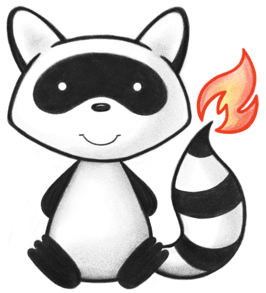
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Source material shall capture information on the taxonomic and anatomical origins as well as the fraction of a material that can result in or can be modified to form a substance. This set of data elements shall be used to define polymer substances isolated from biological matrices. Taxonomic and anatomical origins shall be described using a controlled vocabulary as required. This information is captured for naturally derived polymers ( . starch) and structurally diverse substances. For Organisms belonging to the Kingdom Plantae the Substance level defines the fresh material of a single species or infraspecies, the Herbal Drug and the Herbal preparation. For Herbal preparations, the fraction information will be captured at the Substance information level and additional information for herbal extracts will be captured at the Specified Substance Group 1 information level. See for further explanation the Substance Class: Structurally Diverse and the herbal annex. 052 */ 053@ResourceDef(name="SubstanceSourceMaterial", profile="http://hl7.org/fhir/StructureDefinition/SubstanceSourceMaterial") 054public class SubstanceSourceMaterial extends DomainResource { 055 056 @Block() 057 public static class SubstanceSourceMaterialFractionDescriptionComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * This element is capturing information about the fraction of a plant part, or human plasma for fractionation. 060 */ 061 @Child(name = "fraction", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="This element is capturing information about the fraction of a plant part, or human plasma for fractionation", formalDefinition="This element is capturing information about the fraction of a plant part, or human plasma for fractionation." ) 063 protected StringType fraction; 064 065 /** 066 * The specific type of the material constituting the component. For Herbal preparations the particulars of the extracts (liquid/dry) is described in Specified Substance Group 1. 067 */ 068 @Child(name = "materialType", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 069 @Description(shortDefinition="The specific type of the material constituting the component. For Herbal preparations the particulars of the extracts (liquid/dry) is described in Specified Substance Group 1", formalDefinition="The specific type of the material constituting the component. For Herbal preparations the particulars of the extracts (liquid/dry) is described in Specified Substance Group 1." ) 070 protected CodeableConcept materialType; 071 072 private static final long serialVersionUID = -1118226733L; 073 074 /** 075 * Constructor 076 */ 077 public SubstanceSourceMaterialFractionDescriptionComponent() { 078 super(); 079 } 080 081 /** 082 * @return {@link #fraction} (This element is capturing information about the fraction of a plant part, or human plasma for fractionation.). This is the underlying object with id, value and extensions. The accessor "getFraction" gives direct access to the value 083 */ 084 public StringType getFractionElement() { 085 if (this.fraction == null) 086 if (Configuration.errorOnAutoCreate()) 087 throw new Error("Attempt to auto-create SubstanceSourceMaterialFractionDescriptionComponent.fraction"); 088 else if (Configuration.doAutoCreate()) 089 this.fraction = new StringType(); // bb 090 return this.fraction; 091 } 092 093 public boolean hasFractionElement() { 094 return this.fraction != null && !this.fraction.isEmpty(); 095 } 096 097 public boolean hasFraction() { 098 return this.fraction != null && !this.fraction.isEmpty(); 099 } 100 101 /** 102 * @param value {@link #fraction} (This element is capturing information about the fraction of a plant part, or human plasma for fractionation.). This is the underlying object with id, value and extensions. The accessor "getFraction" gives direct access to the value 103 */ 104 public SubstanceSourceMaterialFractionDescriptionComponent setFractionElement(StringType value) { 105 this.fraction = value; 106 return this; 107 } 108 109 /** 110 * @return This element is capturing information about the fraction of a plant part, or human plasma for fractionation. 111 */ 112 public String getFraction() { 113 return this.fraction == null ? null : this.fraction.getValue(); 114 } 115 116 /** 117 * @param value This element is capturing information about the fraction of a plant part, or human plasma for fractionation. 118 */ 119 public SubstanceSourceMaterialFractionDescriptionComponent setFraction(String value) { 120 if (Utilities.noString(value)) 121 this.fraction = null; 122 else { 123 if (this.fraction == null) 124 this.fraction = new StringType(); 125 this.fraction.setValue(value); 126 } 127 return this; 128 } 129 130 /** 131 * @return {@link #materialType} (The specific type of the material constituting the component. For Herbal preparations the particulars of the extracts (liquid/dry) is described in Specified Substance Group 1.) 132 */ 133 public CodeableConcept getMaterialType() { 134 if (this.materialType == null) 135 if (Configuration.errorOnAutoCreate()) 136 throw new Error("Attempt to auto-create SubstanceSourceMaterialFractionDescriptionComponent.materialType"); 137 else if (Configuration.doAutoCreate()) 138 this.materialType = new CodeableConcept(); // cc 139 return this.materialType; 140 } 141 142 public boolean hasMaterialType() { 143 return this.materialType != null && !this.materialType.isEmpty(); 144 } 145 146 /** 147 * @param value {@link #materialType} (The specific type of the material constituting the component. For Herbal preparations the particulars of the extracts (liquid/dry) is described in Specified Substance Group 1.) 148 */ 149 public SubstanceSourceMaterialFractionDescriptionComponent setMaterialType(CodeableConcept value) { 150 this.materialType = value; 151 return this; 152 } 153 154 protected void listChildren(List<Property> children) { 155 super.listChildren(children); 156 children.add(new Property("fraction", "string", "This element is capturing information about the fraction of a plant part, or human plasma for fractionation.", 0, 1, fraction)); 157 children.add(new Property("materialType", "CodeableConcept", "The specific type of the material constituting the component. For Herbal preparations the particulars of the extracts (liquid/dry) is described in Specified Substance Group 1.", 0, 1, materialType)); 158 } 159 160 @Override 161 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 162 switch (_hash) { 163 case -1653751294: /*fraction*/ return new Property("fraction", "string", "This element is capturing information about the fraction of a plant part, or human plasma for fractionation.", 0, 1, fraction); 164 case -2115601151: /*materialType*/ return new Property("materialType", "CodeableConcept", "The specific type of the material constituting the component. For Herbal preparations the particulars of the extracts (liquid/dry) is described in Specified Substance Group 1.", 0, 1, materialType); 165 default: return super.getNamedProperty(_hash, _name, _checkValid); 166 } 167 168 } 169 170 @Override 171 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 172 switch (hash) { 173 case -1653751294: /*fraction*/ return this.fraction == null ? new Base[0] : new Base[] {this.fraction}; // StringType 174 case -2115601151: /*materialType*/ return this.materialType == null ? new Base[0] : new Base[] {this.materialType}; // CodeableConcept 175 default: return super.getProperty(hash, name, checkValid); 176 } 177 178 } 179 180 @Override 181 public Base setProperty(int hash, String name, Base value) throws FHIRException { 182 switch (hash) { 183 case -1653751294: // fraction 184 this.fraction = TypeConvertor.castToString(value); // StringType 185 return value; 186 case -2115601151: // materialType 187 this.materialType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 188 return value; 189 default: return super.setProperty(hash, name, value); 190 } 191 192 } 193 194 @Override 195 public Base setProperty(String name, Base value) throws FHIRException { 196 if (name.equals("fraction")) { 197 this.fraction = TypeConvertor.castToString(value); // StringType 198 } else if (name.equals("materialType")) { 199 this.materialType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 200 } else 201 return super.setProperty(name, value); 202 return value; 203 } 204 205 @Override 206 public void removeChild(String name, Base value) throws FHIRException { 207 if (name.equals("fraction")) { 208 this.fraction = null; 209 } else if (name.equals("materialType")) { 210 this.materialType = null; 211 } else 212 super.removeChild(name, value); 213 214 } 215 216 @Override 217 public Base makeProperty(int hash, String name) throws FHIRException { 218 switch (hash) { 219 case -1653751294: return getFractionElement(); 220 case -2115601151: return getMaterialType(); 221 default: return super.makeProperty(hash, name); 222 } 223 224 } 225 226 @Override 227 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 228 switch (hash) { 229 case -1653751294: /*fraction*/ return new String[] {"string"}; 230 case -2115601151: /*materialType*/ return new String[] {"CodeableConcept"}; 231 default: return super.getTypesForProperty(hash, name); 232 } 233 234 } 235 236 @Override 237 public Base addChild(String name) throws FHIRException { 238 if (name.equals("fraction")) { 239 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSourceMaterial.fractionDescription.fraction"); 240 } 241 else if (name.equals("materialType")) { 242 this.materialType = new CodeableConcept(); 243 return this.materialType; 244 } 245 else 246 return super.addChild(name); 247 } 248 249 public SubstanceSourceMaterialFractionDescriptionComponent copy() { 250 SubstanceSourceMaterialFractionDescriptionComponent dst = new SubstanceSourceMaterialFractionDescriptionComponent(); 251 copyValues(dst); 252 return dst; 253 } 254 255 public void copyValues(SubstanceSourceMaterialFractionDescriptionComponent dst) { 256 super.copyValues(dst); 257 dst.fraction = fraction == null ? null : fraction.copy(); 258 dst.materialType = materialType == null ? null : materialType.copy(); 259 } 260 261 @Override 262 public boolean equalsDeep(Base other_) { 263 if (!super.equalsDeep(other_)) 264 return false; 265 if (!(other_ instanceof SubstanceSourceMaterialFractionDescriptionComponent)) 266 return false; 267 SubstanceSourceMaterialFractionDescriptionComponent o = (SubstanceSourceMaterialFractionDescriptionComponent) other_; 268 return compareDeep(fraction, o.fraction, true) && compareDeep(materialType, o.materialType, true) 269 ; 270 } 271 272 @Override 273 public boolean equalsShallow(Base other_) { 274 if (!super.equalsShallow(other_)) 275 return false; 276 if (!(other_ instanceof SubstanceSourceMaterialFractionDescriptionComponent)) 277 return false; 278 SubstanceSourceMaterialFractionDescriptionComponent o = (SubstanceSourceMaterialFractionDescriptionComponent) other_; 279 return compareValues(fraction, o.fraction, true); 280 } 281 282 public boolean isEmpty() { 283 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(fraction, materialType); 284 } 285 286 public String fhirType() { 287 return "SubstanceSourceMaterial.fractionDescription"; 288 289 } 290 291 } 292 293 @Block() 294 public static class SubstanceSourceMaterialOrganismComponent extends BackboneElement implements IBaseBackboneElement { 295 /** 296 * The family of an organism shall be specified. 297 */ 298 @Child(name = "family", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 299 @Description(shortDefinition="The family of an organism shall be specified", formalDefinition="The family of an organism shall be specified." ) 300 protected CodeableConcept family; 301 302 /** 303 * The genus of an organism shall be specified; refers to the Latin epithet of the genus element of the plant/animal scientific name; it is present in names for genera, species and infraspecies. 304 */ 305 @Child(name = "genus", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 306 @Description(shortDefinition="The genus of an organism shall be specified; refers to the Latin epithet of the genus element of the plant/animal scientific name; it is present in names for genera, species and infraspecies", formalDefinition="The genus of an organism shall be specified; refers to the Latin epithet of the genus element of the plant/animal scientific name; it is present in names for genera, species and infraspecies." ) 307 protected CodeableConcept genus; 308 309 /** 310 * The species of an organism shall be specified; refers to the Latin epithet of the species of the plant/animal; it is present in names for species and infraspecies. 311 */ 312 @Child(name = "species", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 313 @Description(shortDefinition="The species of an organism shall be specified; refers to the Latin epithet of the species of the plant/animal; it is present in names for species and infraspecies", formalDefinition="The species of an organism shall be specified; refers to the Latin epithet of the species of the plant/animal; it is present in names for species and infraspecies." ) 314 protected CodeableConcept species; 315 316 /** 317 * The Intraspecific type of an organism shall be specified. 318 */ 319 @Child(name = "intraspecificType", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 320 @Description(shortDefinition="The Intraspecific type of an organism shall be specified", formalDefinition="The Intraspecific type of an organism shall be specified." ) 321 protected CodeableConcept intraspecificType; 322 323 /** 324 * The intraspecific description of an organism shall be specified based on a controlled vocabulary. For Influenza Vaccine, the intraspecific description shall contain the syntax of the antigen in line with the WHO convention. 325 */ 326 @Child(name = "intraspecificDescription", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 327 @Description(shortDefinition="The intraspecific description of an organism shall be specified based on a controlled vocabulary. For Influenza Vaccine, the intraspecific description shall contain the syntax of the antigen in line with the WHO convention", formalDefinition="The intraspecific description of an organism shall be specified based on a controlled vocabulary. For Influenza Vaccine, the intraspecific description shall contain the syntax of the antigen in line with the WHO convention." ) 328 protected StringType intraspecificDescription; 329 330 /** 331 * 4.9.13.6.1 Author type (Conditional). 332 */ 333 @Child(name = "author", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 334 @Description(shortDefinition="4.9.13.6.1 Author type (Conditional)", formalDefinition="4.9.13.6.1 Author type (Conditional)." ) 335 protected List<SubstanceSourceMaterialOrganismAuthorComponent> author; 336 337 /** 338 * 4.9.13.8.1 Hybrid species maternal organism ID (Optional). 339 */ 340 @Child(name = "hybrid", type = {}, order=7, min=0, max=1, modifier=false, summary=true) 341 @Description(shortDefinition="4.9.13.8.1 Hybrid species maternal organism ID (Optional)", formalDefinition="4.9.13.8.1 Hybrid species maternal organism ID (Optional)." ) 342 protected SubstanceSourceMaterialOrganismHybridComponent hybrid; 343 344 /** 345 * 4.9.13.7.1 Kingdom (Conditional). 346 */ 347 @Child(name = "organismGeneral", type = {}, order=8, min=0, max=1, modifier=false, summary=true) 348 @Description(shortDefinition="4.9.13.7.1 Kingdom (Conditional)", formalDefinition="4.9.13.7.1 Kingdom (Conditional)." ) 349 protected SubstanceSourceMaterialOrganismOrganismGeneralComponent organismGeneral; 350 351 private static final long serialVersionUID = 941648312L; 352 353 /** 354 * Constructor 355 */ 356 public SubstanceSourceMaterialOrganismComponent() { 357 super(); 358 } 359 360 /** 361 * @return {@link #family} (The family of an organism shall be specified.) 362 */ 363 public CodeableConcept getFamily() { 364 if (this.family == null) 365 if (Configuration.errorOnAutoCreate()) 366 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismComponent.family"); 367 else if (Configuration.doAutoCreate()) 368 this.family = new CodeableConcept(); // cc 369 return this.family; 370 } 371 372 public boolean hasFamily() { 373 return this.family != null && !this.family.isEmpty(); 374 } 375 376 /** 377 * @param value {@link #family} (The family of an organism shall be specified.) 378 */ 379 public SubstanceSourceMaterialOrganismComponent setFamily(CodeableConcept value) { 380 this.family = value; 381 return this; 382 } 383 384 /** 385 * @return {@link #genus} (The genus of an organism shall be specified; refers to the Latin epithet of the genus element of the plant/animal scientific name; it is present in names for genera, species and infraspecies.) 386 */ 387 public CodeableConcept getGenus() { 388 if (this.genus == null) 389 if (Configuration.errorOnAutoCreate()) 390 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismComponent.genus"); 391 else if (Configuration.doAutoCreate()) 392 this.genus = new CodeableConcept(); // cc 393 return this.genus; 394 } 395 396 public boolean hasGenus() { 397 return this.genus != null && !this.genus.isEmpty(); 398 } 399 400 /** 401 * @param value {@link #genus} (The genus of an organism shall be specified; refers to the Latin epithet of the genus element of the plant/animal scientific name; it is present in names for genera, species and infraspecies.) 402 */ 403 public SubstanceSourceMaterialOrganismComponent setGenus(CodeableConcept value) { 404 this.genus = value; 405 return this; 406 } 407 408 /** 409 * @return {@link #species} (The species of an organism shall be specified; refers to the Latin epithet of the species of the plant/animal; it is present in names for species and infraspecies.) 410 */ 411 public CodeableConcept getSpecies() { 412 if (this.species == null) 413 if (Configuration.errorOnAutoCreate()) 414 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismComponent.species"); 415 else if (Configuration.doAutoCreate()) 416 this.species = new CodeableConcept(); // cc 417 return this.species; 418 } 419 420 public boolean hasSpecies() { 421 return this.species != null && !this.species.isEmpty(); 422 } 423 424 /** 425 * @param value {@link #species} (The species of an organism shall be specified; refers to the Latin epithet of the species of the plant/animal; it is present in names for species and infraspecies.) 426 */ 427 public SubstanceSourceMaterialOrganismComponent setSpecies(CodeableConcept value) { 428 this.species = value; 429 return this; 430 } 431 432 /** 433 * @return {@link #intraspecificType} (The Intraspecific type of an organism shall be specified.) 