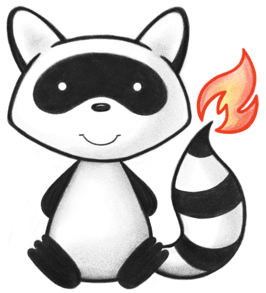
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.ChildOrder; 045import ca.uhn.fhir.model.api.annotation.DatatypeDef; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.Block; 048 049/** 050 * Timing Type: Specifies an event that may occur multiple times. Timing schedules are used to record when things are planned, expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds, and may be used for reporting the schedule to which past regular activities were carried out. 051 */ 052@DatatypeDef(name="Timing") 053public class Timing extends BackboneType implements ICompositeType { 054 055 public enum EventTiming { 056 /** 057 * Event occurs during the morning. The exact time is unspecified and established by institution convention or patient interpretation. 058 */ 059 MORN, 060 /** 061 * Event occurs during the early morning. The exact time is unspecified and established by institution convention or patient interpretation. 062 */ 063 MORN_EARLY, 064 /** 065 * Event occurs during the late morning. The exact time is unspecified and established by institution convention or patient interpretation. 066 */ 067 MORN_LATE, 068 /** 069 * Event occurs around 12:00pm. The exact time is unspecified and established by institution convention or patient interpretation. 070 */ 071 NOON, 072 /** 073 * Event occurs during the afternoon. The exact time is unspecified and established by institution convention or patient interpretation. 074 */ 075 AFT, 076 /** 077 * Event occurs during the early afternoon. The exact time is unspecified and established by institution convention or patient interpretation. 078 */ 079 AFT_EARLY, 080 /** 081 * Event occurs during the late afternoon. The exact time is unspecified and established by institution convention or patient interpretation. 082 */ 083 AFT_LATE, 084 /** 085 * Event occurs during the evening. The exact time is unspecified and established by institution convention or patient interpretation. 086 */ 087 EVE, 088 /** 089 * Event occurs during the early evening. The exact time is unspecified and established by institution convention or patient interpretation. 090 */ 091 EVE_EARLY, 092 /** 093 * Event occurs during the late evening. The exact time is unspecified and established by institution convention or patient interpretation. 094 */ 095 EVE_LATE, 096 /** 097 * Event occurs during the night. The exact time is unspecified and established by institution convention or patient interpretation. 098 */ 099 NIGHT, 100 /** 101 * Event occurs [offset] after subject goes to sleep. The exact time is unspecified and established by institution convention or patient interpretation. 102 */ 103 PHS, 104 /** 105 * Event occurs a single time (with no repetitions) as soon as possible after the scheduled or actual start of the overall event. 106 */ 107 IMD, 108 /** 109 * 110 */ 111 HS, 112 /** 113 * 114 */ 115 WAKE, 116 /** 117 * 118 */ 119 C, 120 /** 121 * 122 */ 123 CM, 124 /** 125 * 126 */ 127 CD, 128 /** 129 * 130 */ 131 CV, 132 /** 133 * 134 */ 135 AC, 136 /** 137 * 138 */ 139 ACM, 140 /** 141 * 142 */ 143 ACD, 144 /** 145 * 146 */ 147 ACV, 148 /** 149 * 150 */ 151 PC, 152 /** 153 * 154 */ 155 PCM, 156 /** 157 * 158 */ 159 PCD, 160 /** 161 * 162 */ 163 PCV, 164 /** 165 * added to help the parsers with the generic types 166 */ 167 NULL; 168 public static EventTiming fromCode(String codeString) throws FHIRException { 169 if (codeString == null || "".equals(codeString)) 170 return null; 171 if ("MORN".equals(codeString)) 172 return MORN; 173 if ("MORN.early".equals(codeString)) 174 return MORN_EARLY; 175 if ("MORN.late".equals(codeString)) 176 return MORN_LATE; 177 if ("NOON".equals(codeString)) 178 return NOON; 179 if ("AFT".equals(codeString)) 180 return AFT; 181 if ("AFT.early".equals(codeString)) 182 return AFT_EARLY; 183 if ("AFT.late".equals(codeString)) 184 return AFT_LATE; 185 if ("EVE".equals(codeString)) 186 return EVE; 187 if ("EVE.early".equals(codeString)) 188 return EVE_EARLY; 189 if ("EVE.late".equals(codeString)) 190 return EVE_LATE; 191 if ("NIGHT".equals(codeString)) 192 return NIGHT; 193 if ("PHS".equals(codeString)) 194 return PHS; 195 if ("IMD".equals(codeString)) 196 return IMD; 197 if ("HS".equals(codeString)) 198 return HS; 199 if ("WAKE".equals(codeString)) 200 return WAKE; 201 if ("C".equals(codeString)) 202 return C; 203 if ("CM".equals(codeString)) 204 return CM; 205 if ("CD".equals(codeString)) 206 return CD; 207 if ("CV".equals(codeString)) 208 return CV; 209 if ("AC".equals(codeString)) 210 return AC; 211 if ("ACM".equals(codeString)) 212 return ACM; 213 if ("ACD".equals(codeString)) 214 return ACD; 215 if ("ACV".equals(codeString)) 216 return ACV; 217 if ("PC".equals(codeString)) 218 return PC; 219 if ("PCM".equals(codeString)) 220 return PCM; 221 if ("PCD".equals(codeString)) 222 return PCD; 223 if ("PCV".equals(codeString)) 224 return PCV; 225 if (Configuration.isAcceptInvalidEnums()) 226 return null; 227 else 228 throw new FHIRException("Unknown EventTiming code '"+codeString+"'"); 229 } 230 public String toCode() { 231 switch (this) { 232 case MORN: return "MORN"; 233 case MORN_EARLY: return "MORN.early"; 234 case MORN_LATE: return "MORN.late"; 235 case NOON: return "NOON"; 236 case AFT: return "AFT"; 237 case AFT_EARLY: return "AFT.early"; 238 case AFT_LATE: return "AFT.late"; 239 case EVE: return "EVE"; 240 case EVE_EARLY: return "EVE.early"; 241 case EVE_LATE: return "EVE.late"; 242 case NIGHT: return "NIGHT"; 243 case PHS: return "PHS"; 244 case IMD: return "IMD"; 245 case HS: return "HS"; 246 case WAKE: return "WAKE"; 247 case C: return "C"; 248 case CM: return "CM"; 249 case CD: return "CD"; 250 case CV: return "CV"; 251 case AC: return "AC"; 252 case ACM: return "ACM"; 253 case ACD: return "ACD"; 254 case ACV: return "ACV"; 255 case PC: return "PC"; 256 case PCM: return "PCM"; 257 case PCD: return "PCD"; 258 case PCV: return "PCV"; 259 case NULL: return null; 260 default: return "?"; 261 } 262 } 263 public String getSystem() { 264 switch (this) { 265 case MORN: return "http://hl7.org/fhir/event-timing"; 266 case MORN_EARLY: return "http://hl7.org/fhir/event-timing"; 267 case MORN_LATE: return "http://hl7.org/fhir/event-timing"; 268 case NOON: return "http://hl7.org/fhir/event-timing"; 269 case AFT: return "http://hl7.org/fhir/event-timing"; 270 case AFT_EARLY: return "http://hl7.org/fhir/event-timing"; 271 case AFT_LATE: return "http://hl7.org/fhir/event-timing"; 272 case EVE: return "http://hl7.org/fhir/event-timing"; 273 case EVE_EARLY: return "http://hl7.org/fhir/event-timing"; 274 case EVE_LATE: return "http://hl7.org/fhir/event-timing"; 275 case NIGHT: return "http://hl7.org/fhir/event-timing"; 276 case PHS: return "http://hl7.org/fhir/event-timing"; 277 case IMD: return "http://hl7.org/fhir/event-timing"; 278 case HS: return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 279 case WAKE: return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 280 case C: return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 281 case CM: return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 282 case CD: return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 283 case CV: return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 284 case AC: return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 285 case ACM: return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 286 case ACD: return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 287 case ACV: return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 288 case PC: return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 289 case PCM: return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 290 case PCD: return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 291 case PCV: return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 292 case NULL: return null; 293 default: return "?"; 294 } 295 } 296 public String getDefinition() { 297 switch (this) { 298 case MORN: return "Event occurs during the morning. The exact time is unspecified and established by institution convention or patient interpretation."; 299 case MORN_EARLY: return "Event occurs during the early morning. The exact time is unspecified and established by institution convention or patient interpretation."; 300 case MORN_LATE: return "Event occurs during the late morning. The exact time is unspecified and established by institution convention or patient interpretation."; 301 case NOON: return "Event occurs around 12:00pm. The exact time is unspecified and established by institution convention or patient interpretation."; 302 case AFT: return "Event occurs during the afternoon. The exact time is unspecified and established by institution convention or patient interpretation."; 303 case AFT_EARLY: return "Event occurs during the early afternoon. The exact time is unspecified and established by institution convention or patient interpretation."; 304 case AFT_LATE: return "Event occurs during the late afternoon. The exact time is unspecified and established by institution convention or patient interpretation."; 305 case EVE: return "Event occurs during the evening. The exact time is unspecified and established by institution convention or patient interpretation."; 306 case EVE_EARLY: return "Event occurs during the early evening. The exact time is unspecified and established by institution convention or patient interpretation."; 307 case EVE_LATE: return "Event occurs during the late evening. The exact time is unspecified and established by institution convention or patient interpretation."; 308 case NIGHT: return "Event occurs during the night. The exact time is unspecified and established by institution convention or patient interpretation."; 309 case PHS: return "Event occurs [offset] after subject goes to sleep. The exact time is unspecified and established by institution convention or patient interpretation."; 310 case IMD: return "Event occurs a single time (with no repetitions) as soon as possible after the scheduled or actual start of the overall event."; 311 case HS: return ""; 312 case WAKE: return ""; 313 case C: return ""; 314 case CM: return ""; 315 case CD: return ""; 316 case CV: return ""; 317 case AC: return ""; 318 case ACM: return ""; 319 case ACD: return ""; 320 case ACV: return ""; 321 case PC: return ""; 322 case PCM: return ""; 323 case PCD: return ""; 324 case PCV: return ""; 325 case NULL: return null; 326 default: return "?"; 327 } 328 } 329 public String getDisplay() { 330 switch (this) { 331 case MORN: return "Morning"; 332 case MORN_EARLY: return "Early Morning"; 333 case MORN_LATE: return "Late Morning"; 334 case NOON: return "Noon"; 335 case AFT: return "Afternoon"; 336 case AFT_EARLY: return "Early Afternoon"; 337 case AFT_LATE: return "Late Afternoon"; 338 case EVE: return "Evening"; 339 case EVE_EARLY: return "Early Evening"; 340 case EVE_LATE: return "Late Evening"; 341 case NIGHT: return "Night"; 342 case PHS: return "After Sleep"; 343 case IMD: return "Immediate"; 344 case HS: return "HS"; 345 case WAKE: return "WAKE"; 346 case C: return "C"; 347 case CM: return "CM"; 348 case CD: return "CD"; 349 case CV: return "CV"; 350 case AC: return "AC"; 351 case ACM: return "ACM"; 352 case ACD: return "ACD"; 353 case ACV: return "ACV"; 354 case PC: return "PC"; 355 case PCM: return "PCM"; 356 case PCD: return "PCD"; 357 case PCV: return "PCV"; 358 case NULL: return null; 359 default: return "?"; 360 } 361 } 362 } 363 364 public static class EventTimingEnumFactory implements EnumFactory<EventTiming> { 365 public EventTiming fromCode(String codeString) throws IllegalArgumentException { 366 if (codeString == null || "".equals(codeString)) 367 if (codeString == null || "".equals(codeString)) 368 return null; 369 if ("MORN".equals(codeString)) 370 return EventTiming.MORN; 371 if ("MORN.early".equals(codeString)) 372 return EventTiming.MORN_EARLY; 373 if ("MORN.late".equals(codeString)) 374 return EventTiming.MORN_LATE; 375 if ("NOON".equals(codeString)) 376 return EventTiming.NOON; 377 if ("AFT".equals(codeString)) 378 return EventTiming.AFT; 379 if ("AFT.early".equals(codeString)) 380 return EventTiming.AFT_EARLY; 381 if ("AFT.late".equals(codeString)) 382 return EventTiming.AFT_LATE; 383 if ("EVE".equals(codeString)) 384 return EventTiming.EVE; 385 if ("EVE.early".equals(codeString)) 386 return EventTiming.EVE_EARLY; 387 if ("EVE.late".equals(codeString)) 388 return EventTiming.EVE_LATE; 389 if ("NIGHT".equals(codeString)) 390 return EventTiming.NIGHT; 391 if ("PHS".equals(codeString)) 392 return EventTiming.PHS; 393 if ("IMD".equals(codeString)) 394 return EventTiming.IMD; 395 if ("HS".equals(codeString)) 396 return EventTiming.HS; 397 if ("WAKE".equals(codeString)) 398 return EventTiming.WAKE; 399 if ("C".equals(codeString)) 400 return EventTiming.C; 401 if ("CM".equals(codeString)) 402 return EventTiming.CM; 403 if ("CD".equals(codeString)) 404 return EventTiming.CD; 405 if ("CV".equals(codeString)) 406 return EventTiming.CV; 407 if ("AC".equals(codeString)) 408 return EventTiming.AC; 409 if ("ACM".equals(codeString)) 410 return EventTiming.ACM; 411 if ("ACD".equals(codeString)) 412 return EventTiming.ACD; 413 if ("ACV".equals(codeString)) 414 return EventTiming.ACV; 415 if ("PC".equals(codeString)) 416 return EventTiming.PC; 417 if ("PCM".equals(codeString)) 418 return EventTiming.PCM; 419 if ("PCD".equals(codeString)) 420 return EventTiming.PCD; 421 if ("PCV".equals(codeString)) 422 return EventTiming.PCV; 423 throw new IllegalArgumentException("Unknown EventTiming code '"+codeString+"'"); 424 } 425 public Enumeration<EventTiming> fromType(PrimitiveType<?