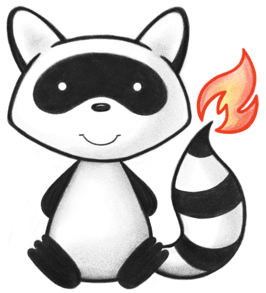
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A SubstanceProtein is defined as a single unit of a linear amino acid sequence, or a combination of subunits that are either covalently linked or have a defined invariant stoichiometric relationship. This includes all synthetic, recombinant and purified SubstanceProteins of defined sequence, whether the use is therapeutic or prophylactic. This set of elements will be used to describe albumins, coagulation factors, cytokines, growth factors, peptide/SubstanceProtein hormones, enzymes, toxins, toxoids, recombinant vaccines, and immunomodulators. 052 */ 053@ResourceDef(name="SubstanceProtein", profile="http://hl7.org/fhir/StructureDefinition/SubstanceProtein") 054public class SubstanceProtein extends DomainResource { 055 056 @Block() 057 public static class SubstanceProteinSubunitComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Index of primary sequences of amino acids linked through peptide bonds in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts. 060 */ 061 @Child(name = "subunit", type = {IntegerType.class}, order=1, min=0, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="Index of primary sequences of amino acids linked through peptide bonds in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts", formalDefinition="Index of primary sequences of amino acids linked through peptide bonds in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts." ) 063 protected IntegerType subunit; 064 065 /** 066 * The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence. 067 */ 068 @Child(name = "sequence", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 069 @Description(shortDefinition="The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence", formalDefinition="The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence." ) 070 protected StringType sequence; 071 072 /** 073 * Length of linear sequences of amino acids contained in the subunit. 074 */ 075 @Child(name = "length", type = {IntegerType.class}, order=3, min=0, max=1, modifier=false, summary=true) 076 @Description(shortDefinition="Length of linear sequences of amino acids contained in the subunit", formalDefinition="Length of linear sequences of amino acids contained in the subunit." ) 077 protected IntegerType length; 078 079 /** 080 * The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence. 081 */ 082 @Child(name = "sequenceAttachment", type = {Attachment.class}, order=4, min=0, max=1, modifier=false, summary=true) 083 @Description(shortDefinition="The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence", formalDefinition="The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence." ) 084 protected Attachment sequenceAttachment; 085 086 /** 087 * Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID. 088 */ 089 @Child(name = "nTerminalModificationId", type = {Identifier.class}, order=5, min=0, max=1, modifier=false, summary=true) 090 @Description(shortDefinition="Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID", formalDefinition="Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID." ) 091 protected Identifier nTerminalModificationId; 092 093 /** 094 * The name of the fragment modified at the N-terminal of the SubstanceProtein shall be specified. 095 */ 096 @Child(name = "nTerminalModification", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 097 @Description(shortDefinition="The name of the fragment modified at the N-terminal of the SubstanceProtein shall be specified", formalDefinition="The name of the fragment modified at the N-terminal of the SubstanceProtein shall be specified." ) 098 protected StringType nTerminalModification; 099 100 /** 101 * Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID. 102 */ 103 @Child(name = "cTerminalModificationId", type = {Identifier.class}, order=7, min=0, max=1, modifier=false, summary=true) 104 @Description(shortDefinition="Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID", formalDefinition="Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID." ) 105 protected Identifier cTerminalModificationId; 106 107 /** 108 * The modification at the C-terminal shall be specified. 109 */ 110 @Child(name = "cTerminalModification", type = {StringType.class}, order=8, min=0, max=1, modifier=false, summary=true) 111 @Description(shortDefinition="The modification at the C-terminal shall be specified", formalDefinition="The modification at the C-terminal shall be specified." ) 112 protected StringType cTerminalModification; 113 114 private static final long serialVersionUID = 99973841L; 115 116 /** 117 * Constructor 118 */ 119 public SubstanceProteinSubunitComponent() { 120 super(); 121 } 122 123 /** 124 * @return {@link #subunit} (Index of primary sequences of amino acids linked through peptide bonds in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts.). This is the underlying object with id, value and extensions. The accessor "getSubunit" gives direct access to the value 125 */ 126 public IntegerType getSubunitElement() { 127 if (this.subunit == null) 128 if (Configuration.errorOnAutoCreate()) 129 throw new Error("Attempt to auto-create SubstanceProteinSubunitComponent.subunit"); 130 else if (Configuration.doAutoCreate()) 131 this.subunit = new IntegerType(); // bb 132 return this.subunit; 133 } 134 135 public boolean hasSubunitElement() { 136 return this.subunit != null && !this.subunit.isEmpty(); 137 } 138 139 public boolean hasSubunit() { 140 return this.subunit != null && !this.subunit.isEmpty(); 141 } 142 143 /** 144 * @param value {@link #subunit} (Index of primary sequences of amino acids linked through peptide bonds in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts.). This is the underlying object with id, value and extensions. The accessor "getSubunit" gives direct access to the value 145 */ 146 public SubstanceProteinSubunitComponent setSubunitElement(IntegerType value) { 147 this.subunit = value; 148 return this; 149 } 150 151 /** 152 * @return Index of primary sequences of amino acids linked through peptide bonds in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts. 153 */ 154 public int getSubunit() { 155 return this.subunit == null || this.subunit.isEmpty() ? 