564 4565 /** 4566 * @return Returns a reference to <code>this</code> for easy method chaining 4567 */ 4568 public SubstanceDefinitionRelationshipComponent setSource(List<Reference> theSource) { 4569 this.source = theSource; 4570 return this; 4571 } 4572 4573 public boolean hasSource() { 4574 if (this.source == null) 4575 return false; 4576 for (Reference item : this.source) 4577 if (!item.isEmpty()) 4578 return true; 4579 return false; 4580 } 4581 4582 public Reference addSource() { //3 4583 Reference t = new Reference(); 4584 if (this.source == null) 4585 this.source = new ArrayList<Reference>(); 4586 this.source.add(t); 4587 return t; 4588 } 4589 4590 public SubstanceDefinitionRelationshipComponent addSource(Reference t) { //3 4591 if (t == null) 4592 return this; 4593 if (this.source == null) 4594 this.source = new ArrayList<Reference>(); 4595 this.source.add(t); 4596 return this; 4597 } 4598 4599 /** 4600 * @return The first repetition of repeating field {@link #source}, creating it if it does not already exist {3} 4601 */ 4602 public Reference getSourceFirstRep() { 4603 if (getSource().isEmpty()) { 4604 addSource(); 4605 } 4606 return getSource().get(0); 4607 } 4608 4609 protected void listChildren(List<Property> children) { 4610 super.listChildren(children); 4611 children.add(new Property("substanceDefinition[x]", "Reference(SubstanceDefinition)|CodeableConcept", "A pointer to another substance, as a resource or just a representational code.", 0, 1, substanceDefinition)); 4612 children.add(new Property("type", "CodeableConcept", "For example \"salt to parent\", \"active moiety\", \"starting material\", \"polymorph\", \"impurity of\".", 0, 1, type)); 4613 children.add(new Property("isDefining", "boolean", "For example where an enzyme strongly bonds with a particular substance, this is a defining relationship for that enzyme, out of several possible substance relationships.", 0, 1, isDefining)); 4614 children.add(new Property("amount[x]", "Quantity|Ratio|string", "A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other.", 0, 1, amount)); 4615 children.add(new Property("ratioHighLimitAmount", "Ratio", "For use when the numeric has an uncertain range.", 0, 1, ratioHighLimitAmount)); 4616 children.add(new Property("comparator", "CodeableConcept", "An operator for the amount, for example \"average\", \"approximately\", \"less than\".", 0, 1, comparator)); 4617 children.add(new Property("source", "Reference(DocumentReference)", "Supporting literature.", 0, java.lang.Integer.MAX_VALUE, source)); 4618 } 4619 4620 @Override 4621 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4622 switch (_hash) { 4623 case -1767011651: /*substanceDefinition[x]*/ return new Property("substanceDefinition[x]", "Reference(SubstanceDefinition)|CodeableConcept", "A pointer to another substance, as a resource or just a representational code.", 0, 1, substanceDefinition); 4624 case 718195427: /*substanceDefinition*/ return new Property("substanceDefinition[x]", "Reference(SubstanceDefinition)|CodeableConcept", "A pointer to another substance, as a resource or just a representational code.", 0, 1, substanceDefinition); 4625 case -308206680: /*substanceDefinitionReference*/ return new Property("substanceDefinition[x]", "Reference(SubstanceDefinition)", "A pointer to another substance, as a resource or just a representational code.", 0, 1, substanceDefinition); 4626 case -132490690: /*substanceDefinitionCodeableConcept*/ return new Property("substanceDefinition[x]", "CodeableConcept", "A pointer to another substance, as a resource or just a representational code.", 0, 1, substanceDefinition); 4627 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "For example \"salt to parent\", \"active moiety\", \"starting material\", \"polymorph\", \"impurity of\".", 0, 1, type); 4628 case -141812990: /*isDefining*/ return new Property("isDefining", "boolean", "For example where an enzyme strongly bonds with a particular substance, this is a defining relationship for that enzyme, out of several possible substance relationships.", 0, 1, isDefining); 4629 case 646780200: /*amount[x]*/ return new Property("amount[x]", "Quantity|Ratio|string", "A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other.", 0, 1, amount); 4630 case -1413853096: /*amount*/ return new Property("amount[x]", "Quantity|Ratio|string", "A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other.", 0, 1, amount); 4631 case 1664303363: /*amountQuantity*/ return new Property("amount[x]", "Quantity", "A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other.", 0, 1, amount); 4632 case -1223457133: /*amountRatio*/ return new Property("amount[x]", "Ratio", "A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other.", 0, 1, amount); 4633 case 773651081: /*amountString*/ return new Property("amount[x]", "string", "A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other.", 0, 1, amount); 4634 case -1708404378: /*ratioHighLimitAmount*/ return new Property("ratioHighLimitAmount", "Ratio", "For use when the numeric has an uncertain range.", 0, 1, ratioHighLimitAmount); 4635 case -844673834: /*comparator*/ return new Property("comparator", "CodeableConcept", "An operator for the amount, for example \"average\", \"approximately\", \"less than\".", 0, 1, comparator); 4636 case -896505829: /*source*/ return new Property("source", "Reference(DocumentReference)", "Supporting literature.", 0, java.lang.Integer.MAX_VALUE, source); 4637 default: return super.getNamedProperty(_hash, _name, _checkValid); 4638 } 4639 4640 } 4641 4642 @Override 4643 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4644 switch (hash) { 4645 case 718195427: /*substanceDefinition*/ return this.substanceDefinition == null ? new Base[0] : new Base[] {this.substanceDefinition}; // DataType 4646 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 4647 case -141812990: /*isDefining*/ return this.isDefining == null ? new Base[0] : new Base[] {this.isDefining}; // BooleanType 4648 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // DataType 4649 case -1708404378: /*ratioHighLimitAmount*/ return this.ratioHighLimitAmount == null ? new Base[0] : new Base[] {this.ratioHighLimitAmount}; // Ratio 4650 case -844673834: /*comparator*/ return this.comparator == null ? new Base[0] : new Base[] {this.comparator}; // CodeableConcept 4651 case -896505829: /*source*/ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 4652 default: return super.getProperty(hash, name, checkValid); 4653 } 4654 4655 } 4656 4657 @Override 4658 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4659 switch (hash) { 4660 case 718195427: // substanceDefinition 4661 this.substanceDefinition = TypeConvertor.castToType(value); // DataType 4662 return value; 4663 case 3575610: // type 4664 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4665 return value; 4666 case -141812990: // isDefining 4667 this.isDefining = TypeConvertor.castToBoolean(value); // BooleanType 4668 return value; 4669 case -1413853096: // amount 4670 this.amount = TypeConvertor.castToType(value); // DataType 4671 return value; 4672 case -1708404378: // ratioHighLimitAmount 4673 this.ratioHighLimitAmount = TypeConvertor.castToRatio(value); // Ratio 4674 return value; 4675 case -844673834: // comparator 4676 this.comparator = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4677 return value; 4678 case -896505829: // source 4679 this.getSource().add(TypeConvertor.castToReference(value)); // Reference 4680 return value; 4681 default: return super.setProperty(hash, name, value); 4682 } 4683 4684 } 4685 4686 @Override 4687 public Base setProperty(String name, Base value) throws FHIRException { 4688 if (name.equals("substanceDefinition[x]")) { 4689 this.substanceDefinition = TypeConvertor.castToType(value); // DataType 4690 } else if (name.equals("type")) { 4691 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4692 } else if (name.equals("isDefining")) { 4693 this.isDefining = TypeConvertor.castToBoolean(value); // BooleanType 4694 } else if (name.equals("amount[x]")) { 4695 this.amount = TypeConvertor.castToType(value); // DataType 4696 } else if (name.equals("ratioHighLimitAmount")) { 4697 this.ratioHighLimitAmount = TypeConvertor.castToRatio(value); // Ratio 4698 } else if (name.equals("comparator")) { 4699 this.comparator = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4700 } else if (name.equals("source")) { 4701 this.getSource().add(TypeConvertor.castToReference(value)); 4702 } else 4703 return super.setProperty(name, value); 4704 return value; 4705 } 4706 4707 @Override 4708 public void removeChild(String name, Base value) throws FHIRException { 4709 if (name.equals("substanceDefinition[x]")) { 4710 this.substanceDefinition = null; 4711 } else if (name.equals("type")) { 4712 this.type = null; 4713 } else if (name.equals("isDefining")) { 4714 this.isDefining = null; 4715 } else if (name.equals("amount[x]")) { 4716 this.amount = null; 4717 } else if (name.equals("ratioHighLimitAmount")) { 4718 this.ratioHighLimitAmount = null; 4719 } else if (name.equals("comparator")) { 4720 this.comparator = null; 4721 } else if (name.equals("source")) { 4722 this.getSource().remove(value); 4723 } else 4724 super.removeChild(name, value); 4725 4726 } 4727 4728 @Override 4729 public Base makeProperty(int hash, String name) throws FHIRException { 4730 switch (hash) { 4731 case -1767011651: return getSubstanceDefinition(); 4732 case 718195427: return getSubstanceDefinition(); 4733 case 3575610: return getType(); 4734 case -141812990: return getIsDefiningElement(); 4735 case 646780200: return getAmount(); 4736 case -1413853096: return getAmount(); 4737 case -1708404378: return getRatioHighLimitAmount(); 4738 case -844673834: return getComparator(); 4739 case -896505829: return addSource(); 4740 default: return super.makeProperty(hash, name); 4741 } 4742 4743 } 4744 4745 @Override 4746 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4747 switch (hash) { 4748 case 718195427: /*substanceDefinition*/ return new String[] {"Reference", "CodeableConcept"}; 4749 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 4750 case -141812990: /*isDefining*/ return new String[] {"boolean"}; 4751 case -1413853096: /*amount*/ return new String[] {"Quantity", "Ratio", "string"}; 4752 case -1708404378: /*ratioHighLimitAmount*/ return new String[] {"Ratio"}; 4753 case -844673834: /*comparator*/ return new String[] {"CodeableConcept"}; 4754 case -896505829: /*source*/ return new String[] {"Reference"}; 4755 default: return super.getTypesForProperty(hash, name); 4756 } 4757 4758 } 4759 4760 @Override 4761 public Base addChild(String name) throws FHIRException { 4762 if (name.equals("substanceDefinitionReference")) { 4763 this.substanceDefinition = new Reference(); 4764 return this.substanceDefinition; 4765 } 4766 else if (name.equals("substanceDefinitionCodeableConcept")) { 4767 this.substanceDefinition = new CodeableConcept(); 4768 return this.substanceDefinition; 4769 } 4770 else if (name.equals("type")) { 4771 this.type = new CodeableConcept(); 4772 return this.type; 4773 } 4774 else if (name.equals("isDefining")) { 4775 throw new FHIRException("Cannot call addChild on a singleton property SubstanceDefinition.relationship.isDefining"); 4776 } 4777 else if (name.equals("amountQuantity")) { 4778 this.amount = new Quantity(); 4779 return this.amount; 4780 } 4781 else if (name.equals("amountRatio")) { 4782 this.amount = new Ratio(); 4783 return this.amount; 4784 } 4785 else if (name.equals("amountString")) { 4786 this.amount = new StringType(); 4787 return this.amount; 4788 } 4789 else if (name.equals("ratioHighLimitAmount")) { 4790 this.ratioHighLimitAmount = new Ratio(); 4791 return this.ratioHighLimitAmount; 4792 } 4793 else if (name.equals("comparator")) { 4794 this.comparator = new CodeableConcept(); 4795 return this.comparator; 4796 } 4797 else if (name.equals("source")) { 4798 return addSource(); 4799 } 4800 else 4801 return super.addChild(name); 4802 } 4803 4804 public SubstanceDefinitionRelationshipComponent copy() { 4805 SubstanceDefinitionRelationshipComponent dst = new SubstanceDefinitionRelationshipComponent(); 4806 copyValues(dst); 4807 return dst; 4808 } 4809 4810 public void copyValues(SubstanceDefinitionRelationshipComponent dst) { 4811 super.copyValues(dst); 4812 dst.substanceDefinition = substanceDefinition == null ? null : substanceDefinition.copy(); 4813 dst.type = type == null ? null : type.copy(); 4814 dst.isDefining = isDefining == null ? null : isDefining.copy(); 4815 dst.amount = amount == null ? null : amount.copy(); 4816 dst.ratioHighLimitAmount = ratioHighLimitAmount == null ? null : ratioHighLimitAmount.copy(); 4817 dst.comparator = comparator == null ? null : comparator.copy(); 4818 if (source != null) { 4819 dst.source = new ArrayList<Reference>(); 4820 for (Reference i : source) 4821 dst.source.add(i.copy()); 4822 }; 4823 } 4824 4825 @Override 4826 public boolean equalsDeep(Base other_) { 4827 if (!super.equalsDeep(other_)) 4828 return false; 4829 if (!(other_ instanceof SubstanceDefinitionRelationshipComponent)) 4830 return false; 4831 SubstanceDefinitionRelationshipComponent o = (SubstanceDefinitionRelationshipComponent) other_; 4832 return compareDeep(substanceDefinition, o.substanceDefinition, true) && compareDeep(type, o.type, true) 4833 && compareDeep(isDefining, o.isDefining, true) && compareDeep(amount, o.amount, true) && compareDeep(ratioHighLimitAmount, o.ratioHighLimitAmount, true) 4834 && compareDeep(comparator, o.comparator, true) && compareDeep(source, o.source, true); 4835 } 4836 4837 @Override 4838 public boolean equalsShallow(Base other_) { 4839 if (!super.equalsShallow(other_)) 4840 return false; 4841 if (!