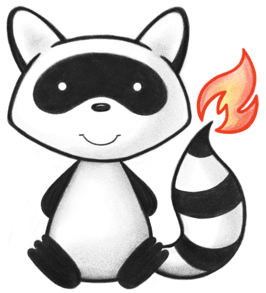
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A TerminologyCapabilities resource documents a set of capabilities (behaviors) of a FHIR Terminology Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation. 052 */ 053@ResourceDef(name="TerminologyCapabilities", profile="http://hl7.org/fhir/StructureDefinition/TerminologyCapabilities") 054public class TerminologyCapabilities extends CanonicalResource { 055 056 public enum CodeSearchSupport { 057 /** 058 * The search for code on ValueSet returns ValueSet resources where the code is included in the extensional definition of the ValueSet. 059 */ 060 INCOMPOSE, 061 /** 062 * The search for code on ValueSet returns ValueSet resources where the code is contained in the ValueSet expansion. 063 */ 064 INEXPANSION, 065 /** 066 * The search for code on ValueSet returns ValueSet resources where the code is included in the extensional definition or contained in the ValueSet expansion. 067 */ 068 INCOMPOSEOREXPANSION, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static CodeSearchSupport fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("in-compose".equals(codeString)) 077 return INCOMPOSE; 078 if ("in-expansion".equals(codeString)) 079 return INEXPANSION; 080 if ("in-compose-or-expansion".equals(codeString)) 081 return INCOMPOSEOREXPANSION; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown CodeSearchSupport code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case INCOMPOSE: return "in-compose"; 090 case INEXPANSION: return "in-expansion"; 091 case INCOMPOSEOREXPANSION: return "in-compose-or-expansion"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case INCOMPOSE: return "http://hl7.org/fhir/code-search-support"; 099 case INEXPANSION: return "http://hl7.org/fhir/code-search-support"; 100 case INCOMPOSEOREXPANSION: return "http://hl7.org/fhir/code-search-support"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case INCOMPOSE: return "The search for code on ValueSet returns ValueSet resources where the code is included in the extensional definition of the ValueSet."; 108 case INEXPANSION: return "The search for code on ValueSet returns ValueSet resources where the code is contained in the ValueSet expansion."; 109 case INCOMPOSEOREXPANSION: return "The search for code on ValueSet returns ValueSet resources where the code is included in the extensional definition or contained in the ValueSet expansion."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case INCOMPOSE: return "In Compose"; 117 case INEXPANSION: return "In Expansion"; 118 case INCOMPOSEOREXPANSION: return "In Compose Or Expansion"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class CodeSearchSupportEnumFactory implements EnumFactory<CodeSearchSupport> { 126 public CodeSearchSupport fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("in-compose".equals(codeString)) 131 return CodeSearchSupport.INCOMPOSE; 132 if ("in-expansion".equals(codeString)) 133 return CodeSearchSupport.INEXPANSION; 134 if ("in-compose-or-expansion".equals(codeString)) 135 return CodeSearchSupport.INCOMPOSEOREXPANSION; 136 throw new IllegalArgumentException("Unknown CodeSearchSupport code '"+codeString+"'"); 137 } 138 public Enumeration<CodeSearchSupport> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<CodeSearchSupport>(this, CodeSearchSupport.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<CodeSearchSupport>(this, CodeSearchSupport.NULL, code); 146 if ("in-compose".equals(codeString)) 147 return new Enumeration<CodeSearchSupport>(this, CodeSearchSupport.INCOMPOSE, code); 148 if ("in-expansion".equals(codeString)) 149 return new Enumeration<CodeSearchSupport>(this, CodeSearchSupport.INEXPANSION, code); 150 if ("in-compose-or-expansion".equals(codeString)) 151 return new Enumeration<CodeSearchSupport>(this, CodeSearchSupport.INCOMPOSEOREXPANSION, code); 152 throw new FHIRException("Unknown CodeSearchSupport code '"+codeString+"'"); 153 } 154 public String toCode(CodeSearchSupport code) { 155 if (code == CodeSearchSupport.NULL) 156 return null; 157 if (code == CodeSearchSupport.INCOMPOSE) 158 return "in-compose"; 159 if (code == CodeSearchSupport.INEXPANSION) 160 return "in-expansion"; 161 if (code == CodeSearchSupport.INCOMPOSEOREXPANSION) 162 return "in-compose-or-expansion"; 163 return "?"; 164 } 165 public String toSystem(CodeSearchSupport code) { 166 return code.getSystem(); 167 } 168 } 169 170 @Block() 171 public static class TerminologyCapabilitiesSoftwareComponent extends BackboneElement implements IBaseBackboneElement { 172 /** 173 * Name the software is known by. 174 */ 175 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 176 @Description(shortDefinition="A name the software is known by", formalDefinition="Name the software is known by." ) 177 protected StringType name; 178 179 /** 180 * The version identifier for the software covered by this statement. 181 */ 182 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 183 @Description(shortDefinition="Version covered by this statement", formalDefinition="The version identifier for the software covered by this statement." ) 184 protected StringType version; 185 186 private static final long serialVersionUID = -790299911L; 187 188 /** 189 * Constructor 190 */ 191 public TerminologyCapabilitiesSoftwareComponent() { 192 super(); 193 } 194 195 /** 196 * Constructor 197 */ 198 public TerminologyCapabilitiesSoftwareComponent(String name) { 199 super(); 200 this.setName(name); 201 } 202 203 /** 204 * @return {@link #name} (Name the software is known by.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 205 */ 206 public StringType getNameElement() { 207 if (this.name == null) 208 if (Configuration.errorOnAutoCreate()) 209 throw new Error("Attempt to auto-create TerminologyCapabilitiesSoftwareComponent.name"); 210 else if (Configuration.doAutoCreate()) 211 this.name = new StringType(); // bb 212 return this.name; 213 } 214 215 public boolean hasNameElement() { 216 return this.name != null && !this.name.isEmpty(); 217 } 218 219 public boolean hasName() { 220 return this.name != null && !this.name.isEmpty(); 221 } 222 223 /** 224 * @param value {@link #name} (Name the software is known by.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 225 */ 226 public TerminologyCapabilitiesSoftwareComponent setNameElement(StringType value) { 227 this.name = value; 228 return this; 229 } 230 231 /** 232 * @return Name the software is known by. 233 */ 234 public String getName() { 235 return this.name == null ? null : this.name.getValue(); 236 } 237 238 /** 239 * @param value Name the software is known by. 240 */ 241 public TerminologyCapabilitiesSoftwareComponent setName(String value) { 242 if (this.name == null) 243 this.name = new StringType(); 244 this.name.setValue(value); 245 return this; 246 } 247 248 /** 249 * @return {@link #version} (The version identifier for the software covered by this statement.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 250 */ 251 public StringType getVersionElement() { 252 if (this.version == null) 253 if (Configuration.errorOnAutoCreate()) 254 throw new Error("Attempt to auto-create TerminologyCapabilitiesSoftwareComponent.version"); 255 else if (Configuration.doAutoCreate()) 256 this.version = new StringType(); // bb 257 return this.version; 258 } 259 260 public boolean hasVersionElement() { 261 return this.version != null && !this.version.isEmpty(); 262 } 263 264 public boolean hasVersion() { 265 return this.version != null && !this.version.isEmpty(); 266 } 267 268 /** 269 * @param value {@link #version} (The version identifier for the software covered by this statement.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 270 */ 271 public TerminologyCapabilitiesSoftwareComponent setVersionElement(StringType value) { 272 this.version = value; 273 return this; 274 } 275 276 /** 277 * @return The version identifier for the software covered by this statement. 278 */ 279 public String getVersion() { 280 return this.version == null ? null : this.version.getValue(); 281 } 282 283 /** 284 * @param value The version identifier for the software covered by this statement. 285 */ 286 public TerminologyCapabilitiesSoftwareComponent setVersion(String value) { 287 if (Utilities.noString(value)) 288 this.version = null; 289 else { 290 if (this.version == null) 291 this.version = new StringType(); 292 this.version.setValue(value); 293 } 294 return this; 295 } 296 297 protected void listChildren(List<Property> children) { 298 super.listChildren(children); 299 children.add(new Property("name", "string", "Name the software is known by.", 0, 1, name)); 300 children.add(new Property("version", "string", "The version identifier for the software covered by this statement.", 0, 1, version)); 301 } 302 303 @Override 304 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 305 switch (_hash) { 306 case 3373707: /*name*/ return new Property("name", "string", "Name the software is known by.", 0, 1, name); 307 case 351608024: /*version*/ return new Property("version", "string", "The version identifier for the software covered by this statement.", 0, 1, version); 308 default: return super.getNamedProperty(_hash, _name, _checkValid); 309 } 310 311 } 312 313 @Override 314 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 315 switch (hash) { 316 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 317 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 318 default: return super.getProperty(hash, name, checkValid); 319 } 320 321 } 322 323 @Override 324 public Base setProperty(int hash, String name, Base value) throws FHIRException { 325 switch (hash) { 326 case 3373707: // name 327 this.name = TypeConvertor.castToString(value); // StringType 328 return value; 329 case 351608024: // version 330 this.version = TypeConvertor.castToString(value); // StringType 331 return value; 332 default: return super.setProperty(hash, name, value); 333 } 334 335 } 336 337 @Override 338 public Base setProperty(String name, Base value) throws FHIRException { 339 if (name.equals("name")) { 340 this.name = TypeConvertor.castToString(value); // StringType 341 } else if (name.equals("version")) { 342 this.version = TypeConvertor.castToString(value); // StringType 343 } else 344 return super.setProperty(name, value); 345 return value; 346 } 347 348 @Override 349 public void removeChild(String name, Base value) throws FHIRException { 350 if (name.equals("name")) { 351 this.name = null; 352 } else if (name.equals("version")) { 353 this.version = null; 354 } else 355 super.removeChild(name, value); 356 357 } 358 359 @Override 360 public Base makeProperty(int hash, String name) throws FHIRException { 361 switch (hash) { 362 case 3373707: return getNameElement(); 363 case 351608024: return getVersionElement(); 364 default: return super.makeProperty(hash, name); 365 } 366 367 } 368 369 @Override 370 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 371 switch (hash) { 372 case 3373707: /*name*/ return new String[] {"string"}; 373 case 351608024: /*version*/ return new String[] {"string"}; 374 default: return super.getTypesForProperty(hash, name); 375 } 376 377 } 378 379 @Override 380 public Base addChild(String name) throws FHIRException { 381 if (name.equals("name")) { 382 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.software.name"); 383 } 384 else if (name.equals("version")) { 385 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.software.version"); 386 } 387 else 388 return super.addChild(name); 389 } 390 391 public TerminologyCapabilitiesSoftwareComponent copy() { 392 TerminologyCapabilitiesSoftwareComponent dst = new TerminologyCapabilitiesSoftwareComponent(); 393 copyValues(dst); 394 return dst; 395 } 396 397 public void copyValues(TerminologyCapabilitiesSoftwareComponent dst) { 398 super.copyValues(dst); 399 dst.name = name == null ? null : name.copy(); 400 dst.version = version == null ? null : version.copy(); 401 } 402 403 @Override 404 public boolean equalsDeep(Base other_) { 405 if (!super.equalsDeep(other_)) 406 return false; 407 if (!(other_ instanceof TerminologyCapabilitiesSoftwareComponent)) 408 return false; 409 TerminologyCapabilitiesSoftwareComponent o = (TerminologyCapabilitiesSoftwareComponent) other_; 410 return compareDeep(name, o.name, true) && compareDeep(version, o.version, true); 411 } 412 413 @Override 414 public boolean equalsShallow(Base other_) { 415 if (!super.equalsShallow(other_)) 416 return false; 417 if (!(other_ instanceof TerminologyCapabilitiesSoftwareComponent)) 418 return false; 419 TerminologyCapabilitiesSoftwareComponent o = (TerminologyCapabilitiesSoftwareComponent) other_; 420 return compareValues(name, o.name, true) && compareValues(version, o.version, true); 421 } 422 423 public boolean isEmpty() { 424 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, version); 425 } 426 427 public String fhirType() { 428 return "TerminologyCapabilities.software"; 429 430 } 431 432 } 433 434 @Block() 435 public static class TerminologyCapabilitiesImplementationComponent extends BackboneElement implements IBaseBackboneElement { 436 /** 437 * Information about the specific installation that this terminology capability statement relates to. 438 */ 439 @Child(name = "description", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 440 @Description(shortDefinition="Describes this specific instance", formalDefinition="Information about the specific installation that this terminology capability statement relates to." ) 441 protected StringType description; 442 443 /** 444 * An absolute base URL for the implementation. 445 */ 446 @Child(name = "url", type = {UrlType.class}, order=2, min=0, max=1, modifier=false, summary=true) 447 @Description(shortDefinition="Base URL for the implementation", formalDefinition="An absolute base URL for the implementation." ) 448 protected UrlType url; 449 450 private static final long serialVersionUID = 98009649L; 451 452 /** 453 * Constructor 454 */ 455 public TerminologyCapabilitiesImplementationComponent() { 456 super(); 457 } 458 459 /** 460 * Constructor 461 */ 462 public TerminologyCapabilitiesImplementationComponent(String description) { 463 super(); 464 this.setDescription(description); 465 } 466 467 /** 468 * @return {@link #description} (Information about the specific installation that this terminology capability statement relates to.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 469 */ 470 public StringType getDescriptionElement() { 471 if (this.description == null) 472 if (Configuration.errorOnAutoCreate()) 473 throw new Error("Attempt to auto-create TerminologyCapabilitiesImplementationComponent.description"); 474 else if (Configuration.doAutoCreate()) 475 this.description = new StringType(); // bb 476 return this.description; 477 } 478 479 public boolean hasDescriptionElement() { 480 return this.description != null && !this.description.isEmpty(); 481 } 482 483 public boolean hasDescription() { 484 return this.description != null && !this.description.isEmpty(); 485 } 486 487 /** 488 * @param value {@link #description} (Information about the specific installation that this terminology capability statement relates to.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 489 */ 490 public TerminologyCapabilitiesImplementationComponent setDescriptionElement(StringType value) { 491 this.description = value; 492 return this; 493 } 494 495 /** 496 * @return Information about the specific installation that this terminology capability statement relates to. 497 */ 498 public String getDescription() { 499 return this.description == null ? null : this.description.getValue(); 500 } 501 502 /** 503 * @param value Information about the specific installation that this terminology capability statement relates to. 504 */ 505 public TerminologyCapabilitiesImplementationComponent setDescription(String value) { 506 if (this.description == null) 507 this.description = new StringType(); 508 this.description.setValue(value); 509 return this; 510 } 511 512 /** 513 * @return {@link #url} (An absolute base URL for the implementation.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 514 */ 515 public UrlType getUrlElement() { 516 if (this.url == null) 517 if (Configuration.errorOnAutoCreate()) 518 throw new Error("Attempt to auto-create TerminologyCapabilitiesImplementationComponent.url"); 519 else if (Configuration.doAutoCreate()) 520 this.url = new UrlType(); // bb 521 return this.url; 522 } 523 524 public boolean hasUrlElement() { 525 return this.url != null && !this.url.isEmpty(); 526 } 527 528 public boolean hasUrl() { 529 return this.url != null && !this.url.isEmpty(); 530 } 531 532 /** 533 * @param value {@link #url} (An absolute base URL for the implementation.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 534 */ 535 public TerminologyCapabilitiesImplementationComponent setUrlElement(UrlType value) { 536 this.url = value; 537 return this; 538 } 539 540 /** 541 * @return An absolute base URL for the implementation. 542 */ 543 public String getUrl() { 544 return this.url == null ? null : this.url.getValue(); 545 } 546 547 /** 548 * @param value An absolute base URL for the implementation. 549 */ 550 public TerminologyCapabilitiesImplementationComponent setUrl(String value) { 551 if (Utilities.noString(value)) 552 this.url = null; 553 else { 554 if (this.url == null) 555 this.url = new UrlType(); 556 this.url.setValue(value); 557 } 558 return this; 559 } 560 561 protected void listChildren(List<Property> children) { 562 super.listChildren(children); 563 children.add(new Property("description", "string", "Information about the specific installation that this terminology capability statement relates to.", 0, 1, description)); 564 children.add(new Property("url", "url", "An absolute base URL for the implementation.", 0, 1, url)); 565 } 566 567 @Override 568 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 569 switch (_hash) { 570 case -1724546052: /*description*/ return new Property("description", "string", "Information about the specific installation that this terminology capability statement relates to.", 0, 1, description); 571 case 116079: /*url*/ return new Property("url", "url", "An absolute base URL for the implementation.", 0, 1, url); 572 default: return super.getNamedProperty(_hash, _name, _checkValid); 573 } 574 575 } 576 577 @Override 578 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 579 switch (hash) { 580 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 581 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UrlType 582 default: return super.getProperty(hash, name, checkValid); 583 } 584 585 } 586 587 @Override 588 public Base setProperty(int hash, String name, Base value) throws FHIRException { 589 switch (hash) { 590 case -1724546052: // description 591 this.description = TypeConvertor.castToString(value); // StringType 592 return value; 593 case 116079: // url 594 this.url = TypeConvertor.castToUrl(value); // UrlType 595 return value; 596 default: return super.setProperty(hash, name, value); 597 } 598 599 } 600 601 @Override 602 public Base setProperty(String name, Base value) throws FHIRException { 603 if (name.equals("description")) { 604 this.description = TypeConvertor.castToString(value); // StringType 605 } else if (name.equals("url")) { 606 this.url = TypeConvertor.castToUrl(value); // UrlType 607 } else 608 return super.setProperty(name, value); 609 return value; 610 } 611 612 @Override 613 public void removeChild(String name, Base value) throws FHIRException { 614 if (name.equals("description")) { 615 this.description = null; 616 } else if (name.equals("url")) { 617 this.url = null; 618 } else 619 super.removeChild(name, value); 620 621 } 622 623 @Override 624 public Base makeProperty(int hash, String name) throws FHIRException { 625 switch (hash) { 626 case -1724546052: return getDescriptionElement(); 627 case 116079: return getUrlElement(); 628 default: return super.makeProperty(hash, name); 629 } 630 631 } 632 633 @Override 634 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 635 switch (hash) { 636 case -1724546052: /*description*/ return new String[] {"string"}; 637 case 116079: /*url*/ return new String[] {"url"}; 638 default: return super.getTypesForProperty(hash, name); 639 } 640 641 } 642 643 @Override 644 public Base addChild(String name) throws FHIRException { 645 if (name.equals("description")) { 646 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.implementation.description"); 647 } 648 else if (name.equals("url")) { 649 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.implementation.url"); 650 } 651 else 652 return super.addChild(name); 653 } 654 655 public TerminologyCapabilitiesImplementationComponent copy() { 656 TerminologyCapabilitiesImplementationComponent dst = new TerminologyCapabilitiesImplementationComponent(); 657 copyValues(dst); 658 return dst; 659 } 660 661 public void copyValues(TerminologyCapabilitiesImplementationComponent dst) { 662 super.copyValues(dst); 663 dst.description = description == null ? null : description.copy(); 664 dst.url = url == null ? null : url.copy(); 665 } 666 667 @Override 668 public boolean equalsDeep(Base other_) { 669 if (!super.equalsDeep(other_)) 670 return false; 671 if (!(other_ instanceof TerminologyCapabilitiesImplementationComponent)) 672 return false; 673 TerminologyCapabilitiesImplementationComponent o = (TerminologyCapabilitiesImplementationComponent) other_; 674 return compareDeep(description, o.description, true) && compareDeep(url, o.url, true); 675 } 676 677 @Override 678 public boolean equalsShallow(Base other_) { 679 if (!super.equalsShallow(other_)) 680 return false; 681 if (!(other_ instanceof TerminologyCapabilitiesImplementationComponent)) 682 return false; 683 TerminologyCapabilitiesImplementationComponent o = (TerminologyCapabilitiesImplementationComponent) other_; 684 return compareValues(description, o.description, true) && compareValues(url, o.url, true); 685 } 686 687 public boolean isEmpty() { 688 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, url); 689 } 690 691 public String fhirType() { 692 return "TerminologyCapabilities.implementation"; 693 694 } 695 696 } 697 698 @Block() 699 public static class TerminologyCapabilitiesCodeSystemComponent extends BackboneElement implements IBaseBackboneElement { 700 /** 701 * Canonical identifier for the code system, represented as a URI. 702 */ 703 @Child(name = "uri", type = {CanonicalType.class}, order=1, min=0, max=1, modifier=false, summary=false) 704 @Description(shortDefinition="Canonical identifier for the code system, represented as a URI", formalDefinition="Canonical identifier for the code system, represented as a URI." ) 705 protected CanonicalType uri; 706 707 /** 708 * For the code system, a list of versions that are supported by the server. 709 */ 710 @Child(name = "version", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 711 @Description(shortDefinition="Version of Code System supported", formalDefinition="For the code system, a list of versions that are supported by the server." ) 712 protected List<TerminologyCapabilitiesCodeSystemVersionComponent> version; 713 714 /** 715 * The extent of the content of the code system (the concepts and codes it defines) are represented in this resource instance. 716 */ 717 @Child(name = "content", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=true) 718 @Description(shortDefinition="not-present | example | fragment | complete | supplement", formalDefinition="The extent of the content of the code system (the concepts and codes it defines) are represented in this resource instance." ) 719 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/codesystem-content-mode") 720 protected Enumeration<CodeSystemContentMode> content; 721 722 /** 723 * True if subsumption is supported for this version of the code system. 724 */ 725 @Child(name = "subsumption", type = {BooleanType.class}, order=4, min=0, max=1, modifier=false, summary=false) 726 @Description(shortDefinition="Whether subsumption is supported", formalDefinition="True if subsumption is supported for this version of the code system." ) 727 protected BooleanType subsumption; 728 729 private static final long serialVersionUID = 1589208601L; 730 731 /** 732 * Constructor 733 */ 734 public TerminologyCapabilitiesCodeSystemComponent() { 735 super(); 736 } 737 738 /** 739 * Constructor 740 */ 741 public TerminologyCapabilitiesCodeSystemComponent(CodeSystemContentMode content) { 742 super(); 743 this.setContent(content); 744 } 745 746 /** 747 * @return {@link #uri} (Canonical identifier for the code system, represented as a URI.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 748 */ 749 public CanonicalType getUriElement() { 750 if (this.uri == null) 751 if (Configuration.errorOnAutoCreate()) 752 throw new Error("Attempt to auto-create TerminologyCapabilitiesCodeSystemComponent.uri"); 753 else if (Configuration.doAutoCreate()) 754 this.uri = new CanonicalType(); // bb 755 return this.uri; 756 } 757 758 public boolean hasUriElement() { 759 return this.uri != null && !this.uri.isEmpty(); 760 } 761 762 public boolean hasUri() { 763 return this.uri != null && !this.uri.isEmpty(); 764 } 765 766 /** 767 * @param value {@link #uri} (Canonical identifier for the code system, represented as a URI.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 768 */ 769 public TerminologyCapabilitiesCodeSystemComponent setUriElement(CanonicalType value) { 770 this.uri = value; 771 return this; 772 } 773 774 /** 775 * @return Canonical identifier for the code system, represented as a URI. 776 */ 777 public String getUri() { 778 return this.uri == null ? null : this.uri.getValue(); 779 } 780 781 /** 782 * @param value Canonical identifier for the code system, represented as a URI. 783 */ 784 public TerminologyCapabilitiesCodeSystemComponent setUri(String value) { 785 if (Utilities.noString(value)) 786 this.uri = null; 787 else { 788 if (this.uri == null) 789 this.uri = new CanonicalType(); 790 this.uri.setValue(value); 791 } 792 return this; 793 } 794 795 /** 796 * @return {@link #version} (For the code system, a list of versions that are supported by the server.) 797 */ 798 public List<TerminologyCapabilitiesCodeSystemVersionComponent> getVersion() { 799 if (this.version == null) 800 this.version = new ArrayList<TerminologyCapabilitiesCodeSystemVersionComponent>(); 801 return this.version; 802 } 803 804 /** 805 * @return Returns a reference to <code>this</code> for easy method chaining 806 */ 807 public TerminologyCapabilitiesCodeSystemComponent setVersion(List<TerminologyCapabilitiesCodeSystemVersionComponent> theVersion) { 808 this.version = theVersion; 809 return this; 810 } 811 812 public boolean hasVersion() { 813 if (this.version == null) 814 return false; 815 for (TerminologyCapabilitiesCodeSystemVersionComponent item : this.version) 816 if (!item.isEmpty()) 817 return true; 818 return false; 819 } 820 821 public TerminologyCapabilitiesCodeSystemVersionComponent addVersion() { //3 822 TerminologyCapabilitiesCodeSystemVersionComponent t = new TerminologyCapabilitiesCodeSystemVersionComponent(); 823 if (this.version == null) 824 this.version = new ArrayList<TerminologyCapabilitiesCodeSystemVersionComponent>(); 825 this.version.add(t); 826 return t; 827 } 828 829 public TerminologyCapabilitiesCodeSystemComponent addVersion(TerminologyCapabilitiesCodeSystemVersionComponent t) { //3 830 if (t == null) 831 return this; 832 if (this.version == null) 833 this.version = new ArrayList<TerminologyCapabilitiesCodeSystemVersionComponent>(); 834 this.version.add(t); 835 return this; 836 } 837 838 /** 839 * @return The first repetition of repeating field {@link #version}, creating it if it does not already exist {3} 840 */ 841 public TerminologyCapabilitiesCodeSystemVersionComponent getVersionFirstRep() { 842 if (getVersion().isEmpty()) { 843 addVersion(); 844 } 845 return getVersion().get(0); 846 } 847 848 /** 849 * @return {@link #content} (The extent of the content of the code system (the concepts and codes it defines) are represented in this resource instance.). This is the underlying object with id, value and extensions. The accessor "getContent" gives direct access to the value 850 */ 851 public Enumeration<CodeSystemContentMode> getContentElement() { 852 if (this.content == null) 853 if (Configuration.errorOnAutoCreate()) 854 throw new Error("Attempt to auto-create TerminologyCapabilitiesCodeSystemComponent.content"); 855 else if (Configuration.doAutoCreate()) 856 this.content = new Enumeration<CodeSystemContentMode>(new CodeSystemContentModeEnumFactory()); // bb 857 return this.content; 858 } 859 860 public boolean hasContentElement() { 861 return this.content != null && !this.content.isEmpty(); 862 } 863 864 public boolean hasContent() { 865 return this.content != null && !this.content.isEmpty(); 866 } 867 868 /** 869 * @param value {@link #content} (The extent of the content of the code system (the concepts and codes it defines) are represented in this resource instance.). This is the underlying object with id, value and extensions. The accessor "getContent" gives direct access to the value 870 */ 871 public TerminologyCapabilitiesCodeSystemComponent setContentElement(Enumeration<CodeSystemContentMode> value) { 872 this.content = value; 873 return this; 874 } 875 876 /** 877 * @return The extent of the content of the code system (the concepts and codes it defines) are represented in this resource instance. 878 */ 879 public CodeSystemContentMode getContent() { 880 return this.content == null ? null : this.content.getValue(); 881 } 882 883 /** 884 * @param value The extent of the content of the code system (the concepts and codes it defines) are represented in this resource instance. 885 */ 886 public TerminologyCapabilitiesCodeSystemComponent setContent(CodeSystemContentMode value) { 887 if (this.content == null) 888 this.content = new Enumeration<CodeSystemContentMode>(new CodeSystemContentModeEnumFactory()); 889 this.content.setValue(value); 890 return this; 891 } 892 893 /** 894 * @return {@link #subsumption} (True if subsumption is supported for this version of the code system.). This is the underlying object with id, value and extensions. The accessor "getSubsumption" gives direct access to the value 895 */ 896 public BooleanType getSubsumptionElement() { 897 if (this.subsumption == null) 898 if (Configuration.errorOnAutoCreate()) 899 throw new Error("Attempt to auto-create TerminologyCapabilitiesCodeSystemComponent.subsumption"); 900 else if (Configuration.doAutoCreate()) 901 this.subsumption = new BooleanType(); // bb 902 return this.subsumption; 903 } 904 905 public boolean hasSubsumptionElement() { 906 return this.subsumption != null && !this.subsumption.isEmpty(); 907 } 908 909 public boolean hasSubsumption() { 910 return this.subsumption != null && !this.subsumption.isEmpty(); 911 } 912 913 /** 914 * @param value {@link #subsumption} (True if subsumption is supported for this version of the code system.). This is the underlying object with id, value and extensions. The accessor "getSubsumption" gives direct access to the value 915 */ 916 public TerminologyCapabilitiesCodeSystemComponent setSubsumptionElement(BooleanType value) { 917 this.subsumption = value; 918 return this; 919 } 920 921 /** 922 * @return True if subsumption is supported for this version of the code system. 923 */ 924 public boolean getSubsumption() { 925 return this.subsumption == null || this.subsumption.isEmpty() ? false : this.subsumption.getValue(); 926 } 927 928 /** 929 * @param value True if subsumption is supported for this version of the code system. 930 */ 931 public TerminologyCapabilitiesCodeSystemComponent setSubsumption(boolean value) { 932 if (this.subsumption == null) 933 this.subsumption = new BooleanType(); 934 this.subsumption.setValue(value); 935 return this; 936 } 937 938 protected void listChildren(List<Property> children) { 939 super.listChildren(children); 940 children.add(new Property("uri", "canonical(CodeSystem)", "Canonical identifier for the code system, represented as a URI.", 0, 1, uri)); 941 children.add(new Property("version", "", "For the code system, a list of versions that are supported by the server.", 0, java.lang.Integer.MAX_VALUE, version)); 942 children.add(new Property("content", "code", "The extent of the content of the code system (the concepts and codes it defines) are represented in this resource instance.", 0, 1, content)); 943 children.add(new Property("subsumption", "boolean", "True if subsumption is supported for this version of the code system.", 0, 1, subsumption)); 944 } 945 946 @Override 947 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 948 switch (_hash) { 949 case 116076: /*uri*/ return new Property("uri", "canonical(CodeSystem)", "Canonical identifier for the code system, represented as a URI.", 0, 1, uri); 950 case 351608024: /*version*/ return new Property("version", "", "For the code system, a list of versions that are supported by the server.", 0, java.lang.Integer.MAX_VALUE, version); 951 case 951530617: /*content*/ return new Property("content", "code", "The extent of the content of the code system (the concepts and codes it defines) are represented in this resource instance.", 0, 1, content); 952 case -499084711: /*subsumption*/ return new Property("subsumption", "boolean", "True if subsumption is supported for this version of the code system.", 0, 1, subsumption); 953 default: return super.getNamedProperty(_hash, _name, _checkValid); 954 } 955 956 } 957 958 @Override 959 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 960 switch (hash) { 961 case 116076: /*uri*/ return this.uri == null ? new Base[0] : new Base[] {this.uri}; // CanonicalType 962 case 351608024: /*version*/ return this.version == null ? new Base[0] : this.version.toArray(new Base[this.version.size()]); // TerminologyCapabilitiesCodeSystemVersionComponent 963 case 951530617: /*content*/ return this.content == null ? new Base[0] : new Base[] {this.content}; // Enumeration<CodeSystemContentMode> 964 case -499084711: /*subsumption*/ return this.subsumption == null ? new Base[0] : new Base[] {this.subsumption}; // BooleanType 965 default: return super.getProperty(hash, name, checkValid); 966 } 967 968 } 969 970 @Override 971 public Base setProperty(int hash, String name, Base value) throws FHIRException { 972 switch (hash) { 973 case 116076: // uri 974 this.uri = TypeConvertor.castToCanonical(value); // CanonicalType 975 return value; 976 case 351608024: // version 977 this.getVersion().add((TerminologyCapabilitiesCodeSystemVersionComponent) value); // TerminologyCapabilitiesCodeSystemVersionComponent 978 return value; 979 case 951530617: // content 980 value = new CodeSystemContentModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 981 this.content = (Enumeration) value; // Enumeration<CodeSystemContentMode> 982 return value; 983 case -499084711: // subsumption 984 this.subsumption = TypeConvertor.castToBoolean(value); // BooleanType 985 return value; 986 default: return super.setProperty(hash, name, value); 987 } 988 989 } 990 991 @Override 992 public Base setProperty(String name, Base value) throws FHIRException { 993 if (name.equals("uri")) { 994 this.uri = TypeConvertor.castToCanonical(value); // CanonicalType 995 } else if (name.equals("version")) { 996 this.getVersion().add((TerminologyCapabilitiesCodeSystemVersionComponent) value); 997 } else if (name.equals("content")) { 998 value = new CodeSystemContentModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 999 this.content = (Enumeration) value; // Enumeration<CodeSystemContentMode> 1000 } else if (name.equals("subsumption")) { 1001 this.subsumption = TypeConvertor.castToBoolean(value); // BooleanType 1002 } else 1003 return super.setProperty(name, value); 1004 return value; 1005 } 1006 1007 @Override 1008 public void removeChild(String name, Base value) throws FHIRException { 1009 if (name.equals("uri")) { 1010 this.uri = null; 1011 } else if (name.equals("version")) { 1012 this.getVersion().remove((TerminologyCapabilitiesCodeSystemVersionComponent) value); 1013 } else if (name.equals("content")) { 1014 value = new CodeSystemContentModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1015 this.content = (Enumeration) value; // Enumeration<CodeSystemContentMode> 1016 } else if (name.equals("subsumption")) { 1017 this.subsumption = null; 1018 } else 1019 super.removeChild(name, value); 1020 1021 } 1022 1023 @Override 1024 public Base makeProperty(int hash, String name) throws FHIRException { 1025 switch (hash) { 1026 case 116076: return getUriElement(); 1027 case 351608024: return addVersion(); 1028 case 951530617: return getContentElement(); 1029 case -499084711: return getSubsumptionElement(); 1030 default: return super.makeProperty(hash, name); 1031 } 1032 1033 } 1034 1035 @Override 1036 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1037 switch (hash) { 1038 case 116076: /*uri*/ return new String[] {"canonical"}; 1039 case 351608024: /*version*/ return new String[] {}; 1040 case 951530617: /*content*/ return new String[] {"code"}; 1041 case -499084711: /*subsumption*/ return new String[] {"boolean"}; 1042 default: return super.getTypesForProperty(hash, name); 1043 } 1044 1045 } 1046 1047 @Override 1048 public Base addChild(String name) throws FHIRException { 1049 if (name.equals("uri")) { 1050 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.codeSystem.uri"); 1051 } 1052 else if (name.equals("version")) { 1053 return addVersion(); 1054 } 1055 else if (name.equals("content")) { 1056 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.codeSystem.content"); 1057 } 1058 else if (name.equals("subsumption")) { 1059 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.codeSystem.subsumption"); 1060 } 1061 else 1062 return super.addChild(name); 1063 } 1064 1065 public TerminologyCapabilitiesCodeSystemComponent copy() { 1066 TerminologyCapabilitiesCodeSystemComponent dst = new TerminologyCapabilitiesCodeSystemComponent(); 1067 copyValues(dst); 1068 return dst; 1069 } 1070 1071 public void copyValues(TerminologyCapabilitiesCodeSystemComponent dst) { 1072 super.copyValues(dst); 1073 dst.uri = uri == null ? null : uri.copy(); 1074 if (version != null) { 1075 dst.version = new ArrayList<TerminologyCapabilitiesCodeSystemVersionComponent>(); 1076 for (TerminologyCapabilitiesCodeSystemVersionComponent i : version) 1077 dst.version.add(i.copy()); 1078 }; 1079 dst.content = content == null ? null : content.copy(); 1080 dst.subsumption = subsumption == null ? null : subsumption.copy(); 1081 } 1082 1083 @Override 1084 public boolean equalsDeep(Base other_) { 1085 if (!super.equalsDeep(other_)) 1086 return false; 1087 if (!