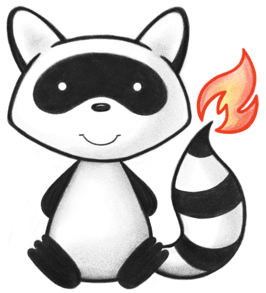
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Nucleic acids are defined by three distinct elements: the base, sugar and linkage. Individual substance/moiety IDs will be created for each of these elements. The nucleotide sequence will be always entered in the 5?-3? direction. 052 */ 053@ResourceDef(name="SubstanceNucleicAcid", profile="http://hl7.org/fhir/StructureDefinition/SubstanceNucleicAcid") 054public class SubstanceNucleicAcid extends DomainResource { 055 056 @Block() 057 public static class SubstanceNucleicAcidSubunitComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Index of linear sequences of nucleic acids in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts. 060 */ 061 @Child(name = "subunit", type = {IntegerType.class}, order=1, min=0, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="Index of linear sequences of nucleic acids in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts", formalDefinition="Index of linear sequences of nucleic acids in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts." ) 063 protected IntegerType subunit; 064 065 /** 066 * Actual nucleotide sequence notation from 5' to 3' end using standard single letter codes. In addition to the base sequence, sugar and type of phosphate or non-phosphate linkage should also be captured. 067 */ 068 @Child(name = "sequence", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 069 @Description(shortDefinition="Actual nucleotide sequence notation from 5' to 3' end using standard single letter codes. In addition to the base sequence, sugar and type of phosphate or non-phosphate linkage should also be captured", formalDefinition="Actual nucleotide sequence notation from 5' to 3' end using standard single letter codes. In addition to the base sequence, sugar and type of phosphate or non-phosphate linkage should also be captured." ) 070 protected StringType sequence; 071 072 /** 073 * The length of the sequence shall be captured. 074 */ 075 @Child(name = "length", type = {IntegerType.class}, order=3, min=0, max=1, modifier=false, summary=true) 076 @Description(shortDefinition="The length of the sequence shall be captured", formalDefinition="The length of the sequence shall be captured." ) 077 protected IntegerType length; 078 079 /** 080 * (TBC). 081 */ 082 @Child(name = "sequenceAttachment", type = {Attachment.class}, order=4, min=0, max=1, modifier=false, summary=true) 083 @Description(shortDefinition="(TBC)", formalDefinition="(TBC)." ) 084 protected Attachment sequenceAttachment; 085 086 /** 087 * The nucleotide present at the 5? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the first position in the sequence. A separate representation would be redundant. 088 */ 089 @Child(name = "fivePrime", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 090 @Description(shortDefinition="The nucleotide present at the 5? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the first position in the sequence. A separate representation would be redundant", formalDefinition="The nucleotide present at the 5? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the first position in the sequence. A separate representation would be redundant." ) 091 protected CodeableConcept fivePrime; 092 093 /** 094 * The nucleotide present at the 3? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the last position in the sequence. A separate representation would be redundant. 095 */ 096 @Child(name = "threePrime", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=true) 097 @Description(shortDefinition="The nucleotide present at the 3? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the last position in the sequence. A separate representation would be redundant", formalDefinition="The nucleotide present at the 3? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the last position in the sequence. A separate representation would be redundant." ) 098 protected CodeableConcept threePrime; 099 100 /** 101 * The linkages between sugar residues will also be captured. 102 */ 103 @Child(name = "linkage", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 104 @Description(shortDefinition="The linkages between sugar residues will also be captured", formalDefinition="The linkages between sugar residues will also be captured." ) 105 protected List<SubstanceNucleicAcidSubunitLinkageComponent> linkage; 106 107 /** 108 * 5.3.6.8.1 Sugar ID (Mandatory). 109 */ 110 @Child(name = "sugar", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 111 @Description(shortDefinition="5.3.6.8.1 Sugar ID (Mandatory)", formalDefinition="5.3.6.8.1 Sugar ID (Mandatory)." ) 112 protected List<SubstanceNucleicAcidSubunitSugarComponent> sugar; 113 114 private static final long serialVersionUID = 1835593659L; 115 116 /** 117 * Constructor 118 */ 119 public SubstanceNucleicAcidSubunitComponent() { 120 super(); 121 } 122 123 /** 124 * @return {@link #subunit} (Index of linear sequences of nucleic acids in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts.). This is the underlying object with id, value and extensions. The accessor "getSubunit" gives direct access to the value 125 */ 126 public IntegerType getSubunitElement() { 127 if (this.subunit == null) 128 if (Configuration.errorOnAutoCreate()) 129 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitComponent.subunit"); 130 else if (Configuration.doAutoCreate()) 131 this.subunit = new IntegerType(); // bb 132 return this.subunit; 133 } 134 135 public boolean hasSubunitElement() { 136 return this.subunit != null && !this.subunit.isEmpty(); 137 } 138 139 public boolean hasSubunit() { 140 return this.subunit != null && !this.subunit.isEmpty(); 141 } 142 143 /** 144 * @param value {@link #subunit} (Index of linear sequences of nucleic acids in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts.). This is the underlying object with id, value and extensions. The accessor "getSubunit" gives direct access to the value 145 */ 146 public SubstanceNucleicAcidSubunitComponent setSubunitElement(IntegerType value) { 147 this.subunit = value; 148 return this; 149 } 150 151 /** 152 * @return Index of linear sequences of nucleic acids in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts. 153 */ 154 public int getSubunit() { 155 return this.subunit == null || this.subunit.isEmpty() ? 0 : this.subunit.getValue(); 156 } 157 158 /** 159 * @param value Index of linear sequences of nucleic acids in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts. 160 */ 161 public SubstanceNucleicAcidSubunitComponent setSubunit(int value) { 162 if (this.subunit == null) 163 this.subunit = new IntegerType(); 164 this.subunit.setValue(value); 165 return this; 166 } 167 168 /** 169 * @return {@link #sequence} (Actual nucleotide sequence notation from 5' to 3' end using standard single letter codes. In addition to the base sequence, sugar and type of phosphate or non-phosphate linkage should also be captured.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 170 */ 171 public StringType getSequenceElement() { 172 if (this.sequence == null) 173 if (Configuration.errorOnAutoCreate()) 174 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitComponent.sequence"); 175 else if (Configuration.doAutoCreate()) 176 this.sequence = new StringType(); // bb 177 return this.sequence; 178 } 179 180 public boolean hasSequenceElement() { 181 return this.sequence != null && !this.sequence.isEmpty(); 182 } 183 184 public boolean hasSequence() { 185 return this.sequence != null && !this.sequence.isEmpty(); 186 } 187 188 /** 189 * @param value {@link #sequence} (Actual nucleotide sequence notation from 5' to 3' end using standard single letter codes. In addition to the base sequence, sugar and type of phosphate or non-phosphate linkage should also be captured.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 190 */ 191 public SubstanceNucleicAcidSubunitComponent setSequenceElement(StringType value) { 192 this.sequence = value; 193 return this; 194 } 195 196 /** 197 * @return Actual nucleotide sequence notation from 5' to 3' end using standard single letter codes. In addition to the base sequence, sugar and type of phosphate or non-phosphate linkage should also be captured. 198 */ 199 public String getSequence() { 200 return this.sequence == null ? null : this.sequence.getValue(); 201 } 202 203 /** 204 * @param value Actual nucleotide sequence notation from 5' to 3' end using standard single letter codes. In addition to the base sequence, sugar and type of phosphate or non-phosphate linkage should also be captured. 205 */ 206 public SubstanceNucleicAcidSubunitComponent setSequence(String value) { 207 if (Utilities.noString(value)) 208 this.sequence = null; 209 else { 210 if (this.sequence == null) 211 this.sequence = new StringType(); 212 this.sequence.setValue(value); 213 } 214 return this; 215 } 216 217 /** 218 * @return {@link #length} (The length of the sequence shall be captured.). This is the underlying object with id, value and extensions. The accessor "getLength" gives direct access to the value 219 */ 220 public IntegerType getLengthElement() { 221 if (this.length == null) 222 if (Configuration.errorOnAutoCreate()) 223 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitComponent.length"); 224 else if (Configuration.doAutoCreate()) 225 this.length = new IntegerType(); // bb 226 return this.length; 227 } 228 229 public boolean hasLengthElement() { 230 return this.length != null && !this.length.isEmpty(); 231 } 232 233 public boolean hasLength() { 234 return this.length != null && !this.length.isEmpty(); 235 } 236 237 /** 238 * @param value {@link #length} (The length of the sequence shall be captured.). This is the underlying object with id, value and extensions. The accessor "getLength" gives direct access to the value 239 */ 240 public SubstanceNucleicAcidSubunitComponent setLengthElement(IntegerType value) { 241 this.length = value; 242 return this; 243 } 244 245 /** 246 * @return The length of the sequence shall be captured. 247 */ 248 public int getLength() { 249 return this.length == null || this.length.isEmpty() ? 0 : this.length.getValue(); 250 } 251 252 /** 253 * @param value The length of the sequence shall be captured. 254 */ 255 public SubstanceNucleicAcidSubunitComponent setLength(int value) { 256 if (this.length == null) 257 this.length = new IntegerType(); 258 this.length.setValue(value); 259 return this; 260 } 261 262 /** 263 * @return {@link #sequenceAttachment} ((TBC).) 