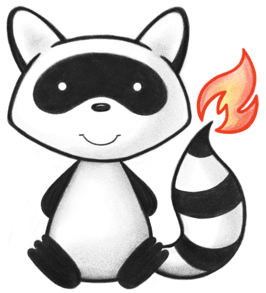
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A record of a non-patient specific request for a medication, substance, device, certain types of biologically derived product, and nutrition product used in the healthcare setting. 052 */ 053@ResourceDef(name="SupplyRequest", profile="http://hl7.org/fhir/StructureDefinition/SupplyRequest") 054public class SupplyRequest extends DomainResource { 055 056 public enum SupplyRequestStatus { 057 /** 058 * The request has been created but is not yet complete or ready for action. 059 */ 060 DRAFT, 061 /** 062 * The request is ready to be acted upon. 063 */ 064 ACTIVE, 065 /** 066 * The authorization/request to act has been temporarily withdrawn but is expected to resume in the future. 067 */ 068 SUSPENDED, 069 /** 070 * The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur. 071 */ 072 CANCELLED, 073 /** 074 * Activity against the request has been sufficiently completed to the satisfaction of the requester. 075 */ 076 COMPLETED, 077 /** 078 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".). 079 */ 080 ENTEREDINERROR, 081 /** 082 * The authoring/source system does not know which of the status values currently applies for this observation. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which. 083 */ 084 UNKNOWN, 085 /** 086 * added to help the parsers with the generic types 087 */ 088 NULL; 089 public static SupplyRequestStatus fromCode(String codeString) throws FHIRException { 090 if (codeString == null || "".equals(codeString)) 091 return null; 092 if ("draft".equals(codeString)) 093 return DRAFT; 094 if ("active".equals(codeString)) 095 return ACTIVE; 096 if ("suspended".equals(codeString)) 097 return SUSPENDED; 098 if ("cancelled".equals(codeString)) 099 return CANCELLED; 100 if ("completed".equals(codeString)) 101 return COMPLETED; 102 if ("entered-in-error".equals(codeString)) 103 return ENTEREDINERROR; 104 if ("unknown".equals(codeString)) 105 return UNKNOWN; 106 if (Configuration.isAcceptInvalidEnums()) 107 return null; 108 else 109 throw new FHIRException("Unknown SupplyRequestStatus code '"+codeString+"'"); 110 } 111 public String toCode() { 112 switch (this) { 113 case DRAFT: return "draft"; 114 case ACTIVE: return "active"; 115 case SUSPENDED: return "suspended"; 116 case CANCELLED: return "cancelled"; 117 case COMPLETED: return "completed"; 118 case ENTEREDINERROR: return "entered-in-error"; 119 case UNKNOWN: return "unknown"; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getSystem() { 125 switch (this) { 126 case DRAFT: return "http://hl7.org/fhir/supplyrequest-status"; 127 case ACTIVE: return "http://hl7.org/fhir/supplyrequest-status"; 128 case SUSPENDED: return "http://hl7.org/fhir/supplyrequest-status"; 129 case CANCELLED: return "http://hl7.org/fhir/supplyrequest-status"; 130 case COMPLETED: return "http://hl7.org/fhir/supplyrequest-status"; 131 case ENTEREDINERROR: return "http://hl7.org/fhir/supplyrequest-status"; 132 case UNKNOWN: return "http://hl7.org/fhir/supplyrequest-status"; 133 case NULL: return null; 134 default: return "?"; 135 } 136 } 137 public String getDefinition() { 138 switch (this) { 139 case DRAFT: return "The request has been created but is not yet complete or ready for action."; 140 case ACTIVE: return "The request is ready to be acted upon."; 141 case SUSPENDED: return "The authorization/request to act has been temporarily withdrawn but is expected to resume in the future."; 142 case CANCELLED: return "The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur."; 143 case COMPLETED: return "Activity against the request has been sufficiently completed to the satisfaction of the requester."; 144 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 145 case UNKNOWN: return "The authoring/source system does not know which of the status values currently applies for this observation. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 146 case NULL: return null; 147 default: return "?"; 148 } 149 } 150 public String getDisplay() { 151 switch (this) { 152 case DRAFT: return "Draft"; 153 case ACTIVE: return "Active"; 154 case SUSPENDED: return "Suspended"; 155 case CANCELLED: return "Cancelled"; 156 case COMPLETED: return "Completed"; 157 case ENTEREDINERROR: return "Entered in Error"; 158 case UNKNOWN: return "Unknown"; 159 case NULL: return null; 160 default: return "?"; 161 } 162 } 163 } 164 165 public static class SupplyRequestStatusEnumFactory implements EnumFactory<SupplyRequestStatus> { 166 public SupplyRequestStatus fromCode(String codeString) throws IllegalArgumentException { 167 if (codeString == null || "".equals(codeString)) 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("draft".equals(codeString)) 171 return SupplyRequestStatus.DRAFT; 172 if ("active".equals(codeString)) 173 return SupplyRequestStatus.ACTIVE; 174 if ("suspended".equals(codeString)) 175 return SupplyRequestStatus.SUSPENDED; 176 if ("cancelled".equals(codeString)) 177 return SupplyRequestStatus.CANCELLED; 178 if ("completed".equals(codeString)) 179 return SupplyRequestStatus.COMPLETED; 180 if ("entered-in-error".equals(codeString)) 181 return SupplyRequestStatus.ENTEREDINERROR; 182 if ("unknown".equals(codeString)) 183 return SupplyRequestStatus.UNKNOWN; 184 throw new IllegalArgumentException("Unknown SupplyRequestStatus code '"+codeString+"'"); 185 } 186 public Enumeration<SupplyRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 187 if (code == null) 188 return null; 189 if (code.isEmpty()) 190 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.NULL, code); 191 String codeString = ((PrimitiveType) code).asStringValue(); 192 if (codeString == null || "".equals(codeString)) 193 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.NULL, code); 194 if ("draft".equals(codeString)) 195 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.DRAFT, code); 196 if ("active".equals(codeString)) 197 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.ACTIVE, code); 198 if ("suspended".equals(codeString)) 199 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.SUSPENDED, code); 200 if ("cancelled".equals(codeString)) 201 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.CANCELLED, code); 202 if ("completed".equals(codeString)) 203 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.COMPLETED, code); 204 if ("entered-in-error".equals(codeString)) 205 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.ENTEREDINERROR, code); 206 if ("unknown".equals(codeString)) 207 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.UNKNOWN, code); 208 throw new FHIRException("Unknown SupplyRequestStatus code '"+codeString+"'"); 209 } 210 public String toCode(SupplyRequestStatus code) { 211 if (code == SupplyRequestStatus.NULL) 212 return null; 213 if (code == SupplyRequestStatus.DRAFT) 214 return "draft"; 215 if (code == SupplyRequestStatus.ACTIVE) 216 return "active"; 217 if (code == SupplyRequestStatus.SUSPENDED) 218 return "suspended"; 219 if (code == SupplyRequestStatus.CANCELLED) 220 return "cancelled"; 221 if (code == SupplyRequestStatus.COMPLETED) 222 return "completed"; 223 if (code == SupplyRequestStatus.ENTEREDINERROR) 224 return "entered-in-error"; 225 if (code == SupplyRequestStatus.UNKNOWN) 226 return "unknown"; 227 return "?"; 228 } 229 public String toSystem(SupplyRequestStatus code) { 230 return code.getSystem(); 231 } 232 } 233 234 @Block() 235 public static class SupplyRequestParameterComponent extends BackboneElement implements IBaseBackboneElement { 236 /** 237 * A code or string that identifies the device detail being asserted. 238 */ 239 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 240 @Description(shortDefinition="Item detail", formalDefinition="A code or string that identifies the device detail being asserted." ) 241 protected CodeableConcept code; 242 243 /** 244 * The value of the device detail. 245 */ 246 @Child(name = "value", type = {CodeableConcept.class, Quantity.class, Range.class, BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 247 @Description(shortDefinition="Value of detail", formalDefinition="The value of the device detail." ) 248 protected DataType value; 249 250 private static final long serialVersionUID = -1950789033L; 251 252 /** 253 * Constructor 254 */ 255 public SupplyRequestParameterComponent() { 256 super(); 257 } 258 259 /** 260 * @return {@link #code} (A code or string that identifies the device detail being asserted.) 261 */ 262 public CodeableConcept getCode() { 263 if (this.code == null) 264 if (Configuration.errorOnAutoCreate()) 265 throw new Error("Attempt to auto-create SupplyRequestParameterComponent.code"); 266 else if (Configuration.doAutoCreate()) 267 this.code = new CodeableConcept(); // cc 268 return this.code; 269 } 270 271 public boolean hasCode() { 272 return this.code != null && !this.code.isEmpty(); 273 } 274 275 /** 276 * @param value {@link #code} (A code or string that identifies the device detail being asserted.) 277 */ 278 public SupplyRequestParameterComponent setCode(CodeableConcept value) { 279 this.code = value; 280 return this; 281 } 282 283 /** 284 * @return {@link #value} (The value of the device detail.) 285 */ 286 public DataType getValue() { 287 return this.value; 288 } 289 290 /** 291 * @return {@link #value} (The value of the device detail.) 292 */ 293 public CodeableConcept getValueCodeableConcept() throws FHIRException { 294 if (this.value == null) 295 this.value = new CodeableConcept(); 296 if (!(this.value instanceof CodeableConcept)) 297 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 298 return (CodeableConcept) this.value; 299 } 300 301 public boolean hasValueCodeableConcept() { 302 return this != null && this.value instanceof CodeableConcept; 303 } 304 305 /** 306 * @return {@link #value} (The value of the device detail.) 307 */ 308 public Quantity getValueQuantity() throws FHIRException { 309 if (this.value == null) 310 this.value = new Quantity(); 311 if (!(this.value instanceof Quantity)) 312 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 313 return (Quantity) this.value; 314 } 315 316 public boolean hasValueQuantity() { 317 return this != null && this.value instanceof Quantity; 318 } 319 320 /** 321 * @return {@link #value} (The value of the device detail.) 322 */ 323 public Range getValueRange() throws FHIRException { 324 if (this.value == null) 325 this.value = new Range(); 326 if (!(this.value instanceof Range)) 327 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 328 return (Range) this.value; 329 } 330 331 public boolean hasValueRange() { 332 return this != null && this.value instanceof Range; 333 } 334 335 /** 336 * @return {@link #value} (The value of the device detail.) 337 */ 338 public BooleanType getValueBooleanType() throws FHIRException { 339 if (this.value == null) 340 this.value = new BooleanType(); 341 if (!(this.value instanceof BooleanType)) 342 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 343 return (BooleanType) this.value; 344 } 345 346 public boolean hasValueBooleanType() { 347 return this != null && this.value instanceof BooleanType; 348 } 349 350 public boolean hasValue() { 351 return this.value != null && !this.value.isEmpty(); 352 } 353 354 /** 355 * @param value {@link #value} (The value of the device detail.) 356 */ 357 public SupplyRequestParameterComponent setValue(DataType value) { 358 if (value != null && !