(other_ instanceof TerminologyCapabilitiesCodeSystemComponent)) 1088 return false; 1089 TerminologyCapabilitiesCodeSystemComponent o = (TerminologyCapabilitiesCodeSystemComponent) other_; 1090 return compareDeep(uri, o.uri, true) && compareDeep(version, o.version, true) && compareDeep(content, o.content, true) 1091 && compareDeep(subsumption, o.subsumption, true); 1092 } 1093 1094 @Override 1095 public boolean equalsShallow(Base other_) { 1096 if (!super.equalsShallow(other_)) 1097 return false; 1098 if (!(other_ instanceof TerminologyCapabilitiesCodeSystemComponent)) 1099 return false; 1100 TerminologyCapabilitiesCodeSystemComponent o = (TerminologyCapabilitiesCodeSystemComponent) other_; 1101 return compareValues(uri, o.uri, true) && compareValues(content, o.content, true) && compareValues(subsumption, o.subsumption, true) 1102 ; 1103 } 1104 1105 public boolean isEmpty() { 1106 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(uri, version, content, subsumption 1107 ); 1108 } 1109 1110 public String fhirType() { 1111 return "TerminologyCapabilities.codeSystem"; 1112 1113 } 1114 1115 } 1116 1117 @Block() 1118 public static class TerminologyCapabilitiesCodeSystemVersionComponent extends BackboneElement implements IBaseBackboneElement { 1119 /** 1120 * For version-less code systems, there should be a single version with no identifier. 1121 */ 1122 @Child(name = "code", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1123 @Description(shortDefinition="Version identifier for this version", formalDefinition="For version-less code systems, there should be a single version with no identifier." ) 1124 protected StringType code; 1125 1126 /** 1127 * If this is the default version for this code system. 1128 */ 1129 @Child(name = "isDefault", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1130 @Description(shortDefinition="If this is the default version for this code system", formalDefinition="If this is the default version for this code system." ) 1131 protected BooleanType isDefault; 1132 1133 /** 1134 * If the compositional grammar defined by the code system is supported. 1135 */ 1136 @Child(name = "compositional", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1137 @Description(shortDefinition="If compositional grammar is supported", formalDefinition="If the compositional grammar defined by the code system is supported." ) 1138 protected BooleanType compositional; 1139 1140 /** 1141 * Language Displays supported. 1142 */ 1143 @Child(name = "language", type = {CodeType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1144 @Description(shortDefinition="Language Displays supported", formalDefinition="Language Displays supported." ) 1145 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 1146 protected List<Enumeration<CommonLanguages>> language; 1147 1148 /** 1149 * Filter Properties supported. 1150 */ 1151 @Child(name = "filter", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1152 @Description(shortDefinition="Filter Properties supported", formalDefinition="Filter Properties supported." ) 1153 protected List<TerminologyCapabilitiesCodeSystemVersionFilterComponent> filter; 1154 1155 /** 1156 * Properties supported for $lookup. 1157 */ 1158 @Child(name = "property", type = {CodeType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1159 @Description(shortDefinition="Properties supported for $lookup", formalDefinition="Properties supported for $lookup." ) 1160 protected List<CodeType> property; 1161 1162 private static final long serialVersionUID = 658198795L; 1163 1164 /** 1165 * Constructor 1166 */ 1167 public TerminologyCapabilitiesCodeSystemVersionComponent() { 1168 super(); 1169 } 1170 1171 /** 1172 * @return {@link #code} (For version-less code systems, there should be a single version with no identifier.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1173 */ 1174 public StringType getCodeElement() { 1175 if (this.code == null) 1176 if (Configuration.errorOnAutoCreate()) 1177 throw new Error("Attempt to auto-create TerminologyCapabilitiesCodeSystemVersionComponent.code"); 1178 else if (Configuration.doAutoCreate()) 1179 this.code = new StringType(); // bb 1180 return this.code; 1181 } 1182 1183 public boolean hasCodeElement() { 1184 return this.code != null && !this.code.isEmpty(); 1185 } 1186 1187 public boolean hasCode() { 1188 return this.code != null && !this.code.isEmpty(); 1189 } 1190 1191 /** 1192 * @param value {@link #code} (For version-less code systems, there should be a single version with no identifier.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1193 */ 1194 public TerminologyCapabilitiesCodeSystemVersionComponent setCodeElement(StringType value) { 1195 this.code = value; 1196 return this; 1197 } 1198 1199 /** 1200 * @return For version-less code systems, there should be a single version with no identifier. 1201 */ 1202 public String getCode() { 1203 return this.code == null ? null : this.code.getValue(); 1204 } 1205 1206 /** 1207 * @param value For version-less code systems, there should be a single version with no identifier. 1208 */ 1209 public TerminologyCapabilitiesCodeSystemVersionComponent setCode(String value) { 1210 if (Utilities.noString(value)) 1211 this.code = null; 1212 else { 1213 if (this.code == null) 1214 this.code = new StringType(); 1215 this.code.setValue(value); 1216 } 1217 return this; 1218 } 1219 1220 /** 1221 * @return {@link #isDefault} (If this is the default version for this code system.). This is the underlying object with id, value and extensions. The accessor "getIsDefault" gives direct access to the value 1222 */ 1223 public BooleanType getIsDefaultElement() { 1224 if (this.isDefault == null) 1225 if (Configuration.errorOnAutoCreate()) 1226 throw new Error("Attempt to auto-create TerminologyCapabilitiesCodeSystemVersionComponent.isDefault"); 1227 else if (Configuration.doAutoCreate()) 1228 this.isDefault = new BooleanType(); // bb 1229 return this.isDefault; 1230 } 1231 1232 public boolean hasIsDefaultElement() { 1233 return this.isDefault != null && !this.isDefault.isEmpty(); 1234 } 1235 1236 public boolean hasIsDefault() { 1237 return this.isDefault != null && !this.isDefault.isEmpty(); 1238 } 1239 1240 /** 1241 * @param value {@link #isDefault} (If this is the default version for this code system.). This is the underlying object with id, value and extensions. The accessor "getIsDefault" gives direct access to the value 1242 */ 1243 public TerminologyCapabilitiesCodeSystemVersionComponent setIsDefaultElement(BooleanType value) { 1244 this.isDefault = value; 1245 return this; 1246 } 1247 1248 /** 1249 * @return If this is the default version for this code system. 1250 */ 1251 public boolean getIsDefault() { 1252 return this.isDefault == null || this.isDefault.isEmpty() ? false : this.isDefault.getValue(); 1253 } 1254 1255 /** 1256 * @param value If this is the default version for this code system. 1257 */ 1258 public TerminologyCapabilitiesCodeSystemVersionComponent setIsDefault(boolean value) { 1259 if (this.isDefault == null) 1260 this.isDefault = new BooleanType(); 1261 this.isDefault.setValue(value); 1262 return this; 1263 } 1264 1265 /** 1266 * @return {@link #compositional} (If the compositional grammar defined by the code system is supported.). This is the underlying object with id, value and extensions. The accessor "getCompositional" gives direct access to the value 1267 */ 1268 public BooleanType getCompositionalElement() { 1269 if (this.compositional == null) 1270 if (Configuration.errorOnAutoCreate()) 1271 throw new Error("Attempt to auto-create TerminologyCapabilitiesCodeSystemVersionComponent.compositional"); 1272 else if (Configuration.doAutoCreate()) 1273 this.compositional = new BooleanType(); // bb 1274 return this.compositional; 1275 } 1276 1277 public boolean hasCompositionalElement() { 1278 return this.compositional != null && !this.compositional.isEmpty(); 1279 } 1280 1281 public boolean hasCompositional() { 1282 return this.compositional != null && !this.compositional.isEmpty(); 1283 } 1284 1285 /** 1286 * @param value {@link #compositional} (If the compositional grammar defined by the code system is supported.). This is the underlying object with id, value and extensions. The accessor "getCompositional" gives direct access to the value 1287 */ 1288 public TerminologyCapabilitiesCodeSystemVersionComponent setCompositionalElement(BooleanType value) { 1289 this.compositional = value; 1290 return this; 1291 } 1292 1293 /** 1294 * @return If the compositional grammar defined by the code system is supported. 1295 */ 1296 public boolean getCompositional() { 1297 return this.compositional == null || this.compositional.isEmpty() ? false : this.compositional.getValue(); 1298 } 1299 1300 /** 1301 * @param value If the compositional grammar defined by the code system is supported. 1302 */ 1303 public TerminologyCapabilitiesCodeSystemVersionComponent setCompositional(boolean value) { 1304 if (this.compositional == null) 1305 this.compositional = new BooleanType(); 1306 this.compositional.setValue(value); 1307 return this; 1308 } 1309 1310 /** 1311 * @return {@link #language} (Language Displays supported.) 1312 */ 1313 public List<Enumeration<CommonLanguages>> getLanguage() { 1314 if (this.language == null) 1315 this.language = new ArrayList<Enumeration<CommonLanguages>>(); 1316 return this.language; 1317 } 1318 1319 /** 1320 * @return Returns a reference to <code>this</code> for easy method chaining 1321 */ 1322 public TerminologyCapabilitiesCodeSystemVersionComponent setLanguage(List<Enumeration<CommonLanguages>> theLanguage) { 1323 this.language = theLanguage; 1324 return this; 1325 } 1326 1327 public boolean hasLanguage() { 1328 if (this.language == null) 1329 return false; 1330 for (Enumeration<CommonLanguages> item : this.language) 1331 if (!item.isEmpty()) 1332 return true; 1333 return false; 1334 } 1335 1336 /** 1337 * @return {@link #language} (Language Displays supported.) 1338 */ 1339 public Enumeration<CommonLanguages> addLanguageElement() {//2 1340 Enumeration<CommonLanguages> t = new Enumeration<CommonLanguages>(new CommonLanguagesEnumFactory()); 1341 if (this.language == null) 1342 this.language = new ArrayList<Enumeration<CommonLanguages>>(); 1343 this.language.add(t); 1344 return t; 1345 } 1346 1347 /** 1348 * @param value {@link #language} (Language Displays supported.) 1349 */ 1350 public TerminologyCapabilitiesCodeSystemVersionComponent addLanguage(CommonLanguages value) { //1 1351 Enumeration<CommonLanguages> t = new Enumeration<CommonLanguages>(new CommonLanguagesEnumFactory()); 1352 t.setValue(value); 1353 if (this.language == null) 1354 this.language = new ArrayList<Enumeration<CommonLanguages>>(); 1355 this.language.add(t); 1356 return this; 1357 } 1358 1359 /** 1360 * @param value {@link #language} (Language Displays supported.) 1361 */ 1362 public boolean hasLanguage(CommonLanguages value) { 1363 if (this.language == null) 1364 return false; 1365 for (Enumeration<CommonLanguages> v : this.language) 1366 if (v.getValue().equals(value)) // code 1367 return true; 1368 return false; 1369 } 1370 1371 /** 1372 * @return {@link #filter} (Filter Properties supported.) 1373 */ 1374 public List<TerminologyCapabilitiesCodeSystemVersionFilterComponent> getFilter() { 1375 if (this.filter == null) 1376 this.filter = new ArrayList<TerminologyCapabilitiesCodeSystemVersionFilterComponent>(); 1377 return this.filter; 1378 } 1379 1380 /** 1381 * @return Returns a reference to <code>this</code> for easy method chaining 1382 */ 1383 public TerminologyCapabilitiesCodeSystemVersionComponent setFilter(List<TerminologyCapabilitiesCodeSystemVersionFilterComponent> theFilter) { 1384 this.filter = theFilter; 1385 return this; 1386 } 1387 1388 public boolean hasFilter() { 1389 if (this.filter == null) 1390 return false; 1391 for (TerminologyCapabilitiesCodeSystemVersionFilterComponent item : this.filter) 1392 if (!item.isEmpty()) 1393 return true; 1394 return false; 1395 } 1396 1397 public TerminologyCapabilitiesCodeSystemVersionFilterComponent addFilter() { //3 1398 TerminologyCapabilitiesCodeSystemVersionFilterComponent t = new TerminologyCapabilitiesCodeSystemVersionFilterComponent(); 1399 if (this.filter == null) 1400 this.filter = new ArrayList<TerminologyCapabilitiesCodeSystemVersionFilterComponent>(); 1401 this.filter.add(t); 1402 return t; 1403 } 1404 1405 public TerminologyCapabilitiesCodeSystemVersionComponent addFilter(TerminologyCapabilitiesCodeSystemVersionFilterComponent t) { //3 1406 if (t == null) 1407 return this; 1408 if (this.filter == null) 1409 this.filter = new ArrayList<TerminologyCapabilitiesCodeSystemVersionFilterComponent>(); 1410 this.filter.add(t); 1411 return this; 1412 } 1413 1414 /** 1415 * @return The first repetition of repeating field {@link #filter}, creating it if it does not already exist {3} 1416 */ 1417 public TerminologyCapabilitiesCodeSystemVersionFilterComponent getFilterFirstRep() { 1418 if (getFilter().isEmpty()) { 1419 addFilter(); 1420 } 1421 return getFilter().get(0); 1422 } 1423 1424 /** 1425 * @return {@link #property} (Properties supported for $lookup.) 1426 */ 1427 public List<CodeType> getProperty() { 1428 if (this.property == null) 1429 this.property = new ArrayList<CodeType>(); 1430 return this.property; 1431 } 1432 1433 /** 1434 * @return Returns a reference to <code>this</code> for easy method chaining 1435 */ 1436 public TerminologyCapabilitiesCodeSystemVersionComponent setProperty(List<CodeType> theProperty) { 1437 this.property = theProperty; 1438 return this; 1439 } 1440 1441 public boolean hasProperty() { 1442 if (this.property == null) 1443 return false; 1444 for (CodeType item : this.property) 1445 if (!item.isEmpty()) 1446 return true; 1447 return false; 1448 } 1449 1450 /** 1451 * @return {@link #property} (Properties supported for $lookup.) 1452 */ 1453 public CodeType addPropertyElement() {//2 1454 CodeType t = new CodeType(); 1455 if (this.property == null) 1456 this.property = new ArrayList<CodeType>(); 1457 this.property.add(t); 1458 return t; 1459 } 1460 1461 /** 1462 * @param value {@link #property} (Properties supported for $lookup.) 1463 */ 1464 public TerminologyCapabilitiesCodeSystemVersionComponent addProperty(String value) { //1 1465 CodeType t = new CodeType(); 1466 t.setValue(value); 1467 if (this.property == null) 1468 this.property = new ArrayList<CodeType>(); 1469 this.property.add(t); 1470 return this; 1471 } 1472 1473 /** 1474 * @param value {@link #property} (Properties supported for $lookup.) 1475 */ 1476 public boolean hasProperty(String value) { 1477 if (this.property == null) 1478 return false; 1479 for (CodeType v : this.property) 1480 if (v.getValue().equals(value)) // code 1481 return true; 1482 return false; 1483 } 1484 1485 protected void listChildren(List<Property> children) { 1486 super.listChildren(children); 1487 children.add(new Property("code", "string", "For version-less code systems, there should be a single version with no identifier.", 0, 1, code)); 1488 children.add(new Property("isDefault", "boolean", "If this is the default version for this code system.", 0, 1, isDefault)); 1489 children.add(new Property("compositional", "boolean", "If the compositional grammar defined by the code system is supported.", 0, 1, compositional)); 1490 children.add(new Property("language", "code", "Language Displays supported.", 0, java.lang.Integer.MAX_VALUE, language)); 1491 children.add(new Property("filter", "", "Filter Properties supported.", 0, java.lang.Integer.MAX_VALUE, filter)); 1492 children.add(new Property("property", "code", "Properties supported for $lookup.", 0, java.lang.Integer.MAX_VALUE, property)); 1493 } 1494 1495 @Override 1496 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1497 switch (_hash) { 1498 case 3059181: /*code*/ return new Property("code", "string", "For version-less code systems, there should be a single version with no identifier.", 0, 1, code); 1499 case 965025207: /*isDefault*/ return new Property("isDefault", "boolean", "If this is the default version for this code system.", 0, 1, isDefault); 1500 case 1248023381: /*compositional*/ return new Property("compositional", "boolean", "If the compositional grammar defined by the code system is supported.", 0, 1, compositional); 1501 case -1613589672: /*language*/ return new Property("language", "code", "Language Displays supported.", 0, java.lang.Integer.MAX_VALUE, language); 1502 case -1274492040: /*filter*/ return new Property("filter", "", "Filter Properties supported.", 0, java.lang.Integer.MAX_VALUE, filter); 1503 case -993141291: /*property*/ return new Property("property", "code", "Properties supported for $lookup.", 0, java.lang.Integer.MAX_VALUE, property); 1504 default: return super.getNamedProperty(_hash, _name, _checkValid); 1505 } 1506 1507 } 1508 1509 @Override 1510 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1511 switch (hash) { 1512 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // StringType 1513 case 965025207: /*isDefault*/ return this.isDefault == null ? new Base[0] : new Base[] {this.isDefault}; // BooleanType 1514 case 1248023381: /*compositional*/ return this.compositional == null ? new Base[0] : new Base[] {this.compositional}; // BooleanType 1515 case -1613589672: /*language*/ return this.language == null ? new Base[0] : this.language.toArray(new Base[this.language.size()]); // Enumeration<CommonLanguages> 1516 case -1274492040: /*filter*/ return this.filter == null ? new Base[0] : this.filter.toArray(new Base[this.filter.size()]); // TerminologyCapabilitiesCodeSystemVersionFilterComponent 1517 case -993141291: /*property*/ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // CodeType 1518 default: return super.getProperty(hash, name, checkValid); 1519 } 1520 1521 } 1522 1523 @Override 1524 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1525 switch (hash) { 1526 case 3059181: // code 1527 this.code = TypeConvertor.castToString(value); // StringType 1528 return value; 1529 case 965025207: // isDefault 1530 this.isDefault = TypeConvertor.castToBoolean(value); // BooleanType 1531 return value; 1532 case 1248023381: // compositional 1533 this.compositional = TypeConvertor.castToBoolean(value); // BooleanType 1534 return value; 1535 case -1613589672: // language 1536 value = new CommonLanguagesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1537 this.getLanguage().add((Enumeration) value); // Enumeration<CommonLanguages> 1538 return value; 1539 case -1274492040: // filter 1540 this.getFilter().add((TerminologyCapabilitiesCodeSystemVersionFilterComponent) value); // TerminologyCapabilitiesCodeSystemVersionFilterComponent 1541 return value; 1542 case -993141291: // property 1543 this.getProperty().add(TypeConvertor.castToCode(value)); // CodeType 1544 return value; 1545 default: return super.setProperty(hash, name, value); 1546 } 1547 1548 } 1549 1550 @Override 1551 public Base setProperty(String name, Base value) throws FHIRException { 1552 if (name.equals("code")) { 1553 this.code = TypeConvertor.castToString(value); // StringType 1554 } else if (name.equals("isDefault")) { 1555 this.isDefault = TypeConvertor.castToBoolean(value); // BooleanType 1556 } else if (name.equals("compositional")) { 1557 this.compositional = TypeConvertor.castToBoolean(value); // BooleanType 1558 } else if (name.equals("language")) { 1559 value = new CommonLanguagesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1560 this.getLanguage().add((Enumeration) value); 1561 } else if (name.equals("filter")) { 1562 this.getFilter().add((TerminologyCapabilitiesCodeSystemVersionFilterComponent) value); 1563 } else if (name.equals("property")) { 1564 this.getProperty().add(TypeConvertor.castToCode(value)); 1565 } else 1566 return super.setProperty(name, value); 1567 return value; 1568 } 1569 1570 @Override 1571 public void removeChild(String name, Base value) throws FHIRException { 1572 if (name.equals("code")) { 1573 this.code = null; 1574 } else if (name.equals("isDefault")) { 1575 this.isDefault = null; 1576 } else if (name.equals("compositional")) { 1577 this.compositional = null; 1578 } else if (name.equals("language")) { 1579 value = new CommonLanguagesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1580 this.getLanguage().remove((Enumeration) value); 1581 } else if (name.equals("filter")) { 1582 this.getFilter().remove((TerminologyCapabilitiesCodeSystemVersionFilterComponent) value); 1583 } else if (name.equals("property")) { 1584 this.getProperty().remove(value); 1585 } else 1586 super.removeChild(name, value); 1587 1588 } 1589 1590 @Override 1591 public Base makeProperty(int hash, String name) throws FHIRException { 1592 switch (hash) { 1593 case 3059181: return getCodeElement(); 1594 case 965025207: return getIsDefaultElement(); 1595 case 1248023381: return getCompositionalElement(); 1596 case -1613589672: return addLanguageElement(); 1597 case -1274492040: return addFilter(); 1598 case -993141291: return addPropertyElement(); 1599 default: return super.makeProperty(hash, name); 1600 } 1601 1602 } 1603 1604 @Override 1605 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1606 switch (hash) { 1607 case 3059181: /*code*/ return new String[] {"string"}; 1608 case 965025207: /*isDefault*/ return new String[] {"boolean"}; 1609 case 1248023381: /*compositional*/ return new String[] {"boolean"}; 1610 case -1613589672: /*language*/ return new String[] {"code"}; 1611 case -1274492040: /*filter*/ return new String[] {}; 1612 case -993141291: /*property*/ return new String[] {"code"}; 1613 default: return super.getTypesForProperty(hash, name); 1614 } 1615 1616 } 1617 1618 @Override 1619 public Base addChild(String name) throws FHIRException { 1620 if (name.equals("code")) { 1621 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.codeSystem.version.code"); 1622 } 1623 else if (name.equals("isDefault")) { 1624 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.codeSystem.version.isDefault"); 1625 } 1626 else if (name.equals("compositional")) { 1627 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.codeSystem.version.compositional"); 1628 } 1629 else if (name.equals("language")) { 1630 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.codeSystem.version.language"); 1631 } 1632 else if (name.equals("filter")) { 1633 return addFilter(); 1634 } 1635 else if (name.equals("property")) { 1636 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.codeSystem.version.property"); 1637 } 1638 else 1639 return super.addChild(name); 1640 } 1641 1642 public TerminologyCapabilitiesCodeSystemVersionComponent copy() { 1643 TerminologyCapabilitiesCodeSystemVersionComponent dst = new TerminologyCapabilitiesCodeSystemVersionComponent(); 1644 copyValues(dst); 1645 return dst; 1646 } 1647 1648 public void copyValues(TerminologyCapabilitiesCodeSystemVersionComponent dst) { 1649 super.copyValues(dst); 1650 dst.code = code == null ? null : code.copy(); 1651 dst.isDefault = isDefault == null ? null : isDefault.copy(); 1652 dst.compositional = compositional == null ? null : compositional.copy(); 1653 if (language != null) { 1654 dst.language = new ArrayList<Enumeration<CommonLanguages>>(); 1655 for (Enumeration<CommonLanguages> i : language) 1656 dst.language.add(i.copy()); 1657 }; 1658 if (filter != null) { 1659 dst.filter = new ArrayList<TerminologyCapabilitiesCodeSystemVersionFilterComponent>(); 1660 for (TerminologyCapabilitiesCodeSystemVersionFilterComponent i : filter) 1661 dst.filter.add(i.copy()); 1662 }; 1663 if (property != null) { 1664 dst.property = new ArrayList<CodeType>(); 1665 for (CodeType i : property) 1666 dst.property.add(i.copy()); 1667 }; 1668 } 1669 1670 @Override 1671 public boolean equalsDeep(Base other_) { 1672 if (!super.equalsDeep(other_)) 1673 return false; 1674 if (!(other_ instanceof TerminologyCapabilitiesCodeSystemVersionComponent)) 1675 return false; 1676 TerminologyCapabilitiesCodeSystemVersionComponent o = (TerminologyCapabilitiesCodeSystemVersionComponent) other_; 1677 return compareDeep(code, o.code, true) && compareDeep(isDefault, o.isDefault, true) && compareDeep(compositional, o.compositional, true) 1678 && compareDeep(language, o.language, true) && compareDeep(filter, o.filter, true) && compareDeep(property, o.property, true) 1679 ; 1680 } 1681 1682 @Override 1683 public boolean equalsShallow(Base other_) { 1684 if (!super.equalsShallow(other_)) 1685 return false; 1686 if (!(other_ instanceof TerminologyCapabilitiesCodeSystemVersionComponent)) 1687 return false; 1688 TerminologyCapabilitiesCodeSystemVersionComponent o = (TerminologyCapabilitiesCodeSystemVersionComponent) other_; 1689 return compareValues(code, o.code, true) && compareValues(isDefault, o.isDefault, true) && compareValues(compositional, o.compositional, true) 1690 && compareValues(language, o.language, true) && compareValues(property, o.property, true); 1691 } 1692 1693 public boolean isEmpty() { 1694 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, isDefault, compositional 1695 , language, filter, property); 1696 } 1697 1698 public String fhirType() { 1699 return "TerminologyCapabilities.codeSystem.version"; 1700 1701 } 1702 1703 } 1704 1705 @Block() 1706 public static class TerminologyCapabilitiesCodeSystemVersionFilterComponent extends BackboneElement implements IBaseBackboneElement { 1707 /** 1708 * Code of the property supported. 1709 */ 1710 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1711 @Description(shortDefinition="Code of the property supported", formalDefinition="Code of the property supported." ) 1712 protected CodeType code; 1713 1714 /** 1715 * Operations supported for the property. 1716 */ 1717 @Child(name = "op", type = {CodeType.class}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1718 @Description(shortDefinition="Operations supported for the property", formalDefinition="Operations supported for the property." ) 1719 protected List<CodeType> op; 1720 1721 private static final long serialVersionUID = -489160282L; 1722 1723 /** 1724 * Constructor 1725 */ 1726 public TerminologyCapabilitiesCodeSystemVersionFilterComponent() { 1727 super(); 1728 } 1729 1730 /** 1731 * Constructor 1732 */ 1733 public TerminologyCapabilitiesCodeSystemVersionFilterComponent(String code, String op) { 1734 super(); 1735 this.setCode(code); 1736 this.addOp(op); 1737 } 1738 1739 /** 1740 * @return {@link #code} (Code of the property supported.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1741 */ 1742 public CodeType getCodeElement() { 1743 if (this.code == null) 1744 if (Configuration.errorOnAutoCreate()) 1745 throw new Error("Attempt to auto-create TerminologyCapabilitiesCodeSystemVersionFilterComponent.code"); 1746 else if (Configuration.doAutoCreate()) 1747 this.code = new CodeType(); // bb 1748 return this.code; 1749 } 1750 1751 public boolean hasCodeElement() { 1752 return this.code != null && !this.code.isEmpty(); 1753 } 1754 1755 public boolean hasCode() { 1756 return this.code != null && !this.code.isEmpty(); 1757 } 1758 1759 /** 1760 * @param value {@link #code} (Code of the property supported.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1761 */ 1762 public TerminologyCapabilitiesCodeSystemVersionFilterComponent setCodeElement(CodeType value) { 1763 this.code = value; 1764 return this; 1765 } 1766 1767 /** 1768 * @return Code of the property supported. 1769 */ 1770 public String getCode() { 1771 return this.code == null ? null : this.code.getValue(); 1772 } 1773 1774 /** 1775 * @param value Code of the property supported. 1776 */ 1777 public TerminologyCapabilitiesCodeSystemVersionFilterComponent setCode(String value) { 1778 if (this.code == null) 1779 this.code = new CodeType(); 1780 this.code.setValue(value); 1781 return this; 1782 } 1783 1784 /** 1785 * @return {@link #op} (Operations supported for the property.) 1786 */ 1787 public List<CodeType> getOp() { 1788 if (this.op == null) 1789 this.op = new ArrayList<CodeType>(); 1790 return this.op; 1791 } 1792 1793 /** 1794 * @return Returns a reference to <code>this</code> for easy method chaining 1795 */ 1796 public TerminologyCapabilitiesCodeSystemVersionFilterComponent setOp(List<CodeType> theOp) { 1797 this.op = theOp; 1798 return this; 1799 } 1800 1801 public boolean hasOp() { 1802 if (this.op == null) 1803 return false; 1804 for (CodeType item : this.op) 1805 if (!item.isEmpty()) 1806 return true; 1807 return false; 1808 } 1809 1810 /** 1811 * @return {@link #op} (Operations supported for the property.) 1812 */ 1813 public CodeType addOpElement() {//2 1814 CodeType t = new CodeType(); 1815 if (this.op == null) 1816 this.op = new ArrayList<CodeType>(); 1817 this.op.add(t); 1818 return t; 1819 } 1820 1821 /** 1822 * @param value {@link #op} (Operations supported for the property.) 1823 */ 1824 public TerminologyCapabilitiesCodeSystemVersionFilterComponent addOp(String value) { //1 1825 CodeType t = new CodeType(); 1826 t.setValue(value); 1827 if (this.op == null) 1828 this.op = new ArrayList<CodeType>(); 1829 this.op.add(t); 1830 return this; 1831 } 1832 1833 /** 1834 * @param value {@link #op} (Operations supported for the property.) 1835 */ 1836 public boolean hasOp(String value) { 1837 if (this.op == null) 1838 return false; 1839 for (CodeType v : this.op) 1840 if (v.getValue().equals(value)) // code 1841 return true; 1842 return false; 1843 } 1844 1845 protected void listChildren(List<Property> children) { 1846 super.listChildren(children); 1847 children.add(new Property("code", "code", "Code of the property supported.", 0, 1, code)); 1848 children.add(new Property("op", "code", "Operations supported for the property.", 0, java.lang.Integer.MAX_VALUE, op)); 1849 } 1850 1851 @Override 1852 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1853 switch (_hash) { 1854 case 3059181: /*code*/ return new Property("code", "code", "Code of the property supported.", 0, 1, code); 1855 case 3553: /*op*/ return new Property("op", "code", "Operations supported for the property.", 0, java.lang.Integer.MAX_VALUE, op); 1856 default: return super.getNamedProperty(_hash, _name, _checkValid); 1857 } 1858 1859 } 1860 1861 @Override 1862 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1863 switch (hash) { 1864 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 1865 case 3553: /*op*/ return this.op == null ? new Base[0] : this.op.toArray(new Base[this.op.size()]); // CodeType 1866 default: return super.getProperty(hash, name, checkValid); 1867 } 1868 1869 } 1870 1871 @Override 1872 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1873 switch (hash) { 1874 case 3059181: // code 1875 this.code = TypeConvertor.castToCode(value); // CodeType 1876 return value; 1877 case 3553: // op 1878 this.getOp().add(TypeConvertor.castToCode(value)); // CodeType 1879 return value; 1880 default: return super.setProperty(hash, name, value); 1881 } 1882 1883 } 1884 1885 @Override 1886 public Base setProperty(String name, Base value) throws FHIRException { 1887 if (name.equals("code")) { 1888 this.code = TypeConvertor.castToCode(value); // CodeType 1889 } else if (name.equals("op")) { 1890 this.getOp().add(TypeConvertor.castToCode(value)); 1891 } else 1892 return super.setProperty(name, value); 1893 return value; 1894 } 1895 1896 @Override 1897 public void removeChild(String name, Base value) throws FHIRException { 1898 if (name.equals("code")) { 1899 this.code = null; 1900 } else if (name.equals("op")) { 1901 this.getOp().remove(value); 1902 } else 1903 super.removeChild(name, value); 1904 1905 } 1906 1907 @Override 1908 public Base makeProperty(int hash, String name) throws FHIRException { 1909 switch (hash) { 1910 case 3059181: return getCodeElement(); 1911 case 3553: return addOpElement(); 1912 default: return super.makeProperty(hash, name); 1913 } 1914 1915 } 1916 1917 @Override 1918 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1919 switch (hash) { 1920 case 3059181: /*code*/ return new String[] {"code"}; 1921 case 3553: /*op*/ return new String[] {"code"}; 1922 default: return super.getTypesForProperty(hash, name); 1923 } 1924 1925 } 1926 1927 @Override 1928 public Base addChild(String name) throws FHIRException { 1929 if (name.equals("code")) { 1930 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.codeSystem.version.filter.code"); 1931 } 1932 else if (name.equals("op")) { 1933 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.codeSystem.version.filter.op"); 1934 } 1935 else 1936 return super.addChild(name); 1937 } 1938 1939 public TerminologyCapabilitiesCodeSystemVersionFilterComponent copy() { 1940 TerminologyCapabilitiesCodeSystemVersionFilterComponent dst = new TerminologyCapabilitiesCodeSystemVersionFilterComponent(); 1941 copyValues(dst); 1942 return dst; 1943 } 1944 1945 public void copyValues(TerminologyCapabilitiesCodeSystemVersionFilterComponent dst) { 1946 super.copyValues(dst); 1947 dst.code = code == null ? null : code.copy(); 1948 if (op != null) { 1949 dst.op = new ArrayList<CodeType>(); 1950 for (CodeType i : op) 1951 dst.op.add(i.copy()); 1952 }; 1953 } 1954 1955 @Override 1956 public boolean equalsDeep(Base other_) { 1957 if (!super.equalsDeep(other_)) 1958 return false; 1959 if (!