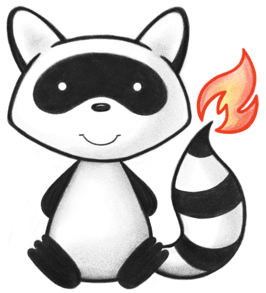
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A plan for executing testing on an artifact or specifications 052 */ 053@ResourceDef(name="TestPlan", profile="http://hl7.org/fhir/StructureDefinition/TestPlan") 054public class TestPlan extends CanonicalResource { 055 056 @Block() 057 public static class TestPlanDependencyComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * A textual description of the criterium - what is needed for the dependency to be considered met. 060 */ 061 @Child(name = "description", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="Description of the dependency criterium", formalDefinition="A textual description of the criterium - what is needed for the dependency to be considered met." ) 063 protected MarkdownType description; 064 065 /** 066 * Predecessor test plans - those that are expected to be successfully performed as a dependency for the execution of this test plan. 067 */ 068 @Child(name = "predecessor", type = {Reference.class}, order=2, min=0, max=1, modifier=false, summary=false) 069 @Description(shortDefinition="Link to predecessor test plans", formalDefinition="Predecessor test plans - those that are expected to be successfully performed as a dependency for the execution of this test plan." ) 070 protected Reference predecessor; 071 072 private static final long serialVersionUID = 1630757943L; 073 074 /** 075 * Constructor 076 */ 077 public TestPlanDependencyComponent() { 078 super(); 079 } 080 081 /** 082 * @return {@link #description} (A textual description of the criterium - what is needed for the dependency to be considered met.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 083 */ 084 public MarkdownType getDescriptionElement() { 085 if (this.description == null) 086 if (Configuration.errorOnAutoCreate()) 087 throw new Error("Attempt to auto-create TestPlanDependencyComponent.description"); 088 else if (Configuration.doAutoCreate()) 089 this.description = new MarkdownType(); // bb 090 return this.description; 091 } 092 093 public boolean hasDescriptionElement() { 094 return this.description != null && !this.description.isEmpty(); 095 } 096 097 public boolean hasDescription() { 098 return this.description != null && !this.description.isEmpty(); 099 } 100 101 /** 102 * @param value {@link #description} (A textual description of the criterium - what is needed for the dependency to be considered met.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 103 */ 104 public TestPlanDependencyComponent setDescriptionElement(MarkdownType value) { 105 this.description = value; 106 return this; 107 } 108 109 /** 110 * @return A textual description of the criterium - what is needed for the dependency to be considered met. 111 */ 112 public String getDescription() { 113 return this.description == null ? null : this.description.getValue(); 114 } 115 116 /** 117 * @param value A textual description of the criterium - what is needed for the dependency to be considered met. 118 */ 119 public TestPlanDependencyComponent setDescription(String value) { 120 if (Utilities.noString(value)) 121 this.description = null; 122 else { 123 if (this.description == null) 124 this.description = new MarkdownType(); 125 this.description.setValue(value); 126 } 127 return this; 128 } 129 130 /** 131 * @return {@link #predecessor} (Predecessor test plans - those that are expected to be successfully performed as a dependency for the execution of this test plan.) 132 */ 133 public Reference getPredecessor() { 134 if (this.predecessor == null) 135 if (Configuration.errorOnAutoCreate()) 136 throw new Error("Attempt to auto-create TestPlanDependencyComponent.predecessor"); 137 else if (Configuration.doAutoCreate()) 138 this.predecessor = new Reference(); // cc 139 return this.predecessor; 140 } 141 142 public boolean hasPredecessor() { 143 return this.predecessor != null && !this.predecessor.isEmpty(); 144 } 145 146 /** 147 * @param value {@link #predecessor} (Predecessor test plans - those that are expected to be successfully performed as a dependency for the execution of this test plan.) 148 */ 149 public TestPlanDependencyComponent setPredecessor(Reference value) { 150 this.predecessor = value; 151 return this; 152 } 153 154 protected void listChildren(List<Property> children) { 155 super.listChildren(children); 156 children.add(new Property("description", "markdown", "A textual description of the criterium - what is needed for the dependency to be considered met.", 0, 1, description)); 157 children.add(new Property("predecessor", "Reference", "Predecessor test plans - those that are expected to be successfully performed as a dependency for the execution of this test plan.", 0, 1, predecessor)); 158 } 159 160 @Override 161 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 162 switch (_hash) { 163 case -1724546052: /*description*/ return new Property("description", "markdown", "A textual description of the criterium - what is needed for the dependency to be considered met.", 0, 1, description); 164 case -1925032183: /*predecessor*/ return new Property("predecessor", "Reference", "Predecessor test plans - those that are expected to be successfully performed as a dependency for the execution of this test plan.", 0, 1, predecessor); 165 default: return super.getNamedProperty(_hash, _name, _checkValid); 166 } 167 168 } 169 170 @Override 171 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 172 switch (hash) { 173 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 174 case -1925032183: /*predecessor*/ return this.predecessor == null ? new Base[0] : new Base[] {this.predecessor}; // Reference 175 default: return super.getProperty(hash, name, checkValid); 176 } 177 178 } 179 180 @Override 181 public Base setProperty(int hash, String name, Base value) throws FHIRException { 182 switch (hash) { 183 case -1724546052: // description 184 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 185 return value; 186 case -1925032183: // predecessor 187 this.predecessor = TypeConvertor.castToReference(value); // Reference 188 return value; 189 default: return super.setProperty(hash, name, value); 190 } 191 192 } 193 194 @Override 195 public Base setProperty(String name, Base value) throws FHIRException { 196 if (name.equals("description")) { 197 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 198 } else if (name.equals("predecessor")) { 199 this.predecessor = TypeConvertor.castToReference(value); // Reference 200 } else 201 return super.setProperty(name, value); 202 return value; 203 } 204 205 @Override 206 public void removeChild(String name, Base value) throws FHIRException { 207 if (name.equals("description")) { 208 this.description = null; 209 } else if (name.equals("predecessor")) { 210 this.predecessor = null; 211 } else 212 super.removeChild(name, value); 213 214 } 215 216 @Override 217 public Base makeProperty(int hash, String name) throws FHIRException { 218 switch (hash) { 219 case -1724546052: return getDescriptionElement(); 220 case -1925032183: return getPredecessor(); 221 default: return super.makeProperty(hash, name); 222 } 223 224 } 225 226 @Override 227 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 228 switch (hash) { 229 case -1724546052: /*description*/ return new String[] {"markdown"}; 230 case -1925032183: /*predecessor*/ return new String[] {"Reference"}; 231 default: return super.getTypesForProperty(hash, name); 232 } 233 234 } 235 236 @Override 237 public Base addChild(String name) throws FHIRException { 238 if (name.equals("description")) { 239 throw new FHIRException("Cannot call addChild on a singleton property TestPlan.dependency.description"); 240 } 241 else if (name.equals("predecessor")) { 242 this.predecessor = new Reference(); 243 return this.predecessor; 244 } 245 else 246 return super.addChild(name); 247 } 248 249 public TestPlanDependencyComponent copy() { 250 TestPlanDependencyComponent dst = new TestPlanDependencyComponent(); 251 copyValues(dst); 252 return dst; 253 } 254 255 public void copyValues(TestPlanDependencyComponent dst) { 256 super.copyValues(dst); 257 dst.description = description == null ? null : description.copy(); 258 dst.predecessor = predecessor == null ? null : predecessor.copy(); 259 } 260 261 @Override 262 public boolean equalsDeep(Base other_) { 263 if (!super.equalsDeep(other_)) 264 return false; 265 if (!(other_ instanceof TestPlanDependencyComponent)) 266 return false; 267 TestPlanDependencyComponent o = (TestPlanDependencyComponent) other_; 268 return compareDeep(description, o.description, true) && compareDeep(predecessor, o.predecessor, true) 269 ; 270 } 271 272 @Override 273 public boolean equalsShallow(Base other_) { 274 if (!super.equalsShallow(other_)) 275 return false; 276 if (!(other_ instanceof TestPlanDependencyComponent)) 277 return false; 278 TestPlanDependencyComponent o = (TestPlanDependencyComponent) other_; 279 return compareValues(description, o.description, true); 280 } 281 282 public boolean isEmpty() { 283 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, predecessor); 284 } 285 286 public String fhirType() { 287 return "TestPlan.dependency"; 288 289 } 290 291 } 292 293 @Block() 294 public static class TestPlanTestCaseComponent extends BackboneElement implements IBaseBackboneElement { 295 /** 296 * Sequence of test case - an ordinal number that indicates the order for the present test case in the test plan. 297 */ 298 @Child(name = "sequence", type = {IntegerType.class}, order=1, min=0, max=1, modifier=false, summary=false) 299 @Description(shortDefinition="Sequence of test case in the test plan", formalDefinition="Sequence of test case - an ordinal number that indicates the order for the present test case in the test plan." ) 300 protected IntegerType sequence; 301 302 /** 303 * The scope or artifact covered by the case, when the individual test case is associated with a testable artifact. 304 */ 305 @Child(name = "scope", type = {Reference.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 306 @Description(shortDefinition="The scope or artifact covered by the case", formalDefinition="The scope or artifact covered by the case, when the individual test case is associated with a testable artifact." ) 307 protected List<Reference> scope; 308 309 /** 310 * The required criteria to execute the test case - e.g. preconditions, previous tests. 311 */ 312 @Child(name = "dependency", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 313 @Description(shortDefinition="Required criteria to execute the test case", formalDefinition="The required criteria to execute the test case - e.g. preconditions, previous tests." ) 314 protected List<TestCaseDependencyComponent> dependency; 315 316 /** 317 * The actual test to be executed. 318 */ 319 @Child(name = "testRun", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 320 @Description(shortDefinition="The actual test to be executed", formalDefinition="The actual test to be executed." ) 321 protected List<TestPlanTestCaseTestRunComponent> testRun; 322 323 /** 324 * The test data used in the test case. 325 */ 326 @Child(name = "testData", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 327 @Description(shortDefinition="The test data used in the test case", formalDefinition="The test data used in the test case." ) 328 protected List<TestPlanTestCaseTestDataComponent> testData; 329 330 /** 331 * The test assertions - the expectations of test results from the execution of the test case. 332 */ 333 @Child(name = "assertion", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 334 @Description(shortDefinition="Test assertions or expectations", formalDefinition="The test assertions - the expectations of test results from the execution of the test case." ) 335 protected List<TestPlanTestCaseAssertionComponent> assertion; 336 337 private static final long serialVersionUID = 1605296611L; 338 339 /** 340 * Constructor 341 */ 342 public TestPlanTestCaseComponent() { 343 super(); 344 } 345 346 /** 347 * @return {@link #sequence} (Sequence of test case - an ordinal number that indicates the order for the present test case in the test plan.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 348 */ 349 public IntegerType getSequenceElement() { 350 if (this.sequence == null) 351 if (Configuration.errorOnAutoCreate()) 352 throw new Error("Attempt to auto-create TestPlanTestCaseComponent.sequence"); 353 else if (Configuration.doAutoCreate()) 354 this.sequence = new IntegerType(); // bb 355 return this.sequence; 356 } 357 358 public boolean hasSequenceElement() { 359 return this.sequence != null && !this.sequence.isEmpty(); 360 } 361 362 public boolean hasSequence() { 363 return this.sequence != null && !this.sequence.isEmpty(); 364 } 365 366 /** 367 * @param value {@link #sequence} (Sequence of test case - an ordinal number that indicates the order for the present test case in the test plan.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 368 */ 369 public TestPlanTestCaseComponent setSequenceElement(IntegerType value) { 370 this.sequence = value; 371 return this; 372 } 373 374 /** 375 * @return Sequence of test case - an ordinal number that indicates the order for the present test case in the test plan. 376 */ 377 public int getSequence() { 378 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 379 } 380 381 /** 382 * @param value Sequence of test case - an ordinal number that indicates the order for the present test case in the test plan. 383 */ 384 public TestPlanTestCaseComponent setSequence(int value) { 385 if (this.sequence == null) 386 this.sequence = new IntegerType(); 387 this.sequence.setValue(value); 388 return this; 389 } 390 391 /** 392 * @return {@link #scope} (The scope or artifact covered by the case, when the individual test case is associated with a testable artifact.) 393 */ 394 public List<Reference> getScope() { 395 if (this.scope == null) 396 this.scope = new ArrayList<Reference>(); 397 return this.scope; 398 } 399 400 /** 401 * @return Returns a reference to <code>this</code> for easy method chaining 402 */ 403 public TestPlanTestCaseComponent setScope(List<Reference> theScope) { 404 this.scope = theScope; 405 return this; 406 } 407 408 public boolean hasScope() { 409 if (this.scope == null) 410 return false; 411 for (Reference item : this.scope) 412 if (!item.isEmpty()) 413 return true; 414 return false; 415 } 416 417 public Reference addScope() { //3 418 Reference t = new Reference(); 419 if (this.scope == null) 420 this.scope = new ArrayList<Reference>(); 421 this.scope.add(t); 422 return t; 423 } 424 425 public TestPlanTestCaseComponent addScope(Reference t) { //3 426 if (t == null) 427 return this; 428 if (this.scope == null) 429 this.scope = new ArrayList<Reference>(); 430 this.scope.add(t); 431 return this; 432 } 433 434 /** 435 * @return The first repetition of repeating field {@link #scope}, creating it if it does not already exist {3} 436 */ 437 public Reference getScopeFirstRep() { 438 if (getScope().isEmpty()) { 439 addScope(); 440 } 441 return getScope().get(0); 442 } 443 444 /** 445 * @return {@link #dependency} (The required criteria to execute the test case - e.g. preconditions, previous tests.) 446 */ 447 public List<TestCaseDependencyComponent> getDependency() { 448 if (this.dependency == null) 449 this.dependency = new ArrayList<TestCaseDependencyComponent>(); 450 return this.dependency; 451 } 452 453 /** 454 * @return Returns a reference to <code>this</code> for easy method chaining 455 */ 456 public TestPlanTestCaseComponent setDependency(List<TestCaseDependencyComponent> theDependency) { 457 this.dependency = theDependency; 458 return this; 459 } 460 461 public boolean hasDependency() { 462 if (this.dependency == null) 463 return false; 464 for (TestCaseDependencyComponent item : this.dependency) 465 if (!item.isEmpty()) 466 return true; 467 return false; 468 } 469 470 public TestCaseDependencyComponent addDependency() { //3 471 TestCaseDependencyComponent t = new TestCaseDependencyComponent(); 472 if (this.dependency == null) 473 this.dependency = new ArrayList<TestCaseDependencyComponent>(); 474 this.dependency.add(t); 475 return t; 476 } 477 478 public TestPlanTestCaseComponent addDependency(TestCaseDependencyComponent t) { //3 479 if (t == null) 480 return this; 481 if (this.dependency == null) 482 this.dependency = new ArrayList<TestCaseDependencyComponent>(); 483 this.dependency.add(t); 484 return this; 485 } 486 487 /** 488 * @return The first repetition of repeating field {@link #dependency}, creating it if it does not already exist {3} 489 */ 490 public TestCaseDependencyComponent getDependencyFirstRep() { 491 if (getDependency().isEmpty()) { 492 addDependency(); 493 } 494 return getDependency().get(0); 495 } 496 497 /** 498 * @return {@link #testRun} (The actual test to be executed.) 499 */ 500 public List<TestPlanTestCaseTestRunComponent> getTestRun() { 501 if (this.testRun == null) 502 this.testRun = new ArrayList<TestPlanTestCaseTestRunComponent>(); 503 return this.testRun; 504 } 505 506 /** 507 * @return Returns a reference to <code>this</code> for easy method chaining 508 */ 509 public TestPlanTestCaseComponent setTestRun(List<TestPlanTestCaseTestRunComponent> theTestRun) { 510 this.testRun = theTestRun; 511 return this; 512 } 513 514 public boolean hasTestRun() { 515 if (this.testRun == null) 516 return false; 517 for (TestPlanTestCaseTestRunComponent item : this.testRun) 518 if (!item.isEmpty()) 519 return true; 520 return false; 521 } 522 523 public TestPlanTestCaseTestRunComponent addTestRun() { //3 524 TestPlanTestCaseTestRunComponent t = new TestPlanTestCaseTestRunComponent(); 525 if (this.testRun == null) 526 this.testRun = new ArrayList<TestPlanTestCaseTestRunComponent>(); 527 this.testRun.add(t); 528 return t; 529 } 530 531 public TestPlanTestCaseComponent addTestRun(TestPlanTestCaseTestRunComponent t) { //3 532 if (t == null) 533 return this; 534 if (this.testRun == null) 535 this.testRun = new ArrayList<TestPlanTestCaseTestRunComponent>(); 536 this.testRun.add(t); 537 return this; 538 } 539 540 /** 541 * @return The first repetition of repeating field {@link #testRun}, creating it if it does not already exist {3} 542 */ 543 public TestPlanTestCaseTestRunComponent getTestRunFirstRep() { 544 if (getTestRun().isEmpty()) { 545 addTestRun(); 546 } 547 return getTestRun().get(0); 548 } 549 550 /** 551 * @return {@link #testData} (The test data used in the test case.) 552 */ 553 public List<TestPlanTestCaseTestDataComponent> getTestData() { 554 if (this.testData == null) 555 this.testData = new ArrayList<TestPlanTestCaseTestDataComponent>(); 556 return this.testData; 557 } 558 559 /** 560 * @return Returns a reference to <code>this</code> for easy method chaining 561 */ 562 public TestPlanTestCaseComponent setTestData(List<TestPlanTestCaseTestDataComponent> theTestData) { 563 this.testData = theTestData; 564 return this; 565 } 566 567 public boolean hasTestData() { 568 if (this.testData == null) 569 return false; 570 for (TestPlanTestCaseTestDataComponent item : this.testData) 571 if (!item.isEmpty()) 572 return true; 573 return false; 574 } 575 576 public TestPlanTestCaseTestDataComponent addTestData() { //3 577 TestPlanTestCaseTestDataComponent t = new TestPlanTestCaseTestDataComponent(); 578 if (this.testData == null) 579 this.testData = new ArrayList<TestPlanTestCaseTestDataComponent>(); 580 this.testData.add(t); 581 return t; 582 } 583 584 public TestPlanTestCaseComponent addTestData(TestPlanTestCaseTestDataComponent t) { //3 585 if (t == null) 586 return this; 587 if (this.testData == null) 588 this.testData = new ArrayList<TestPlanTestCaseTestDataComponent>(); 589 this.testData.add(t); 590 return this; 591 } 592 593 /** 594 * @return The first repetition of repeating field {@link #testData}, creating it if it does not already exist {3} 595 */ 596 public TestPlanTestCaseTestDataComponent getTestDataFirstRep() { 597 if (getTestData().isEmpty()) { 598 addTestData(); 599 } 600 return getTestData().get(0); 601 } 602 603 /** 604 * @return {@link #assertion} (The test assertions - the expectations of test results from the execution of the test case.) 605 */ 606 public List<TestPlanTestCaseAssertionComponent> getAssertion() { 607 if (this.assertion == null) 608 this.assertion = new ArrayList<TestPlanTestCaseAssertionComponent>(); 609 return this.assertion; 610 } 611 612 /** 613 * @return Returns a reference to <code>this</code> for easy method chaining 614 */ 615 public TestPlanTestCaseComponent setAssertion(List<TestPlanTestCaseAssertionComponent> theAssertion) { 616 this.assertion = theAssertion; 617 return this; 618 } 619 620 public boolean hasAssertion() { 621 if (this.assertion == null) 622 return false; 623 for (TestPlanTestCaseAssertionComponent item : this.assertion) 624 if (!item.isEmpty()) 625 return true; 626 return false; 627 } 628 629 public TestPlanTestCaseAssertionComponent addAssertion() { //3 630 TestPlanTestCaseAssertionComponent t = new TestPlanTestCaseAssertionComponent(); 631 if (this.assertion == null) 632 this.assertion = new ArrayList<TestPlanTestCaseAssertionComponent>(); 633 this.assertion.add(t); 634 return t; 635 } 636 637 public TestPlanTestCaseComponent addAssertion(TestPlanTestCaseAssertionComponent t) { //3 638 if (t == null) 639 return this; 640 if (this.assertion == null) 641 this.assertion = new ArrayList<TestPlanTestCaseAssertionComponent>(); 642 this.assertion.add(t); 643 return this; 644 } 645 646 /** 647 * @return The first repetition of repeating field {@link #assertion}, creating it if it does not already exist {3} 648 */ 649 public TestPlanTestCaseAssertionComponent getAssertionFirstRep() { 650 if (getAssertion().isEmpty()) { 651 addAssertion(); 652 } 653 return getAssertion().get(0); 654 } 655 656 protected void listChildren(List<Property> children) { 