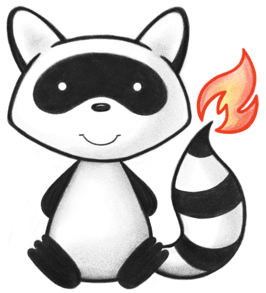
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Record of delivery of what is supplied. 052 */ 053@ResourceDef(name="SupplyDelivery", profile="http://hl7.org/fhir/StructureDefinition/SupplyDelivery") 054public class SupplyDelivery extends DomainResource { 055 056 public enum SupplyDeliveryStatus { 057 /** 058 * Supply has been requested, but not delivered. 059 */ 060 INPROGRESS, 061 /** 062 * Supply has been delivered (\"completed\"). 063 */ 064 COMPLETED, 065 /** 066 * Delivery was not completed. 067 */ 068 ABANDONED, 069 /** 070 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"abandoned\" rather than \"entered-in-error\".). 071 */ 072 ENTEREDINERROR, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static SupplyDeliveryStatus fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("in-progress".equals(codeString)) 081 return INPROGRESS; 082 if ("completed".equals(codeString)) 083 return COMPLETED; 084 if ("abandoned".equals(codeString)) 085 return ABANDONED; 086 if ("entered-in-error".equals(codeString)) 087 return ENTEREDINERROR; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown SupplyDeliveryStatus code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case INPROGRESS: return "in-progress"; 096 case COMPLETED: return "completed"; 097 case ABANDONED: return "abandoned"; 098 case ENTEREDINERROR: return "entered-in-error"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case INPROGRESS: return "http://hl7.org/fhir/supplydelivery-status"; 106 case COMPLETED: return "http://hl7.org/fhir/supplydelivery-status"; 107 case ABANDONED: return "http://hl7.org/fhir/supplydelivery-status"; 108 case ENTEREDINERROR: return "http://hl7.org/fhir/supplydelivery-status"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case INPROGRESS: return "Supply has been requested, but not delivered."; 116 case COMPLETED: return "Supply has been delivered (\"completed\")."; 117 case ABANDONED: return "Delivery was not completed."; 118 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"abandoned\" rather than \"entered-in-error\".)."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case INPROGRESS: return "In Progress"; 126 case COMPLETED: return "Delivered"; 127 case ABANDONED: return "Abandoned"; 128 case ENTEREDINERROR: return "Entered In Error"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class SupplyDeliveryStatusEnumFactory implements EnumFactory<SupplyDeliveryStatus> { 136 public SupplyDeliveryStatus fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("in-progress".equals(codeString)) 141 return SupplyDeliveryStatus.INPROGRESS; 142 if ("completed".equals(codeString)) 143 return SupplyDeliveryStatus.COMPLETED; 144 if ("abandoned".equals(codeString)) 145 return SupplyDeliveryStatus.ABANDONED; 146 if ("entered-in-error".equals(codeString)) 147 return SupplyDeliveryStatus.ENTEREDINERROR; 148 throw new IllegalArgumentException("Unknown SupplyDeliveryStatus code '"+codeString+"'"); 149 } 150 public Enumeration<SupplyDeliveryStatus> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.NULL, code); 155 String codeString = ((PrimitiveType) code).asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.NULL, code); 158 if ("in-progress".equals(codeString)) 159 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.INPROGRESS, code); 160 if ("completed".equals(codeString)) 161 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.COMPLETED, code); 162 if ("abandoned".equals(codeString)) 163 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.ABANDONED, code); 164 if ("entered-in-error".equals(codeString)) 165 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.ENTEREDINERROR, code); 166 throw new FHIRException("Unknown SupplyDeliveryStatus code '"+codeString+"'"); 167 } 168 public String toCode(SupplyDeliveryStatus code) { 169 if (code == SupplyDeliveryStatus.INPROGRESS) 170 return "in-progress"; 171 if (code == SupplyDeliveryStatus.COMPLETED) 172 return "completed"; 173 if (code == SupplyDeliveryStatus.ABANDONED) 174 return "abandoned"; 175 if (code == SupplyDeliveryStatus.ENTEREDINERROR) 176 return "entered-in-error"; 177 return "?"; 178 } 179 public String toSystem(SupplyDeliveryStatus code) { 180 return code.getSystem(); 181 } 182 } 183 184 @Block() 185 public static class SupplyDeliverySuppliedItemComponent extends BackboneElement implements IBaseBackboneElement { 186 /** 187 * The amount of the item that has been supplied. Unit of measure may be included. 188 */ 189 @Child(name = "quantity", type = {Quantity.class}, order=1, min=0, max=1, modifier=false, summary=false) 190 @Description(shortDefinition="Amount supplied", formalDefinition="The amount of the item that has been supplied. Unit of measure may be included." ) 191 protected Quantity quantity; 192 193 /** 194 * Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list. 195 */ 196 @Child(name = "item", type = {CodeableConcept.class, Medication.class, Substance.class, Device.class, BiologicallyDerivedProduct.class, NutritionProduct.class, InventoryItem.class}, order=2, min=0, max=1, modifier=false, summary=false) 197 @Description(shortDefinition="Medication, Substance, Device or Biologically Derived Product supplied", formalDefinition="Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list." ) 198 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/supplydelivery-supplyitemtype") 199 protected DataType item; 200 201 private static final long serialVersionUID = -615919419L; 202 203 /** 204 * Constructor 205 */ 206 public SupplyDeliverySuppliedItemComponent() { 207 super(); 208 } 209 210 /** 211 * @return {@link #quantity} (The amount of the item that has been supplied. Unit of measure may be included.) 212 */ 213 public Quantity getQuantity() { 214 if (this.quantity == null) 215 if (Configuration.errorOnAutoCreate()) 216 throw new Error("Attempt to auto-create SupplyDeliverySuppliedItemComponent.quantity"); 217 else if (Configuration.doAutoCreate()) 218 this.quantity = new Quantity(); // cc 219 return this.quantity; 220 } 221 222 public boolean hasQuantity() { 223 return this.quantity != null && !this.quantity.isEmpty(); 224 } 225 226 /** 227 * @param value {@link #quantity} (The amount of the item that has been supplied. Unit of measure may be included.) 228 */ 229 public SupplyDeliverySuppliedItemComponent setQuantity(Quantity value) { 230 this.quantity = value; 231 return this; 232 } 233 234 /** 235 * @return {@link #item} (Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.) 236 */ 237 public DataType getItem() { 238 return this.item; 239 } 240 241 /** 242 * @return {@link #item} (Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.) 243 */ 244 public CodeableConcept getItemCodeableConcept() throws FHIRException { 245 if (this.item == null) 246 this.item = new CodeableConcept(); 247 if (!(this.item instanceof CodeableConcept)) 248 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.item.getClass().getName()+" was encountered"); 249 return (CodeableConcept) this.item; 250 } 251 252 public boolean hasItemCodeableConcept() { 253 return this != null && this.item instanceof CodeableConcept; 254 } 255 256 /** 257 * @return {@link #item} (Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.) 258 */ 259 public Reference getItemReference() throws FHIRException { 260 if (this.item == null) 261 this.item = new Reference(); 262 if (!(this.item instanceof Reference)) 263 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.item.getClass().getName()+" was encountered"); 264 return (Reference) this.item; 265 } 266 267 public boolean hasItemReference() { 268 return this != null && this.item instanceof Reference; 269 } 270 271 public boolean hasItem() { 272 return this.item != null && !this.item.isEmpty(); 273 } 274 275 /** 276 * @param value {@link #item} (Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.) 