(value instanceof CodeableConcept || value instanceof Quantity || value instanceof Range || value instanceof BooleanType)) 359 throw new FHIRException("Not the right type for SupplyRequest.parameter.value[x]: "+value.fhirType()); 360 this.value = value; 361 return this; 362 } 363 364 protected void listChildren(List<Property> children) { 365 super.listChildren(children); 366 children.add(new Property("code", "CodeableConcept", "A code or string that identifies the device detail being asserted.", 0, 1, code)); 367 children.add(new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", "The value of the device detail.", 0, 1, value)); 368 } 369 370 @Override 371 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 372 switch (_hash) { 373 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code or string that identifies the device detail being asserted.", 0, 1, code); 374 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", "The value of the device detail.", 0, 1, value); 375 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", "The value of the device detail.", 0, 1, value); 376 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The value of the device detail.", 0, 1, value); 377 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The value of the device detail.", 0, 1, value); 378 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The value of the device detail.", 0, 1, value); 379 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The value of the device detail.", 0, 1, value); 380 default: return super.getNamedProperty(_hash, _name, _checkValid); 381 } 382 383 } 384 385 @Override 386 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 387 switch (hash) { 388 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 389 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 390 default: return super.getProperty(hash, name, checkValid); 391 } 392 393 } 394 395 @Override 396 public Base setProperty(int hash, String name, Base value) throws FHIRException { 397 switch (hash) { 398 case 3059181: // code 399 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 400 return value; 401 case 111972721: // value 402 this.value = TypeConvertor.castToType(value); // DataType 403 return value; 404 default: return super.setProperty(hash, name, value); 405 } 406 407 } 408 409 @Override 410 public Base setProperty(String name, Base value) throws FHIRException { 411 if (name.equals("code")) { 412 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 413 } else if (name.equals("value[x]")) { 414 this.value = TypeConvertor.castToType(value); // DataType 415 } else 416 return super.setProperty(name, value); 417 return value; 418 } 419 420 @Override 421 public void removeChild(String name, Base value) throws FHIRException { 422 if (name.equals("code")) { 423 this.code = null; 424 } else if (name.equals("value[x]")) { 425 this.value = null; 426 } else 427 super.removeChild(name, value); 428 429 } 430 431 @Override 432 public Base makeProperty(int hash, String name) throws FHIRException { 433 switch (hash) { 434 case 3059181: return getCode(); 435 case -1410166417: return getValue(); 436 case 111972721: return getValue(); 437 default: return super.makeProperty(hash, name); 438 } 439 440 } 441 442 @Override 443 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 444 switch (hash) { 445 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 446 case 111972721: /*value*/ return new String[] {"CodeableConcept", "Quantity", "Range", "boolean"}; 447 default: return super.getTypesForProperty(hash, name); 448 } 449 450 } 451 452 @Override 453 public Base addChild(String name) throws FHIRException { 454 if (name.equals("code")) { 455 this.code = new CodeableConcept(); 456 return this.code; 457 } 458 else if (name.equals("valueCodeableConcept")) { 459 this.value = new CodeableConcept(); 460 return this.value; 461 } 462 else if (name.equals("valueQuantity")) { 463 this.value = new Quantity(); 464 return this.value; 465 } 466 else if (name.equals("valueRange")) { 467 this.value = new Range(); 468 return this.value; 469 } 470 else if (name.equals("valueBoolean")) { 471 this.value = new BooleanType(); 472 return this.value; 473 } 474 else 475 return super.addChild(name); 476 } 477 478 public SupplyRequestParameterComponent copy() { 479 SupplyRequestParameterComponent dst = new SupplyRequestParameterComponent(); 480 copyValues(dst); 481 return dst; 482 } 483 484 public void copyValues(SupplyRequestParameterComponent dst) { 485 super.copyValues(dst); 486 dst.code = code == null ? null : code.copy(); 487 dst.value = value == null ? null : value.copy(); 488 } 489 490 @Override 491 public boolean equalsDeep(Base other_) { 492 if (!super.equalsDeep(other_)) 493 return false; 494 if (!(other_ instanceof SupplyRequestParameterComponent)) 495 return false; 496 SupplyRequestParameterComponent o = (SupplyRequestParameterComponent) other_; 497 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 498 } 499 500 @Override 501 public boolean equalsShallow(Base other_) { 502 if (!super.equalsShallow(other_)) 503 return false; 504 if (!(other_ instanceof SupplyRequestParameterComponent)) 505 return false; 506 SupplyRequestParameterComponent o = (SupplyRequestParameterComponent) other_; 507 return true; 508 } 509 510 public boolean isEmpty() { 511 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value); 512 } 513 514 public String fhirType() { 515 return "SupplyRequest.parameter"; 516 517 } 518 519 } 520 521 /** 522 * Business identifiers assigned to this SupplyRequest by the author and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server. 523 */ 524 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 525 @Description(shortDefinition="Business Identifier for SupplyRequest", formalDefinition="Business identifiers assigned to this SupplyRequest by the author and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server." ) 526 protected List<Identifier> identifier; 527 528 /** 529 * Status of the supply request. 530 */ 531 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 532 @Description(shortDefinition="draft | active | suspended +", formalDefinition="Status of the supply request." ) 533 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/supplyrequest-status") 534 protected Enumeration<SupplyRequestStatus> status; 535 536 /** 537 * Plan/proposal/order fulfilled by this request. 538 */ 539 @Child(name = "basedOn", type = {Reference.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 540 @Description(shortDefinition="What other request is fulfilled by this supply request", formalDefinition="Plan/proposal/order fulfilled by this request." ) 541 protected List<Reference> basedOn; 542 543 /** 544 * Category of supply, e.g. central, non-stock, etc. This is used to support work flows associated with the supply process. 545 */ 546 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 547 @Description(shortDefinition="The kind of supply (central, non-stock, etc.)", formalDefinition="Category of supply, e.g. central, non-stock, etc. This is used to support work flows associated with the supply process." ) 548 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/supplyrequest-kind") 549 protected CodeableConcept category; 550 551 /** 552 * Indicates how quickly this SupplyRequest should be addressed with respect to other requests. 553 */ 554 @Child(name = "priority", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 555 @Description(shortDefinition="routine | urgent | asap | stat", formalDefinition="Indicates how quickly this SupplyRequest should be addressed with respect to other requests." ) 556 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 557 protected Enumeration<RequestPriority> priority; 558 559 /** 560 * The patient to whom the supply will be given or for whom they will be used. 561 */ 562 @Child(name = "deliverFor", type = {Patient.class}, order=5, min=0, max=1, modifier=false, summary=false) 563 @Description(shortDefinition="The patient for who the supply request is for", formalDefinition="The patient to whom the supply will be given or for whom they will be used." ) 564 protected Reference deliverFor; 565 566 /** 567 * The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list. 568 */ 569 @Child(name = "item", type = {CodeableReference.class}, order=6, min=1, max=1, modifier=false, summary=true) 570 @Description(shortDefinition="Medication, Substance, or Device requested to be supplied", formalDefinition="The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list." ) 571 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/supply-item") 572 protected CodeableReference item; 573 574 /** 575 * The amount that is being ordered of the indicated item. 576 */ 577 @Child(name = "quantity", type = {Quantity.class}, order=7, min=1, max=1, modifier=false, summary=true) 578 @Description(shortDefinition="The requested amount of the item indicated", formalDefinition="The amount that is being ordered of the indicated item." ) 579 protected Quantity quantity; 580 581 /** 582 * Specific parameters for the ordered item. For example, the size of the indicated item. 583 */ 584 @Child(name = "parameter", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 585 @Description(shortDefinition="Ordered item details", formalDefinition="Specific parameters for the ordered item. For example, the size of the indicated item." ) 586 protected List<SupplyRequestParameterComponent> parameter; 587 588 /** 589 * When the request should be fulfilled. 590 */ 591 @Child(name = "occurrence", type = {DateTimeType.class, Period.class, Timing.class}, order=9, min=0, max=1, modifier=false, summary=true) 592 @Description(shortDefinition="When the request should be fulfilled", formalDefinition="When the request should be fulfilled." ) 593 protected DataType occurrence; 594 595 /** 596 * When the request was made. 597 */ 598 @Child(name = "authoredOn", type = {DateTimeType.class}, order=10, min=0, max=1, modifier=false, summary=true) 599 @Description(shortDefinition="When the request was made", formalDefinition="When the request was made." ) 600 protected DateTimeType authoredOn; 601 602 /** 603 * The device, practitioner, etc. who initiated the request. 604 */ 605 @Child(name = "requester", type = {Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, RelatedPerson.class, Device.class, CareTeam.class}, order=11, min=0, max=1, modifier=false, summary=true) 606 @Description(shortDefinition="Individual making the request", formalDefinition="The device, practitioner, etc. who initiated the request." ) 607 protected Reference requester; 608 609 /** 610 * Who is intended to fulfill the request. 611 */ 612 @Child(name = "supplier", type = {Organization.class, HealthcareService.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 613 @Description(shortDefinition="Who is intended to fulfill the request", formalDefinition="Who is intended to fulfill the request." ) 614 protected List<Reference> supplier; 615 616 /** 617 * The reason why the supply item was requested. 618 */ 619 @Child(name = "reason", type = {CodeableReference.