264 */ 265 public Attachment getSequenceAttachment() { 266 if (this.sequenceAttachment == null) 267 if (Configuration.errorOnAutoCreate()) 268 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitComponent.sequenceAttachment"); 269 else if (Configuration.doAutoCreate()) 270 this.sequenceAttachment = new Attachment(); // cc 271 return this.sequenceAttachment; 272 } 273 274 public boolean hasSequenceAttachment() { 275 return this.sequenceAttachment != null && !this.sequenceAttachment.isEmpty(); 276 } 277 278 /** 279 * @param value {@link #sequenceAttachment} ((TBC).) 280 */ 281 public SubstanceNucleicAcidSubunitComponent setSequenceAttachment(Attachment value) { 282 this.sequenceAttachment = value; 283 return this; 284 } 285 286 /** 287 * @return {@link #fivePrime} (The nucleotide present at the 5? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the first position in the sequence. A separate representation would be redundant.) 288 */ 289 public CodeableConcept getFivePrime() { 290 if (this.fivePrime == null) 291 if (Configuration.errorOnAutoCreate()) 292 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitComponent.fivePrime"); 293 else if (Configuration.doAutoCreate()) 294 this.fivePrime = new CodeableConcept(); // cc 295 return this.fivePrime; 296 } 297 298 public boolean hasFivePrime() { 299 return this.fivePrime != null && !this.fivePrime.isEmpty(); 300 } 301 302 /** 303 * @param value {@link #fivePrime} (The nucleotide present at the 5? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the first position in the sequence. A separate representation would be redundant.) 304 */ 305 public SubstanceNucleicAcidSubunitComponent setFivePrime(CodeableConcept value) { 306 this.fivePrime = value; 307 return this; 308 } 309 310 /** 311 * @return {@link #threePrime} (The nucleotide present at the 3? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the last position in the sequence. A separate representation would be redundant.) 312 */ 313 public CodeableConcept getThreePrime() { 314 if (this.threePrime == null) 315 if (Configuration.errorOnAutoCreate()) 316 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitComponent.threePrime"); 317 else if (Configuration.doAutoCreate()) 318 this.threePrime = new CodeableConcept(); // cc 319 return this.threePrime; 320 } 321 322 public boolean hasThreePrime() { 323 return this.threePrime != null && !this.threePrime.isEmpty(); 324 } 325 326 /** 327 * @param value {@link #threePrime} (The nucleotide present at the 3? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the last position in the sequence. A separate representation would be redundant.) 328 */ 329 public SubstanceNucleicAcidSubunitComponent setThreePrime(CodeableConcept value) { 330 this.threePrime = value; 331 return this; 332 } 333 334 /** 335 * @return {@link #linkage} (The linkages between sugar residues will also be captured.) 336 */ 337 public List<SubstanceNucleicAcidSubunitLinkageComponent> getLinkage() { 338 if (this.linkage == null) 339 this.linkage = new ArrayList<SubstanceNucleicAcidSubunitLinkageComponent>(); 340 return this.linkage; 341 } 342 343 /** 344 * @return Returns a reference to <code>this</code> for easy method chaining 345 */ 346 public SubstanceNucleicAcidSubunitComponent setLinkage(List<SubstanceNucleicAcidSubunitLinkageComponent> theLinkage) { 347 this.linkage = theLinkage; 348 return this; 349 } 350 351 public boolean hasLinkage() { 352 if (this.linkage == null) 353 return false; 354 for (SubstanceNucleicAcidSubunitLinkageComponent item : this.linkage) 355 if (!item.isEmpty()) 356 return true; 357 return false; 358 } 359 360 public SubstanceNucleicAcidSubunitLinkageComponent addLinkage() { //3 361 SubstanceNucleicAcidSubunitLinkageComponent t = new SubstanceNucleicAcidSubunitLinkageComponent(); 362 if (this.linkage == null) 363 this.linkage = new ArrayList<SubstanceNucleicAcidSubunitLinkageComponent>(); 364 this.linkage.add(t); 365 return t; 366 } 367 368 public SubstanceNucleicAcidSubunitComponent addLinkage(SubstanceNucleicAcidSubunitLinkageComponent t) { //3 369 if (t == null) 370 return this; 371 if (this.linkage == null) 372 this.linkage = new ArrayList<SubstanceNucleicAcidSubunitLinkageComponent>(); 373 this.linkage.add(t); 374 return this; 375 } 376 377 /** 378 * @return The first repetition of repeating field {@link #linkage}, creating it if it does not already exist {3} 379 */ 380 public SubstanceNucleicAcidSubunitLinkageComponent getLinkageFirstRep() { 381 if (getLinkage().isEmpty()) { 382 addLinkage(); 383 } 384 return getLinkage().get(0); 385 } 386 387 /** 388 * @return {@link #sugar} (5.3.6.8.1 Sugar ID (Mandatory).) 389 */ 390 public List<SubstanceNucleicAcidSubunitSugarComponent> getSugar() { 391 if (this.sugar == null) 392 this.sugar = new ArrayList<SubstanceNucleicAcidSubunitSugarComponent>(); 393 return this.sugar; 394 } 395 396 /** 397 * @return Returns a reference to <code>this</code> for easy method chaining 398 */ 399 public SubstanceNucleicAcidSubunitComponent setSugar(List<SubstanceNucleicAcidSubunitSugarComponent> theSugar) { 400 this.sugar = theSugar; 401 return this; 402 } 403 404 public boolean hasSugar() { 405 if (this.sugar == null) 406 return false; 407 for (SubstanceNucleicAcidSubunitSugarComponent item : this.sugar) 408 if (!item.isEmpty()) 409 return true; 410 return false; 411 } 412 413 public SubstanceNucleicAcidSubunitSugarComponent addSugar() { //3 414 SubstanceNucleicAcidSubunitSugarComponent t = new SubstanceNucleicAcidSubunitSugarComponent(); 415 if (this.sugar == null) 416 this.sugar = new ArrayList<SubstanceNucleicAcidSubunitSugarComponent>(); 417 this.sugar.add(t); 418 return t; 419 } 420 421 public SubstanceNucleicAcidSubunitComponent addSugar(SubstanceNucleicAcidSubunitSugarComponent t) { //3 422 if (t == null) 423 return this; 424 if (this.sugar == null) 425 this.sugar = new ArrayList<SubstanceNucleicAcidSubunitSugarComponent>(); 426 this.sugar.add(t); 427 return this; 428 } 429 430 /** 431 * @return The first repetition of repeating field {@link #sugar}, creating it if it does not already exist {3} 432 */ 433 public SubstanceNucleicAcidSubunitSugarComponent getSugarFirstRep() { 434 if (getSugar().isEmpty()) { 435 addSugar(); 436 } 437 return getSugar().get(0); 438 } 439 440 protected void listChildren(List<Property> children) { 441 super.listChildren(children); 442 children.add(new Property("subunit", "integer", "Index of linear sequences of nucleic acids in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts.", 0, 1, subunit)); 443 children.add(new Property("sequence", "string", "Actual nucleotide sequence notation from 5' to 3' end using standard single letter codes. In addition to the base sequence, sugar and type of phosphate or non-phosphate linkage should also be captured.", 0, 1, sequence)); 444 children.add(new Property("length", "integer", "The length of the sequence shall be captured.", 0, 1, length)); 445 children.add(new Property("sequenceAttachment", "Attachment", "(TBC).", 0, 1, sequenceAttachment)); 446 children.add(new Property("fivePrime", "CodeableConcept", "The nucleotide present at the 5? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the first position in the sequence. A separate representation would be redundant.", 0, 1, fivePrime)); 447 children.add(new Property("threePrime", "CodeableConcept", "The nucleotide present at the 3? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the last position in the sequence. A separate representation would be redundant.", 0, 1, threePrime)); 448 children.add(new Property("linkage", "", "The linkages between sugar residues will also be captured.", 0, java.lang.Integer.MAX_VALUE, linkage)); 449 children.add(new Property("sugar", "", "5.3.6.8.1 Sugar ID (Mandatory).", 0, java.lang.Integer.MAX_VALUE, sugar)); 450 } 451 452 @Override 453 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 454 switch (_hash) { 455 case -1867548732: /*subunit*/ return new Property("subunit", "integer", "Index of linear sequences of nucleic acids in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts.", 0, 1, subunit); 456 case 1349547969: /*sequence*/ return new Property("sequence", "string", "Actual nucleotide sequence notation from 5' to 3' end using standard single letter codes. In addition to the base sequence, sugar and type of phosphate or non-phosphate linkage should also be captured.", 0, 1, sequence); 457 case -1106363674: /*length*/ return new Property("length", "integer", "The length of the sequence shall be captured.", 0, 1, length); 458 case 364621764: /*sequenceAttachment*/ return new Property("sequenceAttachment", "Attachment", "(TBC).", 0, 1, sequenceAttachment); 459 case -1045091603: /*fivePrime*/ return new Property("fivePrime", "CodeableConcept", "The nucleotide present at the 5? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the first position in the sequence. A separate representation would be redundant.", 0, 1, fivePrime); 460 case -1088032895: /*threePrime*/ return new Property("threePrime", "CodeableConcept", "The nucleotide present at the 3? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the last position in the sequence. A separate representation would be redundant.", 0, 1, threePrime); 461 case 177082053: /*linkage*/ return new Property("linkage", "", "The linkages between sugar residues will also be captured.", 0, java.lang.Integer.MAX_VALUE, linkage); 462 case 109792566: /*sugar*/ return new Property("sugar", "", "5.3.6.8.1 Sugar ID (Mandatory).", 0, java.lang.Integer.MAX_VALUE, sugar); 463 default: return super.getNamedProperty(_hash, _name, _checkValid); 464 } 465 466 } 467 468 @Override 469 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 470 switch (hash) { 471 case -1867548732: /*subunit*/ return this.subunit == null ? new Base[0] : new Base[] {this.subunit}; // IntegerType 472 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // StringType 473 case -1106363674: /*length*/ return this.length == null ? new Base[0] : new Base[] {this.length}; // IntegerType 474 case 364621764: /*sequenceAttachment*/ return this.sequenceAttachment == null ? new Base[0] : new Base[] {this.sequenceAttachment}; // Attachment 475 case -1045091603: /*fivePrime*/ return this.fivePrime == null ? new Base[0] : new Base[] {this.fivePrime}; // CodeableConcept 476 case -1088032895: /*threePrime*/ return this.threePrime == null ? new Base[0] : new Base[] {this.threePrime}; // CodeableConcept 477 case 177082053: /*linkage*/ return this.linkage == null ? new Base[0] : this.linkage.toArray(new Base[this.linkage.size()]); // SubstanceNucleicAcidSubunitLinkageComponent 478 case 109792566: /*sugar*/ return this.sugar == null ? new Base[0] : this.sugar.toArray(new Base[this.sugar.size()]); // SubstanceNucleicAcidSubunitSugarComponent 479 default: return super.getProperty(hash, name, checkValid); 480 } 481 482 } 483 484 @Override 485 public Base setProperty(int hash, String name, Base value) throws FHIRException { 486 switch (hash) { 487 case -1867548732: // subunit 488 this.subunit = TypeConvertor.castToInteger(value); // IntegerType 489 return value; 490 case 1349547969: // sequence 491 this.sequence = TypeConvertor.castToString(value); // StringType 492 return value; 493 case -1106363674: // length 494 this.length = TypeConvertor.castToInteger(value); // IntegerType 495 return value; 496 case 364621764: // sequenceAttachment 497 this.sequenceAttachment = TypeConvertor.castToAttachment(value); // Attachment 498 return value; 499 case -1045091603: // fivePrime 500 this.fivePrime = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 501 return value; 502 case -1088032895: // threePrime 503 this.threePrime = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 504 return value; 505 case 177082053: // linkage 506 this.getLinkage().add((SubstanceNucleicAcidSubunitLinkageComponent) value); // SubstanceNucleicAcidSubunitLinkageComponent 507 return value; 508 case 109792566: // sugar 509 this.getSugar().add((SubstanceNucleicAcidSubunitSugarComponent) value); // SubstanceNucleicAcidSubunitSugarComponent 510 return value; 511 default: return super.setProperty(hash, name, value); 512 } 513 514 } 515 516 @Override 517 public Base setProperty(String name, Base value) throws FHIRException { 518 if (name.equals("subunit")) { 519 this.subunit = TypeConvertor.castToInteger(value); // IntegerType 520 } else if (name.equals("sequence")) { 521 this.sequence = TypeConvertor.castToString(value); // StringType 522 } else if (name.equals("length")) { 523 this.length = TypeConvertor.castToInteger(value); // IntegerType 524 } else if (name.equals("sequenceAttachment")) { 525 this.sequenceAttachment = TypeConvertor.castToAttachment(value); // Attachment 526 } else if (name.equals("fivePrime")) { 527 this.fivePrime = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 528 } else if (name.equals("threePrime")) { 529 this.threePrime = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 530 } else if (name.equals("linkage")) { 531 this.getLinkage().add((SubstanceNucleicAcidSubunitLinkageComponent) value); 532 } else if (name.equals("sugar")) { 533 this.getSugar().add((SubstanceNucleicAcidSubunitSugarComponent) value); 534 } else 535 return super.setProperty(name, value); 536 return value; 537 } 538 539 @Override 540 public void removeChild(String name, Base value) throws FHIRException { 541 if (name.equals("subunit")) { 542 this.subunit = null; 543 } else if (name.equals("sequence")) { 544 this.sequence = null; 545 } else if (name.equals("length")) { 546 this.length = null; 547 } else if (name.equals("sequenceAttachment")) { 548 this.sequenceAttachment = null; 549 } else if (name.equals("fivePrime")) { 550 this.fivePrime = null; 551 } else if (name.equals("threePrime")) { 552 this.threePrime = null; 553 } else if (name.equals("linkage")) { 554 this.getLinkage().remove((SubstanceNucleicAcidSubunitLinkageComponent) value); 555 } else if (name.equals("sugar")) { 556 this.getSugar().remove((SubstanceNucleicAcidSubunitSugarComponent) value); 557 } else 558 super.removeChild(name, value); 559 560 } 561 562 @Override 563 public Base makeProperty(int hash, String name) throws FHIRException { 564 switch (hash) { 565 case -1867548732: return getSubunitElement(); 566 case 1349547969: return getSequenceElement(); 567 case -1106363674: return getLengthElement(); 568 case 364621764: return getSequenceAttachment(); 569 case -1045091603: return getFivePrime(); 570 case -1088032895: return getThreePrime(); 571 case 177082053: return addLinkage(); 572 case 109792566: return addSugar(); 573 default: return super.makeProperty(hash, name); 574 } 575 576 } 577 578 @Override 579 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 580 switch (hash) { 581 case -1867548732: /*subunit*/ return new String[] {"integer"}; 582 case 1349547969: /*sequence*/ return new String[] {"string"}; 583 case -1106363674: /*length*/ return new String[] {"integer"}; 584 case 364621764: /*sequenceAttachment*/ return new String[] {"Attachment"}; 585 case -1045091603: /*fivePrime*/ return new String[] {"CodeableConcept"}; 586 case -1088032895: /*threePrime*/ return new String[] {"CodeableConcept"}; 587 case 177082053: /*linkage*/ return new String[] {}; 588 case 109792566: /*sugar*/ return new String[] {}; 589 default: return super.getTypesForProperty(hash, name); 590 } 591 592 } 593 594 @Override 595 public Base addChild(String name) throws FHIRException { 596 if (name.equals("subunit")) { 597 throw new FHIRException("Cannot call addChild on a singleton property SubstanceNucleicAcid.subunit.subunit"); 598 } 599 else if (name.equals("sequence")) { 600 throw new FHIRException("Cannot call addChild on a singleton property SubstanceNucleicAcid.subunit.sequence"); 601 } 602 else if (name.equals("length")) { 603 throw new FHIRException("Cannot call addChild on a singleton property SubstanceNucleicAcid.subunit.length"); 604 } 605 else if (name.equals("sequenceAttachment")) { 606 this.sequenceAttachment = new Attachment(); 607 return this.sequenceAttachment; 608 } 609 else if (name.equals("fivePrime")) { 610 this.fivePrime = new CodeableConcept(); 611 return this.fivePrime; 612 } 613 else if (name.equals("threePrime")) { 614 this.threePrime = new CodeableConcept(); 615 return this.threePrime; 616 } 617 else if (name.equals("linkage")) { 618 return addLinkage(); 619 } 620 else if (name.equals("sugar")) { 621 return addSugar(); 622 } 623 else 624 return super.addChild(name); 625 } 626 627 public SubstanceNucleicAcidSubunitComponent copy() { 628 SubstanceNucleicAcidSubunitComponent dst = new SubstanceNucleicAcidSubunitComponent(); 629 copyValues(dst); 630 return dst; 631 } 632 633 public void copyValues(SubstanceNucleicAcidSubunitComponent dst) { 634 super.copyValues(dst); 635 dst.subunit = subunit == null ? null : subunit.copy(); 636 dst.sequence = sequence == null ? null : sequence.copy(); 637 dst.length = length == null ? null : length.copy(); 638 dst.sequenceAttachment = sequenceAttachment == null ? null : sequenceAttachment.copy(); 639 dst.fivePrime = fivePrime == null ? null : fivePrime.copy(); 640 dst.threePrime = threePrime == null ? null : threePrime.copy(); 641 if (linkage != null) { 642 dst.linkage = new ArrayList<SubstanceNucleicAcidSubunitLinkageComponent>(); 643 for (SubstanceNucleicAcidSubunitLinkageComponent i : linkage) 644 dst.linkage.add(i.copy()); 645 }; 646 if (sugar != null) { 647 dst.sugar = new ArrayList<SubstanceNucleicAcidSubunitSugarComponent>(); 648 for (SubstanceNucleicAcidSubunitSugarComponent i : sugar) 649 dst.sugar.add(i.copy()); 650 }; 651 } 652 653 @Override 654 public boolean equalsDeep(Base other_) { 655 if (!super.equalsDeep(other_)) 656 return false; 657 if (!(other_ instanceof SubstanceNucleicAcidSubunitComponent)) 658 return false; 659 SubstanceNucleicAcidSubunitComponent o = (SubstanceNucleicAcidSubunitComponent) other_; 660 return compareDeep(subunit, o.subunit, true) && compareDeep(sequence, o.sequence, true) && compareDeep(length, o.length, true) 661 && compareDeep(sequenceAttachment, o.sequenceAttachment, true) && compareDeep(fivePrime, o.fivePrime, true) 662 && compareDeep(threePrime, o.threePrime, true) && compareDeep(linkage, o.linkage, true) && compareDeep(sugar, o.sugar, true) 663 ; 664 } 665 666 @Override 667 public boolean equalsShallow(Base other_) { 668 if (!super.equalsShallow(other_)) 669 return false; 670 if (!(other_ instanceof SubstanceNucleicAcidSubunitComponent)) 671 return false; 672 SubstanceNucleicAcidSubunitComponent o = (SubstanceNucleicAcidSubunitComponent) other_; 673 return compareValues(subunit, o.subunit, true) && compareValues(sequence, o.sequence, true) && compareValues(length, o.length, true) 674 ; 675 } 676 677 public boolean isEmpty() { 678 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(subunit, sequence, length 679 , sequenceAttachment, fivePrime, threePrime, linkage, sugar); 680 } 681 682 public String fhirType() { 683 return "SubstanceNucleicAcid.subunit"; 684 685 } 686 687 } 688 689 @Block() 690 public static class SubstanceNucleicAcidSubunitLinkageComponent extends BackboneElement implements IBaseBackboneElement { 691 /** 692 * The entity that links the sugar residues together should also be captured for nearly all naturally occurring nucleic acid the linkage is a phosphate group. For many synthetic oligonucleotides phosphorothioate linkages are often seen. Linkage connectivity is assumed to be 3?-5?. If the linkage is either 3?-3? or 5?-5? this should be specified. 693 */ 694 @Child(name = "connectivity", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 695 @Description(shortDefinition="The entity that links the sugar residues together should also be captured for nearly all naturally occurring nucleic acid the linkage is a phosphate group. For many synthetic oligonucleotides phosphorothioate linkages are often seen. Linkage connectivity is assumed to be 3?-5?. If the linkage is either 3?-3? or 5?-5? this should be specified", formalDefinition="The entity that links the sugar residues together should also be captured for nearly all naturally occurring nucleic acid the linkage is a phosphate group. For many synthetic oligonucleotides phosphorothioate linkages are often seen. Linkage connectivity is assumed to be 3?-5?. If the linkage is either 3?-3? or 5?-5? this should be specified." ) 696 protected StringType connectivity; 697 698 /** 699 * Each linkage will be registered as a fragment and have an ID. 700 */ 701 @Child(name = "identifier", type = {Identifier.class}, order=2, min=0, max=1, modifier=false, summary=true) 702 @Description(shortDefinition="Each linkage will be registered as a fragment and have an ID", formalDefinition="Each linkage will be registered as a fragment and have an ID." ) 703 protected Identifier identifier; 704 705 /** 706 * Each linkage will be registered as a fragment and have at least one name. A single name shall be assigned to each linkage. 707 */ 708 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 709 @Description(shortDefinition="Each linkage will be registered as a fragment and have at least one name. A single name shall be assigned to each linkage", formalDefinition="Each linkage will be registered as a fragment and have at least one name. A single name shall be assigned to each linkage." ) 710 protected StringType name; 711 712 /** 713 * Residues shall be captured as described in 5.3.6.8.3. 