> code) throws FHIRException { 426 if (code == null) 427 return null; 428 if (code.isEmpty()) 429 return new Enumeration<EventTiming>(this, EventTiming.NULL, code); 430 String codeString = ((PrimitiveType) code).asStringValue(); 431 if (codeString == null || "".equals(codeString)) 432 return new Enumeration<EventTiming>(this, EventTiming.NULL, code); 433 if ("MORN".equals(codeString)) 434 return new Enumeration<EventTiming>(this, EventTiming.MORN, code); 435 if ("MORN.early".equals(codeString)) 436 return new Enumeration<EventTiming>(this, EventTiming.MORN_EARLY, code); 437 if ("MORN.late".equals(codeString)) 438 return new Enumeration<EventTiming>(this, EventTiming.MORN_LATE, code); 439 if ("NOON".equals(codeString)) 440 return new Enumeration<EventTiming>(this, EventTiming.NOON, code); 441 if ("AFT".equals(codeString)) 442 return new Enumeration<EventTiming>(this, EventTiming.AFT, code); 443 if ("AFT.early".equals(codeString)) 444 return new Enumeration<EventTiming>(this, EventTiming.AFT_EARLY, code); 445 if ("AFT.late".equals(codeString)) 446 return new Enumeration<EventTiming>(this, EventTiming.AFT_LATE, code); 447 if ("EVE".equals(codeString)) 448 return new Enumeration<EventTiming>(this, EventTiming.EVE, code); 449 if ("EVE.early".equals(codeString)) 450 return new Enumeration<EventTiming>(this, EventTiming.EVE_EARLY, code); 451 if ("EVE.late".equals(codeString)) 452 return new Enumeration<EventTiming>(this, EventTiming.EVE_LATE, code); 453 if ("NIGHT".equals(codeString)) 454 return new Enumeration<EventTiming>(this, EventTiming.NIGHT, code); 455 if ("PHS".equals(codeString)) 456 return new Enumeration<EventTiming>(this, EventTiming.PHS, code); 457 if ("IMD".equals(codeString)) 458 return new Enumeration<EventTiming>(this, EventTiming.IMD, code); 459 if ("HS".equals(codeString)) 460 return new Enumeration<EventTiming>(this, EventTiming.HS, code); 461 if ("WAKE".equals(codeString)) 462 return new Enumeration<EventTiming>(this, EventTiming.WAKE, code); 463 if ("C".equals(codeString)) 464 return new Enumeration<EventTiming>(this, EventTiming.C, code); 465 if ("CM".equals(codeString)) 466 return new Enumeration<EventTiming>(this, EventTiming.CM, code); 467 if ("CD".equals(codeString)) 468 return new Enumeration<EventTiming>(this, EventTiming.CD, code); 469 if ("CV".equals(codeString)) 470 return new Enumeration<EventTiming>(this, EventTiming.CV, code); 471 if ("AC".equals(codeString)) 472 return new Enumeration<EventTiming>(this, EventTiming.AC, code); 473 if ("ACM".equals(codeString)) 474 return new Enumeration<EventTiming>(this, EventTiming.ACM, code); 475 if ("ACD".equals(codeString)) 476 return new Enumeration<EventTiming>(this, EventTiming.ACD, code); 477 if ("ACV".equals(codeString)) 478 return new Enumeration<EventTiming>(this, EventTiming.ACV, code); 479 if ("PC".equals(codeString)) 480 return new Enumeration<EventTiming>(this, EventTiming.PC, code); 481 if ("PCM".equals(codeString)) 482 return new Enumeration<EventTiming>(this, EventTiming.PCM, code); 483 if ("PCD".equals(codeString)) 484 return new Enumeration<EventTiming>(this, EventTiming.PCD, code); 485 if ("PCV".equals(codeString)) 486 return new Enumeration<EventTiming>(this, EventTiming.PCV, code); 487 throw new FHIRException("Unknown EventTiming code '"+codeString+"'"); 488 } 489 public String toCode(EventTiming code) { 490 if (code == EventTiming.NULL) 491 return null; 492 if (code == EventTiming.MORN) 493 return "MORN"; 494 if (code == EventTiming.MORN_EARLY) 495 return "MORN.early"; 496 if (code == EventTiming.MORN_LATE) 497 return "MORN.late"; 498 if (code == EventTiming.NOON) 499 return "NOON"; 500 if (code == EventTiming.AFT) 501 return "AFT"; 502 if (code == EventTiming.AFT_EARLY) 503 return "AFT.early"; 504 if (code == EventTiming.AFT_LATE) 505 return "AFT.late"; 506 if (code == EventTiming.EVE) 507 return "EVE"; 508 if (code == EventTiming.EVE_EARLY) 509 return "EVE.early"; 510 if (code == EventTiming.EVE_LATE) 511 return "EVE.late"; 512 if (code == EventTiming.NIGHT) 513 return "NIGHT"; 514 if (code == EventTiming.PHS) 515 return "PHS"; 516 if (code == EventTiming.IMD) 517 return "IMD"; 518 if (code == EventTiming.HS) 519 return "HS"; 520 if (code == EventTiming.WAKE) 521 return "WAKE"; 522 if (code == EventTiming.C) 523 return "C"; 524 if (code == EventTiming.CM) 525 return "CM"; 526 if (code == EventTiming.CD) 527 return "CD"; 528 if (code == EventTiming.CV) 529 return "CV"; 530 if (code == EventTiming.AC) 531 return "AC"; 532 if (code == EventTiming.ACM) 533 return "ACM"; 534 if (code == EventTiming.ACD) 535 return "ACD"; 536 if (code == EventTiming.ACV) 537 return "ACV"; 538 if (code == EventTiming.PC) 539 return "PC"; 540 if (code == EventTiming.PCM) 541 return "PCM"; 542 if (code == EventTiming.PCD) 543 return "PCD"; 544 if (code == EventTiming.PCV) 545 return "PCV"; 546 return "?"; 547 } 548 public String toSystem(EventTiming code) { 549 return code.getSystem(); 550 } 551 } 552 553 public enum UnitsOfTime { 554 /** 555 * 556 */ 557 S, 558 /** 559 * 560 */ 561 MIN, 562 /** 563 * 564 */ 565 H, 566 /** 567 * 568 */ 569 D, 570 /** 571 * 572 */ 573 WK, 574 /** 575 * 576 */ 577 MO, 578 /** 579 * 580 */ 581 A, 582 /** 583 * added to help the parsers with the generic types 584 */ 585 NULL; 586 public static UnitsOfTime fromCode(String codeString) throws FHIRException { 587 if (codeString == null || "".equals(codeString)) 588 return null; 589 if ("s".equals(codeString)) 590 return S; 591 if ("min".equals(codeString)) 592 return MIN; 593 if ("h".equals(codeString)) 594 return H; 595 if ("d".equals(codeString)) 596 return D; 597 if ("wk".equals(codeString)) 598 return WK; 599 if ("mo".equals(codeString)) 600 return MO; 601 if ("a".equals(codeString)) 602 return A; 603 if (Configuration.isAcceptInvalidEnums()) 604 return null; 605 else 606 throw new FHIRException("Unknown UnitsOfTime code '"+codeString+"'"); 607 } 608 public String toCode() { 609 switch (this) { 610 case S: return "s"; 611 case MIN: return "min"; 612 case H: return "h"; 613 case D: return "d"; 614 case WK: return "wk"; 615 case MO: return "mo"; 616 case A: return "a"; 617 case NULL: return null; 618 default: return "?"; 619 } 620 } 621 public String getSystem() { 622 switch (this) { 623 case S: return "http://unitsofmeasure.org"; 624 case MIN: return "http://unitsofmeasure.org"; 625 case H: return "http://unitsofmeasure.org"; 626 case D: return "http://unitsofmeasure.org"; 627 case WK: return "http://unitsofmeasure.org"; 628 case MO: return "http://unitsofmeasure.org"; 629 case A: return "http://unitsofmeasure.org"; 630 case NULL: return null; 631 default: return "?"; 632 } 633 } 634 public String getDefinition() { 635 switch (this) { 636 case S: return ""; 637 case MIN: return ""; 638 case H: return ""; 639 case D: return ""; 640 case WK: return ""; 641 case MO: return ""; 642 case A: return ""; 643 case NULL: return null; 644 default: return "?"; 645 } 646 } 647 public String getDisplay() { 648 switch (this) { 649 case S: return "?"; 650 case MIN: return "??"; 651 case H: return "??"; 652 case D: return "?"; 653 case WK: return "??"; 654 case MO: return "?"; 655 case A: return "?"; 656 case NULL: return null; 657 default: return "?"; 658 } 659 } 660 } 661 662 public static class UnitsOfTimeEnumFactory implements EnumFactory<UnitsOfTime> { 663 public UnitsOfTime fromCode(String codeString) throws IllegalArgumentException { 664 if (codeString == null || "".equals(codeString)) 665 if (codeString == null || "".equals(codeString)) 666 return null; 667 if ("s".equals(codeString)) 668 return UnitsOfTime.S; 669 if ("min".equals(codeString)) 670 return UnitsOfTime.MIN; 671 if ("h".equals(codeString)) 672 return UnitsOfTime.H; 673 if ("d".equals(codeString)) 674 return UnitsOfTime.D; 675 if ("wk".equals(codeString)) 676 return UnitsOfTime.WK; 677 if ("mo".equals(codeString)) 678 return UnitsOfTime.MO; 679 if ("a".equals(codeString)) 680 return UnitsOfTime.A; 681 throw new IllegalArgumentException("Unknown UnitsOfTime code '"+codeString+"'"); 682 } 683 public Enumeration<UnitsOfTime> fromType(PrimitiveType<?> code) throws FHIRException { 684 if (code == null) 685 return null; 686 if (code.isEmpty()) 687 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.NULL, code); 688 String codeString = ((PrimitiveType) code).asStringValue(); 689 if (codeString == null || "".equals(codeString)) 690 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.NULL, code); 691 if ("s".equals(codeString)) 692 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.S, code); 693 if ("min".equals(codeString)) 694 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.MIN, code); 695 if ("h".equals(codeString)) 696 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.H, code); 697 if ("d".equals(codeString)) 698 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.D, code); 699 if ("wk".equals(codeString)) 700 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.WK, code); 701 if ("mo".equals(codeString)) 702 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.MO, code); 703 if ("a".equals(codeString)) 704 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.A, code); 705 throw new FHIRException("Unknown UnitsOfTime code '"+codeString+"'"); 706 } 707 public String toCode(UnitsOfTime code) { 708 if (code == UnitsOfTime.NULL) 709 return null; 710 if (code == UnitsOfTime.S) 711 return "s"; 712 if (code == UnitsOfTime.MIN) 713 return "min"; 714 if (code == UnitsOfTime.H) 715 return "h"; 716 if (code == UnitsOfTime.D) 717 return "d"; 718 if (code == UnitsOfTime.WK) 719 return "wk"; 720 if (code == UnitsOfTime.MO) 721 return "mo"; 722 if (code == UnitsOfTime.A) 723 return "a"; 724 return "?"; 725 } 726 public String toSystem(UnitsOfTime code) { 727 return code.getSystem(); 728 } 729 } 730 731 @Block() 732 public static class TimingRepeatComponent extends Element implements IBaseDatatypeElement { 733 /** 734 * Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule. 735 */ 736 @Child(name = "bounds", type = {Duration.class, Range.class, Period.class}, order=1, min=0, max=1, modifier=false, summary=true) 737 @Description(shortDefinition="Length/Range of lengths, or (Start and/or end) limits", formalDefinition="Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule." ) 738 protected DataType bounds; 739 740 /** 741 * A total count of the desired number of repetitions across the duration of the entire timing specification. If countMax is present, this element indicates the lower bound of the allowed range of count values. 742 */ 743 @Child(name = "count", type = {PositiveIntType.class}, order=2, min=0, max=1, modifier=false, summary=true) 744 @Description(shortDefinition="Number of times to repeat", formalDefinition="A total count of the desired number of repetitions across the duration of the entire timing specification. If countMax is present, this element indicates the lower bound of the allowed range of count values." ) 745 protected PositiveIntType count; 746 747 /** 748 * If present, indicates that the count is a range - so to perform the action between [count] and [countMax] times. 749 */ 750 @Child(name = "countMax", type = {PositiveIntType.class}, order=3, min=0, max=1, modifier=false, summary=true) 751 @Description(shortDefinition="Maximum number of times to repeat", formalDefinition="If present, indicates that the count is a range - so to perform the action between [count] and [countMax] times." ) 752 protected PositiveIntType countMax; 753 754 /** 755 * How long this thing happens for when it happens. If durationMax is present, this element indicates the lower bound of the allowed range of the duration. 756 */ 757 @Child(name = "duration", type = {DecimalType.class}, order=4, min=0, max=1, modifier=false, summary=true) 758 @Description(shortDefinition="How long when it happens", formalDefinition="How long this thing happens for when it happens. If durationMax is present, this element indicates the lower bound of the allowed range of the duration." ) 759 protected DecimalType duration; 760 761 /** 762 * If present, indicates that the duration is a range - so to perform the action between [duration] and [durationMax] time length. 