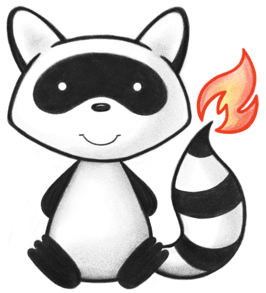
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Properties of a substance specific to it being a polymer. 052 */ 053@ResourceDef(name="SubstancePolymer", profile="http://hl7.org/fhir/StructureDefinition/SubstancePolymer") 054public class SubstancePolymer extends DomainResource { 055 056 @Block() 057 public static class SubstancePolymerMonomerSetComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Captures the type of ratio to the entire polymer, e.g. Monomer/Polymer ratio, SRU/Polymer Ratio. 060 */ 061 @Child(name = "ratioType", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="Captures the type of ratio to the entire polymer, e.g. Monomer/Polymer ratio, SRU/Polymer Ratio", formalDefinition="Captures the type of ratio to the entire polymer, e.g. Monomer/Polymer ratio, SRU/Polymer Ratio." ) 063 protected CodeableConcept ratioType; 064 065 /** 066 * The starting materials - monomer(s) used in the synthesis of the polymer. 067 */ 068 @Child(name = "startingMaterial", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 069 @Description(shortDefinition="The starting materials - monomer(s) used in the synthesis of the polymer", formalDefinition="The starting materials - monomer(s) used in the synthesis of the polymer." ) 070 protected List<SubstancePolymerMonomerSetStartingMaterialComponent> startingMaterial; 071 072 private static final long serialVersionUID = -933825014L; 073 074 /** 075 * Constructor 076 */ 077 public SubstancePolymerMonomerSetComponent() { 078 super(); 079 } 080 081 /** 082 * @return {@link #ratioType} (Captures the type of ratio to the entire polymer, e.g. Monomer/Polymer ratio, SRU/Polymer Ratio.) 083 */ 084 public CodeableConcept getRatioType() { 085 if (this.ratioType == null) 086 if (Configuration.errorOnAutoCreate()) 087 throw new Error("Attempt to auto-create SubstancePolymerMonomerSetComponent.ratioType"); 088 else if (Configuration.doAutoCreate()) 089 this.ratioType = new CodeableConcept(); // cc 090 return this.ratioType; 091 } 092 093 public boolean hasRatioType() { 094 return this.ratioType != null && !this.ratioType.isEmpty(); 095 } 096 097 /** 098 * @param value {@link #ratioType} (Captures the type of ratio to the entire polymer, e.g. Monomer/Polymer ratio, SRU/Polymer Ratio.) 099 */ 100 public SubstancePolymerMonomerSetComponent setRatioType(CodeableConcept value) { 101 this.ratioType = value; 102 return this; 103 } 104 105 /** 106 * @return {@link #startingMaterial} (The starting materials - monomer(s) used in the synthesis of the polymer.) 107 */ 108 public List<SubstancePolymerMonomerSetStartingMaterialComponent> getStartingMaterial() { 109 if (this.startingMaterial == null) 110 this.startingMaterial = new ArrayList<SubstancePolymerMonomerSetStartingMaterialComponent>(); 111 return this.startingMaterial; 112 } 113 114 /** 115 * @return Returns a reference to <code>this</code> for easy method chaining 116 */ 117 public SubstancePolymerMonomerSetComponent setStartingMaterial(List<SubstancePolymerMonomerSetStartingMaterialComponent> theStartingMaterial) { 118 this.startingMaterial = theStartingMaterial; 119 return this; 120 } 121 122 public boolean hasStartingMaterial() { 123 if (this.startingMaterial == null) 124 return false; 125 for (SubstancePolymerMonomerSetStartingMaterialComponent item : this.startingMaterial) 126 if (!item.isEmpty()) 127 return true; 128 return false; 129 } 130 131 public SubstancePolymerMonomerSetStartingMaterialComponent addStartingMaterial() { //3 132 SubstancePolymerMonomerSetStartingMaterialComponent t = new SubstancePolymerMonomerSetStartingMaterialComponent(); 133 if (this.startingMaterial == null) 134 this.startingMaterial = new ArrayList<SubstancePolymerMonomerSetStartingMaterialComponent>(); 135 this.startingMaterial.add(t); 136 return t; 137 } 138 139 public SubstancePolymerMonomerSetComponent addStartingMaterial(SubstancePolymerMonomerSetStartingMaterialComponent t) { //3 140 if (t == null) 141 return this; 142 if (this.startingMaterial == null) 143 this.startingMaterial = new ArrayList<SubstancePolymerMonomerSetStartingMaterialComponent>(); 144 this.startingMaterial.add(t); 145 return this; 146 } 147 148 /** 149 * @return The first repetition of repeating field {@link #startingMaterial}, creating it if it does not already exist {3} 150 */ 151 public SubstancePolymerMonomerSetStartingMaterialComponent getStartingMaterialFirstRep() { 152 if (getStartingMaterial().isEmpty()) { 153 addStartingMaterial(); 154 } 155 return getStartingMaterial().get(0); 156 } 157 158 protected void listChildren(List<Property> children) { 159 super.listChildren(children); 160 children.add(new Property("ratioType", "CodeableConcept", "Captures the type of ratio to the entire polymer, e.g. Monomer/Polymer ratio, SRU/Polymer Ratio.", 0, 1, ratioType)); 161 children.add(new Property("startingMaterial", "", "The starting materials - monomer(s) used in the synthesis of the polymer.", 0, java.lang.Integer.MAX_VALUE, startingMaterial)); 162 } 163 164 @Override 165 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 166 switch (_hash) { 167 case 344937957: /*ratioType*/ return new Property("ratioType", "CodeableConcept", "Captures the type of ratio to the entire polymer, e.g. Monomer/Polymer ratio, SRU/Polymer Ratio.", 0, 1, ratioType); 168 case 442919303: /*startingMaterial*/ return new Property("startingMaterial", "", "The starting materials - monomer(s) used in the synthesis of the polymer.", 0, java.lang.Integer.MAX_VALUE, startingMaterial); 169 default: return super.getNamedProperty(_hash, _name, _checkValid); 170 } 171 172 } 173 174 @Override 175 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 176 switch (hash) { 177 case 344937957: /*ratioType*/ return this.ratioType == null ? new Base[0] : new Base[] {this.ratioType}; // CodeableConcept 178 case 442919303: /*startingMaterial*/ return this.startingMaterial == null ? new Base[0] : this.startingMaterial.toArray(new Base[this.startingMaterial.size()]); // SubstancePolymerMonomerSetStartingMaterialComponent 179 default: return super.getProperty(hash, name, checkValid); 180 } 181 182 } 183 184 @Override 185 public Base setProperty(int hash, String name, Base value) throws FHIRException { 186 switch (hash) { 187 case 344937957: // ratioType 188 this.ratioType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 189 return value; 190 case 442919303: // startingMaterial 191 this.getStartingMaterial().add((SubstancePolymerMonomerSetStartingMaterialComponent) value); // SubstancePolymerMonomerSetStartingMaterialComponent 192 return value; 193 default: return super.setProperty(hash, name, value); 194 } 195 196 } 197 198 @Override 199 public Base setProperty(String name, Base value) throws FHIRException { 200 if (name.equals("ratioType")) { 201 this.ratioType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 202 } else if (name.equals("startingMaterial")) { 203 this.getStartingMaterial().add((SubstancePolymerMonomerSetStartingMaterialComponent) value); 204 } else 205 return super.setProperty(name, value); 206 return value; 207 } 208 209 @Override 210 public void removeChild(String name, Base value) throws FHIRException { 211 if (name.equals("ratioType")) { 212 this.ratioType = null; 213 } else if (name.equals("startingMaterial")) { 214 this.getStartingMaterial().remove((SubstancePolymerMonomerSetStartingMaterialComponent) value); 215 } else 216 super.removeChild(name, value); 217 218 } 219 220 @Override 221 public Base makeProperty(int hash, String name) throws FHIRException { 222 switch (hash) { 223 case 344937957: return getRatioType(); 224 case 442919303: return addStartingMaterial(); 225 default: return super.makeProperty(hash, name); 226 } 227 228 } 229 230 @Override 231 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 232 switch (hash) { 233 case 344937957: /*ratioType*/ return new String[] {"CodeableConcept"}; 234 case 442919303: /*startingMaterial*/ return new String[] {}; 235 default: return super.getTypesForProperty(hash, name); 236 } 237 238 } 239 240 @Override 241 public Base addChild(String name) throws FHIRException { 242 if (name.equals("ratioType")) { 243 this.ratioType = new CodeableConcept(); 244 return this.ratioType; 245 } 246 else if (name.equals("startingMaterial")) { 247 return addStartingMaterial(); 248 } 249 else 250 return super.addChild(name); 251 } 252 253 public SubstancePolymerMonomerSetComponent copy() { 254 SubstancePolymerMonomerSetComponent dst = new SubstancePolymerMonomerSetComponent(); 255 copyValues(dst); 256 return dst; 257 } 258 259 public void copyValues(SubstancePolymerMonomerSetComponent dst) { 260 super.copyValues(dst); 261 dst.ratioType = ratioType == null ? null : ratioType.copy(); 262 if (startingMaterial != null) { 263 dst.startingMaterial = new ArrayList<SubstancePolymerMonomerSetStartingMaterialComponent>(); 264 for (SubstancePolymerMonomerSetStartingMaterialComponent i : startingMaterial) 265 dst.startingMaterial.add(i.copy()); 266 }; 267 } 268 269 @Override 270 public boolean equalsDeep(Base other_) { 271 if (!super.equalsDeep(other_)) 272 return false; 273 if (!(other_ instanceof SubstancePolymerMonomerSetComponent)) 274 return false; 275 SubstancePolymerMonomerSetComponent o = (SubstancePolymerMonomerSetComponent) other_; 276 return compareDeep(ratioType, o.ratioType, true) && compareDeep(startingMaterial, o.startingMaterial, true) 277 ; 278 } 279 280 @Override 281 public boolean equalsShallow(Base other_) { 282 if (!super.equalsShallow(other_)) 283 return false; 284 if (!(other_ instanceof SubstancePolymerMonomerSetComponent)) 285 return false; 286 SubstancePolymerMonomerSetComponent o = (SubstancePolymerMonomerSetComponent) other_; 287 return true; 288 } 289 290 public boolean isEmpty() { 291 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(ratioType, startingMaterial 292 ); 293 } 294 295 public String fhirType() { 296 return "SubstancePolymer.monomerSet"; 297 298 } 299 300 } 301 302 @Block() 303 public static class SubstancePolymerMonomerSetStartingMaterialComponent extends BackboneElement implements IBaseBackboneElement { 304 /** 305 * The type of substance for this starting material. 306 */ 307 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 308 @Description(shortDefinition="The type of substance for this starting material", formalDefinition="The type of substance for this starting material." ) 309 protected CodeableConcept code; 310 311 /** 312 * Substance high level category, e.g. chemical substance. 313 */ 314 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 315 @Description(shortDefinition="Substance high level category, e.g. chemical substance", formalDefinition="Substance high level category, e.g. chemical substance." ) 316 protected CodeableConcept category; 317 318 /** 319 * Used to specify whether the attribute described is a defining element for the unique identification of the polymer. 320 */ 321 @Child(name = "isDefining", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=true) 322 @Description(shortDefinition="Used to specify whether the attribute described is a defining element for the unique identification of the polymer", formalDefinition="Used to specify whether the attribute described is a defining element for the unique identification of the polymer." ) 323 protected BooleanType isDefining; 324 325 /** 326 * A percentage. 327 */ 328 @Child(name = "amount", type = {Quantity.class}, order=4, min=0, max=1, modifier=false, summary=true) 329 @Description(shortDefinition="A percentage", formalDefinition="A percentage." ) 330 protected Quantity amount; 331 332 private static final long serialVersionUID = -1199515148L; 333 334 /** 335 * Constructor 336 */ 337 public SubstancePolymerMonomerSetStartingMaterialComponent() { 338 super(); 339 } 340 341 /** 342 * @return {@link #code} (The type of substance for this starting material.) 343 */ 344 public CodeableConcept getCode() { 345 if (this.code == null) 346 if (Configuration.errorOnAutoCreate()) 347 throw new Error("Attempt to auto-create SubstancePolymerMonomerSetStartingMaterialComponent.code"); 348 else if (Configuration.doAutoCreate()) 349 this.code = new CodeableConcept(); // cc 350 return this.code; 351 } 352 353 public boolean hasCode() { 354 return this.code != null && !this.code.isEmpty(); 355 } 356 357 /** 358 * @param value {@link #code} (The type of substance for this starting material.) 359 */ 360 public SubstancePolymerMonomerSetStartingMaterialComponent setCode(CodeableConcept value) { 361 this.code = value; 362 return this; 363 } 364 365 /** 366 * @return {@link #category} (Substance high level category, e.g. chemical substance.) 367 */ 368 public CodeableConcept getCategory() { 369 if (this.category == null) 370 if (Configuration.errorOnAutoCreate()) 371 throw new Error("Attempt to auto-create SubstancePolymerMonomerSetStartingMaterialComponent.category"); 372 else if (Configuration.doAutoCreate()) 373 this.category = new CodeableConcept(); // cc 374 return this.category; 375 } 376 377 public boolean hasCategory() { 378 return this.category != null && !this.category.isEmpty(); 379 } 380 381 /** 382 * @param value {@link #category} (Substance high level category, e.g. chemical substance.) 