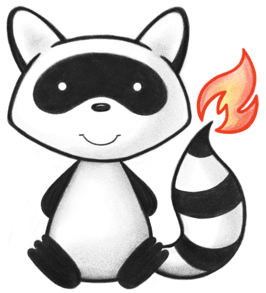
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.Block; 050 051/** 052 * A summary of information based on the results of executing a TestScript. 053 */ 054@ResourceDef(name="TestReport", profile="http://hl7.org/fhir/StructureDefinition/TestReport") 055public class TestReport extends DomainResource { 056 057 public enum TestReportActionResult { 058 /** 059 * The action was successful. 060 */ 061 PASS, 062 /** 063 * The action was skipped. 064 */ 065 SKIP, 066 /** 067 * The action failed. 068 */ 069 FAIL, 070 /** 071 * The action passed but with warnings. 072 */ 073 WARNING, 074 /** 075 * The action encountered a fatal error and the engine was unable to process. 076 */ 077 ERROR, 078 /** 079 * added to help the parsers with the generic types 080 */ 081 NULL; 082 public static TestReportActionResult fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("pass".equals(codeString)) 086 return PASS; 087 if ("skip".equals(codeString)) 088 return SKIP; 089 if ("fail".equals(codeString)) 090 return FAIL; 091 if ("warning".equals(codeString)) 092 return WARNING; 093 if ("error".equals(codeString)) 094 return ERROR; 095 if (Configuration.isAcceptInvalidEnums()) 096 return null; 097 else 098 throw new FHIRException("Unknown TestReportActionResult code '"+codeString+"'"); 099 } 100 public String toCode() { 101 switch (this) { 102 case PASS: return "pass"; 103 case SKIP: return "skip"; 104 case FAIL: return "fail"; 105 case WARNING: return "warning"; 106 case ERROR: return "error"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 public String getSystem() { 112 switch (this) { 113 case PASS: return "http://hl7.org/fhir/report-action-result-codes"; 114 case SKIP: return "http://hl7.org/fhir/report-action-result-codes"; 115 case FAIL: return "http://hl7.org/fhir/report-action-result-codes"; 116 case WARNING: return "http://hl7.org/fhir/report-action-result-codes"; 117 case ERROR: return "http://hl7.org/fhir/report-action-result-codes"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDefinition() { 123 switch (this) { 124 case PASS: return "The action was successful."; 125 case SKIP: return "The action was skipped."; 126 case FAIL: return "The action failed."; 127 case WARNING: return "The action passed but with warnings."; 128 case ERROR: return "The action encountered a fatal error and the engine was unable to process."; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 public String getDisplay() { 134 switch (this) { 135 case PASS: return "Pass"; 136 case SKIP: return "Skip"; 137 case FAIL: return "Fail"; 138 case WARNING: return "Warning"; 139 case ERROR: return "Error"; 140 case NULL: return null; 141 default: return "?"; 142 } 143 } 144 } 145 146 public static class TestReportActionResultEnumFactory implements EnumFactory<TestReportActionResult> { 147 public TestReportActionResult fromCode(String codeString) throws IllegalArgumentException { 148 if (codeString == null || "".equals(codeString)) 149 if (codeString == null || "".equals(codeString)) 150 return null; 151 if ("pass".equals(codeString)) 152 return TestReportActionResult.PASS; 153 if ("skip".equals(codeString)) 154 return TestReportActionResult.SKIP; 155 if ("fail".equals(codeString)) 156 return TestReportActionResult.FAIL; 157 if ("warning".equals(codeString)) 158 return TestReportActionResult.WARNING; 159 if ("error".equals(codeString)) 160 return TestReportActionResult.ERROR; 161 throw new IllegalArgumentException("Unknown TestReportActionResult code '"+codeString+"'"); 162 } 163 public Enumeration<TestReportActionResult> fromType(PrimitiveType<?> code) throws FHIRException { 164 if (code == null) 165 return null; 166 if (code.isEmpty()) 167 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.NULL, code); 168 String codeString = ((PrimitiveType) code).asStringValue(); 169 if (codeString == null || "".equals(codeString)) 170 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.NULL, code); 171 if ("pass".equals(codeString)) 172 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.PASS, code); 173 if ("skip".equals(codeString)) 174 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.SKIP, code); 175 if ("fail".equals(codeString)) 176 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.FAIL, code); 177 if ("warning".equals(codeString)) 178 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.WARNING, code); 179 if ("error".equals(codeString)) 180 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.ERROR, code); 181 throw new FHIRException("Unknown TestReportActionResult code '"+codeString+"'"); 182 } 183 public String toCode(TestReportActionResult code) { 184 if (code == TestReportActionResult.NULL) 185 return null; 186 if (code == TestReportActionResult.PASS) 187 return "pass"; 188 if (code == TestReportActionResult.SKIP) 189 return "skip"; 190 if (code == TestReportActionResult.FAIL) 191 return "fail"; 192 if (code == TestReportActionResult.WARNING) 193 return "warning"; 194 if (code == TestReportActionResult.ERROR) 195 return "error"; 196 return "?"; 197 } 198 public String toSystem(TestReportActionResult code) { 199 return code.getSystem(); 200 } 201 } 202 203 public enum TestReportParticipantType { 204 /** 205 * The test execution engine. 206 */ 207 TESTENGINE, 208 /** 209 * A FHIR Client. 210 */ 211 CLIENT, 212 /** 213 * A FHIR Server. 214 */ 215 SERVER, 216 /** 217 * added to help the parsers with the generic types 218 */ 219 NULL; 220 public static TestReportParticipantType fromCode(String codeString) throws FHIRException { 221 if (codeString == null || "".equals(codeString)) 222 return null; 223 if ("test-engine".equals(codeString)) 224 return TESTENGINE; 225 if ("client".equals(codeString)) 226 return CLIENT; 227 if ("server".equals(codeString)) 228 return SERVER; 229 if (Configuration.isAcceptInvalidEnums()) 230 return null; 231 else 232 throw new FHIRException("Unknown TestReportParticipantType code '"+codeString+"'"); 233 } 234 public String toCode() { 235 switch (this) { 236 case TESTENGINE: return "test-engine"; 237 case CLIENT: return "client"; 238 case SERVER: return "server"; 239 case NULL: return null; 240 default: return "?"; 241 } 242 } 243 public String getSystem() { 244 switch (this) { 245 case TESTENGINE: return "http://hl7.org/fhir/report-participant-type"; 246 case CLIENT: return "http://hl7.org/fhir/report-participant-type"; 247 case SERVER: return "http://hl7.org/fhir/report-participant-type"; 248 case NULL: return null; 249 default: return "?"; 250 } 251 } 252 public String getDefinition() { 253 switch (this) { 254 case TESTENGINE: return "The test execution engine."; 255 case CLIENT: return "A FHIR Client."; 256 case SERVER: return "A FHIR Server."; 257 case NULL: return null; 258 default: return "?"; 259 } 260 } 261 public String getDisplay() { 262 switch (this) { 263 case TESTENGINE: return "Test Engine"; 264 case CLIENT: return "Client"; 265 case SERVER: return "Server"; 266 case NULL: return null; 267 default: return "?"; 268 } 269 } 270 } 271 272 public static class TestReportParticipantTypeEnumFactory implements EnumFactory<TestReportParticipantType> { 273 public TestReportParticipantType fromCode(String codeString) throws IllegalArgumentException { 274 if (codeString == null || "".equals(codeString)) 275 if (codeString == null || "".equals(codeString)) 276 return null; 277 if ("test-engine".equals(codeString)) 278 return TestReportParticipantType.TESTENGINE; 279 if ("client".equals(codeString)) 280 return TestReportParticipantType.CLIENT; 281 if ("server".equals(codeString)) 282 return TestReportParticipantType.SERVER; 283 throw new IllegalArgumentException("Unknown TestReportParticipantType code '"+codeString+"'"); 284 } 285 public Enumeration<TestReportParticipantType> fromType(PrimitiveType<?> code) throws FHIRException { 286 if (code == null) 287 return null; 288 if (code.isEmpty()) 289 return new Enumeration<TestReportParticipantType>(this, TestReportParticipantType.NULL, code); 290 String codeString = ((PrimitiveType) code).asStringValue(); 291 if (codeString == null || "".equals(codeString)) 292 return new Enumeration<TestReportParticipantType>(this, TestReportParticipantType.NULL, code); 293 if ("test-engine".equals(codeString)) 294 return new Enumeration<TestReportParticipantType>(this, TestReportParticipantType.TESTENGINE, code); 295 if ("client".equals(codeString)) 296 return new Enumeration<TestReportParticipantType>(this, TestReportParticipantType.CLIENT, code); 297 if ("server".equals(codeString)) 298 return new Enumeration<TestReportParticipantType>(this, TestReportParticipantType.SERVER, code); 299 throw new FHIRException("Unknown TestReportParticipantType code '"+codeString+"'"); 300 } 301 public String toCode(TestReportParticipantType code) { 302 if (code == TestReportParticipantType.NULL) 303 return null; 304 if (code == TestReportParticipantType.TESTENGINE) 305 return "test-engine"; 306 if (code == TestReportParticipantType.CLIENT) 307 return "client"; 308 if (code == TestReportParticipantType.SERVER) 309 return "server"; 310 return "?"; 311 } 312 public String toSystem(TestReportParticipantType code) { 313 return code.getSystem(); 314 } 315 } 316 317 public enum TestReportResult { 318 /** 319 * All test operations successfully passed all asserts. 320 */ 321 PASS, 322 /** 323 * One or more test operations failed one or more asserts. 324 */ 325 FAIL, 326 /** 327 * One or more test operations is pending execution completion. 328 */ 329 PENDING, 330 /** 331 * added to help the parsers with the generic types 332 */ 333 NULL; 334 public static TestReportResult fromCode(String codeString) throws FHIRException { 335 if (codeString == null || "".equals(codeString)) 336 return null; 337 if ("pass".equals(codeString)) 338 return PASS; 339 if ("fail".equals(codeString)) 340 return FAIL; 341 if ("pending".equals(codeString)) 342 return PENDING; 343 if (Configuration.isAcceptInvalidEnums()) 344 return null; 345 else 346 throw new FHIRException("Unknown TestReportResult code '"+codeString+"'"); 347 } 348 public String toCode() { 349 switch (this) { 350 case PASS: return "pass"; 351 case FAIL: return "fail"; 352 case PENDING: return "pending"; 353 case NULL: return null; 354 default: return "?"; 355 } 356 } 357 public String getSystem() { 358 switch (this) { 359 case PASS: return "http://hl7.org/fhir/report-result-codes"; 360 case FAIL: return "http://hl7.org/fhir/report-result-codes"; 361 case PENDING: return "http://hl7.org/fhir/report-result-codes"; 362 case NULL: return null; 363 default: return "?"; 364 } 365 } 366 public String getDefinition() { 367 switch (this) { 368 case PASS: return "All test operations successfully passed all asserts."; 369 case FAIL: return "One or more test operations failed one or more asserts."; 370 case PENDING: return "One or more test operations is pending execution completion."; 371 case NULL: return null; 372 default: return "?"; 373 } 374 } 375 public String getDisplay() { 376 switch (this) { 377 case PASS: return "Pass"; 378 case FAIL: return "Fail"; 379 case PENDING: return "Pending"; 380 case NULL: return null; 381 default: return "?"; 382 } 383 } 384 } 385 386 public static class TestReportResultEnumFactory implements EnumFactory<TestReportResult> { 387 public TestReportResult fromCode(String codeString) throws IllegalArgumentException { 388 if (codeString == null || "".equals(codeString)) 389 if (codeString == null || "".equals(codeString)) 390 return null; 391 if ("pass".equals(codeString)) 392 return TestReportResult.PASS; 393 if ("fail".equals(codeString)) 394 return TestReportResult.FAIL; 395 if ("pending".equals(codeString)) 396 return TestReportResult.PENDING; 397 throw new IllegalArgumentException("Unknown TestReportResult code '"+codeString+"'"); 398 } 399 public Enumeration<TestReportResult> fromType(PrimitiveType<?> code) throws FHIRException { 400 if (code == null) 401 return null; 402 if (code.isEmpty()) 403 return new Enumeration<TestReportResult>(this, TestReportResult.NULL, code); 404 String codeString = ((PrimitiveType) code).asStringValue(); 405 if (codeString == null || "".equals(codeString)) 406 return new Enumeration<TestReportResult>(this, TestReportResult.NULL, code); 407 if ("pass".equals(codeString)) 408 return new Enumeration<TestReportResult>(this, TestReportResult.PASS, code); 409 if ("fail".equals(codeString)) 410 return new Enumeration<TestReportResult>(this, TestReportResult.FAIL, code); 411 if ("pending".equals(codeString)) 412 return new Enumeration<TestReportResult>(this, TestReportResult.PENDING, code); 413 throw new FHIRException("Unknown TestReportResult code '"+codeString+"'"); 414 } 415 public String toCode(TestReportResult code) { 416 if (code == TestReportResult.NULL) 417 return null; 418 if (code == TestReportResult.PASS) 419 return "pass"; 420 if (code == TestReportResult.FAIL) 421 return "fail"; 422 if (code == TestReportResult.PENDING) 423 return "pending"; 424 return "?"; 425 } 426 public String toSystem(TestReportResult code) { 427 return code.getSystem(); 428 } 429 } 430 431 public enum TestReportStatus { 432 /** 433 * All test operations have completed. 434 */ 435 COMPLETED, 436 /** 437 * A test operations is currently executing. 438 */ 439 INPROGRESS, 440 /** 441 * A test operation is waiting for an external client request. 442 */ 443 WAITING, 444 /** 445 * The test script execution was manually stopped. 446 */ 447 STOPPED, 448 /** 449 * This test report was entered or created in error. 450 */ 451 ENTEREDINERROR, 452 /** 453 * added to help the parsers with the generic types 454 */ 455 NULL; 456 public static TestReportStatus fromCode(String codeString) throws FHIRException { 457 if (codeString == null || "".equals(codeString)) 458 return null; 459 if ("completed".equals(codeString)) 460 return COMPLETED; 461 if ("in-progress".equals(codeString)) 462 return INPROGRESS; 463 if ("waiting".equals(codeString)) 464 return WAITING; 465 if ("stopped".equals(codeString)) 466 return STOPPED; 467 if ("entered-in-error".equals(codeString)) 468 return ENTEREDINERROR; 469 if (Configuration.isAcceptInvalidEnums()) 470 return null; 471 else 472 throw new FHIRException("Unknown TestReportStatus code '"+codeString+"'"); 473 } 474 public String toCode() { 475 switch (this) { 476 case COMPLETED: return "completed"; 477 case INPROGRESS: return "in-progress"; 478 case WAITING: return "waiting"; 479 case STOPPED: return "stopped"; 480 case ENTEREDINERROR: return "entered-in-error"; 481 case NULL: return null; 482 default: return "?"; 483 } 484 } 485 public String getSystem() { 486 switch (this) { 487 case COMPLETED: return "http://hl7.org/fhir/report-status-codes"; 488 case INPROGRESS: return "http://hl7.org/fhir/report-status-codes"; 489 case WAITING: return "http://hl7.org/fhir/report-status-codes"; 490 case STOPPED: return "http://hl7.org/fhir/report-status-codes"; 491 case ENTEREDINERROR: return "http://hl7.org/fhir/report-status-codes"; 492 case NULL: return null; 493 default: return "?"; 494 } 495 } 496 public String getDefinition() { 497 switch (this) { 498 case COMPLETED: return "All test operations have completed."; 499 case INPROGRESS: return "A test operations is currently executing."; 500 case WAITING: return "A test operation is waiting for an external client request."; 501 case STOPPED: return "The test script execution was manually stopped."; 502 case ENTEREDINERROR: return "This test report was entered or created in error."; 503 case NULL: return null; 504 default: return "?"; 505 } 506 } 507 public String getDisplay() { 508 switch (this) { 509 case COMPLETED: return "Completed"; 510 case INPROGRESS: return "In Progress"; 511 case WAITING: return "Waiting"; 512 case STOPPED: return "Stopped"; 513 case ENTEREDINERROR: return "Entered In Error"; 514 case NULL: return null; 515 default: return "?"; 516 } 517 } 518 } 519 520 public static class TestReportStatusEnumFactory implements EnumFactory<TestReportStatus> { 521 public TestReportStatus fromCode(String codeString) throws IllegalArgumentException { 522 if (codeString == null || "".equals(codeString)) 523 if (codeString == null || "".equals(codeString)) 524 return null; 525 if ("completed".equals(codeString)) 526 return TestReportStatus.COMPLETED; 527 if ("in-progress".equals(codeString)) 528 return TestReportStatus.INPROGRESS; 529 if ("waiting".equals(codeString)) 530 return TestReportStatus.WAITING; 531 if ("stopped".equals(codeString)) 532 return TestReportStatus.STOPPED; 533 if ("entered-in-error".equals(codeString)) 534 return TestReportStatus.ENTEREDINERROR; 535 throw new IllegalArgumentException("Unknown TestReportStatus code '"+codeString+"'"); 536 } 537 public Enumeration<TestReportStatus> fromType(PrimitiveType<?> code) throws FHIRException { 538 if (code == null) 539 return null; 540 if (code.isEmpty()) 541 return new Enumeration<TestReportStatus>(this, TestReportStatus.NULL, code); 542 String codeString = ((PrimitiveType) code).asStringValue(); 543 if (codeString == null || "".equals(codeString)) 544 return new Enumeration<TestReportStatus>(this, TestReportStatus.NULL, code); 545 if ("completed".equals(codeString)) 546 return new Enumeration<TestReportStatus>(this, TestReportStatus.COMPLETED, code); 547 if ("in-progress".equals(codeString)) 548 return new Enumeration<TestReportStatus>(this, TestReportStatus.INPROGRESS, code); 549 if ("waiting".equals(codeString)) 550 return new Enumeration<TestReportStatus>(this, TestReportStatus.WAITING, code); 551 if ("stopped".equals(codeString)) 552 return new Enumeration<TestReportStatus>(this, TestReportStatus.STOPPED, code); 553 if ("entered-in-error".equals(codeString)) 554 return new Enumeration<TestReportStatus>(this, TestReportStatus.ENTEREDINERROR, code); 555 throw new FHIRException("Unknown TestReportStatus code '"+codeString+"'"); 556 } 557 public String toCode(TestReportStatus code) { 558 if (code == TestReportStatus.NULL) 559 return null; 560 if (code == TestReportStatus.COMPLETED) 561 return "completed"; 562 if (code == TestReportStatus.INPROGRESS) 563 return "in-progress"; 564 if (code == TestReportStatus.WAITING) 565 return "waiting"; 566 if (code == TestReportStatus.STOPPED) 567 return "stopped"; 568 if (code == TestReportStatus.ENTEREDINERROR) 569 return "entered-in-error"; 570 return "?"; 571 } 572 public String toSystem(TestReportStatus code) { 573 return code.getSystem(); 574 } 575 } 576 577 @Block() 578 public static class TestReportParticipantComponent extends BackboneElement implements IBaseBackboneElement { 579 /** 580 * The type of participant. 581 */ 582 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 583 @Description(shortDefinition="test-engine | client | server", formalDefinition="The type of participant." ) 584 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/report-participant-type") 585 protected Enumeration<TestReportParticipantType> type; 586 587 /** 588 * The uri of the participant. An absolute URL is preferred. 589 */ 590 @Child(name = "uri", type = {UriType.class}, order=2, min=1, max=1, modifier=false, summary=false) 591 @Description(shortDefinition="The uri of the participant. An absolute URL is preferred", formalDefinition="The uri of the participant. An absolute URL is preferred." ) 592 protected UriType uri; 593 594 /** 595 * The display name of the participant. 