(other_ instanceof SubstanceDefinitionRelationshipComponent)) 4842 return false; 4843 SubstanceDefinitionRelationshipComponent o = (SubstanceDefinitionRelationshipComponent) other_; 4844 return compareValues(isDefining, o.isDefining, true); 4845 } 4846 4847 public boolean isEmpty() { 4848 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(substanceDefinition, type 4849 , isDefining, amount, ratioHighLimitAmount, comparator, source); 4850 } 4851 4852 public String fhirType() { 4853 return "SubstanceDefinition.relationship"; 4854 4855 } 4856 4857 } 4858 4859 @Block() 4860 public static class SubstanceDefinitionSourceMaterialComponent extends BackboneElement implements IBaseBackboneElement { 4861 /** 4862 * A classification that provides the origin of the raw material. Example: cat hair would be an Animal source type. 4863 */ 4864 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 4865 @Description(shortDefinition="Classification of the origin of the raw material. e.g. cat hair is an Animal source type", formalDefinition="A classification that provides the origin of the raw material. Example: cat hair would be an Animal source type." ) 4866 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-source-material-type") 4867 protected CodeableConcept type; 4868 4869 /** 4870 * The genus of an organism, typically referring to the Latin epithet of the genus element of the plant/animal scientific name. 4871 */ 4872 @Child(name = "genus", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 4873 @Description(shortDefinition="The genus of an organism e.g. the Latin epithet of the plant/animal scientific name", formalDefinition="The genus of an organism, typically referring to the Latin epithet of the genus element of the plant/animal scientific name." ) 4874 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-source-material-genus") 4875 protected CodeableConcept genus; 4876 4877 /** 4878 * The species of an organism, typically referring to the Latin epithet of the species of the plant/animal. 4879 */ 4880 @Child(name = "species", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 4881 @Description(shortDefinition="The species of an organism e.g. the Latin epithet of the species of the plant/animal", formalDefinition="The species of an organism, typically referring to the Latin epithet of the species of the plant/animal." ) 4882 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-source-material-species") 4883 protected CodeableConcept species; 4884 4885 /** 4886 * An anatomical origin of the source material within an organism. 4887 */ 4888 @Child(name = "part", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 4889 @Description(shortDefinition="An anatomical origin of the source material within an organism", formalDefinition="An anatomical origin of the source material within an organism." ) 4890 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-source-material-part") 4891 protected CodeableConcept part; 4892 4893 /** 4894 * The country or countries where the material is harvested. 4895 */ 4896 @Child(name = "countryOfOrigin", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4897 @Description(shortDefinition="The country or countries where the material is harvested", formalDefinition="The country or countries where the material is harvested." ) 4898 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/country") 4899 protected List<CodeableConcept> countryOfOrigin; 4900 4901 private static final long serialVersionUID = 569352795L; 4902 4903 /** 4904 * Constructor 4905 */ 4906 public SubstanceDefinitionSourceMaterialComponent() { 4907 super(); 4908 } 4909 4910 /** 4911 * @return {@link #type} (A classification that provides the origin of the raw material. Example: cat hair would be an Animal source type.) 4912 */ 4913 public CodeableConcept getType() { 4914 if (this.type == null) 4915 if (Configuration.errorOnAutoCreate()) 4916 throw new Error("Attempt to auto-create SubstanceDefinitionSourceMaterialComponent.type"); 4917 else if (Configuration.doAutoCreate()) 4918 this.type = new CodeableConcept(); // cc 4919 return this.type; 4920 } 4921 4922 public boolean hasType() { 4923 return this.type != null && !this.type.isEmpty(); 4924 } 4925 4926 /** 4927 * @param value {@link #type} (A classification that provides the origin of the raw material. Example: cat hair would be an Animal source type.) 4928 */ 4929 public SubstanceDefinitionSourceMaterialComponent setType(CodeableConcept value) { 4930 this.type = value; 4931 return this; 4932 } 4933 4934 /** 4935 * @return {@link #genus} (The genus of an organism, typically referring to the Latin epithet of the genus element of the plant/animal scientific name.) 4936 */ 4937 public CodeableConcept getGenus() { 4938 if (this.genus == null) 4939 if (Configuration.errorOnAutoCreate()) 4940 throw new Error("Attempt to auto-create SubstanceDefinitionSourceMaterialComponent.genus"); 4941 else if (Configuration.doAutoCreate()) 4942 this.genus = new CodeableConcept(); // cc 4943 return this.genus; 4944 } 4945 4946 public boolean hasGenus() { 4947 return this.genus != null && !this.genus.isEmpty(); 4948 } 4949 4950 /** 4951 * @param value {@link #genus} (The genus of an organism, typically referring to the Latin epithet of the genus element of the plant/animal scientific name.) 4952 */ 4953 public SubstanceDefinitionSourceMaterialComponent setGenus(CodeableConcept value) { 4954 this.genus = value; 4955 return this; 4956 } 4957 4958 /** 4959 * @return {@link #species} (The species of an organism, typically referring to the Latin epithet of the species of the plant/animal.) 4960 */ 4961 public CodeableConcept getSpecies() { 4962 if (this.species == null) 4963 if (Configuration.errorOnAutoCreate()) 4964 throw new Error("Attempt to auto-create SubstanceDefinitionSourceMaterialComponent.species"); 4965 else if (Configuration.doAutoCreate()) 4966 this.species = new CodeableConcept(); // cc 4967 return this.species; 4968 } 4969 4970 public boolean hasSpecies() { 4971 return this.species != null && !this.species.isEmpty(); 4972 } 4973 4974 /** 4975 * @param value {@link #species} (The species of an organism, typically referring to the Latin epithet of the species of the plant/animal.) 4976 */ 4977 public SubstanceDefinitionSourceMaterialComponent setSpecies(CodeableConcept value) { 4978 this.species = value; 4979 return this; 4980 } 4981 4982 /** 4983 * @return {@link #part} (An anatomical origin of the source material within an organism.) 4984 */ 4985 public CodeableConcept getPart() { 4986 if (this.part == null) 4987 if (Configuration.errorOnAutoCreate()) 4988 throw new Error("Attempt to auto-create SubstanceDefinitionSourceMaterialComponent.part"); 4989 else if (Configuration.doAutoCreate()) 4990 this.part = new CodeableConcept(); // cc 4991 return this.part; 4992 } 4993 4994 public boolean hasPart() { 4995 return this.part != null && !this.part.isEmpty(); 4996 } 4997 4998 /** 4999 * @param value {@link #part} (An anatomical origin of the source material within an organism.) 5000 */ 5001 public SubstanceDefinitionSourceMaterialComponent setPart(CodeableConcept value) { 5002 this.part = value; 5003 return this; 5004 } 5005 5006 /** 5007 * @return {@link #countryOfOrigin} (The country or countries where the material is harvested.) 5008 */ 5009 public List<CodeableConcept> getCountryOfOrigin() { 5010 if (this.countryOfOrigin == null) 5011 this.countryOfOrigin = new ArrayList<CodeableConcept>(); 5012 return this.countryOfOrigin; 5013 } 5014 5015 /** 5016 * @return Returns a reference to <code>this</code> for easy method chaining 5017 */ 5018 public SubstanceDefinitionSourceMaterialComponent setCountryOfOrigin(List<CodeableConcept> theCountryOfOrigin) { 5019 this.countryOfOrigin = theCountryOfOrigin; 5020 return this; 5021 } 5022 5023 public boolean hasCountryOfOrigin() { 5024 if (this.countryOfOrigin == null) 5025 return false; 5026 for (CodeableConcept item : this.countryOfOrigin) 5027 if (!item.isEmpty()) 5028 return true; 5029 return false; 5030 } 5031 5032 public CodeableConcept addCountryOfOrigin() { //3 5033 CodeableConcept t = new CodeableConcept(); 5034 if (this.countryOfOrigin == null) 5035 this.countryOfOrigin = new ArrayList<CodeableConcept>(); 5036 this.countryOfOrigin.add(t); 5037 return t; 5038 } 5039 5040 public SubstanceDefinitionSourceMaterialComponent addCountryOfOrigin(CodeableConcept t) { //3 5041 if (t == null) 5042 return this; 5043 if (this.countryOfOrigin == null) 5044 this.countryOfOrigin = new ArrayList<CodeableConcept>(); 5045 this.countryOfOrigin.add(t); 5046 return this; 5047 } 5048 5049 /** 5050 * @return The first repetition of repeating field {@link #countryOfOrigin}, creating it if it does not already exist {3} 5051 */ 5052 public CodeableConcept getCountryOfOriginFirstRep() { 5053 if (getCountryOfOrigin().isEmpty()) { 5054 addCountryOfOrigin(); 5055 } 5056 return getCountryOfOrigin().get(0); 5057 } 5058 5059 protected void listChildren(List<Property> children) { 5060 super.listChildren(children); 5061 children.add(new Property("type", "CodeableConcept", "A classification that provides the origin of the raw material. Example: cat hair would be an Animal source type.", 0, 1, type)); 5062 children.add(new Property("genus", "CodeableConcept", "The genus of an organism, typically referring to the Latin epithet of the genus element of the plant/animal scientific name.", 0, 1, genus)); 5063 children.add(new Property("species", "CodeableConcept", "The species of an organism, typically referring to the Latin epithet of the species of the plant/animal.", 0, 1, species)); 5064 children.add(new Property("part", "CodeableConcept", "An anatomical origin of the source material within an organism.", 0, 1, part)); 5065 children.add(new Property("countryOfOrigin", "CodeableConcept", "The country or countries where the material is harvested.", 0, java.lang.Integer.MAX_VALUE, countryOfOrigin)); 5066 } 5067 5068 @Override 5069 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5070 switch (_hash) { 5071 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A classification that provides the origin of the raw material. Example: cat hair would be an Animal source type.", 0, 1, type); 5072 case 98241006: /*genus*/ return new Property("genus", "CodeableConcept", "The genus of an organism, typically referring to the Latin epithet of the genus element of the plant/animal scientific name.", 0, 1, genus); 5073 case -2008465092: /*species*/ return new Property("species", "CodeableConcept", "The species of an organism, typically referring to the Latin epithet of the species of the plant/animal.", 0, 1, species); 5074 case 3433459: /*part*/ return new Property("part", "CodeableConcept", "An anatomical origin of the source material within an organism.", 0, 1, part); 5075 case 57176467: /*countryOfOrigin*/ return new Property("countryOfOrigin", "CodeableConcept", "The country or countries where the material is harvested.", 0, java.lang.Integer.MAX_VALUE, countryOfOrigin); 5076 default: return super.getNamedProperty(_hash, _name, _checkValid); 5077 } 5078 5079 } 5080 5081 @Override 5082 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5083 switch (hash) { 5084 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 5085 case 98241006: /*genus*/ return this.genus == null ? new Base[0] : new Base[] {this.genus}; // CodeableConcept 5086 case -2008465092: /*species*/ return this.species == null ? new Base[0] : new Base[] {this.species}; // CodeableConcept 5087 case 3433459: /*part*/ return this.part == null ? new Base[0] : new Base[] {this.part}; // CodeableConcept 5088 case 57176467: /*countryOfOrigin*/ return this.countryOfOrigin == null ? new Base[0] : this.countryOfOrigin.toArray(new Base[this.countryOfOrigin.size()]); // CodeableConcept 5089 default: return super.getProperty(hash, name, checkValid); 5090 } 5091 5092 } 5093 5094 @Override 5095 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5096 switch (hash) { 5097 case 3575610: // type 5098 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5099 return value; 5100 case 98241006: // genus 5101 this.genus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5102 return value; 5103 case -2008465092: // species 5104 this.species = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5105 return value; 5106 case 3433459: // part 5107 this.part = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5108 return value; 5109 case 57176467: // countryOfOrigin 5110 this.getCountryOfOrigin().