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 620 @Description(shortDefinition="The reason why the supply item was requested", formalDefinition="The reason why the supply item was requested." ) 621 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/supplyrequest-reason") 622 protected List<CodeableReference> reason; 623 624 /** 625 * Where the supply is expected to come from. 626 */ 627 @Child(name = "deliverFrom", type = {Organization.class, Location.class}, order=14, min=0, max=1, modifier=false, summary=false) 628 @Description(shortDefinition="The origin of the supply", formalDefinition="Where the supply is expected to come from." ) 629 protected Reference deliverFrom; 630 631 /** 632 * Where the supply is destined to go. 633 */ 634 @Child(name = "deliverTo", type = {Organization.class, Location.class, Patient.class, RelatedPerson.class}, order=15, min=0, max=1, modifier=false, summary=false) 635 @Description(shortDefinition="The destination of the supply", formalDefinition="Where the supply is destined to go." ) 636 protected Reference deliverTo; 637 638 private static final long serialVersionUID = -1701051976L; 639 640 /** 641 * Constructor 642 */ 643 public SupplyRequest() { 644 super(); 645 } 646 647 /** 648 * Constructor 649 */ 650 public SupplyRequest(CodeableReference item, Quantity quantity) { 651 super(); 652 this.setItem(item); 653 this.setQuantity(quantity); 654 } 655 656 /** 657 * @return {@link #identifier} (Business identifiers assigned to this SupplyRequest by the author and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.) 658 */ 659 public List<Identifier> getIdentifier() { 660 if (this.identifier == null) 661 this.identifier = new ArrayList<Identifier>(); 662 return this.identifier; 663 } 664 665 /** 666 * @return Returns a reference to <code>this</code> for easy method chaining 667 */ 668 public SupplyRequest setIdentifier(List<Identifier> theIdentifier) { 669 this.identifier = theIdentifier; 670 return this; 671 } 672 673 public boolean hasIdentifier() { 674 if (this.identifier == null) 675 return false; 676 for (Identifier item : this.identifier) 677 if (!item.isEmpty()) 678 return true; 679 return false; 680 } 681 682 public Identifier addIdentifier() { //3 683 Identifier t = new Identifier(); 684 if (this.identifier == null) 685 this.identifier = new ArrayList<Identifier>(); 686 this.identifier.add(t); 687 return t; 688 } 689 690 public SupplyRequest addIdentifier(Identifier t) { //3 691 if (t == null) 692 return this; 693 if (this.identifier == null) 694 this.identifier = new ArrayList<Identifier>(); 695 this.identifier.add(t); 696 return this; 697 } 698 699 /** 700 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 701 */ 702 public Identifier getIdentifierFirstRep() { 703 if (getIdentifier().isEmpty()) { 704 addIdentifier(); 705 } 706 return getIdentifier().get(0); 707 } 708 709 /** 710 * @return {@link #status} (Status of the supply request.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 711 */ 712 public Enumeration<SupplyRequestStatus> getStatusElement() { 713 if (this.status == null) 714 if (Configuration.errorOnAutoCreate()) 715 throw new Error("Attempt to auto-create SupplyRequest.status"); 716 else if (Configuration.doAutoCreate()) 717 this.status = new Enumeration<SupplyRequestStatus>(new SupplyRequestStatusEnumFactory()); // bb 718 return this.status; 719 } 720 721 public boolean hasStatusElement() { 722 return this.status != null && !this.status.isEmpty(); 723 } 724 725 public boolean hasStatus() { 726 return this.status != null && !this.status.isEmpty(); 727 } 728 729 /** 730 * @param value {@link #status} (Status of the supply request.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 731 */ 732 public SupplyRequest setStatusElement(Enumeration<SupplyRequestStatus> value) { 733 this.status = value; 734 return this; 735 } 736 737 /** 738 * @return Status of the supply request. 739 */ 740 public SupplyRequestStatus getStatus() { 741 return this.status == null ? null : this.status.getValue(); 742 } 743 744 /** 745 * @param value Status of the supply request. 746 */ 747 public SupplyRequest setStatus(SupplyRequestStatus value) { 748 if (value == null) 749 this.status = null; 750 else { 751 if (this.status == null) 752 this.status = new Enumeration<SupplyRequestStatus>(new SupplyRequestStatusEnumFactory()); 753 this.status.setValue(value); 754 } 755 return this; 756 } 757 758 /** 759 * @return {@link #basedOn} (Plan/proposal/order fulfilled by this request.) 760 */ 761 public List<Reference> getBasedOn() { 762 if (this.basedOn == null) 763 this.basedOn = new ArrayList<Reference>(); 764 return this.basedOn; 765 } 766 767 /** 768 * @return Returns a reference to <code>this</code> for easy method chaining 769 */ 770 public SupplyRequest setBasedOn(List<Reference> theBasedOn) { 771 this.basedOn = theBasedOn; 772 return this; 773 } 774 775 public boolean hasBasedOn() { 776 if (this.basedOn == null) 777 return false; 778 for (Reference item : this.basedOn) 779 if (!item.isEmpty()) 780 return true; 781 return false; 782 } 783 784 public Reference addBasedOn() { //3 785 Reference t = new Reference(); 786 if (this.basedOn == null) 787 this.basedOn = new ArrayList<Reference>(); 788 this.basedOn.add(t); 789 return t; 790 } 791 792 public SupplyRequest addBasedOn(Reference t) { //3 793 if (t == null) 794 return this; 795 if (this.basedOn == null) 796 this.basedOn = new ArrayList<Reference>(); 797 this.basedOn.add(t); 798 return this; 799 } 800 801 /** 802 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 803 */ 804 public Reference getBasedOnFirstRep() { 805 if (getBasedOn().isEmpty()) { 806 addBasedOn(); 807 } 808 return getBasedOn().get(0); 809 } 810 811 /** 812 * @return {@link #category} (Category of supply, e.g. central, non-stock, etc. This is used to support work flows associated with the supply process.) 813 */ 814 public CodeableConcept getCategory() { 815 if (this.category == null) 816 if (Configuration.errorOnAutoCreate()) 817 throw new Error("Attempt to auto-create SupplyRequest.category"); 818 else if (Configuration.doAutoCreate()) 819 this.category = new CodeableConcept(); // cc 820 return this.category; 821 } 822 823 public boolean hasCategory() { 824 return this.category != null && !this.category.isEmpty(); 825 } 826 827 /** 828 * @param value {@link #category} (Category of supply, e.g. central, non-stock, etc. This is used to support work flows associated with the supply process.) 829 */ 830 public SupplyRequest setCategory(CodeableConcept value) { 831 this.category = value; 832 return this; 833 } 834 835 /** 836 * @return {@link #priority} (Indicates how quickly this SupplyRequest should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 837 */ 838 public Enumeration<RequestPriority> getPriorityElement() { 839 if (this.priority == null) 840 if (Configuration.errorOnAutoCreate()) 841 throw new Error("Attempt to auto-create SupplyRequest.priority"); 842 else if (Configuration.doAutoCreate()) 843 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 844 return this.priority; 845 } 846 847 public boolean hasPriorityElement() { 848 return this.priority != null && !this.priority.isEmpty(); 849 } 850 851 public boolean hasPriority() { 852 return this.priority != null && !this.priority.isEmpty(); 853 } 854 855 /** 856 * @param value {@link #priority} (Indicates how quickly this SupplyRequest should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 857 */ 858 public SupplyRequest setPriorityElement(Enumeration<RequestPriority> value) { 859 this.priority = value; 860 return this; 861 } 862 863 /** 864 * @return Indicates how quickly this SupplyRequest should be addressed with respect to other requests. 865 */ 866 public RequestPriority getPriority() { 867 return this.priority == null ? null : this.priority.getValue(); 868 } 869 870 /** 871 * @param value Indicates how quickly this SupplyRequest should be addressed with respect to other requests. 872 */ 873 public SupplyRequest setPriority(RequestPriority value) { 874 if (value == null) 875 this.priority = null; 876 else { 877 if (this.priority == null) 878 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 879 this.priority.setValue(value); 880 } 881 return this; 882 } 883 884 /** 885 * @return {@link #deliverFor} (The patient to whom the supply will be given or for whom they will be used.) 886 */ 887 public Reference getDeliverFor() { 888 if (this.deliverFor == null) 889 if (Configuration.errorOnAutoCreate()) 890 throw new Error("Attempt to auto-create SupplyRequest.deliverFor"); 891 else if (Configuration.doAutoCreate()) 892 this.deliverFor = new Reference(); // cc 893 return this.deliverFor; 894 } 895 896 public boolean hasDeliverFor() { 897 return this.deliverFor != null && !this.deliverFor.isEmpty(); 898 } 899 900 /** 901 * @param value {@link #deliverFor} (The patient to whom the supply will be given or for whom they will be used.) 902 */ 903 public SupplyRequest setDeliverFor(Reference value) { 904 this.deliverFor = value; 905 return this; 906 } 907 908 /** 909 * @return {@link #item} (The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.) 910 */ 911 public CodeableReference getItem() { 912 if (this.item == null) 913 if (Configuration.errorOnAutoCreate()) 914 throw new Error("Attempt to auto-create SupplyRequest.item"); 915 else if (Configuration.doAutoCreate()) 916 this.item = new CodeableReference(); // cc 917 return this.item; 918 } 919 920 public boolean hasItem() { 921 return this.item != null && !this.item.isEmpty(); 922 } 923 924 /** 925 * @param value {@link #item} (The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.) 926 */ 927 public SupplyRequest setItem(CodeableReference value) { 928 this.item = value; 929 return this; 930 } 931 932 /** 933 * @return {@link #quantity} (The amount that is being ordered of the indicated item.) 934 */ 935 public Quantity getQuantity() { 936 if (this.quantity == null) 937 if (Configuration.errorOnAutoCreate()) 938 throw new Error("Attempt to auto-create SupplyRequest.quantity"); 939 else if (Configuration.doAutoCreate()) 940 this.quantity = new Quantity(); // cc 941 return this.quantity; 942 } 943 944 public boolean hasQuantity() { 945 return this.quantity != null && !this.quantity.isEmpty(); 946 } 947 948 /** 949 * @param value {@link #quantity} (The amount that is being ordered of the indicated item.) 950 */ 951 public SupplyRequest setQuantity(Quantity value) { 952 this.quantity = value; 953 return this; 954 } 955 956 /** 957 * @return {@link #parameter} (Specific parameters for the ordered item. For example, the size of the indicated item.) 958 */ 959 public List<SupplyRequestParameterComponent> getParameter() { 960 if (this.parameter == null) 961 this.parameter = new ArrayList<SupplyRequestParameterComponent>(); 962 return this.parameter; 963 } 964 965 /** 966 * @return Returns a reference to <code>this</code> for easy method chaining 967 */ 968 public SupplyRequest setParameter(List<SupplyRequestParameterComponent> theParameter) { 969 this.parameter = theParameter; 970 return this; 971 } 972 973 public boolean hasParameter() { 974 if (this.parameter == null) 975 return false; 976 for (SupplyRequestParameterComponent item : this.parameter) 977 if (!item.isEmpty()) 978 return true; 979 return false; 980 } 981 982 public SupplyRequestParameterComponent addParameter() { //3 983 SupplyRequestParameterComponent t = new SupplyRequestParameterComponent(); 984 if (this.parameter == null) 985 this.parameter = new ArrayList<SupplyRequestParameterComponent>(); 986 this.parameter.add(t); 987 return t; 988 } 989 990 public SupplyRequest addParameter(SupplyRequestParameterComponent t) { //3 991 if (t == null) 992 return this; 993 if (this.parameter == null) 994 this.parameter = new ArrayList<SupplyRequestParameterComponent>(); 995 this.parameter.add(t); 996 return this; 997 } 998 999 /** 1000 * @return The first repetition of repeating field {@link #parameter}, creating it if it does not already exist {3} 1001 */ 1002 public SupplyRequestParameterComponent getParameterFirstRep() { 1003 if (getParameter().isEmpty()) { 1004 addParameter(); 1005 } 1006 return getParameter().get(0); 1007 } 1008 1009 /** 1010 * @return {@link #occurrence} (When the request should be fulfilled.) 