434 */ 435 public CodeableConcept getIntraspecificType() { 436 if (this.intraspecificType == null) 437 if (Configuration.errorOnAutoCreate()) 438 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismComponent.intraspecificType"); 439 else if (Configuration.doAutoCreate()) 440 this.intraspecificType = new CodeableConcept(); // cc 441 return this.intraspecificType; 442 } 443 444 public boolean hasIntraspecificType() { 445 return this.intraspecificType != null && !this.intraspecificType.isEmpty(); 446 } 447 448 /** 449 * @param value {@link #intraspecificType} (The Intraspecific type of an organism shall be specified.) 450 */ 451 public SubstanceSourceMaterialOrganismComponent setIntraspecificType(CodeableConcept value) { 452 this.intraspecificType = value; 453 return this; 454 } 455 456 /** 457 * @return {@link #intraspecificDescription} (The intraspecific description of an organism shall be specified based on a controlled vocabulary. For Influenza Vaccine, the intraspecific description shall contain the syntax of the antigen in line with the WHO convention.). This is the underlying object with id, value and extensions. The accessor "getIntraspecificDescription" gives direct access to the value 458 */ 459 public StringType getIntraspecificDescriptionElement() { 460 if (this.intraspecificDescription == null) 461 if (Configuration.errorOnAutoCreate()) 462 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismComponent.intraspecificDescription"); 463 else if (Configuration.doAutoCreate()) 464 this.intraspecificDescription = new StringType(); // bb 465 return this.intraspecificDescription; 466 } 467 468 public boolean hasIntraspecificDescriptionElement() { 469 return this.intraspecificDescription != null && !this.intraspecificDescription.isEmpty(); 470 } 471 472 public boolean hasIntraspecificDescription() { 473 return this.intraspecificDescription != null && !this.intraspecificDescription.isEmpty(); 474 } 475 476 /** 477 * @param value {@link #intraspecificDescription} (The intraspecific description of an organism shall be specified based on a controlled vocabulary. For Influenza Vaccine, the intraspecific description shall contain the syntax of the antigen in line with the WHO convention.). This is the underlying object with id, value and extensions. The accessor "getIntraspecificDescription" gives direct access to the value 478 */ 479 public SubstanceSourceMaterialOrganismComponent setIntraspecificDescriptionElement(StringType value) { 480 this.intraspecificDescription = value; 481 return this; 482 } 483 484 /** 485 * @return The intraspecific description of an organism shall be specified based on a controlled vocabulary. For Influenza Vaccine, the intraspecific description shall contain the syntax of the antigen in line with the WHO convention. 486 */ 487 public String getIntraspecificDescription() { 488 return this.intraspecificDescription == null ? null : this.intraspecificDescription.getValue(); 489 } 490 491 /** 492 * @param value The intraspecific description of an organism shall be specified based on a controlled vocabulary. For Influenza Vaccine, the intraspecific description shall contain the syntax of the antigen in line with the WHO convention. 493 */ 494 public SubstanceSourceMaterialOrganismComponent setIntraspecificDescription(String value) { 495 if (Utilities.noString(value)) 496 this.intraspecificDescription = null; 497 else { 498 if (this.intraspecificDescription == null) 499 this.intraspecificDescription = new StringType(); 500 this.intraspecificDescription.setValue(value); 501 } 502 return this; 503 } 504 505 /** 506 * @return {@link #author} (4.9.13.6.1 Author type (Conditional).) 507 */ 508 public List<SubstanceSourceMaterialOrganismAuthorComponent> getAuthor() { 509 if (this.author == null) 510 this.author = new ArrayList<SubstanceSourceMaterialOrganismAuthorComponent>(); 511 return this.author; 512 } 513 514 /** 515 * @return Returns a reference to <code>this</code> for easy method chaining 516 */ 517 public SubstanceSourceMaterialOrganismComponent setAuthor(List<SubstanceSourceMaterialOrganismAuthorComponent> theAuthor) { 518 this.author = theAuthor; 519 return this; 520 } 521 522 public boolean hasAuthor() { 523 if (this.author == null) 524 return false; 525 for (SubstanceSourceMaterialOrganismAuthorComponent item : this.author) 526 if (!item.isEmpty()) 527 return true; 528 return false; 529 } 530 531 public SubstanceSourceMaterialOrganismAuthorComponent addAuthor() { //3 532 SubstanceSourceMaterialOrganismAuthorComponent t = new SubstanceSourceMaterialOrganismAuthorComponent(); 533 if (this.author == null) 534 this.author = new ArrayList<SubstanceSourceMaterialOrganismAuthorComponent>(); 535 this.author.add(t); 536 return t; 537 } 538 539 public SubstanceSourceMaterialOrganismComponent addAuthor(SubstanceSourceMaterialOrganismAuthorComponent t) { //3 540 if (t == null) 541 return this; 542 if (this.author == null) 543 this.author = new ArrayList<SubstanceSourceMaterialOrganismAuthorComponent>(); 544 this.author.add(t); 545 return this; 546 } 547 548 /** 549 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 550 */ 551 public SubstanceSourceMaterialOrganismAuthorComponent getAuthorFirstRep() { 552 if (getAuthor().isEmpty()) { 553 addAuthor(); 554 } 555 return getAuthor().get(0); 556 } 557 558 /** 559 * @return {@link #hybrid} (4.9.13.8.1 Hybrid species maternal organism ID (Optional).) 560 */ 561 public SubstanceSourceMaterialOrganismHybridComponent getHybrid() { 562 if (this.hybrid == null) 563 if (Configuration.errorOnAutoCreate()) 564 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismComponent.hybrid"); 565 else if (Configuration.doAutoCreate()) 566 this.hybrid = new SubstanceSourceMaterialOrganismHybridComponent(); // cc 567 return this.hybrid; 568 } 569 570 public boolean hasHybrid() { 571 return this.hybrid != null && !this.hybrid.isEmpty(); 572 } 573 574 /** 575 * @param value {@link #hybrid} (4.9.13.8.1 Hybrid species maternal organism ID (Optional).) 576 */ 577 public SubstanceSourceMaterialOrganismComponent setHybrid(SubstanceSourceMaterialOrganismHybridComponent value) { 578 this.hybrid = value; 579 return this; 580 } 581 582 /** 583 * @return {@link #organismGeneral} (4.9.13.7.1 Kingdom (Conditional).) 584 */ 585 public SubstanceSourceMaterialOrganismOrganismGeneralComponent getOrganismGeneral() { 586 if (this.organismGeneral == null) 587 if (Configuration.errorOnAutoCreate()) 588 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismComponent.organismGeneral"); 589 else if (Configuration.doAutoCreate()) 590 this.organismGeneral = new SubstanceSourceMaterialOrganismOrganismGeneralComponent(); // cc 591 return this.organismGeneral; 592 } 593 594 public boolean hasOrganismGeneral() { 595 return this.organismGeneral != null && !this.organismGeneral.isEmpty(); 596 } 597 598 /** 599 * @param value {@link #organismGeneral} (4.9.13.7.1 Kingdom (Conditional).) 600 */ 601 public SubstanceSourceMaterialOrganismComponent setOrganismGeneral(SubstanceSourceMaterialOrganismOrganismGeneralComponent value) { 602 this.organismGeneral = value; 603 return this; 604 } 605 606 protected void listChildren(List<Property> children) { 607 super.listChildren(children); 608 children.add(new Property("family", "CodeableConcept", "The family of an organism shall be specified.", 0, 1, family)); 609 children.add(new Property("genus", "CodeableConcept", "The genus of an organism shall be specified; refers to the Latin epithet of the genus element of the plant/animal scientific name; it is present in names for genera, species and infraspecies.", 0, 1, genus)); 610 children.add(new Property("species", "CodeableConcept", "The species of an organism shall be specified; refers to the Latin epithet of the species of the plant/animal; it is present in names for species and infraspecies.", 0, 1, species)); 611 children.add(new Property("intraspecificType", "CodeableConcept", "The Intraspecific type of an organism shall be specified.", 0, 1, intraspecificType)); 612 children.add(new Property("intraspecificDescription", "string", "The intraspecific description of an organism shall be specified based on a controlled vocabulary. For Influenza Vaccine, the intraspecific description shall contain the syntax of the antigen in line with the WHO convention.", 0, 1, intraspecificDescription)); 613 children.add(new Property("author", "", "4.9.13.6.1 Author type (Conditional).", 0, java.lang.Integer.MAX_VALUE, author)); 614 children.add(new Property("hybrid", "", "4.9.13.8.1 Hybrid species maternal organism ID (Optional).", 0, 1, hybrid)); 615 children.add(new Property("organismGeneral", "", "4.9.13.7.1 Kingdom (Conditional).", 0, 1, organismGeneral)); 616 } 617 618 @Override 619 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 620 switch (_hash) { 621 case -1281860764: /*family*/ return new Property("family", "CodeableConcept", "The family of an organism shall be specified.", 0, 1, family); 622 case 98241006: /*genus*/ return new Property("genus", "CodeableConcept", "The genus of an organism shall be specified; refers to the Latin epithet of the genus element of the plant/animal scientific name; it is present in names for genera, species and infraspecies.", 0, 1, genus); 623 case -2008465092: /*species*/ return new Property("species", "CodeableConcept", "The species of an organism shall be specified; refers to the Latin epithet of the species of the plant/animal; it is present in names for species and infraspecies.", 0, 1, species); 624 case 1717161194: /*intraspecificType*/ return new Property("intraspecificType", "CodeableConcept", "The Intraspecific type of an organism shall be specified.", 0, 1, intraspecificType); 625 case -1473085364: /*intraspecificDescription*/ return new Property("intraspecificDescription", "string", "The intraspecific description of an organism shall be specified based on a controlled vocabulary. For Influenza Vaccine, the intraspecific description shall contain the syntax of the antigen in line with the WHO convention.", 0, 1, intraspecificDescription); 626 case -1406328437: /*author*/ return new Property("author", "", "4.9.13.6.1 Author type (Conditional).", 0, java.lang.Integer.MAX_VALUE, author); 627 case -1202757124: /*hybrid*/ return new Property("hybrid", "", "4.9.13.8.1 Hybrid species maternal organism ID (Optional).", 0, 1, hybrid); 628 case -865996874: /*organismGeneral*/ return new Property("organismGeneral", "", "4.9.13.7.1 Kingdom (Conditional).", 0, 1, organismGeneral); 629 default: return super.getNamedProperty(_hash, _name, _checkValid); 630 } 631 632 } 633 634 @Override 635 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 636 switch (hash) { 637 case -1281860764: /*family*/ return this.family == null ? new Base[0] : new Base[] {this.family}; // CodeableConcept 638 case 98241006: /*genus*/ return this.genus == null ? new Base[0] : new Base[] {this.genus}; // CodeableConcept 639 case -2008465092: /*species*/ return this.species == null ? new Base[0] : new Base[] {this.species}; // CodeableConcept 640 case 1717161194: /*intraspecificType*/ return this.intraspecificType == null ? new Base[0] : new Base[] {this.intraspecificType}; // CodeableConcept 641 case -1473085364: /*intraspecificDescription*/ return this.intraspecificDescription == null ? new Base[0] : new Base[] {this.intraspecificDescription}; // StringType 642 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // SubstanceSourceMaterialOrganismAuthorComponent 643 case -1202757124: /*hybrid*/ return this.hybrid == null ? new Base[0] : new Base[] {this.hybrid}; // SubstanceSourceMaterialOrganismHybridComponent 644 case -865996874: /*organismGeneral*/ return this.organismGeneral == null ? new Base[0] : new Base[] {this.organismGeneral}; // SubstanceSourceMaterialOrganismOrganismGeneralComponent 645 default: return super.getProperty(hash, name, checkValid); 646 } 647 648 } 649 650 @Override 651 public Base setProperty(int hash, String name, Base value) throws FHIRException { 652 switch (hash) { 653 case -1281860764: // family 654 this.family = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 655 return value; 656 case 98241006: // genus 657 this.genus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 658 return value; 659 case -2008465092: // species 660 this.species = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 661 return value; 662 case 1717161194: // intraspecificType 663 this.intraspecificType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 664 return value; 665 case -1473085364: // intraspecificDescription 666 this.intraspecificDescription = TypeConvertor.castToString(value); // StringType 667 return value; 668 case -1406328437: // author 669 this.getAuthor().add((SubstanceSourceMaterialOrganismAuthorComponent) value); // SubstanceSourceMaterialOrganismAuthorComponent 670 return value; 671 case -1202757124: // hybrid 672 this.hybrid = (SubstanceSourceMaterialOrganismHybridComponent) value; // SubstanceSourceMaterialOrganismHybridComponent 673 return value; 674 case -865996874: // organismGeneral 675 this.organismGeneral = (SubstanceSourceMaterialOrganismOrganismGeneralComponent) value; // SubstanceSourceMaterialOrganismOrganismGeneralComponent 676 return value; 677 default: return super.setProperty(hash, name, value); 678 } 679 680 } 681 682 @Override 683 public Base setProperty(String name, Base value) throws FHIRException { 684 if (name.equals("family")) { 685 this.family = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 686 } else if (name.equals("genus")) { 687 this.genus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 688 } else if (name.equals("species")) { 689 this.species = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 690 } else if (name.equals("intraspecificType")) { 691 this.intraspecificType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 692 } else if (name.equals("intraspecificDescription")) { 693 this.intraspecificDescription = TypeConvertor.castToString(value); // StringType 694 } else if (name.equals("author")) { 695 this.getAuthor().add((SubstanceSourceMaterialOrganismAuthorComponent) value); 696 } else if (name.equals("hybrid")) { 697 this.hybrid = (SubstanceSourceMaterialOrganismHybridComponent) value; // SubstanceSourceMaterialOrganismHybridComponent 698 } else if (name.equals("organismGeneral")) { 699 this.organismGeneral = (SubstanceSourceMaterialOrganismOrganismGeneralComponent) value; // SubstanceSourceMaterialOrganismOrganismGeneralComponent 700 } else 701 return super.setProperty(name, value); 702 return value; 703 } 704 705 @Override 706 public void removeChild(String name, Base value) throws FHIRException { 707 if (name.equals("family")) { 708 this.family = null; 709 } else if (name.equals("genus")) { 710 this.genus = null; 711 } else if (name.equals("species")) { 712 this.species = null; 713 } else if (name.equals("intraspecificType")) { 714 this.intraspecificType = null; 715 } else if (name.equals("intraspecificDescription")) { 716 this.intraspecificDescription = null; 717 } else if (name.equals("author")) { 718 this.getAuthor().remove((SubstanceSourceMaterialOrganismAuthorComponent) value); 719 } else if (name.equals("hybrid")) { 720 this.hybrid = (SubstanceSourceMaterialOrganismHybridComponent) value; // SubstanceSourceMaterialOrganismHybridComponent 721 } else if (name.equals("organismGeneral")) { 722 this.organismGeneral = (SubstanceSourceMaterialOrganismOrganismGeneralComponent) value; // SubstanceSourceMaterialOrganismOrganismGeneralComponent 723 } else 724 super.removeChild(name, value); 725 726 } 727 728 @Override 729 public Base makeProperty(int hash, String name) throws FHIRException { 730 switch (hash) { 731 case -1281860764: return getFamily(); 732 case 98241006: return getGenus(); 733 case -2008465092: return getSpecies(); 734 case 1717161194: return getIntraspecificType(); 735 case -1473085364: return getIntraspecificDescriptionElement(); 736 case -1406328437: return addAuthor(); 737 case -1202757124: return getHybrid(); 738 case -865996874: return getOrganismGeneral(); 739 default: return super.makeProperty(hash, name); 740 } 741 742 } 743 744 @Override 745 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 746 switch (hash) { 747 case -1281860764: /*family*/ return new String[] {"CodeableConcept"}; 748 case 98241006: /*genus*/ return new String[] {"CodeableConcept"}; 749 case -2008465092: /*species*/ return new String[] {"CodeableConcept"}; 750 case 1717161194: /*intraspecificType*/ return new String[] {"CodeableConcept"}; 751 case -1473085364: /*intraspecificDescription*/ return new String[] {"string"}; 752 case -1406328437: /*author*/ return new String[] {}; 753 case -1202757124: /*hybrid*/ return new String[] {}; 754 case -865996874: /*organismGeneral*/ return new String[] {}; 755 default: return super.getTypesForProperty(hash, name); 756 } 757 758 } 759 760 @Override 761 public Base addChild(String name) throws FHIRException { 762 if (name.equals("family")) { 763 this.family = new CodeableConcept(); 764 return this.family; 765 } 766 else if (name.equals("genus")) { 767 this.genus = new CodeableConcept(); 768 return this.genus; 769 } 770 else if (name.equals("species")) { 771 this.species = new CodeableConcept(); 772 return this.species; 773 } 774 else if (name.equals("intraspecificType")) { 775 this.intraspecificType = new CodeableConcept(); 776 return this.intraspecificType; 777 } 778 else if (name.equals("intraspecificDescription")) { 779 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSourceMaterial.organism.intraspecificDescription"); 780 } 781 else if (name.equals("author")) { 782 return addAuthor(); 783 } 784 else if (name.equals("hybrid")) { 785 this.hybrid = new SubstanceSourceMaterialOrganismHybridComponent(); 786 return this.hybrid; 787 } 788 else if (name.equals("organismGeneral")) { 789 this.organismGeneral = new SubstanceSourceMaterialOrganismOrganismGeneralComponent(); 790 return this.organismGeneral; 791 } 792 else 793 return super.addChild(name); 794 } 795 796 public SubstanceSourceMaterialOrganismComponent copy() { 797 SubstanceSourceMaterialOrganismComponent dst = new SubstanceSourceMaterialOrganismComponent(); 798 copyValues(dst); 799 return dst; 800 } 801 802 public void copyValues(SubstanceSourceMaterialOrganismComponent dst) { 803 super.copyValues(dst); 804 dst.family = family == null ? null : family.copy(); 805 dst.genus = genus == null ? null : genus.copy(); 806 dst.species = species == null ? null : species.copy(); 807 dst.intraspecificType = intraspecificType == null ? null : intraspecificType.copy(); 808 dst.intraspecificDescription = intraspecificDescription == null ? null : intraspecificDescription.copy(); 809 if (author != null) { 810 dst.author = new ArrayList<SubstanceSourceMaterialOrganismAuthorComponent>(); 811 for (SubstanceSourceMaterialOrganismAuthorComponent i : author) 812 dst.author.add(i.copy()); 813 }; 814 dst.hybrid = hybrid == null ? null : hybrid.copy(); 815 dst.organismGeneral = organismGeneral == null ? null : organismGeneral.copy(); 816 } 817 818 @Override 819 public boolean equalsDeep(Base other_) { 820 if (!super.equalsDeep(other_)) 821 return false; 822 if (!