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5111 return value; 5112 default: return super.setProperty(hash, name, value); 5113 } 5114 5115 } 5116 5117 @Override 5118 public Base setProperty(String name, Base value) throws FHIRException { 5119 if (name.equals("type")) { 5120 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5121 } else if (name.equals("genus")) { 5122 this.genus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5123 } else if (name.equals("species")) { 5124 this.species = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5125 } else if (name.equals("part")) { 5126 this.part = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5127 } else if (name.equals("countryOfOrigin")) { 5128 this.getCountryOfOrigin().add(TypeConvertor.castToCodeableConcept(value)); 5129 } else 5130 return super.setProperty(name, value); 5131 return value; 5132 } 5133 5134 @Override 5135 public void removeChild(String name, Base value) throws FHIRException { 5136 if (name.equals("type")) { 5137 this.type = null; 5138 } else if (name.equals("genus")) { 5139 this.genus = null; 5140 } else if (name.equals("species")) { 5141 this.species = null; 5142 } else if (name.equals("part")) { 5143 this.part = null; 5144 } else if (name.equals("countryOfOrigin")) { 5145 this.getCountryOfOrigin().remove(value); 5146 } else 5147 super.removeChild(name, value); 5148 5149 } 5150 5151 @Override 5152 public Base makeProperty(int hash, String name) throws FHIRException { 5153 switch (hash) { 5154 case 3575610: return getType(); 5155 case 98241006: return getGenus(); 5156 case -2008465092: return getSpecies(); 5157 case 3433459: return getPart(); 5158 case 57176467: return addCountryOfOrigin(); 5159 default: return super.makeProperty(hash, name); 5160 } 5161 5162 } 5163 5164 @Override 5165 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5166 switch (hash) { 5167 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 5168 case 98241006: /*genus*/ return new String[] {"CodeableConcept"}; 5169 case -2008465092: /*species*/ return new String[] {"CodeableConcept"}; 5170 case 3433459: /*part*/ return new String[] {"CodeableConcept"}; 5171 case 57176467: /*countryOfOrigin*/ return new String[] {"CodeableConcept"}; 5172 default: return super.getTypesForProperty(hash, name); 5173 } 5174 5175 } 5176 5177 @Override 5178 public Base addChild(String name) throws FHIRException { 5179 if (name.equals("type")) { 5180 this.type = new CodeableConcept(); 5181 return this.type; 5182 } 5183 else if (name.equals("genus")) { 5184 this.genus = new CodeableConcept(); 5185 return this.genus; 5186 } 5187 else if (name.equals("species")) { 5188 this.species = new CodeableConcept(); 5189 return this.species; 5190 } 5191 else if (name.equals("part")) { 5192 this.part = new CodeableConcept(); 5193 return this.part; 5194 } 5195 else if (name.equals("countryOfOrigin")) { 5196 return addCountryOfOrigin(); 5197 } 5198 else 5199 return super.addChild(name); 5200 } 5201 5202 public SubstanceDefinitionSourceMaterialComponent copy() { 5203 SubstanceDefinitionSourceMaterialComponent dst = new SubstanceDefinitionSourceMaterialComponent(); 5204 copyValues(dst); 5205 return dst; 5206 } 5207 5208 public void copyValues(SubstanceDefinitionSourceMaterialComponent dst) { 5209 super.copyValues(dst); 5210 dst.type = type == null ? null : type.copy(); 5211 dst.genus = genus == null ? null : genus.copy(); 5212 dst.species = species == null ? null : species.copy(); 5213 dst.part = part == null ? null : part.copy(); 5214 if (countryOfOrigin != null) { 5215 dst.countryOfOrigin = new ArrayList<CodeableConcept>(); 5216 for (CodeableConcept i : countryOfOrigin) 5217 dst.countryOfOrigin.add(i.copy()); 5218 }; 5219 } 5220 5221 @Override 5222 public boolean equalsDeep(Base other_) { 5223 if (!super.equalsDeep(other_)) 5224 return false; 5225 if (!(other_ instanceof SubstanceDefinitionSourceMaterialComponent)) 5226 return false; 5227 SubstanceDefinitionSourceMaterialComponent o = (SubstanceDefinitionSourceMaterialComponent) other_; 5228 return compareDeep(type, o.type, true) && compareDeep(genus, o.genus, true) && compareDeep(species, o.species, true) 5229 && compareDeep(part, o.part, true) && compareDeep(countryOfOrigin, o.countryOfOrigin, true); 5230 } 5231 5232 @Override 5233 public boolean equalsShallow(Base other_) { 5234 if (!super.equalsShallow(other_)) 5235 return false; 5236 if (!(other_ instanceof SubstanceDefinitionSourceMaterialComponent)) 5237 return false; 5238 SubstanceDefinitionSourceMaterialComponent o = (SubstanceDefinitionSourceMaterialComponent) other_; 5239 return true; 5240 } 5241 5242 public boolean isEmpty() { 5243 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, genus, species, part 5244 , countryOfOrigin); 5245 } 5246 5247 public String fhirType() { 5248 return "SubstanceDefinition.sourceMaterial"; 5249 5250 } 5251 5252 } 5253 5254 /** 5255 * Identifier by which this substance is known. 5256 */ 5257 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5258 @Description(shortDefinition="Identifier by which this substance is known", formalDefinition="Identifier by which this substance is known." ) 5259 protected List<Identifier> identifier; 5260 5261 /** 5262 * A business level version identifier of the substance. 5263 */ 5264 @Child(name = "version", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 5265 @Description(shortDefinition="A business level version identifier of the substance", formalDefinition="A business level version identifier of the substance." ) 5266 protected StringType version; 5267 5268 /** 5269 * Status of substance within the catalogue e.g. active, retired. 5270 */ 5271 @Child(name = "status", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 5272 @Description(shortDefinition="Status of substance within the catalogue e.g. active, retired", formalDefinition="Status of substance within the catalogue e.g. active, retired." ) 5273 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 5274 protected CodeableConcept status; 5275 5276 /** 5277 * A high level categorization, e.g. polymer or nucleic acid, or food, chemical, biological, or a lower level such as the general types of polymer (linear or branch chain) or type of impurity (process related or contaminant). 5278 */ 5279 @Child(name = "classification", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5280 @Description(shortDefinition="A categorization, high level e.g. polymer or nucleic acid, or food, chemical, biological, or lower e.g. polymer linear or branch chain, or type of impurity", formalDefinition="A high level categorization, e.g. polymer or nucleic acid, or food, chemical, biological, or a lower level such as the general types of polymer (linear or branch chain) or type of impurity (process related or contaminant)." ) 5281 protected List<CodeableConcept> classification; 5282 5283 /** 5284 * If the substance applies to human or veterinary use. 5285 */ 5286 @Child(name = "domain", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 5287 @Description(shortDefinition="If the substance applies to human or veterinary use", formalDefinition="If the substance applies to human or veterinary use." ) 5288 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicinal-product-domain") 5289 protected CodeableConcept domain; 5290 5291 /** 5292 * The quality standard, established benchmark, to which substance complies (e.g. USP/NF, Ph. Eur, JP, BP, Company Standard). 5293 */ 5294 @Child(name = "grade", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5295 @Description(shortDefinition="The quality standard, established benchmark, to which substance complies (e.g. USP/NF, BP)", formalDefinition="The quality standard, established benchmark, to which substance complies (e.g. USP/NF, Ph. Eur, JP, BP, Company Standard)." ) 5296 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-grade") 5297 protected List<CodeableConcept> grade; 5298 5299 /** 5300 * Textual description of the substance. 5301 */ 5302 @Child(name = "description", type = {MarkdownType.class}, order=6, min=0, max=1, modifier=false, summary=true) 5303 @Description(shortDefinition="Textual description of the substance", formalDefinition="Textual description of the substance." ) 5304 protected MarkdownType description; 5305 5306 /** 5307 * Supporting literature. 5308 */ 5309 @Child(name = "informationSource", type = {Citation.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5310 @Description(shortDefinition="Supporting literature", formalDefinition="Supporting literature." ) 5311 protected List<Reference> informationSource; 5312 5313 /** 5314 * Textual comment about the substance's catalogue or registry record. 5315 */ 5316 @Child(name = "note", type = {Annotation.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5317 @Description(shortDefinition="Textual comment about the substance's catalogue or registry record", formalDefinition="Textual comment about the substance's catalogue or registry record." ) 5318 protected List<Annotation> note; 5319 5320 /** 5321 * The entity that creates, makes, produces or fabricates the substance. This is a set of potential manufacturers but is not necessarily comprehensive. 5322 */ 5323 @Child(name = "manufacturer", type = {Organization.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5324 @Description(shortDefinition="The entity that creates, makes, produces or fabricates the substance", formalDefinition="The entity that creates, makes, produces or fabricates the substance. This is a set of potential manufacturers but is not necessarily comprehensive." ) 5325 protected List<Reference> manufacturer; 5326 5327 /** 5328 * An entity that is the source for the substance. It may be different from the manufacturer. Supplier is synonymous to a distributor. 5329 */ 5330 @Child(name = "supplier", type = {Organization.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5331 @Description(shortDefinition="An entity that is the source for the substance. It may be different from the manufacturer", formalDefinition="An entity that is the source for the substance. It may be different from the manufacturer. Supplier is synonymous to a distributor." ) 5332 protected List<Reference> supplier; 5333 5334 /** 5335 * Moiety, for structural modifications. 5336 */ 5337 @Child(name = "moiety", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5338 @Description(shortDefinition="Moiety, for structural modifications", formalDefinition="Moiety, for structural modifications." ) 5339 protected List<SubstanceDefinitionMoietyComponent> moiety; 5340 5341 /** 5342 * General specifications for this substance. 5343 */ 5344 @Child(name = "characterization", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5345 @Description(shortDefinition="General specifications for this substance", formalDefinition="General specifications for this substance." ) 5346 protected List<SubstanceDefinitionCharacterizationComponent> characterization; 5347 5348 /** 5349 * General specifications for this substance. 5350 */ 5351 @Child(name = "property", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5352 @Description(shortDefinition="General specifications for this substance", formalDefinition="General specifications for this substance." ) 5353 protected List<SubstanceDefinitionPropertyComponent> property; 5354 5355 /** 5356 * General information detailing this substance. 5357 */ 5358 @Child(name = "referenceInformation", type = {SubstanceReferenceInformation.class}, order=14, min=0, max=1, modifier=false, summary=true) 5359 @Description(shortDefinition="General information detailing this substance", formalDefinition="General information detailing this substance." ) 5360 protected Reference referenceInformation; 5361 5362 /** 5363 * The average mass of a molecule of a compound compared to 1/12 the mass of carbon 12 and calculated as the sum of the atomic weights of the constituent atoms. 5364 */ 5365 @Child(name = "molecularWeight", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5366 @Description(shortDefinition="The average mass of a molecule of a compound", formalDefinition="The average mass of a molecule of a compound compared to 1/12 the mass of carbon 12 and calculated as the sum of the atomic weights of the constituent atoms." ) 5367 protected List<SubstanceDefinitionMolecularWeightComponent> molecularWeight; 5368 5369 /** 5370 * Structural information. 5371 */ 5372 @Child(name = "structure", type = {}, order=16, min=0, max=1, modifier=false, summary=true) 5373 @Description(shortDefinition="Structural information", formalDefinition="Structural information." ) 5374 protected SubstanceDefinitionStructureComponent structure; 5375 5376 /** 5377 * Codes associated with the substance. 5378 */ 5379 @Child(name = "code", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5380 @Description(shortDefinition="Codes associated with the substance", formalDefinition="Codes associated with the substance." ) 5381 protected List<SubstanceDefinitionCodeComponent> code; 5382 5383 /** 5384 * Names applicable to this substance. 5385 */ 5386 @Child(name = "name", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5387 @Description(shortDefinition="Names applicable to this substance", formalDefinition="Names applicable to this substance." ) 5388 protected List<SubstanceDefinitionNameComponent> name; 5389 5390 /** 5391 * A link between this substance and another, with details of the relationship. 5392 */ 5393 @Child(name = "relationship", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5394 @Description(shortDefinition="A link between this substance and another", formalDefinition="A link between this substance and another, with details of the relationship." ) 5395 protected List<SubstanceDefinitionRelationshipComponent> relationship; 5396 5397 /** 5398 * Data items specific to nucleic acids. 5399 */ 5400 @Child(name = "nucleicAcid", type = {SubstanceNucleicAcid.class}, order=20, min=0, max=1, modifier=false, summary=true) 5401 @Description(shortDefinition="Data items specific to nucleic acids", formalDefinition="Data items specific to nucleic acids." ) 5402 protected Reference nucleicAcid; 5403 5404 /** 5405 * Data items specific to polymers. 5406 */ 5407 @Child(name = "polymer", type = {SubstancePolymer.class}, order=21, min=0, max=1, modifier=false, summary=true) 5408 @Description(shortDefinition="Data items specific to polymers", formalDefinition="Data items specific to polymers." ) 5409 protected Reference polymer; 5410 5411 /** 5412 * Data items specific to proteins. 5413 */ 5414 @Child(name = "protein", type = {SubstanceProtein.class}, order=22, min=0, max=1, modifier=false, summary=true) 5415 @Description(shortDefinition="Data items specific to proteins", formalDefinition="Data items specific to proteins." ) 5416 protected Reference protein; 5417 5418 /** 5419 * Material or taxonomic/anatomical source for the substance. 5420 */ 5421 @Child(name = "sourceMaterial", type = {}, order=23, min=0, max=1, modifier=false, summary=true) 5422 @Description(shortDefinition="Material or taxonomic/anatomical source", formalDefinition="Material or taxonomic/anatomical source for the substance." ) 5423 protected SubstanceDefinitionSourceMaterialComponent sourceMaterial; 5424 5425 private static final long serialVersionUID = -206769887L; 5426 5427 /** 5428 * Constructor 5429 */ 5430 public SubstanceDefinition() { 5431 super(); 5432 } 5433 5434 /** 5435 * @return {@link #identifier} (Identifier by which this substance is known.) 