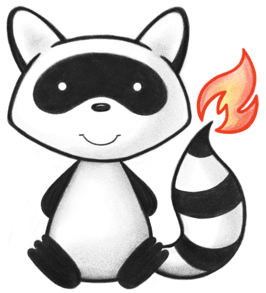
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A task to be performed. 052 */ 053@ResourceDef(name="Task", profile="http://hl7.org/fhir/StructureDefinition/Task") 054public class Task extends DomainResource { 055 056 public enum TaskIntent { 057 /** 058 * The intent is not known. When dealing with Task, it's not always known (or relevant) how the task was initiated - i.e. whether it was proposed, planned, ordered or just done spontaneously. 059 */ 060 UNKNOWN, 061 /** 062 * The request is a suggestion made by someone/something that does not have an intention to ensure it occurs and without providing an authorization to act. 063 */ 064 PROPOSAL, 065 /** 066 * The request represents an intention to ensure something occurs without providing an authorization for others to act. 067 */ 068 PLAN, 069 /** 070 * The request represents a request/demand and authorization for action by the requestor. 071 */ 072 ORDER, 073 /** 074 * The request represents an original authorization for action. 075 */ 076 ORIGINALORDER, 077 /** 078 * The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization. 079 */ 080 REFLEXORDER, 081 /** 082 * The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order. 083 */ 084 FILLERORDER, 085 /** 086 * An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug. 087 */ 088 INSTANCEORDER, 089 /** 090 * The request represents a component or option for a RequestOrchestration that establishes timing, conditionality and/or other constraints among a set of requests. Refer to [[[RequestOrchestration]]] for additional information on how this status is used. 091 */ 092 OPTION, 093 /** 094 * added to help the parsers with the generic types 095 */ 096 NULL; 097 public static TaskIntent fromCode(String codeString) throws FHIRException { 098 if (codeString == null || "".equals(codeString)) 099 return null; 100 if ("unknown".equals(codeString)) 101 return UNKNOWN; 102 if ("proposal".equals(codeString)) 103 return PROPOSAL; 104 if ("plan".equals(codeString)) 105 return PLAN; 106 if ("order".equals(codeString)) 107 return ORDER; 108 if ("original-order".equals(codeString)) 109 return ORIGINALORDER; 110 if ("reflex-order".equals(codeString)) 111 return REFLEXORDER; 112 if ("filler-order".equals(codeString)) 113 return FILLERORDER; 114 if ("instance-order".equals(codeString)) 115 return INSTANCEORDER; 116 if ("option".equals(codeString)) 117 return OPTION; 118 if (Configuration.isAcceptInvalidEnums()) 119 return null; 120 else 121 throw new FHIRException("Unknown TaskIntent code '"+codeString+"'"); 122 } 123 public String toCode() { 124 switch (this) { 125 case UNKNOWN: return "unknown"; 126 case PROPOSAL: return "proposal"; 127 case PLAN: return "plan"; 128 case ORDER: return "order"; 129 case ORIGINALORDER: return "original-order"; 130 case REFLEXORDER: return "reflex-order"; 131 case FILLERORDER: return "filler-order"; 132 case INSTANCEORDER: return "instance-order"; 133 case OPTION: return "option"; 134 case NULL: return null; 135 default: return "?"; 136 } 137 } 138 public String getSystem() { 139 switch (this) { 140 case UNKNOWN: return "http://hl7.org/fhir/task-intent"; 141 case PROPOSAL: return "http://hl7.org/fhir/request-intent"; 142 case PLAN: return "http://hl7.org/fhir/request-intent"; 143 case ORDER: return "http://hl7.org/fhir/request-intent"; 144 case ORIGINALORDER: return "http://hl7.org/fhir/request-intent"; 145 case REFLEXORDER: return "http://hl7.org/fhir/request-intent"; 146 case FILLERORDER: return "http://hl7.org/fhir/request-intent"; 147 case INSTANCEORDER: return "http://hl7.org/fhir/request-intent"; 148 case OPTION: return "http://hl7.org/fhir/request-intent"; 149 case NULL: return null; 150 default: return "?"; 151 } 152 } 153 public String getDefinition() { 154 switch (this) { 155 case UNKNOWN: return "The intent is not known. When dealing with Task, it's not always known (or relevant) how the task was initiated - i.e. whether it was proposed, planned, ordered or just done spontaneously."; 156 case PROPOSAL: return "The request is a suggestion made by someone/something that does not have an intention to ensure it occurs and without providing an authorization to act."; 157 case PLAN: return "The request represents an intention to ensure something occurs without providing an authorization for others to act."; 158 case ORDER: return "The request represents a request/demand and authorization for action by the requestor."; 159 case ORIGINALORDER: return "The request represents an original authorization for action."; 160 case REFLEXORDER: return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization."; 161 case FILLERORDER: return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order."; 162 case INSTANCEORDER: return "An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug."; 163 case OPTION: return "The request represents a component or option for a RequestOrchestration that establishes timing, conditionality and/or other constraints among a set of requests. Refer to [[[RequestOrchestration]]] for additional information on how this status is used."; 164 case NULL: return null; 165 default: return "?"; 166 } 167 } 168 public String getDisplay() { 169 switch (this) { 170 case UNKNOWN: return "Unknown"; 171 case PROPOSAL: return "Proposal"; 172 case PLAN: return "Plan"; 173 case ORDER: return "Order"; 174 case ORIGINALORDER: return "Original Order"; 175 case REFLEXORDER: return "Reflex Order"; 176 case FILLERORDER: return "Filler Order"; 177 case INSTANCEORDER: return "Instance Order"; 178 case OPTION: return "Option"; 179 case NULL: return null; 180 default: return "?"; 181 } 182 } 183 } 184 185 public static class TaskIntentEnumFactory implements EnumFactory<TaskIntent> { 186 public TaskIntent fromCode(String codeString) throws IllegalArgumentException { 187 if (codeString == null || "".equals(codeString)) 188 if (codeString == null || "".equals(codeString)) 189 return null; 190 if ("unknown".equals(codeString)) 191 return TaskIntent.UNKNOWN; 192 if ("proposal".equals(codeString)) 193 return TaskIntent.PROPOSAL; 194 if ("plan".equals(codeString)) 195 return TaskIntent.PLAN; 196 if ("order".equals(codeString)) 197 return TaskIntent.ORDER; 198 if ("original-order".equals(codeString)) 199 return TaskIntent.ORIGINALORDER; 200 if ("reflex-order".equals(codeString)) 201 return TaskIntent.REFLEXORDER; 202 if ("filler-order".equals(codeString)) 203 return TaskIntent.FILLERORDER; 204 if ("instance-order".equals(codeString)) 205 return TaskIntent.INSTANCEORDER; 206 if ("option".equals(codeString)) 207 return TaskIntent.OPTION; 208 throw new IllegalArgumentException("Unknown TaskIntent code '"+codeString+"'"); 209 } 210 public Enumeration<TaskIntent> fromType(PrimitiveType<?> code) throws FHIRException { 211 if (code == null) 212 return null; 213 if (code.isEmpty()) 214 return new Enumeration<TaskIntent>(this, TaskIntent.NULL, code); 215 String codeString = ((PrimitiveType) code).asStringValue(); 216 if (codeString == null || "".equals(codeString)) 217 return new Enumeration<TaskIntent>(this, TaskIntent.NULL, code); 218 if ("unknown".equals(codeString)) 219 return new Enumeration<TaskIntent>(this, TaskIntent.UNKNOWN, code); 220 if ("proposal".equals(codeString)) 221 return new Enumeration<TaskIntent>(this, TaskIntent.PROPOSAL, code); 222 if ("plan".equals(codeString)) 223 return new Enumeration<TaskIntent>(this, TaskIntent.PLAN, code); 224 if ("order".equals(codeString)) 225 return new Enumeration<TaskIntent>(this, TaskIntent.ORDER, code); 226 if ("original-order".equals(codeString)) 227 return new Enumeration<TaskIntent>(this, TaskIntent.ORIGINALORDER, code); 228 if ("reflex-order".equals(codeString)) 229 return new Enumeration<TaskIntent>(this, TaskIntent.REFLEXORDER, code); 230 if ("filler-order".equals(codeString)) 231 return new Enumeration<TaskIntent>(this, TaskIntent.FILLERORDER, code); 232 if ("instance-order".equals(codeString)) 233 return new Enumeration<TaskIntent>(this, TaskIntent.INSTANCEORDER, code); 234 if ("option".equals(codeString)) 235 return new Enumeration<TaskIntent>(this, TaskIntent.OPTION, code); 236 throw new FHIRException("Unknown TaskIntent code '"+codeString+"'"); 237 } 238 public String toCode(TaskIntent code) { 239 if (code == TaskIntent.NULL) 240 return null; 241 if (code == TaskIntent.UNKNOWN) 242 return "unknown"; 243 if (code == TaskIntent.PROPOSAL) 244 return "proposal"; 245 if (code == TaskIntent.PLAN) 246 return "plan"; 247 if (code == TaskIntent.ORDER) 248 return "order"; 249 if (code == TaskIntent.ORIGINALORDER) 250 return "original-order"; 251 if (code == TaskIntent.REFLEXORDER) 252 return "reflex-order"; 253 if (code == TaskIntent.FILLERORDER) 254 return "filler-order"; 255 if (code == TaskIntent.INSTANCEORDER) 256 return "instance-order"; 257 if (code == TaskIntent.OPTION) 258 return "option"; 259 return "?"; 260 } 261 public String toSystem(TaskIntent code) { 262 return code.getSystem(); 263 } 264 } 265 266 public enum TaskStatus { 267 /** 268 * The task is not yet ready to be acted upon. 269 */ 270 DRAFT, 271 /** 272 * The task is ready to be acted upon and action is sought. 273 */ 274 REQUESTED, 275 /** 276 * A potential performer has claimed ownership of the task and is evaluating whether to perform it. 277 */ 278 RECEIVED, 279 /** 280 * The potential performer has agreed to execute the task but has not yet started work. 281 */ 282 ACCEPTED, 283 /** 284 * The potential performer who claimed ownership of the task has decided not to execute it prior to performing any action. 285 */ 286 REJECTED, 287 /** 288 * The task is ready to be performed, but no action has yet been taken. Used in place of requested/received/accepted/rejected when request assignment and acceptance is a given. 289 */ 290 READY, 291 /** 292 * The task was not completed. 293 */ 294 CANCELLED, 295 /** 296 * The task has been started but is not yet complete. 297 */ 298 INPROGRESS, 299 /** 300 * The task has been started but work has been paused. 301 */ 302 ONHOLD, 303 /** 304 * The task was attempted but could not be completed due to some error. 305 */ 306 FAILED, 307 /** 308 * The task has been completed. 309 */ 310 COMPLETED, 311 /** 312 * The task should never have existed and is retained only because of the possibility it may have used. 313 */ 314 ENTEREDINERROR, 315 /** 316 * added to help the parsers with the generic types 317 */ 318 NULL; 319 public static TaskStatus fromCode(String codeString) throws FHIRException { 320 if (codeString == null || "".equals(codeString)) 321 return null; 322 if ("draft".equals(codeString)) 323 return DRAFT; 324 if ("requested".equals(codeString)) 325 return REQUESTED; 326 if ("received".equals(codeString)) 327 return RECEIVED; 328 if ("accepted".equals(codeString)) 329 return ACCEPTED; 330 if ("rejected".equals(codeString)) 331 return REJECTED; 332 if ("ready".equals(codeString)) 333 return READY; 334 if ("cancelled".equals(codeString)) 335 return CANCELLED; 336 if ("in-progress".equals(codeString)) 337 return INPROGRESS; 338 if ("on-hold".equals(codeString)) 339 return ONHOLD; 340 if ("failed".equals(codeString)) 341 return FAILED; 342 if ("completed".equals(codeString)) 343 return COMPLETED; 344 if ("entered-in-error".equals(codeString)) 345 return ENTEREDINERROR; 346 if (Configuration.isAcceptInvalidEnums()) 347 return null; 348 else 349 throw new FHIRException("Unknown TaskStatus code '"+codeString+"'"); 350 } 351 public String toCode() { 352 switch (this) { 353 case DRAFT: return "draft"; 354 case REQUESTED: return "requested"; 355 case RECEIVED: return "received"; 356 case ACCEPTED: return "accepted"; 357 case REJECTED: return "rejected"; 358 case READY: return "ready"; 359 case CANCELLED: return "cancelled"; 360 case INPROGRESS: return "in-progress"; 361 case ONHOLD: return "on-hold"; 362 case FAILED: return "failed"; 363 case COMPLETED: return "completed"; 364 case ENTEREDINERROR: return "entered-in-error"; 365 case NULL: return null; 366 default: return "?"; 367 } 368 } 369 public String getSystem() { 370 switch (this) { 371 case DRAFT: return "http://hl7.org/fhir/task-status"; 372 case REQUESTED: return "http://hl7.org/fhir/task-status"; 373 case RECEIVED: return "http://hl7.org/fhir/task-status"; 374 case ACCEPTED: return "http://hl7.org/fhir/task-status"; 375 case REJECTED: return "http://hl7.org/fhir/task-status"; 376 case READY: return "http://hl7.org/fhir/task-status"; 377 case CANCELLED: return "http://hl7.org/fhir/task-status"; 378 case INPROGRESS: return "http://hl7.org/fhir/task-status"; 379 case ONHOLD: return "http://hl7.org/fhir/task-status"; 380 case FAILED: return "http://hl7.org/fhir/task-status"; 381 case COMPLETED: return "http://hl7.org/fhir/task-status"; 382 case ENTEREDINERROR: return "http://hl7.org/fhir/task-status"; 383 case NULL: return null; 384 default: return "?"; 385 } 386 } 387 public String getDefinition() { 388 switch (this) { 389 case DRAFT: return "The task is not yet ready to be acted upon."; 390 case REQUESTED: return "The task is ready to be acted upon and action is sought."; 391 case RECEIVED: return "A potential performer has claimed ownership of the task and is evaluating whether to perform it."; 392 case ACCEPTED: return "The potential performer has agreed to execute the task but has not yet started work."; 393 case REJECTED: return "The potential performer who claimed ownership of the task has decided not to execute it prior to performing any action."; 394 case READY: return "The task is ready to be performed, but no action has yet been taken. Used in place of requested/received/accepted/rejected when request assignment and acceptance is a given."; 395 case CANCELLED: return "The task was not completed."; 396 case INPROGRESS: return "The task has been started but is not yet complete."; 397 case ONHOLD: return "The task has been started but work has been paused."; 398 case FAILED: return "The task was attempted but could not be completed due to some error."; 399 case COMPLETED: return "The task has been completed."; 400 case ENTEREDINERROR: return "The task should never have existed and is retained only because of the possibility it may have used."; 401 case NULL: return null; 402 default: return "?"; 403 } 404 } 405 public String getDisplay() { 406 switch (this) { 407 case DRAFT: return "Draft"; 408 case REQUESTED: return "Requested"; 409 case RECEIVED: return "Received"; 410 case ACCEPTED: return "Accepted"; 411 case REJECTED: return "Rejected"; 412 case READY: return "Ready"; 413 case CANCELLED: return "Cancelled"; 414 case INPROGRESS: return "In Progress"; 415 case ONHOLD: return "On Hold"; 416 case FAILED: return "Failed"; 417 case COMPLETED: return "Completed"; 418 case ENTEREDINERROR: return "Entered in Error"; 419 case NULL: return null; 420 default: return "?"; 421 } 422 } 423 } 424 425 public static class TaskStatusEnumFactory implements EnumFactory<TaskStatus> { 426 public TaskStatus fromCode(String codeString) throws IllegalArgumentException { 427 if (codeString == null || "".equals(codeString)) 428 if (codeString == null || "".equals(codeString)) 429 return null; 430 if ("draft".equals(codeString)) 431 return TaskStatus.DRAFT; 432 if ("requested".equals(codeString)) 433 return TaskStatus.REQUESTED; 434 if ("received".equals(codeString)) 435 return TaskStatus.RECEIVED; 436 if ("accepted".equals(codeString)) 437 return TaskStatus.ACCEPTED; 438 if ("rejected".equals(codeString)) 439 return TaskStatus.REJECTED; 440 if ("ready".equals(codeString)) 441 return TaskStatus.READY; 442 if ("cancelled".equals(codeString)) 443 return TaskStatus.CANCELLED; 444 if ("in-progress".equals(codeString)) 445 return TaskStatus.INPROGRESS; 446 if ("on-hold".equals(codeString)) 447 return TaskStatus.ONHOLD; 448 if ("failed".equals(codeString)) 449 return TaskStatus.FAILED; 450 if ("completed".equals(codeString)) 451 return TaskStatus.COMPLETED; 452 if ("entered-in-error".equals(codeString)) 453 return TaskStatus.ENTEREDINERROR; 454 throw new IllegalArgumentException("Unknown TaskStatus code '"+codeString+"'"); 455 } 456 public Enumeration<TaskStatus> fromType(PrimitiveType<?> code) throws FHIRException { 457 if (code == null) 458 return null; 459 if (code.isEmpty()) 460 return new Enumeration<TaskStatus>(this, TaskStatus.NULL, code); 461 String codeString = ((PrimitiveType) code).asStringValue(); 462 if (codeString == null || "".equals(codeString)) 463 return new Enumeration<TaskStatus>(this, TaskStatus.NULL, code); 464 if ("draft".equals(codeString)) 465 return new Enumeration<TaskStatus>(this, TaskStatus.DRAFT, code); 466 if ("requested".equals(codeString)) 467 return new Enumeration<TaskStatus>(this, TaskStatus.REQUESTED, code); 468 if ("received".equals(codeString)) 469 return new Enumeration<TaskStatus>(this, TaskStatus.RECEIVED, code); 470 if ("accepted".equals(codeString)) 471 return new Enumeration<TaskStatus>(this, TaskStatus.ACCEPTED, code); 472 if ("rejected".equals(codeString)) 473 return new Enumeration<TaskStatus>(this, TaskStatus.REJECTED, code); 474 if ("ready".equals(codeString)) 475 return new Enumeration<TaskStatus>(this, TaskStatus.READY, code); 476 if ("cancelled".equals(codeString)) 477 return new Enumeration<TaskStatus>(this, TaskStatus.CANCELLED, code); 478 if ("in-progress".equals(codeString)) 479 return new Enumeration<TaskStatus>(this, TaskStatus.INPROGRESS, code); 480 if ("on-hold".equals(codeString)) 481 return new Enumeration<TaskStatus>(this, TaskStatus.ONHOLD, code); 482 if ("failed".equals(codeString)) 483 return new Enumeration<TaskStatus>(this, TaskStatus.FAILED, code); 484 if ("completed".equals(codeString)) 485 return new Enumeration<TaskStatus>(this, TaskStatus.COMPLETED, code); 486 if ("entered-in-error".equals(codeString)) 487 return new Enumeration<TaskStatus>(this, TaskStatus.ENTEREDINERROR, code); 488 throw new FHIRException("Unknown TaskStatus code '"+codeString+"'"); 489 } 490 public String toCode(TaskStatus code) { 491 if (code == TaskStatus.NULL) 492 return null; 493 if (code == TaskStatus.DRAFT) 494 return "draft"; 495 if (code == TaskStatus.REQUESTED) 496 return "requested"; 497 if (code == TaskStatus.RECEIVED) 498 return "received"; 499 if (code == TaskStatus.ACCEPTED) 500 return "accepted"; 501 if (code == TaskStatus.REJECTED) 502 return "rejected"; 503 if (code == TaskStatus.READY) 504 return "ready"; 505 if (code == TaskStatus.CANCELLED) 506 return "cancelled"; 507 if (code == TaskStatus.INPROGRESS) 508 return "in-progress"; 509 if (code == TaskStatus.ONHOLD) 510 return "on-hold"; 511 if (code == TaskStatus.FAILED) 512 return "failed"; 513 if (code == TaskStatus.COMPLETED) 514 return "completed"; 515 if (code == TaskStatus.ENTEREDINERROR) 516 return "entered-in-error"; 517 return "?"; 518 } 519 public String toSystem(TaskStatus code) { 520 return code.getSystem(); 521 } 522 } 523 524 @Block() 525 public static class TaskPerformerComponent extends BackboneElement implements IBaseBackboneElement { 526 /** 527 * A code or description of the performer of the task. 528 */ 529 @Child(name = "function", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 530 @Description(shortDefinition="Type of performance", formalDefinition="A code or description of the performer of the task." ) 531 protected CodeableConcept function; 532 533 /** 534 * The actor or entity who performed the task. 535 */ 536 @Child(name = "actor", type = {Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, Patient.class, RelatedPerson.class}, order=2, min=1, max=1, modifier=false, summary=true) 537 @Description(shortDefinition="Who performed the task", formalDefinition="The actor or entity who performed the task." ) 538 protected Reference actor; 539 540 private static final long serialVersionUID = -576943815L; 541 542 /** 543 * Constructor 544 */ 545 public TaskPerformerComponent() { 546 super(); 547 } 548 549 /** 550 * Constructor 551 */ 552 public TaskPerformerComponent(Reference actor) { 553 super(); 554 this.setActor(actor); 555 } 556 557 /** 558 * @return {@link #function} (A code or description of the performer of the task.) 559 */ 560 public CodeableConcept getFunction() { 561 if (this.function == null) 562 if (Configuration.errorOnAutoCreate()) 563 throw new Error("Attempt to auto-create TaskPerformerComponent.function"); 564 else if (Configuration.doAutoCreate()) 565 this.function = new CodeableConcept(); // cc 566 return this.function; 567 } 568 569 public boolean hasFunction() { 570 return this.function != null && !this.function.isEmpty(); 571 } 572 573 /** 574 * @param value {@link #function} (A code or description of the performer of the task.) 575 */ 576 public TaskPerformerComponent setFunction(CodeableConcept value) { 577 this.function = value; 578 return this; 579 } 580 581 /** 582 * @return {@link #actor} (The actor or entity who performed the task.) 583 */ 584 public Reference getActor() { 585 if (this.actor == null) 586 if (Configuration.errorOnAutoCreate()) 587 throw new Error("Attempt to auto-create TaskPerformerComponent.actor"); 588 else if (Configuration.doAutoCreate()) 589 this.actor = new Reference(); // cc 590 return this.actor; 591 } 592 593 public boolean hasActor() { 594 return this.actor != null && !this.actor.isEmpty(); 595 } 596 597 /** 598 * @param value {@link #actor} (The actor or entity who performed the task.) 599 */ 600 public TaskPerformerComponent setActor(Reference value) { 601 this.actor = value; 602 return this; 603 } 604 605 protected void listChildren(List<Property> children) { 606 super.listChildren(children); 607 children.add(new Property("function", "CodeableConcept", "A code or description of the performer of the task.", 0, 1, function)); 608 children.add(new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|RelatedPerson)", "The actor or entity who performed the task.", 0, 1, actor)); 609 } 610 611 @Override 612 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 613 switch (_hash) { 614 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "A code or description of the performer of the task.", 0, 1, function); 615 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|RelatedPerson)", "The actor or entity who performed the task.", 0, 1, actor); 616 default: return super.getNamedProperty(_hash, _name, _checkValid); 617 } 618 619 } 620 621 @Override 622 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 623 switch (hash) { 624 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 625 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 626 default: return super.getProperty(hash, name, checkValid); 627 } 628 629 } 630 631 @Override 632 public Base setProperty(int hash, String name, Base value) throws FHIRException { 633 switch (hash) { 634 case 1380938712: // function 635 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 636 return value; 637 case 92645877: // actor 638 this.actor = TypeConvertor.castToReference(value); // Reference 639 return value; 640 default: return super.setProperty(hash, name, value); 641 } 642 643 } 644 645 @Override 646 public Base setProperty(String name, Base value) throws FHIRException { 647 if (name.equals("function")) { 648 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 649 } else if (name.equals("actor")) { 650 this.actor = TypeConvertor.castToReference(value); // Reference 651 } else 652 return super.setProperty(name, value); 653 return value; 654 } 655 656 @Override 657 public void removeChild(String name, Base value) throws FHIRException { 658 if (name.equals("function")) { 659 this.function = null; 660 } else if (name.equals("actor")) { 661 this.actor = null; 662 } else 663 super.removeChild(name, value); 664 665 } 666 667 @Override 668 public Base makeProperty(int hash, String name) throws FHIRException { 669 switch (hash) { 670 case 1380938712: return getFunction(); 671 case 92645877: return getActor(); 672 default: return super.makeProperty(hash, name); 673 } 674 675 } 676 677 @Override 678 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 679 switch (hash) { 680 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 681 case 92645877: /*actor*/ return new String[] {"Reference"}; 682 default: return super.getTypesForProperty(hash, name); 683 } 684 685 } 686 687 @Override 688 public Base addChild(String name) throws FHIRException { 689 if (name.equals("function")) { 690 this.function = new CodeableConcept(); 691 return this.function; 692 } 693 else if (name.equals("actor")) { 694 this.actor = new Reference(); 695 return this.actor; 696 } 697 else 698 return super.addChild(name); 699 } 700 701 public TaskPerformerComponent copy() { 702 TaskPerformerComponent dst = new TaskPerformerComponent(); 703 copyValues(dst); 704 return dst; 705 } 706 707 public void copyValues(TaskPerformerComponent dst) { 708 super.copyValues(dst); 709 dst.function = function == null ? null : function.copy(); 710 dst.actor = actor == null ? null : actor.copy(); 711 } 712 713 @Override 714 public boolean equalsDeep(Base other_) { 715 if (!super.equalsDeep(other_)) 716 return false; 717 if (!(other_ instanceof TaskPerformerComponent)) 718 return false; 719 TaskPerformerComponent o = (TaskPerformerComponent) other_; 720 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 721 } 722 723 @Override 724 public boolean equalsShallow(Base other_) { 725 if (!super.equalsShallow(other_)) 726 return false; 727 if (!(other_ instanceof TaskPerformerComponent)) 728 return false; 729 TaskPerformerComponent o = (TaskPerformerComponent) other_; 730 return true; 731 } 732 733 public boolean isEmpty() { 734 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 735 } 736 737 public String fhirType() { 738 return "Task.performer"; 739 740 } 741 742 } 743 744 @Block() 745 public static class TaskRestrictionComponent extends BackboneElement implements IBaseBackboneElement { 746 /** 747 * Indicates the number of times the requested action should occur. 748 */ 749 @Child(name = "repetitions", type = {PositiveIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 750 @Description(shortDefinition="How many times to repeat", formalDefinition="Indicates the number of times the requested action should occur." ) 751 protected PositiveIntType repetitions; 752 753 /** 754 * The time-period for which fulfillment is sought. This must fall within the overall time period authorized in the referenced request. E.g. ServiceRequest.occurance[x]. 755 */ 756 @Child(name = "period", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=false) 757 @Description(shortDefinition="When fulfillment is sought", formalDefinition="The time-period for which fulfillment is sought. This must fall within the overall time period authorized in the referenced request. E.g. ServiceRequest.occurance[x]." ) 758 protected Period period; 759 760 /** 761 * For requests that are targeted to more than one potential recipient/target, to identify who is fulfillment is sought for. 762 */ 763 @Child(name = "recipient", type = {Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, Group.class, Organization.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 764 @Description(shortDefinition="For whom is fulfillment sought?", formalDefinition="For requests that are targeted to more than one potential recipient/target, to identify who is fulfillment is sought for." ) 765 protected List<Reference> recipient; 766 767 private static final long serialVersionUID = 1673996066L; 768 769 /** 770 * Constructor 771 */ 772 public TaskRestrictionComponent() { 773 super(); 774 } 775 776 /** 777 * @return {@link #repetitions} (Indicates the number of times the requested action should occur.). This is the underlying object with id, value and extensions. The accessor "getRepetitions" gives direct access to the value 778 */ 779 public PositiveIntType getRepetitionsElement() { 780 if (this.repetitions == null) 781 if (Configuration.errorOnAutoCreate()) 782 throw new Error("Attempt to auto-create TaskRestrictionComponent.repetitions"); 783 else if (Configuration.doAutoCreate()) 784 this.repetitions = new PositiveIntType(); // bb 785 return this.repetitions; 786 } 787 788 public boolean hasRepetitionsElement() { 789 return this.repetitions != null && !this.repetitions.isEmpty(); 790 } 791 792 public boolean hasRepetitions() { 793 return this.repetitions != null && !this.repetitions.isEmpty(); 794 } 795 796 /** 797 * @param value {@link #repetitions} (Indicates the number of times the requested action should occur.). This is the underlying object with id, value and extensions. The accessor "getRepetitions" gives direct access to the value 798 */ 799 public TaskRestrictionComponent setRepetitionsElement(PositiveIntType value) { 800 this.repetitions = value; 801 return this; 802 } 803 804 /** 805 * @return Indicates the number of times the requested action should occur. 806 */ 807 public int getRepetitions() { 808 return this.repetitions == null || this.repetitions.isEmpty() ? 0 : this.repetitions.getValue(); 809 } 810 811 /** 812 * @param value Indicates the number of times the requested action should occur. 813 */ 814 public TaskRestrictionComponent setRepetitions(int value) { 815 if (this.repetitions == null) 816 this.repetitions = new PositiveIntType(); 817 this.repetitions.setValue(value); 818 return this; 819 } 820 821 /** 822 * @return {@link #period} (The time-period for which fulfillment is sought. This must fall within the overall time period authorized in the referenced request. E.g. ServiceRequest.occurance[x].) 823 */ 824 public Period getPeriod() { 825 if (this.period == null) 826 if (Configuration.errorOnAutoCreate()) 827 throw new Error("Attempt to auto-create TaskRestrictionComponent.period"); 828 else if (Configuration.doAutoCreate()) 829 this.period = new Period(); // cc 830 return this.period; 831 } 832 833 public boolean hasPeriod() { 834 return this.period != null && !this.period.isEmpty(); 835 } 836 837 /** 838 * @param value {@link #period} (The time-period for which fulfillment is sought. This must fall within the overall time period authorized in the referenced request. E.g. ServiceRequest.occurance[x].) 839 */ 840 public TaskRestrictionComponent setPeriod(Period value) { 841 this.period = value; 842 return this; 843 } 844 845 /** 846 * @return {@link #recipient} (For requests that are targeted to more than one potential recipient/target, to identify who is fulfillment is sought for.) 847 */ 848 public List<Reference> getRecipient() { 849 if (this.recipient == null) 850 this.recipient = new ArrayList<Reference>(); 851 return this.recipient; 852 } 853 854 /** 855 * @return Returns a reference to <code>this</code> for easy method chaining 856 */ 857 public TaskRestrictionComponent setRecipient(List<Reference> theRecipient) { 858 this.recipient = theRecipient; 859 return this; 860 } 861 862 public boolean hasRecipient() { 863 if (this.recipient == null) 864 return false; 865 for (Reference item : this.recipient) 866 if (!item.isEmpty()) 867 return true; 868 return false; 869 } 870 871 public Reference addRecipient() { //3 872 Reference t = new Reference(); 873 if (this.recipient == null) 874 this.recipient = new ArrayList<Reference>(); 875 this.recipient.add(t); 876 return t; 877 } 878 879 public TaskRestrictionComponent addRecipient(Reference t) { //3 880 if (t == null) 881 return this; 882 if (this.recipient == null) 883 this.recipient = new ArrayList<Reference>(); 884 this.recipient.add(t); 885 return this; 886 } 887 888 /** 889 * @return The first repetition of repeating field {@link #recipient}, creating it if it does not already exist {3} 890 */ 891 public Reference getRecipientFirstRep() { 892 if (getRecipient().isEmpty()) { 893 addRecipient(); 894 } 895 return getRecipient().get(0); 896 } 897 898 protected void listChildren(List<Property> children) { 899 super.listChildren(children); 900 children.add(new Property("repetitions", "positiveInt", "Indicates the number of times the requested action should occur.", 0, 1, repetitions)); 901 children.add(new Property("period", "Period", "The time-period for which fulfillment is sought. This must fall within the overall time period authorized in the referenced request. E.g. ServiceRequest.occurance[x].", 0, 1, period)); 902 children.add(new Property("recipient", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Group|Organization)", "For requests that are targeted to more than one potential recipient/target, to identify who is fulfillment is sought for.", 0, java.lang.Integer.MAX_VALUE, recipient)); 903 } 904 905 @Override 906 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 907 switch (_hash) { 908 case 984367650: /*repetitions*/ return new Property("repetitions", "positiveInt", "Indicates the number of times the requested action should occur.", 0, 1, repetitions); 909 case -991726143: /*period*/ return new Property("period", "Period", "The time-period for which fulfillment is sought. This must fall within the overall time period authorized in the referenced request. E.g. ServiceRequest.occurance[x].", 0, 1, period); 910 case 820081177: /*recipient*/ return new Property("recipient", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Group|Organization)", "For requests that are targeted to more than one potential recipient/target, to identify who is fulfillment is sought for.", 0, java.lang.Integer.MAX_VALUE, recipient); 911 default: return super.getNamedProperty(_hash, _name, _checkValid); 912 } 913 914 } 915 916 @Override 917 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 918 switch (hash) { 919 case 984367650: /*repetitions*/ return this.repetitions == null ? new Base[0] : new Base[] {this.repetitions}; // PositiveIntType 920 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 921 case 820081177: /*recipient*/ return this.recipient == null ? new Base[0] : this.recipient.toArray(new Base[this.recipient.size()]); // Reference 922 default: return super.getProperty(hash, name, checkValid); 923 } 924 925 } 926 927 @Override 928 public Base setProperty(int hash, String name, Base value) throws FHIRException { 929 switch (hash) { 930 case 984367650: // repetitions 931 this.repetitions = TypeConvertor.castToPositiveInt(value); // PositiveIntType 932 return value; 933 case -991726143: // period 934 this.period = TypeConvertor.castToPeriod(value); // Period 935 return value; 936 case 820081177: // recipient 937 this.getRecipient().add(TypeConvertor.castToReference(value)); // Reference 938 return value; 939 default: return super.setProperty(hash, name, value); 940 } 941 942 } 943 944 @Override 945 public Base setProperty(String name, Base value) throws FHIRException { 946 if (name.equals("repetitions")) { 947 this.repetitions = TypeConvertor.castToPositiveInt(value); // PositiveIntType 948 } else if (name.equals("period")) { 949 this.period = TypeConvertor.castToPeriod(value); // Period 950 } else if (name.equals("recipient")) { 951 this.getRecipient().add(TypeConvertor.castToReference(value)); 952 } else 953 return super.setProperty(name, value); 954 return value; 955 } 956 957 @Override 958 public void removeChild(String name, Base value) throws FHIRException { 959 if (name.equals("repetitions")) { 960 this.repetitions = null; 961 } else if (name.equals("period")) { 962 this.period = null; 963 } else if (name.equals("recipient")) { 964 this.getRecipient().remove(value); 965 } else 966 super.removeChild(name, value); 967 968 } 969 970 @Override 971 public Base makeProperty(int hash, String name) throws FHIRException { 972 switch (hash) { 973 case 984367650: return getRepetitionsElement(); 974 case -991726143: return getPeriod(); 975 case 820081177: return addRecipient(); 976 default: return super.makeProperty(hash, name); 977 } 978 979 } 980 981 @Override 982 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 983 switch (hash) { 984 case 984367650: /*repetitions*/ return new String[] {"positiveInt"}; 985 case -991726143: /*period*/ return new String[] {"Period"}; 986 case 820081177: /*recipient*/ return new String[] {"Reference"}; 987 default: return super.getTypesForProperty(hash, name); 988 } 989 990 } 991 992 @Override 993 public Base addChild(String name) throws FHIRException { 994 if (name.equals("repetitions")) { 995 throw new FHIRException("Cannot call addChild on a singleton property Task.restriction.repetitions"); 996 } 997 else if (name.equals("period")) { 998 this.period = new Period(); 999 return this.period; 1000 } 1001 else if (name.equals("recipient")) { 1002 return addRecipient(); 1003 } 1004 else 1005 return super.addChild(name); 1006 } 1007 1008 public TaskRestrictionComponent copy() { 1009 TaskRestrictionComponent dst = new TaskRestrictionComponent(); 1010 copyValues(dst); 1011 return dst; 1012 } 1013 1014 public void copyValues(TaskRestrictionComponent dst) { 1015 super.copyValues(dst); 1016 dst.repetitions = repetitions == null ? null : repetitions.copy(); 1017 dst.period = period == null ? null : period.copy(); 1018 if (recipient != null) { 1019 dst.recipient = new ArrayList<Reference>(); 1020 for (Reference i : recipient) 1021 dst.recipient.add(i.copy()); 1022 }; 1023 } 1024 1025 @Override 1026 public boolean equalsDeep(Base other_) { 1027 if (!super.equalsDeep(other_)) 1028 return false; 1029 if (!