763 */ 764 @Child(name = "durationMax", type = {DecimalType.class}, order=5, min=0, max=1, modifier=false, summary=true) 765 @Description(shortDefinition="How long when it happens (Max)", formalDefinition="If present, indicates that the duration is a range - so to perform the action between [duration] and [durationMax] time length." ) 766 protected DecimalType durationMax; 767 768 /** 769 * The units of time for the duration, in UCUM units 770Normal practice is to use the 'mo' code as a calendar month when calculating the next occurrence. 771 */ 772 @Child(name = "durationUnit", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 773 @Description(shortDefinition="s | min | h | d | wk | mo | a - unit of time (UCUM)", formalDefinition="The units of time for the duration, in UCUM units\nNormal practice is to use the 'mo' code as a calendar month when calculating the next occurrence." ) 774 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/units-of-time") 775 protected Enumeration<UnitsOfTime> durationUnit; 776 777 /** 778 * The number of times to repeat the action within the specified period. If frequencyMax is present, this element indicates the lower bound of the allowed range of the frequency. 779 */ 780 @Child(name = "frequency", type = {PositiveIntType.class}, order=7, min=0, max=1, modifier=false, summary=true) 781 @Description(shortDefinition="Indicates the number of repetitions that should occur within a period. I.e. Event occurs frequency times per period", formalDefinition="The number of times to repeat the action within the specified period. If frequencyMax is present, this element indicates the lower bound of the allowed range of the frequency." ) 782 protected PositiveIntType frequency; 783 784 /** 785 * If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range. 786 */ 787 @Child(name = "frequencyMax", type = {PositiveIntType.class}, order=8, min=0, max=1, modifier=false, summary=true) 788 @Description(shortDefinition="Event occurs up to frequencyMax times per period", formalDefinition="If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range." ) 789 protected PositiveIntType frequencyMax; 790 791 /** 792 * Indicates the duration of time over which repetitions are to occur; e.g. to express "3 times per day", 3 would be the frequency and "1 day" would be the period. If periodMax is present, this element indicates the lower bound of the allowed range of the period length. 793 */ 794 @Child(name = "period", type = {DecimalType.class}, order=9, min=0, max=1, modifier=false, summary=true) 795 @Description(shortDefinition="The duration to which the frequency applies. I.e. Event occurs frequency times per period", formalDefinition="Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period. If periodMax is present, this element indicates the lower bound of the allowed range of the period length." ) 796 protected DecimalType period; 797 798 /** 799 * If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as "do this once every 3-5 days. 800 */ 801 @Child(name = "periodMax", type = {DecimalType.class}, order=10, min=0, max=1, modifier=false, summary=true) 802 @Description(shortDefinition="Upper limit of period (3-4 hours)", formalDefinition="If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days." ) 803 protected DecimalType periodMax; 804 805 /** 806 * The units of time for the period in UCUM units 807Normal practice is to use the 'mo' code as a calendar month when calculating the next occurrence. 808 */ 809 @Child(name = "periodUnit", type = {CodeType.class}, order=11, min=0, max=1, modifier=false, summary=true) 810 @Description(shortDefinition="s | min | h | d | wk | mo | a - unit of time (UCUM)", formalDefinition="The units of time for the period in UCUM units\nNormal practice is to use the 'mo' code as a calendar month when calculating the next occurrence." ) 811 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/units-of-time") 812 protected Enumeration<UnitsOfTime> periodUnit; 813 814 /** 815 * If one or more days of week is provided, then the action happens only on the specified day(s). 816 */ 817 @Child(name = "dayOfWeek", type = {CodeType.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 818 @Description(shortDefinition="mon | tue | wed | thu | fri | sat | sun", formalDefinition="If one or more days of week is provided, then the action happens only on the specified day(s)." ) 819 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/days-of-week") 820 protected List<Enumeration<DaysOfWeek>> dayOfWeek; 821 822 /** 823 * Specified time of day for action to take place. 824 */ 825 @Child(name = "timeOfDay", type = {TimeType.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 826 @Description(shortDefinition="Time of day for action", formalDefinition="Specified time of day for action to take place." ) 827 protected List<TimeType> timeOfDay; 828 829 /** 830 * An approximate time period during the day, potentially linked to an event of daily living that indicates when the action should occur. 831 */ 832 @Child(name = "when", type = {CodeType.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 833 @Description(shortDefinition="Code for time period of occurrence", formalDefinition="An approximate time period during the day, potentially linked to an event of daily living that indicates when the action should occur." ) 834 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/event-timing") 835 protected List<Enumeration<EventTiming>> when; 836 837 /** 838 * The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event. 839 */ 840 @Child(name = "offset", type = {UnsignedIntType.class}, order=15, min=0, max=1, modifier=false, summary=true) 841 @Description(shortDefinition="Minutes from event (before or after)", formalDefinition="The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event." ) 842 protected UnsignedIntType offset; 843 844 private static final long serialVersionUID = -122116223L; 845 846 /** 847 * Constructor 848 */ 849 public TimingRepeatComponent() { 850 super(); 851 } 852 853 /** 854 * @return {@link #bounds} (Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.) 855 */ 856 public DataType getBounds() { 857 return this.bounds; 858 } 859 860 /** 861 * @return {@link #bounds} (Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.) 862 */ 863 public Duration getBoundsDuration() throws FHIRException { 864 if (this.bounds == null) 865 this.bounds = new Duration(); 866 if (!(this.bounds instanceof Duration)) 867 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.bounds.getClass().getName()+" was encountered"); 868 return (Duration) this.bounds; 869 } 870 871 public boolean hasBoundsDuration() { 872 return this != null && this.bounds instanceof Duration; 873 } 874 875 /** 876 * @return {@link #bounds} (Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.) 877 */ 878 public Range getBoundsRange() throws FHIRException { 879 if (this.bounds == null) 880 this.bounds = new Range(); 881 if (!(this.bounds instanceof Range)) 882 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.bounds.getClass().getName()+" was encountered"); 883 return (Range) this.bounds; 884 } 885 886 public boolean hasBoundsRange() { 887 return this != null && this.bounds instanceof Range; 888 } 889 890 /** 891 * @return {@link #bounds} (Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.) 892 */ 893 public Period getBoundsPeriod() throws FHIRException { 894 if (this.bounds == null) 895 this.bounds = new Period(); 896 if (!(this.bounds instanceof Period)) 897 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.bounds.getClass().getName()+" was encountered"); 898 return (Period) this.bounds; 899 } 900 901 public boolean hasBoundsPeriod() { 902 return this != null && this.bounds instanceof Period; 903 } 904 905 public boolean hasBounds() { 906 return this.bounds != null && !this.bounds.isEmpty(); 907 } 908 909 /** 910 * @param value {@link #bounds} (Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.) 911 */ 912 public TimingRepeatComponent setBounds(DataType value) { 913 if (value != null && !(value instanceof Duration || value instanceof Range || value instanceof Period)) 914 throw new FHIRException("Not the right type for Timing.repeat.bounds[x]: "+value.fhirType()); 915 this.bounds = value; 916 return this; 917 } 918 919 /** 920 * @return {@link #count} (A total count of the desired number of repetitions across the duration of the entire timing specification. If countMax is present, this element indicates the lower bound of the allowed range of count values.). This is the underlying object with id, value and extensions. The accessor "getCount" gives direct access to the value 921 */ 922 public PositiveIntType getCountElement() { 923 if (this.count == null) 924 if (Configuration.errorOnAutoCreate()) 925 throw new Error("Attempt to auto-create TimingRepeatComponent.count"); 926 else if (Configuration.doAutoCreate()) 927 this.count = new PositiveIntType(); // bb 928 return this.count; 929 } 930 931 public boolean hasCountElement() { 932 return this.count != null && !this.count.isEmpty(); 933 } 934 935 public boolean hasCount() { 936 return this.count != null && !this.count.isEmpty(); 937 } 938 939 /** 940 * @param value {@link #count} (A total count of the desired number of repetitions across the duration of the entire timing specification. If countMax is present, this element indicates the lower bound of the allowed range of count values.). This is the underlying object with id, value and extensions. The accessor "getCount" gives direct access to the value 941 */ 942 public TimingRepeatComponent setCountElement(PositiveIntType value) { 943 this.count = value; 944 return this; 945 } 946 947 /** 948 * @return A total count of the desired number of repetitions across the duration of the entire timing specification. If countMax is present, this element indicates the lower bound of the allowed range of count values. 949 */ 950 public int getCount() { 951 return this.count == null || this.count.isEmpty() ? 0 : this.count.getValue(); 952 } 953 954 /** 955 * @param value A total count of the desired number of repetitions across the duration of the entire timing specification. If countMax is present, this element indicates the lower bound of the allowed range of count values. 956 */ 957 public TimingRepeatComponent setCount(int value) { 958 if (this.count == null) 959 this.count = new PositiveIntType(); 960 this.count.setValue(value); 961 return this; 962 } 963 964 /** 965 * @return {@link #countMax} (If present, indicates that the count is a range - so to perform the action between [count] and [countMax] times.). This is the underlying object with id, value and extensions. The accessor "getCountMax" gives direct access to the value 966 */ 967 public PositiveIntType getCountMaxElement() { 968 if (this.countMax == null) 969 if (Configuration.errorOnAutoCreate()) 970 throw new Error("Attempt to auto-create TimingRepeatComponent.countMax"); 971 else if (Configuration.doAutoCreate()) 972 this.countMax = new PositiveIntType(); // bb 973 return this.countMax; 974 } 975 976 public boolean hasCountMaxElement() { 977 return this.countMax != null && !this.countMax.isEmpty(); 978 } 979 980 public boolean hasCountMax() { 981 return this.countMax != null && !this.countMax.isEmpty(); 982 } 983 984 /** 985 * @param value {@link #countMax} (If present, indicates that the count is a range - so to perform the action between [count] and [countMax] times.). This is the underlying object with id, value and extensions. The accessor "getCountMax" gives direct access to the value 986 */ 987 public TimingRepeatComponent setCountMaxElement(PositiveIntType value) { 988 this.countMax = value; 989 return this; 990 } 991 992 /** 993 * @return If present, indicates that the count is a range - so to perform the action between [count] and [countMax] times. 994 */ 995 public int getCountMax() { 996 return this.countMax == null || this.countMax.isEmpty() ? 0 : this.countMax.getValue(); 997 } 998 999 /** 1000 * @param value If present, indicates that the count is a range - so to perform the action between [count] and [countMax] times. 