0 : this.subunit.getValue(); 156 } 157 158 /** 159 * @param value Index of primary sequences of amino acids linked through peptide bonds in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts. 160 */ 161 public SubstanceProteinSubunitComponent setSubunit(int value) { 162 if (this.subunit == null) 163 this.subunit = new IntegerType(); 164 this.subunit.setValue(value); 165 return this; 166 } 167 168 /** 169 * @return {@link #sequence} (The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 170 */ 171 public StringType getSequenceElement() { 172 if (this.sequence == null) 173 if (Configuration.errorOnAutoCreate()) 174 throw new Error("Attempt to auto-create SubstanceProteinSubunitComponent.sequence"); 175 else if (Configuration.doAutoCreate()) 176 this.sequence = new StringType(); // bb 177 return this.sequence; 178 } 179 180 public boolean hasSequenceElement() { 181 return this.sequence != null && !this.sequence.isEmpty(); 182 } 183 184 public boolean hasSequence() { 185 return this.sequence != null && !this.sequence.isEmpty(); 186 } 187 188 /** 189 * @param value {@link #sequence} (The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 190 */ 191 public SubstanceProteinSubunitComponent setSequenceElement(StringType value) { 192 this.sequence = value; 193 return this; 194 } 195 196 /** 197 * @return The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence. 198 */ 199 public String getSequence() { 200 return this.sequence == null ? null : this.sequence.getValue(); 201 } 202 203 /** 204 * @param value The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence. 205 */ 206 public SubstanceProteinSubunitComponent setSequence(String value) { 207 if (Utilities.noString(value)) 208 this.sequence = null; 209 else { 210 if (this.sequence == null) 211 this.sequence = new StringType(); 212 this.sequence.setValue(value); 213 } 214 return this; 215 } 216 217 /** 218 * @return {@link #length} (Length of linear sequences of amino acids contained in the subunit.). This is the underlying object with id, value and extensions. The accessor "getLength" gives direct access to the value 219 */ 220 public IntegerType getLengthElement() { 221 if (this.length == null) 222 if (Configuration.errorOnAutoCreate()) 223 throw new Error("Attempt to auto-create SubstanceProteinSubunitComponent.length"); 224 else if (Configuration.doAutoCreate()) 225 this.length = new IntegerType(); // bb 226 return this.length; 227 } 228 229 public boolean hasLengthElement() { 230 return this.length != null && !this.length.isEmpty(); 231 } 232 233 public boolean hasLength() { 234 return this.length != null && !this.length.isEmpty(); 235 } 236 237 /** 238 * @param value {@link #length} (Length of linear sequences of amino acids contained in the subunit.). This is the underlying object with id, value and extensions. The accessor "getLength" gives direct access to the value 239 */ 240 public SubstanceProteinSubunitComponent setLengthElement(IntegerType value) { 241 this.length = value; 242 return this; 243 } 244 245 /** 246 * @return Length of linear sequences of amino acids contained in the subunit. 247 */ 248 public int getLength() { 249 return this.length == null || this.length.isEmpty() ? 0 : this.length.getValue(); 250 } 251 252 /** 253 * @param value Length of linear sequences of amino acids contained in the subunit. 254 */ 255 public SubstanceProteinSubunitComponent setLength(int value) { 256 if (this.length == null) 257 this.length = new IntegerType(); 258 this.length.setValue(value); 259 return this; 260 } 261 262 /** 263 * @return {@link #sequenceAttachment} (The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence.) 264 */ 265 public Attachment getSequenceAttachment() { 266 if (this.sequenceAttachment == null) 267 if (Configuration.errorOnAutoCreate()) 268 throw new Error("Attempt to auto-create SubstanceProteinSubunitComponent.sequenceAttachment"); 269 else if (Configuration.doAutoCreate()) 270 this.sequenceAttachment = new Attachment(); // cc 271 return this.sequenceAttachment; 272 } 273 274 public boolean hasSequenceAttachment() { 275 return this.sequenceAttachment != null && !this.sequenceAttachment.isEmpty(); 276 } 277 278 /** 279 * @param value {@link #sequenceAttachment} (The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence.) 280 */ 281 public SubstanceProteinSubunitComponent setSequenceAttachment(Attachment value) { 282 this.sequenceAttachment = value; 283 return this; 284 } 285 286 /** 287 * @return {@link #nTerminalModificationId} (Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID.) 288 */ 289 public Identifier getNTerminalModificationId() { 290 if (this.nTerminalModificationId == null) 291 if (Configuration.errorOnAutoCreate()) 292 throw new Error("Attempt to auto-create SubstanceProteinSubunitComponent.nTerminalModificationId"); 293 else if (Configuration.doAutoCreate()) 294 this.nTerminalModificationId = new Identifier(); // cc 295 return this.nTerminalModificationId; 296 } 297 298 public boolean hasNTerminalModificationId() { 299 return this.nTerminalModificationId != null && !this.nTerminalModificationId.isEmpty(); 300 } 301 302 /** 303 * @param value {@link #nTerminalModificationId} (Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID.) 304 */ 305 public SubstanceProteinSubunitComponent setNTerminalModificationId(Identifier value) { 306 this.nTerminalModificationId = value; 307 return this; 308 } 309 310 /** 311 * @return {@link #nTerminalModification} (The name of the fragment modified at the N-terminal of the SubstanceProtein shall be specified.). This is the underlying object with id, value and extensions. The accessor "getNTerminalModification" gives direct access to the value 312 */ 313 public StringType getNTerminalModificationElement() { 314 if (this.nTerminalModification == null) 315 if (Configuration.errorOnAutoCreate()) 316 throw new Error("Attempt to auto-create SubstanceProteinSubunitComponent.nTerminalModification"); 317 else if (Configuration.doAutoCreate()) 318 this.nTerminalModification = new StringType(); // bb 319 return this.nTerminalModification; 320 } 321 322 public boolean hasNTerminalModificationElement() { 323 return this.nTerminalModification != null && !this.nTerminalModification.isEmpty(); 324 } 325 326 public boolean hasNTerminalModification() { 327 return this.nTerminalModification != null && !this.nTerminalModification.isEmpty(); 328 } 329 330 /** 331 * @param value {@link #nTerminalModification} (The name of the fragment modified at the N-terminal of the SubstanceProtein shall be specified.). This is the underlying object with id, value and extensions. The accessor "getNTerminalModification" gives direct access to the value 332 */ 333 public SubstanceProteinSubunitComponent setNTerminalModificationElement(StringType value) { 334 this.nTerminalModification = value; 335 return this; 336 } 337 338 /** 339 * @return The name of the fragment modified at the N-terminal of the SubstanceProtein shall be specified. 340 */ 341 public String getNTerminalModification() { 342 return this.nTerminalModification == null ? null : this.nTerminalModification.getValue(); 343 } 344 345 /** 346 * @param value The name of the fragment modified at the N-terminal of the SubstanceProtein shall be specified. 