(other_ instanceof SubstanceSourceMaterialOrganismComponent)) 823 return false; 824 SubstanceSourceMaterialOrganismComponent o = (SubstanceSourceMaterialOrganismComponent) other_; 825 return compareDeep(family, o.family, true) && compareDeep(genus, o.genus, true) && compareDeep(species, o.species, true) 826 && compareDeep(intraspecificType, o.intraspecificType, true) && compareDeep(intraspecificDescription, o.intraspecificDescription, true) 827 && compareDeep(author, o.author, true) && compareDeep(hybrid, o.hybrid, true) && compareDeep(organismGeneral, o.organismGeneral, true) 828 ; 829 } 830 831 @Override 832 public boolean equalsShallow(Base other_) { 833 if (!super.equalsShallow(other_)) 834 return false; 835 if (!(other_ instanceof SubstanceSourceMaterialOrganismComponent)) 836 return false; 837 SubstanceSourceMaterialOrganismComponent o = (SubstanceSourceMaterialOrganismComponent) other_; 838 return compareValues(intraspecificDescription, o.intraspecificDescription, true); 839 } 840 841 public boolean isEmpty() { 842 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(family, genus, species, intraspecificType 843 , intraspecificDescription, author, hybrid, organismGeneral); 844 } 845 846 public String fhirType() { 847 return "SubstanceSourceMaterial.organism"; 848 849 } 850 851 } 852 853 @Block() 854 public static class SubstanceSourceMaterialOrganismAuthorComponent extends BackboneElement implements IBaseBackboneElement { 855 /** 856 * The type of author of an organism species shall be specified. The parenthetical author of an organism species refers to the first author who published the plant/animal name (of any rank). The primary author of an organism species refers to the first author(s), who validly published the plant/animal name. 857 */ 858 @Child(name = "authorType", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 859 @Description(shortDefinition="The type of author of an organism species shall be specified. The parenthetical author of an organism species refers to the first author who published the plant/animal name (of any rank). The primary author of an organism species refers to the first author(s), who validly published the plant/animal name", formalDefinition="The type of author of an organism species shall be specified. The parenthetical author of an organism species refers to the first author who published the plant/animal name (of any rank). The primary author of an organism species refers to the first author(s), who validly published the plant/animal name." ) 860 protected CodeableConcept authorType; 861 862 /** 863 * The author of an organism species shall be specified. The author year of an organism shall also be specified when applicable; refers to the year in which the first author(s) published the infraspecific plant/animal name (of any rank). 864 */ 865 @Child(name = "authorDescription", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 866 @Description(shortDefinition="The author of an organism species shall be specified. The author year of an organism shall also be specified when applicable; refers to the year in which the first author(s) published the infraspecific plant/animal name (of any rank)", formalDefinition="The author of an organism species shall be specified. The author year of an organism shall also be specified when applicable; refers to the year in which the first author(s) published the infraspecific plant/animal name (of any rank)." ) 867 protected StringType authorDescription; 868 869 private static final long serialVersionUID = 1429770120L; 870 871 /** 872 * Constructor 873 */ 874 public SubstanceSourceMaterialOrganismAuthorComponent() { 875 super(); 876 } 877 878 /** 879 * @return {@link #authorType} (The type of author of an organism species shall be specified. The parenthetical author of an organism species refers to the first author who published the plant/animal name (of any rank). The primary author of an organism species refers to the first author(s), who validly published the plant/animal name.) 880 */ 881 public CodeableConcept getAuthorType() { 882 if (this.authorType == null) 883 if (Configuration.errorOnAutoCreate()) 884 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismAuthorComponent.authorType"); 885 else if (Configuration.doAutoCreate()) 886 this.authorType = new CodeableConcept(); // cc 887 return this.authorType; 888 } 889 890 public boolean hasAuthorType() { 891 return this.authorType != null && !this.authorType.isEmpty(); 892 } 893 894 /** 895 * @param value {@link #authorType} (The type of author of an organism species shall be specified. The parenthetical author of an organism species refers to the first author who published the plant/animal name (of any rank). The primary author of an organism species refers to the first author(s), who validly published the plant/animal name.) 896 */ 897 public SubstanceSourceMaterialOrganismAuthorComponent setAuthorType(CodeableConcept value) { 898 this.authorType = value; 899 return this; 900 } 901 902 /** 903 * @return {@link #authorDescription} (The author of an organism species shall be specified. The author year of an organism shall also be specified when applicable; refers to the year in which the first author(s) published the infraspecific plant/animal name (of any rank).). This is the underlying object with id, value and extensions. The accessor "getAuthorDescription" gives direct access to the value 904 */ 905 public StringType getAuthorDescriptionElement() { 906 if (this.authorDescription == null) 907 if (Configuration.errorOnAutoCreate()) 908 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismAuthorComponent.authorDescription"); 909 else if (Configuration.doAutoCreate()) 910 this.authorDescription = new StringType(); // bb 911 return this.authorDescription; 912 } 913 914 public boolean hasAuthorDescriptionElement() { 915 return this.authorDescription != null && !this.authorDescription.isEmpty(); 916 } 917 918 public boolean hasAuthorDescription() { 919 return this.authorDescription != null && !this.authorDescription.isEmpty(); 920 } 921 922 /** 923 * @param value {@link #authorDescription} (The author of an organism species shall be specified. The author year of an organism shall also be specified when applicable; refers to the year in which the first author(s) published the infraspecific plant/animal name (of any rank).). This is the underlying object with id, value and extensions. The accessor "getAuthorDescription" gives direct access to the value 924 */ 925 public SubstanceSourceMaterialOrganismAuthorComponent setAuthorDescriptionElement(StringType value) { 926 this.authorDescription = value; 927 return this; 928 } 929 930 /** 931 * @return The author of an organism species shall be specified. The author year of an organism shall also be specified when applicable; refers to the year in which the first author(s) published the infraspecific plant/animal name (of any rank). 932 */ 933 public String getAuthorDescription() { 934 return this.authorDescription == null ? null : this.authorDescription.getValue(); 935 } 936 937 /** 938 * @param value The author of an organism species shall be specified. The author year of an organism shall also be specified when applicable; refers to the year in which the first author(s) published the infraspecific plant/animal name (of any rank). 939 */ 940 public SubstanceSourceMaterialOrganismAuthorComponent setAuthorDescription(String value) { 941 if (Utilities.noString(value)) 942 this.authorDescription = null; 943 else { 944 if (this.authorDescription == null) 945 this.authorDescription = new StringType(); 946 this.authorDescription.setValue(value); 947 } 948 return this; 949 } 950 951 protected void listChildren(List<Property> children) { 952 super.listChildren(children); 953 children.add(new Property("authorType", "CodeableConcept", "The type of author of an organism species shall be specified. The parenthetical author of an organism species refers to the first author who published the plant/animal name (of any rank). The primary author of an organism species refers to the first author(s), who validly published the plant/animal name.", 0, 1, authorType)); 954 children.add(new Property("authorDescription", "string", "The author of an organism species shall be specified. The author year of an organism shall also be specified when applicable; refers to the year in which the first author(s) published the infraspecific plant/animal name (of any rank).", 0, 1, authorDescription)); 955 } 956 957 @Override 958 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 959 switch (_hash) { 960 case -1501337755: /*authorType*/ return new Property("authorType", "CodeableConcept", "The type of author of an organism species shall be specified. The parenthetical author of an organism species refers to the first author who published the plant/animal name (of any rank). The primary author of an organism species refers to the first author(s), who validly published the plant/animal name.", 0, 1, authorType); 961 case -166185615: /*authorDescription*/ return new Property("authorDescription", "string", "The author of an organism species shall be specified. The author year of an organism shall also be specified when applicable; refers to the year in which the first author(s) published the infraspecific plant/animal name (of any rank).", 0, 1, authorDescription); 962 default: return super.getNamedProperty(_hash, _name, _checkValid); 963 } 964 965 } 966 967 @Override 968 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 969 switch (hash) { 970 case -1501337755: /*authorType*/ return this.authorType == null ? new Base[0] : new Base[] {this.authorType}; // CodeableConcept 971 case -166185615: /*authorDescription*/ return this.authorDescription == null ? new Base[0] : new Base[] {this.authorDescription}; // StringType 972 default: return super.getProperty(hash, name, checkValid); 973 } 974 975 } 976 977 @Override 978 public Base setProperty(int hash, String name, Base value) throws FHIRException { 979 switch (hash) { 980 case -1501337755: // authorType 981 this.authorType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 982 return value; 983 case -166185615: // authorDescription 984 this.authorDescription = TypeConvertor.castToString(value); // StringType 985 return value; 986 default: return super.setProperty(hash, name, value); 987 } 988 989 } 990 991 @Override 992 public Base setProperty(String name, Base value) throws FHIRException { 993 if (name.equals("authorType")) { 994 this.authorType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 995 } else if (name.equals("authorDescription")) { 996 this.authorDescription = TypeConvertor.castToString(value); // StringType 997 } else 998 return super.setProperty(name, value); 999 return value; 1000 } 1001 1002 @Override 1003 public void removeChild(String name, Base value) throws FHIRException { 1004 if (name.equals("authorType")) { 1005 this.authorType = null; 1006 } else if (name.equals("authorDescription")) { 1007 this.authorDescription = null; 1008 } else 1009 super.removeChild(name, value); 1010 1011 } 1012 1013 @Override 1014 public Base makeProperty(int hash, String name) throws FHIRException { 1015 switch (hash) { 1016 case -1501337755: return getAuthorType(); 1017 case -166185615: return getAuthorDescriptionElement(); 1018 default: return super.makeProperty(hash, name); 1019 } 1020 1021 } 1022 1023 @Override 1024 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1025 switch (hash) { 1026 case -1501337755: /*authorType*/ return new String[] {"CodeableConcept"}; 1027 case -166185615: /*authorDescription*/ return new String[] {"string"}; 1028 default: return super.getTypesForProperty(hash, name); 1029 } 1030 1031 } 1032 1033 @Override 1034 public Base addChild(String name) throws FHIRException { 1035 if (name.equals("authorType")) { 1036 this.authorType = new CodeableConcept(); 1037 return this.authorType; 1038 } 1039 else if (name.equals("authorDescription")) { 1040 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSourceMaterial.organism.author.authorDescription"); 1041 } 1042 else 1043 return super.addChild(name); 1044 } 1045 1046 public SubstanceSourceMaterialOrganismAuthorComponent copy() { 1047 SubstanceSourceMaterialOrganismAuthorComponent dst = new SubstanceSourceMaterialOrganismAuthorComponent(); 1048 copyValues(dst); 1049 return dst; 1050 } 1051 1052 public void copyValues(SubstanceSourceMaterialOrganismAuthorComponent dst) { 1053 super.copyValues(dst); 1054 dst.authorType = authorType == null ? null : authorType.copy(); 1055 dst.authorDescription = authorDescription == null ? null : authorDescription.copy(); 1056 } 1057 1058 @Override 1059 public boolean equalsDeep(Base other_) { 1060 if (!super.equalsDeep(other_)) 1061 return false; 1062 if (!(other_ instanceof SubstanceSourceMaterialOrganismAuthorComponent)) 1063 return false; 1064 SubstanceSourceMaterialOrganismAuthorComponent o = (SubstanceSourceMaterialOrganismAuthorComponent) other_; 1065 return compareDeep(authorType, o.authorType, true) && compareDeep(authorDescription, o.authorDescription, true) 1066 ; 1067 } 1068 1069 @Override 1070 public boolean equalsShallow(Base other_) { 1071 if (!super.equalsShallow(other_)) 1072 return false; 1073 if (!(other_ instanceof SubstanceSourceMaterialOrganismAuthorComponent)) 1074 return false; 1075 SubstanceSourceMaterialOrganismAuthorComponent o = (SubstanceSourceMaterialOrganismAuthorComponent) other_; 1076 return compareValues(authorDescription, o.authorDescription, true); 1077 } 1078 1079 public boolean isEmpty() { 1080 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(authorType, authorDescription 1081 ); 1082 } 1083 1084 public String fhirType() { 1085 return "SubstanceSourceMaterial.organism.author"; 1086 1087 } 1088 1089 } 1090 1091 @Block() 1092 public static class SubstanceSourceMaterialOrganismHybridComponent extends BackboneElement implements IBaseBackboneElement { 1093 /** 1094 * The identifier of the maternal species constituting the hybrid organism shall be specified based on a controlled vocabulary. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal. 1095 */ 1096 @Child(name = "maternalOrganismId", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1097 @Description(shortDefinition="The identifier of the maternal species constituting the hybrid organism shall be specified based on a controlled vocabulary. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal", formalDefinition="The identifier of the maternal species constituting the hybrid organism shall be specified based on a controlled vocabulary. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal." ) 1098 protected StringType maternalOrganismId; 1099 1100 /** 1101 * The name of the maternal species constituting the hybrid organism shall be specified. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal. 1102 */ 1103 @Child(name = "maternalOrganismName", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1104 @Description(shortDefinition="The name of the maternal species constituting the hybrid organism shall be specified. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal", formalDefinition="The name of the maternal species constituting the hybrid organism shall be specified. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal." ) 1105 protected StringType maternalOrganismName; 1106 1107 /** 1108 * The identifier of the paternal species constituting the hybrid organism shall be specified based on a controlled vocabulary. 1109 */ 1110 @Child(name = "paternalOrganismId", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1111 @Description(shortDefinition="The identifier of the paternal species constituting the hybrid organism shall be specified based on a controlled vocabulary", formalDefinition="The identifier of the paternal species constituting the hybrid organism shall be specified based on a controlled vocabulary." ) 1112 protected StringType paternalOrganismId; 1113 1114 /** 1115 * The name of the paternal species constituting the hybrid organism shall be specified. 1116 */ 1117 @Child(name = "paternalOrganismName", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1118 @Description(shortDefinition="The name of the paternal species constituting the hybrid organism shall be specified", formalDefinition="The name of the paternal species constituting the hybrid organism shall be specified." ) 1119 protected StringType paternalOrganismName; 1120 1121 /** 1122 * The hybrid type of an organism shall be specified. 1123 */ 1124 @Child(name = "hybridType", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 1125 @Description(shortDefinition="The hybrid type of an organism shall be specified", formalDefinition="The hybrid type of an organism shall be specified." ) 1126 protected CodeableConcept hybridType; 1127 1128 private static final long serialVersionUID = 1981189787L; 1129 1130 /** 1131 * Constructor 1132 */ 1133 public SubstanceSourceMaterialOrganismHybridComponent() { 1134 super(); 1135 } 1136 1137 /** 1138 * @return {@link #maternalOrganismId} (The identifier of the maternal species constituting the hybrid organism shall be specified based on a controlled vocabulary. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal.). This is the underlying object with id, value and extensions. The accessor "getMaternalOrganismId" gives direct access to the value 1139 */ 1140 public StringType getMaternalOrganismIdElement() { 1141 if (this.maternalOrganismId == null) 1142 if (Configuration.errorOnAutoCreate()) 1143 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismHybridComponent.maternalOrganismId"); 1144 else if (Configuration.doAutoCreate()) 1145 this.maternalOrganismId = new StringType(); // bb 1146 return this.maternalOrganismId; 1147 } 1148 1149 public boolean hasMaternalOrganismIdElement() { 1150 return this.maternalOrganismId != null && !this.maternalOrganismId.isEmpty(); 1151 } 1152 1153 public boolean hasMaternalOrganismId() { 1154 return this.maternalOrganismId != null && !this.maternalOrganismId.isEmpty(); 1155 } 1156 1157 /** 1158 * @param value {@link #maternalOrganismId} (The identifier of the maternal species constituting the hybrid organism shall be specified based on a controlled vocabulary. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal.). This is the underlying object with id, value and extensions. The accessor "getMaternalOrganismId" gives direct access to the value 1159 */ 1160 public SubstanceSourceMaterialOrganismHybridComponent setMaternalOrganismIdElement(StringType value) { 1161 this.maternalOrganismId = value; 1162 return this; 1163 } 1164 1165 /** 1166 * @return The identifier of the maternal species constituting the hybrid organism shall be specified based on a controlled vocabulary. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal. 1167 */ 1168 public String getMaternalOrganismId() { 1169 return this.maternalOrganismId == null ? null : this.maternalOrganismId.getValue(); 1170 } 1171 1172 /** 1173 * @param value The identifier of the maternal species constituting the hybrid organism shall be specified based on a controlled vocabulary. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal. 1174 */ 1175 public SubstanceSourceMaterialOrganismHybridComponent setMaternalOrganismId(String value) { 1176 if (Utilities.noString(value)) 1177 this.maternalOrganismId = null; 1178 else { 1179 if (this.maternalOrganismId == null) 1180 this.maternalOrganismId = new StringType(); 1181 this.maternalOrganismId.setValue(value); 1182 } 1183 return this; 1184 } 1185 1186 /** 1187 * @return {@link #maternalOrganismName} (The name of the maternal species constituting the hybrid organism shall be specified. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal.). This is the underlying object with id, value and extensions. The accessor "getMaternalOrganismName" gives direct access to the value 1188 */ 1189 public StringType getMaternalOrganismNameElement() { 1190 if (this.maternalOrganismName == null) 1191 if (Configuration.errorOnAutoCreate()) 1192 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismHybridComponent.maternalOrganismName"); 1193 else if (Configuration.doAutoCreate()) 1194 this.maternalOrganismName = new StringType(); // bb 1195 return this.maternalOrganismName; 1196 } 1197 1198 public boolean hasMaternalOrganismNameElement() { 1199 return this.maternalOrganismName != null && !this.maternalOrganismName.isEmpty(); 1200 } 1201 1202 public boolean hasMaternalOrganismName() { 1203 return this.maternalOrganismName != null && !this.maternalOrganismName.isEmpty(); 1204 } 1205 1206 /** 1207 * @param value {@link #maternalOrganismName} (The name of the maternal species constituting the hybrid organism shall be specified. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal.). This is the underlying object with id, value and extensions. The accessor "getMaternalOrganismName" gives direct access to the value 1208 */ 1209 public SubstanceSourceMaterialOrganismHybridComponent setMaternalOrganismNameElement(StringType value) { 1210 this.maternalOrganismName = value; 1211 return this; 1212 } 1213 1214 /** 1215 * @return The name of the maternal species constituting the hybrid organism shall be specified. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal. 1216 */ 1217 public String getMaternalOrganismName() { 1218 return this.maternalOrganismName == null ? null : this.maternalOrganismName.getValue(); 1219 } 1220 1221 /** 1222 * @param value The name of the maternal species constituting the hybrid organism shall be specified. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal. 1223 */ 1224 public SubstanceSourceMaterialOrganismHybridComponent setMaternalOrganismName(String value) { 1225 if (Utilities.noString(value)) 1226 this.maternalOrganismName = null; 1227 else { 1228 if (this.maternalOrganismName == null) 1229 this.maternalOrganismName = new StringType(); 1230 this.maternalOrganismName.setValue(value); 1231 } 1232 return this; 1233 } 1234 1235 /** 1236 * @return {@link #paternalOrganismId} (The identifier of the paternal species constituting the hybrid organism shall be specified based on a controlled vocabulary.). This is the underlying object with id, value and extensions. The accessor "getPaternalOrganismId" gives direct access to the value 1237 */ 1238 public StringType getPaternalOrganismIdElement() { 1239 if (this.paternalOrganismId == null) 1240 if (Configuration.errorOnAutoCreate()) 1241 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismHybridComponent.paternalOrganismId"); 1242 else if (Configuration.doAutoCreate()) 1243 this.paternalOrganismId = new StringType(); // bb 1244 return this.paternalOrganismId; 1245 } 1246 1247 public boolean hasPaternalOrganismIdElement() { 1248 return this.paternalOrganismId != null && !this.paternalOrganismId.isEmpty(); 1249 } 1250 1251 public boolean hasPaternalOrganismId() { 1252 return this.paternalOrganismId != null && !this.paternalOrganismId.isEmpty(); 1253 } 1254 1255 /** 1256 * @param value {@link #paternalOrganismId} (The identifier of the paternal species constituting the hybrid organism shall be specified based on a controlled vocabulary.). This is the underlying object with id, value and extensions. The accessor "getPaternalOrganismId" gives direct access to the value 1257 */ 1258 public SubstanceSourceMaterialOrganismHybridComponent setPaternalOrganismIdElement(StringType value) { 1259 this.paternalOrganismId = value; 1260 return this; 1261 } 1262 1263 /** 1264 * @return The identifier of the paternal species constituting the hybrid organism shall be specified based on a controlled vocabulary. 1265 */ 1266 public String getPaternalOrganismId() { 1267 return this.paternalOrganismId == null ? null : this.paternalOrganismId.getValue(); 1268 } 1269 1270 /** 1271 * @param value The identifier of the paternal species constituting the hybrid organism shall be specified based on a controlled vocabulary. 1272 */ 1273 public SubstanceSourceMaterialOrganismHybridComponent setPaternalOrganismId(String value) { 1274 if (Utilities.noString(value)) 1275 this.paternalOrganismId = null; 1276 else { 1277 if (this.paternalOrganismId == null) 1278 this.paternalOrganismId = new StringType(); 1279 this.paternalOrganismId.setValue(value); 1280 } 1281 return this; 1282 } 1283 1284 /** 1285 * @return {@link #paternalOrganismName} (The name of the paternal species constituting the hybrid organism shall be specified.). This is the underlying object with id, value and extensions. The accessor "getPaternalOrganismName" gives direct access to the value 1286 */ 1287 public StringType getPaternalOrganismNameElement() { 1288 if (this.paternalOrganismName == null) 1289 if (Configuration.errorOnAutoCreate()) 1290 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismHybridComponent.paternalOrganismName"); 1291 else if (Configuration.doAutoCreate()) 1292 this.paternalOrganismName = new StringType(); // bb 1293 return this.paternalOrganismName; 1294 } 1295 1296 public boolean hasPaternalOrganismNameElement() { 1297 return this.paternalOrganismName != null && !this.paternalOrganismName.isEmpty(); 1298 } 1299 1300 public boolean hasPaternalOrganismName() { 1301 return this.paternalOrganismName != null && !this.paternalOrganismName.isEmpty(); 1302 } 1303 1304 /** 1305 * @param value {@link #paternalOrganismName} (The name of the paternal species constituting the hybrid organism shall be specified.). This is the underlying object with id, value and extensions. The accessor "getPaternalOrganismName" gives direct access to the value 1306 */ 1307 public SubstanceSourceMaterialOrganismHybridComponent setPaternalOrganismNameElement(StringType value) { 1308 this.paternalOrganismName = value; 1309 return this; 1310 } 1311 1312 /** 1313 * @return The name of the paternal species constituting the hybrid organism shall be specified. 1314 */ 1315 public String getPaternalOrganismName() { 1316 return this.paternalOrganismName == null ? null : this.paternalOrganismName.getValue(); 1317 } 1318 1319 /** 1320 * @param value The name of the paternal species constituting the hybrid organism shall be specified. 1321 */ 1322 public SubstanceSourceMaterialOrganismHybridComponent setPaternalOrganismName(String value) { 1323 if (Utilities.noString(value)) 1324 this.paternalOrganismName = null; 1325 else { 1326 if (this.paternalOrganismName == null) 1327 this.paternalOrganismName = new StringType(); 1328 this.paternalOrganismName.setValue(value); 1329 } 1330 return this; 1331 } 1332 1333 /** 1334 * @return {@link #hybridType} (The hybrid type of an organism shall be specified.) 1335 */ 1336 public CodeableConcept getHybridType() { 1337 if (this.hybridType == null) 1338 if (Configuration.errorOnAutoCreate()) 1339 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismHybridComponent.hybridType"); 1340 else if (Configuration.doAutoCreate()) 1341 this.hybridType = new CodeableConcept(); // cc 1342 return this.hybridType; 1343 } 1344 1345 public boolean hasHybridType() { 1346 return this.hybridType != null && !this.hybridType.isEmpty(); 1347 } 1348 1349 /** 1350 * @param value {@link #hybridType} (The hybrid type of an organism shall be specified.) 1351 */ 1352 public SubstanceSourceMaterialOrganismHybridComponent setHybridType(CodeableConcept value) { 1353 this.hybridType = value; 1354 return this; 1355 } 1356 1357 protected void listChildren(List<Property> children) { 1358 super.listChildren(children); 1359 children.add(new Property("maternalOrganismId", "string", "The identifier of the maternal species constituting the hybrid organism shall be specified based on a controlled vocabulary. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal.", 0, 1, maternalOrganismId)); 1360 children.add(new Property("maternalOrganismName", "string", "The name of the maternal species constituting the hybrid organism shall be specified. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal.", 0, 1, maternalOrganismName)); 1361 children.add(new Property("paternalOrganismId", "string", "The identifier of the paternal species constituting the hybrid organism shall be specified based on a controlled vocabulary.", 0, 1, paternalOrganismId)); 1362 children.add(new Property("paternalOrganismName", "string", "The name of the paternal species constituting the hybrid organism shall be specified.", 0, 1, paternalOrganismName)); 1363 children.add(new Property("hybridType", "CodeableConcept", "The hybrid type of an organism shall be specified.", 0, 1, hybridType)); 1364 } 1365 1366 @Override 1367 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1368 switch (_hash) { 1369 case -1179977063: /*maternalOrganismId*/ return new Property("maternalOrganismId", "string", "The identifier of the maternal species constituting the hybrid organism shall be specified based on a controlled vocabulary. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal.", 0, 1, maternalOrganismId); 1370 case -86441847: /*maternalOrganismName*/ return new Property("maternalOrganismName", "string", "The name of the maternal species constituting the hybrid organism shall be specified. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal.", 0, 1, maternalOrganismName); 1371 case 123773174: /*paternalOrganismId*/ return new Property("paternalOrganismId", "string", "The identifier of the paternal species constituting the hybrid organism shall be specified based on a controlled vocabulary.", 0, 1, paternalOrganismId); 1372 case -1312914522: /*paternalOrganismName*/ return new Property("paternalOrganismName", "string", "The name of the paternal species constituting the hybrid organism shall be specified.", 0, 1, paternalOrganismName); 1373 case 1572734806: /*hybridType*/ return new Property("hybridType", "CodeableConcept", "The hybrid type of an organism shall be specified.", 0, 1, hybridType); 1374 default: return super.getNamedProperty(_hash, _name, _checkValid); 1375 } 1376 1377 } 1378 1379 @Override 1380 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1381 switch (hash) { 1382 case -1179977063: /*maternalOrganismId*/ return this.maternalOrganismId == null ? new Base[0] : new Base[] {this.maternalOrganismId}; // StringType 1383 case -86441847: /*maternalOrganismName*/ return this.maternalOrganismName == null ? new Base[0] : new Base[] {this.maternalOrganismName}; // StringType 1384 case 123773174: /*paternalOrganismId*/ return this.paternalOrganismId == null ? new Base[0] : new Base[] {this.paternalOrganismId}; // StringType 1385 case -1312914522: /*paternalOrganismName*/ return this.paternalOrganismName == null ? new Base[0] : new Base[] {this.paternalOrganismName}; // StringType 1386 case 1572734806: /*hybridType*/ return this.hybridType == null ? new Base[0] : new Base[] {this.hybridType}; // CodeableConcept 1387 default: return super.getProperty(hash, name, checkValid); 1388 } 1389 1390 } 1391 1392 @Override 1393 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1394 switch (hash) { 1395 case -1179977063: // maternalOrganismId 1396 this.maternalOrganismId = TypeConvertor.castToString(value); // StringType 1397 return value; 1398 case -86441847: // maternalOrganismName 1399 this.maternalOrganismName = TypeConvertor.castToString(value); // StringType 1400 return value; 1401 case 123773174: // paternalOrganismId 1402 this.paternalOrganismId = TypeConvertor.castToString(value); // StringType 1403 return value; 1404 case -1312914522: // paternalOrganismName 1405 this.paternalOrganismName = TypeConvertor.castToString(value); // StringType 1406 return value; 1407 case 1572734806: // hybridType 1408 this.hybridType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1409 return value; 1410 default: return super.setProperty(hash, name, value); 1411 } 1412 1413 } 1414 1415 @Override 1416 public Base setProperty(String name, Base value) throws FHIRException { 1417 if (name.equals("maternalOrganismId")) { 1418 this.maternalOrganismId = TypeConvertor.castToString(value); // StringType 1419 } else if (name.equals("maternalOrganismName")) { 1420 this.maternalOrganismName = TypeConvertor.castToString(value); // StringType 1421 } else if (name.equals("paternalOrganismId")) { 1422 this.paternalOrganismId = TypeConvertor.castToString(value); // StringType 1423 } else if (name.equals("paternalOrganismName")) { 1424 this.paternalOrganismName = TypeConvertor.castToString(value); // StringType 1425 } else if (name.equals("hybridType")) { 1426 this.hybridType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1427 } else 1428 return super.setProperty(name, value); 1429 return value; 1430 } 1431 1432 @Override 1433 public void removeChild(String name, Base value) throws FHIRException { 1434 if (name.equals("maternalOrganismId")) { 1435 this.maternalOrganismId = null; 1436 } else if (name.equals("maternalOrganismName")) { 1437 this.maternalOrganismName = null; 1438 } else if (name.equals("paternalOrganismId")) { 1439 this.paternalOrganismId = null; 1440 } else if (name.equals("paternalOrganismName")) { 1441 this.paternalOrganismName = null; 1442 } else if (name.equals("hybridType")) { 1443 this.hybridType = null; 1444 } else 1445 super.removeChild(name, value); 1446 1447 } 1448 1449 @Override 1450 public Base makeProperty(int hash, String name) throws FHIRException { 1451 switch (hash) { 1452 case -1179977063: return getMaternalOrganismIdElement(); 1453 case -86441847: return getMaternalOrganismNameElement(); 1454 case 123773174: return getPaternalOrganismIdElement(); 1455 case -1312914522: return getPaternalOrganismNameElement(); 1456 case 1572734806: return getHybridType(); 1457 default: return super.makeProperty(hash, name); 1458 } 1459 1460 } 1461 1462 @Override 1463 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1464 switch (hash) { 1465 case -1179977063: /*maternalOrganismId*/ return new String[] {"string"}; 1466 case -86441847: /*maternalOrganismName*/ return new String[] {"string"}; 1467 case 123773174: /*paternalOrganismId*/ return new String[] {"string"}; 1468 case -1312914522: /*paternalOrganismName*/ return new String[] {"string"}; 1469 case 1572734806: /*hybridType*/ return new String[] {"CodeableConcept"}; 1470 default: return super.getTypesForProperty(hash, name); 1471 } 1472 1473 } 1474 1475 @Override 1476 public Base addChild(String name) throws FHIRException { 1477 if (name.equals("maternalOrganismId")) { 1478 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSourceMaterial.organism.hybrid.maternalOrganismId"); 1479 } 1480 else if (name.equals("maternalOrganismName")) { 1481 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSourceMaterial.organism.hybrid.maternalOrganismName"); 1482 } 1483 else if (name.equals("paternalOrganismId")) { 1484 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSourceMaterial.organism.hybrid.paternalOrganismId"); 1485 } 1486 else if (name.equals("paternalOrganismName")) { 1487 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSourceMaterial.organism.hybrid.paternalOrganismName"); 1488 } 1489 else if (name.equals("hybridType")) { 1490 this.hybridType = new CodeableConcept(); 1491 return this.hybridType; 1492 } 1493 else 1494 return super.addChild(name); 1495 } 1496 1497 public SubstanceSourceMaterialOrganismHybridComponent copy() { 1498 SubstanceSourceMaterialOrganismHybridComponent dst = new SubstanceSourceMaterialOrganismHybridComponent(); 1499 copyValues(dst); 1500 return dst; 1501 } 1502 1503 public void copyValues(SubstanceSourceMaterialOrganismHybridComponent dst) { 1504 super.copyValues(dst); 1505 dst.maternalOrganismId = maternalOrganismId == null ? null : maternalOrganismId.copy(); 1506 dst.maternalOrganismName = maternalOrganismName == null ? null : maternalOrganismName.copy(); 1507 dst.paternalOrganismId = paternalOrganismId == null ? null : paternalOrganismId.copy(); 1508 dst.paternalOrganismName = paternalOrganismName == null ? null : paternalOrganismName.copy(); 1509 dst.hybridType = hybridType == null ? null : hybridType.copy(); 1510 } 1511 1512 @Override 1513 public boolean equalsDeep(Base other_) { 1514 if (!super.equalsDeep(other_)) 1515 return false; 1516 if (!(other_ instanceof SubstanceSourceMaterialOrganismHybridComponent)) 1517 return false; 1518 SubstanceSourceMaterialOrganismHybridComponent o = (SubstanceSourceMaterialOrganismHybridComponent) other_; 1519 return compareDeep(maternalOrganismId, o.maternalOrganismId, true) && compareDeep(maternalOrganismName, o.maternalOrganismName, true) 1520 && compareDeep(paternalOrganismId, o.paternalOrganismId, true) && compareDeep(paternalOrganismName, o.paternalOrganismName, true) 1521 && compareDeep(hybridType, o.hybridType, true); 1522 } 1523 1524 @Override 1525 public boolean equalsShallow(Base other_) { 1526 if (!super.equalsShallow(other_)) 1527 return false; 1528 if (!(other_ instanceof SubstanceSourceMaterialOrganismHybridComponent)) 1529 return false; 1530 SubstanceSourceMaterialOrganismHybridComponent o = (SubstanceSourceMaterialOrganismHybridComponent) other_; 1531 return compareValues(maternalOrganismId, o.maternalOrganismId, true) && compareValues(maternalOrganismName, o.maternalOrganismName, true) 1532 && compareValues(paternalOrganismId, o.paternalOrganismId, true) && compareValues(paternalOrganismName, o.paternalOrganismName, true) 1533 ; 1534 } 1535 1536 public boolean isEmpty() { 1537 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(maternalOrganismId, maternalOrganismName 1538 , paternalOrganismId, paternalOrganismName, hybridType); 1539 } 1540 1541 public String fhirType() { 1542 return "SubstanceSourceMaterial.organism.hybrid"; 1543 1544 } 1545 1546 } 1547 1548 @Block() 1549 public static class SubstanceSourceMaterialOrganismOrganismGeneralComponent extends BackboneElement implements IBaseBackboneElement { 1550 /** 1551 * The kingdom of an organism shall be specified. 