5436 */ 5437 public List<Identifier> getIdentifier() { 5438 if (this.identifier == null) 5439 this.identifier = new ArrayList<Identifier>(); 5440 return this.identifier; 5441 } 5442 5443 /** 5444 * @return Returns a reference to <code>this</code> for easy method chaining 5445 */ 5446 public SubstanceDefinition setIdentifier(List<Identifier> theIdentifier) { 5447 this.identifier = theIdentifier; 5448 return this; 5449 } 5450 5451 public boolean hasIdentifier() { 5452 if (this.identifier == null) 5453 return false; 5454 for (Identifier item : this.identifier) 5455 if (!item.isEmpty()) 5456 return true; 5457 return false; 5458 } 5459 5460 public Identifier addIdentifier() { //3 5461 Identifier t = new Identifier(); 5462 if (this.identifier == null) 5463 this.identifier = new ArrayList<Identifier>(); 5464 this.identifier.add(t); 5465 return t; 5466 } 5467 5468 public SubstanceDefinition addIdentifier(Identifier t) { //3 5469 if (t == null) 5470 return this; 5471 if (this.identifier == null) 5472 this.identifier = new ArrayList<Identifier>(); 5473 this.identifier.add(t); 5474 return this; 5475 } 5476 5477 /** 5478 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 5479 */ 5480 public Identifier getIdentifierFirstRep() { 5481 if (getIdentifier().isEmpty()) { 5482 addIdentifier(); 5483 } 5484 return getIdentifier().get(0); 5485 } 5486 5487 /** 5488 * @return {@link #version} (A business level version identifier of the substance.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 5489 */ 5490 public StringType getVersionElement() { 5491 if (this.version == null) 5492 if (Configuration.errorOnAutoCreate()) 5493 throw new Error("Attempt to auto-create SubstanceDefinition.version"); 5494 else if (Configuration.doAutoCreate()) 5495 this.version = new StringType(); // bb 5496 return this.version; 5497 } 5498 5499 public boolean hasVersionElement() { 5500 return this.version != null && !this.version.isEmpty(); 5501 } 5502 5503 public boolean hasVersion() { 5504 return this.version != null && !this.version.isEmpty(); 5505 } 5506 5507 /** 5508 * @param value {@link #version} (A business level version identifier of the substance.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 5509 */ 5510 public SubstanceDefinition setVersionElement(StringType value) { 5511 this.version = value; 5512 return this; 5513 } 5514 5515 /** 5516 * @return A business level version identifier of the substance. 5517 */ 5518 public String getVersion() { 5519 return this.version == null ? null : this.version.getValue(); 5520 } 5521 5522 /** 5523 * @param value A business level version identifier of the substance. 5524 */ 5525 public SubstanceDefinition setVersion(String value) { 5526 if (Utilities.noString(value)) 5527 this.version = null; 5528 else { 5529 if (this.version == null) 5530 this.version = new StringType(); 5531 this.version.setValue(value); 5532 } 5533 return this; 5534 } 5535 5536 /** 5537 * @return {@link #status} (Status of substance within the catalogue e.g. active, retired.) 5538 */ 5539 public CodeableConcept getStatus() { 5540 if (this.status == null) 5541 if (Configuration.errorOnAutoCreate()) 5542 throw new Error("Attempt to auto-create SubstanceDefinition.status"); 5543 else if (Configuration.doAutoCreate()) 5544 this.status = new CodeableConcept(); // cc 5545 return this.status; 5546 } 5547 5548 public boolean hasStatus() { 5549 return this.status != null && !this.status.isEmpty(); 5550 } 5551 5552 /** 5553 * @param value {@link #status} (Status of substance within the catalogue e.g. active, retired.) 5554 */ 5555 public SubstanceDefinition setStatus(CodeableConcept value) { 5556 this.status = value; 5557 return this; 5558 } 5559 5560 /** 5561 * @return {@link #classification} (A high level categorization, e.g. polymer or nucleic acid, or food, chemical, biological, or a lower level such as the general types of polymer (linear or branch chain) or type of impurity (process related or contaminant).) 5562 */ 5563 public List<CodeableConcept> getClassification() { 5564 if (this.classification == null) 5565 this.classification = new ArrayList<CodeableConcept>(); 5566 return this.classification; 5567 } 5568 5569 /** 5570 * @return Returns a reference to <code>this</code> for easy method chaining 5571 */ 5572 public SubstanceDefinition setClassification(List<CodeableConcept> theClassification) { 5573 this.classification = theClassification; 5574 return this; 5575 } 5576 5577 public boolean hasClassification() { 5578 if (this.classification == null) 5579 return false; 5580 for (CodeableConcept item : this.classification) 5581 if (!item.isEmpty()) 5582 return true; 5583 return false; 5584 } 5585 5586 public CodeableConcept addClassification() { //3 5587 CodeableConcept t = new CodeableConcept(); 5588 if (this.classification == null) 5589 this.classification = new ArrayList<CodeableConcept>(); 5590 this.classification.add(t); 5591 return t; 5592 } 5593 5594 public SubstanceDefinition addClassification(CodeableConcept t) { //3 5595 if (t == null) 5596 return this; 5597 if (this.classification == null) 5598 this.classification = new ArrayList<CodeableConcept>(); 5599 this.classification.add(t); 5600 return this; 5601 } 5602 5603 /** 5604 * @return The first repetition of repeating field {@link #classification}, creating it if it does not already exist {3} 5605 */ 5606 public CodeableConcept getClassificationFirstRep() { 5607 if (getClassification().isEmpty()) { 5608 addClassification(); 5609 } 5610 return getClassification().get(0); 5611 } 5612 5613 /** 5614 * @return {@link #domain} (If the substance applies to human or veterinary use.) 5615 */ 5616 public CodeableConcept getDomain() { 5617 if (this.domain == null) 5618 if (Configuration.errorOnAutoCreate()) 5619 throw new Error("Attempt to auto-create SubstanceDefinition.domain"); 5620 else if (Configuration.doAutoCreate()) 5621 this.domain = new CodeableConcept(); // cc 5622 return this.domain; 5623 } 5624 5625 public boolean hasDomain() { 5626 return this.domain != null && !this.domain.isEmpty(); 5627 } 5628 5629 /** 5630 * @param value {@link #domain} (If the substance applies to human or veterinary use.) 5631 */ 5632 public SubstanceDefinition setDomain(CodeableConcept value) { 5633 this.domain = value; 5634 return this; 5635 } 5636 5637 /** 5638 * @return {@link #grade} (The quality standard, established benchmark, to which substance complies (e.g. USP/NF, Ph. Eur, JP, BP, Company Standard).) 5639 */ 5640 public List<CodeableConcept> getGrade() { 5641 if (this.grade == null) 5642 this.grade = new ArrayList<CodeableConcept>(); 5643 return this.grade; 5644 } 5645 5646 /** 5647 * @return Returns a reference to <code>this</code> for easy method chaining 5648 */ 5649 public SubstanceDefinition setGrade(List<CodeableConcept> theGrade) { 5650 this.grade = theGrade; 5651 return this; 5652 } 5653 5654 public boolean hasGrade() { 5655 if (this.grade == null) 5656 return false; 5657 for (CodeableConcept item : this.grade) 5658 if (!item.isEmpty()) 5659 return true; 5660 return false; 5661 } 5662 5663 public CodeableConcept addGrade() { //3 5664 CodeableConcept t = new CodeableConcept(); 5665 if (this.grade == null) 5666 this.grade = new ArrayList<CodeableConcept>(); 5667 this.grade.add(t); 5668 return t; 5669 } 5670 5671 public SubstanceDefinition addGrade(CodeableConcept t) { //3 5672 if (t == null) 5673 return this; 5674 if (this.grade == null) 5675 this.grade = new ArrayList<CodeableConcept>(); 5676 this.grade.add(t); 5677 return this; 5678 } 5679 5680 /** 5681 * @return The first repetition of repeating field {@link #grade}, creating it if it does not already exist {3} 5682 */ 5683 public CodeableConcept getGradeFirstRep() { 5684 if (getGrade().isEmpty()) { 5685 addGrade(); 5686 } 5687 return getGrade().get(0); 5688 } 5689 5690 /** 5691 * @return {@link #description} (Textual description of the substance.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 5692 */ 5693 public MarkdownType getDescriptionElement() { 5694 if (this.description == null) 5695 if (Configuration.errorOnAutoCreate()) 5696 throw new Error("Attempt to auto-create SubstanceDefinition.description"); 5697 else if (Configuration.doAutoCreate()) 5698 this.description = new MarkdownType(); // bb 5699 return this.description; 5700 } 5701 5702 public boolean hasDescriptionElement() { 5703 return this.description != null && !this.description.isEmpty(); 5704 } 5705 5706 public boolean hasDescription() { 5707 return this.description != null && !this.description.isEmpty(); 5708 } 5709 5710 /** 5711 * @param value {@link #description} (Textual description of the substance.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 5712 */ 5713 public SubstanceDefinition setDescriptionElement(MarkdownType value) { 5714 this.description = value; 5715 return this; 5716 } 5717 5718 /** 5719 * @return Textual description of the substance. 5720 */ 5721 public String getDescription() { 5722 return this.description == null ? null : this.description.getValue(); 5723 } 5724 5725 /** 5726 * @param value Textual description of the substance. 5727 */ 5728 public SubstanceDefinition setDescription(String value) { 5729 if (Utilities.noString(value)) 5730 this.description = null; 5731 else { 5732 if (this.description == null) 5733 this.description = new MarkdownType(); 5734 this.description.setValue(value); 5735 } 5736 return this; 5737 } 5738 5739 /** 5740 * @return {@link #informationSource} (Supporting literature.) 5741 */ 5742 public List<Reference> getInformationSource() { 5743 if (this.informationSource == null) 5744 this.informationSource = new ArrayList<Reference>(); 5745 return this.informationSource; 5746 } 5747 5748 /** 5749 * @return Returns a reference to <code>this</code> for easy method chaining 5750 */ 5751 public SubstanceDefinition setInformationSource(List<Reference> theInformationSource) { 5752 this.informationSource = theInformationSource; 5753 return this; 5754 } 5755 5756 public boolean hasInformationSource() { 5757 if (this.informationSource == null) 5758 return false; 5759 for (Reference item : this.informationSource) 5760 if (!item.isEmpty()) 5761 return true; 5762 return false; 5763 } 5764 5765 public Reference addInformationSource() { //3 5766 Reference t = new Reference(); 5767 if (this.informationSource == null) 5768 this.informationSource = new ArrayList<Reference>(); 5769 this.informationSource.add(t); 5770 return t; 5771 } 5772 5773 public SubstanceDefinition addInformationSource(Reference t) { //3 5774 if (t == null) 5775 return this; 5776 if (this.informationSource == null) 5777 this.informationSource = new ArrayList<Reference>(); 5778 this.informationSource.add(t); 5779 return this; 5780 } 5781 5782 /** 5783 * @return The first repetition of repeating field {@link #informationSource}, creating it if it does not already exist {3} 5784 */ 5785 public Reference getInformationSourceFirstRep() { 5786 if (getInformationSource().isEmpty()) { 5787 addInformationSource(); 5788 } 5789 return getInformationSource().get(0); 5790 } 5791 5792 /** 5793 * @return {@link #note} (Textual comment about the substance's catalogue or registry record.) 5794 */ 5795 public List<Annotation> getNote() { 5796 if (this.note == null) 5797 this.note = new ArrayList<Annotation>(); 5798 return this.note; 5799 } 5800 5801 /** 5802 * @return Returns a reference to <code>this</code> for easy method chaining 5803 */ 5804 public SubstanceDefinition setNote(List<Annotation> theNote) { 5805 this.note = theNote; 5806 return this; 5807 } 5808 5809 public boolean hasNote() { 5810 if (this.note == null) 5811 return false; 5812 for (Annotation item : this.note) 5813 if (!item.isEmpty()) 5814 return true; 5815 return false; 5816 } 5817 5818 public Annotation addNote() { //3 5819 Annotation t = new Annotation(); 5820 if (this.note == null) 5821 this.note = new ArrayList<Annotation>(); 5822 this.note.add(t); 5823 return t; 5824 } 5825 5826 public SubstanceDefinition addNote(Annotation t) { //3 5827 if (t == null) 5828 return this; 5829 if (this.note == null) 5830 this.note = new ArrayList<Annotation>(); 5831 this.note.add(t); 5832 return this; 5833 } 5834 5835 /** 5836 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 5837 */ 5838 public Annotation getNoteFirstRep() { 5839 if (getNote().isEmpty()) { 5840 addNote(); 5841 } 5842 return getNote().get(0); 5843 } 5844 5845 /** 5846 * @return {@link #manufacturer} (The entity that creates, makes, produces or fabricates the substance. This is a set of potential manufacturers but is not necessarily comprehensive.) 5847 */ 5848 public List<Reference> getManufacturer() { 5849 if (this.manufacturer == null) 5850 this.manufacturer = new ArrayList<Reference>(); 5851 return this.manufacturer; 5852 } 5853 5854 /** 5855 * @return Returns a reference to <code>this</code> for easy method chaining 5856 */ 5857 public SubstanceDefinition setManufacturer(List<Reference> theManufacturer) { 5858 this.manufacturer = theManufacturer; 5859 return this; 5860 } 5861 5862 public boolean hasManufacturer() { 5863 if (this.manufacturer == null) 5864 return false; 5865 for (Reference item : this.manufacturer) 5866 if (!item.isEmpty()) 5867 return true; 5868 return false; 5869 } 5870 5871 public Reference addManufacturer() { //3 5872 Reference t = new Reference(); 5873 if (this.manufacturer == null) 5874 this.manufacturer = new ArrayList<Reference>(); 5875 this.manufacturer.add(t); 5876 return t; 5877 } 5878 5879 public SubstanceDefinition addManufacturer(Reference t) { //3 5880 if (t == null) 5881 return this; 5882 if (this.manufacturer == null) 5883 this.manufacturer = new ArrayList<Reference>(); 5884 this.manufacturer.add(t); 5885 return this; 5886 } 5887 5888 /** 5889 * @return The first repetition of repeating field {@link #manufacturer}, creating it if it does not already exist {3} 5890 */ 5891 public Reference getManufacturerFirstRep() { 5892 if (getManufacturer().isEmpty()) { 5893 addManufacturer(); 5894 } 5895 return getManufacturer().get(0); 5896 } 5897 5898 /** 5899 * @return {@link #supplier} (An entity that is the source for the substance. It may be different from the manufacturer. Supplier is synonymous to a distributor.) 