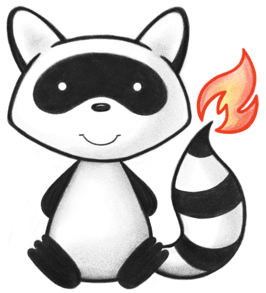
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Record of delivery of what is supplied. 052 */ 053@ResourceDef(name="SupplyDelivery", profile="http://hl7.org/fhir/StructureDefinition/SupplyDelivery") 054public class SupplyDelivery extends DomainResource { 055 056 public enum SupplyDeliveryStatus { 057 /** 058 * Supply has been requested, but not delivered. 059 */ 060 INPROGRESS, 061 /** 062 * Supply has been delivered (\"completed\"). 063 */ 064 COMPLETED, 065 /** 066 * Delivery was not completed. 067 */ 068 ABANDONED, 069 /** 070 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"abandoned\" rather than \"entered-in-error\".). 071 */ 072 ENTEREDINERROR, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static SupplyDeliveryStatus fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("in-progress".equals(codeString)) 081 return INPROGRESS; 082 if ("completed".equals(codeString)) 083 return COMPLETED; 084 if ("abandoned".equals(codeString)) 085 return ABANDONED; 086 if ("entered-in-error".equals(codeString)) 087 return ENTEREDINERROR; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown SupplyDeliveryStatus code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case INPROGRESS: return "in-progress"; 096 case COMPLETED: return "completed"; 097 case ABANDONED: return "abandoned"; 098 case ENTEREDINERROR: return "entered-in-error"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case INPROGRESS: return "http://hl7.org/fhir/supplydelivery-status"; 106 case COMPLETED: return "http://hl7.org/fhir/supplydelivery-status"; 107 case ABANDONED: return "http://hl7.org/fhir/supplydelivery-status"; 108 case ENTEREDINERROR: return "http://hl7.org/fhir/supplydelivery-status"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case INPROGRESS: return "Supply has been requested, but not delivered."; 116 case COMPLETED: return "Supply has been delivered (\"completed\")."; 117 case ABANDONED: return "Delivery was not completed."; 118 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"abandoned\" rather than \"entered-in-error\".)."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case INPROGRESS: return "In Progress"; 126 case COMPLETED: return "Delivered"; 127 case ABANDONED: return "Abandoned"; 128 case ENTEREDINERROR: return "Entered In Error"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class SupplyDeliveryStatusEnumFactory implements EnumFactory<SupplyDeliveryStatus> { 136 public SupplyDeliveryStatus fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("in-progress".equals(codeString)) 141 return SupplyDeliveryStatus.INPROGRESS; 142 if ("completed".equals(codeString)) 143 return SupplyDeliveryStatus.COMPLETED; 144 if ("abandoned".equals(codeString)) 145 return SupplyDeliveryStatus.ABANDONED; 146 if ("entered-in-error".equals(codeString)) 147 return SupplyDeliveryStatus.ENTEREDINERROR; 148 throw new IllegalArgumentException("Unknown SupplyDeliveryStatus code '"+codeString+"'"); 149 } 150 public Enumeration<SupplyDeliveryStatus> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.NULL, code); 155 String codeString = ((PrimitiveType) code).asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.NULL, code); 158 if ("in-progress".equals(codeString)) 159 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.INPROGRESS, code); 160 if ("completed".equals(codeString)) 161 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.COMPLETED, code); 162 if ("abandoned".equals(codeString)) 163 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.ABANDONED, code); 164 if ("entered-in-error".equals(codeString)) 165 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.ENTEREDINERROR, code); 166 throw new FHIRException("Unknown SupplyDeliveryStatus code '"+codeString+"'"); 167 } 168 public String toCode(SupplyDeliveryStatus code) { 169 if (code == SupplyDeliveryStatus.NULL) 170 return null; 171 if (code == SupplyDeliveryStatus.INPROGRESS) 172 return "in-progress"; 173 if (code == SupplyDeliveryStatus.COMPLETED) 174 return "completed"; 175 if (code == SupplyDeliveryStatus.ABANDONED) 176 return "abandoned"; 177 if (code == SupplyDeliveryStatus.ENTEREDINERROR) 178 return "entered-in-error"; 179 return "?"; 180 } 181 public String toSystem(SupplyDeliveryStatus code) { 182 return code.getSystem(); 183 } 184 } 185 186 @Block() 187 public static class SupplyDeliverySuppliedItemComponent extends BackboneElement implements IBaseBackboneElement { 188 /** 189 * The amount of the item that has been supplied. Unit of measure may be included. 190 */ 191 @Child(name = "quantity", type = {Quantity.class}, order=1, min=0, max=1, modifier=false, summary=false) 192 @Description(shortDefinition="Amount supplied", formalDefinition="The amount of the item that has been supplied. Unit of measure may be included." ) 193 protected Quantity quantity; 194 195 /** 196 * Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list. 197 */ 198 @Child(name = "item", type = {CodeableConcept.class, Medication.class, Substance.class, Device.class, BiologicallyDerivedProduct.class, NutritionProduct.class, InventoryItem.class}, order=2, min=0, max=1, modifier=false, summary=false) 199 @Description(shortDefinition="Medication, Substance, Device or Biologically Derived Product supplied", formalDefinition="Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list." ) 200 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/supplydelivery-supplyitemtype") 201 protected DataType item; 202 203 private static final long serialVersionUID = -615919419L; 204 205 /** 206 * Constructor 207 */ 208 public SupplyDeliverySuppliedItemComponent() { 209 super(); 210 } 211 212 /** 213 * @return {@link #quantity} (The amount of the item that has been supplied. Unit of measure may be included.) 214 */ 215 public Quantity getQuantity() { 216 if (this.quantity == null) 217 if (Configuration.errorOnAutoCreate()) 218 throw new Error("Attempt to auto-create SupplyDeliverySuppliedItemComponent.quantity"); 219 else if (Configuration.doAutoCreate()) 220 this.quantity = new Quantity(); // cc 221 return this.quantity; 222 } 223 224 public boolean hasQuantity() { 225 return this.quantity != null && !this.quantity.isEmpty(); 226 } 227 228 /** 229 * @param value {@link #quantity} (The amount of the item that has been supplied. Unit of measure may be included.) 230 */ 231 public SupplyDeliverySuppliedItemComponent setQuantity(Quantity value) { 232 this.quantity = value; 233 return this; 234 } 235 236 /** 237 * @return {@link #item} (Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.) 238 */ 239 public DataType getItem() { 240 return this.item; 241 } 242 243 /** 244 * @return {@link #item} (Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.) 245 */ 246 public CodeableConcept getItemCodeableConcept() throws FHIRException { 247 if (this.item == null) 248 this.item = new CodeableConcept(); 249 if (!(this.item instanceof CodeableConcept)) 250 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.item.getClass().getName()+" was encountered"); 251 return (CodeableConcept) this.item; 252 } 253 254 public boolean hasItemCodeableConcept() { 255 return this != null && this.item instanceof CodeableConcept; 256 } 257 258 /** 259 * @return {@link #item} (Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.) 260 */ 261 public Reference getItemReference() throws FHIRException { 262 if (this.item == null) 263 this.item = new Reference(); 264 if (!(this.item instanceof Reference)) 265 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.item.getClass().getName()+" was encountered"); 266 return (Reference) this.item; 267 } 268 269 public boolean hasItemReference() { 270 return this != null && this.item instanceof Reference; 271 } 272 273 public boolean hasItem() { 274 return this.item != null && !this.item.isEmpty(); 275 } 276 277 /** 278 * @param value {@link #item} (Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.) 