(other_ instanceof TaskRestrictionComponent)) 1030 return false; 1031 TaskRestrictionComponent o = (TaskRestrictionComponent) other_; 1032 return compareDeep(repetitions, o.repetitions, true) && compareDeep(period, o.period, true) && compareDeep(recipient, o.recipient, true) 1033 ; 1034 } 1035 1036 @Override 1037 public boolean equalsShallow(Base other_) { 1038 if (!super.equalsShallow(other_)) 1039 return false; 1040 if (!(other_ instanceof TaskRestrictionComponent)) 1041 return false; 1042 TaskRestrictionComponent o = (TaskRestrictionComponent) other_; 1043 return compareValues(repetitions, o.repetitions, true); 1044 } 1045 1046 public boolean isEmpty() { 1047 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(repetitions, period, recipient 1048 ); 1049 } 1050 1051 public String fhirType() { 1052 return "Task.restriction"; 1053 1054 } 1055 1056 } 1057 1058 @Block() 1059 public static class TaskInputComponent extends BackboneElement implements IBaseBackboneElement { 1060 /** 1061 * A code or description indicating how the input is intended to be used as part of the task execution. 1062 */ 1063 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1064 @Description(shortDefinition="Label for the input", formalDefinition="A code or description indicating how the input is intended to be used as part of the task execution." ) 1065 protected CodeableConcept type; 1066 1067 /** 1068 * The value of the input parameter as a basic type. 1069 */ 1070 @Child(name = "value", type = {Base64BinaryType.class, BooleanType.class, CanonicalType.class, CodeType.class, DateType.class, DateTimeType.class, DecimalType.class, IdType.class, InstantType.class, IntegerType.class, Integer64Type.class, MarkdownType.class, OidType.class, PositiveIntType.class, StringType.class, TimeType.class, UnsignedIntType.class, UriType.class, UrlType.class, UuidType.class, Address.class, Age.class, Annotation.class, Attachment.class, CodeableConcept.class, CodeableReference.class, Coding.class, ContactPoint.class, Count.class, Distance.class, Duration.class, HumanName.class, Identifier.class, Money.class, Period.class, Quantity.class, Range.class, Ratio.class, RatioRange.class, Reference.class, SampledData.class, Signature.class, Timing.class, ContactDetail.class, DataRequirement.class, Expression.class, ParameterDefinition.class, RelatedArtifact.class, TriggerDefinition.class, UsageContext.class, Availability.class, ExtendedContactDetail.class, Dosage.class, Meta.class}, order=2, min=1, max=1, modifier=false, summary=false) 1071 @Description(shortDefinition="Content to use in performing the task", formalDefinition="The value of the input parameter as a basic type." ) 1072 protected DataType value; 1073 1074 private static final long serialVersionUID = -1659186716L; 1075 1076 /** 1077 * Constructor 1078 */ 1079 public TaskInputComponent() { 1080 super(); 1081 } 1082 1083 /** 1084 * Constructor 1085 */ 1086 public TaskInputComponent(CodeableConcept type, DataType value) { 1087 super(); 1088 this.setType(type); 1089 this.setValue(value); 1090 } 1091 1092 /** 1093 * @return {@link #type} (A code or description indicating how the input is intended to be used as part of the task execution.) 1094 */ 1095 public CodeableConcept getType() { 1096 if (this.type == null) 1097 if (Configuration.errorOnAutoCreate()) 1098 throw new Error("Attempt to auto-create TaskInputComponent.type"); 1099 else if (Configuration.doAutoCreate()) 1100 this.type = new CodeableConcept(); // cc 1101 return this.type; 1102 } 1103 1104 public boolean hasType() { 1105 return this.type != null && !this.type.isEmpty(); 1106 } 1107 1108 /** 1109 * @param value {@link #type} (A code or description indicating how the input is intended to be used as part of the task execution.) 1110 */ 1111 public TaskInputComponent setType(CodeableConcept value) { 1112 this.type = value; 1113 return this; 1114 } 1115 1116 /** 1117 * @return {@link #value} (The value of the input parameter as a basic type.) 1118 */ 1119 public DataType getValue() { 1120 return this.value; 1121 } 1122 1123 /** 1124 * @return {@link #value} (The value of the input parameter as a basic type.) 1125 */ 1126 public Base64BinaryType getValueBase64BinaryType() throws FHIRException { 1127 if (this.value == null) 1128 this.value = new Base64BinaryType(); 1129 if (!(this.value instanceof Base64BinaryType)) 1130 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but "+this.value.getClass().getName()+" was encountered"); 1131 return (Base64BinaryType) this.value; 1132 } 1133 1134 public boolean hasValueBase64BinaryType() { 1135 return this != null && this.value instanceof Base64BinaryType; 1136 } 1137 1138 /** 1139 * @return {@link #value} (The value of the input parameter as a basic type.) 1140 */ 1141 public BooleanType getValueBooleanType() throws FHIRException { 1142 if (this.value == null) 1143 this.value = new BooleanType(); 1144 if (!(this.value instanceof BooleanType)) 1145 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 1146 return (BooleanType) this.value; 1147 } 1148 1149 public boolean hasValueBooleanType() { 1150 return this != null && this.value instanceof BooleanType; 1151 } 1152 1153 /** 1154 * @return {@link #value} (The value of the input parameter as a basic type.) 1155 */ 1156 public CanonicalType getValueCanonicalType() throws FHIRException { 1157 if (this.value == null) 1158 this.value = new CanonicalType(); 1159 if (!(this.value instanceof CanonicalType)) 1160 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.value.getClass().getName()+" was encountered"); 1161 return (CanonicalType) this.value; 1162 } 1163 1164 public boolean hasValueCanonicalType() { 1165 return this != null && this.value instanceof CanonicalType; 1166 } 1167 1168 /** 1169 * @return {@link #value} (The value of the input parameter as a basic type.) 1170 */ 1171 public CodeType getValueCodeType() throws FHIRException { 1172 if (this.value == null) 1173 this.value = new CodeType(); 1174 if (!(this.value instanceof CodeType)) 1175 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.value.getClass().getName()+" was encountered"); 1176 return (CodeType) this.value; 1177 } 1178 1179 public boolean hasValueCodeType() { 1180 return this != null && this.value instanceof CodeType; 1181 } 1182 1183 /** 1184 * @return {@link #value} (The value of the input parameter as a basic type.) 1185 */ 1186 public DateType getValueDateType() throws FHIRException { 1187 if (this.value == null) 1188 this.value = new DateType(); 1189 if (!(this.value instanceof DateType)) 1190 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 1191 return (DateType) this.value; 1192 } 1193 1194 public boolean hasValueDateType() { 1195 return this != null && this.value instanceof DateType; 1196 } 1197 1198 /** 1199 * @return {@link #value} (The value of the input parameter as a basic type.) 1200 */ 1201 public DateTimeType getValueDateTimeType() throws FHIRException { 1202 if (this.value == null) 1203 this.value = new DateTimeType(); 1204 if (!(this.value instanceof DateTimeType)) 1205 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 1206 return (DateTimeType) this.value; 1207 } 1208 1209 public boolean hasValueDateTimeType() { 1210 return this != null && this.value instanceof DateTimeType; 1211 } 1212 1213 /** 1214 * @return {@link #value} (The value of the input parameter as a basic type.) 1215 */ 1216 public DecimalType getValueDecimalType() throws FHIRException { 1217 if (this.value == null) 1218 this.value = new DecimalType(); 1219 if (!(this.value instanceof DecimalType)) 1220 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 1221 return (DecimalType) this.value; 1222 } 1223 1224 public boolean hasValueDecimalType() { 1225 return this != null && this.value instanceof DecimalType; 1226 } 1227 1228 /** 1229 * @return {@link #value} (The value of the input parameter as a basic type.) 1230 */ 1231 public IdType getValueIdType() throws FHIRException { 1232 if (this.value == null) 1233 this.value = new IdType(); 1234 if (!(this.value instanceof IdType)) 1235 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.value.getClass().getName()+" was encountered"); 1236 return (IdType) this.value; 1237 } 1238 1239 public boolean hasValueIdType() { 1240 return this != null && this.value instanceof IdType; 1241 } 1242 1243 /** 1244 * @return {@link #value} (The value of the input parameter as a basic type.) 1245 */ 1246 public InstantType getValueInstantType() throws FHIRException { 1247 if (this.value == null) 1248 this.value = new InstantType(); 1249 if (!(this.value instanceof InstantType)) 1250 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.value.getClass().getName()+" was encountered"); 1251 return (InstantType) this.value; 1252 } 1253 1254 public boolean hasValueInstantType() { 1255 return this != null && this.value instanceof InstantType; 1256 } 1257 1258 /** 1259 * @return {@link #value} (The value of the input parameter as a basic type.) 1260 */ 1261 public IntegerType getValueIntegerType() throws FHIRException { 1262 if (this.value == null) 1263 this.value = new IntegerType(); 1264 if (!(this.value instanceof IntegerType)) 1265 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 1266 return (IntegerType) this.value; 1267 } 1268 1269 public boolean hasValueIntegerType() { 1270 return this != null && this.value instanceof IntegerType; 1271 } 1272 1273 /** 1274 * @return {@link #value} (The value of the input parameter as a basic type.) 1275 */ 1276 public Integer64Type getValueInteger64Type() throws FHIRException { 1277 if (this.value == null) 1278 this.value = new Integer64Type(); 1279 if (!(this.value instanceof Integer64Type)) 1280 throw new FHIRException("Type mismatch: the type Integer64Type was expected, but "+this.value.getClass().getName()+" was encountered"); 1281 return (Integer64Type) this.value; 1282 } 1283 1284 public boolean hasValueInteger64Type() { 1285 return this != null && this.value instanceof Integer64Type; 1286 } 1287 1288 /** 1289 * @return {@link #value} (The value of the input parameter as a basic type.) 1290 */ 1291 public MarkdownType getValueMarkdownType() throws FHIRException { 1292 if (this.value == null) 1293 this.value = new MarkdownType(); 1294 if (!(this.value instanceof MarkdownType)) 1295 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.value.getClass().getName()+" was encountered"); 1296 return (MarkdownType) this.value; 1297 } 1298 1299 public boolean hasValueMarkdownType() { 1300 return this != null && this.value instanceof MarkdownType; 1301 } 1302 1303 /** 1304 * @return {@link #value} (The value of the input parameter as a basic type.) 1305 */ 1306 public OidType getValueOidType() throws FHIRException { 1307 if (this.value == null) 1308 this.value = new OidType(); 1309 if (!(this.value instanceof OidType)) 1310 throw new FHIRException("Type mismatch: the type OidType was expected, but "+this.value.getClass().getName()+" was encountered"); 1311 return (OidType) this.value; 1312 } 1313 1314 public boolean hasValueOidType() { 1315 return this != null && this.value instanceof OidType; 1316 } 1317 1318 /** 1319 * @return {@link #value} (The value of the input parameter as a basic type.) 1320 */ 1321 public PositiveIntType getValuePositiveIntType() throws FHIRException { 1322 if (this.value == null) 1323 this.value = new PositiveIntType(); 1324 if (!(this.value instanceof PositiveIntType)) 1325 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.value.getClass().getName()+" was encountered"); 1326 return (PositiveIntType) this.value; 1327 } 1328 1329 public boolean hasValuePositiveIntType() { 1330 return this != null && this.value instanceof PositiveIntType; 1331 } 1332 1333 /** 1334 * @return {@link #value} (The value of the input parameter as a basic type.) 1335 */ 1336 public StringType getValueStringType() throws FHIRException { 1337 if (this.value == null) 1338 this.value = new StringType(); 1339 if (!(this.value instanceof StringType)) 1340 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 1341 return (StringType) this.value; 1342 } 1343 1344 public boolean hasValueStringType() { 1345 return this != null && this.value instanceof StringType; 1346 } 1347 1348 /** 1349 * @return {@link #value} (The value of the input parameter as a basic type.) 1350 */ 1351 public TimeType getValueTimeType() throws FHIRException { 1352 if (this.value == null) 1353 this.value = new TimeType(); 1354 if (!(this.value instanceof TimeType)) 1355 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 1356 return (TimeType) this.value; 1357 } 1358 1359 public boolean hasValueTimeType() { 1360 return this != null && this.value instanceof TimeType; 1361 } 1362 1363 /** 1364 * @return {@link #value} (The value of the input parameter as a basic type.) 1365 */ 1366 public UnsignedIntType getValueUnsignedIntType() throws FHIRException { 1367 if (this.value == null) 1368 this.value = new UnsignedIntType(); 1369 if (!(this.value instanceof UnsignedIntType)) 1370 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.value.getClass().getName()+" was encountered"); 1371 return (UnsignedIntType) this.value; 1372 } 1373 1374 public boolean hasValueUnsignedIntType() { 1375 return this != null && this.value instanceof UnsignedIntType; 1376 } 1377 1378 /** 1379 * @return {@link #value} (The value of the input parameter as a basic type.) 1380 */ 1381 public UriType getValueUriType() throws FHIRException { 1382 if (this.value == null) 1383 this.value = new UriType(); 1384 if (!(this.value instanceof UriType)) 1385 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.value.getClass().getName()+" was encountered"); 1386 return (UriType) this.value; 1387 } 1388 1389 public boolean hasValueUriType() { 1390 return this != null && this.value instanceof UriType; 1391 } 1392 1393 /** 1394 * @return {@link #value} (The value of the input parameter as a basic type.) 1395 */ 1396 public UrlType getValueUrlType() throws FHIRException { 1397 if (this.value == null) 1398 this.value = new UrlType(); 1399 if (!(this.value instanceof UrlType)) 1400 throw new FHIRException("Type mismatch: the type UrlType was expected, but "+this.value.getClass().getName()+" was encountered"); 1401 return (UrlType) this.value; 1402 } 1403 1404 public boolean hasValueUrlType() { 1405 return this != null && this.value instanceof UrlType; 1406 } 1407 1408 /** 1409 * @return {@link #value} (The value of the input parameter as a basic type.) 1410 */ 1411 public UuidType getValueUuidType() throws FHIRException { 1412 if (this.value == null) 1413 this.value = new UuidType(); 1414 if (!(this.value instanceof UuidType)) 1415 throw new FHIRException("Type mismatch: the type UuidType was expected, but "+this.value.getClass().getName()+" was encountered"); 1416 return (UuidType) this.value; 1417 } 1418 1419 public boolean hasValueUuidType() { 1420 return this != null && this.value instanceof UuidType; 1421 } 1422 1423 /** 1424 * @return {@link #value} (The value of the input parameter as a basic type.) 1425 */ 1426 public Address getValueAddress() throws FHIRException { 1427 if (this.value == null) 1428 this.value = new Address(); 1429 if (!(this.value instanceof Address)) 1430 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.value.getClass().getName()+" was encountered"); 1431 return (Address) this.value; 1432 } 1433 1434 public boolean hasValueAddress() { 1435 return this != null && this.value instanceof Address; 1436 } 1437 1438 /** 1439 * @return {@link #value} (The value of the input parameter as a basic type.) 1440 */ 1441 public Age getValueAge() throws FHIRException { 1442 if (this.value == null) 1443 this.value = new Age(); 1444 if (!(this.value instanceof Age)) 1445 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.value.getClass().getName()+" was encountered"); 1446 return (Age) this.value; 1447 } 1448 1449 public boolean hasValueAge() { 1450 return this != null && this.value instanceof Age; 1451 } 1452 1453 /** 1454 * @return {@link #value} (The value of the input parameter as a basic type.) 1455 */ 1456 public Annotation getValueAnnotation() throws FHIRException { 1457 if (this.value == null) 1458 this.value = new Annotation(); 1459 if (!(this.value instanceof Annotation)) 1460 throw new FHIRException("Type mismatch: the type Annotation was expected, but "+this.value.getClass().getName()+" was encountered"); 1461 return (Annotation) this.value; 1462 } 1463 1464 public boolean hasValueAnnotation() { 1465 return this != null && this.value instanceof Annotation; 1466 } 1467 1468 /** 1469 * @return {@link #value} (The value of the input parameter as a basic type.) 1470 */ 1471 public Attachment getValueAttachment() throws FHIRException { 1472 if (this.value == null) 1473 this.value = new Attachment(); 1474 if (!(this.value instanceof Attachment)) 1475 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 1476 return (Attachment) this.value; 1477 } 1478 1479 public boolean hasValueAttachment() { 1480 return this != null && this.value instanceof Attachment; 1481 } 1482 1483 /** 1484 * @return {@link #value} (The value of the input parameter as a basic type.) 1485 */ 1486 public CodeableConcept getValueCodeableConcept() throws FHIRException { 1487 if (this.value == null) 1488 this.value = new CodeableConcept(); 1489 if (!(this.value instanceof CodeableConcept)) 1490 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 1491 return (CodeableConcept) this.value; 1492 } 1493 1494 public boolean hasValueCodeableConcept() { 1495 return this != null && this.value instanceof CodeableConcept; 1496 } 1497 1498 /** 1499 * @return {@link #value} (The value of the input parameter as a basic type.) 1500 */ 1501 public CodeableReference getValueCodeableReference() throws FHIRException { 1502 if (this.value == null) 1503 this.value = new CodeableReference(); 1504 if (!(this.value instanceof CodeableReference)) 1505 throw new FHIRException("Type mismatch: the type CodeableReference was expected, but "+this.value.getClass().getName()+" was encountered"); 1506 return (CodeableReference) this.value; 1507 } 1508 1509 public boolean hasValueCodeableReference() { 1510 return this != null && this.value instanceof CodeableReference; 1511 } 1512 1513 /** 1514 * @return {@link #value} (The value of the input parameter as a basic type.) 1515 */ 1516 public Coding getValueCoding() throws FHIRException { 1517 if (this.value == null) 1518 this.value = new Coding(); 1519 if (!(this.value instanceof Coding)) 1520 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 1521 return (Coding) this.value; 1522 } 1523 1524 public boolean hasValueCoding() { 1525 return this != null && this.value instanceof Coding; 1526 } 1527 1528 /** 1529 * @return {@link #value} (The value of the input parameter as a basic type.) 1530 */ 1531 public ContactPoint getValueContactPoint() throws FHIRException { 1532 if (this.value == null) 1533 this.value = new ContactPoint(); 1534 if (!(this.value instanceof ContactPoint)) 1535 throw new FHIRException("Type mismatch: the type ContactPoint was expected, but "+this.value.getClass().getName()+" was encountered"); 1536 return (ContactPoint) this.value; 1537 } 1538 1539 public boolean hasValueContactPoint() { 1540 return this != null && this.value instanceof ContactPoint; 1541 } 1542 1543 /** 1544 * @return {@link #value} (The value of the input parameter as a basic type.) 1545 */ 1546 public Count getValueCount() throws FHIRException { 1547 if (this.value == null) 1548 this.value = new Count(); 1549 if (!(this.value instanceof Count)) 1550 throw new FHIRException("Type mismatch: the type Count was expected, but "+this.value.getClass().getName()+" was encountered"); 1551 return (Count) this.value; 1552 } 1553 1554 public boolean hasValueCount() { 1555 return this != null && this.value instanceof Count; 1556 } 1557 1558 /** 1559 * @return {@link #value} (The value of the input parameter as a basic type.) 1560 */ 1561 public Distance getValueDistance() throws FHIRException { 1562 if (this.value == null) 1563 this.value = new Distance(); 1564 if (!(this.value instanceof Distance)) 1565 throw new FHIRException("Type mismatch: the type Distance was expected, but "+this.value.getClass().getName()+" was encountered"); 1566 return (Distance) this.value; 1567 } 1568 1569 public boolean hasValueDistance() { 1570 return this != null && this.value instanceof Distance; 1571 } 1572 1573 /** 1574 * @return {@link #value} (The value of the input parameter as a basic type.) 1575 */ 1576 public Duration getValueDuration() throws FHIRException { 1577 if (this.value == null) 1578 this.value = new Duration(); 1579 if (!(this.value instanceof Duration)) 1580 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.value.getClass().getName()+" was encountered"); 1581 return (Duration) this.value; 1582 } 1583 1584 public boolean hasValueDuration() { 1585 return this != null && this.value instanceof Duration; 1586 } 1587 1588 /** 1589 * @return {@link #value} (The value of the input parameter as a basic type.) 1590 */ 1591 public HumanName getValueHumanName() throws FHIRException { 1592 if (this.value == null) 1593 this.value = new HumanName(); 1594 if (!(this.value instanceof HumanName)) 1595 throw new FHIRException("Type mismatch: the type HumanName was expected, but "+this.value.getClass().getName()+" was encountered"); 1596 return (HumanName) this.value; 1597 } 1598 1599 public boolean hasValueHumanName() { 1600 return this != null && this.value instanceof HumanName; 1601 } 1602 1603 /** 1604 * @return {@link #value} (The value of the input parameter as a basic type.) 1605 */ 1606 public Identifier getValueIdentifier() throws FHIRException { 1607 if (this.value == null) 1608 this.value = new Identifier(); 1609 if (!(this.value instanceof Identifier)) 1610 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.value.getClass().getName()+" was encountered"); 1611 return (Identifier) this.value; 1612 } 1613 1614 public boolean hasValueIdentifier() { 1615 return this != null && this.value instanceof Identifier; 1616 } 1617 1618 /** 1619 * @return {@link #value} (The value of the input parameter as a basic type.) 1620 */ 1621 public Money getValueMoney() throws FHIRException { 1622 if (this.value == null) 1623 this.value = new Money(); 1624 if (!(this.value instanceof Money)) 1625 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.value.getClass().getName()+" was encountered"); 1626 return (Money) this.value; 1627 } 1628 1629 public boolean hasValueMoney() { 1630 return this != null && this.value instanceof Money; 1631 } 1632 1633 /** 1634 * @return {@link #value} (The value of the input parameter as a basic type.) 1635 */ 1636 public Period getValuePeriod() throws FHIRException { 1637 if (this.value == null) 1638 this.value = new Period(); 1639 if (!(this.value instanceof Period)) 1640 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.value.getClass().getName()+" was encountered"); 1641 return (Period) this.value; 1642 } 1643 1644 public boolean hasValuePeriod() { 1645 return this != null && this.value instanceof Period; 1646 } 1647 1648 /** 1649 * @return {@link #value} (The value of the input parameter as a basic type.) 1650 */ 1651 public Quantity getValueQuantity() throws FHIRException { 1652 if (this.value == null) 1653 this.value = new Quantity(); 1654 if (!(this.value instanceof Quantity)) 1655 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1656 return (Quantity) this.value; 1657 } 1658 1659 public boolean hasValueQuantity() { 1660 return this != null && this.value instanceof Quantity; 1661 } 1662 1663 /** 1664 * @return {@link #value} (The value of the input parameter as a basic type.) 1665 */ 1666 public Range getValueRange() throws FHIRException { 1667 if (this.value == null) 1668 this.value = new Range(); 1669 if (!(this.value instanceof Range)) 1670 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 1671 return (Range) this.value; 1672 } 1673 1674 public boolean hasValueRange() { 1675 return this != null && this.value instanceof Range; 1676 } 1677 1678 /** 1679 * @return {@link #value} (The value of the input parameter as a basic type.) 1680 */ 1681 public Ratio getValueRatio() throws FHIRException { 1682 if (this.value == null) 1683 this.value = new Ratio(); 1684 if (!(this.value instanceof Ratio)) 1685 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.value.getClass().getName()+" was encountered"); 1686 return (Ratio) this.value; 1687 } 1688 1689 public boolean hasValueRatio() { 1690 return this != null && this.value instanceof Ratio; 1691 } 1692 1693 /** 1694 * @return {@link #value} (The value of the input parameter as a basic type.) 1695 */ 1696 public RatioRange getValueRatioRange() throws FHIRException { 1697 if (this.value == null) 1698 this.value = new RatioRange(); 1699 if (!(this.value instanceof RatioRange)) 1700 throw new FHIRException("Type mismatch: the type RatioRange was expected, but "+this.value.getClass().getName()+" was encountered"); 1701 return (RatioRange) this.value; 1702 } 1703 1704 public boolean hasValueRatioRange() { 1705 return this != null && this.value instanceof RatioRange; 1706 } 1707 1708 /** 1709 * @return {@link #value} (The value of the input parameter as a basic type.) 1710 */ 1711 public Reference getValueReference() throws FHIRException { 1712 if (this.value == null) 1713 this.value = new Reference(); 1714 if (!(this.value instanceof Reference)) 1715 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 1716 return (Reference) this.value; 1717 } 1718 1719 public boolean hasValueReference() { 1720 return this != null && this.value instanceof Reference; 1721 } 1722 1723 /** 1724 * @return {@link #value} (The value of the input parameter as a basic type.) 1725 */ 1726 public SampledData getValueSampledData() throws FHIRException { 1727 if (this.value == null) 1728 this.value = new SampledData(); 1729 if (!(this.value instanceof SampledData)) 1730 throw new FHIRException("Type mismatch: the type SampledData was expected, but "+this.value.getClass().getName()+" was encountered"); 1731 return (SampledData) this.value; 1732 } 1733 1734 public boolean hasValueSampledData() { 1735 return this != null && this.value instanceof SampledData; 1736 } 1737 1738 /** 1739 * @return {@link #value} (The value of the input parameter as a basic type.) 1740 */ 1741 public Signature getValueSignature() throws FHIRException { 1742 if (this.value == null) 1743 this.value = new Signature(); 1744 if (!(this.value instanceof Signature)) 1745 throw new FHIRException("Type mismatch: the type Signature was expected, but "+this.value.getClass().getName()+" was encountered"); 1746 return (Signature) this.value; 1747 } 1748 1749 public boolean hasValueSignature() { 1750 return this != null && this.value instanceof Signature; 1751 } 1752 1753 /** 1754 * @return {@link #value} (The value of the input parameter as a basic type.) 1755 */ 1756 public Timing getValueTiming() throws FHIRException { 1757 if (this.value == null) 1758 this.value = new Timing(); 1759 if (!(this.value instanceof Timing)) 1760 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.value.getClass().getName()+" was encountered"); 1761 return (Timing) this.value; 1762 } 1763 1764 public boolean hasValueTiming() { 1765 return this != null && this.value instanceof Timing; 1766 } 1767 1768 /** 1769 * @return {@link #value} (The value of the input parameter as a basic type.) 1770 */ 1771 public ContactDetail getValueContactDetail() throws FHIRException { 1772 if (this.value == null) 1773 this.value = new ContactDetail(); 1774 if (!(this.value instanceof ContactDetail)) 1775 throw new FHIRException("Type mismatch: the type ContactDetail was expected, but "+this.value.getClass().getName()+" was encountered"); 1776 return (ContactDetail) this.value; 1777 } 1778 1779 public boolean hasValueContactDetail() { 1780 return this != null && this.value instanceof ContactDetail; 1781 } 1782 1783 /** 1784 * @return {@link #value} (The value of the input parameter as a basic type.) 1785 */ 1786 public DataRequirement getValueDataRequirement() throws FHIRException { 1787 if (this.value == null) 1788 this.value = new DataRequirement(); 1789 if (!(this.value instanceof DataRequirement)) 1790 throw new FHIRException("Type mismatch: the type DataRequirement was expected, but "+this.value.getClass().getName()+" was encountered"); 1791 return (DataRequirement) this.value; 1792 } 1793 1794 public boolean hasValueDataRequirement() { 1795 return this != null && this.value instanceof DataRequirement; 1796 } 1797 1798 /** 1799 * @return {@link #value} (The value of the input parameter as a basic type.) 1800 */ 1801 public Expression getValueExpression() throws FHIRException { 1802 if (this.value == null) 1803 this.value = new Expression(); 1804 if (!(this.value instanceof Expression)) 1805 throw new FHIRException("Type mismatch: the type Expression was expected, but "+this.value.getClass().getName()+" was encountered"); 1806 return (Expression) this.value; 1807 } 1808 1809 public boolean hasValueExpression() { 1810 return this != null && this.value instanceof Expression; 1811 } 1812 1813 /** 1814 * @return {@link #value} (The value of the input parameter as a basic type.) 1815 */ 1816 public ParameterDefinition getValueParameterDefinition() throws FHIRException { 1817 if (this.value == null) 1818 this.value = new ParameterDefinition(); 1819 if (!(this.value instanceof ParameterDefinition)) 1820 throw new FHIRException("Type mismatch: the type ParameterDefinition was expected, but "+this.value.getClass().getName()+" was encountered"); 1821 return (ParameterDefinition) this.value; 1822 } 1823 1824 public boolean hasValueParameterDefinition() { 1825 return this != null && this.value instanceof ParameterDefinition; 1826 } 1827 1828 /** 1829 * @return {@link #value} (The value of the input parameter as a basic type.) 1830 */ 1831 public RelatedArtifact getValueRelatedArtifact() throws FHIRException { 1832 if (this.value == null) 1833 this.value = new RelatedArtifact(); 1834 if (!(this.value instanceof RelatedArtifact)) 1835 throw new FHIRException("Type mismatch: the type RelatedArtifact was expected, but "+this.value.getClass().getName()+" was encountered"); 1836 return (RelatedArtifact) this.value; 1837 } 1838 1839 public boolean hasValueRelatedArtifact() { 1840 return this != null && this.value instanceof RelatedArtifact; 1841 } 1842 1843 /** 1844 * @return {@link #value} (The value of the input parameter as a basic type.) 1845 */ 1846 public TriggerDefinition getValueTriggerDefinition() throws FHIRException { 1847 if (this.value == null) 1848 this.value = new TriggerDefinition(); 1849 if (!(this.value instanceof TriggerDefinition)) 1850 throw new FHIRException("Type mismatch: the type TriggerDefinition was expected, but "+this.value.getClass().getName()+" was encountered"); 1851 return (TriggerDefinition) this.value; 1852 } 1853 1854 public boolean hasValueTriggerDefinition() { 1855 return this != null && this.value instanceof TriggerDefinition; 1856 } 1857 1858 /** 1859 * @return {@link #value} (The value of the input parameter as a basic type.) 1860 */ 1861 public UsageContext getValueUsageContext() throws FHIRException { 1862 if (this.value == null) 1863 this.value = new UsageContext(); 1864 if (!(this.value instanceof UsageContext)) 1865 throw new FHIRException("Type mismatch: the type UsageContext was expected, but "+this.value.getClass().getName()+" was encountered"); 1866 return (UsageContext) this.value; 1867 } 1868 1869 public boolean hasValueUsageContext() { 1870 return this != null && this.value instanceof UsageContext; 1871 } 1872 1873 /** 1874 * @return {@link #value} (The value of the input parameter as a basic type.) 1875 */ 1876 public Availability getValueAvailability() throws FHIRException { 1877 if (this.value == null) 1878 this.value = new Availability(); 1879 if (!(this.value instanceof Availability)) 1880 throw new FHIRException("Type mismatch: the type Availability was expected, but "+this.value.getClass().getName()+" was encountered"); 1881 return (Availability) this.value; 1882 } 1883 1884 public boolean hasValueAvailability() { 1885 return this != null && this.value instanceof Availability; 1886 } 1887 1888 /** 1889 * @return {@link #value} (The value of the input parameter as a basic type.) 1890 */ 1891 public ExtendedContactDetail getValueExtendedContactDetail() throws FHIRException { 1892 if (this.value == null) 1893 this.value = new ExtendedContactDetail(); 1894 if (!(this.value instanceof ExtendedContactDetail)) 1895 throw new FHIRException("Type mismatch: the type ExtendedContactDetail was expected, but "+this.value.getClass().getName()+" was encountered"); 1896 return (ExtendedContactDetail) this.value; 1897 } 1898 1899 public boolean hasValueExtendedContactDetail() { 1900 return this != null && this.value instanceof ExtendedContactDetail; 1901 } 1902 1903 /** 1904 * @return {@link #value} (The value of the input parameter as a basic type.) 1905 */ 1906 public Dosage getValueDosage() throws FHIRException { 1907 if (this.value == null) 1908 this.value = new Dosage(); 1909 if (!(this.value instanceof Dosage)) 1910 throw new FHIRException("Type mismatch: the type Dosage was expected, but "+this.value.getClass().getName()+" was encountered"); 1911 return (Dosage) this.value; 1912 } 1913 1914 public boolean hasValueDosage() { 1915 return this != null && this.value instanceof Dosage; 1916 } 1917 1918 /** 1919 * @return {@link #value} (The value of the input parameter as a basic type.) 1920 */ 1921 public Meta getValueMeta() throws FHIRException { 1922 if (this.value == null) 1923 this.value = new Meta(); 1924 if (!(this.value instanceof Meta)) 1925 throw new FHIRException("Type mismatch: the type Meta was expected, but "+this.value.getClass().getName()+" was encountered"); 1926 return (Meta) this.value; 1927 } 1928 1929 public boolean hasValueMeta() { 1930 return this != null && this.value instanceof Meta; 1931 } 1932 1933 public boolean hasValue() { 1934 return this.value != null && !this.value.isEmpty(); 1935 } 1936 1937 /** 1938 * @param value {@link #value} (The value of the input parameter as a basic type.) 1939 */ 1940 public TaskInputComponent setValue(DataType value) { 1941 if (value != null && !