714 */ 715 @Child(name = "residueSite", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 716 @Description(shortDefinition="Residues shall be captured as described in 5.3.6.8.3", formalDefinition="Residues shall be captured as described in 5.3.6.8.3." ) 717 protected StringType residueSite; 718 719 private static final long serialVersionUID = 1392155799L; 720 721 /** 722 * Constructor 723 */ 724 public SubstanceNucleicAcidSubunitLinkageComponent() { 725 super(); 726 } 727 728 /** 729 * @return {@link #connectivity} (The entity that links the sugar residues together should also be captured for nearly all naturally occurring nucleic acid the linkage is a phosphate group. For many synthetic oligonucleotides phosphorothioate linkages are often seen. Linkage connectivity is assumed to be 3?-5?. If the linkage is either 3?-3? or 5?-5? this should be specified.). This is the underlying object with id, value and extensions. The accessor "getConnectivity" gives direct access to the value 730 */ 731 public StringType getConnectivityElement() { 732 if (this.connectivity == null) 733 if (Configuration.errorOnAutoCreate()) 734 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitLinkageComponent.connectivity"); 735 else if (Configuration.doAutoCreate()) 736 this.connectivity = new StringType(); // bb 737 return this.connectivity; 738 } 739 740 public boolean hasConnectivityElement() { 741 return this.connectivity != null && !this.connectivity.isEmpty(); 742 } 743 744 public boolean hasConnectivity() { 745 return this.connectivity != null && !this.connectivity.isEmpty(); 746 } 747 748 /** 749 * @param value {@link #connectivity} (The entity that links the sugar residues together should also be captured for nearly all naturally occurring nucleic acid the linkage is a phosphate group. For many synthetic oligonucleotides phosphorothioate linkages are often seen. Linkage connectivity is assumed to be 3?-5?. If the linkage is either 3?-3? or 5?-5? this should be specified.). This is the underlying object with id, value and extensions. The accessor "getConnectivity" gives direct access to the value 750 */ 751 public SubstanceNucleicAcidSubunitLinkageComponent setConnectivityElement(StringType value) { 752 this.connectivity = value; 753 return this; 754 } 755 756 /** 757 * @return The entity that links the sugar residues together should also be captured for nearly all naturally occurring nucleic acid the linkage is a phosphate group. For many synthetic oligonucleotides phosphorothioate linkages are often seen. Linkage connectivity is assumed to be 3?-5?. If the linkage is either 3?-3? or 5?-5? this should be specified. 758 */ 759 public String getConnectivity() { 760 return this.connectivity == null ? null : this.connectivity.getValue(); 761 } 762 763 /** 764 * @param value The entity that links the sugar residues together should also be captured for nearly all naturally occurring nucleic acid the linkage is a phosphate group. For many synthetic oligonucleotides phosphorothioate linkages are often seen. Linkage connectivity is assumed to be 3?-5?. If the linkage is either 3?-3? or 5?-5? this should be specified. 765 */ 766 public SubstanceNucleicAcidSubunitLinkageComponent setConnectivity(String value) { 767 if (Utilities.noString(value)) 768 this.connectivity = null; 769 else { 770 if (this.connectivity == null) 771 this.connectivity = new StringType(); 772 this.connectivity.setValue(value); 773 } 774 return this; 775 } 776 777 /** 778 * @return {@link #identifier} (Each linkage will be registered as a fragment and have an ID.) 779 */ 780 public Identifier getIdentifier() { 781 if (this.identifier == null) 782 if (Configuration.errorOnAutoCreate()) 783 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitLinkageComponent.identifier"); 784 else if (Configuration.doAutoCreate()) 785 this.identifier = new Identifier(); // cc 786 return this.identifier; 787 } 788 789 public boolean hasIdentifier() { 790 return this.identifier != null && !this.identifier.isEmpty(); 791 } 792 793 /** 794 * @param value {@link #identifier} (Each linkage will be registered as a fragment and have an ID.) 795 */ 796 public SubstanceNucleicAcidSubunitLinkageComponent setIdentifier(Identifier value) { 797 this.identifier = value; 798 return this; 799 } 800 801 /** 802 * @return {@link #name} (Each linkage will be registered as a fragment and have at least one name. A single name shall be assigned to each linkage.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 803 */ 804 public StringType getNameElement() { 805 if (this.name == null) 806 if (Configuration.errorOnAutoCreate()) 807 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitLinkageComponent.name"); 808 else if (Configuration.doAutoCreate()) 809 this.name = new StringType(); // bb 810 return this.name; 811 } 812 813 public boolean hasNameElement() { 814 return this.name != null && !this.name.isEmpty(); 815 } 816 817 public boolean hasName() { 818 return this.name != null && !this.name.isEmpty(); 819 } 820 821 /** 822 * @param value {@link #name} (Each linkage will be registered as a fragment and have at least one name. A single name shall be assigned to each linkage.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 823 */ 824 public SubstanceNucleicAcidSubunitLinkageComponent setNameElement(StringType value) { 825 this.name = value; 826 return this; 827 } 828 829 /** 830 * @return Each linkage will be registered as a fragment and have at least one name. A single name shall be assigned to each linkage. 831 */ 832 public String getName() { 833 return this.name == null ? null : this.name.getValue(); 834 } 835 836 /** 837 * @param value Each linkage will be registered as a fragment and have at least one name. A single name shall be assigned to each linkage. 838 */ 839 public SubstanceNucleicAcidSubunitLinkageComponent setName(String value) { 840 if (Utilities.noString(value)) 841 this.name = null; 842 else { 843 if (this.name == null) 844 this.name = new StringType(); 845 this.name.setValue(value); 846 } 847 return this; 848 } 849 850 /** 851 * @return {@link #residueSite} (Residues shall be captured as described in 5.3.6.8.3.). This is the underlying object with id, value and extensions. The accessor "getResidueSite" gives direct access to the value 852 */ 853 public StringType getResidueSiteElement() { 854 if (this.residueSite == null) 855 if (Configuration.errorOnAutoCreate()) 856 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitLinkageComponent.residueSite"); 857 else if (Configuration.doAutoCreate()) 858 this.residueSite = new StringType(); // bb 859 return this.residueSite; 860 } 861 862 public boolean hasResidueSiteElement() { 863 return this.residueSite != null && !this.residueSite.isEmpty(); 864 } 865 866 public boolean hasResidueSite() { 867 return this.residueSite != null && !this.residueSite.isEmpty(); 868 } 869 870 /** 871 * @param value {@link #residueSite} (Residues shall be captured as described in 5.3.6.8.3.). This is the underlying object with id, value and extensions. The accessor "getResidueSite" gives direct access to the value 872 */ 873 public SubstanceNucleicAcidSubunitLinkageComponent setResidueSiteElement(StringType value) { 874 this.residueSite = value; 875 return this; 876 } 877 878 /** 879 * @return Residues shall be captured as described in 5.3.6.8.3. 880 */ 881 public String getResidueSite() { 882 return this.residueSite == null ? null : this.residueSite.getValue(); 883 } 884 885 /** 886 * @param value Residues shall be captured as described in 5.3.6.8.3. 887 */ 888 public SubstanceNucleicAcidSubunitLinkageComponent setResidueSite(String value) { 889 if (Utilities.noString(value)) 890 this.residueSite = null; 891 else { 892 if (this.residueSite == null) 893 this.residueSite = new StringType(); 894 this.residueSite.setValue(value); 895 } 896 return this; 897 } 898 899 protected void listChildren(List<Property> children) { 900 super.listChildren(children); 901 children.add(new Property("connectivity", "string", "The entity that links the sugar residues together should also be captured for nearly all naturally occurring nucleic acid the linkage is a phosphate group. For many synthetic oligonucleotides phosphorothioate linkages are often seen. Linkage connectivity is assumed to be 3?-5?. If the linkage is either 3?-3? or 5?-5? this should be specified.", 0, 1, connectivity)); 902 children.add(new Property("identifier", "Identifier", "Each linkage will be registered as a fragment and have an ID.", 0, 1, identifier)); 903 children.add(new Property("name", "string", "Each linkage will be registered as a fragment and have at least one name. A single name shall be assigned to each linkage.", 0, 1, name)); 904 children.add(new Property("residueSite", "string", "Residues shall be captured as described in 5.3.6.8.3.", 0, 1, residueSite)); 905 } 906 907 @Override 908 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 909 switch (_hash) { 910 case 1923312055: /*connectivity*/ return new Property("connectivity", "string", "The entity that links the sugar residues together should also be captured for nearly all naturally occurring nucleic acid the linkage is a phosphate group. For many synthetic oligonucleotides phosphorothioate linkages are often seen. Linkage connectivity is assumed to be 3?-5?. If the linkage is either 3?-3? or 5?