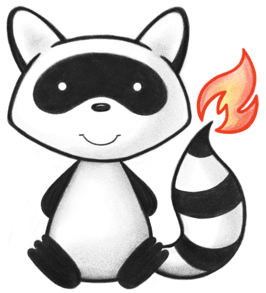
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.Block; 050 051/** 052 * A summary of information based on the results of executing a TestScript. 053 */ 054@ResourceDef(name="TestReport", profile="http://hl7.org/fhir/StructureDefinition/TestReport") 055public class TestReport extends DomainResource { 056 057 public enum TestReportActionResult { 058 /** 059 * The action was successful. 060 */ 061 PASS, 062 /** 063 * The action was skipped. 064 */ 065 SKIP, 066 /** 067 * The action failed. 068 */ 069 FAIL, 070 /** 071 * The action passed but with warnings. 072 */ 073 WARNING, 074 /** 075 * The action encountered a fatal error and the engine was unable to process. 076 */ 077 ERROR, 078 /** 079 * added to help the parsers with the generic types 080 */ 081 NULL; 082 public static TestReportActionResult fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("pass".equals(codeString)) 086 return PASS; 087 if ("skip".equals(codeString)) 088 return SKIP; 089 if ("fail".equals(codeString)) 090 return FAIL; 091 if ("warning".equals(codeString)) 092 return WARNING; 093 if ("error".equals(codeString)) 094 return ERROR; 095 if (Configuration.isAcceptInvalidEnums()) 096 return null; 097 else 098 throw new FHIRException("Unknown TestReportActionResult code '"+codeString+"'"); 099 } 100 public String toCode() { 101 switch (this) { 102 case PASS: return "pass"; 103 case SKIP: return "skip"; 104 case FAIL: return "fail"; 105 case WARNING: return "warning"; 106 case ERROR: return "error"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 public String getSystem() { 112 switch (this) { 113 case PASS: return "http://hl7.org/fhir/report-action-result-codes"; 114 case SKIP: return "http://hl7.org/fhir/report-action-result-codes"; 115 case FAIL: return "http://hl7.org/fhir/report-action-result-codes"; 116 case WARNING: return "http://hl7.org/fhir/report-action-result-codes"; 117 case ERROR: return "http://hl7.org/fhir/report-action-result-codes"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDefinition() { 123 switch (this) { 124 case PASS: return "The action was successful."; 125 case SKIP: return "The action was skipped."; 126 case FAIL: return "The action failed."; 127 case WARNING: return "The action passed but with warnings."; 128 case ERROR: return "The action encountered a fatal error and the engine was unable to process."; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 public String getDisplay() { 134 switch (this) { 135 case PASS: return "Pass"; 136 case SKIP: return "Skip"; 137 case FAIL: return "Fail"; 138 case WARNING: return "Warning"; 139 case ERROR: return "Error"; 140 case NULL: return null; 141 default: return "?"; 142 } 143 } 144 } 145 146 public static class TestReportActionResultEnumFactory implements EnumFactory<TestReportActionResult> { 147 public TestReportActionResult fromCode(String codeString) throws IllegalArgumentException { 148 if (codeString == null || "".equals(codeString)) 149 if (codeString == null || "".equals(codeString)) 150 return null; 151 if ("pass".equals(codeString)) 152 return TestReportActionResult.PASS; 153 if ("skip".equals(codeString)) 154 return TestReportActionResult.SKIP; 155 if ("fail".equals(codeString)) 156 return TestReportActionResult.FAIL; 157 if ("warning".equals(codeString)) 158 return TestReportActionResult.WARNING; 159 if ("error".equals(codeString)) 160 return TestReportActionResult.ERROR; 161 throw new IllegalArgumentException("Unknown TestReportActionResult code '"+codeString+"'"); 162 } 163 public Enumeration<TestReportActionResult> fromType(PrimitiveType<?> code) throws FHIRException { 164 if (code == null) 165 return null; 166 if (code.isEmpty()) 167 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.NULL, code); 168 String codeString = ((PrimitiveType) code).asStringValue(); 169 if (codeString == null || "".equals(codeString)) 170 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.NULL, code); 171 if ("pass".equals(codeString)) 172 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.PASS, code); 173 if ("skip".equals(codeString)) 174 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.SKIP, code); 175 if ("fail".equals(codeString)) 176 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.FAIL, code); 177 if ("warning".equals(codeString)) 178 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.WARNING, code); 179 if ("error".equals(codeString)) 180 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.ERROR, code); 181 throw new FHIRException("Unknown TestReportActionResult code '"+codeString+"'"); 182 } 183 public String toCode(TestReportActionResult code) { 184 if (code == TestReportActionResult.PASS) 185 return "pass"; 186 if (code == TestReportActionResult.SKIP) 187 return "skip"; 188 if (code == TestReportActionResult.FAIL) 189 return "fail"; 190 if (code == TestReportActionResult.WARNING) 191 return "warning"; 192 if (code == TestReportActionResult.ERROR) 193 return "error"; 194 return "?"; 195 } 196 public String toSystem(TestReportActionResult code) { 197 return code.getSystem(); 198 } 199 } 200 201 public enum TestReportParticipantType { 202 /** 203 * The test execution engine. 204 */ 205 TESTENGINE, 206 /** 207 * A FHIR Client. 208 */ 209 CLIENT, 210 /** 211 * A FHIR Server. 212 */ 213 SERVER, 214 /** 215 * added to help the parsers with the generic types 216 */ 217 NULL; 218 public static TestReportParticipantType fromCode(String codeString) throws FHIRException { 219 if (codeString == null || "".equals(codeString)) 220 return null; 221 if ("test-engine".equals(codeString)) 222 return TESTENGINE; 223 if ("client".equals(codeString)) 224 return CLIENT; 225 if ("server".equals(codeString)) 226 return SERVER; 227 if (Configuration.isAcceptInvalidEnums()) 228 return null; 229 else 230 throw new FHIRException("Unknown TestReportParticipantType code '"+codeString+"'"); 231 } 232 public String toCode() { 233 switch (this) { 234 case TESTENGINE: return "test-engine"; 235 case CLIENT: return "client"; 236 case SERVER: return "server"; 237 case NULL: return null; 238 default: return "?"; 239 } 240 } 241 public String getSystem() { 242 switch (this) { 243 case TESTENGINE: return "http://hl7.org/fhir/report-participant-type"; 244 case CLIENT: return "http://hl7.org/fhir/report-participant-type"; 245 case SERVER: return "http://hl7.org/fhir/report-participant-type"; 246 case NULL: return null; 247 default: return "?"; 248 } 249 } 250 public String getDefinition() { 251 switch (this) { 252 case TESTENGINE: return "The test execution engine."; 253 case CLIENT: return "A FHIR Client."; 254 case SERVER: return "A FHIR Server."; 255 case NULL: return null; 256 default: return "?"; 257 } 258 } 259 public String getDisplay() { 260 switch (this) { 261 case TESTENGINE: return "Test Engine"; 262 case CLIENT: return "Client"; 263 case SERVER: return "Server"; 264 case NULL: return null; 265 default: return "?"; 266 } 267 } 268 } 269 270 public static class TestReportParticipantTypeEnumFactory implements EnumFactory<TestReportParticipantType> { 271 public TestReportParticipantType fromCode(String codeString) throws IllegalArgumentException { 272 if (codeString == null || "".equals(codeString)) 273 if (codeString == null || "".equals(codeString)) 274 return null; 275 if ("test-engine".equals(codeString)) 276 return TestReportParticipantType.TESTENGINE; 277 if ("client".equals(codeString)) 278 return TestReportParticipantType.CLIENT; 279 if ("server".equals(codeString)) 280 return TestReportParticipantType.SERVER; 281 throw new IllegalArgumentException("Unknown TestReportParticipantType code '"+codeString+"'"); 282 } 283 public Enumeration<TestReportParticipantType> fromType(PrimitiveType<?> code) throws FHIRException { 284 if (code == null) 285 return null; 286 if (code.isEmpty()) 287 return new Enumeration<TestReportParticipantType>(this, TestReportParticipantType.NULL, code); 288 String codeString = ((PrimitiveType) code).asStringValue(); 289 if (codeString == null || "".equals(codeString)) 290 return new Enumeration<TestReportParticipantType>(this, TestReportParticipantType.NULL, code); 291 if ("test-engine".equals(codeString)) 292 return new Enumeration<TestReportParticipantType>(this, TestReportParticipantType.TESTENGINE, code); 293 if ("client".equals(codeString)) 294 return new Enumeration<TestReportParticipantType>(this, TestReportParticipantType.CLIENT, code); 295 if ("server".equals(codeString)) 296 return new Enumeration<TestReportParticipantType>(this, TestReportParticipantType.SERVER, code); 297 throw new FHIRException("Unknown TestReportParticipantType code '"+codeString+"'"); 298 } 299 public String toCode(TestReportParticipantType code) { 300 if (code == TestReportParticipantType.TESTENGINE) 301 return "test-engine"; 302 if (code == TestReportParticipantType.CLIENT) 303 return "client"; 304 if (code == TestReportParticipantType.SERVER) 305 return "server"; 306 return "?"; 307 } 308 public String toSystem(TestReportParticipantType code) { 309 return code.getSystem(); 310 } 311 } 312 313 public enum TestReportResult { 314 /** 315 * All test operations successfully passed all asserts. 316 */ 317 PASS, 318 /** 319 * One or more test operations failed one or more asserts. 320 */ 321 FAIL, 322 /** 323 * One or more test operations is pending execution completion. 324 */ 325 PENDING, 326 /** 327 * added to help the parsers with the generic types 328 */ 329 NULL; 330 public static TestReportResult fromCode(String codeString) throws FHIRException { 331 if (codeString == null || "".equals(codeString)) 332 return null; 333 if ("pass".equals(codeString)) 334 return PASS; 335 if ("fail".equals(codeString)) 336 return FAIL; 337 if ("pending".equals(codeString)) 338 return PENDING; 339 if (Configuration.isAcceptInvalidEnums()) 340 return null; 341 else 342 throw new FHIRException("Unknown TestReportResult code '"+codeString+"'"); 343 } 344 public String toCode() { 345 switch (this) { 346 case PASS: return "pass"; 347 case FAIL: return "fail"; 348 case PENDING: return "pending"; 349 case NULL: return null; 350 default: return "?"; 351 } 352 } 353 public String getSystem() { 354 switch (this) { 355 case PASS: return "http://hl7.org/fhir/report-result-codes"; 356 case FAIL: return "http://hl7.org/fhir/report-result-codes"; 357 case PENDING: return "http://hl7.org/fhir/report-result-codes"; 358 case NULL: return null; 359 default: return "?"; 360 } 361 } 362 public String getDefinition() { 363 switch (this) { 364 case PASS: return "All test operations successfully passed all asserts."; 365 case FAIL: return "One or more test operations failed one or more asserts."; 366 case PENDING: return "One or more test operations is pending execution completion."; 367 case NULL: return null; 368 default: return "?"; 369 } 370 } 371 public String getDisplay() { 372 switch (this) { 373 case PASS: return "Pass"; 374 case FAIL: return "Fail"; 375 case PENDING: return "Pending"; 376 case NULL: return null; 377 default: return "?"; 378 } 379 } 380 } 381 382 public static class TestReportResultEnumFactory implements EnumFactory<TestReportResult> { 383 public TestReportResult fromCode(String codeString) throws IllegalArgumentException { 384 if (codeString == null || "".equals(codeString)) 385 if (codeString == null || "".equals(codeString)) 386 return null; 387 if ("pass".equals(codeString)) 388 return TestReportResult.PASS; 389 if ("fail".equals(codeString)) 390 return TestReportResult.FAIL; 391 if ("pending".equals(codeString)) 392 return TestReportResult.PENDING; 393 throw new IllegalArgumentException("Unknown TestReportResult code '"+codeString+"'"); 394 } 395 public Enumeration<TestReportResult> fromType(PrimitiveType<?> code) throws FHIRException { 396 if (code == null) 397 return null; 398 if (code.isEmpty()) 399 return new Enumeration<TestReportResult>(this, TestReportResult.NULL, code); 400 String codeString = ((PrimitiveType) code).asStringValue(); 401 if (codeString == null || "".equals(codeString)) 402 return new Enumeration<TestReportResult>(this, TestReportResult.NULL, code); 403 if ("pass".equals(codeString)) 404 return new Enumeration<TestReportResult>(this, TestReportResult.PASS, code); 405 if ("fail".equals(codeString)) 406 return new Enumeration<TestReportResult>(this, TestReportResult.FAIL, code); 407 if ("pending".equals(codeString)) 408 return new Enumeration<TestReportResult>(this, TestReportResult.PENDING, code); 409 throw new FHIRException("Unknown TestReportResult code '"+codeString+"'"); 410 } 411 public String toCode(TestReportResult code) { 412 if (code == TestReportResult.PASS) 413 return "pass"; 414 if (code == TestReportResult.FAIL) 415 return "fail"; 416 if (code == TestReportResult.PENDING) 417 return "pending"; 418 return "?"; 419 } 420 public String toSystem(TestReportResult code) { 421 return code.getSystem(); 422 } 423 } 424 425 public enum TestReportStatus { 426 /** 427 * All test operations have completed. 428 */ 429 COMPLETED, 430 /** 431 * A test operations is currently executing. 432 */ 433 INPROGRESS, 434 /** 435 * A test operation is waiting for an external client request. 436 */ 437 WAITING, 438 /** 439 * The test script execution was manually stopped. 440 */ 441 STOPPED, 442 /** 443 * This test report was entered or created in error. 444 */ 445 ENTEREDINERROR, 446 /** 447 * added to help the parsers with the generic types 448 */ 449 NULL; 450 public static TestReportStatus fromCode(String codeString) throws FHIRException { 451 if (codeString == null || "".equals(codeString)) 452 return null; 453 if ("completed".equals(codeString)) 454 return COMPLETED; 455 if ("in-progress".equals(codeString)) 456 return INPROGRESS; 457 if ("waiting".equals(codeString)) 458 return WAITING; 459 if ("stopped".equals(codeString)) 460 return STOPPED; 461 if ("entered-in-error".equals(codeString)) 462 return ENTEREDINERROR; 463 if (Configuration.isAcceptInvalidEnums()) 464 return null; 465 else 466 throw new FHIRException("Unknown TestReportStatus code '"+codeString+"'"); 467 } 468 public String toCode() { 469 switch (this) { 470 case COMPLETED: return "completed"; 471 case INPROGRESS: return "in-progress"; 472 case WAITING: return "waiting"; 473 case STOPPED: return "stopped"; 474 case ENTEREDINERROR: return "entered-in-error"; 475 case NULL: return null; 476 default: return "?"; 477 } 478 } 479 public String getSystem() { 480 switch (this) { 481 case COMPLETED: return "http://hl7.org/fhir/report-status-codes"; 482 case INPROGRESS: return "http://hl7.org/fhir/report-status-codes"; 483 case WAITING: return "http://hl7.org/fhir/report-status-codes"; 484 case STOPPED: return "http://hl7.org/fhir/report-status-codes"; 485 case ENTEREDINERROR: return "http://hl7.org/fhir/report-status-codes"; 486 case NULL: return null; 487 default: return "?"; 488 } 489 } 490 public String getDefinition() { 491 switch (this) { 492 case COMPLETED: return "All test operations have completed."; 493 case INPROGRESS: return "A test operations is currently executing."; 494 case WAITING: return "A test operation is waiting for an external client request."; 495 case STOPPED: return "The test script execution was manually stopped."; 496 case ENTEREDINERROR: return "This test report was entered or created in error."; 497 case NULL: return null; 498 default: return "?"; 499 } 500 } 501 public String getDisplay() { 502 switch (this) { 503 case COMPLETED: return "Completed"; 504 case INPROGRESS: return "In Progress"; 505 case WAITING: return "Waiting"; 506 case STOPPED: return "Stopped"; 507 case ENTEREDINERROR: return "Entered In Error"; 508 case NULL: return null; 509 default: return "?"; 510 } 511 } 512 } 513 514 public static class TestReportStatusEnumFactory implements EnumFactory<TestReportStatus> { 515 public TestReportStatus fromCode(String codeString) throws IllegalArgumentException { 516 if (codeString == null || "".equals(codeString)) 517 if (codeString == null || "".equals(codeString)) 518 return null; 519 if ("completed".equals(codeString)) 520 return TestReportStatus.COMPLETED; 521 if ("in-progress".equals(codeString)) 522 return TestReportStatus.INPROGRESS; 523 if ("waiting".equals(codeString)) 524 return TestReportStatus.WAITING; 525 if ("stopped".equals(codeString)) 526 return TestReportStatus.STOPPED; 527 if ("entered-in-error".equals(codeString)) 528 return TestReportStatus.ENTEREDINERROR; 529 throw new IllegalArgumentException("Unknown TestReportStatus code '"+codeString+"'"); 530 } 531 public Enumeration<TestReportStatus> fromType(PrimitiveType<?> code) throws FHIRException { 532 if (code == null) 533 return null; 534 if (code.isEmpty()) 535 return new Enumeration<TestReportStatus>(this, TestReportStatus.NULL, code); 536 String codeString = ((PrimitiveType) code).asStringValue(); 537 if (codeString == null || "".equals(codeString)) 538 return new Enumeration<TestReportStatus>(this, TestReportStatus.NULL, code); 539 if ("completed".equals(codeString)) 540 return new Enumeration<TestReportStatus>(this, TestReportStatus.COMPLETED, code); 541 if ("in-progress".equals(codeString)) 542 return new Enumeration<TestReportStatus>(this, TestReportStatus.INPROGRESS, code); 543 if ("waiting".equals(codeString)) 544 return new Enumeration<TestReportStatus>(this, TestReportStatus.WAITING, code); 545 if ("stopped".equals(codeString)) 546 return new Enumeration<TestReportStatus>(this, TestReportStatus.STOPPED, code); 547 if ("entered-in-error".equals(codeString)) 548 return new Enumeration<TestReportStatus>(this, TestReportStatus.ENTEREDINERROR, code); 549 throw new FHIRException("Unknown TestReportStatus code '"+codeString+"'"); 550 } 551 public String toCode(TestReportStatus code) { 552 if (code == TestReportStatus.COMPLETED) 553 return "completed"; 554 if (code == TestReportStatus.INPROGRESS) 555 return "in-progress"; 556 if (code == TestReportStatus.WAITING) 557 return "waiting"; 558 if (code == TestReportStatus.STOPPED) 559 return "stopped"; 560 if (code == TestReportStatus.ENTEREDINERROR) 561 return "entered-in-error"; 562 return "?"; 563 } 564 public String toSystem(TestReportStatus code) { 565 return code.getSystem(); 566 } 567 } 568 569 @Block() 570 public static class TestReportParticipantComponent extends BackboneElement implements IBaseBackboneElement { 571 /** 572 * The type of participant. 573 */ 574 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 575 @Description(shortDefinition="test-engine | client | server", formalDefinition="The type of participant." ) 576 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/report-participant-type") 577 protected Enumeration<TestReportParticipantType> type; 578 579 /** 580 * The uri of the participant. An absolute URL is preferred. 581 */ 582 @Child(name = "uri", type = {UriType.class}, order=2, min=1, max=1, modifier=false, summary=false) 583 @Description(shortDefinition="The uri of the participant. An absolute URL is preferred", formalDefinition="The uri of the participant. An absolute URL is preferred." ) 584 protected UriType uri; 585 586 /** 587 * The display name of the participant. 588 */ 589 @Child(name = "display", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 590 @Description(shortDefinition="The display name of the participant", formalDefinition="The display name of the participant." ) 591 protected StringType display; 592 593 private static final long serialVersionUID = 577488357L; 594 595 /** 596 * Constructor 597 */ 598 public TestReportParticipantComponent() { 599 super(); 600 } 601 602 /** 603 * Constructor 604 */ 605 public TestReportParticipantComponent(TestReportParticipantType type, String uri) { 606 super(); 607 this.setType(type); 608 this.setUri(uri); 609 } 610 611 /** 612 * @return {@link #type} (The type of participant.