5900 */ 5901 public List<Reference> getSupplier() { 5902 if (this.supplier == null) 5903 this.supplier = new ArrayList<Reference>(); 5904 return this.supplier; 5905 } 5906 5907 /** 5908 * @return Returns a reference to <code>this</code> for easy method chaining 5909 */ 5910 public SubstanceDefinition setSupplier(List<Reference> theSupplier) { 5911 this.supplier = theSupplier; 5912 return this; 5913 } 5914 5915 public boolean hasSupplier() { 5916 if (this.supplier == null) 5917 return false; 5918 for (Reference item : this.supplier) 5919 if (!item.isEmpty()) 5920 return true; 5921 return false; 5922 } 5923 5924 public Reference addSupplier() { //3 5925 Reference t = new Reference(); 5926 if (this.supplier == null) 5927 this.supplier = new ArrayList<Reference>(); 5928 this.supplier.add(t); 5929 return t; 5930 } 5931 5932 public SubstanceDefinition addSupplier(Reference t) { //3 5933 if (t == null) 5934 return this; 5935 if (this.supplier == null) 5936 this.supplier = new ArrayList<Reference>(); 5937 this.supplier.add(t); 5938 return this; 5939 } 5940 5941 /** 5942 * @return The first repetition of repeating field {@link #supplier}, creating it if it does not already exist {3} 5943 */ 5944 public Reference getSupplierFirstRep() { 5945 if (getSupplier().isEmpty()) { 5946 addSupplier(); 5947 } 5948 return getSupplier().get(0); 5949 } 5950 5951 /** 5952 * @return {@link #moiety} (Moiety, for structural modifications.) 5953 */ 5954 public List<SubstanceDefinitionMoietyComponent> getMoiety() { 5955 if (this.moiety == null) 5956 this.moiety = new ArrayList<SubstanceDefinitionMoietyComponent>(); 5957 return this.moiety; 5958 } 5959 5960 /** 5961 * @return Returns a reference to <code>this</code> for easy method chaining 5962 */ 5963 public SubstanceDefinition setMoiety(List<SubstanceDefinitionMoietyComponent> theMoiety) { 5964 this.moiety = theMoiety; 5965 return this; 5966 } 5967 5968 public boolean hasMoiety() { 5969 if (this.moiety == null) 5970 return false; 5971 for (SubstanceDefinitionMoietyComponent item : this.moiety) 5972 if (!item.isEmpty()) 5973 return true; 5974 return false; 5975 } 5976 5977 public SubstanceDefinitionMoietyComponent addMoiety() { //3 5978 SubstanceDefinitionMoietyComponent t = new SubstanceDefinitionMoietyComponent(); 5979 if (this.moiety == null) 5980 this.moiety = new ArrayList<SubstanceDefinitionMoietyComponent>(); 5981 this.moiety.add(t); 5982 return t; 5983 } 5984 5985 public SubstanceDefinition addMoiety(SubstanceDefinitionMoietyComponent t) { //3 5986 if (t == null) 5987 return this; 5988 if (this.moiety == null) 5989 this.moiety = new ArrayList<SubstanceDefinitionMoietyComponent>(); 5990 this.moiety.add(t); 5991 return this; 5992 } 5993 5994 /** 5995 * @return The first repetition of repeating field {@link #moiety}, creating it if it does not already exist {3} 5996 */ 5997 public SubstanceDefinitionMoietyComponent getMoietyFirstRep() { 5998 if (getMoiety().isEmpty()) { 5999 addMoiety(); 6000 } 6001 return getMoiety().get(0); 6002 } 6003 6004 /** 6005 * @return {@link #characterization} (General specifications for this substance.) 6006 */ 6007 public List<SubstanceDefinitionCharacterizationComponent> getCharacterization() { 6008 if (this.characterization == null) 6009 this.characterization = new ArrayList<SubstanceDefinitionCharacterizationComponent>(); 6010 return this.characterization; 6011 } 6012 6013 /** 6014 * @return Returns a reference to <code>this</code> for easy method chaining 6015 */ 6016 public SubstanceDefinition setCharacterization(List<SubstanceDefinitionCharacterizationComponent> theCharacterization) { 6017 this.characterization = theCharacterization; 6018 return this; 6019 } 6020 6021 public boolean hasCharacterization() { 6022 if (this.characterization == null) 6023 return false; 6024 for (SubstanceDefinitionCharacterizationComponent item : this.characterization) 6025 if (!item.isEmpty()) 6026 return true; 6027 return false; 6028 } 6029 6030 public SubstanceDefinitionCharacterizationComponent addCharacterization() { //3 6031 SubstanceDefinitionCharacterizationComponent t = new SubstanceDefinitionCharacterizationComponent(); 6032 if (this.characterization == null) 6033 this.characterization = new ArrayList<SubstanceDefinitionCharacterizationComponent>(); 6034 this.characterization.add(t); 6035 return t; 6036 } 6037 6038 public SubstanceDefinition addCharacterization(SubstanceDefinitionCharacterizationComponent t) { //3 6039 if (t == null) 6040 return this; 6041 if (this.characterization == null) 6042 this.characterization = new ArrayList<SubstanceDefinitionCharacterizationComponent>(); 6043 this.characterization.add(t); 6044 return this; 6045 } 6046 6047 /** 6048 * @return The first repetition of repeating field {@link #characterization}, creating it if it does not already exist {3} 6049 */ 6050 public SubstanceDefinitionCharacterizationComponent getCharacterizationFirstRep() { 6051 if (getCharacterization().isEmpty()) { 6052 addCharacterization(); 6053 } 6054 return getCharacterization().get(0); 6055 } 6056 6057 /** 6058 * @return {@link #property} (General specifications for this substance.) 6059 */ 6060 public List<SubstanceDefinitionPropertyComponent> getProperty() { 6061 if (this.property == null) 6062 this.property = new ArrayList<SubstanceDefinitionPropertyComponent>(); 6063 return this.property; 6064 } 6065 6066 /** 6067 * @return Returns a reference to <code>this</code> for easy method chaining 6068 */ 6069 public SubstanceDefinition setProperty(List<SubstanceDefinitionPropertyComponent> theProperty) { 6070 this.property = theProperty; 6071 return this; 6072 } 6073 6074 public boolean hasProperty() { 6075 if (this.property == null) 6076 return false; 6077 for (SubstanceDefinitionPropertyComponent item : this.property) 6078 if (!item.isEmpty()) 6079 return true; 6080 return false; 6081 } 6082 6083 public SubstanceDefinitionPropertyComponent addProperty() { //3 6084 SubstanceDefinitionPropertyComponent t = new SubstanceDefinitionPropertyComponent(); 6085 if (this.property == null) 6086 this.property = new ArrayList<SubstanceDefinitionPropertyComponent>(); 6087 this.property.add(t); 6088 return t; 6089 } 6090 6091 public SubstanceDefinition addProperty(SubstanceDefinitionPropertyComponent t) { //3 6092 if (t == null) 6093 return this; 6094 if (this.property == null) 6095 this.property = new ArrayList<SubstanceDefinitionPropertyComponent>(); 6096 this.property.add(t); 6097 return this; 6098 } 6099 6100 /** 6101 * @return The first repetition of repeating field {@link #property}, creating it if it does not already exist {3} 6102 */ 6103 public SubstanceDefinitionPropertyComponent getPropertyFirstRep() { 6104 if (getProperty().isEmpty()) { 6105 addProperty(); 6106 } 6107 return getProperty().get(0); 6108 } 6109 6110 /** 6111 * @return {@link #referenceInformation} (General information detailing this substance.) 6112 */ 6113 public Reference getReferenceInformation() { 6114 if (this.referenceInformation == null) 6115 if (Configuration.errorOnAutoCreate()) 6116 throw new Error("Attempt to auto-create SubstanceDefinition.referenceInformation"); 6117 else if (Configuration.doAutoCreate()) 6118 this.referenceInformation = new Reference(); // cc 6119 return this.referenceInformation; 6120 } 6121 6122 public boolean hasReferenceInformation() { 6123 return this.referenceInformation != null && !this.referenceInformation.isEmpty(); 6124 } 6125 6126 /** 6127 * @param value {@link #referenceInformation} (General information detailing this substance.) 6128 */ 6129 public SubstanceDefinition setReferenceInformation(Reference value) { 6130 this.referenceInformation = value; 6131 return this; 6132 } 6133 6134 /** 6135 * @return {@link #molecularWeight} (The average mass of a molecule of a compound compared to 1/12 the mass of carbon 12 and calculated as the sum of the atomic weights of the constituent atoms.) 6136 */ 6137 public List<SubstanceDefinitionMolecularWeightComponent> getMolecularWeight() { 6138 if (this.molecularWeight == null) 6139 this.molecularWeight = new ArrayList<SubstanceDefinitionMolecularWeightComponent>(); 6140 return this.molecularWeight; 6141 } 6142 6143 /** 6144 * @return Returns a reference to <code>this</code> for easy method chaining 6145 */ 6146 public SubstanceDefinition setMolecularWeight(List<SubstanceDefinitionMolecularWeightComponent> theMolecularWeight) { 6147 this.molecularWeight = theMolecularWeight; 6148 return this; 6149 } 6150 6151 public boolean hasMolecularWeight() { 6152 if (this.molecularWeight == null) 6153 return false; 6154 for (SubstanceDefinitionMolecularWeightComponent item : this.molecularWeight) 6155 if (!item.isEmpty()) 6156 return true; 6157 return false; 6158 } 6159 6160 public SubstanceDefinitionMolecularWeightComponent addMolecularWeight() { //3 6161 SubstanceDefinitionMolecularWeightComponent t = new SubstanceDefinitionMolecularWeightComponent(); 6162 if (this.molecularWeight == null) 6163 this.molecularWeight = new ArrayList<SubstanceDefinitionMolecularWeightComponent>(); 6164 this.molecularWeight.add(t); 6165 return t; 6166 } 6167 6168 public SubstanceDefinition addMolecularWeight(SubstanceDefinitionMolecularWeightComponent t) { //3 6169 if (t == null) 6170 return this; 6171 if (this.molecularWeight == null) 6172 this.molecularWeight = new ArrayList<SubstanceDefinitionMolecularWeightComponent>(); 6173 this.molecularWeight.add(t); 6174 return this; 6175 } 6176 6177 /** 6178 * @return The first repetition of repeating field {@link #molecularWeight}, creating it if it does not already exist {3} 6179 */ 6180 public SubstanceDefinitionMolecularWeightComponent getMolecularWeightFirstRep() { 6181 if (getMolecularWeight().isEmpty()) { 6182 addMolecularWeight(); 6183 } 6184 return getMolecularWeight().get(0); 6185 } 6186 6187 /** 6188 * @return {@link #structure} (Structural information.) 6189 */ 6190 public SubstanceDefinitionStructureComponent getStructure() { 6191 if (this.structure == null) 6192 if (Configuration.errorOnAutoCreate()) 6193 throw new Error("Attempt to auto-create SubstanceDefinition.structure"); 6194 else if (Configuration.doAutoCreate()) 6195 this.structure = new SubstanceDefinitionStructureComponent(); // cc 6196 return this.structure; 6197 } 6198 6199 public boolean hasStructure() { 6200 return this.structure != null && !this.structure.isEmpty(); 6201 } 6202 6203 /** 6204 * @param value {@link #structure} (Structural information.) 6205 */ 6206 public SubstanceDefinition setStructure(SubstanceDefinitionStructureComponent value) { 6207 this.structure = value; 6208 return this; 6209 } 6210 6211 /** 6212 * @return {@link #code} (Codes associated with the substance.) 6213 */ 6214 public List<SubstanceDefinitionCodeComponent> getCode() { 6215 if (this.code == null) 6216 this.code = new ArrayList<SubstanceDefinitionCodeComponent>(); 6217 return this.code; 6218 } 6219 6220 /** 6221 * @return Returns a reference to <code>this</code> for easy method chaining 6222 */ 6223 public SubstanceDefinition setCode(List<SubstanceDefinitionCodeComponent> theCode) { 6224 this.code = theCode; 6225 return this; 6226 } 6227 6228 public boolean hasCode() { 6229 if (this.code == null) 6230 return false; 6231 for (SubstanceDefinitionCodeComponent item : this.code) 6232 if (!item.isEmpty()) 6233 return true; 6234 return false; 6235 } 6236 6237 public SubstanceDefinitionCodeComponent addCode() { //3 6238 SubstanceDefinitionCodeComponent t = new SubstanceDefinitionCodeComponent(); 6239 if (this.code == null) 6240 this.code = new ArrayList<SubstanceDefinitionCodeComponent>(); 6241 this.code.add(t); 6242 return t; 6243 } 6244 6245 public SubstanceDefinition addCode(SubstanceDefinitionCodeComponent t) { //3 6246 if (t == null) 6247 return this; 6248 if (this.code == null) 6249 this.code = new ArrayList<SubstanceDefinitionCodeComponent>(); 6250 this.code.add(t); 6251 return this; 6252 } 6253 6254 /** 6255 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist {3} 6256 */ 6257 public SubstanceDefinitionCodeComponent getCodeFirstRep() { 6258 if (getCode().isEmpty()) { 6259 addCode(); 6260 } 6261 return getCode().get(0); 6262 } 6263 6264 /** 6265 * @return {@link #name} (Names applicable to this substance.) 6266 */ 6267 public List<SubstanceDefinitionNameComponent> getName() { 6268 if (this.name == null) 6269 this.name = new ArrayList<SubstanceDefinitionNameComponent>(); 6270 return this.name; 6271 } 6272 6273 /** 6274 * @return Returns a reference to <code>this</code> for easy method chaining 6275 */ 6276 public SubstanceDefinition setName(List<SubstanceDefinitionNameComponent> theName) { 6277 this.name = theName; 6278 return this; 6279 } 6280 6281 public boolean hasName() { 6282 if (this.name == null) 6283 return false; 6284 for (SubstanceDefinitionNameComponent item : this.name) 6285 if (!item.isEmpty()) 6286 return true; 6287 return false; 6288 } 6289 6290 public SubstanceDefinitionNameComponent addName() { //3 6291 SubstanceDefinitionNameComponent t = new SubstanceDefinitionNameComponent(); 6292 if (this.name == null) 6293 this.name = new ArrayList<SubstanceDefinitionNameComponent>(); 6294 this.name.add(t); 6295 return t; 6296 } 6297 6298 public SubstanceDefinition addName(SubstanceDefinitionNameComponent t) { //3 6299 if (t == null) 6300 return this; 6301 if (this.name == null) 6302 this.name = new ArrayList<SubstanceDefinitionNameComponent>(); 6303 this.name.add(t); 6304 return this; 6305 } 6306 6307 /** 6308 * @return The first repetition of repeating field {@link #name}, creating it if it does not already exist {3} 6309 */ 6310 public SubstanceDefinitionNameComponent getNameFirstRep() { 6311 if (getName().isEmpty()) { 6312 addName(); 6313 } 6314 return getName().get(0); 6315 } 6316 6317 /** 6318 * @return {@link #relationship} (A link between this substance and another, with details of the relationship.) 