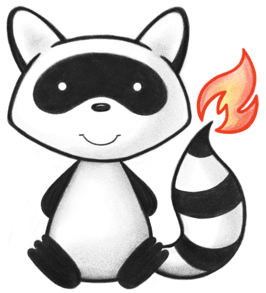
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A structured set of tests against a FHIR server or client implementation to determine compliance against the FHIR specification. 052 */ 053@ResourceDef(name="TestScript", profile="http://hl7.org/fhir/StructureDefinition/TestScript") 054public class TestScript extends CanonicalResource { 055 056 public enum AssertionDirectionType { 057 /** 058 * The assertion is evaluated on the response. This is the default value. 059 */ 060 RESPONSE, 061 /** 062 * The assertion is evaluated on the request. 063 */ 064 REQUEST, 065 /** 066 * added to help the parsers with the generic types 067 */ 068 NULL; 069 public static AssertionDirectionType fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("response".equals(codeString)) 073 return RESPONSE; 074 if ("request".equals(codeString)) 075 return REQUEST; 076 if (Configuration.isAcceptInvalidEnums()) 077 return null; 078 else 079 throw new FHIRException("Unknown AssertionDirectionType code '"+codeString+"'"); 080 } 081 public String toCode() { 082 switch (this) { 083 case RESPONSE: return "response"; 084 case REQUEST: return "request"; 085 case NULL: return null; 086 default: return "?"; 087 } 088 } 089 public String getSystem() { 090 switch (this) { 091 case RESPONSE: return "http://hl7.org/fhir/assert-direction-codes"; 092 case REQUEST: return "http://hl7.org/fhir/assert-direction-codes"; 093 case NULL: return null; 094 default: return "?"; 095 } 096 } 097 public String getDefinition() { 098 switch (this) { 099 case RESPONSE: return "The assertion is evaluated on the response. This is the default value."; 100 case REQUEST: return "The assertion is evaluated on the request."; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDisplay() { 106 switch (this) { 107 case RESPONSE: return "response"; 108 case REQUEST: return "request"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 } 114 115 public static class AssertionDirectionTypeEnumFactory implements EnumFactory<AssertionDirectionType> { 116 public AssertionDirectionType fromCode(String codeString) throws IllegalArgumentException { 117 if (codeString == null || "".equals(codeString)) 118 if (codeString == null || "".equals(codeString)) 119 return null; 120 if ("response".equals(codeString)) 121 return AssertionDirectionType.RESPONSE; 122 if ("request".equals(codeString)) 123 return AssertionDirectionType.REQUEST; 124 throw new IllegalArgumentException("Unknown AssertionDirectionType code '"+codeString+"'"); 125 } 126 public Enumeration<AssertionDirectionType> fromType(PrimitiveType<?> code) throws FHIRException { 127 if (code == null) 128 return null; 129 if (code.isEmpty()) 130 return new Enumeration<AssertionDirectionType>(this, AssertionDirectionType.NULL, code); 131 String codeString = ((PrimitiveType) code).asStringValue(); 132 if (codeString == null || "".equals(codeString)) 133 return new Enumeration<AssertionDirectionType>(this, AssertionDirectionType.NULL, code); 134 if ("response".equals(codeString)) 135 return new Enumeration<AssertionDirectionType>(this, AssertionDirectionType.RESPONSE, code); 136 if ("request".equals(codeString)) 137 return new Enumeration<AssertionDirectionType>(this, AssertionDirectionType.REQUEST, code); 138 throw new FHIRException("Unknown AssertionDirectionType code '"+codeString+"'"); 139 } 140 public String toCode(AssertionDirectionType code) { 141 if (code == AssertionDirectionType.NULL) 142 return null; 143 if (code == AssertionDirectionType.RESPONSE) 144 return "response"; 145 if (code == AssertionDirectionType.REQUEST) 146 return "request"; 147 return "?"; 148 } 149 public String toSystem(AssertionDirectionType code) { 150 return code.getSystem(); 151 } 152 } 153 154 public enum AssertionManualCompletionType { 155 /** 156 * Mark the currently waiting test failed and proceed with the next assert if the stopTestOnFail is false or the next test in the TestScript if the stopTestOnFail is true. 157 */ 158 FAIL, 159 /** 160 * Mark the currently waiting test passed (if the test is not failed already) and proceed with the next action in the TestScript. 161 */ 162 PASS, 163 /** 164 * Mark this assert as skipped and proceed with the next action in the TestScript. 165 */ 166 SKIP, 167 /** 168 * Stop execution of this TestScript. The overall status of this TestScript is evaluated based on the status of the completed tests. 169 */ 170 STOP, 171 /** 172 * added to help the parsers with the generic types 173 */ 174 NULL; 175 public static AssertionManualCompletionType fromCode(String codeString) throws FHIRException { 176 if (codeString == null || "".equals(codeString)) 177 return null; 178 if ("fail".equals(codeString)) 179 return FAIL; 180 if ("pass".equals(codeString)) 181 return PASS; 182 if ("skip".equals(codeString)) 183 return SKIP; 184 if ("stop".equals(codeString)) 185 return STOP; 186 if (Configuration.isAcceptInvalidEnums()) 187 return null; 188 else 189 throw new FHIRException("Unknown AssertionManualCompletionType code '"+codeString+"'"); 190 } 191 public String toCode() { 192 switch (this) { 193 case FAIL: return "fail"; 194 case PASS: return "pass"; 195 case SKIP: return "skip"; 196 case STOP: return "stop"; 197 case NULL: return null; 198 default: return "?"; 199 } 200 } 201 public String getSystem() { 202 switch (this) { 203 case FAIL: return "http://hl7.org/fhir/assert-manual-completion-codes"; 204 case PASS: return "http://hl7.org/fhir/assert-manual-completion-codes"; 205 case SKIP: return "http://hl7.org/fhir/assert-manual-completion-codes"; 206 case STOP: return "http://hl7.org/fhir/assert-manual-completion-codes"; 207 case NULL: return null; 208 default: return "?"; 209 } 210 } 211 public String getDefinition() { 212 switch (this) { 213 case FAIL: return "Mark the currently waiting test failed and proceed with the next assert if the stopTestOnFail is false or the next test in the TestScript if the stopTestOnFail is true."; 214 case PASS: return "Mark the currently waiting test passed (if the test is not failed already) and proceed with the next action in the TestScript."; 215 case SKIP: return "Mark this assert as skipped and proceed with the next action in the TestScript."; 216 case STOP: return "Stop execution of this TestScript. The overall status of this TestScript is evaluated based on the status of the completed tests."; 217 case NULL: return null; 218 default: return "?"; 219 } 220 } 221 public String getDisplay() { 222 switch (this) { 223 case FAIL: return "Fail"; 224 case PASS: return "Pass"; 225 case SKIP: return "Skip"; 226 case STOP: return "Stop"; 227 case NULL: return null; 228 default: return "?"; 229 } 230 } 231 } 232 233 public static class AssertionManualCompletionTypeEnumFactory implements EnumFactory<AssertionManualCompletionType> { 234 public AssertionManualCompletionType fromCode(String codeString) throws IllegalArgumentException { 235 if (codeString == null || "".equals(codeString)) 236 if (codeString == null || "".equals(codeString)) 237 return null; 238 if ("fail".equals(codeString)) 239 return AssertionManualCompletionType.FAIL; 240 if ("pass".equals(codeString)) 241 return AssertionManualCompletionType.PASS; 242 if ("skip".equals(codeString)) 243 return AssertionManualCompletionType.SKIP; 244 if ("stop".equals(codeString)) 245 return AssertionManualCompletionType.STOP; 246 throw new IllegalArgumentException("Unknown AssertionManualCompletionType code '"+codeString+"'"); 247 } 248 public Enumeration<AssertionManualCompletionType> fromType(PrimitiveType<?> code) throws FHIRException { 249 if (code == null) 250 return null; 251 if (code.isEmpty()) 252 return new Enumeration<AssertionManualCompletionType>(this, AssertionManualCompletionType.NULL, code); 253 String codeString = ((PrimitiveType) code).asStringValue(); 254 if (codeString == null || "".equals(codeString)) 255 return new Enumeration<AssertionManualCompletionType>(this, AssertionManualCompletionType.NULL, code); 256 if ("fail".equals(codeString)) 257 return new Enumeration<AssertionManualCompletionType>(this, AssertionManualCompletionType.FAIL, code); 258 if ("pass".equals(codeString)) 259 return new Enumeration<AssertionManualCompletionType>(this, AssertionManualCompletionType.PASS, code); 260 if ("skip".equals(codeString)) 261 return new Enumeration<AssertionManualCompletionType>(this, AssertionManualCompletionType.SKIP, code); 262 if ("stop".equals(codeString)) 263 return new Enumeration<AssertionManualCompletionType>(this, AssertionManualCompletionType.STOP, code); 264 throw new FHIRException("Unknown AssertionManualCompletionType code '"+codeString+"'"); 265 } 266 public String toCode(AssertionManualCompletionType code) { 267 if (code == AssertionManualCompletionType.NULL) 268 return null; 269 if (code == AssertionManualCompletionType.FAIL) 270 return "fail"; 271 if (code == AssertionManualCompletionType.PASS) 272 return "pass"; 273 if (code == AssertionManualCompletionType.SKIP) 274 return "skip"; 275 if (code == AssertionManualCompletionType.STOP) 276 return "stop"; 277 return "?"; 278 } 279 public String toSystem(AssertionManualCompletionType code) { 280 return code.getSystem(); 281 } 282 } 283 284 public enum AssertionOperatorType { 285 /** 286 * Default value. Equals comparison. 287 */ 288 EQUALS, 289 /** 290 * Not equals comparison. 291 */ 292 NOTEQUALS, 293 /** 294 * Compare value within a known set of values. 295 */ 296 IN, 297 /** 298 * Compare value not within a known set of values. 299 */ 300 NOTIN, 301 /** 302 * Compare value to be greater than a known value. 303 */ 304 GREATERTHAN, 305 /** 306 * Compare value to be less than a known value. 307 */ 308 LESSTHAN, 309 /** 310 * Compare value is empty. 311 */ 312 EMPTY, 313 /** 314 * Compare value is not empty. 315 */ 316 NOTEMPTY, 317 /** 318 * Compare value string contains a known value. 319 */ 320 CONTAINS, 321 /** 322 * Compare value string does not contain a known value. 323 */ 324 NOTCONTAINS, 325 /** 326 * Evaluate the FHIRPath expression as a boolean condition. 327 */ 328 EVAL, 329 /** 330 * Manually evaluate the condition described by this assert. The test engine SHALL pause and provide an input mechanism to set the outcome of this assert to 'pass', 'fail', 'skip' or 'stop'. 331 */ 332 MANUALEVAL, 333 /** 334 * added to help the parsers with the generic types 335 */ 336 NULL; 337 public static AssertionOperatorType fromCode(String codeString) throws FHIRException { 338 if (codeString == null || "".equals(codeString)) 339 return null; 340 if ("equals".equals(codeString)) 341 return EQUALS; 342 if ("notEquals".equals(codeString)) 343 return NOTEQUALS; 344 if ("in".equals(codeString)) 345 return IN; 346 if ("notIn".equals(codeString)) 347 return NOTIN; 348 if ("greaterThan".equals(codeString)) 349 return GREATERTHAN; 350 if ("lessThan".equals(codeString)) 351 return LESSTHAN; 352 if ("empty".equals(codeString)) 353 return EMPTY; 354 if ("notEmpty".equals(codeString)) 355 return NOTEMPTY; 356 if ("contains".equals(codeString)) 357 return CONTAINS; 358 if ("notContains".equals(codeString)) 359 return NOTCONTAINS; 360 if ("eval".equals(codeString)) 361 return EVAL; 362 if ("manualEval".equals(codeString)) 363 return MANUALEVAL; 364 if (Configuration.isAcceptInvalidEnums()) 365 return null; 366 else 367 throw new FHIRException("Unknown AssertionOperatorType code '"+codeString+"'"); 368 } 369 public String toCode() { 370 switch (this) { 371 case EQUALS: return "equals"; 372 case NOTEQUALS: return "notEquals"; 373 case IN: return "in"; 374 case NOTIN: return "notIn"; 375 case GREATERTHAN: return "greaterThan"; 376 case LESSTHAN: return "lessThan"; 377 case EMPTY: return "empty"; 378 case NOTEMPTY: return "notEmpty"; 379 case CONTAINS: return "contains"; 380 case NOTCONTAINS: return "notContains"; 381 case EVAL: return "eval"; 382 case MANUALEVAL: return "manualEval"; 383 case NULL: return null; 384 default: return "?"; 385 } 386 } 387 public String getSystem() { 388 switch (this) { 389 case EQUALS: return "http://hl7.org/fhir/assert-operator-codes"; 390 case NOTEQUALS: return "http://hl7.org/fhir/assert-operator-codes"; 391 case IN: return "http://hl7.org/fhir/assert-operator-codes"; 392 case NOTIN: return "http://hl7.org/fhir/assert-operator-codes"; 393 case GREATERTHAN: return "http://hl7.org/fhir/assert-operator-codes"; 394 case LESSTHAN: return "http://hl7.org/fhir/assert-operator-codes"; 395 case EMPTY: return "http://hl7.org/fhir/assert-operator-codes"; 396 case NOTEMPTY: return "http://hl7.org/fhir/assert-operator-codes"; 397 case CONTAINS: return "http://hl7.org/fhir/assert-operator-codes"; 398 case NOTCONTAINS: return "http://hl7.org/fhir/assert-operator-codes"; 399 case EVAL: return "http://hl7.org/fhir/assert-operator-codes"; 400 case MANUALEVAL: return "http://hl7.org/fhir/assert-operator-codes"; 401 case NULL: return null; 402 default: return "?"; 403 } 404 } 405 public String getDefinition() { 406 switch (this) { 407 case EQUALS: return "Default value. Equals comparison."; 408 case NOTEQUALS: return "Not equals comparison."; 409 case IN: return "Compare value within a known set of values."; 410 case NOTIN: return "Compare value not within a known set of values."; 411 case GREATERTHAN: return "Compare value to be greater than a known value."; 412 case LESSTHAN: return "Compare value to be less than a known value."; 413 case EMPTY: return "Compare value is empty."; 414 case NOTEMPTY: return "Compare value is not empty."; 415 case CONTAINS: return "Compare value string contains a known value."; 416 case NOTCONTAINS: return "Compare value string does not contain a known value."; 417 case EVAL: return "Evaluate the FHIRPath expression as a boolean condition."; 418 case MANUALEVAL: return "Manually evaluate the condition described by this assert. The test engine SHALL pause and provide an input mechanism to set the outcome of this assert to 'pass', 'fail', 'skip' or 'stop'."; 419 case NULL: return null; 420 default: return "?"; 421 } 422 } 423 public String getDisplay() { 424 switch (this) { 425 case EQUALS: return "equals"; 426 case NOTEQUALS: return "notEquals"; 427 case IN: return "in"; 428 case NOTIN: return "notIn"; 429 case GREATERTHAN: return "greaterThan"; 430 case LESSTHAN: return "lessThan"; 431 case EMPTY: return "empty"; 432 case NOTEMPTY: return "notEmpty"; 433 case CONTAINS: return "contains"; 434 case NOTCONTAINS: return "notContains"; 435 case EVAL: return "evaluate"; 436 case MANUALEVAL: return "manualEvaluate"; 437 case NULL: return null; 438 default: return "?"; 439 } 440 } 441 } 442 443 public static class AssertionOperatorTypeEnumFactory implements EnumFactory<AssertionOperatorType> { 444 public AssertionOperatorType fromCode(String codeString) throws IllegalArgumentException { 445 if (codeString == null || "".equals(codeString)) 446 if (codeString == null || "".equals(codeString)) 447 return null; 448 if ("equals".equals(codeString)) 449 return AssertionOperatorType.EQUALS; 450 if ("notEquals".equals(codeString)) 451 return AssertionOperatorType.NOTEQUALS; 452 if ("in".equals(codeString)) 453 return AssertionOperatorType.IN; 454 if ("notIn".equals(codeString)) 455 return AssertionOperatorType.NOTIN; 456 if ("greaterThan".equals(codeString)) 457 return AssertionOperatorType.GREATERTHAN; 458 if ("lessThan".equals(codeString)) 459 return AssertionOperatorType.LESSTHAN; 460 if ("empty".equals(codeString)) 461 return AssertionOperatorType.EMPTY; 462 if ("notEmpty".equals(codeString)) 463 return AssertionOperatorType.NOTEMPTY; 464 if ("contains".equals(codeString)) 465 return AssertionOperatorType.CONTAINS; 466 if ("notContains".equals(codeString)) 467 return AssertionOperatorType.NOTCONTAINS; 468 if ("eval".equals(codeString)) 469 return AssertionOperatorType.EVAL; 470 if ("manualEval".equals(codeString)) 471 return AssertionOperatorType.MANUALEVAL; 472 throw new IllegalArgumentException("Unknown AssertionOperatorType code '"+codeString+"'"); 473 } 474 public Enumeration<AssertionOperatorType> fromType(PrimitiveType<?> code) throws FHIRException { 475 if (code == null) 476 return null; 477 if (code.isEmpty()) 478 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.NULL, code); 479 String codeString = ((PrimitiveType) code).asStringValue(); 480 if (codeString == null || "".equals(codeString)) 481 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.NULL, code); 482 if ("equals".equals(codeString)) 483 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.EQUALS, code); 484 if ("notEquals".equals(codeString)) 485 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.NOTEQUALS, code); 486 if ("in".equals(codeString)) 487 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.IN, code); 488 if ("notIn".equals(codeString)) 489 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.NOTIN, code); 490 if ("greaterThan".equals(codeString)) 491 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.GREATERTHAN, code); 492 if ("lessThan".equals(codeString)) 493 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.LESSTHAN, code); 494 if ("empty".equals(codeString)) 495 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.EMPTY, code); 496 if ("notEmpty".equals(codeString)) 497 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.NOTEMPTY, code); 498 if ("contains".equals(codeString)) 499 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.CONTAINS, code); 500 if ("notContains".equals(codeString)) 501 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.NOTCONTAINS, code); 502 if ("eval".equals(codeString)) 503 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.EVAL, code); 504 if ("manualEval".equals(codeString)) 505 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.MANUALEVAL, code); 506 throw new FHIRException("Unknown AssertionOperatorType code '"+codeString+"'"); 507 } 508 public String toCode(AssertionOperatorType code) { 509 if (code == AssertionOperatorType.NULL) 510 return null; 511 if (code == AssertionOperatorType.EQUALS) 512 return "equals"; 513 if (code == AssertionOperatorType.NOTEQUALS) 514 return "notEquals"; 515 if (code == AssertionOperatorType.IN) 516 return "in"; 517 if (code == AssertionOperatorType.NOTIN) 518 return "notIn"; 519 if (code == AssertionOperatorType.GREATERTHAN) 520 return "greaterThan"; 521 if (code == AssertionOperatorType.LESSTHAN) 522 return "lessThan"; 523 if (code == AssertionOperatorType.EMPTY) 524 return "empty"; 525 if (code == AssertionOperatorType.NOTEMPTY) 526 return "notEmpty"; 527 if (code == AssertionOperatorType.CONTAINS) 528 return "contains"; 529 if (code == AssertionOperatorType.NOTCONTAINS) 530 return "notContains"; 531 if (code == AssertionOperatorType.EVAL) 532 return "eval"; 533 if (code == AssertionOperatorType.MANUALEVAL) 534 return "manualEval"; 535 return "?"; 536 } 537 public String toSystem(AssertionOperatorType code) { 538 return code.getSystem(); 539 } 540 } 541 542 public enum AssertionResponseTypes { 543 /** 544 * Response code is 100. 545 */ 546 CONTINUE, 547 /** 548 * Response code is 101. 549 */ 550 SWITCHINGPROTOCOLS, 551 /** 552 * Response code is 200. 553 */ 554 OKAY, 555 /** 556 * Response code is 201. 557 */ 558 CREATED, 559 /** 560 * Response code is 202. 561 */ 562 ACCEPTED, 563 /** 564 * Response code is 203. 565 */ 566 NONAUTHORITATIVEINFORMATION, 567 /** 568 * Response code is 204. 569 */ 570 NOCONTENT, 571 /** 572 * Response code is 205. 573 */ 574 RESETCONTENT, 575 /** 576 * Response code is 206. 577 */ 578 PARTIALCONTENT, 579 /** 580 * Response code is 300. 581 */ 582 MULTIPLECHOICES, 583 /** 584 * Response code is 301. 585 */ 586 MOVEDPERMANENTLY, 587 /** 588 * Response code is 302. 589 */ 590 FOUND, 591 /** 592 * Response code is 303. 593 */ 594 SEEOTHER, 595 /** 596 * Response code is 304. 597 */ 598 NOTMODIFIED, 599 /** 600 * Response code is 305. 601 */ 602 USEPROXY, 603 /** 604 * Response code is 307. 605 */ 606 TEMPORARYREDIRECT, 607 /** 608 * Response code is 308. 609 */ 610 PERMANENTREDIRECT, 611 /** 612 * Response code is 400. 613 */ 614 BADREQUEST, 615 /** 616 * Response code is 401. 617 */ 618 UNAUTHORIZED, 619 /** 620 * Response code is 402. 621 */ 622 PAYMENTREQUIRED, 623 /** 624 * Response code is 403. 625 */ 626 FORBIDDEN, 627 /** 628 * Response code is 404. 629 */ 630 NOTFOUND, 631 /** 632 * Response code is 405. 633 */ 634 METHODNOTALLOWED, 635 /** 636 * Response code is 406. 637 */ 638 NOTACCEPTABLE, 639 /** 640 * Response code is 407. 641 */ 642 PROXYAUTHENTICATIONREQUIRED, 643 /** 644 * Response code is 408. 645 */ 646 REQUESTTIMEOUT, 647 /** 648 * Response code is 409. 649 */ 650 CONFLICT, 651 /** 652 * Response code is 410. 653 */ 654 GONE, 655 /** 656 * Response code is 411. 657 */ 658 LENGTHREQUIRED, 659 /** 660 * Response code is 412. 661 */ 662 PRECONDITIONFAILED, 663 /** 664 * Response code is 413. 665 */ 666 CONTENTTOOLARGE, 667 /** 668 * Response code is 414. 669 */ 670 URITOOLONG, 671 /** 672 * Response code is 415. 673 */ 674 UNSUPPORTEDMEDIATYPE, 675 /** 676 * Response code is 416. 677 */ 678 RANGENOTSATISFIABLE, 679 /** 680 * Response code is 417. 681 */ 682 EXPECTATIONFAILED, 683 /** 684 * Response code is 421. 685 */ 686 MISDIRECTEDREQUEST, 687 /** 688 * Response code is 422. 689 */ 690 UNPROCESSABLECONTENT, 691 /** 692 * Response code is 426. 693 */ 694 UPGRADEREQUIRED, 695 /** 696 * Response code is 500. 697 */ 698 INTERNALSERVERERROR, 699 /** 700 * Response code is 501. 701 */ 702 NOTIMPLEMENTED, 703 /** 704 * Response code is 502. 705 */ 706 BADGATEWAY, 707 /** 708 * Response code is 503. 709 */ 710 SERVICEUNAVAILABLE, 711 /** 712 * Response code is 504. 713 */ 714 GATEWAYTIMEOUT, 715 /** 716 * Response code is 505. 717 */ 718 HTTPVERSIONNOTSUPPORTED, 719 /** 720 * added to help the parsers with the generic types 721 */ 722 NULL; 723 public static AssertionResponseTypes fromCode(String codeString) throws FHIRException { 724 if (codeString == null || "".equals(codeString)) 725 return null; 726 if ("continue".equals(codeString)) 727 return CONTINUE; 728 if ("switchingProtocols".equals(codeString)) 729 return SWITCHINGPROTOCOLS; 730 if ("okay".equals(codeString)) 731 return OKAY; 732 if ("created".equals(codeString)) 733 return CREATED; 734 if ("accepted".equals(codeString)) 735 return ACCEPTED; 736 if ("nonAuthoritativeInformation".equals(codeString)) 737 return NONAUTHORITATIVEINFORMATION; 738 if ("noContent".equals(codeString)) 739 return NOCONTENT; 740 if ("resetContent".equals(codeString)) 741 return RESETCONTENT; 742 if ("partialContent".equals(codeString)) 743 return PARTIALCONTENT; 744 if ("multipleChoices".equals(codeString)) 745 return MULTIPLECHOICES; 746 if ("movedPermanently".equals(codeString)) 747 return MOVEDPERMANENTLY; 748 if ("found".equals(codeString)) 749 return FOUND; 750 if ("seeOther".equals(codeString)) 751 return SEEOTHER; 752 if ("notModified".equals(codeString)) 753 return NOTMODIFIED; 754 if ("useProxy".equals(codeString)) 755 return USEPROXY; 756 if ("temporaryRedirect".equals(codeString)) 757 return TEMPORARYREDIRECT; 758 if ("permanentRedirect".equals(codeString)) 759 return PERMANENTREDIRECT; 760 if ("badRequest".equals(codeString)) 761 return BADREQUEST; 762 if ("unauthorized".equals(codeString)) 763 return UNAUTHORIZED; 764 if ("paymentRequired".equals(codeString)) 765 return PAYMENTREQUIRED; 766 if ("forbidden".equals(codeString)) 767 return FORBIDDEN; 768 if ("notFound".equals(codeString)) 769 return NOTFOUND; 770 if ("methodNotAllowed".equals(codeString)) 771 return METHODNOTALLOWED; 772 if ("notAcceptable".equals(codeString)) 773 return NOTACCEPTABLE; 774 if ("proxyAuthenticationRequired".equals(codeString)) 775 return PROXYAUTHENTICATIONREQUIRED; 776 if ("requestTimeout".equals(codeString)) 777 return REQUESTTIMEOUT; 778 if ("conflict".equals(codeString)) 779 return CONFLICT; 780 if ("gone".equals(codeString)) 781 return GONE; 782 if ("lengthRequired".equals(codeString)) 783 return LENGTHREQUIRED; 784 if ("preconditionFailed".equals(codeString)) 785 return PRECONDITIONFAILED; 786 if ("contentTooLarge".equals(codeString)) 787 return CONTENTTOOLARGE; 788 if ("uriTooLong".equals(codeString)) 789 return URITOOLONG; 790 if ("unsupportedMediaType".equals(codeString)) 791 return UNSUPPORTEDMEDIATYPE; 792 if ("rangeNotSatisfiable".equals(codeString)) 793 return RANGENOTSATISFIABLE; 794 if ("expectationFailed".equals(codeString)) 795 return EXPECTATIONFAILED; 796 if ("misdirectedRequest".equals(codeString)) 797 return MISDIRECTEDREQUEST; 798 if ("unprocessableContent".equals(codeString)) 799 return UNPROCESSABLECONTENT; 800 if ("upgradeRequired".equals(codeString)) 801 return UPGRADEREQUIRED; 802 if ("internalServerError".equals(codeString)) 803 return INTERNALSERVERERROR; 804 if ("notImplemented".equals(codeString)) 805 return NOTIMPLEMENTED; 806 if ("badGateway".equals(codeString)) 807 return BADGATEWAY; 808 if ("serviceUnavailable".equals(codeString)) 809 return SERVICEUNAVAILABLE; 810 if ("gatewayTimeout".equals(codeString)) 811 return GATEWAYTIMEOUT; 812 if ("httpVersionNotSupported".equals(codeString)) 813 return HTTPVERSIONNOTSUPPORTED; 814 if (Configuration.isAcceptInvalidEnums()) 815 return null; 816 else 817 throw new FHIRException("Unknown AssertionResponseTypes code '"+codeString+"'"); 818 } 819 public String toCode() { 820 switch (this) { 821 case CONTINUE: return "continue"; 822 case SWITCHINGPROTOCOLS: return "switchingProtocols"; 823 case OKAY: return "okay"; 824 case CREATED: return "created"; 825 case ACCEPTED: return "accepted"; 826 case NONAUTHORITATIVEINFORMATION: return "nonAuthoritativeInformation"; 827 case NOCONTENT: return "noContent"; 828 case RESETCONTENT: return "resetContent"; 829 case PARTIALCONTENT: return "partialContent"; 830 case MULTIPLECHOICES: return "multipleChoices"; 831 case MOVEDPERMANENTLY: return "movedPermanently"; 832 case FOUND: return "found"; 833 case SEEOTHER: return "seeOther"; 834 case NOTMODIFIED: return "notModified"; 835 case USEPROXY: return "useProxy"; 836 case TEMPORARYREDIRECT: return "temporaryRedirect"; 837 case PERMANENTREDIRECT: return "permanentRedirect"; 838 case BADREQUEST: return "badRequest"; 839 case UNAUTHORIZED: return "unauthorized"; 840 case PAYMENTREQUIRED: return "paymentRequired"; 841 case FORBIDDEN: return "forbidden"; 842 case NOTFOUND: return "notFound"; 843 case METHODNOTALLOWED: return "methodNotAllowed"; 844 case NOTACCEPTABLE: return "notAcceptable"; 845 case PROXYAUTHENTICATIONREQUIRED: return "proxyAuthenticationRequired"; 846 case REQUESTTIMEOUT: return "requestTimeout"; 847 case CONFLICT: return "conflict"; 848 case GONE: return "gone"; 849 case LENGTHREQUIRED: return "lengthRequired"; 850 case PRECONDITIONFAILED: return "preconditionFailed"; 851 case CONTENTTOOLARGE: return "contentTooLarge"; 852 case URITOOLONG: return "uriTooLong"; 853 case UNSUPPORTEDMEDIATYPE: return "unsupportedMediaType"; 854 case RANGENOTSATISFIABLE: return "rangeNotSatisfiable"; 855 case EXPECTATIONFAILED: return "expectationFailed"; 856 case MISDIRECTEDREQUEST: return "misdirectedRequest"; 857 case UNPROCESSABLECONTENT: return "unprocessableContent"; 858 case UPGRADEREQUIRED: return "upgradeRequired"; 859 case INTERNALSERVERERROR: return "internalServerError"; 860 case NOTIMPLEMENTED: return "notImplemented"; 861 case BADGATEWAY: return "badGateway"; 862 case SERVICEUNAVAILABLE: return "serviceUnavailable"; 863 case GATEWAYTIMEOUT: return "gatewayTimeout"; 864 case HTTPVERSIONNOTSUPPORTED: return "httpVersionNotSupported"; 865 case NULL: return null; 866 default: return "?"; 867 } 868 } 869 public String getSystem() { 870 switch (this) { 871 case CONTINUE: return "http://hl7.org/fhir/assert-response-code-types"; 872 case SWITCHINGPROTOCOLS: return "http://hl7.org/fhir/assert-response-code-types"; 873 case OKAY: return "http://hl7.org/fhir/assert-response-code-types"; 874 case CREATED: return "http://hl7.org/fhir/assert-response-code-types"; 875 case ACCEPTED: return "http://hl7.org/fhir/assert-response-code-types"; 876 case NONAUTHORITATIVEINFORMATION: return "http://hl7.org/fhir/assert-response-code-types"; 877 case NOCONTENT: return "http://hl7.org/fhir/assert-response-code-types"; 878 case RESETCONTENT: return "http://hl7.org/fhir/assert-response-code-types"; 879 case PARTIALCONTENT: return "http://hl7.org/fhir/assert-response-code-types"; 880 case MULTIPLECHOICES: return "http://hl7.org/fhir/assert-response-code-types"; 881 case MOVEDPERMANENTLY: return "http://hl7.org/fhir/assert-response-code-types"; 882 case FOUND: return "http://hl7.org/fhir/assert-response-code-types"; 883 case SEEOTHER: return "http://hl7.org/fhir/assert-response-code-types"; 884 case NOTMODIFIED: return "http://hl7.org/fhir/assert-response-code-types"; 885 case USEPROXY: return "http://hl7.org/fhir/assert-response-code-types"; 886 case TEMPORARYREDIRECT: return "http://hl7.org/fhir/assert-response-code-types"; 887 case PERMANENTREDIRECT: return "http://hl7.org/fhir/assert-response-code-types"; 888 case BADREQUEST: return "http://hl7.org/fhir/assert-response-code-types"; 889 case UNAUTHORIZED: return "http://hl7.org/fhir/assert-response-code-types"; 890 case PAYMENTREQUIRED: return "http://hl7.org/fhir/assert-response-code-types"; 891 case FORBIDDEN: return "http://hl7.org/fhir/assert-response-code-types"; 892 case NOTFOUND: return "http://hl7.org/fhir/assert-response-code-types"; 893 case METHODNOTALLOWED: return "http://hl7.org/fhir/assert-response-code-types"; 894 case NOTACCEPTABLE: return "http://hl7.org/fhir/assert-response-code-types"; 895 case PROXYAUTHENTICATIONREQUIRED: return "http://hl7.org/fhir/assert-response-code-types"; 896 case REQUESTTIMEOUT: return "http://hl7.org/fhir/assert-response-code-types"; 897 case CONFLICT: return "http://hl7.org/fhir/assert-response-code-types"; 898 case GONE: return "http://hl7.org/fhir/assert-response-code-types"; 899 case LENGTHREQUIRED: return "http://hl7.org/fhir/assert-response-code-types"; 900 case PRECONDITIONFAILED: return "http://hl7.org/fhir/assert-response-code-types"; 901 case CONTENTTOOLARGE: return "http://hl7.org/fhir/assert-response-code-types"; 902 case URITOOLONG: return "http://hl7.org/fhir/assert-response-code-types"; 903 case UNSUPPORTEDMEDIATYPE: return "http://hl7.org/fhir/assert-response-code-types"; 904 case RANGENOTSATISFIABLE: return "http://hl7.org/fhir/assert-response-code-types"; 905 case EXPECTATIONFAILED: return "http://hl7.org/fhir/assert-response-code-types"; 906 case MISDIRECTEDREQUEST: return "http://hl7.org/fhir/assert-response-code-types"; 907 case UNPROCESSABLECONTENT: return "http://hl7.org/fhir/assert-response-code-types"; 908 case UPGRADEREQUIRED: return "http://hl7.org/fhir/assert-response-code-types"; 909 case INTERNALSERVERERROR: return "http://hl7.org/fhir/assert-response-code-types"; 910 case NOTIMPLEMENTED: return "http://hl7.org/fhir/assert-response-code-types"; 911 case BADGATEWAY: return "http://hl7.org/fhir/assert-response-code-types"; 912 case SERVICEUNAVAILABLE: return "http://hl7.org/fhir/assert-response-code-types"; 913 case GATEWAYTIMEOUT: return "http://hl7.org/fhir/assert-response-code-types"; 914 case HTTPVERSIONNOTSUPPORTED: return "http://hl7.org/fhir/assert-response-code-types"; 915 case NULL: return null; 916 default: return "?"; 917 } 918 } 919 public String getDefinition() { 920 switch (this) { 921 case CONTINUE: return "Response code is 100."; 922 case SWITCHINGPROTOCOLS: return "Response code is 101."; 923 case OKAY: return "Response code is 200."; 924 case CREATED: return "Response code is 201."; 925 case ACCEPTED: return "Response code is 202."; 926 case NONAUTHORITATIVEINFORMATION: return "Response code is 203."; 927 case NOCONTENT: return "Response code is 204."; 928 case RESETCONTENT: return "Response code is 205."; 929 case PARTIALCONTENT: return "Response code is 206."; 930 case MULTIPLECHOICES: return "Response code is 300."; 931 case MOVEDPERMANENTLY: return "Response code is 301."; 932 case FOUND: return "Response code is 302."; 933 case SEEOTHER: return "Response code is 303."; 934 case NOTMODIFIED: return "Response code is 304."; 935 case USEPROXY: return "Response code is 305."; 936 case TEMPORARYREDIRECT: return "Response code is 307."; 937 case PERMANENTREDIRECT: return "Response code is 308."; 938 case BADREQUEST: return "Response code is 400."; 939 case UNAUTHORIZED: return "Response code is 401."; 940 case PAYMENTREQUIRED: return "Response code is 402."; 941 case FORBIDDEN: return "Response code is 403."; 942 case NOTFOUND: return "Response code is 404."; 943 case METHODNOTALLOWED: return "Response code is 405."; 944 case NOTACCEPTABLE: return "Response code is 406."; 945 case PROXYAUTHENTICATIONREQUIRED: return "Response code is 407."; 946 case REQUESTTIMEOUT: return "Response code is 408."; 947 case CONFLICT: return "Response code is 409."; 948 case GONE: return "Response code is 410."; 949 case LENGTHREQUIRED: return "Response code is 411."; 950 case PRECONDITIONFAILED: return "Response code is 412."; 951 case CONTENTTOOLARGE: return "Response code is 413."; 952 case URITOOLONG: return "Response code is 414."; 953 case UNSUPPORTEDMEDIATYPE: return "Response code is 415."; 954 case RANGENOTSATISFIABLE: return "Response code is 416."; 955 case EXPECTATIONFAILED: return "Response code is 417."; 956 case MISDIRECTEDREQUEST: return "Response code is 421."; 957 case UNPROCESSABLECONTENT: return "Response code is 422."; 958 case UPGRADEREQUIRED: return "Response code is 426."; 959 case INTERNALSERVERERROR: return "Response code is 500."; 960 case NOTIMPLEMENTED: return "Response code is 501."; 961 case BADGATEWAY: return "Response code is 502."; 962 case SERVICEUNAVAILABLE: return "Response code is 503."; 963 case GATEWAYTIMEOUT: return "Response code is 504."; 964 case HTTPVERSIONNOTSUPPORTED: return "Response code is 505."; 965 case NULL: return null; 966 default: return "?"; 967 } 968 } 969 public String getDisplay() { 970 switch (this) { 971 case CONTINUE: return "Continue"; 972 case SWITCHINGPROTOCOLS: return "Switching Protocols"; 973 case OKAY: return "OK"; 974 case CREATED: return "Created"; 975 case ACCEPTED: return "Accepted"; 976 case NONAUTHORITATIVEINFORMATION: return "Non-Authoritative Information"; 977 case NOCONTENT: return "No Content"; 978 case RESETCONTENT: return "Reset Content"; 979 case PARTIALCONTENT: return "Partial Content"; 980 case MULTIPLECHOICES: return "Multiple Choices"; 981 case MOVEDPERMANENTLY: return "Moved Permanently"; 982 case FOUND: return "Found"; 983 case SEEOTHER: return "See Other"; 984 case NOTMODIFIED: return "Not Modified"; 985 case USEPROXY: return "Use Proxy"; 986 case TEMPORARYREDIRECT: return "Temporary Redirect"; 987 case PERMANENTREDIRECT: return "Permanent Redirect"; 988 case BADREQUEST: return "Bad Request"; 989 case UNAUTHORIZED: return "Unauthorized"; 990 case PAYMENTREQUIRED: return "Payment Required"; 991 case FORBIDDEN: return "Forbidden"; 992 case NOTFOUND: return "Not Found"; 993 case METHODNOTALLOWED: return "Method Not Allowed"; 994 case NOTACCEPTABLE: return "Not Acceptable"; 995 case PROXYAUTHENTICATIONREQUIRED: return "Proxy Authentication Required"; 996 case REQUESTTIMEOUT: return "Request Timeout"; 997 case CONFLICT: return "Conflict"; 998 case GONE: return "Gone"; 999 case LENGTHREQUIRED: return "Length Required"; 1000 case PRECONDITIONFAILED: return "Precondition Failed"; 1001 case CONTENTTOOLARGE: return "Content Too Large"; 1002 case URITOOLONG: return "URI Too Long"; 1003 case UNSUPPORTEDMEDIATYPE: return "Unsupported Media Type"; 1004 case RANGENOTSATISFIABLE: return "Range Not Satisfiable"; 1005 case EXPECTATIONFAILED: return "Expectation Failed"; 1006 case MISDIRECTEDREQUEST: return "Misdirected Request"; 1007 case UNPROCESSABLECONTENT: return "Unprocessable Content"; 1008 case UPGRADEREQUIRED: return "Upgrade Required"; 1009 case INTERNALSERVERERROR: return "Internal Server Error"; 1010 case NOTIMPLEMENTED: return "Not Implemented"; 1011 case BADGATEWAY: return "Bad Gateway"; 1012 case SERVICEUNAVAILABLE: return "Service Unavailable"; 1013 case GATEWAYTIMEOUT: return "Gateway Timeout"; 1014 case HTTPVERSIONNOTSUPPORTED: return "HTTP Version Not Supported"; 1015 case NULL: return null; 1016 default: return "?"; 1017 } 1018 } 1019 } 1020 1021 public static class AssertionResponseTypesEnumFactory implements EnumFactory<AssertionResponseTypes> { 1022 public AssertionResponseTypes fromCode(String codeString) throws IllegalArgumentException { 1023 if (codeString == null || "".equals(codeString)) 1024 if (codeString == null || "".equals(codeString)) 1025 return null; 1026 if ("continue".equals(codeString)) 1027 return AssertionResponseTypes.CONTINUE; 1028 if ("switchingProtocols".equals(codeString)) 1029 return AssertionResponseTypes.SWITCHINGPROTOCOLS; 1030 if ("okay".equals(codeString)) 1031 return AssertionResponseTypes.OKAY; 1032 if ("created".equals(codeString)) 1033 return AssertionResponseTypes.CREATED; 1034 if ("accepted".equals(codeString)) 1035 return AssertionResponseTypes.ACCEPTED; 1036 if ("nonAuthoritativeInformation".equals(codeString)) 1037 return AssertionResponseTypes.NONAUTHORITATIVEINFORMATION; 1038 if ("noContent".equals(codeString)) 1039 return AssertionResponseTypes.NOCONTENT; 1040 if ("resetContent".equals(codeString)) 1041 return AssertionResponseTypes.RESETCONTENT; 1042 if ("partialContent".equals(codeString)) 1043 return AssertionResponseTypes.PARTIALCONTENT; 1044 if ("multipleChoices".equals(codeString)) 1045 return AssertionResponseTypes.MULTIPLECHOICES; 1046 if ("movedPermanently".equals(codeString)) 1047 return AssertionResponseTypes.MOVEDPERMANENTLY; 1048 if ("found".equals(codeString)) 1049 return AssertionResponseTypes.FOUND; 1050 if ("seeOther".equals(codeString)) 1051 return AssertionResponseTypes.SEEOTHER; 1052 if ("notModified".equals(codeString)) 1053 return AssertionResponseTypes.NOTMODIFIED; 1054 if ("useProxy".equals(codeString)) 1055 return AssertionResponseTypes.USEPROXY; 1056 if ("temporaryRedirect".equals(codeString)) 1057 return AssertionResponseTypes.TEMPORARYREDIRECT; 1058 if ("permanentRedirect".equals(codeString)) 1059 return AssertionResponseTypes.PERMANENTREDIRECT; 1060 if ("badRequest".equals(codeString)) 1061 return AssertionResponseTypes.BADREQUEST; 1062 if ("unauthorized".equals(codeString)) 1063 return AssertionResponseTypes.UNAUTHORIZED; 1064 if ("paymentRequired".equals(codeString)) 1065 return AssertionResponseTypes.PAYMENTREQUIRED; 1066 if ("forbidden".equals(codeString)) 1067 return AssertionResponseTypes.FORBIDDEN; 1068 if ("notFound".equals(codeString)) 1069 return AssertionResponseTypes.NOTFOUND; 1070 if ("methodNotAllowed".equals(codeString)) 1071 return AssertionResponseTypes.METHODNOTALLOWED; 1072 if ("notAcceptable".equals(codeString)) 1073 return AssertionResponseTypes.NOTACCEPTABLE; 1074 if ("proxyAuthenticationRequired".equals(codeString)) 1075 return AssertionResponseTypes.PROXYAUTHENTICATIONREQUIRED; 1076 if ("requestTimeout".equals(codeString)) 1077 return AssertionResponseTypes.REQUESTTIMEOUT; 1078 if ("conflict".equals(codeString)) 1079 return AssertionResponseTypes.CONFLICT; 1080 if ("gone".equals(codeString)) 1081 return AssertionResponseTypes.GONE; 1082 if ("lengthRequired".equals(codeString)) 1083 return AssertionResponseTypes.LENGTHREQUIRED; 1084 if ("preconditionFailed".equals(codeString)) 1085 return AssertionResponseTypes.PRECONDITIONFAILED; 1086 if ("contentTooLarge".equals(codeString)) 1087 return AssertionResponseTypes.CONTENTTOOLARGE; 1088 if ("uriTooLong".equals(codeString)) 1089 return AssertionResponseTypes.URITOOLONG; 1090 if ("unsupportedMediaType".equals(codeString)) 1091 return AssertionResponseTypes.UNSUPPORTEDMEDIATYPE; 1092 if ("rangeNotSatisfiable".equals(codeString)) 1093 return AssertionResponseTypes.RANGENOTSATISFIABLE; 1094 if ("expectationFailed".equals(codeString)) 1095 return AssertionResponseTypes.EXPECTATIONFAILED; 1096 if ("misdirectedRequest".equals(codeString)) 1097 return AssertionResponseTypes.MISDIRECTEDREQUEST; 1098 if ("unprocessableContent".equals(codeString)) 1099 return AssertionResponseTypes.UNPROCESSABLECONTENT; 1100 if ("upgradeRequired".equals(codeString)) 1101 return AssertionResponseTypes.UPGRADEREQUIRED; 1102 if ("internalServerError".equals(codeString)) 1103 return AssertionResponseTypes.INTERNALSERVERERROR; 1104 if ("notImplemented".equals(codeString)) 1105 return AssertionResponseTypes.NOTIMPLEMENTED; 1106 if ("badGateway".equals(codeString)) 1107 return AssertionResponseTypes.BADGATEWAY; 1108 if ("serviceUnavailable".equals(codeString)) 1109 return AssertionResponseTypes.SERVICEUNAVAILABLE; 1110 if ("gatewayTimeout".equals(codeString)) 1111 return AssertionResponseTypes.GATEWAYTIMEOUT; 1112 if ("httpVersionNotSupported".equals(codeString)) 1113 return AssertionResponseTypes.HTTPVERSIONNOTSUPPORTED; 1114 throw new IllegalArgumentException("Unknown AssertionResponseTypes code '"+codeString+"'"); 1115 } 1116 public Enumeration<AssertionResponseTypes> fromType(PrimitiveType<?> code) throws FHIRException { 1117 if (code == null) 1118 return null; 1119 if (code.isEmpty()) 1120 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.NULL, code); 1121 String codeString = ((PrimitiveType) code).asStringValue(); 1122 if (codeString == null || "".equals(codeString)) 1123 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.NULL, code); 1124 if ("continue".equals(codeString)) 1125 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.CONTINUE, code); 1126 if ("switchingProtocols".equals(codeString)) 1127 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.SWITCHINGPROTOCOLS, code); 1128 if ("okay".equals(codeString)) 1129 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.OKAY, code); 1130 if ("created".equals(codeString)) 1131 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.CREATED, code); 1132 if ("accepted".equals(codeString)) 1133 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.ACCEPTED, code); 1134 if ("nonAuthoritativeInformation".equals(codeString)) 1135 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.NONAUTHORITATIVEINFORMATION, code); 1136 if ("noContent".equals(codeString)) 1137 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.NOCONTENT, code); 1138 if ("resetContent".equals(codeString)) 1139 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.RESETCONTENT, code); 1140 if ("partialContent".equals(codeString)) 1141 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.PARTIALCONTENT, code); 1142 if ("multipleChoices".equals(codeString)) 1143 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.MULTIPLECHOICES, code); 1144 if ("movedPermanently".equals(codeString)) 1145 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.MOVEDPERMANENTLY, code); 1146 if ("found".equals(codeString)) 1147 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.FOUND, code); 1148 if ("seeOther".equals(codeString)) 1149 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.SEEOTHER, code); 1150 if ("notModified".equals(codeString)) 1151 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.NOTMODIFIED, code); 1152 if ("useProxy".equals(codeString)) 1153 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.USEPROXY, code); 1154 if ("temporaryRedirect".equals(codeString)) 1155 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.TEMPORARYREDIRECT, code); 1156 if ("permanentRedirect".equals(codeString)) 1157 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.PERMANENTREDIRECT, code); 1158 if ("badRequest".equals(codeString)) 1159 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.BADREQUEST, code); 1160 if ("unauthorized".equals(codeString)) 1161 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.UNAUTHORIZED, code); 1162 if ("paymentRequired".equals(codeString)) 1163 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.PAYMENTREQUIRED, code); 1164 if ("forbidden".equals(codeString)) 1165 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.FORBIDDEN, code); 1166 if ("notFound".equals(codeString)) 1167 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.NOTFOUND, code); 1168 if ("methodNotAllowed".equals(codeString)) 1169 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.METHODNOTALLOWED, code); 1170 if ("notAcceptable".equals(codeString)) 1171 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.NOTACCEPTABLE, code); 1172 if ("proxyAuthenticationRequired".equals(codeString)) 1173 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.PROXYAUTHENTICATIONREQUIRED, code); 1174 if ("requestTimeout".equals(codeString)) 1175 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.REQUESTTIMEOUT, code); 1176 if ("conflict".equals(codeString)) 1177 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.CONFLICT, code); 1178 if ("gone".equals(codeString)) 1179 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.GONE, code); 1180 if ("lengthRequired".equals(codeString)) 1181 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.LENGTHREQUIRED, code); 1182 if ("preconditionFailed".equals(codeString)) 1183 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.PRECONDITIONFAILED, code); 1184 if ("contentTooLarge".equals(codeString)) 1185 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.CONTENTTOOLARGE, code); 1186 if ("uriTooLong".equals(codeString)) 1187 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.URITOOLONG, code); 1188 if ("unsupportedMediaType".equals(codeString)) 1189 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.UNSUPPORTEDMEDIATYPE, code); 1190 if ("rangeNotSatisfiable".equals(codeString)) 1191 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.RANGENOTSATISFIABLE, code); 1192 if ("expectationFailed".equals(codeString)) 1193 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.EXPECTATIONFAILED, code); 1194 if ("misdirectedRequest".equals(codeString)) 1195 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.MISDIRECTEDREQUEST, code); 1196 if ("unprocessableContent".equals(codeString)) 1197 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.UNPROCESSABLECONTENT, code); 1198 if ("upgradeRequired".equals(codeString)) 1199 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.UPGRADEREQUIRED, code); 1200 if ("internalServerError".equals(codeString)) 1201 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.INTERNALSERVERERROR, code); 1202 if ("notImplemented".equals(codeString)) 1203 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.NOTIMPLEMENTED, code); 1204 if ("badGateway".equals(codeString)) 1205 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.BADGATEWAY, code); 1206 if ("serviceUnavailable".equals(codeString)) 1207 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.SERVICEUNAVAILABLE, code); 1208 if ("gatewayTimeout".equals(codeString)) 1209 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.GATEWAYTIMEOUT, code); 1210 if ("httpVersionNotSupported".equals(codeString)) 1211 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.HTTPVERSIONNOTSUPPORTED, code); 1212 throw new FHIRException("Unknown AssertionResponseTypes code '"+codeString+"'"); 1213 } 1214 public String toCode(AssertionResponseTypes code) { 1215 if (code == AssertionResponseTypes.NULL) 1216 return null; 1217 if (code == AssertionResponseTypes.CONTINUE) 1218 return "continue"; 1219 if (code == AssertionResponseTypes.SWITCHINGPROTOCOLS) 1220 return "switchingProtocols"; 1221 if (code == AssertionResponseTypes.OKAY) 1222 return "okay"; 1223 if (code == AssertionResponseTypes.CREATED) 1224 return "created"; 1225 if (code == AssertionResponseTypes.ACCEPTED) 1226 return "accepted"; 1227 if (code == AssertionResponseTypes.NONAUTHORITATIVEINFORMATION) 1228 return "nonAuthoritativeInformation"; 1229 if (code == AssertionResponseTypes.NOCONTENT) 1230 return "noContent"; 1231 if (code == AssertionResponseTypes.RESETCONTENT) 1232 return "resetContent"; 1233 if (code == AssertionResponseTypes.PARTIALCONTENT) 1234 return "partialContent"; 1235 if (code == AssertionResponseTypes.MULTIPLECHOICES) 1236 return "multipleChoices"; 1237 if (code == AssertionResponseTypes.MOVEDPERMANENTLY) 1238 return "movedPermanently"; 1239 if (code == AssertionResponseTypes.FOUND) 1240 return "found"; 1241 if (code == AssertionResponseTypes.SEEOTHER) 1242 return "seeOther"; 1243 if (code == AssertionResponseTypes.NOTMODIFIED) 1244 return "notModified"; 1245 if (code == AssertionResponseTypes.USEPROXY) 1246 return "useProxy"; 1247 if (code == AssertionResponseTypes.TEMPORARYREDIRECT) 1248 return "temporaryRedirect"; 1249 if (code == AssertionResponseTypes.PERMANENTREDIRECT) 1250 return "permanentRedirect"; 1251 if (code == AssertionResponseTypes.BADREQUEST) 1252 return "badRequest"; 1253 if (code == AssertionResponseTypes.UNAUTHORIZED) 1254 return "unauthorized"; 1255 if (code == AssertionResponseTypes.PAYMENTREQUIRED) 1256 return "paymentRequired"; 1257 if (code == AssertionResponseTypes.FORBIDDEN) 1258 return "forbidden"; 1259 if (code == AssertionResponseTypes.NOTFOUND) 1260 return "notFound"; 1261 if (code == AssertionResponseTypes.METHODNOTALLOWED) 1262 return "methodNotAllowed"; 1263 if (code == AssertionResponseTypes.NOTACCEPTABLE) 1264 return "notAcceptable"; 1265 if (code == AssertionResponseTypes.PROXYAUTHENTICATIONREQUIRED) 1266 return "proxyAuthenticationRequired"; 1267 if (code == AssertionResponseTypes.REQUESTTIMEOUT) 1268 return "requestTimeout"; 1269 if (code == AssertionResponseTypes.CONFLICT) 1270 return "conflict"; 1271 if (code == AssertionResponseTypes.GONE) 1272 return "gone"; 1273 if (code == AssertionResponseTypes.LENGTHREQUIRED) 1274 return "lengthRequired"; 1275 if (code == AssertionResponseTypes.PRECONDITIONFAILED) 1276 return "preconditionFailed"; 1277 if (code == AssertionResponseTypes.CONTENTTOOLARGE) 1278 return "contentTooLarge"; 1279 if (code == AssertionResponseTypes.URITOOLONG) 1280 return "uriTooLong"; 1281 if (code == AssertionResponseTypes.UNSUPPORTEDMEDIATYPE) 1282 return "unsupportedMediaType"; 1283 if (code == AssertionResponseTypes.RANGENOTSATISFIABLE) 1284 return "rangeNotSatisfiable"; 1285 if (code == AssertionResponseTypes.EXPECTATIONFAILED) 1286 return "expectationFailed"; 1287 if (code == AssertionResponseTypes.MISDIRECTEDREQUEST) 1288 return "misdirectedRequest"; 1289 if (code == AssertionResponseTypes.UNPROCESSABLECONTENT) 1290 return "unprocessableContent"; 1291 if (code == AssertionResponseTypes.UPGRADEREQUIRED) 1292 return "upgradeRequired"; 1293 if (code == AssertionResponseTypes.INTERNALSERVERERROR) 1294 return "internalServerError"; 1295 if (code == AssertionResponseTypes.NOTIMPLEMENTED) 1296 return "notImplemented"; 1297 if (code == AssertionResponseTypes.BADGATEWAY) 1298 return "badGateway"; 1299 if (code == AssertionResponseTypes.SERVICEUNAVAILABLE) 1300 return "serviceUnavailable"; 1301 if (code == AssertionResponseTypes.GATEWAYTIMEOUT) 1302 return "gatewayTimeout"; 1303 if (code == AssertionResponseTypes.HTTPVERSIONNOTSUPPORTED) 1304 return "httpVersionNotSupported"; 1305 return "?"; 1306 } 1307 public String toSystem(AssertionResponseTypes code) { 1308 return code.getSystem(); 1309 } 1310 } 1311 1312 public enum TestScriptRequestMethodCode { 1313 /** 1314 * HTTP DELETE operation. 1315 */ 1316 DELETE, 1317 /** 1318 * HTTP GET operation. 1319 */ 1320 GET, 1321 /** 1322 * HTTP OPTIONS operation. 1323 */ 1324 OPTIONS, 1325 /** 1326 * HTTP PATCH operation. 1327 */ 1328 PATCH, 1329 /** 1330 * HTTP POST operation. 1331 */ 1332 POST, 1333 /** 1334 * HTTP PUT operation. 1335 */ 1336 PUT, 1337 /** 1338 * HTTP HEAD operation. 1339 */ 1340 HEAD, 1341 /** 1342 * added to help the parsers with the generic types 1343 */ 1344 NULL; 1345 public static TestScriptRequestMethodCode fromCode(String codeString) throws FHIRException { 1346 if (codeString == null || "".equals(codeString)) 1347 return null; 1348 if ("delete".equals(codeString)) 1349 return DELETE; 1350 if ("get".equals(codeString)) 1351 return GET; 1352 if ("options".equals(codeString)) 1353 return OPTIONS; 1354 if ("patch".equals(codeString)) 1355 return PATCH; 1356 if ("post".equals(codeString)) 1357 return POST; 1358 if ("put".equals(codeString)) 1359 return PUT; 1360 if ("head".equals(codeString)) 1361 return HEAD; 1362 if (Configuration.isAcceptInvalidEnums()) 1363 return null; 1364 else 1365 throw new FHIRException("Unknown TestScriptRequestMethodCode code '"+codeString+"'"); 1366 } 1367 public String toCode() { 1368 switch (this) { 1369 case DELETE: return "delete"; 1370 case GET: return "get"; 1371 case OPTIONS: return "options"; 1372 case PATCH: return "patch"; 1373 case POST: return "post"; 1374 case PUT: return "put"; 1375 case HEAD: return "head"; 1376 case NULL: return null; 1377 default: return "?"; 1378 } 1379 } 1380 public String getSystem() { 1381 switch (this) { 1382 case DELETE: return "http://hl7.org/fhir/http-operations"; 1383 case GET: return "http://hl7.org/fhir/http-operations"; 1384 case OPTIONS: return "http://hl7.org/fhir/http-operations"; 1385 case PATCH: return "http://hl7.org/fhir/http-operations"; 1386 case POST: return "http://hl7.org/fhir/http-operations"; 1387 case PUT: return "http://hl7.org/fhir/http-operations"; 1388 case HEAD: return "http://hl7.org/fhir/http-operations"; 1389 case NULL: return null; 1390 default: return "?"; 1391 } 1392 } 1393 public String getDefinition() { 1394 switch (this) { 1395 case DELETE: return "HTTP DELETE operation."; 1396 case GET: return "HTTP GET operation."; 1397 case OPTIONS: return "HTTP OPTIONS operation."; 1398 case PATCH: return "HTTP PATCH operation."; 1399 case POST: return "HTTP POST operation."; 1400 case PUT: return "HTTP PUT operation."; 1401 case HEAD: return "HTTP HEAD operation."; 1402 case NULL: return null; 1403 default: return "?"; 1404 } 1405 } 1406 public String getDisplay() { 1407 switch (this) { 1408 case DELETE: return "DELETE"; 1409 case GET: return "GET"; 1410 case OPTIONS: return "OPTIONS"; 1411 case PATCH: return "PATCH"; 1412 case POST: return "POST"; 1413 case PUT: return "PUT"; 1414 case HEAD: return "HEAD"; 1415 case NULL: return null; 1416 default: return "?"; 1417 } 1418 } 1419 } 1420 1421 public static class TestScriptRequestMethodCodeEnumFactory implements EnumFactory<TestScriptRequestMethodCode> { 1422 public TestScriptRequestMethodCode fromCode(String codeString) throws IllegalArgumentException { 1423 if (codeString == null || "".equals(codeString)) 1424 if (codeString == null || "".equals(codeString)) 1425 return null; 1426 if ("delete".equals(codeString)) 1427 return TestScriptRequestMethodCode.DELETE; 1428 if ("get".equals(codeString)) 1429 return TestScriptRequestMethodCode.GET; 1430 if ("options".equals(codeString)) 1431 return TestScriptRequestMethodCode.OPTIONS; 1432 if ("patch".equals(codeString)) 1433 return TestScriptRequestMethodCode.PATCH; 1434 if ("post".equals(codeString)) 1435 return TestScriptRequestMethodCode.POST; 1436 if ("put".equals(codeString)) 1437 return TestScriptRequestMethodCode.PUT; 1438 if ("head".equals(codeString)) 1439 return TestScriptRequestMethodCode.HEAD; 1440 throw new IllegalArgumentException("Unknown TestScriptRequestMethodCode code '"+codeString+"'"); 1441 } 1442 public Enumeration<TestScriptRequestMethodCode> fromType(PrimitiveType<?> code) throws FHIRException { 1443 if (code == null) 1444 return null; 1445 if (code.isEmpty()) 1446 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.NULL, code); 1447 String codeString = ((PrimitiveType) code).asStringValue(); 1448 if (codeString == null || "".equals(codeString)) 1449 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.NULL, code); 1450 if ("delete".equals(codeString)) 1451 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.DELETE, code); 1452 if ("get".equals(codeString)) 1453 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.GET, code); 1454 if ("options".equals(codeString)) 1455 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.OPTIONS, code); 1456 if ("patch".equals(codeString)) 1457 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.PATCH, code); 1458 if ("post".equals(codeString)) 1459 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.POST, code); 1460 if ("put".equals(codeString)) 1461 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.PUT, code); 1462 if ("head".equals(codeString)) 1463 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.HEAD, code); 1464 throw new FHIRException("Unknown TestScriptRequestMethodCode code '"+codeString+"'"); 1465 } 1466 public String toCode(TestScriptRequestMethodCode code) { 1467 if (code == TestScriptRequestMethodCode.NULL) 1468 return null; 1469 if (code == TestScriptRequestMethodCode.DELETE) 1470 return "delete"; 1471 if (code == TestScriptRequestMethodCode.GET) 1472 return "get"; 1473 if (code == TestScriptRequestMethodCode.OPTIONS) 1474 return "options"; 1475 if (code == TestScriptRequestMethodCode.PATCH) 1476 return "patch"; 1477 if (code == TestScriptRequestMethodCode.POST) 1478 return "post"; 1479 if (code == TestScriptRequestMethodCode.PUT) 1480 return "put"; 1481 if (code == TestScriptRequestMethodCode.HEAD) 1482 return "head"; 1483 return "?"; 1484 } 1485 public String toSystem(TestScriptRequestMethodCode code) { 1486 return code.getSystem(); 1487 } 1488 } 1489 1490 @Block() 1491 public static class TestScriptOriginComponent extends BackboneElement implements IBaseBackboneElement { 1492 /** 1493 * Abstract name given to an origin server in this test script. The name is provided as a number starting at 1. 1494 */ 1495 @Child(name = "index", type = {IntegerType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1496 @Description(shortDefinition="The index of the abstract origin server starting at 1", formalDefinition="Abstract name given to an origin server in this test script. The name is provided as a number starting at 1." ) 1497 protected IntegerType index; 1498 1499 /** 1500 * The type of origin profile the test system supports. 1501 */ 1502 @Child(name = "profile", type = {Coding.class}, order=2, min=1, max=1, modifier=false, summary=false) 1503 @Description(shortDefinition="FHIR-Client | FHIR-SDC-FormFiller", formalDefinition="The type of origin profile the test system supports." ) 1504 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/testscript-profile-origin-types") 1505 protected Coding profile; 1506 1507 /** 1508 * The explicit url path of the origin server used in this test script. 1509 */ 1510 @Child(name = "url", type = {UrlType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1511 @Description(shortDefinition="The url path of the origin server", formalDefinition="The explicit url path of the origin server used in this test script." ) 1512 protected UrlType url; 1513 1514 private static final long serialVersionUID = -188072923L; 1515 1516 /** 1517 * Constructor 1518 */ 1519 public TestScriptOriginComponent() { 1520 super(); 1521 } 1522 1523 /** 1524 * Constructor 1525 */ 1526 public TestScriptOriginComponent(int index, Coding profile) { 1527 super(); 1528 this.setIndex(index); 1529 this.setProfile(profile); 1530 } 1531 1532 /** 1533 * @return {@link #index} (Abstract name given to an origin server in this test script. The name is provided as a number starting at 1.). This is the underlying object with id, value and extensions. The accessor "getIndex" gives direct access to the value 1534 */ 1535 public IntegerType getIndexElement() { 1536 if (this.index == null) 1537 if (Configuration.errorOnAutoCreate()) 1538 throw new Error("Attempt to auto-create TestScriptOriginComponent.index"); 1539 else if (Configuration.doAutoCreate()) 1540 this.index = new IntegerType(); // bb 1541 return this.index; 1542 } 1543 1544 public boolean hasIndexElement() { 1545 return this.index != null && !this.index.isEmpty(); 1546 } 1547 1548 public boolean hasIndex() { 1549 return this.index != null && !this.index.isEmpty(); 1550 } 1551 1552 /** 1553 * @param value {@link #index} (Abstract name given to an origin server in this test script. The name is provided as a number starting at 1.). This is the underlying object with id, value and extensions. The accessor "getIndex" gives direct access to the value 1554 */ 1555 public TestScriptOriginComponent setIndexElement(IntegerType value) { 1556 this.index = value; 1557 return this; 1558 } 1559 1560 /** 1561 * @return Abstract name given to an origin server in this test script. The name is provided as a number starting at 1. 1562 */ 1563 public int getIndex() { 1564 return this.index == null || this.index.isEmpty() ? 0 : this.index.getValue(); 1565 } 1566 1567 /** 1568 * @param value Abstract name given to an origin server in this test script. The name is provided as a number starting at 1. 1569 */ 1570 public TestScriptOriginComponent setIndex(int value) { 1571 if (this.index == null) 1572 this.index = new IntegerType(); 1573 this.index.setValue(value); 1574 return this; 1575 } 1576 1577 /** 1578 * @return {@link #profile} (The type of origin profile the test system supports.) 1579 */ 1580 public Coding getProfile() { 1581 if (this.profile == null) 1582 if (Configuration.errorOnAutoCreate()) 1583 throw new Error("Attempt to auto-create TestScriptOriginComponent.profile"); 1584 else if (Configuration.doAutoCreate()) 1585 this.profile = new Coding(); // cc 1586 return this.profile; 1587 } 1588 1589 public boolean hasProfile() { 1590 return this.profile != null && !this.profile.isEmpty(); 1591 } 1592 1593 /** 1594 * @param value {@link #profile} (The type of origin profile the test system supports.) 1595 */ 1596 public TestScriptOriginComponent setProfile(Coding value) { 1597 this.profile = value; 1598 return this; 1599 } 1600 1601 /** 1602 * @return {@link #url} (The explicit url path of the origin server used in this test script.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1603 */ 1604 public UrlType getUrlElement() { 1605 if (this.url == null) 1606 if (Configuration.errorOnAutoCreate()) 1607 throw new Error("Attempt to auto-create TestScriptOriginComponent.url"); 1608 else if (Configuration.doAutoCreate()) 1609 this.url = new UrlType(); // bb 1610 return this.url; 1611 } 1612 1613 public boolean hasUrlElement() { 1614 return this.url != null && !this.url.isEmpty(); 1615 } 1616 1617 public boolean hasUrl() { 1618 return this.url != null && !this.url.isEmpty(); 1619 } 1620 1621 /** 1622 * @param value {@link #url} (The explicit url path of the origin server used in this test script.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1623 */ 1624 public TestScriptOriginComponent setUrlElement(UrlType value) { 1625 this.url = value; 1626 return this; 1627 } 1628 1629 /** 1630 * @return The explicit url path of the origin server used in this test script. 1631 */ 1632 public String getUrl() { 1633 return this.url == null ? null : this.url.getValue(); 1634 } 1635 1636 /** 1637 * @param value The explicit url path of the origin server used in this test script. 1638 */ 1639 public TestScriptOriginComponent setUrl(String value) { 1640 if (Utilities.noString(value)) 1641 this.url = null; 1642 else { 1643 if (this.url == null) 1644 this.url = new UrlType(); 1645 this.url.setValue(value); 1646 } 1647 return this; 1648 } 1649 1650 protected void listChildren(List<Property> children) { 1651 super.listChildren(children); 1652 children.add(new Property("index", "integer", "Abstract name given to an origin server in this test script. The name is provided as a number starting at 1.", 0, 1, index)); 1653 children.add(new Property("profile", "Coding", "The type of origin profile the test system supports.", 0, 1, profile)); 1654 children.add(new Property("url", "url", "The explicit url path of the origin server used in this test script.", 0, 1, url)); 1655 } 1656 1657 @Override 1658 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1659 switch (_hash) { 1660 case 100346066: /*index*/ return new Property("index", "integer", "Abstract name given to an origin server in this test script. The name is provided as a number starting at 1.", 0, 1, index); 1661 case -309425751: /*profile*/ return new Property("profile", "Coding", "The type of origin profile the test system supports.", 0, 1, profile); 1662 case 116079: /*url*/ return new Property("url", "url", "The explicit url path of the origin server used in this test script.", 0, 1, url); 1663 default: return super.getNamedProperty(_hash, _name, _checkValid); 1664 } 1665 1666 } 1667 1668 @Override 1669 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1670 switch (hash) { 1671 case 100346066: /*index*/ return this.index == null ? new Base[0] : new Base[] {this.index}; // IntegerType 1672 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // Coding 1673 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UrlType 1674 default: return super.getProperty(hash, name, checkValid); 1675 } 1676 1677 } 1678 1679 @Override 1680 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1681 switch (hash) { 1682 case 100346066: // index 1683 this.index = TypeConvertor.castToInteger(value); // IntegerType 1684 return value; 1685 case -309425751: // profile 1686 this.profile = TypeConvertor.castToCoding(value); // Coding 1687 return value; 1688 case 116079: // url 1689 this.url = TypeConvertor.castToUrl(value); // UrlType 1690 return value; 1691 default: return super.setProperty(hash, name, value); 1692 } 1693 1694 } 1695 1696 @Override 1697 public Base setProperty(String name, Base value) throws FHIRException { 1698 if (name.equals("index")) { 1699 this.index = TypeConvertor.castToInteger(value); // IntegerType 1700 } else if (name.equals("profile")) { 1701 this.profile = TypeConvertor.castToCoding(value); // Coding 1702 } else if (name.equals("url")) { 1703 this.url = TypeConvertor.castToUrl(value); // UrlType 1704 } else 1705 return super.setProperty(name, value); 1706 return value; 1707 } 1708 1709 @Override 1710 public void removeChild(String name, Base value) throws FHIRException { 1711 if (name.equals("index")) { 1712 this.index = null; 1713 } else if (name.equals("profile")) { 1714 this.profile = null; 1715 } else if (name.equals("url")) { 1716 this.url = null; 1717 } else 1718 super.removeChild(name, value); 1719 1720 } 1721 1722 @Override 1723 public Base makeProperty(int hash, String name) throws FHIRException { 1724 switch (hash) { 1725 case 100346066: return getIndexElement(); 1726 case -309425751: return getProfile(); 1727 case 116079: return getUrlElement(); 1728 default: return super.makeProperty(hash, name); 1729 } 1730 1731 } 1732 1733 @Override 1734 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1735 switch (hash) { 1736 case 100346066: /*index*/ return new String[] {"integer"}; 1737 case -309425751: /*profile*/ return new String[] {"Coding"}; 1738 case 116079: /*url*/ return new String[] {"url"}; 1739 default: return super.getTypesForProperty(hash, name); 1740 } 1741 1742 } 1743 1744 @Override 1745 public Base addChild(String name) throws FHIRException { 1746 if (name.equals("index")) { 1747 throw new FHIRException("Cannot call addChild on a singleton property TestScript.origin.index"); 1748 } 1749 else if (name.equals("profile")) { 1750 this.profile = new Coding(); 1751 return this.profile; 1752 } 1753 else if (name.equals("url")) { 1754 throw new FHIRException("Cannot call addChild on a singleton property TestScript.origin.url"); 1755 } 1756 else 1757 return super.addChild(name); 1758 } 1759 1760 public TestScriptOriginComponent copy() { 1761 TestScriptOriginComponent dst = new TestScriptOriginComponent(); 1762 copyValues(dst); 1763 return dst; 1764 } 1765 1766 public void copyValues(TestScriptOriginComponent dst) { 1767 super.copyValues(dst); 1768 dst.index = index == null ? null : index.copy(); 1769 dst.profile = profile == null ? null : profile.copy(); 1770 dst.url = url == null ? null : url.copy(); 1771 } 1772 1773 @Override 1774 public boolean equalsDeep(Base other_) { 1775 if (!super.equalsDeep(other_)) 1776 return false; 1777 if (!(other_ instanceof TestScriptOriginComponent)) 1778 return false; 1779 TestScriptOriginComponent o = (TestScriptOriginComponent) other_; 1780 return compareDeep(index, o.index, true) && compareDeep(profile, o.profile, true) && compareDeep(url, o.url, true) 1781 ; 1782 } 1783 1784 @Override 1785 public boolean equalsShallow(Base other_) { 1786 if (!super.equalsShallow(other_)) 1787 return false; 1788 if (!(other_ instanceof TestScriptOriginComponent)) 1789 return false; 1790 TestScriptOriginComponent o = (TestScriptOriginComponent) other_; 1791 return compareValues(index, o.index, true) && compareValues(url, o.url, true); 1792 } 1793 1794 public boolean isEmpty() { 1795 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(index, profile, url); 1796 } 1797 1798 public String fhirType() { 1799 return "TestScript.origin"; 1800 1801 } 1802 1803 } 1804 1805 @Block() 1806 public static class TestScriptDestinationComponent extends BackboneElement implements IBaseBackboneElement { 1807 /** 1808 * Abstract name given to a destination server in this test script. The name is provided as a number starting at 1. 1809 */ 1810 @Child(name = "index", type = {IntegerType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1811 @Description(shortDefinition="The index of the abstract destination server starting at 1", formalDefinition="Abstract name given to a destination server in this test script. The name is provided as a number starting at 1." ) 1812 protected IntegerType index; 1813 1814 /** 1815 * The type of destination profile the test system supports. 1816 */ 1817 @Child(name = "profile", type = {Coding.class}, order=2, min=1, max=1, modifier=false, summary=false) 1818 @Description(shortDefinition="FHIR-Server | FHIR-SDC-FormManager | FHIR-SDC-FormReceiver | FHIR-SDC-FormProcessor", formalDefinition="The type of destination profile the test system supports." ) 1819 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/testscript-profile-destination-types") 1820 protected Coding profile; 1821 1822 /** 1823 * The explicit url path of the destination server used in this test script. 1824 */ 1825 @Child(name = "url", type = {UrlType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1826 @Description(shortDefinition="The url path of the destination server", formalDefinition="The explicit url path of the destination server used in this test script." ) 1827 protected UrlType url; 1828 1829 private static final long serialVersionUID = -188072923L; 1830 1831 /** 1832 * Constructor 1833 */ 1834 public TestScriptDestinationComponent() { 1835 super(); 1836 } 1837 1838 /** 1839 * Constructor 1840 */ 1841 public TestScriptDestinationComponent(int index, Coding profile) { 1842 super(); 1843 this.setIndex(index); 1844 this.setProfile(profile); 1845 } 1846 1847 /** 1848 * @return {@link #index} (Abstract name given to a destination server in this test script. The name is provided as a number starting at 1.). This is the underlying object with id, value and extensions. The accessor "getIndex" gives direct access to the value 1849 */ 1850 public IntegerType getIndexElement() { 1851 if (this.index == null) 1852 if (Configuration.errorOnAutoCreate()) 1853 throw new Error("Attempt to auto-create TestScriptDestinationComponent.index"); 1854 else if (Configuration.doAutoCreate()) 1855 this.index = new IntegerType(); // bb 1856 return this.index; 1857 } 1858 1859 public boolean hasIndexElement() { 1860 return this.index != null && !this.index.isEmpty(); 1861 } 1862 1863 public boolean hasIndex() { 1864 return this.index != null && !this.index.isEmpty(); 1865 } 1866 1867 /** 1868 * @param value {@link #index} (Abstract name given to a destination server in this test script. The name is provided as a number starting at 1.). This is the underlying object with id, value and extensions. The accessor "getIndex" gives direct access to the value 1869 */ 1870 public TestScriptDestinationComponent setIndexElement(IntegerType value) { 1871 this.index = value; 1872 return this; 1873 } 1874 1875 /** 1876 * @return Abstract name given to a destination server in this test script. The name is provided as a number starting at 1. 