(other_ instanceof TerminologyCapabilitiesCodeSystemVersionFilterComponent)) 1960 return false; 1961 TerminologyCapabilitiesCodeSystemVersionFilterComponent o = (TerminologyCapabilitiesCodeSystemVersionFilterComponent) other_; 1962 return compareDeep(code, o.code, true) && compareDeep(op, o.op, true); 1963 } 1964 1965 @Override 1966 public boolean equalsShallow(Base other_) { 1967 if (!super.equalsShallow(other_)) 1968 return false; 1969 if (!(other_ instanceof TerminologyCapabilitiesCodeSystemVersionFilterComponent)) 1970 return false; 1971 TerminologyCapabilitiesCodeSystemVersionFilterComponent o = (TerminologyCapabilitiesCodeSystemVersionFilterComponent) other_; 1972 return compareValues(code, o.code, true) && compareValues(op, o.op, true); 1973 } 1974 1975 public boolean isEmpty() { 1976 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, op); 1977 } 1978 1979 public String fhirType() { 1980 return "TerminologyCapabilities.codeSystem.version.filter"; 1981 1982 } 1983 1984 } 1985 1986 @Block() 1987 public static class TerminologyCapabilitiesExpansionComponent extends BackboneElement implements IBaseBackboneElement { 1988 /** 1989 * Whether the server can return nested value sets. 1990 */ 1991 @Child(name = "hierarchical", type = {BooleanType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1992 @Description(shortDefinition="Whether the server can return nested value sets", formalDefinition="Whether the server can return nested value sets." ) 1993 protected BooleanType hierarchical; 1994 1995 /** 1996 * Whether the server supports paging on expansion. 1997 */ 1998 @Child(name = "paging", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1999 @Description(shortDefinition="Whether the server supports paging on expansion", formalDefinition="Whether the server supports paging on expansion." ) 2000 protected BooleanType paging; 2001 2002 /** 2003 * True if requests for incomplete expansions are allowed. 2004 */ 2005 @Child(name = "incomplete", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2006 @Description(shortDefinition="Allow request for incomplete expansions?", formalDefinition="True if requests for incomplete expansions are allowed." ) 2007 protected BooleanType incomplete; 2008 2009 /** 2010 * Supported expansion parameter. 2011 */ 2012 @Child(name = "parameter", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2013 @Description(shortDefinition="Supported expansion parameter", formalDefinition="Supported expansion parameter." ) 2014 protected List<TerminologyCapabilitiesExpansionParameterComponent> parameter; 2015 2016 /** 2017 * Documentation about text searching works. 2018 */ 2019 @Child(name = "textFilter", type = {MarkdownType.class}, order=5, min=0, max=1, modifier=false, summary=false) 2020 @Description(shortDefinition="Documentation about text searching works", formalDefinition="Documentation about text searching works." ) 2021 protected MarkdownType textFilter; 2022 2023 private static final long serialVersionUID = -1011350616L; 2024 2025 /** 2026 * Constructor 2027 */ 2028 public TerminologyCapabilitiesExpansionComponent() { 2029 super(); 2030 } 2031 2032 /** 2033 * @return {@link #hierarchical} (Whether the server can return nested value sets.). This is the underlying object with id, value and extensions. The accessor "getHierarchical" gives direct access to the value 2034 */ 2035 public BooleanType getHierarchicalElement() { 2036 if (this.hierarchical == null) 2037 if (Configuration.errorOnAutoCreate()) 2038 throw new Error("Attempt to auto-create TerminologyCapabilitiesExpansionComponent.hierarchical"); 2039 else if (Configuration.doAutoCreate()) 2040 this.hierarchical = new BooleanType(); // bb 2041 return this.hierarchical; 2042 } 2043 2044 public boolean hasHierarchicalElement() { 2045 return this.hierarchical != null && !this.hierarchical.isEmpty(); 2046 } 2047 2048 public boolean hasHierarchical() { 2049 return this.hierarchical != null && !this.hierarchical.isEmpty(); 2050 } 2051 2052 /** 2053 * @param value {@link #hierarchical} (Whether the server can return nested value sets.). This is the underlying object with id, value and extensions. The accessor "getHierarchical" gives direct access to the value 2054 */ 2055 public TerminologyCapabilitiesExpansionComponent setHierarchicalElement(BooleanType value) { 2056 this.hierarchical = value; 2057 return this; 2058 } 2059 2060 /** 2061 * @return Whether the server can return nested value sets. 2062 */ 2063 public boolean getHierarchical() { 2064 return this.hierarchical == null || this.hierarchical.isEmpty() ? false : this.hierarchical.getValue(); 2065 } 2066 2067 /** 2068 * @param value Whether the server can return nested value sets. 2069 */ 2070 public TerminologyCapabilitiesExpansionComponent setHierarchical(boolean value) { 2071 if (this.hierarchical == null) 2072 this.hierarchical = new BooleanType(); 2073 this.hierarchical.setValue(value); 2074 return this; 2075 } 2076 2077 /** 2078 * @return {@link #paging} (Whether the server supports paging on expansion.). This is the underlying object with id, value and extensions. The accessor "getPaging" gives direct access to the value 2079 */ 2080 public BooleanType getPagingElement() { 2081 if (this.paging == null) 2082 if (Configuration.errorOnAutoCreate()) 2083 throw new Error("Attempt to auto-create TerminologyCapabilitiesExpansionComponent.paging"); 2084 else if (Configuration.doAutoCreate()) 2085 this.paging = new BooleanType(); // bb 2086 return this.paging; 2087 } 2088 2089 public boolean hasPagingElement() { 2090 return this.paging != null && !this.paging.isEmpty(); 2091 } 2092 2093 public boolean hasPaging() { 2094 return this.paging != null && !this.paging.isEmpty(); 2095 } 2096 2097 /** 2098 * @param value {@link #paging} (Whether the server supports paging on expansion.). This is the underlying object with id, value and extensions. The accessor "getPaging" gives direct access to the value 2099 */ 2100 public TerminologyCapabilitiesExpansionComponent setPagingElement(BooleanType value) { 2101 this.paging = value; 2102 return this; 2103 } 2104 2105 /** 2106 * @return Whether the server supports paging on expansion. 2107 */ 2108 public boolean getPaging() { 2109 return this.paging == null || this.paging.isEmpty() ? false : this.paging.getValue(); 2110 } 2111 2112 /** 2113 * @param value Whether the server supports paging on expansion. 2114 */ 2115 public TerminologyCapabilitiesExpansionComponent setPaging(boolean value) { 2116 if (this.paging == null) 2117 this.paging = new BooleanType(); 2118 this.paging.setValue(value); 2119 return this; 2120 } 2121 2122 /** 2123 * @return {@link #incomplete} (True if requests for incomplete expansions are allowed.). This is the underlying object with id, value and extensions. The accessor "getIncomplete" gives direct access to the value 2124 */ 2125 public BooleanType getIncompleteElement() { 2126 if (this.incomplete == null) 2127 if (Configuration.errorOnAutoCreate()) 2128 throw new Error("Attempt to auto-create TerminologyCapabilitiesExpansionComponent.incomplete"); 2129 else if (Configuration.doAutoCreate()) 2130 this.incomplete = new BooleanType(); // bb 2131 return this.incomplete; 2132 } 2133 2134 public boolean hasIncompleteElement() { 2135 return this.incomplete != null && !this.incomplete.isEmpty(); 2136 } 2137 2138 public boolean hasIncomplete() { 2139 return this.incomplete != null && !this.incomplete.isEmpty(); 2140 } 2141 2142 /** 2143 * @param value {@link #incomplete} (True if requests for incomplete expansions are allowed.). This is the underlying object with id, value and extensions. The accessor "getIncomplete" gives direct access to the value 2144 */ 2145 public TerminologyCapabilitiesExpansionComponent setIncompleteElement(BooleanType value) { 2146 this.incomplete = value; 2147 return this; 2148 } 2149 2150 /** 2151 * @return True if requests for incomplete expansions are allowed. 2152 */ 2153 public boolean getIncomplete() { 2154 return this.incomplete == null || this.incomplete.isEmpty() ? false : this.incomplete.getValue(); 2155 } 2156 2157 /** 2158 * @param value True if requests for incomplete expansions are allowed. 2159 */ 2160 public TerminologyCapabilitiesExpansionComponent setIncomplete(boolean value) { 2161 if (this.incomplete == null) 2162 this.incomplete = new BooleanType(); 2163 this.incomplete.setValue(value); 2164 return this; 2165 } 2166 2167 /** 2168 * @return {@link #parameter} (Supported expansion parameter.) 2169 */ 2170 public List<TerminologyCapabilitiesExpansionParameterComponent> getParameter() { 2171 if (this.parameter == null) 2172 this.parameter = new ArrayList<TerminologyCapabilitiesExpansionParameterComponent>(); 2173 return this.parameter; 2174 } 2175 2176 /** 2177 * @return Returns a reference to <code>this</code> for easy method chaining 2178 */ 2179 public TerminologyCapabilitiesExpansionComponent setParameter(List<TerminologyCapabilitiesExpansionParameterComponent> theParameter) { 2180 this.parameter = theParameter; 2181 return this; 2182 } 2183 2184 public boolean hasParameter() { 2185 if (this.parameter == null) 2186 return false; 2187 for (TerminologyCapabilitiesExpansionParameterComponent item : this.parameter) 2188 if (!item.isEmpty()) 2189 return true; 2190 return false; 2191 } 2192 2193 public TerminologyCapabilitiesExpansionParameterComponent addParameter() { //3 2194 TerminologyCapabilitiesExpansionParameterComponent t = new TerminologyCapabilitiesExpansionParameterComponent(); 2195 if (this.parameter == null) 2196 this.parameter = new ArrayList<TerminologyCapabilitiesExpansionParameterComponent>(); 2197 this.parameter.add(t); 2198 return t; 2199 } 2200 2201 public TerminologyCapabilitiesExpansionComponent addParameter(TerminologyCapabilitiesExpansionParameterComponent t) { //3 2202 if (t == null) 2203 return this; 2204 if (this.parameter == null) 2205 this.parameter = new ArrayList<TerminologyCapabilitiesExpansionParameterComponent>(); 2206 this.parameter.add(t); 2207 return this; 2208 } 2209 2210 /** 2211 * @return The first repetition of repeating field {@link #parameter}, creating it if it does not already exist {3} 2212 */ 2213 public TerminologyCapabilitiesExpansionParameterComponent getParameterFirstRep() { 2214 if (getParameter().isEmpty()) { 2215 addParameter(); 2216 } 2217 return getParameter().get(0); 2218 } 2219 2220 /** 2221 * @return {@link #textFilter} (Documentation about text searching works.). This is the underlying object with id, value and extensions. The accessor "getTextFilter" gives direct access to the value 2222 */ 2223 public MarkdownType getTextFilterElement() { 2224 if (this.textFilter == null) 2225 if (Configuration.errorOnAutoCreate()) 2226 throw new Error("Attempt to auto-create TerminologyCapabilitiesExpansionComponent.textFilter"); 2227 else if (Configuration.doAutoCreate()) 2228 this.textFilter = new MarkdownType(); // bb 2229 return this.textFilter; 2230 } 2231 2232 public boolean hasTextFilterElement() { 2233 return this.textFilter != null && !this.textFilter.isEmpty(); 2234 } 2235 2236 public boolean hasTextFilter() { 2237 return this.textFilter != null && !this.textFilter.isEmpty(); 2238 } 2239 2240 /** 2241 * @param value {@link #textFilter} (Documentation about text searching works.). This is the underlying object with id, value and extensions. The accessor "getTextFilter" gives direct access to the value 2242 */ 2243 public TerminologyCapabilitiesExpansionComponent setTextFilterElement(MarkdownType value) { 2244 this.textFilter = value; 2245 return this; 2246 } 2247 2248 /** 2249 * @return Documentation about text searching works. 2250 */ 2251 public String getTextFilter() { 2252 return this.textFilter == null ? null : this.textFilter.getValue(); 2253 } 2254 2255 /** 2256 * @param value Documentation about text searching works. 2257 */ 2258 public TerminologyCapabilitiesExpansionComponent setTextFilter(String value) { 2259 if (Utilities.noString(value)) 2260 this.textFilter = null; 2261 else { 2262 if (this.textFilter == null) 2263 this.textFilter = new MarkdownType(); 2264 this.textFilter.setValue(value); 2265 } 2266 return this; 2267 } 2268 2269 protected void listChildren(List<Property> children) { 2270 super.listChildren(children); 2271 children.add(new Property("hierarchical", "boolean", "Whether the server can return nested value sets.", 0, 1, hierarchical)); 2272 children.add(new Property("paging", "boolean", "Whether the server supports paging on expansion.", 0, 1, paging)); 2273 children.add(new Property("incomplete", "boolean", "True if requests for incomplete expansions are allowed.", 0, 1, incomplete)); 2274 children.add(new Property("parameter", "", "Supported expansion parameter.", 0, java.lang.Integer.MAX_VALUE, parameter)); 2275 children.add(new Property("textFilter", "markdown", "Documentation about text searching works.", 0, 1, textFilter)); 2276 } 2277 2278 @Override 2279 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2280 switch (_hash) { 2281 case 857636745: /*hierarchical*/ return new Property("hierarchical", "boolean", "Whether the server can return nested value sets.", 0, 1, hierarchical); 2282 case -995747956: /*paging*/ return new Property("paging", "boolean", "Whether the server supports paging on expansion.", 0, 1, paging); 2283 case -1010022050: /*incomplete*/ return new Property("incomplete", "boolean", "True if requests for incomplete expansions are allowed.", 0, 1, incomplete); 2284 case 1954460585: /*parameter*/ return new Property("parameter", "", "Supported expansion parameter.", 0, java.lang.Integer.MAX_VALUE, parameter); 2285 case 1469359877: /*textFilter*/ return new Property("textFilter", "markdown", "Documentation about text searching works.", 0, 1, textFilter); 2286 default: return super.getNamedProperty(_hash, _name, _checkValid); 2287 } 2288 2289 } 2290 2291 @Override 2292 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2293 switch (hash) { 2294 case 857636745: /*hierarchical*/ return this.hierarchical == null ? new Base[0] : new Base[] {this.hierarchical}; // BooleanType 2295 case -995747956: /*paging*/ return this.paging == null ? new Base[0] : new Base[] {this.paging}; // BooleanType 2296 case -1010022050: /*incomplete*/ return this.incomplete == null ? new Base[0] : new Base[] {this.incomplete}; // BooleanType 2297 case 1954460585: /*parameter*/ return this.parameter == null ? new Base[0] : this.parameter.toArray(new Base[this.parameter.size()]); // TerminologyCapabilitiesExpansionParameterComponent 2298 case 1469359877: /*textFilter*/ return this.textFilter == null ? new Base[0] : new Base[] {this.textFilter}; // MarkdownType 2299 default: return super.getProperty(hash, name, checkValid); 2300 } 2301 2302 } 2303 2304 @Override 2305 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2306 switch (hash) { 2307 case 857636745: // hierarchical 2308 this.hierarchical = TypeConvertor.castToBoolean(value); // BooleanType 2309 return value; 2310 case -995747956: // paging 2311 this.paging = TypeConvertor.castToBoolean(value); // BooleanType 2312 return value; 2313 case -1010022050: // incomplete 2314 this.incomplete = TypeConvertor.castToBoolean(value); // BooleanType 2315 return value; 2316 case 1954460585: // parameter 2317 this.getParameter().add((TerminologyCapabilitiesExpansionParameterComponent) value); // TerminologyCapabilitiesExpansionParameterComponent 2318 return value; 2319 case 1469359877: // textFilter 2320 this.textFilter = TypeConvertor.castToMarkdown(value); // MarkdownType 2321 return value; 2322 default: return super.setProperty(hash, name, value); 2323 } 2324 2325 } 2326 2327 @Override 2328 public Base setProperty(String name, Base value) throws FHIRException { 2329 if (name.equals("hierarchical")) { 2330 this.hierarchical = TypeConvertor.castToBoolean(value); // BooleanType 2331 } else if (name.equals("paging")) { 2332 this.paging = TypeConvertor.castToBoolean(value); // BooleanType 2333 } else if (name.equals("incomplete")) { 2334 this.incomplete = TypeConvertor.castToBoolean(value); // BooleanType 2335 } else if (name.equals("parameter")) { 2336 this.getParameter().add((TerminologyCapabilitiesExpansionParameterComponent) value); 2337 } else if (name.equals("textFilter")) { 2338 this.textFilter = TypeConvertor.castToMarkdown(value); // MarkdownType 2339 } else 2340 return super.setProperty(name, value); 2341 return value; 2342 } 2343 2344 @Override 2345 public void removeChild(String name, Base value) throws FHIRException { 2346 if (name.equals("hierarchical")) { 2347 this.hierarchical = null; 2348 } else if (name.equals("paging")) { 2349 this.paging = null; 2350 } else if (name.equals("incomplete")) { 2351 this.incomplete = null; 2352 } else if (name.equals("parameter")) { 2353 this.getParameter().remove((TerminologyCapabilitiesExpansionParameterComponent) value); 2354 } else if (name.equals("textFilter")) { 2355 this.textFilter = null; 2356 } else 2357 super.removeChild(name, value); 2358 2359 } 2360 2361 @Override 2362 public Base makeProperty(int hash, String name) throws FHIRException { 2363 switch (hash) { 2364 case 857636745: return getHierarchicalElement(); 2365 case -995747956: return getPagingElement(); 2366 case -1010022050: return getIncompleteElement(); 2367 case 1954460585: return addParameter(); 2368 case 1469359877: return getTextFilterElement(); 2369 default: return super.makeProperty(hash, name); 2370 } 2371 2372 } 2373 2374 @Override 2375 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2376 switch (hash) { 2377 case 857636745: /*hierarchical*/ return new String[] {"boolean"}; 2378 case -995747956: /*paging*/ return new String[] {"boolean"}; 2379 case -1010022050: /*incomplete*/ return new String[] {"boolean"}; 2380 case 1954460585: /*parameter*/ return new String[] {}; 2381 case 1469359877: /*textFilter*/ return new String[] {"markdown"}; 2382 default: return super.getTypesForProperty(hash, name); 2383 } 2384 2385 } 2386 2387 @Override 2388 public Base addChild(String name) throws FHIRException { 2389 if (name.equals("hierarchical")) { 2390 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.expansion.hierarchical"); 2391 } 2392 else if (name.equals("paging")) { 2393 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.expansion.paging"); 2394 } 2395 else if (name.equals("incomplete")) { 2396 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.expansion.incomplete"); 2397 } 2398 else if (name.equals("parameter")) { 2399 return addParameter(); 2400 } 2401 else if (name.equals("textFilter")) { 2402 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.expansion.textFilter"); 2403 } 2404 else 2405 return super.addChild(name); 2406 } 2407 2408 public TerminologyCapabilitiesExpansionComponent copy() { 2409 TerminologyCapabilitiesExpansionComponent dst = new TerminologyCapabilitiesExpansionComponent(); 2410 copyValues(dst); 2411 return dst; 2412 } 2413 2414 public void copyValues(TerminologyCapabilitiesExpansionComponent dst) { 2415 super.copyValues(dst); 2416 dst.hierarchical = hierarchical == null ? null : hierarchical.copy(); 2417 dst.paging = paging == null ? null : paging.copy(); 2418 dst.incomplete = incomplete == null ? null : incomplete.copy(); 2419 if (parameter != null) { 2420 dst.parameter = new ArrayList<TerminologyCapabilitiesExpansionParameterComponent>(); 2421 for (TerminologyCapabilitiesExpansionParameterComponent i : parameter) 2422 dst.parameter.add(i.copy()); 2423 }; 2424 dst.textFilter = textFilter == null ? null : textFilter.copy(); 2425 } 2426 2427 @Override 2428 public boolean equalsDeep(Base other_) { 2429 if (!super.equalsDeep(other_)) 2430 return false; 2431 if (!(other_ instanceof TerminologyCapabilitiesExpansionComponent)) 2432 return false; 2433 TerminologyCapabilitiesExpansionComponent o = (TerminologyCapabilitiesExpansionComponent) other_; 2434 return compareDeep(hierarchical, o.hierarchical, true) && compareDeep(paging, o.paging, true) && compareDeep(incomplete, o.incomplete, true) 2435 && compareDeep(parameter, o.parameter, true) && compareDeep(textFilter, o.textFilter, true); 2436 } 2437 2438 @Override 2439 public boolean equalsShallow(Base other_) { 2440 if (!super.equalsShallow(other_)) 2441 return false; 2442 if (!(other_ instanceof TerminologyCapabilitiesExpansionComponent)) 2443 return false; 2444 TerminologyCapabilitiesExpansionComponent o = (TerminologyCapabilitiesExpansionComponent) other_; 2445 return compareValues(hierarchical, o.hierarchical, true) && compareValues(paging, o.paging, true) && compareValues(incomplete, o.incomplete, true) 2446 && compareValues(textFilter, o.textFilter, true); 2447 } 2448 2449 public boolean isEmpty() { 2450 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(hierarchical, paging, incomplete 2451 , parameter, textFilter); 2452 } 2453 2454 public String fhirType() { 2455 return "TerminologyCapabilities.expansion"; 2456 2457 } 2458 2459 } 2460 2461 @Block() 2462 public static class TerminologyCapabilitiesExpansionParameterComponent extends BackboneElement implements IBaseBackboneElement { 2463 /** 2464 * Name of the supported expansion parameter. 2465 */ 2466 @Child(name = "name", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2467 @Description(shortDefinition="Name of the supported expansion parameter", formalDefinition="Name of the supported expansion parameter." ) 2468 protected CodeType name; 2469 2470 /** 2471 * Description of support for parameter. 2472 */ 2473 @Child(name = "documentation", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2474 @Description(shortDefinition="Description of support for parameter", formalDefinition="Description of support for parameter." ) 2475 protected StringType documentation; 2476 2477 private static final long serialVersionUID = -1703372741L; 2478 2479 /** 2480 * Constructor 2481 */ 2482 public TerminologyCapabilitiesExpansionParameterComponent() { 2483 super(); 2484 } 2485 2486 /** 2487 * Constructor 2488 */ 2489 public TerminologyCapabilitiesExpansionParameterComponent(String name) { 2490 super(); 2491 this.setName(name); 2492 } 2493 2494 /** 2495 * @return {@link #name} (Name of the supported expansion parameter.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2496 */ 2497 public CodeType getNameElement() { 2498 if (this.name == null) 2499 if (Configuration.errorOnAutoCreate()) 2500 throw new Error("Attempt to auto-create TerminologyCapabilitiesExpansionParameterComponent.name"); 2501 else if (Configuration.doAutoCreate()) 2502 this.name = new CodeType(); // bb 2503 return this.name; 2504 } 2505 2506 public boolean hasNameElement() { 2507 return this.name != null && !this.name.isEmpty(); 2508 } 2509 2510 public boolean hasName() { 2511 return this.name != null && !this.name.isEmpty(); 2512 } 2513 2514 /** 2515 * @param value {@link #name} (Name of the supported expansion parameter.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2516 */ 2517 public TerminologyCapabilitiesExpansionParameterComponent setNameElement(CodeType value) { 2518 this.name = value; 2519 return this; 2520 } 2521 2522 /** 2523 * @return Name of the supported expansion parameter. 2524 */ 2525 public String getName() { 2526 return this.name == null ? null : this.name.getValue(); 2527 } 2528 2529 /** 2530 * @param value Name of the supported expansion parameter. 2531 */ 2532 public TerminologyCapabilitiesExpansionParameterComponent setName(String value) { 2533 if (this.name == null) 2534 this.name = new CodeType(); 2535 this.name.setValue(value); 2536 return this; 2537 } 2538 2539 /** 2540 * @return {@link #documentation} (Description of support for parameter.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 2541 */ 2542 public StringType getDocumentationElement() { 2543 if (this.documentation == null) 2544 if (Configuration.errorOnAutoCreate()) 2545 throw new Error("Attempt to auto-create TerminologyCapabilitiesExpansionParameterComponent.documentation"); 2546 else if (Configuration.doAutoCreate()) 2547 this.documentation = new StringType(); // bb 2548 return this.documentation; 2549 } 2550 2551 public boolean hasDocumentationElement() { 2552 return this.documentation != null && !this.documentation.isEmpty(); 2553 } 2554 2555 public boolean hasDocumentation() { 2556 return this.documentation != null && !this.documentation.isEmpty(); 2557 } 2558 2559 /** 2560 * @param value {@link #documentation} (Description of support for parameter.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 2561 */ 2562 public TerminologyCapabilitiesExpansionParameterComponent setDocumentationElement(StringType value) { 2563 this.documentation = value; 2564 return this; 2565 } 2566 2567 /** 2568 * @return Description of support for parameter. 2569 */ 2570 public String getDocumentation() { 2571 return this.documentation == null ? null : this.documentation.getValue(); 2572 } 2573 2574 /** 2575 * @param value Description of support for parameter. 2576 */ 2577 public TerminologyCapabilitiesExpansionParameterComponent setDocumentation(String value) { 2578 if (Utilities.noString(value)) 2579 this.documentation = null; 2580 else { 2581 if (this.documentation == null) 2582 this.documentation = new StringType(); 2583 this.documentation.setValue(value); 2584 } 2585 return this; 2586 } 2587 2588 protected void listChildren(List<Property> children) { 2589 super.listChildren(children); 2590 children.add(new Property("name", "code", "Name of the supported expansion parameter.", 0, 1, name)); 2591 children.add(new Property("documentation", "string", "Description of support for parameter.", 0, 1, documentation)); 2592 } 2593 2594 @Override 2595 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2596 switch (_hash) { 2597 case 3373707: /*name*/ return new Property("name", "code", "Name of the supported expansion parameter.", 0, 1, name); 2598 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Description of support for parameter.", 0, 1, documentation); 2599 default: return super.getNamedProperty(_hash, _name, _checkValid); 2600 } 2601 2602 } 2603 2604 @Override 2605 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2606 switch (hash) { 2607 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // CodeType 2608 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 2609 default: return super.getProperty(hash, name, checkValid); 2610 } 2611 2612 } 2613 2614 @Override 2615 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2616 switch (hash) { 2617 case 3373707: // name 2618 this.name = TypeConvertor.castToCode(value); // CodeType 2619 return value; 2620 case 1587405498: // documentation 2621 this.documentation = TypeConvertor.castToString(value); // StringType 2622 return value; 2623 default: return super.setProperty(hash, name, value); 2624 } 2625 2626 } 2627 2628 @Override 2629 public Base setProperty(String name, Base value) throws FHIRException { 2630 if (name.equals("name")) { 2631 this.name = TypeConvertor.castToCode(value); // CodeType 2632 } else if (name.equals("documentation")) { 2633 this.documentation = TypeConvertor.castToString(value); // StringType 2634 } else 2635 return super.setProperty(name, value); 2636 return value; 2637 } 2638 2639 @Override 2640 public void removeChild(String name, Base value) throws FHIRException { 2641 if (name.equals("name")) { 2642 this.name = null; 2643 } else if (name.equals("documentation")) { 2644 this.documentation = null; 2645 } else 2646 super.removeChild(name, value); 2647 2648 } 2649 2650 @Override 2651 public Base makeProperty(int hash, String name) throws FHIRException { 2652 switch (hash) { 2653 case 3373707: return getNameElement(); 2654 case 1587405498: return getDocumentationElement(); 2655 default: return super.makeProperty(hash, name); 2656 } 2657 2658 } 2659 2660 @Override 2661 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2662 switch (hash) { 2663 case 3373707: /*name*/ return new String[] {"code"}; 2664 case 1587405498: /*documentation*/ return new String[] {"string"}; 2665 default: return super.getTypesForProperty(hash, name); 2666 } 2667 2668 } 2669 2670 @Override 2671 public Base addChild(String name) throws FHIRException { 2672 if (name.equals("name")) { 2673 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.expansion.parameter.name"); 2674 } 2675 else if (name.equals("documentation")) { 2676 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.expansion.parameter.documentation"); 2677 } 2678 else 2679 return super.addChild(name); 2680 } 2681 2682 public TerminologyCapabilitiesExpansionParameterComponent copy() { 2683 TerminologyCapabilitiesExpansionParameterComponent dst = new TerminologyCapabilitiesExpansionParameterComponent(); 2684 copyValues(dst); 2685 return dst; 2686 } 2687 2688 public void copyValues(TerminologyCapabilitiesExpansionParameterComponent dst) { 2689 super.copyValues(dst); 2690 dst.name = name == null ? null : name.copy(); 2691 dst.documentation = documentation == null ? null : documentation.copy(); 2692 } 2693 2694 @Override 2695 public boolean equalsDeep(Base other_) { 2696 if (!super.equalsDeep(other_)) 2697 return false; 2698 if (!(other_ instanceof TerminologyCapabilitiesExpansionParameterComponent)) 2699 return false; 2700 TerminologyCapabilitiesExpansionParameterComponent o = (TerminologyCapabilitiesExpansionParameterComponent) other_; 2701 return compareDeep(name, o.name, true) && compareDeep(documentation, o.documentation, true); 2702 } 2703 2704 @Override 2705 public boolean equalsShallow(Base other_) { 2706 if (!super.equalsShallow(other_)) 2707 return false; 2708 if (!(other_ instanceof TerminologyCapabilitiesExpansionParameterComponent)) 2709 return false; 2710 TerminologyCapabilitiesExpansionParameterComponent o = (TerminologyCapabilitiesExpansionParameterComponent) other_; 2711 return compareValues(name, o.name, true) && compareValues(documentation, o.documentation, true); 2712 } 2713 2714 public boolean isEmpty() { 2715 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, documentation); 2716 } 2717 2718 public String fhirType() { 2719 return "TerminologyCapabilities.expansion.parameter"; 2720 2721 } 2722 2723 } 2724 2725 @Block() 2726 public static class TerminologyCapabilitiesValidateCodeComponent extends BackboneElement implements IBaseBackboneElement { 2727 /** 2728 * Whether translations are validated. 2729 */ 2730 @Child(name = "translations", type = {BooleanType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2731 @Description(shortDefinition="Whether translations are validated", formalDefinition="Whether translations are validated." ) 2732 protected BooleanType translations; 2733 2734 private static final long serialVersionUID = -1212814906L; 2735 2736 /** 2737 * Constructor 2738 */ 2739 public TerminologyCapabilitiesValidateCodeComponent() { 2740 super(); 2741 } 2742 2743 /** 2744 * Constructor 2745 */ 2746 public TerminologyCapabilitiesValidateCodeComponent(boolean translations) { 2747 super(); 2748 this.setTranslations(translations); 2749 } 2750 2751 /** 2752 * @return {@link #translations} (Whether translations are validated.). This is the underlying object with id, value and extensions. The accessor "getTranslations" gives direct access to the value 2753 */ 2754 public BooleanType getTranslationsElement() { 2755 if (this.translations == null) 2756 if (Configuration.errorOnAutoCreate()) 2757 throw new Error("Attempt to auto-create TerminologyCapabilitiesValidateCodeComponent.translations"); 2758 else if (Configuration.doAutoCreate()) 2759 this.translations = new BooleanType(); // bb 2760 return this.translations; 2761 } 2762 2763 public boolean hasTranslationsElement() { 2764 return this.translations != null && !this.translations.isEmpty(); 2765 } 2766 2767 public boolean hasTranslations() { 2768 return this.translations != null && !this.translations.isEmpty(); 2769 } 2770 2771 /** 2772 * @param value {@link #translations} (Whether translations are validated.). This is the underlying object with id, value and extensions. The accessor "getTranslations" gives direct access to the value 2773 */ 2774 public TerminologyCapabilitiesValidateCodeComponent setTranslationsElement(BooleanType value) { 2775 this.translations = value; 2776 return this; 2777 } 2778 2779 /** 2780 * @return Whether translations are validated. 2781 */ 2782 public boolean getTranslations() { 2783 return this.translations == null || this.translations.isEmpty() ? false : this.translations.getValue(); 2784 } 2785 2786 /** 2787 * @param value Whether translations are validated. 2788 */ 2789 public TerminologyCapabilitiesValidateCodeComponent setTranslations(boolean value) { 2790 if (this.translations == null) 2791 this.translations = new BooleanType(); 2792 this.translations.setValue(value); 2793 return this; 2794 } 2795 2796 protected void listChildren(List<Property> children) { 2797 super.listChildren(children); 2798 children.add(new Property("translations", "boolean", "Whether translations are validated.", 0, 1, translations)); 2799 } 2800 2801 @Override 2802 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2803 switch (_hash) { 2804 case -1225497630: /*translations*/ return new Property("translations", "boolean", "Whether translations are validated.", 0, 1, translations); 2805 default: return super.getNamedProperty(_hash, _name, _checkValid); 2806 } 2807 2808 } 2809 2810 @Override 2811 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2812 switch (hash) { 2813 case -1225497630: /*translations*/ return this.translations == null ? new Base[0] : new Base[] {this.translations}; // BooleanType 2814 default: return super.getProperty(hash, name, checkValid); 2815 } 2816 2817 } 2818 2819 @Override 2820 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2821 switch (hash) { 2822 case -1225497630: // translations 2823 this.translations = TypeConvertor.castToBoolean(value); // BooleanType 2824 return value; 2825 default: return super.setProperty(hash, name, value); 2826 } 2827 2828 } 2829 2830 @Override 2831 public Base setProperty(String name, Base value) throws FHIRException { 2832 if (name.equals("translations")) { 2833 this.translations = TypeConvertor.castToBoolean(value); // BooleanType 2834 } else 2835 return super.setProperty(name, value); 2836 return value; 2837 } 2838 2839 @Override 2840 public void removeChild(String name, Base value) throws FHIRException { 2841 if (name.equals("translations")) { 2842 this.translations = null; 2843 } else 2844 super.removeChild(name, value); 2845 2846 } 2847 2848 @Override 2849 public Base makeProperty(int hash, String name) throws FHIRException { 2850 switch (hash) { 2851 case -1225497630: return getTranslationsElement(); 2852 default: return super.makeProperty(hash, name); 2853 } 2854 2855 } 2856 2857 @Override 2858 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2859 switch (hash) { 2860 case -1225497630: /*translations*/ return new String[] {"boolean"}; 2861 default: return super.getTypesForProperty(hash, name); 2862 } 2863 2864 } 2865 2866 @Override 2867 public Base addChild(String name) throws FHIRException { 2868 if (name.equals("translations")) { 2869 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.validateCode.translations"); 2870 } 2871 else 2872 return super.addChild(name); 2873 } 2874 2875 public TerminologyCapabilitiesValidateCodeComponent copy() { 2876 TerminologyCapabilitiesValidateCodeComponent dst = new TerminologyCapabilitiesValidateCodeComponent(); 2877 copyValues(dst); 2878 return dst; 2879 } 2880 2881 public void copyValues(TerminologyCapabilitiesValidateCodeComponent dst) { 2882 super.copyValues(dst); 2883 dst.translations = translations == null ? null : translations.copy(); 2884 } 2885 2886 @Override 2887 public boolean equalsDeep(Base other_) { 2888 if (!super.equalsDeep(other_)) 2889 return false; 2890 if (!