1011 */ 1012 public DataType getOccurrence() { 1013 return this.occurrence; 1014 } 1015 1016 /** 1017 * @return {@link #occurrence} (When the request should be fulfilled.) 1018 */ 1019 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1020 if (this.occurrence == null) 1021 this.occurrence = new DateTimeType(); 1022 if (!(this.occurrence instanceof DateTimeType)) 1023 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1024 return (DateTimeType) this.occurrence; 1025 } 1026 1027 public boolean hasOccurrenceDateTimeType() { 1028 return this != null && this.occurrence instanceof DateTimeType; 1029 } 1030 1031 /** 1032 * @return {@link #occurrence} (When the request should be fulfilled.) 1033 */ 1034 public Period getOccurrencePeriod() throws FHIRException { 1035 if (this.occurrence == null) 1036 this.occurrence = new Period(); 1037 if (!(this.occurrence instanceof Period)) 1038 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1039 return (Period) this.occurrence; 1040 } 1041 1042 public boolean hasOccurrencePeriod() { 1043 return this != null && this.occurrence instanceof Period; 1044 } 1045 1046 /** 1047 * @return {@link #occurrence} (When the request should be fulfilled.) 1048 */ 1049 public Timing getOccurrenceTiming() throws FHIRException { 1050 if (this.occurrence == null) 1051 this.occurrence = new Timing(); 1052 if (!(this.occurrence instanceof Timing)) 1053 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1054 return (Timing) this.occurrence; 1055 } 1056 1057 public boolean hasOccurrenceTiming() { 1058 return this != null && this.occurrence instanceof Timing; 1059 } 1060 1061 public boolean hasOccurrence() { 1062 return this.occurrence != null && !this.occurrence.isEmpty(); 1063 } 1064 1065 /** 1066 * @param value {@link #occurrence} (When the request should be fulfilled.) 1067 */ 1068 public SupplyRequest setOccurrence(DataType value) { 1069 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 1070 throw new FHIRException("Not the right type for SupplyRequest.occurrence[x]: "+value.fhirType()); 1071 this.occurrence = value; 1072 return this; 1073 } 1074 1075 /** 1076 * @return {@link #authoredOn} (When the request was made.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1077 */ 1078 public DateTimeType getAuthoredOnElement() { 1079 if (this.authoredOn == null) 1080 if (Configuration.errorOnAutoCreate()) 1081 throw new Error("Attempt to auto-create SupplyRequest.authoredOn"); 1082 else if (Configuration.doAutoCreate()) 1083 this.authoredOn = new DateTimeType(); // bb 1084 return this.authoredOn; 1085 } 1086 1087 public boolean hasAuthoredOnElement() { 1088 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1089 } 1090 1091 public boolean hasAuthoredOn() { 1092 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1093 } 1094 1095 /** 1096 * @param value {@link #authoredOn} (When the request was made.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1097 */ 1098 public SupplyRequest setAuthoredOnElement(DateTimeType value) { 1099 this.authoredOn = value; 1100 return this; 1101 } 1102 1103 /** 1104 * @return When the request was made. 1105 */ 1106 public Date getAuthoredOn() { 1107 return this.authoredOn == null ? null : this.authoredOn.getValue(); 1108 } 1109 1110 /** 1111 * @param value When the request was made. 1112 */ 1113 public SupplyRequest setAuthoredOn(Date value) { 1114 if (value == null) 1115 this.authoredOn = null; 1116 else { 1117 if (this.authoredOn == null) 1118 this.authoredOn = new DateTimeType(); 1119 this.authoredOn.setValue(value); 1120 } 1121 return this; 1122 } 1123 1124 /** 1125 * @return {@link #requester} (The device, practitioner, etc. who initiated the request.) 1126 */ 1127 public Reference getRequester() { 1128 if (this.requester == null) 1129 if (Configuration.errorOnAutoCreate()) 1130 throw new Error("Attempt to auto-create SupplyRequest.requester"); 1131 else if (Configuration.doAutoCreate()) 1132 this.requester = new Reference(); // cc 1133 return this.requester; 1134 } 1135 1136 public boolean hasRequester() { 1137 return this.requester != null && !this.requester.isEmpty(); 1138 } 1139 1140 /** 1141 * @param value {@link #requester} (The device, practitioner, etc. who initiated the request.) 1142 */ 1143 public SupplyRequest setRequester(Reference value) { 1144 this.requester = value; 1145 return this; 1146 } 1147 1148 /** 1149 * @return {@link #supplier} (Who is intended to fulfill the request.) 1150 */ 1151 public List<Reference> getSupplier() { 1152 if (this.supplier == null) 1153 this.supplier = new ArrayList<Reference>(); 1154 return this.supplier; 1155 } 1156 1157 /** 1158 * @return Returns a reference to <code>this</code> for easy method chaining 1159 */ 1160 public SupplyRequest setSupplier(List<Reference> theSupplier) { 1161 this.supplier = theSupplier; 1162 return this; 1163 } 1164 1165 public boolean hasSupplier() { 1166 if (this.supplier == null) 1167 return false; 1168 for (Reference item : this.supplier) 1169 if (!item.isEmpty()) 1170 return true; 1171 return false; 1172 } 1173 1174 public Reference addSupplier() { //3 1175 Reference t = new Reference(); 1176 if (this.supplier == null) 1177 this.supplier = new ArrayList<Reference>(); 1178 this.supplier.add(t); 1179 return t; 1180 } 1181 1182 public SupplyRequest addSupplier(Reference t) { //3 1183 if (t == null) 1184 return this; 1185 if (this.supplier == null) 1186 this.supplier = new ArrayList<Reference>(); 1187 this.supplier.add(t); 1188 return this; 1189 } 1190 1191 /** 1192 * @return The first repetition of repeating field {@link #supplier}, creating it if it does not already exist {3} 1193 */ 1194 public Reference getSupplierFirstRep() { 1195 if (getSupplier().isEmpty()) { 1196 addSupplier(); 1197 } 1198 return getSupplier().get(0); 1199 } 1200 1201 /** 1202 * @return {@link #reason} (The reason why the supply item was requested.) 1203 */ 1204 public List<CodeableReference> getReason() { 1205 if (this.reason == null) 1206 this.reason = new ArrayList<CodeableReference>(); 1207 return this.reason; 1208 } 1209 1210 /** 1211 * @return Returns a reference to <code>this</code> for easy method chaining 1212 */ 1213 public SupplyRequest setReason(List<CodeableReference> theReason) { 1214 this.reason = theReason; 1215 return this; 1216 } 1217 1218 public boolean hasReason() { 1219 if (this.reason == null) 1220 return false; 1221 for (CodeableReference item : this.reason) 1222 if (!item.isEmpty()) 1223 return true; 1224 return false; 1225 } 1226 1227 public CodeableReference addReason() { //3 1228 CodeableReference t = new CodeableReference(); 1229 if (this.reason == null) 1230 this.reason = new ArrayList<CodeableReference>(); 1231 this.reason.add(t); 1232 return t; 1233 } 1234 1235 public SupplyRequest addReason(CodeableReference t) { //3 1236 if (t == null) 1237 return this; 1238 if (this.reason == null) 1239 this.reason = new ArrayList<CodeableReference>(); 1240 this.reason.add(t); 1241 return this; 1242 } 1243 1244 /** 1245 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 1246 */ 1247 public CodeableReference getReasonFirstRep() { 1248 if (getReason().isEmpty()) { 1249 addReason(); 1250 } 1251 return getReason().get(0); 1252 } 1253 1254 /** 1255 * @return {@link #deliverFrom} (Where the supply is expected to come from.) 1256 */ 1257 public Reference getDeliverFrom() { 1258 if (this.deliverFrom == null) 1259 if (Configuration.errorOnAutoCreate()) 1260 throw new Error("Attempt to auto-create SupplyRequest.deliverFrom"); 1261 else if (Configuration.doAutoCreate()) 1262 this.deliverFrom = new Reference(); // cc 1263 return this.deliverFrom; 1264 } 1265 1266 public boolean hasDeliverFrom() { 1267 return this.deliverFrom != null && !this.deliverFrom.isEmpty(); 1268 } 1269 1270 /** 1271 * @param value {@link #deliverFrom} (Where the supply is expected to come from.) 1272 */ 1273 public SupplyRequest setDeliverFrom(Reference value) { 1274 this.deliverFrom = value; 1275 return this; 1276 } 1277 1278 /** 1279 * @return {@link #deliverTo} (Where the supply is destined to go.) 1280 */ 1281 public Reference getDeliverTo() { 1282 if (this.deliverTo == null) 1283 if (Configuration.errorOnAutoCreate()) 1284 throw new Error("Attempt to auto-create SupplyRequest.deliverTo"); 1285 else if (Configuration.doAutoCreate()) 1286 this.deliverTo = new Reference(); // cc 1287 return this.deliverTo; 1288 } 1289 1290 public boolean hasDeliverTo() { 1291 return this.deliverTo != null && !this.deliverTo.isEmpty(); 1292 } 1293 1294 /** 1295 * @param value {@link #deliverTo} (Where the supply is destined to go.) 1296 */ 1297 public SupplyRequest setDeliverTo(Reference value) { 1298 this.deliverTo = value; 1299 return this; 1300 } 1301 1302 protected void listChildren(List<Property> children) { 1303 super.listChildren(children); 1304 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this SupplyRequest by the author and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1305 children.add(new Property("status", "code", "Status of the supply request.", 0, 1, status)); 1306 children.add(new Property("basedOn", "Reference(Any)", "Plan/proposal/order fulfilled by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1307 children.add(new Property("category", "CodeableConcept", "Category of supply, e.g. central, non-stock, etc. This is used to support work flows associated with the supply process.", 0, 1, category)); 1308 children.add(new Property("priority", "code", "Indicates how quickly this SupplyRequest should be addressed with respect to other requests.", 0, 1, priority)); 1309 children.add(new Property("deliverFor", "Reference(Patient)", "The patient to whom the supply will be given or for whom they will be used.", 0, 1, deliverFor)); 1310 children.add(new Property("item", "CodeableReference(Medication|Substance|Device|DeviceDefinition|BiologicallyDerivedProduct|NutritionProduct|InventoryItem)", "The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item)); 1311 children.add(new Property("quantity", "Quantity", "The amount that is being ordered of the indicated item.", 0, 1, quantity)); 1312 children.add(new Property("parameter", "", "Specific parameters for the ordered item. For example, the size of the indicated item.", 0, java.lang.Integer.MAX_VALUE, parameter)); 1313 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "When the request should be fulfilled.", 0, 1, occurrence)); 1314 children.add(new Property("authoredOn", "dateTime", "When the request was made.", 0, 1, authoredOn)); 1315 children.add(new Property("requester", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device|CareTeam)", "The device, practitioner, etc. who initiated the request.", 0, 1, requester)); 1316 children.add(new Property("supplier", "Reference(Organization|HealthcareService)", "Who is intended to fulfill the request.", 0, java.lang.Integer.MAX_VALUE, supplier)); 1317 children.add(new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport|DocumentReference)", "The reason why the supply item was requested.", 0, java.lang.Integer.MAX_VALUE, reason)); 1318 children.add(new Property("deliverFrom", "Reference(Organization|Location)", "Where the supply is expected to come from.", 0, 1, deliverFrom)); 1319 children.add(new Property("deliverTo", "Reference(Organization|Location|Patient|RelatedPerson)", "Where the supply is destined to go.", 0, 1, deliverTo)); 1320 } 1321 1322 @Override 1323 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1324 switch (_hash) { 1325 