383 */ 384 public SubstancePolymerMonomerSetStartingMaterialComponent setCategory(CodeableConcept value) { 385 this.category = value; 386 return this; 387 } 388 389 /** 390 * @return {@link #isDefining} (Used to specify whether the attribute described is a defining element for the unique identification of the polymer.). This is the underlying object with id, value and extensions. The accessor "getIsDefining" gives direct access to the value 391 */ 392 public BooleanType getIsDefiningElement() { 393 if (this.isDefining == null) 394 if (Configuration.errorOnAutoCreate()) 395 throw new Error("Attempt to auto-create SubstancePolymerMonomerSetStartingMaterialComponent.isDefining"); 396 else if (Configuration.doAutoCreate()) 397 this.isDefining = new BooleanType(); // bb 398 return this.isDefining; 399 } 400 401 public boolean hasIsDefiningElement() { 402 return this.isDefining != null && !this.isDefining.isEmpty(); 403 } 404 405 public boolean hasIsDefining() { 406 return this.isDefining != null && !this.isDefining.isEmpty(); 407 } 408 409 /** 410 * @param value {@link #isDefining} (Used to specify whether the attribute described is a defining element for the unique identification of the polymer.). This is the underlying object with id, value and extensions. The accessor "getIsDefining" gives direct access to the value 411 */ 412 public SubstancePolymerMonomerSetStartingMaterialComponent setIsDefiningElement(BooleanType value) { 413 this.isDefining = value; 414 return this; 415 } 416 417 /** 418 * @return Used to specify whether the attribute described is a defining element for the unique identification of the polymer. 419 */ 420 public boolean getIsDefining() { 421 return this.isDefining == null || this.isDefining.isEmpty() ? false : this.isDefining.getValue(); 422 } 423 424 /** 425 * @param value Used to specify whether the attribute described is a defining element for the unique identification of the polymer. 426 */ 427 public SubstancePolymerMonomerSetStartingMaterialComponent setIsDefining(boolean value) { 428 if (this.isDefining == null) 429 this.isDefining = new BooleanType(); 430 this.isDefining.setValue(value); 431 return this; 432 } 433 434 /** 435 * @return {@link #amount} (A percentage.) 436 */ 437 public Quantity getAmount() { 438 if (this.amount == null) 439 if (Configuration.errorOnAutoCreate()) 440 throw new Error("Attempt to auto-create SubstancePolymerMonomerSetStartingMaterialComponent.amount"); 441 else if (Configuration.doAutoCreate()) 442 this.amount = new Quantity(); // cc 443 return this.amount; 444 } 445 446 public boolean hasAmount() { 447 return this.amount != null && !this.amount.isEmpty(); 448 } 449 450 /** 451 * @param value {@link #amount} (A percentage.) 452 */ 453 public SubstancePolymerMonomerSetStartingMaterialComponent setAmount(Quantity value) { 454 this.amount = value; 455 return this; 456 } 457 458 protected void listChildren(List<Property> children) { 459 super.listChildren(children); 460 children.add(new Property("code", "CodeableConcept", "The type of substance for this starting material.", 0, 1, code)); 461 children.add(new Property("category", "CodeableConcept", "Substance high level category, e.g. chemical substance.", 0, 1, category)); 462 children.add(new Property("isDefining", "boolean", "Used to specify whether the attribute described is a defining element for the unique identification of the polymer.", 0, 1, isDefining)); 463 children.add(new Property("amount", "Quantity", "A percentage.", 0, 1, amount)); 464 } 465 466 @Override 467 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 468 switch (_hash) { 469 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The type of substance for this starting material.", 0, 1, code); 470 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Substance high level category, e.g. chemical substance.", 0, 1, category); 471 case -141812990: /*isDefining*/ return new Property("isDefining", "boolean", "Used to specify whether the attribute described is a defining element for the unique identification of the polymer.", 0, 1, isDefining); 472 case -1413853096: /*amount*/ return new Property("amount", "Quantity", "A percentage.", 0, 1, amount); 473 default: return super.getNamedProperty(_hash, _name, _checkValid); 474 } 475 476 } 477 478 @Override 479 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 480 switch (hash) { 481 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 482 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 483 case -141812990: /*isDefining*/ return this.isDefining == null ? new Base[0] : new Base[] {this.isDefining}; // BooleanType 484 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Quantity 485 default: return super.getProperty(hash, name, checkValid); 486 } 487 488 } 489 490 @Override 491 public Base setProperty(int hash, String name, Base value) throws FHIRException { 492 switch (hash) { 493 case 3059181: // code 494 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 495 return value; 496 case 50511102: // category 497 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 498 return value; 499 case -141812990: // isDefining 500 this.isDefining = TypeConvertor.castToBoolean(value); // BooleanType 501 return value; 502 case -1413853096: // amount 503 this.amount = TypeConvertor.castToQuantity(value); // Quantity 504 return value; 505 default: return super.setProperty(hash, name, value); 506 } 507 508 } 509 510 @Override 511 public Base setProperty(String name, Base value) throws FHIRException { 512 if (name.equals("code")) { 513 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 514 } else if (name.equals("category")) { 515 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 516 } else if (name.equals("isDefining")) { 517 this.isDefining = TypeConvertor.castToBoolean(value); // BooleanType 518 } else if (name.equals("amount")) { 519 this.amount = TypeConvertor.castToQuantity(value); // Quantity 520 } else 521 return super.setProperty(name, value); 522 return value; 523 } 524 525 @Override 526 public void removeChild(String name, Base value) throws FHIRException { 527 if (name.equals("code")) { 528 this.code = null; 529 } else if (name.equals("category")) { 530 this.category = null; 531 } else if (name.equals("isDefining")) { 532 this.isDefining = null; 533 } else if (name.equals("amount")) { 534 this.amount = null; 535 } else 536 super.removeChild(name, value); 537 538 } 539 540 @Override 541 public Base makeProperty(int hash, String name) throws FHIRException { 542 switch (hash) { 543 case 3059181: return getCode(); 544 case 50511102: return getCategory(); 545 case -141812990: return getIsDefiningElement(); 546 case -1413853096: return getAmount(); 547 default: return super.makeProperty(hash, name); 548 } 549 550 } 551 552 @Override 553 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 554 switch (hash) { 555 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 556 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 557 case -141812990: /*isDefining*/ return new String[] {"boolean"}; 558 case -1413853096: /*amount*/ return new String[] {"Quantity"}; 559 default: return super.getTypesForProperty(hash, name); 560 } 561 562 } 563 564 @Override 565 public Base addChild(String name) throws FHIRException { 566 if (name.equals("code")) { 567 this.code = new CodeableConcept(); 568 return this.code; 569 } 570 else if (name.equals("category")) { 571 this.category = new CodeableConcept(); 572 return this.category; 573 } 574 else if (name.equals("isDefining")) { 575 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.monomerSet.startingMaterial.isDefining"); 576 } 577 else if (name.equals("amount")) { 578 this.amount = new Quantity(); 579 return this.amount; 580 } 581 else 582 return super.addChild(name); 583 } 584 585 public SubstancePolymerMonomerSetStartingMaterialComponent copy() { 586 SubstancePolymerMonomerSetStartingMaterialComponent dst = new SubstancePolymerMonomerSetStartingMaterialComponent(); 587 copyValues(dst); 588 return dst; 589 } 590 591 public void copyValues(SubstancePolymerMonomerSetStartingMaterialComponent dst) { 592 super.copyValues(dst); 593 dst.code = code == null ? null : code.copy(); 594 dst.category = category == null ? null : category.copy(); 595 dst.isDefining = isDefining == null ? null : isDefining.copy(); 596 dst.amount = amount == null ? null : amount.copy(); 597 } 598 599 @Override 600 public boolean equalsDeep(Base other_) { 601 if (!super.equalsDeep(other_)) 602 return false; 603 if (!(other_ instanceof SubstancePolymerMonomerSetStartingMaterialComponent)) 604 return false; 605 SubstancePolymerMonomerSetStartingMaterialComponent o = (SubstancePolymerMonomerSetStartingMaterialComponent) other_; 606 return compareDeep(code, o.code, true) && compareDeep(category, o.category, true) && compareDeep(isDefining, o.isDefining, true) 607 && compareDeep(amount, o.amount, true); 608 } 609 610 @Override 611 public boolean equalsShallow(Base other_) { 612 if (!super.equalsShallow(other_)) 613 return false; 614 if (!(other_ instanceof SubstancePolymerMonomerSetStartingMaterialComponent)) 615 return false; 616 SubstancePolymerMonomerSetStartingMaterialComponent o = (SubstancePolymerMonomerSetStartingMaterialComponent) other_; 617 return compareValues(isDefining, o.isDefining, true); 618 } 619 620 public boolean isEmpty() { 621 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, category, isDefining 622 , amount); 623 } 624 625 public String fhirType() { 626 return "SubstancePolymer.monomerSet.startingMaterial"; 627 628 } 629 630 } 631 632 @Block() 633 public static class SubstancePolymerRepeatComponent extends BackboneElement implements IBaseBackboneElement { 634 /** 635 * A representation of an (average) molecular formula from a polymer. 636 */ 637 @Child(name = "averageMolecularFormula", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 638 @Description(shortDefinition="A representation of an (average) molecular formula from a polymer", formalDefinition="A representation of an (average) molecular formula from a polymer." ) 639 protected StringType averageMolecularFormula; 640 641 /** 642 * How the quantitative amount of Structural Repeat Units is captured (e.g. Exact, Numeric, Average). 643 */ 644 @Child(name = "repeatUnitAmountType", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 645 @Description(shortDefinition="How the quantitative amount of Structural Repeat Units is captured (e.g. Exact, Numeric, Average)", formalDefinition="How the quantitative amount of Structural Repeat Units is captured (e.g. Exact, Numeric, Average)." ) 646 protected CodeableConcept repeatUnitAmountType; 647 648 /** 649 * An SRU - Structural Repeat Unit. 650 */ 651 @Child(name = "repeatUnit", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 652 @Description(shortDefinition="An SRU - Structural Repeat Unit", formalDefinition="An SRU - Structural Repeat Unit." ) 653 protected List<SubstancePolymerRepeatRepeatUnitComponent> repeatUnit; 654 655 private static final long serialVersionUID = -366644176L; 656 657 /** 658 * Constructor 659 */ 660 public SubstancePolymerRepeatComponent() { 661 super(); 662 } 663 664 /** 665 * @return {@link #averageMolecularFormula} (A representation of an (average) molecular formula from a polymer.). This is the underlying object with id, value and extensions. The accessor "getAverageMolecularFormula" gives direct access to the value 666 */ 667 public StringType getAverageMolecularFormulaElement() { 668 if (this.averageMolecularFormula == null) 669 if (Configuration.errorOnAutoCreate()) 670 throw new Error("Attempt to auto-create SubstancePolymerRepeatComponent.averageMolecularFormula"); 671 else if (Configuration.doAutoCreate()) 672 this.averageMolecularFormula = new StringType(); // bb 673 return this.averageMolecularFormula; 674 } 675 676 public boolean hasAverageMolecularFormulaElement() { 677 return this.averageMolecularFormula != null && !this.averageMolecularFormula.isEmpty(); 678 } 679 680 public boolean hasAverageMolecularFormula() { 681 return this.averageMolecularFormula != null && !this.averageMolecularFormula.isEmpty(); 682 } 683 684 /** 685 * @param value {@link #averageMolecularFormula} (A representation of an (average) molecular formula from a polymer.). This is the underlying object with id, value and extensions. The accessor "getAverageMolecularFormula" gives direct access to the value 686 */ 687 public SubstancePolymerRepeatComponent setAverageMolecularFormulaElement(StringType value) { 688 this.averageMolecularFormula = value; 689 return this; 690 } 691 692 /** 693 * @return A representation of an (average) molecular formula from a polymer. 694 */ 695 public String getAverageMolecularFormula() { 696 return this.averageMolecularFormula == null ? null : this.averageMolecularFormula.getValue(); 697 } 698 699 /** 700 * @param value A representation of an (average) molecular formula from a polymer. 701 */ 702 public SubstancePolymerRepeatComponent setAverageMolecularFormula(String value) { 703 if (Utilities.noString(value)) 704 this.averageMolecularFormula = null; 705 else { 706 if (this.averageMolecularFormula == null) 707 this.averageMolecularFormula = new StringType(); 708 this.averageMolecularFormula.setValue(value); 709 } 710 return this; 711 } 712 713 /** 714 * @return {@link #repeatUnitAmountType} (How the quantitative amount of Structural Repeat Units is captured (e.g. Exact, Numeric, Average).) 715 */ 716 public CodeableConcept getRepeatUnitAmountType() { 717 if (this.repeatUnitAmountType == null) 718 if (Configuration.errorOnAutoCreate()) 719 throw new Error("Attempt to auto-create SubstancePolymerRepeatComponent.repeatUnitAmountType"); 720 else if (Configuration.doAutoCreate()) 721 this.repeatUnitAmountType = new CodeableConcept(); // cc 722 return this.repeatUnitAmountType; 723 } 724 725 public boolean hasRepeatUnitAmountType() { 726 return this.repeatUnitAmountType != null && !this.repeatUnitAmountType.isEmpty(); 727 } 728 729 /** 730 * @param value {@link #repeatUnitAmountType} (How the quantitative amount of Structural Repeat Units is captured (e.g. Exact, Numeric, Average).) 