(other_ instanceof TerminologyCapabilitiesValidateCodeComponent)) 2891 return false; 2892 TerminologyCapabilitiesValidateCodeComponent o = (TerminologyCapabilitiesValidateCodeComponent) other_; 2893 return compareDeep(translations, o.translations, true); 2894 } 2895 2896 @Override 2897 public boolean equalsShallow(Base other_) { 2898 if (!super.equalsShallow(other_)) 2899 return false; 2900 if (!(other_ instanceof TerminologyCapabilitiesValidateCodeComponent)) 2901 return false; 2902 TerminologyCapabilitiesValidateCodeComponent o = (TerminologyCapabilitiesValidateCodeComponent) other_; 2903 return compareValues(translations, o.translations, true); 2904 } 2905 2906 public boolean isEmpty() { 2907 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(translations); 2908 } 2909 2910 public String fhirType() { 2911 return "TerminologyCapabilities.validateCode"; 2912 2913 } 2914 2915 } 2916 2917 @Block() 2918 public static class TerminologyCapabilitiesTranslationComponent extends BackboneElement implements IBaseBackboneElement { 2919 /** 2920 * Whether the client must identify the map. 2921 */ 2922 @Child(name = "needsMap", type = {BooleanType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2923 @Description(shortDefinition="Whether the client must identify the map", formalDefinition="Whether the client must identify the map." ) 2924 protected BooleanType needsMap; 2925 2926 private static final long serialVersionUID = -1727843575L; 2927 2928 /** 2929 * Constructor 2930 */ 2931 public TerminologyCapabilitiesTranslationComponent() { 2932 super(); 2933 } 2934 2935 /** 2936 * Constructor 2937 */ 2938 public TerminologyCapabilitiesTranslationComponent(boolean needsMap) { 2939 super(); 2940 this.setNeedsMap(needsMap); 2941 } 2942 2943 /** 2944 * @return {@link #needsMap} (Whether the client must identify the map.). This is the underlying object with id, value and extensions. The accessor "getNeedsMap" gives direct access to the value 2945 */ 2946 public BooleanType getNeedsMapElement() { 2947 if (this.needsMap == null) 2948 if (Configuration.errorOnAutoCreate()) 2949 throw new Error("Attempt to auto-create TerminologyCapabilitiesTranslationComponent.needsMap"); 2950 else if (Configuration.doAutoCreate()) 2951 this.needsMap = new BooleanType(); // bb 2952 return this.needsMap; 2953 } 2954 2955 public boolean hasNeedsMapElement() { 2956 return this.needsMap != null && !this.needsMap.isEmpty(); 2957 } 2958 2959 public boolean hasNeedsMap() { 2960 return this.needsMap != null && !this.needsMap.isEmpty(); 2961 } 2962 2963 /** 2964 * @param value {@link #needsMap} (Whether the client must identify the map.). This is the underlying object with id, value and extensions. The accessor "getNeedsMap" gives direct access to the value 2965 */ 2966 public TerminologyCapabilitiesTranslationComponent setNeedsMapElement(BooleanType value) { 2967 this.needsMap = value; 2968 return this; 2969 } 2970 2971 /** 2972 * @return Whether the client must identify the map. 2973 */ 2974 public boolean getNeedsMap() { 2975 return this.needsMap == null || this.needsMap.isEmpty() ? false : this.needsMap.getValue(); 2976 } 2977 2978 /** 2979 * @param value Whether the client must identify the map. 2980 */ 2981 public TerminologyCapabilitiesTranslationComponent setNeedsMap(boolean value) { 2982 if (this.needsMap == null) 2983 this.needsMap = new BooleanType(); 2984 this.needsMap.setValue(value); 2985 return this; 2986 } 2987 2988 protected void listChildren(List<Property> children) { 2989 super.listChildren(children); 2990 children.add(new Property("needsMap", "boolean", "Whether the client must identify the map.", 0, 1, needsMap)); 2991 } 2992 2993 @Override 2994 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2995 switch (_hash) { 2996 case 866566527: /*needsMap*/ return new Property("needsMap", "boolean", "Whether the client must identify the map.", 0, 1, needsMap); 2997 default: return super.getNamedProperty(_hash, _name, _checkValid); 2998 } 2999 3000 } 3001 3002 @Override 3003 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3004 switch (hash) { 3005 case 866566527: /*needsMap*/ return this.needsMap == null ? new Base[0] : new Base[] {this.needsMap}; // BooleanType 3006 default: return super.getProperty(hash, name, checkValid); 3007 } 3008 3009 } 3010 3011 @Override 3012 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3013 switch (hash) { 3014 case 866566527: // needsMap 3015 this.needsMap = TypeConvertor.castToBoolean(value); // BooleanType 3016 return value; 3017 default: return super.setProperty(hash, name, value); 3018 } 3019 3020 } 3021 3022 @Override 3023 public Base setProperty(String name, Base value) throws FHIRException { 3024 if (name.equals("needsMap")) { 3025 this.needsMap = TypeConvertor.castToBoolean(value); // BooleanType 3026 } else 3027 return super.setProperty(name, value); 3028 return value; 3029 } 3030 3031 @Override 3032 public void removeChild(String name, Base value) throws FHIRException { 3033 if (name.equals("needsMap")) { 3034 this.needsMap = null; 3035 } else 3036 super.removeChild(name, value); 3037 3038 } 3039 3040 @Override 3041 public Base makeProperty(int hash, String name) throws FHIRException { 3042 switch (hash) { 3043 case 866566527: return getNeedsMapElement(); 3044 default: return super.makeProperty(hash, name); 3045 } 3046 3047 } 3048 3049 @Override 3050 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3051 switch (hash) { 3052 case 866566527: /*needsMap*/ return new String[] {"boolean"}; 3053 default: return super.getTypesForProperty(hash, name); 3054 } 3055 3056 } 3057 3058 @Override 3059 public Base addChild(String name) throws FHIRException { 3060 if (name.equals("needsMap")) { 3061 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.translation.needsMap"); 3062 } 3063 else 3064 return super.addChild(name); 3065 } 3066 3067 public TerminologyCapabilitiesTranslationComponent copy() { 3068 TerminologyCapabilitiesTranslationComponent dst = new TerminologyCapabilitiesTranslationComponent(); 3069 copyValues(dst); 3070 return dst; 3071 } 3072 3073 public void copyValues(TerminologyCapabilitiesTranslationComponent dst) { 3074 super.copyValues(dst); 3075 dst.needsMap = needsMap == null ? null : needsMap.copy(); 3076 } 3077 3078 @Override 3079 public boolean equalsDeep(Base other_) { 3080 if (!super.equalsDeep(other_)) 3081 return false; 3082 if (!(other_ instanceof TerminologyCapabilitiesTranslationComponent)) 3083 return false; 3084 TerminologyCapabilitiesTranslationComponent o = (TerminologyCapabilitiesTranslationComponent) other_; 3085 return compareDeep(needsMap, o.needsMap, true); 3086 } 3087 3088 @Override 3089 public boolean equalsShallow(Base other_) { 3090 if (!super.equalsShallow(other_)) 3091 return false; 3092 if (!(other_ instanceof TerminologyCapabilitiesTranslationComponent)) 3093 return false; 3094 TerminologyCapabilitiesTranslationComponent o = (TerminologyCapabilitiesTranslationComponent) other_; 3095 return compareValues(needsMap, o.needsMap, true); 3096 } 3097 3098 public boolean isEmpty() { 3099 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(needsMap); 3100 } 3101 3102 public String fhirType() { 3103 return "TerminologyCapabilities.translation"; 3104 3105 } 3106 3107 } 3108 3109 @Block() 3110 public static class TerminologyCapabilitiesClosureComponent extends BackboneElement implements IBaseBackboneElement { 3111 /** 3112 * If cross-system closure is supported. 3113 */ 3114 @Child(name = "translation", type = {BooleanType.class}, order=1, min=0, max=1, modifier=false, summary=false) 3115 @Description(shortDefinition="If cross-system closure is supported", formalDefinition="If cross-system closure is supported." ) 3116 protected BooleanType translation; 3117 3118 private static final long serialVersionUID = 1900484343L; 3119 3120 /** 3121 * Constructor 3122 */ 3123 public TerminologyCapabilitiesClosureComponent() { 3124 super(); 3125 } 3126 3127 /** 3128 * @return {@link #translation} (If cross-system closure is supported.). This is the underlying object with id, value and extensions. The accessor "getTranslation" gives direct access to the value 3129 */ 3130 public BooleanType getTranslationElement() { 3131 if (this.translation == null) 3132 if (Configuration.errorOnAutoCreate()) 3133 throw new Error("Attempt to auto-create TerminologyCapabilitiesClosureComponent.translation"); 3134 else if (Configuration.doAutoCreate()) 3135 this.translation = new BooleanType(); // bb 3136 return this.translation; 3137 } 3138 3139 public boolean hasTranslationElement() { 3140 return this.translation != null && !this.translation.isEmpty(); 3141 } 3142 3143 public boolean hasTranslation() { 3144 return this.translation != null && !this.translation.isEmpty(); 3145 } 3146 3147 /** 3148 * @param value {@link #translation} (If cross-system closure is supported.). This is the underlying object with id, value and extensions. The accessor "getTranslation" gives direct access to the value 3149 */ 3150 public TerminologyCapabilitiesClosureComponent setTranslationElement(BooleanType value) { 3151 this.translation = value; 3152 return this; 3153 } 3154 3155 /** 3156 * @return If cross-system closure is supported. 3157 */ 3158 public boolean getTranslation() { 3159 return this.translation == null || this.translation.isEmpty() ? false : this.translation.getValue(); 3160 } 3161 3162 /** 3163 * @param value If cross-system closure is supported. 3164 */ 3165 public TerminologyCapabilitiesClosureComponent setTranslation(boolean value) { 3166 if (this.translation == null) 3167 this.translation = new BooleanType(); 3168 this.translation.setValue(value); 3169 return this; 3170 } 3171 3172 protected void listChildren(List<Property> children) { 3173 super.listChildren(children); 3174 children.add(new Property("translation", "boolean", "If cross-system closure is supported.", 0, 1, translation)); 3175 } 3176 3177 @Override 3178 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3179 switch (_hash) { 3180 case -1840647503: /*translation*/ return new Property("translation", "boolean", "If cross-system closure is supported.", 0, 1, translation); 3181 default: return super.getNamedProperty(_hash, _name, _checkValid); 3182 } 3183 3184 } 3185 3186 @Override 3187 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3188 switch (hash) { 3189 case -1840647503: /*translation*/ return this.translation == null ? new Base[0] : new Base[] {this.translation}; // BooleanType 3190 default: return super.getProperty(hash, name, checkValid); 3191 } 3192 3193 } 3194 3195 @Override 3196 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3197 switch (hash) { 3198 case -1840647503: // translation 3199 this.translation = TypeConvertor.castToBoolean(value); // BooleanType 3200 return value; 3201 default: return super.setProperty(hash, name, value); 3202 } 3203 3204 } 3205 3206 @Override 3207 public Base setProperty(String name, Base value) throws FHIRException { 3208 if (name.equals("translation")) { 3209 this.translation = TypeConvertor.castToBoolean(value); // BooleanType 3210 } else 3211 return super.setProperty(name, value); 3212 return value; 3213 } 3214 3215 @Override 3216 public void removeChild(String name, Base value) throws FHIRException { 3217 if (name.equals("translation")) { 3218 this.translation = null; 3219 } else 3220 super.removeChild(name, value); 3221 3222 } 3223 3224 @Override 3225 public Base makeProperty(int hash, String name) throws FHIRException { 3226 switch (hash) { 3227 case -1840647503: return getTranslationElement(); 3228 default: return super.makeProperty(hash, name); 3229 } 3230 3231 } 3232 3233 @Override 3234 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3235 switch (hash) { 3236 case -1840647503: /*translation*/ return new String[] {"boolean"}; 3237 default: return super.getTypesForProperty(hash, name); 3238 } 3239 3240 } 3241 3242 @Override 3243 public Base addChild(String name) throws FHIRException { 3244 if (name.equals("translation")) { 3245 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.closure.translation"); 3246 } 3247 else 3248 return super.addChild(name); 3249 } 3250 3251 public TerminologyCapabilitiesClosureComponent copy() { 3252 TerminologyCapabilitiesClosureComponent dst = new TerminologyCapabilitiesClosureComponent(); 3253 copyValues(dst); 3254 return dst; 3255 } 3256 3257 public void copyValues(TerminologyCapabilitiesClosureComponent dst) { 3258 super.copyValues(dst); 3259 dst.translation = translation == null ? null : translation.copy(); 3260 } 3261 3262 @Override 3263 public boolean equalsDeep(Base other_) { 3264 if (!super.equalsDeep(other_)) 3265 return false; 3266 if (!(other_ instanceof TerminologyCapabilitiesClosureComponent)) 3267 return false; 3268 TerminologyCapabilitiesClosureComponent o = (TerminologyCapabilitiesClosureComponent) other_; 3269 return compareDeep(translation, o.translation, true); 3270 } 3271 3272 @Override 3273 public boolean equalsShallow(Base other_) { 3274 if (!super.equalsShallow(other_)) 3275 return false; 3276 if (!(other_ instanceof TerminologyCapabilitiesClosureComponent)) 3277 return false; 3278 TerminologyCapabilitiesClosureComponent o = (TerminologyCapabilitiesClosureComponent) other_; 3279 return compareValues(translation, o.translation, true); 3280 } 3281 3282 public boolean isEmpty() { 3283 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(translation); 3284 } 3285 3286 public String fhirType() { 3287 return "TerminologyCapabilities.closure"; 3288 3289 } 3290 3291 } 3292 3293 /** 3294 * An absolute URI that is used to identify this terminology capabilities when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this terminology capabilities is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the terminology capabilities is stored on different servers. 3295 */ 3296 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 3297 @Description(shortDefinition="Canonical identifier for this terminology capabilities, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this terminology capabilities when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this terminology capabilities is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the terminology capabilities is stored on different servers." ) 3298 protected UriType url; 3299 3300 /** 3301 * A formal identifier that is used to identify this terminology capabilities when it is represented in other formats, or referenced in a specification, model, design or an instance. 3302 */ 3303 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3304 @Description(shortDefinition="Additional identifier for the terminology capabilities", formalDefinition="A formal identifier that is used to identify this terminology capabilities when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 3305 protected List<Identifier> identifier; 3306 3307 /** 3308 * The identifier that is used to identify this version of the terminology capabilities when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the terminology capabilities author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 3309 */ 3310 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 3311 @Description(shortDefinition="Business version of the terminology capabilities", formalDefinition="The identifier that is used to identify this version of the terminology capabilities when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the terminology capabilities author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 3312 protected StringType version; 3313 3314 /** 3315 * Indicates the mechanism used to compare versions to determine which is more current. 3316 */ 3317 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 3318 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 3319 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 3320 protected DataType versionAlgorithm; 3321 3322 /** 3323 * A natural language name identifying the terminology capabilities. This name should be usable as an identifier for the module by machine processing applications such as code generation. 3324 */ 3325 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 3326 @Description(shortDefinition="Name for this terminology capabilities (computer friendly)", formalDefinition="A natural language name identifying the terminology capabilities. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 3327 protected StringType name; 3328 3329 /** 3330 * A short, descriptive, user-friendly title for the terminology capabilities. 3331 */ 3332 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 3333 @Description(shortDefinition="Name for this terminology capabilities (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the terminology capabilities." ) 3334 protected StringType title; 3335 3336 /** 3337 * The status of this terminology capabilities. Enables tracking the life-cycle of the content. 3338 */ 3339 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 3340 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this terminology capabilities. Enables tracking the life-cycle of the content." ) 3341 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 3342 protected Enumeration<PublicationStatus> status; 3343 3344 /** 3345 * A Boolean value to indicate that this terminology capabilities is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 3346 */ 3347 @Child(name = "experimental", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 3348 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this terminology capabilities is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 3349 protected BooleanType experimental; 3350 3351 /** 3352 * The date (and optionally time) when the terminology capabilities was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the terminology capabilities changes. 3353 */ 3354 @Child(name = "date", type = {DateTimeType.class}, order=8, min=1, max=1, modifier=false, summary=true) 3355 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the terminology capabilities was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the terminology capabilities changes." ) 3356 protected DateTimeType date; 3357 3358 /** 3359 * The name of the organization or individual responsible for the release and ongoing maintenance of the terminology capabilities. 3360 */ 3361 @Child(name = "publisher", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 3362 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the terminology capabilities." ) 3363 protected StringType publisher; 3364 3365 /** 3366 * Contact details to assist a user in finding and communicating with the publisher. 3367 */ 3368 @Child(name = "contact", type = {ContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3369 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 3370 protected List<ContactDetail> contact; 3371 3372 /** 3373 * A free text natural language description of the terminology capabilities from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP. 3374 */ 3375 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 3376 @Description(shortDefinition="Natural language description of the terminology capabilities", formalDefinition="A free text natural language description of the terminology capabilities from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP." ) 3377 protected MarkdownType description; 3378 3379 /** 3380 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate terminology capabilities instances. 3381 */ 3382 @Child(name = "useContext", type = {UsageContext.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3383 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate terminology capabilities instances." ) 3384 protected List<UsageContext> useContext; 3385 3386 /** 3387 * A legal or geographic region in which the terminology capabilities is intended to be used. 3388 */ 3389 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3390 @Description(shortDefinition="Intended jurisdiction for terminology capabilities (if applicable)", formalDefinition="A legal or geographic region in which the terminology capabilities is intended to be used." ) 3391 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 3392 protected List<CodeableConcept> jurisdiction; 3393 3394 /** 3395 * Explanation of why this terminology capabilities is needed and why it has been designed as it has. 3396 */ 3397 @Child(name = "purpose", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 3398 @Description(shortDefinition="Why this terminology capabilities is defined", formalDefinition="Explanation of why this terminology capabilities is needed and why it has been designed as it has." ) 3399 protected MarkdownType purpose; 3400 3401 /** 3402 * A copyright statement relating to the terminology capabilities and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the terminology capabilities. 3403 */ 3404 @Child(name = "copyright", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=true) 3405 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the terminology capabilities and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the terminology capabilities." ) 3406 protected MarkdownType copyright; 3407 3408 /** 3409 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3410 */ 3411 @Child(name = "copyrightLabel", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 3412 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 3413 protected StringType copyrightLabel; 3414 3415 /** 3416 * The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase). 3417 */ 3418 @Child(name = "kind", type = {CodeType.class}, order=17, min=1, max=1, modifier=false, summary=true) 3419 @Description(shortDefinition="instance | capability | requirements", formalDefinition="The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase)." ) 3420 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/capability-statement-kind") 3421 protected Enumeration<CapabilityStatementKind> kind; 3422 3423 /** 3424 * Software that is covered by this terminology capability statement. It is used when the statement describes the capabilities of a particular software version, independent of an installation. 3425 */ 3426 @Child(name = "software", type = {}, order=18, min=0, max=1, modifier=false, summary=true) 3427 @Description(shortDefinition="Software that is covered by this terminology capability statement", formalDefinition="Software that is covered by this terminology capability statement. It is used when the statement describes the capabilities of a particular software version, independent of an installation." ) 3428 protected TerminologyCapabilitiesSoftwareComponent software; 3429 3430 /** 3431 * Identifies a specific implementation instance that is described by the terminology capability statement - i.e. a particular installation, rather than the capabilities of a software program. 3432 */ 3433 @Child(name = "implementation", type = {}, order=19, min=0, max=1, modifier=false, summary=true) 3434 @Description(shortDefinition="If this describes a specific instance", formalDefinition="Identifies a specific implementation instance that is described by the terminology capability statement - i.e. a particular installation, rather than the capabilities of a software program." ) 3435 protected TerminologyCapabilitiesImplementationComponent implementation; 3436 3437 /** 3438 * Whether the server supports lockedDate. 3439 */ 3440 @Child(name = "lockedDate", type = {BooleanType.class}, order=20, min=0, max=1, modifier=false, summary=true) 3441 @Description(shortDefinition="Whether lockedDate is supported", formalDefinition="Whether the server supports lockedDate." ) 3442 protected BooleanType lockedDate; 3443 3444 /** 3445 * Identifies a code system that is supported by the server. If there is a no code system URL, then this declares the general assumptions a client can make about support for any CodeSystem resource. 3446 */ 3447 @Child(name = "codeSystem", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3448 @Description(shortDefinition="A code system supported by the server", formalDefinition="Identifies a code system that is supported by the server. If there is a no code system URL, then this declares the general assumptions a client can make about support for any CodeSystem resource." ) 3449 protected List<TerminologyCapabilitiesCodeSystemComponent> codeSystem; 3450 3451 /** 3452 * Information about the [ValueSet/$expand](valueset-operation-expand.html) operation. 3453 */ 3454 @Child(name = "expansion", type = {}, order=22, min=0, max=1, modifier=false, summary=false) 3455 @Description(shortDefinition="Information about the [ValueSet/$expand](valueset-operation-expand.html) operation", formalDefinition="Information about the [ValueSet/$expand](valueset-operation-expand.html) operation." ) 3456 protected TerminologyCapabilitiesExpansionComponent expansion; 3457 3458 /** 3459 * The degree to which the server supports the code search parameter on ValueSet, if it is supported. 3460 */ 3461 @Child(name = "codeSearch", type = {CodeType.class}, order=23, min=0, max=1, modifier=false, summary=false) 3462 @Description(shortDefinition="in-compose | in-expansion | in-compose-or-expansion", formalDefinition="The degree to which the server supports the code search parameter on ValueSet, if it is supported." ) 3463 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/code-search-support") 3464 protected Enumeration<CodeSearchSupport> codeSearch; 3465 3466 /** 3467 * Information about the [ValueSet/$validate-code](valueset-operation-validate-code.html) operation. 3468 */ 3469 @Child(name = "validateCode", type = {}, order=24, min=0, max=1, modifier=false, summary=false) 3470 @Description(shortDefinition="Information about the [ValueSet/$validate-code](valueset-operation-validate-code.html) operation", formalDefinition="Information about the [ValueSet/$validate-code](valueset-operation-validate-code.html) operation." ) 3471 protected TerminologyCapabilitiesValidateCodeComponent validateCode; 3472 3473 /** 3474 * Information about the [ConceptMap/$translate](conceptmap-operation-translate.html) operation. 3475 */ 3476 @Child(name = "translation", type = {}, order=25, min=0, max=1, modifier=false, summary=false) 3477 @Description(shortDefinition="Information about the [ConceptMap/$translate](conceptmap-operation-translate.html) operation", formalDefinition="Information about the [ConceptMap/$translate](conceptmap-operation-translate.html) operation." ) 3478 protected TerminologyCapabilitiesTranslationComponent translation; 3479 3480 /** 3481 * Whether the $closure operation is supported. 3482 */ 3483 @Child(name = "closure", type = {}, order=26, min=0, max=1, modifier=false, summary=false) 3484 @Description(shortDefinition="Information about the [ConceptMap/$closure](conceptmap-operation-closure.html) operation", formalDefinition="Whether the $closure operation is supported." ) 3485 protected TerminologyCapabilitiesClosureComponent closure; 3486 3487 private static final long serialVersionUID = 179684544L; 3488 3489 /** 3490 * Constructor 3491 */ 3492 public TerminologyCapabilities() { 3493 super(); 3494 } 3495 3496 /** 3497 * Constructor 3498 */ 3499 public TerminologyCapabilities(PublicationStatus status, Date date, CapabilityStatementKind kind) { 3500 super(); 3501 this.setStatus(status); 3502 this.setDate(date); 3503 this.setKind(kind); 3504 } 3505 3506 /** 3507 * @return {@link #url} (An absolute URI that is used to identify this terminology capabilities when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this terminology capabilities is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the terminology capabilities is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 3508 */ 3509 public UriType getUrlElement() { 3510 if (this.url == null) 3511 if (Configuration.errorOnAutoCreate()) 3512 throw new Error("Attempt to auto-create TerminologyCapabilities.url"); 3513 else if (Configuration.doAutoCreate()) 3514 this.url = new UriType(); // bb 3515 return this.url; 3516 } 3517 3518 public boolean hasUrlElement() { 3519 return this.url != null && !this.url.isEmpty(); 3520 } 3521 3522 public boolean hasUrl() { 3523 return this.url != null && !this.url.isEmpty(); 3524 } 3525 3526 /** 3527 * @param value {@link #url} (An absolute URI that is used to identify this terminology capabilities when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this terminology capabilities is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the terminology capabilities is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 3528 */ 3529 public TerminologyCapabilities setUrlElement(UriType value) { 3530 this.url = value; 3531 return this; 3532 } 3533 3534 /** 3535 * @return An absolute URI that is used to identify this terminology capabilities when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this terminology capabilities is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the terminology capabilities is stored on different servers. 3536 */ 3537 public String getUrl() { 3538 return this.url == null ? null : this.url.getValue(); 3539 } 3540 3541 /** 3542 * @param value An absolute URI that is used to identify this terminology capabilities when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this terminology capabilities is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the terminology capabilities is stored on different servers. 3543 */ 3544 public TerminologyCapabilities setUrl(String value) { 3545 if (Utilities.noString(value)) 3546 this.url = null; 3547 else { 3548 if (this.url == null) 3549 this.url = new UriType(); 3550 this.url.setValue(value); 3551 } 3552 return this; 3553 } 3554 3555 /** 3556 * @return {@link #identifier} (A formal identifier that is used to identify this terminology capabilities when it is represented in other formats, or referenced in a specification, model, design or an instance.) 3557 */ 3558 public List<Identifier> getIdentifier() { 3559 if (this.identifier == null) 3560 this.identifier = new ArrayList<Identifier>(); 3561 return this.identifier; 3562 } 3563 3564 /** 3565 * @return Returns a reference to <code>this</code> for easy method chaining 3566 */ 3567 public TerminologyCapabilities setIdentifier(List<Identifier> theIdentifier) { 3568 this.identifier = theIdentifier; 3569 return this; 3570 } 3571 3572 public boolean hasIdentifier() { 3573 if (this.identifier == null) 3574 return false; 3575 for (Identifier item : this.identifier) 3576 if (!item.isEmpty()) 3577 return true; 3578 return false; 3579 } 3580 3581 public Identifier addIdentifier() { //3 3582 Identifier t = new Identifier(); 3583 if (this.identifier == null) 3584 this.identifier = new ArrayList<Identifier>(); 3585 this.identifier.add(t); 3586 return t; 3587 } 3588 3589 public TerminologyCapabilities addIdentifier(Identifier t) { //3 3590 if (t == null) 3591 return this; 3592 if (this.identifier == null) 3593 this.identifier = new ArrayList<Identifier>(); 3594 this.identifier.add(t); 3595 return this; 3596 } 3597 3598 /** 3599 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 3600 */ 3601 public Identifier getIdentifierFirstRep() { 3602 if (getIdentifier().isEmpty()) { 3603 addIdentifier(); 3604 } 3605 return getIdentifier().get(0); 3606 } 3607 3608 /** 3609 * @return {@link #version} (The identifier that is used to identify this version of the terminology capabilities when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the terminology capabilities author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3610 */ 3611 public StringType getVersionElement() { 3612 if (this.version == null) 3613 if (Configuration.errorOnAutoCreate()) 3614 throw new Error("Attempt to auto-create TerminologyCapabilities.version"); 3615 else if (Configuration.doAutoCreate()) 3616 this.version = new StringType(); // bb 3617 return this.version; 3618 } 3619 3620 public boolean hasVersionElement() { 3621 return this.version != null && !this.version.isEmpty(); 3622 } 3623 3624 public boolean hasVersion() { 3625 return this.version != null && !this.version.isEmpty(); 3626 } 3627 3628 /** 3629 * @param value {@link #version} (The identifier that is used to identify this version of the terminology capabilities when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the terminology capabilities author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3630 */ 3631 public TerminologyCapabilities setVersionElement(StringType value) { 3632 this.version = value; 3633 return this; 3634 } 3635 3636 /** 3637 * @return The identifier that is used to identify this version of the terminology capabilities when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the terminology capabilities author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 3638 */ 3639 public String getVersion() { 3640 return this.version == null ? null : this.version.getValue(); 3641 } 3642 3643 /** 3644 * @param value The identifier that is used to identify this version of the terminology capabilities when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the terminology capabilities author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 3645 */ 3646 public TerminologyCapabilities setVersion(String value) { 3647 if (Utilities.noString(value)) 3648 this.version = null; 3649 else { 3650 if (this.version == null) 3651 this.version = new StringType(); 3652 this.version.setValue(value); 3653 } 3654 return this; 3655 } 3656 3657 /** 3658 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3659 */ 3660 public DataType getVersionAlgorithm() { 3661 return this.versionAlgorithm; 3662 } 3663 3664 /** 3665 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3666 */ 3667 public StringType getVersionAlgorithmStringType() throws FHIRException { 3668 if (this.versionAlgorithm == null) 3669 this.versionAlgorithm = new StringType(); 3670 if (!(this.versionAlgorithm instanceof StringType)) 3671 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 3672 return (StringType) this.versionAlgorithm; 3673 } 3674 3675 public boolean hasVersionAlgorithmStringType() { 3676 return this != null && this.versionAlgorithm instanceof StringType; 3677 } 3678 3679 /** 3680 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3681 */ 3682 public Coding getVersionAlgorithmCoding() throws FHIRException { 3683 if (this.versionAlgorithm == null) 3684 this.versionAlgorithm = new Coding(); 3685 if (!