657 super.listChildren(children); 658 children.add(new Property("sequence", "integer", "Sequence of test case - an ordinal number that indicates the order for the present test case in the test plan.", 0, 1, sequence)); 659 children.add(new Property("scope", "Reference", "The scope or artifact covered by the case, when the individual test case is associated with a testable artifact.", 0, java.lang.Integer.MAX_VALUE, scope)); 660 children.add(new Property("dependency", "", "The required criteria to execute the test case - e.g. preconditions, previous tests.", 0, java.lang.Integer.MAX_VALUE, dependency)); 661 children.add(new Property("testRun", "", "The actual test to be executed.", 0, java.lang.Integer.MAX_VALUE, testRun)); 662 children.add(new Property("testData", "", "The test data used in the test case.", 0, java.lang.Integer.MAX_VALUE, testData)); 663 children.add(new Property("assertion", "", "The test assertions - the expectations of test results from the execution of the test case.", 0, java.lang.Integer.MAX_VALUE, assertion)); 664 } 665 666 @Override 667 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 668 switch (_hash) { 669 case 1349547969: /*sequence*/ return new Property("sequence", "integer", "Sequence of test case - an ordinal number that indicates the order for the present test case in the test plan.", 0, 1, sequence); 670 case 109264468: /*scope*/ return new Property("scope", "Reference", "The scope or artifact covered by the case, when the individual test case is associated with a testable artifact.", 0, java.lang.Integer.MAX_VALUE, scope); 671 case -26291381: /*dependency*/ return new Property("dependency", "", "The required criteria to execute the test case - e.g. preconditions, previous tests.", 0, java.lang.Integer.MAX_VALUE, dependency); 672 case -1422467943: /*testRun*/ return new Property("testRun", "", "The actual test to be executed.", 0, java.lang.Integer.MAX_VALUE, testRun); 673 case -1147269284: /*testData*/ return new Property("testData", "", "The test data used in the test case.", 0, java.lang.Integer.MAX_VALUE, testData); 674 case 1314395906: /*assertion*/ return new Property("assertion", "", "The test assertions - the expectations of test results from the execution of the test case.", 0, java.lang.Integer.MAX_VALUE, assertion); 675 default: return super.getNamedProperty(_hash, _name, _checkValid); 676 } 677 678 } 679 680 @Override 681 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 682 switch (hash) { 683 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // IntegerType 684 case 109264468: /*scope*/ return this.scope == null ? new Base[0] : this.scope.toArray(new Base[this.scope.size()]); // Reference 685 case -26291381: /*dependency*/ return this.dependency == null ? new Base[0] : this.dependency.toArray(new Base[this.dependency.size()]); // TestCaseDependencyComponent 686 case -1422467943: /*testRun*/ return this.testRun == null ? new Base[0] : this.testRun.toArray(new Base[this.testRun.size()]); // TestPlanTestCaseTestRunComponent 687 case -1147269284: /*testData*/ return this.testData == null ? new Base[0] : this.testData.toArray(new Base[this.testData.size()]); // TestPlanTestCaseTestDataComponent 688 case 1314395906: /*assertion*/ return this.assertion == null ? new Base[0] : this.assertion.toArray(new Base[this.assertion.size()]); // TestPlanTestCaseAssertionComponent 689 default: return super.getProperty(hash, name, checkValid); 690 } 691 692 } 693 694 @Override 695 public Base setProperty(int hash, String name, Base value) throws FHIRException { 696 switch (hash) { 697 case 1349547969: // sequence 698 this.sequence = TypeConvertor.castToInteger(value); // IntegerType 699 return value; 700 case 109264468: // scope 701 this.getScope().add(TypeConvertor.castToReference(value)); // Reference 702 return value; 703 case -26291381: // dependency 704 this.getDependency().add((TestCaseDependencyComponent) value); // TestCaseDependencyComponent 705 return value; 706 case -1422467943: // testRun 707 this.getTestRun().add((TestPlanTestCaseTestRunComponent) value); // TestPlanTestCaseTestRunComponent 708 return value; 709 case -1147269284: // testData 710 this.getTestData().add((TestPlanTestCaseTestDataComponent) value); // TestPlanTestCaseTestDataComponent 711 return value; 712 case 1314395906: // assertion 713 this.getAssertion().add((TestPlanTestCaseAssertionComponent) value); // TestPlanTestCaseAssertionComponent 714 return value; 715 default: return super.setProperty(hash, name, value); 716 } 717 718 } 719 720 @Override 721 public Base setProperty(String name, Base value) throws FHIRException { 722 if (name.equals("sequence")) { 723 this.sequence = TypeConvertor.castToInteger(value); // IntegerType 724 } else if (name.equals("scope")) { 725 this.getScope().add(TypeConvertor.castToReference(value)); 726 } else if (name.equals("dependency")) { 727 this.getDependency().add((TestCaseDependencyComponent) value); 728 } else if (name.equals("testRun")) { 729 this.getTestRun().add((TestPlanTestCaseTestRunComponent) value); 730 } else if (name.equals("testData")) { 731 this.getTestData().add((TestPlanTestCaseTestDataComponent) value); 732 } else if (name.equals("assertion")) { 733 this.getAssertion().add((TestPlanTestCaseAssertionComponent) value); 734 } else 735 return super.setProperty(name, value); 736 return value; 737 } 738 739 @Override 740 public void removeChild(String name, Base value) throws FHIRException { 741 if (name.equals("sequence")) { 742 this.sequence = null; 743 } else if (name.equals("scope")) { 744 this.getScope().remove(value); 745 } else if (name.equals("dependency")) { 746 this.getDependency().remove((TestCaseDependencyComponent) value); 747 } else if (name.equals("testRun")) { 748 this.getTestRun().remove((TestPlanTestCaseTestRunComponent) value); 749 } else if (name.equals("testData")) { 750 this.getTestData().remove((TestPlanTestCaseTestDataComponent) value); 751 } else if (name.equals("assertion")) { 752 this.getAssertion().remove((TestPlanTestCaseAssertionComponent) value); 753 } else 754 super.removeChild(name, value); 755 756 } 757 758 @Override 759 public Base makeProperty(int hash, String name) throws FHIRException { 760 switch (hash) { 761 case 1349547969: return getSequenceElement(); 762 case 109264468: return addScope(); 763 case -26291381: return addDependency(); 764 case -1422467943: return addTestRun(); 765 case -1147269284: return addTestData(); 766 case 1314395906: return addAssertion(); 767 default: return super.makeProperty(hash, name); 768 } 769 770 } 771 772 @Override 773 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 774 switch (hash) { 775 case 1349547969: /*sequence*/ return new String[] {"integer"}; 776 case 109264468: /*scope*/ return new String[] {"Reference"}; 777 case -26291381: /*dependency*/ return new String[] {}; 778 case -1422467943: /*testRun*/ return new String[] {}; 779 case -1147269284: /*testData*/ return new String[] {}; 780 case 1314395906: /*assertion*/ return new String[] {}; 781 default: return super.getTypesForProperty(hash, name); 782 } 783 784 } 785 786 @Override 787 public Base addChild(String name) throws FHIRException { 788 if (name.equals("sequence")) { 789 throw new FHIRException("Cannot call addChild on a singleton property TestPlan.testCase.sequence"); 790 } 791 else if (name.equals("scope")) { 792 return addScope(); 793 } 794 else if (name.equals("dependency")) { 795 return addDependency(); 796 } 797 else if (name.equals("testRun")) { 798 return addTestRun(); 799 } 800 else if (name.equals("testData")) { 801 return addTestData(); 802 } 803 else if (name.equals("assertion")) { 804 return addAssertion(); 805 } 806 else 807 return super.addChild(name); 808 } 809 810 public TestPlanTestCaseComponent copy() { 811 TestPlanTestCaseComponent dst = new TestPlanTestCaseComponent(); 812 copyValues(dst); 813 return dst; 814 } 815 816 public void copyValues(TestPlanTestCaseComponent dst) { 817 super.copyValues(dst); 818 dst.sequence = sequence == null ? null : sequence.copy(); 819 if (scope != null) { 820 dst.scope = new ArrayList<Reference>(); 821 for (Reference i : scope) 822 dst.scope.add(i.copy()); 823 }; 824 if (dependency != null) { 825 dst.dependency = new ArrayList<TestCaseDependencyComponent>(); 826 for (TestCaseDependencyComponent i : dependency) 827 dst.dependency.add(i.copy()); 828 }; 829 if (testRun != null) { 830 dst.testRun = new ArrayList<TestPlanTestCaseTestRunComponent>(); 831 for (TestPlanTestCaseTestRunComponent i : testRun) 832 dst.testRun.add(i.copy()); 833 }; 834 if (testData != null) { 835 dst.testData = new ArrayList<TestPlanTestCaseTestDataComponent>(); 836 for (TestPlanTestCaseTestDataComponent i : testData) 837 dst.testData.add(i.copy()); 838 }; 839 if (assertion != null) { 840 dst.assertion = new ArrayList<TestPlanTestCaseAssertionComponent>(); 841 for (TestPlanTestCaseAssertionComponent i : assertion) 842 dst.assertion.add(i.copy()); 843 }; 844 } 845 846 @Override 847 public boolean equalsDeep(Base other_) { 848 if (!super.equalsDeep(other_)) 849 return false; 850 if (!(other_ instanceof TestPlanTestCaseComponent)) 851 return false; 852 TestPlanTestCaseComponent o = (TestPlanTestCaseComponent) other_; 853 return compareDeep(sequence, o.sequence, true) && compareDeep(scope, o.scope, true) && compareDeep(dependency, o.dependency, true) 854 && compareDeep(testRun, o.testRun, true) && compareDeep(testData, o.testData, true) && compareDeep(assertion, o.assertion, true) 855 ; 856 } 857 858 @Override 859 public boolean equalsShallow(Base other_) { 860 if (!super.equalsShallow(other_)) 861 return false; 862 if (!(other_ instanceof TestPlanTestCaseComponent)) 863 return false; 864 TestPlanTestCaseComponent o = (TestPlanTestCaseComponent) other_; 865 return compareValues(sequence, o.sequence, true); 866 } 867 868 public boolean isEmpty() { 869 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, scope, dependency 870 , testRun, testData, assertion); 871 } 872 873 public String fhirType() { 874 return "TestPlan.testCase"; 875 876 } 877 878 } 879 880 @Block() 881 public static class TestCaseDependencyComponent extends BackboneElement implements IBaseBackboneElement { 882 /** 883 * Description of the criteria. 884 */ 885 @Child(name = "description", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 886 @Description(shortDefinition="Description of the criteria", formalDefinition="Description of the criteria." ) 887 protected MarkdownType description; 888 889 /** 890 * Link to predecessor test plans. 891 */ 892 @Child(name = "predecessor", type = {Reference.class}, order=2, min=0, max=1, modifier=false, summary=false) 893 @Description(shortDefinition="Link to predecessor test plans", formalDefinition="Link to predecessor test plans." ) 894 protected Reference predecessor; 895 896 private static final long serialVersionUID = 1630757943L; 897 898 /** 899 * Constructor 900 */ 901 public TestCaseDependencyComponent() { 902 super(); 903 } 904 905 /** 906 * @return {@link #description} (Description of the criteria.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 907 */ 908 public MarkdownType getDescriptionElement() { 909 if (this.description == null) 910 if (Configuration.errorOnAutoCreate()) 911 throw new Error("Attempt to auto-create TestCaseDependencyComponent.description"); 912 else if (Configuration.doAutoCreate()) 913 this.description = new MarkdownType(); // bb 914 return this.description; 915 } 916 917 public boolean hasDescriptionElement() { 918 return this.description != null && !this.description.isEmpty(); 919 } 920 921 public boolean hasDescription() { 922 return this.description != null && !this.description.isEmpty(); 923 } 924 925 /** 926 * @param value {@link #description} (Description of the criteria.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 927 */ 928 public TestCaseDependencyComponent setDescriptionElement(MarkdownType value) { 929 this.description = value; 930 return this; 931 } 932 933 /** 934 * @return Description of the criteria. 935 */ 936 public String getDescription() { 937 return this.description == null ? null : this.description.getValue(); 938 } 939 940 /** 941 * @param value Description of the criteria. 942 */ 943 public TestCaseDependencyComponent setDescription(String value) { 944 if (Utilities.noString(value)) 945 this.description = null; 946 else { 947 if (this.description == null) 948 this.description = new MarkdownType(); 949 this.description.setValue(value); 950 } 951 return this; 952 } 953 954 /** 955 * @return {@link #predecessor} (Link to predecessor test plans.) 956 */ 957 public Reference getPredecessor() { 958 if (this.predecessor == null) 959 if (Configuration.errorOnAutoCreate()) 960 throw new Error("Attempt to auto-create TestCaseDependencyComponent.predecessor"); 961 else if (Configuration.doAutoCreate()) 962 this.predecessor = new Reference(); // cc 963 return this.predecessor; 964 } 965 966 public boolean hasPredecessor() { 967 return this.predecessor != null && !this.predecessor.isEmpty(); 968 } 969 970 /** 971 * @param value {@link #predecessor} (Link to predecessor test plans.) 972 */ 973 public TestCaseDependencyComponent setPredecessor(Reference value) { 974 this.predecessor = value; 975 return this; 976 } 977 978 protected void listChildren(List<Property> children) { 979 super.listChildren(children); 980 children.add(new Property("description", "markdown", "Description of the criteria.", 0, 1, description)); 981 children.add(new Property("predecessor", "Reference", "Link to predecessor test plans.", 0, 1, predecessor)); 982 } 983 984 @Override 985 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 986 switch (_hash) { 987 case -1724546052: /*description*/ return new Property("description", "markdown", "Description of the criteria.", 0, 1, description); 988 case -1925032183: /*predecessor*/ return new Property("predecessor", "Reference", "Link to predecessor test plans.", 0, 1, predecessor); 989 default: return super.getNamedProperty(_hash, _name, _checkValid); 990 } 991 992 } 993 994 @Override 995 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 996 switch (hash) { 997 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 998 case -1925032183: /*predecessor*/ return this.predecessor == null ? new Base[0] : new Base[] {this.predecessor}; // Reference 999 default: return super.getProperty(hash, name, checkValid); 1000 } 1001 1002 } 1003 1004 @Override 1005 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1006 switch (hash) { 1007 case -1724546052: // description 1008 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1009 return value; 1010 case -1925032183: // predecessor 1011 this.predecessor = TypeConvertor.castToReference(value); // Reference 1012 return value; 1013 default: return super.setProperty(hash, name, value); 1014 } 1015 1016 } 1017 1018 @Override 1019 public Base setProperty(String name, Base value) throws FHIRException { 1020 if (name.equals("description")) { 1021 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1022 } else if (name.equals("predecessor")) { 1023 this.predecessor = TypeConvertor.castToReference(value); // Reference 1024 } else 1025 return super.setProperty(name, value); 1026 return value; 1027 } 1028 1029 @Override 1030 public void removeChild(String name, Base value) throws FHIRException { 1031 if (name.equals("description")) { 1032 this.description = null; 1033 } else if (name.equals("predecessor")) { 1034 this.predecessor = null; 1035 } else 1036 super.removeChild(name, value); 1037 1038 } 1039 1040 @Override 1041 public Base makeProperty(int hash, String name) throws FHIRException { 1042 switch (hash) { 1043 case -1724546052: return getDescriptionElement(); 1044 case -1925032183: return getPredecessor(); 1045 default: return super.makeProperty(hash, name); 1046 } 1047 1048 } 1049 1050 @Override 1051 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1052 switch (hash) { 1053 case -1724546052: /*description*/ return new String[] {"markdown"}; 1054 case -1925032183: /*predecessor*/ return new String[] {"Reference"}; 1055 default: return super.getTypesForProperty(hash, name); 1056 } 1057 1058 } 1059 1060 @Override 1061 public Base addChild(String name) throws FHIRException { 1062 if (name.equals("description")) { 1063 throw new FHIRException("Cannot call addChild on a singleton property TestPlan.testCase.dependency.description"); 1064 } 1065 else if (name.equals("predecessor")) { 1066 this.predecessor = new Reference(); 1067 return this.predecessor; 1068 } 1069 else 1070 return super.addChild(name); 1071 } 1072 1073 public TestCaseDependencyComponent copy() { 1074 TestCaseDependencyComponent dst = new TestCaseDependencyComponent(); 1075 copyValues(dst); 1076 return dst; 1077 } 1078 1079 public void copyValues(TestCaseDependencyComponent dst) { 1080 super.copyValues(dst); 1081 dst.description = description == null ? null : description.copy(); 1082 dst.predecessor = predecessor == null ? null : predecessor.copy(); 1083 } 1084 1085 @Override 1086 public boolean equalsDeep(Base other_) { 1087 if (!super.equalsDeep(other_)) 1088 return false; 1089 if (!(other_ instanceof TestCaseDependencyComponent)) 1090 return false; 1091 TestCaseDependencyComponent o = (TestCaseDependencyComponent) other_; 1092 return compareDeep(description, o.description, true) && compareDeep(predecessor, o.predecessor, true) 1093 ; 1094 } 1095 1096 @Override 1097 public boolean equalsShallow(Base other_) { 1098 if (!super.equalsShallow(other_)) 1099 return false; 1100 if (!(other_ instanceof TestCaseDependencyComponent)) 1101 return false; 1102 TestCaseDependencyComponent o = (TestCaseDependencyComponent) other_; 1103 return compareValues(description, o.description, true); 1104 } 1105 1106 public boolean isEmpty() { 1107 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, predecessor); 1108 } 1109 1110 public String fhirType() { 1111 return "TestPlan.testCase.dependency"; 1112 1113 } 1114 1115 } 1116 1117 @Block() 1118 public static class TestPlanTestCaseTestRunComponent extends BackboneElement implements IBaseBackboneElement { 1119 /** 1120 * The narrative description of the tests. 1121 */ 1122 @Child(name = "narrative", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1123 @Description(shortDefinition="The narrative description of the tests", formalDefinition="The narrative description of the tests." ) 1124 protected MarkdownType narrative; 1125 1126 /** 1127 * The test cases in a structured language e.g. gherkin, Postman, or FHIR TestScript. 1128 */ 1129 @Child(name = "script", type = {}, order=2, min=0, max=1, modifier=false, summary=false) 1130 @Description(shortDefinition="The test cases in a structured language e.g. gherkin, Postman, or FHIR TestScript", formalDefinition="The test cases in a structured language e.g. gherkin, Postman, or FHIR TestScript." ) 1131 protected TestPlanTestCaseTestRunScriptComponent script; 1132 1133 private static final long serialVersionUID = -763780736L; 1134 1135 /** 1136 * Constructor 1137 */ 1138 public TestPlanTestCaseTestRunComponent() { 1139 super(); 1140 } 1141 1142 /** 1143 * @return {@link #narrative} (The narrative description of the tests.). This is the underlying object with id, value and extensions. The accessor "getNarrative" gives direct access to the value 1144 */ 1145 public MarkdownType getNarrativeElement() { 1146 if (this.narrative == null) 1147 if (Configuration.errorOnAutoCreate()) 1148 throw new Error("Attempt to auto-create TestPlanTestCaseTestRunComponent.narrative"); 1149 else if (Configuration.doAutoCreate()) 1150 this.narrative = new MarkdownType(); // bb 1151 return this.narrative; 1152 } 1153 1154 public boolean hasNarrativeElement() { 1155 return this.narrative != null && !this.narrative.isEmpty(); 1156 } 1157 1158 public boolean hasNarrative() { 1159 return this.narrative != null && !this.narrative.isEmpty(); 1160 } 1161 1162 /** 1163 * @param value {@link #narrative} (The narrative description of the tests.). This is the underlying object with id, value and extensions. The accessor "getNarrative" gives direct access to the value 1164 */ 1165 public TestPlanTestCaseTestRunComponent setNarrativeElement(MarkdownType value) { 1166 this.narrative = value; 1167 return this; 1168 } 1169 1170 /** 1171 * @return The narrative description of the tests. 1172 */ 1173 public String getNarrative() { 1174 return this.narrative == null ? null : this.narrative.getValue(); 1175 } 1176 1177 /** 1178 * @param value The narrative description of the tests. 1179 */ 1180 public TestPlanTestCaseTestRunComponent setNarrative(String value) { 1181 if (Utilities.noString(value)) 1182 this.narrative = null; 1183 else { 1184 if (this.narrative == null) 1185 this.narrative = new MarkdownType(); 1186 this.narrative.setValue(value); 1187 } 1188 return this; 1189 } 1190 1191 /** 1192 * @return {@link #script} (The test cases in a structured language e.g. gherkin, Postman, or FHIR TestScript.) 1193 */ 1194 public TestPlanTestCaseTestRunScriptComponent getScript() { 1195 if (this.script == null) 1196 if (Configuration.errorOnAutoCreate()) 1197 throw new Error("Attempt to auto-create TestPlanTestCaseTestRunComponent.script"); 1198 else if (Configuration.doAutoCreate()) 1199 this.script = new TestPlanTestCaseTestRunScriptComponent(); // cc 1200 return this.script; 1201 } 1202 1203 public boolean hasScript() { 1204 return this.script != null && !this.script.isEmpty(); 1205 } 1206 1207 /** 1208 * @param value {@link #script} (The test cases in a structured language e.g. gherkin, Postman, or FHIR TestScript.) 