277 */ 278 public SupplyDeliverySuppliedItemComponent setItem(DataType value) { 279 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 280 throw new FHIRException("Not the right type for SupplyDelivery.suppliedItem.item[x]: "+value.fhirType()); 281 this.item = value; 282 return this; 283 } 284 285 protected void listChildren(List<Property> children) { 286 super.listChildren(children); 287 children.add(new Property("quantity", "Quantity", "The amount of the item that has been supplied. Unit of measure may be included.", 0, 1, quantity)); 288 children.add(new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device|BiologicallyDerivedProduct|NutritionProduct|InventoryItem)", "Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item)); 289 } 290 291 @Override 292 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 293 switch (_hash) { 294 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The amount of the item that has been supplied. Unit of measure may be included.", 0, 1, quantity); 295 case 2116201613: /*item[x]*/ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device|BiologicallyDerivedProduct|NutritionProduct|InventoryItem)", "Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item); 296 case 3242771: /*item*/ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device|BiologicallyDerivedProduct|NutritionProduct|InventoryItem)", "Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item); 297 case 106644494: /*itemCodeableConcept*/ return new Property("item[x]", "CodeableConcept", "Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item); 298 case 1376364920: /*itemReference*/ return new Property("item[x]", "Reference(Medication|Substance|Device|BiologicallyDerivedProduct|NutritionProduct|InventoryItem)", "Identifies the medication, substance, device or biologically derived product being supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item); 299 default: return super.getNamedProperty(_hash, _name, _checkValid); 300 } 301 302 } 303 304 @Override 305 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 306 switch (hash) { 307 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 308 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // DataType 309 default: return super.getProperty(hash, name, checkValid); 310 } 311 312 } 313 314 @Override 315 public Base setProperty(int hash, String name, Base value) throws FHIRException { 316 switch (hash) { 317 case -1285004149: // quantity 318 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 319 return value; 320 case 3242771: // item 321 this.item = TypeConvertor.castToType(value); // DataType 322 return value; 323 default: return super.setProperty(hash, name, value); 324 } 325 326 } 327 328 @Override 329 public Base setProperty(String name, Base value) throws FHIRException { 330 if (name.equals("quantity")) { 331 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 332 } else if (name.equals("item[x]")) { 333 this.item = TypeConvertor.castToType(value); // DataType 334 } else 335 return super.setProperty(name, value); 336 return value; 337 } 338 339 @Override 340 public void removeChild(String name, Base value) throws FHIRException { 341 if (name.equals("quantity")) { 342 this.quantity = null; 343 } else if (name.equals("item[x]")) { 344 this.item = null; 345 } else 346 super.removeChild(name, value); 347 348 } 349 350 @Override 351 public Base makeProperty(int hash, String name) throws FHIRException { 352 switch (hash) { 353 case -1285004149: return getQuantity(); 354 case 2116201613: return getItem(); 355 case 3242771: return getItem(); 356 default: return super.makeProperty(hash, name); 357 } 358 359 } 360 361 @Override 362 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 363 switch (hash) { 364 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 365 case 3242771: /*item*/ return new String[] {"CodeableConcept", "Reference"}; 366 default: return super.getTypesForProperty(hash, name); 367 } 368 369 } 370 371 @Override 372 public Base addChild(String name) throws FHIRException { 373 if (name.equals("quantity")) { 374 this.quantity = new Quantity(); 375 return this.quantity; 376 } 377 else if (name.equals("itemCodeableConcept")) { 378 this.item = new CodeableConcept(); 379 return this.item; 380 } 381 else if (name.equals("itemReference")) { 382 this.item = new Reference(); 383 return this.item; 384 } 385 else 386 return super.addChild(name); 387 } 388 389 public SupplyDeliverySuppliedItemComponent copy() { 390 SupplyDeliverySuppliedItemComponent dst = new SupplyDeliverySuppliedItemComponent(); 391 copyValues(dst); 392 return dst; 393 } 394 395 public void copyValues(SupplyDeliverySuppliedItemComponent dst) { 396 super.copyValues(dst); 397 dst.quantity = quantity == null ? null : quantity.copy(); 398 dst.item = item == null ? null : item.copy(); 399 } 400 401 @Override 402 public boolean equalsDeep(Base other_) { 403 if (!super.equalsDeep(other_)) 404 return false; 405 if (!(other_ instanceof SupplyDeliverySuppliedItemComponent)) 406 return false; 407 SupplyDeliverySuppliedItemComponent o = (SupplyDeliverySuppliedItemComponent) other_; 408 return compareDeep(quantity, o.quantity, true) && compareDeep(item, o.item, true); 409 } 410 411 @Override 412 public boolean equalsShallow(Base other_) { 413 if (!super.equalsShallow(other_)) 414 return false; 415 if (!(other_ instanceof SupplyDeliverySuppliedItemComponent)) 416 return false; 417 SupplyDeliverySuppliedItemComponent o = (SupplyDeliverySuppliedItemComponent) other_; 418 return true; 419 } 420 421 public boolean isEmpty() { 422 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(quantity, item); 423 } 424 425 public String fhirType() { 426 return "SupplyDelivery.suppliedItem"; 427 428 } 429 430 } 431 432 /** 433 * Identifier for the supply delivery event that is used to identify it across multiple disparate systems. 434 */ 435 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 436 @Description(shortDefinition="External identifier", formalDefinition="Identifier for the supply delivery event that is used to identify it across multiple disparate systems." ) 437 protected List<Identifier> identifier; 438 439 /** 440 * A plan, proposal or order that is fulfilled in whole or in part by this event. 441 */ 442 @Child(name = "basedOn", type = {SupplyRequest.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 443 @Description(shortDefinition="Fulfills plan, proposal or order", formalDefinition="A plan, proposal or order that is fulfilled in whole or in part by this event." ) 444 protected List<Reference> basedOn; 445 446 /** 447 * A larger event of which this particular event is a component or step. 448 */ 449 @Child(name = "partOf", type = {SupplyDelivery.class, Contract.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 450 @Description(shortDefinition="Part of referenced event", formalDefinition="A larger event of which this particular event is a component or step." ) 451 protected List<Reference> partOf; 452 453 /** 454 * A code specifying the state of the dispense event. 455 */ 456 @Child(name = "status", type = {CodeType.class}, order=3, min=0, max=1, modifier=true, summary=true) 457 @Description(shortDefinition="in-progress | completed | abandoned | entered-in-error", formalDefinition="A code specifying the state of the dispense event." ) 458 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/supplydelivery-status") 459 protected Enumeration<SupplyDeliveryStatus> status; 460 461 /** 462 * A link to a resource representing the person whom the delivered item is for. 463 */ 464 @Child(name = "patient", type = {Patient.class}, order=4, min=0, max=1, modifier=false, summary=false) 465 @Description(shortDefinition="Patient for whom the item is supplied", formalDefinition="A link to a resource representing the person whom the delivered item is for." ) 466 protected Reference patient; 467 468 /** 469 * Indicates the type of supply being provided. Examples include: Medication, Device, Biologically Derived Product. 470 */ 471 @Child(name = "type", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 472 @Description(shortDefinition="Category of supply event", formalDefinition="Indicates the type of supply being provided. Examples include: Medication, Device, Biologically Derived Product." ) 473 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/supplydelivery-supplyitemtype") 474 protected CodeableConcept type; 475 476 /** 477 * The item that is being delivered or has been supplied. 478 */ 479 @Child(name = "suppliedItem", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 480 @Description(shortDefinition="The item that is delivered or supplied", formalDefinition="The item that is being delivered or has been supplied." ) 481 protected List<SupplyDeliverySuppliedItemComponent> suppliedItem; 482 483 /** 484 * The date or time(s) the activity occurred. 485 */ 486 @Child(name = "occurrence", type = {DateTimeType.class, Period.class, Timing.class}, order=7, min=0, max=1, modifier=false, summary=true) 487 @Description(shortDefinition="When event occurred", formalDefinition="The date or time(s) the activity occurred." ) 488 protected DataType occurrence; 489 490 /** 491 * The individual or organization responsible for supplying the delivery. 492 */ 493 @Child(name = "supplier", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=8, min=0, max=1, modifier=false, summary=false) 494 @Description(shortDefinition="The item supplier", formalDefinition="The individual or organization responsible for supplying the delivery." ) 495 protected Reference supplier; 496 497 /** 498 * Identification of the facility/location where the delivery was shipped to. 499 */ 500 @Child(name = "destination", type = {Location.class}, order=9, min=0, max=1, modifier=false, summary=false) 501 @Description(shortDefinition="Where the delivery was sent", formalDefinition="Identification of the facility/location where the delivery was shipped to." ) 502 protected Reference destination; 503 504 /** 505 * Identifies the individual or organization that received the delivery. 