279 */ 280 public SupplyDeliverySuppliedItemComponent setItem(DataType value) { 281 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 282 throw new FHIRException("Not the right type for SupplyDelivery.suppliedItem.item[x]: "+value.fhirType()); 283 this.item = value; 284 return this; 285 } 286 287 protected void listChildren(List<Property> children) { 288 super.listChildren(children); 289 children.add(new Property("quantity", "Quantity", "The amount of the item that has been supplied. Unit of measure may be included.", 0, 1, quantity)); 290 children.add(new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device|BiologicallyDerivedProduct|NutritionProduct|InventoryItem)", "Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item)); 291 } 292 293 @Override 294 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 295 switch (_hash) { 296 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The amount of the item that has been supplied. Unit of measure may be included.", 0, 1, quantity); 297 case 2116201613: /*item[x]*/ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device|BiologicallyDerivedProduct|NutritionProduct|InventoryItem)", "Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item); 298 case 3242771: /*item*/ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device|BiologicallyDerivedProduct|NutritionProduct|InventoryItem)", "Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item); 299 case 106644494: /*itemCodeableConcept*/ return new Property("item[x]", "CodeableConcept", "Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item); 300 case 1376364920: /*itemReference*/ return new Property("item[x]", "Reference(Medication|Substance|Device|BiologicallyDerivedProduct|NutritionProduct|InventoryItem)", "Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item); 301 default: return super.getNamedProperty(_hash, _name, _checkValid); 302 } 303 304 } 305 306 @Override 307 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 308 switch (hash) { 309 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 310 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // DataType 311 default: return super.getProperty(hash, name, checkValid); 312 } 313 314 } 315 316 @Override 317 public Base setProperty(int hash, String name, Base value) throws FHIRException { 318 switch (hash) { 319 case -1285004149: // quantity 320 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 321 return value; 322 case 3242771: // item 323 this.item = TypeConvertor.castToType(value); // DataType 324 return value; 325 default: return super.setProperty(hash, name, value); 326 } 327 328 } 329 330 @Override 331 public Base setProperty(String name, Base value) throws FHIRException { 332 if (name.equals("quantity")) { 333 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 334 } else if (name.equals("item[x]")) { 335 this.item = TypeConvertor.castToType(value); // DataType 336 } else 337 return super.setProperty(name, value); 338 return value; 339 } 340 341 @Override 342 public void removeChild(String name, Base value) throws FHIRException { 343 if (name.equals("quantity")) { 344 this.quantity = null; 345 } else if (name.equals("item[x]")) { 346 this.item = null; 347 } else 348 super.removeChild(name, value); 349 350 } 351 352 @Override 353 public Base makeProperty(int hash, String name) throws FHIRException { 354 switch (hash) { 355 case -1285004149: return getQuantity(); 356 case 2116201613: return getItem(); 357 case 3242771: return getItem(); 358 default: return super.makeProperty(hash, name); 359 } 360 361 } 362 363 @Override 364 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 365 switch (hash) { 366 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 367 case 3242771: /*item*/ return new String[] {"CodeableConcept", "Reference"}; 368 default: return super.getTypesForProperty(hash, name); 369 } 370 371 } 372 373 @Override 374 public Base addChild(String name) throws FHIRException { 375 if (name.equals("quantity")) { 376 this.quantity = new Quantity(); 377 return this.quantity; 378 } 379 else if (name.equals("itemCodeableConcept")) { 380 this.item = new CodeableConcept(); 381 return this.item; 382 } 383 else if (name.equals("itemReference")) { 384 this.item = new Reference(); 385 return this.item; 386 } 387 else 388 return super.addChild(name); 389 } 390 391 public SupplyDeliverySuppliedItemComponent copy() { 392 SupplyDeliverySuppliedItemComponent dst = new SupplyDeliverySuppliedItemComponent(); 393 copyValues(dst); 394 return dst; 395 } 396 397 public void copyValues(SupplyDeliverySuppliedItemComponent dst) { 398 super.copyValues(dst); 399 dst.quantity = quantity == null ? null : quantity.copy(); 400 dst.item = item == null ? null : item.copy(); 401 } 402 403 @Override 404 public boolean equalsDeep(Base other_) { 405 if (!super.equalsDeep(other_)) 406 return false; 407 if (!(other_ instanceof SupplyDeliverySuppliedItemComponent)) 408 return false; 409 SupplyDeliverySuppliedItemComponent o = (SupplyDeliverySuppliedItemComponent) other_; 410 return compareDeep(quantity, o.quantity, true) && compareDeep(item, o.item, true); 411 } 412 413 @Override 414 public boolean equalsShallow(Base other_) { 415 if (!super.equalsShallow(other_)) 416 return false; 417 if (!(other_ instanceof SupplyDeliverySuppliedItemComponent)) 418 return false; 419 SupplyDeliverySuppliedItemComponent o = (SupplyDeliverySuppliedItemComponent) other_; 420 return true; 421 } 422 423 public boolean isEmpty() { 424 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(quantity, item); 425 } 426 427 public String fhirType() { 428 return "SupplyDelivery.suppliedItem"; 429 430 } 431 432 } 433 434 /** 435 * Identifier for the supply delivery event that is used to identify it across multiple disparate systems. 436 */ 437 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 438 @Description(shortDefinition="External identifier", formalDefinition="Identifier for the supply delivery event that is used to identify it across multiple disparate systems." ) 439 protected List<Identifier> identifier; 440 441 /** 442 * A plan, proposal or order that is fulfilled in whole or in part by this event. 443 */ 444 @Child(name = "basedOn", type = {SupplyRequest.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 445 @Description(shortDefinition="Fulfills plan, proposal or order", formalDefinition="A plan, proposal or order that is fulfilled in whole or in part by this event." ) 446 protected List<Reference> basedOn; 447 448 /** 449 * A larger event of which this particular event is a component or step. 450 */ 451 @Child(name = "partOf", type = {SupplyDelivery.class, Contract.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 452 @Description(shortDefinition="Part of referenced event", formalDefinition="A larger event of which this particular event is a component or step." ) 453 protected List<Reference> partOf; 454 455 /** 456 * A code specifying the state of the dispense event. 457 */ 458 @Child(name = "status", type = {CodeType.class}, order=3, min=0, max=1, modifier=true, summary=true) 459 @Description(shortDefinition="in-progress | completed | abandoned | entered-in-error", formalDefinition="A code specifying the state of the dispense event." ) 460 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/supplydelivery-status") 461 protected Enumeration<SupplyDeliveryStatus> status; 462 463 /** 464 * A link to a resource representing the person whom the delivered item is for. 465 */ 466 @Child(name = "patient", type = {Patient.class}, order=4, min=0, max=1, modifier=false, summary=false) 467 @Description(shortDefinition="Patient for whom the item is supplied", formalDefinition="A link to a resource representing the person whom the delivered item is for." ) 468 protected Reference patient; 469 470 /** 471 * Indicates the type of supply being provided. Examples include: Medication, Device, Biologically Derived Product. 472 */ 473 @Child(name = "type", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 474 @Description(shortDefinition="Category of supply event", formalDefinition="Indicates the type of supply being provided. Examples include: Medication, Device, Biologically Derived Product." ) 475 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/supplydelivery-supplyitemtype") 476 protected CodeableConcept type; 477 478 /** 479 * The item that is being delivered or has been supplied. 480 */ 481 @Child(name = "suppliedItem", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 482 @Description(shortDefinition="The item that is delivered or supplied", formalDefinition="The item that is being delivered or has been supplied." ) 483 protected List<SupplyDeliverySuppliedItemComponent> suppliedItem; 484 485 /** 486 * The date or time(s) the activity occurred. 487 */ 488 @Child(name = "occurrence", type = {DateTimeType.class, Period.class, Timing.class}, order=7, min=0, max=1, modifier=false, summary=true) 489 @Description(shortDefinition="When event occurred", formalDefinition="The date or time(s) the activity occurred." ) 490 protected DataType occurrence; 491 492 /** 493 * The individual or organization responsible for supplying the delivery. 494 */ 495 @Child(name = "supplier", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=8, min=0, max=1, modifier=false, summary=false) 496 @Description(shortDefinition="The item supplier", formalDefinition="The individual or organization responsible for supplying the delivery." ) 497 protected Reference supplier; 498 499 /** 500 * Identification of the facility/location where the delivery was shipped to. 501 */ 502 @Child(name = "destination", type = {Location.class}, order=9, min=0, max=1, modifier=false, summary=false) 503 @Description(shortDefinition="Where the delivery was sent", formalDefinition="Identification of the facility/location where the delivery was shipped to." ) 504 protected Reference destination; 505 506 /** 507 * Identifies the individual or organization that received the delivery. 