596 */ 597 @Child(name = "display", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 598 @Description(shortDefinition="The display name of the participant", formalDefinition="The display name of the participant." ) 599 protected StringType display; 600 601 private static final long serialVersionUID = 577488357L; 602 603 /** 604 * Constructor 605 */ 606 public TestReportParticipantComponent() { 607 super(); 608 } 609 610 /** 611 * Constructor 612 */ 613 public TestReportParticipantComponent(TestReportParticipantType type, String uri) { 614 super(); 615 this.setType(type); 616 this.setUri(uri); 617 } 618 619 /** 620 * @return {@link #type} (The type of participant.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 621 */ 622 public Enumeration<TestReportParticipantType> getTypeElement() { 623 if (this.type == null) 624 if (Configuration.errorOnAutoCreate()) 625 throw new Error("Attempt to auto-create TestReportParticipantComponent.type"); 626 else if (Configuration.doAutoCreate()) 627 this.type = new Enumeration<TestReportParticipantType>(new TestReportParticipantTypeEnumFactory()); // bb 628 return this.type; 629 } 630 631 public boolean hasTypeElement() { 632 return this.type != null && !this.type.isEmpty(); 633 } 634 635 public boolean hasType() { 636 return this.type != null && !this.type.isEmpty(); 637 } 638 639 /** 640 * @param value {@link #type} (The type of participant.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 641 */ 642 public TestReportParticipantComponent setTypeElement(Enumeration<TestReportParticipantType> value) { 643 this.type = value; 644 return this; 645 } 646 647 /** 648 * @return The type of participant. 649 */ 650 public TestReportParticipantType getType() { 651 return this.type == null ? null : this.type.getValue(); 652 } 653 654 /** 655 * @param value The type of participant. 656 */ 657 public TestReportParticipantComponent setType(TestReportParticipantType value) { 658 if (this.type == null) 659 this.type = new Enumeration<TestReportParticipantType>(new TestReportParticipantTypeEnumFactory()); 660 this.type.setValue(value); 661 return this; 662 } 663 664 /** 665 * @return {@link #uri} (The uri of the participant. An absolute URL is preferred.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 666 */ 667 public UriType getUriElement() { 668 if (this.uri == null) 669 if (Configuration.errorOnAutoCreate()) 670 throw new Error("Attempt to auto-create TestReportParticipantComponent.uri"); 671 else if (Configuration.doAutoCreate()) 672 this.uri = new UriType(); // bb 673 return this.uri; 674 } 675 676 public boolean hasUriElement() { 677 return this.uri != null && !this.uri.isEmpty(); 678 } 679 680 public boolean hasUri() { 681 return this.uri != null && !this.uri.isEmpty(); 682 } 683 684 /** 685 * @param value {@link #uri} (The uri of the participant. An absolute URL is preferred.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 686 */ 687 public TestReportParticipantComponent setUriElement(UriType value) { 688 this.uri = value; 689 return this; 690 } 691 692 /** 693 * @return The uri of the participant. An absolute URL is preferred. 694 */ 695 public String getUri() { 696 return this.uri == null ? null : this.uri.getValue(); 697 } 698 699 /** 700 * @param value The uri of the participant. An absolute URL is preferred. 701 */ 702 public TestReportParticipantComponent setUri(String value) { 703 if (this.uri == null) 704 this.uri = new UriType(); 705 this.uri.setValue(value); 706 return this; 707 } 708 709 /** 710 * @return {@link #display} (The display name of the participant.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 711 */ 712 public StringType getDisplayElement() { 713 if (this.display == null) 714 if (Configuration.errorOnAutoCreate()) 715 throw new Error("Attempt to auto-create TestReportParticipantComponent.display"); 716 else if (Configuration.doAutoCreate()) 717 this.display = new StringType(); // bb 718 return this.display; 719 } 720 721 public boolean hasDisplayElement() { 722 return this.display != null && !this.display.isEmpty(); 723 } 724 725 public boolean hasDisplay() { 726 return this.display != null && !this.display.isEmpty(); 727 } 728 729 /** 730 * @param value {@link #display} (The display name of the participant.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 731 */ 732 public TestReportParticipantComponent setDisplayElement(StringType value) { 733 this.display = value; 734 return this; 735 } 736 737 /** 738 * @return The display name of the participant. 739 */ 740 public String getDisplay() { 741 return this.display == null ? null : this.display.getValue(); 742 } 743 744 /** 745 * @param value The display name of the participant. 746 */ 747 public TestReportParticipantComponent setDisplay(String value) { 748 if (Utilities.noString(value)) 749 this.display = null; 750 else { 751 if (this.display == null) 752 this.display = new StringType(); 753 this.display.setValue(value); 754 } 755 return this; 756 } 757 758 protected void listChildren(List<Property> children) { 759 super.listChildren(children); 760 children.add(new Property("type", "code", "The type of participant.", 0, 1, type)); 761 children.add(new Property("uri", "uri", "The uri of the participant. An absolute URL is preferred.", 0, 1, uri)); 762 children.add(new Property("display", "string", "The display name of the participant.", 0, 1, display)); 763 } 764 765 @Override 766 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 767 switch (_hash) { 768 case 3575610: /*type*/ return new Property("type", "code", "The type of participant.", 0, 1, type); 769 case 116076: /*uri*/ return new Property("uri", "uri", "The uri of the participant. An absolute URL is preferred.", 0, 1, uri); 770 case 1671764162: /*display*/ return new Property("display", "string", "The display name of the participant.", 0, 1, display); 771 default: return super.getNamedProperty(_hash, _name, _checkValid); 772 } 773 774 } 775 776 @Override 777 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 778 switch (hash) { 779 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<TestReportParticipantType> 780 case 116076: /*uri*/ return this.uri == null ? new Base[0] : new Base[] {this.uri}; // UriType 781 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 782 default: return super.getProperty(hash, name, checkValid); 783 } 784 785 } 786 787 @Override 788 public Base setProperty(int hash, String name, Base value) throws FHIRException { 789 switch (hash) { 790 case 3575610: // type 791 value = new TestReportParticipantTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 792 this.type = (Enumeration) value; // Enumeration<TestReportParticipantType> 793 return value; 794 case 116076: // uri 795 this.uri = TypeConvertor.castToUri(value); // UriType 796 return value; 797 case 1671764162: // display 798 this.display = TypeConvertor.castToString(value); // StringType 799 return value; 800 default: return super.setProperty(hash, name, value); 801 } 802 803 } 804 805 @Override 806 public Base setProperty(String name, Base value) throws FHIRException { 807 if (name.equals("type")) { 808 value = new TestReportParticipantTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 809 this.type = (Enumeration) value; // Enumeration<TestReportParticipantType> 810 } else if (name.equals("uri")) { 811 this.uri = TypeConvertor.castToUri(value); // UriType 812 } else if (name.equals("display")) { 813 this.display = TypeConvertor.castToString(value); // StringType 814 } else 815 return super.setProperty(name, value); 816 return value; 817 } 818 819 @Override 820 public void removeChild(String name, Base value) throws FHIRException { 821 if (name.equals("type")) { 822 value = new TestReportParticipantTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 823 this.type = (Enumeration) value; // Enumeration<TestReportParticipantType> 824 } else if (name.equals("uri")) { 825 this.uri = null; 826 } else if (name.equals("display")) { 827 this.display = null; 828 } else 829 super.removeChild(name, value); 830 831 } 832 833 @Override 834 public Base makeProperty(int hash, String name) throws FHIRException { 835 switch (hash) { 836 case 3575610: return getTypeElement(); 837 case 116076: return getUriElement(); 838 case 1671764162: return getDisplayElement(); 839 default: return super.makeProperty(hash, name); 840 } 841 842 } 843 844 @Override 845 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 846 switch (hash) { 847 case 3575610: /*type*/ return new String[] {"code"}; 848 case 116076: /*uri*/ return new String[] {"uri"}; 849 case 1671764162: /*display*/ return new String[] {"string"}; 850 default: return super.getTypesForProperty(hash, name); 851 } 852 853 } 854 855 @Override 856 public Base addChild(String name) throws FHIRException { 857 if (name.equals("type")) { 858 throw new FHIRException("Cannot call addChild on a singleton property TestReport.participant.type"); 859 } 860 else if (name.equals("uri")) { 861 throw new FHIRException("Cannot call addChild on a singleton property TestReport.participant.uri"); 862 } 863 else if (name.equals("display")) { 864 throw new FHIRException("Cannot call addChild on a singleton property TestReport.participant.display"); 865 } 866 else 867 return super.addChild(name); 868 } 869 870 public TestReportParticipantComponent copy() { 871 TestReportParticipantComponent dst = new TestReportParticipantComponent(); 872 copyValues(dst); 873 return dst; 874 } 875 876 public void copyValues(TestReportParticipantComponent dst) { 877 super.copyValues(dst); 878 dst.type = type == null ? null : type.copy(); 879 dst.uri = uri == null ? null : uri.copy(); 880 dst.display = display == null ? null : display.copy(); 881 } 882 883 @Override 884 public boolean equalsDeep(Base other_) { 885 if (!super.equalsDeep(other_)) 886 return false; 887 if (!(other_ instanceof TestReportParticipantComponent)) 888 return false; 889 TestReportParticipantComponent o = (TestReportParticipantComponent) other_; 890 return compareDeep(type, o.type, true) && compareDeep(uri, o.uri, true) && compareDeep(display, o.display, true) 891 ; 892 } 893 894 @Override 895 public boolean equalsShallow(Base other_) { 896 if (!super.equalsShallow(other_)) 897 return false; 898 if (!(other_ instanceof TestReportParticipantComponent)) 899 return false; 900 TestReportParticipantComponent o = (TestReportParticipantComponent) other_; 901 return compareValues(type, o.type, true) && compareValues(uri, o.uri, true) && compareValues(display, o.display, true) 902 ; 903 } 904 905 public boolean isEmpty() { 906 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, uri, display); 907 } 908 909 public String fhirType() { 910 return "TestReport.participant"; 911 912 } 913 914 } 915 916 @Block() 917 public static class TestReportSetupComponent extends BackboneElement implements IBaseBackboneElement { 918 /** 919 * Action would contain either an operation or an assertion. 920 */ 921 @Child(name = "action", type = {}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 922 @Description(shortDefinition="A setup operation or assert that was executed", formalDefinition="Action would contain either an operation or an assertion." ) 923 protected List<SetupActionComponent> action; 924 925 private static final long serialVersionUID = -123374486L; 926 927 /** 928 * Constructor 929 */ 930 public TestReportSetupComponent() { 931 super(); 932 } 933 934 /** 935 * Constructor 936 */ 937 public TestReportSetupComponent(SetupActionComponent action) { 938 super(); 939 this.addAction(action); 940 } 941 942 /** 943 * @return {@link #action} (Action would contain either an operation or an assertion.) 944 */ 945 public List<SetupActionComponent> getAction() { 946 if (this.action == null) 947 this.action = new ArrayList<SetupActionComponent>(); 948 return this.action; 949 } 950 951 /** 952 * @return Returns a reference to <code>this</code> for easy method chaining 953 */ 954 public TestReportSetupComponent setAction(List<SetupActionComponent> theAction) { 955 this.action = theAction; 956 return this; 957 } 958 959 public boolean hasAction() { 960 if (this.action == null) 961 return false; 962 for (SetupActionComponent item : this.action) 963 if (!item.isEmpty()) 964 return true; 965 return false; 966 } 967 968 public SetupActionComponent addAction() { //3 969 SetupActionComponent t = new SetupActionComponent(); 970 if (this.action == null) 971 this.action = new ArrayList<SetupActionComponent>(); 972 this.action.add(t); 973 return t; 974 } 975 976 public TestReportSetupComponent addAction(SetupActionComponent t) { //3 977 if (t == null) 978 return this; 979 if (this.action == null) 980 this.action = new ArrayList<SetupActionComponent>(); 981 this.action.add(t); 982 return this; 983 } 984 985 /** 986 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist {3} 987 */ 988 public SetupActionComponent getActionFirstRep() { 989 if (getAction().isEmpty()) { 990 addAction(); 991 } 992 return getAction().get(0); 993 } 994 995 protected void listChildren(List<Property> children) { 996 super.listChildren(children); 997 children.add(new Property("action", "", "Action would contain either an operation or an assertion.", 0, java.lang.Integer.MAX_VALUE, action)); 998 } 999 1000 @Override 1001 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1002 switch (_hash) { 1003 case -1422950858: /*action*/ return new Property("action", "", "Action would contain either an operation or an assertion.", 0, java.lang.Integer.MAX_VALUE, action); 1004 default: return super.getNamedProperty(_hash, _name, _checkValid); 1005 } 1006 1007 } 1008 1009 @Override 1010 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1011 switch (hash) { 1012 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // SetupActionComponent 1013 default: return super.getProperty(hash, name, checkValid); 1014 } 1015 1016 } 1017 1018 @Override 1019 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1020 switch (hash) { 1021 case -1422950858: // action 1022 this.getAction().add((SetupActionComponent) value); // SetupActionComponent 1023 return value; 1024 default: return super.setProperty(hash, name, value); 1025 } 1026 1027 } 1028 1029 @Override 1030 public Base setProperty(String name, Base value) throws FHIRException { 1031 if (name.equals("action")) { 1032 this.getAction().add((SetupActionComponent) value); 1033 } else 1034 return super.setProperty(name, value); 1035 return value; 1036 } 1037 1038 @Override 1039 public void removeChild(String name, Base value) throws FHIRException { 1040 if (name.equals("action")) { 1041 this.getAction().remove((SetupActionComponent) value); 1042 } else 1043 super.removeChild(name, value); 1044 1045 } 1046 1047 @Override 1048 public Base makeProperty(int hash, String name) throws FHIRException { 1049 switch (hash) { 1050 case -1422950858: return addAction(); 1051 default: return super.makeProperty(hash, name); 1052 } 1053 1054 } 1055 1056 @Override 1057 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1058 switch (hash) { 1059 case -1422950858: /*action*/ return new String[] {}; 1060 default: return super.getTypesForProperty(hash, name); 1061 } 1062 1063 } 1064 1065 @Override 1066 public Base addChild(String name) throws FHIRException { 1067 if (name.equals("action")) { 1068 return addAction(); 1069 } 1070 else 1071 return super.addChild(name); 1072 } 1073 1074 public TestReportSetupComponent copy() { 1075 TestReportSetupComponent dst = new TestReportSetupComponent(); 1076 copyValues(dst); 1077 return dst; 1078 } 1079 1080 public void copyValues(TestReportSetupComponent dst) { 1081 super.copyValues(dst); 1082 if (action != null) { 1083 dst.action = new ArrayList<SetupActionComponent>(); 1084 for (SetupActionComponent i : action) 1085 dst.action.add(i.copy()); 1086 }; 1087 } 1088 1089 @Override 1090 public boolean equalsDeep(Base other_) { 1091 if (!super.equalsDeep(other_)) 1092 return false; 1093 if (!(other_ instanceof TestReportSetupComponent)) 1094 return false; 1095 TestReportSetupComponent o = (TestReportSetupComponent) other_; 1096 return compareDeep(action, o.action, true); 1097 } 1098 1099 @Override 1100 public boolean equalsShallow(Base other_) { 1101 if (!super.equalsShallow(other_)) 1102 return false; 1103 if (!(other_ instanceof TestReportSetupComponent)) 1104 return false; 1105 TestReportSetupComponent o = (TestReportSetupComponent) other_; 1106 return true; 1107 } 1108 1109 public boolean isEmpty() { 1110 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action); 1111 } 1112 1113 public String fhirType() { 1114 return "TestReport.setup"; 1115 1116 } 1117 1118 } 1119 1120 @Block() 1121 public static class SetupActionComponent extends BackboneElement implements IBaseBackboneElement { 1122 /** 1123 * The operation performed. 1124 */ 1125 @Child(name = "operation", type = {}, order=1, min=0, max=1, modifier=false, summary=false) 1126 @Description(shortDefinition="The operation to perform", formalDefinition="The operation performed." ) 1127 protected SetupActionOperationComponent operation; 1128 1129 /** 1130 * The results of the assertion performed on the previous operations. 1131 */ 1132 @Child(name = "assert", type = {}, order=2, min=0, max=1, modifier=false, summary=false) 1133 @Description(shortDefinition="The assertion to perform", formalDefinition="The results of the assertion performed on the previous operations." ) 1134 protected SetupActionAssertComponent assert_; 1135 1136 private static final long serialVersionUID = -252088305L; 1137 1138 /** 1139 * Constructor 1140 */ 1141 public SetupActionComponent() { 1142 super(); 1143 } 1144 1145 /** 1146 * @return {@link #operation} (The operation performed.) 1147 */ 1148 public SetupActionOperationComponent getOperation() { 1149 if (this.operation == null) 1150 if (Configuration.errorOnAutoCreate()) 1151 throw new Error("Attempt to auto-create SetupActionComponent.operation"); 1152 else if (Configuration.doAutoCreate()) 1153 this.operation = new SetupActionOperationComponent(); // cc 1154 return this.operation; 1155 } 1156 1157 public boolean hasOperation() { 1158 return this.operation != null && !this.operation.isEmpty(); 1159 } 1160 1161 /** 1162 * @param value {@link #operation} (The operation performed.) 1163 */ 1164 public SetupActionComponent setOperation(SetupActionOperationComponent value) { 1165 this.operation = value; 1166 return this; 1167 } 1168 1169 /** 1170 * @return {@link #assert_} (The results of the assertion performed on the previous operations.) 1171 */ 1172 public SetupActionAssertComponent getAssert() { 1173 if (this.assert_ == null) 1174 if (Configuration.errorOnAutoCreate()) 1175 throw new Error("Attempt to auto-create SetupActionComponent.assert_"); 1176 else if (Configuration.doAutoCreate()) 1177 this.assert_ = new SetupActionAssertComponent(); // cc 1178 return this.assert_; 1179 } 1180 1181 public boolean hasAssert() { 1182 return this.assert_ != null && !this.assert_.isEmpty(); 1183 } 1184 1185 /** 1186 * @param value {@link #assert_} (The results of the assertion performed on the previous operations.) 