1877 */ 1878 public int getIndex() { 1879 return this.index == null || this.index.isEmpty() ? 0 : this.index.getValue(); 1880 } 1881 1882 /** 1883 * @param value Abstract name given to a destination server in this test script. The name is provided as a number starting at 1. 1884 */ 1885 public TestScriptDestinationComponent setIndex(int value) { 1886 if (this.index == null) 1887 this.index = new IntegerType(); 1888 this.index.setValue(value); 1889 return this; 1890 } 1891 1892 /** 1893 * @return {@link #profile} (The type of destination profile the test system supports.) 1894 */ 1895 public Coding getProfile() { 1896 if (this.profile == null) 1897 if (Configuration.errorOnAutoCreate()) 1898 throw new Error("Attempt to auto-create TestScriptDestinationComponent.profile"); 1899 else if (Configuration.doAutoCreate()) 1900 this.profile = new Coding(); // cc 1901 return this.profile; 1902 } 1903 1904 public boolean hasProfile() { 1905 return this.profile != null && !this.profile.isEmpty(); 1906 } 1907 1908 /** 1909 * @param value {@link #profile} (The type of destination profile the test system supports.) 1910 */ 1911 public TestScriptDestinationComponent setProfile(Coding value) { 1912 this.profile = value; 1913 return this; 1914 } 1915 1916 /** 1917 * @return {@link #url} (The explicit url path of the destination server used in this test script.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1918 */ 1919 public UrlType getUrlElement() { 1920 if (this.url == null) 1921 if (Configuration.errorOnAutoCreate()) 1922 throw new Error("Attempt to auto-create TestScriptDestinationComponent.url"); 1923 else if (Configuration.doAutoCreate()) 1924 this.url = new UrlType(); // bb 1925 return this.url; 1926 } 1927 1928 public boolean hasUrlElement() { 1929 return this.url != null && !this.url.isEmpty(); 1930 } 1931 1932 public boolean hasUrl() { 1933 return this.url != null && !this.url.isEmpty(); 1934 } 1935 1936 /** 1937 * @param value {@link #url} (The explicit url path of the destination server used in this test script.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1938 */ 1939 public TestScriptDestinationComponent setUrlElement(UrlType value) { 1940 this.url = value; 1941 return this; 1942 } 1943 1944 /** 1945 * @return The explicit url path of the destination server used in this test script. 1946 */ 1947 public String getUrl() { 1948 return this.url == null ? null : this.url.getValue(); 1949 } 1950 1951 /** 1952 * @param value The explicit url path of the destination server used in this test script. 1953 */ 1954 public TestScriptDestinationComponent setUrl(String value) { 1955 if (Utilities.noString(value)) 1956 this.url = null; 1957 else { 1958 if (this.url == null) 1959 this.url = new UrlType(); 1960 this.url.setValue(value); 1961 } 1962 return this; 1963 } 1964 1965 protected void listChildren(List<Property> children) { 1966 super.listChildren(children); 1967 children.add(new Property("index", "integer", "Abstract name given to a destination server in this test script. The name is provided as a number starting at 1.", 0, 1, index)); 1968 children.add(new Property("profile", "Coding", "The type of destination profile the test system supports.", 0, 1, profile)); 1969 children.add(new Property("url", "url", "The explicit url path of the destination server used in this test script.", 0, 1, url)); 1970 } 1971 1972 @Override 1973 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1974 switch (_hash) { 1975 case 100346066: /*index*/ return new Property("index", "integer", "Abstract name given to a destination server in this test script. The name is provided as a number starting at 1.", 0, 1, index); 1976 case -309425751: /*profile*/ return new Property("profile", "Coding", "The type of destination profile the test system supports.", 0, 1, profile); 1977 case 116079: /*url*/ return new Property("url", "url", "The explicit url path of the destination server used in this test script.", 0, 1, url); 1978 default: return super.getNamedProperty(_hash, _name, _checkValid); 1979 } 1980 1981 } 1982 1983 @Override 1984 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1985 switch (hash) { 1986 case 100346066: /*index*/ return this.index == null ? new Base[0] : new Base[] {this.index}; // IntegerType 1987 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // Coding 1988 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UrlType 1989 default: return super.getProperty(hash, name, checkValid); 1990 } 1991 1992 } 1993 1994 @Override 1995 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1996 switch (hash) { 1997 case 100346066: // index 1998 this.index = TypeConvertor.castToInteger(value); // IntegerType 1999 return value; 2000 case -309425751: // profile 2001 this.profile = TypeConvertor.castToCoding(value); // Coding 2002 return value; 2003 case 116079: // url 2004 this.url = TypeConvertor.castToUrl(value); // UrlType 2005 return value; 2006 default: return super.setProperty(hash, name, value); 2007 } 2008 2009 } 2010 2011 @Override 2012 public Base setProperty(String name, Base value) throws FHIRException { 2013 if (name.equals("index")) { 2014 this.index = TypeConvertor.castToInteger(value); // IntegerType 2015 } else if (name.equals("profile")) { 2016 this.profile = TypeConvertor.castToCoding(value); // Coding 2017 } else if (name.equals("url")) { 2018 this.url = TypeConvertor.castToUrl(value); // UrlType 2019 } else 2020 return super.setProperty(name, value); 2021 return value; 2022 } 2023 2024 @Override 2025 public void removeChild(String name, Base value) throws FHIRException { 2026 if (name.equals("index")) { 2027 this.index = null; 2028 } else if (name.equals("profile")) { 2029 this.profile = null; 2030 } else if (name.equals("url")) { 2031 this.url = null; 2032 } else 2033 super.removeChild(name, value); 2034 2035 } 2036 2037 @Override 2038 public Base makeProperty(int hash, String name) throws FHIRException { 2039 switch (hash) { 2040 case 100346066: return getIndexElement(); 2041 case -309425751: return getProfile(); 2042 case 116079: return getUrlElement(); 2043 default: return super.makeProperty(hash, name); 2044 } 2045 2046 } 2047 2048 @Override 2049 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2050 switch (hash) { 2051 case 100346066: /*index*/ return new String[] {"integer"}; 2052 case -309425751: /*profile*/ return new String[] {"Coding"}; 2053 case 116079: /*url*/ return new String[] {"url"}; 2054 default: return super.getTypesForProperty(hash, name); 2055 } 2056 2057 } 2058 2059 @Override 2060 public Base addChild(String name) throws FHIRException { 2061 if (name.equals("index")) { 2062 throw new FHIRException("Cannot call addChild on a singleton property TestScript.destination.index"); 2063 } 2064 else if (name.equals("profile")) { 2065 this.profile = new Coding(); 2066 return this.profile; 2067 } 2068 else if (name.equals("url")) { 2069 throw new FHIRException("Cannot call addChild on a singleton property TestScript.destination.url"); 2070 } 2071 else 2072 return super.addChild(name); 2073 } 2074 2075 public TestScriptDestinationComponent copy() { 2076 TestScriptDestinationComponent dst = new TestScriptDestinationComponent(); 2077 copyValues(dst); 2078 return dst; 2079 } 2080 2081 public void copyValues(TestScriptDestinationComponent dst) { 2082 super.copyValues(dst); 2083 dst.index = index == null ? null : index.copy(); 2084 dst.profile = profile == null ? null : profile.copy(); 2085 dst.url = url == null ? null : url.copy(); 2086 } 2087 2088 @Override 2089 public boolean equalsDeep(Base other_) { 2090 if (!super.equalsDeep(other_)) 2091 return false; 2092 if (!(other_ instanceof TestScriptDestinationComponent)) 2093 return false; 2094 TestScriptDestinationComponent o = (TestScriptDestinationComponent) other_; 2095 return compareDeep(index, o.index, true) && compareDeep(profile, o.profile, true) && compareDeep(url, o.url, true) 2096 ; 2097 } 2098 2099 @Override 2100 public boolean equalsShallow(Base other_) { 2101 if (!super.equalsShallow(other_)) 2102 return false; 2103 if (!(other_ instanceof TestScriptDestinationComponent)) 2104 return false; 2105 TestScriptDestinationComponent o = (TestScriptDestinationComponent) other_; 2106 return compareValues(index, o.index, true) && compareValues(url, o.url, true); 2107 } 2108 2109 public boolean isEmpty() { 2110 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(index, profile, url); 2111 } 2112 2113 public String fhirType() { 2114 return "TestScript.destination"; 2115 2116 } 2117 2118 } 2119 2120 @Block() 2121 public static class TestScriptMetadataComponent extends BackboneElement implements IBaseBackboneElement { 2122 /** 2123 * A link to the FHIR specification that this test is covering. 2124 */ 2125 @Child(name = "link", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2126 @Description(shortDefinition="Links to the FHIR specification", formalDefinition="A link to the FHIR specification that this test is covering." ) 2127 protected List<TestScriptMetadataLinkComponent> link; 2128 2129 /** 2130 * Capabilities that must exist and are assumed to function correctly on the FHIR server being tested. 2131 */ 2132 @Child(name = "capability", type = {}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2133 @Description(shortDefinition="Capabilities that are assumed to function correctly on the FHIR server being tested", formalDefinition="Capabilities that must exist and are assumed to function correctly on the FHIR server being tested." ) 2134 protected List<TestScriptMetadataCapabilityComponent> capability; 2135 2136 private static final long serialVersionUID = 745183328L; 2137 2138 /** 2139 * Constructor 2140 */ 2141 public TestScriptMetadataComponent() { 2142 super(); 2143 } 2144 2145 /** 2146 * Constructor 2147 */ 2148 public TestScriptMetadataComponent(TestScriptMetadataCapabilityComponent capability) { 2149 super(); 2150 this.addCapability(capability); 2151 } 2152 2153 /** 2154 * @return {@link #link} (A link to the FHIR specification that this test is covering.) 2155 */ 2156 public List<TestScriptMetadataLinkComponent> getLink() { 2157 if (this.link == null) 2158 this.link = new ArrayList<TestScriptMetadataLinkComponent>(); 2159 return this.link; 2160 } 2161 2162 /** 2163 * @return Returns a reference to <code>this</code> for easy method chaining 2164 */ 2165 public TestScriptMetadataComponent setLink(List<TestScriptMetadataLinkComponent> theLink) { 2166 this.link = theLink; 2167 return this; 2168 } 2169 2170 public boolean hasLink() { 2171 if (this.link == null) 2172 return false; 2173 for (TestScriptMetadataLinkComponent item : this.link) 2174 if (!item.isEmpty()) 2175 return true; 2176 return false; 2177 } 2178 2179 public TestScriptMetadataLinkComponent addLink() { //3 2180 TestScriptMetadataLinkComponent t = new TestScriptMetadataLinkComponent(); 2181 if (this.link == null) 2182 this.link = new ArrayList<TestScriptMetadataLinkComponent>(); 2183 this.link.add(t); 2184 return t; 2185 } 2186 2187 public TestScriptMetadataComponent addLink(TestScriptMetadataLinkComponent t) { //3 2188 if (t == null) 2189 return this; 2190 if (this.link == null) 2191 this.link = new ArrayList<TestScriptMetadataLinkComponent>(); 2192 this.link.add(t); 2193 return this; 2194 } 2195 2196 /** 2197 * @return The first repetition of repeating field {@link #link}, creating it if it does not already exist {3} 2198 */ 2199 public TestScriptMetadataLinkComponent getLinkFirstRep() { 2200 if (getLink().isEmpty()) { 2201 addLink(); 2202 } 2203 return getLink().get(0); 2204 } 2205 2206 /** 2207 * @return {@link #capability} (Capabilities that must exist and are assumed to function correctly on the FHIR server being tested.) 2208 */ 2209 public List<TestScriptMetadataCapabilityComponent> getCapability() { 2210 if (this.capability == null) 2211 this.capability = new ArrayList<TestScriptMetadataCapabilityComponent>(); 2212 return this.capability; 2213 } 2214 2215 /** 2216 * @return Returns a reference to <code>this</code> for easy method chaining 2217 */ 2218 public TestScriptMetadataComponent setCapability(List<TestScriptMetadataCapabilityComponent> theCapability) { 2219 this.capability = theCapability; 2220 return this; 2221 } 2222 2223 public boolean hasCapability() { 2224 if (this.capability == null) 2225 return false; 2226 for (TestScriptMetadataCapabilityComponent item : this.capability) 2227 if (!item.isEmpty()) 2228 return true; 2229 return false; 2230 } 2231 2232 public TestScriptMetadataCapabilityComponent addCapability() { //3 2233 TestScriptMetadataCapabilityComponent t = new TestScriptMetadataCapabilityComponent(); 2234 if (this.capability == null) 2235 this.capability = new ArrayList<TestScriptMetadataCapabilityComponent>(); 2236 this.capability.add(t); 2237 return t; 2238 } 2239 2240 public TestScriptMetadataComponent addCapability(TestScriptMetadataCapabilityComponent t) { //3 2241 if (t == null) 2242 return this; 2243 if (this.capability == null) 2244 this.capability = new ArrayList<TestScriptMetadataCapabilityComponent>(); 2245 this.capability.add(t); 2246 return this; 2247 } 2248 2249 /** 2250 * @return The first repetition of repeating field {@link #capability}, creating it if it does not already exist {3} 2251 */ 2252 public TestScriptMetadataCapabilityComponent getCapabilityFirstRep() { 2253 if (getCapability().isEmpty()) { 2254 addCapability(); 2255 } 2256 return getCapability().get(0); 2257 } 2258 2259 protected void listChildren(List<Property> children) { 2260 super.listChildren(children); 2261 children.add(new Property("link", "", "A link to the FHIR specification that this test is covering.", 0, java.lang.Integer.MAX_VALUE, link)); 2262 children.add(new Property("capability", "", "Capabilities that must exist and are assumed to function correctly on the FHIR server being tested.", 0, java.lang.Integer.MAX_VALUE, capability)); 2263 } 2264 2265 @Override 2266 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2267 switch (_hash) { 2268 case 3321850: /*link*/ return new Property("link", "", "A link to the FHIR specification that this test is covering.", 0, java.lang.Integer.MAX_VALUE, link); 2269 case -783669992: /*capability*/ return new Property("capability", "", "Capabilities that must exist and are assumed to function correctly on the FHIR server being tested.", 0, java.lang.Integer.MAX_VALUE, capability); 2270 default: return super.getNamedProperty(_hash, _name, _checkValid); 2271 } 2272 2273 } 2274 2275 @Override 2276 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2277 switch (hash) { 2278 case 3321850: /*link*/ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // TestScriptMetadataLinkComponent 2279 case -783669992: /*capability*/ return this.capability == null ? new Base[0] : this.capability.toArray(new Base[this.capability.size()]); // TestScriptMetadataCapabilityComponent 2280 default: return super.getProperty(hash, name, checkValid); 2281 } 2282 2283 } 2284 2285 @Override 2286 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2287 switch (hash) { 2288 case 3321850: // link 2289 this.getLink().add((TestScriptMetadataLinkComponent) value); // TestScriptMetadataLinkComponent 2290 return value; 2291 case -783669992: // capability 2292 this.getCapability().add((TestScriptMetadataCapabilityComponent) value); // TestScriptMetadataCapabilityComponent 2293 return value; 2294 default: return super.setProperty(hash, name, value); 2295 } 2296 2297 } 2298 2299 @Override 2300 public Base setProperty(String name, Base value) throws FHIRException { 2301 if (name.equals("link")) { 2302 this.getLink().add((TestScriptMetadataLinkComponent) value); 2303 } else if (name.equals("capability")) { 2304 this.getCapability().add((TestScriptMetadataCapabilityComponent) value); 2305 } else 2306 return super.setProperty(name, value); 2307 return value; 2308 } 2309 2310 @Override 2311 public void removeChild(String name, Base value) throws FHIRException { 2312 if (name.equals("link")) { 2313 this.getLink().remove((TestScriptMetadataLinkComponent) value); 2314 } else if (name.equals("capability")) { 2315 this.getCapability().remove((TestScriptMetadataCapabilityComponent) value); 2316 } else 2317 super.removeChild(name, value); 2318 2319 } 2320 2321 @Override 2322 public Base makeProperty(int hash, String name) throws FHIRException { 2323 switch (hash) { 2324 case 3321850: return addLink(); 2325 case -783669992: return addCapability(); 2326 default: return super.makeProperty(hash, name); 2327 } 2328 2329 } 2330 2331 @Override 2332 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2333 switch (hash) { 2334 case 3321850: /*link*/ return new String[] {}; 2335 case -783669992: /*capability*/ return new String[] {}; 2336 default: return super.getTypesForProperty(hash, name); 2337 } 2338 2339 } 2340 2341 @Override 2342 public Base addChild(String name) throws FHIRException { 2343 if (name.equals("link")) { 2344 return addLink(); 2345 } 2346 else if (name.equals("capability")) { 2347 return addCapability(); 2348 } 2349 else 2350 return super.addChild(name); 2351 } 2352 2353 public TestScriptMetadataComponent copy() { 2354 TestScriptMetadataComponent dst = new TestScriptMetadataComponent(); 2355 copyValues(dst); 2356 return dst; 2357 } 2358 2359 public void copyValues(TestScriptMetadataComponent dst) { 2360 super.copyValues(dst); 2361 if (link != null) { 2362 dst.link = new ArrayList<TestScriptMetadataLinkComponent>(); 2363 for (TestScriptMetadataLinkComponent i : link) 2364 dst.link.add(i.copy()); 2365 }; 2366 if (capability != null) { 2367 dst.capability = new ArrayList<TestScriptMetadataCapabilityComponent>(); 2368 for (TestScriptMetadataCapabilityComponent i : capability) 2369 dst.capability.add(i.copy()); 2370 }; 2371 } 2372 2373 @Override 2374 public boolean equalsDeep(Base other_) { 2375 if (!super.equalsDeep(other_)) 2376 return false; 2377 if (!(other_ instanceof TestScriptMetadataComponent)) 2378 return false; 2379 TestScriptMetadataComponent o = (TestScriptMetadataComponent) other_; 2380 return compareDeep(link, o.link, true) && compareDeep(capability, o.capability, true); 2381 } 2382 2383 @Override 2384 public boolean equalsShallow(Base other_) { 2385 if (!super.equalsShallow(other_)) 2386 return false; 2387 if (!(other_ instanceof TestScriptMetadataComponent)) 2388 return false; 2389 TestScriptMetadataComponent o = (TestScriptMetadataComponent) other_; 2390 return true; 2391 } 2392 2393 public boolean isEmpty() { 2394 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(link, capability); 2395 } 2396 2397 public String fhirType() { 2398 return "TestScript.metadata"; 2399 2400 } 2401 2402 } 2403 2404 @Block() 2405 public static class TestScriptMetadataLinkComponent extends BackboneElement implements IBaseBackboneElement { 2406 /** 2407 * URL to a particular requirement or feature within the FHIR specification. 2408 */ 2409 @Child(name = "url", type = {UriType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2410 @Description(shortDefinition="URL to the specification", formalDefinition="URL to a particular requirement or feature within the FHIR specification." ) 2411 protected UriType url; 2412 2413 /** 2414 * Short description of the link. 2415 */ 2416 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2417 @Description(shortDefinition="Short description", formalDefinition="Short description of the link." ) 2418 protected StringType description; 2419 2420 private static final long serialVersionUID = 213372298L; 2421 2422 /** 2423 * Constructor 2424 */ 2425 public TestScriptMetadataLinkComponent() { 2426 super(); 2427 } 2428 2429 /** 2430 * Constructor 2431 */ 2432 public TestScriptMetadataLinkComponent(String url) { 2433 super(); 2434 this.setUrl(url); 2435 } 2436 2437 /** 2438 * @return {@link #url} (URL to a particular requirement or feature within the FHIR specification.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2439 */ 2440 public UriType getUrlElement() { 2441 if (this.url == null) 2442 if (Configuration.errorOnAutoCreate()) 2443 throw new Error("Attempt to auto-create TestScriptMetadataLinkComponent.url"); 2444 else if (Configuration.doAutoCreate()) 2445 this.url = new UriType(); // bb 2446 return this.url; 2447 } 2448 2449 public boolean hasUrlElement() { 2450 return this.url != null && !this.url.isEmpty(); 2451 } 2452 2453 public boolean hasUrl() { 2454 return this.url != null && !this.url.isEmpty(); 2455 } 2456 2457 /** 2458 * @param value {@link #url} (URL to a particular requirement or feature within the FHIR specification.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2459 */ 2460 public TestScriptMetadataLinkComponent setUrlElement(UriType value) { 2461 this.url = value; 2462 return this; 2463 } 2464 2465 /** 2466 * @return URL to a particular requirement or feature within the FHIR specification. 2467 */ 2468 public String getUrl() { 2469 return this.url == null ? null : this.url.getValue(); 2470 } 2471 2472 /** 2473 * @param value URL to a particular requirement or feature within the FHIR specification. 2474 */ 2475 public TestScriptMetadataLinkComponent setUrl(String value) { 2476 if (this.url == null) 2477 this.url = new UriType(); 2478 this.url.setValue(value); 2479 return this; 2480 } 2481 2482 /** 2483 * @return {@link #description} (Short description of the link.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2484 */ 2485 public StringType getDescriptionElement() { 2486 if (this.description == null) 2487 if (Configuration.errorOnAutoCreate()) 2488 throw new Error("Attempt to auto-create TestScriptMetadataLinkComponent.description"); 2489 else if (Configuration.doAutoCreate()) 2490 this.description = new StringType(); // bb 2491 return this.description; 2492 } 2493 2494 public boolean hasDescriptionElement() { 2495 return this.description != null && !this.description.isEmpty(); 2496 } 2497 2498 public boolean hasDescription() { 2499 return this.description != null && !this.description.isEmpty(); 2500 } 2501 2502 /** 2503 * @param value {@link #description} (Short description of the link.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2504 */ 2505 public TestScriptMetadataLinkComponent setDescriptionElement(StringType value) { 2506 this.description = value; 2507 return this; 2508 } 2509 2510 /** 2511 * @return Short description of the link. 2512 */ 2513 public String getDescription() { 2514 return this.description == null ? null : this.description.getValue(); 2515 } 2516 2517 /** 2518 * @param value Short description of the link. 2519 */ 2520 public TestScriptMetadataLinkComponent setDescription(String value) { 2521 if (Utilities.noString(value)) 2522 this.description = null; 2523 else { 2524 if (this.description == null) 2525 this.description = new StringType(); 2526 this.description.setValue(value); 2527 } 2528 return this; 2529 } 2530 2531 protected void listChildren(List<Property> children) { 2532 super.listChildren(children); 2533 children.add(new Property("url", "uri", "URL to a particular requirement or feature within the FHIR specification.", 0, 1, url)); 2534 children.add(new Property("description", "string", "Short description of the link.", 0, 1, description)); 2535 } 2536 2537 @Override 2538 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2539 switch (_hash) { 2540 case 116079: /*url*/ return new Property("url", "uri", "URL to a particular requirement or feature within the FHIR specification.", 0, 1, url); 2541 case -1724546052: /*description*/ return new Property("description", "string", "Short description of the link.", 0, 1, description); 2542 default: return super.getNamedProperty(_hash, _name, _checkValid); 2543 } 2544 2545 } 2546 2547 @Override 2548 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2549 switch (hash) { 2550 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2551 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 2552 default: return super.getProperty(hash, name, checkValid); 2553 } 2554 2555 } 2556 2557 @Override 2558 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2559 switch (hash) { 2560 case 116079: // url 2561 this.url = TypeConvertor.castToUri(value); // UriType 2562 return value; 2563 case -1724546052: // description 2564 this.description = TypeConvertor.castToString(value); // StringType 2565 return value; 2566 default: return super.setProperty(hash, name, value); 2567 } 2568 2569 } 2570 2571 @Override 2572 public Base setProperty(String name, Base value) throws FHIRException { 2573 if (name.equals("url")) { 2574 this.url = TypeConvertor.castToUri(value); // UriType 2575 } else if (name.equals("description")) { 2576 this.description = TypeConvertor.castToString(value); // StringType 2577 } else 2578 return super.setProperty(name, value); 2579 return value; 2580 } 2581 2582 @Override 2583 public void removeChild(String name, Base value) throws FHIRException { 2584 if (name.equals("url")) { 2585 this.url = null; 2586 } else if (name.equals("description")) { 2587 this.description = null; 2588 } else 2589 super.removeChild(name, value); 2590 2591 } 2592 2593 @Override 2594 public Base makeProperty(int hash, String name) throws FHIRException { 2595 switch (hash) { 2596 case 116079: return getUrlElement(); 2597 case -1724546052: return getDescriptionElement(); 2598 default: return super.makeProperty(hash, name); 2599 } 2600 2601 } 2602 2603 @Override 2604 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2605 switch (hash) { 2606 case 116079: /*url*/ return new String[] {"uri"}; 2607 case -1724546052: /*description*/ return new String[] {"string"}; 2608 default: return super.getTypesForProperty(hash, name); 2609 } 2610 2611 } 2612 2613 @Override 2614 public Base addChild(String name) throws FHIRException { 2615 if (name.equals("url")) { 2616 throw new FHIRException("Cannot call addChild on a singleton property TestScript.metadata.link.url"); 2617 } 2618 else if (name.equals("description")) { 2619 throw new FHIRException("Cannot call addChild on a singleton property TestScript.metadata.link.description"); 2620 } 2621 else 2622 return super.addChild(name); 2623 } 2624 2625 public TestScriptMetadataLinkComponent copy() { 2626 TestScriptMetadataLinkComponent dst = new TestScriptMetadataLinkComponent(); 2627 copyValues(dst); 2628 return dst; 2629 } 2630 2631 public void copyValues(TestScriptMetadataLinkComponent dst) { 2632 super.copyValues(dst); 2633 dst.url = url == null ? null : url.copy(); 2634 dst.description = description == null ? null : description.copy(); 2635 } 2636 2637 @Override 2638 public boolean equalsDeep(Base other_) { 2639 if (!super.equalsDeep(other_)) 2640 return false; 2641 if (!(other_ instanceof TestScriptMetadataLinkComponent)) 2642 return false; 2643 TestScriptMetadataLinkComponent o = (TestScriptMetadataLinkComponent) other_; 2644 return compareDeep(url, o.url, true) && compareDeep(description, o.description, true); 2645 } 2646 2647 @Override 2648 public boolean equalsShallow(Base other_) { 2649 if (!super.equalsShallow(other_)) 2650 return false; 2651 if (!(other_ instanceof TestScriptMetadataLinkComponent)) 2652 return false; 2653 TestScriptMetadataLinkComponent o = (TestScriptMetadataLinkComponent) other_; 2654 return compareValues(url, o.url, true) && compareValues(description, o.description, true); 2655 } 2656 2657 public boolean isEmpty() { 2658 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, description); 2659 } 2660 2661 public String fhirType() { 2662 return "TestScript.metadata.link"; 2663 2664 } 2665 2666 } 2667 2668 @Block() 2669 public static class TestScriptMetadataCapabilityComponent extends BackboneElement implements IBaseBackboneElement { 2670 /** 2671 * Whether or not the test execution will require the given capabilities of the server in order for this test script to execute. 2672 */ 2673 @Child(name = "required", type = {BooleanType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2674 @Description(shortDefinition="Are the capabilities required?", formalDefinition="Whether or not the test execution will require the given capabilities of the server in order for this test script to execute." ) 2675 protected BooleanType required; 2676 2677 /** 2678 * Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute. 2679 */ 2680 @Child(name = "validated", type = {BooleanType.class}, order=2, min=1, max=1, modifier=false, summary=false) 2681 @Description(shortDefinition="Are the capabilities validated?", formalDefinition="Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute." ) 2682 protected BooleanType validated; 2683 2684 /** 2685 * Description of the capabilities that this test script is requiring the server to support. 2686 */ 2687 @Child(name = "description", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2688 @Description(shortDefinition="The expected capabilities of the server", formalDefinition="Description of the capabilities that this test script is requiring the server to support." ) 2689 protected StringType description; 2690 2691 /** 2692 * Which origin server these requirements apply to. 2693 */ 2694 @Child(name = "origin", type = {IntegerType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2695 @Description(shortDefinition="Which origin server these requirements apply to", formalDefinition="Which origin server these requirements apply to." ) 2696 protected List<IntegerType> origin; 2697 2698 /** 2699 * Which server these requirements apply to. 2700 */ 2701 @Child(name = "destination", type = {IntegerType.class}, order=5, min=0, max=1, modifier=false, summary=false) 2702 @Description(shortDefinition="Which server these requirements apply to", formalDefinition="Which server these requirements apply to." ) 2703 protected IntegerType destination; 2704 2705 /** 2706 * Links to the FHIR specification that describes this interaction and the resources involved in more detail. 2707 */ 2708 @Child(name = "link", type = {UriType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2709 @Description(shortDefinition="Links to the FHIR specification", formalDefinition="Links to the FHIR specification that describes this interaction and the resources involved in more detail." ) 2710 protected List<UriType> link; 2711 2712 /** 2713 * Minimum capabilities required of server for test script to execute successfully. If server does not meet at a minimum the referenced capability statement, then all tests in this script are skipped. 2714 */ 2715 @Child(name = "capabilities", type = {CanonicalType.class}, order=7, min=1, max=1, modifier=false, summary=false) 2716 @Description(shortDefinition="Required Capability Statement", formalDefinition="Minimum capabilities required of server for test script to execute successfully. If server does not meet at a minimum the referenced capability statement, then all tests in this script are skipped." ) 2717 protected CanonicalType capabilities; 2718 2719 private static final long serialVersionUID = -1368199288L; 2720 2721 /** 2722 * Constructor 2723 */ 2724 public TestScriptMetadataCapabilityComponent() { 2725 super(); 2726 } 2727 2728 /** 2729 * Constructor 2730 */ 2731 public TestScriptMetadataCapabilityComponent(boolean required, boolean validated, String capabilities) { 2732 super(); 2733 this.setRequired(required); 2734 this.setValidated(validated); 2735 this.setCapabilities(capabilities); 2736 } 2737 2738 /** 2739 * @return {@link #required} (Whether or not the test execution will require the given capabilities of the server in order for this test script to execute.). This is the underlying object with id, value and extensions. The accessor "getRequired" gives direct access to the value 2740 */ 2741 public BooleanType getRequiredElement() { 2742 if (this.required == null) 2743 if (Configuration.errorOnAutoCreate()) 2744 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.required"); 2745 else if (Configuration.doAutoCreate()) 2746 this.required = new BooleanType(); // bb 2747 return this.required; 2748 } 2749 2750 public boolean hasRequiredElement() { 2751 return this.required != null && !this.required.isEmpty(); 2752 } 2753 2754 public boolean hasRequired() { 2755 return this.required != null && !this.required.isEmpty(); 2756 } 2757 2758 /** 2759 * @param value {@link #required} (Whether or not the test execution will require the given capabilities of the server in order for this test script to execute.). This is the underlying object with id, value and extensions. The accessor "getRequired" gives direct access to the value 2760 */ 2761 public TestScriptMetadataCapabilityComponent setRequiredElement(BooleanType value) { 2762 this.required = value; 2763 return this; 2764 } 2765 2766 /** 2767 * @return Whether or not the test execution will require the given capabilities of the server in order for this test script to execute. 2768 */ 2769 public boolean getRequired() { 2770 return this.required == null || this.required.isEmpty() ? false : this.required.getValue(); 2771 } 2772 2773 /** 2774 * @param value Whether or not the test execution will require the given capabilities of the server in order for this test script to execute. 2775 */ 2776 public TestScriptMetadataCapabilityComponent setRequired(boolean value) { 2777 if (this.required == null) 2778 this.required = new BooleanType(); 2779 this.required.setValue(value); 2780 return this; 2781 } 2782 2783 /** 2784 * @return {@link #validated} (Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute.). This is the underlying object with id, value and extensions. The accessor "getValidated" gives direct access to the value 2785 */ 2786 public BooleanType getValidatedElement() { 2787 if (this.validated == null) 2788 if (Configuration.errorOnAutoCreate()) 2789 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.validated"); 2790 else if (Configuration.doAutoCreate()) 2791 this.validated = new BooleanType(); // bb 2792 return this.validated; 2793 } 2794 2795 public boolean hasValidatedElement() { 2796 return this.validated != null && !this.validated.isEmpty(); 2797 } 2798 2799 public boolean hasValidated() { 2800 return this.validated != null && !this.validated.isEmpty(); 2801 } 2802 2803 /** 2804 * @param value {@link #validated} (Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute.). This is the underlying object with id, value and extensions. The accessor "getValidated" gives direct access to the value 2805 */ 2806 public TestScriptMetadataCapabilityComponent setValidatedElement(BooleanType value) { 2807 this.validated = value; 2808 return this; 2809 } 2810 2811 /** 2812 * @return Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute. 2813 */ 2814 public boolean getValidated() { 2815 return this.validated == null || this.validated.isEmpty() ? false : this.validated.getValue(); 2816 } 2817 2818 /** 2819 * @param value Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute. 2820 */ 2821 public TestScriptMetadataCapabilityComponent setValidated(boolean value) { 2822 if (this.validated == null) 2823 this.validated = new BooleanType(); 2824 this.validated.setValue(value); 2825 return this; 2826 } 2827 2828 /** 2829 * @return {@link #description} (Description of the capabilities that this test script is requiring the server to support.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2830 */ 2831 public StringType getDescriptionElement() { 2832 if (this.description == null) 2833 if (Configuration.errorOnAutoCreate()) 2834 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.description"); 2835 else if (Configuration.doAutoCreate()) 2836 this.description = new StringType(); // bb 2837 return this.description; 2838 } 2839 2840 public boolean hasDescriptionElement() { 2841 return this.description != null && !this.description.isEmpty(); 2842 } 2843 2844 public boolean hasDescription() { 2845 return this.description != null && !this.description.isEmpty(); 2846 } 2847 2848 /** 2849 * @param value {@link #description} (Description of the capabilities that this test script is requiring the server to support.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2850 */ 2851 public TestScriptMetadataCapabilityComponent setDescriptionElement(StringType value) { 2852 this.description = value; 2853 return this; 2854 } 2855 2856 /** 2857 * @return Description of the capabilities that this test script is requiring the server to support. 2858 */ 2859 public String getDescription() { 2860 return this.description == null ? null : this.description.getValue(); 2861 } 2862 2863 /** 2864 * @param value Description of the capabilities that this test script is requiring the server to support. 2865 */ 2866 public TestScriptMetadataCapabilityComponent setDescription(String value) { 2867 if (Utilities.noString(value)) 2868 this.description = null; 2869 else { 2870 if (this.description == null) 2871 this.description = new StringType(); 2872 this.description.setValue(value); 2873 } 2874 return this; 2875 } 2876 2877 /** 2878 * @return {@link #origin} (Which origin server these requirements apply to.) 2879 */ 2880 public List<IntegerType> getOrigin() { 2881 if (this.origin == null) 2882 this.origin = new ArrayList<IntegerType>(); 2883 return this.origin; 2884 } 2885 2886 /** 2887 * @return Returns a reference to <code>this</code> for easy method chaining 2888 */ 2889 public TestScriptMetadataCapabilityComponent setOrigin(List<IntegerType> theOrigin) { 2890 this.origin = theOrigin; 2891 return this; 2892 } 2893 2894 public boolean hasOrigin() { 2895 if (this.origin == null) 2896 return false; 2897 for (IntegerType item : this.origin) 2898 if (!item.isEmpty()) 2899 return true; 2900 return false; 2901 } 2902 2903 /** 2904 * @return {@link #origin} (Which origin server these requirements apply to.) 2905 */ 2906 public IntegerType addOriginElement() {//2 2907 IntegerType t = new IntegerType(); 2908 if (this.origin == null) 2909 this.origin = new ArrayList<IntegerType>(); 2910 this.origin.add(t); 2911 return t; 2912 } 2913 2914 /** 2915 * @param value {@link #origin} (Which origin server these requirements apply to.) 2916 */ 2917 public TestScriptMetadataCapabilityComponent addOrigin(int value) { //1 2918 IntegerType t = new IntegerType(); 2919 t.setValue(value); 2920 if (this.origin == null) 2921 this.origin = new ArrayList<IntegerType>(); 2922 this.origin.add(t); 2923 return this; 2924 } 2925 2926 /** 2927 * @param value {@link #origin} (Which origin server these requirements apply to.) 2928 */ 2929 public boolean hasOrigin(int value) { 2930 if (this.origin == null) 2931 return false; 2932 for (IntegerType v : this.origin) 2933 if (v.getValue().equals(value)) // integer 2934 return true; 2935 return false; 2936 } 2937 2938 /** 2939 * @return {@link #destination} (Which server these requirements apply to.). This is the underlying object with id, value and extensions. The accessor "getDestination" gives direct access to the value 2940 */ 2941 public IntegerType getDestinationElement() { 2942 if (this.destination == null) 2943 if (Configuration.errorOnAutoCreate()) 2944 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.destination"); 2945 else if (Configuration.doAutoCreate()) 2946 this.destination = new IntegerType(); // bb 2947 return this.destination; 2948 } 2949 2950 public boolean hasDestinationElement() { 2951 return this.destination != null && !this.destination.isEmpty(); 2952 } 2953 2954 public boolean hasDestination() { 2955 return this.destination != null && !this.destination.isEmpty(); 2956 } 2957 2958 /** 2959 * @param value {@link #destination} (Which server these requirements apply to.). This is the underlying object with id, value and extensions. The accessor "getDestination" gives direct access to the value 2960 */ 2961 public TestScriptMetadataCapabilityComponent setDestinationElement(IntegerType value) { 2962 this.destination = value; 2963 return this; 2964 } 2965 2966 /** 2967 * @return Which server these requirements apply to. 2968 */ 2969 public int getDestination() { 2970 return this.destination == null || this.destination.isEmpty() ? 0 : this.destination.getValue(); 2971 } 2972 2973 /** 2974 * @param value Which server these requirements apply to. 2975 */ 2976 public TestScriptMetadataCapabilityComponent setDestination(int value) { 2977 if (this.destination == null) 2978 this.destination = new IntegerType(); 2979 this.destination.setValue(value); 2980 return this; 2981 } 2982 2983 /** 2984 * @return {@link #link} (Links to the FHIR specification that describes this interaction and the resources involved in more detail.) 2985 */ 2986 public List<UriType> getLink() { 2987 if (this.link == null) 2988 this.link = new ArrayList<UriType>(); 2989 return this.link; 2990 } 2991 2992 /** 2993 * @return Returns a reference to <code>this</code> for easy method chaining 2994 */ 2995 public TestScriptMetadataCapabilityComponent setLink(List<UriType> theLink) { 2996 this.link = theLink; 2997 return this; 2998 } 2999 3000 public boolean hasLink() { 3001 if (this.link == null) 3002 return false; 3003 for (UriType item : this.link) 3004 if (!item.isEmpty()) 3005 return true; 3006 return false; 3007 } 3008 3009 /** 3010 * @return {@link #link} (Links to the FHIR specification that describes this interaction and the resources involved in more detail.) 3011 */ 3012 public UriType addLinkElement() {//2 3013 UriType t = new UriType(); 3014 if (this.link == null) 3015 this.link = new ArrayList<UriType>(); 3016 this.link.add(t); 3017 return t; 3018 } 3019 3020 /** 3021 * @param value {@link #link} (Links to the FHIR specification that describes this interaction and the resources involved in more detail.) 3022 */ 3023 public TestScriptMetadataCapabilityComponent addLink(String value) { //1 3024 UriType t = new UriType(); 3025 t.setValue(value); 3026 if (this.link == null) 3027 this.link = new ArrayList<UriType>(); 3028 this.link.add(t); 3029 return this; 3030 } 3031 3032 /** 3033 * @param value {@link #link} (Links to the FHIR specification that describes this interaction and the resources involved in more detail.) 3034 */ 3035 public boolean hasLink(String value) { 3036 if (this.link == null) 3037 return false; 3038 for (UriType v : this.link) 3039 if (v.getValue().equals(value)) // uri 3040 return true; 3041 return false; 3042 } 3043 3044 /** 3045 * @return {@link #capabilities} (Minimum capabilities required of server for test script to execute successfully. If server does not meet at a minimum the referenced capability statement, then all tests in this script are skipped.). This is the underlying object with id, value and extensions. The accessor "getCapabilities" gives direct access to the value 3046 */ 3047 public CanonicalType getCapabilitiesElement() { 3048 if (this.capabilities == null) 3049 if (Configuration.errorOnAutoCreate()) 3050 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.capabilities"); 3051 else if (Configuration.doAutoCreate()) 3052 this.capabilities = new CanonicalType(); // bb 3053 return this.capabilities; 3054 } 3055 3056 public boolean hasCapabilitiesElement() { 3057 return this.capabilities != null && !this.capabilities.isEmpty(); 3058 } 3059 3060 public boolean hasCapabilities() { 3061 return this.capabilities != null && !this.capabilities.isEmpty(); 3062 } 3063 3064 /** 3065 * @param value {@link #capabilities} (Minimum capabilities required of server for test script to execute successfully. If server does not meet at a minimum the referenced capability statement, then all tests in this script are skipped.). This is the underlying object with id, value and extensions. The accessor "getCapabilities" gives direct access to the value 3066 */ 3067 public TestScriptMetadataCapabilityComponent setCapabilitiesElement(CanonicalType value) { 3068 this.capabilities = value; 3069 return this; 3070 } 3071 3072 /** 3073 * @return Minimum capabilities required of server for test script to execute successfully. If server does not meet at a minimum the referenced capability statement, then all tests in this script are skipped. 3074 */ 3075 public String getCapabilities() { 3076 return this.capabilities == null ? null : this.capabilities.getValue(); 3077 } 3078 3079 /** 3080 * @param value Minimum capabilities required of server for test script to execute successfully. If server does not meet at a minimum the referenced capability statement, then all tests in this script are skipped. 3081 */ 3082 public TestScriptMetadataCapabilityComponent setCapabilities(String value) { 3083 if (this.capabilities == null) 3084 this.capabilities = new CanonicalType(); 3085 this.capabilities.setValue(value); 3086 return this; 3087 } 3088 3089 protected void listChildren(List<Property> children) { 3090 super.listChildren(children); 3091 children.add(new Property("required", "boolean", "Whether or not the test execution will require the given capabilities of the server in order for this test script to execute.", 0, 1, required)); 3092 children.add(new Property("validated", "boolean", "Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute.", 0, 1, validated)); 3093 children.add(new Property("description", "string", "Description of the capabilities that this test script is requiring the server to support.", 0, 1, description)); 3094 children.add(new Property("origin", "integer", "Which origin server these requirements apply to.", 0, java.lang.Integer.MAX_VALUE, origin)); 3095 children.add(new Property("destination", "integer", "Which server these requirements apply to.", 0, 1, destination)); 3096 children.add(new Property("link", "uri", "Links to the FHIR specification that describes this interaction and the resources involved in more detail.", 0, java.lang.Integer.MAX_VALUE, link)); 3097 children.add(new Property("capabilities", "canonical(CapabilityStatement)", "Minimum capabilities required of server for test script to execute successfully. If server does not meet at a minimum the referenced capability statement, then all tests in this script are skipped.", 0, 1, capabilities)); 3098 } 3099 3100 @Override 3101 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3102 switch (_hash) { 3103 case -393139297: /*required*/ return new Property("required", "boolean", "Whether or not the test execution will require the given capabilities of the server in order for this test script to execute.", 0, 1, required); 3104 case -1109784050: /*validated*/ return new Property("validated", "boolean", "Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute.", 0, 1, validated); 3105 case -1724546052: /*description*/ return new Property("description", "string", "Description of the capabilities that this test script is requiring the server to support.", 0, 1, description); 3106 case -1008619738: /*origin*/ return new Property("origin", "integer", "Which origin server these requirements apply to.", 0, java.lang.Integer.MAX_VALUE, origin); 3107 case -1429847026: /*destination*/ return new Property("destination", "integer", "Which server these requirements apply to.", 0, 1, destination); 3108 case 3321850: /*link*/ return new Property("link", "uri", "Links to the FHIR specification that describes this interaction and the resources involved in more detail.", 0, java.lang.Integer.MAX_VALUE, link); 3109 case -1487597642: /*capabilities*/ return new Property("capabilities", "canonical(CapabilityStatement)", "Minimum capabilities required of server for test script to execute successfully. If server does not meet at a minimum the referenced capability statement, then all tests in this script are skipped.", 0, 1, capabilities); 3110 default: return super.getNamedProperty(_hash, _name, _checkValid); 3111 } 3112 3113 } 3114 3115 @Override 3116 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3117 switch (hash) { 3118 case -393139297: /*required*/ return this.required == null ? new Base[0] : new Base[] {this.required}; // BooleanType 3119 case -1109784050: /*validated*/ return this.validated == null ? new Base[0] : new Base[] {this.validated}; // BooleanType 3120 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 3121 case -1008619738: /*origin*/ return this.origin == null ? new Base[0] : this.origin.toArray(new Base[this.origin.size()]); // IntegerType 3122 case -1429847026: /*destination*/ return this.destination == null ? new Base[0] : new Base[] {this.destination}; // IntegerType 3123 case 3321850: /*link*/ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // UriType 3124 case -1487597642: /*capabilities*/ return this.capabilities == null ? new Base[0] : new Base[] {this.capabilities}; // CanonicalType 3125 default: return super.getProperty(hash, name, checkValid); 3126 } 3127 3128 } 3129 3130 @Override 3131 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3132 switch (hash) { 3133 case -393139297: // required 3134 this.required = TypeConvertor.castToBoolean(value); // BooleanType 3135 return value; 3136 case -1109784050: // validated 3137 this.validated = TypeConvertor.castToBoolean(value); // BooleanType 3138 return value; 3139 case -1724546052: // description 3140 this.description = TypeConvertor.castToString(value); // StringType 3141 return value; 3142 case -1008619738: // origin 3143 this.getOrigin().add(TypeConvertor.castToInteger(value)); // IntegerType 3144 return value; 3145 case -1429847026: // destination 3146 this.destination = TypeConvertor.castToInteger(value); // IntegerType 3147 return value; 3148 case 3321850: // link 3149 this.getLink().add(TypeConvertor.castToUri(value)); // UriType 3150 return value; 3151 case -1487597642: // capabilities 3152 this.capabilities = TypeConvertor.castToCanonical(value); // CanonicalType 3153 return value; 3154 default: return super.setProperty(hash, name, value); 3155 } 3156 3157 } 3158 3159 @Override 3160 public Base setProperty(String name, Base value) throws FHIRException { 3161 if (name.equals("required")) { 3162 this.required = TypeConvertor.castToBoolean(value); // BooleanType 3163 } else if (name.equals("validated")) { 3164 this.validated = TypeConvertor.castToBoolean(value); // BooleanType 3165 } else if (name.equals("description")) { 3166 this.description = TypeConvertor.castToString(value); // StringType 3167 } else if (name.equals("origin")) { 3168 this.getOrigin().add(TypeConvertor.castToInteger(value)); 3169 } else if (name.equals("destination")) { 3170 this.destination = TypeConvertor.castToInteger(value); // IntegerType 3171 } else if (name.equals("link")) { 3172 this.getLink().add(TypeConvertor.castToUri(value)); 3173 } else if (name.equals("capabilities")) { 3174 this.capabilities = TypeConvertor.castToCanonical(value); // CanonicalType 3175 } else 3176 return super.setProperty(name, value); 3177 return value; 3178 } 3179 3180 @Override 3181 public void removeChild(String name, Base value) throws FHIRException { 3182 if (name.equals("required")) { 3183 this.required = null; 3184 } else if (name.equals("validated")) { 3185 this.validated = null; 3186 } else if (name.equals("description")) { 3187 this.description = null; 3188 } else if (name.equals("origin")) { 3189 this.getOrigin().remove(value); 3190 } else if (name.equals("destination")) { 3191 this.destination = null; 3192 } else if (name.equals("link")) { 3193 this.getLink().remove(value); 3194 } else if (name.equals("capabilities")) { 3195 this.capabilities = null; 3196 } else 3197 super.removeChild(name, value); 3198 3199 } 3200 3201 @Override 3202 public Base makeProperty(int hash, String name) throws FHIRException { 3203 switch (hash) { 3204 case -393139297: return getRequiredElement(); 3205 case -1109784050: return getValidatedElement(); 3206 case -1724546052: return getDescriptionElement(); 3207 case -1008619738: return addOriginElement(); 3208 case -1429847026: return getDestinationElement(); 3209 case 3321850: return addLinkElement(); 3210 case -1487597642: return getCapabilitiesElement(); 3211 default: return super.makeProperty(hash, name); 3212 } 3213 3214 } 3215 3216 @Override 3217 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3218 switch (hash) { 3219 case -393139297: /*required*/ return new String[] {"boolean"}; 3220 case -1109784050: /*validated*/ return new String[] {"boolean"}; 3221 case -1724546052: /*description*/ return new String[] {"string"}; 3222 case -1008619738: /*origin*/ return new String[] {"integer"}; 3223 case -1429847026: /*destination*/ return new String[] {"integer"}; 3224 case 3321850: /*link*/ return new String[] {"uri"}; 3225 case -1487597642: /*capabilities*/ return new String[] {"canonical"}; 3226 default: return super.getTypesForProperty(hash, name); 3227 } 3228 3229 } 3230 3231 @Override 3232 public Base addChild(String name) throws FHIRException { 3233 if (name.equals("required")) { 3234 throw new FHIRException("Cannot call addChild on a singleton property TestScript.metadata.capability.required"); 3235 } 3236 else if (name.equals("validated")) { 3237 throw new FHIRException("Cannot call addChild on a singleton property TestScript.metadata.capability.validated"); 3238 } 3239 else if (name.equals("description")) { 3240 throw new FHIRException("Cannot call addChild on a singleton property TestScript.metadata.capability.description"); 3241 } 3242 else if (name.equals("origin")) { 3243 throw new FHIRException("Cannot call addChild on a singleton property TestScript.metadata.capability.origin"); 3244 } 3245 else if (name.equals("destination")) { 3246 throw new FHIRException("Cannot call addChild on a singleton property TestScript.metadata.capability.destination"); 3247 } 3248 else if (name.equals("link")) { 3249 throw new FHIRException("Cannot call addChild on a singleton property TestScript.metadata.capability.link"); 3250 } 3251 else if (name.equals("capabilities")) { 3252 throw new FHIRException("Cannot call addChild on a singleton property TestScript.metadata.capability.capabilities"); 3253 } 3254 else 3255 return super.addChild(name); 3256 } 3257 3258 public TestScriptMetadataCapabilityComponent copy() { 3259 TestScriptMetadataCapabilityComponent dst = new TestScriptMetadataCapabilityComponent(); 3260 copyValues(dst); 3261 return dst; 3262 } 3263 3264 public void copyValues(TestScriptMetadataCapabilityComponent dst) { 3265 super.copyValues(dst); 3266 dst.required = required == null ? null : required.copy(); 3267 dst.validated = validated == null ? null : validated.copy(); 3268 dst.description = description == null ? null : description.copy(); 3269 if (origin != null) { 3270 dst.origin = new ArrayList<IntegerType>(); 3271 for (IntegerType i : origin) 3272 dst.origin.add(i.copy()); 3273 }; 3274 dst.destination = destination == null ? null : destination.copy(); 3275 if (link != null) { 3276 dst.link = new ArrayList<UriType>(); 3277 for (UriType i : link) 3278 dst.link.add(i.copy()); 3279 }; 3280 dst.capabilities = capabilities == null ? null : capabilities.copy(); 3281 } 3282 3283 @Override 3284 public boolean equalsDeep(Base other_) { 3285 if (!super.equalsDeep(other_)) 3286 return false; 3287 if (!(other_ instanceof TestScriptMetadataCapabilityComponent)) 3288 return false; 3289 TestScriptMetadataCapabilityComponent o = (TestScriptMetadataCapabilityComponent) other_; 3290 return compareDeep(required, o.required, true) && compareDeep(validated, o.validated, true) && compareDeep(description, o.description, true) 3291 && compareDeep(origin, o.origin, true) && compareDeep(destination, o.destination, true) && compareDeep(link, o.link, true) 3292 && compareDeep(capabilities, o.capabilities, true); 3293 } 3294 3295 @Override 3296 public boolean equalsShallow(Base other_) { 3297 if (!super.equalsShallow(other_)) 3298 return false; 3299 if (!(other_ instanceof TestScriptMetadataCapabilityComponent)) 3300 return false; 3301 TestScriptMetadataCapabilityComponent o = (TestScriptMetadataCapabilityComponent) other_; 3302 return compareValues(required, o.required, true) && compareValues(validated, o.validated, true) && compareValues(description, o.description, true) 3303 && compareValues(origin, o.origin, true) && compareValues(destination, o.destination, true) && compareValues(link, o.link, true) 3304 && compareValues(capabilities, o.capabilities, true); 3305 } 3306 3307 public boolean isEmpty() { 3308 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(required, validated, description 3309 , origin, destination, link, capabilities); 3310 } 3311 3312 public String fhirType() { 3313 return "TestScript.metadata.capability"; 3314 3315 } 3316 3317 } 3318 3319 @Block() 3320 public static class TestScriptScopeComponent extends BackboneElement implements IBaseBackboneElement { 3321 /** 3322 * The specific conformance artifact being tested. The canonical reference can be version-specific. 3323 */ 3324 @Child(name = "artifact", type = {CanonicalType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3325 @Description(shortDefinition="The specific conformance artifact being tested", formalDefinition="The specific conformance artifact being tested. The canonical reference can be version-specific." ) 3326 protected CanonicalType artifact; 3327 3328 /** 3329 * The expectation of whether the test must pass for the system to be considered conformant with the artifact: required - all tests are expected to pass, optional - all test are expected to pass but non-pass status may be allowed, strict - all tests are expected to pass and warnings are treated as a failure. 3330 */ 3331 @Child(name = "conformance", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 3332 @Description(shortDefinition="required | optional | strict", formalDefinition="The expectation of whether the test must pass for the system to be considered conformant with the artifact: required - all tests are expected to pass, optional - all test are expected to pass but non-pass status may be allowed, strict - all tests are expected to pass and warnings are treated as a failure." ) 3333 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/testscript-scope-conformance-codes") 3334 protected CodeableConcept conformance; 3335 3336 /** 3337 * The phase of testing for this artifact: unit - development / implementation phase, integration - internal system to system phase, production - live system to system phase (Note, this may involve pii/phi data). 3338 */ 3339 @Child(name = "phase", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 3340 @Description(shortDefinition="unit | integration | production", formalDefinition="The phase of testing for this artifact: unit - development / implementation phase, integration - internal system to system phase, production - live system to system phase (Note, this may involve pii/phi data)." ) 3341 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/testscript-scope-phase-codes") 3342 protected CodeableConcept phase; 3343 3344 private static final long serialVersionUID = 1236847076L; 3345 3346 /** 3347 * Constructor 3348 */ 3349 public TestScriptScopeComponent() { 3350 super(); 3351 } 3352 3353 /** 3354 * Constructor 3355 */ 3356 public TestScriptScopeComponent(String artifact) { 3357 super(); 3358 this.setArtifact(artifact); 3359 } 3360 3361 /** 3362 * @return {@link #artifact} (The specific conformance artifact being tested. The canonical reference can be version-specific.). This is the underlying object with id, value and extensions. The accessor "getArtifact" gives direct access to the value 3363 */ 3364 public CanonicalType getArtifactElement() { 3365 if (this.artifact == null) 3366 if (Configuration.errorOnAutoCreate()) 3367 throw new Error("Attempt to auto-create TestScriptScopeComponent.artifact"); 3368 else if (Configuration.doAutoCreate()) 3369 this.artifact = new CanonicalType(); // bb 3370 return this.artifact; 3371 } 3372 3373 public boolean hasArtifactElement() { 3374 return this.artifact != null && !this.artifact.isEmpty(); 3375 } 3376 3377 public boolean hasArtifact() { 3378 return this.artifact != null && !this.artifact.isEmpty(); 3379 } 3380 3381 /** 3382 * @param value {@link #artifact} (The specific conformance artifact being tested. The canonical reference can be version-specific.). This is the underlying object with id, value and extensions. The accessor "getArtifact" gives direct access to the value 3383 */ 3384 public TestScriptScopeComponent setArtifactElement(CanonicalType value) { 3385 this.artifact = value; 3386 return this; 3387 } 3388 3389 /** 3390 * @return The specific conformance artifact being tested. The canonical reference can be version-specific. 3391 */ 3392 public String getArtifact() { 3393 return this.artifact == null ? null : this.artifact.getValue(); 3394 } 3395 3396 /** 3397 * @param value The specific conformance artifact being tested. The canonical reference can be version-specific. 3398 */ 3399 public TestScriptScopeComponent setArtifact(String value) { 3400 if (this.artifact == null) 3401 this.artifact = new CanonicalType(); 3402 this.artifact.setValue(value); 3403 return this; 3404 } 3405 3406 /** 3407 * @return {@link #conformance} (The expectation of whether the test must pass for the system to be considered conformant with the artifact: required - all tests are expected to pass, optional - all test are expected to pass but non-pass status may be allowed, strict - all tests are expected to pass and warnings are treated as a failure.) 3408 */ 3409 public CodeableConcept getConformance() { 3410 if (this.conformance == null) 3411 if (Configuration.errorOnAutoCreate()) 3412 throw new Error("Attempt to auto-create TestScriptScopeComponent.conformance"); 3413 else if (Configuration.doAutoCreate()) 3414 this.conformance = new CodeableConcept(); // cc 3415 return this.conformance; 3416 } 3417 3418 public boolean hasConformance() { 3419 return this.conformance != null && !this.conformance.isEmpty(); 3420 } 3421 3422 /** 3423 * @param value {@link #conformance} (The expectation of whether the test must pass for the system to be considered conformant with the artifact: required - all tests are expected to pass, optional - all test are expected to pass but non-pass status may be allowed, strict - all tests are expected to pass and warnings are treated as a failure.) 3424 */ 3425 public TestScriptScopeComponent setConformance(CodeableConcept value) { 3426 this.conformance = value; 3427 return this; 3428 } 3429 3430 /** 3431 * @return {@link #phase} (The phase of testing for this artifact: unit - development / implementation phase, integration - internal system to system phase, production - live system to system phase (Note, this may involve pii/phi data).) 3432 */ 3433 public CodeableConcept getPhase() { 3434 if (this.phase == null) 3435 if (Configuration.errorOnAutoCreate()) 3436 throw new Error("Attempt to auto-create TestScriptScopeComponent.phase"); 3437 else if (Configuration.doAutoCreate()) 3438 this.phase = new CodeableConcept(); // cc 3439 return this.phase; 3440 } 3441 3442 public boolean hasPhase() { 3443 return this.phase != null && !this.phase.isEmpty(); 3444 } 3445 3446 /** 3447 * @param value {@link #phase} (The phase of testing for this artifact: unit - development / implementation phase, integration - internal system to system phase, production - live system to system phase (Note, this may involve pii/phi data).) 3448 */ 3449 public TestScriptScopeComponent setPhase(CodeableConcept value) { 3450 this.phase = value; 3451 return this; 3452 } 3453 3454 protected void listChildren(List<Property> children) { 3455 super.listChildren(children); 3456 children.add(new Property("artifact", "canonical(Any)", "The specific conformance artifact being tested. The canonical reference can be version-specific.", 0, 1, artifact)); 3457 children.add(new Property("conformance", "CodeableConcept", "The expectation of whether the test must pass for the system to be considered conformant with the artifact: required - all tests are expected to pass, optional - all test are expected to pass but non-pass status may be allowed, strict - all tests are expected to pass and warnings are treated as a failure.", 0, 1, conformance)); 3458 children.add(new Property("phase", "CodeableConcept", "The phase of testing for this artifact: unit - development / implementation phase, integration - internal system to system phase, production - live system to system phase (Note, this may involve pii/phi data).", 0, 1, phase)); 3459 } 3460 3461 @Override 3462 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3463 switch (_hash) { 3464 case -1228798510: /*artifact*/ return new Property("artifact", "canonical(Any)", "The specific conformance artifact being tested. The canonical reference can be version-specific.", 0, 1, artifact); 3465 case 1374858133: /*conformance*/ return new Property("conformance", "CodeableConcept", "The expectation of whether the test must pass for the system to be considered conformant with the artifact: required - all tests are expected to pass, optional - all test are expected to pass but non-pass status may be allowed, strict - all tests are expected to pass and warnings are treated as a failure.", 0, 1, conformance); 3466 case 106629499: /*phase*/ return new Property("phase", "CodeableConcept", "The phase of testing for this artifact: unit - development / implementation phase, integration - internal system to system phase, production - live system to system phase (Note, this may involve pii/phi data).", 0, 1, phase); 3467 default: return super.getNamedProperty(_hash, _name, _checkValid); 3468 } 3469 3470 } 3471 3472 @Override 3473 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3474 switch (hash) { 3475 case -1228798510: /*artifact*/ return this.artifact == null ? new Base[0] : new Base[] {this.artifact}; // CanonicalType 3476 case 1374858133: /*conformance*/ return this.conformance == null ? new Base[0] : new Base[] {this.conformance}; // CodeableConcept 3477 case 106629499: /*phase*/ return this.phase == null ? new Base[0] : new Base[] {this.phase}; // CodeableConcept 3478 default: return super.getProperty(hash, name, checkValid); 3479 } 3480 3481 } 3482 3483 @Override 3484 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3485 switch (hash) { 3486 case -1228798510: // artifact 3487 this.artifact = TypeConvertor.castToCanonical(value); // CanonicalType 3488 return value; 3489 case 1374858133: // conformance 3490 this.conformance = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3491 return value; 3492 case 106629499: // phase 3493 this.phase = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3494 return value; 3495 default: return super.setProperty(hash, name, value); 3496 } 3497 3498 } 3499 3500 @Override 3501 public Base setProperty(String name, Base value) throws FHIRException { 3502 if (name.equals("artifact")) { 3503 this.artifact = TypeConvertor.castToCanonical(value); // CanonicalType 3504 } else if (name.equals("conformance")) { 3505 this.conformance = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3506 } else if (name.equals("phase")) { 3507 this.phase = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3508 } else 3509 return super.setProperty(name, value); 3510 return value; 3511 } 3512 3513 @Override 3514 public void removeChild(String name, Base value) throws FHIRException { 3515 if (name.equals("artifact")) { 3516 this.artifact = null; 3517 } else if (name.equals("conformance")) { 3518 this.conformance = null; 3519 } else if (name.equals("phase")) { 3520 this.phase = null; 3521 } else 3522 super.removeChild(name, value); 3523 3524 } 3525 3526 @Override 3527 public Base makeProperty(int hash, String name) throws FHIRException { 3528 switch (hash) { 3529 case -1228798510: return getArtifactElement(); 3530 case 1374858133: return getConformance(); 3531 case 106629499: return getPhase(); 3532 default: return super.makeProperty(hash, name); 3533 } 3534 3535 } 3536 3537 @Override 3538 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3539 switch (hash) { 3540 case -1228798510: /*artifact*/ return new String[] {"canonical"}; 3541 case 1374858133: /*conformance*/ return new String[] {"CodeableConcept"}; 3542 case 106629499: /*phase*/ return new String[] {"CodeableConcept"}; 3543 default: return super.getTypesForProperty(hash, name); 3544 } 3545 3546 } 3547 3548 @Override 3549 public Base addChild(String name) throws FHIRException { 3550 if (name.equals("artifact")) { 3551 throw new FHIRException("Cannot call addChild on a singleton property TestScript.scope.artifact"); 3552 } 3553 else if (name.equals("conformance")) { 3554 this.conformance = new CodeableConcept(); 3555 return this.conformance; 3556 } 3557 else if (name.equals("phase")) { 3558 this.phase = new CodeableConcept(); 3559 return this.phase; 3560 } 3561 else 3562 return super.addChild(name); 3563 } 3564 3565 public TestScriptScopeComponent copy() { 3566 TestScriptScopeComponent dst = new TestScriptScopeComponent(); 3567 copyValues(dst); 3568 return dst; 3569 } 3570 3571 public void copyValues(TestScriptScopeComponent dst) { 3572 super.copyValues(dst); 3573 dst.artifact = artifact == null ? null : artifact.copy(); 3574 dst.conformance = conformance == null ? null : conformance.copy(); 3575 dst.phase = phase == null ? null : phase.copy(); 3576 } 3577 3578 @Override 3579 public boolean equalsDeep(Base other_) { 3580 if (!super.equalsDeep(other_)) 3581 return false; 3582 if (!(other_ instanceof TestScriptScopeComponent)) 3583 return false; 3584 TestScriptScopeComponent o = (TestScriptScopeComponent) other_; 3585 return compareDeep(artifact, o.artifact, true) && compareDeep(conformance, o.conformance, true) 3586 && compareDeep(phase, o.phase, true); 3587 } 3588 3589 @Override 3590 public boolean equalsShallow(Base other_) { 3591 if (!super.equalsShallow(other_)) 3592 return false; 3593 if (!(other_ instanceof TestScriptScopeComponent)) 3594 return false; 3595 TestScriptScopeComponent o = (TestScriptScopeComponent) other_; 3596 return compareValues(artifact, o.artifact, true); 3597 } 3598 3599 public boolean isEmpty() { 3600 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(artifact, conformance, phase 3601 ); 3602 } 3603 3604 public String fhirType() { 3605 return "TestScript.scope"; 3606 3607 } 3608 3609 } 3610 3611 @Block() 3612 public static class TestScriptFixtureComponent extends BackboneElement implements IBaseBackboneElement { 3613 /** 3614 * Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section. 3615 */ 3616 @Child(name = "autocreate", type = {BooleanType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3617 @Description(shortDefinition="Whether or not to implicitly create the fixture during setup", formalDefinition="Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section." ) 3618 protected BooleanType autocreate; 3619 3620 /** 3621 * Whether or not to implicitly delete the fixture during teardown. If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section. 