508 */ 509 @Child(name = "receiver", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 510 @Description(shortDefinition="Who received the delivery", formalDefinition="Identifies the individual or organization that received the delivery." ) 511 protected List<Reference> receiver; 512 513 private static final long serialVersionUID = -734856482L; 514 515 /** 516 * Constructor 517 */ 518 public SupplyDelivery() { 519 super(); 520 } 521 522 /** 523 * @return {@link #identifier} (Identifier for the supply delivery event that is used to identify it across multiple disparate systems.) 524 */ 525 public List<Identifier> getIdentifier() { 526 if (this.identifier == null) 527 this.identifier = new ArrayList<Identifier>(); 528 return this.identifier; 529 } 530 531 /** 532 * @return Returns a reference to <code>this</code> for easy method chaining 533 */ 534 public SupplyDelivery setIdentifier(List<Identifier> theIdentifier) { 535 this.identifier = theIdentifier; 536 return this; 537 } 538 539 public boolean hasIdentifier() { 540 if (this.identifier == null) 541 return false; 542 for (Identifier item : this.identifier) 543 if (!item.isEmpty()) 544 return true; 545 return false; 546 } 547 548 public Identifier addIdentifier() { //3 549 Identifier t = new Identifier(); 550 if (this.identifier == null) 551 this.identifier = new ArrayList<Identifier>(); 552 this.identifier.add(t); 553 return t; 554 } 555 556 public SupplyDelivery addIdentifier(Identifier t) { //3 557 if (t == null) 558 return this; 559 if (this.identifier == null) 560 this.identifier = new ArrayList<Identifier>(); 561 this.identifier.add(t); 562 return this; 563 } 564 565 /** 566 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 567 */ 568 public Identifier getIdentifierFirstRep() { 569 if (getIdentifier().isEmpty()) { 570 addIdentifier(); 571 } 572 return getIdentifier().get(0); 573 } 574 575 /** 576 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in whole or in part by this event.) 577 */ 578 public List<Reference> getBasedOn() { 579 if (this.basedOn == null) 580 this.basedOn = new ArrayList<Reference>(); 581 return this.basedOn; 582 } 583 584 /** 585 * @return Returns a reference to <code>this</code> for easy method chaining 586 */ 587 public SupplyDelivery setBasedOn(List<Reference> theBasedOn) { 588 this.basedOn = theBasedOn; 589 return this; 590 } 591 592 public boolean hasBasedOn() { 593 if (this.basedOn == null) 594 return false; 595 for (Reference item : this.basedOn) 596 if (!item.isEmpty()) 597 return true; 598 return false; 599 } 600 601 public Reference addBasedOn() { //3 602 Reference t = new Reference(); 603 if (this.basedOn == null) 604 this.basedOn = new ArrayList<Reference>(); 605 this.basedOn.add(t); 606 return t; 607 } 608 609 public SupplyDelivery addBasedOn(Reference t) { //3 610 if (t == null) 611 return this; 612 if (this.basedOn == null) 613 this.basedOn = new ArrayList<Reference>(); 614 this.basedOn.add(t); 615 return this; 616 } 617 618 /** 619 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 620 */ 621 public Reference getBasedOnFirstRep() { 622 if (getBasedOn().isEmpty()) { 623 addBasedOn(); 624 } 625 return getBasedOn().get(0); 626 } 627 628 /** 629 * @return {@link #partOf} (A larger event of which this particular event is a component or step.) 630 */ 631 public List<Reference> getPartOf() { 632 if (this.partOf == null) 633 this.partOf = new ArrayList<Reference>(); 634 return this.partOf; 635 } 636 637 /** 638 * @return Returns a reference to <code>this</code> for easy method chaining 639 */ 640 public SupplyDelivery setPartOf(List<Reference> thePartOf) { 641 this.partOf = thePartOf; 642 return this; 643 } 644 645 public boolean hasPartOf() { 646 if (this.partOf == null) 647 return false; 648 for (Reference item : this.partOf) 649 if (!item.isEmpty()) 650 return true; 651 return false; 652 } 653 654 public Reference addPartOf() { //3 655 Reference t = new Reference(); 656 if (this.partOf == null) 657 this.partOf = new ArrayList<Reference>(); 658 this.partOf.add(t); 659 return t; 660 } 661 662 public SupplyDelivery addPartOf(Reference t) { //3 663 if (t == null) 664 return this; 665 if (this.partOf == null) 666 this.partOf = new ArrayList<Reference>(); 667 this.partOf.add(t); 668 return this; 669 } 670 671 /** 672 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 673 */ 674 public Reference getPartOfFirstRep() { 675 if (getPartOf().isEmpty()) { 676 addPartOf(); 677 } 678 return getPartOf().get(0); 679 } 680 681 /** 682 * @return {@link #status} (A code specifying the state of the dispense event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 683 */ 684 public Enumeration<SupplyDeliveryStatus> getStatusElement() { 685 if (this.status == null) 686 if (Configuration.errorOnAutoCreate()) 687 throw new Error("Attempt to auto-create SupplyDelivery.status"); 688 else if (Configuration.doAutoCreate()) 689 this.status = new Enumeration<SupplyDeliveryStatus>(new SupplyDeliveryStatusEnumFactory()); // bb 690 return this.status; 691 } 692 693 public boolean hasStatusElement() { 694 return this.status != null && !this.status.isEmpty(); 695 } 696 697 public boolean hasStatus() { 698 return this.status != null && !this.status.isEmpty(); 699 } 700 701 /** 702 * @param value {@link #status} (A code specifying the state of the dispense event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 703 */ 704 public SupplyDelivery setStatusElement(Enumeration<SupplyDeliveryStatus> value) { 705 this.status = value; 706 return this; 707 } 708 709 /** 710 * @return A code specifying the state of the dispense event. 711 */ 712 public SupplyDeliveryStatus getStatus() { 713 return this.status == null ? null : this.status.getValue(); 714 } 715 716 /** 717 * @param value A code specifying the state of the dispense event. 718 */ 719 public SupplyDelivery setStatus(SupplyDeliveryStatus value) { 720 if (value == null) 721 this.status = null; 722 else { 723 if (this.status == null) 724 this.status = new Enumeration<SupplyDeliveryStatus>(new SupplyDeliveryStatusEnumFactory()); 725 this.status.setValue(value); 726 } 727 return this; 728 } 729 730 /** 731 * @return {@link #patient} (A link to a resource representing the person whom the delivered item is for.) 732 */ 733 public Reference getPatient() { 734 if (this.patient == null) 735 if (Configuration.errorOnAutoCreate()) 736 throw new Error("Attempt to auto-create SupplyDelivery.patient"); 737 else if (Configuration.doAutoCreate()) 738 this.patient = new Reference(); // cc 739 return this.patient; 740 } 741 742 public boolean hasPatient() { 743 return this.patient != null && !this.patient.isEmpty(); 744 } 745 746 /** 747 * @param value {@link #patient} (A link to a resource representing the person whom the delivered item is for.) 748 */ 749 public SupplyDelivery setPatient(Reference value) { 750 this.patient = value; 751 return this; 752 } 753 754 /** 755 * @return {@link #type} (Indicates the type of supply being provided. Examples include: Medication, Device, Biologically Derived Product.) 756 */ 757 public CodeableConcept getType() { 758 if (this.type == null) 759 if (Configuration.errorOnAutoCreate()) 760 throw new Error("Attempt to auto-create SupplyDelivery.type"); 761 else if (Configuration.doAutoCreate()) 762 this.type = new CodeableConcept(); // cc 763 return this.type; 764 } 765 766 public boolean hasType() { 767 return this.type != null && !this.type.isEmpty(); 768 } 769 770 /** 771 * @param value {@link #type} (Indicates the type of supply being provided. Examples include: Medication, Device, Biologically Derived Product.) 772 */ 773 public SupplyDelivery setType(CodeableConcept value) { 774 this.type = value; 775 return this; 776 } 777 778 /** 779 * @return {@link #suppliedItem} (The item that is being delivered or has been supplied.) 