1187 */ 1188 public SetupActionComponent setAssert(SetupActionAssertComponent value) { 1189 this.assert_ = value; 1190 return this; 1191 } 1192 1193 protected void listChildren(List<Property> children) { 1194 super.listChildren(children); 1195 children.add(new Property("operation", "", "The operation performed.", 0, 1, operation)); 1196 children.add(new Property("assert", "", "The results of the assertion performed on the previous operations.", 0, 1, assert_)); 1197 } 1198 1199 @Override 1200 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1201 switch (_hash) { 1202 case 1662702951: /*operation*/ return new Property("operation", "", "The operation performed.", 0, 1, operation); 1203 case -1408208058: /*assert*/ return new Property("assert", "", "The results of the assertion performed on the previous operations.", 0, 1, assert_); 1204 default: return super.getNamedProperty(_hash, _name, _checkValid); 1205 } 1206 1207 } 1208 1209 @Override 1210 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1211 switch (hash) { 1212 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : new Base[] {this.operation}; // SetupActionOperationComponent 1213 case -1408208058: /*assert*/ return this.assert_ == null ? new Base[0] : new Base[] {this.assert_}; // SetupActionAssertComponent 1214 default: return super.getProperty(hash, name, checkValid); 1215 } 1216 1217 } 1218 1219 @Override 1220 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1221 switch (hash) { 1222 case 1662702951: // operation 1223 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 1224 return value; 1225 case -1408208058: // assert 1226 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 1227 return value; 1228 default: return super.setProperty(hash, name, value); 1229 } 1230 1231 } 1232 1233 @Override 1234 public Base setProperty(String name, Base value) throws FHIRException { 1235 if (name.equals("operation")) { 1236 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 1237 } else if (name.equals("assert")) { 1238 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 1239 } else 1240 return super.setProperty(name, value); 1241 return value; 1242 } 1243 1244 @Override 1245 public void removeChild(String name, Base value) throws FHIRException { 1246 if (name.equals("operation")) { 1247 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 1248 } else if (name.equals("assert")) { 1249 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 1250 } else 1251 super.removeChild(name, value); 1252 1253 } 1254 1255 @Override 1256 public Base makeProperty(int hash, String name) throws FHIRException { 1257 switch (hash) { 1258 case 1662702951: return getOperation(); 1259 case -1408208058: return getAssert(); 1260 default: return super.makeProperty(hash, name); 1261 } 1262 1263 } 1264 1265 @Override 1266 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1267 switch (hash) { 1268 case 1662702951: /*operation*/ return new String[] {}; 1269 case -1408208058: /*assert*/ return new String[] {}; 1270 default: return super.getTypesForProperty(hash, name); 1271 } 1272 1273 } 1274 1275 @Override 1276 public Base addChild(String name) throws FHIRException { 1277 if (name.equals("operation")) { 1278 this.operation = new SetupActionOperationComponent(); 1279 return this.operation; 1280 } 1281 else if (name.equals("assert")) { 1282 this.assert_ = new SetupActionAssertComponent(); 1283 return this.assert_; 1284 } 1285 else 1286 return super.addChild(name); 1287 } 1288 1289 public SetupActionComponent copy() { 1290 SetupActionComponent dst = new SetupActionComponent(); 1291 copyValues(dst); 1292 return dst; 1293 } 1294 1295 public void copyValues(SetupActionComponent dst) { 1296 super.copyValues(dst); 1297 dst.operation = operation == null ? null : operation.copy(); 1298 dst.assert_ = assert_ == null ? null : assert_.copy(); 1299 } 1300 1301 @Override 1302 public boolean equalsDeep(Base other_) { 1303 if (!super.equalsDeep(other_)) 1304 return false; 1305 if (!(other_ instanceof SetupActionComponent)) 1306 return false; 1307 SetupActionComponent o = (SetupActionComponent) other_; 1308 return compareDeep(operation, o.operation, true) && compareDeep(assert_, o.assert_, true); 1309 } 1310 1311 @Override 1312 public boolean equalsShallow(Base other_) { 1313 if (!super.equalsShallow(other_)) 1314 return false; 1315 if (!(other_ instanceof SetupActionComponent)) 1316 return false; 1317 SetupActionComponent o = (SetupActionComponent) other_; 1318 return true; 1319 } 1320 1321 public boolean isEmpty() { 1322 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation, assert_); 1323 } 1324 1325 public String fhirType() { 1326 return "TestReport.setup.action"; 1327 1328 } 1329 1330 } 1331 1332 @Block() 1333 public static class SetupActionOperationComponent extends BackboneElement implements IBaseBackboneElement { 1334 /** 1335 * The result of this operation. 1336 */ 1337 @Child(name = "result", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1338 @Description(shortDefinition="pass | skip | fail | warning | error", formalDefinition="The result of this operation." ) 1339 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/report-action-result-codes") 1340 protected Enumeration<TestReportActionResult> result; 1341 1342 /** 1343 * An explanatory message associated with the result. 1344 */ 1345 @Child(name = "message", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1346 @Description(shortDefinition="A message associated with the result", formalDefinition="An explanatory message associated with the result." ) 1347 protected MarkdownType message; 1348 1349 /** 1350 * A link to further details on the result. 1351 */ 1352 @Child(name = "detail", type = {UriType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1353 @Description(shortDefinition="A link to further details on the result", formalDefinition="A link to further details on the result." ) 1354 protected UriType detail; 1355 1356 private static final long serialVersionUID = 269088798L; 1357 1358 /** 1359 * Constructor 1360 */ 1361 public SetupActionOperationComponent() { 1362 super(); 1363 } 1364 1365 /** 1366 * Constructor 1367 */ 1368 public SetupActionOperationComponent(TestReportActionResult result) { 1369 super(); 1370 this.setResult(result); 1371 } 1372 1373 /** 1374 * @return {@link #result} (The result of this operation.). This is the underlying object with id, value and extensions. The accessor "getResult" gives direct access to the value 1375 */ 1376 public Enumeration<TestReportActionResult> getResultElement() { 1377 if (this.result == null) 1378 if (Configuration.errorOnAutoCreate()) 1379 throw new Error("Attempt to auto-create SetupActionOperationComponent.result"); 1380 else if (Configuration.doAutoCreate()) 1381 this.result = new Enumeration<TestReportActionResult>(new TestReportActionResultEnumFactory()); // bb 1382 return this.result; 1383 } 1384 1385 public boolean hasResultElement() { 1386 return this.result != null && !this.result.isEmpty(); 1387 } 1388 1389 public boolean hasResult() { 1390 return this.result != null && !this.result.isEmpty(); 1391 } 1392 1393 /** 1394 * @param value {@link #result} (The result of this operation.). This is the underlying object with id, value and extensions. The accessor "getResult" gives direct access to the value 1395 */ 1396 public SetupActionOperationComponent setResultElement(Enumeration<TestReportActionResult> value) { 1397 this.result = value; 1398 return this; 1399 } 1400 1401 /** 1402 * @return The result of this operation. 1403 */ 1404 public TestReportActionResult getResult() { 1405 return this.result == null ? null : this.result.getValue(); 1406 } 1407 1408 /** 1409 * @param value The result of this operation. 1410 */ 1411 public SetupActionOperationComponent setResult(TestReportActionResult value) { 1412 if (this.result == null) 1413 this.result = new Enumeration<TestReportActionResult>(new TestReportActionResultEnumFactory()); 1414 this.result.setValue(value); 1415 return this; 1416 } 1417 1418 /** 1419 * @return {@link #message} (An explanatory message associated with the result.). This is the underlying object with id, value and extensions. The accessor "getMessage" gives direct access to the value 1420 */ 1421 public MarkdownType getMessageElement() { 1422 if (this.message == null) 1423 if (Configuration.errorOnAutoCreate()) 1424 throw new Error("Attempt to auto-create SetupActionOperationComponent.message"); 1425 else if (Configuration.doAutoCreate()) 1426 this.message = new MarkdownType(); // bb 1427 return this.message; 1428 } 1429 1430 public boolean hasMessageElement() { 1431 return this.message != null && !this.message.isEmpty(); 1432 } 1433 1434 public boolean hasMessage() { 1435 return this.message != null && !this.message.isEmpty(); 1436 } 1437 1438 /** 1439 * @param value {@link #message} (An explanatory message associated with the result.). This is the underlying object with id, value and extensions. The accessor "getMessage" gives direct access to the value 1440 */ 1441 public SetupActionOperationComponent setMessageElement(MarkdownType value) { 1442 this.message = value; 1443 return this; 1444 } 1445 1446 /** 1447 * @return An explanatory message associated with the result. 1448 */ 1449 public String getMessage() { 1450 return this.message == null ? null : this.message.getValue(); 1451 } 1452 1453 /** 1454 * @param value An explanatory message associated with the result. 1455 */ 1456 public SetupActionOperationComponent setMessage(String value) { 1457 if (Utilities.noString(value)) 1458 this.message = null; 1459 else { 1460 if (this.message == null) 1461 this.message = new MarkdownType(); 1462 this.message.setValue(value); 1463 } 1464 return this; 1465 } 1466 1467 /** 1468 * @return {@link #detail} (A link to further details on the result.). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value 1469 */ 1470 public UriType getDetailElement() { 1471 if (this.detail == null) 1472 if (Configuration.errorOnAutoCreate()) 1473 throw new Error("Attempt to auto-create SetupActionOperationComponent.detail"); 1474 else if (Configuration.doAutoCreate()) 1475 this.detail = new UriType(); // bb 1476 return this.detail; 1477 } 1478 1479 public boolean hasDetailElement() { 1480 return this.detail != null && !this.detail.isEmpty(); 1481 } 1482 1483 public boolean hasDetail() { 1484 return this.detail != null && !this.detail.isEmpty(); 1485 } 1486 1487 /** 1488 * @param value {@link #detail} (A link to further details on the result.). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value 1489 */ 1490 public SetupActionOperationComponent setDetailElement(UriType value) { 1491 this.detail = value; 1492 return this; 1493 } 1494 1495 /** 1496 * @return A link to further details on the result. 1497 */ 1498 public String getDetail() { 1499 return this.detail == null ? null : this.detail.getValue(); 1500 } 1501 1502 /** 1503 * @param value A link to further details on the result. 1504 */ 1505 public SetupActionOperationComponent setDetail(String value) { 1506 if (Utilities.noString(value)) 1507 this.detail = null; 1508 else { 1509 if (this.detail == null) 1510 this.detail = new UriType(); 1511 this.detail.setValue(value); 1512 } 1513 return this; 1514 } 1515 1516 protected void listChildren(List<Property> children) { 1517 super.listChildren(children); 1518 children.add(new Property("result", "code", "The result of this operation.", 0, 1, result)); 1519 children.add(new Property("message", "markdown", "An explanatory message associated with the result.", 0, 1, message)); 1520 children.add(new Property("detail", "uri", "A link to further details on the result.", 0, 1, detail)); 1521 } 1522 1523 @Override 1524 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1525 switch (_hash) { 1526 case -934426595: /*result*/ return new Property("result", "code", "The result of this operation.", 0, 1, result); 1527 case 954925063: /*message*/ return new Property("message", "markdown", "An explanatory message associated with the result.", 0, 1, message); 1528 case -1335224239: /*detail*/ return new Property("detail", "uri", "A link to further details on the result.", 0, 1, detail); 1529 default: return super.getNamedProperty(_hash, _name, _checkValid); 1530 } 1531 1532 } 1533 1534 @Override 1535 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1536 switch (hash) { 1537 case -934426595: /*result*/ return this.result == null ? new Base[0] : new Base[] {this.result}; // Enumeration<TestReportActionResult> 1538 case 954925063: /*message*/ return this.message == null ? new Base[0] : new Base[] {this.message}; // MarkdownType 1539 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : new Base[] {this.detail}; // UriType 1540 default: return super.getProperty(hash, name, checkValid); 1541 } 1542 1543 } 1544 1545 @Override 1546 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1547 switch (hash) { 1548 case -934426595: // result 1549 value = new TestReportActionResultEnumFactory().fromType(TypeConvertor.castToCode(value)); 1550 this.result = (Enumeration) value; // Enumeration<TestReportActionResult> 1551 return value; 1552 case 954925063: // message 1553 this.message = TypeConvertor.castToMarkdown(value); // MarkdownType 1554 return value; 1555 case -1335224239: // detail 1556 this.detail = TypeConvertor.castToUri(value); // UriType 1557 return value; 1558 default: return super.setProperty(hash, name, value); 1559 } 1560 1561 } 1562 1563 @Override 1564 public Base setProperty(String name, Base value) throws FHIRException { 1565 if (name.equals("result")) { 1566 value = new TestReportActionResultEnumFactory().fromType(TypeConvertor.castToCode(value)); 1567 this.result = (Enumeration) value; // Enumeration<TestReportActionResult> 1568 } else if (name.equals("message")) { 1569 this.message = TypeConvertor.castToMarkdown(value); // MarkdownType 1570 } else if (name.equals("detail")) { 1571 this.detail = TypeConvertor.castToUri(value); // UriType 1572 } else 1573 return super.setProperty(name, value); 1574 return value; 1575 } 1576 1577 @Override 1578 public void removeChild(String name, Base value) throws FHIRException { 1579 if (name.equals("result")) { 1580 value = new TestReportActionResultEnumFactory().fromType(TypeConvertor.castToCode(value)); 1581 this.result = (Enumeration) value; // Enumeration<TestReportActionResult> 1582 } else if (name.equals("message")) { 1583 this.message = null; 1584 } else if (name.equals("detail")) { 1585 this.detail = null; 1586 } else 1587 super.removeChild(name, value); 1588 1589 } 1590 1591 @Override 1592 public Base makeProperty(int hash, String name) throws FHIRException { 1593 switch (hash) { 1594 case -934426595: return getResultElement(); 1595 case 954925063: return getMessageElement(); 1596 case -1335224239: return getDetailElement(); 1597 default: return super.makeProperty(hash, name); 1598 } 1599 1600 } 1601 1602 @Override 1603 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1604 switch (hash) { 1605 case -934426595: /*result*/ return new String[] {"code"}; 1606 case 954925063: /*message*/ return new String[] {"markdown"}; 1607 case -1335224239: /*detail*/ return new String[] {"uri"}; 1608 default: return super.getTypesForProperty(hash, name); 1609 } 1610 1611 } 1612 1613 @Override 1614 public Base addChild(String name) throws FHIRException { 1615 if (name.equals("result")) { 1616 throw new FHIRException("Cannot call addChild on a singleton property TestReport.setup.action.operation.result"); 1617 } 1618 else if (name.equals("message")) { 1619 throw new FHIRException("Cannot call addChild on a singleton property TestReport.setup.action.operation.message"); 1620 } 1621 else if (name.equals("detail")) { 1622 throw new FHIRException("Cannot call addChild on a singleton property TestReport.setup.action.operation.detail"); 1623 } 1624 else 1625 return super.addChild(name); 1626 } 1627 1628 public SetupActionOperationComponent copy() { 1629 SetupActionOperationComponent dst = new SetupActionOperationComponent(); 1630 copyValues(dst); 1631 return dst; 1632 } 1633 1634 public void copyValues(SetupActionOperationComponent dst) { 1635 super.copyValues(dst); 1636 dst.result = result == null ? null : result.copy(); 1637 dst.message = message == null ? null : message.copy(); 1638 dst.detail = detail == null ? null : detail.copy(); 1639 } 1640 1641 @Override 1642 public boolean equalsDeep(Base other_) { 1643 if (!super.equalsDeep(other_)) 1644 return false; 1645 if (!(other_ instanceof SetupActionOperationComponent)) 1646 return false; 1647 SetupActionOperationComponent o = (SetupActionOperationComponent) other_; 1648 return compareDeep(result, o.result, true) && compareDeep(message, o.message, true) && compareDeep(detail, o.detail, true) 1649 ; 1650 } 1651 1652 @Override 1653 public boolean equalsShallow(Base other_) { 1654 if (!super.equalsShallow(other_)) 1655 return false; 1656 if (!(other_ instanceof SetupActionOperationComponent)) 1657 return false; 1658 SetupActionOperationComponent o = (SetupActionOperationComponent) other_; 1659 return compareValues(result, o.result, true) && compareValues(message, o.message, true) && compareValues(detail, o.detail, true) 1660 ; 1661 } 1662 1663 public boolean isEmpty() { 1664 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(result, message, detail 1665 ); 1666 } 1667 1668 public String fhirType() { 1669 return "TestReport.setup.action.operation"; 1670 1671 } 1672 1673 } 1674 1675 @Block() 1676 public static class SetupActionAssertComponent extends BackboneElement implements IBaseBackboneElement { 1677 /** 1678 * The result of this assertion. 1679 */ 1680 @Child(name = "result", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1681 @Description(shortDefinition="pass | skip | fail | warning | error", formalDefinition="The result of this assertion." ) 1682 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/report-action-result-codes") 1683 protected Enumeration<TestReportActionResult> result; 1684 1685 /** 1686 * An explanatory message associated with the result. 1687 */ 1688 @Child(name = "message", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1689 @Description(shortDefinition="A message associated with the result", formalDefinition="An explanatory message associated with the result." ) 1690 protected MarkdownType message; 1691 1692 /** 1693 * A link to further details on the result. 1694 */ 1695 @Child(name = "detail", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1696 @Description(shortDefinition="A link to further details on the result", formalDefinition="A link to further details on the result." ) 1697 protected StringType detail; 1698 1699 /** 1700 * Links or references providing traceability to the testing requirements for this assert. 1701 */ 1702 @Child(name = "requirement", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1703 @Description(shortDefinition="Links or references to the testing requirements", formalDefinition="Links or references providing traceability to the testing requirements for this assert." ) 1704 protected List<SetupActionAssertRequirementComponent> requirement; 1705 1706 private static final long serialVersionUID = -132618689L; 1707 1708 /** 1709 * Constructor 1710 */ 1711 public SetupActionAssertComponent() { 1712 super(); 1713 } 1714 1715 /** 1716 * Constructor 1717 */ 1718 public SetupActionAssertComponent(TestReportActionResult result) { 1719 super(); 1720 this.setResult(result); 1721 } 1722 1723 /** 1724 * @return {@link #result} (The result of this assertion.). This is the underlying object with id, value and extensions. The accessor "getResult" gives direct access to the value 1725 */ 1726 public Enumeration<TestReportActionResult> getResultElement() { 1727 if (this.result == null) 1728 if (Configuration.errorOnAutoCreate()) 1729 throw new Error("Attempt to auto-create SetupActionAssertComponent.result"); 1730 else if (Configuration.doAutoCreate()) 1731 this.result = new Enumeration<TestReportActionResult>(new TestReportActionResultEnumFactory()); // bb 1732 return this.result; 1733 } 1734 1735 public boolean hasResultElement() { 1736 return this.result != null && !this.result.isEmpty(); 1737 } 1738 1739 public boolean hasResult() { 1740 return this.result != null && !this.result.isEmpty(); 1741 } 1742 1743 /** 1744 * @param value {@link #result} (The result of this assertion.). This is the underlying object with id, value and extensions. The accessor "getResult" gives direct access to the value 1745 */ 1746 public SetupActionAssertComponent setResultElement(Enumeration<TestReportActionResult> value) { 1747 this.result = value; 1748 return this; 1749 } 1750 1751 /** 1752 * @return The result of this assertion. 