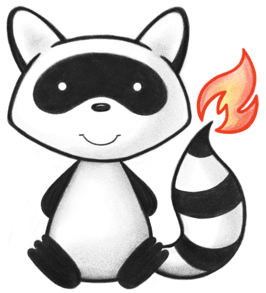
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Todo. 052 */ 053@ResourceDef(name="SubstanceReferenceInformation", profile="http://hl7.org/fhir/StructureDefinition/SubstanceReferenceInformation") 054public class SubstanceReferenceInformation extends DomainResource { 055 056 @Block() 057 public static class SubstanceReferenceInformationGeneComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Todo. 060 */ 061 @Child(name = "geneSequenceOrigin", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="Todo", formalDefinition="Todo." ) 063 protected CodeableConcept geneSequenceOrigin; 064 065 /** 066 * Todo. 067 */ 068 @Child(name = "gene", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 069 @Description(shortDefinition="Todo", formalDefinition="Todo." ) 070 protected CodeableConcept gene; 071 072 /** 073 * Todo. 074 */ 075 @Child(name = "source", type = {DocumentReference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 076 @Description(shortDefinition="Todo", formalDefinition="Todo." ) 077 protected List<Reference> source; 078 079 private static final long serialVersionUID = -1733820939L; 080 081 /** 082 * Constructor 083 */ 084 public SubstanceReferenceInformationGeneComponent() { 085 super(); 086 } 087 088 /** 089 * @return {@link #geneSequenceOrigin} (Todo.) 090 */ 091 public CodeableConcept getGeneSequenceOrigin() { 092 if (this.geneSequenceOrigin == null) 093 if (Configuration.errorOnAutoCreate()) 094 throw new Error("Attempt to auto-create SubstanceReferenceInformationGeneComponent.geneSequenceOrigin"); 095 else if (Configuration.doAutoCreate()) 096 this.geneSequenceOrigin = new CodeableConcept(); // cc 097 return this.geneSequenceOrigin; 098 } 099 100 public boolean hasGeneSequenceOrigin() { 101 return this.geneSequenceOrigin != null && !this.geneSequenceOrigin.isEmpty(); 102 } 103 104 /** 105 * @param value {@link #geneSequenceOrigin} (Todo.) 106 */ 107 public SubstanceReferenceInformationGeneComponent setGeneSequenceOrigin(CodeableConcept value) { 108 this.geneSequenceOrigin = value; 109 return this; 110 } 111 112 /** 113 * @return {@link #gene} (Todo.) 114 */ 115 public CodeableConcept getGene() { 116 if (this.gene == null) 117 if (Configuration.errorOnAutoCreate()) 118 throw new Error("Attempt to auto-create SubstanceReferenceInformationGeneComponent.gene"); 119 else if (Configuration.doAutoCreate()) 120 this.gene = new CodeableConcept(); // cc 121 return this.gene; 122 } 123 124 public boolean hasGene() { 125 return this.gene != null && !this.gene.isEmpty(); 126 } 127 128 /** 129 * @param value {@link #gene} (Todo.) 130 */ 131 public SubstanceReferenceInformationGeneComponent setGene(CodeableConcept value) { 132 this.gene = value; 133 return this; 134 } 135 136 /** 137 * @return {@link #source} (Todo.) 138 */ 139 public List<Reference> getSource() { 140 if (this.source == null) 141 this.source = new ArrayList<Reference>(); 142 return this.source; 143 } 144 145 /** 146 * @return Returns a reference to <code>this</code> for easy method chaining 147 */ 148 public SubstanceReferenceInformationGeneComponent setSource(List<Reference> theSource) { 149 this.source = theSource; 150 return this; 151 } 152 153 public boolean hasSource() { 154 if (this.source == null) 155 return false; 156 for (Reference item : this.source) 157 if (!item.isEmpty()) 158 return true; 159 return false; 160 } 161 162 public Reference addSource() { //3 163 Reference t = new Reference(); 164 if (this.source == null) 165 this.source = new ArrayList<Reference>(); 166 this.source.add(t); 167 return t; 168 } 169 170 public SubstanceReferenceInformationGeneComponent addSource(Reference t) { //3 171 if (t == null) 172 return this; 173 if (this.source == null) 174 this.source = new ArrayList<Reference>(); 175 this.source.add(t); 176 return this; 177 } 178 179 /** 180 * @return The first repetition of repeating field {@link #source}, creating it if it does not already exist {3} 181 */ 182 public Reference getSourceFirstRep() { 183 if (getSource().isEmpty()) { 184 addSource(); 185 } 186 return getSource().get(0); 187 } 188 189 protected void listChildren(List<Property> children) { 190 super.listChildren(children); 191 children.add(new Property("geneSequenceOrigin", "CodeableConcept", "Todo.", 0, 1, geneSequenceOrigin)); 192 children.add(new Property("gene", "CodeableConcept", "Todo.", 0, 1, gene)); 193 children.add(new Property("source", "Reference(DocumentReference)", "Todo.", 0, java.lang.Integer.MAX_VALUE, source)); 194 } 195 196 @Override 197 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 198 switch (_hash) { 199 case -1089463108: /*geneSequenceOrigin*/ return new Property("geneSequenceOrigin", "CodeableConcept", "Todo.", 0, 1, geneSequenceOrigin); 200 case 3169045: /*gene*/ return new Property("gene", "CodeableConcept", "Todo.", 0, 1, gene); 201 case -896505829: /*source*/ return new Property("source", "Reference(DocumentReference)", "Todo.", 0, java.lang.Integer.MAX_VALUE, source); 202 default: return super.getNamedProperty(_hash, _name, _checkValid); 203 } 204 205 } 206 207 @Override 208 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 209 switch (hash) { 210 case -1089463108: /*geneSequenceOrigin*/ return this.geneSequenceOrigin == null ? new Base[0] : new Base[] {this.geneSequenceOrigin}; // CodeableConcept 211 case 3169045: /*gene*/ return this.gene == null ? new Base[0] : new Base[] {this.gene}; // CodeableConcept 212 case -896505829: /*source*/ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 213 default: return super.getProperty(hash, name, checkValid); 214 } 215 216 } 217 218 @Override 219 public Base setProperty(int hash, String name, Base value) throws FHIRException { 220 switch (hash) { 221 case -1089463108: // geneSequenceOrigin 222 this.geneSequenceOrigin = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 223 return value; 224 case 3169045: // gene 225 this.gene = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 226 return value; 227 case -896505829: // source 228 this.getSource().add(TypeConvertor.castToReference(value)); // Reference 229 return value; 230 default: return super.setProperty(hash, name, value); 231 } 232 233 } 234 235 @Override 236 public Base setProperty(String name, Base value) throws FHIRException { 237 if (name.equals("geneSequenceOrigin")) { 238 this.geneSequenceOrigin = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 239 } else if (name.equals("gene")) { 240 this.gene = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 241 } else if (name.equals("source")) { 242 this.getSource().add(TypeConvertor.castToReference(value)); 243 } else 244 return super.setProperty(name, value); 245 return value; 246 } 247 248 @Override 249 public void removeChild(String name, Base value) throws FHIRException { 250 if (name.equals("geneSequenceOrigin")) { 251 this.geneSequenceOrigin = null; 252 } else if (name.equals("gene")) { 253 this.gene = null; 254 } else if (name.equals("source")) { 