1001 */ 1002 public TimingRepeatComponent setCountMax(int value) { 1003 if (this.countMax == null) 1004 this.countMax = new PositiveIntType(); 1005 this.countMax.setValue(value); 1006 return this; 1007 } 1008 1009 /** 1010 * @return {@link #duration} (How long this thing happens for when it happens. If durationMax is present, this element indicates the lower bound of the allowed range of the duration.). This is the underlying object with id, value and extensions. The accessor "getDuration" gives direct access to the value 1011 */ 1012 public DecimalType getDurationElement() { 1013 if (this.duration == null) 1014 if (Configuration.errorOnAutoCreate()) 1015 throw new Error("Attempt to auto-create TimingRepeatComponent.duration"); 1016 else if (Configuration.doAutoCreate()) 1017 this.duration = new DecimalType(); // bb 1018 return this.duration; 1019 } 1020 1021 public boolean hasDurationElement() { 1022 return this.duration != null && !this.duration.isEmpty(); 1023 } 1024 1025 public boolean hasDuration() { 1026 return this.duration != null && !this.duration.isEmpty(); 1027 } 1028 1029 /** 1030 * @param value {@link #duration} (How long this thing happens for when it happens. If durationMax is present, this element indicates the lower bound of the allowed range of the duration.). This is the underlying object with id, value and extensions. The accessor "getDuration" gives direct access to the value 1031 */ 1032 public TimingRepeatComponent setDurationElement(DecimalType value) { 1033 this.duration = value; 1034 return this; 1035 } 1036 1037 /** 1038 * @return How long this thing happens for when it happens. If durationMax is present, this element indicates the lower bound of the allowed range of the duration. 1039 */ 1040 public BigDecimal getDuration() { 1041 return this.duration == null ? null : this.duration.getValue(); 1042 } 1043 1044 /** 1045 * @param value How long this thing happens for when it happens. If durationMax is present, this element indicates the lower bound of the allowed range of the duration. 1046 */ 1047 public TimingRepeatComponent setDuration(BigDecimal value) { 1048 if (value == null) 1049 this.duration = null; 1050 else { 1051 if (this.duration == null) 1052 this.duration = new DecimalType(); 1053 this.duration.setValue(value); 1054 } 1055 return this; 1056 } 1057 1058 /** 1059 * @param value How long this thing happens for when it happens. If durationMax is present, this element indicates the lower bound of the allowed range of the duration. 1060 */ 1061 public TimingRepeatComponent setDuration(long value) { 1062 this.duration = new DecimalType(); 1063 this.duration.setValue(value); 1064 return this; 1065 } 1066 1067 /** 1068 * @param value How long this thing happens for when it happens. If durationMax is present, this element indicates the lower bound of the allowed range of the duration. 1069 */ 1070 public TimingRepeatComponent setDuration(double value) { 1071 this.duration = new DecimalType(); 1072 this.duration.setValue(value); 1073 return this; 1074 } 1075 1076 /** 1077 * @return {@link #durationMax} (If present, indicates that the duration is a range - so to perform the action between [duration] and [durationMax] time length.). This is the underlying object with id, value and extensions. The accessor "getDurationMax" gives direct access to the value 1078 */ 1079 public DecimalType getDurationMaxElement() { 1080 if (this.durationMax == null) 1081 if (Configuration.errorOnAutoCreate()) 1082 throw new Error("Attempt to auto-create TimingRepeatComponent.durationMax"); 1083 else if (Configuration.doAutoCreate()) 1084 this.durationMax = new DecimalType(); // bb 1085 return this.durationMax; 1086 } 1087 1088 public boolean hasDurationMaxElement() { 1089 return this.durationMax != null && !this.durationMax.isEmpty(); 1090 } 1091 1092 public boolean hasDurationMax() { 1093 return this.durationMax != null && !this.durationMax.isEmpty(); 1094 } 1095 1096 /** 1097 * @param value {@link #durationMax} (If present, indicates that the duration is a range - so to perform the action between [duration] and [durationMax] time length.). This is the underlying object with id, value and extensions. The accessor "getDurationMax" gives direct access to the value 1098 */ 1099 public TimingRepeatComponent setDurationMaxElement(DecimalType value) { 1100 this.durationMax = value; 1101 return this; 1102 } 1103 1104 /** 1105 * @return If present, indicates that the duration is a range - so to perform the action between [duration] and [durationMax] time length. 1106 */ 1107 public BigDecimal getDurationMax() { 1108 return this.durationMax == null ? null : this.durationMax.getValue(); 1109 } 1110 1111 /** 1112 * @param value If present, indicates that the duration is a range - so to perform the action between [duration] and [durationMax] time length. 1113 */ 1114 public TimingRepeatComponent setDurationMax(BigDecimal value) { 1115 if (value == null) 1116 this.durationMax = null; 1117 else { 1118 if (this.durationMax == null) 1119 this.durationMax = new DecimalType(); 1120 this.durationMax.setValue(value); 1121 } 1122 return this; 1123 } 1124 1125 /** 1126 * @param value If present, indicates that the duration is a range - so to perform the action between [duration] and [durationMax] time length. 1127 */ 1128 public TimingRepeatComponent setDurationMax(long value) { 1129 this.durationMax = new DecimalType(); 1130 this.durationMax.setValue(value); 1131 return this; 1132 } 1133 1134 /** 1135 * @param value If present, indicates that the duration is a range - so to perform the action between [duration] and [durationMax] time length. 1136 */ 1137 public TimingRepeatComponent setDurationMax(double value) { 1138 this.durationMax = new DecimalType(); 1139 this.durationMax.setValue(value); 1140 return this; 1141 } 1142 1143 /** 1144 * @return {@link #durationUnit} (The units of time for the duration, in UCUM units 1145Normal practice is to use the 'mo' code as a calendar month when calculating the next occurrence.). This is the underlying object with id, value and extensions. The accessor "getDurationUnit" gives direct access to the value 1146 */ 1147 public Enumeration<UnitsOfTime> getDurationUnitElement() { 1148 if (this.durationUnit == null) 1149 if (Configuration.errorOnAutoCreate()) 1150 throw new Error("Attempt to auto-create TimingRepeatComponent.durationUnit"); 1151 else if (Configuration.doAutoCreate()) 1152 this.durationUnit = new Enumeration<UnitsOfTime>(new UnitsOfTimeEnumFactory()); // bb 1153 return this.durationUnit; 1154 } 1155 1156 public boolean hasDurationUnitElement() { 1157 return this.durationUnit != null && !this.durationUnit.isEmpty(); 1158 } 1159 1160 public boolean hasDurationUnit() { 1161 return this.durationUnit != null && !this.durationUnit.isEmpty(); 1162 } 1163 1164 /** 1165 * @param value {@link #durationUnit} (The units of time for the duration, in UCUM units 1166Normal practice is to use the 'mo' code as a calendar month when calculating the next occurrence.). This is the underlying object with id, value and extensions. The accessor "getDurationUnit" gives direct access to the value 1167 */ 1168 public TimingRepeatComponent setDurationUnitElement(Enumeration<UnitsOfTime> value) { 1169 this.durationUnit = value; 1170 return this; 1171 } 1172 1173 /** 1174 * @return The units of time for the duration, in UCUM units 1175Normal practice is to use the 'mo' code as a calendar month when calculating the next occurrence. 1176 */ 1177 public UnitsOfTime getDurationUnit() { 1178 return this.durationUnit == null ? null : this.durationUnit.getValue(); 1179 } 1180 1181 /** 1182 * @param value The units of time for the duration, in UCUM units 1183Normal practice is to use the 'mo' code as a calendar month when calculating the next occurrence. 1184 */ 1185 public TimingRepeatComponent setDurationUnit(UnitsOfTime value) { 1186 if (value == null) 1187 this.durationUnit = null; 1188 else { 1189 if (this.durationUnit == null) 1190 this.durationUnit = new Enumeration<UnitsOfTime>(new UnitsOfTimeEnumFactory()); 1191 this.durationUnit.setValue(value); 1192 } 1193 return this; 1194 } 1195 1196 /** 1197 * @return {@link #frequency} (The number of times to repeat the action within the specified period. If frequencyMax is present, this element indicates the lower bound of the allowed range of the frequency.). This is the underlying object with id, value and extensions. The accessor "getFrequency" gives direct access to the value 1198 */ 1199 public PositiveIntType getFrequencyElement() { 1200 if (this.frequency == null) 1201 if (Configuration.errorOnAutoCreate()) 1202 throw new Error("Attempt to auto-create TimingRepeatComponent.frequency"); 1203 else if (Configuration.doAutoCreate()) 1204 this.frequency = new PositiveIntType(); // bb 1205 return this.frequency; 1206 } 1207 1208 public boolean hasFrequencyElement() { 1209 return this.frequency != null && !this.frequency.isEmpty(); 1210 } 1211 1212 public boolean hasFrequency() { 1213 return this.frequency != null && !this.frequency.isEmpty(); 1214 } 1215 1216 /** 1217 * @param value {@link #frequency} (The number of times to repeat the action within the specified period. If frequencyMax is present, this element indicates the lower bound of the allowed range of the frequency.). This is the underlying object with id, value and extensions. The accessor "getFrequency" gives direct access to the value 1218 */ 1219 public TimingRepeatComponent setFrequencyElement(PositiveIntType value) { 1220 this.frequency = value; 1221 return this; 1222 } 1223 1224 /** 1225 * @return The number of times to repeat the action within the specified period. If frequencyMax is present, this element indicates the lower bound of the allowed range of the frequency. 1226 */ 1227 public int getFrequency() { 1228 return this.frequency == null || this.frequency.isEmpty() ? 0 : this.frequency.getValue(); 1229 } 1230 1231 /** 1232 * @param value The number of times to repeat the action within the specified period. If frequencyMax is present, this element indicates the lower bound of the allowed range of the frequency. 1233 */ 1234 public TimingRepeatComponent setFrequency(int value) { 1235 if (this.frequency == null) 1236 this.frequency = new PositiveIntType(); 1237 this.frequency.setValue(value); 1238 return this; 1239 } 1240 1241 /** 1242 * @return {@link #frequencyMax} (If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range.). This is the underlying object with id, value and extensions. The accessor "getFrequencyMax" gives direct access to the value 1243 */ 1244 public PositiveIntType getFrequencyMaxElement() { 1245 if (this.frequencyMax == null) 1246 if (Configuration.errorOnAutoCreate()) 1247 throw new Error("Attempt to auto-create TimingRepeatComponent.frequencyMax"); 1248 else if (Configuration.doAutoCreate()) 1249 this.frequencyMax = new PositiveIntType(); // bb 1250 return this.frequencyMax; 1251 } 1252 1253 public boolean hasFrequencyMaxElement() { 1254 return this.frequencyMax != null && !this.frequencyMax.isEmpty(); 1255 } 1256 1257 public boolean hasFrequencyMax() { 1258 return this.frequencyMax != null && !this.frequencyMax.isEmpty(); 1259 } 1260 1261 /** 1262 * @param value {@link #frequencyMax} (If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range.). This is the underlying object with id, value and extensions. The accessor "getFrequencyMax" gives direct access to the value 1263 */ 1264 public TimingRepeatComponent setFrequencyMaxElement(PositiveIntType value) { 1265 this.frequencyMax = value; 1266 return this; 1267 } 1268 1269 /** 1270 * @return If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range. 1271 */ 1272 public int getFrequencyMax() { 1273 return this.frequencyMax == null || this.frequencyMax.isEmpty() ? 0 : this.frequencyMax.getValue(); 1274 } 1275 1276 /** 1277 * @param value If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range. 1278 */ 1279 public TimingRepeatComponent setFrequencyMax(int value) { 1280 if (this.frequencyMax == null) 1281 this.frequencyMax = new PositiveIntType(); 1282 this.frequencyMax.setValue(value); 1283 return this; 1284 } 1285 1286 /** 1287 * @return {@link #period} (Indicates the duration of time over which repetitions are to occur; e.g. to express "3 times per day", 3 would be the frequency and "1 day" would be the period. If periodMax is present, this element indicates the lower bound of the allowed range of the period length.). This is the underlying object with id, value and extensions. The accessor "getPeriod" gives direct access to the value 1288 */ 1289 public DecimalType getPeriodElement() { 1290 if (this.period == null) 1291 if (Configuration.errorOnAutoCreate()) 1292 throw new Error("Attempt to auto-create TimingRepeatComponent.period"); 1293 else if (Configuration.doAutoCreate()) 1294 this.period = new DecimalType(); // bb 1295 return this.period; 1296 } 1297 1298 public boolean hasPeriodElement() { 1299 return this.period != null && !this.period.isEmpty(); 1300 } 1301 1302 public boolean hasPeriod() { 1303 return this.period != null && !this.period.isEmpty(); 1304 } 1305 1306 /** 1307 * @param value {@link #period} (Indicates the duration of time over which repetitions are to occur; e.g. to express "3 times per day", 3 would be the frequency and "1 day" would be the period. If periodMax is present, this element indicates the lower bound of the allowed range of the period length.). This is the underlying object with id, value and extensions. The accessor "getPeriod" gives direct access to the value 1308 */ 1309 public TimingRepeatComponent setPeriodElement(DecimalType value) { 1310 this.period = value; 1311 return this; 1312 } 1313 1314 /** 1315 * @return Indicates the duration of time over which repetitions are to occur; e.g. to express "3 times per day", 3 would be the frequency and "1 day" would be the period. If periodMax is present, this element indicates the lower bound of the allowed range of the period length. 