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this SupplyRequest by the author and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 1326 case -892481550: /*status*/ return new Property("status", "code", "Status of the supply request.", 0, 1, status); 1327 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(Any)", "Plan/proposal/order fulfilled by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1328 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Category of supply, e.g. central, non-stock, etc. This is used to support work flows associated with the supply process.", 0, 1, category); 1329 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly this SupplyRequest should be addressed with respect to other requests.", 0, 1, priority); 1330 case 1077755236: /*deliverFor*/ return new Property("deliverFor", "Reference(Patient)", "The patient to whom the supply will be given or for whom they will be used.", 0, 1, deliverFor); 1331 case 3242771: /*item*/ return new Property("item", "CodeableReference(Medication|Substance|Device|DeviceDefinition|BiologicallyDerivedProduct|NutritionProduct|InventoryItem)", "The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item); 1332 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The amount that is being ordered of the indicated item.", 0, 1, quantity); 1333 case 1954460585: /*parameter*/ return new Property("parameter", "", "Specific parameters for the ordered item. For example, the size of the indicated item.", 0, java.lang.Integer.MAX_VALUE, parameter); 1334 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "When the request should be fulfilled.", 0, 1, occurrence); 1335 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "When the request should be fulfilled.", 0, 1, occurrence); 1336 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime", "When the request should be fulfilled.", 0, 1, occurrence); 1337 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "Period", "When the request should be fulfilled.", 0, 1, occurrence); 1338 case 1515218299: /*occurrenceTiming*/ return new Property("occurrence[x]", "Timing", "When the request should be fulfilled.", 0, 1, occurrence); 1339 case -1500852503: /*authoredOn*/ return new Property("authoredOn", "dateTime", "When the request was made.", 0, 1, authoredOn); 1340 case 693933948: /*requester*/ return new Property("requester", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device|CareTeam)", "The device, practitioner, etc. who initiated the request.", 0, 1, requester); 1341 case -1663305268: /*supplier*/ return new Property("supplier", "Reference(Organization|HealthcareService)", "Who is intended to fulfill the request.", 0, java.lang.Integer.MAX_VALUE, supplier); 1342 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport|DocumentReference)", "The reason why the supply item was requested.", 0, java.lang.Integer.MAX_VALUE, reason); 1343 case -949323153: /*deliverFrom*/ return new Property("deliverFrom", "Reference(Organization|Location)", "Where the supply is expected to come from.", 0, 1, deliverFrom); 1344 case -242327936: /*deliverTo*/ return new Property("deliverTo", "Reference(Organization|Location|Patient|RelatedPerson)", "Where the supply is destined to go.", 0, 1, deliverTo); 1345 default: return super.getNamedProperty(_hash, _name, _checkValid); 1346 } 1347 1348 } 1349 1350 @Override 1351 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1352 switch (hash) { 1353 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1354 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<SupplyRequestStatus> 1355 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1356 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1357 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<RequestPriority> 1358 case 1077755236: /*deliverFor*/ return this.deliverFor == null ? new Base[0] : new Base[] {this.deliverFor}; // Reference 1359 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // CodeableReference 1360 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 1361 case 1954460585: /*parameter*/ return this.parameter == null ? new Base[0] : this.parameter.toArray(new Base[this.parameter.size()]); // SupplyRequestParameterComponent 1362 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // DataType 1363 case -1500852503: /*authoredOn*/ return this.authoredOn == null ? new Base[0] : new Base[] {this.authoredOn}; // DateTimeType 1364 case 693933948: /*requester*/ return this.requester == null ? new Base[0] : new Base[] {this.requester}; // Reference 1365 case -1663305268: /*supplier*/ return this.supplier == null ? new Base[0] : this.supplier.toArray(new Base[this.supplier.size()]); // Reference 1366 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 1367 case -949323153: /*deliverFrom*/ return this.deliverFrom == null ? new Base[0] : new Base[] {this.deliverFrom}; // Reference 1368 case -242327936: /*deliverTo*/ return this.deliverTo == null ? new Base[0] : new Base[] {this.deliverTo}; // Reference 1369 default: return super.getProperty(hash, name, checkValid); 1370 } 1371 1372 } 1373 1374 @Override 1375 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1376 switch (hash) { 1377 case -1618432855: // identifier 1378 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1379 return value; 1380 case -892481550: // status 1381 value = new SupplyRequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1382 this.status = (Enumeration) value; // Enumeration<SupplyRequestStatus> 1383 return value; 1384 case -332612366: // basedOn 1385 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 1386 return value; 1387 case 50511102: // category 1388 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1389 return value; 1390 case -1165461084: // priority 1391 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1392 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1393 return value; 1394 case 1077755236: // deliverFor 1395 this.deliverFor = TypeConvertor.castToReference(value); // Reference 1396 return value; 1397 case 3242771: // item 1398 this.item = TypeConvertor.castToCodeableReference(value); // CodeableReference 1399 return value; 1400 case -1285004149: // quantity 1401 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1402 return value; 1403 case 1954460585: // parameter 1404 this.getParameter().add((SupplyRequestParameterComponent) value); // SupplyRequestParameterComponent 1405 return value; 1406 case 1687874001: // occurrence 1407 this.occurrence = TypeConvertor.castToType(value); // DataType 1408 return value; 1409 case -1500852503: // authoredOn 1410 this.authoredOn = TypeConvertor.castToDateTime(value); // DateTimeType 1411 return value; 1412 case 693933948: // requester 1413 this.requester = TypeConvertor.castToReference(value); // Reference 1414 return value; 1415 case -1663305268: // supplier 1416 this.getSupplier().add(TypeConvertor.castToReference(value)); // Reference 1417 return value; 1418 case -934964668: // reason 1419 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1420 return value; 1421 case -949323153: // deliverFrom 1422 this.deliverFrom = TypeConvertor.castToReference(value); // Reference 1423 return value; 1424 case -242327936: // deliverTo 1425 this.deliverTo = TypeConvertor.castToReference(value); // Reference 1426 return value; 1427 default: return super.setProperty(hash, name, value); 1428 } 1429 1430 } 1431 1432 @Override 1433 public Base setProperty(String name, Base value) throws FHIRException { 1434 if (name.equals("identifier")) { 1435 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1436 } else if (name.equals("status")) { 1437 value = new SupplyRequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1438 this.status = (Enumeration) value; // Enumeration<SupplyRequestStatus> 1439 } else if (name.equals("basedOn")) { 1440 this.getBasedOn().add(TypeConvertor.castToReference(value)); 1441 } else if (name.equals("category")) { 1442 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1443 } else if (name.equals("priority")) { 1444 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1445 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1446 } else if (name.equals("deliverFor")) { 1447 this.deliverFor = TypeConvertor.castToReference(value); // Reference 1448 } else if (name.equals("item")) { 1449 this.item = TypeConvertor.castToCodeableReference(value); // CodeableReference 1450 } else if (name.equals("quantity")) { 1451 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 1452 } else if (name.equals("parameter")) { 1453 this.getParameter().add((SupplyRequestParameterComponent) value); 1454 } else if (name.equals("occurrence[x]")) { 1455 this.occurrence = TypeConvertor.castToType(value); // DataType 1456 } else if (name.equals("authoredOn")) { 1457 this.authoredOn = TypeConvertor.castToDateTime(value); // DateTimeType 1458 } else if (name.equals("requester")) { 1459 this.requester = TypeConvertor.castToReference(value); // Reference 1460 } else if (name.equals("supplier")) { 1461 this.getSupplier().add(TypeConvertor.castToReference(value)); 1462 } else if (name.equals("reason")) { 1463 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 1464 } else if (name.equals("deliverFrom")) { 1465 this.deliverFrom = TypeConvertor.castToReference(value); // Reference 1466 } else if (name.equals("deliverTo")) { 1467 this.deliverTo = TypeConvertor.castToReference(value); // Reference 1468 } else 1469 return super.setProperty(name, value); 1470 return value; 1471 } 1472 1473 @Override 1474 public void removeChild(String name, Base value) throws FHIRException { 1475 if (name.equals("identifier")) { 1476 this.getIdentifier().remove(value); 1477 } else if (name.equals("status")) { 1478 value = new SupplyRequestStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1479 this.status = (Enumeration) value; // Enumeration<SupplyRequestStatus> 1480 } else if (name.equals("basedOn")) { 1481 this.getBasedOn().remove(value); 1482 } else if (name.equals("category")) { 1483 this.category = null; 1484 } else if (name.equals("priority")) { 1485 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 1486 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1487 } else if (name.equals("deliverFor")) { 1488 this.deliverFor = null; 1489 } else if (name.equals("item")) { 1490 this.item = null; 1491 } else if (name.equals("quantity")) { 1492 this.quantity = null; 1493 } else if (name.equals("parameter")) { 1494 this.getParameter().remove((SupplyRequestParameterComponent) value); 1495 } else if (name.equals("occurrence[x]")) { 1496 this.occurrence = null; 1497 } else if (name.equals("authoredOn")) { 1498 this.authoredOn = null; 1499 } else if (name.equals("requester")) { 1500 this.requester = null; 1501 } else if (name.equals("supplier")) { 1502 this.getSupplier().remove(value); 1503 } else if (name.equals("reason")) { 1504 this.getReason().remove(value); 1505 } else if (name.equals("deliverFrom")) { 1506 this.deliverFrom = null; 1507 } else if (name.equals("deliverTo")) { 1508 this.deliverTo = null; 1509 } else 1510 super.removeChild(name, value); 1511 1512 } 1513 1514 @Override 1515 public Base makeProperty(int hash, String name) throws FHIRException { 1516 switch (hash) { 1517 case -1618432855: return addIdentifier(); 1518 case -892481550: return getStatusElement(); 1519 case -332612366: return addBasedOn(); 1520 case 50511102: return getCategory(); 1521 case -1165461084: return getPriorityElement(); 1522 case 1077755236: return getDeliverFor(); 1523 case 3242771: return getItem(); 1524 case -1285004149: return