731 */ 732 public SubstancePolymerRepeatComponent setRepeatUnitAmountType(CodeableConcept value) { 733 this.repeatUnitAmountType = value; 734 return this; 735 } 736 737 /** 738 * @return {@link #repeatUnit} (An SRU - Structural Repeat Unit.) 739 */ 740 public List<SubstancePolymerRepeatRepeatUnitComponent> getRepeatUnit() { 741 if (this.repeatUnit == null) 742 this.repeatUnit = new ArrayList<SubstancePolymerRepeatRepeatUnitComponent>(); 743 return this.repeatUnit; 744 } 745 746 /** 747 * @return Returns a reference to <code>this</code> for easy method chaining 748 */ 749 public SubstancePolymerRepeatComponent setRepeatUnit(List<SubstancePolymerRepeatRepeatUnitComponent> theRepeatUnit) { 750 this.repeatUnit = theRepeatUnit; 751 return this; 752 } 753 754 public boolean hasRepeatUnit() { 755 if (this.repeatUnit == null) 756 return false; 757 for (SubstancePolymerRepeatRepeatUnitComponent item : this.repeatUnit) 758 if (!item.isEmpty()) 759 return true; 760 return false; 761 } 762 763 public SubstancePolymerRepeatRepeatUnitComponent addRepeatUnit() { //3 764 SubstancePolymerRepeatRepeatUnitComponent t = new SubstancePolymerRepeatRepeatUnitComponent(); 765 if (this.repeatUnit == null) 766 this.repeatUnit = new ArrayList<SubstancePolymerRepeatRepeatUnitComponent>(); 767 this.repeatUnit.add(t); 768 return t; 769 } 770 771 public SubstancePolymerRepeatComponent addRepeatUnit(SubstancePolymerRepeatRepeatUnitComponent t) { //3 772 if (t == null) 773 return this; 774 if (this.repeatUnit == null) 775 this.repeatUnit = new ArrayList<SubstancePolymerRepeatRepeatUnitComponent>(); 776 this.repeatUnit.add(t); 777 return this; 778 } 779 780 /** 781 * @return The first repetition of repeating field {@link #repeatUnit}, creating it if it does not already exist {3} 782 */ 783 public SubstancePolymerRepeatRepeatUnitComponent getRepeatUnitFirstRep() { 784 if (getRepeatUnit().isEmpty()) { 785 addRepeatUnit(); 786 } 787 return getRepeatUnit().get(0); 788 } 789 790 protected void listChildren(List<Property> children) { 791 super.listChildren(children); 792 children.add(new Property("averageMolecularFormula", "string", "A representation of an (average) molecular formula from a polymer.", 0, 1, averageMolecularFormula)); 793 children.add(new Property("repeatUnitAmountType", "CodeableConcept", "How the quantitative amount of Structural Repeat Units is captured (e.g. Exact, Numeric, Average).", 0, 1, repeatUnitAmountType)); 794 children.add(new Property("repeatUnit", "", "An SRU - Structural Repeat Unit.", 0, java.lang.Integer.MAX_VALUE, repeatUnit)); 795 } 796 797 @Override 798 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 799 switch (_hash) { 800 case 111461715: /*averageMolecularFormula*/ return new Property("averageMolecularFormula", "string", "A representation of an (average) molecular formula from a polymer.", 0, 1, averageMolecularFormula); 801 case -1994025263: /*repeatUnitAmountType*/ return new Property("repeatUnitAmountType", "CodeableConcept", "How the quantitative amount of Structural Repeat Units is captured (e.g. Exact, Numeric, Average).", 0, 1, repeatUnitAmountType); 802 case 1159607743: /*repeatUnit*/ return new Property("repeatUnit", "", "An SRU - Structural Repeat Unit.", 0, java.lang.Integer.MAX_VALUE, repeatUnit); 803 default: return super.getNamedProperty(_hash, _name, _checkValid); 804 } 805 806 } 807 808 @Override 809 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 810 switch (hash) { 811 case 111461715: /*averageMolecularFormula*/ return this.averageMolecularFormula == null ? new Base[0] : new Base[] {this.averageMolecularFormula}; // StringType 812 case -1994025263: /*repeatUnitAmountType*/ return this.repeatUnitAmountType == null ? new Base[0] : new Base[] {this.repeatUnitAmountType}; // CodeableConcept 813 case 1159607743: /*repeatUnit*/ return this.repeatUnit == null ? new Base[0] : this.repeatUnit.toArray(new Base[this.repeatUnit.size()]); // SubstancePolymerRepeatRepeatUnitComponent 814 default: return super.getProperty(hash, name, checkValid); 815 } 816 817 } 818 819 @Override 820 public Base setProperty(int hash, String name, Base value) throws FHIRException { 821 switch (hash) { 822 case 111461715: // averageMolecularFormula 823 this.averageMolecularFormula = TypeConvertor.castToString(value); // StringType 824 return value; 825 case -1994025263: // repeatUnitAmountType 826 this.repeatUnitAmountType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 827 return value; 828 case 1159607743: // repeatUnit 829 this.getRepeatUnit().add((SubstancePolymerRepeatRepeatUnitComponent) value); // SubstancePolymerRepeatRepeatUnitComponent 830 return value; 831 default: return super.setProperty(hash, name, value); 832 } 833 834 } 835 836 @Override 837 public Base setProperty(String name, Base value) throws FHIRException { 838 if (name.equals("averageMolecularFormula")) { 839 this.averageMolecularFormula = TypeConvertor.castToString(value); // StringType 840 } else if (name.equals("repeatUnitAmountType")) { 841 this.repeatUnitAmountType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 842 } else if (name.equals("repeatUnit")) { 843 this.getRepeatUnit().add((SubstancePolymerRepeatRepeatUnitComponent) value); 844 } else 845 return super.setProperty(name, value); 846 return value; 847 } 848 849 @Override 850 public void removeChild(String name, Base value) throws FHIRException { 851 if (name.equals("averageMolecularFormula")) { 852 this.averageMolecularFormula = null; 853 } else if (name.equals("repeatUnitAmountType")) { 854 this.repeatUnitAmountType = null; 855 } else if (name.equals("repeatUnit")) { 856 this.getRepeatUnit().remove((SubstancePolymerRepeatRepeatUnitComponent) value); 857 } else 858 super.removeChild(name, value); 859 860 } 861 862 @Override 863 public Base makeProperty(int hash, String name) throws FHIRException { 864 switch (hash) { 865 case 111461715: return getAverageMolecularFormulaElement(); 866 case -1994025263: return getRepeatUnitAmountType(); 867 case 1159607743: return addRepeatUnit(); 868 default: return super.makeProperty(hash, name); 869 } 870 871 } 872 873 @Override 874 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 875 switch (hash) { 876 case 111461715: /*averageMolecularFormula*/ return new String[] {"string"}; 877 case -1994025263: /*repeatUnitAmountType*/ return new String[] {"CodeableConcept"}; 878 case 1159607743: /*repeatUnit*/ return new String[] {}; 879 default: return super.getTypesForProperty(hash, name); 880 } 881 882 } 883 884 @Override 885 public Base addChild(String name) throws FHIRException { 886 if (name.equals("averageMolecularFormula")) { 887 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.repeat.averageMolecularFormula"); 888 } 889 else if (name.equals("repeatUnitAmountType")) { 890 this.repeatUnitAmountType = new CodeableConcept(); 891 return this.repeatUnitAmountType; 892 } 893 else if (name.equals("repeatUnit")) { 894 return addRepeatUnit(); 895 } 896 else 897 return super.addChild(name); 898 } 899 900 public SubstancePolymerRepeatComponent copy() { 901 SubstancePolymerRepeatComponent dst = new SubstancePolymerRepeatComponent(); 902 copyValues(dst); 903 return dst; 904 } 905 906 public void copyValues(SubstancePolymerRepeatComponent dst) { 907 super.copyValues(dst); 908 dst.averageMolecularFormula = averageMolecularFormula == null ? null : averageMolecularFormula.copy(); 909 dst.repeatUnitAmountType = repeatUnitAmountType == null ? null : repeatUnitAmountType.copy(); 910 if (repeatUnit != null) { 911 dst.repeatUnit = new ArrayList<SubstancePolymerRepeatRepeatUnitComponent>(); 912 for (SubstancePolymerRepeatRepeatUnitComponent i : repeatUnit) 913 dst.repeatUnit.add(i.copy()); 914 }; 915 } 916 917 @Override 918 public boolean equalsDeep(Base other_) { 919 if (!super.equalsDeep(other_)) 920 return false; 921 if (!(other_ instanceof SubstancePolymerRepeatComponent)) 922 return false; 923 SubstancePolymerRepeatComponent o = (SubstancePolymerRepeatComponent) other_; 924 return compareDeep(averageMolecularFormula, o.averageMolecularFormula, true) && compareDeep(repeatUnitAmountType, o.repeatUnitAmountType, true) 925 && compareDeep(repeatUnit, o.repeatUnit, true); 926 } 927 928 @Override 929 public boolean equalsShallow(Base other_) { 930 if (!super.equalsShallow(other_)) 931 return false; 932 if (!(other_ instanceof SubstancePolymerRepeatComponent)) 933 return false; 934 SubstancePolymerRepeatComponent o = (SubstancePolymerRepeatComponent) other_; 935 return compareValues(averageMolecularFormula, o.averageMolecularFormula, true); 936 } 937 938 public boolean isEmpty() { 939 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(averageMolecularFormula, repeatUnitAmountType 940 , repeatUnit); 941 } 942 943 public String fhirType() { 944 return "SubstancePolymer.repeat"; 945 946 } 947 948 } 949 950 @Block() 951 public static class SubstancePolymerRepeatRepeatUnitComponent extends BackboneElement implements IBaseBackboneElement { 952 /** 953 * Structural repeat units are essential elements for defining polymers. 954 */ 955 @Child(name = "unit", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 956 @Description(shortDefinition="Structural repeat units are essential elements for defining polymers", formalDefinition="Structural repeat units are essential elements for defining polymers." ) 957 protected StringType unit; 958 959 /** 960 * The orientation of the polymerisation, e.g. head-tail, head-head, random. 961 */ 962 @Child(name = "orientation", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 963 @Description(shortDefinition="The orientation of the polymerisation, e.g. head-tail, head-head, random", formalDefinition="The orientation of the polymerisation, e.g. head-tail, head-head, random." ) 964 protected CodeableConcept orientation; 965 966 /** 967 * Number of repeats of this unit. 968 */ 969 @Child(name = "amount", type = {IntegerType.class}, order=3, min=0, max=1, modifier=false, summary=true) 970 @Description(shortDefinition="Number of repeats of this unit", formalDefinition="Number of repeats of this unit." ) 971 protected IntegerType amount; 972 973 /** 974 * Applies to homopolymer and block co-polymers where the degree of polymerisation within a block can be described. 975 */ 976 @Child(name = "degreeOfPolymerisation", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 977 @Description(shortDefinition="Applies to homopolymer and block co-polymers where the degree of polymerisation within a block can be described", formalDefinition="Applies to homopolymer and block co-polymers where the degree of polymerisation within a block can be described." ) 978 protected List<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent> degreeOfPolymerisation; 979 980 /** 981 * A graphical structure for this SRU. 982 */ 983 @Child(name = "structuralRepresentation", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 984 @Description(shortDefinition="A graphical structure for this SRU", formalDefinition="A graphical structure for this SRU." ) 985 protected List<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent> structuralRepresentation; 986 987 private static final long serialVersionUID = 727054044L; 988 989 /** 990 * Constructor 991 */ 992 public SubstancePolymerRepeatRepeatUnitComponent() { 993 super(); 994 } 995 996 /** 997 * @return {@link #unit} (Structural repeat units are essential elements for defining polymers.). This is the underlying object with id, value and extensions. The accessor "getUnit" gives direct access to the value 998 */ 999 public StringType getUnitElement() { 1000 if (this.unit == null) 1001 if (Configuration.errorOnAutoCreate()) 1002 throw new Error("Attempt to auto-create SubstancePolymerRepeatRepeatUnitComponent.unit"); 1003 else if (Configuration.doAutoCreate()) 1004 this.unit = new StringType(); // bb 1005 return this.unit; 1006 } 1007 1008 public boolean hasUnitElement() { 1009 return this.unit != null && !this.unit.isEmpty(); 1010 } 1011 1012 public boolean hasUnit() { 1013 return this.unit != null && !this.unit.isEmpty(); 1014 } 1015 1016 /** 1017 * @param value {@link #unit} (Structural repeat units are essential elements for defining polymers.). This is the underlying object with id, value and extensions. The accessor "getUnit" gives direct access to the value 1018 */ 1019 public SubstancePolymerRepeatRepeatUnitComponent setUnitElement(StringType value) { 1020 this.unit = value; 1021 return this; 1022 } 1023 1024 /** 1025 * @return Structural repeat units are essential elements for defining polymers. 1026 */ 1027 public String getUnit() { 1028 return this.unit == null ? null : this.unit.getValue(); 1029 } 1030 1031 /** 1032 * @param value Structural repeat units are essential elements for defining polymers. 1033 */ 1034 public SubstancePolymerRepeatRepeatUnitComponent setUnit(String value) { 1035 if (Utilities.noString(value)) 1036 this.unit = null; 1037 else { 1038 if (this.unit == null) 1039 this.unit = new StringType(); 1040 this.unit.setValue(value); 1041 } 1042 return this; 1043 } 1044 1045 /** 1046 * @return {@link #orientation} (The orientation of the polymerisation, e.g. head-tail, head-head, random.) 1047 */ 1048 public CodeableConcept getOrientation() { 1049 if (this.orientation == null) 1050 if (Configuration.errorOnAutoCreate()) 1051 throw new Error("Attempt to auto-create SubstancePolymerRepeatRepeatUnitComponent.orientation"); 1052 else if (Configuration.doAutoCreate()) 1053 this.orientation = new CodeableConcept(); // cc 1054 return this.orientation; 1055 } 1056 1057 public boolean hasOrientation() { 1058 return this.orientation != null && !this.orientation.isEmpty(); 1059 } 1060 1061 /** 1062 * @param value {@link #orientation} (The orientation of the polymerisation, e.g. head-tail, head-head, random.) 