3622 */ 3623 @Child(name = "autodelete", type = {BooleanType.class}, order=2, min=1, max=1, modifier=false, summary=false) 3624 @Description(shortDefinition="Whether or not to implicitly delete the fixture during teardown", formalDefinition="Whether or not to implicitly delete the fixture during teardown. If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section." ) 3625 protected BooleanType autodelete; 3626 3627 /** 3628 * Reference to the resource (containing the contents of the resource needed for operations). This is allowed to be a Parameters resource. 3629 */ 3630 @Child(name = "resource", type = {Reference.class}, order=3, min=0, max=1, modifier=false, summary=false) 3631 @Description(shortDefinition="Reference of the resource", formalDefinition="Reference to the resource (containing the contents of the resource needed for operations). This is allowed to be a Parameters resource." ) 3632 protected Reference resource; 3633 3634 private static final long serialVersionUID = 672117234L; 3635 3636 /** 3637 * Constructor 3638 */ 3639 public TestScriptFixtureComponent() { 3640 super(); 3641 } 3642 3643 /** 3644 * Constructor 3645 */ 3646 public TestScriptFixtureComponent(boolean autocreate, boolean autodelete) { 3647 super(); 3648 this.setAutocreate(autocreate); 3649 this.setAutodelete(autodelete); 3650 } 3651 3652 /** 3653 * @return {@link #autocreate} (Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section.). This is the underlying object with id, value and extensions. The accessor "getAutocreate" gives direct access to the value 3654 */ 3655 public BooleanType getAutocreateElement() { 3656 if (this.autocreate == null) 3657 if (Configuration.errorOnAutoCreate()) 3658 throw new Error("Attempt to auto-create TestScriptFixtureComponent.autocreate"); 3659 else if (Configuration.doAutoCreate()) 3660 this.autocreate = new BooleanType(); // bb 3661 return this.autocreate; 3662 } 3663 3664 public boolean hasAutocreateElement() { 3665 return this.autocreate != null && !this.autocreate.isEmpty(); 3666 } 3667 3668 public boolean hasAutocreate() { 3669 return this.autocreate != null && !this.autocreate.isEmpty(); 3670 } 3671 3672 /** 3673 * @param value {@link #autocreate} (Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section.). This is the underlying object with id, value and extensions. The accessor "getAutocreate" gives direct access to the value 3674 */ 3675 public TestScriptFixtureComponent setAutocreateElement(BooleanType value) { 3676 this.autocreate = value; 3677 return this; 3678 } 3679 3680 /** 3681 * @return Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section. 3682 */ 3683 public boolean getAutocreate() { 3684 return this.autocreate == null || this.autocreate.isEmpty() ? false : this.autocreate.getValue(); 3685 } 3686 3687 /** 3688 * @param value Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section. 3689 */ 3690 public TestScriptFixtureComponent setAutocreate(boolean value) { 3691 if (this.autocreate == null) 3692 this.autocreate = new BooleanType(); 3693 this.autocreate.setValue(value); 3694 return this; 3695 } 3696 3697 /** 3698 * @return {@link #autodelete} (Whether or not to implicitly delete the fixture during teardown. If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section.). This is the underlying object with id, value and extensions. The accessor "getAutodelete" gives direct access to the value 3699 */ 3700 public BooleanType getAutodeleteElement() { 3701 if (this.autodelete == null) 3702 if (Configuration.errorOnAutoCreate()) 3703 throw new Error("Attempt to auto-create TestScriptFixtureComponent.autodelete"); 3704 else if (Configuration.doAutoCreate()) 3705 this.autodelete = new BooleanType(); // bb 3706 return this.autodelete; 3707 } 3708 3709 public boolean hasAutodeleteElement() { 3710 return this.autodelete != null && !this.autodelete.isEmpty(); 3711 } 3712 3713 public boolean hasAutodelete() { 3714 return this.autodelete != null && !this.autodelete.isEmpty(); 3715 } 3716 3717 /** 3718 * @param value {@link #autodelete} (Whether or not to implicitly delete the fixture during teardown. If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section.). This is the underlying object with id, value and extensions. The accessor "getAutodelete" gives direct access to the value 3719 */ 3720 public TestScriptFixtureComponent setAutodeleteElement(BooleanType value) { 3721 this.autodelete = value; 3722 return this; 3723 } 3724 3725 /** 3726 * @return Whether or not to implicitly delete the fixture during teardown. If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section. 3727 */ 3728 public boolean getAutodelete() { 3729 return this.autodelete == null || this.autodelete.isEmpty() ? false : this.autodelete.getValue(); 3730 } 3731 3732 /** 3733 * @param value Whether or not to implicitly delete the fixture during teardown. If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section. 3734 */ 3735 public TestScriptFixtureComponent setAutodelete(boolean value) { 3736 if (this.autodelete == null) 3737 this.autodelete = new BooleanType(); 3738 this.autodelete.setValue(value); 3739 return this; 3740 } 3741 3742 /** 3743 * @return {@link #resource} (Reference to the resource (containing the contents of the resource needed for operations). This is allowed to be a Parameters resource.) 3744 */ 3745 public Reference getResource() { 3746 if (this.resource == null) 3747 if (Configuration.errorOnAutoCreate()) 3748 throw new Error("Attempt to auto-create TestScriptFixtureComponent.resource"); 3749 else if (Configuration.doAutoCreate()) 3750 this.resource = new Reference(); // cc 3751 return this.resource; 3752 } 3753 3754 public boolean hasResource() { 3755 return this.resource != null && !this.resource.isEmpty(); 3756 } 3757 3758 /** 3759 * @param value {@link #resource} (Reference to the resource (containing the contents of the resource needed for operations). This is allowed to be a Parameters resource.) 3760 */ 3761 public TestScriptFixtureComponent setResource(Reference value) { 3762 this.resource = value; 3763 return this; 3764 } 3765 3766 protected void listChildren(List<Property> children) { 3767 super.listChildren(children); 3768 children.add(new Property("autocreate", "boolean", "Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section.", 0, 1, autocreate)); 3769 children.add(new Property("autodelete", "boolean", "Whether or not to implicitly delete the fixture during teardown. If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section.", 0, 1, autodelete)); 3770 children.add(new Property("resource", "Reference(Any)", "Reference to the resource (containing the contents of the resource needed for operations). This is allowed to be a Parameters resource.", 0, 1, resource)); 3771 } 3772 3773 @Override 3774 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3775 switch (_hash) { 3776 case 73154411: /*autocreate*/ return new Property("autocreate", "boolean", "Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section.", 0, 1, autocreate); 3777 case 89990170: /*autodelete*/ return new Property("autodelete", "boolean", "Whether or not to implicitly delete the fixture during teardown. If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section.", 0, 1, autodelete); 3778 case -341064690: /*resource*/ return new Property("resource", "Reference(Any)", "Reference to the resource (containing the contents of the resource needed for operations). This is allowed to be a Parameters resource.", 0, 1, resource); 3779 default: return super.getNamedProperty(_hash, _name, _checkValid); 3780 } 3781 3782 } 3783 3784 @Override 3785 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3786 switch (hash) { 3787 case 73154411: /*autocreate*/ return this.autocreate == null ? new Base[0] : new Base[] {this.autocreate}; // BooleanType 3788 case 89990170: /*autodelete*/ return this.autodelete == null ? new Base[0] : new Base[] {this.autodelete}; // BooleanType 3789 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // Reference 3790 default: return super.getProperty(hash, name, checkValid); 3791 } 3792 3793 } 3794 3795 @Override 3796 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3797 switch (hash) { 3798 case 73154411: // autocreate 3799 this.autocreate = TypeConvertor.castToBoolean(value); // BooleanType 3800 return value; 3801 case 89990170: // autodelete 3802 this.autodelete = TypeConvertor.castToBoolean(value); // BooleanType 3803 return value; 3804 case -341064690: // resource 3805 this.resource = TypeConvertor.castToReference(value); // Reference 3806 return value; 3807 default: return super.setProperty(hash, name, value); 3808 } 3809 3810 } 3811 3812 @Override 3813 public Base setProperty(String name, Base value) throws FHIRException { 3814 if (name.equals("autocreate")) { 3815 this.autocreate = TypeConvertor.castToBoolean(value); // BooleanType 3816 } else if (name.equals("autodelete")) { 3817 this.autodelete = TypeConvertor.castToBoolean(value); // BooleanType 3818 } else if (name.equals("resource")) { 3819 this.resource = TypeConvertor.castToReference(value); // Reference 3820 } else 3821 return super.setProperty(name, value); 3822 return value; 3823 } 3824 3825 @Override 3826 public void removeChild(String name, Base value) throws FHIRException { 3827 if (name.equals("autocreate")) { 3828 this.autocreate = null; 3829 } else if (name.equals("autodelete")) { 3830 this.autodelete = null; 3831 } else if (name.equals("resource")) { 3832 this.resource = null; 3833 } else 3834 super.removeChild(name, value); 3835 3836 } 3837 3838 @Override 3839 public Base makeProperty(int hash, String name) throws FHIRException { 3840 switch (hash) { 3841 case 73154411: return getAutocreateElement(); 3842 case 89990170: return getAutodeleteElement(); 3843 case -341064690: return getResource(); 3844 default: return super.makeProperty(hash, name); 3845 } 3846 3847 } 3848 3849 @Override 3850 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3851 switch (hash) { 3852 case 73154411: /*autocreate*/ return new String[] {"boolean"}; 3853 case 89990170: /*autodelete*/ return new String[] {"boolean"}; 3854 case -341064690: /*resource*/ return new String[] {"Reference"}; 3855 default: return super.getTypesForProperty(hash, name); 3856 } 3857 3858 } 3859 3860 @Override 3861 public Base addChild(String name) throws FHIRException { 3862 if (name.equals("autocreate")) { 3863 throw new FHIRException("Cannot call addChild on a singleton property TestScript.fixture.autocreate"); 3864 } 3865 else if (name.equals("autodelete")) { 3866 throw new FHIRException("Cannot call addChild on a singleton property TestScript.fixture.autodelete"); 3867 } 3868 else if (name.equals("resource")) { 3869 this.resource = new Reference(); 3870 return this.resource; 3871 } 3872 else 3873 return super.addChild(name); 3874 } 3875 3876 public TestScriptFixtureComponent copy() { 3877 TestScriptFixtureComponent dst = new TestScriptFixtureComponent(); 3878 copyValues(dst); 3879 return dst; 3880 } 3881 3882 public void copyValues(TestScriptFixtureComponent dst) { 3883 super.copyValues(dst); 3884 dst.autocreate = autocreate == null ? null : autocreate.copy(); 3885 dst.autodelete = autodelete == null ? null : autodelete.copy(); 3886 dst.resource = resource == null ? null : resource.copy(); 3887 } 3888 3889 @Override 3890 public boolean equalsDeep(Base other_) { 3891 if (!super.equalsDeep(other_)) 3892 return false; 3893 if (!(other_ instanceof TestScriptFixtureComponent)) 3894 return false; 3895 TestScriptFixtureComponent o = (TestScriptFixtureComponent) other_; 3896 return compareDeep(autocreate, o.autocreate, true) && compareDeep(autodelete, o.autodelete, true) 3897 && compareDeep(resource, o.resource, true); 3898 } 3899 3900 @Override 3901 public boolean equalsShallow(Base other_) { 3902 if (!super.equalsShallow(other_)) 3903 return false; 3904 if (!(other_ instanceof TestScriptFixtureComponent)) 3905 return false; 3906 TestScriptFixtureComponent o = (TestScriptFixtureComponent) other_; 3907 return compareValues(autocreate, o.autocreate, true) && compareValues(autodelete, o.autodelete, true) 3908 ; 3909 } 3910 3911 public boolean isEmpty() { 3912 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(autocreate, autodelete, resource 3913 ); 3914 } 3915 3916 public String fhirType() { 3917 return "TestScript.fixture"; 3918 3919 } 3920 3921 } 3922 3923 @Block() 3924 public static class TestScriptVariableComponent extends BackboneElement implements IBaseBackboneElement { 3925 /** 3926 * Descriptive name for this variable. 3927 */ 3928 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3929 @Description(shortDefinition="Descriptive name for this variable", formalDefinition="Descriptive name for this variable." ) 3930 protected StringType name; 3931 3932 /** 3933 * A default, hard-coded, or user-defined value for this variable. 3934 */ 3935 @Child(name = "defaultValue", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 3936 @Description(shortDefinition="Default, hard-coded, or user-defined value for this variable", formalDefinition="A default, hard-coded, or user-defined value for this variable." ) 3937 protected StringType defaultValue; 3938 3939 /** 3940 * A free text natural language description of the variable and its purpose. 3941 */ 3942 @Child(name = "description", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 3943 @Description(shortDefinition="Natural language description of the variable", formalDefinition="A free text natural language description of the variable and its purpose." ) 3944 protected StringType description; 3945 3946 /** 3947 * The FHIRPath expression for a specific value to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified. 3948 */ 3949 @Child(name = "expression", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 3950 @Description(shortDefinition="The FHIRPath expression against the fixture body", formalDefinition="The FHIRPath expression for a specific value to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified." ) 3951 protected StringType expression; 3952 3953 /** 3954 * Will be used to grab the HTTP header field value from the headers that sourceId is pointing to. 3955 */ 3956 @Child(name = "headerField", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 3957 @Description(shortDefinition="HTTP header field name for source", formalDefinition="Will be used to grab the HTTP header field value from the headers that sourceId is pointing to." ) 3958 protected StringType headerField; 3959 3960 /** 3961 * Displayable text string with hint help information to the user when entering a default value. 3962 */ 3963 @Child(name = "hint", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 3964 @Description(shortDefinition="Hint help text for default value to enter", formalDefinition="Displayable text string with hint help information to the user when entering a default value." ) 3965 protected StringType hint; 3966 3967 /** 3968 * XPath or JSONPath to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified. 3969 */ 3970 @Child(name = "path", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=false) 3971 @Description(shortDefinition="XPath or JSONPath against the fixture body", formalDefinition="XPath or JSONPath to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified." ) 3972 protected StringType path; 3973 3974 /** 3975 * Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable. 3976 */ 3977 @Child(name = "sourceId", type = {IdType.class}, order=8, min=0, max=1, modifier=false, summary=false) 3978 @Description(shortDefinition="Fixture Id of source expression or headerField within this variable", formalDefinition="Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable." ) 3979 protected IdType sourceId; 3980 3981 private static final long serialVersionUID = -1592325432L; 3982 3983 /** 3984 * Constructor 3985 */ 3986 public TestScriptVariableComponent() { 3987 super(); 3988 } 3989 3990 /** 3991 * Constructor 3992 */ 3993 public TestScriptVariableComponent(String name) { 3994 super(); 3995 this.setName(name); 3996 } 3997 3998 /** 3999 * @return {@link #name} (Descriptive name for this variable.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 4000 */ 4001 public StringType getNameElement() { 4002 if (this.name == null) 4003 if (Configuration.errorOnAutoCreate()) 4004 throw new Error("Attempt to auto-create TestScriptVariableComponent.name"); 4005 else if (Configuration.doAutoCreate()) 4006 this.name = new StringType(); // bb 4007 return this.name; 4008 } 4009 4010 public boolean hasNameElement() { 4011 return this.name != null && !this.name.isEmpty(); 4012 } 4013 4014 public boolean hasName() { 4015 return this.name != null && !this.name.isEmpty(); 4016 } 4017 4018 /** 4019 * @param value {@link #name} (Descriptive name for this variable.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 4020 */ 4021 public TestScriptVariableComponent setNameElement(StringType value) { 4022 this.name = value; 4023 return this; 4024 } 4025 4026 /** 4027 * @return Descriptive name for this variable. 4028 */ 4029 public String getName() { 4030 return this.name == null ? null : this.name.getValue(); 4031 } 4032 4033 /** 4034 * @param value Descriptive name for this variable. 4035 */ 4036 public TestScriptVariableComponent setName(String value) { 4037 if (this.name == null) 4038 this.name = new StringType(); 4039 this.name.setValue(value); 4040 return this; 4041 } 4042 4043 /** 4044 * @return {@link #defaultValue} (A default, hard-coded, or user-defined value for this variable.). This is the underlying object with id, value and extensions. The accessor "getDefaultValue" gives direct access to the value 4045 */ 4046 public StringType getDefaultValueElement() { 4047 if (this.defaultValue == null) 4048 if (Configuration.errorOnAutoCreate()) 4049 throw new Error("Attempt to auto-create TestScriptVariableComponent.defaultValue"); 4050 else if (Configuration.doAutoCreate()) 4051 this.defaultValue = new StringType(); // bb 4052 return this.defaultValue; 4053 } 4054 4055 public boolean hasDefaultValueElement() { 4056 return this.defaultValue != null && !this.defaultValue.isEmpty(); 4057 } 4058 4059 public boolean hasDefaultValue() { 4060 return this.defaultValue != null && !this.defaultValue.isEmpty(); 4061 } 4062 4063 /** 4064 * @param value {@link #defaultValue} (A default, hard-coded, or user-defined value for this variable.). This is the underlying object with id, value and extensions. The accessor "getDefaultValue" gives direct access to the value 4065 */ 4066 public TestScriptVariableComponent setDefaultValueElement(StringType value) { 4067 this.defaultValue = value; 4068 return this; 4069 } 4070 4071 /** 4072 * @return A default, hard-coded, or user-defined value for this variable. 4073 */ 4074 public String getDefaultValue() { 4075 return this.defaultValue == null ? null : this.defaultValue.getValue(); 4076 } 4077 4078 /** 4079 * @param value A default, hard-coded, or user-defined value for this variable. 4080 */ 4081 public TestScriptVariableComponent setDefaultValue(String value) { 4082 if (Utilities.noString(value)) 4083 this.defaultValue = null; 4084 else { 4085 if (this.defaultValue == null) 4086 this.defaultValue = new StringType(); 4087 this.defaultValue.setValue(value); 4088 } 4089 return this; 4090 } 4091 4092 /** 4093 * @return {@link #description} (A free text natural language description of the variable and its purpose.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4094 */ 4095 public StringType getDescriptionElement() { 4096 if (this.description == null) 4097 if (Configuration.errorOnAutoCreate()) 4098 throw new Error("Attempt to auto-create TestScriptVariableComponent.description"); 4099 else if (Configuration.doAutoCreate()) 4100 this.description = new StringType(); // bb 4101 return this.description; 4102 } 4103 4104 public boolean hasDescriptionElement() { 4105 return this.description != null && !this.description.isEmpty(); 4106 } 4107 4108 public boolean hasDescription() { 4109 return this.description != null && !this.description.isEmpty(); 4110 } 4111 4112 /** 4113 * @param value {@link #description} (A free text natural language description of the variable and its purpose.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4114 */ 4115 public TestScriptVariableComponent setDescriptionElement(StringType value) { 4116 this.description = value; 4117 return this; 4118 } 4119 4120 /** 4121 * @return A free text natural language description of the variable and its purpose. 4122 */ 4123 public String getDescription() { 4124 return this.description == null ? null : this.description.getValue(); 4125 } 4126 4127 /** 4128 * @param value A free text natural language description of the variable and its purpose. 4129 */ 4130 public TestScriptVariableComponent setDescription(String value) { 4131 if (Utilities.noString(value)) 4132 this.description = null; 4133 else { 4134 if (this.description == null) 4135 this.description = new StringType(); 4136 this.description.setValue(value); 4137 } 4138 return this; 4139 } 4140 4141 /** 4142 * @return {@link #expression} (The FHIRPath expression for a specific value to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 4143 */ 4144 public StringType getExpressionElement() { 4145 if (this.expression == null) 4146 if (Configuration.errorOnAutoCreate()) 4147 throw new Error("Attempt to auto-create TestScriptVariableComponent.expression"); 4148 else if (Configuration.doAutoCreate()) 4149 this.expression = new StringType(); // bb 4150 return this.expression; 4151 } 4152 4153 public boolean hasExpressionElement() { 4154 return this.expression != null && !this.expression.isEmpty(); 4155 } 4156 4157 public boolean hasExpression() { 4158 return this.expression != null && !this.expression.isEmpty(); 4159 } 4160 4161 /** 4162 * @param value {@link #expression} (The FHIRPath expression for a specific value to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 4163 */ 4164 public TestScriptVariableComponent setExpressionElement(StringType value) { 4165 this.expression = value; 4166 return this; 4167 } 4168 4169 /** 4170 * @return The FHIRPath expression for a specific value to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified. 4171 */ 4172 public String getExpression() { 4173 return this.expression == null ? null : this.expression.getValue(); 4174 } 4175 4176 /** 4177 * @param value The FHIRPath expression for a specific value to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified. 4178 */ 4179 public TestScriptVariableComponent setExpression(String value) { 4180 if (Utilities.noString(value)) 4181 this.expression = null; 4182 else { 4183 if (this.expression == null) 4184 this.expression = new StringType(); 4185 this.expression.setValue(value); 4186 } 4187 return this; 4188 } 4189 4190 /** 4191 * @return {@link #headerField} (Will be used to grab the HTTP header field value from the headers that sourceId is pointing to.). This is the underlying object with id, value and extensions. The accessor "getHeaderField" gives direct access to the value 4192 */ 4193 public StringType getHeaderFieldElement() { 4194 if (this.headerField == null) 4195 if (Configuration.errorOnAutoCreate()) 4196 throw new Error("Attempt to auto-create TestScriptVariableComponent.headerField"); 4197 else if (Configuration.doAutoCreate()) 4198 this.headerField = new StringType(); // bb 4199 return this.headerField; 4200 } 4201 4202 public boolean hasHeaderFieldElement() { 4203 return this.headerField != null && !this.headerField.isEmpty(); 4204 } 4205 4206 public boolean hasHeaderField() { 4207 return this.headerField != null && !this.headerField.isEmpty(); 4208 } 4209 4210 /** 4211 * @param value {@link #headerField} (Will be used to grab the HTTP header field value from the headers that sourceId is pointing to.). This is the underlying object with id, value and extensions. The accessor "getHeaderField" gives direct access to the value 4212 */ 4213 public TestScriptVariableComponent setHeaderFieldElement(StringType value) { 4214 this.headerField = value; 4215 return this; 4216 } 4217 4218 /** 4219 * @return Will be used to grab the HTTP header field value from the headers that sourceId is pointing to. 4220 */ 4221 public String getHeaderField() { 4222 return this.headerField == null ? null : this.headerField.getValue(); 4223 } 4224 4225 /** 4226 * @param value Will be used to grab the HTTP header field value from the headers that sourceId is pointing to. 4227 */ 4228 public TestScriptVariableComponent setHeaderField(String value) { 4229 if (Utilities.noString(value)) 4230 this.headerField = null; 4231 else { 4232 if (this.headerField == null) 4233 this.headerField = new StringType(); 4234 this.headerField.setValue(value); 4235 } 4236 return this; 4237 } 4238 4239 /** 4240 * @return {@link #hint} (Displayable text string with hint help information to the user when entering a default value.). This is the underlying object with id, value and extensions. The accessor "getHint" gives direct access to the value 4241 */ 4242 public StringType getHintElement() { 4243 if (this.hint == null) 4244 if (Configuration.errorOnAutoCreate()) 4245 throw new Error("Attempt to auto-create TestScriptVariableComponent.hint"); 4246 else if (Configuration.doAutoCreate()) 4247 this.hint = new StringType(); // bb 4248 return this.hint; 4249 } 4250 4251 public boolean hasHintElement() { 4252 return this.hint != null && !this.hint.isEmpty(); 4253 } 4254 4255 public boolean hasHint() { 4256 return this.hint != null && !this.hint.isEmpty(); 4257 } 4258 4259 /** 4260 * @param value {@link #hint} (Displayable text string with hint help information to the user when entering a default value.). This is the underlying object with id, value and extensions. The accessor "getHint" gives direct access to the value 4261 */ 4262 public TestScriptVariableComponent setHintElement(StringType value) { 4263 this.hint = value; 4264 return this; 4265 } 4266 4267 /** 4268 * @return Displayable text string with hint help information to the user when entering a default value. 4269 */ 4270 public String getHint() { 4271 return this.hint == null ? null : this.hint.getValue(); 4272 } 4273 4274 /** 4275 * @param value Displayable text string with hint help information to the user when entering a default value. 4276 */ 4277 public TestScriptVariableComponent setHint(String value) { 4278 if (Utilities.noString(value)) 4279 this.hint = null; 4280 else { 4281 if (this.hint == null) 4282 this.hint = new StringType(); 4283 this.hint.setValue(value); 4284 } 4285 return this; 4286 } 4287 4288 /** 4289 * @return {@link #path} (XPath or JSONPath to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 4290 */ 4291 public StringType getPathElement() { 4292 if (this.path == null) 4293 if (Configuration.errorOnAutoCreate()) 4294 throw new Error("Attempt to auto-create TestScriptVariableComponent.path"); 4295 else if (Configuration.doAutoCreate()) 4296 this.path = new StringType(); // bb 4297 return this.path; 4298 } 4299 4300 public boolean hasPathElement() { 4301 return this.path != null && !this.path.isEmpty(); 4302 } 4303 4304 public boolean hasPath() { 4305 return this.path != null && !this.path.isEmpty(); 4306 } 4307 4308 /** 4309 * @param value {@link #path} (XPath or JSONPath to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 4310 */ 4311 public TestScriptVariableComponent setPathElement(StringType value) { 4312 this.path = value; 4313 return this; 4314 } 4315 4316 /** 4317 * @return XPath or JSONPath to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified. 4318 */ 4319 public String getPath() { 4320 return this.path == null ? null : this.path.getValue(); 4321 } 4322 4323 /** 4324 * @param value XPath or JSONPath to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified. 4325 */ 4326 public TestScriptVariableComponent setPath(String value) { 4327 if (Utilities.noString(value)) 4328 this.path = null; 4329 else { 4330 if (this.path == null) 4331 this.path = new StringType(); 4332 this.path.setValue(value); 4333 } 4334 return this; 4335 } 4336 4337 /** 4338 * @return {@link #sourceId} (Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable.). This is the underlying object with id, value and extensions. The accessor "getSourceId" gives direct access to the value 4339 */ 4340 public IdType getSourceIdElement() { 4341 if (this.sourceId == null) 4342 if (Configuration.errorOnAutoCreate()) 4343 throw new Error("Attempt to auto-create TestScriptVariableComponent.sourceId"); 4344 else if (Configuration.doAutoCreate()) 4345 this.sourceId = new IdType(); // bb 4346 return this.sourceId; 4347 } 4348 4349 public boolean hasSourceIdElement() { 4350 return this.sourceId != null && !this.sourceId.isEmpty(); 4351 } 4352 4353 public boolean hasSourceId() { 4354 return this.sourceId != null && !this.sourceId.isEmpty(); 4355 } 4356 4357 /** 4358 * @param value {@link #sourceId} (Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable.). This is the underlying object with id, value and extensions. The accessor "getSourceId" gives direct access to the value 4359 */ 4360 public TestScriptVariableComponent setSourceIdElement(IdType value) { 4361 this.sourceId = value; 4362 return this; 4363 } 4364 4365 /** 4366 * @return Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable. 4367 */ 4368 public String getSourceId() { 4369 return this.sourceId == null ? null : this.sourceId.getValue(); 4370 } 4371 4372 /** 4373 * @param value Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable. 4374 */ 4375 public TestScriptVariableComponent setSourceId(String value) { 4376 if (Utilities.noString(value)) 4377 this.sourceId = null; 4378 else { 4379 if (this.sourceId == null) 4380 this.sourceId = new IdType(); 4381 this.sourceId.setValue(value); 4382 } 4383 return this; 4384 } 4385 4386 protected void listChildren(List<Property> children) { 4387 super.listChildren(children); 4388 children.add(new Property("name", "string", "Descriptive name for this variable.", 0, 1, name)); 4389 children.add(new Property("defaultValue", "string", "A default, hard-coded, or user-defined value for this variable.", 0, 1, defaultValue)); 4390 children.add(new Property("description", "string", "A free text natural language description of the variable and its purpose.", 0, 1, description)); 4391 children.add(new Property("expression", "string", "The FHIRPath expression for a specific value to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.", 0, 1, expression)); 4392 children.add(new Property("headerField", "string", "Will be used to grab the HTTP header field value from the headers that sourceId is pointing to.", 0, 1, headerField)); 4393 children.add(new Property("hint", "string", "Displayable text string with hint help information to the user when entering a default value.", 0, 1, hint)); 4394 children.add(new Property("path", "string", "XPath or JSONPath to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.", 0, 1, path)); 4395 children.add(new Property("sourceId", "id", "Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable.", 0, 1, sourceId)); 4396 } 4397 4398 @Override 4399 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4400 switch (_hash) { 4401 case 3373707: /*name*/ return new Property("name", "string", "Descriptive name for this variable.", 0, 1, name); 4402 case -659125328: /*defaultValue*/ return new Property("defaultValue", "string", "A default, hard-coded, or user-defined value for this variable.", 0, 1, defaultValue); 4403 case -1724546052: /*description*/ return new Property("description", "string", "A free text natural language description of the variable and its purpose.", 0, 1, description); 4404 case -1795452264: /*expression*/ return new Property("expression", "string", "The FHIRPath expression for a specific value to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.", 0, 1, expression); 4405 case 1160732269: /*headerField*/ return new Property("headerField", "string", "Will be used to grab the HTTP header field value from the headers that sourceId is pointing to.", 0, 1, headerField); 4406 case 3202695: /*hint*/ return new Property("hint", "string", "Displayable text string with hint help information to the user when entering a default value.", 0, 1, hint); 4407 case 3433509: /*path*/ return new Property("path", "string", "XPath or JSONPath to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.", 0, 1, path); 4408 case 1746327190: /*sourceId*/ return new Property("sourceId", "id", "Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable.", 0, 1, sourceId); 4409 default: return super.getNamedProperty(_hash, _name, _checkValid); 4410 } 4411 4412 } 4413 4414 @Override 4415 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4416 switch (hash) { 4417 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 4418 case -659125328: /*defaultValue*/ return this.defaultValue == null ? new Base[0] : new Base[] {this.defaultValue}; // StringType 4419 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 4420 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 4421 case 1160732269: /*headerField*/ return this.headerField == null ? new Base[0] : new Base[] {this.headerField}; // StringType 4422 case 3202695: /*hint*/ return this.hint == null ? new Base[0] : new Base[] {this.hint}; // StringType 4423 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 4424 case 1746327190: /*sourceId*/ return this.sourceId == null ? new Base[0] : new Base[] {this.sourceId}; // IdType 4425 default: return super.getProperty(hash, name, checkValid); 4426 } 4427 4428 } 4429 4430 @Override 4431 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4432 switch (hash) { 4433 case 3373707: // name 4434 this.name = TypeConvertor.castToString(value); // StringType 4435 return value; 4436 case -659125328: // defaultValue 4437 this.defaultValue = TypeConvertor.castToString(value); // StringType 4438 return value; 4439 case -1724546052: // description 4440 this.description = TypeConvertor.castToString(value); // StringType 4441 return value; 4442 case -1795452264: // expression 4443 this.expression = TypeConvertor.castToString(value); // StringType 4444 return value; 4445 case 1160732269: // headerField 4446 this.headerField = TypeConvertor.castToString(value); // StringType 4447 return value; 4448 case 3202695: // hint 4449 this.hint = TypeConvertor.castToString(value); // StringType 4450 return value; 4451 case 3433509: // path 4452 this.path = TypeConvertor.castToString(value); // StringType 4453 return value; 4454 case 1746327190: // sourceId 4455 this.sourceId = TypeConvertor.castToId(value); // IdType 4456 return value; 4457 default: return super.setProperty(hash, name, value); 4458 } 4459 4460 } 4461 4462 @Override 4463 public Base setProperty(String name, Base value) throws FHIRException { 4464 if (name.equals("name")) { 4465 this.name = TypeConvertor.castToString(value); // StringType 4466 } else if (name.equals("defaultValue")) { 4467 this.defaultValue = TypeConvertor.castToString(value); // StringType 4468 } else if (name.equals("description")) { 4469 this.description = TypeConvertor.castToString(value); // StringType 4470 } else if (name.equals("expression")) { 4471 this.expression = TypeConvertor.castToString(value); // StringType 4472 } else if (name.equals("headerField")) { 4473 this.headerField = TypeConvertor.castToString(value); // StringType 4474 } else if (name.equals("hint")) { 4475 this.hint = TypeConvertor.castToString(value); // StringType 4476 } else if (name.equals("path")) { 4477 this.path = TypeConvertor.castToString(value); // StringType 4478 } else if (name.equals("sourceId")) { 4479 this.sourceId = TypeConvertor.castToId(value); // IdType 4480 } else 4481 return super.setProperty(name, value); 4482 return value; 4483 } 4484 4485 @Override 4486 public void removeChild(String name, Base value) throws FHIRException { 4487 if (name.equals("name")) { 4488 this.name = null; 4489 } else if (name.equals("defaultValue")) { 4490 this.defaultValue = null; 4491 } else if (name.equals("description")) { 4492 this.description = null; 4493 } else if (name.equals("expression")) { 4494 this.expression = null; 4495 } else if (name.equals("headerField")) { 4496 this.headerField = null; 4497 } else if (name.equals("hint")) { 4498 this.hint = null; 4499 } else if (name.equals("path")) { 4500 this.path = null; 4501 } else if (name.equals("sourceId")) { 4502 this.sourceId = null; 4503 } else 4504 super.removeChild(name, value); 4505 4506 } 4507 4508 @Override 4509 public Base makeProperty(int hash, String name) throws FHIRException { 4510 switch (hash) { 4511 case 3373707: return getNameElement(); 4512 case -659125328: return getDefaultValueElement(); 4513 case -1724546052: return getDescriptionElement(); 4514 case -1795452264: return getExpressionElement(); 4515 case 1160732269: return getHeaderFieldElement(); 4516 case 3202695: return getHintElement(); 4517 case 3433509: return getPathElement(); 4518 case 1746327190: return getSourceIdElement(); 4519 default: return super.makeProperty(hash, name); 4520 } 4521 4522 } 4523 4524 @Override 4525 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4526 switch (hash) { 4527 case 3373707: /*name*/ return new String[] {"string"}; 4528 case -659125328: /*defaultValue*/ return new String[] {"string"}; 4529 case -1724546052: /*description*/ return new String[] {"string"}; 4530 case -1795452264: /*expression*/ return new String[] {"string"}; 4531 case 1160732269: /*headerField*/ return new String[] {"string"}; 4532 case 3202695: /*hint*/ return new String[] {"string"}; 4533 case 3433509: /*path*/ return new String[] {"string"}; 4534 case 1746327190: /*sourceId*/ return new String[] {"id"}; 4535 default: return super.getTypesForProperty(hash, name); 4536 } 4537 4538 } 4539 4540 @Override 4541 public Base addChild(String name) throws FHIRException { 4542 if (name.equals("name")) { 4543 throw new FHIRException("Cannot call addChild on a singleton property TestScript.variable.name"); 4544 } 4545 else if (name.equals("defaultValue")) { 4546 throw new FHIRException("Cannot call addChild on a singleton property TestScript.variable.defaultValue"); 4547 } 4548 else if (name.equals("description")) { 4549 throw new FHIRException("Cannot call addChild on a singleton property TestScript.variable.description"); 4550 } 4551 else if (name.equals("expression")) { 4552 throw new FHIRException("Cannot call addChild on a singleton property TestScript.variable.expression"); 4553 } 4554 else if (name.equals("headerField")) { 4555 throw new FHIRException("Cannot call addChild on a singleton property TestScript.variable.headerField"); 4556 } 4557 else if (name.equals("hint")) { 4558 throw new FHIRException("Cannot call addChild on a singleton property TestScript.variable.hint"); 4559 } 4560 else if (name.equals("path")) { 4561 throw new FHIRException("Cannot call addChild on a singleton property TestScript.variable.path"); 4562 } 4563 else if (name.equals("sourceId")) { 4564 throw new FHIRException("Cannot call addChild on a singleton property TestScript.variable.sourceId"); 4565 } 4566 else 4567 return super.addChild(name); 4568 } 4569 4570 public TestScriptVariableComponent copy() { 4571 TestScriptVariableComponent dst = new TestScriptVariableComponent(); 4572 copyValues(dst); 4573 return dst; 4574 } 4575 4576 public void copyValues(TestScriptVariableComponent dst) { 4577 super.copyValues(dst); 4578 dst.name = name == null ? null : name.copy(); 4579 dst.defaultValue = defaultValue == null ? null : defaultValue.copy(); 4580 dst.description = description == null ? null : description.copy(); 4581 dst.expression = expression == null ? null : expression.copy(); 4582 dst.headerField = headerField == null ? null : headerField.copy(); 4583 dst.hint = hint == null ? null : hint.copy(); 4584 dst.path = path == null ? null : path.copy(); 4585 dst.sourceId = sourceId == null ? null : sourceId.copy(); 4586 } 4587 4588 @Override 4589 public boolean equalsDeep(Base other_) { 4590 if (!super.equalsDeep(other_)) 4591 return false; 4592 if (!(other_ instanceof TestScriptVariableComponent)) 4593 return false; 4594 TestScriptVariableComponent o = (TestScriptVariableComponent) other_; 4595 return compareDeep(name, o.name, true) && compareDeep(defaultValue, o.defaultValue, true) && compareDeep(description, o.description, true) 4596 && compareDeep(expression, o.expression, true) && compareDeep(headerField, o.headerField, true) 4597 && compareDeep(hint, o.hint, true) && compareDeep(path, o.path, true) && compareDeep(sourceId, o.sourceId, true) 4598 ; 4599 } 4600 4601 @Override 4602 public boolean equalsShallow(Base other_) { 4603 if (!super.equalsShallow(other_)) 4604 return false; 4605 if (!(other_ instanceof TestScriptVariableComponent)) 4606 return false; 4607 TestScriptVariableComponent o = (TestScriptVariableComponent) other_; 4608 return compareValues(name, o.name, true) && compareValues(defaultValue, o.defaultValue, true) && compareValues(description, o.description, true) 4609 && compareValues(expression, o.expression, true) && compareValues(headerField, o.headerField, true) 4610 && compareValues(hint, o.hint, true) && compareValues(path, o.path, true) && compareValues(sourceId, o.sourceId, true) 4611 ; 4612 } 4613 4614 public boolean isEmpty() { 4615 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, defaultValue, description 4616 , expression, headerField, hint, path, sourceId); 4617 } 4618 4619 public String fhirType() { 4620 return "TestScript.variable"; 4621 4622 } 4623 4624 } 4625 4626 @Block() 4627 public static class TestScriptSetupComponent extends BackboneElement implements IBaseBackboneElement { 4628 /** 4629 * Action would contain either an operation or an assertion. 4630 */ 4631 @Child(name = "action", type = {}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4632 @Description(shortDefinition="A setup operation or assert to perform", formalDefinition="Action would contain either an operation or an assertion." ) 4633 protected List<SetupActionComponent> action; 4634 4635 private static final long serialVersionUID = -123374486L; 4636 4637 /** 4638 * Constructor 4639 */ 4640 public TestScriptSetupComponent() { 4641 super(); 4642 } 4643 4644 /** 4645 * Constructor 4646 */ 4647 public TestScriptSetupComponent(SetupActionComponent action) { 4648 super(); 4649 this.addAction(action); 4650 } 4651 4652 /** 4653 * @return {@link #action} (Action would contain either an operation or an assertion.) 4654 */ 4655 public List<SetupActionComponent> getAction() { 4656 if (this.action == null) 4657 this.action = new ArrayList<SetupActionComponent>(); 4658 return this.action; 4659 } 4660 4661 /** 4662 * @return Returns a reference to <code>this</code> for easy method chaining 4663 */ 4664 public TestScriptSetupComponent setAction(List<SetupActionComponent> theAction) { 4665 this.action = theAction; 4666 return this; 4667 } 4668 4669 public boolean hasAction() { 4670 if (this.action == null) 4671 return false; 4672 for (SetupActionComponent item : this.action) 4673 if (!item.isEmpty()) 4674 return true; 4675 return false; 4676 } 4677 4678 public SetupActionComponent addAction() { //3 4679 SetupActionComponent t = new SetupActionComponent(); 4680 if (this.action == null) 4681 this.action = new ArrayList<SetupActionComponent>(); 4682 this.action.add(t); 4683 return t; 4684 } 4685 4686 public TestScriptSetupComponent addAction(SetupActionComponent t) { //3 4687 if (t == null) 4688 return this; 4689 if (this.action == null) 4690 this.action = new ArrayList<SetupActionComponent>(); 4691 this.action.add(t); 4692 return this; 4693 } 4694 4695 /** 4696 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist {3} 4697 */ 4698 public SetupActionComponent getActionFirstRep() { 4699 if (getAction().isEmpty()) { 4700 addAction(); 4701 } 4702 return getAction().get(0); 4703 } 4704 4705 protected void listChildren(List<Property> children) { 4706 super.listChildren(children); 4707 children.add(new Property("action", "", "Action would contain either an operation or an assertion.", 0, java.lang.Integer.MAX_VALUE, action)); 4708 } 4709 4710 @Override 4711 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4712 switch (_hash) { 4713 case -1422950858: /*action*/ return new Property("action", "", "Action would contain either an operation or an assertion.", 0, java.lang.Integer.MAX_VALUE, action); 4714 default: return super.getNamedProperty(_hash, _name, _checkValid); 4715 } 4716 4717 } 4718 4719 @Override 4720 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4721 switch (hash) { 4722 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // SetupActionComponent 4723 default: return super.getProperty(hash, name, checkValid); 4724 } 4725 4726 } 4727 4728 @Override 4729 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4730 switch (hash) { 4731 case -1422950858: // action 4732 this.getAction().add((SetupActionComponent) value); // SetupActionComponent 4733 return value; 4734 default: return super.setProperty(hash, name, value); 4735 } 4736 4737 } 4738 4739 @Override 4740 public Base setProperty(String name, Base value) throws FHIRException { 4741 if (name.equals("action")) { 4742 this.getAction().add((SetupActionComponent) value); 4743 } else 4744 return super.setProperty(name, value); 4745 return value; 4746 } 4747 4748 @Override 4749 public void removeChild(String name, Base value) throws FHIRException { 4750 if (name.equals("action")) { 4751 this.getAction().remove((SetupActionComponent) value); 4752 } else 4753 super.removeChild(name, value); 4754 4755 } 4756 4757 @Override 4758 public Base makeProperty(int hash, String name) throws FHIRException { 4759 switch (hash) { 4760 case -1422950858: return addAction(); 4761 default: return super.makeProperty(hash, name); 4762 } 4763 4764 } 4765 4766 @Override 4767 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4768 switch (hash) { 4769 case -1422950858: /*action*/ return new String[] {}; 4770 default: return super.getTypesForProperty(hash, name); 4771 } 4772 4773 } 4774 4775 @Override 4776 public Base addChild(String name) throws FHIRException { 4777 if (name.equals("action")) { 4778 return addAction(); 4779 } 4780 else 4781 return super.addChild(name); 4782 } 4783 4784 public TestScriptSetupComponent copy() { 4785 TestScriptSetupComponent dst = new TestScriptSetupComponent(); 4786 copyValues(dst); 4787 return dst; 4788 } 4789 4790 public void copyValues(TestScriptSetupComponent dst) { 4791 super.copyValues(dst); 4792 if (action != null) { 4793 dst.action = new ArrayList<SetupActionComponent>(); 4794 for (SetupActionComponent i : action) 4795 dst.action.add(i.copy()); 4796 }; 4797 } 4798 4799 @Override 4800 public boolean equalsDeep(Base other_) { 4801 if (!super.equalsDeep(other_)) 4802 return false; 4803 if (!(other_ instanceof TestScriptSetupComponent)) 4804 return false; 4805 TestScriptSetupComponent o = (TestScriptSetupComponent) other_; 4806 return compareDeep(action, o.action, true); 4807 } 4808 4809 @Override 4810 public boolean equalsShallow(Base other_) { 4811 if (!super.equalsShallow(other_)) 4812 return false; 4813 if (!(other_ instanceof TestScriptSetupComponent)) 4814 return false; 4815 TestScriptSetupComponent o = (TestScriptSetupComponent) other_; 4816 return true; 4817 } 4818 4819 public boolean isEmpty() { 4820 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action); 4821 } 4822 4823 public String fhirType() { 4824 return "TestScript.setup"; 4825 4826 } 4827 4828 } 4829 4830 @Block() 4831 public static class SetupActionComponent extends BackboneElement implements IBaseBackboneElement { 4832 /** 4833 * The operation to perform. 4834 */ 4835 @Child(name = "operation", type = {}, order=1, min=0, max=1, modifier=false, summary=false) 4836 @Description(shortDefinition="The setup operation to perform", formalDefinition="The operation to perform." ) 4837 protected SetupActionOperationComponent operation; 4838 4839 /** 4840 * Evaluates the results of previous operations to determine if the server under test behaves appropriately. 4841 */ 4842 @Child(name = "assert", type = {}, order=2, min=0, max=1, modifier=false, summary=false) 4843 @Description(shortDefinition="The assertion to perform", formalDefinition="Evaluates the results of previous operations to determine if the server under test behaves appropriately." ) 4844 protected SetupActionAssertComponent assert_; 4845 4846 private static final long serialVersionUID = -252088305L; 4847 4848 /** 4849 * Constructor 4850 */ 4851 public SetupActionComponent() { 4852 super(); 4853 } 4854 4855 /** 4856 * @return {@link #operation} (The operation to perform.) 4857 */ 4858 public SetupActionOperationComponent getOperation() { 4859 if (this.operation == null) 4860 if (Configuration.errorOnAutoCreate()) 4861 throw new Error("Attempt to auto-create SetupActionComponent.operation"); 4862 else if (Configuration.doAutoCreate()) 4863 this.operation = new SetupActionOperationComponent(); // cc 4864 return this.operation; 4865 } 4866 4867 public boolean hasOperation() { 4868 return this.operation != null && !this.operation.isEmpty(); 4869 } 4870 4871 /** 4872 * @param value {@link #operation} (The operation to perform.) 4873 */ 4874 public SetupActionComponent setOperation(SetupActionOperationComponent value) { 4875 this.operation = value; 4876 return this; 4877 } 4878 4879 /** 4880 * @return {@link #assert_} (Evaluates the results of previous operations to determine if the server under test behaves appropriately.) 4881 */ 4882 public SetupActionAssertComponent getAssert() { 4883 if (this.assert_ == null) 4884 if (Configuration.errorOnAutoCreate()) 4885 throw new Error("Attempt to auto-create SetupActionComponent.assert_"); 4886 else if (Configuration.doAutoCreate()) 4887 this.assert_ = new SetupActionAssertComponent(); // cc 4888 return this.assert_; 4889 } 4890 4891 public boolean hasAssert() { 4892 return this.assert_ != null && !this.assert_.isEmpty(); 4893 } 4894 4895 /** 4896 * @param value {@link #assert_} (Evaluates the results of previous operations to determine if the server under test behaves appropriately.) 4897 */ 4898 public SetupActionComponent setAssert(SetupActionAssertComponent value) { 4899 this.assert_ = value; 4900 return this; 4901 } 4902 4903 protected void listChildren(List<Property> children) { 4904 super.listChildren(children); 4905 children.add(new Property("operation", "", "The operation to perform.", 0, 1, operation)); 4906 children.add(new Property("assert", "", "Evaluates the results of previous operations to determine if the server under test behaves appropriately.", 0, 1, assert_)); 4907 } 4908 4909 @Override 4910 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4911 switch (_hash) { 4912 case 1662702951: /*operation*/ return new Property("operation", "", "The operation to perform.", 0, 1, operation); 4913 case -1408208058: /*assert*/ return new Property("assert", "", "Evaluates the results of previous operations to determine if the server under test behaves appropriately.", 0, 1, assert_); 4914 default: return super.getNamedProperty(_hash, _name, _checkValid); 4915 } 4916 4917 } 4918 4919 @Override 4920 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4921 switch (hash) { 4922 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : new Base[] {this.operation}; // SetupActionOperationComponent 4923 case -1408208058: /*assert*/ return this.assert_ == null ? new Base[0] : new Base[] {this.assert_}; // SetupActionAssertComponent 4924 default: return super.getProperty(hash, name, checkValid); 4925 } 4926 4927 } 4928 4929 @Override 4930 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4931 switch (hash) { 4932 case 1662702951: // operation 4933 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 4934 return value; 4935 case -1408208058: // assert 4936 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 4937 return value; 4938 default: return super.setProperty(hash, name, value); 4939 } 4940 4941 } 4942 4943 @Override 4944 public Base setProperty(String name, Base value) throws FHIRException { 4945 if (name.equals("operation")) { 4946 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 4947 } else if (name.equals("assert")) { 4948 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 4949 } else 4950 return super.setProperty(name, value); 4951 return value; 4952 } 4953 4954 @Override 4955 public void removeChild(String name, Base value) throws FHIRException { 4956 if (name.equals("operation")) { 4957 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 4958 } else if (name.equals("assert")) { 4959 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 4960 } else 4961 super.removeChild(name, value); 4962 4963 } 4964 4965 @Override 4966 public Base makeProperty(int hash, String name) throws FHIRException { 4967 switch (hash) { 4968 case 1662702951: return getOperation(); 4969 case -1408208058: return getAssert(); 4970 default: return super.makeProperty(hash, name); 4971 } 4972 4973 } 4974 4975 @Override 4976 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4977 switch (hash) { 4978 case 1662702951: /*operation*/ return new String[] {}; 4979 case -1408208058: /*assert*/ return new String[] {}; 4980 default: return super.getTypesForProperty(hash, name); 4981 } 4982 4983 } 4984 4985 @Override 4986 public Base addChild(String name) throws FHIRException { 4987 if (name.equals("operation")) { 4988 this.operation = new SetupActionOperationComponent(); 4989 return this.operation; 4990 } 4991 else if (name.equals("assert")) { 4992 this.assert_ = new SetupActionAssertComponent(); 4993 return this.assert_; 4994 } 4995 else 4996 return super.addChild(name); 4997 } 4998 4999 public SetupActionComponent copy() { 5000 SetupActionComponent dst = new SetupActionComponent(); 5001 copyValues(dst); 5002 return dst; 5003 } 5004 5005 public void copyValues(SetupActionComponent dst) { 5006 super.copyValues(dst); 5007 dst.operation = operation == null ? null : operation.copy(); 5008 dst.assert_ = assert_ == null ? null : assert_.copy(); 5009 } 5010 5011 @Override 5012 public boolean equalsDeep(Base other_) { 5013 if (!super.equalsDeep(other_)) 5014 return false; 5015 if (!(other_ instanceof SetupActionComponent)) 5016 return false; 5017 SetupActionComponent o = (SetupActionComponent) other_; 5018 return compareDeep(operation, o.operation, true) && compareDeep(assert_, o.assert_, true); 5019 } 5020 5021 @Override 5022 public boolean equalsShallow(Base other_) { 5023 if (!super.equalsShallow(other_)) 5024 return false; 5025 if (!(other_ instanceof SetupActionComponent)) 5026 return false; 5027 SetupActionComponent o = (SetupActionComponent) other_; 5028 return true; 5029 } 5030 5031 public boolean isEmpty() { 5032 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation, assert_); 5033 } 5034 5035 public String fhirType() { 5036 return "TestScript.setup.action"; 5037 5038 } 5039 5040 } 5041 5042 @Block() 5043 public static class SetupActionOperationComponent extends BackboneElement implements IBaseBackboneElement { 5044 /** 5045 * Server interaction or operation type. 5046 */ 5047 @Child(name = "type", type = {Coding.class}, order=1, min=0, max=1, modifier=false, summary=false) 5048 @Description(shortDefinition="The operation code type that will be executed", formalDefinition="Server interaction or operation type." ) 5049 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/testscript-operation-codes") 5050 protected Coding type; 5051 5052 /** 5053 * The type of the FHIR resource. See the [resource list](resourcelist.html). Data type of uri is needed when non-HL7 artifacts are identified. 5054 */ 5055 @Child(name = "resource", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 5056 @Description(shortDefinition="Resource type", formalDefinition="The type of the FHIR resource. See the [resource list](resourcelist.html). Data type of uri is needed when non-HL7 artifacts are identified." ) 5057 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/concrete-fhir-types") 5058 protected UriType resource; 5059 5060 /** 5061 * The label would be used for tracking/logging purposes by test engines. 5062 */ 5063 @Child(name = "label", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 5064 @Description(shortDefinition="Tracking/logging operation label", formalDefinition="The label would be used for tracking/logging purposes by test engines." ) 5065 protected StringType label; 5066 5067 /** 5068 * The description would be used by test engines for tracking and reporting purposes. 5069 */ 5070 @Child(name = "description", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 5071 @Description(shortDefinition="Tracking/reporting operation description", formalDefinition="The description would be used by test engines for tracking and reporting purposes." ) 5072 protected StringType description; 5073 5074 /** 5075 * The mime-type to use for RESTful operation in the 'Accept' header. 5076 */ 5077 @Child(name = "accept", type = {CodeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 5078 @Description(shortDefinition="Mime type to accept in the payload of the response, with charset etc", formalDefinition="The mime-type to use for RESTful operation in the 'Accept' header." ) 5079 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/mimetypes") 5080 protected CodeType accept; 5081 5082 /** 5083 * The mime-type to use for RESTful operation in the 'Content-Type' header. 5084 */ 5085 @Child(name = "contentType", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 5086 @Description(shortDefinition="Mime type of the request payload contents, with charset etc", formalDefinition="The mime-type to use for RESTful operation in the 'Content-Type' header." ) 5087 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/mimetypes") 5088 protected CodeType contentType; 5089 5090 /** 5091 * The server where the request message is destined for. Must be one of the server numbers listed in TestScript.destination section. 5092 */ 5093 @Child(name = "destination", type = {IntegerType.class}, order=7, min=0, max=1, modifier=false, summary=false) 5094 @Description(shortDefinition="Server responding to the request", formalDefinition="The server where the request message is destined for. Must be one of the server numbers listed in TestScript.destination section." ) 5095 protected IntegerType destination; 5096 5097 /** 5098 * Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths. 5099 */ 5100 @Child(name = "encodeRequestUrl", type = {BooleanType.class}, order=8, min=1, max=1, modifier=false, summary=false) 5101 @Description(shortDefinition="Whether or not to send the request url in encoded format", formalDefinition="Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths." ) 5102 protected BooleanType encodeRequestUrl; 5103 5104 /** 5105 * The HTTP method the test engine MUST use for this operation regardless of any other operation details. 5106 */ 5107 @Child(name = "method", type = {CodeType.class}, order=9, min=0, max=1, modifier=false, summary=false) 5108 @Description(shortDefinition="delete | get | options | patch | post | put | head", formalDefinition="The HTTP method the test engine MUST use for this operation regardless of any other operation details." ) 5109 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/http-operations") 5110 protected Enumeration<TestScriptRequestMethodCode> method; 5111 5112 /** 5113 * The server where the request message originates from. Must be one of the server numbers listed in TestScript.origin section. 5114 */ 5115 @Child(name = "origin", type = {IntegerType.class}, order=10, min=0, max=1, modifier=false, summary=false) 5116 @Description(shortDefinition="Server initiating the request", formalDefinition="The server where the request message originates from. Must be one of the server numbers listed in TestScript.origin section." ) 5117 protected IntegerType origin; 5118 5119 /** 5120 * Path plus parameters after [type]. Used to set parts of the request URL explicitly. 5121 */ 5122 @Child(name = "params", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=false) 5123 @Description(shortDefinition="Explicitly defined path parameters", formalDefinition="Path plus parameters after [type]. Used to set parts of the request URL explicitly." ) 5124 protected StringType params; 5125 5126 /** 5127 * Header elements would be used to set HTTP headers. 5128 */ 5129 @Child(name = "requestHeader", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5130 @Description(shortDefinition="Each operation can have one or more header elements", formalDefinition="Header elements would be used to set HTTP headers." ) 5131 protected List<SetupActionOperationRequestHeaderComponent> requestHeader; 5132 5133 /** 5134 * The fixture id (maybe new) to map to the request. 5135 */ 5136 @Child(name = "requestId", type = {IdType.class}, order=13, min=0, max=1, modifier=false, summary=false) 5137 @Description(shortDefinition="Fixture Id of mapped request", formalDefinition="The fixture id (maybe new) to map to the request." ) 5138 protected IdType requestId; 5139 5140 /** 5141 * The fixture id (maybe new) to map to the response. 5142 */ 5143 @Child(name = "responseId", type = {IdType.class}, order=14, min=0, max=1, modifier=false, summary=false) 5144 @Description(shortDefinition="Fixture Id of mapped response", formalDefinition="The fixture id (maybe new) to map to the response." ) 5145 protected IdType responseId; 5146 5147 /** 5148 * The id of the fixture used as the body of a PUT or POST request. 5149 */ 5150 @Child(name = "sourceId", type = {IdType.class}, order=15, min=0, max=1, modifier=false, summary=false) 5151 @Description(shortDefinition="Fixture Id of body for PUT and POST requests", formalDefinition="The id of the fixture used as the body of a PUT or POST request." ) 5152 protected IdType sourceId; 5153 5154 /** 5155 * Id of fixture used for extracting the [id], [type], and [vid] for GET requests. 5156 */ 5157 @Child(name = "targetId", type = {IdType.class}, order=16, min=0, max=1, modifier=false, summary=false) 5158 @Description(shortDefinition="Id of fixture used for extracting the [id], [type], and [vid] for GET requests", formalDefinition="Id of fixture used for extracting the [id], [type], and [vid] for GET requests." ) 5159 protected IdType targetId; 5160 5161 /** 5162 * Complete request URL. 5163 */ 5164 @Child(name = "url", type = {StringType.class}, order=17, min=0, max=1, modifier=false, summary=false) 5165 @Description(shortDefinition="Request URL", formalDefinition="Complete request URL." ) 5166 protected StringType url; 5167 5168 private static final long serialVersionUID = 308704897L; 5169 5170 /** 5171 * Constructor 5172 */ 5173 public SetupActionOperationComponent() { 5174 super(); 5175 } 5176 5177 /** 5178 * Constructor 5179 */ 5180 public SetupActionOperationComponent(boolean encodeRequestUrl) { 5181 super(); 5182 this.setEncodeRequestUrl(encodeRequestUrl); 5183 } 5184 5185 /** 5186 * @return {@link #type} (Server interaction or operation type.) 5187 */ 5188 public Coding getType() { 5189 if (this.type == null) 5190 if (Configuration.errorOnAutoCreate()) 5191 throw new Error("Attempt to auto-create SetupActionOperationComponent.type"); 5192 else if (Configuration.doAutoCreate()) 5193 this.type = new Coding(); // cc 5194 return this.type; 5195 } 5196 5197 public boolean hasType() { 5198 return this.type != null && !this.type.isEmpty(); 5199 } 5200 5201 /** 5202 * @param value {@link #type} (Server interaction or operation type.) 5203 */ 5204 public SetupActionOperationComponent setType(Coding value) { 5205 this.type = value; 5206 return this; 5207 } 5208 5209 /** 5210 * @return {@link #resource} (The type of the FHIR resource. See the [resource list](resourcelist.html). Data type of uri is needed when non-HL7 artifacts are identified.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 5211 */ 5212 public UriType getResourceElement() { 5213 if (this.resource == null) 5214 if (Configuration.errorOnAutoCreate()) 5215 throw new Error("Attempt to auto-create SetupActionOperationComponent.resource"); 5216 else if (Configuration.doAutoCreate()) 5217 this.resource = new UriType(); // bb 5218 return this.resource; 5219 } 5220 5221 public boolean hasResourceElement() { 5222 return this.resource != null && !this.resource.isEmpty(); 5223 } 5224 5225 public boolean hasResource() { 5226 return this.resource != null && !this.resource.isEmpty(); 5227 } 5228 5229 /** 5230 * @param value {@link #resource} (The type of the FHIR resource. See the [resource list](resourcelist.html). Data type of uri is needed when non-HL7 artifacts are identified.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 5231 */ 5232 public SetupActionOperationComponent setResourceElement(UriType value) { 5233 this.resource = value; 5234 return this; 5235 } 5236 5237 /** 5238 * @return The type of the FHIR resource. See the [resource list](resourcelist.html). Data type of uri is needed when non-HL7 artifacts are identified. 5239 */ 5240 public String getResource() { 5241 return this.resource == null ? null : this.resource.getValue(); 5242 } 5243 5244 /** 5245 * @param value The type of the FHIR resource. See the [resource list](resourcelist.html). Data type of uri is needed when non-HL7 artifacts are identified. 5246 */ 5247 public SetupActionOperationComponent setResource(String value) { 5248 if (Utilities.noString(value)) 5249 this.resource = null; 5250 else { 5251 if (this.resource == null) 5252 this.resource = new UriType(); 5253 this.resource.setValue(value); 5254 } 5255 return this; 5256 } 5257 5258 /** 5259 * @return {@link #label} (The label would be used for tracking/logging purposes by test engines.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 5260 */ 5261 public StringType getLabelElement() { 5262 if (this.label == null) 5263 if (Configuration.errorOnAutoCreate()) 5264 throw new Error("Attempt to auto-create SetupActionOperationComponent.label"); 5265 else if (Configuration.doAutoCreate()) 5266 this.label = new StringType(); // bb 5267 return this.label; 5268 } 5269 5270 public boolean hasLabelElement() { 5271 return this.label != null && !this.label.isEmpty(); 5272 } 5273 5274 public boolean hasLabel() { 5275 return this.label != null && !this.label.isEmpty(); 5276 } 5277 5278 /** 5279 * @param value {@link #label} (The label would be used for tracking/logging purposes by test engines.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 5280 */ 5281 public SetupActionOperationComponent setLabelElement(StringType value) { 5282 this.label = value; 5283 return this; 5284 } 5285 5286 /** 5287 * @return The label would be used for tracking/logging purposes by test engines. 5288 */ 5289 public String getLabel() { 5290 return this.label == null ? null : this.label.getValue(); 5291 } 5292 5293 /** 5294 * @param value The label would be used for tracking/logging purposes by test engines. 5295 */ 5296 public SetupActionOperationComponent setLabel(String value) { 5297 if (Utilities.noString(value)) 5298 this.label = null; 5299 else { 5300 if (this.label == null) 5301 this.label = new StringType(); 5302 this.label.setValue(value); 5303 } 5304 return this; 5305 } 5306 5307 /** 5308 * @return {@link #description} (The description would be used by test engines for tracking and reporting purposes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 5309 */ 5310 public StringType getDescriptionElement() { 5311 if (this.description == null) 5312 if (Configuration.errorOnAutoCreate()) 5313 throw new Error("Attempt to auto-create SetupActionOperationComponent.description"); 5314 else if (Configuration.doAutoCreate()) 5315 this.description = new StringType(); // bb 5316 return this.description; 5317 } 5318 5319 public boolean hasDescriptionElement() { 5320 return this.description != null && !this.description.isEmpty(); 5321 } 5322 5323 public boolean hasDescription() { 5324 return this.description != null && !this.description.isEmpty(); 5325 } 5326 5327 /** 5328 * @param value {@link #description} (The description would be used by test engines for tracking and reporting purposes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 5329 */ 5330 public SetupActionOperationComponent setDescriptionElement(StringType value) { 5331 this.description = value; 5332 return this; 5333 } 5334 5335 /** 5336 * @return The description would be used by test engines for tracking and reporting purposes. 5337 */ 5338 public String getDescription() { 5339 return this.description == null ? null : this.description.getValue(); 5340 } 5341 5342 /** 5343 * @param value The description would be used by test engines for tracking and reporting purposes. 5344 */ 5345 public SetupActionOperationComponent setDescription(String value) { 5346 if (Utilities.noString(value)) 5347 this.description = null; 5348 else { 5349 if (this.description == null) 5350 this.description = new StringType(); 5351 this.description.setValue(value); 5352 } 5353 return this; 5354 } 5355 5356 /** 5357 * @return {@link #accept} (The mime-type to use for RESTful operation in the 'Accept' header.). This is the underlying object with id, value and extensions. The accessor "getAccept" gives direct access to the value 5358 */ 5359 public CodeType getAcceptElement() { 5360 if (this.accept == null) 5361 if (Configuration.errorOnAutoCreate()) 5362 throw new Error("Attempt to auto-create SetupActionOperationComponent.accept"); 5363 else if (Configuration.doAutoCreate()) 5364 this.accept = new CodeType(); // bb 5365 return this.accept; 5366 } 5367 5368 public boolean hasAcceptElement() { 5369 return this.accept != null && !this.accept.isEmpty(); 5370 } 5371 5372 public boolean hasAccept() { 5373 return this.accept != null && !this.accept.isEmpty(); 5374 } 5375 5376 /** 5377 * @param value {@link #accept} (The mime-type to use for RESTful operation in the 'Accept' header.). This is the underlying object with id, value and extensions. The accessor "getAccept" gives direct access to the value 5378 */ 5379 public SetupActionOperationComponent setAcceptElement(CodeType value) { 5380 this.accept = value; 5381 return this; 5382 } 5383 5384 /** 5385 * @return The mime-type to use for RESTful operation in the 'Accept' header. 5386 */ 5387 public String getAccept() { 5388 return this.accept == null ? null : this.accept.getValue(); 5389 } 5390 5391 /** 5392 * @param value The mime-type to use for RESTful operation in the 'Accept' header. 5393 */ 5394 public SetupActionOperationComponent setAccept(String value) { 5395 if (Utilities.noString(value)) 5396 this.accept = null; 5397 else { 5398 if (this.accept == null) 5399 this.accept = new CodeType(); 5400 this.accept.setValue(value); 5401 } 5402 return this; 5403 } 5404 5405 /** 5406 * @return {@link #contentType} (The mime-type to use for RESTful operation in the 'Content-Type' header.). This is the underlying object with id, value and extensions. The accessor "getContentType" gives direct access to the value 5407 */ 5408 public CodeType getContentTypeElement() { 5409 if (this.contentType == null) 5410 if (Configuration.errorOnAutoCreate()) 5411 throw new Error("Attempt to auto-create SetupActionOperationComponent.contentType"); 5412 else if (Configuration.doAutoCreate()) 5413 this.contentType = new CodeType(); // bb 5414 return this.contentType; 5415 } 5416 5417 public boolean hasContentTypeElement() { 5418 return this.contentType != null && !this.contentType.isEmpty(); 5419 } 5420 5421 public boolean hasContentType() { 5422 return this.contentType != null && !this.contentType.isEmpty(); 5423 } 5424 5425 /** 5426 * @param value {@link #contentType} (The mime-type to use for RESTful operation in the 'Content-Type' header.). This is the underlying object with id, value and extensions. The accessor "getContentType" gives direct access to the value 5427 */ 5428 public SetupActionOperationComponent setContentTypeElement(CodeType value) { 5429 this.contentType = value; 5430 return this; 5431 } 5432 5433 /** 5434 * @return The mime-type to use for RESTful operation in the 'Content-Type' header. 