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 613 */ 614 public Enumeration<TestReportParticipantType> getTypeElement() { 615 if (this.type == null) 616 if (Configuration.errorOnAutoCreate()) 617 throw new Error("Attempt to auto-create TestReportParticipantComponent.type"); 618 else if (Configuration.doAutoCreate()) 619 this.type = new Enumeration<TestReportParticipantType>(new TestReportParticipantTypeEnumFactory()); // bb 620 return this.type; 621 } 622 623 public boolean hasTypeElement() { 624 return this.type != null && !this.type.isEmpty(); 625 } 626 627 public boolean hasType() { 628 return this.type != null && !this.type.isEmpty(); 629 } 630 631 /** 632 * @param value {@link #type} (The type of participant.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 633 */ 634 public TestReportParticipantComponent setTypeElement(Enumeration<TestReportParticipantType> value) { 635 this.type = value; 636 return this; 637 } 638 639 /** 640 * @return The type of participant. 641 */ 642 public TestReportParticipantType getType() { 643 return this.type == null ? null : this.type.getValue(); 644 } 645 646 /** 647 * @param value The type of participant. 648 */ 649 public TestReportParticipantComponent setType(TestReportParticipantType value) { 650 if (this.type == null) 651 this.type = new Enumeration<TestReportParticipantType>(new TestReportParticipantTypeEnumFactory()); 652 this.type.setValue(value); 653 return this; 654 } 655 656 /** 657 * @return {@link #uri} (The uri of the participant. An absolute URL is preferred.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 658 */ 659 public UriType getUriElement() { 660 if (this.uri == null) 661 if (Configuration.errorOnAutoCreate()) 662 throw new Error("Attempt to auto-create TestReportParticipantComponent.uri"); 663 else if (Configuration.doAutoCreate()) 664 this.uri = new UriType(); // bb 665 return this.uri; 666 } 667 668 public boolean hasUriElement() { 669 return this.uri != null && !this.uri.isEmpty(); 670 } 671 672 public boolean hasUri() { 673 return this.uri != null && !this.uri.isEmpty(); 674 } 675 676 /** 677 * @param value {@link #uri} (The uri of the participant. An absolute URL is preferred.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 678 */ 679 public TestReportParticipantComponent setUriElement(UriType value) { 680 this.uri = value; 681 return this; 682 } 683 684 /** 685 * @return The uri of the participant. An absolute URL is preferred. 686 */ 687 public String getUri() { 688 return this.uri == null ? null : this.uri.getValue(); 689 } 690 691 /** 692 * @param value The uri of the participant. An absolute URL is preferred. 693 */ 694 public TestReportParticipantComponent setUri(String value) { 695 if (this.uri == null) 696 this.uri = new UriType(); 697 this.uri.setValue(value); 698 return this; 699 } 700 701 /** 702 * @return {@link #display} (The display name of the participant.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 703 */ 704 public StringType getDisplayElement() { 705 if (this.display == null) 706 if (Configuration.errorOnAutoCreate()) 707 throw new Error("Attempt to auto-create TestReportParticipantComponent.display"); 708 else if (Configuration.doAutoCreate()) 709 this.display = new StringType(); // bb 710 return this.display; 711 } 712 713 public boolean hasDisplayElement() { 714 return this.display != null && !this.display.isEmpty(); 715 } 716 717 public boolean hasDisplay() { 718 return this.display != null && !this.display.isEmpty(); 719 } 720 721 /** 722 * @param value {@link #display} (The display name of the participant.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 723 */ 724 public TestReportParticipantComponent setDisplayElement(StringType value) { 725 this.display = value; 726 return this; 727 } 728 729 /** 730 * @return The display name of the participant. 731 */ 732 public String getDisplay() { 733 return this.display == null ? null : this.display.getValue(); 734 } 735 736 /** 737 * @param value The display name of the participant. 738 */ 739 public TestReportParticipantComponent setDisplay(String value) { 740 if (Utilities.noString(value)) 741 this.display = null; 742 else { 743 if (this.display == null) 744 this.display = new StringType(); 745 this.display.setValue(value); 746 } 747 return this; 748 } 749 750 protected void listChildren(List<Property> children) { 751 super.listChildren(children); 752 children.add(new Property("type", "code", "The type of participant.", 0, 1, type)); 753 children.add(new Property("uri", "uri", "The uri of the participant. An absolute URL is preferred.", 0, 1, uri)); 754 children.add(new Property("display", "string", "The display name of the participant.", 0, 1, display)); 755 } 756 757 @Override 758 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 759 switch (_hash) { 760 case 3575610: /*type*/ return new Property("type", "code", "The type of participant.", 0, 1, type); 761 case 116076: /*uri*/ return new Property("uri", "uri", "The uri of the participant. An absolute URL is preferred.", 0, 1, uri); 762 case 1671764162: /*display*/ return new Property("display", "string", "The display name of the participant.", 0, 1, display); 763 default: return super.getNamedProperty(_hash, _name, _checkValid); 764 } 765 766 } 767 768 @Override 769 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 770 switch (hash) { 771 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<TestReportParticipantType> 772 case 116076: /*uri*/ return this.uri == null ? new Base[0] : new Base[] {this.uri}; // UriType 773 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 774 default: return super.getProperty(hash, name, checkValid); 775 } 776 777 } 778 779 @Override 780 public Base setProperty(int hash, String name, Base value) throws FHIRException { 781 switch (hash) { 782 case 3575610: // type 783 value = new TestReportParticipantTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 784 this.type = (Enumeration) value; // Enumeration<TestReportParticipantType> 785 return value; 786 case 116076: // uri 787 this.uri = TypeConvertor.castToUri(value); // UriType 788 return value; 789 case 1671764162: // display 790 this.display = TypeConvertor.castToString(value); // StringType 791 return value; 792 default: return super.setProperty(hash, name, value); 793 } 794 795 } 796 797 @Override 798 public Base setProperty(String name, Base value) throws FHIRException { 799 if (name.equals("type")) { 800 value = new TestReportParticipantTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 801 this.type = (Enumeration) value; // Enumeration<TestReportParticipantType> 802 } else if (name.equals("uri")) { 803 this.uri = TypeConvertor.castToUri(value); // UriType 804 } else if (name.equals("display")) { 805 this.display = TypeConvertor.castToString(value); // StringType 806 } else 807 return super.setProperty(name, value); 808 return value; 809 } 810 811 @Override 812 public void removeChild(String name, Base value) throws FHIRException { 813 if (name.equals("type")) { 814 value = new TestReportParticipantTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 815 this.type = (Enumeration) value; // Enumeration<TestReportParticipantType> 816 } else if (name.equals("uri")) { 817 this.uri = null; 818 } else if (name.equals("display")) { 819 this.display = null; 820 } else 821 super.removeChild(name, value); 822 823 } 824 825 @Override 826 public Base makeProperty(int hash, String name) throws FHIRException { 827 switch (hash) { 828 case 3575610: return getTypeElement(); 829 case 116076: return getUriElement(); 830 case 1671764162: return getDisplayElement(); 831 default: return super.makeProperty(hash, name); 832 } 833 834 } 835 836 @Override 837 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 838 switch (hash) { 839 case 3575610: /*type*/ return new String[] {"code"}; 840 case 116076: /*uri*/ return new String[] {"uri"}; 841 case 1671764162: /*display*/ return new String[] {"string"}; 842 default: return super.getTypesForProperty(hash, name); 843 } 844 845 } 846 847 @Override 848 public Base addChild(String name) throws FHIRException { 849 if (name.equals("type")) { 850 throw new FHIRException("Cannot call addChild on a singleton property TestReport.participant.type"); 851 } 852 else if (name.equals("uri")) { 853 throw new FHIRException("Cannot call addChild on a singleton property TestReport.participant.uri"); 854 } 855 else if (name.equals("display")) { 856 throw new FHIRException("Cannot call addChild on a singleton property TestReport.participant.display"); 857 } 858 else 859 return super.addChild(name); 860 } 861 862 public TestReportParticipantComponent copy() { 863 TestReportParticipantComponent dst = new TestReportParticipantComponent(); 864 copyValues(dst); 865 return dst; 866 } 867 868 public void copyValues(TestReportParticipantComponent dst) { 869 super.copyValues(dst); 870 dst.type = type == null ? null : type.copy(); 871 dst.uri = uri == null ? null : uri.copy(); 872 dst.display = display == null ? null : display.copy(); 873 } 874 875 @Override 876 public boolean equalsDeep(Base other_) { 877 if (!super.equalsDeep(other_)) 878 return false; 879 if (!(other_ instanceof TestReportParticipantComponent)) 880 return false; 881 TestReportParticipantComponent o = (TestReportParticipantComponent) other_; 882 return compareDeep(type, o.type, true) && compareDeep(uri, o.uri, true) && compareDeep(display, o.display, true) 883 ; 884 } 885 886 @Override 887 public boolean equalsShallow(Base other_) { 888 if (!super.equalsShallow(other_)) 889 return false; 890 if (!(other_ instanceof TestReportParticipantComponent)) 891 return false; 892 TestReportParticipantComponent o = (TestReportParticipantComponent) other_; 893 return compareValues(type, o.type, true) && compareValues(uri, o.uri, true) && compareValues(display, o.display, true) 894 ; 895 } 896 897 public boolean isEmpty() { 898 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, uri, display); 899 } 900 901 public String fhirType() { 902 return "TestReport.participant"; 903 904 } 905 906 } 907 908 @Block() 909 public static class TestReportSetupComponent extends BackboneElement implements IBaseBackboneElement { 910 /** 911 * Action would contain either an operation or an assertion. 912 */ 913 @Child(name = "action", type = {}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 914 @Description(shortDefinition="A setup operation or assert that was executed", formalDefinition="Action would contain either an operation or an assertion." ) 915 protected List<SetupActionComponent> action; 916 917 private static final long serialVersionUID = -123374486L; 918 919 /** 920 * Constructor 921 */ 922 public TestReportSetupComponent() { 923 super(); 924 } 925 926 /** 927 * Constructor 928 */ 929 public TestReportSetupComponent(SetupActionComponent action) { 930 super(); 931 this.addAction(action); 932 } 933 934 /** 935 * @return {@link #action} (Action would contain either an operation or an assertion.) 936 */ 937 public List<SetupActionComponent> getAction() { 938 if (this.action == null) 939 this.action = new ArrayList<SetupActionComponent>(); 940 return this.action; 941 } 942 943 /** 944 * @return Returns a reference to <code>this</code> for easy method chaining 945 */ 946 public TestReportSetupComponent setAction(List<SetupActionComponent> theAction) { 947 this.action = theAction; 948 return this; 949 } 950 951 public boolean hasAction() { 952 if (this.action == null) 953 return false; 954 for (SetupActionComponent item : this.action) 955 if (!item.isEmpty()) 956 return true; 957 return false; 958 } 959 960 public SetupActionComponent addAction() { //3 961 SetupActionComponent t = new SetupActionComponent(); 962 if (this.action == null) 963 this.action = new ArrayList<SetupActionComponent>(); 964 this.action.add(t); 965 return t; 966 } 967 968 public TestReportSetupComponent addAction(SetupActionComponent t) { //3 969 if (t == null) 970 return this; 971 if (this.action == null) 972 this.action = new ArrayList<SetupActionComponent>(); 973 this.action.add(t); 974 return this; 975 } 976 977 /** 978 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist {3} 979 */ 980 public SetupActionComponent getActionFirstRep() { 981 if (getAction().isEmpty()) { 982 addAction(); 983 } 984 return getAction().get(0); 985 } 986 987 protected void listChildren(List<Property> children) { 988 super.listChildren(children); 989 children.add(new Property("action", "", "Action would contain either an operation or an assertion.", 0, java.lang.Integer.MAX_VALUE, action)); 990 } 991 992 @Override 993 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 994 switch (_hash) { 995 case -1422950858: /*action*/ return new Property("action", "", "Action would contain either an operation or an assertion.", 0, java.lang.Integer.MAX_VALUE, action); 996 default: return super.getNamedProperty(_hash, _name, _checkValid); 997 } 998 999 } 1000 1001 @Override 1002 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1003 switch (hash) { 1004 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // SetupActionComponent 1005 default: return super.getProperty(hash, name, checkValid); 1006 } 1007 1008 } 1009 1010 @Override 1011 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1012 switch (hash) { 1013 case -1422950858: // action 1014 this.getAction().add((SetupActionComponent) value); // SetupActionComponent 1015 return value; 1016 default: return super.setProperty(hash, name, value); 1017 } 1018 1019 } 1020 1021 @Override 1022 public Base setProperty(String name, Base value) throws FHIRException { 1023 if (name.equals("action")) { 1024 this.getAction().add((SetupActionComponent) value); 1025 } else 1026 return super.setProperty(name, value); 1027 return value; 1028 } 1029 1030 @Override 1031 public void removeChild(String name, Base value) throws FHIRException { 1032 if (name.equals("action")) { 1033 this.getAction().remove((SetupActionComponent) value); 1034 } else 1035 super.removeChild(name, value); 1036 1037 } 1038 1039 @Override 1040 public Base makeProperty(int hash, String name) throws FHIRException { 1041 switch (hash) { 1042 case -1422950858: return addAction(); 1043 default: return super.makeProperty(hash, name); 1044 } 1045 1046 } 1047 1048 @Override 1049 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1050 switch (hash) { 1051 case -1422950858: /*action*/ return new String[] {}; 1052 default: return super.getTypesForProperty(hash, name); 1053 } 1054 1055 } 1056 1057 @Override 1058 public Base addChild(String name) throws FHIRException { 1059 if (name.equals("action")) { 1060 return addAction(); 1061 } 1062 else 1063 return super.addChild(name); 1064 } 1065 1066 public TestReportSetupComponent copy() { 1067 TestReportSetupComponent dst = new TestReportSetupComponent(); 1068 copyValues(dst); 1069 return dst; 1070 } 1071 1072 public void copyValues(TestReportSetupComponent dst) { 1073 super.copyValues(dst); 1074 if (action != null) { 1075 dst.action = new ArrayList<SetupActionComponent>(); 1076 for (SetupActionComponent i : action) 1077 dst.action.add(i.copy()); 1078 }; 1079 } 1080 1081 @Override 1082 public boolean equalsDeep(Base other_) { 1083 if (!super.equalsDeep(other_)) 1084 return false; 1085 if (!(other_ instanceof TestReportSetupComponent)) 1086 return false; 1087 TestReportSetupComponent o = (TestReportSetupComponent) other_; 1088 return compareDeep(action, o.action, true); 1089 } 1090 1091 @Override 1092 public boolean equalsShallow(Base other_) { 1093 if (!super.equalsShallow(other_)) 1094 return false; 1095 if (!(other_ instanceof TestReportSetupComponent)) 1096 return false; 1097 TestReportSetupComponent o = (TestReportSetupComponent) other_; 1098 return true; 1099 } 1100 1101 public boolean isEmpty() { 1102 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action); 1103 } 1104 1105 public String fhirType() { 1106 return "TestReport.setup"; 1107 1108 } 1109 1110 } 1111 1112 @Block() 1113 public static class SetupActionComponent extends BackboneElement implements IBaseBackboneElement { 1114 /** 1115 * The operation performed. 1116 */ 1117 @Child(name = "operation", type = {}, order=1, min=0, max=1, modifier=false, summary=false) 1118 @Description(shortDefinition="The operation to perform", formalDefinition="The operation performed." ) 1119 protected SetupActionOperationComponent operation; 1120 1121 /** 1122 * The results of the assertion performed on the previous operations. 1123 */ 1124 @Child(name = "assert", type = {}, order=2, min=0, max=1, modifier=false, summary=false) 1125 @Description(shortDefinition="The assertion to perform", formalDefinition="The results of the assertion performed on the previous operations." ) 1126 protected SetupActionAssertComponent assert_; 1127 1128 private static final long serialVersionUID = -252088305L; 1129 1130 /** 1131 * Constructor 1132 */ 1133 public SetupActionComponent() { 1134 super(); 1135 } 1136 1137 /** 1138 * @return {@link #operation} (The operation performed.) 1139 */ 1140 public SetupActionOperationComponent getOperation() { 1141 if (this.operation == null) 1142 if (Configuration.errorOnAutoCreate()) 1143 throw new Error("Attempt to auto-create SetupActionComponent.operation"); 1144 else if (Configuration.doAutoCreate()) 1145 this.operation = new SetupActionOperationComponent(); // cc 1146 return this.operation; 1147 } 1148 1149 public boolean hasOperation() { 1150 return this.operation != null && !this.operation.isEmpty(); 1151 } 1152 1153 /** 1154 * @param value {@link #operation} (The operation performed.) 1155 */ 1156 public SetupActionComponent setOperation(SetupActionOperationComponent value) { 1157 this.operation = value; 1158 return this; 1159 } 1160 1161 /** 1162 * @return {@link #assert_} (The results of the assertion performed on the previous operations.) 1163 */ 1164 public SetupActionAssertComponent getAssert() { 1165 if (this.assert_ == null) 1166 if (Configuration.errorOnAutoCreate()) 1167 throw new Error("Attempt to auto-create SetupActionComponent.assert_"); 1168 else if (Configuration.doAutoCreate()) 1169 this.assert_ = new SetupActionAssertComponent(); // cc 1170 return this.assert_; 1171 } 1172 1173 public boolean hasAssert() { 1174 return this.assert_ != null && !this.assert_.isEmpty(); 1175 } 1176 1177 /** 1178 * @param value {@link #assert_} (The results of the assertion performed on the previous operations.) 