506 */ 507 @Child(name = "receiver", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 508 @Description(shortDefinition="Who received the delivery", formalDefinition="Identifies the individual or organization that received the delivery." ) 509 protected List<Reference> receiver; 510 511 private static final long serialVersionUID = -734856482L; 512 513 /** 514 * Constructor 515 */ 516 public SupplyDelivery() { 517 super(); 518 } 519 520 /** 521 * @return {@link #identifier} (Identifier for the supply delivery event that is used to identify it across multiple disparate systems.) 522 */ 523 public List<Identifier> getIdentifier() { 524 if (this.identifier == null) 525 this.identifier = new ArrayList<Identifier>(); 526 return this.identifier; 527 } 528 529 /** 530 * @return Returns a reference to <code>this</code> for easy method chaining 531 */ 532 public SupplyDelivery setIdentifier(List<Identifier> theIdentifier) { 533 this.identifier = theIdentifier; 534 return this; 535 } 536 537 public boolean hasIdentifier() { 538 if (this.identifier == null) 539 return false; 540 for (Identifier item : this.identifier) 541 if (!item.isEmpty()) 542 return true; 543 return false; 544 } 545 546 public Identifier addIdentifier() { //3 547 Identifier t = new Identifier(); 548 if (this.identifier == null) 549 this.identifier = new ArrayList<Identifier>(); 550 this.identifier.add(t); 551 return t; 552 } 553 554 public SupplyDelivery addIdentifier(Identifier t) { //3 555 if (t == null) 556 return this; 557 if (this.identifier == null) 558 this.identifier = new ArrayList<Identifier>(); 559 this.identifier.add(t); 560 return this; 561 } 562 563 /** 564 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 565 */ 566 public Identifier getIdentifierFirstRep() { 567 if (getIdentifier().isEmpty()) { 568 addIdentifier(); 569 } 570 return getIdentifier().get(0); 571 } 572 573 /** 574 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in whole or in part by this event.) 575 */ 576 public List<Reference> getBasedOn() { 577 if (this.basedOn == null) 578 this.basedOn = new ArrayList<Reference>(); 579 return this.basedOn; 580 } 581 582 /** 583 * @return Returns a reference to <code>this</code> for easy method chaining 584 */ 585 public SupplyDelivery setBasedOn(List<Reference> theBasedOn) { 586 this.basedOn = theBasedOn; 587 return this; 588 } 589 590 public boolean hasBasedOn() { 591 if (this.basedOn == null) 592 return false; 593 for (Reference item : this.basedOn) 594 if (!item.isEmpty()) 595 return true; 596 return false; 597 } 598 599 public Reference addBasedOn() { //3 600 Reference t = new Reference(); 601 if (this.basedOn == null) 602 this.basedOn = new ArrayList<Reference>(); 603 this.basedOn.add(t); 604 return t; 605 } 606 607 public SupplyDelivery addBasedOn(Reference t) { //3 608 if (t == null) 609 return this; 610 if (this.basedOn == null) 611 this.basedOn = new ArrayList<Reference>(); 612 this.basedOn.add(t); 613 return this; 614 } 615 616 /** 617 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 618 */ 619 public Reference getBasedOnFirstRep() { 620 if (getBasedOn().isEmpty()) { 621 addBasedOn(); 622 } 623 return getBasedOn().get(0); 624 } 625 626 /** 627 * @return {@link #partOf} (A larger event of which this particular event is a component or step.) 628 */ 629 public List<Reference> getPartOf() { 630 if (this.partOf == null) 631 this.partOf = new ArrayList<Reference>(); 632 return this.partOf; 633 } 634 635 /** 636 * @return Returns a reference to <code>this</code> for easy method chaining 637 */ 638 public SupplyDelivery setPartOf(List<Reference> thePartOf) { 639 this.partOf = thePartOf; 640 return this; 641 } 642 643 public boolean hasPartOf() { 644 if (this.partOf == null) 645 return false; 646 for (Reference item : this.partOf) 647 if (!item.isEmpty()) 648 return true; 649 return false; 650 } 651 652 public Reference addPartOf() { //3 653 Reference t = new Reference(); 654 if (this.partOf == null) 655 this.partOf = new ArrayList<Reference>(); 656 this.partOf.add(t); 657 return t; 658 } 659 660 public SupplyDelivery addPartOf(Reference t) { //3 661 if (t == null) 662 return this; 663 if (this.partOf == null) 664 this.partOf = new ArrayList<Reference>(); 665 this.partOf.add(t); 666 return this; 667 } 668 669 /** 670 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 671 */ 672 public Reference getPartOfFirstRep() { 673 if (getPartOf().isEmpty()) { 674 addPartOf(); 675 } 676 return getPartOf().get(0); 677 } 678 679 /** 680 * @return {@link #status} (A code specifying the state of the dispense event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 681 */ 682 public Enumeration<SupplyDeliveryStatus> getStatusElement() { 683 if (this.status == null) 684 if (Configuration.errorOnAutoCreate()) 685 throw new Error("Attempt to auto-create SupplyDelivery.status"); 686 else if (Configuration.doAutoCreate()) 687 this.status = new Enumeration<SupplyDeliveryStatus>(new SupplyDeliveryStatusEnumFactory()); // bb 688 return this.status; 689 } 690 691 public boolean hasStatusElement() { 692 return this.status != null && !this.status.isEmpty(); 693 } 694 695 public boolean hasStatus() { 696 return this.status != null && !this.status.isEmpty(); 697 } 698 699 /** 700 * @param value {@link #status} (A code specifying the state of the dispense event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 701 */ 702 public SupplyDelivery setStatusElement(Enumeration<SupplyDeliveryStatus> value) { 703 this.status = value; 704 return this; 705 } 706 707 /** 708 * @return A code specifying the state of the dispense event. 709 */ 710 public SupplyDeliveryStatus getStatus() { 711 return this.status == null ? null : this.status.getValue(); 712 } 713 714 /** 715 * @param value A code specifying the state of the dispense event. 716 */ 717 public SupplyDelivery setStatus(SupplyDeliveryStatus value) { 718 if (value == null) 719 this.status = null; 720 else { 721 if (this.status == null) 722 this.status = new Enumeration<SupplyDeliveryStatus>(new SupplyDeliveryStatusEnumFactory()); 723 this.status.setValue(value); 724 } 725 return this; 726 } 727 728 /** 729 * @return {@link #patient} (A link to a resource representing the person whom the delivered item is for.) 730 */ 731 public Reference getPatient() { 732 if (this.patient == null) 733 if (Configuration.errorOnAutoCreate()) 734 throw new Error("Attempt to auto-create SupplyDelivery.patient"); 735 else if (Configuration.doAutoCreate()) 736 this.patient = new Reference(); // cc 737 return this.patient; 738 } 739 740 public boolean hasPatient() { 741 return this.patient != null && !this.patient.isEmpty(); 742 } 743 744 /** 745 * @param value {@link #patient} (A link to a resource representing the person whom the delivered item is for.) 746 */ 747 public SupplyDelivery setPatient(Reference value) { 748 this.patient = value; 749 return this; 750 } 751 752 /** 753 * @return {@link #type} (Indicates the type of supply being provided. Examples include: Medication, Device, Biologically Derived Product.) 754 */ 755 public CodeableConcept getType() { 756 if (this.type == null) 757 if (Configuration.errorOnAutoCreate()) 758 throw new Error("Attempt to auto-create SupplyDelivery.type"); 759 else if (Configuration.doAutoCreate()) 760 this.type = new CodeableConcept(); // cc 761 return this.type; 762 } 763 764 public boolean hasType() { 765 return this.type != null && !this.type.isEmpty(); 766 } 767 768 /** 769 * @param value {@link #type} (Indicates the type of supply being provided. Examples include: Medication, Device, Biologically Derived Product.) 770 */ 771 public SupplyDelivery setType(CodeableConcept value) { 772 this.type = value; 773 return this; 774 } 775 776 /** 777 * @return {@link #suppliedItem} (The item that is being delivered or has been supplied.) 