1552 */ 1553 @Child(name = "kingdom", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 1554 @Description(shortDefinition="The kingdom of an organism shall be specified", formalDefinition="The kingdom of an organism shall be specified." ) 1555 protected CodeableConcept kingdom; 1556 1557 /** 1558 * The phylum of an organism shall be specified. 1559 */ 1560 @Child(name = "phylum", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 1561 @Description(shortDefinition="The phylum of an organism shall be specified", formalDefinition="The phylum of an organism shall be specified." ) 1562 protected CodeableConcept phylum; 1563 1564 /** 1565 * The class of an organism shall be specified. 1566 */ 1567 @Child(name = "class", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 1568 @Description(shortDefinition="The class of an organism shall be specified", formalDefinition="The class of an organism shall be specified." ) 1569 protected CodeableConcept class_; 1570 1571 /** 1572 * The order of an organism shall be specified,. 1573 */ 1574 @Child(name = "order", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 1575 @Description(shortDefinition="The order of an organism shall be specified,", formalDefinition="The order of an organism shall be specified,." ) 1576 protected CodeableConcept order; 1577 1578 private static final long serialVersionUID = 659838613L; 1579 1580 /** 1581 * Constructor 1582 */ 1583 public SubstanceSourceMaterialOrganismOrganismGeneralComponent() { 1584 super(); 1585 } 1586 1587 /** 1588 * @return {@link #kingdom} (The kingdom of an organism shall be specified.) 1589 */ 1590 public CodeableConcept getKingdom() { 1591 if (this.kingdom == null) 1592 if (Configuration.errorOnAutoCreate()) 1593 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismOrganismGeneralComponent.kingdom"); 1594 else if (Configuration.doAutoCreate()) 1595 this.kingdom = new CodeableConcept(); // cc 1596 return this.kingdom; 1597 } 1598 1599 public boolean hasKingdom() { 1600 return this.kingdom != null && !this.kingdom.isEmpty(); 1601 } 1602 1603 /** 1604 * @param value {@link #kingdom} (The kingdom of an organism shall be specified.) 1605 */ 1606 public SubstanceSourceMaterialOrganismOrganismGeneralComponent setKingdom(CodeableConcept value) { 1607 this.kingdom = value; 1608 return this; 1609 } 1610 1611 /** 1612 * @return {@link #phylum} (The phylum of an organism shall be specified.) 1613 */ 1614 public CodeableConcept getPhylum() { 1615 if (this.phylum == null) 1616 if (Configuration.errorOnAutoCreate()) 1617 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismOrganismGeneralComponent.phylum"); 1618 else if (Configuration.doAutoCreate()) 1619 this.phylum = new CodeableConcept(); // cc 1620 return this.phylum; 1621 } 1622 1623 public boolean hasPhylum() { 1624 return this.phylum != null && !this.phylum.isEmpty(); 1625 } 1626 1627 /** 1628 * @param value {@link #phylum} (The phylum of an organism shall be specified.) 1629 */ 1630 public SubstanceSourceMaterialOrganismOrganismGeneralComponent setPhylum(CodeableConcept value) { 1631 this.phylum = value; 1632 return this; 1633 } 1634 1635 /** 1636 * @return {@link #class_} (The class of an organism shall be specified.) 1637 */ 1638 public CodeableConcept getClass_() { 1639 if (this.class_ == null) 1640 if (Configuration.errorOnAutoCreate()) 1641 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismOrganismGeneralComponent.class_"); 1642 else if (Configuration.doAutoCreate()) 1643 this.class_ = new CodeableConcept(); // cc 1644 return this.class_; 1645 } 1646 1647 public boolean hasClass_() { 1648 return this.class_ != null && !this.class_.isEmpty(); 1649 } 1650 1651 /** 1652 * @param value {@link #class_} (The class of an organism shall be specified.) 1653 */ 1654 public SubstanceSourceMaterialOrganismOrganismGeneralComponent setClass_(CodeableConcept value) { 1655 this.class_ = value; 1656 return this; 1657 } 1658 1659 /** 1660 * @return {@link #order} (The order of an organism shall be specified,.) 1661 */ 1662 public CodeableConcept getOrder() { 1663 if (this.order == null) 1664 if (Configuration.errorOnAutoCreate()) 1665 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismOrganismGeneralComponent.order"); 1666 else if (Configuration.doAutoCreate()) 1667 this.order = new CodeableConcept(); // cc 1668 return this.order; 1669 } 1670 1671 public boolean hasOrder() { 1672 return this.order != null && !this.order.isEmpty(); 1673 } 1674 1675 /** 1676 * @param value {@link #order} (The order of an organism shall be specified,.) 1677 */ 1678 public SubstanceSourceMaterialOrganismOrganismGeneralComponent setOrder(CodeableConcept value) { 1679 this.order = value; 1680 return this; 1681 } 1682 1683 protected void listChildren(List<Property> children) { 1684 super.listChildren(children); 1685 children.add(new Property("kingdom", "CodeableConcept", "The kingdom of an organism shall be specified.", 0, 1, kingdom)); 1686 children.add(new Property("phylum", "CodeableConcept", "The phylum of an organism shall be specified.", 0, 1, phylum)); 1687 children.add(new Property("class", "CodeableConcept", "The class of an organism shall be specified.", 0, 1, class_)); 1688 children.add(new Property("order", "CodeableConcept", "The order of an organism shall be specified,.", 0, 1, order)); 1689 } 1690 1691 @Override 1692 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1693 switch (_hash) { 1694 case -710537653: /*kingdom*/ return new Property("kingdom", "CodeableConcept", "The kingdom of an organism shall be specified.", 0, 1, kingdom); 1695 case -988743965: /*phylum*/ return new Property("phylum", "CodeableConcept", "The phylum of an organism shall be specified.", 0, 1, phylum); 1696 case 94742904: /*class*/ return new Property("class", "CodeableConcept", "The class of an organism shall be specified.", 0, 1, class_); 1697 case 106006350: /*order*/ return new Property("order", "CodeableConcept", "The order of an organism shall be specified,.", 0, 1, order); 1698 default: return super.getNamedProperty(_hash, _name, _checkValid); 1699 } 1700 1701 } 1702 1703 @Override 1704 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1705 switch (hash) { 1706 case -710537653: /*kingdom*/ return this.kingdom == null ? new Base[0] : new Base[] {this.kingdom}; // CodeableConcept 1707 case -988743965: /*phylum*/ return this.phylum == null ? new Base[0] : new Base[] {this.phylum}; // CodeableConcept 1708 case 94742904: /*class*/ return this.class_ == null ? new Base[0] : new Base[] {this.class_}; // CodeableConcept 1709 case 106006350: /*order*/ return this.order == null ? new Base[0] : new Base[] {this.order}; // CodeableConcept 1710 default: return super.getProperty(hash, name, checkValid); 1711 } 1712 1713 } 1714 1715 @Override 1716 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1717 switch (hash) { 1718 case -710537653: // kingdom 1719 this.kingdom = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1720 return value; 1721 case -988743965: // phylum 1722 this.phylum = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1723 return value; 1724 case 94742904: // class 1725 this.class_ = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1726 return value; 1727 case 106006350: // order 1728 this.order = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1729 return value; 1730 default: return super.setProperty(hash, name, value); 1731 } 1732 1733 } 1734 1735 @Override 1736 public Base setProperty(String name, Base value) throws FHIRException { 1737 if (name.equals("kingdom")) { 1738 this.kingdom = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1739 } else if (name.equals("phylum")) { 1740 this.phylum = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1741 } else if (name.equals("class")) { 1742 this.class_ = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1743 } else if (name.equals("order")) { 1744 this.order = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1745 } else 1746 return super.setProperty(name, value); 1747 return value; 1748 } 1749 1750 @Override 1751 public void removeChild(String name, Base value) throws FHIRException { 1752 if (name.equals("kingdom")) { 1753 this.kingdom = null; 1754 } else if (name.equals("phylum")) { 1755 this.phylum = null; 1756 } else if (name.equals("class")) { 1757 this.class_ = null; 1758 } else if (name.equals("order")) { 1759 this.order = null; 1760 } else 1761 super.removeChild(name, value); 1762 1763 } 1764 1765 @Override 1766 public Base makeProperty(int hash, String name) throws FHIRException { 1767 switch (hash) { 1768 case -710537653: return getKingdom(); 1769 case -988743965: return getPhylum(); 1770 case 94742904: return getClass_(); 1771 case 106006350: return getOrder(); 1772 default: return super.makeProperty(hash, name); 1773 } 1774 1775 } 1776 1777 @Override 1778 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1779 switch (hash) { 1780 case -710537653: /*kingdom*/ return new String[] {"CodeableConcept"}; 1781 case -988743965: /*phylum*/ return new String[] {"CodeableConcept"}; 1782 case 94742904: /*class*/ return new String[] {"CodeableConcept"}; 1783 case 106006350: /*order*/ return new String[] {"CodeableConcept"}; 1784 default: return super.getTypesForProperty(hash, name); 1785 } 1786 1787 } 1788 1789 @Override 1790 public Base addChild(String name) throws FHIRException { 1791 if (name.equals("kingdom")) { 1792 this.kingdom = new CodeableConcept(); 1793 return this.kingdom; 1794 } 1795 else if (name.equals("phylum")) { 1796 this.phylum = new CodeableConcept(); 1797 return this.phylum; 1798 } 1799 else if (name.equals("class")) { 1800 this.class_ = new CodeableConcept(); 1801 return this.class_; 1802 } 1803 else if (name.equals("order")) { 1804 this.order = new CodeableConcept(); 1805 return this.order; 1806 } 1807 else 1808 return super.addChild(name); 1809 } 1810 1811 public SubstanceSourceMaterialOrganismOrganismGeneralComponent copy() { 1812 SubstanceSourceMaterialOrganismOrganismGeneralComponent dst = new SubstanceSourceMaterialOrganismOrganismGeneralComponent(); 1813 copyValues(dst); 1814 return dst; 1815 } 1816 1817 public void copyValues(SubstanceSourceMaterialOrganismOrganismGeneralComponent dst) { 1818 super.copyValues(dst); 1819 dst.kingdom = kingdom == null ? null : kingdom.copy(); 1820 dst.phylum = phylum == null ? null : phylum.copy(); 1821 dst.class_ = class_ == null ? null : class_.copy(); 1822 dst.order = order == null ? null : order.copy(); 1823 } 1824 1825 @Override 1826 public boolean equalsDeep(Base other_) { 1827 if (!super.equalsDeep(other_)) 1828 return false; 1829 if (!(other_ instanceof SubstanceSourceMaterialOrganismOrganismGeneralComponent)) 1830 return false; 1831 SubstanceSourceMaterialOrganismOrganismGeneralComponent o = (SubstanceSourceMaterialOrganismOrganismGeneralComponent) other_; 1832 return compareDeep(kingdom, o.kingdom, true) && compareDeep(phylum, o.phylum, true) && compareDeep(class_, o.class_, true) 1833 && compareDeep(order, o.order, true); 1834 } 1835 1836 @Override 1837 public boolean equalsShallow(Base other_) { 1838 if (!super.equalsShallow(other_)) 1839 return false; 1840 if (!(other_ instanceof SubstanceSourceMaterialOrganismOrganismGeneralComponent)) 1841 return false; 1842 SubstanceSourceMaterialOrganismOrganismGeneralComponent o = (SubstanceSourceMaterialOrganismOrganismGeneralComponent) other_; 1843 return true; 1844 } 1845 1846 public boolean isEmpty() { 1847 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(kingdom, phylum, class_ 1848 , order); 1849 } 1850 1851 public String fhirType() { 1852 return "SubstanceSourceMaterial.organism.organismGeneral"; 1853 1854 } 1855 1856 } 1857 1858 @Block() 1859 public static class SubstanceSourceMaterialPartDescriptionComponent extends BackboneElement implements IBaseBackboneElement { 1860 /** 1861 * Entity of anatomical origin of source material within an organism. 1862 */ 1863 @Child(name = "part", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 1864 @Description(shortDefinition="Entity of anatomical origin of source material within an organism", formalDefinition="Entity of anatomical origin of source material within an organism." ) 1865 protected CodeableConcept part; 1866 1867 /** 1868 * The detailed anatomic location when the part can be extracted from different anatomical locations of the organism. Multiple alternative locations may apply. 1869 */ 1870 @Child(name = "partLocation", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 1871 @Description(shortDefinition="The detailed anatomic location when the part can be extracted from different anatomical locations of the organism. Multiple alternative locations may apply", formalDefinition="The detailed anatomic location when the part can be extracted from different anatomical locations of the organism. Multiple alternative locations may apply." ) 1872 protected CodeableConcept partLocation; 1873 1874 private static final long serialVersionUID = 308875915L; 1875 1876 /** 1877 * Constructor 1878 */ 1879 public SubstanceSourceMaterialPartDescriptionComponent() { 1880 super(); 1881 } 1882 1883 /** 1884 * @return {@link #part} (Entity of anatomical origin of source material within an organism.) 1885 */ 1886 public CodeableConcept getPart() { 1887 if (this.part == null) 1888 if (Configuration.errorOnAutoCreate()) 1889 throw new Error("Attempt to auto-create SubstanceSourceMaterialPartDescriptionComponent.part"); 1890 else if (Configuration.doAutoCreate()) 1891 this.part = new CodeableConcept(); // cc 1892 return this.part; 1893 } 1894 1895 public boolean hasPart() { 1896 return this.part != null && !this.part.isEmpty(); 1897 } 1898 1899 /** 1900 * @param value {@link #part} (Entity of anatomical origin of source material within an organism.) 1901 */ 1902 public SubstanceSourceMaterialPartDescriptionComponent setPart(CodeableConcept value) { 1903 this.part = value; 1904 return this; 1905 } 1906 1907 /** 1908 * @return {@link #partLocation} (The detailed anatomic location when the part can be extracted from different anatomical locations of the organism. Multiple alternative locations may apply.) 1909 */ 1910 public CodeableConcept getPartLocation() { 1911 if (this.partLocation == null) 1912 if (Configuration.errorOnAutoCreate()) 1913 throw new Error("Attempt to auto-create SubstanceSourceMaterialPartDescriptionComponent.partLocation"); 1914 else if (Configuration.doAutoCreate()) 1915 this.partLocation = new CodeableConcept(); // cc 1916 return this.partLocation; 1917 } 1918 1919 public boolean hasPartLocation() { 1920 return this.partLocation != null && !this.partLocation.isEmpty(); 1921 } 1922 1923 /** 1924 * @param value {@link #partLocation} (The detailed anatomic location when the part can be extracted from different anatomical locations of the organism. Multiple alternative locations may apply.) 1925 */ 1926 public SubstanceSourceMaterialPartDescriptionComponent setPartLocation(CodeableConcept value) { 1927 this.partLocation = value; 1928 return this; 1929 } 1930 1931 protected void listChildren(List<Property> children) { 1932 super.listChildren(children); 1933 children.add(new Property("part", "CodeableConcept", "Entity of anatomical origin of source material within an organism.", 0, 1, part)); 1934 children.add(new Property("partLocation", "CodeableConcept", "The detailed anatomic location when the part can be extracted from different anatomical locations of the organism. Multiple alternative locations may apply.", 0, 1, partLocation)); 1935 } 1936 1937 @Override 1938 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1939 switch (_hash) { 1940 case 3433459: /*part*/ return new Property("part", "CodeableConcept", "Entity of anatomical origin of source material within an organism.", 0, 1, part); 1941 case 893437128: /*partLocation*/ return new Property("partLocation", "CodeableConcept", "The detailed anatomic location when the part can be extracted from different anatomical locations of the organism. Multiple alternative locations may apply.", 0, 1, partLocation); 1942 default: return super.getNamedProperty(_hash, _name, _checkValid); 1943 } 1944 1945 } 1946 1947 @Override 1948 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1949 switch (hash) { 1950 case 3433459: /*part*/ return this.part == null ? new Base[0] : new Base[] {this.part}; // CodeableConcept 1951 case 893437128: /*partLocation*/ return this.partLocation == null ? new Base[0] : new Base[] {this.partLocation}; // CodeableConcept 1952 default: return super.getProperty(hash, name, checkValid); 1953 } 1954 1955 } 1956 1957 @Override 1958 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1959 switch (hash) { 1960 case 3433459: // part 1961 this.part = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1962 return value; 1963 case 893437128: // partLocation 1964 this.partLocation = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1965 return value; 1966 default: return super.setProperty(hash, name, value); 1967 } 1968 1969 } 1970 1971 @Override 1972 public Base setProperty(String name, Base value) throws FHIRException { 1973 if (name.equals("part")) { 1974 this.part = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1975 } else if (name.equals("partLocation")) { 1976 this.partLocation = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1977 } else 1978 return super.setProperty(name, value); 1979 return value; 1980 } 1981 1982 @Override 1983 public void removeChild(String name, Base value) throws FHIRException { 1984 if (name.equals("part")) { 1985 this.part = null; 1986 } else if (name.equals("partLocation")) { 1987 this.partLocation = null; 1988 } else 1989 super.removeChild(name, value); 1990 1991 } 1992 1993 @Override 1994 public Base makeProperty(int hash, String name) throws FHIRException { 1995 switch (hash) { 1996 case 3433459: return getPart(); 1997 case 893437128: return getPartLocation(); 1998 default: return super.makeProperty(hash, name); 1999 } 2000 2001 } 2002 2003 @Override 2004 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2005 switch (hash) { 2006 case 3433459: /*part*/ return new String[] {"CodeableConcept"}; 2007 case 893437128: /*partLocation*/ return new String[] {"CodeableConcept"}; 2008 default: return super.getTypesForProperty(hash, name); 2009 } 2010 2011 } 2012 2013 @Override 2014 public Base addChild(String name) throws FHIRException { 2015 if (name.equals("part")) { 2016 this.part = new CodeableConcept(); 2017 return this.part; 2018 } 2019 else if (name.equals("partLocation")) { 2020 this.partLocation = new CodeableConcept(); 2021 return this.partLocation; 2022 } 2023 else 2024 return super.addChild(name); 2025 } 2026 2027 public SubstanceSourceMaterialPartDescriptionComponent copy() { 2028 SubstanceSourceMaterialPartDescriptionComponent dst = new SubstanceSourceMaterialPartDescriptionComponent(); 2029 copyValues(dst); 2030 return dst; 2031 } 2032 2033 public void copyValues(SubstanceSourceMaterialPartDescriptionComponent dst) { 2034 super.copyValues(dst); 2035 dst.part = part == null ? null : part.copy(); 2036 dst.partLocation = partLocation == null ? null : partLocation.copy(); 2037 } 2038 2039 @Override 2040 public boolean equalsDeep(Base other_) { 2041 if (!super.equalsDeep(other_)) 2042 return false; 2043 if (!