255 this.getSource().remove(value); 256 } else 257 super.removeChild(name, value); 258 259 } 260 261 @Override 262 public Base makeProperty(int hash, String name) throws FHIRException { 263 switch (hash) { 264 case -1089463108: return getGeneSequenceOrigin(); 265 case 3169045: return getGene(); 266 case -896505829: return addSource(); 267 default: return super.makeProperty(hash, name); 268 } 269 270 } 271 272 @Override 273 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 274 switch (hash) { 275 case -1089463108: /*geneSequenceOrigin*/ return new String[] {"CodeableConcept"}; 276 case 3169045: /*gene*/ return new String[] {"CodeableConcept"}; 277 case -896505829: /*source*/ return new String[] {"Reference"}; 278 default: return super.getTypesForProperty(hash, name); 279 } 280 281 } 282 283 @Override 284 public Base addChild(String name) throws FHIRException { 285 if (name.equals("geneSequenceOrigin")) { 286 this.geneSequenceOrigin = new CodeableConcept(); 287 return this.geneSequenceOrigin; 288 } 289 else if (name.equals("gene")) { 290 this.gene = new CodeableConcept(); 291 return this.gene; 292 } 293 else if (name.equals("source")) { 294 return addSource(); 295 } 296 else 297 return super.addChild(name); 298 } 299 300 public SubstanceReferenceInformationGeneComponent copy() { 301 SubstanceReferenceInformationGeneComponent dst = new SubstanceReferenceInformationGeneComponent(); 302 copyValues(dst); 303 return dst; 304 } 305 306 public void copyValues(SubstanceReferenceInformationGeneComponent dst) { 307 super.copyValues(dst); 308 dst.geneSequenceOrigin = geneSequenceOrigin == null ? null : geneSequenceOrigin.copy(); 309 dst.gene = gene == null ? null : gene.copy(); 310 if (source != null) { 311 dst.source = new ArrayList<Reference>(); 312 for (Reference i : source) 313 dst.source.add(i.copy()); 314 }; 315 } 316 317 @Override 318 public boolean equalsDeep(Base other_) { 319 if (!super.equalsDeep(other_)) 320 return false; 321 if (!(other_ instanceof SubstanceReferenceInformationGeneComponent)) 322 return false; 323 SubstanceReferenceInformationGeneComponent o = (SubstanceReferenceInformationGeneComponent) other_; 324 return compareDeep(geneSequenceOrigin, o.geneSequenceOrigin, true) && compareDeep(gene, o.gene, true) 325 && compareDeep(source, o.source, true); 326 } 327 328 @Override 329 public boolean equalsShallow(Base other_) { 330 if (!super.equalsShallow(other_)) 331 return false; 332 if (!(other_ instanceof SubstanceReferenceInformationGeneComponent)) 333 return false; 334 SubstanceReferenceInformationGeneComponent o = (SubstanceReferenceInformationGeneComponent) other_; 335 return true; 336 } 337 338 public boolean isEmpty() { 339 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(geneSequenceOrigin, gene, source 340 ); 341 } 342 343 public String fhirType() { 344 return "SubstanceReferenceInformation.gene"; 345 346 } 347 348 } 349 350 @Block() 351 public static class SubstanceReferenceInformationGeneElementComponent extends BackboneElement implements IBaseBackboneElement { 352 /** 353 * Todo. 354 */ 355 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 356 @Description(shortDefinition="Todo", formalDefinition="Todo." ) 357 protected CodeableConcept type; 358 359 /** 360 * Todo. 361 */ 362 @Child(name = "element", type = {Identifier.class}, order=2, min=0, max=1, modifier=false, summary=true) 363 @Description(shortDefinition="Todo", formalDefinition="Todo." ) 364 protected Identifier element; 365 366 /** 367 * Todo. 368 */ 369 @Child(name = "source", type = {DocumentReference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 370 @Description(shortDefinition="Todo", formalDefinition="Todo." ) 371 protected List<Reference> source; 372 373 private static final long serialVersionUID = 261257288L; 374 375 /** 376 * Constructor 377 */ 378 public SubstanceReferenceInformationGeneElementComponent() { 379 super(); 380 } 381 382 /** 383 * @return {@link #type} (Todo.) 384 */ 385 public CodeableConcept getType() { 386 if (this.type == null) 387 if (Configuration.errorOnAutoCreate()) 388 throw new Error("Attempt to auto-create SubstanceReferenceInformationGeneElementComponent.type"); 389 else if (Configuration.doAutoCreate()) 390 this.type = new CodeableConcept(); // cc 391 return this.type; 392 } 393 394 public boolean hasType() { 395 return this.type != null && !this.type.isEmpty(); 396 } 397 398 /** 399 * @param value {@link #type} (Todo.) 400 */ 401 public SubstanceReferenceInformationGeneElementComponent setType(CodeableConcept value) { 402 this.type = value; 403 return this; 404 } 405 406 /** 407 * @return {@link #element} (Todo.) 408 */ 409 public Identifier getElement() { 410 if (this.element == null) 411 if (Configuration.errorOnAutoCreate()) 412 throw new Error("Attempt to auto-create SubstanceReferenceInformationGeneElementComponent.element"); 413 else if (Configuration.doAutoCreate()) 414 this.element = new Identifier(); // cc 415 return this.element; 416 } 417 418 public boolean hasElement() { 419 return this.element != null && !this.element.isEmpty(); 420 } 421 422 /** 423 * @param value {@link #element} (Todo.) 424 */ 425 public SubstanceReferenceInformationGeneElementComponent setElement(Identifier value) { 426 this.element = value; 427 return this; 428 } 429 430 /** 431 * @return {@link #source} (Todo.) 432 */ 433 public List<Reference> getSource() { 434 if (this.source == null) 435 this.source = new ArrayList<Reference>(); 436 return this.source; 437 } 438 439 /** 440 * @return Returns a reference to <code>this</code> for easy method chaining 441 */ 442 public SubstanceReferenceInformationGeneElementComponent setSource(List<Reference> theSource) { 443 this.source = theSource; 444 return this; 445 } 446 447 public boolean hasSource() { 448 if (this.source == null) 449 return false; 450 for (Reference item : this.source) 451 if (!item.isEmpty()) 452 return true; 453 return false; 454 } 455 456 public Reference addSource() { //3 457 Reference t = new Reference(); 458 if (this.source == null) 459 this.source = new ArrayList<Reference>(); 460 this.source.add(t); 461 return t; 462 } 463 464 public SubstanceReferenceInformationGeneElementComponent addSource(Reference t) { //3 465 if (t == null) 466 return this; 467 if (this.source == null) 468 this.source = new ArrayList<Reference>(); 469 this.source.add(t); 470 return this; 471 } 472 473 /** 474 * @return The first repetition of repeating field {@link #source}, creating it if it does not already exist {3} 475 */ 476 public Reference getSourceFirstRep() { 477 if (getSource().isEmpty()) { 478 addSource(); 479 } 480 return getSource().get(0); 481 } 482 483 protected void listChildren(List<Property> children) { 484 super.listChildren(children); 485 children.add(new Property("type", "CodeableConcept", "Todo.", 0, 1, type)); 486 children.add(new Property("element", "Identifier", "Todo.", 0, 1, element)); 487 children.add(new Property("source", "Reference(DocumentReference)", "Todo.", 