778 */ 779 public List<SupplyDeliverySuppliedItemComponent> getSuppliedItem() { 780 if (this.suppliedItem == null) 781 this.suppliedItem = new ArrayList<SupplyDeliverySuppliedItemComponent>(); 782 return this.suppliedItem; 783 } 784 785 /** 786 * @return Returns a reference to <code>this</code> for easy method chaining 787 */ 788 public SupplyDelivery setSuppliedItem(List<SupplyDeliverySuppliedItemComponent> theSuppliedItem) { 789 this.suppliedItem = theSuppliedItem; 790 return this; 791 } 792 793 public boolean hasSuppliedItem() { 794 if (this.suppliedItem == null) 795 return false; 796 for (SupplyDeliverySuppliedItemComponent item : this.suppliedItem) 797 if (!item.isEmpty()) 798 return true; 799 return false; 800 } 801 802 public SupplyDeliverySuppliedItemComponent addSuppliedItem() { //3 803 SupplyDeliverySuppliedItemComponent t = new SupplyDeliverySuppliedItemComponent(); 804 if (this.suppliedItem == null) 805 this.suppliedItem = new ArrayList<SupplyDeliverySuppliedItemComponent>(); 806 this.suppliedItem.add(t); 807 return t; 808 } 809 810 public SupplyDelivery addSuppliedItem(SupplyDeliverySuppliedItemComponent t) { //3 811 if (t == null) 812 return this; 813 if (this.suppliedItem == null) 814 this.suppliedItem = new ArrayList<SupplyDeliverySuppliedItemComponent>(); 815 this.suppliedItem.add(t); 816 return this; 817 } 818 819 /** 820 * @return The first repetition of repeating field {@link #suppliedItem}, creating it if it does not already exist {3} 821 */ 822 public SupplyDeliverySuppliedItemComponent getSuppliedItemFirstRep() { 823 if (getSuppliedItem().isEmpty()) { 824 addSuppliedItem(); 825 } 826 return getSuppliedItem().get(0); 827 } 828 829 /** 830 * @return {@link #occurrence} (The date or time(s) the activity occurred.) 831 */ 832 public DataType getOccurrence() { 833 return this.occurrence; 834 } 835 836 /** 837 * @return {@link #occurrence} (The date or time(s) the activity occurred.) 838 */ 839 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 840 if (this.occurrence == null) 841 this.occurrence = new DateTimeType(); 842 if (!(this.occurrence instanceof DateTimeType)) 843 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 844 return (DateTimeType) this.occurrence; 845 } 846 847 public boolean hasOccurrenceDateTimeType() { 848 return this != null && this.occurrence instanceof DateTimeType; 849 } 850 851 /** 852 * @return {@link #occurrence} (The date or time(s) the activity occurred.) 853 */ 854 public Period getOccurrencePeriod() throws FHIRException { 855 if (this.occurrence == null) 856 this.occurrence = new Period(); 857 if (!(this.occurrence instanceof Period)) 858 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 859 return (Period) this.occurrence; 860 } 861 862 public boolean hasOccurrencePeriod() { 863 return this != null && this.occurrence instanceof Period; 864 } 865 866 /** 867 * @return {@link #occurrence} (The date or time(s) the activity occurred.) 868 */ 869 public Timing getOccurrenceTiming() throws FHIRException { 870 if (this.occurrence == null) 871 this.occurrence = new Timing(); 872 if (!(this.occurrence instanceof Timing)) 873 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 874 return (Timing) this.occurrence; 875 } 876 877 public boolean hasOccurrenceTiming() { 878 return this != null && this.occurrence instanceof Timing; 879 } 880 881 public boolean hasOccurrence() { 882 return this.occurrence != null && !this.occurrence.isEmpty(); 883 } 884 885 /** 886 * @param value {@link #occurrence} (The date or time(s) the activity occurred.) 887 */ 888 public SupplyDelivery setOccurrence(DataType value) { 889 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 890 throw new FHIRException("Not the right type for SupplyDelivery.occurrence[x]: "+value.fhirType()); 891 this.occurrence = value; 892 return this; 893 } 894 895 /** 896 * @return {@link #supplier} (The individual or organization responsible for supplying the delivery.) 897 */ 898 public Reference getSupplier() { 899 if (this.supplier == null) 900 if (Configuration.errorOnAutoCreate()) 901 throw new Error("Attempt to auto-create SupplyDelivery.supplier"); 902 else if (Configuration.doAutoCreate()) 903 this.supplier = new Reference(); // cc 904 return this.supplier; 905 } 906 907 public boolean hasSupplier() { 908 return this.supplier != null && !this.supplier.isEmpty(); 909 } 910 911 /** 912 * @param value {@link #supplier} (The individual or organization responsible for supplying the delivery.) 913 */ 914 public SupplyDelivery setSupplier(Reference value) { 915 this.supplier = value; 916 return this; 917 } 918 919 /** 920 * @return {@link #destination} (Identification of the facility/location where the delivery was shipped to.) 921 */ 922 public Reference getDestination() { 923 if (this.destination == null) 924 if (Configuration.errorOnAutoCreate()) 925 throw new Error("Attempt to auto-create SupplyDelivery.destination"); 926 else if (Configuration.doAutoCreate()) 927 this.destination = new Reference(); // cc 928 return this.destination; 929 } 930 931 public boolean hasDestination() { 932 return this.destination != null && !this.destination.isEmpty(); 933 } 934 935 /** 936 * @param value {@link #destination} (Identification of the facility/location where the delivery was shipped to.) 937 */ 938 public SupplyDelivery setDestination(Reference value) { 939 this.destination = value; 940 return this; 941 } 942 943 /** 944 * @return {@link #receiver} (Identifies the individual or organization that received the delivery.) 945 */ 946 public List<Reference> getReceiver() { 947 if (this.receiver == null) 948 this.receiver = new ArrayList<Reference>(); 949 return this.receiver; 950 } 951 952 /** 953 * @return Returns a reference to <code>this</code> for easy method chaining 954 */ 955 public SupplyDelivery setReceiver(List<Reference> theReceiver) { 956 this.receiver = theReceiver; 957 return this; 958 } 959 960 public boolean hasReceiver() { 961 if (this.receiver == null) 962 return false; 963 for (Reference item : this.receiver) 964 if (!item.isEmpty()) 965 return true; 966 return false; 967 } 968 969 public Reference addReceiver() { //3 970 Reference t = new Reference(); 971 if (this.receiver == null) 972 this.receiver = new ArrayList<Reference>(); 973 this.receiver.add(t); 974 return t; 975 } 976 977 public SupplyDelivery addReceiver(Reference t) { //3 978 if (t == null) 979 return this; 980 if (this.receiver == null) 981 this.receiver = new ArrayList<Reference>(); 982 this.receiver.add(t); 983 return this; 984 } 985 986 /** 987 * @return The first repetition of repeating field {@link #receiver}, creating it if it does not already exist {3} 988 */ 989 public Reference getReceiverFirstRep() { 990 if (getReceiver().isEmpty()) { 991 addReceiver(); 992 } 993 return getReceiver().get(0); 994 } 995 996 protected void listChildren(List<Property> children) { 997 super.listChildren(children); 998 children.add(new Property("identifier", "Identifier", "Identifier for the supply delivery event that is used to identify it across multiple disparate systems.", 0, java.lang.Integer.MAX_VALUE, identifier)); 999 children.add(new Property("basedOn", "Reference(SupplyRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this event.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1000 children.add(new Property("partOf", "Reference(SupplyDelivery|Contract)", "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 1001 children.add(new Property("status", "code", "A code specifying the state of the dispense event.", 0, 1, status)); 1002 children.add(new Property("patient", "Reference(Patient)", "A link to a resource representing the person whom the delivered item is for.", 0, 1, patient)); 1003 children.add(new Property("type", "CodeableConcept", "Indicates the type of supply being provided. Examples include: Medication, Device, Biologically Derived Product.", 0, 1, type)); 1004 children.add(new Property("suppliedItem", "", "The item that is being delivered or has been supplied.", 0, java.lang.Integer.MAX_VALUE, suppliedItem)); 1005 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "The date or time(s) the activity occurred.", 0, 1, occurrence)); 1006 children.add(new Property("supplier", "Reference(Practitioner|PractitionerRole|Organization)", "The individual or organization responsible for supplying the delivery.", 0, 1, supplier)); 1007 children.add(new Property("destination", "Reference(Location)", "Identification of the facility/location where the delivery was shipped to.", 0, 1, destination)); 1008 children.add(new Property("receiver", "Reference(Practitioner|PractitionerRole|Organization)", "Identifies the individual or organization that received the delivery.", 0, java.lang.Integer.MAX_VALUE, receiver)); 1009 } 1010 1011 @Override 1012 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1013 switch (_hash) { 1014 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier for the supply delivery event that is used to identify it across multiple disparate systems.", 0, java.lang.Integer.MAX_VALUE, identifier); 1015 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(SupplyRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this event.