6319 */ 6320 public List<SubstanceDefinitionRelationshipComponent> getRelationship() { 6321 if (this.relationship == null) 6322 this.relationship = new ArrayList<SubstanceDefinitionRelationshipComponent>(); 6323 return this.relationship; 6324 } 6325 6326 /** 6327 * @return Returns a reference to <code>this</code> for easy method chaining 6328 */ 6329 public SubstanceDefinition setRelationship(List<SubstanceDefinitionRelationshipComponent> theRelationship) { 6330 this.relationship = theRelationship; 6331 return this; 6332 } 6333 6334 public boolean hasRelationship() { 6335 if (this.relationship == null) 6336 return false; 6337 for (SubstanceDefinitionRelationshipComponent item : this.relationship) 6338 if (!item.isEmpty()) 6339 return true; 6340 return false; 6341 } 6342 6343 public SubstanceDefinitionRelationshipComponent addRelationship() { //3 6344 SubstanceDefinitionRelationshipComponent t = new SubstanceDefinitionRelationshipComponent(); 6345 if (this.relationship == null) 6346 this.relationship = new ArrayList<SubstanceDefinitionRelationshipComponent>(); 6347 this.relationship.add(t); 6348 return t; 6349 } 6350 6351 public SubstanceDefinition addRelationship(SubstanceDefinitionRelationshipComponent t) { //3 6352 if (t == null) 6353 return this; 6354 if (this.relationship == null) 6355 this.relationship = new ArrayList<SubstanceDefinitionRelationshipComponent>(); 6356 this.relationship.add(t); 6357 return this; 6358 } 6359 6360 /** 6361 * @return The first repetition of repeating field {@link #relationship}, creating it if it does not already exist {3} 6362 */ 6363 public SubstanceDefinitionRelationshipComponent getRelationshipFirstRep() { 6364 if (getRelationship().isEmpty()) { 6365 addRelationship(); 6366 } 6367 return getRelationship().get(0); 6368 } 6369 6370 /** 6371 * @return {@link #nucleicAcid} (Data items specific to nucleic acids.) 6372 */ 6373 public Reference getNucleicAcid() { 6374 if (this.nucleicAcid == null) 6375 if (Configuration.errorOnAutoCreate()) 6376 throw new Error("Attempt to auto-create SubstanceDefinition.nucleicAcid"); 6377 else if (Configuration.doAutoCreate()) 6378 this.nucleicAcid = new Reference(); // cc 6379 return this.nucleicAcid; 6380 } 6381 6382 public boolean hasNucleicAcid() { 6383 return this.nucleicAcid != null && !this.nucleicAcid.isEmpty(); 6384 } 6385 6386 /** 6387 * @param value {@link #nucleicAcid} (Data items specific to nucleic acids.) 6388 */ 6389 public SubstanceDefinition setNucleicAcid(Reference value) { 6390 this.nucleicAcid = value; 6391 return this; 6392 } 6393 6394 /** 6395 * @return {@link #polymer} (Data items specific to polymers.) 6396 */ 6397 public Reference getPolymer() { 6398 if (this.polymer == null) 6399 if (Configuration.errorOnAutoCreate()) 6400 throw new Error("Attempt to auto-create SubstanceDefinition.polymer"); 6401 else if (Configuration.doAutoCreate()) 6402 this.polymer = new Reference(); // cc 6403 return this.polymer; 6404 } 6405 6406 public boolean hasPolymer() { 6407 return this.polymer != null && !this.polymer.isEmpty(); 6408 } 6409 6410 /** 6411 * @param value {@link #polymer} (Data items specific to polymers.) 6412 */ 6413 public SubstanceDefinition setPolymer(Reference value) { 6414 this.polymer = value; 6415 return this; 6416 } 6417 6418 /** 6419 * @return {@link #protein} (Data items specific to proteins.) 6420 */ 6421 public Reference getProtein() { 6422 if (this.protein == null) 6423 if (Configuration.errorOnAutoCreate()) 6424 throw new Error("Attempt to auto-create SubstanceDefinition.protein"); 6425 else if (Configuration.doAutoCreate()) 6426 this.protein = new Reference(); // cc 6427 return this.protein; 6428 } 6429 6430 public boolean hasProtein() { 6431 return this.protein != null && !this.protein.isEmpty(); 6432 } 6433 6434 /** 6435 * @param value {@link #protein} (Data items specific to proteins.) 6436 */ 6437 public SubstanceDefinition setProtein(Reference value) { 6438 this.protein = value; 6439 return this; 6440 } 6441 6442 /** 6443 * @return {@link #sourceMaterial} (Material or taxonomic/anatomical source for the substance.) 6444 */ 6445 public SubstanceDefinitionSourceMaterialComponent getSourceMaterial() { 6446 if (this.sourceMaterial == null) 6447 if (Configuration.errorOnAutoCreate()) 6448 throw new Error("Attempt to auto-create SubstanceDefinition.sourceMaterial"); 6449 else if (Configuration.doAutoCreate()) 6450 this.sourceMaterial = new SubstanceDefinitionSourceMaterialComponent(); // cc 6451 return this.sourceMaterial; 6452 } 6453 6454 public boolean hasSourceMaterial() { 6455 return this.sourceMaterial != null && !this.sourceMaterial.isEmpty(); 6456 } 6457 6458 /** 6459 * @param value {@link #sourceMaterial} (Material or taxonomic/anatomical source for the substance.) 6460 */ 6461 public SubstanceDefinition setSourceMaterial(SubstanceDefinitionSourceMaterialComponent value) { 6462 this.sourceMaterial = value; 6463 return this; 6464 } 6465 6466 protected void listChildren(List<Property> children) { 6467 super.listChildren(children); 6468 children.add(new Property("identifier", "Identifier", "Identifier by which this substance is known.", 0, java.lang.Integer.MAX_VALUE, identifier)); 6469 children.add(new Property("version", "string", "A business level version identifier of the substance.", 0, 1, version)); 6470 children.add(new Property("status", "CodeableConcept", "Status of substance within the catalogue e.g. active, retired.", 0, 1, status)); 6471 children.add(new Property("classification", "CodeableConcept", "A high level categorization, e.g. polymer or nucleic acid, or food, chemical, biological, or a lower level such as the general types of polymer (linear or branch chain) or type of impurity (process related or contaminant).", 0, java.lang.Integer.MAX_VALUE, classification)); 6472 children.add(new Property("domain", "CodeableConcept", "If the substance applies to human or veterinary use.", 0, 1, domain)); 6473 children.add(new Property("grade", "CodeableConcept", "The quality standard, established benchmark, to which substance complies (e.g. USP/NF, Ph. Eur, JP, BP, Company Standard).", 0, java.lang.Integer.MAX_VALUE, grade)); 6474 children.add(new Property("description", "markdown", "Textual description of the substance.", 0, 1, description)); 6475 children.add(new Property("informationSource", "Reference(Citation)", "Supporting literature.", 0, java.lang.Integer.MAX_VALUE, informationSource)); 6476 children.add(new Property("note", "Annotation", "Textual comment about the substance's catalogue or registry record.", 0, java.lang.Integer.MAX_VALUE, note)); 6477 children.add(new Property("manufacturer", "Reference(Organization)", "The entity that creates, makes, produces or fabricates the substance. This is a set of potential manufacturers but is not necessarily comprehensive.", 0, java.lang.Integer.MAX_VALUE, manufacturer)); 6478 children.add(new Property("supplier", "Reference(Organization)", "An entity that is the source for the substance. It may be different from the manufacturer. Supplier is synonymous to a distributor.", 0, java.lang.Integer.MAX_VALUE, supplier)); 6479 children.add(new Property("moiety", "", "Moiety, for structural modifications.", 0, java.lang.Integer.MAX_VALUE, moiety)); 6480 children.add(new Property("characterization", "", "General specifications for this substance.", 0, java.lang.Integer.MAX_VALUE, characterization)); 6481 children.add(new Property("property", "", "General specifications for this substance.", 0, java.lang.Integer.MAX_VALUE, property)); 6482 children.add(new Property("referenceInformation", "Reference(SubstanceReferenceInformation)", "General information detailing this substance.", 0, 1, referenceInformation)); 6483 children.add(new Property("molecularWeight", "", "The average mass of a molecule of a compound compared to 1/12 the mass of carbon 12 and calculated as the sum of the atomic weights of the constituent atoms.", 0, java.lang.Integer.MAX_VALUE, molecularWeight)); 6484 children.add(new Property("structure", "", "Structural information.", 0, 1, structure)); 6485 children.add(new Property("code", "", "Codes associated with the substance.", 0, java.lang.Integer.MAX_VALUE, code)); 6486 children.add(new Property("name", "", "Names applicable to this substance.", 0, java.lang.Integer.MAX_VALUE, name)); 6487 children.add(new Property("relationship", "", "A link between this substance and another, with details of the relationship.", 0, java.lang.Integer.MAX_VALUE, relationship)); 6488 children.add(new Property("nucleicAcid", "Reference(SubstanceNucleicAcid)", "Data items specific to nucleic acids.", 0, 1, nucleicAcid)); 6489 children.add(new Property("polymer", "Reference(SubstancePolymer)", "Data items specific to polymers.", 0, 1, polymer)); 6490 children.add(new Property("protein", "Reference(SubstanceProtein)", "Data items specific to proteins.", 0, 1, protein)); 6491 children.add(new Property("sourceMaterial", "", "Material or taxonomic/anatomical source for the substance.", 0, 1, sourceMaterial)); 6492 } 6493 6494 @Override 6495 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6496 switch (_hash) { 6497 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier by which this substance is known.", 0, java.lang.Integer.MAX_VALUE, identifier); 6498 case 351608024: /*version*/ return new Property("version", "string", "A business level version identifier of the substance.", 0, 1, version); 6499 case -892481550: /*status*/ return new Property("status", "CodeableConcept", "Status of substance within the catalogue e.g. active, retired.", 0, 1, status); 6500 case 382350310: /*classification*/ return new Property("classification", "CodeableConcept", "A high level categorization, e.g. polymer or nucleic acid, or food, chemical, biological, or a lower level such as the general types of polymer (linear or branch chain) or type of impurity (process related or contaminant).", 0, java.lang.Integer.MAX_VALUE, classification); 6501 case -1326197564: /*domain*/ return new Property("domain", "CodeableConcept", "If the substance applies to human or veterinary use.", 0, 1, domain); 6502 case 98615255: /*grade*/ return new Property("grade", "CodeableConcept", "The quality standard, established benchmark, to which substance complies (e.g. USP/NF, Ph. Eur, JP, BP, Company Standard).", 0, java.lang.Integer.MAX_VALUE, grade); 6503 case -1724546052: /*description*/ return new Property("description", "markdown", "Textual description of the substance.", 0, 1, description); 6504 case -2123220889: /*informationSource*/ return new Property("informationSource", "Reference(Citation)", "Supporting literature.", 0, java.lang.Integer.MAX_VALUE, informationSource); 6505 case 3387378: /*note*/ return new Property("note", "Annotation", "Textual comment about the substance's catalogue or registry record.", 0, java.lang.Integer.MAX_VALUE, note); 6506 case -1969347631: /*manufacturer*/ return new Property("manufacturer", "Reference(Organization)", "The entity that creates, makes, produces or fabricates the substance. This is a set of potential manufacturers but is not necessarily comprehensive.", 0, java.lang.Integer.MAX_VALUE, manufacturer); 6507 case -1663305268: /*supplier*/ return new Property("supplier", "Reference(Organization)", "An entity that is the source for the substance. It may be different from the manufacturer. Supplier is synonymous to a distributor.", 0, java.lang.Integer.MAX_VALUE, supplier); 6508 case -1068650173: /*moiety*/ return new Property("moiety", "", "Moiety, for structural modifications.", 0, java.lang.Integer.MAX_VALUE, moiety); 6509 case 23517467: /*characterization*/ return new Property("characterization", "", "General specifications for this substance.", 0, java.lang.Integer.MAX_VALUE, characterization); 6510 case -993141291: /*property*/ return new Property("property", "", "General specifications for this substance.", 0, java.lang.Integer.MAX_VALUE, property); 6511 case -2117930783: /*referenceInformation*/ return new Property("referenceInformation", "Reference(SubstanceReferenceInformation)", "General information detailing this substance.", 0, 1, referenceInformation); 6512 case 635625672: /*molecularWeight*/ return new Property("molecularWeight", "", "The average mass of a molecule of a compound compared to 1/12 the mass of carbon 12 and calculated as the sum of the atomic weights of the constituent atoms.", 0, java.lang.Integer.MAX_VALUE, molecularWeight); 6513 case 144518515: /*structure*/ return new Property("structure", "", "Structural information.", 0, 1, structure); 6514 case 3059181: /*code*/ return new Property("code", "", "Codes associated with the substance.", 0, java.lang.Integer.MAX_VALUE, code); 6515 case 3373707: /*name*/ return new Property("name", "", "Names applicable to this substance.", 0, java.lang.Integer.MAX_VALUE, name); 6516 case -261851592: /*relationship*/ return new Property("relationship", "", "A link between this substance and another, with details of the relationship.", 0, java.lang.Integer.MAX_VALUE, relationship); 6517 case 1625275180: /*nucleicAcid*/ return new Property("nucleicAcid", "Reference(SubstanceNucleicAcid)", "Data items specific to nucleic acids.", 0, 1, nucleicAcid); 6518 case -397514098: /*polymer*/ return new Property("polymer", "Reference(SubstancePolymer)", "Data items specific to polymers.", 0, 1, polymer); 6519 case -309012605: /*protein*/ return new Property("protein", "Reference(SubstanceProtein)", "Data items specific to proteins.", 0, 1, protein); 6520 case -1064442270: /*sourceMaterial*/ return new Property("sourceMaterial", "", "Material or taxonomic/anatomical source for the substance.", 0, 1, sourceMaterial); 6521 default: return super.getNamedProperty(_hash, _name, _checkValid); 6522 } 6523 6524 } 6525 6526 @Override 6527 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6528 switch (hash) { 6529 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 6530 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 6531 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // CodeableConcept 6532 case 382350310: /*classification*/ return this.classification == null ? new Base[0] : this.classification.toArray(new