(value instanceof Base64BinaryType || value instanceof BooleanType || value instanceof CanonicalType || value instanceof CodeType || value instanceof DateType || value instanceof DateTimeType || value instanceof DecimalType || value instanceof IdType || value instanceof InstantType || value instanceof IntegerType || value instanceof Integer64Type || value instanceof MarkdownType || value instanceof OidType || value instanceof PositiveIntType || value instanceof StringType || value instanceof TimeType || value instanceof UnsignedIntType || value instanceof UriType || value instanceof UrlType || value instanceof UuidType || value instanceof Address || value instanceof Age || value instanceof Annotation || value instanceof Attachment || value instanceof CodeableConcept || value instanceof CodeableReference || value instanceof Coding || value instanceof ContactPoint || value instanceof Count || value instanceof Distance || value instanceof Duration || value instanceof HumanName || value instanceof Identifier || value instanceof Money || value instanceof Period || value instanceof Quantity || value instanceof Range || value instanceof Ratio || value instanceof RatioRange || value instanceof Reference || value instanceof SampledData || value instanceof Signature || value instanceof Timing || value instanceof ContactDetail || value instanceof DataRequirement || value instanceof Expression || value instanceof ParameterDefinition || value instanceof RelatedArtifact || value instanceof TriggerDefinition || value instanceof UsageContext || value instanceof Availability || value instanceof ExtendedContactDetail || value instanceof Dosage || value instanceof Meta)) 1942 throw new FHIRException("Not the right type for Task.input.value[x]: "+value.fhirType()); 1943 this.value = value; 1944 return this; 1945 } 1946 1947 protected void listChildren(List<Property> children) { 1948 super.listChildren(children); 1949 children.add(new Property("type", "CodeableConcept", "A code or description indicating how the input is intended to be used as part of the task execution.", 0, 1, type)); 1950 children.add(new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The value of the input parameter as a basic type.", 0, 1, value)); 1951 } 1952 1953 @Override 1954 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1955 switch (_hash) { 1956 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A code or description indicating how the input is intended to be used as part of the task execution.", 0, 1, type); 1957 case -1410166417: /*value[x]*/ return new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The value of the input parameter as a basic type.", 0, 1, value); 1958 case 111972721: /*value*/ return new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The value of the input parameter as a basic type.", 0, 1, value); 1959 case -1535024575: /*valueBase64Binary*/ return new Property("value[x]", "base64Binary", "The value of the input parameter as a basic type.", 0, 1, value); 1960 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The value of the input parameter as a basic type.", 0, 1, value); 1961 case -786218365: /*valueCanonical*/ return new Property("value[x]", "canonical", "The value of the input parameter as a basic type.", 0, 1, value); 1962 case -766209282: /*valueCode*/ return new Property("value[x]", "code", "The value of the input parameter as a basic type.", 0, 1, value); 1963 case -766192449: /*valueDate*/ return new Property("value[x]", "date", "The value of the input parameter as a basic type.", 0, 1, value); 1964 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The value of the input parameter as a basic type.", 0, 1, value); 1965 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "The value of the input parameter as a basic type.", 0, 1, value); 1966 case 231604844: /*valueId*/ return new Property("value[x]", "id", "The value of the input parameter as a basic type.", 0, 1, value); 1967 case -1668687056: /*valueInstant*/ return new Property("value[x]", "instant", "The value of the input parameter as a basic type.", 0, 1, value); 1968 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The value of the input parameter as a basic type.", 0, 1, value); 1969 case -1122120181: /*valueInteger64*/ return new Property("value[x]", "integer64", "The value of the input parameter as a basic type.", 0, 1, value); 1970 case -497880704: /*valueMarkdown*/ return new Property("value[x]", "markdown", "The value of the input parameter as a basic type.", 0, 1, value); 1971 case -1410178407: /*valueOid*/ return new Property("value[x]", "oid", "The value of the input parameter as a basic type.", 0, 1, value); 1972 case -1249932027: /*valuePositiveInt*/ return new Property("value[x]", "positiveInt", "The value of the input parameter as a basic type.", 0, 1, value); 1973 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The value of the input parameter as a basic type.", 0, 1, value); 1974 case -765708322: /*valueTime*/ return new Property("value[x]", "time", "The value of the input parameter as a basic type.", 0, 1, value); 1975 case 26529417: /*valueUnsignedInt*/ return new Property("value[x]", "unsignedInt", "The value of the input parameter as a basic type.", 0, 1, value); 1976 case -1410172357: /*valueUri*/ return new Property("value[x]", "uri", "The value of the input parameter as a basic type.", 0, 1, value); 1977 case -1410172354: /*valueUrl*/ return new Property("value[x]", "url", "The value of the input parameter as a basic type.", 0, 1, value); 1978 case -765667124: /*valueUuid*/ return new Property("value[x]", "uuid", "The value of the input parameter as a basic type.", 0, 1, value); 1979 case -478981821: /*valueAddress*/ return new Property("value[x]", "Address", "The value of the input parameter as a basic type.", 0, 1, value); 1980 case -1410191922: /*valueAge*/ return new Property("value[x]", "Age", "The value of the input parameter as a basic type.", 0, 1, value); 1981 case -67108992: /*valueAnnotation*/ return new Property("value[x]", "Annotation", "The value of the input parameter as a basic type.", 0, 1, value); 1982 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "The value of the input parameter as a basic type.", 0, 1, value); 1983 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The value of the input parameter as a basic type.", 0, 1, value); 1984 case -257955629: /*valueCodeableReference*/ return new Property("value[x]", "CodeableReference", "The value of the input parameter as a basic type.", 0, 1, value); 1985 case -1887705029: /*valueCoding*/ return new Property("value[x]", "Coding", "The value of the input parameter as a basic type.", 0, 1, value); 1986 case 944904545: /*valueContactPoint*/ return new Property("value[x]", "ContactPoint", "The value of the input parameter as a basic type.", 0, 1, value); 1987 case 2017332766: /*valueCount*/ return new Property("value[x]", "Count", "The value of the input parameter as a basic type.", 0, 1, value); 1988 case -456359802: /*valueDistance*/ return new Property("value[x]", "Distance", "The value of the input parameter as a basic type.", 0, 1, value); 1989 case 1558135333: /*valueDuration*/ return new Property("value[x]", "Duration", "The value of the input parameter as a basic type.", 0, 1, value); 1990 case -2026205465: /*valueHumanName*/ return new Property("value[x]", "HumanName", "The value of the input parameter as a basic type.", 0, 1, value); 1991 case -130498310: /*valueIdentifier*/ return new Property("value[x]", "Identifier", "The value of the input parameter as a basic type.", 0, 1, value); 1992 case 2026560975: /*valueMoney*/ return new Property("value[x]", "Money", "The value of the input parameter as a basic type.", 0, 1, value); 1993 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "Period", "The value of the input parameter as a basic type.", 0, 1, value); 1994 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The value of the input parameter as a basic type.", 0, 1, value); 1995 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The value of the input parameter as a basic type.", 0, 1, value); 1996 case 2030767386: /*valueRatio*/ return new Property("value[x]", "Ratio", "The value of the input parameter as a basic type.", 0, 1, value); 1997 case -706454461: /*valueRatioRange*/ return new Property("value[x]", "RatioRange", "The value of the input parameter as a basic type.", 0, 1, value); 1998 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference", "The value of the input parameter as a basic type.", 0, 1, value); 1999 case -962229101: /*valueSampledData*/ return new Property("value[x]", "SampledData", "The value of the input parameter as a basic type.", 0, 1, value); 2000 case -540985785: /*valueSignature*/ return new Property("value[x]", "Signature", "The value of the input parameter as a basic type.", 0, 1, value); 2001 case -1406282469: /*valueTiming*/ return new Property("value[x]", "Timing", "The value of the input parameter as a basic type.", 0, 1, value); 2002 case -1125200224: /*valueContactDetail*/ return new Property("value[x]", "ContactDetail", "The value of the input parameter as a basic type.", 0, 1, value); 2003 case 1710554248: /*valueDataRequirement*/ return new Property("value[x]", "DataRequirement", "The value of the input parameter as a basic type.", 0, 1, value); 2004 case -307517719: /*valueExpression*/ return new Property("value[x]", "Expression", "The value of the input parameter as a basic type.", 0, 1, value); 2005 case 1387478187: /*valueParameterDefinition*/ return new Property("value[x]", "ParameterDefinition", "The value of the input parameter as a basic type.", 0, 1, value); 2006 case 1748214124: /*valueRelatedArtifact*/ return new Property("value[x]", "RelatedArtifact", "The value of the input parameter as a basic type.", 0, 1, value); 2007 case 976830394: /*valueTriggerDefinition*/ return new Property("value[x]", "TriggerDefinition", "The value of the input parameter as a basic type.", 0, 1, value); 2008 case 588000479: /*valueUsageContext*/ return new Property("value[x]", "UsageContext", "The value of the input parameter as a basic type.", 0, 1, value); 2009 case 1678530924: /*valueAvailability*/ return new Property("value[x]", "Availability", "The value of the input parameter as a basic type.", 0, 1, value); 2010 case -1567222041: /*valueExtendedContactDetail*/ return new Property("value[x]", "ExtendedContactDetail", "The value of the input parameter as a basic type.", 0, 1, value); 2011 case -1858636920: /*valueDosage*/ return new Property("value[x]", "Dosage", "The value of the input parameter as a basic type.", 0, 1, value); 2012 case -765920490: /*valueMeta*/ return new Property("value[x]", "Meta", "The value of the input parameter as a basic type.", 0, 1, value); 2013 default: return super.getNamedProperty(_hash, _name, _checkValid); 2014 } 2015 2016 } 2017 2018 @Override 2019 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2020 switch (hash) { 2021 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2022 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 2023 default: return super.getProperty(hash, name, checkValid); 2024 } 2025 2026 } 2027 2028 @Override 2029 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2030 switch (hash) { 2031 case 3575610: // type 2032 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2033 return value; 2034 case 111972721: // value 2035 this.value = TypeConvertor.castToType(value); // DataType 2036 return value; 2037 default: return super.setProperty(hash, name, value); 2038 } 2039 2040 } 2041 2042 @Override 2043 public Base setProperty(String name, Base value) throws FHIRException { 2044 if (name.equals("type")) { 2045 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2046 } else if (name.equals("value[x]")) { 2047 this.value = TypeConvertor.castToType(value); // DataType 2048 } else 2049 return super.setProperty(name, value); 2050 return value; 2051 } 2052 2053 @Override 2054 public void removeChild(String name, Base value) throws FHIRException { 2055 if (name.equals("type")) { 2056 this.type = null; 2057 } else if (name.equals("value[x]")) { 2058 this.value = null; 2059 } else 2060 super.removeChild(name, value); 2061 2062 } 2063 2064 @Override 2065 public Base makeProperty(int hash, String name) throws FHIRException { 2066 switch (hash) { 2067 case 3575610: return getType(); 2068 case -1410166417: return getValue(); 2069 case 111972721: return getValue(); 2070 default: return super.makeProperty(hash, name); 2071 } 2072 2073 } 2074 2075 @Override 2076 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2077 switch (hash) { 2078 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2079 case 111972721: /*value*/ return new String[] {"base64Binary", "boolean", "canonical", "code", "date", "dateTime", "decimal", "id", "instant", "integer", "integer64", "markdown", "oid", "positiveInt", "string", "time", "unsignedInt", "uri", "url", "uuid", "Address", "Age", "Annotation", "Attachment", "CodeableConcept", "CodeableReference", "Coding", "ContactPoint", "Count", "Distance", "Duration", "HumanName", "Identifier", "Money", "Period", "Quantity", "Range", "Ratio", "RatioRange", "Reference", "SampledData", "Signature", "Timing", "ContactDetail", "DataRequirement", "Expression", "ParameterDefinition", "RelatedArtifact", "TriggerDefinition", "UsageContext", "Availability", "ExtendedContactDetail", "Dosage", "Meta"}; 2080 default: return super.getTypesForProperty(hash, name); 2081 } 2082 2083 } 2084 2085 @Override 2086 public Base addChild(String name) throws FHIRException { 2087 if (name.equals("type")) { 2088 this.type = new CodeableConcept(); 2089 return this.type; 2090 } 2091 else if (name.equals("valueBase64Binary")) { 2092 this.value = new Base64BinaryType(); 2093 return this.value; 2094 } 2095 else if (name.equals("valueBoolean")) { 2096 this.value = new BooleanType(); 2097 return this.value; 2098 } 2099 else if (name.equals("valueCanonical")) { 2100 this.value = new CanonicalType(); 2101 return this.value; 2102 } 2103 else if (name.equals("valueCode")) { 2104 this.value = new CodeType(); 2105 return this.value; 2106 } 2107 else if (name.equals("valueDate")) { 2108 this.value = new DateType(); 2109 return this.value; 2110 } 2111 else if (name.equals("valueDateTime")) { 2112 this.value = new DateTimeType(); 2113 return this.value; 2114 } 2115 else if (name.equals("valueDecimal")) { 2116 this.value = new DecimalType(); 2117 return this.value; 2118 } 2119 else if (name.equals("valueId")) { 2120 this.value = new IdType(); 2121 return this.value; 2122 } 2123 else if (name.equals("valueInstant")) { 2124 this.value = new InstantType(); 2125 return this.value; 2126 } 2127 else if (name.equals("valueInteger")) { 2128 this.value = new IntegerType(); 2129 return this.value; 2130 } 2131 else if (name.equals("valueInteger64")) { 2132 this.value = new Integer64Type(); 2133 return this.value; 2134 } 2135 else if (name.equals("valueMarkdown")) { 2136 this.value = new MarkdownType(); 2137 return this.value; 2138 } 2139 else if (name.equals("valueOid")) { 2140 this.value = new OidType(); 2141 return this.value; 2142 } 2143 else if (name.equals("valuePositiveInt")) { 2144 this.value = new PositiveIntType(); 2145 return this.value; 2146 } 2147 else if (name.equals("valueString")) { 2148 this.value = new StringType(); 2149 return this.value; 2150 } 2151 else if (name.equals("valueTime")) { 2152 this.value = new TimeType(); 2153 return this.value; 2154 } 2155 else if (name.equals("valueUnsignedInt")) { 2156 this.value = new UnsignedIntType(); 2157 return this.value; 2158 } 2159 else if (name.equals("valueUri")) { 2160 this.value = new UriType(); 2161 return this.value; 2162 } 2163 else if (name.equals("valueUrl")) { 2164 this.value = new UrlType(); 2165 return this.value; 2166 } 2167 else if (name.equals("valueUuid")) { 2168 this.value = new UuidType(); 2169 return this.value; 2170 } 2171 else if (name.equals("valueAddress")) { 2172 this.value = new Address(); 2173 return this.value; 2174 } 2175 else if (name.equals("valueAge")) { 2176 this.value = new Age(); 2177 return this.value; 2178 } 2179 else if (name.equals("valueAnnotation")) { 2180 this.value = new Annotation(); 2181 return this.value; 2182 } 2183 else if (name.equals("valueAttachment")) { 2184 this.value = new Attachment(); 2185 return this.value; 2186 } 2187 else if (name.equals("valueCodeableConcept")) { 2188 this.value = new CodeableConcept(); 2189 return this.value; 2190 } 2191 else if (name.equals("valueCodeableReference")) { 2192 this.value = new CodeableReference(); 2193 return this.value; 2194 } 2195 else if (name.equals("valueCoding")) { 2196 this.value = new Coding(); 2197 return this.value; 2198 } 2199 else if (name.equals("valueContactPoint")) { 2200 this.value = new ContactPoint(); 2201 return this.value; 2202 } 2203 else if (name.equals("valueCount")) { 2204 this.value = new Count(); 2205 return this.value; 2206 } 2207 else if (name.equals("valueDistance")) { 2208 this.value = new Distance(); 2209 return this.value; 2210 } 2211 else if (name.equals("valueDuration")) { 2212 this.value = new Duration(); 2213 return this.value; 2214 } 2215 else if (name.equals("valueHumanName")) { 2216 this.value = new HumanName(); 2217 return this.value; 2218 } 2219 else if (name.equals("valueIdentifier")) { 2220 this.value = new Identifier(); 2221 return this.value; 2222 } 2223 else if (name.equals("valueMoney")) { 2224 this.value = new Money(); 2225 return this.value; 2226 } 2227 else if (name.equals("valuePeriod")) { 2228 this.value = new Period(); 2229 return this.value; 2230 } 2231 else if (name.equals("valueQuantity")) { 2232 this.value = new Quantity(); 2233 return this.value; 2234 } 2235 else if (name.equals("valueRange")) { 2236 this.value = new Range(); 2237 return this.value; 2238 } 2239 else if (name.equals("valueRatio")) { 2240 this.value = new Ratio(); 2241 return this.value; 2242 } 2243 else if (name.equals("valueRatioRange")) { 2244 this.value = new RatioRange(); 2245 return this.value; 2246 } 2247 else if (name.equals("valueReference")) { 2248 this.value = new Reference(); 2249 return this.value; 2250 } 2251 else if (name.equals("valueSampledData")) { 2252 this.value = new SampledData(); 2253 return this.value; 2254 } 2255 else if (name.equals("valueSignature")) { 2256 this.value = new Signature(); 2257 return this.value; 2258 } 2259 else if (name.equals("valueTiming")) { 2260 this.value = new Timing(); 2261 return this.value; 2262 } 2263 else if (name.equals("valueContactDetail")) { 2264 this.value = new ContactDetail(); 2265 return this.value; 2266 } 2267 else if (name.equals("valueDataRequirement")) { 2268 this.value = new DataRequirement(); 2269 return this.value; 2270 } 2271 else if (name.equals("valueExpression")) { 2272 this.value = new Expression(); 2273 return this.value; 2274 } 2275 else if (name.equals("valueParameterDefinition")) { 2276 this.value = new ParameterDefinition(); 2277 return this.value; 2278 } 2279 else if (name.equals("valueRelatedArtifact")) { 2280 this.value = new RelatedArtifact(); 2281 return this.value; 2282 } 2283 else if (name.equals("valueTriggerDefinition")) { 2284 this.value = new TriggerDefinition(); 2285 return this.value; 2286 } 2287 else if (name.equals("valueUsageContext")) { 2288 this.value = new UsageContext(); 2289 return this.value; 2290 } 2291 else if (name.equals("valueAvailability")) { 2292 this.value = new Availability(); 2293 return this.value; 2294 } 2295 else if (name.equals("valueExtendedContactDetail")) { 2296 this.value = new ExtendedContactDetail(); 2297 return this.value; 2298 } 2299 else if (name.equals("valueDosage")) { 2300 this.value = new Dosage(); 2301 return this.value; 2302 } 2303 else if (name.equals("valueMeta")) { 2304 this.value = new Meta(); 2305 return this.value; 2306 } 2307 else 2308 return super.addChild(name); 2309 } 2310 2311 public TaskInputComponent copy() { 2312 TaskInputComponent dst = new TaskInputComponent(); 2313 copyValues(dst); 2314 return dst; 2315 } 2316 2317 public void copyValues(TaskInputComponent dst) { 2318 super.copyValues(dst); 2319 dst.type = type == null ? null : type.copy(); 2320 dst.value = value == null ? null : value.copy(); 2321 } 2322 2323 @Override 2324 public boolean equalsDeep(Base other_) { 2325 if (!super.equalsDeep(other_)) 2326 return false; 2327 if (!(other_ instanceof TaskInputComponent)) 2328 return false; 2329 TaskInputComponent o = (TaskInputComponent) other_; 2330 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 2331 } 2332 2333 @Override 2334 public boolean equalsShallow(Base other_) { 2335 if (!super.equalsShallow(other_)) 2336 return false; 2337 if (!(other_ instanceof TaskInputComponent)) 2338 return false; 2339 TaskInputComponent o = (TaskInputComponent) other_; 2340 return true; 2341 } 2342 2343 public boolean isEmpty() { 2344 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 2345 } 2346 2347 public String fhirType() { 2348 return "Task.input"; 2349 2350 } 2351 2352 } 2353 2354 @Block() 2355 public static class TaskOutputComponent extends BackboneElement implements IBaseBackboneElement { 2356 /** 2357 * The name of the Output parameter. 2358 */ 2359 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 2360 @Description(shortDefinition="Label for output", formalDefinition="The name of the Output parameter." ) 2361 protected CodeableConcept type; 2362 2363 /** 2364 * The value of the Output parameter as a basic type. 2365 */ 2366 @Child(name = "value", type = {Base64BinaryType.class, BooleanType.class, CanonicalType.class, CodeType.class, DateType.class, DateTimeType.class, DecimalType.class, IdType.class, InstantType.class, IntegerType.class, Integer64Type.class, MarkdownType.class, OidType.class, PositiveIntType.class, StringType.class, TimeType.class, UnsignedIntType.class, UriType.class, UrlType.class, UuidType.class, Address.class, Age.class, Annotation.class, Attachment.class, CodeableConcept.class, CodeableReference.class, Coding.class, ContactPoint.class, Count.class, Distance.class, Duration.class, HumanName.class, Identifier.class, Money.class, Period.class, Quantity.class, Range.class, Ratio.class, RatioRange.class, Reference.class, SampledData.class, Signature.class, Timing.class, ContactDetail.class, DataRequirement.class, Expression.class, ParameterDefinition.class, RelatedArtifact.class, TriggerDefinition.class, UsageContext.class, Availability.class, ExtendedContactDetail.class, Dosage.class, Meta.class}, order=2, min=1, max=1, modifier=false, summary=false) 2367 @Description(shortDefinition="Result of output", formalDefinition="The value of the Output parameter as a basic type." ) 2368 protected DataType value; 2369 2370 private static final long serialVersionUID = -1659186716L; 2371 2372 /** 2373 * Constructor 2374 */ 2375 public TaskOutputComponent() { 2376 super(); 2377 } 2378 2379 /** 2380 * Constructor 2381 */ 2382 public TaskOutputComponent(CodeableConcept type, DataType value) { 2383 super(); 2384 this.setType(type); 2385 this.setValue(value); 2386 } 2387 2388 /** 2389 * @return {@link #type} (The name of the Output parameter.) 2390 */ 2391 public CodeableConcept getType() { 2392 if (this.type == null) 2393 if (Configuration.errorOnAutoCreate()) 2394 throw new Error("Attempt to auto-create TaskOutputComponent.type"); 2395 else if (Configuration.doAutoCreate()) 2396 this.type = new CodeableConcept(); // cc 2397 return this.type; 2398 } 2399 2400 public boolean hasType() { 2401 return this.type != null && !this.type.isEmpty(); 2402 } 2403 2404 /** 2405 * @param value {@link #type} (The name of the Output parameter.) 2406 */ 2407 public TaskOutputComponent setType(CodeableConcept value) { 2408 this.type = value; 2409 return this; 2410 } 2411 2412 /** 2413 * @return {@link #value} (The value of the Output parameter as a basic type.) 2414 */ 2415 public DataType getValue() { 2416 return this.value; 2417 } 2418 2419 /** 2420 * @return {@link #value} (The value of the Output parameter as a basic type.) 2421 */ 2422 public Base64BinaryType getValueBase64BinaryType() throws FHIRException { 2423 if (this.value == null) 2424 this.value = new Base64BinaryType(); 2425 if (!(this.value instanceof Base64BinaryType)) 2426 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but "+this.value.getClass().getName()+" was encountered"); 2427 return (Base64BinaryType) this.value; 2428 } 2429 2430 public boolean hasValueBase64BinaryType() { 2431 return this != null && this.value instanceof Base64BinaryType; 2432 } 2433 2434 /** 2435 * @return {@link #value} (The value of the Output parameter as a basic type.) 2436 */ 2437 public BooleanType getValueBooleanType() throws FHIRException { 2438 if (this.value == null) 2439 this.value = new BooleanType(); 2440 if (!(this.value instanceof BooleanType)) 2441 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 2442 return (BooleanType) this.value; 2443 } 2444 2445 public boolean hasValueBooleanType() { 2446 return this != null && this.value instanceof BooleanType; 2447 } 2448 2449 /** 2450 * @return {@link #value} (The value of the Output parameter as a basic type.) 2451 */ 2452 public CanonicalType getValueCanonicalType() throws FHIRException { 2453 if (this.value == null) 2454 this.value = new CanonicalType(); 2455 if (!(this.value instanceof CanonicalType)) 2456 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.value.getClass().getName()+" was encountered"); 2457 return (CanonicalType) this.value; 2458 } 2459 2460 public boolean hasValueCanonicalType() { 2461 return this != null && this.value instanceof CanonicalType; 2462 } 2463 2464 /** 2465 * @return {@link #value} (The value of the Output parameter as a basic type.) 2466 */ 2467 public CodeType getValueCodeType() throws FHIRException { 2468 if (this.value == null) 2469 this.value = new CodeType(); 2470 if (!(this.value instanceof CodeType)) 2471 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2472 return (CodeType) this.value; 2473 } 2474 2475 public boolean hasValueCodeType() { 2476 return this != null && this.value instanceof CodeType; 2477 } 2478 2479 /** 2480 * @return {@link #value} (The value of the Output parameter as a basic type.) 2481 */ 2482 public DateType getValueDateType() throws FHIRException { 2483 if (this.value == null) 2484 this.value = new DateType(); 2485 if (!(this.value instanceof DateType)) 2486 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 2487 return (DateType) this.value; 2488 } 2489 2490 public boolean hasValueDateType() { 2491 return this != null && this.value instanceof DateType; 2492 } 2493 2494 /** 2495 * @return {@link #value} (The value of the Output parameter as a basic type.) 2496 */ 2497 public DateTimeType getValueDateTimeType() throws FHIRException { 2498 if (this.value == null) 2499 this.value = new DateTimeType(); 2500 if (!(this.value instanceof DateTimeType)) 2501 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2502 return (DateTimeType) this.value; 2503 } 2504 2505 public boolean hasValueDateTimeType() { 2506 return this != null && this.value instanceof DateTimeType; 2507 } 2508 2509 /** 2510 * @return {@link #value} (The value of the Output parameter as a basic type.) 2511 */ 2512 public DecimalType getValueDecimalType() throws FHIRException { 2513 if (this.value == null) 2514 this.value = new DecimalType(); 2515 if (!(this.value instanceof DecimalType)) 2516 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 2517 return (DecimalType) this.value; 2518 } 2519 2520 public boolean hasValueDecimalType() { 2521 return this != null && this.value instanceof DecimalType; 2522 } 2523 2524 /** 2525 * @return {@link #value} (The value of the Output parameter as a basic type.) 2526 */ 2527 public IdType getValueIdType() throws FHIRException { 2528 if (this.value == null) 2529 this.value = new IdType(); 2530 if (!(this.value instanceof IdType)) 2531 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.value.getClass().getName()+" was encountered"); 2532 return (IdType) this.value; 2533 } 2534 2535 public boolean hasValueIdType() { 2536 return this != null && this.value instanceof IdType; 2537 } 2538 2539 /** 2540 * @return {@link #value} (The value of the Output parameter as a basic type.) 2541 */ 2542 public InstantType getValueInstantType() throws FHIRException { 2543 if (this.value == null) 2544 this.value = new InstantType(); 2545 if (!(this.value instanceof InstantType)) 2546 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.value.getClass().getName()+" was encountered"); 2547 return (InstantType) this.value; 2548 } 2549 2550 public boolean hasValueInstantType() { 2551 return this != null && this.value instanceof InstantType; 2552 } 2553 2554 /** 2555 * @return {@link #value} (The value of the Output parameter as a basic type.) 2556 */ 2557 public IntegerType getValueIntegerType() throws FHIRException { 2558 if (this.value == null) 2559 this.value = new IntegerType(); 2560 if (!(this.value instanceof IntegerType)) 2561 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 2562 return (IntegerType) this.value; 2563 } 2564 2565 public boolean hasValueIntegerType() { 2566 return this != null && this.value instanceof IntegerType; 2567 } 2568 2569 /** 2570 * @return {@link #value} (The value of the Output parameter as a basic type.) 2571 */ 2572 public Integer64Type getValueInteger64Type() throws FHIRException { 2573 if (this.value == null) 2574 this.value = new Integer64Type(); 2575 if (!(this.value instanceof Integer64Type)) 2576 throw new FHIRException("Type mismatch: the type Integer64Type was expected, but "+this.value.getClass().getName()+" was encountered"); 2577 return (Integer64Type) this.value; 2578 } 2579 2580 public boolean hasValueInteger64Type() { 2581 return this != null && this.value instanceof Integer64Type; 2582 } 2583 2584 /** 2585 * @return {@link #value} (The value of the Output parameter as a basic type.) 2586 */ 2587 public MarkdownType getValueMarkdownType() throws FHIRException { 2588 if (this.value == null) 2589 this.value = new MarkdownType(); 2590 if (!(this.value instanceof MarkdownType)) 2591 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.value.getClass().getName()+" was encountered"); 2592 return (MarkdownType) this.value; 2593 } 2594 2595 public boolean hasValueMarkdownType() { 2596 return this != null && this.value instanceof MarkdownType; 2597 } 2598 2599 /** 2600 * @return {@link #value} (The value of the Output parameter as a basic type.) 2601 */ 2602 public OidType getValueOidType() throws FHIRException { 2603 if (this.value == null) 2604 this.value = new OidType(); 2605 if (!(this.value instanceof OidType)) 2606 throw new FHIRException("Type mismatch: the type OidType was expected, but "+this.value.getClass().getName()+" was encountered"); 2607 return (OidType) this.value; 2608 } 2609 2610 public boolean hasValueOidType() { 2611 return this != null && this.value instanceof OidType; 2612 } 2613 2614 /** 2615 * @return {@link #value} (The value of the Output parameter as a basic type.) 2616 */ 2617 public PositiveIntType getValuePositiveIntType() throws FHIRException { 2618 if (this.value == null) 2619 this.value = new PositiveIntType(); 2620 if (!(this.value instanceof PositiveIntType)) 2621 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.value.getClass().getName()+" was encountered"); 2622 return (PositiveIntType) this.value; 2623 } 2624 2625 public boolean hasValuePositiveIntType() { 2626 return this != null && this.value instanceof PositiveIntType; 2627 } 2628 2629 /** 2630 * @return {@link #value} (The value of the Output parameter as a basic type.) 2631 */ 2632 public StringType getValueStringType() throws FHIRException { 2633 if (this.value == null) 2634 this.value = new StringType(); 2635 if (!(this.value instanceof StringType)) 2636 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 2637 return (StringType) this.value; 2638 } 2639 2640 public boolean hasValueStringType() { 2641 return this != null && this.value instanceof StringType; 2642 } 2643 2644 /** 2645 * @return {@link #value} (The value of the Output parameter as a basic type.) 2646 */ 2647 public TimeType getValueTimeType() throws FHIRException { 2648 if (this.value == null) 2649 this.value = new TimeType(); 2650 if (!(this.value instanceof TimeType)) 2651 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2652 return (TimeType) this.value; 2653 } 2654 2655 public boolean hasValueTimeType() { 2656 return this != null && this.value instanceof TimeType; 2657 } 2658 2659 /** 2660 * @return {@link #value} (The value of the Output parameter as a basic type.) 2661 */ 2662 public UnsignedIntType getValueUnsignedIntType() throws FHIRException { 2663 if (this.value == null) 2664 this.value = new UnsignedIntType(); 2665 if (!(this.value instanceof UnsignedIntType)) 2666 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.value.getClass().getName()+" was encountered"); 2667 return (UnsignedIntType) this.value; 2668 } 2669 2670 public boolean hasValueUnsignedIntType() { 2671 return this != null && this.value instanceof UnsignedIntType; 2672 } 2673 2674 /** 2675 * @return {@link #value} (The value of the Output parameter as a basic type.) 2676 */ 2677 public UriType getValueUriType() throws FHIRException { 2678 if (this.value == null) 2679 this.value = new UriType(); 2680 if (!(this.value instanceof UriType)) 2681 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.value.getClass().getName()+" was encountered"); 2682 return (UriType) this.value; 2683 } 2684 2685 public boolean hasValueUriType() { 2686 return this != null && this.value instanceof UriType; 2687 } 2688 2689 /** 2690 * @return {@link #value} (The value of the Output parameter as a basic type.) 2691 */ 2692 public UrlType getValueUrlType() throws FHIRException { 2693 if (this.value == null) 2694 this.value = new UrlType(); 2695 if (!(this.value instanceof UrlType)) 2696 throw new FHIRException("Type mismatch: the type UrlType was expected, but "+this.value.getClass().getName()+" was encountered"); 2697 return (UrlType) this.value; 2698 } 2699 2700 public boolean hasValueUrlType() { 2701 return this != null && this.value instanceof UrlType; 2702 } 2703 2704 /** 2705 * @return {@link #value} (The value of the Output parameter as a basic type.) 2706 */ 2707 public UuidType getValueUuidType() throws FHIRException { 2708 if (this.value == null) 2709 this.value = new UuidType(); 2710 if (!(this.value instanceof UuidType)) 2711 throw new FHIRException("Type mismatch: the type UuidType was expected, but "+this.value.getClass().getName()+" was encountered"); 2712 return (UuidType) this.value; 2713 } 2714 2715 public boolean hasValueUuidType() { 2716 return this != null && this.value instanceof UuidType; 2717 } 2718 2719 /** 2720 * @return {@link #value} (The value of the Output parameter as a basic type.) 2721 */ 2722 public Address getValueAddress() throws FHIRException { 2723 if (this.value == null) 2724 this.value = new Address(); 2725 if (!(this.value instanceof Address)) 2726 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.value.getClass().getName()+" was encountered"); 2727 return (Address) this.value; 2728 } 2729 2730 public boolean hasValueAddress() { 2731 return this != null && this.value instanceof Address; 2732 } 2733 2734 /** 2735 * @return {@link #value} (The value of the Output parameter as a basic type.) 2736 */ 2737 public Age getValueAge() throws FHIRException { 2738 if (this.value == null) 2739 this.value = new Age(); 2740 if (!(this.value instanceof Age)) 2741 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.value.getClass().getName()+" was encountered"); 2742 return (Age) this.value; 2743 } 2744 2745 public boolean hasValueAge() { 2746 return this != null && this.value instanceof Age; 2747 } 2748 2749 /** 2750 * @return {@link #value} (The value of the Output parameter as a basic type.) 2751 */ 2752 public Annotation getValueAnnotation() throws FHIRException { 2753 if (this.value == null) 2754 this.value = new Annotation(); 2755 if (!(this.value instanceof Annotation)) 2756 throw new FHIRException("Type mismatch: the type Annotation was expected, but "+this.value.getClass().getName()+" was encountered"); 2757 return (Annotation) this.value; 2758 } 2759 2760 public boolean hasValueAnnotation() { 2761 return this != null && this.value instanceof Annotation; 2762 } 2763 2764 /** 2765 * @return {@link #value} (The value of the Output parameter as a basic type.) 2766 */ 2767 public Attachment getValueAttachment() throws FHIRException { 2768 if (this.value == null) 2769 this.value = new Attachment(); 2770 if (!(this.value instanceof Attachment)) 2771 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 2772 return (Attachment) this.value; 2773 } 2774 2775 public boolean hasValueAttachment() { 2776 return this != null && this.value instanceof Attachment; 2777 } 2778 2779 /** 2780 * @return {@link #value} (The value of the Output parameter as a basic type.) 2781 */ 2782 public CodeableConcept getValueCodeableConcept() throws FHIRException { 2783 if (this.value == null) 2784 this.value = new CodeableConcept(); 2785 if (!(this.value instanceof CodeableConcept)) 2786 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 2787 return (CodeableConcept) this.value; 2788 } 2789 2790 public boolean hasValueCodeableConcept() { 2791 return this != null && this.value instanceof CodeableConcept; 2792 } 2793 2794 /** 2795 * @return {@link #value} (The value of the Output parameter as a basic type.) 2796 */ 2797 public CodeableReference getValueCodeableReference() throws FHIRException { 2798 if (this.value == null) 2799 this.value = new CodeableReference(); 2800 if (!