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1016 case -995410646: /*partOf*/ return new Property("partOf", "Reference(SupplyDelivery|Contract)", "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 1017 case -892481550: /*status*/ return new Property("status", "code", "A code specifying the state of the dispense event.", 0, 1, status); 1018 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "A link to a resource representing the person whom the delivered item is for.", 0, 1, patient); 1019 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Indicates the type of supply being provided. Examples include: Medication, Device, Biologically Derived Product.", 0, 1, type); 1020 case 1993333233: /*suppliedItem*/ return new Property("suppliedItem", "", "The item that is being delivered or has been supplied.", 0, java.lang.Integer.MAX_VALUE, suppliedItem); 1021 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date or time(s) the activity occurred.", 0, 1, occurrence); 1022 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date or time(s) the activity occurred.", 0, 1, occurrence); 1023 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime", "The date or time(s) the activity occurred.", 0, 1, occurrence); 1024 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "Period", "The date or time(s) the activity occurred.", 0, 1, occurrence); 1025 case 1515218299: /*occurrenceTiming*/ return new Property("occurrence[x]", "Timing", "The date or time(s) the activity occurred.", 0, 1, occurrence); 1026 case -1663305268: /*supplier*/ return new Property("supplier", "Reference(Practitioner|PractitionerRole|Organization)", "The individual or organization responsible for supplying the delivery.", 0, 1, supplier); 1027 case -1429847026: /*destination*/ return new Property("destination", "Reference(Location)", "Identification of the facility/location where the delivery was shipped to.", 0, 1, destination); 1028 case -808719889: /*receiver*/ return new Property("receiver", "Reference(Practitioner|PractitionerRole|Organization)", "Identifies the individual or organization that received the delivery.", 0, java.lang.Integer.MAX_VALUE, receiver); 1029 default: return super.getNamedProperty(_hash, _name, _checkValid); 1030 } 1031 1032 } 1033 1034 @Override 1035 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1036 switch (hash) { 1037 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1038 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1039 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1040 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<SupplyDeliveryStatus> 1041 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 1042 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1043 case 1993333233: /*suppliedItem*/ return this.suppliedItem == null ? new Base[0] : this.suppliedItem.toArray(new Base[this.suppliedItem.size()]); // SupplyDeliverySuppliedItemComponent 1044 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // DataType 1045 case -1663305268: /*supplier*/ return this.supplier == null ? new Base[0] : new Base[] {this.supplier}; // Reference 1046 case -1429847026: /*destination*/ return this.destination == null ? new Base[0] : new Base[] {this.destination}; // Reference 1047 case -808719889: /*receiver*/ return this.receiver == null ? new Base[0] : this.receiver.toArray(new Base[this.receiver.size()]); // Reference 1048 default: return super.getProperty(hash, name, checkValid); 1049 } 1050 1051 } 1052 1053 @Override 1054 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1055 switch (hash) { 1056 case -1618432855: // identifier 1057 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1058 return value; 1059 case -332612366: // basedOn 1060 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 1061 return value; 1062 case -995410646: // partOf 1063 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 1064 return value; 1065 case -892481550: // status 1066 value = new SupplyDeliveryStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1067 this.status = (Enumeration) value; // Enumeration<SupplyDeliveryStatus> 1068 return value; 1069 case -791418107: // patient 1070 this.patient = TypeConvertor.castToReference(value); // Reference 1071 return value; 1072 case 3575610: // type 1073 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1074 return value; 1075 case 1993333233: // suppliedItem 1076 this.getSuppliedItem().add((SupplyDeliverySuppliedItemComponent) value); // SupplyDeliverySuppliedItemComponent 1077 return value; 1078 case 1687874001: // occurrence 1079 this.occurrence = TypeConvertor.castToType(value); // DataType 1080 return value; 1081 case -1663305268: // supplier 1082 this.supplier = TypeConvertor.castToReference(value); // Reference 1083 return value; 1084 case -1429847026: // destination 1085 this.destination = TypeConvertor.castToReference(value); // Reference 1086 return value; 1087 case -808719889: // receiver 1088 this.getReceiver().add(TypeConvertor.castToReference(value)); // Reference 1089 return value; 1090 default: return super.setProperty(hash, name, value); 1091 } 1092 1093 } 1094 1095 @Override 1096 public Base setProperty(String name, Base value) throws FHIRException { 1097 if (name.equals("identifier")) { 1098 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1099 } else if (name.equals("basedOn")) { 1100 this.getBasedOn().add(TypeConvertor.castToReference(value)); 1101 } else if (name.equals("partOf")) { 1102 this.getPartOf().add(TypeConvertor.castToReference(value)); 1103 } else if (name.equals("status")) { 1104 value = new SupplyDeliveryStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1105 this.status = (Enumeration) value; // Enumeration<SupplyDeliveryStatus> 1106 } else if (name.equals("patient")) { 1107 this.patient = TypeConvertor.castToReference(value); // Reference 1108 } else if (name.equals("type")) { 1109 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1110 } else if (name.equals("suppliedItem")) { 1111 this.getSuppliedItem().add((SupplyDeliverySuppliedItemComponent) value); 1112 } else if (name.equals("occurrence[x]")) { 1113 this.occurrence = TypeConvertor.castToType(value); // DataType 1114 } else if (name.equals("supplier")) { 1115 this.supplier = TypeConvertor.castToReference(value); // Reference 1116 } else if (name.equals("destination")) { 1117 this.destination = TypeConvertor.castToReference(value); // Reference 1118 } else if (name.equals("receiver")) { 1119 this.getReceiver().add(TypeConvertor.castToReference(value)); 1120 } else 1121 return super.setProperty(name, value); 1122 return value; 1123 } 1124 1125 @Override 1126 public void removeChild(String name, Base value) throws FHIRException { 1127 if (name.equals("identifier")) { 1128 this.getIdentifier().remove(value); 1129 } else if (name.equals("basedOn")) { 1130 this.getBasedOn().remove(value); 1131 } else if (name.equals("partOf")) { 1132 this.getPartOf().remove(value); 1133 } else if (name.equals("status")) { 1134 value = new SupplyDeliveryStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1135 this.status = (Enumeration) value; // Enumeration<SupplyDeliveryStatus> 1136 } else if (name.equals("patient")) { 1137 this.patient = null; 1138 } else if (name.equals("type")) { 1139 this.type = null; 1140 } else if (name.equals("suppliedItem")) { 1141 this.getSuppliedItem().remove((SupplyDeliverySuppliedItemComponent) value); 1142 } else if (name.equals("occurrence[x]")) { 1143 this.occurrence = null; 1144 } else if (name.equals("supplier")) { 1145 this.supplier = null; 1146 } else if (name.equals("destination")) { 1147 this.destination = null; 1148 } else if (name.equals("receiver")) { 1149 this.getReceiver().remove(value); 1150 } else 1151 super.removeChild(name, value); 1152 1153 } 1154 1155 @Override 1156 public Base makeProperty(int hash, String name) throws FHIRException { 1157 switch (hash) { 1158 case -1618432855: return addIdentifier(); 1159 case -332612366: return addBasedOn(); 1160 case -995410646: return addPartOf(); 1161 case -892481550: return getStatusElement(); 1162 case -791418107: return getPatient(); 1163 case 3575610: return getType(); 1164 case 1993333233: return addSuppliedItem(); 1165 case -2022646513: return getOccurrence(); 1166 case 1687874001: return getOccurrence(); 1167 case -1663305268: return getSupplier(); 1168 case -1429847026: return getDestination(); 1169 case -808719889: return addReceiver(); 1170 default: return super.makeProperty(hash, name); 1171 } 1172 1173 } 1174 1175 @Override 1176 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1177 switch (hash) { 1178 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1179 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1180 case -995410646: /*partOf*/ return new String[] {"Reference"}; 1181 case -892481550: /*status*/ return new String[] {"code"}; 1182 case -791418107: /*patient*/ return