347 */ 348 public SubstanceProteinSubunitComponent setNTerminalModification(String value) { 349 if (Utilities.noString(value)) 350 this.nTerminalModification = null; 351 else { 352 if (this.nTerminalModification == null) 353 this.nTerminalModification = new StringType(); 354 this.nTerminalModification.setValue(value); 355 } 356 return this; 357 } 358 359 /** 360 * @return {@link #cTerminalModificationId} (Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID.) 361 */ 362 public Identifier getCTerminalModificationId() { 363 if (this.cTerminalModificationId == null) 364 if (Configuration.errorOnAutoCreate()) 365 throw new Error("Attempt to auto-create SubstanceProteinSubunitComponent.cTerminalModificationId"); 366 else if (Configuration.doAutoCreate()) 367 this.cTerminalModificationId = new Identifier(); // cc 368 return this.cTerminalModificationId; 369 } 370 371 public boolean hasCTerminalModificationId() { 372 return this.cTerminalModificationId != null && !this.cTerminalModificationId.isEmpty(); 373 } 374 375 /** 376 * @param value {@link #cTerminalModificationId} (Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID.) 377 */ 378 public SubstanceProteinSubunitComponent setCTerminalModificationId(Identifier value) { 379 this.cTerminalModificationId = value; 380 return this; 381 } 382 383 /** 384 * @return {@link #cTerminalModification} (The modification at the C-terminal shall be specified.). This is the underlying object with id, value and extensions. The accessor "getCTerminalModification" gives direct access to the value 385 */ 386 public StringType getCTerminalModificationElement() { 387 if (this.cTerminalModification == null) 388 if (Configuration.errorOnAutoCreate()) 389 throw new Error("Attempt to auto-create SubstanceProteinSubunitComponent.cTerminalModification"); 390 else if (Configuration.doAutoCreate()) 391 this.cTerminalModification = new StringType(); // bb 392 return this.cTerminalModification; 393 } 394 395 public boolean hasCTerminalModificationElement() { 396 return this.cTerminalModification != null && !this.cTerminalModification.isEmpty(); 397 } 398 399 public boolean hasCTerminalModification() { 400 return this.cTerminalModification != null && !this.cTerminalModification.isEmpty(); 401 } 402 403 /** 404 * @param value {@link #cTerminalModification} (The modification at the C-terminal shall be specified.). This is the underlying object with id, value and extensions. The accessor "getCTerminalModification" gives direct access to the value 405 */ 406 public SubstanceProteinSubunitComponent setCTerminalModificationElement(StringType value) { 407 this.cTerminalModification = value; 408 return this; 409 } 410 411 /** 412 * @return The modification at the C-terminal shall be specified. 413 */ 414 public String getCTerminalModification() { 415 return this.cTerminalModification == null ? null : this.cTerminalModification.getValue(); 416 } 417 418 /** 419 * @param value The modification at the C-terminal shall be specified. 420 */ 421 public SubstanceProteinSubunitComponent setCTerminalModification(String value) { 422 if (Utilities.noString(value)) 423 this.cTerminalModification = null; 424 else { 425 if (this.cTerminalModification == null) 426 this.cTerminalModification = new StringType(); 427 this.cTerminalModification.setValue(value); 428 } 429 return this; 430 } 431 432 protected void listChildren(List<Property> children) { 433 super.listChildren(children); 434 children.add(new Property("subunit", "integer", "Index of primary sequences of amino acids linked through peptide bonds in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts.", 0, 1, subunit)); 435 children.add(new Property("sequence", "string", "The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence.", 0, 1, sequence)); 436 children.add(new Property("length", "integer", "Length of linear sequences of amino acids contained in the subunit.", 0, 1, length)); 437 children.add(new Property("sequenceAttachment", "Attachment", "The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence.", 0, 1, sequenceAttachment)); 438 children.add(new Property("nTerminalModificationId", "Identifier", "Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID.", 0, 1, nTerminalModificationId)); 439 children.add(new Property("nTerminalModification", "string", "The name of the fragment modified at the N-terminal of the SubstanceProtein shall be specified.", 0, 1, nTerminalModification)); 440 children.add(new Property("cTerminalModificationId", "Identifier", "Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID.", 0, 1, cTerminalModificationId)); 441 children.add(new Property("cTerminalModification", "string", "The modification at the C-terminal shall be specified.", 0, 1, cTerminalModification)); 442 } 443 444 @Override 445 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 446 switch (_hash) { 447 case -1867548732: /*subunit*/ return new Property("subunit", "integer", "Index of primary sequences of amino acids linked through peptide bonds in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts.", 0, 1, subunit); 448 case 1349547969: /*sequence*/ return new Property("sequence", "string", "The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence.", 0, 1, sequence); 449 case -1106363674: /*length*/ return new Property("length", "integer", "Length of linear sequences of amino acids contained in the subunit.", 0, 1, length); 450 case 364621764: /*sequenceAttachment*/ return new Property("sequenceAttachment", "Attachment", "The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence.", 0, 1, sequenceAttachment); 451 case -182796415: /*nTerminalModificationId*/ return new Property("nTerminalModificationId", "Identifier", "Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID.", 0, 1, nTerminalModificationId); 452 case -1497395258: /*nTerminalModification*/ return new Property("nTerminalModification", "string", "The name of the fragment modified at the N-terminal of the SubstanceProtein shall be specified.", 0, 1, nTerminalModification); 453 case -990303818: /*cTerminalModificationId*/ return new Property("cTerminalModificationId", "Identifier", "Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID.", 0, 1, cTerminalModificationId); 454 case 472711995: /*cTerminalModification*/ return new Property("cTerminalModification", "string", "The modification at the C-terminal shall be specified.", 0, 1, cTerminalModification); 455 default: return super.getNamedProperty(_hash, _name, _checkValid); 456 } 457 458 } 459 460 @Override 461 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 462 switch (hash) { 463 case -1867548732: /*subunit*/ return this.subunit == null ? new Base[0] : new Base[] {this.subunit}; // IntegerType 464 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // StringType 465 case -1106363674: /*length*/ return this.length == null ? new Base[0] : new Base[] {this.length}; // IntegerType 466 case 364621764: /*sequenceAttachment*/ return this.sequenceAttachment == null ? new Base[0] : new Base[] {this.sequenceAttachment}; // Attachment 467 case -182796415: /*nTerminalModificationId*/ return this.nTerminalModificationId == null ? new Base[0] : new Base[] {this.nTerminalModificationId}; // Identifier 468 case -1497395258: /*nTerminalModification*/ return this.nTerminalModification == null ? new Base[0] : new Base[] {this.nTerminalModification}; // StringType 469 case -990303818: /*cTerminalModificationId*/ return this.cTerminalModificationId == null ? new Base[0] : new Base[] {this.cTerminalModificationId}; // Identifier 470 case 472711995: /*cTerminalModification*/ return this.cTerminalModification == null ? new Base[0] : new Base[] {this.cTerminalModification}; // StringType 471 default: return super.getProperty(hash, name, checkValid); 472 } 473 474 } 475 476 @Override 477 public Base setProperty(int hash, String name, Base value) throws FHIRException { 478 switch (hash) { 479 case -1867548732: // subunit 480 this.subunit = TypeConvertor.castToInteger(value); // IntegerType 481 return value; 482 case 1349547969: // sequence 483 this.sequence = TypeConvertor.castToString(value); // StringType 484 return value; 485 case -1106363674: // length 486 this.length = TypeConvertor.castToInteger(value); // IntegerType 487 return value; 488 case 364621764: // sequenceAttachment 489 this.sequenceAttachment = TypeConvertor.castToAttachment(value); // Attachment 490 return value; 491 case -182796415: // nTerminalModificationId 492 this.nTerminalModificationId = TypeConvertor.castToIdentifier(value); // Identifier 493 return value; 494 case -1497395258: // nTerminalModification 495 this.nTerminalModification = TypeConvertor.castToString(value); // StringType 496 return value; 497 case -990303818: // cTerminalModificationId 498 this.cTerminalModificationId = TypeConvertor.castToIdentifier(value); // Identifier 499 return value; 500 case 472711995: // cTerminalModification 501 this.cTerminalModification = TypeConvertor.castToString(value); // StringType 502 return value; 503 default: return super.setProperty(hash, name, value); 504 } 505 506 } 507 508 @Override 509 public Base setProperty(String name, Base value) throws FHIRException { 510 if (name.equals("subunit")) { 511 this.subunit = TypeConvertor.castToInteger(value); // IntegerType 512 } else if (name.equals("sequence")) { 513 this.sequence = TypeConvertor.castToString(value); // StringType 514 } else if (name.equals("length")) { 515 this.length = TypeConvertor.castToInteger(value); // IntegerType 516 } else if (name.equals("sequenceAttachment")) { 517 this.sequenceAttachment = TypeConvertor.castToAttachment(value); // Attachment 518 } else if (name.equals("nTerminalModificationId")) { 519 this.nTerminalModificationId = TypeConvertor.castToIdentifier(value); // Identifier 520 } else if (name.equals("nTerminalModification")) { 521 this.nTerminalModification = TypeConvertor.castToString(value); // StringType 522 } else if (name.equals("cTerminalModificationId")) { 523 this.cTerminalModificationId = TypeConvertor.castToIdentifier(value); // Identifier 524 } else if (name.equals("cTerminalModification")) { 525 this.cTerminalModification = TypeConvertor.castToString(value); // StringType 526 } else 527 return super.setProperty(name, value); 528 return value; 529 } 530 531 @Override 532 public void removeChild(String name, Base value) throws FHIRException { 533 if (name.equals("subunit")) { 534 this.subunit = null; 535 } else if (name.equals("sequence")) { 536 this.sequence = null; 537 } else if (name.equals("length")) { 538 this.length = null; 539 } else if (name.equals("sequenceAttachment")) { 540 this.sequenceAttachment = null; 541 } else if (name.equals("nTerminalModificationId")) { 542 this.nTerminalModificationId = null; 543 } else if (name.equals("nTerminalModification")) { 544 this.nTerminalModification = null; 545 } else if (name.equals("cTerminalModificationId")) { 546 this.cTerminalModificationId = null; 547 } else if (name.equals("cTerminalModification")) { 548 this.cTerminalModification = null; 549 } else 550 super.removeChild(name, value); 551 552 } 553 554 @Override 555 public Base makeProperty(int hash, String name) throws FHIRException { 556 switch (hash) { 557 case -1867548732: return getSubunitElement(); 558 case 1349547969: return getSequenceElement(); 559 case -1106363674: return getLengthElement(); 560 case 364621764: return getSequenceAttachment(); 561 case -182796415: return getNTerminalModificationId(); 562 case -1497395258: return getNTerminalModificationElement(); 563 case -990303818: return getCTerminalModificationId(); 564 case 472711995: return getCTerminalModificationElement(); 565 default: return super.makeProperty(hash, name); 566 } 567 568 } 569 570 @Override 571 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 572 switch (hash) { 573 case -1867548732: /*subunit*/ return new String[] {"integer"}; 574 case 1349547969: /*sequence*/ return new String[] {"string"}; 575 case -1106363674: /*length*/ return new String[] {"integer"}; 576 case 364621764: /*sequenceAttachment*/ return new String[] {"Attachment"}; 577 case -182796415: /*nTerminalModificationId*/ return new String[] {"Identifier"}; 578 case -1497395258: /*nTerminalModification*/ return new String[] {"string"}; 579 case -990303818: /*cTerminalModificationId*/ return new String[] {"Identifier"}; 580 case 472711995: /*cTerminalModification*/ return new String[] {"string"}; 581 default: return super.getTypesForProperty(hash, name); 582 } 583 584 } 585 586 @Override 587 public Base addChild(String name) throws FHIRException { 588 if (name.equals("subunit")) { 589 throw new FHIRException("Cannot call addChild on a singleton property SubstanceProtein.subunit.subunit"); 590 } 591 else if (name.equals("sequence")) { 592 throw new FHIRException("Cannot call addChild on a singleton property SubstanceProtein.subunit.sequence"); 593 } 594 else if (name.equals("length")) { 595 throw new FHIRException("Cannot call addChild on a singleton property SubstanceProtein.subunit.length"); 596 } 597 else if (name.equals("sequenceAttachment")) { 598 this.sequenceAttachment = new Attachment(); 599 return this.sequenceAttachment; 600 } 601 else if (name.equals("nTerminalModificationId")) { 602 this.nTerminalModificationId = new Identifier(); 603 return this.nTerminalModificationId; 604 } 605 else if (name.equals("nTerminalModification")) { 606 throw new FHIRException("Cannot call addChild on a singleton property SubstanceProtein.subunit.nTerminalModification"); 607 } 608 else if (name.equals("cTerminalModificationId")) { 609 this.cTerminalModificationId = new Identifier(); 610 return this.cTerminalModificationId; 611 } 612 else if (name.equals("cTerminalModification")) { 613 throw new