1209 */ 1210 public TestPlanTestCaseTestRunComponent setScript(TestPlanTestCaseTestRunScriptComponent value) { 1211 this.script = value; 1212 return this; 1213 } 1214 1215 protected void listChildren(List<Property> children) { 1216 super.listChildren(children); 1217 children.add(new Property("narrative", "markdown", "The narrative description of the tests.", 0, 1, narrative)); 1218 children.add(new Property("script", "", "The test cases in a structured language e.g. gherkin, Postman, or FHIR TestScript.", 0, 1, script)); 1219 } 1220 1221 @Override 1222 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1223 switch (_hash) { 1224 case 1750452338: /*narrative*/ return new Property("narrative", "markdown", "The narrative description of the tests.", 0, 1, narrative); 1225 case -907685685: /*script*/ return new Property("script", "", "The test cases in a structured language e.g. gherkin, Postman, or FHIR TestScript.", 0, 1, script); 1226 default: return super.getNamedProperty(_hash, _name, _checkValid); 1227 } 1228 1229 } 1230 1231 @Override 1232 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1233 switch (hash) { 1234 case 1750452338: /*narrative*/ return this.narrative == null ? new Base[0] : new Base[] {this.narrative}; // MarkdownType 1235 case -907685685: /*script*/ return this.script == null ? new Base[0] : new Base[] {this.script}; // TestPlanTestCaseTestRunScriptComponent 1236 default: return super.getProperty(hash, name, checkValid); 1237 } 1238 1239 } 1240 1241 @Override 1242 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1243 switch (hash) { 1244 case 1750452338: // narrative 1245 this.narrative = TypeConvertor.castToMarkdown(value); // MarkdownType 1246 return value; 1247 case -907685685: // script 1248 this.script = (TestPlanTestCaseTestRunScriptComponent) value; // TestPlanTestCaseTestRunScriptComponent 1249 return value; 1250 default: return super.setProperty(hash, name, value); 1251 } 1252 1253 } 1254 1255 @Override 1256 public Base setProperty(String name, Base value) throws FHIRException { 1257 if (name.equals("narrative")) { 1258 this.narrative = TypeConvertor.castToMarkdown(value); // MarkdownType 1259 } else if (name.equals("script")) { 1260 this.script = (TestPlanTestCaseTestRunScriptComponent) value; // TestPlanTestCaseTestRunScriptComponent 1261 } else 1262 return super.setProperty(name, value); 1263 return value; 1264 } 1265 1266 @Override 1267 public void removeChild(String name, Base value) throws FHIRException { 1268 if (name.equals("narrative")) { 1269 this.narrative = null; 1270 } else if (name.equals("script")) { 1271 this.script = (TestPlanTestCaseTestRunScriptComponent) value; // TestPlanTestCaseTestRunScriptComponent 1272 } else 1273 super.removeChild(name, value); 1274 1275 } 1276 1277 @Override 1278 public Base makeProperty(int hash, String name) throws FHIRException { 1279 switch (hash) { 1280 case 1750452338: return getNarrativeElement(); 1281 case -907685685: return getScript(); 1282 default: return super.makeProperty(hash, name); 1283 } 1284 1285 } 1286 1287 @Override 1288 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1289 switch (hash) { 1290 case 1750452338: /*narrative*/ return new String[] {"markdown"}; 1291 case -907685685: /*script*/ return new String[] {}; 1292 default: return super.getTypesForProperty(hash, name); 1293 } 1294 1295 } 1296 1297 @Override 1298 public Base addChild(String name) throws FHIRException { 1299 if (name.equals("narrative")) { 1300 throw new FHIRException("Cannot call addChild on a singleton property TestPlan.testCase.testRun.narrative"); 1301 } 1302 else if (name.equals("script")) { 1303 this.script = new TestPlanTestCaseTestRunScriptComponent(); 1304 return this.script; 1305 } 1306 else 1307 return super.addChild(name); 1308 } 1309 1310 public TestPlanTestCaseTestRunComponent copy() { 1311 TestPlanTestCaseTestRunComponent dst = new TestPlanTestCaseTestRunComponent(); 1312 copyValues(dst); 1313 return dst; 1314 } 1315 1316 public void copyValues(TestPlanTestCaseTestRunComponent dst) { 1317 super.copyValues(dst); 1318 dst.narrative = narrative == null ? null : narrative.copy(); 1319 dst.script = script == null ? null : script.copy(); 1320 } 1321 1322 @Override 1323 public boolean equalsDeep(Base other_) { 1324 if (!super.equalsDeep(other_)) 1325 return false; 1326 if (!(other_ instanceof TestPlanTestCaseTestRunComponent)) 1327 return false; 1328 TestPlanTestCaseTestRunComponent o = (TestPlanTestCaseTestRunComponent) other_; 1329 return compareDeep(narrative, o.narrative, true) && compareDeep(script, o.script, true); 1330 } 1331 1332 @Override 1333 public boolean equalsShallow(Base other_) { 1334 if (!super.equalsShallow(other_)) 1335 return false; 1336 if (!(other_ instanceof TestPlanTestCaseTestRunComponent)) 1337 return false; 1338 TestPlanTestCaseTestRunComponent o = (TestPlanTestCaseTestRunComponent) other_; 1339 return compareValues(narrative, o.narrative, true); 1340 } 1341 1342 public boolean isEmpty() { 1343 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(narrative, script); 1344 } 1345 1346 public String fhirType() { 1347 return "TestPlan.testCase.testRun"; 1348 1349 } 1350 1351 } 1352 1353 @Block() 1354 public static class TestPlanTestCaseTestRunScriptComponent extends BackboneElement implements IBaseBackboneElement { 1355 /** 1356 * The language for the test cases e.g. 'gherkin', 'testscript'. 1357 */ 1358 @Child(name = "language", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1359 @Description(shortDefinition="The language for the test cases e.g. 'gherkin', 'testscript'", formalDefinition="The language for the test cases e.g. 'gherkin', 'testscript'." ) 1360 protected CodeableConcept language; 1361 1362 /** 1363 * The actual content of the cases - references to TestScripts or externally defined content. 1364 */ 1365 @Child(name = "source", type = {StringType.class, Reference.class}, order=2, min=0, max=1, modifier=false, summary=false) 1366 @Description(shortDefinition="The actual content of the cases - references to TestScripts or externally defined content", formalDefinition="The actual content of the cases - references to TestScripts or externally defined content." ) 1367 protected DataType source; 1368 1369 private static final long serialVersionUID = 1308596610L; 1370 1371 /** 1372 * Constructor 1373 */ 1374 public TestPlanTestCaseTestRunScriptComponent() { 1375 super(); 1376 } 1377 1378 /** 1379 * @return {@link #language} (The language for the test cases e.g. 'gherkin', 'testscript'.) 1380 */ 1381 public CodeableConcept getLanguage() { 1382 if (this.language == null) 1383 if (Configuration.errorOnAutoCreate()) 1384 throw new Error("Attempt to auto-create TestPlanTestCaseTestRunScriptComponent.language"); 1385 else if (Configuration.doAutoCreate()) 1386 this.language = new CodeableConcept(); // cc 1387 return this.language; 1388 } 1389 1390 public boolean hasLanguage() { 1391 return this.language != null && !this.language.isEmpty(); 1392 } 1393 1394 /** 1395 * @param value {@link #language} (The language for the test cases e.g. 'gherkin', 'testscript'.) 1396 */ 1397 public TestPlanTestCaseTestRunScriptComponent setLanguage(CodeableConcept value) { 1398 this.language = value; 1399 return this; 1400 } 1401 1402 /** 1403 * @return {@link #source} (The actual content of the cases - references to TestScripts or externally defined content.) 1404 */ 1405 public DataType getSource() { 1406 return this.source; 1407 } 1408 1409 /** 1410 * @return {@link #source} (The actual content of the cases - references to TestScripts or externally defined content.) 1411 */ 1412 public StringType getSourceStringType() throws FHIRException { 1413 if (this.source == null) 1414 this.source = new StringType(); 1415 if (!(this.source instanceof StringType)) 1416 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.source.getClass().getName()+" was encountered"); 1417 return (StringType) this.source; 1418 } 1419 1420 public boolean hasSourceStringType() { 1421 return this.source instanceof StringType; 1422 } 1423 1424 /** 1425 * @return {@link #source} (The actual content of the cases - references to TestScripts or externally defined content.) 1426 */ 1427 public Reference getSourceReference() throws FHIRException { 1428 if (this.source == null) 1429 this.source = new Reference(); 1430 if (!(this.source instanceof Reference)) 1431 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.source.getClass().getName()+" was encountered"); 1432 return (Reference) this.source; 1433 } 1434 1435 public boolean hasSourceReference() { 1436 return this.source instanceof Reference; 1437 } 1438 1439 public boolean hasSource() { 1440 return this.source != null && !this.source.isEmpty(); 1441 } 1442 1443 /** 1444 * @param value {@link #source} (The actual content of the cases - references to TestScripts or externally defined content.) 1445 */ 1446 public TestPlanTestCaseTestRunScriptComponent setSource(DataType value) { 1447 if (value != null && !(value instanceof StringType || value instanceof Reference)) 1448 throw new FHIRException("Not the right type for TestPlan.testCase.testRun.script.source[x]: "+value.fhirType()); 1449 this.source = value; 1450 return this; 1451 } 1452 1453 protected void listChildren(List<Property> children) { 1454 super.listChildren(children); 1455 children.add(new Property("language", "CodeableConcept", "The language for the test cases e.g. 'gherkin', 'testscript'.", 0, 1, language)); 1456 children.add(new Property("source[x]", "string|Reference", "The actual content of the cases - references to TestScripts or externally defined content.", 0, 1, source)); 1457 } 1458 1459 @Override 1460 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1461 switch (_hash) { 1462 case -1613589672: /*language*/ return new Property("language", "CodeableConcept", "The language for the test cases e.g. 'gherkin', 'testscript'.", 0, 1, language); 1463 case -1698413947: /*source[x]*/ return new Property("source[x]", "string|Reference", "The actual content of the cases - references to TestScripts or externally defined content.", 0, 1, source); 1464 case -896505829: /*source*/ return new Property("source[x]", "string|Reference", "The actual content of the cases - references to TestScripts or externally defined content.", 0, 1, source); 1465 case 1327821836: /*sourceString*/ return new Property("source[x]", "string", "The actual content of the cases - references to TestScripts or externally defined content.", 0, 1, source); 1466 case -244259472: /*sourceReference*/ return new Property("source[x]", "Reference", "The actual content of the cases - references to TestScripts or externally defined content.", 0, 1, source); 1467 default: return super.getNamedProperty(_hash, _name, _checkValid); 1468 } 1469 1470 } 1471 1472 @Override 1473 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1474 switch (hash) { 1475 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeableConcept 1476 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // DataType 1477 default: return super.getProperty(hash, name, checkValid); 1478 } 1479 1480 } 1481 1482 @Override 1483 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1484 switch (hash) { 1485 case -1613589672: // language 1486 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1487 return value; 1488 case -896505829: // source 1489 this.source = TypeConvertor.castToType(value); // DataType 1490 return value; 1491 default: return super.setProperty(hash, name, value); 1492 } 1493 1494 } 1495 1496 @Override 1497 public Base setProperty(String name, Base value) throws FHIRException { 1498 if (name.equals("language")) { 1499 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1500 } else if (name.equals("source[x]")) { 1501 this.source = TypeConvertor.castToType(value); // DataType 1502 } else 1503 return super.setProperty(name, value); 1504 return value; 1505 } 1506 1507 @Override 1508 public void removeChild(String name, Base value) throws FHIRException { 1509 if (name.equals("language")) { 1510 this.language = null; 1511 } else if (name.equals("source[x]")) { 1512 this.source = null; 1513 } else 1514 super.removeChild(name, value); 1515 1516 } 1517 1518 @Override 1519 public Base makeProperty(int hash, String name) throws FHIRException { 1520 switch (hash) { 1521 case -1613589672: return getLanguage(); 1522 case -1698413947: return getSource(); 1523 case -896505829: return getSource(); 1524 default: return super.makeProperty(hash, name); 1525 } 1526 1527 } 1528 1529 @Override 1530 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1531 switch (hash) { 1532 case -1613589672: /*language*/ return new String[] {"CodeableConcept"}; 1533 case -896505829: /*source*/ return new String[] {"string", "Reference"}; 1534 default: return super.getTypesForProperty(hash, name); 1535 } 1536 1537 } 1538 1539 @Override 1540 public Base addChild(String name) throws FHIRException { 1541 if (name.equals("language")) { 1542 this.language = new CodeableConcept(); 1543 return this.language; 1544 } 1545 else if (name.equals("sourceString")) { 1546 this.source = new StringType(); 1547 return this.source; 1548 } 1549 else if (name.equals("sourceReference")) { 1550 this.source = new Reference(); 1551 return this.source; 1552 } 1553 else 1554 return super.addChild(name); 1555 } 1556 1557 public TestPlanTestCaseTestRunScriptComponent copy() { 1558 TestPlanTestCaseTestRunScriptComponent dst = new TestPlanTestCaseTestRunScriptComponent(); 1559 copyValues(dst); 1560 return dst; 1561 } 1562 1563 public void copyValues(TestPlanTestCaseTestRunScriptComponent dst) { 1564 super.copyValues(dst); 1565 dst.language = language == null ? null : language.copy(); 1566 dst.source = source == null ? null : source.copy(); 1567 } 1568 1569 @Override 1570 public boolean equalsDeep(Base other_) { 1571 if (!super.equalsDeep(other_)) 1572 return false; 1573 if (!(other_ instanceof TestPlanTestCaseTestRunScriptComponent)) 1574 return false; 1575 TestPlanTestCaseTestRunScriptComponent o = (TestPlanTestCaseTestRunScriptComponent) other_; 1576 return compareDeep(language, o.language, true) && compareDeep(source, o.source, true); 1577 } 1578 1579 @Override 1580 public boolean equalsShallow(Base other_) { 1581 if (!super.equalsShallow(other_)) 1582 return false; 1583 if (!(other_ instanceof TestPlanTestCaseTestRunScriptComponent)) 1584 return false; 1585 TestPlanTestCaseTestRunScriptComponent o = (TestPlanTestCaseTestRunScriptComponent) other_; 1586 return true; 1587 } 1588 1589 public boolean isEmpty() { 1590 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, source); 1591 } 1592 1593 public String fhirType() { 1594 return "TestPlan.testCase.testRun.script"; 1595 1596 } 1597 1598 } 1599 1600 @Block() 1601 public static class TestPlanTestCaseTestDataComponent extends BackboneElement implements IBaseBackboneElement { 1602 /** 1603 * The type of test data description, e.g. 'synthea'. 1604 */ 1605 @Child(name = "type", type = {Coding.class}, order=1, min=1, max=1, modifier=false, summary=false) 1606 @Description(shortDefinition="The type of test data description, e.g. 'synthea'", formalDefinition="The type of test data description, e.g. 'synthea'." ) 1607 protected Coding type; 1608 1609 /** 1610 * The actual test resources when they exist. 1611 */ 1612 @Child(name = "content", type = {Reference.class}, order=2, min=0, max=1, modifier=false, summary=false) 1613 @Description(shortDefinition="The actual test resources when they exist", formalDefinition="The actual test resources when they exist." ) 1614 protected Reference content; 1615 1616 /** 1617 * Pointer to a definition of test resources - narrative or structured e.g. synthetic data generation, etc. 1618 */ 1619 @Child(name = "source", type = {StringType.class, Reference.class}, order=3, min=0, max=1, modifier=false, summary=false) 1620 @Description(shortDefinition="Pointer to a definition of test resources - narrative or structured e.g. synthetic data generation, etc", formalDefinition="Pointer to a definition of test resources - narrative or structured e.g. synthetic data generation, etc." ) 1621 protected DataType source; 1622 1623 private static final long serialVersionUID = -300912813L; 1624 1625 /** 1626 * Constructor 1627 */ 1628 public TestPlanTestCaseTestDataComponent() { 1629 super(); 1630 } 1631 1632 /** 1633 * Constructor 1634 */ 1635 public TestPlanTestCaseTestDataComponent(Coding type) { 1636 super(); 1637 this.setType(type); 1638 } 1639 1640 /** 1641 * @return {@link #type} (The type of test data description, e.g. 'synthea'.) 1642 */ 1643 public Coding getType() { 1644 if (this.type == null) 1645 if (Configuration.errorOnAutoCreate()) 1646 throw new Error("Attempt to auto-create TestPlanTestCaseTestDataComponent.type"); 1647 else if (Configuration.doAutoCreate()) 1648 this.type = new Coding(); // cc 1649 return this.type; 1650 } 1651 1652 public boolean hasType() { 1653 return this.type != null && !this.type.isEmpty(); 1654 } 1655 1656 /** 1657 * @param value {@link #type} (The type of test data description, e.g. 'synthea'.) 1658 */ 1659 public TestPlanTestCaseTestDataComponent setType(Coding value) { 1660 this.type = value; 1661 return this; 1662 } 1663 1664 /** 1665 * @return {@link #content} (The actual test resources when they exist.) 1666 */ 1667 public Reference getContent() { 1668 if (this.content == null) 1669 if (Configuration.errorOnAutoCreate()) 1670 throw new Error("Attempt to auto-create TestPlanTestCaseTestDataComponent.content"); 1671 else if (Configuration.doAutoCreate()) 1672 this.content = new Reference(); // cc 1673 return this.content; 1674 } 1675 1676 public boolean hasContent() { 1677 return this.content != null && !this.content.isEmpty(); 1678 } 1679 1680 /** 1681 * @param value {@link #content} (The actual test resources when they exist.) 1682 */ 1683 public TestPlanTestCaseTestDataComponent setContent(Reference value) { 1684 this.content = value; 1685 return this; 1686 } 1687 1688 /** 1689 * @return {@link #source} (Pointer to a definition of test resources - narrative or structured e.g. synthetic data generation, etc.) 1690 */ 1691 public DataType getSource() { 1692 return this.source; 1693 } 1694 1695 /** 1696 * @return {@link #source} (Pointer to a definition of test resources - narrative or structured e.g. synthetic data generation, etc.) 1697 */ 1698 public StringType getSourceStringType() throws FHIRException { 1699 if (this.source == null) 1700 this.source = new StringType(); 1701 if (!(this.source instanceof StringType)) 1702 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.source.getClass().getName()+" was encountered"); 1703 return (StringType) this.source; 1704 } 1705 1706 public boolean hasSourceStringType() { 1707 return this.source instanceof StringType; 1708 } 1709 1710 /** 1711 * @return {@link #source} (Pointer to a definition of test resources - narrative or structured e.g. synthetic data generation, etc.) 1712 */ 1713 public Reference getSourceReference() throws FHIRException { 1714 if (this.source == null) 1715 this.source = new Reference(); 1716 if (!(this.source instanceof Reference)) 1717 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.source.getClass().getName()+" was encountered"); 1718 return (Reference) this.source; 1719 } 1720 1721 public boolean hasSourceReference() { 1722 return this.source instanceof Reference; 1723 } 1724 1725 public boolean hasSource() { 1726 return this.source != null && !this.source.isEmpty(); 1727 } 1728 1729 /** 1730 * @param value {@link #source} (Pointer to a definition of test resources - narrative or structured e.g. synthetic data generation, etc.) 1731 */ 1732 public TestPlanTestCaseTestDataComponent setSource(DataType value) { 1733 if (value != null && !(value instanceof StringType || value instanceof Reference)) 1734 throw new FHIRException("Not the right type for TestPlan.testCase.testData.source[x]: "+value.fhirType()); 1735 this.source = value; 1736 return this; 1737 } 1738 1739 protected void listChildren(List<Property> children) { 1740 super.listChildren(children); 1741 children.add(new Property("type", "Coding", "The type of test data description, e.g. 'synthea'.", 0, 1, type)); 1742 children.add(new Property("content", "Reference", "The actual test resources when they exist.", 0, 1, content)); 1743 children.add(new Property("source[x]", "string|Reference", "Pointer to a definition of test resources - narrative or structured e.g. synthetic data generation, etc.", 0, 1, source)); 1744 } 1745 1746 @Override 1747 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1748 switch (_hash) { 1749 case 3575610: /*type*/ return new Property("type", "Coding", "The type of test data description, e.g. 