new String[] {"Reference"}; 1183 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1184 case 1993333233: /*suppliedItem*/ return new String[] {}; 1185 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period", "Timing"}; 1186 case -1663305268: /*supplier*/ return new String[] {"Reference"}; 1187 case -1429847026: /*destination*/ return new String[] {"Reference"}; 1188 case -808719889: /*receiver*/ return new String[] {"Reference"}; 1189 default: return super.getTypesForProperty(hash, name); 1190 } 1191 1192 } 1193 1194 @Override 1195 public Base addChild(String name) throws FHIRException { 1196 if (name.equals("identifier")) { 1197 return addIdentifier(); 1198 } 1199 else if (name.equals("basedOn")) { 1200 return addBasedOn(); 1201 } 1202 else if (name.equals("partOf")) { 1203 return addPartOf(); 1204 } 1205 else if (name.equals("status")) { 1206 throw new FHIRException("Cannot call addChild on a singleton property SupplyDelivery.status"); 1207 } 1208 else if (name.equals("patient")) { 1209 this.patient = new Reference(); 1210 return this.patient; 1211 } 1212 else if (name.equals("type")) { 1213 this.type = new CodeableConcept(); 1214 return this.type; 1215 } 1216 else if (name.equals("suppliedItem")) { 1217 return addSuppliedItem(); 1218 } 1219 else if (name.equals("occurrenceDateTime")) { 1220 this.occurrence = new DateTimeType(); 1221 return this.occurrence; 1222 } 1223 else if (name.equals("occurrencePeriod")) { 1224 this.occurrence = new Period(); 1225 return this.occurrence; 1226 } 1227 else if (name.equals("occurrenceTiming")) { 1228 this.occurrence = new Timing(); 1229 return this.occurrence; 1230 } 1231 else if (name.equals("supplier")) { 1232 this.supplier = new Reference(); 1233 return this.supplier; 1234 } 1235 else if (name.equals("destination")) { 1236 this.destination = new Reference(); 1237 return this.destination; 1238 } 1239 else if (name.equals("receiver")) { 1240 return addReceiver(); 1241 } 1242 else 1243 return super.addChild(name); 1244 } 1245 1246 public String fhirType() { 1247 return "SupplyDelivery"; 1248 1249 } 1250 1251 public SupplyDelivery copy() { 1252 SupplyDelivery dst = new SupplyDelivery(); 1253 copyValues(dst); 1254 return dst; 1255 } 1256 1257 public void copyValues(SupplyDelivery dst) { 1258 super.copyValues(dst); 1259 if (identifier != null) { 1260 dst.identifier = new ArrayList<Identifier>(); 1261 for (Identifier i : identifier) 1262 dst.identifier.add(i.copy()); 1263 }; 1264 if (basedOn != null) { 1265 dst.basedOn = new ArrayList<Reference>(); 1266 for (Reference i : basedOn) 1267 dst.basedOn.add(i.copy()); 1268 }; 1269 if (partOf != null) { 1270 dst.partOf = new ArrayList<Reference>(); 1271 for (Reference i : partOf) 1272 dst.partOf.add(i.copy()); 1273 }; 1274 dst.status = status == null ? null : status.copy(); 1275 dst.patient = patient == null ? null : patient.copy(); 1276 dst.type = type == null ? null : type.copy(); 1277 if (suppliedItem != null) { 1278 dst.suppliedItem = new ArrayList<SupplyDeliverySuppliedItemComponent>(); 1279 for (SupplyDeliverySuppliedItemComponent i : suppliedItem) 1280 dst.suppliedItem.add(i.copy()); 1281 }; 1282 dst.occurrence = occurrence == null ? null : occurrence.copy(); 1283 dst.supplier = supplier == null ? null : supplier.copy(); 1284 dst.destination = destination == null ? null : destination.copy(); 1285 if (receiver != null) { 1286 dst.receiver = new ArrayList<Reference>(); 1287 for (Reference i : receiver) 1288 dst.receiver.add(i.copy()); 1289 }; 1290 } 1291 1292 protected SupplyDelivery typedCopy() { 1293 return copy(); 1294 } 1295 1296 @Override 1297 public boolean equalsDeep(Base other_) { 1298 if (!super.equalsDeep(other_)) 1299 return false; 1300 if (!(other_ instanceof SupplyDelivery)) 1301 return false; 1302 SupplyDelivery o = (SupplyDelivery) other_; 1303 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(partOf, o.partOf, true) 1304 && compareDeep(status, o.status, true) && compareDeep(patient, o.patient, true) && compareDeep(type, o.type, true) 1305 && compareDeep(suppliedItem, o.suppliedItem, true) && compareDeep(occurrence, o.occurrence, true) 1306 && compareDeep(supplier, o.supplier, true) && compareDeep(destination, o.destination, true) && compareDeep(receiver, o.receiver, true) 1307 ; 1308 } 1309 1310 @Override 1311 public boolean equalsShallow(Base other_) { 1312 if (!super.equalsShallow(other_)) 1313 return false; 1314 if (!(other_ instanceof SupplyDelivery)) 1315 return false; 1316 SupplyDelivery o = (SupplyDelivery) other_; 1317 return compareValues(status, o.status, true); 1318 } 1319 1320 public boolean isEmpty() { 1321 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, partOf 1322 , status, patient, type, suppliedItem, occurrence, supplier, destination, receiver 1323 ); 1324 } 1325 1326 @Override 1327 public ResourceType getResourceType() { 1328 return ResourceType.SupplyDelivery; 1329 } 1330 1331 /** 1332 * Search parameter: <b>receiver</b> 1333 * <p> 1334 * Description: <b>Who collected the Supply</b><br> 1335 * Type: <b>reference</b><br> 1336 * Path: <b>SupplyDelivery.receiver</b><br> 1337 * </p> 1338 */ 1339 @SearchParamDefinition(name="receiver", path="SupplyDelivery.receiver", description="Who collected the Supply", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 1340 public static final String SP_RECEIVER = "receiver"; 1341 /** 1342 * <b>Fluent Client</b> search parameter constant for <b>receiver</b> 1343 * <p> 1344 * Description: <b>Who collected the Supply</b><br> 1345 * Type: <b>reference</b><br> 1346 * Path: <b>SupplyDelivery.receiver</b><br> 1347 * </p> 1348 */ 1349 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECEIVER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RECEIVER); 1350 1351/** 1352 * Constant for fluent queries to be used to add include statements. Specifies 1353 * the path value of "<b>SupplyDelivery:receiver</b>". 1354 */ 1355 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECEIVER = new ca.uhn.fhir.model.api.Include("SupplyDelivery:receiver").toLocked(); 1356 1357 /** 1358 * Search parameter: <b>status</b> 1359 * <p> 1360 * Description: <b>in-progress | completed | abandoned | entered-in-error</b><br> 1361 * Type: <b>token</b><br> 1362 * Path: <b>SupplyDelivery.status</b><br> 1363 * </p> 1364 */ 1365 @SearchParamDefinition(name="status", path="SupplyDelivery.status", description="in-progress | completed | abandoned | entered-in-error", type="token" ) 1366 public static final String SP_STATUS = "status"; 1367 /** 1368 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1369 * <p> 1370 * Description: <b>in-progress | completed | abandoned | entered-in-error</b><br> 1371 * Type: <b>token</b><br> 1372 * Path: <b>SupplyDelivery.status</b><br> 1373 * </p> 1374 */ 1375 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1376 1377 /** 1378 * Search parameter: <b>supplier</b> 1379 * <p> 1380 * Description: <b>Dispenser</b><br> 1381 * Type: <b>reference</b><br> 1382 * Path: <b>SupplyDelivery.supplier</b><br> 1383 * </p> 1384 */ 1385 @SearchParamDefinition(name="supplier", path="SupplyDelivery.supplier", description="Dispenser", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 1386 public static final String SP_SUPPLIER = "supplier"; 1387 /** 1388 * <b>Fluent Client</b> search parameter constant for <b>supplier</b> 1389 * <p> 1390 * Description: <b>Dispenser</b><br> 1391 * Type: <b>reference</b><br> 1392 * Path: <b>SupplyDelivery.supplier</b><br> 1393 * </p> 1394 */ 1395 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPLIER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUPPLIER); 1396 1397/** 1398 * Constant for fluent queries to be used to add include statements. Specifies 1399 * the path value of "<b>SupplyDelivery:supplier</b>". 