-5? this should be specified.", 0, 1, connectivity); 911 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Each linkage will be registered as a fragment and have an ID.", 0, 1, identifier); 912 case 3373707: /*name*/ return new Property("name", "string", "Each linkage will be registered as a fragment and have at least one name. A single name shall be assigned to each linkage.", 0, 1, name); 913 case 1547124594: /*residueSite*/ return new Property("residueSite", "string", "Residues shall be captured as described in 5.3.6.8.3.", 0, 1, residueSite); 914 default: return super.getNamedProperty(_hash, _name, _checkValid); 915 } 916 917 } 918 919 @Override 920 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 921 switch (hash) { 922 case 1923312055: /*connectivity*/ return this.connectivity == null ? new Base[0] : new Base[] {this.connectivity}; // StringType 923 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 924 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 925 case 1547124594: /*residueSite*/ return this.residueSite == null ? new Base[0] : new Base[] {this.residueSite}; // StringType 926 default: return super.getProperty(hash, name, checkValid); 927 } 928 929 } 930 931 @Override 932 public Base setProperty(int hash, String name, Base value) throws FHIRException { 933 switch (hash) { 934 case 1923312055: // connectivity 935 this.connectivity = TypeConvertor.castToString(value); // StringType 936 return value; 937 case -1618432855: // identifier 938 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 939 return value; 940 case 3373707: // name 941 this.name = TypeConvertor.castToString(value); // StringType 942 return value; 943 case 1547124594: // residueSite 944 this.residueSite = TypeConvertor.castToString(value); // StringType 945 return value; 946 default: return super.setProperty(hash, name, value); 947 } 948 949 } 950 951 @Override 952 public Base setProperty(String name, Base value) throws FHIRException { 953 if (name.equals("connectivity")) { 954 this.connectivity = TypeConvertor.castToString(value); // StringType 955 } else if (name.equals("identifier")) { 956 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 957 } else if (name.equals("name")) { 958 this.name = TypeConvertor.castToString(value); // StringType 959 } else if (name.equals("residueSite")) { 960 this.residueSite = TypeConvertor.castToString(value); // StringType 961 } else 962 return super.setProperty(name, value); 963 return value; 964 } 965 966 @Override 967 public void removeChild(String name, Base value) throws FHIRException { 968 if (name.equals("connectivity")) { 969 this.connectivity = null; 970 } else if (name.equals("identifier")) { 971 this.identifier = null; 972 } else if (name.equals("name")) { 973 this.name = null; 974 } else if (name.equals("residueSite")) { 975 this.residueSite = null; 976 } else 977 super.removeChild(name, value); 978 979 } 980 981 @Override 982 public Base makeProperty(int hash, String name) throws FHIRException { 983 switch (hash) { 984 case 1923312055: return getConnectivityElement(); 985 case -1618432855: return getIdentifier(); 986 case 3373707: return getNameElement(); 987 case 1547124594: return getResidueSiteElement(); 988 default: return super.makeProperty(hash, name); 989 } 990 991 } 992 993 @Override 994 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 995 switch (hash) { 996 case 1923312055: /*connectivity*/ return new String[] {"string"}; 997 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 998 case 3373707: /*name*/ return new String[] {"string"}; 999 case 1547124594: /*residueSite*/ return new String[] {"string"}; 1000 default: return super.getTypesForProperty(hash, name); 1001 } 1002 1003 } 1004 1005 @Override 1006 public Base addChild(String name) throws FHIRException { 1007 if (name.equals("connectivity")) { 1008 throw new FHIRException("Cannot call addChild on a singleton property SubstanceNucleicAcid.subunit.linkage.connectivity"); 1009 } 1010 else if (name.equals("identifier")) { 1011 this.identifier = new Identifier(); 1012 return this.identifier; 1013 } 1014 else if (name.equals("name")) { 1015 throw new FHIRException("Cannot call addChild on a singleton property SubstanceNucleicAcid.subunit.linkage.name"); 1016 } 1017 else if (name.equals("residueSite")) { 1018 throw new FHIRException("Cannot call addChild on a singleton property SubstanceNucleicAcid.subunit.linkage.residueSite"); 1019 } 1020 else 1021 return super.addChild(name); 1022 } 1023 1024 public SubstanceNucleicAcidSubunitLinkageComponent copy() { 1025 SubstanceNucleicAcidSubunitLinkageComponent dst = new SubstanceNucleicAcidSubunitLinkageComponent(); 1026 copyValues(dst); 1027 return dst; 1028 } 1029 1030 public void copyValues(SubstanceNucleicAcidSubunitLinkageComponent dst) { 1031 super.copyValues(dst); 1032 dst.connectivity = connectivity == null ? null : connectivity.copy(); 1033 dst.identifier = identifier == null ? null : identifier.copy(); 1034 dst.name = name == null ? null : name.copy(); 1035 dst.residueSite = residueSite == null ? null : residueSite.copy(); 1036 } 1037 1038 @Override 1039 public boolean equalsDeep(Base other_) { 1040 if (!super.equalsDeep(other_)) 1041 return false; 1042 if (!(other_ instanceof SubstanceNucleicAcidSubunitLinkageComponent)) 1043 return false; 1044 SubstanceNucleicAcidSubunitLinkageComponent o = (SubstanceNucleicAcidSubunitLinkageComponent) other_; 1045 return compareDeep(connectivity, o.connectivity, true) && compareDeep(identifier, o.identifier, true) 1046 && compareDeep(name, o.name, true) && compareDeep(residueSite, o.residueSite, true); 1047 } 1048 1049 @Override 1050 public boolean equalsShallow(Base other_) { 1051 if (!super.equalsShallow(other_)) 1052 return false; 1053 if (!(other_ instanceof SubstanceNucleicAcidSubunitLinkageComponent)) 1054 return false; 1055 SubstanceNucleicAcidSubunitLinkageComponent o = (SubstanceNucleicAcidSubunitLinkageComponent) other_; 1056 return compareValues(connectivity, o.connectivity, true) && compareValues(name, o.name, true) && compareValues(residueSite, o.residueSite, true) 1057 ; 1058 } 1059 1060 public boolean isEmpty() { 1061 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(connectivity, identifier, name 1062 , residueSite); 1063 } 1064 1065 public String fhirType() { 1066 return "SubstanceNucleicAcid.subunit.linkage"; 1067 1068 } 1069 1070 } 1071 1072 @Block() 1073 public static class SubstanceNucleicAcidSubunitSugarComponent extends BackboneElement implements IBaseBackboneElement { 1074 /** 1075 * The Substance ID of the sugar or sugar-like component that make up the nucleotide. 1076 */ 1077 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 1078 @Description(shortDefinition="The Substance ID of the sugar or sugar-like component that make up the nucleotide", formalDefinition="The Substance ID of the sugar or sugar-like component that make up the nucleotide." ) 1079 protected Identifier identifier; 1080 1081 /** 1082 * The name of the sugar or sugar-like component that make up the nucleotide. 1083 */ 1084 @Child(name = "name", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1085 @Description(shortDefinition="The name of the sugar or sugar-like component that make up the nucleotide", formalDefinition="The name of the sugar or sugar-like component that make up the nucleotide." ) 1086 protected StringType name; 1087 1088 /** 1089 * The residues that contain a given sugar will be captured. The order of given residues will be captured in the 5?-3?direction consistent with the base sequences listed above. 1090 */ 1091 @Child(name = "residueSite", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1092 @Description(shortDefinition="The residues that contain a given sugar will be captured. The order of given residues will be captured in the 5?-3?direction consistent with the base sequences listed above", formalDefinition="The residues that contain a given sugar will be captured. The order of given residues will be captured in the 5?-3?direction consistent with the base sequences listed above." ) 1093 protected StringType residueSite; 1094 1095 private static final long serialVersionUID = 1933713781L; 1096 1097 /** 1098 * Constructor 1099 */ 1100 public SubstanceNucleicAcidSubunitSugarComponent() { 1101 super(); 1102 } 1103 1104 /** 1105 * @return {@link #identifier} (The Substance ID of the sugar or sugar-like component that make up the nucleotide.) 1106 */ 1107 public Identifier getIdentifier() { 1108 if (this.identifier == null) 1109 if (Configuration.errorOnAutoCreate()) 1110 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitSugarComponent.identifier"); 1111 else if (Configuration.doAutoCreate()) 1112 this.identifier = new Identifier(); // cc 1113 return this.identifier; 1114 } 1115 1116 public boolean hasIdentifier() { 1117 return this.identifier != null && !this.identifier.isEmpty(); 1118 } 1119 1120 /** 1121 * @param value {@link #identifier} (The Substance ID of the sugar or sugar-like component that make up the nucleotide.) 1122 */ 1123 public SubstanceNucleicAcidSubunitSugarComponent setIdentifier(Identifier value) { 1124 this.identifier = value; 1125 return this; 1126 } 1127 1128 /** 1129 * @return {@link #name} (The name of the sugar or sugar-like component that make up the nucleotide.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1130 */ 1131 public StringType getNameElement() { 1132 if (this.name == null) 1133 if (Configuration.errorOnAutoCreate()) 1134 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitSugarComponent.name"); 1135 else if (Configuration.doAutoCreate()) 1136 this.name = new StringType(); // bb 1137 return this.name; 1138 } 1139 1140 public boolean hasNameElement() { 1141 return this.name != null && !this.name.isEmpty(); 1142 } 1143 1144 public boolean hasName() { 1145 return this.name != null && !this.name.isEmpty(); 1146 } 1147 1148 /** 1149 * @param value {@link #name} (The name of the sugar or sugar-like component that make up the nucleotide.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1150 */ 1151 public SubstanceNucleicAcidSubunitSugarComponent setNameElement(StringType value) { 1152 this.name = value; 1153 return this; 1154 } 1155 1156 /** 1157 * @return The name of the sugar or sugar-like component that make up the nucleotide. 1158 */ 1159 public String getName() { 1160 return this.name == null ? null : this.name.getValue(); 1161 } 1162 1163 /** 1164 * @param value The name of the sugar or sugar-like component that make up the nucleotide. 1165 */ 1166 public SubstanceNucleicAcidSubunitSugarComponent setName(String value) { 1167 if (Utilities.noString(value)) 1168 this.name = null; 1169 else { 1170 if (this.name == null) 1171 this.name = new StringType(); 1172 this.name.setValue(value); 1173 } 1174 return this; 1175 } 1176 1177 /** 1178 * @return {@link #residueSite} (The residues that contain a given sugar will be captured. The order of given residues will be captured in the 5?-3?direction consistent with the base sequences listed above.). This is the underlying object with id, value and extensions. The accessor "getResidueSite" gives direct access to the value 1179 */ 1180 public StringType getResidueSiteElement() { 1181 if (this.residueSite == null) 1182 if (Configuration.errorOnAutoCreate()) 1183 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitSugarComponent.residueSite"); 1184 else if (Configuration.doAutoCreate()) 1185 this.residueSite = new StringType(); // bb 1186 return this.residueSite; 1187 } 1188 1189 public boolean hasResidueSiteElement() { 1190 return this.residueSite != null && !this.residueSite.isEmpty(); 1191 } 1192 1193 public boolean hasResidueSite() { 1194 return this.residueSite != null && !this.residueSite.isEmpty(); 1195 } 1196 1197 /** 1198 * @param value {@link #residueSite} (The residues that contain a given sugar will be captured. The order of given residues will be captured in the 5?