1063 */ 1064 public SubstancePolymerRepeatRepeatUnitComponent setOrientation(CodeableConcept value) { 1065 this.orientation = value; 1066 return this; 1067 } 1068 1069 /** 1070 * @return {@link #amount} (Number of repeats of this unit.). This is the underlying object with id, value and extensions. The accessor "getAmount" gives direct access to the value 1071 */ 1072 public IntegerType getAmountElement() { 1073 if (this.amount == null) 1074 if (Configuration.errorOnAutoCreate()) 1075 throw new Error("Attempt to auto-create SubstancePolymerRepeatRepeatUnitComponent.amount"); 1076 else if (Configuration.doAutoCreate()) 1077 this.amount = new IntegerType(); // bb 1078 return this.amount; 1079 } 1080 1081 public boolean hasAmountElement() { 1082 return this.amount != null && !this.amount.isEmpty(); 1083 } 1084 1085 public boolean hasAmount() { 1086 return this.amount != null && !this.amount.isEmpty(); 1087 } 1088 1089 /** 1090 * @param value {@link #amount} (Number of repeats of this unit.). This is the underlying object with id, value and extensions. The accessor "getAmount" gives direct access to the value 1091 */ 1092 public SubstancePolymerRepeatRepeatUnitComponent setAmountElement(IntegerType value) { 1093 this.amount = value; 1094 return this; 1095 } 1096 1097 /** 1098 * @return Number of repeats of this unit. 1099 */ 1100 public int getAmount() { 1101 return this.amount == null || this.amount.isEmpty() ? 0 : this.amount.getValue(); 1102 } 1103 1104 /** 1105 * @param value Number of repeats of this unit. 1106 */ 1107 public SubstancePolymerRepeatRepeatUnitComponent setAmount(int value) { 1108 if (this.amount == null) 1109 this.amount = new IntegerType(); 1110 this.amount.setValue(value); 1111 return this; 1112 } 1113 1114 /** 1115 * @return {@link #degreeOfPolymerisation} (Applies to homopolymer and block co-polymers where the degree of polymerisation within a block can be described.) 1116 */ 1117 public List<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent> getDegreeOfPolymerisation() { 1118 if (this.degreeOfPolymerisation == null) 1119 this.degreeOfPolymerisation = new ArrayList<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent>(); 1120 return this.degreeOfPolymerisation; 1121 } 1122 1123 /** 1124 * @return Returns a reference to <code>this</code> for easy method chaining 1125 */ 1126 public SubstancePolymerRepeatRepeatUnitComponent setDegreeOfPolymerisation(List<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent> theDegreeOfPolymerisation) { 1127 this.degreeOfPolymerisation = theDegreeOfPolymerisation; 1128 return this; 1129 } 1130 1131 public boolean hasDegreeOfPolymerisation() { 1132 if (this.degreeOfPolymerisation == null) 1133 return false; 1134 for (SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent item : this.degreeOfPolymerisation) 1135 if (!item.isEmpty()) 1136 return true; 1137 return false; 1138 } 1139 1140 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent addDegreeOfPolymerisation() { //3 1141 SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent t = new SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent(); 1142 if (this.degreeOfPolymerisation == null) 1143 this.degreeOfPolymerisation = new ArrayList<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent>(); 1144 this.degreeOfPolymerisation.add(t); 1145 return t; 1146 } 1147 1148 public SubstancePolymerRepeatRepeatUnitComponent addDegreeOfPolymerisation(SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent t) { //3 1149 if (t == null) 1150 return this; 1151 if (this.degreeOfPolymerisation == null) 1152 this.degreeOfPolymerisation = new ArrayList<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent>(); 1153 this.degreeOfPolymerisation.add(t); 1154 return this; 1155 } 1156 1157 /** 1158 * @return The first repetition of repeating field {@link #degreeOfPolymerisation}, creating it if it does not already exist {3} 1159 */ 1160 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent getDegreeOfPolymerisationFirstRep() { 1161 if (getDegreeOfPolymerisation().isEmpty()) { 1162 addDegreeOfPolymerisation(); 1163 } 1164 return getDegreeOfPolymerisation().get(0); 1165 } 1166 1167 /** 1168 * @return {@link #structuralRepresentation} (A graphical structure for this SRU.) 1169 */ 1170 public List<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent> getStructuralRepresentation() { 1171 if (this.structuralRepresentation == null) 1172 this.structuralRepresentation = new ArrayList<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent>(); 1173 return this.structuralRepresentation; 1174 } 1175 1176 /** 1177 * @return Returns a reference to <code>this</code> for easy method chaining 1178 */ 1179 public SubstancePolymerRepeatRepeatUnitComponent setStructuralRepresentation(List<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent> theStructuralRepresentation) { 1180 this.structuralRepresentation = theStructuralRepresentation; 1181 return this; 1182 } 1183 1184 public boolean hasStructuralRepresentation() { 1185 if (this.structuralRepresentation == null) 1186 return false; 1187 for (SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent item : this.structuralRepresentation) 1188 if (!item.isEmpty()) 1189 return true; 1190 return false; 1191 } 1192 1193 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent addStructuralRepresentation() { //3 1194 SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent t = new SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent(); 1195 if (this.structuralRepresentation == null) 1196 this.structuralRepresentation = new ArrayList<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent>(); 1197 this.structuralRepresentation.add(t); 1198 return t; 1199 } 1200 1201 public SubstancePolymerRepeatRepeatUnitComponent addStructuralRepresentation(SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent t) { //3 1202 if (t == null) 1203 return this; 1204 if (this.structuralRepresentation == null) 1205 this.structuralRepresentation = new ArrayList<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent>(); 1206 this.structuralRepresentation.add(t); 1207 return this; 1208 } 1209 1210 /** 1211 * @return The first repetition of repeating field {@link #structuralRepresentation}, creating it if it does not already exist {3} 1212 */ 1213 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent getStructuralRepresentationFirstRep() { 1214 if (getStructuralRepresentation().isEmpty()) { 1215 addStructuralRepresentation(); 1216 } 1217 return getStructuralRepresentation().get(0); 1218 } 1219 1220 protected void listChildren(List<Property> children) { 1221 super.listChildren(children); 1222 children.add(new Property("unit", "string", "Structural repeat units are essential elements for defining polymers.", 0, 1, unit)); 1223 children.add(new Property("orientation", "CodeableConcept", "The orientation of the polymerisation, e.g. head-tail, head-head, random.", 0, 1, orientation)); 1224 children.add(new Property("amount", "integer", "Number of repeats of this unit.", 0, 1, amount)); 1225 children.add(new Property("degreeOfPolymerisation", "", "Applies to homopolymer and block co-polymers where the degree of polymerisation within a block can be described.", 0, java.lang.Integer.MAX_VALUE, degreeOfPolymerisation)); 1226 children.add(new Property("structuralRepresentation", "", "A graphical structure for this SRU.", 0, java.lang.Integer.MAX_VALUE, structuralRepresentation)); 1227 } 1228 1229 @Override 1230 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1231 switch (_hash) { 1232 case 3594628: /*unit*/ return new Property("unit", "string", "Structural repeat units are essential elements for defining polymers.", 0, 1, unit); 1233 case -1439500848: /*orientation*/ return new Property("orientation", "CodeableConcept", "The orientation of the polymerisation, e.g. head-tail, head-head, random.", 0, 1, orientation); 1234 case -1413853096: /*amount*/ return new Property("amount", "integer", "Number of repeats of this unit.", 0, 1, amount); 1235 case -159251872: /*degreeOfPolymerisation*/ return new Property("degreeOfPolymerisation", "", "Applies to homopolymer and block co-polymers where the degree of polymerisation within a block can be described.", 0, java.lang.Integer.MAX_VALUE, degreeOfPolymerisation); 1236 case 14311178: /*structuralRepresentation*/ return new Property("structuralRepresentation", "", "A graphical structure for this SRU.", 0, java.lang.Integer.MAX_VALUE, structuralRepresentation); 1237 default: return super.getNamedProperty(_hash, _name, _checkValid); 1238 } 1239 1240 } 1241 1242 @Override 1243 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1244 switch (hash) { 1245 case 3594628: /*unit*/ return this.unit == null ? new Base[0] : new Base[] {this.unit}; // StringType 1246 case -1439500848: /*orientation*/ return this.orientation == null ? new Base[0] : new Base[] {this.orientation}; // CodeableConcept 1247 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // IntegerType 1248 case -159251872: /*degreeOfPolymerisation*/ return this.degreeOfPolymerisation == null ? new Base[0] : this.degreeOfPolymerisation.toArray(new Base[this.degreeOfPolymerisation.size()]); // SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent 1249 case 14311178: /*structuralRepresentation*/ return this.structuralRepresentation == null ? new Base[0] : this.structuralRepresentation.toArray(new Base[this.structuralRepresentation.size()]); // SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent 1250 default: return super.getProperty(hash, name, checkValid); 1251 } 1252 1253 } 1254 1255 @Override 1256 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1257 switch (hash) { 1258 case 3594628: // unit 1259 this.unit = TypeConvertor.castToString(value); // StringType 1260 return value; 1261 case -1439500848: // orientation 1262 this.orientation = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1263 return value; 1264 case -1413853096: // amount 1265 this.amount = TypeConvertor.castToInteger(value); // IntegerType 1266 return value; 1267 case -159251872: // degreeOfPolymerisation 1268 this.getDegreeOfPolymerisation().add((SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent) value); // SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent 1269 return value; 1270 case 14311178: // structuralRepresentation 1271 this.getStructuralRepresentation().add((SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent) value); // SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent 1272 return value; 1273 default: return super.setProperty(hash, name, value); 1274 } 1275 1276 } 1277 1278 @Override 1279 public Base setProperty(String name, Base value) throws FHIRException { 1280 if (name.equals("unit")) { 1281 this.unit = TypeConvertor.castToString(value); // StringType 1282 } else if (name.equals("orientation")) { 1283 this.orientation = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1284 } else if (name.equals("amount")) { 1285 this.amount = TypeConvertor.castToInteger(value); // IntegerType 1286 } else if (name.equals("degreeOfPolymerisation")) { 1287 this.getDegreeOfPolymerisation().add((SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent) value); 1288 } else if (name.equals("structuralRepresentation")) { 1289 this.getStructuralRepresentation().add((SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent) value); 1290 } else 1291 return super.setProperty(name, value); 1292 return value; 1293 } 1294 1295 @Override 1296 public void removeChild(String name, Base value) throws FHIRException { 1297 if (name.equals("unit")) { 1298 this.unit = null; 1299 } else if (name.equals("orientation")) { 1300 this.orientation = null; 1301 } else if (name.equals("amount")) { 1302 this.amount = null; 1303 } else if (name.equals("degreeOfPolymerisation")) { 1304 this.getDegreeOfPolymerisation().remove((SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent) value); 1305 } else if (name.equals("structuralRepresentation")) { 1306 this.getStructuralRepresentation().remove((SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent) value); 1307 } else 1308 super.removeChild(name, value); 1309 1310 } 1311 1312 @Override 1313 public Base makeProperty(int hash, String name) throws FHIRException { 1314 switch (hash) { 1315 case 3594628: return getUnitElement(); 1316 case -1439500848: return getOrientation(); 1317 case -1413853096: return getAmountElement(); 1318 case -159251872: return addDegreeOfPolymerisation(); 1319 case 14311178: return addStructuralRepresentation(); 1320 default: return super.makeProperty(hash, name); 1321 } 1322 1323 } 1324 1325 @Override 1326 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1327 switch (hash) { 1328 case 3594628: /*unit*/ return new String[] {"string"}; 1329 case -1439500848: /*orientation*/ return new String[] {"CodeableConcept"}; 1330 case -1413853096: /*amount*/ return new String[] {"integer"}; 1331 case -159251872: /*degreeOfPolymerisation*/ return new String[] {}; 1332 case 14311178: /*structuralRepresentation*/ return new String[] {}; 1333 default: return super.getTypesForProperty(hash, name); 1334 } 1335 1336 } 1337 1338 @Override 1339 public Base addChild(String name) throws FHIRException { 1340 if (name.equals("unit")) { 1341 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.repeat.repeatUnit.unit"); 1342 } 1343 else if (name.equals("orientation")) { 1344 this.orientation = new CodeableConcept(); 1345 return this.orientation; 1346 } 1347 else if (name.equals("amount")) { 1348 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.repeat.repeatUnit.amount"); 1349 } 1350 else if (name.equals("degreeOfPolymerisation")) { 1351 return addDegreeOfPolymerisation(); 1352 } 1353 else if (name.equals("structuralRepresentation")) { 1354 return addStructuralRepresentation(); 1355 } 1356 else 1357 return super.addChild(name); 1358 } 1359 1360 public SubstancePolymerRepeatRepeatUnitComponent copy() { 1361 SubstancePolymerRepeatRepeatUnitComponent dst = new SubstancePolymerRepeatRepeatUnitComponent(); 1362 copyValues(dst); 1363 return dst; 1364 } 1365 1366 public void copyValues(SubstancePolymerRepeatRepeatUnitComponent dst) { 1367 super.copyValues(dst); 1368 dst.unit = unit == null ? null : unit.copy(); 1369 dst.orientation = orientation == null ? null : orientation.copy(); 1370 dst.amount = amount == null ? null : amount.copy(); 1371 if (degreeOfPolymerisation != null) { 1372 dst.degreeOfPolymerisation = new ArrayList<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent>(); 1373 for (SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent i : degreeOfPolymerisation) 1374 dst.degreeOfPolymerisation.add(i.copy()); 1375 }; 1376 if (structuralRepresentation != null) { 1377 dst.structuralRepresentation = new ArrayList<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent>(); 1378 for (SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent i : structuralRepresentation) 1379 dst.structuralRepresentation.add(i.copy()); 1380 }; 1381 } 1382 1383 @Override 1384 public boolean equalsDeep(Base other_) { 1385 if (!super.equalsDeep(other_)) 1386 return false; 1387 if (!