1400 */ 1401 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPLIER = new ca.uhn.fhir.model.api.Include("SupplyDelivery:supplier").toLocked(); 1402 1403 /** 1404 * Search parameter: <b>identifier</b> 1405 * <p> 1406 * Description: <b>Multiple Resources: 1407 1408* [Account](account.html): Account number 1409* [AdverseEvent](adverseevent.html): Business identifier for the event 1410* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1411* [Appointment](appointment.html): An Identifier of the Appointment 1412* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1413* [Basic](basic.html): Business identifier 1414* [BodyStructure](bodystructure.html): Bodystructure identifier 1415* [CarePlan](careplan.html): External Ids for this plan 1416* [CareTeam](careteam.html): External Ids for this team 1417* [ChargeItem](chargeitem.html): Business Identifier for item 1418* [Claim](claim.html): The primary identifier of the financial resource 1419* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1420* [ClinicalImpression](clinicalimpression.html): Business identifier 1421* [Communication](communication.html): Unique identifier 1422* [CommunicationRequest](communicationrequest.html): Unique identifier 1423* [Composition](composition.html): Version-independent identifier for the Composition 1424* [Condition](condition.html): A unique identifier of the condition record 1425* [Consent](consent.html): Identifier for this record (external references) 1426* [Contract](contract.html): The identity of the contract 1427* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1428* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1429* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1430* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1431* [DeviceRequest](devicerequest.html): Business identifier for request/order 1432* [DeviceUsage](deviceusage.html): Search by identifier 1433* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1434* [DocumentReference](documentreference.html): Identifier of the attachment binary 1435* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1436* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1437* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1438* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1439* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1440* [Flag](flag.html): Business identifier 1441* [Goal](goal.html): External Ids for this goal 1442* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1443* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1444* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1445* [Immunization](immunization.html): Business identifier 1446* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1447* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1448* [Invoice](invoice.html): Business Identifier for item 1449* [List](list.html): Business identifier 1450* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1451* [Medication](medication.html): Returns medications with this external identifier 1452* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1453* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1454* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1455* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1456* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1457* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1458* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1459* [Observation](observation.html): The unique id for a particular observation 1460* [Person](person.html): A person Identifier 1461* [Procedure](procedure.html): A unique identifier for a procedure 1462* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1463* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1464* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1465* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1466* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1467* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1468* [Specimen](specimen.html): The unique identifier associated with the specimen 1469* [SupplyDelivery](supplydelivery.html): External identifier 1470* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1471* [Task](task.html): Search for a task instance by its business identifier 1472* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1473</b><br> 1474 * Type: <b>token</b><br> 1475 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1476 * </p> 1477 */ 1478 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 1479 public static final String SP_IDENTIFIER = "identifier"; 1480 /** 1481 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1482 * <p> 1483 * Description: <b>Multiple Resources: 1484 1485* [Account](account.html): Account number 1486* [AdverseEvent](adverseevent.html): Business identifier for the event 1487* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1488* [Appointment](appointment.html): An Identifier of the Appointment 1489* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1490* [Basic](basic.html): Business identifier 1491* [BodyStructure](bodystructure.html): Bodystructure identifier 1492* [CarePlan](careplan.html): External Ids for this plan 1493* [CareTeam](careteam.html): External Ids for this team 1494* [ChargeItem](chargeitem.html): Business Identifier for item 1495* [Claim](claim.html): The primary identifier of the financial resource 1496* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1497* [ClinicalImpression](clinicalimpression.html): Business identifier 1498* [Communication](communication.html): Unique identifier 1499* [CommunicationRequest](communicationrequest.html): Unique identifier 1500* [Composition](composition.html): Version-independent identifier for the Composition 1501* [Condition](condition.html): A unique identifier of the condition record 1502* [Consent](consent.html): Identifier for this record (external references) 1503* [Contract](contract.html): The identity of the contract 1504* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1505* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1506* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1507* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1508* [DeviceRequest](devicerequest.html): Business identifier for request/order 1509* [DeviceUsage](deviceusage.html): Search by identifier 1510* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1511* [DocumentReference](documentreference.html): Identifier of the attachment binary 1512* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1513* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1514* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1515* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1516* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1517* [Flag](flag.html): Business identifier 1518* [Goal](goal.html): External Ids for this goal 1519* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1520* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1521* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1522* [Immunization](immunization.html): Business identifier 1523* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1524* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1525* [Invoice](invoice.html): Business Identifier for item 1526* [List](list.html): Business identifier 1527* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1528* [Medication](medication.html): Returns medications with this external identifier 1529* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1530* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1531* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1532* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1533* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1534* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1535* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1536* [Observation](observation.html): The unique id for a particular observation 1537* [Person](person.html): A person Identifier 1538* [Procedure](procedure.html): A unique identifier for a procedure 1539* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1540* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1541* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1542* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1543* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1544* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1545* [Specimen](specimen.html): The unique identifier associated with the specimen 1546* [SupplyDelivery](supplydelivery.html): External identifier 1547* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1548* [Task](task.html): Search for a task instance by its business identifier 1549* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1550</b><br> 1551 * Type: <b>token</b><br> 1552 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1553 * </p> 1554 */ 1555 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1556 1557 /** 1558 * Search parameter: <b>patient</b> 1559 * <p> 1560 * Description: <b>Multiple Resources: 1561 1562* [Account](account.html): The entity that caused the expenses 1563* [AdverseEvent](adverseevent.html): Subject impacted by event 1564* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 1565* [Appointment](appointment.html): One of the individuals of the appointment is this patient 1566* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 1567* [AuditEvent](auditevent.html): Where the activity involved patient data 1568* [Basic](basic.html): Identifies the focus of this resource 1569* [BodyStructure](bodystructure.html): Who this is about 1570* [CarePlan](careplan.html): Who the care plan is for 1571* [CareTeam](careteam.html): Who care team is for 1572* [ChargeItem](chargeitem.html): Individual service was done for/to 1573* [Claim](claim.html): Patient receiving the products or services 1574* [ClaimResponse](claimresponse.html): The subject of care 1575* [ClinicalImpression](clinicalimpression.html): Patient assessed 1576* [Communication](communication.html): Focus of message 1577* [CommunicationRequest](communicationrequest.html): Focus of message 1578* [Composition](composition.html): Who and/or what the composition is about 1579* [Condition](condition.html): Who has the condition? 