-3?direction consistent with the base sequences listed above.). This is the underlying object with id, value and extensions. The accessor "getResidueSite" gives direct access to the value 1199 */ 1200 public SubstanceNucleicAcidSubunitSugarComponent setResidueSiteElement(StringType value) { 1201 this.residueSite = value; 1202 return this; 1203 } 1204 1205 /** 1206 * @return The residues that contain a given sugar will be captured. The order of given residues will be captured in the 5?-3?direction consistent with the base sequences listed above. 1207 */ 1208 public String getResidueSite() { 1209 return this.residueSite == null ? null : this.residueSite.getValue(); 1210 } 1211 1212 /** 1213 * @param value The residues that contain a given sugar will be captured. The order of given residues will be captured in the 5?-3?direction consistent with the base sequences listed above. 1214 */ 1215 public SubstanceNucleicAcidSubunitSugarComponent setResidueSite(String value) { 1216 if (Utilities.noString(value)) 1217 this.residueSite = null; 1218 else { 1219 if (this.residueSite == null) 1220 this.residueSite = new StringType(); 1221 this.residueSite.setValue(value); 1222 } 1223 return this; 1224 } 1225 1226 protected void listChildren(List<Property> children) { 1227 super.listChildren(children); 1228 children.add(new Property("identifier", "Identifier", "The Substance ID of the sugar or sugar-like component that make up the nucleotide.", 0, 1, identifier)); 1229 children.add(new Property("name", "string", "The name of the sugar or sugar-like component that make up the nucleotide.", 0, 1, name)); 1230 children.add(new Property("residueSite", "string", "The residues that contain a given sugar will be captured. The order of given residues will be captured in the 5?-3?direction consistent with the base sequences listed above.", 0, 1, residueSite)); 1231 } 1232 1233 @Override 1234 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1235 switch (_hash) { 1236 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The Substance ID of the sugar or sugar-like component that make up the nucleotide.", 0, 1, identifier); 1237 case 3373707: /*name*/ return new Property("name", "string", "The name of the sugar or sugar-like component that make up the nucleotide.", 0, 1, name); 1238 case 1547124594: /*residueSite*/ return new Property("residueSite", "string", "The residues that contain a given sugar will be captured. The order of given residues will be captured in the 5?-3?direction consistent with the base sequences listed above.", 0, 1, residueSite); 1239 default: return super.getNamedProperty(_hash, _name, _checkValid); 1240 } 1241 1242 } 1243 1244 @Override 1245 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1246 switch (hash) { 1247 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1248 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1249 case 1547124594: /*residueSite*/ return this.residueSite == null ? new Base[0] : new Base[] {this.residueSite}; // StringType 1250 default: return super.getProperty(hash, name, checkValid); 1251 } 1252 1253 } 1254 1255 @Override 1256 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1257 switch (hash) { 1258 case -1618432855: // identifier 1259 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 1260 return value; 1261 case 3373707: // name 1262 this.name = TypeConvertor.castToString(value); // StringType 1263 return value; 1264 case 1547124594: // residueSite 1265 this.residueSite = TypeConvertor.castToString(value); // StringType 1266 return value; 1267 default: return super.setProperty(hash, name, value); 1268 } 1269 1270 } 1271 1272 @Override 1273 public Base setProperty(String name, Base value) throws FHIRException { 1274 if (name.equals("identifier")) { 1275 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 1276 } else if (name.equals("name")) { 1277 this.name = TypeConvertor.castToString(value); // StringType 1278 } else if (name.equals("residueSite")) { 1279 this.residueSite = TypeConvertor.castToString(value); // StringType 1280 } else 1281 return super.setProperty(name, value); 1282 return value; 1283 } 1284 1285 @Override 1286 public void removeChild(String name, Base value) throws FHIRException { 1287 if (name.equals("identifier")) { 1288 this.identifier = null; 1289 } else if (name.equals("name")) { 1290 this.name = null; 1291 } else if (name.equals("residueSite")) { 1292 this.residueSite = null; 1293 } else 1294 super.removeChild(name, value); 1295 1296 } 1297 1298 @Override 1299 public Base makeProperty(int hash, String name) throws FHIRException { 1300 switch (hash) { 1301 case -1618432855: return getIdentifier(); 1302 case 3373707: return getNameElement(); 1303 case 1547124594: return getResidueSiteElement(); 1304 default: return super.makeProperty(hash, name); 1305 } 1306 1307 } 1308 1309 @Override 1310 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1311 switch (hash) { 1312 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1313 case 3373707: /*name*/ return new String[] {"string"}; 1314 case 1547124594: /*residueSite*/ return new String[] {"string"}; 1315 default: return super.getTypesForProperty(hash, name); 1316 } 1317 1318 } 1319 1320 @Override 1321 public Base addChild(String name) throws FHIRException { 1322 if (name.equals("identifier")) { 1323 this.identifier = new Identifier(); 1324 return this.identifier; 1325 } 1326 else if (name.equals("name")) { 1327 throw new FHIRException("Cannot call addChild on a singleton property SubstanceNucleicAcid.subunit.sugar.name"); 1328 } 1329 else if (name.equals("residueSite")) { 1330 throw new FHIRException("Cannot call addChild on a singleton property SubstanceNucleicAcid.subunit.sugar.residueSite"); 1331 } 1332 else 1333 return super.addChild(name); 1334 } 1335 1336 public SubstanceNucleicAcidSubunitSugarComponent copy() { 1337 SubstanceNucleicAcidSubunitSugarComponent dst = new SubstanceNucleicAcidSubunitSugarComponent(); 1338 copyValues(dst); 1339 return dst; 1340 } 1341 1342 public void copyValues(SubstanceNucleicAcidSubunitSugarComponent dst) { 1343 super.copyValues(dst); 1344 dst.identifier = identifier == null ? null : identifier.copy(); 1345 dst.name = name == null ? null : name.copy(); 1346 dst.residueSite = residueSite == null ? null : residueSite.copy(); 1347 } 1348 1349 @Override 1350 public boolean equalsDeep(Base other_) { 1351 if (!super.equalsDeep(other_)) 1352 return false; 1353 if (!(other_ instanceof SubstanceNucleicAcidSubunitSugarComponent)) 1354 return false; 1355 SubstanceNucleicAcidSubunitSugarComponent o = (SubstanceNucleicAcidSubunitSugarComponent) other_; 1356 return compareDeep(identifier, o.identifier, true) && compareDeep(name, o.name, true) && compareDeep(residueSite, o.residueSite, true) 1357 ; 1358 } 1359 1360 @Override 1361 public boolean equalsShallow(Base other_) { 1362 if (!super.equalsShallow(other_)) 1363 return false; 1364 if (!(other_ instanceof SubstanceNucleicAcidSubunitSugarComponent)) 1365 return false; 1366 SubstanceNucleicAcidSubunitSugarComponent o = (SubstanceNucleicAcidSubunitSugarComponent) other_; 1367 return compareValues(name, o.name, true) && compareValues(residueSite, o.residueSite, true); 1368 } 1369 1370 public boolean isEmpty() { 1371 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, name, residueSite 1372 ); 1373 } 1374 1375 public String fhirType() { 1376 return "SubstanceNucleicAcid.subunit.sugar"; 1377 1378 } 1379 1380 } 1381 1382 /** 1383 * The type of the sequence shall be specified based on a controlled vocabulary. 1384 */ 1385 @Child(name = "sequenceType", type = {CodeableConcept.class}, order=0, min=0, max=1, modifier=false, summary=true) 1386 @Description(shortDefinition="The type of the sequence shall be specified based on a controlled vocabulary", formalDefinition="The type of the sequence shall be specified based on a controlled vocabulary." ) 1387 protected CodeableConcept sequenceType; 1388 1389 /** 1390 * The number of linear sequences of nucleotides linked through phosphodiester bonds shall be described. Subunits would be strands of nucleic acids that are tightly associated typically through Watson-Crick base pairing. NOTE: If not specified in the reference source, the assumption is that there is 1 subunit. 1391 */ 1392 @Child(name = "numberOfSubunits", type = {IntegerType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1393 @Description(shortDefinition="The number of linear sequences of nucleotides linked through phosphodiester bonds shall be described. Subunits would be strands of nucleic acids that are tightly associated typically through Watson-Crick base pairing. NOTE: If not specified in the reference source, the assumption is that there is 1 subunit", formalDefinition="The number of linear sequences of nucleotides linked through phosphodiester bonds shall be described. Subunits would be strands of nucleic acids that are tightly associated typically through Watson-Crick base pairing. NOTE: If not specified in the reference source, the assumption is that there is 1 subunit." ) 1394 protected IntegerType numberOfSubunits; 1395 1396 /** 1397 * The area of hybridisation shall be described if applicable for double stranded RNA or DNA. The number associated with the subunit followed by the number associated to the residue shall be specified in increasing order. The underscore ?? shall be used as separator as follows: ?Subunitnumber Residue?. 1398 */ 1399 @Child(name = "areaOfHybridisation", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1400 @Description(shortDefinition="The area of hybridisation shall be described if applicable for double stranded RNA or DNA. The number associated with the subunit followed by the number associated to the residue shall be specified in increasing order. The underscore ?? shall be used as separator as follows: ?Subunitnumber Residue?", formalDefinition="The area of hybridisation shall be described if applicable for double stranded RNA or DNA. The number associated with the subunit followed by the number associated to the residue shall be specified in increasing order. The underscore ?? shall be used as separator as follows: ?Subunitnumber Residue?." ) 1401 protected StringType areaOfHybridisation; 1402 1403 /** 1404 * (TBC). 