'synthea'.", 0, 1, type); 1750 case 951530617: /*content*/ return new Property("content", "Reference", "The actual test resources when they exist.", 0, 1, content); 1751 case -1698413947: /*source[x]*/ return new Property("source[x]", "string|Reference", "Pointer to a definition of test resources - narrative or structured e.g. synthetic data generation, etc.", 0, 1, source); 1752 case -896505829: /*source*/ return new Property("source[x]", "string|Reference", "Pointer to a definition of test resources - narrative or structured e.g. synthetic data generation, etc.", 0, 1, source); 1753 case 1327821836: /*sourceString*/ return new Property("source[x]", "string", "Pointer to a definition of test resources - narrative or structured e.g. synthetic data generation, etc.", 0, 1, source); 1754 case -244259472: /*sourceReference*/ return new Property("source[x]", "Reference", "Pointer to a definition of test resources - narrative or structured e.g. synthetic data generation, etc.", 0, 1, source); 1755 default: return super.getNamedProperty(_hash, _name, _checkValid); 1756 } 1757 1758 } 1759 1760 @Override 1761 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1762 switch (hash) { 1763 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Coding 1764 case 951530617: /*content*/ return this.content == null ? new Base[0] : new Base[] {this.content}; // Reference 1765 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // DataType 1766 default: return super.getProperty(hash, name, checkValid); 1767 } 1768 1769 } 1770 1771 @Override 1772 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1773 switch (hash) { 1774 case 3575610: // type 1775 this.type = TypeConvertor.castToCoding(value); // Coding 1776 return value; 1777 case 951530617: // content 1778 this.content = TypeConvertor.castToReference(value); // Reference 1779 return value; 1780 case -896505829: // source 1781 this.source = TypeConvertor.castToType(value); // DataType 1782 return value; 1783 default: return super.setProperty(hash, name, value); 1784 } 1785 1786 } 1787 1788 @Override 1789 public Base setProperty(String name, Base value) throws FHIRException { 1790 if (name.equals("type")) { 1791 this.type = TypeConvertor.castToCoding(value); // Coding 1792 } else if (name.equals("content")) { 1793 this.content = TypeConvertor.castToReference(value); // Reference 1794 } else if (name.equals("source[x]")) { 1795 this.source = TypeConvertor.castToType(value); // DataType 1796 } else 1797 return super.setProperty(name, value); 1798 return value; 1799 } 1800 1801 @Override 1802 public void removeChild(String name, Base value) throws FHIRException { 1803 if (name.equals("type")) { 1804 this.type = null; 1805 } else if (name.equals("content")) { 1806 this.content = null; 1807 } else if (name.equals("source[x]")) { 1808 this.source = null; 1809 } else 1810 super.removeChild(name, value); 1811 1812 } 1813 1814 @Override 1815 public Base makeProperty(int hash, String name) throws FHIRException { 1816 switch (hash) { 1817 case 3575610: return getType(); 1818 case 951530617: return getContent(); 1819 case -1698413947: return getSource(); 1820 case -896505829: return getSource(); 1821 default: return super.makeProperty(hash, name); 1822 } 1823 1824 } 1825 1826 @Override 1827 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1828 switch (hash) { 1829 case 3575610: /*type*/ return new String[] {"Coding"}; 1830 case 951530617: /*content*/ return new String[] {"Reference"}; 1831 case -896505829: /*source*/ return new String[] {"string", "Reference"}; 1832 default: return super.getTypesForProperty(hash, name); 1833 } 1834 1835 } 1836 1837 @Override 1838 public Base addChild(String name) throws FHIRException { 1839 if (name.equals("type")) { 1840 this.type = new Coding(); 1841 return this.type; 1842 } 1843 else if (name.equals("content")) { 1844 this.content = new Reference(); 1845 return this.content; 1846 } 1847 else if (name.equals("sourceString")) { 1848 this.source = new StringType(); 1849 return this.source; 1850 } 1851 else if (name.equals("sourceReference")) { 1852 this.source = new Reference(); 1853 return this.source; 1854 } 1855 else 1856 return super.addChild(name); 1857 } 1858 1859 public TestPlanTestCaseTestDataComponent copy() { 1860 TestPlanTestCaseTestDataComponent dst = new TestPlanTestCaseTestDataComponent(); 1861 copyValues(dst); 1862 return dst; 1863 } 1864 1865 public void copyValues(TestPlanTestCaseTestDataComponent dst) { 1866 super.copyValues(dst); 1867 dst.type = type == null ? null : type.copy(); 1868 dst.content = content == null ? null : content.copy(); 1869 dst.source = source == null ? null : source.copy(); 1870 } 1871 1872 @Override 1873 public boolean equalsDeep(Base other_) { 1874 if (!super.equalsDeep(other_)) 1875 return false; 1876 if (!(other_ instanceof TestPlanTestCaseTestDataComponent)) 1877 return false; 1878 TestPlanTestCaseTestDataComponent o = (TestPlanTestCaseTestDataComponent) other_; 1879 return compareDeep(type, o.type, true) && compareDeep(content, o.content, true) && compareDeep(source, o.source, true) 1880 ; 1881 } 1882 1883 @Override 1884 public boolean equalsShallow(Base other_) { 1885 if (!super.equalsShallow(other_)) 1886 return false; 1887 if (!(other_ instanceof TestPlanTestCaseTestDataComponent)) 1888 return false; 1889 TestPlanTestCaseTestDataComponent o = (TestPlanTestCaseTestDataComponent) other_; 1890 return true; 1891 } 1892 1893 public boolean isEmpty() { 1894 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, content, source); 1895 } 1896 1897 public String fhirType() { 1898 return "TestPlan.testCase.testData"; 1899 1900 } 1901 1902 } 1903 1904 @Block() 1905 public static class TestPlanTestCaseAssertionComponent extends BackboneElement implements IBaseBackboneElement { 1906 /** 1907 * The test assertion type - this can be used to group assertions as 'required' or 'optional', or can be used for other classification of the assertion. 1908 */ 1909 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1910 @Description(shortDefinition="Assertion type - for example 'informative' or 'required' ", formalDefinition="The test assertion type - this can be used to group assertions as 'required' or 'optional', or can be used for other classification of the assertion." ) 1911 protected List<CodeableConcept> type; 1912 1913 /** 1914 * The focus or object of the assertion i.e. a resource. 1915 */ 1916 @Child(name = "object", type = {CodeableReference.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1917 @Description(shortDefinition="The focus or object of the assertion", formalDefinition="The focus or object of the assertion i.e. a resource." ) 1918 protected List<CodeableReference> object; 1919 1920 /** 1921 * The test assertion - the expected outcome from the test case execution. 1922 */ 1923 @Child(name = "result", type = {CodeableReference.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1924 @Description(shortDefinition="The actual result assertion", formalDefinition="The test assertion - the expected outcome from the test case execution." ) 1925 protected List<CodeableReference> result; 1926 1927 private static final long serialVersionUID = 481177705L; 1928 1929 /** 1930 * Constructor 1931 */ 1932 public TestPlanTestCaseAssertionComponent() { 1933 super(); 1934 } 1935 1936 /** 1937 * @return {@link #type} (The test assertion type - this can be used to group assertions as 'required' or 'optional', or can be used for other classification of the assertion.) 1938 */ 1939 public List<CodeableConcept> getType() { 1940 if (this.type == null) 1941 this.type = new ArrayList<CodeableConcept>(); 1942 return this.type; 1943 } 1944 1945 /** 1946 * @return Returns a reference to <code>this</code> for easy method chaining 1947 */ 1948 public TestPlanTestCaseAssertionComponent setType(List<CodeableConcept> theType) { 1949 this.type = theType; 1950 return this; 1951 } 1952 1953 public boolean hasType() { 1954 if (this.type == null) 1955 return false; 1956 for (CodeableConcept item : this.type) 1957 if (!item.isEmpty()) 1958 return true; 1959 return false; 1960 } 1961 1962 public CodeableConcept addType() { //3 1963 CodeableConcept t = new CodeableConcept(); 1964 if (this.type == null) 1965 this.type = new ArrayList<CodeableConcept>(); 1966 this.type.add(t); 1967 return t; 1968 } 1969 1970 public TestPlanTestCaseAssertionComponent addType(CodeableConcept t) { //3 1971 if (t == null) 1972 return this; 1973 if (this.type == null) 1974 this.type = new ArrayList<CodeableConcept>(); 1975 this.type.add(t); 1976 return this; 1977 } 1978 1979 /** 1980 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 1981 */ 1982 public CodeableConcept getTypeFirstRep() { 1983 if (getType().isEmpty()) { 1984 addType(); 1985 } 1986 return getType().get(0); 1987 } 1988 1989 /** 1990 * @return {@link #object} (The focus or object of the assertion i.e. a resource.) 1991 */ 1992 public List<CodeableReference> getObject() { 1993 if (this.object == null) 1994 this.object = new ArrayList<CodeableReference>(); 1995 return this.object; 1996 } 1997 1998 /** 1999 * @return Returns a reference to <code>this</code> for easy method chaining 2000 */ 2001 public TestPlanTestCaseAssertionComponent setObject(List<CodeableReference> theObject) { 2002 this.object = theObject; 2003 return this; 2004 } 2005 2006 public boolean hasObject() { 2007 if (this.object == null) 2008 return false; 2009 for (CodeableReference item : this.object) 2010 if (!item.isEmpty()) 2011 return true; 2012 return false; 2013 } 2014 2015 public CodeableReference addObject() { //3 2016 CodeableReference t = new CodeableReference(); 2017 if (this.object == null) 2018 this.object = new ArrayList<CodeableReference>(); 2019 this.object.add(t); 2020 return t; 2021 } 2022 2023 public TestPlanTestCaseAssertionComponent addObject(CodeableReference t) { //3 2024 if (t == null) 2025 return this; 2026 if (this.object == null) 2027 this.object = new ArrayList<CodeableReference>(); 2028 this.object.add(t); 2029 return this; 2030 } 2031 2032 /** 2033 * @return The first repetition of repeating field {@link #object}, creating it if it does not already exist {3} 2034 */ 2035 public CodeableReference getObjectFirstRep() { 2036 if (getObject().isEmpty()) { 2037 addObject(); 2038 } 2039 return getObject().get(0); 2040 } 2041 2042 /** 2043 * @return {@link #result} (The test assertion - the expected outcome from the test case execution.) 2044 */ 2045 public List<CodeableReference> getResult() { 2046 if (this.result == null) 2047 this.result = new ArrayList<CodeableReference>(); 2048 return this.result; 2049 } 2050 2051 /** 2052 * @return Returns a reference to <code>this</code> for easy method chaining 2053 */ 2054 public TestPlanTestCaseAssertionComponent setResult(List<CodeableReference> theResult) { 2055 this.result = theResult; 2056 return this; 2057 } 2058 2059 public boolean hasResult() { 2060 if (this.result == null) 2061 return false; 2062 for (CodeableReference item : this.result) 2063 if (!item.isEmpty()) 2064 return true; 2065 return false; 2066 } 2067 2068 public CodeableReference addResult() { //3 2069 CodeableReference t = new CodeableReference(); 2070 if (this.result == null) 2071 this.result = new ArrayList<CodeableReference>(); 2072 this.result.add(t); 2073 return t; 2074 } 2075 2076 public TestPlanTestCaseAssertionComponent addResult(CodeableReference t) { //3 2077 if (t == null) 2078 return this; 2079 if (this.result == null) 2080 this.result = new ArrayList<CodeableReference>(); 2081 this.result.add(t); 2082 return this; 2083 } 2084 2085 /** 2086 * @return The first repetition of repeating field {@link #result}, creating it if it does not already exist {3} 2087 */ 2088 public CodeableReference getResultFirstRep() { 2089 if (getResult().isEmpty()) { 2090 addResult(); 2091 } 2092 return getResult().get(0); 2093 } 2094 2095 protected void listChildren(List<Property> children) { 2096 super.listChildren(children); 2097 children.add(new Property("type", "CodeableConcept", "The test assertion type - this can be used to group assertions as 'required' or 'optional', or can be used for other classification of the assertion.", 0, java.lang.Integer.MAX_VALUE, type)); 2098 children.add(new Property("object", "CodeableReference", "The focus or object of the assertion i.e. a resource.", 0, java.lang.Integer.MAX_VALUE, object)); 2099 children.add(new Property("result", "CodeableReference", "The test assertion - the expected outcome from the test case execution.", 0, java.lang.Integer.MAX_VALUE, result)); 2100 } 2101 2102 @Override 2103 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2104 switch (_hash) { 2105 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The test assertion type - this can be used to group assertions as 'required' or 'optional', or can be used for other classification of the assertion.", 0, java.lang.Integer.MAX_VALUE, type); 2106 case -1023368385: /*object*/ return new Property("object", "CodeableReference", "The focus or object of the assertion i.e. a resource.", 0, java.lang.Integer.MAX_VALUE, object); 2107 case -934426595: /*result*/ return new Property("result", "CodeableReference", "The test assertion - the expected outcome from the test case execution.", 0, java.lang.Integer.MAX_VALUE, result); 2108 default: return super.getNamedProperty(_hash, _name, _checkValid); 2109 } 2110 2111 } 2112 2113 @Override 2114 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2115 switch (hash) { 2116 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 2117 case -1023368385: /*object*/ return this.object == null ? new Base[0] : this.object.toArray(new Base[this.object.size()]); // CodeableReference 2118 case -934426595: /*result*/ return this.result == null ? new Base[0] : this.result.toArray(new Base[this.result.size()]); // CodeableReference 2119 default: return super.getProperty(hash, name, checkValid); 2120 } 2121 2122 } 2123 2124 @Override 2125 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2126 switch (hash) { 2127 case 3575610: // type 2128 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2129 return value; 2130 case -1023368385: // object 2131 this.getObject().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 2132 return value; 2133 case -934426595: // result 2134 this.getResult().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 2135 return value; 2136 default: return super.setProperty(hash, name, value); 2137 } 2138 2139 } 2140 2141 @Override 2142 public Base setProperty(String name, Base value) throws FHIRException { 2143 if (name.equals("type")) { 2144 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 2145 } else if (name.equals("object")) { 2146 this.getObject().add(TypeConvertor.castToCodeableReference(value)); 2147 } else if (name.equals("result")) { 2148 this.getResult().add(TypeConvertor.castToCodeableReference(value)); 2149 } else 2150 return super.setProperty(name, value); 2151 return value; 2152 } 2153 2154 @Override 2155 public void removeChild(String name, Base value) throws FHIRException { 2156 if (name.equals("type")) { 2157 this.getType().remove(value); 2158 } else if (name.equals("object")) { 2159 this.getObject().remove(value); 2160 } else if (name.equals("result")) { 2161 this.getResult().remove(value); 2162 } else 2163 super.removeChild(name, value); 2164 2165 } 2166 2167 @Override 2168 public Base makeProperty(int hash, String name) throws FHIRException { 2169 switch (hash) { 2170 case 3575610: return addType(); 2171 case -1023368385: return addObject(); 2172 case -934426595: return addResult(); 2173 default: return super.makeProperty(hash, name); 2174 } 2175 2176 } 2177 2178 @Override 2179 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2180 switch (hash) { 2181 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2182 case -1023368385: /*object*/ return new String[] {"CodeableReference"}; 2183 case -934426595: /*result*/ return new String[] {"CodeableReference"}; 2184 default: return super.getTypesForProperty(hash, name); 2185 } 2186 2187 } 2188 2189 @Override 2190 public Base addChild(String name) throws FHIRException { 2191 if (name.equals("type")) { 2192 return addType(); 2193 } 2194 else if (name.equals("object")) { 2195 return addObject(); 2196 } 2197 else if (name.equals("result")) { 2198 return addResult(); 2199 } 2200 else 2201 return super.addChild(name); 2202 } 2203 2204 public TestPlanTestCaseAssertionComponent copy() { 2205 TestPlanTestCaseAssertionComponent dst = new TestPlanTestCaseAssertionComponent(); 2206 copyValues(dst); 2207 return dst; 2208 } 2209 2210 public void copyValues(TestPlanTestCaseAssertionComponent dst) { 2211 super.copyValues(dst); 2212 if (type != null) { 2213 dst.type = new ArrayList<CodeableConcept>(); 2214 for (CodeableConcept i : type) 2215 dst.type.add(i.copy()); 2216 }; 2217 if (object != null) { 2218 dst.object = new ArrayList<CodeableReference>(); 2219 for (CodeableReference i : object) 2220 dst.object.add(i.copy()); 2221 }; 2222 if (result != null) { 2223 dst.result = new ArrayList<CodeableReference>(); 2224 for (CodeableReference i : result) 2225 dst.result.add(i.copy()); 2226 }; 2227 } 2228 2229 @Override 2230 public boolean equalsDeep(Base other_) { 2231 if (!super.equalsDeep(other_)) 2232 return false; 2233 if (!(other_ instanceof TestPlanTestCaseAssertionComponent)) 2234 return false; 2235 TestPlanTestCaseAssertionComponent o = (TestPlanTestCaseAssertionComponent) other_; 2236 return compareDeep(type, o.type, true) && compareDeep(object, o.object, true) && compareDeep(result, o.result, true) 2237 ; 2238 } 2239 2240 @Override 2241 public boolean equalsShallow(Base other_) { 2242 if (!super.equalsShallow(other_)) 2243 return false; 2244 if (!(other_ instanceof TestPlanTestCaseAssertionComponent)) 2245 return false; 2246 TestPlanTestCaseAssertionComponent o = (TestPlanTestCaseAssertionComponent) other_; 2247 return true; 2248 } 2249 2250 public boolean isEmpty() { 2251 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, object, result); 2252 } 2253 2254 public String fhirType() { 2255 return "TestPlan.testCase.assertion"; 2256 2257 } 2258 2259 } 2260 2261 /** 2262 * An absolute URI that is used to identify this test plan when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this test plan is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the test plan is stored on different servers. 2263 */ 2264 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 2265 @Description(shortDefinition="Canonical identifier for this test plan, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this test plan when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this test plan is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the test plan is stored on different servers." ) 2266 protected UriType url; 2267 2268 /** 2269 * A formal identifier that is used to identify this test plan when it is represented in other formats, or referenced in a specification, model, design or an instance. 2270 */ 2271 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2272 @Description(shortDefinition="Business identifier identifier for the test plan", formalDefinition="A formal identifier that is used to identify this test plan when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 2273 protected List<Identifier> identifier; 2274 2275 /** 2276 * The identifier that is used to identify this version of the test plan when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test plan author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 2277 */ 2278 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2279 @Description(shortDefinition="Business version of the test plan", formalDefinition="The identifier that is used to identify this version of the test plan when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test plan author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 2280 protected StringType version; 2281 2282 /** 2283 * Indicates the mechanism used to compare versions to determine which is more current. 2284 */ 2285 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 2286 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 2287 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 2288 protected DataType versionAlgorithm; 2289 2290 /** 2291 * A natural language name identifying the test plan. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2292 */ 2293 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 2294 @Description(shortDefinition="Name for this test plan (computer friendly)", formalDefinition="A natural language name identifying the test plan. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 2295 protected StringType name; 2296 2297 /** 2298 * A short, descriptive, user-friendly title for the test plan. 2299 */ 2300 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 2301 @Description(shortDefinition="Name for this test plan (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the test plan." ) 2302 protected StringType title; 2303 2304 /** 2305 * The status of this test plan. Enables tracking the life-cycle of the content. 2306 */ 2307 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 2308 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this test plan. Enables tracking the life-cycle of the content." ) 2309 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 2310 protected Enumeration<PublicationStatus> status; 2311 2312 /** 2313 * A Boolean value to indicate that this test plan is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 2314 */ 2315 @Child(name = "experimental", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 2316 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this test plan is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 2317 protected BooleanType experimental; 2318 2319 /** 2320 * The date (and optionally time) when the test plan was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test plan changes. 2321 */ 2322 @Child(name = "date", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 2323 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the test plan was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test plan changes." ) 2324 protected DateTimeType date; 2325 2326 /** 2327 * The name of the organization or individual responsible for the release and ongoing maintenance of the test plan. 2328 */ 2329 @Child(name = "publisher", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 2330 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the test plan." ) 2331 protected StringType publisher; 2332 2333 /** 2334 * Contact details to assist a user in finding and communicating with the publisher. 2335 */ 2336 @Child(name = "contact", type = {ContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2337 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 2338 protected List<ContactDetail> contact; 2339 2340 /** 2341 * A free text natural language description of the test plan from a consumer's perspective. 2342 */ 2343 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 2344 @Description(shortDefinition="Natural language description of the test plan", formalDefinition="A free text natural language description of the test plan from a consumer's perspective." ) 2345 protected MarkdownType description; 2346 2347 /** 2348 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate test plan instances. 