1316 */ 1317 public BigDecimal getPeriod() { 1318 return this.period == null ? null : this.period.getValue(); 1319 } 1320 1321 /** 1322 * @param value Indicates the duration of time over which repetitions are to occur; e.g. to express "3 times per day", 3 would be the frequency and "1 day" would be the period. If periodMax is present, this element indicates the lower bound of the allowed range of the period length. 1323 */ 1324 public TimingRepeatComponent setPeriod(BigDecimal value) { 1325 if (value == null) 1326 this.period = null; 1327 else { 1328 if (this.period == null) 1329 this.period = new DecimalType(); 1330 this.period.setValue(value); 1331 } 1332 return this; 1333 } 1334 1335 /** 1336 * @param value Indicates the duration of time over which repetitions are to occur; e.g. to express "3 times per day", 3 would be the frequency and "1 day" would be the period. If periodMax is present, this element indicates the lower bound of the allowed range of the period length. 1337 */ 1338 public TimingRepeatComponent setPeriod(long value) { 1339 this.period = new DecimalType(); 1340 this.period.setValue(value); 1341 return this; 1342 } 1343 1344 /** 1345 * @param value Indicates the duration of time over which repetitions are to occur; e.g. to express "3 times per day", 3 would be the frequency and "1 day" would be the period. If periodMax is present, this element indicates the lower bound of the allowed range of the period length. 1346 */ 1347 public TimingRepeatComponent setPeriod(double value) { 1348 this.period = new DecimalType(); 1349 this.period.setValue(value); 1350 return this; 1351 } 1352 1353 /** 1354 * @return {@link #periodMax} (If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as "do this once every 3-5 days.). This is the underlying object with id, value and extensions. The accessor "getPeriodMax" gives direct access to the value 1355 */ 1356 public DecimalType getPeriodMaxElement() { 1357 if (this.periodMax == null) 1358 if (Configuration.errorOnAutoCreate()) 1359 throw new Error("Attempt to auto-create TimingRepeatComponent.periodMax"); 1360 else if (Configuration.doAutoCreate()) 1361 this.periodMax = new DecimalType(); // bb 1362 return this.periodMax; 1363 } 1364 1365 public boolean hasPeriodMaxElement() { 1366 return this.periodMax != null && !this.periodMax.isEmpty(); 1367 } 1368 1369 public boolean hasPeriodMax() { 1370 return this.periodMax != null && !this.periodMax.isEmpty(); 1371 } 1372 1373 /** 1374 * @param value {@link #periodMax} (If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as "do this once every 3-5 days.). This is the underlying object with id, value and extensions. The accessor "getPeriodMax" gives direct access to the value 1375 */ 1376 public TimingRepeatComponent setPeriodMaxElement(DecimalType value) { 1377 this.periodMax = value; 1378 return this; 1379 } 1380 1381 /** 1382 * @return If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as "do this once every 3-5 days. 1383 */ 1384 public BigDecimal getPeriodMax() { 1385 return this.periodMax == null ? null : this.periodMax.getValue(); 1386 } 1387 1388 /** 1389 * @param value If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as "do this once every 3-5 days. 1390 */ 1391 public TimingRepeatComponent setPeriodMax(BigDecimal value) { 1392 if (value == null) 1393 this.periodMax = null; 1394 else { 1395 if (this.periodMax == null) 1396 this.periodMax = new DecimalType(); 1397 this.periodMax.setValue(value); 1398 } 1399 return this; 1400 } 1401 1402 /** 1403 * @param value If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as "do this once every 3-5 days. 1404 */ 1405 public TimingRepeatComponent setPeriodMax(long value) { 1406 this.periodMax = new DecimalType(); 1407 this.periodMax.setValue(value); 1408 return this; 1409 } 1410 1411 /** 1412 * @param value If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as "do this once every 3-5 days. 1413 */ 1414 public TimingRepeatComponent setPeriodMax(double value) { 1415 this.periodMax = new DecimalType(); 1416 this.periodMax.setValue(value); 1417 return this; 1418 } 1419 1420 /** 1421 * @return {@link #periodUnit} (The units of time for the period in UCUM units 1422Normal practice is to use the 'mo' code as a calendar month when calculating the next occurrence.). This is the underlying object with id, value and extensions. The accessor "getPeriodUnit" gives direct access to the value 1423 */ 1424 public Enumeration<UnitsOfTime> getPeriodUnitElement() { 1425 if (this.periodUnit == null) 1426 if (Configuration.errorOnAutoCreate()) 1427 throw new Error("Attempt to auto-create TimingRepeatComponent.periodUnit"); 1428 else if (Configuration.doAutoCreate()) 1429 this.periodUnit = new Enumeration<UnitsOfTime>(new UnitsOfTimeEnumFactory()); // bb 1430 return this.periodUnit; 1431 } 1432 1433 public boolean hasPeriodUnitElement() { 1434 return this.periodUnit != null && !this.periodUnit.isEmpty(); 1435 } 1436 1437 public boolean hasPeriodUnit() { 1438 return this.periodUnit != null && !this.periodUnit.isEmpty(); 1439 } 1440 1441 /** 1442 * @param value {@link #periodUnit} (The units of time for the period in UCUM units 1443Normal practice is to use the 'mo' code as a calendar month when calculating the next occurrence.). This is the underlying object with id, value and extensions. The accessor "getPeriodUnit" gives direct access to the value 1444 */ 1445 public TimingRepeatComponent setPeriodUnitElement(Enumeration<UnitsOfTime> value) { 1446 this.periodUnit = value; 1447 return this; 1448 } 1449 1450 /** 1451 * @return The units of time for the period in UCUM units 1452Normal practice is to use the 'mo' code as a calendar month when calculating the next occurrence. 1453 */ 1454 public UnitsOfTime getPeriodUnit() { 1455 return this.periodUnit == null ? null : this.periodUnit.getValue(); 1456 } 1457 1458 /** 1459 * @param value The units of time for the period in UCUM units 1460Normal practice is to use the 'mo' code as a calendar month when calculating the next occurrence. 1461 */ 1462 public TimingRepeatComponent setPeriodUnit(UnitsOfTime value) { 1463 if (value == null) 1464 this.periodUnit = null; 1465 else { 1466 if (this.periodUnit == null) 1467 this.periodUnit = new Enumeration<UnitsOfTime>(new UnitsOfTimeEnumFactory()); 1468 this.periodUnit.setValue(value); 1469 } 1470 return this; 1471 } 1472 1473 /** 1474 * @return {@link #dayOfWeek} (If one or more days of week is provided, then the action happens only on the specified day(s).) 1475 */ 1476 public List<Enumeration<DaysOfWeek>> getDayOfWeek() { 1477 if (this.dayOfWeek == null) 1478 this.dayOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 1479 return this.dayOfWeek; 1480 } 1481 1482 /** 1483 * @return Returns a reference to <code>this</code> for easy method chaining 1484 */ 1485 public TimingRepeatComponent setDayOfWeek(List<Enumeration<DaysOfWeek>> theDayOfWeek) { 1486 this.dayOfWeek = theDayOfWeek; 1487 return this; 1488 } 1489 1490 public boolean hasDayOfWeek() { 1491 if (this.dayOfWeek == null) 1492 return false; 1493 for (Enumeration<DaysOfWeek> item : this.dayOfWeek) 1494 if (!item.isEmpty()) 1495 return true; 1496 return false; 1497 } 1498 1499 /** 1500 * @return {@link #dayOfWeek} (If one or more days of week is provided, then the action happens only on the specified day(s).) 1501 */ 1502 public Enumeration<DaysOfWeek> addDayOfWeekElement() {//2 1503 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 1504 if (this.dayOfWeek == null) 1505 this.dayOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 1506 this.dayOfWeek.add(t); 1507 return t; 1508 } 1509 1510 /** 1511 * @param value {@link #dayOfWeek} (If one or more days of week is provided, then the action happens only on the specified day(s).) 1512 */ 1513 public TimingRepeatComponent addDayOfWeek(DaysOfWeek value) { //1 1514 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 1515 t.setValue(value); 1516 if (this.dayOfWeek == null) 1517 this.dayOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 1518 this.dayOfWeek.add(t); 1519 return this; 1520 } 1521 1522 /** 1523 * @param value {@link #dayOfWeek} (If one or more days of week is provided, then the action happens only on the specified day(s).) 1524 */ 1525 public boolean hasDayOfWeek(DaysOfWeek value) { 1526 if (this.dayOfWeek == null) 1527 return false; 1528 for (Enumeration<DaysOfWeek> v : this.dayOfWeek) 1529 if (v.getValue().equals(value)) // code 1530 return true; 1531 return false; 1532 } 1533 1534 /** 1535 * @return {@link #timeOfDay} (Specified time of day for action to take place.) 1536 */ 1537 public List<TimeType> getTimeOfDay() { 1538 if (this.timeOfDay == null) 1539 this.timeOfDay = new ArrayList<TimeType>(); 1540 return this.timeOfDay; 1541 } 1542 1543 /** 1544 * @return Returns a reference to <code>this</code> for easy method chaining 1545 */ 1546 public TimingRepeatComponent setTimeOfDay(List<TimeType> theTimeOfDay) { 1547 this.timeOfDay = theTimeOfDay; 1548 return this; 1549 } 1550 1551 public boolean hasTimeOfDay() { 1552 if (this.timeOfDay == null) 1553 return false; 1554 for (TimeType item : this.timeOfDay) 1555 if (!item.isEmpty()) 1556 return true; 1557 return false; 1558 } 1559 1560 /** 1561 * @return {@link #timeOfDay} (Specified time of day for action to take place.) 1562 */ 1563 public TimeType addTimeOfDayElement() {//2 1564 TimeType t = new TimeType(); 1565 if (this.timeOfDay == null) 1566 this.timeOfDay = new ArrayList<TimeType>(); 1567 this.timeOfDay.add(t); 1568 return t; 1569 } 1570 1571 /** 1572 * @param value {@link #timeOfDay} (Specified time of day for action to take place.) 1573 */ 1574 public TimingRepeatComponent addTimeOfDay(String value) { //1 1575 TimeType t = new TimeType(); 1576 t.setValue(value); 1577 if (this.timeOfDay == null) 1578 this.timeOfDay = new ArrayList<TimeType>(); 1579 this.timeOfDay.add(t); 1580 return this; 1581 } 1582 1583 /** 1584 * @param value {@link #timeOfDay} (Specified time of day for action to take place.) 1585 */ 1586 public boolean hasTimeOfDay(String value) { 1587 if (this.timeOfDay == null) 1588 return false; 1589 for (TimeType v : this.timeOfDay) 1590 if (v.getValue().equals(value)) // time 1591 return true; 1592 return false; 1593 } 1594 1595 /** 1596 * @return {@link #when} (An approximate time period during the day, potentially linked to an event of daily living that indicates when the action should occur.) 1597 */ 1598 public List<Enumeration<EventTiming>> getWhen() { 1599 if (this.when == null) 1600 this.when = new ArrayList<Enumeration<EventTiming>>(); 1601 return this.when; 1602 } 1603 1604 /** 1605 * @return Returns a reference to <code>this</code> for easy method chaining 1606 */ 1607 public TimingRepeatComponent setWhen(List<Enumeration<EventTiming>> theWhen) { 1608 this.when = theWhen; 1609 return this; 1610 } 1611 1612 public boolean hasWhen() { 1613 if (this.when == null) 1614 return false; 1615 for (Enumeration<EventTiming> item : this.when) 1616 if (!item.isEmpty()) 1617 return true; 1618 return false; 1619 } 1620 1621 /** 1622 * @return {@link #when} (An approximate time period during the day, potentially linked to an event of daily living that indicates when the action should occur.) 1623 */ 1624 public Enumeration<EventTiming> addWhenElement() {//2 1625 Enumeration<EventTiming> t = new Enumeration<EventTiming>(new EventTimingEnumFactory()); 1626 if (this.when == null) 1627 this.when = new ArrayList<Enumeration<EventTiming>>(); 1628 this.when.add(t); 1629 return t; 1630 } 1631 1632 /** 1633 * @param value {@link #when} (An approximate time period during the day, potentially linked to an event of daily living that indicates when the action should occur.) 1634 */ 1635 public TimingRepeatComponent addWhen(EventTiming value) { //1 1636 Enumeration<EventTiming> t = new Enumeration<EventTiming>(new EventTimingEnumFactory()); 1637 t.setValue(value); 1638 if (this.when == null) 1639 this.when = new ArrayList<Enumeration<EventTiming>>(); 1640 this.when.add(t); 1641 return this; 1642 } 1643 1644 /** 1645 * @param value {@link #when} (An approximate time period during the day, potentially linked to an event of daily living that indicates when the action should occur.) 