780 */ 781 public List<SupplyDeliverySuppliedItemComponent> getSuppliedItem() { 782 if (this.suppliedItem == null) 783 this.suppliedItem = new ArrayList<SupplyDeliverySuppliedItemComponent>(); 784 return this.suppliedItem; 785 } 786 787 /** 788 * @return Returns a reference to <code>this</code> for easy method chaining 789 */ 790 public SupplyDelivery setSuppliedItem(List<SupplyDeliverySuppliedItemComponent> theSuppliedItem) { 791 this.suppliedItem = theSuppliedItem; 792 return this; 793 } 794 795 public boolean hasSuppliedItem() { 796 if (this.suppliedItem == null) 797 return false; 798 for (SupplyDeliverySuppliedItemComponent item : this.suppliedItem) 799 if (!item.isEmpty()) 800 return true; 801 return false; 802 } 803 804 public SupplyDeliverySuppliedItemComponent addSuppliedItem() { //3 805 SupplyDeliverySuppliedItemComponent t = new SupplyDeliverySuppliedItemComponent(); 806 if (this.suppliedItem == null) 807 this.suppliedItem = new ArrayList<SupplyDeliverySuppliedItemComponent>(); 808 this.suppliedItem.add(t); 809 return t; 810 } 811 812 public SupplyDelivery addSuppliedItem(SupplyDeliverySuppliedItemComponent t) { //3 813 if (t == null) 814 return this; 815 if (this.suppliedItem == null) 816 this.suppliedItem = new ArrayList<SupplyDeliverySuppliedItemComponent>(); 817 this.suppliedItem.add(t); 818 return this; 819 } 820 821 /** 822 * @return The first repetition of repeating field {@link #suppliedItem}, creating it if it does not already exist {3} 823 */ 824 public SupplyDeliverySuppliedItemComponent getSuppliedItemFirstRep() { 825 if (getSuppliedItem().isEmpty()) { 826 addSuppliedItem(); 827 } 828 return getSuppliedItem().get(0); 829 } 830 831 /** 832 * @return {@link #occurrence} (The date or time(s) the activity occurred.) 833 */ 834 public DataType getOccurrence() { 835 return this.occurrence; 836 } 837 838 /** 839 * @return {@link #occurrence} (The date or time(s) the activity occurred.) 840 */ 841 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 842 if (this.occurrence == null) 843 this.occurrence = new DateTimeType(); 844 if (!(this.occurrence instanceof DateTimeType)) 845 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 846 return (DateTimeType) this.occurrence; 847 } 848 849 public boolean hasOccurrenceDateTimeType() { 850 return this != null && this.occurrence instanceof DateTimeType; 851 } 852 853 /** 854 * @return {@link #occurrence} (The date or time(s) the activity occurred.) 855 */ 856 public Period getOccurrencePeriod() throws FHIRException { 857 if (this.occurrence == null) 858 this.occurrence = new Period(); 859 if (!(this.occurrence instanceof Period)) 860 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 861 return (Period) this.occurrence; 862 } 863 864 public boolean hasOccurrencePeriod() { 865 return this != null && this.occurrence instanceof Period; 866 } 867 868 /** 869 * @return {@link #occurrence} (The date or time(s) the activity occurred.) 870 */ 871 public Timing getOccurrenceTiming() throws FHIRException { 872 if (this.occurrence == null) 873 this.occurrence = new Timing(); 874 if (!(this.occurrence instanceof Timing)) 875 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 876 return (Timing) this.occurrence; 877 } 878 879 public boolean hasOccurrenceTiming() { 880 return this != null && this.occurrence instanceof Timing; 881 } 882 883 public boolean hasOccurrence() { 884 return this.occurrence != null && !this.occurrence.isEmpty(); 885 } 886 887 /** 888 * @param value {@link #occurrence} (The date or time(s) the activity occurred.) 889 */ 890 public SupplyDelivery setOccurrence(DataType value) { 891 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 892 throw new FHIRException("Not the right type for SupplyDelivery.occurrence[x]: "+value.fhirType()); 893 this.occurrence = value; 894 return this; 895 } 896 897 /** 898 * @return {@link #supplier} (The individual or organization responsible for supplying the delivery.) 899 */ 900 public Reference getSupplier() { 901 if (this.supplier == null) 902 if (Configuration.errorOnAutoCreate()) 903 throw new Error("Attempt to auto-create SupplyDelivery.supplier"); 904 else if (Configuration.doAutoCreate()) 905 this.supplier = new Reference(); // cc 906 return this.supplier; 907 } 908 909 public boolean hasSupplier() { 910 return this.supplier != null && !this.supplier.isEmpty(); 911 } 912 913 /** 914 * @param value {@link #supplier} (The individual or organization responsible for supplying the delivery.) 915 */ 916 public SupplyDelivery setSupplier(Reference value) { 917 this.supplier = value; 918 return this; 919 } 920 921 /** 922 * @return {@link #destination} (Identification of the facility/location where the delivery was shipped to.) 923 */ 924 public Reference getDestination() { 925 if (this.destination == null) 926 if (Configuration.errorOnAutoCreate()) 927 throw new Error("Attempt to auto-create SupplyDelivery.destination"); 928 else if (Configuration.doAutoCreate()) 929 this.destination = new Reference(); // cc 930 return this.destination; 931 } 932 933 public boolean hasDestination() { 934 return this.destination != null && !this.destination.isEmpty(); 935 } 936 937 /** 938 * @param value {@link #destination} (Identification of the facility/location where the delivery was shipped to.) 939 */ 940 public SupplyDelivery setDestination(Reference value) { 941 this.destination = value; 942 return this; 943 } 944 945 /** 946 * @return {@link #receiver} (Identifies the individual or organization that received the delivery.) 947 */ 948 public List<Reference> getReceiver() { 949 if (this.receiver == null) 950 this.receiver = new ArrayList<Reference>(); 951 return this.receiver; 952 } 953 954 /** 955 * @return Returns a reference to <code>this</code> for easy method chaining 956 */ 957 public SupplyDelivery setReceiver(List<Reference> theReceiver) { 958 this.receiver = theReceiver; 959 return this; 960 } 961 962 public boolean hasReceiver() { 963 if (this.receiver == null) 964 return false; 965 for (Reference item : this.receiver) 966 if (!item.isEmpty()) 967 return true; 968 return false; 969 } 970 971 public Reference addReceiver() { //3 972 Reference t = new Reference(); 973 if (this.receiver == null) 974 this.receiver = new ArrayList<Reference>(); 975 this.receiver.add(t); 976 return t; 977 } 978 979 public SupplyDelivery addReceiver(Reference t) { //3 980 if (t == null) 981 return this; 982 if (this.receiver == null) 983 this.receiver = new ArrayList<Reference>(); 984 this.receiver.add(t); 985 return this; 986 } 987 988 /** 989 * @return The first repetition of repeating field {@link #receiver}, creating it if it does not already exist {3} 990 */ 991 public Reference getReceiverFirstRep() { 992 if (getReceiver().isEmpty()) { 993 addReceiver(); 994 } 995 return getReceiver().get(0); 996 } 997 998 protected void listChildren(List<Property> children) { 999 super.listChildren(children); 1000 children.add(new Property("identifier", "Identifier", "Identifier for the supply delivery event that is used to identify it across multiple disparate systems.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1001 children.add(new Property("basedOn", "Reference(SupplyRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this event.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1002 children.add(new Property("partOf", "Reference(SupplyDelivery|Contract)", "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 1003 children.add(new Property("status", "code", "A code specifying the state of the dispense event.", 0, 1, status)); 1004 children.add(new Property("patient", "Reference(Patient)", "A link to a resource representing the person whom the delivered item is for.", 0, 1, patient)); 1005 children.add(new Property("type", "CodeableConcept", "Indicates the type of supply being provided. Examples include: Medication, Device, Biologically Derived Product.", 0, 1, type)); 1006 children.add(new Property("suppliedItem", "", "The item that is being delivered or has been supplied.", 0, java.lang.Integer.MAX_VALUE, suppliedItem)); 1007 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "The date or time(s) the activity occurred.", 0, 1, occurrence)); 1008 children.add(new Property("supplier", "Reference(Practitioner|PractitionerRole|Organization)", "The individual or organization responsible for supplying the delivery.", 0, 1, supplier)); 1009 children.add(new Property("destination", "Reference(Location)", "Identification of the facility/location where the delivery was shipped to.", 0, 1, destination)); 1010 children.add(new Property("receiver", "Reference(Practitioner|PractitionerRole|Organization)", "Identifies the individual or organization that received the delivery.", 0, java.lang.Integer.MAX_VALUE, receiver)); 1011 } 1012 1013 @Override 1014 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1015 switch (_hash) { 1016 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier for the supply delivery event that is used to identify it across multiple disparate systems.", 0, java.lang.Integer.MAX_VALUE, identifier); 1017 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(SupplyRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this event.