(this.value instanceof CodeableReference)) 2801 throw new FHIRException("Type mismatch: the type CodeableReference was expected, but "+this.value.getClass().getName()+" was encountered"); 2802 return (CodeableReference) this.value; 2803 } 2804 2805 public boolean hasValueCodeableReference() { 2806 return this != null && this.value instanceof CodeableReference; 2807 } 2808 2809 /** 2810 * @return {@link #value} (The value of the Output parameter as a basic type.) 2811 */ 2812 public Coding getValueCoding() throws FHIRException { 2813 if (this.value == null) 2814 this.value = new Coding(); 2815 if (!(this.value instanceof Coding)) 2816 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 2817 return (Coding) this.value; 2818 } 2819 2820 public boolean hasValueCoding() { 2821 return this != null && this.value instanceof Coding; 2822 } 2823 2824 /** 2825 * @return {@link #value} (The value of the Output parameter as a basic type.) 2826 */ 2827 public ContactPoint getValueContactPoint() throws FHIRException { 2828 if (this.value == null) 2829 this.value = new ContactPoint(); 2830 if (!(this.value instanceof ContactPoint)) 2831 throw new FHIRException("Type mismatch: the type ContactPoint was expected, but "+this.value.getClass().getName()+" was encountered"); 2832 return (ContactPoint) this.value; 2833 } 2834 2835 public boolean hasValueContactPoint() { 2836 return this != null && this.value instanceof ContactPoint; 2837 } 2838 2839 /** 2840 * @return {@link #value} (The value of the Output parameter as a basic type.) 2841 */ 2842 public Count getValueCount() throws FHIRException { 2843 if (this.value == null) 2844 this.value = new Count(); 2845 if (!(this.value instanceof Count)) 2846 throw new FHIRException("Type mismatch: the type Count was expected, but "+this.value.getClass().getName()+" was encountered"); 2847 return (Count) this.value; 2848 } 2849 2850 public boolean hasValueCount() { 2851 return this != null && this.value instanceof Count; 2852 } 2853 2854 /** 2855 * @return {@link #value} (The value of the Output parameter as a basic type.) 2856 */ 2857 public Distance getValueDistance() throws FHIRException { 2858 if (this.value == null) 2859 this.value = new Distance(); 2860 if (!(this.value instanceof Distance)) 2861 throw new FHIRException("Type mismatch: the type Distance was expected, but "+this.value.getClass().getName()+" was encountered"); 2862 return (Distance) this.value; 2863 } 2864 2865 public boolean hasValueDistance() { 2866 return this != null && this.value instanceof Distance; 2867 } 2868 2869 /** 2870 * @return {@link #value} (The value of the Output parameter as a basic type.) 2871 */ 2872 public Duration getValueDuration() throws FHIRException { 2873 if (this.value == null) 2874 this.value = new Duration(); 2875 if (!(this.value instanceof Duration)) 2876 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.value.getClass().getName()+" was encountered"); 2877 return (Duration) this.value; 2878 } 2879 2880 public boolean hasValueDuration() { 2881 return this != null && this.value instanceof Duration; 2882 } 2883 2884 /** 2885 * @return {@link #value} (The value of the Output parameter as a basic type.) 2886 */ 2887 public HumanName getValueHumanName() throws FHIRException { 2888 if (this.value == null) 2889 this.value = new HumanName(); 2890 if (!(this.value instanceof HumanName)) 2891 throw new FHIRException("Type mismatch: the type HumanName was expected, but "+this.value.getClass().getName()+" was encountered"); 2892 return (HumanName) this.value; 2893 } 2894 2895 public boolean hasValueHumanName() { 2896 return this != null && this.value instanceof HumanName; 2897 } 2898 2899 /** 2900 * @return {@link #value} (The value of the Output parameter as a basic type.) 2901 */ 2902 public Identifier getValueIdentifier() throws FHIRException { 2903 if (this.value == null) 2904 this.value = new Identifier(); 2905 if (!(this.value instanceof Identifier)) 2906 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.value.getClass().getName()+" was encountered"); 2907 return (Identifier) this.value; 2908 } 2909 2910 public boolean hasValueIdentifier() { 2911 return this != null && this.value instanceof Identifier; 2912 } 2913 2914 /** 2915 * @return {@link #value} (The value of the Output parameter as a basic type.) 2916 */ 2917 public Money getValueMoney() throws FHIRException { 2918 if (this.value == null) 2919 this.value = new Money(); 2920 if (!(this.value instanceof Money)) 2921 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.value.getClass().getName()+" was encountered"); 2922 return (Money) this.value; 2923 } 2924 2925 public boolean hasValueMoney() { 2926 return this != null && this.value instanceof Money; 2927 } 2928 2929 /** 2930 * @return {@link #value} (The value of the Output parameter as a basic type.) 2931 */ 2932 public Period getValuePeriod() throws FHIRException { 2933 if (this.value == null) 2934 this.value = new Period(); 2935 if (!(this.value instanceof Period)) 2936 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.value.getClass().getName()+" was encountered"); 2937 return (Period) this.value; 2938 } 2939 2940 public boolean hasValuePeriod() { 2941 return this != null && this.value instanceof Period; 2942 } 2943 2944 /** 2945 * @return {@link #value} (The value of the Output parameter as a basic type.) 2946 */ 2947 public Quantity getValueQuantity() throws FHIRException { 2948 if (this.value == null) 2949 this.value = new Quantity(); 2950 if (!(this.value instanceof Quantity)) 2951 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 2952 return (Quantity) this.value; 2953 } 2954 2955 public boolean hasValueQuantity() { 2956 return this != null && this.value instanceof Quantity; 2957 } 2958 2959 /** 2960 * @return {@link #value} (The value of the Output parameter as a basic type.) 2961 */ 2962 public Range getValueRange() throws FHIRException { 2963 if (this.value == null) 2964 this.value = new Range(); 2965 if (!(this.value instanceof Range)) 2966 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 2967 return (Range) this.value; 2968 } 2969 2970 public boolean hasValueRange() { 2971 return this != null && this.value instanceof Range; 2972 } 2973 2974 /** 2975 * @return {@link #value} (The value of the Output parameter as a basic type.) 2976 */ 2977 public Ratio getValueRatio() throws FHIRException { 2978 if (this.value == null) 2979 this.value = new Ratio(); 2980 if (!(this.value instanceof Ratio)) 2981 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.value.getClass().getName()+" was encountered"); 2982 return (Ratio) this.value; 2983 } 2984 2985 public boolean hasValueRatio() { 2986 return this != null && this.value instanceof Ratio; 2987 } 2988 2989 /** 2990 * @return {@link #value} (The value of the Output parameter as a basic type.) 2991 */ 2992 public RatioRange getValueRatioRange() throws FHIRException { 2993 if (this.value == null) 2994 this.value = new RatioRange(); 2995 if (!(this.value instanceof RatioRange)) 2996 throw new FHIRException("Type mismatch: the type RatioRange was expected, but "+this.value.getClass().getName()+" was encountered"); 2997 return (RatioRange) this.value; 2998 } 2999 3000 public boolean hasValueRatioRange() { 3001 return this != null && this.value instanceof RatioRange; 3002 } 3003 3004 /** 3005 * @return {@link #value} (The value of the Output parameter as a basic type.) 3006 */ 3007 public Reference getValueReference() throws FHIRException { 3008 if (this.value == null) 3009 this.value = new Reference(); 3010 if (!(this.value instanceof Reference)) 3011 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 3012 return (Reference) this.value; 3013 } 3014 3015 public boolean hasValueReference() { 3016 return this != null && this.value instanceof Reference; 3017 } 3018 3019 /** 3020 * @return {@link #value} (The value of the Output parameter as a basic type.) 3021 */ 3022 public SampledData getValueSampledData() throws FHIRException { 3023 if (this.value == null) 3024 this.value = new SampledData(); 3025 if (!(this.value instanceof SampledData)) 3026 throw new FHIRException("Type mismatch: the type SampledData was expected, but "+this.value.getClass().getName()+" was encountered"); 3027 return (SampledData) this.value; 3028 } 3029 3030 public boolean hasValueSampledData() { 3031 return this != null && this.value instanceof SampledData; 3032 } 3033 3034 /** 3035 * @return {@link #value} (The value of the Output parameter as a basic type.) 3036 */ 3037 public Signature getValueSignature() throws FHIRException { 3038 if (this.value == null) 3039 this.value = new Signature(); 3040 if (!(this.value instanceof Signature)) 3041 throw new FHIRException("Type mismatch: the type Signature was expected, but "+this.value.getClass().getName()+" was encountered"); 3042 return (Signature) this.value; 3043 } 3044 3045 public boolean hasValueSignature() { 3046 return this != null && this.value instanceof Signature; 3047 } 3048 3049 /** 3050 * @return {@link #value} (The value of the Output parameter as a basic type.) 3051 */ 3052 public Timing getValueTiming() throws FHIRException { 3053 if (this.value == null) 3054 this.value = new Timing(); 3055 if (!(this.value instanceof Timing)) 3056 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.value.getClass().getName()+" was encountered"); 3057 return (Timing) this.value; 3058 } 3059 3060 public boolean hasValueTiming() { 3061 return this != null && this.value instanceof Timing; 3062 } 3063 3064 /** 3065 * @return {@link #value} (The value of the Output parameter as a basic type.) 3066 */ 3067 public ContactDetail getValueContactDetail() throws FHIRException { 3068 if (this.value == null) 3069 this.value = new ContactDetail(); 3070 if (!(this.value instanceof ContactDetail)) 3071 throw new FHIRException("Type mismatch: the type ContactDetail was expected, but "+this.value.getClass().getName()+" was encountered"); 3072 return (ContactDetail) this.value; 3073 } 3074 3075 public boolean hasValueContactDetail() { 3076 return this != null && this.value instanceof ContactDetail; 3077 } 3078 3079 /** 3080 * @return {@link #value} (The value of the Output parameter as a basic type.) 3081 */ 3082 public DataRequirement getValueDataRequirement() throws FHIRException { 3083 if (this.value == null) 3084 this.value = new DataRequirement(); 3085 if (!(this.value instanceof DataRequirement)) 3086 throw new FHIRException("Type mismatch: the type DataRequirement was expected, but "+this.value.getClass().getName()+" was encountered"); 3087 return (DataRequirement) this.value; 3088 } 3089 3090 public boolean hasValueDataRequirement() { 3091 return this != null && this.value instanceof DataRequirement; 3092 } 3093 3094 /** 3095 * @return {@link #value} (The value of the Output parameter as a basic type.) 3096 */ 3097 public Expression getValueExpression() throws FHIRException { 3098 if (this.value == null) 3099 this.value = new Expression(); 3100 if (!(this.value instanceof Expression)) 3101 throw new FHIRException("Type mismatch: the type Expression was expected, but "+this.value.getClass().getName()+" was encountered"); 3102 return (Expression) this.value; 3103 } 3104 3105 public boolean hasValueExpression() { 3106 return this != null && this.value instanceof Expression; 3107 } 3108 3109 /** 3110 * @return {@link #value} (The value of the Output parameter as a basic type.) 3111 */ 3112 public ParameterDefinition getValueParameterDefinition() throws FHIRException { 3113 if (this.value == null) 3114 this.value = new ParameterDefinition(); 3115 if (!(this.value instanceof ParameterDefinition)) 3116 throw new FHIRException("Type mismatch: the type ParameterDefinition was expected, but "+this.value.getClass().getName()+" was encountered"); 3117 return (ParameterDefinition) this.value; 3118 } 3119 3120 public boolean hasValueParameterDefinition() { 3121 return this != null && this.value instanceof ParameterDefinition; 3122 } 3123 3124 /** 3125 * @return {@link #value} (The value of the Output parameter as a basic type.) 3126 */ 3127 public RelatedArtifact getValueRelatedArtifact() throws FHIRException { 3128 if (this.value == null) 3129 this.value = new RelatedArtifact(); 3130 if (!(this.value instanceof RelatedArtifact)) 3131 throw new FHIRException("Type mismatch: the type RelatedArtifact was expected, but "+this.value.getClass().getName()+" was encountered"); 3132 return (RelatedArtifact) this.value; 3133 } 3134 3135 public boolean hasValueRelatedArtifact() { 3136 return this != null && this.value instanceof RelatedArtifact; 3137 } 3138 3139 /** 3140 * @return {@link #value} (The value of the Output parameter as a basic type.) 3141 */ 3142 public TriggerDefinition getValueTriggerDefinition() throws FHIRException { 3143 if (this.value == null) 3144 this.value = new TriggerDefinition(); 3145 if (!(this.value instanceof TriggerDefinition)) 3146 throw new FHIRException("Type mismatch: the type TriggerDefinition was expected, but "+this.value.getClass().getName()+" was encountered"); 3147 return (TriggerDefinition) this.value; 3148 } 3149 3150 public boolean hasValueTriggerDefinition() { 3151 return this != null && this.value instanceof TriggerDefinition; 3152 } 3153 3154 /** 3155 * @return {@link #value} (The value of the Output parameter as a basic type.) 3156 */ 3157 public UsageContext getValueUsageContext() throws FHIRException { 3158 if (this.value == null) 3159 this.value = new UsageContext(); 3160 if (!(this.value instanceof UsageContext)) 3161 throw new FHIRException("Type mismatch: the type UsageContext was expected, but "+this.value.getClass().getName()+" was encountered"); 3162 return (UsageContext) this.value; 3163 } 3164 3165 public boolean hasValueUsageContext() { 3166 return this != null && this.value instanceof UsageContext; 3167 } 3168 3169 /** 3170 * @return {@link #value} (The value of the Output parameter as a basic type.) 3171 */ 3172 public Availability getValueAvailability() throws FHIRException { 3173 if (this.value == null) 3174 this.value = new Availability(); 3175 if (!(this.value instanceof Availability)) 3176 throw new FHIRException("Type mismatch: the type Availability was expected, but "+this.value.getClass().getName()+" was encountered"); 3177 return (Availability) this.value; 3178 } 3179 3180 public boolean hasValueAvailability() { 3181 return this != null && this.value instanceof Availability; 3182 } 3183 3184 /** 3185 * @return {@link #value} (The value of the Output parameter as a basic type.) 3186 */ 3187 public ExtendedContactDetail getValueExtendedContactDetail() throws FHIRException { 3188 if (this.value == null) 3189 this.value = new ExtendedContactDetail(); 3190 if (!(this.value instanceof ExtendedContactDetail)) 3191 throw new FHIRException("Type mismatch: the type ExtendedContactDetail was expected, but "+this.value.getClass().getName()+" was encountered"); 3192 return (ExtendedContactDetail) this.value; 3193 } 3194 3195 public boolean hasValueExtendedContactDetail() { 3196 return this != null && this.value instanceof ExtendedContactDetail; 3197 } 3198 3199 /** 3200 * @return {@link #value} (The value of the Output parameter as a basic type.) 3201 */ 3202 public Dosage getValueDosage() throws FHIRException { 3203 if (this.value == null) 3204 this.value = new Dosage(); 3205 if (!(this.value instanceof Dosage)) 3206 throw new FHIRException("Type mismatch: the type Dosage was expected, but "+this.value.getClass().getName()+" was encountered"); 3207 return (Dosage) this.value; 3208 } 3209 3210 public boolean hasValueDosage() { 3211 return this != null && this.value instanceof Dosage; 3212 } 3213 3214 /** 3215 * @return {@link #value} (The value of the Output parameter as a basic type.) 3216 */ 3217 public Meta getValueMeta() throws FHIRException { 3218 if (this.value == null) 3219 this.value = new Meta(); 3220 if (!(this.value instanceof Meta)) 3221 throw new FHIRException("Type mismatch: the type Meta was expected, but "+this.value.getClass().getName()+" was encountered"); 3222 return (Meta) this.value; 3223 } 3224 3225 public boolean hasValueMeta() { 3226 return this != null && this.value instanceof Meta; 3227 } 3228 3229 public boolean hasValue() { 3230 return this.value != null && !this.value.isEmpty(); 3231 } 3232 3233 /** 3234 * @param value {@link #value} (The value of the Output parameter as a basic type.) 3235 */ 3236 public TaskOutputComponent setValue(DataType value) { 3237 if (value != null && !(value instanceof Base64BinaryType || value instanceof BooleanType || value instanceof CanonicalType || value instanceof CodeType || value instanceof DateType || value instanceof DateTimeType || value instanceof DecimalType || value instanceof IdType || value instanceof InstantType || value instanceof IntegerType || value instanceof Integer64Type || value instanceof MarkdownType || value instanceof OidType || value instanceof PositiveIntType || value instanceof StringType || value instanceof TimeType || value instanceof UnsignedIntType || value instanceof UriType || value instanceof UrlType || value instanceof UuidType || value instanceof Address || value instanceof Age || value instanceof Annotation || value instanceof Attachment || value instanceof CodeableConcept || value instanceof CodeableReference || value instanceof Coding || value instanceof ContactPoint || value instanceof Count || value instanceof Distance || value instanceof Duration || value instanceof HumanName || value instanceof Identifier || value instanceof Money || value instanceof Period || value instanceof Quantity || value instanceof Range || value instanceof Ratio || value instanceof RatioRange || value instanceof Reference || value instanceof SampledData || value instanceof Signature || value instanceof Timing || value instanceof ContactDetail || value instanceof DataRequirement || value instanceof Expression || value instanceof ParameterDefinition || value instanceof RelatedArtifact || value instanceof TriggerDefinition || value instanceof UsageContext || value instanceof Availability || value instanceof ExtendedContactDetail || value instanceof Dosage || value instanceof Meta)) 3238 throw new FHIRException("Not the right type for Task.output.value[x]: "+value.fhirType()); 3239 this.value = value; 3240 return this; 3241 } 3242 3243 protected void listChildren(List<Property> children) { 3244 super.listChildren(children); 3245 children.add(new Property("type", "CodeableConcept", "The name of the Output parameter.", 0, 1, type)); 3246 children.add(new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The value of the Output parameter as a basic type.", 0, 1, value)); 3247 } 3248 3249 @Override 3250 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3251 switch (_hash) { 3252 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The name of the Output parameter.", 0, 1, type); 3253 case -1410166417: /*value[x]*/ return new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The value of the Output parameter as a basic type.", 0, 1, value); 3254 case 111972721: /*value*/ return new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "The value of the Output parameter as a basic type.", 0, 1, value); 3255 case -1535024575: /*valueBase64Binary*/ return new Property("value[x]", "base64Binary", "The value of the Output parameter as a basic type.", 0, 1, value); 3256 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The value of the Output parameter as a basic type.", 0, 1, value); 3257 case -786218365: /*valueCanonical*/ return new Property("value[x]", "canonical", "The value of the Output parameter as a basic type.", 0, 1, value); 3258 case -766209282: /*valueCode*/ return new Property("value[x]", "code", "The value of the Output parameter as a basic type.", 0, 1, value); 3259 case -766192449: /*valueDate*/ return new Property("value[x]", "date", "The value of the Output parameter as a basic type.", 0, 1, value); 3260 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The value of the Output parameter as a basic type.", 0, 1, value); 3261 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "The value of the Output parameter as a basic type.", 0, 1, value); 3262 case 231604844: /*valueId*/ return new Property("value[x]", "id", "The value of the Output parameter as a basic type.", 0, 1, value); 3263 case -1668687056: /*valueInstant*/ return new Property("value[x]", "instant", "The value of the Output parameter as a basic type.", 0, 1, value); 3264 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The value of the Output parameter as a basic type.", 0, 1, value); 3265 case -1122120181: /*valueInteger64*/ return new Property("value[x]", "integer64", "The value of the Output parameter as a basic type.", 0, 1, value); 3266 case -497880704: /*valueMarkdown*/ return new Property("value[x]", "markdown", "The value of the Output parameter as a basic type.", 0, 1, value); 3267 case -1410178407: /*valueOid*/ return new Property("value[x]", "oid", "The value of the Output parameter as a basic type.", 0, 1, value); 3268 case -1249932027: /*valuePositiveInt*/ return new Property("value[x]", "positiveInt", "The value of the Output parameter as a basic type.", 0, 1, value); 3269 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The value of the Output parameter as a basic type.", 0, 1, value); 3270 case -765708322: /*valueTime*/ return new Property("value[x]", "time", "The value of the Output parameter as a basic type.", 0, 1, value); 3271 case 26529417: /*valueUnsignedInt*/ return new Property("value[x]", "unsignedInt", "The value of the Output parameter as a basic type.", 0, 1, value); 3272 case -1410172357: /*valueUri*/ return new Property("value[x]", "uri", "The value of the Output parameter as a basic type.", 0, 1, value); 3273 case -1410172354: /*valueUrl*/ return new Property("value[x]", "url", "The value of the Output parameter as a basic type.", 0, 1, value); 3274 case -765667124: /*valueUuid*/ return new Property("value[x]", "uuid", "The value of the Output parameter as a basic type.", 0, 1, value); 3275 case -478981821: /*valueAddress*/ return new Property("value[x]", "Address", "The value of the Output parameter as a basic type.", 0, 1, value); 3276 case -1410191922: /*valueAge*/ return new Property("value[x]", "Age", "The value of the Output parameter as a basic type.", 0, 1, value); 3277 case -67108992: /*valueAnnotation*/ return new Property("value[x]", "Annotation", "The value of the Output parameter as a basic type.", 0, 1, value); 3278 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "The value of the Output parameter as a basic type.", 0, 1, value); 3279 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The value of the Output parameter as a basic type.", 0, 1, value); 3280 case -257955629: /*valueCodeableReference*/ return new Property("value[x]", "CodeableReference", "The value of the Output parameter as a basic type.", 0, 1, value); 3281 case -1887705029: /*valueCoding*/ return new Property("value[x]", "Coding", "The value of the Output parameter as a basic type.", 0, 1, value); 3282 case 944904545: /*valueContactPoint*/ return new Property("value[x]", "ContactPoint", "The value of the Output parameter as a basic type.", 0, 1, value); 3283 case 2017332766: /*valueCount*/ return new Property("value[x]", "Count", "The value of the Output parameter as a basic type.", 0, 1, value); 3284 case -456359802: /*valueDistance*/ return new Property("value[x]", "Distance", "The value of the Output parameter as a basic type.", 0, 1, value); 3285 case 1558135333: /*valueDuration*/ return new Property("value[x]", "Duration", "The value of the Output parameter as a basic type.", 0, 1, value); 3286 case -2026205465: /*valueHumanName*/ return new Property("value[x]", "HumanName", "The value of the Output parameter as a basic type.", 0, 1, value); 3287 case -130498310: /*valueIdentifier*/ return new Property("value[x]", "Identifier", "The value of the Output parameter as a basic type.", 0, 1, value); 3288 case 2026560975: /*valueMoney*/ return new Property("value[x]", "Money", "The value of the Output parameter as a basic type.", 0, 1, value); 3289 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "Period", "The value of the Output parameter as a basic type.", 0, 1, value); 3290 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The value of the Output parameter as a basic type.", 0, 1, value); 3291 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The value of the Output parameter as a basic type.", 0, 1, value); 3292 case 2030767386: /*valueRatio*/ return new Property("value[x]", "Ratio", "The value of the Output parameter as a basic type.", 0, 1, value); 3293 case -706454461: /*valueRatioRange*/ return new Property("value[x]", "RatioRange", "The value of the Output parameter as a basic type.", 0, 1, value); 3294 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference", "The value of the Output parameter as a basic type.", 0, 1, value); 3295 case -962229101: /*valueSampledData*/ return new Property("value[x]", "SampledData", "The value of the Output parameter as a basic type.", 0, 1, value); 3296 case -540985785: /*valueSignature*/ return new Property("value[x]", "Signature", "The value of the Output parameter as a basic type.", 0, 1, value); 3297 case -1406282469: /*valueTiming*/ return new Property("value[x]", "Timing", "The value of the Output parameter as a basic type.", 0, 1, value); 3298 case -1125200224: /*valueContactDetail*/ return new Property("value[x]", "ContactDetail", "The value of the Output parameter as a basic type.", 0, 1, value); 3299 case 1710554248: /*valueDataRequirement*/ return new Property("value[x]", "DataRequirement", "The value of the Output parameter as a basic type.", 0, 1, value); 3300 case -307517719: /*valueExpression*/ return new Property("value[x]", "Expression", "The value of the Output parameter as a basic type.", 0, 1, value); 3301 case 1387478187: /*valueParameterDefinition*/ return new Property("value[x]", "ParameterDefinition", "The value of the Output parameter as a basic type.", 0, 1, value); 3302 case 1748214124: /*valueRelatedArtifact*/ return new Property("value[x]", "RelatedArtifact", "The value of the Output parameter as a basic type.", 0, 1, value); 3303 case 976830394: /*valueTriggerDefinition*/ return new Property("value[x]", "TriggerDefinition", "The value of the Output parameter as a basic type.", 0, 1, value); 3304 case 588000479: /*valueUsageContext*/ return new Property("value[x]", "UsageContext", "The value of the Output parameter as a basic type.", 0, 1, value); 3305 case 1678530924: /*valueAvailability*/ return new Property("value[x]", "Availability", "The value of the Output parameter as a basic type.", 0, 1, value); 3306 case -1567222041: /*valueExtendedContactDetail*/ return new Property("value[x]", "ExtendedContactDetail", "The value of the Output parameter as a basic type.", 0, 1, value); 3307 case -1858636920: /*valueDosage*/ return new Property("value[x]", "Dosage", "The value of the Output parameter as a basic type.", 0, 1, value); 3308 case -765920490: /*valueMeta*/ return new Property("value[x]", "Meta", "The value of the Output parameter as a basic type.", 0, 1, value); 3309 default: return super.getNamedProperty(_hash, _name, _checkValid); 3310 } 3311 3312 } 3313 3314 @Override 3315 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3316 switch (hash) { 3317 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3318 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 3319 default: return super.getProperty(hash, name, checkValid); 3320 } 3321 3322 } 3323 3324 @Override 3325 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3326 switch (hash) { 3327 case 3575610: // type 3328 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3329 return value; 3330 case 111972721: // value 3331 this.value = TypeConvertor.castToType(value); // DataType 3332 return value; 3333 default: return super.setProperty(hash, name, value); 3334 } 3335 3336 } 3337 3338 @Override 3339 public Base setProperty(String name, Base value) throws FHIRException { 3340 if (name.equals("type")) { 3341 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3342 } else if (name.equals("value[x]")) { 3343 this.value = TypeConvertor.castToType(value); // DataType 3344 } else 3345 return super.setProperty(name, value); 3346 return value; 3347 } 3348 3349 @Override 3350 public void removeChild(String name, Base value) throws FHIRException { 3351 if (name.equals("type")) { 3352 this.type = null; 3353 } else if (name.equals("value[x]")) { 3354 this.value = null; 3355 } else 3356 super.removeChild(name, value); 3357 3358 } 3359 3360 @Override 3361 public Base makeProperty(int hash, String name) throws FHIRException { 3362 switch (hash) { 3363 case 3575610: return getType(); 3364 case -1410166417: return getValue(); 3365 case 111972721: return getValue(); 3366 default: return super.makeProperty(hash, name); 3367 } 3368 3369 } 3370 3371 @Override 3372 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3373 switch (hash) { 3374 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3375 case 111972721: /*value*/ return new String[] {"base64Binary", "boolean", "canonical", "code", "date", "dateTime", "decimal", "id", "instant", "integer", "integer64", "markdown", "oid", "positiveInt", "string", "time", "unsignedInt", "uri", "url", "uuid", "Address", "Age", "Annotation", "Attachment", "CodeableConcept", "CodeableReference", "Coding", "ContactPoint", "Count", "Distance", "Duration", "HumanName", "Identifier", "Money", "Period", "Quantity", "Range", "Ratio", "RatioRange", "Reference", "SampledData", "Signature", "Timing", "ContactDetail", "DataRequirement", "Expression", "ParameterDefinition", "RelatedArtifact", "TriggerDefinition", "UsageContext", "Availability", "ExtendedContactDetail", "Dosage", "Meta"}; 3376 default: return super.getTypesForProperty(hash, name); 3377 } 3378 3379 } 3380 3381 @Override 3382 public Base addChild(String name) throws FHIRException { 3383 if (name.equals("type")) { 3384 this.type = new CodeableConcept(); 3385 return this.type; 3386 } 3387 else if (name.equals("valueBase64Binary")) { 3388 this.value = new Base64BinaryType(); 3389 return this.value; 3390 } 3391 else if (name.equals("valueBoolean")) { 3392 this.value = new BooleanType(); 3393 return this.value; 3394 } 3395 else if (name.equals("valueCanonical")) { 3396 this.value = new CanonicalType(); 3397 return this.value; 3398 } 3399 else if (name.equals("valueCode")) { 3400 this.value = new CodeType(); 3401 return this.value; 3402 } 3403 else if (name.equals("valueDate")) { 3404 this.value = new DateType(); 3405 return this.value; 3406 } 3407 else if (name.equals("valueDateTime")) { 3408 this.value = new DateTimeType(); 3409 return this.value; 3410 } 3411 else if (name.equals("valueDecimal")) { 3412 this.value = new DecimalType(); 3413 return this.value; 3414 } 3415 else if (name.equals("valueId")) { 3416 this.value = new IdType(); 3417 return this.value; 3418 } 3419 else if (name.equals("valueInstant")) { 3420 this.value = new InstantType(); 3421 return this.value; 3422 } 3423 else if (name.equals("valueInteger")) { 3424 this.value = new IntegerType(); 3425 return this.value; 3426 } 3427 else if (name.equals("valueInteger64")) { 3428 this.value = new Integer64Type(); 3429 return this.value; 3430 } 3431 else if (name.equals("valueMarkdown")) { 3432 this.value = new MarkdownType(); 3433 return this.value; 3434 } 3435 else if (name.equals("valueOid")) { 3436 this.value = new OidType(); 3437 return this.value; 3438 } 3439 else if (name.equals("valuePositiveInt")) { 3440 this.value = new PositiveIntType(); 3441 return this.value; 3442 } 3443 else if (name.equals("valueString")) { 3444 this.value = new StringType(); 3445 return this.value; 3446 } 3447 else if (name.equals("valueTime")) { 3448 this.value = new TimeType(); 3449 return this.value; 3450 } 3451 else if (name.equals("valueUnsignedInt")) { 3452 this.value = new UnsignedIntType(); 3453 return this.value; 3454 } 3455 else if (name.equals("valueUri")) { 3456 this.value = new UriType(); 3457 return this.value; 3458 } 3459 else if (name.equals("valueUrl")) { 3460 this.value = new UrlType(); 3461 return this.value; 3462 } 3463 else if (name.equals("valueUuid")) { 3464 this.value = new UuidType(); 3465 return this.value; 3466 } 3467 else if (name.equals("valueAddress")) { 3468 this.value = new Address(); 3469 return this.value; 3470 } 3471 else if (name.equals("valueAge")) { 3472 this.value = new Age(); 3473 return this.value; 3474 } 3475 else if (name.equals("valueAnnotation")) { 3476 this.value = new Annotation(); 3477 return this.value; 3478 } 3479 else if (name.equals("valueAttachment")) { 3480 this.value = new Attachment(); 3481 return this.value; 3482 } 3483 else if (name.equals("valueCodeableConcept")) { 3484 this.value = new CodeableConcept(); 3485 return this.value; 3486 } 3487 else if (name.equals("valueCodeableReference")) { 3488 this.value = new CodeableReference(); 3489 return this.value; 3490 } 3491 else if (name.equals("valueCoding")) { 3492 this.value = new Coding(); 3493 return this.value; 3494 } 3495 else if (name.equals("valueContactPoint")) { 3496 this.value = new ContactPoint(); 3497 return this.value; 3498 } 3499 else if (name.equals("valueCount")) { 3500 this.value = new Count(); 3501 return this.value; 3502 } 3503 else if (name.equals("valueDistance")) { 3504 this.value = new Distance(); 3505 return this.value; 3506 } 3507 else if (name.equals("valueDuration")) { 3508 this.value = new Duration(); 3509 return this.value; 3510 } 3511 else if (name.equals("valueHumanName")) { 3512 this.value = new HumanName(); 3513 return this.value; 3514 } 3515 else if (name.equals("valueIdentifier")) { 3516 this.value = new Identifier(); 3517 return this.value; 3518 } 3519 else if (name.equals("valueMoney")) { 3520 this.value = new Money(); 3521 return this.value; 3522 } 3523 else if (name.equals("valuePeriod")) { 3524 this.value = new Period(); 3525 return this.value; 3526 } 3527 else if (name.equals("valueQuantity")) { 3528 this.value = new Quantity(); 3529 return this.value; 3530 } 3531 else if (name.equals("valueRange")) { 3532 this.value = new Range(); 3533 return this.value; 3534 } 3535 else if (name.equals("valueRatio")) { 3536 this.value = new Ratio(); 3537 return this.value; 3538 } 3539 else if (name.equals("valueRatioRange")) { 3540 this.value = new RatioRange(); 3541 return this.value; 3542 } 3543 else if (name.equals("valueReference")) { 3544 this.value = new Reference(); 3545 return this.value; 3546 } 3547 else if (name.equals("valueSampledData")) { 3548 this.value = new SampledData(); 3549 return this.value; 3550 } 3551 else if (name.equals("valueSignature")) { 3552 this.value = new Signature(); 3553 return this.value; 3554 } 3555 else if (name.equals("valueTiming")) { 3556 this.value = new Timing(); 3557 return this.value; 3558 } 3559 else if (name.equals("valueContactDetail")) { 3560 this.value = new ContactDetail(); 3561 return this.value; 3562 } 3563 else if (name.equals("valueDataRequirement")) { 3564 this.value = new DataRequirement(); 3565 return this.value; 3566 } 3567 else if (name.equals("valueExpression")) { 3568 this.value = new Expression(); 3569 return this.value; 3570 } 3571 else if (name.equals("valueParameterDefinition")) { 3572 this.value = new ParameterDefinition(); 3573 return this.value; 3574 } 3575 else if (name.equals("valueRelatedArtifact")) { 3576 this.value = new RelatedArtifact(); 3577 return this.value; 3578 } 3579 else if (name.equals("valueTriggerDefinition")) { 3580 this.value = new TriggerDefinition(); 3581 return this.value; 3582 } 3583 else if (name.equals("valueUsageContext")) { 3584 this.value = new UsageContext(); 3585 return this.value; 3586 } 3587 else if (name.equals("valueAvailability")) { 3588 this.value = new Availability(); 3589 return this.value; 3590 } 3591 else if (name.equals("valueExtendedContactDetail")) { 3592 this.value = new ExtendedContactDetail(); 3593 return this.value; 3594 } 3595 else if (name.equals("valueDosage")) { 3596 this.value = new Dosage(); 3597 return this.value; 3598 } 3599 else if (name.equals("valueMeta")) { 3600 this.value = new Meta(); 3601 return this.value; 3602 } 3603 else 3604 return super.addChild(name); 3605 } 3606 3607 public TaskOutputComponent copy() { 3608 TaskOutputComponent dst = new TaskOutputComponent(); 3609 copyValues(dst); 3610 return dst; 3611 } 3612 3613 public void copyValues(TaskOutputComponent dst) { 3614 super.copyValues(dst); 3615 dst.type = type == null ? null : type.copy(); 3616 dst.value = value == null ? null : value.copy(); 3617 } 3618 3619 @Override 3620 public boolean equalsDeep(Base other_) { 3621 if (!super.equalsDeep(other_)) 3622 return false; 3623 if (!