0, java.lang.Integer.MAX_VALUE, source)); 488 } 489 490 @Override 491 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 492 switch (_hash) { 493 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Todo.", 0, 1, type); 494 case -1662836996: /*element*/ return new Property("element", "Identifier", "Todo.", 0, 1, element); 495 case -896505829: /*source*/ return new Property("source", "Reference(DocumentReference)", "Todo.", 0, java.lang.Integer.MAX_VALUE, source); 496 default: return super.getNamedProperty(_hash, _name, _checkValid); 497 } 498 499 } 500 501 @Override 502 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 503 switch (hash) { 504 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 505 case -1662836996: /*element*/ return this.element == null ? new Base[0] : new Base[] {this.element}; // Identifier 506 case -896505829: /*source*/ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 507 default: return super.getProperty(hash, name, checkValid); 508 } 509 510 } 511 512 @Override 513 public Base setProperty(int hash, String name, Base value) throws FHIRException { 514 switch (hash) { 515 case 3575610: // type 516 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 517 return value; 518 case -1662836996: // element 519 this.element = TypeConvertor.castToIdentifier(value); // Identifier 520 return value; 521 case -896505829: // source 522 this.getSource().add(TypeConvertor.castToReference(value)); // Reference 523 return value; 524 default: return super.setProperty(hash, name, value); 525 } 526 527 } 528 529 @Override 530 public Base setProperty(String name, Base value) throws FHIRException { 531 if (name.equals("type")) { 532 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 533 } else if (name.equals("element")) { 534 this.element = TypeConvertor.castToIdentifier(value); // Identifier 535 } else if (name.equals("source")) { 536 this.getSource().add(TypeConvertor.castToReference(value)); 537 } else 538 return super.setProperty(name, value); 539 return value; 540 } 541 542 @Override 543 public void removeChild(String name, Base value) throws FHIRException { 544 if (name.equals("type")) { 545 this.type = null; 546 } else if (name.equals("element")) { 547 this.element = null; 548 } else if (name.equals("source")) { 549 this.getSource().remove(value); 550 } else 551 super.removeChild(name, value); 552 553 } 554 555 @Override 556 public Base makeProperty(int hash, String name) throws FHIRException { 557 switch (hash) { 558 case 3575610: return getType(); 559 case -1662836996: return getElement(); 560 case -896505829: return addSource(); 561 default: return super.makeProperty(hash, name); 562 } 563 564 } 565 566 @Override 567 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 568 switch (hash) { 569 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 570 case -1662836996: /*element*/ return new String[] {"Identifier"}; 571 case -896505829: /*source*/ return new String[] {"Reference"}; 572 default: return super.getTypesForProperty(hash, name); 573 } 574 575 } 576 577 @Override 578 public Base addChild(String name) throws FHIRException { 579 if (name.equals("type")) { 580 this.type = new CodeableConcept(); 581 return this.type; 582 } 583 else if (name.equals("element")) { 584 this.element = new Identifier(); 585 return this.element; 586 } 587 else if (name.equals("source")) { 588 return addSource(); 589 } 590 else 591 return super.addChild(name); 592 } 593 594 public SubstanceReferenceInformationGeneElementComponent copy() { 595 SubstanceReferenceInformationGeneElementComponent dst = new SubstanceReferenceInformationGeneElementComponent(); 596 copyValues(dst); 597 return dst; 598 } 599 600 public void copyValues(SubstanceReferenceInformationGeneElementComponent dst) { 601 super.copyValues(dst); 602 dst.type = type == null ? null : type.copy(); 603 dst.element = element == null ? null : element.copy(); 604 if (source != null) { 605 dst.source = new ArrayList<Reference>(); 606 for (Reference i : source) 607 dst.source.add(i.copy()); 608 }; 609 } 610 611 @Override 612 public boolean equalsDeep(Base other_) { 613 if (!super.equalsDeep(other_)) 614 return false; 615 if (!(other_ instanceof SubstanceReferenceInformationGeneElementComponent)) 616 return false; 617 SubstanceReferenceInformationGeneElementComponent o = (SubstanceReferenceInformationGeneElementComponent) other_; 618 return compareDeep(type, o.type, true) && compareDeep(element, o.element, true) && compareDeep(source, o.source, true) 619 ; 620 } 621 622 @Override 623 public boolean equalsShallow(Base other_) { 624 if (!super.equalsShallow(other_)) 625 return false; 626 if (!(other_ instanceof SubstanceReferenceInformationGeneElementComponent)) 627 return false; 628 SubstanceReferenceInformationGeneElementComponent o = (SubstanceReferenceInformationGeneElementComponent) other_; 629 return true; 630 } 631 632 public boolean isEmpty() { 633 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, element, source); 634 } 635 636 public String fhirType() { 637 return "SubstanceReferenceInformation.geneElement"; 638 639 } 640 641 } 642 643 @Block() 644 public static class SubstanceReferenceInformationTargetComponent extends BackboneElement implements IBaseBackboneElement { 645 /** 646 * Todo. 647 */ 648 @Child(name = "target", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 649 @Description(shortDefinition="Todo", formalDefinition="Todo." ) 650 protected Identifier target; 651 652 /** 653 * Todo. 654 */ 655 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 656 @Description(shortDefinition="Todo", formalDefinition="Todo." ) 657 protected CodeableConcept type; 658 659 /** 660 * Todo. 661 */ 662 @Child(name = "interaction", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 663 @Description(shortDefinition="Todo", formalDefinition="Todo." ) 664 protected CodeableConcept interaction; 665 666 /** 667 * Todo. 668 */ 669 @Child(name = "organism", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 670 @Description(shortDefinition="Todo", formalDefinition="Todo." ) 671 protected CodeableConcept organism; 672 673 /** 674 * Todo. 675 */ 676 @Child(name = "organismType", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 677 @Description(shortDefinition="Todo", formalDefinition="Todo." ) 678 protected CodeableConcept organismType; 679 680 /** 681 * Todo. 682 */ 683 @Child(name = "amount", type = {Quantity.class, Range.class, StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 684 @Description(shortDefinition="Todo", formalDefinition="Todo." ) 685 protected DataType amount; 686 687 /** 688 * Todo. 689 */ 690 @Child(name = "amountType", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=true) 691 @Description(shortDefinition="Todo", formalDefinition="Todo." ) 692 protected CodeableConcept amountType; 693 694 /** 695 * Todo. 696 */ 697 @Child(name = "source", type = {DocumentReference.