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1018 case -995410646: /*partOf*/ return new Property("partOf", "Reference(SupplyDelivery|Contract)", "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 1019 case -892481550: /*status*/ return new Property("status", "code", "A code specifying the state of the dispense event.", 0, 1, status); 1020 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "A link to a resource representing the person whom the delivered item is for.", 0, 1, patient); 1021 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Indicates the type of supply being provided. Examples include: Medication, Device, Biologically Derived Product.", 0, 1, type); 1022 case 1993333233: /*suppliedItem*/ return new Property("suppliedItem", "", "The item that is being delivered or has been supplied.", 0, java.lang.Integer.MAX_VALUE, suppliedItem); 1023 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date or time(s) the activity occurred.", 0, 1, occurrence); 1024 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date or time(s) the activity occurred.", 0, 1, occurrence); 1025 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime", "The date or time(s) the activity occurred.", 0, 1, occurrence); 1026 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "Period", "The date or time(s) the activity occurred.", 0, 1, occurrence); 1027 case 1515218299: /*occurrenceTiming*/ return new Property("occurrence[x]", "Timing", "The date or time(s) the activity occurred.", 0, 1, occurrence); 1028 case -1663305268: /*supplier*/ return new Property("supplier", "Reference(Practitioner|PractitionerRole|Organization)", "The individual or organization responsible for supplying the delivery.", 0, 1, supplier); 1029 case -1429847026: /*destination*/ return new Property("destination", "Reference(Location)", "Identification of the facility/location where the delivery was shipped to.", 0, 1, destination); 1030 case -808719889: /*receiver*/ return new Property("receiver", "Reference(Practitioner|PractitionerRole|Organization)", "Identifies the individual or organization that received the delivery.", 0, java.lang.Integer.MAX_VALUE, receiver); 1031 default: return super.getNamedProperty(_hash, _name, _checkValid); 1032 } 1033 1034 } 1035 1036 @Override 1037 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1038 switch (hash) { 1039 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1040 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1041 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1042 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<SupplyDeliveryStatus> 1043 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 1044 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1045 case 1993333233: /*suppliedItem*/ return this.suppliedItem == null ? new Base[0] : this.suppliedItem.toArray(new Base[this.suppliedItem.size()]); // SupplyDeliverySuppliedItemComponent 1046 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // DataType 1047 case -1663305268: /*supplier*/ return this.supplier == null ? new Base[0] : new Base[] {this.supplier}; // Reference 1048 case -1429847026: /*destination*/ return this.destination == null ? new Base[0] : new Base[] {this.destination}; // Reference 1049 case -808719889: /*receiver*/ return this.receiver == null ? new Base[0] : this.receiver.toArray(new Base[this.receiver.size()]); // Reference 1050 default: return super.getProperty(hash, name, checkValid); 1051 } 1052 1053 } 1054 1055 @Override 1056 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1057 switch (hash) { 1058 case -1618432855: // identifier 1059 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1060 return value; 1061 case -332612366: // basedOn 1062 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 1063 return value; 1064 case -995410646: // partOf 1065 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 1066 return value; 1067 case -892481550: // status 1068 value = new SupplyDeliveryStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1069 this.status = (Enumeration) value; // Enumeration<SupplyDeliveryStatus> 1070 return value; 1071 case -791418107: // patient 1072 this.patient = TypeConvertor.castToReference(value); // Reference 1073 return value; 1074 case 3575610: // type 1075 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1076 return value; 1077 case 1993333233: // suppliedItem 1078 this.getSuppliedItem().add((SupplyDeliverySuppliedItemComponent) value); // SupplyDeliverySuppliedItemComponent 1079 return value; 1080 case 1687874001: // occurrence 1081 this.occurrence = TypeConvertor.castToType(value); // DataType 1082 return value; 1083 case -1663305268: // supplier 1084 this.supplier = TypeConvertor.castToReference(value); // Reference 1085 return value; 1086 case -1429847026: // destination 1087 this.destination = TypeConvertor.castToReference(value); // Reference 1088 return value; 1089 case -808719889: // receiver 1090 this.getReceiver().add(TypeConvertor.castToReference(value)); // Reference 1091 return value; 1092 default: return super.setProperty(hash, name, value); 1093 } 1094 1095 } 1096 1097 @Override 1098 public Base setProperty(String name, Base value) throws FHIRException { 1099 if (name.equals("identifier")) { 1100 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1101 } else if (name.equals("basedOn")) { 1102 this.getBasedOn().add(TypeConvertor.castToReference(value)); 1103 } else if (name.equals("partOf")) { 1104 this.getPartOf().add(TypeConvertor.castToReference(value)); 1105 } else if (name.equals("status")) { 1106 value = new SupplyDeliveryStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1107 this.status = (Enumeration) value; // Enumeration<SupplyDeliveryStatus> 1108 } else if (name.equals("patient")) { 1109 this.patient = TypeConvertor.castToReference(value); // Reference 1110 } else if (name.equals("type")) { 1111 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1112 } else if (name.equals("suppliedItem")) { 1113 this.getSuppliedItem().add((SupplyDeliverySuppliedItemComponent) value); 1114 } else if (name.equals("occurrence[x]")) { 1115 this.occurrence = TypeConvertor.castToType(value); // DataType 1116 } else if (name.equals("supplier")) { 1117 this.supplier = TypeConvertor.castToReference(value); // Reference 1118 } else if (name.equals("destination")) { 1119 this.destination = TypeConvertor.castToReference(value); // Reference 1120 } else if (name.equals("receiver")) { 1121 this.getReceiver().add(TypeConvertor.castToReference(value)); 1122 } else 1123 return super.setProperty(name, value); 1124 return value; 1125 } 1126 1127 @Override 1128 public void removeChild(String name, Base value) throws FHIRException { 1129 if (name.equals("identifier")) { 1130 this.getIdentifier().remove(value); 1131 } else if (name.equals("basedOn")) { 1132 this.getBasedOn().remove(value); 1133 } else if (name.equals("partOf")) { 1134 this.getPartOf().remove(value); 1135 } else if (name.equals("status")) { 1136 value = new SupplyDeliveryStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1137 this.status = (Enumeration) value; // Enumeration<SupplyDeliveryStatus> 1138 } else if (name.equals("patient")) { 1139 this.patient = null; 1140 } else if (name.equals("type")) { 1141 this.type = null; 1142 } else if (name.equals("suppliedItem")) { 1143 this.getSuppliedItem().remove((SupplyDeliverySuppliedItemComponent) value); 1144 } else if (name.equals("occurrence[x]")) { 1145 this.occurrence = null; 1146 } else if (name.equals("supplier")) { 1147 this.supplier = null; 1148 } else if (name.equals("destination")) { 1149 this.destination = null; 1150 } else if (name.equals("receiver")) { 1151 this.getReceiver().remove(value); 1152 } else 1153 super.removeChild(name, value); 1154 1155 } 1156 1157 @Override 1158 public Base makeProperty(int hash, String name) throws FHIRException { 1159 switch (hash) { 1160 case -1618432855: return addIdentifier(); 1161 case -332612366: return addBasedOn(); 1162 case -995410646: return addPartOf(); 1163 case -892481550: return getStatusElement(); 1164 case -791418107: return getPatient(); 1165 case 3575610: return getType(); 1166 case 1993333233: return addSuppliedItem(); 1167 case -2022646513: return getOccurrence(); 1168 case 1687874001: return getOccurrence(); 1169 case -1663305268: return getSupplier(); 1170 case -1429847026: return getDestination(); 1171 case -808719889: return addReceiver(); 1172 default: return super.makeProperty(hash, name); 1173 } 1174 1175 } 1176 1177 @Override 1178 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1179 switch (hash) { 1180 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1181 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1182 case -995410646: /*partOf*/ return new String[] {"Reference"}; 1183 case -892481550: /*status*/ return new String[] {"code"}; 1184 case -791418107: /*patient*/ return new