5435 */ 5436 public String getContentType() { 5437 return this.contentType == null ? null : this.contentType.getValue(); 5438 } 5439 5440 /** 5441 * @param value The mime-type to use for RESTful operation in the 'Content-Type' header. 5442 */ 5443 public SetupActionOperationComponent setContentType(String value) { 5444 if (Utilities.noString(value)) 5445 this.contentType = null; 5446 else { 5447 if (this.contentType == null) 5448 this.contentType = new CodeType(); 5449 this.contentType.setValue(value); 5450 } 5451 return this; 5452 } 5453 5454 /** 5455 * @return {@link #destination} (The server where the request message is destined for. Must be one of the server numbers listed in TestScript.destination section.). This is the underlying object with id, value and extensions. The accessor "getDestination" gives direct access to the value 5456 */ 5457 public IntegerType getDestinationElement() { 5458 if (this.destination == null) 5459 if (Configuration.errorOnAutoCreate()) 5460 throw new Error("Attempt to auto-create SetupActionOperationComponent.destination"); 5461 else if (Configuration.doAutoCreate()) 5462 this.destination = new IntegerType(); // bb 5463 return this.destination; 5464 } 5465 5466 public boolean hasDestinationElement() { 5467 return this.destination != null && !this.destination.isEmpty(); 5468 } 5469 5470 public boolean hasDestination() { 5471 return this.destination != null && !this.destination.isEmpty(); 5472 } 5473 5474 /** 5475 * @param value {@link #destination} (The server where the request message is destined for. Must be one of the server numbers listed in TestScript.destination section.). This is the underlying object with id, value and extensions. The accessor "getDestination" gives direct access to the value 5476 */ 5477 public SetupActionOperationComponent setDestinationElement(IntegerType value) { 5478 this.destination = value; 5479 return this; 5480 } 5481 5482 /** 5483 * @return The server where the request message is destined for. Must be one of the server numbers listed in TestScript.destination section. 5484 */ 5485 public int getDestination() { 5486 return this.destination == null || this.destination.isEmpty() ? 0 : this.destination.getValue(); 5487 } 5488 5489 /** 5490 * @param value The server where the request message is destined for. Must be one of the server numbers listed in TestScript.destination section. 5491 */ 5492 public SetupActionOperationComponent setDestination(int value) { 5493 if (this.destination == null) 5494 this.destination = new IntegerType(); 5495 this.destination.setValue(value); 5496 return this; 5497 } 5498 5499 /** 5500 * @return {@link #encodeRequestUrl} (Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths.). This is the underlying object with id, value and extensions. The accessor "getEncodeRequestUrl" gives direct access to the value 5501 */ 5502 public BooleanType getEncodeRequestUrlElement() { 5503 if (this.encodeRequestUrl == null) 5504 if (Configuration.errorOnAutoCreate()) 5505 throw new Error("Attempt to auto-create SetupActionOperationComponent.encodeRequestUrl"); 5506 else if (Configuration.doAutoCreate()) 5507 this.encodeRequestUrl = new BooleanType(); // bb 5508 return this.encodeRequestUrl; 5509 } 5510 5511 public boolean hasEncodeRequestUrlElement() { 5512 return this.encodeRequestUrl != null && !this.encodeRequestUrl.isEmpty(); 5513 } 5514 5515 public boolean hasEncodeRequestUrl() { 5516 return this.encodeRequestUrl != null && !this.encodeRequestUrl.isEmpty(); 5517 } 5518 5519 /** 5520 * @param value {@link #encodeRequestUrl} (Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths.). This is the underlying object with id, value and extensions. The accessor "getEncodeRequestUrl" gives direct access to the value 5521 */ 5522 public SetupActionOperationComponent setEncodeRequestUrlElement(BooleanType value) { 5523 this.encodeRequestUrl = value; 5524 return this; 5525 } 5526 5527 /** 5528 * @return Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths. 5529 */ 5530 public boolean getEncodeRequestUrl() { 5531 return this.encodeRequestUrl == null || this.encodeRequestUrl.isEmpty() ? false : this.encodeRequestUrl.getValue(); 5532 } 5533 5534 /** 5535 * @param value Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths. 5536 */ 5537 public SetupActionOperationComponent setEncodeRequestUrl(boolean value) { 5538 if (this.encodeRequestUrl == null) 5539 this.encodeRequestUrl = new BooleanType(); 5540 this.encodeRequestUrl.setValue(value); 5541 return this; 5542 } 5543 5544 /** 5545 * @return {@link #method} (The HTTP method the test engine MUST use for this operation regardless of any other operation details.). This is the underlying object with id, value and extensions. The accessor "getMethod" gives direct access to the value 5546 */ 5547 public Enumeration<TestScriptRequestMethodCode> getMethodElement() { 5548 if (this.method == null) 5549 if (Configuration.errorOnAutoCreate()) 5550 throw new Error("Attempt to auto-create SetupActionOperationComponent.method"); 5551 else if (Configuration.doAutoCreate()) 5552 this.method = new Enumeration<TestScriptRequestMethodCode>(new TestScriptRequestMethodCodeEnumFactory()); // bb 5553 return this.method; 5554 } 5555 5556 public boolean hasMethodElement() { 5557 return this.method != null && !this.method.isEmpty(); 5558 } 5559 5560 public boolean hasMethod() { 5561 return this.method != null && !this.method.isEmpty(); 5562 } 5563 5564 /** 5565 * @param value {@link #method} (The HTTP method the test engine MUST use for this operation regardless of any other operation details.). This is the underlying object with id, value and extensions. The accessor "getMethod" gives direct access to the value 5566 */ 5567 public SetupActionOperationComponent setMethodElement(Enumeration<TestScriptRequestMethodCode> value) { 5568 this.method = value; 5569 return this; 5570 } 5571 5572 /** 5573 * @return The HTTP method the test engine MUST use for this operation regardless of any other operation details. 5574 */ 5575 public TestScriptRequestMethodCode getMethod() { 5576 return this.method == null ? null : this.method.getValue(); 5577 } 5578 5579 /** 5580 * @param value The HTTP method the test engine MUST use for this operation regardless of any other operation details. 5581 */ 5582 public SetupActionOperationComponent setMethod(TestScriptRequestMethodCode value) { 5583 if (value == null) 5584 this.method = null; 5585 else { 5586 if (this.method == null) 5587 this.method = new Enumeration<TestScriptRequestMethodCode>(new TestScriptRequestMethodCodeEnumFactory()); 5588 this.method.setValue(value); 5589 } 5590 return this; 5591 } 5592 5593 /** 5594 * @return {@link #origin} (The server where the request message originates from. Must be one of the server numbers listed in TestScript.origin section.). This is the underlying object with id, value and extensions. The accessor "getOrigin" gives direct access to the value 5595 */ 5596 public IntegerType getOriginElement() { 5597 if (this.origin == null) 5598 if (Configuration.errorOnAutoCreate()) 5599 throw new Error("Attempt to auto-create SetupActionOperationComponent.origin"); 5600 else if (Configuration.doAutoCreate()) 5601 this.origin = new IntegerType(); // bb 5602 return this.origin; 5603 } 5604 5605 public boolean hasOriginElement() { 5606 return this.origin != null && !this.origin.isEmpty(); 5607 } 5608 5609 public boolean hasOrigin() { 5610 return this.origin != null && !this.origin.isEmpty(); 5611 } 5612 5613 /** 5614 * @param value {@link #origin} (The server where the request message originates from. Must be one of the server numbers listed in TestScript.origin section.). This is the underlying object with id, value and extensions. The accessor "getOrigin" gives direct access to the value 5615 */ 5616 public SetupActionOperationComponent setOriginElement(IntegerType value) { 5617 this.origin = value; 5618 return this; 5619 } 5620 5621 /** 5622 * @return The server where the request message originates from. Must be one of the server numbers listed in TestScript.origin section. 5623 */ 5624 public int getOrigin() { 5625 return this.origin == null || this.origin.isEmpty() ? 0 : this.origin.getValue(); 5626 } 5627 5628 /** 5629 * @param value The server where the request message originates from. Must be one of the server numbers listed in TestScript.origin section. 5630 */ 5631 public SetupActionOperationComponent setOrigin(int value) { 5632 if (this.origin == null) 5633 this.origin = new IntegerType(); 5634 this.origin.setValue(value); 5635 return this; 5636 } 5637 5638 /** 5639 * @return {@link #params} (Path plus parameters after [type]. Used to set parts of the request URL explicitly.). This is the underlying object with id, value and extensions. The accessor "getParams" gives direct access to the value 5640 */ 5641 public StringType getParamsElement() { 5642 if (this.params == null) 5643 if (Configuration.errorOnAutoCreate()) 5644 throw new Error("Attempt to auto-create SetupActionOperationComponent.params"); 5645 else if (Configuration.doAutoCreate()) 5646 this.params = new StringType(); // bb 5647 return this.params; 5648 } 5649 5650 public boolean hasParamsElement() { 5651 return this.params != null && !this.params.isEmpty(); 5652 } 5653 5654 public boolean hasParams() { 5655 return this.params != null && !this.params.isEmpty(); 5656 } 5657 5658 /** 5659 * @param value {@link #params} (Path plus parameters after [type]. Used to set parts of the request URL explicitly.). This is the underlying object with id, value and extensions. The accessor "getParams" gives direct access to the value 5660 */ 5661 public SetupActionOperationComponent setParamsElement(StringType value) { 5662 this.params = value; 5663 return this; 5664 } 5665 5666 /** 5667 * @return Path plus parameters after [type]. Used to set parts of the request URL explicitly. 5668 */ 5669 public String getParams() { 5670 return this.params == null ? null : this.params.getValue(); 5671 } 5672 5673 /** 5674 * @param value Path plus parameters after [type]. Used to set parts of the request URL explicitly. 5675 */ 5676 public SetupActionOperationComponent setParams(String value) { 5677 if (Utilities.noString(value)) 5678 this.params = null; 5679 else { 5680 if (this.params == null) 5681 this.params = new StringType(); 5682 this.params.setValue(value); 5683 } 5684 return this; 5685 } 5686 5687 /** 5688 * @return {@link #requestHeader} (Header elements would be used to set HTTP headers.) 5689 */ 5690 public List<SetupActionOperationRequestHeaderComponent> getRequestHeader() { 5691 if (this.requestHeader == null) 5692 this.requestHeader = new ArrayList<SetupActionOperationRequestHeaderComponent>(); 5693 return this.requestHeader; 5694 } 5695 5696 /** 5697 * @return Returns a reference to <code>this</code> for easy method chaining 5698 */ 5699 public SetupActionOperationComponent setRequestHeader(List<SetupActionOperationRequestHeaderComponent> theRequestHeader) { 5700 this.requestHeader = theRequestHeader; 5701 return this; 5702 } 5703 5704 public boolean hasRequestHeader() { 5705 if (this.requestHeader == null) 5706 return false; 5707 for (SetupActionOperationRequestHeaderComponent item : this.requestHeader) 5708 if (!item.isEmpty()) 5709 return true; 5710 return false; 5711 } 5712 5713 public SetupActionOperationRequestHeaderComponent addRequestHeader() { //3 5714 SetupActionOperationRequestHeaderComponent t = new SetupActionOperationRequestHeaderComponent(); 5715 if (this.requestHeader == null) 5716 this.requestHeader = new ArrayList<SetupActionOperationRequestHeaderComponent>(); 5717 this.requestHeader.add(t); 5718 return t; 5719 } 5720 5721 public SetupActionOperationComponent addRequestHeader(SetupActionOperationRequestHeaderComponent t) { //3 5722 if (t == null) 5723 return this; 5724 if (this.requestHeader == null) 5725 this.requestHeader = new ArrayList<SetupActionOperationRequestHeaderComponent>(); 5726 this.requestHeader.add(t); 5727 return this; 5728 } 5729 5730 /** 5731 * @return The first repetition of repeating field {@link #requestHeader}, creating it if it does not already exist {3} 5732 */ 5733 public SetupActionOperationRequestHeaderComponent getRequestHeaderFirstRep() { 5734 if (getRequestHeader().isEmpty()) { 5735 addRequestHeader(); 5736 } 5737 return getRequestHeader().get(0); 5738 } 5739 5740 /** 5741 * @return {@link #requestId} (The fixture id (maybe new) to map to the request.). This is the underlying object with id, value and extensions. The accessor "getRequestId" gives direct access to the value 5742 */ 5743 public IdType getRequestIdElement() { 5744 if (this.requestId == null) 5745 if (Configuration.errorOnAutoCreate()) 5746 throw new Error("Attempt to auto-create SetupActionOperationComponent.requestId"); 5747 else if (Configuration.doAutoCreate()) 5748 this.requestId = new IdType(); // bb 5749 return this.requestId; 5750 } 5751 5752 public boolean hasRequestIdElement() { 5753 return this.requestId != null && !this.requestId.isEmpty(); 5754 } 5755 5756 public boolean hasRequestId() { 5757 return this.requestId != null && !this.requestId.isEmpty(); 5758 } 5759 5760 /** 5761 * @param value {@link #requestId} (The fixture id (maybe new) to map to the request.). This is the underlying object with id, value and extensions. The accessor "getRequestId" gives direct access to the value 5762 */ 5763 public SetupActionOperationComponent setRequestIdElement(IdType value) { 5764 this.requestId = value; 5765 return this; 5766 } 5767 5768 /** 5769 * @return The fixture id (maybe new) to map to the request. 5770 */ 5771 public String getRequestId() { 5772 return this.requestId == null ? null : this.requestId.getValue(); 5773 } 5774 5775 /** 5776 * @param value The fixture id (maybe new) to map to the request. 5777 */ 5778 public SetupActionOperationComponent setRequestId(String value) { 5779 if (Utilities.noString(value)) 5780 this.requestId = null; 5781 else { 5782 if (this.requestId == null) 5783 this.requestId = new IdType(); 5784 this.requestId.setValue(value); 5785 } 5786 return this; 5787 } 5788 5789 /** 5790 * @return {@link #responseId} (The fixture id (maybe new) to map to the response.). This is the underlying object with id, value and extensions. The accessor "getResponseId" gives direct access to the value 5791 */ 5792 public IdType getResponseIdElement() { 5793 if (this.responseId == null) 5794 if (Configuration.errorOnAutoCreate()) 5795 throw new Error("Attempt to auto-create SetupActionOperationComponent.responseId"); 5796 else if (Configuration.doAutoCreate()) 5797 this.responseId = new IdType(); // bb 5798 return this.responseId; 5799 } 5800 5801 public boolean hasResponseIdElement() { 5802 return this.responseId != null && !this.responseId.isEmpty(); 5803 } 5804 5805 public boolean hasResponseId() { 5806 return this.responseId != null && !this.responseId.isEmpty(); 5807 } 5808 5809 /** 5810 * @param value {@link #responseId} (The fixture id (maybe new) to map to the response.). This is the underlying object with id, value and extensions. The accessor "getResponseId" gives direct access to the value 5811 */ 5812 public SetupActionOperationComponent setResponseIdElement(IdType value) { 5813 this.responseId = value; 5814 return this; 5815 } 5816 5817 /** 5818 * @return The fixture id (maybe new) to map to the response. 5819 */ 5820 public String getResponseId() { 5821 return this.responseId == null ? null : this.responseId.getValue(); 5822 } 5823 5824 /** 5825 * @param value The fixture id (maybe new) to map to the response. 5826 */ 5827 public SetupActionOperationComponent setResponseId(String value) { 5828 if (Utilities.noString(value)) 5829 this.responseId = null; 5830 else { 5831 if (this.responseId == null) 5832 this.responseId = new IdType(); 5833 this.responseId.setValue(value); 5834 } 5835 return this; 5836 } 5837 5838 /** 5839 * @return {@link #sourceId} (The id of the fixture used as the body of a PUT or POST request.). This is the underlying object with id, value and extensions. The accessor "getSourceId" gives direct access to the value 5840 */ 5841 public IdType getSourceIdElement() { 5842 if (this.sourceId == null) 5843 if (Configuration.errorOnAutoCreate()) 5844 throw new Error("Attempt to auto-create SetupActionOperationComponent.sourceId"); 5845 else if (Configuration.doAutoCreate()) 5846 this.sourceId = new IdType(); // bb 5847 return this.sourceId; 5848 } 5849 5850 public boolean hasSourceIdElement() { 5851 return this.sourceId != null && !this.sourceId.isEmpty(); 5852 } 5853 5854 public boolean hasSourceId() { 5855 return this.sourceId != null && !this.sourceId.isEmpty(); 5856 } 5857 5858 /** 5859 * @param value {@link #sourceId} (The id of the fixture used as the body of a PUT or POST request.). This is the underlying object with id, value and extensions. The accessor "getSourceId" gives direct access to the value 5860 */ 5861 public SetupActionOperationComponent setSourceIdElement(IdType value) { 5862 this.sourceId = value; 5863 return this; 5864 } 5865 5866 /** 5867 * @return The id of the fixture used as the body of a PUT or POST request. 5868 */ 5869 public String getSourceId() { 5870 return this.sourceId == null ? null : this.sourceId.getValue(); 5871 } 5872 5873 /** 5874 * @param value The id of the fixture used as the body of a PUT or POST request. 5875 */ 5876 public SetupActionOperationComponent setSourceId(String value) { 5877 if (Utilities.noString(value)) 5878 this.sourceId = null; 5879 else { 5880 if (this.sourceId == null) 5881 this.sourceId = new IdType(); 5882 this.sourceId.setValue(value); 5883 } 5884 return this; 5885 } 5886 5887 /** 5888 * @return {@link #targetId} (Id of fixture used for extracting the [id], [type], and [vid] for GET requests.). This is the underlying object with id, value and extensions. The accessor "getTargetId" gives direct access to the value 5889 */ 5890 public IdType getTargetIdElement() { 5891 if (this.targetId == null) 5892 if (Configuration.errorOnAutoCreate()) 5893 throw new Error("Attempt to auto-create SetupActionOperationComponent.targetId"); 5894 else if (Configuration.doAutoCreate()) 5895 this.targetId = new IdType(); // bb 5896 return this.targetId; 5897 } 5898 5899 public boolean hasTargetIdElement() { 5900 return this.targetId != null && !this.targetId.isEmpty(); 5901 } 5902 5903 public boolean hasTargetId() { 5904 return this.targetId != null && !this.targetId.isEmpty(); 5905 } 5906 5907 /** 5908 * @param value {@link #targetId} (Id of fixture used for extracting the [id], [type], and [vid] for GET requests.). This is the underlying object with id, value and extensions. The accessor "getTargetId" gives direct access to the value 5909 */ 5910 public SetupActionOperationComponent setTargetIdElement(IdType value) { 5911 this.targetId = value; 5912 return this; 5913 } 5914 5915 /** 5916 * @return Id of fixture used for extracting the [id], [type], and [vid] for GET requests. 5917 */ 5918 public String getTargetId() { 5919 return this.targetId == null ? null : this.targetId.getValue(); 5920 } 5921 5922 /** 5923 * @param value Id of fixture used for extracting the [id], [type], and [vid] for GET requests. 5924 */ 5925 public SetupActionOperationComponent setTargetId(String value) { 5926 if (Utilities.noString(value)) 5927 this.targetId = null; 5928 else { 5929 if (this.targetId == null) 5930 this.targetId = new IdType(); 5931 this.targetId.setValue(value); 5932 } 5933 return this; 5934 } 5935 5936 /** 5937 * @return {@link #url} (Complete request URL.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 5938 */ 5939 public StringType getUrlElement() { 5940 if (this.url == null) 5941 if (Configuration.errorOnAutoCreate()) 5942 throw new Error("Attempt to auto-create SetupActionOperationComponent.url"); 5943 else if (Configuration.doAutoCreate()) 5944 this.url = new StringType(); // bb 5945 return this.url; 5946 } 5947 5948 public boolean hasUrlElement() { 5949 return this.url != null && !this.url.isEmpty(); 5950 } 5951 5952 public boolean hasUrl() { 5953 return this.url != null && !this.url.isEmpty(); 5954 } 5955 5956 /** 5957 * @param value {@link #url} (Complete request URL.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 5958 */ 5959 public SetupActionOperationComponent setUrlElement(StringType value) { 5960 this.url = value; 5961 return this; 5962 } 5963 5964 /** 5965 * @return Complete request URL. 5966 */ 5967 public String getUrl() { 5968 return this.url == null ? null : this.url.getValue(); 5969 } 5970 5971 /** 5972 * @param value Complete request URL. 5973 */ 5974 public SetupActionOperationComponent setUrl(String value) { 5975 if (Utilities.noString(value)) 5976 this.url = null; 5977 else { 5978 if (this.url == null) 5979 this.url = new StringType(); 5980 this.url.setValue(value); 5981 } 5982 return this; 5983 } 5984 5985 protected void listChildren(List<Property> children) { 5986 super.listChildren(children); 5987 children.add(new Property("type", "Coding", "Server interaction or operation type.", 0, 1, type)); 5988 children.add(new Property("resource", "uri", "The type of the FHIR resource. See the [resource list](resourcelist.html). Data type of uri is needed when non-HL7 artifacts are identified.", 0, 1, resource)); 5989 children.add(new Property("label", "string", "The label would be used for tracking/logging purposes by test engines.", 0, 1, label)); 5990 children.add(new Property("description", "string", "The description would be used by test engines for tracking and reporting purposes.", 0, 1, description)); 5991 children.add(new Property("accept", "code", "The mime-type to use for RESTful operation in the 'Accept' header.", 0, 1, accept)); 5992 children.add(new Property("contentType", "code", "The mime-type to use for RESTful operation in the 'Content-Type' header.", 0, 1, contentType)); 5993 children.add(new Property("destination", "integer", "The server where the request message is destined for. Must be one of the server numbers listed in TestScript.destination section.", 0, 1, destination)); 5994 children.add(new Property("encodeRequestUrl", "boolean", "Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths.", 0, 1, encodeRequestUrl)); 5995 children.add(new Property("method", "code", "The HTTP method the test engine MUST use for this operation regardless of any other operation details.", 0, 1, method)); 5996 children.add(new Property("origin", "integer", "The server where the request message originates from. Must be one of the server numbers listed in TestScript.origin section.", 0, 1, origin)); 5997 children.add(new Property("params", "string", "Path plus parameters after [type]. Used to set parts of the request URL explicitly.", 0, 1, params)); 5998 children.add(new Property("requestHeader", "", "Header elements would be used to set HTTP headers.", 0, java.lang.Integer.MAX_VALUE, requestHeader)); 5999 children.add(new Property("requestId", "id", "The fixture id (maybe new) to map to the request.", 0, 1, requestId)); 6000 children.add(new Property("responseId", "id", "The fixture id (maybe new) to map to the response.", 0, 1, responseId)); 6001 children.add(new Property("sourceId", "id", "The id of the fixture used as the body of a PUT or POST request.", 0, 1, sourceId)); 6002 children.add(new Property("targetId", "id", "Id of fixture used for extracting the [id], [type], and [vid] for GET requests.", 0, 1, targetId)); 6003 children.add(new Property("url", "string", "Complete request URL.", 0, 1, url)); 6004 } 6005 6006 @Override 6007 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6008 switch (_hash) { 6009 case 3575610: /*type*/ return new Property("type", "Coding", "Server interaction or operation type.", 0, 1, type); 6010 case -341064690: /*resource*/ return new Property("resource", "uri", "The type of the FHIR resource. See the [resource list](resourcelist.html). Data type of uri is needed when non-HL7 artifacts are identified.", 0, 1, resource); 6011 case 102727412: /*label*/ return new Property("label", "string", "The label would be used for tracking/logging purposes by test engines.", 0, 1, label); 6012 case -1724546052: /*description*/ return new Property("description", "string", "The description would be used by test engines for tracking and reporting purposes.", 0, 1, description); 6013 case -1423461112: /*accept*/ return new Property("accept", "code", "The mime-type to use for RESTful operation in the 'Accept' header.", 0, 1, accept); 6014 case -389131437: /*contentType*/ return new Property("contentType", "code", "The mime-type to use for RESTful operation in the 'Content-Type' header.", 0, 1, contentType); 6015 case -1429847026: /*destination*/ return new Property("destination", "integer", "The server where the request message is destined for. Must be one of the server numbers listed in TestScript.destination section.", 0, 1, destination); 6016 case -1760554218: /*encodeRequestUrl*/ return new Property("encodeRequestUrl", "boolean", "Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths.", 0, 1, encodeRequestUrl); 6017 case -1077554975: /*method*/ return new Property("method", "code", "The HTTP method the test engine MUST use for this operation regardless of any other operation details.", 0, 1, method); 6018 case -1008619738: /*origin*/ return new Property("origin", "integer", "The server where the request message originates from. Must be one of the server numbers listed in TestScript.origin section.", 0, 1, origin); 6019 case -995427962: /*params*/ return new Property("params", "string", "Path plus parameters after [type]. Used to set parts of the request URL explicitly.", 0, 1, params); 6020 case 1074158076: /*requestHeader*/ return new Property("requestHeader", "", "Header elements would be used to set HTTP headers.", 0, java.lang.Integer.MAX_VALUE, requestHeader); 6021 case 693933066: /*requestId*/ return new Property("requestId", "id", "The fixture id (maybe new) to map to the request.", 0, 1, requestId); 6022 case -633138884: /*responseId*/ return new Property("responseId", "id", "The fixture id (maybe new) to map to the response.", 0, 1, responseId); 6023 case 1746327190: /*sourceId*/ return new Property("sourceId", "id", "The id of the fixture used as the body of a PUT or POST request.", 0, 1, sourceId); 6024 case -441951604: /*targetId*/ return new Property("targetId", "id", "Id of fixture used for extracting the [id], [type], and [vid] for GET requests.", 0, 1, targetId); 6025 case 116079: /*url*/ return new Property("url", "string", "Complete request URL.", 0, 1, url); 6026 default: return super.getNamedProperty(_hash, _name, _checkValid); 6027 } 6028 6029 } 6030 6031 @Override 6032 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6033 switch (hash) { 6034 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Coding 6035 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // UriType 6036 case 102727412: /*label*/ return this.label == null ? new Base[0] : new Base[] {this.label}; // StringType 6037 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 6038 case -1423461112: /*accept*/ return this.accept == null ? new Base[0] : new Base[] {this.accept}; // CodeType 6039 case -389131437: /*contentType*/ return this.contentType == null ? new Base[0] : new Base[] {this.contentType}; // CodeType 6040 case -1429847026: /*destination*/ return this.destination == null ? new Base[0] : new Base[] {this.destination}; // IntegerType 6041 case -1760554218: /*encodeRequestUrl*/ return this.encodeRequestUrl == null ? new Base[0] : new Base[] {this.encodeRequestUrl}; // BooleanType 6042 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // Enumeration<TestScriptRequestMethodCode> 6043 case -1008619738: /*origin*/ return this.origin == null ? new Base[0] : new Base[] {this.origin}; // IntegerType 6044 case -995427962: /*params*/ return this.params == null ? new Base[0] : new Base[] {this.params}; // StringType 6045 case 1074158076: /*requestHeader*/ return this.requestHeader == null ? new Base[0] : this.requestHeader.toArray(new Base[this.requestHeader.size()]); // SetupActionOperationRequestHeaderComponent 6046 case 693933066: /*requestId*/ return this.requestId == null ? new Base[0] : new Base[] {this.requestId}; // IdType 6047 case -633138884: /*responseId*/ return this.responseId == null ? new Base[0] : new Base[] {this.responseId}; // IdType 6048 case 1746327190: /*sourceId*/ return this.sourceId == null ? new Base[0] : new Base[] {this.sourceId}; // IdType 6049 case -441951604: /*targetId*/ return this.targetId == null ? new Base[0] : new Base[] {this.targetId}; // IdType 6050 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // StringType 6051 default: return super.getProperty(hash, name, checkValid); 6052 } 6053 6054 } 6055 6056 @Override 6057 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6058 switch (hash) { 6059 case 3575610: // type 6060 this.type = TypeConvertor.castToCoding(value); // Coding 6061 return value; 6062 case -341064690: // resource 6063 this.resource = TypeConvertor.castToUri(value); // UriType 6064 return value; 6065 case 102727412: // label 6066 this.label = TypeConvertor.castToString(value); // StringType 6067 return value; 6068 case -1724546052: // description 6069 this.description = TypeConvertor.castToString(value); // StringType 6070 return value; 6071 case -1423461112: // accept 6072 this.accept = TypeConvertor.castToCode(value); // CodeType 6073 return value; 6074 case -389131437: // contentType 6075 this.contentType = TypeConvertor.castToCode(value); // CodeType 6076 return value; 6077 case -1429847026: // destination 6078 this.destination = TypeConvertor.castToInteger(value); // IntegerType 6079 return value; 6080 case -1760554218: // encodeRequestUrl 6081 this.encodeRequestUrl = TypeConvertor.castToBoolean(value); // BooleanType 6082 return value; 6083 case -1077554975: // method 6084 value = new TestScriptRequestMethodCodeEnumFactory().fromType(TypeConvertor.castToCode(value)); 6085 this.method = (Enumeration) value; // Enumeration<TestScriptRequestMethodCode> 6086 return value; 6087 case -1008619738: // origin 6088 this.origin = TypeConvertor.castToInteger(value); // IntegerType 6089 return value; 6090 case -995427962: // params 6091 this.params = TypeConvertor.castToString(value); // StringType 6092 return value; 6093 case 1074158076: // requestHeader 6094 this.getRequestHeader().add((SetupActionOperationRequestHeaderComponent) value); // SetupActionOperationRequestHeaderComponent 6095 return value; 6096 case 693933066: // requestId 6097 this.requestId = TypeConvertor.castToId(value); // IdType 6098 return value; 6099 case -633138884: // responseId 6100 this.responseId = TypeConvertor.castToId(value); // IdType 6101 return value; 6102 case 1746327190: // sourceId 6103 this.sourceId = TypeConvertor.castToId(value); // IdType 6104 return value; 6105 case -441951604: // targetId 6106 this.targetId = TypeConvertor.castToId(value); // IdType 6107 return value; 6108 case 116079: // url 6109 this.url = TypeConvertor.castToString(value); // StringType 6110 return value; 6111 default: return super.setProperty(hash, name, value); 6112 } 6113 6114 } 6115 6116 @Override 6117 public Base setProperty(String name, Base value) throws FHIRException { 6118 if (name.equals("type")) { 6119 this.type = TypeConvertor.castToCoding(value); // Coding 6120 } else if (name.equals("resource")) { 6121 this.resource = TypeConvertor.castToUri(value); // UriType 6122 } else if (name.equals("label")) { 6123 this.label = TypeConvertor.castToString(value); // StringType 6124 } else if (name.equals("description")) { 6125 this.description = TypeConvertor.castToString(value); // StringType 6126 } else if (name.equals("accept")) { 6127 this.accept = TypeConvertor.castToCode(value); // CodeType 6128 } else if (name.equals("contentType")) { 6129 this.contentType = TypeConvertor.castToCode(value); // CodeType 6130 } else if (name.equals("destination")) { 6131 this.destination = TypeConvertor.castToInteger(value); // IntegerType 6132 } else if (name.equals("encodeRequestUrl")) { 6133 this.encodeRequestUrl = TypeConvertor.castToBoolean(value); // BooleanType 6134 } else if (name.equals("method")) { 6135 value = new TestScriptRequestMethodCodeEnumFactory().fromType(TypeConvertor.castToCode(value)); 6136 this.method = (Enumeration) value; // Enumeration<TestScriptRequestMethodCode> 6137 } else if (name.equals("origin")) { 6138 this.origin = TypeConvertor.castToInteger(value); // IntegerType 6139 } else if (name.equals("params")) { 6140 this.params = TypeConvertor.castToString(value); // StringType 6141 } else if (name.equals("requestHeader")) { 6142 this.getRequestHeader().add((SetupActionOperationRequestHeaderComponent) value); 6143 } else if (name.equals("requestId")) { 6144 this.requestId = TypeConvertor.castToId(value); // IdType 6145 } else if (name.equals("responseId")) { 6146 this.responseId = TypeConvertor.castToId(value); // IdType 6147 } else if (name.equals("sourceId")) { 6148 this.sourceId = TypeConvertor.castToId(value); // IdType 6149 } else if (name.equals("targetId")) { 6150 this.targetId = TypeConvertor.castToId(value); // IdType 6151 } else if (name.equals("url")) { 6152 this.url = TypeConvertor.castToString(value); // StringType 6153 } else 6154 return super.setProperty(name, value); 6155 return value; 6156 } 6157 6158 @Override 6159 public void removeChild(String name, Base value) throws FHIRException { 6160 if (name.equals("type")) { 6161 this.type = null; 6162 } else if (name.equals("resource")) { 6163 this.resource = null; 6164 } else if (name.equals("label")) { 6165 this.label = null; 6166 } else if (name.equals("description")) { 6167 this.description = null; 6168 } else if (name.equals("accept")) { 6169 this.accept = null; 6170 } else if (name.equals("contentType")) { 6171 this.contentType = null; 6172 } else if (name.equals("destination")) { 6173 this.destination = null; 6174 } else if (name.equals("encodeRequestUrl")) { 6175 this.encodeRequestUrl = null; 6176 } else if (name.equals("method")) { 6177 value = new TestScriptRequestMethodCodeEnumFactory().fromType(TypeConvertor.castToCode(value)); 6178 this.method = (Enumeration) value; // Enumeration<TestScriptRequestMethodCode> 6179 } else if (name.equals("origin")) { 6180 this.origin = null; 6181 } else if (name.equals("params")) { 6182 this.params = null; 6183 } else if (name.equals("requestHeader")) { 6184 this.getRequestHeader().remove((SetupActionOperationRequestHeaderComponent) value); 6185 } else if (name.equals("requestId")) { 6186 this.requestId = null; 6187 } else if (name.equals("responseId")) { 6188 this.responseId = null; 6189 } else if (name.equals("sourceId")) { 6190 this.sourceId = null; 6191 } else if (name.equals("targetId")) { 6192 this.targetId = null; 6193 } else if (name.equals("url")) { 6194 this.url = null; 6195 } else 6196 super.removeChild(name, value); 6197 6198 } 6199 6200 @Override 6201 public Base makeProperty(int hash, String name) throws FHIRException { 6202 switch (hash) { 6203 case 3575610: return getType(); 6204 case -341064690: return getResourceElement(); 6205 case 102727412: return getLabelElement(); 6206 case -1724546052: return getDescriptionElement(); 6207 case -1423461112: return getAcceptElement(); 6208 case -389131437: return getContentTypeElement(); 6209 case -1429847026: return getDestinationElement(); 6210 case -1760554218: return getEncodeRequestUrlElement(); 6211 case -1077554975: return getMethodElement(); 6212 case -1008619738: return getOriginElement(); 6213 case -995427962: return getParamsElement(); 6214 case 1074158076: return addRequestHeader(); 6215 case 693933066: return getRequestIdElement(); 6216 case -633138884: return getResponseIdElement(); 6217 case 1746327190: return getSourceIdElement(); 6218 case -441951604: return getTargetIdElement(); 6219 case 116079: return getUrlElement(); 6220 default: return super.makeProperty(hash, name); 6221 } 6222 6223 } 6224 6225 @Override 6226 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6227 switch (hash) { 6228 case 3575610: /*type*/ return new String[] {"Coding"}; 6229 case -341064690: /*resource*/ return new String[] {"uri"}; 6230 case 102727412: /*label*/ return new String[] {"string"}; 6231 case -1724546052: /*description*/ return new String[] {"string"}; 6232 case -1423461112: /*accept*/ return new String[] {"code"}; 6233 case -389131437: /*contentType*/ return new String[] {"code"}; 6234 case -1429847026: /*destination*/ return new String[] {"integer"}; 6235 case -1760554218: /*encodeRequestUrl*/ return new String[] {"boolean"}; 6236 case -1077554975: /*method*/ return new String[] {"code"}; 6237 case -1008619738: /*origin*/ return new String[] {"integer"}; 6238 case -995427962: /*params*/ return new String[] {"string"}; 6239 case 1074158076: /*requestHeader*/ return new String[] {}; 6240 case 693933066: /*requestId*/ return new String[] {"id"}; 6241 case -633138884: /*responseId*/ return new String[] {"id"}; 6242 case 1746327190: /*sourceId*/ return new String[] {"id"}; 6243 case -441951604: /*targetId*/ return new String[] {"id"}; 6244 case 116079: /*url*/ return new String[] {"string"}; 6245 default: return super.getTypesForProperty(hash, name); 6246 } 6247 6248 } 6249 6250 @Override 6251 public Base addChild(String name) throws FHIRException { 6252 if (name.equals("type")) { 6253 this.type = new Coding(); 6254 return this.type; 6255 } 6256 else if (name.equals("resource")) { 6257 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.operation.resource"); 6258 } 6259 else if (name.equals("label")) { 6260 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.operation.label"); 6261 } 6262 else if (name.equals("description")) { 6263 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.operation.description"); 6264 } 6265 else if (name.equals("accept")) { 6266 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.operation.accept"); 6267 } 6268 else if (name.equals("contentType")) { 6269 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.operation.contentType"); 6270 } 6271 else if (name.equals("destination")) { 6272 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.operation.destination"); 6273 } 6274 else if (name.equals("encodeRequestUrl")) { 6275 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.operation.encodeRequestUrl"); 6276 } 6277 else if (name.equals("method")) { 6278 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.operation.method"); 6279 } 6280 else if (name.equals("origin")) { 6281 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.operation.origin"); 6282 } 6283 else if (name.equals("params")) { 6284 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.operation.params"); 6285 } 6286 else if (name.equals("requestHeader")) { 6287 return addRequestHeader(); 6288 } 6289 else if (name.equals("requestId")) { 6290 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.operation.requestId"); 6291 } 6292 else if (name.equals("responseId")) { 6293 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.operation.responseId"); 6294 } 6295 else if (name.equals("sourceId")) { 6296 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.operation.sourceId"); 6297 } 6298 else if (name.equals("targetId")) { 6299 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.operation.targetId"); 6300 } 6301 else if (name.equals("url")) { 6302 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.operation.url"); 6303 } 6304 else 6305 return super.addChild(name); 6306 } 6307 6308 public SetupActionOperationComponent copy() { 6309 SetupActionOperationComponent dst = new SetupActionOperationComponent(); 6310 copyValues(dst); 6311 return dst; 6312 } 6313 6314 public void copyValues(SetupActionOperationComponent dst) { 6315 super.copyValues(dst); 6316 dst.type = type == null ? null : type.copy(); 6317 dst.resource = resource == null ? null : resource.copy(); 6318 dst.label = label == null ? null : label.copy(); 6319 dst.description = description == null ? null : description.copy(); 6320 dst.accept = accept == null ? null : accept.copy(); 6321 dst.contentType = contentType == null ? null : contentType.copy(); 6322 dst.destination = destination == null ? null : destination.copy(); 6323 dst.encodeRequestUrl = encodeRequestUrl == null ? null : encodeRequestUrl.copy(); 6324 dst.method = method == null ? null : method.copy(); 6325 dst.origin = origin == null ? null : origin.copy(); 6326 dst.params = params == null ? null : params.copy(); 6327 if (requestHeader != null) { 6328 dst.requestHeader = new ArrayList<SetupActionOperationRequestHeaderComponent>(); 6329 for (SetupActionOperationRequestHeaderComponent i : requestHeader) 6330 dst.requestHeader.add(i.copy()); 6331 }; 6332 dst.requestId = requestId == null ? null : requestId.copy(); 6333 dst.responseId = responseId == null ? null : responseId.copy(); 6334 dst.sourceId = sourceId == null ? null : sourceId.copy(); 6335 dst.targetId = targetId == null ? null : targetId.copy(); 6336 dst.url = url == null ? null : url.copy(); 6337 } 6338 6339 @Override 6340 public boolean equalsDeep(Base other_) { 6341 if (!super.equalsDeep(other_)) 6342 return false; 6343 if (!(other_ instanceof SetupActionOperationComponent)) 6344 return false; 6345 SetupActionOperationComponent o = (SetupActionOperationComponent) other_; 6346 return compareDeep(type, o.type, true) && compareDeep(resource, o.resource, true) && compareDeep(label, o.label, true) 6347 && compareDeep(description, o.description, true) && compareDeep(accept, o.accept, true) && compareDeep(contentType, o.contentType, true) 6348 && compareDeep(destination, o.destination, true) && compareDeep(encodeRequestUrl, o.encodeRequestUrl, true) 6349 && compareDeep(method, o.method, true) && compareDeep(origin, o.origin, true) && compareDeep(params, o.params, true) 6350 && compareDeep(requestHeader, o.requestHeader, true) && compareDeep(requestId, o.requestId, true) 6351 && compareDeep(responseId, o.responseId, true) && compareDeep(sourceId, o.sourceId, true) && compareDeep(targetId, o.targetId, true) 6352 && compareDeep(url, o.url, true); 6353 } 6354 6355 @Override 6356 public boolean equalsShallow(Base other_) { 6357 if (!super.equalsShallow(other_)) 6358 return false; 6359 if (!(other_ instanceof SetupActionOperationComponent)) 6360 return false; 6361 SetupActionOperationComponent o = (SetupActionOperationComponent) other_; 6362 return compareValues(resource, o.resource, true) && compareValues(label, o.label, true) && compareValues(description, o.description, true) 6363 && compareValues(accept, o.accept, true) && compareValues(contentType, o.contentType, true) && compareValues(destination, o.destination, true) 6364 && compareValues(encodeRequestUrl, o.encodeRequestUrl, true) && compareValues(method, o.method, true) 6365 && compareValues(origin, o.origin, true) && compareValues(params, o.params, true) && compareValues(requestId, o.requestId, true) 6366 && compareValues(responseId, o.responseId, true) && compareValues(sourceId, o.sourceId, true) && compareValues(targetId, o.targetId, true) 6367 && compareValues(url, o.url, true); 6368 } 6369 6370 public boolean isEmpty() { 6371 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, resource, label, description 6372 , accept, contentType, destination, encodeRequestUrl, method, origin, params, requestHeader 6373 , requestId, responseId, sourceId, targetId, url); 6374 } 6375 6376 public String fhirType() { 6377 return "TestScript.setup.action.operation"; 6378 6379 } 6380 6381 } 6382 6383 @Block() 6384 public static class SetupActionOperationRequestHeaderComponent extends BackboneElement implements IBaseBackboneElement { 6385 /** 6386 * The HTTP header field e.g. "Accept". 6387 */ 6388 @Child(name = "field", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 6389 @Description(shortDefinition="HTTP header field name", formalDefinition="The HTTP header field e.g. \"Accept\"." ) 6390 protected StringType field; 6391 6392 /** 6393 * The value of the header e.g. "application/fhir+xml". 6394 */ 6395 @Child(name = "value", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 6396 @Description(shortDefinition="HTTP headerfield value", formalDefinition="The value of the header e.g. \"application/fhir+xml\"." ) 6397 protected StringType value; 6398 6399 private static final long serialVersionUID = 274395337L; 6400 6401 /** 6402 * Constructor 6403 */ 6404 public SetupActionOperationRequestHeaderComponent() { 6405 super(); 6406 } 6407 6408 /** 6409 * Constructor 6410 */ 6411 public SetupActionOperationRequestHeaderComponent(String field, String value) { 6412 super(); 6413 this.setField(field); 6414 this.setValue(value); 6415 } 6416 6417 /** 6418 * @return {@link #field} (The HTTP header field e.g. "Accept".). This is the underlying object with id, value and extensions. The accessor "getField" gives direct access to the value 6419 */ 6420 public StringType getFieldElement() { 6421 if (this.field == null) 6422 if (Configuration.errorOnAutoCreate()) 6423 throw new Error("Attempt to auto-create SetupActionOperationRequestHeaderComponent.field"); 6424 else if (Configuration.doAutoCreate()) 6425 this.field = new StringType(); // bb 6426 return this.field; 6427 } 6428 6429 public boolean hasFieldElement() { 6430 return this.field != null && !this.field.isEmpty(); 6431 } 6432 6433 public boolean hasField() { 6434 return this.field != null && !this.field.isEmpty(); 6435 } 6436 6437 /** 6438 * @param value {@link #field} (The HTTP header field e.g. "Accept".). This is the underlying object with id, value and extensions. The accessor "getField" gives direct access to the value 6439 */ 6440 public SetupActionOperationRequestHeaderComponent setFieldElement(StringType value) { 6441 this.field = value; 6442 return this; 6443 } 6444 6445 /** 6446 * @return The HTTP header field e.g. "Accept". 6447 */ 6448 public String getField() { 6449 return this.field == null ? null : this.field.getValue(); 6450 } 6451 6452 /** 6453 * @param value The HTTP header field e.g. "Accept". 6454 */ 6455 public SetupActionOperationRequestHeaderComponent setField(String value) { 6456 if (this.field == null) 6457 this.field = new StringType(); 6458 this.field.setValue(value); 6459 return this; 6460 } 6461 6462 /** 6463 * @return {@link #value} (The value of the header e.g. "application/fhir+xml".). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 6464 */ 6465 public StringType getValueElement() { 6466 if (this.value == null) 6467 if (Configuration.errorOnAutoCreate()) 6468 throw new Error("Attempt to auto-create SetupActionOperationRequestHeaderComponent.value"); 6469 else if (Configuration.doAutoCreate()) 6470 this.value = new StringType(); // bb 6471 return this.value; 6472 } 6473 6474 public boolean hasValueElement() { 6475 return this.value != null && !this.value.isEmpty(); 6476 } 6477 6478 public boolean hasValue() { 6479 return this.value != null && !this.value.isEmpty(); 6480 } 6481 6482 /** 6483 * @param value {@link #value} (The value of the header e.g. "application/fhir+xml".). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 6484 */ 6485 public SetupActionOperationRequestHeaderComponent setValueElement(StringType value) { 6486 this.value = value; 6487 return this; 6488 } 6489 6490 /** 6491 * @return The value of the header e.g. "application/fhir+xml". 6492 */ 6493 public String getValue() { 6494 return this.value == null ? null : this.value.getValue(); 6495 } 6496 6497 /** 6498 * @param value The value of the header e.g. "application/fhir+xml". 6499 */ 6500 public SetupActionOperationRequestHeaderComponent setValue(String value) { 6501 if (this.value == null) 6502 this.value = new StringType(); 6503 this.value.setValue(value); 6504 return this; 6505 } 6506 6507 protected void listChildren(List<Property> children) { 6508 super.listChildren(children); 6509 children.add(new Property("field", "string", "The HTTP header field e.g. \"Accept\".", 0, 1, field)); 6510 children.add(new Property("value", "string", "The value of the header e.g. \"application/fhir+xml\".", 0, 1, value)); 6511 } 6512 6513 @Override 6514 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6515 switch (_hash) { 6516 case 97427706: /*field*/ return new Property("field", "string", "The HTTP header field e.g. \"Accept\".", 0, 1, field); 6517 case 111972721: /*value*/ return new Property("value", "string", "The value of the header e.g. \"application/fhir+xml\".", 0, 1, value); 6518 default: return super.getNamedProperty(_hash, _name, _checkValid); 6519 } 6520 6521 } 6522 6523 @Override 6524 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6525 switch (hash) { 6526 case 97427706: /*field*/ return this.field == null ? new Base[0] : new Base[] {this.field}; // StringType 6527 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 6528 default: return super.getProperty(hash, name, checkValid); 6529 } 6530 6531 } 6532 6533 @Override 6534 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6535 switch (hash) { 6536 case 97427706: // field 6537 this.field = TypeConvertor.castToString(value); // StringType 6538 return value; 6539 case 111972721: // value 6540 this.value = TypeConvertor.castToString(value); // StringType 6541 return value; 6542 default: return super.setProperty(hash, name, value); 6543 } 6544 6545 } 6546 6547 @Override 6548 public Base setProperty(String name, Base value) throws FHIRException { 6549 if (name.equals("field")) { 6550 this.field = TypeConvertor.castToString(value); // StringType 6551 } else if (name.equals("value")) { 6552 this.value = TypeConvertor.castToString(value); // StringType 6553 } else 6554 return super.setProperty(name, value); 6555 return value; 6556 } 6557 6558 @Override 6559 public void removeChild(String name, Base value) throws FHIRException { 6560 if (name.equals("field")) { 6561 this.field = null; 6562 } else if (name.equals("value")) { 6563 this.value = null; 6564 } else 6565 super.removeChild(name, value); 6566 6567 } 6568 6569 @Override 6570 public Base makeProperty(int hash, String name) throws FHIRException { 6571 switch (hash) { 6572 case 97427706: return getFieldElement(); 6573 case 111972721: return getValueElement(); 6574 default: return super.makeProperty(hash, name); 6575 } 6576 6577 } 6578 6579 @Override 6580 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6581 switch (hash) { 6582 case 97427706: /*field*/ return new String[] {"string"}; 6583 case 111972721: /*value*/ return new String[] {"string"}; 6584 default: return super.getTypesForProperty(hash, name); 6585 } 6586 6587 } 6588 6589 @Override 6590 public Base addChild(String name) throws FHIRException { 6591 if (name.equals("field")) { 6592 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.operation.requestHeader.field"); 6593 } 6594 else if (name.equals("value")) { 6595 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.operation.requestHeader.value"); 6596 } 6597 else 6598 return super.addChild(name); 6599 } 6600 6601 public SetupActionOperationRequestHeaderComponent copy() { 6602 SetupActionOperationRequestHeaderComponent dst = new SetupActionOperationRequestHeaderComponent(); 6603 copyValues(dst); 6604 return dst; 6605 } 6606 6607 public void copyValues(SetupActionOperationRequestHeaderComponent dst) { 6608 super.copyValues(dst); 6609 dst.field = field == null ? null : field.copy(); 6610 dst.value = value == null ? null : value.copy(); 6611 } 6612 6613 @Override 6614 public boolean equalsDeep(Base other_) { 6615 if (!super.equalsDeep(other_)) 6616 return false; 6617 if (!(other_ instanceof SetupActionOperationRequestHeaderComponent)) 6618 return false; 6619 SetupActionOperationRequestHeaderComponent o = (SetupActionOperationRequestHeaderComponent) other_; 6620 return compareDeep(field, o.field, true) && compareDeep(value, o.value, true); 6621 } 6622 6623 @Override 6624 public boolean equalsShallow(Base other_) { 6625 if (!super.equalsShallow(other_)) 6626 return false; 6627 if (!(other_ instanceof SetupActionOperationRequestHeaderComponent)) 6628 return false; 6629 SetupActionOperationRequestHeaderComponent o = (SetupActionOperationRequestHeaderComponent) other_; 6630 return compareValues(field, o.field, true) && compareValues(value, o.value, true); 6631 } 6632 6633 public boolean isEmpty() { 6634 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(field, value); 6635 } 6636 6637 public String fhirType() { 6638 return "TestScript.setup.action.operation.requestHeader"; 6639 6640 } 6641 6642 } 6643 6644 @Block() 6645 public static class SetupActionAssertComponent extends BackboneElement implements IBaseBackboneElement { 6646 /** 6647 * The label would be used for tracking/logging purposes by test engines. 6648 */ 6649 @Child(name = "label", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 6650 @Description(shortDefinition="Tracking/logging assertion label", formalDefinition="The label would be used for tracking/logging purposes by test engines." ) 6651 protected StringType label; 6652 6653 /** 6654 * The description would be used by test engines for tracking and reporting purposes. 6655 */ 6656 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 6657 @Description(shortDefinition="Tracking/reporting assertion description", formalDefinition="The description would be used by test engines for tracking and reporting purposes." ) 6658 protected StringType description; 6659 6660 /** 6661 * The direction to use for the assertion. 6662 */ 6663 @Child(name = "direction", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 6664 @Description(shortDefinition="response | request", formalDefinition="The direction to use for the assertion." ) 6665 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/assert-direction-codes") 6666 protected Enumeration<AssertionDirectionType> direction; 6667 6668 /** 6669 * Id of the source fixture used as the contents to be evaluated by either the "source/expression" or "sourceId/path" definition. 6670 */ 6671 @Child(name = "compareToSourceId", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 6672 @Description(shortDefinition="Id of the source fixture to be evaluated", formalDefinition="Id of the source fixture used as the contents to be evaluated by either the \"source/expression\" or \"sourceId/path\" definition." ) 6673 protected StringType compareToSourceId; 6674 6675 /** 6676 * The FHIRPath expression for a specific value to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both. 6677 */ 6678 @Child(name = "compareToSourceExpression", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 6679 @Description(shortDefinition="The FHIRPath expression to evaluate against the source fixture", formalDefinition="The FHIRPath expression for a specific value to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both." ) 6680 protected StringType compareToSourceExpression; 6681 6682 /** 6683 * XPath or JSONPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both. 6684 */ 6685 @Child(name = "compareToSourcePath", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 6686 @Description(shortDefinition="XPath or JSONPath expression to evaluate against the source fixture", formalDefinition="XPath or JSONPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both." ) 6687 protected StringType compareToSourcePath; 6688 6689 /** 6690 * The mime-type contents to compare against the request or response message 'Content-Type' header. 6691 */ 6692 @Child(name = "contentType", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=false) 6693 @Description(shortDefinition="Mime type to compare against the 'Content-Type' header", formalDefinition="The mime-type contents to compare against the request or response message 'Content-Type' header." ) 6694 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/mimetypes") 6695 protected CodeType contentType; 6696 6697 /** 6698 * The default manual completion outcome applied to this assertion. 6699 */ 6700 @Child(name = "defaultManualCompletion", type = {CodeType.class}, order=8, min=0, max=1, modifier=false, summary=false) 6701 @Description(shortDefinition="fail | pass | skip | stop", formalDefinition="The default manual completion outcome applied to this assertion." ) 6702 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/assert-manual-completion-codes") 6703 protected Enumeration<AssertionManualCompletionType> defaultManualCompletion; 6704 6705 /** 6706 * The FHIRPath expression to be evaluated against the request or response message contents - HTTP headers and payload. 6707 */ 6708 @Child(name = "expression", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=false) 6709 @Description(shortDefinition="The FHIRPath expression to be evaluated", formalDefinition="The FHIRPath expression to be evaluated against the request or response message contents - HTTP headers and payload." ) 6710 protected StringType expression; 6711 6712 /** 6713 * The HTTP header field name e.g. 'Location'. 6714 */ 6715 @Child(name = "headerField", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=false) 6716 @Description(shortDefinition="HTTP header field name", formalDefinition="The HTTP header field name e.g. 'Location'." ) 6717 protected StringType headerField; 6718 6719 /** 6720 * The ID of a fixture. Asserts that the response contains at a minimum the fixture specified by minimumId. 6721 */ 6722 @Child(name = "minimumId", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=false) 6723 @Description(shortDefinition="Fixture Id of minimum content resource", formalDefinition="The ID of a fixture. Asserts that the response contains at a minimum the fixture specified by minimumId." ) 6724 protected StringType minimumId; 6725 6726 /** 6727 * Whether or not the test execution performs validation on the bundle navigation links. 6728 */ 6729 @Child(name = "navigationLinks", type = {BooleanType.class}, order=12, min=0, max=1, modifier=false, summary=false) 6730 @Description(shortDefinition="Perform validation on navigation links?", formalDefinition="Whether or not the test execution performs validation on the bundle navigation links." ) 6731 protected BooleanType navigationLinks; 6732 6733 /** 6734 * The operator type defines the conditional behavior of the assert. 6735 */ 6736 @Child(name = "operator", type = {CodeType.class}, order=13, min=0, max=1, modifier=false, summary=false) 6737 @Description(shortDefinition="equals | notEquals | in | notIn | greaterThan | lessThan | empty | notEmpty | contains | notContains | eval | manualEval", formalDefinition="The operator type defines the conditional behavior of the assert." ) 6738 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/assert-operator-codes") 6739 protected Enumeration<AssertionOperatorType> operator; 6740 6741 /** 6742 * The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server. 6743 */ 6744 @Child(name = "path", type = {StringType.class}, order=14, min=0, max=1, modifier=false, summary=false) 6745 @Description(shortDefinition="XPath or JSONPath expression", formalDefinition="The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server." ) 6746 protected StringType path; 6747 6748 /** 6749 * The request method or HTTP operation code to compare against that used by the client system under test. 6750 */ 6751 @Child(name = "requestMethod", type = {CodeType.class}, order=15, min=0, max=1, modifier=false, summary=false) 6752 @Description(shortDefinition="delete | get | options | patch | post | put | head", formalDefinition="The request method or HTTP operation code to compare against that used by the client system under test." ) 6753 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/http-operations") 6754 protected Enumeration<TestScriptRequestMethodCode> requestMethod; 6755 6756 /** 6757 * The value to use in a comparison against the request URL path string. 6758 */ 6759 @Child(name = "requestURL", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 6760 @Description(shortDefinition="Request URL comparison value", formalDefinition="The value to use in a comparison against the request URL path string." ) 6761 protected StringType requestURL; 6762 6763 /** 6764 * The type of the resource. See the [resource list](resourcelist.html). 6765 */ 6766 @Child(name = "resource", type = {UriType.class}, order=17, min=0, max=1, modifier=false, summary=false) 6767 @Description(shortDefinition="Resource type", formalDefinition="The type of the resource. See the [resource list](resourcelist.html)." ) 6768 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/concrete-fhir-types") 6769 protected UriType resource; 6770 6771 /** 6772 * continue | switchingProtocols | okay | created | accepted | nonAuthoritativeInformation | noContent | resetContent | partialContent | multipleChoices | movedPermanently | found | seeOther | notModified | useProxy | temporaryRedirect | permanentRedirect | badRequest | unauthorized | paymentRequired | forbidden | notFound | methodNotAllowed | notAcceptable | proxyAuthenticationRequired | requestTimeout | conflict | gone | lengthRequired | preconditionFailed | contentTooLarge | uriTooLong | unsupportedMediaType | rangeNotSatisfiable | expectationFailed | misdirectedRequest | unprocessableContent | upgradeRequired | internalServerError | notImplemented | badGateway | serviceUnavailable | gatewayTimeout | httpVersionNotSupported. 6773 */ 6774 @Child(name = "response", type = {CodeType.class}, order=18, min=0, max=1, modifier=false, summary=false) 6775 @Description(shortDefinition="continue | switchingProtocols | okay | created | accepted | nonAuthoritativeInformation | noContent | resetContent | partialContent | multipleChoices | movedPermanently | found | seeOther | notModified | useProxy | temporaryRedirect | permanentRedirect | badRequest | unauthorized | paymentRequired | forbidden | notFound | methodNotAllowed | notAcceptable | proxyAuthenticationRequired | requestTimeout | conflict | gone | lengthRequired | preconditionFailed | contentTooLarge | uriTooLong | unsupportedMediaType | rangeNotSatisfiable | expectationFailed | misdirectedRequest | unprocessableContent | upgradeRequired | internalServerError | notImplemented | badGateway | serviceUnavailable | gatewayTimeout | httpVersionNotSupported", formalDefinition="continue | switchingProtocols | okay | created | accepted | nonAuthoritativeInformation | noContent | resetContent | partialContent | multipleChoices | movedPermanently | found | seeOther | notModified | useProxy | temporaryRedirect | permanentRedirect | badRequest | unauthorized | paymentRequired | forbidden | notFound | methodNotAllowed | notAcceptable | proxyAuthenticationRequired | requestTimeout | conflict | gone | lengthRequired | preconditionFailed | contentTooLarge | uriTooLong | unsupportedMediaType | rangeNotSatisfiable | expectationFailed | misdirectedRequest | unprocessableContent | upgradeRequired | internalServerError | notImplemented | badGateway | serviceUnavailable | gatewayTimeout | httpVersionNotSupported." ) 6776 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/assert-response-code-types") 6777 protected Enumeration<AssertionResponseTypes> response; 6778 6779 /** 6780 * The value of the HTTP response code to be tested. 6781 */ 6782 @Child(name = "responseCode", type = {StringType.class}, order=19, min=0, max=1, modifier=false, summary=false) 6783 @Description(shortDefinition="HTTP response code to test", formalDefinition="The value of the HTTP response code to be tested." ) 6784 protected StringType responseCode; 6785 6786 /** 6787 * Fixture to evaluate the XPath/JSONPath expression or the headerField against. 6788 */ 6789 @Child(name = "sourceId", type = {IdType.class}, order=20, min=0, max=1, modifier=false, summary=false) 6790 @Description(shortDefinition="Fixture Id of source expression or headerField", formalDefinition="Fixture to evaluate the XPath/JSONPath expression or the headerField against." ) 6791 protected IdType sourceId; 6792 6793 /** 6794 * Whether or not the current test execution will stop on failure for this assert. 6795 */ 6796 @Child(name = "stopTestOnFail", type = {BooleanType.class}, order=21, min=1, max=1, modifier=false, summary=false) 6797 @Description(shortDefinition="If this assert fails, will the current test execution stop?", formalDefinition="Whether or not the current test execution will stop on failure for this assert." ) 6798 protected BooleanType stopTestOnFail; 6799 6800 /** 6801 * The ID of the Profile to validate against. 6802 */ 6803 @Child(name = "validateProfileId", type = {IdType.class}, order=22, min=0, max=1, modifier=false, summary=false) 6804 @Description(shortDefinition="Profile Id of validation profile reference", formalDefinition="The ID of the Profile to validate against." ) 6805 protected IdType validateProfileId; 6806 6807 /** 6808 * The value to compare to. 6809 */ 6810 @Child(name = "value", type = {StringType.class}, order=23, min=0, max=1, modifier=false, summary=false) 6811 @Description(shortDefinition="The value to compare to", formalDefinition="The value to compare to." ) 6812 protected StringType value; 6813 6814 /** 6815 * Whether or not the test execution will produce a warning only on error for this assert. 6816 */ 6817 @Child(name = "warningOnly", type = {BooleanType.class}, order=24, min=1, max=1, modifier=false, summary=false) 6818 @Description(shortDefinition="Will this assert produce a warning only on error?", formalDefinition="Whether or not the test execution will produce a warning only on error for this assert." ) 6819 protected BooleanType warningOnly; 6820 6821 /** 6822 * Links or references providing traceability to the testing requirements for this assert. 6823 */ 6824 @Child(name = "requirement", type = {}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6825 @Description(shortDefinition="Links or references to the testing requirements", formalDefinition="Links or references providing traceability to the testing requirements for this assert." ) 6826 protected List<SetupActionAssertRequirementComponent> requirement; 6827 6828 private static final long serialVersionUID = -1269963728L; 6829 6830 /** 6831 * Constructor 6832 */ 6833 public SetupActionAssertComponent() { 6834 super(); 6835 } 6836 6837 /** 6838 * Constructor 6839 */ 6840 public SetupActionAssertComponent(boolean stopTestOnFail, boolean warningOnly) { 6841 super(); 6842 this.setStopTestOnFail(stopTestOnFail); 6843 this.setWarningOnly(warningOnly); 6844 } 6845 6846 /** 6847 * @return {@link #label} (The label would be used for tracking/logging purposes by test engines.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 6848 */ 6849 public StringType getLabelElement() { 6850 if (this.label == null) 6851 if (Configuration.errorOnAutoCreate()) 6852 throw new Error("Attempt to auto-create SetupActionAssertComponent.label"); 6853 else if (Configuration.doAutoCreate()) 6854 this.label = new StringType(); // bb 6855 return this.label; 6856 } 6857 6858 public boolean hasLabelElement() { 6859 return this.label != null && !this.label.isEmpty(); 6860 } 6861 6862 public boolean hasLabel() { 6863 return this.label != null && !this.label.isEmpty(); 6864 } 6865 6866 /** 6867 * @param value {@link #label} (The label would be used for tracking/logging purposes by test engines.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 6868 */ 6869 public SetupActionAssertComponent setLabelElement(StringType value) { 6870 this.label = value; 6871 return this; 6872 } 6873 6874 /** 6875 * @return The label would be used for tracking/logging purposes by test engines. 6876 */ 6877 public String getLabel() { 6878 return this.label == null ? null : this.label.getValue(); 6879 } 6880 6881 /** 6882 * @param value The label would be used for tracking/logging purposes by test engines. 6883 */ 6884 public SetupActionAssertComponent setLabel(String value) { 6885 if (Utilities.noString(value)) 6886 this.label = null; 6887 else { 6888 if (this.label == null) 6889 this.label = new StringType(); 6890 this.label.setValue(value); 6891 } 6892 return this; 6893 } 6894 6895 /** 6896 * @return {@link #description} (The description would be used by test engines for tracking and reporting purposes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 6897 */ 6898 public StringType getDescriptionElement() { 6899 if (this.description == null) 6900 if (Configuration.errorOnAutoCreate()) 6901 throw new Error("Attempt to auto-create SetupActionAssertComponent.description"); 6902 else if (Configuration.doAutoCreate()) 6903 this.description = new StringType(); // bb 6904 return this.description; 6905 } 6906 6907 public boolean hasDescriptionElement() { 6908 return this.description != null && !this.description.isEmpty(); 6909 } 6910 6911 public boolean hasDescription() { 6912 return this.description != null && !this.description.isEmpty(); 6913 } 6914 6915 /** 6916 * @param value {@link #description} (The description would be used by test engines for tracking and reporting purposes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 6917 */ 6918 public SetupActionAssertComponent setDescriptionElement(StringType value) { 6919 this.description = value; 6920 return this; 6921 } 6922 6923 /** 6924 * @return The description would be used by test engines for tracking and reporting purposes. 6925 */ 6926 public String getDescription() { 6927 return this.description == null ? null : this.description.getValue(); 6928 } 6929 6930 /** 6931 * @param value The description would be used by test engines for tracking and reporting purposes. 6932 */ 6933 public SetupActionAssertComponent setDescription(String value) { 6934 if (Utilities.noString(value)) 6935 this.description = null; 6936 else { 6937 if (this.description == null) 6938 this.description = new StringType(); 6939 this.description.setValue(value); 6940 } 6941 return this; 6942 } 6943 6944 /** 6945 * @return {@link #direction} (The direction to use for the assertion.). This is the underlying object with id, value and extensions. The accessor "getDirection" gives direct access to the value 6946 */ 6947 public Enumeration<AssertionDirectionType> getDirectionElement() { 6948 if (this.direction == null) 6949 if (Configuration.errorOnAutoCreate()) 6950 throw new Error("Attempt to auto-create SetupActionAssertComponent.direction"); 6951 else if (Configuration.doAutoCreate()) 6952 this.direction = new Enumeration<AssertionDirectionType>(new AssertionDirectionTypeEnumFactory()); // bb 6953 return this.direction; 6954 } 6955 6956 public boolean hasDirectionElement() { 6957 return this.direction != null && !this.direction.isEmpty(); 6958 } 6959 6960 public boolean hasDirection() { 6961 return this.direction != null && !this.direction.isEmpty(); 6962 } 6963 6964 /** 6965 * @param value {@link #direction} (The direction to use for the assertion.). This is the underlying object with id, value and extensions. The accessor "getDirection" gives direct access to the value 6966 */ 6967 public SetupActionAssertComponent setDirectionElement(Enumeration<AssertionDirectionType> value) { 6968 this.direction = value; 6969 return this; 6970 } 6971 6972 /** 6973 * @return The direction to use for the assertion. 