(other_ instanceof SubstancePolymerRepeatRepeatUnitComponent)) 1388 return false; 1389 SubstancePolymerRepeatRepeatUnitComponent o = (SubstancePolymerRepeatRepeatUnitComponent) other_; 1390 return compareDeep(unit, o.unit, true) && compareDeep(orientation, o.orientation, true) && compareDeep(amount, o.amount, true) 1391 && compareDeep(degreeOfPolymerisation, o.degreeOfPolymerisation, true) && compareDeep(structuralRepresentation, o.structuralRepresentation, true) 1392 ; 1393 } 1394 1395 @Override 1396 public boolean equalsShallow(Base other_) { 1397 if (!super.equalsShallow(other_)) 1398 return false; 1399 if (!(other_ instanceof SubstancePolymerRepeatRepeatUnitComponent)) 1400 return false; 1401 SubstancePolymerRepeatRepeatUnitComponent o = (SubstancePolymerRepeatRepeatUnitComponent) other_; 1402 return compareValues(unit, o.unit, true) && compareValues(amount, o.amount, true); 1403 } 1404 1405 public boolean isEmpty() { 1406 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(unit, orientation, amount 1407 , degreeOfPolymerisation, structuralRepresentation); 1408 } 1409 1410 public String fhirType() { 1411 return "SubstancePolymer.repeat.repeatUnit"; 1412 1413 } 1414 1415 } 1416 1417 @Block() 1418 public static class SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent extends BackboneElement implements IBaseBackboneElement { 1419 /** 1420 * The type of the degree of polymerisation shall be described, e.g. SRU/Polymer Ratio. 1421 */ 1422 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 1423 @Description(shortDefinition="The type of the degree of polymerisation shall be described, e.g. SRU/Polymer Ratio", formalDefinition="The type of the degree of polymerisation shall be described, e.g. SRU/Polymer Ratio." ) 1424 protected CodeableConcept type; 1425 1426 /** 1427 * An average amount of polymerisation. 1428 */ 1429 @Child(name = "average", type = {IntegerType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1430 @Description(shortDefinition="An average amount of polymerisation", formalDefinition="An average amount of polymerisation." ) 1431 protected IntegerType average; 1432 1433 /** 1434 * A low expected limit of the amount. 1435 */ 1436 @Child(name = "low", type = {IntegerType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1437 @Description(shortDefinition="A low expected limit of the amount", formalDefinition="A low expected limit of the amount." ) 1438 protected IntegerType low; 1439 1440 /** 1441 * A high expected limit of the amount. 1442 */ 1443 @Child(name = "high", type = {IntegerType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1444 @Description(shortDefinition="A high expected limit of the amount", formalDefinition="A high expected limit of the amount." ) 1445 protected IntegerType high; 1446 1447 private static final long serialVersionUID = -1950663748L; 1448 1449 /** 1450 * Constructor 1451 */ 1452 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent() { 1453 super(); 1454 } 1455 1456 /** 1457 * @return {@link #type} (The type of the degree of polymerisation shall be described, e.g. SRU/Polymer Ratio.) 1458 */ 1459 public CodeableConcept getType() { 1460 if (this.type == null) 1461 if (Configuration.errorOnAutoCreate()) 1462 throw new Error("Attempt to auto-create SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent.type"); 1463 else if (Configuration.doAutoCreate()) 1464 this.type = new CodeableConcept(); // cc 1465 return this.type; 1466 } 1467 1468 public boolean hasType() { 1469 return this.type != null && !this.type.isEmpty(); 1470 } 1471 1472 /** 1473 * @param value {@link #type} (The type of the degree of polymerisation shall be described, e.g. SRU/Polymer Ratio.) 1474 */ 1475 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent setType(CodeableConcept value) { 1476 this.type = value; 1477 return this; 1478 } 1479 1480 /** 1481 * @return {@link #average} (An average amount of polymerisation.). This is the underlying object with id, value and extensions. The accessor "getAverage" gives direct access to the value 1482 */ 1483 public IntegerType getAverageElement() { 1484 if (this.average == null) 1485 if (Configuration.errorOnAutoCreate()) 1486 throw new Error("Attempt to auto-create SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent.average"); 1487 else if (Configuration.doAutoCreate()) 1488 this.average = new IntegerType(); // bb 1489 return this.average; 1490 } 1491 1492 public boolean hasAverageElement() { 1493 return this.average != null && !this.average.isEmpty(); 1494 } 1495 1496 public boolean hasAverage() { 1497 return this.average != null && !this.average.isEmpty(); 1498 } 1499 1500 /** 1501 * @param value {@link #average} (An average amount of polymerisation.). This is the underlying object with id, value and extensions. The accessor "getAverage" gives direct access to the value 1502 */ 1503 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent setAverageElement(IntegerType value) { 1504 this.average = value; 1505 return this; 1506 } 1507 1508 /** 1509 * @return An average amount of polymerisation. 1510 */ 1511 public int getAverage() { 1512 return this.average == null || this.average.isEmpty() ? 0 : this.average.getValue(); 1513 } 1514 1515 /** 1516 * @param value An average amount of polymerisation. 1517 */ 1518 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent setAverage(int value) { 1519 if (this.average == null) 1520 this.average = new IntegerType(); 1521 this.average.setValue(value); 1522 return this; 1523 } 1524 1525 /** 1526 * @return {@link #low} (A low expected limit of the amount.). This is the underlying object with id, value and extensions. The accessor "getLow" gives direct access to the value 1527 */ 1528 public IntegerType getLowElement() { 1529 if (this.low == null) 1530 if (Configuration.errorOnAutoCreate()) 1531 throw new Error("Attempt to auto-create SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent.low"); 1532 else if (Configuration.doAutoCreate()) 1533 this.low = new IntegerType(); // bb 1534 return this.low; 1535 } 1536 1537 public boolean hasLowElement() { 1538 return this.low != null && !this.low.isEmpty(); 1539 } 1540 1541 public boolean hasLow() { 1542 return this.low != null && !this.low.isEmpty(); 1543 } 1544 1545 /** 1546 * @param value {@link #low} (A low expected limit of the amount.). This is the underlying object with id, value and extensions. The accessor "getLow" gives direct access to the value 1547 */ 1548 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent setLowElement(IntegerType value) { 1549 this.low = value; 1550 return this; 1551 } 1552 1553 /** 1554 * @return A low expected limit of the amount. 1555 */ 1556 public int getLow() { 1557 return this.low == null || this.low.isEmpty() ? 0 : this.low.getValue(); 1558 } 1559 1560 /** 1561 * @param value A low expected limit of the amount. 1562 */ 1563 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent setLow(int value) { 1564 if (this.low == null) 1565 this.low = new IntegerType(); 1566 this.low.setValue(value); 1567 return this; 1568 } 1569 1570 /** 1571 * @return {@link #high} (A high expected limit of the amount.). This is the underlying object with id, value and extensions. The accessor "getHigh" gives direct access to the value 1572 */ 1573 public IntegerType getHighElement() { 1574 if (this.high == null) 1575 if (Configuration.errorOnAutoCreate()) 1576 throw new Error("Attempt to auto-create SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent.high"); 1577 else if (Configuration.doAutoCreate()) 1578 this.high = new IntegerType(); // bb 1579 return this.high; 1580 } 1581 1582 public boolean hasHighElement() { 1583 return this.high != null && !this.high.isEmpty(); 1584 } 1585 1586 public boolean hasHigh() { 1587 return this.high != null && !this.high.isEmpty(); 1588 } 1589 1590 /** 1591 * @param value {@link #high} (A high expected limit of the amount.). This is the underlying object with id, value and extensions. The accessor "getHigh" gives direct access to the value 1592 */ 1593 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent setHighElement(IntegerType value) { 1594 this.high = value; 1595 return this; 1596 } 1597 1598 /** 1599 * @return A high expected limit of the amount. 1600 */ 1601 public int getHigh() { 1602 return this.high == null || this.high.isEmpty() ? 0 : this.high.getValue(); 1603 } 1604 1605 /** 1606 * @param value A high expected limit of the amount. 1607 */ 1608 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent setHigh(int value) { 1609 if (this.high == null) 1610 this.high = new IntegerType(); 1611 this.high.setValue(value); 1612 return this; 1613 } 1614 1615 protected void listChildren(List<Property> children) { 1616 super.listChildren(children); 1617 children.add(new Property("type", "CodeableConcept", "The type of the degree of polymerisation shall be described, e.g. SRU/Polymer Ratio.", 0, 1, type)); 1618 children.add(new Property("average", "integer", "An average amount of polymerisation.", 0, 1, average)); 1619 children.add(new Property("low", "integer", "A low expected limit of the amount.", 0, 1, low)); 1620 children.add(new Property("high", "integer", "A high expected limit of the amount.", 0, 1, high)); 1621 } 1622 1623 @Override 1624 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1625 switch (_hash) { 1626 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of the degree of polymerisation shall be described, e.g. SRU/Polymer Ratio.", 0, 1, type); 1627 case -631448035: /*average*/ return new Property("average", "integer", "An average amount of polymerisation.", 0, 1, average); 1628 case 107348: /*low*/ return new Property("low", "integer", "A low expected limit of the amount.", 0, 1, low); 1629 case 3202466: /*high*/ return new Property("high", "integer", "A high expected limit of the amount.", 0, 1, high); 1630 default: return super.getNamedProperty(_hash, _name, _checkValid); 1631 } 1632 1633 } 1634 1635 @Override 1636 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1637 switch (hash) { 1638 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1639 case -631448035: /*average*/ return this.average == null ? new Base[0] : new Base[] {this.average}; // IntegerType 1640 case 107348: /*low*/ return this.low == null ? new Base[0] : new Base[] {this.low}; // IntegerType 1641 case 3202466: /*high*/ return this.high == null ? new Base[0] : new Base[] {this.high}; // IntegerType 1642 default: return super.getProperty(hash, name, checkValid); 1643 } 1644 1645 } 1646 1647 @Override 1648 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1649 switch (hash) { 1650 case 3575610: // type 1651 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1652 return value; 1653 case -631448035: // average 1654 this.average = TypeConvertor.castToInteger(value); // IntegerType 1655 return value; 1656 case 107348: // low 1657 this.low = TypeConvertor.castToInteger(value); // IntegerType 1658 return value; 1659 case 3202466: // high 1660 this.high = TypeConvertor.castToInteger(value); // IntegerType 1661 return value; 1662 default: return super.setProperty(hash, name, value); 1663 } 1664 1665 } 1666 1667 @Override 1668 public Base setProperty(String name, Base value) throws FHIRException { 1669 if (name.equals("type")) { 1670 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1671 } else if (name.equals("average")) { 1672 this.average = TypeConvertor.castToInteger(value); // IntegerType 1673 } else if (name.equals("low")) { 1674 this.low = TypeConvertor.castToInteger(value); // IntegerType 1675 } else if (name.equals("high")) { 1676 this.high = TypeConvertor.castToInteger(value); // IntegerType 1677 } else 1678 return super.setProperty(name, value); 1679 return value; 1680 } 1681 1682 @Override 1683 public void removeChild(String name, Base value) throws FHIRException { 1684 if (name.equals("type")) { 1685 this.type = null; 1686 } else if (name.equals("average")) { 1687 this.average = null; 1688 } else if (name.equals("low")) { 1689 this.low = null; 1690 } else if (name.equals("high")) { 1691 this.high = null; 1692 } else 1693 super.removeChild(name, value); 1694 1695 } 1696 1697 @Override 1698 public Base makeProperty(int hash, String name) throws FHIRException { 1699 switch (hash) { 1700 case 3575610: return getType(); 1701 case -631448035: return getAverageElement(); 1702 case 107348: return getLowElement(); 1703 case 3202466: return getHighElement(); 1704 default: return super.makeProperty(hash, name); 1705 } 1706 1707 } 1708 1709 @Override 1710 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1711 switch (hash) { 1712 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1713 case -631448035: /*average*/ return new String[] {"integer"}; 1714 case 107348: /*low*/ return new String[] {"integer"}; 1715 case 3202466: /*high*/ return new String[] {"integer"}; 1716 default: return super.getTypesForProperty(hash, name); 1717 } 1718 1719 } 1720 1721 @Override 1722 public Base addChild(String name) throws FHIRException { 1723 if (name.equals("type")) { 1724 this.type = new CodeableConcept(); 1725 return this.type; 1726 } 1727 else if (name.equals("average")) { 1728 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.repeat.repeatUnit.degreeOfPolymerisation.average"); 1729 } 1730 else if (name.equals("low")) { 1731 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.repeat.repeatUnit.degreeOfPolymerisation.low"); 1732 } 1733 else if (name.equals("high")) { 1734 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.repeat.repeatUnit.degreeOfPolymerisation.high"); 1735 } 1736 else 1737 return super.addChild(name); 1738 } 1739 1740 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent copy() { 1741 SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent dst = new SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent(); 1742 copyValues(dst); 1743 return dst; 1744 } 1745 1746 public void copyValues(SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent dst) { 1747 super.copyValues(dst); 1748 dst.type = type == null ? null : type.copy(); 1749 dst.average = average == null ? null : average.copy(); 1750 dst.low = low == null ? null : low.copy(); 1751 dst.high = high == null ? null : high.copy(); 1752 } 1753 1754 @Override 1755 public boolean equalsDeep(Base other_) { 1756 if (!super.equalsDeep(other_)) 1757 return false; 1758 if (!