1753 */ 1754 public TestReportActionResult getResult() { 1755 return this.result == null ? null : this.result.getValue(); 1756 } 1757 1758 /** 1759 * @param value The result of this assertion. 1760 */ 1761 public SetupActionAssertComponent setResult(TestReportActionResult value) { 1762 if (this.result == null) 1763 this.result = new Enumeration<TestReportActionResult>(new TestReportActionResultEnumFactory()); 1764 this.result.setValue(value); 1765 return this; 1766 } 1767 1768 /** 1769 * @return {@link #message} (An explanatory message associated with the result.). This is the underlying object with id, value and extensions. The accessor "getMessage" gives direct access to the value 1770 */ 1771 public MarkdownType getMessageElement() { 1772 if (this.message == null) 1773 if (Configuration.errorOnAutoCreate()) 1774 throw new Error("Attempt to auto-create SetupActionAssertComponent.message"); 1775 else if (Configuration.doAutoCreate()) 1776 this.message = new MarkdownType(); // bb 1777 return this.message; 1778 } 1779 1780 public boolean hasMessageElement() { 1781 return this.message != null && !this.message.isEmpty(); 1782 } 1783 1784 public boolean hasMessage() { 1785 return this.message != null && !this.message.isEmpty(); 1786 } 1787 1788 /** 1789 * @param value {@link #message} (An explanatory message associated with the result.). This is the underlying object with id, value and extensions. The accessor "getMessage" gives direct access to the value 1790 */ 1791 public SetupActionAssertComponent setMessageElement(MarkdownType value) { 1792 this.message = value; 1793 return this; 1794 } 1795 1796 /** 1797 * @return An explanatory message associated with the result. 1798 */ 1799 public String getMessage() { 1800 return this.message == null ? null : this.message.getValue(); 1801 } 1802 1803 /** 1804 * @param value An explanatory message associated with the result. 1805 */ 1806 public SetupActionAssertComponent setMessage(String value) { 1807 if (Utilities.noString(value)) 1808 this.message = null; 1809 else { 1810 if (this.message == null) 1811 this.message = new MarkdownType(); 1812 this.message.setValue(value); 1813 } 1814 return this; 1815 } 1816 1817 /** 1818 * @return {@link #detail} (A link to further details on the result.). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value 1819 */ 1820 public StringType getDetailElement() { 1821 if (this.detail == null) 1822 if (Configuration.errorOnAutoCreate()) 1823 throw new Error("Attempt to auto-create SetupActionAssertComponent.detail"); 1824 else if (Configuration.doAutoCreate()) 1825 this.detail = new StringType(); // bb 1826 return this.detail; 1827 } 1828 1829 public boolean hasDetailElement() { 1830 return this.detail != null && !this.detail.isEmpty(); 1831 } 1832 1833 public boolean hasDetail() { 1834 return this.detail != null && !this.detail.isEmpty(); 1835 } 1836 1837 /** 1838 * @param value {@link #detail} (A link to further details on the result.). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value 1839 */ 1840 public SetupActionAssertComponent setDetailElement(StringType value) { 1841 this.detail = value; 1842 return this; 1843 } 1844 1845 /** 1846 * @return A link to further details on the result. 1847 */ 1848 public String getDetail() { 1849 return this.detail == null ? null : this.detail.getValue(); 1850 } 1851 1852 /** 1853 * @param value A link to further details on the result. 1854 */ 1855 public SetupActionAssertComponent setDetail(String value) { 1856 if (Utilities.noString(value)) 1857 this.detail = null; 1858 else { 1859 if (this.detail == null) 1860 this.detail = new StringType(); 1861 this.detail.setValue(value); 1862 } 1863 return this; 1864 } 1865 1866 /** 1867 * @return {@link #requirement} (Links or references providing traceability to the testing requirements for this assert.) 1868 */ 1869 public List<SetupActionAssertRequirementComponent> getRequirement() { 1870 if (this.requirement == null) 1871 this.requirement = new ArrayList<SetupActionAssertRequirementComponent>(); 1872 return this.requirement; 1873 } 1874 1875 /** 1876 * @return Returns a reference to <code>this</code> for easy method chaining 1877 */ 1878 public SetupActionAssertComponent setRequirement(List<SetupActionAssertRequirementComponent> theRequirement) { 1879 this.requirement = theRequirement; 1880 return this; 1881 } 1882 1883 public boolean hasRequirement() { 1884 if (this.requirement == null) 1885 return false; 1886 for (SetupActionAssertRequirementComponent item : this.requirement) 1887 if (!item.isEmpty()) 1888 return true; 1889 return false; 1890 } 1891 1892 public SetupActionAssertRequirementComponent addRequirement() { //3 1893 SetupActionAssertRequirementComponent t = new SetupActionAssertRequirementComponent(); 1894 if (this.requirement == null) 1895 this.requirement = new ArrayList<SetupActionAssertRequirementComponent>(); 1896 this.requirement.add(t); 1897 return t; 1898 } 1899 1900 public SetupActionAssertComponent addRequirement(SetupActionAssertRequirementComponent t) { //3 1901 if (t == null) 1902 return this; 1903 if (this.requirement == null) 1904 this.requirement = new ArrayList<SetupActionAssertRequirementComponent>(); 1905 this.requirement.add(t); 1906 return this; 1907 } 1908 1909 /** 1910 * @return The first repetition of repeating field {@link #requirement}, creating it if it does not already exist {3} 1911 */ 1912 public SetupActionAssertRequirementComponent getRequirementFirstRep() { 1913 if (getRequirement().isEmpty()) { 1914 addRequirement(); 1915 } 1916 return getRequirement().get(0); 1917 } 1918 1919 protected void listChildren(List<Property> children) { 1920 super.listChildren(children); 1921 children.add(new Property("result", "code", "The result of this assertion.", 0, 1, result)); 1922 children.add(new Property("message", "markdown", "An explanatory message associated with the result.", 0, 1, message)); 1923 children.add(new Property("detail", "string", "A link to further details on the result.", 0, 1, detail)); 1924 children.add(new Property("requirement", "", "Links or references providing traceability to the testing requirements for this assert.", 0, java.lang.Integer.MAX_VALUE, requirement)); 1925 } 1926 1927 @Override 1928 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1929 switch (_hash) { 1930 case -934426595: /*result*/ return new Property("result", "code", "The result of this assertion.", 0, 1, result); 1931 case 954925063: /*message*/ return new Property("message", "markdown", "An explanatory message associated with the result.", 0, 1, message); 1932 case -1335224239: /*detail*/ return new Property("detail", "string", "A link to further details on the result.", 0, 1, detail); 1933 case 363387971: /*requirement*/ return new Property("requirement", "", "Links or references providing traceability to the testing requirements for this assert.", 0, java.lang.Integer.MAX_VALUE, requirement); 1934 default: return super.getNamedProperty(_hash, _name, _checkValid); 1935 } 1936 1937 } 1938 1939 @Override 1940 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1941 switch (hash) { 1942 case -934426595: /*result*/ return this.result == null ? new Base[0] : new Base[] {this.result}; // Enumeration<TestReportActionResult> 1943 case 954925063: /*message*/ return this.message == null ? new Base[0] : new Base[] {this.message}; // MarkdownType 1944 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : new Base[] {this.detail}; // StringType 1945 case 363387971: /*requirement*/ return this.requirement == null ? new Base[0] : this.requirement.toArray(new Base[this.requirement.size()]); // SetupActionAssertRequirementComponent 1946 default: return super.getProperty(hash, name, checkValid); 1947 } 1948 1949 } 1950 1951 @Override 1952 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1953 switch (hash) { 1954 case -934426595: // result 1955 value = new TestReportActionResultEnumFactory().fromType(TypeConvertor.castToCode(value)); 1956 this.result = (Enumeration) value; // Enumeration<TestReportActionResult> 1957 return value; 1958 case 954925063: // message 1959 this.message = TypeConvertor.castToMarkdown(value); // MarkdownType 1960 return value; 1961 case -1335224239: // detail 1962 this.detail = TypeConvertor.castToString(value); // StringType 1963 return value; 1964 case 363387971: // requirement 1965 this.getRequirement().add((SetupActionAssertRequirementComponent) value); // SetupActionAssertRequirementComponent 1966 return value; 1967 default: return super.setProperty(hash, name, value); 1968 } 1969 1970 } 1971 1972 @Override 1973 public Base setProperty(String name, Base value) throws FHIRException { 1974 if (name.equals("result")) { 1975 value = new TestReportActionResultEnumFactory().fromType(TypeConvertor.castToCode(value)); 1976 this.result = (Enumeration) value; // Enumeration<TestReportActionResult> 1977 } else if (name.equals("message")) { 1978 this.message = TypeConvertor.castToMarkdown(value); // MarkdownType 1979 } else if (name.equals("detail")) { 1980 this.detail = TypeConvertor.castToString(value); // StringType 1981 } else if (name.equals("requirement")) { 1982 this.getRequirement().add((SetupActionAssertRequirementComponent) value); 1983 } else 1984 return super.setProperty(name, value); 1985 return value; 1986 } 1987 1988 @Override 1989 public void removeChild(String name, Base value) throws FHIRException { 1990 if (name.equals("result")) { 1991 value = new TestReportActionResultEnumFactory().fromType(TypeConvertor.castToCode(value)); 1992 this.result = (Enumeration) value; // Enumeration<TestReportActionResult> 1993 } else if (name.equals("message")) { 1994 this.message = null; 1995 } else if (name.equals("detail")) { 1996 this.detail = null; 1997 } else if (name.equals("requirement")) { 1998 this.getRequirement().remove((SetupActionAssertRequirementComponent) value); 1999 } else 2000 super.removeChild(name, value); 2001 2002 } 2003 2004 @Override 2005 public Base makeProperty(int hash, String name) throws FHIRException { 2006 switch (hash) { 2007 case -934426595: return getResultElement(); 2008 case 954925063: return getMessageElement(); 2009 case -1335224239: return getDetailElement(); 2010 case 363387971: return addRequirement(); 2011 default: return super.makeProperty(hash, name); 2012 } 2013 2014 } 2015 2016 @Override 2017 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2018 switch (hash) { 2019 case -934426595: /*result*/ return new String[] {"code"}; 2020 case 954925063: /*message*/ return new String[] {"markdown"}; 2021 case -1335224239: /*detail*/ return new String[] {"string"}; 2022 case 363387971: /*requirement*/ return new String[] {}; 2023 default: return super.getTypesForProperty(hash, name); 2024 } 2025 2026 } 2027 2028 @Override 2029 public Base addChild(String name) throws FHIRException { 2030 if (name.equals("result")) { 2031 throw new FHIRException("Cannot call addChild on a singleton property TestReport.setup.action.assert.result"); 2032 } 2033 else if (name.equals("message")) { 2034 throw new FHIRException("Cannot call addChild on a singleton property TestReport.setup.action.assert.message"); 2035 } 2036 else if (name.equals("detail")) { 2037 throw new FHIRException("Cannot call addChild on a singleton property TestReport.setup.action.assert.detail"); 2038 } 2039 else if (name.equals("requirement")) { 2040 return addRequirement(); 2041 } 2042 else 2043 return super.addChild(name); 2044 } 2045 2046 public SetupActionAssertComponent copy() { 2047 SetupActionAssertComponent dst = new SetupActionAssertComponent(); 2048 copyValues(dst); 2049 return dst; 2050 } 2051 2052 public void copyValues(SetupActionAssertComponent dst) { 2053 super.copyValues(dst); 2054 dst.result = result == null ? null : result.copy(); 2055 dst.message = message == null ? null : message.copy(); 2056 dst.detail = detail == null ? null : detail.copy(); 2057 if (requirement != null) { 2058 dst.requirement = new ArrayList<SetupActionAssertRequirementComponent>(); 2059 for (SetupActionAssertRequirementComponent i : requirement) 2060 dst.requirement.add(i.copy()); 2061 }; 2062 } 2063 2064 @Override 2065 public boolean equalsDeep(Base other_) { 2066 if (!super.equalsDeep(other_)) 2067 return false; 2068 if (!(other_ instanceof SetupActionAssertComponent)) 2069 return false; 2070 SetupActionAssertComponent o = (SetupActionAssertComponent) other_; 2071 return compareDeep(result, o.result, true) && compareDeep(message, o.message, true) && compareDeep(detail, o.detail, true) 2072 && compareDeep(requirement, o.requirement, true); 2073 } 2074 2075 @Override 2076 public boolean equalsShallow(Base other_) { 2077 if (!super.equalsShallow(other_)) 2078 return false; 2079 if (!(other_ instanceof SetupActionAssertComponent)) 2080 return false; 2081 SetupActionAssertComponent o = (SetupActionAssertComponent) other_; 2082 return compareValues(result, o.result, true) && compareValues(message, o.message, true) && compareValues(detail, o.detail, true) 2083 ; 2084 } 2085 2086 public boolean isEmpty() { 2087 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(result, message, detail 2088 , requirement); 2089 } 2090 2091 public String fhirType() { 2092 return "TestReport.setup.action.assert"; 2093 2094 } 2095 2096 } 2097 2098 @Block() 2099 public static class SetupActionAssertRequirementComponent extends BackboneElement implements IBaseBackboneElement { 2100 /** 2101 * Link or reference providing traceability to the testing requirement for this test. 2102 */ 2103 @Child(name = "link", type = {UriType.class, CanonicalType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2104 @Description(shortDefinition="Link or reference to the testing requirement", formalDefinition="Link or reference providing traceability to the testing requirement for this test." ) 2105 protected DataType link; 2106 2107 private static final long serialVersionUID = -91187948L; 2108 2109 /** 2110 * Constructor 2111 */ 2112 public SetupActionAssertRequirementComponent() { 2113 super(); 2114 } 2115 2116 /** 2117 * @return {@link #link} (Link or reference providing traceability to the testing requirement for this test.) 2118 */ 2119 public DataType getLink() { 2120 return this.link; 2121 } 2122 2123 /** 2124 * @return {@link #link} (Link or reference providing traceability to the testing requirement for this test.) 2125 */ 2126 public UriType getLinkUriType() throws FHIRException { 2127 if (this.link == null) 2128 this.link = new UriType(); 2129 if (!(this.link instanceof UriType)) 2130 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.link.getClass().getName()+" was encountered"); 2131 return (UriType) this.link; 2132 } 2133 2134 public boolean hasLinkUriType() { 2135 return this != null && this.link instanceof UriType; 2136 } 2137 2138 /** 2139 * @return {@link #link} (Link or reference providing traceability to the testing requirement for this test.) 2140 */ 2141 public CanonicalType getLinkCanonicalType() throws FHIRException { 2142 if (this.link == null) 2143 this.link = new CanonicalType(); 2144 if (!(this.link instanceof CanonicalType)) 2145 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.link.getClass().getName()+" was encountered"); 2146 return (CanonicalType) this.link; 2147 } 2148 2149 public boolean hasLinkCanonicalType() { 2150 return this != null && this.link instanceof CanonicalType; 2151 } 2152 2153 public boolean hasLink() { 2154 return this.link != null && !this.link.isEmpty(); 2155 } 2156 2157 /** 2158 * @param value {@link #link} (Link or reference providing traceability to the testing requirement for this test.) 2159 */ 2160 public SetupActionAssertRequirementComponent setLink(DataType value) { 2161 if (value != null && !(value instanceof UriType || value instanceof CanonicalType)) 2162 throw new FHIRException("Not the right type for TestReport.setup.action.assert.requirement.link[x]: "+value.fhirType()); 2163 this.link = value; 2164 return this; 2165 } 2166 2167 protected void listChildren(List<Property> children) { 2168 super.listChildren(children); 2169 children.add(new Property("link[x]", "uri|canonical(Requirements)", "Link or reference providing traceability to the testing requirement for this test.", 0, 1, link)); 2170 } 2171 2172 @Override 2173 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2174 switch (_hash) { 2175 case 177076806: /*link[x]*/ return new Property("link[x]", "uri|canonical(Requirements)", "Link or reference providing traceability to the testing requirement for this test.", 0, 1, link); 2176 case 3321850: /*link*/ return new Property("link[x]", "uri|canonical(Requirements)", "Link or reference providing traceability to the testing requirement for this test.", 0, 1, link); 2177 case 177070866: /*linkUri*/ return new Property("link[x]", "uri", "Link or reference providing traceability to the testing requirement for this test.", 0, 1, link); 2178 case -2064880102: /*linkCanonical*/ return new Property("link[x]", "canonical(Requirements)", "Link or reference providing traceability to the testing requirement for this test.", 0, 1, link); 2179 default: return super.getNamedProperty(_hash, _name, _checkValid); 2180 } 2181 2182 } 2183 2184 @Override 2185 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2186 switch (hash) { 2187 case 3321850: /*link*/ return this.link == null ? new Base[0] : new Base[] {this.link}; // DataType 2188 default: return super.getProperty(hash, name, checkValid); 2189 } 2190 2191 } 2192 2193 @Override 2194 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2195 switch (hash) { 2196 case 3321850: // link 2197 this.link = TypeConvertor.castToType(value); // DataType 2198 return value; 2199 default: return super.setProperty(hash, name, value); 2200 } 2201 2202 } 2203 2204 @Override 2205 public Base setProperty(String name, Base value) throws FHIRException { 2206 if (name.equals("link[x]")) { 2207 this.link = TypeConvertor.castToType(value); // DataType 2208 } else 2209 return super.setProperty(name, value); 2210 return value; 2211 } 2212 2213 @Override 2214 public void removeChild(String name, Base value) throws FHIRException { 2215 if (name.equals("link[x]")) { 2216 this.link = null; 2217 } else 2218 super.removeChild(name, value); 2219 2220 } 2221 2222 @Override 2223 public Base makeProperty(int hash, String name) throws FHIRException { 2224 switch (hash) { 2225 case 177076806: return getLink(); 2226 case 3321850: return getLink(); 2227 default: return super.makeProperty(hash, name); 2228 } 2229 2230 } 2231 2232 @Override 2233 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2234 switch (hash) { 2235 case 3321850: /*link*/ return new String[] {"uri", "canonical"}; 2236 default: return super.getTypesForProperty(hash, name); 2237 } 2238 2239 } 2240 2241 @Override 2242 public Base addChild(String name) throws FHIRException { 2243 if (name.equals("linkUri")) { 2244 this.link = new UriType(); 2245 return this.link; 2246 } 2247 else if (name.equals("linkCanonical")) { 2248 this.link = new CanonicalType(); 2249 return this.link; 2250 } 2251 else 2252 return super.addChild(name); 2253 } 2254 2255 public SetupActionAssertRequirementComponent copy() { 2256 SetupActionAssertRequirementComponent dst = new SetupActionAssertRequirementComponent(); 2257 copyValues(dst); 2258 return dst; 2259 } 2260 2261 public void copyValues(SetupActionAssertRequirementComponent dst) { 2262 super.copyValues(dst); 2263 dst.link = link == null ? null : link.copy(); 2264 } 2265 2266 @Override 2267 public boolean equalsDeep(Base other_) { 2268 if (!super.equalsDeep(other_)) 2269 return false; 2270 if (!