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 698 @Description(shortDefinition="Todo", formalDefinition="Todo." ) 699 protected List<Reference> source; 700 701 private static final long serialVersionUID = 819518021L; 702 703 /** 704 * Constructor 705 */ 706 public SubstanceReferenceInformationTargetComponent() { 707 super(); 708 } 709 710 /** 711 * @return {@link #target} (Todo.) 712 */ 713 public Identifier getTarget() { 714 if (this.target == null) 715 if (Configuration.errorOnAutoCreate()) 716 throw new Error("Attempt to auto-create SubstanceReferenceInformationTargetComponent.target"); 717 else if (Configuration.doAutoCreate()) 718 this.target = new Identifier(); // cc 719 return this.target; 720 } 721 722 public boolean hasTarget() { 723 return this.target != null && !this.target.isEmpty(); 724 } 725 726 /** 727 * @param value {@link #target} (Todo.) 728 */ 729 public SubstanceReferenceInformationTargetComponent setTarget(Identifier value) { 730 this.target = value; 731 return this; 732 } 733 734 /** 735 * @return {@link #type} (Todo.) 736 */ 737 public CodeableConcept getType() { 738 if (this.type == null) 739 if (Configuration.errorOnAutoCreate()) 740 throw new Error("Attempt to auto-create SubstanceReferenceInformationTargetComponent.type"); 741 else if (Configuration.doAutoCreate()) 742 this.type = new CodeableConcept(); // cc 743 return this.type; 744 } 745 746 public boolean hasType() { 747 return this.type != null && !this.type.isEmpty(); 748 } 749 750 /** 751 * @param value {@link #type} (Todo.) 752 */ 753 public SubstanceReferenceInformationTargetComponent setType(CodeableConcept value) { 754 this.type = value; 755 return this; 756 } 757 758 /** 759 * @return {@link #interaction} (Todo.) 760 */ 761 public CodeableConcept getInteraction() { 762 if (this.interaction == null) 763 if (Configuration.errorOnAutoCreate()) 764 throw new Error("Attempt to auto-create SubstanceReferenceInformationTargetComponent.interaction"); 765 else if (Configuration.doAutoCreate()) 766 this.interaction = new CodeableConcept(); // cc 767 return this.interaction; 768 } 769 770 public boolean hasInteraction() { 771 return this.interaction != null && !this.interaction.isEmpty(); 772 } 773 774 /** 775 * @param value {@link #interaction} (Todo.) 776 */ 777 public SubstanceReferenceInformationTargetComponent setInteraction(CodeableConcept value) { 778 this.interaction = value; 779 return this; 780 } 781 782 /** 783 * @return {@link #organism} (Todo.) 784 */ 785 public CodeableConcept getOrganism() { 786 if (this.organism == null) 787 if (Configuration.errorOnAutoCreate()) 788 throw new Error("Attempt to auto-create SubstanceReferenceInformationTargetComponent.organism"); 789 else if (Configuration.doAutoCreate()) 790 this.organism = new CodeableConcept(); // cc 791 return this.organism; 792 } 793 794 public boolean hasOrganism() { 795 return this.organism != null && !this.organism.isEmpty(); 796 } 797 798 /** 799 * @param value {@link #organism} (Todo.) 800 */ 801 public SubstanceReferenceInformationTargetComponent setOrganism(CodeableConcept value) { 802 this.organism = value; 803 return this; 804 } 805 806 /** 807 * @return {@link #organismType} (Todo.) 808 */ 809 public CodeableConcept getOrganismType() { 810 if (this.organismType == null) 811 if (Configuration.errorOnAutoCreate()) 812 throw new Error("Attempt to auto-create SubstanceReferenceInformationTargetComponent.organismType"); 813 else if (Configuration.doAutoCreate()) 814 this.organismType = new CodeableConcept(); // cc 815 return this.organismType; 816 } 817 818 public boolean hasOrganismType() { 819 return this.organismType != null && !this.organismType.isEmpty(); 820 } 821 822 /** 823 * @param value {@link #organismType} (Todo.) 824 */ 825 public SubstanceReferenceInformationTargetComponent setOrganismType(CodeableConcept value) { 826 this.organismType = value; 827 return this; 828 } 829 830 /** 831 * @return {@link #amount} (Todo.) 832 */ 833 public DataType getAmount() { 834 return this.amount; 835 } 836 837 /** 838 * @return {@link #amount} (Todo.) 839 */ 840 public Quantity getAmountQuantity() throws FHIRException { 841 if (this.amount == null) 842 this.amount = new Quantity(); 843 if (!(this.amount instanceof Quantity)) 844 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.amount.getClass().getName()+" was encountered"); 845 return (Quantity) this.amount; 846 } 847 848 public boolean hasAmountQuantity() { 849 return this != null && this.amount instanceof Quantity; 850 } 851 852 /** 853 * @return {@link #amount} (Todo.) 854 */ 855 public Range getAmountRange() throws FHIRException { 856 if (this.amount == null) 857 this.amount = new Range(); 858 if (!(this.amount instanceof Range)) 859 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.amount.getClass().getName()+" was encountered"); 860 return (Range) this.amount; 861 } 862 863 public boolean hasAmountRange() { 864 return this != null && this.amount instanceof Range; 865 } 866 867 /** 868 * @return {@link #amount} (Todo.) 869 */ 870 public StringType getAmountStringType() throws FHIRException { 871 if (this.amount == null) 872 this.amount = new StringType(); 873 if (!(this.amount instanceof StringType)) 874 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.amount.getClass().getName()+" was encountered"); 875 return (StringType) this.amount; 876 } 877 878 public boolean hasAmountStringType() { 879 return this != null && this.amount instanceof StringType; 880 } 881 882 public boolean hasAmount() { 883 return this.amount != null && !this.amount.isEmpty(); 884 } 885 886 /** 887 * @param value {@link #amount} (Todo.) 888 */ 889 public SubstanceReferenceInformationTargetComponent setAmount(DataType value) { 890 if (value != null && !(value instanceof Quantity || value instanceof Range || value instanceof StringType)) 891 throw new FHIRException("Not the right type for SubstanceReferenceInformation.target.amount[x]: "+value.fhirType()); 892 this.amount = value; 893 return this; 894 } 895 896 /** 897 * @return {@link #amountType} (Todo.) 898 */ 899 public CodeableConcept getAmountType() { 900 if (this.amountType == null) 901 if (Configuration.errorOnAutoCreate()) 902 throw new Error("Attempt to auto-create SubstanceReferenceInformationTargetComponent.amountType"); 903 else if (Configuration.doAutoCreate()) 904 this.amountType = new CodeableConcept(); // cc 905 return this.amountType; 906 } 907 908 public boolean hasAmountType() { 909 return this.amountType != null && !this.amountType.isEmpty(); 910 } 911 912 /** 913 * @param value {@link #amountType} (Todo.) 914 */ 915 public SubstanceReferenceInformationTargetComponent setAmountType(CodeableConcept value) { 916 this.amountType = value; 917 return this; 918 } 919 920 /** 921 * @return {@link #source} (Todo.) 922 */ 923 public List<Reference> getSource() { 924 if (this.source == null) 925 this.source = new ArrayList<Reference>(); 926 return this.source; 927 } 928 929 /** 930 * @return Returns a reference to <code>this</code> for easy method chaining 931 */ 932 