Base[this.classification.size()]); // CodeableConcept 6533 case -1326197564: /*domain*/ return this.domain == null ? new Base[0] : new Base[] {this.domain}; // CodeableConcept 6534 case 98615255: /*grade*/ return this.grade == null ? new Base[0] : this.grade.toArray(new Base[this.grade.size()]); // CodeableConcept 6535 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 6536 case -2123220889: /*informationSource*/ return this.informationSource == null ? new Base[0] : this.informationSource.toArray(new Base[this.informationSource.size()]); // Reference 6537 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 6538 case -1969347631: /*manufacturer*/ return this.manufacturer == null ? new Base[0] : this.manufacturer.toArray(new Base[this.manufacturer.size()]); // Reference 6539 case -1663305268: /*supplier*/ return this.supplier == null ? new Base[0] : this.supplier.toArray(new Base[this.supplier.size()]); // Reference 6540 case -1068650173: /*moiety*/ return this.moiety == null ? new Base[0] : this.moiety.toArray(new Base[this.moiety.size()]); // SubstanceDefinitionMoietyComponent 6541 case 23517467: /*characterization*/ return this.characterization == null ? new Base[0] : this.characterization.toArray(new Base[this.characterization.size()]); // SubstanceDefinitionCharacterizationComponent 6542 case -993141291: /*property*/ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // SubstanceDefinitionPropertyComponent 6543 case -2117930783: /*referenceInformation*/ return this.referenceInformation == null ? new Base[0] : new Base[] {this.referenceInformation}; // Reference 6544 case 635625672: /*molecularWeight*/ return this.molecularWeight == null ? new Base[0] : this.molecularWeight.toArray(new Base[this.molecularWeight.size()]); // SubstanceDefinitionMolecularWeightComponent 6545 case 144518515: /*structure*/ return this.structure == null ? new Base[0] : new Base[] {this.structure}; // SubstanceDefinitionStructureComponent 6546 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // SubstanceDefinitionCodeComponent 6547 case 3373707: /*name*/ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // SubstanceDefinitionNameComponent 6548 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : this.relationship.toArray(new Base[this.relationship.size()]); // SubstanceDefinitionRelationshipComponent 6549 case 1625275180: /*nucleicAcid*/ return this.nucleicAcid == null ? new Base[0] : new Base[] {this.nucleicAcid}; // Reference 6550 case -397514098: /*polymer*/ return this.polymer == null ? new Base[0] : new Base[] {this.polymer}; // Reference 6551 case -309012605: /*protein*/ return this.protein == null ? new Base[0] : new Base[] {this.protein}; // Reference 6552 case -1064442270: /*sourceMaterial*/ return this.sourceMaterial == null ? new Base[0] : new Base[] {this.sourceMaterial}; // SubstanceDefinitionSourceMaterialComponent 6553 default: return super.getProperty(hash, name, checkValid); 6554 } 6555 6556 } 6557 6558 @Override 6559 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6560 switch (hash) { 6561 case -1618432855: // identifier 6562 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 6563 return value; 6564 case 351608024: // version 6565 this.version = TypeConvertor.castToString(value); // StringType 6566 return value; 6567 case -892481550: // status 6568 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6569 return value; 6570 case 382350310: // classification 6571 this.getClassification().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 6572 return value; 6573 case -1326197564: // domain 6574 this.domain = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6575 return value; 6576 case 98615255: // grade 6577 this.getGrade().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 6578 return value; 6579 case -1724546052: // description 6580 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 6581 return value; 6582 case -2123220889: // informationSource 6583 this.getInformationSource().add(TypeConvertor.castToReference(value)); // Reference 6584 return value; 6585 case 3387378: // note 6586 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 6587 return value; 6588 case -1969347631: // manufacturer 6589 this.getManufacturer().add(TypeConvertor.castToReference(value)); // Reference 6590 return value; 6591 case -1663305268: // supplier 6592 this.getSupplier().add(TypeConvertor.castToReference(value)); // Reference 6593 return value; 6594 case -1068650173: // moiety 6595 this.getMoiety().add((SubstanceDefinitionMoietyComponent) value); // SubstanceDefinitionMoietyComponent 6596 return value; 6597 case 23517467: // characterization 6598 this.getCharacterization().add((SubstanceDefinitionCharacterizationComponent) value); // SubstanceDefinitionCharacterizationComponent 6599 return value; 6600 case -993141291: // property 6601 this.getProperty().add((SubstanceDefinitionPropertyComponent) value); // SubstanceDefinitionPropertyComponent 6602 return value; 6603 case -2117930783: // referenceInformation 6604 this.referenceInformation = TypeConvertor.castToReference(value); // Reference 6605 return value; 6606 case 635625672: // molecularWeight 6607 this.getMolecularWeight().add((SubstanceDefinitionMolecularWeightComponent) value); // SubstanceDefinitionMolecularWeightComponent 6608 return value; 6609 case 144518515: // structure 6610 this.structure = (SubstanceDefinitionStructureComponent) value; // SubstanceDefinitionStructureComponent 6611 return value; 6612 case 3059181: // code 6613 this.getCode().add((SubstanceDefinitionCodeComponent) value); // SubstanceDefinitionCodeComponent 6614 return value; 6615 case 3373707: // name 6616 this.getName().add((SubstanceDefinitionNameComponent) value); // SubstanceDefinitionNameComponent 6617 return value; 6618 case -261851592: // relationship 6619 this.getRelationship().add((SubstanceDefinitionRelationshipComponent) value); // SubstanceDefinitionRelationshipComponent 6620 return value; 6621 case 1625275180: // nucleicAcid 6622 this.nucleicAcid = TypeConvertor.castToReference(value); // Reference 6623 return value; 6624 case -397514098: // polymer 6625 this.polymer = TypeConvertor.castToReference(value); // Reference 6626 return value; 6627 case -309012605: // protein 6628 this.protein = TypeConvertor.castToReference(value); // Reference 6629 return value; 6630 case -1064442270: // sourceMaterial 6631 this.sourceMaterial = (SubstanceDefinitionSourceMaterialComponent) value; // SubstanceDefinitionSourceMaterialComponent 6632 return value; 6633 default: return super.setProperty(hash, name, value); 6634 } 6635 6636 } 6637 6638 @Override 6639 public Base setProperty(String name, Base value) throws FHIRException { 6640 if (name.equals("identifier")) { 6641 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 6642 } else if (name.equals("version")) { 6643 this.version = TypeConvertor.castToString(value); // StringType 6644 } else if (name.equals("status")) { 6645 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6646 } else if (name.equals("classification")) { 6647 this.getClassification().add(TypeConvertor.castToCodeableConcept(value)); 6648 } else if (name.equals("domain")) { 6649 this.domain = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6650 } else if (name.equals("grade")) { 6651 this.getGrade().add(TypeConvertor.castToCodeableConcept(value)); 6652 } else if (name.equals("description")) { 6653 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 6654 } else if (name.equals("informationSource")) { 6655 this.getInformationSource().add(TypeConvertor.castToReference(value)); 6656 } else if (name.equals("note")) { 6657 this.getNote().add(TypeConvertor.castToAnnotation(value)); 6658 } else if (name.equals("manufacturer")) { 6659 this.getManufacturer().add(TypeConvertor.castToReference(value)); 6660 } else if (name.equals("supplier")) { 6661 this.getSupplier().add(TypeConvertor.castToReference(value)); 6662 } else if (name.equals("moiety")) { 6663 this.getMoiety().add((SubstanceDefinitionMoietyComponent) value); 6664 } else if (name.equals("characterization")) { 6665 this.getCharacterization().add((SubstanceDefinitionCharacterizationComponent) value); 6666 } else if (name.equals("property")) { 6667 this.getProperty().add((SubstanceDefinitionPropertyComponent) value); 6668 } else if (name.equals("referenceInformation")) { 6669 this.referenceInformation = TypeConvertor.castToReference(value); // Reference 6670 } else if (name.equals("molecularWeight")) { 6671 this.getMolecularWeight().add((SubstanceDefinitionMolecularWeightComponent) value); 6672 } else if (name.equals("structure")) { 6673 this.structure = (SubstanceDefinitionStructureComponent) value; // SubstanceDefinitionStructureComponent 6674 } else if (name.equals("code")) { 6675 this.getCode().add((SubstanceDefinitionCodeComponent) value); 6676 } else if (name.equals("name")) { 6677 this.getName().add((SubstanceDefinitionNameComponent) value); 6678 } else if (name.equals("relationship")) { 6679 this.getRelationship().add((SubstanceDefinitionRelationshipComponent) value); 6680 } else if (name.equals("nucleicAcid")) { 6681 this.nucleicAcid = TypeConvertor.castToReference(value); // Reference 6682 } else if (name.equals("polymer")) { 6683 this.polymer = TypeConvertor.castToReference(value); // Reference 6684 } else if (name.equals("protein")) { 6685 this.protein = TypeConvertor.castToReference(value); // Reference 6686 } else if (name.equals("sourceMaterial")) { 6687 this.sourceMaterial = (SubstanceDefinitionSourceMaterialComponent) value; // SubstanceDefinitionSourceMaterialComponent 6688 } else 6689 return super.setProperty(name, value); 6690 return value; 6691 } 6692 6693 @Override 6694 public void removeChild(String name, Base value) throws FHIRException { 6695 if (name.equals("identifier")) { 6696 this.getIdentifier().remove(value); 6697 } else if (name.equals("version")) { 6698 this.version = null; 6699 } else if (name.equals("status")) { 6700 this.status = null; 6701 } else if (name.equals("classification")) { 6702 this.getClassification().remove(value); 6703 } else if (name.equals("domain")) { 6704 this.domain = null; 6705 } else if (name.equals("grade")) { 6706 this.getGrade().remove(value); 6707 } else if (name.equals("description")) { 6708 this.description = null; 6709 } else if (name.equals("informationSource")) { 6710 this.getInformationSource().remove(value); 6711 } else if (name.equals("note")) { 6712 this.getNote().remove(value); 6713 } else if (name.equals("manufacturer")) { 6714 this.getManufacturer().remove(value); 6715 } else if (name.equals("supplier")) { 6716 this.getSupplier().remove(value); 6717 } else if (name.equals("moiety")) { 6718 this.getMoiety().remove((SubstanceDefinitionMoietyComponent) value); 6719 } else if (name.equals("characterization")) { 6720 this.getCharacterization().remove((SubstanceDefinitionCharacterizationComponent) value); 6721 } else if (name.equals("property")) { 6722 this.getProperty().remove((SubstanceDefinitionPropertyComponent) value); 6723 } else if (name.equals("referenceInformation")) { 6724 this.referenceInformation = null; 6725 } else if (name.equals("molecularWeight")) { 6726 this.getMolecularWeight().remove((SubstanceDefinitionMolecularWeightComponent) value); 6727 } else if (name.equals("structure")) { 6728 this.structure = (SubstanceDefinitionStructureComponent) value; // SubstanceDefinitionStructureComponent 6729 } else if (name.equals("code")) { 6730 this.getCode().remove((SubstanceDefinitionCodeComponent) value); 6731 } else if (name.equals("name")) { 6732 this.getName().remove((SubstanceDefinitionNameComponent) value); 6733 } else if (name.equals("relationship")) { 6734 this.getRelationship().remove((SubstanceDefinitionRelationshipComponent) value); 6735 } else if (name.equals("nucleicAcid")) { 6736 this.nucleicAcid = null; 6737 } else if (name.equals("polymer")) { 6738 this.polymer = null; 6739 } else if (name.equals("protein")) { 6740 this.protein = null; 6741 } else if (name.equals("sourceMaterial")) { 6742 this.sourceMaterial = (SubstanceDefinitionSourceMaterialComponent) value; // SubstanceDefinitionSourceMaterialComponent 6743 } else 6744 super.removeChild(name, value); 6745 6746 } 6747 6748 @Override 6749 public Base makeProperty(int hash, String name) throws FHIRException { 6750 switch (hash) { 6751 case -1618432855: return addIdentifier(); 6752 case 351608024: return getVersionElement(); 6753 case -892481550: return getStatus(); 6754 case 382350310: return addClassification(); 6755 case -1326197564: return getDomain(); 6756 case 98615255: return addGrade(); 6757 case -1724546052: return getDescriptionElement(); 6758 case -2123220889: return addInformationSource(); 6759 case 3387378: return addNote(); 6760 case -1969347631: return addManufacturer(); 6761 case -1663305268: return addSupplier(); 6762 case -1068650173: return addMoiety(); 6763 case 23517467: return addCharacterization(); 6764 case -993141291: return addProperty(); 6765 case -2117930783: return getReferenceInformation(); 6766 case 635625672: return addMolecularWeight(); 6767 case 144518515: return getStructure(); 6768 case 3059181: return addCode(); 6769 case 3373707: return addName(); 6770 case -261851592: return addRelationship(); 6771 case 1625275180: return getNucleicAcid(); 6772 case -397514098: return getPolymer(); 6773 case -309012605: return getProtein(); 6774 case -1064442270: return getSourceMaterial(); 6775 default: return super.makeProperty(hash, name); 6776 } 6777 6778 } 6779 6780 @Override 6781 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6782 switch (hash) { 6783 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 6784 case 351608024: /*version*/ return new String[] {"string"}; 6785 case -892481550: /*status*/ return new String[] {"CodeableConcept"}; 6786 case 382350310: /*classification*/ return new String[] {"CodeableConcept"}; 6787 case -1326197564: /*domain*/ return new String[] {"CodeableConcept"}; 6788 case 98615255: /*grade*/ return new String[] {"CodeableConcept"}; 