getQuantity(); 1525 case 1954460585: return addParameter(); 1526 case -2022646513: return getOccurrence(); 1527 case 1687874001: return getOccurrence(); 1528 case -1500852503: return getAuthoredOnElement(); 1529 case 693933948: return getRequester(); 1530 case -1663305268: return addSupplier(); 1531 case -934964668: return addReason(); 1532 case -949323153: return getDeliverFrom(); 1533 case -242327936: return getDeliverTo(); 1534 default: return super.makeProperty(hash, name); 1535 } 1536 1537 } 1538 1539 @Override 1540 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1541 switch (hash) { 1542 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1543 case -892481550: /*status*/ return new String[] {"code"}; 1544 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1545 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1546 case -1165461084: /*priority*/ return new String[] {"code"}; 1547 case 1077755236: /*deliverFor*/ return new String[] {"Reference"}; 1548 case 3242771: /*item*/ return new String[] {"CodeableReference"}; 1549 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 1550 case 1954460585: /*parameter*/ return new String[] {}; 1551 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period", "Timing"}; 1552 case -1500852503: /*authoredOn*/ return new String[] {"dateTime"}; 1553 case 693933948: /*requester*/ return new String[] {"Reference"}; 1554 case -1663305268: /*supplier*/ return new String[] {"Reference"}; 1555 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 1556 case -949323153: /*deliverFrom*/ return new String[] {"Reference"}; 1557 case -242327936: /*deliverTo*/ return new String[] {"Reference"}; 1558 default: return super.getTypesForProperty(hash, name); 1559 } 1560 1561 } 1562 1563 @Override 1564 public Base addChild(String name) throws FHIRException { 1565 if (name.equals("identifier")) { 1566 return addIdentifier(); 1567 } 1568 else if (name.equals("status")) { 1569 throw new FHIRException("Cannot call addChild on a singleton property SupplyRequest.status"); 1570 } 1571 else if (name.equals("basedOn")) { 1572 return addBasedOn(); 1573 } 1574 else if (name.equals("category")) { 1575 this.category = new CodeableConcept(); 1576 return this.category; 1577 } 1578 else if (name.equals("priority")) { 1579 throw new FHIRException("Cannot call addChild on a singleton property SupplyRequest.priority"); 1580 } 1581 else if (name.equals("deliverFor")) { 1582 this.deliverFor = new Reference(); 1583 return this.deliverFor; 1584 } 1585 else if (name.equals("item")) { 1586 this.item = new CodeableReference(); 1587 return this.item; 1588 } 1589 else if (name.equals("quantity")) { 1590 this.quantity = new Quantity(); 1591 return this.quantity; 1592 } 1593 else if (name.equals("parameter")) { 1594 return addParameter(); 1595 } 1596 else if (name.equals("occurrenceDateTime")) { 1597 this.occurrence = new DateTimeType(); 1598 return this.occurrence; 1599 } 1600 else if (name.equals("occurrencePeriod")) { 1601 this.occurrence = new Period(); 1602 return this.occurrence; 1603 } 1604 else if (name.equals("occurrenceTiming")) { 1605 this.occurrence = new Timing(); 1606 return this.occurrence; 1607 } 1608 else if (name.equals("authoredOn")) { 1609 throw new FHIRException("Cannot call addChild on a singleton property SupplyRequest.authoredOn"); 1610 } 1611 else if (name.equals("requester")) { 1612 this.requester = new Reference(); 1613 return this.requester; 1614 } 1615 else if (name.equals("supplier")) { 1616 return addSupplier(); 1617 } 1618 else if (name.equals("reason")) { 1619 return addReason(); 1620 } 1621 else if (name.equals("deliverFrom")) { 1622 this.deliverFrom = new Reference(); 1623 return this.deliverFrom; 1624 } 1625 else if (name.equals("deliverTo")) { 1626 this.deliverTo = new Reference(); 1627 return this.deliverTo; 1628 } 1629 else 1630 return super.addChild(name); 1631 } 1632 1633 public String fhirType() { 1634 return "SupplyRequest"; 1635 1636 } 1637 1638 public SupplyRequest copy() { 1639 SupplyRequest dst = new SupplyRequest(); 1640 copyValues(dst); 1641 return dst; 1642 } 1643 1644 public void copyValues(SupplyRequest dst) { 1645 super.copyValues(dst); 1646 if (identifier != null) { 1647 dst.identifier = new ArrayList<Identifier>(); 1648 for (Identifier i : identifier) 1649 dst.identifier.add(i.copy()); 1650 }; 1651 dst.status = status == null ? null : status.copy(); 1652 if (basedOn != null) { 1653 dst.basedOn = new ArrayList<Reference>(); 1654 for (Reference i : basedOn) 1655 dst.basedOn.add(i.copy()); 1656 }; 1657 dst.category = category == null ? null : category.copy(); 1658 dst.priority = priority == null ? null : priority.copy(); 1659 dst.deliverFor = deliverFor == null ? null : deliverFor.copy(); 1660 dst.item = item == null ? null : item.copy(); 1661 dst.quantity = quantity == null ? null : quantity.copy(); 1662 if (parameter != null) { 1663 dst.parameter = new ArrayList<SupplyRequestParameterComponent>(); 1664 for (SupplyRequestParameterComponent i : parameter) 1665 dst.parameter.add(i.copy()); 1666 }; 1667 dst.occurrence = occurrence == null ? null : occurrence.copy(); 1668 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 1669 dst.requester = requester == null ? null : requester.copy(); 1670 if (supplier != null) { 1671 dst.supplier = new ArrayList<Reference>(); 1672 for (Reference i : supplier) 1673 dst.supplier.add(i.copy()); 1674 }; 1675 if (reason != null) { 1676 dst.reason = new ArrayList<CodeableReference>(); 1677 for (CodeableReference i : reason) 1678 dst.reason.add(i.copy()); 1679 }; 1680 dst.deliverFrom = deliverFrom == null ? null : deliverFrom.copy(); 1681 dst.deliverTo = deliverTo == null ? null : deliverTo.copy(); 1682 } 1683 1684 protected SupplyRequest typedCopy() { 1685 return copy(); 1686 } 1687 1688 @Override 1689 public boolean equalsDeep(Base other_) { 1690 if (!super.equalsDeep(other_)) 1691 return false; 1692 if (!(other_ instanceof SupplyRequest)) 1693 return false; 1694 SupplyRequest o = (SupplyRequest) other_; 1695 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(basedOn, o.basedOn, true) 1696 && compareDeep(category, o.category, true) && compareDeep(priority, o.priority, true) && compareDeep(deliverFor, o.deliverFor, true) 1697 && compareDeep(item, o.item, true) && compareDeep(quantity, o.quantity, true) && compareDeep(parameter, o.parameter, true) 1698 && compareDeep(occurrence, o.occurrence, true) && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(requester, o.requester, true) 1699 && compareDeep(supplier, o.supplier, true) && compareDeep(reason, o.reason, true) && compareDeep(deliverFrom, o.deliverFrom, true) 1700 && compareDeep(deliverTo, o.deliverTo, true); 1701 } 1702 1703 @Override 1704 public boolean equalsShallow(Base other_) { 1705 if (!super.equalsShallow(other_)) 1706 return false; 1707 if (!(other_ instanceof SupplyRequest)) 1708 return false; 1709 SupplyRequest o = (SupplyRequest) other_; 1710 return compareValues(status, o.status, true) && compareValues(priority, o.priority, true) && compareValues(authoredOn, o.authoredOn, true) 1711 ; 1712 } 1713 1714 public boolean isEmpty() { 1715 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, basedOn 1716 , category, priority, deliverFor, item, quantity, parameter, occurrence, authoredOn 1717 , requester, supplier, reason, deliverFrom, deliverTo); 1718 } 1719 1720 @Override 1721 public ResourceType getResourceType() { 1722 return ResourceType.SupplyRequest; 1723 } 1724 1725 /** 1726 * Search parameter: <b>category</b> 1727 * <p> 1728 * Description: <b>The kind of supply (central, non-stock, etc.)</b><br> 1729 * Type: <b>token</b><br> 1730 * Path: <b>SupplyRequest.category</b><br> 1731 * </p> 1732 */ 1733 @SearchParamDefinition(name="category", path="SupplyRequest.category", description="The kind of supply (central, non-stock, etc.)", type="token" ) 1734 public static final String SP_CATEGORY = "category"; 1735 /** 1736 * <b>Fluent Client</b> search parameter constant for <b>category</b> 1737 * <p> 1738 * Description: <b>The kind of supply (central, non-stock, etc.)</b><br> 1739 * Type: <b>token</b><br> 1740 * Path: <b>SupplyRequest.category</b><br> 1741 * </p> 1742 */ 1743 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 1744 1745 /** 1746 * Search parameter: <b>requester</b> 1747 * <p> 1748 * Description: <b>Individual making the request</b><br> 1749 * Type: <b>reference</b><br> 1750 * Path: <b>SupplyRequest.requester</b><br> 1751 * </p> 1752 */ 1753 @SearchParamDefinition(name="requester", path="SupplyRequest.requester", description="Individual making the request", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={CareTeam.class, Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 1754 public static final String SP_REQUESTER = "requester"; 1755 /** 1756 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 1757 * <p> 1758 * Description: <b>Individual making the request</b><br> 1759 * Type: <b>reference</b><br> 1760 * Path: <b>SupplyRequest.requester</b><br> 1761 * </p> 1762 */ 1763 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTER); 1764 1765/** 1766 * Constant for fluent queries to be used to add include statements. Specifies 1767 * the path value of "<b>SupplyRequest:requester</b>". 1768 */ 1769 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include("SupplyRequest:requester").toLocked(); 1770 1771 /** 1772 * Search parameter: <b>status</b> 1773 * <p> 1774 * Description: <b>draft | active | suspended +</b><br> 1775 * Type: <b>token</b><br> 1776 * Path: <b>SupplyRequest.status</b><br> 1777 * </p> 1778 */ 1779 @SearchParamDefinition(name="status", path="SupplyRequest.status", description="draft | active | suspended +", type="token" ) 1780 public static final String SP_STATUS = "status"; 1781 /** 1782 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1783 * <p> 1784 * Description: <b>draft | active | suspended +</b><br> 1785 * Type: <b>token</b><br> 1786 * Path: <b>SupplyRequest.status</b><br> 1787 * </p> 1788 */ 1789 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1790 1791 /** 1792 * Search parameter: <b>subject</b> 1793 * <p> 1794 * Description: <b>The destination of the supply</b><br> 1795 * Type: <b>reference</b><br> 1796 * Path: <b>SupplyRequest.deliverTo</b><br> 1797 * </p> 1798 */ 1799 @SearchParamDefinition(name="subject", path="SupplyRequest.deliverTo", description="The destination of the supply", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Location.class, Organization.class, Patient.class, RelatedPerson.class } ) 1800 public static final String SP_SUBJECT = "subject"; 1801 /** 1802 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1803 * <p> 1804 * Description: <b>The destination of the supply</b><br> 1805 * Type: <b>reference</b><br> 1806 * Path: <b>SupplyRequest.deliverTo</b><br> 1807 * </p> 1808 */ 1809 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1810 1811/** 1812 * Constant for fluent queries to be used to add include statements. Specifies 1813 * the path value of "<b>SupplyRequest:subject</b>". 