1580* [Consent](consent.html): Who the consent applies to 1581* [Contract](contract.html): The identity of the subject of the contract (if a patient) 1582* [Coverage](coverage.html): Retrieve coverages for a patient 1583* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 1584* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 1585* [DetectedIssue](detectedissue.html): Associated patient 1586* [DeviceRequest](devicerequest.html): Individual the service is ordered for 1587* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 1588* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 1589* [DocumentReference](documentreference.html): Who/what is the subject of the document 1590* [Encounter](encounter.html): The patient present at the encounter 1591* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 1592* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 1593* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 1594* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 1595* [Flag](flag.html): The identity of a subject to list flags for 1596* [Goal](goal.html): Who this goal is intended for 1597* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 1598* [ImagingSelection](imagingselection.html): Who the study is about 1599* [ImagingStudy](imagingstudy.html): Who the study is about 1600* [Immunization](immunization.html): The patient for the vaccination record 1601* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 1602* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 1603* [Invoice](invoice.html): Recipient(s) of goods and services 1604* [List](list.html): If all resources have the same subject 1605* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 1606* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 1607* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 1608* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 1609* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 1610* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 1611* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 1612* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 1613* [Observation](observation.html): The subject that the observation is about (if patient) 1614* [Person](person.html): The Person links to this Patient 1615* [Procedure](procedure.html): Search by subject - a patient 1616* [Provenance](provenance.html): Where the activity involved patient data 1617* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 1618* [RelatedPerson](relatedperson.html): The patient this related person is related to 1619* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 1620* [ResearchSubject](researchsubject.html): Who or what is part of study 1621* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 1622* [ServiceRequest](servicerequest.html): Search by subject - a patient 1623* [Specimen](specimen.html): The patient the specimen comes from 1624* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 1625* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 1626* [Task](task.html): Search by patient 1627* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 1628</b><br> 1629 * Type: <b>reference</b><br> 1630 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 1631 * </p> 1632 */ 1633 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 1634 public static final String SP_PATIENT = "patient"; 1635 /** 1636 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1637 * <p> 1638 * Description: <b>Multiple Resources: 1639 1640* [Account](account.html): The entity that caused the expenses 1641* [AdverseEvent](adverseevent.html): Subject impacted by event 1642* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 1643* [Appointment](appointment.html): One of the individuals of the appointment is this patient 1644* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 1645* [AuditEvent](auditevent.html): Where the activity involved patient data 1646* [Basic](basic.html): Identifies the focus of this resource 1647* [BodyStructure](bodystructure.html): Who this is about 1648* [CarePlan](careplan.html): Who the care plan is for 1649* [CareTeam](careteam.html): Who care team is for 1650* [ChargeItem](chargeitem.html): Individual service was done for/to 1651* [Claim](claim.html): Patient receiving the products or services 1652* [ClaimResponse](claimresponse.html): The subject of care 1653* [ClinicalImpression](clinicalimpression.html): Patient assessed 1654* [Communication](communication.html): Focus of message 1655* [CommunicationRequest](communicationrequest.html): Focus of message 1656* [Composition](composition.html): Who and/or what the composition is about 1657* [Condition](condition.html): Who has the condition? 1658* [Consent](consent.html): Who the consent applies to 1659* [Contract](contract.html): The identity of the subject of the contract (if a patient) 1660* [Coverage](coverage.html): Retrieve coverages for a patient 1661* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 1662* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 1663* [DetectedIssue](detectedissue.html): Associated patient 1664* [DeviceRequest](devicerequest.html): Individual the service is ordered for 1665* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 1666* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 1667* [DocumentReference](documentreference.html): Who/what is the subject of the document 1668* [Encounter](encounter.html): The patient present at the encounter 1669* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 1670* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 1671* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 1672* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 1673* [Flag](flag.html): The identity of a subject to list flags for 1674* [Goal](goal.html): Who this goal is intended for 1675* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 1676* [ImagingSelection](imagingselection.html): Who the study is about 1677* [ImagingStudy](imagingstudy.html): Who the study is about 1678* [Immunization](immunization.html): The patient for the vaccination record 1679* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 1680* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 1681* [Invoice](invoice.html): Recipient(s) of goods and services 1682* [List](list.html): If all resources have the same subject 1683* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 1684* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 1685* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 1686* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 1687* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 1688* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 1689* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 1690* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 1691* [Observation](observation.html): The subject that the observation is about (if patient) 1692* [Person](person.html): The Person links to this Patient 1693* [Procedure](procedure.html): Search by subject - a patient 1694* [Provenance](provenance.html): Where the activity involved patient data 1695* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 1696* [RelatedPerson](relatedperson.html): The patient this related person is related to 1697* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 1698* [ResearchSubject](researchsubject.html): Who or what is part of study 1699* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 1700* [ServiceRequest](servicerequest.html): Search by subject - a patient 1701* [Specimen](specimen.html): The patient the specimen comes from 1702* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 1703* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 1704* [Task](task.html): Search by patient 1705* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 1706</b><br> 1707 * Type: <b>reference</b><br> 1708 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 1709 * </p> 1710 */ 1711 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1712 1713/** 1714 * Constant for fluent queries to be used to add include statements. Specifies 1715 * the path value of "<b>SupplyDelivery:patient</b>". 1716 */ 1717 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("SupplyDelivery:patient").toLocked(); 1718 1719 1720} 1721