6789 case -1724546052: /*description*/ return new String[] {"markdown"}; 6790 case -2123220889: /*informationSource*/ return new String[] {"Reference"}; 6791 case 3387378: /*note*/ return new String[] {"Annotation"}; 6792 case -1969347631: /*manufacturer*/ return new String[] {"Reference"}; 6793 case -1663305268: /*supplier*/ return new String[] {"Reference"}; 6794 case -1068650173: /*moiety*/ return new String[] {}; 6795 case 23517467: /*characterization*/ return new String[] {}; 6796 case -993141291: /*property*/ return new String[] {}; 6797 case -2117930783: /*referenceInformation*/ return new String[] {"Reference"}; 6798 case 635625672: /*molecularWeight*/ return new String[] {}; 6799 case 144518515: /*structure*/ return new String[] {}; 6800 case 3059181: /*code*/ return new String[] {}; 6801 case 3373707: /*name*/ return new String[] {}; 6802 case -261851592: /*relationship*/ return new String[] {}; 6803 case 1625275180: /*nucleicAcid*/ return new String[] {"Reference"}; 6804 case -397514098: /*polymer*/ return new String[] {"Reference"}; 6805 case -309012605: /*protein*/ return new String[] {"Reference"}; 6806 case -1064442270: /*sourceMaterial*/ return new String[] {}; 6807 default: return super.getTypesForProperty(hash, name); 6808 } 6809 6810 } 6811 6812 @Override 6813 public Base addChild(String name) throws FHIRException { 6814 if (name.equals("identifier")) { 6815 return addIdentifier(); 6816 } 6817 else if (name.equals("version")) { 6818 throw new FHIRException("Cannot call addChild on a singleton property SubstanceDefinition.version"); 6819 } 6820 else if (name.equals("status")) { 6821 this.status = new CodeableConcept(); 6822 return this.status; 6823 } 6824 else if (name.equals("classification")) { 6825 return addClassification(); 6826 } 6827 else if (name.equals("domain")) { 6828 this.domain = new CodeableConcept(); 6829 return this.domain; 6830 } 6831 else if (name.equals("grade")) { 6832 return addGrade(); 6833 } 6834 else if (name.equals("description")) { 6835 throw new FHIRException("Cannot call addChild on a singleton property SubstanceDefinition.description"); 6836 } 6837 else if (name.equals("informationSource")) { 6838 return addInformationSource(); 6839 } 6840 else if (name.equals("note")) { 6841 return addNote(); 6842 } 6843 else if (name.equals("manufacturer")) { 6844 return addManufacturer(); 6845 } 6846 else if (name.equals("supplier")) { 6847 return addSupplier(); 6848 } 6849 else if (name.equals("moiety")) { 6850 return addMoiety(); 6851 } 6852 else if (name.equals("characterization")) { 6853 return addCharacterization(); 6854 } 6855 else if (name.equals("property")) { 6856 return addProperty(); 6857 } 6858 else if (name.equals("referenceInformation")) { 6859 this.referenceInformation = new Reference(); 6860 return this.referenceInformation; 6861 } 6862 else if (name.equals("molecularWeight")) { 6863 return addMolecularWeight(); 6864 } 6865 else if (name.equals("structure")) { 6866 this.structure = new SubstanceDefinitionStructureComponent(); 6867 return this.structure; 6868 } 6869 else if (name.equals("code")) { 6870 return addCode(); 6871 } 6872 else if (name.equals("name")) { 6873 return addName(); 6874 } 6875 else if (name.equals("relationship")) { 6876 return addRelationship(); 6877 } 6878 else if (name.equals("nucleicAcid")) { 6879 this.nucleicAcid = new Reference(); 6880 return this.nucleicAcid; 6881 } 6882 else if (name.equals("polymer")) { 6883 this.polymer = new Reference(); 6884 return this.polymer; 6885 } 6886 else if (name.equals("protein")) { 6887 this.protein = new Reference(); 6888 return this.protein; 6889 } 6890 else if (name.equals("sourceMaterial")) { 6891 this.sourceMaterial = new SubstanceDefinitionSourceMaterialComponent(); 6892 return this.sourceMaterial; 6893 } 6894 else 6895 return super.addChild(name); 6896 } 6897 6898 public String fhirType() { 6899 return "SubstanceDefinition"; 6900 6901 } 6902 6903 public SubstanceDefinition copy() { 6904 SubstanceDefinition dst = new SubstanceDefinition(); 6905 copyValues(dst); 6906 return dst; 6907 } 6908 6909 public void copyValues(SubstanceDefinition dst) { 6910 super.copyValues(dst); 6911 if (identifier != null) { 6912 dst.identifier = new ArrayList<Identifier>(); 6913 for (Identifier i : identifier) 6914 dst.identifier.add(i.copy()); 6915 }; 6916 dst.version = version == null ? null : version.copy(); 6917 dst.status = status == null ? null : status.copy(); 6918 if (classification != null) { 6919 dst.classification = new ArrayList<CodeableConcept>(); 6920 for (CodeableConcept i : classification) 6921 dst.classification.add(i.copy()); 6922 }; 6923 dst.domain = domain == null ? null : domain.copy(); 6924 if (grade != null) { 6925 dst.grade = new ArrayList<CodeableConcept>(); 6926 for (CodeableConcept i : grade) 6927 dst.grade.add(i.copy()); 6928 }; 6929 dst.description = description == null ? null : description.copy(); 6930 if (informationSource != null) { 6931 dst.informationSource = new ArrayList<Reference>(); 6932 for (Reference i : informationSource) 6933 dst.informationSource.add(i.copy()); 6934 }; 6935 if (note != null) { 6936 dst.note = new ArrayList<Annotation>(); 6937 for (Annotation i : note) 6938 dst.note.add(i.copy()); 6939 }; 6940 if (manufacturer != null) { 6941 dst.manufacturer = new ArrayList<Reference>(); 6942 for (Reference i : manufacturer) 6943 dst.manufacturer.add(i.copy()); 6944 }; 6945 if (supplier != null) { 6946 dst.supplier = new ArrayList<Reference>(); 6947 for (Reference i : supplier) 6948 dst.supplier.add(i.copy()); 6949 }; 6950 if (moiety != null) { 6951 dst.moiety = new ArrayList<SubstanceDefinitionMoietyComponent>(); 6952 for (SubstanceDefinitionMoietyComponent i : moiety) 6953 dst.moiety.add(i.copy()); 6954 }; 6955 if (characterization != null) { 6956 dst.characterization = new ArrayList<SubstanceDefinitionCharacterizationComponent>(); 6957 for (SubstanceDefinitionCharacterizationComponent i : characterization) 6958 dst.characterization.add(i.copy()); 6959 }; 6960 if (property != null) { 6961 dst.property = new ArrayList<SubstanceDefinitionPropertyComponent>(); 6962 for (SubstanceDefinitionPropertyComponent i : property) 6963 dst.property.add(i.copy()); 6964 }; 6965 dst.referenceInformation = referenceInformation == null ? null : referenceInformation.copy(); 6966 if (molecularWeight != null) { 6967 dst.molecularWeight = new ArrayList<SubstanceDefinitionMolecularWeightComponent>(); 6968 for (SubstanceDefinitionMolecularWeightComponent i : molecularWeight) 6969 dst.molecularWeight.add(i.copy()); 6970 }; 6971 dst.structure = structure == null ? null : structure.copy(); 6972 if (code != null) { 6973 dst.code = new ArrayList<SubstanceDefinitionCodeComponent>(); 6974 for (SubstanceDefinitionCodeComponent i : code) 6975 dst.code.add(i.copy()); 6976 }; 6977 if (name != null) { 6978 dst.name = new ArrayList<SubstanceDefinitionNameComponent>(); 6979 for (SubstanceDefinitionNameComponent i : name) 6980 dst.name.add(i.copy()); 6981 }; 6982 if (relationship != null) { 6983 dst.relationship = new ArrayList<SubstanceDefinitionRelationshipComponent>(); 6984 for (SubstanceDefinitionRelationshipComponent i : relationship) 6985 dst.relationship.add(i.copy()); 6986 }; 6987 dst.nucleicAcid = nucleicAcid == null ? null : nucleicAcid.copy(); 6988 dst.polymer = polymer == null ? null : polymer.copy(); 6989 dst.protein = protein == null ? null : protein.copy(); 6990 dst.sourceMaterial = sourceMaterial == null ? null : sourceMaterial.copy(); 6991 } 6992 6993 protected SubstanceDefinition typedCopy() { 6994 return copy(); 6995 } 6996 6997 @Override 6998 public boolean equalsDeep(Base other_) { 6999 if (!super.equalsDeep(other_)) 7000 return false; 7001 if (!(other_ instanceof SubstanceDefinition)) 7002 return false; 7003 SubstanceDefinition o = (SubstanceDefinition) other_; 7004 return compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) && compareDeep(status, o.status, true) 7005 && compareDeep(classification, o.classification, true) && compareDeep(domain, o.domain, true) && compareDeep(grade, o.grade, true) 7006 && compareDeep(description, o.description, true) && compareDeep(informationSource, o.informationSource, true) 7007 && compareDeep(note, o.note, true) && compareDeep(manufacturer, o.manufacturer, true) && compareDeep(supplier, o.supplier, true) 7008 && compareDeep(moiety, o.moiety, true) && compareDeep(characterization, o.characterization, true) 7009 && compareDeep(property, o.property, true) && compareDeep(referenceInformation, o.referenceInformation, true) 7010 && compareDeep(molecularWeight, o.molecularWeight, true) && compareDeep(structure, o.structure, true) 7011 && compareDeep(code, o.code, true) && compareDeep(name, o.name, true) && compareDeep(relationship, o.relationship, true) 7012 && compareDeep(nucleicAcid, o.nucleicAcid, true) && compareDeep(polymer, o.polymer, true) && compareDeep(protein, o.protein, true) 7013 && compareDeep(sourceMaterial, o.sourceMaterial, true); 7014 } 7015 7016 @Override 7017 public boolean equalsShallow(Base other_) { 7018 if (!super.equalsShallow(other_)) 7019 return false; 7020 if (!(other_ instanceof SubstanceDefinition)) 7021 return false; 7022 SubstanceDefinition o = (SubstanceDefinition) other_; 7023 return compareValues(version, o.version, true) && compareValues(description, o.description, true); 7024 } 7025 7026 public boolean isEmpty() { 7027 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, version, status 7028 , classification, domain, grade, description, informationSource, note, manufacturer 7029 , supplier, moiety, characterization, property, referenceInformation, molecularWeight 7030 , structure, code, name, relationship, nucleicAcid, polymer, protein, sourceMaterial 7031 ); 7032 } 7033 7034 @Override 7035 public ResourceType getResourceType() { 7036 return ResourceType.SubstanceDefinition; 7037 } 7038 7039 /** 7040 * Search parameter: <b>classification</b> 7041 * <p> 7042 * Description: <b>High or low level categorization, e.g. polymer vs. nucleic acid or linear vs. branch chain</b><br> 7043 * Type: <b>token</b><br> 7044 * Path: <b>SubstanceDefinition.classification</b><br> 7045 * </p> 7046 */ 7047 @SearchParamDefinition(name="classification", path="SubstanceDefinition.classification", description="High or low level categorization, e.g. polymer vs. nucleic acid or linear vs. branch chain", type="token" ) 7048 public static final String SP_CLASSIFICATION = "classification"; 7049 /** 7050 * <b>Fluent Client</b> search parameter constant for <b>classification</b> 7051 * <p> 7052 * Description: <b>High or low level categorization, e.g. polymer vs. nucleic acid or linear vs. branch chain</b><br> 7053 * Type: <b>token</b><br> 7054 * Path: <b>SubstanceDefinition.classification</b><br> 7055 * </p> 7056 */ 7057 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASSIFICATION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CLASSIFICATION); 7058 7059 /** 7060 * Search parameter: <b>code</b> 7061 * <p> 7062 * Description: <b>The specific code</b><br> 7063 * Type: <b>token</b><br> 7064 * Path: <b>SubstanceDefinition.code.code</b><br> 7065 * </p> 7066 */ 7067 @SearchParamDefinition(name="code", path="SubstanceDefinition.code.code", description="The specific code", type="token" ) 7068 public static final String SP_CODE = "code"; 7069 /** 7070 * <b>Fluent Client</b> search parameter constant for <b>code</b> 7071 * <p> 7072 * Description: <b>The specific code</b><br> 7073 * Type: <b>token</b><br> 7074 * Path: <b>SubstanceDefinition.code.code</b><br> 7075 * </p> 7076 */ 7077 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 7078 7079 /** 7080 * Search parameter: <b>domain</b> 7081 * <p> 7082 * Description: <b>If the substance applies to only human or veterinary use</b><br> 7083 * Type: <b>token</b><br> 7084 * Path: <b>SubstanceDefinition.domain</b><br> 7085 * </p> 7086 */ 7087 @SearchParamDefinition(name="domain", path="SubstanceDefinition.domain", description="If the substance applies to only human or veterinary use", type="token" ) 7088 public static final String SP_DOMAIN = "domain"; 7089 /** 7090 * <b>Fluent Client</b> search parameter constant for <b>domain</b> 7091 * <p> 7092 * Description: <b>If the substance applies to only human or veterinary use</b><br> 7093 * Type: <b>token</b><br> 7094 * Path: <b>SubstanceDefinition.domain</b><br> 7095 * </p> 7096 */ 7097 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DOMAIN = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DOMAIN); 7098 7099 /** 7100 * Search parameter: <b>identifier</b> 7101 * <p> 7102 * Description: <b>Identifier by which this substance is known</b><br> 7103 * Type: <b>token</b><br> 7104 * Path: <b>SubstanceDefinition.identifier</b><br> 7105 * </p> 7106 */ 7107 @SearchParamDefinition(name="identifier", path="SubstanceDefinition.identifier", description="Identifier by which this substance is known", type="token" ) 7108 public static final String SP_IDENTIFIER = "identifier"; 7109 /** 7110 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 7111 * <p> 7112 * Description: <b>Identifier by which this substance is known</b><br> 7113 * Type: <b>token</b><br> 7114 * Path: <b>SubstanceDefinition.identifier</b><br> 7115 * </p> 7116 */ 7117 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 7118 7119 /** 7120 * Search parameter: <b>name</b> 7121 * <p> 7122 * Description: <b>The actual name</b><br> 7123 * Type: <b>string</b><br> 7124 * Path: <b>SubstanceDefinition.name.name</b><br> 7125 * </p> 7126 */ 7127 @SearchParamDefinition(name="name", path="SubstanceDefinition.name.name", description="The actual name", type="string" ) 7128 public static final String SP_NAME = "name"; 7129 /** 7130 * <b>Fluent Client</b> search parameter constant for <b>name</b> 7131 * <p> 7132 * Description: <b>The actual name</b><br> 7133 * Type: <b>string</b><br> 7134 * Path: <b>SubstanceDefinition.name.name</b><br> 7135 * </p> 7136 */ 7137 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 7138 7139 7140} 7141