1646 */ 1647 public boolean hasWhen(EventTiming value) { 1648 if (this.when == null) 1649 return false; 1650 for (Enumeration<EventTiming> v : this.when) 1651 if (v.getValue().equals(value)) // code 1652 return true; 1653 return false; 1654 } 1655 1656 /** 1657 * @return {@link #offset} (The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event.). This is the underlying object with id, value and extensions. The accessor "getOffset" gives direct access to the value 1658 */ 1659 public UnsignedIntType getOffsetElement() { 1660 if (this.offset == null) 1661 if (Configuration.errorOnAutoCreate()) 1662 throw new Error("Attempt to auto-create TimingRepeatComponent.offset"); 1663 else if (Configuration.doAutoCreate()) 1664 this.offset = new UnsignedIntType(); // bb 1665 return this.offset; 1666 } 1667 1668 public boolean hasOffsetElement() { 1669 return this.offset != null && !this.offset.isEmpty(); 1670 } 1671 1672 public boolean hasOffset() { 1673 return this.offset != null && !this.offset.isEmpty(); 1674 } 1675 1676 /** 1677 * @param value {@link #offset} (The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event.). This is the underlying object with id, value and extensions. The accessor "getOffset" gives direct access to the value 1678 */ 1679 public TimingRepeatComponent setOffsetElement(UnsignedIntType value) { 1680 this.offset = value; 1681 return this; 1682 } 1683 1684 /** 1685 * @return The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event. 1686 */ 1687 public int getOffset() { 1688 return this.offset == null || this.offset.isEmpty() ? 0 : this.offset.getValue(); 1689 } 1690 1691 /** 1692 * @param value The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event. 1693 */ 1694 public TimingRepeatComponent setOffset(int value) { 1695 if (this.offset == null) 1696 this.offset = new UnsignedIntType(); 1697 this.offset.setValue(value); 1698 return this; 1699 } 1700 1701 protected void listChildren(List<Property> children) { 1702 super.listChildren(children); 1703 children.add(new Property("bounds[x]", "Duration|Range|Period", "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 0, 1, bounds)); 1704 children.add(new Property("count", "positiveInt", "A total count of the desired number of repetitions across the duration of the entire timing specification. If countMax is present, this element indicates the lower bound of the allowed range of count values.", 0, 1, count)); 1705 children.add(new Property("countMax", "positiveInt", "If present, indicates that the count is a range - so to perform the action between [count] and [countMax] times.", 0, 1, countMax)); 1706 children.add(new Property("duration", "decimal", "How long this thing happens for when it happens. If durationMax is present, this element indicates the lower bound of the allowed range of the duration.", 0, 1, duration)); 1707 children.add(new Property("durationMax", "decimal", "If present, indicates that the duration is a range - so to perform the action between [duration] and [durationMax] time length.", 0, 1, durationMax)); 1708 children.add(new Property("durationUnit", "code", "The units of time for the duration, in UCUM units\nNormal practice is to use the 'mo' code as a calendar month when calculating the next occurrence.", 0, 1, durationUnit)); 1709 children.add(new Property("frequency", "positiveInt", "The number of times to repeat the action within the specified period. If frequencyMax is present, this element indicates the lower bound of the allowed range of the frequency.", 0, 1, frequency)); 1710 children.add(new Property("frequencyMax", "positiveInt", "If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range.", 0, 1, frequencyMax)); 1711 children.add(new Property("period", "decimal", "Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period. If periodMax is present, this element indicates the lower bound of the allowed range of the period length.", 0, 1, period)); 1712 children.add(new Property("periodMax", "decimal", "If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days.", 0, 1, periodMax)); 1713 children.add(new Property("periodUnit", "code", "The units of time for the period in UCUM units\nNormal practice is to use the 'mo' code as a calendar month when calculating the next occurrence.", 0, 1, periodUnit)); 1714 children.add(new Property("dayOfWeek", "code", "If one or more days of week is provided, then the action happens only on the specified day(s).", 0, java.lang.Integer.MAX_VALUE, dayOfWeek)); 1715 children.add(new Property("timeOfDay", "time", "Specified time of day for action to take place.", 0, java.lang.Integer.MAX_VALUE, timeOfDay)); 1716 children.add(new Property("when", "code", "An approximate time period during the day, potentially linked to an event of daily living that indicates when the action should occur.", 0, java.lang.Integer.MAX_VALUE, when)); 1717 children.add(new Property("offset", "unsignedInt", "The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event.", 0, 1, offset)); 1718 } 1719 1720 @Override 1721 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1722 switch (_hash) { 1723 case -1149635157: /*bounds[x]*/ return new Property("bounds[x]", "Duration|Range|Period", "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 0, 1, bounds); 1724 case -1383205195: /*bounds*/ return new Property("bounds[x]", "Duration|Range|Period", "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 0, 1, bounds); 1725 case -189193367: /*boundsDuration*/ return new Property("bounds[x]", "Duration", "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 0, 1, bounds); 1726 case -1001768056: /*boundsRange*/ return new Property("bounds[x]", "Range", "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 0, 1, bounds); 1727 case -1043481386: /*boundsPeriod*/ return new Property("bounds[x]", "Period", "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 0, 1, bounds); 1728 case 94851343: /*count*/ return new Property("count", "positiveInt", "A total count of the desired number of repetitions across the duration of the entire timing specification. If countMax is present, this element indicates the lower bound of the allowed range of count values.", 0, 1, count); 1729 case -372044331: /*countMax*/ return new Property("countMax", "positiveInt", "If present, indicates that the count is a range - so to perform the action between [count] and [countMax] times.", 0, 1, countMax); 1730 case -1992012396: /*duration*/ return new Property("duration", "decimal", "How long this thing happens for when it happens. If durationMax is present, this element indicates the lower bound of the allowed range of the duration.", 0, 1, duration); 1731 case -478083280: /*durationMax*/ return new Property("durationMax", "decimal", "If present, indicates that the duration is a range - so to perform the action between [duration] and [durationMax] time length.", 0, 1, durationMax); 1732 case -1935429320: /*durationUnit*/ return new Property("durationUnit", "code", "The units of time for the duration, in UCUM units\nNormal practice is to use the 'mo' code as a calendar month when calculating the next occurrence.", 0, 1, durationUnit); 1733 case -70023844: /*frequency*/ return new Property("frequency", "positiveInt", "The number of times to repeat the action within the specified period. If frequencyMax is present, this element indicates the lower bound of the allowed range of the frequency.", 0, 1, frequency); 1734 case 1273846376: /*frequencyMax*/ return new Property("frequencyMax", "positiveInt", "If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range.", 0, 1, frequencyMax); 1735 case -991726143: /*period*/ return new Property("period", "decimal", "Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period. If periodMax is present, this element indicates the lower bound of the allowed range of the period length.", 0, 1, period); 1736 case 566580195: /*periodMax*/ return new Property("periodMax", "decimal", "If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days.", 0, 1, periodMax); 1737 case 384367333: /*periodUnit*/ return new Property("periodUnit", "code", "The units of time for the period in UCUM units\nNormal practice is to use the 'mo' code as a calendar month when calculating the next occurrence.", 0, 1, periodUnit); 1738 case -730552025: /*dayOfWeek*/ return new Property("dayOfWeek", "code", "If one or more days of week is provided, then the action happens only on the specified day(s).", 0, java.lang.Integer.MAX_VALUE, dayOfWeek); 1739 case 21434232: /*timeOfDay*/ return new Property("timeOfDay", "time", "Specified time of day for action to take place.", 0, java.lang.Integer.MAX_VALUE, timeOfDay); 1740 case 3648314: /*when*/ return new Property("when", "code", "An approximate time period during the day, potentially linked to an event of daily living that indicates when the action should occur.", 0, java.lang.Integer.MAX_VALUE, when); 1741 case -1019779949: /*offset*/ return new Property("offset", "unsignedInt", "The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event.", 0, 1, offset); 1742 default: return super.getNamedProperty(_hash, _name, _checkValid); 1743 } 1744 1745 } 1746 1747 @Override 1748 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1749 switch (hash) { 1750 case -1383205195: /*bounds*/ return this.bounds == null ? new Base[0] : new Base[] {this.bounds}; // DataType 1751 case 94851343: /*count*/ return this.count == null ? new Base[0] : new Base[] {this.count}; // PositiveIntType 1752 case -372044331: /*countMax*/ return this.countMax == null ? new Base[0] : new Base[] {this.countMax}; // PositiveIntType 1753 case -1992012396: /*duration*/ return this.duration == null ? new Base[0] : new Base[] {this.duration}; // DecimalType 1754 case -478083280: /*durationMax*/ return this.durationMax == null ? new Base[0] : new Base[] {this.durationMax}; // DecimalType 1755 case -1935429320: /*durationUnit*/ return this.durationUnit == null ? new Base[0] : new Base[] {this.durationUnit}; // Enumeration<UnitsOfTime> 1756 case -70023844: /*frequency*/ return this.frequency == null ? new Base[0] : new Base[] {this.frequency}; // PositiveIntType 1757 case 1273846376: /*frequencyMax*/ return this.frequencyMax == null ? new Base[0] : new Base[] {this.frequencyMax}; // PositiveIntType 1758 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // DecimalType 1759 case 566580195: /*periodMax*/ return this.periodMax == null ? new Base[0] : new Base[] {this.periodMax}; // DecimalType 1760 case 384367333: /*periodUnit*/ return this.periodUnit == null ? new Base[0] : new Base[] {this.periodUnit}; // Enumeration<UnitsOfTime> 1761 case -730552025: /*dayOfWeek*/ return this.dayOfWeek == null ? new Base[0] : this.dayOfWeek.toArray(new Base[this.dayOfWeek.size()]); // Enumeration<DaysOfWeek> 1762 case 21434232: /*timeOfDay*/ return this.timeOfDay == null ? new Base[0] : this.timeOfDay.toArray(new Base[this.timeOfDay.size()]); // TimeType 1763 case 3648314: /*when*/ return this.when == null ? new Base[0] : this.when.toArray(new Base[this.when.size()]); // Enumeration<EventTiming> 1764 case -1019779949: /*offset*/ return this.offset == null ? new Base[0] : new Base[] {this.offset}; // UnsignedIntType 1765 default: return super.getProperty(hash, name, checkValid); 1766 } 1767 1768 } 1769 1770 @Override 1771 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1772 switch (hash) { 1773 case -1383205195: // bounds 1774 this.bounds = TypeConvertor.castToType(value); // DataType 1775 return value; 1776 case 94851343: // count 1777 this.count = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1778 return value; 1779 case -372044331: // countMax 1780 this.countMax = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1781 return value; 1782 case -1992012396: // duration 1783 this.duration = TypeConvertor.castToDecimal(value); // DecimalType 1784 return value; 1785 case -478083280: // durationMax 1786 this.durationMax = TypeConvertor.castToDecimal(value); // DecimalType 1787 return value; 1788 case -1935429320: // durationUnit 1789 value = new UnitsOfTimeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1790 this.durationUnit = (Enumeration) value; // Enumeration<UnitsOfTime> 1791 return value; 1792 case -70023844: // frequency 1793 this.frequency = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1794 return value; 1795 case 1273846376: // frequencyMax 1796 this.frequencyMax = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1797 return value; 1798 case -991726143: // period 1799 this.period = TypeConvertor.castToDecimal(value); // DecimalType 1800 return value; 1801 case 566580195: // periodMax 1802 this.periodMax = TypeConvertor.castToDecimal(value); // DecimalType 1803 return value; 1804 case 384367333: // periodUnit 1805 value = new UnitsOfTimeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1806 this.periodUnit = (Enumeration) value; // Enumeration<UnitsOfTime> 1807 return value; 1808 case -730552025: // dayOfWeek 1809 value = new DaysOfWeekEnumFactory().fromType(TypeConvertor.castToCode(value)); 1810 this.getDayOfWeek().add((Enumeration) value); // Enumeration<DaysOfWeek> 1811 return value; 1812 case 21434232: // timeOfDay 1813 this.getTimeOfDay().add(TypeConvertor.castToTime(value)); // TimeType 1814 return value; 1815 case 3648314: // when 1816 value = new EventTimingEnumFactory().fromType(TypeConvertor.castToCode(value)); 1817 this.getWhen().add((Enumeration) value); // Enumeration<EventTiming> 1818 return value; 1819 case -1019779949: // offset 1820 this.offset = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1821 return value; 1822 default: return super.setProperty(hash, name, value); 1823 } 1824 1825 } 1826 1827 @Override 1828 public Base setProperty(String name, Base value) throws FHIRException { 1829 if (name.equals("bounds[x]")) { 1830 this.bounds = TypeConvertor.castToType(value); // DataType 1831 } else if (name.equals("count")) { 1832 this.count = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1833 } else if (name.equals("countMax")) { 1834 this.countMax = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1835 } else if (name.equals("duration")) { 1836 this.duration = TypeConvertor.castToDecimal(value); // DecimalType 1837 } else if (name.equals("durationMax")) { 1838 this.durationMax = TypeConvertor.castToDecimal(value); // DecimalType 1839 } else if (name.equals("durationUnit")) { 1840 value = new UnitsOfTimeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1841 this.durationUnit = (Enumeration) value; // Enumeration<UnitsOfTime> 1842 } else if (name.equals("frequency")) { 1843 this.frequency = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1844 } else if (name.equals("frequencyMax")) { 1845 this.frequencyMax = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1846 } else if (name.equals("period")) { 1847 this.period = TypeConvertor.castToDecimal(value); // DecimalType 1848 } else if (name.equals("periodMax")) { 1849 this.periodMax = TypeConvertor.castToDecimal(value); // DecimalType 1850 } else if (name.equals("periodUnit")) { 1851 value = new UnitsOfTimeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1852 this.periodUnit = (Enumeration) value; // Enumeration<UnitsOfTime> 1853 } else if (name.equals("dayOfWeek")) { 1854 value = new DaysOfWeekEnumFactory().fromType(TypeConvertor.castToCode(value)); 1855 this.getDayOfWeek().add((Enumeration) value); 1856 } else if (name.equals("timeOfDay")) { 1857 this.getTimeOfDay().add(TypeConvertor.castToTime(value)); 1858 } else if (name.equals("when")) { 1859 value = new EventTimingEnumFactory().fromType(TypeConvertor.castToCode(value)); 1860 this.getWhen().add((Enumeration) value); 1861 } else if (name.equals("offset")) { 1862 this.offset = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1863 } else 1864 return super.setProperty(name, value); 1865 return value; 1866 } 1867 1868 @Override 1869 public void removeChild(String name, Base value) throws FHIRException { 1870 if (name.equals("bounds[x]")) { 1871 this.bounds = null; 1872 } else if (name.equals("count")) { 1873 this.count = null; 1874 } else if (name.equals("countMax")) { 1875 this.countMax = null; 1876 } else if (name.equals("duration")) { 1877 this.duration = null; 1878 } else if (name.equals("durationMax")) { 1879 this.durationMax = null; 1880 } else if (name.equals("durationUnit")) { 1881 value = new UnitsOfTimeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1882 this.durationUnit = (Enumeration) value; // Enumeration<UnitsOfTime> 1883 } else if (name.equals("frequency")) { 1884 this.frequency = null; 1885 } else if (name.equals("frequencyMax")) { 1886 this.frequencyMax = null; 1887 } else if (name.equals("period")) { 1888 this.period = null; 1889 } else if (name.equals("periodMax")) { 1890 this.periodMax = null; 1891 } else if (name.equals("periodUnit")) { 1892 value = new UnitsOfTimeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1893 this.periodUnit = (Enumeration) value; // Enumeration<UnitsOfTime> 1894 } else if (name.equals("dayOfWeek")) { 1895 value = new DaysOfWeekEnumFactory().fromType(TypeConvertor.castToCode(value)); 1896 this.getDayOfWeek().remove((Enumeration) value); 1897 } else if (name.equals("timeOfDay")) { 1898 this.getTimeOfDay().remove(value); 1899 } else if (name.equals("when")) { 1900 value = new EventTimingEnumFactory().fromType(TypeConvertor.castToCode(value)); 1901 this.getWhen().remove((Enumeration) value); 1902 } else if (name.equals("offset")) { 1903 this.offset = null; 1904 } else 1905 super.removeChild(name, value); 1906 1907 } 1908 1909 @Override 1910 public Base makeProperty(int hash, String name) throws FHIRException { 1911 switch (hash) { 1912 case -1149635157: return getBounds(); 1913 case -1383205195: return getBounds(); 1914 case 94851343: return getCountElement(); 1915 case -372044331: return getCountMaxElement(); 1916 case -1992012396: return getDurationElement(); 1917 case -478083280: return getDurationMaxElement(); 1918 case -1935429320: return getDurationUnitElement(); 1919 case -70023844: return getFrequencyElement(); 1920 case 1273846376: return getFrequencyMaxElement(); 1921 case -991726143: return getPeriodElement(); 1922 case 566580195: return getPeriodMaxElement(); 1923 case 384367333: return getPeriodUnitElement(); 1924 case -730552025: return addDayOfWeekElement(); 1925 case 21434232: return addTimeOfDayElement(); 1926 case 3648314: return addWhenElement(); 1927 case -1019779949: return getOffsetElement(); 1928 default: return super.makeProperty(hash, name); 1929 } 1930 1931 } 1932 1933 @Override 1934 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1935 switch (hash) { 1936 case -1383205195: /*bounds*/ return new String[] {"Duration", "Range", "Period"}; 1937 case 94851343: /*count*/ return new String[] {"positiveInt"}; 1938 case -372044331: /*countMax*/ return new String[] {"positiveInt"}; 1939 case -1992012396: /*duration*/ return new String[] {"decimal"}; 1940 case -478083280: /*durationMax*/ return new String[] {"decimal"}; 1941 case -1935429320: /*durationUnit*/ return new String[] {"code"}; 1942 case -70023844: /*frequency*/ return new String[] {"positiveInt"}; 1943 case 1273846376: /*frequencyMax*/ return new String[] {"positiveInt"}; 1944 case -991726143: /*period*/ return new String[] {"decimal"}; 1945 case 566580195: /*periodMax*/ return new String[] {"decimal"}; 1946 case 384367333: /*periodUnit*/ return new String[] {"code"}; 1947 case -730552025: /*dayOfWeek*/ return new String[] {"code"}; 1948 case 21434232: /*timeOfDay*/ return new String[] {"time"}; 1949 case 3648314: /*when*/ return new String[] {"code"}; 1950 case -1019779949: /*offset*/ return new String[] {"unsignedInt"}; 1951 default: return super.getTypesForProperty(hash, name); 1952 } 1953 1954 } 1955 1956 @Override 1957 public Base addChild(String name) throws FHIRException { 1958 if (name.equals("boundsDuration")) { 1959 this.bounds = new Duration(); 1960 return this.bounds; 1961 } 1962 else if (name.equals("boundsRange")) { 1963 this.bounds = new Range(); 1964 return this.bounds; 1965 } 1966 else if (name.equals("boundsPeriod")) { 1967 this.bounds = new Period(); 1968 return this.bounds; 1969 } 1970 else if (name.equals("count")) { 1971 throw new FHIRException("Cannot call addChild on a singleton property Timing.repeat.count"); 1972 } 1973 else if (name.equals("countMax")) { 1974 throw new FHIRException("Cannot call addChild on a singleton property Timing.repeat.countMax"); 1975 } 1976 else if (name.equals("duration")) { 1977 throw new FHIRException("Cannot call addChild on a singleton property Timing.repeat.duration"); 1978 } 1979 else if (name.equals("durationMax")) { 1980 throw new FHIRException("Cannot call addChild on a singleton property Timing.repeat.durationMax"); 1981 } 1982 else if (name.equals("durationUnit")) { 1983 throw new FHIRException("Cannot call addChild on a singleton property Timing.repeat.durationUnit"); 1984 } 1985 else if (name.equals("frequency")) { 1986 throw new FHIRException("Cannot call addChild on a singleton property Timing.repeat.frequency"); 1987 } 1988 else if (name.equals("frequencyMax")) { 1989 throw new FHIRException("Cannot call addChild on a singleton property Timing.repeat.frequencyMax"); 1990 } 1991 else if (name.equals("period")) { 1992 throw new FHIRException("Cannot call addChild on a singleton property Timing.repeat.period"); 1993 } 1994 else if (name.equals("periodMax")) { 1995 throw new FHIRException("Cannot call addChild on a singleton property Timing.repeat.periodMax"); 1996 } 1997 else if (name.equals("periodUnit")) { 1998 throw new FHIRException("Cannot call addChild on a singleton property Timing.repeat.periodUnit"); 1999 } 2000 else if (name.equals("dayOfWeek")) { 2001 throw new FHIRException("Cannot call addChild on a singleton property Timing.repeat.dayOfWeek"); 2002 } 2003 else if (name.equals("timeOfDay")) { 2004 throw new FHIRException("Cannot call addChild on a singleton property Timing.repeat.timeOfDay"); 2005 } 2006 else if (name.equals("when")) { 2007 throw new FHIRException("Cannot call addChild on a singleton property Timing.repeat.when"); 2008 } 2009 else if (name.equals("offset")) { 2010 throw new FHIRException("Cannot call addChild on a singleton property Timing.repeat.offset"); 2011 } 2012 else 2013 return super.addChild(name); 2014 } 2015 2016 public TimingRepeatComponent copy() { 2017 TimingRepeatComponent dst = new TimingRepeatComponent(); 2018 copyValues(dst); 2019 return dst; 2020 } 2021 2022 public void copyValues(TimingRepeatComponent dst) { 2023 super.copyValues(dst); 2024 dst.bounds = bounds == null ? null : bounds.copy(); 2025 dst.count = count == null ? null : count.copy(); 2026 dst.countMax = countMax == null ? null : countMax.copy(); 2027 dst.duration = duration == null ? null : duration.copy(); 2028 dst.durationMax = durationMax == null ? null : durationMax.copy(); 2029 dst.durationUnit = durationUnit == null ? null : durationUnit.copy(); 2030 dst.frequency = frequency == null ? null : frequency.copy(); 2031 dst.frequencyMax = frequencyMax == null ? null : frequencyMax.copy(); 2032 dst.period = period == null ? null : period.copy(); 2033 dst.periodMax = periodMax == null ? null : periodMax.copy(); 2034 dst.periodUnit = periodUnit == null ? null : periodUnit.copy(); 2035 if (dayOfWeek != null) { 2036 dst.dayOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 2037 for (Enumeration<DaysOfWeek> i : dayOfWeek) 2038 dst.dayOfWeek.add(i.copy()); 2039 }; 2040 if (timeOfDay != null) { 2041 dst.timeOfDay = new ArrayList<TimeType>(); 2042 for (TimeType i : timeOfDay) 2043 dst.timeOfDay.add(i.copy()); 2044 }; 2045 if (when != null) { 2046 dst.when = new ArrayList<Enumeration<EventTiming>>(); 2047 for (Enumeration<EventTiming> i : when) 2048 dst.when.add(i.copy()); 2049 }; 2050 dst.offset = offset == null ? null : offset.copy(); 2051 } 2052 2053 @Override 2054 public boolean equalsDeep(Base other_) { 2055 if (!super.equalsDeep(other_)) 2056 return false; 2057 if (!(other_ instanceof TimingRepeatComponent)) 2058 return false; 2059 TimingRepeatComponent o = (TimingRepeatComponent) other_; 2060 return compareDeep(bounds, o.bounds, true) && compareDeep(count, o.count, true) && compareDeep(countMax, o.countMax, true) 2061 && compareDeep(duration, o.duration, true) && compareDeep(durationMax, o.durationMax, true) && compareDeep(durationUnit, o.durationUnit, true) 2062 && compareDeep(frequency, o.frequency, true) && compareDeep(frequencyMax, o.frequencyMax, true) 2063 && compareDeep(period, o.period, true) && compareDeep(periodMax, o.periodMax, true) && compareDeep(periodUnit, o.periodUnit, true) 2064 && compareDeep(dayOfWeek, o.dayOfWeek, true) && compareDeep(timeOfDay, o.timeOfDay, true) && compareDeep(when, o.when, true) 2065 && compareDeep(offset, o.offset, true); 2066 } 2067 2068 @Override 2069 public boolean equalsShallow(Base other_) { 2070 if (!super.equalsShallow(other_)) 2071 return false; 2072 if (!(other_ instanceof TimingRepeatComponent)) 2073 return false; 2074 TimingRepeatComponent o = (TimingRepeatComponent) other_; 2075 return compareValues(count, o.count, true) && compareValues(countMax, o.countMax, true) && compareValues(duration, o.duration, true) 2076 && compareValues(durationMax, o.durationMax, true) && compareValues(durationUnit, o.durationUnit, true) 2077 && compareValues(frequency, o.frequency, true) && compareValues(frequencyMax, o.frequencyMax, true) 2078 && compareValues(period, o.period, true) && compareValues(periodMax, o.periodMax, true) && compareValues(periodUnit, o.periodUnit, true) 2079 && compareValues(dayOfWeek, o.dayOfWeek, true) && compareValues(timeOfDay, o.timeOfDay, true) && compareValues(when, o.when, true) 2080 && compareValues(offset, o.offset, true); 2081 } 2082 2083 public boolean isEmpty() { 2084 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(bounds, count, countMax 2085 , duration, durationMax, durationUnit, frequency, frequencyMax, period, periodMax 2086 , periodUnit, dayOfWeek, timeOfDay, when, offset); 2087 } 2088 2089 public String fhirType() { 2090 return "Timing.repeat"; 2091 2092 } 2093 2094 } 2095 2096 /** 2097 * Identifies specific times when the event occurs. 