FHIRException("Cannot call addChild on a singleton property SubstanceProtein.subunit.cTerminalModification"); 614 } 615 else 616 return super.addChild(name); 617 } 618 619 public SubstanceProteinSubunitComponent copy() { 620 SubstanceProteinSubunitComponent dst = new SubstanceProteinSubunitComponent(); 621 copyValues(dst); 622 return dst; 623 } 624 625 public void copyValues(SubstanceProteinSubunitComponent dst) { 626 super.copyValues(dst); 627 dst.subunit = subunit == null ? null : subunit.copy(); 628 dst.sequence = sequence == null ? null : sequence.copy(); 629 dst.length = length == null ? null : length.copy(); 630 dst.sequenceAttachment = sequenceAttachment == null ? null : sequenceAttachment.copy(); 631 dst.nTerminalModificationId = nTerminalModificationId == null ? null : nTerminalModificationId.copy(); 632 dst.nTerminalModification = nTerminalModification == null ? null : nTerminalModification.copy(); 633 dst.cTerminalModificationId = cTerminalModificationId == null ? null : cTerminalModificationId.copy(); 634 dst.cTerminalModification = cTerminalModification == null ? null : cTerminalModification.copy(); 635 } 636 637 @Override 638 public boolean equalsDeep(Base other_) { 639 if (!super.equalsDeep(other_)) 640 return false; 641 if (!(other_ instanceof SubstanceProteinSubunitComponent)) 642 return false; 643 SubstanceProteinSubunitComponent o = (SubstanceProteinSubunitComponent) other_; 644 return compareDeep(subunit, o.subunit, true) && compareDeep(sequence, o.sequence, true) && compareDeep(length, o.length, true) 645 && compareDeep(sequenceAttachment, o.sequenceAttachment, true) && compareDeep(nTerminalModificationId, o.nTerminalModificationId, true) 646 && compareDeep(nTerminalModification, o.nTerminalModification, true) && compareDeep(cTerminalModificationId, o.cTerminalModificationId, true) 647 && compareDeep(cTerminalModification, o.cTerminalModification, true); 648 } 649 650 @Override 651 public boolean equalsShallow(Base other_) { 652 if (!super.equalsShallow(other_)) 653 return false; 654 if (!(other_ instanceof SubstanceProteinSubunitComponent)) 655 return false; 656 SubstanceProteinSubunitComponent o = (SubstanceProteinSubunitComponent) other_; 657 return compareValues(subunit, o.subunit, true) && compareValues(sequence, o.sequence, true) && compareValues(length, o.length, true) 658 && compareValues(nTerminalModification, o.nTerminalModification, true) && compareValues(cTerminalModification, o.cTerminalModification, true) 659 ; 660 } 661 662 public boolean isEmpty() { 663 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(subunit, sequence, length 664 , sequenceAttachment, nTerminalModificationId, nTerminalModification, cTerminalModificationId 665 , cTerminalModification); 666 } 667 668 public String fhirType() { 669 return "SubstanceProtein.subunit"; 670 671 } 672 673 } 674 675 /** 676 * The SubstanceProtein descriptive elements will only be used when a complete or partial amino acid sequence is available or derivable from a nucleic acid sequence. 677 */ 678 @Child(name = "sequenceType", type = {CodeableConcept.class}, order=0, min=0, max=1, modifier=false, summary=true) 679 @Description(shortDefinition="The SubstanceProtein descriptive elements will only be used when a complete or partial amino acid sequence is available or derivable from a nucleic acid sequence", formalDefinition="The SubstanceProtein descriptive elements will only be used when a complete or partial amino acid sequence is available or derivable from a nucleic acid sequence." ) 680 protected CodeableConcept sequenceType; 681 682 /** 683 * Number of linear sequences of amino acids linked through peptide bonds. The number of subunits constituting the SubstanceProtein shall be described. It is possible that the number of subunits can be variable. 684 */ 685 @Child(name = "numberOfSubunits", type = {IntegerType.class}, order=1, min=0, max=1, modifier=false, summary=true) 686 @Description(shortDefinition="Number of linear sequences of amino acids linked through peptide bonds. The number of subunits constituting the SubstanceProtein shall be described. It is possible that the number of subunits can be variable", formalDefinition="Number of linear sequences of amino acids linked through peptide bonds. The number of subunits constituting the SubstanceProtein shall be described. It is possible that the number of subunits can be variable." ) 687 protected IntegerType numberOfSubunits; 688 689 /** 690 * The disulphide bond between two cysteine residues either on the same subunit or on two different subunits shall be described. The position of the disulfide bonds in the SubstanceProtein shall be listed in increasing order of subunit number and position within subunit followed by the abbreviation of the amino acids involved. The disulfide linkage positions shall actually contain the amino acid Cysteine at the respective positions. 691 */ 692 @Child(name = "disulfideLinkage", type = {StringType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 693 @Description(shortDefinition="The disulphide bond between two cysteine residues either on the same subunit or on two different subunits shall be described. The position of the disulfide bonds in the SubstanceProtein shall be listed in increasing order of subunit number and position within subunit followed by the abbreviation of the amino acids involved. The disulfide linkage positions shall actually contain the amino acid Cysteine at the respective positions", formalDefinition="The disulphide bond between two cysteine residues either on the same subunit or on two different subunits shall be described. The position of the disulfide bonds in the SubstanceProtein shall be listed in increasing order of subunit number and position within subunit followed by the abbreviation of the amino acids involved. The disulfide linkage positions shall actually contain the amino acid Cysteine at the respective positions." ) 694 protected List<StringType> disulfideLinkage; 695 696 /** 697 * This subclause refers to the description of each subunit constituting the SubstanceProtein. A subunit is a linear sequence of amino acids linked through peptide bonds. The Subunit information shall be provided when the finished SubstanceProtein is a complex of multiple sequences; subunits are not used to delineate domains within a single sequence. Subunits are listed in order of decreasing length; sequences of the same length will be ordered by decreasing molecular weight; subunits that have identical sequences will be repeated multiple times. 