(other_ instanceof SetupActionAssertRequirementComponent)) 2271 return false; 2272 SetupActionAssertRequirementComponent o = (SetupActionAssertRequirementComponent) other_; 2273 return compareDeep(link, o.link, true); 2274 } 2275 2276 @Override 2277 public boolean equalsShallow(Base other_) { 2278 if (!super.equalsShallow(other_)) 2279 return false; 2280 if (!(other_ instanceof SetupActionAssertRequirementComponent)) 2281 return false; 2282 SetupActionAssertRequirementComponent o = (SetupActionAssertRequirementComponent) other_; 2283 return true; 2284 } 2285 2286 public boolean isEmpty() { 2287 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(link); 2288 } 2289 2290 public String fhirType() { 2291 return "TestReport.setup.action.assert.requirement"; 2292 2293 } 2294 2295 } 2296 2297 @Block() 2298 public static class TestReportTestComponent extends BackboneElement implements IBaseBackboneElement { 2299 /** 2300 * The name of this test used for tracking/logging purposes by test engines. 2301 */ 2302 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2303 @Description(shortDefinition="Tracking/logging name of this test", formalDefinition="The name of this test used for tracking/logging purposes by test engines." ) 2304 protected StringType name; 2305 2306 /** 2307 * A short description of the test used by test engines for tracking and reporting purposes. 2308 */ 2309 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2310 @Description(shortDefinition="Tracking/reporting short description of the test", formalDefinition="A short description of the test used by test engines for tracking and reporting purposes." ) 2311 protected StringType description; 2312 2313 /** 2314 * Action would contain either an operation or an assertion. 2315 */ 2316 @Child(name = "action", type = {}, order=3, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2317 @Description(shortDefinition="A test operation or assert that was performed", formalDefinition="Action would contain either an operation or an assertion." ) 2318 protected List<TestActionComponent> action; 2319 2320 private static final long serialVersionUID = -865006110L; 2321 2322 /** 2323 * Constructor 2324 */ 2325 public TestReportTestComponent() { 2326 super(); 2327 } 2328 2329 /** 2330 * Constructor 2331 */ 2332 public TestReportTestComponent(TestActionComponent action) { 2333 super(); 2334 this.addAction(action); 2335 } 2336 2337 /** 2338 * @return {@link #name} (The name of this test used for tracking/logging purposes by test engines.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2339 */ 2340 public StringType getNameElement() { 2341 if (this.name == null) 2342 if (Configuration.errorOnAutoCreate()) 2343 throw new Error("Attempt to auto-create TestReportTestComponent.name"); 2344 else if (Configuration.doAutoCreate()) 2345 this.name = new StringType(); // bb 2346 return this.name; 2347 } 2348 2349 public boolean hasNameElement() { 2350 return this.name != null && !this.name.isEmpty(); 2351 } 2352 2353 public boolean hasName() { 2354 return this.name != null && !this.name.isEmpty(); 2355 } 2356 2357 /** 2358 * @param value {@link #name} (The name of this test used for tracking/logging purposes by test engines.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2359 */ 2360 public TestReportTestComponent setNameElement(StringType value) { 2361 this.name = value; 2362 return this; 2363 } 2364 2365 /** 2366 * @return The name of this test used for tracking/logging purposes by test engines. 2367 */ 2368 public String getName() { 2369 return this.name == null ? null : this.name.getValue(); 2370 } 2371 2372 /** 2373 * @param value The name of this test used for tracking/logging purposes by test engines. 2374 */ 2375 public TestReportTestComponent setName(String value) { 2376 if (Utilities.noString(value)) 2377 this.name = null; 2378 else { 2379 if (this.name == null) 2380 this.name = new StringType(); 2381 this.name.setValue(value); 2382 } 2383 return this; 2384 } 2385 2386 /** 2387 * @return {@link #description} (A short description of the test used by test engines for tracking and reporting purposes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2388 */ 2389 public StringType getDescriptionElement() { 2390 if (this.description == null) 2391 if (Configuration.errorOnAutoCreate()) 2392 throw new Error("Attempt to auto-create TestReportTestComponent.description"); 2393 else if (Configuration.doAutoCreate()) 2394 this.description = new StringType(); // bb 2395 return this.description; 2396 } 2397 2398 public boolean hasDescriptionElement() { 2399 return this.description != null && !this.description.isEmpty(); 2400 } 2401 2402 public boolean hasDescription() { 2403 return this.description != null && !this.description.isEmpty(); 2404 } 2405 2406 /** 2407 * @param value {@link #description} (A short description of the test used by test engines for tracking and reporting purposes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2408 */ 2409 public TestReportTestComponent setDescriptionElement(StringType value) { 2410 this.description = value; 2411 return this; 2412 } 2413 2414 /** 2415 * @return A short description of the test used by test engines for tracking and reporting purposes. 2416 */ 2417 public String getDescription() { 2418 return this.description == null ? null : this.description.getValue(); 2419 } 2420 2421 /** 2422 * @param value A short description of the test used by test engines for tracking and reporting purposes. 2423 */ 2424 public TestReportTestComponent setDescription(String value) { 2425 if (Utilities.noString(value)) 2426 this.description = null; 2427 else { 2428 if (this.description == null) 2429 this.description = new StringType(); 2430 this.description.setValue(value); 2431 } 2432 return this; 2433 } 2434 2435 /** 2436 * @return {@link #action} (Action would contain either an operation or an assertion.) 2437 */ 2438 public List<TestActionComponent> getAction() { 2439 if (this.action == null) 2440 this.action = new ArrayList<TestActionComponent>(); 2441 return this.action; 2442 } 2443 2444 /** 2445 * @return Returns a reference to <code>this</code> for easy method chaining 2446 */ 2447 public TestReportTestComponent setAction(List<TestActionComponent> theAction) { 2448 this.action = theAction; 2449 return this; 2450 } 2451 2452 public boolean hasAction() { 2453 if (this.action == null) 2454 return false; 2455 for (TestActionComponent item : this.action) 2456 if (!item.isEmpty()) 2457 return true; 2458 return false; 2459 } 2460 2461 public TestActionComponent addAction() { //3 2462 TestActionComponent t = new TestActionComponent(); 2463 if (this.action == null) 2464 this.action = new ArrayList<TestActionComponent>(); 2465 this.action.add(t); 2466 return t; 2467 } 2468 2469 public TestReportTestComponent addAction(TestActionComponent t) { //3 2470 if (t == null) 2471 return this; 2472 if (this.action == null) 2473 this.action = new ArrayList<TestActionComponent>(); 2474 this.action.add(t); 2475 return this; 2476 } 2477 2478 /** 2479 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist {3} 2480 */ 2481 public TestActionComponent getActionFirstRep() { 2482 if (getAction().isEmpty()) { 2483 addAction(); 2484 } 2485 return getAction().get(0); 2486 } 2487 2488 protected void listChildren(List<Property> children) { 2489 super.listChildren(children); 2490 children.add(new Property("name", "string", "The name of this test used for tracking/logging purposes by test engines.", 0, 1, name)); 2491 children.add(new Property("description", "string", "A short description of the test used by test engines for tracking and reporting purposes.", 0, 1, description)); 2492 children.add(new Property("action", "", "Action would contain either an operation or an assertion.", 0, java.lang.Integer.MAX_VALUE, action)); 2493 } 2494 2495 @Override 2496 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2497 switch (_hash) { 2498 case 3373707: /*name*/ return new Property("name", "string", "The name of this test used for tracking/logging purposes by test engines.", 0, 1, name); 2499 case -1724546052: /*description*/ return new Property("description", "string", "A short description of the test used by test engines for tracking and reporting purposes.", 0, 1, description); 2500 case -1422950858: /*action*/ return new Property("action", "", "Action would contain either an operation or an assertion.", 0, java.lang.Integer.MAX_VALUE, action); 2501 default: return super.getNamedProperty(_hash, _name, _checkValid); 2502 } 2503 2504 } 2505 2506 @Override 2507 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2508 switch (hash) { 2509 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2510 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 2511 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // TestActionComponent 2512 default: return super.getProperty(hash, name, checkValid); 2513 } 2514 2515 } 2516 2517 @Override 2518 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2519 switch (hash) { 2520 case 3373707: // name 2521 this.name = TypeConvertor.castToString(value); // StringType 2522 return value; 2523 case -1724546052: // description 2524 this.description = TypeConvertor.castToString(value); // StringType 2525 return value; 2526 case -1422950858: // action 2527 this.getAction().add((TestActionComponent) value); // TestActionComponent 2528 return value; 2529 default: return super.setProperty(hash, name, value); 2530 } 2531 2532 } 2533 2534 @Override 2535 public Base setProperty(String name, Base value) throws FHIRException { 2536 if (name.equals("name")) { 2537 this.name = TypeConvertor.castToString(value); // StringType 2538 } else if (name.equals("description")) { 2539 this.description = TypeConvertor.castToString(value); // StringType 2540 } else if (name.equals("action")) { 2541 this.getAction().add((TestActionComponent) value); 2542 } else 2543 return super.setProperty(name, value); 2544 return value; 2545 } 2546 2547 @Override 2548 public void removeChild(String name, Base value) throws FHIRException { 2549 if (name.equals("name")) { 2550 this.name = null; 2551 } else if (name.equals("description")) { 2552 this.description = null; 2553 } else if (name.equals("action")) { 2554 this.getAction().remove((TestActionComponent) value); 2555 } else 2556 super.removeChild(name, value); 2557 2558 } 2559 2560 @Override 2561 public Base makeProperty(int hash, String name) throws FHIRException { 2562 switch (hash) { 2563 case 3373707: return getNameElement(); 2564 case -1724546052: return getDescriptionElement(); 2565 case -1422950858: return addAction(); 2566 default: return super.makeProperty(hash, name); 2567 } 2568 2569 } 2570 2571 @Override 2572 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2573 switch (hash) { 2574 case 3373707: /*name*/ return new String[] {"string"}; 2575 case -1724546052: /*description*/ return new String[] {"string"}; 2576 case -1422950858: /*action*/ return new String[] {}; 2577 default: return super.getTypesForProperty(hash, name); 2578 } 2579 2580 } 2581 2582 @Override 2583 public Base addChild(String name) throws FHIRException { 2584 if (name.equals("name")) { 2585 throw new FHIRException("Cannot call addChild on a singleton property TestReport.test.name"); 2586 } 2587 else if (name.equals("description")) { 2588 throw new FHIRException("Cannot call addChild on a singleton property TestReport.test.description"); 2589 } 2590 else if (name.equals("action")) { 2591 return addAction(); 2592 } 2593 else 2594 return super.addChild(name); 2595 } 2596 2597 public TestReportTestComponent copy() { 2598 TestReportTestComponent dst = new TestReportTestComponent(); 2599 copyValues(dst); 2600 return dst; 2601 } 2602 2603 public void copyValues(TestReportTestComponent dst) { 2604 super.copyValues(dst); 2605 dst.name = name == null ? null : name.copy(); 2606 dst.description = description == null ? null : description.copy(); 2607 if (action != null) { 2608 dst.action = new ArrayList<TestActionComponent>(); 2609 for (TestActionComponent i : action) 2610 dst.action.add(i.copy()); 2611 }; 2612 } 2613 2614 @Override 2615 public boolean equalsDeep(Base other_) { 2616 if (!super.equalsDeep(other_)) 2617 return false; 2618 if (!(other_ instanceof TestReportTestComponent)) 2619 return false; 2620 TestReportTestComponent o = (TestReportTestComponent) other_; 2621 return compareDeep(name, o.name, true) && compareDeep(description, o.description, true) && compareDeep(action, o.action, true) 2622 ; 2623 } 2624 2625 @Override 2626 public boolean equalsShallow(Base other_) { 2627 if (!super.equalsShallow(other_)) 2628 return false; 2629 if (!(other_ instanceof TestReportTestComponent)) 2630 return false; 2631 TestReportTestComponent o = (TestReportTestComponent) other_; 2632 return compareValues(name, o.name, true) && compareValues(description, o.description, true); 2633 } 2634 2635 public boolean isEmpty() { 2636 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, description, action 2637 ); 2638 } 2639 2640 public String fhirType() { 2641 return "TestReport.test"; 2642 2643 } 2644 2645 } 2646 2647 @Block() 2648 public static class TestActionComponent extends BackboneElement implements IBaseBackboneElement { 2649 /** 2650 * An operation would involve a REST request to a server. 2651 */ 2652 @Child(name = "operation", type = {SetupActionOperationComponent.class}, order=1, min=0, max=1, modifier=false, summary=false) 2653 @Description(shortDefinition="The operation performed", formalDefinition="An operation would involve a REST request to a server." ) 2654 protected SetupActionOperationComponent operation; 2655 2656 /** 2657 * The results of the assertion performed on the previous operations. 2658 */ 2659 @Child(name = "assert", type = {SetupActionAssertComponent.class}, order=2, min=0, max=1, modifier=false, summary=false) 2660 @Description(shortDefinition="The assertion performed", formalDefinition="The results of the assertion performed on the previous operations." ) 2661 protected SetupActionAssertComponent assert_; 2662 2663 private static final long serialVersionUID = -252088305L; 2664 2665 /** 2666 * Constructor 2667 */ 2668 public TestActionComponent() { 2669 super(); 2670 } 2671 2672 /** 2673 * @return {@link #operation} (An operation would involve a REST request to a server.) 2674 */ 2675 public SetupActionOperationComponent getOperation() { 2676 if (this.operation == null) 2677 if (Configuration.errorOnAutoCreate()) 2678 throw new Error("Attempt to auto-create TestActionComponent.operation"); 2679 else if (Configuration.doAutoCreate()) 2680 this.operation = new SetupActionOperationComponent(); // cc 2681 return this.operation; 2682 } 2683 2684 public boolean hasOperation() { 2685 return this.operation != null && !this.operation.isEmpty(); 2686 } 2687 2688 /** 2689 * @param value {@link #operation} (An operation would involve a REST request to a server.) 2690 */ 2691 public TestActionComponent setOperation(SetupActionOperationComponent value) { 2692 this.operation = value; 2693 return this; 2694 } 2695 2696 /** 2697 * @return {@link #assert_} (The results of the assertion performed on the previous operations.) 2698 */ 2699 public SetupActionAssertComponent getAssert() { 2700 if (this.assert_ == null) 2701 if (Configuration.errorOnAutoCreate()) 2702 throw new Error("Attempt to auto-create TestActionComponent.assert_"); 2703 else if (Configuration.doAutoCreate()) 2704 this.assert_ = new SetupActionAssertComponent(); // cc 2705 return this.assert_; 2706 } 2707 2708 public boolean hasAssert() { 2709 return this.assert_ != null && !this.assert_.isEmpty(); 2710 } 2711 2712 /** 2713 * @param value {@link #assert_} (The results of the assertion performed on the previous operations.) 2714 */ 2715 public TestActionComponent setAssert(SetupActionAssertComponent value) { 2716 this.assert_ = value; 2717 return this; 2718 } 2719 2720 protected void listChildren(List<Property> children) { 2721 super.listChildren(children); 2722 children.add(new Property("operation", "@TestReport.setup.action.operation", "An operation would involve a REST request to a server.", 0, 1, operation)); 2723 children.add(new Property("assert", "@TestReport.setup.action.assert", "The results of the assertion performed on the previous operations.", 0, 1, assert_)); 2724 } 2725 2726 @Override 2727 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2728 switch (_hash) { 2729 case 1662702951: /*operation*/ return new Property("operation", "@TestReport.setup.action.operation", "An operation would involve a REST request to a server.", 0, 1, operation); 2730 case -1408208058: /*assert*/ return new Property("assert", "@TestReport.setup.action.assert", "The results of the assertion performed on the previous operations.", 0, 1, assert_); 2731 default: return super.getNamedProperty(_hash, _name, _checkValid); 2732 } 2733 2734 } 2735 2736 @Override 2737 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2738 switch (hash) { 2739 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : new Base[] {this.operation}; // SetupActionOperationComponent 2740 case -1408208058: /*assert*/ return this.assert_ == null ? new Base[0] : new Base[] {this.assert_}; // SetupActionAssertComponent 2741 default: return super.getProperty(hash, name, checkValid); 2742 } 2743 2744 } 2745 2746 @Override 2747 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2748 switch (hash) { 2749 case 1662702951: // operation 2750 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 2751 return value; 2752 case -1408208058: // assert 2753 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 2754 return value; 2755 default: return super.setProperty(hash, name, value); 2756 } 2757 2758 } 2759 2760 @Override 2761 public Base setProperty(String name, Base value) throws FHIRException { 2762 if (name.equals("operation")) { 2763 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 2764 } else if (name.equals("assert")) { 2765 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 2766 } else 2767 return super.setProperty(name, value); 2768 return value; 2769 } 2770 2771 @Override 2772 public void removeChild(String name, Base value) throws FHIRException { 2773 if (name.equals("operation")) { 2774 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 2775 } else if (name.equals("assert")) { 2776 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 2777 } else 2778 super.removeChild(name, value); 2779 2780 } 2781 2782 @Override 2783 public Base makeProperty(int hash, String name) throws FHIRException { 2784 switch (hash) { 2785 case 1662702951: return getOperation(); 2786 case -1408208058: return getAssert(); 2787 default: return super.makeProperty(hash, name); 2788 } 2789 2790 } 2791 2792 @Override 2793 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2794 switch (hash) { 2795 case 1662702951: /*operation*/ return new String[] {"@TestReport.setup.action.operation"}; 2796 case -1408208058: /*assert*/ return new String[] {"@TestReport.setup.action.assert"}; 2797 default: return super.getTypesForProperty(hash, name); 2798 } 2799 2800 } 2801 2802 @Override 2803 public Base addChild(String name) throws FHIRException { 2804 if (name.equals("operation")) { 2805 this.operation = new SetupActionOperationComponent(); 2806 return this.operation; 2807 } 2808 else if (name.equals("assert")) { 2809 this.assert_ = new SetupActionAssertComponent(); 2810 return this.assert_; 2811 } 2812 else 2813 return super.addChild(name); 2814 } 2815 2816 public TestActionComponent copy() { 2817 TestActionComponent dst = new TestActionComponent(); 2818 copyValues(dst); 2819 return dst; 2820 } 2821 2822 public void copyValues(TestActionComponent dst) { 2823 super.copyValues(dst); 2824 dst.operation = operation == null ? null : operation.copy(); 2825 dst.assert_ = assert_ == null ? null : assert_.copy(); 2826 } 2827 2828 @Override 2829 public boolean equalsDeep(Base other_) { 2830 if (!super.equalsDeep(other_)) 2831 return false; 2832 if (!