1179 */ 1180 public SetupActionComponent setAssert(SetupActionAssertComponent value) { 1181 this.assert_ = value; 1182 return this; 1183 } 1184 1185 protected void listChildren(List<Property> children) { 1186 super.listChildren(children); 1187 children.add(new Property("operation", "", "The operation performed.", 0, 1, operation)); 1188 children.add(new Property("assert", "", "The results of the assertion performed on the previous operations.", 0, 1, assert_)); 1189 } 1190 1191 @Override 1192 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1193 switch (_hash) { 1194 case 1662702951: /*operation*/ return new Property("operation", "", "The operation performed.", 0, 1, operation); 1195 case -1408208058: /*assert*/ return new Property("assert", "", "The results of the assertion performed on the previous operations.", 0, 1, assert_); 1196 default: return super.getNamedProperty(_hash, _name, _checkValid); 1197 } 1198 1199 } 1200 1201 @Override 1202 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1203 switch (hash) { 1204 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : new Base[] {this.operation}; // SetupActionOperationComponent 1205 case -1408208058: /*assert*/ return this.assert_ == null ? new Base[0] : new Base[] {this.assert_}; // SetupActionAssertComponent 1206 default: return super.getProperty(hash, name, checkValid); 1207 } 1208 1209 } 1210 1211 @Override 1212 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1213 switch (hash) { 1214 case 1662702951: // operation 1215 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 1216 return value; 1217 case -1408208058: // assert 1218 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 1219 return value; 1220 default: return super.setProperty(hash, name, value); 1221 } 1222 1223 } 1224 1225 @Override 1226 public Base setProperty(String name, Base value) throws FHIRException { 1227 if (name.equals("operation")) { 1228 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 1229 } else if (name.equals("assert")) { 1230 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 1231 } else 1232 return super.setProperty(name, value); 1233 return value; 1234 } 1235 1236 @Override 1237 public void removeChild(String name, Base value) throws FHIRException { 1238 if (name.equals("operation")) { 1239 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 1240 } else if (name.equals("assert")) { 1241 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 1242 } else 1243 super.removeChild(name, value); 1244 1245 } 1246 1247 @Override 1248 public Base makeProperty(int hash, String name) throws FHIRException { 1249 switch (hash) { 1250 case 1662702951: return getOperation(); 1251 case -1408208058: return getAssert(); 1252 default: return super.makeProperty(hash, name); 1253 } 1254 1255 } 1256 1257 @Override 1258 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1259 switch (hash) { 1260 case 1662702951: /*operation*/ return new String[] {}; 1261 case -1408208058: /*assert*/ return new String[] {}; 1262 default: return super.getTypesForProperty(hash, name); 1263 } 1264 1265 } 1266 1267 @Override 1268 public Base addChild(String name) throws FHIRException { 1269 if (name.equals("operation")) { 1270 this.operation = new SetupActionOperationComponent(); 1271 return this.operation; 1272 } 1273 else if (name.equals("assert")) { 1274 this.assert_ = new SetupActionAssertComponent(); 1275 return this.assert_; 1276 } 1277 else 1278 return super.addChild(name); 1279 } 1280 1281 public SetupActionComponent copy() { 1282 SetupActionComponent dst = new SetupActionComponent(); 1283 copyValues(dst); 1284 return dst; 1285 } 1286 1287 public void copyValues(SetupActionComponent dst) { 1288 super.copyValues(dst); 1289 dst.operation = operation == null ? null : operation.copy(); 1290 dst.assert_ = assert_ == null ? null : assert_.copy(); 1291 } 1292 1293 @Override 1294 public boolean equalsDeep(Base other_) { 1295 if (!super.equalsDeep(other_)) 1296 return false; 1297 if (!(other_ instanceof SetupActionComponent)) 1298 return false; 1299 SetupActionComponent o = (SetupActionComponent) other_; 1300 return compareDeep(operation, o.operation, true) && compareDeep(assert_, o.assert_, true); 1301 } 1302 1303 @Override 1304 public boolean equalsShallow(Base other_) { 1305 if (!super.equalsShallow(other_)) 1306 return false; 1307 if (!(other_ instanceof SetupActionComponent)) 1308 return false; 1309 SetupActionComponent o = (SetupActionComponent) other_; 1310 return true; 1311 } 1312 1313 public boolean isEmpty() { 1314 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation, assert_); 1315 } 1316 1317 public String fhirType() { 1318 return "TestReport.setup.action"; 1319 1320 } 1321 1322 } 1323 1324 @Block() 1325 public static class SetupActionOperationComponent extends BackboneElement implements IBaseBackboneElement { 1326 /** 1327 * The result of this operation. 1328 */ 1329 @Child(name = "result", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1330 @Description(shortDefinition="pass | skip | fail | warning | error", formalDefinition="The result of this operation." ) 1331 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/report-action-result-codes") 1332 protected Enumeration<TestReportActionResult> result; 1333 1334 /** 1335 * An explanatory message associated with the result. 1336 */ 1337 @Child(name = "message", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1338 @Description(shortDefinition="A message associated with the result", formalDefinition="An explanatory message associated with the result." ) 1339 protected MarkdownType message; 1340 1341 /** 1342 * A link to further details on the result. 1343 */ 1344 @Child(name = "detail", type = {UriType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1345 @Description(shortDefinition="A link to further details on the result", formalDefinition="A link to further details on the result." ) 1346 protected UriType detail; 1347 1348 private static final long serialVersionUID = 269088798L; 1349 1350 /** 1351 * Constructor 1352 */ 1353 public SetupActionOperationComponent() { 1354 super(); 1355 } 1356 1357 /** 1358 * Constructor 1359 */ 1360 public SetupActionOperationComponent(TestReportActionResult result) { 1361 super(); 1362 this.setResult(result); 1363 } 1364 1365 /** 1366 * @return {@link #result} (The result of this operation.). This is the underlying object with id, value and extensions. The accessor "getResult" gives direct access to the value 1367 */ 1368 public Enumeration<TestReportActionResult> getResultElement() { 1369 if (this.result == null) 1370 if (Configuration.errorOnAutoCreate()) 1371 throw new Error("Attempt to auto-create SetupActionOperationComponent.result"); 1372 else if (Configuration.doAutoCreate()) 1373 this.result = new Enumeration<TestReportActionResult>(new TestReportActionResultEnumFactory()); // bb 1374 return this.result; 1375 } 1376 1377 public boolean hasResultElement() { 1378 return this.result != null && !this.result.isEmpty(); 1379 } 1380 1381 public boolean hasResult() { 1382 return this.result != null && !this.result.isEmpty(); 1383 } 1384 1385 /** 1386 * @param value {@link #result} (The result of this operation.). This is the underlying object with id, value and extensions. The accessor "getResult" gives direct access to the value 1387 */ 1388 public SetupActionOperationComponent setResultElement(Enumeration<TestReportActionResult> value) { 1389 this.result = value; 1390 return this; 1391 } 1392 1393 /** 1394 * @return The result of this operation. 1395 */ 1396 public TestReportActionResult getResult() { 1397 return this.result == null ? null : this.result.getValue(); 1398 } 1399 1400 /** 1401 * @param value The result of this operation. 1402 */ 1403 public SetupActionOperationComponent setResult(TestReportActionResult value) { 1404 if (this.result == null) 1405 this.result = new Enumeration<TestReportActionResult>(new TestReportActionResultEnumFactory()); 1406 this.result.setValue(value); 1407 return this; 1408 } 1409 1410 /** 1411 * @return {@link #message} (An explanatory message associated with the result.). This is the underlying object with id, value and extensions. The accessor "getMessage" gives direct access to the value 1412 */ 1413 public MarkdownType getMessageElement() { 1414 if (this.message == null) 1415 if (Configuration.errorOnAutoCreate()) 1416 throw new Error("Attempt to auto-create SetupActionOperationComponent.message"); 1417 else if (Configuration.doAutoCreate()) 1418 this.message = new MarkdownType(); // bb 1419 return this.message; 1420 } 1421 1422 public boolean hasMessageElement() { 1423 return this.message != null && !this.message.isEmpty(); 1424 } 1425 1426 public boolean hasMessage() { 1427 return this.message != null && !this.message.isEmpty(); 1428 } 1429 1430 /** 1431 * @param value {@link #message} (An explanatory message associated with the result.). This is the underlying object with id, value and extensions. The accessor "getMessage" gives direct access to the value 1432 */ 1433 public SetupActionOperationComponent setMessageElement(MarkdownType value) { 1434 this.message = value; 1435 return this; 1436 } 1437 1438 /** 1439 * @return An explanatory message associated with the result. 1440 */ 1441 public String getMessage() { 1442 return this.message == null ? null : this.message.getValue(); 1443 } 1444 1445 /** 1446 * @param value An explanatory message associated with the result. 1447 */ 1448 public SetupActionOperationComponent setMessage(String value) { 1449 if (Utilities.noString(value)) 1450 this.message = null; 1451 else { 1452 if (this.message == null) 1453 this.message = new MarkdownType(); 1454 this.message.setValue(value); 1455 } 1456 return this; 1457 } 1458 1459 /** 1460 * @return {@link #detail} (A link to further details on the result.). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value 1461 */ 1462 public UriType getDetailElement() { 1463 if (this.detail == null) 1464 if (Configuration.errorOnAutoCreate()) 1465 throw new Error("Attempt to auto-create SetupActionOperationComponent.detail"); 1466 else if (Configuration.doAutoCreate()) 1467 this.detail = new UriType(); // bb 1468 return this.detail; 1469 } 1470 1471 public boolean hasDetailElement() { 1472 return this.detail != null && !this.detail.isEmpty(); 1473 } 1474 1475 public boolean hasDetail() { 1476 return this.detail != null && !this.detail.isEmpty(); 1477 } 1478 1479 /** 1480 * @param value {@link #detail} (A link to further details on the result.). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value 1481 */ 1482 public SetupActionOperationComponent setDetailElement(UriType value) { 1483 this.detail = value; 1484 return this; 1485 } 1486 1487 /** 1488 * @return A link to further details on the result. 1489 */ 1490 public String getDetail() { 1491 return this.detail == null ? null : this.detail.getValue(); 1492 } 1493 1494 /** 1495 * @param value A link to further details on the result. 1496 */ 1497 public SetupActionOperationComponent setDetail(String value) { 1498 if (Utilities.noString(value)) 1499 this.detail = null; 1500 else { 1501 if (this.detail == null) 1502 this.detail = new UriType(); 1503 this.detail.setValue(value); 1504 } 1505 return this; 1506 } 1507 1508 protected void listChildren(List<Property> children) { 1509 super.listChildren(children); 1510 children.add(new Property("result", "code", "The result of this operation.", 0, 1, result)); 1511 children.add(new Property("message", "markdown", "An explanatory message associated with the result.", 0, 1, message)); 1512 children.add(new Property("detail", "uri", "A link to further details on the result.", 0, 1, detail)); 1513 } 1514 1515 @Override 1516 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1517 switch (_hash) { 1518 case -934426595: /*result*/ return new Property("result", "code", "The result of this operation.", 0, 1, result); 1519 case 954925063: /*message*/ return new Property("message", "markdown", "An explanatory message associated with the result.", 0, 1, message); 1520 case -1335224239: /*detail*/ return new Property("detail", "uri", "A link to further details on the result.", 0, 1, detail); 1521 default: return super.getNamedProperty(_hash, _name, _checkValid); 1522 } 1523 1524 } 1525 1526 @Override 1527 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1528 switch (hash) { 1529 case -934426595: /*result*/ return this.result == null ? new Base[0] : new Base[] {this.result}; // Enumeration<TestReportActionResult> 1530 case 954925063: /*message*/ return this.message == null ? new Base[0] : new Base[] {this.message}; // MarkdownType 1531 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : new Base[] {this.detail}; // UriType 1532 default: return super.getProperty(hash, name, checkValid); 1533 } 1534 1535 } 1536 1537 @Override 1538 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1539 switch (hash) { 1540 case -934426595: // result 1541 value = new TestReportActionResultEnumFactory().fromType(TypeConvertor.castToCode(value)); 1542 this.result = (Enumeration) value; // Enumeration<TestReportActionResult> 1543 return value; 1544 case 954925063: // message 1545 this.message = TypeConvertor.castToMarkdown(value); // MarkdownType 1546 return value; 1547 case -1335224239: // detail 1548 this.detail = TypeConvertor.castToUri(value); // UriType 1549 return value; 1550 default: return super.setProperty(hash, name, value); 1551 } 1552 1553 } 1554 1555 @Override 1556 public Base setProperty(String name, Base value) throws FHIRException { 1557 if (name.equals("result")) { 1558 value = new TestReportActionResultEnumFactory().fromType(TypeConvertor.castToCode(value)); 1559 this.result = (Enumeration) value; // Enumeration<TestReportActionResult> 1560 } else if (name.equals("message")) { 1561 this.message = TypeConvertor.castToMarkdown(value); // MarkdownType 1562 } else if (name.equals("detail")) { 1563 this.detail = TypeConvertor.castToUri(value); // UriType 1564 } else 1565 return super.setProperty(name, value); 1566 return value; 1567 } 1568 1569 @Override 1570 public void removeChild(String name, Base value) throws FHIRException { 1571 if (name.equals("result")) { 1572 value = new TestReportActionResultEnumFactory().fromType(TypeConvertor.castToCode(value)); 1573 this.result = (Enumeration) value; // Enumeration<TestReportActionResult> 1574 } else if (name.equals("message")) { 1575 this.message = null; 1576 } else if (name.equals("detail")) { 1577 this.detail = null; 1578 } else 1579 super.removeChild(name, value); 1580 1581 } 1582 1583 @Override 1584 public Base makeProperty(int hash, String name) throws FHIRException { 1585 switch (hash) { 1586 case -934426595: return getResultElement(); 1587 case 954925063: return getMessageElement(); 1588 case -1335224239: return getDetailElement(); 1589 default: return super.makeProperty(hash, name); 1590 } 1591 1592 } 1593 1594 @Override 1595 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1596 switch (hash) { 1597 case -934426595: /*result*/ return new String[] {"code"}; 1598 case 954925063: /*message*/ return new String[] {"markdown"}; 1599 case -1335224239: /*detail*/ return new String[] {"uri"}; 1600 default: return super.getTypesForProperty(hash, name); 1601 } 1602 1603 } 1604 1605 @Override 1606 public Base addChild(String name) throws FHIRException { 1607 if (name.equals("result")) { 1608 throw new FHIRException("Cannot call addChild on a singleton property TestReport.setup.action.operation.result"); 1609 } 1610 else if (name.equals("message")) { 1611 throw new FHIRException("Cannot call addChild on a singleton property TestReport.setup.action.operation.message"); 1612 } 1613 else if (name.equals("detail")) { 1614 throw new FHIRException("Cannot call addChild on a singleton property TestReport.setup.action.operation.detail"); 1615 } 1616 else 1617 return super.addChild(name); 1618 } 1619 1620 public SetupActionOperationComponent copy() { 1621 SetupActionOperationComponent dst = new SetupActionOperationComponent(); 1622 copyValues(dst); 1623 return dst; 1624 } 1625 1626 public void copyValues(SetupActionOperationComponent dst) { 1627 super.copyValues(dst); 1628 dst.result = result == null ? null : result.copy(); 1629 dst.message = message == null ? null : message.copy(); 1630 dst.detail = detail == null ? null : detail.copy(); 1631 } 1632 1633 @Override 1634 public boolean equalsDeep(Base other_) { 1635 if (!super.equalsDeep(other_)) 1636 return false; 1637 if (!(other_ instanceof SetupActionOperationComponent)) 1638 return false; 1639 SetupActionOperationComponent o = (SetupActionOperationComponent) other_; 1640 return compareDeep(result, o.result, true) && compareDeep(message, o.message, true) && compareDeep(detail, o.detail, true) 1641 ; 1642 } 1643 1644 @Override 1645 public boolean equalsShallow(Base other_) { 1646 if (!super.equalsShallow(other_)) 1647 return false; 1648 if (!(other_ instanceof SetupActionOperationComponent)) 1649 return false; 1650 SetupActionOperationComponent o = (SetupActionOperationComponent) other_; 1651 return compareValues(result, o.result, true) && compareValues(message, o.message, true) && compareValues(detail, o.detail, true) 1652 ; 1653 } 1654 1655 public boolean isEmpty() { 1656 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(result, message, detail 1657 ); 1658 } 1659 1660 public String fhirType() { 1661 return "TestReport.setup.action.operation"; 1662 1663 } 1664 1665 } 1666 1667 @Block() 1668 public static class SetupActionAssertComponent extends BackboneElement implements IBaseBackboneElement { 1669 /** 1670 * The result of this assertion. 1671 */ 1672 @Child(name = "result", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1673 @Description(shortDefinition="pass | skip | fail | warning | error", formalDefinition="The result of this assertion." ) 1674 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/report-action-result-codes") 1675 protected Enumeration<TestReportActionResult> result; 1676 1677 /** 1678 * An explanatory message associated with the result. 1679 */ 1680 @Child(name = "message", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1681 @Description(shortDefinition="A message associated with the result", formalDefinition="An explanatory message associated with the result." ) 1682 protected MarkdownType message; 1683 1684 /** 1685 * A link to further details on the result. 1686 */ 1687 @Child(name = "detail", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1688 @Description(shortDefinition="A link to further details on the result", formalDefinition="A link to further details on the result." ) 1689 protected StringType detail; 1690 1691 /** 1692 * Links or references providing traceability to the testing requirements for this assert. 1693 */ 1694 @Child(name = "requirement", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1695 @Description(shortDefinition="Links or references to the testing requirements", formalDefinition="Links or references providing traceability to the testing requirements for this assert." ) 1696 protected List<SetupActionAssertRequirementComponent> requirement; 1697 1698 private static final long serialVersionUID = -132618689L; 1699 1700 /** 1701 * Constructor 1702 */ 1703 public SetupActionAssertComponent() { 1704 super(); 1705 } 1706 1707 /** 1708 * Constructor 1709 */ 1710 public SetupActionAssertComponent(TestReportActionResult result) { 1711 super(); 1712 this.setResult(result); 1713 } 1714 1715 /** 1716 * @return {@link #result} (The result of this assertion.). This is the underlying object with id, value and extensions. The accessor "getResult" gives direct access to the value 1717 */ 1718 public Enumeration<TestReportActionResult> getResultElement() { 1719 if (this.result == null) 1720 if (Configuration.errorOnAutoCreate()) 1721 throw new Error("Attempt to auto-create SetupActionAssertComponent.result"); 1722 else if (Configuration.doAutoCreate()) 1723 this.result = new Enumeration<TestReportActionResult>(new TestReportActionResultEnumFactory()); // bb 1724 return this.result; 1725 } 1726 1727 public boolean hasResultElement() { 1728 return this.result != null && !this.result.isEmpty(); 1729 } 1730 1731 public boolean hasResult() { 1732 return this.result != null && !this.result.isEmpty(); 1733 } 1734 1735 /** 1736 * @param value {@link #result} (The result of this assertion.). This is the underlying object with id, value and extensions. The accessor "getResult" gives direct access to the value 1737 */ 1738 public SetupActionAssertComponent setResultElement(Enumeration<TestReportActionResult> value) { 1739 this.result = value; 1740 return this; 1741 } 1742 1743 /** 1744 * @return The result of this assertion. 