2349 */ 2350 @Child(name = "useContext", type = {UsageContext.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2351 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate test plan instances." ) 2352 protected List<UsageContext> useContext; 2353 2354 /** 2355 * A legal or geographic region in which the test plan is intended to be used. 2356 */ 2357 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2358 @Description(shortDefinition="Intended jurisdiction where the test plan applies (if applicable)", formalDefinition="A legal or geographic region in which the test plan is intended to be used." ) 2359 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 2360 protected List<CodeableConcept> jurisdiction; 2361 2362 /** 2363 * Explanation of why this test plan is needed and why it has been designed as it has. 2364 */ 2365 @Child(name = "purpose", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 2366 @Description(shortDefinition="Why this test plan is defined", formalDefinition="Explanation of why this test plan is needed and why it has been designed as it has." ) 2367 protected MarkdownType purpose; 2368 2369 /** 2370 * A copyright statement relating to the test plan and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test plan. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element. 2371 */ 2372 @Child(name = "copyright", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 2373 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the test plan and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test plan. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element." ) 2374 protected MarkdownType copyright; 2375 2376 /** 2377 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2378 */ 2379 @Child(name = "copyrightLabel", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 2380 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 2381 protected StringType copyrightLabel; 2382 2383 /** 2384 * The category of the Test Plan - can be acceptance, unit, performance, etc. 2385 */ 2386 @Child(name = "category", type = {CodeableConcept.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2387 @Description(shortDefinition="The category of the Test Plan - can be acceptance, unit, performance", formalDefinition="The category of the Test Plan - can be acceptance, unit, performance, etc." ) 2388 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/testscript-scope-phase-codes") 2389 protected List<CodeableConcept> category; 2390 2391 /** 2392 * What is being tested with this Test Plan - a conformance resource, or narrative criteria, or an external reference... 2393 */ 2394 @Child(name = "scope", type = {Reference.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2395 @Description(shortDefinition="What is being tested with this Test Plan - a conformance resource, or narrative criteria, or an external reference", formalDefinition="What is being tested with this Test Plan - a conformance resource, or narrative criteria, or an external reference..." ) 2396 protected List<Reference> scope; 2397 2398 /** 2399 * A description of test tools to be used in the test plan. 2400 */ 2401 @Child(name = "testTools", type = {MarkdownType.class}, order=19, min=0, max=1, modifier=false, summary=false) 2402 @Description(shortDefinition="A description of test tools to be used in the test plan - narrative for now", formalDefinition="A description of test tools to be used in the test plan." ) 2403 protected MarkdownType testTools; 2404 2405 /** 2406 * The required criteria to execute the test plan - e.g. preconditions, previous tests... 2407 */ 2408 @Child(name = "dependency", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2409 @Description(shortDefinition="The required criteria to execute the test plan - e.g. preconditions, previous tests", formalDefinition="The required criteria to execute the test plan - e.g. preconditions, previous tests..." ) 2410 protected List<TestPlanDependencyComponent> dependency; 2411 2412 /** 2413 * The threshold or criteria for the test plan to be considered successfully executed - narrative. 2414 */ 2415 @Child(name = "exitCriteria", type = {MarkdownType.class}, order=21, min=0, max=1, modifier=false, summary=false) 2416 @Description(shortDefinition="The threshold or criteria for the test plan to be considered successfully executed - narrative", formalDefinition="The threshold or criteria for the test plan to be considered successfully executed - narrative." ) 2417 protected MarkdownType exitCriteria; 2418 2419 /** 2420 * The individual test cases that are part of this plan, when they they are made explicit. 2421 */ 2422 @Child(name = "testCase", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2423 @Description(shortDefinition="The test cases that constitute this plan", formalDefinition="The individual test cases that are part of this plan, when they they are made explicit." ) 2424 protected List<TestPlanTestCaseComponent> testCase; 2425 2426 private static final long serialVersionUID = 235546950L; 2427 2428 /** 2429 * Constructor 2430 */ 2431 public TestPlan() { 2432 super(); 2433 } 2434 2435 /** 2436 * Constructor 2437 */ 2438 public TestPlan(PublicationStatus status) { 2439 super(); 2440 this.setStatus(status); 2441 } 2442 2443 /** 2444 * @return {@link #url} (An absolute URI that is used to identify this test plan when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this test plan is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the test plan is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2445 */ 2446 public UriType getUrlElement() { 2447 if (this.url == null) 2448 if (Configuration.errorOnAutoCreate()) 2449 throw new Error("Attempt to auto-create TestPlan.url"); 2450 else if (Configuration.doAutoCreate()) 2451 this.url = new UriType(); // bb 2452 return this.url; 2453 } 2454 2455 public boolean hasUrlElement() { 2456 return this.url != null && !this.url.isEmpty(); 2457 } 2458 2459 public boolean hasUrl() { 2460 return this.url != null && !this.url.isEmpty(); 2461 } 2462 2463 /** 2464 * @param value {@link #url} (An absolute URI that is used to identify this test plan when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this test plan is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the test plan is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2465 */ 2466 public TestPlan setUrlElement(UriType value) { 2467 this.url = value; 2468 return this; 2469 } 2470 2471 /** 2472 * @return An absolute URI that is used to identify this test plan when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this test plan is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the test plan is stored on different servers. 2473 */ 2474 public String getUrl() { 2475 return this.url == null ? null : this.url.getValue(); 2476 } 2477 2478 /** 2479 * @param value An absolute URI that is used to identify this test plan when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this test plan is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the test plan is stored on different servers. 2480 */ 2481 public TestPlan setUrl(String value) { 2482 if (Utilities.noString(value)) 2483 this.url = null; 2484 else { 2485 if (this.url == null) 2486 this.url = new UriType(); 2487 this.url.setValue(value); 2488 } 2489 return this; 2490 } 2491 2492 /** 2493 * @return {@link #identifier} (A formal identifier that is used to identify this test plan when it is represented in other formats, or referenced in a specification, model, design or an instance.) 2494 */ 2495 public List<Identifier> getIdentifier() { 2496 if (this.identifier == null) 2497 this.identifier = new ArrayList<Identifier>(); 2498 return this.identifier; 2499 } 2500 2501 /** 2502 * @return Returns a reference to <code>this</code> for easy method chaining 2503 */ 2504 public TestPlan setIdentifier(List<Identifier> theIdentifier) { 2505 this.identifier = theIdentifier; 2506 return this; 2507 } 2508 2509 public boolean hasIdentifier() { 2510 if (this.identifier == null) 2511 return false; 2512 for (Identifier item : this.identifier) 2513 if (!item.isEmpty()) 2514 return true; 2515 return false; 2516 } 2517 2518 public Identifier addIdentifier() { //3 2519 Identifier t = new Identifier(); 2520 if (this.identifier == null) 2521 this.identifier = new ArrayList<Identifier>(); 2522 this.identifier.add(t); 2523 return t; 2524 } 2525 2526 public TestPlan addIdentifier(Identifier t) { //3 2527 if (t == null) 2528 return this; 2529 if (this.identifier == null) 2530 this.identifier = new ArrayList<Identifier>(); 2531 this.identifier.add(t); 2532 return this; 2533 } 2534 2535 /** 2536 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2537 */ 2538 public Identifier getIdentifierFirstRep() { 2539 if (getIdentifier().isEmpty()) { 2540 addIdentifier(); 2541 } 2542 return getIdentifier().get(0); 2543 } 2544 2545 /** 2546 * @return {@link #version} (The identifier that is used to identify this version of the test plan when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test plan author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2547 */ 2548 public StringType getVersionElement() { 2549 if (this.version == null) 2550 if (Configuration.errorOnAutoCreate()) 2551 throw new Error("Attempt to auto-create TestPlan.version"); 2552 else if (Configuration.doAutoCreate()) 2553 this.version = new StringType(); // bb 2554 return this.version; 2555 } 2556 2557 public boolean hasVersionElement() { 2558 return this.version != null && !this.version.isEmpty(); 2559 } 2560 2561 public boolean hasVersion() { 2562 return this.version != null && !this.version.isEmpty(); 2563 } 2564 2565 /** 2566 * @param value {@link #version} (The identifier that is used to identify this version of the test plan when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test plan author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2567 */ 2568 public TestPlan setVersionElement(StringType value) { 2569 this.version = value; 2570 return this; 2571 } 2572 2573 /** 2574 * @return The identifier that is used to identify this version of the test plan when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test plan author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 2575 */ 2576 public String getVersion() { 2577 return this.version == null ? null : this.version.getValue(); 2578 } 2579 2580 /** 2581 * @param value The identifier that is used to identify this version of the test plan when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test plan author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 2582 */ 2583 public TestPlan setVersion(String value) { 2584 if (Utilities.noString(value)) 2585 this.version = null; 2586 else { 2587 if (this.version == null) 2588 this.version = new StringType(); 2589 this.version.setValue(value); 2590 } 2591 return this; 2592 } 2593 2594 /** 2595 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2596 */ 2597 public DataType getVersionAlgorithm() { 2598 return this.versionAlgorithm; 2599 } 2600 2601 /** 2602 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2603 */ 2604 public StringType getVersionAlgorithmStringType() throws FHIRException { 2605 if (this.versionAlgorithm == null) 2606 this.versionAlgorithm = new StringType(); 2607 if (!(this.versionAlgorithm instanceof StringType)) 2608 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 2609 return (StringType) this.versionAlgorithm; 2610 } 2611 2612 public boolean hasVersionAlgorithmStringType() { 2613 return this.versionAlgorithm instanceof StringType; 2614 } 2615 2616 /** 2617 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2618 */ 2619 public Coding getVersionAlgorithmCoding() throws FHIRException { 2620 if (this.versionAlgorithm == null) 2621 this.versionAlgorithm = new Coding(); 2622 if (!(this.versionAlgorithm instanceof Coding)) 2623 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 2624 return (Coding) this.versionAlgorithm; 2625 } 2626 2627 public boolean hasVersionAlgorithmCoding() { 2628 return this.versionAlgorithm instanceof Coding; 2629 } 2630 2631 public boolean hasVersionAlgorithm() { 2632 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 2633 } 2634 2635 /** 2636 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2637 */ 2638 public TestPlan setVersionAlgorithm(DataType value) { 2639 if (value != null && !(value instanceof StringType || value instanceof Coding)) 2640 throw new FHIRException("Not the right type for TestPlan.versionAlgorithm[x]: "+value.fhirType()); 2641 this.versionAlgorithm = value; 2642 return this; 2643 } 2644 2645 /** 2646 * @return {@link #name} (A natural language name identifying the test plan. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2647 */ 2648 public StringType getNameElement() { 2649 if (this.name == null) 2650 if (Configuration.errorOnAutoCreate()) 2651 throw new Error("Attempt to auto-create TestPlan.name"); 2652 else if (Configuration.doAutoCreate()) 2653 this.name = new StringType(); // bb 2654 return this.name; 2655 } 2656 2657 public boolean hasNameElement() { 2658 return this.name != null && !this.name.isEmpty(); 2659 } 2660 2661 public boolean hasName() { 2662 return this.name != null && !this.name.isEmpty(); 2663 } 2664 2665 /** 2666 * @param value {@link #name} (A natural language name identifying the test plan. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2667 */ 2668 public TestPlan setNameElement(StringType value) { 2669 this.name = value; 2670 return this; 2671 } 2672 2673 /** 2674 * @return A natural language name identifying the test plan. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2675 */ 2676 public String getName() { 2677 return this.name == null ? null : this.name.getValue(); 2678 } 2679 2680 /** 2681 * @param value A natural language name identifying the test plan. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2682 */ 2683 public TestPlan setName(String value) { 2684 if (Utilities.noString(value)) 2685 this.name = null; 2686 else { 2687 if (this.name == null) 2688 this.name = new StringType(); 2689 this.name.setValue(value); 2690 } 2691 return this; 2692 } 2693 2694 /** 2695 * @return {@link #title} (A short, descriptive, user-friendly title for the test plan.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2696 */ 2697 public StringType getTitleElement() { 2698 if (this.title == null) 2699 if (Configuration.errorOnAutoCreate()) 2700 throw new Error("Attempt to auto-create TestPlan.title"); 2701 else if (Configuration.doAutoCreate()) 2702 this.title = new StringType(); // bb 2703 return this.title; 2704 } 2705 2706 public boolean hasTitleElement() { 2707 return this.title != null && !this.title.isEmpty(); 2708 } 2709 2710 public boolean hasTitle() { 2711 return this.title != null && !this.title.isEmpty(); 2712 } 2713 2714 /** 2715 * @param value {@link #title} (A short, descriptive, user-friendly title for the test plan.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2716 */ 2717 public TestPlan setTitleElement(StringType value) { 2718 this.title = value; 2719 return this; 2720 } 2721 2722 /** 2723 * @return A short, descriptive, user-friendly title for the test plan. 2724 */ 2725 public String getTitle() { 2726 return this.title == null ? null : this.title.getValue(); 2727 } 2728 2729 /** 2730 * @param value A short, descriptive, user-friendly title for the test plan. 2731 */ 2732 public TestPlan setTitle(String value) { 2733 if (Utilities.noString(value)) 2734 this.title = null; 2735 else { 2736 if (this.title == null) 2737 this.title = new StringType(); 2738 this.title.setValue(value); 2739 } 2740 return this; 2741 } 2742 2743 /** 2744 * @return {@link #status} (The status of this test plan. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2745 */ 2746 public Enumeration<PublicationStatus> getStatusElement() { 2747 if (this.status == null) 2748 if (Configuration.errorOnAutoCreate()) 2749 throw new Error("Attempt to auto-create TestPlan.status"); 2750 else if (Configuration.doAutoCreate()) 2751 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2752 return this.status; 2753 } 2754 2755 public boolean hasStatusElement() { 2756 return this.status != null && !this.status.isEmpty(); 2757 } 2758 2759 public boolean hasStatus() { 2760 return this.status != null && !this.status.isEmpty(); 2761 } 2762 2763 /** 2764 * @param value {@link #status} (The status of this test plan. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2765 */ 2766 public TestPlan setStatusElement(Enumeration<PublicationStatus> value) { 2767 this.status = value; 2768 return this; 2769 } 2770 2771 /** 2772 * @return The status of this test plan. Enables tracking the life-cycle of the content. 2773 */ 2774 public PublicationStatus getStatus() { 2775 return this.status == null ? null : this.status.getValue(); 2776 } 2777 2778 /** 2779 * @param value The status of this test plan. Enables tracking the life-cycle of the content. 2780 */ 2781 public TestPlan setStatus(PublicationStatus value) { 2782 if (this.status == null) 2783 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2784 this.status.setValue(value); 2785 return this; 2786 } 2787 2788 /** 2789 * @return {@link #experimental} (A Boolean value to indicate that this test plan is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2790 */ 2791 public BooleanType getExperimentalElement() { 2792 if (this.experimental == null) 2793 if (Configuration.errorOnAutoCreate()) 2794 throw new Error("Attempt to auto-create TestPlan.experimental"); 2795 else if (Configuration.doAutoCreate()) 2796 this.experimental = new BooleanType(); // bb 2797 return this.experimental; 2798 } 2799 2800 public boolean hasExperimentalElement() { 2801 return this.experimental != null && !this.experimental.isEmpty(); 2802 } 2803 2804 public boolean hasExperimental() { 2805 return this.experimental != null && !this.experimental.isEmpty(); 2806 } 2807 2808 /** 2809 * @param value {@link #experimental} (A Boolean value to indicate that this test plan is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2810 */ 2811 public TestPlan setExperimentalElement(BooleanType value) { 2812 this.experimental = value; 2813 return this; 2814 } 2815 2816 /** 2817 * @return A Boolean value to indicate that this test plan is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 2818 */ 2819 public boolean getExperimental() { 2820 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2821 } 2822 2823 /** 2824 * @param value A Boolean value to indicate that this test plan is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 2825 */ 2826 public TestPlan setExperimental(boolean value) { 2827 if (this.experimental == null) 2828 this.experimental = new BooleanType(); 2829 this.experimental.setValue(value); 2830 return this; 2831 } 2832 2833 /** 2834 * @return {@link #date} (The date (and optionally time) when the test plan was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test plan changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2835 */ 2836 public DateTimeType getDateElement() { 2837 if (this.date == null) 2838 if (Configuration.errorOnAutoCreate()) 2839 throw new Error("Attempt to auto-create TestPlan.date"); 2840 else if (Configuration.doAutoCreate()) 2841 this.date = new DateTimeType(); // bb 2842 return this.date; 2843 } 2844 2845 public boolean hasDateElement() { 2846 return this.date != null && !this.date.isEmpty(); 2847 } 2848 2849 public boolean hasDate() { 2850 return this.date != null && !this.date.isEmpty(); 2851 } 2852 2853 /** 2854 * @param value {@link #date} (The date (and optionally time) when the test plan was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test plan changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2855 */ 2856 public TestPlan setDateElement(DateTimeType value) { 2857 this.date = value; 2858 return this; 2859 } 2860 2861 /** 2862 * @return The date (and optionally time) when the test plan was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test plan changes. 2863 */ 2864 public Date getDate() { 2865 return this.date == null ? null : this.date.getValue(); 2866 } 2867 2868 /** 2869 * @param value The date (and optionally time) when the test plan was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test plan changes. 2870 */ 2871 public TestPlan setDate(Date value) { 2872 if (value == null) 2873 this.date = null; 2874 else { 2875 if (this.date == null) 2876 this.date = new DateTimeType(); 2877 this.date.setValue(value); 2878 } 2879 return this; 2880 } 2881 2882 /** 2883 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the test plan.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2884 */ 2885 public StringType getPublisherElement() { 2886 if (this.publisher == null) 2887 if (Configuration.errorOnAutoCreate()) 2888 throw new Error("Attempt to auto-create TestPlan.publisher"); 2889 else if (Configuration.doAutoCreate()) 2890 this.publisher = new StringType(); // bb 2891 return this.publisher; 2892 } 2893 2894 public boolean hasPublisherElement() { 2895 return this.publisher != null && !this.publisher.isEmpty(); 2896 } 2897 2898 public boolean hasPublisher() { 2899 return this.publisher != null && !this.publisher.isEmpty(); 2900 } 2901 2902 /** 2903 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the test plan.