(this.versionAlgorithm instanceof Coding)) 3686 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 3687 return (Coding) this.versionAlgorithm; 3688 } 3689 3690 public boolean hasVersionAlgorithmCoding() { 3691 return this != null && this.versionAlgorithm instanceof Coding; 3692 } 3693 3694 public boolean hasVersionAlgorithm() { 3695 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 3696 } 3697 3698 /** 3699 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3700 */ 3701 public TerminologyCapabilities setVersionAlgorithm(DataType value) { 3702 if (value != null && !(value instanceof StringType || value instanceof Coding)) 3703 throw new FHIRException("Not the right type for TerminologyCapabilities.versionAlgorithm[x]: "+value.fhirType()); 3704 this.versionAlgorithm = value; 3705 return this; 3706 } 3707 3708 /** 3709 * @return {@link #name} (A natural language name identifying the terminology capabilities. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3710 */ 3711 public StringType getNameElement() { 3712 if (this.name == null) 3713 if (Configuration.errorOnAutoCreate()) 3714 throw new Error("Attempt to auto-create TerminologyCapabilities.name"); 3715 else if (Configuration.doAutoCreate()) 3716 this.name = new StringType(); // bb 3717 return this.name; 3718 } 3719 3720 public boolean hasNameElement() { 3721 return this.name != null && !this.name.isEmpty(); 3722 } 3723 3724 public boolean hasName() { 3725 return this.name != null && !this.name.isEmpty(); 3726 } 3727 3728 /** 3729 * @param value {@link #name} (A natural language name identifying the terminology capabilities. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3730 */ 3731 public TerminologyCapabilities setNameElement(StringType value) { 3732 this.name = value; 3733 return this; 3734 } 3735 3736 /** 3737 * @return A natural language name identifying the terminology capabilities. This name should be usable as an identifier for the module by machine processing applications such as code generation. 3738 */ 3739 public String getName() { 3740 return this.name == null ? null : this.name.getValue(); 3741 } 3742 3743 /** 3744 * @param value A natural language name identifying the terminology capabilities. This name should be usable as an identifier for the module by machine processing applications such as code generation. 3745 */ 3746 public TerminologyCapabilities setName(String value) { 3747 if (Utilities.noString(value)) 3748 this.name = null; 3749 else { 3750 if (this.name == null) 3751 this.name = new StringType(); 3752 this.name.setValue(value); 3753 } 3754 return this; 3755 } 3756 3757 /** 3758 * @return {@link #title} (A short, descriptive, user-friendly title for the terminology capabilities.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3759 */ 3760 public StringType getTitleElement() { 3761 if (this.title == null) 3762 if (Configuration.errorOnAutoCreate()) 3763 throw new Error("Attempt to auto-create TerminologyCapabilities.title"); 3764 else if (Configuration.doAutoCreate()) 3765 this.title = new StringType(); // bb 3766 return this.title; 3767 } 3768 3769 public boolean hasTitleElement() { 3770 return this.title != null && !this.title.isEmpty(); 3771 } 3772 3773 public boolean hasTitle() { 3774 return this.title != null && !this.title.isEmpty(); 3775 } 3776 3777 /** 3778 * @param value {@link #title} (A short, descriptive, user-friendly title for the terminology capabilities.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3779 */ 3780 public TerminologyCapabilities setTitleElement(StringType value) { 3781 this.title = value; 3782 return this; 3783 } 3784 3785 /** 3786 * @return A short, descriptive, user-friendly title for the terminology capabilities. 3787 */ 3788 public String getTitle() { 3789 return this.title == null ? null : this.title.getValue(); 3790 } 3791 3792 /** 3793 * @param value A short, descriptive, user-friendly title for the terminology capabilities. 3794 */ 3795 public TerminologyCapabilities setTitle(String value) { 3796 if (Utilities.noString(value)) 3797 this.title = null; 3798 else { 3799 if (this.title == null) 3800 this.title = new StringType(); 3801 this.title.setValue(value); 3802 } 3803 return this; 3804 } 3805 3806 /** 3807 * @return {@link #status} (The status of this terminology capabilities. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3808 */ 3809 public Enumeration<PublicationStatus> getStatusElement() { 3810 if (this.status == null) 3811 if (Configuration.errorOnAutoCreate()) 3812 throw new Error("Attempt to auto-create TerminologyCapabilities.status"); 3813 else if (Configuration.doAutoCreate()) 3814 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 3815 return this.status; 3816 } 3817 3818 public boolean hasStatusElement() { 3819 return this.status != null && !this.status.isEmpty(); 3820 } 3821 3822 public boolean hasStatus() { 3823 return this.status != null && !this.status.isEmpty(); 3824 } 3825 3826 /** 3827 * @param value {@link #status} (The status of this terminology capabilities. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3828 */ 3829 public TerminologyCapabilities setStatusElement(Enumeration<PublicationStatus> value) { 3830 this.status = value; 3831 return this; 3832 } 3833 3834 /** 3835 * @return The status of this terminology capabilities. Enables tracking the life-cycle of the content. 3836 */ 3837 public PublicationStatus getStatus() { 3838 return this.status == null ? null : this.status.getValue(); 3839 } 3840 3841 /** 3842 * @param value The status of this terminology capabilities. Enables tracking the life-cycle of the content. 3843 */ 3844 public TerminologyCapabilities setStatus(PublicationStatus value) { 3845 if (this.status == null) 3846 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 3847 this.status.setValue(value); 3848 return this; 3849 } 3850 3851 /** 3852 * @return {@link #experimental} (A Boolean value to indicate that this terminology capabilities is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3853 */ 3854 public BooleanType getExperimentalElement() { 3855 if (this.experimental == null) 3856 if (Configuration.errorOnAutoCreate()) 3857 throw new Error("Attempt to auto-create TerminologyCapabilities.experimental"); 3858 else if (Configuration.doAutoCreate()) 3859 this.experimental = new BooleanType(); // bb 3860 return this.experimental; 3861 } 3862 3863 public boolean hasExperimentalElement() { 3864 return this.experimental != null && !this.experimental.isEmpty(); 3865 } 3866 3867 public boolean hasExperimental() { 3868 return this.experimental != null && !this.experimental.isEmpty(); 3869 } 3870 3871 /** 3872 * @param value {@link #experimental} (A Boolean value to indicate that this terminology capabilities is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3873 */ 3874 public TerminologyCapabilities setExperimentalElement(BooleanType value) { 3875 this.experimental = value; 3876 return this; 3877 } 3878 3879 /** 3880 * @return A Boolean value to indicate that this terminology capabilities is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 3881 */ 3882 public boolean getExperimental() { 3883 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 3884 } 3885 3886 /** 3887 * @param value A Boolean value to indicate that this terminology capabilities is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 3888 */ 3889 public TerminologyCapabilities setExperimental(boolean value) { 3890 if (this.experimental == null) 3891 this.experimental = new BooleanType(); 3892 this.experimental.setValue(value); 3893 return this; 3894 } 3895 3896 /** 3897 * @return {@link #date} (The date (and optionally time) when the terminology capabilities was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the terminology capabilities changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3898 */ 3899 public DateTimeType getDateElement() { 3900 if (this.date == null) 3901 if (Configuration.errorOnAutoCreate()) 3902 throw new Error("Attempt to auto-create TerminologyCapabilities.date"); 3903 else if (Configuration.doAutoCreate()) 3904 this.date = new DateTimeType(); // bb 3905 return this.date; 3906 } 3907 3908 public boolean hasDateElement() { 3909 return this.date != null && !this.date.isEmpty(); 3910 } 3911 3912 public boolean hasDate() { 3913 return this.date != null && !this.date.isEmpty(); 3914 } 3915 3916 /** 3917 * @param value {@link #date} (The date (and optionally time) when the terminology capabilities was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the terminology capabilities changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3918 */ 3919 public TerminologyCapabilities setDateElement(DateTimeType value) { 3920 this.date = value; 3921 return this; 3922 } 3923 3924 /** 3925 * @return The date (and optionally time) when the terminology capabilities was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the terminology capabilities changes. 3926 */ 3927 public Date getDate() { 3928 return this.date == null ? null : this.date.getValue(); 3929 } 3930 3931 /** 3932 * @param value The date (and optionally time) when the terminology capabilities was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the terminology capabilities changes. 3933 */ 3934 public TerminologyCapabilities setDate(Date value) { 3935 if (this.date == null) 3936 this.date = new DateTimeType(); 3937 this.date.setValue(value); 3938 return this; 3939 } 3940 3941 /** 3942 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the terminology capabilities.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3943 */ 3944 public StringType getPublisherElement() { 3945 if (this.publisher == null) 3946 if (Configuration.errorOnAutoCreate()) 3947 throw new Error("Attempt to auto-create TerminologyCapabilities.publisher"); 3948 else if (Configuration.doAutoCreate()) 3949 this.publisher = new StringType(); // bb 3950 return this.publisher; 3951 } 3952 3953 public boolean hasPublisherElement() { 3954 return this.publisher != null && !this.publisher.isEmpty(); 3955 } 3956 3957 public boolean hasPublisher() { 3958 return this.publisher != null && !this.publisher.isEmpty(); 3959 } 3960 3961 /** 3962 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the terminology capabilities.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 3963 */ 3964 public TerminologyCapabilities setPublisherElement(StringType value) { 3965 this.publisher = value; 3966 return this; 3967 } 3968 3969 /** 3970 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the terminology capabilities. 3971 */ 3972 public String getPublisher() { 3973 return this.publisher == null ? null : this.publisher.getValue(); 3974 } 3975 3976 /** 3977 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the terminology capabilities. 3978 */ 3979 public TerminologyCapabilities setPublisher(String value) { 3980 if (Utilities.noString(value)) 3981 this.publisher = null; 3982 else { 3983 if (this.publisher == null) 3984 this.publisher = new StringType(); 3985 this.publisher.setValue(value); 3986 } 3987 return this; 3988 } 3989 3990 /** 3991 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 3992 */ 3993 public List<ContactDetail> getContact() { 3994 if (this.contact == null) 3995 this.contact = new ArrayList<ContactDetail>(); 3996 return this.contact; 3997 } 3998 3999 /** 4000 * @return Returns a reference to <code>this</code> for easy method chaining 4001 */ 4002 public TerminologyCapabilities setContact(List<ContactDetail> theContact) { 4003 this.contact = theContact; 4004 return this; 4005 } 4006 4007 public boolean hasContact() { 4008 if (this.contact == null) 4009 return false; 4010 for (ContactDetail item : this.contact) 4011 if (!item.isEmpty()) 4012 return true; 4013 return false; 4014 } 4015 4016 public ContactDetail addContact() { //3 4017 ContactDetail t = new ContactDetail(); 4018 if (this.contact == null) 4019 this.contact = new ArrayList<ContactDetail>(); 4020 this.contact.add(t); 4021 return t; 4022 } 4023 4024 public TerminologyCapabilities addContact(ContactDetail t) { //3 4025 if (t == null) 4026 return this; 4027 if (this.contact == null) 4028 this.contact = new ArrayList<ContactDetail>(); 4029 this.contact.add(t); 4030 return this; 4031 } 4032 4033 /** 4034 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 4035 */ 4036 public ContactDetail getContactFirstRep() { 4037 if (getContact().isEmpty()) { 4038 addContact(); 4039 } 4040 return getContact().get(0); 4041 } 4042 4043 /** 4044 * @return {@link #description} (A free text natural language description of the terminology capabilities from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4045 */ 4046 public MarkdownType getDescriptionElement() { 4047 if (this.description == null) 4048 if (Configuration.errorOnAutoCreate()) 4049 throw new Error("Attempt to auto-create TerminologyCapabilities.description"); 4050 else if (Configuration.doAutoCreate()) 4051 this.description = new MarkdownType(); // bb 4052 return this.description; 4053 } 4054 4055 public boolean hasDescriptionElement() { 4056 return this.description != null && !this.description.isEmpty(); 4057 } 4058 4059 public boolean hasDescription() { 4060 return this.description != null && !this.description.isEmpty(); 4061 } 4062 4063 /** 4064 * @param value {@link #description} (A free text natural language description of the terminology capabilities from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4065 */ 4066 public TerminologyCapabilities setDescriptionElement(MarkdownType value) { 4067 this.description = value; 4068 return this; 4069 } 4070 4071 /** 4072 * @return A free text natural language description of the terminology capabilities from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP. 4073 */ 4074 public String getDescription() { 4075 return this.description == null ? null : this.description.getValue(); 4076 } 4077 4078 /** 4079 * @param value A free text natural language description of the terminology capabilities from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP. 4080 */ 4081 public TerminologyCapabilities setDescription(String value) { 4082 if (Utilities.noString(value)) 4083 this.description = null; 4084 else { 4085 if (this.description == null) 4086 this.description = new MarkdownType(); 4087 this.description.setValue(value); 4088 } 4089 return this; 4090 } 4091 4092 /** 4093 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate terminology capabilities instances.) 4094 */ 4095 public List<UsageContext> getUseContext() { 4096 if (this.useContext == null) 4097 this.useContext = new ArrayList<UsageContext>(); 4098 return this.useContext; 4099 } 4100 4101 /** 4102 * @return Returns a reference to <code>this</code> for easy method chaining 4103 */ 4104 public TerminologyCapabilities setUseContext(List<UsageContext> theUseContext) { 4105 this.useContext = theUseContext; 4106 return this; 4107 } 4108 4109 public boolean hasUseContext() { 4110 if (this.useContext == null) 4111 return false; 4112 for (UsageContext item : this.useContext) 4113 if (!item.isEmpty()) 4114 return true; 4115 return false; 4116 } 4117 4118 public UsageContext addUseContext() { //3 4119 UsageContext t = new UsageContext(); 4120 if (this.useContext == null) 4121 this.useContext = new ArrayList<UsageContext>(); 4122 this.useContext.add(t); 4123 return t; 4124 } 4125 4126 public TerminologyCapabilities addUseContext(UsageContext t) { //3 4127 if (t == null) 4128 return this; 4129 if (this.useContext == null) 4130 this.useContext = new ArrayList<UsageContext>(); 4131 this.useContext.add(t); 4132 return this; 4133 } 4134 4135 /** 4136 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 4137 */ 4138 public UsageContext getUseContextFirstRep() { 4139 if (getUseContext().isEmpty()) { 4140 addUseContext(); 4141 } 4142 return getUseContext().get(0); 4143 } 4144 4145 /** 4146 * @return {@link #jurisdiction} (A legal or geographic region in which the terminology capabilities is intended to be used.) 4147 */ 4148 public List<CodeableConcept> getJurisdiction() { 4149 if (this.jurisdiction == null) 4150 this.jurisdiction = new ArrayList<CodeableConcept>(); 4151 return this.jurisdiction; 4152 } 4153 4154 /** 4155 * @return Returns a reference to <code>this</code> for easy method chaining 4156 */ 4157 public TerminologyCapabilities setJurisdiction(List<CodeableConcept> theJurisdiction) { 4158 this.jurisdiction = theJurisdiction; 4159 return this; 4160 } 4161 4162 public boolean hasJurisdiction() { 4163 if (this.jurisdiction == null) 4164 return false; 4165 for (CodeableConcept item : this.jurisdiction) 4166 if (!item.isEmpty()) 4167 return true; 4168 return false; 4169 } 4170 4171 public CodeableConcept addJurisdiction() { //3 4172 CodeableConcept t = new CodeableConcept(); 4173 if (this.jurisdiction == null) 4174 this.jurisdiction = new ArrayList<CodeableConcept>(); 4175 this.jurisdiction.add(t); 4176 return t; 4177 } 4178 4179 public TerminologyCapabilities addJurisdiction(CodeableConcept t) { //3 4180 if (t == null) 4181 return this; 4182 if (this.jurisdiction == null) 4183 this.jurisdiction = new ArrayList<CodeableConcept>(); 4184 this.jurisdiction.add(t); 4185 return this; 4186 } 4187 4188 /** 4189 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 4190 */ 4191 public CodeableConcept getJurisdictionFirstRep() { 4192 if (getJurisdiction().isEmpty()) { 4193 addJurisdiction(); 4194 } 4195 return getJurisdiction().get(0); 4196 } 4197 4198 /** 4199 * @return {@link #purpose} (Explanation of why this terminology capabilities is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 4200 */ 4201 public MarkdownType getPurposeElement() { 4202 if (this.purpose == null) 4203 if (Configuration.errorOnAutoCreate()) 4204 throw new Error("Attempt to auto-create TerminologyCapabilities.purpose"); 4205 else if (Configuration.doAutoCreate()) 4206 this.purpose = new MarkdownType(); // bb 4207 return this.purpose; 4208 } 4209 4210 public boolean hasPurposeElement() { 4211 return this.purpose != null && !this.purpose.isEmpty(); 4212 } 4213 4214 public boolean hasPurpose() { 4215 return this.purpose != null && !this.purpose.isEmpty(); 4216 } 4217 4218 /** 4219 * @param value {@link #purpose} (Explanation of why this terminology capabilities is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 4220 */ 4221 public TerminologyCapabilities setPurposeElement(MarkdownType value) { 4222 this.purpose = value; 4223 return this; 4224 } 4225 4226 /** 4227 * @return Explanation of why this terminology capabilities is needed and why it has been designed as it has. 4228 */ 4229 public String getPurpose() { 4230 return this.purpose == null ? null : this.purpose.getValue(); 4231 } 4232 4233 /** 4234 * @param value Explanation of why this terminology capabilities is needed and why it has been designed as it has. 4235 */ 4236 public TerminologyCapabilities setPurpose(String value) { 4237 if (Utilities.noString(value)) 4238 this.purpose = null; 4239 else { 4240 if (this.purpose == null) 4241 this.purpose = new MarkdownType(); 4242 this.purpose.setValue(value); 4243 } 4244 return this; 4245 } 4246 4247 /** 4248 * @return {@link #copyright} (A copyright statement relating to the terminology capabilities and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the terminology capabilities.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 4249 */ 4250 public MarkdownType getCopyrightElement() { 4251 if (this.copyright == null) 4252 if (Configuration.errorOnAutoCreate()) 4253 throw new Error("Attempt to auto-create TerminologyCapabilities.copyright"); 4254 else if (Configuration.doAutoCreate()) 4255 this.copyright = new MarkdownType(); // bb 4256 return this.copyright; 4257 } 4258 4259 public boolean hasCopyrightElement() { 4260 return this.copyright != null && !this.copyright.isEmpty(); 4261 } 4262 4263 public boolean hasCopyright() { 4264 return this.copyright != null && !this.copyright.isEmpty(); 4265 } 4266 4267 /** 4268 * @param value {@link #copyright} (A copyright statement relating to the terminology capabilities and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the terminology capabilities.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 4269 */ 4270 public TerminologyCapabilities setCopyrightElement(MarkdownType value) { 4271 this.copyright = value; 4272 return this; 4273 } 4274 4275 /** 4276 * @return A copyright statement relating to the terminology capabilities and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the terminology capabilities. 4277 */ 4278 public String getCopyright() { 4279 return this.copyright == null ? null : this.copyright.getValue(); 4280 } 4281 4282 /** 4283 * @param value A copyright statement relating to the terminology capabilities and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the terminology capabilities. 4284 */ 4285 public TerminologyCapabilities setCopyright(String value) { 4286 if (Utilities.noString(value)) 4287 this.copyright = null; 4288 else { 4289 if (this.copyright == null) 4290 this.copyright = new MarkdownType(); 4291 this.copyright.setValue(value); 4292 } 4293 return this; 4294 } 4295 4296 /** 4297 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 4298 */ 4299 public StringType getCopyrightLabelElement() { 4300 if (this.copyrightLabel == null) 4301 if (Configuration.errorOnAutoCreate()) 4302 throw new Error("Attempt to auto-create TerminologyCapabilities.copyrightLabel"); 4303 else if (Configuration.doAutoCreate()) 4304 this.copyrightLabel = new StringType(); // bb 4305 return this.copyrightLabel; 4306 } 4307 4308 public boolean hasCopyrightLabelElement() { 4309 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 4310 } 4311 4312 public boolean hasCopyrightLabel() { 4313 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 4314 } 4315 4316 /** 4317 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 4318 */ 4319 public TerminologyCapabilities setCopyrightLabelElement(StringType value) { 4320 this.copyrightLabel = value; 4321 return this; 4322 } 4323 4324 /** 4325 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 4326 */ 4327 public String getCopyrightLabel() { 4328 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 4329 } 4330 4331 /** 4332 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 4333 */ 4334 public TerminologyCapabilities setCopyrightLabel(String value) { 4335 if (Utilities.noString(value)) 4336 this.copyrightLabel = null; 4337 else { 4338 if (this.copyrightLabel == null) 4339 this.copyrightLabel = new StringType(); 4340 this.copyrightLabel.setValue(value); 4341 } 4342 return this; 4343 } 4344 4345 /** 4346 * @return {@link #kind} (The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase).). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 4347 */ 4348 public Enumeration<CapabilityStatementKind> getKindElement() { 4349 if (this.kind == null) 4350 if (Configuration.errorOnAutoCreate()) 4351 throw new Error("Attempt to auto-create TerminologyCapabilities.kind"); 4352 else if (Configuration.doAutoCreate()) 4353 this.kind = new Enumeration<CapabilityStatementKind>(new CapabilityStatementKindEnumFactory()); // bb 4354 return this.kind; 4355 } 4356 4357 public boolean hasKindElement() { 4358 return this.kind != null && !this.kind.isEmpty(); 4359 } 4360 4361 public boolean hasKind() { 4362 return this.kind != null && !this.kind.isEmpty(); 4363 } 4364 4365 /** 4366 * @param value {@link #kind} (The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase).). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 4367 */ 4368 public TerminologyCapabilities setKindElement(Enumeration<CapabilityStatementKind> value) { 4369 this.kind = value; 4370 return this; 4371 } 4372 4373 /** 4374 * @return The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase). 4375 */ 4376 public CapabilityStatementKind getKind() { 4377 return this.kind == null ? null : this.kind.getValue(); 4378 } 4379 4380 /** 4381 * @param value The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase). 4382 */ 4383 public TerminologyCapabilities setKind(CapabilityStatementKind value) { 4384 if (this.kind == null) 4385 this.kind = new Enumeration<CapabilityStatementKind>(new CapabilityStatementKindEnumFactory()); 4386 this.kind.setValue(value); 4387 return this; 4388 } 4389 4390 /** 4391 * @return {@link #software} (Software that is covered by this terminology capability statement. It is used when the statement describes the capabilities of a particular software version, independent of an installation.) 4392 */ 4393 public TerminologyCapabilitiesSoftwareComponent getSoftware() { 4394 if (this.software == null) 4395 if (Configuration.errorOnAutoCreate()) 4396 throw new Error("Attempt to auto-create TerminologyCapabilities.software"); 4397 else if (Configuration.doAutoCreate()) 4398 this.software = new TerminologyCapabilitiesSoftwareComponent(); // cc 4399 return this.software; 4400 } 4401 4402 public boolean hasSoftware() { 4403 return this.software != null && !this.software.isEmpty(); 4404 } 4405 4406 /** 4407 * @param value {@link #software} (Software that is covered by this terminology capability statement. It is used when the statement describes the capabilities of a particular software version, independent of an installation.) 4408 */ 4409 public TerminologyCapabilities setSoftware(TerminologyCapabilitiesSoftwareComponent value) { 4410 this.software = value; 4411 return this; 4412 } 4413 4414 /** 4415 * @return {@link #implementation} (Identifies a specific implementation instance that is described by the terminology capability statement - i.e. a particular installation, rather than the capabilities of a software program.) 4416 */ 4417 public TerminologyCapabilitiesImplementationComponent getImplementation() { 4418 if (this.implementation == null) 4419 if (Configuration.errorOnAutoCreate()) 4420 throw new Error("Attempt to auto-create TerminologyCapabilities.implementation"); 4421 else if (Configuration.doAutoCreate()) 4422 this.implementation = new TerminologyCapabilitiesImplementationComponent(); // cc 4423 return this.implementation; 4424 } 4425 4426 public boolean hasImplementation() { 4427 return this.implementation != null && !this.implementation.isEmpty(); 4428 } 4429 4430 /** 4431 * @param value {@link #implementation} (Identifies a specific implementation instance that is described by the terminology capability statement - i.e. a particular installation, rather than the capabilities of a software program.) 4432 */ 4433 public TerminologyCapabilities setImplementation(TerminologyCapabilitiesImplementationComponent value) { 4434 this.implementation = value; 4435 return this; 4436 } 4437 4438 /** 4439 * @return {@link #lockedDate} (Whether the server supports lockedDate.). This is the underlying object with id, value and extensions. The accessor "getLockedDate" gives direct access to the value 4440 */ 4441 public BooleanType getLockedDateElement() { 4442 if (this.lockedDate == null) 4443 if (Configuration.errorOnAutoCreate()) 4444 throw new Error("Attempt to auto-create TerminologyCapabilities.lockedDate"); 4445 else if (Configuration.doAutoCreate()) 4446 this.lockedDate = new BooleanType(); // bb 4447 return this.lockedDate; 4448 } 4449 4450 public boolean hasLockedDateElement() { 4451 return this.lockedDate != null && !this.lockedDate.isEmpty(); 4452 } 4453 4454 public boolean hasLockedDate() { 4455 return this.lockedDate != null && !this.lockedDate.isEmpty(); 4456 } 4457 4458 /** 4459 * @param value {@link #lockedDate} (Whether the server supports lockedDate.). This is the underlying object with id, value and extensions. The accessor "getLockedDate" gives direct access to the value 4460 */ 4461 public TerminologyCapabilities setLockedDateElement(BooleanType value) { 4462 this.lockedDate = value; 4463 return this; 4464 } 4465 4466 /** 4467 * @return Whether the server supports lockedDate. 4468 */ 4469 public boolean getLockedDate() { 4470 return this.lockedDate == null || this.lockedDate.isEmpty() ? false : this.lockedDate.getValue(); 4471 } 4472 4473 /** 4474 * @param value Whether the server supports lockedDate. 4475 */ 4476 public TerminologyCapabilities setLockedDate(boolean value) { 4477 if (this.lockedDate == null) 4478 this.lockedDate = new BooleanType(); 4479 this.lockedDate.setValue(value); 4480 return this; 4481 } 4482 4483 /** 4484 * @return {@link #codeSystem} (Identifies a code system that is supported by the server. If there is a no code system URL, then this declares the general assumptions a client can make about support for any CodeSystem resource.) 4485 */ 4486 public List<TerminologyCapabilitiesCodeSystemComponent> getCodeSystem() { 4487 if (this.codeSystem == null) 4488 this.codeSystem = new ArrayList<TerminologyCapabilitiesCodeSystemComponent>(); 4489 return this.codeSystem; 4490 } 4491 4492 /** 4493 * @return Returns a reference to <code>this</code> for easy method chaining 4494 */ 4495 public TerminologyCapabilities setCodeSystem(List<TerminologyCapabilitiesCodeSystemComponent> theCodeSystem) { 4496 this.codeSystem = theCodeSystem; 4497 return this; 4498 } 4499 4500 public boolean hasCodeSystem() { 4501 if (this.codeSystem == null) 4502 return false; 4503 for (TerminologyCapabilitiesCodeSystemComponent item : this.codeSystem) 4504 if (!item.isEmpty()) 4505 return true; 4506 return false; 4507 } 4508 4509 public TerminologyCapabilitiesCodeSystemComponent addCodeSystem() { //3 4510 TerminologyCapabilitiesCodeSystemComponent t = new TerminologyCapabilitiesCodeSystemComponent(); 4511 if (this.codeSystem == null) 4512 this.codeSystem = new ArrayList<TerminologyCapabilitiesCodeSystemComponent>(); 4513 this.codeSystem.add(t); 4514 return t; 4515 } 4516 4517 public TerminologyCapabilities addCodeSystem(TerminologyCapabilitiesCodeSystemComponent t) { //3 4518 if (t == null) 4519 return this; 4520 if (this.codeSystem == null) 4521 this.codeSystem = new ArrayList<TerminologyCapabilitiesCodeSystemComponent>(); 4522 this.codeSystem.add(t); 4523 return this; 4524 } 4525 4526 /** 4527 * @return The first repetition of repeating field {@link #codeSystem}, creating it if it does not already exist {3} 4528 */ 4529 public TerminologyCapabilitiesCodeSystemComponent getCodeSystemFirstRep() { 4530 if (getCodeSystem().isEmpty()) { 4531 addCodeSystem(); 4532 } 4533 return getCodeSystem().get(0); 4534 } 4535 4536 /** 4537 * @return {@link #expansion} (Information about the [ValueSet/$expand](valueset-operation-expand.html) operation.) 4538 */ 4539 public TerminologyCapabilitiesExpansionComponent getExpansion() { 4540 if (this.expansion == null) 4541 if (Configuration.errorOnAutoCreate()) 4542 throw new Error("Attempt to auto-create TerminologyCapabilities.expansion"); 4543 else if (Configuration.doAutoCreate()) 4544 this.expansion = new TerminologyCapabilitiesExpansionComponent(); // cc 4545 return this.expansion; 4546 } 4547 4548 public boolean hasExpansion() { 4549 return this.expansion != null && !this.expansion.isEmpty(); 4550 } 4551 4552 /** 4553 * @param value {@link #expansion} (Information about the [ValueSet/$expand](valueset-operation-expand.html) operation.) 4554 */ 4555 public TerminologyCapabilities setExpansion(TerminologyCapabilitiesExpansionComponent value) { 4556 this.expansion = value; 4557 return this; 4558 } 4559 4560 /** 4561 * @return {@link #codeSearch} (The degree to which the server supports the code search parameter on ValueSet, if it is supported.). This is the underlying object with id, value and extensions. The accessor "getCodeSearch" gives direct access to the value 4562 */ 4563 public Enumeration<CodeSearchSupport> getCodeSearchElement() { 4564 if (this.codeSearch == null) 4565 if (Configuration.errorOnAutoCreate()) 4566 throw new Error("Attempt to auto-create TerminologyCapabilities.codeSearch"); 4567 else if (Configuration.doAutoCreate()) 4568 this.codeSearch = new Enumeration<CodeSearchSupport>(new CodeSearchSupportEnumFactory()); // bb 4569 return this.codeSearch; 4570 } 4571 4572 public boolean hasCodeSearchElement() { 4573 return this.codeSearch != null && !this.codeSearch.isEmpty(); 4574 } 4575 4576 public boolean hasCodeSearch() { 4577 return this.codeSearch != null && !this.codeSearch.isEmpty(); 4578 } 4579 4580 /** 4581 * @param value {@link #codeSearch} (The degree to which the server supports the code search parameter on ValueSet, if it is supported.). This is the underlying object with id, value and extensions. The accessor "getCodeSearch" gives direct access to the value 4582 */ 4583 public TerminologyCapabilities setCodeSearchElement(Enumeration<CodeSearchSupport> value) { 4584 this.codeSearch = value; 4585 return this; 4586 } 4587 4588 /** 4589 * @return The degree to which the server supports the code search parameter on ValueSet, if it is supported. 4590 */ 4591 public CodeSearchSupport getCodeSearch() { 4592 return this.codeSearch == null ? null : this.codeSearch.getValue(); 4593 } 4594 4595 /** 4596 * @param value The degree to which the server supports the code search parameter on ValueSet, if it is supported. 4597 */ 4598 public TerminologyCapabilities setCodeSearch(CodeSearchSupport value) { 4599 if (value == null) 4600 this.codeSearch = null; 4601 else { 4602 if (this.codeSearch == null) 4603 this.codeSearch = new Enumeration<CodeSearchSupport>(new CodeSearchSupportEnumFactory()); 4604 this.codeSearch.setValue(value); 4605 } 4606 return this; 4607 } 4608 4609 /** 4610 * @return {@link #validateCode} (Information about the [ValueSet/$validate-code](valueset-operation-validate-code.html) operation.) 4611 */ 4612 public TerminologyCapabilitiesValidateCodeComponent getValidateCode() { 4613 if (this.validateCode == null) 4614 if (Configuration.errorOnAutoCreate()) 4615 throw new Error("Attempt to auto-create TerminologyCapabilities.validateCode"); 4616 else if (Configuration.doAutoCreate()) 4617 this.validateCode = new TerminologyCapabilitiesValidateCodeComponent(); // cc 4618 return this.validateCode; 4619 } 4620 4621 public boolean hasValidateCode() { 4622 return this.validateCode != null && !this.validateCode.isEmpty(); 4623 } 4624 4625 /** 4626 * @param value {@link #validateCode} (Information about the [ValueSet/$validate-code](valueset-operation-validate-code.html) operation.) 4627 */ 4628 public TerminologyCapabilities setValidateCode(TerminologyCapabilitiesValidateCodeComponent value) { 4629 this.validateCode = value; 4630 return this; 4631 } 4632 4633 /** 4634 * @return {@link #translation} (Information about the [ConceptMap/$translate](conceptmap-operation-translate.html) operation.) 4635 */ 4636 public TerminologyCapabilitiesTranslationComponent getTranslation() { 4637 if (this.translation == null) 4638 if (Configuration.errorOnAutoCreate()) 4639 throw new Error("Attempt to auto-create TerminologyCapabilities.translation"); 4640 else if (Configuration.doAutoCreate()) 4641 this.translation = new TerminologyCapabilitiesTranslationComponent(); // cc 4642 return this.translation; 4643 } 4644 4645 public boolean hasTranslation() { 4646 return this.translation != null && !this.translation.isEmpty(); 4647 } 4648 4649 /** 4650 * @param value {@link #translation} (Information about the [ConceptMap/$translate](conceptmap-operation-translate.html) operation.) 4651 */ 4652 public TerminologyCapabilities setTranslation(TerminologyCapabilitiesTranslationComponent value) { 4653 this.translation = value; 4654 return this; 4655 } 4656 4657 /** 4658 * @return {@link #closure} (Whether the $closure operation is supported.) 