1745 */ 1746 public TestReportActionResult getResult() { 1747 return this.result == null ? null : this.result.getValue(); 1748 } 1749 1750 /** 1751 * @param value The result of this assertion. 1752 */ 1753 public SetupActionAssertComponent setResult(TestReportActionResult value) { 1754 if (this.result == null) 1755 this.result = new Enumeration<TestReportActionResult>(new TestReportActionResultEnumFactory()); 1756 this.result.setValue(value); 1757 return this; 1758 } 1759 1760 /** 1761 * @return {@link #message} (An explanatory message associated with the result.). This is the underlying object with id, value and extensions. The accessor "getMessage" gives direct access to the value 1762 */ 1763 public MarkdownType getMessageElement() { 1764 if (this.message == null) 1765 if (Configuration.errorOnAutoCreate()) 1766 throw new Error("Attempt to auto-create SetupActionAssertComponent.message"); 1767 else if (Configuration.doAutoCreate()) 1768 this.message = new MarkdownType(); // bb 1769 return this.message; 1770 } 1771 1772 public boolean hasMessageElement() { 1773 return this.message != null && !this.message.isEmpty(); 1774 } 1775 1776 public boolean hasMessage() { 1777 return this.message != null && !this.message.isEmpty(); 1778 } 1779 1780 /** 1781 * @param value {@link #message} (An explanatory message associated with the result.). This is the underlying object with id, value and extensions. The accessor "getMessage" gives direct access to the value 1782 */ 1783 public SetupActionAssertComponent setMessageElement(MarkdownType value) { 1784 this.message = value; 1785 return this; 1786 } 1787 1788 /** 1789 * @return An explanatory message associated with the result. 1790 */ 1791 public String getMessage() { 1792 return this.message == null ? null : this.message.getValue(); 1793 } 1794 1795 /** 1796 * @param value An explanatory message associated with the result. 1797 */ 1798 public SetupActionAssertComponent setMessage(String value) { 1799 if (Utilities.noString(value)) 1800 this.message = null; 1801 else { 1802 if (this.message == null) 1803 this.message = new MarkdownType(); 1804 this.message.setValue(value); 1805 } 1806 return this; 1807 } 1808 1809 /** 1810 * @return {@link #detail} (A link to further details on the result.). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value 1811 */ 1812 public StringType getDetailElement() { 1813 if (this.detail == null) 1814 if (Configuration.errorOnAutoCreate()) 1815 throw new Error("Attempt to auto-create SetupActionAssertComponent.detail"); 1816 else if (Configuration.doAutoCreate()) 1817 this.detail = new StringType(); // bb 1818 return this.detail; 1819 } 1820 1821 public boolean hasDetailElement() { 1822 return this.detail != null && !this.detail.isEmpty(); 1823 } 1824 1825 public boolean hasDetail() { 1826 return this.detail != null && !this.detail.isEmpty(); 1827 } 1828 1829 /** 1830 * @param value {@link #detail} (A link to further details on the result.). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value 1831 */ 1832 public SetupActionAssertComponent setDetailElement(StringType value) { 1833 this.detail = value; 1834 return this; 1835 } 1836 1837 /** 1838 * @return A link to further details on the result. 1839 */ 1840 public String getDetail() { 1841 return this.detail == null ? null : this.detail.getValue(); 1842 } 1843 1844 /** 1845 * @param value A link to further details on the result. 1846 */ 1847 public SetupActionAssertComponent setDetail(String value) { 1848 if (Utilities.noString(value)) 1849 this.detail = null; 1850 else { 1851 if (this.detail == null) 1852 this.detail = new StringType(); 1853 this.detail.setValue(value); 1854 } 1855 return this; 1856 } 1857 1858 /** 1859 * @return {@link #requirement} (Links or references providing traceability to the testing requirements for this assert.) 1860 */ 1861 public List<SetupActionAssertRequirementComponent> getRequirement() { 1862 if (this.requirement == null) 1863 this.requirement = new ArrayList<SetupActionAssertRequirementComponent>(); 1864 return this.requirement; 1865 } 1866 1867 /** 1868 * @return Returns a reference to <code>this</code> for easy method chaining 1869 */ 1870 public SetupActionAssertComponent setRequirement(List<SetupActionAssertRequirementComponent> theRequirement) { 1871 this.requirement = theRequirement; 1872 return this; 1873 } 1874 1875 public boolean hasRequirement() { 1876 if (this.requirement == null) 1877 return false; 1878 for (SetupActionAssertRequirementComponent item : this.requirement) 1879 if (!item.isEmpty()) 1880 return true; 1881 return false; 1882 } 1883 1884 public SetupActionAssertRequirementComponent addRequirement() { //3 1885 SetupActionAssertRequirementComponent t = new SetupActionAssertRequirementComponent(); 1886 if (this.requirement == null) 1887 this.requirement = new ArrayList<SetupActionAssertRequirementComponent>(); 1888 this.requirement.add(t); 1889 return t; 1890 } 1891 1892 public SetupActionAssertComponent addRequirement(SetupActionAssertRequirementComponent t) { //3 1893 if (t == null) 1894 return this; 1895 if (this.requirement == null) 1896 this.requirement = new ArrayList<SetupActionAssertRequirementComponent>(); 1897 this.requirement.add(t); 1898 return this; 1899 } 1900 1901 /** 1902 * @return The first repetition of repeating field {@link #requirement}, creating it if it does not already exist {3} 1903 */ 1904 public SetupActionAssertRequirementComponent getRequirementFirstRep() { 1905 if (getRequirement().isEmpty()) { 1906 addRequirement(); 1907 } 1908 return getRequirement().get(0); 1909 } 1910 1911 protected void listChildren(List<Property> children) { 1912 super.listChildren(children); 1913 children.add(new Property("result", "code", "The result of this assertion.", 0, 1, result)); 1914 children.add(new Property("message", "markdown", "An explanatory message associated with the result.", 0, 1, message)); 1915 children.add(new Property("detail", "string", "A link to further details on the result.", 0, 1, detail)); 1916 children.add(new Property("requirement", "", "Links or references providing traceability to the testing requirements for this assert.", 0, java.lang.Integer.MAX_VALUE, requirement)); 1917 } 1918 1919 @Override 1920 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1921 switch (_hash) { 1922 case -934426595: /*result*/ return new Property("result", "code", "The result of this assertion.", 0, 1, result); 1923 case 954925063: /*message*/ return new Property("message", "markdown", "An explanatory message associated with the result.", 0, 1, message); 1924 case -1335224239: /*detail*/ return new Property("detail", "string", "A link to further details on the result.", 0, 1, detail); 1925 case 363387971: /*requirement*/ return new Property("requirement", "", "Links or references providing traceability to the testing requirements for this assert.", 0, java.lang.Integer.MAX_VALUE, requirement); 1926 default: return super.getNamedProperty(_hash, _name, _checkValid); 1927 } 1928 1929 } 1930 1931 @Override 1932 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1933 switch (hash) { 1934 case -934426595: /*result*/ return this.result == null ? new Base[0] : new Base[] {this.result}; // Enumeration<TestReportActionResult> 1935 case 954925063: /*message*/ return this.message == null ? new Base[0] : new Base[] {this.message}; // MarkdownType 1936 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : new Base[] {this.detail}; // StringType 1937 case 363387971: /*requirement*/ return this.requirement == null ? new Base[0] : this.requirement.toArray(new Base[this.requirement.size()]); // SetupActionAssertRequirementComponent 1938 default: return super.getProperty(hash, name, checkValid); 1939 } 1940 1941 } 1942 1943 @Override 1944 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1945 switch (hash) { 1946 case -934426595: // result 1947 value = new TestReportActionResultEnumFactory().fromType(TypeConvertor.castToCode(value)); 1948 this.result = (Enumeration) value; // Enumeration<TestReportActionResult> 1949 return value; 1950 case 954925063: // message 1951 this.message = TypeConvertor.castToMarkdown(value); // MarkdownType 1952 return value; 1953 case -1335224239: // detail 1954 this.detail = TypeConvertor.castToString(value); // StringType 1955 return value; 1956 case 363387971: // requirement 1957 this.getRequirement().add((SetupActionAssertRequirementComponent) value); // SetupActionAssertRequirementComponent 1958 return value; 1959 default: return super.setProperty(hash, name, value); 1960 } 1961 1962 } 1963 1964 @Override 1965 public Base setProperty(String name, Base value) throws FHIRException { 1966 if (name.equals("result")) { 1967 value = new TestReportActionResultEnumFactory().fromType(TypeConvertor.castToCode(value)); 1968 this.result = (Enumeration) value; // Enumeration<TestReportActionResult> 1969 } else if (name.equals("message")) { 1970 this.message = TypeConvertor.castToMarkdown(value); // MarkdownType 1971 } else if (name.equals("detail")) { 1972 this.detail = TypeConvertor.castToString(value); // StringType 1973 } else if (name.equals("requirement")) { 1974 this.getRequirement().add((SetupActionAssertRequirementComponent) value); 1975 } else 1976 return super.setProperty(name, value); 1977 return value; 1978 } 1979 1980 @Override 1981 public void removeChild(String name, Base value) throws FHIRException { 1982 if (name.equals("result")) { 1983 value = new TestReportActionResultEnumFactory().fromType(TypeConvertor.castToCode(value)); 1984 this.result = (Enumeration) value; // Enumeration<TestReportActionResult> 1985 } else if (name.equals("message")) { 1986 this.message = null; 1987 } else if (name.equals("detail")) { 1988 this.detail = null; 1989 } else if (name.equals("requirement")) { 1990 this.getRequirement().remove((SetupActionAssertRequirementComponent) value); 1991 } else 1992 super.removeChild(name, value); 1993 1994 } 1995 1996 @Override 1997 public Base makeProperty(int hash, String name) throws FHIRException { 1998 switch (hash) { 1999 case -934426595: return getResultElement(); 2000 case 954925063: return getMessageElement(); 2001 case -1335224239: return getDetailElement(); 2002 case 363387971: return addRequirement(); 2003 default: return super.makeProperty(hash, name); 2004 } 2005 2006 } 2007 2008 @Override 2009 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2010 switch (hash) { 2011 case -934426595: /*result*/ return new String[] {"code"}; 2012 case 954925063: /*message*/ return new String[] {"markdown"}; 2013 case -1335224239: /*detail*/ return new String[] {"string"}; 2014 case 363387971: /*requirement*/ return new String[] {}; 2015 default: return super.getTypesForProperty(hash, name); 2016 } 2017 2018 } 2019 2020 @Override 2021 public Base addChild(String name) throws FHIRException { 2022 if (name.equals("result")) { 2023 throw new FHIRException("Cannot call addChild on a singleton property TestReport.setup.action.assert.result"); 2024 } 2025 else if (name.equals("message")) { 2026 throw new FHIRException("Cannot call addChild on a singleton property TestReport.setup.action.assert.message"); 2027 } 2028 else if (name.equals("detail")) { 2029 throw new FHIRException("Cannot call addChild on a singleton property TestReport.setup.action.assert.detail"); 2030 } 2031 else if (name.equals("requirement")) { 2032 return addRequirement(); 2033 } 2034 else 2035 return super.addChild(name); 2036 } 2037 2038 public SetupActionAssertComponent copy() { 2039 SetupActionAssertComponent dst = new SetupActionAssertComponent(); 2040 copyValues(dst); 2041 return dst; 2042 } 2043 2044 public void copyValues(SetupActionAssertComponent dst) { 2045 super.copyValues(dst); 2046 dst.result = result == null ? null : result.copy(); 2047 dst.message = message == null ? null : message.copy(); 2048 dst.detail = detail == null ? null : detail.copy(); 2049 if (requirement != null) { 2050 dst.requirement = new ArrayList<SetupActionAssertRequirementComponent>(); 2051 for (SetupActionAssertRequirementComponent i : requirement) 2052 dst.requirement.add(i.copy()); 2053 }; 2054 } 2055 2056 @Override 2057 public boolean equalsDeep(Base other_) { 2058 if (!super.equalsDeep(other_)) 2059 return false; 2060 if (!(other_ instanceof SetupActionAssertComponent)) 2061 return false; 2062 SetupActionAssertComponent o = (SetupActionAssertComponent) other_; 2063 return compareDeep(result, o.result, true) && compareDeep(message, o.message, true) && compareDeep(detail, o.detail, true) 2064 && compareDeep(requirement, o.requirement, true); 2065 } 2066 2067 @Override 2068 public boolean equalsShallow(Base other_) { 2069 if (!super.equalsShallow(other_)) 2070 return false; 2071 if (!(other_ instanceof SetupActionAssertComponent)) 2072 return false; 2073 SetupActionAssertComponent o = (SetupActionAssertComponent) other_; 2074 return compareValues(result, o.result, true) && compareValues(message, o.message, true) && compareValues(detail, o.detail, true) 2075 ; 2076 } 2077 2078 public boolean isEmpty() { 2079 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(result, message, detail 2080 , requirement); 2081 } 2082 2083 public String fhirType() { 2084 return "TestReport.setup.action.assert"; 2085 2086 } 2087 2088 } 2089 2090 @Block() 2091 public static class SetupActionAssertRequirementComponent extends BackboneElement implements IBaseBackboneElement { 2092 /** 2093 * Link or reference providing traceability to the testing requirement for this test. 2094 */ 2095 @Child(name = "link", type = {UriType.class, CanonicalType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2096 @Description(shortDefinition="Link or reference to the testing requirement", formalDefinition="Link or reference providing traceability to the testing requirement for this test." ) 2097 protected DataType link; 2098 2099 private static final long serialVersionUID = -91187948L; 2100 2101 /** 2102 * Constructor 2103 */ 2104 public SetupActionAssertRequirementComponent() { 2105 super(); 2106 } 2107 2108 /** 2109 * @return {@link #link} (Link or reference providing traceability to the testing requirement for this test.) 2110 */ 2111 public DataType getLink() { 2112 return this.link; 2113 } 2114 2115 /** 2116 * @return {@link #link} (Link or reference providing traceability to the testing requirement for this test.) 2117 */ 2118 public UriType getLinkUriType() throws FHIRException { 2119 if (this.link == null) 2120 this.link = new UriType(); 2121 if (!(this.link instanceof UriType)) 2122 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.link.getClass().getName()+" was encountered"); 2123 return (UriType) this.link; 2124 } 2125 2126 public boolean hasLinkUriType() { 2127 return this != null && this.link instanceof UriType; 2128 } 2129 2130 /** 2131 * @return {@link #link} (Link or reference providing traceability to the testing requirement for this test.) 2132 */ 2133 public CanonicalType getLinkCanonicalType() throws FHIRException { 2134 if (this.link == null) 2135 this.link = new CanonicalType(); 2136 if (!(this.link instanceof CanonicalType)) 2137 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.link.getClass().getName()+" was encountered"); 2138 return (CanonicalType) this.link; 2139 } 2140 2141 public boolean hasLinkCanonicalType() { 2142 return this != null && this.link instanceof CanonicalType; 2143 } 2144 2145 public boolean hasLink() { 2146 return this.link != null && !this.link.isEmpty(); 2147 } 2148 2149 /** 2150 * @param value {@link #link} (Link or reference providing traceability to the testing requirement for this test.) 2151 */ 2152 public SetupActionAssertRequirementComponent setLink(DataType value) { 2153 if (value != null && !(value instanceof UriType || value instanceof CanonicalType)) 2154 throw new FHIRException("Not the right type for TestReport.setup.action.assert.requirement.link[x]: "+value.fhirType()); 2155 this.link = value; 2156 return this; 2157 } 2158 2159 protected void listChildren(List<Property> children) { 2160 super.listChildren(children); 2161 children.add(new Property("link[x]", "uri|canonical(Requirements)", "Link or reference providing traceability to the testing requirement for this test.", 0, 1, link)); 2162 } 2163 2164 @Override 2165 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2166 switch (_hash) { 2167 case 177076806: /*link[x]*/ return new Property("link[x]", "uri|canonical(Requirements)", "Link or reference providing traceability to the testing requirement for this test.", 0, 1, link); 2168 case 3321850: /*link*/ return new Property("link[x]", "uri|canonical(Requirements)", "Link or reference providing traceability to the testing requirement for this test.", 0, 1, link); 2169 case 177070866: /*linkUri*/ return new Property("link[x]", "uri", "Link or reference providing traceability to the testing requirement for this test.", 0, 1, link); 2170 case -2064880102: /*linkCanonical*/ return new Property("link[x]", "canonical(Requirements)", "Link or reference providing traceability to the testing requirement for this test.", 0, 1, link); 2171 default: return super.getNamedProperty(_hash, _name, _checkValid); 2172 } 2173 2174 } 2175 2176 @Override 2177 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2178 switch (hash) { 2179 case 3321850: /*link*/ return this.link == null ? new Base[0] : new Base[] {this.link}; // DataType 2180 default: return super.getProperty(hash, name, checkValid); 2181 } 2182 2183 } 2184 2185 @Override 2186 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2187 switch (hash) { 2188 case 3321850: // link 2189 this.link = TypeConvertor.castToType(value); // DataType 2190 return value; 2191 default: return super.setProperty(hash, name, value); 2192 } 2193 2194 } 2195 2196 @Override 2197 public Base setProperty(String name, Base value) throws FHIRException { 2198 if (name.equals("link[x]")) { 2199 this.link = TypeConvertor.castToType(value); // DataType 2200 } else 2201 return super.setProperty(name, value); 2202 return value; 2203 } 2204 2205 @Override 2206 public void removeChild(String name, Base value) throws FHIRException { 2207 if (name.equals("link[x]")) { 2208 this.link = null; 2209 } else 2210 super.removeChild(name, value); 2211 2212 } 2213 2214 @Override 2215 public Base makeProperty(int hash, String name) throws FHIRException { 2216 switch (hash) { 2217 case 177076806: return getLink(); 2218 case 3321850: return getLink(); 2219 default: return super.makeProperty(hash, name); 2220 } 2221 2222 } 2223 2224 @Override 2225 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2226 switch (hash) { 2227 case 3321850: /*link*/ return new String[] {"uri", "canonical"}; 2228 default: return super.getTypesForProperty(hash, name); 2229 } 2230 2231 } 2232 2233 @Override 2234 public Base addChild(String name) throws FHIRException { 2235 if (name.equals("linkUri")) { 2236 this.link = new UriType(); 2237 return this.link; 2238 } 2239 else if (name.equals("linkCanonical")) { 2240 this.link = new CanonicalType(); 2241 return this.link; 2242 } 2243 else 2244 return super.addChild(name); 2245 } 2246 2247 public SetupActionAssertRequirementComponent copy() { 2248 SetupActionAssertRequirementComponent dst = new SetupActionAssertRequirementComponent(); 2249 copyValues(dst); 2250 return dst; 2251 } 2252 2253 public void copyValues(SetupActionAssertRequirementComponent dst) { 2254 super.copyValues(dst); 2255 dst.link = link == null ? null : link.copy(); 2256 } 2257 2258 @Override 2259 public boolean equalsDeep(Base other_) { 2260 if (!super.equalsDeep(other_)) 2261 return false; 2262 if (!