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2904 */ 2905 public TestPlan setPublisherElement(StringType value) { 2906 this.publisher = value; 2907 return this; 2908 } 2909 2910 /** 2911 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the test plan. 2912 */ 2913 public String getPublisher() { 2914 return this.publisher == null ? null : this.publisher.getValue(); 2915 } 2916 2917 /** 2918 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the test plan. 2919 */ 2920 public TestPlan setPublisher(String value) { 2921 if (Utilities.noString(value)) 2922 this.publisher = null; 2923 else { 2924 if (this.publisher == null) 2925 this.publisher = new StringType(); 2926 this.publisher.setValue(value); 2927 } 2928 return this; 2929 } 2930 2931 /** 2932 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 2933 */ 2934 public List<ContactDetail> getContact() { 2935 if (this.contact == null) 2936 this.contact = new ArrayList<ContactDetail>(); 2937 return this.contact; 2938 } 2939 2940 /** 2941 * @return Returns a reference to <code>this</code> for easy method chaining 2942 */ 2943 public TestPlan setContact(List<ContactDetail> theContact) { 2944 this.contact = theContact; 2945 return this; 2946 } 2947 2948 public boolean hasContact() { 2949 if (this.contact == null) 2950 return false; 2951 for (ContactDetail item : this.contact) 2952 if (!item.isEmpty()) 2953 return true; 2954 return false; 2955 } 2956 2957 public ContactDetail addContact() { //3 2958 ContactDetail t = new ContactDetail(); 2959 if (this.contact == null) 2960 this.contact = new ArrayList<ContactDetail>(); 2961 this.contact.add(t); 2962 return t; 2963 } 2964 2965 public TestPlan addContact(ContactDetail t) { //3 2966 if (t == null) 2967 return this; 2968 if (this.contact == null) 2969 this.contact = new ArrayList<ContactDetail>(); 2970 this.contact.add(t); 2971 return this; 2972 } 2973 2974 /** 2975 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 2976 */ 2977 public ContactDetail getContactFirstRep() { 2978 if (getContact().isEmpty()) { 2979 addContact(); 2980 } 2981 return getContact().get(0); 2982 } 2983 2984 /** 2985 * @return {@link #description} (A free text natural language description of the test plan from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2986 */ 2987 public MarkdownType getDescriptionElement() { 2988 if (this.description == null) 2989 if (Configuration.errorOnAutoCreate()) 2990 throw new Error("Attempt to auto-create TestPlan.description"); 2991 else if (Configuration.doAutoCreate()) 2992 this.description = new MarkdownType(); // bb 2993 return this.description; 2994 } 2995 2996 public boolean hasDescriptionElement() { 2997 return this.description != null && !this.description.isEmpty(); 2998 } 2999 3000 public boolean hasDescription() { 3001 return this.description != null && !this.description.isEmpty(); 3002 } 3003 3004 /** 3005 * @param value {@link #description} (A free text natural language description of the test plan from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3006 */ 3007 public TestPlan setDescriptionElement(MarkdownType value) { 3008 this.description = value; 3009 return this; 3010 } 3011 3012 /** 3013 * @return A free text natural language description of the test plan from a consumer's perspective. 3014 */ 3015 public String getDescription() { 3016 return this.description == null ? null : this.description.getValue(); 3017 } 3018 3019 /** 3020 * @param value A free text natural language description of the test plan from a consumer's perspective. 3021 */ 3022 public TestPlan setDescription(String value) { 3023 if (Utilities.noString(value)) 3024 this.description = null; 3025 else { 3026 if (this.description == null) 3027 this.description = new MarkdownType(); 3028 this.description.setValue(value); 3029 } 3030 return this; 3031 } 3032 3033 /** 3034 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate test plan instances.) 3035 */ 3036 public List<UsageContext> getUseContext() { 3037 if (this.useContext == null) 3038 this.useContext = new ArrayList<UsageContext>(); 3039 return this.useContext; 3040 } 3041 3042 /** 3043 * @return Returns a reference to <code>this</code> for easy method chaining 3044 */ 3045 public TestPlan setUseContext(List<UsageContext> theUseContext) { 3046 this.useContext = theUseContext; 3047 return this; 3048 } 3049 3050 public boolean hasUseContext() { 3051 if (this.useContext == null) 3052 return false; 3053 for (UsageContext item : this.useContext) 3054 if (!item.isEmpty()) 3055 return true; 3056 return false; 3057 } 3058 3059 public UsageContext addUseContext() { //3 3060 UsageContext t = new UsageContext(); 3061 if (this.useContext == null) 3062 this.useContext = new ArrayList<UsageContext>(); 3063 this.useContext.add(t); 3064 return t; 3065 } 3066 3067 public TestPlan addUseContext(UsageContext t) { //3 3068 if (t == null) 3069 return this; 3070 if (this.useContext == null) 3071 this.useContext = new ArrayList<UsageContext>(); 3072 this.useContext.add(t); 3073 return this; 3074 } 3075 3076 /** 3077 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 3078 */ 3079 public UsageContext getUseContextFirstRep() { 3080 if (getUseContext().isEmpty()) { 3081 addUseContext(); 3082 } 3083 return getUseContext().get(0); 3084 } 3085 3086 /** 3087 * @return {@link #jurisdiction} (A legal or geographic region in which the test plan is intended to be used.) 3088 */ 3089 public List<CodeableConcept> getJurisdiction() { 3090 if (this.jurisdiction == null) 3091 this.jurisdiction = new ArrayList<CodeableConcept>(); 3092 return this.jurisdiction; 3093 } 3094 3095 /** 3096 * @return Returns a reference to <code>this</code> for easy method chaining 3097 */ 3098 public TestPlan setJurisdiction(List<CodeableConcept> theJurisdiction) { 3099 this.jurisdiction = theJurisdiction; 3100 return this; 3101 } 3102 3103 public boolean hasJurisdiction() { 3104 if (this.jurisdiction == null) 3105 return false; 3106 for (CodeableConcept item : this.jurisdiction) 3107 if (!item.isEmpty()) 3108 return true; 3109 return false; 3110 } 3111 3112 public CodeableConcept addJurisdiction() { //3 3113 CodeableConcept t = new CodeableConcept(); 3114 if (this.jurisdiction == null) 3115 this.jurisdiction = new ArrayList<CodeableConcept>(); 3116 this.jurisdiction.add(t); 3117 return t; 3118 } 3119 3120 public TestPlan addJurisdiction(CodeableConcept t) { //3 3121 if (t == null) 3122 return this; 3123 if (this.jurisdiction == null) 3124 this.jurisdiction = new ArrayList<CodeableConcept>(); 3125 this.jurisdiction.add(t); 3126 return this; 3127 } 3128 3129 /** 3130 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 3131 */ 3132 public CodeableConcept getJurisdictionFirstRep() { 3133 if (getJurisdiction().isEmpty()) { 3134 addJurisdiction(); 3135 } 3136 return getJurisdiction().get(0); 3137 } 3138 3139 /** 3140 * @return {@link #purpose} (Explanation of why this test plan is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3141 */ 3142 public MarkdownType getPurposeElement() { 3143 if (this.purpose == null) 3144 if (Configuration.errorOnAutoCreate()) 3145 throw new Error("Attempt to auto-create TestPlan.purpose"); 3146 else if (Configuration.doAutoCreate()) 3147 this.purpose = new MarkdownType(); // bb 3148 return this.purpose; 3149 } 3150 3151 public boolean hasPurposeElement() { 3152 return this.purpose != null && !this.purpose.isEmpty(); 3153 } 3154 3155 public boolean hasPurpose() { 3156 return this.purpose != null && !this.purpose.isEmpty(); 3157 } 3158 3159 /** 3160 * @param value {@link #purpose} (Explanation of why this test plan is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 3161 */ 3162 public TestPlan setPurposeElement(MarkdownType value) { 3163 this.purpose = value; 3164 return this; 3165 } 3166 3167 /** 3168 * @return Explanation of why this test plan is needed and why it has been designed as it has. 3169 */ 3170 public String getPurpose() { 3171 return this.purpose == null ? null : this.purpose.getValue(); 3172 } 3173 3174 /** 3175 * @param value Explanation of why this test plan is needed and why it has been designed as it has. 3176 */ 3177 public TestPlan setPurpose(String value) { 3178 if (Utilities.noString(value)) 3179 this.purpose = null; 3180 else { 3181 if (this.purpose == null) 3182 this.purpose = new MarkdownType(); 3183 this.purpose.setValue(value); 3184 } 3185 return this; 3186 } 3187 3188 /** 3189 * @return {@link #copyright} (A copyright statement relating to the test plan and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test plan. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3190 */ 3191 public MarkdownType getCopyrightElement() { 3192 if (this.copyright == null) 3193 if (Configuration.errorOnAutoCreate()) 3194 throw new Error("Attempt to auto-create TestPlan.copyright"); 3195 else if (Configuration.doAutoCreate()) 3196 this.copyright = new MarkdownType(); // bb 3197 return this.copyright; 3198 } 3199 3200 public boolean hasCopyrightElement() { 3201 return this.copyright != null && !this.copyright.isEmpty(); 3202 } 3203 3204 public boolean hasCopyright() { 3205 return this.copyright != null && !this.copyright.isEmpty(); 3206 } 3207 3208 /** 3209 * @param value {@link #copyright} (A copyright statement relating to the test plan and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test plan. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 3210 */ 3211 public TestPlan setCopyrightElement(MarkdownType value) { 3212 this.copyright = value; 3213 return this; 3214 } 3215 3216 /** 3217 * @return A copyright statement relating to the test plan and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test plan. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element. 3218 */ 3219 public String getCopyright() { 3220 return this.copyright == null ? null : this.copyright.getValue(); 3221 } 3222 3223 /** 3224 * @param value A copyright statement relating to the test plan and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test plan. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element. 3225 */ 3226 public TestPlan setCopyright(String value) { 3227 if (Utilities.noString(value)) 3228 this.copyright = null; 3229 else { 3230 if (this.copyright == null) 3231 this.copyright = new MarkdownType(); 3232 this.copyright.setValue(value); 3233 } 3234 return this; 3235 } 3236 3237 /** 3238 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 3239 */ 3240 public StringType getCopyrightLabelElement() { 3241 if (this.copyrightLabel == null) 3242 if (Configuration.errorOnAutoCreate()) 3243 throw new Error("Attempt to auto-create TestPlan.copyrightLabel"); 3244 else if (Configuration.doAutoCreate()) 3245 this.copyrightLabel = new StringType(); // bb 3246 return this.copyrightLabel; 3247 } 3248 3249 public boolean hasCopyrightLabelElement() { 3250 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 3251 } 3252 3253 public boolean hasCopyrightLabel() { 3254 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 3255 } 3256 3257 /** 3258 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 3259 */ 3260 public TestPlan setCopyrightLabelElement(StringType value) { 3261 this.copyrightLabel = value; 3262 return this; 3263 } 3264 3265 /** 3266 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3267 */ 3268 public String getCopyrightLabel() { 3269 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 3270 } 3271 3272 /** 3273 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3274 */ 3275 public TestPlan setCopyrightLabel(String value) { 3276 if (Utilities.noString(value)) 3277 this.copyrightLabel = null; 3278 else { 3279 if (this.copyrightLabel == null) 3280 this.copyrightLabel = new StringType(); 3281 this.copyrightLabel.setValue(value); 3282 } 3283 return this; 3284 } 3285 3286 /** 3287 * @return {@link #category} (The category of the Test Plan - can be acceptance, unit, performance, etc.) 3288 */ 3289 public List<CodeableConcept> getCategory() { 3290 if (this.category == null) 3291 this.category = new ArrayList<CodeableConcept>(); 3292 return this.category; 3293 } 3294 3295 /** 3296 * @return Returns a reference to <code>this</code> for easy method chaining 3297 */ 3298 public TestPlan setCategory(List<CodeableConcept> theCategory) { 3299 this.category = theCategory; 3300 return this; 3301 } 3302 3303 public boolean hasCategory() { 3304 if (this.category == null) 3305 return false; 3306 for (CodeableConcept item : this.category) 3307 if (!item.isEmpty()) 3308 return true; 3309 return false; 3310 } 3311 3312 public CodeableConcept addCategory() { //3 3313 CodeableConcept t = new CodeableConcept(); 3314 if (this.category == null) 3315 this.category = new ArrayList<CodeableConcept>(); 3316 this.category.add(t); 3317 return t; 3318 } 3319 3320 public TestPlan addCategory(CodeableConcept t) { //3 3321 if (t == null) 3322 return this; 3323 if (this.category == null) 3324 this.category = new ArrayList<CodeableConcept>(); 3325 this.category.add(t); 3326 return this; 3327 } 3328 3329 /** 3330 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 3331 */ 3332 public CodeableConcept getCategoryFirstRep() { 3333 if (getCategory().isEmpty()) { 3334 addCategory(); 3335 } 3336 return getCategory().get(0); 3337 } 3338 3339 /** 3340 * @return {@link #scope} (What is being tested with this Test Plan - a conformance resource, or narrative criteria, or an external reference...) 3341 */ 3342 public List<Reference> getScope() { 3343 if (this.scope == null) 3344 this.scope = new ArrayList<Reference>(); 3345 return this.scope; 3346 } 3347 3348 /** 3349 * @return Returns a reference to <code>this</code> for easy method chaining 3350 */ 3351 public TestPlan setScope(List<Reference> theScope) { 3352 this.scope = theScope; 3353 return this; 3354 } 3355 3356 public boolean hasScope() { 3357 if (this.scope == null) 3358 return false; 3359 for (Reference item : this.scope) 3360 if (!item.isEmpty()) 3361 return true; 3362 return false; 3363 } 3364 3365 public Reference addScope() { //3 3366 Reference t = new Reference(); 3367 if (this.scope == null) 3368 this.scope = new ArrayList<Reference>(); 3369 this.scope.add(t); 3370 return t; 3371 } 3372 3373 public TestPlan addScope(Reference t) { //3 3374 if (t == null) 3375 return this; 3376 if (this.scope == null) 3377 this.scope = new ArrayList<Reference>(); 3378 this.scope.add(t); 3379 return this; 3380 } 3381 3382 /** 3383 * @return The first repetition of repeating field {@link #scope}, creating it if it does not already exist {3} 3384 */ 3385 public Reference getScopeFirstRep() { 3386 if (getScope().isEmpty()) { 3387 addScope(); 3388 } 3389 return getScope().get(0); 3390 } 3391 3392 /** 3393 * @return {@link #testTools} (A description of test tools to be used in the test plan.). This is the underlying object with id, value and extensions. The accessor "getTestTools" gives direct access to the value 3394 */ 3395 public MarkdownType getTestToolsElement() { 3396 if (this.testTools == null) 3397 if (Configuration.errorOnAutoCreate()) 3398 throw new Error("Attempt to auto-create TestPlan.testTools"); 3399 else if (Configuration.doAutoCreate()) 3400 this.testTools = new MarkdownType(); // bb 3401 return this.testTools; 3402 } 3403 3404 public boolean hasTestToolsElement() { 3405 return this.testTools != null && !this.testTools.isEmpty(); 3406 } 3407 3408 public boolean hasTestTools() { 3409 return this.testTools != null && !this.testTools.isEmpty(); 3410 } 3411 3412 /** 3413 * @param value {@link #testTools} (A description of test tools to be used in the test plan.). This is the underlying object with id, value and extensions. The accessor "getTestTools" gives direct access to the value 3414 */ 3415 public TestPlan setTestToolsElement(MarkdownType value) { 3416 this.testTools = value; 3417 return this; 3418 } 3419 3420 /** 3421 * @return A description of test tools to be used in the test plan. 3422 */ 3423 public String getTestTools() { 3424 return this.testTools == null ? null : this.testTools.getValue(); 3425 } 3426 3427 /** 3428 * @param value A description of test tools to be used in the test plan. 3429 */ 3430 public TestPlan setTestTools(String value) { 3431 if (Utilities.noString(value)) 3432 this.testTools = null; 3433 else { 3434 if (this.testTools == null) 3435 this.testTools = new MarkdownType(); 3436 this.testTools.setValue(value); 3437 } 3438 return this; 3439 } 3440 3441 /** 3442 * @return {@link #dependency} (The required criteria to execute the test plan - e.g. preconditions, previous tests...) 3443 */ 3444 public List<TestPlanDependencyComponent> getDependency() { 3445 if (this.dependency == null) 3446 this.dependency = new ArrayList<TestPlanDependencyComponent>(); 3447 return this.dependency; 3448 } 3449 3450 /** 3451 * @return Returns a reference to <code>this</code> for easy method chaining 3452 */ 3453 public TestPlan setDependency(List<TestPlanDependencyComponent> theDependency) { 3454 this.dependency = theDependency; 3455 return this; 3456 } 3457 3458 public boolean hasDependency() { 3459 if (this.dependency == null) 3460 return false; 3461 for (TestPlanDependencyComponent item : this.dependency) 3462 if (!item.isEmpty()) 3463 return true; 3464 return false; 3465 } 3466 3467 public TestPlanDependencyComponent addDependency() { //3 3468 TestPlanDependencyComponent t = new TestPlanDependencyComponent(); 3469 if (this.dependency == null) 3470 this.dependency = new ArrayList<TestPlanDependencyComponent>(); 3471 this.dependency.add(t); 3472 return t; 3473 } 3474 3475 public TestPlan addDependency(TestPlanDependencyComponent t) { //3 3476 if (t == null) 3477 return this; 3478 if (this.dependency == null) 3479 this.dependency = new ArrayList<TestPlanDependencyComponent>(); 3480 this.dependency.add(t); 3481 return this; 3482 } 3483 3484 /** 3485 * @return The first repetition of repeating field {@link #dependency}, creating it if it does not already exist {3} 3486 */ 3487 public TestPlanDependencyComponent getDependencyFirstRep() { 3488 if (getDependency().isEmpty()) { 3489 addDependency(); 3490 } 3491 return getDependency().get(0); 3492 } 3493 3494 /** 3495 * @return {@link #exitCriteria} (The threshold or criteria for the test plan to be considered successfully executed - narrative.). This is the underlying object with id, value and extensions. The accessor "getExitCriteria" gives direct access to the value 3496 */ 3497 public MarkdownType getExitCriteriaElement() { 3498 if (this.exitCriteria == null) 3499 if (Configuration.errorOnAutoCreate()) 3500 throw new Error("Attempt to auto-create TestPlan.exitCriteria"); 3501 else if (Configuration.doAutoCreate()) 3502 this.exitCriteria = new MarkdownType(); // bb 3503 return this.exitCriteria; 3504 } 3505 3506 public boolean hasExitCriteriaElement() { 3507 return this.exitCriteria != null && !this.exitCriteria.isEmpty(); 3508 } 3509 3510 public boolean hasExitCriteria() { 3511 return this.exitCriteria != null && !this.exitCriteria.isEmpty(); 3512 } 3513 3514 /** 3515 * @param value {@link #exitCriteria} (The threshold or criteria for the test plan to be considered successfully executed - narrative.). This is the underlying object with id, value and extensions. The accessor "getExitCriteria" gives direct access to the value 3516 */ 3517 public TestPlan setExitCriteriaElement(MarkdownType value) { 3518 this.exitCriteria = value; 3519 return this; 3520 } 3521 3522 /** 3523 * @return The threshold or criteria for the test plan to be considered successfully executed - narrative. 3524 */ 3525 public String getExitCriteria() { 3526 return this.exitCriteria == null ? null : this.exitCriteria.getValue(); 3527 } 3528 3529 /** 3530 * @param value The threshold or criteria for the test plan to be considered successfully executed - narrative. 3531 */ 3532 public TestPlan setExitCriteria(String value) { 3533 if (Utilities.noString(value)) 3534 this.exitCriteria = null; 3535 else { 3536 if (this.exitCriteria == null) 3537 this.exitCriteria = new MarkdownType(); 3538 this.exitCriteria.setValue(value); 3539 } 3540 return this; 3541 } 3542 3543 /** 3544 * @return {@link #testCase} (The individual test cases that are part of this plan, when they they are made explicit.) 3545 */ 3546 public List<TestPlanTestCaseComponent> getTestCase() { 3547 if (this.testCase == null) 3548 this.testCase = new ArrayList<TestPlanTestCaseComponent>(); 3549 return this.testCase; 3550 } 3551 3552 /** 3553 * @return Returns a reference to <code>this</code> for easy method chaining 3554 */ 3555 public TestPlan setTestCase(List<TestPlanTestCaseComponent> theTestCase) { 3556 this.testCase = theTestCase; 3557 return this; 3558 } 3559 3560 public boolean hasTestCase() { 3561 if (this.testCase == null) 3562 return false; 3563 for (TestPlanTestCaseComponent item : this.testCase) 3564 if (!item.isEmpty()) 3565 return true; 3566 return false; 3567 } 3568 3569 public TestPlanTestCaseComponent addTestCase() { //3 3570 TestPlanTestCaseComponent t = new TestPlanTestCaseComponent(); 3571 if (this.testCase == null) 3572 this.testCase = new ArrayList<TestPlanTestCaseComponent>(); 3573 this.testCase.add(t); 3574 return t; 3575 } 3576 3577 public TestPlan addTestCase(TestPlanTestCaseComponent t) { //3 3578 if (t == null) 3579 return this; 3580 if (this.testCase == null) 3581 this.testCase = new ArrayList<TestPlanTestCaseComponent>(); 3582 this.testCase.add(t); 3583 return this; 3584 } 3585 3586 /** 3587 * @return The first repetition of repeating field {@link #testCase}, creating it if it does not already exist {3} 3588 */ 3589 public TestPlanTestCaseComponent getTestCaseFirstRep() { 3590 if (getTestCase().isEmpty()) { 3591 addTestCase(); 3592 } 3593 return getTestCase().get(0); 3594 } 3595 3596 protected void listChildren(List<Property> children) { 3597 super.listChildren(children); 3598 children.add(new Property("url", "uri", "An absolute URI that is used to identify this test plan when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this test plan is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the test plan is stored on different servers.", 0, 1, url)); 3599 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this test plan when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3600 children.add(new Property("version", "string", "The identifier that is used to identify this version of the test plan when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test plan author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 3601 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 3602 children.add(new Property("name", "string", "A natural language name identifying the test plan. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 3603 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the test plan.", 0, 1, title)); 3604 children.add(new Property("status", "code", "The status of this test plan. Enables tracking the life-cycle of the content.", 0, 1, status)); 3605 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this test plan is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 3606 children.add(new Property("date", "dateTime", "The date (and optionally time) when the test plan was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test plan changes.", 0, 1, date)); 3607 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the test plan.", 0, 1, publisher)); 3608 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 3609 children.add(new Property("description", "markdown", "A free text natural language description of the test plan from a consumer's perspective.", 0, 1, description)); 3610 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate test plan instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 3611 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the test plan is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 3612 children.add(new Property("purpose", "markdown", "Explanation of why this test plan is needed and why it has been designed as it has.", 0, 1, purpose)); 3613 children.add(new Property("copyright", "markdown", "A copyright statement relating to the test plan and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test plan. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element.", 0, 1, copyright)); 3614 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 3615 children.add(new Property("category", "CodeableConcept", "The category of the Test Plan - can be acceptance, unit, performance, etc.", 0, java.lang.Integer.MAX_VALUE, category)); 3616 children.add(new Property("scope", "Reference", "What is being tested with this Test Plan - a conformance resource, or narrative criteria, or an external reference...", 0, java.lang.Integer.MAX_VALUE, scope)); 3617 children.add(new Property("testTools", "markdown", "A description of test tools to be used in the test plan.", 0, 1, testTools)); 3618 children.add(new Property("dependency", "", "The required criteria to execute the test plan - e.g. preconditions, previous tests...", 0, java.lang.Integer.MAX_VALUE, dependency)); 3619 children.add(new Property("exitCriteria", "markdown", "The threshold or criteria for the test plan to be considered successfully executed - narrative.", 0, 1, exitCriteria)); 3620 children.add(new Property("testCase", "", "The individual test cases that are part of this plan, when they they are made explicit.", 0, java.lang.Integer.MAX_VALUE, testCase)); 3621 } 3622 3623 @Override 3624 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3625 switch (_hash) { 3626 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this test plan when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this test plan is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the test plan is stored on different servers.", 0, 1, url); 3627 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this test plan when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 3628 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the test plan when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test plan author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 3629 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3630 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3631 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3632 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3633 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the test plan. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 3634 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the test plan.", 0, 1, title); 3635 case -892481550: /*status*/ return new Property("status", "code", "The status of this test plan. Enables tracking the life-cycle of the content.", 0, 1, status); 3636 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this test plan is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 3637 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the test plan was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test plan changes.", 0, 1, date); 3638 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the test plan.", 0, 1, publisher); 3639 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 3640 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the test plan from a consumer's perspective.", 0, 1, description); 3641 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate test plan instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 3642 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the test plan is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 3643 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this test plan is needed and why it has been designed as it has.", 0, 1, purpose); 3644 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the test plan and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test plan. The short copyright declaration (e.g. (c) '2015+ xyz organization' should be sent in the copyrightLabel element.", 0, 1, copyright); 3645 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 3646 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "The category of the Test Plan - can be acceptance, unit, performance, etc.", 0, java.lang.Integer.MAX_VALUE, category); 3647 case 109264468: /*scope*/ return new Property("scope", "Reference", "What is being tested with this Test Plan - a conformance resource, or narrative criteria, or an external reference...", 0, java.lang.Integer.MAX_VALUE, scope); 3648 case -1190420375: /*testTools*/ return new Property("testTools", "markdown", "A description of test tools to be used in the test plan.", 0, 1, testTools); 3649 case -26291381: /*dependency*/ return new Property("dependency", "", "The required criteria to execute the test plan - e.g. preconditions, previous tests...", 0, java.lang.Integer.MAX_VALUE, dependency); 3650 case -1382023523: /*exitCriteria*/ return new Property("exitCriteria", "markdown", "The threshold or criteria for the test plan to be considered successfully executed - narrative.", 0, 1, exitCriteria); 3651 case -1147299102: /*testCase*/ return new Property("testCase", "", "The individual test cases that are part of this plan, when they they are made explicit.", 0, java.lang.Integer.MAX_VALUE, testCase); 3652 default: return super.getNamedProperty(_hash, _name, _checkValid); 3653 } 3654 3655 } 3656 3657 @Override 3658 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3659 switch (hash) { 3660 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 3661 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3662 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 3663 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 3664 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3665 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 3666 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 3667 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 3668 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 3669 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 3670 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3671 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3672 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3673 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3674 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 3675 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 3676 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 3677 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3678 case 109264468: /*scope*/ return this.scope == null ? new Base[0] : this.scope.toArray(new Base[this.scope.size()]); // Reference 3679 case -1190420375: /*testTools*/ return this.testTools == null ? new Base[0] : new Base[] {this.testTools}; // MarkdownType 3680 case -26291381: /*dependency*/ return this.dependency == null ? new Base[0] : this.dependency.toArray(new Base[this.dependency.size()]); // TestPlanDependencyComponent 3681 case -1382023523: /*exitCriteria*/ return this.exitCriteria == null ? new Base[0] : new Base[] {this.exitCriteria}; // MarkdownType 3682 case -1147299102: /*testCase*/ return this.testCase == null ? new Base[0] : this.testCase.toArray(new Base[this.testCase.size()]); // TestPlanTestCaseComponent 3683 default: return super.getProperty(hash, name, checkValid); 3684 } 3685 3686 } 3687 3688 @Override 3689 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3690 switch (hash) { 3691 case 116079: // url 3692 this.url = TypeConvertor.castToUri(value); // UriType 3693 return value; 3694 case -1618432855: // identifier 3695 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3696 return value; 3697 case 351608024: // version 3698 this.version = TypeConvertor.castToString(value); // StringType 3699 return value; 3700 case 1508158071: // versionAlgorithm 3701 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 3702 return value; 3703 case 3373707: // name 3704 this.name = TypeConvertor.castToString(value); // StringType 3705 return value; 3706 case 110371416: // title 3707 this.title = TypeConvertor.castToString(value); // StringType 3708 return value; 3709 case -892481550: // status 3710 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3711 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3712 return value; 3713 case -404562712: // experimental 3714 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 3715 return value; 3716 case 3076014: // date 3717 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 3718 return value; 3719 case 1447404028: // publisher 3720 this.publisher = TypeConvertor.castToString(value); // StringType 3721 return value; 3722 case 951526432: // contact 3723 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 3724 return value; 3725 case -1724546052: // description 3726 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3727 return value; 3728 case -669707736: // useContext 3729 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 3730 return value; 3731 case -507075711: // jurisdiction 3732 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3733 return value; 3734 case -220463842: // purpose 3735 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 3736 return value; 3737 case 1522889671: // copyright 3738 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 3739 return value; 3740 case 765157229: // copyrightLabel 3741 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 3742 return value; 3743 case 50511102: // category 3744 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3745 return value; 3746 case 109264468: // scope 3747 this.getScope().add(TypeConvertor.castToReference(value)); // Reference 3748 return value; 3749 case -1190420375: // testTools 3750 this.testTools = TypeConvertor.castToMarkdown(value); // MarkdownType 3751 return value; 3752 case -26291381: // dependency 3753 this.getDependency().add((TestPlanDependencyComponent) value); // TestPlanDependencyComponent 3754 return value; 3755 case -1382023523: // exitCriteria 3756 this.exitCriteria = TypeConvertor.castToMarkdown(value); // MarkdownType 3757 return value; 3758 case -1147299102: // testCase 3759 this.getTestCase().add((TestPlanTestCaseComponent) value); // TestPlanTestCaseComponent 3760 return value; 3761 default: return super.setProperty(hash, name, value); 3762 } 3763 3764 } 3765 3766 @Override 3767 public Base setProperty(String name, Base value) throws FHIRException { 3768 if (name.equals("url")) { 3769 this.url = TypeConvertor.castToUri(value); // UriType 3770 } else if (name.equals("identifier")) { 3771 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3772 } else if (name.equals("version")) { 3773 this.version = TypeConvertor.castToString(value); // StringType 3774 } else if (name.equals("versionAlgorithm[x]")) { 3775 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 3776 } else if (name.equals("name")) { 3777 this.name = TypeConvertor.castToString(value); // StringType 3778 } else if (name.equals("title")) { 3779 this.title = TypeConvertor.castToString(value); // StringType 3780 } else if (name.equals("status")) { 3781 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3782 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3783 } else if (name.equals("experimental")) { 3784 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 3785 } else if (name.equals("date")) { 3786 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 3787 } else if (name.equals("publisher")) { 3788 this.publisher = TypeConvertor.castToString(value); // StringType 3789 } else if (name.equals("contact")) { 3790 this.getContact().add(TypeConvertor.castToContactDetail(value)); 3791 } else if (name.equals("description")) { 3792 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3793 } else if (name.equals("useContext")) { 3794 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 3795 } else if (name.equals("jurisdiction")) { 3796 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 3797 } else if (name.equals("purpose")) { 3798 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 3799 } else if (name.equals("copyright")) { 3800 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 3801 } else if (name.equals("copyrightLabel")) { 3802 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 3803 } else if (name.equals("category")) { 3804 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 3805 } else if (name.equals("scope")) { 3806 this.getScope().add(TypeConvertor.castToReference(value)); 3807 } else if (name.equals("testTools")) { 3808 this.testTools = TypeConvertor.castToMarkdown(value); // MarkdownType 3809 } else if (name.equals("dependency")) { 3810 this.getDependency().add((TestPlanDependencyComponent) value); 3811 } else if (name.equals("exitCriteria")) { 3812 this.exitCriteria = TypeConvertor.castToMarkdown(value); // MarkdownType 3813 } else if (name.equals("testCase")) { 3814 this.getTestCase().add((TestPlanTestCaseComponent) value); 3815 } else 3816 return super.setProperty(name, value); 3817 return value; 3818 } 3819 3820 @Override 3821 public void removeChild(String name, Base value) throws FHIRException { 3822 if (name.equals("url")) { 3823 this.url = null; 3824 } else if (name.equals("identifier")) { 3825 this.getIdentifier().remove(value); 3826 } else if (name.equals("version")) { 3827 this.version = null; 3828 } else if (name.equals("versionAlgorithm[x]")) { 3829 this.versionAlgorithm = null; 3830 } else if (name.equals("name")) { 3831 this.name = null; 3832 } else if (name.equals("title")) { 3833 this.title = null; 3834 } else if (name.equals("status")) { 3835 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3836 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3837 } else if (name.equals("experimental")) { 3838 this.experimental = null; 3839 } else if (name.equals("date")) { 3840 this.date = null; 3841 } else if (name.equals("publisher")) { 3842 this.publisher = null; 3843 } else if (name.equals("contact")) { 3844 this.getContact().remove(value); 3845 } else if (name.equals("description")) { 3846 this.description = null; 3847 } else if (name.equals("useContext")) { 3848 this.getUseContext().remove(value); 3849 } else if (name.equals("jurisdiction")) { 3850 this.getJurisdiction().remove(value); 3851 } else if (name.equals("purpose")) { 3852 this.purpose = null; 3853 } else if (name.equals("copyright")) { 3854 this.copyright = null; 3855 } else if (name.equals("copyrightLabel")) { 3856 this.copyrightLabel = null; 3857 } else if (name.equals("category")) { 3858 this.getCategory().remove(value); 3859 } else if (name.equals("scope")) { 3860 this.getScope().remove(value); 3861 } else if (name.equals("testTools")) { 3862 this.testTools = null; 3863 } else if (name.equals("dependency")) { 3864 this.getDependency().remove((TestPlanDependencyComponent) value); 3865 } else if (name.equals("exitCriteria")) { 3866 this.exitCriteria = null; 3867 } else if (name.equals("testCase")) { 3868 this.getTestCase().remove((TestPlanTestCaseComponent) value); 3869 } else 3870 super.removeChild(name, value); 3871 3872 } 3873 3874 @Override 3875 public Base makeProperty(int hash, String name) throws FHIRException { 3876 switch (hash) { 3877 case 116079: return getUrlElement(); 3878 case -1618432855: return addIdentifier(); 3879 case 351608024: return getVersionElement(); 3880 case -115699031: return getVersionAlgorithm(); 3881 case 1508158071: return getVersionAlgorithm(); 3882 case 3373707: return getNameElement(); 3883 case 110371416: return getTitleElement(); 3884 case -892481550: return getStatusElement(); 3885 case -404562712: return getExperimentalElement(); 3886 case 3076014: return getDateElement(); 3887 case 1447404028: return getPublisherElement(); 3888 case 951526432: return addContact(); 3889 case -1724546052: return getDescriptionElement(); 3890 case -669707736: return addUseContext(); 3891 case -507075711: return addJurisdiction(); 3892 case -220463842: return getPurposeElement(); 3893 case 1522889671: return getCopyrightElement(); 3894 case 765157229: return getCopyrightLabelElement(); 3895 case 50511102: return addCategory(); 3896 case 109264468: return addScope(); 3897 case -1190420375: return getTestToolsElement(); 3898 case -26291381: return addDependency(); 3899 case -1382023523: return getExitCriteriaElement(); 3900 case -1147299102: return addTestCase(); 3901 default: return super.makeProperty(hash, name); 3902 } 3903 3904 } 3905 3906 @Override 3907 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3908 switch (hash) { 3909 case 116079: /*url*/ return new String[] {"uri"}; 3910 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3911 case 351608024: /*version*/ return new String[] {"string"}; 3912 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 3913 case 3373707: /*name*/ return new String[] {"string"}; 3914 case 110371416: /*title*/ return new String[] {"string"}; 3915 case -892481550: /*status*/ return new String[] {"code"}; 3916 case -404562712: /*experimental*/ return new String[] {"boolean"}; 3917 case 3076014: /*date*/ return new String[] {"dateTime"}; 3918 case 1447404028: /*publisher*/ return new String[] {"string"}; 3919 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 3920 case -1724546052: /*description*/ return new String[] {"markdown"}; 3921 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 3922 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 3923 case -220463842: /*purpose*/ return new String[] {"markdown"}; 3924 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 3925 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 3926 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3927 case 109264468: /*scope*/ return new String[] {"Reference"}; 3928 case -1190420375: /*testTools*/ return new String[] {"markdown"}; 3929 case -26291381: /*dependency*/ return new String[] {}; 3930 case -1382023523: /*exitCriteria*/ return new String[] {"markdown"}; 3931 case -1147299102: /*testCase*/ return new String[] {}; 3932 default: return super.getTypesForProperty(hash, name); 3933 } 3934 3935 } 3936 3937 @Override 3938 public Base addChild(String name) throws FHIRException { 3939 if (name.equals("url")) { 3940 throw new FHIRException("Cannot call addChild on a singleton property TestPlan.url"); 3941 } 3942 else if (name.equals("identifier")) { 3943 return addIdentifier(); 3944 } 3945 else if (name.equals("version")) { 3946 throw new FHIRException("Cannot call addChild on a singleton property TestPlan.version"); 3947 } 3948 else if (name.equals("versionAlgorithmString")) { 3949 this.versionAlgorithm = new StringType(); 3950 return this.versionAlgorithm; 3951 } 3952 else if (name.equals("versionAlgorithmCoding")) { 3953 this.versionAlgorithm = new Coding(); 3954 return this.versionAlgorithm; 3955 } 3956 else if (name.equals("name")) { 3957 throw new FHIRException("Cannot call addChild on a singleton property TestPlan.name"); 3958 } 3959 else if (name.equals("title")) { 3960 throw new FHIRException("Cannot call addChild on a singleton property TestPlan.title"); 3961 } 3962 else if (name.equals("status")) { 3963 throw new FHIRException("Cannot call addChild on a singleton property TestPlan.status"); 3964 } 3965 else if (name.equals("experimental")) { 3966 throw new FHIRException("Cannot call addChild on a singleton property TestPlan.experimental"); 3967 } 3968 else if (name.equals("date")) { 3969 throw new FHIRException("Cannot call addChild on a singleton property TestPlan.date"); 3970 } 3971 else if (name.equals("publisher")) { 3972 throw new FHIRException("Cannot call addChild on a singleton property TestPlan.publisher"); 3973 } 3974 else if (name.equals("contact")) { 3975 return addContact(); 3976 } 3977 else if (name.equals("description")) { 3978 throw new FHIRException("Cannot call addChild on a singleton property TestPlan.description"); 3979 } 3980 else if (name.equals("useContext")) { 3981 return addUseContext(); 3982 } 3983 else if (name.equals("jurisdiction")) { 3984 return addJurisdiction(); 3985 } 3986 else if (name.equals("purpose")) { 3987 throw new FHIRException("Cannot call addChild on a singleton property TestPlan.purpose"); 3988 } 3989 else if (name.equals("copyright")) { 3990 throw new FHIRException("Cannot call addChild on a singleton property TestPlan.copyright"); 3991 } 3992 else if (name.equals("copyrightLabel")) { 3993 throw new FHIRException("Cannot call addChild on a singleton property