String[] {"Reference"}; 1185 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1186 case 1993333233: /*suppliedItem*/ return new String[] {}; 1187 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period", "Timing"}; 1188 case -1663305268: /*supplier*/ return new String[] {"Reference"}; 1189 case -1429847026: /*destination*/ return new String[] {"Reference"}; 1190 case -808719889: /*receiver*/ return new String[] {"Reference"}; 1191 default: return super.getTypesForProperty(hash, name); 1192 } 1193 1194 } 1195 1196 @Override 1197 public Base addChild(String name) throws FHIRException { 1198 if (name.equals("identifier")) { 1199 return addIdentifier(); 1200 } 1201 else if (name.equals("basedOn")) { 1202 return addBasedOn(); 1203 } 1204 else if (name.equals("partOf")) { 1205 return addPartOf(); 1206 } 1207 else if (name.equals("status")) { 1208 throw new FHIRException("Cannot call addChild on a singleton property SupplyDelivery.status"); 1209 } 1210 else if (name.equals("patient")) { 1211 this.patient = new Reference(); 1212 return this.patient; 1213 } 1214 else if (name.equals("type")) { 1215 this.type = new CodeableConcept(); 1216 return this.type; 1217 } 1218 else if (name.equals("suppliedItem")) { 1219 return addSuppliedItem(); 1220 } 1221 else if (name.equals("occurrenceDateTime")) { 1222 this.occurrence = new DateTimeType(); 1223 return this.occurrence; 1224 } 1225 else if (name.equals("occurrencePeriod")) { 1226 this.occurrence = new Period(); 1227 return this.occurrence; 1228 } 1229 else if (name.equals("occurrenceTiming")) { 1230 this.occurrence = new Timing(); 1231 return this.occurrence; 1232 } 1233 else if (name.equals("supplier")) { 1234 this.supplier = new Reference(); 1235 return this.supplier; 1236 } 1237 else if (name.equals("destination")) { 1238 this.destination = new Reference(); 1239 return this.destination; 1240 } 1241 else if (name.equals("receiver")) { 1242 return addReceiver(); 1243 } 1244 else 1245 return super.addChild(name); 1246 } 1247 1248 public String fhirType() { 1249 return "SupplyDelivery"; 1250 1251 } 1252 1253 public SupplyDelivery copy() { 1254 SupplyDelivery dst = new SupplyDelivery(); 1255 copyValues(dst); 1256 return dst; 1257 } 1258 1259 public void copyValues(SupplyDelivery dst) { 1260 super.copyValues(dst); 1261 if (identifier != null) { 1262 dst.identifier = new ArrayList<Identifier>(); 1263 for (Identifier i : identifier) 1264 dst.identifier.add(i.copy()); 1265 }; 1266 if (basedOn != null) { 1267 dst.basedOn = new ArrayList<Reference>(); 1268 for (Reference i : basedOn) 1269 dst.basedOn.add(i.copy()); 1270 }; 1271 if (partOf != null) { 1272 dst.partOf = new ArrayList<Reference>(); 1273 for (Reference i : partOf) 1274 dst.partOf.add(i.copy()); 1275 }; 1276 dst.status = status == null ? null : status.copy(); 1277 dst.patient = patient == null ? null : patient.copy(); 1278 dst.type = type == null ? null : type.copy(); 1279 if (suppliedItem != null) { 1280 dst.suppliedItem = new ArrayList<SupplyDeliverySuppliedItemComponent>(); 1281 for (SupplyDeliverySuppliedItemComponent i : suppliedItem) 1282 dst.suppliedItem.add(i.copy()); 1283 }; 1284 dst.occurrence = occurrence == null ? null : occurrence.copy(); 1285 dst.supplier = supplier == null ? null : supplier.copy(); 1286 dst.destination = destination == null ? null : destination.copy(); 1287 if (receiver != null) { 1288 dst.receiver = new ArrayList<Reference>(); 1289 for (Reference i : receiver) 1290 dst.receiver.add(i.copy()); 1291 }; 1292 } 1293 1294 protected SupplyDelivery typedCopy() { 1295 return copy(); 1296 } 1297 1298 @Override 1299 public boolean equalsDeep(Base other_) { 1300 if (!super.equalsDeep(other_)) 1301 return false; 1302 if (!(other_ instanceof SupplyDelivery)) 1303 return false; 1304 SupplyDelivery o = (SupplyDelivery) other_; 1305 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(partOf, o.partOf, true) 1306 && compareDeep(status, o.status, true) && compareDeep(patient, o.patient, true) && compareDeep(type, o.type, true) 1307 && compareDeep(suppliedItem, o.suppliedItem, true) && compareDeep(occurrence, o.occurrence, true) 1308 && compareDeep(supplier, o.supplier, true) && compareDeep(destination, o.destination, true) && compareDeep(receiver, o.receiver, true) 1309 ; 1310 } 1311 1312 @Override 1313 public boolean equalsShallow(Base other_) { 1314 if (!super.equalsShallow(other_)) 1315 return false; 1316 if (!(other_ instanceof SupplyDelivery)) 1317 return false; 1318 SupplyDelivery o = (SupplyDelivery) other_; 1319 return compareValues(status, o.status, true); 1320 } 1321 1322 public boolean isEmpty() { 1323 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, partOf 1324 , status, patient, type, suppliedItem, occurrence, supplier, destination, receiver 1325 ); 1326 } 1327 1328 @Override 1329 public ResourceType getResourceType() { 1330 return ResourceType.SupplyDelivery; 1331 } 1332 1333 /** 1334 * Search parameter: <b>receiver</b> 1335 * <p> 1336 * Description: <b>Who collected the Supply</b><br> 1337 * Type: <b>reference</b><br> 1338 * Path: <b>SupplyDelivery.receiver</b><br> 1339 * </p> 1340 */ 1341 @SearchParamDefinition(name="receiver", path="SupplyDelivery.receiver", description="Who collected the Supply", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 1342 public static final String SP_RECEIVER = "receiver"; 1343 /** 1344 * <b>Fluent Client</b> search parameter constant for <b>receiver</b> 1345 * <p> 1346 * Description: <b>Who collected the Supply</b><br> 1347 * Type: <b>reference</b><br> 1348 * Path: <b>SupplyDelivery.receiver</b><br> 1349 * </p> 1350 */ 1351 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECEIVER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RECEIVER); 1352 1353/** 1354 * Constant for fluent queries to be used to add include statements. Specifies 1355 * the path value of "<b>SupplyDelivery:receiver</b>". 1356 */ 1357 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECEIVER = new ca.uhn.fhir.model.api.Include("SupplyDelivery:receiver").toLocked(); 1358 1359 /** 1360 * Search parameter: <b>status</b> 1361 * <p> 1362 * Description: <b>in-progress | completed | abandoned | entered-in-error</b><br> 1363 * Type: <b>token</b><br> 1364 * Path: <b>SupplyDelivery.status</b><br> 1365 * </p> 1366 */ 1367 @SearchParamDefinition(name="status", path="SupplyDelivery.status", description="in-progress | completed | abandoned | entered-in-error", type="token" ) 1368 public static final String SP_STATUS = "status"; 1369 /** 1370 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1371 * <p> 1372 * Description: <b>in-progress | completed | abandoned | entered-in-error</b><br> 1373 * Type: <b>token</b><br> 1374 * Path: <b>SupplyDelivery.status</b><br> 1375 * </p> 1376 */ 1377 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1378 1379 /** 1380 * Search parameter: <b>supplier</b> 1381 * <p> 1382 * Description: <b>Dispenser</b><br> 1383 * Type: <b>reference</b><br> 1384 * Path: <b>SupplyDelivery.supplier</b><br> 1385 * </p> 1386 */ 1387 @SearchParamDefinition(name="supplier", path="SupplyDelivery.supplier", description="Dispenser", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 1388 public static final String SP_SUPPLIER = "supplier"; 1389 /** 1390 * <b>Fluent Client</b> search parameter constant for <b>supplier</b> 1391 * <p> 1392 * Description: <b>Dispenser</b><br> 1393 * Type: <b>reference</b><br> 1394 * Path: <b>SupplyDelivery.supplier</b><br> 1395 * </p> 1396 */ 1397 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPLIER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUPPLIER); 1398 1399/** 1400 * Constant for fluent queries to be used to add include statements. Specifies 1401 * the path value of "<b>SupplyDelivery:supplier</b>". 