(other_ instanceof SetupActionAssertRequirementComponent)) 2263 return false; 2264 SetupActionAssertRequirementComponent o = (SetupActionAssertRequirementComponent) other_; 2265 return compareDeep(link, o.link, true); 2266 } 2267 2268 @Override 2269 public boolean equalsShallow(Base other_) { 2270 if (!super.equalsShallow(other_)) 2271 return false; 2272 if (!(other_ instanceof SetupActionAssertRequirementComponent)) 2273 return false; 2274 SetupActionAssertRequirementComponent o = (SetupActionAssertRequirementComponent) other_; 2275 return true; 2276 } 2277 2278 public boolean isEmpty() { 2279 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(link); 2280 } 2281 2282 public String fhirType() { 2283 return "TestReport.setup.action.assert.requirement"; 2284 2285 } 2286 2287 } 2288 2289 @Block() 2290 public static class TestReportTestComponent extends BackboneElement implements IBaseBackboneElement { 2291 /** 2292 * The name of this test used for tracking/logging purposes by test engines. 2293 */ 2294 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2295 @Description(shortDefinition="Tracking/logging name of this test", formalDefinition="The name of this test used for tracking/logging purposes by test engines." ) 2296 protected StringType name; 2297 2298 /** 2299 * A short description of the test used by test engines for tracking and reporting purposes. 2300 */ 2301 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2302 @Description(shortDefinition="Tracking/reporting short description of the test", formalDefinition="A short description of the test used by test engines for tracking and reporting purposes." ) 2303 protected StringType description; 2304 2305 /** 2306 * Action would contain either an operation or an assertion. 2307 */ 2308 @Child(name = "action", type = {}, order=3, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2309 @Description(shortDefinition="A test operation or assert that was performed", formalDefinition="Action would contain either an operation or an assertion." ) 2310 protected List<TestActionComponent> action; 2311 2312 private static final long serialVersionUID = -865006110L; 2313 2314 /** 2315 * Constructor 2316 */ 2317 public TestReportTestComponent() { 2318 super(); 2319 } 2320 2321 /** 2322 * Constructor 2323 */ 2324 public TestReportTestComponent(TestActionComponent action) { 2325 super(); 2326 this.addAction(action); 2327 } 2328 2329 /** 2330 * @return {@link #name} (The name of this test used for tracking/logging purposes by test engines.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2331 */ 2332 public StringType getNameElement() { 2333 if (this.name == null) 2334 if (Configuration.errorOnAutoCreate()) 2335 throw new Error("Attempt to auto-create TestReportTestComponent.name"); 2336 else if (Configuration.doAutoCreate()) 2337 this.name = new StringType(); // bb 2338 return this.name; 2339 } 2340 2341 public boolean hasNameElement() { 2342 return this.name != null && !this.name.isEmpty(); 2343 } 2344 2345 public boolean hasName() { 2346 return this.name != null && !this.name.isEmpty(); 2347 } 2348 2349 /** 2350 * @param value {@link #name} (The name of this test used for tracking/logging purposes by test engines.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2351 */ 2352 public TestReportTestComponent setNameElement(StringType value) { 2353 this.name = value; 2354 return this; 2355 } 2356 2357 /** 2358 * @return The name of this test used for tracking/logging purposes by test engines. 2359 */ 2360 public String getName() { 2361 return this.name == null ? null : this.name.getValue(); 2362 } 2363 2364 /** 2365 * @param value The name of this test used for tracking/logging purposes by test engines. 2366 */ 2367 public TestReportTestComponent setName(String value) { 2368 if (Utilities.noString(value)) 2369 this.name = null; 2370 else { 2371 if (this.name == null) 2372 this.name = new StringType(); 2373 this.name.setValue(value); 2374 } 2375 return this; 2376 } 2377 2378 /** 2379 * @return {@link #description} (A short description of the test used by test engines for tracking and reporting purposes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2380 */ 2381 public StringType getDescriptionElement() { 2382 if (this.description == null) 2383 if (Configuration.errorOnAutoCreate()) 2384 throw new Error("Attempt to auto-create TestReportTestComponent.description"); 2385 else if (Configuration.doAutoCreate()) 2386 this.description = new StringType(); // bb 2387 return this.description; 2388 } 2389 2390 public boolean hasDescriptionElement() { 2391 return this.description != null && !this.description.isEmpty(); 2392 } 2393 2394 public boolean hasDescription() { 2395 return this.description != null && !this.description.isEmpty(); 2396 } 2397 2398 /** 2399 * @param value {@link #description} (A short description of the test used by test engines for tracking and reporting purposes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2400 */ 2401 public TestReportTestComponent setDescriptionElement(StringType value) { 2402 this.description = value; 2403 return this; 2404 } 2405 2406 /** 2407 * @return A short description of the test used by test engines for tracking and reporting purposes. 2408 */ 2409 public String getDescription() { 2410 return this.description == null ? null : this.description.getValue(); 2411 } 2412 2413 /** 2414 * @param value A short description of the test used by test engines for tracking and reporting purposes. 2415 */ 2416 public TestReportTestComponent setDescription(String value) { 2417 if (Utilities.noString(value)) 2418 this.description = null; 2419 else { 2420 if (this.description == null) 2421 this.description = new StringType(); 2422 this.description.setValue(value); 2423 } 2424 return this; 2425 } 2426 2427 /** 2428 * @return {@link #action} (Action would contain either an operation or an assertion.) 2429 */ 2430 public List<TestActionComponent> getAction() { 2431 if (this.action == null) 2432 this.action = new ArrayList<TestActionComponent>(); 2433 return this.action; 2434 } 2435 2436 /** 2437 * @return Returns a reference to <code>this</code> for easy method chaining 2438 */ 2439 public TestReportTestComponent setAction(List<TestActionComponent> theAction) { 2440 this.action = theAction; 2441 return this; 2442 } 2443 2444 public boolean hasAction() { 2445 if (this.action == null) 2446 return false; 2447 for (TestActionComponent item : this.action) 2448 if (!item.isEmpty()) 2449 return true; 2450 return false; 2451 } 2452 2453 public TestActionComponent addAction() { //3 2454 TestActionComponent t = new TestActionComponent(); 2455 if (this.action == null) 2456 this.action = new ArrayList<TestActionComponent>(); 2457 this.action.add(t); 2458 return t; 2459 } 2460 2461 public TestReportTestComponent addAction(TestActionComponent t) { //3 2462 if (t == null) 2463 return this; 2464 if (this.action == null) 2465 this.action = new ArrayList<TestActionComponent>(); 2466 this.action.add(t); 2467 return this; 2468 } 2469 2470 /** 2471 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist {3} 2472 */ 2473 public TestActionComponent getActionFirstRep() { 2474 if (getAction().isEmpty()) { 2475 addAction(); 2476 } 2477 return getAction().get(0); 2478 } 2479 2480 protected void listChildren(List<Property> children) { 2481 super.listChildren(children); 2482 children.add(new Property("name", "string", "The name of this test used for tracking/logging purposes by test engines.", 0, 1, name)); 2483 children.add(new Property("description", "string", "A short description of the test used by test engines for tracking and reporting purposes.", 0, 1, description)); 2484 children.add(new Property("action", "", "Action would contain either an operation or an assertion.", 0, java.lang.Integer.MAX_VALUE, action)); 2485 } 2486 2487 @Override 2488 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2489 switch (_hash) { 2490 case 3373707: /*name*/ return new Property("name", "string", "The name of this test used for tracking/logging purposes by test engines.", 0, 1, name); 2491 case -1724546052: /*description*/ return new Property("description", "string", "A short description of the test used by test engines for tracking and reporting purposes.", 0, 1, description); 2492 case -1422950858: /*action*/ return new Property("action", "", "Action would contain either an operation or an assertion.", 0, java.lang.Integer.MAX_VALUE, action); 2493 default: return super.getNamedProperty(_hash, _name, _checkValid); 2494 } 2495 2496 } 2497 2498 @Override 2499 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2500 switch (hash) { 2501 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2502 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 2503 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // TestActionComponent 2504 default: return super.getProperty(hash, name, checkValid); 2505 } 2506 2507 } 2508 2509 @Override 2510 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2511 switch (hash) { 2512 case 3373707: // name 2513 this.name = TypeConvertor.castToString(value); // StringType 2514 return value; 2515 case -1724546052: // description 2516 this.description = TypeConvertor.castToString(value); // StringType 2517 return value; 2518 case -1422950858: // action 2519 this.getAction().add((TestActionComponent) value); // TestActionComponent 2520 return value; 2521 default: return super.setProperty(hash, name, value); 2522 } 2523 2524 } 2525 2526 @Override 2527 public Base setProperty(String name, Base value) throws FHIRException { 2528 if (name.equals("name")) { 2529 this.name = TypeConvertor.castToString(value); // StringType 2530 } else if (name.equals("description")) { 2531 this.description = TypeConvertor.castToString(value); // StringType 2532 } else if (name.equals("action")) { 2533 this.getAction().add((TestActionComponent) value); 2534 } else 2535 return super.setProperty(name, value); 2536 return value; 2537 } 2538 2539 @Override 2540 public void removeChild(String name, Base value) throws FHIRException { 2541 if (name.equals("name")) { 2542 this.name = null; 2543 } else if (name.equals("description")) { 2544 this.description = null; 2545 } else if (name.equals("action")) { 2546 this.getAction().remove((TestActionComponent) value); 2547 } else 2548 super.removeChild(name, value); 2549 2550 } 2551 2552 @Override 2553 public Base makeProperty(int hash, String name) throws FHIRException { 2554 switch (hash) { 2555 case 3373707: return getNameElement(); 2556 case -1724546052: return getDescriptionElement(); 2557 case -1422950858: return addAction(); 2558 default: return super.makeProperty(hash, name); 2559 } 2560 2561 } 2562 2563 @Override 2564 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2565 switch (hash) { 2566 case 3373707: /*name*/ return new String[] {"string"}; 2567 case -1724546052: /*description*/ return new String[] {"string"}; 2568 case -1422950858: /*action*/ return new String[] {}; 2569 default: return super.getTypesForProperty(hash, name); 2570 } 2571 2572 } 2573 2574 @Override 2575 public Base addChild(String name) throws FHIRException { 2576 if (name.equals("name")) { 2577 throw new FHIRException("Cannot call addChild on a singleton property TestReport.test.name"); 2578 } 2579 else if (name.equals("description")) { 2580 throw new FHIRException("Cannot call addChild on a singleton property TestReport.test.description"); 2581 } 2582 else if (name.equals("action")) { 2583 return addAction(); 2584 } 2585 else 2586 return super.addChild(name); 2587 } 2588 2589 public TestReportTestComponent copy() { 2590 TestReportTestComponent dst = new TestReportTestComponent(); 2591 copyValues(dst); 2592 return dst; 2593 } 2594 2595 public void copyValues(TestReportTestComponent dst) { 2596 super.copyValues(dst); 2597 dst.name = name == null ? null : name.copy(); 2598 dst.description = description == null ? null : description.copy(); 2599 if (action != null) { 2600 dst.action = new ArrayList<TestActionComponent>(); 2601 for (TestActionComponent i : action) 2602 dst.action.add(i.copy()); 2603 }; 2604 } 2605 2606 @Override 2607 public boolean equalsDeep(Base other_) { 2608 if (!super.equalsDeep(other_)) 2609 return false; 2610 if (!(other_ instanceof TestReportTestComponent)) 2611 return false; 2612 TestReportTestComponent o = (TestReportTestComponent) other_; 2613 return compareDeep(name, o.name, true) && compareDeep(description, o.description, true) && compareDeep(action, o.action, true) 2614 ; 2615 } 2616 2617 @Override 2618 public boolean equalsShallow(Base other_) { 2619 if (!super.equalsShallow(other_)) 2620 return false; 2621 if (!(other_ instanceof TestReportTestComponent)) 2622 return false; 2623 TestReportTestComponent o = (TestReportTestComponent) other_; 2624 return compareValues(name, o.name, true) && compareValues(description, o.description, true); 2625 } 2626 2627 public boolean isEmpty() { 2628 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, description, action 2629 ); 2630 } 2631 2632 public String fhirType() { 2633 return "TestReport.test"; 2634 2635 } 2636 2637 } 2638 2639 @Block() 2640 public static class TestActionComponent extends BackboneElement implements IBaseBackboneElement { 2641 /** 2642 * An operation would involve a REST request to a server. 2643 */ 2644 @Child(name = "operation", type = {SetupActionOperationComponent.class}, order=1, min=0, max=1, modifier=false, summary=false) 2645 @Description(shortDefinition="The operation performed", formalDefinition="An operation would involve a REST request to a server." ) 2646 protected SetupActionOperationComponent operation; 2647 2648 /** 2649 * The results of the assertion performed on the previous operations. 2650 */ 2651 @Child(name = "assert", type = {SetupActionAssertComponent.class}, order=2, min=0, max=1, modifier=false, summary=false) 2652 @Description(shortDefinition="The assertion performed", formalDefinition="The results of the assertion performed on the previous operations." ) 2653 protected SetupActionAssertComponent assert_; 2654 2655 private static final long serialVersionUID = -252088305L; 2656 2657 /** 2658 * Constructor 2659 */ 2660 public TestActionComponent() { 2661 super(); 2662 } 2663 2664 /** 2665 * @return {@link #operation} (An operation would involve a REST request to a server.) 2666 */ 2667 public SetupActionOperationComponent getOperation() { 2668 if (this.operation == null) 2669 if (Configuration.errorOnAutoCreate()) 2670 throw new Error("Attempt to auto-create TestActionComponent.operation"); 2671 else if (Configuration.doAutoCreate()) 2672 this.operation = new SetupActionOperationComponent(); // cc 2673 return this.operation; 2674 } 2675 2676 public boolean hasOperation() { 2677 return this.operation != null && !this.operation.isEmpty(); 2678 } 2679 2680 /** 2681 * @param value {@link #operation} (An operation would involve a REST request to a server.) 2682 */ 2683 public TestActionComponent setOperation(SetupActionOperationComponent value) { 2684 this.operation = value; 2685 return this; 2686 } 2687 2688 /** 2689 * @return {@link #assert_} (The results of the assertion performed on the previous operations.) 2690 */ 2691 public SetupActionAssertComponent getAssert() { 2692 if (this.assert_ == null) 2693 if (Configuration.errorOnAutoCreate()) 2694 throw new Error("Attempt to auto-create TestActionComponent.assert_"); 2695 else if (Configuration.doAutoCreate()) 2696 this.assert_ = new SetupActionAssertComponent(); // cc 2697 return this.assert_; 2698 } 2699 2700 public boolean hasAssert() { 2701 return this.assert_ != null && !this.assert_.isEmpty(); 2702 } 2703 2704 /** 2705 * @param value {@link #assert_} (The results of the assertion performed on the previous operations.) 2706 */ 2707 public TestActionComponent setAssert(SetupActionAssertComponent value) { 2708 this.assert_ = value; 2709 return this; 2710 } 2711 2712 protected void listChildren(List<Property> children) { 2713 super.listChildren(children); 2714 children.add(new Property("operation", "@TestReport.setup.action.operation", "An operation would involve a REST request to a server.", 0, 1, operation)); 2715 children.add(new Property("assert", "@TestReport.setup.action.assert", "The results of the assertion performed on the previous operations.", 0, 1, assert_)); 2716 } 2717 2718 @Override 2719 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2720 switch (_hash) { 2721 case 1662702951: /*operation*/ return new Property("operation", "@TestReport.setup.action.operation", "An operation would involve a REST request to a server.", 0, 1, operation); 2722 case -1408208058: /*assert*/ return new Property("assert", "@TestReport.setup.action.assert", "The results of the assertion performed on the previous operations.", 0, 1, assert_); 2723 default: return super.getNamedProperty(_hash, _name, _checkValid); 2724 } 2725 2726 } 2727 2728 @Override 2729 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2730 switch (hash) { 2731 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : new Base[] {this.operation}; // SetupActionOperationComponent 2732 case -1408208058: /*assert*/ return this.assert_ == null ? new Base[0] : new Base[] {this.assert_}; // SetupActionAssertComponent 2733 default: return super.getProperty(hash, name, checkValid); 2734 } 2735 2736 } 2737 2738 @Override 2739 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2740 switch (hash) { 2741 case 1662702951: // operation 2742 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 2743 return value; 2744 case -1408208058: // assert 2745 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 2746 return value; 2747 default: return super.setProperty(hash, name, value); 2748 } 2749 2750 } 2751 2752 @Override 2753 public Base setProperty(String name, Base value) throws FHIRException { 2754 if (name.equals("operation")) { 2755 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 2756 } else if (name.equals("assert")) { 2757 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 2758 } else 2759 return super.setProperty(name, value); 2760 return value; 2761 } 2762 2763 @Override 2764 public void removeChild(String name, Base value) throws FHIRException { 2765 if (name.equals("operation")) { 2766 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 2767 } else if (name.equals("assert")) { 2768 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 2769 } else 2770 super.removeChild(name, value); 2771 2772 } 2773 2774 @Override 2775 public Base makeProperty(int hash, String name) throws FHIRException { 2776 switch (hash) { 2777 case 1662702951: return getOperation(); 2778 case -1408208058: return getAssert(); 2779 default: return super.makeProperty(hash, name); 2780 } 2781 2782 } 2783 2784 @Override 2785 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2786 switch (hash) { 2787 case 1662702951: /*operation*/ return new String[] {"@TestReport.setup.action.operation"}; 2788 case -1408208058: /*assert*/ return new String[] {"@TestReport.setup.action.assert"}; 2789 default: return super.getTypesForProperty(hash, name); 2790 } 2791 2792 } 2793 2794 @Override 2795 public Base addChild(String name) throws FHIRException { 2796 if (name.equals("operation")) { 2797 this.operation = new SetupActionOperationComponent(); 2798 return this.operation; 2799 } 2800 else if (name.equals("assert")) { 2801 this.assert_ = new SetupActionAssertComponent(); 2802 return this.assert_; 2803 } 2804 else 2805 return super.addChild(name); 2806 } 2807 2808 public TestActionComponent copy() { 2809 TestActionComponent dst = new TestActionComponent(); 2810 copyValues(dst); 2811 return dst; 2812 } 2813 2814 public void copyValues(TestActionComponent dst) { 2815 super.copyValues(dst); 2816 dst.operation = operation == null ? null : operation.copy(); 2817 dst.assert_ = assert_ == null ? null : assert_.copy(); 2818 } 2819 2820 @Override 2821 public boolean equalsDeep(Base other_) { 2822 if (!super.equalsDeep(other_)) 2823 return false; 2824 if (!