1405 */ 1406 @Child(name = "oligoNucleotideType", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 1407 @Description(shortDefinition="(TBC)", formalDefinition="(TBC)." ) 1408 protected CodeableConcept oligoNucleotideType; 1409 1410 /** 1411 * Subunits are listed in order of decreasing length; sequences of the same length will be ordered by molecular weight; subunits that have identical sequences will be repeated multiple times. 1412 */ 1413 @Child(name = "subunit", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1414 @Description(shortDefinition="Subunits are listed in order of decreasing length; sequences of the same length will be ordered by molecular weight; subunits that have identical sequences will be repeated multiple times", formalDefinition="Subunits are listed in order of decreasing length; sequences of the same length will be ordered by molecular weight; subunits that have identical sequences will be repeated multiple times." ) 1415 protected List<SubstanceNucleicAcidSubunitComponent> subunit; 1416 1417 private static final long serialVersionUID = -1906822433L; 1418 1419 /** 1420 * Constructor 1421 */ 1422 public SubstanceNucleicAcid() { 1423 super(); 1424 } 1425 1426 /** 1427 * @return {@link #sequenceType} (The type of the sequence shall be specified based on a controlled vocabulary.) 1428 */ 1429 public CodeableConcept getSequenceType() { 1430 if (this.sequenceType == null) 1431 if (Configuration.errorOnAutoCreate()) 1432 throw new Error("Attempt to auto-create SubstanceNucleicAcid.sequenceType"); 1433 else if (Configuration.doAutoCreate()) 1434 this.sequenceType = new CodeableConcept(); // cc 1435 return this.sequenceType; 1436 } 1437 1438 public boolean hasSequenceType() { 1439 return this.sequenceType != null && !this.sequenceType.isEmpty(); 1440 } 1441 1442 /** 1443 * @param value {@link #sequenceType} (The type of the sequence shall be specified based on a controlled vocabulary.) 1444 */ 1445 public SubstanceNucleicAcid setSequenceType(CodeableConcept value) { 1446 this.sequenceType = value; 1447 return this; 1448 } 1449 1450 /** 1451 * @return {@link #numberOfSubunits} (The number of linear sequences of nucleotides linked through phosphodiester bonds shall be described. Subunits would be strands of nucleic acids that are tightly associated typically through Watson-Crick base pairing. NOTE: If not specified in the reference source, the assumption is that there is 1 subunit.). This is the underlying object with id, value and extensions. The accessor "getNumberOfSubunits" gives direct access to the value 1452 */ 1453 public IntegerType getNumberOfSubunitsElement() { 1454 if (this.numberOfSubunits == null) 1455 if (Configuration.errorOnAutoCreate()) 1456 throw new Error("Attempt to auto-create SubstanceNucleicAcid.numberOfSubunits"); 1457 else if (Configuration.doAutoCreate()) 1458 this.numberOfSubunits = new IntegerType(); // bb 1459 return this.numberOfSubunits; 1460 } 1461 1462 public boolean hasNumberOfSubunitsElement() { 1463 return this.numberOfSubunits != null && !this.numberOfSubunits.isEmpty(); 1464 } 1465 1466 public boolean hasNumberOfSubunits() { 1467 return this.numberOfSubunits != null && !this.numberOfSubunits.isEmpty(); 1468 } 1469 1470 /** 1471 * @param value {@link #numberOfSubunits} (The number of linear sequences of nucleotides linked through phosphodiester bonds shall be described. Subunits would be strands of nucleic acids that are tightly associated typically through Watson-Crick base pairing. NOTE: If not specified in the reference source, the assumption is that there is 1 subunit.). This is the underlying object with id, value and extensions. The accessor "getNumberOfSubunits" gives direct access to the value 1472 */ 1473 public SubstanceNucleicAcid setNumberOfSubunitsElement(IntegerType value) { 1474 this.numberOfSubunits = value; 1475 return this; 1476 } 1477 1478 /** 1479 * @return The number of linear sequences of nucleotides linked through phosphodiester bonds shall be described. Subunits would be strands of nucleic acids that are tightly associated typically through Watson-Crick base pairing. NOTE: If not specified in the reference source, the assumption is that there is 1 subunit. 1480 */ 1481 public int getNumberOfSubunits() { 1482 return this.numberOfSubunits == null || this.numberOfSubunits.isEmpty() ? 0 : this.numberOfSubunits.getValue(); 1483 } 1484 1485 /** 1486 * @param value The number of linear sequences of nucleotides linked through phosphodiester bonds shall be described. Subunits would be strands of nucleic acids that are tightly associated typically through Watson-Crick base pairing. NOTE: If not specified in the reference source, the assumption is that there is 1 subunit. 1487 */ 1488 public SubstanceNucleicAcid setNumberOfSubunits(int value) { 1489 if (this.numberOfSubunits == null) 1490 this.numberOfSubunits = new IntegerType(); 1491 this.numberOfSubunits.setValue(value); 1492 return this; 1493 } 1494 1495 /** 1496 * @return {@link #areaOfHybridisation} (The area of hybridisation shall be described if applicable for double stranded RNA or DNA. The number associated with the subunit followed by the number associated to the residue shall be specified in increasing order. The underscore ?? shall be used as separator as follows: ?Subunitnumber Residue?.). This is the underlying object with id, value and extensions. The accessor "getAreaOfHybridisation" gives direct access to the value 1497 */ 1498 public StringType getAreaOfHybridisationElement() { 1499 if (this.areaOfHybridisation == null) 1500 if (Configuration.errorOnAutoCreate()) 1501 throw new Error("Attempt to auto-create SubstanceNucleicAcid.areaOfHybridisation"); 1502 else if (Configuration.doAutoCreate()) 1503 this.areaOfHybridisation = new StringType(); // bb 1504 return this.areaOfHybridisation; 1505 } 1506 1507 public boolean hasAreaOfHybridisationElement() { 1508 return this.areaOfHybridisation != null && !this.areaOfHybridisation.isEmpty(); 1509 } 1510 1511 public boolean hasAreaOfHybridisation() { 1512 return this.areaOfHybridisation != null && !this.areaOfHybridisation.isEmpty(); 1513 } 1514 1515 /** 1516 * @param value {@link #areaOfHybridisation} (The area of hybridisation shall be described if applicable for double stranded RNA or DNA. The number associated with the subunit followed by the number associated to the residue shall be specified in increasing order. The underscore ?? shall be used as separator as follows: ?Subunitnumber Residue?.). This is the underlying object with id, value and extensions. The accessor "getAreaOfHybridisation" gives direct access to the value 1517 */ 1518 public SubstanceNucleicAcid setAreaOfHybridisationElement(StringType value) { 1519 this.areaOfHybridisation = value; 1520 return this; 1521 } 1522 1523 /** 1524 * @return The area of hybridisation shall be described if applicable for double stranded RNA or DNA. The number associated with the subunit followed by the number associated to the residue shall be specified in increasing order. The underscore ?? shall be used as separator as follows: ?Subunitnumber Residue?. 1525 */ 1526 public String getAreaOfHybridisation() { 1527 return this.areaOfHybridisation == null ? null : this.areaOfHybridisation.getValue(); 1528 } 1529 1530 /** 1531 * @param value The area of hybridisation shall be described if applicable for double stranded RNA or DNA. The number associated with the subunit followed by the number associated to the residue shall be specified in increasing order. The underscore ?? shall be used as separator as follows: ?Subunitnumber Residue?. 1532 */ 1533 public SubstanceNucleicAcid setAreaOfHybridisation(String value) { 1534 if (Utilities.noString(value)) 1535 this.areaOfHybridisation = null; 1536 else { 1537 if (this.areaOfHybridisation == null) 1538 this.areaOfHybridisation = new StringType(); 1539 this.areaOfHybridisation.setValue(value); 1540 } 1541 return this; 1542 } 1543 1544 /** 1545 * @return {@link #oligoNucleotideType} ((TBC).) 1546 */ 1547 public CodeableConcept getOligoNucleotideType() { 1548 if (this.oligoNucleotideType == null) 1549 if (Configuration.errorOnAutoCreate()) 1550 throw new Error("Attempt to auto-create SubstanceNucleicAcid.oligoNucleotideType"); 1551 else if (Configuration.doAutoCreate()) 1552 this.oligoNucleotideType = new CodeableConcept(); // cc 1553 return this.oligoNucleotideType; 1554 } 1555 1556 public boolean hasOligoNucleotideType() { 1557 return this.oligoNucleotideType != null && !this.oligoNucleotideType.isEmpty(); 1558 } 1559 1560 /** 1561 * @param value {@link #oligoNucleotideType} ((TBC).) 1562 */ 1563 public SubstanceNucleicAcid setOligoNucleotideType(CodeableConcept value) { 1564 this.oligoNucleotideType = value; 1565 return this; 1566 } 1567 1568 /** 1569 * @return {@link #subunit} (Subunits are listed in order of decreasing length; sequences of the same length will be ordered by molecular weight; subunits that have identical sequences will be repeated multiple times.) 1570 */ 1571 public List<SubstanceNucleicAcidSubunitComponent> getSubunit() { 1572 if (this.subunit == null) 1573 this.subunit = new ArrayList<SubstanceNucleicAcidSubunitComponent>(); 1574 return this.subunit; 1575 } 1576 1577 /** 1578 * @return Returns a reference to <code>this</code> for easy method chaining 1579 */ 1580 public SubstanceNucleicAcid setSubunit(List<SubstanceNucleicAcidSubunitComponent> theSubunit) { 1581 this.subunit = theSubunit; 1582 return this; 1583 } 1584 1585 public boolean hasSubunit() { 1586 if (this.subunit == null) 1587 return false; 1588 for (SubstanceNucleicAcidSubunitComponent item : this.subunit) 1589 if (!item.isEmpty()) 1590 return true; 1591 return false; 1592 } 1593 1594 public SubstanceNucleicAcidSubunitComponent addSubunit() { //3 1595 SubstanceNucleicAcidSubunitComponent t = new SubstanceNucleicAcidSubunitComponent(); 1596 if (this.subunit == null) 1597 this.subunit = new ArrayList<SubstanceNucleicAcidSubunitComponent>(); 1598 this.subunit.add(t); 1599 return t; 1600 } 1601 1602 public SubstanceNucleicAcid addSubunit(SubstanceNucleicAcidSubunitComponent t) { //3 1603 if (t == null) 1604 return this; 1605 if (this.subunit == null) 1606 this.subunit = new ArrayList<SubstanceNucleicAcidSubunitComponent>(); 1607 this.subunit.add(t); 1608 return this; 1609 } 1610 1611 /** 1612 * @return The first repetition of repeating field {@link #subunit}, creating it if it does not already exist {3} 1613 */ 1614 public SubstanceNucleicAcidSubunitComponent getSubunitFirstRep() { 1615 if (getSubunit().isEmpty()) { 1616 addSubunit(); 1617 } 1618 return getSubunit().get(0); 1619 } 