1402 */ 1403 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPLIER = new ca.uhn.fhir.model.api.Include("SupplyDelivery:supplier").toLocked(); 1404 1405 /** 1406 * Search parameter: <b>identifier</b> 1407 * <p> 1408 * Description: <b>Multiple Resources: 1409 1410* [Account](account.html): Account number 1411* [AdverseEvent](adverseevent.html): Business identifier for the event 1412* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1413* [Appointment](appointment.html): An Identifier of the Appointment 1414* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1415* [Basic](basic.html): Business identifier 1416* [BodyStructure](bodystructure.html): Bodystructure identifier 1417* [CarePlan](careplan.html): External Ids for this plan 1418* [CareTeam](careteam.html): External Ids for this team 1419* [ChargeItem](chargeitem.html): Business Identifier for item 1420* [Claim](claim.html): The primary identifier of the financial resource 1421* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1422* [ClinicalImpression](clinicalimpression.html): Business identifier 1423* [Communication](communication.html): Unique identifier 1424* [CommunicationRequest](communicationrequest.html): Unique identifier 1425* [Composition](composition.html): Version-independent identifier for the Composition 1426* [Condition](condition.html): A unique identifier of the condition record 1427* [Consent](consent.html): Identifier for this record (external references) 1428* [Contract](contract.html): The identity of the contract 1429* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1430* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1431* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1432* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1433* [DeviceRequest](devicerequest.html): Business identifier for request/order 1434* [DeviceUsage](deviceusage.html): Search by identifier 1435* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1436* [DocumentReference](documentreference.html): Identifier of the attachment binary 1437* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1438* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1439* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1440* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1441* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1442* [Flag](flag.html): Business identifier 1443* [Goal](goal.html): External Ids for this goal 1444* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1445* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1446* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1447* [Immunization](immunization.html): Business identifier 1448* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1449* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1450* [Invoice](invoice.html): Business Identifier for item 1451* [List](list.html): Business identifier 1452* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1453* [Medication](medication.html): Returns medications with this external identifier 1454* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1455* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1456* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1457* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1458* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1459* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1460* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1461* [Observation](observation.html): The unique id for a particular observation 1462* [Person](person.html): A person Identifier 1463* [Procedure](procedure.html): A unique identifier for a procedure 1464* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1465* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1466* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1467* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1468* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1469* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1470* [Specimen](specimen.html): The unique identifier associated with the specimen 1471* [SupplyDelivery](supplydelivery.html): External identifier 1472* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1473* [Task](task.html): Search for a task instance by its business identifier 1474* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1475</b><br> 1476 * Type: <b>token</b><br> 1477 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1478 * </p> 1479 */ 1480 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 1481 public static final String SP_IDENTIFIER = "identifier"; 1482 /** 1483 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1484 * <p> 1485 * Description: <b>Multiple Resources: 1486 1487* [Account](account.html): Account number 1488* [AdverseEvent](adverseevent.html): Business identifier for the event 1489* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1490* [Appointment](appointment.html): An Identifier of the Appointment 1491* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1492* [Basic](basic.html): Business identifier 1493* [BodyStructure](bodystructure.html): Bodystructure identifier 1494* [CarePlan](careplan.html): External Ids for this plan 1495* [CareTeam](careteam.html): External Ids for this team 1496* [ChargeItem](chargeitem.html): Business Identifier for item 1497* [Claim](claim.html): The primary identifier of the financial resource 1498* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1499* [ClinicalImpression](clinicalimpression.html): Business identifier 1500* [Communication](communication.html): Unique identifier 1501* [CommunicationRequest](communicationrequest.html): Unique identifier 1502* [Composition](composition.html): Version-independent identifier for the Composition 1503* [Condition](condition.html): A unique identifier of the condition record 1504* [Consent](consent.html): Identifier for this record (external references) 1505* [Contract](contract.html): The identity of the contract 1506* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1507* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1508* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1509* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1510* [DeviceRequest](devicerequest.html): Business identifier for request/order 1511* [DeviceUsage](deviceusage.html): Search by identifier 1512* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1513* [DocumentReference](documentreference.html): Identifier of the attachment binary 1514* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1515* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1516* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1517* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1518* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1519* [Flag](flag.html): Business identifier 1520* [Goal](goal.html): External Ids for this goal 1521* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1522* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1523* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1524* [Immunization](immunization.html): Business identifier 1525* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1526* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1527* [Invoice](invoice.html): Business Identifier for item 1528* [List](list.html): Business identifier 1529* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1530* [Medication](medication.html): Returns medications with this external identifier 1531* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1532* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1533* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1534* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1535* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1536* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1537* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1538* [Observation](observation.html): The unique id for a particular observation 1539* [Person](person.html): A person Identifier 1540* [Procedure](procedure.html): A unique identifier for a procedure 1541* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1542* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1543* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1544* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1545* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1546* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1547* [Specimen](specimen.html): The unique identifier associated with the specimen 1548* [SupplyDelivery](supplydelivery.html): External identifier 1549* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1550* [Task](task.html): Search for a task instance by its business identifier 1551* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1552</b><br> 1553 * Type: <b>token</b><br> 1554 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1555 * </p> 1556 */ 1557 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1558 1559 /** 1560 * Search parameter: <b>patient</b> 1561 * <p> 1562 * Description: <b>Multiple Resources: 1563 1564* [Account](account.html): The entity that caused the expenses 1565* [AdverseEvent](adverseevent.html): Subject impacted by event 1566* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 1567* [Appointment](appointment.html): One of the individuals of the appointment is this patient 1568* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 1569* [AuditEvent](auditevent.html): Where the activity involved patient data 1570* [Basic](basic.html): Identifies the focus of this resource 1571* [BodyStructure](bodystructure.html): Who this is about 1572* [CarePlan](careplan.html): Who the care plan is for 1573* [CareTeam](careteam.html): Who care team is for 1574* [ChargeItem](chargeitem.html): Individual service was done for/to 1575* [Claim](claim.html): Patient receiving the products or services 1576* [ClaimResponse](claimresponse.html): The subject of care 1577* [ClinicalImpression](clinicalimpression.html): Patient assessed 1578* [Communication](communication.html): Focus of message 1579* [CommunicationRequest](communicationrequest.html): Focus of message 1580* [Composition](composition.html): Who and/or what the composition is about 1581* [Condition](condition.html): Who has the condition? 