4659 */ 4660 public TerminologyCapabilitiesClosureComponent getClosure() { 4661 if (this.closure == null) 4662 if (Configuration.errorOnAutoCreate()) 4663 throw new Error("Attempt to auto-create TerminologyCapabilities.closure"); 4664 else if (Configuration.doAutoCreate()) 4665 this.closure = new TerminologyCapabilitiesClosureComponent(); // cc 4666 return this.closure; 4667 } 4668 4669 public boolean hasClosure() { 4670 return this.closure != null && !this.closure.isEmpty(); 4671 } 4672 4673 /** 4674 * @param value {@link #closure} (Whether the $closure operation is supported.) 4675 */ 4676 public TerminologyCapabilities setClosure(TerminologyCapabilitiesClosureComponent value) { 4677 this.closure = value; 4678 return this; 4679 } 4680 4681 protected void listChildren(List<Property> children) { 4682 super.listChildren(children); 4683 children.add(new Property("url", "uri", "An absolute URI that is used to identify this terminology capabilities when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this terminology capabilities is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the terminology capabilities is stored on different servers.", 0, 1, url)); 4684 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this terminology capabilities when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 4685 children.add(new Property("version", "string", "The identifier that is used to identify this version of the terminology capabilities when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the terminology capabilities author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 4686 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 4687 children.add(new Property("name", "string", "A natural language name identifying the terminology capabilities. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 4688 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the terminology capabilities.", 0, 1, title)); 4689 children.add(new Property("status", "code", "The status of this terminology capabilities. Enables tracking the life-cycle of the content.", 0, 1, status)); 4690 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this terminology capabilities is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 4691 children.add(new Property("date", "dateTime", "The date (and optionally time) when the terminology capabilities was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the terminology capabilities changes.", 0, 1, date)); 4692 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the terminology capabilities.", 0, 1, publisher)); 4693 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 4694 children.add(new Property("description", "markdown", "A free text natural language description of the terminology capabilities from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.", 0, 1, description)); 4695 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate terminology capabilities instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 4696 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the terminology capabilities is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 4697 children.add(new Property("purpose", "markdown", "Explanation of why this terminology capabilities is needed and why it has been designed as it has.", 0, 1, purpose)); 4698 children.add(new Property("copyright", "markdown", "A copyright statement relating to the terminology capabilities and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the terminology capabilities.", 0, 1, copyright)); 4699 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 4700 children.add(new Property("kind", "code", "The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase).", 0, 1, kind)); 4701 children.add(new Property("software", "", "Software that is covered by this terminology capability statement. It is used when the statement describes the capabilities of a particular software version, independent of an installation.", 0, 1, software)); 4702 children.add(new Property("implementation", "", "Identifies a specific implementation instance that is described by the terminology capability statement - i.e. a particular installation, rather than the capabilities of a software program.", 0, 1, implementation)); 4703 children.add(new Property("lockedDate", "boolean", "Whether the server supports lockedDate.", 0, 1, lockedDate)); 4704 children.add(new Property("codeSystem", "", "Identifies a code system that is supported by the server. If there is a no code system URL, then this declares the general assumptions a client can make about support for any CodeSystem resource.", 0, java.lang.Integer.MAX_VALUE, codeSystem)); 4705 children.add(new Property("expansion", "", "Information about the [ValueSet/$expand](valueset-operation-expand.html) operation.", 0, 1, expansion)); 4706 children.add(new Property("codeSearch", "code", "The degree to which the server supports the code search parameter on ValueSet, if it is supported.", 0, 1, codeSearch)); 4707 children.add(new Property("validateCode", "", "Information about the [ValueSet/$validate-code](valueset-operation-validate-code.html) operation.", 0, 1, validateCode)); 4708 children.add(new Property("translation", "", "Information about the [ConceptMap/$translate](conceptmap-operation-translate.html) operation.", 0, 1, translation)); 4709 children.add(new Property("closure", "", "Whether the $closure operation is supported.", 0, 1, closure)); 4710 } 4711 4712 @Override 4713 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4714 switch (_hash) { 4715 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this terminology capabilities when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this terminology capabilities is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the terminology capabilities is stored on different servers.", 0, 1, url); 4716 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this terminology capabilities when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 4717 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the terminology capabilities when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the terminology capabilities author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 4718 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 4719 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 4720 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 4721 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 4722 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the terminology capabilities. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 4723 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the terminology capabilities.", 0, 1, title); 4724 case -892481550: /*status*/ return new Property("status", "code", "The status of this terminology capabilities. Enables tracking the life-cycle of the content.", 0, 1, status); 4725 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this terminology capabilities is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 4726 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the terminology capabilities was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the terminology capabilities changes.", 0, 1, date); 4727 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the terminology capabilities.", 0, 1, publisher); 4728 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 4729 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the terminology capabilities from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.", 0, 1, description); 4730 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate terminology capabilities instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 4731 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the terminology capabilities is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 4732 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this terminology capabilities is needed and why it has been designed as it has.", 0, 1, purpose); 4733 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the terminology capabilities and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the terminology capabilities.", 0, 1, copyright); 4734 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 4735 case 3292052: /*kind*/ return new Property("kind", "code", "The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase).", 0, 1, kind); 4736 case 1319330215: /*software*/ return new Property("software", "", "Software that is covered by this terminology capability statement. It is used when the statement describes the capabilities of a particular software version, independent of an installation.", 0, 1, software); 4737 case 1683336114: /*implementation*/ return new Property("implementation", "", "Identifies a specific implementation instance that is described by the terminology capability statement - i.e. a particular installation, rather than the capabilities of a software program.", 0, 1, implementation); 4738 case 1391591896: /*lockedDate*/ return new Property("lockedDate", "boolean", "Whether the server supports lockedDate.", 0, 1, lockedDate); 4739 case -916511108: /*codeSystem*/ return new Property("codeSystem", "", "Identifies a code system that is supported by the server. If there is a no code system URL, then this declares the general assumptions a client can make about support for any CodeSystem resource.", 0, java.lang.Integer.MAX_VALUE, codeSystem); 4740 case 17878207: /*expansion*/ return new Property("expansion", "", "Information about the [ValueSet/$expand](valueset-operation-expand.html) operation.", 0, 1, expansion); 4741 case -935519755: /*codeSearch*/ return new Property("codeSearch", "code", "The degree to which the server supports the code search parameter on ValueSet, if it is supported.", 0, 1, codeSearch); 4742 case 1080737827: /*validateCode*/ return new Property("validateCode", "", "Information about the [ValueSet/$validate-code](valueset-operation-validate-code.html) operation.", 0, 1, validateCode); 4743 case -1840647503: /*translation*/ return new Property("translation", "", "Information about the [ConceptMap/$translate](conceptmap-operation-translate.html) operation.", 0, 1, translation); 4744 case 866552379: /*closure*/ return new Property("closure", "", "Whether the $closure operation is supported.", 0, 1, closure); 4745 default: return super.getNamedProperty(_hash, _name, _checkValid); 4746 } 4747 4748 } 4749 4750 @Override 4751 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4752 switch (hash) { 4753 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 4754 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4755 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 4756 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 4757 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 4758 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 4759 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 4760 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 4761 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 4762 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 4763 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 4764 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 4765 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 4766 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 4767 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 4768 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 4769 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 4770 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // Enumeration<CapabilityStatementKind> 4771 case 1319330215: /*software*/ return this.software == null ? new Base[0] : new Base[] {this.software}; // TerminologyCapabilitiesSoftwareComponent 4772 case 1683336114: /*implementation*/ return this.implementation == null ? new Base[0] : new Base[] {this.implementation}; // TerminologyCapabilitiesImplementationComponent 4773 case 1391591896: /*lockedDate*/ return this.lockedDate == null ? new Base[0] : new Base[] {this.lockedDate}; // BooleanType 4774 case -916511108: /*codeSystem*/ return this.codeSystem == null ? new Base[0] : this.codeSystem.toArray(new Base[this.codeSystem.size()]); // TerminologyCapabilitiesCodeSystemComponent 4775 case 17878207: /*expansion*/ return this.expansion == null ? new Base[0] : new Base[] {this.expansion}; // TerminologyCapabilitiesExpansionComponent 4776 case -935519755: /*codeSearch*/ return this.codeSearch == null ? new Base[0] : new Base[] {this.codeSearch}; // Enumeration<CodeSearchSupport> 4777 case 1080737827: /*validateCode*/ return this.validateCode == null ? new Base[0] : new Base[] {this.validateCode}; // TerminologyCapabilitiesValidateCodeComponent 4778 case -1840647503: /*translation*/ return this.translation == null ? new Base[0] : new Base[] {this.translation}; // TerminologyCapabilitiesTranslationComponent 4779 case 866552379: /*closure*/ return this.closure == null ? new Base[0] : new Base[] {this.closure}; // TerminologyCapabilitiesClosureComponent 4780 default: return super.getProperty(hash, name, checkValid); 4781 } 4782 4783 } 4784 4785 @Override 4786 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4787 switch (hash) { 4788 case 116079: // url 4789 this.url = TypeConvertor.castToUri(value); // UriType 4790 return value; 4791 case -1618432855: // identifier 4792 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 4793 return value; 4794 case 351608024: // version 4795 this.version = TypeConvertor.castToString(value); // StringType 4796 return value; 4797 case 1508158071: // versionAlgorithm 4798 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 4799 return value; 4800 case 3373707: // name 4801 this.name = TypeConvertor.castToString(value); // StringType 4802 return value; 4803 case 110371416: // title 4804 this.title = TypeConvertor.castToString(value); // StringType 4805 return value; 4806 case -892481550: // status 4807 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4808 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4809 return value; 4810 case -404562712: // experimental 4811 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 4812 return value; 4813 case 3076014: // date 4814 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4815 return value; 4816 case 1447404028: // publisher 4817 this.publisher = TypeConvertor.castToString(value); // StringType 4818 return value; 4819 case 951526432: // contact 4820 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 4821 return value; 4822 case -1724546052: // description 4823 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4824 return value; 4825 case -669707736: // useContext 4826 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 4827 return value; 4828 case -507075711: // jurisdiction 4829 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4830 return value; 4831 case -220463842: // purpose 4832 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 4833 return value; 4834 case 1522889671: // copyright 4835 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 4836 return value; 4837 case 765157229: // copyrightLabel 4838 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 4839 return value; 4840 case 3292052: // kind 4841 value = new CapabilityStatementKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 4842 this.kind = (Enumeration) value; // Enumeration<CapabilityStatementKind> 4843 return value; 4844 case 1319330215: // software 4845 this.software = (TerminologyCapabilitiesSoftwareComponent) value; // TerminologyCapabilitiesSoftwareComponent 4846 return value; 4847 case 1683336114: // implementation 4848 this.implementation = (TerminologyCapabilitiesImplementationComponent) value; // TerminologyCapabilitiesImplementationComponent 4849 return value; 4850 case 1391591896: // lockedDate 4851 this.lockedDate = TypeConvertor.castToBoolean(value); // BooleanType 4852 return value; 4853 case -916511108: // codeSystem 4854 this.getCodeSystem().add((TerminologyCapabilitiesCodeSystemComponent) value); // TerminologyCapabilitiesCodeSystemComponent 4855 return value; 4856 case 17878207: // expansion 4857 this.expansion = (TerminologyCapabilitiesExpansionComponent) value; // TerminologyCapabilitiesExpansionComponent 4858 return value; 4859 case -935519755: // codeSearch 4860 value = new CodeSearchSupportEnumFactory().fromType(TypeConvertor.castToCode(value)); 4861 this.codeSearch = (Enumeration) value; // Enumeration<CodeSearchSupport> 4862 return value; 4863 case 1080737827: // validateCode 4864 this.validateCode = (TerminologyCapabilitiesValidateCodeComponent) value; // TerminologyCapabilitiesValidateCodeComponent 4865 return value; 4866 case -1840647503: // translation 4867 this.translation = (TerminologyCapabilitiesTranslationComponent) value; // TerminologyCapabilitiesTranslationComponent 4868 return value; 4869 case 866552379: // closure 4870 this.closure = (TerminologyCapabilitiesClosureComponent) value; // TerminologyCapabilitiesClosureComponent 4871 return value; 4872 default: return super.setProperty(hash, name, value); 4873 } 4874 4875 } 4876 4877 @Override 4878 public Base setProperty(String name, Base value) throws FHIRException { 4879 if (name.equals("url")) { 4880 this.url = TypeConvertor.castToUri(value); // UriType 4881 } else if (name.equals("identifier")) { 4882 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 4883 } else if (name.equals("version")) { 4884 this.version = TypeConvertor.castToString(value); // StringType 4885 } else if (name.equals("versionAlgorithm[x]")) { 4886 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 4887 } else if (name.equals("name")) { 4888 this.name = TypeConvertor.castToString(value); // StringType 4889 } else if (name.equals("title")) { 4890 this.title = TypeConvertor.castToString(value); // StringType 4891 } else if (name.equals("status")) { 4892 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4893 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4894 } else if (name.equals("experimental")) { 4895 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 4896 } else if (name.equals("date")) { 4897 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4898 } else if (name.equals("publisher")) { 4899 this.publisher = TypeConvertor.castToString(value); // StringType 4900 } else if (name.equals("contact")) { 4901 this.getContact().add(TypeConvertor.castToContactDetail(value)); 4902 } else if (name.equals("description")) { 4903 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 4904 } else if (name.equals("useContext")) { 4905 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 4906 } else if (name.equals("jurisdiction")) { 4907 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 4908 } else if (name.equals("purpose")) { 4909 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 4910 } else if (name.equals("copyright")) { 4911 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 4912 } else if (name.equals("copyrightLabel")) { 4913 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 4914 } else if (name.equals("kind")) { 4915 value = new CapabilityStatementKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 4916 this.kind = (Enumeration) value; // Enumeration<CapabilityStatementKind> 4917 } else if (name.equals("software")) { 4918 this.software = (TerminologyCapabilitiesSoftwareComponent) value; // TerminologyCapabilitiesSoftwareComponent 4919 } else if (name.equals("implementation")) { 4920 this.implementation = (TerminologyCapabilitiesImplementationComponent) value; // TerminologyCapabilitiesImplementationComponent 4921 } else if (name.equals("lockedDate")) { 4922 this.lockedDate = TypeConvertor.castToBoolean(value); // BooleanType 4923 } else if (name.equals("codeSystem")) { 4924 this.getCodeSystem().add((TerminologyCapabilitiesCodeSystemComponent) value); 4925 } else if (name.equals("expansion")) { 4926 this.expansion = (TerminologyCapabilitiesExpansionComponent) value; // TerminologyCapabilitiesExpansionComponent 4927 } else if (name.equals("codeSearch")) { 4928 value = new CodeSearchSupportEnumFactory().fromType(TypeConvertor.castToCode(value)); 4929 this.codeSearch = (Enumeration) value; // Enumeration<CodeSearchSupport> 4930 } else if (name.equals("validateCode")) { 4931 this.validateCode = (TerminologyCapabilitiesValidateCodeComponent) value; // TerminologyCapabilitiesValidateCodeComponent 4932 } else if (name.equals("translation")) { 4933 this.translation = (TerminologyCapabilitiesTranslationComponent) value; // TerminologyCapabilitiesTranslationComponent 4934 } else if (name.equals("closure")) { 4935 this.closure = (TerminologyCapabilitiesClosureComponent) value; // TerminologyCapabilitiesClosureComponent 4936 } else 4937 return super.setProperty(name, value); 4938 return value; 4939 } 4940 4941 @Override 4942 public void removeChild(String name, Base value) throws FHIRException { 4943 if (name.equals("url")) { 4944 this.url = null; 4945 } else if (name.equals("identifier")) { 4946 this.getIdentifier().remove(value); 4947 } else if (name.equals("version")) { 4948 this.version = null; 4949 } else if (name.equals("versionAlgorithm[x]")) { 4950 this.versionAlgorithm = null; 4951 } else if (name.equals("name")) { 4952 this.name = null; 4953 } else if (name.equals("title")) { 4954 this.title = null; 4955 } else if (name.equals("status")) { 4956 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4957 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4958 } else if (name.equals("experimental")) { 4959 this.experimental = null; 4960 } else if (name.equals("date")) { 4961 this.date = null; 4962 } else if (name.equals("publisher")) { 4963 this.publisher = null; 4964 } else if (name.equals("contact")) { 4965 this.getContact().remove(value); 4966 } else if (name.equals("description")) { 4967 this.description = null; 4968 } else if (name.equals("useContext")) { 4969 this.getUseContext().remove(value); 4970 } else if (name.equals("jurisdiction")) { 4971 this.getJurisdiction().remove(value); 4972 } else if (name.equals("purpose")) { 4973 this.purpose = null; 4974 } else if (name.equals("copyright")) { 4975 this.copyright = null; 4976 } else if (name.equals("copyrightLabel")) { 4977 this.copyrightLabel = null; 4978 } else if (name.equals("kind")) { 4979 value = new CapabilityStatementKindEnumFactory().fromType(TypeConvertor.castToCode(value)); 4980 this.kind = (Enumeration) value; // Enumeration<CapabilityStatementKind> 4981 } else if (name.equals("software")) { 4982 this.software = (TerminologyCapabilitiesSoftwareComponent) value; // TerminologyCapabilitiesSoftwareComponent 4983 } else if (name.equals("implementation")) { 4984 this.implementation = (TerminologyCapabilitiesImplementationComponent) value; // TerminologyCapabilitiesImplementationComponent 4985 } else if (name.equals("lockedDate")) { 4986 this.lockedDate = null; 4987 } else if (name.equals("codeSystem")) { 4988 this.getCodeSystem().remove((TerminologyCapabilitiesCodeSystemComponent) value); 4989 } else if (name.equals("expansion")) { 4990 this.expansion = (TerminologyCapabilitiesExpansionComponent) value; // TerminologyCapabilitiesExpansionComponent 4991 } else if (name.equals("codeSearch")) { 4992 value = new CodeSearchSupportEnumFactory().fromType(TypeConvertor.castToCode(value)); 4993 this.codeSearch = (Enumeration) value; // Enumeration<CodeSearchSupport> 4994 } else if (name.equals("validateCode")) { 4995 this.validateCode = (TerminologyCapabilitiesValidateCodeComponent) value; // TerminologyCapabilitiesValidateCodeComponent 4996 } else if (name.equals("translation")) { 4997 this.translation = (TerminologyCapabilitiesTranslationComponent) value; // TerminologyCapabilitiesTranslationComponent 4998 } else if (name.equals("closure")) { 4999 this.closure = (TerminologyCapabilitiesClosureComponent) value; // TerminologyCapabilitiesClosureComponent 5000 } else 5001 super.removeChild(name, value); 5002 5003 } 5004 5005 @Override 5006 public Base makeProperty(int hash, String name) throws FHIRException { 5007 switch (hash) { 5008 case 116079: return getUrlElement(); 5009 case -1618432855: return addIdentifier(); 5010 case 351608024: return getVersionElement(); 5011 case -115699031: return getVersionAlgorithm(); 5012 case 1508158071: return getVersionAlgorithm(); 5013 case 3373707: return getNameElement(); 5014 case 110371416: return getTitleElement(); 5015 case -892481550: return getStatusElement(); 5016 case -404562712: return getExperimentalElement(); 5017 case 3076014: return getDateElement(); 5018 case 1447404028: return getPublisherElement(); 5019 case 951526432: return addContact(); 5020 case -1724546052: return getDescriptionElement(); 5021 case -669707736: return addUseContext(); 5022 case -507075711: return addJurisdiction(); 5023 case -220463842: return getPurposeElement(); 5024 case 1522889671: return getCopyrightElement(); 5025 case 765157229: return getCopyrightLabelElement(); 5026 case 3292052: return getKindElement(); 5027 case 1319330215: return getSoftware(); 5028 case 1683336114: return getImplementation(); 5029 case 1391591896: return getLockedDateElement(); 5030 case -916511108: return addCodeSystem(); 5031 case 17878207: return getExpansion(); 5032 case -935519755: return getCodeSearchElement(); 5033 case 1080737827: return getValidateCode(); 5034 case -1840647503: return getTranslation(); 5035 case 866552379: return getClosure(); 5036 default: return super.makeProperty(hash, name); 5037 } 5038 5039 } 5040 5041 @Override 5042 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5043 switch (hash) { 5044 case 116079: /*url*/ return new String[] {"uri"}; 5045 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 5046 case 351608024: /*version*/ return new String[] {"string"}; 5047 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 5048 case 3373707: /*name*/ return new String[] {"string"}; 5049 case 110371416: /*title*/ return new String[] {"string"}; 5050 case -892481550: /*status*/ return new String[] {"code"}; 5051 case -404562712: /*experimental*/ return new String[] {"boolean"}; 5052 case 3076014: /*date*/ return new String[] {"dateTime"}; 5053 case 1447404028: /*publisher*/ return new String[] {"string"}; 5054 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 5055 case -1724546052: /*description*/ return new String[] {"markdown"}; 5056 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 5057 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 5058 case -220463842: /*purpose*/ return new String[] {"markdown"}; 5059 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 5060 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 5061 case 3292052: /*kind*/ return new String[] {"code"}; 5062 case 1319330215: /*software*/ return new String[] {}; 5063 case 1683336114: /*implementation*/ return new String[] {}; 5064 case 1391591896: /*lockedDate*/ return new String[] {"boolean"}; 5065 case -916511108: /*codeSystem*/ return new String[] {}; 5066 case 17878207: /*expansion*/ return new String[] {}; 5067 case -935519755: /*codeSearch*/ return new String[] {"code"}; 5068 case 1080737827: /*validateCode*/ return new String[] {}; 5069 case -1840647503: /*translation*/ return new String[] {}; 5070 case 866552379: /*closure*/ return new String[] {}; 5071 default: return super.getTypesForProperty(hash, name); 5072 } 5073 5074 } 5075 5076 @Override 5077 public Base addChild(String name) throws FHIRException { 5078 if (name.equals("url")) { 5079 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.url"); 5080 } 5081 else if (name.equals("identifier")) { 5082 return addIdentifier(); 5083 } 5084 else if (name.equals("version")) { 5085 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.version"); 5086 } 5087 else if (name.equals("versionAlgorithmString")) { 5088 this.versionAlgorithm = new StringType(); 5089 return this.versionAlgorithm; 5090 } 5091 else if (name.equals("versionAlgorithmCoding")) { 5092 this.versionAlgorithm = new Coding(); 5093 return this.versionAlgorithm; 5094 } 5095 else if (name.equals("name")) { 5096 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.name"); 5097 } 5098 else if (name.equals("title")) { 5099 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.title"); 5100 } 5101 else if (name.equals("status")) { 5102 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.status"); 5103 } 5104 else if (name.equals("experimental")) { 5105 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.experimental"); 5106 } 5107 else if (name.equals("date")) { 5108 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.date"); 5109 } 5110 else if (name.equals("publisher")) { 5111 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.publisher"); 5112 } 5113 else if (name.equals("contact")) { 5114 return addContact(); 5115 } 5116 else if (name.equals("description")) { 5117 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.description"); 5118 } 5119 else if (name.equals("useContext")) { 5120 return addUseContext(); 5121 } 5122 else if (name.equals("jurisdiction")) { 5123 return addJurisdiction(); 5124 } 5125 else if (name.equals("purpose")) { 5126 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.purpose"); 5127 } 5128 else if (name.equals("copyright")) { 5129 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.copyright"); 5130 } 5131 else if (name.equals("copyrightLabel")) { 5132 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.copyrightLabel"); 5133 } 5134 else if (name.equals("kind")) { 5135 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.kind"); 5136 } 5137 else if (name.equals("software")) { 5138 this.software = new TerminologyCapabilitiesSoftwareComponent(); 5139 return this.software; 5140 } 5141 else if (name.equals("implementation")) { 5142 this.implementation = new TerminologyCapabilitiesImplementationComponent(); 5143 return this.implementation; 5144 } 5145 else if (name.equals("lockedDate")) { 5146 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.lockedDate"); 5147 } 5148 else if (name.equals("codeSystem")) { 5149 return addCodeSystem(); 5150 } 5151 else if (name.equals("expansion")) { 5152 this.expansion = new TerminologyCapabilitiesExpansionComponent(); 5153 return this.expansion; 5154 } 5155 else if (name.equals("codeSearch")) { 5156 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.codeSearch"); 5157 } 5158 else if (name.equals("validateCode")) { 5159 this.validateCode = new TerminologyCapabilitiesValidateCodeComponent(); 5160 return this.validateCode; 5161 } 5162 else if (name.equals("translation")) { 5163 this.translation = new TerminologyCapabilitiesTranslationComponent(); 5164 return this.translation; 5165 } 5166 else if (name.equals("closure")) { 5167 this.closure = new TerminologyCapabilitiesClosureComponent(); 5168 return this.closure; 5169 } 5170 else 5171 return super.addChild(name); 5172 } 5173 5174 public String fhirType() { 5175 return "TerminologyCapabilities"; 5176 5177 } 5178 5179 public TerminologyCapabilities copy() { 5180 TerminologyCapabilities dst = new TerminologyCapabilities(); 5181 copyValues(dst); 5182 return dst; 5183 } 5184 5185 public void copyValues(TerminologyCapabilities dst) { 5186 super.copyValues(dst); 5187 dst.url = url == null ? null : url.copy(); 5188 if (identifier != null) { 5189 dst.identifier = new ArrayList<Identifier>(); 5190 for (Identifier i : identifier) 5191 dst.identifier.add(i.copy()); 5192 }; 5193 dst.version = version == null ? null : version.copy(); 5194 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 5195 dst.name = name == null ? null : name.copy(); 5196 dst.title = title == null ? null : title.copy(); 5197 dst.status = status == null ? null : status.copy(); 5198 dst.experimental = experimental == null ? null : experimental.copy(); 5199 dst.date = date == null ? null : date.copy(); 5200 dst.publisher = publisher == null ? null : publisher.copy(); 5201 if (contact != null) { 5202 dst.contact = new ArrayList<ContactDetail>(); 5203 for (ContactDetail i : contact) 5204 dst.contact.add(i.copy()); 5205 }; 5206 dst.description = description == null ? null : description.copy(); 5207 if (useContext != null) { 5208 dst.useContext = new ArrayList<UsageContext>(); 5209 for (UsageContext i : useContext) 5210 dst.useContext.add(i.copy()); 5211 }; 5212 if (jurisdiction != null) { 5213 dst.jurisdiction = new ArrayList<CodeableConcept>(); 5214 for (CodeableConcept i : jurisdiction) 5215 dst.jurisdiction.add(i.copy()); 5216 }; 5217 dst.purpose = purpose == null ? null : purpose.copy(); 5218 dst.copyright = copyright == null ? null : copyright.copy(); 5219 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 5220 dst.kind = kind == null ? null : kind.copy(); 5221 dst.software = software == null ? null : software.copy(); 5222 dst.implementation = implementation == null ? null : implementation.copy(); 5223 dst.lockedDate = lockedDate == null ? null : lockedDate.copy(); 5224 if (codeSystem != null) { 5225 dst.codeSystem = new ArrayList<TerminologyCapabilitiesCodeSystemComponent>(); 5226 for (TerminologyCapabilitiesCodeSystemComponent i : codeSystem) 5227 dst.codeSystem.add(i.copy()); 5228 }; 5229 dst.expansion = expansion == null ? null : expansion.copy(); 5230 dst.codeSearch = codeSearch == null ? null : codeSearch.copy(); 5231 dst.validateCode = validateCode == null ? null : validateCode.copy(); 5232 dst.translation = translation == null ? null : translation.copy(); 5233 dst.closure = closure == null ? null : closure.copy(); 5234 } 5235 5236 protected TerminologyCapabilities typedCopy() { 5237 return copy(); 5238 } 5239 5240 @Override 5241 public boolean equalsDeep(Base other_) { 5242 if (!super.equalsDeep(other_)) 5243 return false; 5244 if (!(other_ instanceof TerminologyCapabilities)) 5245 return false; 5246 TerminologyCapabilities o = (TerminologyCapabilities) other_; 5247 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 5248 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 5249 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 5250 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 5251 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 5252 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 5253 && compareDeep(kind, o.kind, true) && compareDeep(software, o.software, true) && compareDeep(implementation, o.implementation, true) 5254 && compareDeep(lockedDate, o.lockedDate, true) && compareDeep(codeSystem, o.codeSystem, true) && compareDeep(expansion, o.expansion, true) 5255 && compareDeep(codeSearch, o.codeSearch, true) && compareDeep(validateCode, o.validateCode, true) 5256 && compareDeep(translation, o.translation, true) && compareDeep(closure, o.closure, true); 5257 } 5258 5259 @Override 5260 public boolean equalsShallow(Base other_) { 5261 if (!super.equalsShallow(other_)) 5262 return false; 5263 if (!