(other_ instanceof SubstanceSourceMaterialPartDescriptionComponent)) 2044 return false; 2045 SubstanceSourceMaterialPartDescriptionComponent o = (SubstanceSourceMaterialPartDescriptionComponent) other_; 2046 return compareDeep(part, o.part, true) && compareDeep(partLocation, o.partLocation, true); 2047 } 2048 2049 @Override 2050 public boolean equalsShallow(Base other_) { 2051 if (!super.equalsShallow(other_)) 2052 return false; 2053 if (!(other_ instanceof SubstanceSourceMaterialPartDescriptionComponent)) 2054 return false; 2055 SubstanceSourceMaterialPartDescriptionComponent o = (SubstanceSourceMaterialPartDescriptionComponent) other_; 2056 return true; 2057 } 2058 2059 public boolean isEmpty() { 2060 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(part, partLocation); 2061 } 2062 2063 public String fhirType() { 2064 return "SubstanceSourceMaterial.partDescription"; 2065 2066 } 2067 2068 } 2069 2070 /** 2071 * General high level classification of the source material specific to the origin of the material. 2072 */ 2073 @Child(name = "sourceMaterialClass", type = {CodeableConcept.class}, order=0, min=0, max=1, modifier=false, summary=true) 2074 @Description(shortDefinition="General high level classification of the source material specific to the origin of the material", formalDefinition="General high level classification of the source material specific to the origin of the material." ) 2075 protected CodeableConcept sourceMaterialClass; 2076 2077 /** 2078 * The type of the source material shall be specified based on a controlled vocabulary. For vaccines, this subclause refers to the class of infectious agent. 2079 */ 2080 @Child(name = "sourceMaterialType", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 2081 @Description(shortDefinition="The type of the source material shall be specified based on a controlled vocabulary. For vaccines, this subclause refers to the class of infectious agent", formalDefinition="The type of the source material shall be specified based on a controlled vocabulary. For vaccines, this subclause refers to the class of infectious agent." ) 2082 protected CodeableConcept sourceMaterialType; 2083 2084 /** 2085 * The state of the source material when extracted. 2086 */ 2087 @Child(name = "sourceMaterialState", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 2088 @Description(shortDefinition="The state of the source material when extracted", formalDefinition="The state of the source material when extracted." ) 2089 protected CodeableConcept sourceMaterialState; 2090 2091 /** 2092 * The unique identifier associated with the source material parent organism shall be specified. 2093 */ 2094 @Child(name = "organismId", type = {Identifier.class}, order=3, min=0, max=1, modifier=false, summary=true) 2095 @Description(shortDefinition="The unique identifier associated with the source material parent organism shall be specified", formalDefinition="The unique identifier associated with the source material parent organism shall be specified." ) 2096 protected Identifier organismId; 2097 2098 /** 2099 * The organism accepted Scientific name shall be provided based on the organism taxonomy. 2100 */ 2101 @Child(name = "organismName", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 2102 @Description(shortDefinition="The organism accepted Scientific name shall be provided based on the organism taxonomy", formalDefinition="The organism accepted Scientific name shall be provided based on the organism taxonomy." ) 2103 protected StringType organismName; 2104 2105 /** 2106 * The parent of the herbal drug Ginkgo biloba, Leaf is the substance ID of the substance (fresh) of Ginkgo biloba L. or Ginkgo biloba L. (Whole plant). 2107 */ 2108 @Child(name = "parentSubstanceId", type = {Identifier.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2109 @Description(shortDefinition="The parent of the herbal drug Ginkgo biloba, Leaf is the substance ID of the substance (fresh) of Ginkgo biloba L. or Ginkgo biloba L. (Whole plant)", formalDefinition="The parent of the herbal drug Ginkgo biloba, Leaf is the substance ID of the substance (fresh) of Ginkgo biloba L. or Ginkgo biloba L. (Whole plant)." ) 2110 protected List<Identifier> parentSubstanceId; 2111 2112 /** 2113 * The parent substance of the Herbal Drug, or Herbal preparation. 2114 */ 2115 @Child(name = "parentSubstanceName", type = {StringType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2116 @Description(shortDefinition="The parent substance of the Herbal Drug, or Herbal preparation", formalDefinition="The parent substance of the Herbal Drug, or Herbal preparation." ) 2117 protected List<StringType> parentSubstanceName; 2118 2119 /** 2120 * The country where the plant material is harvested or the countries where the plasma is sourced from as laid down in accordance with the Plasma Master File. For ?Plasma-derived substances? the attribute country of origin provides information about the countries used for the manufacturing of the Cryopoor plama or Crioprecipitate. 2121 */ 2122 @Child(name = "countryOfOrigin", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2123 @Description(shortDefinition="The country where the plant material is harvested or the countries where the plasma is sourced from as laid down in accordance with the Plasma Master File. For ?Plasma-derived substances? the attribute country of origin provides information about the countries used for the manufacturing of the Cryopoor plama or Crioprecipitate", formalDefinition="The country where the plant material is harvested or the countries where the plasma is sourced from as laid down in accordance with the Plasma Master File. For ?Plasma-derived substances? the attribute country of origin provides information about the countries used for the manufacturing of the Cryopoor plama or Crioprecipitate." ) 2124 protected List<CodeableConcept> countryOfOrigin; 2125 2126 /** 2127 * The place/region where the plant is harvested or the places/regions where the animal source material has its habitat. 2128 */ 2129 @Child(name = "geographicalLocation", type = {StringType.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2130 @Description(shortDefinition="The place/region where the plant is harvested or the places/regions where the animal source material has its habitat", formalDefinition="The place/region where the plant is harvested or the places/regions where the animal source material has its habitat." ) 2131 protected List<StringType> geographicalLocation; 2132 2133 /** 2134 * Stage of life for animals, plants, insects and microorganisms. This information shall be provided only when the substance is significantly different in these stages (e.g. foetal bovine serum). 2135 */ 2136 @Child(name = "developmentStage", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=true) 2137 @Description(shortDefinition="Stage of life for animals, plants, insects and microorganisms. This information shall be provided only when the substance is significantly different in these stages (e.g. foetal bovine serum)", formalDefinition="Stage of life for animals, plants, insects and microorganisms. This information shall be provided only when the substance is significantly different in these stages (e.g. foetal bovine serum)." ) 2138 protected CodeableConcept developmentStage; 2139 2140 /** 2141 * Many complex materials are fractions of parts of plants, animals, or minerals. Fraction elements are often necessary to define both Substances and Specified Group 1 Substances. For substances derived from Plants, fraction information will be captured at the Substance information level ( . Oils, Juices and Exudates). Additional information for Extracts, such as extraction solvent composition, will be captured at the Specified Substance Group 1 information level. For plasma-derived products fraction information will be captured at the Substance and the Specified Substance Group 1 levels. 2142 */ 2143 @Child(name = "fractionDescription", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2144 @Description(shortDefinition="Many complex materials are fractions of parts of plants, animals, or minerals. Fraction elements are often necessary to define both Substances and Specified Group 1 Substances. For substances derived from Plants, fraction information will be captured at the Substance information level ( . Oils, Juices and Exudates). Additional information for Extracts, such as extraction solvent composition, will be captured at the Specified Substance Group 1 information level. For plasma-derived products fraction information will be captured at the Substance and the Specified Substance Group 1 levels", formalDefinition="Many complex materials are fractions of parts of plants, animals, or minerals. Fraction elements are often necessary to define both Substances and Specified Group 1 Substances. For substances derived from Plants, fraction information will be captured at the Substance information level ( . Oils, Juices and Exudates). Additional information for Extracts, such as extraction solvent composition, will be captured at the Specified Substance Group 1 information level. For plasma-derived products fraction information will be captured at the Substance and the Specified Substance Group 1 levels." ) 2145 protected List<SubstanceSourceMaterialFractionDescriptionComponent> fractionDescription; 2146 2147 /** 2148 * This subclause describes the organism which the substance is derived from. For vaccines, the parent organism shall be specified based on these subclause elements. As an example, full taxonomy will be described for the Substance Name: ., Leaf. 2149 */ 2150 @Child(name = "organism", type = {}, order=11, min=0, max=1, modifier=false, summary=true) 2151 @Description(shortDefinition="This subclause describes the organism which the substance is derived from. For vaccines, the parent organism shall be specified based on these subclause elements. As an example, full taxonomy will be described for the Substance Name: ., Leaf", formalDefinition="This subclause describes the organism which the substance is derived from. For vaccines, the parent organism shall be specified based on these subclause elements. As an example, full taxonomy will be described for the Substance Name: ., Leaf." ) 2152 protected SubstanceSourceMaterialOrganismComponent organism; 2153 2154 /** 2155 * To do. 2156 */ 2157 @Child(name = "partDescription", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2158 @Description(shortDefinition="To do", formalDefinition="To do." ) 2159 protected List<SubstanceSourceMaterialPartDescriptionComponent> partDescription; 2160 2161 private static final long serialVersionUID = 442657667L; 2162 2163 /** 2164 * Constructor 2165 */ 2166 public SubstanceSourceMaterial() { 2167 super(); 2168 } 2169 2170 /** 2171 * @return {@link #sourceMaterialClass} (General high level classification of the source material specific to the origin of the material.) 2172 */ 2173 public CodeableConcept getSourceMaterialClass() { 2174 if (this.sourceMaterialClass == null) 2175 if (Configuration.errorOnAutoCreate()) 2176 throw new Error("Attempt to auto-create SubstanceSourceMaterial.sourceMaterialClass"); 2177 else if (Configuration.doAutoCreate()) 2178 this.sourceMaterialClass = new CodeableConcept(); // cc 2179 return this.sourceMaterialClass; 2180 } 2181 2182 public boolean hasSourceMaterialClass() { 2183 return this.sourceMaterialClass != null && !this.sourceMaterialClass.isEmpty(); 2184 } 2185 2186 /** 2187 * @param value {@link #sourceMaterialClass} (General high level classification of the source material specific to the origin of the material.) 2188 */ 2189 public SubstanceSourceMaterial setSourceMaterialClass(CodeableConcept value) { 2190 this.sourceMaterialClass = value; 2191 return this; 2192 } 2193 2194 /** 2195 * @return {@link #sourceMaterialType} (The type of the source material shall be specified based on a controlled vocabulary. For vaccines, this subclause refers to the class of infectious agent.) 2196 */ 2197 public CodeableConcept getSourceMaterialType() { 2198 if (this.sourceMaterialType == null) 2199 if (Configuration.errorOnAutoCreate()) 2200 throw new Error("Attempt to auto-create SubstanceSourceMaterial.sourceMaterialType"); 2201 else if (Configuration.doAutoCreate()) 2202 this.sourceMaterialType = new CodeableConcept(); // cc 2203 return this.sourceMaterialType; 2204 } 2205 2206 public boolean hasSourceMaterialType() { 2207 return this.sourceMaterialType != null && !this.sourceMaterialType.isEmpty(); 2208 } 2209 2210 /** 2211 * @param value {@link #sourceMaterialType} (The type of the source material shall be specified based on a controlled vocabulary. For vaccines, this subclause refers to the class of infectious agent.) 2212 */ 2213 public SubstanceSourceMaterial setSourceMaterialType(CodeableConcept value) { 2214 this.sourceMaterialType = value; 2215 return this; 2216 } 2217 2218 /** 2219 * @return {@link #sourceMaterialState} (The state of the source material when extracted.) 2220 */ 2221 public CodeableConcept getSourceMaterialState() { 2222 if (this.sourceMaterialState == null) 2223 if (Configuration.errorOnAutoCreate()) 2224 throw new Error("Attempt to auto-create SubstanceSourceMaterial.sourceMaterialState"); 2225 else if (Configuration.doAutoCreate()) 2226 this.sourceMaterialState = new CodeableConcept(); // cc 2227 return this.sourceMaterialState; 2228 } 2229 2230 public boolean hasSourceMaterialState() { 2231 return this.sourceMaterialState != null && !this.sourceMaterialState.isEmpty(); 2232 } 2233 2234 /** 2235 * @param value {@link #sourceMaterialState} (The state of the source material when extracted.) 2236 */ 2237 public SubstanceSourceMaterial setSourceMaterialState(CodeableConcept value) { 2238 this.sourceMaterialState = value; 2239 return this; 2240 } 2241 2242 /** 2243 * @return {@link #organismId} (The unique identifier associated with the source material parent organism shall be specified.) 2244 */ 2245 public Identifier getOrganismId() { 2246 if (this.organismId == null) 2247 if (Configuration.errorOnAutoCreate()) 2248 throw new Error("Attempt to auto-create SubstanceSourceMaterial.organismId"); 2249 else if (Configuration.doAutoCreate()) 2250 this.organismId = new Identifier(); // cc 2251 return this.organismId; 2252 } 2253 2254 public boolean hasOrganismId() { 2255 return this.organismId != null && !this.organismId.isEmpty(); 2256 } 2257 2258 /** 2259 * @param value {@link #organismId} (The unique identifier associated with the source material parent organism shall be specified.) 2260 */ 2261 public SubstanceSourceMaterial setOrganismId(Identifier value) { 2262 this.organismId = value; 2263 return this; 2264 } 2265 2266 /** 2267 * @return {@link #organismName} (The organism accepted Scientific name shall be provided based on the organism taxonomy.). This is the underlying object with id, value and extensions. The accessor "getOrganismName" gives direct access to the value 2268 */ 2269 public StringType getOrganismNameElement() { 2270 if (this.organismName == null) 2271 if (Configuration.errorOnAutoCreate()) 2272 throw new Error("Attempt to auto-create SubstanceSourceMaterial.organismName"); 2273 else if (Configuration.doAutoCreate()) 2274 this.organismName = new StringType(); // bb 2275 return this.organismName; 2276 } 2277 2278 public boolean hasOrganismNameElement() { 2279 return this.organismName != null && !this.organismName.isEmpty(); 2280 } 2281 2282 public boolean hasOrganismName() { 2283 return this.organismName != null && !this.organismName.isEmpty(); 2284 } 2285 2286 /** 2287 * @param value {@link #organismName} (The organism accepted Scientific name shall be provided based on the organism taxonomy.). This is the underlying object with id, value and extensions. The accessor "getOrganismName" gives direct access to the value 2288 */ 2289 public SubstanceSourceMaterial setOrganismNameElement(StringType value) { 2290 this.organismName = value; 2291 return this; 2292 } 2293 2294 /** 2295 * @return The organism accepted Scientific name shall be provided based on the organism taxonomy. 2296 */ 2297 public String getOrganismName() { 2298 return this.organismName == null ? null : this.organismName.getValue(); 2299 } 2300 2301 /** 2302 * @param value The organism accepted Scientific name shall be provided based on the organism taxonomy. 2303 */ 2304 public SubstanceSourceMaterial setOrganismName(String value) { 2305 if (Utilities.noString(value)) 2306 this.organismName = null; 2307 else { 2308 if (this.organismName == null) 2309 this.organismName = new StringType(); 2310 this.organismName.setValue(value); 2311 } 2312 return this; 2313 } 2314 2315 /** 2316 * @return {@link #parentSubstanceId} (The parent of the herbal drug Ginkgo biloba, Leaf is the substance ID of the substance (fresh) of Ginkgo biloba L. or Ginkgo biloba L. (Whole plant).) 2317 */ 2318 public List<Identifier> getParentSubstanceId() { 2319 if (this.parentSubstanceId == null) 2320 this.parentSubstanceId = new ArrayList<Identifier>(); 2321 return this.parentSubstanceId; 2322 } 2323 2324 /** 2325 * @return Returns a reference to <code>this</code> for easy method chaining 2326 */ 2327 public SubstanceSourceMaterial setParentSubstanceId(List<Identifier> theParentSubstanceId) { 2328 this.parentSubstanceId = theParentSubstanceId; 2329 return this; 2330 } 2331 2332 public boolean hasParentSubstanceId() { 2333 if (this.parentSubstanceId == null) 2334 return false; 2335 for (Identifier item : this.parentSubstanceId) 2336 if (!item.isEmpty()) 2337 return true; 2338 return false; 2339 } 2340 2341 public Identifier addParentSubstanceId() { //3 2342 Identifier t = new Identifier(); 2343 if (this.parentSubstanceId == null) 2344 this.parentSubstanceId = new ArrayList<Identifier>(); 2345 this.parentSubstanceId.add(t); 2346 return t; 2347 } 2348 2349 public SubstanceSourceMaterial addParentSubstanceId(Identifier t) { //3 2350 if (t == null) 2351 return this; 2352 if (this.parentSubstanceId == null) 2353 this.parentSubstanceId = new ArrayList<Identifier>(); 2354 this.parentSubstanceId.add(t); 2355 return this; 2356 } 2357 2358 /** 2359 * @return The first repetition of repeating field {@link #parentSubstanceId}, creating it if it does not already exist {3} 2360 */ 2361 public Identifier getParentSubstanceIdFirstRep() { 2362 if (getParentSubstanceId().isEmpty()) { 2363 addParentSubstanceId(); 2364 } 2365 return getParentSubstanceId().get(0); 2366 } 2367 2368 /** 2369 * @return {@link #parentSubstanceName} (The parent substance of the Herbal Drug, or Herbal preparation.) 