6974 */ 6975 public AssertionDirectionType getDirection() { 6976 return this.direction == null ? null : this.direction.getValue(); 6977 } 6978 6979 /** 6980 * @param value The direction to use for the assertion. 6981 */ 6982 public SetupActionAssertComponent setDirection(AssertionDirectionType value) { 6983 if (value == null) 6984 this.direction = null; 6985 else { 6986 if (this.direction == null) 6987 this.direction = new Enumeration<AssertionDirectionType>(new AssertionDirectionTypeEnumFactory()); 6988 this.direction.setValue(value); 6989 } 6990 return this; 6991 } 6992 6993 /** 6994 * @return {@link #compareToSourceId} (Id of the source fixture used as the contents to be evaluated by either the "source/expression" or "sourceId/path" definition.). This is the underlying object with id, value and extensions. The accessor "getCompareToSourceId" gives direct access to the value 6995 */ 6996 public StringType getCompareToSourceIdElement() { 6997 if (this.compareToSourceId == null) 6998 if (Configuration.errorOnAutoCreate()) 6999 throw new Error("Attempt to auto-create SetupActionAssertComponent.compareToSourceId"); 7000 else if (Configuration.doAutoCreate()) 7001 this.compareToSourceId = new StringType(); // bb 7002 return this.compareToSourceId; 7003 } 7004 7005 public boolean hasCompareToSourceIdElement() { 7006 return this.compareToSourceId != null && !this.compareToSourceId.isEmpty(); 7007 } 7008 7009 public boolean hasCompareToSourceId() { 7010 return this.compareToSourceId != null && !this.compareToSourceId.isEmpty(); 7011 } 7012 7013 /** 7014 * @param value {@link #compareToSourceId} (Id of the source fixture used as the contents to be evaluated by either the "source/expression" or "sourceId/path" definition.). This is the underlying object with id, value and extensions. The accessor "getCompareToSourceId" gives direct access to the value 7015 */ 7016 public SetupActionAssertComponent setCompareToSourceIdElement(StringType value) { 7017 this.compareToSourceId = value; 7018 return this; 7019 } 7020 7021 /** 7022 * @return Id of the source fixture used as the contents to be evaluated by either the "source/expression" or "sourceId/path" definition. 7023 */ 7024 public String getCompareToSourceId() { 7025 return this.compareToSourceId == null ? null : this.compareToSourceId.getValue(); 7026 } 7027 7028 /** 7029 * @param value Id of the source fixture used as the contents to be evaluated by either the "source/expression" or "sourceId/path" definition. 7030 */ 7031 public SetupActionAssertComponent setCompareToSourceId(String value) { 7032 if (Utilities.noString(value)) 7033 this.compareToSourceId = null; 7034 else { 7035 if (this.compareToSourceId == null) 7036 this.compareToSourceId = new StringType(); 7037 this.compareToSourceId.setValue(value); 7038 } 7039 return this; 7040 } 7041 7042 /** 7043 * @return {@link #compareToSourceExpression} (The FHIRPath expression for a specific value to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.). This is the underlying object with id, value and extensions. The accessor "getCompareToSourceExpression" gives direct access to the value 7044 */ 7045 public StringType getCompareToSourceExpressionElement() { 7046 if (this.compareToSourceExpression == null) 7047 if (Configuration.errorOnAutoCreate()) 7048 throw new Error("Attempt to auto-create SetupActionAssertComponent.compareToSourceExpression"); 7049 else if (Configuration.doAutoCreate()) 7050 this.compareToSourceExpression = new StringType(); // bb 7051 return this.compareToSourceExpression; 7052 } 7053 7054 public boolean hasCompareToSourceExpressionElement() { 7055 return this.compareToSourceExpression != null && !this.compareToSourceExpression.isEmpty(); 7056 } 7057 7058 public boolean hasCompareToSourceExpression() { 7059 return this.compareToSourceExpression != null && !this.compareToSourceExpression.isEmpty(); 7060 } 7061 7062 /** 7063 * @param value {@link #compareToSourceExpression} (The FHIRPath expression for a specific value to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.). This is the underlying object with id, value and extensions. The accessor "getCompareToSourceExpression" gives direct access to the value 7064 */ 7065 public SetupActionAssertComponent setCompareToSourceExpressionElement(StringType value) { 7066 this.compareToSourceExpression = value; 7067 return this; 7068 } 7069 7070 /** 7071 * @return The FHIRPath expression for a specific value to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both. 7072 */ 7073 public String getCompareToSourceExpression() { 7074 return this.compareToSourceExpression == null ? null : this.compareToSourceExpression.getValue(); 7075 } 7076 7077 /** 7078 * @param value The FHIRPath expression for a specific value to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both. 7079 */ 7080 public SetupActionAssertComponent setCompareToSourceExpression(String value) { 7081 if (Utilities.noString(value)) 7082 this.compareToSourceExpression = null; 7083 else { 7084 if (this.compareToSourceExpression == null) 7085 this.compareToSourceExpression = new StringType(); 7086 this.compareToSourceExpression.setValue(value); 7087 } 7088 return this; 7089 } 7090 7091 /** 7092 * @return {@link #compareToSourcePath} (XPath or JSONPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.). This is the underlying object with id, value and extensions. The accessor "getCompareToSourcePath" gives direct access to the value 7093 */ 7094 public StringType getCompareToSourcePathElement() { 7095 if (this.compareToSourcePath == null) 7096 if (Configuration.errorOnAutoCreate()) 7097 throw new Error("Attempt to auto-create SetupActionAssertComponent.compareToSourcePath"); 7098 else if (Configuration.doAutoCreate()) 7099 this.compareToSourcePath = new StringType(); // bb 7100 return this.compareToSourcePath; 7101 } 7102 7103 public boolean hasCompareToSourcePathElement() { 7104 return this.compareToSourcePath != null && !this.compareToSourcePath.isEmpty(); 7105 } 7106 7107 public boolean hasCompareToSourcePath() { 7108 return this.compareToSourcePath != null && !this.compareToSourcePath.isEmpty(); 7109 } 7110 7111 /** 7112 * @param value {@link #compareToSourcePath} (XPath or JSONPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.). This is the underlying object with id, value and extensions. The accessor "getCompareToSourcePath" gives direct access to the value 7113 */ 7114 public SetupActionAssertComponent setCompareToSourcePathElement(StringType value) { 7115 this.compareToSourcePath = value; 7116 return this; 7117 } 7118 7119 /** 7120 * @return XPath or JSONPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both. 7121 */ 7122 public String getCompareToSourcePath() { 7123 return this.compareToSourcePath == null ? null : this.compareToSourcePath.getValue(); 7124 } 7125 7126 /** 7127 * @param value XPath or JSONPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both. 7128 */ 7129 public SetupActionAssertComponent setCompareToSourcePath(String value) { 7130 if (Utilities.noString(value)) 7131 this.compareToSourcePath = null; 7132 else { 7133 if (this.compareToSourcePath == null) 7134 this.compareToSourcePath = new StringType(); 7135 this.compareToSourcePath.setValue(value); 7136 } 7137 return this; 7138 } 7139 7140 /** 7141 * @return {@link #contentType} (The mime-type contents to compare against the request or response message 'Content-Type' header.). This is the underlying object with id, value and extensions. The accessor "getContentType" gives direct access to the value 7142 */ 7143 public CodeType getContentTypeElement() { 7144 if (this.contentType == null) 7145 if (Configuration.errorOnAutoCreate()) 7146 throw new Error("Attempt to auto-create SetupActionAssertComponent.contentType"); 7147 else if (Configuration.doAutoCreate()) 7148 this.contentType = new CodeType(); // bb 7149 return this.contentType; 7150 } 7151 7152 public boolean hasContentTypeElement() { 7153 return this.contentType != null && !this.contentType.isEmpty(); 7154 } 7155 7156 public boolean hasContentType() { 7157 return this.contentType != null && !this.contentType.isEmpty(); 7158 } 7159 7160 /** 7161 * @param value {@link #contentType} (The mime-type contents to compare against the request or response message 'Content-Type' header.). This is the underlying object with id, value and extensions. The accessor "getContentType" gives direct access to the value 7162 */ 7163 public SetupActionAssertComponent setContentTypeElement(CodeType value) { 7164 this.contentType = value; 7165 return this; 7166 } 7167 7168 /** 7169 * @return The mime-type contents to compare against the request or response message 'Content-Type' header. 7170 */ 7171 public String getContentType() { 7172 return this.contentType == null ? null : this.contentType.getValue(); 7173 } 7174 7175 /** 7176 * @param value The mime-type contents to compare against the request or response message 'Content-Type' header. 7177 */ 7178 public SetupActionAssertComponent setContentType(String value) { 7179 if (Utilities.noString(value)) 7180 this.contentType = null; 7181 else { 7182 if (this.contentType == null) 7183 this.contentType = new CodeType(); 7184 this.contentType.setValue(value); 7185 } 7186 return this; 7187 } 7188 7189 /** 7190 * @return {@link #defaultManualCompletion} (The default manual completion outcome applied to this assertion.). This is the underlying object with id, value and extensions. The accessor "getDefaultManualCompletion" gives direct access to the value 7191 */ 7192 public Enumeration<AssertionManualCompletionType> getDefaultManualCompletionElement() { 7193 if (this.defaultManualCompletion == null) 7194 if (Configuration.errorOnAutoCreate()) 7195 throw new Error("Attempt to auto-create SetupActionAssertComponent.defaultManualCompletion"); 7196 else if (Configuration.doAutoCreate()) 7197 this.defaultManualCompletion = new Enumeration<AssertionManualCompletionType>(new AssertionManualCompletionTypeEnumFactory()); // bb 7198 return this.defaultManualCompletion; 7199 } 7200 7201 public boolean hasDefaultManualCompletionElement() { 7202 return this.defaultManualCompletion != null && !this.defaultManualCompletion.isEmpty(); 7203 } 7204 7205 public boolean hasDefaultManualCompletion() { 7206 return this.defaultManualCompletion != null && !this.defaultManualCompletion.isEmpty(); 7207 } 7208 7209 /** 7210 * @param value {@link #defaultManualCompletion} (The default manual completion outcome applied to this assertion.). This is the underlying object with id, value and extensions. The accessor "getDefaultManualCompletion" gives direct access to the value 7211 */ 7212 public SetupActionAssertComponent setDefaultManualCompletionElement(Enumeration<AssertionManualCompletionType> value) { 7213 this.defaultManualCompletion = value; 7214 return this; 7215 } 7216 7217 /** 7218 * @return The default manual completion outcome applied to this assertion. 7219 */ 7220 public AssertionManualCompletionType getDefaultManualCompletion() { 7221 return this.defaultManualCompletion == null ? null : this.defaultManualCompletion.getValue(); 7222 } 7223 7224 /** 7225 * @param value The default manual completion outcome applied to this assertion. 7226 */ 7227 public SetupActionAssertComponent setDefaultManualCompletion(AssertionManualCompletionType value) { 7228 if (value == null) 7229 this.defaultManualCompletion = null; 7230 else { 7231 if (this.defaultManualCompletion == null) 7232 this.defaultManualCompletion = new Enumeration<AssertionManualCompletionType>(new AssertionManualCompletionTypeEnumFactory()); 7233 this.defaultManualCompletion.setValue(value); 7234 } 7235 return this; 7236 } 7237 7238 /** 7239 * @return {@link #expression} (The FHIRPath expression to be evaluated against the request or response message contents - HTTP headers and payload.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 7240 */ 7241 public StringType getExpressionElement() { 7242 if (this.expression == null) 7243 if (Configuration.errorOnAutoCreate()) 7244 throw new Error("Attempt to auto-create SetupActionAssertComponent.expression"); 7245 else if (Configuration.doAutoCreate()) 7246 this.expression = new StringType(); // bb 7247 return this.expression; 7248 } 7249 7250 public boolean hasExpressionElement() { 7251 return this.expression != null && !this.expression.isEmpty(); 7252 } 7253 7254 public boolean hasExpression() { 7255 return this.expression != null && !this.expression.isEmpty(); 7256 } 7257 7258 /** 7259 * @param value {@link #expression} (The FHIRPath expression to be evaluated against the request or response message contents - HTTP headers and payload.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 7260 */ 7261 public SetupActionAssertComponent setExpressionElement(StringType value) { 7262 this.expression = value; 7263 return this; 7264 } 7265 7266 /** 7267 * @return The FHIRPath expression to be evaluated against the request or response message contents - HTTP headers and payload. 7268 */ 7269 public String getExpression() { 7270 return this.expression == null ? null : this.expression.getValue(); 7271 } 7272 7273 /** 7274 * @param value The FHIRPath expression to be evaluated against the request or response message contents - HTTP headers and payload. 7275 */ 7276 public SetupActionAssertComponent setExpression(String value) { 7277 if (Utilities.noString(value)) 7278 this.expression = null; 7279 else { 7280 if (this.expression == null) 7281 this.expression = new StringType(); 7282 this.expression.setValue(value); 7283 } 7284 return this; 7285 } 7286 7287 /** 7288 * @return {@link #headerField} (The HTTP header field name e.g. 'Location'.). This is the underlying object with id, value and extensions. The accessor "getHeaderField" gives direct access to the value 7289 */ 7290 public StringType getHeaderFieldElement() { 7291 if (this.headerField == null) 7292 if (Configuration.errorOnAutoCreate()) 7293 throw new Error("Attempt to auto-create SetupActionAssertComponent.headerField"); 7294 else if (Configuration.doAutoCreate()) 7295 this.headerField = new StringType(); // bb 7296 return this.headerField; 7297 } 7298 7299 public boolean hasHeaderFieldElement() { 7300 return this.headerField != null && !this.headerField.isEmpty(); 7301 } 7302 7303 public boolean hasHeaderField() { 7304 return this.headerField != null && !this.headerField.isEmpty(); 7305 } 7306 7307 /** 7308 * @param value {@link #headerField} (The HTTP header field name e.g. 'Location'.). This is the underlying object with id, value and extensions. The accessor "getHeaderField" gives direct access to the value 7309 */ 7310 public SetupActionAssertComponent setHeaderFieldElement(StringType value) { 7311 this.headerField = value; 7312 return this; 7313 } 7314 7315 /** 7316 * @return The HTTP header field name e.g. 'Location'. 7317 */ 7318 public String getHeaderField() { 7319 return this.headerField == null ? null : this.headerField.getValue(); 7320 } 7321 7322 /** 7323 * @param value The HTTP header field name e.g. 'Location'. 7324 */ 7325 public SetupActionAssertComponent setHeaderField(String value) { 7326 if (Utilities.noString(value)) 7327 this.headerField = null; 7328 else { 7329 if (this.headerField == null) 7330 this.headerField = new StringType(); 7331 this.headerField.setValue(value); 7332 } 7333 return this; 7334 } 7335 7336 /** 7337 * @return {@link #minimumId} (The ID of a fixture. Asserts that the response contains at a minimum the fixture specified by minimumId.). This is the underlying object with id, value and extensions. The accessor "getMinimumId" gives direct access to the value 7338 */ 7339 public StringType getMinimumIdElement() { 7340 if (this.minimumId == null) 7341 if (Configuration.errorOnAutoCreate()) 7342 throw new Error("Attempt to auto-create SetupActionAssertComponent.minimumId"); 7343 else if (Configuration.doAutoCreate()) 7344 this.minimumId = new StringType(); // bb 7345 return this.minimumId; 7346 } 7347 7348 public boolean hasMinimumIdElement() { 7349 return this.minimumId != null && !this.minimumId.isEmpty(); 7350 } 7351 7352 public boolean hasMinimumId() { 7353 return this.minimumId != null && !this.minimumId.isEmpty(); 7354 } 7355 7356 /** 7357 * @param value {@link #minimumId} (The ID of a fixture. Asserts that the response contains at a minimum the fixture specified by minimumId.). This is the underlying object with id, value and extensions. The accessor "getMinimumId" gives direct access to the value 7358 */ 7359 public SetupActionAssertComponent setMinimumIdElement(StringType value) { 7360 this.minimumId = value; 7361 return this; 7362 } 7363 7364 /** 7365 * @return The ID of a fixture. Asserts that the response contains at a minimum the fixture specified by minimumId. 7366 */ 7367 public String getMinimumId() { 7368 return this.minimumId == null ? null : this.minimumId.getValue(); 7369 } 7370 7371 /** 7372 * @param value The ID of a fixture. Asserts that the response contains at a minimum the fixture specified by minimumId. 7373 */ 7374 public SetupActionAssertComponent setMinimumId(String value) { 7375 if (Utilities.noString(value)) 7376 this.minimumId = null; 7377 else { 7378 if (this.minimumId == null) 7379 this.minimumId = new StringType(); 7380 this.minimumId.setValue(value); 7381 } 7382 return this; 7383 } 7384 7385 /** 7386 * @return {@link #navigationLinks} (Whether or not the test execution performs validation on the bundle navigation links.). This is the underlying object with id, value and extensions. The accessor "getNavigationLinks" gives direct access to the value 7387 */ 7388 public BooleanType getNavigationLinksElement() { 7389 if (this.navigationLinks == null) 7390 if (Configuration.errorOnAutoCreate()) 7391 throw new Error("Attempt to auto-create SetupActionAssertComponent.navigationLinks"); 7392 else if (Configuration.doAutoCreate()) 7393 this.navigationLinks = new BooleanType(); // bb 7394 return this.navigationLinks; 7395 } 7396 7397 public boolean hasNavigationLinksElement() { 7398 return this.navigationLinks != null && !this.navigationLinks.isEmpty(); 7399 } 7400 7401 public boolean hasNavigationLinks() { 7402 return this.navigationLinks != null && !this.navigationLinks.isEmpty(); 7403 } 7404 7405 /** 7406 * @param value {@link #navigationLinks} (Whether or not the test execution performs validation on the bundle navigation links.). This is the underlying object with id, value and extensions. The accessor "getNavigationLinks" gives direct access to the value 7407 */ 7408 public SetupActionAssertComponent setNavigationLinksElement(BooleanType value) { 7409 this.navigationLinks = value; 7410 return this; 7411 } 7412 7413 /** 7414 * @return Whether or not the test execution performs validation on the bundle navigation links. 7415 */ 7416 public boolean getNavigationLinks() { 7417 return this.navigationLinks == null || this.navigationLinks.isEmpty() ? false : this.navigationLinks.getValue(); 7418 } 7419 7420 /** 7421 * @param value Whether or not the test execution performs validation on the bundle navigation links. 7422 */ 7423 public SetupActionAssertComponent setNavigationLinks(boolean value) { 7424 if (this.navigationLinks == null) 7425 this.navigationLinks = new BooleanType(); 7426 this.navigationLinks.setValue(value); 7427 return this; 7428 } 7429 7430 /** 7431 * @return {@link #operator} (The operator type defines the conditional behavior of the assert.). This is the underlying object with id, value and extensions. The accessor "getOperator" gives direct access to the value 7432 */ 7433 public Enumeration<AssertionOperatorType> getOperatorElement() { 7434 if (this.operator == null) 7435 if (Configuration.errorOnAutoCreate()) 7436 throw new Error("Attempt to auto-create SetupActionAssertComponent.operator"); 7437 else if (Configuration.doAutoCreate()) 7438 this.operator = new Enumeration<AssertionOperatorType>(new AssertionOperatorTypeEnumFactory()); // bb 7439 return this.operator; 7440 } 7441 7442 public boolean hasOperatorElement() { 7443 return this.operator != null && !this.operator.isEmpty(); 7444 } 7445 7446 public boolean hasOperator() { 7447 return this.operator != null && !this.operator.isEmpty(); 7448 } 7449 7450 /** 7451 * @param value {@link #operator} (The operator type defines the conditional behavior of the assert.). This is the underlying object with id, value and extensions. The accessor "getOperator" gives direct access to the value 7452 */ 7453 public SetupActionAssertComponent setOperatorElement(Enumeration<AssertionOperatorType> value) { 7454 this.operator = value; 7455 return this; 7456 } 7457 7458 /** 7459 * @return The operator type defines the conditional behavior of the assert. 7460 */ 7461 public AssertionOperatorType getOperator() { 7462 return this.operator == null ? null : this.operator.getValue(); 7463 } 7464 7465 /** 7466 * @param value The operator type defines the conditional behavior of the assert. 7467 */ 7468 public SetupActionAssertComponent setOperator(AssertionOperatorType value) { 7469 if (value == null) 7470 this.operator = null; 7471 else { 7472 if (this.operator == null) 7473 this.operator = new Enumeration<AssertionOperatorType>(new AssertionOperatorTypeEnumFactory()); 7474 this.operator.setValue(value); 7475 } 7476 return this; 7477 } 7478 7479 /** 7480 * @return {@link #path} (The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 7481 */ 7482 public StringType getPathElement() { 7483 if (this.path == null) 7484 if (Configuration.errorOnAutoCreate()) 7485 throw new Error("Attempt to auto-create SetupActionAssertComponent.path"); 7486 else if (Configuration.doAutoCreate()) 7487 this.path = new StringType(); // bb 7488 return this.path; 7489 } 7490 7491 public boolean hasPathElement() { 7492 return this.path != null && !this.path.isEmpty(); 7493 } 7494 7495 public boolean hasPath() { 7496 return this.path != null && !this.path.isEmpty(); 7497 } 7498 7499 /** 7500 * @param value {@link #path} (The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 7501 */ 7502 public SetupActionAssertComponent setPathElement(StringType value) { 7503 this.path = value; 7504 return this; 7505 } 7506 7507 /** 7508 * @return The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server. 7509 */ 7510 public String getPath() { 7511 return this.path == null ? null : this.path.getValue(); 7512 } 7513 7514 /** 7515 * @param value The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server. 7516 */ 7517 public SetupActionAssertComponent setPath(String value) { 7518 if (Utilities.noString(value)) 7519 this.path = null; 7520 else { 7521 if (this.path == null) 7522 this.path = new StringType(); 7523 this.path.setValue(value); 7524 } 7525 return this; 7526 } 7527 7528 /** 7529 * @return {@link #requestMethod} (The request method or HTTP operation code to compare against that used by the client system under test.). This is the underlying object with id, value and extensions. The accessor "getRequestMethod" gives direct access to the value 7530 */ 7531 public Enumeration<TestScriptRequestMethodCode> getRequestMethodElement() { 7532 if (this.requestMethod == null) 7533 if (Configuration.errorOnAutoCreate()) 7534 throw new Error("Attempt to auto-create SetupActionAssertComponent.requestMethod"); 7535 else if (Configuration.doAutoCreate()) 7536 this.requestMethod = new Enumeration<TestScriptRequestMethodCode>(new TestScriptRequestMethodCodeEnumFactory()); // bb 7537 return this.requestMethod; 7538 } 7539 7540 public boolean hasRequestMethodElement() { 7541 return this.requestMethod != null && !this.requestMethod.isEmpty(); 7542 } 7543 7544 public boolean hasRequestMethod() { 7545 return this.requestMethod != null && !this.requestMethod.isEmpty(); 7546 } 7547 7548 /** 7549 * @param value {@link #requestMethod} (The request method or HTTP operation code to compare against that used by the client system under test.). This is the underlying object with id, value and extensions. The accessor "getRequestMethod" gives direct access to the value 7550 */ 7551 public SetupActionAssertComponent setRequestMethodElement(Enumeration<TestScriptRequestMethodCode> value) { 7552 this.requestMethod = value; 7553 return this; 7554 } 7555 7556 /** 7557 * @return The request method or HTTP operation code to compare against that used by the client system under test. 7558 */ 7559 public TestScriptRequestMethodCode getRequestMethod() { 7560 return this.requestMethod == null ? null : this.requestMethod.getValue(); 7561 } 7562 7563 /** 7564 * @param value The request method or HTTP operation code to compare against that used by the client system under test. 7565 */ 7566 public SetupActionAssertComponent setRequestMethod(TestScriptRequestMethodCode value) { 7567 if (value == null) 7568 this.requestMethod = null; 7569 else { 7570 if (this.requestMethod == null) 7571 this.requestMethod = new Enumeration<TestScriptRequestMethodCode>(new TestScriptRequestMethodCodeEnumFactory()); 7572 this.requestMethod.setValue(value); 7573 } 7574 return this; 7575 } 7576 7577 /** 7578 * @return {@link #requestURL} (The value to use in a comparison against the request URL path string.). This is the underlying object with id, value and extensions. The accessor "getRequestURL" gives direct access to the value 7579 */ 7580 public StringType getRequestURLElement() { 7581 if (this.requestURL == null) 7582 if (Configuration.errorOnAutoCreate()) 7583 throw new Error("Attempt to auto-create SetupActionAssertComponent.requestURL"); 7584 else if (Configuration.doAutoCreate()) 7585 this.requestURL = new StringType(); // bb 7586 return this.requestURL; 7587 } 7588 7589 public boolean hasRequestURLElement() { 7590 return this.requestURL != null && !this.requestURL.isEmpty(); 7591 } 7592 7593 public boolean hasRequestURL() { 7594 return this.requestURL != null && !this.requestURL.isEmpty(); 7595 } 7596 7597 /** 7598 * @param value {@link #requestURL} (The value to use in a comparison against the request URL path string.). This is the underlying object with id, value and extensions. The accessor "getRequestURL" gives direct access to the value 7599 */ 7600 public SetupActionAssertComponent setRequestURLElement(StringType value) { 7601 this.requestURL = value; 7602 return this; 7603 } 7604 7605 /** 7606 * @return The value to use in a comparison against the request URL path string. 7607 */ 7608 public String getRequestURL() { 7609 return this.requestURL == null ? null : this.requestURL.getValue(); 7610 } 7611 7612 /** 7613 * @param value The value to use in a comparison against the request URL path string. 7614 */ 7615 public SetupActionAssertComponent setRequestURL(String value) { 7616 if (Utilities.noString(value)) 7617 this.requestURL = null; 7618 else { 7619 if (this.requestURL == null) 7620 this.requestURL = new StringType(); 7621 this.requestURL.setValue(value); 7622 } 7623 return this; 7624 } 7625 7626 /** 7627 * @return {@link #resource} (The type of the resource. See the [resource list](resourcelist.html).). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 7628 */ 7629 public UriType getResourceElement() { 7630 if (this.resource == null) 7631 if (Configuration.errorOnAutoCreate()) 7632 throw new Error("Attempt to auto-create SetupActionAssertComponent.resource"); 7633 else if (Configuration.doAutoCreate()) 7634 this.resource = new UriType(); // bb 7635 return this.resource; 7636 } 7637 7638 public boolean hasResourceElement() { 7639 return this.resource != null && !this.resource.isEmpty(); 7640 } 7641 7642 public boolean hasResource() { 7643 return this.resource != null && !this.resource.isEmpty(); 7644 } 7645 7646 /** 7647 * @param value {@link #resource} (The type of the resource. See the [resource list](resourcelist.html).). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 7648 */ 7649 public SetupActionAssertComponent setResourceElement(UriType value) { 7650 this.resource = value; 7651 return this; 7652 } 7653 7654 /** 7655 * @return The type of the resource. See the [resource list](resourcelist.html). 7656 */ 7657 public String getResource() { 7658 return this.resource == null ? null : this.resource.getValue(); 7659 } 7660 7661 /** 7662 * @param value The type of the resource. See the [resource list](resourcelist.html). 7663 */ 7664 public SetupActionAssertComponent setResource(String value) { 7665 if (Utilities.noString(value)) 7666 this.resource = null; 7667 else { 7668 if (this.resource == null) 7669 this.resource = new UriType(); 7670 this.resource.setValue(value); 7671 } 7672 return this; 7673 } 7674 7675 /** 7676 * @return {@link #response} (continue | switchingProtocols | okay | created | accepted | nonAuthoritativeInformation | noContent | resetContent | partialContent | multipleChoices | movedPermanently | found | seeOther | notModified | useProxy | temporaryRedirect | permanentRedirect | badRequest | unauthorized | paymentRequired | forbidden | notFound | methodNotAllowed | notAcceptable | proxyAuthenticationRequired | requestTimeout | conflict | gone | lengthRequired | preconditionFailed | contentTooLarge | uriTooLong | unsupportedMediaType | rangeNotSatisfiable | expectationFailed | misdirectedRequest | unprocessableContent | upgradeRequired | internalServerError | notImplemented | badGateway | serviceUnavailable | gatewayTimeout | httpVersionNotSupported.). This is the underlying object with id, value and extensions. The accessor "getResponse" gives direct access to the value 7677 */ 7678 public Enumeration<AssertionResponseTypes> getResponseElement() { 7679 if (this.response == null) 7680 if (Configuration.errorOnAutoCreate()) 7681 throw new Error("Attempt to auto-create SetupActionAssertComponent.response"); 7682 else if (Configuration.doAutoCreate()) 7683 this.response = new Enumeration<AssertionResponseTypes>(new AssertionResponseTypesEnumFactory()); // bb 7684 return this.response; 7685 } 7686 7687 public boolean hasResponseElement() { 7688 return this.response != null && !this.response.isEmpty(); 7689 } 7690 7691 public boolean hasResponse() { 7692 return this.response != null && !this.response.isEmpty(); 7693 } 7694 7695 /** 7696 * @param value {@link #response} (continue | switchingProtocols | okay | created | accepted | nonAuthoritativeInformation | noContent | resetContent | partialContent | multipleChoices | movedPermanently | found | seeOther | notModified | useProxy | temporaryRedirect | permanentRedirect | badRequest | unauthorized | paymentRequired | forbidden | notFound | methodNotAllowed | notAcceptable | proxyAuthenticationRequired | requestTimeout | conflict | gone | lengthRequired | preconditionFailed | contentTooLarge | uriTooLong | unsupportedMediaType | rangeNotSatisfiable | expectationFailed | misdirectedRequest | unprocessableContent | upgradeRequired | internalServerError | notImplemented | badGateway | serviceUnavailable | gatewayTimeout | httpVersionNotSupported.). This is the underlying object with id, value and extensions. The accessor "getResponse" gives direct access to the value 7697 */ 7698 public SetupActionAssertComponent setResponseElement(Enumeration<AssertionResponseTypes> value) { 7699 this.response = value; 7700 return this; 7701 } 7702 7703 /** 7704 * @return continue | switchingProtocols | okay | created | accepted | nonAuthoritativeInformation | noContent | resetContent | partialContent | multipleChoices | movedPermanently | found | seeOther | notModified | useProxy | temporaryRedirect | permanentRedirect | badRequest | unauthorized | paymentRequired | forbidden | notFound | methodNotAllowed | notAcceptable | proxyAuthenticationRequired | requestTimeout | conflict | gone | lengthRequired | preconditionFailed | contentTooLarge | uriTooLong | unsupportedMediaType | rangeNotSatisfiable | expectationFailed | misdirectedRequest | unprocessableContent | upgradeRequired | internalServerError | notImplemented | badGateway | serviceUnavailable | gatewayTimeout | httpVersionNotSupported. 7705 */ 7706 public AssertionResponseTypes getResponse() { 7707 return this.response == null ? null : this.response.getValue(); 7708 } 7709 7710 /** 7711 * @param value continue | switchingProtocols | okay | created | accepted | nonAuthoritativeInformation | noContent | resetContent | partialContent | multipleChoices | movedPermanently | found | seeOther | notModified | useProxy | temporaryRedirect | permanentRedirect | badRequest | unauthorized | paymentRequired | forbidden | notFound | methodNotAllowed | notAcceptable | proxyAuthenticationRequired | requestTimeout | conflict | gone | lengthRequired | preconditionFailed | contentTooLarge | uriTooLong | unsupportedMediaType | rangeNotSatisfiable | expectationFailed | misdirectedRequest | unprocessableContent | upgradeRequired | internalServerError | notImplemented | badGateway | serviceUnavailable | gatewayTimeout | httpVersionNotSupported. 7712 */ 7713 public SetupActionAssertComponent setResponse(AssertionResponseTypes value) { 7714 if (value == null) 7715 this.response = null; 7716 else { 7717 if (this.response == null) 7718 this.response = new Enumeration<AssertionResponseTypes>(new AssertionResponseTypesEnumFactory()); 7719 this.response.setValue(value); 7720 } 7721 return this; 7722 } 7723 7724 /** 7725 * @return {@link #responseCode} (The value of the HTTP response code to be tested.). This is the underlying object with id, value and extensions. The accessor "getResponseCode" gives direct access to the value 7726 */ 7727 public StringType getResponseCodeElement() { 7728 if (this.responseCode == null) 7729 if (Configuration.errorOnAutoCreate()) 7730 throw new Error("Attempt to auto-create SetupActionAssertComponent.responseCode"); 7731 else if (Configuration.doAutoCreate()) 7732 this.responseCode = new StringType(); // bb 7733 return this.responseCode; 7734 } 7735 7736 public boolean hasResponseCodeElement() { 7737 return this.responseCode != null && !this.responseCode.isEmpty(); 7738 } 7739 7740 public boolean hasResponseCode() { 7741 return this.responseCode != null && !this.responseCode.isEmpty(); 7742 } 7743 7744 /** 7745 * @param value {@link #responseCode} (The value of the HTTP response code to be tested.). This is the underlying object with id, value and extensions. The accessor "getResponseCode" gives direct access to the value 7746 */ 7747 public SetupActionAssertComponent setResponseCodeElement(StringType value) { 7748 this.responseCode = value; 7749 return this; 7750 } 7751 7752 /** 7753 * @return The value of the HTTP response code to be tested. 7754 */ 7755 public String getResponseCode() { 7756 return this.responseCode == null ? null : this.responseCode.getValue(); 7757 } 7758 7759 /** 7760 * @param value The value of the HTTP response code to be tested. 7761 */ 7762 public SetupActionAssertComponent setResponseCode(String value) { 7763 if (Utilities.noString(value)) 7764 this.responseCode = null; 7765 else { 7766 if (this.responseCode == null) 7767 this.responseCode = new StringType(); 7768 this.responseCode.setValue(value); 7769 } 7770 return this; 7771 } 7772 7773 /** 7774 * @return {@link #sourceId} (Fixture to evaluate the XPath/JSONPath expression or the headerField against.). This is the underlying object with id, value and extensions. The accessor "getSourceId" gives direct access to the value 7775 */ 7776 public IdType getSourceIdElement() { 7777 if (this.sourceId == null) 7778 if (Configuration.errorOnAutoCreate()) 7779 throw new Error("Attempt to auto-create SetupActionAssertComponent.sourceId"); 7780 else if (Configuration.doAutoCreate()) 7781 this.sourceId = new IdType(); // bb 7782 return this.sourceId; 7783 } 7784 7785 public boolean hasSourceIdElement() { 7786 return this.sourceId != null && !this.sourceId.isEmpty(); 7787 } 7788 7789 public boolean hasSourceId() { 7790 return this.sourceId != null && !this.sourceId.isEmpty(); 7791 } 7792 7793 /** 7794 * @param value {@link #sourceId} (Fixture to evaluate the XPath/JSONPath expression or the headerField against.). This is the underlying object with id, value and extensions. The accessor "getSourceId" gives direct access to the value 7795 */ 7796 public SetupActionAssertComponent setSourceIdElement(IdType value) { 7797 this.sourceId = value; 7798 return this; 7799 } 7800 7801 /** 7802 * @return Fixture to evaluate the XPath/JSONPath expression or the headerField against. 7803 */ 7804 public String getSourceId() { 7805 return this.sourceId == null ? null : this.sourceId.getValue(); 7806 } 7807 7808 /** 7809 * @param value Fixture to evaluate the XPath/JSONPath expression or the headerField against. 7810 */ 7811 public SetupActionAssertComponent setSourceId(String value) { 7812 if (Utilities.noString(value)) 7813 this.sourceId = null; 7814 else { 7815 if (this.sourceId == null) 7816 this.sourceId = new IdType(); 7817 this.sourceId.setValue(value); 7818 } 7819 return this; 7820 } 7821 7822 /** 7823 * @return {@link #stopTestOnFail} (Whether or not the current test execution will stop on failure for this assert.). This is the underlying object with id, value and extensions. The accessor "getStopTestOnFail" gives direct access to the value 7824 */ 7825 public BooleanType getStopTestOnFailElement() { 7826 if (this.stopTestOnFail == null) 7827 if (Configuration.errorOnAutoCreate()) 7828 throw new Error("Attempt to auto-create SetupActionAssertComponent.stopTestOnFail"); 7829 else if (Configuration.doAutoCreate()) 7830 this.stopTestOnFail = new BooleanType(); // bb 7831 return this.stopTestOnFail; 7832 } 7833 7834 public boolean hasStopTestOnFailElement() { 7835 return this.stopTestOnFail != null && !this.stopTestOnFail.isEmpty(); 7836 } 7837 7838 public boolean hasStopTestOnFail() { 7839 return this.stopTestOnFail != null && !this.stopTestOnFail.isEmpty(); 7840 } 7841 7842 /** 7843 * @param value {@link #stopTestOnFail} (Whether or not the current test execution will stop on failure for this assert.). This is the underlying object with id, value and extensions. The accessor "getStopTestOnFail" gives direct access to the value 7844 */ 7845 public SetupActionAssertComponent setStopTestOnFailElement(BooleanType value) { 7846 this.stopTestOnFail = value; 7847 return this; 7848 } 7849 7850 /** 7851 * @return Whether or not the current test execution will stop on failure for this assert. 7852 */ 7853 public boolean getStopTestOnFail() { 7854 return this.stopTestOnFail == null || this.stopTestOnFail.isEmpty() ? false : this.stopTestOnFail.getValue(); 7855 } 7856 7857 /** 7858 * @param value Whether or not the current test execution will stop on failure for this assert. 7859 */ 7860 public SetupActionAssertComponent setStopTestOnFail(boolean value) { 7861 if (this.stopTestOnFail == null) 7862 this.stopTestOnFail = new BooleanType(); 7863 this.stopTestOnFail.setValue(value); 7864 return this; 7865 } 7866 7867 /** 7868 * @return {@link #validateProfileId} (The ID of the Profile to validate against.). This is the underlying object with id, value and extensions. The accessor "getValidateProfileId" gives direct access to the value 7869 */ 7870 public IdType getValidateProfileIdElement() { 7871 if (this.validateProfileId == null) 7872 if (Configuration.errorOnAutoCreate()) 7873 throw new Error("Attempt to auto-create SetupActionAssertComponent.validateProfileId"); 7874 else if (Configuration.doAutoCreate()) 7875 this.validateProfileId = new IdType(); // bb 7876 return this.validateProfileId; 7877 } 7878 7879 public boolean hasValidateProfileIdElement() { 7880 return this.validateProfileId != null && !this.validateProfileId.isEmpty(); 7881 } 7882 7883 public boolean hasValidateProfileId() { 7884 return this.validateProfileId != null && !this.validateProfileId.isEmpty(); 7885 } 7886 7887 /** 7888 * @param value {@link #validateProfileId} (The ID of the Profile to validate against.). This is the underlying object with id, value and extensions. The accessor "getValidateProfileId" gives direct access to the value 7889 */ 7890 public SetupActionAssertComponent setValidateProfileIdElement(IdType value) { 7891 this.validateProfileId = value; 7892 return this; 7893 } 7894 7895 /** 7896 * @return The ID of the Profile to validate against. 7897 */ 7898 public String getValidateProfileId() { 7899 return this.validateProfileId == null ? null : this.validateProfileId.getValue(); 7900 } 7901 7902 /** 7903 * @param value The ID of the Profile to validate against. 7904 */ 7905 public SetupActionAssertComponent setValidateProfileId(String value) { 7906 if (Utilities.noString(value)) 7907 this.validateProfileId = null; 7908 else { 7909 if (this.validateProfileId == null) 7910 this.validateProfileId = new IdType(); 7911 this.validateProfileId.setValue(value); 7912 } 7913 return this; 7914 } 7915 7916 /** 7917 * @return {@link #value} (The value to compare to.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 7918 */ 7919 public StringType getValueElement() { 7920 if (this.value == null) 7921 if (Configuration.errorOnAutoCreate()) 7922 throw new Error("Attempt to auto-create SetupActionAssertComponent.value"); 7923 else if (Configuration.doAutoCreate()) 7924 this.value = new StringType(); // bb 7925 return this.value; 7926 } 7927 7928 public boolean hasValueElement() { 7929 return this.value != null && !this.value.isEmpty(); 7930 } 7931 7932 public boolean hasValue() { 7933 return this.value != null && !this.value.isEmpty(); 7934 } 7935 7936 /** 7937 * @param value {@link #value} (The value to compare to.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 7938 */ 7939 public SetupActionAssertComponent setValueElement(StringType value) { 7940 this.value = value; 7941 return this; 7942 } 7943 7944 /** 7945 * @return The value to compare to. 7946 */ 7947 public String getValue() { 7948 return this.value == null ? null : this.value.getValue(); 7949 } 7950 7951 /** 7952 * @param value The value to compare to. 7953 */ 7954 public SetupActionAssertComponent setValue(String value) { 7955 if (Utilities.noString(value)) 7956 this.value = null; 7957 else { 7958 if (this.value == null) 7959 this.value = new StringType(); 7960 this.value.setValue(value); 7961 } 7962 return this; 7963 } 7964 7965 /** 7966 * @return {@link #warningOnly} (Whether or not the test execution will produce a warning only on error for this assert.). This is the underlying object with id, value and extensions. The accessor "getWarningOnly" gives direct access to the value 7967 */ 7968 public BooleanType getWarningOnlyElement() { 7969 if (this.warningOnly == null) 7970 if (Configuration.errorOnAutoCreate()) 7971 throw new Error("Attempt to auto-create SetupActionAssertComponent.warningOnly"); 7972 else if (Configuration.doAutoCreate()) 7973 this.warningOnly = new BooleanType(); // bb 7974 return this.warningOnly; 7975 } 7976 7977 public boolean hasWarningOnlyElement() { 7978 return this.warningOnly != null && !this.warningOnly.isEmpty(); 7979 } 7980 7981 public boolean hasWarningOnly() { 7982 return this.warningOnly != null && !this.warningOnly.isEmpty(); 7983 } 7984 7985 /** 7986 * @param value {@link #warningOnly} (Whether or not the test execution will produce a warning only on error for this assert.). This is the underlying object with id, value and extensions. The accessor "getWarningOnly" gives direct access to the value 7987 */ 7988 public SetupActionAssertComponent setWarningOnlyElement(BooleanType value) { 7989 this.warningOnly = value; 7990 return this; 7991 } 7992 7993 /** 7994 * @return Whether or not the test execution will produce a warning only on error for this assert. 7995 */ 7996 public boolean getWarningOnly() { 7997 return this.warningOnly == null || this.warningOnly.isEmpty() ? false : this.warningOnly.getValue(); 7998 } 7999 8000 /** 8001 * @param value Whether or not the test execution will produce a warning only on error for this assert. 8002 */ 8003 public SetupActionAssertComponent setWarningOnly(boolean value) { 8004 if (this.warningOnly == null) 8005 this.warningOnly = new BooleanType(); 8006 this.warningOnly.setValue(value); 8007 return this; 8008 } 8009 8010 /** 8011 * @return {@link #requirement} (Links or references providing traceability to the testing requirements for this assert.) 8012 */ 8013 public List<SetupActionAssertRequirementComponent> getRequirement() { 8014 if (this.requirement == null) 8015 this.requirement = new ArrayList<SetupActionAssertRequirementComponent>(); 8016 return this.requirement; 8017 } 8018 8019 /** 8020 * @return Returns a reference to <code>this</code> for easy method chaining 8021 */ 8022 public SetupActionAssertComponent setRequirement(List<SetupActionAssertRequirementComponent> theRequirement) { 8023 this.requirement = theRequirement; 8024 return this; 8025 } 8026 8027 public boolean hasRequirement() { 8028 if (this.requirement == null) 8029 return false; 8030 for (SetupActionAssertRequirementComponent item : this.requirement) 8031 if (!item.isEmpty()) 8032 return true; 8033 return false; 8034 } 8035 8036 public SetupActionAssertRequirementComponent addRequirement() { //3 8037 SetupActionAssertRequirementComponent t = new SetupActionAssertRequirementComponent(); 8038 if (this.requirement == null) 8039 this.requirement = new ArrayList<SetupActionAssertRequirementComponent>(); 8040 this.requirement.add(t); 8041 return t; 8042 } 8043 8044 public SetupActionAssertComponent addRequirement(SetupActionAssertRequirementComponent t) { //3 8045 if (t == null) 8046 return this; 8047 if (this.requirement == null) 8048 this.requirement = new ArrayList<SetupActionAssertRequirementComponent>(); 8049 this.requirement.add(t); 8050 return this; 8051 } 8052 8053 /** 8054 * @return The first repetition of repeating field {@link #requirement}, creating it if it does not already exist {3} 8055 */ 8056 public SetupActionAssertRequirementComponent getRequirementFirstRep() { 8057 if (getRequirement().isEmpty()) { 8058 addRequirement(); 8059 } 8060 return getRequirement().get(0); 8061 } 8062 8063 protected void listChildren(List<Property> children) { 8064 super.listChildren(children); 8065 children.add(new Property("label", "string", "The label would be used for tracking/logging purposes by test engines.", 0, 1, label)); 8066 children.add(new Property("description", "string", "The description would be used by test engines for tracking and reporting purposes.", 0, 1, description)); 8067 children.add(new Property("direction", "code", "The direction to use for the assertion.", 0, 1, direction)); 8068 children.add(new Property("compareToSourceId", "string", "Id of the source fixture used as the contents to be evaluated by either the \"source/expression\" or \"sourceId/path\" definition.", 0, 1, compareToSourceId)); 8069 children.add(new Property("compareToSourceExpression", "string", "The FHIRPath expression for a specific value to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.", 0, 1, compareToSourceExpression)); 8070 children.add(new Property("compareToSourcePath", "string", "XPath or JSONPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.", 0, 1, compareToSourcePath)); 8071 children.add(new Property("contentType", "code", "The mime-type contents to compare against the request or response message 'Content-Type' header.", 0, 1, contentType)); 8072 children.add(new Property("defaultManualCompletion", "code", "The default manual completion outcome applied to this assertion.", 0, 1, defaultManualCompletion)); 8073 children.add(new Property("expression", "string", "The FHIRPath expression to be evaluated against the request or response message contents - HTTP headers and payload.", 0, 1, expression)); 8074 children.add(new Property("headerField", "string", "The HTTP header field name e.g. 'Location'.", 0, 1, headerField)); 8075 children.add(new Property("minimumId", "string", "The ID of a fixture. Asserts that the response contains at a minimum the fixture specified by minimumId.", 0, 1, minimumId)); 8076 children.add(new Property("navigationLinks", "boolean", "Whether or not the test execution performs validation on the bundle navigation links.", 0, 1, navigationLinks)); 8077 children.add(new Property("operator", "code", "The operator type defines the conditional behavior of the assert.", 0, 1, operator)); 8078 children.add(new Property("path", "string", "The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server.", 0, 1, path)); 8079 children.add(new Property("requestMethod", "code", "The request method or HTTP operation code to compare against that used by the client system under test.", 0, 1, requestMethod)); 8080 children.add(new Property("requestURL", "string", "The value to use in a comparison against the request URL path string.", 0, 1, requestURL)); 8081 children.add(new Property("resource", "uri", "The type of the resource. See the [resource list](resourcelist.html).", 0, 1, resource)); 8082 children.add(new Property("response", "code", "continue | switchingProtocols | okay | created | accepted | nonAuthoritativeInformation | noContent | resetContent | partialContent | multipleChoices | movedPermanently | found | seeOther | notModified | useProxy | temporaryRedirect | permanentRedirect | badRequest | unauthorized | paymentRequired | forbidden | notFound | methodNotAllowed | notAcceptable | proxyAuthenticationRequired | requestTimeout | conflict | gone | lengthRequired | preconditionFailed | contentTooLarge | uriTooLong | unsupportedMediaType | rangeNotSatisfiable | expectationFailed | misdirectedRequest | unprocessableContent | upgradeRequired | internalServerError | notImplemented | badGateway | serviceUnavailable | gatewayTimeout | httpVersionNotSupported.", 0, 1, response)); 8083 children.add(new Property("responseCode", "string", "The value of the HTTP response code to be tested.", 0, 1, responseCode)); 8084 children.add(new Property("sourceId", "id", "Fixture to evaluate the XPath/JSONPath expression or the headerField against.", 0, 1, sourceId)); 8085 children.add(new Property("stopTestOnFail", "boolean", "Whether or not the current test execution will stop on failure for this assert.", 0, 1, stopTestOnFail)); 8086 children.add(new Property("validateProfileId", "id", "The ID of the Profile to validate against.", 0, 1, validateProfileId)); 8087 children.add(new Property("value", "string", "The value to compare to.", 0, 1, value)); 8088 children.add(new Property("warningOnly", "boolean", "Whether or not the test execution will produce a warning only on error for this assert.", 0, 1, warningOnly)); 8089 children.add(new Property("requirement", "", "Links or references providing traceability to the testing requirements for this assert.", 0, java.lang.Integer.MAX_VALUE, requirement)); 8090 } 8091 8092 @Override 8093 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8094 switch (_hash) { 8095 case 102727412: /*label*/ return new Property("label", "string", "The label would be used for tracking/logging purposes by test engines.", 0, 1, label); 8096 case -1724546052: /*description*/ return new Property("description", "string", "The description would be used by test engines for tracking and reporting purposes.", 0, 1, description); 8097 case -962590849: /*direction*/ return new Property("direction", "code", "The direction to use for the assertion.", 0, 1, direction); 8098 case 2081856758: /*compareToSourceId*/ return new Property("compareToSourceId", "string", "Id of the source fixture used as the contents to be evaluated by either the \"source/expression\" or \"sourceId/path\" definition.", 0, 1, compareToSourceId); 8099 case -1415702669: /*compareToSourceExpression*/ return new Property("compareToSourceExpression", "string", "The FHIRPath expression for a specific value to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.", 0, 1, compareToSourceExpression); 8100 case -790206144: /*compareToSourcePath*/ return new Property("compareToSourcePath", "string", "XPath or JSONPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.", 0, 1, compareToSourcePath); 8101 case -389131437: /*contentType*/ return new Property("contentType", "code", "The mime-type contents to compare against the request or response message 'Content-Type' header.", 0, 1, contentType); 8102 case 1448452259: /*defaultManualCompletion*/ return new Property("defaultManualCompletion", "code", "The default manual completion outcome applied to this assertion.", 0, 1, defaultManualCompletion); 8103 case -1795452264: /*expression*/ return new Property("expression", "string", "The FHIRPath expression to be evaluated against the request or response message contents - HTTP headers and payload.", 0, 1, expression); 8104 case 1160732269: /*headerField*/ return new Property("headerField", "string", "The HTTP header field name e.g. 'Location'.", 0, 1, headerField); 8105 case 818925001: /*minimumId*/ return new Property("minimumId", "string", "The ID of a fixture. Asserts that the response contains at a minimum the fixture specified by minimumId.", 0, 1, minimumId); 8106 case 1001488901: /*navigationLinks*/ return new Property("navigationLinks", "boolean", "Whether or not the test execution performs validation on the bundle navigation links.", 0, 1, navigationLinks); 8107 case -500553564: /*operator*/ return new Property("operator", "code", "The operator type defines the conditional behavior of the assert.", 0, 1, operator); 8108 case 3433509: /*path*/ return new Property("path", "string", "The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server.", 0, 1, path); 8109 case 1217874000: /*requestMethod*/ return new Property("requestMethod", "code", "The request method or HTTP operation code to compare against that used by the client system under test.", 0, 1, requestMethod); 8110 case 37099616: /*requestURL*/ return new Property("requestURL", "string", "The value to use in a comparison against the request URL path string.", 0, 1, requestURL); 8111 case -341064690: /*resource*/ return new Property("resource", "uri", "The type of the resource. See the [resource list](resourcelist.html).", 0, 1, resource); 8112 case -340323263: /*response*/ return new Property("response", "code", "continue | switchingProtocols | okay | created | accepted | nonAuthoritativeInformation | noContent | resetContent | partialContent | multipleChoices | movedPermanently | found | seeOther | notModified | useProxy | temporaryRedirect | permanentRedirect | badRequest | unauthorized | paymentRequired | forbidden | notFound | methodNotAllowed | notAcceptable | proxyAuthenticationRequired | requestTimeout | conflict | gone | lengthRequired | preconditionFailed | contentTooLarge | uriTooLong | unsupportedMediaType | rangeNotSatisfiable | expectationFailed | misdirectedRequest | unprocessableContent | upgradeRequired | internalServerError | notImplemented | badGateway | serviceUnavailable | gatewayTimeout | httpVersionNotSupported.", 0, 1, response); 8113 case 1438723534: /*responseCode*/ return new Property("responseCode", "string", "The value of the HTTP response code to be tested.", 0, 1, responseCode); 8114 case 1746327190: /*sourceId*/ return new Property("sourceId", "id", "Fixture to evaluate the XPath/JSONPath expression or the headerField against.", 0, 1, sourceId); 8115 case -626616719: /*stopTestOnFail*/ return new Property("stopTestOnFail", "boolean", "Whether or not the current test execution will stop on failure for this assert.", 0, 1, stopTestOnFail); 8116 case 1555541038: /*validateProfileId*/ return new Property("validateProfileId", "id", "The ID of the Profile to validate against.", 0, 1, validateProfileId); 8117 case 111972721: /*value*/ return new Property("value", "string", "The value to compare to.", 0, 1, value); 8118 case -481159832: /*warningOnly*/ return new Property("warningOnly", "boolean", "Whether or not the test execution will produce a warning only on error for this assert.", 0, 1, warningOnly); 8119 case 363387971: /*requirement*/ return new Property("requirement", "", "Links or references providing traceability to the testing requirements for this assert.", 0, java.lang.Integer.MAX_VALUE, requirement); 8120 default: return super.getNamedProperty(_hash, _name, _checkValid); 8121 } 8122 8123 } 8124 8125 @Override 8126 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8127 switch (hash) { 8128 case 102727412: /*label*/ return this.label == null ? new Base[0] : new Base[] {this.label}; // StringType 8129 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 8130 case -962590849: /*direction*/ return this.direction == null ? new Base[0] : new Base[] {this.direction}; // Enumeration<AssertionDirectionType> 8131 case 2081856758: /*compareToSourceId*/ return this.compareToSourceId == null ? new Base[0] : new Base[] {this.compareToSourceId}; // StringType 8132 case -1415702669: /*compareToSourceExpression*/ return this.compareToSourceExpression == null ? new Base[0] : new Base[] {this.compareToSourceExpression}; // StringType 8133 case -790206144: /*compareToSourcePath*/ return this.compareToSourcePath == null ? new Base[0] : new Base[] {this.compareToSourcePath}; // StringType 8134 case -389131437: /*contentType*/ return this.contentType == null ? new Base[0] : new Base[] {this.contentType}; // CodeType 8135 case 1448452259: /*defaultManualCompletion*/ return this.defaultManualCompletion == null ? new Base[0] : new Base[] {this.defaultManualCompletion}; // Enumeration<AssertionManualCompletionType> 8136 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 8137 case 1160732269: /*headerField*/ return this.headerField == null ? new Base[0] : new Base[] {this.headerField}; // StringType 8138 case 818925001: /*minimumId*/ return this.minimumId == null ? new Base[0] : new Base[] {this.minimumId}; // StringType 8139 case 1001488901: /*navigationLinks*/ return this.navigationLinks == null ? new Base[0] : new Base[] {this.navigationLinks}; // BooleanType 8140 case -500553564: /*operator*/ return this.operator == null ? new Base[0] : new Base[] {this.operator}; // Enumeration<AssertionOperatorType> 8141 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 8142 case 1217874000: /*requestMethod*/ return this.requestMethod == null ? new Base[0] : new Base[] {this.requestMethod}; // Enumeration<TestScriptRequestMethodCode> 8143 case 37099616: /*requestURL*/ return this.requestURL == null ? new Base[0] : new Base[] {this.requestURL}; // StringType 8144 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // UriType 8145 case -340323263: /*response*/ return this.response == null ? new Base[0] : new Base[] {this.response}; // Enumeration<AssertionResponseTypes> 8146 case 1438723534: /*responseCode*/ return this.responseCode == null ? new Base[0] : new Base[] {this.responseCode}; // StringType 8147 case 1746327190: /*sourceId*/ return this.sourceId == null ? new Base[0] : new Base[] {this.sourceId}; // IdType 8148 case -626616719: /*stopTestOnFail*/ return this.stopTestOnFail == null ? new Base[0] : new Base[] {this.stopTestOnFail}; // BooleanType 8149 case 1555541038: /*validateProfileId*/ return this.validateProfileId == null ? new Base[0] : new Base[] {this.validateProfileId}; // IdType 8150 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 8151 case -481159832: /*warningOnly*/ return this.warningOnly == null ? new Base[0] : new Base[] {this.warningOnly}; // BooleanType 8152 case 363387971: /*requirement*/ return this.requirement == null ? new Base[0] : this.requirement.toArray(new Base[this.requirement.size()]); // SetupActionAssertRequirementComponent 8153 default: return super.getProperty(hash, name, checkValid); 8154 } 8155 8156 } 8157 8158 @Override 8159 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8160 switch (hash) { 8161 case 102727412: // label 8162 this.label = TypeConvertor.castToString(value); // StringType 8163 return value; 8164 case -1724546052: // description 8165 this.description = TypeConvertor.castToString(value); // StringType 8166 return value; 8167 case -962590849: // direction 8168 value = new AssertionDirectionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 8169 this.direction = (Enumeration) value; // Enumeration<AssertionDirectionType> 8170 return value; 8171 case 2081856758: // compareToSourceId 8172 this.compareToSourceId = TypeConvertor.castToString(value); // StringType 8173 return value; 8174 case -1415702669: // compareToSourceExpression 8175 this.compareToSourceExpression = TypeConvertor.castToString(value); // StringType 8176 return value; 8177 case -790206144: // compareToSourcePath 8178 this.compareToSourcePath = TypeConvertor.castToString(value); // StringType 8179 return value; 8180 case -389131437: // contentType 8181 this.contentType = TypeConvertor.castToCode(value); // CodeType 8182 return value; 8183 case 1448452259: // defaultManualCompletion 8184 value = new AssertionManualCompletionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 8185 this.defaultManualCompletion = (Enumeration) value; // Enumeration<AssertionManualCompletionType> 8186 return value; 8187 case -1795452264: // expression 8188 this.expression = TypeConvertor.castToString(value); // StringType 8189 return value; 8190 case 1160732269: // headerField 8191 this.headerField = TypeConvertor.castToString(value); // StringType 8192 return value; 8193 case 818925001: // minimumId 8194 this.minimumId = TypeConvertor.castToString(value); // StringType 8195 return value; 8196 case 1001488901: // navigationLinks 8197 this.navigationLinks = TypeConvertor.castToBoolean(value); // BooleanType 8198 return value; 8199 case -500553564: // operator 8200 value = new AssertionOperatorTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 8201 this.operator = (Enumeration) value; // Enumeration<AssertionOperatorType> 8202 return value; 8203 case 3433509: // path 8204 this.path = TypeConvertor.castToString(value); // StringType 8205 return value; 8206 case 1217874000: // requestMethod 8207 value = new TestScriptRequestMethodCodeEnumFactory().fromType(TypeConvertor.castToCode(value)); 8208 this.requestMethod = (Enumeration) value; // Enumeration<TestScriptRequestMethodCode> 8209 return value; 8210 case 37099616: // requestURL 8211 this.requestURL = TypeConvertor.castToString(value); // StringType 8212 return value; 8213 case -341064690: // resource 8214 this.resource = TypeConvertor.castToUri(value); // UriType 8215 return value; 8216 case -340323263: // response 8217 value = new AssertionResponseTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 8218 this.response = (Enumeration) value; // Enumeration<AssertionResponseTypes> 8219 return value; 8220 case 1438723534: // responseCode 8221 this.responseCode = TypeConvertor.castToString(value); // StringType 8222 return value; 8223 case 1746327190: // sourceId 8224 this.sourceId = TypeConvertor.castToId(value); // IdType 8225 return value; 8226 case -626616719: // stopTestOnFail 8227 this.stopTestOnFail = TypeConvertor.castToBoolean(value); // BooleanType 8228 return value; 8229 case 1555541038: // validateProfileId 8230 this.validateProfileId = TypeConvertor.castToId(value); // IdType 8231 return value; 8232 case 111972721: // value 8233 this.value = TypeConvertor.castToString(value); // StringType 8234 return value; 8235 case -481159832: // warningOnly 8236 this.warningOnly = TypeConvertor.castToBoolean(value); // BooleanType 8237 return value; 8238 case 363387971: // requirement 8239 this.getRequirement().add((SetupActionAssertRequirementComponent) value); // SetupActionAssertRequirementComponent 8240 return value; 8241 default: return super.setProperty(hash, name, value); 8242 } 8243 8244 } 8245 8246 @Override 8247 public Base setProperty(String name, Base value) throws FHIRException { 8248 if (name.equals("label")) { 8249 this.label = TypeConvertor.castToString(value); // StringType 8250 } else if (name.equals("description")) { 8251 this.description = TypeConvertor.castToString(value); // StringType 8252 } else if (name.equals("direction")) { 8253 value = new AssertionDirectionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 8254 this.direction = (Enumeration) value; // Enumeration<AssertionDirectionType> 8255 } else if (name.equals("compareToSourceId")) { 8256 this.compareToSourceId = TypeConvertor.castToString(value); // StringType 8257 } else if (name.equals("compareToSourceExpression")) { 8258 this.compareToSourceExpression = TypeConvertor.castToString(value); // StringType 8259 } else if (name.equals("compareToSourcePath")) { 8260 this.compareToSourcePath = TypeConvertor.castToString(value); // StringType 8261 } else if (name.equals("contentType")) { 8262 this.contentType = TypeConvertor.castToCode(value); // CodeType 8263 } else if (name.equals("defaultManualCompletion")) { 8264 value = new AssertionManualCompletionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 8265 this.defaultManualCompletion = (Enumeration) value; // Enumeration<AssertionManualCompletionType> 8266 } else if (name.equals("expression")) { 8267 this.expression = TypeConvertor.castToString(value); // StringType 8268 } else if (name.equals("headerField")) { 8269 this.headerField = TypeConvertor.castToString(value); // StringType 8270 } else if (name.equals("minimumId")) { 8271 this.minimumId = TypeConvertor.castToString(value); // StringType 8272 } else if (name.equals("navigationLinks")) { 8273 this.navigationLinks = TypeConvertor.castToBoolean(value); // BooleanType 8274 } else if (name.equals("operator")) { 8275 value = new AssertionOperatorTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 8276 this.operator = (Enumeration) value; // Enumeration<AssertionOperatorType> 8277 } else if (name.equals("path")) { 8278 this.path = TypeConvertor.castToString(value); // StringType 8279 } else if (name.equals("requestMethod")) { 8280 value = new TestScriptRequestMethodCodeEnumFactory().fromType(TypeConvertor.castToCode(value)); 8281 this.requestMethod = (Enumeration) value; // Enumeration<TestScriptRequestMethodCode> 8282 } else if (name.equals("requestURL")) { 8283 this.requestURL = TypeConvertor.castToString(value); // StringType 8284 } else if (name.equals("resource")) { 8285 this.resource = TypeConvertor.castToUri(value); // UriType 8286 } else if (name.equals("response")) { 8287 value = new AssertionResponseTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 8288 this.response = (Enumeration) value; // Enumeration<AssertionResponseTypes> 8289 } else if (name.equals("responseCode")) { 8290 this.responseCode = TypeConvertor.castToString(value); // StringType 8291 } else if (name.equals("sourceId")) { 8292 this.sourceId = TypeConvertor.castToId(value); // IdType 8293 } else if (name.equals("stopTestOnFail")) { 8294 this.stopTestOnFail = TypeConvertor.castToBoolean(value); // BooleanType 8295 } else if (name.equals("validateProfileId")) { 8296 this.validateProfileId = TypeConvertor.castToId(value); // IdType 8297 } else if (name.equals("value")) { 8298 this.value = TypeConvertor.castToString(value); // StringType 8299 } else if (name.equals("warningOnly")) { 8300 this.warningOnly = TypeConvertor.castToBoolean(value); // BooleanType 8301 } else if (name.equals("requirement")) { 8302 this.getRequirement().add((SetupActionAssertRequirementComponent) value); 8303 } else 8304 return super.setProperty(name, value); 8305 return value; 8306 } 8307 8308 @Override 8309 public void removeChild(String name, Base value) throws FHIRException { 8310 if (name.equals("label")) { 8311 this.label = null; 8312 } else if (name.equals("description")) { 8313 this.description = null; 8314 } else if (name.equals("direction")) { 8315 value = new AssertionDirectionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 8316 this.direction = (Enumeration) value; // Enumeration<AssertionDirectionType> 8317 } else if (name.equals("compareToSourceId")) { 8318 this.compareToSourceId = null; 8319 } else if (name.equals("compareToSourceExpression")) { 8320 this.compareToSourceExpression = null; 8321 } else if (name.equals("compareToSourcePath")) { 8322 this.compareToSourcePath = null; 8323 } else if (name.equals("contentType")) { 8324 this.contentType = null; 8325 } else if (name.equals("defaultManualCompletion")) { 8326 value = new AssertionManualCompletionTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 8327 this.defaultManualCompletion = (Enumeration) value; // Enumeration<AssertionManualCompletionType> 8328 } else if (name.equals("expression")) { 8329 this.expression = null; 8330 } else if (name.equals("headerField")) { 8331 this.headerField = null; 8332 } else if (name.equals("minimumId")) { 8333 this.minimumId = null; 8334 } else if (name.equals("navigationLinks")) { 8335 this.navigationLinks = null; 8336 } else if (name.equals("operator")) { 8337 value = new AssertionOperatorTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 8338 this.operator = (Enumeration) value; // Enumeration<AssertionOperatorType> 8339 } else if (name.equals("path")) { 8340 this.path = null; 8341 } else if (name.equals("requestMethod")) { 8342 value = new TestScriptRequestMethodCodeEnumFactory().fromType(TypeConvertor.castToCode(value)); 8343 this.requestMethod = (Enumeration) value; // Enumeration<TestScriptRequestMethodCode> 8344 } else if (name.equals("requestURL")) { 8345 this.requestURL = null; 8346 } else if (name.equals("resource")) { 8347 this.resource = null; 8348 } else if (name.equals("response")) { 8349 value = new AssertionResponseTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 8350 this.response = (Enumeration) value; // Enumeration<AssertionResponseTypes> 8351 } else if (name.equals("responseCode")) { 8352 this.responseCode = null; 8353 } else if (name.equals("sourceId")) { 8354 this.sourceId = null; 8355 } else if (name.equals("stopTestOnFail")) { 8356 this.stopTestOnFail = null; 8357 } else if (name.equals("validateProfileId")) { 8358 this.validateProfileId = null; 8359 } else if (name.equals("value")) { 8360 this.value = null; 8361 } else if (name.equals("warningOnly")) { 8362 this.warningOnly = null; 8363 } else if (name.equals("requirement")) { 8364 this.getRequirement().remove((SetupActionAssertRequirementComponent) value); 8365 } else 8366 super.removeChild(name, value); 8367 8368 } 8369 8370 @Override 8371 public Base makeProperty(int hash, String name) throws FHIRException { 8372 switch (hash) { 8373 case 102727412: return getLabelElement(); 8374 case -1724546052: return getDescriptionElement(); 8375 case -962590849: return getDirectionElement(); 8376 case 2081856758: return getCompareToSourceIdElement(); 8377 case -1415702669: return getCompareToSourceExpressionElement(); 8378 case -790206144: return getCompareToSourcePathElement(); 8379 case -389131437: return getContentTypeElement(); 8380 case 1448452259: return getDefaultManualCompletionElement(); 8381 case -1795452264: return getExpressionElement(); 8382 case 1160732269: return getHeaderFieldElement(); 8383 case 818925001: return getMinimumIdElement(); 8384 case 1001488901: return getNavigationLinksElement(); 8385 case -500553564: return getOperatorElement(); 8386 case 3433509: return getPathElement(); 8387 case 1217874000: return getRequestMethodElement(); 8388 case 37099616: return getRequestURLElement(); 8389 case -341064690: return getResourceElement(); 8390 case -340323263: return getResponseElement(); 8391 case 1438723534: return getResponseCodeElement(); 8392 case 1746327190: return getSourceIdElement(); 8393 case -626616719: return getStopTestOnFailElement(); 8394 case 1555541038: return getValidateProfileIdElement(); 8395 case 111972721: return getValueElement(); 8396 case -481159832: return getWarningOnlyElement(); 8397 case 363387971: return addRequirement(); 8398 default: return super.makeProperty(hash, name); 8399 } 8400 8401 } 8402 8403 @Override 8404 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8405 switch (hash) { 8406 case 102727412: /*label*/ return new String[] {"string"}; 8407 case -1724546052: /*description*/ return new String[] {"string"}; 8408 case -962590849: /*direction*/ return new String[] {"code"}; 8409 case 2081856758: /*compareToSourceId*/ return new String[] {"string"}; 8410 case -1415702669: /*compareToSourceExpression*/ return new String[] {"string"}; 8411 case -790206144: /*compareToSourcePath*/ return new String[] {"string"}; 8412 case -389131437: /*contentType*/ return new String[] {"code"}; 8413 case 1448452259: /*defaultManualCompletion*/ return new String[] {"code"}; 8414 case -1795452264: /*expression*/ return new String[] {"string"}; 8415 case 1160732269: /*headerField*/ return new String[] {"string"}; 8416 case 818925001: /*minimumId*/ return new String[] {"string"}; 8417 case 1001488901: /*navigationLinks*/ return new String[] {"boolean"}; 8418 case -500553564: /*operator*/ return new String[] {"code"}; 8419 case 3433509: /*path*/ return new String[] {"string"}; 8420 case 1217874000: /*requestMethod*/ return new String[] {"code"}; 8421 case 37099616: /*requestURL*/ return new String[] {"string"}; 8422 case -341064690: /*resource*/ return new String[] {"uri"}; 8423 case -340323263: /*response*/ return new String[] {"code"}; 8424 case 1438723534: /*responseCode*/ return new String[] {"string"}; 8425 case 1746327190: /*sourceId*/ return new String[] {"id"}; 8426 case -626616719: /*stopTestOnFail*/ return new String[] {"boolean"}; 8427 case 1555541038: /*validateProfileId*/ return new String[] {"id"}; 8428 case 111972721: /*value*/ return new String[] {"string"}; 8429 case -481159832: /*warningOnly*/ return new String[] {"boolean"}; 8430 case 363387971: /*requirement*/ return new String[] {}; 8431 default: return super.getTypesForProperty(hash, name); 8432 } 8433 8434 } 8435 8436 @Override 8437 public Base addChild(String name) throws FHIRException { 8438 if (name.equals("label")) { 8439 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.label"); 8440 } 8441 else if (name.equals("description")) { 8442 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.description"); 8443 } 8444 else if (name.equals("direction")) { 8445 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.direction"); 8446 } 8447 else if (name.equals("compareToSourceId")) { 8448 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.compareToSourceId"); 8449 } 8450 else if (name.equals("compareToSourceExpression")) { 8451 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.compareToSourceExpression"); 8452 } 8453 else if (name.equals("compareToSourcePath")) { 8454 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.compareToSourcePath"); 8455 } 8456 else if (name.equals("contentType")) { 8457 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.contentType"); 8458 } 8459 else if (name.equals("defaultManualCompletion")) { 8460 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.defaultManualCompletion"); 8461 } 8462 else if (name.equals("expression")) { 8463 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.expression"); 8464 } 8465 else if (name.equals("headerField")) { 8466 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.headerField"); 8467 } 8468 else if (name.equals("minimumId")) { 8469 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.minimumId"); 8470 } 8471 else if (name.equals("navigationLinks")) { 8472 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.navigationLinks"); 8473 } 8474 else if (name.equals("operator")) { 8475 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.operator"); 8476 } 8477 else if (name.equals("path")) { 8478 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.path"); 8479 } 8480 else if (name.equals("requestMethod")) { 8481 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.requestMethod"); 8482 } 8483 else if (name.equals("requestURL")) { 8484 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.requestURL"); 8485 } 8486 else if (name.equals("resource")) { 8487 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.resource"); 8488 } 8489 else if (name.equals("response")) { 8490 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.response"); 8491 } 8492 else if (name.equals("responseCode")) { 8493 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.responseCode"); 8494 } 8495 else if (name.equals("sourceId")) { 8496 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.sourceId"); 8497 } 8498 else if (name.equals("stopTestOnFail")) { 8499 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.stopTestOnFail"); 8500 } 8501 else if (name.equals("validateProfileId")) { 8502 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.validateProfileId"); 8503 } 8504 else if (name.equals("value")) { 8505 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.value"); 8506 } 8507 else if (name.equals("warningOnly")) { 8508 throw new FHIRException("Cannot call addChild on a singleton property TestScript.setup.action.assert.warningOnly"); 8509 } 8510 else if (name.equals("requirement")) { 8511 return addRequirement(); 8512 } 8513 else 8514 return super.addChild(name); 8515 } 8516 8517 public SetupActionAssertComponent copy() { 8518 SetupActionAssertComponent dst = new SetupActionAssertComponent(); 8519 copyValues(dst); 8520 return dst; 8521 } 8522 8523 public void copyValues(SetupActionAssertComponent dst) { 8524 super.copyValues(dst); 8525 dst.label = label == null ? null : label.copy(); 8526 dst.description = description == null ? null : description.copy(); 8527 dst.direction = direction == null ? null : direction.copy(); 8528 dst.compareToSourceId = compareToSourceId == null ? null : compareToSourceId.copy(); 8529 dst.compareToSourceExpression = compareToSourceExpression == null ? null : compareToSourceExpression.copy(); 8530 dst.compareToSourcePath = compareToSourcePath == null ? null : compareToSourcePath.copy(); 8531 dst.contentType = contentType == null ? null : contentType.copy(); 8532 dst.defaultManualCompletion = defaultManualCompletion == null ? null : defaultManualCompletion.copy(); 8533 dst.expression = expression == null ? null : expression.copy(); 8534 dst.headerField = headerField == null ? null : headerField.copy(); 8535 dst.minimumId = minimumId == null ? null : minimumId.copy(); 8536 dst.navigationLinks = navigationLinks == null ? null : navigationLinks.copy(); 8537 dst.operator = operator == null ? null : operator.copy(); 8538 dst.path = path == null ? null : path.copy(); 8539 dst.requestMethod = requestMethod == null ? null : requestMethod.copy(); 8540 dst.requestURL = requestURL == null ? null : requestURL.copy(); 8541 dst.resource = resource == null ? null : resource.copy(); 8542 dst.response = response == null ? null : response.copy(); 8543 dst.responseCode = responseCode == null ? null : responseCode.copy(); 8544 dst.sourceId = sourceId == null ? null : sourceId.copy(); 8545 dst.stopTestOnFail = stopTestOnFail == null ? null : stopTestOnFail.copy(); 8546 dst.validateProfileId = validateProfileId == null ? null : validateProfileId.copy(); 8547 dst.value = value == null ? null : value.copy(); 8548 dst.warningOnly = warningOnly == null ? null : warningOnly.copy(); 8549 if (requirement != null) { 8550 dst.requirement = new ArrayList<SetupActionAssertRequirementComponent>(); 8551 for (SetupActionAssertRequirementComponent i : requirement) 8552 dst.requirement.add(i.copy()); 8553 }; 8554 } 8555 8556 @Override 8557 public boolean equalsDeep(Base other_) { 8558 if (!super.equalsDeep(other_)) 8559 return false; 8560 if (!