(other_ instanceof TestActionComponent)) 2833 return false; 2834 TestActionComponent o = (TestActionComponent) other_; 2835 return compareDeep(operation, o.operation, true) && compareDeep(assert_, o.assert_, true); 2836 } 2837 2838 @Override 2839 public boolean equalsShallow(Base other_) { 2840 if (!super.equalsShallow(other_)) 2841 return false; 2842 if (!(other_ instanceof TestActionComponent)) 2843 return false; 2844 TestActionComponent o = (TestActionComponent) other_; 2845 return true; 2846 } 2847 2848 public boolean isEmpty() { 2849 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation, assert_); 2850 } 2851 2852 public String fhirType() { 2853 return "TestReport.test.action"; 2854 2855 } 2856 2857 } 2858 2859 @Block() 2860 public static class TestReportTeardownComponent extends BackboneElement implements IBaseBackboneElement { 2861 /** 2862 * The teardown action will only contain an operation. 2863 */ 2864 @Child(name = "action", type = {}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2865 @Description(shortDefinition="One or more teardown operations performed", formalDefinition="The teardown action will only contain an operation." ) 2866 protected List<TeardownActionComponent> action; 2867 2868 private static final long serialVersionUID = 1168638089L; 2869 2870 /** 2871 * Constructor 2872 */ 2873 public TestReportTeardownComponent() { 2874 super(); 2875 } 2876 2877 /** 2878 * Constructor 2879 */ 2880 public TestReportTeardownComponent(TeardownActionComponent action) { 2881 super(); 2882 this.addAction(action); 2883 } 2884 2885 /** 2886 * @return {@link #action} (The teardown action will only contain an operation.) 2887 */ 2888 public List<TeardownActionComponent> getAction() { 2889 if (this.action == null) 2890 this.action = new ArrayList<TeardownActionComponent>(); 2891 return this.action; 2892 } 2893 2894 /** 2895 * @return Returns a reference to <code>this</code> for easy method chaining 2896 */ 2897 public TestReportTeardownComponent setAction(List<TeardownActionComponent> theAction) { 2898 this.action = theAction; 2899 return this; 2900 } 2901 2902 public boolean hasAction() { 2903 if (this.action == null) 2904 return false; 2905 for (TeardownActionComponent item : this.action) 2906 if (!item.isEmpty()) 2907 return true; 2908 return false; 2909 } 2910 2911 public TeardownActionComponent addAction() { //3 2912 TeardownActionComponent t = new TeardownActionComponent(); 2913 if (this.action == null) 2914 this.action = new ArrayList<TeardownActionComponent>(); 2915 this.action.add(t); 2916 return t; 2917 } 2918 2919 public TestReportTeardownComponent addAction(TeardownActionComponent t) { //3 2920 if (t == null) 2921 return this; 2922 if (this.action == null) 2923 this.action = new ArrayList<TeardownActionComponent>(); 2924 this.action.add(t); 2925 return this; 2926 } 2927 2928 /** 2929 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist {3} 2930 */ 2931 public TeardownActionComponent getActionFirstRep() { 2932 if (getAction().isEmpty()) { 2933 addAction(); 2934 } 2935 return getAction().get(0); 2936 } 2937 2938 protected void listChildren(List<Property> children) { 2939 super.listChildren(children); 2940 children.add(new Property("action", "", "The teardown action will only contain an operation.", 0, java.lang.Integer.MAX_VALUE, action)); 2941 } 2942 2943 @Override 2944 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2945 switch (_hash) { 2946 case -1422950858: /*action*/ return new Property("action", "", "The teardown action will only contain an operation.", 0, java.lang.Integer.MAX_VALUE, action); 2947 default: return super.getNamedProperty(_hash, _name, _checkValid); 2948 } 2949 2950 } 2951 2952 @Override 2953 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2954 switch (hash) { 2955 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // TeardownActionComponent 2956 default: return super.getProperty(hash, name, checkValid); 2957 } 2958 2959 } 2960 2961 @Override 2962 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2963 switch (hash) { 2964 case -1422950858: // action 2965 this.getAction().add((TeardownActionComponent) value); // TeardownActionComponent 2966 return value; 2967 default: return super.setProperty(hash, name, value); 2968 } 2969 2970 } 2971 2972 @Override 2973 public Base setProperty(String name, Base value) throws FHIRException { 2974 if (name.equals("action")) { 2975 this.getAction().add((TeardownActionComponent) value); 2976 } else 2977 return super.setProperty(name, value); 2978 return value; 2979 } 2980 2981 @Override 2982 public void removeChild(String name, Base value) throws FHIRException { 2983 if (name.equals("action")) { 2984 this.getAction().remove((TeardownActionComponent) value); 2985 } else 2986 super.removeChild(name, value); 2987 2988 } 2989 2990 @Override 2991 public Base makeProperty(int hash, String name) throws FHIRException { 2992 switch (hash) { 2993 case -1422950858: return addAction(); 2994 default: return super.makeProperty(hash, name); 2995 } 2996 2997 } 2998 2999 @Override 3000 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3001 switch (hash) { 3002 case -1422950858: /*action*/ return new String[] {}; 3003 default: return super.getTypesForProperty(hash, name); 3004 } 3005 3006 } 3007 3008 @Override 3009 public Base addChild(String name) throws FHIRException { 3010 if (name.equals("action")) { 3011 return addAction(); 3012 } 3013 else 3014 return super.addChild(name); 3015 } 3016 3017 public TestReportTeardownComponent copy() { 3018 TestReportTeardownComponent dst = new TestReportTeardownComponent(); 3019 copyValues(dst); 3020 return dst; 3021 } 3022 3023 public void copyValues(TestReportTeardownComponent dst) { 3024 super.copyValues(dst); 3025 if (action != null) { 3026 dst.action = new ArrayList<TeardownActionComponent>(); 3027 for (TeardownActionComponent i : action) 3028 dst.action.add(i.copy()); 3029 }; 3030 } 3031 3032 @Override 3033 public boolean equalsDeep(Base other_) { 3034 if (!super.equalsDeep(other_)) 3035 return false; 3036 if (!(other_ instanceof TestReportTeardownComponent)) 3037 return false; 3038 TestReportTeardownComponent o = (TestReportTeardownComponent) other_; 3039 return compareDeep(action, o.action, true); 3040 } 3041 3042 @Override 3043 public boolean equalsShallow(Base other_) { 3044 if (!super.equalsShallow(other_)) 3045 return false; 3046 if (!(other_ instanceof TestReportTeardownComponent)) 3047 return false; 3048 TestReportTeardownComponent o = (TestReportTeardownComponent) other_; 3049 return true; 3050 } 3051 3052 public boolean isEmpty() { 3053 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action); 3054 } 3055 3056 public String fhirType() { 3057 return "TestReport.teardown"; 3058 3059 } 3060 3061 } 3062 3063 @Block() 3064 public static class TeardownActionComponent extends BackboneElement implements IBaseBackboneElement { 3065 /** 3066 * An operation would involve a REST request to a server. 3067 */ 3068 @Child(name = "operation", type = {SetupActionOperationComponent.class}, order=1, min=1, max=1, modifier=false, summary=false) 3069 @Description(shortDefinition="The teardown operation performed", formalDefinition="An operation would involve a REST request to a server." ) 3070 protected SetupActionOperationComponent operation; 3071 3072 private static final long serialVersionUID = -1099598054L; 3073 3074 /** 3075 * Constructor 3076 */ 3077 public TeardownActionComponent() { 3078 super(); 3079 } 3080 3081 /** 3082 * Constructor 3083 */ 3084 public TeardownActionComponent(SetupActionOperationComponent operation) { 3085 super(); 3086 this.setOperation(operation); 3087 } 3088 3089 /** 3090 * @return {@link #operation} (An operation would involve a REST request to a server.) 3091 */ 3092 public SetupActionOperationComponent getOperation() { 3093 if (this.operation == null) 3094 if (Configuration.errorOnAutoCreate()) 3095 throw new Error("Attempt to auto-create TeardownActionComponent.operation"); 3096 else if (Configuration.doAutoCreate()) 3097 this.operation = new SetupActionOperationComponent(); // cc 3098 return this.operation; 3099 } 3100 3101 public boolean hasOperation() { 3102 return this.operation != null && !this.operation.isEmpty(); 3103 } 3104 3105 /** 3106 * @param value {@link #operation} (An operation would involve a REST request to a server.) 3107 */ 3108 public TeardownActionComponent setOperation(SetupActionOperationComponent value) { 3109 this.operation = value; 3110 return this; 3111 } 3112 3113 protected void listChildren(List<Property> children) { 3114 super.listChildren(children); 3115 children.add(new Property("operation", "@TestReport.setup.action.operation", "An operation would involve a REST request to a server.", 0, 1, operation)); 3116 } 3117 3118 @Override 3119 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3120 switch (_hash) { 3121 case 1662702951: /*operation*/ return new Property("operation", "@TestReport.setup.action.operation", "An operation would involve a REST request to a server.", 0, 1, operation); 3122 default: return super.getNamedProperty(_hash, _name, _checkValid); 3123 } 3124 3125 } 3126 3127 @Override 3128 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3129 switch (hash) { 3130 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : new Base[] {this.operation}; // SetupActionOperationComponent 3131 default: return super.getProperty(hash, name, checkValid); 3132 } 3133 3134 } 3135 3136 @Override 3137 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3138 switch (hash) { 3139 case 1662702951: // operation 3140 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 3141 return value; 3142 default: return super.setProperty(hash, name, value); 3143 } 3144 3145 } 3146 3147 @Override 3148 public Base setProperty(String name, Base value) throws FHIRException { 3149 if (name.equals("operation")) { 3150 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 3151 } else 3152 return super.setProperty(name, value); 3153 return value; 3154 } 3155 3156 @Override 3157 public void removeChild(String name, Base value) throws FHIRException { 3158 if (name.equals("operation")) { 3159 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 3160 } else 3161 super.removeChild(name, value); 3162 3163 } 3164 3165 @Override 3166 public Base makeProperty(int hash, String name) throws FHIRException { 3167 switch (hash) { 3168 case 1662702951: return getOperation(); 3169 default: return super.makeProperty(hash, name); 3170 } 3171 3172 } 3173 3174 @Override 3175 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3176 switch (hash) { 3177 case 1662702951: /*operation*/ return new String[] {"@TestReport.setup.action.operation"}; 3178 default: return super.getTypesForProperty(hash, name); 3179 } 3180 3181 } 3182 3183 @Override 3184 public Base addChild(String name) throws FHIRException { 3185 if (name.equals("operation")) { 3186 this.operation = new SetupActionOperationComponent(); 3187 return this.operation; 3188 } 3189 else 3190 return super.addChild(name); 3191 } 3192 3193 public TeardownActionComponent copy() { 3194 TeardownActionComponent dst = new TeardownActionComponent(); 3195 copyValues(dst); 3196 return dst; 3197 } 3198 3199 public void copyValues(TeardownActionComponent dst) { 3200 super.copyValues(dst); 3201 dst.operation = operation == null ? null : operation.copy(); 3202 } 3203 3204 @Override 3205 public boolean equalsDeep(Base other_) { 3206 if (!super.equalsDeep(other_)) 3207 return false; 3208 if (!(other_ instanceof TeardownActionComponent)) 3209 return false; 3210 TeardownActionComponent o = (TeardownActionComponent) other_; 3211 return compareDeep(operation, o.operation, true); 3212 } 3213 3214 @Override 3215 public boolean equalsShallow(Base other_) { 3216 if (!super.equalsShallow(other_)) 3217 return false; 3218 if (!(other_ instanceof TeardownActionComponent)) 3219 return false; 3220 TeardownActionComponent o = (TeardownActionComponent) other_; 3221 return true; 3222 } 3223 3224 public boolean isEmpty() { 3225 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation); 3226 } 3227 3228 public String fhirType() { 3229 return "TestReport.teardown.action"; 3230 3231 } 3232 3233 } 3234 3235 /** 3236 * Identifier for the TestReport assigned for external purposes outside the context of FHIR. 3237 */ 3238 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 3239 @Description(shortDefinition="External identifier", formalDefinition="Identifier for the TestReport assigned for external purposes outside the context of FHIR." ) 3240 protected Identifier identifier; 3241 3242 /** 3243 * A free text natural language name identifying the executed TestReport. 3244 */ 3245 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 3246 @Description(shortDefinition="Informal name of the executed TestReport", formalDefinition="A free text natural language name identifying the executed TestReport." ) 3247 protected StringType name; 3248 3249 /** 3250 * The current state of this test report. 3251 */ 3252 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 3253 @Description(shortDefinition="completed | in-progress | waiting | stopped | entered-in-error", formalDefinition="The current state of this test report." ) 3254 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/report-status-codes") 3255 protected Enumeration<TestReportStatus> status; 3256 3257 /** 3258 * Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`. 3259 */ 3260 @Child(name = "testScript", type = {CanonicalType.class}, order=3, min=1, max=1, modifier=false, summary=true) 3261 @Description(shortDefinition="Canonical URL to the version-specific TestScript that was executed to produce this TestReport", formalDefinition="Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`." ) 3262 protected CanonicalType testScript; 3263 3264 /** 3265 * The overall result from the execution of the TestScript. 3266 */ 3267 @Child(name = "result", type = {CodeType.class}, order=4, min=1, max=1, modifier=false, summary=true) 3268 @Description(shortDefinition="pass | fail | pending", formalDefinition="The overall result from the execution of the TestScript." ) 3269 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/report-result-codes") 3270 protected Enumeration<TestReportResult> result; 3271 3272 /** 3273 * The final score (percentage of tests passed) resulting from the execution of the TestScript. 3274 */ 3275 @Child(name = "score", type = {DecimalType.class}, order=5, min=0, max=1, modifier=false, summary=true) 3276 @Description(shortDefinition="The final score (percentage of tests passed) resulting from the execution of the TestScript", formalDefinition="The final score (percentage of tests passed) resulting from the execution of the TestScript." ) 3277 protected DecimalType score; 3278 3279 /** 3280 * Name of the tester producing this report (Organization or individual). 3281 */ 3282 @Child(name = "tester", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 3283 @Description(shortDefinition="Name of the tester producing this report (Organization or individual)", formalDefinition="Name of the tester producing this report (Organization or individual)." ) 3284 protected StringType tester; 3285 3286 /** 3287 * When the TestScript was executed and this TestReport was generated. 3288 */ 3289 @Child(name = "issued", type = {DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 3290 @Description(shortDefinition="When the TestScript was executed and this TestReport was generated", formalDefinition="When the TestScript was executed and this TestReport was generated." ) 3291 protected DateTimeType issued; 3292 3293 /** 3294 * A participant in the test execution, either the execution engine, a client, or a server. 3295 */ 3296 @Child(name = "participant", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3297 @Description(shortDefinition="A participant in the test execution, either the execution engine, a client, or a server", formalDefinition="A participant in the test execution, either the execution engine, a client, or a server." ) 3298 protected List<TestReportParticipantComponent> participant; 3299 3300 /** 3301 * The results of the series of required setup operations before the tests were executed. 3302 */ 3303 @Child(name = "setup", type = {}, order=9, min=0, max=1, modifier=false, summary=false) 3304 @Description(shortDefinition="The results of the series of required setup operations before the tests were executed", formalDefinition="The results of the series of required setup operations before the tests were executed." ) 3305 protected TestReportSetupComponent setup; 3306 3307 /** 3308 * A test executed from the test script. 3309 */ 3310 @Child(name = "test", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3311 @Description(shortDefinition="A test executed from the test script", formalDefinition="A test executed from the test script." ) 3312 protected List<TestReportTestComponent> test; 3313 3314 /** 3315 * The results of the series of operations required to clean up after all the tests were executed (successfully or otherwise). 3316 */ 3317 @Child(name = "teardown", type = {}, order=11, min=0, max=1, modifier=false, summary=false) 3318 @Description(shortDefinition="The results of running the series of required clean up steps", formalDefinition="The results of the series of operations required to clean up after all the tests were executed (successfully or otherwise)." ) 3319 protected TestReportTeardownComponent teardown; 3320 3321 private static final long serialVersionUID = -1863229334L; 3322 3323 /** 3324 * Constructor 3325 */ 3326 public TestReport() { 3327 super(); 3328 } 3329 3330 /** 3331 * Constructor 3332 */ 3333 public TestReport(TestReportStatus status, String testScript, TestReportResult result) { 3334 super(); 3335 this.setStatus(status); 3336 this.setTestScript(testScript); 3337 this.setResult(result); 3338 } 3339 3340 /** 3341 * @return {@link #identifier} (Identifier for the TestReport assigned for external purposes outside the context of FHIR.) 3342 */ 3343 public Identifier getIdentifier() { 3344 if (this.identifier == null) 3345 if (Configuration.errorOnAutoCreate()) 3346 throw new Error("Attempt to auto-create TestReport.identifier"); 3347 else if (Configuration.doAutoCreate()) 3348 this.identifier = new Identifier(); // cc 3349 return this.identifier; 3350 } 3351 3352 public boolean hasIdentifier() { 3353 return this.identifier != null && !this.identifier.isEmpty(); 3354 } 3355 3356 /** 3357 * @param value {@link #identifier} (Identifier for the TestReport assigned for external purposes outside the context of FHIR.) 3358 */ 3359 public TestReport setIdentifier(Identifier value) { 3360 this.identifier = value; 3361 return this; 3362 } 3363 3364 /** 3365 * @return {@link #name} (A free text natural language name identifying the executed TestReport.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3366 */ 3367 public StringType getNameElement() { 3368 if (this.name == null) 3369 if (Configuration.errorOnAutoCreate()) 3370 throw new Error("Attempt to auto-create TestReport.name"); 3371 else if (Configuration.doAutoCreate()) 3372 this.name = new StringType(); // bb 3373 return this.name; 3374 } 3375 3376 public boolean hasNameElement() { 3377 return this.name != null && !this.name.isEmpty(); 3378 } 3379 3380 public boolean hasName() { 3381 return this.name != null && !this.name.isEmpty(); 3382 } 3383 3384 /** 3385 * @param value {@link #name} (A free text natural language name identifying the executed TestReport.