(other_ instanceof TaskOutputComponent)) 3624 return false; 3625 TaskOutputComponent o = (TaskOutputComponent) other_; 3626 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 3627 } 3628 3629 @Override 3630 public boolean equalsShallow(Base other_) { 3631 if (!super.equalsShallow(other_)) 3632 return false; 3633 if (!(other_ instanceof TaskOutputComponent)) 3634 return false; 3635 TaskOutputComponent o = (TaskOutputComponent) other_; 3636 return true; 3637 } 3638 3639 public boolean isEmpty() { 3640 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 3641 } 3642 3643 public String fhirType() { 3644 return "Task.output"; 3645 3646 } 3647 3648 } 3649 3650 /** 3651 * The business identifier for this task. 3652 */ 3653 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3654 @Description(shortDefinition="Task Instance Identifier", formalDefinition="The business identifier for this task." ) 3655 protected List<Identifier> identifier; 3656 3657 /** 3658 * The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task. 3659 */ 3660 @Child(name = "instantiatesCanonical", type = {CanonicalType.class}, order=1, min=0, max=1, modifier=false, summary=true) 3661 @Description(shortDefinition="Formal definition of task", formalDefinition="The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task." ) 3662 protected CanonicalType instantiatesCanonical; 3663 3664 /** 3665 * The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task. 3666 */ 3667 @Child(name = "instantiatesUri", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=true) 3668 @Description(shortDefinition="Formal definition of task", formalDefinition="The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task." ) 3669 protected UriType instantiatesUri; 3670 3671 /** 3672 * BasedOn refers to a higher-level authorization that triggered the creation of the task. It references a "request" resource such as a ServiceRequest, MedicationRequest, CarePlan, etc. which is distinct from the "request" resource the task is seeking to fulfill. This latter resource is referenced by focus. For example, based on a CarePlan (= basedOn), a task is created to fulfill a ServiceRequest ( = focus ) to collect a specimen from a patient. 3673 */ 3674 @Child(name = "basedOn", type = {Reference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3675 @Description(shortDefinition="Request fulfilled by this task", formalDefinition="BasedOn refers to a higher-level authorization that triggered the creation of the task. It references a \"request\" resource such as a ServiceRequest, MedicationRequest, CarePlan, etc. which is distinct from the \"request\" resource the task is seeking to fulfill. This latter resource is referenced by focus. For example, based on a CarePlan (= basedOn), a task is created to fulfill a ServiceRequest ( = focus ) to collect a specimen from a patient." ) 3676 protected List<Reference> basedOn; 3677 3678 /** 3679 * A shared identifier common to multiple independent Task and Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time. 3680 */ 3681 @Child(name = "groupIdentifier", type = {Identifier.class}, order=4, min=0, max=1, modifier=false, summary=true) 3682 @Description(shortDefinition="Requisition or grouper id", formalDefinition="A shared identifier common to multiple independent Task and Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time." ) 3683 protected Identifier groupIdentifier; 3684 3685 /** 3686 * Task that this particular task is part of. 3687 */ 3688 @Child(name = "partOf", type = {Task.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3689 @Description(shortDefinition="Composite task", formalDefinition="Task that this particular task is part of." ) 3690 protected List<Reference> partOf; 3691 3692 /** 3693 * The current status of the task. 3694 */ 3695 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 3696 @Description(shortDefinition="draft | requested | received | accepted | +", formalDefinition="The current status of the task." ) 3697 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/task-status") 3698 protected Enumeration<TaskStatus> status; 3699 3700 /** 3701 * An explanation as to why this task is held, failed, was refused, etc. 3702 */ 3703 @Child(name = "statusReason", type = {CodeableReference.class}, order=7, min=0, max=1, modifier=false, summary=true) 3704 @Description(shortDefinition="Reason for current status", formalDefinition="An explanation as to why this task is held, failed, was refused, etc." ) 3705 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/task-status-reason") 3706 protected CodeableReference statusReason; 3707 3708 /** 3709 * Contains business-specific nuances of the business state. 3710 */ 3711 @Child(name = "businessStatus", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=true) 3712 @Description(shortDefinition="E.g. \"Specimen collected\", \"IV prepped\"", formalDefinition="Contains business-specific nuances of the business state." ) 3713 protected CodeableConcept businessStatus; 3714 3715 /** 3716 * Indicates the "level" of actionability associated with the Task, i.e. i+R[9]Cs this a proposed task, a planned task, an actionable task, etc. 3717 */ 3718 @Child(name = "intent", type = {CodeType.class}, order=9, min=1, max=1, modifier=false, summary=true) 3719 @Description(shortDefinition="unknown | proposal | plan | order | original-order | reflex-order | filler-order | instance-order | option", formalDefinition="Indicates the \"level\" of actionability associated with the Task, i.e. i+R[9]Cs this a proposed task, a planned task, an actionable task, etc." ) 3720 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/task-intent") 3721 protected Enumeration<TaskIntent> intent; 3722 3723 /** 3724 * Indicates how quickly the Task should be addressed with respect to other requests. 3725 */ 3726 @Child(name = "priority", type = {CodeType.class}, order=10, min=0, max=1, modifier=false, summary=false) 3727 @Description(shortDefinition="routine | urgent | asap | stat", formalDefinition="Indicates how quickly the Task should be addressed with respect to other requests." ) 3728 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 3729 protected Enumeration<RequestPriority> priority; 3730 3731 /** 3732 * If true indicates that the Task is asking for the specified action to *not* occur. 3733 */ 3734 @Child(name = "doNotPerform", type = {BooleanType.class}, order=11, min=0, max=1, modifier=true, summary=true) 3735 @Description(shortDefinition="True if Task is prohibiting action", formalDefinition="If true indicates that the Task is asking for the specified action to *not* occur." ) 3736 protected BooleanType doNotPerform; 3737 3738 /** 3739 * A name or code (or both) briefly describing what the task involves. 3740 */ 3741 @Child(name = "code", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=false, summary=true) 3742 @Description(shortDefinition="Task Type", formalDefinition="A name or code (or both) briefly describing what the task involves." ) 3743 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/task-code") 3744 protected CodeableConcept code; 3745 3746 /** 3747 * A free-text description of what is to be performed. 3748 */ 3749 @Child(name = "description", type = {StringType.class}, order=13, min=0, max=1, modifier=false, summary=true) 3750 @Description(shortDefinition="Human-readable explanation of task", formalDefinition="A free-text description of what is to be performed." ) 3751 protected StringType description; 3752 3753 /** 3754 * The request being fulfilled or the resource being manipulated (changed, suspended, etc.) by this task. 3755 */ 3756 @Child(name = "focus", type = {Reference.class}, order=14, min=0, max=1, modifier=false, summary=true) 3757 @Description(shortDefinition="What task is acting on", formalDefinition="The request being fulfilled or the resource being manipulated (changed, suspended, etc.) by this task." ) 3758 protected Reference focus; 3759 3760 /** 3761 * The entity who benefits from the performance of the service specified in the task (e.g., the patient). 3762 */ 3763 @Child(name = "for", type = {Reference.class}, order=15, min=0, max=1, modifier=false, summary=true) 3764 @Description(shortDefinition="Beneficiary of the Task", formalDefinition="The entity who benefits from the performance of the service specified in the task (e.g., the patient)." ) 3765 protected Reference for_; 3766 3767 /** 3768 * The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created. 3769 */ 3770 @Child(name = "encounter", type = {Encounter.class}, order=16, min=0, max=1, modifier=false, summary=true) 3771 @Description(shortDefinition="Healthcare event during which this task originated", formalDefinition="The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created." ) 3772 protected Reference encounter; 3773 3774 /** 3775 * Indicates the start and/or end of the period of time when completion of the task is desired to take place. 3776 */ 3777 @Child(name = "requestedPeriod", type = {Period.class}, order=17, min=0, max=1, modifier=false, summary=true) 3778 @Description(shortDefinition="When the task should be performed", formalDefinition="Indicates the start and/or end of the period of time when completion of the task is desired to take place." ) 3779 protected Period requestedPeriod; 3780 3781 /** 3782 * Identifies the time action was first taken against the task (start) and/or the time final action was taken against the task prior to marking it as completed (end). 3783 */ 3784 @Child(name = "executionPeriod", type = {Period.class}, order=18, min=0, max=1, modifier=false, summary=true) 3785 @Description(shortDefinition="Start and end time of execution", formalDefinition="Identifies the time action was first taken against the task (start) and/or the time final action was taken against the task prior to marking it as completed (end)." ) 3786 protected Period executionPeriod; 3787 3788 /** 3789 * The date and time this task was created. 3790 */ 3791 @Child(name = "authoredOn", type = {DateTimeType.class}, order=19, min=0, max=1, modifier=false, summary=false) 3792 @Description(shortDefinition="Task Creation Date", formalDefinition="The date and time this task was created." ) 3793 protected DateTimeType authoredOn; 3794 3795 /** 3796 * The date and time of last modification to this task. 3797 */ 3798 @Child(name = "lastModified", type = {DateTimeType.class}, order=20, min=0, max=1, modifier=false, summary=true) 3799 @Description(shortDefinition="Task Last Modified Date", formalDefinition="The date and time of last modification to this task." ) 3800 protected DateTimeType lastModified; 3801 3802 /** 3803 * The creator of the task. 3804 */ 3805 @Child(name = "requester", type = {Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class}, order=21, min=0, max=1, modifier=false, summary=true) 3806 @Description(shortDefinition="Who is asking for task to be done", formalDefinition="The creator of the task." ) 3807 protected Reference requester; 3808 3809 /** 3810 * The kind of participant or specific participant that should perform the task. 3811 */ 3812 @Child(name = "requestedPerformer", type = {CodeableReference.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3813 @Description(shortDefinition="Who should perform Task", formalDefinition="The kind of participant or specific participant that should perform the task." ) 3814 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/performer-role") 3815 protected List<CodeableReference> requestedPerformer; 3816 3817 /** 3818 * Party responsible for managing task execution. 3819 */ 3820 @Child(name = "owner", type = {Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, Patient.class, RelatedPerson.class}, order=23, min=0, max=1, modifier=false, summary=true) 3821 @Description(shortDefinition="Responsible individual", formalDefinition="Party responsible for managing task execution." ) 3822 protected Reference owner; 3823 3824 /** 3825 * The entity who performed the requested task. 3826 */ 3827 @Child(name = "performer", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3828 @Description(shortDefinition="Who or what performed the task", formalDefinition="The entity who performed the requested task." ) 3829 protected List<TaskPerformerComponent> performer; 3830 3831 /** 3832 * Principal physical location where this task is performed. 3833 */ 3834 @Child(name = "location", type = {Location.class}, order=25, min=0, max=1, modifier=false, summary=true) 3835 @Description(shortDefinition="Where task occurs", formalDefinition="Principal physical location where this task is performed." ) 3836 protected Reference location; 3837 3838 /** 3839 * A description, code, or reference indicating why this task needs to be performed. 3840 */ 3841 @Child(name = "reason", type = {CodeableReference.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3842 @Description(shortDefinition="Why task is needed", formalDefinition="A description, code, or reference indicating why this task needs to be performed." ) 3843 protected List<CodeableReference> reason; 3844 3845 /** 3846 * Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be relevant to the Task. 3847 */ 3848 @Child(name = "insurance", type = {Coverage.class, ClaimResponse.class}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3849 @Description(shortDefinition="Associated insurance coverage", formalDefinition="Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be relevant to the Task." ) 3850 protected List<Reference> insurance; 3851 3852 /** 3853 * Free-text information captured about the task as it progresses. 3854 */ 3855 @Child(name = "note", type = {Annotation.class}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3856 @Description(shortDefinition="Comments made about the task", formalDefinition="Free-text information captured about the task as it progresses." ) 3857 protected List<Annotation> note; 3858 3859 /** 3860 * Links to Provenance records for past versions of this Task that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the task. 3861 */ 3862 @Child(name = "relevantHistory", type = {Provenance.class}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3863 @Description(shortDefinition="Key events in history of the Task", formalDefinition="Links to Provenance records for past versions of this Task that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the task." ) 3864 protected List<Reference> relevantHistory; 3865 3866 /** 3867 * If the Task.focus is a request resource and the task is seeking fulfillment (i.e. is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned. 3868 */ 3869 @Child(name = "restriction", type = {}, order=30, min=0, max=1, modifier=false, summary=false) 3870 @Description(shortDefinition="Constraints on fulfillment tasks", formalDefinition="If the Task.focus is a request resource and the task is seeking fulfillment (i.e. is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned." ) 3871 protected TaskRestrictionComponent restriction; 3872 3873 /** 3874 * Additional information that may be needed in the execution of the task. 3875 */ 3876 @Child(name = "input", type = {}, order=31, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3877 @Description(shortDefinition="Information used to perform task", formalDefinition="Additional information that may be needed in the execution of the task." ) 3878 protected List<TaskInputComponent> input; 3879 3880 /** 3881 * Outputs produced by the Task. 3882 */ 3883 @Child(name = "output", type = {}, order=32, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3884 @Description(shortDefinition="Information produced as part of task", formalDefinition="Outputs produced by the Task." ) 3885 protected List<TaskOutputComponent> output; 3886 3887 private static final long serialVersionUID = 1363715195L; 3888 3889 /** 3890 * Constructor 3891 */ 3892 public Task() { 3893 super(); 3894 } 3895 3896 /** 3897 * Constructor 3898 */ 3899 public Task(TaskStatus status, TaskIntent intent) { 3900 super(); 3901 this.setStatus(status); 3902 this.setIntent(intent); 3903 } 3904 3905 /** 3906 * @return {@link #identifier} (The business identifier for this task.) 3907 */ 3908 public List<Identifier> getIdentifier() { 3909 if (this.identifier == null) 3910 this.identifier = new ArrayList<Identifier>(); 3911 return this.identifier; 3912 } 3913 3914 /** 3915 * @return Returns a reference to <code>this</code> for easy method chaining 3916 */ 3917 public Task setIdentifier(List<Identifier> theIdentifier) { 3918 this.identifier = theIdentifier; 3919 return this; 3920 } 3921 3922 public boolean hasIdentifier() { 3923 if (this.identifier == null) 3924 return false; 3925 for (Identifier item : this.identifier) 3926 if (!item.isEmpty()) 3927 return true; 3928 return false; 3929 } 3930 3931 public Identifier addIdentifier() { //3 3932 Identifier t = new Identifier(); 3933 if (this.identifier == null) 3934 this.identifier = new ArrayList<Identifier>(); 3935 this.identifier.add(t); 3936 return t; 3937 } 3938 3939 public Task addIdentifier(Identifier t) { //3 3940 if (t == null) 3941 return this; 3942 if (this.identifier == null) 3943 this.identifier = new ArrayList<Identifier>(); 3944 this.identifier.add(t); 3945 return this; 3946 } 3947 3948 /** 3949 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 3950 */ 3951 public Identifier getIdentifierFirstRep() { 3952 if (getIdentifier().isEmpty()) { 3953 addIdentifier(); 3954 } 3955 return getIdentifier().get(0); 3956 } 3957 3958 /** 3959 * @return {@link #instantiatesCanonical} (The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task.). This is the underlying object with id, value and extensions. The accessor "getInstantiatesCanonical" gives direct access to the value 3960 */ 3961 public CanonicalType getInstantiatesCanonicalElement() { 3962 if (this.instantiatesCanonical == null) 3963 if (Configuration.errorOnAutoCreate()) 3964 throw new Error("Attempt to auto-create Task.instantiatesCanonical"); 3965 else if (Configuration.doAutoCreate()) 3966 this.instantiatesCanonical = new CanonicalType(); // bb 3967 return this.instantiatesCanonical; 3968 } 3969 3970 public boolean hasInstantiatesCanonicalElement() { 3971 return this.instantiatesCanonical != null && !this.instantiatesCanonical.isEmpty(); 3972 } 3973 3974 public boolean hasInstantiatesCanonical() { 3975 return this.instantiatesCanonical != null && !this.instantiatesCanonical.isEmpty(); 3976 } 3977 3978 /** 3979 * @param value {@link #instantiatesCanonical} (The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task.). This is the underlying object with id, value and extensions. The accessor "getInstantiatesCanonical" gives direct access to the value 3980 */ 3981 public Task setInstantiatesCanonicalElement(CanonicalType value) { 3982 this.instantiatesCanonical = value; 3983 return this; 3984 } 3985 3986 /** 3987 * @return The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task. 3988 */ 3989 public String getInstantiatesCanonical() { 3990 return this.instantiatesCanonical == null ? null : this.instantiatesCanonical.getValue(); 3991 } 3992 3993 /** 3994 * @param value The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task. 3995 */ 3996 public Task setInstantiatesCanonical(String value) { 3997 if (Utilities.noString(value)) 3998 this.instantiatesCanonical = null; 3999 else { 4000 if (this.instantiatesCanonical == null) 4001 this.instantiatesCanonical = new CanonicalType(); 4002 this.instantiatesCanonical.setValue(value); 4003 } 4004 return this; 4005 } 4006 4007 /** 4008 * @return {@link #instantiatesUri} (The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task.). This is the underlying object with id, value and extensions. The accessor "getInstantiatesUri" gives direct access to the value 4009 */ 4010 public UriType getInstantiatesUriElement() { 4011 if (this.instantiatesUri == null) 4012 if (Configuration.errorOnAutoCreate()) 4013 throw new Error("Attempt to auto-create Task.instantiatesUri"); 4014 else if (Configuration.doAutoCreate()) 4015 this.instantiatesUri = new UriType(); // bb 4016 return this.instantiatesUri; 4017 } 4018 4019 public boolean hasInstantiatesUriElement() { 4020 return this.instantiatesUri != null && !this.instantiatesUri.isEmpty(); 4021 } 4022 4023 public boolean hasInstantiatesUri() { 4024 return this.instantiatesUri != null && !this.instantiatesUri.isEmpty(); 4025 } 4026 4027 /** 4028 * @param value {@link #instantiatesUri} (The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task.). This is the underlying object with id, value and extensions. The accessor "getInstantiatesUri" gives direct access to the value 4029 */ 4030 public Task setInstantiatesUriElement(UriType value) { 4031 this.instantiatesUri = value; 4032 return this; 4033 } 4034 4035 /** 4036 * @return The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task. 4037 */ 4038 public String getInstantiatesUri() { 4039 return this.instantiatesUri == null ? null : this.instantiatesUri.getValue(); 4040 } 4041 4042 /** 4043 * @param value The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task. 4044 */ 4045 public Task setInstantiatesUri(String value) { 4046 if (Utilities.noString(value)) 4047 this.instantiatesUri = null; 4048 else { 4049 if (this.instantiatesUri == null) 4050 this.instantiatesUri = new UriType(); 4051 this.instantiatesUri.setValue(value); 4052 } 4053 return this; 4054 } 4055 4056 /** 4057 * @return {@link #basedOn} (BasedOn refers to a higher-level authorization that triggered the creation of the task. It references a "request" resource such as a ServiceRequest, MedicationRequest, CarePlan, etc. which is distinct from the "request" resource the task is seeking to fulfill. This latter resource is referenced by focus. For example, based on a CarePlan (= basedOn), a task is created to fulfill a ServiceRequest ( = focus ) to collect a specimen from a patient.) 4058 */ 4059 public List<Reference> getBasedOn() { 4060 if (this.basedOn == null) 4061 this.basedOn = new ArrayList<Reference>(); 4062 return this.basedOn; 4063 } 4064 4065 /** 4066 * @return Returns a reference to <code>this</code> for easy method chaining 4067 */ 4068 public Task setBasedOn(List<Reference> theBasedOn) { 4069 this.basedOn = theBasedOn; 4070 return this; 4071 } 4072 4073 public boolean hasBasedOn() { 4074 if (this.basedOn == null) 4075 return false; 4076 for (Reference item : this.basedOn) 4077 if (!item.isEmpty()) 4078 return true; 4079 return false; 4080 } 4081 4082 public Reference addBasedOn() { //3 4083 Reference t = new Reference(); 4084 if (this.basedOn == null) 4085 this.basedOn = new ArrayList<Reference>(); 4086 this.basedOn.add(t); 4087 return t; 4088 } 4089 4090 public Task addBasedOn(Reference t) { //3 4091 if (t == null) 4092 return this; 4093 if (this.basedOn == null) 4094 this.basedOn = new ArrayList<Reference>(); 4095 this.basedOn.add(t); 4096 return this; 4097 } 4098 4099 /** 4100 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 4101 */ 4102 public Reference getBasedOnFirstRep() { 4103 if (getBasedOn().isEmpty()) { 4104 addBasedOn(); 4105 } 4106 return getBasedOn().get(0); 4107 } 4108 4109 /** 4110 * @return {@link #groupIdentifier} (A shared identifier common to multiple independent Task and Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.) 4111 */ 4112 public Identifier getGroupIdentifier() { 4113 if (this.groupIdentifier == null) 4114 if (Configuration.errorOnAutoCreate()) 4115 throw new Error("Attempt to auto-create Task.groupIdentifier"); 4116 else if (Configuration.doAutoCreate()) 4117 this.groupIdentifier = new Identifier(); // cc 4118 return this.groupIdentifier; 4119 } 4120 4121 public boolean hasGroupIdentifier() { 4122 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 4123 } 4124 4125 /** 4126 * @param value {@link #groupIdentifier} (A shared identifier common to multiple independent Task and Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.) 4127 */ 4128 public Task setGroupIdentifier(Identifier value) { 4129 this.groupIdentifier = value; 4130 return this; 4131 } 4132 4133 /** 4134 * @return {@link #partOf} (Task that this particular task is part of.) 4135 */ 4136 public List<Reference> getPartOf() { 4137 if (this.partOf == null) 4138 this.partOf = new ArrayList<Reference>(); 4139 return this.partOf; 4140 } 4141 4142 /** 4143 * @return Returns a reference to <code>this</code> for easy method chaining 4144 */ 4145 public Task setPartOf(List<Reference> thePartOf) { 4146 this.partOf = thePartOf; 4147 return this; 4148 } 4149 4150 public boolean hasPartOf() { 4151 if (this.partOf == null) 4152 return false; 4153 for (Reference item : this.partOf) 4154 if (!item.isEmpty()) 4155 return true; 4156 return false; 4157 } 4158 4159 public Reference addPartOf() { //3 4160 Reference t = new Reference(); 4161 if (this.partOf == null) 4162 this.partOf = new ArrayList<Reference>(); 4163 this.partOf.add(t); 4164 return t; 4165 } 4166 4167 public Task addPartOf(Reference t) { //3 4168 if (t == null) 4169 return this; 4170 if (this.partOf == null) 4171 this.partOf = new ArrayList<Reference>(); 4172 this.partOf.add(t); 4173 return this; 4174 } 4175 4176 /** 4177 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 4178 */ 4179 public Reference getPartOfFirstRep() { 4180 if (getPartOf().isEmpty()) { 4181 addPartOf(); 4182 } 4183 return getPartOf().get(0); 4184 } 4185 4186 /** 4187 * @return {@link #status} (The current status of the task.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4188 */ 4189 public Enumeration<TaskStatus> getStatusElement() { 4190 if (this.status == null) 4191 if (Configuration.errorOnAutoCreate()) 4192 throw new Error("Attempt to auto-create Task.status"); 4193 else if (Configuration.doAutoCreate()) 4194 this.status = new Enumeration<TaskStatus>(new TaskStatusEnumFactory()); // bb 4195 return this.status; 4196 } 4197 4198 public boolean hasStatusElement() { 4199 return this.status != null && !this.status.isEmpty(); 4200 } 4201 4202 public boolean hasStatus() { 4203 return this.status != null && !this.status.isEmpty(); 4204 } 4205 4206 /** 4207 * @param value {@link #status} (The current status of the task.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4208 */ 4209 public Task setStatusElement(Enumeration<TaskStatus> value) { 4210 this.status = value; 4211 return this; 4212 } 4213 4214 /** 4215 * @return The current status of the task. 4216 */ 4217 public TaskStatus getStatus() { 4218 return this.status == null ? null : this.status.getValue(); 4219 } 4220 4221 /** 4222 * @param value The current status of the task. 4223 */ 4224 public Task setStatus(TaskStatus value) { 4225 if (this.status == null) 4226 this.status = new Enumeration<TaskStatus>(new TaskStatusEnumFactory()); 4227 this.status.setValue(value); 4228 return this; 4229 } 4230 4231 /** 4232 * @return {@link #statusReason} (An explanation as to why this task is held, failed, was refused, etc.) 4233 */ 4234 public CodeableReference getStatusReason() { 4235 if (this.statusReason == null) 4236 if (Configuration.errorOnAutoCreate()) 4237 throw new Error("Attempt to auto-create Task.statusReason"); 4238 else if (Configuration.doAutoCreate()) 4239 this.statusReason = new CodeableReference(); // cc 4240 return this.statusReason; 4241 } 4242 4243 public boolean hasStatusReason() { 4244 return this.statusReason != null && !this.statusReason.isEmpty(); 4245 } 4246 4247 /** 4248 * @param value {@link #statusReason} (An explanation as to why this task is held, failed, was refused, etc.) 4249 */ 4250 public Task setStatusReason(CodeableReference value) { 4251 this.statusReason = value; 4252 return this; 4253 } 4254 4255 /** 4256 * @return {@link #businessStatus} (Contains business-specific nuances of the business state.) 4257 */ 4258 public CodeableConcept getBusinessStatus() { 4259 if (this.businessStatus == null) 4260 if (Configuration.errorOnAutoCreate()) 4261 throw new Error("Attempt to auto-create Task.businessStatus"); 4262 else if (Configuration.doAutoCreate()) 4263 this.businessStatus = new CodeableConcept(); // cc 4264 return this.businessStatus; 4265 } 4266 4267 public boolean hasBusinessStatus() { 4268 return this.businessStatus != null && !this.businessStatus.isEmpty(); 4269 } 4270 4271 /** 4272 * @param value {@link #businessStatus} (Contains business-specific nuances of the business state.) 4273 */ 4274 public Task setBusinessStatus(CodeableConcept value) { 4275 this.businessStatus = value; 4276 return this; 4277 } 4278 4279 /** 4280 * @return {@link #intent} (Indicates the "level" of actionability associated with the Task, i.e. i+R[9]Cs this a proposed task, a planned task, an actionable task, etc.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 4281 */ 4282 public Enumeration<TaskIntent> getIntentElement() { 4283 if (this.intent == null) 4284 if (Configuration.errorOnAutoCreate()) 4285 throw new Error("Attempt to auto-create Task.intent"); 4286 else if (Configuration.doAutoCreate()) 4287 this.intent = new Enumeration<TaskIntent>(new TaskIntentEnumFactory()); // bb 4288 return this.intent; 4289 } 4290 4291 public boolean hasIntentElement() { 4292 return this.intent != null && !this.intent.isEmpty(); 4293 } 4294 4295 public boolean hasIntent() { 4296 return this.intent != null && !this.intent.isEmpty(); 4297 } 4298 4299 /** 4300 * @param value {@link #intent} (Indicates the "level" of actionability associated with the Task, i.e. i+R[9]Cs this a proposed task, a planned task, an actionable task, etc.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 4301 */ 4302 public Task setIntentElement(Enumeration<TaskIntent> value) { 4303 this.intent = value; 4304 return this; 4305 } 4306 4307 /** 4308 * @return Indicates the "level" of actionability associated with the Task, i.e. i+R[9]Cs this a proposed task, a planned task, an actionable task, etc. 4309 */ 4310 public TaskIntent getIntent() { 4311 return this.intent == null ? null : this.intent.getValue(); 4312 } 4313 4314 /** 4315 * @param value Indicates the "level" of actionability associated with the Task, i.e. i+R[9]Cs this a proposed task, a planned task, an actionable task, etc. 4316 */ 4317 public Task setIntent(TaskIntent value) { 4318 if (this.intent == null) 4319 this.intent = new Enumeration<TaskIntent>(new TaskIntentEnumFactory()); 4320 this.intent.setValue(value); 4321 return this; 4322 } 4323 4324 /** 4325 * @return {@link #priority} (Indicates how quickly the Task should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 4326 */ 4327 public Enumeration<RequestPriority> getPriorityElement() { 4328 if (this.priority == null) 4329 if (Configuration.errorOnAutoCreate()) 4330 throw new Error("Attempt to auto-create Task.priority"); 4331 else if (Configuration.doAutoCreate()) 4332 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 4333 return this.priority; 4334 } 4335 4336 public boolean hasPriorityElement() { 4337 return this.priority != null && !this.priority.isEmpty(); 4338 } 4339 4340 public boolean hasPriority() { 4341 return this.priority != null && !this.priority.isEmpty(); 4342 } 4343 4344 /** 4345 * @param value {@link #priority} (Indicates how quickly the Task should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 4346 */ 4347 public Task setPriorityElement(Enumeration<RequestPriority> value) { 4348 this.priority = value; 4349 return this; 4350 } 4351 4352 /** 4353 * @return Indicates how quickly the Task should be addressed with respect to other requests. 4354 */ 4355 public RequestPriority getPriority() { 4356 return this.priority == null ? null : this.priority.getValue(); 4357 } 4358 4359 /** 4360 * @param value Indicates how quickly the Task should be addressed with respect to other requests. 4361 */ 4362 public Task setPriority(RequestPriority value) { 4363 if (value == null) 4364 this.priority = null; 4365 else { 4366 if (this.priority == null) 4367 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 4368 this.priority.setValue(value); 4369 } 4370 return this; 4371 } 4372 4373 /** 4374 * @return {@link #doNotPerform} (If true indicates that the Task is asking for the specified action to *not* occur.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 4375 */ 4376 public BooleanType getDoNotPerformElement() { 4377 if (this.doNotPerform == null) 4378 if (Configuration.errorOnAutoCreate()) 4379 throw new Error("Attempt to auto-create Task.doNotPerform"); 4380 else if (Configuration.doAutoCreate()) 4381 this.doNotPerform = new BooleanType(); // bb 4382 return this.doNotPerform; 4383 } 4384 4385 public boolean hasDoNotPerformElement() { 4386 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 4387 } 4388 4389 public boolean hasDoNotPerform() { 4390 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 4391 } 4392 4393 /** 4394 * @param value {@link #doNotPerform} (If true indicates that the Task is asking for the specified action to *not* occur.). This is the underlying object with id, value and extensions. The accessor "getDoNotPerform" gives direct access to the value 4395 */ 4396 public Task setDoNotPerformElement(BooleanType value) { 4397 this.doNotPerform = value; 4398 return this; 4399 } 4400 4401 /** 4402 * @return If true indicates that the Task is asking for the specified action to *not* occur. 4403 */ 4404 public boolean getDoNotPerform() { 4405 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 4406 } 4407 4408 /** 4409 * @param value If true indicates that the Task is asking for the specified action to *not* occur. 4410 */ 4411 public Task setDoNotPerform(boolean value) { 4412 if (this.doNotPerform == null) 4413 this.doNotPerform = new BooleanType(); 4414 this.doNotPerform.setValue(value); 4415 return this; 4416 } 4417 4418 /** 4419 * @return {@link #code} (A name or code (or both) briefly describing what the task involves.) 4420 */ 4421 public CodeableConcept getCode() { 4422 if (this.code == null) 4423 if (Configuration.errorOnAutoCreate()) 4424 throw new Error("Attempt to auto-create Task.code"); 4425 else if (Configuration.doAutoCreate()) 4426 this.code = new CodeableConcept(); // cc 4427 return this.code; 4428 } 4429 4430 public boolean hasCode() { 4431 return this.code != null && !this.code.isEmpty(); 4432 } 4433 4434 /** 4435 * @param value {@link #code} (A name or code (or both) briefly describing what the task involves.) 4436 */ 4437 public Task setCode(CodeableConcept value) { 4438 this.code = value; 4439 return this; 4440 } 4441 4442 /** 4443 * @return {@link #description} (A free-text description of what is to be performed.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4444 */ 4445 public StringType getDescriptionElement() { 4446 if (this.description == null) 4447 if (Configuration.errorOnAutoCreate()) 4448 throw new Error("Attempt to auto-create Task.description"); 4449 else if (Configuration.doAutoCreate()) 4450 this.description = new StringType(); // bb 4451 return this.description; 4452 } 4453 4454 public boolean hasDescriptionElement() { 4455 return this.description != null && !this.description.isEmpty(); 4456 } 4457 4458 public boolean hasDescription() { 4459 return this.description != null && !this.description.isEmpty(); 4460 } 4461 4462 /** 4463 * @param value {@link #description} (A free-text description of what is to be performed.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4464 */ 4465 public Task setDescriptionElement(StringType value) { 4466 this.description = value; 4467 return this; 4468 } 4469 4470 /** 4471 * @return A free-text description of what is to be performed. 4472 */ 4473 public String getDescription() { 4474 return this.description == null ? null : this.description.getValue(); 4475 } 4476 4477 /** 4478 * @param value A free-text description of what is to be performed. 4479 */ 4480 public Task setDescription(String value) { 4481 if (Utilities.noString(value)) 4482 this.description = null; 4483 else { 4484 if (this.description == null) 4485 this.description = new StringType(); 4486 this.description.setValue(value); 4487 } 4488 return this; 4489 } 4490 4491 /** 4492 * @return {@link #focus} (The request being fulfilled or the resource being manipulated (changed, suspended, etc.) by this task.) 4493 */ 4494 public Reference getFocus() { 4495 if (this.focus == null) 4496 if (Configuration.errorOnAutoCreate()) 4497 throw new Error("Attempt to auto-create Task.focus"); 4498 else if (Configuration.doAutoCreate()) 4499 this.focus = new Reference(); // cc 4500 return this.focus; 4501 } 4502 4503 public boolean hasFocus() { 4504 return this.focus != null && !this.focus.isEmpty(); 4505 } 4506 4507 /** 4508 * @param value {@link #focus} (The request being fulfilled or the resource being manipulated (changed, suspended, etc.) by this task.) 4509 */ 4510 public Task setFocus(Reference value) { 4511 this.focus = value; 4512 return this; 4513 } 4514 4515 /** 4516 * @return {@link #for_} (The entity who benefits from the performance of the service specified in the task (e.g., the patient).) 