(other_ instanceof TestActionComponent)) 2825 return false; 2826 TestActionComponent o = (TestActionComponent) other_; 2827 return compareDeep(operation, o.operation, true) && compareDeep(assert_, o.assert_, true); 2828 } 2829 2830 @Override 2831 public boolean equalsShallow(Base other_) { 2832 if (!super.equalsShallow(other_)) 2833 return false; 2834 if (!(other_ instanceof TestActionComponent)) 2835 return false; 2836 TestActionComponent o = (TestActionComponent) other_; 2837 return true; 2838 } 2839 2840 public boolean isEmpty() { 2841 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation, assert_); 2842 } 2843 2844 public String fhirType() { 2845 return "TestReport.test.action"; 2846 2847 } 2848 2849 } 2850 2851 @Block() 2852 public static class TestReportTeardownComponent extends BackboneElement implements IBaseBackboneElement { 2853 /** 2854 * The teardown action will only contain an operation. 2855 */ 2856 @Child(name = "action", type = {}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2857 @Description(shortDefinition="One or more teardown operations performed", formalDefinition="The teardown action will only contain an operation." ) 2858 protected List<TeardownActionComponent> action; 2859 2860 private static final long serialVersionUID = 1168638089L; 2861 2862 /** 2863 * Constructor 2864 */ 2865 public TestReportTeardownComponent() { 2866 super(); 2867 } 2868 2869 /** 2870 * Constructor 2871 */ 2872 public TestReportTeardownComponent(TeardownActionComponent action) { 2873 super(); 2874 this.addAction(action); 2875 } 2876 2877 /** 2878 * @return {@link #action} (The teardown action will only contain an operation.) 2879 */ 2880 public List<TeardownActionComponent> getAction() { 2881 if (this.action == null) 2882 this.action = new ArrayList<TeardownActionComponent>(); 2883 return this.action; 2884 } 2885 2886 /** 2887 * @return Returns a reference to <code>this</code> for easy method chaining 2888 */ 2889 public TestReportTeardownComponent setAction(List<TeardownActionComponent> theAction) { 2890 this.action = theAction; 2891 return this; 2892 } 2893 2894 public boolean hasAction() { 2895 if (this.action == null) 2896 return false; 2897 for (TeardownActionComponent item : this.action) 2898 if (!item.isEmpty()) 2899 return true; 2900 return false; 2901 } 2902 2903 public TeardownActionComponent addAction() { //3 2904 TeardownActionComponent t = new TeardownActionComponent(); 2905 if (this.action == null) 2906 this.action = new ArrayList<TeardownActionComponent>(); 2907 this.action.add(t); 2908 return t; 2909 } 2910 2911 public TestReportTeardownComponent addAction(TeardownActionComponent t) { //3 2912 if (t == null) 2913 return this; 2914 if (this.action == null) 2915 this.action = new ArrayList<TeardownActionComponent>(); 2916 this.action.add(t); 2917 return this; 2918 } 2919 2920 /** 2921 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist {3} 2922 */ 2923 public TeardownActionComponent getActionFirstRep() { 2924 if (getAction().isEmpty()) { 2925 addAction(); 2926 } 2927 return getAction().get(0); 2928 } 2929 2930 protected void listChildren(List<Property> children) { 2931 super.listChildren(children); 2932 children.add(new Property("action", "", "The teardown action will only contain an operation.", 0, java.lang.Integer.MAX_VALUE, action)); 2933 } 2934 2935 @Override 2936 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2937 switch (_hash) { 2938 case -1422950858: /*action*/ return new Property("action", "", "The teardown action will only contain an operation.", 0, java.lang.Integer.MAX_VALUE, action); 2939 default: return super.getNamedProperty(_hash, _name, _checkValid); 2940 } 2941 2942 } 2943 2944 @Override 2945 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2946 switch (hash) { 2947 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // TeardownActionComponent 2948 default: return super.getProperty(hash, name, checkValid); 2949 } 2950 2951 } 2952 2953 @Override 2954 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2955 switch (hash) { 2956 case -1422950858: // action 2957 this.getAction().add((TeardownActionComponent) value); // TeardownActionComponent 2958 return value; 2959 default: return super.setProperty(hash, name, value); 2960 } 2961 2962 } 2963 2964 @Override 2965 public Base setProperty(String name, Base value) throws FHIRException { 2966 if (name.equals("action")) { 2967 this.getAction().add((TeardownActionComponent) value); 2968 } else 2969 return super.setProperty(name, value); 2970 return value; 2971 } 2972 2973 @Override 2974 public void removeChild(String name, Base value) throws FHIRException { 2975 if (name.equals("action")) { 2976 this.getAction().remove((TeardownActionComponent) value); 2977 } else 2978 super.removeChild(name, value); 2979 2980 } 2981 2982 @Override 2983 public Base makeProperty(int hash, String name) throws FHIRException { 2984 switch (hash) { 2985 case -1422950858: return addAction(); 2986 default: return super.makeProperty(hash, name); 2987 } 2988 2989 } 2990 2991 @Override 2992 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2993 switch (hash) { 2994 case -1422950858: /*action*/ return new String[] {}; 2995 default: return super.getTypesForProperty(hash, name); 2996 } 2997 2998 } 2999 3000 @Override 3001 public Base addChild(String name) throws FHIRException { 3002 if (name.equals("action")) { 3003 return addAction(); 3004 } 3005 else 3006 return super.addChild(name); 3007 } 3008 3009 public TestReportTeardownComponent copy() { 3010 TestReportTeardownComponent dst = new TestReportTeardownComponent(); 3011 copyValues(dst); 3012 return dst; 3013 } 3014 3015 public void copyValues(TestReportTeardownComponent dst) { 3016 super.copyValues(dst); 3017 if (action != null) { 3018 dst.action = new ArrayList<TeardownActionComponent>(); 3019 for (TeardownActionComponent i : action) 3020 dst.action.add(i.copy()); 3021 }; 3022 } 3023 3024 @Override 3025 public boolean equalsDeep(Base other_) { 3026 if (!super.equalsDeep(other_)) 3027 return false; 3028 if (!(other_ instanceof TestReportTeardownComponent)) 3029 return false; 3030 TestReportTeardownComponent o = (TestReportTeardownComponent) other_; 3031 return compareDeep(action, o.action, true); 3032 } 3033 3034 @Override 3035 public boolean equalsShallow(Base other_) { 3036 if (!super.equalsShallow(other_)) 3037 return false; 3038 if (!(other_ instanceof TestReportTeardownComponent)) 3039 return false; 3040 TestReportTeardownComponent o = (TestReportTeardownComponent) other_; 3041 return true; 3042 } 3043 3044 public boolean isEmpty() { 3045 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action); 3046 } 3047 3048 public String fhirType() { 3049 return "TestReport.teardown"; 3050 3051 } 3052 3053 } 3054 3055 @Block() 3056 public static class TeardownActionComponent extends BackboneElement implements IBaseBackboneElement { 3057 /** 3058 * An operation would involve a REST request to a server. 3059 */ 3060 @Child(name = "operation", type = {SetupActionOperationComponent.class}, order=1, min=1, max=1, modifier=false, summary=false) 3061 @Description(shortDefinition="The teardown operation performed", formalDefinition="An operation would involve a REST request to a server." ) 3062 protected SetupActionOperationComponent operation; 3063 3064 private static final long serialVersionUID = -1099598054L; 3065 3066 /** 3067 * Constructor 3068 */ 3069 public TeardownActionComponent() { 3070 super(); 3071 } 3072 3073 /** 3074 * Constructor 3075 */ 3076 public TeardownActionComponent(SetupActionOperationComponent operation) { 3077 super(); 3078 this.setOperation(operation); 3079 } 3080 3081 /** 3082 * @return {@link #operation} (An operation would involve a REST request to a server.) 3083 */ 3084 public SetupActionOperationComponent getOperation() { 3085 if (this.operation == null) 3086 if (Configuration.errorOnAutoCreate()) 3087 throw new Error("Attempt to auto-create TeardownActionComponent.operation"); 3088 else if (Configuration.doAutoCreate()) 3089 this.operation = new SetupActionOperationComponent(); // cc 3090 return this.operation; 3091 } 3092 3093 public boolean hasOperation() { 3094 return this.operation != null && !this.operation.isEmpty(); 3095 } 3096 3097 /** 3098 * @param value {@link #operation} (An operation would involve a REST request to a server.) 3099 */ 3100 public TeardownActionComponent setOperation(SetupActionOperationComponent value) { 3101 this.operation = value; 3102 return this; 3103 } 3104 3105 protected void listChildren(List<Property> children) { 3106 super.listChildren(children); 3107 children.add(new Property("operation", "@TestReport.setup.action.operation", "An operation would involve a REST request to a server.", 0, 1, operation)); 3108 } 3109 3110 @Override 3111 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3112 switch (_hash) { 3113 case 1662702951: /*operation*/ return new Property("operation", "@TestReport.setup.action.operation", "An operation would involve a REST request to a server.", 0, 1, operation); 3114 default: return super.getNamedProperty(_hash, _name, _checkValid); 3115 } 3116 3117 } 3118 3119 @Override 3120 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3121 switch (hash) { 3122 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : new Base[] {this.operation}; // SetupActionOperationComponent 3123 default: return super.getProperty(hash, name, checkValid); 3124 } 3125 3126 } 3127 3128 @Override 3129 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3130 switch (hash) { 3131 case 1662702951: // operation 3132 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 3133 return value; 3134 default: return super.setProperty(hash, name, value); 3135 } 3136 3137 } 3138 3139 @Override 3140 public Base setProperty(String name, Base value) throws FHIRException { 3141 if (name.equals("operation")) { 3142 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 3143 } else 3144 return super.setProperty(name, value); 3145 return value; 3146 } 3147 3148 @Override 3149 public void removeChild(String name, Base value) throws FHIRException { 3150 if (name.equals("operation")) { 3151 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 3152 } else 3153 super.removeChild(name, value); 3154 3155 } 3156 3157 @Override 3158 public Base makeProperty(int hash, String name) throws FHIRException { 3159 switch (hash) { 3160 case 1662702951: return getOperation(); 3161 default: return super.makeProperty(hash, name); 3162 } 3163 3164 } 3165 3166 @Override 3167 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3168 switch (hash) { 3169 case 1662702951: /*operation*/ return new String[] {"@TestReport.setup.action.operation"}; 3170 default: return super.getTypesForProperty(hash, name); 3171 } 3172 3173 } 3174 3175 @Override 3176 public Base addChild(String name) throws FHIRException { 3177 if (name.equals("operation")) { 3178 this.operation = new SetupActionOperationComponent(); 3179 return this.operation; 3180 } 3181 else 3182 return super.addChild(name); 3183 } 3184 3185 public TeardownActionComponent copy() { 3186 TeardownActionComponent dst = new TeardownActionComponent(); 3187 copyValues(dst); 3188 return dst; 3189 } 3190 3191 public void copyValues(TeardownActionComponent dst) { 3192 super.copyValues(dst); 3193 dst.operation = operation == null ? null : operation.copy(); 3194 } 3195 3196 @Override 3197 public boolean equalsDeep(Base other_) { 3198 if (!super.equalsDeep(other_)) 3199 return false; 3200 if (!(other_ instanceof TeardownActionComponent)) 3201 return false; 3202 TeardownActionComponent o = (TeardownActionComponent) other_; 3203 return compareDeep(operation, o.operation, true); 3204 } 3205 3206 @Override 3207 public boolean equalsShallow(Base other_) { 3208 if (!super.equalsShallow(other_)) 3209 return false; 3210 if (!(other_ instanceof TeardownActionComponent)) 3211 return false; 3212 TeardownActionComponent o = (TeardownActionComponent) other_; 3213 return true; 3214 } 3215 3216 public boolean isEmpty() { 3217 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation); 3218 } 3219 3220 public String fhirType() { 3221 return "TestReport.teardown.action"; 3222 3223 } 3224 3225 } 3226 3227 /** 3228 * Identifier for the TestReport assigned for external purposes outside the context of FHIR. 3229 */ 3230 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 3231 @Description(shortDefinition="External identifier", formalDefinition="Identifier for the TestReport assigned for external purposes outside the context of FHIR." ) 3232 protected Identifier identifier; 3233 3234 /** 3235 * A free text natural language name identifying the executed TestReport. 3236 */ 3237 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 3238 @Description(shortDefinition="Informal name of the executed TestReport", formalDefinition="A free text natural language name identifying the executed TestReport." ) 3239 protected StringType name; 3240 3241 /** 3242 * The current state of this test report. 3243 */ 3244 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 3245 @Description(shortDefinition="completed | in-progress | waiting | stopped | entered-in-error", formalDefinition="The current state of this test report." ) 3246 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/report-status-codes") 3247 protected Enumeration<TestReportStatus> status; 3248 3249 /** 3250 * Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`. 3251 */ 3252 @Child(name = "testScript", type = {CanonicalType.class}, order=3, min=1, max=1, modifier=false, summary=true) 3253 @Description(shortDefinition="Canonical URL to the version-specific TestScript that was executed to produce this TestReport", formalDefinition="Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`." ) 3254 protected CanonicalType testScript; 3255 3256 /** 3257 * The overall result from the execution of the TestScript. 3258 */ 3259 @Child(name = "result", type = {CodeType.class}, order=4, min=1, max=1, modifier=false, summary=true) 3260 @Description(shortDefinition="pass | fail | pending", formalDefinition="The overall result from the execution of the TestScript." ) 3261 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/report-result-codes") 3262 protected Enumeration<TestReportResult> result; 3263 3264 /** 3265 * The final score (percentage of tests passed) resulting from the execution of the TestScript. 3266 */ 3267 @Child(name = "score", type = {DecimalType.class}, order=5, min=0, max=1, modifier=false, summary=true) 3268 @Description(shortDefinition="The final score (percentage of tests passed) resulting from the execution of the TestScript", formalDefinition="The final score (percentage of tests passed) resulting from the execution of the TestScript." ) 3269 protected DecimalType score; 3270 3271 /** 3272 * Name of the tester producing this report (Organization or individual). 3273 */ 3274 @Child(name = "tester", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 3275 @Description(shortDefinition="Name of the tester producing this report (Organization or individual)", formalDefinition="Name of the tester producing this report (Organization or individual)." ) 3276 protected StringType tester; 3277 3278 /** 3279 * When the TestScript was executed and this TestReport was generated. 3280 */ 3281 @Child(name = "issued", type = {DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 3282 @Description(shortDefinition="When the TestScript was executed and this TestReport was generated", formalDefinition="When the TestScript was executed and this TestReport was generated." ) 3283 protected DateTimeType issued; 3284 3285 /** 3286 * A participant in the test execution, either the execution engine, a client, or a server. 3287 */ 3288 @Child(name = "participant", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3289 @Description(shortDefinition="A participant in the test execution, either the execution engine, a client, or a server", formalDefinition="A participant in the test execution, either the execution engine, a client, or a server." ) 3290 protected List<TestReportParticipantComponent> participant; 3291 3292 /** 3293 * The results of the series of required setup operations before the tests were executed. 3294 */ 3295 @Child(name = "setup", type = {}, order=9, min=0, max=1, modifier=false, summary=false) 3296 @Description(shortDefinition="The results of the series of required setup operations before the tests were executed", formalDefinition="The results of the series of required setup operations before the tests were executed." ) 3297 protected TestReportSetupComponent setup; 3298 3299 /** 3300 * A test executed from the test script. 3301 */ 3302 @Child(name = "test", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3303 @Description(shortDefinition="A test executed from the test script", formalDefinition="A test executed from the test script." ) 3304 protected List<TestReportTestComponent> test; 3305 3306 /** 3307 * The results of the series of operations required to clean up after all the tests were executed (successfully or otherwise). 3308 */ 3309 @Child(name = "teardown", type = {}, order=11, min=0, max=1, modifier=false, summary=false) 3310 @Description(shortDefinition="The results of running the series of required clean up steps", formalDefinition="The results of the series of operations required to clean up after all the tests were executed (successfully or otherwise)." ) 3311 protected TestReportTeardownComponent teardown; 3312 3313 private static final long serialVersionUID = -1863229334L; 3314 3315 /** 3316 * Constructor 3317 */ 3318 public TestReport() { 3319 super(); 3320 } 3321 3322 /** 3323 * Constructor 3324 */ 3325 public TestReport(TestReportStatus status, String testScript, TestReportResult result) { 3326 super(); 3327 this.setStatus(status); 3328 this.setTestScript(testScript); 3329 this.setResult(result); 3330 } 3331 3332 /** 3333 * @return {@link #identifier} (Identifier for the TestReport assigned for external purposes outside the context of FHIR.) 3334 */ 3335 public Identifier getIdentifier() { 3336 if (this.identifier == null) 3337 if (Configuration.errorOnAutoCreate()) 3338 throw new Error("Attempt to auto-create TestReport.identifier"); 3339 else if (Configuration.doAutoCreate()) 3340 this.identifier = new Identifier(); // cc 3341 return this.identifier; 3342 } 3343 3344 public boolean hasIdentifier() { 3345 return this.identifier != null && !this.identifier.isEmpty(); 3346 } 3347 3348 /** 3349 * @param value {@link #identifier} (Identifier for the TestReport assigned for external purposes outside the context of FHIR.) 3350 */ 3351 public TestReport setIdentifier(Identifier value) { 3352 this.identifier = value; 3353 return this; 3354 } 3355 3356 /** 3357 * @return {@link #name} (A free text natural language name identifying the executed TestReport.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3358 */ 3359 public StringType getNameElement() { 3360 if (this.name == null) 3361 if (Configuration.errorOnAutoCreate()) 3362 throw new Error("Attempt to auto-create TestReport.name"); 3363 else if (Configuration.doAutoCreate()) 3364 this.name = new StringType(); // bb 3365 return this.name; 3366 } 3367 3368 public boolean hasNameElement() { 3369 return this.name != null && !this.name.isEmpty(); 3370 } 3371 3372 public boolean hasName() { 3373 return this.name != null && !this.name.isEmpty(); 3374 } 3375 3376 /** 3377 * @param value {@link #name} (A free text natural language name identifying the executed TestReport.