698 */ 699 @Child(name = "subunit", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 700 @Description(shortDefinition="This subclause refers to the description of each subunit constituting the SubstanceProtein. A subunit is a linear sequence of amino acids linked through peptide bonds. The Subunit information shall be provided when the finished SubstanceProtein is a complex of multiple sequences; subunits are not used to delineate domains within a single sequence. Subunits are listed in order of decreasing length; sequences of the same length will be ordered by decreasing molecular weight; subunits that have identical sequences will be repeated multiple times", formalDefinition="This subclause refers to the description of each subunit constituting the SubstanceProtein. A subunit is a linear sequence of amino acids linked through peptide bonds. The Subunit information shall be provided when the finished SubstanceProtein is a complex of multiple sequences; subunits are not used to delineate domains within a single sequence. Subunits are listed in order of decreasing length; sequences of the same length will be ordered by decreasing molecular weight; subunits that have identical sequences will be repeated multiple times." ) 701 protected List<SubstanceProteinSubunitComponent> subunit; 702 703 private static final long serialVersionUID = 469786856L; 704 705 /** 706 * Constructor 707 */ 708 public SubstanceProtein() { 709 super(); 710 } 711 712 /** 713 * @return {@link #sequenceType} (The SubstanceProtein descriptive elements will only be used when a complete or partial amino acid sequence is available or derivable from a nucleic acid sequence.) 714 */ 715 public CodeableConcept getSequenceType() { 716 if (this.sequenceType == null) 717 if (Configuration.errorOnAutoCreate()) 718 throw new Error("Attempt to auto-create SubstanceProtein.sequenceType"); 719 else if (Configuration.doAutoCreate()) 720 this.sequenceType = new CodeableConcept(); // cc 721 return this.sequenceType; 722 } 723 724 public boolean hasSequenceType() { 725 return this.sequenceType != null && !this.sequenceType.isEmpty(); 726 } 727 728 /** 729 * @param value {@link #sequenceType} (The SubstanceProtein descriptive elements will only be used when a complete or partial amino acid sequence is available or derivable from a nucleic acid sequence.) 730 */ 731 public SubstanceProtein setSequenceType(CodeableConcept value) { 732 this.sequenceType = value; 733 return this; 734 } 735 736 /** 737 * @return {@link #numberOfSubunits} (Number of linear sequences of amino acids linked through peptide bonds. The number of subunits constituting the SubstanceProtein shall be described. It is possible that the number of subunits can be variable.). This is the underlying object with id, value and extensions. The accessor "getNumberOfSubunits" gives direct access to the value 738 */ 739 public IntegerType getNumberOfSubunitsElement() { 740 if (this.numberOfSubunits == null) 741 if (Configuration.errorOnAutoCreate()) 742 throw new Error("Attempt to auto-create SubstanceProtein.numberOfSubunits"); 743 else if (Configuration.doAutoCreate()) 744 this.numberOfSubunits = new IntegerType(); // bb 745 return this.numberOfSubunits; 746 } 747 748 public boolean hasNumberOfSubunitsElement() { 749 return this.numberOfSubunits != null && !this.numberOfSubunits.isEmpty(); 750 } 751 752 public boolean hasNumberOfSubunits() { 753 return this.numberOfSubunits != null && !this.numberOfSubunits.isEmpty(); 754 } 755 756 /** 757 * @param value {@link #numberOfSubunits} (Number of linear sequences of amino acids linked through peptide bonds. The number of subunits constituting the SubstanceProtein shall be described. It is possible that the number of subunits can be variable.). This is the underlying object with id, value and extensions. The accessor "getNumberOfSubunits" gives direct access to the value 758 */ 759 public SubstanceProtein setNumberOfSubunitsElement(IntegerType value) { 760 this.numberOfSubunits = value; 761 return this; 762 } 763 764 /** 765 * @return Number of linear sequences of amino acids linked through peptide bonds. The number of subunits constituting the SubstanceProtein shall be described. It is possible that the number of subunits can be variable. 766 */ 767 public int getNumberOfSubunits() { 768 return this.numberOfSubunits == null || this.numberOfSubunits.isEmpty() ? 0 : this.numberOfSubunits.getValue(); 769 } 770 771 /** 772 * @param value Number of linear sequences of amino acids linked through peptide bonds. The number of subunits constituting the SubstanceProtein shall be described. It is possible that the number of subunits can be variable. 773 */ 774 public SubstanceProtein setNumberOfSubunits(int value) { 775 if (this.numberOfSubunits == null) 776 this.numberOfSubunits = new IntegerType(); 777 this.numberOfSubunits.setValue(value); 778 return this; 779 } 780 781 /** 782 * @return {@link #disulfideLinkage} (The disulphide bond between two cysteine residues either on the same subunit or on two different subunits shall be described. The position of the disulfide bonds in the SubstanceProtein shall be listed in increasing order of subunit number and position within subunit followed by the abbreviation of the amino acids involved. The disulfide linkage positions shall actually contain the amino acid Cysteine at the respective positions.) 783 */ 784 public List<StringType> getDisulfideLinkage() { 785 if (this.disulfideLinkage == null) 786 this.disulfideLinkage = new ArrayList<StringType>(); 787 return this.disulfideLinkage; 788 } 789 790 /** 791 * @return Returns a reference to <code>this</code> for easy method chaining 792 */ 793 public SubstanceProtein setDisulfideLinkage(List<StringType> theDisulfideLinkage) { 794 this.disulfideLinkage = theDisulfideLinkage; 795 return this; 796 } 797 798 public boolean hasDisulfideLinkage() { 799 if (this.disulfideLinkage == null) 800 return false; 801 for (StringType item : this.disulfideLinkage) 802 if (!item.isEmpty()) 803 return true; 804 return false; 805 } 806 807 /** 808 * @return {@link #disulfideLinkage} (The disulphide bond between two cysteine residues either on the same subunit or on two different subunits shall be described. The position of the disulfide bonds in the SubstanceProtein shall be listed in increasing order of subunit number and position within subunit followed by the abbreviation of the amino acids involved. The disulfide linkage positions shall actually contain the amino acid Cysteine at the respective positions.) 809 */ 810 public StringType addDisulfideLinkageElement() {//2 811 StringType t = new StringType(); 812 if (this.disulfideLinkage == null) 813 this.disulfideLinkage = new ArrayList<StringType>(); 814 this.disulfideLinkage.add(t); 815 return t; 816 } 817 818 /** 819 * @param value {@link #disulfideLinkage} (The disulphide bond between two cysteine residues either on the same subunit or on two different subunits shall be described. The position of the disulfide bonds in the SubstanceProtein shall be listed in increasing order of subunit number and position within subunit followed by the abbreviation of the amino acids involved. The disulfide linkage positions shall actually contain the amino acid Cysteine at the respective positions.) 