TestPlan.copyrightLabel"); 3994 } 3995 else if (name.equals("category")) { 3996 return addCategory(); 3997 } 3998 else if (name.equals("scope")) { 3999 return addScope(); 4000 } 4001 else if (name.equals("testTools")) { 4002 throw new FHIRException("Cannot call addChild on a singleton property TestPlan.testTools"); 4003 } 4004 else if (name.equals("dependency")) { 4005 return addDependency(); 4006 } 4007 else if (name.equals("exitCriteria")) { 4008 throw new FHIRException("Cannot call addChild on a singleton property TestPlan.exitCriteria"); 4009 } 4010 else if (name.equals("testCase")) { 4011 return addTestCase(); 4012 } 4013 else 4014 return super.addChild(name); 4015 } 4016 4017 public String fhirType() { 4018 return "TestPlan"; 4019 4020 } 4021 4022 public TestPlan copy() { 4023 TestPlan dst = new TestPlan(); 4024 copyValues(dst); 4025 return dst; 4026 } 4027 4028 public void copyValues(TestPlan dst) { 4029 super.copyValues(dst); 4030 dst.url = url == null ? null : url.copy(); 4031 if (identifier != null) { 4032 dst.identifier = new ArrayList<Identifier>(); 4033 for (Identifier i : identifier) 4034 dst.identifier.add(i.copy()); 4035 }; 4036 dst.version = version == null ? null : version.copy(); 4037 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 4038 dst.name = name == null ? null : name.copy(); 4039 dst.title = title == null ? null : title.copy(); 4040 dst.status = status == null ? null : status.copy(); 4041 dst.experimental = experimental == null ? null : experimental.copy(); 4042 dst.date = date == null ? null : date.copy(); 4043 dst.publisher = publisher == null ? null : publisher.copy(); 4044 if (contact != null) { 4045 dst.contact = new ArrayList<ContactDetail>(); 4046 for (ContactDetail i : contact) 4047 dst.contact.add(i.copy()); 4048 }; 4049 dst.description = description == null ? null : description.copy(); 4050 if (useContext != null) { 4051 dst.useContext = new ArrayList<UsageContext>(); 4052 for (UsageContext i : useContext) 4053 dst.useContext.add(i.copy()); 4054 }; 4055 if (jurisdiction != null) { 4056 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4057 for (CodeableConcept i : jurisdiction) 4058 dst.jurisdiction.add(i.copy()); 4059 }; 4060 dst.purpose = purpose == null ? null : purpose.copy(); 4061 dst.copyright = copyright == null ? null : copyright.copy(); 4062 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 4063 if (category != null) { 4064 dst.category = new ArrayList<CodeableConcept>(); 4065 for (CodeableConcept i : category) 4066 dst.category.add(i.copy()); 4067 }; 4068 if (scope != null) { 4069 dst.scope = new ArrayList<Reference>(); 4070 for (Reference i : scope) 4071 dst.scope.add(i.copy()); 4072 }; 4073 dst.testTools = testTools == null ? null : testTools.copy(); 4074 if (dependency != null) { 4075 dst.dependency = new ArrayList<TestPlanDependencyComponent>(); 4076 for (TestPlanDependencyComponent i : dependency) 4077 dst.dependency.add(i.copy()); 4078 }; 4079 dst.exitCriteria = exitCriteria == null ? null : exitCriteria.copy(); 4080 if (testCase != null) { 4081 dst.testCase = new ArrayList<TestPlanTestCaseComponent>(); 4082 for (TestPlanTestCaseComponent i : testCase) 4083 dst.testCase.add(i.copy()); 4084 }; 4085 } 4086 4087 protected TestPlan typedCopy() { 4088 return copy(); 4089 } 4090 4091 @Override 4092 public boolean equalsDeep(Base other_) { 4093 if (!super.equalsDeep(other_)) 4094 return false; 4095 if (!(other_ instanceof TestPlan)) 4096 return false; 4097 TestPlan o = (TestPlan) other_; 4098 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 4099 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 4100 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 4101 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 4102 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 4103 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 4104 && compareDeep(category, o.category, true) && compareDeep(scope, o.scope, true) && compareDeep(testTools, o.testTools, true) 4105 && compareDeep(dependency, o.dependency, true) && compareDeep(exitCriteria, o.exitCriteria, true) 4106 && compareDeep(testCase, o.testCase, true); 4107 } 4108 4109 @Override 4110 public boolean equalsShallow(Base other_) { 4111 if (!super.equalsShallow(other_)) 4112 return false; 4113 if (!(other_ instanceof TestPlan)) 4114 return false; 4115 TestPlan o = (TestPlan) other_; 4116 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 4117 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 4118 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 4119 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 4120 && compareValues(testTools, o.testTools, true) && compareValues(exitCriteria, o.exitCriteria, true) 4121 ; 4122 } 4123 4124 public boolean isEmpty() { 4125 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 4126 , versionAlgorithm, name, title, status, experimental, date, publisher, contact 4127 , description, useContext, jurisdiction, purpose, copyright, copyrightLabel, category 4128 , scope, testTools, dependency, exitCriteria, testCase); 4129 } 4130 4131 @Override 4132 public ResourceType getResourceType() { 4133 return ResourceType.TestPlan; 4134 } 4135 4136 /** 4137 * Search parameter: <b>identifier</b> 4138 * <p> 4139 * Description: <b>Multiple Resources: 4140 4141* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4142* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4143* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4144* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4145* [Citation](citation.html): External identifier for the citation 4146* [CodeSystem](codesystem.html): External identifier for the code system 4147* [ConceptMap](conceptmap.html): External identifier for the concept map 4148* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4149* [EventDefinition](eventdefinition.html): External identifier for the event definition 4150* [Evidence](evidence.html): External identifier for the evidence 4151* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4152* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4153* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4154* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4155* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4156* [Library](library.html): External identifier for the library 4157* [Measure](measure.html): External identifier for the measure 4158* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4159* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4160* [NamingSystem](namingsystem.html): External identifier for the naming system 4161* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4162* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4163* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4164* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4165* [Requirements](requirements.html): External identifier for the requirements 4166* [SearchParameter](searchparameter.html): External identifier for the search parameter 4167* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4168* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4169* [StructureMap](structuremap.html): External identifier for the structure map 4170* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4171* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4172* [TestPlan](testplan.html): An identifier for the test plan 4173* [TestScript](testscript.html): External identifier for the test script 4174* [ValueSet](valueset.html): External identifier for the value set 4175</b><br> 4176 * Type: <b>token</b><br> 4177 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4178 * </p> 4179 */ 4180 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 4181 public static final String SP_IDENTIFIER = "identifier"; 4182 /** 4183 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4184 * <p> 4185 * Description: <b>Multiple Resources: 4186 4187* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4188* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4189* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4190* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4191* [Citation](citation.html): External identifier for the citation 4192* [CodeSystem](codesystem.html): External identifier for the code system 4193* [ConceptMap](conceptmap.html): External identifier for the concept map 4194* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4195* [EventDefinition](eventdefinition.html): External identifier for the event definition 4196* [Evidence](evidence.html): External identifier for the evidence 4197* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4198* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4199* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4200* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4201* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4202* [Library](library.html): External identifier for the library 4203* [Measure](measure.html): External identifier for the measure 4204* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4205* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4206* [NamingSystem](namingsystem.html): External identifier for the naming system 4207* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4208* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4209* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4210* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4211* [Requirements](requirements.html): External identifier for the requirements 4212* [SearchParameter](searchparameter.html): External identifier for the search parameter 4213* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4214* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4215* [StructureMap](structuremap.html): External identifier for the structure map 4216* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4217* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4218* [TestPlan](testplan.html): An identifier for the test plan 4219* [TestScript](testscript.html): External identifier for the test script 4220* [ValueSet](valueset.html): External identifier for the value set 4221</b><br> 4222 * Type: <b>token</b><br> 4223 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4224 * </p> 4225 */ 4226 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4227 4228 /** 4229 * Search parameter: <b>status</b> 4230 * <p> 4231 * Description: <b>Multiple Resources: 4232 4233* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4234* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4235* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4236* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4237* [Citation](citation.html): The current status of the citation 4238* [CodeSystem](codesystem.html): The current status of the code system 4239* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4240* [ConceptMap](conceptmap.html): The current status of the concept map 4241* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4242* [EventDefinition](eventdefinition.html): The current status of the event definition 4243* [Evidence](evidence.html): The current status of the evidence 4244* [EvidenceReport](evidencereport.html): The current status of the evidence report 4245* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4246* [ExampleScenario](examplescenario.html): The current status of the example scenario 4247* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4248* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4249* [Library](library.html): The current status of the library 4250* [Measure](measure.html): The current status of the measure 4251* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4252* [MessageDefinition](messagedefinition.html): The current status of the message definition 4253* [NamingSystem](namingsystem.html): The current status of the naming system 4254* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4255* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4256* [PlanDefinition](plandefinition.html): The current status of the plan definition 4257* [Questionnaire](questionnaire.html): The current status of the questionnaire 4258* [Requirements](requirements.html): The current status of the requirements 4259* [SearchParameter](searchparameter.html): The current status of the search parameter 4260* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4261* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4262* [StructureMap](structuremap.html): The current status of the structure map 4263* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4264* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4265* [TestPlan](testplan.html): The current status of the test plan 4266* [TestScript](testscript.html): The current status of the test script 4267* [ValueSet](valueset.html): The current status of the value set 4268</b><br> 4269 * Type: <b>token</b><br> 4270 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4271 * </p> 4272 */ 4273 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 4274 public static final String SP_STATUS = "status"; 4275 /** 4276 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4277 * <p> 4278 * Description: <b>Multiple Resources: 4279 4280* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4281* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4282* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4283* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4284* [Citation](citation.html): The current status of the citation 4285* [CodeSystem](codesystem.html): The current status of the code system 4286* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4287* [ConceptMap](conceptmap.html): The current status of the concept map 4288* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4289* [EventDefinition](eventdefinition.html): The current status of the event definition 4290* [Evidence](evidence.html): The current status of the evidence 4291* [EvidenceReport](evidencereport.html): The current status of the evidence report 4292* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4293* [ExampleScenario](examplescenario.html): The current status of the example scenario 4294* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4295* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4296* [Library](library.html): The current status of the library 4297* [Measure](measure.html): The current status of the measure 4298* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4299* [MessageDefinition](messagedefinition.html): The current status of the message definition 4300* [NamingSystem](namingsystem.html): The current status of the naming system 4301* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4302* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4303* [PlanDefinition](plandefinition.html): The current status of the plan definition 4304* [Questionnaire](questionnaire.html): The current status of the questionnaire 4305* [Requirements](requirements.html): The current status of the requirements 4306* [SearchParameter](searchparameter.html): The current status of the search parameter 4307* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4308* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4309* [StructureMap](structuremap.html): The current status of the structure map 4310* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4311* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4312* [TestPlan](testplan.html): The current status of the test plan 4313* [TestScript](testscript.html): The current status of the test script 4314* [ValueSet](valueset.html): The current status of the value set 4315</b><br> 4316 * Type: <b>token</b><br> 4317 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4318 * </p> 4319 */ 4320 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4321 4322 /** 4323 * Search parameter: <b>url</b> 4324 * <p> 4325 * Description: <b>Multiple Resources: 4326 4327* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4328* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4329* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4330* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4331* [Citation](citation.html): The uri that identifies the citation 4332* [CodeSystem](codesystem.html): The uri that identifies the code system 4333* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4334* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4335* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4336* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4337* [Evidence](evidence.html): The uri that identifies the evidence 4338* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4339* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4340* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4341* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4342* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4343* [Library](library.html): The uri that identifies the library 4344* [Measure](measure.html): The uri that identifies the measure 4345* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4346* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4347* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4348* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4349* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4350* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4351* [Requirements](requirements.html): The uri that identifies the requirements 4352* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4353* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4354* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4355* [StructureMap](structuremap.html): The uri that identifies the structure map 4356* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4357* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4358* [TestPlan](testplan.html): The uri that identifies the test plan 4359* [TestScript](testscript.html): The uri that identifies the test script 4360* [ValueSet](valueset.html): The uri that identifies the value set 4361</b><br> 4362 * Type: <b>uri</b><br> 4363 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4364 * </p> 4365 */ 4366 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 4367 public static final String SP_URL = "url"; 4368 /** 4369 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4370 * <p> 4371 * Description: <b>Multiple Resources: 4372 4373* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4374* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4375* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4376* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4377* [Citation](citation.html): The uri that identifies the citation 4378* [CodeSystem](codesystem.html): The uri that identifies the code system 4379* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4380* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4381* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4382* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4383* [Evidence](evidence.html): The uri that identifies the evidence 4384* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4385* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4386* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4387* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4388* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4389* [Library](library.html): The uri that identifies the library 4390* [Measure](measure.html): The uri that identifies the measure 4391* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4392* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4393* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4394* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4395* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4396* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4397* [Requirements](requirements.html): The uri that identifies the requirements 4398* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4399* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4400* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4401* [StructureMap](structuremap.html): The uri that identifies the structure map 4402* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4403* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4404* [TestPlan](testplan.html): The uri that identifies the test plan 4405* [TestScript](testscript.html): The uri that identifies the test script 4406* [ValueSet](valueset.html): The uri that identifies the value set 4407</b><br> 4408 * Type: <b>uri</b><br> 4409 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4410 * </p> 4411 */ 4412 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4413 4414 /** 4415 * Search parameter: <b>scope</b> 4416 * <p> 4417 * Description: <b>The scope that is to be tested with this test plan</b><br> 4418 * Type: <b>reference</b><br> 4419 * Path: <b>TestPlan.scope</b><br> 4420 * </p> 4421 */ 4422 @SearchParamDefinition(name="scope", path="TestPlan.scope", description="The scope that is to be tested with this test plan", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 4423 public static final String SP_SCOPE = "scope"; 4424 /** 4425 * <b>Fluent Client</b> search parameter constant for <b>scope</b> 4426 * <p> 4427 * Description: <b>The scope that is to be tested with this test plan</b><br> 4428 * Type: <b>reference</b><br> 4429 * Path: <b>TestPlan.scope</b><br> 4430 * </p> 4431 */ 4432 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SCOPE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SCOPE); 4433 4434/** 4435 * Constant for fluent queries to be used to add include statements. Specifies 4436 * the path value of "<b>TestPlan:scope</b>". 4437 */ 4438 public static final ca.uhn.fhir.model.api.Include INCLUDE_SCOPE = new ca.uhn.fhir.model.api.Include("TestPlan:scope").toLocked(); 4439 4440 4441} 4442