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3386 */ 3387 public TestReport setNameElement(StringType value) { 3388 this.name = value; 3389 return this; 3390 } 3391 3392 /** 3393 * @return A free text natural language name identifying the executed TestReport. 3394 */ 3395 public String getName() { 3396 return this.name == null ? null : this.name.getValue(); 3397 } 3398 3399 /** 3400 * @param value A free text natural language name identifying the executed TestReport. 3401 */ 3402 public TestReport setName(String value) { 3403 if (Utilities.noString(value)) 3404 this.name = null; 3405 else { 3406 if (this.name == null) 3407 this.name = new StringType(); 3408 this.name.setValue(value); 3409 } 3410 return this; 3411 } 3412 3413 /** 3414 * @return {@link #status} (The current state of this test report.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3415 */ 3416 public Enumeration<TestReportStatus> getStatusElement() { 3417 if (this.status == null) 3418 if (Configuration.errorOnAutoCreate()) 3419 throw new Error("Attempt to auto-create TestReport.status"); 3420 else if (Configuration.doAutoCreate()) 3421 this.status = new Enumeration<TestReportStatus>(new TestReportStatusEnumFactory()); // bb 3422 return this.status; 3423 } 3424 3425 public boolean hasStatusElement() { 3426 return this.status != null && !this.status.isEmpty(); 3427 } 3428 3429 public boolean hasStatus() { 3430 return this.status != null && !this.status.isEmpty(); 3431 } 3432 3433 /** 3434 * @param value {@link #status} (The current state of this test report.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3435 */ 3436 public TestReport setStatusElement(Enumeration<TestReportStatus> value) { 3437 this.status = value; 3438 return this; 3439 } 3440 3441 /** 3442 * @return The current state of this test report. 3443 */ 3444 public TestReportStatus getStatus() { 3445 return this.status == null ? null : this.status.getValue(); 3446 } 3447 3448 /** 3449 * @param value The current state of this test report. 3450 */ 3451 public TestReport setStatus(TestReportStatus value) { 3452 if (this.status == null) 3453 this.status = new Enumeration<TestReportStatus>(new TestReportStatusEnumFactory()); 3454 this.status.setValue(value); 3455 return this; 3456 } 3457 3458 /** 3459 * @return {@link #testScript} (Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`.). This is the underlying object with id, value and extensions. The accessor "getTestScript" gives direct access to the value 3460 */ 3461 public CanonicalType getTestScriptElement() { 3462 if (this.testScript == null) 3463 if (Configuration.errorOnAutoCreate()) 3464 throw new Error("Attempt to auto-create TestReport.testScript"); 3465 else if (Configuration.doAutoCreate()) 3466 this.testScript = new CanonicalType(); // bb 3467 return this.testScript; 3468 } 3469 3470 public boolean hasTestScriptElement() { 3471 return this.testScript != null && !this.testScript.isEmpty(); 3472 } 3473 3474 public boolean hasTestScript() { 3475 return this.testScript != null && !this.testScript.isEmpty(); 3476 } 3477 3478 /** 3479 * @param value {@link #testScript} (Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`.). This is the underlying object with id, value and extensions. The accessor "getTestScript" gives direct access to the value 3480 */ 3481 public TestReport setTestScriptElement(CanonicalType value) { 3482 this.testScript = value; 3483 return this; 3484 } 3485 3486 /** 3487 * @return Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`. 3488 */ 3489 public String getTestScript() { 3490 return this.testScript == null ? null : this.testScript.getValue(); 3491 } 3492 3493 /** 3494 * @param value Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`. 3495 */ 3496 public TestReport setTestScript(String value) { 3497 if (this.testScript == null) 3498 this.testScript = new CanonicalType(); 3499 this.testScript.setValue(value); 3500 return this; 3501 } 3502 3503 /** 3504 * @return {@link #result} (The overall result from the execution of the TestScript.). This is the underlying object with id, value and extensions. The accessor "getResult" gives direct access to the value 3505 */ 3506 public Enumeration<TestReportResult> getResultElement() { 3507 if (this.result == null) 3508 if (Configuration.errorOnAutoCreate()) 3509 throw new Error("Attempt to auto-create TestReport.result"); 3510 else if (Configuration.doAutoCreate()) 3511 this.result = new Enumeration<TestReportResult>(new TestReportResultEnumFactory()); // bb 3512 return this.result; 3513 } 3514 3515 public boolean hasResultElement() { 3516 return this.result != null && !this.result.isEmpty(); 3517 } 3518 3519 public boolean hasResult() { 3520 return this.result != null && !this.result.isEmpty(); 3521 } 3522 3523 /** 3524 * @param value {@link #result} (The overall result from the execution of the TestScript.). This is the underlying object with id, value and extensions. The accessor "getResult" gives direct access to the value 3525 */ 3526 public TestReport setResultElement(Enumeration<TestReportResult> value) { 3527 this.result = value; 3528 return this; 3529 } 3530 3531 /** 3532 * @return The overall result from the execution of the TestScript. 3533 */ 3534 public TestReportResult getResult() { 3535 return this.result == null ? null : this.result.getValue(); 3536 } 3537 3538 /** 3539 * @param value The overall result from the execution of the TestScript. 3540 */ 3541 public TestReport setResult(TestReportResult value) { 3542 if (this.result == null) 3543 this.result = new Enumeration<TestReportResult>(new TestReportResultEnumFactory()); 3544 this.result.setValue(value); 3545 return this; 3546 } 3547 3548 /** 3549 * @return {@link #score} (The final score (percentage of tests passed) resulting from the execution of the TestScript.). This is the underlying object with id, value and extensions. The accessor "getScore" gives direct access to the value 3550 */ 3551 public DecimalType getScoreElement() { 3552 if (this.score == null) 3553 if (Configuration.errorOnAutoCreate()) 3554 throw new Error("Attempt to auto-create TestReport.score"); 3555 else if (Configuration.doAutoCreate()) 3556 this.score = new DecimalType(); // bb 3557 return this.score; 3558 } 3559 3560 public boolean hasScoreElement() { 3561 return this.score != null && !this.score.isEmpty(); 3562 } 3563 3564 public boolean hasScore() { 3565 return this.score != null && !this.score.isEmpty(); 3566 } 3567 3568 /** 3569 * @param value {@link #score} (The final score (percentage of tests passed) resulting from the execution of the TestScript.). This is the underlying object with id, value and extensions. The accessor "getScore" gives direct access to the value 3570 */ 3571 public TestReport setScoreElement(DecimalType value) { 3572 this.score = value; 3573 return this; 3574 } 3575 3576 /** 3577 * @return The final score (percentage of tests passed) resulting from the execution of the TestScript. 3578 */ 3579 public BigDecimal getScore() { 3580 return this.score == null ? null : this.score.getValue(); 3581 } 3582 3583 /** 3584 * @param value The final score (percentage of tests passed) resulting from the execution of the TestScript. 3585 */ 3586 public TestReport setScore(BigDecimal value) { 3587 if (value == null) 3588 this.score = null; 3589 else { 3590 if (this.score == null) 3591 this.score = new DecimalType(); 3592 this.score.setValue(value); 3593 } 3594 return this; 3595 } 3596 3597 /** 3598 * @param value The final score (percentage of tests passed) resulting from the execution of the TestScript. 3599 */ 3600 public TestReport setScore(long value) { 3601 this.score = new DecimalType(); 3602 this.score.setValue(value); 3603 return this; 3604 } 3605 3606 /** 3607 * @param value The final score (percentage of tests passed) resulting from the execution of the TestScript. 3608 */ 3609 public TestReport setScore(double value) { 3610 this.score = new DecimalType(); 3611 this.score.setValue(value); 3612 return this; 3613 } 3614 3615 /** 3616 * @return {@link #tester} (Name of the tester producing this report (Organization or individual).). This is the underlying object with id, value and extensions. The accessor "getTester" gives direct access to the value 3617 */ 3618 public StringType getTesterElement() { 3619 if (this.tester == null) 3620 if (Configuration.errorOnAutoCreate()) 3621 throw new Error("Attempt to auto-create TestReport.tester"); 3622 else if (Configuration.doAutoCreate()) 3623 this.tester = new StringType(); // bb 3624 return this.tester; 3625 } 3626 3627 public boolean hasTesterElement() { 3628 return this.tester != null && !this.tester.isEmpty(); 3629 } 3630 3631 public boolean hasTester() { 3632 return this.tester != null && !this.tester.isEmpty(); 3633 } 3634 3635 /** 3636 * @param value {@link #tester} (Name of the tester producing this report (Organization or individual).). This is the underlying object with id, value and extensions. The accessor "getTester" gives direct access to the value 3637 */ 3638 public TestReport setTesterElement(StringType value) { 3639 this.tester = value; 3640 return this; 3641 } 3642 3643 /** 3644 * @return Name of the tester producing this report (Organization or individual). 3645 */ 3646 public String getTester() { 3647 return this.tester == null ? null : this.tester.getValue(); 3648 } 3649 3650 /** 3651 * @param value Name of the tester producing this report (Organization or individual). 3652 */ 3653 public TestReport setTester(String value) { 3654 if (Utilities.noString(value)) 3655 this.tester = null; 3656 else { 3657 if (this.tester == null) 3658 this.tester = new StringType(); 3659 this.tester.setValue(value); 3660 } 3661 return this; 3662 } 3663 3664 /** 3665 * @return {@link #issued} (When the TestScript was executed and this TestReport was generated.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 3666 */ 3667 public DateTimeType getIssuedElement() { 3668 if (this.issued == null) 3669 if (Configuration.errorOnAutoCreate()) 3670 throw new Error("Attempt to auto-create TestReport.issued"); 3671 else if (Configuration.doAutoCreate()) 3672 this.issued = new DateTimeType(); // bb 3673 return this.issued; 3674 } 3675 3676 public boolean hasIssuedElement() { 3677 return this.issued != null && !this.issued.isEmpty(); 3678 } 3679 3680 public boolean hasIssued() { 3681 return this.issued != null && !this.issued.isEmpty(); 3682 } 3683 3684 /** 3685 * @param value {@link #issued} (When the TestScript was executed and this TestReport was generated.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 3686 */ 3687 public TestReport setIssuedElement(DateTimeType value) { 3688 this.issued = value; 3689 return this; 3690 } 3691 3692 /** 3693 * @return When the TestScript was executed and this TestReport was generated. 3694 */ 3695 public Date getIssued() { 3696 return this.issued == null ? null : this.issued.getValue(); 3697 } 3698 3699 /** 3700 * @param value When the TestScript was executed and this TestReport was generated. 3701 */ 3702 public TestReport setIssued(Date value) { 3703 if (value == null) 3704 this.issued = null; 3705 else { 3706 if (this.issued == null) 3707 this.issued = new DateTimeType(); 3708 this.issued.setValue(value); 3709 } 3710 return this; 3711 } 3712 3713 /** 3714 * @return {@link #participant} (A participant in the test execution, either the execution engine, a client, or a server.) 3715 */ 3716 public List<TestReportParticipantComponent> getParticipant() { 3717 if (this.participant == null) 3718 this.participant = new ArrayList<TestReportParticipantComponent>(); 3719 return this.participant; 3720 } 3721 3722 /** 3723 * @return Returns a reference to <code>this</code> for easy method chaining 3724 */ 3725 public TestReport setParticipant(List<TestReportParticipantComponent> theParticipant) { 3726 this.participant = theParticipant; 3727 return this; 3728 } 3729 3730 public boolean hasParticipant() { 3731 if (this.participant == null) 3732 return false; 3733 for (TestReportParticipantComponent item : this.participant) 3734 if (!item.isEmpty()) 3735 return true; 3736 return false; 3737 } 3738 3739 public TestReportParticipantComponent addParticipant() { //3 3740 TestReportParticipantComponent t = new TestReportParticipantComponent(); 3741 if (this.participant == null) 3742 this.participant = new ArrayList<TestReportParticipantComponent>(); 3743 this.participant.add(t); 3744 return t; 3745 } 3746 3747 public TestReport addParticipant(TestReportParticipantComponent t) { //3 3748 if (t == null) 3749 return this; 3750 if (this.participant == null) 3751 this.participant = new ArrayList<TestReportParticipantComponent>(); 3752 this.participant.add(t); 3753 return this; 3754 } 3755 3756 /** 3757 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist {3} 3758 */ 3759 public TestReportParticipantComponent getParticipantFirstRep() { 3760 if (getParticipant().isEmpty()) { 3761 addParticipant(); 3762 } 3763 return getParticipant().get(0); 3764 } 3765 3766 /** 3767 * @return {@link #setup} (The results of the series of required setup operations before the tests were executed.) 3768 */ 3769 public TestReportSetupComponent getSetup() { 3770 if (this.setup == null) 3771 if (Configuration.errorOnAutoCreate()) 3772 throw new Error("Attempt to auto-create TestReport.setup"); 3773 else if (Configuration.doAutoCreate()) 3774 this.setup = new TestReportSetupComponent(); // cc 3775 return this.setup; 3776 } 3777 3778 public boolean hasSetup() { 3779 return this.setup != null && !this.setup.isEmpty(); 3780 } 3781 3782 /** 3783 * @param value {@link #setup} (The results of the series of required setup operations before the tests were executed.) 3784 */ 3785 public TestReport setSetup(TestReportSetupComponent value) { 3786 this.setup = value; 3787 return this; 3788 } 3789 3790 /** 3791 * @return {@link #test} (A test executed from the test script.) 3792 */ 3793 public List<TestReportTestComponent> getTest() { 3794 if (this.test == null) 3795 this.test = new ArrayList<TestReportTestComponent>(); 3796 return this.test; 3797 } 3798 3799 /** 3800 * @return Returns a reference to <code>this</code> for easy method chaining 3801 */ 3802 public TestReport setTest(List<TestReportTestComponent> theTest) { 3803 this.test = theTest; 3804 return this; 3805 } 3806 3807 public boolean hasTest() { 3808 if (this.test == null) 3809 return false; 3810 for (TestReportTestComponent item : this.test) 3811 if (!item.isEmpty()) 3812 return true; 3813 return false; 3814 } 3815 3816 public TestReportTestComponent addTest() { //3 3817 TestReportTestComponent t = new TestReportTestComponent(); 3818 if (this.test == null) 3819 this.test = new ArrayList<TestReportTestComponent>(); 3820 this.test.add(t); 3821 return t; 3822 } 3823 3824 public TestReport addTest(TestReportTestComponent t) { //3 3825 if (t == null) 3826 return this; 3827 if (this.test == null) 3828 this.test = new ArrayList<TestReportTestComponent>(); 3829 this.test.add(t); 3830 return this; 3831 } 3832 3833 /** 3834 * @return The first repetition of repeating field {@link #test}, creating it if it does not already exist {3} 3835 */ 3836 public TestReportTestComponent getTestFirstRep() { 3837 if (getTest().isEmpty()) { 3838 addTest(); 3839 } 3840 return getTest().get(0); 3841 } 3842 3843 /** 3844 * @return {@link #teardown} (The results of the series of operations required to clean up after all the tests were executed (successfully or otherwise).) 3845 */ 3846 public TestReportTeardownComponent getTeardown() { 3847 if (this.teardown == null) 3848 if (Configuration.errorOnAutoCreate()) 3849 throw new Error("Attempt to auto-create TestReport.teardown"); 3850 else if (Configuration.doAutoCreate()) 3851 this.teardown = new TestReportTeardownComponent(); // cc 3852 return this.teardown; 3853 } 3854 3855 public boolean hasTeardown() { 3856 return this.teardown != null && !this.teardown.isEmpty(); 3857 } 3858 3859 /** 3860 * @param value {@link #teardown} (The results of the series of operations required to clean up after all the tests were executed (successfully or otherwise).) 3861 */ 3862 public TestReport setTeardown(TestReportTeardownComponent value) { 3863 this.teardown = value; 3864 return this; 3865 } 3866 3867 protected void listChildren(List<Property> children) { 3868 super.listChildren(children); 3869 children.add(new Property("identifier", "Identifier", "Identifier for the TestReport assigned for external purposes outside the context of FHIR.", 0, 1, identifier)); 3870 children.add(new Property("name", "string", "A free text natural language name identifying the executed TestReport.", 0, 1, name)); 3871 children.add(new Property("status", "code", "The current state of this test report.", 0, 1, status)); 3872 children.add(new Property("testScript", "canonical(TestScript)", "Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`.", 0, 1, testScript)); 3873 children.add(new Property("result", "code", "The overall result from the execution of the TestScript.", 0, 1, result)); 3874 children.add(new Property("score", "decimal", "The final score (percentage of tests passed) resulting from the execution of the TestScript.", 0, 1, score)); 3875 children.add(new Property("tester", "string", "Name of the tester producing this report (Organization or individual).", 0, 1, tester)); 3876 children.add(new Property("issued", "dateTime", "When the TestScript was executed and this TestReport was generated.", 0, 1, issued)); 3877 children.add(new Property("participant", "", "A participant in the test execution, either the execution engine, a client, or a server.", 0, java.lang.Integer.MAX_VALUE, participant)); 3878 children.add(new Property("setup", "", "The results of the series of required setup operations before the tests were executed.", 0, 1, setup)); 3879 children.add(new Property("test", "", "A test executed from the test script.", 0, java.lang.Integer.MAX_VALUE, test)); 3880 children.add(new Property("teardown", "", "The results of the series of operations required to clean up after all the tests were executed (successfully or otherwise).", 0, 1, teardown)); 3881 } 3882 3883 @Override 3884 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3885 switch (_hash) { 3886 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier for the TestReport assigned for external purposes outside the context of FHIR.", 0, 1, identifier); 3887 case 3373707: /*name*/ return new Property("name", "string", "A free text natural language name identifying the executed TestReport.", 0, 1, name); 3888 case -892481550: /*status*/ return new Property("status", "code", "The current state of this test report.", 0, 1, status); 3889 case 1712049149: /*testScript*/ return new Property("testScript", "canonical(TestScript)", "Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`.", 0, 1, testScript); 3890 case -934426595: /*result*/ return new Property("result", "code", "The overall result from the execution of the TestScript.", 0, 1, result); 3891 case 109264530: /*score*/ return new Property("score", "decimal", "The final score (percentage of tests passed) resulting from the execution of the TestScript.", 0, 1, score); 3892 case -877169473: /*tester*/ return new Property("tester", "string", "Name of the tester producing this report (Organization or individual).", 0, 1, tester); 3893 case -1179159893: /*issued*/ return new