1582* [Consent](consent.html): Who the consent applies to 1583* [Contract](contract.html): The identity of the subject of the contract (if a patient) 1584* [Coverage](coverage.html): Retrieve coverages for a patient 1585* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 1586* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 1587* [DetectedIssue](detectedissue.html): Associated patient 1588* [DeviceRequest](devicerequest.html): Individual the service is ordered for 1589* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 1590* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 1591* [DocumentReference](documentreference.html): Who/what is the subject of the document 1592* [Encounter](encounter.html): The patient present at the encounter 1593* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 1594* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 1595* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 1596* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 1597* [Flag](flag.html): The identity of a subject to list flags for 1598* [Goal](goal.html): Who this goal is intended for 1599* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 1600* [ImagingSelection](imagingselection.html): Who the study is about 1601* [ImagingStudy](imagingstudy.html): Who the study is about 1602* [Immunization](immunization.html): The patient for the vaccination record 1603* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 1604* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 1605* [Invoice](invoice.html): Recipient(s) of goods and services 1606* [List](list.html): If all resources have the same subject 1607* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 1608* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 1609* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 1610* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 1611* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 1612* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 1613* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 1614* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 1615* [Observation](observation.html): The subject that the observation is about (if patient) 1616* [Person](person.html): The Person links to this Patient 1617* [Procedure](procedure.html): Search by subject - a patient 1618* [Provenance](provenance.html): Where the activity involved patient data 1619* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 1620* [RelatedPerson](relatedperson.html): The patient this related person is related to 1621* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 1622* [ResearchSubject](researchsubject.html): Who or what is part of study 1623* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 1624* [ServiceRequest](servicerequest.html): Search by subject - a patient 1625* [Specimen](specimen.html): The patient the specimen comes from 1626* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 1627* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 1628* [Task](task.html): Search by patient 1629* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 1630</b><br> 1631 * Type: <b>reference</b><br> 1632 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 1633 * </p> 1634 */ 1635 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 1636 public static final String SP_PATIENT = "patient"; 1637 /** 1638 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1639 * <p> 1640 * Description: <b>Multiple Resources: 1641 1642* [Account](account.html): The entity that caused the expenses 1643* [AdverseEvent](adverseevent.html): Subject impacted by event 1644* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 1645* [Appointment](appointment.html): One of the individuals of the appointment is this patient 1646* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 1647* [AuditEvent](auditevent.html): Where the activity involved patient data 1648* [Basic](basic.html): Identifies the focus of this resource 1649* [BodyStructure](bodystructure.html): Who this is about 1650* [CarePlan](careplan.html): Who the care plan is for 1651* [CareTeam](careteam.html): Who care team is for 1652* [ChargeItem](chargeitem.html): Individual service was done for/to 1653* [Claim](claim.html): Patient receiving the products or services 1654* [ClaimResponse](claimresponse.html): The subject of care 1655* [ClinicalImpression](clinicalimpression.html): Patient assessed 1656* [Communication](communication.html): Focus of message 1657* [CommunicationRequest](communicationrequest.html): Focus of message 1658* [Composition](composition.html): Who and/or what the composition is about 1659* [Condition](condition.html): Who has the condition? 1660* [Consent](consent.html): Who the consent applies to 1661* [Contract](contract.html): The identity of the subject of the contract (if a patient) 1662* [Coverage](coverage.html): Retrieve coverages for a patient 1663* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 1664* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 1665* [DetectedIssue](detectedissue.html): Associated patient 1666* [DeviceRequest](devicerequest.html): Individual the service is ordered for 1667* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 1668* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 1669* [DocumentReference](documentreference.html): Who/what is the subject of the document 1670* [Encounter](encounter.html): The patient present at the encounter 1671* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 1672* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 1673* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 1674* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 1675* [Flag](flag.html): The identity of a subject to list flags for 1676* [Goal](goal.html): Who this goal is intended for 1677* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 1678* [ImagingSelection](imagingselection.html): Who the study is about 1679* [ImagingStudy](imagingstudy.html): Who the study is about 1680* [Immunization](immunization.html): The patient for the vaccination record 1681* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 1682* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 1683* [Invoice](invoice.html): Recipient(s) of goods and services 1684* [List](list.html): If all resources have the same subject 1685* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 1686* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 1687* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 1688* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 1689* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 1690* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 1691* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 1692* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 1693* [Observation](observation.html): The subject that the observation is about (if patient) 1694* [Person](person.html): The Person links to this Patient 1695* [Procedure](procedure.html): Search by subject - a patient 1696* [Provenance](provenance.html): Where the activity involved patient data 1697* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 1698* [RelatedPerson](relatedperson.html): The patient this related person is related to 1699* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 1700* [ResearchSubject](researchsubject.html): Who or what is part of study 1701* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 1702* [ServiceRequest](servicerequest.html): Search by subject - a patient 1703* [Specimen](specimen.html): The patient the specimen comes from 1704* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 1705* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 1706* [Task](task.html): Search by patient 1707* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 1708</b><br> 1709 * Type: <b>reference</b><br> 1710 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 1711 * </p> 1712 */ 1713 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1714 1715/** 1716 * Constant for fluent queries to be used to add include statements. Specifies 1717 * the path value of "<b>SupplyDelivery:patient</b>". 1718 */ 1719 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("SupplyDelivery:patient").toLocked(); 1720 1721 1722} 1723