2370 */ 2371 public List<StringType> getParentSubstanceName() { 2372 if (this.parentSubstanceName == null) 2373 this.parentSubstanceName = new ArrayList<StringType>(); 2374 return this.parentSubstanceName; 2375 } 2376 2377 /** 2378 * @return Returns a reference to <code>this</code> for easy method chaining 2379 */ 2380 public SubstanceSourceMaterial setParentSubstanceName(List<StringType> theParentSubstanceName) { 2381 this.parentSubstanceName = theParentSubstanceName; 2382 return this; 2383 } 2384 2385 public boolean hasParentSubstanceName() { 2386 if (this.parentSubstanceName == null) 2387 return false; 2388 for (StringType item : this.parentSubstanceName) 2389 if (!item.isEmpty()) 2390 return true; 2391 return false; 2392 } 2393 2394 /** 2395 * @return {@link #parentSubstanceName} (The parent substance of the Herbal Drug, or Herbal preparation.) 2396 */ 2397 public StringType addParentSubstanceNameElement() {//2 2398 StringType t = new StringType(); 2399 if (this.parentSubstanceName == null) 2400 this.parentSubstanceName = new ArrayList<StringType>(); 2401 this.parentSubstanceName.add(t); 2402 return t; 2403 } 2404 2405 /** 2406 * @param value {@link #parentSubstanceName} (The parent substance of the Herbal Drug, or Herbal preparation.) 2407 */ 2408 public SubstanceSourceMaterial addParentSubstanceName(String value) { //1 2409 StringType t = new StringType(); 2410 t.setValue(value); 2411 if (this.parentSubstanceName == null) 2412 this.parentSubstanceName = new ArrayList<StringType>(); 2413 this.parentSubstanceName.add(t); 2414 return this; 2415 } 2416 2417 /** 2418 * @param value {@link #parentSubstanceName} (The parent substance of the Herbal Drug, or Herbal preparation.) 2419 */ 2420 public boolean hasParentSubstanceName(String value) { 2421 if (this.parentSubstanceName == null) 2422 return false; 2423 for (StringType v : this.parentSubstanceName) 2424 if (v.getValue().equals(value)) // string 2425 return true; 2426 return false; 2427 } 2428 2429 /** 2430 * @return {@link #countryOfOrigin} (The country where the plant material is harvested or the countries where the plasma is sourced from as laid down in accordance with the Plasma Master File. For ?Plasma-derived substances? the attribute country of origin provides information about the countries used for the manufacturing of the Cryopoor plama or Crioprecipitate.) 2431 */ 2432 public List<CodeableConcept> getCountryOfOrigin() { 2433 if (this.countryOfOrigin == null) 2434 this.countryOfOrigin = new ArrayList<CodeableConcept>(); 2435 return this.countryOfOrigin; 2436 } 2437 2438 /** 2439 * @return Returns a reference to <code>this</code> for easy method chaining 2440 */ 2441 public SubstanceSourceMaterial setCountryOfOrigin(List<CodeableConcept> theCountryOfOrigin) { 2442 this.countryOfOrigin = theCountryOfOrigin; 2443 return this; 2444 } 2445 2446 public boolean hasCountryOfOrigin() { 2447 if (this.countryOfOrigin == null) 2448 return false; 2449 for (CodeableConcept item : this.countryOfOrigin) 2450 if (!item.isEmpty()) 2451 return true; 2452 return false; 2453 } 2454 2455 public CodeableConcept addCountryOfOrigin() { //3 2456 CodeableConcept t = new CodeableConcept(); 2457 if (this.countryOfOrigin == null) 2458 this.countryOfOrigin = new ArrayList<CodeableConcept>(); 2459 this.countryOfOrigin.add(t); 2460 return t; 2461 } 2462 2463 public SubstanceSourceMaterial addCountryOfOrigin(CodeableConcept t) { //3 2464 if (t == null) 2465 return this; 2466 if (this.countryOfOrigin == null) 2467 this.countryOfOrigin = new ArrayList<CodeableConcept>(); 2468 this.countryOfOrigin.add(t); 2469 return this; 2470 } 2471 2472 /** 2473 * @return The first repetition of repeating field {@link #countryOfOrigin}, creating it if it does not already exist {3} 2474 */ 2475 public CodeableConcept getCountryOfOriginFirstRep() { 2476 if (getCountryOfOrigin().isEmpty()) { 2477 addCountryOfOrigin(); 2478 } 2479 return getCountryOfOrigin().get(0); 2480 } 2481 2482 /** 2483 * @return {@link #geographicalLocation} (The place/region where the plant is harvested or the places/regions where the animal source material has its habitat.) 2484 */ 2485 public List<StringType> getGeographicalLocation() { 2486 if (this.geographicalLocation == null) 2487 this.geographicalLocation = new ArrayList<StringType>(); 2488 return this.geographicalLocation; 2489 } 2490 2491 /** 2492 * @return Returns a reference to <code>this</code> for easy method chaining 2493 */ 2494 public SubstanceSourceMaterial setGeographicalLocation(List<StringType> theGeographicalLocation) { 2495 this.geographicalLocation = theGeographicalLocation; 2496 return this; 2497 } 2498 2499 public boolean hasGeographicalLocation() { 2500 if (this.geographicalLocation == null) 2501 return false; 2502 for (StringType item : this.geographicalLocation) 2503 if (!item.isEmpty()) 2504 return true; 2505 return false; 2506 } 2507 2508 /** 2509 * @return {@link #geographicalLocation} (The place/region where the plant is harvested or the places/regions where the animal source material has its habitat.) 2510 */ 2511 public StringType addGeographicalLocationElement() {//2 2512 StringType t = new StringType(); 2513 if (this.geographicalLocation == null) 2514 this.geographicalLocation = new ArrayList<StringType>(); 2515 this.geographicalLocation.add(t); 2516 return t; 2517 } 2518 2519 /** 2520 * @param value {@link #geographicalLocation} (The place/region where the plant is harvested or the places/regions where the animal source material has its habitat.) 2521 */ 2522 public SubstanceSourceMaterial addGeographicalLocation(String value) { //1 2523 StringType t = new StringType(); 2524 t.setValue(value); 2525 if (this.geographicalLocation == null) 2526 this.geographicalLocation = new ArrayList<StringType>(); 2527 this.geographicalLocation.add(t); 2528 return this; 2529 } 2530 2531 /** 2532 * @param value {@link #geographicalLocation} (The place/region where the plant is harvested or the places/regions where the animal source material has its habitat.) 2533 */ 2534 public boolean hasGeographicalLocation(String value) { 2535 if (this.geographicalLocation == null) 2536 return false; 2537 for (StringType v : this.geographicalLocation) 2538 if (v.getValue().equals(value)) // string 2539 return true; 2540 return false; 2541 } 2542 2543 /** 2544 * @return {@link #developmentStage} (Stage of life for animals, plants, insects and microorganisms. This information shall be provided only when the substance is significantly different in these stages (e.g. foetal bovine serum).) 2545 */ 2546 public CodeableConcept getDevelopmentStage() { 2547 if (this.developmentStage == null) 2548 if (Configuration.errorOnAutoCreate()) 2549 throw new Error("Attempt to auto-create SubstanceSourceMaterial.developmentStage"); 2550 else if (Configuration.doAutoCreate()) 2551 this.developmentStage = new CodeableConcept(); // cc 2552 return this.developmentStage; 2553 } 2554 2555 public boolean hasDevelopmentStage() { 2556 return this.developmentStage != null && !this.developmentStage.isEmpty(); 2557 } 2558 2559 /** 2560 * @param value {@link #developmentStage} (Stage of life for animals, plants, insects and microorganisms. This information shall be provided only when the substance is significantly different in these stages (e.g. foetal bovine serum).) 2561 */ 2562 public SubstanceSourceMaterial setDevelopmentStage(CodeableConcept value) { 2563 this.developmentStage = value; 2564 return this; 2565 } 2566 2567 /** 2568 * @return {@link #fractionDescription} (Many complex materials are fractions of parts of plants, animals, or minerals. Fraction elements are often necessary to define both Substances and Specified Group 1 Substances. For substances derived from Plants, fraction information will be captured at the Substance information level ( . Oils, Juices and Exudates). Additional information for Extracts, such as extraction solvent composition, will be captured at the Specified Substance Group 1 information level. For plasma-derived products fraction information will be captured at the Substance and the Specified Substance Group 1 levels.) 2569 */ 2570 public List<SubstanceSourceMaterialFractionDescriptionComponent> getFractionDescription() { 2571 if (this.fractionDescription == null) 2572 this.fractionDescription = new ArrayList<SubstanceSourceMaterialFractionDescriptionComponent>(); 2573 return this.fractionDescription; 2574 } 2575 2576 /** 2577 * @return Returns a reference to <code>this</code> for easy method chaining 2578 */ 2579 public SubstanceSourceMaterial setFractionDescription(List<SubstanceSourceMaterialFractionDescriptionComponent> theFractionDescription) { 2580 this.fractionDescription = theFractionDescription; 2581 return this; 2582 } 2583 2584 public boolean hasFractionDescription() { 2585 if (this.fractionDescription == null) 2586 return false; 2587 for (SubstanceSourceMaterialFractionDescriptionComponent item : this.fractionDescription) 2588 if (!item.isEmpty()) 2589 return true; 2590 return false; 2591 } 2592 2593 public SubstanceSourceMaterialFractionDescriptionComponent addFractionDescription() { //3 2594 SubstanceSourceMaterialFractionDescriptionComponent t = new SubstanceSourceMaterialFractionDescriptionComponent(); 2595 if (this.fractionDescription == null) 2596 this.fractionDescription = new ArrayList<SubstanceSourceMaterialFractionDescriptionComponent>(); 2597 this.fractionDescription.add(t); 2598 return t; 2599 } 2600 2601 public SubstanceSourceMaterial addFractionDescription(SubstanceSourceMaterialFractionDescriptionComponent t) { //3 2602 if (t == null) 2603 return this; 2604 if (this.fractionDescription == null) 2605 this.fractionDescription = new ArrayList<SubstanceSourceMaterialFractionDescriptionComponent>(); 2606 this.fractionDescription.add(t); 2607 return this; 2608 } 2609 2610 /** 2611 * @return The first repetition of repeating field {@link #fractionDescription}, creating it if it does not already exist {3} 2612 */ 2613 public SubstanceSourceMaterialFractionDescriptionComponent getFractionDescriptionFirstRep() { 2614 if (getFractionDescription().isEmpty()) { 2615 addFractionDescription(); 2616 } 2617 return getFractionDescription().get(0); 2618 } 2619 2620 /** 2621 * @return {@link #organism} (This subclause describes the organism which the substance is derived from. For vaccines, the parent organism shall be specified based on these subclause elements. As an example, full taxonomy will be described for the Substance Name: ., Leaf.) 2622 */ 2623 public SubstanceSourceMaterialOrganismComponent getOrganism() { 2624 if (this.organism == null) 2625 if (Configuration.errorOnAutoCreate()) 2626 throw new Error("Attempt to auto-create SubstanceSourceMaterial.organism"); 2627 else if (Configuration.doAutoCreate()) 2628 this.organism = new SubstanceSourceMaterialOrganismComponent(); // cc 2629 return this.organism; 2630 } 2631 2632 public boolean hasOrganism() { 2633 return this.organism != null && !this.organism.isEmpty(); 2634 } 2635 2636 /** 2637 * @param value {@link #organism} (This subclause describes the organism which the substance is derived from. For vaccines, the parent organism shall be specified based on these subclause elements. As an example, full taxonomy will be described for the Substance Name: ., Leaf.) 2638 */ 2639 public SubstanceSourceMaterial setOrganism(SubstanceSourceMaterialOrganismComponent value) { 2640 this.organism = value; 2641 return this; 2642 } 2643 2644 /** 2645 * @return {@link #partDescription} (To do.) 2646 */ 2647 public List<SubstanceSourceMaterialPartDescriptionComponent> getPartDescription() { 2648 if (this.partDescription == null) 2649 this.partDescription = new ArrayList<SubstanceSourceMaterialPartDescriptionComponent>(); 2650 return this.partDescription; 2651 } 2652 2653 /** 2654 * @return Returns a reference to <code>this</code> for easy method chaining 2655 */ 2656 public SubstanceSourceMaterial setPartDescription(List<SubstanceSourceMaterialPartDescriptionComponent> thePartDescription) { 2657 this.partDescription = thePartDescription; 2658 return this; 2659 } 2660 2661 public boolean hasPartDescription() { 2662 if (this.partDescription == null) 2663 return false; 2664 for (SubstanceSourceMaterialPartDescriptionComponent item : this.partDescription) 2665 if (!item.isEmpty()) 2666 return true; 2667 return false; 2668 } 2669 2670 public SubstanceSourceMaterialPartDescriptionComponent addPartDescription() { //3 2671 SubstanceSourceMaterialPartDescriptionComponent t = new SubstanceSourceMaterialPartDescriptionComponent(); 2672 if (this.partDescription == null) 2673 this.partDescription = new ArrayList<SubstanceSourceMaterialPartDescriptionComponent>(); 2674 this.partDescription.add(t); 2675 return t; 2676 } 2677 2678 public SubstanceSourceMaterial addPartDescription(SubstanceSourceMaterialPartDescriptionComponent t) { //3 2679 if (t == null) 2680 return this; 2681 if (this.partDescription == null) 2682 this.partDescription = new ArrayList<SubstanceSourceMaterialPartDescriptionComponent>(); 2683 this.partDescription.add(t); 2684 return this; 2685 } 2686 2687 /** 2688 * @return The first repetition of repeating field {@link #partDescription}, creating it if it does not already exist {3} 2689 */ 2690 public SubstanceSourceMaterialPartDescriptionComponent getPartDescriptionFirstRep() { 2691 if (getPartDescription().isEmpty()) { 2692 addPartDescription(); 2693 } 2694 return getPartDescription().get(0); 2695 } 2696 2697 protected void listChildren(List<Property> children) { 2698 super.listChildren(children); 2699 children.add(new Property("sourceMaterialClass", "CodeableConcept", "General high level classification of the source material specific to the origin of the material.", 0, 1, sourceMaterialClass)); 2700 children.add(new Property("sourceMaterialType", "CodeableConcept", "The type of the source material shall be specified based on a controlled vocabulary. For vaccines, this subclause refers to the class of infectious agent.", 0, 1, sourceMaterialType)); 2701 children.add(new Property("sourceMaterialState", "CodeableConcept", "The state of the source material when extracted.", 0, 1, sourceMaterialState)); 2702 children.add(new Property("organismId", "Identifier", "The unique identifier associated with the source material parent organism shall be specified.", 0, 1, organismId)); 2703 children.add(new Property("organismName", "string", "The organism accepted Scientific name shall be provided based on the organism taxonomy.", 0, 1, organismName)); 2704 children.add(new Property("parentSubstanceId", "Identifier", "The parent of the herbal drug Ginkgo biloba, Leaf is the substance ID of the substance (fresh) of Ginkgo biloba L. or Ginkgo biloba L. (Whole plant).", 0, java.lang.Integer.MAX_VALUE, parentSubstanceId)); 2705 children.add(new Property("parentSubstanceName", "string", "The parent substance of the Herbal Drug, or Herbal preparation.", 0, java.lang.Integer.MAX_VALUE, parentSubstanceName)); 2706 children.add(new Property("countryOfOrigin", "CodeableConcept", "The country where the plant material is harvested or the countries where the plasma is sourced from as laid down in accordance with the Plasma Master File. For ?Plasma-derived substances? the attribute country of origin provides information about the countries used for the manufacturing of the Cryopoor plama or Crioprecipitate.", 0, java.lang.Integer.MAX_VALUE, countryOfOrigin)); 2707 children.add(new Property("geographicalLocation", "string", "The place/region where the plant is harvested or the places/regions where the animal source material has its habitat.", 0, java.lang.Integer.MAX_VALUE, geographicalLocation)); 2708 children.add(new Property("developmentStage", "CodeableConcept", "Stage of life for animals, plants, insects and microorganisms. This information shall be provided only when the substance is significantly different in these stages (e.g. foetal bovine serum).", 0, 1, developmentStage)); 2709 children.add(new Property("fractionDescription", "", "Many complex materials are fractions of parts of plants, animals, or minerals. Fraction elements are often necessary to define both Substances and Specified Group 1 Substances. For substances derived from Plants, fraction information will be captured at the Substance information level ( . Oils, Juices and Exudates). Additional information for Extracts, such as extraction solvent composition, will be captured at the Specified Substance Group 1 information level. For plasma-derived products fraction information will be captured at the Substance and the Specified Substance Group 1 levels.", 0, java.lang.Integer.MAX_VALUE, fractionDescription)); 2710 children.add(new Property("organism", "", "This subclause describes the organism which the substance is derived from. For vaccines, the parent organism shall be specified based on these subclause elements. As an example, full taxonomy will be described for the Substance Name: ., Leaf.", 0, 1, organism)); 2711 children.add(new Property("partDescription", "", "To do.", 0, java.lang.Integer.MAX_VALUE, partDescription)); 2712 } 2713 2714 @Override 2715 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2716 switch (_hash) { 2717 case -1253081034: /*sourceMaterialClass*/ return new Property("sourceMaterialClass", "CodeableConcept", "General high level classification of the source material specific to the origin of the material.", 0, 1, sourceMaterialClass); 2718 case 1622665404: /*sourceMaterialType*/ return new Property("sourceMaterialType", "CodeableConcept", "The type of the source material shall be specified based on a controlled vocabulary. For vaccines, this subclause refers to the class of infectious agent.", 0, 1, sourceMaterialType); 2719 case -1238066353: /*sourceMaterialState*/ return new Property("sourceMaterialState", "CodeableConcept", "The state of the source material when extracted.", 0, 1, sourceMaterialState); 2720 case -1965449843: /*organismId*/ return new Property("organismId", "Identifier", "The unique identifier associated with the source material parent organism shall be specified.", 0, 1, organismId); 2721 case 988460669: /*organismName*/ return new Property("organismName", "string", "The organism accepted Scientific name shall be provided based on the organism taxonomy.", 0, 1, organismName); 2722 case -675437663: /*parentSubstanceId*/ return new Property("parentSubstanceId", "Identifier", "The parent of the herbal drug Ginkgo biloba, Leaf is the substance ID of the substance (fresh) of Ginkgo biloba L. or Ginkgo biloba L. (Whole plant).", 0, java.lang.Integer.MAX_VALUE, parentSubstanceId); 2723 case -555382895: /*parentSubstanceName*/ return new