1814 */ 1815 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("SupplyRequest:subject").toLocked(); 1816 1817 /** 1818 * Search parameter: <b>supplier</b> 1819 * <p> 1820 * Description: <b>Who is intended to fulfill the request</b><br> 1821 * Type: <b>reference</b><br> 1822 * Path: <b>SupplyRequest.supplier</b><br> 1823 * </p> 1824 */ 1825 @SearchParamDefinition(name="supplier", path="SupplyRequest.supplier", description="Who is intended to fulfill the request", type="reference", target={HealthcareService.class, Organization.class } ) 1826 public static final String SP_SUPPLIER = "supplier"; 1827 /** 1828 * <b>Fluent Client</b> search parameter constant for <b>supplier</b> 1829 * <p> 1830 * Description: <b>Who is intended to fulfill the request</b><br> 1831 * Type: <b>reference</b><br> 1832 * Path: <b>SupplyRequest.supplier</b><br> 1833 * </p> 1834 */ 1835 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPLIER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUPPLIER); 1836 1837/** 1838 * Constant for fluent queries to be used to add include statements. Specifies 1839 * the path value of "<b>SupplyRequest:supplier</b>". 1840 */ 1841 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPLIER = new ca.uhn.fhir.model.api.Include("SupplyRequest:supplier").toLocked(); 1842 1843 /** 1844 * Search parameter: <b>date</b> 1845 * <p> 1846 * Description: <b>Multiple Resources: 1847 1848* [AdverseEvent](adverseevent.html): When the event occurred 1849* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 1850* [Appointment](appointment.html): Appointment date/time. 1851* [AuditEvent](auditevent.html): Time when the event was recorded 1852* [CarePlan](careplan.html): Time period plan covers 1853* [CareTeam](careteam.html): A date within the coverage time period. 1854* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 1855* [Composition](composition.html): Composition editing time 1856* [Consent](consent.html): When consent was agreed to 1857* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 1858* [DocumentReference](documentreference.html): When this document reference was created 1859* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 1860* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 1861* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 1862* [Flag](flag.html): Time period when flag is active 1863* [Immunization](immunization.html): Vaccination (non)-Administration Date 1864* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 1865* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 1866* [Invoice](invoice.html): Invoice date / posting date 1867* [List](list.html): When the list was prepared 1868* [MeasureReport](measurereport.html): The date of the measure report 1869* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 1870* [Observation](observation.html): Clinically relevant time/time-period for observation 1871* [Procedure](procedure.html): When the procedure occurred or is occurring 1872* [ResearchSubject](researchsubject.html): Start and end of participation 1873* [RiskAssessment](riskassessment.html): When was assessment made? 1874* [SupplyRequest](supplyrequest.html): When the request was made 1875</b><br> 1876 * Type: <b>date</b><br> 1877 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 1878 * </p> 1879 */ 1880 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 1881 public static final String SP_DATE = "date"; 1882 /** 1883 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1884 * <p> 1885 * Description: <b>Multiple Resources: 1886 1887* [AdverseEvent](adverseevent.html): When the event occurred 1888* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 1889* [Appointment](appointment.html): Appointment date/time. 1890* [AuditEvent](auditevent.html): Time when the event was recorded 1891* [CarePlan](careplan.html): Time period plan covers 1892* [CareTeam](careteam.html): A date within the coverage time period. 1893* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 1894* [Composition](composition.html): Composition editing time 1895* [Consent](consent.html): When consent was agreed to 1896* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 1897* [DocumentReference](documentreference.html): When this document reference was created 1898* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 1899* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 1900* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 1901* [Flag](flag.html): Time period when flag is active 1902* [Immunization](immunization.html): Vaccination (non)-Administration Date 1903* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 1904* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 1905* [Invoice](invoice.html): Invoice date / posting date 1906* [List](list.html): When the list was prepared 1907* [MeasureReport](measurereport.html): The date of the measure report 1908* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 1909* [Observation](observation.html): Clinically relevant time/time-period for observation 1910* [Procedure](procedure.html): When the procedure occurred or is occurring 1911* [ResearchSubject](researchsubject.html): Start and end of participation 1912* [RiskAssessment](riskassessment.html): When was assessment made? 1913* [SupplyRequest](supplyrequest.html): When the request was made 1914</b><br> 1915 * Type: <b>date</b><br> 1916 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 1917 * </p> 1918 */ 1919 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1920 1921 /** 1922 * Search parameter: <b>identifier</b> 1923 * <p> 1924 * Description: <b>Multiple Resources: 1925 1926* [Account](account.html): Account number 1927* [AdverseEvent](adverseevent.html): Business identifier for the event 1928* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1929* [Appointment](appointment.html): An Identifier of the Appointment 1930* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1931* [Basic](basic.html): Business identifier 1932* [BodyStructure](bodystructure.html): Bodystructure identifier 1933* [CarePlan](careplan.html): External Ids for this plan 1934* [CareTeam](careteam.html): External Ids for this team 1935* [ChargeItem](chargeitem.html): Business Identifier for item 1936* [Claim](claim.html): The primary identifier of the financial resource 1937* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1938* [ClinicalImpression](clinicalimpression.html): Business identifier 1939* [Communication](communication.html): Unique identifier 1940* [CommunicationRequest](communicationrequest.html): Unique identifier 1941* [Composition](composition.html): Version-independent identifier for the Composition 1942* [Condition](condition.html): A unique identifier of the condition record 1943* [Consent](consent.html): Identifier for this record (external references) 1944* [Contract](contract.html): The identity of the contract 1945* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1946* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1947* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1948* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1949* [DeviceRequest](devicerequest.html): Business identifier for request/order 1950* [DeviceUsage](deviceusage.html): Search by identifier 1951* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1952* [DocumentReference](documentreference.html): Identifier of the attachment binary 1953* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1954* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1955* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1956* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1957* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1958* [Flag](flag.html): Business identifier 1959* [Goal](goal.html): External Ids for this goal 1960* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1961* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1962* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1963* [Immunization](immunization.html): Business identifier 1964* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1965* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1966* [Invoice](invoice.html): Business Identifier for item 1967* [List](list.html): Business identifier 1968* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1969* [Medication](medication.html): Returns medications with this external identifier 1970* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1971* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1972* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1973* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1974* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1975* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1976* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1977* [Observation](observation.html): The unique id for a particular observation 1978* [Person](person.html): A person Identifier 1979* [Procedure](procedure.html): A unique identifier for a procedure 1980* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1981* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1982* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1983* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1984* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1985* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1986* [Specimen](specimen.html): The unique identifier associated with the specimen 1987* [SupplyDelivery](supplydelivery.html): External identifier 1988* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1989* [Task](task.html): Search for a task instance by its business identifier 1990* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1991</b><br> 1992 * Type: <b>token</b><br> 1993 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1994 * </p> 1995 */ 1996 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 1997 public static final String SP_IDENTIFIER = "identifier"; 1998 /** 1999 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2000 * <p> 2001 * Description: <b>Multiple Resources: 2002 2003* [Account](account.html): Account number 2004* [AdverseEvent](adverseevent.html): Business identifier for the event 2005* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2006* [Appointment](appointment.html): An Identifier of the Appointment 2007* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2008* [Basic](basic.html): Business identifier 2009* [BodyStructure](bodystructure.html): Bodystructure identifier 2010* [CarePlan](careplan.html): External Ids for this plan 2011* [CareTeam](careteam.html): External Ids for this team 2012* [ChargeItem](chargeitem.html): Business Identifier for item 2013* [Claim](claim.html): The primary identifier of the financial resource 2014* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2015* [ClinicalImpression](clinicalimpression.html): Business identifier 2016* [Communication](communication.html): Unique identifier 2017* [CommunicationRequest](communicationrequest.html): Unique identifier 2018* [Composition](composition.html): Version-independent identifier for the Composition 2019* [Condition](condition.html): A unique identifier of the condition record 2020* [Consent](consent.html): Identifier for this record (external references) 2021* [Contract](contract.html): The identity of the contract 2022* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2023* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2024* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2025* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2026* [DeviceRequest](devicerequest.html): Business identifier for request/order 2027* [DeviceUsage](deviceusage.html): Search by identifier 2028* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2029* [DocumentReference](documentreference.html): Identifier of the attachment binary 2030* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2031* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2032* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2033* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2034* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2035* [Flag](flag.html): Business