1620 1621 protected void listChildren(List<Property> children) { 1622 super.listChildren(children); 1623 children.add(new Property("sequenceType", "CodeableConcept", "The type of the sequence shall be specified based on a controlled vocabulary.", 0, 1, sequenceType)); 1624 children.add(new Property("numberOfSubunits", "integer", "The number of linear sequences of nucleotides linked through phosphodiester bonds shall be described. Subunits would be strands of nucleic acids that are tightly associated typically through Watson-Crick base pairing. NOTE: If not specified in the reference source, the assumption is that there is 1 subunit.", 0, 1, numberOfSubunits)); 1625 children.add(new Property("areaOfHybridisation", "string", "The area of hybridisation shall be described if applicable for double stranded RNA or DNA. The number associated with the subunit followed by the number associated to the residue shall be specified in increasing order. The underscore ?? shall be used as separator as follows: ?Subunitnumber Residue?.", 0, 1, areaOfHybridisation)); 1626 children.add(new Property("oligoNucleotideType", "CodeableConcept", "(TBC).", 0, 1, oligoNucleotideType)); 1627 children.add(new Property("subunit", "", "Subunits are listed in order of decreasing length; sequences of the same length will be ordered by molecular weight; subunits that have identical sequences will be repeated multiple times.", 0, java.lang.Integer.MAX_VALUE, subunit)); 1628 } 1629 1630 @Override 1631 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1632 switch (_hash) { 1633 case 807711387: /*sequenceType*/ return new Property("sequenceType", "CodeableConcept", "The type of the sequence shall be specified based on a controlled vocabulary.", 0, 1, sequenceType); 1634 case -847111089: /*numberOfSubunits*/ return new Property("numberOfSubunits", "integer", "The number of linear sequences of nucleotides linked through phosphodiester bonds shall be described. Subunits would be strands of nucleic acids that are tightly associated typically through Watson-Crick base pairing. NOTE: If not specified in the reference source, the assumption is that there is 1 subunit.", 0, 1, numberOfSubunits); 1635 case -617269845: /*areaOfHybridisation*/ return new Property("areaOfHybridisation", "string", "The area of hybridisation shall be described if applicable for double stranded RNA or DNA. The number associated with the subunit followed by the number associated to the residue shall be specified in increasing order. The underscore ?? shall be used as separator as follows: ?Subunitnumber Residue?.", 0, 1, areaOfHybridisation); 1636 case -1526251938: /*oligoNucleotideType*/ return new Property("oligoNucleotideType", "CodeableConcept", "(TBC).", 0, 1, oligoNucleotideType); 1637 case -1867548732: /*subunit*/ return new Property("subunit", "", "Subunits are listed in order of decreasing length; sequences of the same length will be ordered by molecular weight; subunits that have identical sequences will be repeated multiple times.", 0, java.lang.Integer.MAX_VALUE, subunit); 1638 default: return super.getNamedProperty(_hash, _name, _checkValid); 1639 } 1640 1641 } 1642 1643 @Override 1644 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1645 switch (hash) { 1646 case 807711387: /*sequenceType*/ return this.sequenceType == null ? new Base[0] : new Base[] {this.sequenceType}; // CodeableConcept 1647 case -847111089: /*numberOfSubunits*/ return this.numberOfSubunits == null ? new Base[0] : new Base[] {this.numberOfSubunits}; // IntegerType 1648 case -617269845: /*areaOfHybridisation*/ return this.areaOfHybridisation == null ? new Base[0] : new Base[] {this.areaOfHybridisation}; // StringType 1649 case -1526251938: /*oligoNucleotideType*/ return this.oligoNucleotideType == null ? new Base[0] : new Base[] {this.oligoNucleotideType}; // CodeableConcept 1650 case -1867548732: /*subunit*/ return this.subunit == null ? new Base[0] : this.subunit.toArray(new Base[this.subunit.size()]); // SubstanceNucleicAcidSubunitComponent 1651 default: return super.getProperty(hash, name, checkValid); 1652 } 1653 1654 } 1655 1656 @Override 1657 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1658 switch (hash) { 1659 case 807711387: // sequenceType 1660 this.sequenceType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1661 return value; 1662 case -847111089: // numberOfSubunits 1663 this.numberOfSubunits = TypeConvertor.castToInteger(value); // IntegerType 1664 return value; 1665 case -617269845: // areaOfHybridisation 1666 this.areaOfHybridisation = TypeConvertor.castToString(value); // StringType 1667 return value; 1668 case -1526251938: // oligoNucleotideType 1669 this.oligoNucleotideType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1670 return value; 1671 case -1867548732: // subunit 1672 this.getSubunit().add((SubstanceNucleicAcidSubunitComponent) value); // SubstanceNucleicAcidSubunitComponent 1673 return value; 1674 default: return super.setProperty(hash, name, value); 1675 } 1676 1677 } 1678 1679 @Override 1680 public Base setProperty(String name, Base value) throws FHIRException { 1681 if (name.equals("sequenceType")) { 1682 this.sequenceType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1683 } else if (name.equals("numberOfSubunits")) { 1684 this.numberOfSubunits = TypeConvertor.castToInteger(value); // IntegerType 1685 } else if (name.equals("areaOfHybridisation")) { 1686 this.areaOfHybridisation = TypeConvertor.castToString(value); // StringType 1687 } else if (name.equals("oligoNucleotideType")) { 1688 this.oligoNucleotideType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1689 } else if (name.equals("subunit")) { 1690 this.getSubunit().add((SubstanceNucleicAcidSubunitComponent) value); 1691 } else 1692 return super.setProperty(name, value); 1693 return value; 1694 } 1695 1696 @Override 1697 public void removeChild(String name, Base value) throws FHIRException { 1698 if (name.equals("sequenceType")) { 1699 this.sequenceType = null; 1700 } else if (name.equals("numberOfSubunits")) { 1701 this.numberOfSubunits = null; 1702 } else if (name.equals("areaOfHybridisation")) { 1703 this.areaOfHybridisation = null; 1704 } else if (name.equals("oligoNucleotideType")) { 1705 this.oligoNucleotideType = null; 1706 } else if (name.equals("subunit")) { 1707 this.getSubunit().remove((SubstanceNucleicAcidSubunitComponent) value); 1708 } else 1709 super.removeChild(name, value); 1710 1711 } 1712 1713 @Override 1714 public Base makeProperty(int hash, String name) throws FHIRException { 1715 switch (hash) { 1716 case 807711387: return getSequenceType(); 1717 case -847111089: return getNumberOfSubunitsElement(); 1718 case -617269845: return getAreaOfHybridisationElement(); 1719 case -1526251938: return getOligoNucleotideType(); 1720 case -1867548732: return addSubunit(); 1721 default: return super.makeProperty(hash, name); 1722 } 1723 1724 } 1725 1726 @Override 1727 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1728 switch (hash) { 1729 case 807711387: /*sequenceType*/ return new String[] {"CodeableConcept"}; 1730 case -847111089: /*numberOfSubunits*/ return new String[] {"integer"}; 1731 case -617269845: /*areaOfHybridisation*/ return new String[] {"string"}; 1732 case -1526251938: /*oligoNucleotideType*/ return new String[] {"CodeableConcept"}; 1733 case -1867548732: /*subunit*/ return new String[] {}; 1734 default: return super.getTypesForProperty(hash, name); 1735 } 1736 1737 } 1738 1739 @Override 1740 public Base addChild(String name) throws FHIRException { 1741 if (name.equals("sequenceType")) { 1742 this.sequenceType = new CodeableConcept(); 1743 return this.sequenceType; 1744 } 1745 else if (name.equals("numberOfSubunits")) { 1746 throw new FHIRException("Cannot call addChild on a singleton property SubstanceNucleicAcid.numberOfSubunits"); 1747 } 1748 else if (name.equals("areaOfHybridisation")) { 1749 throw new FHIRException("Cannot call addChild on a singleton property SubstanceNucleicAcid.areaOfHybridisation"); 1750 } 1751 else if (name.equals("oligoNucleotideType")) { 1752 this.oligoNucleotideType = new CodeableConcept(); 1753 return this.oligoNucleotideType; 1754 } 1755 else if (name.equals("subunit")) { 1756 return addSubunit(); 1757 } 1758 else 1759 return super.addChild(name); 1760 } 1761 1762 public String fhirType() { 1763 return "SubstanceNucleicAcid"; 1764 1765 } 1766 1767 public SubstanceNucleicAcid copy() { 1768 SubstanceNucleicAcid dst = new SubstanceNucleicAcid(); 1769 copyValues(dst); 1770 return dst; 1771 } 1772 1773 public void copyValues(SubstanceNucleicAcid dst) { 1774 super.copyValues(dst); 1775 dst.sequenceType = sequenceType == null ? null : sequenceType.copy(); 1776 dst.numberOfSubunits = numberOfSubunits == null ? null : numberOfSubunits.copy(); 1777 dst.areaOfHybridisation = areaOfHybridisation == null ? null : areaOfHybridisation.copy(); 1778 dst.oligoNucleotideType = oligoNucleotideType == null ? null : oligoNucleotideType.copy(); 1779 if (subunit != null) { 1780 dst.subunit = new ArrayList<SubstanceNucleicAcidSubunitComponent>(); 1781 for (SubstanceNucleicAcidSubunitComponent i : subunit) 1782 dst.subunit.add(i.copy()); 1783 }; 1784 } 1785 1786 protected SubstanceNucleicAcid typedCopy() { 1787 return copy(); 1788 } 1789 1790 @Override 1791 public boolean equalsDeep(Base other_) { 1792 if (!super.equalsDeep(other_)) 1793 return false; 1794 if (!(other_ instanceof SubstanceNucleicAcid)) 1795 return false; 1796 SubstanceNucleicAcid o = (SubstanceNucleicAcid) other_; 1797 return compareDeep(sequenceType, o.sequenceType, true) && compareDeep(numberOfSubunits, o.numberOfSubunits, true) 1798 && compareDeep(areaOfHybridisation, o.areaOfHybridisation, true) && compareDeep(oligoNucleotideType, o.oligoNucleotideType, true) 1799 && compareDeep(subunit, o.subunit, true); 1800 } 1801 1802 @Override 1803 public boolean equalsShallow(Base other_) { 1804 if (!super.equalsShallow(other_)) 1805 return false; 1806 if (!(other_ instanceof SubstanceNucleicAcid)) 1807 return false; 1808 SubstanceNucleicAcid o = (SubstanceNucleicAcid) other_; 1809 return compareValues(numberOfSubunits, o.numberOfSubunits, true) && compareValues(areaOfHybridisation, o.areaOfHybridisation, true) 1810 ; 1811 } 1812 1813 public boolean isEmpty() { 1814 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequenceType, numberOfSubunits 1815 , areaOfHybridisation, oligoNucleotideType, subunit); 1816 } 1817 1818 @Override 1819 public ResourceType getResourceType() { 1820 return ResourceType.SubstanceNucleicAcid; 1821 } 1822 1823 1824} 1825