(other_ instanceof SetupActionAssertComponent)) 8561 return false; 8562 SetupActionAssertComponent o = (SetupActionAssertComponent) other_; 8563 return compareDeep(label, o.label, true) && compareDeep(description, o.description, true) && compareDeep(direction, o.direction, true) 8564 && compareDeep(compareToSourceId, o.compareToSourceId, true) && compareDeep(compareToSourceExpression, o.compareToSourceExpression, true) 8565 && compareDeep(compareToSourcePath, o.compareToSourcePath, true) && compareDeep(contentType, o.contentType, true) 8566 && compareDeep(defaultManualCompletion, o.defaultManualCompletion, true) && compareDeep(expression, o.expression, true) 8567 && compareDeep(headerField, o.headerField, true) && compareDeep(minimumId, o.minimumId, true) && compareDeep(navigationLinks, o.navigationLinks, true) 8568 && compareDeep(operator, o.operator, true) && compareDeep(path, o.path, true) && compareDeep(requestMethod, o.requestMethod, true) 8569 && compareDeep(requestURL, o.requestURL, true) && compareDeep(resource, o.resource, true) && compareDeep(response, o.response, true) 8570 && compareDeep(responseCode, o.responseCode, true) && compareDeep(sourceId, o.sourceId, true) && compareDeep(stopTestOnFail, o.stopTestOnFail, true) 8571 && compareDeep(validateProfileId, o.validateProfileId, true) && compareDeep(value, o.value, true) 8572 && compareDeep(warningOnly, o.warningOnly, true) && compareDeep(requirement, o.requirement, true) 8573 ; 8574 } 8575 8576 @Override 8577 public boolean equalsShallow(Base other_) { 8578 if (!super.equalsShallow(other_)) 8579 return false; 8580 if (!(other_ instanceof SetupActionAssertComponent)) 8581 return false; 8582 SetupActionAssertComponent o = (SetupActionAssertComponent) other_; 8583 return compareValues(label, o.label, true) && compareValues(description, o.description, true) && compareValues(direction, o.direction, true) 8584 && compareValues(compareToSourceId, o.compareToSourceId, true) && compareValues(compareToSourceExpression, o.compareToSourceExpression, true) 8585 && compareValues(compareToSourcePath, o.compareToSourcePath, true) && compareValues(contentType, o.contentType, true) 8586 && compareValues(defaultManualCompletion, o.defaultManualCompletion, true) && compareValues(expression, o.expression, true) 8587 && compareValues(headerField, o.headerField, true) && compareValues(minimumId, o.minimumId, true) && compareValues(navigationLinks, o.navigationLinks, true) 8588 && compareValues(operator, o.operator, true) && compareValues(path, o.path, true) && compareValues(requestMethod, o.requestMethod, true) 8589 && compareValues(requestURL, o.requestURL, true) && compareValues(resource, o.resource, true) && compareValues(response, o.response, true) 8590 && compareValues(responseCode, o.responseCode, true) && compareValues(sourceId, o.sourceId, true) && compareValues(stopTestOnFail, o.stopTestOnFail, true) 8591 && compareValues(validateProfileId, o.validateProfileId, true) && compareValues(value, o.value, true) 8592 && compareValues(warningOnly, o.warningOnly, true); 8593 } 8594 8595 public boolean isEmpty() { 8596 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(label, description, direction 8597 , compareToSourceId, compareToSourceExpression, compareToSourcePath, contentType, defaultManualCompletion 8598 , expression, headerField, minimumId, navigationLinks, operator, path, requestMethod 8599 , requestURL, resource, response, responseCode, sourceId, stopTestOnFail, validateProfileId 8600 , value, warningOnly, requirement); 8601 } 8602 8603 public String fhirType() { 8604 return "TestScript.setup.action.assert"; 8605 8606 } 8607 8608 } 8609 8610 @Block() 8611 public static class SetupActionAssertRequirementComponent extends BackboneElement implements IBaseBackboneElement { 8612 /** 8613 * Link or reference providing traceability to the testing requirement for this test. 8614 */ 8615 @Child(name = "link", type = {UriType.class, CanonicalType.class}, order=1, min=0, max=1, modifier=false, summary=false) 8616 @Description(shortDefinition="Link or reference to the testing requirement", formalDefinition="Link or reference providing traceability to the testing requirement for this test." ) 8617 protected DataType link; 8618 8619 private static final long serialVersionUID = -91187948L; 8620 8621 /** 8622 * Constructor 8623 */ 8624 public SetupActionAssertRequirementComponent() { 8625 super(); 8626 } 8627 8628 /** 8629 * @return {@link #link} (Link or reference providing traceability to the testing requirement for this test.) 8630 */ 8631 public DataType getLink() { 8632 return this.link; 8633 } 8634 8635 /** 8636 * @return {@link #link} (Link or reference providing traceability to the testing requirement for this test.) 8637 */ 8638 public UriType getLinkUriType() throws FHIRException { 8639 if (this.link == null) 8640 this.link = new UriType(); 8641 if (!(this.link instanceof UriType)) 8642 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.link.getClass().getName()+" was encountered"); 8643 return (UriType) this.link; 8644 } 8645 8646 public boolean hasLinkUriType() { 8647 return this.link instanceof UriType; 8648 } 8649 8650 /** 8651 * @return {@link #link} (Link or reference providing traceability to the testing requirement for this test.) 8652 */ 8653 public CanonicalType getLinkCanonicalType() throws FHIRException { 8654 if (this.link == null) 8655 this.link = new CanonicalType(); 8656 if (!(this.link instanceof CanonicalType)) 8657 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.link.getClass().getName()+" was encountered"); 8658 return (CanonicalType) this.link; 8659 } 8660 8661 public boolean hasLinkCanonicalType() { 8662 return this.link instanceof CanonicalType; 8663 } 8664 8665 public boolean hasLink() { 8666 return this.link != null && !this.link.isEmpty(); 8667 } 8668 8669 /** 8670 * @param value {@link #link} (Link or reference providing traceability to the testing requirement for this test.) 8671 */ 8672 public SetupActionAssertRequirementComponent setLink(DataType value) { 8673 if (value != null && !(value instanceof UriType || value instanceof CanonicalType)) 8674 throw new FHIRException("Not the right type for TestScript.setup.action.assert.requirement.link[x]: "+value.fhirType()); 8675 this.link = value; 8676 return this; 8677 } 8678 8679 protected void listChildren(List<Property> children) { 8680 super.listChildren(children); 8681 children.add(new Property("link[x]", "uri|canonical(Requirements)", "Link or reference providing traceability to the testing requirement for this test.", 0, 1, link)); 8682 } 8683 8684 @Override 8685 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8686 switch (_hash) { 8687 case 177076806: /*link[x]*/ return new Property("link[x]", "uri|canonical(Requirements)", "Link or reference providing traceability to the testing requirement for this test.", 0, 1, link); 8688 case 3321850: /*link*/ return new Property("link[x]", "uri|canonical(Requirements)", "Link or reference providing traceability to the testing requirement for this test.", 0, 1, link); 8689 case 177070866: /*linkUri*/ return new Property("link[x]", "uri", "Link or reference providing traceability to the testing requirement for this test.", 0, 1, link); 8690 case -2064880102: /*linkCanonical*/ return new Property("link[x]", "canonical(Requirements)", "Link or reference providing traceability to the testing requirement for this test.", 0, 1, link); 8691 default: return super.getNamedProperty(_hash, _name, _checkValid); 8692 } 8693 8694 } 8695 8696 @Override 8697 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8698 switch (hash) { 8699 case 3321850: /*link*/ return this.link == null ? new Base[0] : new Base[] {this.link}; // DataType 8700 default: return super.getProperty(hash, name, checkValid); 8701 } 8702 8703 } 8704 8705 @Override 8706 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8707 switch (hash) { 8708 case 3321850: // link 8709 this.link = TypeConvertor.castToType(value); // DataType 8710 return value; 8711 default: return super.setProperty(hash, name, value); 8712 } 8713 8714 } 8715 8716 @Override 8717 public Base setProperty(String name, Base value) throws FHIRException { 8718 if (name.equals("link[x]")) { 8719 this.link = TypeConvertor.castToType(value); // DataType 8720 } else 8721 return super.setProperty(name, value); 8722 return value; 8723 } 8724 8725 @Override 8726 public void removeChild(String name, Base value) throws FHIRException { 8727 if (name.equals("link[x]")) { 8728 this.link = null; 8729 } else 8730 super.removeChild(name, value); 8731 8732 } 8733 8734 @Override 8735 public Base makeProperty(int hash, String name) throws FHIRException { 8736 switch (hash) { 8737 case 177076806: return getLink(); 8738 case 3321850: return getLink(); 8739 default: return super.makeProperty(hash, name); 8740 } 8741 8742 } 8743 8744 @Override 8745 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8746 switch (hash) { 8747 case 3321850: /*link*/ return new String[] {"uri", "canonical"}; 8748 default: return super.getTypesForProperty(hash, name); 8749 } 8750 8751 } 8752 8753 @Override 8754 public Base addChild(String name) throws FHIRException { 8755 if (name.equals("linkUri")) { 8756 this.link = new UriType(); 8757 return this.link; 8758 } 8759 else if (name.equals("linkCanonical")) { 8760 this.link = new CanonicalType(); 8761 return this.link; 8762 } 8763 else 8764 return super.addChild(name); 8765 } 8766 8767 public SetupActionAssertRequirementComponent copy() { 8768 SetupActionAssertRequirementComponent dst = new SetupActionAssertRequirementComponent(); 8769 copyValues(dst); 8770 return dst; 8771 } 8772 8773 public void copyValues(SetupActionAssertRequirementComponent dst) { 8774 super.copyValues(dst); 8775 dst.link = link == null ? null : link.copy(); 8776 } 8777 8778 @Override 8779 public boolean equalsDeep(Base other_) { 8780 if (!super.equalsDeep(other_)) 8781 return false; 8782 if (!(other_ instanceof SetupActionAssertRequirementComponent)) 8783 return false; 8784 SetupActionAssertRequirementComponent o = (SetupActionAssertRequirementComponent) other_; 8785 return compareDeep(link, o.link, true); 8786 } 8787 8788 @Override 8789 public boolean equalsShallow(Base other_) { 8790 if (!super.equalsShallow(other_)) 8791 return false; 8792 if (!(other_ instanceof SetupActionAssertRequirementComponent)) 8793 return false; 8794 SetupActionAssertRequirementComponent o = (SetupActionAssertRequirementComponent) other_; 8795 return true; 8796 } 8797 8798 public boolean isEmpty() { 8799 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(link); 8800 } 8801 8802 public String fhirType() { 8803 return "TestScript.setup.action.assert.requirement"; 8804 8805 } 8806 8807 } 8808 8809 @Block() 8810 public static class TestScriptTestComponent extends BackboneElement implements IBaseBackboneElement { 8811 /** 8812 * The name of this test used for tracking/logging purposes by test engines. 8813 */ 8814 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 8815 @Description(shortDefinition="Tracking/logging name of this test", formalDefinition="The name of this test used for tracking/logging purposes by test engines." ) 8816 protected StringType name; 8817 8818 /** 8819 * A short description of the test used by test engines for tracking and reporting purposes. 8820 */ 8821 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 8822 @Description(shortDefinition="Tracking/reporting short description of the test", formalDefinition="A short description of the test used by test engines for tracking and reporting purposes." ) 8823 protected StringType description; 8824 8825 /** 8826 * Action would contain either an operation or an assertion. 8827 */ 8828 @Child(name = "action", type = {}, order=3, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8829 @Description(shortDefinition="A test operation or assert to perform", formalDefinition="Action would contain either an operation or an assertion." ) 8830 protected List<TestActionComponent> action; 8831 8832 private static final long serialVersionUID = -865006110L; 8833 8834 /** 8835 * Constructor 8836 */ 8837 public TestScriptTestComponent() { 8838 super(); 8839 } 8840 8841 /** 8842 * Constructor 8843 */ 8844 public TestScriptTestComponent(TestActionComponent action) { 8845 super(); 8846 this.addAction(action); 8847 } 8848 8849 /** 8850 * @return {@link #name} (The name of this test used for tracking/logging purposes by test engines.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 8851 */ 8852 public StringType getNameElement() { 8853 if (this.name == null) 8854 if (Configuration.errorOnAutoCreate()) 8855 throw new Error("Attempt to auto-create TestScriptTestComponent.name"); 8856 else if (Configuration.doAutoCreate()) 8857 this.name = new StringType(); // bb 8858 return this.name; 8859 } 8860 8861 public boolean hasNameElement() { 8862 return this.name != null && !this.name.isEmpty(); 8863 } 8864 8865 public boolean hasName() { 8866 return this.name != null && !this.name.isEmpty(); 8867 } 8868 8869 /** 8870 * @param value {@link #name} (The name of this test used for tracking/logging purposes by test engines.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 8871 */ 8872 public TestScriptTestComponent setNameElement(StringType value) { 8873 this.name = value; 8874 return this; 8875 } 8876 8877 /** 8878 * @return The name of this test used for tracking/logging purposes by test engines. 8879 */ 8880 public String getName() { 8881 return this.name == null ? null : this.name.getValue(); 8882 } 8883 8884 /** 8885 * @param value The name of this test used for tracking/logging purposes by test engines. 8886 */ 8887 public TestScriptTestComponent setName(String value) { 8888 if (Utilities.noString(value)) 8889 this.name = null; 8890 else { 8891 if (this.name == null) 8892 this.name = new StringType(); 8893 this.name.setValue(value); 8894 } 8895 return this; 8896 } 8897 8898 /** 8899 * @return {@link #description} (A short description of the test used by test engines for tracking and reporting purposes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 8900 */ 8901 public StringType getDescriptionElement() { 8902 if (this.description == null) 8903 if (Configuration.errorOnAutoCreate()) 8904 throw new Error("Attempt to auto-create TestScriptTestComponent.description"); 8905 else if (Configuration.doAutoCreate()) 8906 this.description = new StringType(); // bb 8907 return this.description; 8908 } 8909 8910 public boolean hasDescriptionElement() { 8911 return this.description != null && !this.description.isEmpty(); 8912 } 8913 8914 public boolean hasDescription() { 8915 return this.description != null && !this.description.isEmpty(); 8916 } 8917 8918 /** 8919 * @param value {@link #description} (A short description of the test used by test engines for tracking and reporting purposes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 8920 */ 8921 public TestScriptTestComponent setDescriptionElement(StringType value) { 8922 this.description = value; 8923 return this; 8924 } 8925 8926 /** 8927 * @return A short description of the test used by test engines for tracking and reporting purposes. 8928 */ 8929 public String getDescription() { 8930 return this.description == null ? null : this.description.getValue(); 8931 } 8932 8933 /** 8934 * @param value A short description of the test used by test engines for tracking and reporting purposes. 8935 */ 8936 public TestScriptTestComponent setDescription(String value) { 8937 if (Utilities.noString(value)) 8938 this.description = null; 8939 else { 8940 if (this.description == null) 8941 this.description = new StringType(); 8942 this.description.setValue(value); 8943 } 8944 return this; 8945 } 8946 8947 /** 8948 * @return {@link #action} (Action would contain either an operation or an assertion.) 8949 */ 8950 public List<TestActionComponent> getAction() { 8951 if (this.action == null) 8952 this.action = new ArrayList<TestActionComponent>(); 8953 return this.action; 8954 } 8955 8956 /** 8957 * @return Returns a reference to <code>this</code> for easy method chaining 8958 */ 8959 public TestScriptTestComponent setAction(List<TestActionComponent> theAction) { 8960 this.action = theAction; 8961 return this; 8962 } 8963 8964 public boolean hasAction() { 8965 if (this.action == null) 8966 return false; 8967 for (TestActionComponent item : this.action) 8968 if (!item.isEmpty()) 8969 return true; 8970 return false; 8971 } 8972 8973 public TestActionComponent addAction() { //3 8974 TestActionComponent t = new TestActionComponent(); 8975 if (this.action == null) 8976 this.action = new ArrayList<TestActionComponent>(); 8977 this.action.add(t); 8978 return t; 8979 } 8980 8981 public TestScriptTestComponent addAction(TestActionComponent t) { //3 8982 if (t == null) 8983 return this; 8984 if (this.action == null) 8985 this.action = new ArrayList<TestActionComponent>(); 8986 this.action.add(t); 8987 return this; 8988 } 8989 8990 /** 8991 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist {3} 8992 */ 8993 public TestActionComponent getActionFirstRep() { 8994 if (getAction().isEmpty()) { 8995 addAction(); 8996 } 8997 return getAction().get(0); 8998 } 8999 9000 protected void listChildren(List<Property> children) { 9001 super.listChildren(children); 9002 children.add(new Property("name", "string", "The name of this test used for tracking/logging purposes by test engines.", 0, 1, name)); 9003 children.add(new Property("description", "string", "A short description of the test used by test engines for tracking and reporting purposes.", 0, 1, description)); 9004 children.add(new Property("action", "", "Action would contain either an operation or an assertion.", 0, java.lang.Integer.MAX_VALUE, action)); 9005 } 9006 9007 @Override 9008 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9009 switch (_hash) { 9010 case 3373707: /*name*/ return new Property("name", "string", "The name of this test used for tracking/logging purposes by test engines.", 0, 1, name); 9011 case -1724546052: /*description*/ return new Property("description", "string", "A short description of the test used by test engines for tracking and reporting purposes.", 0, 1, description); 9012 case -1422950858: /*action*/ return new Property("action", "", "Action would contain either an operation or an assertion.", 0, java.lang.Integer.MAX_VALUE, action); 9013 default: return super.getNamedProperty(_hash, _name, _checkValid); 9014 } 9015 9016 } 9017 9018 @Override 9019 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9020 switch (hash) { 9021 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 9022 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 9023 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // TestActionComponent 9024 default: return super.getProperty(hash, name, checkValid); 9025 } 9026 9027 } 9028 9029 @Override 9030 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9031 switch (hash) { 9032 case 3373707: // name 9033 this.name = TypeConvertor.castToString(value); // StringType 9034 return value; 9035 case -1724546052: // description 9036 this.description = TypeConvertor.castToString(value); // StringType 9037 return value; 9038 case -1422950858: // action 9039 this.getAction().add((TestActionComponent) value); // TestActionComponent 9040 return value; 9041 default: return super.setProperty(hash, name, value); 9042 } 9043 9044 } 9045 9046 @Override 9047 public Base setProperty(String name, Base value) throws FHIRException { 9048 if (name.equals("name")) { 9049 this.name = TypeConvertor.castToString(value); // StringType 9050 } else if (name.equals("description")) { 9051 this.description = TypeConvertor.castToString(value); // StringType 9052 } else if (name.equals("action")) { 9053 this.getAction().add((TestActionComponent) value); 9054 } else 9055 return super.setProperty(name, value); 9056 return value; 9057 } 9058 9059 @Override 9060 public void removeChild(String name, Base value) throws FHIRException { 9061 if (name.equals("name")) { 9062 this.name = null; 9063 } else if (name.equals("description")) { 9064 this.description = null; 9065 } else if (name.equals("action")) { 9066 this.getAction().remove((TestActionComponent) value); 9067 } else 9068 super.removeChild(name, value); 9069 9070 } 9071 9072 @Override 9073 public Base makeProperty(int hash, String name) throws FHIRException { 9074 switch (hash) { 9075 case 3373707: return getNameElement(); 9076 case -1724546052: return getDescriptionElement(); 9077 case -1422950858: return addAction(); 9078 default: return super.makeProperty(hash, name); 9079 } 9080 9081 } 9082 9083 @Override 9084 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9085 switch (hash) { 9086 case 3373707: /*name*/ return new String[] {"string"}; 9087 case -1724546052: /*description*/ return new String[] {"string"}; 9088 case -1422950858: /*action*/ return new String[] {}; 9089 default: return super.getTypesForProperty(hash, name); 9090 } 9091 9092 } 9093 9094 @Override 9095 public Base addChild(String name) throws FHIRException { 9096 if (name.equals("name")) { 9097 throw new FHIRException("Cannot call addChild on a singleton property TestScript.test.name"); 9098 } 9099 else if (name.equals("description")) { 9100 throw new FHIRException("Cannot call addChild on a singleton property TestScript.test.description"); 9101 } 9102 else if (name.equals("action")) { 9103 return addAction(); 9104 } 9105 else 9106 return super.addChild(name); 9107 } 9108 9109 public TestScriptTestComponent copy() { 9110 TestScriptTestComponent dst = new TestScriptTestComponent(); 9111 copyValues(dst); 9112 return dst; 9113 } 9114 9115 public void copyValues(TestScriptTestComponent dst) { 9116 super.copyValues(dst); 9117 dst.name = name == null ? null : name.copy(); 9118 dst.description = description == null ? null : description.copy(); 9119 if (action != null) { 9120 dst.action = new ArrayList<TestActionComponent>(); 9121 for (TestActionComponent i : action) 9122 dst.action.add(i.copy()); 9123 }; 9124 } 9125 9126 @Override 9127 public boolean equalsDeep(Base other_) { 9128 if (!super.equalsDeep(other_)) 9129 return false; 9130 if (!(other_ instanceof TestScriptTestComponent)) 9131 return false; 9132 TestScriptTestComponent o = (TestScriptTestComponent) other_; 9133 return compareDeep(name, o.name, true) && compareDeep(description, o.description, true) && compareDeep(action, o.action, true) 9134 ; 9135 } 9136 9137 @Override 9138 public boolean equalsShallow(Base other_) { 9139 if (!super.equalsShallow(other_)) 9140 return false; 9141 if (!(other_ instanceof TestScriptTestComponent)) 9142 return false; 9143 TestScriptTestComponent o = (TestScriptTestComponent) other_; 9144 return compareValues(name, o.name, true) && compareValues(description, o.description, true); 9145 } 9146 9147 public boolean isEmpty() { 9148 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, description, action 9149 ); 9150 } 9151 9152 public String fhirType() { 9153 return "TestScript.test"; 9154 9155 } 9156 9157 } 9158 9159 @Block() 9160 public static class TestActionComponent extends BackboneElement implements IBaseBackboneElement { 9161 /** 9162 * An operation would involve a REST request to a server. 9163 */ 9164 @Child(name = "operation", type = {SetupActionOperationComponent.class}, order=1, min=0, max=1, modifier=false, summary=false) 9165 @Description(shortDefinition="The setup operation to perform", formalDefinition="An operation would involve a REST request to a server." ) 9166 protected SetupActionOperationComponent operation; 9167 9168 /** 9169 * Evaluates the results of previous operations to determine if the server under test behaves appropriately. 9170 */ 9171 @Child(name = "assert", type = {SetupActionAssertComponent.class}, order=2, min=0, max=1, modifier=false, summary=false) 9172 @Description(shortDefinition="The setup assertion to perform", formalDefinition="Evaluates the results of previous operations to determine if the server under test behaves appropriately." ) 9173 protected SetupActionAssertComponent assert_; 9174 9175 private static final long serialVersionUID = -252088305L; 9176 9177 /** 9178 * Constructor 9179 */ 9180 public TestActionComponent() { 9181 super(); 9182 } 9183 9184 /** 9185 * @return {@link #operation} (An operation would involve a REST request to a server.) 9186 */ 9187 public SetupActionOperationComponent getOperation() { 9188 if (this.operation == null) 9189 if (Configuration.errorOnAutoCreate()) 9190 throw new Error("Attempt to auto-create TestActionComponent.operation"); 9191 else if (Configuration.doAutoCreate()) 9192 this.operation = new SetupActionOperationComponent(); // cc 9193 return this.operation; 9194 } 9195 9196 public boolean hasOperation() { 9197 return this.operation != null && !this.operation.isEmpty(); 9198 } 9199 9200 /** 9201 * @param value {@link #operation} (An operation would involve a REST request to a server.) 9202 */ 9203 public TestActionComponent setOperation(SetupActionOperationComponent value) { 9204 this.operation = value; 9205 return this; 9206 } 9207 9208 /** 9209 * @return {@link #assert_} (Evaluates the results of previous operations to determine if the server under test behaves appropriately.) 9210 */ 9211 public SetupActionAssertComponent getAssert() { 9212 if (this.assert_ == null) 9213 if (Configuration.errorOnAutoCreate()) 9214 throw new Error("Attempt to auto-create TestActionComponent.assert_"); 9215 else if (Configuration.doAutoCreate()) 9216 this.assert_ = new SetupActionAssertComponent(); // cc 9217 return this.assert_; 9218 } 9219 9220 public boolean hasAssert() { 9221 return this.assert_ != null && !this.assert_.isEmpty(); 9222 } 9223 9224 /** 9225 * @param value {@link #assert_} (Evaluates the results of previous operations to determine if the server under test behaves appropriately.) 9226 */ 9227 public TestActionComponent setAssert(SetupActionAssertComponent value) { 9228 this.assert_ = value; 9229 return this; 9230 } 9231 9232 protected void listChildren(List<Property> children) { 9233 super.listChildren(children); 9234 children.add(new Property("operation", "@TestScript.setup.action.operation", "An operation would involve a REST request to a server.", 0, 1, operation)); 9235 children.add(new Property("assert", "@TestScript.setup.action.assert", "Evaluates the results of previous operations to determine if the server under test behaves appropriately.", 0, 1, assert_)); 9236 } 9237 9238 @Override 9239 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9240 switch (_hash) { 9241 case 1662702951: /*operation*/ return new Property("operation", "@TestScript.setup.action.operation", "An operation would involve a REST request to a server.", 0, 1, operation); 9242 case -1408208058: /*assert*/ return new Property("assert", "@TestScript.setup.action.assert", "Evaluates the results of previous operations to determine if the server under test behaves appropriately.", 0, 1, assert_); 9243 default: return super.getNamedProperty(_hash, _name, _checkValid); 9244 } 9245 9246 } 9247 9248 @Override 9249 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9250 switch (hash) { 9251 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : new Base[] {this.operation}; // SetupActionOperationComponent 9252 case -1408208058: /*assert*/ return this.assert_ == null ? new Base[0] : new Base[] {this.assert_}; // SetupActionAssertComponent 9253 default: return super.getProperty(hash, name, checkValid); 9254 } 9255 9256 } 9257 9258 @Override 9259 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9260 switch (hash) { 9261 case 1662702951: // operation 9262 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 9263 return value; 9264 case -1408208058: // assert 9265 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 9266 return value; 9267 default: return super.setProperty(hash, name, value); 9268 } 9269 9270 } 9271 9272 @Override 9273 public Base setProperty(String name, Base value) throws FHIRException { 9274 if (name.equals("operation")) { 9275 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 9276 } else if (name.equals("assert")) { 9277 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 9278 } else 9279 return super.setProperty(name, value); 9280 return value; 9281 } 9282 9283 @Override 9284 public void removeChild(String name, Base value) throws FHIRException { 9285 if (name.equals("operation")) { 9286 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 9287 } else if (name.equals("assert")) { 9288 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 9289 } else 9290 super.removeChild(name, value); 9291 9292 } 9293 9294 @Override 9295 public Base makeProperty(int hash, String name) throws FHIRException { 9296 switch (hash) { 9297 case 1662702951: return getOperation(); 9298 case -1408208058: return getAssert(); 9299 default: return super.makeProperty(hash, name); 9300 } 9301 9302 } 9303 9304 @Override 9305 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9306 switch (hash) { 9307 case 1662702951: /*operation*/ return new String[] {"@TestScript.setup.action.operation"}; 9308 case -1408208058: /*assert*/ return new String[] {"@TestScript.setup.action.assert"}; 9309 default: return super.getTypesForProperty(hash, name); 9310 } 9311 9312 } 9313 9314 @Override 9315 public Base addChild(String name) throws FHIRException { 9316 if (name.equals("operation")) { 9317 this.operation = new SetupActionOperationComponent(); 9318 return this.operation; 9319 } 9320 else if (name.equals("assert")) { 9321 this.assert_ = new SetupActionAssertComponent(); 9322 return this.assert_; 9323 } 9324 else 9325 return super.addChild(name); 9326 } 9327 9328 public TestActionComponent copy() { 9329 TestActionComponent dst = new TestActionComponent(); 9330 copyValues(dst); 9331 return dst; 9332 } 9333 9334 public void copyValues(TestActionComponent dst) { 9335 super.copyValues(dst); 9336 dst.operation = operation == null ? null : operation.copy(); 9337 dst.assert_ = assert_ == null ? null : assert_.copy(); 9338 } 9339 9340 @Override 9341 public boolean equalsDeep(Base other_) { 9342 if (!super.equalsDeep(other_)) 9343 return false; 9344 if (!(other_ instanceof TestActionComponent)) 9345 return false; 9346 TestActionComponent o = (TestActionComponent) other_; 9347 return compareDeep(operation, o.operation, true) && compareDeep(assert_, o.assert_, true); 9348 } 9349 9350 @Override 9351 public boolean equalsShallow(Base other_) { 9352 if (!super.equalsShallow(other_)) 9353 return false; 9354 if (!(other_ instanceof TestActionComponent)) 9355 return false; 9356 TestActionComponent o = (TestActionComponent) other_; 9357 return true; 9358 } 9359 9360 public boolean isEmpty() { 9361 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation, assert_); 9362 } 9363 9364 public String fhirType() { 9365 return "TestScript.test.action"; 9366 9367 } 9368 9369 } 9370 9371 @Block() 9372 public static class TestScriptTeardownComponent extends BackboneElement implements IBaseBackboneElement { 9373 /** 9374 * The teardown action will only contain an operation. 9375 */ 9376 @Child(name = "action", type = {}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9377 @Description(shortDefinition="One or more teardown operations to perform", formalDefinition="The teardown action will only contain an operation." ) 9378 protected List<TeardownActionComponent> action; 9379 9380 private static final long serialVersionUID = 1168638089L; 9381 9382 /** 9383 * Constructor 9384 */ 9385 public TestScriptTeardownComponent() { 9386 super(); 9387 } 9388 9389 /** 9390 * Constructor 9391 */ 9392 public TestScriptTeardownComponent(TeardownActionComponent action) { 9393 super(); 9394 this.addAction(action); 9395 } 9396 9397 /** 9398 * @return {@link #action} (The teardown action will only contain an operation.) 9399 */ 9400 public List<TeardownActionComponent> getAction() { 9401 if (this.action == null) 9402 this.action = new ArrayList<TeardownActionComponent>(); 9403 return this.action; 9404 } 9405 9406 /** 9407 * @return Returns a reference to <code>this</code> for easy method chaining 9408 */ 9409 public TestScriptTeardownComponent setAction(List<TeardownActionComponent> theAction) { 9410 this.action = theAction; 9411 return this; 9412 } 9413 9414 public boolean hasAction() { 9415 if (this.action == null) 9416 return false; 9417 for (TeardownActionComponent item : this.action) 9418 if (!item.isEmpty()) 9419 return true; 9420 return false; 9421 } 9422 9423 public TeardownActionComponent addAction() { //3 9424 TeardownActionComponent t = new TeardownActionComponent(); 9425 if (this.action == null) 9426 this.action = new ArrayList<TeardownActionComponent>(); 9427 this.action.add(t); 9428 return t; 9429 } 9430 9431 public TestScriptTeardownComponent addAction(TeardownActionComponent t) { //3 9432 if (t == null) 9433 return this; 9434 if (this.action == null) 9435 this.action = new ArrayList<TeardownActionComponent>(); 9436 this.action.add(t); 9437 return this; 9438 } 9439 9440 /** 9441 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist {3} 9442 */ 9443 public TeardownActionComponent getActionFirstRep() { 9444 if (getAction().isEmpty()) { 9445 addAction(); 9446 } 9447 return getAction().get(0); 9448 } 9449 9450 protected void listChildren(List<Property> children) { 9451 super.listChildren(children); 9452 children.add(new Property("action", "", "The teardown action will only contain an operation.", 0, java.lang.Integer.MAX_VALUE, action)); 9453 } 9454 9455 @Override 9456 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9457 switch (_hash) { 9458 case -1422950858: /*action*/ return new Property("action", "", "The teardown action will only contain an operation.", 0, java.lang.Integer.MAX_VALUE, action); 9459 default: return super.getNamedProperty(_hash, _name, _checkValid); 9460 } 9461 9462 } 9463 9464 @Override 9465 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9466 switch (hash) { 9467 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // TeardownActionComponent 9468 default: return super.getProperty(hash, name, checkValid); 9469 } 9470 9471 } 9472 9473 @Override 9474 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9475 switch (hash) { 9476 case -1422950858: // action 9477 this.getAction().add((TeardownActionComponent) value); // TeardownActionComponent 9478 return value; 9479 default: return super.setProperty(hash, name, value); 9480 } 9481 9482 } 9483 9484 @Override 9485 public Base setProperty(String name, Base value) throws FHIRException { 9486 if (name.equals("action")) { 9487 this.getAction().add((TeardownActionComponent) value); 9488 } else 9489 return super.setProperty(name, value); 9490 return value; 9491 } 9492 9493 @Override 9494 public void removeChild(String name, Base value) throws FHIRException { 9495 if (name.equals("action")) { 9496 this.getAction().remove((TeardownActionComponent) value); 9497 } else 9498 super.removeChild(name, value); 9499 9500 } 9501 9502 @Override 9503 public Base makeProperty(int hash, String name) throws FHIRException { 9504 switch (hash) { 9505 case -1422950858: return addAction(); 9506 default: return super.makeProperty(hash, name); 9507 } 9508 9509 } 9510 9511 @Override 9512 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9513 switch (hash) { 9514 case -1422950858: /*action*/ return new String[] {}; 9515 default: return super.getTypesForProperty(hash, name); 9516 } 9517 9518 } 9519 9520 @Override 9521 public Base addChild(String name) throws FHIRException { 9522 if (name.equals("action")) { 9523 return addAction(); 9524 } 9525 else 9526 return super.addChild(name); 9527 } 9528 9529 public TestScriptTeardownComponent copy() { 9530 TestScriptTeardownComponent dst = new TestScriptTeardownComponent(); 9531 copyValues(dst); 9532 return dst; 9533 } 9534 9535 public void copyValues(TestScriptTeardownComponent dst) { 9536 super.copyValues(dst); 9537 if (action != null) { 9538 dst.action = new ArrayList<TeardownActionComponent>(); 9539 for (TeardownActionComponent i : action) 9540 dst.action.add(i.copy()); 9541 }; 9542 } 9543 9544 @Override 9545 public boolean equalsDeep(Base other_) { 9546 if (!super.equalsDeep(other_)) 9547 return false; 9548 if (!(other_ instanceof TestScriptTeardownComponent)) 9549 return false; 9550 TestScriptTeardownComponent o = (TestScriptTeardownComponent) other_; 9551 return compareDeep(action, o.action, true); 9552 } 9553 9554 @Override 9555 public boolean equalsShallow(Base other_) { 9556 if (!super.equalsShallow(other_)) 9557 return false; 9558 if (!(other_ instanceof TestScriptTeardownComponent)) 9559 return false; 9560 TestScriptTeardownComponent o = (TestScriptTeardownComponent) other_; 9561 return true; 9562 } 9563 9564 public boolean isEmpty() { 9565 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action); 9566 } 9567 9568 public String fhirType() { 9569 return "TestScript.teardown"; 9570 9571 } 9572 9573 } 9574 9575 @Block() 9576 public static class TeardownActionComponent extends BackboneElement implements IBaseBackboneElement { 9577 /** 9578 * An operation would involve a REST request to a server. 9579 */ 9580 @Child(name = "operation", type = {SetupActionOperationComponent.class}, order=1, min=1, max=1, modifier=false, summary=false) 9581 @Description(shortDefinition="The teardown operation to perform", formalDefinition="An operation would involve a REST request to a server." ) 9582 protected SetupActionOperationComponent operation; 9583 9584 private static final long serialVersionUID = -1099598054L; 9585 9586 /** 9587 * Constructor 9588 */ 9589 public TeardownActionComponent() { 9590 super(); 9591 } 9592 9593 /** 9594 * Constructor 9595 */ 9596 public TeardownActionComponent(SetupActionOperationComponent operation) { 9597 super(); 9598 this.setOperation(operation); 9599 } 9600 9601 /** 9602 * @return {@link #operation} (An operation would involve a REST request to a server.) 9603 */ 9604 public SetupActionOperationComponent getOperation() { 9605 if (this.operation == null) 9606 if (Configuration.errorOnAutoCreate()) 9607 throw new Error("Attempt to auto-create TeardownActionComponent.operation"); 9608 else if (Configuration.doAutoCreate()) 9609 this.operation = new SetupActionOperationComponent(); // cc 9610 return this.operation; 9611 } 9612 9613 public boolean hasOperation() { 9614 return this.operation != null && !this.operation.isEmpty(); 9615 } 9616 9617 /** 9618 * @param value {@link #operation} (An operation would involve a REST request to a server.) 9619 */ 9620 public TeardownActionComponent setOperation(SetupActionOperationComponent value) { 9621 this.operation = value; 9622 return this; 9623 } 9624 9625 protected void listChildren(List<Property> children) { 9626 super.listChildren(children); 9627 children.add(new Property("operation", "@TestScript.setup.action.operation", "An operation would involve a REST request to a server.", 0, 1, operation)); 9628 } 9629 9630 @Override 9631 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9632 switch (_hash) { 9633 case 1662702951: /*operation*/ return new Property("operation", "@TestScript.setup.action.operation", "An operation would involve a REST request to a server.", 0, 1, operation); 9634 default: return super.getNamedProperty(_hash, _name, _checkValid); 9635 } 9636 9637 } 9638 9639 @Override 9640 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9641 switch (hash) { 9642 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : new Base[] {this.operation}; // SetupActionOperationComponent 9643 default: return super.getProperty(hash, name, checkValid); 9644 } 9645 9646 } 9647 9648 @Override 9649 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9650 switch (hash) { 9651 case 1662702951: // operation 9652 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 9653 return value; 9654 default: return super.setProperty(hash, name, value); 9655 } 9656 9657 } 9658 9659 @Override 9660 public Base setProperty(String name, Base value) throws FHIRException { 9661 if (name.equals("operation")) { 9662 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 9663 } else 9664 return super.setProperty(name, value); 9665 return value; 9666 } 9667 9668 @Override 9669 public void removeChild(String name, Base value) throws FHIRException { 9670 if (name.equals("operation")) { 9671 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 9672 } else 9673 super.removeChild(name, value); 9674 9675 } 9676 9677 @Override 9678 public Base makeProperty(int hash, String name) throws FHIRException { 9679 switch (hash) { 9680 case 1662702951: return getOperation(); 9681 default: return super.makeProperty(hash, name); 9682 } 9683 9684 } 9685 9686 @Override 9687 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9688 switch (hash) { 9689 case 1662702951: /*operation*/ return new String[] {"@TestScript.setup.action.operation"}; 9690 default: return super.getTypesForProperty(hash, name); 9691 } 9692 9693 } 9694 9695 @Override 9696 public Base addChild(String name) throws FHIRException { 9697 if (name.equals("operation")) { 9698 this.operation = new SetupActionOperationComponent(); 9699 return this.operation; 9700 } 9701 else 9702 return super.addChild(name); 9703 } 9704 9705 public TeardownActionComponent copy() { 9706 TeardownActionComponent dst = new TeardownActionComponent(); 9707 copyValues(dst); 9708 return dst; 9709 } 9710 9711 public void copyValues(TeardownActionComponent dst) { 9712 super.copyValues(dst); 9713 dst.operation = operation == null ? null : operation.copy(); 9714 } 9715 9716 @Override 9717 public boolean equalsDeep(Base other_) { 9718 if (!super.equalsDeep(other_)) 9719 return false; 9720 if (!(other_ instanceof TeardownActionComponent)) 9721 return false; 9722 TeardownActionComponent o = (TeardownActionComponent) other_; 9723 return compareDeep(operation, o.operation, true); 9724 } 9725 9726 @Override 9727 public boolean equalsShallow(Base other_) { 9728 if (!super.equalsShallow(other_)) 9729 return false; 9730 if (!(other_ instanceof TeardownActionComponent)) 9731 return false; 9732 TeardownActionComponent o = (TeardownActionComponent) other_; 9733 return true; 9734 } 9735 9736 public boolean isEmpty() { 9737 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation); 9738 } 9739 9740 public String fhirType() { 9741 return "TestScript.teardown.action"; 9742 9743 } 9744 9745 } 9746 9747 /** 9748 * An absolute URI that is used to identify this test script when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this test script is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the test script is stored on different servers. 9749 */ 9750 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 9751 @Description(shortDefinition="Canonical identifier for this test script, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this test script when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this test script is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the test script is stored on different servers." ) 9752 protected UriType url; 9753 9754 /** 9755 * A formal identifier that is used to identify this test script when it is represented in other formats, or referenced in a specification, model, design or an instance. 9756 */ 9757 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 9758 @Description(shortDefinition="Additional identifier for the test script", formalDefinition="A formal identifier that is used to identify this test script when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 9759 protected List<Identifier> identifier; 9760 9761 /** 9762 * The identifier that is used to identify this version of the test script when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test script author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 9763 */ 9764 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 9765 @Description(shortDefinition="Business version of the test script", formalDefinition="The identifier that is used to identify this version of the test script when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test script author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 9766 protected StringType version; 9767 9768 /** 9769 * Indicates the mechanism used to compare versions to determine which is more current. 9770 */ 9771 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 9772 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 9773 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 9774 protected DataType versionAlgorithm; 9775 9776 /** 9777 * A natural language name identifying the test script. This name should be usable as an identifier for the module by machine processing applications such as code generation. 9778 */ 9779 @Child(name = "name", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=true) 9780 @Description(shortDefinition="Name for this test script (computer friendly)", formalDefinition="A natural language name identifying the test script. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 9781 protected StringType name; 9782 9783 /** 9784 * A short, descriptive, user-friendly title for the test script. 9785 */ 9786 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 9787 @Description(shortDefinition="Name for this test script (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the test script." ) 9788 protected StringType title; 9789 9790 /** 9791 * The status of this test script. Enables tracking the life-cycle of the content. 9792 */ 9793 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 9794 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this test script. Enables tracking the life-cycle of the content." ) 9795 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 9796 protected Enumeration<PublicationStatus> status; 9797 9798 /** 9799 * A Boolean value to indicate that this test script is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 9800 */ 9801 @Child(name = "experimental", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 9802 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this test script is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 9803 protected BooleanType experimental; 9804 9805 /** 9806 * The date (and optionally time) when the test script was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test script changes. 9807 */ 9808 @Child(name = "date", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 9809 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the test script was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test script changes." ) 9810 protected DateTimeType date; 9811 9812 /** 9813 * The name of the organization or individual responsible for the release and ongoing maintenance of the test script. 9814 */ 9815 @Child(name = "publisher", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 9816 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the test script." ) 9817 protected StringType publisher; 9818 9819 /** 9820 * Contact details to assist a user in finding and communicating with the publisher. 9821 */ 9822 @Child(name = "contact", type = {ContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 9823 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 9824 protected List<ContactDetail> contact; 9825 9826 /** 9827 * A free text natural language description of the test script from a consumer's perspective. 9828 */ 9829 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 9830 @Description(shortDefinition="Natural language description of the test script", formalDefinition="A free text natural language description of the test script from a consumer's perspective." ) 9831 protected MarkdownType description; 9832 9833 /** 9834 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate test script instances. 9835 */ 9836 @Child(name = "useContext", type = {UsageContext.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 9837 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate test script instances." ) 9838 protected List<UsageContext> useContext; 9839 9840 /** 9841 * A legal or geographic region in which the test script is intended to be used. 9842 */ 9843 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 9844 @Description(shortDefinition="Intended jurisdiction for test script (if applicable)", formalDefinition="A legal or geographic region in which the test script is intended to be used." ) 9845 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 9846 protected List<CodeableConcept> jurisdiction; 9847 9848 /** 9849 * Explanation of why this test script is needed and why it has been designed as it has. 9850 */ 9851 @Child(name = "purpose", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 9852 @Description(shortDefinition="Why this test script is defined", formalDefinition="Explanation of why this test script is needed and why it has been designed as it has." ) 9853 protected MarkdownType purpose; 9854 9855 /** 9856 * A copyright statement relating to the test script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test script. 9857 */ 9858 @Child(name = "copyright", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 9859 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the test script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test script." ) 9860 protected MarkdownType copyright; 9861 9862 /** 9863 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 9864 */ 9865 @Child(name = "copyrightLabel", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 9866 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 9867 protected StringType copyrightLabel; 9868 9869 /** 9870 * An abstract server used in operations within this test script in the origin element. 9871 */ 9872 @Child(name = "origin", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9873 @Description(shortDefinition="An abstract server representing a client or sender in a message exchange", formalDefinition="An abstract server used in operations within this test script in the origin element." ) 9874 protected List<TestScriptOriginComponent> origin; 9875 9876 /** 9877 * An abstract server used in operations within this test script in the destination element. 9878 */ 9879 @Child(name = "destination", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9880 @Description(shortDefinition="An abstract server representing a destination or receiver in a message exchange", formalDefinition="An abstract server used in operations within this test script in the destination element." ) 9881 protected List<TestScriptDestinationComponent> destination; 9882 9883 /** 9884 * The required capability must exist and are assumed to function correctly on the FHIR server being tested. 9885 */ 9886 @Child(name = "metadata", type = {}, order=19, min=0, max=1, modifier=false, summary=false) 9887 @Description(shortDefinition="Required capability that is assumed to function correctly on the FHIR server being tested", formalDefinition="The required capability must exist and are assumed to function correctly on the FHIR server being tested." ) 9888 protected TestScriptMetadataComponent metadata; 9889 9890 /** 9891 * The scope indicates a conformance artifact that is tested by the test(s) within this test case and the expectation of the test outcome(s) as well as the intended test phase inclusion. 9892 */ 9893 @Child(name = "scope", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9894 @Description(shortDefinition="Indication of the artifact(s) that are tested by this test case", formalDefinition="The scope indicates a conformance artifact that is tested by the test(s) within this test case and the expectation of the test outcome(s) as well as the intended test phase inclusion." ) 9895 protected List<TestScriptScopeComponent> scope; 9896 9897 /** 9898 * Fixture in the test script - by reference (uri). All fixtures are required for the test script to execute. 9899 */ 9900 @Child(name = "fixture", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9901 @Description(shortDefinition="Fixture in the test script - by reference (uri)", formalDefinition="Fixture in the test script - by reference (uri). All fixtures are required for the test script to execute." ) 9902 protected List<TestScriptFixtureComponent> fixture; 9903 9904 /** 9905 * Reference to the profile to be used for validation. 9906 */ 9907 @Child(name = "profile", type = {CanonicalType.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9908 @Description(shortDefinition="Reference of the validation profile", formalDefinition="Reference to the profile to be used for validation." ) 9909 protected List<CanonicalType> profile; 9910 9911 /** 9912 * Variable is set based either on element value in response body or on header field value in the response headers. 9913 */ 9914 @Child(name = "variable", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9915 @Description(shortDefinition="Placeholder for evaluated elements", formalDefinition="Variable is set based either on element value in response body or on header field value in the response headers." ) 9916 protected List<TestScriptVariableComponent> variable; 9917 9918 /** 9919 * A series of required setup operations before tests are executed. 9920 */ 9921 @Child(name = "setup", type = {}, order=24, min=0, max=1, modifier=false, summary=false) 9922 @Description(shortDefinition="A series of required setup operations before tests are executed", formalDefinition="A series of required setup operations before tests are executed." ) 9923 protected TestScriptSetupComponent setup; 9924 9925 /** 9926 * A test in this script. 9927 */ 9928 @Child(name = "test", type = {}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9929 @Description(shortDefinition="A test in this script", formalDefinition="A test in this script." ) 9930 protected List<TestScriptTestComponent> test; 9931 9932 /** 9933 * A series of operations required to clean up after all the tests are executed (successfully or otherwise). 9934 */ 9935 @Child(name = "teardown", type = {}, order=26, min=0, max=1, modifier=false, summary=false) 9936 @Description(shortDefinition="A series of required clean up steps", formalDefinition="A series of operations required to clean up after all the tests are executed (successfully or otherwise)." ) 9937 protected TestScriptTeardownComponent teardown; 9938 9939 private static final long serialVersionUID = 1566648997L; 9940 9941 /** 9942 * Constructor 9943 */ 9944 public TestScript() { 9945 super(); 9946 } 9947 9948 /** 9949 * Constructor 9950 */ 9951 public TestScript(String name, PublicationStatus status) { 9952 super(); 9953 this.setName(name); 9954 this.setStatus(status); 9955 } 9956 9957 /** 9958 * @return {@link #url} (An absolute URI that is used to identify this test script when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this test script is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the test script is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 9959 */ 9960 public UriType getUrlElement() { 9961 if (this.url == null) 9962 if (Configuration.errorOnAutoCreate()) 9963 throw new Error("Attempt to auto-create TestScript.url"); 9964 else if (Configuration.doAutoCreate()) 9965 this.url = new UriType(); // bb 9966 return this.url; 9967 } 9968 9969 public boolean hasUrlElement() { 9970 return this.url != null && !this.url.isEmpty(); 9971 } 9972 9973 public boolean hasUrl() { 9974 return this.url != null && !this.url.isEmpty(); 9975 } 9976 9977 /** 9978 * @param value {@link #url} (An absolute URI that is used to identify this test script when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this test script is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the test script is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 9979 */ 9980 public TestScript setUrlElement(UriType value) { 9981 this.url = value; 9982 return this; 9983 } 9984 9985 /** 9986 * @return An absolute URI that is used to identify this test script when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this test script is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the test script is stored on different servers. 9987 */ 9988 public String getUrl() { 9989 return this.url == null ? null : this.url.getValue(); 9990 } 9991 9992 /** 9993 * @param value An absolute URI that is used to identify this test script when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this test script is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the test script is stored on different servers. 9994 */ 9995 public TestScript setUrl(String value) { 9996 if (Utilities.noString(value)) 9997 this.url = null; 9998 else { 9999 if (this.url == null) 10000 this.url = new UriType(); 10001 this.url.setValue(value); 10002 } 10003 return this; 10004 } 10005 10006 /** 10007 * @return {@link #identifier} (A formal identifier that is used to identify this test script when it is represented in other formats, or referenced in a specification, model, design or an instance.) 10008 */ 10009 public List<Identifier> getIdentifier() { 10010 if (this.identifier == null) 10011 this.identifier = new ArrayList<Identifier>(); 10012 return this.identifier; 10013 } 10014 10015 /** 10016 * @return Returns a reference to <code>this</code> for easy method chaining 10017 */ 10018 public TestScript setIdentifier(List<Identifier> theIdentifier) { 10019 this.identifier = theIdentifier; 10020 return this; 10021 } 10022 10023 public boolean hasIdentifier() { 10024 if (this.identifier == null) 10025 return false; 10026 for (Identifier item : this.identifier) 10027 if (!item.isEmpty()) 10028 return true; 10029 return false; 10030 } 10031 10032 public Identifier addIdentifier() { //3 10033 Identifier t = new Identifier(); 10034 if (this.identifier == null) 10035 this.identifier = new ArrayList<Identifier>(); 10036 this.identifier.add(t); 10037 return t; 10038 } 10039 10040 public TestScript addIdentifier(Identifier t) { //3 10041 if (t == null) 10042 return this; 10043 if (this.identifier == null) 10044 this.identifier = new ArrayList<Identifier>(); 10045 this.identifier.add(t); 10046 return this; 10047 } 10048 10049 /** 10050 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 10051 */ 10052 public Identifier getIdentifierFirstRep() { 10053 if (getIdentifier().isEmpty()) { 10054 addIdentifier(); 10055 } 10056 return getIdentifier().get(0); 10057 } 10058 10059 /** 10060 * @return {@link #version} (The identifier that is used to identify this version of the test script when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test script author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 10061 */ 10062 public StringType getVersionElement() { 10063 if (this.version == null) 10064 if (Configuration.errorOnAutoCreate()) 10065 throw new Error("Attempt to auto-create TestScript.version"); 10066 else if (Configuration.doAutoCreate()) 10067 this.version = new StringType(); // bb 10068 return this.version; 10069 } 10070 10071 public boolean hasVersionElement() { 10072 return this.version != null && !this.version.isEmpty(); 10073 } 10074 10075 public boolean hasVersion() { 10076 return this.version != null && !this.version.isEmpty(); 10077 } 10078 10079 /** 10080 * @param value {@link #version} (The identifier that is used to identify this version of the test script when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test script author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 10081 */ 10082 public TestScript setVersionElement(StringType value) { 10083 this.version = value; 10084 return this; 10085 } 10086 10087 /** 10088 * @return The identifier that is used to identify this version of the test script when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test script author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 10089 */ 10090 public String getVersion() { 10091 return this.version == null ? null : this.version.getValue(); 10092 } 10093 10094 /** 10095 * @param value The identifier that is used to identify this version of the test script when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test script author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 10096 */ 10097 public TestScript setVersion(String value) { 10098 if (Utilities.noString(value)) 10099 this.version = null; 10100 else { 10101 if (this.version == null) 10102 this.version = new StringType(); 10103 this.version.setValue(value); 10104 } 10105 return this; 10106 } 10107 10108 /** 10109 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 10110 */ 10111 public DataType getVersionAlgorithm() { 10112 return this.versionAlgorithm; 10113 } 10114 10115 /** 10116 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 10117 */ 10118 public StringType getVersionAlgorithmStringType() throws FHIRException { 10119 if (this.versionAlgorithm == null) 10120 this.versionAlgorithm = new StringType(); 10121 if (!(this.versionAlgorithm instanceof StringType)) 10122 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 10123 return (StringType) this.versionAlgorithm; 10124 } 10125 10126 public boolean hasVersionAlgorithmStringType() { 10127 return this.versionAlgorithm instanceof StringType; 10128 } 10129 10130 /** 10131 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 10132 */ 10133 public Coding getVersionAlgorithmCoding() throws FHIRException { 10134 if (this.versionAlgorithm == null) 10135 this.versionAlgorithm = new Coding(); 10136 if (!(this.versionAlgorithm instanceof Coding)) 10137 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 10138 return (Coding) this.versionAlgorithm; 10139 } 10140 10141 public boolean hasVersionAlgorithmCoding() { 10142 return this.versionAlgorithm instanceof Coding; 10143 } 10144 10145 public boolean hasVersionAlgorithm() { 10146 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 10147 } 10148 10149 /** 10150 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 10151 */ 10152 public TestScript setVersionAlgorithm(DataType value) { 10153 if (value != null && !(value instanceof StringType || value instanceof Coding)) 10154 throw new FHIRException("Not the right type for TestScript.versionAlgorithm[x]: "+value.fhirType()); 10155 this.versionAlgorithm = value; 10156 return this; 10157 } 10158 10159 /** 10160 * @return {@link #name} (A natural language name identifying the test script. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 10161 */ 10162 public StringType getNameElement() { 10163 if (this.name == null) 10164 if (Configuration.errorOnAutoCreate()) 10165 throw new Error("Attempt to auto-create TestScript.name"); 10166 else if (Configuration.doAutoCreate()) 10167 this.name = new StringType(); // bb 10168 return this.name; 10169 } 10170 10171 public boolean hasNameElement() { 10172 return this.name != null && !this.name.isEmpty(); 10173 } 10174 10175 public boolean hasName() { 10176 return this.name != null && !this.name.isEmpty(); 10177 } 10178 10179 /** 10180 * @param value {@link #name} (A natural language name identifying the test script. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 10181 */ 10182 public TestScript setNameElement(StringType value) { 10183 this.name = value; 10184 return this; 10185 } 10186 10187 /** 10188 * @return A natural language name identifying the test script. This name should be usable as an identifier for the module by machine processing applications such as code generation. 