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3378 */ 3379 public TestReport setNameElement(StringType value) { 3380 this.name = value; 3381 return this; 3382 } 3383 3384 /** 3385 * @return A free text natural language name identifying the executed TestReport. 3386 */ 3387 public String getName() { 3388 return this.name == null ? null : this.name.getValue(); 3389 } 3390 3391 /** 3392 * @param value A free text natural language name identifying the executed TestReport. 3393 */ 3394 public TestReport setName(String value) { 3395 if (Utilities.noString(value)) 3396 this.name = null; 3397 else { 3398 if (this.name == null) 3399 this.name = new StringType(); 3400 this.name.setValue(value); 3401 } 3402 return this; 3403 } 3404 3405 /** 3406 * @return {@link #status} (The current state of this test report.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3407 */ 3408 public Enumeration<TestReportStatus> getStatusElement() { 3409 if (this.status == null) 3410 if (Configuration.errorOnAutoCreate()) 3411 throw new Error("Attempt to auto-create TestReport.status"); 3412 else if (Configuration.doAutoCreate()) 3413 this.status = new Enumeration<TestReportStatus>(new TestReportStatusEnumFactory()); // bb 3414 return this.status; 3415 } 3416 3417 public boolean hasStatusElement() { 3418 return this.status != null && !this.status.isEmpty(); 3419 } 3420 3421 public boolean hasStatus() { 3422 return this.status != null && !this.status.isEmpty(); 3423 } 3424 3425 /** 3426 * @param value {@link #status} (The current state of this test report.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3427 */ 3428 public TestReport setStatusElement(Enumeration<TestReportStatus> value) { 3429 this.status = value; 3430 return this; 3431 } 3432 3433 /** 3434 * @return The current state of this test report. 3435 */ 3436 public TestReportStatus getStatus() { 3437 return this.status == null ? null : this.status.getValue(); 3438 } 3439 3440 /** 3441 * @param value The current state of this test report. 3442 */ 3443 public TestReport setStatus(TestReportStatus value) { 3444 if (this.status == null) 3445 this.status = new Enumeration<TestReportStatus>(new TestReportStatusEnumFactory()); 3446 this.status.setValue(value); 3447 return this; 3448 } 3449 3450 /** 3451 * @return {@link #testScript} (Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`.). This is the underlying object with id, value and extensions. The accessor "getTestScript" gives direct access to the value 3452 */ 3453 public CanonicalType getTestScriptElement() { 3454 if (this.testScript == null) 3455 if (Configuration.errorOnAutoCreate()) 3456 throw new Error("Attempt to auto-create TestReport.testScript"); 3457 else if (Configuration.doAutoCreate()) 3458 this.testScript = new CanonicalType(); // bb 3459 return this.testScript; 3460 } 3461 3462 public boolean hasTestScriptElement() { 3463 return this.testScript != null && !this.testScript.isEmpty(); 3464 } 3465 3466 public boolean hasTestScript() { 3467 return this.testScript != null && !this.testScript.isEmpty(); 3468 } 3469 3470 /** 3471 * @param value {@link #testScript} (Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`.). This is the underlying object with id, value and extensions. The accessor "getTestScript" gives direct access to the value 3472 */ 3473 public TestReport setTestScriptElement(CanonicalType value) { 3474 this.testScript = value; 3475 return this; 3476 } 3477 3478 /** 3479 * @return Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`. 3480 */ 3481 public String getTestScript() { 3482 return this.testScript == null ? null : this.testScript.getValue(); 3483 } 3484 3485 /** 3486 * @param value Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`. 3487 */ 3488 public TestReport setTestScript(String value) { 3489 if (this.testScript == null) 3490 this.testScript = new CanonicalType(); 3491 this.testScript.setValue(value); 3492 return this; 3493 } 3494 3495 /** 3496 * @return {@link #result} (The overall result from the execution of the TestScript.). This is the underlying object with id, value and extensions. The accessor "getResult" gives direct access to the value 3497 */ 3498 public Enumeration<TestReportResult> getResultElement() { 3499 if (this.result == null) 3500 if (Configuration.errorOnAutoCreate()) 3501 throw new Error("Attempt to auto-create TestReport.result"); 3502 else if (Configuration.doAutoCreate()) 3503 this.result = new Enumeration<TestReportResult>(new TestReportResultEnumFactory()); // bb 3504 return this.result; 3505 } 3506 3507 public boolean hasResultElement() { 3508 return this.result != null && !this.result.isEmpty(); 3509 } 3510 3511 public boolean hasResult() { 3512 return this.result != null && !this.result.isEmpty(); 3513 } 3514 3515 /** 3516 * @param value {@link #result} (The overall result from the execution of the TestScript.). This is the underlying object with id, value and extensions. The accessor "getResult" gives direct access to the value 3517 */ 3518 public TestReport setResultElement(Enumeration<TestReportResult> value) { 3519 this.result = value; 3520 return this; 3521 } 3522 3523 /** 3524 * @return The overall result from the execution of the TestScript. 3525 */ 3526 public TestReportResult getResult() { 3527 return this.result == null ? null : this.result.getValue(); 3528 } 3529 3530 /** 3531 * @param value The overall result from the execution of the TestScript. 3532 */ 3533 public TestReport setResult(TestReportResult value) { 3534 if (this.result == null) 3535 this.result = new Enumeration<TestReportResult>(new TestReportResultEnumFactory()); 3536 this.result.setValue(value); 3537 return this; 3538 } 3539 3540 /** 3541 * @return {@link #score} (The final score (percentage of tests passed) resulting from the execution of the TestScript.). This is the underlying object with id, value and extensions. The accessor "getScore" gives direct access to the value 3542 */ 3543 public DecimalType getScoreElement() { 3544 if (this.score == null) 3545 if (Configuration.errorOnAutoCreate()) 3546 throw new Error("Attempt to auto-create TestReport.score"); 3547 else if (Configuration.doAutoCreate()) 3548 this.score = new DecimalType(); // bb 3549 return this.score; 3550 } 3551 3552 public boolean hasScoreElement() { 3553 return this.score != null && !this.score.isEmpty(); 3554 } 3555 3556 public boolean hasScore() { 3557 return this.score != null && !this.score.isEmpty(); 3558 } 3559 3560 /** 3561 * @param value {@link #score} (The final score (percentage of tests passed) resulting from the execution of the TestScript.). This is the underlying object with id, value and extensions. The accessor "getScore" gives direct access to the value 3562 */ 3563 public TestReport setScoreElement(DecimalType value) { 3564 this.score = value; 3565 return this; 3566 } 3567 3568 /** 3569 * @return The final score (percentage of tests passed) resulting from the execution of the TestScript. 3570 */ 3571 public BigDecimal getScore() { 3572 return this.score == null ? null : this.score.getValue(); 3573 } 3574 3575 /** 3576 * @param value The final score (percentage of tests passed) resulting from the execution of the TestScript. 3577 */ 3578 public TestReport setScore(BigDecimal value) { 3579 if (value == null) 3580 this.score = null; 3581 else { 3582 if (this.score == null) 3583 this.score = new DecimalType(); 3584 this.score.setValue(value); 3585 } 3586 return this; 3587 } 3588 3589 /** 3590 * @param value The final score (percentage of tests passed) resulting from the execution of the TestScript. 3591 */ 3592 public TestReport setScore(long value) { 3593 this.score = new DecimalType(); 3594 this.score.setValue(value); 3595 return this; 3596 } 3597 3598 /** 3599 * @param value The final score (percentage of tests passed) resulting from the execution of the TestScript. 3600 */ 3601 public TestReport setScore(double value) { 3602 this.score = new DecimalType(); 3603 this.score.setValue(value); 3604 return this; 3605 } 3606 3607 /** 3608 * @return {@link #tester} (Name of the tester producing this report (Organization or individual).). This is the underlying object with id, value and extensions. The accessor "getTester" gives direct access to the value 3609 */ 3610 public StringType getTesterElement() { 3611 if (this.tester == null) 3612 if (Configuration.errorOnAutoCreate()) 3613 throw new Error("Attempt to auto-create TestReport.tester"); 3614 else if (Configuration.doAutoCreate()) 3615 this.tester = new StringType(); // bb 3616 return this.tester; 3617 } 3618 3619 public boolean hasTesterElement() { 3620 return this.tester != null && !this.tester.isEmpty(); 3621 } 3622 3623 public boolean hasTester() { 3624 return this.tester != null && !this.tester.isEmpty(); 3625 } 3626 3627 /** 3628 * @param value {@link #tester} (Name of the tester producing this report (Organization or individual).). This is the underlying object with id, value and extensions. The accessor "getTester" gives direct access to the value 3629 */ 3630 public TestReport setTesterElement(StringType value) { 3631 this.tester = value; 3632 return this; 3633 } 3634 3635 /** 3636 * @return Name of the tester producing this report (Organization or individual). 3637 */ 3638 public String getTester() { 3639 return this.tester == null ? null : this.tester.getValue(); 3640 } 3641 3642 /** 3643 * @param value Name of the tester producing this report (Organization or individual). 3644 */ 3645 public TestReport setTester(String value) { 3646 if (Utilities.noString(value)) 3647 this.tester = null; 3648 else { 3649 if (this.tester == null) 3650 this.tester = new StringType(); 3651 this.tester.setValue(value); 3652 } 3653 return this; 3654 } 3655 3656 /** 3657 * @return {@link #issued} (When the TestScript was executed and this TestReport was generated.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 3658 */ 3659 public DateTimeType getIssuedElement() { 3660 if (this.issued == null) 3661 if (Configuration.errorOnAutoCreate()) 3662 throw new Error("Attempt to auto-create TestReport.issued"); 3663 else if (Configuration.doAutoCreate()) 3664 this.issued = new DateTimeType(); // bb 3665 return this.issued; 3666 } 3667 3668 public boolean hasIssuedElement() { 3669 return this.issued != null && !this.issued.isEmpty(); 3670 } 3671 3672 public boolean hasIssued() { 3673 return this.issued != null && !this.issued.isEmpty(); 3674 } 3675 3676 /** 3677 * @param value {@link #issued} (When the TestScript was executed and this TestReport was generated.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 3678 */ 3679 public TestReport setIssuedElement(DateTimeType value) { 3680 this.issued = value; 3681 return this; 3682 } 3683 3684 /** 3685 * @return When the TestScript was executed and this TestReport was generated. 3686 */ 3687 public Date getIssued() { 3688 return this.issued == null ? null : this.issued.getValue(); 3689 } 3690 3691 /** 3692 * @param value When the TestScript was executed and this TestReport was generated. 3693 */ 3694 public TestReport setIssued(Date value) { 3695 if (value == null) 3696 this.issued = null; 3697 else { 3698 if (this.issued == null) 3699 this.issued = new DateTimeType(); 3700 this.issued.setValue(value); 3701 } 3702 return this; 3703 } 3704 3705 /** 3706 * @return {@link #participant} (A participant in the test execution, either the execution engine, a client, or a server.) 3707 */ 3708 public List<TestReportParticipantComponent> getParticipant() { 3709 if (this.participant == null) 3710 this.participant = new ArrayList<TestReportParticipantComponent>(); 3711 return this.participant; 3712 } 3713 3714 /** 3715 * @return Returns a reference to <code>this</code> for easy method chaining 3716 */ 3717 public TestReport setParticipant(List<TestReportParticipantComponent> theParticipant) { 3718 this.participant = theParticipant; 3719 return this; 3720 } 3721 3722 public boolean hasParticipant() { 3723 if (this.participant == null) 3724 return false; 3725 for (TestReportParticipantComponent item : this.participant) 3726 if (!item.isEmpty()) 3727 return true; 3728 return false; 3729 } 3730 3731 public TestReportParticipantComponent addParticipant() { //3 3732 TestReportParticipantComponent t = new TestReportParticipantComponent(); 3733 if (this.participant == null) 3734 this.participant = new ArrayList<TestReportParticipantComponent>(); 3735 this.participant.add(t); 3736 return t; 3737 } 3738 3739 public TestReport addParticipant(TestReportParticipantComponent t) { //3 3740 if (t == null) 3741 return this; 3742 if (this.participant == null) 3743 this.participant = new ArrayList<TestReportParticipantComponent>(); 3744 this.participant.add(t); 3745 return this; 3746 } 3747 3748 /** 3749 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist {3} 3750 */ 3751 public TestReportParticipantComponent getParticipantFirstRep() { 3752 if (getParticipant().isEmpty()) { 3753 addParticipant(); 3754 } 3755 return getParticipant().get(0); 3756 } 3757 3758 /** 3759 * @return {@link #setup} (The results of the series of required setup operations before the tests were executed.) 3760 */ 3761 public TestReportSetupComponent getSetup() { 3762 if (this.setup == null) 3763 if (Configuration.errorOnAutoCreate()) 3764 throw new Error("Attempt to auto-create TestReport.setup"); 3765 else if (Configuration.doAutoCreate()) 3766 this.setup = new TestReportSetupComponent(); // cc 3767 return this.setup; 3768 } 3769 3770 public boolean hasSetup() { 3771 return this.setup != null && !this.setup.isEmpty(); 3772 } 3773 3774 /** 3775 * @param value {@link #setup} (The results of the series of required setup operations before the tests were executed.) 3776 */ 3777 public TestReport setSetup(TestReportSetupComponent value) { 3778 this.setup = value; 3779 return this; 3780 } 3781 3782 /** 3783 * @return {@link #test} (A test executed from the test script.) 3784 */ 3785 public List<TestReportTestComponent> getTest() { 3786 if (this.test == null) 3787 this.test = new ArrayList<TestReportTestComponent>(); 3788 return this.test; 3789 } 3790 3791 /** 3792 * @return Returns a reference to <code>this</code> for easy method chaining 3793 */ 3794 public TestReport setTest(List<TestReportTestComponent> theTest) { 3795 this.test = theTest; 3796 return this; 3797 } 3798 3799 public boolean hasTest() { 3800 if (this.test == null) 3801 return false; 3802 for (TestReportTestComponent item : this.test) 3803 if (!item.isEmpty()) 3804 return true; 3805 return false; 3806 } 3807 3808 public TestReportTestComponent addTest() { //3 3809 TestReportTestComponent t = new TestReportTestComponent(); 3810 if (this.test == null) 3811 this.test = new ArrayList<TestReportTestComponent>(); 3812 this.test.add(t); 3813 return t; 3814 } 3815 3816 public TestReport addTest(TestReportTestComponent t) { //3 3817 if (t == null) 3818 return this; 3819 if (this.test == null) 3820 this.test = new ArrayList<TestReportTestComponent>(); 3821 this.test.add(t); 3822 return this; 3823 } 3824 3825 /** 3826 * @return The first repetition of repeating field {@link #test}, creating it if it does not already exist {3} 3827 */ 3828 public TestReportTestComponent getTestFirstRep() { 3829 if (getTest().isEmpty()) { 3830 addTest(); 3831 } 3832 return getTest().get(0); 3833 } 3834 3835 /** 3836 * @return {@link #teardown} (The results of the series of operations required to clean up after all the tests were executed (successfully or otherwise).) 3837 */ 3838 public TestReportTeardownComponent getTeardown() { 3839 if (this.teardown == null) 3840 if (Configuration.errorOnAutoCreate()) 3841 throw new Error("Attempt to auto-create TestReport.teardown"); 3842 else if (Configuration.doAutoCreate()) 3843 this.teardown = new TestReportTeardownComponent(); // cc 3844 return this.teardown; 3845 } 3846 3847 public boolean hasTeardown() { 3848 return this.teardown != null && !this.teardown.isEmpty(); 3849 } 3850 3851 /** 3852 * @param value {@link #teardown} (The results of the series of operations required to clean up after all the tests were executed (successfully or otherwise).) 3853 */ 3854 public TestReport setTeardown(TestReportTeardownComponent value) { 3855 this.teardown = value; 3856 return this; 3857 } 3858 3859 protected void listChildren(List<Property> children) { 3860 super.listChildren(children); 3861 children.add(new Property("identifier", "Identifier", "Identifier for the TestReport assigned for external purposes outside the context of FHIR.", 0, 1, identifier)); 3862 children.add(new Property("name", "string", "A free text natural language name identifying the executed TestReport.", 0, 1, name)); 3863 children.add(new Property("status", "code", "The current state of this test report.", 0, 1, status)); 3864 children.add(new Property("testScript", "canonical(TestScript)", "Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`.", 0, 1, testScript)); 3865 children.add(new Property("result", "code", "The overall result from the execution of the TestScript.", 0, 1, result)); 3866 children.add(new Property("score", "decimal", "The final score (percentage of tests passed) resulting from the execution of the TestScript.", 0, 1, score)); 3867 children.add(new Property("tester", "string", "Name of the tester producing this report (Organization or individual).", 0, 1, tester)); 3868 children.add(new Property("issued", "dateTime", "When the TestScript was executed and this TestReport was generated.", 0, 1, issued)); 3869 children.add(new Property("participant", "", "A participant in the test execution, either the execution engine, a client, or a server.", 0, java.lang.Integer.MAX_VALUE, participant)); 3870 children.add(new Property("setup", "", "The results of the series of required setup operations before the tests were executed.", 0, 1, setup)); 3871 children.add(new Property("test", "", "A test executed from the test script.", 0, java.lang.Integer.MAX_VALUE, test)); 3872 children.add(new Property("teardown", "", "The results of the series of operations required to clean up after all the tests were executed (successfully or otherwise).", 0, 1, teardown)); 3873 } 3874 3875 @Override 3876 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3877 switch (_hash) { 3878 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier for the TestReport assigned for external purposes outside the context of FHIR.", 0, 1, identifier); 3879 case 3373707: /*name*/ return new Property("name", "string", "A free text natural language name identifying the executed TestReport.", 0, 1, name); 3880 case -892481550: /*status*/ return new Property("status", "code", "The current state of this test report.", 0, 1, status); 3881 case 1712049149: /*testScript*/ return new Property("testScript", "canonical(TestScript)", "Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`.", 0, 1, testScript); 3882 case -934426595: /*result*/ return new Property("result", "code", "The overall result from the execution of the TestScript.", 0, 1, result); 3883 case 109264530: /*score*/ return new Property("score", "decimal", "The final score (percentage of tests passed) resulting from the execution of the TestScript.", 0, 1, score); 3884 case -877169473: /*tester*/ return new Property("tester", "string", "Name of the tester producing this report (Organization or individual).", 0, 1, tester); 3885 case -1179159893: /*issued*/ return new Property("issued", "dateTime", "When