4517 */ 4518 public Reference getFor() { 4519 if (this.for_ == null) 4520 if (Configuration.errorOnAutoCreate()) 4521 throw new Error("Attempt to auto-create Task.for_"); 4522 else if (Configuration.doAutoCreate()) 4523 this.for_ = new Reference(); // cc 4524 return this.for_; 4525 } 4526 4527 public boolean hasFor() { 4528 return this.for_ != null && !this.for_.isEmpty(); 4529 } 4530 4531 /** 4532 * @param value {@link #for_} (The entity who benefits from the performance of the service specified in the task (e.g., the patient).) 4533 */ 4534 public Task setFor(Reference value) { 4535 this.for_ = value; 4536 return this; 4537 } 4538 4539 /** 4540 * @return {@link #encounter} (The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created.) 4541 */ 4542 public Reference getEncounter() { 4543 if (this.encounter == null) 4544 if (Configuration.errorOnAutoCreate()) 4545 throw new Error("Attempt to auto-create Task.encounter"); 4546 else if (Configuration.doAutoCreate()) 4547 this.encounter = new Reference(); // cc 4548 return this.encounter; 4549 } 4550 4551 public boolean hasEncounter() { 4552 return this.encounter != null && !this.encounter.isEmpty(); 4553 } 4554 4555 /** 4556 * @param value {@link #encounter} (The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created.) 4557 */ 4558 public Task setEncounter(Reference value) { 4559 this.encounter = value; 4560 return this; 4561 } 4562 4563 /** 4564 * @return {@link #requestedPeriod} (Indicates the start and/or end of the period of time when completion of the task is desired to take place.) 4565 */ 4566 public Period getRequestedPeriod() { 4567 if (this.requestedPeriod == null) 4568 if (Configuration.errorOnAutoCreate()) 4569 throw new Error("Attempt to auto-create Task.requestedPeriod"); 4570 else if (Configuration.doAutoCreate()) 4571 this.requestedPeriod = new Period(); // cc 4572 return this.requestedPeriod; 4573 } 4574 4575 public boolean hasRequestedPeriod() { 4576 return this.requestedPeriod != null && !this.requestedPeriod.isEmpty(); 4577 } 4578 4579 /** 4580 * @param value {@link #requestedPeriod} (Indicates the start and/or end of the period of time when completion of the task is desired to take place.) 4581 */ 4582 public Task setRequestedPeriod(Period value) { 4583 this.requestedPeriod = value; 4584 return this; 4585 } 4586 4587 /** 4588 * @return {@link #executionPeriod} (Identifies the time action was first taken against the task (start) and/or the time final action was taken against the task prior to marking it as completed (end).) 4589 */ 4590 public Period getExecutionPeriod() { 4591 if (this.executionPeriod == null) 4592 if (Configuration.errorOnAutoCreate()) 4593 throw new Error("Attempt to auto-create Task.executionPeriod"); 4594 else if (Configuration.doAutoCreate()) 4595 this.executionPeriod = new Period(); // cc 4596 return this.executionPeriod; 4597 } 4598 4599 public boolean hasExecutionPeriod() { 4600 return this.executionPeriod != null && !this.executionPeriod.isEmpty(); 4601 } 4602 4603 /** 4604 * @param value {@link #executionPeriod} (Identifies the time action was first taken against the task (start) and/or the time final action was taken against the task prior to marking it as completed (end).) 4605 */ 4606 public Task setExecutionPeriod(Period value) { 4607 this.executionPeriod = value; 4608 return this; 4609 } 4610 4611 /** 4612 * @return {@link #authoredOn} (The date and time this task was created.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 4613 */ 4614 public DateTimeType getAuthoredOnElement() { 4615 if (this.authoredOn == null) 4616 if (Configuration.errorOnAutoCreate()) 4617 throw new Error("Attempt to auto-create Task.authoredOn"); 4618 else if (Configuration.doAutoCreate()) 4619 this.authoredOn = new DateTimeType(); // bb 4620 return this.authoredOn; 4621 } 4622 4623 public boolean hasAuthoredOnElement() { 4624 return this.authoredOn != null && !this.authoredOn.isEmpty(); 4625 } 4626 4627 public boolean hasAuthoredOn() { 4628 return this.authoredOn != null && !this.authoredOn.isEmpty(); 4629 } 4630 4631 /** 4632 * @param value {@link #authoredOn} (The date and time this task was created.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 4633 */ 4634 public Task setAuthoredOnElement(DateTimeType value) { 4635 this.authoredOn = value; 4636 return this; 4637 } 4638 4639 /** 4640 * @return The date and time this task was created. 4641 */ 4642 public Date getAuthoredOn() { 4643 return this.authoredOn == null ? null : this.authoredOn.getValue(); 4644 } 4645 4646 /** 4647 * @param value The date and time this task was created. 4648 */ 4649 public Task setAuthoredOn(Date value) { 4650 if (value == null) 4651 this.authoredOn = null; 4652 else { 4653 if (this.authoredOn == null) 4654 this.authoredOn = new DateTimeType(); 4655 this.authoredOn.setValue(value); 4656 } 4657 return this; 4658 } 4659 4660 /** 4661 * @return {@link #lastModified} (The date and time of last modification to this task.). This is the underlying object with id, value and extensions. The accessor "getLastModified" gives direct access to the value 4662 */ 4663 public DateTimeType getLastModifiedElement() { 4664 if (this.lastModified == null) 4665 if (Configuration.errorOnAutoCreate()) 4666 throw new Error("Attempt to auto-create Task.lastModified"); 4667 else if (Configuration.doAutoCreate()) 4668 this.lastModified = new DateTimeType(); // bb 4669 return this.lastModified; 4670 } 4671 4672 public boolean hasLastModifiedElement() { 4673 return this.lastModified != null && !this.lastModified.isEmpty(); 4674 } 4675 4676 public boolean hasLastModified() { 4677 return this.lastModified != null && !this.lastModified.isEmpty(); 4678 } 4679 4680 /** 4681 * @param value {@link #lastModified} (The date and time of last modification to this task.). This is the underlying object with id, value and extensions. The accessor "getLastModified" gives direct access to the value 4682 */ 4683 public Task setLastModifiedElement(DateTimeType value) { 4684 this.lastModified = value; 4685 return this; 4686 } 4687 4688 /** 4689 * @return The date and time of last modification to this task. 4690 */ 4691 public Date getLastModified() { 4692 return this.lastModified == null ? null : this.lastModified.getValue(); 4693 } 4694 4695 /** 4696 * @param value The date and time of last modification to this task. 4697 */ 4698 public Task setLastModified(Date value) { 4699 if (value == null) 4700 this.lastModified = null; 4701 else { 4702 if (this.lastModified == null) 4703 this.lastModified = new DateTimeType(); 4704 this.lastModified.setValue(value); 4705 } 4706 return this; 4707 } 4708 4709 /** 4710 * @return {@link #requester} (The creator of the task.) 4711 */ 4712 public Reference getRequester() { 4713 if (this.requester == null) 4714 if (Configuration.errorOnAutoCreate()) 4715 throw new Error("Attempt to auto-create Task.requester"); 4716 else if (Configuration.doAutoCreate()) 4717 this.requester = new Reference(); // cc 4718 return this.requester; 4719 } 4720 4721 public boolean hasRequester() { 4722 return this.requester != null && !this.requester.isEmpty(); 4723 } 4724 4725 /** 4726 * @param value {@link #requester} (The creator of the task.) 4727 */ 4728 public Task setRequester(Reference value) { 4729 this.requester = value; 4730 return this; 4731 } 4732 4733 /** 4734 * @return {@link #requestedPerformer} (The kind of participant or specific participant that should perform the task.) 4735 */ 4736 public List<CodeableReference> getRequestedPerformer() { 4737 if (this.requestedPerformer == null) 4738 this.requestedPerformer = new ArrayList<CodeableReference>(); 4739 return this.requestedPerformer; 4740 } 4741 4742 /** 4743 * @return Returns a reference to <code>this</code> for easy method chaining 4744 */ 4745 public Task setRequestedPerformer(List<CodeableReference> theRequestedPerformer) { 4746 this.requestedPerformer = theRequestedPerformer; 4747 return this; 4748 } 4749 4750 public boolean hasRequestedPerformer() { 4751 if (this.requestedPerformer == null) 4752 return false; 4753 for (CodeableReference item : this.requestedPerformer) 4754 if (!item.isEmpty()) 4755 return true; 4756 return false; 4757 } 4758 4759 public CodeableReference addRequestedPerformer() { //3 4760 CodeableReference t = new CodeableReference(); 4761 if (this.requestedPerformer == null) 4762 this.requestedPerformer = new ArrayList<CodeableReference>(); 4763 this.requestedPerformer.add(t); 4764 return t; 4765 } 4766 4767 public Task addRequestedPerformer(CodeableReference t) { //3 4768 if (t == null) 4769 return this; 4770 if (this.requestedPerformer == null) 4771 this.requestedPerformer = new ArrayList<CodeableReference>(); 4772 this.requestedPerformer.add(t); 4773 return this; 4774 } 4775 4776 /** 4777 * @return The first repetition of repeating field {@link #requestedPerformer}, creating it if it does not already exist {3} 4778 */ 4779 public CodeableReference getRequestedPerformerFirstRep() { 4780 if (getRequestedPerformer().isEmpty()) { 4781 addRequestedPerformer(); 4782 } 4783 return getRequestedPerformer().get(0); 4784 } 4785 4786 /** 4787 * @return {@link #owner} (Party responsible for managing task execution.) 4788 */ 4789 public Reference getOwner() { 4790 if (this.owner == null) 4791 if (Configuration.errorOnAutoCreate()) 4792 throw new Error("Attempt to auto-create Task.owner"); 4793 else if (Configuration.doAutoCreate()) 4794 this.owner = new Reference(); // cc 4795 return this.owner; 4796 } 4797 4798 public boolean hasOwner() { 4799 return this.owner != null && !this.owner.isEmpty(); 4800 } 4801 4802 /** 4803 * @param value {@link #owner} (Party responsible for managing task execution.) 4804 */ 4805 public Task setOwner(Reference value) { 4806 this.owner = value; 4807 return this; 4808 } 4809 4810 /** 4811 * @return {@link #performer} (The entity who performed the requested task.) 4812 */ 4813 public List<TaskPerformerComponent> getPerformer() { 4814 if (this.performer == null) 4815 this.performer = new ArrayList<TaskPerformerComponent>(); 4816 return this.performer; 4817 } 4818 4819 /** 4820 * @return Returns a reference to <code>this</code> for easy method chaining 4821 */ 4822 public Task setPerformer(List<TaskPerformerComponent> thePerformer) { 4823 this.performer = thePerformer; 4824 return this; 4825 } 4826 4827 public boolean hasPerformer() { 4828 if (this.performer == null) 4829 return false; 4830 for (TaskPerformerComponent item : this.performer) 4831 if (!item.isEmpty()) 4832 return true; 4833 return false; 4834 } 4835 4836 public TaskPerformerComponent addPerformer() { //3 4837 TaskPerformerComponent t = new TaskPerformerComponent(); 4838 if (this.performer == null) 4839 this.performer = new ArrayList<TaskPerformerComponent>(); 4840 this.performer.add(t); 4841 return t; 4842 } 4843 4844 public Task addPerformer(TaskPerformerComponent t) { //3 4845 if (t == null) 4846 return this; 4847 if (this.performer == null) 4848 this.performer = new ArrayList<TaskPerformerComponent>(); 4849 this.performer.add(t); 4850 return this; 4851 } 4852 4853 /** 4854 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist {3} 4855 */ 4856 public TaskPerformerComponent getPerformerFirstRep() { 4857 if (getPerformer().isEmpty()) { 4858 addPerformer(); 4859 } 4860 return getPerformer().get(0); 4861 } 4862 4863 /** 4864 * @return {@link #location} (Principal physical location where this task is performed.) 4865 */ 4866 public Reference getLocation() { 4867 if (this.location == null) 4868 if (Configuration.errorOnAutoCreate()) 4869 throw new Error("Attempt to auto-create Task.location"); 4870 else if (Configuration.doAutoCreate()) 4871 this.location = new Reference(); // cc 4872 return this.location; 4873 } 4874 4875 public boolean hasLocation() { 4876 return this.location != null && !this.location.isEmpty(); 4877 } 4878 4879 /** 4880 * @param value {@link #location} (Principal physical location where this task is performed.) 4881 */ 4882 public Task setLocation(Reference value) { 4883 this.location = value; 4884 return this; 4885 } 4886 4887 /** 4888 * @return {@link #reason} (A description, code, or reference indicating why this task needs to be performed.) 4889 */ 4890 public List<CodeableReference> getReason() { 4891 if (this.reason == null) 4892 this.reason = new ArrayList<CodeableReference>(); 4893 return this.reason; 4894 } 4895 4896 /** 4897 * @return Returns a reference to <code>this</code> for easy method chaining 4898 */ 4899 public Task setReason(List<CodeableReference> theReason) { 4900 this.reason = theReason; 4901 return this; 4902 } 4903 4904 public boolean hasReason() { 4905 if (this.reason == null) 4906 return false; 4907 for (CodeableReference item : this.reason) 4908 if (!item.isEmpty()) 4909 return true; 4910 return false; 4911 } 4912 4913 public CodeableReference addReason() { //3 4914 CodeableReference t = new CodeableReference(); 4915 if (this.reason == null) 4916 this.reason = new ArrayList<CodeableReference>(); 4917 this.reason.add(t); 4918 return t; 4919 } 4920 4921 public Task addReason(CodeableReference t) { //3 4922 if (t == null) 4923 return this; 4924 if (this.reason == null) 4925 this.reason = new ArrayList<CodeableReference>(); 4926 this.reason.add(t); 4927 return this; 4928 } 4929 4930 /** 4931 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 4932 */ 4933 public CodeableReference getReasonFirstRep() { 4934 if (getReason().isEmpty()) { 4935 addReason(); 4936 } 4937 return getReason().get(0); 4938 } 4939 4940 /** 4941 * @return {@link #insurance} (Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be relevant to the Task.) 4942 */ 4943 public List<Reference> getInsurance() { 4944 if (this.insurance == null) 4945 this.insurance = new ArrayList<Reference>(); 4946 return this.insurance; 4947 } 4948 4949 /** 4950 * @return Returns a reference to <code>this</code> for easy method chaining 4951 */ 4952 public Task setInsurance(List<Reference> theInsurance) { 4953 this.insurance = theInsurance; 4954 return this; 4955 } 4956 4957 public boolean hasInsurance() { 4958 if (this.insurance == null) 4959 return false; 4960 for (Reference item : this.insurance) 4961 if (!item.isEmpty()) 4962 return true; 4963 return false; 4964 } 4965 4966 public Reference addInsurance() { //3 4967 Reference t = new Reference(); 4968 if (this.insurance == null) 4969 this.insurance = new ArrayList<Reference>(); 4970 this.insurance.add(t); 4971 return t; 4972 } 4973 4974 public Task addInsurance(Reference t) { //3 4975 if (t == null) 4976 return this; 4977 if (this.insurance == null) 4978 this.insurance = new ArrayList<Reference>(); 4979 this.insurance.add(t); 4980 return this; 4981 } 4982 4983 /** 4984 * @return The first repetition of repeating field {@link #insurance}, creating it if it does not already exist {3} 4985 */ 4986 public Reference getInsuranceFirstRep() { 4987 if (getInsurance().isEmpty()) { 4988 addInsurance(); 4989 } 4990 return getInsurance().get(0); 4991 } 4992 4993 /** 4994 * @return {@link #note} (Free-text information captured about the task as it progresses.) 4995 */ 4996 public List<Annotation> getNote() { 4997 if (this.note == null) 4998 this.note = new ArrayList<Annotation>(); 4999 return this.note; 5000 } 5001 5002 /** 5003 * @return Returns a reference to <code>this</code> for easy method chaining 5004 */ 5005 public Task setNote(List<Annotation> theNote) { 5006 this.note = theNote; 5007 return this; 5008 } 5009 5010 public boolean hasNote() { 5011 if (this.note == null) 5012 return false; 5013 for (Annotation item : this.note) 5014 if (!item.isEmpty()) 5015 return true; 5016 return false; 5017 } 5018 5019 public Annotation addNote() { //3 5020 Annotation t = new Annotation(); 5021 if (this.note == null) 5022 this.note = new ArrayList<Annotation>(); 5023 this.note.add(t); 5024 return t; 5025 } 5026 5027 public Task addNote(Annotation t) { //3 5028 if (t == null) 5029 return this; 5030 if (this.note == null) 5031 this.note = new ArrayList<Annotation>(); 5032 this.note.add(t); 5033 return this; 5034 } 5035 5036 /** 5037 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 5038 */ 5039 public Annotation getNoteFirstRep() { 5040 if (getNote().isEmpty()) { 5041 addNote(); 5042 } 5043 return getNote().get(0); 5044 } 5045 5046 /** 5047 * @return {@link #relevantHistory} (Links to Provenance records for past versions of this Task that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the task.) 5048 */ 5049 public List<Reference> getRelevantHistory() { 5050 if (this.relevantHistory == null) 5051 this.relevantHistory = new ArrayList<Reference>(); 5052 return this.relevantHistory; 5053 } 5054 5055 /** 5056 * @return Returns a reference to <code>this</code> for easy method chaining 5057 */ 5058 public Task setRelevantHistory(List<Reference> theRelevantHistory) { 5059 this.relevantHistory = theRelevantHistory; 5060 return this; 5061 } 5062 5063 public boolean hasRelevantHistory() { 5064 if (this.relevantHistory == null) 5065 return false; 5066 for (Reference item : this.relevantHistory) 5067 if (!item.isEmpty()) 5068 return true; 5069 return false; 5070 } 5071 5072 public Reference addRelevantHistory() { //3 5073 Reference t = new Reference(); 5074 if (this.relevantHistory == null) 5075 this.relevantHistory = new ArrayList<Reference>(); 5076 this.relevantHistory.add(t); 5077 return t; 5078 } 5079 5080 public Task addRelevantHistory(Reference t) { //3 5081 if (t == null) 5082 return this; 5083 if (this.relevantHistory == null) 5084 this.relevantHistory = new ArrayList<Reference>(); 5085 this.relevantHistory.add(t); 5086 return this; 5087 } 5088 5089 /** 5090 * @return The first repetition of repeating field {@link #relevantHistory}, creating it if it does not already exist {3} 5091 */ 5092 public Reference getRelevantHistoryFirstRep() { 5093 if (getRelevantHistory().isEmpty()) { 5094 addRelevantHistory(); 5095 } 5096 return getRelevantHistory().get(0); 5097 } 5098 5099 /** 5100 * @return {@link #restriction} (If the Task.focus is a request resource and the task is seeking fulfillment (i.e. is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned.) 5101 */ 5102 public TaskRestrictionComponent getRestriction() { 5103 if (this.restriction == null) 5104 if (Configuration.errorOnAutoCreate()) 5105 throw new Error("Attempt to auto-create Task.restriction"); 5106 else if (Configuration.doAutoCreate()) 5107 this.restriction = new TaskRestrictionComponent(); // cc 5108 return this.restriction; 5109 } 5110 5111 public boolean hasRestriction() { 5112 return this.restriction != null && !this.restriction.isEmpty(); 5113 } 5114 5115 /** 5116 * @param value {@link #restriction} (If the Task.focus is a request resource and the task is seeking fulfillment (i.e. is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned.) 5117 */ 5118 public Task setRestriction(TaskRestrictionComponent value) { 5119 this.restriction = value; 5120 return this; 5121 } 5122 5123 /** 5124 * @return {@link #input} (Additional information that may be needed in the execution of the task.) 5125 */ 5126 public List<TaskInputComponent> getInput() { 5127 if (this.input == null) 5128 this.input = new ArrayList<TaskInputComponent>(); 5129 return this.input; 5130 } 5131 5132 /** 5133 * @return Returns a reference to <code>this</code> for easy method chaining 5134 */ 5135 public Task setInput(List<TaskInputComponent> theInput) { 5136 this.input = theInput; 5137 return this; 5138 } 5139 5140 public boolean hasInput() { 5141 if (this.input == null) 5142 return false; 5143 for (TaskInputComponent item : this.input) 5144 if (!item.isEmpty()) 5145 return true; 5146 return false; 5147 } 5148 5149 public TaskInputComponent addInput() { //3 5150 TaskInputComponent t = new TaskInputComponent(); 5151 if (this.input == null) 5152 this.input = new ArrayList<TaskInputComponent>(); 5153 this.input.add(t); 5154 return t; 5155 } 5156 5157 public Task addInput(TaskInputComponent t) { //3 5158 if (t == null) 5159 return this; 5160 if (this.input == null) 5161 this.input = new ArrayList<TaskInputComponent>(); 5162 this.input.add(t); 5163 return this; 5164 } 5165 5166 /** 5167 * @return The first repetition of repeating field {@link #input}, creating it if it does not already exist {3} 5168 */ 5169 public TaskInputComponent getInputFirstRep() { 5170 if (getInput().isEmpty()) { 5171 addInput(); 5172 } 5173 return getInput().get(0); 5174 } 5175 5176 /** 5177 * @return {@link #output} (Outputs produced by the Task.) 5178 */ 5179 public List<TaskOutputComponent> getOutput() { 5180 if (this.output == null) 5181 this.output = new ArrayList<TaskOutputComponent>(); 5182 return this.output; 5183 } 5184 5185 /** 5186 * @return Returns a reference to <code>this</code> for easy method chaining 5187 */ 5188 public Task setOutput(List<TaskOutputComponent> theOutput) { 5189 this.output = theOutput; 5190 return this; 5191 } 5192 5193 public boolean hasOutput() { 5194 if (this.output == null) 5195 return false; 5196 for (TaskOutputComponent item : this.output) 5197 if (!item.isEmpty()) 5198 return true; 5199 return false; 5200 } 5201 5202 public TaskOutputComponent addOutput() { //3 5203 TaskOutputComponent t = new TaskOutputComponent(); 5204 if (this.output == null) 5205 this.output = new ArrayList<TaskOutputComponent>(); 5206 this.output.add(t); 5207 return t; 5208 } 5209 5210 public Task addOutput(TaskOutputComponent t) { //3 5211 if (t == null) 5212 return this; 5213 if (this.output == null) 5214 this.output = new ArrayList<TaskOutputComponent>(); 5215 this.output.add(t); 5216 return this; 5217 } 5218 5219 /** 5220 * @return The first repetition of repeating field {@link #output}, creating it if it does not already exist {3} 5221 */ 5222 public TaskOutputComponent getOutputFirstRep() { 5223 if (getOutput().isEmpty()) { 5224 addOutput(); 5225 } 5226 return getOutput().get(0); 5227 } 5228 5229 protected void listChildren(List<Property> children) { 5230 super.listChildren(children); 5231 children.add(new Property("identifier", "Identifier", "The business identifier for this task.", 0, java.lang.Integer.MAX_VALUE, identifier)); 5232 children.add(new Property("instantiatesCanonical", "canonical(ActivityDefinition)", "The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task.", 0, 1, instantiatesCanonical)); 5233 children.add(new Property("instantiatesUri", "uri", "The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task.", 0, 1, instantiatesUri)); 5234 children.add(new Property("basedOn", "Reference(Any)", "BasedOn refers to a higher-level authorization that triggered the creation of the task. It references a \"request\" resource such as a ServiceRequest, MedicationRequest, CarePlan, etc. which is distinct from the \"request\" resource the task is seeking to fulfill. This latter resource is referenced by focus. For example, based on a CarePlan (= basedOn), a task is created to fulfill a ServiceRequest ( = focus ) to collect a specimen from a patient.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 5235 children.add(new Property("groupIdentifier", "Identifier", "A shared identifier common to multiple independent Task and Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.", 0, 1, groupIdentifier)); 5236 children.add(new Property("partOf", "Reference(Task)", "Task that this particular task is part of.", 0, java.lang.Integer.MAX_VALUE, partOf)); 5237 children.add(new Property("status", "code", "The current status of the task.", 0, 1, status)); 5238 children.add(new Property("statusReason", "CodeableReference", "An explanation as to why this task is held, failed, was refused, etc.", 0, 1, statusReason)); 5239 children.add(new Property("businessStatus", "CodeableConcept", "Contains business-specific nuances of the business state.", 0, 1, businessStatus)); 5240 children.add(new Property("intent", "code", "Indicates the \"level\" of actionability associated with the Task, i.e. i+R[9]Cs this a proposed task, a planned task, an actionable task, etc.", 0, 1, intent)); 5241 children.add(new Property("priority", "code", "Indicates how quickly the Task should be addressed with respect to other requests.", 0, 1, priority)); 5242 children.add(new Property("doNotPerform", "boolean", "If true indicates that the Task is asking for the specified action to *not* occur.", 0, 1, doNotPerform)); 5243 children.add(new Property("code", "CodeableConcept", "A name or code (or both) briefly describing what the task involves.", 0, 1, code)); 5244 children.add(new Property("description", "string", "A free-text description of what is to be performed.", 0, 1, description)); 5245 children.add(new Property("focus", "Reference(Any)", "The request being fulfilled or the resource being manipulated (changed, suspended, etc.) by this task.", 0, 1, focus)); 5246 children.add(new Property("for", "Reference(Any)", "The entity who benefits from the performance of the service specified in the task (e.g., the patient).", 0, 1, for_)); 5247 children.add(new Property("encounter", "Reference(Encounter)", "The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created.", 0, 1, encounter)); 5248 children.add(new Property("requestedPeriod", "Period", "Indicates the start and/or end of the period of time when completion of the task is desired to take place.", 0, 1, requestedPeriod)); 5249 children.add(new Property("executionPeriod", "Period", "Identifies the time action was first taken against the task (start) and/or the time final action was taken against the task prior to marking it as completed (end).", 0, 1, executionPeriod)); 5250 children.add(new Property("authoredOn", "dateTime", "The date and time this task was created.", 0, 1, authoredOn)); 5251 children.add(new Property("lastModified", "dateTime", "The date and time of last modification to this task.", 0, 1, lastModified)); 5252 children.add(new Property("requester", "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "The creator of the task.", 0, 1, requester)); 5253 children.add(new Property("requestedPerformer", "CodeableReference(Practitioner|PractitionerRole|Organization|CareTeam|HealthcareService|Patient|Device|RelatedPerson)", "The kind of participant or specific participant that should perform the task.", 0, java.lang.Integer.MAX_VALUE, requestedPerformer)); 5254 children.add(new Property("owner", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|RelatedPerson)", "Party responsible for managing task execution.", 0, 1, owner)); 5255 children.add(new Property("performer", "", "The entity who performed the requested task.", 0, java.lang.Integer.MAX_VALUE, performer)); 5256 children.add(new Property("location", "Reference(Location)", "Principal physical location where this task is performed.", 0, 1, location)); 5257 children.add(new Property("reason", "CodeableReference", "A description, code, or reference indicating why this task needs to be performed.", 0, java.lang.Integer.MAX_VALUE, reason)); 5258 children.add(new Property("insurance", "Reference(Coverage|ClaimResponse)", "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be relevant to the Task.", 0, java.lang.Integer.MAX_VALUE, insurance)); 5259 children.add(new Property("note", "Annotation", "Free-text information captured about the task as it progresses.", 0, java.lang.Integer.MAX_VALUE, note)); 5260 children.add(new Property("relevantHistory", "Reference(Provenance)", "Links to Provenance records for past versions of this Task that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the task.", 0, java.lang.Integer.MAX_VALUE, relevantHistory)); 5261 children.add(new Property("restriction", "", "If the Task.focus is a request resource and the task is seeking fulfillment (i.e. is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned.", 0, 1, restriction)); 5262 children.add(new Property("input", "", "Additional information that may be needed in the execution of the task.", 0, java.lang.Integer.MAX_VALUE, input)); 5263 children.add(new Property("output", "", "Outputs produced by the Task.", 0, java.lang.Integer.MAX_VALUE, output)); 5264 } 5265 5266 @Override 5267 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5268 switch (_hash) { 5269 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The business identifier for this task.", 0, java.lang.Integer.MAX_VALUE, identifier); 5270 case 8911915: /*instantiatesCanonical*/ return new Property("instantiatesCanonical", "canonical(ActivityDefinition)", "The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task.", 0, 1, instantiatesCanonical); 5271 case -1926393373: /*instantiatesUri*/ return new Property("instantiatesUri", "uri", "The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task.", 0, 1, instantiatesUri); 5272 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(Any)", "BasedOn refers to a higher-level authorization that triggered the creation of the task. It references a \"request\" resource such as a ServiceRequest, MedicationRequest, CarePlan, etc. which is distinct from the \"request\" resource the task is seeking to fulfill. This latter resource is referenced by focus. For example, based on a CarePlan (= basedOn), a task is created to fulfill a ServiceRequest ( = focus ) to collect a specimen from a patient.", 0, java.lang.Integer.MAX_VALUE, basedOn); 5273 case -445338488: /*groupIdentifier*/ return new Property("groupIdentifier", "Identifier", "A shared identifier common to multiple independent Task and Request instances that were activated/authorized more or less simultaneously by a single author. The presence of the same identifier on each request ties those requests together and may have business ramifications in terms of reporting of results, billing, etc. E.g. a requisition number shared by a set of lab tests ordered together, or a prescription number shared by all meds ordered at one time.", 0, 1, groupIdentifier); 5274 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Task)", "Task that this particular task is part of.", 0, java.lang.Integer.MAX_VALUE, partOf); 5275 case -892481550: /*status*/ return new Property("status", "code", "The current status of the task.", 0, 1, status); 5276 case 2051346646: /*statusReason*/ return new Property("statusReason", "CodeableReference", "An explanation as to why this task is held, failed, was refused, etc.", 0, 1, statusReason); 5277 case 2008591314: /*businessStatus*/ return new Property("businessStatus", "CodeableConcept", "Contains business-specific nuances of the business state.", 0, 1, businessStatus); 5278 case -1183762788: /*intent*/ return new Property("intent", "code", "Indicates the \"level\" of actionability associated with the Task, i.e. i+R[9]Cs this a proposed task, a planned task, an actionable task, etc.", 0, 1, intent); 5279 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly the Task should be addressed with respect to other requests.", 0, 1, priority); 5280 case -1788508167: /*doNotPerform*/ return new Property("doNotPerform", "boolean", "If true indicates that the Task is asking for the specified action to *not* occur.", 0, 1, doNotPerform); 5281 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A name or code (or both) briefly describing what the task involves.", 0, 1, code); 5282 case -1724546052: /*description*/ return new Property("description", "string", "A free-text description of what is to be performed.", 0, 1, description); 5283 case 97604824: /*focus*/ return new Property("focus", "Reference(Any)", "The request being fulfilled or the resource being manipulated (changed, suspended, etc.) by this task.", 0, 1, focus); 5284 case 101577: /*for*/ return new Property("for", "Reference(Any)", "The entity who benefits from the performance of the service specified in the task (e.g., the patient).", 0, 1, for_); 5285 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created.", 0, 1, encounter); 5286 case -897241393: /*requestedPeriod*/ return new Property("requestedPeriod", "Period", "Indicates the start and/or end of the period of time when completion of the task is desired to take place.", 0, 1, requestedPeriod); 5287 case 1218624249: /*executionPeriod*/ return new Property("executionPeriod", "Period", "Identifies the time action was first taken against the task (start) and/or the time final action was taken against the task prior to marking it as completed (end).", 0, 1, executionPeriod); 5288 case -1500852503: /*authoredOn*/ return new Property("authoredOn", "dateTime", "The date and time this task was created.", 0, 1, authoredOn); 5289 case 1959003007: /*lastModified*/ return new Property("lastModified", "dateTime", "The date and time of last modification to this task.", 0, 1, lastModified); 5290 case 693933948: /*requester*/ return new Property("requester", "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "The creator of the task.", 0, 1, requester); 5291 case 2072749056: /*requestedPerformer*/ return new Property("requestedPerformer", "CodeableReference(Practitioner|PractitionerRole|Organization|CareTeam|HealthcareService|Patient|Device|RelatedPerson)", "The kind of participant or specific participant that should perform the task.", 0, java.lang.Integer.MAX_VALUE, requestedPerformer); 5292 case 106164915: /*owner*/ return new Property("owner", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|RelatedPerson)", "Party responsible for managing task execution.", 0, 1, owner); 5293 case 481140686: /*performer*/ return new Property("performer", "", "The entity who performed the requested task.", 0, java.lang.Integer.MAX_VALUE, performer); 5294 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "Principal physical location where this task is performed.", 0, 1, location); 5295 case -934964668: /*reason*/ return new Property("reason", "CodeableReference", "A description, code, or reference indicating why this task needs to be performed.", 0, java.lang.Integer.MAX_VALUE, reason); 5296 case 73049818: /*insurance*/ return new Property("insurance", "Reference(Coverage|ClaimResponse)", "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be relevant to the Task.", 0, java.lang.Integer.MAX_VALUE, insurance); 5297 case 3387378: /*note*/ return new Property("note", "Annotation", "Free-text information captured about the task as it progresses.", 0, java.lang.Integer.MAX_VALUE, note); 5298 case 1538891575: /*relevantHistory*/ return new Property("relevantHistory", "Reference(Provenance)", "Links to Provenance records for past versions of this Task that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the task.", 0, java.lang.Integer.MAX_VALUE, relevantHistory); 5299 case -1561062452: /*restriction*/ return new Property("restriction", "", "If the Task.focus is a request resource and the task is seeking fulfillment (i.e. is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned.", 0, 1, restriction); 5300 case 100358090: /*input*/ return new Property("input", "", "Additional information that may be needed in the execution of the task.", 0, java.lang.Integer.MAX_VALUE, input); 5301 case -1005512447: /*output*/ return new Property("output", "", "Outputs produced by the Task.", 0, java.lang.Integer.MAX_VALUE, output); 5302 default: return super.getNamedProperty(_hash, _name, _checkValid); 5303 } 5304 5305 } 5306 5307 @Override 5308 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5309 switch (hash) { 5310 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 5311 case 8911915: /*instantiatesCanonical*/ return this.instantiatesCanonical == null ? new Base[0] : new Base[] {this.instantiatesCanonical}; // CanonicalType 5312 case -1926393373: /*instantiatesUri*/ return this.instantiatesUri == null ? new Base[0] : new Base[] {this.instantiatesUri}; // UriType 5313 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 5314 case -445338488: /*groupIdentifier*/ return this.groupIdentifier == null ? new Base[0] : new Base[] {this.groupIdentifier}; // Identifier 5315 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 5316 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<TaskStatus> 5317 case 2051346646: /*statusReason*/ return this.statusReason == null ? new Base[0] : new Base[] {this.statusReason}; // CodeableReference 5318 case 2008591314: /*businessStatus*/ return this.businessStatus == null ? new Base[0] : new Base[] {this.businessStatus}; // CodeableConcept 5319 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // Enumeration<TaskIntent> 5320 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<RequestPriority> 5321 case -1788508167: /*doNotPerform*/ return this.doNotPerform == null ? new Base[0] : new Base[] {this.doNotPerform}; // BooleanType 5322 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 5323 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 5324 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : new Base[] {this.focus}; // Reference 5325 case 101577: /*for*/ return this.for_ == null ? new Base[0] : new Base[] {this.for_}; // Reference 5326 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 5327 case -897241393: /*requestedPeriod*/ return this.requestedPeriod == null ? new Base[0] : new Base[] {this.requestedPeriod}; // Period 5328 case 1218624249: /*executionPeriod*/ return this.executionPeriod == null ? new Base[0] : new Base[] {this.executionPeriod}; // Period 5329 case -1500852503: /*authoredOn*/ return this.authoredOn == null ? new Base[0] : new Base[] {this.authoredOn}; // DateTimeType 5330 case 1959003007: /*lastModified*/ return this.lastModified == null ? new Base[0] : new Base[] {this.lastModified}; // DateTimeType 5331 case 693933948: /*requester*/ return this.requester == null ? new Base[0] : new Base[] {this.requester}; // Reference 5332 case 2072749056: /*requestedPerformer*/ return this.requestedPerformer == null ? new Base[0] : this.requestedPerformer.toArray(new Base[this.requestedPerformer.size()]); // CodeableReference 5333 case 106164915: /*owner*/ return this.owner == null ? new Base[0] : new Base[] {this.owner}; // Reference 5334 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // TaskPerformerComponent 5335 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 5336 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 5337 case 73049818: /*insurance*/ return this.insurance == null ? new Base[0] : this.insurance.toArray(new Base[this.insurance.size()]); // Reference 5338 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 5339 case 1538891575: /*relevantHistory*/ return this.relevantHistory == null ? new Base[0] : this.relevantHistory.toArray(new Base[this.relevantHistory.size()]); // Reference 5340 case -1561062452: /*restriction*/ return this.restriction == null ? new Base[0] : new Base[] {this.restriction}; // TaskRestrictionComponent 5341 case 100358090: /*input*/ return this.input == null ? new Base[0] : this.input.toArray(new Base[this.input.size()]); // TaskInputComponent 5342 case -1005512447: /*output*/ return this.output == null ? new Base[0] : this.output.toArray(new Base[this.output.size()]); // TaskOutputComponent 5343 default: return super.getProperty(hash, name, checkValid); 5344 } 5345 5346 } 5347 5348 @Override 5349 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5350 switch (hash) { 5351 case -1618432855: // identifier 5352 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 5353 return value; 5354 case 8911915: // instantiatesCanonical 5355 this.instantiatesCanonical = TypeConvertor.castToCanonical(value); // CanonicalType 5356 return value; 5357 case -1926393373: // instantiatesUri 5358 this.instantiatesUri = TypeConvertor.castToUri(value); // UriType 5359 return value; 5360 case -332612366: // basedOn 5361 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 5362 return value; 5363 case -445338488: // groupIdentifier 5364 this.groupIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 5365 return value; 5366 case -995410646: // partOf 5367 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 5368 return value; 5369 case -892481550: // status 5370 value = new TaskStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5371 this.status = (Enumeration) value; // Enumeration<TaskStatus> 5372 return value; 5373 case 2051346646: // statusReason 5374 this.statusReason = TypeConvertor.castToCodeableReference(value); // CodeableReference 5375 return value; 5376 case 2008591314: // businessStatus 5377 this.businessStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5378 return value; 5379 case -1183762788: // intent 5380 value = new TaskIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 5381 this.intent = (Enumeration) value; // Enumeration<TaskIntent> 5382 return value; 5383 case -1165461084: // priority 5384 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 5385 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 5386 return value; 5387 case -1788508167: // doNotPerform 5388 this.doNotPerform = TypeConvertor.castToBoolean(value); // BooleanType 5389 return value; 5390 case 3059181: // code 5391 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5392 return value; 5393 case -1724546052: // description 5394 this.description = TypeConvertor.castToString(value); // StringType 5395 return value; 5396 case 97604824: // focus 5397 this.focus = TypeConvertor.castToReference(value); // Reference 5398 return value; 5399 case 101577: // for 5400 this.for_ = TypeConvertor.castToReference(value); // Reference 5401 return value; 5402 case 1524132147: // encounter 5403 this.encounter = TypeConvertor.castToReference(value); // Reference 5404 return value; 5405 case -897241393: // requestedPeriod 5406 this.requestedPeriod = TypeConvertor.castToPeriod(value); // Period 5407 return value; 5408 case 1218624249: // executionPeriod 5409 this.executionPeriod = TypeConvertor.castToPeriod(value); // Period 5410 return value; 5411 case -1500852503: // authoredOn 5412 this.authoredOn = TypeConvertor.castToDateTime(value); // DateTimeType 5413 return value; 5414 case 1959003007: // lastModified 5415 this.lastModified = TypeConvertor.castToDateTime(value); // DateTimeType 5416 return value; 5417 case 693933948: // requester 5418 this.requester = TypeConvertor.castToReference(value); // Reference 5419 return value; 5420 case 2072749056: // requestedPerformer 5421 this.getRequestedPerformer().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 5422 return value; 5423 case 106164915: // owner 5424 this.owner = TypeConvertor.castToReference(value); // Reference 5425 return value; 5426 case 481140686: // performer 5427 this.getPerformer().add((TaskPerformerComponent) value); // TaskPerformerComponent 5428 return value; 5429 case 1901043637: // location 5430 this.location = TypeConvertor.castToReference(value); // Reference 5431 return value; 5432 case -934964668: // reason 5433 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 5434 return value; 5435 case 73049818: // insurance 5436 this.getInsurance().add(TypeConvertor.castToReference(value)); // Reference 5437 return value; 5438 case 3387378: // note 5439 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 5440 return value; 5441 case 1538891575: // relevantHistory 5442 this.getRelevantHistory().add(TypeConvertor.castToReference(value)); // Reference 5443 return value; 5444 case -1561062452: // restriction 5445 this.restriction = (TaskRestrictionComponent) value; // TaskRestrictionComponent 5446 return value; 5447 case 100358090: // input 5448 this.getInput().add((TaskInputComponent) value); // TaskInputComponent 5449 return value; 5450 case -1005512447: // output 5451 this.getOutput().add((TaskOutputComponent) value); // TaskOutputComponent 5452 return value; 5453 default: return super.setProperty(hash, name, value); 5454 } 5455 5456 } 5457 5458 @Override 5459 public Base setProperty(String name, Base value) throws FHIRException { 5460 if (name.equals("identifier")) { 5461 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 5462 } else if (name.equals("instantiatesCanonical")) { 5463 this.instantiatesCanonical = TypeConvertor.castToCanonical(value); // CanonicalType 5464 } else if (name.equals("instantiatesUri")) { 5465 this.instantiatesUri = TypeConvertor.castToUri(value); // UriType 5466 } else if (name.equals("basedOn")) { 5467 this.getBasedOn().add(TypeConvertor.castToReference(value)); 5468 } else if (name.equals("groupIdentifier")) { 5469 this.groupIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 5470 } else if (name.equals("partOf")) { 5471 this.getPartOf().add(TypeConvertor.castToReference(value)); 5472 } else if (name.equals("status")) { 5473 value = new TaskStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5474 this.status = (Enumeration) value; // Enumeration<TaskStatus> 5475 } else if (name.equals("statusReason")) { 5476 this.statusReason = TypeConvertor.castToCodeableReference(value); // CodeableReference 5477 } else if (name.equals("businessStatus")) { 5478 this.businessStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5479 } else if (name.equals("intent")) { 5480 value = new TaskIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 5481 this.intent = (Enumeration) value; // Enumeration<TaskIntent> 5482 } else if (name.equals("priority")) { 5483 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 5484 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 5485 } else if (name.equals("doNotPerform")) { 5486 this.doNotPerform = TypeConvertor.castToBoolean(value); // BooleanType 5487 } else if (name.equals("code")) { 5488 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5489 } else if (name.equals("description")) { 5490 this.description = TypeConvertor.castToString(value); // StringType 5491 } else if (name.equals("focus")) { 5492 this.focus = TypeConvertor.castToReference(value); // Reference 5493 } else if (name.equals("for")) { 5494 this.for_ = TypeConvertor.castToReference(value); // Reference 5495 } else if (name.equals("encounter")) { 5496 this.encounter = TypeConvertor.castToReference(value); // Reference 5497 } else if (name.equals("requestedPeriod")) { 5498 this.requestedPeriod = TypeConvertor.castToPeriod(value); // Period 5499 } else if (name.equals("executionPeriod")) { 5500 this.executionPeriod = TypeConvertor.castToPeriod(value); // Period 5501 } else if (name.equals("authoredOn")) { 5502 this.authoredOn = TypeConvertor.castToDateTime(value); // DateTimeType 5503 } else if (name.equals("lastModified")) { 5504 this.lastModified = TypeConvertor.castToDateTime(value); // DateTimeType 5505 } else if (name.equals("requester")) { 5506 this.requester = TypeConvertor.castToReference(value); // Reference 5507 } else if (name.equals("requestedPerformer")) { 5508 this.getRequestedPerformer().add(TypeConvertor.castToCodeableReference(value)); 5509 } else if (name.equals("owner")) { 5510 this.owner = TypeConvertor.castToReference(value); // Reference 5511 } else if (name.equals("performer")) { 5512 this.getPerformer().add((TaskPerformerComponent) value); 5513 } else if (name.equals("location")) { 5514 this.location = TypeConvertor.castToReference(value); // Reference 5515 } else if (name.equals("reason")) { 5516 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 5517 } else if (name.equals("insurance")) { 5518 this.getInsurance().add(TypeConvertor.castToReference(value)); 5519 } else if (name.equals("note")) { 5520 this.getNote().add(TypeConvertor.castToAnnotation(value)); 5521 } else if (name.equals("relevantHistory")) { 5522 this.getRelevantHistory().add(TypeConvertor.castToReference(value)); 5523 } else if (name.equals("restriction")) { 5524 this.restriction = (TaskRestrictionComponent) value; // TaskRestrictionComponent 5525 } else if (name.equals("input")) { 5526 this.getInput().add((TaskInputComponent) value); 5527 } else if (name.equals("output")) { 5528 this.getOutput().add((TaskOutputComponent) value); 5529 } else 5530 return super.setProperty(name, value); 5531 return value; 5532 } 5533 5534 @Override 5535 public void removeChild(String name, Base value) throws FHIRException { 5536 if (name.equals("identifier")) { 5537 this.getIdentifier().remove(value); 5538 } else if (name.equals("instantiatesCanonical")) { 5539 this.instantiatesCanonical = null; 5540 } else if (name.equals("instantiatesUri")) { 5541 this.instantiatesUri = null; 5542 } else if (name.equals("basedOn")) { 5543 this.getBasedOn().remove(value); 5544 } else if (name.equals("groupIdentifier")) { 5545 this.groupIdentifier = null; 5546 } else if (name.equals("partOf")) { 5547 this.getPartOf().remove(value); 5548 } else if (name.equals("status")) { 5549 value = new TaskStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5550 this.status = (Enumeration) value; // Enumeration<TaskStatus> 5551 } else if (name.equals("statusReason")) { 5552 this.statusReason = null; 5553 } else if (name.equals("businessStatus")) { 5554 this.businessStatus = null; 5555 } else if (name.equals("intent")) { 5556 value = new TaskIntentEnumFactory().fromType(TypeConvertor.castToCode(value)); 5557 this.intent = (Enumeration) value; // Enumeration<TaskIntent> 5558 } else if (name.equals("priority")) { 5559 value = new RequestPriorityEnumFactory().fromType(TypeConvertor.castToCode(value)); 5560 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 5561 } else if (name.equals("doNotPerform")) { 5562 this.doNotPerform = null; 5563 } else if (name.equals("code")) { 5564 this.code = null; 5565 } else if (name.equals("description")) { 5566 this.description = null; 5567 } else if (name.equals("focus")) { 5568 this.focus = null; 5569 } else if (name.equals("for")) { 5570 this.for_ = null; 5571 } else if (name.equals("encounter")) { 5572 this.encounter = null; 5573 } else if (name.equals("requestedPeriod")) { 5574 this.requestedPeriod = null; 5575 } else if (name.equals("executionPeriod")) { 5576 this.executionPeriod = null; 5577 } else if (name.equals("authoredOn")) { 5578 this.authoredOn = null; 5579 } else if (name.equals("lastModified")) { 5580 this.lastModified = null; 5581 } else if (name.equals("requester")) { 5582 this.requester = null; 5583 } else if (name.equals("requestedPerformer")) { 5584 this.getRequestedPerformer().remove(value); 5585 } else if (name.equals("owner")) { 5586 this.owner = null; 5587 } else if (name.equals("performer")) { 5588 this.getPerformer().remove((TaskPerformerComponent) value); 5589 } else if (name.equals("location")) { 5590 this.location = null; 5591 } else if (name.equals("reason")) { 5592 this.getReason().remove(value); 5593 } else if (name.equals("insurance")) { 5594 this.getInsurance().remove(value); 5595 } else if (name.equals("note")) { 5596 this.getNote().remove(value); 5597 } else if (name.equals("relevantHistory")) { 5598 this.getRelevantHistory().remove(value); 5599 } else if (name.equals("restriction")) { 5600 this.restriction = (TaskRestrictionComponent) value; // TaskRestrictionComponent 5601 } else if (name.equals("input")) { 5602 this.getInput().remove((TaskInputComponent) value); 5603 } else if (name.equals("output")) { 5604 this.getOutput().remove((TaskOutputComponent) value); 5605 } else 5606 super.removeChild(name, value); 5607 5608 } 5609 5610 @Override 5611 public Base makeProperty(int hash, String name) throws FHIRException { 5612 switch (hash) { 5613 case -1618432855: return addIdentifier(); 5614 case 8911915: return getInstantiatesCanonicalElement(); 5615 case -1926393373: return getInstantiatesUriElement(); 5616 case -332612366: return addBasedOn(); 5617 case -445338488: return getGroupIdentifier(); 5618 case -995410646: return addPartOf(); 5619 case -892481550: return getStatusElement(); 5620 case 2051346646: return getStatusReason(); 5621 case 2008591314: return getBusinessStatus(); 5622 case -1183762788: return getIntentElement(); 5623 case -1165461084: return getPriorityElement(); 5624 case -1788508167: return getDoNotPerformElement(); 5625 case 3059181: return getCode(); 5626 case -1724546052: return getDescriptionElement(); 5627 case 97604824: return getFocus(); 5628 case 101577: return getFor(); 5629 case 1524132147: return getEncounter(); 5630 case -897241393: return getRequestedPeriod(); 5631 case 1218624249: return getExecutionPeriod(); 5632 case -1500852503: return getAuthoredOnElement(); 5633 case 1959003007: return getLastModifiedElement(); 5634 case 693933948: return getRequester(); 5635 case 2072749056: return addRequestedPerformer(); 5636 case 106164915: return getOwner(); 5637 case 481140686: return addPerformer(); 5638 case 1901043637: return getLocation(); 5639 case -934964668: return addReason(); 5640 case 73049818: return addInsurance(); 5641 case 3387378: return addNote(); 5642 case 1538891575: return addRelevantHistory(); 5643 case -1561062452: return getRestriction(); 5644 case 100358090: return addInput(); 5645 case -1005512447: return addOutput(); 5646 default: return super.makeProperty(hash, name); 5647 } 5648 5649 } 5650 5651 @Override 5652 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5653 switch (hash) { 5654 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 5655 case 8911915: /*instantiatesCanonical*/ return new String[] {"canonical"}; 5656 case -1926393373: /*instantiatesUri*/ return new String[] {"uri"}; 5657 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 5658 case -445338488: /*groupIdentifier*/ return new String[] {"Identifier"}; 5659 case -995410646: /*partOf*/ return new String[] {"Reference"}; 5660 case -892481550: /*status*/ return new String[] {"code"}; 5661 case 2051346646: /*statusReason*/ return new String[] {"CodeableReference"}; 5662 case 2008591314: /*businessStatus*/ return new String[] {"CodeableConcept"}; 5663 case -1183762788: /*intent*/ return new String[] {"code"}; 5664 case -1165461084: /*priority*/ return new String[] {"code"}; 5665 case -1788508167: /*doNotPerform*/ return new String[] {"boolean"}; 5666 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 5667 case -1724546052: /*description*/ return new String[] {"string"}; 5668 case 97604824: /*focus*/ return new String[] {"Reference"}; 5669 case 101577: /*for*/ return new String[] {"Reference"}; 5670 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 5671 case -897241393: /*requestedPeriod*/ return new String[] {"Period"}; 5672 case 1218624249: /*executionPeriod*/ return new String[] {"Period"}; 5673 case -1500852503: /*authoredOn*/ return new String[] {"dateTime"}; 5674 case 1959003007: /*lastModified*/ return new String[] {"dateTime"}; 5675 case 693933948: /*requester*/ return new String[] {"Reference"}; 5676 case 2072749056: /*requestedPerformer*/ return new String[] {"CodeableReference"}; 5677 case 106164915: /*owner*/ return new String[] {"Reference"}; 5678 case 481140686: /*performer*/ return new String[] {}; 5679 case 1901043637: /*location*/ return new String[] {"Reference"}; 5680 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 5681 case 73049818: /*insurance*/ return new String[] {"Reference"}; 5682 case 3387378: /*note*/ return new String[] {"Annotation"}; 5683 case 1538891575: /*relevantHistory*/ return new String[] {"Reference"}; 5684 case -1561062452: /*restriction*/ return new String[] {}; 5685 case 100358090: /*input*/ return new String[] {}; 5686 case -1005512447: /*output*/ return new String[] {}; 5687 default: return super.getTypesForProperty(hash, name); 5688 } 5689 5690 } 5691 5692 @Override 5693 public Base addChild(String name) throws FHIRException { 5694 if (name.equals("identifier")) { 5695 return addIdentifier(); 5696 } 5697 else if (name.equals("instantiatesCanonical")) { 5698 throw new FHIRException("Cannot call addChild on a singleton property Task.instantiatesCanonical"); 5699 } 5700 else if (name.equals("instantiatesUri")) { 5701 throw new FHIRException("Cannot call addChild on a singleton property Task.instantiatesUri"); 5702 } 5703 else if (name.equals("basedOn")) { 5704 return addBasedOn(); 5705 } 5706 else if (name.equals("groupIdentifier")) { 5707 this.groupIdentifier = new Identifier(); 5708 return this.groupIdentifier; 5709 } 5710 else if (name.equals("partOf")) { 5711 return addPartOf(); 5712 } 5713 else if (name.equals("status")) { 5714 throw new FHIRException("Cannot call addChild on a singleton property Task.status"); 5715 } 5716 else if (name.equals("statusReason")) { 5717 this.statusReason = new CodeableReference(); 5718 return this.statusReason; 5719 } 5720 else if (name.equals("businessStatus")) { 5721 this.businessStatus = new CodeableConcept(); 5722 return this.businessStatus; 5723 } 5724 else if (name.equals("intent")) { 5725 throw new FHIRException("Cannot call addChild on a singleton property Task.intent"); 5726 } 5727 else if (name.equals("priority")) { 5728 throw new FHIRException("Cannot call addChild on a singleton property Task.priority"); 5729 } 5730 else if (name.equals("doNotPerform")) { 5731 throw new FHIRException("Cannot call addChild on a singleton property Task.doNotPerform"); 5732 } 5733 else if (name.equals("code")) { 5734 this.code = new CodeableConcept(); 5735 return this.code; 5736 } 5737 else if (name.equals("description")) { 5738 throw new FHIRException("Cannot call addChild on a singleton property Task.description"); 5739 } 5740 else if (name.equals("focus")) { 5741 this.focus = new Reference(); 5742 return this.focus; 5743 } 5744 else if (name.equals("for")) { 5745 this.for_ = new Reference(); 5746 return this.for_; 5747 } 5748 else if (name.equals("encounter")) { 5749 this.encounter = new Reference(); 5750 return this.encounter; 5751 } 5752 else if (name.equals("requestedPeriod")) { 5753 this.requestedPeriod = new Period(); 5754 return this.requestedPeriod; 5755 } 5756 else if (name.equals("executionPeriod")) { 5757 this.executionPeriod = new Period(); 5758 return this.executionPeriod; 5759 } 5760 else if (name.equals("authoredOn")) { 5761 throw new FHIRException("Cannot call addChild on a singleton property Task.authoredOn"); 5762 } 5763 else if (name.equals("lastModified")) { 5764 throw new FHIRException("Cannot call addChild on a singleton property Task.lastModified"); 5765 } 5766 else if (name.equals("requester")) { 5767 this.requester = new Reference(); 5768 return this.requester; 5769 } 5770 else if (name.equals("requestedPerformer")) { 5771 return addRequestedPerformer(); 5772 } 5773 else if (name.equals("owner")) { 5774 this.owner = new Reference(); 5775 return this.owner; 5776 } 5777 else if (name.equals("performer")) { 5778 return addPerformer(); 5779 } 5780 else if (name.equals("location")) { 5781 this.location = new Reference(); 5782 return this.location; 5783 } 5784 else if (name.equals("reason")) { 5785 return addReason(); 5786 } 5787 else if (name.equals("insurance")) { 5788 return addInsurance(); 5789 } 5790 else if (name.equals("note")) { 5791 return addNote(); 5792 } 5793 else if (name.equals("relevantHistory")) { 5794 return addRelevantHistory(); 5795 } 5796 else if (name.equals("restriction")) { 5797 this.restriction = new TaskRestrictionComponent(); 5798 return this.restriction; 5799 } 5800 else if (name.equals("input")) { 5801 return addInput(); 5802 } 5803 else if (name.equals("output")) { 5804 return addOutput(); 5805 } 5806 else 5807 return super.addChild(name); 5808 } 5809 5810 public String fhirType() { 5811 return "Task"; 5812 5813 } 5814 5815 public Task copy() { 5816 Task dst = new Task(); 5817 copyValues(dst); 5818 return dst; 5819 } 5820 5821 public void copyValues(Task dst) { 5822 super.copyValues(dst); 5823 if (identifier != null) { 5824 dst.identifier = new ArrayList<Identifier>(); 5825 for (Identifier i : identifier) 5826 dst.identifier.add(i.copy()); 5827 }; 5828 dst.instantiatesCanonical = instantiatesCanonical == null ? null : instantiatesCanonical.copy(); 5829 dst.instantiatesUri = instantiatesUri == null ? null : instantiatesUri.copy(); 5830 if (basedOn != null) { 5831 dst.basedOn = new ArrayList<Reference>(); 5832 for (Reference i : basedOn) 5833 dst.basedOn.add(i.copy()); 5834 }; 5835 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 5836 if (partOf != null) { 5837 dst.partOf = new ArrayList<Reference>(); 5838 for (Reference i : partOf) 5839 dst.partOf.add(i.copy()); 5840 }; 5841 dst.status = status == null ? null : status.copy(); 5842 dst.statusReason = statusReason == null ? null : statusReason.copy(); 5843 dst.businessStatus = businessStatus == null ? null : businessStatus.copy(); 5844 dst.intent = intent == null ? null : intent.copy(); 5845 dst.priority = priority == null ? null : priority.copy(); 5846 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 5847 dst.code = code == null ? null : code.copy(); 5848 dst.description = description == null ? null : description.copy(); 5849 dst.focus = focus == null ? null : focus.copy(); 5850 dst.for_ = for_ == null ? null : for_.copy(); 5851 dst.encounter = encounter == null ? null : encounter.copy(); 5852 dst.requestedPeriod = requestedPeriod == null ? null : requestedPeriod.copy(); 5853 dst.executionPeriod = executionPeriod == null ? null : executionPeriod.copy(); 5854 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 5855 dst.lastModified = lastModified == null ? null : lastModified.copy(); 5856 dst.requester = requester == null ? null : requester.copy(); 5857 if (requestedPerformer != null) { 5858 dst.requestedPerformer = new ArrayList<CodeableReference>(); 5859 for (CodeableReference i : requestedPerformer) 5860 dst.requestedPerformer.add(i.copy()); 5861 }; 5862 dst.owner = owner == null ? null : owner.copy(); 5863 if (performer != null) { 5864 dst.performer = new ArrayList<TaskPerformerComponent>(); 5865 for (TaskPerformerComponent i : performer) 5866 dst.performer.add(i.copy()); 5867 }; 5868 dst.location = location == null ? null : location.copy(); 5869 if (reason != null) { 5870 dst.reason = new ArrayList<CodeableReference>(); 5871 for (CodeableReference i : reason) 5872 dst.reason.add(i.copy()); 5873 }; 5874 if (insurance != null) { 5875 dst.insurance = new ArrayList<Reference>(); 5876 for (Reference i : insurance) 5877 dst.insurance.add(i.copy()); 5878 }; 5879 if (note != null) { 5880 dst.note = new ArrayList<Annotation>(); 5881 for (Annotation i : note) 5882 dst.note.add(i.copy()); 5883 }; 5884 if (relevantHistory != null) { 5885 dst.relevantHistory = new ArrayList<Reference>(); 5886 for (Reference i : relevantHistory) 5887 dst.relevantHistory.add(i.copy()); 5888 }; 5889 dst.restriction = restriction == null ? null : restriction.copy(); 5890 if (input != null) { 5891 dst.input = new ArrayList<TaskInputComponent>(); 5892 for (TaskInputComponent i : input) 5893 dst.input.add(i.copy()); 5894 }; 5895 if (output != null) { 5896 dst.output = new ArrayList<TaskOutputComponent>(); 5897 for (TaskOutputComponent i : output) 5898 dst.output.add(i.copy()); 5899 }; 5900 } 5901 5902 protected Task typedCopy() { 5903 return copy(); 5904 } 5905 5906 @Override 5907 public boolean equalsDeep(Base other_) { 5908 if (!super.equalsDeep(other_)) 5909 return false; 5910 if (!(other_ instanceof Task)) 5911 return false; 5912 Task o = (Task) other_; 5913 return compareDeep(identifier, o.identifier, true) && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 5914 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(basedOn, o.basedOn, true) 5915 && compareDeep(groupIdentifier, o.groupIdentifier, true) && compareDeep(partOf, o.partOf, true) 5916 && compareDeep(status, o.status, true) && compareDeep(statusReason, o.statusReason, true) && compareDeep(businessStatus, o.businessStatus, true) 5917 && compareDeep(intent, o.intent, true) && compareDeep(priority, o.priority, true) && compareDeep(doNotPerform, o.doNotPerform, true) 5918 && compareDeep(code, o.code, true) && compareDeep(description, o.description, true) && compareDeep(focus, o.focus, true) 5919 && compareDeep(for_, o.for_, true) && compareDeep(encounter, o.encounter, true) && compareDeep(requestedPeriod, o.requestedPeriod, true) 5920 && compareDeep(executionPeriod, o.executionPeriod, true) && compareDeep(authoredOn, o.authoredOn, true) 5921 && compareDeep(lastModified, o.lastModified, true) && compareDeep(requester, o.requester, true) 5922 && compareDeep(requestedPerformer, o.requestedPerformer, true) && compareDeep(owner, o.owner, true) 5923 && compareDeep(performer, o.performer, true) && compareDeep(location, o.location, true) && compareDeep(reason, o.reason, true) 5924 && compareDeep(insurance, o.insurance, true) && compareDeep(note, o.note, true) && compareDeep(relevantHistory, o.relevantHistory, true) 5925 && compareDeep(restriction, o.restriction, true) && compareDeep(input, o.input, true) && compareDeep(output, o.output, true) 5926 ; 5927 } 5928 5929 @Override 5930 public boolean equalsShallow(Base other_) { 5931 if (!super.equalsShallow(other_)) 5932 return false; 5933 if (!(other_ instanceof Task)) 5934 return false; 5935 Task o = (Task) other_; 5936 return compareValues(instantiatesCanonical, o.instantiatesCanonical, true) && compareValues(instantiatesUri, o.instantiatesUri, true) 5937 && compareValues(status, o.status, true) && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) 5938 && compareValues(doNotPerform, o.doNotPerform, true) && compareValues(description, o.description, true) 5939 && compareValues(authoredOn, o.authoredOn, true) && compareValues(lastModified, o.lastModified, true) 5940 ; 5941 } 5942 5943 public boolean isEmpty() { 5944 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical 5945 , instantiatesUri, basedOn, groupIdentifier, partOf, status, statusReason, businessStatus 5946 , intent, priority, doNotPerform, code, description, focus, for_, encounter 5947 , requestedPeriod, executionPeriod, authoredOn, lastModified, requester, requestedPerformer 5948 , owner, performer, location, reason, insurance, note, relevantHistory, restriction 5949 , input, output); 5950 } 5951 5952 @Override 5953 public ResourceType getResourceType() { 5954 return ResourceType.Task; 5955 } 5956 5957 /** 5958 * Search parameter: <b>actor</b> 5959 * <p> 5960 * Description: <b>Search by specific performer.</b><br> 5961 * Type: <b>reference</b><br> 5962 * Path: <b>Task.performer.actor</b><br> 5963 * </p> 5964 */ 5965 @SearchParamDefinition(name="actor", path="Task.performer.actor", description="Search by specific performer.", type="reference", target={CareTeam.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 5966 public static final String SP_ACTOR = "actor"; 5967 /** 5968 * <b>Fluent Client</b> search parameter constant for <b>actor</b> 5969 * <p> 5970 * Description: <b>Search by specific performer.</b><br> 5971 * Type: <b>reference</b><br> 5972 * Path: <b>Task.performer.actor</b><br> 5973 * </p> 5974 */ 5975 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACTOR); 5976 5977/** 5978 * Constant for fluent queries to be used to add include statements. Specifies 5979 * the path value of "<b>Task:actor</b>". 5980 */ 5981 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTOR = new ca.uhn.fhir.model.api.Include("Task:actor").toLocked(); 5982 5983 /** 5984 * Search parameter: <b>authored-on</b> 5985 * <p> 5986 * Description: <b>Search by creation date</b><br> 5987 * Type: <b>date</b><br> 5988 * Path: <b>Task.authoredOn</b><br> 5989 * </p> 5990 */ 5991 @SearchParamDefinition(name="authored-on", path="Task.authoredOn", description="Search by creation date", type="date" ) 5992 public static final String SP_AUTHORED_ON = "authored-on"; 5993 /** 5994 * <b>Fluent Client</b> search parameter constant for <b>authored-on</b> 5995 * <p> 5996 * Description: <b>Search by creation date</b><br> 5997 * Type: <b>date</b><br> 5998 * Path: <b>Task.authoredOn</b><br> 5999 * </p> 6000 */ 6001 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED_ON = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHORED_ON); 6002 6003 /** 6004 * Search parameter: <b>based-on</b> 6005 * <p> 6006 * Description: <b>Search by requests this task is based on</b><br> 6007 * Type: <b>reference</b><br> 6008 * Path: <b>Task.basedOn</b><br> 6009 * </p> 6010 */ 6011 @SearchParamDefinition(name="based-on", path="Task.basedOn", description="Search by requests this task is based on", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6012 public static final String SP_BASED_ON = "based-on"; 6013 /** 6014 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 6015 * <p> 6016 * Description: <b>Search by requests this task is based on</b><br> 6017 * Type: <b>reference</b><br> 6018 * Path: <b>Task.basedOn</b><br> 6019 * </p> 6020 */ 6021 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 6022 6023/** 6024 * Constant for fluent queries to be used to add include statements. Specifies 6025 * the path value of "<b>Task:based-on</b>". 6026 */ 6027 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("Task:based-on").toLocked(); 6028 6029 /** 6030 * Search parameter: <b>business-status</b> 6031 * <p> 6032 * Description: <b>Search by business status</b><br> 6033 * Type: <b>token</b><br> 6034 * Path: <b>Task.businessStatus</b><br> 6035 * </p> 6036 */ 6037 @SearchParamDefinition(name="business-status", path="Task.businessStatus", description="Search by business status", type="token" ) 6038 public static final String SP_BUSINESS_STATUS = "business-status"; 6039 /** 6040 * <b>Fluent Client</b> search parameter constant for <b>business-status</b> 6041 * <p> 6042 * Description: <b>Search by business status</b><br> 6043 * Type: <b>token</b><br> 6044 * Path: <b>Task.businessStatus</b><br> 6045 * </p> 6046 */ 6047 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BUSINESS_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BUSINESS_STATUS); 6048 6049 /** 6050 * Search parameter: <b>focus</b> 6051 * <p> 6052 * Description: <b>Search by task focus</b><br> 6053 * Type: <b>reference</b><br> 6054 * Path: <b>Task.focus</b><br> 6055 * </p> 6056 */ 6057 @SearchParamDefinition(name="focus", path="Task.focus", description="Search by task focus", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6058 public static final String SP_FOCUS = "focus"; 6059 /** 6060 * <b>Fluent Client</b> search parameter constant for <b>focus</b> 6061 * <p> 6062 * Description: <b>Search by task focus</b><br> 6063 * Type: <b>reference</b><br> 6064 * Path: <b>Task.focus</b><br> 6065 * </p> 6066 */ 6067 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FOCUS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FOCUS); 6068 6069/** 6070 * Constant for fluent queries to be used to add include statements. Specifies 6071 * the path value of "<b>Task:focus</b>". 6072 */ 6073 public static final ca.uhn.fhir.model.api.Include INCLUDE_FOCUS = new ca.uhn.fhir.model.api.Include("Task:focus").toLocked(); 6074 6075 /** 6076 * Search parameter: <b>group-identifier</b> 6077 * <p> 6078 * Description: <b>Search by group identifier</b><br> 6079 * Type: <b>token</b><br> 6080 * Path: <b>Task.groupIdentifier</b><br> 6081 * </p> 6082 */ 6083 @SearchParamDefinition(name="group-identifier", path="Task.groupIdentifier", description="Search by group identifier", type="token" ) 6084 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 6085 /** 6086 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 6087 * <p> 6088 * Description: <b>Search by group identifier</b><br> 6089 * Type: <b>token</b><br> 6090 * Path: <b>Task.groupIdentifier</b><br> 6091 * </p> 6092 */ 6093 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GROUP_IDENTIFIER); 6094 6095 /** 6096 * Search parameter: <b>intent</b> 6097 * <p> 6098 * Description: <b>Search by task intent</b><br> 6099 * Type: <b>token</b><br> 6100 * Path: <b>Task.intent</b><br> 6101 * </p> 6102 */ 6103 @SearchParamDefinition(name="intent", path="Task.intent", description="Search by task intent", type="token" ) 6104 public static final String SP_INTENT = "intent"; 6105 /** 6106 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 6107 * <p> 6108 * Description: <b>Search by task intent</b><br> 6109 * Type: <b>token</b><br> 6110 * Path: <b>Task.intent</b><br> 6111 * </p> 6112 */ 6113 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INTENT); 6114 6115 /** 6116 * Search parameter: <b>modified</b> 6117 * <p> 6118 * Description: <b>Search by last modification date</b><br> 6119 * Type: <b>date</b><br> 6120 * Path: <b>Task.lastModified</b><br> 6121 * </p> 6122 */ 6123 @SearchParamDefinition(name="modified", path="Task.lastModified", description="Search by last modification date", type="date" ) 6124 public static final String SP_MODIFIED = "modified"; 6125 /** 6126 * <b>Fluent Client</b> search parameter constant for <b>modified</b> 6127 * <p> 6128 * Description: <b>Search by last modification date</b><br> 6129 * Type: <b>date</b><br> 6130 * Path: <b>Task.lastModified</b><br> 6131 * </p> 6132 */ 6133 public static final ca.uhn.fhir.rest.gclient.DateClientParam MODIFIED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_MODIFIED); 6134 6135 /** 6136 * Search parameter: <b>output</b> 6137 * <p> 6138 * Description: <b>Search by task output</b><br> 6139 * Type: <b>reference</b><br> 6140 * Path: <b>Task.output.value.ofType(Reference)</b><br> 6141 * </p> 6142 */ 6143 @SearchParamDefinition(name="output", path="Task.output.value.ofType(Reference)", description="Search by task output", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6144 public static final String SP_OUTPUT = "output"; 6145 /** 6146 * <b>Fluent Client</b> search parameter constant for <b>output</b> 6147 * <p> 6148 * Description: <b>Search by task output</b><br> 6149 * Type: <b>reference</b><br> 6150 * Path: <b>Task.output.value.ofType(Reference)</b><br> 6151 * </p> 6152 */ 6153 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OUTPUT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_OUTPUT); 6154 6155/** 6156 * Constant for fluent queries to be used to add include statements. Specifies 6157 * the path value of "<b>Task:output</b>". 