(other_ instanceof SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent)) 1759 return false; 1760 SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent o = (SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent) other_; 1761 return compareDeep(type, o.type, true) && compareDeep(average, o.average, true) && compareDeep(low, o.low, true) 1762 && compareDeep(high, o.high, true); 1763 } 1764 1765 @Override 1766 public boolean equalsShallow(Base other_) { 1767 if (!super.equalsShallow(other_)) 1768 return false; 1769 if (!(other_ instanceof SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent)) 1770 return false; 1771 SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent o = (SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent) other_; 1772 return compareValues(average, o.average, true) && compareValues(low, o.low, true) && compareValues(high, o.high, true) 1773 ; 1774 } 1775 1776 public boolean isEmpty() { 1777 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, average, low, high 1778 ); 1779 } 1780 1781 public String fhirType() { 1782 return "SubstancePolymer.repeat.repeatUnit.degreeOfPolymerisation"; 1783 1784 } 1785 1786 } 1787 1788 @Block() 1789 public static class SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent extends BackboneElement implements IBaseBackboneElement { 1790 /** 1791 * The type of structure (e.g. Full, Partial, Representative). 1792 */ 1793 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 1794 @Description(shortDefinition="The type of structure (e.g. Full, Partial, Representative)", formalDefinition="The type of structure (e.g. Full, Partial, Representative)." ) 1795 protected CodeableConcept type; 1796 1797 /** 1798 * The structural representation as text string in a standard format e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF. 1799 */ 1800 @Child(name = "representation", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1801 @Description(shortDefinition="The structural representation as text string in a standard format e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF", formalDefinition="The structural representation as text string in a standard format e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF." ) 1802 protected StringType representation; 1803 1804 /** 1805 * The format of the representation e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF. 1806 */ 1807 @Child(name = "format", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 1808 @Description(shortDefinition="The format of the representation e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF", formalDefinition="The format of the representation e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF." ) 1809 protected CodeableConcept format; 1810 1811 /** 1812 * An attached file with the structural representation. 1813 */ 1814 @Child(name = "attachment", type = {Attachment.class}, order=4, min=0, max=1, modifier=false, summary=true) 1815 @Description(shortDefinition="An attached file with the structural representation", formalDefinition="An attached file with the structural representation." ) 1816 protected Attachment attachment; 1817 1818 private static final long serialVersionUID = -1385695515L; 1819 1820 /** 1821 * Constructor 1822 */ 1823 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent() { 1824 super(); 1825 } 1826 1827 /** 1828 * @return {@link #type} (The type of structure (e.g. Full, Partial, Representative).) 1829 */ 1830 public CodeableConcept getType() { 1831 if (this.type == null) 1832 if (Configuration.errorOnAutoCreate()) 1833 throw new Error("Attempt to auto-create SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent.type"); 1834 else if (Configuration.doAutoCreate()) 1835 this.type = new CodeableConcept(); // cc 1836 return this.type; 1837 } 1838 1839 public boolean hasType() { 1840 return this.type != null && !this.type.isEmpty(); 1841 } 1842 1843 /** 1844 * @param value {@link #type} (The type of structure (e.g. Full, Partial, Representative).) 1845 */ 1846 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent setType(CodeableConcept value) { 1847 this.type = value; 1848 return this; 1849 } 1850 1851 /** 1852 * @return {@link #representation} (The structural representation as text string in a standard format e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF.). This is the underlying object with id, value and extensions. The accessor "getRepresentation" gives direct access to the value 1853 */ 1854 public StringType getRepresentationElement() { 1855 if (this.representation == null) 1856 if (Configuration.errorOnAutoCreate()) 1857 throw new Error("Attempt to auto-create SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent.representation"); 1858 else if (Configuration.doAutoCreate()) 1859 this.representation = new StringType(); // bb 1860 return this.representation; 1861 } 1862 1863 public boolean hasRepresentationElement() { 1864 return this.representation != null && !this.representation.isEmpty(); 1865 } 1866 1867 public boolean hasRepresentation() { 1868 return this.representation != null && !this.representation.isEmpty(); 1869 } 1870 1871 /** 1872 * @param value {@link #representation} (The structural representation as text string in a standard format e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF.). This is the underlying object with id, value and extensions. The accessor "getRepresentation" gives direct access to the value 1873 */ 1874 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent setRepresentationElement(StringType value) { 1875 this.representation = value; 1876 return this; 1877 } 1878 1879 /** 1880 * @return The structural representation as text string in a standard format e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF. 1881 */ 1882 public String getRepresentation() { 1883 return this.representation == null ? null : this.representation.getValue(); 1884 } 1885 1886 /** 1887 * @param value The structural representation as text string in a standard format e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF. 1888 */ 1889 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent setRepresentation(String value) { 1890 if (Utilities.noString(value)) 1891 this.representation = null; 1892 else { 1893 if (this.representation == null) 1894 this.representation = new StringType(); 1895 this.representation.setValue(value); 1896 } 1897 return this; 1898 } 1899 1900 /** 1901 * @return {@link #format} (The format of the representation e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF.) 1902 */ 1903 public CodeableConcept getFormat() { 1904 if (this.format == null) 1905 if (Configuration.errorOnAutoCreate()) 1906 throw new Error("Attempt to auto-create SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent.format"); 1907 else if (Configuration.doAutoCreate()) 1908 this.format = new CodeableConcept(); // cc 1909 return this.format; 1910 } 1911 1912 public boolean hasFormat() { 1913 return this.format != null && !this.format.isEmpty(); 1914 } 1915 1916 /** 1917 * @param value {@link #format} (The format of the representation e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF.) 1918 */ 1919 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent setFormat(CodeableConcept value) { 1920 this.format = value; 1921 return this; 1922 } 1923 1924 /** 1925 * @return {@link #attachment} (An attached file with the structural representation.) 1926 */ 1927 public Attachment getAttachment() { 1928 if (this.attachment == null) 1929 if (Configuration.errorOnAutoCreate()) 1930 throw new Error("Attempt to auto-create SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent.attachment"); 1931 else if (Configuration.doAutoCreate()) 1932 this.attachment = new Attachment(); // cc 1933 return this.attachment; 1934 } 1935 1936 public boolean hasAttachment() { 1937 return this.attachment != null && !this.attachment.isEmpty(); 1938 } 1939 1940 /** 1941 * @param value {@link #attachment} (An attached file with the structural representation.) 1942 */ 1943 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent setAttachment(Attachment value) { 1944 this.attachment = value; 1945 return this; 1946 } 1947 1948 protected void listChildren(List<Property> children) { 1949 super.listChildren(children); 1950 children.add(new Property("type", "CodeableConcept", "The type of structure (e.g. Full, Partial, Representative).", 0, 1, type)); 1951 children.add(new Property("representation", "string", "The structural representation as text string in a standard format e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF.", 0, 1, representation)); 1952 children.add(new Property("format", "CodeableConcept", "The format of the representation e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF.", 0, 1, format)); 1953 children.add(new Property("attachment", "Attachment", "An attached file with the structural representation.", 0, 1, attachment)); 1954 } 1955 1956 @Override 1957 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1958 switch (_hash) { 1959 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of structure (e.g. Full, Partial, Representative).", 0, 1, type); 1960 case -671065907: /*representation*/ return new Property("representation", "string", "The structural representation as text string in a standard format e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF.", 0, 1, representation); 1961 case -1268779017: /*format*/ return new Property("format", "CodeableConcept", "The format of the representation e.g. InChI, SMILES, MOLFILE, CDX, SDF, PDB, mmCIF.", 0, 1, format); 1962 case -1963501277: /*attachment*/ return new Property("attachment", "Attachment", "An attached file with the structural representation.", 0, 1, attachment); 1963 default: return super.getNamedProperty(_hash, _name, _checkValid); 1964 } 1965 1966 } 1967 1968 @Override 1969 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1970 switch (hash) { 1971 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1972 case -671065907: /*representation*/ return this.representation == null ? new Base[0] : new Base[] {this.representation}; // StringType 1973 case -1268779017: /*format*/ return this.format == null ? new Base[0] : new Base[] {this.format}; // CodeableConcept 1974 case -1963501277: /*attachment*/ return this.attachment == null ? new Base[0] : new Base[] {this.attachment}; // Attachment 1975 default: return super.getProperty(hash, name, checkValid); 1976 } 1977 1978 } 1979 1980 @Override 1981 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1982 switch (hash) { 1983 case 3575610: // type 1984 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1985 return value; 1986 case -671065907: // representation 1987 this.representation = TypeConvertor.castToString(value); // StringType 1988 return value; 1989 case -1268779017: // format 1990 this.format = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1991 return value; 1992 case -1963501277: // attachment 1993 this.attachment = TypeConvertor.castToAttachment(value); // Attachment 1994 return value; 1995 default: return super.setProperty(hash, name, value); 1996 } 1997 1998 } 1999 2000 @Override 2001 public Base setProperty(String name, Base value) throws FHIRException { 2002 if (name.equals("type")) { 2003 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2004 } else if (name.equals("representation")) { 2005 this.representation = TypeConvertor.castToString(value); // StringType 2006 } else if (name.equals("format")) { 2007 this.format = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2008 } else if (name.equals("attachment")) { 2009 this.attachment = TypeConvertor.castToAttachment(value); // Attachment 2010 } else 2011 return super.setProperty(name, value); 2012 return value; 2013 } 2014 2015 @Override 2016 public void removeChild(String name, Base value) throws FHIRException { 2017 if (name.equals("type")) { 2018 this.type = null; 2019 } else if (name.equals("representation")) { 2020 this.representation = null; 2021 } else if (name.equals("format")) { 2022 this.format = null; 2023 } else if (name.equals("attachment")) { 2024 this.attachment = null; 2025 } else 2026 super.removeChild(name, value); 2027 2028 } 2029 2030 @Override 2031 public Base makeProperty(int hash, String name) throws FHIRException { 2032 switch (hash) { 2033 case 3575610: return getType(); 2034 case -671065907: return getRepresentationElement(); 2035 case -1268779017: return getFormat(); 2036 case -1963501277: return getAttachment(); 2037 default: return super.makeProperty(hash, name); 2038 } 2039 2040 } 2041 2042 @Override 2043 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2044 switch (hash) { 2045 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2046 case -671065907: /*representation*/ return new String[] {"string"}; 2047 case -1268779017: /*format*/ return new String[] {"CodeableConcept"}; 2048 case -1963501277: /*attachment*/ return new String[] {"Attachment"}; 2049 default: return super.getTypesForProperty(hash, name); 2050 } 2051 2052 } 2053 2054 @Override 2055 public Base addChild(String name) throws FHIRException { 2056 if (name.equals("type")) { 2057 this.type = new CodeableConcept(); 2058 return this.type; 2059 } 2060 else if (name.equals("representation")) { 2061 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.repeat.repeatUnit.structuralRepresentation.representation"); 2062 } 2063 else if (name.equals("format")) { 2064 this.format = new CodeableConcept(); 2065 return this.format; 2066 } 2067 else if (name.equals("attachment")) { 2068 this.attachment = new Attachment(); 2069 return this.attachment; 2070 } 2071 else 2072 return super.addChild(name); 2073 } 2074 2075 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent copy() { 2076 SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent dst = new SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent(); 2077 copyValues(dst); 2078 return dst; 2079 } 2080 2081 public void copyValues(SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent dst) { 2082 super.copyValues(dst); 2083 dst.type = type == null ? null : type.copy(); 2084 dst.representation = representation == null ? null : representation.copy(); 2085 dst.format = format == null ? null : format.copy(); 2086 dst.attachment = attachment == null ? null : attachment.copy(); 2087 } 2088 2089 @Override 2090 public boolean equalsDeep(Base other_) { 2091 if (!super.equalsDeep(other_)) 2092 return false; 2093 if (!(other_ instanceof SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent)) 2094 return false; 2095 SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent o = (SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent) other_; 2096 return compareDeep(type, o.type, true) && compareDeep(representation, o.representation, true) && compareDeep(format, o.format, true) 2097 && compareDeep(attachment, o.attachment, true); 2098 } 2099 2100 @Override 2101 public boolean equalsShallow(Base other_) { 2102 if (!super.equalsShallow(other_)) 2103 return false; 2104 if (!(other_ instanceof SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent)) 2105 return false; 2106 SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent o = (SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent) other_; 2107 return compareValues(representation, o.representation, true); 2108 } 2109 2110 public boolean isEmpty() { 2111 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, representation, format 2112 , attachment); 2113 } 2114 2115 public String fhirType() { 2116 return "SubstancePolymer.repeat.repeatUnit.structuralRepresentation"; 2117 2118 } 2119 2120 } 2121 2122 /** 2123 * A business idenfier for this polymer, but typically this is handled by a SubstanceDefinition identifier. 2124 */ 2125 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 2126 @Description(shortDefinition="A business idenfier for this polymer, but typically this is handled by a SubstanceDefinition identifier", formalDefinition="A business idenfier for this polymer, but typically this is handled by a SubstanceDefinition identifier." ) 2127 protected Identifier identifier; 2128 2129 /** 2130 * Overall type of the polymer. 2131 */ 2132 @Child(name = "class", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 2133 @Description(shortDefinition="Overall type of the polymer", formalDefinition="Overall type of the polymer." ) 2134 protected CodeableConcept class_; 2135 2136 /** 2137 * Polymer geometry, e.g. linear, branched, cross-linked, network or dendritic. 2138 */ 2139 @Child(name = "geometry", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 2140 @Description(shortDefinition="Polymer geometry, e.g. linear, branched, cross-linked, network or dendritic", formalDefinition="Polymer geometry, e.g. linear, branched, cross-linked, network or dendritic." ) 2141 protected CodeableConcept geometry; 2142 2143 /** 2144 * Descrtibes the copolymer sequence type (polymer connectivity). 2145 */ 2146 @Child(name = "copolymerConnectivity", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2147 @Description(shortDefinition="Descrtibes the copolymer sequence type (polymer connectivity)", formalDefinition="Descrtibes the copolymer sequence type (polymer connectivity)." ) 2148 protected List<CodeableConcept> copolymerConnectivity; 2149 2150 /** 2151 * Todo - this is intended to connect to a repeating full modification structure, also used by Protein and Nucleic Acid . String is just a placeholder. 2152 */ 2153 @Child(name = "modification", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 2154 @Description(shortDefinition="Todo - this is intended to connect to a repeating full modification structure, also used by Protein and Nucleic Acid . String is just a placeholder", formalDefinition="Todo - this is intended to connect to a repeating full modification structure, also used by Protein and Nucleic Acid . String is just a placeholder." ) 2155 protected StringType modification; 2156 2157 /** 2158 * Todo. 2159 */ 2160 @Child(name = "monomerSet", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2161 @Description(shortDefinition="Todo", formalDefinition="Todo." ) 2162 protected List<SubstancePolymerMonomerSetComponent> monomerSet; 2163 2164 /** 2165 * Specifies and quantifies the repeated units and their configuration. 2166 */ 2167 @Child(name = "repeat", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2168 @Description(shortDefinition="Specifies and quantifies the repeated units and their configuration", formalDefinition="Specifies and quantifies the repeated units and their configuration." ) 2169 protected List<SubstancePolymerRepeatComponent> repeat; 2170 2171 private static final long serialVersionUID = 11937102L; 2172 2173 /** 2174 * Constructor 2175 */ 2176 public SubstancePolymer() { 2177 super(); 2178 } 2179 2180 /** 2181 * @return {@link #identifier} (A business idenfier for this polymer, but typically this is handled by a SubstanceDefinition identifier.) 2182 */ 2183 public Identifier getIdentifier() { 2184 if (this.identifier == null) 2185 if (Configuration.errorOnAutoCreate()) 2186 throw new Error("Attempt to auto-create SubstancePolymer.identifier"); 2187 else if (Configuration.doAutoCreate()) 2188 this.identifier = new Identifier(); // cc 2189 return this.identifier; 2190 } 2191 2192 public boolean hasIdentifier() { 2193 return this.identifier != null && !this.identifier.isEmpty(); 2194 } 2195 2196 /** 2197 * @param value {@link #identifier} (A business idenfier for this polymer, but typically this is handled by a SubstanceDefinition identifier.) 2198 */ 2199 public SubstancePolymer setIdentifier(Identifier value) { 2200 this.identifier = value; 2201 return this; 2202 } 2203 2204 /** 2205 * @return {@link #class_} (Overall type of the polymer.) 2206 */ 2207 public CodeableConcept getClass_() { 2208 if (this.class_ == null) 2209 if (Configuration.errorOnAutoCreate()) 2210 throw new Error("Attempt to auto-create SubstancePolymer.class_"); 2211 else if (Configuration.doAutoCreate()) 2212 this.class_ = new CodeableConcept(); // cc 2213 return this.class_; 2214 } 2215 2216 public boolean hasClass_() { 2217 return this.class_ != null && !this.class_.isEmpty(); 2218 } 2219 2220 /** 2221 * @param value {@link #class_} (Overall type of the polymer.) 2222 */ 2223 public SubstancePolymer setClass_(CodeableConcept value) { 2224 this.class_ = value; 2225 return this; 2226 } 2227 2228 /** 2229 * @return {@link #geometry} (Polymer geometry, e.g. linear, branched, cross-linked, network or dendritic.) 2230 */ 2231 public CodeableConcept getGeometry() { 2232 if (this.geometry == null) 2233 if (Configuration.errorOnAutoCreate()) 2234 throw new Error("Attempt to auto-create SubstancePolymer.geometry"); 2235 else if (Configuration.doAutoCreate()) 2236 this.geometry = new CodeableConcept(); // cc 2237 return this.geometry; 2238 } 2239 2240 public boolean hasGeometry() { 2241 return this.geometry != null && !this.geometry.isEmpty(); 2242 } 2243 2244 /** 2245 * @param value {@link #geometry} (Polymer geometry, e.g. linear, branched, cross-linked, network or dendritic.) 2246 */ 2247 public SubstancePolymer setGeometry(CodeableConcept value) { 2248 this.geometry = value; 2249 return this; 2250 } 2251 2252 /** 2253 * @return {@link #copolymerConnectivity} (Descrtibes the copolymer sequence type (polymer connectivity).) 2254 */ 2255 public List<CodeableConcept> getCopolymerConnectivity() { 2256 if (this.copolymerConnectivity == null) 2257 this.copolymerConnectivity = new ArrayList<CodeableConcept>(); 2258 return this.copolymerConnectivity; 2259 } 2260 2261 /** 2262 * @return Returns a reference to <code>this</code> for easy method chaining 2263 */ 2264 public SubstancePolymer setCopolymerConnectivity(List<CodeableConcept> theCopolymerConnectivity) { 2265 this.copolymerConnectivity = theCopolymerConnectivity; 2266 return this; 2267 } 2268 2269 public boolean hasCopolymerConnectivity() { 2270 if (this.copolymerConnectivity == null) 2271 return false; 2272 for (CodeableConcept item : this.copolymerConnectivity) 2273 if (!item.isEmpty()) 2274 return true; 2275 return false; 2276 } 2277 2278 public CodeableConcept addCopolymerConnectivity() { //3 2279 CodeableConcept t = new CodeableConcept(); 2280 if (this.copolymerConnectivity == null) 2281 this.copolymerConnectivity = new ArrayList<CodeableConcept>(); 2282 this.copolymerConnectivity.add(t); 2283 return t; 2284 } 2285 2286 public SubstancePolymer addCopolymerConnectivity(CodeableConcept t) { //3 2287 if (t == null) 2288 return this; 2289 if (this.copolymerConnectivity == null) 2290 this.copolymerConnectivity = new ArrayList<CodeableConcept>(); 2291 this.copolymerConnectivity.add(t); 2292 return this; 2293 } 2294 2295 /** 2296 * @return The first repetition of repeating field {@link #copolymerConnectivity}, creating it if it does not already exist {3} 2297 */ 2298 public CodeableConcept getCopolymerConnectivityFirstRep() { 2299 if (getCopolymerConnectivity().isEmpty()) { 2300 addCopolymerConnectivity(); 2301 } 2302 return getCopolymerConnectivity().get(0); 2303 } 2304 2305 /** 2306 * @return {@link #modification} (Todo - this is intended to connect to a repeating full modification structure, also used by Protein and Nucleic Acid . String is just a placeholder.). This is the underlying object with id, value and extensions. The accessor "getModification" gives direct access to the value 2307 */ 2308 public StringType getModificationElement() { 2309 if (this.modification == null) 2310 if (Configuration.errorOnAutoCreate()) 2311 throw new Error("Attempt to auto-create SubstancePolymer.modification"); 2312 else if (Configuration.doAutoCreate()) 2313 this.modification = new StringType(); // bb 2314 return this.modification; 2315 } 2316 2317 public boolean hasModificationElement() { 2318 return this.modification != null && !this.modification.isEmpty(); 2319 } 2320 2321 public boolean hasModification() { 2322 return this.modification != null && !this.modification.isEmpty(); 2323 } 2324 2325 /** 2326 * @param value {@link #modification} (Todo - this is intended to connect to a repeating full modification structure, also used by Protein and Nucleic Acid . String is just a placeholder.). This is the underlying object with id, value and extensions. The accessor "getModification" gives direct access to the value 2327 */ 2328 public SubstancePolymer setModificationElement(StringType value) { 2329 this.modification = value; 2330 return this; 2331 } 2332 2333 /** 2334 * @return Todo - this is intended to connect to a repeating full modification structure, also used by Protein and Nucleic Acid . String is just a placeholder. 2335 */ 2336 public String getModification() { 2337 return this.modification == null ? null : this.modification.getValue(); 2338 } 2339 2340 /** 2341 * @param value Todo - this is intended to connect to a repeating full modification structure, also used by Protein and Nucleic Acid . String is just a placeholder. 2342 */ 2343 public SubstancePolymer setModification(String value) { 2344 if (Utilities.noString(value)) 2345 this.modification = null; 2346 else { 2347 if (this.modification == null) 2348 this.modification = new StringType(); 2349 this.modification.setValue(value); 2350 } 2351 return this; 2352 } 2353 2354 /** 2355 * @return {@link #monomerSet} (Todo.) 2356 */ 2357 public List<SubstancePolymerMonomerSetComponent> getMonomerSet() { 2358 if (this.monomerSet == null) 2359 this.monomerSet = new ArrayList<SubstancePolymerMonomerSetComponent>(); 2360 return this.monomerSet; 2361 } 2362 2363 /** 2364 * @return Returns a reference to <code>this</code> for easy method chaining 2365 */ 2366 public SubstancePolymer setMonomerSet(List<SubstancePolymerMonomerSetComponent> theMonomerSet) { 2367 this.monomerSet = theMonomerSet; 2368 return this; 2369 } 2370 2371 public boolean hasMonomerSet() { 2372 if (this.monomerSet == null) 2373 return false; 2374 for (SubstancePolymerMonomerSetComponent item : this.monomerSet) 2375 if (!item.isEmpty()) 2376 return true; 2377 return false; 2378 } 2379 2380 public SubstancePolymerMonomerSetComponent addMonomerSet() { //3 2381 SubstancePolymerMonomerSetComponent t = new SubstancePolymerMonomerSetComponent(); 2382 if (this.monomerSet == null) 2383 this.monomerSet = new ArrayList<SubstancePolymerMonomerSetComponent>(); 2384 this.monomerSet.add(t); 2385 return t; 2386 } 2387 2388 public SubstancePolymer addMonomerSet(SubstancePolymerMonomerSetComponent t) { //3 2389 if (t == null) 2390 return this; 2391 if (this.monomerSet == null) 2392 this.monomerSet = new ArrayList<SubstancePolymerMonomerSetComponent>(); 2393 this.monomerSet.add(t); 2394 return this; 2395 } 2396 2397 /** 2398 * @return The first repetition of repeating field {@link #monomerSet}, creating it if it does not already exist {3} 2399 */ 2400 public SubstancePolymerMonomerSetComponent getMonomerSetFirstRep() { 2401 if (getMonomerSet().isEmpty()) { 2402 addMonomerSet(); 2403 } 2404 return getMonomerSet().get(0); 2405 } 2406 2407 /** 2408 * @return {@link #repeat} (Specifies and quantifies the repeated units and their configuration.) 