10189 */ 10190 public String getName() { 10191 return this.name == null ? null : this.name.getValue(); 10192 } 10193 10194 /** 10195 * @param value A natural language name identifying the test script. This name should be usable as an identifier for the module by machine processing applications such as code generation. 10196 */ 10197 public TestScript setName(String value) { 10198 if (this.name == null) 10199 this.name = new StringType(); 10200 this.name.setValue(value); 10201 return this; 10202 } 10203 10204 /** 10205 * @return {@link #title} (A short, descriptive, user-friendly title for the test script.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 10206 */ 10207 public StringType getTitleElement() { 10208 if (this.title == null) 10209 if (Configuration.errorOnAutoCreate()) 10210 throw new Error("Attempt to auto-create TestScript.title"); 10211 else if (Configuration.doAutoCreate()) 10212 this.title = new StringType(); // bb 10213 return this.title; 10214 } 10215 10216 public boolean hasTitleElement() { 10217 return this.title != null && !this.title.isEmpty(); 10218 } 10219 10220 public boolean hasTitle() { 10221 return this.title != null && !this.title.isEmpty(); 10222 } 10223 10224 /** 10225 * @param value {@link #title} (A short, descriptive, user-friendly title for the test script.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 10226 */ 10227 public TestScript setTitleElement(StringType value) { 10228 this.title = value; 10229 return this; 10230 } 10231 10232 /** 10233 * @return A short, descriptive, user-friendly title for the test script. 10234 */ 10235 public String getTitle() { 10236 return this.title == null ? null : this.title.getValue(); 10237 } 10238 10239 /** 10240 * @param value A short, descriptive, user-friendly title for the test script. 10241 */ 10242 public TestScript setTitle(String value) { 10243 if (Utilities.noString(value)) 10244 this.title = null; 10245 else { 10246 if (this.title == null) 10247 this.title = new StringType(); 10248 this.title.setValue(value); 10249 } 10250 return this; 10251 } 10252 10253 /** 10254 * @return {@link #status} (The status of this test script. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 10255 */ 10256 public Enumeration<PublicationStatus> getStatusElement() { 10257 if (this.status == null) 10258 if (Configuration.errorOnAutoCreate()) 10259 throw new Error("Attempt to auto-create TestScript.status"); 10260 else if (Configuration.doAutoCreate()) 10261 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 10262 return this.status; 10263 } 10264 10265 public boolean hasStatusElement() { 10266 return this.status != null && !this.status.isEmpty(); 10267 } 10268 10269 public boolean hasStatus() { 10270 return this.status != null && !this.status.isEmpty(); 10271 } 10272 10273 /** 10274 * @param value {@link #status} (The status of this test script. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 10275 */ 10276 public TestScript setStatusElement(Enumeration<PublicationStatus> value) { 10277 this.status = value; 10278 return this; 10279 } 10280 10281 /** 10282 * @return The status of this test script. Enables tracking the life-cycle of the content. 10283 */ 10284 public PublicationStatus getStatus() { 10285 return this.status == null ? null : this.status.getValue(); 10286 } 10287 10288 /** 10289 * @param value The status of this test script. Enables tracking the life-cycle of the content. 10290 */ 10291 public TestScript setStatus(PublicationStatus value) { 10292 if (this.status == null) 10293 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 10294 this.status.setValue(value); 10295 return this; 10296 } 10297 10298 /** 10299 * @return {@link #experimental} (A Boolean value to indicate that this test script is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 10300 */ 10301 public BooleanType getExperimentalElement() { 10302 if (this.experimental == null) 10303 if (Configuration.errorOnAutoCreate()) 10304 throw new Error("Attempt to auto-create TestScript.experimental"); 10305 else if (Configuration.doAutoCreate()) 10306 this.experimental = new BooleanType(); // bb 10307 return this.experimental; 10308 } 10309 10310 public boolean hasExperimentalElement() { 10311 return this.experimental != null && !this.experimental.isEmpty(); 10312 } 10313 10314 public boolean hasExperimental() { 10315 return this.experimental != null && !this.experimental.isEmpty(); 10316 } 10317 10318 /** 10319 * @param value {@link #experimental} (A Boolean value to indicate that this test script is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 10320 */ 10321 public TestScript setExperimentalElement(BooleanType value) { 10322 this.experimental = value; 10323 return this; 10324 } 10325 10326 /** 10327 * @return A Boolean value to indicate that this test script is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 10328 */ 10329 public boolean getExperimental() { 10330 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 10331 } 10332 10333 /** 10334 * @param value A Boolean value to indicate that this test script is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 10335 */ 10336 public TestScript setExperimental(boolean value) { 10337 if (this.experimental == null) 10338 this.experimental = new BooleanType(); 10339 this.experimental.setValue(value); 10340 return this; 10341 } 10342 10343 /** 10344 * @return {@link #date} (The date (and optionally time) when the test script was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test script changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 10345 */ 10346 public DateTimeType getDateElement() { 10347 if (this.date == null) 10348 if (Configuration.errorOnAutoCreate()) 10349 throw new Error("Attempt to auto-create TestScript.date"); 10350 else if (Configuration.doAutoCreate()) 10351 this.date = new DateTimeType(); // bb 10352 return this.date; 10353 } 10354 10355 public boolean hasDateElement() { 10356 return this.date != null && !this.date.isEmpty(); 10357 } 10358 10359 public boolean hasDate() { 10360 return this.date != null && !this.date.isEmpty(); 10361 } 10362 10363 /** 10364 * @param value {@link #date} (The date (and optionally time) when the test script was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test script changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 10365 */ 10366 public TestScript setDateElement(DateTimeType value) { 10367 this.date = value; 10368 return this; 10369 } 10370 10371 /** 10372 * @return The date (and optionally time) when the test script was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test script changes. 10373 */ 10374 public Date getDate() { 10375 return this.date == null ? null : this.date.getValue(); 10376 } 10377 10378 /** 10379 * @param value The date (and optionally time) when the test script was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test script changes. 10380 */ 10381 public TestScript setDate(Date value) { 10382 if (value == null) 10383 this.date = null; 10384 else { 10385 if (this.date == null) 10386 this.date = new DateTimeType(); 10387 this.date.setValue(value); 10388 } 10389 return this; 10390 } 10391 10392 /** 10393 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the test script.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 10394 */ 10395 public StringType getPublisherElement() { 10396 if (this.publisher == null) 10397 if (Configuration.errorOnAutoCreate()) 10398 throw new Error("Attempt to auto-create TestScript.publisher"); 10399 else if (Configuration.doAutoCreate()) 10400 this.publisher = new StringType(); // bb 10401 return this.publisher; 10402 } 10403 10404 public boolean hasPublisherElement() { 10405 return this.publisher != null && !this.publisher.isEmpty(); 10406 } 10407 10408 public boolean hasPublisher() { 10409 return this.publisher != null && !this.publisher.isEmpty(); 10410 } 10411 10412 /** 10413 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the test script.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 10414 */ 10415 public TestScript setPublisherElement(StringType value) { 10416 this.publisher = value; 10417 return this; 10418 } 10419 10420 /** 10421 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the test script. 10422 */ 10423 public String getPublisher() { 10424 return this.publisher == null ? null : this.publisher.getValue(); 10425 } 10426 10427 /** 10428 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the test script. 10429 */ 10430 public TestScript setPublisher(String value) { 10431 if (Utilities.noString(value)) 10432 this.publisher = null; 10433 else { 10434 if (this.publisher == null) 10435 this.publisher = new StringType(); 10436 this.publisher.setValue(value); 10437 } 10438 return this; 10439 } 10440 10441 /** 10442 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 10443 */ 10444 public List<ContactDetail> getContact() { 10445 if (this.contact == null) 10446 this.contact = new ArrayList<ContactDetail>(); 10447 return this.contact; 10448 } 10449 10450 /** 10451 * @return Returns a reference to <code>this</code> for easy method chaining 10452 */ 10453 public TestScript setContact(List<ContactDetail> theContact) { 10454 this.contact = theContact; 10455 return this; 10456 } 10457 10458 public boolean hasContact() { 10459 if (this.contact == null) 10460 return false; 10461 for (ContactDetail item : this.contact) 10462 if (!item.isEmpty()) 10463 return true; 10464 return false; 10465 } 10466 10467 public ContactDetail addContact() { //3 10468 ContactDetail t = new ContactDetail(); 10469 if (this.contact == null) 10470 this.contact = new ArrayList<ContactDetail>(); 10471 this.contact.add(t); 10472 return t; 10473 } 10474 10475 public TestScript addContact(ContactDetail t) { //3 10476 if (t == null) 10477 return this; 10478 if (this.contact == null) 10479 this.contact = new ArrayList<ContactDetail>(); 10480 this.contact.add(t); 10481 return this; 10482 } 10483 10484 /** 10485 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 10486 */ 10487 public ContactDetail getContactFirstRep() { 10488 if (getContact().isEmpty()) { 10489 addContact(); 10490 } 10491 return getContact().get(0); 10492 } 10493 10494 /** 10495 * @return {@link #description} (A free text natural language description of the test script from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 10496 */ 10497 public MarkdownType getDescriptionElement() { 10498 if (this.description == null) 10499 if (Configuration.errorOnAutoCreate()) 10500 throw new Error("Attempt to auto-create TestScript.description"); 10501 else if (Configuration.doAutoCreate()) 10502 this.description = new MarkdownType(); // bb 10503 return this.description; 10504 } 10505 10506 public boolean hasDescriptionElement() { 10507 return this.description != null && !this.description.isEmpty(); 10508 } 10509 10510 public boolean hasDescription() { 10511 return this.description != null && !this.description.isEmpty(); 10512 } 10513 10514 /** 10515 * @param value {@link #description} (A free text natural language description of the test script from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 10516 */ 10517 public TestScript setDescriptionElement(MarkdownType value) { 10518 this.description = value; 10519 return this; 10520 } 10521 10522 /** 10523 * @return A free text natural language description of the test script from a consumer's perspective. 10524 */ 10525 public String getDescription() { 10526 return this.description == null ? null : this.description.getValue(); 10527 } 10528 10529 /** 10530 * @param value A free text natural language description of the test script from a consumer's perspective. 10531 */ 10532 public TestScript setDescription(String value) { 10533 if (Utilities.noString(value)) 10534 this.description = null; 10535 else { 10536 if (this.description == null) 10537 this.description = new MarkdownType(); 10538 this.description.setValue(value); 10539 } 10540 return this; 10541 } 10542 10543 /** 10544 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate test script instances.) 10545 */ 10546 public List<UsageContext> getUseContext() { 10547 if (this.useContext == null) 10548 this.useContext = new ArrayList<UsageContext>(); 10549 return this.useContext; 10550 } 10551 10552 /** 10553 * @return Returns a reference to <code>this</code> for easy method chaining 10554 */ 10555 public TestScript setUseContext(List<UsageContext> theUseContext) { 10556 this.useContext = theUseContext; 10557 return this; 10558 } 10559 10560 public boolean hasUseContext() { 10561 if (this.useContext == null) 10562 return false; 10563 for (UsageContext item : this.useContext) 10564 if (!item.isEmpty()) 10565 return true; 10566 return false; 10567 } 10568 10569 public UsageContext addUseContext() { //3 10570 UsageContext t = new UsageContext(); 10571 if (this.useContext == null) 10572 this.useContext = new ArrayList<UsageContext>(); 10573 this.useContext.add(t); 10574 return t; 10575 } 10576 10577 public TestScript addUseContext(UsageContext t) { //3 10578 if (t == null) 10579 return this; 10580 if (this.useContext == null) 10581 this.useContext = new ArrayList<UsageContext>(); 10582 this.useContext.add(t); 10583 return this; 10584 } 10585 10586 /** 10587 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 10588 */ 10589 public UsageContext getUseContextFirstRep() { 10590 if (getUseContext().isEmpty()) { 10591 addUseContext(); 10592 } 10593 return getUseContext().get(0); 10594 } 10595 10596 /** 10597 * @return {@link #jurisdiction} (A legal or geographic region in which the test script is intended to be used.) 10598 */ 10599 public List<CodeableConcept> getJurisdiction() { 10600 if (this.jurisdiction == null) 10601 this.jurisdiction = new ArrayList<CodeableConcept>(); 10602 return this.jurisdiction; 10603 } 10604 10605 /** 10606 * @return Returns a reference to <code>this</code> for easy method chaining 10607 */ 10608 public TestScript setJurisdiction(List<CodeableConcept> theJurisdiction) { 10609 this.jurisdiction = theJurisdiction; 10610 return this; 10611 } 10612 10613 public boolean hasJurisdiction() { 10614 if (this.jurisdiction == null) 10615 return false; 10616 for (CodeableConcept item : this.jurisdiction) 10617 if (!item.isEmpty()) 10618 return true; 10619 return false; 10620 } 10621 10622 public CodeableConcept addJurisdiction() { //3 10623 CodeableConcept t = new CodeableConcept(); 10624 if (this.jurisdiction == null) 10625 this.jurisdiction = new ArrayList<CodeableConcept>(); 10626 this.jurisdiction.add(t); 10627 return t; 10628 } 10629 10630 public TestScript addJurisdiction(CodeableConcept t) { //3 10631 if (t == null) 10632 return this; 10633 if (this.jurisdiction == null) 10634 this.jurisdiction = new ArrayList<CodeableConcept>(); 10635 this.jurisdiction.add(t); 10636 return this; 10637 } 10638 10639 /** 10640 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 10641 */ 10642 public CodeableConcept getJurisdictionFirstRep() { 10643 if (getJurisdiction().isEmpty()) { 10644 addJurisdiction(); 10645 } 10646 return getJurisdiction().get(0); 10647 } 10648 10649 /** 10650 * @return {@link #purpose} (Explanation of why this test script is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 10651 */ 10652 public MarkdownType getPurposeElement() { 10653 if (this.purpose == null) 10654 if (Configuration.errorOnAutoCreate()) 10655 throw new Error("Attempt to auto-create TestScript.purpose"); 10656 else if (Configuration.doAutoCreate()) 10657 this.purpose = new MarkdownType(); // bb 10658 return this.purpose; 10659 } 10660 10661 public boolean hasPurposeElement() { 10662 return this.purpose != null && !this.purpose.isEmpty(); 10663 } 10664 10665 public boolean hasPurpose() { 10666 return this.purpose != null && !this.purpose.isEmpty(); 10667 } 10668 10669 /** 10670 * @param value {@link #purpose} (Explanation of why this test script is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 10671 */ 10672 public TestScript setPurposeElement(MarkdownType value) { 10673 this.purpose = value; 10674 return this; 10675 } 10676 10677 /** 10678 * @return Explanation of why this test script is needed and why it has been designed as it has. 10679 */ 10680 public String getPurpose() { 10681 return this.purpose == null ? null : this.purpose.getValue(); 10682 } 10683 10684 /** 10685 * @param value Explanation of why this test script is needed and why it has been designed as it has. 10686 */ 10687 public TestScript setPurpose(String value) { 10688 if (Utilities.noString(value)) 10689 this.purpose = null; 10690 else { 10691 if (this.purpose == null) 10692 this.purpose = new MarkdownType(); 10693 this.purpose.setValue(value); 10694 } 10695 return this; 10696 } 10697 10698 /** 10699 * @return {@link #copyright} (A copyright statement relating to the test script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test script.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 10700 */ 10701 public MarkdownType getCopyrightElement() { 10702 if (this.copyright == null) 10703 if (Configuration.errorOnAutoCreate()) 10704 throw new Error("Attempt to auto-create TestScript.copyright"); 10705 else if (Configuration.doAutoCreate()) 10706 this.copyright = new MarkdownType(); // bb 10707 return this.copyright; 10708 } 10709 10710 public boolean hasCopyrightElement() { 10711 return this.copyright != null && !this.copyright.isEmpty(); 10712 } 10713 10714 public boolean hasCopyright() { 10715 return this.copyright != null && !this.copyright.isEmpty(); 10716 } 10717 10718 /** 10719 * @param value {@link #copyright} (A copyright statement relating to the test script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test script.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 10720 */ 10721 public TestScript setCopyrightElement(MarkdownType value) { 10722 this.copyright = value; 10723 return this; 10724 } 10725 10726 /** 10727 * @return A copyright statement relating to the test script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test script. 10728 */ 10729 public String getCopyright() { 10730 return this.copyright == null ? null : this.copyright.getValue(); 10731 } 10732 10733 /** 10734 * @param value A copyright statement relating to the test script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test script. 10735 */ 10736 public TestScript setCopyright(String value) { 10737 if (Utilities.noString(value)) 10738 this.copyright = null; 10739 else { 10740 if (this.copyright == null) 10741 this.copyright = new MarkdownType(); 10742 this.copyright.setValue(value); 10743 } 10744 return this; 10745 } 10746 10747 /** 10748 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 10749 */ 10750 public StringType getCopyrightLabelElement() { 10751 if (this.copyrightLabel == null) 10752 if (Configuration.errorOnAutoCreate()) 10753 throw new Error("Attempt to auto-create TestScript.copyrightLabel"); 10754 else if (Configuration.doAutoCreate()) 10755 this.copyrightLabel = new StringType(); // bb 10756 return this.copyrightLabel; 10757 } 10758 10759 public boolean hasCopyrightLabelElement() { 10760 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 10761 } 10762 10763 public boolean hasCopyrightLabel() { 10764 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 10765 } 10766 10767 /** 10768 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 10769 */ 10770 public TestScript setCopyrightLabelElement(StringType value) { 10771 this.copyrightLabel = value; 10772 return this; 10773 } 10774 10775 /** 10776 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 10777 */ 10778 public String getCopyrightLabel() { 10779 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 10780 } 10781 10782 /** 10783 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 10784 */ 10785 public TestScript setCopyrightLabel(String value) { 10786 if (Utilities.noString(value)) 10787 this.copyrightLabel = null; 10788 else { 10789 if (this.copyrightLabel == null) 10790 this.copyrightLabel = new StringType(); 10791 this.copyrightLabel.setValue(value); 10792 } 10793 return this; 10794 } 10795 10796 /** 10797 * @return {@link #origin} (An abstract server used in operations within this test script in the origin element.) 10798 */ 10799 public List<TestScriptOriginComponent> getOrigin() { 10800 if (this.origin == null) 10801 this.origin = new ArrayList<TestScriptOriginComponent>(); 10802 return this.origin; 10803 } 10804 10805 /** 10806 * @return Returns a reference to <code>this</code> for easy method chaining 10807 */ 10808 public TestScript setOrigin(List<TestScriptOriginComponent> theOrigin) { 10809 this.origin = theOrigin; 10810 return this; 10811 } 10812 10813 public boolean hasOrigin() { 10814 if (this.origin == null) 10815 return false; 10816 for (TestScriptOriginComponent item : this.origin) 10817 if (!item.isEmpty()) 10818 return true; 10819 return false; 10820 } 10821 10822 public TestScriptOriginComponent addOrigin() { //3 10823 TestScriptOriginComponent t = new TestScriptOriginComponent(); 10824 if (this.origin == null) 10825 this.origin = new ArrayList<TestScriptOriginComponent>(); 10826 this.origin.add(t); 10827 return t; 10828 } 10829 10830 public TestScript addOrigin(TestScriptOriginComponent t) { //3 10831 if (t == null) 10832 return this; 10833 if (this.origin == null) 10834 this.origin = new ArrayList<TestScriptOriginComponent>(); 10835 this.origin.add(t); 10836 return this; 10837 } 10838 10839 /** 10840 * @return The first repetition of repeating field {@link #origin}, creating it if it does not already exist {3} 10841 */ 10842 public TestScriptOriginComponent getOriginFirstRep() { 10843 if (getOrigin().isEmpty()) { 10844 addOrigin(); 10845 } 10846 return getOrigin().get(0); 10847 } 10848 10849 /** 10850 * @return {@link #destination} (An abstract server used in operations within this test script in the destination element.) 10851 */ 10852 public List<TestScriptDestinationComponent> getDestination() { 10853 if (this.destination == null) 10854 this.destination = new ArrayList<TestScriptDestinationComponent>(); 10855 return this.destination; 10856 } 10857 10858 /** 10859 * @return Returns a reference to <code>this</code> for easy method chaining 10860 */ 10861 public TestScript setDestination(List<TestScriptDestinationComponent> theDestination) { 10862 this.destination = theDestination; 10863 return this; 10864 } 10865 10866 public boolean hasDestination() { 10867 if (this.destination == null) 10868 return false; 10869 for (TestScriptDestinationComponent item : this.destination) 10870 if (!item.isEmpty()) 10871 return true; 10872 return false; 10873 } 10874 10875 public TestScriptDestinationComponent addDestination() { //3 10876 TestScriptDestinationComponent t = new TestScriptDestinationComponent(); 10877 if (this.destination == null) 10878 this.destination = new ArrayList<TestScriptDestinationComponent>(); 10879 this.destination.add(t); 10880 return t; 10881 } 10882 10883 public TestScript addDestination(TestScriptDestinationComponent t) { //3 10884 if (t == null) 10885 return this; 10886 if (this.destination == null) 10887 this.destination = new ArrayList<TestScriptDestinationComponent>(); 10888 this.destination.add(t); 10889 return this; 10890 } 10891 10892 /** 10893 * @return The first repetition of repeating field {@link #destination}, creating it if it does not already exist {3} 10894 */ 10895 public TestScriptDestinationComponent getDestinationFirstRep() { 10896 if (getDestination().isEmpty()) { 10897 addDestination(); 10898 } 10899 return getDestination().get(0); 10900 } 10901 10902 /** 10903 * @return {@link #metadata} (The required capability must exist and are assumed to function correctly on the FHIR server being tested.) 10904 */ 10905 public TestScriptMetadataComponent getMetadata() { 10906 if (this.metadata == null) 10907 if (Configuration.errorOnAutoCreate()) 10908 throw new Error("Attempt to auto-create TestScript.metadata"); 10909 else if (Configuration.doAutoCreate()) 10910 this.metadata = new TestScriptMetadataComponent(); // cc 10911 return this.metadata; 10912 } 10913 10914 public boolean hasMetadata() { 10915 return this.metadata != null && !this.metadata.isEmpty(); 10916 } 10917 10918 /** 10919 * @param value {@link #metadata} (The required capability must exist and are assumed to function correctly on the FHIR server being tested.) 10920 */ 10921 public TestScript setMetadata(TestScriptMetadataComponent value) { 10922 this.metadata = value; 10923 return this; 10924 } 10925 10926 /** 10927 * @return {@link #scope} (The scope indicates a conformance artifact that is tested by the test(s) within this test case and the expectation of the test outcome(s) as well as the intended test phase inclusion.) 10928 */ 10929 public List<TestScriptScopeComponent> getScope() { 10930 if (this.scope == null) 10931 this.scope = new ArrayList<TestScriptScopeComponent>(); 10932 return this.scope; 10933 } 10934 10935 /** 10936 * @return Returns a reference to <code>this</code> for easy method chaining 10937 */ 10938 public TestScript setScope(List<TestScriptScopeComponent> theScope) { 10939 this.scope = theScope; 10940 return this; 10941 } 10942 10943 public boolean hasScope() { 10944 if (this.scope == null) 10945 return false; 10946 for (TestScriptScopeComponent item : this.scope) 10947 if (!item.isEmpty()) 10948 return true; 10949 return false; 10950 } 10951 10952 public TestScriptScopeComponent addScope() { //3 10953 TestScriptScopeComponent t = new TestScriptScopeComponent(); 10954 if (this.scope == null) 10955 this.scope = new ArrayList<TestScriptScopeComponent>(); 10956 this.scope.add(t); 10957 return t; 10958 } 10959 10960 public TestScript addScope(TestScriptScopeComponent t) { //3 10961 if (t == null) 10962 return this; 10963 if (this.scope == null) 10964 this.scope = new ArrayList<TestScriptScopeComponent>(); 10965 this.scope.add(t); 10966 return this; 10967 } 10968 10969 /** 10970 * @return The first repetition of repeating field {@link #scope}, creating it if it does not already exist {3} 10971 */ 10972 public TestScriptScopeComponent getScopeFirstRep() { 10973 if (getScope().isEmpty()) { 10974 addScope(); 10975 } 10976 return getScope().get(0); 10977 } 10978 10979 /** 10980 * @return {@link #fixture} (Fixture in the test script - by reference (uri). All fixtures are required for the test script to execute.) 10981 */ 10982 public List<TestScriptFixtureComponent> getFixture() { 10983 if (this.fixture == null) 10984 this.fixture = new ArrayList<TestScriptFixtureComponent>(); 10985 return this.fixture; 10986 } 10987 10988 /** 10989 * @return Returns a reference to <code>this</code> for easy method chaining 10990 */ 10991 public TestScript setFixture(List<TestScriptFixtureComponent> theFixture) { 10992 this.fixture = theFixture; 10993 return this; 10994 } 10995 10996 public boolean hasFixture() { 10997 if (this.fixture == null) 10998 return false; 10999 for (TestScriptFixtureComponent item : this.fixture) 11000 if (!item.isEmpty()) 11001 return true; 11002 return false; 11003 } 11004 11005 public TestScriptFixtureComponent addFixture() { //3 11006 TestScriptFixtureComponent t = new TestScriptFixtureComponent(); 11007 if (this.fixture == null) 11008 this.fixture = new ArrayList<TestScriptFixtureComponent>(); 11009 this.fixture.add(t); 11010 return t; 11011 } 11012 11013 public TestScript addFixture(TestScriptFixtureComponent t) { //3 11014 if (t == null) 11015 return this; 11016 if (this.fixture == null) 11017 this.fixture = new ArrayList<TestScriptFixtureComponent>(); 11018 this.fixture.add(t); 11019 return this; 11020 } 11021 11022 /** 11023 * @return The first repetition of repeating field {@link #fixture}, creating it if it does not already exist {3} 11024 */ 11025 public TestScriptFixtureComponent getFixtureFirstRep() { 11026 if (getFixture().isEmpty()) { 11027 addFixture(); 11028 } 11029 return getFixture().get(0); 11030 } 11031 11032 /** 11033 * @return {@link #profile} (Reference to the profile to be used for validation.) 11034 */ 11035 public List<CanonicalType> getProfile() { 11036 if (this.profile == null) 11037 this.profile = new ArrayList<CanonicalType>(); 11038 return this.profile; 11039 } 11040 11041 /** 11042 * @return Returns a reference to <code>this</code> for easy method chaining 11043 */ 11044 public TestScript setProfile(List<CanonicalType> theProfile) { 11045 this.profile = theProfile; 11046 return this; 11047 } 11048 11049 public boolean hasProfile() { 11050 if (this.profile == null) 11051 return false; 11052 for (CanonicalType item : this.profile) 11053 if (!item.isEmpty()) 11054 return true; 11055 return false; 11056 } 11057 11058 /** 11059 * @return {@link #profile} (Reference to the profile to be used for validation.) 11060 */ 11061 public CanonicalType addProfileElement() {//2 11062 CanonicalType t = new CanonicalType(); 11063 if (this.profile == null) 11064 this.profile = new ArrayList<CanonicalType>(); 11065 this.profile.add(t); 11066 return t; 11067 } 11068 11069 /** 11070 * @param value {@link #profile} (Reference to the profile to be used for validation.) 11071 */ 11072 public TestScript addProfile(String value) { //1 11073 CanonicalType t = new CanonicalType(); 11074 t.setValue(value); 11075 if (this.profile == null) 11076 this.profile = new ArrayList<CanonicalType>(); 11077 this.profile.add(t); 11078 return this; 11079 } 11080 11081 /** 11082 * @param value {@link #profile} (Reference to the profile to be used for validation.) 11083 */ 11084 public boolean hasProfile(String value) { 11085 if (this.profile == null) 11086 return false; 11087 for (CanonicalType v : this.profile) 11088 if (v.getValue().equals(value)) // canonical 11089 return true; 11090 return false; 11091 } 11092 11093 /** 11094 * @return {@link #variable} (Variable is set based either on element value in response body or on header field value in the response headers.) 11095 */ 11096 public List<TestScriptVariableComponent> getVariable() { 11097 if (this.variable == null) 11098 this.variable = new ArrayList<TestScriptVariableComponent>(); 11099 return this.variable; 11100 } 11101 11102 /** 11103 * @return Returns a reference to <code>this</code> for easy method chaining 11104 */ 11105 public TestScript setVariable(List<TestScriptVariableComponent> theVariable) { 11106 this.variable = theVariable; 11107 return this; 11108 } 11109 11110 public boolean hasVariable() { 11111 if (this.variable == null) 11112 return false; 11113 for (TestScriptVariableComponent item : this.variable) 11114 if (!item.isEmpty()) 11115 return true; 11116 return false; 11117 } 11118 11119 public TestScriptVariableComponent addVariable() { //3 11120 TestScriptVariableComponent t = new TestScriptVariableComponent(); 11121 if (this.variable == null) 11122 this.variable = new ArrayList<TestScriptVariableComponent>(); 11123 this.variable.add(t); 11124 return t; 11125 } 11126 11127 public TestScript addVariable(TestScriptVariableComponent t) { //3 11128 if (t == null) 11129 return this; 11130 if (this.variable == null) 11131 this.variable = new ArrayList<TestScriptVariableComponent>(); 11132 this.variable.add(t); 11133 return this; 11134 } 11135 11136 /** 11137 * @return The first repetition of repeating field {@link #variable}, creating it if it does not already exist {3} 11138 */ 11139 public TestScriptVariableComponent getVariableFirstRep() { 11140 if (getVariable().isEmpty()) { 11141 addVariable(); 11142 } 11143 return getVariable().get(0); 11144 } 11145 11146 /** 11147 * @return {@link #setup} (A series of required setup operations before tests are executed.) 11148 */ 11149 public TestScriptSetupComponent getSetup() { 11150 if (this.setup == null) 11151 if (Configuration.errorOnAutoCreate()) 11152 throw new Error("Attempt to auto-create TestScript.setup"); 11153 else if (Configuration.doAutoCreate()) 11154 this.setup = new TestScriptSetupComponent(); // cc 11155 return this.setup; 11156 } 11157 11158 public boolean hasSetup() { 11159 return this.setup != null && !this.setup.isEmpty(); 11160 } 11161 11162 /** 11163 * @param value {@link #setup} (A series of required setup operations before tests are executed.) 11164 */ 11165 public TestScript setSetup(TestScriptSetupComponent value) { 11166 this.setup = value; 11167 return this; 11168 } 11169 11170 /** 11171 * @return {@link #test} (A test in this script.) 11172 */ 11173 public List<TestScriptTestComponent> getTest() { 11174 if (this.test == null) 11175 this.test = new ArrayList<TestScriptTestComponent>(); 11176 return this.test; 11177 } 11178 11179 /** 11180 * @return Returns a reference to <code>this</code> for easy method chaining 11181 */ 11182 public TestScript setTest(List<TestScriptTestComponent> theTest) { 11183 this.test = theTest; 11184 return this; 11185 } 11186 11187 public boolean hasTest() { 11188 if (this.test == null) 11189 return false; 11190 for (TestScriptTestComponent item : this.test) 11191 if (!item.isEmpty()) 11192 return true; 11193 return false; 11194 } 11195 11196 public TestScriptTestComponent addTest() { //3 11197 TestScriptTestComponent t = new TestScriptTestComponent(); 11198 if (this.test == null) 11199 this.test = new ArrayList<TestScriptTestComponent>(); 11200 this.test.add(t); 11201 return t; 11202 } 11203 11204 public TestScript addTest(TestScriptTestComponent t) { //3 11205 if (t == null) 11206 return this; 11207 if (this.test == null) 11208 this.test = new ArrayList<TestScriptTestComponent>(); 11209 this.test.add(t); 11210 return this; 11211 } 11212 11213 /** 11214 * @return The first repetition of repeating field {@link #test}, creating it if it does not already exist {3} 11215 */ 11216 public TestScriptTestComponent getTestFirstRep() { 11217 if (getTest().isEmpty()) { 11218 addTest(); 11219 } 11220 return getTest().get(0); 11221 } 11222 11223 /** 11224 * @return {@link #teardown} (A series of operations required to clean up after all the tests are executed (successfully or otherwise).) 11225 */ 11226 public TestScriptTeardownComponent getTeardown() { 11227 if (this.teardown == null) 11228 if (Configuration.errorOnAutoCreate()) 11229 throw new Error("Attempt to auto-create TestScript.teardown"); 11230 else if (Configuration.doAutoCreate()) 11231 this.teardown = new TestScriptTeardownComponent(); // cc 11232 return this.teardown; 11233 } 11234 11235 public boolean hasTeardown() { 11236 return this.teardown != null && !this.teardown.isEmpty(); 11237 } 11238 11239 /** 11240 * @param value {@link #teardown} (A series of operations required to clean up after all the tests are executed (successfully or otherwise).) 11241 */ 11242 public TestScript setTeardown(TestScriptTeardownComponent value) { 11243 this.teardown = value; 11244 return this; 11245 } 11246 11247 protected void listChildren(List<Property> children) { 11248 super.listChildren(children); 11249 children.add(new Property("url", "uri", "An absolute URI that is used to identify this test script when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this test script is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the test script is stored on different servers.", 0, 1, url)); 11250 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this test script when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 11251 children.add(new Property("version", "string", "The identifier that is used to identify this version of the test script when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test script author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 11252 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 11253 children.add(new Property("name", "string", "A natural language name identifying the test script. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 11254 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the test script.", 0, 1, title)); 11255 children.add(new Property("status", "code", "The status of this test script. Enables tracking the life-cycle of the content.", 0, 1, status)); 11256 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this test script is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 11257 children.add(new Property("date", "dateTime", "The date (and optionally time) when the test script was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test script changes.", 0, 1, date)); 11258 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the test script.", 0, 1, publisher)); 11259 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 11260 children.add(new Property("description", "markdown", "A free text natural language description of the test script from a consumer's perspective.", 0, 1, description)); 11261 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate test script instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 11262 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the test script is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 11263 children.add(new Property("purpose", "markdown", "Explanation of why this test script is needed and why it has been designed as it has.", 0, 1, purpose)); 11264 children.add(new Property("copyright", "markdown", "A copyright statement relating to the test script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test script.", 0, 1, copyright)); 11265 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 11266 children.add(new Property("origin", "", "An abstract server used in operations within this test script in the origin element.", 0, java.lang.Integer.MAX_VALUE, origin)); 11267 children.add(new Property("destination", "", "An abstract server used in operations within this test script in the destination element.", 0, java.lang.Integer.MAX_VALUE, destination)); 11268 children.add(new Property("metadata", "", "The required capability must exist and are assumed to function correctly on the FHIR server being tested.", 0, 1, metadata)); 11269 children.add(new Property("scope", "", "The scope indicates a conformance artifact that is tested by the test(s) within this test case and the expectation of the test outcome(s) as well as the intended test phase inclusion.", 0, java.lang.Integer.MAX_VALUE, scope)); 11270 children.add(new Property("fixture", "", "Fixture in the test script - by reference (uri). All fixtures are required for the test script to execute.", 0, java.lang.Integer.MAX_VALUE, fixture)); 11271 children.add(new Property("profile", "canonical(StructureDefinition)", "Reference to the profile to be used for validation.", 0, java.lang.Integer.MAX_VALUE, profile)); 11272 children.add(new Property("variable", "", "Variable is set based either on element value in response body or on header field value in the response headers.", 0, java.lang.Integer.MAX_VALUE, variable)); 11273 children.add(new Property("setup", "", "A series of required setup operations before tests are executed.", 0, 1, setup)); 11274 children.add(new Property("test", "", "A test in this script.", 0, java.lang.Integer.MAX_VALUE, test)); 11275 children.add(new Property("teardown", "", "A series of operations required to clean up after all the tests are executed (successfully or otherwise).", 0, 1, teardown)); 11276 } 11277 11278 @Override 11279 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 11280 switch (_hash) { 11281 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this test script when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this test script is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the test script is stored on different servers.", 0, 1, url); 11282 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this test script when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 11283 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the test script when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test script author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 11284 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 11285 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 11286 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 11287 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 11288 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the test script. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 11289 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the test script.", 0, 1, title); 11290 case -892481550: /*status*/ return new Property("status", "code", "The status of this test script. Enables tracking the life-cycle of the content.", 0, 1, status); 11291 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this test script is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 11292 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the test script was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test script changes.", 0, 1, date); 11293 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the test script.", 0, 1, publisher); 11294 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 11295 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the test script from a consumer's perspective.", 0, 1, description); 11296 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate test script instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 11297 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the test script is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 11298 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this test script is needed and why it has been designed as it has.", 0, 1, purpose); 11299 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the test script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test script.", 0, 1, copyright); 11300 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 11301 case -1008619738: /*origin*/ return new Property("origin", "", "An abstract server used in operations within this test script in the origin element.", 0, java.lang.Integer.MAX_VALUE, origin); 11302 case -1429847026: /*destination*/ return new Property("destination", "", "An abstract server used in operations within this test script in the destination element.", 0, java.lang.Integer.MAX_VALUE, destination); 11303 case -450004177: /*metadata*/ return new Property("metadata", "", "The required capability must exist and are assumed to function correctly on the FHIR server being tested.", 0, 1, metadata); 11304 case 109264468: /*scope*/ return new Property("scope", "", "The scope indicates a conformance artifact that is tested by the test(s) within this test case and the expectation of the test outcome(s) as well as the intended test phase inclusion.", 0, java.lang.Integer.MAX_VALUE, scope); 11305 case -843449847: /*fixture*/ return new Property("fixture", "", "Fixture in the test script - by reference (uri). All fixtures are required for the test script to execute.", 0, java.lang.Integer.MAX_VALUE, fixture); 11306 case -309425751: /*profile*/ return new Property("profile", "canonical(StructureDefinition)", "Reference to the profile to be used for validation.", 0, java.lang.Integer.MAX_VALUE, profile); 11307 case -1249586564: /*variable*/ return new Property("variable", "", "Variable is set based either on element value in response body or on header field value in the response headers.", 0, java.lang.Integer.MAX_VALUE, variable); 11308 case 109329021: /*setup*/ return new Property("setup", "", "A series of required setup operations before tests are executed.", 0, 1, setup); 11309 case 3556498: /*test*/ return new Property("test", "", "A test in this script.", 0, java.lang.Integer.MAX_VALUE, test); 11310 case -1663474172: /*teardown*/ return new Property("teardown", "", "A series of operations required to clean up after all the tests are executed (successfully or otherwise).", 0, 1, teardown); 11311 default: return super.getNamedProperty(_hash, _name, _checkValid); 11312 } 11313 11314 } 11315 11316 @Override 11317 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 11318 switch (hash) { 11319 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 11320 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 11321 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 11322 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 11323 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 11324 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 11325 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 11326 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 11327 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 11328 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 11329 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 11330 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 11331 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 11332 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 11333 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 11334 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 11335 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 11336 case -1008619738: /*origin*/ return this.origin == null ? new Base[0] : this.origin.toArray(new Base[this.origin.size()]); // TestScriptOriginComponent 11337 case -1429847026: /*destination*/ return this.destination == null ? new Base[0] : this.destination.toArray(new Base[this.destination.size()]); // TestScriptDestinationComponent 11338 case -450004177: /*metadata*/ return this.metadata == null ? new Base[0] : new Base[] {this.metadata}; // TestScriptMetadataComponent 11339 case 109264468: /*scope*/ return this.scope == null ? new Base[0] : this.scope.toArray(new Base[this.scope.size()]); // TestScriptScopeComponent 11340 case -843449847: /*fixture*/ return this.fixture == null ? new Base[0] : this.fixture.toArray(new Base[this.fixture.size()]); // TestScriptFixtureComponent 11341 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : this.profile.toArray(new Base[this.profile.size()]); // CanonicalType 11342 case -1249586564: /*variable*/ return this.variable == null ? new Base[0] : this.variable.toArray(new Base[this.variable.size()]); // TestScriptVariableComponent 11343 case 109329021: /*setup*/ return this.setup == null ? new Base[0] : new Base[] {this.setup}; // TestScriptSetupComponent 11344 case 3556498: /*test*/ return this.test == null ? new Base[0] : this.test.toArray(new Base[this.test.size()]); // TestScriptTestComponent 11345 case -1663474172: /*teardown*/ return this.teardown == null ? new Base[0] : new Base[] {this.teardown}; // TestScriptTeardownComponent 11346 default: return super.getProperty(hash, name, checkValid); 11347 } 11348 11349 } 11350 11351 @Override 11352 public Base setProperty(int hash, String name, Base value) throws FHIRException { 11353 switch (hash) { 11354 case 116079: // url 11355 this.url = TypeConvertor.castToUri(value); // UriType 11356 return value; 11357 case -1618432855: // identifier 11358 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 11359 return value; 11360 case 351608024: // version 11361 this.version = TypeConvertor.castToString(value); // StringType 11362 return value; 11363 case 1508158071: // versionAlgorithm 11364 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 11365 return value; 11366 case 3373707: // name 11367 this.name = TypeConvertor.castToString(value); // StringType 11368 return value; 11369 case 110371416: // title 11370 this.title = TypeConvertor.castToString(value); // StringType 11371 return value; 11372 case -892481550: // status 11373 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 11374 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 11375 return value; 11376 case -404562712: // experimental 11377 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 11378 return value; 11379 case 3076014: // date 11380 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 11381 return value; 11382 case 1447404028: // publisher 11383 this.publisher = TypeConvertor.castToString(value); // StringType 11384 return value; 11385 case 951526432: // contact 11386 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 11387 return value; 11388 case -1724546052: // description 11389 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 11390 return value; 11391 case -669707736: // useContext 11392 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 11393 return value; 11394 case -507075711: // jurisdiction 11395 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 11396 return value; 11397 case -220463842: // purpose 11398 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 11399 return value; 11400 case 1522889671: // copyright 11401 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 11402 return value; 11403 case 765157229: // copyrightLabel 11404 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 11405 return value; 11406 case -1008619738: // origin 11407 this.getOrigin().add((TestScriptOriginComponent) value); // TestScriptOriginComponent 11408 return value; 11409 case -1429847026: // destination 11410 this.getDestination().add((TestScriptDestinationComponent) value); // TestScriptDestinationComponent 11411 return value; 11412 case -450004177: // metadata 11413 this.metadata = (TestScriptMetadataComponent) value; // TestScriptMetadataComponent 11414 return value; 11415 case 109264468: // scope 11416 this.getScope().add((TestScriptScopeComponent) value); // TestScriptScopeComponent 11417 return value; 11418 case -843449847: // fixture 11419 this.getFixture().add((TestScriptFixtureComponent) value); // TestScriptFixtureComponent 11420 return value; 11421 case -309425751: // profile 11422 this.getProfile().add(TypeConvertor.castToCanonical(value)); // CanonicalType 11423 return value; 11424 case -1249586564: // variable 11425 this.getVariable().add((TestScriptVariableComponent) value); // TestScriptVariableComponent 11426 return value; 11427 case 109329021: // setup 11428 this.setup = (TestScriptSetupComponent) value; // TestScriptSetupComponent 11429 return value; 11430 case 3556498: // test 11431 this.getTest().add((TestScriptTestComponent) value); // TestScriptTestComponent 11432 return value; 11433 case -1663474172: // teardown 11434 this.teardown = (TestScriptTeardownComponent) value; // TestScriptTeardownComponent 11435 return value; 11436 default: return super.setProperty(hash, name, value); 11437 } 11438 11439 } 11440 11441 @Override 11442 public Base setProperty(String name, Base value) throws FHIRException { 11443 if (name.equals("url")) { 11444 this.url = TypeConvertor.castToUri(value); // UriType 11445 } else if (name.equals("identifier")) { 11446 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 11447 } else if (name.equals("version")) { 11448 this.version = TypeConvertor.castToString(value); // StringType 11449 } else if (name.equals("versionAlgorithm[x]")) { 11450 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 11451 } else if (name.equals("name")) { 11452 this.name = TypeConvertor.castToString(value); // StringType 11453 } else if (name.equals("title")) { 11454 this.title = TypeConvertor.castToString(value); // StringType 11455 } else if (name.equals("status")) { 11456 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 11457 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 11458 } else if (name.equals("experimental")) { 11459 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 11460 } else if (name.equals("date")) { 11461 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 11462 } else if (name.equals("publisher")) { 11463 this.publisher = TypeConvertor.castToString(value); // StringType 11464 } else if (name.equals("contact")) { 11465 this.getContact().add(TypeConvertor.castToContactDetail(value)); 11466 } else if (name.equals("description")) { 11467 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 11468 } else if (name.equals("useContext")) { 11469 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 11470 } else if (name.equals("jurisdiction")) { 11471 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 11472 } else if (name.equals("purpose")) { 11473 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 11474 } else if (name.equals("copyright")) { 11475 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 11476 } else if (name.equals("copyrightLabel")) { 11477 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 11478 } else if (name.equals("origin")) { 11479 this.getOrigin().add((TestScriptOriginComponent) value); 11480 } else if (name.equals("destination")) { 11481 this.getDestination().add((TestScriptDestinationComponent) value); 11482 } else if (name.equals("metadata")) { 11483 this.metadata = (TestScriptMetadataComponent) value; // TestScriptMetadataComponent 11484 } else if (name.equals("scope")) { 11485 this.getScope().add((TestScriptScopeComponent) value); 11486 } else if (name.equals("fixture")) { 11487 this.getFixture().add((TestScriptFixtureComponent) value); 11488 } else if (name.equals("profile")) { 11489 this.getProfile().add(TypeConvertor.castToCanonical(value)); 11490 } else if (name.equals("variable")) { 11491 this.getVariable().add((TestScriptVariableComponent) value); 11492 } else if (name.equals("setup")) { 11493 this.setup = (TestScriptSetupComponent) value; // TestScriptSetupComponent 11494 } else if (name.equals("test")) { 11495 this.getTest().add((TestScriptTestComponent) value); 11496 } else if (name.equals("teardown")) { 11497 this.teardown = (TestScriptTeardownComponent) value; // TestScriptTeardownComponent 11498 } else 11499 return super.setProperty(name, value); 11500 return value; 11501 } 11502 11503 @Override 11504 public void removeChild(String name, Base value) throws FHIRException { 11505 if (name.equals("url")) { 11506 this.url = null; 11507 } else if (name.equals("identifier")) { 11508 this.getIdentifier().remove(value); 11509 } else if (name.equals("version")) { 11510 this.version = null; 11511 } else if (name.equals("versionAlgorithm[x]")) { 11512 this.versionAlgorithm = null; 11513 } else if (name.equals("name")) { 11514 this.name = null; 11515 } else if (name.equals("title")) { 11516 this.title = null; 11517 } else if (name.equals("status")) { 11518 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 11519 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 11520 } else if (name.equals("experimental")) { 11521 this.experimental = null; 11522 } else if (name.equals("date")) { 11523 this.date = null; 11524 } else if (name.equals("publisher")) { 11525 this.publisher = null; 11526 } else if (name.equals("contact")) { 11527 this.getContact().remove(value); 11528 } else if (name.equals("description")) { 11529 this.description = null; 11530 } else if (name.equals("useContext")) { 11531 this.getUseContext().remove(value); 11532 } else if (name.equals("jurisdiction")) { 11533 this.getJurisdiction().remove(value); 11534 } else if (name.equals("purpose")) { 11535 this.purpose = null; 11536 } else if (name.equals("copyright")) { 11537 this.copyright = null; 11538 } else if (name.equals("copyrightLabel")) { 11539 this.copyrightLabel = null; 11540 } else if (name.equals("origin")) { 11541 this.getOrigin().remove((TestScriptOriginComponent) value); 11542 } else if (name.equals("destination")) { 11543 this.getDestination().remove((TestScriptDestinationComponent) value); 11544 } else if (name.equals("metadata")) { 11545 this.metadata = (TestScriptMetadataComponent) value; // TestScriptMetadataComponent 11546 } else if (name.equals("scope")) { 11547 this.getScope().remove((TestScriptScopeComponent) value); 11548 } else if (name.equals("fixture")) { 11549 this.getFixture().remove((TestScriptFixtureComponent) value); 11550 } else if (name.equals("profile")) { 11551 this.getProfile().remove(value); 11552 } else if (name.equals("variable")) { 11553 this.getVariable().remove((TestScriptVariableComponent) value); 11554 } else if (name.equals("setup")) { 11555 this.setup = (TestScriptSetupComponent) value; // TestScriptSetupComponent 11556 } else if (name.equals("test")) { 11557 this.getTest().remove((TestScriptTestComponent) value); 11558 } else if (name.equals("teardown")) { 11559 this.teardown = (TestScriptTeardownComponent) value; // TestScriptTeardownComponent 11560 } else 11561 super.removeChild(name, value); 11562 11563 } 11564 11565 @Override 11566 public Base makeProperty(int hash, String name) throws FHIRException { 11567 switch (hash) { 11568 case 116079: return getUrlElement(); 11569 case -1618432855: return addIdentifier(); 11570 case 351608024: return getVersionElement(); 11571 case -115699031: return getVersionAlgorithm(); 11572 case 1508158071: return getVersionAlgorithm(); 11573 case 3373707: return getNameElement(); 11574 case 110371416: return getTitleElement(); 11575 case -892481550: return getStatusElement(); 11576 case -404562712: return getExperimentalElement(); 11577 case 3076014: return getDateElement(); 11578 case 1447404028: return getPublisherElement(); 11579 case 951526432: return addContact(); 11580 case -1724546052: return getDescriptionElement(); 11581 case -669707736: return addUseContext(); 11582 case -507075711: return addJurisdiction(); 11583 case -220463842: return getPurposeElement(); 11584 case 1522889671: return getCopyrightElement(); 11585 case 765157229: return getCopyrightLabelElement(); 11586 case -1008619738: return addOrigin(); 11587 case -1429847026: return addDestination(); 11588 case -450004177: return getMetadata(); 11589 case 109264468: return addScope(); 11590 case -843449847: return addFixture(); 11591 case -309425751: return addProfileElement(); 11592 case -1249586564: return addVariable(); 11593 case 109329021: return getSetup(); 11594 case 3556498: return addTest(); 11595 case -1663474172: return getTeardown(); 11596 default: return super.makeProperty(hash, name); 11597 } 11598 11599 } 11600 11601 @Override 11602 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 11603 switch (hash) { 11604 case 116079: /*url*/ return new String[] {"uri"}; 11605 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 11606 case 351608024: /*version*/ return new String[] {"string"}; 11607 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 11608 case 3373707: /*name*/ return new String[] {"string"}; 11609 case 110371416: /*title*/ return new String[] {"string"}; 11610 case -892481550: /*status*/ return new String[] {"code"}; 11611 case -404562712: /*experimental*/ return new String[] {"boolean"}; 11612 case 3076014: /*date*/ return new String[] {"dateTime"}; 11613 case 1447404028: /*publisher*/ return new String[] {"string"}; 11614 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 11615 case -1724546052: /*description*/ return new String[] {"markdown"}; 11616 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 11617 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 11618 case -220463842: /*purpose*/ return new String[] {"markdown"}; 11619 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 11620 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 11621 case -1008619738: /*origin*/ return new String[] {}; 11622 case -1429847026: /*destination*/ return new String[] {}; 11623 case -450004177: /*metadata*/ return new String[] {}; 11624 case 109264468: /*scope*/ return new String[] {}; 11625 case -843449847: /*fixture*/ return new String[] {}; 11626 case -309425751: /*profile*/ return new String[] {"canonical"}; 11627 case -1249586564: /*variable*/ return new String[] {}; 11628 case 109329021: /*setup*/ return new String[] {}; 11629 case 3556498: /*test*/ return new String[] {}; 11630 case -1663474172: /*teardown*/ return new String[] {}; 11631 default: return super.getTypesForProperty(hash, name); 11632 } 11633 11634 } 11635 11636 @Override 11637 public Base addChild(String name) throws FHIRException { 11638 if (name.equals("url")) { 11639 throw new FHIRException("Cannot call addChild on a singleton property TestScript.url"); 11640 } 11641 else if (name.equals("identifier")) { 11642 return addIdentifier(); 11643 } 11644 else if (name.equals("version")) { 11645 throw new FHIRException("Cannot call addChild on a singleton property TestScript.version"); 11646 } 11647 else if (name.equals("versionAlgorithmString")) { 11648 this.versionAlgorithm = new StringType(); 11649 return this.versionAlgorithm; 11650 } 11651 else if (name.equals("versionAlgorithmCoding")) { 11652 this.versionAlgorithm = new Coding(); 11653 return this.versionAlgorithm; 11654 } 11655 else if (name.equals("name")) { 11656 throw new FHIRException("Cannot call addChild on a singleton property TestScript.name"); 11657 } 11658 else if (name.equals("title")) { 11659 throw new FHIRException("Cannot call addChild on a singleton property TestScript.title"); 11660 } 11661 else if (name.equals("status")) { 11662 throw new FHIRException("Cannot call addChild on a singleton property TestScript.status"); 11663 } 11664 else if (name.equals("experimental")) { 11665 throw new FHIRException("Cannot call addChild on a singleton property TestScript.experimental"); 11666 } 11667 else if (name.equals("date")) { 11668 throw new FHIRException("Cannot call addChild on a singleton property TestScript.date"); 11669 } 11670 else if (name.equals("publisher")) { 11671 throw new FHIRException("Cannot call addChild on a singleton property TestScript.publisher"); 11672 } 11673 else if (name.equals("contact")) { 11674 return addContact(); 11675 } 11676 else if (name.equals("description")) { 11677 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 11678 } 11679 else if (name.equals("useContext")) { 11680 return addUseContext(); 11681 } 11682 else if (name.equals("jurisdiction")) { 11683 return addJurisdiction(); 11684 } 11685 else if (name.equals("purpose")) { 11686 throw new FHIRException("Cannot call addChild on a singleton property TestScript.purpose"); 11687 } 11688 else if (name.equals("copyright")) { 11689 throw new FHIRException("Cannot call addChild on a singleton property TestScript.copyright"); 11690 } 11691 else if (name.equals("copyrightLabel")) { 11692 throw new FHIRException("Cannot call addChild on a singleton property TestScript.copyrightLabel"); 11693 } 11694 else if (name.equals("origin")) { 11695 return addOrigin(); 11696 } 11697 else if (name.equals("destination")) { 11698 return addDestination(); 11699 } 11700 else if (name.equals("metadata")) { 11701 this.metadata = new TestScriptMetadataComponent(); 11702 return this.metadata; 11703 } 11704 else if (name.equals("scope")) { 11705 return addScope(); 11706 } 11707 else if (name.equals("fixture")) { 11708 return addFixture(); 11709 } 11710 else if (name.equals("profile")) { 11711 throw new FHIRException("Cannot call addChild on a singleton property TestScript.profile"); 11712 } 11713 else if (name.equals("variable")) { 11714 return addVariable(); 11715 } 11716 else if (name.equals("setup")) { 11717 this.setup = new TestScriptSetupComponent(); 11718 return this.setup; 11719 } 11720 else if (name.equals("test")) { 11721 return addTest(); 11722 } 11723 else if (name.equals("teardown")) { 11724 this.teardown = new TestScriptTeardownComponent(); 11725 return this.teardown; 11726 } 11727 else 11728 return super.addChild(name); 11729 } 11730 11731 public String fhirType() { 11732 return "TestScript"; 11733 11734 } 11735 11736 public TestScript copy() { 11737 TestScript dst = new TestScript(); 11738 copyValues(dst); 11739 return dst; 11740 } 11741 11742 public void copyValues(TestScript dst) { 11743 super.copyValues(dst); 11744 dst.url = url == null ? null : url.copy(); 11745 if (identifier != null) { 11746 dst.identifier = new ArrayList<Identifier>(); 11747 for (Identifier i : identifier) 11748 dst.identifier.add(i.copy()); 11749 }; 11750 dst.version = version == null ? null : version.copy(); 11751 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 11752 dst.name = name == null ? null : name.copy(); 11753 dst.title = title == null ? null : title.copy(); 11754 dst.status = status == null ? null : status.copy(); 11755 dst.experimental = experimental == null ? null : experimental.copy(); 11756 dst.date = date == null ? null : date.copy(); 11757 dst.publisher = publisher == null ? null : publisher.copy(); 11758 if (contact != null) { 11759 dst.contact = new ArrayList<ContactDetail>(); 11760 for (ContactDetail i : contact) 11761 dst.contact.add(i.copy()); 11762 }; 11763 dst.description = description == null ? null : description.copy(); 11764 if (useContext != null) { 11765 dst.useContext = new ArrayList<UsageContext>(); 11766 for (UsageContext i : useContext) 11767 dst.useContext.add(i.copy()); 11768 }; 11769 if (jurisdiction != null) { 11770 dst.jurisdiction = new ArrayList<CodeableConcept>(); 11771 for (CodeableConcept i : jurisdiction) 11772 dst.jurisdiction.add(i.copy()); 11773 }; 11774 dst.purpose = purpose == null ? null : purpose.copy(); 11775 dst.copyright = copyright == null ? null : copyright.copy(); 11776 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 11777 if (origin != null) { 11778 dst.origin = new ArrayList<TestScriptOriginComponent>(); 11779 for (TestScriptOriginComponent i : origin) 11780 dst.origin.add(i.copy()); 11781 }; 11782 if (destination != null) { 11783 dst.destination = new ArrayList<TestScriptDestinationComponent>(); 11784 for (TestScriptDestinationComponent i : destination) 11785 dst.destination.add(i.copy()); 11786 }; 11787 dst.metadata = metadata == null ? null : metadata.copy(); 11788 if (scope != null) { 11789 dst.scope = new ArrayList<TestScriptScopeComponent>(); 11790 for (TestScriptScopeComponent i : scope) 11791 dst.scope.add(i.copy()); 11792 }; 11793 if (fixture != null) { 11794 dst.fixture = new ArrayList<TestScriptFixtureComponent>(); 11795 for (TestScriptFixtureComponent i : fixture) 11796 dst.fixture.add(i.copy()); 11797 }; 11798 if (profile != null) { 11799 dst.profile = new ArrayList<CanonicalType>(); 11800 for (CanonicalType i : profile) 11801 dst.profile.add(i.copy()); 11802 }; 11803 if (variable != null) { 11804 dst.variable = new ArrayList<TestScriptVariableComponent>(); 11805 for (TestScriptVariableComponent i : variable) 11806 dst.variable.add(i.copy()); 11807 }; 11808 dst.setup = setup == null ? null : setup.copy(); 11809 if (test != null) { 11810 dst.test = new ArrayList<TestScriptTestComponent>(); 11811 for (TestScriptTestComponent i : test) 11812 dst.test.add(i.copy()); 11813 }; 11814 dst.teardown = teardown == null ? null : teardown.copy(); 11815 } 11816 11817 protected TestScript typedCopy() { 11818 return copy(); 11819 } 11820 11821 @Override 11822 public boolean equalsDeep(Base other_) { 11823 if (!super.equalsDeep(other_)) 11824 return false; 11825 if (!(other_ instanceof TestScript)) 11826 return false; 11827 TestScript o = (TestScript) other_; 11828 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 11829 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 11830 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 11831 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 11832 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 11833 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 11834 && compareDeep(origin, o.origin, true) && compareDeep(destination, o.destination, true) && compareDeep(metadata, o.metadata, true) 11835 && compareDeep(scope, o.scope, true) && compareDeep(fixture, o.fixture, true) && compareDeep(profile, o.profile, true) 11836 && compareDeep(variable, o.variable, true) && compareDeep(setup, o.setup, true) && compareDeep(test, o.test, true) 11837 && compareDeep(teardown, o.teardown, true); 11838 } 11839 11840 @Override 11841 public boolean equalsShallow(Base other_) { 11842 if (!super.equalsShallow(other_)) 11843 return false; 11844 if (!