820 */ 821 public SubstanceProtein addDisulfideLinkage(String value) { //1 822 StringType t = new StringType(); 823 t.setValue(value); 824 if (this.disulfideLinkage == null) 825 this.disulfideLinkage = new ArrayList<StringType>(); 826 this.disulfideLinkage.add(t); 827 return this; 828 } 829 830 /** 831 * @param value {@link #disulfideLinkage} (The disulphide bond between two cysteine residues either on the same subunit or on two different subunits shall be described. The position of the disulfide bonds in the SubstanceProtein shall be listed in increasing order of subunit number and position within subunit followed by the abbreviation of the amino acids involved. The disulfide linkage positions shall actually contain the amino acid Cysteine at the respective positions.) 832 */ 833 public boolean hasDisulfideLinkage(String value) { 834 if (this.disulfideLinkage == null) 835 return false; 836 for (StringType v : this.disulfideLinkage) 837 if (v.getValue().equals(value)) // string 838 return true; 839 return false; 840 } 841 842 /** 843 * @return {@link #subunit} (This subclause refers to the description of each subunit constituting the SubstanceProtein. A subunit is a linear sequence of amino acids linked through peptide bonds. The Subunit information shall be provided when the finished SubstanceProtein is a complex of multiple sequences; subunits are not used to delineate domains within a single sequence. Subunits are listed in order of decreasing length; sequences of the same length will be ordered by decreasing molecular weight; subunits that have identical sequences will be repeated multiple times.) 844 */ 845 public List<SubstanceProteinSubunitComponent> getSubunit() { 846 if (this.subunit == null) 847 this.subunit = new ArrayList<SubstanceProteinSubunitComponent>(); 848 return this.subunit; 849 } 850 851 /** 852 * @return Returns a reference to <code>this</code> for easy method chaining 853 */ 854 public SubstanceProtein setSubunit(List<SubstanceProteinSubunitComponent> theSubunit) { 855 this.subunit = theSubunit; 856 return this; 857 } 858 859 public boolean hasSubunit() { 860 if (this.subunit == null) 861 return false; 862 for (SubstanceProteinSubunitComponent item : this.subunit) 863 if (!item.isEmpty()) 864 return true; 865 return false; 866 } 867 868 public SubstanceProteinSubunitComponent addSubunit() { //3 869 SubstanceProteinSubunitComponent t = new SubstanceProteinSubunitComponent(); 870 if (this.subunit == null) 871 this.subunit = new ArrayList<SubstanceProteinSubunitComponent>(); 872 this.subunit.add(t); 873 return t; 874 } 875 876 public SubstanceProtein addSubunit(SubstanceProteinSubunitComponent t) { //3 877 if (t == null) 878 return this; 879 if (this.subunit == null) 880 this.subunit = new ArrayList<SubstanceProteinSubunitComponent>(); 881 this.subunit.add(t); 882 return this; 883 } 884 885 /** 886 * @return The first repetition of repeating field {@link #subunit}, creating it if it does not already exist {3} 887 */ 888 public SubstanceProteinSubunitComponent getSubunitFirstRep() { 889 if (getSubunit().isEmpty()) { 890 addSubunit(); 891 } 892 return getSubunit().get(0); 893 } 894 895 protected void listChildren(List<Property> children) { 896 super.listChildren(children); 897 children.add(new Property("sequenceType", "CodeableConcept", "The SubstanceProtein descriptive elements will only be used when a complete or partial amino acid sequence is available or derivable from a nucleic acid sequence.", 0, 1, sequenceType)); 898 children.add(new Property("numberOfSubunits", "integer", "Number of linear sequences of amino acids linked through peptide bonds. The number of subunits constituting the SubstanceProtein shall be described. It is possible that the number of subunits can be variable.", 0, 1, numberOfSubunits)); 899 children.add(new Property("disulfideLinkage", "string", "The disulphide bond between two cysteine residues either on the same subunit or on two different subunits shall be described. The position of the disulfide bonds in the SubstanceProtein shall be listed in increasing order of subunit number and position within subunit followed by the abbreviation of the amino acids involved. The disulfide linkage positions shall actually contain the amino acid Cysteine at the respective positions.", 0, java.lang.Integer.MAX_VALUE, disulfideLinkage)); 900 children.add(new Property("subunit", "", "This subclause refers to the description of each subunit constituting the SubstanceProtein. A subunit is a linear sequence of amino acids linked through peptide bonds. The Subunit information shall be provided when the finished SubstanceProtein is a complex of multiple sequences; subunits are not used to delineate domains within a single sequence. Subunits are listed in order of decreasing length; sequences of the same length will be ordered by decreasing molecular weight; subunits that have identical sequences will be repeated multiple times.", 0, java.lang.Integer.MAX_VALUE, subunit)); 901 } 902 903 @Override 904 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 905 switch (_hash) { 906 case 807711387: /*sequenceType*/ return new Property("sequenceType", "CodeableConcept", "The SubstanceProtein descriptive elements will only be used when a complete or partial amino acid sequence is available or derivable from a nucleic acid sequence.", 0, 1, sequenceType); 907 case -847111089: /*numberOfSubunits*/ return new Property("numberOfSubunits", "integer", "Number of linear sequences of amino acids linked through peptide bonds. The number of subunits constituting the SubstanceProtein shall be described. It is possible that the number of subunits can be variable.", 0, 1, numberOfSubunits); 908 case -1996102436: /*disulfideLinkage*/ return new Property("disulfideLinkage", "string", "The disulphide bond between two cysteine residues either on the same subunit or on two different subunits shall be described. The position of the disulfide bonds in the SubstanceProtein shall be listed in increasing order of subunit number and position within subunit followed by the abbreviation of the amino acids involved. The disulfide linkage positions shall actually contain the amino acid Cysteine at the respective positions.", 0, java.lang.Integer.MAX_VALUE, disulfideLinkage); 909 case -1867548732: /*subunit*/ return new Property("subunit", "", "This subclause refers to the description of each subunit constituting the SubstanceProtein. A subunit is a linear sequence of amino acids linked through peptide bonds. The Subunit information shall be provided when the