(other_ instanceof TerminologyCapabilities)) 5264 return false; 5265 TerminologyCapabilities o = (TerminologyCapabilities) other_; 5266 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 5267 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 5268 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 5269 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 5270 && compareValues(kind, o.kind, true) && compareValues(lockedDate, o.lockedDate, true) && compareValues(codeSearch, o.codeSearch, true) 5271 ; 5272 } 5273 5274 public boolean isEmpty() { 5275 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 5276 , versionAlgorithm, name, title, status, experimental, date, publisher, contact 5277 , description, useContext, jurisdiction, purpose, copyright, copyrightLabel, kind 5278 , software, implementation, lockedDate, codeSystem, expansion, codeSearch, validateCode 5279 , translation, closure); 5280 } 5281 5282 @Override 5283 public ResourceType getResourceType() { 5284 return ResourceType.TerminologyCapabilities; 5285 } 5286 5287 /** 5288 * Search parameter: <b>context-quantity</b> 5289 * <p> 5290 * Description: <b>Multiple Resources: 5291 5292* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 5293* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 5294* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 5295* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 5296* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 5297* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 5298* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 5299* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 5300* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 5301* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 5302* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 5303* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 5304* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 5305* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 5306* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 5307* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 5308* [Library](library.html): A quantity- or range-valued use context assigned to the library 5309* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 5310* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 5311* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 5312* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 5313* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 5314* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 5315* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 5316* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 5317* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 5318* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 5319* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 5320* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 5321* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 5322</b><br> 5323 * Type: <b>quantity</b><br> 5324 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 5325 * </p> 5326 */ 5327 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 5328 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 5329 /** 5330 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 5331 * <p> 5332 * Description: <b>Multiple Resources: 5333 5334* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 5335* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 5336* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 5337* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 5338* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 5339* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 5340* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 5341* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 5342* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 5343* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 5344* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 5345* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 5346* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 5347* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 5348* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 5349* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 5350* [Library](library.html): A quantity- or range-valued use context assigned to the library 5351* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 5352* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 5353* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 5354* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 5355* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 5356* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 5357* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 5358* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 5359* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 5360* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 5361* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 5362* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 5363* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 5364</b><br> 5365 * Type: <b>quantity</b><br> 5366 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 5367 * </p> 5368 */ 5369 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 5370 5371 /** 5372 * Search parameter: <b>context-type-quantity</b> 5373 * <p> 5374 * Description: <b>Multiple Resources: 5375 5376* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 5377* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 5378* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 5379* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 5380* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 5381* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 5382* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 5383* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 5384* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 5385* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 5386* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 5387* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 5388* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 5389* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 5390* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 5391* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 5392* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 5393* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 5394* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 5395* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 5396* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 5397* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 5398* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 5399* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 5400* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 5401* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 5402* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 5403* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 5404* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 5405* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 5406</b><br> 5407 * Type: <b>composite</b><br> 5408 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5409 * </p> 5410 */ 5411 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 5412 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 5413 /** 5414 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 5415 * <p> 5416 * Description: <b>Multiple Resources: 5417 5418* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 5419* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 5420* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 5421* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 5422* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 5423* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 5424* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 5425* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 5426* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 5427* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 5428* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 5429* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 5430* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 5431* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 5432* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 5433* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 5434* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 5435* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 5436* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 5437* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 5438* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 5439* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 5440* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 5441* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 5442* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 5443* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 5444* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 5445* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 5446* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 5447* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 5448</b><br> 5449 * Type: <b>composite</b><br> 5450 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5451 * </p> 5452 */ 5453 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 5454 5455 /** 5456 * Search parameter: <b>context-type-value</b> 5457 * <p> 5458 * Description: <b>Multiple Resources: 5459 5460* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 5461* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 5462* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 5463* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 5464* [Citation](citation.html): A use context type and value assigned to the citation 5465* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 5466* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 5467* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 5468* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 5469* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 5470* [Evidence](evidence.html): A use context type and value assigned to the evidence 5471* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 5472* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 5473* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 5474* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 5475* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 5476* [Library](library.html): A use context type and value assigned to the library 5477* [Measure](measure.html): A use context type and value assigned to the measure 5478* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 5479* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 5480* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 5481* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 5482* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 5483* [Requirements](requirements.html): A use context type and value assigned to the requirements 5484* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 5485* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 5486* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 5487* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 5488* [TestScript](testscript.html): A use context type and value assigned to the test script 5489* [ValueSet](valueset.html): A use context type and value assigned to the value set 5490</b><br> 5491 * Type: <b>composite</b><br> 5492 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5493 * </p> 5494 */ 5495 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 5496 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 5497 /** 5498 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 5499 * <p> 5500 * Description: <b>Multiple Resources: 5501 5502* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 5503* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 5504* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 5505* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 5506* [Citation](citation.html): A use context type and value assigned to the citation 5507* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 5508* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 5509* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 5510* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 5511* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 5512* [Evidence](evidence.html): A use context type and value assigned to the evidence 5513* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 5514* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 5515* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 5516* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 5517* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 5518* [Library](library.html): A use context type and value assigned to the library 5519* [Measure](measure.html): A use context type and value assigned to the measure 5520* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 5521* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 5522* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 5523* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 5524* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 5525* [Requirements](requirements.html): A use context type and value assigned to the requirements 5526* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 5527* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 5528* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 5529* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 5530* [TestScript](testscript.html): A use context type and value assigned to the test script 5531* [ValueSet](valueset.html): A use context type and value assigned to the value set 5532</b><br> 5533 * Type: <b>composite</b><br> 5534 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5535 * </p> 5536 */ 5537 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 5538 5539 /** 5540 * Search parameter: <b>context-type</b> 5541 * <p> 5542 * Description: <b>Multiple Resources: 5543 5544* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 5545* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 5546* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 5547* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 5548* [Citation](citation.html): A type of use context assigned to the citation 5549* [CodeSystem](codesystem.html): A type of use context assigned to the code system 5550* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 5551* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 5552* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 5553* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 5554* [Evidence](evidence.html): A type of use context assigned to the evidence 5555* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 5556* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 5557* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 5558* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 5559* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 5560* [Library](library.html): A type of use context assigned to the library 5561* [Measure](measure.html): A type of use context assigned to the measure 5562* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 5563* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 5564* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 5565* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 5566* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 5567* [Requirements](requirements.html): A type of use context assigned to the requirements 5568* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 5569* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 5570* [StructureMap](structuremap.html): A type of use context assigned to the structure map 5571* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 5572* [TestScript](testscript.html): A type of use context assigned to the test script 5573* [ValueSet](valueset.html): A type of use context assigned to the value set 5574</b><br> 5575 * Type: <b>token</b><br> 5576 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 5577 * </p> 5578 */ 5579 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 5580 public static final String SP_CONTEXT_TYPE = "context-type"; 5581 /** 5582 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 5583 * <p> 5584 * Description: <b>Multiple Resources: 5585 5586* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 5587* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 5588* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 5589* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 5590* [Citation](citation.html): A type of use context assigned to the citation 5591* [CodeSystem](codesystem.html): A type of use context assigned to the code system 5592* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 5593* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 5594* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 5595* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 5596* [Evidence](evidence.html): A type of use context assigned to the evidence 5597* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 5598* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 5599* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 5600* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 5601* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 5602* [Library](library.html): A type of use context assigned to the library 5603* [Measure](measure.html): A type of use context assigned to the measure 5604* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 5605* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 5606* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 5607* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 5608* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 5609* [Requirements](requirements.html): A type of use context assigned to the requirements 5610* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 5611* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 5612* [StructureMap](structuremap.html): A type of use context assigned to the structure map 5613* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 5614* [TestScript](testscript.html): A type of use context assigned to the test script 5615* [ValueSet](valueset.html): A type of use context assigned to the value set 5616</b><br> 5617 * Type: <b>token</b><br> 5618 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 5619 * </p> 5620 */ 5621 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 5622 5623 /** 5624 * Search parameter: <b>context</b> 5625 * <p> 5626 * Description: <b>Multiple Resources: 5627 5628* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 5629* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 5630* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 5631* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 5632* [Citation](citation.html): A use context assigned to the citation 5633* [CodeSystem](codesystem.html): A use context assigned to the code system 5634* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 5635* [ConceptMap](conceptmap.html): A use context assigned to the concept map 5636* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 5637* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 5638* [Evidence](evidence.html): A use context assigned to the evidence 5639* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 5640* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 5641* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 5642* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 5643* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 5644* [Library](library.html): A use context assigned to the library 5645* [Measure](measure.html): A use context assigned to the measure 5646* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 5647* [NamingSystem](namingsystem.html): A use context assigned to the naming system 5648* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 5649* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 5650* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 5651* [Requirements](requirements.html): A use context assigned to the requirements 5652* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 5653* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 5654* [StructureMap](structuremap.html): A use context assigned to the structure map 5655* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 5656* [TestScript](testscript.html): A use context assigned to the test script 5657* [ValueSet](valueset.html): A use context assigned to the value set 5658</b><br> 5659 * Type: <b>token</b><br> 5660 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 5661 * </p> 5662 */ 5663 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 5664 public static final String SP_CONTEXT = "context"; 5665 /** 5666 * <b>Fluent Client</b> search parameter constant for <b>context</b> 5667 * <p> 5668 * Description: <b>Multiple Resources: 5669 5670* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 5671* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 5672* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 5673* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 5674* [Citation](citation.html): A use context assigned to the citation 5675* [CodeSystem](codesystem.html): A use context assigned to the code system 5676* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 5677* [ConceptMap](conceptmap.html): A use context assigned to the concept map 5678* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 5679* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 5680* [Evidence](evidence.html): A use context assigned to the evidence 5681* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 5682* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 5683* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 5684* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 5685* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 5686* [Library](library.html): A use context assigned to the library 5687* [Measure](measure.html): A use context assigned to the measure 5688* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 5689* [NamingSystem](namingsystem.html): A use context assigned to the naming system 5690* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 5691* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 5692* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 5693* [Requirements](requirements.html): A use context assigned to the requirements 5694* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 5695* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 5696* [StructureMap](structuremap.html): A use context assigned to the structure map 5697* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 5698* [TestScript](testscript.html): A use context assigned to the test script 5699* [ValueSet](valueset.html): A use context assigned to the value set 5700</b><br> 5701 * Type: <b>token</b><br> 5702 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 5703 * </p> 5704 */ 5705 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 5706 5707 /** 5708 * Search parameter: <b>date</b> 5709 * <p> 5710 * Description: <b>Multiple Resources: 5711 5712* [ActivityDefinition](activitydefinition.html): The activity definition publication date 5713* [ActorDefinition](actordefinition.html): The Actor Definition publication date 5714* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 5715* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 5716* [Citation](citation.html): The citation publication date 5717* [CodeSystem](codesystem.html): The code system publication date 5718* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 5719* [ConceptMap](conceptmap.html): The concept map publication date 5720* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 5721* [EventDefinition](eventdefinition.html): The event definition publication date 5722* [Evidence](evidence.html): The evidence publication date 5723* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 5724* [ExampleScenario](examplescenario.html): The example scenario publication date 5725* [GraphDefinition](graphdefinition.html): The graph definition publication date 5726* [ImplementationGuide](implementationguide.html): The implementation guide publication date 5727* [Library](library.html): The library publication date 5728* [Measure](measure.html): The measure publication date 5729* [MessageDefinition](messagedefinition.html): The message definition publication date 5730* [NamingSystem](namingsystem.html): The naming system publication date 5731* [OperationDefinition](operationdefinition.html): The operation definition publication date 5732* [PlanDefinition](plandefinition.html): The plan definition publication date 5733* [Questionnaire](questionnaire.html): The questionnaire publication date 5734* [Requirements](requirements.html): The requirements publication date 5735* [SearchParameter](searchparameter.html): The search parameter publication date 5736* [StructureDefinition](structuredefinition.html): The structure definition publication date 5737* [StructureMap](structuremap.html): The structure map publication date 5738* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 5739* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 5740* [TestScript](testscript.html): The test script publication date 5741* [ValueSet](valueset.html): The value set publication date 5742</b><br> 5743 * Type: <b>date</b><br> 5744 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 5745 * </p> 5746 */ 5747 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 5748 public static final String SP_DATE = "date"; 5749 /** 5750 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5751 * <p> 5752 * Description: <b>Multiple Resources: 5753 5754* [ActivityDefinition](activitydefinition.html): The activity definition publication date 5755* [ActorDefinition](actordefinition.html): The Actor Definition publication date 5756* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 5757* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 5758* [Citation](citation.html): The citation publication date 5759* [CodeSystem](codesystem.html): The code system publication date 5760* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 5761* [ConceptMap](conceptmap.html): The concept map publication date 5762* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 5763* [EventDefinition](eventdefinition.html): The event definition publication date 5764* [Evidence](evidence.html): The evidence publication date 5765* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 5766* [ExampleScenario](examplescenario.html): The example scenario publication date 5767* [GraphDefinition](graphdefinition.html): The graph definition publication date 5768* [ImplementationGuide](implementationguide.html): The implementation guide publication date 5769* [Library](library.html): The library publication date 5770* [Measure](measure.html): The measure publication date 5771* [MessageDefinition](messagedefinition.html): The message definition publication date 5772* [NamingSystem](namingsystem.html): The naming system publication date 5773* [OperationDefinition](operationdefinition.html): The operation definition publication date 5774* [PlanDefinition](plandefinition.html): The plan definition publication date 5775* [Questionnaire](questionnaire.html): The questionnaire publication date 5776* [Requirements](requirements.html): The requirements publication date 5777* [SearchParameter](searchparameter.html): The search parameter publication date 5778* [StructureDefinition](structuredefinition.html): The structure definition publication date 5779* [StructureMap](structuremap.html): The structure map publication date 5780* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 5781* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 5782* [TestScript](testscript.html): The test script publication date 5783* [ValueSet](valueset.html): The value set publication date 5784</b><br> 5785 * Type: <b>date</b><br> 5786 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 5787 * </p> 5788 */ 5789 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 5790 5791 /** 5792 * Search parameter: <b>description</b> 5793 * <p> 5794 * Description: <b>Multiple Resources: 5795 5796* [ActivityDefinition](activitydefinition.html): The description of the activity definition 5797* [ActorDefinition](actordefinition.html): The description of the Actor Definition 5798* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 5799* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 5800* [Citation](citation.html): The description of the citation 5801* [CodeSystem](codesystem.html): The description of the code system 5802* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 5803* [ConceptMap](conceptmap.html): The description of the concept map 5804* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 5805* [EventDefinition](eventdefinition.html): The description of the event definition 5806* [Evidence](evidence.html): The description of the evidence 5807* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 5808* [GraphDefinition](graphdefinition.html): The description of the graph definition 5809* [ImplementationGuide](implementationguide.html): The description of the implementation guide 5810* [Library](library.html): The description of the library 5811* [Measure](measure.html): The description of the measure 5812* [MessageDefinition](messagedefinition.html): The description of the message definition 5813* [NamingSystem](namingsystem.html): The description of the naming system 5814* [OperationDefinition](operationdefinition.html): The description of the operation definition 5815* [PlanDefinition](plandefinition.html): The description of the plan definition 5816* [Questionnaire](questionnaire.html): The description of the questionnaire 5817* [Requirements](requirements.html): The description of the requirements 5818* [SearchParameter](searchparameter.html): The description of the search parameter 5819* [StructureDefinition](structuredefinition.html): The description of the structure definition 5820* [StructureMap](structuremap.html): The description of the structure map 5821* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 5822* [TestScript](testscript.html): The description of the test script 5823* [ValueSet](valueset.html): The description of the value set 5824</b><br> 5825 * Type: <b>string</b><br> 5826 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 5827 * </p> 5828 */ 5829 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 5830 public static final String SP_DESCRIPTION = "description"; 5831 /** 5832 * <b>Fluent Client</b> search parameter constant for <b>description</b> 5833 * <p> 5834 * Description: <b>Multiple Resources: 5835 5836* [ActivityDefinition](activitydefinition.html): The description of the activity definition 5837* [ActorDefinition](actordefinition.html): The description of the Actor Definition 5838* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 5839* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 5840* [Citation](citation.html): The description of the citation 5841* [CodeSystem](codesystem.html): The description of the code system 5842* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 5843* [ConceptMap](conceptmap.html): The description of the concept map 5844* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 5845* [EventDefinition](eventdefinition.html): The description of the event definition 5846* [Evidence](evidence.html): The description of the evidence 5847* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 5848* [GraphDefinition](graphdefinition.html): The description of the graph definition 5849* [ImplementationGuide](implementationguide.html): The description of the implementation guide 5850* [Library](library.html): The description of the library 5851* [Measure](measure.html): The description of the measure 5852* [MessageDefinition](messagedefinition.html): The description of the message definition 5853* [NamingSystem](namingsystem.html): The description of the naming system 5854* [OperationDefinition](operationdefinition.html): The description of the operation definition 5855* [PlanDefinition](plandefinition.html): The description of the plan definition 5856* [Questionnaire](questionnaire.html): The description of the questionnaire 5857* [Requirements](requirements.html): The description of the requirements 5858* [SearchParameter](searchparameter.html): The description of the search parameter 5859* [StructureDefinition](structuredefinition.html): The description of the structure definition 5860* [StructureMap](structuremap.html): The description of the structure map 5861* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 5862* [TestScript](testscript.html): The description of the test script 5863* [ValueSet](valueset.html): The description of the value set 5864</b><br> 5865 * Type: <b>string</b><br> 5866 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 5867 * </p> 5868 */ 5869 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 5870 5871 /** 5872 * Search parameter: <b>identifier</b> 5873 * <p> 5874 * Description: <b>Multiple Resources: 5875 5876* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 5877* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 5878* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 5879* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 5880* [Citation](citation.html): External identifier for the citation 5881* [CodeSystem](codesystem.html): External identifier for the code system 5882* [ConceptMap](conceptmap.html): External identifier for the concept map 5883* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 5884* [EventDefinition](eventdefinition.html): External identifier for the event definition 5885* [Evidence](evidence.html): External identifier for the evidence 5886* [EvidenceReport](evidencereport.html): External identifier for the evidence report 5887* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 5888* [ExampleScenario](examplescenario.html): External identifier for the example scenario 5889* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 5890* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 5891* [Library](library.html): External identifier for the library 5892* [Measure](measure.html): External identifier for the measure 5893* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 5894* [MessageDefinition](messagedefinition.html): External identifier for the message definition 5895* [NamingSystem](namingsystem.html): External identifier for the naming system 5896* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 5897* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 5898* [PlanDefinition](plandefinition.html): External identifier for the plan definition 5899* [Questionnaire](questionnaire.html): External identifier for the questionnaire 5900* [Requirements](requirements.html): External identifier for the requirements 5901* [SearchParameter](searchparameter.html): External identifier for the search parameter 5902* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 5903* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 5904* [StructureMap](structuremap.html): External identifier for the structure map 5905* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 5906* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 5907* [TestPlan](testplan.html): An identifier for the test plan 5908* [TestScript](testscript.html): External identifier for the test script 5909* [ValueSet](valueset.html): External identifier for the value set 5910</b><br> 5911 * Type: <b>token</b><br> 5912 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 5913 * </p> 5914 */ 5915 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 5916 public