the TestScript was executed and this TestReport was generated.", 0, 1, issued); 3886 case 767422259: /*participant*/ return new Property("participant", "", "A participant in the test execution, either the execution engine, a client, or a server.", 0, java.lang.Integer.MAX_VALUE, participant); 3887 case 109329021: /*setup*/ return new Property("setup", "", "The results of the series of required setup operations before the tests were executed.", 0, 1, setup); 3888 case 3556498: /*test*/ return new Property("test", "", "A test executed from the test script.", 0, java.lang.Integer.MAX_VALUE, test); 3889 case -1663474172: /*teardown*/ return new Property("teardown", "", "The results of the series of operations required to clean up after all the tests were executed (successfully or otherwise).", 0, 1, teardown); 3890 default: return super.getNamedProperty(_hash, _name, _checkValid); 3891 } 3892 3893 } 3894 3895 @Override 3896 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3897 switch (hash) { 3898 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 3899 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3900 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<TestReportStatus> 3901 case 1712049149: /*testScript*/ return this.testScript == null ? new Base[0] : new Base[] {this.testScript}; // CanonicalType 3902 case -934426595: /*result*/ return this.result == null ? new Base[0] : new Base[] {this.result}; // Enumeration<TestReportResult> 3903 case 109264530: /*score*/ return this.score == null ? new Base[0] : new Base[] {this.score}; // DecimalType 3904 case -877169473: /*tester*/ return this.tester == null ? new Base[0] : new Base[] {this.tester}; // StringType 3905 case -1179159893: /*issued*/ return this.issued == null ? new Base[0] : new Base[] {this.issued}; // DateTimeType 3906 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // TestReportParticipantComponent 3907 case 109329021: /*setup*/ return this.setup == null ? new Base[0] : new Base[] {this.setup}; // TestReportSetupComponent 3908 case 3556498: /*test*/ return this.test == null ? new Base[0] : this.test.toArray(new Base[this.test.size()]); // TestReportTestComponent 3909 case -1663474172: /*teardown*/ return this.teardown == null ? new Base[0] : new Base[] {this.teardown}; // TestReportTeardownComponent 3910 default: return super.getProperty(hash, name, checkValid); 3911 } 3912 3913 } 3914 3915 @Override 3916 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3917 switch (hash) { 3918 case -1618432855: // identifier 3919 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 3920 return value; 3921 case 3373707: // name 3922 this.name = TypeConvertor.castToString(value); // StringType 3923 return value; 3924 case -892481550: // status 3925 value = new TestReportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3926 this.status = (Enumeration) value; // Enumeration<TestReportStatus> 3927 return value; 3928 case 1712049149: // testScript 3929 this.testScript = TypeConvertor.castToCanonical(value); // CanonicalType 3930 return value; 3931 case -934426595: // result 3932 value = new TestReportResultEnumFactory().fromType(TypeConvertor.castToCode(value)); 3933 this.result = (Enumeration) value; // Enumeration<TestReportResult> 3934 return value; 3935 case 109264530: // score 3936 this.score = TypeConvertor.castToDecimal(value); // DecimalType 3937 return value; 3938 case -877169473: // tester 3939 this.tester = TypeConvertor.castToString(value); // StringType 3940 return value; 3941 case -1179159893: // issued 3942 this.issued = TypeConvertor.castToDateTime(value); // DateTimeType 3943 return value; 3944 case 767422259: // participant 3945 this.getParticipant().add((TestReportParticipantComponent) value); // TestReportParticipantComponent 3946 return value; 3947 case 109329021: // setup 3948 this.setup = (TestReportSetupComponent) value; // TestReportSetupComponent 3949 return value; 3950 case 3556498: // test 3951 this.getTest().add((TestReportTestComponent) value); // TestReportTestComponent 3952 return value; 3953 case -1663474172: // teardown 3954 this.teardown = (TestReportTeardownComponent) value; // TestReportTeardownComponent 3955 return value; 3956 default: return super.setProperty(hash, name, value); 3957 } 3958 3959 } 3960 3961 @Override 3962 public Base setProperty(String name, Base value) throws FHIRException { 3963 if (name.equals("identifier")) { 3964 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 3965 } else if (name.equals("name")) { 3966 this.name = TypeConvertor.castToString(value); // StringType 3967 } else if (name.equals("status")) { 3968 value = new TestReportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3969 this.status = (Enumeration) value; // Enumeration<TestReportStatus> 3970 } else if (name.equals("testScript")) { 3971 this.testScript = TypeConvertor.castToCanonical(value); // CanonicalType 3972 } else if (name.equals("result")) { 3973 value = new TestReportResultEnumFactory().fromType(TypeConvertor.castToCode(value)); 3974 this.result = (Enumeration) value; // Enumeration<TestReportResult> 3975 } else if (name.equals("score")) { 3976 this.score = TypeConvertor.castToDecimal(value); // DecimalType 3977 } else if (name.equals("tester")) { 3978 this.tester = TypeConvertor.castToString(value); // StringType 3979 } else if (name.equals("issued")) { 3980 this.issued = TypeConvertor.castToDateTime(value); // DateTimeType 3981 } else if (name.equals("participant")) { 3982 this.getParticipant().add((TestReportParticipantComponent) value); 3983 } else if (name.equals("setup")) { 3984 this.setup = (TestReportSetupComponent) value; // TestReportSetupComponent 3985 } else if (name.equals("test")) { 3986 this.getTest().add((TestReportTestComponent) value); 3987 } else if (name.equals("teardown")) { 3988 this.teardown = (TestReportTeardownComponent) value; // TestReportTeardownComponent 3989 } else 3990 return super.setProperty(name, value); 3991 return value; 3992 } 3993 3994 @Override 3995 public void removeChild(String name, Base value) throws FHIRException { 3996 if (name.equals("identifier")) { 3997 this.identifier = null; 3998 } else if (name.equals("name")) { 3999 this.name = null; 4000 } else if (name.equals("status")) { 4001 value = new TestReportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4002 this.status = (Enumeration) value; // Enumeration<TestReportStatus> 4003 } else if (name.equals("testScript")) { 4004 this.testScript = null; 4005 } else if (name.equals("result")) { 4006 value = new TestReportResultEnumFactory().fromType(TypeConvertor.castToCode(value)); 4007 this.result = (Enumeration) value; // Enumeration<TestReportResult> 4008 } else if (name.equals("score")) { 4009 this.score = null; 4010 } else if (name.equals("tester")) { 4011 this.tester = null; 4012 } else if (name.equals("issued")) { 4013 this.issued = null; 4014 } else if (name.equals("participant")) { 4015 this.getParticipant().remove((TestReportParticipantComponent) value); 4016 } else if (name.equals("setup")) { 4017 this.setup = (TestReportSetupComponent) value; // TestReportSetupComponent 4018 } else if (name.equals("test")) { 4019 this.getTest().remove((TestReportTestComponent) value); 4020 } else if (name.equals("teardown")) { 4021 this.teardown = (TestReportTeardownComponent) value; // TestReportTeardownComponent 4022 } else 4023 super.removeChild(name, value); 4024 4025 } 4026 4027 @Override 4028 public Base makeProperty(int hash, String name) throws FHIRException { 4029 switch (hash) { 4030 case -1618432855: return getIdentifier(); 4031 case 3373707: return getNameElement(); 4032 case -892481550: return getStatusElement(); 4033 case 1712049149: return getTestScriptElement(); 4034 case -934426595: return getResultElement(); 4035 case 109264530: return getScoreElement(); 4036 case -877169473: return getTesterElement(); 4037 case -1179159893: return getIssuedElement(); 4038 case 767422259: return addParticipant(); 4039 case 109329021: return getSetup(); 4040 case 3556498: return addTest(); 4041 case -1663474172: return getTeardown(); 4042 default: return super.makeProperty(hash, name); 4043 } 4044 4045 } 4046 4047 @Override 4048 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4049 switch (hash) { 4050 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4051 case 3373707: /*name*/ return new String[] {"string"}; 4052 case -892481550: /*status*/ return new String[] {"code"}; 4053 case 1712049149: /*testScript*/ return new String[] {"canonical"}; 4054 case -934426595: /*result*/ return new String[] {"code"}; 4055 case 109264530: /*score*/ return new String[] {"decimal"}; 4056 case -877169473: /*tester*/ return new String[] {"string"}; 4057 case -1179159893: /*issued*/ return new String[] {"dateTime"}; 4058 case 767422259: /*participant*/ return new String[] {}; 4059 case 109329021: /*setup*/ return new String[] {}; 4060 case 3556498: /*test*/ return new String[] {}; 4061 case -1663474172: /*teardown*/ return new String[] {}; 4062 default: return super.getTypesForProperty(hash, name); 4063 } 4064 4065 } 4066 4067 @Override 4068 public Base addChild(String name) throws FHIRException { 4069 if (name.equals("identifier")) { 4070 this.identifier = new Identifier(); 4071 return this.identifier; 4072 } 4073 else if (name.equals("name")) { 4074 throw new FHIRException("Cannot call addChild on a singleton property TestReport.name"); 4075 } 4076 else if (name.equals("status")) { 4077 throw new FHIRException("Cannot call addChild on a singleton property TestReport.status"); 4078 } 4079 else if (name.equals("testScript")) { 4080 throw new FHIRException("Cannot call addChild on a singleton property TestReport.testScript"); 4081 } 4082 else if (name.equals("result")) { 4083 throw new FHIRException("Cannot call addChild on a singleton property TestReport.result"); 4084 } 4085 else if (name.equals("score")) { 4086 throw new FHIRException("Cannot call addChild on a singleton property TestReport.score"); 4087 } 4088 else if (name.equals("tester")) { 4089 throw new FHIRException("Cannot call addChild on a singleton property TestReport.tester"); 4090 } 4091 else if (name.equals("issued")) { 4092 throw new FHIRException("Cannot call addChild on a singleton property TestReport.issued"); 4093 } 4094 else if (name.equals("participant")) { 4095 return addParticipant(); 4096 } 4097 else if (name.equals("setup")) { 4098 this.setup = new TestReportSetupComponent(); 4099 return this.setup; 4100 } 4101 else if (name.equals("test")) { 4102 return addTest(); 4103 } 4104 else if (name.equals("teardown")) { 4105 this.teardown = new TestReportTeardownComponent(); 4106 return this.teardown; 4107 } 4108 else 4109 return super.addChild(name); 4110 } 4111 4112 public String fhirType() { 4113 return "TestReport"; 4114 4115 } 4116 4117 public TestReport copy() { 4118 TestReport dst = new TestReport(); 4119 copyValues(dst); 4120 return dst; 4121 } 4122 4123 public void copyValues(TestReport dst) { 4124 super.copyValues(dst); 4125 dst.identifier = identifier == null ? null : identifier.copy(); 4126 dst.name = name == null ? null : name.copy(); 4127 dst.status = status == null ? null : status.copy(); 4128 dst.testScript = testScript == null ? null : testScript.copy(); 4129 dst.result = result == null ? null : result.copy(); 4130 dst.score = score == null ? null : score.copy(); 4131 dst.tester = tester == null ? null : tester.copy(); 4132 dst.issued = issued == null ? null : issued.copy(); 4133 if (participant != null) { 4134 dst.participant = new ArrayList<TestReportParticipantComponent>(); 4135 for (TestReportParticipantComponent i : participant) 4136 dst.participant.add(i.copy()); 4137 }; 4138 dst.setup = setup == null ? null : setup.copy(); 4139 if (test != null) { 4140 dst.test = new ArrayList<TestReportTestComponent>(); 4141 for (TestReportTestComponent i : test) 4142 dst.test.add(i.copy()); 4143 }; 4144 dst.teardown = teardown == null ? null : teardown.copy(); 4145 } 4146 4147 protected TestReport typedCopy() { 4148 return copy(); 4149 } 4150 4151 @Override 4152 public boolean equalsDeep(Base other_) { 4153 if (!super.equalsDeep(other_)) 4154 return false; 4155 if (!(other_ instanceof TestReport)) 4156 return false; 4157 TestReport o = (TestReport) other_; 4158 return compareDeep(identifier, o.identifier, true) && compareDeep(name, o.name, true) && compareDeep(status, o.status, true) 4159 && compareDeep(testScript, o.testScript, true) && compareDeep(result, o.result, true) && compareDeep(score, o.score, true) 4160 && compareDeep(tester, o.tester, true) && compareDeep(issued, o.issued, true) && compareDeep(participant, o.participant, true) 4161 && compareDeep(setup, o.setup, true) && compareDeep(test, o.test, true) && compareDeep(teardown, o.teardown, true) 4162 ; 4163 } 4164 4165 @Override 4166 public boolean equalsShallow(Base other_) { 4167 if (!super.equalsShallow(other_)) 4168 return false; 4169 if (!(other_ instanceof TestReport)) 4170 return false; 4171 TestReport o = (TestReport) other_; 4172 return compareValues(name, o.name, true) && compareValues(status, o.status, true) && compareValues(testScript, o.testScript, true) 4173 && compareValues(result, o.result, true) && compareValues(score, o.score, true) && compareValues(tester, o.tester, true) 4174 && compareValues(issued, o.issued, true); 4175 } 4176 4177 public boolean isEmpty() { 4178 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, name, status 4179 , testScript, result, score, tester, issued, participant, setup, test, teardown 4180 ); 4181 } 4182 4183 @Override 4184 public ResourceType getResourceType() { 4185 return ResourceType.TestReport; 4186 } 4187 4188 /** 4189 * Search parameter: <b>identifier</b> 4190 * <p> 4191 * Description: <b>An external identifier for the test report</b><br> 4192 * Type: <b>token</b><br> 4193 * Path: <b>TestReport.identifier</b><br> 4194 * </p> 4195 */ 4196 @SearchParamDefinition(name="identifier", path="TestReport.identifier", description="An external identifier for the test report", type="token" ) 4197 public static final String SP_IDENTIFIER = "identifier"; 4198 /** 4199 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4200 * <p> 4201 * Description: <b>An external identifier for the test report</b><br> 4202 * Type: <b>token</b><br> 4203 * Path: <b>TestReport.identifier</b><br> 4204 * </p> 4205 */ 4206 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4207 4208 /** 4209 * Search parameter: <b>issued</b> 4210 * <p> 4211 * Description: <b>The test report generation date</b><br> 4212 * Type: <b>date</b><br> 4213 * Path: <b>TestReport.issued</b><br> 4214 * </p> 4215 */ 4216 @SearchParamDefinition(name="issued", path="TestReport.issued", description="The test report generation date", type="date" ) 4217 public static final String SP_ISSUED = "issued"; 4218 /** 4219 * <b>Fluent Client</b> search parameter constant for <b>issued</b> 4220 * <p> 4221 * Description: <b>The test report generation date</b><br> 4222 * Type: <b>date</b><br> 4223 * Path: <b>TestReport.issued</b><br> 4224 * </p> 4225 */ 4226 public static final ca.uhn.fhir.rest.gclient.DateClientParam ISSUED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_ISSUED); 4227 4228 /** 4229 * Search parameter: <b>participant</b> 4230 * <p> 4231 * Description: <b>The reference to a participant in the test execution</b><br> 4232 * Type: <b>uri</b><br> 4233 * Path: <b>TestReport.participant.uri</b><br> 4234 * </p> 4235 */ 4236 @SearchParamDefinition(name="participant", path="TestReport.participant.uri", description="The reference to a participant in the test execution", type="uri" ) 4237 public static final String SP_PARTICIPANT = "participant"; 4238 /** 4239 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 4240 * <p> 4241 * Description: <b>The reference to a participant in the test execution</b><br> 4242 * Type: <b>uri</b><br> 4243 * Path: <b>TestReport.participant.uri</b><br> 4244 * </p> 4245 */ 4246 public static final ca.uhn.fhir.rest.gclient.UriClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_PARTICIPANT); 4247 4248 /** 4249 * Search parameter: <b>result</b> 4250 * <p> 4251 * Description: <b>The result disposition of the test execution</b><br> 4252 * Type: <b>token</b><br> 4253 * Path: <b>TestReport.result</b><br> 4254 * </p> 4255 */ 4256 @SearchParamDefinition(name="result", path="TestReport.result", description="The result disposition of the test execution", type="token" ) 4257 public static final String SP_RESULT = "result"; 4258 /** 4259 * <b>Fluent Client</b> search parameter constant for <b>result</b> 4260 * <p> 4261 * Description: <b>The result disposition of the test execution</b><br> 4262 * Type: <b>token</b><br> 4263 * Path: <b>TestReport.result</b><br> 4264 * </p> 4265 */ 4266 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RESULT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_RESULT); 4267 4268 /** 4269 * Search parameter: <b>status</b> 4270 * <p> 4271 * Description: <b>The current status of the test report</b><br> 4272 * Type: <b>token</b><br> 4273 * Path: <b>TestReport.status</b><br> 4274 * </p> 4275 */ 4276 @SearchParamDefinition(name="status", path="TestReport.status", description="The current status of the test report", type="token" ) 4277 public static final String SP_STATUS = "status"; 4278 /** 4279 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4280 * <p> 4281 * Description: <b>The current status of the test report</b><br> 4282 * Type: <b>token</b><br> 4283 * Path: <b>TestReport.status</b><br> 4284 * </p> 4285 */ 4286 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4287 4288 /** 4289 * Search parameter: <b>tester</b> 4290 * <p> 4291 * Description: <b>The name of the testing organization</b><br> 4292 * Type: <b>string</b><br> 4293 * Path: <b>TestReport.tester</b><br> 4294 * </p> 4295 */ 4296 @SearchParamDefinition(name="tester", path="TestReport.tester", description="The name of the testing organization", type="string" ) 4297 public static final String SP_TESTER = "tester"; 4298 /** 4299 * <b>Fluent Client</b> search parameter constant for <b>tester</b> 4300 * <p> 4301 * Description: <b>The name of the testing organization</b><br> 4302 * Type: <b>string</b><br> 4303 * Path: <b>TestReport.tester</b><br> 4304 * </p> 4305 */ 4306 public static final ca.uhn.fhir.rest.gclient.StringClientParam TESTER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TESTER); 4307 4308 /** 4309 * Search parameter: <b>testscript</b> 4310 * <p> 4311 * Description: <b>The test script executed to produce this report</b><br> 4312 * Type: <b>reference</b><br> 4313 * Path: <b>TestReport.testScript</b><br> 4314 * </p> 4315 */ 4316 @SearchParamDefinition(name="testscript", path="TestReport.testScript", description="The test script executed to produce this report", type="reference", target={TestScript.class } ) 4317 public static final String SP_TESTSCRIPT = "testscript"; 4318 /** 4319 * <b>Fluent Client</b> search parameter constant for <b>testscript</b> 4320 * <p> 4321 * Description: <b>The test script executed to produce this report</b><br> 4322 * Type: <b>reference</b><br> 4323 * Path: <b>TestReport.testScript</b><br> 4324 * </p> 4325 */ 4326 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TESTSCRIPT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_TESTSCRIPT); 4327 4328/** 4329 * Constant for fluent queries to be used to add include statements. Specifies 4330 * the path value of "<b>TestReport:testscript</b>". 4331 */ 4332 public static final ca.uhn.fhir.model.api.Include INCLUDE_TESTSCRIPT = new ca.uhn.fhir.model.api.Include("TestReport:testscript").toLocked(); 4333 4334 4335} 4336