identifier 2036* [Goal](goal.html): External Ids for this goal 2037* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2038* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2039* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2040* [Immunization](immunization.html): Business identifier 2041* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2042* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2043* [Invoice](invoice.html): Business Identifier for item 2044* [List](list.html): Business identifier 2045* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2046* [Medication](medication.html): Returns medications with this external identifier 2047* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2048* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2049* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2050* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2051* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2052* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2053* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2054* [Observation](observation.html): The unique id for a particular observation 2055* [Person](person.html): A person Identifier 2056* [Procedure](procedure.html): A unique identifier for a procedure 2057* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2058* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2059* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2060* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2061* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2062* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2063* [Specimen](specimen.html): The unique identifier associated with the specimen 2064* [SupplyDelivery](supplydelivery.html): External identifier 2065* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2066* [Task](task.html): Search for a task instance by its business identifier 2067* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2068</b><br> 2069 * Type: <b>token</b><br> 2070 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2071 * </p> 2072 */ 2073 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2074 2075 /** 2076 * Search parameter: <b>patient</b> 2077 * <p> 2078 * Description: <b>Multiple Resources: 2079 2080* [Account](account.html): The entity that caused the expenses 2081* [AdverseEvent](adverseevent.html): Subject impacted by event 2082* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2083* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2084* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2085* [AuditEvent](auditevent.html): Where the activity involved patient data 2086* [Basic](basic.html): Identifies the focus of this resource 2087* [BodyStructure](bodystructure.html): Who this is about 2088* [CarePlan](careplan.html): Who the care plan is for 2089* [CareTeam](careteam.html): Who care team is for 2090* [ChargeItem](chargeitem.html): Individual service was done for/to 2091* [Claim](claim.html): Patient receiving the products or services 2092* [ClaimResponse](claimresponse.html): The subject of care 2093* [ClinicalImpression](clinicalimpression.html): Patient assessed 2094* [Communication](communication.html): Focus of message 2095* [CommunicationRequest](communicationrequest.html): Focus of message 2096* [Composition](composition.html): Who and/or what the composition is about 2097* [Condition](condition.html): Who has the condition? 2098* [Consent](consent.html): Who the consent applies to 2099* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2100* [Coverage](coverage.html): Retrieve coverages for a patient 2101* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2102* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2103* [DetectedIssue](detectedissue.html): Associated patient 2104* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2105* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2106* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2107* [DocumentReference](documentreference.html): Who/what is the subject of the document 2108* [Encounter](encounter.html): The patient present at the encounter 2109* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2110* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2111* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2112* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2113* [Flag](flag.html): The identity of a subject to list flags for 2114* [Goal](goal.html): Who this goal is intended for 2115* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2116* [ImagingSelection](imagingselection.html): Who the study is about 2117* [ImagingStudy](imagingstudy.html): Who the study is about 2118* [Immunization](immunization.html): The patient for the vaccination record 2119* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2120* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2121* [Invoice](invoice.html): Recipient(s) of goods and services 2122* [List](list.html): If all resources have the same subject 2123* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2124* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2125* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2126* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2127* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2128* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2129* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2130* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2131* [Observation](observation.html): The subject that the observation is about (if patient) 2132* [Person](person.html): The Person links to this Patient 2133* [Procedure](procedure.html): Search by subject - a patient 2134* [Provenance](provenance.html): Where the activity involved patient data 2135* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2136* [RelatedPerson](relatedperson.html): The patient this related person is related to 2137* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2138* [ResearchSubject](researchsubject.html): Who or what is part of study 2139* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2140* [ServiceRequest](servicerequest.html): Search by subject - a patient 2141* [Specimen](specimen.html): The patient the specimen comes from 2142* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2143* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2144* [Task](task.html): Search by patient 2145* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2146</b><br> 2147 * Type: <b>reference</b><br> 2148 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2149 * </p> 2150 */ 2151 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 2152 public static final String SP_PATIENT = "patient"; 2153 /** 2154 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2155 * <p> 2156 * Description: <b>Multiple Resources: 2157 2158* [Account](account.html): The entity that caused the expenses 2159* [AdverseEvent](adverseevent.html): Subject impacted by event 2160* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2161* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2162* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2163* [AuditEvent](auditevent.html): Where the activity involved patient data 2164* [Basic](basic.html): Identifies the focus of this resource 2165* [BodyStructure](bodystructure.html): Who this is about 2166* [CarePlan](careplan.html): Who the care plan is for 2167* [CareTeam](careteam.html): Who care team is for 2168* [ChargeItem](chargeitem.html): Individual service was done for/to 2169* [Claim](claim.html): Patient receiving the products or services 2170* [ClaimResponse](claimresponse.html): The subject of care 2171* [ClinicalImpression](clinicalimpression.html): Patient assessed 2172* [Communication](communication.html): Focus of message 2173* [CommunicationRequest](communicationrequest.html): Focus of message 2174* [Composition](composition.html): Who and/or what the composition is about 2175* [Condition](condition.html): Who has the condition? 2176* [Consent](consent.html): Who the consent applies to 2177* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2178* [Coverage](coverage.html): Retrieve coverages for a patient 2179* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2180* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2181* [DetectedIssue](detectedissue.html): Associated patient 2182* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2183* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2184* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2185* [DocumentReference](documentreference.html): Who/what is the subject of the document 2186* [Encounter](encounter.html): The patient present at the encounter 2187* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2188* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2189* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2190* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2191* [Flag](flag.html): The identity of a subject to list flags for 2192* [Goal](goal.html): Who this goal is intended for 2193* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2194* [ImagingSelection](imagingselection.html): Who the study is about 2195* [ImagingStudy](imagingstudy.html): Who the study is about 2196* [Immunization](immunization.html): The patient for the vaccination record 2197* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2198* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2199* [Invoice](invoice.html): Recipient(s) of goods and services 2200* [List](list.html): If all resources have the same subject 2201* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2202* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2203* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2204* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2205* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2206* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2207* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2208* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2209* [Observation](observation.html): The subject that the observation is about (if patient) 2210* [Person](person.html): The Person links to this Patient 2211* [Procedure](procedure.html): Search by subject - a patient 2212* [Provenance](provenance.html): Where the activity involved patient data 2213* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2214* [RelatedPerson](relatedperson.html): The patient this related person is related to 2215* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2216* [ResearchSubject](researchsubject.html): Who or what is part of study 2217* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2218* [ServiceRequest](servicerequest.html): Search by subject - a patient 2219* [Specimen](specimen.html): The patient the specimen comes from 2220* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2221* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2222* [Task](task.html): Search by patient 2223* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2224</b><br> 2225 * Type: <b>reference</b><br> 2226 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2227 * </p> 2228 */ 2229 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2230 2231/** 2232 * Constant for fluent queries to be used to add include statements. Specifies 2233 * the path value of "<b>SupplyRequest:patient</b>". 2234 */ 2235 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("SupplyRequest:patient").toLocked(); 2236 2237 2238} 2239