public SubstanceReferenceInformationTargetComponent setSource(List<Reference> theSource) { 933 this.source = theSource; 934 return this; 935 } 936 937 public boolean hasSource() { 938 if (this.source == null) 939 return false; 940 for (Reference item : this.source) 941 if (!item.isEmpty()) 942 return true; 943 return false; 944 } 945 946 public Reference addSource() { //3 947 Reference t = new Reference(); 948 if (this.source == null) 949 this.source = new ArrayList<Reference>(); 950 this.source.add(t); 951 return t; 952 } 953 954 public SubstanceReferenceInformationTargetComponent addSource(Reference t) { //3 955 if (t == null) 956 return this; 957 if (this.source == null) 958 this.source = new ArrayList<Reference>(); 959 this.source.add(t); 960 return this; 961 } 962 963 /** 964 * @return The first repetition of repeating field {@link #source}, creating it if it does not already exist {3} 965 */ 966 public Reference getSourceFirstRep() { 967 if (getSource().isEmpty()) { 968 addSource(); 969 } 970 return getSource().get(0); 971 } 972 973 protected void listChildren(List<Property> children) { 974 super.listChildren(children); 975 children.add(new Property("target", "Identifier", "Todo.", 0, 1, target)); 976 children.add(new Property("type", "CodeableConcept", "Todo.", 0, 1, type)); 977 children.add(new Property("interaction", "CodeableConcept", "Todo.", 0, 1, interaction)); 978 children.add(new Property("organism", "CodeableConcept", "Todo.", 0, 1, organism)); 979 children.add(new Property("organismType", "CodeableConcept", "Todo.", 0, 1, organismType)); 980 children.add(new Property("amount[x]", "Quantity|Range|string", "Todo.", 0, 1, amount)); 981 children.add(new Property("amountType", "CodeableConcept", "Todo.", 0, 1, amountType)); 982 children.add(new Property("source", "Reference(DocumentReference)", "Todo.", 0, java.lang.Integer.MAX_VALUE, source)); 983 } 984 985 @Override 986 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 987 switch (_hash) { 988 case -880905839: /*target*/ return new Property("target", "Identifier", "Todo.", 0, 1, target); 989 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Todo.", 0, 1, type); 990 case 1844104722: /*interaction*/ return new Property("interaction", "CodeableConcept", "Todo.", 0, 1, interaction); 991 case 1316389074: /*organism*/ return new Property("organism", "CodeableConcept", "Todo.", 0, 1, organism); 992 case 988662572: /*organismType*/ return new Property("organismType", "CodeableConcept", "Todo.", 0, 1, organismType); 993 case 646780200: /*amount[x]*/ return new Property("amount[x]", "Quantity|Range|string", "Todo.", 0, 1, amount); 994 case -1413853096: /*amount*/ return new Property("amount[x]", "Quantity|Range|string", "Todo.", 0, 1, amount); 995 case 1664303363: /*amountQuantity*/ return new Property("amount[x]", "Quantity", "Todo.", 0, 1, amount); 996 case -1223462971: /*amountRange*/ return new Property("amount[x]", "Range", "Todo.", 0, 1, amount); 997 case 773651081: /*amountString*/ return new Property("amount[x]", "string", "Todo.", 0, 1, amount); 998 case -1424857166: /*amountType*/ return new Property("amountType", "CodeableConcept", "Todo.", 0, 1, amountType); 999 case -896505829: /*source*/ return new Property("source", "Reference(DocumentReference)", "Todo.", 0, java.lang.Integer.MAX_VALUE, source); 1000 default: return super.getNamedProperty(_hash, _name, _checkValid); 1001 } 1002 1003 } 1004 1005 @Override 1006 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1007 switch (hash) { 1008 case -880905839: /*target*/ return this.target == null ? new Base[0] : new Base[] {this.target}; // Identifier 1009 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1010 case 1844104722: /*interaction*/ return this.interaction == null ? new Base[0] : new Base[] {this.interaction}; // CodeableConcept 1011 case 1316389074: /*organism*/ return this.organism == null ? new Base[0] : new Base[] {this.organism}; // CodeableConcept 1012 case 988662572: /*organismType*/ return this.organismType == null ? new Base[0] : new Base[] {this.organismType}; // CodeableConcept 1013 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // DataType 1014 case -1424857166: /*amountType*/ return this.amountType == null ? new Base[0] : new Base[] {this.amountType}; // CodeableConcept 1015 case -896505829: /*source*/ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 1016 default: return super.getProperty(hash, name, checkValid); 1017 } 1018 1019 } 1020 1021 @Override 1022 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1023 switch (hash) { 1024 case -880905839: // target 1025 this.target = TypeConvertor.castToIdentifier(value); // Identifier 1026 return value; 1027 case 3575610: // type 1028 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1029 return value; 1030 case 1844104722: // interaction 1031 this.interaction = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1032 return value; 1033 case 1316389074: // organism 1034 this.organism = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1035 return value; 1036 case 988662572: // organismType 1037 this.organismType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1038 return value; 1039 case -1413853096: // amount 1040 this.amount = TypeConvertor.castToType(value); // DataType 1041 return value; 1042 case -1424857166: // amountType 1043 this.amountType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1044 return value; 1045 case -896505829: // source 1046 this.getSource().add(TypeConvertor.castToReference(value)); // Reference 1047 return value; 1048 default: return super.setProperty(hash, name, value); 1049 } 1050 1051 } 1052 1053 @Override 1054 public Base setProperty(String name, Base value) throws FHIRException { 1055 if (name.equals("target")) { 1056 this.target = TypeConvertor.castToIdentifier(value); // Identifier 1057 } else if (name.equals("type")) { 1058 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1059 } else if (name.equals("interaction")) { 1060 this.interaction = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1061 } else if (name.equals("organism")) { 1062 this.organism = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1063 } else if (name.equals("organismType")) { 1064 this.organismType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1065 } else if (name.equals("amount[x]")) { 1066 this.amount = TypeConvertor.castToType(value); // DataType 1067 } else if (name.equals("amountType")) { 1068 this.amountType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1069 } else if (name.equals("source")) { 1070 this.getSource().add(TypeConvertor.castToReference(value)); 1071 } else 1072 return super.setProperty(name, value); 1073 return value; 1074 } 1075 1076 @Override 1077 public void removeChild(String name, Base value) throws FHIRException { 1078 if (name.equals("target")) { 1079 this.target = null; 1080 } else if (name.equals("type")) { 1081 this.type = null; 1082 } else if (name.equals("interaction")) { 1083 