2098 */ 2099 @Child(name = "event", type = {DateTimeType.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2100 @Description(shortDefinition="When the event occurs", formalDefinition="Identifies specific times when the event occurs." ) 2101 protected List<DateTimeType> event; 2102 2103 /** 2104 * A set of rules that describe when the event is scheduled. 2105 */ 2106 @Child(name = "repeat", type = {}, order=1, min=0, max=1, modifier=false, summary=true) 2107 @Description(shortDefinition="When the event is to occur", formalDefinition="A set of rules that describe when the event is scheduled." ) 2108 protected TimingRepeatComponent repeat; 2109 2110 /** 2111 * A code for the timing schedule (or just text in code.text). Some codes such as BID are ubiquitous, but many institutions define their own additional codes. If a code is provided, the code is understood to be a complete statement of whatever is specified in the structured timing data, and either the code or the data may be used to interpret the Timing, with the exception that .repeat.bounds still applies over the code (and is not contained in the code). 2112 */ 2113 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 2114 @Description(shortDefinition="C | BID | TID | QID | AM | PM | QD | QOD | +", formalDefinition="A code for the timing schedule (or just text in code.text). Some codes such as BID are ubiquitous, but many institutions define their own additional codes. If a code is provided, the code is understood to be a complete statement of whatever is specified in the structured timing data, and either the code or the data may be used to interpret the Timing, with the exception that .repeat.bounds still applies over the code (and is not contained in the code)." ) 2115 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/timing-abbreviation") 2116 protected CodeableConcept code; 2117 2118 private static final long serialVersionUID = 791565112L; 2119 2120 /** 2121 * Constructor 2122 */ 2123 public Timing() { 2124 super(); 2125 } 2126 2127 /** 2128 * @return {@link #event} (Identifies specific times when the event occurs.) 2129 */ 2130 public List<DateTimeType> getEvent() { 2131 if (this.event == null) 2132 this.event = new ArrayList<DateTimeType>(); 2133 return this.event; 2134 } 2135 2136 /** 2137 * @return Returns a reference to <code>this</code> for easy method chaining 2138 */ 2139 public Timing setEvent(List<DateTimeType> theEvent) { 2140 this.event = theEvent; 2141 return this; 2142 } 2143 2144 public boolean hasEvent() { 2145 if (this.event == null) 2146 return false; 2147 for (DateTimeType item : this.event) 2148 if (!item.isEmpty()) 2149 return true; 2150 return false; 2151 } 2152 2153 /** 2154 * @return {@link #event} (Identifies specific times when the event occurs.) 2155 */ 2156 public DateTimeType addEventElement() {//2 2157 DateTimeType t = new DateTimeType(); 2158 if (this.event == null) 2159 this.event = new ArrayList<DateTimeType>(); 2160 this.event.add(t); 2161 return t; 2162 } 2163 2164 /** 2165 * @param value {@link #event} (Identifies specific times when the event occurs.) 2166 */ 2167 public Timing addEvent(Date value) { //1 2168 DateTimeType t = new DateTimeType(); 2169 t.setValue(value); 2170 if (this.event == null) 2171 this.event = new ArrayList<DateTimeType>(); 2172 this.event.add(t); 2173 return this; 2174 } 2175 2176 /** 2177 * @param value {@link #event} (Identifies specific times when the event occurs.) 2178 */ 2179 public boolean hasEvent(Date value) { 2180 if (this.event == null) 2181 return false; 2182 for (DateTimeType v : this.event) 2183 if (v.getValue().equals(value)) // dateTime 2184 return true; 2185 return false; 2186 } 2187 2188 /** 2189 * @return {@link #repeat} (A set of rules that describe when the event is scheduled.) 2190 */ 2191 public TimingRepeatComponent getRepeat() { 2192 if (this.repeat == null) 2193 if (Configuration.errorOnAutoCreate()) 2194 throw new Error("Attempt to auto-create Timing.repeat"); 2195 else if (Configuration.doAutoCreate()) 2196 this.repeat = new TimingRepeatComponent(); // cc 2197 return this.repeat; 2198 } 2199 2200 public boolean hasRepeat() { 2201 return this.repeat != null && !this.repeat.isEmpty(); 2202 } 2203 2204 /** 2205 * @param value {@link #repeat} (A set of rules that describe when the event is scheduled.) 2206 */ 2207 public Timing setRepeat(TimingRepeatComponent value) { 2208 this.repeat = value; 2209 return this; 2210 } 2211 2212 /** 2213 * @return {@link #code} (A code for the timing schedule (or just text in code.text). Some codes such as BID are ubiquitous, but many institutions define their own additional codes. If a code is provided, the code is understood to be a complete statement of whatever is specified in the structured timing data, and either the code or the data may be used to interpret the Timing, with the exception that .repeat.bounds still applies over the code (and is not contained in the code).) 2214 */ 2215 public CodeableConcept getCode() { 2216 if (this.code == null) 2217 if (Configuration.errorOnAutoCreate()) 2218 throw new Error("Attempt to auto-create Timing.code"); 2219 else if (Configuration.doAutoCreate()) 2220 this.code = new CodeableConcept(); // cc 2221 return this.code; 2222 } 2223 2224 public boolean hasCode() { 2225 return this.code != null && !this.code.isEmpty(); 2226 } 2227 2228 /** 2229 * @param value {@link #code} (A code for the timing schedule (or just text in code.text). Some codes such as BID are ubiquitous, but many institutions define their own additional codes. If a code is provided, the code is understood to be a complete statement of whatever is specified in the structured timing data, and either the code or the data may be used to interpret the Timing, with the exception that .repeat.bounds still applies over the code (and is not contained in the code).) 2230 */ 2231 public Timing setCode(CodeableConcept value) { 2232 this.code = value; 2233 return this; 2234 } 2235 2236 protected void listChildren(List<Property> children) { 2237 super.listChildren(children); 2238 children.add(new Property("event", "dateTime", "Identifies specific times when the event occurs.", 0, java.lang.Integer.MAX_VALUE, event)); 2239 children.add(new Property("repeat", "", "A set of rules that describe when the event is scheduled.", 0, 1, repeat)); 2240 children.add(new Property("code", "CodeableConcept", "A code for the timing schedule (or just text in code.text). Some codes such as BID are ubiquitous, but many institutions define their own additional codes. If a code is provided, the code is understood to be a complete statement of whatever is specified in the structured timing data, and either the code or the data may be used to interpret the Timing, with the exception that .repeat.bounds still applies over the code (and is not contained in the code).", 0, 1, code)); 2241 } 2242 2243 @Override 2244 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2245 switch (_hash) { 2246 case 96891546: /*event*/ return new Property("event", "dateTime", "Identifies specific times when the event occurs.", 0, java.lang.Integer.MAX_VALUE, event); 2247 case -934531685: /*repeat*/ return new Property("repeat", "", "A set of rules that describe when the event is scheduled.", 0, 1, repeat); 2248 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code for the timing schedule (or just text in code.text). Some codes such as BID are ubiquitous, but many institutions define their own additional codes. If a code is provided, the code is understood to be a complete statement of whatever is specified in the structured timing data, and either the code or the data may be used to interpret the Timing, with the exception that .repeat.bounds still applies over the code (and is not contained in the code).", 0, 1, code); 2249 default: return super.getNamedProperty(_hash, _name, _checkValid); 2250 } 2251 2252 } 2253 2254 @Override 2255 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2256 switch (hash) { 2257 case 96891546: /*event*/ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // DateTimeType 2258 case -934531685: /*repeat*/ return this.repeat == null ? new Base[0] : new Base[] {this.repeat}; // TimingRepeatComponent 2259 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2260 default: return super.getProperty(hash, name, checkValid); 2261 } 2262 2263 } 2264 2265 @Override 2266 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2267 switch (hash) { 2268 case 96891546: // event 2269 this.getEvent().add(TypeConvertor.castToDateTime(value)); // DateTimeType 2270 return value; 2271 case -934531685: // repeat 2272 this.repeat = (TimingRepeatComponent) value; // TimingRepeatComponent 2273 return value; 2274 case 3059181: // code 2275 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2276 return value; 2277 default: return super.setProperty(hash, name, value); 2278 } 2279 2280 } 2281 2282 @Override 2283 public Base setProperty(String name, Base value) throws FHIRException { 2284 if (name.equals("event")) { 2285 this.getEvent().add(TypeConvertor.castToDateTime(value)); 2286 } else if (name.equals("repeat")) { 2287 this.repeat = (TimingRepeatComponent) value; // TimingRepeatComponent 2288 } else if (name.equals("code")) { 2289 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2290 } else 2291 return super.setProperty(name, value); 2292 return value; 2293 } 2294 2295 @Override 2296 public void removeChild(String name, Base value) throws FHIRException { 2297 if (name.equals("event")) { 2298 this.getEvent().remove(value); 2299 } else if (name.equals("repeat")) { 2300 this.repeat = (TimingRepeatComponent) value; // TimingRepeatComponent 2301 } else if (name.equals("code")) { 2302 this.code = null; 2303 } else 2304 super.removeChild(name, value); 2305 2306 } 2307 2308 @Override 2309 public Base makeProperty(int hash, String name) throws FHIRException { 2310 switch (hash) { 2311 case 96891546: return addEventElement(); 2312 case -934531685: return getRepeat(); 2313 case 3059181: return getCode(); 2314 default: return super.makeProperty(hash, name); 2315 } 2316 2317 } 2318 2319 @Override 2320 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2321 switch (hash) { 2322 case 96891546: /*event*/ return new String[] {"dateTime"}; 2323 case -934531685: /*repeat*/ return new String[] {}; 2324 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2325 default: return super.getTypesForProperty(hash, name); 2326 } 2327 2328 } 2329 2330 @Override 2331 public Base addChild(String name) throws FHIRException { 2332 if (name.equals("event")) { 2333 throw new FHIRException("Cannot call addChild on a singleton property Timing.event"); 2334 } 2335 else if (name.equals("repeat")) { 2336 this.repeat = new TimingRepeatComponent(); 2337 return this.repeat; 2338 } 2339 else if (name.equals("code")) { 2340 this.code = new CodeableConcept(); 2341 return this.code; 2342 } 2343 else 2344 return super.addChild(name); 2345 } 2346 2347 public String fhirType() { 2348 return "Timing"; 2349 2350 } 2351 2352 public Timing copy() { 2353 Timing dst = new Timing(); 2354 copyValues(dst); 2355 return dst; 2356 } 2357 2358 public void copyValues(Timing dst) { 2359 super.copyValues(dst); 2360 if (event != null) { 2361 dst.event = new ArrayList<DateTimeType>(); 2362 for (DateTimeType i : event) 2363 dst.event.add(i.copy()); 2364 }; 2365 dst.repeat = repeat == null ? null : repeat.copy(); 2366 dst.code = code == null ? null : code.copy(); 2367 } 2368 2369 protected Timing typedCopy() { 2370 return copy(); 2371 } 2372 2373 @Override 2374 public boolean equalsDeep(Base other_) { 2375 if (!super.equalsDeep(other_)) 2376 return false; 2377 if (!(other_ instanceof Timing)) 2378 return false; 2379 Timing o = (Timing) other_; 2380 return compareDeep(event, o.event, true) && compareDeep(repeat, o.repeat, true) && compareDeep(code, o.code, true) 2381 ; 2382 } 2383 2384 @Override 2385 public boolean equalsShallow(Base other_) { 2386 if (!super.equalsShallow(other_)) 2387 return false; 2388 if (!(other_ instanceof Timing)) 2389 return false; 2390 Timing o = (Timing) other_; 2391 return compareValues(event, o.event, true); 2392 } 2393 2394 public boolean isEmpty() { 2395 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(event, repeat, code); 2396 } 2397 2398 2399} 2400