Property("issued", "dateTime", "When the TestScript was executed and this TestReport was generated.", 0, 1, issued); 3894 case 767422259: /*participant*/ return new Property("participant", "", "A participant in the test execution, either the execution engine, a client, or a server.", 0, java.lang.Integer.MAX_VALUE, participant); 3895 case 109329021: /*setup*/ return new Property("setup", "", "The results of the series of required setup operations before the tests were executed.", 0, 1, setup); 3896 case 3556498: /*test*/ return new Property("test", "", "A test executed from the test script.", 0, java.lang.Integer.MAX_VALUE, test); 3897 case -1663474172: /*teardown*/ return new Property("teardown", "", "The results of the series of operations required to clean up after all the tests were executed (successfully or otherwise).", 0, 1, teardown); 3898 default: return super.getNamedProperty(_hash, _name, _checkValid); 3899 } 3900 3901 } 3902 3903 @Override 3904 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3905 switch (hash) { 3906 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 3907 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3908 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<TestReportStatus> 3909 case 1712049149: /*testScript*/ return this.testScript == null ? new Base[0] : new Base[] {this.testScript}; // CanonicalType 3910 case -934426595: /*result*/ return this.result == null ? new Base[0] : new Base[] {this.result}; // Enumeration<TestReportResult> 3911 case 109264530: /*score*/ return this.score == null ? new Base[0] : new Base[] {this.score}; // DecimalType 3912 case -877169473: /*tester*/ return this.tester == null ? new Base[0] : new Base[] {this.tester}; // StringType 3913 case -1179159893: /*issued*/ return this.issued == null ? new Base[0] : new Base[] {this.issued}; // DateTimeType 3914 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // TestReportParticipantComponent 3915 case 109329021: /*setup*/ return this.setup == null ? new Base[0] : new Base[] {this.setup}; // TestReportSetupComponent 3916 case 3556498: /*test*/ return this.test == null ? new Base[0] : this.test.toArray(new Base[this.test.size()]); // TestReportTestComponent 3917 case -1663474172: /*teardown*/ return this.teardown == null ? new Base[0] : new Base[] {this.teardown}; // TestReportTeardownComponent 3918 default: return super.getProperty(hash, name, checkValid); 3919 } 3920 3921 } 3922 3923 @Override 3924 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3925 switch (hash) { 3926 case -1618432855: // identifier 3927 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 3928 return value; 3929 case 3373707: // name 3930 this.name = TypeConvertor.castToString(value); // StringType 3931 return value; 3932 case -892481550: // status 3933 value = new TestReportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3934 this.status = (Enumeration) value; // Enumeration<TestReportStatus> 3935 return value; 3936 case 1712049149: // testScript 3937 this.testScript = TypeConvertor.castToCanonical(value); // CanonicalType 3938 return value; 3939 case -934426595: // result 3940 value = new TestReportResultEnumFactory().fromType(TypeConvertor.castToCode(value)); 3941 this.result = (Enumeration) value; // Enumeration<TestReportResult> 3942 return value; 3943 case 109264530: // score 3944 this.score = TypeConvertor.castToDecimal(value); // DecimalType 3945 return value; 3946 case -877169473: // tester 3947 this.tester = TypeConvertor.castToString(value); // StringType 3948 return value; 3949 case -1179159893: // issued 3950 this.issued = TypeConvertor.castToDateTime(value); // DateTimeType 3951 return value; 3952 case 767422259: // participant 3953 this.getParticipant().add((TestReportParticipantComponent) value); // TestReportParticipantComponent 3954 return value; 3955 case 109329021: // setup 3956 this.setup = (TestReportSetupComponent) value; // TestReportSetupComponent 3957 return value; 3958 case 3556498: // test 3959 this.getTest().add((TestReportTestComponent) value); // TestReportTestComponent 3960 return value; 3961 case -1663474172: // teardown 3962 this.teardown = (TestReportTeardownComponent) value; // TestReportTeardownComponent 3963 return value; 3964 default: return super.setProperty(hash, name, value); 3965 } 3966 3967 } 3968 3969 @Override 3970 public Base setProperty(String name, Base value) throws FHIRException { 3971 if (name.equals("identifier")) { 3972 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 3973 } else if (name.equals("name")) { 3974 this.name = TypeConvertor.castToString(value); // StringType 3975 } else if (name.equals("status")) { 3976 value = new TestReportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3977 this.status = (Enumeration) value; // Enumeration<TestReportStatus> 3978 } else if (name.equals("testScript")) { 3979 this.testScript = TypeConvertor.castToCanonical(value); // CanonicalType 3980 } else if (name.equals("result")) { 3981 value = new TestReportResultEnumFactory().fromType(TypeConvertor.castToCode(value)); 3982 this.result = (Enumeration) value; // Enumeration<TestReportResult> 3983 } else if (name.equals("score")) { 3984 this.score = TypeConvertor.castToDecimal(value); // DecimalType 3985 } else if (name.equals("tester")) { 3986 this.tester = TypeConvertor.castToString(value); // StringType 3987 } else if (name.equals("issued")) { 3988 this.issued = TypeConvertor.castToDateTime(value); // DateTimeType 3989 } else if (name.equals("participant")) { 3990 this.getParticipant().add((TestReportParticipantComponent) value); 3991 } else if (name.equals("setup")) { 3992 this.setup = (TestReportSetupComponent) value; // TestReportSetupComponent 3993 } else if (name.equals("test")) { 3994 this.getTest().add((TestReportTestComponent) value); 3995 } else if (name.equals("teardown")) { 3996 this.teardown = (TestReportTeardownComponent) value; // TestReportTeardownComponent 3997 } else 3998 return super.setProperty(name, value); 3999 return value; 4000 } 4001 4002 @Override 4003 public void removeChild(String name, Base value) throws FHIRException { 4004 if (name.equals("identifier")) { 4005 this.identifier = null; 4006 } else if (name.equals("name")) { 4007 this.name = null; 4008 } else if (name.equals("status")) { 4009 value = new TestReportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4010 this.status = (Enumeration) value; // Enumeration<TestReportStatus> 4011 } else if (name.equals("testScript")) { 4012 this.testScript = null; 4013 } else if (name.equals("result")) { 4014 value = new TestReportResultEnumFactory().fromType(TypeConvertor.castToCode(value)); 4015 this.result = (Enumeration) value; // Enumeration<TestReportResult> 4016 } else if (name.equals("score")) { 4017 this.score = null; 4018 } else if (name.equals("tester")) { 4019 this.tester = null; 4020 } else if (name.equals("issued")) { 4021 this.issued = null; 4022 } else if (name.equals("participant")) { 4023 this.getParticipant().remove((TestReportParticipantComponent) value); 4024 } else if (name.equals("setup")) { 4025 this.setup = (TestReportSetupComponent) value; // TestReportSetupComponent 4026 } else if (name.equals("test")) { 4027 this.getTest().remove((TestReportTestComponent) value); 4028 } else if (name.equals("teardown")) { 4029 this.teardown = (TestReportTeardownComponent) value; // TestReportTeardownComponent 4030 } else 4031 super.removeChild(name, value); 4032 4033 } 4034 4035 @Override 4036 public Base makeProperty(int hash, String name) throws FHIRException { 4037 switch (hash) { 4038 case -1618432855: return getIdentifier(); 4039 case 3373707: return getNameElement(); 4040 case -892481550: return getStatusElement(); 4041 case 1712049149: return getTestScriptElement(); 4042 case -934426595: return getResultElement(); 4043 case 109264530: return getScoreElement(); 4044 case -877169473: return getTesterElement(); 4045 case -1179159893: return getIssuedElement(); 4046 case 767422259: return addParticipant(); 4047 case 109329021: return getSetup(); 4048 case 3556498: return addTest(); 4049 case -1663474172: return getTeardown(); 4050 default: return super.makeProperty(hash, name); 4051 } 4052 4053 } 4054 4055 @Override 4056 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4057 switch (hash) { 4058 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4059 case 3373707: /*name*/ return new String[] {"string"}; 4060 case -892481550: /*status*/ return new String[] {"code"}; 4061 case 1712049149: /*testScript*/ return new String[] {"canonical"}; 4062 case -934426595: /*result*/ return new String[] {"code"}; 4063 case 109264530: /*score*/ return new String[] {"decimal"}; 4064 case -877169473: /*tester*/ return new String[] {"string"}; 4065 case -1179159893: /*issued*/ return new String[] {"dateTime"}; 4066 case 767422259: /*participant*/ return new String[] {}; 4067 case 109329021: /*setup*/ return new String[] {}; 4068 case 3556498: /*test*/ return new String[] {}; 4069 case -1663474172: /*teardown*/ return new String[] {}; 4070 default: return super.getTypesForProperty(hash, name); 4071 } 4072 4073 } 4074 4075 @Override 4076 public Base addChild(String name) throws FHIRException { 4077 if (name.equals("identifier")) { 4078 this.identifier = new Identifier(); 4079 return this.identifier; 4080 } 4081 else if (name.equals("name")) { 4082 throw new FHIRException("Cannot call addChild on a singleton property TestReport.name"); 4083 } 4084 else if (name.equals("status")) { 4085 throw new FHIRException("Cannot call addChild on a singleton property TestReport.status"); 4086 } 4087 else if (name.equals("testScript")) { 4088 throw new FHIRException("Cannot call addChild on a singleton property TestReport.testScript"); 4089 } 4090 else if (name.equals("result")) { 4091 throw new FHIRException("Cannot call addChild on a singleton property TestReport.result"); 4092 } 4093 else if (name.equals("score")) { 4094 throw new FHIRException("Cannot call addChild on a singleton property TestReport.score"); 4095 } 4096 else if (name.equals("tester")) { 4097 throw new FHIRException("Cannot call addChild on a singleton property TestReport.tester"); 4098 } 4099 else if (name.equals("issued")) { 4100 throw new FHIRException("Cannot call addChild on a singleton property TestReport.issued"); 4101 } 4102 else if (name.equals("participant")) { 4103 return addParticipant(); 4104 } 4105 else if (name.equals("setup")) { 4106 this.setup = new TestReportSetupComponent(); 4107 return this.setup; 4108 } 4109 else if (name.equals("test")) { 4110 return addTest(); 4111 } 4112 else if (name.equals("teardown")) { 4113 this.teardown = new TestReportTeardownComponent(); 4114 return this.teardown; 4115 } 4116 else 4117 return super.addChild(name); 4118 } 4119 4120 public String fhirType() { 4121 return "TestReport"; 4122 4123 } 4124 4125 public TestReport copy() { 4126 TestReport dst = new TestReport(); 4127 copyValues(dst); 4128 return dst; 4129 } 4130 4131 public void copyValues(TestReport dst) { 4132 super.copyValues(dst); 4133 dst.identifier = identifier == null ? null : identifier.copy(); 4134 dst.name = name == null ? null : name.copy(); 4135 dst.status = status == null ? null : status.copy(); 4136 dst.testScript = testScript == null ? null : testScript.copy(); 4137 dst.result = result == null ? null : result.copy(); 4138 dst.score = score == null ? null : score.copy(); 4139 dst.tester = tester == null ? null : tester.copy(); 4140 dst.issued = issued == null ? null : issued.copy(); 4141 if (participant != null) { 4142 dst.participant = new ArrayList<TestReportParticipantComponent>(); 4143 for (TestReportParticipantComponent i : participant) 4144 dst.participant.add(i.copy()); 4145 }; 4146 dst.setup = setup == null ? null : setup.copy(); 4147 if (test != null) { 4148 dst.test = new ArrayList<TestReportTestComponent>(); 4149 for (TestReportTestComponent i : test) 4150 dst.test.add(i.copy()); 4151 }; 4152 dst.teardown = teardown == null ? null : teardown.copy(); 4153 } 4154 4155 protected TestReport typedCopy() { 4156 return copy(); 4157 } 4158 4159 @Override 4160 public boolean equalsDeep(Base other_) { 4161 if (!super.equalsDeep(other_)) 4162 return false; 4163 if (!(other_ instanceof TestReport)) 4164 return false; 4165 TestReport o = (TestReport) other_; 4166 return compareDeep(identifier, o.identifier, true) && compareDeep(name, o.name, true) && compareDeep(status, o.status, true) 4167 && compareDeep(testScript, o.testScript, true) && compareDeep(result, o.result, true) && compareDeep(score, o.score, true) 4168 && compareDeep(tester, o.tester, true) && compareDeep(issued, o.issued, true) && compareDeep(participant, o.participant, true) 4169 && compareDeep(setup, o.setup, true) && compareDeep(test, o.test, true) && compareDeep(teardown, o.teardown, true) 4170 ; 4171 } 4172 4173 @Override 4174 public boolean equalsShallow(Base other_) { 4175 if (!super.equalsShallow(other_)) 4176 return false; 4177 if (!(other_ instanceof TestReport)) 4178 return false; 4179 TestReport o = (TestReport) other_; 4180 return compareValues(name, o.name, true) && compareValues(status, o.status, true) && compareValues(testScript, o.testScript, true) 4181 && compareValues(result, o.result, true) && compareValues(score, o.score, true) && compareValues(tester, o.tester, true) 4182 && compareValues(issued, o.issued, true); 4183 } 4184 4185 public boolean isEmpty() { 4186 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, name, status 4187 , testScript, result, score, tester, issued, participant, setup, test, teardown 4188 ); 4189 } 4190 4191 @Override 4192 public ResourceType getResourceType() { 4193 return ResourceType.TestReport; 4194 } 4195 4196 /** 4197 * Search parameter: <b>identifier</b> 4198 * <p> 4199 * Description: <b>An external identifier for the test report</b><br> 4200 * Type: <b>token</b><br> 4201 * Path: <b>TestReport.identifier</b><br> 4202 * </p> 4203 */ 4204 @SearchParamDefinition(name="identifier", path="TestReport.identifier", description="An external identifier for the test report", type="token" ) 4205 public static final String SP_IDENTIFIER = "identifier"; 4206 /** 4207 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4208 * <p> 4209 * Description: <b>An external identifier for the test report</b><br> 4210 * Type: <b>token</b><br> 4211 * Path: <b>TestReport.identifier</b><br> 4212 * </p> 4213 */ 4214 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4215 4216 /** 4217 * Search parameter: <b>issued</b> 4218 * <p> 4219 * Description: <b>The test report generation date</b><br> 4220 * Type: <b>date</b><br> 4221 * Path: <b>TestReport.issued</b><br> 4222 * </p> 4223 */ 4224 @SearchParamDefinition(name="issued", path="TestReport.issued", description="The test report generation date", type="date" ) 4225 public static final String SP_ISSUED = "issued"; 4226 /** 4227 * <b>Fluent Client</b> search parameter constant for <b>issued</b> 4228 * <p> 4229 * Description: <b>The test report generation date</b><br> 4230 * Type: <b>date</b><br> 4231 * Path: <b>TestReport.issued</b><br> 4232 * </p> 4233 */ 4234 public static final ca.uhn.fhir.rest.gclient.DateClientParam ISSUED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_ISSUED); 4235 4236 /** 4237 * Search parameter: <b>participant</b> 4238 * <p> 4239 * Description: <b>The reference to a participant in the test execution</b><br> 4240 * Type: <b>uri</b><br> 4241 * Path: <b>TestReport.participant.uri</b><br> 4242 * </p> 4243 */ 4244 @SearchParamDefinition(name="participant", path="TestReport.participant.uri", description="The reference to a participant in the test execution", type="uri" ) 4245 public static final String SP_PARTICIPANT = "participant"; 4246 /** 4247 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 4248 * <p> 4249 * Description: <b>The reference to a participant in the test execution</b><br> 4250 * Type: <b>uri</b><br> 4251 * Path: <b>TestReport.participant.uri</b><br> 4252 * </p> 4253 */ 4254 public static final ca.uhn.fhir.rest.gclient.UriClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_PARTICIPANT); 4255 4256 /** 4257 * Search parameter: <b>result</b> 4258 * <p> 4259 * Description: <b>The result disposition of the test execution</b><br> 4260 * Type: <b>token</b><br> 4261 * Path: <b>TestReport.result</b><br> 4262 * </p> 4263 */ 4264 @SearchParamDefinition(name="result", path="TestReport.result", description="The result disposition of the test execution", type="token" ) 4265 public static final String SP_RESULT = "result"; 4266 /** 4267 * <b>Fluent Client</b> search parameter constant for <b>result</b> 4268 * <p> 4269 * Description: <b>The result disposition of the test execution</b><br> 4270 * Type: <b>token</b><br> 4271 * Path: <b>TestReport.result</b><br> 4272 * </p> 4273 */ 4274 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RESULT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_RESULT); 4275 4276 /** 4277 * Search parameter: <b>status</b> 4278 * <p> 4279 * Description: <b>The current status of the test report</b><br> 4280 * Type: <b>token</b><br> 4281 * Path: <b>TestReport.status</b><br> 4282 * </p> 4283 */ 4284 @SearchParamDefinition(name="status", path="TestReport.status", description="The current status of the test report", type="token" ) 4285 public static final String SP_STATUS = "status"; 4286 /** 4287 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4288 * <p> 4289 * Description: <b>The current status of the test report</b><br> 4290 * Type: <b>token</b><br> 4291 * Path: <b>TestReport.status</b><br> 4292 * </p> 4293 */ 4294 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4295 4296 /** 4297 * Search parameter: <b>tester</b> 4298 * <p> 4299 * Description: <b>The name of the testing organization</b><br> 4300 * Type: <b>string</b><br> 4301 * Path: <b>TestReport.tester</b><br> 4302 * </p> 4303 */ 4304 @SearchParamDefinition(name="tester", path="TestReport.tester", description="The name of the testing organization", type="string" ) 4305 public static final String SP_TESTER = "tester"; 4306 /** 4307 * <b>Fluent Client</b> search parameter constant for <b>tester</b> 4308 * <p> 4309 * Description: <b>The name of the testing organization</b><br> 4310 * Type: <b>string</b><br> 4311 * Path: <b>TestReport.tester</b><br> 4312 * </p> 4313 */ 4314 public static final ca.uhn.fhir.rest.gclient.StringClientParam TESTER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TESTER); 4315 4316 /** 4317 * Search parameter: <b>testscript</b> 4318 * <p> 4319 * Description: <b>The test script executed to produce this report</b><br> 4320 * Type: <b>reference</b><br> 4321 * Path: <b>TestReport.testScript</b><br> 4322 * </p> 4323 */ 4324 @SearchParamDefinition(name="testscript", path="TestReport.testScript", description="The test script executed to produce this report", type="reference", target={TestScript.class } ) 4325 public static final String SP_TESTSCRIPT = "testscript"; 4326 /** 4327 * <b>Fluent Client</b> search parameter constant for <b>testscript</b> 4328 * <p> 4329 * Description: <b>The test script executed to produce this report</b><br> 4330 * Type: <b>reference</b><br> 4331 * Path: <b>TestReport.testScript</b><br> 4332 * </p> 4333 */ 4334 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TESTSCRIPT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_TESTSCRIPT); 4335 4336/** 4337 * Constant for fluent queries to be used to add include statements. Specifies 4338 * the path value of "<b>TestReport:testscript</b>". 4339 */ 4340 public static final ca.uhn.fhir.model.api.Include INCLUDE_TESTSCRIPT = new ca.uhn.fhir.model.api.Include("TestReport:testscript").toLocked(); 4341 4342 4343} 4344