this.interaction = null; 1084 } else if (name.equals("organism")) { 1085 this.organism = null; 1086 } else if (name.equals("organismType")) { 1087 this.organismType = null; 1088 } else if (name.equals("amount[x]")) { 1089 this.amount = null; 1090 } else if (name.equals("amountType")) { 1091 this.amountType = null; 1092 } else if (name.equals("source")) { 1093 this.getSource().remove(value); 1094 } else 1095 super.removeChild(name, value); 1096 1097 } 1098 1099 @Override 1100 public Base makeProperty(int hash, String name) throws FHIRException { 1101 switch (hash) { 1102 case -880905839: return getTarget(); 1103 case 3575610: return getType(); 1104 case 1844104722: return getInteraction(); 1105 case 1316389074: return getOrganism(); 1106 case 988662572: return getOrganismType(); 1107 case 646780200: return getAmount(); 1108 case -1413853096: return getAmount(); 1109 case -1424857166: return getAmountType(); 1110 case -896505829: return addSource(); 1111 default: return super.makeProperty(hash, name); 1112 } 1113 1114 } 1115 1116 @Override 1117 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1118 switch (hash) { 1119 case -880905839: /*target*/ return new String[] {"Identifier"}; 1120 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1121 case 1844104722: /*interaction*/ return new String[] {"CodeableConcept"}; 1122 case 1316389074: /*organism*/ return new String[] {"CodeableConcept"}; 1123 case 988662572: /*organismType*/ return new String[] {"CodeableConcept"}; 1124 case -1413853096: /*amount*/ return new String[] {"Quantity", "Range", "string"}; 1125 case -1424857166: /*amountType*/ return new String[] {"CodeableConcept"}; 1126 case -896505829: /*source*/ return new String[] {"Reference"}; 1127 default: return super.getTypesForProperty(hash, name); 1128 } 1129 1130 } 1131 1132 @Override 1133 public Base addChild(String name) throws FHIRException { 1134 if (name.equals("target")) { 1135 this.target = new Identifier(); 1136 return this.target; 1137 } 1138 else if (name.equals("type")) { 1139 this.type = new CodeableConcept(); 1140 return this.type; 1141 } 1142 else if (name.equals("interaction")) { 1143 this.interaction = new CodeableConcept(); 1144 return this.interaction; 1145 } 1146 else if (name.equals("organism")) { 1147 this.organism = new CodeableConcept(); 1148 return this.organism; 1149 } 1150 else if (name.equals("organismType")) { 1151 this.organismType = new CodeableConcept(); 1152 return this.organismType; 1153 } 1154 else if (name.equals("amountQuantity")) { 1155 this.amount = new Quantity(); 1156 return this.amount; 1157 } 1158 else if (name.equals("amountRange")) { 1159 this.amount = new Range(); 1160 return this.amount; 1161 } 1162 else if (name.equals("amountString")) { 1163 this.amount = new StringType(); 1164 return this.amount; 1165 } 1166 else if (name.equals("amountType")) { 1167 this.amountType = new CodeableConcept(); 1168 return this.amountType; 1169 } 1170 else if (name.equals("source")) { 1171 return addSource(); 1172 } 1173 else 1174 return super.addChild(name); 1175 } 1176 1177 public SubstanceReferenceInformationTargetComponent copy() { 1178 SubstanceReferenceInformationTargetComponent dst = new SubstanceReferenceInformationTargetComponent(); 1179 copyValues(dst); 1180 return dst; 1181 } 1182 1183 public void copyValues(SubstanceReferenceInformationTargetComponent dst) { 1184 super.copyValues(dst); 1185 dst.target = target == null ? null : target.copy(); 1186 dst.type = type == null ? null : type.copy(); 1187 dst.interaction = interaction == null ? null : interaction.copy(); 1188 dst.organism = organism == null ? null : organism.copy(); 1189 dst.organismType = organismType == null ? null : organismType.copy(); 1190 dst.amount = amount == null ? null : amount.copy(); 1191 dst.amountType = amountType == null ? null : amountType.copy(); 1192 if (source != null) { 1193 dst.source = new ArrayList<Reference>(); 1194 for (Reference i : source) 1195 dst.source.add(i.copy()); 1196 }; 1197 } 1198 1199 @Override 1200 public boolean equalsDeep(Base other_) { 1201 if (!super.equalsDeep(other_)) 1202 return false; 1203 if (!(other_ instanceof SubstanceReferenceInformationTargetComponent)) 1204 return false; 1205 SubstanceReferenceInformationTargetComponent o = (SubstanceReferenceInformationTargetComponent) other_; 1206 return compareDeep(target, o.target, true) && compareDeep(type, o.type, true) && compareDeep(interaction, o.interaction, true) 1207 && compareDeep(organism, o.organism, true) && compareDeep(organismType, o.organismType, true) && compareDeep(amount, o.amount, true) 1208 && compareDeep(amountType, o.amountType, true) && compareDeep(source, o.source, true); 1209 } 1210 1211 @Override 1212 public boolean equalsShallow(Base other_) { 1213 if (!super.equalsShallow(other_)) 1214 return false; 1215 if (!(other_ instanceof SubstanceReferenceInformationTargetComponent)) 1216 return false; 1217 SubstanceReferenceInformationTargetComponent o = (SubstanceReferenceInformationTargetComponent) other_; 1218 return true; 1219 } 1220 1221 public boolean isEmpty() { 1222 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(target, type, interaction 1223 , organism, organismType, amount, amountType, source); 1224 } 1225 1226 public String fhirType() { 1227 return "SubstanceReferenceInformation.target"; 1228 1229 } 1230 1231 } 1232 1233 /** 1234 * Todo. 1235 */ 1236 @Child(name = "comment", type = {StringType.class}, order=0, min=0, max=1, modifier=false, summary=true) 1237 @Description(shortDefinition="Todo", formalDefinition="Todo." ) 1238 protected StringType comment; 1239 1240 /** 1241 * Todo. 1242 */ 1243 @Child(name = "gene", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1244 @Description(shortDefinition="Todo", formalDefinition="Todo." ) 1245 protected List<SubstanceReferenceInformationGeneComponent> gene; 1246 1247 /** 1248 * Todo. 1249 */ 1250 @Child(name = "geneElement", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1251 @Description(shortDefinition="Todo", formalDefinition="Todo." ) 1252 protected List<SubstanceReferenceInformationGeneElementComponent> geneElement; 1253 1254 /** 1255 * Todo. 1256 */ 1257 @Child(name = "target", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1258 @Description(shortDefinition="Todo", formalDefinition="Todo." ) 1259 protected List<SubstanceReferenceInformationTargetComponent> target; 1260 1261 private static final long serialVersionUID = -496299386L; 1262 1263 /** 1264 * Constructor 1265 */ 1266 public SubstanceReferenceInformation() { 1267 super(); 1268 } 1269 1270 /** 1271 * @return {@link #comment} (Todo.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 1272 */ 1273 public StringType getCommentElement() { 1274 if (this.comment == null) 1275 if (Configuration.errorOnAutoCreate()) 1276 throw new Error("Attempt to auto-create SubstanceReferenceInformation.comment"); 1277 else if (Configuration.doAutoCreate()) 1278 this.comment = new StringType(); // bb 1279 return this.comment; 1280 } 1281 1282 public boolean hasCommentElement() { 1283 return this.comment != null && !this.comment.isEmpty(); 1284 } 1285 1286 public boolean hasComment() { 1287 return this.comment != null && !this.comment.isEmpty(); 1288 } 1289 1290 /** 1291 * @param value {@link #comment} (Todo.