2409 */ 2410 public List<SubstancePolymerRepeatComponent> getRepeat() { 2411 if (this.repeat == null) 2412 this.repeat = new ArrayList<SubstancePolymerRepeatComponent>(); 2413 return this.repeat; 2414 } 2415 2416 /** 2417 * @return Returns a reference to <code>this</code> for easy method chaining 2418 */ 2419 public SubstancePolymer setRepeat(List<SubstancePolymerRepeatComponent> theRepeat) { 2420 this.repeat = theRepeat; 2421 return this; 2422 } 2423 2424 public boolean hasRepeat() { 2425 if (this.repeat == null) 2426 return false; 2427 for (SubstancePolymerRepeatComponent item : this.repeat) 2428 if (!item.isEmpty()) 2429 return true; 2430 return false; 2431 } 2432 2433 public SubstancePolymerRepeatComponent addRepeat() { //3 2434 SubstancePolymerRepeatComponent t = new SubstancePolymerRepeatComponent(); 2435 if (this.repeat == null) 2436 this.repeat = new ArrayList<SubstancePolymerRepeatComponent>(); 2437 this.repeat.add(t); 2438 return t; 2439 } 2440 2441 public SubstancePolymer addRepeat(SubstancePolymerRepeatComponent t) { //3 2442 if (t == null) 2443 return this; 2444 if (this.repeat == null) 2445 this.repeat = new ArrayList<SubstancePolymerRepeatComponent>(); 2446 this.repeat.add(t); 2447 return this; 2448 } 2449 2450 /** 2451 * @return The first repetition of repeating field {@link #repeat}, creating it if it does not already exist {3} 2452 */ 2453 public SubstancePolymerRepeatComponent getRepeatFirstRep() { 2454 if (getRepeat().isEmpty()) { 2455 addRepeat(); 2456 } 2457 return getRepeat().get(0); 2458 } 2459 2460 protected void listChildren(List<Property> children) { 2461 super.listChildren(children); 2462 children.add(new Property("identifier", "Identifier", "A business idenfier for this polymer, but typically this is handled by a SubstanceDefinition identifier.", 0, 1, identifier)); 2463 children.add(new Property("class", "CodeableConcept", "Overall type of the polymer.", 0, 1, class_)); 2464 children.add(new Property("geometry", "CodeableConcept", "Polymer geometry, e.g. linear, branched, cross-linked, network or dendritic.", 0, 1, geometry)); 2465 children.add(new Property("copolymerConnectivity", "CodeableConcept", "Descrtibes the copolymer sequence type (polymer connectivity).", 0, java.lang.Integer.MAX_VALUE, copolymerConnectivity)); 2466 children.add(new Property("modification", "string", "Todo - this is intended to connect to a repeating full modification structure, also used by Protein and Nucleic Acid . String is just a placeholder.", 0, 1, modification)); 2467 children.add(new Property("monomerSet", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, monomerSet)); 2468 children.add(new Property("repeat", "", "Specifies and quantifies the repeated units and their configuration.", 0, java.lang.Integer.MAX_VALUE, repeat)); 2469 } 2470 2471 @Override 2472 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2473 switch (_hash) { 2474 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A business idenfier for this polymer, but typically this is handled by a SubstanceDefinition identifier.", 0, 1, identifier); 2475 case 94742904: /*class*/ return new Property("class", "CodeableConcept", "Overall type of the polymer.", 0, 1, class_); 2476 case 1846020210: /*geometry*/ return new Property("geometry", "CodeableConcept", "Polymer geometry, e.g. linear, branched, cross-linked, network or dendritic.", 0, 1, geometry); 2477 case 997107577: /*copolymerConnectivity*/ return new Property("copolymerConnectivity", "CodeableConcept", "Descrtibes the copolymer sequence type (polymer connectivity).", 0, java.lang.Integer.MAX_VALUE, copolymerConnectivity); 2478 case -684600932: /*modification*/ return new Property("modification", "string", "Todo - this is intended to connect to a repeating full modification structure, also used by Protein and Nucleic Acid . String is just a placeholder.", 0, 1, modification); 2479 case -1622483765: /*monomerSet*/ return new Property("monomerSet", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, monomerSet); 2480 case -934531685: /*repeat*/ return new Property("repeat", "", "Specifies and quantifies the repeated units and their configuration.", 0, java.lang.Integer.MAX_VALUE, repeat); 2481 default: return super.getNamedProperty(_hash, _name, _checkValid); 2482 } 2483 2484 } 2485 2486 @Override 2487 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2488 switch (hash) { 2489 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 2490 case 94742904: /*class*/ return this.class_ == null ? new Base[0] : new Base[] {this.class_}; // CodeableConcept 2491 case 1846020210: /*geometry*/ return this.geometry == null ? new Base[0] : new Base[] {this.geometry}; // CodeableConcept 2492 case 997107577: /*copolymerConnectivity*/ return this.copolymerConnectivity == null ? new Base[0] : this.copolymerConnectivity.toArray(new Base[this.copolymerConnectivity.size()]); // CodeableConcept 2493 case -684600932: /*modification*/ return this.modification == null ? new Base[0] : new Base[] {this.modification}; // StringType 2494 case -1622483765: /*monomerSet*/ return this.monomerSet == null ? new Base[0] : this.monomerSet.toArray(new Base[this.monomerSet.size()]); // SubstancePolymerMonomerSetComponent 2495 case -934531685: /*repeat*/ return this.repeat == null ? new Base[0] : this.repeat.toArray(new Base[this.repeat.size()]); // SubstancePolymerRepeatComponent 2496 default: return super.getProperty(hash, name, checkValid); 2497 } 2498 2499 } 2500 2501 @Override 2502 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2503 switch (hash) { 2504 case -1618432855: // identifier 2505 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 2506 return value; 2507 case 94742904: // class 2508 this.class_ = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2509 return value; 2510 case 1846020210: // geometry 2511 this.geometry = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2512 return value; 2513 case 997107577: // copolymerConnectivity 2514 this.getCopolymerConnectivity().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2515 return value; 2516 case -684600932: // modification 2517 this.modification = TypeConvertor.castToString(value); // StringType 2518 return value; 2519 case -1622483765: // monomerSet 2520 this.getMonomerSet().add((SubstancePolymerMonomerSetComponent) value); // SubstancePolymerMonomerSetComponent 2521 return value; 2522 case -934531685: // repeat 2523 this.getRepeat().add((SubstancePolymerRepeatComponent) value); // SubstancePolymerRepeatComponent 2524 return value; 2525 default: return super.setProperty(hash, name, value); 2526 } 2527 2528 } 2529 2530 @Override 2531 public Base setProperty(String name, Base value) throws FHIRException { 2532 if (name.equals("identifier")) { 2533 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 2534 } else if (name.equals("class")) { 2535 this.class_ = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2536 } else if (name.equals("geometry")) { 2537 this.geometry = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2538 } else if (name.equals("copolymerConnectivity")) { 2539 this.getCopolymerConnectivity().add(TypeConvertor.castToCodeableConcept(value)); 2540 } else if (name.equals("modification")) { 2541 this.modification = TypeConvertor.castToString(value); // StringType 2542 } else if (name.equals("monomerSet")) { 2543 this.getMonomerSet().add((SubstancePolymerMonomerSetComponent) value); 2544 } else if (name.equals("repeat")) { 2545 this.getRepeat().add((SubstancePolymerRepeatComponent) value); 2546 } else 2547 return super.setProperty(name, value); 2548 return value; 2549 } 2550 2551 @Override 2552 public void removeChild(String name, Base value) throws FHIRException { 2553 if (name.equals("identifier")) { 2554 this.identifier = null; 2555 } else if (name.equals("class")) { 2556 this.class_ = null; 2557 } else if (name.equals("geometry")) { 2558 this.geometry = null; 2559 } else if (name.equals("copolymerConnectivity")) { 2560 this.getCopolymerConnectivity().remove(value); 2561 } else if (name.equals("modification")) { 2562 this.modification = null; 2563 } else if (name.equals("monomerSet")) { 2564 this.getMonomerSet().remove((SubstancePolymerMonomerSetComponent) value); 2565 } else if (name.equals("repeat")) { 2566 this.getRepeat().remove((SubstancePolymerRepeatComponent) value); 2567 } else 2568 super.removeChild(name, value); 2569 2570 } 2571 2572 @Override 2573 public Base makeProperty(int hash, String name) throws FHIRException { 2574 switch (hash) { 2575 case -1618432855: return getIdentifier(); 2576 case 94742904: return getClass_(); 2577 case 1846020210: return getGeometry(); 2578 case 997107577: return addCopolymerConnectivity(); 2579 case -684600932: return getModificationElement(); 2580 case -1622483765: return addMonomerSet(); 2581 case -934531685: return addRepeat(); 2582 default: return super.makeProperty(hash, name); 2583 } 2584 2585 } 2586 2587 @Override 2588 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2589 switch (hash) { 2590 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2591 case 94742904: /*class*/ return new String[] {"CodeableConcept"}; 2592 case 1846020210: /*geometry*/ return new String[] {"CodeableConcept"}; 2593 case 997107577: /*copolymerConnectivity*/ return new String[] {"CodeableConcept"}; 2594 case -684600932: /*modification*/ return new String[] {"string"}; 2595 case -1622483765: /*monomerSet*/ return new String[] {}; 2596 case -934531685: /*repeat*/ return new String[] {}; 2597 default: return super.getTypesForProperty(hash, name); 2598 } 2599 2600 } 2601 2602 @Override 2603 public Base addChild(String name) throws FHIRException { 2604 if (name.equals("identifier")) { 2605 this.identifier = new Identifier(); 2606 return this.identifier; 2607 } 2608 else if (name.equals("class")) { 2609 this.class_ = new CodeableConcept(); 2610 return this.class_; 2611 } 2612 else if (name.equals("geometry")) { 2613 this.geometry = new CodeableConcept(); 2614 return this.geometry; 2615 } 2616 else if (name.equals("copolymerConnectivity")) { 2617 return addCopolymerConnectivity(); 2618 } 2619 else if (name.equals("modification")) { 2620 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.modification"); 2621 } 2622 else if (name.equals("monomerSet")) { 2623 return addMonomerSet(); 2624 } 2625 else if (name.equals("repeat")) { 2626 return addRepeat(); 2627 } 2628 else 2629 return super.addChild(name); 2630 } 2631 2632 public String fhirType() { 2633 return "SubstancePolymer"; 2634 2635 } 2636 2637 public SubstancePolymer copy() { 2638 SubstancePolymer dst = new SubstancePolymer(); 2639 copyValues(dst); 2640 return dst; 2641 } 2642 2643 public void copyValues(SubstancePolymer dst) { 2644 super.copyValues(dst); 2645 dst.identifier = identifier == null ? null : identifier.copy(); 2646 dst.class_ = class_ == null ? null : class_.copy(); 2647 dst.geometry = geometry == null ? null : geometry.copy(); 2648 if (copolymerConnectivity != null) { 2649 dst.copolymerConnectivity = new ArrayList<CodeableConcept>(); 2650 for (CodeableConcept i : copolymerConnectivity) 2651 dst.copolymerConnectivity.add(i.copy()); 2652 }; 2653 dst.modification = modification == null ? null : modification.copy(); 2654 if (monomerSet != null) { 2655 dst.monomerSet = new ArrayList<SubstancePolymerMonomerSetComponent>(); 2656 for (SubstancePolymerMonomerSetComponent i : monomerSet) 2657 dst.monomerSet.add(i.copy()); 2658 }; 2659 if (repeat != null) { 2660 dst.repeat = new ArrayList<SubstancePolymerRepeatComponent>(); 2661 for (SubstancePolymerRepeatComponent i : repeat) 2662 dst.repeat.add(i.copy()); 2663 }; 2664 } 2665 2666 protected SubstancePolymer typedCopy() { 2667 return copy(); 2668 } 2669 2670 @Override 2671 public boolean equalsDeep(Base other_) { 2672 if (!super.equalsDeep(other_)) 2673 return false; 2674 if (!(other_ instanceof SubstancePolymer)) 2675 return false; 2676 SubstancePolymer o = (SubstancePolymer) other_; 2677 return compareDeep(identifier, o.identifier, true) && compareDeep(class_, o.class_, true) && compareDeep(geometry, o.geometry, true) 2678 && compareDeep(copolymerConnectivity, o.copolymerConnectivity, true) && compareDeep(modification, o.modification, true) 2679 && compareDeep(monomerSet, o.monomerSet, true) && compareDeep(repeat, o.repeat, true); 2680 } 2681 2682 @Override 2683 public boolean equalsShallow(Base other_) { 2684 if (!super.equalsShallow(other_)) 2685 return false; 2686 if (!(other_ instanceof SubstancePolymer)) 2687 return false; 2688 SubstancePolymer o = (SubstancePolymer) other_; 2689 return compareValues(modification, o.modification, true); 2690 } 2691 2692 public boolean isEmpty() { 2693 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, class_, geometry 2694 , copolymerConnectivity, modification, monomerSet, repeat); 2695 } 2696 2697 @Override 2698 public ResourceType getResourceType() { 2699 return ResourceType.SubstancePolymer; 2700 } 2701 2702 2703} 2704