6158 */ 6159 public static final ca.uhn.fhir.model.api.Include INCLUDE_OUTPUT = new ca.uhn.fhir.model.api.Include("Task:output").toLocked(); 6160 6161 /** 6162 * Search parameter: <b>owner</b> 6163 * <p> 6164 * Description: <b>Search by task owner</b><br> 6165 * Type: <b>reference</b><br> 6166 * Path: <b>Task.owner</b><br> 6167 * </p> 6168 */ 6169 @SearchParamDefinition(name="owner", path="Task.owner", description="Search by task owner", type="reference", target={CareTeam.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 6170 public static final String SP_OWNER = "owner"; 6171 /** 6172 * <b>Fluent Client</b> search parameter constant for <b>owner</b> 6173 * <p> 6174 * Description: <b>Search by task owner</b><br> 6175 * Type: <b>reference</b><br> 6176 * Path: <b>Task.owner</b><br> 6177 * </p> 6178 */ 6179 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OWNER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_OWNER); 6180 6181/** 6182 * Constant for fluent queries to be used to add include statements. Specifies 6183 * the path value of "<b>Task:owner</b>". 6184 */ 6185 public static final ca.uhn.fhir.model.api.Include INCLUDE_OWNER = new ca.uhn.fhir.model.api.Include("Task:owner").toLocked(); 6186 6187 /** 6188 * Search parameter: <b>part-of</b> 6189 * <p> 6190 * Description: <b>Search by task this task is part of</b><br> 6191 * Type: <b>reference</b><br> 6192 * Path: <b>Task.partOf</b><br> 6193 * </p> 6194 */ 6195 @SearchParamDefinition(name="part-of", path="Task.partOf", description="Search by task this task is part of", type="reference", target={Task.class } ) 6196 public static final String SP_PART_OF = "part-of"; 6197 /** 6198 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 6199 * <p> 6200 * Description: <b>Search by task this task is part of</b><br> 6201 * Type: <b>reference</b><br> 6202 * Path: <b>Task.partOf</b><br> 6203 * </p> 6204 */ 6205 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PART_OF); 6206 6207/** 6208 * Constant for fluent queries to be used to add include statements. Specifies 6209 * the path value of "<b>Task:part-of</b>". 6210 */ 6211 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("Task:part-of").toLocked(); 6212 6213 /** 6214 * Search parameter: <b>performer</b> 6215 * <p> 6216 * Description: <b>Search by recommended type of performer (e.g., Requester, Performer, Scheduler).</b><br> 6217 * Type: <b>token</b><br> 6218 * Path: <b>Task.requestedPerformer.concept</b><br> 6219 * </p> 6220 */ 6221 @SearchParamDefinition(name="performer", path="Task.requestedPerformer.concept", description="Search by recommended type of performer (e.g., Requester, Performer, Scheduler).", type="token" ) 6222 public static final String SP_PERFORMER = "performer"; 6223 /** 6224 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 6225 * <p> 6226 * Description: <b>Search by recommended type of performer (e.g., Requester, Performer, Scheduler).</b><br> 6227 * Type: <b>token</b><br> 6228 * Path: <b>Task.requestedPerformer.concept</b><br> 6229 * </p> 6230 */ 6231 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PERFORMER); 6232 6233 /** 6234 * Search parameter: <b>period</b> 6235 * <p> 6236 * Description: <b>Search by period Task is/was underway</b><br> 6237 * Type: <b>date</b><br> 6238 * Path: <b>Task.executionPeriod</b><br> 6239 * </p> 6240 */ 6241 @SearchParamDefinition(name="period", path="Task.executionPeriod", description="Search by period Task is/was underway", type="date" ) 6242 public static final String SP_PERIOD = "period"; 6243 /** 6244 * <b>Fluent Client</b> search parameter constant for <b>period</b> 6245 * <p> 6246 * Description: <b>Search by period Task is/was underway</b><br> 6247 * Type: <b>date</b><br> 6248 * Path: <b>Task.executionPeriod</b><br> 6249 * </p> 6250 */ 6251 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_PERIOD); 6252 6253 /** 6254 * Search parameter: <b>priority</b> 6255 * <p> 6256 * Description: <b>Search by task priority</b><br> 6257 * Type: <b>token</b><br> 6258 * Path: <b>Task.priority</b><br> 6259 * </p> 6260 */ 6261 @SearchParamDefinition(name="priority", path="Task.priority", description="Search by task priority", type="token" ) 6262 public static final String SP_PRIORITY = "priority"; 6263 /** 6264 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 6265 * <p> 6266 * Description: <b>Search by task priority</b><br> 6267 * Type: <b>token</b><br> 6268 * Path: <b>Task.priority</b><br> 6269 * </p> 6270 */ 6271 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRIORITY); 6272 6273 /** 6274 * Search parameter: <b>requestedperformer-reference</b> 6275 * <p> 6276 * Description: <b>Search by specific requested performer.</b><br> 6277 * Type: <b>reference</b><br> 6278 * Path: <b>Task.requestedPerformer.reference</b><br> 6279 * </p> 6280 */ 6281 @SearchParamDefinition(name="requestedperformer-reference", path="Task.requestedPerformer.reference", description="Search by specific requested performer.", type="reference", target={CareTeam.class, Device.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 6282 public static final String SP_REQUESTEDPERFORMER_REFERENCE = "requestedperformer-reference"; 6283 /** 6284 * <b>Fluent Client</b> search parameter constant for <b>requestedperformer-reference</b> 6285 * <p> 6286 * Description: <b>Search by specific requested performer.</b><br> 6287 * Type: <b>reference</b><br> 6288 * Path: <b>Task.requestedPerformer.reference</b><br> 6289 * </p> 6290 */ 6291 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTEDPERFORMER_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTEDPERFORMER_REFERENCE); 6292 6293/** 6294 * Constant for fluent queries to be used to add include statements. Specifies 6295 * the path value of "<b>Task:requestedperformer-reference</b>". 6296 */ 6297 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTEDPERFORMER_REFERENCE = new ca.uhn.fhir.model.api.Include("Task:requestedperformer-reference").toLocked(); 6298 6299 /** 6300 * Search parameter: <b>requester</b> 6301 * <p> 6302 * Description: <b>Search by task requester</b><br> 6303 * Type: <b>reference</b><br> 6304 * Path: <b>Task.requester</b><br> 6305 * </p> 6306 */ 6307 @SearchParamDefinition(name="requester", path="Task.requester", description="Search by task requester", type="reference", target={Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 6308 public static final String SP_REQUESTER = "requester"; 6309 /** 6310 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 6311 * <p> 6312 * Description: <b>Search by task requester</b><br> 6313 * Type: <b>reference</b><br> 6314 * Path: <b>Task.requester</b><br> 6315 * </p> 6316 */ 6317 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTER); 6318 6319/** 6320 * Constant for fluent queries to be used to add include statements. Specifies 6321 * the path value of "<b>Task:requester</b>". 6322 */ 6323 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include("Task:requester").toLocked(); 6324 6325 /** 6326 * Search parameter: <b>status</b> 6327 * <p> 6328 * Description: <b>Search by task status</b><br> 6329 * Type: <b>token</b><br> 6330 * Path: <b>Task.status</b><br> 6331 * </p> 6332 */ 6333 @SearchParamDefinition(name="status", path="Task.status", description="Search by task status", type="token" ) 6334 public static final String SP_STATUS = "status"; 6335 /** 6336 * <b>Fluent Client</b> search parameter constant for <b>status</b> 6337 * <p> 6338 * Description: <b>Search by task status</b><br> 6339 * Type: <b>token</b><br> 6340 * Path: <b>Task.status</b><br> 6341 * </p> 6342 */ 6343 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 6344 6345 /** 6346 * Search parameter: <b>subject</b> 6347 * <p> 6348 * Description: <b>Search by subject</b><br> 6349 * Type: <b>reference</b><br> 6350 * Path: <b>Task.for</b><br> 6351 * </p> 6352 */ 6353 @SearchParamDefinition(name="subject", path="Task.for", description="Search by subject", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 6354 public static final String SP_SUBJECT = "subject"; 6355 /** 6356 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 6357 * <p> 6358 * Description: <b>Search by subject</b><br> 6359 * Type: <b>reference</b><br> 6360 * Path: <b>Task.for</b><br> 6361 * </p> 6362 */ 6363 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 6364 6365/** 6366 * Constant for fluent queries to be used to add include statements. Specifies 6367 * the path value of "<b>Task:subject</b>". 6368 */ 6369 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Task:subject").toLocked(); 6370 6371 /** 6372 * Search parameter: <b>code</b> 6373 * <p> 6374 * Description: <b>Multiple Resources: 6375 6376* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 6377* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 6378* [AuditEvent](auditevent.html): More specific code for the event 6379* [Basic](basic.html): Kind of Resource 6380* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 6381* [Condition](condition.html): Code for the condition 6382* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 6383* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 6384* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 6385* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 6386* [ImagingSelection](imagingselection.html): The imaging selection status 6387* [List](list.html): What the purpose of this list is 6388* [Medication](medication.html): Returns medications for a specific code 6389* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 6390* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 6391* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 6392* [MedicationStatement](medicationstatement.html): Return statements of this medication code 6393* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 6394* [Observation](observation.html): The code of the observation type 6395* [Procedure](procedure.html): A code to identify a procedure 6396* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 6397* [Task](task.html): Search by task code 6398</b><br> 6399 * Type: <b>token</b><br> 6400 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 6401 * </p> 6402 */ 6403 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 6404 public static final String SP_CODE = "code"; 6405 /** 6406 * <b>Fluent Client</b> search parameter constant for <b>code</b> 6407 * <p> 6408 * Description: <b>Multiple Resources: 6409 6410* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 6411* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 6412* [AuditEvent](auditevent.html): More specific code for the event 6413* [Basic](basic.html): Kind of Resource 6414* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 6415* [Condition](condition.html): Code for the condition 6416* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 6417* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 6418* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 6419* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 6420* [ImagingSelection](imagingselection.html): The imaging selection status 6421* [List](list.html): What the purpose of this list is 6422* [Medication](medication.html): Returns medications for a specific code 6423* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 6424* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 6425* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 6426* [MedicationStatement](medicationstatement.html): Return statements of this medication code 6427* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 6428* [Observation](observation.html): The code of the observation type 6429* [Procedure](procedure.html): A code to identify a procedure 6430* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 6431* [Task](task.html): Search by task code 6432</b><br> 6433 * Type: <b>token</b><br> 6434 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 6435 * </p> 6436 */ 6437 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 6438 6439 /** 6440 * Search parameter: <b>encounter</b> 6441 * <p> 6442 * Description: <b>Multiple Resources: 6443 6444* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 6445* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 6446* [ChargeItem](chargeitem.html): Encounter associated with event 6447* [Claim](claim.html): Encounters associated with a billed line item 6448* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 6449* [Communication](communication.html): The Encounter during which this Communication was created 6450* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 6451* [Composition](composition.html): Context of the Composition 6452* [Condition](condition.html): The Encounter during which this Condition was created 6453* [DeviceRequest](devicerequest.html): Encounter during which request was created 6454* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 6455* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 6456* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 6457* [Flag](flag.html): Alert relevant during encounter 6458* [ImagingStudy](imagingstudy.html): The context of the study 6459* [List](list.html): Context in which list created 6460* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 6461* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 6462* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 6463* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 6464* [Observation](observation.html): Encounter related to the observation 6465* [Procedure](procedure.html): The Encounter during which this Procedure was created 6466* [Provenance](provenance.html): Encounter related to the Provenance 6467* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 6468* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 6469* [RiskAssessment](riskassessment.html): Where was assessment performed? 6470* [ServiceRequest](servicerequest.html): An encounter in which this request is made 6471* [Task](task.html): Search by encounter 6472* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 6473</b><br> 6474 * Type: <b>reference</b><br> 6475 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 6476 * </p> 6477 */ 6478 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", target={Encounter.class } ) 6479 public static final String SP_ENCOUNTER = "encounter"; 6480 /** 6481 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 6482 * <p> 6483 * Description: <b>Multiple Resources: 6484 6485* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 6486* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 6487* [ChargeItem](chargeitem.html): Encounter associated with event 6488* [Claim](claim.html): Encounters associated with a billed line item 6489* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 6490* [Communication](communication.html): The Encounter during which this Communication was created 6491* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 6492* [Composition](composition.html): Context of the Composition 6493* [Condition](condition.html): The Encounter during which this Condition was created 6494* [DeviceRequest](devicerequest.html): Encounter during which request was created 6495* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 6496* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 6497* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 6498* [Flag](flag.html): Alert relevant during encounter 6499* [ImagingStudy](imagingstudy.html): The context of the study 6500* [List](list.html): Context in which list created 6501* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 6502* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 6503* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 6504* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 6505* [Observation](observation.html): Encounter related to the observation 6506* [Procedure](procedure.html): The Encounter during which this Procedure was created 6507* [Provenance](provenance.html): Encounter related to the Provenance 6508* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 6509* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 6510* [RiskAssessment](riskassessment.html): Where was assessment performed? 6511* [ServiceRequest](servicerequest.html): An encounter in which this request is made 6512* [Task](task.html): Search by encounter 6513* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 6514</b><br> 6515 * Type: <b>reference</b><br> 6516 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 6517 * </p> 6518 */ 6519 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 6520 6521/** 6522 * Constant for fluent queries to be used to add include statements. Specifies 6523 * the path value of "<b>Task:encounter</b>". 6524 */ 6525 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("Task:encounter").toLocked(); 6526 6527 /** 6528 * Search parameter: <b>identifier</b> 6529 * <p> 6530 * Description: <b>Multiple Resources: 6531 6532* [Account](account.html): Account number 6533* [AdverseEvent](adverseevent.html): Business identifier for the event 6534* [AllergyIntolerance](allergyintolerance.html): External ids for this item 6535* [Appointment](appointment.html): An Identifier of the Appointment 6536* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 6537* [Basic](basic.html): Business identifier 6538* [BodyStructure](bodystructure.html): Bodystructure identifier 6539* [CarePlan](careplan.html): External Ids for this plan 6540* [CareTeam](careteam.html): External Ids for this team 6541* [ChargeItem](chargeitem.html): Business Identifier for item 6542* [Claim](claim.html): The primary identifier of the financial resource 6543* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 6544* [ClinicalImpression](clinicalimpression.html): Business identifier 6545* [Communication](communication.html): Unique identifier 6546* [CommunicationRequest](communicationrequest.html): Unique identifier 6547* [Composition](composition.html): Version-independent identifier for the Composition 6548* [Condition](condition.html): A unique identifier of the condition record 6549* [Consent](consent.html): Identifier for this record (external references) 6550* [Contract](contract.html): The identity of the contract 6551* [Coverage](coverage.html): The primary identifier of the insured and the coverage 6552* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 6553* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 6554* [DetectedIssue](detectedissue.html): Unique id for the detected issue 6555* [DeviceRequest](devicerequest.html): Business identifier for request/order 6556* [DeviceUsage](deviceusage.html): Search by identifier 6557* [DiagnosticReport](diagnosticreport.html): An identifier for the report 6558* [DocumentReference](documentreference.html): Identifier of the attachment binary 6559* [Encounter](encounter.html): Identifier(s) by which this encounter is known 6560* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 6561* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 6562* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 6563* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 6564* [Flag](flag.html): Business identifier 6565* [Goal](goal.html): External Ids for this goal 6566* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 6567* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 6568* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 6569* [Immunization](immunization.html): Business identifier 6570* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 6571* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 6572* [Invoice](invoice.html): Business Identifier for item 6573* [List](list.html): Business identifier 6574* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 6575* [Medication](medication.html): Returns medications with this external identifier 6576* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 6577* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 6578* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 6579* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 6580* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 6581* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 6582* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 6583* [Observation](observation.html): The unique id for a particular observation 6584* [Person](person.html): A person Identifier 6585* [Procedure](procedure.html): A unique identifier for a procedure 6586* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 6587* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 6588* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 6589* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 6590* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 6591* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 6592* [Specimen](specimen.html): The unique identifier associated with the specimen 6593* [SupplyDelivery](supplydelivery.html): External identifier 6594* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 6595* [Task](task.html): Search for a task instance by its business identifier 6596* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 6597</b><br> 6598 * Type: <b>token</b><br> 6599 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 6600 * </p> 6601 */ 6602 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 6603 public static final String SP_IDENTIFIER = "identifier"; 6604 /** 6605 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 6606 * <p> 6607 * Description: <b>Multiple Resources: 6608 6609* [Account](account.html): Account number 6610* [AdverseEvent](adverseevent.html): Business identifier for the event 6611* [AllergyIntolerance](allergyintolerance.html): External ids for this item 6612* [Appointment](appointment.html): An Identifier of the Appointment 6613* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 6614* [Basic](basic.html): Business identifier 6615* [BodyStructure](bodystructure.html): Bodystructure identifier 6616* [CarePlan](careplan.html): External Ids for this plan 6617* [CareTeam](careteam.html): External Ids for this team 6618* [ChargeItem](chargeitem.html): Business Identifier for item 6619* [Claim](claim.html): The primary identifier of the financial resource 6620* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 6621* [ClinicalImpression](clinicalimpression.html): Business identifier 6622* [Communication](communication.html): Unique identifier 6623* [CommunicationRequest](communicationrequest.html): Unique identifier 6624* [Composition](composition.html): Version-independent identifier for the Composition 6625* [Condition](condition.html): A unique identifier of the condition record 6626* [Consent](consent.html): Identifier for this record (external references) 6627* [Contract](contract.html): The identity of the contract 6628* [Coverage](coverage.html): The primary identifier of the insured and the coverage 6629* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 6630* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 6631* [DetectedIssue](detectedissue.html): Unique id for the detected issue 6632* [DeviceRequest](devicerequest.html): Business identifier for request/order 6633* [DeviceUsage](deviceusage.html): Search by identifier 6634* [DiagnosticReport](diagnosticreport.html): An identifier for the report 6635* [DocumentReference](documentreference.html): Identifier of the attachment binary 6636* [Encounter](encounter.html): Identifier(s) by which this encounter is known 6637* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 6638* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 6639* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 6640* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 6641* [Flag](flag.html): Business identifier 6642* [Goal](goal.html): External Ids for this goal 6643* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 6644* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 6645* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 6646* [Immunization](immunization.html): Business identifier 6647* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 6648* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 6649* [Invoice](invoice.html): Business Identifier for item 6650* [List](list.html): Business identifier 6651* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 6652* [Medication](medication.html): Returns medications with this external identifier 6653* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 6654* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 6655* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 6656* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 6657* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 6658* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 6659* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 6660* [Observation](observation.html): The unique id for a particular observation 6661* [Person](person.html): A person Identifier 6662* [Procedure](procedure.html): A unique identifier for a procedure 6663* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 6664* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 6665* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 6666* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 6667* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 6668* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 6669* [Specimen](specimen.html): The unique identifier associated with the specimen 6670* [SupplyDelivery](supplydelivery.html): External identifier 6671* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 6672* [Task](task.html): Search for a task instance by its business identifier 6673* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 6674</b><br> 6675 * Type: <b>token</b><br> 6676 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 6677 * </p> 6678 */ 6679 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 6680 6681 /** 6682 * Search parameter: <b>patient</b> 6683 * <p> 6684 * Description: <b>Multiple Resources: 6685 6686* [Account](account.html): The entity that caused the expenses 6687* [AdverseEvent](adverseevent.html): Subject impacted by event 6688* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 6689* [Appointment](appointment.html): One of the individuals of the appointment is this patient 6690* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 6691* [AuditEvent](auditevent.html): Where the activity involved patient data 6692* [Basic](basic.html): Identifies the focus of this resource 6693* [BodyStructure](bodystructure.html): Who this is about 6694* [CarePlan](careplan.html): Who the care plan is for 6695* [CareTeam](careteam.html): Who care team is for 6696* [ChargeItem](chargeitem.html): Individual service was done for/to 6697* [Claim](claim.html): Patient receiving the products or services 6698* [ClaimResponse](claimresponse.html): The subject of care 6699* [ClinicalImpression](clinicalimpression.html): Patient assessed 6700* [Communication](communication.html): Focus of message 6701* [CommunicationRequest](communicationrequest.html): Focus of message 6702* [Composition](composition.html): Who and/or what the composition is about 6703* [Condition](condition.html): Who has the condition? 6704* [Consent](consent.html): Who the consent applies to 6705* [Contract](contract.html): The identity of the subject of the contract (if a patient) 6706* [Coverage](coverage.html): Retrieve coverages for a patient 6707* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 6708* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 6709* [DetectedIssue](detectedissue.html): Associated patient 6710* [DeviceRequest](devicerequest.html): Individual the service is ordered for 6711* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 6712* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 6713* [DocumentReference](documentreference.html): Who/what is the subject of the document 6714* [Encounter](encounter.html): The patient present at the encounter 6715* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 6716* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 6717* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 6718* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 6719* [Flag](flag.html): The identity of a subject to list flags for 6720* [Goal](goal.html): Who this goal is intended for 6721* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 6722* [ImagingSelection](imagingselection.html): Who the study is about 6723* [ImagingStudy](imagingstudy.html): Who the study is about 6724* [Immunization](immunization.html): The patient for the vaccination record 6725* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 6726* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 6727* [Invoice](invoice.html): Recipient(s) of goods and services 6728* [List](list.html): If all resources have the same subject 6729* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 6730* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 6731* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 6732* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 6733* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 6734* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 6735* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 6736* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 6737* [Observation](observation.html): The subject that the observation is about (if patient) 6738* [Person](person.html): The Person links to this Patient 6739* [Procedure](procedure.html): Search by subject - a patient 6740* [Provenance](provenance.html): Where the activity involved patient data 6741* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 6742* [RelatedPerson](relatedperson.html): The patient this related person is related to 6743* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 6744* [ResearchSubject](researchsubject.html): Who or what is part of study 6745* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 6746* [ServiceRequest](servicerequest.html): Search by subject - a patient 6747* [Specimen](specimen.html): The patient the specimen comes from 6748* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 6749* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 6750* [Task](task.html): Search by patient 6751* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 6752</b><br> 6753 * Type: <b>reference</b><br> 6754 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 6755 * </p> 6756 */ 6757 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 6758 public static final String SP_PATIENT = "patient"; 6759 /** 6760 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 6761 * <p> 6762 * Description: <b>Multiple Resources: 6763 6764* [Account](account.html): The entity that caused the expenses 6765* [AdverseEvent](adverseevent.html): Subject impacted by event 6766* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 6767* [Appointment](appointment.html): One of the individuals of the appointment is this patient 6768* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 6769* [AuditEvent](auditevent.html): Where the activity involved patient data 6770* [Basic](basic.html): Identifies the focus of this resource 6771* [BodyStructure](bodystructure.html): Who this is about 6772* [CarePlan](careplan.html): Who the care plan is for 6773* [CareTeam](careteam.html): Who care team is for 6774* [ChargeItem](chargeitem.html): Individual service was done for/to 6775* [Claim](claim.html): Patient receiving the products or services 6776* [ClaimResponse](claimresponse.html): The subject of care 6777* [ClinicalImpression](clinicalimpression.html): Patient assessed 6778* [Communication](communication.html): Focus of message 6779* [CommunicationRequest](communicationrequest.html): Focus of message 6780* [Composition](composition.html): Who and/or what the composition is about 6781* [Condition](condition.html): Who has the condition? 6782* [Consent](consent.html): Who the consent applies to 6783* [Contract](contract.html): The identity of the subject of the contract (if a patient) 6784* [Coverage](coverage.html): Retrieve coverages for a patient 6785* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 6786* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 6787* [DetectedIssue](detectedissue.html): Associated patient 6788* [DeviceRequest](devicerequest.html): Individual the service is ordered for 6789* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 6790* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 6791* [DocumentReference](documentreference.html): Who/what is the subject of the document 6792* [Encounter](encounter.html): The patient present at the encounter 6793* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 6794* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 6795* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 6796* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 6797* [Flag](flag.html): The identity of a subject to list flags for 6798* [Goal](goal.html): Who this goal is intended for 6799* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 6800* [ImagingSelection](imagingselection.html): Who the study is about 6801* [ImagingStudy](imagingstudy.html): Who the study is about 6802* [Immunization](immunization.html): The patient for the vaccination record 6803* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 6804* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 6805* [Invoice](invoice.html): Recipient(s) of goods and services 6806* [List](list.html): If all resources have the same subject 6807* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 6808* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 6809* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 6810* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 6811* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 6812* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 6813* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 6814* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 6815* [Observation](observation.html): The subject that the observation is about (if patient) 6816* [Person](person.html): The Person links to this Patient 6817* [Procedure](procedure.html): Search by subject - a patient 6818* [Provenance](provenance.html): Where the activity involved patient data 6819* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 6820* [RelatedPerson](relatedperson.html): The patient this related person is related to 6821* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 6822* [ResearchSubject](researchsubject.html): Who or what is part of study 6823* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 6824* [ServiceRequest](servicerequest.html): Search by subject - a patient 6825* [Specimen](specimen.html): The patient the specimen comes from 6826* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 6827* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 6828* [Task](task.html): Search by patient 6829* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 6830</b><br> 6831 * Type: <b>reference</b><br> 6832 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 6833 * </p> 6834 */ 6835 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 6836 6837/** 6838 * Constant for fluent queries to be used to add include statements. Specifies 6839 * the path value of "<b>Task:patient</b>". 6840 */ 6841 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Task:patient").toLocked(); 6842 6843 6844} 6845