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 1292 */ 1293 public SubstanceReferenceInformation setCommentElement(StringType value) { 1294 this.comment = value; 1295 return this; 1296 } 1297 1298 /** 1299 * @return Todo. 1300 */ 1301 public String getComment() { 1302 return this.comment == null ? null : this.comment.getValue(); 1303 } 1304 1305 /** 1306 * @param value Todo. 1307 */ 1308 public SubstanceReferenceInformation setComment(String value) { 1309 if (Utilities.noString(value)) 1310 this.comment = null; 1311 else { 1312 if (this.comment == null) 1313 this.comment = new StringType(); 1314 this.comment.setValue(value); 1315 } 1316 return this; 1317 } 1318 1319 /** 1320 * @return {@link #gene} (Todo.) 1321 */ 1322 public List<SubstanceReferenceInformationGeneComponent> getGene() { 1323 if (this.gene == null) 1324 this.gene = new ArrayList<SubstanceReferenceInformationGeneComponent>(); 1325 return this.gene; 1326 } 1327 1328 /** 1329 * @return Returns a reference to <code>this</code> for easy method chaining 1330 */ 1331 public SubstanceReferenceInformation setGene(List<SubstanceReferenceInformationGeneComponent> theGene) { 1332 this.gene = theGene; 1333 return this; 1334 } 1335 1336 public boolean hasGene() { 1337 if (this.gene == null) 1338 return false; 1339 for (SubstanceReferenceInformationGeneComponent item : this.gene) 1340 if (!item.isEmpty()) 1341 return true; 1342 return false; 1343 } 1344 1345 public SubstanceReferenceInformationGeneComponent addGene() { //3 1346 SubstanceReferenceInformationGeneComponent t = new SubstanceReferenceInformationGeneComponent(); 1347 if (this.gene == null) 1348 this.gene = new ArrayList<SubstanceReferenceInformationGeneComponent>(); 1349 this.gene.add(t); 1350 return t; 1351 } 1352 1353 public SubstanceReferenceInformation addGene(SubstanceReferenceInformationGeneComponent t) { //3 1354 if (t == null) 1355 return this; 1356 if (this.gene == null) 1357 this.gene = new ArrayList<SubstanceReferenceInformationGeneComponent>(); 1358 this.gene.add(t); 1359 return this; 1360 } 1361 1362 /** 1363 * @return The first repetition of repeating field {@link #gene}, creating it if it does not already exist {3} 1364 */ 1365 public SubstanceReferenceInformationGeneComponent getGeneFirstRep() { 1366 if (getGene().isEmpty()) { 1367 addGene(); 1368 } 1369 return getGene().get(0); 1370 } 1371 1372 /** 1373 * @return {@link #geneElement} (Todo.) 1374 */ 1375 public List<SubstanceReferenceInformationGeneElementComponent> getGeneElement() { 1376 if (this.geneElement == null) 1377 this.geneElement = new ArrayList<SubstanceReferenceInformationGeneElementComponent>(); 1378 return this.geneElement; 1379 } 1380 1381 /** 1382 * @return Returns a reference to <code>this</code> for easy method chaining 1383 */ 1384 public SubstanceReferenceInformation setGeneElement(List<SubstanceReferenceInformationGeneElementComponent> theGeneElement) { 1385 this.geneElement = theGeneElement; 1386 return this; 1387 } 1388 1389 public boolean hasGeneElement() { 1390 if (this.geneElement == null) 1391 return false; 1392 for (SubstanceReferenceInformationGeneElementComponent item : this.geneElement) 1393 if (!item.isEmpty()) 1394 return true; 1395 return false; 1396 } 1397 1398 public SubstanceReferenceInformationGeneElementComponent addGeneElement() { //3 1399 SubstanceReferenceInformationGeneElementComponent t = new SubstanceReferenceInformationGeneElementComponent(); 1400 if (this.geneElement == null) 1401 this.geneElement = new ArrayList<SubstanceReferenceInformationGeneElementComponent>(); 1402 this.geneElement.add(t); 1403 return t; 1404 } 1405 1406 public SubstanceReferenceInformation addGeneElement(SubstanceReferenceInformationGeneElementComponent t) { //3 1407 if (t == null) 1408 return this; 1409 if (this.geneElement == null) 1410 this.geneElement = new ArrayList<SubstanceReferenceInformationGeneElementComponent>(); 1411 this.geneElement.add(t); 1412 return this; 1413 } 1414 1415 /** 1416 * @return The first repetition of repeating field {@link #geneElement}, creating it if it does not already exist {3} 1417 */ 1418 public SubstanceReferenceInformationGeneElementComponent getGeneElementFirstRep() { 1419 if (getGeneElement().isEmpty()) { 1420 addGeneElement(); 1421 } 1422 return getGeneElement().get(0); 1423 } 1424 1425 /** 1426 * @return {@link #target} (Todo.) 1427 */ 1428 public List<SubstanceReferenceInformationTargetComponent> getTarget() { 1429 if (this.target == null) 1430 this.target = new ArrayList<SubstanceReferenceInformationTargetComponent>(); 1431 return this.target; 1432 } 1433 1434 /** 1435 * @return Returns a reference to <code>this</code> for easy method chaining 1436 */ 1437 public SubstanceReferenceInformation setTarget(List<SubstanceReferenceInformationTargetComponent> theTarget) { 1438 this.target = theTarget; 1439 return this; 1440 } 1441 1442 public boolean hasTarget() { 1443 if (this.target == null) 1444 return false; 1445 for (SubstanceReferenceInformationTargetComponent item : this.target) 1446 if (!item.isEmpty()) 1447 return true; 1448 return false; 1449 } 1450 1451 public SubstanceReferenceInformationTargetComponent addTarget() { //3 1452 SubstanceReferenceInformationTargetComponent t = new SubstanceReferenceInformationTargetComponent(); 1453 if (this.target == null) 1454 this.target = new ArrayList<SubstanceReferenceInformationTargetComponent>(); 1455 this.target.add(t); 1456 return t; 1457 } 1458 1459 public SubstanceReferenceInformation addTarget(SubstanceReferenceInformationTargetComponent t) { //3 1460 if (t == null) 1461 return this; 1462 if (this.target == null) 1463 this.target = new ArrayList<SubstanceReferenceInformationTargetComponent>(); 1464 this.target.add(t); 1465 return this; 1466 } 1467 1468 /** 1469 * @return The first repetition of repeating field {@link #target}, creating it if it does not already exist {3} 1470 */ 1471 public SubstanceReferenceInformationTargetComponent getTargetFirstRep() { 1472 if (getTarget().isEmpty()) { 1473 addTarget(); 1474 } 1475 return getTarget().get(0); 1476 } 1477 1478 protected void listChildren(List<Property> children) { 1479 super.listChildren(children); 1480 children.add(new Property("comment", "string", "Todo.", 0, 1, comment)); 1481 children.add(new Property("gene", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, gene)); 1482 children.add(new Property("geneElement", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, geneElement)); 1483 children.add(new Property("target", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, target)); 1484 } 1485 1486 @Override 1487 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1488 switch (_hash) { 1489 case 950398559: /*comment*/ return new Property("comment", "string", "Todo.", 0, 1, comment); 1490 case 3169045: /*gene*/ return new Property("gene", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, gene); 1491 case -94918105: /*geneElement*/ return new Property("geneElement", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, geneElement); 1492 case -880905839: /*target*/ return new Property("target", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, target); 1493 default: return super.getNamedProperty(_hash, _name, _checkValid); 1494 } 1495 1496 } 1497 1498 @Override 1499 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1500 switch (hash) { 1501 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 1502 case 3169045: /*gene*/ return this.gene == null ? new Base[0] : this.gene.toArray(new Base[this.gene.size()]); // SubstanceReferenceInformationGeneComponent 1503 case -94918105: /*geneElement*/ return this.geneElement == null ? new Base[0] : this.geneElement.toArray(new Base[this.geneElement.size()]); // SubstanceReferenceInformationGeneElementComponent 1504 case -880905839: /*target*/ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // SubstanceReferenceInformationTargetComponent 1505 default: return super.getProperty(hash, name, checkValid); 1506 } 1507 1508 } 1509 1510 @Override 1511 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1512 switch (hash) { 1513 case 950398559: // comment 1514 this.comment = TypeConvertor.castToString(value); // StringType 1515 return value; 1516 case 3169045: // gene 1517 this.getGene().add((SubstanceReferenceInformationGeneComponent) value); // SubstanceReferenceInformationGeneComponent 1518 return value; 1519 case -94918105: // geneElement 1520 this.getGeneElement().add((SubstanceReferenceInformationGeneElementComponent) value); // SubstanceReferenceInformationGeneElementComponent 1521 return value; 1522 case -880905839: // target 1523 this.getTarget().add((SubstanceReferenceInformationTargetComponent) value); // SubstanceReferenceInformationTargetComponent 1524 return value; 1525 default: return super.setProperty(hash, name, value); 1526 } 1527 1528 } 1529 1530 @Override 1531 public Base setProperty(String name, Base value) throws FHIRException { 1532 if (name.equals("comment")) { 1533 this.comment = TypeConvertor.castToString(value); // StringType 1534 } else if (name.equals("gene")) { 1535 this.getGene().add((SubstanceReferenceInformationGeneComponent) value); 1536 } else if (name.equals("geneElement")) { 1537 this.getGeneElement().add((SubstanceReferenceInformationGeneElementComponent) value); 1538 } else if (name.equals("target")) { 1539 this.getTarget().add((SubstanceReferenceInformationTargetComponent) value); 1540 } else 1541 return super.setProperty(name, value); 1542 return value; 1543 } 1544 1545 @Override 1546 public void removeChild(String name, Base value) throws FHIRException { 1547 if (name.equals("comment")) { 1548 this.comment = null; 1549 } else if (name.equals("gene")) { 1550 this.getGene().remove((SubstanceReferenceInformationGeneComponent) value); 1551 } else if (name.equals("geneElement")) { 1552 this.getGeneElement().remove((SubstanceReferenceInformationGeneElementComponent) value); 1553 } else if (name.equals("target")) { 1554 this.getTarget().remove((SubstanceReferenceInformationTargetComponent) value); 1555 } else 1556 super.removeChild(name, value); 1557 1558 } 1559 1560 @Override 1561 public Base makeProperty(int hash, String name) throws FHIRException { 1562 switch (hash) { 1563 case 950398559: return getCommentElement(); 1564 case 3169045: return addGene(); 1565 case -94918105: return addGeneElement(); 1566 case -880905839: return addTarget(); 1567 default: return super.makeProperty(hash, name); 1568 } 1569 1570 } 1571 1572 @Override 1573 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1574 switch (hash) { 1575 case 950398559: /*comment*/ return new String[] {"string"}; 1576 case 3169045: /*gene*/ return new String[] {}; 1577 case -94918105: /*geneElement*/ return new String[] {}; 1578 case -880905839: /*target*/ return new String[] {}; 1579 default: return super.getTypesForProperty(hash, name); 1580 } 1581 1582 } 1583 1584 @Override 1585 public Base addChild(String name) throws FHIRException { 1586 if (name.equals("comment")) { 1587 throw new FHIRException("Cannot call addChild on a singleton property SubstanceReferenceInformation.comment"); 1588 } 1589 else if (name.equals("gene")) { 1590 return addGene(); 1591 } 1592 else if (name.equals("geneElement")) { 1593 return addGeneElement(); 1594 } 1595 else if (name.equals("target")) { 1596 return addTarget(); 1597 } 1598 else 1599 return super.addChild(name); 1600 } 1601 1602 public String fhirType() { 1603 return "SubstanceReferenceInformation"; 1604 1605 } 1606 1607 public SubstanceReferenceInformation copy() { 1608 SubstanceReferenceInformation dst = new SubstanceReferenceInformation(); 1609 copyValues(dst); 1610 return dst; 1611 } 1612 1613 public void copyValues(SubstanceReferenceInformation dst) { 1614 super.copyValues(dst); 1615 dst.comment = comment == null ? null : comment.copy(); 1616 if (gene != null) { 1617 dst.gene = new ArrayList<SubstanceReferenceInformationGeneComponent>(); 1618 for (SubstanceReferenceInformationGeneComponent i : gene) 1619 dst.gene.add(i.copy()); 1620 }; 1621 if (geneElement != null) { 1622 dst.geneElement = new ArrayList<SubstanceReferenceInformationGeneElementComponent>(); 1623 for (SubstanceReferenceInformationGeneElementComponent i : geneElement) 1624 dst.geneElement.add(i.copy()); 1625 }; 1626 if (target != null) { 1627 dst.target = new ArrayList<SubstanceReferenceInformationTargetComponent>(); 1628 for (SubstanceReferenceInformationTargetComponent i : target) 1629 dst.target.add(i.copy()); 1630 }; 1631 } 1632 1633 protected SubstanceReferenceInformation typedCopy() { 1634 return copy(); 1635 } 1636 1637 @Override 1638 public boolean equalsDeep(Base other_) { 1639 if (!super.equalsDeep(other_)) 1640 return false; 1641 if (!(other_ instanceof SubstanceReferenceInformation)) 1642 return false; 1643 SubstanceReferenceInformation o = (SubstanceReferenceInformation) other_; 1644 return compareDeep(comment, o.comment, true) && compareDeep(gene, o.gene, true) && compareDeep(geneElement, o.geneElement, true) 1645 && compareDeep(target, o.target, true); 1646 } 1647 1648 @Override 1649 public boolean equalsShallow(Base other_) { 1650 if (!super.equalsShallow(other_)) 1651 return false; 1652 if (!(other_ instanceof SubstanceReferenceInformation)) 1653 return false; 1654 SubstanceReferenceInformation o = (SubstanceReferenceInformation) other_; 1655 return compareValues(comment, o.comment, true); 1656 } 1657 1658 public boolean isEmpty() { 1659 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(comment, gene, geneElement 1660 , target); 1661 } 1662 1663 @Override 1664 public ResourceType getResourceType() { 1665 return ResourceType.SubstanceReferenceInformation; 1666 } 1667 1668 1669} 1670