(other_ instanceof TestScript)) 11845 return false; 11846 TestScript o = (TestScript) other_; 11847 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 11848 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 11849 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 11850 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 11851 && compareValues(profile, o.profile, true); 11852 } 11853 11854 public boolean isEmpty() { 11855 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 11856 , versionAlgorithm, name, title, status, experimental, date, publisher, contact 11857 , description, useContext, jurisdiction, purpose, copyright, copyrightLabel, origin 11858 , destination, metadata, scope, fixture, profile, variable, setup, test, teardown 11859 ); 11860 } 11861 11862 @Override 11863 public ResourceType getResourceType() { 11864 return ResourceType.TestScript; 11865 } 11866 11867 /** 11868 * Search parameter: <b>context-quantity</b> 11869 * <p> 11870 * Description: <b>Multiple Resources: 11871 11872* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 11873* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 11874* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 11875* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 11876* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 11877* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 11878* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 11879* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 11880* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 11881* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 11882* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 11883* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 11884* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 11885* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 11886* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 11887* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 11888* [Library](library.html): A quantity- or range-valued use context assigned to the library 11889* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 11890* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 11891* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 11892* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 11893* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 11894* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 11895* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 11896* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 11897* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 11898* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 11899* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 11900* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 11901* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 11902</b><br> 11903 * Type: <b>quantity</b><br> 11904 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 11905 * </p> 11906 */ 11907 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 11908 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 11909 /** 11910 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 11911 * <p> 11912 * Description: <b>Multiple Resources: 11913 11914* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 11915* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 11916* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 11917* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 11918* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 11919* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 11920* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 11921* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 11922* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 11923* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 11924* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 11925* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 11926* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 11927* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 11928* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 11929* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 11930* [Library](library.html): A quantity- or range-valued use context assigned to the library 11931* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 11932* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 11933* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 11934* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 11935* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 11936* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 11937* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 11938* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 11939* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 11940* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 11941* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 11942* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 11943* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 11944</b><br> 11945 * Type: <b>quantity</b><br> 11946 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 11947 * </p> 11948 */ 11949 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 11950 11951 /** 11952 * Search parameter: <b>context-type-quantity</b> 11953 * <p> 11954 * Description: <b>Multiple Resources: 11955 11956* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 11957* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 11958* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 11959* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 11960* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 11961* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 11962* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 11963* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 11964* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 11965* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 11966* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 11967* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 11968* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 11969* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 11970* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 11971* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 11972* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 11973* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 11974* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 11975* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 11976* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 11977* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 11978* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 11979* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 11980* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 11981* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 11982* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 11983* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 11984* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 11985* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 11986</b><br> 11987 * Type: <b>composite</b><br> 11988 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 11989 * </p> 11990 */ 11991 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 11992 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 11993 /** 11994 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 11995 * <p> 11996 * Description: <b>Multiple Resources: 11997 11998* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 11999* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 12000* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 12001* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 12002* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 12003* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 12004* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 12005* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 12006* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 12007* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 12008* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 12009* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 12010* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 12011* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 12012* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 12013* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 12014* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 12015* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 12016* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 12017* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 12018* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 12019* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 12020* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 12021* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 12022* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 12023* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 12024* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 12025* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 12026* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 12027* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 12028</b><br> 12029 * Type: <b>composite</b><br> 12030 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 12031 * </p> 12032 */ 12033 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 12034 12035 /** 12036 * Search parameter: <b>context-type-value</b> 12037 * <p> 12038 * Description: <b>Multiple Resources: 12039 12040* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 12041* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 12042* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 12043* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 12044* [Citation](citation.html): A use context type and value assigned to the citation 12045* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 12046* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 12047* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 12048* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 12049* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 12050* [Evidence](evidence.html): A use context type and value assigned to the evidence 12051* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 12052* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 12053* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 12054* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 12055* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 12056* [Library](library.html): A use context type and value assigned to the library 12057* [Measure](measure.html): A use context type and value assigned to the measure 12058* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 12059* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 12060* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 12061* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 12062* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 12063* [Requirements](requirements.html): A use context type and value assigned to the requirements 12064* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 12065* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 12066* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 12067* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 12068* [TestScript](testscript.html): A use context type and value assigned to the test script 12069* [ValueSet](valueset.html): A use context type and value assigned to the value set 12070</b><br> 12071 * Type: <b>composite</b><br> 12072 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 12073 * </p> 12074 */ 12075 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 12076 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 12077 /** 12078 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 12079 * <p> 12080 * Description: <b>Multiple Resources: 12081 12082* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 12083* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 12084* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 12085* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 12086* [Citation](citation.html): A use context type and value assigned to the citation 12087* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 12088* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 12089* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 12090* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 12091* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 12092* [Evidence](evidence.html): A use context type and value assigned to the evidence 12093* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 12094* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 12095* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 12096* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 12097* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 12098* [Library](library.html): A use context type and value assigned to the library 12099* [Measure](measure.html): A use context type and value assigned to the measure 12100* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 12101* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 12102* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 12103* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 12104* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 12105* [Requirements](requirements.html): A use context type and value assigned to the requirements 12106* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 12107* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 12108* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 12109* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 12110* [TestScript](testscript.html): A use context type and value assigned to the test script 12111* [ValueSet](valueset.html): A use context type and value assigned to the value set 12112</b><br> 12113 * Type: <b>composite</b><br> 12114 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 12115 * </p> 12116 */ 12117 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 12118 12119 /** 12120 * Search parameter: <b>context-type</b> 12121 * <p> 12122 * Description: <b>Multiple Resources: 12123 12124* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 12125* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 12126* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 12127* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 12128* [Citation](citation.html): A type of use context assigned to the citation 12129* [CodeSystem](codesystem.html): A type of use context assigned to the code system 12130* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 12131* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 12132* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 12133* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 12134* [Evidence](evidence.html): A type of use context assigned to the evidence 12135* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 12136* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 12137* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 12138* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 12139* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 12140* [Library](library.html): A type of use context assigned to the library 12141* [Measure](measure.html): A type of use context assigned to the measure 12142* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 12143* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 12144* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 12145* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 12146* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 12147* [Requirements](requirements.html): A type of use context assigned to the requirements 12148* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 12149* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 12150* [StructureMap](structuremap.html): A type of use context assigned to the structure map 12151* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 12152* [TestScript](testscript.html): A type of use context assigned to the test script 12153* [ValueSet](valueset.html): A type of use context assigned to the value set 12154</b><br> 12155 * Type: <b>token</b><br> 12156 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 12157 * </p> 12158 */ 12159 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 12160 public static final String SP_CONTEXT_TYPE = "context-type"; 12161 /** 12162 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 12163 * <p> 12164 * Description: <b>Multiple Resources: 12165 12166* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 12167* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 12168* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 12169* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 12170* [Citation](citation.html): A type of use context assigned to the citation 12171* [CodeSystem](codesystem.html): A type of use context assigned to the code system 12172* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 12173* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 12174* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 12175* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 12176* [Evidence](evidence.html): A type of use context assigned to the evidence 12177* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 12178* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 12179* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 12180* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 12181* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 12182* [Library](library.html): A type of use context assigned to the library 12183* [Measure](measure.html): A type of use context assigned to the measure 12184* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 12185* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 12186* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 12187* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 12188* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 12189* [Requirements](requirements.html): A type of use context assigned to the requirements 12190* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 12191* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 12192* [StructureMap](structuremap.html): A type of use context assigned to the structure map 12193* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 12194* [TestScript](testscript.html): A type of use context assigned to the test script 12195* [ValueSet](valueset.html): A type of use context assigned to the value set 12196</b><br> 12197 * Type: <b>token</b><br> 12198 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 12199 * </p> 12200 */ 12201 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 12202 12203 /** 12204 * Search parameter: <b>context</b> 12205 * <p> 12206 * Description: <b>Multiple Resources: 12207 12208* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 12209* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 12210* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 12211* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 12212* [Citation](citation.html): A use context assigned to the citation 12213* [CodeSystem](codesystem.html): A use context assigned to the code system 12214* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 12215* [ConceptMap](conceptmap.html): A use context assigned to the concept map 12216* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 12217* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 12218* [Evidence](evidence.html): A use context assigned to the evidence 12219* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 12220* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 12221* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 12222* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 12223* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 12224* [Library](library.html): A use context assigned to the library 12225* [Measure](measure.html): A use context assigned to the measure 12226* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 12227* [NamingSystem](namingsystem.html): A use context assigned to the naming system 12228* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 12229* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 12230* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 12231* [Requirements](requirements.html): A use context assigned to the requirements 12232* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 12233* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 12234* [StructureMap](structuremap.html): A use context assigned to the structure map 12235* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 12236* [TestScript](testscript.html): A use context assigned to the test script 12237* [ValueSet](valueset.html): A use context assigned to the value set 12238</b><br> 12239 * Type: <b>token</b><br> 12240 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 12241 * </p> 12242 */ 12243 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 12244 public static final String SP_CONTEXT = "context"; 12245 /** 12246 * <b>Fluent Client</b> search parameter constant for <b>context</b> 12247 * <p> 12248 * Description: <b>Multiple Resources: 12249 12250* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 12251* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 12252* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 12253* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 12254* [Citation](citation.html): A use context assigned to the citation 12255* [CodeSystem](codesystem.html): A use context assigned to the code system 12256* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 12257* [ConceptMap](conceptmap.html): A use context assigned to the concept map 12258* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 12259* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 12260* [Evidence](evidence.html): A use context assigned to the evidence 12261* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 12262* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 12263* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 12264* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 12265* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 12266* [Library](library.html): A use context assigned to the library 12267* [Measure](measure.html): A use context assigned to the measure 12268* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 12269* [NamingSystem](namingsystem.html): A use context assigned to the naming system 12270* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 12271* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 12272* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 12273* [Requirements](requirements.html): A use context assigned to the requirements 12274* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 12275* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 12276* [StructureMap](structuremap.html): A use context assigned to the structure map 12277* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 12278* [TestScript](testscript.html): A use context assigned to the test script 12279* [ValueSet](valueset.html): A use context assigned to the value set 12280</b><br> 12281 * Type: <b>token</b><br> 12282 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 12283 * </p> 12284 */ 12285 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 12286 12287 /** 12288 * Search parameter: <b>date</b> 12289 * <p> 12290 * Description: <b>Multiple Resources: 12291 12292* [ActivityDefinition](activitydefinition.html): The activity definition publication date 12293* [ActorDefinition](actordefinition.html): The Actor Definition publication date 12294* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 12295* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 12296* [Citation](citation.html): The citation publication date 12297* [CodeSystem](codesystem.html): The code system publication date 12298* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 12299* [ConceptMap](conceptmap.html): The concept map publication date 12300* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 12301* [EventDefinition](eventdefinition.html): The event definition publication date 12302* [Evidence](evidence.html): The evidence publication date 12303* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 12304* [ExampleScenario](examplescenario.html): The example scenario publication date 12305* [GraphDefinition](graphdefinition.html): The graph definition publication date 12306* [ImplementationGuide](implementationguide.html): The implementation guide publication date 12307* [Library](library.html): The library publication date 12308* [Measure](measure.html): The measure publication date 12309* [MessageDefinition](messagedefinition.html): The message definition publication date 12310* [NamingSystem](namingsystem.html): The naming system publication date 12311* [OperationDefinition](operationdefinition.html): The operation definition publication date 12312* [PlanDefinition](plandefinition.html): The plan definition publication date 12313* [Questionnaire](questionnaire.html): The questionnaire publication date 12314* [Requirements](requirements.html): The requirements publication date 12315* [SearchParameter](searchparameter.html): The search parameter publication date 12316* [StructureDefinition](structuredefinition.html): The structure definition publication date 12317* [StructureMap](structuremap.html): The structure map publication date 12318* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 12319* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 12320* [TestScript](testscript.html): The test script publication date 12321* [ValueSet](valueset.html): The value set publication date 12322</b><br> 12323 * Type: <b>date</b><br> 12324 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 12325 * </p> 12326 */ 12327 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 12328 public static final String SP_DATE = "date"; 12329 /** 12330 * <b>Fluent Client</b> search parameter constant for <b>date</b> 12331 * <p> 12332 * Description: <b>Multiple Resources: 12333 12334* [ActivityDefinition](activitydefinition.html): The activity definition publication date 12335* [ActorDefinition](actordefinition.html): The Actor Definition publication date 12336* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 12337* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 12338* [Citation](citation.html): The citation publication date 12339* [CodeSystem](codesystem.html): The code system publication date 12340* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 12341* [ConceptMap](conceptmap.html): The concept map publication date 12342* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 12343* [EventDefinition](eventdefinition.html): The event definition publication date 12344* [Evidence](evidence.html): The evidence publication date 12345* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 12346* [ExampleScenario](examplescenario.html): The example scenario publication date 12347* [GraphDefinition](graphdefinition.html): The graph definition publication date 12348* [ImplementationGuide](implementationguide.html): The implementation guide publication date 12349* [Library](library.html): The library publication date 12350* [Measure](measure.html): The measure publication date 12351* [MessageDefinition](messagedefinition.html): The message definition publication date 12352* [NamingSystem](namingsystem.html): The naming system publication date 12353* [OperationDefinition](operationdefinition.html): The operation definition publication date 12354* [PlanDefinition](plandefinition.html): The plan definition publication date 12355* [Questionnaire](questionnaire.html): The questionnaire publication date 12356* [Requirements](requirements.html): The requirements publication date 12357* [SearchParameter](searchparameter.html): The search parameter publication date 12358* [StructureDefinition](structuredefinition.html): The structure definition publication date 12359* [StructureMap](structuremap.html): The structure map publication date 12360* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 12361* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 12362* [TestScript](testscript.html): The test script publication date 12363* [ValueSet](valueset.html): The value set publication date 12364</b><br> 12365 * Type: <b>date</b><br> 12366 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 12367 * </p> 12368 */ 12369 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 12370 12371 /** 12372 * Search parameter: <b>description</b> 12373 * <p> 12374 * Description: <b>Multiple Resources: 12375 12376* [ActivityDefinition](activitydefinition.html): The description of the activity definition 12377* [ActorDefinition](actordefinition.html): The description of the Actor Definition 12378* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 12379* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 12380* [Citation](citation.html): The description of the citation 12381* [CodeSystem](codesystem.html): The description of the code system 12382* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 12383* [ConceptMap](conceptmap.html): The description of the concept map 12384* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 12385* [EventDefinition](eventdefinition.html): The description of the event definition 12386* [Evidence](evidence.html): The description of the evidence 12387* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 12388* [GraphDefinition](graphdefinition.html): The description of the graph definition 12389* [ImplementationGuide](implementationguide.html): The description of the implementation guide 12390* [Library](library.html): The description of the library 12391* [Measure](measure.html): The description of the measure 12392* [MessageDefinition](messagedefinition.html): The description of the message definition 12393* [NamingSystem](namingsystem.html): The description of the naming system 12394* [OperationDefinition](operationdefinition.html): The description of the operation definition 12395* [PlanDefinition](plandefinition.html): The description of the plan definition 12396* [Questionnaire](questionnaire.html): The description of the questionnaire 12397* [Requirements](requirements.html): The description of the requirements 12398* [SearchParameter](searchparameter.html): The description of the search parameter 12399* [StructureDefinition](structuredefinition.html): The description of the structure definition 12400* [StructureMap](structuremap.html): The description of the structure map 12401* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 12402* [TestScript](testscript.html): The description of the test script 12403* [ValueSet](valueset.html): The description of the value set 12404</b><br> 12405 * Type: <b>string</b><br> 12406 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 12407 * </p> 12408 */ 12409 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 12410 public static final String SP_DESCRIPTION = "description"; 12411 /** 12412 * <b>Fluent Client</b> search parameter constant for <b>description</b> 12413 * <p> 12414 * Description: <b>Multiple Resources: 12415 12416* [ActivityDefinition](activitydefinition.html): The description of the activity definition 12417* [ActorDefinition](actordefinition.html): The description of the Actor Definition 12418* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 12419* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 12420* [Citation](citation.html): The description of the citation 12421* [CodeSystem](codesystem.html): The description of the code system 12422* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 12423* [ConceptMap](conceptmap.html): The description of the concept map 12424* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 12425* [EventDefinition](eventdefinition.html): The description of the event definition 12426* [Evidence](evidence.html): The description of the evidence 12427* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 12428* [GraphDefinition](graphdefinition.html): The description of the graph definition 12429* [ImplementationGuide](implementationguide.html): The description of the implementation guide 12430* [Library](library.html): The description of the library 12431* [Measure](measure.html): The description of the measure 12432* [MessageDefinition](messagedefinition.html): The description of the message definition 12433* [NamingSystem](namingsystem.html): The description of the naming system 12434* [OperationDefinition](operationdefinition.html): The description of the operation definition 12435* [PlanDefinition](plandefinition.html): The description of the plan definition 12436* [Questionnaire](questionnaire.html): The description of the questionnaire 12437* [Requirements](requirements.html): The description of the requirements 12438* [SearchParameter](searchparameter.html): The description of the search parameter 12439* [StructureDefinition](structuredefinition.html): The description of the structure definition 12440* [StructureMap](structuremap.html): The description of the structure map 12441* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 12442* [TestScript](testscript.html): The description of the test script 12443* [ValueSet](valueset.html): The description of the value set 12444</b><br> 12445 * Type: <b>string</b><br> 12446 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 12447 * </p> 12448 */ 12449 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 12450 12451 /** 12452 * Search parameter: <b>identifier</b> 12453 * <p> 12454 * Description: <b>Multiple Resources: 12455 12456* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 12457* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 12458* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 12459* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 12460* [Citation](citation.html): External identifier for the citation 12461* [CodeSystem](codesystem.html): External identifier for the code system 12462* [ConceptMap](conceptmap.html): External identifier for the concept map 12463* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 12464* [EventDefinition](eventdefinition.html): External identifier for the event definition 12465* [Evidence](evidence.html): External identifier for the evidence 12466* [EvidenceReport](evidencereport.html): External identifier for the evidence report 12467* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 12468* [ExampleScenario](examplescenario.html): External identifier for the example scenario 12469* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 12470* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 12471* [Library](library.html): External identifier for the library 12472* [Measure](measure.html): External identifier for the measure 12473* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 12474* [MessageDefinition](messagedefinition.html): External identifier for the message definition 12475* [NamingSystem](namingsystem.html): External identifier for the naming system 12476* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 12477* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 12478* [PlanDefinition](plandefinition.html): External identifier for the plan definition 12479* [Questionnaire](questionnaire.html): External identifier for the questionnaire 12480* [Requirements](requirements.html): External identifier for the requirements 12481* [SearchParameter](searchparameter.html): External identifier for the search parameter 12482* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 12483* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 12484* [StructureMap](structuremap.html): External identifier for the structure map 12485* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 12486* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 12487* [TestPlan](testplan.html): An identifier for the test plan 12488* [TestScript](testscript.html): External identifier for the test script 12489* [ValueSet](valueset.html): External identifier for the value set 12490</b><br> 12491 * Type: <b>token</b><br> 12492 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 12493 * </p> 12494 */ 12495 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 12496 public static final String SP_IDENTIFIER = "identifier"; 12497 /** 12498 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 12499 * <p> 12500 * Description: <b>Multiple Resources: 12501 12502* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 12503* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 12504* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 12505* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 12506* [Citation](citation.html): External identifier for the citation 12507* [CodeSystem](codesystem.html): External identifier for the code system 12508* [ConceptMap](conceptmap.html): External identifier for the concept map 12509* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 12510* [EventDefinition](eventdefinition.html): External identifier for the event definition 12511* [Evidence](evidence.html): External identifier for the evidence 12512* [EvidenceReport](evidencereport.html): External identifier for the evidence report 12513* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 12514* [ExampleScenario](examplescenario.html): External identifier for the example scenario 12515* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 12516* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 12517* [Library](library.html): External identifier for the library 12518* [Measure](measure.html): External identifier for the measure 12519* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 12520* [MessageDefinition](messagedefinition.html): External identifier for the message definition 12521* [NamingSystem](namingsystem.html): External identifier for the naming system 12522* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 12523* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 12524* [PlanDefinition](plandefinition.html): External identifier for the plan definition 12525* [Questionnaire](questionnaire.html): External identifier for the questionnaire 12526* [Requirements](requirements.html): External identifier for the requirements 12527* [SearchParameter](searchparameter.html): External identifier for the search parameter 12528* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 12529* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 12530* [StructureMap](structuremap.html): External identifier for the structure map 12531* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 12532* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 12533* [TestPlan](testplan.html): An identifier for the test plan 12534* [TestScript](testscript.html): External identifier for the test script 12535* [ValueSet](valueset.html): External identifier for the value set 12536</b><br> 12537 * Type: <b>token</b><br> 12538 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 12539 * </p> 12540 */ 12541 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 12542 12543 /** 12544 * Search parameter: <b>jurisdiction</b> 12545 * <p> 12546 * Description: <b>Multiple Resources: 12547 12548* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 12549* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 12550* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 12551* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 12552* [Citation](citation.html): Intended jurisdiction for the citation 12553* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 12554* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 12555* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 12556* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 12557* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 12558* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 12559* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 12560* [Library](library.html): Intended jurisdiction for the library 12561* [Measure](measure.html): Intended jurisdiction for the measure 12562* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 12563* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 12564* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 12565* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 12566* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 12567* [Requirements](requirements.html): Intended jurisdiction for the requirements 12568* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 12569* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 12570* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 12571* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 12572* [TestScript](testscript.html): Intended jurisdiction for the test script 12573* [ValueSet](valueset.html): Intended jurisdiction for the value set 12574</b><br> 12575 * Type: <b>token</b><br> 12576 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 12577 * </p> 12578 */ 12579 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 12580 public static final String SP_JURISDICTION = "jurisdiction"; 12581 /** 12582 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 12583 * <p> 12584 * Description: <b>Multiple Resources: 12585 12586* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 12587* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 12588* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 12589* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 12590* [Citation](citation.html): Intended jurisdiction for the citation 12591* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 12592* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 12593* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 12594* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 12595* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 12596* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 12597* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 12598* [Library](library.html): Intended jurisdiction for the library 12599* [Measure](measure.html): Intended jurisdiction for the measure 12600* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 12601* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 12602* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 12603* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 12604* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 12605* [Requirements](requirements.html): Intended jurisdiction for the requirements 12606* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 12607* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 12608* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 12609* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 12610* [TestScript](testscript.html): Intended jurisdiction for the test script 12611* [ValueSet](valueset.html): Intended jurisdiction for the value set 12612</b><br> 12613 * Type: <b>token</b><br> 12614 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 12615 * </p> 12616 */ 12617 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 12618 12619 /** 12620 * Search parameter: <b>name</b> 12621 * <p> 12622 * Description: <b>Multiple Resources: 12623 12624* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 12625* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 12626* [Citation](citation.html): Computationally friendly name of the citation 12627* [CodeSystem](codesystem.html): Computationally friendly name of the code system 12628* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 12629* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 12630* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 12631* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 12632* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 12633* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 12634* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 12635* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 12636* [Library](library.html): Computationally friendly name of the library 12637* [Measure](measure.html): Computationally friendly name of the measure 12638* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 12639* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 12640* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 12641* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 12642* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 12643* [Requirements](requirements.html): Computationally friendly name of the requirements 12644* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 12645* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 12646* [StructureMap](structuremap.html): Computationally friendly name of the structure map 12647* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 12648* [TestScript](testscript.html): Computationally friendly name of the test script 12649* [ValueSet](valueset.html): Computationally friendly name of the value set 12650</b><br> 12651 * Type: <b>string</b><br> 12652 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 12653 * </p> 12654 */ 12655 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 12656 public static final String SP_NAME = "name"; 12657 /** 12658 * <b>Fluent Client</b> search parameter constant for <b>name</b> 12659 * <p> 12660 * Description: <b>Multiple Resources: 12661 12662* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 12663* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 12664* [Citation](citation.html): Computationally friendly name of the citation 12665* [CodeSystem](codesystem.html): Computationally friendly name of the code system 12666* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 12667* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 12668* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 12669* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 12670* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 12671* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 12672* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 12673* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 12674* [Library](library.html): Computationally friendly name of the library 12675* [Measure](measure.html): Computationally friendly name of the measure 12676* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 12677* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 12678* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 12679* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 12680* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 12681* [Requirements](requirements.html): Computationally friendly name of the requirements 12682* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 12683* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 12684* [StructureMap](structuremap.html): Computationally friendly name of the structure map 12685* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 12686* [TestScript](testscript.html): Computationally friendly name of the test script 12687* [ValueSet](valueset.html): Computationally friendly name of the value set 12688</b><br> 12689 * Type: <b>string</b><br> 12690 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 12691 * </p> 12692 */ 12693 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 12694 12695 /** 12696 * Search parameter: <b>publisher</b> 12697 * <p> 12698 * Description: <b>Multiple Resources: 12699 12700* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 12701* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 12702* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 12703* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 12704* [Citation](citation.html): Name of the publisher of the citation 12705* [CodeSystem](codesystem.html): Name of the publisher of the code system 12706* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 12707* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 12708* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 12709* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 12710* [Evidence](evidence.html): Name of the publisher of the evidence 12711* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 12712* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 12713* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 12714* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 12715* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 12716* [Library](library.html): Name of the publisher of the library 12717* [Measure](measure.html): Name of the publisher of the measure 12718* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 12719* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 12720* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 12721* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 12722* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 12723* [Requirements](requirements.html): Name of the publisher of the requirements 12724* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 12725* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 12726* [StructureMap](structuremap.html): Name of the publisher of the structure map 12727* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 12728* [TestScript](testscript.html): Name of the publisher of the test script 12729* [ValueSet](valueset.html): Name of the publisher of the value set 12730</b><br> 12731 * Type: <b>string</b><br> 12732 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 12733 * </p> 12734 */ 12735 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 12736 public static final String SP_PUBLISHER = "publisher"; 12737 /** 12738 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 12739 * <p> 12740 * Description: <b>Multiple Resources: 12741 12742* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 12743* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 12744* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 12745* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 12746* [Citation](citation.html): Name of the publisher of the citation 12747* [CodeSystem](codesystem.html): Name of the publisher of the code system 12748* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 12749* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 12750* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 12751* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 12752* [Evidence](evidence.html): Name of the publisher of the evidence 12753* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 12754* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 12755* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 12756* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 12757* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 12758* [Library](library.html): Name of the publisher of the library 12759* [Measure](measure.html): Name of the publisher of the measure 12760* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 12761* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 12762* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 12763* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 12764* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 12765* [Requirements](requirements.html): Name of the publisher of the requirements 12766* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 12767* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 12768* [StructureMap](structuremap.html): Name of the publisher of the structure map 12769* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 12770* [TestScript](testscript.html): Name of the publisher of the test script 12771* [ValueSet](valueset.html): Name of the publisher of the value set 12772</b><br> 12773 * Type: <b>string</b><br> 12774 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 12775 * </p> 12776 */ 12777 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 12778 12779 /** 12780 * Search parameter: <b>status</b> 12781 * <p> 12782 * Description: <b>Multiple Resources: 12783 12784* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 12785* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 12786* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 12787* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 12788* [Citation](citation.html): The current status of the citation 12789* [CodeSystem](codesystem.html): The current status of the code system 12790* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 12791* [ConceptMap](conceptmap.html): The current status of the concept map 12792* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 12793* [EventDefinition](eventdefinition.html): The current status of the event definition 12794* [Evidence](evidence.html): The current status of the evidence 12795* [EvidenceReport](evidencereport.html): The current status of the evidence report 12796* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 12797* [ExampleScenario](examplescenario.html): The current status of the example scenario 12798* [GraphDefinition](graphdefinition.html): The current status of the graph definition 12799* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 12800* [Library](library.html): The current status of the library 12801* [Measure](measure.html): The current status of the measure 12802* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 12803* [MessageDefinition](messagedefinition.html): The current status of the message definition 12804* [NamingSystem](namingsystem.html): The current status of the naming system 12805* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 12806* [OperationDefinition](operationdefinition.html): The current status of the operation definition 12807* [PlanDefinition](plandefinition.html): The current status of the plan definition 12808* [Questionnaire](questionnaire.html): The current status of the questionnaire 12809* [Requirements](requirements.html): The current status of the requirements 12810* [SearchParameter](searchparameter.html): The current status of the search parameter 12811* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 12812* [StructureDefinition](structuredefinition.html): The current status of the structure definition 12813* [StructureMap](structuremap.html): The current status of the structure map 12814* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 12815* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 12816* [TestPlan](testplan.html): The current status of the test plan 12817* [TestScript](testscript.html): The current status of the test script 12818* [ValueSet](valueset.html): The current status of the value set 12819</b><br> 12820 * Type: <b>token</b><br> 12821 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 12822 * </p> 12823 */ 12824 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 12825 public static final String SP_STATUS = "status"; 12826 /** 12827 * <b>Fluent Client</b> search parameter constant for <b>status</b> 12828 * <p> 12829 * Description: <b>Multiple Resources: 12830 12831* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 12832* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 12833* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 12834* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 12835* [Citation](citation.html): The current status of the citation 12836* [CodeSystem](codesystem.html): The current status of the code system 12837* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 12838* [ConceptMap](conceptmap.html): The current status of the concept map 12839* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 12840* [EventDefinition](eventdefinition.html): The current status of the event definition 12841* [Evidence](evidence.html): The current status of the evidence 12842* [EvidenceReport](evidencereport.html): The current status of the evidence report 12843* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 12844* [ExampleScenario](examplescenario.html): The current status of the example scenario 12845* [GraphDefinition](graphdefinition.html): The current status of the graph definition 12846* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 12847* [Library](library.html): The current status of the library 12848* [Measure](measure.html): The current status of the measure 12849* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 12850* [MessageDefinition](messagedefinition.html): The current status of the message definition 12851* [NamingSystem](namingsystem.html): The current status of the naming system 12852* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 12853* [OperationDefinition](operationdefinition.html): The current status of the operation definition 12854* [PlanDefinition](plandefinition.html): The current status of the plan definition 12855* [Questionnaire](questionnaire.html): The current status of the questionnaire 12856* [Requirements](requirements.html): The current status of the requirements 12857* [SearchParameter](searchparameter.html): The current status of the search parameter 12858* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 12859* [StructureDefinition](structuredefinition.html): The current status of the structure definition 12860* [StructureMap](structuremap.html): The current status of the structure map 12861* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 12862* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 12863* [TestPlan](testplan.html): The current status of the test plan 12864* [TestScript](testscript.html): The current status of the test script 12865* [ValueSet](valueset.html): The current status of the value set 12866</b><br> 12867 * Type: <b>token</b><br> 12868 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 12869 * </p> 12870 */ 12871 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 12872 12873 /** 12874 * Search parameter: <b>title</b> 12875 * <p> 12876 * Description: <b>Multiple Resources: 12877 12878* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 12879* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 12880* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 12881* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 12882* [Citation](citation.html): The human-friendly name of the citation 12883* [CodeSystem](codesystem.html): The human-friendly name of the code system 12884* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 12885* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 12886* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 12887* [Evidence](evidence.html): The human-friendly name of the evidence 12888* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 12889* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 12890* [Library](library.html): The human-friendly name of the library 12891* [Measure](measure.html): The human-friendly name of the measure 12892* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 12893* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 12894* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 12895* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 12896* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 12897* [Requirements](requirements.html): The human-friendly name of the requirements 12898* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 12899* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 12900* [StructureMap](structuremap.html): The human-friendly name of the structure map 12901* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 12902* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 12903* [TestScript](testscript.html): The human-friendly name of the test script 12904* [ValueSet](valueset.html): The human-friendly name of the value set 12905</b><br> 12906 * Type: <b>string</b><br> 12907 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 12908 * </p> 12909 */ 12910 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 12911 public static final String SP_TITLE = "title"; 12912 /** 12913 * <b>Fluent Client</b> search parameter constant for <b>title</b> 12914 * <p> 12915 * Description: <b>Multiple Resources: 12916 12917* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 12918* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 12919* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 12920* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 12921* [Citation](citation.html): The human-friendly name of the citation 12922* [CodeSystem](codesystem.html): The human-friendly name of the code system 12923* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 12924* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 12925* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 12926* [Evidence](evidence.html): The human-friendly name of the evidence 12927* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 12928* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 12929* [Library](library.html): The human-friendly name of the library 12930* [Measure](measure.html): The human-friendly name of the measure 12931* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 12932* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 12933* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 12934* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 12935* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 12936* [Requirements](requirements.html): The human-friendly name of the requirements 12937* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 12938* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 12939* [StructureMap](structuremap.html): The human-friendly name of the structure map 12940* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 12941* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 12942* [TestScript](testscript.html): The human-friendly name of the test script 12943* [ValueSet](valueset.html): The human-friendly name of the value set 12944</b><br> 12945 * Type: <b>string</b><br> 12946 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 12947 * </p> 12948 */ 12949 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 12950 12951 /** 12952 * Search parameter: <b>url</b> 12953 * <p> 12954 * Description: <b>Multiple Resources: 12955 12956* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 12957* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 12958* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 12959* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 12960* [Citation](citation.html): The uri that identifies the citation 12961* [CodeSystem](codesystem.html): The uri that identifies the code system 12962* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 12963* [ConceptMap](conceptmap.html): The URI that identifies the concept map 12964* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 12965* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 12966* [Evidence](evidence.html): The uri that identifies the evidence 12967* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 12968* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 12969* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 12970* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 12971* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 12972* [Library](library.html): The uri that identifies the library 12973* [Measure](measure.html): The uri that identifies the measure 12974* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 12975* [NamingSystem](namingsystem.html): The uri that identifies the naming system 12976* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 12977* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 12978* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 12979* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 12980* [Requirements](requirements.html): The uri that identifies the requirements 12981* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 12982* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 12983* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 12984* [StructureMap](structuremap.html): The uri that identifies the structure map 12985* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 12986* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 12987* [TestPlan](testplan.html): The uri that identifies the test plan 12988* [TestScript](testscript.html): The uri that identifies the test script 12989* [ValueSet](valueset.html): The uri that identifies the value set 12990</b><br> 12991 * Type: <b>uri</b><br> 12992 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 12993 * </p> 12994 */ 12995 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 12996 public static final String SP_URL = "url"; 12997 /** 12998 * <b>Fluent Client</b> search parameter constant for <b>url</b> 12999 * <p> 13000 * Description: <b>Multiple Resources: 13001 13002* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 13003* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 13004* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 13005* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 13006* [Citation](citation.html): The uri that identifies the citation 13007* [CodeSystem](codesystem.html): The uri that identifies the code system 13008* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 13009* [ConceptMap](conceptmap.html): The URI that identifies the concept map 13010* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 13011* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 13012* [Evidence](evidence.html): The uri that identifies the evidence 13013* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 13014* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 13015* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 13016* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 13017* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 13018* [Library](library.html): The uri that identifies the library 13019* [Measure](measure.html): The uri that identifies the measure 13020* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 13021* [NamingSystem](namingsystem.html): The uri that identifies the naming system 13022* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 13023* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 13024* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 13025* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 13026* [Requirements](requirements.html): The uri that identifies the requirements 13027* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 13028* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 13029* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 13030* [StructureMap](structuremap.html): The uri that identifies the structure map 13031* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 13032* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 13033* [TestPlan](testplan.html): The uri that identifies the test plan 13034* [TestScript](testscript.html): The uri that identifies the test script 13035* [ValueSet](valueset.html): The uri that identifies the value set 13036</b><br> 13037 * Type: <b>uri</b><br> 13038 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 13039 * </p> 13040 */ 13041 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 13042 13043 /** 13044 * Search parameter: <b>version</b> 13045 * <p> 13046 * Description: <b>Multiple Resources: 13047 13048* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 13049* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 13050* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 13051* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 13052* [Citation](citation.html): The business version of the citation 13053* [CodeSystem](codesystem.html): The business version of the code system 13054* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 13055* [ConceptMap](conceptmap.html): The business version of the concept map 13056* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 13057* [EventDefinition](eventdefinition.html): The business version of the event definition 13058* [Evidence](evidence.html): The business version of the evidence 13059* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 13060* [ExampleScenario](examplescenario.html): The business version of the example scenario 13061* [GraphDefinition](graphdefinition.html): The business version of the graph definition 13062* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 13063* [Library](library.html): The business version of the library 13064* [Measure](measure.html): The business version of the measure 13065* [MessageDefinition](messagedefinition.html): The business version of the message definition 13066* [NamingSystem](namingsystem.html): The business version of the naming system 13067* [OperationDefinition](operationdefinition.html): The business version of the operation definition 13068* [PlanDefinition](plandefinition.html): The business version of the plan definition 13069* [Questionnaire](questionnaire.html): The business version of the questionnaire 13070* [Requirements](requirements.html): The business version of the requirements 13071* [SearchParameter](searchparameter.html): The business version of the search parameter 13072* [StructureDefinition](structuredefinition.html): The business version of the structure definition 13073* [StructureMap](structuremap.html): The business version of the structure map 13074* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 13075* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 13076* [TestScript](testscript.html): The business version of the test script 13077* [ValueSet](valueset.html): The business version of the value set 13078</b><br> 13079 * Type: <b>token</b><br> 13080 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 13081 * </p> 13082 */ 13083 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 13084 public static final String SP_VERSION = "version"; 13085 /** 13086 * <b>Fluent Client</b> search parameter constant for <b>version</b> 13087 * <p> 13088 * Description: <b>Multiple Resources: 13089 13090* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 13091* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 13092* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 13093* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 13094* [Citation](citation.html): The business version of the citation 13095* [CodeSystem](codesystem.html): The business version of the code system 13096* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 13097* [ConceptMap](conceptmap.html): The business version of the concept map 13098* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 13099* [EventDefinition](eventdefinition.html): The business version of the event definition 13100* [Evidence](evidence.html): The business version of the evidence 13101* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 13102* [ExampleScenario](examplescenario.html): The business version of the example scenario 13103* [GraphDefinition](graphdefinition.html): The business version of the graph definition 13104* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 13105* [Library](library.html): The business version of the library 13106* [Measure](measure.html): The business version of the measure 13107* [MessageDefinition](messagedefinition.html): The business version of the message definition 13108* [NamingSystem](namingsystem.html): The business version of the naming system 13109* [OperationDefinition](operationdefinition.html): The business version of the operation definition 13110* [PlanDefinition](plandefinition.html): The business version of the plan definition 13111* [Questionnaire](questionnaire.html): The business version of the questionnaire 13112* [Requirements](requirements.html): The business version of the requirements 13113* [SearchParameter](searchparameter.html): The business version of the search parameter 13114* [StructureDefinition](structuredefinition.html): The business version of the structure definition 13115* [StructureMap](structuremap.html): The business version of the structure map 13116* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 13117* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 13118* [TestScript](testscript.html): The business version of the test script 13119* [ValueSet](valueset.html): The business version of the value set 13120</b><br> 13121 * Type: <b>token</b><br> 13122 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 13123 * </p> 13124 */ 13125 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 13126 13127 /** 13128 * Search parameter: <b>scope-artifact</b> 13129 * <p> 13130 * Description: <b>The artifact under test</b><br> 13131 * Type: <b>reference</b><br> 13132 * Path: <b>TestScript.scope.artifact</b><br> 13133 * </p> 13134 */ 13135 @SearchParamDefinition(name="scope-artifact", path="TestScript.scope.artifact", description="The artifact under test", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 13136 public static final String SP_SCOPE_ARTIFACT = "scope-artifact"; 13137 /** 13138 * <b>Fluent Client</b> search parameter constant for <b>scope-artifact</b> 13139 * <p> 13140 * Description: <b>The artifact under test</b><br> 13141 * Type: <b>reference</b><br> 13142 * Path: <b>TestScript.scope.artifact</b><br> 13143 * </p> 13144 */ 13145 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SCOPE_ARTIFACT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SCOPE_ARTIFACT); 13146 13147/** 13148 * Constant for fluent queries to be used to add include statements. Specifies 13149 * the path value of "<b>TestScript:scope-artifact</b>". 13150 */ 13151 public static final ca.uhn.fhir.model.api.Include INCLUDE_SCOPE_ARTIFACT = new ca.uhn.fhir.model.api.Include("TestScript:scope-artifact").toLocked(); 13152 13153 /** 13154 * Search parameter: <b>testscript-capability</b> 13155 * <p> 13156 * Description: <b>TestScript required and validated capability</b><br> 13157 * Type: <b>string</b><br> 13158 * Path: <b>TestScript.metadata.capability.description</b><br> 13159 * </p> 13160 */ 13161 @SearchParamDefinition(name="testscript-capability", path="TestScript.metadata.capability.description", description="TestScript required and validated capability", type="string" ) 13162 public static final String SP_TESTSCRIPT_CAPABILITY = "testscript-capability"; 13163 /** 13164 * <b>Fluent Client</b> search parameter constant for <b>testscript-capability</b> 13165 * <p> 13166 * Description: <b>TestScript required and validated capability</b><br> 13167 * Type: <b>string</b><br> 13168 * Path: <b>TestScript.metadata.capability.description</b><br> 13169 * </p> 13170 */ 13171 public static final ca.uhn.fhir.rest.gclient.StringClientParam TESTSCRIPT_CAPABILITY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TESTSCRIPT_CAPABILITY); 13172 13173 13174} 13175