finished SubstanceProtein is a complex of multiple sequences; subunits are not used to delineate domains within a single sequence. Subunits are listed in order of decreasing length; sequences of the same length will be ordered by decreasing molecular weight; subunits that have identical sequences will be repeated multiple times.", 0, java.lang.Integer.MAX_VALUE, subunit); 910 default: return super.getNamedProperty(_hash, _name, _checkValid); 911 } 912 913 } 914 915 @Override 916 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 917 switch (hash) { 918 case 807711387: /*sequenceType*/ return this.sequenceType == null ? new Base[0] : new Base[] {this.sequenceType}; // CodeableConcept 919 case -847111089: /*numberOfSubunits*/ return this.numberOfSubunits == null ? new Base[0] : new Base[] {this.numberOfSubunits}; // IntegerType 920 case -1996102436: /*disulfideLinkage*/ return this.disulfideLinkage == null ? new Base[0] : this.disulfideLinkage.toArray(new Base[this.disulfideLinkage.size()]); // StringType 921 case -1867548732: /*subunit*/ return this.subunit == null ? new Base[0] : this.subunit.toArray(new Base[this.subunit.size()]); // SubstanceProteinSubunitComponent 922 default: return super.getProperty(hash, name, checkValid); 923 } 924 925 } 926 927 @Override 928 public Base setProperty(int hash, String name, Base value) throws FHIRException { 929 switch (hash) { 930 case 807711387: // sequenceType 931 this.sequenceType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 932 return value; 933 case -847111089: // numberOfSubunits 934 this.numberOfSubunits = TypeConvertor.castToInteger(value); // IntegerType 935 return value; 936 case -1996102436: // disulfideLinkage 937 this.getDisulfideLinkage().add(TypeConvertor.castToString(value)); // StringType 938 return value; 939 case -1867548732: // subunit 940 this.getSubunit().add((SubstanceProteinSubunitComponent) value); // SubstanceProteinSubunitComponent 941 return value; 942 default: return super.setProperty(hash, name, value); 943 } 944 945 } 946 947 @Override 948 public Base setProperty(String name, Base value) throws FHIRException { 949 if (name.equals("sequenceType")) { 950 this.sequenceType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 951 } else if (name.equals("numberOfSubunits")) { 952 this.numberOfSubunits = TypeConvertor.castToInteger(value); // IntegerType 953 } else if (name.equals("disulfideLinkage")) { 954 this.getDisulfideLinkage().add(TypeConvertor.castToString(value)); 955 } else if (name.equals("subunit")) { 956 this.getSubunit().add((SubstanceProteinSubunitComponent) value); 957 } else 958 return super.setProperty(name, value); 959 return value; 960 } 961 962 @Override 963 public void removeChild(String name, Base value) throws FHIRException { 964 if (name.equals("sequenceType")) { 965 this.sequenceType = null; 966 } else if (name.equals("numberOfSubunits")) { 967 this.numberOfSubunits = null; 968 } else if (name.equals("disulfideLinkage")) { 969 this.getDisulfideLinkage().remove(value); 970 } else if (name.equals("subunit")) { 971 this.getSubunit().remove((SubstanceProteinSubunitComponent) value); 972 } else 973 super.removeChild(name, value); 974 975 } 976 977 @Override 978 public Base makeProperty(int hash, String name) throws FHIRException { 979 switch (hash) { 980 case 807711387: return getSequenceType(); 981 case -847111089: return getNumberOfSubunitsElement(); 982 case -1996102436: return addDisulfideLinkageElement(); 983 case -1867548732: return addSubunit(); 984 default: return super.makeProperty(hash, name); 985 } 986 987 } 988 989 @Override 990 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 991 switch (hash) { 992 case 807711387: /*sequenceType*/ return new String[] {"CodeableConcept"}; 993 case -847111089: /*numberOfSubunits*/ return new String[] {"integer"}; 994 case -1996102436: /*disulfideLinkage*/ return new String[] {"string"}; 995 case -1867548732: /*subunit*/ return new String[] {}; 996 default: return super.getTypesForProperty(hash, name); 997 } 998 999 } 1000 1001 @Override 1002 public Base addChild(String name) throws FHIRException { 1003 if (name.equals("sequenceType")) { 1004 this.sequenceType = new CodeableConcept(); 1005 return this.sequenceType; 1006 } 1007 else if (name.equals("numberOfSubunits")) { 1008 throw new FHIRException("Cannot call addChild on a singleton property SubstanceProtein.numberOfSubunits"); 1009 } 1010 else if (name.equals("disulfideLinkage")) { 1011 throw new FHIRException("Cannot call addChild on a singleton property SubstanceProtein.disulfideLinkage"); 1012 } 1013 else if (name.equals("subunit")) { 1014 return addSubunit(); 1015 } 1016 else 1017 return super.addChild(name); 1018 } 1019 1020 public String fhirType() { 1021 return "SubstanceProtein"; 1022 1023 } 1024 1025 public SubstanceProtein copy() { 1026 SubstanceProtein dst = new SubstanceProtein(); 1027 copyValues(dst); 1028 return dst; 1029 } 1030 1031 public void copyValues(SubstanceProtein dst) { 1032 super.copyValues(dst); 1033 dst.sequenceType = sequenceType == null ? null : sequenceType.copy(); 1034 dst.numberOfSubunits = numberOfSubunits == null ? null : numberOfSubunits.copy(); 1035 if (disulfideLinkage != null) { 1036 dst.disulfideLinkage = new ArrayList<StringType>(); 1037 for (StringType i : disulfideLinkage) 1038 dst.disulfideLinkage.add(i.copy()); 1039 }; 1040 if (subunit != null) { 1041 dst.subunit = new ArrayList<SubstanceProteinSubunitComponent>(); 1042 for (SubstanceProteinSubunitComponent i : subunit) 1043 dst.subunit.add(i.copy()); 1044 }; 1045 } 1046 1047 protected SubstanceProtein typedCopy() { 1048 return copy(); 1049 } 1050 1051 @Override 1052 public boolean equalsDeep(Base other_) { 1053 if (!super.equalsDeep(other_)) 1054 return false; 1055 if (!(other_ instanceof SubstanceProtein)) 1056 return false; 1057 SubstanceProtein o = (SubstanceProtein) other_; 1058 return compareDeep(sequenceType, o.sequenceType, true) && compareDeep(numberOfSubunits, o.numberOfSubunits, true) 1059 && compareDeep(disulfideLinkage, o.disulfideLinkage, true) && compareDeep(subunit, o.subunit, true) 1060 ; 1061 } 1062 1063 @Override 1064 public boolean equalsShallow(Base other_) { 1065 if (!super.equalsShallow(other_)) 1066 return false; 1067 if (!(other_ instanceof SubstanceProtein)) 1068 return false; 1069 SubstanceProtein o = (SubstanceProtein) other_; 1070 return compareValues(numberOfSubunits, o.numberOfSubunits, true) && compareValues(disulfideLinkage, o.disulfideLinkage, true) 1071 ; 1072 } 1073 1074 public boolean isEmpty() { 1075 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequenceType, numberOfSubunits 1076 , disulfideLinkage, subunit); 1077 } 1078 1079 @Override 1080 public ResourceType getResourceType() { 1081 return ResourceType.SubstanceProtein; 1082 } 1083 1084 1085} 1086