static final String SP_IDENTIFIER = "identifier"; 5917 /** 5918 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5919 * <p> 5920 * Description: <b>Multiple Resources: 5921 5922* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 5923* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 5924* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 5925* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 5926* [Citation](citation.html): External identifier for the citation 5927* [CodeSystem](codesystem.html): External identifier for the code system 5928* [ConceptMap](conceptmap.html): External identifier for the concept map 5929* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 5930* [EventDefinition](eventdefinition.html): External identifier for the event definition 5931* [Evidence](evidence.html): External identifier for the evidence 5932* [EvidenceReport](evidencereport.html): External identifier for the evidence report 5933* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 5934* [ExampleScenario](examplescenario.html): External identifier for the example scenario 5935* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 5936* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 5937* [Library](library.html): External identifier for the library 5938* [Measure](measure.html): External identifier for the measure 5939* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 5940* [MessageDefinition](messagedefinition.html): External identifier for the message definition 5941* [NamingSystem](namingsystem.html): External identifier for the naming system 5942* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 5943* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 5944* [PlanDefinition](plandefinition.html): External identifier for the plan definition 5945* [Questionnaire](questionnaire.html): External identifier for the questionnaire 5946* [Requirements](requirements.html): External identifier for the requirements 5947* [SearchParameter](searchparameter.html): External identifier for the search parameter 5948* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 5949* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 5950* [StructureMap](structuremap.html): External identifier for the structure map 5951* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 5952* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 5953* [TestPlan](testplan.html): An identifier for the test plan 5954* [TestScript](testscript.html): External identifier for the test script 5955* [ValueSet](valueset.html): External identifier for the value set 5956</b><br> 5957 * Type: <b>token</b><br> 5958 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 5959 * </p> 5960 */ 5961 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 5962 5963 /** 5964 * Search parameter: <b>jurisdiction</b> 5965 * <p> 5966 * Description: <b>Multiple Resources: 5967 5968* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 5969* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 5970* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 5971* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 5972* [Citation](citation.html): Intended jurisdiction for the citation 5973* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 5974* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 5975* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 5976* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 5977* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 5978* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 5979* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 5980* [Library](library.html): Intended jurisdiction for the library 5981* [Measure](measure.html): Intended jurisdiction for the measure 5982* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 5983* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 5984* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 5985* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 5986* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 5987* [Requirements](requirements.html): Intended jurisdiction for the requirements 5988* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 5989* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 5990* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 5991* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 5992* [TestScript](testscript.html): Intended jurisdiction for the test script 5993* [ValueSet](valueset.html): Intended jurisdiction for the value set 5994</b><br> 5995 * Type: <b>token</b><br> 5996 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 5997 * </p> 5998 */ 5999 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 6000 public static final String SP_JURISDICTION = "jurisdiction"; 6001 /** 6002 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 6003 * <p> 6004 * Description: <b>Multiple Resources: 6005 6006* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 6007* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 6008* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 6009* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 6010* [Citation](citation.html): Intended jurisdiction for the citation 6011* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 6012* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 6013* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 6014* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 6015* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 6016* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 6017* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 6018* [Library](library.html): Intended jurisdiction for the library 6019* [Measure](measure.html): Intended jurisdiction for the measure 6020* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 6021* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 6022* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 6023* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 6024* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 6025* [Requirements](requirements.html): Intended jurisdiction for the requirements 6026* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 6027* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 6028* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 6029* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 6030* [TestScript](testscript.html): Intended jurisdiction for the test script 6031* [ValueSet](valueset.html): Intended jurisdiction for the value set 6032</b><br> 6033 * Type: <b>token</b><br> 6034 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 6035 * </p> 6036 */ 6037 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 6038 6039 /** 6040 * Search parameter: <b>name</b> 6041 * <p> 6042 * Description: <b>Multiple Resources: 6043 6044* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 6045* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 6046* [Citation](citation.html): Computationally friendly name of the citation 6047* [CodeSystem](codesystem.html): Computationally friendly name of the code system 6048* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 6049* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 6050* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 6051* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 6052* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 6053* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 6054* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 6055* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 6056* [Library](library.html): Computationally friendly name of the library 6057* [Measure](measure.html): Computationally friendly name of the measure 6058* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 6059* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 6060* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 6061* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 6062* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 6063* [Requirements](requirements.html): Computationally friendly name of the requirements 6064* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 6065* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 6066* [StructureMap](structuremap.html): Computationally friendly name of the structure map 6067* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 6068* [TestScript](testscript.html): Computationally friendly name of the test script 6069* [ValueSet](valueset.html): Computationally friendly name of the value set 6070</b><br> 6071 * Type: <b>string</b><br> 6072 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 6073 * </p> 6074 */ 6075 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 6076 public static final String SP_NAME = "name"; 6077 /** 6078 * <b>Fluent Client</b> search parameter constant for <b>name</b> 6079 * <p> 6080 * Description: <b>Multiple Resources: 6081 6082* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 6083* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 6084* [Citation](citation.html): Computationally friendly name of the citation 6085* [CodeSystem](codesystem.html): Computationally friendly name of the code system 6086* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 6087* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 6088* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 6089* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 6090* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 6091* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 6092* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 6093* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 6094* [Library](library.html): Computationally friendly name of the library 6095* [Measure](measure.html): Computationally friendly name of the measure 6096* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 6097* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 6098* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 6099* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 6100* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 6101* [Requirements](requirements.html): Computationally friendly name of the requirements 6102* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 6103* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 6104* [StructureMap](structuremap.html): Computationally friendly name of the structure map 6105* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 6106* [TestScript](testscript.html): Computationally friendly name of the test script 6107* [ValueSet](valueset.html): Computationally friendly name of the value set 6108</b><br> 6109 * Type: <b>string</b><br> 6110 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 6111 * </p> 6112 */ 6113 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 6114 6115 /** 6116 * Search parameter: <b>publisher</b> 6117 * <p> 6118 * Description: <b>Multiple Resources: 6119 6120* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 6121* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 6122* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 6123* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 6124* [Citation](citation.html): Name of the publisher of the citation 6125* [CodeSystem](codesystem.html): Name of the publisher of the code system 6126* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 6127* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 6128* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 6129* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 6130* [Evidence](evidence.html): Name of the publisher of the evidence 6131* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 6132* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 6133* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 6134* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 6135* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 6136* [Library](library.html): Name of the publisher of the library 6137* [Measure](measure.html): Name of the publisher of the measure 6138* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 6139* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 6140* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 6141* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 6142* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 6143* [Requirements](requirements.html): Name of the publisher of the requirements 6144* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 6145* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 6146* [StructureMap](structuremap.html): Name of the publisher of the structure map 6147* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 6148* [TestScript](testscript.html): Name of the publisher of the test script 6149* [ValueSet](valueset.html): Name of the publisher of the value set 6150</b><br> 6151 * Type: <b>string</b><br> 6152 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 6153 * </p> 6154 */ 6155 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 6156 public static final String SP_PUBLISHER = "publisher"; 6157 /** 6158 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 6159 * <p> 6160 * Description: <b>Multiple Resources: 6161 6162* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 6163* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 6164* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 6165* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 6166* [Citation](citation.html): Name of the publisher of the citation 6167* [CodeSystem](codesystem.html): Name of the publisher of the code system 6168* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 6169* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 6170* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 6171* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 6172* [Evidence](evidence.html): Name of the publisher of the evidence 6173* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 6174* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 6175* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 6176* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 6177* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 6178* [Library](library.html): Name of the publisher of the library 6179* [Measure](measure.html): Name of the publisher of the measure 6180* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 6181* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 6182* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 6183* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 6184* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 6185* [Requirements](requirements.html): Name of the publisher of the requirements 6186* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 6187* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 6188* [StructureMap](structuremap.html): Name of the publisher of the structure map 6189* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 6190* [TestScript](testscript.html): Name of the publisher of the test script 6191* [ValueSet](valueset.html): Name of the publisher of the value set 6192</b><br> 6193 * Type: <b>string</b><br> 6194 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 6195 * </p> 6196 */ 6197 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 6198 6199 /** 6200 * Search parameter: <b>status</b> 6201 * <p> 6202 * Description: <b>Multiple Resources: 6203 6204* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 6205* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 6206* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 6207* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 6208* [Citation](citation.html): The current status of the citation 6209* [CodeSystem](codesystem.html): The current status of the code system 6210* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 6211* [ConceptMap](conceptmap.html): The current status of the concept map 6212* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 6213* [EventDefinition](eventdefinition.html): The current status of the event definition 6214* [Evidence](evidence.html): The current status of the evidence 6215* [EvidenceReport](evidencereport.html): The current status of the evidence report 6216* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 6217* [ExampleScenario](examplescenario.html): The current status of the example scenario 6218* [GraphDefinition](graphdefinition.html): The current status of the graph definition 6219* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 6220* [Library](library.html): The current status of the library 6221* [Measure](measure.html): The current status of the measure 6222* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 6223* [MessageDefinition](messagedefinition.html): The current status of the message definition 6224* [NamingSystem](namingsystem.html): The current status of the naming system 6225* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 6226* [OperationDefinition](operationdefinition.html): The current status of the operation definition 6227* [PlanDefinition](plandefinition.html): The current status of the plan definition 6228* [Questionnaire](questionnaire.html): The current status of the questionnaire 6229* [Requirements](requirements.html): The current status of the requirements 6230* [SearchParameter](searchparameter.html): The current status of the search parameter 6231* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 6232* [StructureDefinition](structuredefinition.html): The current status of the structure definition 6233* [StructureMap](structuremap.html): The current status of the structure map 6234* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 6235* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 6236* [TestPlan](testplan.html): The current status of the test plan 6237* [TestScript](testscript.html): The current status of the test script 6238* [ValueSet](valueset.html): The current status of the value set 6239</b><br> 6240 * Type: <b>token</b><br> 6241 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 6242 * </p> 6243 */ 6244 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 6245 public static final String SP_STATUS = "status"; 6246 /** 6247 * <b>Fluent Client</b> search parameter constant for <b>status</b> 6248 * <p> 6249 * Description: <b>Multiple Resources: 6250 6251* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 6252* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 6253* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 6254* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 6255* [Citation](citation.html): The current status of the citation 6256* [CodeSystem](codesystem.html): The current status of the code system 6257* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 6258* [ConceptMap](conceptmap.html): The current status of the concept map 6259* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 6260* [EventDefinition](eventdefinition.html): The current status of the event definition 6261* [Evidence](evidence.html): The current status of the evidence 6262* [EvidenceReport](evidencereport.html): The current status of the evidence report 6263* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 6264* [ExampleScenario](examplescenario.html): The current status of the example scenario 6265* [GraphDefinition](graphdefinition.html): The current status of the graph definition 6266* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 6267* [Library](library.html): The current status of the library 6268* [Measure](measure.html): The current status of the measure 6269* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 6270* [MessageDefinition](messagedefinition.html): The current status of the message definition 6271* [NamingSystem](namingsystem.html): The current status of the naming system 6272* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 6273* [OperationDefinition](operationdefinition.html): The current status of the operation definition 6274* [PlanDefinition](plandefinition.html): The current status of the plan definition 6275* [Questionnaire](questionnaire.html): The current status of the questionnaire 6276* [Requirements](requirements.html): The current status of the requirements 6277* [SearchParameter](searchparameter.html): The current status of the search parameter 6278* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 6279* [StructureDefinition](structuredefinition.html): The current status of the structure definition 6280* [StructureMap](structuremap.html): The current status of the structure map 6281* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 6282* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 6283* [TestPlan](testplan.html): The current status of the test plan 6284* [TestScript](testscript.html): The current status of the test script 6285* [ValueSet](valueset.html): The current status of the value set 6286</b><br> 6287 * Type: <b>token</b><br> 6288 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 6289 * </p> 6290 */ 6291 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 6292 6293 /** 6294 * Search parameter: <b>title</b> 6295 * <p> 6296 * Description: <b>Multiple Resources: 6297 6298* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 6299* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 6300* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 6301* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 6302* [Citation](citation.html): The human-friendly name of the citation 6303* [CodeSystem](codesystem.html): The human-friendly name of the code system 6304* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 6305* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 6306* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 6307* [Evidence](evidence.html): The human-friendly name of the evidence 6308* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 6309* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 6310* [Library](library.html): The human-friendly name of the library 6311* [Measure](measure.html): The human-friendly name of the measure 6312* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 6313* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 6314* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 6315* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 6316* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 6317* [Requirements](requirements.html): The human-friendly name of the requirements 6318* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 6319* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 6320* [StructureMap](structuremap.html): The human-friendly name of the structure map 6321* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 6322* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 6323* [TestScript](testscript.html): The human-friendly name of the test script 6324* [ValueSet](valueset.html): The human-friendly name of the value set 6325</b><br> 6326 * Type: <b>string</b><br> 6327 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 6328 * </p> 6329 */ 6330 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 6331 public static final String SP_TITLE = "title"; 6332 /** 6333 * <b>Fluent Client</b> search parameter constant for <b>title</b> 6334 * <p> 6335 * Description: <b>Multiple Resources: 6336 6337* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 6338* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 6339* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 6340* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 6341* [Citation](citation.html): The human-friendly name of the citation 6342* [CodeSystem](codesystem.html): The human-friendly name of the code system 6343* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 6344* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 6345* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 6346* [Evidence](evidence.html): The human-friendly name of the evidence 6347* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 6348* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 6349* [Library](library.html): The human-friendly name of the library 6350* [Measure](measure.html): The human-friendly name of the measure 6351* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 6352* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 6353* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 6354* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 6355* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 6356* [Requirements](requirements.html): The human-friendly name of the requirements 6357* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 6358* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 6359* [StructureMap](structuremap.html): The human-friendly name of the structure map 6360* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 6361* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 6362* [TestScript](testscript.html): The human-friendly name of the test script 6363* [ValueSet](valueset.html): The human-friendly name of the value set 6364</b><br> 6365 * Type: <b>string</b><br> 6366 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 6367 * </p> 6368 */ 6369 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 6370 6371 /** 6372 * Search parameter: <b>url</b> 6373 * <p> 6374 * Description: <b>Multiple Resources: 6375 6376* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 6377* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 6378* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 6379* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 6380* [Citation](citation.html): The uri that identifies the citation 6381* [CodeSystem](codesystem.html): The uri that identifies the code system 6382* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 6383* [ConceptMap](conceptmap.html): The URI that identifies the concept map 6384* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 6385* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 6386* [Evidence](evidence.html): The uri that identifies the evidence 6387* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 6388* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 6389* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 6390* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 6391* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 6392* [Library](library.html): The uri that identifies the library 6393* [Measure](measure.html): The uri that identifies the measure 6394* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 6395* [NamingSystem](namingsystem.html): The uri that identifies the naming system 6396* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 6397* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 6398* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 6399* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 6400* [Requirements](requirements.html): The uri that identifies the requirements 6401* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 6402* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 6403* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 6404* [StructureMap](structuremap.html): The uri that identifies the structure map 6405* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 6406* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 6407* [TestPlan](testplan.html): The uri that identifies the test plan 6408* [TestScript](testscript.html): The uri that identifies the test script 6409* [ValueSet](valueset.html): The uri that identifies the value set 6410</b><br> 6411 * Type: <b>uri</b><br> 6412 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 6413 * </p> 6414 */ 6415 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 6416 public static final String SP_URL = "url"; 6417 /** 6418 * <b>Fluent Client</b> search parameter constant for <b>url</b> 6419 * <p> 6420 * Description: <b>Multiple Resources: 6421 6422* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 6423* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 6424* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 6425* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 6426* [Citation](citation.html): The uri that identifies the citation 6427* [CodeSystem](codesystem.html): The uri that identifies the code system 6428* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 6429* [ConceptMap](conceptmap.html): The URI that identifies the concept map 6430* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 6431* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 6432* [Evidence](evidence.html): The uri that identifies the evidence 6433* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 6434* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 6435* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 6436* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 6437* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 6438* [Library](library.html): The uri that identifies the library 6439* [Measure](measure.html): The uri that identifies the measure 6440* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 6441* [NamingSystem](namingsystem.html): The uri that identifies the naming system 6442* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 6443* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 6444* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 6445* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 6446* [Requirements](requirements.html): The uri that identifies the requirements 6447* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 6448* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 6449* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 6450* [StructureMap](structuremap.html): The uri that identifies the structure map 6451* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 6452* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 6453* [TestPlan](testplan.html): The uri that identifies the test plan 6454* [TestScript](testscript.html): The uri that identifies the test script 6455* [ValueSet](valueset.html): The uri that identifies the value set 6456</b><br> 6457 * Type: <b>uri</b><br> 6458 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 6459 * </p> 6460 */ 6461 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 6462 6463 /** 6464 * Search parameter: <b>version</b> 6465 * <p> 6466 * Description: <b>Multiple Resources: 6467 6468* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 6469* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 6470* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 6471* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 6472* [Citation](citation.html): The business version of the citation 6473* [CodeSystem](codesystem.html): The business version of the code system 6474* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 6475* [ConceptMap](conceptmap.html): The business version of the concept map 6476* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 6477* [EventDefinition](eventdefinition.html): The business version of the event definition 6478* [Evidence](evidence.html): The business version of the evidence 6479* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 6480* [ExampleScenario](examplescenario.html): The business version of the example scenario 6481* [GraphDefinition](graphdefinition.html): The business version of the graph definition 6482* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 6483* [Library](library.html): The business version of the library 6484* [Measure](measure.html): The business version of the measure 6485* [MessageDefinition](messagedefinition.html): The business version of the message definition 6486* [NamingSystem](namingsystem.html): The business version of the naming system 6487* [OperationDefinition](operationdefinition.html): The business version of the operation definition 6488* [PlanDefinition](plandefinition.html): The business version of the plan definition 6489* [Questionnaire](questionnaire.html): The business version of the questionnaire 6490* [Requirements](requirements.html): The business version of the requirements 6491* [SearchParameter](searchparameter.html): The business version of the search parameter 6492* [StructureDefinition](structuredefinition.html): The business version of the structure definition 6493* [StructureMap](structuremap.html): The business version of the structure map 6494* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 6495* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 6496* [TestScript](testscript.html): The business version of the test script 6497* [ValueSet](valueset.html): The business version of the value set 6498</b><br> 6499 * Type: <b>token</b><br> 6500 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 6501 * </p> 6502 */ 6503 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 6504 public static final String SP_VERSION = "version"; 6505 /** 6506 * <b>Fluent Client</b> search parameter constant for <b>version</b> 6507 * <p> 6508 * Description: <b>Multiple Resources: 6509 6510* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 6511* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 6512* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 6513* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 6514* [Citation](citation.html): The business version of the citation 6515* [CodeSystem](codesystem.html): The business version of the code system 6516* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 6517* [ConceptMap](conceptmap.html): The business version of the concept map 6518* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 6519* [EventDefinition](eventdefinition.html): The business version of the event definition 6520* [Evidence](evidence.html): The business version of the evidence 6521* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 6522* [ExampleScenario](examplescenario.html): The business version of the example scenario 6523* [GraphDefinition](graphdefinition.html): The business version of the graph definition 6524* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 6525* [Library](library.html): The business version of the library 6526* [Measure](measure.html): The business version of the measure 6527* [MessageDefinition](messagedefinition.html): The business version of the message definition 6528* [NamingSystem](namingsystem.html): The business version of the naming system 6529* [OperationDefinition](operationdefinition.html): The business version of the operation definition 6530* [PlanDefinition](plandefinition.html): The business version of the plan definition 6531* [Questionnaire](questionnaire.html): The business version of the questionnaire 6532* [Requirements](requirements.html): The business version of the requirements 6533* [SearchParameter](searchparameter.html): The business version of the search parameter 6534* [StructureDefinition](structuredefinition.html): The business version of the structure definition 6535* [StructureMap](structuremap.html): The business version of the structure map 6536* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 6537* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 6538* [TestScript](testscript.html): The business version of the test script 6539* [ValueSet](valueset.html): The business version of the value set 6540</b><br> 6541 * Type: <b>token</b><br> 6542 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 6543 * </p> 6544 */ 6545 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 6546 6547 6548} 6549