Property("parentSubstanceName", "string", "The parent substance of the Herbal Drug, or Herbal preparation.", 0, java.lang.Integer.MAX_VALUE, parentSubstanceName); 2724 case 57176467: /*countryOfOrigin*/ return new Property("countryOfOrigin", "CodeableConcept", "The country where the plant material is harvested or the countries where the plasma is sourced from as laid down in accordance with the Plasma Master File. For ?Plasma-derived substances? the attribute country of origin provides information about the countries used for the manufacturing of the Cryopoor plama or Crioprecipitate.", 0, java.lang.Integer.MAX_VALUE, countryOfOrigin); 2725 case -1988836681: /*geographicalLocation*/ return new Property("geographicalLocation", "string", "The place/region where the plant is harvested or the places/regions where the animal source material has its habitat.", 0, java.lang.Integer.MAX_VALUE, geographicalLocation); 2726 case 391529091: /*developmentStage*/ return new Property("developmentStage", "CodeableConcept", "Stage of life for animals, plants, insects and microorganisms. This information shall be provided only when the substance is significantly different in these stages (e.g. foetal bovine serum).", 0, 1, developmentStage); 2727 case 1472689306: /*fractionDescription*/ return new Property("fractionDescription", "", "Many complex materials are fractions of parts of plants, animals, or minerals. Fraction elements are often necessary to define both Substances and Specified Group 1 Substances. For substances derived from Plants, fraction information will be captured at the Substance information level ( . Oils, Juices and Exudates). Additional information for Extracts, such as extraction solvent composition, will be captured at the Specified Substance Group 1 information level. For plasma-derived products fraction information will be captured at the Substance and the Specified Substance Group 1 levels.", 0, java.lang.Integer.MAX_VALUE, fractionDescription); 2728 case 1316389074: /*organism*/ return new Property("organism", "", "This subclause describes the organism which the substance is derived from. For vaccines, the parent organism shall be specified based on these subclause elements. As an example, full taxonomy will be described for the Substance Name: ., Leaf.", 0, 1, organism); 2729 case -1803623927: /*partDescription*/ return new Property("partDescription", "", "To do.", 0, java.lang.Integer.MAX_VALUE, partDescription); 2730 default: return super.getNamedProperty(_hash, _name, _checkValid); 2731 } 2732 2733 } 2734 2735 @Override 2736 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2737 switch (hash) { 2738 case -1253081034: /*sourceMaterialClass*/ return this.sourceMaterialClass == null ? new Base[0] : new Base[] {this.sourceMaterialClass}; // CodeableConcept 2739 case 1622665404: /*sourceMaterialType*/ return this.sourceMaterialType == null ? new Base[0] : new Base[] {this.sourceMaterialType}; // CodeableConcept 2740 case -1238066353: /*sourceMaterialState*/ return this.sourceMaterialState == null ? new Base[0] : new Base[] {this.sourceMaterialState}; // CodeableConcept 2741 case -1965449843: /*organismId*/ return this.organismId == null ? new Base[0] : new Base[] {this.organismId}; // Identifier 2742 case 988460669: /*organismName*/ return this.organismName == null ? new Base[0] : new Base[] {this.organismName}; // StringType 2743 case -675437663: /*parentSubstanceId*/ return this.parentSubstanceId == null ? new Base[0] : this.parentSubstanceId.toArray(new Base[this.parentSubstanceId.size()]); // Identifier 2744 case -555382895: /*parentSubstanceName*/ return this.parentSubstanceName == null ? new Base[0] : this.parentSubstanceName.toArray(new Base[this.parentSubstanceName.size()]); // StringType 2745 case 57176467: /*countryOfOrigin*/ return this.countryOfOrigin == null ? new Base[0] : this.countryOfOrigin.toArray(new Base[this.countryOfOrigin.size()]); // CodeableConcept 2746 case -1988836681: /*geographicalLocation*/ return this.geographicalLocation == null ? new Base[0] : this.geographicalLocation.toArray(new Base[this.geographicalLocation.size()]); // StringType 2747 case 391529091: /*developmentStage*/ return this.developmentStage == null ? new Base[0] : new Base[] {this.developmentStage}; // CodeableConcept 2748 case 1472689306: /*fractionDescription*/ return this.fractionDescription == null ? new Base[0] : this.fractionDescription.toArray(new Base[this.fractionDescription.size()]); // SubstanceSourceMaterialFractionDescriptionComponent 2749 case 1316389074: /*organism*/ return this.organism == null ? new Base[0] : new Base[] {this.organism}; // SubstanceSourceMaterialOrganismComponent 2750 case -1803623927: /*partDescription*/ return this.partDescription == null ? new Base[0] : this.partDescription.toArray(new Base[this.partDescription.size()]); // SubstanceSourceMaterialPartDescriptionComponent 2751 default: return super.getProperty(hash, name, checkValid); 2752 } 2753 2754 } 2755 2756 @Override 2757 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2758 switch (hash) { 2759 case -1253081034: // sourceMaterialClass 2760 this.sourceMaterialClass = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2761 return value; 2762 case 1622665404: // sourceMaterialType 2763 this.sourceMaterialType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2764 return value; 2765 case -1238066353: // sourceMaterialState 2766 this.sourceMaterialState = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2767 return value; 2768 case -1965449843: // organismId 2769 this.organismId = TypeConvertor.castToIdentifier(value); // Identifier 2770 return value; 2771 case 988460669: // organismName 2772 this.organismName = TypeConvertor.castToString(value); // StringType 2773 return value; 2774 case -675437663: // parentSubstanceId 2775 this.getParentSubstanceId().add(TypeConvertor.castToIdentifier(value)); // Identifier 2776 return value; 2777 case -555382895: // parentSubstanceName 2778 this.getParentSubstanceName().add(TypeConvertor.castToString(value)); // StringType 2779 return value; 2780 case 57176467: // countryOfOrigin 2781 this.getCountryOfOrigin().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2782 return value; 2783 case -1988836681: // geographicalLocation 2784 this.getGeographicalLocation().add(TypeConvertor.castToString(value)); // StringType 2785 return value; 2786 case 391529091: // developmentStage 2787 this.developmentStage = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2788 return value; 2789 case 1472689306: // fractionDescription 2790 this.getFractionDescription().add((SubstanceSourceMaterialFractionDescriptionComponent) value); // SubstanceSourceMaterialFractionDescriptionComponent 2791 return value; 2792 case 1316389074: // organism 2793 this.organism = (SubstanceSourceMaterialOrganismComponent) value; // SubstanceSourceMaterialOrganismComponent 2794 return value; 2795 case -1803623927: // partDescription 2796 this.getPartDescription().add((SubstanceSourceMaterialPartDescriptionComponent) value); // SubstanceSourceMaterialPartDescriptionComponent 2797 return value; 2798 default: return super.setProperty(hash, name, value); 2799 } 2800 2801 } 2802 2803 @Override 2804 public Base setProperty(String name, Base value) throws FHIRException { 2805 if (name.equals("sourceMaterialClass")) { 2806 this.sourceMaterialClass = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2807 } else if (name.equals("sourceMaterialType")) { 2808 this.sourceMaterialType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2809 } else if (name.equals("sourceMaterialState")) { 2810 this.sourceMaterialState = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2811 } else if (name.equals("organismId")) { 2812 this.organismId = TypeConvertor.castToIdentifier(value); // Identifier 2813 } else if (name.equals("organismName")) { 2814 this.organismName = TypeConvertor.castToString(value); // StringType 2815 } else if (name.equals("parentSubstanceId")) { 2816 this.getParentSubstanceId().add(TypeConvertor.castToIdentifier(value)); 2817 } else if (name.equals("parentSubstanceName")) { 2818 this.getParentSubstanceName().add(TypeConvertor.castToString(value)); 2819 } else if (name.equals("countryOfOrigin")) { 2820 this.getCountryOfOrigin().add(TypeConvertor.castToCodeableConcept(value)); 2821 } else if (name.equals("geographicalLocation")) { 2822 this.getGeographicalLocation().add(TypeConvertor.castToString(value)); 2823 } else if (name.equals("developmentStage")) { 2824 this.developmentStage = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2825 } else if (name.equals("fractionDescription")) { 2826 this.getFractionDescription().add((SubstanceSourceMaterialFractionDescriptionComponent) value); 2827 } else if (name.equals("organism")) { 2828 this.organism = (SubstanceSourceMaterialOrganismComponent) value; // SubstanceSourceMaterialOrganismComponent 2829 } else if (name.equals("partDescription")) { 2830 this.getPartDescription().add((SubstanceSourceMaterialPartDescriptionComponent) value); 2831 } else 2832 return super.setProperty(name, value); 2833 return value; 2834 } 2835 2836 @Override 2837 public void removeChild(String name, Base value) throws FHIRException { 2838 if (name.equals("sourceMaterialClass")) { 2839 this.sourceMaterialClass = null; 2840 } else if (name.equals("sourceMaterialType")) { 2841 this.sourceMaterialType = null; 2842 } else if (name.equals("sourceMaterialState")) { 2843 this.sourceMaterialState = null; 2844 } else if (name.equals("organismId")) { 2845 this.organismId = null; 2846 } else if (name.equals("organismName")) { 2847 this.organismName = null; 2848 } else if (name.equals("parentSubstanceId")) { 2849 this.getParentSubstanceId().remove(value); 2850 } else if (name.equals("parentSubstanceName")) { 2851 this.getParentSubstanceName().remove(value); 2852 } else if (name.equals("countryOfOrigin")) { 2853 this.getCountryOfOrigin().remove(value); 2854 } else if (name.equals("geographicalLocation")) { 2855 this.getGeographicalLocation().remove(value); 2856 } else if (name.equals("developmentStage")) { 2857 this.developmentStage = null; 2858 } else if (name.equals("fractionDescription")) { 2859 this.getFractionDescription().remove((SubstanceSourceMaterialFractionDescriptionComponent) value); 2860 } else if (name.equals("organism")) { 2861 this.organism = (SubstanceSourceMaterialOrganismComponent) value; // SubstanceSourceMaterialOrganismComponent 2862 } else if (name.equals("partDescription")) { 2863 this.getPartDescription().remove((SubstanceSourceMaterialPartDescriptionComponent) value); 2864 } else 2865 super.removeChild(name, value); 2866 2867 } 2868 2869 @Override 2870 public Base makeProperty(int hash, String name) throws FHIRException { 2871 switch (hash) { 2872 case -1253081034: return getSourceMaterialClass(); 2873 case 1622665404: return getSourceMaterialType(); 2874 case -1238066353: return getSourceMaterialState(); 2875 case -1965449843: return getOrganismId(); 2876 case 988460669: return getOrganismNameElement(); 2877 case -675437663: return addParentSubstanceId(); 2878 case -555382895: return addParentSubstanceNameElement(); 2879 case 57176467: return addCountryOfOrigin(); 2880 case -1988836681: return addGeographicalLocationElement(); 2881 case 391529091: return getDevelopmentStage(); 2882 case 1472689306: return addFractionDescription(); 2883 case 1316389074: return getOrganism(); 2884 case -1803623927: return addPartDescription(); 2885 default: return super.makeProperty(hash, name); 2886 } 2887 2888 } 2889 2890 @Override 2891 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2892 switch (hash) { 2893 case -1253081034: /*sourceMaterialClass*/ return new String[] {"CodeableConcept"}; 2894 case 1622665404: /*sourceMaterialType*/ return new String[] {"CodeableConcept"}; 2895 case -1238066353: /*sourceMaterialState*/ return new String[] {"CodeableConcept"}; 2896 case -1965449843: /*organismId*/ return new String[] {"Identifier"}; 2897 case 988460669: /*organismName*/ return new String[] {"string"}; 2898 case -675437663: /*parentSubstanceId*/ return new String[] {"Identifier"}; 2899 case -555382895: /*parentSubstanceName*/ return new String[] {"string"}; 2900 case 57176467: /*countryOfOrigin*/ return new String[] {"CodeableConcept"}; 2901 case -1988836681: /*geographicalLocation*/ return new String[] {"string"}; 2902 case 391529091: /*developmentStage*/ return new String[] {"CodeableConcept"}; 2903 case 1472689306: /*fractionDescription*/ return new String[] {}; 2904 case 1316389074: /*organism*/ return new String[] {}; 2905 case -1803623927: /*partDescription*/ return new String[] {}; 2906 default: return super.getTypesForProperty(hash, name); 2907 } 2908 2909 } 2910 2911 @Override 2912 public Base addChild(String name) throws FHIRException { 2913 if (name.equals("sourceMaterialClass")) { 2914 this.sourceMaterialClass = new CodeableConcept(); 2915 return this.sourceMaterialClass; 2916 } 2917 else if (name.equals("sourceMaterialType")) { 2918 this.sourceMaterialType = new CodeableConcept(); 2919 return this.sourceMaterialType; 2920 } 2921 else if (name.equals("sourceMaterialState")) { 2922 this.sourceMaterialState = new CodeableConcept(); 2923 return this.sourceMaterialState; 2924 } 2925 else if (name.equals("organismId")) { 2926 this.organismId = new Identifier(); 2927 return this.organismId; 2928 } 2929 else if (name.equals("organismName")) { 2930 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSourceMaterial.organismName"); 2931 } 2932 else if (name.equals("parentSubstanceId")) { 2933 return addParentSubstanceId(); 2934 } 2935 else if (name.equals("parentSubstanceName")) { 2936 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSourceMaterial.parentSubstanceName"); 2937 } 2938 else if (name.equals("countryOfOrigin")) { 2939 return addCountryOfOrigin(); 2940 } 2941 else if (name.equals("geographicalLocation")) { 2942 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSourceMaterial.geographicalLocation"); 2943 } 2944 else if (name.equals("developmentStage")) { 2945 this.developmentStage = new CodeableConcept(); 2946 return this.developmentStage; 2947 } 2948 else if (name.equals("fractionDescription")) { 2949 return addFractionDescription(); 2950 } 2951 else if (name.equals("organism")) { 2952 this.organism = new SubstanceSourceMaterialOrganismComponent(); 2953 return this.organism; 2954 } 2955 else if (name.equals("partDescription")) { 2956 return addPartDescription(); 2957 } 2958 else 2959 return super.addChild(name); 2960 } 2961 2962 public String fhirType() { 2963 return "SubstanceSourceMaterial"; 2964 2965 } 2966 2967 public SubstanceSourceMaterial copy() { 2968 SubstanceSourceMaterial dst = new SubstanceSourceMaterial(); 2969 copyValues(dst); 2970 return dst; 2971 } 2972 2973 public void copyValues(SubstanceSourceMaterial dst) { 2974 super.copyValues(dst); 2975 dst.sourceMaterialClass = sourceMaterialClass == null ? null : sourceMaterialClass.copy(); 2976 dst.sourceMaterialType = sourceMaterialType == null ? null : sourceMaterialType.copy(); 2977 dst.sourceMaterialState = sourceMaterialState == null ? null : sourceMaterialState.copy(); 2978 dst.organismId = organismId == null ? null : organismId.copy(); 2979 dst.organismName = organismName == null ? null : organismName.copy(); 2980 if (parentSubstanceId != null) { 2981 dst.parentSubstanceId = new ArrayList<Identifier>(); 2982 for (Identifier i : parentSubstanceId) 2983 dst.parentSubstanceId.add(i.copy()); 2984 }; 2985 if (parentSubstanceName != null) { 2986 dst.parentSubstanceName = new ArrayList<StringType>(); 2987 for (StringType i : parentSubstanceName) 2988 dst.parentSubstanceName.add(i.copy()); 2989 }; 2990 if (countryOfOrigin != null) { 2991 dst.countryOfOrigin = new ArrayList<CodeableConcept>(); 2992 for (CodeableConcept i : countryOfOrigin) 2993 dst.countryOfOrigin.add(i.copy()); 2994 }; 2995 if (geographicalLocation != null) { 2996 dst.geographicalLocation = new ArrayList<StringType>(); 2997 for (StringType i : geographicalLocation) 2998 dst.geographicalLocation.add(i.copy()); 2999 }; 3000 dst.developmentStage = developmentStage == null ? null : developmentStage.copy(); 3001 if (fractionDescription != null) { 3002 dst.fractionDescription = new ArrayList<SubstanceSourceMaterialFractionDescriptionComponent>(); 3003 for (SubstanceSourceMaterialFractionDescriptionComponent i : fractionDescription) 3004 dst.fractionDescription.add(i.copy()); 3005 }; 3006 dst.organism = organism == null ? null : organism.copy(); 3007 if (partDescription != null) { 3008 dst.partDescription = new ArrayList<SubstanceSourceMaterialPartDescriptionComponent>(); 3009 for (SubstanceSourceMaterialPartDescriptionComponent i : partDescription) 3010 dst.partDescription.add(i.copy()); 3011 }; 3012 } 3013 3014 protected SubstanceSourceMaterial typedCopy() { 3015 return copy(); 3016 } 3017 3018 @Override 3019 public boolean equalsDeep(Base other_) { 3020 if (!super.equalsDeep(other_)) 3021 return false; 3022 if (!(other_ instanceof SubstanceSourceMaterial)) 3023 return false; 3024 SubstanceSourceMaterial o = (SubstanceSourceMaterial) other_; 3025 return compareDeep(sourceMaterialClass, o.sourceMaterialClass, true) && compareDeep(sourceMaterialType, o.sourceMaterialType, true) 3026 && compareDeep(sourceMaterialState, o.sourceMaterialState, true) && compareDeep(organismId, o.organismId, true) 3027 && compareDeep(organismName, o.organismName, true) && compareDeep(parentSubstanceId, o.parentSubstanceId, true) 3028 && compareDeep(parentSubstanceName, o.parentSubstanceName, true) && compareDeep(countryOfOrigin, o.countryOfOrigin, true) 3029 && compareDeep(geographicalLocation, o.geographicalLocation, true) && compareDeep(developmentStage, o.developmentStage, true) 3030 && compareDeep(fractionDescription, o.fractionDescription, true) && compareDeep(organism, o.organism, true) 3031 && compareDeep(partDescription, o.partDescription, true); 3032 } 3033 3034 @Override 3035 public boolean equalsShallow(Base other_) { 3036 if (!super.equalsShallow(other_)) 3037 return false; 3038 if (!(other_ instanceof SubstanceSourceMaterial)) 3039 return false; 3040 SubstanceSourceMaterial o = (SubstanceSourceMaterial) other_; 3041 return compareValues(organismName, o.organismName, true) && compareValues(parentSubstanceName, o.parentSubstanceName, true) 3042 && compareValues(geographicalLocation, o.geographicalLocation, true); 3043 } 3044 3045 public boolean isEmpty() { 3046 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sourceMaterialClass, sourceMaterialType 3047 , sourceMaterialState, organismId, organismName, parentSubstanceId, parentSubstanceName 3048 , countryOfOrigin, geographicalLocation, developmentStage, fractionDescription, organism 3049 , partDescription); 3050 } 3051 3052 @Override 3053 public ResourceType getResourceType() { 3054 return ResourceType.SubstanceSourceMaterial; 3055 } 3056 3057 3058} 3059